code
stringlengths 501
5.19M
| package
stringlengths 2
81
| path
stringlengths 9
304
| filename
stringlengths 4
145
|
---|---|---|---|
export default Decimal;
export namespace Decimal {
export type Constructor = typeof Decimal;
export type Instance = Decimal;
export type Rounding = 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8;
export type Modulo = Rounding | 9;
export type Value = string | number | Decimal;
// http://mikemcl.github.io/decimal.js/#constructor-properties
export interface Config {
precision?: number;
rounding?: Rounding;
toExpNeg?: number;
toExpPos?: number;
minE?: number;
maxE?: number;
crypto?: boolean;
modulo?: Modulo;
defaults?: boolean;
}
}
export declare class Decimal {
readonly d: number[];
readonly e: number;
readonly s: number;
private readonly name: string;
constructor(n: Decimal.Value);
absoluteValue(): Decimal;
abs(): Decimal;
ceil(): Decimal;
comparedTo(n: Decimal.Value): number;
cmp(n: Decimal.Value): number;
cosine(): Decimal;
cos(): Decimal;
cubeRoot(): Decimal;
cbrt(): Decimal;
decimalPlaces(): number;
dp(): number;
dividedBy(n: Decimal.Value): Decimal;
div(n: Decimal.Value): Decimal;
dividedToIntegerBy(n: Decimal.Value): Decimal;
divToInt(n: Decimal.Value): Decimal;
equals(n: Decimal.Value): boolean;
eq(n: Decimal.Value): boolean;
floor(): Decimal;
greaterThan(n: Decimal.Value): boolean;
gt(n: Decimal.Value): boolean;
greaterThanOrEqualTo(n: Decimal.Value): boolean;
gte(n: Decimal.Value): boolean;
hyperbolicCosine(): Decimal;
cosh(): Decimal;
hyperbolicSine(): Decimal;
sinh(): Decimal;
hyperbolicTangent(): Decimal;
tanh(): Decimal;
inverseCosine(): Decimal;
acos(): Decimal;
inverseHyperbolicCosine(): Decimal;
acosh(): Decimal;
inverseHyperbolicSine(): Decimal;
asinh(): Decimal;
inverseHyperbolicTangent(): Decimal;
atanh(): Decimal;
inverseSine(): Decimal;
asin(): Decimal;
inverseTangent(): Decimal;
atan(): Decimal;
isFinite(): boolean;
isInteger(): boolean;
isInt(): boolean;
isNaN(): boolean;
isNegative(): boolean;
isNeg(): boolean;
isPositive(): boolean;
isPos(): boolean;
isZero(): boolean;
lessThan(n: Decimal.Value): boolean;
lt(n: Decimal.Value): boolean;
lessThanOrEqualTo(n: Decimal.Value): boolean;
lte(n: Decimal.Value): boolean;
logarithm(n?: Decimal.Value): Decimal;
log(n?: Decimal.Value): Decimal;
minus(n: Decimal.Value): Decimal;
sub(n: Decimal.Value): Decimal;
modulo(n: Decimal.Value): Decimal;
mod(n: Decimal.Value): Decimal;
naturalExponential(): Decimal;
exp(): Decimal;
naturalLogarithm(): Decimal;
ln(): Decimal;
negated(): Decimal;
neg(): Decimal;
plus(n: Decimal.Value): Decimal;
add(n: Decimal.Value): Decimal;
precision(includeZeros?: boolean): number;
sd(includeZeros?: boolean): number;
round(): Decimal;
sine() : Decimal;
sin() : Decimal;
squareRoot(): Decimal;
sqrt(): Decimal;
tangent() : Decimal;
tan() : Decimal;
times(n: Decimal.Value): Decimal;
mul(n: Decimal.Value) : Decimal;
toBinary(significantDigits?: number): string;
toBinary(significantDigits: number, rounding: Decimal.Rounding): string;
toDecimalPlaces(decimalPlaces?: number): Decimal;
toDecimalPlaces(decimalPlaces: number, rounding: Decimal.Rounding): Decimal;
toDP(decimalPlaces?: number): Decimal;
toDP(decimalPlaces: number, rounding: Decimal.Rounding): Decimal;
toExponential(decimalPlaces?: number): string;
toExponential(decimalPlaces: number, rounding: Decimal.Rounding): string;
toFixed(decimalPlaces?: number): string;
toFixed(decimalPlaces: number, rounding: Decimal.Rounding): string;
toFraction(max_denominator?: Decimal.Value): Decimal[];
toHexadecimal(significantDigits?: number): string;
toHexadecimal(significantDigits: number, rounding: Decimal.Rounding): string;
toHex(significantDigits?: number): string;
toHex(significantDigits: number, rounding?: Decimal.Rounding): string;
toJSON(): string;
toNearest(n: Decimal.Value, rounding?: Decimal.Rounding): Decimal;
toNumber(): number;
toOctal(significantDigits?: number): string;
toOctal(significantDigits: number, rounding: Decimal.Rounding): string;
toPower(n: Decimal.Value): Decimal;
pow(n: Decimal.Value): Decimal;
toPrecision(significantDigits?: number): string;
toPrecision(significantDigits: number, rounding: Decimal.Rounding): string;
toSignificantDigits(significantDigits?: number): Decimal;
toSignificantDigits(significantDigits: number, rounding: Decimal.Rounding): Decimal;
toSD(significantDigits?: number): Decimal;
toSD(significantDigits: number, rounding: Decimal.Rounding): Decimal;
toString(): string;
truncated(): Decimal;
trunc(): Decimal;
valueOf(): string;
static abs(n: Decimal.Value): Decimal;
static acos(n: Decimal.Value): Decimal;
static acosh(n: Decimal.Value): Decimal;
static add(x: Decimal.Value, y: Decimal.Value): Decimal;
static asin(n: Decimal.Value): Decimal;
static asinh(n: Decimal.Value): Decimal;
static atan(n: Decimal.Value): Decimal;
static atanh(n: Decimal.Value): Decimal;
static atan2(y: Decimal.Value, x: Decimal.Value): Decimal;
static cbrt(n: Decimal.Value): Decimal;
static ceil(n: Decimal.Value): Decimal;
static clone(object?: Decimal.Config): Decimal.Constructor;
static config(object: Decimal.Config): Decimal.Constructor;
static cos(n: Decimal.Value): Decimal;
static cosh(n: Decimal.Value): Decimal;
static div(x: Decimal.Value, y: Decimal.Value): Decimal;
static exp(n: Decimal.Value): Decimal;
static floor(n: Decimal.Value): Decimal;
static hypot(...n: Decimal.Value[]): Decimal;
static isDecimal(object: any): boolean
static ln(n: Decimal.Value): Decimal;
static log(n: Decimal.Value, base?: Decimal.Value): Decimal;
static log2(n: Decimal.Value): Decimal;
static log10(n: Decimal.Value): Decimal;
static max(...n: Decimal.Value[]): Decimal;
static min(...n: Decimal.Value[]): Decimal;
static mod(x: Decimal.Value, y: Decimal.Value): Decimal;
static mul(x: Decimal.Value, y: Decimal.Value): Decimal;
static noConflict(): Decimal.Constructor; // Browser only
static pow(base: Decimal.Value, exponent: Decimal.Value): Decimal;
static random(significantDigits?: number): Decimal;
static round(n: Decimal.Value): Decimal;
static set(object: Decimal.Config): Decimal.Constructor;
static sign(n: Decimal.Value): Decimal;
static sin(n: Decimal.Value): Decimal;
static sinh(n: Decimal.Value): Decimal;
static sqrt(n: Decimal.Value): Decimal;
static sub(x: Decimal.Value, y: Decimal.Value): Decimal;
static tan(n: Decimal.Value): Decimal;
static tanh(n: Decimal.Value): Decimal;
static trunc(n: Decimal.Value): Decimal;
static readonly default?: Decimal.Constructor;
static readonly Decimal?: Decimal.Constructor;
static readonly precision: number;
static readonly rounding: Decimal.Rounding;
static readonly toExpNeg: number;
static readonly toExpPos: number;
static readonly minE: number;
static readonly maxE: number;
static readonly crypto: boolean;
static readonly modulo: Decimal.Modulo;
static readonly ROUND_UP: 0;
static readonly ROUND_DOWN: 1;
static readonly ROUND_CEIL: 2;
static readonly ROUND_FLOOR: 3;
static readonly ROUND_HALF_UP: 4;
static readonly ROUND_HALF_DOWN: 5;
static readonly ROUND_HALF_EVEN: 6;
static readonly ROUND_HALF_CEIL: 7;
static readonly ROUND_HALF_FLOOR: 8;
static readonly EUCLID: 9;
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/decimal.d.ts | decimal.d.ts |
The MIT Licence.
Copyright (c) 2019 Michael Mclaughlin
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/LICENCE.md | LICENCE.md |
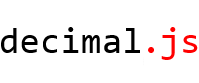
An arbitrary-precision Decimal type for JavaScript.
[](https://travis-ci.org/MikeMcl/decimal.js)
[](https://cdnjs.com/libraries/decimal.js)
<br>
## Features
- Integers and floats
- Simple but full-featured API
- Replicates many of the methods of JavaScript's `Number.prototype` and `Math` objects
- Also handles hexadecimal, binary and octal values
- Faster, smaller, and perhaps easier to use than JavaScript versions of Java's BigDecimal
- No dependencies
- Wide platform compatibility: uses JavaScript 1.5 (ECMAScript 3) features only
- Comprehensive [documentation](http://mikemcl.github.io/decimal.js/) and test set
- Includes a TypeScript declaration file: *decimal.d.ts*

The library is similar to [bignumber.js](https://github.com/MikeMcl/bignumber.js/), but here
precision is specified in terms of significant digits rather than decimal places, and all
calculations are rounded to the precision (similar to Python's decimal module) rather than just
those involving division.
This library also adds the trigonometric functions, among others, and supports non-integer powers,
which makes it a significantly larger library than *bignumber.js* and the even smaller
[big.js](https://github.com/MikeMcl/big.js/).
For a lighter version of this library without the trigonometric functions see [decimal.js-light](https://github.com/MikeMcl/decimal.js-light/).
## Load
The library is the single JavaScript file *decimal.js* (or minified, *decimal.min.js*).
Browser:
```html
<script src='path/to/decimal.js'></script>
```
[Node.js](http://nodejs.org):
```bash
$ npm install --save decimal.js
```
```js
var Decimal = require('decimal.js');
```
ES6 module (*decimal.mjs*):
```js
//import Decimal from 'decimal.js';
import {Decimal} from 'decimal.js';
```
AMD loader libraries such as [requireJS](http://requirejs.org/):
```js
require(['decimal'], function(Decimal) {
// Use Decimal here in local scope. No global Decimal.
});
```
## Use
*In all examples below, `var`, semicolons and `toString` calls are not shown.
If a commented-out value is in quotes it means `toString` has been called on the preceding expression.*
The library exports a single function object, `Decimal`, the constructor of Decimal instances.
It accepts a value of type number, string or Decimal.
```js
x = new Decimal(123.4567)
y = new Decimal('123456.7e-3')
z = new Decimal(x)
x.equals(y) && y.equals(z) && x.equals(z) // true
```
A value can also be in binary, hexadecimal or octal if the appropriate prefix is included.
```js
x = new Decimal('0xff.f') // '255.9375'
y = new Decimal('0b10101100') // '172'
z = x.plus(y) // '427.9375'
z.toBinary() // '0b110101011.1111'
z.toBinary(13) // '0b1.101010111111p+8'
```
Using binary exponential notation to create a Decimal with the value of `Number.MAX_VALUE`:
```js
x = new Decimal('0b1.1111111111111111111111111111111111111111111111111111p+1023')
```
A Decimal is immutable in the sense that it is not changed by its methods.
```js
0.3 - 0.1 // 0.19999999999999998
x = new Decimal(0.3)
x.minus(0.1) // '0.2'
x // '0.3'
```
The methods that return a Decimal can be chained.
```js
x.dividedBy(y).plus(z).times(9).floor()
x.times('1.23456780123456789e+9').plus(9876.5432321).dividedBy('4444562598.111772').ceil()
```
Many method names have a shorter alias.
```js
x.squareRoot().dividedBy(y).toPower(3).equals(x.sqrt().div(y).pow(3)) // true
x.cmp(y.mod(z).neg()) == 1 && x.comparedTo(y.modulo(z).negated()) == 1 // true
```
Like JavaScript's Number type, there are `toExponential`, `toFixed` and `toPrecision` methods,
```js
x = new Decimal(255.5)
x.toExponential(5) // '2.55500e+2'
x.toFixed(5) // '255.50000'
x.toPrecision(5) // '255.50'
```
and almost all of the methods of JavaScript's Math object are also replicated.
```js
Decimal.sqrt('6.98372465832e+9823') // '8.3568682281821340204e+4911'
Decimal.pow(2, 0.0979843) // '1.0702770511687781839'
```
There are `isNaN` and `isFinite` methods, as `NaN` and `Infinity` are valid `Decimal` values,
```js
x = new Decimal(NaN) // 'NaN'
y = new Decimal(Infinity) // 'Infinity'
x.isNaN() && !y.isNaN() && !x.isFinite() && !y.isFinite() // true
```
and a `toFraction` method with an optional *maximum denominator* argument
```js
z = new Decimal(355)
pi = z.dividedBy(113) // '3.1415929204'
pi.toFraction() // [ '7853982301', '2500000000' ]
pi.toFraction(1000) // [ '355', '113' ]
```
All calculations are rounded according to the number of significant digits and rounding mode
specified by the `precision` and `rounding` properties of the Decimal constructor.
For advanced usage, multiple Decimal constructors can be created, each with their own independent configuration which
applies to all Decimal numbers created from it.
```js
// Set the precision and rounding of the default Decimal constructor
Decimal.set({ precision: 5, rounding: 4 })
// Create another Decimal constructor, optionally passing in a configuration object
Decimal9 = Decimal.clone({ precision: 9, rounding: 1 })
x = new Decimal(5)
y = new Decimal9(5)
x.div(3) // '1.6667'
y.div(3) // '1.66666666'
```
The value of a Decimal is stored in a floating point format in terms of its digits, exponent and sign.
```js
x = new Decimal(-12345.67);
x.d // [ 12345, 6700000 ] digits (base 10000000)
x.e // 4 exponent (base 10)
x.s // -1 sign
```
For further information see the [API](http://mikemcl.github.io/decimal.js/) reference in the *doc* directory.
## Test
The library can be tested using Node.js or a browser.
The *test* directory contains the file *test.js* which runs all the tests when executed by Node,
and the file *test.html* which runs all the tests when opened in a browser.
To run all the tests, from a command-line at the root directory using npm
```bash
$ npm test
```
or at the *test* directory using Node
```bash
$ node test
```
Each separate test module can also be executed individually, for example, at the *test/modules* directory
```bash
$ node toFraction
```
## Build
For Node, if [uglify-js](https://github.com/mishoo/UglifyJS2) is installed
```bash
npm install uglify-js -g
```
then
```bash
npm run build
```
will create *decimal.min.js*, and a source map will also be added to the *doc* directory.
## Licence
MIT.
See *LICENCE.md*
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/README.md | README.md |
export default Decimal;
export namespace Decimal {
export type Config = DecimalConfig;
export type Constructor = DecimalConstructor;
export type Instance = DecimalInstance;
export type Modulo = DecimalModulo;
export type Rounding = DecimalRounding;
export type Value = DecimalValue;
}
declare global {
const Decimal: DecimalConstructor;
type Decimal = DecimalInstance;
namespace Decimal {
type Config = DecimalConfig;
type Constructor = DecimalConstructor;
type Instance = DecimalInstance;
type Modulo = DecimalModulo;
type Rounding = DecimalRounding;
type Value = DecimalValue;
}
}
type DecimalInstance = Decimal;
type DecimalConstructor = typeof Decimal;
type DecimalValue = string | number | Decimal;
type DecimalRounding = 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8;
type DecimalModulo = DecimalRounding | 9;
// http://mikemcl.github.io/decimal.js/#constructor-properties
interface DecimalConfig {
precision?: number;
rounding?: DecimalRounding;
toExpNeg?: number;
toExpPos?: number;
minE?: number;
maxE?: number;
crypto?: boolean;
modulo?: DecimalModulo;
defaults?: boolean;
}
export declare class Decimal {
readonly d: number[];
readonly e: number;
readonly s: number;
private readonly name: string;
constructor(n: DecimalValue);
absoluteValue(): Decimal;
abs(): Decimal;
ceil(): Decimal;
comparedTo(n: DecimalValue): number;
cmp(n: DecimalValue): number;
cosine(): Decimal;
cos(): Decimal;
cubeRoot(): Decimal;
cbrt(): Decimal;
decimalPlaces(): number;
dp(): number;
dividedBy(n: DecimalValue): Decimal;
div(n: DecimalValue): Decimal;
dividedToIntegerBy(n: DecimalValue): Decimal;
divToInt(n: DecimalValue): Decimal;
equals(n: DecimalValue): boolean;
eq(n: DecimalValue): boolean;
floor(): Decimal;
greaterThan(n: DecimalValue): boolean;
gt(n: DecimalValue): boolean;
greaterThanOrEqualTo(n: DecimalValue): boolean;
gte(n: DecimalValue): boolean;
hyperbolicCosine(): Decimal;
cosh(): Decimal;
hyperbolicSine(): Decimal;
sinh(): Decimal;
hyperbolicTangent(): Decimal;
tanh(): Decimal;
inverseCosine(): Decimal;
acos(): Decimal;
inverseHyperbolicCosine(): Decimal;
acosh(): Decimal;
inverseHyperbolicSine(): Decimal;
asinh(): Decimal;
inverseHyperbolicTangent(): Decimal;
atanh(): Decimal;
inverseSine(): Decimal;
asin(): Decimal;
inverseTangent(): Decimal;
atan(): Decimal;
isFinite(): boolean;
isInteger(): boolean;
isInt(): boolean;
isNaN(): boolean;
isNegative(): boolean;
isNeg(): boolean;
isPositive(): boolean;
isPos(): boolean;
isZero(): boolean;
lessThan(n: DecimalValue): boolean;
lt(n: DecimalValue): boolean;
lessThanOrEqualTo(n: DecimalValue): boolean;
lte(n: DecimalValue): boolean;
logarithm(n?: DecimalValue): Decimal;
log(n?: DecimalValue): Decimal;
minus(n: DecimalValue): Decimal;
sub(n: DecimalValue): Decimal;
modulo(n: DecimalValue): Decimal;
mod(n: DecimalValue): Decimal;
naturalExponential(): Decimal;
exp(): Decimal;
naturalLogarithm(): Decimal;
ln(): Decimal;
negated(): Decimal;
neg(): Decimal;
plus(n: DecimalValue): Decimal;
add(n: DecimalValue): Decimal;
precision(includeZeros?: boolean): number;
sd(includeZeros?: boolean): number;
round(): Decimal;
sine() : Decimal;
sin() : Decimal;
squareRoot(): Decimal;
sqrt(): Decimal;
tangent() : Decimal;
tan() : Decimal;
times(n: DecimalValue): Decimal;
mul(n: DecimalValue) : Decimal;
toBinary(significantDigits?: number): string;
toBinary(significantDigits: number, rounding: DecimalRounding): string;
toDecimalPlaces(decimalPlaces?: number): Decimal;
toDecimalPlaces(decimalPlaces: number, rounding: DecimalRounding): Decimal;
toDP(decimalPlaces?: number): Decimal;
toDP(decimalPlaces: number, rounding: DecimalRounding): Decimal;
toExponential(decimalPlaces?: number): string;
toExponential(decimalPlaces: number, rounding: DecimalRounding): string;
toFixed(decimalPlaces?: number): string;
toFixed(decimalPlaces: number, rounding: DecimalRounding): string;
toFraction(max_denominator?: DecimalValue): Decimal[];
toHexadecimal(significantDigits?: number): string;
toHexadecimal(significantDigits: number, rounding: DecimalRounding): string;
toHex(significantDigits?: number): string;
toHex(significantDigits: number, rounding?: DecimalRounding): string;
toJSON(): string;
toNearest(n: DecimalValue, rounding?: DecimalRounding): Decimal;
toNumber(): number;
toOctal(significantDigits?: number): string;
toOctal(significantDigits: number, rounding: DecimalRounding): string;
toPower(n: DecimalValue): Decimal;
pow(n: DecimalValue): Decimal;
toPrecision(significantDigits?: number): string;
toPrecision(significantDigits: number, rounding: DecimalRounding): string;
toSignificantDigits(significantDigits?: number): Decimal;
toSignificantDigits(significantDigits: number, rounding: DecimalRounding): Decimal;
toSD(significantDigits?: number): Decimal;
toSD(significantDigits: number, rounding: DecimalRounding): Decimal;
toString(): string;
truncated(): Decimal;
trunc(): Decimal;
valueOf(): string;
static abs(n: DecimalValue): Decimal;
static acos(n: DecimalValue): Decimal;
static acosh(n: DecimalValue): Decimal;
static add(x: DecimalValue, y: DecimalValue): Decimal;
static asin(n: DecimalValue): Decimal;
static asinh(n: DecimalValue): Decimal;
static atan(n: DecimalValue): Decimal;
static atanh(n: DecimalValue): Decimal;
static atan2(y: DecimalValue, x: DecimalValue): Decimal;
static cbrt(n: DecimalValue): Decimal;
static ceil(n: DecimalValue): Decimal;
static clone(object?: DecimalConfig): DecimalConstructor;
static config(object: DecimalConfig): DecimalConstructor;
static cos(n: DecimalValue): Decimal;
static cosh(n: DecimalValue): Decimal;
static div(x: DecimalValue, y: DecimalValue): Decimal;
static exp(n: DecimalValue): Decimal;
static floor(n: DecimalValue): Decimal;
static hypot(...n: DecimalValue[]): Decimal;
static isDecimal(object: any): boolean
static ln(n: DecimalValue): Decimal;
static log(n: DecimalValue, base?: DecimalValue): Decimal;
static log2(n: DecimalValue): Decimal;
static log10(n: DecimalValue): Decimal;
static max(...n: DecimalValue[]): Decimal;
static min(...n: DecimalValue[]): Decimal;
static mod(x: DecimalValue, y: DecimalValue): Decimal;
static mul(x: DecimalValue, y: DecimalValue): Decimal;
static noConflict(): DecimalConstructor; // Browser only
static pow(base: DecimalValue, exponent: DecimalValue): Decimal;
static random(significantDigits?: number): Decimal;
static round(n: DecimalValue): Decimal;
static set(object: DecimalConfig): DecimalConstructor;
static sign(n: DecimalValue): Decimal;
static sin(n: DecimalValue): Decimal;
static sinh(n: DecimalValue): Decimal;
static sqrt(n: DecimalValue): Decimal;
static sub(x: DecimalValue, y: DecimalValue): Decimal;
static tan(n: DecimalValue): Decimal;
static tanh(n: DecimalValue): Decimal;
static trunc(n: DecimalValue): Decimal;
static readonly default?: DecimalConstructor;
static readonly Decimal?: DecimalConstructor;
static readonly precision: number;
static readonly rounding: DecimalRounding;
static readonly toExpNeg: number;
static readonly toExpPos: number;
static readonly minE: number;
static readonly maxE: number;
static readonly crypto: boolean;
static readonly modulo: DecimalModulo;
static readonly ROUND_UP: 0;
static readonly ROUND_DOWN: 1;
static readonly ROUND_CEIL: 2;
static readonly ROUND_FLOOR: 3;
static readonly ROUND_HALF_UP: 4;
static readonly ROUND_HALF_DOWN: 5;
static readonly ROUND_HALF_EVEN: 6;
static readonly ROUND_HALF_CEIL: 7;
static readonly ROUND_HALF_FLOOR: 8;
static readonly EUCLID: 9;
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/decimal.global.d.ts | decimal.global.d.ts |
!function(n){"use strict";var h,R,e,o,u=9e15,g=1e9,m="0123456789abcdef",t="2.3025850929940456840179914546843642076011014886287729760333279009675726096773524802359972050895982983419677840422862486334095254650828067566662873690987816894829072083255546808437998948262331985283935053089653777326288461633662222876982198867465436674744042432743651550489343149393914796194044002221051017141748003688084012647080685567743216228355220114804663715659121373450747856947683463616792101806445070648000277502684916746550586856935673420670581136429224554405758925724208241314695689016758940256776311356919292033376587141660230105703089634572075440370847469940168269282808481184289314848524948644871927809676271275775397027668605952496716674183485704422507197965004714951050492214776567636938662976979522110718264549734772662425709429322582798502585509785265383207606726317164309505995087807523710333101197857547331541421808427543863591778117054309827482385045648019095610299291824318237525357709750539565187697510374970888692180205189339507238539205144634197265287286965110862571492198849978748873771345686209167058",r="3.1415926535897932384626433832795028841971693993751058209749445923078164062862089986280348253421170679821480865132823066470938446095505822317253594081284811174502841027019385211055596446229489549303819644288109756659334461284756482337867831652712019091456485669234603486104543266482133936072602491412737245870066063155881748815209209628292540917153643678925903600113305305488204665213841469519415116094330572703657595919530921861173819326117931051185480744623799627495673518857527248912279381830119491298336733624406566430860213949463952247371907021798609437027705392171762931767523846748184676694051320005681271452635608277857713427577896091736371787214684409012249534301465495853710507922796892589235420199561121290219608640344181598136297747713099605187072113499999983729780499510597317328160963185950244594553469083026425223082533446850352619311881710100031378387528865875332083814206171776691473035982534904287554687311595628638823537875937519577818577805321712268066130019278766111959092164201989380952572010654858632789",c={precision:20,rounding:4,modulo:1,toExpNeg:-7,toExpPos:21,minE:-u,maxE:u,crypto:!1},N=!0,f="[DecimalError] ",w=f+"Invalid argument: ",s=f+"Precision limit exceeded",a=f+"crypto unavailable",L=Math.floor,v=Math.pow,l=/^0b([01]+(\.[01]*)?|\.[01]+)(p[+-]?\d+)?$/i,d=/^0x([0-9a-f]+(\.[0-9a-f]*)?|\.[0-9a-f]+)(p[+-]?\d+)?$/i,p=/^0o([0-7]+(\.[0-7]*)?|\.[0-7]+)(p[+-]?\d+)?$/i,b=/^(\d+(\.\d*)?|\.\d+)(e[+-]?\d+)?$/i,T=1e7,U=7,E=t.length-1,x=r.length-1,y={name:"[object Decimal]"};function M(n){var e,i,t,r=n.length-1,s="",o=n[0];if(0<r){for(s+=o,e=1;e<r;e++)t=n[e]+"",(i=U-t.length)&&(s+=C(i)),s+=t;o=n[e],(i=U-(t=o+"").length)&&(s+=C(i))}else if(0===o)return"0";for(;o%10==0;)o/=10;return s+o}function q(n,e,i){if(n!==~~n||n<e||i<n)throw Error(w+n)}function O(n,e,i,t){var r,s,o;for(s=n[0];10<=s;s/=10)--e;return--e<0?(e+=U,r=0):(r=Math.ceil((e+1)/U),e%=U),s=v(10,U-e),o=n[r]%s|0,null==t?e<3?(0==e?o=o/100|0:1==e&&(o=o/10|0),i<4&&99999==o||3<i&&49999==o||5e4==o||0==o):(i<4&&o+1==s||3<i&&o+1==s/2)&&(n[r+1]/s/100|0)==v(10,e-2)-1||(o==s/2||0==o)&&0==(n[r+1]/s/100|0):e<4?(0==e?o=o/1e3|0:1==e?o=o/100|0:2==e&&(o=o/10|0),(t||i<4)&&9999==o||!t&&3<i&&4999==o):((t||i<4)&&o+1==s||!t&&3<i&&o+1==s/2)&&(n[r+1]/s/1e3|0)==v(10,e-3)-1}function D(n,e,i){for(var t,r,s=[0],o=0,u=n.length;o<u;){for(r=s.length;r--;)s[r]*=e;for(s[0]+=m.indexOf(n.charAt(o++)),t=0;t<s.length;t++)s[t]>i-1&&(void 0===s[t+1]&&(s[t+1]=0),s[t+1]+=s[t]/i|0,s[t]%=i)}return s.reverse()}y.absoluteValue=y.abs=function(){var n=new this.constructor(this);return n.s<0&&(n.s=1),_(n)},y.ceil=function(){return _(new this.constructor(this),this.e+1,2)},y.comparedTo=y.cmp=function(n){var e,i,t,r,s=this,o=s.d,u=(n=new s.constructor(n)).d,c=s.s,f=n.s;if(!o||!u)return c&&f?c!==f?c:o===u?0:!o^c<0?1:-1:NaN;if(!o[0]||!u[0])return o[0]?c:u[0]?-f:0;if(c!==f)return c;if(s.e!==n.e)return s.e>n.e^c<0?1:-1;for(e=0,i=(t=o.length)<(r=u.length)?t:r;e<i;++e)if(o[e]!==u[e])return o[e]>u[e]^c<0?1:-1;return t===r?0:r<t^c<0?1:-1},y.cosine=y.cos=function(){var n,e,i=this,t=i.constructor;return i.d?i.d[0]?(n=t.precision,e=t.rounding,t.precision=n+Math.max(i.e,i.sd())+U,t.rounding=1,i=function(n,e){var i,t,r=e.d.length;t=r<32?(i=Math.ceil(r/3),(1/J(4,i)).toString()):(i=16,"2.3283064365386962890625e-10");n.precision+=i,e=W(n,1,e.times(t),new n(1));for(var s=i;s--;){var o=e.times(e);e=o.times(o).minus(o).times(8).plus(1)}return n.precision-=i,e}(t,z(t,i)),t.precision=n,t.rounding=e,_(2==o||3==o?i.neg():i,n,e,!0)):new t(1):new t(NaN)},y.cubeRoot=y.cbrt=function(){var n,e,i,t,r,s,o,u,c,f,a=this,h=a.constructor;if(!a.isFinite()||a.isZero())return new h(a);for(N=!1,(s=a.s*v(a.s*a,1/3))&&Math.abs(s)!=1/0?t=new h(s.toString()):(i=M(a.d),(s=((n=a.e)-i.length+1)%3)&&(i+=1==s||-2==s?"0":"00"),s=v(i,1/3),n=L((n+1)/3)-(n%3==(n<0?-1:2)),(t=new h(i=s==1/0?"5e"+n:(i=s.toExponential()).slice(0,i.indexOf("e")+1)+n)).s=a.s),o=(n=h.precision)+3;;)if(f=(c=(u=t).times(u).times(u)).plus(a),t=F(f.plus(a).times(u),f.plus(c),o+2,1),M(u.d).slice(0,o)===(i=M(t.d)).slice(0,o)){if("9999"!=(i=i.slice(o-3,o+1))&&(r||"4999"!=i)){+i&&(+i.slice(1)||"5"!=i.charAt(0))||(_(t,n+1,1),e=!t.times(t).times(t).eq(a));break}if(!r&&(_(u,n+1,0),u.times(u).times(u).eq(a))){t=u;break}o+=4,r=1}return N=!0,_(t,n,h.rounding,e)},y.decimalPlaces=y.dp=function(){var n,e=this.d,i=NaN;if(e){if(i=((n=e.length-1)-L(this.e/U))*U,n=e[n])for(;n%10==0;n/=10)i--;i<0&&(i=0)}return i},y.dividedBy=y.div=function(n){return F(this,new this.constructor(n))},y.dividedToIntegerBy=y.divToInt=function(n){var e=this.constructor;return _(F(this,new e(n),0,1,1),e.precision,e.rounding)},y.equals=y.eq=function(n){return 0===this.cmp(n)},y.floor=function(){return _(new this.constructor(this),this.e+1,3)},y.greaterThan=y.gt=function(n){return 0<this.cmp(n)},y.greaterThanOrEqualTo=y.gte=function(n){var e=this.cmp(n);return 1==e||0===e},y.hyperbolicCosine=y.cosh=function(){var n,e,i,t,r,s=this,o=s.constructor,u=new o(1);if(!s.isFinite())return new o(s.s?1/0:NaN);if(s.isZero())return u;i=o.precision,t=o.rounding,o.precision=i+Math.max(s.e,s.sd())+4,o.rounding=1,e=(r=s.d.length)<32?(1/J(4,n=Math.ceil(r/3))).toString():(n=16,"2.3283064365386962890625e-10"),s=W(o,1,s.times(e),new o(1),!0);for(var c,f=n,a=new o(8);f--;)c=s.times(s),s=u.minus(c.times(a.minus(c.times(a))));return _(s,o.precision=i,o.rounding=t,!0)},y.hyperbolicSine=y.sinh=function(){var n,e,i,t,r=this,s=r.constructor;if(!r.isFinite()||r.isZero())return new s(r);if(e=s.precision,i=s.rounding,s.precision=e+Math.max(r.e,r.sd())+4,s.rounding=1,(t=r.d.length)<3)r=W(s,2,r,r,!0);else{n=16<(n=1.4*Math.sqrt(t))?16:0|n,r=W(s,2,r=r.times(1/J(5,n)),r,!0);for(var o,u=new s(5),c=new s(16),f=new s(20);n--;)o=r.times(r),r=r.times(u.plus(o.times(c.times(o).plus(f))))}return _(r,s.precision=e,s.rounding=i,!0)},y.hyperbolicTangent=y.tanh=function(){var n,e,i=this,t=i.constructor;return i.isFinite()?i.isZero()?new t(i):(n=t.precision,e=t.rounding,t.precision=n+7,t.rounding=1,F(i.sinh(),i.cosh(),t.precision=n,t.rounding=e)):new t(i.s)},y.inverseCosine=y.acos=function(){var n,e=this,i=e.constructor,t=e.abs().cmp(1),r=i.precision,s=i.rounding;return-1!==t?0===t?e.isNeg()?P(i,r,s):new i(0):new i(NaN):e.isZero()?P(i,r+4,s).times(.5):(i.precision=r+6,i.rounding=1,e=e.asin(),n=P(i,r+4,s).times(.5),i.precision=r,i.rounding=s,n.minus(e))},y.inverseHyperbolicCosine=y.acosh=function(){var n,e,i=this,t=i.constructor;return i.lte(1)?new t(i.eq(1)?0:NaN):i.isFinite()?(n=t.precision,e=t.rounding,t.precision=n+Math.max(Math.abs(i.e),i.sd())+4,t.rounding=1,N=!1,i=i.times(i).minus(1).sqrt().plus(i),N=!0,t.precision=n,t.rounding=e,i.ln()):new t(i)},y.inverseHyperbolicSine=y.asinh=function(){var n,e,i=this,t=i.constructor;return!i.isFinite()||i.isZero()?new t(i):(n=t.precision,e=t.rounding,t.precision=n+2*Math.max(Math.abs(i.e),i.sd())+6,t.rounding=1,N=!1,i=i.times(i).plus(1).sqrt().plus(i),N=!0,t.precision=n,t.rounding=e,i.ln())},y.inverseHyperbolicTangent=y.atanh=function(){var n,e,i,t,r=this,s=r.constructor;return r.isFinite()?0<=r.e?new s(r.abs().eq(1)?r.s/0:r.isZero()?r:NaN):(n=s.precision,e=s.rounding,t=r.sd(),Math.max(t,n)<2*-r.e-1?_(new s(r),n,e,!0):(s.precision=i=t-r.e,r=F(r.plus(1),new s(1).minus(r),i+n,1),s.precision=n+4,s.rounding=1,r=r.ln(),s.precision=n,s.rounding=e,r.times(.5))):new s(NaN)},y.inverseSine=y.asin=function(){var n,e,i,t,r=this,s=r.constructor;return r.isZero()?new s(r):(e=r.abs().cmp(1),i=s.precision,t=s.rounding,-1!==e?0===e?((n=P(s,i+4,t).times(.5)).s=r.s,n):new s(NaN):(s.precision=i+6,s.rounding=1,r=r.div(new s(1).minus(r.times(r)).sqrt().plus(1)).atan(),s.precision=i,s.rounding=t,r.times(2)))},y.inverseTangent=y.atan=function(){var n,e,i,t,r,s,o,u,c,f=this,a=f.constructor,h=a.precision,l=a.rounding;if(f.isFinite()){if(f.isZero())return new a(f);if(f.abs().eq(1)&&h+4<=x)return(o=P(a,h+4,l).times(.25)).s=f.s,o}else{if(!f.s)return new a(NaN);if(h+4<=x)return(o=P(a,h+4,l).times(.5)).s=f.s,o}for(a.precision=u=h+10,a.rounding=1,n=i=Math.min(28,u/U+2|0);n;--n)f=f.div(f.times(f).plus(1).sqrt().plus(1));for(N=!1,e=Math.ceil(u/U),t=1,c=f.times(f),o=new a(f),r=f;-1!==n;)if(r=r.times(c),s=o.minus(r.div(t+=2)),r=r.times(c),void 0!==(o=s.plus(r.div(t+=2))).d[e])for(n=e;o.d[n]===s.d[n]&&n--;);return i&&(o=o.times(2<<i-1)),N=!0,_(o,a.precision=h,a.rounding=l,!0)},y.isFinite=function(){return!!this.d},y.isInteger=y.isInt=function(){return!!this.d&&L(this.e/U)>this.d.length-2},y.isNaN=function(){return!this.s},y.isNegative=y.isNeg=function(){return this.s<0},y.isPositive=y.isPos=function(){return 0<this.s},y.isZero=function(){return!!this.d&&0===this.d[0]},y.lessThan=y.lt=function(n){return this.cmp(n)<0},y.lessThanOrEqualTo=y.lte=function(n){return this.cmp(n)<1},y.logarithm=y.log=function(n){var e,i,t,r,s,o,u,c,f=this,a=f.constructor,h=a.precision,l=a.rounding;if(null==n)n=new a(10),e=!0;else{if(i=(n=new a(n)).d,n.s<0||!i||!i[0]||n.eq(1))return new a(NaN);e=n.eq(10)}if(i=f.d,f.s<0||!i||!i[0]||f.eq(1))return new a(i&&!i[0]?-1/0:1!=f.s?NaN:i?0:1/0);if(e)if(1<i.length)s=!0;else{for(r=i[0];r%10==0;)r/=10;s=1!==r}if(N=!1,o=V(f,u=h+5),t=e?Z(a,u+10):V(n,u),O((c=F(o,t,u,1)).d,r=h,l))do{if(o=V(f,u+=10),t=e?Z(a,u+10):V(n,u),c=F(o,t,u,1),!s){+M(c.d).slice(r+1,r+15)+1==1e14&&(c=_(c,h+1,0));break}}while(O(c.d,r+=10,l));return N=!0,_(c,h,l)},y.minus=y.sub=function(n){var e,i,t,r,s,o,u,c,f,a,h,l,d=this,p=d.constructor;if(n=new p(n),!d.d||!n.d)return d.s&&n.s?d.d?n.s=-n.s:n=new p(n.d||d.s!==n.s?d:NaN):n=new p(NaN),n;if(d.s!=n.s)return n.s=-n.s,d.plus(n);if(f=d.d,l=n.d,u=p.precision,c=p.rounding,!f[0]||!l[0]){if(l[0])n.s=-n.s;else{if(!f[0])return new p(3===c?-0:0);n=new p(d)}return N?_(n,u,c):n}if(i=L(n.e/U),a=L(d.e/U),f=f.slice(),s=a-i){for(o=(h=s<0)?(e=f,s=-s,l.length):(e=l,i=a,f.length),(t=Math.max(Math.ceil(u/U),o)+2)<s&&(s=t,e.length=1),e.reverse(),t=s;t--;)e.push(0);e.reverse()}else{for((h=(t=f.length)<(o=l.length))&&(o=t),t=0;t<o;t++)if(f[t]!=l[t]){h=f[t]<l[t];break}s=0}for(h&&(e=f,f=l,l=e,n.s=-n.s),o=f.length,t=l.length-o;0<t;--t)f[o++]=0;for(t=l.length;s<t;){if(f[--t]<l[t]){for(r=t;r&&0===f[--r];)f[r]=T-1;--f[r],f[t]+=T}f[t]-=l[t]}for(;0===f[--o];)f.pop();for(;0===f[0];f.shift())--i;return f[0]?(n.d=f,n.e=S(f,i),N?_(n,u,c):n):new p(3===c?-0:0)},y.modulo=y.mod=function(n){var e,i=this,t=i.constructor;return n=new t(n),!i.d||!n.s||n.d&&!n.d[0]?new t(NaN):!n.d||i.d&&!i.d[0]?_(new t(i),t.precision,t.rounding):(N=!1,9==t.modulo?(e=F(i,n.abs(),0,3,1)).s*=n.s:e=F(i,n,0,t.modulo,1),e=e.times(n),N=!0,i.minus(e))},y.naturalExponential=y.exp=function(){return B(this)},y.naturalLogarithm=y.ln=function(){return V(this)},y.negated=y.neg=function(){var n=new this.constructor(this);return n.s=-n.s,_(n)},y.plus=y.add=function(n){var e,i,t,r,s,o,u,c,f,a,h=this,l=h.constructor;if(n=new l(n),!h.d||!n.d)return h.s&&n.s?h.d||(n=new l(n.d||h.s===n.s?h:NaN)):n=new l(NaN),n;if(h.s!=n.s)return n.s=-n.s,h.minus(n);if(f=h.d,a=n.d,u=l.precision,c=l.rounding,!f[0]||!a[0])return a[0]||(n=new l(h)),N?_(n,u,c):n;if(s=L(h.e/U),t=L(n.e/U),f=f.slice(),r=s-t){for((o=(o=r<0?(i=f,r=-r,a.length):(i=a,t=s,f.length))<(s=Math.ceil(u/U))?s+1:o+1)<r&&(r=o,i.length=1),i.reverse();r--;)i.push(0);i.reverse()}for((o=f.length)-(r=a.length)<0&&(r=o,i=a,a=f,f=i),e=0;r;)e=(f[--r]=f[r]+a[r]+e)/T|0,f[r]%=T;for(e&&(f.unshift(e),++t),o=f.length;0==f[--o];)f.pop();return n.d=f,n.e=S(f,t),N?_(n,u,c):n},y.precision=y.sd=function(n){var e;if(void 0!==n&&n!==!!n&&1!==n&&0!==n)throw Error(w+n);return this.d?(e=k(this.d),n&&this.e+1>e&&(e=this.e+1)):e=NaN,e},y.round=function(){var n=this.constructor;return _(new n(this),this.e+1,n.rounding)},y.sine=y.sin=function(){var n,e,i=this,t=i.constructor;return i.isFinite()?i.isZero()?new t(i):(n=t.precision,e=t.rounding,t.precision=n+Math.max(i.e,i.sd())+U,t.rounding=1,i=function(n,e){var i,t=e.d.length;if(t<3)return W(n,2,e,e);i=16<(i=1.4*Math.sqrt(t))?16:0|i,e=e.times(1/J(5,i)),e=W(n,2,e,e);for(var r,s=new n(5),o=new n(16),u=new n(20);i--;)r=e.times(e),e=e.times(s.plus(r.times(o.times(r).minus(u))));return e}(t,z(t,i)),t.precision=n,t.rounding=e,_(2<o?i.neg():i,n,e,!0)):new t(NaN)},y.squareRoot=y.sqrt=function(){var n,e,i,t,r,s,o=this,u=o.d,c=o.e,f=o.s,a=o.constructor;if(1!==f||!u||!u[0])return new a(!f||f<0&&(!u||u[0])?NaN:u?o:1/0);for(N=!1,t=0==(f=Math.sqrt(+o))||f==1/0?(((e=M(u)).length+c)%2==0&&(e+="0"),f=Math.sqrt(e),c=L((c+1)/2)-(c<0||c%2),new a(e=f==1/0?"1e"+c:(e=f.toExponential()).slice(0,e.indexOf("e")+1)+c)):new a(f.toString()),i=(c=a.precision)+3;;)if(t=(s=t).plus(F(o,s,i+2,1)).times(.5),M(s.d).slice(0,i)===(e=M(t.d)).slice(0,i)){if("9999"!=(e=e.slice(i-3,i+1))&&(r||"4999"!=e)){+e&&(+e.slice(1)||"5"!=e.charAt(0))||(_(t,c+1,1),n=!t.times(t).eq(o));break}if(!r&&(_(s,c+1,0),s.times(s).eq(o))){t=s;break}i+=4,r=1}return N=!0,_(t,c,a.rounding,n)},y.tangent=y.tan=function(){var n,e,i=this,t=i.constructor;return i.isFinite()?i.isZero()?new t(i):(n=t.precision,e=t.rounding,t.precision=n+10,t.rounding=1,(i=i.sin()).s=1,i=F(i,new t(1).minus(i.times(i)).sqrt(),n+10,0),t.precision=n,t.rounding=e,_(2==o||4==o?i.neg():i,n,e,!0)):new t(NaN)},y.times=y.mul=function(n){var e,i,t,r,s,o,u,c,f,a=this.constructor,h=this.d,l=(n=new a(n)).d;if(n.s*=this.s,!(h&&h[0]&&l&&l[0]))return new a(!n.s||h&&!h[0]&&!l||l&&!l[0]&&!h?NaN:h&&l?0*n.s:n.s/0);for(i=L(this.e/U)+L(n.e/U),(c=h.length)<(f=l.length)&&(s=h,h=l,l=s,o=c,c=f,f=o),s=[],t=o=c+f;t--;)s.push(0);for(t=f;0<=--t;){for(e=0,r=c+t;t<r;)u=s[r]+l[t]*h[r-t-1]+e,s[r--]=u%T|0,e=u/T|0;s[r]=(s[r]+e)%T|0}for(;!s[--o];)s.pop();return e?++i:s.shift(),n.d=s,n.e=S(s,i),N?_(n,a.precision,a.rounding):n},y.toBinary=function(n,e){return G(this,2,n,e)},y.toDecimalPlaces=y.toDP=function(n,e){var i=this,t=i.constructor;return i=new t(i),void 0===n?i:(q(n,0,g),void 0===e?e=t.rounding:q(e,0,8),_(i,n+i.e+1,e))},y.toExponential=function(n,e){var i,t=this,r=t.constructor;return i=void 0===n?A(t,!0):(q(n,0,g),void 0===e?e=r.rounding:q(e,0,8),A(t=_(new r(t),n+1,e),!0,n+1)),t.isNeg()&&!t.isZero()?"-"+i:i},y.toFixed=function(n,e){var i,t,r=this,s=r.constructor;return i=void 0===n?A(r):(q(n,0,g),void 0===e?e=s.rounding:q(e,0,8),A(t=_(new s(r),n+r.e+1,e),!1,n+t.e+1)),r.isNeg()&&!r.isZero()?"-"+i:i},y.toFraction=function(n){var e,i,t,r,s,o,u,c,f,a,h,l,d=this,p=d.d,g=d.constructor;if(!p)return new g(d);if(f=i=new g(1),o=(s=(e=new g(t=c=new g(0))).e=k(p)-d.e-1)%U,e.d[0]=v(10,o<0?U+o:o),null==n)n=0<s?e:f;else{if(!(u=new g(n)).isInt()||u.lt(f))throw Error(w+u);n=u.gt(e)?0<s?e:f:u}for(N=!1,u=new g(M(p)),a=g.precision,g.precision=s=p.length*U*2;h=F(u,e,0,1,1),1!=(r=i.plus(h.times(t))).cmp(n);)i=t,t=r,r=f,f=c.plus(h.times(r)),c=r,r=e,e=u.minus(h.times(r)),u=r;return r=F(n.minus(i),t,0,1,1),c=c.plus(r.times(f)),i=i.plus(r.times(t)),c.s=f.s=d.s,l=F(f,t,s,1).minus(d).abs().cmp(F(c,i,s,1).minus(d).abs())<1?[f,t]:[c,i],g.precision=a,N=!0,l},y.toHexadecimal=y.toHex=function(n,e){return G(this,16,n,e)},y.toNearest=function(n,e){var i=this,t=i.constructor;if(i=new t(i),null==n){if(!i.d)return i;n=new t(1),e=t.rounding}else{if(n=new t(n),void 0===e?e=t.rounding:q(e,0,8),!i.d)return n.s?i:n;if(!n.d)return n.s&&(n.s=i.s),n}return n.d[0]?(N=!1,i=F(i,n,0,e,1).times(n),N=!0,_(i)):(n.s=i.s,i=n),i},y.toNumber=function(){return+this},y.toOctal=function(n,e){return G(this,8,n,e)},y.toPower=y.pow=function(n){var e,i,t,r,s,o,u=this,c=u.constructor,f=+(n=new c(n));if(!(u.d&&n.d&&u.d[0]&&n.d[0]))return new c(v(+u,f));if((u=new c(u)).eq(1))return u;if(t=c.precision,s=c.rounding,n.eq(1))return _(u,t,s);if((e=L(n.e/U))>=n.d.length-1&&(i=f<0?-f:f)<=9007199254740991)return r=I(c,u,i,t),n.s<0?new c(1).div(r):_(r,t,s);if((o=u.s)<0){if(e<n.d.length-1)return new c(NaN);if(0==(1&n.d[e])&&(o=1),0==u.e&&1==u.d[0]&&1==u.d.length)return u.s=o,u}return(e=0!=(i=v(+u,f))&&isFinite(i)?new c(i+"").e:L(f*(Math.log("0."+M(u.d))/Math.LN10+u.e+1)))>c.maxE+1||e<c.minE-1?new c(0<e?o/0:0):(N=!1,c.rounding=u.s=1,i=Math.min(12,(e+"").length),(r=B(n.times(V(u,t+i)),t)).d&&O((r=_(r,t+5,1)).d,t,s)&&(e=t+10,+M((r=_(B(n.times(V(u,e+i)),e),e+5,1)).d).slice(t+1,t+15)+1==1e14&&(r=_(r,t+1,0))),r.s=o,N=!0,_(r,t,c.rounding=s))},y.toPrecision=function(n,e){var i,t=this,r=t.constructor;return i=void 0===n?A(t,t.e<=r.toExpNeg||t.e>=r.toExpPos):(q(n,1,g),void 0===e?e=r.rounding:q(e,0,8),A(t=_(new r(t),n,e),n<=t.e||t.e<=r.toExpNeg,n)),t.isNeg()&&!t.isZero()?"-"+i:i},y.toSignificantDigits=y.toSD=function(n,e){var i=this.constructor;return void 0===n?(n=i.precision,e=i.rounding):(q(n,1,g),void 0===e?e=i.rounding:q(e,0,8)),_(new i(this),n,e)},y.toString=function(){var n=this,e=n.constructor,i=A(n,n.e<=e.toExpNeg||n.e>=e.toExpPos);return n.isNeg()&&!n.isZero()?"-"+i:i},y.truncated=y.trunc=function(){return _(new this.constructor(this),this.e+1,1)},y.valueOf=y.toJSON=function(){var n=this,e=n.constructor,i=A(n,n.e<=e.toExpNeg||n.e>=e.toExpPos);return n.isNeg()?"-"+i:i};var F=function(){function S(n,e,i){var t,r=0,s=n.length;for(n=n.slice();s--;)t=n[s]*e+r,n[s]=t%i|0,r=t/i|0;return r&&n.unshift(r),n}function Z(n,e,i,t){var r,s;if(i!=t)s=t<i?1:-1;else for(r=s=0;r<i;r++)if(n[r]!=e[r]){s=n[r]>e[r]?1:-1;break}return s}function P(n,e,i,t){for(var r=0;i--;)n[i]-=r,r=n[i]<e[i]?1:0,n[i]=r*t+n[i]-e[i];for(;!n[0]&&1<n.length;)n.shift()}return function(n,e,i,t,r,s){var o,u,c,f,a,h,l,d,p,g,m,w,v,N,b,E,x,y,M,q,O=n.constructor,D=n.s==e.s?1:-1,F=n.d,A=e.d;if(!(F&&F[0]&&A&&A[0]))return new O(n.s&&e.s&&(F?!A||F[0]!=A[0]:A)?F&&0==F[0]||!A?0*D:D/0:NaN);for(u=s?(a=1,n.e-e.e):(s=T,a=U,L(n.e/a)-L(e.e/a)),M=A.length,x=F.length,g=(p=new O(D)).d=[],c=0;A[c]==(F[c]||0);c++);if(A[c]>(F[c]||0)&&u--,null==i?(N=i=O.precision,t=O.rounding):N=r?i+(n.e-e.e)+1:i,N<0)g.push(1),h=!0;else{if(N=N/a+2|0,c=0,1==M){for(A=A[f=0],N++;(c<x||f)&&N--;c++)b=f*s+(F[c]||0),g[c]=b/A|0,f=b%A|0;h=f||c<x}else{for(1<(f=s/(A[0]+1)|0)&&(A=S(A,f,s),F=S(F,f,s),M=A.length,x=F.length),E=M,w=(m=F.slice(0,M)).length;w<M;)m[w++]=0;for((q=A.slice()).unshift(0),y=A[0],A[1]>=s/2&&++y;f=0,(o=Z(A,m,M,w))<0?(v=m[0],M!=w&&(v=v*s+(m[1]||0)),1<(f=v/y|0)?(s<=f&&(f=s-1),1==(o=Z(l=S(A,f,s),m,d=l.length,w=m.length))&&(f--,P(l,M<d?q:A,d,s))):(0==f&&(o=f=1),l=A.slice()),(d=l.length)<w&&l.unshift(0),P(m,l,w,s),-1==o&&(o=Z(A,m,M,w=m.length))<1&&(f++,P(m,M<w?q:A,w,s)),w=m.length):0===o&&(f++,m=[0]),g[c++]=f,o&&m[0]?m[w++]=F[E]||0:(m=[F[E]],w=1),(E++<x||void 0!==m[0])&&N--;);h=void 0!==m[0]}g[0]||g.shift()}if(1==a)p.e=u,R=h;else{for(c=1,f=g[0];10<=f;f/=10)c++;p.e=c+u*a-1,_(p,r?i+p.e+1:i,t,h)}return p}}();function _(n,e,i,t){var r,s,o,u,c,f,a,h,l,d=n.constructor;n:if(null!=e){if(!(h=n.d))return n;for(r=1,u=h[0];10<=u;u/=10)r++;if((s=e-r)<0)s+=U,o=e,c=(a=h[l=0])/v(10,r-o-1)%10|0;else if(l=Math.ceil((s+1)/U),(u=h.length)<=l){if(!t)break n;for(;u++<=l;)h.push(0);a=c=0,o=(s%=U)-U+(r=1)}else{for(a=u=h[l],r=1;10<=u;u/=10)r++;c=(o=(s%=U)-U+r)<0?0:a/v(10,r-o-1)%10|0}if(t=t||e<0||void 0!==h[l+1]||(o<0?a:a%v(10,r-o-1)),f=i<4?(c||t)&&(0==i||i==(n.s<0?3:2)):5<c||5==c&&(4==i||t||6==i&&(0<s?0<o?a/v(10,r-o):0:h[l-1])%10&1||i==(n.s<0?8:7)),e<1||!h[0])return h.length=0,f?(e-=n.e+1,h[0]=v(10,(U-e%U)%U),n.e=-e||0):h[0]=n.e=0,n;if(0==s?(h.length=l,u=1,l--):(h.length=l+1,u=v(10,U-s),h[l]=0<o?(a/v(10,r-o)%v(10,o)|0)*u:0),f)for(;;){if(0==l){for(s=1,o=h[0];10<=o;o/=10)s++;for(o=h[0]+=u,u=1;10<=o;o/=10)u++;s!=u&&(n.e++,h[0]==T&&(h[0]=1));break}if(h[l]+=u,h[l]!=T)break;h[l--]=0,u=1}for(s=h.length;0===h[--s];)h.pop()}return N&&(n.e>d.maxE?(n.d=null,n.e=NaN):n.e<d.minE&&(n.e=0,n.d=[0])),n}function A(n,e,i){if(!n.isFinite())return j(n);var t,r=n.e,s=M(n.d),o=s.length;return e?(i&&0<(t=i-o)?s=s.charAt(0)+"."+s.slice(1)+C(t):1<o&&(s=s.charAt(0)+"."+s.slice(1)),s=s+(n.e<0?"e":"e+")+n.e):r<0?(s="0."+C(-r-1)+s,i&&0<(t=i-o)&&(s+=C(t))):o<=r?(s+=C(r+1-o),i&&0<(t=i-r-1)&&(s=s+"."+C(t))):((t=r+1)<o&&(s=s.slice(0,t)+"."+s.slice(t)),i&&0<(t=i-o)&&(r+1===o&&(s+="."),s+=C(t))),s}function S(n,e){var i=n[0];for(e*=U;10<=i;i/=10)e++;return e}function Z(n,e,i){if(E<e)throw N=!0,i&&(n.precision=i),Error(s);return _(new n(t),e,1,!0)}function P(n,e,i){if(x<e)throw Error(s);return _(new n(r),e,i,!0)}function k(n){var e=n.length-1,i=e*U+1;if(e=n[e]){for(;e%10==0;e/=10)i--;for(e=n[0];10<=e;e/=10)i++}return i}function C(n){for(var e="";n--;)e+="0";return e}function I(n,e,i,t){var r,s=new n(1),o=Math.ceil(t/U+4);for(N=!1;;){if(i%2&&K((s=s.times(e)).d,o)&&(r=!0),0===(i=L(i/2))){i=s.d.length-1,r&&0===s.d[i]&&++s.d[i];break}K((e=e.times(e)).d,o)}return N=!0,s}function H(n){return 1&n.d[n.d.length-1]}function i(n,e,i){for(var t,r=new n(e[0]),s=0;++s<e.length;){if(!(t=new n(e[s])).s){r=t;break}r[i](t)&&(r=t)}return r}function B(n,e){var i,t,r,s,o,u,c,f=0,a=0,h=0,l=n.constructor,d=l.rounding,p=l.precision;if(!n.d||!n.d[0]||17<n.e)return new l(n.d?n.d[0]?n.s<0?0:1/0:1:n.s?n.s<0?0:n:NaN);for(c=null==e?(N=!1,p):e,u=new l(.03125);-2<n.e;)n=n.times(u),h+=5;for(c+=t=Math.log(v(2,h))/Math.LN10*2+5|0,i=s=o=new l(1),l.precision=c;;){if(s=_(s.times(n),c,1),i=i.times(++a),M((u=o.plus(F(s,i,c,1))).d).slice(0,c)===M(o.d).slice(0,c)){for(r=h;r--;)o=_(o.times(o),c,1);if(null!=e)return l.precision=p,o;if(!(f<3&&O(o.d,c-t,d,f)))return _(o,l.precision=p,d,N=!0);l.precision=c+=10,i=s=u=new l(1),a=0,f++}o=u}}function V(n,e){var i,t,r,s,o,u,c,f,a,h,l,d=1,p=n,g=p.d,m=p.constructor,w=m.rounding,v=m.precision;if(p.s<0||!g||!g[0]||!p.e&&1==g[0]&&1==g.length)return new m(g&&!g[0]?-1/0:1!=p.s?NaN:g?0:p);if(a=null==e?(N=!1,v):e,m.precision=a+=10,t=(i=M(g)).charAt(0),!(Math.abs(s=p.e)<15e14))return f=Z(m,a+2,v).times(s+""),p=V(new m(t+"."+i.slice(1)),a-10).plus(f),m.precision=v,null==e?_(p,v,w,N=!0):p;for(;t<7&&1!=t||1==t&&3<i.charAt(1);)t=(i=M((p=p.times(n)).d)).charAt(0),d++;for(s=p.e,1<t?(p=new m("0."+i),s++):p=new m(t+"."+i.slice(1)),c=o=p=F((h=p).minus(1),p.plus(1),a,1),l=_(p.times(p),a,1),r=3;;){if(o=_(o.times(l),a,1),M((f=c.plus(F(o,new m(r),a,1))).d).slice(0,a)===M(c.d).slice(0,a)){if(c=c.times(2),0!==s&&(c=c.plus(Z(m,a+2,v).times(s+""))),c=F(c,new m(d),a,1),null!=e)return m.precision=v,c;if(!O(c.d,a-10,w,u))return _(c,m.precision=v,w,N=!0);m.precision=a+=10,f=o=p=F(h.minus(1),h.plus(1),a,1),l=_(p.times(p),a,1),r=u=1}c=f,r+=2}}function j(n){return String(n.s*n.s/0)}function $(n,e){var i,t,r;for(-1<(i=e.indexOf("."))&&(e=e.replace(".","")),0<(t=e.search(/e/i))?(i<0&&(i=t),i+=+e.slice(t+1),e=e.substring(0,t)):i<0&&(i=e.length),t=0;48===e.charCodeAt(t);t++);for(r=e.length;48===e.charCodeAt(r-1);--r);if(e=e.slice(t,r)){if(r-=t,n.e=i=i-t-1,n.d=[],t=(i+1)%U,i<0&&(t+=U),t<r){for(t&&n.d.push(+e.slice(0,t)),r-=U;t<r;)n.d.push(+e.slice(t,t+=U));e=e.slice(t),t=U-e.length}else t-=r;for(;t--;)e+="0";n.d.push(+e),N&&(n.e>n.constructor.maxE?(n.d=null,n.e=NaN):n.e<n.constructor.minE&&(n.e=0,n.d=[0]))}else n.e=0,n.d=[0];return n}function W(n,e,i,t,r){var s,o,u,c,f=n.precision,a=Math.ceil(f/U);for(N=!1,c=i.times(i),u=new n(t);;){if(o=F(u.times(c),new n(e++*e++),f,1),u=r?t.plus(o):t.minus(o),t=F(o.times(c),new n(e++*e++),f,1),void 0!==(o=u.plus(t)).d[a]){for(s=a;o.d[s]===u.d[s]&&s--;);if(-1==s)break}s=u,u=t,t=o,o=s,0}return N=!0,o.d.length=a+1,o}function J(n,e){for(var i=n;--e;)i*=n;return i}function z(n,e){var i,t=e.s<0,r=P(n,n.precision,1),s=r.times(.5);if((e=e.abs()).lte(s))return o=t?4:1,e;if((i=e.divToInt(r)).isZero())o=t?3:2;else{if((e=e.minus(i.times(r))).lte(s))return o=H(i)?t?2:3:t?4:1,e;o=H(i)?t?1:4:t?3:2}return e.minus(r).abs()}function G(n,e,i,t){var r,s,o,u,c,f,a,h,l,d=n.constructor,p=void 0!==i;if(p?(q(i,1,g),void 0===t?t=d.rounding:q(t,0,8)):(i=d.precision,t=d.rounding),n.isFinite()){for(p?(r=2,16==e?i=4*i-3:8==e&&(i=3*i-2)):r=e,0<=(o=(a=A(n)).indexOf("."))&&(a=a.replace(".",""),(l=new d(1)).e=a.length-o,l.d=D(A(l),10,r),l.e=l.d.length),s=c=(h=D(a,10,r)).length;0==h[--c];)h.pop();if(h[0]){if(o<0?s--:((n=new d(n)).d=h,n.e=s,h=(n=F(n,l,i,t,0,r)).d,s=n.e,f=R),o=h[i],u=r/2,f=f||void 0!==h[i+1],f=t<4?(void 0!==o||f)&&(0===t||t===(n.s<0?3:2)):u<o||o===u&&(4===t||f||6===t&&1&h[i-1]||t===(n.s<0?8:7)),h.length=i,f)for(;++h[--i]>r-1;)h[i]=0,i||(++s,h.unshift(1));for(c=h.length;!h[c-1];--c);for(o=0,a="";o<c;o++)a+=m.charAt(h[o]);if(p){if(1<c)if(16==e||8==e){for(o=16==e?4:3,--c;c%o;c++)a+="0";for(c=(h=D(a,r,e)).length;!h[c-1];--c);for(o=1,a="1.";o<c;o++)a+=m.charAt(h[o])}else a=a.charAt(0)+"."+a.slice(1);a=a+(s<0?"p":"p+")+s}else if(s<0){for(;++s;)a="0"+a;a="0."+a}else if(++s>c)for(s-=c;s--;)a+="0";else s<c&&(a=a.slice(0,s)+"."+a.slice(s))}else a=p?"0p+0":"0";a=(16==e?"0x":2==e?"0b":8==e?"0o":"")+a}else a=j(n);return n.s<0?"-"+a:a}function K(n,e){if(n.length>e)return n.length=e,!0}function Q(n){return new this(n).abs()}function X(n){return new this(n).acos()}function Y(n){return new this(n).acosh()}function nn(n,e){return new this(n).plus(e)}function en(n){return new this(n).asin()}function tn(n){return new this(n).asinh()}function rn(n){return new this(n).atan()}function sn(n){return new this(n).atanh()}function on(n,e){n=new this(n),e=new this(e);var i,t=this.precision,r=this.rounding,s=t+4;return n.s&&e.s?n.d||e.d?!e.d||n.isZero()?(i=e.s<0?P(this,t,r):new this(0)).s=n.s:!n.d||e.isZero()?(i=P(this,s,1).times(.5)).s=n.s:i=e.s<0?(this.precision=s,this.rounding=1,i=this.atan(F(n,e,s,1)),e=P(this,s,1),this.precision=t,this.rounding=r,n.s<0?i.minus(e):i.plus(e)):this.atan(F(n,e,s,1)):(i=P(this,s,1).times(0<e.s?.25:.75)).s=n.s:i=new this(NaN),i}function un(n){return new this(n).cbrt()}function cn(n){return _(n=new this(n),n.e+1,2)}function fn(n){if(!n||"object"!=typeof n)throw Error(f+"Object expected");var e,i,t,r=!0===n.defaults,s=["precision",1,g,"rounding",0,8,"toExpNeg",-u,0,"toExpPos",0,u,"maxE",0,u,"minE",-u,0,"modulo",0,9];for(e=0;e<s.length;e+=3)if(i=s[e],r&&(this[i]=c[i]),void 0!==(t=n[i])){if(!(L(t)===t&&s[e+1]<=t&&t<=s[e+2]))throw Error(w+i+": "+t);this[i]=t}if(i="crypto",r&&(this[i]=c[i]),void 0!==(t=n[i])){if(!0!==t&&!1!==t&&0!==t&&1!==t)throw Error(w+i+": "+t);if(t){if("undefined"==typeof crypto||!crypto||!crypto.getRandomValues&&!crypto.randomBytes)throw Error(a);this[i]=!0}else this[i]=!1}return this}function an(n){return new this(n).cos()}function hn(n){return new this(n).cosh()}function ln(n,e){return new this(n).div(e)}function dn(n){return new this(n).exp()}function pn(n){return _(n=new this(n),n.e+1,3)}function gn(){var n,e,i=new this(0);for(N=!1,n=0;n<arguments.length;)if((e=new this(arguments[n++])).d)i.d&&(i=i.plus(e.times(e)));else{if(e.s)return N=!0,new this(1/0);i=e}return N=!0,i.sqrt()}function mn(n){return n instanceof h||n&&"[object Decimal]"===n.name||!1}function wn(n){return new this(n).ln()}function vn(n,e){return new this(n).log(e)}function Nn(n){return new this(n).log(2)}function bn(n){return new this(n).log(10)}function En(){return i(this,arguments,"lt")}function xn(){return i(this,arguments,"gt")}function yn(n,e){return new this(n).mod(e)}function Mn(n,e){return new this(n).mul(e)}function qn(n,e){return new this(n).pow(e)}function On(n){var e,i,t,r,s=0,o=new this(1),u=[];if(void 0===n?n=this.precision:q(n,1,g),t=Math.ceil(n/U),this.crypto)if(crypto.getRandomValues)for(e=crypto.getRandomValues(new Uint32Array(t));s<t;)429e7<=(r=e[s])?e[s]=crypto.getRandomValues(new Uint32Array(1))[0]:u[s++]=r%1e7;else{if(!crypto.randomBytes)throw Error(a);for(e=crypto.randomBytes(t*=4);s<t;)214e7<=(r=e[s]+(e[s+1]<<8)+(e[s+2]<<16)+((127&e[s+3])<<24))?crypto.randomBytes(4).copy(e,s):(u.push(r%1e7),s+=4);s=t/4}else for(;s<t;)u[s++]=1e7*Math.random()|0;for(t=u[--s],n%=U,t&&n&&(r=v(10,U-n),u[s]=(t/r|0)*r);0===u[s];s--)u.pop();if(s<0)u=[i=0];else{for(i=-1;0===u[0];i-=U)u.shift();for(t=1,r=u[0];10<=r;r/=10)t++;t<U&&(i-=U-t)}return o.e=i,o.d=u,o}function Dn(n){return _(n=new this(n),n.e+1,this.rounding)}function Fn(n){return(n=new this(n)).d?n.d[0]?n.s:0*n.s:n.s||NaN}function An(n){return new this(n).sin()}function Sn(n){return new this(n).sinh()}function Zn(n){return new this(n).sqrt()}function Pn(n,e){return new this(n).sub(e)}function Rn(n){return new this(n).tan()}function Ln(n){return new this(n).tanh()}function Tn(n){return _(n=new this(n),n.e+1,1)}(h=function n(e){var i,t,r;function s(n){var e,i,t,r=this;if(!(r instanceof s))return new s(n);if(n instanceof(r.constructor=s))return r.s=n.s,void(N?!n.d||n.e>s.maxE?(r.e=NaN,r.d=null):n.e<s.minE?(r.e=0,r.d=[0]):(r.e=n.e,r.d=n.d.slice()):(r.e=n.e,r.d=n.d?n.d.slice():n.d));if("number"==(t=typeof n)){if(0===n)return r.s=1/n<0?-1:1,r.e=0,void(r.d=[0]);if(r.s=n<0?(n=-n,-1):1,n===~~n&&n<1e7){for(e=0,i=n;10<=i;i/=10)e++;return void(r.d=N?s.maxE<e?(r.e=NaN,null):e<s.minE?[r.e=0]:(r.e=e,[n]):(r.e=e,[n]))}return 0*n!=0?(n||(r.s=NaN),r.e=NaN,void(r.d=null)):$(r,n.toString())}if("string"!==t)throw Error(w+n);return 45===(i=n.charCodeAt(0))?(n=n.slice(1),r.s=-1):(43===i&&(n=n.slice(1)),r.s=1),b.test(n)?$(r,n):function(n,e){var i,t,r,s,o,u,c,f,a;if("Infinity"===e||"NaN"===e)return+e||(n.s=NaN),n.e=NaN,n.d=null,n;if(d.test(e))i=16,e=e.toLowerCase();else if(l.test(e))i=2;else{if(!p.test(e))throw Error(w+e);i=8}for(o=0<=(s=(e=0<(s=e.search(/p/i))?(c=+e.slice(s+1),e.substring(2,s)):e.slice(2)).indexOf(".")),t=n.constructor,o&&(s=(u=(e=e.replace(".","")).length)-s,r=I(t,new t(i),s,2*s)),s=a=(f=D(e,i,T)).length-1;0===f[s];--s)f.pop();return s<0?new t(0*n.s):(n.e=S(f,a),n.d=f,N=!1,o&&(n=F(n,r,4*u)),c&&(n=n.times(Math.abs(c)<54?v(2,c):h.pow(2,c))),N=!0,n)}(r,n)}if(s.prototype=y,s.ROUND_UP=0,s.ROUND_DOWN=1,s.ROUND_CEIL=2,s.ROUND_FLOOR=3,s.ROUND_HALF_UP=4,s.ROUND_HALF_DOWN=5,s.ROUND_HALF_EVEN=6,s.ROUND_HALF_CEIL=7,s.ROUND_HALF_FLOOR=8,s.EUCLID=9,s.config=s.set=fn,s.clone=n,s.isDecimal=mn,s.abs=Q,s.acos=X,s.acosh=Y,s.add=nn,s.asin=en,s.asinh=tn,s.atan=rn,s.atanh=sn,s.atan2=on,s.cbrt=un,s.ceil=cn,s.cos=an,s.cosh=hn,s.div=ln,s.exp=dn,s.floor=pn,s.hypot=gn,s.ln=wn,s.log=vn,s.log10=bn,s.log2=Nn,s.max=En,s.min=xn,s.mod=yn,s.mul=Mn,s.pow=qn,s.random=On,s.round=Dn,s.sign=Fn,s.sin=An,s.sinh=Sn,s.sqrt=Zn,s.sub=Pn,s.tan=Rn,s.tanh=Ln,s.trunc=Tn,void 0===e&&(e={}),e&&!0!==e.defaults)for(r=["precision","rounding","toExpNeg","toExpPos","maxE","minE","modulo","crypto"],i=0;i<r.length;)e.hasOwnProperty(t=r[i++])||(e[t]=this[t]);return s.config(e),s}(c)).default=h.Decimal=h,t=new h(t),r=new h(r),"function"==typeof define&&define.amd?define(function(){return h}):"undefined"!=typeof module&&module.exports?("function"==typeof Symbol&&"symbol"==typeof Symbol.iterator&&(y[Symbol.for("nodejs.util.inspect.custom")]=y.toString,y[Symbol.toStringTag]="Decimal"),module.exports=h):(n||(n="undefined"!=typeof self&&self&&self.self==self?self:window),e=n.Decimal,h.noConflict=function(){return n.Decimal=e,h},n.Decimal=h)}(this);
//# sourceMappingURL=decimal.min.js.map | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/decimal.min.js | decimal.min.js |
#### 10.2.0
* 08/05/2019
* #128 Workaround V8 `Math.pow` change.
* #93 Accept `+` prefix when parsing string values.
* #129 Fix typo.
#### 10.1.1
* 27/02/2019
* Check `Symbol` properly.
#### 10.1.0
* 26/02/2019
* #122 Add custom `util.inspect()` function.
* Add `Symbol.toStringTag`.
* #121 Constructor: add range check for arguments of type number and Decimal.
* Remove premable from uglifyjs build script.
* Move *decimal.min.js.map* to root directory.
#### 10.0.2
* 13/12/2018
* #114 Remove soureMappingURL from *decimal.min.js*.
* Remove *bower.json*.
#### 10.0.1
* 24/05/2018
* Add `browser` field to *package.json*.
#### 10.0.0
* 10/03/2018
* #88 `toNearest` to return the nearest multiple in the direction of the rounding mode.
* #82 #91 `const` to `var`.
* Add trigonometric precision limit explanantion to documentation.
* Put global ts definitions in separate file (see *bignumber.js* #143).
#### 9.0.1
* 15/12/2017
* #80 Typings: correct return type.
#### 9.0.0
* 14/12/2017
* #78 Typings: remove `toFormat`.
#### 8.0.0
* 10/12/2017
* Correct typings: `toFraction` returns `Decimal[]`.
* Type-checking: add `Decimal.isDecimal` method.
* Enable configuration reset with `defaults: true`.
* Add named export, Decimal, to *decimal.mjs*.
#### 7.5.1
* 03/12/2017
* Remove typo.
#### 7.5.0
* 03/12/2017
* Use TypeScript declarations outside modules.
#### 7.4.0
* 25/11/2017
* Add TypeScript typings.
#### 7.3.0
* 26/09/2017
* Rename *decimal.es6.js* to *decimal.mjs*.
* Amend *.travis.yml*.
#### 7.2.4
* 09/09/2017
* Update docs regarding `global.crypto`.
* Fix `import` issues.
#### 7.2.3
* 27/06/2017
* Bugfix: #58 `pow` sometimes throws when result is `Infinity`.
#### 7.2.2
* 25/06/2017
* Bugfix: #57 Powers of -1 for integers over `Number.MAX_SAFE_INTEGER`.
#### 7.2.1
* 04/05/2017
* Fix *README* badges.
#### 7.2.0
* 09/04/2017
* Add *decimal.es6.js*
#### 7.1.2
* 05/04/2017
* `Decimal.default` to `Decimal['default']` IE8 issue
#### 7.1.1
* 10/01/2017
* Remove duplicated for-loop
* Minor refactoring
#### 7.1.0
* 09/11/2016
* Support ES6 imports.
#### 7.0.0
* 09/11/2016
* Remove `require('crypto')` - leave it to the user
* Default `Decimal.crypto` to `false`
* Add `Decimal.set` as `Decimal.config` alias
#### 6.0.0
* 30/06/2016
* Removed base-88 serialization format
* Amended `toJSON` and removed `Decimal.fromJSON` accordingly
#### 5.0.8
* 09/03/2016
* Add newline to single test results
* Correct year
#### 5.0.7
* 29/02/2016
* Add decimal.js-light link
* Remove outdated example from docs
#### 5.0.6
* 22/02/2016
* Add bower.json
#### 5.0.5
* 20/02/2016
* Bugfix: #26 wrong precision applied
#### 5.0.4
* 14/02/2016
* Bugfix: #26 clone
#### 5.0.3
* 06/02/2016
* Refactor tests
#### 5.0.2
* 05/02/2016
* Added immutability tests
* Minor *decimal.js* clean-up
#### 5.0.1
* 28/01/2016
* Bugfix: #20 cos mutates value
* Add pi info to docs
#### 5.0.0
* 25/01/2016
* Added trigonometric functions and `cubeRoot` method
* Added most of JavaScript's `Math` object methods as Decimal methods
* Added `toBinary`, `toHexadecimal` and `toOctal` methods
* Added `isPositive` method
* Removed the 15 significant digit limit for numbers
* `toFraction` now returns an array of two Decimals, not two strings
* String values containing whitespace or a plus sign are no longer accepted
* `valueOf` now returns `'-0'` for minus zero
* `comparedTo` now returns `NaN` not `null` for comparisons with `NaN`
* `Decimal.max` and `Decimal.min` no longer accept an array
* The Decimal constructor and `toString` no longer accept a base argument
* Binary, hexadecimal and octal prefixes are now recognised for string values
* Removed `Decimal.errors` configuration property
* Removed `toFormat` method
* Removed `Decimal.ONE`
* Renamed `exponential` method to `naturalExponential`
* Renamed `Decimal.constructor` method to `Decimal.clone`
* Simplified error handling and amended error messages
* Refactored the test suite
* `Decimal.crypto` is now `undefined` by default, and the `crypto` object will be used if available
* Major internal refactoring
* Removed *bower.json*
#### 4.0.2
* 20/02/2015 Add bower.json. Add source map. Amend travis CI. Amend doc/comments
#### 4.0.1
* 11/12/2014 Assign correct constructor when duplicating a Decimal
#### 4.0.0
* 10/11/2014 `toFormat` amended to use `Decimal.format` object for more flexible configuration
#### 3.0.1
* 8/06/2014 Surround crypto require in try catch. See issue #5
#### 3.0.0
* 4/06/2014 `random` simplified. Major internal changes mean the properties of a Decimal must now be considered read-only
#### 2.1.0
* 4/06/2014 Amend UMD
#### 2.0.3
* 8/05/2014 Fix NaN toNumber
#### 2.0.2
* 30/04/2014 Correct doc links
#### 2.0.1
* 10/04/2014 Update npmignore
#### 2.0.0
* 10/04/2014 Add `toSignificantDigits`
* Remove `toInteger`
* No arguments to `ceil`, `floor`, `round` and `trunc`
#### 1.0.1
* 07/04/2014 Minor documentation clean-up
#### 1.0.0
* 02/04/2014 Initial release
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/decimal.js/CHANGELOG.md | CHANGELOG.md |
# ip-regex [](https://travis-ci.org/sindresorhus/ip-regex)
> Regular expression for matching IP addresses
## Install
```
$ npm install --save ip-regex
```
## Usage
```js
const ipRegex = require('ip-regex');
// Contains an IP address?
ipRegex().test('unicorn 192.168.0.1');
//=> true
// Is an IP address?
ipRegex({exact: true}).test('unicorn 192.168.0.1');
//=> false
ipRegex.v6({exact: true}).test('1:2:3:4:5:6:7:8');
//=> true
'unicorn 192.168.0.1 cake 1:2:3:4:5:6:7:8 rainbow'.match(ipRegex());
//=> ['192.168.0.1', '1:2:3:4:5:6:7:8']
```
## API
### ipRegex([options])
Returns a regex for matching both IPv4 and IPv6.
### ipRegex.v4([options])
Returns a regex for matching IPv4.
### ipRegex.v6([options])
Returns a regex for matching IPv6.
#### options.exact
Type: `boolean`<br>
Default: `false` *(Matches any IP address in a string)*
Only match an exact string. Useful with `RegExp#test()` to check if a string is an IP address.
## Related
- [is-ip](https://github.com/sindresorhus/is-ip) - Check if a string is an IP address
## License
MIT © [Sindre Sorhus](https://sindresorhus.com)
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/ip-regex/readme.md | readme.md |
A library to create readable ```"multipart/form-data"``` streams. Can be used to submit forms and file uploads to other web applications.
The API of this library is inspired by the [XMLHttpRequest-2 FormData Interface][xhr2-fd].
[xhr2-fd]: http://dev.w3.org/2006/webapi/XMLHttpRequest-2/Overview.html#the-formdata-interface
[](https://travis-ci.org/form-data/form-data)
[](https://travis-ci.org/form-data/form-data)
[](https://ci.appveyor.com/project/alexindigo/form-data)
[](https://coveralls.io/github/form-data/form-data?branch=master)
[](https://david-dm.org/form-data/form-data)
[](https://www.bithound.io/github/form-data/form-data)
## Install
```
npm install --save form-data
```
## Usage
In this example we are constructing a form with 3 fields that contain a string,
a buffer and a file stream.
``` javascript
var FormData = require('form-data');
var fs = require('fs');
var form = new FormData();
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_file', fs.createReadStream('/foo/bar.jpg'));
```
Also you can use http-response stream:
``` javascript
var FormData = require('form-data');
var http = require('http');
var form = new FormData();
http.request('http://nodejs.org/images/logo.png', function(response) {
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_logo', response);
});
```
Or @mikeal's [request](https://github.com/request/request) stream:
``` javascript
var FormData = require('form-data');
var request = require('request');
var form = new FormData();
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_logo', request('http://nodejs.org/images/logo.png'));
```
In order to submit this form to a web application, call ```submit(url, [callback])``` method:
``` javascript
form.submit('http://example.org/', function(err, res) {
// res – response object (http.IncomingMessage) //
res.resume();
});
```
For more advanced request manipulations ```submit()``` method returns ```http.ClientRequest``` object, or you can choose from one of the alternative submission methods.
### Custom options
You can provide custom options, such as `maxDataSize`:
``` javascript
var FormData = require('form-data');
var form = new FormData({ maxDataSize: 20971520 });
form.append('my_field', 'my value');
form.append('my_buffer', /* something big */);
```
List of available options could be found in [combined-stream](https://github.com/felixge/node-combined-stream/blob/master/lib/combined_stream.js#L7-L15)
### Alternative submission methods
You can use node's http client interface:
``` javascript
var http = require('http');
var request = http.request({
method: 'post',
host: 'example.org',
path: '/upload',
headers: form.getHeaders()
});
form.pipe(request);
request.on('response', function(res) {
console.log(res.statusCode);
});
```
Or if you would prefer the `'Content-Length'` header to be set for you:
``` javascript
form.submit('example.org/upload', function(err, res) {
console.log(res.statusCode);
});
```
To use custom headers and pre-known length in parts:
``` javascript
var CRLF = '\r\n';
var form = new FormData();
var options = {
header: CRLF + '--' + form.getBoundary() + CRLF + 'X-Custom-Header: 123' + CRLF + CRLF,
knownLength: 1
};
form.append('my_buffer', buffer, options);
form.submit('http://example.com/', function(err, res) {
if (err) throw err;
console.log('Done');
});
```
Form-Data can recognize and fetch all the required information from common types of streams (```fs.readStream```, ```http.response``` and ```mikeal's request```), for some other types of streams you'd need to provide "file"-related information manually:
``` javascript
someModule.stream(function(err, stdout, stderr) {
if (err) throw err;
var form = new FormData();
form.append('file', stdout, {
filename: 'unicycle.jpg', // ... or:
filepath: 'photos/toys/unicycle.jpg',
contentType: 'image/jpeg',
knownLength: 19806
});
form.submit('http://example.com/', function(err, res) {
if (err) throw err;
console.log('Done');
});
});
```
The `filepath` property overrides `filename` and may contain a relative path. This is typically used when uploading [multiple files from a directory](https://wicg.github.io/entries-api/#dom-htmlinputelement-webkitdirectory).
For edge cases, like POST request to URL with query string or to pass HTTP auth credentials, object can be passed to `form.submit()` as first parameter:
``` javascript
form.submit({
host: 'example.com',
path: '/probably.php?extra=params',
auth: 'username:password'
}, function(err, res) {
console.log(res.statusCode);
});
```
In case you need to also send custom HTTP headers with the POST request, you can use the `headers` key in first parameter of `form.submit()`:
``` javascript
form.submit({
host: 'example.com',
path: '/surelynot.php',
headers: {'x-test-header': 'test-header-value'}
}, function(err, res) {
console.log(res.statusCode);
});
```
### Integration with other libraries
#### Request
Form submission using [request](https://github.com/request/request):
```javascript
var formData = {
my_field: 'my_value',
my_file: fs.createReadStream(__dirname + '/unicycle.jpg'),
};
request.post({url:'http://service.com/upload', formData: formData}, function(err, httpResponse, body) {
if (err) {
return console.error('upload failed:', err);
}
console.log('Upload successful! Server responded with:', body);
});
```
For more details see [request readme](https://github.com/request/request#multipartform-data-multipart-form-uploads).
#### node-fetch
You can also submit a form using [node-fetch](https://github.com/bitinn/node-fetch):
```javascript
var form = new FormData();
form.append('a', 1);
fetch('http://example.com', { method: 'POST', body: form })
.then(function(res) {
return res.json();
}).then(function(json) {
console.log(json);
});
```
## Notes
- ```getLengthSync()``` method DOESN'T calculate length for streams, use ```knownLength``` options as workaround.
- Starting version `2.x` FormData has dropped support for `[email protected]`.
## License
Form-Data is released under the [MIT](License) license. | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/form-data/README.md.bak | README.md.bak |
# Form-Data [](https://www.npmjs.com/package/form-data) [](https://gitter.im/form-data/form-data)
A library to create readable ```"multipart/form-data"``` streams. Can be used to submit forms and file uploads to other web applications.
The API of this library is inspired by the [XMLHttpRequest-2 FormData Interface][xhr2-fd].
[xhr2-fd]: http://dev.w3.org/2006/webapi/XMLHttpRequest-2/Overview.html#the-formdata-interface
[](https://travis-ci.org/form-data/form-data)
[](https://travis-ci.org/form-data/form-data)
[](https://ci.appveyor.com/project/alexindigo/form-data)
[](https://coveralls.io/github/form-data/form-data?branch=master)
[](https://david-dm.org/form-data/form-data)
[](https://www.bithound.io/github/form-data/form-data)
## Install
```
npm install --save form-data
```
## Usage
In this example we are constructing a form with 3 fields that contain a string,
a buffer and a file stream.
``` javascript
var FormData = require('form-data');
var fs = require('fs');
var form = new FormData();
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_file', fs.createReadStream('/foo/bar.jpg'));
```
Also you can use http-response stream:
``` javascript
var FormData = require('form-data');
var http = require('http');
var form = new FormData();
http.request('http://nodejs.org/images/logo.png', function(response) {
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_logo', response);
});
```
Or @mikeal's [request](https://github.com/request/request) stream:
``` javascript
var FormData = require('form-data');
var request = require('request');
var form = new FormData();
form.append('my_field', 'my value');
form.append('my_buffer', new Buffer(10));
form.append('my_logo', request('http://nodejs.org/images/logo.png'));
```
In order to submit this form to a web application, call ```submit(url, [callback])``` method:
``` javascript
form.submit('http://example.org/', function(err, res) {
// res – response object (http.IncomingMessage) //
res.resume();
});
```
For more advanced request manipulations ```submit()``` method returns ```http.ClientRequest``` object, or you can choose from one of the alternative submission methods.
### Custom options
You can provide custom options, such as `maxDataSize`:
``` javascript
var FormData = require('form-data');
var form = new FormData({ maxDataSize: 20971520 });
form.append('my_field', 'my value');
form.append('my_buffer', /* something big */);
```
List of available options could be found in [combined-stream](https://github.com/felixge/node-combined-stream/blob/master/lib/combined_stream.js#L7-L15)
### Alternative submission methods
You can use node's http client interface:
``` javascript
var http = require('http');
var request = http.request({
method: 'post',
host: 'example.org',
path: '/upload',
headers: form.getHeaders()
});
form.pipe(request);
request.on('response', function(res) {
console.log(res.statusCode);
});
```
Or if you would prefer the `'Content-Length'` header to be set for you:
``` javascript
form.submit('example.org/upload', function(err, res) {
console.log(res.statusCode);
});
```
To use custom headers and pre-known length in parts:
``` javascript
var CRLF = '\r\n';
var form = new FormData();
var options = {
header: CRLF + '--' + form.getBoundary() + CRLF + 'X-Custom-Header: 123' + CRLF + CRLF,
knownLength: 1
};
form.append('my_buffer', buffer, options);
form.submit('http://example.com/', function(err, res) {
if (err) throw err;
console.log('Done');
});
```
Form-Data can recognize and fetch all the required information from common types of streams (```fs.readStream```, ```http.response``` and ```mikeal's request```), for some other types of streams you'd need to provide "file"-related information manually:
``` javascript
someModule.stream(function(err, stdout, stderr) {
if (err) throw err;
var form = new FormData();
form.append('file', stdout, {
filename: 'unicycle.jpg', // ... or:
filepath: 'photos/toys/unicycle.jpg',
contentType: 'image/jpeg',
knownLength: 19806
});
form.submit('http://example.com/', function(err, res) {
if (err) throw err;
console.log('Done');
});
});
```
The `filepath` property overrides `filename` and may contain a relative path. This is typically used when uploading [multiple files from a directory](https://wicg.github.io/entries-api/#dom-htmlinputelement-webkitdirectory).
For edge cases, like POST request to URL with query string or to pass HTTP auth credentials, object can be passed to `form.submit()` as first parameter:
``` javascript
form.submit({
host: 'example.com',
path: '/probably.php?extra=params',
auth: 'username:password'
}, function(err, res) {
console.log(res.statusCode);
});
```
In case you need to also send custom HTTP headers with the POST request, you can use the `headers` key in first parameter of `form.submit()`:
``` javascript
form.submit({
host: 'example.com',
path: '/surelynot.php',
headers: {'x-test-header': 'test-header-value'}
}, function(err, res) {
console.log(res.statusCode);
});
```
### Integration with other libraries
#### Request
Form submission using [request](https://github.com/request/request):
```javascript
var formData = {
my_field: 'my_value',
my_file: fs.createReadStream(__dirname + '/unicycle.jpg'),
};
request.post({url:'http://service.com/upload', formData: formData}, function(err, httpResponse, body) {
if (err) {
return console.error('upload failed:', err);
}
console.log('Upload successful! Server responded with:', body);
});
```
For more details see [request readme](https://github.com/request/request#multipartform-data-multipart-form-uploads).
#### node-fetch
You can also submit a form using [node-fetch](https://github.com/bitinn/node-fetch):
```javascript
var form = new FormData();
form.append('a', 1);
fetch('http://example.com', { method: 'POST', body: form })
.then(function(res) {
return res.json();
}).then(function(json) {
console.log(json);
});
```
## Notes
- ```getLengthSync()``` method DOESN'T calculate length for streams, use ```knownLength``` options as workaround.
- Starting version `2.x` FormData has dropped support for `[email protected]`.
## License
Form-Data is released under the [MIT](License) license.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/form-data/README.md | README.md |
var CombinedStream = require('combined-stream');
var util = require('util');
var path = require('path');
var http = require('http');
var https = require('https');
var parseUrl = require('url').parse;
var fs = require('fs');
var mime = require('mime-types');
var asynckit = require('asynckit');
var populate = require('form-data/lib/populate.js');
// Public API
module.exports = FormData;
// make it a Stream
util.inherits(FormData, CombinedStream);
/**
* Create readable "multipart/form-data" streams.
* Can be used to submit forms
* and file uploads to other web applications.
*
* @constructor
* @param {Object} options - Properties to be added/overriden for FormData and CombinedStream
*/
function FormData(options) {
if (!(this instanceof FormData)) {
return new FormData();
}
this._overheadLength = 0;
this._valueLength = 0;
this._valuesToMeasure = [];
CombinedStream.call(this);
options = options || {};
for (var option in options) {
this[option] = options[option];
}
}
FormData.LINE_BREAK = '\r\n';
FormData.DEFAULT_CONTENT_TYPE = 'application/octet-stream';
FormData.prototype.append = function(field, value, options) {
options = options || {};
// allow filename as single option
if (typeof options == 'string') {
options = {filename: options};
}
var append = CombinedStream.prototype.append.bind(this);
// all that streamy business can't handle numbers
if (typeof value == 'number') {
value = '' + value;
}
// https://github.com/felixge/node-form-data/issues/38
if (util.isArray(value)) {
// Please convert your array into string
// the way web server expects it
this._error(new Error('Arrays are not supported.'));
return;
}
var header = this._multiPartHeader(field, value, options);
var footer = this._multiPartFooter();
append(header);
append(value);
append(footer);
// pass along options.knownLength
this._trackLength(header, value, options);
};
FormData.prototype._trackLength = function(header, value, options) {
var valueLength = 0;
// used w/ getLengthSync(), when length is known.
// e.g. for streaming directly from a remote server,
// w/ a known file a size, and not wanting to wait for
// incoming file to finish to get its size.
if (options.knownLength != null) {
valueLength += +options.knownLength;
} else if (Buffer.isBuffer(value)) {
valueLength = value.length;
} else if (typeof value === 'string') {
valueLength = Buffer.byteLength(value);
}
this._valueLength += valueLength;
// @check why add CRLF? does this account for custom/multiple CRLFs?
this._overheadLength +=
Buffer.byteLength(header) +
FormData.LINE_BREAK.length;
// empty or either doesn't have path or not an http response
if (!value || ( !value.path && !(value.readable && value.hasOwnProperty('httpVersion')) )) {
return;
}
// no need to bother with the length
if (!options.knownLength) {
this._valuesToMeasure.push(value);
}
};
FormData.prototype._lengthRetriever = function(value, callback) {
if (value.hasOwnProperty('fd')) {
// take read range into a account
// `end` = Infinity –> read file till the end
//
// TODO: Looks like there is bug in Node fs.createReadStream
// it doesn't respect `end` options without `start` options
// Fix it when node fixes it.
// https://github.com/joyent/node/issues/7819
if (value.end != undefined && value.end != Infinity && value.start != undefined) {
// when end specified
// no need to calculate range
// inclusive, starts with 0
callback(null, value.end + 1 - (value.start ? value.start : 0));
// not that fast snoopy
} else {
// still need to fetch file size from fs
fs.stat(value.path, function(err, stat) {
var fileSize;
if (err) {
callback(err);
return;
}
// update final size based on the range options
fileSize = stat.size - (value.start ? value.start : 0);
callback(null, fileSize);
});
}
// or http response
} else if (value.hasOwnProperty('httpVersion')) {
callback(null, +value.headers['content-length']);
// or request stream http://github.com/mikeal/request
} else if (value.hasOwnProperty('httpModule')) {
// wait till response come back
value.on('response', function(response) {
value.pause();
callback(null, +response.headers['content-length']);
});
value.resume();
// something else
} else {
callback('Unknown stream');
}
};
FormData.prototype._multiPartHeader = function(field, value, options) {
// custom header specified (as string)?
// it becomes responsible for boundary
// (e.g. to handle extra CRLFs on .NET servers)
if (typeof options.header == 'string') {
return options.header;
}
var contentDisposition = this._getContentDisposition(value, options);
var contentType = this._getContentType(value, options);
var contents = '';
var headers = {
// add custom disposition as third element or keep it two elements if not
'Content-Disposition': ['form-data', 'name="' + field + '"'].concat(contentDisposition || []),
// if no content type. allow it to be empty array
'Content-Type': [].concat(contentType || [])
};
// allow custom headers.
if (typeof options.header == 'object') {
populate(headers, options.header);
}
var header;
for (var prop in headers) {
if (!headers.hasOwnProperty(prop)) continue;
header = headers[prop];
// skip nullish headers.
if (header == null) {
continue;
}
// convert all headers to arrays.
if (!Array.isArray(header)) {
header = [header];
}
// add non-empty headers.
if (header.length) {
contents += prop + ': ' + header.join('; ') + FormData.LINE_BREAK;
}
}
return '--' + this.getBoundary() + FormData.LINE_BREAK + contents + FormData.LINE_BREAK;
};
FormData.prototype._getContentDisposition = function(value, options) {
var filename
, contentDisposition
;
if (typeof options.filepath === 'string') {
// custom filepath for relative paths
filename = path.normalize(options.filepath).replace(/\\/g, '/');
} else if (options.filename || value.name || value.path) {
// custom filename take precedence
// formidable and the browser add a name property
// fs- and request- streams have path property
filename = path.basename(options.filename || value.name || value.path);
} else if (value.readable && value.hasOwnProperty('httpVersion')) {
// or try http response
filename = path.basename(value.client._httpMessage.path);
}
if (filename) {
contentDisposition = 'filename="' + filename + '"';
}
return contentDisposition;
};
FormData.prototype._getContentType = function(value, options) {
// use custom content-type above all
var contentType = options.contentType;
// or try `name` from formidable, browser
if (!contentType && value.name) {
contentType = mime.lookup(value.name);
}
// or try `path` from fs-, request- streams
if (!contentType && value.path) {
contentType = mime.lookup(value.path);
}
// or if it's http-reponse
if (!contentType && value.readable && value.hasOwnProperty('httpVersion')) {
contentType = value.headers['content-type'];
}
// or guess it from the filepath or filename
if (!contentType && (options.filepath || options.filename)) {
contentType = mime.lookup(options.filepath || options.filename);
}
// fallback to the default content type if `value` is not simple value
if (!contentType && typeof value == 'object') {
contentType = FormData.DEFAULT_CONTENT_TYPE;
}
return contentType;
};
FormData.prototype._multiPartFooter = function() {
return function(next) {
var footer = FormData.LINE_BREAK;
var lastPart = (this._streams.length === 0);
if (lastPart) {
footer += this._lastBoundary();
}
next(footer);
}.bind(this);
};
FormData.prototype._lastBoundary = function() {
return '--' + this.getBoundary() + '--' + FormData.LINE_BREAK;
};
FormData.prototype.getHeaders = function(userHeaders) {
var header;
var formHeaders = {
'content-type': 'multipart/form-data; boundary=' + this.getBoundary()
};
for (header in userHeaders) {
if (userHeaders.hasOwnProperty(header)) {
formHeaders[header.toLowerCase()] = userHeaders[header];
}
}
return formHeaders;
};
FormData.prototype.getBoundary = function() {
if (!this._boundary) {
this._generateBoundary();
}
return this._boundary;
};
FormData.prototype._generateBoundary = function() {
// This generates a 50 character boundary similar to those used by Firefox.
// They are optimized for boyer-moore parsing.
var boundary = '--------------------------';
for (var i = 0; i < 24; i++) {
boundary += Math.floor(Math.random() * 10).toString(16);
}
this._boundary = boundary;
};
// Note: getLengthSync DOESN'T calculate streams length
// As workaround one can calculate file size manually
// and add it as knownLength option
FormData.prototype.getLengthSync = function() {
var knownLength = this._overheadLength + this._valueLength;
// Don't get confused, there are 3 "internal" streams for each keyval pair
// so it basically checks if there is any value added to the form
if (this._streams.length) {
knownLength += this._lastBoundary().length;
}
// https://github.com/form-data/form-data/issues/40
if (!this.hasKnownLength()) {
// Some async length retrievers are present
// therefore synchronous length calculation is false.
// Please use getLength(callback) to get proper length
this._error(new Error('Cannot calculate proper length in synchronous way.'));
}
return knownLength;
};
// Public API to check if length of added values is known
// https://github.com/form-data/form-data/issues/196
// https://github.com/form-data/form-data/issues/262
FormData.prototype.hasKnownLength = function() {
var hasKnownLength = true;
if (this._valuesToMeasure.length) {
hasKnownLength = false;
}
return hasKnownLength;
};
FormData.prototype.getLength = function(cb) {
var knownLength = this._overheadLength + this._valueLength;
if (this._streams.length) {
knownLength += this._lastBoundary().length;
}
if (!this._valuesToMeasure.length) {
process.nextTick(cb.bind(this, null, knownLength));
return;
}
asynckit.parallel(this._valuesToMeasure, this._lengthRetriever, function(err, values) {
if (err) {
cb(err);
return;
}
values.forEach(function(length) {
knownLength += length;
});
cb(null, knownLength);
});
};
FormData.prototype.submit = function(params, cb) {
var request
, options
, defaults = {method: 'post'}
;
// parse provided url if it's string
// or treat it as options object
if (typeof params == 'string') {
params = parseUrl(params);
options = populate({
port: params.port,
path: params.pathname,
host: params.hostname,
protocol: params.protocol
}, defaults);
// use custom params
} else {
options = populate(params, defaults);
// if no port provided use default one
if (!options.port) {
options.port = options.protocol == 'https:' ? 443 : 80;
}
}
// put that good code in getHeaders to some use
options.headers = this.getHeaders(params.headers);
// https if specified, fallback to http in any other case
if (options.protocol == 'https:') {
request = https.request(options);
} else {
request = http.request(options);
}
// get content length and fire away
this.getLength(function(err, length) {
if (err) {
this._error(err);
return;
}
// add content length
request.setHeader('Content-Length', length);
this.pipe(request);
if (cb) {
request.on('error', cb);
request.on('response', cb.bind(this, null));
}
}.bind(this));
return request;
};
FormData.prototype._error = function(err) {
if (!this.error) {
this.error = err;
this.pause();
this.emit('error', err);
}
};
FormData.prototype.toString = function () {
return '[object FormData]';
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/form-data/lib/form_data.js | form_data.js |
var LARGE_ARRAY_SIZE = 200;
/** Used as the `TypeError` message for "Functions" methods. */
var FUNC_ERROR_TEXT = 'Expected a function';
/** Used to stand-in for `undefined` hash values. */
var HASH_UNDEFINED = '__lodash_hash_undefined__';
/** Used to compose bitmasks for comparison styles. */
var UNORDERED_COMPARE_FLAG = 1,
PARTIAL_COMPARE_FLAG = 2;
/** Used as references for various `Number` constants. */
var INFINITY = 1 / 0,
MAX_SAFE_INTEGER = 9007199254740991;
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
funcTag = '[object Function]',
genTag = '[object GeneratorFunction]',
mapTag = '[object Map]',
numberTag = '[object Number]',
objectTag = '[object Object]',
promiseTag = '[object Promise]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
symbolTag = '[object Symbol]',
weakMapTag = '[object WeakMap]';
var arrayBufferTag = '[object ArrayBuffer]',
dataViewTag = '[object DataView]',
float32Tag = '[object Float32Array]',
float64Tag = '[object Float64Array]',
int8Tag = '[object Int8Array]',
int16Tag = '[object Int16Array]',
int32Tag = '[object Int32Array]',
uint8Tag = '[object Uint8Array]',
uint8ClampedTag = '[object Uint8ClampedArray]',
uint16Tag = '[object Uint16Array]',
uint32Tag = '[object Uint32Array]';
/** Used to match property names within property paths. */
var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,
reIsPlainProp = /^\w*$/,
reLeadingDot = /^\./,
rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g;
/**
* Used to match `RegExp`
* [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
*/
var reRegExpChar = /[\\^$.*+?()[\]{}|]/g;
/** Used to match backslashes in property paths. */
var reEscapeChar = /\\(\\)?/g;
/** Used to detect host constructors (Safari). */
var reIsHostCtor = /^\[object .+?Constructor\]$/;
/** Used to detect unsigned integer values. */
var reIsUint = /^(?:0|[1-9]\d*)$/;
/** Used to identify `toStringTag` values of typed arrays. */
var typedArrayTags = {};
typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
typedArrayTags[uint32Tag] = true;
typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
typedArrayTags[dataViewTag] = typedArrayTags[dateTag] =
typedArrayTags[errorTag] = typedArrayTags[funcTag] =
typedArrayTags[mapTag] = typedArrayTags[numberTag] =
typedArrayTags[objectTag] = typedArrayTags[regexpTag] =
typedArrayTags[setTag] = typedArrayTags[stringTag] =
typedArrayTags[weakMapTag] = false;
/** Detect free variable `global` from Node.js. */
var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
/** Detect free variable `self`. */
var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
/** Used as a reference to the global object. */
var root = freeGlobal || freeSelf || Function('return this')();
/** Detect free variable `exports`. */
var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
/** Detect free variable `module`. */
var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
/** Detect the popular CommonJS extension `module.exports`. */
var moduleExports = freeModule && freeModule.exports === freeExports;
/** Detect free variable `process` from Node.js. */
var freeProcess = moduleExports && freeGlobal.process;
/** Used to access faster Node.js helpers. */
var nodeUtil = (function() {
try {
return freeProcess && freeProcess.binding('util');
} catch (e) {}
}());
/* Node.js helper references. */
var nodeIsTypedArray = nodeUtil && nodeUtil.isTypedArray;
/**
* A faster alternative to `Function#apply`, this function invokes `func`
* with the `this` binding of `thisArg` and the arguments of `args`.
*
* @private
* @param {Function} func The function to invoke.
* @param {*} thisArg The `this` binding of `func`.
* @param {Array} args The arguments to invoke `func` with.
* @returns {*} Returns the result of `func`.
*/
function apply(func, thisArg, args) {
switch (args.length) {
case 0: return func.call(thisArg);
case 1: return func.call(thisArg, args[0]);
case 2: return func.call(thisArg, args[0], args[1]);
case 3: return func.call(thisArg, args[0], args[1], args[2]);
}
return func.apply(thisArg, args);
}
/**
* A specialized version of `_.map` for arrays without support for iteratee
* shorthands.
*
* @private
* @param {Array} [array] The array to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
*/
function arrayMap(array, iteratee) {
var index = -1,
length = array ? array.length : 0,
result = Array(length);
while (++index < length) {
result[index] = iteratee(array[index], index, array);
}
return result;
}
/**
* Appends the elements of `values` to `array`.
*
* @private
* @param {Array} array The array to modify.
* @param {Array} values The values to append.
* @returns {Array} Returns `array`.
*/
function arrayPush(array, values) {
var index = -1,
length = values.length,
offset = array.length;
while (++index < length) {
array[offset + index] = values[index];
}
return array;
}
/**
* A specialized version of `_.some` for arrays without support for iteratee
* shorthands.
*
* @private
* @param {Array} [array] The array to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
*/
function arraySome(array, predicate) {
var index = -1,
length = array ? array.length : 0;
while (++index < length) {
if (predicate(array[index], index, array)) {
return true;
}
}
return false;
}
/**
* The base implementation of `_.property` without support for deep paths.
*
* @private
* @param {string} key The key of the property to get.
* @returns {Function} Returns the new accessor function.
*/
function baseProperty(key) {
return function(object) {
return object == null ? undefined : object[key];
};
}
/**
* The base implementation of `_.sortBy` which uses `comparer` to define the
* sort order of `array` and replaces criteria objects with their corresponding
* values.
*
* @private
* @param {Array} array The array to sort.
* @param {Function} comparer The function to define sort order.
* @returns {Array} Returns `array`.
*/
function baseSortBy(array, comparer) {
var length = array.length;
array.sort(comparer);
while (length--) {
array[length] = array[length].value;
}
return array;
}
/**
* The base implementation of `_.times` without support for iteratee shorthands
* or max array length checks.
*
* @private
* @param {number} n The number of times to invoke `iteratee`.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the array of results.
*/
function baseTimes(n, iteratee) {
var index = -1,
result = Array(n);
while (++index < n) {
result[index] = iteratee(index);
}
return result;
}
/**
* The base implementation of `_.unary` without support for storing metadata.
*
* @private
* @param {Function} func The function to cap arguments for.
* @returns {Function} Returns the new capped function.
*/
function baseUnary(func) {
return function(value) {
return func(value);
};
}
/**
* Gets the value at `key` of `object`.
*
* @private
* @param {Object} [object] The object to query.
* @param {string} key The key of the property to get.
* @returns {*} Returns the property value.
*/
function getValue(object, key) {
return object == null ? undefined : object[key];
}
/**
* Checks if `value` is a host object in IE < 9.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a host object, else `false`.
*/
function isHostObject(value) {
// Many host objects are `Object` objects that can coerce to strings
// despite having improperly defined `toString` methods.
var result = false;
if (value != null && typeof value.toString != 'function') {
try {
result = !!(value + '');
} catch (e) {}
}
return result;
}
/**
* Converts `map` to its key-value pairs.
*
* @private
* @param {Object} map The map to convert.
* @returns {Array} Returns the key-value pairs.
*/
function mapToArray(map) {
var index = -1,
result = Array(map.size);
map.forEach(function(value, key) {
result[++index] = [key, value];
});
return result;
}
/**
* Creates a unary function that invokes `func` with its argument transformed.
*
* @private
* @param {Function} func The function to wrap.
* @param {Function} transform The argument transform.
* @returns {Function} Returns the new function.
*/
function overArg(func, transform) {
return function(arg) {
return func(transform(arg));
};
}
/**
* Converts `set` to an array of its values.
*
* @private
* @param {Object} set The set to convert.
* @returns {Array} Returns the values.
*/
function setToArray(set) {
var index = -1,
result = Array(set.size);
set.forEach(function(value) {
result[++index] = value;
});
return result;
}
/** Used for built-in method references. */
var arrayProto = Array.prototype,
funcProto = Function.prototype,
objectProto = Object.prototype;
/** Used to detect overreaching core-js shims. */
var coreJsData = root['__core-js_shared__'];
/** Used to detect methods masquerading as native. */
var maskSrcKey = (function() {
var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || '');
return uid ? ('Symbol(src)_1.' + uid) : '';
}());
/** Used to resolve the decompiled source of functions. */
var funcToString = funcProto.toString;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Used to resolve the
* [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
* of values.
*/
var objectToString = objectProto.toString;
/** Used to detect if a method is native. */
var reIsNative = RegExp('^' +
funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&')
.replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
);
/** Built-in value references. */
var Symbol = root.Symbol,
Uint8Array = root.Uint8Array,
propertyIsEnumerable = objectProto.propertyIsEnumerable,
splice = arrayProto.splice,
spreadableSymbol = Symbol ? Symbol.isConcatSpreadable : undefined;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeKeys = overArg(Object.keys, Object),
nativeMax = Math.max;
/* Built-in method references that are verified to be native. */
var DataView = getNative(root, 'DataView'),
Map = getNative(root, 'Map'),
Promise = getNative(root, 'Promise'),
Set = getNative(root, 'Set'),
WeakMap = getNative(root, 'WeakMap'),
nativeCreate = getNative(Object, 'create');
/** Used to detect maps, sets, and weakmaps. */
var dataViewCtorString = toSource(DataView),
mapCtorString = toSource(Map),
promiseCtorString = toSource(Promise),
setCtorString = toSource(Set),
weakMapCtorString = toSource(WeakMap);
/** Used to convert symbols to primitives and strings. */
var symbolProto = Symbol ? Symbol.prototype : undefined,
symbolValueOf = symbolProto ? symbolProto.valueOf : undefined,
symbolToString = symbolProto ? symbolProto.toString : undefined;
/**
* Creates a hash object.
*
* @private
* @constructor
* @param {Array} [entries] The key-value pairs to cache.
*/
function Hash(entries) {
var index = -1,
length = entries ? entries.length : 0;
this.clear();
while (++index < length) {
var entry = entries[index];
this.set(entry[0], entry[1]);
}
}
/**
* Removes all key-value entries from the hash.
*
* @private
* @name clear
* @memberOf Hash
*/
function hashClear() {
this.__data__ = nativeCreate ? nativeCreate(null) : {};
}
/**
* Removes `key` and its value from the hash.
*
* @private
* @name delete
* @memberOf Hash
* @param {Object} hash The hash to modify.
* @param {string} key The key of the value to remove.
* @returns {boolean} Returns `true` if the entry was removed, else `false`.
*/
function hashDelete(key) {
return this.has(key) && delete this.__data__[key];
}
/**
* Gets the hash value for `key`.
*
* @private
* @name get
* @memberOf Hash
* @param {string} key The key of the value to get.
* @returns {*} Returns the entry value.
*/
function hashGet(key) {
var data = this.__data__;
if (nativeCreate) {
var result = data[key];
return result === HASH_UNDEFINED ? undefined : result;
}
return hasOwnProperty.call(data, key) ? data[key] : undefined;
}
/**
* Checks if a hash value for `key` exists.
*
* @private
* @name has
* @memberOf Hash
* @param {string} key The key of the entry to check.
* @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
*/
function hashHas(key) {
var data = this.__data__;
return nativeCreate ? data[key] !== undefined : hasOwnProperty.call(data, key);
}
/**
* Sets the hash `key` to `value`.
*
* @private
* @name set
* @memberOf Hash
* @param {string} key The key of the value to set.
* @param {*} value The value to set.
* @returns {Object} Returns the hash instance.
*/
function hashSet(key, value) {
var data = this.__data__;
data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value;
return this;
}
// Add methods to `Hash`.
Hash.prototype.clear = hashClear;
Hash.prototype['delete'] = hashDelete;
Hash.prototype.get = hashGet;
Hash.prototype.has = hashHas;
Hash.prototype.set = hashSet;
/**
* Creates an list cache object.
*
* @private
* @constructor
* @param {Array} [entries] The key-value pairs to cache.
*/
function ListCache(entries) {
var index = -1,
length = entries ? entries.length : 0;
this.clear();
while (++index < length) {
var entry = entries[index];
this.set(entry[0], entry[1]);
}
}
/**
* Removes all key-value entries from the list cache.
*
* @private
* @name clear
* @memberOf ListCache
*/
function listCacheClear() {
this.__data__ = [];
}
/**
* Removes `key` and its value from the list cache.
*
* @private
* @name delete
* @memberOf ListCache
* @param {string} key The key of the value to remove.
* @returns {boolean} Returns `true` if the entry was removed, else `false`.
*/
function listCacheDelete(key) {
var data = this.__data__,
index = assocIndexOf(data, key);
if (index < 0) {
return false;
}
var lastIndex = data.length - 1;
if (index == lastIndex) {
data.pop();
} else {
splice.call(data, index, 1);
}
return true;
}
/**
* Gets the list cache value for `key`.
*
* @private
* @name get
* @memberOf ListCache
* @param {string} key The key of the value to get.
* @returns {*} Returns the entry value.
*/
function listCacheGet(key) {
var data = this.__data__,
index = assocIndexOf(data, key);
return index < 0 ? undefined : data[index][1];
}
/**
* Checks if a list cache value for `key` exists.
*
* @private
* @name has
* @memberOf ListCache
* @param {string} key The key of the entry to check.
* @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
*/
function listCacheHas(key) {
return assocIndexOf(this.__data__, key) > -1;
}
/**
* Sets the list cache `key` to `value`.
*
* @private
* @name set
* @memberOf ListCache
* @param {string} key The key of the value to set.
* @param {*} value The value to set.
* @returns {Object} Returns the list cache instance.
*/
function listCacheSet(key, value) {
var data = this.__data__,
index = assocIndexOf(data, key);
if (index < 0) {
data.push([key, value]);
} else {
data[index][1] = value;
}
return this;
}
// Add methods to `ListCache`.
ListCache.prototype.clear = listCacheClear;
ListCache.prototype['delete'] = listCacheDelete;
ListCache.prototype.get = listCacheGet;
ListCache.prototype.has = listCacheHas;
ListCache.prototype.set = listCacheSet;
/**
* Creates a map cache object to store key-value pairs.
*
* @private
* @constructor
* @param {Array} [entries] The key-value pairs to cache.
*/
function MapCache(entries) {
var index = -1,
length = entries ? entries.length : 0;
this.clear();
while (++index < length) {
var entry = entries[index];
this.set(entry[0], entry[1]);
}
}
/**
* Removes all key-value entries from the map.
*
* @private
* @name clear
* @memberOf MapCache
*/
function mapCacheClear() {
this.__data__ = {
'hash': new Hash,
'map': new (Map || ListCache),
'string': new Hash
};
}
/**
* Removes `key` and its value from the map.
*
* @private
* @name delete
* @memberOf MapCache
* @param {string} key The key of the value to remove.
* @returns {boolean} Returns `true` if the entry was removed, else `false`.
*/
function mapCacheDelete(key) {
return getMapData(this, key)['delete'](key);
}
/**
* Gets the map value for `key`.
*
* @private
* @name get
* @memberOf MapCache
* @param {string} key The key of the value to get.
* @returns {*} Returns the entry value.
*/
function mapCacheGet(key) {
return getMapData(this, key).get(key);
}
/**
* Checks if a map value for `key` exists.
*
* @private
* @name has
* @memberOf MapCache
* @param {string} key The key of the entry to check.
* @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
*/
function mapCacheHas(key) {
return getMapData(this, key).has(key);
}
/**
* Sets the map `key` to `value`.
*
* @private
* @name set
* @memberOf MapCache
* @param {string} key The key of the value to set.
* @param {*} value The value to set.
* @returns {Object} Returns the map cache instance.
*/
function mapCacheSet(key, value) {
getMapData(this, key).set(key, value);
return this;
}
// Add methods to `MapCache`.
MapCache.prototype.clear = mapCacheClear;
MapCache.prototype['delete'] = mapCacheDelete;
MapCache.prototype.get = mapCacheGet;
MapCache.prototype.has = mapCacheHas;
MapCache.prototype.set = mapCacheSet;
/**
*
* Creates an array cache object to store unique values.
*
* @private
* @constructor
* @param {Array} [values] The values to cache.
*/
function SetCache(values) {
var index = -1,
length = values ? values.length : 0;
this.__data__ = new MapCache;
while (++index < length) {
this.add(values[index]);
}
}
/**
* Adds `value` to the array cache.
*
* @private
* @name add
* @memberOf SetCache
* @alias push
* @param {*} value The value to cache.
* @returns {Object} Returns the cache instance.
*/
function setCacheAdd(value) {
this.__data__.set(value, HASH_UNDEFINED);
return this;
}
/**
* Checks if `value` is in the array cache.
*
* @private
* @name has
* @memberOf SetCache
* @param {*} value The value to search for.
* @returns {number} Returns `true` if `value` is found, else `false`.
*/
function setCacheHas(value) {
return this.__data__.has(value);
}
// Add methods to `SetCache`.
SetCache.prototype.add = SetCache.prototype.push = setCacheAdd;
SetCache.prototype.has = setCacheHas;
/**
* Creates a stack cache object to store key-value pairs.
*
* @private
* @constructor
* @param {Array} [entries] The key-value pairs to cache.
*/
function Stack(entries) {
this.__data__ = new ListCache(entries);
}
/**
* Removes all key-value entries from the stack.
*
* @private
* @name clear
* @memberOf Stack
*/
function stackClear() {
this.__data__ = new ListCache;
}
/**
* Removes `key` and its value from the stack.
*
* @private
* @name delete
* @memberOf Stack
* @param {string} key The key of the value to remove.
* @returns {boolean} Returns `true` if the entry was removed, else `false`.
*/
function stackDelete(key) {
return this.__data__['delete'](key);
}
/**
* Gets the stack value for `key`.
*
* @private
* @name get
* @memberOf Stack
* @param {string} key The key of the value to get.
* @returns {*} Returns the entry value.
*/
function stackGet(key) {
return this.__data__.get(key);
}
/**
* Checks if a stack value for `key` exists.
*
* @private
* @name has
* @memberOf Stack
* @param {string} key The key of the entry to check.
* @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
*/
function stackHas(key) {
return this.__data__.has(key);
}
/**
* Sets the stack `key` to `value`.
*
* @private
* @name set
* @memberOf Stack
* @param {string} key The key of the value to set.
* @param {*} value The value to set.
* @returns {Object} Returns the stack cache instance.
*/
function stackSet(key, value) {
var cache = this.__data__;
if (cache instanceof ListCache) {
var pairs = cache.__data__;
if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) {
pairs.push([key, value]);
return this;
}
cache = this.__data__ = new MapCache(pairs);
}
cache.set(key, value);
return this;
}
// Add methods to `Stack`.
Stack.prototype.clear = stackClear;
Stack.prototype['delete'] = stackDelete;
Stack.prototype.get = stackGet;
Stack.prototype.has = stackHas;
Stack.prototype.set = stackSet;
/**
* Creates an array of the enumerable property names of the array-like `value`.
*
* @private
* @param {*} value The value to query.
* @param {boolean} inherited Specify returning inherited property names.
* @returns {Array} Returns the array of property names.
*/
function arrayLikeKeys(value, inherited) {
// Safari 8.1 makes `arguments.callee` enumerable in strict mode.
// Safari 9 makes `arguments.length` enumerable in strict mode.
var result = (isArray(value) || isArguments(value))
? baseTimes(value.length, String)
: [];
var length = result.length,
skipIndexes = !!length;
for (var key in value) {
if ((inherited || hasOwnProperty.call(value, key)) &&
!(skipIndexes && (key == 'length' || isIndex(key, length)))) {
result.push(key);
}
}
return result;
}
/**
* Gets the index at which the `key` is found in `array` of key-value pairs.
*
* @private
* @param {Array} array The array to inspect.
* @param {*} key The key to search for.
* @returns {number} Returns the index of the matched value, else `-1`.
*/
function assocIndexOf(array, key) {
var length = array.length;
while (length--) {
if (eq(array[length][0], key)) {
return length;
}
}
return -1;
}
/**
* The base implementation of `_.forEach` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array|Object} Returns `collection`.
*/
var baseEach = createBaseEach(baseForOwn);
/**
* The base implementation of `_.flatten` with support for restricting flattening.
*
* @private
* @param {Array} array The array to flatten.
* @param {number} depth The maximum recursion depth.
* @param {boolean} [predicate=isFlattenable] The function invoked per iteration.
* @param {boolean} [isStrict] Restrict to values that pass `predicate` checks.
* @param {Array} [result=[]] The initial result value.
* @returns {Array} Returns the new flattened array.
*/
function baseFlatten(array, depth, predicate, isStrict, result) {
var index = -1,
length = array.length;
predicate || (predicate = isFlattenable);
result || (result = []);
while (++index < length) {
var value = array[index];
if (depth > 0 && predicate(value)) {
if (depth > 1) {
// Recursively flatten arrays (susceptible to call stack limits).
baseFlatten(value, depth - 1, predicate, isStrict, result);
} else {
arrayPush(result, value);
}
} else if (!isStrict) {
result[result.length] = value;
}
}
return result;
}
/**
* The base implementation of `baseForOwn` which iterates over `object`
* properties returned by `keysFunc` and invokes `iteratee` for each property.
* Iteratee functions may exit iteration early by explicitly returning `false`.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {Function} keysFunc The function to get the keys of `object`.
* @returns {Object} Returns `object`.
*/
var baseFor = createBaseFor();
/**
* The base implementation of `_.forOwn` without support for iteratee shorthands.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Object} Returns `object`.
*/
function baseForOwn(object, iteratee) {
return object && baseFor(object, iteratee, keys);
}
/**
* The base implementation of `_.get` without support for default values.
*
* @private
* @param {Object} object The object to query.
* @param {Array|string} path The path of the property to get.
* @returns {*} Returns the resolved value.
*/
function baseGet(object, path) {
path = isKey(path, object) ? [path] : castPath(path);
var index = 0,
length = path.length;
while (object != null && index < length) {
object = object[toKey(path[index++])];
}
return (index && index == length) ? object : undefined;
}
/**
* The base implementation of `getTag`.
*
* @private
* @param {*} value The value to query.
* @returns {string} Returns the `toStringTag`.
*/
function baseGetTag(value) {
return objectToString.call(value);
}
/**
* The base implementation of `_.hasIn` without support for deep paths.
*
* @private
* @param {Object} [object] The object to query.
* @param {Array|string} key The key to check.
* @returns {boolean} Returns `true` if `key` exists, else `false`.
*/
function baseHasIn(object, key) {
return object != null && key in Object(object);
}
/**
* The base implementation of `_.isEqual` which supports partial comparisons
* and tracks traversed objects.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @param {Function} [customizer] The function to customize comparisons.
* @param {boolean} [bitmask] The bitmask of comparison flags.
* The bitmask may be composed of the following flags:
* 1 - Unordered comparison
* 2 - Partial comparison
* @param {Object} [stack] Tracks traversed `value` and `other` objects.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
*/
function baseIsEqual(value, other, customizer, bitmask, stack) {
if (value === other) {
return true;
}
if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
return value !== value && other !== other;
}
return baseIsEqualDeep(value, other, baseIsEqual, customizer, bitmask, stack);
}
/**
* A specialized version of `baseIsEqual` for arrays and objects which performs
* deep comparisons and tracks traversed objects enabling objects with circular
* references to be compared.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} [customizer] The function to customize comparisons.
* @param {number} [bitmask] The bitmask of comparison flags. See `baseIsEqual`
* for more details.
* @param {Object} [stack] Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function baseIsEqualDeep(object, other, equalFunc, customizer, bitmask, stack) {
var objIsArr = isArray(object),
othIsArr = isArray(other),
objTag = arrayTag,
othTag = arrayTag;
if (!objIsArr) {
objTag = getTag(object);
objTag = objTag == argsTag ? objectTag : objTag;
}
if (!othIsArr) {
othTag = getTag(other);
othTag = othTag == argsTag ? objectTag : othTag;
}
var objIsObj = objTag == objectTag && !isHostObject(object),
othIsObj = othTag == objectTag && !isHostObject(other),
isSameTag = objTag == othTag;
if (isSameTag && !objIsObj) {
stack || (stack = new Stack);
return (objIsArr || isTypedArray(object))
? equalArrays(object, other, equalFunc, customizer, bitmask, stack)
: equalByTag(object, other, objTag, equalFunc, customizer, bitmask, stack);
}
if (!(bitmask & PARTIAL_COMPARE_FLAG)) {
var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
if (objIsWrapped || othIsWrapped) {
var objUnwrapped = objIsWrapped ? object.value() : object,
othUnwrapped = othIsWrapped ? other.value() : other;
stack || (stack = new Stack);
return equalFunc(objUnwrapped, othUnwrapped, customizer, bitmask, stack);
}
}
if (!isSameTag) {
return false;
}
stack || (stack = new Stack);
return equalObjects(object, other, equalFunc, customizer, bitmask, stack);
}
/**
* The base implementation of `_.isMatch` without support for iteratee shorthands.
*
* @private
* @param {Object} object The object to inspect.
* @param {Object} source The object of property values to match.
* @param {Array} matchData The property names, values, and compare flags to match.
* @param {Function} [customizer] The function to customize comparisons.
* @returns {boolean} Returns `true` if `object` is a match, else `false`.
*/
function baseIsMatch(object, source, matchData, customizer) {
var index = matchData.length,
length = index,
noCustomizer = !customizer;
if (object == null) {
return !length;
}
object = Object(object);
while (index--) {
var data = matchData[index];
if ((noCustomizer && data[2])
? data[1] !== object[data[0]]
: !(data[0] in object)
) {
return false;
}
}
while (++index < length) {
data = matchData[index];
var key = data[0],
objValue = object[key],
srcValue = data[1];
if (noCustomizer && data[2]) {
if (objValue === undefined && !(key in object)) {
return false;
}
} else {
var stack = new Stack;
if (customizer) {
var result = customizer(objValue, srcValue, key, object, source, stack);
}
if (!(result === undefined
? baseIsEqual(srcValue, objValue, customizer, UNORDERED_COMPARE_FLAG | PARTIAL_COMPARE_FLAG, stack)
: result
)) {
return false;
}
}
}
return true;
}
/**
* The base implementation of `_.isNative` without bad shim checks.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a native function,
* else `false`.
*/
function baseIsNative(value) {
if (!isObject(value) || isMasked(value)) {
return false;
}
var pattern = (isFunction(value) || isHostObject(value)) ? reIsNative : reIsHostCtor;
return pattern.test(toSource(value));
}
/**
* The base implementation of `_.isTypedArray` without Node.js optimizations.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
*/
function baseIsTypedArray(value) {
return isObjectLike(value) &&
isLength(value.length) && !!typedArrayTags[objectToString.call(value)];
}
/**
* The base implementation of `_.iteratee`.
*
* @private
* @param {*} [value=_.identity] The value to convert to an iteratee.
* @returns {Function} Returns the iteratee.
*/
function baseIteratee(value) {
// Don't store the `typeof` result in a variable to avoid a JIT bug in Safari 9.
// See https://bugs.webkit.org/show_bug.cgi?id=156034 for more details.
if (typeof value == 'function') {
return value;
}
if (value == null) {
return identity;
}
if (typeof value == 'object') {
return isArray(value)
? baseMatchesProperty(value[0], value[1])
: baseMatches(value);
}
return property(value);
}
/**
* The base implementation of `_.keys` which doesn't treat sparse arrays as dense.
*
* @private
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
*/
function baseKeys(object) {
if (!isPrototype(object)) {
return nativeKeys(object);
}
var result = [];
for (var key in Object(object)) {
if (hasOwnProperty.call(object, key) && key != 'constructor') {
result.push(key);
}
}
return result;
}
/**
* The base implementation of `_.map` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
*/
function baseMap(collection, iteratee) {
var index = -1,
result = isArrayLike(collection) ? Array(collection.length) : [];
baseEach(collection, function(value, key, collection) {
result[++index] = iteratee(value, key, collection);
});
return result;
}
/**
* The base implementation of `_.matches` which doesn't clone `source`.
*
* @private
* @param {Object} source The object of property values to match.
* @returns {Function} Returns the new spec function.
*/
function baseMatches(source) {
var matchData = getMatchData(source);
if (matchData.length == 1 && matchData[0][2]) {
return matchesStrictComparable(matchData[0][0], matchData[0][1]);
}
return function(object) {
return object === source || baseIsMatch(object, source, matchData);
};
}
/**
* The base implementation of `_.matchesProperty` which doesn't clone `srcValue`.
*
* @private
* @param {string} path The path of the property to get.
* @param {*} srcValue The value to match.
* @returns {Function} Returns the new spec function.
*/
function baseMatchesProperty(path, srcValue) {
if (isKey(path) && isStrictComparable(srcValue)) {
return matchesStrictComparable(toKey(path), srcValue);
}
return function(object) {
var objValue = get(object, path);
return (objValue === undefined && objValue === srcValue)
? hasIn(object, path)
: baseIsEqual(srcValue, objValue, undefined, UNORDERED_COMPARE_FLAG | PARTIAL_COMPARE_FLAG);
};
}
/**
* The base implementation of `_.orderBy` without param guards.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by.
* @param {string[]} orders The sort orders of `iteratees`.
* @returns {Array} Returns the new sorted array.
*/
function baseOrderBy(collection, iteratees, orders) {
var index = -1;
iteratees = arrayMap(iteratees.length ? iteratees : [identity], baseUnary(baseIteratee));
var result = baseMap(collection, function(value, key, collection) {
var criteria = arrayMap(iteratees, function(iteratee) {
return iteratee(value);
});
return { 'criteria': criteria, 'index': ++index, 'value': value };
});
return baseSortBy(result, function(object, other) {
return compareMultiple(object, other, orders);
});
}
/**
* A specialized version of `baseProperty` which supports deep paths.
*
* @private
* @param {Array|string} path The path of the property to get.
* @returns {Function} Returns the new accessor function.
*/
function basePropertyDeep(path) {
return function(object) {
return baseGet(object, path);
};
}
/**
* The base implementation of `_.rest` which doesn't validate or coerce arguments.
*
* @private
* @param {Function} func The function to apply a rest parameter to.
* @param {number} [start=func.length-1] The start position of the rest parameter.
* @returns {Function} Returns the new function.
*/
function baseRest(func, start) {
start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
return function() {
var args = arguments,
index = -1,
length = nativeMax(args.length - start, 0),
array = Array(length);
while (++index < length) {
array[index] = args[start + index];
}
index = -1;
var otherArgs = Array(start + 1);
while (++index < start) {
otherArgs[index] = args[index];
}
otherArgs[start] = array;
return apply(func, this, otherArgs);
};
}
/**
* The base implementation of `_.toString` which doesn't convert nullish
* values to empty strings.
*
* @private
* @param {*} value The value to process.
* @returns {string} Returns the string.
*/
function baseToString(value) {
// Exit early for strings to avoid a performance hit in some environments.
if (typeof value == 'string') {
return value;
}
if (isSymbol(value)) {
return symbolToString ? symbolToString.call(value) : '';
}
var result = (value + '');
return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
}
/**
* Casts `value` to a path array if it's not one.
*
* @private
* @param {*} value The value to inspect.
* @returns {Array} Returns the cast property path array.
*/
function castPath(value) {
return isArray(value) ? value : stringToPath(value);
}
/**
* Compares values to sort them in ascending order.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {number} Returns the sort order indicator for `value`.
*/
function compareAscending(value, other) {
if (value !== other) {
var valIsDefined = value !== undefined,
valIsNull = value === null,
valIsReflexive = value === value,
valIsSymbol = isSymbol(value);
var othIsDefined = other !== undefined,
othIsNull = other === null,
othIsReflexive = other === other,
othIsSymbol = isSymbol(other);
if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) ||
(valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) ||
(valIsNull && othIsDefined && othIsReflexive) ||
(!valIsDefined && othIsReflexive) ||
!valIsReflexive) {
return 1;
}
if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) ||
(othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) ||
(othIsNull && valIsDefined && valIsReflexive) ||
(!othIsDefined && valIsReflexive) ||
!othIsReflexive) {
return -1;
}
}
return 0;
}
/**
* Used by `_.orderBy` to compare multiple properties of a value to another
* and stable sort them.
*
* If `orders` is unspecified, all values are sorted in ascending order. Otherwise,
* specify an order of "desc" for descending or "asc" for ascending sort order
* of corresponding values.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {boolean[]|string[]} orders The order to sort by for each property.
* @returns {number} Returns the sort order indicator for `object`.
*/
function compareMultiple(object, other, orders) {
var index = -1,
objCriteria = object.criteria,
othCriteria = other.criteria,
length = objCriteria.length,
ordersLength = orders.length;
while (++index < length) {
var result = compareAscending(objCriteria[index], othCriteria[index]);
if (result) {
if (index >= ordersLength) {
return result;
}
var order = orders[index];
return result * (order == 'desc' ? -1 : 1);
}
}
// Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
// that causes it, under certain circumstances, to provide the same value for
// `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247
// for more details.
//
// This also ensures a stable sort in V8 and other engines.
// See https://bugs.chromium.org/p/v8/issues/detail?id=90 for more details.
return object.index - other.index;
}
/**
* Creates a `baseEach` or `baseEachRight` function.
*
* @private
* @param {Function} eachFunc The function to iterate over a collection.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseEach(eachFunc, fromRight) {
return function(collection, iteratee) {
if (collection == null) {
return collection;
}
if (!isArrayLike(collection)) {
return eachFunc(collection, iteratee);
}
var length = collection.length,
index = fromRight ? length : -1,
iterable = Object(collection);
while ((fromRight ? index-- : ++index < length)) {
if (iteratee(iterable[index], index, iterable) === false) {
break;
}
}
return collection;
};
}
/**
* Creates a base function for methods like `_.forIn` and `_.forOwn`.
*
* @private
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseFor(fromRight) {
return function(object, iteratee, keysFunc) {
var index = -1,
iterable = Object(object),
props = keysFunc(object),
length = props.length;
while (length--) {
var key = props[fromRight ? length : ++index];
if (iteratee(iterable[key], key, iterable) === false) {
break;
}
}
return object;
};
}
/**
* A specialized version of `baseIsEqualDeep` for arrays with support for
* partial deep comparisons.
*
* @private
* @param {Array} array The array to compare.
* @param {Array} other The other array to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} customizer The function to customize comparisons.
* @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual`
* for more details.
* @param {Object} stack Tracks traversed `array` and `other` objects.
* @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
*/
function equalArrays(array, other, equalFunc, customizer, bitmask, stack) {
var isPartial = bitmask & PARTIAL_COMPARE_FLAG,
arrLength = array.length,
othLength = other.length;
if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
return false;
}
// Assume cyclic values are equal.
var stacked = stack.get(array);
if (stacked && stack.get(other)) {
return stacked == other;
}
var index = -1,
result = true,
seen = (bitmask & UNORDERED_COMPARE_FLAG) ? new SetCache : undefined;
stack.set(array, other);
stack.set(other, array);
// Ignore non-index properties.
while (++index < arrLength) {
var arrValue = array[index],
othValue = other[index];
if (customizer) {
var compared = isPartial
? customizer(othValue, arrValue, index, other, array, stack)
: customizer(arrValue, othValue, index, array, other, stack);
}
if (compared !== undefined) {
if (compared) {
continue;
}
result = false;
break;
}
// Recursively compare arrays (susceptible to call stack limits).
if (seen) {
if (!arraySome(other, function(othValue, othIndex) {
if (!seen.has(othIndex) &&
(arrValue === othValue || equalFunc(arrValue, othValue, customizer, bitmask, stack))) {
return seen.add(othIndex);
}
})) {
result = false;
break;
}
} else if (!(
arrValue === othValue ||
equalFunc(arrValue, othValue, customizer, bitmask, stack)
)) {
result = false;
break;
}
}
stack['delete'](array);
stack['delete'](other);
return result;
}
/**
* A specialized version of `baseIsEqualDeep` for comparing objects of
* the same `toStringTag`.
*
* **Note:** This function only supports comparing values with tags of
* `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {string} tag The `toStringTag` of the objects to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} customizer The function to customize comparisons.
* @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual`
* for more details.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalByTag(object, other, tag, equalFunc, customizer, bitmask, stack) {
switch (tag) {
case dataViewTag:
if ((object.byteLength != other.byteLength) ||
(object.byteOffset != other.byteOffset)) {
return false;
}
object = object.buffer;
other = other.buffer;
case arrayBufferTag:
if ((object.byteLength != other.byteLength) ||
!equalFunc(new Uint8Array(object), new Uint8Array(other))) {
return false;
}
return true;
case boolTag:
case dateTag:
case numberTag:
// Coerce booleans to `1` or `0` and dates to milliseconds.
// Invalid dates are coerced to `NaN`.
return eq(+object, +other);
case errorTag:
return object.name == other.name && object.message == other.message;
case regexpTag:
case stringTag:
// Coerce regexes to strings and treat strings, primitives and objects,
// as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
// for more details.
return object == (other + '');
case mapTag:
var convert = mapToArray;
case setTag:
var isPartial = bitmask & PARTIAL_COMPARE_FLAG;
convert || (convert = setToArray);
if (object.size != other.size && !isPartial) {
return false;
}
// Assume cyclic values are equal.
var stacked = stack.get(object);
if (stacked) {
return stacked == other;
}
bitmask |= UNORDERED_COMPARE_FLAG;
// Recursively compare objects (susceptible to call stack limits).
stack.set(object, other);
var result = equalArrays(convert(object), convert(other), equalFunc, customizer, bitmask, stack);
stack['delete'](object);
return result;
case symbolTag:
if (symbolValueOf) {
return symbolValueOf.call(object) == symbolValueOf.call(other);
}
}
return false;
}
/**
* A specialized version of `baseIsEqualDeep` for objects with support for
* partial deep comparisons.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} customizer The function to customize comparisons.
* @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual`
* for more details.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalObjects(object, other, equalFunc, customizer, bitmask, stack) {
var isPartial = bitmask & PARTIAL_COMPARE_FLAG,
objProps = keys(object),
objLength = objProps.length,
othProps = keys(other),
othLength = othProps.length;
if (objLength != othLength && !isPartial) {
return false;
}
var index = objLength;
while (index--) {
var key = objProps[index];
if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
return false;
}
}
// Assume cyclic values are equal.
var stacked = stack.get(object);
if (stacked && stack.get(other)) {
return stacked == other;
}
var result = true;
stack.set(object, other);
stack.set(other, object);
var skipCtor = isPartial;
while (++index < objLength) {
key = objProps[index];
var objValue = object[key],
othValue = other[key];
if (customizer) {
var compared = isPartial
? customizer(othValue, objValue, key, other, object, stack)
: customizer(objValue, othValue, key, object, other, stack);
}
// Recursively compare objects (susceptible to call stack limits).
if (!(compared === undefined
? (objValue === othValue || equalFunc(objValue, othValue, customizer, bitmask, stack))
: compared
)) {
result = false;
break;
}
skipCtor || (skipCtor = key == 'constructor');
}
if (result && !skipCtor) {
var objCtor = object.constructor,
othCtor = other.constructor;
// Non `Object` object instances with different constructors are not equal.
if (objCtor != othCtor &&
('constructor' in object && 'constructor' in other) &&
!(typeof objCtor == 'function' && objCtor instanceof objCtor &&
typeof othCtor == 'function' && othCtor instanceof othCtor)) {
result = false;
}
}
stack['delete'](object);
stack['delete'](other);
return result;
}
/**
* Gets the data for `map`.
*
* @private
* @param {Object} map The map to query.
* @param {string} key The reference key.
* @returns {*} Returns the map data.
*/
function getMapData(map, key) {
var data = map.__data__;
return isKeyable(key)
? data[typeof key == 'string' ? 'string' : 'hash']
: data.map;
}
/**
* Gets the property names, values, and compare flags of `object`.
*
* @private
* @param {Object} object The object to query.
* @returns {Array} Returns the match data of `object`.
*/
function getMatchData(object) {
var result = keys(object),
length = result.length;
while (length--) {
var key = result[length],
value = object[key];
result[length] = [key, value, isStrictComparable(value)];
}
return result;
}
/**
* Gets the native function at `key` of `object`.
*
* @private
* @param {Object} object The object to query.
* @param {string} key The key of the method to get.
* @returns {*} Returns the function if it's native, else `undefined`.
*/
function getNative(object, key) {
var value = getValue(object, key);
return baseIsNative(value) ? value : undefined;
}
/**
* Gets the `toStringTag` of `value`.
*
* @private
* @param {*} value The value to query.
* @returns {string} Returns the `toStringTag`.
*/
var getTag = baseGetTag;
// Fallback for data views, maps, sets, and weak maps in IE 11,
// for data views in Edge < 14, and promises in Node.js.
if ((DataView && getTag(new DataView(new ArrayBuffer(1))) != dataViewTag) ||
(Map && getTag(new Map) != mapTag) ||
(Promise && getTag(Promise.resolve()) != promiseTag) ||
(Set && getTag(new Set) != setTag) ||
(WeakMap && getTag(new WeakMap) != weakMapTag)) {
getTag = function(value) {
var result = objectToString.call(value),
Ctor = result == objectTag ? value.constructor : undefined,
ctorString = Ctor ? toSource(Ctor) : undefined;
if (ctorString) {
switch (ctorString) {
case dataViewCtorString: return dataViewTag;
case mapCtorString: return mapTag;
case promiseCtorString: return promiseTag;
case setCtorString: return setTag;
case weakMapCtorString: return weakMapTag;
}
}
return result;
};
}
/**
* Checks if `path` exists on `object`.
*
* @private
* @param {Object} object The object to query.
* @param {Array|string} path The path to check.
* @param {Function} hasFunc The function to check properties.
* @returns {boolean} Returns `true` if `path` exists, else `false`.
*/
function hasPath(object, path, hasFunc) {
path = isKey(path, object) ? [path] : castPath(path);
var result,
index = -1,
length = path.length;
while (++index < length) {
var key = toKey(path[index]);
if (!(result = object != null && hasFunc(object, key))) {
break;
}
object = object[key];
}
if (result) {
return result;
}
var length = object ? object.length : 0;
return !!length && isLength(length) && isIndex(key, length) &&
(isArray(object) || isArguments(object));
}
/**
* Checks if `value` is a flattenable `arguments` object or array.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is flattenable, else `false`.
*/
function isFlattenable(value) {
return isArray(value) || isArguments(value) ||
!!(spreadableSymbol && value && value[spreadableSymbol]);
}
/**
* Checks if `value` is a valid array-like index.
*
* @private
* @param {*} value The value to check.
* @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
* @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
*/
function isIndex(value, length) {
length = length == null ? MAX_SAFE_INTEGER : length;
return !!length &&
(typeof value == 'number' || reIsUint.test(value)) &&
(value > -1 && value % 1 == 0 && value < length);
}
/**
* Checks if the given arguments are from an iteratee call.
*
* @private
* @param {*} value The potential iteratee value argument.
* @param {*} index The potential iteratee index or key argument.
* @param {*} object The potential iteratee object argument.
* @returns {boolean} Returns `true` if the arguments are from an iteratee call,
* else `false`.
*/
function isIterateeCall(value, index, object) {
if (!isObject(object)) {
return false;
}
var type = typeof index;
if (type == 'number'
? (isArrayLike(object) && isIndex(index, object.length))
: (type == 'string' && index in object)
) {
return eq(object[index], value);
}
return false;
}
/**
* Checks if `value` is a property name and not a property path.
*
* @private
* @param {*} value The value to check.
* @param {Object} [object] The object to query keys on.
* @returns {boolean} Returns `true` if `value` is a property name, else `false`.
*/
function isKey(value, object) {
if (isArray(value)) {
return false;
}
var type = typeof value;
if (type == 'number' || type == 'symbol' || type == 'boolean' ||
value == null || isSymbol(value)) {
return true;
}
return reIsPlainProp.test(value) || !reIsDeepProp.test(value) ||
(object != null && value in Object(object));
}
/**
* Checks if `value` is suitable for use as unique object key.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is suitable, else `false`.
*/
function isKeyable(value) {
var type = typeof value;
return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean')
? (value !== '__proto__')
: (value === null);
}
/**
* Checks if `func` has its source masked.
*
* @private
* @param {Function} func The function to check.
* @returns {boolean} Returns `true` if `func` is masked, else `false`.
*/
function isMasked(func) {
return !!maskSrcKey && (maskSrcKey in func);
}
/**
* Checks if `value` is likely a prototype object.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
*/
function isPrototype(value) {
var Ctor = value && value.constructor,
proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
return value === proto;
}
/**
* Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` if suitable for strict
* equality comparisons, else `false`.
*/
function isStrictComparable(value) {
return value === value && !isObject(value);
}
/**
* A specialized version of `matchesProperty` for source values suitable
* for strict equality comparisons, i.e. `===`.
*
* @private
* @param {string} key The key of the property to get.
* @param {*} srcValue The value to match.
* @returns {Function} Returns the new spec function.
*/
function matchesStrictComparable(key, srcValue) {
return function(object) {
if (object == null) {
return false;
}
return object[key] === srcValue &&
(srcValue !== undefined || (key in Object(object)));
};
}
/**
* Converts `string` to a property path array.
*
* @private
* @param {string} string The string to convert.
* @returns {Array} Returns the property path array.
*/
var stringToPath = memoize(function(string) {
string = toString(string);
var result = [];
if (reLeadingDot.test(string)) {
result.push('');
}
string.replace(rePropName, function(match, number, quote, string) {
result.push(quote ? string.replace(reEscapeChar, '$1') : (number || match));
});
return result;
});
/**
* Converts `value` to a string key if it's not a string or symbol.
*
* @private
* @param {*} value The value to inspect.
* @returns {string|symbol} Returns the key.
*/
function toKey(value) {
if (typeof value == 'string' || isSymbol(value)) {
return value;
}
var result = (value + '');
return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
}
/**
* Converts `func` to its source code.
*
* @private
* @param {Function} func The function to process.
* @returns {string} Returns the source code.
*/
function toSource(func) {
if (func != null) {
try {
return funcToString.call(func);
} catch (e) {}
try {
return (func + '');
} catch (e) {}
}
return '';
}
/**
* Creates an array of elements, sorted in ascending order by the results of
* running each element in a collection thru each iteratee. This method
* performs a stable sort, that is, it preserves the original sort order of
* equal elements. The iteratees are invoked with one argument: (value).
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {...(Function|Function[])} [iteratees=[_.identity]]
* The iteratees to sort by.
* @returns {Array} Returns the new sorted array.
* @example
*
* var users = [
* { 'user': 'fred', 'age': 48 },
* { 'user': 'barney', 'age': 36 },
* { 'user': 'fred', 'age': 40 },
* { 'user': 'barney', 'age': 34 }
* ];
*
* _.sortBy(users, function(o) { return o.user; });
* // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]]
*
* _.sortBy(users, ['user', 'age']);
* // => objects for [['barney', 34], ['barney', 36], ['fred', 40], ['fred', 48]]
*
* _.sortBy(users, 'user', function(o) {
* return Math.floor(o.age / 10);
* });
* // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]]
*/
var sortBy = baseRest(function(collection, iteratees) {
if (collection == null) {
return [];
}
var length = iteratees.length;
if (length > 1 && isIterateeCall(collection, iteratees[0], iteratees[1])) {
iteratees = [];
} else if (length > 2 && isIterateeCall(iteratees[0], iteratees[1], iteratees[2])) {
iteratees = [iteratees[0]];
}
return baseOrderBy(collection, baseFlatten(iteratees, 1), []);
});
/**
* Creates a function that memoizes the result of `func`. If `resolver` is
* provided, it determines the cache key for storing the result based on the
* arguments provided to the memoized function. By default, the first argument
* provided to the memoized function is used as the map cache key. The `func`
* is invoked with the `this` binding of the memoized function.
*
* **Note:** The cache is exposed as the `cache` property on the memoized
* function. Its creation may be customized by replacing the `_.memoize.Cache`
* constructor with one whose instances implement the
* [`Map`](http://ecma-international.org/ecma-262/7.0/#sec-properties-of-the-map-prototype-object)
* method interface of `delete`, `get`, `has`, and `set`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to have its output memoized.
* @param {Function} [resolver] The function to resolve the cache key.
* @returns {Function} Returns the new memoized function.
* @example
*
* var object = { 'a': 1, 'b': 2 };
* var other = { 'c': 3, 'd': 4 };
*
* var values = _.memoize(_.values);
* values(object);
* // => [1, 2]
*
* values(other);
* // => [3, 4]
*
* object.a = 2;
* values(object);
* // => [1, 2]
*
* // Modify the result cache.
* values.cache.set(object, ['a', 'b']);
* values(object);
* // => ['a', 'b']
*
* // Replace `_.memoize.Cache`.
* _.memoize.Cache = WeakMap;
*/
function memoize(func, resolver) {
if (typeof func != 'function' || (resolver && typeof resolver != 'function')) {
throw new TypeError(FUNC_ERROR_TEXT);
}
var memoized = function() {
var args = arguments,
key = resolver ? resolver.apply(this, args) : args[0],
cache = memoized.cache;
if (cache.has(key)) {
return cache.get(key);
}
var result = func.apply(this, args);
memoized.cache = cache.set(key, result);
return result;
};
memoized.cache = new (memoize.Cache || MapCache);
return memoized;
}
// Assign cache to `_.memoize`.
memoize.Cache = MapCache;
/**
* Performs a
* [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
* comparison between two values to determine if they are equivalent.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
* @example
*
* var object = { 'a': 1 };
* var other = { 'a': 1 };
*
* _.eq(object, object);
* // => true
*
* _.eq(object, other);
* // => false
*
* _.eq('a', 'a');
* // => true
*
* _.eq('a', Object('a'));
* // => false
*
* _.eq(NaN, NaN);
* // => true
*/
function eq(value, other) {
return value === other || (value !== value && other !== other);
}
/**
* Checks if `value` is likely an `arguments` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an `arguments` object,
* else `false`.
* @example
*
* _.isArguments(function() { return arguments; }());
* // => true
*
* _.isArguments([1, 2, 3]);
* // => false
*/
function isArguments(value) {
// Safari 8.1 makes `arguments.callee` enumerable in strict mode.
return isArrayLikeObject(value) && hasOwnProperty.call(value, 'callee') &&
(!propertyIsEnumerable.call(value, 'callee') || objectToString.call(value) == argsTag);
}
/**
* Checks if `value` is classified as an `Array` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an array, else `false`.
* @example
*
* _.isArray([1, 2, 3]);
* // => true
*
* _.isArray(document.body.children);
* // => false
*
* _.isArray('abc');
* // => false
*
* _.isArray(_.noop);
* // => false
*/
var isArray = Array.isArray;
/**
* Checks if `value` is array-like. A value is considered array-like if it's
* not a function and has a `value.length` that's an integer greater than or
* equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is array-like, else `false`.
* @example
*
* _.isArrayLike([1, 2, 3]);
* // => true
*
* _.isArrayLike(document.body.children);
* // => true
*
* _.isArrayLike('abc');
* // => true
*
* _.isArrayLike(_.noop);
* // => false
*/
function isArrayLike(value) {
return value != null && isLength(value.length) && !isFunction(value);
}
/**
* This method is like `_.isArrayLike` except that it also checks if `value`
* is an object.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an array-like object,
* else `false`.
* @example
*
* _.isArrayLikeObject([1, 2, 3]);
* // => true
*
* _.isArrayLikeObject(document.body.children);
* // => true
*
* _.isArrayLikeObject('abc');
* // => false
*
* _.isArrayLikeObject(_.noop);
* // => false
*/
function isArrayLikeObject(value) {
return isObjectLike(value) && isArrayLike(value);
}
/**
* Checks if `value` is classified as a `Function` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a function, else `false`.
* @example
*
* _.isFunction(_);
* // => true
*
* _.isFunction(/abc/);
* // => false
*/
function isFunction(value) {
// The use of `Object#toString` avoids issues with the `typeof` operator
// in Safari 8-9 which returns 'object' for typed array and other constructors.
var tag = isObject(value) ? objectToString.call(value) : '';
return tag == funcTag || tag == genTag;
}
/**
* Checks if `value` is a valid array-like length.
*
* **Note:** This method is loosely based on
* [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
* @example
*
* _.isLength(3);
* // => true
*
* _.isLength(Number.MIN_VALUE);
* // => false
*
* _.isLength(Infinity);
* // => false
*
* _.isLength('3');
* // => false
*/
function isLength(value) {
return typeof value == 'number' &&
value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
}
/**
* Checks if `value` is the
* [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
* of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an object, else `false`.
* @example
*
* _.isObject({});
* // => true
*
* _.isObject([1, 2, 3]);
* // => true
*
* _.isObject(_.noop);
* // => true
*
* _.isObject(null);
* // => false
*/
function isObject(value) {
var type = typeof value;
return !!value && (type == 'object' || type == 'function');
}
/**
* Checks if `value` is object-like. A value is object-like if it's not `null`
* and has a `typeof` result of "object".
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is object-like, else `false`.
* @example
*
* _.isObjectLike({});
* // => true
*
* _.isObjectLike([1, 2, 3]);
* // => true
*
* _.isObjectLike(_.noop);
* // => false
*
* _.isObjectLike(null);
* // => false
*/
function isObjectLike(value) {
return !!value && typeof value == 'object';
}
/**
* Checks if `value` is classified as a `Symbol` primitive or object.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a symbol, else `false`.
* @example
*
* _.isSymbol(Symbol.iterator);
* // => true
*
* _.isSymbol('abc');
* // => false
*/
function isSymbol(value) {
return typeof value == 'symbol' ||
(isObjectLike(value) && objectToString.call(value) == symbolTag);
}
/**
* Checks if `value` is classified as a typed array.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
* @example
*
* _.isTypedArray(new Uint8Array);
* // => true
*
* _.isTypedArray([]);
* // => false
*/
var isTypedArray = nodeIsTypedArray ? baseUnary(nodeIsTypedArray) : baseIsTypedArray;
/**
* Converts `value` to a string. An empty string is returned for `null`
* and `undefined` values. The sign of `-0` is preserved.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to process.
* @returns {string} Returns the string.
* @example
*
* _.toString(null);
* // => ''
*
* _.toString(-0);
* // => '-0'
*
* _.toString([1, 2, 3]);
* // => '1,2,3'
*/
function toString(value) {
return value == null ? '' : baseToString(value);
}
/**
* Gets the value at `path` of `object`. If the resolved value is
* `undefined`, the `defaultValue` is returned in its place.
*
* @static
* @memberOf _
* @since 3.7.0
* @category Object
* @param {Object} object The object to query.
* @param {Array|string} path The path of the property to get.
* @param {*} [defaultValue] The value returned for `undefined` resolved values.
* @returns {*} Returns the resolved value.
* @example
*
* var object = { 'a': [{ 'b': { 'c': 3 } }] };
*
* _.get(object, 'a[0].b.c');
* // => 3
*
* _.get(object, ['a', '0', 'b', 'c']);
* // => 3
*
* _.get(object, 'a.b.c', 'default');
* // => 'default'
*/
function get(object, path, defaultValue) {
var result = object == null ? undefined : baseGet(object, path);
return result === undefined ? defaultValue : result;
}
/**
* Checks if `path` is a direct or inherited property of `object`.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Object
* @param {Object} object The object to query.
* @param {Array|string} path The path to check.
* @returns {boolean} Returns `true` if `path` exists, else `false`.
* @example
*
* var object = _.create({ 'a': _.create({ 'b': 2 }) });
*
* _.hasIn(object, 'a');
* // => true
*
* _.hasIn(object, 'a.b');
* // => true
*
* _.hasIn(object, ['a', 'b']);
* // => true
*
* _.hasIn(object, 'b');
* // => false
*/
function hasIn(object, path) {
return object != null && hasPath(object, path, baseHasIn);
}
/**
* Creates an array of the own enumerable property names of `object`.
*
* **Note:** Non-object values are coerced to objects. See the
* [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
* for more details.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.keys(new Foo);
* // => ['a', 'b'] (iteration order is not guaranteed)
*
* _.keys('hi');
* // => ['0', '1']
*/
function keys(object) {
return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object);
}
/**
* This method returns the first argument it receives.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @param {*} value Any value.
* @returns {*} Returns `value`.
* @example
*
* var object = { 'a': 1 };
*
* console.log(_.identity(object) === object);
* // => true
*/
function identity(value) {
return value;
}
/**
* Creates a function that returns the value at `path` of a given object.
*
* @static
* @memberOf _
* @since 2.4.0
* @category Util
* @param {Array|string} path The path of the property to get.
* @returns {Function} Returns the new accessor function.
* @example
*
* var objects = [
* { 'a': { 'b': 2 } },
* { 'a': { 'b': 1 } }
* ];
*
* _.map(objects, _.property('a.b'));
* // => [2, 1]
*
* _.map(_.sortBy(objects, _.property(['a', 'b'])), 'a.b');
* // => [1, 2]
*/
function property(path) {
return isKey(path) ? baseProperty(toKey(path)) : basePropertyDeep(path);
}
module.exports = sortBy; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash.sortby/index.js | index.js |
# Parse `data:` URLs
This package helps you parse `data:` URLs [according to the WHATWG Fetch Standard](https://fetch.spec.whatwg.org/#data-urls):
```js
const parseDataURL = require("data-urls");
const textExample = parseDataURL("data:,Hello%2C%20World!");
console.log(textExample.mimeType.toString()); // "text/plain;charset=US-ASCII"
console.log(textExample.body.toString()); // "Hello, World!"
const htmlExample = dataURL("data:text/html,%3Ch1%3EHello%2C%20World!%3C%2Fh1%3E");
console.log(htmlExample.mimeType.toString()); // "text/html"
console.log(htmlExample.body.toString()); // <h1>Hello, World!</h1>
const pngExample = parseDataURL("data:image/png;base64,iVBORw0KGgoAAA" +
"ANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4" +
"//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU" +
"5ErkJggg==");
console.log(pngExample.mimeType.toString()); // "image/png"
console.log(pngExample.body); // <Buffer 89 50 4e 47 0d ... >
```
## API
This package's main module's default export is a function that accepts a string and returns a `{ mimeType, body }` object, or `null` if the result cannot be parsed as a `data:` URL.
- The `mimeType` property is an instance of [whatwg-mimetype](https://www.npmjs.com/package/whatwg-mimetype)'s `MIMEType` class.
- The `body` property is a Node.js [`Buffer`](https://nodejs.org/docs/latest/api/buffer.html) instance.
As shown in the examples above, both of these have useful `toString()` methods for manipulating them as string values. However…
### A word of caution on string decoding
Because Node.js's `Buffer.prototype.toString()` assumes a UTF-8 encoding, simply doing `dataURL.body.toString()` may not work correctly if the `data:` URL's contents were not originally written in UTF-8. This includes if the encoding is "US-ASCII", [aka windows-1252](https://encoding.spec.whatwg.org/#names-and-labels), which is notable for being the default in many cases.
A more complete decoding example would use the [whatwg-encoding](https://www.npmjs.com/package/whatwg-encoding) package as follows:
```js
const parseDataURL = require("data-urls");
const { labelToName, decode } = require("whatwg-encoding");
const dataURL = parseDataURL(arbitraryString);
const encodingName = labelToName(dataURL.mimeType.parameters.get("charset"));
const bodyDecoded = decode(dataURL.body, encodingName);
```
For example, given an `arbitraryString` of `data:,Hello!`, this will produce a `bodyDecoded` of `"Hello!"`, as expected. But given an `arbitraryString` of `"data:,Héllo!"`, this will correctly produce a `bodyDecoded` of `"Héllo!"`, whereas just doing `dataURL.body.toString()` will give back `"Héllo!"`.
In summary, only use `dataURL.body.toString()` when you are very certain your data is inside the ASCII range (i.e. code points within the range U+0000 to U+007F).
### Advanced functionality: parsing from a URL record
If you are using the [whatwg-url](https://github.com/jsdom/whatwg-url) package, you may already have a "URL record" object on hand, as produced by that package's `parseURL` export. In that case, you can use this package's `fromURLRecord` export to save a bit of work:
```js
const { parseURL } = require("whatwg-url");
const dataURLFromURLRecord = require("data-urls").fromURLRecord;
const urlRecord = parseURL("data:,Hello%2C%20World!");
const dataURL = dataURLFromURLRecord(urlRecord);
```
In practice, we expect this functionality only to be used by consumers like [jsdom](https://www.npmjs.com/package/jsdom), which are using these packages at a very low level.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/data-urls/README.md | README.md |
# levn [](https://travis-ci.org/gkz/levn) <a name="levn" />
__Light ECMAScript (JavaScript) Value Notation__
Levn is a library which allows you to parse a string into a JavaScript value based on an expected type. It is meant for short amounts of human entered data (eg. config files, command line arguments).
Levn aims to concisely describe JavaScript values in text, and allow for the extraction and validation of those values. Levn uses [type-check](https://github.com/gkz/type-check) for its type format, and to validate the results. MIT license. Version 0.3.0.
__How is this different than JSON?__ levn is meant to be written by humans only, is (due to the previous point) much more concise, can be validated against supplied types, has regex and date literals, and can easily be extended with custom types. On the other hand, it is probably slower and thus less efficient at transporting large amounts of data, which is fine since this is not its purpose.
npm install levn
For updates on levn, [follow me on twitter](https://twitter.com/gkzahariev).
## Quick Examples
```js
var parse = require('levn').parse;
parse('Number', '2'); // 2
parse('String', '2'); // '2'
parse('String', 'levn'); // 'levn'
parse('String', 'a b'); // 'a b'
parse('Boolean', 'true'); // true
parse('Date', '#2011-11-11#'); // (Date object)
parse('Date', '2011-11-11'); // (Date object)
parse('RegExp', '/[a-z]/gi'); // /[a-z]/gi
parse('RegExp', 're'); // /re/
parse('Int', '2'); // 2
parse('Number | String', 'str'); // 'str'
parse('Number | String', '2'); // 2
parse('[Number]', '[1,2,3]'); // [1,2,3]
parse('(String, Boolean)', '(hi, false)'); // ['hi', false]
parse('{a: String, b: Number}', '{a: str, b: 2}'); // {a: 'str', b: 2}
// at the top level, you can ommit surrounding delimiters
parse('[Number]', '1,2,3'); // [1,2,3]
parse('(String, Boolean)', 'hi, false'); // ['hi', false]
parse('{a: String, b: Number}', 'a: str, b: 2'); // {a: 'str', b: 2}
// wildcard - auto choose type
parse('*', '[hi,(null,[42]),{k: true}]'); // ['hi', [null, [42]], {k: true}]
```
## Usage
`require('levn');` returns an object that exposes three properties. `VERSION` is the current version of the library as a string. `parse` and `parsedTypeParse` are functions.
```js
// parse(type, input, options);
parse('[Number]', '1,2,3'); // [1, 2, 3]
// parsedTypeParse(parsedType, input, options);
var parsedType = require('type-check').parseType('[Number]');
parsedTypeParse(parsedType, '1,2,3'); // [1, 2, 3]
```
### parse(type, input, options)
`parse` casts the string `input` into a JavaScript value according to the specified `type` in the [type format](https://github.com/gkz/type-check#type-format) (and taking account the optional `options`) and returns the resulting JavaScript value.
##### arguments
* type - `String` - the type written in the [type format](https://github.com/gkz/type-check#type-format) which to check against
* input - `String` - the value written in the [levn format](#levn-format)
* options - `Maybe Object` - an optional parameter specifying additional [options](#options)
##### returns
`*` - the resulting JavaScript value
##### example
```js
parse('[Number]', '1,2,3'); // [1, 2, 3]
```
### parsedTypeParse(parsedType, input, options)
`parsedTypeParse` casts the string `input` into a JavaScript value according to the specified `type` which has already been parsed (and taking account the optional `options`) and returns the resulting JavaScript value. You can parse a type using the [type-check](https://github.com/gkz/type-check) library's `parseType` function.
##### arguments
* type - `Object` - the type in the parsed type format which to check against
* input - `String` - the value written in the [levn format](#levn-format)
* options - `Maybe Object` - an optional parameter specifying additional [options](#options)
##### returns
`*` - the resulting JavaScript value
##### example
```js
var parsedType = require('type-check').parseType('[Number]');
parsedTypeParse(parsedType, '1,2,3'); // [1, 2, 3]
```
## Levn Format
Levn can use the type information you provide to choose the appropriate value to produce from the input. For the same input, it will choose a different output value depending on the type provided. For example, `parse('Number', '2')` will produce the number `2`, but `parse('String', '2')` will produce the string `"2"`.
If you do not provide type information, and simply use `*`, levn will parse the input according the unambiguous "explicit" mode, which we will now detail - you can also set the `explicit` option to true manually in the [options](#options).
* `"string"`, `'string'` are parsed as a String, eg. `"a msg"` is `"a msg"`
* `#date#` is parsed as a Date, eg. `#2011-11-11#` is `new Date('2011-11-11')`
* `/regexp/flags` is parsed as a RegExp, eg. `/re/gi` is `/re/gi`
* `undefined`, `null`, `NaN`, `true`, and `false` are all their JavaScript equivalents
* `[element1, element2, etc]` is an Array, and the casting procedure is recursively applied to each element. Eg. `[1,2,3]` is `[1,2,3]`.
* `(element1, element2, etc)` is an tuple, and the casting procedure is recursively applied to each element. Eg. `(1, a)` is `(1, a)` (is `[1, 'a']`).
* `{key1: val1, key2: val2, ...}` is an Object, and the casting procedure is recursively applied to each property. Eg. `{a: 1, b: 2}` is `{a: 1, b: 2}`.
* Any test which does not fall under the above, and which does not contain special characters (`[``]``(``)``{``}``:``,`) is a string, eg. `$12- blah` is `"$12- blah"`.
If you do provide type information, you can make your input more concise as the program already has some information about what it expects. Please see the [type format](https://github.com/gkz/type-check#type-format) section of [type-check](https://github.com/gkz/type-check) for more information about how to specify types. There are some rules about what levn can do with the information:
* If a String is expected, and only a String, all characters of the input (including any special ones) will become part of the output. Eg. `[({})]` is `"[({})]"`, and `"hi"` is `'"hi"'`.
* If a Date is expected, the surrounding `#` can be omitted from date literals. Eg. `2011-11-11` is `new Date('2011-11-11')`.
* If a RegExp is expected, no flags need to be specified, and the regex is not using any of the special characters,the opening and closing `/` can be omitted - this will have the affect of setting the source of the regex to the input. Eg. `regex` is `/regex/`.
* If an Array is expected, and it is the root node (at the top level), the opening `[` and closing `]` can be omitted. Eg. `1,2,3` is `[1,2,3]`.
* If a tuple is expected, and it is the root node (at the top level), the opening `(` and closing `)` can be omitted. Eg. `1, a` is `(1, a)` (is `[1, 'a']`).
* If an Object is expected, and it is the root node (at the top level), the opening `{` and closing `}` can be omitted. Eg `a: 1, b: 2` is `{a: 1, b: 2}`.
If you list multiple types (eg. `Number | String`), it will first attempt to cast to the first type and then validate - if the validation fails it will move on to the next type and so forth, left to right. You must be careful as some types will succeed with any input, such as String. Thus put String at the end of your list. In non-explicit mode, Date and RegExp will succeed with a large variety of input - also be careful with these and list them near the end if not last in your list.
Whitespace between special characters and elements is inconsequential.
## Options
Options is an object. It is an optional parameter to the `parse` and `parsedTypeParse` functions.
### Explicit
A `Boolean`. By default it is `false`.
__Example:__
```js
parse('RegExp', 're', {explicit: false}); // /re/
parse('RegExp', 're', {explicit: true}); // Error: ... does not type check...
parse('RegExp | String', 're', {explicit: true}); // 're'
```
`explicit` sets whether to be in explicit mode or not. Using `*` automatically activates explicit mode. For more information, read the [levn format](#levn-format) section.
### customTypes
An `Object`. Empty `{}` by default.
__Example:__
```js
var options = {
customTypes: {
Even: {
typeOf: 'Number',
validate: function (x) {
return x % 2 === 0;
},
cast: function (x) {
return {type: 'Just', value: parseInt(x)};
}
}
}
}
parse('Even', '2', options); // 2
parse('Even', '3', options); // Error: Value: "3" does not type check...
```
__Another Example:__
```js
function Person(name, age){
this.name = name;
this.age = age;
}
var options = {
customTypes: {
Person: {
typeOf: 'Object',
validate: function (x) {
x instanceof Person;
},
cast: function (value, options, typesCast) {
var name, age;
if ({}.toString.call(value).slice(8, -1) !== 'Object') {
return {type: 'Nothing'};
}
name = typesCast(value.name, [{type: 'String'}], options);
age = typesCast(value.age, [{type: 'Numger'}], options);
return {type: 'Just', value: new Person(name, age)};
}
}
}
parse('Person', '{name: Laura, age: 25}', options); // Person {name: 'Laura', age: 25}
```
`customTypes` is an object whose keys are the name of the types, and whose values are an object with three properties, `typeOf`, `validate`, and `cast`. For more information about `typeOf` and `validate`, please see the [custom types](https://github.com/gkz/type-check#custom-types) section of type-check.
`cast` is a function which receives three arguments, the value under question, options, and the typesCast function. In `cast`, attempt to cast the value into the specified type. If you are successful, return an object in the format `{type: 'Just', value: CAST-VALUE}`, if you know it won't work, return `{type: 'Nothing'}`. You can use the `typesCast` function to cast any child values. Remember to pass `options` to it. In your function you can also check for `options.explicit` and act accordingly.
## Technical About
`levn` is written in [LiveScript](http://livescript.net/) - a language that compiles to JavaScript. It uses [type-check](https://github.com/gkz/type-check) to both parse types and validate values. It also uses the [prelude.ls](http://preludels.com/) library.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/levn/README.md | README.md |
(function(){
var reject, special, tokenRegex;
reject = require('prelude-ls').reject;
function consumeOp(tokens, op){
if (tokens[0] === op) {
return tokens.shift();
} else {
throw new Error("Expected '" + op + "', but got '" + tokens[0] + "' instead in " + JSON.stringify(tokens) + ".");
}
}
function maybeConsumeOp(tokens, op){
if (tokens[0] === op) {
return tokens.shift();
}
}
function consumeList(tokens, delimiters, hasDelimiters){
var result;
if (hasDelimiters) {
consumeOp(tokens, delimiters[0]);
}
result = [];
while (tokens.length && tokens[0] !== delimiters[1]) {
result.push(consumeElement(tokens));
maybeConsumeOp(tokens, ',');
}
if (hasDelimiters) {
consumeOp(tokens, delimiters[1]);
}
return result;
}
function consumeArray(tokens, hasDelimiters){
return consumeList(tokens, ['[', ']'], hasDelimiters);
}
function consumeTuple(tokens, hasDelimiters){
return consumeList(tokens, ['(', ')'], hasDelimiters);
}
function consumeFields(tokens, hasDelimiters){
var result, key;
if (hasDelimiters) {
consumeOp(tokens, '{');
}
result = {};
while (tokens.length && (!hasDelimiters || tokens[0] !== '}')) {
key = tokens.shift();
consumeOp(tokens, ':');
result[key] = consumeElement(tokens);
maybeConsumeOp(tokens, ',');
}
if (hasDelimiters) {
consumeOp(tokens, '}');
}
return result;
}
function consumeElement(tokens){
switch (tokens[0]) {
case '[':
return consumeArray(tokens, true);
case '(':
return consumeTuple(tokens, true);
case '{':
return consumeFields(tokens, true);
default:
return tokens.shift();
}
}
function consumeTopLevel(tokens, types){
var ref$, type, structure, origTokens, result, finalResult, x$, y$;
ref$ = types[0], type = ref$.type, structure = ref$.structure;
origTokens = tokens.concat();
if (types.length === 1 && (structure || (type === 'Array' || type === 'Object'))) {
result = structure === 'array' || type === 'Array'
? consumeArray(tokens, tokens[0] === '[')
: structure === 'tuple'
? consumeTuple(tokens, tokens[0] === '(')
: consumeFields(tokens, tokens[0] === '{');
finalResult = tokens.length ? consumeElement(structure === 'array' || type === 'Array'
? (x$ = origTokens, x$.unshift('['), x$.push(']'), x$)
: (y$ = origTokens, y$.unshift('('), y$.push(')'), y$)) : result;
} else {
finalResult = consumeElement(tokens);
}
if (tokens.length && origTokens.length) {
throw new Error("Unable to parse " + JSON.stringify(origTokens) + " of type " + JSON.stringify(types) + ".");
} else {
return finalResult;
}
}
special = /\[\]\(\)}{:,/.source;
tokenRegex = RegExp('("(?:[^"]|\\\\")*")|(\'(?:[^\']|\\\\\')*\')|(#.*#)|(/(?:\\\\/|[^/])*/[gimy]*)|([' + special + '])|([^\\s' + special + ']+)|\\s*');
module.exports = function(string, types){
var tokens, node;
tokens = reject(function(it){
return !it || /^\s+$/.test(it);
}, string.split(tokenRegex));
node = consumeTopLevel(tokens, types);
if (!node) {
throw new Error("Error parsing '" + string + "'.");
}
return node;
};
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/levn/lib/parse.js | parse.js |
(function(){
var parsedTypeCheck, types, toString$ = {}.toString;
parsedTypeCheck = require('type-check').parsedTypeCheck;
types = {
'*': function(value, options){
switch (toString$.call(value).slice(8, -1)) {
case 'Array':
return typeCast(value, {
type: 'Array'
}, options);
case 'Object':
return typeCast(value, {
type: 'Object'
}, options);
default:
return {
type: 'Just',
value: typesCast(value, [
{
type: 'Undefined'
}, {
type: 'Null'
}, {
type: 'NaN'
}, {
type: 'Boolean'
}, {
type: 'Number'
}, {
type: 'Date'
}, {
type: 'RegExp'
}, {
type: 'Array'
}, {
type: 'Object'
}, {
type: 'String'
}
], (options.explicit = true, options))
};
}
},
Undefined: function(it){
if (it === 'undefined' || it === void 8) {
return {
type: 'Just',
value: void 8
};
} else {
return {
type: 'Nothing'
};
}
},
Null: function(it){
if (it === 'null') {
return {
type: 'Just',
value: null
};
} else {
return {
type: 'Nothing'
};
}
},
NaN: function(it){
if (it === 'NaN') {
return {
type: 'Just',
value: NaN
};
} else {
return {
type: 'Nothing'
};
}
},
Boolean: function(it){
if (it === 'true') {
return {
type: 'Just',
value: true
};
} else if (it === 'false') {
return {
type: 'Just',
value: false
};
} else {
return {
type: 'Nothing'
};
}
},
Number: function(it){
return {
type: 'Just',
value: +it
};
},
Int: function(it){
return {
type: 'Just',
value: +it
};
},
Float: function(it){
return {
type: 'Just',
value: +it
};
},
Date: function(value, options){
var that;
if (that = /^\#([\s\S]*)\#$/.exec(value)) {
return {
type: 'Just',
value: new Date(+that[1] || that[1])
};
} else if (options.explicit) {
return {
type: 'Nothing'
};
} else {
return {
type: 'Just',
value: new Date(+value || value)
};
}
},
RegExp: function(value, options){
var that;
if (that = /^\/([\s\S]*)\/([gimy]*)$/.exec(value)) {
return {
type: 'Just',
value: new RegExp(that[1], that[2])
};
} else if (options.explicit) {
return {
type: 'Nothing'
};
} else {
return {
type: 'Just',
value: new RegExp(value)
};
}
},
Array: function(value, options){
return castArray(value, {
of: [{
type: '*'
}]
}, options);
},
Object: function(value, options){
return castFields(value, {
of: {}
}, options);
},
String: function(it){
var that;
if (toString$.call(it).slice(8, -1) !== 'String') {
return {
type: 'Nothing'
};
}
if (that = it.match(/^'([\s\S]*)'$/)) {
return {
type: 'Just',
value: that[1].replace(/\\'/g, "'")
};
} else if (that = it.match(/^"([\s\S]*)"$/)) {
return {
type: 'Just',
value: that[1].replace(/\\"/g, '"')
};
} else {
return {
type: 'Just',
value: it
};
}
}
};
function castArray(node, type, options){
var typeOf, element;
if (toString$.call(node).slice(8, -1) !== 'Array') {
return {
type: 'Nothing'
};
}
typeOf = type.of;
return {
type: 'Just',
value: (function(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = node).length; i$ < len$; ++i$) {
element = ref$[i$];
results$.push(typesCast(element, typeOf, options));
}
return results$;
}())
};
}
function castTuple(node, type, options){
var result, i, i$, ref$, len$, types, cast;
if (toString$.call(node).slice(8, -1) !== 'Array') {
return {
type: 'Nothing'
};
}
result = [];
i = 0;
for (i$ = 0, len$ = (ref$ = type.of).length; i$ < len$; ++i$) {
types = ref$[i$];
cast = typesCast(node[i], types, options);
if (toString$.call(cast).slice(8, -1) !== 'Undefined') {
result.push(cast);
}
i++;
}
if (node.length <= i) {
return {
type: 'Just',
value: result
};
} else {
return {
type: 'Nothing'
};
}
}
function castFields(node, type, options){
var typeOf, key, value;
if (toString$.call(node).slice(8, -1) !== 'Object') {
return {
type: 'Nothing'
};
}
typeOf = type.of;
return {
type: 'Just',
value: (function(){
var ref$, resultObj$ = {};
for (key in ref$ = node) {
value = ref$[key];
resultObj$[typesCast(key, [{
type: 'String'
}], options)] = typesCast(value, typeOf[key] || [{
type: '*'
}], options);
}
return resultObj$;
}())
};
}
function typeCast(node, typeObj, options){
var type, structure, castFunc, ref$;
type = typeObj.type, structure = typeObj.structure;
if (type) {
castFunc = ((ref$ = options.customTypes[type]) != null ? ref$.cast : void 8) || types[type];
if (!castFunc) {
throw new Error("Type not defined: " + type + ".");
}
return castFunc(node, options, typesCast);
} else {
switch (structure) {
case 'array':
return castArray(node, typeObj, options);
case 'tuple':
return castTuple(node, typeObj, options);
case 'fields':
return castFields(node, typeObj, options);
}
}
}
function typesCast(node, types, options){
var i$, len$, type, ref$, valueType, value;
for (i$ = 0, len$ = types.length; i$ < len$; ++i$) {
type = types[i$];
ref$ = typeCast(node, type, options), valueType = ref$.type, value = ref$.value;
if (valueType === 'Nothing') {
continue;
}
if (parsedTypeCheck([type], value, {
customTypes: options.customTypes
})) {
return value;
}
}
throw new Error("Value " + JSON.stringify(node) + " does not type check against " + JSON.stringify(types) + ".");
}
module.exports = typesCast;
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/levn/lib/cast.js | cast.js |
(function(){
var reject, special, tokenRegex;
reject = require('prelude-ls').reject;
function consumeOp(tokens, op){
if (tokens[0] === op) {
return tokens.shift();
} else {
throw new Error("Expected '" + op + "', but got '" + tokens[0] + "' instead in " + JSON.stringify(tokens) + ".");
}
}
function maybeConsumeOp(tokens, op){
if (tokens[0] === op) {
return tokens.shift();
}
}
function consumeList(tokens, arg$, hasDelimiters){
var open, close, result, untilTest;
open = arg$[0], close = arg$[1];
if (hasDelimiters) {
consumeOp(tokens, open);
}
result = [];
untilTest = "," + (hasDelimiters ? close : '');
while (tokens.length && (hasDelimiters && tokens[0] !== close)) {
result.push(consumeElement(tokens, untilTest));
maybeConsumeOp(tokens, ',');
}
if (hasDelimiters) {
consumeOp(tokens, close);
}
return result;
}
function consumeArray(tokens, hasDelimiters){
return consumeList(tokens, ['[', ']'], hasDelimiters);
}
function consumeTuple(tokens, hasDelimiters){
return consumeList(tokens, ['(', ')'], hasDelimiters);
}
function consumeFields(tokens, hasDelimiters){
var result, untilTest, key;
if (hasDelimiters) {
consumeOp(tokens, '{');
}
result = {};
untilTest = "," + (hasDelimiters ? '}' : '');
while (tokens.length && (!hasDelimiters || tokens[0] !== '}')) {
key = consumeValue(tokens, ':');
consumeOp(tokens, ':');
result[key] = consumeElement(tokens, untilTest);
maybeConsumeOp(tokens, ',');
}
if (hasDelimiters) {
consumeOp(tokens, '}');
}
return result;
}
function consumeValue(tokens, untilTest){
var out;
untilTest == null && (untilTest = '');
out = '';
while (tokens.length && -1 === untilTest.indexOf(tokens[0])) {
out += tokens.shift();
}
return out;
}
function consumeElement(tokens, untilTest){
switch (tokens[0]) {
case '[':
return consumeArray(tokens, true);
case '(':
return consumeTuple(tokens, true);
case '{':
return consumeFields(tokens, true);
default:
return consumeValue(tokens, untilTest);
}
}
function consumeTopLevel(tokens, types, options){
var ref$, type, structure, origTokens, result, finalResult, x$, y$;
ref$ = types[0], type = ref$.type, structure = ref$.structure;
origTokens = tokens.concat();
if (!options.explicit && types.length === 1 && ((!type && structure) || (type === 'Array' || type === 'Object'))) {
result = structure === 'array' || type === 'Array'
? consumeArray(tokens, tokens[0] === '[')
: structure === 'tuple'
? consumeTuple(tokens, tokens[0] === '(')
: consumeFields(tokens, tokens[0] === '{');
finalResult = tokens.length ? consumeElement(structure === 'array' || type === 'Array'
? (x$ = origTokens, x$.unshift('['), x$.push(']'), x$)
: (y$ = origTokens, y$.unshift('('), y$.push(')'), y$)) : result;
} else {
finalResult = consumeElement(tokens);
}
return finalResult;
}
special = /\[\]\(\)}{:,/.source;
tokenRegex = RegExp('("(?:\\\\"|[^"])*")|(\'(?:\\\\\'|[^\'])*\')|(/(?:\\\\/|[^/])*/[a-zA-Z]*)|(#.*#)|([' + special + '])|([^\\s' + special + '](?:\\s*[^\\s' + special + ']+)*)|\\s*');
module.exports = function(types, string, options){
var tokens, node;
options == null && (options = {});
if (!options.explicit && types.length === 1 && types[0].type === 'String') {
return "'" + string.replace(/\\'/g, "\\\\'") + "'";
}
tokens = reject(not$, string.split(tokenRegex));
node = consumeTopLevel(tokens, types, options);
if (!node) {
throw new Error("Error parsing '" + string + "'.");
}
return node;
};
function not$(x){ return !x; }
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/levn/lib/parse-string.js | parse-string.js |
(function(){
var parsedTypeCheck, types, toString$ = {}.toString;
parsedTypeCheck = require('type-check').parsedTypeCheck;
types = {
'*': function(it){
switch (toString$.call(it).slice(8, -1)) {
case 'Array':
return coerceType(it, {
type: 'Array'
});
case 'Object':
return coerceType(it, {
type: 'Object'
});
default:
return {
type: 'Just',
value: coerceTypes(it, [
{
type: 'Undefined'
}, {
type: 'Null'
}, {
type: 'NaN'
}, {
type: 'Boolean'
}, {
type: 'Number'
}, {
type: 'Date'
}, {
type: 'RegExp'
}, {
type: 'Array'
}, {
type: 'Object'
}, {
type: 'String'
}
], {
explicit: true
})
};
}
},
Undefined: function(it){
if (it === 'undefined' || it === void 8) {
return {
type: 'Just',
value: void 8
};
} else {
return {
type: 'Nothing'
};
}
},
Null: function(it){
if (it === 'null') {
return {
type: 'Just',
value: null
};
} else {
return {
type: 'Nothing'
};
}
},
NaN: function(it){
if (it === 'NaN') {
return {
type: 'Just',
value: NaN
};
} else {
return {
type: 'Nothing'
};
}
},
Boolean: function(it){
if (it === 'true') {
return {
type: 'Just',
value: true
};
} else if (it === 'false') {
return {
type: 'Just',
value: false
};
} else {
return {
type: 'Nothing'
};
}
},
Number: function(it){
return {
type: 'Just',
value: +it
};
},
Int: function(it){
return {
type: 'Just',
value: parseInt(it)
};
},
Float: function(it){
return {
type: 'Just',
value: parseFloat(it)
};
},
Date: function(value, options){
var that;
if (that = /^\#(.*)\#$/.exec(value)) {
return {
type: 'Just',
value: new Date(+that[1] || that[1])
};
} else if (options.explicit) {
return {
type: 'Nothing'
};
} else {
return {
type: 'Just',
value: new Date(+value || value)
};
}
},
RegExp: function(value, options){
var that;
if (that = /^\/(.*)\/([gimy]*)$/.exec(value)) {
return {
type: 'Just',
value: new RegExp(that[1], that[2])
};
} else if (options.explicit) {
return {
type: 'Nothing'
};
} else {
return {
type: 'Just',
value: new RegExp(value)
};
}
},
Array: function(it){
return coerceArray(it, {
of: [{
type: '*'
}]
});
},
Object: function(it){
return coerceFields(it, {
of: {}
});
},
String: function(it){
var that;
if (toString$.call(it).slice(8, -1) !== 'String') {
return {
type: 'Nothing'
};
}
if (that = it.match(/^'(.*)'$/)) {
return {
type: 'Just',
value: that[1]
};
} else if (that = it.match(/^"(.*)"$/)) {
return {
type: 'Just',
value: that[1]
};
} else {
return {
type: 'Just',
value: it
};
}
}
};
function coerceArray(node, type){
var typeOf, element;
if (toString$.call(node).slice(8, -1) !== 'Array') {
return {
type: 'Nothing'
};
}
typeOf = type.of;
return {
type: 'Just',
value: (function(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = node).length; i$ < len$; ++i$) {
element = ref$[i$];
results$.push(coerceTypes(element, typeOf));
}
return results$;
}())
};
}
function coerceTuple(node, type){
var result, i$, ref$, len$, i, types, that;
if (toString$.call(node).slice(8, -1) !== 'Array') {
return {
type: 'Nothing'
};
}
result = [];
for (i$ = 0, len$ = (ref$ = type.of).length; i$ < len$; ++i$) {
i = i$;
types = ref$[i$];
if (that = coerceTypes(node[i], types)) {
result.push(that);
}
}
return {
type: 'Just',
value: result
};
}
function coerceFields(node, type){
var typeOf, key, value;
if (toString$.call(node).slice(8, -1) !== 'Object') {
return {
type: 'Nothing'
};
}
typeOf = type.of;
return {
type: 'Just',
value: (function(){
var ref$, results$ = {};
for (key in ref$ = node) {
value = ref$[key];
results$[key] = coerceTypes(value, typeOf[key] || [{
type: '*'
}]);
}
return results$;
}())
};
}
function coerceType(node, typeObj, options){
var type, structure, coerceFunc;
type = typeObj.type, structure = typeObj.structure;
if (type) {
coerceFunc = types[type];
return coerceFunc(node, options);
} else {
switch (structure) {
case 'array':
return coerceArray(node, typeObj);
case 'tuple':
return coerceTuple(node, typeObj);
case 'fields':
return coerceFields(node, typeObj);
}
}
}
function coerceTypes(node, types, options){
var i$, len$, type, ref$, valueType, value;
for (i$ = 0, len$ = types.length; i$ < len$; ++i$) {
type = types[i$];
ref$ = coerceType(node, type, options), valueType = ref$.type, value = ref$.value;
if (valueType === 'Nothing') {
continue;
}
if (parsedTypeCheck([type], value)) {
return value;
}
}
throw new Error("Value " + JSON.stringify(node) + " does not type check against " + JSON.stringify(types) + ".");
}
module.exports = coerceTypes;
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/levn/lib/coerce.js | coerce.js |
# psl (Public Suffix List)
[](https://nodei.co/npm/psl/)
[](https://greenkeeper.io/)
[](https://travis-ci.org/lupomontero/psl)
[](https://david-dm.org/lupomontero/psl#info=devDependencies)
`psl` is a `JavaScript` domain name parser based on the
[Public Suffix List](https://publicsuffix.org/).
This implementation is tested against the
[test data hosted by Mozilla](http://mxr.mozilla.org/mozilla-central/source/netwerk/test/unit/data/test_psl.txt?raw=1)
and kindly provided by [Comodo](https://www.comodo.com/).
Cross browser testing provided by
[<img alt="BrowserStack" width="160" src="./browserstack-logo.svg" />](https://www.browserstack.com/)
## What is the Public Suffix List?
The Public Suffix List is a cross-vendor initiative to provide an accurate list
of domain name suffixes.
The Public Suffix List is an initiative of the Mozilla Project, but is
maintained as a community resource. It is available for use in any software,
but was originally created to meet the needs of browser manufacturers.
A "public suffix" is one under which Internet users can directly register names.
Some examples of public suffixes are ".com", ".co.uk" and "pvt.k12.wy.us". The
Public Suffix List is a list of all known public suffixes.
Source: http://publicsuffix.org
## Installation
### Node.js
```sh
npm install --save psl
```
### Browser
Download [psl.min.js](https://raw.githubusercontent.com/lupomontero/psl/master/dist/psl.min.js)
and include it in a script tag.
```html
<script src="psl.min.js"></script>
```
This script is browserified and wrapped in a [umd](https://github.com/umdjs/umd)
wrapper so you should be able to use it standalone or together with a module
loader.
## API
### `psl.parse(domain)`
Parse domain based on Public Suffix List. Returns an `Object` with the following
properties:
* `tld`: Top level domain (this is the _public suffix_).
* `sld`: Second level domain (the first private part of the domain name).
* `domain`: The domain name is the `sld` + `tld`.
* `subdomain`: Optional parts left of the domain.
#### Example:
```js
var psl = require('psl');
// Parse domain without subdomain
var parsed = psl.parse('google.com');
console.log(parsed.tld); // 'com'
console.log(parsed.sld); // 'google'
console.log(parsed.domain); // 'google.com'
console.log(parsed.subdomain); // null
// Parse domain with subdomain
var parsed = psl.parse('www.google.com');
console.log(parsed.tld); // 'com'
console.log(parsed.sld); // 'google'
console.log(parsed.domain); // 'google.com'
console.log(parsed.subdomain); // 'www'
// Parse domain with nested subdomains
var parsed = psl.parse('a.b.c.d.foo.com');
console.log(parsed.tld); // 'com'
console.log(parsed.sld); // 'foo'
console.log(parsed.domain); // 'foo.com'
console.log(parsed.subdomain); // 'a.b.c.d'
```
### `psl.get(domain)`
Get domain name, `sld` + `tld`. Returns `null` if not valid.
#### Example:
```js
var psl = require('psl');
// null input.
psl.get(null); // null
// Mixed case.
psl.get('COM'); // null
psl.get('example.COM'); // 'example.com'
psl.get('WwW.example.COM'); // 'example.com'
// Unlisted TLD.
psl.get('example'); // null
psl.get('example.example'); // 'example.example'
psl.get('b.example.example'); // 'example.example'
psl.get('a.b.example.example'); // 'example.example'
// TLD with only 1 rule.
psl.get('biz'); // null
psl.get('domain.biz'); // 'domain.biz'
psl.get('b.domain.biz'); // 'domain.biz'
psl.get('a.b.domain.biz'); // 'domain.biz'
// TLD with some 2-level rules.
psl.get('uk.com'); // null);
psl.get('example.uk.com'); // 'example.uk.com');
psl.get('b.example.uk.com'); // 'example.uk.com');
// More complex TLD.
psl.get('c.kobe.jp'); // null
psl.get('b.c.kobe.jp'); // 'b.c.kobe.jp'
psl.get('a.b.c.kobe.jp'); // 'b.c.kobe.jp'
psl.get('city.kobe.jp'); // 'city.kobe.jp'
psl.get('www.city.kobe.jp'); // 'city.kobe.jp'
// IDN labels.
psl.get('食狮.com.cn'); // '食狮.com.cn'
psl.get('食狮.公司.cn'); // '食狮.公司.cn'
psl.get('www.食狮.公司.cn'); // '食狮.公司.cn'
// Same as above, but punycoded.
psl.get('xn--85x722f.com.cn'); // 'xn--85x722f.com.cn'
psl.get('xn--85x722f.xn--55qx5d.cn'); // 'xn--85x722f.xn--55qx5d.cn'
psl.get('www.xn--85x722f.xn--55qx5d.cn'); // 'xn--85x722f.xn--55qx5d.cn'
```
### `psl.isValid(domain)`
Check whether a domain has a valid Public Suffix. Returns a `Boolean` indicating
whether the domain has a valid Public Suffix.
#### Example
```js
var psl = require('psl');
psl.isValid('google.com'); // true
psl.isValid('www.google.com'); // true
psl.isValid('x.yz'); // false
```
## Testing and Building
Test are written using [`mocha`](https://mochajs.org/) and can be
run in two different environments: `node` and `phantomjs`.
```sh
# This will run `eslint`, `mocha` and `karma`.
npm test
# Individual test environments
# Run tests in node only.
./node_modules/.bin/mocha test
# Run tests in phantomjs only.
./node_modules/.bin/karma start ./karma.conf.js --single-run
# Build data (parse raw list) and create dist files
npm run build
```
Feel free to fork if you see possible improvements!
## Acknowledgements
* Mozilla Foundation's [Public Suffix List](https://publicsuffix.org/)
* Thanks to Rob Stradling of [Comodo](https://www.comodo.com/) for providing
test data.
* Inspired by [weppos/publicsuffix-ruby](https://github.com/weppos/publicsuffix-ruby)
## License
The MIT License (MIT)
Copyright (c) 2017 Lupo Montero <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/psl/README.md | README.md |
'use strict';
var Punycode = require('punycode');
var internals = {};
//
// Read rules from file.
//
internals.rules = require('psl/data/rules.json').map(function (rule) {
return {
rule: rule,
suffix: rule.replace(/^(\*\.|\!)/, ''),
punySuffix: -1,
wildcard: rule.charAt(0) === '*',
exception: rule.charAt(0) === '!'
};
});
//
// Check is given string ends with `suffix`.
//
internals.endsWith = function (str, suffix) {
return str.indexOf(suffix, str.length - suffix.length) !== -1;
};
//
// Find rule for a given domain.
//
internals.findRule = function (domain) {
var punyDomain = Punycode.toASCII(domain);
return internals.rules.reduce(function (memo, rule) {
if (rule.punySuffix === -1){
rule.punySuffix = Punycode.toASCII(rule.suffix);
}
if (!internals.endsWith(punyDomain, '.' + rule.punySuffix) && punyDomain !== rule.punySuffix) {
return memo;
}
// This has been commented out as it never seems to run. This is because
// sub tlds always appear after their parents and we never find a shorter
// match.
//if (memo) {
// var memoSuffix = Punycode.toASCII(memo.suffix);
// if (memoSuffix.length >= punySuffix.length) {
// return memo;
// }
//}
return rule;
}, null);
};
//
// Error codes and messages.
//
exports.errorCodes = {
DOMAIN_TOO_SHORT: 'Domain name too short.',
DOMAIN_TOO_LONG: 'Domain name too long. It should be no more than 255 chars.',
LABEL_STARTS_WITH_DASH: 'Domain name label can not start with a dash.',
LABEL_ENDS_WITH_DASH: 'Domain name label can not end with a dash.',
LABEL_TOO_LONG: 'Domain name label should be at most 63 chars long.',
LABEL_TOO_SHORT: 'Domain name label should be at least 1 character long.',
LABEL_INVALID_CHARS: 'Domain name label can only contain alphanumeric characters or dashes.'
};
//
// Validate domain name and throw if not valid.
//
// From wikipedia:
//
// Hostnames are composed of series of labels concatenated with dots, as are all
// domain names. Each label must be between 1 and 63 characters long, and the
// entire hostname (including the delimiting dots) has a maximum of 255 chars.
//
// Allowed chars:
//
// * `a-z`
// * `0-9`
// * `-` but not as a starting or ending character
// * `.` as a separator for the textual portions of a domain name
//
// * http://en.wikipedia.org/wiki/Domain_name
// * http://en.wikipedia.org/wiki/Hostname
//
internals.validate = function (input) {
// Before we can validate we need to take care of IDNs with unicode chars.
var ascii = Punycode.toASCII(input);
if (ascii.length < 1) {
return 'DOMAIN_TOO_SHORT';
}
if (ascii.length > 255) {
return 'DOMAIN_TOO_LONG';
}
// Check each part's length and allowed chars.
var labels = ascii.split('.');
var label;
for (var i = 0; i < labels.length; ++i) {
label = labels[i];
if (!label.length) {
return 'LABEL_TOO_SHORT';
}
if (label.length > 63) {
return 'LABEL_TOO_LONG';
}
if (label.charAt(0) === '-') {
return 'LABEL_STARTS_WITH_DASH';
}
if (label.charAt(label.length - 1) === '-') {
return 'LABEL_ENDS_WITH_DASH';
}
if (!/^[a-z0-9\-]+$/.test(label)) {
return 'LABEL_INVALID_CHARS';
}
}
};
//
// Public API
//
//
// Parse domain.
//
exports.parse = function (input) {
if (typeof input !== 'string') {
throw new TypeError('Domain name must be a string.');
}
// Force domain to lowercase.
var domain = input.slice(0).toLowerCase();
// Handle FQDN.
// TODO: Simply remove trailing dot?
if (domain.charAt(domain.length - 1) === '.') {
domain = domain.slice(0, domain.length - 1);
}
// Validate and sanitise input.
var error = internals.validate(domain);
if (error) {
return {
input: input,
error: {
message: exports.errorCodes[error],
code: error
}
};
}
var parsed = {
input: input,
tld: null,
sld: null,
domain: null,
subdomain: null,
listed: false
};
var domainParts = domain.split('.');
// Non-Internet TLD
if (domainParts[domainParts.length - 1] === 'local') {
return parsed;
}
var handlePunycode = function () {
if (!/xn--/.test(domain)) {
return parsed;
}
if (parsed.domain) {
parsed.domain = Punycode.toASCII(parsed.domain);
}
if (parsed.subdomain) {
parsed.subdomain = Punycode.toASCII(parsed.subdomain);
}
return parsed;
};
var rule = internals.findRule(domain);
// Unlisted tld.
if (!rule) {
if (domainParts.length < 2) {
return parsed;
}
parsed.tld = domainParts.pop();
parsed.sld = domainParts.pop();
parsed.domain = [parsed.sld, parsed.tld].join('.');
if (domainParts.length) {
parsed.subdomain = domainParts.pop();
}
return handlePunycode();
}
// At this point we know the public suffix is listed.
parsed.listed = true;
var tldParts = rule.suffix.split('.');
var privateParts = domainParts.slice(0, domainParts.length - tldParts.length);
if (rule.exception) {
privateParts.push(tldParts.shift());
}
parsed.tld = tldParts.join('.');
if (!privateParts.length) {
return handlePunycode();
}
if (rule.wildcard) {
tldParts.unshift(privateParts.pop());
parsed.tld = tldParts.join('.');
}
if (!privateParts.length) {
return handlePunycode();
}
parsed.sld = privateParts.pop();
parsed.domain = [parsed.sld, parsed.tld].join('.');
if (privateParts.length) {
parsed.subdomain = privateParts.join('.');
}
return handlePunycode();
};
//
// Get domain.
//
exports.get = function (domain) {
if (!domain) {
return null;
}
return exports.parse(domain).domain || null;
};
//
// Check whether domain belongs to a known public suffix.
//
exports.isValid = function (domain) {
var parsed = exports.parse(domain);
return Boolean(parsed.domain && parsed.listed);
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/psl/index.js | index.js |
(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.psl = f()}})(function(){var define,module,exports;return (function(){function r(e,n,t){function o(i,f){if(!n[i]){if(!e[i]){var c="function"==typeof require&&require;if(!f&&c)return c(i,!0);if(u)return u(i,!0);var a=new Error("Cannot find module '"+i+"'");throw a.code="MODULE_NOT_FOUND",a}var p=n[i]={exports:{}};e[i][0].call(p.exports,function(r){var n=e[i][1][r];return o(n||r)},p,p.exports,r,e,n,t)}return n[i].exports}for(var u="function"==typeof require&&require,i=0;i<t.length;i++)o(t[i]);return o}return r})()({1:[function(require,module,exports){
module.exports=[
"ac",
"com.ac",
"edu.ac",
"gov.ac",
"net.ac",
"mil.ac",
"org.ac",
"ad",
"nom.ad",
"ae",
"co.ae",
"net.ae",
"org.ae",
"sch.ae",
"ac.ae",
"gov.ae",
"mil.ae",
"aero",
"accident-investigation.aero",
"accident-prevention.aero",
"aerobatic.aero",
"aeroclub.aero",
"aerodrome.aero",
"agents.aero",
"aircraft.aero",
"airline.aero",
"airport.aero",
"air-surveillance.aero",
"airtraffic.aero",
"air-traffic-control.aero",
"ambulance.aero",
"amusement.aero",
"association.aero",
"author.aero",
"ballooning.aero",
"broker.aero",
"caa.aero",
"cargo.aero",
"catering.aero",
"certification.aero",
"championship.aero",
"charter.aero",
"civilaviation.aero",
"club.aero",
"conference.aero",
"consultant.aero",
"consulting.aero",
"control.aero",
"council.aero",
"crew.aero",
"design.aero",
"dgca.aero",
"educator.aero",
"emergency.aero",
"engine.aero",
"engineer.aero",
"entertainment.aero",
"equipment.aero",
"exchange.aero",
"express.aero",
"federation.aero",
"flight.aero",
"freight.aero",
"fuel.aero",
"gliding.aero",
"government.aero",
"groundhandling.aero",
"group.aero",
"hanggliding.aero",
"homebuilt.aero",
"insurance.aero",
"journal.aero",
"journalist.aero",
"leasing.aero",
"logistics.aero",
"magazine.aero",
"maintenance.aero",
"media.aero",
"microlight.aero",
"modelling.aero",
"navigation.aero",
"parachuting.aero",
"paragliding.aero",
"passenger-association.aero",
"pilot.aero",
"press.aero",
"production.aero",
"recreation.aero",
"repbody.aero",
"res.aero",
"research.aero",
"rotorcraft.aero",
"safety.aero",
"scientist.aero",
"services.aero",
"show.aero",
"skydiving.aero",
"software.aero",
"student.aero",
"trader.aero",
"trading.aero",
"trainer.aero",
"union.aero",
"workinggroup.aero",
"works.aero",
"af",
"gov.af",
"com.af",
"org.af",
"net.af",
"edu.af",
"ag",
"com.ag",
"org.ag",
"net.ag",
"co.ag",
"nom.ag",
"ai",
"off.ai",
"com.ai",
"net.ai",
"org.ai",
"al",
"com.al",
"edu.al",
"gov.al",
"mil.al",
"net.al",
"org.al",
"am",
"co.am",
"com.am",
"commune.am",
"net.am",
"org.am",
"ao",
"ed.ao",
"gv.ao",
"og.ao",
"co.ao",
"pb.ao",
"it.ao",
"aq",
"ar",
"com.ar",
"edu.ar",
"gob.ar",
"gov.ar",
"int.ar",
"mil.ar",
"musica.ar",
"net.ar",
"org.ar",
"tur.ar",
"arpa",
"e164.arpa",
"in-addr.arpa",
"ip6.arpa",
"iris.arpa",
"uri.arpa",
"urn.arpa",
"as",
"gov.as",
"asia",
"at",
"ac.at",
"co.at",
"gv.at",
"or.at",
"au",
"com.au",
"net.au",
"org.au",
"edu.au",
"gov.au",
"asn.au",
"id.au",
"info.au",
"conf.au",
"oz.au",
"act.au",
"nsw.au",
"nt.au",
"qld.au",
"sa.au",
"tas.au",
"vic.au",
"wa.au",
"act.edu.au",
"catholic.edu.au",
"nsw.edu.au",
"nt.edu.au",
"qld.edu.au",
"sa.edu.au",
"tas.edu.au",
"vic.edu.au",
"wa.edu.au",
"qld.gov.au",
"sa.gov.au",
"tas.gov.au",
"vic.gov.au",
"wa.gov.au",
"education.tas.edu.au",
"schools.nsw.edu.au",
"aw",
"com.aw",
"ax",
"az",
"com.az",
"net.az",
"int.az",
"gov.az",
"org.az",
"edu.az",
"info.az",
"pp.az",
"mil.az",
"name.az",
"pro.az",
"biz.az",
"ba",
"com.ba",
"edu.ba",
"gov.ba",
"mil.ba",
"net.ba",
"org.ba",
"bb",
"biz.bb",
"co.bb",
"com.bb",
"edu.bb",
"gov.bb",
"info.bb",
"net.bb",
"org.bb",
"store.bb",
"tv.bb",
"*.bd",
"be",
"ac.be",
"bf",
"gov.bf",
"bg",
"a.bg",
"b.bg",
"c.bg",
"d.bg",
"e.bg",
"f.bg",
"g.bg",
"h.bg",
"i.bg",
"j.bg",
"k.bg",
"l.bg",
"m.bg",
"n.bg",
"o.bg",
"p.bg",
"q.bg",
"r.bg",
"s.bg",
"t.bg",
"u.bg",
"v.bg",
"w.bg",
"x.bg",
"y.bg",
"z.bg",
"0.bg",
"1.bg",
"2.bg",
"3.bg",
"4.bg",
"5.bg",
"6.bg",
"7.bg",
"8.bg",
"9.bg",
"bh",
"com.bh",
"edu.bh",
"net.bh",
"org.bh",
"gov.bh",
"bi",
"co.bi",
"com.bi",
"edu.bi",
"or.bi",
"org.bi",
"biz",
"bj",
"asso.bj",
"barreau.bj",
"gouv.bj",
"bm",
"com.bm",
"edu.bm",
"gov.bm",
"net.bm",
"org.bm",
"bn",
"com.bn",
"edu.bn",
"gov.bn",
"net.bn",
"org.bn",
"bo",
"com.bo",
"edu.bo",
"gob.bo",
"int.bo",
"org.bo",
"net.bo",
"mil.bo",
"tv.bo",
"web.bo",
"academia.bo",
"agro.bo",
"arte.bo",
"blog.bo",
"bolivia.bo",
"ciencia.bo",
"cooperativa.bo",
"democracia.bo",
"deporte.bo",
"ecologia.bo",
"economia.bo",
"empresa.bo",
"indigena.bo",
"industria.bo",
"info.bo",
"medicina.bo",
"movimiento.bo",
"musica.bo",
"natural.bo",
"nombre.bo",
"noticias.bo",
"patria.bo",
"politica.bo",
"profesional.bo",
"plurinacional.bo",
"pueblo.bo",
"revista.bo",
"salud.bo",
"tecnologia.bo",
"tksat.bo",
"transporte.bo",
"wiki.bo",
"br",
"9guacu.br",
"abc.br",
"adm.br",
"adv.br",
"agr.br",
"aju.br",
"am.br",
"anani.br",
"aparecida.br",
"arq.br",
"art.br",
"ato.br",
"b.br",
"barueri.br",
"belem.br",
"bhz.br",
"bio.br",
"blog.br",
"bmd.br",
"boavista.br",
"bsb.br",
"campinagrande.br",
"campinas.br",
"caxias.br",
"cim.br",
"cng.br",
"cnt.br",
"com.br",
"contagem.br",
"coop.br",
"cri.br",
"cuiaba.br",
"curitiba.br",
"def.br",
"ecn.br",
"eco.br",
"edu.br",
"emp.br",
"eng.br",
"esp.br",
"etc.br",
"eti.br",
"far.br",
"feira.br",
"flog.br",
"floripa.br",
"fm.br",
"fnd.br",
"fortal.br",
"fot.br",
"foz.br",
"fst.br",
"g12.br",
"ggf.br",
"goiania.br",
"gov.br",
"ac.gov.br",
"al.gov.br",
"am.gov.br",
"ap.gov.br",
"ba.gov.br",
"ce.gov.br",
"df.gov.br",
"es.gov.br",
"go.gov.br",
"ma.gov.br",
"mg.gov.br",
"ms.gov.br",
"mt.gov.br",
"pa.gov.br",
"pb.gov.br",
"pe.gov.br",
"pi.gov.br",
"pr.gov.br",
"rj.gov.br",
"rn.gov.br",
"ro.gov.br",
"rr.gov.br",
"rs.gov.br",
"sc.gov.br",
"se.gov.br",
"sp.gov.br",
"to.gov.br",
"gru.br",
"imb.br",
"ind.br",
"inf.br",
"jab.br",
"jampa.br",
"jdf.br",
"joinville.br",
"jor.br",
"jus.br",
"leg.br",
"lel.br",
"londrina.br",
"macapa.br",
"maceio.br",
"manaus.br",
"maringa.br",
"mat.br",
"med.br",
"mil.br",
"morena.br",
"mp.br",
"mus.br",
"natal.br",
"net.br",
"niteroi.br",
"*.nom.br",
"not.br",
"ntr.br",
"odo.br",
"ong.br",
"org.br",
"osasco.br",
"palmas.br",
"poa.br",
"ppg.br",
"pro.br",
"psc.br",
"psi.br",
"pvh.br",
"qsl.br",
"radio.br",
"rec.br",
"recife.br",
"ribeirao.br",
"rio.br",
"riobranco.br",
"riopreto.br",
"salvador.br",
"sampa.br",
"santamaria.br",
"santoandre.br",
"saobernardo.br",
"saogonca.br",
"sjc.br",
"slg.br",
"slz.br",
"sorocaba.br",
"srv.br",
"taxi.br",
"tc.br",
"teo.br",
"the.br",
"tmp.br",
"trd.br",
"tur.br",
"tv.br",
"udi.br",
"vet.br",
"vix.br",
"vlog.br",
"wiki.br",
"zlg.br",
"bs",
"com.bs",
"net.bs",
"org.bs",
"edu.bs",
"gov.bs",
"bt",
"com.bt",
"edu.bt",
"gov.bt",
"net.bt",
"org.bt",
"bv",
"bw",
"co.bw",
"org.bw",
"by",
"gov.by",
"mil.by",
"com.by",
"of.by",
"bz",
"com.bz",
"net.bz",
"org.bz",
"edu.bz",
"gov.bz",
"ca",
"ab.ca",
"bc.ca",
"mb.ca",
"nb.ca",
"nf.ca",
"nl.ca",
"ns.ca",
"nt.ca",
"nu.ca",
"on.ca",
"pe.ca",
"qc.ca",
"sk.ca",
"yk.ca",
"gc.ca",
"cat",
"cc",
"cd",
"gov.cd",
"cf",
"cg",
"ch",
"ci",
"org.ci",
"or.ci",
"com.ci",
"co.ci",
"edu.ci",
"ed.ci",
"ac.ci",
"net.ci",
"go.ci",
"asso.ci",
"aéroport.ci",
"int.ci",
"presse.ci",
"md.ci",
"gouv.ci",
"*.ck",
"!www.ck",
"cl",
"aprendemas.cl",
"co.cl",
"gob.cl",
"gov.cl",
"mil.cl",
"cm",
"co.cm",
"com.cm",
"gov.cm",
"net.cm",
"cn",
"ac.cn",
"com.cn",
"edu.cn",
"gov.cn",
"net.cn",
"org.cn",
"mil.cn",
"公司.cn",
"网络.cn",
"網絡.cn",
"ah.cn",
"bj.cn",
"cq.cn",
"fj.cn",
"gd.cn",
"gs.cn",
"gz.cn",
"gx.cn",
"ha.cn",
"hb.cn",
"he.cn",
"hi.cn",
"hl.cn",
"hn.cn",
"jl.cn",
"js.cn",
"jx.cn",
"ln.cn",
"nm.cn",
"nx.cn",
"qh.cn",
"sc.cn",
"sd.cn",
"sh.cn",
"sn.cn",
"sx.cn",
"tj.cn",
"xj.cn",
"xz.cn",
"yn.cn",
"zj.cn",
"hk.cn",
"mo.cn",
"tw.cn",
"co",
"arts.co",
"com.co",
"edu.co",
"firm.co",
"gov.co",
"info.co",
"int.co",
"mil.co",
"net.co",
"nom.co",
"org.co",
"rec.co",
"web.co",
"com",
"coop",
"cr",
"ac.cr",
"co.cr",
"ed.cr",
"fi.cr",
"go.cr",
"or.cr",
"sa.cr",
"cu",
"com.cu",
"edu.cu",
"org.cu",
"net.cu",
"gov.cu",
"inf.cu",
"cv",
"cw",
"com.cw",
"edu.cw",
"net.cw",
"org.cw",
"cx",
"gov.cx",
"cy",
"ac.cy",
"biz.cy",
"com.cy",
"ekloges.cy",
"gov.cy",
"ltd.cy",
"name.cy",
"net.cy",
"org.cy",
"parliament.cy",
"press.cy",
"pro.cy",
"tm.cy",
"cz",
"de",
"dj",
"dk",
"dm",
"com.dm",
"net.dm",
"org.dm",
"edu.dm",
"gov.dm",
"do",
"art.do",
"com.do",
"edu.do",
"gob.do",
"gov.do",
"mil.do",
"net.do",
"org.do",
"sld.do",
"web.do",
"dz",
"com.dz",
"org.dz",
"net.dz",
"gov.dz",
"edu.dz",
"asso.dz",
"pol.dz",
"art.dz",
"ec",
"com.ec",
"info.ec",
"net.ec",
"fin.ec",
"k12.ec",
"med.ec",
"pro.ec",
"org.ec",
"edu.ec",
"gov.ec",
"gob.ec",
"mil.ec",
"edu",
"ee",
"edu.ee",
"gov.ee",
"riik.ee",
"lib.ee",
"med.ee",
"com.ee",
"pri.ee",
"aip.ee",
"org.ee",
"fie.ee",
"eg",
"com.eg",
"edu.eg",
"eun.eg",
"gov.eg",
"mil.eg",
"name.eg",
"net.eg",
"org.eg",
"sci.eg",
"*.er",
"es",
"com.es",
"nom.es",
"org.es",
"gob.es",
"edu.es",
"et",
"com.et",
"gov.et",
"org.et",
"edu.et",
"biz.et",
"name.et",
"info.et",
"net.et",
"eu",
"fi",
"aland.fi",
"fj",
"ac.fj",
"biz.fj",
"com.fj",
"gov.fj",
"info.fj",
"mil.fj",
"name.fj",
"net.fj",
"org.fj",
"pro.fj",
"*.fk",
"fm",
"fo",
"fr",
"asso.fr",
"com.fr",
"gouv.fr",
"nom.fr",
"prd.fr",
"tm.fr",
"aeroport.fr",
"avocat.fr",
"avoues.fr",
"cci.fr",
"chambagri.fr",
"chirurgiens-dentistes.fr",
"experts-comptables.fr",
"geometre-expert.fr",
"greta.fr",
"huissier-justice.fr",
"medecin.fr",
"notaires.fr",
"pharmacien.fr",
"port.fr",
"veterinaire.fr",
"ga",
"gb",
"gd",
"ge",
"com.ge",
"edu.ge",
"gov.ge",
"org.ge",
"mil.ge",
"net.ge",
"pvt.ge",
"gf",
"gg",
"co.gg",
"net.gg",
"org.gg",
"gh",
"com.gh",
"edu.gh",
"gov.gh",
"org.gh",
"mil.gh",
"gi",
"com.gi",
"ltd.gi",
"gov.gi",
"mod.gi",
"edu.gi",
"org.gi",
"gl",
"co.gl",
"com.gl",
"edu.gl",
"net.gl",
"org.gl",
"gm",
"gn",
"ac.gn",
"com.gn",
"edu.gn",
"gov.gn",
"org.gn",
"net.gn",
"gov",
"gp",
"com.gp",
"net.gp",
"mobi.gp",
"edu.gp",
"org.gp",
"asso.gp",
"gq",
"gr",
"com.gr",
"edu.gr",
"net.gr",
"org.gr",
"gov.gr",
"gs",
"gt",
"com.gt",
"edu.gt",
"gob.gt",
"ind.gt",
"mil.gt",
"net.gt",
"org.gt",
"gu",
"com.gu",
"edu.gu",
"gov.gu",
"guam.gu",
"info.gu",
"net.gu",
"org.gu",
"web.gu",
"gw",
"gy",
"co.gy",
"com.gy",
"edu.gy",
"gov.gy",
"net.gy",
"org.gy",
"hk",
"com.hk",
"edu.hk",
"gov.hk",
"idv.hk",
"net.hk",
"org.hk",
"公司.hk",
"教育.hk",
"敎育.hk",
"政府.hk",
"個人.hk",
"个人.hk",
"箇人.hk",
"網络.hk",
"网络.hk",
"组織.hk",
"網絡.hk",
"网絡.hk",
"组织.hk",
"組織.hk",
"組织.hk",
"hm",
"hn",
"com.hn",
"edu.hn",
"org.hn",
"net.hn",
"mil.hn",
"gob.hn",
"hr",
"iz.hr",
"from.hr",
"name.hr",
"com.hr",
"ht",
"com.ht",
"shop.ht",
"firm.ht",
"info.ht",
"adult.ht",
"net.ht",
"pro.ht",
"org.ht",
"med.ht",
"art.ht",
"coop.ht",
"pol.ht",
"asso.ht",
"edu.ht",
"rel.ht",
"gouv.ht",
"perso.ht",
"hu",
"co.hu",
"info.hu",
"org.hu",
"priv.hu",
"sport.hu",
"tm.hu",
"2000.hu",
"agrar.hu",
"bolt.hu",
"casino.hu",
"city.hu",
"erotica.hu",
"erotika.hu",
"film.hu",
"forum.hu",
"games.hu",
"hotel.hu",
"ingatlan.hu",
"jogasz.hu",
"konyvelo.hu",
"lakas.hu",
"media.hu",
"news.hu",
"reklam.hu",
"sex.hu",
"shop.hu",
"suli.hu",
"szex.hu",
"tozsde.hu",
"utazas.hu",
"video.hu",
"id",
"ac.id",
"biz.id",
"co.id",
"desa.id",
"go.id",
"mil.id",
"my.id",
"net.id",
"or.id",
"ponpes.id",
"sch.id",
"web.id",
"ie",
"gov.ie",
"il",
"ac.il",
"co.il",
"gov.il",
"idf.il",
"k12.il",
"muni.il",
"net.il",
"org.il",
"im",
"ac.im",
"co.im",
"com.im",
"ltd.co.im",
"net.im",
"org.im",
"plc.co.im",
"tt.im",
"tv.im",
"in",
"co.in",
"firm.in",
"net.in",
"org.in",
"gen.in",
"ind.in",
"nic.in",
"ac.in",
"edu.in",
"res.in",
"gov.in",
"mil.in",
"info",
"int",
"eu.int",
"io",
"com.io",
"iq",
"gov.iq",
"edu.iq",
"mil.iq",
"com.iq",
"org.iq",
"net.iq",
"ir",
"ac.ir",
"co.ir",
"gov.ir",
"id.ir",
"net.ir",
"org.ir",
"sch.ir",
"ایران.ir",
"ايران.ir",
"is",
"net.is",
"com.is",
"edu.is",
"gov.is",
"org.is",
"int.is",
"it",
"gov.it",
"edu.it",
"abr.it",
"abruzzo.it",
"aosta-valley.it",
"aostavalley.it",
"bas.it",
"basilicata.it",
"cal.it",
"calabria.it",
"cam.it",
"campania.it",
"emilia-romagna.it",
"emiliaromagna.it",
"emr.it",
"friuli-v-giulia.it",
"friuli-ve-giulia.it",
"friuli-vegiulia.it",
"friuli-venezia-giulia.it",
"friuli-veneziagiulia.it",
"friuli-vgiulia.it",
"friuliv-giulia.it",
"friulive-giulia.it",
"friulivegiulia.it",
"friulivenezia-giulia.it",
"friuliveneziagiulia.it",
"friulivgiulia.it",
"fvg.it",
"laz.it",
"lazio.it",
"lig.it",
"liguria.it",
"lom.it",
"lombardia.it",
"lombardy.it",
"lucania.it",
"mar.it",
"marche.it",
"mol.it",
"molise.it",
"piedmont.it",
"piemonte.it",
"pmn.it",
"pug.it",
"puglia.it",
"sar.it",
"sardegna.it",
"sardinia.it",
"sic.it",
"sicilia.it",
"sicily.it",
"taa.it",
"tos.it",
"toscana.it",
"trentin-sud-tirol.it",
"trentin-süd-tirol.it",
"trentin-sudtirol.it",
"trentin-südtirol.it",
"trentin-sued-tirol.it",
"trentin-suedtirol.it",
"trentino-a-adige.it",
"trentino-aadige.it",
"trentino-alto-adige.it",
"trentino-altoadige.it",
"trentino-s-tirol.it",
"trentino-stirol.it",
"trentino-sud-tirol.it",
"trentino-süd-tirol.it",
"trentino-sudtirol.it",
"trentino-südtirol.it",
"trentino-sued-tirol.it",
"trentino-suedtirol.it",
"trentino.it",
"trentinoa-adige.it",
"trentinoaadige.it",
"trentinoalto-adige.it",
"trentinoaltoadige.it",
"trentinos-tirol.it",
"trentinostirol.it",
"trentinosud-tirol.it",
"trentinosüd-tirol.it",
"trentinosudtirol.it",
"trentinosüdtirol.it",
"trentinosued-tirol.it",
"trentinosuedtirol.it",
"trentinsud-tirol.it",
"trentinsüd-tirol.it",
"trentinsudtirol.it",
"trentinsüdtirol.it",
"trentinsued-tirol.it",
"trentinsuedtirol.it",
"tuscany.it",
"umb.it",
"umbria.it",
"val-d-aosta.it",
"val-daosta.it",
"vald-aosta.it",
"valdaosta.it",
"valle-aosta.it",
"valle-d-aosta.it",
"valle-daosta.it",
"valleaosta.it",
"valled-aosta.it",
"valledaosta.it",
"vallee-aoste.it",
"vallée-aoste.it",
"vallee-d-aoste.it",
"vallée-d-aoste.it",
"valleeaoste.it",
"valléeaoste.it",
"valleedaoste.it",
"valléedaoste.it",
"vao.it",
"vda.it",
"ven.it",
"veneto.it",
"ag.it",
"agrigento.it",
"al.it",
"alessandria.it",
"alto-adige.it",
"altoadige.it",
"an.it",
"ancona.it",
"andria-barletta-trani.it",
"andria-trani-barletta.it",
"andriabarlettatrani.it",
"andriatranibarletta.it",
"ao.it",
"aosta.it",
"aoste.it",
"ap.it",
"aq.it",
"aquila.it",
"ar.it",
"arezzo.it",
"ascoli-piceno.it",
"ascolipiceno.it",
"asti.it",
"at.it",
"av.it",
"avellino.it",
"ba.it",
"balsan-sudtirol.it",
"balsan-südtirol.it",
"balsan-suedtirol.it",
"balsan.it",
"bari.it",
"barletta-trani-andria.it",
"barlettatraniandria.it",
"belluno.it",
"benevento.it",
"bergamo.it",
"bg.it",
"bi.it",
"biella.it",
"bl.it",
"bn.it",
"bo.it",
"bologna.it",
"bolzano-altoadige.it",
"bolzano.it",
"bozen-sudtirol.it",
"bozen-südtirol.it",
"bozen-suedtirol.it",
"bozen.it",
"br.it",
"brescia.it",
"brindisi.it",
"bs.it",
"bt.it",
"bulsan-sudtirol.it",
"bulsan-südtirol.it",
"bulsan-suedtirol.it",
"bulsan.it",
"bz.it",
"ca.it",
"cagliari.it",
"caltanissetta.it",
"campidano-medio.it",
"campidanomedio.it",
"campobasso.it",
"carbonia-iglesias.it",
"carboniaiglesias.it",
"carrara-massa.it",
"carraramassa.it",
"caserta.it",
"catania.it",
"catanzaro.it",
"cb.it",
"ce.it",
"cesena-forli.it",
"cesena-forlì.it",
"cesenaforli.it",
"cesenaforlì.it",
"ch.it",
"chieti.it",
"ci.it",
"cl.it",
"cn.it",
"co.it",
"como.it",
"cosenza.it",
"cr.it",
"cremona.it",
"crotone.it",
"cs.it",
"ct.it",
"cuneo.it",
"cz.it",
"dell-ogliastra.it",
"dellogliastra.it",
"en.it",
"enna.it",
"fc.it",
"fe.it",
"fermo.it",
"ferrara.it",
"fg.it",
"fi.it",
"firenze.it",
"florence.it",
"fm.it",
"foggia.it",
"forli-cesena.it",
"forlì-cesena.it",
"forlicesena.it",
"forlìcesena.it",
"fr.it",
"frosinone.it",
"ge.it",
"genoa.it",
"genova.it",
"go.it",
"gorizia.it",
"gr.it",
"grosseto.it",
"iglesias-carbonia.it",
"iglesiascarbonia.it",
"im.it",
"imperia.it",
"is.it",
"isernia.it",
"kr.it",
"la-spezia.it",
"laquila.it",
"laspezia.it",
"latina.it",
"lc.it",
"le.it",
"lecce.it",
"lecco.it",
"li.it",
"livorno.it",
"lo.it",
"lodi.it",
"lt.it",
"lu.it",
"lucca.it",
"macerata.it",
"mantova.it",
"massa-carrara.it",
"massacarrara.it",
"matera.it",
"mb.it",
"mc.it",
"me.it",
"medio-campidano.it",
"mediocampidano.it",
"messina.it",
"mi.it",
"milan.it",
"milano.it",
"mn.it",
"mo.it",
"modena.it",
"monza-brianza.it",
"monza-e-della-brianza.it",
"monza.it",
"monzabrianza.it",
"monzaebrianza.it",
"monzaedellabrianza.it",
"ms.it",
"mt.it",
"na.it",
"naples.it",
"napoli.it",
"no.it",
"novara.it",
"nu.it",
"nuoro.it",
"og.it",
"ogliastra.it",
"olbia-tempio.it",
"olbiatempio.it",
"or.it",
"oristano.it",
"ot.it",
"pa.it",
"padova.it",
"padua.it",
"palermo.it",
"parma.it",
"pavia.it",
"pc.it",
"pd.it",
"pe.it",
"perugia.it",
"pesaro-urbino.it",
"pesarourbino.it",
"pescara.it",
"pg.it",
"pi.it",
"piacenza.it",
"pisa.it",
"pistoia.it",
"pn.it",
"po.it",
"pordenone.it",
"potenza.it",
"pr.it",
"prato.it",
"pt.it",
"pu.it",
"pv.it",
"pz.it",
"ra.it",
"ragusa.it",
"ravenna.it",
"rc.it",
"re.it",
"reggio-calabria.it",
"reggio-emilia.it",
"reggiocalabria.it",
"reggioemilia.it",
"rg.it",
"ri.it",
"rieti.it",
"rimini.it",
"rm.it",
"rn.it",
"ro.it",
"roma.it",
"rome.it",
"rovigo.it",
"sa.it",
"salerno.it",
"sassari.it",
"savona.it",
"si.it",
"siena.it",
"siracusa.it",
"so.it",
"sondrio.it",
"sp.it",
"sr.it",
"ss.it",
"suedtirol.it",
"südtirol.it",
"sv.it",
"ta.it",
"taranto.it",
"te.it",
"tempio-olbia.it",
"tempioolbia.it",
"teramo.it",
"terni.it",
"tn.it",
"to.it",
"torino.it",
"tp.it",
"tr.it",
"trani-andria-barletta.it",
"trani-barletta-andria.it",
"traniandriabarletta.it",
"tranibarlettaandria.it",
"trapani.it",
"trento.it",
"treviso.it",
"trieste.it",
"ts.it",
"turin.it",
"tv.it",
"ud.it",
"udine.it",
"urbino-pesaro.it",
"urbinopesaro.it",
"va.it",
"varese.it",
"vb.it",
"vc.it",
"ve.it",
"venezia.it",
"venice.it",
"verbania.it",
"vercelli.it",
"verona.it",
"vi.it",
"vibo-valentia.it",
"vibovalentia.it",
"vicenza.it",
"viterbo.it",
"vr.it",
"vs.it",
"vt.it",
"vv.it",
"je",
"co.je",
"net.je",
"org.je",
"*.jm",
"jo",
"com.jo",
"org.jo",
"net.jo",
"edu.jo",
"sch.jo",
"gov.jo",
"mil.jo",
"name.jo",
"jobs",
"jp",
"ac.jp",
"ad.jp",
"co.jp",
"ed.jp",
"go.jp",
"gr.jp",
"lg.jp",
"ne.jp",
"or.jp",
"aichi.jp",
"akita.jp",
"aomori.jp",
"chiba.jp",
"ehime.jp",
"fukui.jp",
"fukuoka.jp",
"fukushima.jp",
"gifu.jp",
"gunma.jp",
"hiroshima.jp",
"hokkaido.jp",
"hyogo.jp",
"ibaraki.jp",
"ishikawa.jp",
"iwate.jp",
"kagawa.jp",
"kagoshima.jp",
"kanagawa.jp",
"kochi.jp",
"kumamoto.jp",
"kyoto.jp",
"mie.jp",
"miyagi.jp",
"miyazaki.jp",
"nagano.jp",
"nagasaki.jp",
"nara.jp",
"niigata.jp",
"oita.jp",
"okayama.jp",
"okinawa.jp",
"osaka.jp",
"saga.jp",
"saitama.jp",
"shiga.jp",
"shimane.jp",
"shizuoka.jp",
"tochigi.jp",
"tokushima.jp",
"tokyo.jp",
"tottori.jp",
"toyama.jp",
"wakayama.jp",
"yamagata.jp",
"yamaguchi.jp",
"yamanashi.jp",
"栃木.jp",
"愛知.jp",
"愛媛.jp",
"兵庫.jp",
"熊本.jp",
"茨城.jp",
"北海道.jp",
"千葉.jp",
"和歌山.jp",
"長崎.jp",
"長野.jp",
"新潟.jp",
"青森.jp",
"静岡.jp",
"東京.jp",
"石川.jp",
"埼玉.jp",
"三重.jp",
"京都.jp",
"佐賀.jp",
"大分.jp",
"大阪.jp",
"奈良.jp",
"宮城.jp",
"宮崎.jp",
"富山.jp",
"山口.jp",
"山形.jp",
"山梨.jp",
"岩手.jp",
"岐阜.jp",
"岡山.jp",
"島根.jp",
"広島.jp",
"徳島.jp",
"沖縄.jp",
"滋賀.jp",
"神奈川.jp",
"福井.jp",
"福岡.jp",
"福島.jp",
"秋田.jp",
"群馬.jp",
"香川.jp",
"高知.jp",
"鳥取.jp",
"鹿児島.jp",
"*.kawasaki.jp",
"*.kitakyushu.jp",
"*.kobe.jp",
"*.nagoya.jp",
"*.sapporo.jp",
"*.sendai.jp",
"*.yokohama.jp",
"!city.kawasaki.jp",
"!city.kitakyushu.jp",
"!city.kobe.jp",
"!city.nagoya.jp",
"!city.sapporo.jp",
"!city.sendai.jp",
"!city.yokohama.jp",
"aisai.aichi.jp",
"ama.aichi.jp",
"anjo.aichi.jp",
"asuke.aichi.jp",
"chiryu.aichi.jp",
"chita.aichi.jp",
"fuso.aichi.jp",
"gamagori.aichi.jp",
"handa.aichi.jp",
"hazu.aichi.jp",
"hekinan.aichi.jp",
"higashiura.aichi.jp",
"ichinomiya.aichi.jp",
"inazawa.aichi.jp",
"inuyama.aichi.jp",
"isshiki.aichi.jp",
"iwakura.aichi.jp",
"kanie.aichi.jp",
"kariya.aichi.jp",
"kasugai.aichi.jp",
"kira.aichi.jp",
"kiyosu.aichi.jp",
"komaki.aichi.jp",
"konan.aichi.jp",
"kota.aichi.jp",
"mihama.aichi.jp",
"miyoshi.aichi.jp",
"nishio.aichi.jp",
"nisshin.aichi.jp",
"obu.aichi.jp",
"oguchi.aichi.jp",
"oharu.aichi.jp",
"okazaki.aichi.jp",
"owariasahi.aichi.jp",
"seto.aichi.jp",
"shikatsu.aichi.jp",
"shinshiro.aichi.jp",
"shitara.aichi.jp",
"tahara.aichi.jp",
"takahama.aichi.jp",
"tobishima.aichi.jp",
"toei.aichi.jp",
"togo.aichi.jp",
"tokai.aichi.jp",
"tokoname.aichi.jp",
"toyoake.aichi.jp",
"toyohashi.aichi.jp",
"toyokawa.aichi.jp",
"toyone.aichi.jp",
"toyota.aichi.jp",
"tsushima.aichi.jp",
"yatomi.aichi.jp",
"akita.akita.jp",
"daisen.akita.jp",
"fujisato.akita.jp",
"gojome.akita.jp",
"hachirogata.akita.jp",
"happou.akita.jp",
"higashinaruse.akita.jp",
"honjo.akita.jp",
"honjyo.akita.jp",
"ikawa.akita.jp",
"kamikoani.akita.jp",
"kamioka.akita.jp",
"katagami.akita.jp",
"kazuno.akita.jp",
"kitaakita.akita.jp",
"kosaka.akita.jp",
"kyowa.akita.jp",
"misato.akita.jp",
"mitane.akita.jp",
"moriyoshi.akita.jp",
"nikaho.akita.jp",
"noshiro.akita.jp",
"odate.akita.jp",
"oga.akita.jp",
"ogata.akita.jp",
"semboku.akita.jp",
"yokote.akita.jp",
"yurihonjo.akita.jp",
"aomori.aomori.jp",
"gonohe.aomori.jp",
"hachinohe.aomori.jp",
"hashikami.aomori.jp",
"hiranai.aomori.jp",
"hirosaki.aomori.jp",
"itayanagi.aomori.jp",
"kuroishi.aomori.jp",
"misawa.aomori.jp",
"mutsu.aomori.jp",
"nakadomari.aomori.jp",
"noheji.aomori.jp",
"oirase.aomori.jp",
"owani.aomori.jp",
"rokunohe.aomori.jp",
"sannohe.aomori.jp",
"shichinohe.aomori.jp",
"shingo.aomori.jp",
"takko.aomori.jp",
"towada.aomori.jp",
"tsugaru.aomori.jp",
"tsuruta.aomori.jp",
"abiko.chiba.jp",
"asahi.chiba.jp",
"chonan.chiba.jp",
"chosei.chiba.jp",
"choshi.chiba.jp",
"chuo.chiba.jp",
"funabashi.chiba.jp",
"futtsu.chiba.jp",
"hanamigawa.chiba.jp",
"ichihara.chiba.jp",
"ichikawa.chiba.jp",
"ichinomiya.chiba.jp",
"inzai.chiba.jp",
"isumi.chiba.jp",
"kamagaya.chiba.jp",
"kamogawa.chiba.jp",
"kashiwa.chiba.jp",
"katori.chiba.jp",
"katsuura.chiba.jp",
"kimitsu.chiba.jp",
"kisarazu.chiba.jp",
"kozaki.chiba.jp",
"kujukuri.chiba.jp",
"kyonan.chiba.jp",
"matsudo.chiba.jp",
"midori.chiba.jp",
"mihama.chiba.jp",
"minamiboso.chiba.jp",
"mobara.chiba.jp",
"mutsuzawa.chiba.jp",
"nagara.chiba.jp",
"nagareyama.chiba.jp",
"narashino.chiba.jp",
"narita.chiba.jp",
"noda.chiba.jp",
"oamishirasato.chiba.jp",
"omigawa.chiba.jp",
"onjuku.chiba.jp",
"otaki.chiba.jp",
"sakae.chiba.jp",
"sakura.chiba.jp",
"shimofusa.chiba.jp",
"shirako.chiba.jp",
"shiroi.chiba.jp",
"shisui.chiba.jp",
"sodegaura.chiba.jp",
"sosa.chiba.jp",
"tako.chiba.jp",
"tateyama.chiba.jp",
"togane.chiba.jp",
"tohnosho.chiba.jp",
"tomisato.chiba.jp",
"urayasu.chiba.jp",
"yachimata.chiba.jp",
"yachiyo.chiba.jp",
"yokaichiba.chiba.jp",
"yokoshibahikari.chiba.jp",
"yotsukaido.chiba.jp",
"ainan.ehime.jp",
"honai.ehime.jp",
"ikata.ehime.jp",
"imabari.ehime.jp",
"iyo.ehime.jp",
"kamijima.ehime.jp",
"kihoku.ehime.jp",
"kumakogen.ehime.jp",
"masaki.ehime.jp",
"matsuno.ehime.jp",
"matsuyama.ehime.jp",
"namikata.ehime.jp",
"niihama.ehime.jp",
"ozu.ehime.jp",
"saijo.ehime.jp",
"seiyo.ehime.jp",
"shikokuchuo.ehime.jp",
"tobe.ehime.jp",
"toon.ehime.jp",
"uchiko.ehime.jp",
"uwajima.ehime.jp",
"yawatahama.ehime.jp",
"echizen.fukui.jp",
"eiheiji.fukui.jp",
"fukui.fukui.jp",
"ikeda.fukui.jp",
"katsuyama.fukui.jp",
"mihama.fukui.jp",
"minamiechizen.fukui.jp",
"obama.fukui.jp",
"ohi.fukui.jp",
"ono.fukui.jp",
"sabae.fukui.jp",
"sakai.fukui.jp",
"takahama.fukui.jp",
"tsuruga.fukui.jp",
"wakasa.fukui.jp",
"ashiya.fukuoka.jp",
"buzen.fukuoka.jp",
"chikugo.fukuoka.jp",
"chikuho.fukuoka.jp",
"chikujo.fukuoka.jp",
"chikushino.fukuoka.jp",
"chikuzen.fukuoka.jp",
"chuo.fukuoka.jp",
"dazaifu.fukuoka.jp",
"fukuchi.fukuoka.jp",
"hakata.fukuoka.jp",
"higashi.fukuoka.jp",
"hirokawa.fukuoka.jp",
"hisayama.fukuoka.jp",
"iizuka.fukuoka.jp",
"inatsuki.fukuoka.jp",
"kaho.fukuoka.jp",
"kasuga.fukuoka.jp",
"kasuya.fukuoka.jp",
"kawara.fukuoka.jp",
"keisen.fukuoka.jp",
"koga.fukuoka.jp",
"kurate.fukuoka.jp",
"kurogi.fukuoka.jp",
"kurume.fukuoka.jp",
"minami.fukuoka.jp",
"miyako.fukuoka.jp",
"miyama.fukuoka.jp",
"miyawaka.fukuoka.jp",
"mizumaki.fukuoka.jp",
"munakata.fukuoka.jp",
"nakagawa.fukuoka.jp",
"nakama.fukuoka.jp",
"nishi.fukuoka.jp",
"nogata.fukuoka.jp",
"ogori.fukuoka.jp",
"okagaki.fukuoka.jp",
"okawa.fukuoka.jp",
"oki.fukuoka.jp",
"omuta.fukuoka.jp",
"onga.fukuoka.jp",
"onojo.fukuoka.jp",
"oto.fukuoka.jp",
"saigawa.fukuoka.jp",
"sasaguri.fukuoka.jp",
"shingu.fukuoka.jp",
"shinyoshitomi.fukuoka.jp",
"shonai.fukuoka.jp",
"soeda.fukuoka.jp",
"sue.fukuoka.jp",
"tachiarai.fukuoka.jp",
"tagawa.fukuoka.jp",
"takata.fukuoka.jp",
"toho.fukuoka.jp",
"toyotsu.fukuoka.jp",
"tsuiki.fukuoka.jp",
"ukiha.fukuoka.jp",
"umi.fukuoka.jp",
"usui.fukuoka.jp",
"yamada.fukuoka.jp",
"yame.fukuoka.jp",
"yanagawa.fukuoka.jp",
"yukuhashi.fukuoka.jp",
"aizubange.fukushima.jp",
"aizumisato.fukushima.jp",
"aizuwakamatsu.fukushima.jp",
"asakawa.fukushima.jp",
"bandai.fukushima.jp",
"date.fukushima.jp",
"fukushima.fukushima.jp",
"furudono.fukushima.jp",
"futaba.fukushima.jp",
"hanawa.fukushima.jp",
"higashi.fukushima.jp",
"hirata.fukushima.jp",
"hirono.fukushima.jp",
"iitate.fukushima.jp",
"inawashiro.fukushima.jp",
"ishikawa.fukushima.jp",
"iwaki.fukushima.jp",
"izumizaki.fukushima.jp",
"kagamiishi.fukushima.jp",
"kaneyama.fukushima.jp",
"kawamata.fukushima.jp",
"kitakata.fukushima.jp",
"kitashiobara.fukushima.jp",
"koori.fukushima.jp",
"koriyama.fukushima.jp",
"kunimi.fukushima.jp",
"miharu.fukushima.jp",
"mishima.fukushima.jp",
"namie.fukushima.jp",
"nango.fukushima.jp",
"nishiaizu.fukushima.jp",
"nishigo.fukushima.jp",
"okuma.fukushima.jp",
"omotego.fukushima.jp",
"ono.fukushima.jp",
"otama.fukushima.jp",
"samegawa.fukushima.jp",
"shimogo.fukushima.jp",
"shirakawa.fukushima.jp",
"showa.fukushima.jp",
"soma.fukushima.jp",
"sukagawa.fukushima.jp",
"taishin.fukushima.jp",
"tamakawa.fukushima.jp",
"tanagura.fukushima.jp",
"tenei.fukushima.jp",
"yabuki.fukushima.jp",
"yamato.fukushima.jp",
"yamatsuri.fukushima.jp",
"yanaizu.fukushima.jp",
"yugawa.fukushima.jp",
"anpachi.gifu.jp",
"ena.gifu.jp",
"gifu.gifu.jp",
"ginan.gifu.jp",
"godo.gifu.jp",
"gujo.gifu.jp",
"hashima.gifu.jp",
"hichiso.gifu.jp",
"hida.gifu.jp",
"higashishirakawa.gifu.jp",
"ibigawa.gifu.jp",
"ikeda.gifu.jp",
"kakamigahara.gifu.jp",
"kani.gifu.jp",
"kasahara.gifu.jp",
"kasamatsu.gifu.jp",
"kawaue.gifu.jp",
"kitagata.gifu.jp",
"mino.gifu.jp",
"minokamo.gifu.jp",
"mitake.gifu.jp",
"mizunami.gifu.jp",
"motosu.gifu.jp",
"nakatsugawa.gifu.jp",
"ogaki.gifu.jp",
"sakahogi.gifu.jp",
"seki.gifu.jp",
"sekigahara.gifu.jp",
"shirakawa.gifu.jp",
"tajimi.gifu.jp",
"takayama.gifu.jp",
"tarui.gifu.jp",
"toki.gifu.jp",
"tomika.gifu.jp",
"wanouchi.gifu.jp",
"yamagata.gifu.jp",
"yaotsu.gifu.jp",
"yoro.gifu.jp",
"annaka.gunma.jp",
"chiyoda.gunma.jp",
"fujioka.gunma.jp",
"higashiagatsuma.gunma.jp",
"isesaki.gunma.jp",
"itakura.gunma.jp",
"kanna.gunma.jp",
"kanra.gunma.jp",
"katashina.gunma.jp",
"kawaba.gunma.jp",
"kiryu.gunma.jp",
"kusatsu.gunma.jp",
"maebashi.gunma.jp",
"meiwa.gunma.jp",
"midori.gunma.jp",
"minakami.gunma.jp",
"naganohara.gunma.jp",
"nakanojo.gunma.jp",
"nanmoku.gunma.jp",
"numata.gunma.jp",
"oizumi.gunma.jp",
"ora.gunma.jp",
"ota.gunma.jp",
"shibukawa.gunma.jp",
"shimonita.gunma.jp",
"shinto.gunma.jp",
"showa.gunma.jp",
"takasaki.gunma.jp",
"takayama.gunma.jp",
"tamamura.gunma.jp",
"tatebayashi.gunma.jp",
"tomioka.gunma.jp",
"tsukiyono.gunma.jp",
"tsumagoi.gunma.jp",
"ueno.gunma.jp",
"yoshioka.gunma.jp",
"asaminami.hiroshima.jp",
"daiwa.hiroshima.jp",
"etajima.hiroshima.jp",
"fuchu.hiroshima.jp",
"fukuyama.hiroshima.jp",
"hatsukaichi.hiroshima.jp",
"higashihiroshima.hiroshima.jp",
"hongo.hiroshima.jp",
"jinsekikogen.hiroshima.jp",
"kaita.hiroshima.jp",
"kui.hiroshima.jp",
"kumano.hiroshima.jp",
"kure.hiroshima.jp",
"mihara.hiroshima.jp",
"miyoshi.hiroshima.jp",
"naka.hiroshima.jp",
"onomichi.hiroshima.jp",
"osakikamijima.hiroshima.jp",
"otake.hiroshima.jp",
"saka.hiroshima.jp",
"sera.hiroshima.jp",
"seranishi.hiroshima.jp",
"shinichi.hiroshima.jp",
"shobara.hiroshima.jp",
"takehara.hiroshima.jp",
"abashiri.hokkaido.jp",
"abira.hokkaido.jp",
"aibetsu.hokkaido.jp",
"akabira.hokkaido.jp",
"akkeshi.hokkaido.jp",
"asahikawa.hokkaido.jp",
"ashibetsu.hokkaido.jp",
"ashoro.hokkaido.jp",
"assabu.hokkaido.jp",
"atsuma.hokkaido.jp",
"bibai.hokkaido.jp",
"biei.hokkaido.jp",
"bifuka.hokkaido.jp",
"bihoro.hokkaido.jp",
"biratori.hokkaido.jp",
"chippubetsu.hokkaido.jp",
"chitose.hokkaido.jp",
"date.hokkaido.jp",
"ebetsu.hokkaido.jp",
"embetsu.hokkaido.jp",
"eniwa.hokkaido.jp",
"erimo.hokkaido.jp",
"esan.hokkaido.jp",
"esashi.hokkaido.jp",
"fukagawa.hokkaido.jp",
"fukushima.hokkaido.jp",
"furano.hokkaido.jp",
"furubira.hokkaido.jp",
"haboro.hokkaido.jp",
"hakodate.hokkaido.jp",
"hamatonbetsu.hokkaido.jp",
"hidaka.hokkaido.jp",
"higashikagura.hokkaido.jp",
"higashikawa.hokkaido.jp",
"hiroo.hokkaido.jp",
"hokuryu.hokkaido.jp",
"hokuto.hokkaido.jp",
"honbetsu.hokkaido.jp",
"horokanai.hokkaido.jp",
"horonobe.hokkaido.jp",
"ikeda.hokkaido.jp",
"imakane.hokkaido.jp",
"ishikari.hokkaido.jp",
"iwamizawa.hokkaido.jp",
"iwanai.hokkaido.jp",
"kamifurano.hokkaido.jp",
"kamikawa.hokkaido.jp",
"kamishihoro.hokkaido.jp",
"kamisunagawa.hokkaido.jp",
"kamoenai.hokkaido.jp",
"kayabe.hokkaido.jp",
"kembuchi.hokkaido.jp",
"kikonai.hokkaido.jp",
"kimobetsu.hokkaido.jp",
"kitahiroshima.hokkaido.jp",
"kitami.hokkaido.jp",
"kiyosato.hokkaido.jp",
"koshimizu.hokkaido.jp",
"kunneppu.hokkaido.jp",
"kuriyama.hokkaido.jp",
"kuromatsunai.hokkaido.jp",
"kushiro.hokkaido.jp",
"kutchan.hokkaido.jp",
"kyowa.hokkaido.jp",
"mashike.hokkaido.jp",
"matsumae.hokkaido.jp",
"mikasa.hokkaido.jp",
"minamifurano.hokkaido.jp",
"mombetsu.hokkaido.jp",
"moseushi.hokkaido.jp",
"mukawa.hokkaido.jp",
"muroran.hokkaido.jp",
"naie.hokkaido.jp",
"nakagawa.hokkaido.jp",
"nakasatsunai.hokkaido.jp",
"nakatombetsu.hokkaido.jp",
"nanae.hokkaido.jp",
"nanporo.hokkaido.jp",
"nayoro.hokkaido.jp",
"nemuro.hokkaido.jp",
"niikappu.hokkaido.jp",
"niki.hokkaido.jp",
"nishiokoppe.hokkaido.jp",
"noboribetsu.hokkaido.jp",
"numata.hokkaido.jp",
"obihiro.hokkaido.jp",
"obira.hokkaido.jp",
"oketo.hokkaido.jp",
"okoppe.hokkaido.jp",
"otaru.hokkaido.jp",
"otobe.hokkaido.jp",
"otofuke.hokkaido.jp",
"otoineppu.hokkaido.jp",
"oumu.hokkaido.jp",
"ozora.hokkaido.jp",
"pippu.hokkaido.jp",
"rankoshi.hokkaido.jp",
"rebun.hokkaido.jp",
"rikubetsu.hokkaido.jp",
"rishiri.hokkaido.jp",
"rishirifuji.hokkaido.jp",
"saroma.hokkaido.jp",
"sarufutsu.hokkaido.jp",
"shakotan.hokkaido.jp",
"shari.hokkaido.jp",
"shibecha.hokkaido.jp",
"shibetsu.hokkaido.jp",
"shikabe.hokkaido.jp",
"shikaoi.hokkaido.jp",
"shimamaki.hokkaido.jp",
"shimizu.hokkaido.jp",
"shimokawa.hokkaido.jp",
"shinshinotsu.hokkaido.jp",
"shintoku.hokkaido.jp",
"shiranuka.hokkaido.jp",
"shiraoi.hokkaido.jp",
"shiriuchi.hokkaido.jp",
"sobetsu.hokkaido.jp",
"sunagawa.hokkaido.jp",
"taiki.hokkaido.jp",
"takasu.hokkaido.jp",
"takikawa.hokkaido.jp",
"takinoue.hokkaido.jp",
"teshikaga.hokkaido.jp",
"tobetsu.hokkaido.jp",
"tohma.hokkaido.jp",
"tomakomai.hokkaido.jp",
"tomari.hokkaido.jp",
"toya.hokkaido.jp",
"toyako.hokkaido.jp",
"toyotomi.hokkaido.jp",
"toyoura.hokkaido.jp",
"tsubetsu.hokkaido.jp",
"tsukigata.hokkaido.jp",
"urakawa.hokkaido.jp",
"urausu.hokkaido.jp",
"uryu.hokkaido.jp",
"utashinai.hokkaido.jp",
"wakkanai.hokkaido.jp",
"wassamu.hokkaido.jp",
"yakumo.hokkaido.jp",
"yoichi.hokkaido.jp",
"aioi.hyogo.jp",
"akashi.hyogo.jp",
"ako.hyogo.jp",
"amagasaki.hyogo.jp",
"aogaki.hyogo.jp",
"asago.hyogo.jp",
"ashiya.hyogo.jp",
"awaji.hyogo.jp",
"fukusaki.hyogo.jp",
"goshiki.hyogo.jp",
"harima.hyogo.jp",
"himeji.hyogo.jp",
"ichikawa.hyogo.jp",
"inagawa.hyogo.jp",
"itami.hyogo.jp",
"kakogawa.hyogo.jp",
"kamigori.hyogo.jp",
"kamikawa.hyogo.jp",
"kasai.hyogo.jp",
"kasuga.hyogo.jp",
"kawanishi.hyogo.jp",
"miki.hyogo.jp",
"minamiawaji.hyogo.jp",
"nishinomiya.hyogo.jp",
"nishiwaki.hyogo.jp",
"ono.hyogo.jp",
"sanda.hyogo.jp",
"sannan.hyogo.jp",
"sasayama.hyogo.jp",
"sayo.hyogo.jp",
"shingu.hyogo.jp",
"shinonsen.hyogo.jp",
"shiso.hyogo.jp",
"sumoto.hyogo.jp",
"taishi.hyogo.jp",
"taka.hyogo.jp",
"takarazuka.hyogo.jp",
"takasago.hyogo.jp",
"takino.hyogo.jp",
"tamba.hyogo.jp",
"tatsuno.hyogo.jp",
"toyooka.hyogo.jp",
"yabu.hyogo.jp",
"yashiro.hyogo.jp",
"yoka.hyogo.jp",
"yokawa.hyogo.jp",
"ami.ibaraki.jp",
"asahi.ibaraki.jp",
"bando.ibaraki.jp",
"chikusei.ibaraki.jp",
"daigo.ibaraki.jp",
"fujishiro.ibaraki.jp",
"hitachi.ibaraki.jp",
"hitachinaka.ibaraki.jp",
"hitachiomiya.ibaraki.jp",
"hitachiota.ibaraki.jp",
"ibaraki.ibaraki.jp",
"ina.ibaraki.jp",
"inashiki.ibaraki.jp",
"itako.ibaraki.jp",
"iwama.ibaraki.jp",
"joso.ibaraki.jp",
"kamisu.ibaraki.jp",
"kasama.ibaraki.jp",
"kashima.ibaraki.jp",
"kasumigaura.ibaraki.jp",
"koga.ibaraki.jp",
"miho.ibaraki.jp",
"mito.ibaraki.jp",
"moriya.ibaraki.jp",
"naka.ibaraki.jp",
"namegata.ibaraki.jp",
"oarai.ibaraki.jp",
"ogawa.ibaraki.jp",
"omitama.ibaraki.jp",
"ryugasaki.ibaraki.jp",
"sakai.ibaraki.jp",
"sakuragawa.ibaraki.jp",
"shimodate.ibaraki.jp",
"shimotsuma.ibaraki.jp",
"shirosato.ibaraki.jp",
"sowa.ibaraki.jp",
"suifu.ibaraki.jp",
"takahagi.ibaraki.jp",
"tamatsukuri.ibaraki.jp",
"tokai.ibaraki.jp",
"tomobe.ibaraki.jp",
"tone.ibaraki.jp",
"toride.ibaraki.jp",
"tsuchiura.ibaraki.jp",
"tsukuba.ibaraki.jp",
"uchihara.ibaraki.jp",
"ushiku.ibaraki.jp",
"yachiyo.ibaraki.jp",
"yamagata.ibaraki.jp",
"yawara.ibaraki.jp",
"yuki.ibaraki.jp",
"anamizu.ishikawa.jp",
"hakui.ishikawa.jp",
"hakusan.ishikawa.jp",
"kaga.ishikawa.jp",
"kahoku.ishikawa.jp",
"kanazawa.ishikawa.jp",
"kawakita.ishikawa.jp",
"komatsu.ishikawa.jp",
"nakanoto.ishikawa.jp",
"nanao.ishikawa.jp",
"nomi.ishikawa.jp",
"nonoichi.ishikawa.jp",
"noto.ishikawa.jp",
"shika.ishikawa.jp",
"suzu.ishikawa.jp",
"tsubata.ishikawa.jp",
"tsurugi.ishikawa.jp",
"uchinada.ishikawa.jp",
"wajima.ishikawa.jp",
"fudai.iwate.jp",
"fujisawa.iwate.jp",
"hanamaki.iwate.jp",
"hiraizumi.iwate.jp",
"hirono.iwate.jp",
"ichinohe.iwate.jp",
"ichinoseki.iwate.jp",
"iwaizumi.iwate.jp",
"iwate.iwate.jp",
"joboji.iwate.jp",
"kamaishi.iwate.jp",
"kanegasaki.iwate.jp",
"karumai.iwate.jp",
"kawai.iwate.jp",
"kitakami.iwate.jp",
"kuji.iwate.jp",
"kunohe.iwate.jp",
"kuzumaki.iwate.jp",
"miyako.iwate.jp",
"mizusawa.iwate.jp",
"morioka.iwate.jp",
"ninohe.iwate.jp",
"noda.iwate.jp",
"ofunato.iwate.jp",
"oshu.iwate.jp",
"otsuchi.iwate.jp",
"rikuzentakata.iwate.jp",
"shiwa.iwate.jp",
"shizukuishi.iwate.jp",
"sumita.iwate.jp",
"tanohata.iwate.jp",
"tono.iwate.jp",
"yahaba.iwate.jp",
"yamada.iwate.jp",
"ayagawa.kagawa.jp",
"higashikagawa.kagawa.jp",
"kanonji.kagawa.jp",
"kotohira.kagawa.jp",
"manno.kagawa.jp",
"marugame.kagawa.jp",
"mitoyo.kagawa.jp",
"naoshima.kagawa.jp",
"sanuki.kagawa.jp",
"tadotsu.kagawa.jp",
"takamatsu.kagawa.jp",
"tonosho.kagawa.jp",
"uchinomi.kagawa.jp",
"utazu.kagawa.jp",
"zentsuji.kagawa.jp",
"akune.kagoshima.jp",
"amami.kagoshima.jp",
"hioki.kagoshima.jp",
"isa.kagoshima.jp",
"isen.kagoshima.jp",
"izumi.kagoshima.jp",
"kagoshima.kagoshima.jp",
"kanoya.kagoshima.jp",
"kawanabe.kagoshima.jp",
"kinko.kagoshima.jp",
"kouyama.kagoshima.jp",
"makurazaki.kagoshima.jp",
"matsumoto.kagoshima.jp",
"minamitane.kagoshima.jp",
"nakatane.kagoshima.jp",
"nishinoomote.kagoshima.jp",
"satsumasendai.kagoshima.jp",
"soo.kagoshima.jp",
"tarumizu.kagoshima.jp",
"yusui.kagoshima.jp",
"aikawa.kanagawa.jp",
"atsugi.kanagawa.jp",
"ayase.kanagawa.jp",
"chigasaki.kanagawa.jp",
"ebina.kanagawa.jp",
"fujisawa.kanagawa.jp",
"hadano.kanagawa.jp",
"hakone.kanagawa.jp",
"hiratsuka.kanagawa.jp",
"isehara.kanagawa.jp",
"kaisei.kanagawa.jp",
"kamakura.kanagawa.jp",
"kiyokawa.kanagawa.jp",
"matsuda.kanagawa.jp",
"minamiashigara.kanagawa.jp",
"miura.kanagawa.jp",
"nakai.kanagawa.jp",
"ninomiya.kanagawa.jp",
"odawara.kanagawa.jp",
"oi.kanagawa.jp",
"oiso.kanagawa.jp",
"sagamihara.kanagawa.jp",
"samukawa.kanagawa.jp",
"tsukui.kanagawa.jp",
"yamakita.kanagawa.jp",
"yamato.kanagawa.jp",
"yokosuka.kanagawa.jp",
"yugawara.kanagawa.jp",
"zama.kanagawa.jp",
"zushi.kanagawa.jp",
"aki.kochi.jp",
"geisei.kochi.jp",
"hidaka.kochi.jp",
"higashitsuno.kochi.jp",
"ino.kochi.jp",
"kagami.kochi.jp",
"kami.kochi.jp",
"kitagawa.kochi.jp",
"kochi.kochi.jp",
"mihara.kochi.jp",
"motoyama.kochi.jp",
"muroto.kochi.jp",
"nahari.kochi.jp",
"nakamura.kochi.jp",
"nankoku.kochi.jp",
"nishitosa.kochi.jp",
"niyodogawa.kochi.jp",
"ochi.kochi.jp",
"okawa.kochi.jp",
"otoyo.kochi.jp",
"otsuki.kochi.jp",
"sakawa.kochi.jp",
"sukumo.kochi.jp",
"susaki.kochi.jp",
"tosa.kochi.jp",
"tosashimizu.kochi.jp",
"toyo.kochi.jp",
"tsuno.kochi.jp",
"umaji.kochi.jp",
"yasuda.kochi.jp",
"yusuhara.kochi.jp",
"amakusa.kumamoto.jp",
"arao.kumamoto.jp",
"aso.kumamoto.jp",
"choyo.kumamoto.jp",
"gyokuto.kumamoto.jp",
"kamiamakusa.kumamoto.jp",
"kikuchi.kumamoto.jp",
"kumamoto.kumamoto.jp",
"mashiki.kumamoto.jp",
"mifune.kumamoto.jp",
"minamata.kumamoto.jp",
"minamioguni.kumamoto.jp",
"nagasu.kumamoto.jp",
"nishihara.kumamoto.jp",
"oguni.kumamoto.jp",
"ozu.kumamoto.jp",
"sumoto.kumamoto.jp",
"takamori.kumamoto.jp",
"uki.kumamoto.jp",
"uto.kumamoto.jp",
"yamaga.kumamoto.jp",
"yamato.kumamoto.jp",
"yatsushiro.kumamoto.jp",
"ayabe.kyoto.jp",
"fukuchiyama.kyoto.jp",
"higashiyama.kyoto.jp",
"ide.kyoto.jp",
"ine.kyoto.jp",
"joyo.kyoto.jp",
"kameoka.kyoto.jp",
"kamo.kyoto.jp",
"kita.kyoto.jp",
"kizu.kyoto.jp",
"kumiyama.kyoto.jp",
"kyotamba.kyoto.jp",
"kyotanabe.kyoto.jp",
"kyotango.kyoto.jp",
"maizuru.kyoto.jp",
"minami.kyoto.jp",
"minamiyamashiro.kyoto.jp",
"miyazu.kyoto.jp",
"muko.kyoto.jp",
"nagaokakyo.kyoto.jp",
"nakagyo.kyoto.jp",
"nantan.kyoto.jp",
"oyamazaki.kyoto.jp",
"sakyo.kyoto.jp",
"seika.kyoto.jp",
"tanabe.kyoto.jp",
"uji.kyoto.jp",
"ujitawara.kyoto.jp",
"wazuka.kyoto.jp",
"yamashina.kyoto.jp",
"yawata.kyoto.jp",
"asahi.mie.jp",
"inabe.mie.jp",
"ise.mie.jp",
"kameyama.mie.jp",
"kawagoe.mie.jp",
"kiho.mie.jp",
"kisosaki.mie.jp",
"kiwa.mie.jp",
"komono.mie.jp",
"kumano.mie.jp",
"kuwana.mie.jp",
"matsusaka.mie.jp",
"meiwa.mie.jp",
"mihama.mie.jp",
"minamiise.mie.jp",
"misugi.mie.jp",
"miyama.mie.jp",
"nabari.mie.jp",
"shima.mie.jp",
"suzuka.mie.jp",
"tado.mie.jp",
"taiki.mie.jp",
"taki.mie.jp",
"tamaki.mie.jp",
"toba.mie.jp",
"tsu.mie.jp",
"udono.mie.jp",
"ureshino.mie.jp",
"watarai.mie.jp",
"yokkaichi.mie.jp",
"furukawa.miyagi.jp",
"higashimatsushima.miyagi.jp",
"ishinomaki.miyagi.jp",
"iwanuma.miyagi.jp",
"kakuda.miyagi.jp",
"kami.miyagi.jp",
"kawasaki.miyagi.jp",
"marumori.miyagi.jp",
"matsushima.miyagi.jp",
"minamisanriku.miyagi.jp",
"misato.miyagi.jp",
"murata.miyagi.jp",
"natori.miyagi.jp",
"ogawara.miyagi.jp",
"ohira.miyagi.jp",
"onagawa.miyagi.jp",
"osaki.miyagi.jp",
"rifu.miyagi.jp",
"semine.miyagi.jp",
"shibata.miyagi.jp",
"shichikashuku.miyagi.jp",
"shikama.miyagi.jp",
"shiogama.miyagi.jp",
"shiroishi.miyagi.jp",
"tagajo.miyagi.jp",
"taiwa.miyagi.jp",
"tome.miyagi.jp",
"tomiya.miyagi.jp",
"wakuya.miyagi.jp",
"watari.miyagi.jp",
"yamamoto.miyagi.jp",
"zao.miyagi.jp",
"aya.miyazaki.jp",
"ebino.miyazaki.jp",
"gokase.miyazaki.jp",
"hyuga.miyazaki.jp",
"kadogawa.miyazaki.jp",
"kawaminami.miyazaki.jp",
"kijo.miyazaki.jp",
"kitagawa.miyazaki.jp",
"kitakata.miyazaki.jp",
"kitaura.miyazaki.jp",
"kobayashi.miyazaki.jp",
"kunitomi.miyazaki.jp",
"kushima.miyazaki.jp",
"mimata.miyazaki.jp",
"miyakonojo.miyazaki.jp",
"miyazaki.miyazaki.jp",
"morotsuka.miyazaki.jp",
"nichinan.miyazaki.jp",
"nishimera.miyazaki.jp",
"nobeoka.miyazaki.jp",
"saito.miyazaki.jp",
"shiiba.miyazaki.jp",
"shintomi.miyazaki.jp",
"takaharu.miyazaki.jp",
"takanabe.miyazaki.jp",
"takazaki.miyazaki.jp",
"tsuno.miyazaki.jp",
"achi.nagano.jp",
"agematsu.nagano.jp",
"anan.nagano.jp",
"aoki.nagano.jp",
"asahi.nagano.jp",
"azumino.nagano.jp",
"chikuhoku.nagano.jp",
"chikuma.nagano.jp",
"chino.nagano.jp",
"fujimi.nagano.jp",
"hakuba.nagano.jp",
"hara.nagano.jp",
"hiraya.nagano.jp",
"iida.nagano.jp",
"iijima.nagano.jp",
"iiyama.nagano.jp",
"iizuna.nagano.jp",
"ikeda.nagano.jp",
"ikusaka.nagano.jp",
"ina.nagano.jp",
"karuizawa.nagano.jp",
"kawakami.nagano.jp",
"kiso.nagano.jp",
"kisofukushima.nagano.jp",
"kitaaiki.nagano.jp",
"komagane.nagano.jp",
"komoro.nagano.jp",
"matsukawa.nagano.jp",
"matsumoto.nagano.jp",
"miasa.nagano.jp",
"minamiaiki.nagano.jp",
"minamimaki.nagano.jp",
"minamiminowa.nagano.jp",
"minowa.nagano.jp",
"miyada.nagano.jp",
"miyota.nagano.jp",
"mochizuki.nagano.jp",
"nagano.nagano.jp",
"nagawa.nagano.jp",
"nagiso.nagano.jp",
"nakagawa.nagano.jp",
"nakano.nagano.jp",
"nozawaonsen.nagano.jp",
"obuse.nagano.jp",
"ogawa.nagano.jp",
"okaya.nagano.jp",
"omachi.nagano.jp",
"omi.nagano.jp",
"ookuwa.nagano.jp",
"ooshika.nagano.jp",
"otaki.nagano.jp",
"otari.nagano.jp",
"sakae.nagano.jp",
"sakaki.nagano.jp",
"saku.nagano.jp",
"sakuho.nagano.jp",
"shimosuwa.nagano.jp",
"shinanomachi.nagano.jp",
"shiojiri.nagano.jp",
"suwa.nagano.jp",
"suzaka.nagano.jp",
"takagi.nagano.jp",
"takamori.nagano.jp",
"takayama.nagano.jp",
"tateshina.nagano.jp",
"tatsuno.nagano.jp",
"togakushi.nagano.jp",
"togura.nagano.jp",
"tomi.nagano.jp",
"ueda.nagano.jp",
"wada.nagano.jp",
"yamagata.nagano.jp",
"yamanouchi.nagano.jp",
"yasaka.nagano.jp",
"yasuoka.nagano.jp",
"chijiwa.nagasaki.jp",
"futsu.nagasaki.jp",
"goto.nagasaki.jp",
"hasami.nagasaki.jp",
"hirado.nagasaki.jp",
"iki.nagasaki.jp",
"isahaya.nagasaki.jp",
"kawatana.nagasaki.jp",
"kuchinotsu.nagasaki.jp",
"matsuura.nagasaki.jp",
"nagasaki.nagasaki.jp",
"obama.nagasaki.jp",
"omura.nagasaki.jp",
"oseto.nagasaki.jp",
"saikai.nagasaki.jp",
"sasebo.nagasaki.jp",
"seihi.nagasaki.jp",
"shimabara.nagasaki.jp",
"shinkamigoto.nagasaki.jp",
"togitsu.nagasaki.jp",
"tsushima.nagasaki.jp",
"unzen.nagasaki.jp",
"ando.nara.jp",
"gose.nara.jp",
"heguri.nara.jp",
"higashiyoshino.nara.jp",
"ikaruga.nara.jp",
"ikoma.nara.jp",
"kamikitayama.nara.jp",
"kanmaki.nara.jp",
"kashiba.nara.jp",
"kashihara.nara.jp",
"katsuragi.nara.jp",
"kawai.nara.jp",
"kawakami.nara.jp",
"kawanishi.nara.jp",
"koryo.nara.jp",
"kurotaki.nara.jp",
"mitsue.nara.jp",
"miyake.nara.jp",
"nara.nara.jp",
"nosegawa.nara.jp",
"oji.nara.jp",
"ouda.nara.jp",
"oyodo.nara.jp",
"sakurai.nara.jp",
"sango.nara.jp",
"shimoichi.nara.jp",
"shimokitayama.nara.jp",
"shinjo.nara.jp",
"soni.nara.jp",
"takatori.nara.jp",
"tawaramoto.nara.jp",
"tenkawa.nara.jp",
"tenri.nara.jp",
"uda.nara.jp",
"yamatokoriyama.nara.jp",
"yamatotakada.nara.jp",
"yamazoe.nara.jp",
"yoshino.nara.jp",
"aga.niigata.jp",
"agano.niigata.jp",
"gosen.niigata.jp",
"itoigawa.niigata.jp",
"izumozaki.niigata.jp",
"joetsu.niigata.jp",
"kamo.niigata.jp",
"kariwa.niigata.jp",
"kashiwazaki.niigata.jp",
"minamiuonuma.niigata.jp",
"mitsuke.niigata.jp",
"muika.niigata.jp",
"murakami.niigata.jp",
"myoko.niigata.jp",
"nagaoka.niigata.jp",
"niigata.niigata.jp",
"ojiya.niigata.jp",
"omi.niigata.jp",
"sado.niigata.jp",
"sanjo.niigata.jp",
"seiro.niigata.jp",
"seirou.niigata.jp",
"sekikawa.niigata.jp",
"shibata.niigata.jp",
"tagami.niigata.jp",
"tainai.niigata.jp",
"tochio.niigata.jp",
"tokamachi.niigata.jp",
"tsubame.niigata.jp",
"tsunan.niigata.jp",
"uonuma.niigata.jp",
"yahiko.niigata.jp",
"yoita.niigata.jp",
"yuzawa.niigata.jp",
"beppu.oita.jp",
"bungoono.oita.jp",
"bungotakada.oita.jp",
"hasama.oita.jp",
"hiji.oita.jp",
"himeshima.oita.jp",
"hita.oita.jp",
"kamitsue.oita.jp",
"kokonoe.oita.jp",
"kuju.oita.jp",
"kunisaki.oita.jp",
"kusu.oita.jp",
"oita.oita.jp",
"saiki.oita.jp",
"taketa.oita.jp",
"tsukumi.oita.jp",
"usa.oita.jp",
"usuki.oita.jp",
"yufu.oita.jp",
"akaiwa.okayama.jp",
"asakuchi.okayama.jp",
"bizen.okayama.jp",
"hayashima.okayama.jp",
"ibara.okayama.jp",
"kagamino.okayama.jp",
"kasaoka.okayama.jp",
"kibichuo.okayama.jp",
"kumenan.okayama.jp",
"kurashiki.okayama.jp",
"maniwa.okayama.jp",
"misaki.okayama.jp",
"nagi.okayama.jp",
"niimi.okayama.jp",
"nishiawakura.okayama.jp",
"okayama.okayama.jp",
"satosho.okayama.jp",
"setouchi.okayama.jp",
"shinjo.okayama.jp",
"shoo.okayama.jp",
"soja.okayama.jp",
"takahashi.okayama.jp",
"tamano.okayama.jp",
"tsuyama.okayama.jp",
"wake.okayama.jp",
"yakage.okayama.jp",
"aguni.okinawa.jp",
"ginowan.okinawa.jp",
"ginoza.okinawa.jp",
"gushikami.okinawa.jp",
"haebaru.okinawa.jp",
"higashi.okinawa.jp",
"hirara.okinawa.jp",
"iheya.okinawa.jp",
"ishigaki.okinawa.jp",
"ishikawa.okinawa.jp",
"itoman.okinawa.jp",
"izena.okinawa.jp",
"kadena.okinawa.jp",
"kin.okinawa.jp",
"kitadaito.okinawa.jp",
"kitanakagusuku.okinawa.jp",
"kumejima.okinawa.jp",
"kunigami.okinawa.jp",
"minamidaito.okinawa.jp",
"motobu.okinawa.jp",
"nago.okinawa.jp",
"naha.okinawa.jp",
"nakagusuku.okinawa.jp",
"nakijin.okinawa.jp",
"nanjo.okinawa.jp",
"nishihara.okinawa.jp",
"ogimi.okinawa.jp",
"okinawa.okinawa.jp",
"onna.okinawa.jp",
"shimoji.okinawa.jp",
"taketomi.okinawa.jp",
"tarama.okinawa.jp",
"tokashiki.okinawa.jp",
"tomigusuku.okinawa.jp",
"tonaki.okinawa.jp",
"urasoe.okinawa.jp",
"uruma.okinawa.jp",
"yaese.okinawa.jp",
"yomitan.okinawa.jp",
"yonabaru.okinawa.jp",
"yonaguni.okinawa.jp",
"zamami.okinawa.jp",
"abeno.osaka.jp",
"chihayaakasaka.osaka.jp",
"chuo.osaka.jp",
"daito.osaka.jp",
"fujiidera.osaka.jp",
"habikino.osaka.jp",
"hannan.osaka.jp",
"higashiosaka.osaka.jp",
"higashisumiyoshi.osaka.jp",
"higashiyodogawa.osaka.jp",
"hirakata.osaka.jp",
"ibaraki.osaka.jp",
"ikeda.osaka.jp",
"izumi.osaka.jp",
"izumiotsu.osaka.jp",
"izumisano.osaka.jp",
"kadoma.osaka.jp",
"kaizuka.osaka.jp",
"kanan.osaka.jp",
"kashiwara.osaka.jp",
"katano.osaka.jp",
"kawachinagano.osaka.jp",
"kishiwada.osaka.jp",
"kita.osaka.jp",
"kumatori.osaka.jp",
"matsubara.osaka.jp",
"minato.osaka.jp",
"minoh.osaka.jp",
"misaki.osaka.jp",
"moriguchi.osaka.jp",
"neyagawa.osaka.jp",
"nishi.osaka.jp",
"nose.osaka.jp",
"osakasayama.osaka.jp",
"sakai.osaka.jp",
"sayama.osaka.jp",
"sennan.osaka.jp",
"settsu.osaka.jp",
"shijonawate.osaka.jp",
"shimamoto.osaka.jp",
"suita.osaka.jp",
"tadaoka.osaka.jp",
"taishi.osaka.jp",
"tajiri.osaka.jp",
"takaishi.osaka.jp",
"takatsuki.osaka.jp",
"tondabayashi.osaka.jp",
"toyonaka.osaka.jp",
"toyono.osaka.jp",
"yao.osaka.jp",
"ariake.saga.jp",
"arita.saga.jp",
"fukudomi.saga.jp",
"genkai.saga.jp",
"hamatama.saga.jp",
"hizen.saga.jp",
"imari.saga.jp",
"kamimine.saga.jp",
"kanzaki.saga.jp",
"karatsu.saga.jp",
"kashima.saga.jp",
"kitagata.saga.jp",
"kitahata.saga.jp",
"kiyama.saga.jp",
"kouhoku.saga.jp",
"kyuragi.saga.jp",
"nishiarita.saga.jp",
"ogi.saga.jp",
"omachi.saga.jp",
"ouchi.saga.jp",
"saga.saga.jp",
"shiroishi.saga.jp",
"taku.saga.jp",
"tara.saga.jp",
"tosu.saga.jp",
"yoshinogari.saga.jp",
"arakawa.saitama.jp",
"asaka.saitama.jp",
"chichibu.saitama.jp",
"fujimi.saitama.jp",
"fujimino.saitama.jp",
"fukaya.saitama.jp",
"hanno.saitama.jp",
"hanyu.saitama.jp",
"hasuda.saitama.jp",
"hatogaya.saitama.jp",
"hatoyama.saitama.jp",
"hidaka.saitama.jp",
"higashichichibu.saitama.jp",
"higashimatsuyama.saitama.jp",
"honjo.saitama.jp",
"ina.saitama.jp",
"iruma.saitama.jp",
"iwatsuki.saitama.jp",
"kamiizumi.saitama.jp",
"kamikawa.saitama.jp",
"kamisato.saitama.jp",
"kasukabe.saitama.jp",
"kawagoe.saitama.jp",
"kawaguchi.saitama.jp",
"kawajima.saitama.jp",
"kazo.saitama.jp",
"kitamoto.saitama.jp",
"koshigaya.saitama.jp",
"kounosu.saitama.jp",
"kuki.saitama.jp",
"kumagaya.saitama.jp",
"matsubushi.saitama.jp",
"minano.saitama.jp",
"misato.saitama.jp",
"miyashiro.saitama.jp",
"miyoshi.saitama.jp",
"moroyama.saitama.jp",
"nagatoro.saitama.jp",
"namegawa.saitama.jp",
"niiza.saitama.jp",
"ogano.saitama.jp",
"ogawa.saitama.jp",
"ogose.saitama.jp",
"okegawa.saitama.jp",
"omiya.saitama.jp",
"otaki.saitama.jp",
"ranzan.saitama.jp",
"ryokami.saitama.jp",
"saitama.saitama.jp",
"sakado.saitama.jp",
"satte.saitama.jp",
"sayama.saitama.jp",
"shiki.saitama.jp",
"shiraoka.saitama.jp",
"soka.saitama.jp",
"sugito.saitama.jp",
"toda.saitama.jp",
"tokigawa.saitama.jp",
"tokorozawa.saitama.jp",
"tsurugashima.saitama.jp",
"urawa.saitama.jp",
"warabi.saitama.jp",
"yashio.saitama.jp",
"yokoze.saitama.jp",
"yono.saitama.jp",
"yorii.saitama.jp",
"yoshida.saitama.jp",
"yoshikawa.saitama.jp",
"yoshimi.saitama.jp",
"aisho.shiga.jp",
"gamo.shiga.jp",
"higashiomi.shiga.jp",
"hikone.shiga.jp",
"koka.shiga.jp",
"konan.shiga.jp",
"kosei.shiga.jp",
"koto.shiga.jp",
"kusatsu.shiga.jp",
"maibara.shiga.jp",
"moriyama.shiga.jp",
"nagahama.shiga.jp",
"nishiazai.shiga.jp",
"notogawa.shiga.jp",
"omihachiman.shiga.jp",
"otsu.shiga.jp",
"ritto.shiga.jp",
"ryuoh.shiga.jp",
"takashima.shiga.jp",
"takatsuki.shiga.jp",
"torahime.shiga.jp",
"toyosato.shiga.jp",
"yasu.shiga.jp",
"akagi.shimane.jp",
"ama.shimane.jp",
"gotsu.shimane.jp",
"hamada.shimane.jp",
"higashiizumo.shimane.jp",
"hikawa.shimane.jp",
"hikimi.shimane.jp",
"izumo.shimane.jp",
"kakinoki.shimane.jp",
"masuda.shimane.jp",
"matsue.shimane.jp",
"misato.shimane.jp",
"nishinoshima.shimane.jp",
"ohda.shimane.jp",
"okinoshima.shimane.jp",
"okuizumo.shimane.jp",
"shimane.shimane.jp",
"tamayu.shimane.jp",
"tsuwano.shimane.jp",
"unnan.shimane.jp",
"yakumo.shimane.jp",
"yasugi.shimane.jp",
"yatsuka.shimane.jp",
"arai.shizuoka.jp",
"atami.shizuoka.jp",
"fuji.shizuoka.jp",
"fujieda.shizuoka.jp",
"fujikawa.shizuoka.jp",
"fujinomiya.shizuoka.jp",
"fukuroi.shizuoka.jp",
"gotemba.shizuoka.jp",
"haibara.shizuoka.jp",
"hamamatsu.shizuoka.jp",
"higashiizu.shizuoka.jp",
"ito.shizuoka.jp",
"iwata.shizuoka.jp",
"izu.shizuoka.jp",
"izunokuni.shizuoka.jp",
"kakegawa.shizuoka.jp",
"kannami.shizuoka.jp",
"kawanehon.shizuoka.jp",
"kawazu.shizuoka.jp",
"kikugawa.shizuoka.jp",
"kosai.shizuoka.jp",
"makinohara.shizuoka.jp",
"matsuzaki.shizuoka.jp",
"minamiizu.shizuoka.jp",
"mishima.shizuoka.jp",
"morimachi.shizuoka.jp",
"nishiizu.shizuoka.jp",
"numazu.shizuoka.jp",
"omaezaki.shizuoka.jp",
"shimada.shizuoka.jp",
"shimizu.shizuoka.jp",
"shimoda.shizuoka.jp",
"shizuoka.shizuoka.jp",
"susono.shizuoka.jp",
"yaizu.shizuoka.jp",
"yoshida.shizuoka.jp",
"ashikaga.tochigi.jp",
"bato.tochigi.jp",
"haga.tochigi.jp",
"ichikai.tochigi.jp",
"iwafune.tochigi.jp",
"kaminokawa.tochigi.jp",
"kanuma.tochigi.jp",
"karasuyama.tochigi.jp",
"kuroiso.tochigi.jp",
"mashiko.tochigi.jp",
"mibu.tochigi.jp",
"moka.tochigi.jp",
"motegi.tochigi.jp",
"nasu.tochigi.jp",
"nasushiobara.tochigi.jp",
"nikko.tochigi.jp",
"nishikata.tochigi.jp",
"nogi.tochigi.jp",
"ohira.tochigi.jp",
"ohtawara.tochigi.jp",
"oyama.tochigi.jp",
"sakura.tochigi.jp",
"sano.tochigi.jp",
"shimotsuke.tochigi.jp",
"shioya.tochigi.jp",
"takanezawa.tochigi.jp",
"tochigi.tochigi.jp",
"tsuga.tochigi.jp",
"ujiie.tochigi.jp",
"utsunomiya.tochigi.jp",
"yaita.tochigi.jp",
"aizumi.tokushima.jp",
"anan.tokushima.jp",
"ichiba.tokushima.jp",
"itano.tokushima.jp",
"kainan.tokushima.jp",
"komatsushima.tokushima.jp",
"matsushige.tokushima.jp",
"mima.tokushima.jp",
"minami.tokushima.jp",
"miyoshi.tokushima.jp",
"mugi.tokushima.jp",
"nakagawa.tokushima.jp",
"naruto.tokushima.jp",
"sanagochi.tokushima.jp",
"shishikui.tokushima.jp",
"tokushima.tokushima.jp",
"wajiki.tokushima.jp",
"adachi.tokyo.jp",
"akiruno.tokyo.jp",
"akishima.tokyo.jp",
"aogashima.tokyo.jp",
"arakawa.tokyo.jp",
"bunkyo.tokyo.jp",
"chiyoda.tokyo.jp",
"chofu.tokyo.jp",
"chuo.tokyo.jp",
"edogawa.tokyo.jp",
"fuchu.tokyo.jp",
"fussa.tokyo.jp",
"hachijo.tokyo.jp",
"hachioji.tokyo.jp",
"hamura.tokyo.jp",
"higashikurume.tokyo.jp",
"higashimurayama.tokyo.jp",
"higashiyamato.tokyo.jp",
"hino.tokyo.jp",
"hinode.tokyo.jp",
"hinohara.tokyo.jp",
"inagi.tokyo.jp",
"itabashi.tokyo.jp",
"katsushika.tokyo.jp",
"kita.tokyo.jp",
"kiyose.tokyo.jp",
"kodaira.tokyo.jp",
"koganei.tokyo.jp",
"kokubunji.tokyo.jp",
"komae.tokyo.jp",
"koto.tokyo.jp",
"kouzushima.tokyo.jp",
"kunitachi.tokyo.jp",
"machida.tokyo.jp",
"meguro.tokyo.jp",
"minato.tokyo.jp",
"mitaka.tokyo.jp",
"mizuho.tokyo.jp",
"musashimurayama.tokyo.jp",
"musashino.tokyo.jp",
"nakano.tokyo.jp",
"nerima.tokyo.jp",
"ogasawara.tokyo.jp",
"okutama.tokyo.jp",
"ome.tokyo.jp",
"oshima.tokyo.jp",
"ota.tokyo.jp",
"setagaya.tokyo.jp",
"shibuya.tokyo.jp",
"shinagawa.tokyo.jp",
"shinjuku.tokyo.jp",
"suginami.tokyo.jp",
"sumida.tokyo.jp",
"tachikawa.tokyo.jp",
"taito.tokyo.jp",
"tama.tokyo.jp",
"toshima.tokyo.jp",
"chizu.tottori.jp",
"hino.tottori.jp",
"kawahara.tottori.jp",
"koge.tottori.jp",
"kotoura.tottori.jp",
"misasa.tottori.jp",
"nanbu.tottori.jp",
"nichinan.tottori.jp",
"sakaiminato.tottori.jp",
"tottori.tottori.jp",
"wakasa.tottori.jp",
"yazu.tottori.jp",
"yonago.tottori.jp",
"asahi.toyama.jp",
"fuchu.toyama.jp",
"fukumitsu.toyama.jp",
"funahashi.toyama.jp",
"himi.toyama.jp",
"imizu.toyama.jp",
"inami.toyama.jp",
"johana.toyama.jp",
"kamiichi.toyama.jp",
"kurobe.toyama.jp",
"nakaniikawa.toyama.jp",
"namerikawa.toyama.jp",
"nanto.toyama.jp",
"nyuzen.toyama.jp",
"oyabe.toyama.jp",
"taira.toyama.jp",
"takaoka.toyama.jp",
"tateyama.toyama.jp",
"toga.toyama.jp",
"tonami.toyama.jp",
"toyama.toyama.jp",
"unazuki.toyama.jp",
"uozu.toyama.jp",
"yamada.toyama.jp",
"arida.wakayama.jp",
"aridagawa.wakayama.jp",
"gobo.wakayama.jp",
"hashimoto.wakayama.jp",
"hidaka.wakayama.jp",
"hirogawa.wakayama.jp",
"inami.wakayama.jp",
"iwade.wakayama.jp",
"kainan.wakayama.jp",
"kamitonda.wakayama.jp",
"katsuragi.wakayama.jp",
"kimino.wakayama.jp",
"kinokawa.wakayama.jp",
"kitayama.wakayama.jp",
"koya.wakayama.jp",
"koza.wakayama.jp",
"kozagawa.wakayama.jp",
"kudoyama.wakayama.jp",
"kushimoto.wakayama.jp",
"mihama.wakayama.jp",
"misato.wakayama.jp",
"nachikatsuura.wakayama.jp",
"shingu.wakayama.jp",
"shirahama.wakayama.jp",
"taiji.wakayama.jp",
"tanabe.wakayama.jp",
"wakayama.wakayama.jp",
"yuasa.wakayama.jp",
"yura.wakayama.jp",
"asahi.yamagata.jp",
"funagata.yamagata.jp",
"higashine.yamagata.jp",
"iide.yamagata.jp",
"kahoku.yamagata.jp",
"kaminoyama.yamagata.jp",
"kaneyama.yamagata.jp",
"kawanishi.yamagata.jp",
"mamurogawa.yamagata.jp",
"mikawa.yamagata.jp",
"murayama.yamagata.jp",
"nagai.yamagata.jp",
"nakayama.yamagata.jp",
"nanyo.yamagata.jp",
"nishikawa.yamagata.jp",
"obanazawa.yamagata.jp",
"oe.yamagata.jp",
"oguni.yamagata.jp",
"ohkura.yamagata.jp",
"oishida.yamagata.jp",
"sagae.yamagata.jp",
"sakata.yamagata.jp",
"sakegawa.yamagata.jp",
"shinjo.yamagata.jp",
"shirataka.yamagata.jp",
"shonai.yamagata.jp",
"takahata.yamagata.jp",
"tendo.yamagata.jp",
"tozawa.yamagata.jp",
"tsuruoka.yamagata.jp",
"yamagata.yamagata.jp",
"yamanobe.yamagata.jp",
"yonezawa.yamagata.jp",
"yuza.yamagata.jp",
"abu.yamaguchi.jp",
"hagi.yamaguchi.jp",
"hikari.yamaguchi.jp",
"hofu.yamaguchi.jp",
"iwakuni.yamaguchi.jp",
"kudamatsu.yamaguchi.jp",
"mitou.yamaguchi.jp",
"nagato.yamaguchi.jp",
"oshima.yamaguchi.jp",
"shimonoseki.yamaguchi.jp",
"shunan.yamaguchi.jp",
"tabuse.yamaguchi.jp",
"tokuyama.yamaguchi.jp",
"toyota.yamaguchi.jp",
"ube.yamaguchi.jp",
"yuu.yamaguchi.jp",
"chuo.yamanashi.jp",
"doshi.yamanashi.jp",
"fuefuki.yamanashi.jp",
"fujikawa.yamanashi.jp",
"fujikawaguchiko.yamanashi.jp",
"fujiyoshida.yamanashi.jp",
"hayakawa.yamanashi.jp",
"hokuto.yamanashi.jp",
"ichikawamisato.yamanashi.jp",
"kai.yamanashi.jp",
"kofu.yamanashi.jp",
"koshu.yamanashi.jp",
"kosuge.yamanashi.jp",
"minami-alps.yamanashi.jp",
"minobu.yamanashi.jp",
"nakamichi.yamanashi.jp",
"nanbu.yamanashi.jp",
"narusawa.yamanashi.jp",
"nirasaki.yamanashi.jp",
"nishikatsura.yamanashi.jp",
"oshino.yamanashi.jp",
"otsuki.yamanashi.jp",
"showa.yamanashi.jp",
"tabayama.yamanashi.jp",
"tsuru.yamanashi.jp",
"uenohara.yamanashi.jp",
"yamanakako.yamanashi.jp",
"yamanashi.yamanashi.jp",
"ke",
"ac.ke",
"co.ke",
"go.ke",
"info.ke",
"me.ke",
"mobi.ke",
"ne.ke",
"or.ke",
"sc.ke",
"kg",
"org.kg",
"net.kg",
"com.kg",
"edu.kg",
"gov.kg",
"mil.kg",
"*.kh",
"ki",
"edu.ki",
"biz.ki",
"net.ki",
"org.ki",
"gov.ki",
"info.ki",
"com.ki",
"km",
"org.km",
"nom.km",
"gov.km",
"prd.km",
"tm.km",
"edu.km",
"mil.km",
"ass.km",
"com.km",
"coop.km",
"asso.km",
"presse.km",
"medecin.km",
"notaires.km",
"pharmaciens.km",
"veterinaire.km",
"gouv.km",
"kn",
"net.kn",
"org.kn",
"edu.kn",
"gov.kn",
"kp",
"com.kp",
"edu.kp",
"gov.kp",
"org.kp",
"rep.kp",
"tra.kp",
"kr",
"ac.kr",
"co.kr",
"es.kr",
"go.kr",
"hs.kr",
"kg.kr",
"mil.kr",
"ms.kr",
"ne.kr",
"or.kr",
"pe.kr",
"re.kr",
"sc.kr",
"busan.kr",
"chungbuk.kr",
"chungnam.kr",
"daegu.kr",
"daejeon.kr",
"gangwon.kr",
"gwangju.kr",
"gyeongbuk.kr",
"gyeonggi.kr",
"gyeongnam.kr",
"incheon.kr",
"jeju.kr",
"jeonbuk.kr",
"jeonnam.kr",
"seoul.kr",
"ulsan.kr",
"kw",
"com.kw",
"edu.kw",
"emb.kw",
"gov.kw",
"ind.kw",
"net.kw",
"org.kw",
"ky",
"edu.ky",
"gov.ky",
"com.ky",
"org.ky",
"net.ky",
"kz",
"org.kz",
"edu.kz",
"net.kz",
"gov.kz",
"mil.kz",
"com.kz",
"la",
"int.la",
"net.la",
"info.la",
"edu.la",
"gov.la",
"per.la",
"com.la",
"org.la",
"lb",
"com.lb",
"edu.lb",
"gov.lb",
"net.lb",
"org.lb",
"lc",
"com.lc",
"net.lc",
"co.lc",
"org.lc",
"edu.lc",
"gov.lc",
"li",
"lk",
"gov.lk",
"sch.lk",
"net.lk",
"int.lk",
"com.lk",
"org.lk",
"edu.lk",
"ngo.lk",
"soc.lk",
"web.lk",
"ltd.lk",
"assn.lk",
"grp.lk",
"hotel.lk",
"ac.lk",
"lr",
"com.lr",
"edu.lr",
"gov.lr",
"org.lr",
"net.lr",
"ls",
"ac.ls",
"biz.ls",
"co.ls",
"edu.ls",
"gov.ls",
"info.ls",
"net.ls",
"org.ls",
"sc.ls",
"lt",
"gov.lt",
"lu",
"lv",
"com.lv",
"edu.lv",
"gov.lv",
"org.lv",
"mil.lv",
"id.lv",
"net.lv",
"asn.lv",
"conf.lv",
"ly",
"com.ly",
"net.ly",
"gov.ly",
"plc.ly",
"edu.ly",
"sch.ly",
"med.ly",
"org.ly",
"id.ly",
"ma",
"co.ma",
"net.ma",
"gov.ma",
"org.ma",
"ac.ma",
"press.ma",
"mc",
"tm.mc",
"asso.mc",
"md",
"me",
"co.me",
"net.me",
"org.me",
"edu.me",
"ac.me",
"gov.me",
"its.me",
"priv.me",
"mg",
"org.mg",
"nom.mg",
"gov.mg",
"prd.mg",
"tm.mg",
"edu.mg",
"mil.mg",
"com.mg",
"co.mg",
"mh",
"mil",
"mk",
"com.mk",
"org.mk",
"net.mk",
"edu.mk",
"gov.mk",
"inf.mk",
"name.mk",
"ml",
"com.ml",
"edu.ml",
"gouv.ml",
"gov.ml",
"net.ml",
"org.ml",
"presse.ml",
"*.mm",
"mn",
"gov.mn",
"edu.mn",
"org.mn",
"mo",
"com.mo",
"net.mo",
"org.mo",
"edu.mo",
"gov.mo",
"mobi",
"mp",
"mq",
"mr",
"gov.mr",
"ms",
"com.ms",
"edu.ms",
"gov.ms",
"net.ms",
"org.ms",
"mt",
"com.mt",
"edu.mt",
"net.mt",
"org.mt",
"mu",
"com.mu",
"net.mu",
"org.mu",
"gov.mu",
"ac.mu",
"co.mu",
"or.mu",
"museum",
"academy.museum",
"agriculture.museum",
"air.museum",
"airguard.museum",
"alabama.museum",
"alaska.museum",
"amber.museum",
"ambulance.museum",
"american.museum",
"americana.museum",
"americanantiques.museum",
"americanart.museum",
"amsterdam.museum",
"and.museum",
"annefrank.museum",
"anthro.museum",
"anthropology.museum",
"antiques.museum",
"aquarium.museum",
"arboretum.museum",
"archaeological.museum",
"archaeology.museum",
"architecture.museum",
"art.museum",
"artanddesign.museum",
"artcenter.museum",
"artdeco.museum",
"arteducation.museum",
"artgallery.museum",
"arts.museum",
"artsandcrafts.museum",
"asmatart.museum",
"assassination.museum",
"assisi.museum",
"association.museum",
"astronomy.museum",
"atlanta.museum",
"austin.museum",
"australia.museum",
"automotive.museum",
"aviation.museum",
"axis.museum",
"badajoz.museum",
"baghdad.museum",
"bahn.museum",
"bale.museum",
"baltimore.museum",
"barcelona.museum",
"baseball.museum",
"basel.museum",
"baths.museum",
"bauern.museum",
"beauxarts.museum",
"beeldengeluid.museum",
"bellevue.museum",
"bergbau.museum",
"berkeley.museum",
"berlin.museum",
"bern.museum",
"bible.museum",
"bilbao.museum",
"bill.museum",
"birdart.museum",
"birthplace.museum",
"bonn.museum",
"boston.museum",
"botanical.museum",
"botanicalgarden.museum",
"botanicgarden.museum",
"botany.museum",
"brandywinevalley.museum",
"brasil.museum",
"bristol.museum",
"british.museum",
"britishcolumbia.museum",
"broadcast.museum",
"brunel.museum",
"brussel.museum",
"brussels.museum",
"bruxelles.museum",
"building.museum",
"burghof.museum",
"bus.museum",
"bushey.museum",
"cadaques.museum",
"california.museum",
"cambridge.museum",
"can.museum",
"canada.museum",
"capebreton.museum",
"carrier.museum",
"cartoonart.museum",
"casadelamoneda.museum",
"castle.museum",
"castres.museum",
"celtic.museum",
"center.museum",
"chattanooga.museum",
"cheltenham.museum",
"chesapeakebay.museum",
"chicago.museum",
"children.museum",
"childrens.museum",
"childrensgarden.museum",
"chiropractic.museum",
"chocolate.museum",
"christiansburg.museum",
"cincinnati.museum",
"cinema.museum",
"circus.museum",
"civilisation.museum",
"civilization.museum",
"civilwar.museum",
"clinton.museum",
"clock.museum",
"coal.museum",
"coastaldefence.museum",
"cody.museum",
"coldwar.museum",
"collection.museum",
"colonialwilliamsburg.museum",
"coloradoplateau.museum",
"columbia.museum",
"columbus.museum",
"communication.museum",
"communications.museum",
"community.museum",
"computer.museum",
"computerhistory.museum",
"comunicações.museum",
"contemporary.museum",
"contemporaryart.museum",
"convent.museum",
"copenhagen.museum",
"corporation.museum",
"correios-e-telecomunicações.museum",
"corvette.museum",
"costume.museum",
"countryestate.museum",
"county.museum",
"crafts.museum",
"cranbrook.museum",
"creation.museum",
"cultural.museum",
"culturalcenter.museum",
"culture.museum",
"cyber.museum",
"cymru.museum",
"dali.museum",
"dallas.museum",
"database.museum",
"ddr.museum",
"decorativearts.museum",
"delaware.museum",
"delmenhorst.museum",
"denmark.museum",
"depot.museum",
"design.museum",
"detroit.museum",
"dinosaur.museum",
"discovery.museum",
"dolls.museum",
"donostia.museum",
"durham.museum",
"eastafrica.museum",
"eastcoast.museum",
"education.museum",
"educational.museum",
"egyptian.museum",
"eisenbahn.museum",
"elburg.museum",
"elvendrell.museum",
"embroidery.museum",
"encyclopedic.museum",
"england.museum",
"entomology.museum",
"environment.museum",
"environmentalconservation.museum",
"epilepsy.museum",
"essex.museum",
"estate.museum",
"ethnology.museum",
"exeter.museum",
"exhibition.museum",
"family.museum",
"farm.museum",
"farmequipment.museum",
"farmers.museum",
"farmstead.museum",
"field.museum",
"figueres.museum",
"filatelia.museum",
"film.museum",
"fineart.museum",
"finearts.museum",
"finland.museum",
"flanders.museum",
"florida.museum",
"force.museum",
"fortmissoula.museum",
"fortworth.museum",
"foundation.museum",
"francaise.museum",
"frankfurt.museum",
"franziskaner.museum",
"freemasonry.museum",
"freiburg.museum",
"fribourg.museum",
"frog.museum",
"fundacio.museum",
"furniture.museum",
"gallery.museum",
"garden.museum",
"gateway.museum",
"geelvinck.museum",
"gemological.museum",
"geology.museum",
"georgia.museum",
"giessen.museum",
"glas.museum",
"glass.museum",
"gorge.museum",
"grandrapids.museum",
"graz.museum",
"guernsey.museum",
"halloffame.museum",
"hamburg.museum",
"handson.museum",
"harvestcelebration.museum",
"hawaii.museum",
"health.museum",
"heimatunduhren.museum",
"hellas.museum",
"helsinki.museum",
"hembygdsforbund.museum",
"heritage.museum",
"histoire.museum",
"historical.museum",
"historicalsociety.museum",
"historichouses.museum",
"historisch.museum",
"historisches.museum",
"history.museum",
"historyofscience.museum",
"horology.museum",
"house.museum",
"humanities.museum",
"illustration.museum",
"imageandsound.museum",
"indian.museum",
"indiana.museum",
"indianapolis.museum",
"indianmarket.museum",
"intelligence.museum",
"interactive.museum",
"iraq.museum",
"iron.museum",
"isleofman.museum",
"jamison.museum",
"jefferson.museum",
"jerusalem.museum",
"jewelry.museum",
"jewish.museum",
"jewishart.museum",
"jfk.museum",
"journalism.museum",
"judaica.museum",
"judygarland.museum",
"juedisches.museum",
"juif.museum",
"karate.museum",
"karikatur.museum",
"kids.museum",
"koebenhavn.museum",
"koeln.museum",
"kunst.museum",
"kunstsammlung.museum",
"kunstunddesign.museum",
"labor.museum",
"labour.museum",
"lajolla.museum",
"lancashire.museum",
"landes.museum",
"lans.museum",
"läns.museum",
"larsson.museum",
"lewismiller.museum",
"lincoln.museum",
"linz.museum",
"living.museum",
"livinghistory.museum",
"localhistory.museum",
"london.museum",
"losangeles.museum",
"louvre.museum",
"loyalist.museum",
"lucerne.museum",
"luxembourg.museum",
"luzern.museum",
"mad.museum",
"madrid.museum",
"mallorca.museum",
"manchester.museum",
"mansion.museum",
"mansions.museum",
"manx.museum",
"marburg.museum",
"maritime.museum",
"maritimo.museum",
"maryland.museum",
"marylhurst.museum",
"media.museum",
"medical.museum",
"medizinhistorisches.museum",
"meeres.museum",
"memorial.museum",
"mesaverde.museum",
"michigan.museum",
"midatlantic.museum",
"military.museum",
"mill.museum",
"miners.museum",
"mining.museum",
"minnesota.museum",
"missile.museum",
"missoula.museum",
"modern.museum",
"moma.museum",
"money.museum",
"monmouth.museum",
"monticello.museum",
"montreal.museum",
"moscow.museum",
"motorcycle.museum",
"muenchen.museum",
"muenster.museum",
"mulhouse.museum",
"muncie.museum",
"museet.museum",
"museumcenter.museum",
"museumvereniging.museum",
"music.museum",
"national.museum",
"nationalfirearms.museum",
"nationalheritage.museum",
"nativeamerican.museum",
"naturalhistory.museum",
"naturalhistorymuseum.museum",
"naturalsciences.museum",
"nature.museum",
"naturhistorisches.museum",
"natuurwetenschappen.museum",
"naumburg.museum",
"naval.museum",
"nebraska.museum",
"neues.museum",
"newhampshire.museum",
"newjersey.museum",
"newmexico.museum",
"newport.museum",
"newspaper.museum",
"newyork.museum",
"niepce.museum",
"norfolk.museum",
"north.museum",
"nrw.museum",
"nyc.museum",
"nyny.museum",
"oceanographic.museum",
"oceanographique.museum",
"omaha.museum",
"online.museum",
"ontario.museum",
"openair.museum",
"oregon.museum",
"oregontrail.museum",
"otago.museum",
"oxford.museum",
"pacific.museum",
"paderborn.museum",
"palace.museum",
"paleo.museum",
"palmsprings.museum",
"panama.museum",
"paris.museum",
"pasadena.museum",
"pharmacy.museum",
"philadelphia.museum",
"philadelphiaarea.museum",
"philately.museum",
"phoenix.museum",
"photography.museum",
"pilots.museum",
"pittsburgh.museum",
"planetarium.museum",
"plantation.museum",
"plants.museum",
"plaza.museum",
"portal.museum",
"portland.museum",
"portlligat.museum",
"posts-and-telecommunications.museum",
"preservation.museum",
"presidio.museum",
"press.museum",
"project.museum",
"public.museum",
"pubol.museum",
"quebec.museum",
"railroad.museum",
"railway.museum",
"research.museum",
"resistance.museum",
"riodejaneiro.museum",
"rochester.museum",
"rockart.museum",
"roma.museum",
"russia.museum",
"saintlouis.museum",
"salem.museum",
"salvadordali.museum",
"salzburg.museum",
"sandiego.museum",
"sanfrancisco.museum",
"santabarbara.museum",
"santacruz.museum",
"santafe.museum",
"saskatchewan.museum",
"satx.museum",
"savannahga.museum",
"schlesisches.museum",
"schoenbrunn.museum",
"schokoladen.museum",
"school.museum",
"schweiz.museum",
"science.museum",
"scienceandhistory.museum",
"scienceandindustry.museum",
"sciencecenter.museum",
"sciencecenters.museum",
"science-fiction.museum",
"sciencehistory.museum",
"sciences.museum",
"sciencesnaturelles.museum",
"scotland.museum",
"seaport.museum",
"settlement.museum",
"settlers.museum",
"shell.museum",
"sherbrooke.museum",
"sibenik.museum",
"silk.museum",
"ski.museum",
"skole.museum",
"society.museum",
"sologne.museum",
"soundandvision.museum",
"southcarolina.museum",
"southwest.museum",
"space.museum",
"spy.museum",
"square.museum",
"stadt.museum",
"stalbans.museum",
"starnberg.museum",
"state.museum",
"stateofdelaware.museum",
"station.museum",
"steam.museum",
"steiermark.museum",
"stjohn.museum",
"stockholm.museum",
"stpetersburg.museum",
"stuttgart.museum",
"suisse.museum",
"surgeonshall.museum",
"surrey.museum",
"svizzera.museum",
"sweden.museum",
"sydney.museum",
"tank.museum",
"tcm.museum",
"technology.museum",
"telekommunikation.museum",
"television.museum",
"texas.museum",
"textile.museum",
"theater.museum",
"time.museum",
"timekeeping.museum",
"topology.museum",
"torino.museum",
"touch.museum",
"town.museum",
"transport.museum",
"tree.museum",
"trolley.museum",
"trust.museum",
"trustee.museum",
"uhren.museum",
"ulm.museum",
"undersea.museum",
"university.museum",
"usa.museum",
"usantiques.museum",
"usarts.museum",
"uscountryestate.museum",
"usculture.museum",
"usdecorativearts.museum",
"usgarden.museum",
"ushistory.museum",
"ushuaia.museum",
"uslivinghistory.museum",
"utah.museum",
"uvic.museum",
"valley.museum",
"vantaa.museum",
"versailles.museum",
"viking.museum",
"village.museum",
"virginia.museum",
"virtual.museum",
"virtuel.museum",
"vlaanderen.museum",
"volkenkunde.museum",
"wales.museum",
"wallonie.museum",
"war.museum",
"washingtondc.museum",
"watchandclock.museum",
"watch-and-clock.museum",
"western.museum",
"westfalen.museum",
"whaling.museum",
"wildlife.museum",
"williamsburg.museum",
"windmill.museum",
"workshop.museum",
"york.museum",
"yorkshire.museum",
"yosemite.museum",
"youth.museum",
"zoological.museum",
"zoology.museum",
"ירושלים.museum",
"иком.museum",
"mv",
"aero.mv",
"biz.mv",
"com.mv",
"coop.mv",
"edu.mv",
"gov.mv",
"info.mv",
"int.mv",
"mil.mv",
"museum.mv",
"name.mv",
"net.mv",
"org.mv",
"pro.mv",
"mw",
"ac.mw",
"biz.mw",
"co.mw",
"com.mw",
"coop.mw",
"edu.mw",
"gov.mw",
"int.mw",
"museum.mw",
"net.mw",
"org.mw",
"mx",
"com.mx",
"org.mx",
"gob.mx",
"edu.mx",
"net.mx",
"my",
"com.my",
"net.my",
"org.my",
"gov.my",
"edu.my",
"mil.my",
"name.my",
"mz",
"ac.mz",
"adv.mz",
"co.mz",
"edu.mz",
"gov.mz",
"mil.mz",
"net.mz",
"org.mz",
"na",
"info.na",
"pro.na",
"name.na",
"school.na",
"or.na",
"dr.na",
"us.na",
"mx.na",
"ca.na",
"in.na",
"cc.na",
"tv.na",
"ws.na",
"mobi.na",
"co.na",
"com.na",
"org.na",
"name",
"nc",
"asso.nc",
"nom.nc",
"ne",
"net",
"nf",
"com.nf",
"net.nf",
"per.nf",
"rec.nf",
"web.nf",
"arts.nf",
"firm.nf",
"info.nf",
"other.nf",
"store.nf",
"ng",
"com.ng",
"edu.ng",
"gov.ng",
"i.ng",
"mil.ng",
"mobi.ng",
"name.ng",
"net.ng",
"org.ng",
"sch.ng",
"ni",
"ac.ni",
"biz.ni",
"co.ni",
"com.ni",
"edu.ni",
"gob.ni",
"in.ni",
"info.ni",
"int.ni",
"mil.ni",
"net.ni",
"nom.ni",
"org.ni",
"web.ni",
"nl",
"no",
"fhs.no",
"vgs.no",
"fylkesbibl.no",
"folkebibl.no",
"museum.no",
"idrett.no",
"priv.no",
"mil.no",
"stat.no",
"dep.no",
"kommune.no",
"herad.no",
"aa.no",
"ah.no",
"bu.no",
"fm.no",
"hl.no",
"hm.no",
"jan-mayen.no",
"mr.no",
"nl.no",
"nt.no",
"of.no",
"ol.no",
"oslo.no",
"rl.no",
"sf.no",
"st.no",
"svalbard.no",
"tm.no",
"tr.no",
"va.no",
"vf.no",
"gs.aa.no",
"gs.ah.no",
"gs.bu.no",
"gs.fm.no",
"gs.hl.no",
"gs.hm.no",
"gs.jan-mayen.no",
"gs.mr.no",
"gs.nl.no",
"gs.nt.no",
"gs.of.no",
"gs.ol.no",
"gs.oslo.no",
"gs.rl.no",
"gs.sf.no",
"gs.st.no",
"gs.svalbard.no",
"gs.tm.no",
"gs.tr.no",
"gs.va.no",
"gs.vf.no",
"akrehamn.no",
"åkrehamn.no",
"algard.no",
"ålgård.no",
"arna.no",
"brumunddal.no",
"bryne.no",
"bronnoysund.no",
"brønnøysund.no",
"drobak.no",
"drøbak.no",
"egersund.no",
"fetsund.no",
"floro.no",
"florø.no",
"fredrikstad.no",
"hokksund.no",
"honefoss.no",
"hønefoss.no",
"jessheim.no",
"jorpeland.no",
"jørpeland.no",
"kirkenes.no",
"kopervik.no",
"krokstadelva.no",
"langevag.no",
"langevåg.no",
"leirvik.no",
"mjondalen.no",
"mjøndalen.no",
"mo-i-rana.no",
"mosjoen.no",
"mosjøen.no",
"nesoddtangen.no",
"orkanger.no",
"osoyro.no",
"osøyro.no",
"raholt.no",
"råholt.no",
"sandnessjoen.no",
"sandnessjøen.no",
"skedsmokorset.no",
"slattum.no",
"spjelkavik.no",
"stathelle.no",
"stavern.no",
"stjordalshalsen.no",
"stjørdalshalsen.no",
"tananger.no",
"tranby.no",
"vossevangen.no",
"afjord.no",
"åfjord.no",
"agdenes.no",
"al.no",
"ål.no",
"alesund.no",
"ålesund.no",
"alstahaug.no",
"alta.no",
"áltá.no",
"alaheadju.no",
"álaheadju.no",
"alvdal.no",
"amli.no",
"åmli.no",
"amot.no",
"åmot.no",
"andebu.no",
"andoy.no",
"andøy.no",
"andasuolo.no",
"ardal.no",
"årdal.no",
"aremark.no",
"arendal.no",
"ås.no",
"aseral.no",
"åseral.no",
"asker.no",
"askim.no",
"askvoll.no",
"askoy.no",
"askøy.no",
"asnes.no",
"åsnes.no",
"audnedaln.no",
"aukra.no",
"aure.no",
"aurland.no",
"aurskog-holand.no",
"aurskog-høland.no",
"austevoll.no",
"austrheim.no",
"averoy.no",
"averøy.no",
"balestrand.no",
"ballangen.no",
"balat.no",
"bálát.no",
"balsfjord.no",
"bahccavuotna.no",
"báhccavuotna.no",
"bamble.no",
"bardu.no",
"beardu.no",
"beiarn.no",
"bajddar.no",
"bájddar.no",
"baidar.no",
"báidár.no",
"berg.no",
"bergen.no",
"berlevag.no",
"berlevåg.no",
"bearalvahki.no",
"bearalváhki.no",
"bindal.no",
"birkenes.no",
"bjarkoy.no",
"bjarkøy.no",
"bjerkreim.no",
"bjugn.no",
"bodo.no",
"bodø.no",
"badaddja.no",
"bådåddjå.no",
"budejju.no",
"bokn.no",
"bremanger.no",
"bronnoy.no",
"brønnøy.no",
"bygland.no",
"bykle.no",
"barum.no",
"bærum.no",
"bo.telemark.no",
"bø.telemark.no",
"bo.nordland.no",
"bø.nordland.no",
"bievat.no",
"bievát.no",
"bomlo.no",
"bømlo.no",
"batsfjord.no",
"båtsfjord.no",
"bahcavuotna.no",
"báhcavuotna.no",
"dovre.no",
"drammen.no",
"drangedal.no",
"dyroy.no",
"dyrøy.no",
"donna.no",
"dønna.no",
"eid.no",
"eidfjord.no",
"eidsberg.no",
"eidskog.no",
"eidsvoll.no",
"eigersund.no",
"elverum.no",
"enebakk.no",
"engerdal.no",
"etne.no",
"etnedal.no",
"evenes.no",
"evenassi.no",
"evenášši.no",
"evje-og-hornnes.no",
"farsund.no",
"fauske.no",
"fuossko.no",
"fuoisku.no",
"fedje.no",
"fet.no",
"finnoy.no",
"finnøy.no",
"fitjar.no",
"fjaler.no",
"fjell.no",
"flakstad.no",
"flatanger.no",
"flekkefjord.no",
"flesberg.no",
"flora.no",
"fla.no",
"flå.no",
"folldal.no",
"forsand.no",
"fosnes.no",
"frei.no",
"frogn.no",
"froland.no",
"frosta.no",
"frana.no",
"fræna.no",
"froya.no",
"frøya.no",
"fusa.no",
"fyresdal.no",
"forde.no",
"førde.no",
"gamvik.no",
"gangaviika.no",
"gáŋgaviika.no",
"gaular.no",
"gausdal.no",
"gildeskal.no",
"gildeskål.no",
"giske.no",
"gjemnes.no",
"gjerdrum.no",
"gjerstad.no",
"gjesdal.no",
"gjovik.no",
"gjøvik.no",
"gloppen.no",
"gol.no",
"gran.no",
"grane.no",
"granvin.no",
"gratangen.no",
"grimstad.no",
"grong.no",
"kraanghke.no",
"kråanghke.no",
"grue.no",
"gulen.no",
"hadsel.no",
"halden.no",
"halsa.no",
"hamar.no",
"hamaroy.no",
"habmer.no",
"hábmer.no",
"hapmir.no",
"hápmir.no",
"hammerfest.no",
"hammarfeasta.no",
"hámmárfeasta.no",
"haram.no",
"hareid.no",
"harstad.no",
"hasvik.no",
"aknoluokta.no",
"ákŋoluokta.no",
"hattfjelldal.no",
"aarborte.no",
"haugesund.no",
"hemne.no",
"hemnes.no",
"hemsedal.no",
"heroy.more-og-romsdal.no",
"herøy.møre-og-romsdal.no",
"heroy.nordland.no",
"herøy.nordland.no",
"hitra.no",
"hjartdal.no",
"hjelmeland.no",
"hobol.no",
"hobøl.no",
"hof.no",
"hol.no",
"hole.no",
"holmestrand.no",
"holtalen.no",
"holtålen.no",
"hornindal.no",
"horten.no",
"hurdal.no",
"hurum.no",
"hvaler.no",
"hyllestad.no",
"hagebostad.no",
"hægebostad.no",
"hoyanger.no",
"høyanger.no",
"hoylandet.no",
"høylandet.no",
"ha.no",
"hå.no",
"ibestad.no",
"inderoy.no",
"inderøy.no",
"iveland.no",
"jevnaker.no",
"jondal.no",
"jolster.no",
"jølster.no",
"karasjok.no",
"karasjohka.no",
"kárášjohka.no",
"karlsoy.no",
"galsa.no",
"gálsá.no",
"karmoy.no",
"karmøy.no",
"kautokeino.no",
"guovdageaidnu.no",
"klepp.no",
"klabu.no",
"klæbu.no",
"kongsberg.no",
"kongsvinger.no",
"kragero.no",
"kragerø.no",
"kristiansand.no",
"kristiansund.no",
"krodsherad.no",
"krødsherad.no",
"kvalsund.no",
"rahkkeravju.no",
"ráhkkerávju.no",
"kvam.no",
"kvinesdal.no",
"kvinnherad.no",
"kviteseid.no",
"kvitsoy.no",
"kvitsøy.no",
"kvafjord.no",
"kvæfjord.no",
"giehtavuoatna.no",
"kvanangen.no",
"kvænangen.no",
"navuotna.no",
"návuotna.no",
"kafjord.no",
"kåfjord.no",
"gaivuotna.no",
"gáivuotna.no",
"larvik.no",
"lavangen.no",
"lavagis.no",
"loabat.no",
"loabát.no",
"lebesby.no",
"davvesiida.no",
"leikanger.no",
"leirfjord.no",
"leka.no",
"leksvik.no",
"lenvik.no",
"leangaviika.no",
"leaŋgaviika.no",
"lesja.no",
"levanger.no",
"lier.no",
"lierne.no",
"lillehammer.no",
"lillesand.no",
"lindesnes.no",
"lindas.no",
"lindås.no",
"lom.no",
"loppa.no",
"lahppi.no",
"láhppi.no",
"lund.no",
"lunner.no",
"luroy.no",
"lurøy.no",
"luster.no",
"lyngdal.no",
"lyngen.no",
"ivgu.no",
"lardal.no",
"lerdal.no",
"lærdal.no",
"lodingen.no",
"lødingen.no",
"lorenskog.no",
"lørenskog.no",
"loten.no",
"løten.no",
"malvik.no",
"masoy.no",
"måsøy.no",
"muosat.no",
"muosát.no",
"mandal.no",
"marker.no",
"marnardal.no",
"masfjorden.no",
"meland.no",
"meldal.no",
"melhus.no",
"meloy.no",
"meløy.no",
"meraker.no",
"meråker.no",
"moareke.no",
"moåreke.no",
"midsund.no",
"midtre-gauldal.no",
"modalen.no",
"modum.no",
"molde.no",
"moskenes.no",
"moss.no",
"mosvik.no",
"malselv.no",
"målselv.no",
"malatvuopmi.no",
"málatvuopmi.no",
"namdalseid.no",
"aejrie.no",
"namsos.no",
"namsskogan.no",
"naamesjevuemie.no",
"nååmesjevuemie.no",
"laakesvuemie.no",
"nannestad.no",
"narvik.no",
"narviika.no",
"naustdal.no",
"nedre-eiker.no",
"nes.akershus.no",
"nes.buskerud.no",
"nesna.no",
"nesodden.no",
"nesseby.no",
"unjarga.no",
"unjárga.no",
"nesset.no",
"nissedal.no",
"nittedal.no",
"nord-aurdal.no",
"nord-fron.no",
"nord-odal.no",
"norddal.no",
"nordkapp.no",
"davvenjarga.no",
"davvenjárga.no",
"nordre-land.no",
"nordreisa.no",
"raisa.no",
"ráisa.no",
"nore-og-uvdal.no",
"notodden.no",
"naroy.no",
"nærøy.no",
"notteroy.no",
"nøtterøy.no",
"odda.no",
"oksnes.no",
"øksnes.no",
"oppdal.no",
"oppegard.no",
"oppegård.no",
"orkdal.no",
"orland.no",
"ørland.no",
"orskog.no",
"ørskog.no",
"orsta.no",
"ørsta.no",
"os.hedmark.no",
"os.hordaland.no",
"osen.no",
"osteroy.no",
"osterøy.no",
"ostre-toten.no",
"østre-toten.no",
"overhalla.no",
"ovre-eiker.no",
"øvre-eiker.no",
"oyer.no",
"øyer.no",
"oygarden.no",
"øygarden.no",
"oystre-slidre.no",
"øystre-slidre.no",
"porsanger.no",
"porsangu.no",
"porsáŋgu.no",
"porsgrunn.no",
"radoy.no",
"radøy.no",
"rakkestad.no",
"rana.no",
"ruovat.no",
"randaberg.no",
"rauma.no",
"rendalen.no",
"rennebu.no",
"rennesoy.no",
"rennesøy.no",
"rindal.no",
"ringebu.no",
"ringerike.no",
"ringsaker.no",
"rissa.no",
"risor.no",
"risør.no",
"roan.no",
"rollag.no",
"rygge.no",
"ralingen.no",
"rælingen.no",
"rodoy.no",
"rødøy.no",
"romskog.no",
"rømskog.no",
"roros.no",
"røros.no",
"rost.no",
"røst.no",
"royken.no",
"røyken.no",
"royrvik.no",
"røyrvik.no",
"rade.no",
"råde.no",
"salangen.no",
"siellak.no",
"saltdal.no",
"salat.no",
"sálát.no",
"sálat.no",
"samnanger.no",
"sande.more-og-romsdal.no",
"sande.møre-og-romsdal.no",
"sande.vestfold.no",
"sandefjord.no",
"sandnes.no",
"sandoy.no",
"sandøy.no",
"sarpsborg.no",
"sauda.no",
"sauherad.no",
"sel.no",
"selbu.no",
"selje.no",
"seljord.no",
"sigdal.no",
"siljan.no",
"sirdal.no",
"skaun.no",
"skedsmo.no",
"ski.no",
"skien.no",
"skiptvet.no",
"skjervoy.no",
"skjervøy.no",
"skierva.no",
"skiervá.no",
"skjak.no",
"skjåk.no",
"skodje.no",
"skanland.no",
"skånland.no",
"skanit.no",
"skánit.no",
"smola.no",
"smøla.no",
"snillfjord.no",
"snasa.no",
"snåsa.no",
"snoasa.no",
"snaase.no",
"snåase.no",
"sogndal.no",
"sokndal.no",
"sola.no",
"solund.no",
"songdalen.no",
"sortland.no",
"spydeberg.no",
"stange.no",
"stavanger.no",
"steigen.no",
"steinkjer.no",
"stjordal.no",
"stjørdal.no",
"stokke.no",
"stor-elvdal.no",
"stord.no",
"stordal.no",
"storfjord.no",
"omasvuotna.no",
"strand.no",
"stranda.no",
"stryn.no",
"sula.no",
"suldal.no",
"sund.no",
"sunndal.no",
"surnadal.no",
"sveio.no",
"svelvik.no",
"sykkylven.no",
"sogne.no",
"søgne.no",
"somna.no",
"sømna.no",
"sondre-land.no",
"søndre-land.no",
"sor-aurdal.no",
"sør-aurdal.no",
"sor-fron.no",
"sør-fron.no",
"sor-odal.no",
"sør-odal.no",
"sor-varanger.no",
"sør-varanger.no",
"matta-varjjat.no",
"mátta-várjjat.no",
"sorfold.no",
"sørfold.no",
"sorreisa.no",
"sørreisa.no",
"sorum.no",
"sørum.no",
"tana.no",
"deatnu.no",
"time.no",
"tingvoll.no",
"tinn.no",
"tjeldsund.no",
"dielddanuorri.no",
"tjome.no",
"tjøme.no",
"tokke.no",
"tolga.no",
"torsken.no",
"tranoy.no",
"tranøy.no",
"tromso.no",
"tromsø.no",
"tromsa.no",
"romsa.no",
"trondheim.no",
"troandin.no",
"trysil.no",
"trana.no",
"træna.no",
"trogstad.no",
"trøgstad.no",
"tvedestrand.no",
"tydal.no",
"tynset.no",
"tysfjord.no",
"divtasvuodna.no",
"divttasvuotna.no",
"tysnes.no",
"tysvar.no",
"tysvær.no",
"tonsberg.no",
"tønsberg.no",
"ullensaker.no",
"ullensvang.no",
"ulvik.no",
"utsira.no",
"vadso.no",
"vadsø.no",
"cahcesuolo.no",
"čáhcesuolo.no",
"vaksdal.no",
"valle.no",
"vang.no",
"vanylven.no",
"vardo.no",
"vardø.no",
"varggat.no",
"várggát.no",
"vefsn.no",
"vaapste.no",
"vega.no",
"vegarshei.no",
"vegårshei.no",
"vennesla.no",
"verdal.no",
"verran.no",
"vestby.no",
"vestnes.no",
"vestre-slidre.no",
"vestre-toten.no",
"vestvagoy.no",
"vestvågøy.no",
"vevelstad.no",
"vik.no",
"vikna.no",
"vindafjord.no",
"volda.no",
"voss.no",
"varoy.no",
"værøy.no",
"vagan.no",
"vågan.no",
"voagat.no",
"vagsoy.no",
"vågsøy.no",
"vaga.no",
"vågå.no",
"valer.ostfold.no",
"våler.østfold.no",
"valer.hedmark.no",
"våler.hedmark.no",
"*.np",
"nr",
"biz.nr",
"info.nr",
"gov.nr",
"edu.nr",
"org.nr",
"net.nr",
"com.nr",
"nu",
"nz",
"ac.nz",
"co.nz",
"cri.nz",
"geek.nz",
"gen.nz",
"govt.nz",
"health.nz",
"iwi.nz",
"kiwi.nz",
"maori.nz",
"mil.nz",
"māori.nz",
"net.nz",
"org.nz",
"parliament.nz",
"school.nz",
"om",
"co.om",
"com.om",
"edu.om",
"gov.om",
"med.om",
"museum.om",
"net.om",
"org.om",
"pro.om",
"onion",
"org",
"pa",
"ac.pa",
"gob.pa",
"com.pa",
"org.pa",
"sld.pa",
"edu.pa",
"net.pa",
"ing.pa",
"abo.pa",
"med.pa",
"nom.pa",
"pe",
"edu.pe",
"gob.pe",
"nom.pe",
"mil.pe",
"org.pe",
"com.pe",
"net.pe",
"pf",
"com.pf",
"org.pf",
"edu.pf",
"*.pg",
"ph",
"com.ph",
"net.ph",
"org.ph",
"gov.ph",
"edu.ph",
"ngo.ph",
"mil.ph",
"i.ph",
"pk",
"com.pk",
"net.pk",
"edu.pk",
"org.pk",
"fam.pk",
"biz.pk",
"web.pk",
"gov.pk",
"gob.pk",
"gok.pk",
"gon.pk",
"gop.pk",
"gos.pk",
"info.pk",
"pl",
"com.pl",
"net.pl",
"org.pl",
"aid.pl",
"agro.pl",
"atm.pl",
"auto.pl",
"biz.pl",
"edu.pl",
"gmina.pl",
"gsm.pl",
"info.pl",
"mail.pl",
"miasta.pl",
"media.pl",
"mil.pl",
"nieruchomosci.pl",
"nom.pl",
"pc.pl",
"powiat.pl",
"priv.pl",
"realestate.pl",
"rel.pl",
"sex.pl",
"shop.pl",
"sklep.pl",
"sos.pl",
"szkola.pl",
"targi.pl",
"tm.pl",
"tourism.pl",
"travel.pl",
"turystyka.pl",
"gov.pl",
"ap.gov.pl",
"ic.gov.pl",
"is.gov.pl",
"us.gov.pl",
"kmpsp.gov.pl",
"kppsp.gov.pl",
"kwpsp.gov.pl",
"psp.gov.pl",
"wskr.gov.pl",
"kwp.gov.pl",
"mw.gov.pl",
"ug.gov.pl",
"um.gov.pl",
"umig.gov.pl",
"ugim.gov.pl",
"upow.gov.pl",
"uw.gov.pl",
"starostwo.gov.pl",
"pa.gov.pl",
"po.gov.pl",
"psse.gov.pl",
"pup.gov.pl",
"rzgw.gov.pl",
"sa.gov.pl",
"so.gov.pl",
"sr.gov.pl",
"wsa.gov.pl",
"sko.gov.pl",
"uzs.gov.pl",
"wiih.gov.pl",
"winb.gov.pl",
"pinb.gov.pl",
"wios.gov.pl",
"witd.gov.pl",
"wzmiuw.gov.pl",
"piw.gov.pl",
"wiw.gov.pl",
"griw.gov.pl",
"wif.gov.pl",
"oum.gov.pl",
"sdn.gov.pl",
"zp.gov.pl",
"uppo.gov.pl",
"mup.gov.pl",
"wuoz.gov.pl",
"konsulat.gov.pl",
"oirm.gov.pl",
"augustow.pl",
"babia-gora.pl",
"bedzin.pl",
"beskidy.pl",
"bialowieza.pl",
"bialystok.pl",
"bielawa.pl",
"bieszczady.pl",
"boleslawiec.pl",
"bydgoszcz.pl",
"bytom.pl",
"cieszyn.pl",
"czeladz.pl",
"czest.pl",
"dlugoleka.pl",
"elblag.pl",
"elk.pl",
"glogow.pl",
"gniezno.pl",
"gorlice.pl",
"grajewo.pl",
"ilawa.pl",
"jaworzno.pl",
"jelenia-gora.pl",
"jgora.pl",
"kalisz.pl",
"kazimierz-dolny.pl",
"karpacz.pl",
"kartuzy.pl",
"kaszuby.pl",
"katowice.pl",
"kepno.pl",
"ketrzyn.pl",
"klodzko.pl",
"kobierzyce.pl",
"kolobrzeg.pl",
"konin.pl",
"konskowola.pl",
"kutno.pl",
"lapy.pl",
"lebork.pl",
"legnica.pl",
"lezajsk.pl",
"limanowa.pl",
"lomza.pl",
"lowicz.pl",
"lubin.pl",
"lukow.pl",
"malbork.pl",
"malopolska.pl",
"mazowsze.pl",
"mazury.pl",
"mielec.pl",
"mielno.pl",
"mragowo.pl",
"naklo.pl",
"nowaruda.pl",
"nysa.pl",
"olawa.pl",
"olecko.pl",
"olkusz.pl",
"olsztyn.pl",
"opoczno.pl",
"opole.pl",
"ostroda.pl",
"ostroleka.pl",
"ostrowiec.pl",
"ostrowwlkp.pl",
"pila.pl",
"pisz.pl",
"podhale.pl",
"podlasie.pl",
"polkowice.pl",
"pomorze.pl",
"pomorskie.pl",
"prochowice.pl",
"pruszkow.pl",
"przeworsk.pl",
"pulawy.pl",
"radom.pl",
"rawa-maz.pl",
"rybnik.pl",
"rzeszow.pl",
"sanok.pl",
"sejny.pl",
"slask.pl",
"slupsk.pl",
"sosnowiec.pl",
"stalowa-wola.pl",
"skoczow.pl",
"starachowice.pl",
"stargard.pl",
"suwalki.pl",
"swidnica.pl",
"swiebodzin.pl",
"swinoujscie.pl",
"szczecin.pl",
"szczytno.pl",
"tarnobrzeg.pl",
"tgory.pl",
"turek.pl",
"tychy.pl",
"ustka.pl",
"walbrzych.pl",
"warmia.pl",
"warszawa.pl",
"waw.pl",
"wegrow.pl",
"wielun.pl",
"wlocl.pl",
"wloclawek.pl",
"wodzislaw.pl",
"wolomin.pl",
"wroclaw.pl",
"zachpomor.pl",
"zagan.pl",
"zarow.pl",
"zgora.pl",
"zgorzelec.pl",
"pm",
"pn",
"gov.pn",
"co.pn",
"org.pn",
"edu.pn",
"net.pn",
"post",
"pr",
"com.pr",
"net.pr",
"org.pr",
"gov.pr",
"edu.pr",
"isla.pr",
"pro.pr",
"biz.pr",
"info.pr",
"name.pr",
"est.pr",
"prof.pr",
"ac.pr",
"pro",
"aaa.pro",
"aca.pro",
"acct.pro",
"avocat.pro",
"bar.pro",
"cpa.pro",
"eng.pro",
"jur.pro",
"law.pro",
"med.pro",
"recht.pro",
"ps",
"edu.ps",
"gov.ps",
"sec.ps",
"plo.ps",
"com.ps",
"org.ps",
"net.ps",
"pt",
"net.pt",
"gov.pt",
"org.pt",
"edu.pt",
"int.pt",
"publ.pt",
"com.pt",
"nome.pt",
"pw",
"co.pw",
"ne.pw",
"or.pw",
"ed.pw",
"go.pw",
"belau.pw",
"py",
"com.py",
"coop.py",
"edu.py",
"gov.py",
"mil.py",
"net.py",
"org.py",
"qa",
"com.qa",
"edu.qa",
"gov.qa",
"mil.qa",
"name.qa",
"net.qa",
"org.qa",
"sch.qa",
"re",
"asso.re",
"com.re",
"nom.re",
"ro",
"arts.ro",
"com.ro",
"firm.ro",
"info.ro",
"nom.ro",
"nt.ro",
"org.ro",
"rec.ro",
"store.ro",
"tm.ro",
"www.ro",
"rs",
"ac.rs",
"co.rs",
"edu.rs",
"gov.rs",
"in.rs",
"org.rs",
"ru",
"rw",
"ac.rw",
"co.rw",
"coop.rw",
"gov.rw",
"mil.rw",
"net.rw",
"org.rw",
"sa",
"com.sa",
"net.sa",
"org.sa",
"gov.sa",
"med.sa",
"pub.sa",
"edu.sa",
"sch.sa",
"sb",
"com.sb",
"edu.sb",
"gov.sb",
"net.sb",
"org.sb",
"sc",
"com.sc",
"gov.sc",
"net.sc",
"org.sc",
"edu.sc",
"sd",
"com.sd",
"net.sd",
"org.sd",
"edu.sd",
"med.sd",
"tv.sd",
"gov.sd",
"info.sd",
"se",
"a.se",
"ac.se",
"b.se",
"bd.se",
"brand.se",
"c.se",
"d.se",
"e.se",
"f.se",
"fh.se",
"fhsk.se",
"fhv.se",
"g.se",
"h.se",
"i.se",
"k.se",
"komforb.se",
"kommunalforbund.se",
"komvux.se",
"l.se",
"lanbib.se",
"m.se",
"n.se",
"naturbruksgymn.se",
"o.se",
"org.se",
"p.se",
"parti.se",
"pp.se",
"press.se",
"r.se",
"s.se",
"t.se",
"tm.se",
"u.se",
"w.se",
"x.se",
"y.se",
"z.se",
"sg",
"com.sg",
"net.sg",
"org.sg",
"gov.sg",
"edu.sg",
"per.sg",
"sh",
"com.sh",
"net.sh",
"gov.sh",
"org.sh",
"mil.sh",
"si",
"sj",
"sk",
"sl",
"com.sl",
"net.sl",
"edu.sl",
"gov.sl",
"org.sl",
"sm",
"sn",
"art.sn",
"com.sn",
"edu.sn",
"gouv.sn",
"org.sn",
"perso.sn",
"univ.sn",
"so",
"com.so",
"edu.so",
"gov.so",
"me.so",
"net.so",
"org.so",
"sr",
"ss",
"biz.ss",
"com.ss",
"edu.ss",
"gov.ss",
"net.ss",
"org.ss",
"st",
"co.st",
"com.st",
"consulado.st",
"edu.st",
"embaixada.st",
"gov.st",
"mil.st",
"net.st",
"org.st",
"principe.st",
"saotome.st",
"store.st",
"su",
"sv",
"com.sv",
"edu.sv",
"gob.sv",
"org.sv",
"red.sv",
"sx",
"gov.sx",
"sy",
"edu.sy",
"gov.sy",
"net.sy",
"mil.sy",
"com.sy",
"org.sy",
"sz",
"co.sz",
"ac.sz",
"org.sz",
"tc",
"td",
"tel",
"tf",
"tg",
"th",
"ac.th",
"co.th",
"go.th",
"in.th",
"mi.th",
"net.th",
"or.th",
"tj",
"ac.tj",
"biz.tj",
"co.tj",
"com.tj",
"edu.tj",
"go.tj",
"gov.tj",
"int.tj",
"mil.tj",
"name.tj",
"net.tj",
"nic.tj",
"org.tj",
"test.tj",
"web.tj",
"tk",
"tl",
"gov.tl",
"tm",
"com.tm",
"co.tm",
"org.tm",
"net.tm",
"nom.tm",
"gov.tm",
"mil.tm",
"edu.tm",
"tn",
"com.tn",
"ens.tn",
"fin.tn",
"gov.tn",
"ind.tn",
"intl.tn",
"nat.tn",
"net.tn",
"org.tn",
"info.tn",
"perso.tn",
"tourism.tn",
"edunet.tn",
"rnrt.tn",
"rns.tn",
"rnu.tn",
"mincom.tn",
"agrinet.tn",
"defense.tn",
"turen.tn",
"to",
"com.to",
"gov.to",
"net.to",
"org.to",
"edu.to",
"mil.to",
"tr",
"av.tr",
"bbs.tr",
"bel.tr",
"biz.tr",
"com.tr",
"dr.tr",
"edu.tr",
"gen.tr",
"gov.tr",
"info.tr",
"mil.tr",
"k12.tr",
"kep.tr",
"name.tr",
"net.tr",
"org.tr",
"pol.tr",
"tel.tr",
"tsk.tr",
"tv.tr",
"web.tr",
"nc.tr",
"gov.nc.tr",
"tt",
"co.tt",
"com.tt",
"org.tt",
"net.tt",
"biz.tt",
"info.tt",
"pro.tt",
"int.tt",
"coop.tt",
"jobs.tt",
"mobi.tt",
"travel.tt",
"museum.tt",
"aero.tt",
"name.tt",
"gov.tt",
"edu.tt",
"tv",
"tw",
"edu.tw",
"gov.tw",
"mil.tw",
"com.tw",
"net.tw",
"org.tw",
"idv.tw",
"game.tw",
"ebiz.tw",
"club.tw",
"網路.tw",
"組織.tw",
"商業.tw",
"tz",
"ac.tz",
"co.tz",
"go.tz",
"hotel.tz",
"info.tz",
"me.tz",
"mil.tz",
"mobi.tz",
"ne.tz",
"or.tz",
"sc.tz",
"tv.tz",
"ua",
"com.ua",
"edu.ua",
"gov.ua",
"in.ua",
"net.ua",
"org.ua",
"cherkassy.ua",
"cherkasy.ua",
"chernigov.ua",
"chernihiv.ua",
"chernivtsi.ua",
"chernovtsy.ua",
"ck.ua",
"cn.ua",
"cr.ua",
"crimea.ua",
"cv.ua",
"dn.ua",
"dnepropetrovsk.ua",
"dnipropetrovsk.ua",
"dominic.ua",
"donetsk.ua",
"dp.ua",
"if.ua",
"ivano-frankivsk.ua",
"kh.ua",
"kharkiv.ua",
"kharkov.ua",
"kherson.ua",
"khmelnitskiy.ua",
"khmelnytskyi.ua",
"kiev.ua",
"kirovograd.ua",
"km.ua",
"kr.ua",
"krym.ua",
"ks.ua",
"kv.ua",
"kyiv.ua",
"lg.ua",
"lt.ua",
"lugansk.ua",
"lutsk.ua",
"lv.ua",
"lviv.ua",
"mk.ua",
"mykolaiv.ua",
"nikolaev.ua",
"od.ua",
"odesa.ua",
"odessa.ua",
"pl.ua",
"poltava.ua",
"rivne.ua",
"rovno.ua",
"rv.ua",
"sb.ua",
"sebastopol.ua",
"sevastopol.ua",
"sm.ua",
"sumy.ua",
"te.ua",
"ternopil.ua",
"uz.ua",
"uzhgorod.ua",
"vinnica.ua",
"vinnytsia.ua",
"vn.ua",
"volyn.ua",
"yalta.ua",
"zaporizhzhe.ua",
"zaporizhzhia.ua",
"zhitomir.ua",
"zhytomyr.ua",
"zp.ua",
"zt.ua",
"ug",
"co.ug",
"or.ug",
"ac.ug",
"sc.ug",
"go.ug",
"ne.ug",
"com.ug",
"org.ug",
"uk",
"ac.uk",
"co.uk",
"gov.uk",
"ltd.uk",
"me.uk",
"net.uk",
"nhs.uk",
"org.uk",
"plc.uk",
"police.uk",
"*.sch.uk",
"us",
"dni.us",
"fed.us",
"isa.us",
"kids.us",
"nsn.us",
"ak.us",
"al.us",
"ar.us",
"as.us",
"az.us",
"ca.us",
"co.us",
"ct.us",
"dc.us",
"de.us",
"fl.us",
"ga.us",
"gu.us",
"hi.us",
"ia.us",
"id.us",
"il.us",
"in.us",
"ks.us",
"ky.us",
"la.us",
"ma.us",
"md.us",
"me.us",
"mi.us",
"mn.us",
"mo.us",
"ms.us",
"mt.us",
"nc.us",
"nd.us",
"ne.us",
"nh.us",
"nj.us",
"nm.us",
"nv.us",
"ny.us",
"oh.us",
"ok.us",
"or.us",
"pa.us",
"pr.us",
"ri.us",
"sc.us",
"sd.us",
"tn.us",
"tx.us",
"ut.us",
"vi.us",
"vt.us",
"va.us",
"wa.us",
"wi.us",
"wv.us",
"wy.us",
"k12.ak.us",
"k12.al.us",
"k12.ar.us",
"k12.as.us",
"k12.az.us",
"k12.ca.us",
"k12.co.us",
"k12.ct.us",
"k12.dc.us",
"k12.de.us",
"k12.fl.us",
"k12.ga.us",
"k12.gu.us",
"k12.ia.us",
"k12.id.us",
"k12.il.us",
"k12.in.us",
"k12.ks.us",
"k12.ky.us",
"k12.la.us",
"k12.ma.us",
"k12.md.us",
"k12.me.us",
"k12.mi.us",
"k12.mn.us",
"k12.mo.us",
"k12.ms.us",
"k12.mt.us",
"k12.nc.us",
"k12.ne.us",
"k12.nh.us",
"k12.nj.us",
"k12.nm.us",
"k12.nv.us",
"k12.ny.us",
"k12.oh.us",
"k12.ok.us",
"k12.or.us",
"k12.pa.us",
"k12.pr.us",
"k12.ri.us",
"k12.sc.us",
"k12.tn.us",
"k12.tx.us",
"k12.ut.us",
"k12.vi.us",
"k12.vt.us",
"k12.va.us",
"k12.wa.us",
"k12.wi.us",
"k12.wy.us",
"cc.ak.us",
"cc.al.us",
"cc.ar.us",
"cc.as.us",
"cc.az.us",
"cc.ca.us",
"cc.co.us",
"cc.ct.us",
"cc.dc.us",
"cc.de.us",
"cc.fl.us",
"cc.ga.us",
"cc.gu.us",
"cc.hi.us",
"cc.ia.us",
"cc.id.us",
"cc.il.us",
"cc.in.us",
"cc.ks.us",
"cc.ky.us",
"cc.la.us",
"cc.ma.us",
"cc.md.us",
"cc.me.us",
"cc.mi.us",
"cc.mn.us",
"cc.mo.us",
"cc.ms.us",
"cc.mt.us",
"cc.nc.us",
"cc.nd.us",
"cc.ne.us",
"cc.nh.us",
"cc.nj.us",
"cc.nm.us",
"cc.nv.us",
"cc.ny.us",
"cc.oh.us",
"cc.ok.us",
"cc.or.us",
"cc.pa.us",
"cc.pr.us",
"cc.ri.us",
"cc.sc.us",
"cc.sd.us",
"cc.tn.us",
"cc.tx.us",
"cc.ut.us",
"cc.vi.us",
"cc.vt.us",
"cc.va.us",
"cc.wa.us",
"cc.wi.us",
"cc.wv.us",
"cc.wy.us",
"lib.ak.us",
"lib.al.us",
"lib.ar.us",
"lib.as.us",
"lib.az.us",
"lib.ca.us",
"lib.co.us",
"lib.ct.us",
"lib.dc.us",
"lib.fl.us",
"lib.ga.us",
"lib.gu.us",
"lib.hi.us",
"lib.ia.us",
"lib.id.us",
"lib.il.us",
"lib.in.us",
"lib.ks.us",
"lib.ky.us",
"lib.la.us",
"lib.ma.us",
"lib.md.us",
"lib.me.us",
"lib.mi.us",
"lib.mn.us",
"lib.mo.us",
"lib.ms.us",
"lib.mt.us",
"lib.nc.us",
"lib.nd.us",
"lib.ne.us",
"lib.nh.us",
"lib.nj.us",
"lib.nm.us",
"lib.nv.us",
"lib.ny.us",
"lib.oh.us",
"lib.ok.us",
"lib.or.us",
"lib.pa.us",
"lib.pr.us",
"lib.ri.us",
"lib.sc.us",
"lib.sd.us",
"lib.tn.us",
"lib.tx.us",
"lib.ut.us",
"lib.vi.us",
"lib.vt.us",
"lib.va.us",
"lib.wa.us",
"lib.wi.us",
"lib.wy.us",
"pvt.k12.ma.us",
"chtr.k12.ma.us",
"paroch.k12.ma.us",
"ann-arbor.mi.us",
"cog.mi.us",
"dst.mi.us",
"eaton.mi.us",
"gen.mi.us",
"mus.mi.us",
"tec.mi.us",
"washtenaw.mi.us",
"uy",
"com.uy",
"edu.uy",
"gub.uy",
"mil.uy",
"net.uy",
"org.uy",
"uz",
"co.uz",
"com.uz",
"net.uz",
"org.uz",
"va",
"vc",
"com.vc",
"net.vc",
"org.vc",
"gov.vc",
"mil.vc",
"edu.vc",
"ve",
"arts.ve",
"co.ve",
"com.ve",
"e12.ve",
"edu.ve",
"firm.ve",
"gob.ve",
"gov.ve",
"info.ve",
"int.ve",
"mil.ve",
"net.ve",
"org.ve",
"rec.ve",
"store.ve",
"tec.ve",
"web.ve",
"vg",
"vi",
"co.vi",
"com.vi",
"k12.vi",
"net.vi",
"org.vi",
"vn",
"com.vn",
"net.vn",
"org.vn",
"edu.vn",
"gov.vn",
"int.vn",
"ac.vn",
"biz.vn",
"info.vn",
"name.vn",
"pro.vn",
"health.vn",
"vu",
"com.vu",
"edu.vu",
"net.vu",
"org.vu",
"wf",
"ws",
"com.ws",
"net.ws",
"org.ws",
"gov.ws",
"edu.ws",
"yt",
"امارات",
"հայ",
"বাংলা",
"бг",
"бел",
"中国",
"中國",
"الجزائر",
"مصر",
"ею",
"ευ",
"موريتانيا",
"გე",
"ελ",
"香港",
"公司.香港",
"教育.香港",
"政府.香港",
"個人.香港",
"網絡.香港",
"組織.香港",
"ಭಾರತ",
"ଭାରତ",
"ভাৰত",
"भारतम्",
"भारोत",
"ڀارت",
"ഭാരതം",
"भारत",
"بارت",
"بھارت",
"భారత్",
"ભારત",
"ਭਾਰਤ",
"ভারত",
"இந்தியா",
"ایران",
"ايران",
"عراق",
"الاردن",
"한국",
"қаз",
"ලංකා",
"இலங்கை",
"المغرب",
"мкд",
"мон",
"澳門",
"澳门",
"مليسيا",
"عمان",
"پاکستان",
"پاكستان",
"فلسطين",
"срб",
"пр.срб",
"орг.срб",
"обр.срб",
"од.срб",
"упр.срб",
"ак.срб",
"рф",
"قطر",
"السعودية",
"السعودیة",
"السعودیۃ",
"السعوديه",
"سودان",
"新加坡",
"சிங்கப்பூர்",
"سورية",
"سوريا",
"ไทย",
"ศึกษา.ไทย",
"ธุรกิจ.ไทย",
"รัฐบาล.ไทย",
"ทหาร.ไทย",
"เน็ต.ไทย",
"องค์กร.ไทย",
"تونس",
"台灣",
"台湾",
"臺灣",
"укр",
"اليمن",
"xxx",
"*.ye",
"ac.za",
"agric.za",
"alt.za",
"co.za",
"edu.za",
"gov.za",
"grondar.za",
"law.za",
"mil.za",
"net.za",
"ngo.za",
"nic.za",
"nis.za",
"nom.za",
"org.za",
"school.za",
"tm.za",
"web.za",
"zm",
"ac.zm",
"biz.zm",
"co.zm",
"com.zm",
"edu.zm",
"gov.zm",
"info.zm",
"mil.zm",
"net.zm",
"org.zm",
"sch.zm",
"zw",
"ac.zw",
"co.zw",
"gov.zw",
"mil.zw",
"org.zw",
"aaa",
"aarp",
"abarth",
"abb",
"abbott",
"abbvie",
"abc",
"able",
"abogado",
"abudhabi",
"academy",
"accenture",
"accountant",
"accountants",
"aco",
"actor",
"adac",
"ads",
"adult",
"aeg",
"aetna",
"afamilycompany",
"afl",
"africa",
"agakhan",
"agency",
"aig",
"aigo",
"airbus",
"airforce",
"airtel",
"akdn",
"alfaromeo",
"alibaba",
"alipay",
"allfinanz",
"allstate",
"ally",
"alsace",
"alstom",
"amazon",
"americanexpress",
"americanfamily",
"amex",
"amfam",
"amica",
"amsterdam",
"analytics",
"android",
"anquan",
"anz",
"aol",
"apartments",
"app",
"apple",
"aquarelle",
"arab",
"aramco",
"archi",
"army",
"art",
"arte",
"asda",
"associates",
"athleta",
"attorney",
"auction",
"audi",
"audible",
"audio",
"auspost",
"author",
"auto",
"autos",
"avianca",
"aws",
"axa",
"azure",
"baby",
"baidu",
"banamex",
"bananarepublic",
"band",
"bank",
"bar",
"barcelona",
"barclaycard",
"barclays",
"barefoot",
"bargains",
"baseball",
"basketball",
"bauhaus",
"bayern",
"bbc",
"bbt",
"bbva",
"bcg",
"bcn",
"beats",
"beauty",
"beer",
"bentley",
"berlin",
"best",
"bestbuy",
"bet",
"bharti",
"bible",
"bid",
"bike",
"bing",
"bingo",
"bio",
"black",
"blackfriday",
"blockbuster",
"blog",
"bloomberg",
"blue",
"bms",
"bmw",
"bnpparibas",
"boats",
"boehringer",
"bofa",
"bom",
"bond",
"boo",
"book",
"booking",
"bosch",
"bostik",
"boston",
"bot",
"boutique",
"box",
"bradesco",
"bridgestone",
"broadway",
"broker",
"brother",
"brussels",
"budapest",
"bugatti",
"build",
"builders",
"business",
"buy",
"buzz",
"bzh",
"cab",
"cafe",
"cal",
"call",
"calvinklein",
"cam",
"camera",
"camp",
"cancerresearch",
"canon",
"capetown",
"capital",
"capitalone",
"car",
"caravan",
"cards",
"care",
"career",
"careers",
"cars",
"casa",
"case",
"caseih",
"cash",
"casino",
"catering",
"catholic",
"cba",
"cbn",
"cbre",
"cbs",
"ceb",
"center",
"ceo",
"cern",
"cfa",
"cfd",
"chanel",
"channel",
"charity",
"chase",
"chat",
"cheap",
"chintai",
"christmas",
"chrome",
"church",
"cipriani",
"circle",
"cisco",
"citadel",
"citi",
"citic",
"city",
"cityeats",
"claims",
"cleaning",
"click",
"clinic",
"clinique",
"clothing",
"cloud",
"club",
"clubmed",
"coach",
"codes",
"coffee",
"college",
"cologne",
"comcast",
"commbank",
"community",
"company",
"compare",
"computer",
"comsec",
"condos",
"construction",
"consulting",
"contact",
"contractors",
"cooking",
"cookingchannel",
"cool",
"corsica",
"country",
"coupon",
"coupons",
"courses",
"cpa",
"credit",
"creditcard",
"creditunion",
"cricket",
"crown",
"crs",
"cruise",
"cruises",
"csc",
"cuisinella",
"cymru",
"cyou",
"dabur",
"dad",
"dance",
"data",
"date",
"dating",
"datsun",
"day",
"dclk",
"dds",
"deal",
"dealer",
"deals",
"degree",
"delivery",
"dell",
"deloitte",
"delta",
"democrat",
"dental",
"dentist",
"desi",
"design",
"dev",
"dhl",
"diamonds",
"diet",
"digital",
"direct",
"directory",
"discount",
"discover",
"dish",
"diy",
"dnp",
"docs",
"doctor",
"dog",
"domains",
"dot",
"download",
"drive",
"dtv",
"dubai",
"duck",
"dunlop",
"dupont",
"durban",
"dvag",
"dvr",
"earth",
"eat",
"eco",
"edeka",
"education",
"email",
"emerck",
"energy",
"engineer",
"engineering",
"enterprises",
"epson",
"equipment",
"ericsson",
"erni",
"esq",
"estate",
"esurance",
"etisalat",
"eurovision",
"eus",
"events",
"exchange",
"expert",
"exposed",
"express",
"extraspace",
"fage",
"fail",
"fairwinds",
"faith",
"family",
"fan",
"fans",
"farm",
"farmers",
"fashion",
"fast",
"fedex",
"feedback",
"ferrari",
"ferrero",
"fiat",
"fidelity",
"fido",
"film",
"final",
"finance",
"financial",
"fire",
"firestone",
"firmdale",
"fish",
"fishing",
"fit",
"fitness",
"flickr",
"flights",
"flir",
"florist",
"flowers",
"fly",
"foo",
"food",
"foodnetwork",
"football",
"ford",
"forex",
"forsale",
"forum",
"foundation",
"fox",
"free",
"fresenius",
"frl",
"frogans",
"frontdoor",
"frontier",
"ftr",
"fujitsu",
"fujixerox",
"fun",
"fund",
"furniture",
"futbol",
"fyi",
"gal",
"gallery",
"gallo",
"gallup",
"game",
"games",
"gap",
"garden",
"gay",
"gbiz",
"gdn",
"gea",
"gent",
"genting",
"george",
"ggee",
"gift",
"gifts",
"gives",
"giving",
"glade",
"glass",
"gle",
"global",
"globo",
"gmail",
"gmbh",
"gmo",
"gmx",
"godaddy",
"gold",
"goldpoint",
"golf",
"goo",
"goodyear",
"goog",
"google",
"gop",
"got",
"grainger",
"graphics",
"gratis",
"green",
"gripe",
"grocery",
"group",
"guardian",
"gucci",
"guge",
"guide",
"guitars",
"guru",
"hair",
"hamburg",
"hangout",
"haus",
"hbo",
"hdfc",
"hdfcbank",
"health",
"healthcare",
"help",
"helsinki",
"here",
"hermes",
"hgtv",
"hiphop",
"hisamitsu",
"hitachi",
"hiv",
"hkt",
"hockey",
"holdings",
"holiday",
"homedepot",
"homegoods",
"homes",
"homesense",
"honda",
"horse",
"hospital",
"host",
"hosting",
"hot",
"hoteles",
"hotels",
"hotmail",
"house",
"how",
"hsbc",
"hughes",
"hyatt",
"hyundai",
"ibm",
"icbc",
"ice",
"icu",
"ieee",
"ifm",
"ikano",
"imamat",
"imdb",
"immo",
"immobilien",
"inc",
"industries",
"infiniti",
"ing",
"ink",
"institute",
"insurance",
"insure",
"intel",
"international",
"intuit",
"investments",
"ipiranga",
"irish",
"ismaili",
"ist",
"istanbul",
"itau",
"itv",
"iveco",
"jaguar",
"java",
"jcb",
"jcp",
"jeep",
"jetzt",
"jewelry",
"jio",
"jll",
"jmp",
"jnj",
"joburg",
"jot",
"joy",
"jpmorgan",
"jprs",
"juegos",
"juniper",
"kaufen",
"kddi",
"kerryhotels",
"kerrylogistics",
"kerryproperties",
"kfh",
"kia",
"kim",
"kinder",
"kindle",
"kitchen",
"kiwi",
"koeln",
"komatsu",
"kosher",
"kpmg",
"kpn",
"krd",
"kred",
"kuokgroup",
"kyoto",
"lacaixa",
"lamborghini",
"lamer",
"lancaster",
"lancia",
"land",
"landrover",
"lanxess",
"lasalle",
"lat",
"latino",
"latrobe",
"law",
"lawyer",
"lds",
"lease",
"leclerc",
"lefrak",
"legal",
"lego",
"lexus",
"lgbt",
"lidl",
"life",
"lifeinsurance",
"lifestyle",
"lighting",
"like",
"lilly",
"limited",
"limo",
"lincoln",
"linde",
"link",
"lipsy",
"live",
"living",
"lixil",
"llc",
"llp",
"loan",
"loans",
"locker",
"locus",
"loft",
"lol",
"london",
"lotte",
"lotto",
"love",
"lpl",
"lplfinancial",
"ltd",
"ltda",
"lundbeck",
"lupin",
"luxe",
"luxury",
"macys",
"madrid",
"maif",
"maison",
"makeup",
"man",
"management",
"mango",
"map",
"market",
"marketing",
"markets",
"marriott",
"marshalls",
"maserati",
"mattel",
"mba",
"mckinsey",
"med",
"media",
"meet",
"melbourne",
"meme",
"memorial",
"men",
"menu",
"merckmsd",
"metlife",
"miami",
"microsoft",
"mini",
"mint",
"mit",
"mitsubishi",
"mlb",
"mls",
"mma",
"mobile",
"moda",
"moe",
"moi",
"mom",
"monash",
"money",
"monster",
"mormon",
"mortgage",
"moscow",
"moto",
"motorcycles",
"mov",
"movie",
"msd",
"mtn",
"mtr",
"mutual",
"nab",
"nadex",
"nagoya",
"nationwide",
"natura",
"navy",
"nba",
"nec",
"netbank",
"netflix",
"network",
"neustar",
"new",
"newholland",
"news",
"next",
"nextdirect",
"nexus",
"nfl",
"ngo",
"nhk",
"nico",
"nike",
"nikon",
"ninja",
"nissan",
"nissay",
"nokia",
"northwesternmutual",
"norton",
"now",
"nowruz",
"nowtv",
"nra",
"nrw",
"ntt",
"nyc",
"obi",
"observer",
"off",
"office",
"okinawa",
"olayan",
"olayangroup",
"oldnavy",
"ollo",
"omega",
"one",
"ong",
"onl",
"online",
"onyourside",
"ooo",
"open",
"oracle",
"orange",
"organic",
"origins",
"osaka",
"otsuka",
"ott",
"ovh",
"page",
"panasonic",
"paris",
"pars",
"partners",
"parts",
"party",
"passagens",
"pay",
"pccw",
"pet",
"pfizer",
"pharmacy",
"phd",
"philips",
"phone",
"photo",
"photography",
"photos",
"physio",
"pics",
"pictet",
"pictures",
"pid",
"pin",
"ping",
"pink",
"pioneer",
"pizza",
"place",
"play",
"playstation",
"plumbing",
"plus",
"pnc",
"pohl",
"poker",
"politie",
"porn",
"pramerica",
"praxi",
"press",
"prime",
"prod",
"productions",
"prof",
"progressive",
"promo",
"properties",
"property",
"protection",
"pru",
"prudential",
"pub",
"pwc",
"qpon",
"quebec",
"quest",
"qvc",
"racing",
"radio",
"raid",
"read",
"realestate",
"realtor",
"realty",
"recipes",
"red",
"redstone",
"redumbrella",
"rehab",
"reise",
"reisen",
"reit",
"reliance",
"ren",
"rent",
"rentals",
"repair",
"report",
"republican",
"rest",
"restaurant",
"review",
"reviews",
"rexroth",
"rich",
"richardli",
"ricoh",
"rightathome",
"ril",
"rio",
"rip",
"rmit",
"rocher",
"rocks",
"rodeo",
"rogers",
"room",
"rsvp",
"rugby",
"ruhr",
"run",
"rwe",
"ryukyu",
"saarland",
"safe",
"safety",
"sakura",
"sale",
"salon",
"samsclub",
"samsung",
"sandvik",
"sandvikcoromant",
"sanofi",
"sap",
"sarl",
"sas",
"save",
"saxo",
"sbi",
"sbs",
"sca",
"scb",
"schaeffler",
"schmidt",
"scholarships",
"school",
"schule",
"schwarz",
"science",
"scjohnson",
"scor",
"scot",
"search",
"seat",
"secure",
"security",
"seek",
"select",
"sener",
"services",
"ses",
"seven",
"sew",
"sex",
"sexy",
"sfr",
"shangrila",
"sharp",
"shaw",
"shell",
"shia",
"shiksha",
"shoes",
"shop",
"shopping",
"shouji",
"show",
"showtime",
"shriram",
"silk",
"sina",
"singles",
"site",
"ski",
"skin",
"sky",
"skype",
"sling",
"smart",
"smile",
"sncf",
"soccer",
"social",
"softbank",
"software",
"sohu",
"solar",
"solutions",
"song",
"sony",
"soy",
"spa",
"space",
"sport",
"spot",
"spreadbetting",
"srl",
"stada",
"staples",
"star",
"statebank",
"statefarm",
"stc",
"stcgroup",
"stockholm",
"storage",
"store",
"stream",
"studio",
"study",
"style",
"sucks",
"supplies",
"supply",
"support",
"surf",
"surgery",
"suzuki",
"swatch",
"swiftcover",
"swiss",
"sydney",
"symantec",
"systems",
"tab",
"taipei",
"talk",
"taobao",
"target",
"tatamotors",
"tatar",
"tattoo",
"tax",
"taxi",
"tci",
"tdk",
"team",
"tech",
"technology",
"temasek",
"tennis",
"teva",
"thd",
"theater",
"theatre",
"tiaa",
"tickets",
"tienda",
"tiffany",
"tips",
"tires",
"tirol",
"tjmaxx",
"tjx",
"tkmaxx",
"tmall",
"today",
"tokyo",
"tools",
"top",
"toray",
"toshiba",
"total",
"tours",
"town",
"toyota",
"toys",
"trade",
"trading",
"training",
"travel",
"travelchannel",
"travelers",
"travelersinsurance",
"trust",
"trv",
"tube",
"tui",
"tunes",
"tushu",
"tvs",
"ubank",
"ubs",
"unicom",
"university",
"uno",
"uol",
"ups",
"vacations",
"vana",
"vanguard",
"vegas",
"ventures",
"verisign",
"versicherung",
"vet",
"viajes",
"video",
"vig",
"viking",
"villas",
"vin",
"vip",
"virgin",
"visa",
"vision",
"viva",
"vivo",
"vlaanderen",
"vodka",
"volkswagen",
"volvo",
"vote",
"voting",
"voto",
"voyage",
"vuelos",
"wales",
"walmart",
"walter",
"wang",
"wanggou",
"watch",
"watches",
"weather",
"weatherchannel",
"webcam",
"weber",
"website",
"wed",
"wedding",
"weibo",
"weir",
"whoswho",
"wien",
"wiki",
"williamhill",
"win",
"windows",
"wine",
"winners",
"wme",
"wolterskluwer",
"woodside",
"work",
"works",
"world",
"wow",
"wtc",
"wtf",
"xbox",
"xerox",
"xfinity",
"xihuan",
"xin",
"कॉम",
"セール",
"佛山",
"慈善",
"集团",
"在线",
"大众汽车",
"点看",
"คอม",
"八卦",
"موقع",
"公益",
"公司",
"香格里拉",
"网站",
"移动",
"我爱你",
"москва",
"католик",
"онлайн",
"сайт",
"联通",
"קום",
"时尚",
"微博",
"淡马锡",
"ファッション",
"орг",
"नेट",
"ストア",
"アマゾン",
"삼성",
"商标",
"商店",
"商城",
"дети",
"ポイント",
"新闻",
"工行",
"家電",
"كوم",
"中文网",
"中信",
"娱乐",
"谷歌",
"電訊盈科",
"购物",
"クラウド",
"通販",
"网店",
"संगठन",
"餐厅",
"网络",
"ком",
"亚马逊",
"诺基亚",
"食品",
"飞利浦",
"手表",
"手机",
"ارامكو",
"العليان",
"اتصالات",
"بازار",
"ابوظبي",
"كاثوليك",
"همراه",
"닷컴",
"政府",
"شبكة",
"بيتك",
"عرب",
"机构",
"组织机构",
"健康",
"招聘",
"рус",
"珠宝",
"大拿",
"みんな",
"グーグル",
"世界",
"書籍",
"网址",
"닷넷",
"コム",
"天主教",
"游戏",
"vermögensberater",
"vermögensberatung",
"企业",
"信息",
"嘉里大酒店",
"嘉里",
"广东",
"政务",
"xyz",
"yachts",
"yahoo",
"yamaxun",
"yandex",
"yodobashi",
"yoga",
"yokohama",
"you",
"youtube",
"yun",
"zappos",
"zara",
"zero",
"zip",
"zone",
"zuerich",
"cc.ua",
"inf.ua",
"ltd.ua",
"adobeaemcloud.com",
"adobeaemcloud.net",
"*.dev.adobeaemcloud.com",
"beep.pl",
"barsy.ca",
"*.compute.estate",
"*.alces.network",
"altervista.org",
"alwaysdata.net",
"cloudfront.net",
"*.compute.amazonaws.com",
"*.compute-1.amazonaws.com",
"*.compute.amazonaws.com.cn",
"us-east-1.amazonaws.com",
"cn-north-1.eb.amazonaws.com.cn",
"cn-northwest-1.eb.amazonaws.com.cn",
"elasticbeanstalk.com",
"ap-northeast-1.elasticbeanstalk.com",
"ap-northeast-2.elasticbeanstalk.com",
"ap-northeast-3.elasticbeanstalk.com",
"ap-south-1.elasticbeanstalk.com",
"ap-southeast-1.elasticbeanstalk.com",
"ap-southeast-2.elasticbeanstalk.com",
"ca-central-1.elasticbeanstalk.com",
"eu-central-1.elasticbeanstalk.com",
"eu-west-1.elasticbeanstalk.com",
"eu-west-2.elasticbeanstalk.com",
"eu-west-3.elasticbeanstalk.com",
"sa-east-1.elasticbeanstalk.com",
"us-east-1.elasticbeanstalk.com",
"us-east-2.elasticbeanstalk.com",
"us-gov-west-1.elasticbeanstalk.com",
"us-west-1.elasticbeanstalk.com",
"us-west-2.elasticbeanstalk.com",
"*.elb.amazonaws.com",
"*.elb.amazonaws.com.cn",
"s3.amazonaws.com",
"s3-ap-northeast-1.amazonaws.com",
"s3-ap-northeast-2.amazonaws.com",
"s3-ap-south-1.amazonaws.com",
"s3-ap-southeast-1.amazonaws.com",
"s3-ap-southeast-2.amazonaws.com",
"s3-ca-central-1.amazonaws.com",
"s3-eu-central-1.amazonaws.com",
"s3-eu-west-1.amazonaws.com",
"s3-eu-west-2.amazonaws.com",
"s3-eu-west-3.amazonaws.com",
"s3-external-1.amazonaws.com",
"s3-fips-us-gov-west-1.amazonaws.com",
"s3-sa-east-1.amazonaws.com",
"s3-us-gov-west-1.amazonaws.com",
"s3-us-east-2.amazonaws.com",
"s3-us-west-1.amazonaws.com",
"s3-us-west-2.amazonaws.com",
"s3.ap-northeast-2.amazonaws.com",
"s3.ap-south-1.amazonaws.com",
"s3.cn-north-1.amazonaws.com.cn",
"s3.ca-central-1.amazonaws.com",
"s3.eu-central-1.amazonaws.com",
"s3.eu-west-2.amazonaws.com",
"s3.eu-west-3.amazonaws.com",
"s3.us-east-2.amazonaws.com",
"s3.dualstack.ap-northeast-1.amazonaws.com",
"s3.dualstack.ap-northeast-2.amazonaws.com",
"s3.dualstack.ap-south-1.amazonaws.com",
"s3.dualstack.ap-southeast-1.amazonaws.com",
"s3.dualstack.ap-southeast-2.amazonaws.com",
"s3.dualstack.ca-central-1.amazonaws.com",
"s3.dualstack.eu-central-1.amazonaws.com",
"s3.dualstack.eu-west-1.amazonaws.com",
"s3.dualstack.eu-west-2.amazonaws.com",
"s3.dualstack.eu-west-3.amazonaws.com",
"s3.dualstack.sa-east-1.amazonaws.com",
"s3.dualstack.us-east-1.amazonaws.com",
"s3.dualstack.us-east-2.amazonaws.com",
"s3-website-us-east-1.amazonaws.com",
"s3-website-us-west-1.amazonaws.com",
"s3-website-us-west-2.amazonaws.com",
"s3-website-ap-northeast-1.amazonaws.com",
"s3-website-ap-southeast-1.amazonaws.com",
"s3-website-ap-southeast-2.amazonaws.com",
"s3-website-eu-west-1.amazonaws.com",
"s3-website-sa-east-1.amazonaws.com",
"s3-website.ap-northeast-2.amazonaws.com",
"s3-website.ap-south-1.amazonaws.com",
"s3-website.ca-central-1.amazonaws.com",
"s3-website.eu-central-1.amazonaws.com",
"s3-website.eu-west-2.amazonaws.com",
"s3-website.eu-west-3.amazonaws.com",
"s3-website.us-east-2.amazonaws.com",
"amsw.nl",
"t3l3p0rt.net",
"tele.amune.org",
"apigee.io",
"on-aptible.com",
"user.aseinet.ne.jp",
"gv.vc",
"d.gv.vc",
"user.party.eus",
"pimienta.org",
"poivron.org",
"potager.org",
"sweetpepper.org",
"myasustor.com",
"myfritz.net",
"*.awdev.ca",
"*.advisor.ws",
"b-data.io",
"backplaneapp.io",
"balena-devices.com",
"app.banzaicloud.io",
"betainabox.com",
"bnr.la",
"blackbaudcdn.net",
"boomla.net",
"boxfuse.io",
"square7.ch",
"bplaced.com",
"bplaced.de",
"square7.de",
"bplaced.net",
"square7.net",
"browsersafetymark.io",
"uk0.bigv.io",
"dh.bytemark.co.uk",
"vm.bytemark.co.uk",
"mycd.eu",
"carrd.co",
"crd.co",
"uwu.ai",
"ae.org",
"ar.com",
"br.com",
"cn.com",
"com.de",
"com.se",
"de.com",
"eu.com",
"gb.com",
"gb.net",
"hu.com",
"hu.net",
"jp.net",
"jpn.com",
"kr.com",
"mex.com",
"no.com",
"qc.com",
"ru.com",
"sa.com",
"se.net",
"uk.com",
"uk.net",
"us.com",
"uy.com",
"za.bz",
"za.com",
"africa.com",
"gr.com",
"in.net",
"us.org",
"co.com",
"c.la",
"certmgr.org",
"xenapponazure.com",
"discourse.group",
"discourse.team",
"virtueeldomein.nl",
"cleverapps.io",
"*.lcl.dev",
"*.stg.dev",
"c66.me",
"cloud66.ws",
"cloud66.zone",
"jdevcloud.com",
"wpdevcloud.com",
"cloudaccess.host",
"freesite.host",
"cloudaccess.net",
"cloudcontrolled.com",
"cloudcontrolapp.com",
"cloudera.site",
"trycloudflare.com",
"workers.dev",
"wnext.app",
"co.ca",
"*.otap.co",
"co.cz",
"c.cdn77.org",
"cdn77-ssl.net",
"r.cdn77.net",
"rsc.cdn77.org",
"ssl.origin.cdn77-secure.org",
"cloudns.asia",
"cloudns.biz",
"cloudns.club",
"cloudns.cc",
"cloudns.eu",
"cloudns.in",
"cloudns.info",
"cloudns.org",
"cloudns.pro",
"cloudns.pw",
"cloudns.us",
"cloudeity.net",
"cnpy.gdn",
"co.nl",
"co.no",
"webhosting.be",
"hosting-cluster.nl",
"ac.ru",
"edu.ru",
"gov.ru",
"int.ru",
"mil.ru",
"test.ru",
"dyn.cosidns.de",
"dynamisches-dns.de",
"dnsupdater.de",
"internet-dns.de",
"l-o-g-i-n.de",
"dynamic-dns.info",
"feste-ip.net",
"knx-server.net",
"static-access.net",
"realm.cz",
"*.cryptonomic.net",
"cupcake.is",
"*.customer-oci.com",
"*.oci.customer-oci.com",
"*.ocp.customer-oci.com",
"*.ocs.customer-oci.com",
"cyon.link",
"cyon.site",
"daplie.me",
"localhost.daplie.me",
"dattolocal.com",
"dattorelay.com",
"dattoweb.com",
"mydatto.com",
"dattolocal.net",
"mydatto.net",
"biz.dk",
"co.dk",
"firm.dk",
"reg.dk",
"store.dk",
"*.dapps.earth",
"*.bzz.dapps.earth",
"builtwithdark.com",
"edgestack.me",
"debian.net",
"dedyn.io",
"dnshome.de",
"online.th",
"shop.th",
"drayddns.com",
"dreamhosters.com",
"mydrobo.com",
"drud.io",
"drud.us",
"duckdns.org",
"dy.fi",
"tunk.org",
"dyndns-at-home.com",
"dyndns-at-work.com",
"dyndns-blog.com",
"dyndns-free.com",
"dyndns-home.com",
"dyndns-ip.com",
"dyndns-mail.com",
"dyndns-office.com",
"dyndns-pics.com",
"dyndns-remote.com",
"dyndns-server.com",
"dyndns-web.com",
"dyndns-wiki.com",
"dyndns-work.com",
"dyndns.biz",
"dyndns.info",
"dyndns.org",
"dyndns.tv",
"at-band-camp.net",
"ath.cx",
"barrel-of-knowledge.info",
"barrell-of-knowledge.info",
"better-than.tv",
"blogdns.com",
"blogdns.net",
"blogdns.org",
"blogsite.org",
"boldlygoingnowhere.org",
"broke-it.net",
"buyshouses.net",
"cechire.com",
"dnsalias.com",
"dnsalias.net",
"dnsalias.org",
"dnsdojo.com",
"dnsdojo.net",
"dnsdojo.org",
"does-it.net",
"doesntexist.com",
"doesntexist.org",
"dontexist.com",
"dontexist.net",
"dontexist.org",
"doomdns.com",
"doomdns.org",
"dvrdns.org",
"dyn-o-saur.com",
"dynalias.com",
"dynalias.net",
"dynalias.org",
"dynathome.net",
"dyndns.ws",
"endofinternet.net",
"endofinternet.org",
"endoftheinternet.org",
"est-a-la-maison.com",
"est-a-la-masion.com",
"est-le-patron.com",
"est-mon-blogueur.com",
"for-better.biz",
"for-more.biz",
"for-our.info",
"for-some.biz",
"for-the.biz",
"forgot.her.name",
"forgot.his.name",
"from-ak.com",
"from-al.com",
"from-ar.com",
"from-az.net",
"from-ca.com",
"from-co.net",
"from-ct.com",
"from-dc.com",
"from-de.com",
"from-fl.com",
"from-ga.com",
"from-hi.com",
"from-ia.com",
"from-id.com",
"from-il.com",
"from-in.com",
"from-ks.com",
"from-ky.com",
"from-la.net",
"from-ma.com",
"from-md.com",
"from-me.org",
"from-mi.com",
"from-mn.com",
"from-mo.com",
"from-ms.com",
"from-mt.com",
"from-nc.com",
"from-nd.com",
"from-ne.com",
"from-nh.com",
"from-nj.com",
"from-nm.com",
"from-nv.com",
"from-ny.net",
"from-oh.com",
"from-ok.com",
"from-or.com",
"from-pa.com",
"from-pr.com",
"from-ri.com",
"from-sc.com",
"from-sd.com",
"from-tn.com",
"from-tx.com",
"from-ut.com",
"from-va.com",
"from-vt.com",
"from-wa.com",
"from-wi.com",
"from-wv.com",
"from-wy.com",
"ftpaccess.cc",
"fuettertdasnetz.de",
"game-host.org",
"game-server.cc",
"getmyip.com",
"gets-it.net",
"go.dyndns.org",
"gotdns.com",
"gotdns.org",
"groks-the.info",
"groks-this.info",
"ham-radio-op.net",
"here-for-more.info",
"hobby-site.com",
"hobby-site.org",
"home.dyndns.org",
"homedns.org",
"homeftp.net",
"homeftp.org",
"homeip.net",
"homelinux.com",
"homelinux.net",
"homelinux.org",
"homeunix.com",
"homeunix.net",
"homeunix.org",
"iamallama.com",
"in-the-band.net",
"is-a-anarchist.com",
"is-a-blogger.com",
"is-a-bookkeeper.com",
"is-a-bruinsfan.org",
"is-a-bulls-fan.com",
"is-a-candidate.org",
"is-a-caterer.com",
"is-a-celticsfan.org",
"is-a-chef.com",
"is-a-chef.net",
"is-a-chef.org",
"is-a-conservative.com",
"is-a-cpa.com",
"is-a-cubicle-slave.com",
"is-a-democrat.com",
"is-a-designer.com",
"is-a-doctor.com",
"is-a-financialadvisor.com",
"is-a-geek.com",
"is-a-geek.net",
"is-a-geek.org",
"is-a-green.com",
"is-a-guru.com",
"is-a-hard-worker.com",
"is-a-hunter.com",
"is-a-knight.org",
"is-a-landscaper.com",
"is-a-lawyer.com",
"is-a-liberal.com",
"is-a-libertarian.com",
"is-a-linux-user.org",
"is-a-llama.com",
"is-a-musician.com",
"is-a-nascarfan.com",
"is-a-nurse.com",
"is-a-painter.com",
"is-a-patsfan.org",
"is-a-personaltrainer.com",
"is-a-photographer.com",
"is-a-player.com",
"is-a-republican.com",
"is-a-rockstar.com",
"is-a-socialist.com",
"is-a-soxfan.org",
"is-a-student.com",
"is-a-teacher.com",
"is-a-techie.com",
"is-a-therapist.com",
"is-an-accountant.com",
"is-an-actor.com",
"is-an-actress.com",
"is-an-anarchist.com",
"is-an-artist.com",
"is-an-engineer.com",
"is-an-entertainer.com",
"is-by.us",
"is-certified.com",
"is-found.org",
"is-gone.com",
"is-into-anime.com",
"is-into-cars.com",
"is-into-cartoons.com",
"is-into-games.com",
"is-leet.com",
"is-lost.org",
"is-not-certified.com",
"is-saved.org",
"is-slick.com",
"is-uberleet.com",
"is-very-bad.org",
"is-very-evil.org",
"is-very-good.org",
"is-very-nice.org",
"is-very-sweet.org",
"is-with-theband.com",
"isa-geek.com",
"isa-geek.net",
"isa-geek.org",
"isa-hockeynut.com",
"issmarterthanyou.com",
"isteingeek.de",
"istmein.de",
"kicks-ass.net",
"kicks-ass.org",
"knowsitall.info",
"land-4-sale.us",
"lebtimnetz.de",
"leitungsen.de",
"likes-pie.com",
"likescandy.com",
"merseine.nu",
"mine.nu",
"misconfused.org",
"mypets.ws",
"myphotos.cc",
"neat-url.com",
"office-on-the.net",
"on-the-web.tv",
"podzone.net",
"podzone.org",
"readmyblog.org",
"saves-the-whales.com",
"scrapper-site.net",
"scrapping.cc",
"selfip.biz",
"selfip.com",
"selfip.info",
"selfip.net",
"selfip.org",
"sells-for-less.com",
"sells-for-u.com",
"sells-it.net",
"sellsyourhome.org",
"servebbs.com",
"servebbs.net",
"servebbs.org",
"serveftp.net",
"serveftp.org",
"servegame.org",
"shacknet.nu",
"simple-url.com",
"space-to-rent.com",
"stuff-4-sale.org",
"stuff-4-sale.us",
"teaches-yoga.com",
"thruhere.net",
"traeumtgerade.de",
"webhop.biz",
"webhop.info",
"webhop.net",
"webhop.org",
"worse-than.tv",
"writesthisblog.com",
"ddnss.de",
"dyn.ddnss.de",
"dyndns.ddnss.de",
"dyndns1.de",
"dyn-ip24.de",
"home-webserver.de",
"dyn.home-webserver.de",
"myhome-server.de",
"ddnss.org",
"definima.net",
"definima.io",
"bci.dnstrace.pro",
"ddnsfree.com",
"ddnsgeek.com",
"giize.com",
"gleeze.com",
"kozow.com",
"loseyourip.com",
"ooguy.com",
"theworkpc.com",
"casacam.net",
"dynu.net",
"accesscam.org",
"camdvr.org",
"freeddns.org",
"mywire.org",
"webredirect.org",
"myddns.rocks",
"blogsite.xyz",
"dynv6.net",
"e4.cz",
"en-root.fr",
"mytuleap.com",
"onred.one",
"staging.onred.one",
"enonic.io",
"customer.enonic.io",
"eu.org",
"al.eu.org",
"asso.eu.org",
"at.eu.org",
"au.eu.org",
"be.eu.org",
"bg.eu.org",
"ca.eu.org",
"cd.eu.org",
"ch.eu.org",
"cn.eu.org",
"cy.eu.org",
"cz.eu.org",
"de.eu.org",
"dk.eu.org",
"edu.eu.org",
"ee.eu.org",
"es.eu.org",
"fi.eu.org",
"fr.eu.org",
"gr.eu.org",
"hr.eu.org",
"hu.eu.org",
"ie.eu.org",
"il.eu.org",
"in.eu.org",
"int.eu.org",
"is.eu.org",
"it.eu.org",
"jp.eu.org",
"kr.eu.org",
"lt.eu.org",
"lu.eu.org",
"lv.eu.org",
"mc.eu.org",
"me.eu.org",
"mk.eu.org",
"mt.eu.org",
"my.eu.org",
"net.eu.org",
"ng.eu.org",
"nl.eu.org",
"no.eu.org",
"nz.eu.org",
"paris.eu.org",
"pl.eu.org",
"pt.eu.org",
"q-a.eu.org",
"ro.eu.org",
"ru.eu.org",
"se.eu.org",
"si.eu.org",
"sk.eu.org",
"tr.eu.org",
"uk.eu.org",
"us.eu.org",
"eu-1.evennode.com",
"eu-2.evennode.com",
"eu-3.evennode.com",
"eu-4.evennode.com",
"us-1.evennode.com",
"us-2.evennode.com",
"us-3.evennode.com",
"us-4.evennode.com",
"twmail.cc",
"twmail.net",
"twmail.org",
"mymailer.com.tw",
"url.tw",
"apps.fbsbx.com",
"ru.net",
"adygeya.ru",
"bashkiria.ru",
"bir.ru",
"cbg.ru",
"com.ru",
"dagestan.ru",
"grozny.ru",
"kalmykia.ru",
"kustanai.ru",
"marine.ru",
"mordovia.ru",
"msk.ru",
"mytis.ru",
"nalchik.ru",
"nov.ru",
"pyatigorsk.ru",
"spb.ru",
"vladikavkaz.ru",
"vladimir.ru",
"abkhazia.su",
"adygeya.su",
"aktyubinsk.su",
"arkhangelsk.su",
"armenia.su",
"ashgabad.su",
"azerbaijan.su",
"balashov.su",
"bashkiria.su",
"bryansk.su",
"bukhara.su",
"chimkent.su",
"dagestan.su",
"east-kazakhstan.su",
"exnet.su",
"georgia.su",
"grozny.su",
"ivanovo.su",
"jambyl.su",
"kalmykia.su",
"kaluga.su",
"karacol.su",
"karaganda.su",
"karelia.su",
"khakassia.su",
"krasnodar.su",
"kurgan.su",
"kustanai.su",
"lenug.su",
"mangyshlak.su",
"mordovia.su",
"msk.su",
"murmansk.su",
"nalchik.su",
"navoi.su",
"north-kazakhstan.su",
"nov.su",
"obninsk.su",
"penza.su",
"pokrovsk.su",
"sochi.su",
"spb.su",
"tashkent.su",
"termez.su",
"togliatti.su",
"troitsk.su",
"tselinograd.su",
"tula.su",
"tuva.su",
"vladikavkaz.su",
"vladimir.su",
"vologda.su",
"channelsdvr.net",
"u.channelsdvr.net",
"fastly-terrarium.com",
"fastlylb.net",
"map.fastlylb.net",
"freetls.fastly.net",
"map.fastly.net",
"a.prod.fastly.net",
"global.prod.fastly.net",
"a.ssl.fastly.net",
"b.ssl.fastly.net",
"global.ssl.fastly.net",
"fastpanel.direct",
"fastvps-server.com",
"fhapp.xyz",
"fedorainfracloud.org",
"fedorapeople.org",
"cloud.fedoraproject.org",
"app.os.fedoraproject.org",
"app.os.stg.fedoraproject.org",
"mydobiss.com",
"filegear.me",
"filegear-au.me",
"filegear-de.me",
"filegear-gb.me",
"filegear-ie.me",
"filegear-jp.me",
"filegear-sg.me",
"firebaseapp.com",
"flynnhub.com",
"flynnhosting.net",
"0e.vc",
"freebox-os.com",
"freeboxos.com",
"fbx-os.fr",
"fbxos.fr",
"freebox-os.fr",
"freeboxos.fr",
"freedesktop.org",
"*.futurecms.at",
"*.ex.futurecms.at",
"*.in.futurecms.at",
"futurehosting.at",
"futuremailing.at",
"*.ex.ortsinfo.at",
"*.kunden.ortsinfo.at",
"*.statics.cloud",
"service.gov.uk",
"gehirn.ne.jp",
"usercontent.jp",
"gentapps.com",
"lab.ms",
"github.io",
"githubusercontent.com",
"gitlab.io",
"glitch.me",
"lolipop.io",
"cloudapps.digital",
"london.cloudapps.digital",
"homeoffice.gov.uk",
"ro.im",
"shop.ro",
"goip.de",
"run.app",
"a.run.app",
"web.app",
"*.0emm.com",
"appspot.com",
"*.r.appspot.com",
"blogspot.ae",
"blogspot.al",
"blogspot.am",
"blogspot.ba",
"blogspot.be",
"blogspot.bg",
"blogspot.bj",
"blogspot.ca",
"blogspot.cf",
"blogspot.ch",
"blogspot.cl",
"blogspot.co.at",
"blogspot.co.id",
"blogspot.co.il",
"blogspot.co.ke",
"blogspot.co.nz",
"blogspot.co.uk",
"blogspot.co.za",
"blogspot.com",
"blogspot.com.ar",
"blogspot.com.au",
"blogspot.com.br",
"blogspot.com.by",
"blogspot.com.co",
"blogspot.com.cy",
"blogspot.com.ee",
"blogspot.com.eg",
"blogspot.com.es",
"blogspot.com.mt",
"blogspot.com.ng",
"blogspot.com.tr",
"blogspot.com.uy",
"blogspot.cv",
"blogspot.cz",
"blogspot.de",
"blogspot.dk",
"blogspot.fi",
"blogspot.fr",
"blogspot.gr",
"blogspot.hk",
"blogspot.hr",
"blogspot.hu",
"blogspot.ie",
"blogspot.in",
"blogspot.is",
"blogspot.it",
"blogspot.jp",
"blogspot.kr",
"blogspot.li",
"blogspot.lt",
"blogspot.lu",
"blogspot.md",
"blogspot.mk",
"blogspot.mr",
"blogspot.mx",
"blogspot.my",
"blogspot.nl",
"blogspot.no",
"blogspot.pe",
"blogspot.pt",
"blogspot.qa",
"blogspot.re",
"blogspot.ro",
"blogspot.rs",
"blogspot.ru",
"blogspot.se",
"blogspot.sg",
"blogspot.si",
"blogspot.sk",
"blogspot.sn",
"blogspot.td",
"blogspot.tw",
"blogspot.ug",
"blogspot.vn",
"cloudfunctions.net",
"cloud.goog",
"codespot.com",
"googleapis.com",
"googlecode.com",
"pagespeedmobilizer.com",
"publishproxy.com",
"withgoogle.com",
"withyoutube.com",
"awsmppl.com",
"fin.ci",
"free.hr",
"caa.li",
"ua.rs",
"conf.se",
"hs.zone",
"hs.run",
"hashbang.sh",
"hasura.app",
"hasura-app.io",
"hepforge.org",
"herokuapp.com",
"herokussl.com",
"myravendb.com",
"ravendb.community",
"ravendb.me",
"development.run",
"ravendb.run",
"bpl.biz",
"orx.biz",
"ng.city",
"biz.gl",
"ng.ink",
"col.ng",
"firm.ng",
"gen.ng",
"ltd.ng",
"ngo.ng",
"ng.school",
"sch.so",
"häkkinen.fi",
"*.moonscale.io",
"moonscale.net",
"iki.fi",
"dyn-berlin.de",
"in-berlin.de",
"in-brb.de",
"in-butter.de",
"in-dsl.de",
"in-dsl.net",
"in-dsl.org",
"in-vpn.de",
"in-vpn.net",
"in-vpn.org",
"biz.at",
"info.at",
"info.cx",
"ac.leg.br",
"al.leg.br",
"am.leg.br",
"ap.leg.br",
"ba.leg.br",
"ce.leg.br",
"df.leg.br",
"es.leg.br",
"go.leg.br",
"ma.leg.br",
"mg.leg.br",
"ms.leg.br",
"mt.leg.br",
"pa.leg.br",
"pb.leg.br",
"pe.leg.br",
"pi.leg.br",
"pr.leg.br",
"rj.leg.br",
"rn.leg.br",
"ro.leg.br",
"rr.leg.br",
"rs.leg.br",
"sc.leg.br",
"se.leg.br",
"sp.leg.br",
"to.leg.br",
"pixolino.com",
"ipifony.net",
"mein-iserv.de",
"test-iserv.de",
"iserv.dev",
"iobb.net",
"myjino.ru",
"*.hosting.myjino.ru",
"*.landing.myjino.ru",
"*.spectrum.myjino.ru",
"*.vps.myjino.ru",
"*.triton.zone",
"*.cns.joyent.com",
"js.org",
"kaas.gg",
"khplay.nl",
"keymachine.de",
"kinghost.net",
"uni5.net",
"knightpoint.systems",
"oya.to",
"co.krd",
"edu.krd",
"git-repos.de",
"lcube-server.de",
"svn-repos.de",
"leadpages.co",
"lpages.co",
"lpusercontent.com",
"lelux.site",
"co.business",
"co.education",
"co.events",
"co.financial",
"co.network",
"co.place",
"co.technology",
"app.lmpm.com",
"linkitools.space",
"linkyard.cloud",
"linkyard-cloud.ch",
"members.linode.com",
"nodebalancer.linode.com",
"we.bs",
"loginline.app",
"loginline.dev",
"loginline.io",
"loginline.services",
"loginline.site",
"krasnik.pl",
"leczna.pl",
"lubartow.pl",
"lublin.pl",
"poniatowa.pl",
"swidnik.pl",
"uklugs.org",
"glug.org.uk",
"lug.org.uk",
"lugs.org.uk",
"barsy.bg",
"barsy.co.uk",
"barsyonline.co.uk",
"barsycenter.com",
"barsyonline.com",
"barsy.club",
"barsy.de",
"barsy.eu",
"barsy.in",
"barsy.info",
"barsy.io",
"barsy.me",
"barsy.menu",
"barsy.mobi",
"barsy.net",
"barsy.online",
"barsy.org",
"barsy.pro",
"barsy.pub",
"barsy.shop",
"barsy.site",
"barsy.support",
"barsy.uk",
"*.magentosite.cloud",
"mayfirst.info",
"mayfirst.org",
"hb.cldmail.ru",
"miniserver.com",
"memset.net",
"cloud.metacentrum.cz",
"custom.metacentrum.cz",
"flt.cloud.muni.cz",
"usr.cloud.muni.cz",
"meteorapp.com",
"eu.meteorapp.com",
"co.pl",
"azurecontainer.io",
"azurewebsites.net",
"azure-mobile.net",
"cloudapp.net",
"mozilla-iot.org",
"bmoattachments.org",
"net.ru",
"org.ru",
"pp.ru",
"ui.nabu.casa",
"pony.club",
"of.fashion",
"on.fashion",
"of.football",
"in.london",
"of.london",
"for.men",
"and.mom",
"for.mom",
"for.one",
"for.sale",
"of.work",
"to.work",
"nctu.me",
"bitballoon.com",
"netlify.com",
"4u.com",
"ngrok.io",
"nh-serv.co.uk",
"nfshost.com",
"dnsking.ch",
"mypi.co",
"n4t.co",
"001www.com",
"ddnslive.com",
"myiphost.com",
"forumz.info",
"16-b.it",
"32-b.it",
"64-b.it",
"soundcast.me",
"tcp4.me",
"dnsup.net",
"hicam.net",
"now-dns.net",
"ownip.net",
"vpndns.net",
"dynserv.org",
"now-dns.org",
"x443.pw",
"now-dns.top",
"ntdll.top",
"freeddns.us",
"crafting.xyz",
"zapto.xyz",
"nsupdate.info",
"nerdpol.ovh",
"blogsyte.com",
"brasilia.me",
"cable-modem.org",
"ciscofreak.com",
"collegefan.org",
"couchpotatofries.org",
"damnserver.com",
"ddns.me",
"ditchyourip.com",
"dnsfor.me",
"dnsiskinky.com",
"dvrcam.info",
"dynns.com",
"eating-organic.net",
"fantasyleague.cc",
"geekgalaxy.com",
"golffan.us",
"health-carereform.com",
"homesecuritymac.com",
"homesecuritypc.com",
"hopto.me",
"ilovecollege.info",
"loginto.me",
"mlbfan.org",
"mmafan.biz",
"myactivedirectory.com",
"mydissent.net",
"myeffect.net",
"mymediapc.net",
"mypsx.net",
"mysecuritycamera.com",
"mysecuritycamera.net",
"mysecuritycamera.org",
"net-freaks.com",
"nflfan.org",
"nhlfan.net",
"no-ip.ca",
"no-ip.co.uk",
"no-ip.net",
"noip.us",
"onthewifi.com",
"pgafan.net",
"point2this.com",
"pointto.us",
"privatizehealthinsurance.net",
"quicksytes.com",
"read-books.org",
"securitytactics.com",
"serveexchange.com",
"servehumour.com",
"servep2p.com",
"servesarcasm.com",
"stufftoread.com",
"ufcfan.org",
"unusualperson.com",
"workisboring.com",
"3utilities.com",
"bounceme.net",
"ddns.net",
"ddnsking.com",
"gotdns.ch",
"hopto.org",
"myftp.biz",
"myftp.org",
"myvnc.com",
"no-ip.biz",
"no-ip.info",
"no-ip.org",
"noip.me",
"redirectme.net",
"servebeer.com",
"serveblog.net",
"servecounterstrike.com",
"serveftp.com",
"servegame.com",
"servehalflife.com",
"servehttp.com",
"serveirc.com",
"serveminecraft.net",
"servemp3.com",
"servepics.com",
"servequake.com",
"sytes.net",
"webhop.me",
"zapto.org",
"stage.nodeart.io",
"nodum.co",
"nodum.io",
"pcloud.host",
"nyc.mn",
"nom.ae",
"nom.af",
"nom.ai",
"nom.al",
"nym.by",
"nom.bz",
"nym.bz",
"nom.cl",
"nym.ec",
"nom.gd",
"nom.ge",
"nom.gl",
"nym.gr",
"nom.gt",
"nym.gy",
"nym.hk",
"nom.hn",
"nym.ie",
"nom.im",
"nom.ke",
"nym.kz",
"nym.la",
"nym.lc",
"nom.li",
"nym.li",
"nym.lt",
"nym.lu",
"nom.lv",
"nym.me",
"nom.mk",
"nym.mn",
"nym.mx",
"nom.nu",
"nym.nz",
"nym.pe",
"nym.pt",
"nom.pw",
"nom.qa",
"nym.ro",
"nom.rs",
"nom.si",
"nym.sk",
"nom.st",
"nym.su",
"nym.sx",
"nom.tj",
"nym.tw",
"nom.ug",
"nom.uy",
"nom.vc",
"nom.vg",
"static.observableusercontent.com",
"cya.gg",
"cloudycluster.net",
"nid.io",
"opencraft.hosting",
"operaunite.com",
"skygearapp.com",
"outsystemscloud.com",
"ownprovider.com",
"own.pm",
"ox.rs",
"oy.lc",
"pgfog.com",
"pagefrontapp.com",
"art.pl",
"gliwice.pl",
"krakow.pl",
"poznan.pl",
"wroc.pl",
"zakopane.pl",
"pantheonsite.io",
"gotpantheon.com",
"mypep.link",
"perspecta.cloud",
"on-web.fr",
"*.platform.sh",
"*.platformsh.site",
"dyn53.io",
"co.bn",
"xen.prgmr.com",
"priv.at",
"prvcy.page",
"*.dweb.link",
"protonet.io",
"chirurgiens-dentistes-en-france.fr",
"byen.site",
"pubtls.org",
"qualifioapp.com",
"qbuser.com",
"instantcloud.cn",
"ras.ru",
"qa2.com",
"qcx.io",
"*.sys.qcx.io",
"dev-myqnapcloud.com",
"alpha-myqnapcloud.com",
"myqnapcloud.com",
"*.quipelements.com",
"vapor.cloud",
"vaporcloud.io",
"rackmaze.com",
"rackmaze.net",
"*.on-k3s.io",
"*.on-rancher.cloud",
"*.on-rio.io",
"readthedocs.io",
"rhcloud.com",
"app.render.com",
"onrender.com",
"repl.co",
"repl.run",
"resindevice.io",
"devices.resinstaging.io",
"hzc.io",
"wellbeingzone.eu",
"ptplus.fit",
"wellbeingzone.co.uk",
"git-pages.rit.edu",
"sandcats.io",
"logoip.de",
"logoip.com",
"schokokeks.net",
"gov.scot",
"scrysec.com",
"firewall-gateway.com",
"firewall-gateway.de",
"my-gateway.de",
"my-router.de",
"spdns.de",
"spdns.eu",
"firewall-gateway.net",
"my-firewall.org",
"myfirewall.org",
"spdns.org",
"senseering.net",
"biz.ua",
"co.ua",
"pp.ua",
"shiftedit.io",
"myshopblocks.com",
"shopitsite.com",
"mo-siemens.io",
"1kapp.com",
"appchizi.com",
"applinzi.com",
"sinaapp.com",
"vipsinaapp.com",
"siteleaf.net",
"bounty-full.com",
"alpha.bounty-full.com",
"beta.bounty-full.com",
"stackhero-network.com",
"static.land",
"dev.static.land",
"sites.static.land",
"apps.lair.io",
"*.stolos.io",
"spacekit.io",
"customer.speedpartner.de",
"api.stdlib.com",
"storj.farm",
"utwente.io",
"soc.srcf.net",
"user.srcf.net",
"temp-dns.com",
"applicationcloud.io",
"scapp.io",
"*.s5y.io",
"*.sensiosite.cloud",
"syncloud.it",
"diskstation.me",
"dscloud.biz",
"dscloud.me",
"dscloud.mobi",
"dsmynas.com",
"dsmynas.net",
"dsmynas.org",
"familyds.com",
"familyds.net",
"familyds.org",
"i234.me",
"myds.me",
"synology.me",
"vpnplus.to",
"direct.quickconnect.to",
"taifun-dns.de",
"gda.pl",
"gdansk.pl",
"gdynia.pl",
"med.pl",
"sopot.pl",
"edugit.org",
"telebit.app",
"telebit.io",
"*.telebit.xyz",
"gwiddle.co.uk",
"thingdustdata.com",
"cust.dev.thingdust.io",
"cust.disrec.thingdust.io",
"cust.prod.thingdust.io",
"cust.testing.thingdust.io",
"arvo.network",
"azimuth.network",
"bloxcms.com",
"townnews-staging.com",
"12hp.at",
"2ix.at",
"4lima.at",
"lima-city.at",
"12hp.ch",
"2ix.ch",
"4lima.ch",
"lima-city.ch",
"trafficplex.cloud",
"de.cool",
"12hp.de",
"2ix.de",
"4lima.de",
"lima-city.de",
"1337.pictures",
"clan.rip",
"lima-city.rocks",
"webspace.rocks",
"lima.zone",
"*.transurl.be",
"*.transurl.eu",
"*.transurl.nl",
"tuxfamily.org",
"dd-dns.de",
"diskstation.eu",
"diskstation.org",
"dray-dns.de",
"draydns.de",
"dyn-vpn.de",
"dynvpn.de",
"mein-vigor.de",
"my-vigor.de",
"my-wan.de",
"syno-ds.de",
"synology-diskstation.de",
"synology-ds.de",
"uber.space",
"*.uberspace.de",
"hk.com",
"hk.org",
"ltd.hk",
"inc.hk",
"virtualuser.de",
"virtual-user.de",
"urown.cloud",
"dnsupdate.info",
"lib.de.us",
"2038.io",
"router.management",
"v-info.info",
"voorloper.cloud",
"v.ua",
"wafflecell.com",
"*.webhare.dev",
"wedeploy.io",
"wedeploy.me",
"wedeploy.sh",
"remotewd.com",
"wmflabs.org",
"myforum.community",
"community-pro.de",
"diskussionsbereich.de",
"community-pro.net",
"meinforum.net",
"half.host",
"xnbay.com",
"u2.xnbay.com",
"u2-local.xnbay.com",
"cistron.nl",
"demon.nl",
"xs4all.space",
"yandexcloud.net",
"storage.yandexcloud.net",
"website.yandexcloud.net",
"official.academy",
"yolasite.com",
"ybo.faith",
"yombo.me",
"homelink.one",
"ybo.party",
"ybo.review",
"ybo.science",
"ybo.trade",
"nohost.me",
"noho.st",
"za.net",
"za.org",
"now.sh",
"bss.design",
"basicserver.io",
"virtualserver.io",
"enterprisecloud.nu"
]
},{}],2:[function(require,module,exports){
/*eslint no-var:0, prefer-arrow-callback: 0, object-shorthand: 0 */
'use strict';
var Punycode = require('punycode');
var internals = {};
//
// Read rules from file.
//
internals.rules = require('./data/rules.json').map(function (rule) {
return {
rule: rule,
suffix: rule.replace(/^(\*\.|\!)/, ''),
punySuffix: -1,
wildcard: rule.charAt(0) === '*',
exception: rule.charAt(0) === '!'
};
});
//
// Check is given string ends with `suffix`.
//
internals.endsWith = function (str, suffix) {
return str.indexOf(suffix, str.length - suffix.length) !== -1;
};
//
// Find rule for a given domain.
//
internals.findRule = function (domain) {
var punyDomain = Punycode.toASCII(domain);
return internals.rules.reduce(function (memo, rule) {
if (rule.punySuffix === -1){
rule.punySuffix = Punycode.toASCII(rule.suffix);
}
if (!internals.endsWith(punyDomain, '.' + rule.punySuffix) && punyDomain !== rule.punySuffix) {
return memo;
}
// This has been commented out as it never seems to run. This is because
// sub tlds always appear after their parents and we never find a shorter
// match.
//if (memo) {
// var memoSuffix = Punycode.toASCII(memo.suffix);
// if (memoSuffix.length >= punySuffix.length) {
// return memo;
// }
//}
return rule;
}, null);
};
//
// Error codes and messages.
//
exports.errorCodes = {
DOMAIN_TOO_SHORT: 'Domain name too short.',
DOMAIN_TOO_LONG: 'Domain name too long. It should be no more than 255 chars.',
LABEL_STARTS_WITH_DASH: 'Domain name label can not start with a dash.',
LABEL_ENDS_WITH_DASH: 'Domain name label can not end with a dash.',
LABEL_TOO_LONG: 'Domain name label should be at most 63 chars long.',
LABEL_TOO_SHORT: 'Domain name label should be at least 1 character long.',
LABEL_INVALID_CHARS: 'Domain name label can only contain alphanumeric characters or dashes.'
};
//
// Validate domain name and throw if not valid.
//
// From wikipedia:
//
// Hostnames are composed of series of labels concatenated with dots, as are all
// domain names. Each label must be between 1 and 63 characters long, and the
// entire hostname (including the delimiting dots) has a maximum of 255 chars.
//
// Allowed chars:
//
// * `a-z`
// * `0-9`
// * `-` but not as a starting or ending character
// * `.` as a separator for the textual portions of a domain name
//
// * http://en.wikipedia.org/wiki/Domain_name
// * http://en.wikipedia.org/wiki/Hostname
//
internals.validate = function (input) {
// Before we can validate we need to take care of IDNs with unicode chars.
var ascii = Punycode.toASCII(input);
if (ascii.length < 1) {
return 'DOMAIN_TOO_SHORT';
}
if (ascii.length > 255) {
return 'DOMAIN_TOO_LONG';
}
// Check each part's length and allowed chars.
var labels = ascii.split('.');
var label;
for (var i = 0; i < labels.length; ++i) {
label = labels[i];
if (!label.length) {
return 'LABEL_TOO_SHORT';
}
if (label.length > 63) {
return 'LABEL_TOO_LONG';
}
if (label.charAt(0) === '-') {
return 'LABEL_STARTS_WITH_DASH';
}
if (label.charAt(label.length - 1) === '-') {
return 'LABEL_ENDS_WITH_DASH';
}
if (!/^[a-z0-9\-]+$/.test(label)) {
return 'LABEL_INVALID_CHARS';
}
}
};
//
// Public API
//
//
// Parse domain.
//
exports.parse = function (input) {
if (typeof input !== 'string') {
throw new TypeError('Domain name must be a string.');
}
// Force domain to lowercase.
var domain = input.slice(0).toLowerCase();
// Handle FQDN.
// TODO: Simply remove trailing dot?
if (domain.charAt(domain.length - 1) === '.') {
domain = domain.slice(0, domain.length - 1);
}
// Validate and sanitise input.
var error = internals.validate(domain);
if (error) {
return {
input: input,
error: {
message: exports.errorCodes[error],
code: error
}
};
}
var parsed = {
input: input,
tld: null,
sld: null,
domain: null,
subdomain: null,
listed: false
};
var domainParts = domain.split('.');
// Non-Internet TLD
if (domainParts[domainParts.length - 1] === 'local') {
return parsed;
}
var handlePunycode = function () {
if (!/xn--/.test(domain)) {
return parsed;
}
if (parsed.domain) {
parsed.domain = Punycode.toASCII(parsed.domain);
}
if (parsed.subdomain) {
parsed.subdomain = Punycode.toASCII(parsed.subdomain);
}
return parsed;
};
var rule = internals.findRule(domain);
// Unlisted tld.
if (!rule) {
if (domainParts.length < 2) {
return parsed;
}
parsed.tld = domainParts.pop();
parsed.sld = domainParts.pop();
parsed.domain = [parsed.sld, parsed.tld].join('.');
if (domainParts.length) {
parsed.subdomain = domainParts.pop();
}
return handlePunycode();
}
// At this point we know the public suffix is listed.
parsed.listed = true;
var tldParts = rule.suffix.split('.');
var privateParts = domainParts.slice(0, domainParts.length - tldParts.length);
if (rule.exception) {
privateParts.push(tldParts.shift());
}
parsed.tld = tldParts.join('.');
if (!privateParts.length) {
return handlePunycode();
}
if (rule.wildcard) {
tldParts.unshift(privateParts.pop());
parsed.tld = tldParts.join('.');
}
if (!privateParts.length) {
return handlePunycode();
}
parsed.sld = privateParts.pop();
parsed.domain = [parsed.sld, parsed.tld].join('.');
if (privateParts.length) {
parsed.subdomain = privateParts.join('.');
}
return handlePunycode();
};
//
// Get domain.
//
exports.get = function (domain) {
if (!domain) {
return null;
}
return exports.parse(domain).domain || null;
};
//
// Check whether domain belongs to a known public suffix.
//
exports.isValid = function (domain) {
var parsed = exports.parse(domain);
return Boolean(parsed.domain && parsed.listed);
};
},{"./data/rules.json":1,"punycode":3}],3:[function(require,module,exports){
(function (global){
/*! https://mths.be/punycode v1.4.1 by @mathias */
;(function(root) {
/** Detect free variables */
var freeExports = typeof exports == 'object' && exports &&
!exports.nodeType && exports;
var freeModule = typeof module == 'object' && module &&
!module.nodeType && module;
var freeGlobal = typeof global == 'object' && global;
if (
freeGlobal.global === freeGlobal ||
freeGlobal.window === freeGlobal ||
freeGlobal.self === freeGlobal
) {
root = freeGlobal;
}
/**
* The `punycode` object.
* @name punycode
* @type Object
*/
var punycode,
/** Highest positive signed 32-bit float value */
maxInt = 2147483647, // aka. 0x7FFFFFFF or 2^31-1
/** Bootstring parameters */
base = 36,
tMin = 1,
tMax = 26,
skew = 38,
damp = 700,
initialBias = 72,
initialN = 128, // 0x80
delimiter = '-', // '\x2D'
/** Regular expressions */
regexPunycode = /^xn--/,
regexNonASCII = /[^\x20-\x7E]/, // unprintable ASCII chars + non-ASCII chars
regexSeparators = /[\x2E\u3002\uFF0E\uFF61]/g, // RFC 3490 separators
/** Error messages */
errors = {
'overflow': 'Overflow: input needs wider integers to process',
'not-basic': 'Illegal input >= 0x80 (not a basic code point)',
'invalid-input': 'Invalid input'
},
/** Convenience shortcuts */
baseMinusTMin = base - tMin,
floor = Math.floor,
stringFromCharCode = String.fromCharCode,
/** Temporary variable */
key;
/*--------------------------------------------------------------------------*/
/**
* A generic error utility function.
* @private
* @param {String} type The error type.
* @returns {Error} Throws a `RangeError` with the applicable error message.
*/
function error(type) {
throw new RangeError(errors[type]);
}
/**
* A generic `Array#map` utility function.
* @private
* @param {Array} array The array to iterate over.
* @param {Function} callback The function that gets called for every array
* item.
* @returns {Array} A new array of values returned by the callback function.
*/
function map(array, fn) {
var length = array.length;
var result = [];
while (length--) {
result[length] = fn(array[length]);
}
return result;
}
/**
* A simple `Array#map`-like wrapper to work with domain name strings or email
* addresses.
* @private
* @param {String} domain The domain name or email address.
* @param {Function} callback The function that gets called for every
* character.
* @returns {Array} A new string of characters returned by the callback
* function.
*/
function mapDomain(string, fn) {
var parts = string.split('@');
var result = '';
if (parts.length > 1) {
// In email addresses, only the domain name should be punycoded. Leave
// the local part (i.e. everything up to `@`) intact.
result = parts[0] + '@';
string = parts[1];
}
// Avoid `split(regex)` for IE8 compatibility. See #17.
string = string.replace(regexSeparators, '\x2E');
var labels = string.split('.');
var encoded = map(labels, fn).join('.');
return result + encoded;
}
/**
* Creates an array containing the numeric code points of each Unicode
* character in the string. While JavaScript uses UCS-2 internally,
* this function will convert a pair of surrogate halves (each of which
* UCS-2 exposes as separate characters) into a single code point,
* matching UTF-16.
* @see `punycode.ucs2.encode`
* @see <https://mathiasbynens.be/notes/javascript-encoding>
* @memberOf punycode.ucs2
* @name decode
* @param {String} string The Unicode input string (UCS-2).
* @returns {Array} The new array of code points.
*/
function ucs2decode(string) {
var output = [],
counter = 0,
length = string.length,
value,
extra;
while (counter < length) {
value = string.charCodeAt(counter++);
if (value >= 0xD800 && value <= 0xDBFF && counter < length) {
// high surrogate, and there is a next character
extra = string.charCodeAt(counter++);
if ((extra & 0xFC00) == 0xDC00) { // low surrogate
output.push(((value & 0x3FF) << 10) + (extra & 0x3FF) + 0x10000);
} else {
// unmatched surrogate; only append this code unit, in case the next
// code unit is the high surrogate of a surrogate pair
output.push(value);
counter--;
}
} else {
output.push(value);
}
}
return output;
}
/**
* Creates a string based on an array of numeric code points.
* @see `punycode.ucs2.decode`
* @memberOf punycode.ucs2
* @name encode
* @param {Array} codePoints The array of numeric code points.
* @returns {String} The new Unicode string (UCS-2).
*/
function ucs2encode(array) {
return map(array, function(value) {
var output = '';
if (value > 0xFFFF) {
value -= 0x10000;
output += stringFromCharCode(value >>> 10 & 0x3FF | 0xD800);
value = 0xDC00 | value & 0x3FF;
}
output += stringFromCharCode(value);
return output;
}).join('');
}
/**
* Converts a basic code point into a digit/integer.
* @see `digitToBasic()`
* @private
* @param {Number} codePoint The basic numeric code point value.
* @returns {Number} The numeric value of a basic code point (for use in
* representing integers) in the range `0` to `base - 1`, or `base` if
* the code point does not represent a value.
*/
function basicToDigit(codePoint) {
if (codePoint - 48 < 10) {
return codePoint - 22;
}
if (codePoint - 65 < 26) {
return codePoint - 65;
}
if (codePoint - 97 < 26) {
return codePoint - 97;
}
return base;
}
/**
* Converts a digit/integer into a basic code point.
* @see `basicToDigit()`
* @private
* @param {Number} digit The numeric value of a basic code point.
* @returns {Number} The basic code point whose value (when used for
* representing integers) is `digit`, which needs to be in the range
* `0` to `base - 1`. If `flag` is non-zero, the uppercase form is
* used; else, the lowercase form is used. The behavior is undefined
* if `flag` is non-zero and `digit` has no uppercase form.
*/
function digitToBasic(digit, flag) {
// 0..25 map to ASCII a..z or A..Z
// 26..35 map to ASCII 0..9
return digit + 22 + 75 * (digit < 26) - ((flag != 0) << 5);
}
/**
* Bias adaptation function as per section 3.4 of RFC 3492.
* https://tools.ietf.org/html/rfc3492#section-3.4
* @private
*/
function adapt(delta, numPoints, firstTime) {
var k = 0;
delta = firstTime ? floor(delta / damp) : delta >> 1;
delta += floor(delta / numPoints);
for (/* no initialization */; delta > baseMinusTMin * tMax >> 1; k += base) {
delta = floor(delta / baseMinusTMin);
}
return floor(k + (baseMinusTMin + 1) * delta / (delta + skew));
}
/**
* Converts a Punycode string of ASCII-only symbols to a string of Unicode
* symbols.
* @memberOf punycode
* @param {String} input The Punycode string of ASCII-only symbols.
* @returns {String} The resulting string of Unicode symbols.
*/
function decode(input) {
// Don't use UCS-2
var output = [],
inputLength = input.length,
out,
i = 0,
n = initialN,
bias = initialBias,
basic,
j,
index,
oldi,
w,
k,
digit,
t,
/** Cached calculation results */
baseMinusT;
// Handle the basic code points: let `basic` be the number of input code
// points before the last delimiter, or `0` if there is none, then copy
// the first basic code points to the output.
basic = input.lastIndexOf(delimiter);
if (basic < 0) {
basic = 0;
}
for (j = 0; j < basic; ++j) {
// if it's not a basic code point
if (input.charCodeAt(j) >= 0x80) {
error('not-basic');
}
output.push(input.charCodeAt(j));
}
// Main decoding loop: start just after the last delimiter if any basic code
// points were copied; start at the beginning otherwise.
for (index = basic > 0 ? basic + 1 : 0; index < inputLength; /* no final expression */) {
// `index` is the index of the next character to be consumed.
// Decode a generalized variable-length integer into `delta`,
// which gets added to `i`. The overflow checking is easier
// if we increase `i` as we go, then subtract off its starting
// value at the end to obtain `delta`.
for (oldi = i, w = 1, k = base; /* no condition */; k += base) {
if (index >= inputLength) {
error('invalid-input');
}
digit = basicToDigit(input.charCodeAt(index++));
if (digit >= base || digit > floor((maxInt - i) / w)) {
error('overflow');
}
i += digit * w;
t = k <= bias ? tMin : (k >= bias + tMax ? tMax : k - bias);
if (digit < t) {
break;
}
baseMinusT = base - t;
if (w > floor(maxInt / baseMinusT)) {
error('overflow');
}
w *= baseMinusT;
}
out = output.length + 1;
bias = adapt(i - oldi, out, oldi == 0);
// `i` was supposed to wrap around from `out` to `0`,
// incrementing `n` each time, so we'll fix that now:
if (floor(i / out) > maxInt - n) {
error('overflow');
}
n += floor(i / out);
i %= out;
// Insert `n` at position `i` of the output
output.splice(i++, 0, n);
}
return ucs2encode(output);
}
/**
* Converts a string of Unicode symbols (e.g. a domain name label) to a
* Punycode string of ASCII-only symbols.
* @memberOf punycode
* @param {String} input The string of Unicode symbols.
* @returns {String} The resulting Punycode string of ASCII-only symbols.
*/
function encode(input) {
var n,
delta,
handledCPCount,
basicLength,
bias,
j,
m,
q,
k,
t,
currentValue,
output = [],
/** `inputLength` will hold the number of code points in `input`. */
inputLength,
/** Cached calculation results */
handledCPCountPlusOne,
baseMinusT,
qMinusT;
// Convert the input in UCS-2 to Unicode
input = ucs2decode(input);
// Cache the length
inputLength = input.length;
// Initialize the state
n = initialN;
delta = 0;
bias = initialBias;
// Handle the basic code points
for (j = 0; j < inputLength; ++j) {
currentValue = input[j];
if (currentValue < 0x80) {
output.push(stringFromCharCode(currentValue));
}
}
handledCPCount = basicLength = output.length;
// `handledCPCount` is the number of code points that have been handled;
// `basicLength` is the number of basic code points.
// Finish the basic string - if it is not empty - with a delimiter
if (basicLength) {
output.push(delimiter);
}
// Main encoding loop:
while (handledCPCount < inputLength) {
// All non-basic code points < n have been handled already. Find the next
// larger one:
for (m = maxInt, j = 0; j < inputLength; ++j) {
currentValue = input[j];
if (currentValue >= n && currentValue < m) {
m = currentValue;
}
}
// Increase `delta` enough to advance the decoder's <n,i> state to <m,0>,
// but guard against overflow
handledCPCountPlusOne = handledCPCount + 1;
if (m - n > floor((maxInt - delta) / handledCPCountPlusOne)) {
error('overflow');
}
delta += (m - n) * handledCPCountPlusOne;
n = m;
for (j = 0; j < inputLength; ++j) {
currentValue = input[j];
if (currentValue < n && ++delta > maxInt) {
error('overflow');
}
if (currentValue == n) {
// Represent delta as a generalized variable-length integer
for (q = delta, k = base; /* no condition */; k += base) {
t = k <= bias ? tMin : (k >= bias + tMax ? tMax : k - bias);
if (q < t) {
break;
}
qMinusT = q - t;
baseMinusT = base - t;
output.push(
stringFromCharCode(digitToBasic(t + qMinusT % baseMinusT, 0))
);
q = floor(qMinusT / baseMinusT);
}
output.push(stringFromCharCode(digitToBasic(q, 0)));
bias = adapt(delta, handledCPCountPlusOne, handledCPCount == basicLength);
delta = 0;
++handledCPCount;
}
}
++delta;
++n;
}
return output.join('');
}
/**
* Converts a Punycode string representing a domain name or an email address
* to Unicode. Only the Punycoded parts of the input will be converted, i.e.
* it doesn't matter if you call it on a string that has already been
* converted to Unicode.
* @memberOf punycode
* @param {String} input The Punycoded domain name or email address to
* convert to Unicode.
* @returns {String} The Unicode representation of the given Punycode
* string.
*/
function toUnicode(input) {
return mapDomain(input, function(string) {
return regexPunycode.test(string)
? decode(string.slice(4).toLowerCase())
: string;
});
}
/**
* Converts a Unicode string representing a domain name or an email address to
* Punycode. Only the non-ASCII parts of the domain name will be converted,
* i.e. it doesn't matter if you call it with a domain that's already in
* ASCII.
* @memberOf punycode
* @param {String} input The domain name or email address to convert, as a
* Unicode string.
* @returns {String} The Punycode representation of the given domain name or
* email address.
*/
function toASCII(input) {
return mapDomain(input, function(string) {
return regexNonASCII.test(string)
? 'xn--' + encode(string)
: string;
});
}
/*--------------------------------------------------------------------------*/
/** Define the public API */
punycode = {
/**
* A string representing the current Punycode.js version number.
* @memberOf punycode
* @type String
*/
'version': '1.4.1',
/**
* An object of methods to convert from JavaScript's internal character
* representation (UCS-2) to Unicode code points, and back.
* @see <https://mathiasbynens.be/notes/javascript-encoding>
* @memberOf punycode
* @type Object
*/
'ucs2': {
'decode': ucs2decode,
'encode': ucs2encode
},
'decode': decode,
'encode': encode,
'toASCII': toASCII,
'toUnicode': toUnicode
};
/** Expose `punycode` */
// Some AMD build optimizers, like r.js, check for specific condition patterns
// like the following:
if (
typeof define == 'function' &&
typeof define.amd == 'object' &&
define.amd
) {
define('punycode', function() {
return punycode;
});
} else if (freeExports && freeModule) {
if (module.exports == freeExports) {
// in Node.js, io.js, or RingoJS v0.8.0+
freeModule.exports = punycode;
} else {
// in Narwhal or RingoJS v0.7.0-
for (key in punycode) {
punycode.hasOwnProperty(key) && (freeExports[key] = punycode[key]);
}
}
} else {
// in Rhino or a web browser
root.punycode = punycode;
}
}(this));
}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
},{}]},{},[2])(2)
}); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/psl/dist/psl.js | psl.js |
[![Build Status][travis-svg]][travis-url]
[![dependency status][deps-svg]][deps-url]
[![dev dependency status][dev-deps-svg]][dev-deps-url]
# extend() for Node.js <sup>[![Version Badge][npm-version-png]][npm-url]</sup>
`node-extend` is a port of the classic extend() method from jQuery. It behaves as you expect. It is simple, tried and true.
Notes:
* Since Node.js >= 4,
[`Object.assign`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign)
now offers the same functionality natively (but without the "deep copy" option).
See [ECMAScript 2015 (ES6) in Node.js](https://nodejs.org/en/docs/es6).
* Some native implementations of `Object.assign` in both Node.js and many
browsers (since NPM modules are for the browser too) may not be fully
spec-compliant.
Check [`object.assign`](https://www.npmjs.com/package/object.assign) module for
a compliant candidate.
## Installation
This package is available on [npm][npm-url] as: `extend`
``` sh
npm install extend
```
## Usage
**Syntax:** extend **(** [`deep`], `target`, `object1`, [`objectN`] **)**
*Extend one object with one or more others, returning the modified object.*
**Example:**
``` js
var extend = require('extend');
extend(targetObject, object1, object2);
```
Keep in mind that the target object will be modified, and will be returned from extend().
If a boolean true is specified as the first argument, extend performs a deep copy, recursively copying any objects it finds. Otherwise, the copy will share structure with the original object(s).
Undefined properties are not copied. However, properties inherited from the object's prototype will be copied over.
Warning: passing `false` as the first argument is not supported.
### Arguments
* `deep` *Boolean* (optional)
If set, the merge becomes recursive (i.e. deep copy).
* `target` *Object*
The object to extend.
* `object1` *Object*
The object that will be merged into the first.
* `objectN` *Object* (Optional)
More objects to merge into the first.
## License
`node-extend` is licensed under the [MIT License][mit-license-url].
## Acknowledgements
All credit to the jQuery authors for perfecting this amazing utility.
Ported to Node.js by [Stefan Thomas][github-justmoon] with contributions by [Jonathan Buchanan][github-insin] and [Jordan Harband][github-ljharb].
[travis-svg]: https://travis-ci.org/justmoon/node-extend.svg
[travis-url]: https://travis-ci.org/justmoon/node-extend
[npm-url]: https://npmjs.org/package/extend
[mit-license-url]: http://opensource.org/licenses/MIT
[github-justmoon]: https://github.com/justmoon
[github-insin]: https://github.com/insin
[github-ljharb]: https://github.com/ljharb
[npm-version-png]: http://versionbadg.es/justmoon/node-extend.svg
[deps-svg]: https://david-dm.org/justmoon/node-extend.svg
[deps-url]: https://david-dm.org/justmoon/node-extend
[dev-deps-svg]: https://david-dm.org/justmoon/node-extend/dev-status.svg
[dev-deps-url]: https://david-dm.org/justmoon/node-extend#info=devDependencies
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/extend/README.md | README.md |
'use strict';
var hasOwn = Object.prototype.hasOwnProperty;
var toStr = Object.prototype.toString;
var defineProperty = Object.defineProperty;
var gOPD = Object.getOwnPropertyDescriptor;
var isArray = function isArray(arr) {
if (typeof Array.isArray === 'function') {
return Array.isArray(arr);
}
return toStr.call(arr) === '[object Array]';
};
var isPlainObject = function isPlainObject(obj) {
if (!obj || toStr.call(obj) !== '[object Object]') {
return false;
}
var hasOwnConstructor = hasOwn.call(obj, 'constructor');
var hasIsPrototypeOf = obj.constructor && obj.constructor.prototype && hasOwn.call(obj.constructor.prototype, 'isPrototypeOf');
// Not own constructor property must be Object
if (obj.constructor && !hasOwnConstructor && !hasIsPrototypeOf) {
return false;
}
// Own properties are enumerated firstly, so to speed up,
// if last one is own, then all properties are own.
var key;
for (key in obj) { /**/ }
return typeof key === 'undefined' || hasOwn.call(obj, key);
};
// If name is '__proto__', and Object.defineProperty is available, define __proto__ as an own property on target
var setProperty = function setProperty(target, options) {
if (defineProperty && options.name === '__proto__') {
defineProperty(target, options.name, {
enumerable: true,
configurable: true,
value: options.newValue,
writable: true
});
} else {
target[options.name] = options.newValue;
}
};
// Return undefined instead of __proto__ if '__proto__' is not an own property
var getProperty = function getProperty(obj, name) {
if (name === '__proto__') {
if (!hasOwn.call(obj, name)) {
return void 0;
} else if (gOPD) {
// In early versions of node, obj['__proto__'] is buggy when obj has
// __proto__ as an own property. Object.getOwnPropertyDescriptor() works.
return gOPD(obj, name).value;
}
}
return obj[name];
};
module.exports = function extend() {
var options, name, src, copy, copyIsArray, clone;
var target = arguments[0];
var i = 1;
var length = arguments.length;
var deep = false;
// Handle a deep copy situation
if (typeof target === 'boolean') {
deep = target;
target = arguments[1] || {};
// skip the boolean and the target
i = 2;
}
if (target == null || (typeof target !== 'object' && typeof target !== 'function')) {
target = {};
}
for (; i < length; ++i) {
options = arguments[i];
// Only deal with non-null/undefined values
if (options != null) {
// Extend the base object
for (name in options) {
src = getProperty(target, name);
copy = getProperty(options, name);
// Prevent never-ending loop
if (target !== copy) {
// Recurse if we're merging plain objects or arrays
if (deep && copy && (isPlainObject(copy) || (copyIsArray = isArray(copy)))) {
if (copyIsArray) {
copyIsArray = false;
clone = src && isArray(src) ? src : [];
} else {
clone = src && isPlainObject(src) ? src : {};
}
// Never move original objects, clone them
setProperty(target, { name: name, newValue: extend(deep, clone, copy) });
// Don't bring in undefined values
} else if (typeof copy !== 'undefined') {
setProperty(target, { name: name, newValue: copy });
}
}
}
}
}
// Return the modified object
return target;
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/extend/index.js | index.js |
3.0.2 / 2018-07-19
==================
* [Fix] Prevent merging `__proto__` property (#48)
* [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `tape`
* [Tests] up to `node` `v10.7`, `v9.11`, `v8.11`, `v7.10`, `v6.14`, `v4.9`; use `nvm install-latest-npm`
3.0.1 / 2017-04-27
==================
* [Fix] deep extending should work with a non-object (#46)
* [Dev Deps] update `tape`, `eslint`, `@ljharb/eslint-config`
* [Tests] up to `node` `v7.9`, `v6.10`, `v4.8`; improve matrix
* [Docs] Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG.
* [Docs] Add example to readme (#34)
3.0.0 / 2015-07-01
==================
* [Possible breaking change] Use global "strict" directive (#32)
* [Tests] `int` is an ES3 reserved word
* [Tests] Test up to `io.js` `v2.3`
* [Tests] Add `npm run eslint`
* [Dev Deps] Update `covert`, `jscs`
2.0.1 / 2015-04-25
==================
* Use an inline `isArray` check, for ES3 browsers. (#27)
* Some old browsers fail when an identifier is `toString`
* Test latest `node` and `io.js` versions on `travis-ci`; speed up builds
* Add license info to package.json (#25)
* Update `tape`, `jscs`
* Adding a CHANGELOG
2.0.0 / 2014-10-01
==================
* Increase code coverage to 100%; run code coverage as part of tests
* Add `npm run lint`; Run linter as part of tests
* Remove nodeType and setInterval checks in isPlainObject
* Updating `tape`, `jscs`, `covert`
* General style and README cleanup
1.3.0 / 2014-06-20
==================
* Add component.json for browser support (#18)
* Use SVG for badges in README (#16)
* Updating `tape`, `covert`
* Updating travis-ci to work with multiple node versions
* Fix `deep === false` bug (returning target as {}) (#14)
* Fixing constructor checks in isPlainObject
* Adding additional test coverage
* Adding `npm run coverage`
* Add LICENSE (#13)
* Adding a warning about `false`, per #11
* General style and whitespace cleanup
1.2.1 / 2013-09-14
==================
* Fixing hasOwnProperty bugs that would only have shown up in specific browsers. Fixes #8
* Updating `tape`
1.2.0 / 2013-09-02
==================
* Updating the README: add badges
* Adding a missing variable reference.
* Using `tape` instead of `buster` for tests; add more tests (#7)
* Adding node 0.10 to Travis CI (#6)
* Enabling "npm test" and cleaning up package.json (#5)
* Add Travis CI.
1.1.3 / 2012-12-06
==================
* Added unit tests.
* Ensure extend function is named. (Looks nicer in a stack trace.)
* README cleanup.
1.1.1 / 2012-11-07
==================
* README cleanup.
* Added installation instructions.
* Added a missing semicolon
1.0.0 / 2012-04-08
==================
* Initial commit
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/extend/CHANGELOG.md | CHANGELOG.md |
# json-stringify-safe
Like JSON.stringify, but doesn't throw on circular references.
## Usage
Takes the same arguments as `JSON.stringify`.
```javascript
var stringify = require('json-stringify-safe');
var circularObj = {};
circularObj.circularRef = circularObj;
circularObj.list = [ circularObj, circularObj ];
console.log(stringify(circularObj, null, 2));
```
Output:
```json
{
"circularRef": "[Circular]",
"list": [
"[Circular]",
"[Circular]"
]
}
```
## Details
```
stringify(obj, serializer, indent, decycler)
```
The first three arguments are the same as to JSON.stringify. The last
is an argument that's only used when the object has been seen already.
The default `decycler` function returns the string `'[Circular]'`.
If, for example, you pass in `function(k,v){}` (return nothing) then it
will prune cycles. If you pass in `function(k,v){ return {foo: 'bar'}}`,
then cyclical objects will always be represented as `{"foo":"bar"}` in
the result.
```
stringify.getSerialize(serializer, decycler)
```
Returns a serializer that can be used elsewhere. This is the actual
function that's passed to JSON.stringify.
**Note** that the function returned from `getSerialize` is stateful for now, so
do **not** use it more than once.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/json-stringify-safe/README.md | README.md |
## Unreleased
- Fixes stringify to only take ancestors into account when checking
circularity.
It previously assumed every visited object was circular which led to [false
positives][issue9].
Uses the tiny serializer I wrote for [Must.js][must] a year and a half ago.
- Fixes calling the `replacer` function in the proper context (`thisArg`).
- Fixes calling the `cycleReplacer` function in the proper context (`thisArg`).
- Speeds serializing by a factor of
Big-O(h-my-god-it-linearly-searched-every-object) it had ever seen. Searching
only the ancestors for a circular references speeds up things considerably.
[must]: https://github.com/moll/js-must
[issue9]: https://github.com/isaacs/json-stringify-safe/issues/9
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/json-stringify-safe/CHANGELOG.md | CHANGELOG.md |
# Optionator
<a name="optionator" />
Optionator is a JavaScript/Node.js option parsing and help generation library used by [eslint](http://eslint.org), [Grasp](http://graspjs.com), [LiveScript](http://livescript.net), [esmangle](https://github.com/estools/esmangle), [escodegen](https://github.com/estools/escodegen), and [many more](https://www.npmjs.com/browse/depended/optionator).
For an online demo, check out the [Grasp online demo](http://www.graspjs.com/#demo).
[About](#about) · [Usage](#usage) · [Settings Format](#settings-format) · [Argument Format](#argument-format)
## Why?
The problem with other option parsers, such as `yargs` or `minimist`, is they just accept all input, valid or not.
With Optionator, if you mistype an option, it will give you an error (with a suggestion for what you meant).
If you give the wrong type of argument for an option, it will give you an error rather than supplying the wrong input to your application.
$ cmd --halp
Invalid option '--halp' - perhaps you meant '--help'?
$ cmd --count str
Invalid value for option 'count' - expected type Int, received value: str.
Other helpful features include reformatting the help text based on the size of the console, so that it fits even if the console is narrow, and accepting not just an array (eg. process.argv), but a string or object as well, making things like testing much easier.
## About
Optionator uses [type-check](https://github.com/gkz/type-check) and [levn](https://github.com/gkz/levn) behind the scenes to cast and verify input according the specified types.
MIT license. Version 0.8.3
npm install optionator
For updates on Optionator, [follow me on twitter](https://twitter.com/gkzahariev).
Optionator is a Node.js module, but can be used in the browser as well if packed with webpack/browserify.
## Usage
`require('optionator');` returns a function. It has one property, `VERSION`, the current version of the library as a string. This function is called with an object specifying your options and other information, see the [settings format section](#settings-format). This in turn returns an object with three properties, `parse`, `parseArgv`, `generateHelp`, and `generateHelpForOption`, which are all functions.
```js
var optionator = require('optionator')({
prepend: 'Usage: cmd [options]',
append: 'Version 1.0.0',
options: [{
option: 'help',
alias: 'h',
type: 'Boolean',
description: 'displays help'
}, {
option: 'count',
alias: 'c',
type: 'Int',
description: 'number of things',
example: 'cmd --count 2'
}]
});
var options = optionator.parseArgv(process.argv);
if (options.help) {
console.log(optionator.generateHelp());
}
...
```
### parse(input, parseOptions)
`parse` processes the `input` according to your settings, and returns an object with the results.
##### arguments
* input - `[String] | Object | String` - the input you wish to parse
* parseOptions - `{slice: Int}` - all options optional
- `slice` specifies how much to slice away from the beginning if the input is an array or string - by default `0` for string, `2` for array (works with `process.argv`)
##### returns
`Object` - the parsed options, each key is a camelCase version of the option name (specified in dash-case), and each value is the processed value for that option. Positional values are in an array under the `_` key.
##### example
```js
parse(['node', 't.js', '--count', '2', 'positional']); // {count: 2, _: ['positional']}
parse('--count 2 positional'); // {count: 2, _: ['positional']}
parse({count: 2, _:['positional']}); // {count: 2, _: ['positional']}
```
### parseArgv(input)
`parseArgv` works exactly like `parse`, but only for array input and it slices off the first two elements.
##### arguments
* input - `[String]` - the input you wish to parse
##### returns
See "returns" section in "parse"
##### example
```js
parseArgv(process.argv);
```
### generateHelp(helpOptions)
`generateHelp` produces help text based on your settings.
##### arguments
* helpOptions - `{showHidden: Boolean, interpolate: Object}` - all options optional
- `showHidden` specifies whether to show options with `hidden: true` specified, by default it is `false`
- `interpolate` specify data to be interpolated in `prepend` and `append` text, `{{key}}` is the format - eg. `generateHelp({interpolate:{version: '0.4.2'}})`, will change this `append` text: `Version {{version}}` to `Version 0.4.2`
##### returns
`String` - the generated help text
##### example
```js
generateHelp(); /*
"Usage: cmd [options] positional
-h, --help displays help
-c, --count Int number of things
Version 1.0.0
"*/
```
### generateHelpForOption(optionName)
`generateHelpForOption` produces expanded help text for the specified with `optionName` option. If an `example` was specified for the option, it will be displayed, and if a `longDescription` was specified, it will display that instead of the `description`.
##### arguments
* optionName - `String` - the name of the option to display
##### returns
`String` - the generated help text for the option
##### example
```js
generateHelpForOption('count'); /*
"-c, --count Int
description: number of things
example: cmd --count 2
"*/
```
## Settings Format
When your `require('optionator')`, you get a function that takes in a settings object. This object has the type:
{
prepend: String,
append: String,
options: [{heading: String} | {
option: String,
alias: [String] | String,
type: String,
enum: [String],
default: String,
restPositional: Boolean,
required: Boolean,
overrideRequired: Boolean,
dependsOn: [String] | String,
concatRepeatedArrays: Boolean | (Boolean, Object),
mergeRepeatedObjects: Boolean,
description: String,
longDescription: String,
example: [String] | String
}],
helpStyle: {
aliasSeparator: String,
typeSeparator: String,
descriptionSeparator: String,
initialIndent: Int,
secondaryIndent: Int,
maxPadFactor: Number
},
mutuallyExclusive: [[String | [String]]],
concatRepeatedArrays: Boolean | (Boolean, Object), // deprecated, set in defaults object
mergeRepeatedObjects: Boolean, // deprecated, set in defaults object
positionalAnywhere: Boolean,
typeAliases: Object,
defaults: Object
}
All of the properties are optional (the `Maybe` has been excluded for brevities sake), except for having either `heading: String` or `option: String` in each object in the `options` array.
### Top Level Properties
* `prepend` is an optional string to be placed before the options in the help text
* `append` is an optional string to be placed after the options in the help text
* `options` is a required array specifying your options and headings, the options and headings will be displayed in the order specified
* `helpStyle` is an optional object which enables you to change the default appearance of some aspects of the help text
* `mutuallyExclusive` is an optional array of arrays of either strings or arrays of strings. The top level array is a list of rules, each rule is a list of elements - each element can be either a string (the name of an option), or a list of strings (a group of option names) - there will be an error if more than one element is present
* `concatRepeatedArrays` see description under the "Option Properties" heading - use at the top level is deprecated, if you want to set this for all options, use the `defaults` property
* `mergeRepeatedObjects` see description under the "Option Properties" heading - use at the top level is deprecated, if you want to set this for all options, use the `defaults` property
* `positionalAnywhere` is an optional boolean (defaults to `true`) - when `true` it allows positional arguments anywhere, when `false`, all arguments after the first positional one are taken to be positional as well, even if they look like a flag. For example, with `positionalAnywhere: false`, the arguments `--flag --boom 12 --crack` would have two positional arguments: `12` and `--crack`
* `typeAliases` is an optional object, it allows you to set aliases for types, eg. `{Path: 'String'}` would allow you to use the type `Path` as an alias for the type `String`
* `defaults` is an optional object following the option properties format, which specifies default values for all options. A default will be overridden if manually set. For example, you can do `default: { type: "String" }` to set the default type of all options to `String`, and then override that default in an individual option by setting the `type` property
#### Heading Properties
* `heading` a required string, the name of the heading
#### Option Properties
* `option` the required name of the option - use dash-case, without the leading dashes
* `alias` is an optional string or array of strings which specify any aliases for the option
* `type` is a required string in the [type check](https://github.com/gkz/type-check) [format](https://github.com/gkz/type-check#type-format), this will be used to cast the inputted value and validate it
* `enum` is an optional array of strings, each string will be parsed by [levn](https://github.com/gkz/levn) - the argument value must be one of the resulting values - each potential value must validate against the specified `type`
* `default` is a optional string, which will be parsed by [levn](https://github.com/gkz/levn) and used as the default value if none is set - the value must validate against the specified `type`
* `restPositional` is an optional boolean - if set to `true`, everything after the option will be taken to be a positional argument, even if it looks like a named argument
* `required` is an optional boolean - if set to `true`, the option parsing will fail if the option is not defined
* `overrideRequired` is a optional boolean - if set to `true` and the option is used, and there is another option which is required but not set, it will override the need for the required option and there will be no error - this is useful if you have required options and want to use `--help` or `--version` flags
* `concatRepeatedArrays` is an optional boolean or tuple with boolean and options object (defaults to `false`) - when set to `true` and an option contains an array value and is repeated, the subsequent values for the flag will be appended rather than overwriting the original value - eg. option `g` of type `[String]`: `-g a -g b -g c,d` will result in `['a','b','c','d']`
You can supply an options object by giving the following value: `[true, options]`. The one currently supported option is `oneValuePerFlag`, this only allows one array value per flag. This is useful if your potential values contain a comma.
* `mergeRepeatedObjects` is an optional boolean (defaults to `false`) - when set to `true` and an option contains an object value and is repeated, the subsequent values for the flag will be merged rather than overwriting the original value - eg. option `g` of type `Object`: `-g a:1 -g b:2 -g c:3,d:4` will result in `{a: 1, b: 2, c: 3, d: 4}`
* `dependsOn` is an optional string or array of strings - if simply a string (the name of another option), it will make sure that that other option is set, if an array of strings, depending on whether `'and'` or `'or'` is first, it will either check whether all (`['and', 'option-a', 'option-b']`), or at least one (`['or', 'option-a', 'option-b']`) other options are set
* `description` is an optional string, which will be displayed next to the option in the help text
* `longDescription` is an optional string, it will be displayed instead of the `description` when `generateHelpForOption` is used
* `example` is an optional string or array of strings with example(s) for the option - these will be displayed when `generateHelpForOption` is used
#### Help Style Properties
* `aliasSeparator` is an optional string, separates multiple names from each other - default: ' ,'
* `typeSeparator` is an optional string, separates the type from the names - default: ' '
* `descriptionSeparator` is an optional string , separates the description from the padded name and type - default: ' '
* `initialIndent` is an optional int - the amount of indent for options - default: 2
* `secondaryIndent` is an optional int - the amount of indent if wrapped fully (in addition to the initial indent) - default: 4
* `maxPadFactor` is an optional number - affects the default level of padding for the names/type, it is multiplied by the average of the length of the names/type - default: 1.5
## Argument Format
At the highest level there are two types of arguments: named, and positional.
Name arguments of any length are prefixed with `--` (eg. `--go`), and those of one character may be prefixed with either `--` or `-` (eg. `-g`).
There are two types of named arguments: boolean flags (eg. `--problemo`, `-p`) which take no value and result in a `true` if they are present, the falsey `undefined` if they are not present, or `false` if present and explicitly prefixed with `no` (eg. `--no-problemo`). Named arguments with values (eg. `--tseries 800`, `-t 800`) are the other type. If the option has a type `Boolean` it will automatically be made into a boolean flag. Any other type results in a named argument that takes a value.
For more information about how to properly set types to get the value you want, take a look at the [type check](https://github.com/gkz/type-check) and [levn](https://github.com/gkz/levn) pages.
You can group single character arguments that use a single `-`, however all except the last must be boolean flags (which take no value). The last may be a boolean flag, or an argument which takes a value - eg. `-ba 2` is equivalent to `-b -a 2`.
Positional arguments are all those values which do not fall under the above - they can be anywhere, not just at the end. For example, in `cmd -b one -a 2 two` where `b` is a boolean flag, and `a` has the type `Number`, there are two positional arguments, `one` and `two`.
Everything after an `--` is positional, even if it looks like a named argument.
You may optionally use `=` to separate option names from values, for example: `--count=2`.
If you specify the option `NUM`, then any argument using a single `-` followed by a number will be valid and will set the value of `NUM`. Eg. `-2` will be parsed into `NUM: 2`.
If duplicate named arguments are present, the last one will be taken.
## Technical About
`optionator` is written in [LiveScript](http://livescript.net/) - a language that compiles to JavaScript. It uses [levn](https://github.com/gkz/levn) to cast arguments to their specified type, and uses [type-check](https://github.com/gkz/type-check) to validate values. It also uses the [prelude.ls](http://preludels.com/) library.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/optionator/README.md | README.md |
# 0.8.3
- changes dependency from `wordwrap` to `word-wrap` due to license issue
- update dependencies
# 0.8.2
- fix bug #18 - detect missing value when flag is last item
- update dependencies
# 0.8.1
- update `fast-levenshtein` dependency
# 0.8.0
- update `levn` dependency - supplying a float value to an option with type `Int` now throws an error, instead of silently converting to an `Int`
# 0.7.1
- fix bug with use of `defaults` and `concatRepeatedArrays` or `mergeRepeatedObjects`
# 0.7.0
- added `concatrepeatedarrays` option: `oneValuePerFlag`, only allows one array value per flag
- added `typeAliases` option
- added `parseArgv` which takes an array and parses with the first two items sliced off
- changed enum help style
- bug fixes (#12)
- use of `concatRepeatedArrays` and `mergeRepeatedObjects` at the top level is deprecated, use it as either a per-option option, or set them in the `defaults` object to set them for all objects
# 0.6.0
- added `defaults` lib-option flag, allowing one to set default properties for all options
- added `concatRepeatedArrays` and `mergeRepeatedObjects` as option level properties, allowing you to turn this feature on for specific options only
# 0.5.0
- `Boolean` flags with `default: 'true'`, and no short aliases, will by default show the `--no` version in help
# 0.4.0
- add `mergeRepeatedObjects` setting
# 0.3.0
- add `concatRepeatedArrays` setting
- add `overrideRequired` option setting
- use just Levenshtein string compare algo rather than Levenshtein Damerau to due dependency license issue
# 0.2.2
- bug fixes
# 0.2.1
- improved interpolation
- added changelog
# 0.2.0
- add dependency checks to options - added `dependsOn` as an option property
- add interpolation for `prepend` and `append` text with new `generateHelp` option, `interpolate`
# 0.1.1
- update dependencies
# 0.1.0
- initial release
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/optionator/CHANGELOG.md | CHANGELOG.md |
(function(){
var ref$, id, find, sort, min, max, map, unlines, nameToRaw, dasherize, naturalJoin, wordWrap, wordwrap, getPreText, setHelpStyleDefaults, generateHelpForOption, generateHelp;
ref$ = require('prelude-ls'), id = ref$.id, find = ref$.find, sort = ref$.sort, min = ref$.min, max = ref$.max, map = ref$.map, unlines = ref$.unlines;
ref$ = require('optionator/lib/util'), nameToRaw = ref$.nameToRaw, dasherize = ref$.dasherize, naturalJoin = ref$.naturalJoin;
wordWrap = require('word-wrap');
wordwrap = function(a, b){
var ref$, indent, width;
ref$ = b === undefined
? ['', a - 1]
: [repeatString$(' ', a), b - a - 1], indent = ref$[0], width = ref$[1];
return function(text){
return wordWrap(text, {
indent: indent,
width: width,
trim: true
});
};
};
getPreText = function(option, arg$, maxWidth){
var mainName, shortNames, ref$, longNames, type, description, aliasSeparator, typeSeparator, initialIndent, names, namesString, namesStringLen, typeSeparatorString, typeSeparatorStringLen, wrap;
mainName = option.option, shortNames = (ref$ = option.shortNames) != null
? ref$
: [], longNames = (ref$ = option.longNames) != null
? ref$
: [], type = option.type, description = option.description;
aliasSeparator = arg$.aliasSeparator, typeSeparator = arg$.typeSeparator, initialIndent = arg$.initialIndent;
if (option.negateName) {
mainName = "no-" + mainName;
if (longNames) {
longNames = map(function(it){
return "no-" + it;
}, longNames);
}
}
names = mainName.length === 1
? [mainName].concat(shortNames, longNames)
: shortNames.concat([mainName], longNames);
namesString = map(nameToRaw, names).join(aliasSeparator);
namesStringLen = namesString.length;
typeSeparatorString = mainName === 'NUM' ? '::' : typeSeparator;
typeSeparatorStringLen = typeSeparatorString.length;
if (maxWidth != null && !option.boolean && initialIndent + namesStringLen + typeSeparatorStringLen + type.length > maxWidth) {
wrap = wordwrap(initialIndent + namesStringLen + typeSeparatorStringLen, maxWidth);
return namesString + "" + typeSeparatorString + wrap(type).replace(/^\s+/, '');
} else {
return namesString + "" + (option.boolean
? ''
: typeSeparatorString + "" + type);
}
};
setHelpStyleDefaults = function(helpStyle){
helpStyle.aliasSeparator == null && (helpStyle.aliasSeparator = ', ');
helpStyle.typeSeparator == null && (helpStyle.typeSeparator = ' ');
helpStyle.descriptionSeparator == null && (helpStyle.descriptionSeparator = ' ');
helpStyle.initialIndent == null && (helpStyle.initialIndent = 2);
helpStyle.secondaryIndent == null && (helpStyle.secondaryIndent = 4);
helpStyle.maxPadFactor == null && (helpStyle.maxPadFactor = 1.5);
};
generateHelpForOption = function(getOption, arg$){
var stdout, helpStyle, ref$;
stdout = arg$.stdout, helpStyle = (ref$ = arg$.helpStyle) != null
? ref$
: {};
setHelpStyleDefaults(helpStyle);
return function(optionName){
var maxWidth, wrap, option, e, pre, defaultString, restPositionalString, description, fullDescription, that, preDescription, descriptionString, exampleString, examples, seperator;
maxWidth = stdout != null && stdout.isTTY ? stdout.columns - 1 : null;
wrap = maxWidth ? wordwrap(maxWidth) : id;
try {
option = getOption(dasherize(optionName));
} catch (e$) {
e = e$;
return e.message;
}
pre = getPreText(option, helpStyle);
defaultString = option['default'] && !option.negateName ? "\ndefault: " + option['default'] : '';
restPositionalString = option.restPositional ? 'Everything after this option is considered a positional argument, even if it looks like an option.' : '';
description = option.longDescription || option.description && sentencize(option.description);
fullDescription = description && restPositionalString
? description + " " + restPositionalString
: (that = description || restPositionalString) ? that : '';
preDescription = 'description:';
descriptionString = !fullDescription
? ''
: maxWidth && fullDescription.length - 1 - preDescription.length > maxWidth
? "\n" + preDescription + "\n" + wrap(fullDescription)
: "\n" + preDescription + " " + fullDescription;
exampleString = (that = option.example) ? (examples = [].concat(that), examples.length > 1
? "\nexamples:\n" + unlines(examples)
: "\nexample: " + examples[0]) : '';
seperator = defaultString || descriptionString || exampleString ? "\n" + repeatString$('=', pre.length) : '';
return pre + "" + seperator + defaultString + descriptionString + exampleString;
};
};
generateHelp = function(arg$){
var options, prepend, append, helpStyle, ref$, stdout, aliasSeparator, typeSeparator, descriptionSeparator, maxPadFactor, initialIndent, secondaryIndent;
options = arg$.options, prepend = arg$.prepend, append = arg$.append, helpStyle = (ref$ = arg$.helpStyle) != null
? ref$
: {}, stdout = arg$.stdout;
setHelpStyleDefaults(helpStyle);
aliasSeparator = helpStyle.aliasSeparator, typeSeparator = helpStyle.typeSeparator, descriptionSeparator = helpStyle.descriptionSeparator, maxPadFactor = helpStyle.maxPadFactor, initialIndent = helpStyle.initialIndent, secondaryIndent = helpStyle.secondaryIndent;
return function(arg$){
var ref$, showHidden, interpolate, maxWidth, output, out, data, optionCount, totalPreLen, preLens, i$, len$, item, that, pre, descParts, desc, preLen, sortedPreLens, maxPreLen, preLenMean, x, padAmount, descSepLen, fullWrapCount, partialWrapCount, descLen, totalLen, initialSpace, wrapAllFull, i, wrap;
ref$ = arg$ != null
? arg$
: {}, showHidden = ref$.showHidden, interpolate = ref$.interpolate;
maxWidth = stdout != null && stdout.isTTY ? stdout.columns - 1 : null;
output = [];
out = function(it){
return output.push(it != null ? it : '');
};
if (prepend) {
out(interpolate ? interp(prepend, interpolate) : prepend);
out();
}
data = [];
optionCount = 0;
totalPreLen = 0;
preLens = [];
for (i$ = 0, len$ = (ref$ = options).length; i$ < len$; ++i$) {
item = ref$[i$];
if (showHidden || !item.hidden) {
if (that = item.heading) {
data.push({
type: 'heading',
value: that
});
} else {
pre = getPreText(item, helpStyle, maxWidth);
descParts = [];
if ((that = item.description) != null) {
descParts.push(that);
}
if (that = item['enum']) {
descParts.push("either: " + naturalJoin(that));
}
if (item['default'] && !item.negateName) {
descParts.push("default: " + item['default']);
}
desc = descParts.join(' - ');
data.push({
type: 'option',
pre: pre,
desc: desc,
descLen: desc.length
});
preLen = pre.length;
optionCount++;
totalPreLen += preLen;
preLens.push(preLen);
}
}
}
sortedPreLens = sort(preLens);
maxPreLen = sortedPreLens[sortedPreLens.length - 1];
preLenMean = initialIndent + totalPreLen / optionCount;
x = optionCount > 2 ? min(preLenMean * maxPadFactor, maxPreLen) : maxPreLen;
for (i$ = sortedPreLens.length - 1; i$ >= 0; --i$) {
preLen = sortedPreLens[i$];
if (preLen <= x) {
padAmount = preLen;
break;
}
}
descSepLen = descriptionSeparator.length;
if (maxWidth != null) {
fullWrapCount = 0;
partialWrapCount = 0;
for (i$ = 0, len$ = data.length; i$ < len$; ++i$) {
item = data[i$];
if (item.type === 'option') {
pre = item.pre, desc = item.desc, descLen = item.descLen;
if (descLen === 0) {
item.wrap = 'none';
} else {
preLen = max(padAmount, pre.length) + initialIndent + descSepLen;
totalLen = preLen + descLen;
if (totalLen > maxWidth) {
if (descLen / 2.5 > maxWidth - preLen) {
fullWrapCount++;
item.wrap = 'full';
} else {
partialWrapCount++;
item.wrap = 'partial';
}
} else {
item.wrap = 'none';
}
}
}
}
}
initialSpace = repeatString$(' ', initialIndent);
wrapAllFull = optionCount > 1 && fullWrapCount + partialWrapCount * 0.5 > optionCount * 0.5;
for (i$ = 0, len$ = data.length; i$ < len$; ++i$) {
i = i$;
item = data[i$];
if (item.type === 'heading') {
if (i !== 0) {
out();
}
out(item.value + ":");
} else {
pre = item.pre, desc = item.desc, descLen = item.descLen, wrap = item.wrap;
if (maxWidth != null) {
if (wrapAllFull || wrap === 'full') {
wrap = wordwrap(initialIndent + secondaryIndent, maxWidth);
out(initialSpace + "" + pre + "\n" + wrap(desc));
continue;
} else if (wrap === 'partial') {
wrap = wordwrap(initialIndent + descSepLen + max(padAmount, pre.length), maxWidth);
out(initialSpace + "" + pad(pre, padAmount) + descriptionSeparator + wrap(desc).replace(/^\s+/, ''));
continue;
}
}
if (descLen === 0) {
out(initialSpace + "" + pre);
} else {
out(initialSpace + "" + pad(pre, padAmount) + descriptionSeparator + desc);
}
}
}
if (append) {
out();
out(interpolate ? interp(append, interpolate) : append);
}
return unlines(output);
};
};
function pad(str, num){
var len, padAmount;
len = str.length;
padAmount = num - len;
return str + "" + repeatString$(' ', padAmount > 0 ? padAmount : 0);
}
function sentencize(str){
var first, rest, period;
first = str.charAt(0).toUpperCase();
rest = str.slice(1);
period = /[\.!\?]$/.test(str) ? '' : '.';
return first + "" + rest + period;
}
function interp(string, object){
return string.replace(/{{([a-zA-Z$_][a-zA-Z$_0-9]*)}}/g, function(arg$, key){
var ref$;
return (ref$ = object[key]) != null
? ref$
: "{{" + key + "}}";
});
}
module.exports = {
generateHelp: generateHelp,
generateHelpForOption: generateHelpForOption
};
function repeatString$(str, n){
for (var r = ''; n > 0; (n >>= 1) && (str += str)) if (n & 1) r += str;
return r;
}
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/optionator/lib/help.js | help.js |
(function(){
var VERSION, ref$, id, map, compact, any, groupBy, partition, chars, isItNaN, keys, Obj, camelize, deepIs, closestString, nameToRaw, dasherize, naturalJoin, generateHelp, generateHelpForOption, parsedTypeCheck, parseType, parseLevn, camelizeKeys, parseString, main, toString$ = {}.toString, slice$ = [].slice, arrayFrom$ = Array.from || function(x){return slice$.call(x);};
VERSION = '0.8.3';
ref$ = require('prelude-ls'), id = ref$.id, map = ref$.map, compact = ref$.compact, any = ref$.any, groupBy = ref$.groupBy, partition = ref$.partition, chars = ref$.chars, isItNaN = ref$.isItNaN, keys = ref$.keys, Obj = ref$.Obj, camelize = ref$.camelize;
deepIs = require('deep-is');
ref$ = require('optionator/lib/util'), closestString = ref$.closestString, nameToRaw = ref$.nameToRaw, dasherize = ref$.dasherize, naturalJoin = ref$.naturalJoin;
ref$ = require('optionator/lib/help'), generateHelp = ref$.generateHelp, generateHelpForOption = ref$.generateHelpForOption;
ref$ = require('type-check'), parsedTypeCheck = ref$.parsedTypeCheck, parseType = ref$.parseType;
parseLevn = require('levn').parsedTypeParse;
camelizeKeys = function(obj){
var key, value, resultObj$ = {};
for (key in obj) {
value = obj[key];
resultObj$[camelize(key)] = value;
}
return resultObj$;
};
parseString = function(string){
var assignOpt, regex, replaceRegex, result;
assignOpt = '--?[a-zA-Z][-a-z-A-Z0-9]*=';
regex = RegExp('(?:' + assignOpt + ')?(?:\'(?:\\\\\'|[^\'])+\'|"(?:\\\\"|[^"])+")|[^\'"\\s]+', 'g');
replaceRegex = RegExp('^(' + assignOpt + ')?[\'"]([\\s\\S]*)[\'"]$');
result = map(function(it){
return it.replace(replaceRegex, '$1$2');
}, string.match(regex) || []);
return result;
};
main = function(libOptions){
var opts, defaults, required, traverse, getOption, parse;
opts = {};
defaults = {};
required = [];
if (toString$.call(libOptions.stdout).slice(8, -1) === 'Undefined') {
libOptions.stdout = process.stdout;
}
libOptions.positionalAnywhere == null && (libOptions.positionalAnywhere = true);
libOptions.typeAliases == null && (libOptions.typeAliases = {});
libOptions.defaults == null && (libOptions.defaults = {});
if (libOptions.concatRepeatedArrays != null) {
libOptions.defaults.concatRepeatedArrays = libOptions.concatRepeatedArrays;
}
if (libOptions.mergeRepeatedObjects != null) {
libOptions.defaults.mergeRepeatedObjects = libOptions.mergeRepeatedObjects;
}
traverse = function(options){
var i$, len$, option, name, k, ref$, v, type, that, e, parsedPossibilities, parsedType, j$, len1$, possibility, rawDependsType, dependsOpts, dependsType, cra, alias, shortNames, longNames;
if (toString$.call(options).slice(8, -1) !== 'Array') {
throw new Error('No options defined.');
}
for (i$ = 0, len$ = options.length; i$ < len$; ++i$) {
option = options[i$];
if (option.heading == null) {
name = option.option;
if (opts[name] != null) {
throw new Error("Option '" + name + "' already defined.");
}
for (k in ref$ = libOptions.defaults) {
v = ref$[k];
option[k] == null && (option[k] = v);
}
if (option.type === 'Boolean') {
option.boolean == null && (option.boolean = true);
}
if (option.parsedType == null) {
if (!option.type) {
throw new Error("No type defined for option '" + name + "'.");
}
try {
type = (that = libOptions.typeAliases[option.type]) != null
? that
: option.type;
option.parsedType = parseType(type);
} catch (e$) {
e = e$;
throw new Error("Option '" + name + "': Error parsing type '" + option.type + "': " + e.message);
}
}
if (option['default']) {
try {
defaults[name] = parseLevn(option.parsedType, option['default']);
} catch (e$) {
e = e$;
throw new Error("Option '" + name + "': Error parsing default value '" + option['default'] + "' for type '" + option.type + "': " + e.message);
}
}
if (option['enum'] && !option.parsedPossiblities) {
parsedPossibilities = [];
parsedType = option.parsedType;
for (j$ = 0, len1$ = (ref$ = option['enum']).length; j$ < len1$; ++j$) {
possibility = ref$[j$];
try {
parsedPossibilities.push(parseLevn(parsedType, possibility));
} catch (e$) {
e = e$;
throw new Error("Option '" + name + "': Error parsing enum value '" + possibility + "' for type '" + option.type + "': " + e.message);
}
}
option.parsedPossibilities = parsedPossibilities;
}
if (that = option.dependsOn) {
if (that.length) {
ref$ = [].concat(option.dependsOn), rawDependsType = ref$[0], dependsOpts = slice$.call(ref$, 1);
dependsType = rawDependsType.toLowerCase();
if (dependsOpts.length) {
if (dependsType === 'and' || dependsType === 'or') {
option.dependsOn = [dependsType].concat(arrayFrom$(dependsOpts));
} else {
throw new Error("Option '" + name + "': If you have more than one dependency, you must specify either 'and' or 'or'");
}
} else {
if ((ref$ = dependsType.toLowerCase()) === 'and' || ref$ === 'or') {
option.dependsOn = null;
} else {
option.dependsOn = ['and', rawDependsType];
}
}
} else {
option.dependsOn = null;
}
}
if (option.required) {
required.push(name);
}
opts[name] = option;
if (option.concatRepeatedArrays != null) {
cra = option.concatRepeatedArrays;
if ('Boolean' === toString$.call(cra).slice(8, -1)) {
option.concatRepeatedArrays = [cra, {}];
} else if (cra.length === 1) {
option.concatRepeatedArrays = [cra[0], {}];
} else if (cra.length !== 2) {
throw new Error("Invalid setting for concatRepeatedArrays");
}
}
if (option.alias || option.aliases) {
if (name === 'NUM') {
throw new Error("-NUM option can't have aliases.");
}
if (option.alias) {
option.aliases == null && (option.aliases = [].concat(option.alias));
}
for (j$ = 0, len1$ = (ref$ = option.aliases).length; j$ < len1$; ++j$) {
alias = ref$[j$];
if (opts[alias] != null) {
throw new Error("Option '" + alias + "' already defined.");
}
opts[alias] = option;
}
ref$ = partition(fn$, option.aliases), shortNames = ref$[0], longNames = ref$[1];
option.shortNames == null && (option.shortNames = shortNames);
option.longNames == null && (option.longNames = longNames);
}
if ((!option.aliases || option.shortNames.length === 0) && option.type === 'Boolean' && option['default'] === 'true') {
option.negateName = true;
}
}
}
function fn$(it){
return it.length === 1;
}
};
traverse(libOptions.options);
getOption = function(name){
var opt, possiblyMeant;
opt = opts[name];
if (opt == null) {
possiblyMeant = closestString(keys(opts), name);
throw new Error("Invalid option '" + nameToRaw(name) + "'" + (possiblyMeant ? " - perhaps you meant '" + nameToRaw(possiblyMeant) + "'?" : '.'));
}
return opt;
};
parse = function(input, arg$){
var slice, obj, positional, restPositional, overrideRequired, prop, setValue, setDefaults, checkRequired, mutuallyExclusiveError, checkMutuallyExclusive, checkDependency, checkDependencies, checkProp, args, key, value, option, ref$, i$, len$, arg, that, result, short, argName, usingAssign, val, flags, len, j$, len1$, i, flag, opt, name, valPrime, negated, noedName;
slice = (arg$ != null
? arg$
: {}).slice;
obj = {};
positional = [];
restPositional = false;
overrideRequired = false;
prop = null;
setValue = function(name, value){
var opt, val, cra, e, currentType;
opt = getOption(name);
if (opt.boolean) {
val = value;
} else {
try {
cra = opt.concatRepeatedArrays;
if (cra != null && cra[0] && cra[1].oneValuePerFlag && opt.parsedType.length === 1 && opt.parsedType[0].structure === 'array') {
val = [parseLevn(opt.parsedType[0].of, value)];
} else {
val = parseLevn(opt.parsedType, value);
}
} catch (e$) {
e = e$;
throw new Error("Invalid value for option '" + name + "' - expected type " + opt.type + ", received value: " + value + ".");
}
if (opt['enum'] && !any(function(it){
return deepIs(it, val);
}, opt.parsedPossibilities)) {
throw new Error("Option " + name + ": '" + val + "' not one of " + naturalJoin(opt['enum']) + ".");
}
}
currentType = toString$.call(obj[name]).slice(8, -1);
if (obj[name] != null) {
if (opt.concatRepeatedArrays != null && opt.concatRepeatedArrays[0] && currentType === 'Array') {
obj[name] = obj[name].concat(val);
} else if (opt.mergeRepeatedObjects && currentType === 'Object') {
import$(obj[name], val);
} else {
obj[name] = val;
}
} else {
obj[name] = val;
}
if (opt.restPositional) {
restPositional = true;
}
if (opt.overrideRequired) {
overrideRequired = true;
}
};
setDefaults = function(){
var name, ref$, value;
for (name in ref$ = defaults) {
value = ref$[name];
if (obj[name] == null) {
obj[name] = value;
}
}
};
checkRequired = function(){
var i$, ref$, len$, name;
if (overrideRequired) {
return;
}
for (i$ = 0, len$ = (ref$ = required).length; i$ < len$; ++i$) {
name = ref$[i$];
if (!obj[name]) {
throw new Error("Option " + nameToRaw(name) + " is required.");
}
}
};
mutuallyExclusiveError = function(first, second){
throw new Error("The options " + nameToRaw(first) + " and " + nameToRaw(second) + " are mutually exclusive - you cannot use them at the same time.");
};
checkMutuallyExclusive = function(){
var rules, i$, len$, rule, present, j$, len1$, element, k$, len2$, opt;
rules = libOptions.mutuallyExclusive;
if (!rules) {
return;
}
for (i$ = 0, len$ = rules.length; i$ < len$; ++i$) {
rule = rules[i$];
present = null;
for (j$ = 0, len1$ = rule.length; j$ < len1$; ++j$) {
element = rule[j$];
if (toString$.call(element).slice(8, -1) === 'Array') {
for (k$ = 0, len2$ = element.length; k$ < len2$; ++k$) {
opt = element[k$];
if (opt in obj) {
if (present != null) {
mutuallyExclusiveError(present, opt);
} else {
present = opt;
break;
}
}
}
} else {
if (element in obj) {
if (present != null) {
mutuallyExclusiveError(present, element);
} else {
present = element;
}
}
}
}
}
};
checkDependency = function(option){
var dependsOn, type, targetOptionNames, i$, len$, targetOptionName, targetOption;
dependsOn = option.dependsOn;
if (!dependsOn || option.dependenciesMet) {
return true;
}
type = dependsOn[0], targetOptionNames = slice$.call(dependsOn, 1);
for (i$ = 0, len$ = targetOptionNames.length; i$ < len$; ++i$) {
targetOptionName = targetOptionNames[i$];
targetOption = obj[targetOptionName];
if (targetOption && checkDependency(targetOption)) {
if (type === 'or') {
return true;
}
} else if (type === 'and') {
throw new Error("The option '" + option.option + "' did not have its dependencies met.");
}
}
if (type === 'and') {
return true;
} else {
throw new Error("The option '" + option.option + "' did not meet any of its dependencies.");
}
};
checkDependencies = function(){
var name;
for (name in obj) {
checkDependency(opts[name]);
}
};
checkProp = function(){
if (prop) {
throw new Error("Value for '" + prop + "' of type '" + getOption(prop).type + "' required.");
}
};
switch (toString$.call(input).slice(8, -1)) {
case 'String':
args = parseString(input.slice(slice != null ? slice : 0));
break;
case 'Array':
args = input.slice(slice != null ? slice : 2);
break;
case 'Object':
obj = {};
for (key in input) {
value = input[key];
if (key !== '_') {
option = getOption(dasherize(key));
if (parsedTypeCheck(option.parsedType, value)) {
obj[option.option] = value;
} else {
throw new Error("Option '" + option.option + "': Invalid type for '" + value + "' - expected type '" + option.type + "'.");
}
}
}
checkMutuallyExclusive();
checkDependencies();
setDefaults();
checkRequired();
return ref$ = camelizeKeys(obj), ref$._ = input._ || [], ref$;
default:
throw new Error("Invalid argument to 'parse': " + input + ".");
}
for (i$ = 0, len$ = args.length; i$ < len$; ++i$) {
arg = args[i$];
if (arg === '--') {
restPositional = true;
} else if (restPositional) {
positional.push(arg);
} else {
if (that = arg.match(/^(--?)([a-zA-Z][-a-zA-Z0-9]*)(=)?(.*)?$/)) {
result = that;
checkProp();
short = result[1].length === 1;
argName = result[2];
usingAssign = result[3] != null;
val = result[4];
if (usingAssign && val == null) {
throw new Error("No value for '" + argName + "' specified.");
}
if (short) {
flags = chars(argName);
len = flags.length;
for (j$ = 0, len1$ = flags.length; j$ < len1$; ++j$) {
i = j$;
flag = flags[j$];
opt = getOption(flag);
name = opt.option;
if (restPositional) {
positional.push(flag);
} else if (i === len - 1) {
if (usingAssign) {
valPrime = opt.boolean ? parseLevn([{
type: 'Boolean'
}], val) : val;
setValue(name, valPrime);
} else if (opt.boolean) {
setValue(name, true);
} else {
prop = name;
}
} else if (opt.boolean) {
setValue(name, true);
} else {
throw new Error("Can't set argument '" + flag + "' when not last flag in a group of short flags.");
}
}
} else {
negated = false;
if (that = argName.match(/^no-(.+)$/)) {
negated = true;
noedName = that[1];
opt = getOption(noedName);
} else {
opt = getOption(argName);
}
name = opt.option;
if (opt.boolean) {
valPrime = usingAssign ? parseLevn([{
type: 'Boolean'
}], val) : true;
if (negated) {
setValue(name, !valPrime);
} else {
setValue(name, valPrime);
}
} else {
if (negated) {
throw new Error("Only use 'no-' prefix for Boolean options, not with '" + noedName + "'.");
}
if (usingAssign) {
setValue(name, val);
} else {
prop = name;
}
}
}
} else if (that = arg.match(/^-([0-9]+(?:\.[0-9]+)?)$/)) {
opt = opts.NUM;
if (!opt) {
throw new Error('No -NUM option defined.');
}
setValue(opt.option, that[1]);
} else {
if (prop) {
setValue(prop, arg);
prop = null;
} else {
positional.push(arg);
if (!libOptions.positionalAnywhere) {
restPositional = true;
}
}
}
}
}
checkProp();
checkMutuallyExclusive();
checkDependencies();
setDefaults();
checkRequired();
return ref$ = camelizeKeys(obj), ref$._ = positional, ref$;
};
return {
parse: parse,
parseArgv: function(it){
return parse(it, {
slice: 2
});
},
generateHelp: generateHelp(libOptions),
generateHelpForOption: generateHelpForOption(getOption, libOptions)
};
};
main.VERSION = VERSION;
module.exports = main;
function import$(obj, src){
var own = {}.hasOwnProperty;
for (var key in src) if (own.call(src, key)) obj[key] = src[key];
return obj;
}
}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/optionator/lib/index.js | index.js |
# The MIT License (MIT)
Copyright (c) 2017 Tiancheng "Timothy" Gu and other contributors
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/w3c-hr-time/LICENSE.md | LICENSE.md |
# w3c-hr-time
This module implements the W3C [High Resolution Time Level 2][HR-TIME] specification. It provides exactly three exports:
- `Performance` class
- `getGlobalMonotonicClockMS(): number`
- `clockIsAccurate: boolean`
In all APIs, a "high-resolution timestamp" means a number in milliseconds that may have a fractional part, if the system clock is accurate enough (see "Clock accuracy" section below). It is identical to the [`DOMHighResTimeStamp`][] type in the High Resolution Time spec.
Portability is paramount to this module. It uses only APIs exposed from Node.js core like `Date.now()` and `process.hrtime()` and does not use or require any native modules. It also employs the [browser-process-hrtime][] module for graceful degrades for platforms that do not have `process.hrtime()` (such as browsers).
## `Performance` class
```js
const { Performance } = require("w3c-hr-time");
const performance = new Performance();
console.log(performance.timeOrigin);
// Prints a number close to Date.now() but that may have fractional parts, like
// 1514888563819.351.
console.log(new Date(performance.timeOrigin));
// Prints a date close to new Date(), like 2018-01-02T10:22:43.819Z.
setTimeout(() => {
console.log(performance.now());
// Prints a number close to 5000, like 5008.023059.
}, 5000);
```
Perhaps the most interesting export is the [`Performance`][] class. By constructing the class, you can get an instance quite similar to the `window.performance` object in browsers. Specifically, the following APIs are implemented:
* [`performance.now(): number`][`Performance#now()`] returns the high-resolution duration since the construction of the `Performance` object.
* [`performance.timeOrigin: number`][`Performance#timeOrigin`] is a high-resolution timestamp of the `Performance` object's construction, expressed in [Unix time][].
* [`performance.toJSON(): object`][`Performance#toJSON()`] returns an object with `timeOrigin` property set to the corresponding value of this object. This allows serializing the `Performance` object with `JSON.stringify()`. In browsers, the returned object may contain additional properties such as `navigation` and `timing`. However, those properties are specific to browser navigation and are unsuitable for a Node.js implementation. Furthermore, they are specified not in the High Resolution Time spec but in [Navigation Timing][NAVIGATION-TIMING], and are thereby outside the scope of this module.
### Limitations
This module does not aim for full [Web IDL][WEBIDL] conformance, so things like `performance.toJSON.call({})` will not throw `TypeError`s like it does in browsers. If you need full Web IDL conformance, you may be interested in the [webidl2js][] module.
The `Performance` class provided also does not have `mark()`, `measure()`, `getEntries()`, and such functions. They are specified in other specs than High Resolution Timing, such as [User Timing][USER-TIMING] (marks and measures) and [Performance Timeline][PERFORMANCE-TIMELINE] (entries management). Those specs extend the definition of the `Performance` class defined in High Resolution Timing, and to implement those specs you can extend the `Performance` class exported by this module.
Due to the limitations of the APIs exposed through Node.js, the construction of a `Performance` object may take up to 1 millisecond to gather information for high-resolution `timeOrigin`.
## Global monotonic clock
The High Resolution Time spec defines a [global monotonic clock][] that is "shared by time origin's *[sic]*, is monotonically increasing and not subject to system clock adjustments or system clock skew, and whose reference point is the Unix time."
This module exports a function `getGlobalMonotonicClockMS()` that is the basis of all timing functions used my this module when a monotonic time is required. It returns a high-resolution timestamp whose zero value is at some arbitrary point in the past. (For the current high-resolution timestamp based on the Unix epoch, use `new Performance().timeOrigin` instead.)
```js
const { getGlobalMonotonicClockMS } = require("w3c-hr-time");
const start = getGlobalMonotonicClockMS();
console.log(start);
// Prints a millisecond timestamp based on an arbitrary point in the past, like
// 280249733.012151.
setTimeout(() => {
console.log(getGlobalMonotonicClockMS() - a);
// Prints a number close to 5000, like 5006.156536.
}, 5000);
```
Unlike other functions that return only integer timestamps if the system clock does not provide enough resolution, this function may still return timestamps with fractional parts on those systems with less accurate clocks. See "Clock accuracy" section below for more information.
## Clock accuracy
The High Resolution Time spec [states][HR-TIME §4] that
> A [`DOMHighResTimeStamp`][] *SHOULD* represent a time in milliseconds accurate to 5 microseconds - see [8. Privacy and Security][HR-TIME §8].
>
> If the User Agent is unable to provide a time value accurate to 5 microseconds due to hardware or software constraints, the User Agent can represent a [`DOMHighResTimeStamp`][] as a time in milliseconds accurate to a millisecond.
This module implements this suggestion faithfully. It executes a test at `require()`-time to determine if the system clock (both `Date.now()` and `process.hrtime()`) is accurate enough to 5 microseconds. The result of this test can be accessed through the exported `clockIsAccurate` boolean value.
```js
const { Performance, clockIsAccurate } = require("w3c-hr-time");
const performance = new Performance();
if (!clockIsAccurate) {
console.assert(Number.isInteger(performance.timeOrigin));
console.assert(Number.isInteger(performance.now()));
}
```
If `clockIsAccurate` is false, `performance.timeOrigin` and `performance.now()` are always rounded to millisecond accuracy. `getGlobalMonotonicClockMS()` however is exempt from this requirement due to its best-effort nature, and the fact that it is not an API exposed by the High Resolution Time spec.
## Clock drift
[Clock drift][clock drift] can be observed through system or user clock adjustments -- that is, the speed at which `Date.now()` changes may be faster or slower than real time if there is a pending adjustment to the system clock, for example through NTP synchronizing.
In the spec, the [global monotonic clock][] is defined to be immune to such drifts. Correspondingly, the APIs exposed through this module that are defined using the global monotonic clock such as `performance.now()` and `getGlobalMonotonicClockMS()` are also guaranteed to reflect real time.
For example, if `performance.now()` returns 1000, it is guaranteed that the time of this call is exactly one second since the construction of the `Performance` object. But the difference in `Date.now()`'s value from the construction of the `Performance` object to when `performance.now()` returns 1000 may not be exactly 1000. You may also see `performance.now() - Date.now()` diverge over time as a result of clock drifts.
On the other hand, `performance.timeOrigin` returns the *[Unix time][]* at which the `Performance` object is constructed and relies on the current time exposed through `Date.now()`. That means that it is susceptible to clock drifts that has occurred before the `Performance` object was constructed.
## Supporting w3c-hr-time
The jsdom project (including w3c-hr-time) is a community-driven project maintained by a team of [volunteers](https://github.com/orgs/jsdom/people). You could support us by:
- [Getting professional support for w3c-hr-time](https://tidelift.com/subscription/pkg/npm-w3c-hr-time?utm_source=npm-w3c-hr-time&utm_medium=referral&utm_campaign=readme) as part of a Tidelift subscription. Tidelift helps making open source sustainable for us while giving teams assurances for maintenance, licensing, and security.
- Contributing directly to the project.
## License
This software is licensed under the MIT license. See LICENSE.md file for more detail.
[HR-TIME]: https://w3c.github.io/hr-time/
[NAVIGATION-TIMING]: https://w3c.github.io/navigation-timing/
[PERFORMANCE-TIMELINE]: https://w3c.github.io/performance-timeline/
[USER-TIMING]: https://w3c.github.io/user-timing/
[WEBIDL]: https://heycam.github.io/webidl/
[HR-TIME §4]: https://w3c.github.io/hr-time/#dom-domhighrestimestamp
[HR-TIME §8]: https://w3c.github.io/hr-time/#privacy-security
[`DOMHighResTimeStamp`]: https://w3c.github.io/hr-time/#dom-domhighrestimestamp
[`Performance`]: https://w3c.github.io/hr-time/#dfn-performance
[`Performance#now()`]: https://w3c.github.io/hr-time/#dom-performance-now
[`Performance#timeOrigin`]: https://w3c.github.io/hr-time/#dom-performance-timeorigin
[`Performance#toJSON()`]: https://w3c.github.io/hr-time/#dom-performance-tojson
[browser-process-hrtime]: https://www.npmjs.com/package/browser-process-hrtime
[clock drift]: https://en.wikipedia.org/wiki/Clock_drift
[global monotonic clock]: https://w3c.github.io/hr-time/#dfn-global-monotonic-clock
[Unix time]: https://en.wikipedia.org/wiki/Unix_time
[webidl2js]: https://github.com/jsdom/webidl2js
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/w3c-hr-time/README.md | README.md |
# Change log
## 1.0.2 (2020-03-05)
* Do not allow infinite loops when calculating `hrtime()`'s offset relative to UNIX time. ([#9])
* Add a LICENSE file. ([#3])
* Add Tidelift sponsorship link to README.
## 1.0.1 (2018-01-03)
* Make `performance.toJSON()` return an object with `timeOrigin` property, per [clarifications from specification authors][heycam/webidl#505].
## 1.0.0 (2018-01-02)
* Initial release.
[heycam/webidl#505]: https://github.com/heycam/webidl/pull/505
[#3]: https://github.com/jsdom/w3c-hr-time/issues/3
[#9]: https://github.com/jsdom/w3c-hr-time/issues/9
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/w3c-hr-time/CHANGELOG.md | CHANGELOG.md |
(function () {
'use strict';
var Syntax,
Precedence,
BinaryPrecedence,
SourceNode,
estraverse,
esutils,
base,
indent,
json,
renumber,
hexadecimal,
quotes,
escapeless,
newline,
space,
parentheses,
semicolons,
safeConcatenation,
directive,
extra,
parse,
sourceMap,
sourceCode,
preserveBlankLines,
FORMAT_MINIFY,
FORMAT_DEFAULTS;
estraverse = require('estraverse');
esutils = require('esutils');
Syntax = estraverse.Syntax;
// Generation is done by generateExpression.
function isExpression(node) {
return CodeGenerator.Expression.hasOwnProperty(node.type);
}
// Generation is done by generateStatement.
function isStatement(node) {
return CodeGenerator.Statement.hasOwnProperty(node.type);
}
Precedence = {
Sequence: 0,
Yield: 1,
Assignment: 1,
Conditional: 2,
ArrowFunction: 2,
LogicalOR: 3,
LogicalAND: 4,
BitwiseOR: 5,
BitwiseXOR: 6,
BitwiseAND: 7,
Equality: 8,
Relational: 9,
BitwiseSHIFT: 10,
Additive: 11,
Multiplicative: 12,
Exponentiation: 13,
Await: 14,
Unary: 14,
Postfix: 15,
Call: 16,
New: 17,
TaggedTemplate: 18,
Member: 19,
Primary: 20
};
BinaryPrecedence = {
'||': Precedence.LogicalOR,
'&&': Precedence.LogicalAND,
'|': Precedence.BitwiseOR,
'^': Precedence.BitwiseXOR,
'&': Precedence.BitwiseAND,
'==': Precedence.Equality,
'!=': Precedence.Equality,
'===': Precedence.Equality,
'!==': Precedence.Equality,
'is': Precedence.Equality,
'isnt': Precedence.Equality,
'<': Precedence.Relational,
'>': Precedence.Relational,
'<=': Precedence.Relational,
'>=': Precedence.Relational,
'in': Precedence.Relational,
'instanceof': Precedence.Relational,
'<<': Precedence.BitwiseSHIFT,
'>>': Precedence.BitwiseSHIFT,
'>>>': Precedence.BitwiseSHIFT,
'+': Precedence.Additive,
'-': Precedence.Additive,
'*': Precedence.Multiplicative,
'%': Precedence.Multiplicative,
'/': Precedence.Multiplicative,
'**': Precedence.Exponentiation
};
//Flags
var F_ALLOW_IN = 1,
F_ALLOW_CALL = 1 << 1,
F_ALLOW_UNPARATH_NEW = 1 << 2,
F_FUNC_BODY = 1 << 3,
F_DIRECTIVE_CTX = 1 << 4,
F_SEMICOLON_OPT = 1 << 5;
//Expression flag sets
//NOTE: Flag order:
// F_ALLOW_IN
// F_ALLOW_CALL
// F_ALLOW_UNPARATH_NEW
var E_FTT = F_ALLOW_CALL | F_ALLOW_UNPARATH_NEW,
E_TTF = F_ALLOW_IN | F_ALLOW_CALL,
E_TTT = F_ALLOW_IN | F_ALLOW_CALL | F_ALLOW_UNPARATH_NEW,
E_TFF = F_ALLOW_IN,
E_FFT = F_ALLOW_UNPARATH_NEW,
E_TFT = F_ALLOW_IN | F_ALLOW_UNPARATH_NEW;
//Statement flag sets
//NOTE: Flag order:
// F_ALLOW_IN
// F_FUNC_BODY
// F_DIRECTIVE_CTX
// F_SEMICOLON_OPT
var S_TFFF = F_ALLOW_IN,
S_TFFT = F_ALLOW_IN | F_SEMICOLON_OPT,
S_FFFF = 0x00,
S_TFTF = F_ALLOW_IN | F_DIRECTIVE_CTX,
S_TTFF = F_ALLOW_IN | F_FUNC_BODY;
function getDefaultOptions() {
// default options
return {
indent: null,
base: null,
parse: null,
comment: false,
format: {
indent: {
style: ' ',
base: 0,
adjustMultilineComment: false
},
newline: '\n',
space: ' ',
json: false,
renumber: false,
hexadecimal: false,
quotes: 'single',
escapeless: false,
compact: false,
parentheses: true,
semicolons: true,
safeConcatenation: false,
preserveBlankLines: false
},
moz: {
comprehensionExpressionStartsWithAssignment: false,
starlessGenerator: false
},
sourceMap: null,
sourceMapRoot: null,
sourceMapWithCode: false,
directive: false,
raw: true,
verbatim: null,
sourceCode: null
};
}
function stringRepeat(str, num) {
var result = '';
for (num |= 0; num > 0; num >>>= 1, str += str) {
if (num & 1) {
result += str;
}
}
return result;
}
function hasLineTerminator(str) {
return (/[\r\n]/g).test(str);
}
function endsWithLineTerminator(str) {
var len = str.length;
return len && esutils.code.isLineTerminator(str.charCodeAt(len - 1));
}
function merge(target, override) {
var key;
for (key in override) {
if (override.hasOwnProperty(key)) {
target[key] = override[key];
}
}
return target;
}
function updateDeeply(target, override) {
var key, val;
function isHashObject(target) {
return typeof target === 'object' && target instanceof Object && !(target instanceof RegExp);
}
for (key in override) {
if (override.hasOwnProperty(key)) {
val = override[key];
if (isHashObject(val)) {
if (isHashObject(target[key])) {
updateDeeply(target[key], val);
} else {
target[key] = updateDeeply({}, val);
}
} else {
target[key] = val;
}
}
}
return target;
}
function generateNumber(value) {
var result, point, temp, exponent, pos;
if (value !== value) {
throw new Error('Numeric literal whose value is NaN');
}
if (value < 0 || (value === 0 && 1 / value < 0)) {
throw new Error('Numeric literal whose value is negative');
}
if (value === 1 / 0) {
return json ? 'null' : renumber ? '1e400' : '1e+400';
}
result = '' + value;
if (!renumber || result.length < 3) {
return result;
}
point = result.indexOf('.');
if (!json && result.charCodeAt(0) === 0x30 /* 0 */ && point === 1) {
point = 0;
result = result.slice(1);
}
temp = result;
result = result.replace('e+', 'e');
exponent = 0;
if ((pos = temp.indexOf('e')) > 0) {
exponent = +temp.slice(pos + 1);
temp = temp.slice(0, pos);
}
if (point >= 0) {
exponent -= temp.length - point - 1;
temp = +(temp.slice(0, point) + temp.slice(point + 1)) + '';
}
pos = 0;
while (temp.charCodeAt(temp.length + pos - 1) === 0x30 /* 0 */) {
--pos;
}
if (pos !== 0) {
exponent -= pos;
temp = temp.slice(0, pos);
}
if (exponent !== 0) {
temp += 'e' + exponent;
}
if ((temp.length < result.length ||
(hexadecimal && value > 1e12 && Math.floor(value) === value && (temp = '0x' + value.toString(16)).length < result.length)) &&
+temp === value) {
result = temp;
}
return result;
}
// Generate valid RegExp expression.
// This function is based on https://github.com/Constellation/iv Engine
function escapeRegExpCharacter(ch, previousIsBackslash) {
// not handling '\' and handling \u2028 or \u2029 to unicode escape sequence
if ((ch & ~1) === 0x2028) {
return (previousIsBackslash ? 'u' : '\\u') + ((ch === 0x2028) ? '2028' : '2029');
} else if (ch === 10 || ch === 13) { // \n, \r
return (previousIsBackslash ? '' : '\\') + ((ch === 10) ? 'n' : 'r');
}
return String.fromCharCode(ch);
}
function generateRegExp(reg) {
var match, result, flags, i, iz, ch, characterInBrack, previousIsBackslash;
result = reg.toString();
if (reg.source) {
// extract flag from toString result
match = result.match(/\/([^/]*)$/);
if (!match) {
return result;
}
flags = match[1];
result = '';
characterInBrack = false;
previousIsBackslash = false;
for (i = 0, iz = reg.source.length; i < iz; ++i) {
ch = reg.source.charCodeAt(i);
if (!previousIsBackslash) {
if (characterInBrack) {
if (ch === 93) { // ]
characterInBrack = false;
}
} else {
if (ch === 47) { // /
result += '\\';
} else if (ch === 91) { // [
characterInBrack = true;
}
}
result += escapeRegExpCharacter(ch, previousIsBackslash);
previousIsBackslash = ch === 92; // \
} else {
// if new RegExp("\\\n') is provided, create /\n/
result += escapeRegExpCharacter(ch, previousIsBackslash);
// prevent like /\\[/]/
previousIsBackslash = false;
}
}
return '/' + result + '/' + flags;
}
return result;
}
function escapeAllowedCharacter(code, next) {
var hex;
if (code === 0x08 /* \b */) {
return '\\b';
}
if (code === 0x0C /* \f */) {
return '\\f';
}
if (code === 0x09 /* \t */) {
return '\\t';
}
hex = code.toString(16).toUpperCase();
if (json || code > 0xFF) {
return '\\u' + '0000'.slice(hex.length) + hex;
} else if (code === 0x0000 && !esutils.code.isDecimalDigit(next)) {
return '\\0';
} else if (code === 0x000B /* \v */) { // '\v'
return '\\x0B';
} else {
return '\\x' + '00'.slice(hex.length) + hex;
}
}
function escapeDisallowedCharacter(code) {
if (code === 0x5C /* \ */) {
return '\\\\';
}
if (code === 0x0A /* \n */) {
return '\\n';
}
if (code === 0x0D /* \r */) {
return '\\r';
}
if (code === 0x2028) {
return '\\u2028';
}
if (code === 0x2029) {
return '\\u2029';
}
throw new Error('Incorrectly classified character');
}
function escapeDirective(str) {
var i, iz, code, quote;
quote = quotes === 'double' ? '"' : '\'';
for (i = 0, iz = str.length; i < iz; ++i) {
code = str.charCodeAt(i);
if (code === 0x27 /* ' */) {
quote = '"';
break;
} else if (code === 0x22 /* " */) {
quote = '\'';
break;
} else if (code === 0x5C /* \ */) {
++i;
}
}
return quote + str + quote;
}
function escapeString(str) {
var result = '', i, len, code, singleQuotes = 0, doubleQuotes = 0, single, quote;
for (i = 0, len = str.length; i < len; ++i) {
code = str.charCodeAt(i);
if (code === 0x27 /* ' */) {
++singleQuotes;
} else if (code === 0x22 /* " */) {
++doubleQuotes;
} else if (code === 0x2F /* / */ && json) {
result += '\\';
} else if (esutils.code.isLineTerminator(code) || code === 0x5C /* \ */) {
result += escapeDisallowedCharacter(code);
continue;
} else if (!esutils.code.isIdentifierPartES5(code) && (json && code < 0x20 /* SP */ || !json && !escapeless && (code < 0x20 /* SP */ || code > 0x7E /* ~ */))) {
result += escapeAllowedCharacter(code, str.charCodeAt(i + 1));
continue;
}
result += String.fromCharCode(code);
}
single = !(quotes === 'double' || (quotes === 'auto' && doubleQuotes < singleQuotes));
quote = single ? '\'' : '"';
if (!(single ? singleQuotes : doubleQuotes)) {
return quote + result + quote;
}
str = result;
result = quote;
for (i = 0, len = str.length; i < len; ++i) {
code = str.charCodeAt(i);
if ((code === 0x27 /* ' */ && single) || (code === 0x22 /* " */ && !single)) {
result += '\\';
}
result += String.fromCharCode(code);
}
return result + quote;
}
/**
* flatten an array to a string, where the array can contain
* either strings or nested arrays
*/
function flattenToString(arr) {
var i, iz, elem, result = '';
for (i = 0, iz = arr.length; i < iz; ++i) {
elem = arr[i];
result += Array.isArray(elem) ? flattenToString(elem) : elem;
}
return result;
}
/**
* convert generated to a SourceNode when source maps are enabled.
*/
function toSourceNodeWhenNeeded(generated, node) {
if (!sourceMap) {
// with no source maps, generated is either an
// array or a string. if an array, flatten it.
// if a string, just return it
if (Array.isArray(generated)) {
return flattenToString(generated);
} else {
return generated;
}
}
if (node == null) {
if (generated instanceof SourceNode) {
return generated;
} else {
node = {};
}
}
if (node.loc == null) {
return new SourceNode(null, null, sourceMap, generated, node.name || null);
}
return new SourceNode(node.loc.start.line, node.loc.start.column, (sourceMap === true ? node.loc.source || null : sourceMap), generated, node.name || null);
}
function noEmptySpace() {
return (space) ? space : ' ';
}
function join(left, right) {
var leftSource,
rightSource,
leftCharCode,
rightCharCode;
leftSource = toSourceNodeWhenNeeded(left).toString();
if (leftSource.length === 0) {
return [right];
}
rightSource = toSourceNodeWhenNeeded(right).toString();
if (rightSource.length === 0) {
return [left];
}
leftCharCode = leftSource.charCodeAt(leftSource.length - 1);
rightCharCode = rightSource.charCodeAt(0);
if ((leftCharCode === 0x2B /* + */ || leftCharCode === 0x2D /* - */) && leftCharCode === rightCharCode ||
esutils.code.isIdentifierPartES5(leftCharCode) && esutils.code.isIdentifierPartES5(rightCharCode) ||
leftCharCode === 0x2F /* / */ && rightCharCode === 0x69 /* i */) { // infix word operators all start with `i`
return [left, noEmptySpace(), right];
} else if (esutils.code.isWhiteSpace(leftCharCode) || esutils.code.isLineTerminator(leftCharCode) ||
esutils.code.isWhiteSpace(rightCharCode) || esutils.code.isLineTerminator(rightCharCode)) {
return [left, right];
}
return [left, space, right];
}
function addIndent(stmt) {
return [base, stmt];
}
function withIndent(fn) {
var previousBase;
previousBase = base;
base += indent;
fn(base);
base = previousBase;
}
function calculateSpaces(str) {
var i;
for (i = str.length - 1; i >= 0; --i) {
if (esutils.code.isLineTerminator(str.charCodeAt(i))) {
break;
}
}
return (str.length - 1) - i;
}
function adjustMultilineComment(value, specialBase) {
var array, i, len, line, j, spaces, previousBase, sn;
array = value.split(/\r\n|[\r\n]/);
spaces = Number.MAX_VALUE;
// first line doesn't have indentation
for (i = 1, len = array.length; i < len; ++i) {
line = array[i];
j = 0;
while (j < line.length && esutils.code.isWhiteSpace(line.charCodeAt(j))) {
++j;
}
if (spaces > j) {
spaces = j;
}
}
if (typeof specialBase !== 'undefined') {
// pattern like
// {
// var t = 20; /*
// * this is comment
// */
// }
previousBase = base;
if (array[1][spaces] === '*') {
specialBase += ' ';
}
base = specialBase;
} else {
if (spaces & 1) {
// /*
// *
// */
// If spaces are odd number, above pattern is considered.
// We waste 1 space.
--spaces;
}
previousBase = base;
}
for (i = 1, len = array.length; i < len; ++i) {
sn = toSourceNodeWhenNeeded(addIndent(array[i].slice(spaces)));
array[i] = sourceMap ? sn.join('') : sn;
}
base = previousBase;
return array.join('\n');
}
function generateComment(comment, specialBase) {
if (comment.type === 'Line') {
if (endsWithLineTerminator(comment.value)) {
return '//' + comment.value;
} else {
// Always use LineTerminator
var result = '//' + comment.value;
if (!preserveBlankLines) {
result += '\n';
}
return result;
}
}
if (extra.format.indent.adjustMultilineComment && /[\n\r]/.test(comment.value)) {
return adjustMultilineComment('/*' + comment.value + '*/', specialBase);
}
return '/*' + comment.value + '*/';
}
function addComments(stmt, result) {
var i, len, comment, save, tailingToStatement, specialBase, fragment,
extRange, range, prevRange, prefix, infix, suffix, count;
if (stmt.leadingComments && stmt.leadingComments.length > 0) {
save = result;
if (preserveBlankLines) {
comment = stmt.leadingComments[0];
result = [];
extRange = comment.extendedRange;
range = comment.range;
prefix = sourceCode.substring(extRange[0], range[0]);
count = (prefix.match(/\n/g) || []).length;
if (count > 0) {
result.push(stringRepeat('\n', count));
result.push(addIndent(generateComment(comment)));
} else {
result.push(prefix);
result.push(generateComment(comment));
}
prevRange = range;
for (i = 1, len = stmt.leadingComments.length; i < len; i++) {
comment = stmt.leadingComments[i];
range = comment.range;
infix = sourceCode.substring(prevRange[1], range[0]);
count = (infix.match(/\n/g) || []).length;
result.push(stringRepeat('\n', count));
result.push(addIndent(generateComment(comment)));
prevRange = range;
}
suffix = sourceCode.substring(range[1], extRange[1]);
count = (suffix.match(/\n/g) || []).length;
result.push(stringRepeat('\n', count));
} else {
comment = stmt.leadingComments[0];
result = [];
if (safeConcatenation && stmt.type === Syntax.Program && stmt.body.length === 0) {
result.push('\n');
}
result.push(generateComment(comment));
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push('\n');
}
for (i = 1, len = stmt.leadingComments.length; i < len; ++i) {
comment = stmt.leadingComments[i];
fragment = [generateComment(comment)];
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
fragment.push('\n');
}
result.push(addIndent(fragment));
}
}
result.push(addIndent(save));
}
if (stmt.trailingComments) {
if (preserveBlankLines) {
comment = stmt.trailingComments[0];
extRange = comment.extendedRange;
range = comment.range;
prefix = sourceCode.substring(extRange[0], range[0]);
count = (prefix.match(/\n/g) || []).length;
if (count > 0) {
result.push(stringRepeat('\n', count));
result.push(addIndent(generateComment(comment)));
} else {
result.push(prefix);
result.push(generateComment(comment));
}
} else {
tailingToStatement = !endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString());
specialBase = stringRepeat(' ', calculateSpaces(toSourceNodeWhenNeeded([base, result, indent]).toString()));
for (i = 0, len = stmt.trailingComments.length; i < len; ++i) {
comment = stmt.trailingComments[i];
if (tailingToStatement) {
// We assume target like following script
//
// var t = 20; /**
// * This is comment of t
// */
if (i === 0) {
// first case
result = [result, indent];
} else {
result = [result, specialBase];
}
result.push(generateComment(comment, specialBase));
} else {
result = [result, addIndent(generateComment(comment))];
}
if (i !== len - 1 && !endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result = [result, '\n'];
}
}
}
}
return result;
}
function generateBlankLines(start, end, result) {
var j, newlineCount = 0;
for (j = start; j < end; j++) {
if (sourceCode[j] === '\n') {
newlineCount++;
}
}
for (j = 1; j < newlineCount; j++) {
result.push(newline);
}
}
function parenthesize(text, current, should) {
if (current < should) {
return ['(', text, ')'];
}
return text;
}
function generateVerbatimString(string) {
var i, iz, result;
result = string.split(/\r\n|\n/);
for (i = 1, iz = result.length; i < iz; i++) {
result[i] = newline + base + result[i];
}
return result;
}
function generateVerbatim(expr, precedence) {
var verbatim, result, prec;
verbatim = expr[extra.verbatim];
if (typeof verbatim === 'string') {
result = parenthesize(generateVerbatimString(verbatim), Precedence.Sequence, precedence);
} else {
// verbatim is object
result = generateVerbatimString(verbatim.content);
prec = (verbatim.precedence != null) ? verbatim.precedence : Precedence.Sequence;
result = parenthesize(result, prec, precedence);
}
return toSourceNodeWhenNeeded(result, expr);
}
function CodeGenerator() {
}
// Helpers.
CodeGenerator.prototype.maybeBlock = function(stmt, flags) {
var result, noLeadingComment, that = this;
noLeadingComment = !extra.comment || !stmt.leadingComments;
if (stmt.type === Syntax.BlockStatement && noLeadingComment) {
return [space, this.generateStatement(stmt, flags)];
}
if (stmt.type === Syntax.EmptyStatement && noLeadingComment) {
return ';';
}
withIndent(function () {
result = [
newline,
addIndent(that.generateStatement(stmt, flags))
];
});
return result;
};
CodeGenerator.prototype.maybeBlockSuffix = function (stmt, result) {
var ends = endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString());
if (stmt.type === Syntax.BlockStatement && (!extra.comment || !stmt.leadingComments) && !ends) {
return [result, space];
}
if (ends) {
return [result, base];
}
return [result, newline, base];
};
function generateIdentifier(node) {
return toSourceNodeWhenNeeded(node.name, node);
}
function generateAsyncPrefix(node, spaceRequired) {
return node.async ? 'async' + (spaceRequired ? noEmptySpace() : space) : '';
}
function generateStarSuffix(node) {
var isGenerator = node.generator && !extra.moz.starlessGenerator;
return isGenerator ? '*' + space : '';
}
function generateMethodPrefix(prop) {
var func = prop.value, prefix = '';
if (func.async) {
prefix += generateAsyncPrefix(func, !prop.computed);
}
if (func.generator) {
// avoid space before method name
prefix += generateStarSuffix(func) ? '*' : '';
}
return prefix;
}
CodeGenerator.prototype.generatePattern = function (node, precedence, flags) {
if (node.type === Syntax.Identifier) {
return generateIdentifier(node);
}
return this.generateExpression(node, precedence, flags);
};
CodeGenerator.prototype.generateFunctionParams = function (node) {
var i, iz, result, hasDefault;
hasDefault = false;
if (node.type === Syntax.ArrowFunctionExpression &&
!node.rest && (!node.defaults || node.defaults.length === 0) &&
node.params.length === 1 && node.params[0].type === Syntax.Identifier) {
// arg => { } case
result = [generateAsyncPrefix(node, true), generateIdentifier(node.params[0])];
} else {
result = node.type === Syntax.ArrowFunctionExpression ? [generateAsyncPrefix(node, false)] : [];
result.push('(');
if (node.defaults) {
hasDefault = true;
}
for (i = 0, iz = node.params.length; i < iz; ++i) {
if (hasDefault && node.defaults[i]) {
// Handle default values.
result.push(this.generateAssignment(node.params[i], node.defaults[i], '=', Precedence.Assignment, E_TTT));
} else {
result.push(this.generatePattern(node.params[i], Precedence.Assignment, E_TTT));
}
if (i + 1 < iz) {
result.push(',' + space);
}
}
if (node.rest) {
if (node.params.length) {
result.push(',' + space);
}
result.push('...');
result.push(generateIdentifier(node.rest));
}
result.push(')');
}
return result;
};
CodeGenerator.prototype.generateFunctionBody = function (node) {
var result, expr;
result = this.generateFunctionParams(node);
if (node.type === Syntax.ArrowFunctionExpression) {
result.push(space);
result.push('=>');
}
if (node.expression) {
result.push(space);
expr = this.generateExpression(node.body, Precedence.Assignment, E_TTT);
if (expr.toString().charAt(0) === '{') {
expr = ['(', expr, ')'];
}
result.push(expr);
} else {
result.push(this.maybeBlock(node.body, S_TTFF));
}
return result;
};
CodeGenerator.prototype.generateIterationForStatement = function (operator, stmt, flags) {
var result = ['for' + (stmt.await ? noEmptySpace() + 'await' : '') + space + '('], that = this;
withIndent(function () {
if (stmt.left.type === Syntax.VariableDeclaration) {
withIndent(function () {
result.push(stmt.left.kind + noEmptySpace());
result.push(that.generateStatement(stmt.left.declarations[0], S_FFFF));
});
} else {
result.push(that.generateExpression(stmt.left, Precedence.Call, E_TTT));
}
result = join(result, operator);
result = [join(
result,
that.generateExpression(stmt.right, Precedence.Assignment, E_TTT)
), ')'];
});
result.push(this.maybeBlock(stmt.body, flags));
return result;
};
CodeGenerator.prototype.generatePropertyKey = function (expr, computed) {
var result = [];
if (computed) {
result.push('[');
}
result.push(this.generateExpression(expr, Precedence.Assignment, E_TTT));
if (computed) {
result.push(']');
}
return result;
};
CodeGenerator.prototype.generateAssignment = function (left, right, operator, precedence, flags) {
if (Precedence.Assignment < precedence) {
flags |= F_ALLOW_IN;
}
return parenthesize(
[
this.generateExpression(left, Precedence.Call, flags),
space + operator + space,
this.generateExpression(right, Precedence.Assignment, flags)
],
Precedence.Assignment,
precedence
);
};
CodeGenerator.prototype.semicolon = function (flags) {
if (!semicolons && flags & F_SEMICOLON_OPT) {
return '';
}
return ';';
};
// Statements.
CodeGenerator.Statement = {
BlockStatement: function (stmt, flags) {
var range, content, result = ['{', newline], that = this;
withIndent(function () {
// handle functions without any code
if (stmt.body.length === 0 && preserveBlankLines) {
range = stmt.range;
if (range[1] - range[0] > 2) {
content = sourceCode.substring(range[0] + 1, range[1] - 1);
if (content[0] === '\n') {
result = ['{'];
}
result.push(content);
}
}
var i, iz, fragment, bodyFlags;
bodyFlags = S_TFFF;
if (flags & F_FUNC_BODY) {
bodyFlags |= F_DIRECTIVE_CTX;
}
for (i = 0, iz = stmt.body.length; i < iz; ++i) {
if (preserveBlankLines) {
// handle spaces before the first line
if (i === 0) {
if (stmt.body[0].leadingComments) {
range = stmt.body[0].leadingComments[0].extendedRange;
content = sourceCode.substring(range[0], range[1]);
if (content[0] === '\n') {
result = ['{'];
}
}
if (!stmt.body[0].leadingComments) {
generateBlankLines(stmt.range[0], stmt.body[0].range[0], result);
}
}
// handle spaces between lines
if (i > 0) {
if (!stmt.body[i - 1].trailingComments && !stmt.body[i].leadingComments) {
generateBlankLines(stmt.body[i - 1].range[1], stmt.body[i].range[0], result);
}
}
}
if (i === iz - 1) {
bodyFlags |= F_SEMICOLON_OPT;
}
if (stmt.body[i].leadingComments && preserveBlankLines) {
fragment = that.generateStatement(stmt.body[i], bodyFlags);
} else {
fragment = addIndent(that.generateStatement(stmt.body[i], bodyFlags));
}
result.push(fragment);
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
if (preserveBlankLines && i < iz - 1) {
// don't add a new line if there are leading coments
// in the next statement
if (!stmt.body[i + 1].leadingComments) {
result.push(newline);
}
} else {
result.push(newline);
}
}
if (preserveBlankLines) {
// handle spaces after the last line
if (i === iz - 1) {
if (!stmt.body[i].trailingComments) {
generateBlankLines(stmt.body[i].range[1], stmt.range[1], result);
}
}
}
}
});
result.push(addIndent('}'));
return result;
},
BreakStatement: function (stmt, flags) {
if (stmt.label) {
return 'break ' + stmt.label.name + this.semicolon(flags);
}
return 'break' + this.semicolon(flags);
},
ContinueStatement: function (stmt, flags) {
if (stmt.label) {
return 'continue ' + stmt.label.name + this.semicolon(flags);
}
return 'continue' + this.semicolon(flags);
},
ClassBody: function (stmt, flags) {
var result = [ '{', newline], that = this;
withIndent(function (indent) {
var i, iz;
for (i = 0, iz = stmt.body.length; i < iz; ++i) {
result.push(indent);
result.push(that.generateExpression(stmt.body[i], Precedence.Sequence, E_TTT));
if (i + 1 < iz) {
result.push(newline);
}
}
});
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(base);
result.push('}');
return result;
},
ClassDeclaration: function (stmt, flags) {
var result, fragment;
result = ['class'];
if (stmt.id) {
result = join(result, this.generateExpression(stmt.id, Precedence.Sequence, E_TTT));
}
if (stmt.superClass) {
fragment = join('extends', this.generateExpression(stmt.superClass, Precedence.Unary, E_TTT));
result = join(result, fragment);
}
result.push(space);
result.push(this.generateStatement(stmt.body, S_TFFT));
return result;
},
DirectiveStatement: function (stmt, flags) {
if (extra.raw && stmt.raw) {
return stmt.raw + this.semicolon(flags);
}
return escapeDirective(stmt.directive) + this.semicolon(flags);
},
DoWhileStatement: function (stmt, flags) {
// Because `do 42 while (cond)` is Syntax Error. We need semicolon.
var result = join('do', this.maybeBlock(stmt.body, S_TFFF));
result = this.maybeBlockSuffix(stmt.body, result);
return join(result, [
'while' + space + '(',
this.generateExpression(stmt.test, Precedence.Sequence, E_TTT),
')' + this.semicolon(flags)
]);
},
CatchClause: function (stmt, flags) {
var result, that = this;
withIndent(function () {
var guard;
if (stmt.param) {
result = [
'catch' + space + '(',
that.generateExpression(stmt.param, Precedence.Sequence, E_TTT),
')'
];
if (stmt.guard) {
guard = that.generateExpression(stmt.guard, Precedence.Sequence, E_TTT);
result.splice(2, 0, ' if ', guard);
}
} else {
result = ['catch'];
}
});
result.push(this.maybeBlock(stmt.body, S_TFFF));
return result;
},
DebuggerStatement: function (stmt, flags) {
return 'debugger' + this.semicolon(flags);
},
EmptyStatement: function (stmt, flags) {
return ';';
},
ExportDefaultDeclaration: function (stmt, flags) {
var result = [ 'export' ], bodyFlags;
bodyFlags = (flags & F_SEMICOLON_OPT) ? S_TFFT : S_TFFF;
// export default HoistableDeclaration[Default]
// export default AssignmentExpression[In] ;
result = join(result, 'default');
if (isStatement(stmt.declaration)) {
result = join(result, this.generateStatement(stmt.declaration, bodyFlags));
} else {
result = join(result, this.generateExpression(stmt.declaration, Precedence.Assignment, E_TTT) + this.semicolon(flags));
}
return result;
},
ExportNamedDeclaration: function (stmt, flags) {
var result = [ 'export' ], bodyFlags, that = this;
bodyFlags = (flags & F_SEMICOLON_OPT) ? S_TFFT : S_TFFF;
// export VariableStatement
// export Declaration[Default]
if (stmt.declaration) {
return join(result, this.generateStatement(stmt.declaration, bodyFlags));
}
// export ExportClause[NoReference] FromClause ;
// export ExportClause ;
if (stmt.specifiers) {
if (stmt.specifiers.length === 0) {
result = join(result, '{' + space + '}');
} else if (stmt.specifiers[0].type === Syntax.ExportBatchSpecifier) {
result = join(result, this.generateExpression(stmt.specifiers[0], Precedence.Sequence, E_TTT));
} else {
result = join(result, '{');
withIndent(function (indent) {
var i, iz;
result.push(newline);
for (i = 0, iz = stmt.specifiers.length; i < iz; ++i) {
result.push(indent);
result.push(that.generateExpression(stmt.specifiers[i], Precedence.Sequence, E_TTT));
if (i + 1 < iz) {
result.push(',' + newline);
}
}
});
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(base + '}');
}
if (stmt.source) {
result = join(result, [
'from' + space,
// ModuleSpecifier
this.generateExpression(stmt.source, Precedence.Sequence, E_TTT),
this.semicolon(flags)
]);
} else {
result.push(this.semicolon(flags));
}
}
return result;
},
ExportAllDeclaration: function (stmt, flags) {
// export * FromClause ;
return [
'export' + space,
'*' + space,
'from' + space,
// ModuleSpecifier
this.generateExpression(stmt.source, Precedence.Sequence, E_TTT),
this.semicolon(flags)
];
},
ExpressionStatement: function (stmt, flags) {
var result, fragment;
function isClassPrefixed(fragment) {
var code;
if (fragment.slice(0, 5) !== 'class') {
return false;
}
code = fragment.charCodeAt(5);
return code === 0x7B /* '{' */ || esutils.code.isWhiteSpace(code) || esutils.code.isLineTerminator(code);
}
function isFunctionPrefixed(fragment) {
var code;
if (fragment.slice(0, 8) !== 'function') {
return false;
}
code = fragment.charCodeAt(8);
return code === 0x28 /* '(' */ || esutils.code.isWhiteSpace(code) || code === 0x2A /* '*' */ || esutils.code.isLineTerminator(code);
}
function isAsyncPrefixed(fragment) {
var code, i, iz;
if (fragment.slice(0, 5) !== 'async') {
return false;
}
if (!esutils.code.isWhiteSpace(fragment.charCodeAt(5))) {
return false;
}
for (i = 6, iz = fragment.length; i < iz; ++i) {
if (!esutils.code.isWhiteSpace(fragment.charCodeAt(i))) {
break;
}
}
if (i === iz) {
return false;
}
if (fragment.slice(i, i + 8) !== 'function') {
return false;
}
code = fragment.charCodeAt(i + 8);
return code === 0x28 /* '(' */ || esutils.code.isWhiteSpace(code) || code === 0x2A /* '*' */ || esutils.code.isLineTerminator(code);
}
result = [this.generateExpression(stmt.expression, Precedence.Sequence, E_TTT)];
// 12.4 '{', 'function', 'class' is not allowed in this position.
// wrap expression with parentheses
fragment = toSourceNodeWhenNeeded(result).toString();
if (fragment.charCodeAt(0) === 0x7B /* '{' */ || // ObjectExpression
isClassPrefixed(fragment) ||
isFunctionPrefixed(fragment) ||
isAsyncPrefixed(fragment) ||
(directive && (flags & F_DIRECTIVE_CTX) && stmt.expression.type === Syntax.Literal && typeof stmt.expression.value === 'string')) {
result = ['(', result, ')' + this.semicolon(flags)];
} else {
result.push(this.semicolon(flags));
}
return result;
},
ImportDeclaration: function (stmt, flags) {
// ES6: 15.2.1 valid import declarations:
// - import ImportClause FromClause ;
// - import ModuleSpecifier ;
var result, cursor, that = this;
// If no ImportClause is present,
// this should be `import ModuleSpecifier` so skip `from`
// ModuleSpecifier is StringLiteral.
if (stmt.specifiers.length === 0) {
// import ModuleSpecifier ;
return [
'import',
space,
// ModuleSpecifier
this.generateExpression(stmt.source, Precedence.Sequence, E_TTT),
this.semicolon(flags)
];
}
// import ImportClause FromClause ;
result = [
'import'
];
cursor = 0;
// ImportedBinding
if (stmt.specifiers[cursor].type === Syntax.ImportDefaultSpecifier) {
result = join(result, [
this.generateExpression(stmt.specifiers[cursor], Precedence.Sequence, E_TTT)
]);
++cursor;
}
if (stmt.specifiers[cursor]) {
if (cursor !== 0) {
result.push(',');
}
if (stmt.specifiers[cursor].type === Syntax.ImportNamespaceSpecifier) {
// NameSpaceImport
result = join(result, [
space,
this.generateExpression(stmt.specifiers[cursor], Precedence.Sequence, E_TTT)
]);
} else {
// NamedImports
result.push(space + '{');
if ((stmt.specifiers.length - cursor) === 1) {
// import { ... } from "...";
result.push(space);
result.push(this.generateExpression(stmt.specifiers[cursor], Precedence.Sequence, E_TTT));
result.push(space + '}' + space);
} else {
// import {
// ...,
// ...,
// } from "...";
withIndent(function (indent) {
var i, iz;
result.push(newline);
for (i = cursor, iz = stmt.specifiers.length; i < iz; ++i) {
result.push(indent);
result.push(that.generateExpression(stmt.specifiers[i], Precedence.Sequence, E_TTT));
if (i + 1 < iz) {
result.push(',' + newline);
}
}
});
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(base + '}' + space);
}
}
}
result = join(result, [
'from' + space,
// ModuleSpecifier
this.generateExpression(stmt.source, Precedence.Sequence, E_TTT),
this.semicolon(flags)
]);
return result;
},
VariableDeclarator: function (stmt, flags) {
var itemFlags = (flags & F_ALLOW_IN) ? E_TTT : E_FTT;
if (stmt.init) {
return [
this.generateExpression(stmt.id, Precedence.Assignment, itemFlags),
space,
'=',
space,
this.generateExpression(stmt.init, Precedence.Assignment, itemFlags)
];
}
return this.generatePattern(stmt.id, Precedence.Assignment, itemFlags);
},
VariableDeclaration: function (stmt, flags) {
// VariableDeclarator is typed as Statement,
// but joined with comma (not LineTerminator).
// So if comment is attached to target node, we should specialize.
var result, i, iz, node, bodyFlags, that = this;
result = [ stmt.kind ];
bodyFlags = (flags & F_ALLOW_IN) ? S_TFFF : S_FFFF;
function block() {
node = stmt.declarations[0];
if (extra.comment && node.leadingComments) {
result.push('\n');
result.push(addIndent(that.generateStatement(node, bodyFlags)));
} else {
result.push(noEmptySpace());
result.push(that.generateStatement(node, bodyFlags));
}
for (i = 1, iz = stmt.declarations.length; i < iz; ++i) {
node = stmt.declarations[i];
if (extra.comment && node.leadingComments) {
result.push(',' + newline);
result.push(addIndent(that.generateStatement(node, bodyFlags)));
} else {
result.push(',' + space);
result.push(that.generateStatement(node, bodyFlags));
}
}
}
if (stmt.declarations.length > 1) {
withIndent(block);
} else {
block();
}
result.push(this.semicolon(flags));
return result;
},
ThrowStatement: function (stmt, flags) {
return [join(
'throw',
this.generateExpression(stmt.argument, Precedence.Sequence, E_TTT)
), this.semicolon(flags)];
},
TryStatement: function (stmt, flags) {
var result, i, iz, guardedHandlers;
result = ['try', this.maybeBlock(stmt.block, S_TFFF)];
result = this.maybeBlockSuffix(stmt.block, result);
if (stmt.handlers) {
// old interface
for (i = 0, iz = stmt.handlers.length; i < iz; ++i) {
result = join(result, this.generateStatement(stmt.handlers[i], S_TFFF));
if (stmt.finalizer || i + 1 !== iz) {
result = this.maybeBlockSuffix(stmt.handlers[i].body, result);
}
}
} else {
guardedHandlers = stmt.guardedHandlers || [];
for (i = 0, iz = guardedHandlers.length; i < iz; ++i) {
result = join(result, this.generateStatement(guardedHandlers[i], S_TFFF));
if (stmt.finalizer || i + 1 !== iz) {
result = this.maybeBlockSuffix(guardedHandlers[i].body, result);
}
}
// new interface
if (stmt.handler) {
if (Array.isArray(stmt.handler)) {
for (i = 0, iz = stmt.handler.length; i < iz; ++i) {
result = join(result, this.generateStatement(stmt.handler[i], S_TFFF));
if (stmt.finalizer || i + 1 !== iz) {
result = this.maybeBlockSuffix(stmt.handler[i].body, result);
}
}
} else {
result = join(result, this.generateStatement(stmt.handler, S_TFFF));
if (stmt.finalizer) {
result = this.maybeBlockSuffix(stmt.handler.body, result);
}
}
}
}
if (stmt.finalizer) {
result = join(result, ['finally', this.maybeBlock(stmt.finalizer, S_TFFF)]);
}
return result;
},
SwitchStatement: function (stmt, flags) {
var result, fragment, i, iz, bodyFlags, that = this;
withIndent(function () {
result = [
'switch' + space + '(',
that.generateExpression(stmt.discriminant, Precedence.Sequence, E_TTT),
')' + space + '{' + newline
];
});
if (stmt.cases) {
bodyFlags = S_TFFF;
for (i = 0, iz = stmt.cases.length; i < iz; ++i) {
if (i === iz - 1) {
bodyFlags |= F_SEMICOLON_OPT;
}
fragment = addIndent(this.generateStatement(stmt.cases[i], bodyFlags));
result.push(fragment);
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
result.push(newline);
}
}
}
result.push(addIndent('}'));
return result;
},
SwitchCase: function (stmt, flags) {
var result, fragment, i, iz, bodyFlags, that = this;
withIndent(function () {
if (stmt.test) {
result = [
join('case', that.generateExpression(stmt.test, Precedence.Sequence, E_TTT)),
':'
];
} else {
result = ['default:'];
}
i = 0;
iz = stmt.consequent.length;
if (iz && stmt.consequent[0].type === Syntax.BlockStatement) {
fragment = that.maybeBlock(stmt.consequent[0], S_TFFF);
result.push(fragment);
i = 1;
}
if (i !== iz && !endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
bodyFlags = S_TFFF;
for (; i < iz; ++i) {
if (i === iz - 1 && flags & F_SEMICOLON_OPT) {
bodyFlags |= F_SEMICOLON_OPT;
}
fragment = addIndent(that.generateStatement(stmt.consequent[i], bodyFlags));
result.push(fragment);
if (i + 1 !== iz && !endsWithLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
result.push(newline);
}
}
});
return result;
},
IfStatement: function (stmt, flags) {
var result, bodyFlags, semicolonOptional, that = this;
withIndent(function () {
result = [
'if' + space + '(',
that.generateExpression(stmt.test, Precedence.Sequence, E_TTT),
')'
];
});
semicolonOptional = flags & F_SEMICOLON_OPT;
bodyFlags = S_TFFF;
if (semicolonOptional) {
bodyFlags |= F_SEMICOLON_OPT;
}
if (stmt.alternate) {
result.push(this.maybeBlock(stmt.consequent, S_TFFF));
result = this.maybeBlockSuffix(stmt.consequent, result);
if (stmt.alternate.type === Syntax.IfStatement) {
result = join(result, ['else ', this.generateStatement(stmt.alternate, bodyFlags)]);
} else {
result = join(result, join('else', this.maybeBlock(stmt.alternate, bodyFlags)));
}
} else {
result.push(this.maybeBlock(stmt.consequent, bodyFlags));
}
return result;
},
ForStatement: function (stmt, flags) {
var result, that = this;
withIndent(function () {
result = ['for' + space + '('];
if (stmt.init) {
if (stmt.init.type === Syntax.VariableDeclaration) {
result.push(that.generateStatement(stmt.init, S_FFFF));
} else {
// F_ALLOW_IN becomes false.
result.push(that.generateExpression(stmt.init, Precedence.Sequence, E_FTT));
result.push(';');
}
} else {
result.push(';');
}
if (stmt.test) {
result.push(space);
result.push(that.generateExpression(stmt.test, Precedence.Sequence, E_TTT));
result.push(';');
} else {
result.push(';');
}
if (stmt.update) {
result.push(space);
result.push(that.generateExpression(stmt.update, Precedence.Sequence, E_TTT));
result.push(')');
} else {
result.push(')');
}
});
result.push(this.maybeBlock(stmt.body, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF));
return result;
},
ForInStatement: function (stmt, flags) {
return this.generateIterationForStatement('in', stmt, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF);
},
ForOfStatement: function (stmt, flags) {
return this.generateIterationForStatement('of', stmt, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF);
},
LabeledStatement: function (stmt, flags) {
return [stmt.label.name + ':', this.maybeBlock(stmt.body, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF)];
},
Program: function (stmt, flags) {
var result, fragment, i, iz, bodyFlags;
iz = stmt.body.length;
result = [safeConcatenation && iz > 0 ? '\n' : ''];
bodyFlags = S_TFTF;
for (i = 0; i < iz; ++i) {
if (!safeConcatenation && i === iz - 1) {
bodyFlags |= F_SEMICOLON_OPT;
}
if (preserveBlankLines) {
// handle spaces before the first line
if (i === 0) {
if (!stmt.body[0].leadingComments) {
generateBlankLines(stmt.range[0], stmt.body[i].range[0], result);
}
}
// handle spaces between lines
if (i > 0) {
if (!stmt.body[i - 1].trailingComments && !stmt.body[i].leadingComments) {
generateBlankLines(stmt.body[i - 1].range[1], stmt.body[i].range[0], result);
}
}
}
fragment = addIndent(this.generateStatement(stmt.body[i], bodyFlags));
result.push(fragment);
if (i + 1 < iz && !endsWithLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
if (preserveBlankLines) {
if (!stmt.body[i + 1].leadingComments) {
result.push(newline);
}
} else {
result.push(newline);
}
}
if (preserveBlankLines) {
// handle spaces after the last line
if (i === iz - 1) {
if (!stmt.body[i].trailingComments) {
generateBlankLines(stmt.body[i].range[1], stmt.range[1], result);
}
}
}
}
return result;
},
FunctionDeclaration: function (stmt, flags) {
return [
generateAsyncPrefix(stmt, true),
'function',
generateStarSuffix(stmt) || noEmptySpace(),
stmt.id ? generateIdentifier(stmt.id) : '',
this.generateFunctionBody(stmt)
];
},
ReturnStatement: function (stmt, flags) {
if (stmt.argument) {
return [join(
'return',
this.generateExpression(stmt.argument, Precedence.Sequence, E_TTT)
), this.semicolon(flags)];
}
return ['return' + this.semicolon(flags)];
},
WhileStatement: function (stmt, flags) {
var result, that = this;
withIndent(function () {
result = [
'while' + space + '(',
that.generateExpression(stmt.test, Precedence.Sequence, E_TTT),
')'
];
});
result.push(this.maybeBlock(stmt.body, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF));
return result;
},
WithStatement: function (stmt, flags) {
var result, that = this;
withIndent(function () {
result = [
'with' + space + '(',
that.generateExpression(stmt.object, Precedence.Sequence, E_TTT),
')'
];
});
result.push(this.maybeBlock(stmt.body, flags & F_SEMICOLON_OPT ? S_TFFT : S_TFFF));
return result;
}
};
merge(CodeGenerator.prototype, CodeGenerator.Statement);
// Expressions.
CodeGenerator.Expression = {
SequenceExpression: function (expr, precedence, flags) {
var result, i, iz;
if (Precedence.Sequence < precedence) {
flags |= F_ALLOW_IN;
}
result = [];
for (i = 0, iz = expr.expressions.length; i < iz; ++i) {
result.push(this.generateExpression(expr.expressions[i], Precedence.Assignment, flags));
if (i + 1 < iz) {
result.push(',' + space);
}
}
return parenthesize(result, Precedence.Sequence, precedence);
},
AssignmentExpression: function (expr, precedence, flags) {
return this.generateAssignment(expr.left, expr.right, expr.operator, precedence, flags);
},
ArrowFunctionExpression: function (expr, precedence, flags) {
return parenthesize(this.generateFunctionBody(expr), Precedence.ArrowFunction, precedence);
},
ConditionalExpression: function (expr, precedence, flags) {
if (Precedence.Conditional < precedence) {
flags |= F_ALLOW_IN;
}
return parenthesize(
[
this.generateExpression(expr.test, Precedence.LogicalOR, flags),
space + '?' + space,
this.generateExpression(expr.consequent, Precedence.Assignment, flags),
space + ':' + space,
this.generateExpression(expr.alternate, Precedence.Assignment, flags)
],
Precedence.Conditional,
precedence
);
},
LogicalExpression: function (expr, precedence, flags) {
return this.BinaryExpression(expr, precedence, flags);
},
BinaryExpression: function (expr, precedence, flags) {
var result, leftPrecedence, rightPrecedence, currentPrecedence, fragment, leftSource;
currentPrecedence = BinaryPrecedence[expr.operator];
leftPrecedence = expr.operator === '**' ? Precedence.Postfix : currentPrecedence;
rightPrecedence = expr.operator === '**' ? currentPrecedence : currentPrecedence + 1;
if (currentPrecedence < precedence) {
flags |= F_ALLOW_IN;
}
fragment = this.generateExpression(expr.left, leftPrecedence, flags);
leftSource = fragment.toString();
if (leftSource.charCodeAt(leftSource.length - 1) === 0x2F /* / */ && esutils.code.isIdentifierPartES5(expr.operator.charCodeAt(0))) {
result = [fragment, noEmptySpace(), expr.operator];
} else {
result = join(fragment, expr.operator);
}
fragment = this.generateExpression(expr.right, rightPrecedence, flags);
if (expr.operator === '/' && fragment.toString().charAt(0) === '/' ||
expr.operator.slice(-1) === '<' && fragment.toString().slice(0, 3) === '!--') {
// If '/' concats with '/' or `<` concats with `!--`, it is interpreted as comment start
result.push(noEmptySpace());
result.push(fragment);
} else {
result = join(result, fragment);
}
if (expr.operator === 'in' && !(flags & F_ALLOW_IN)) {
return ['(', result, ')'];
}
return parenthesize(result, currentPrecedence, precedence);
},
CallExpression: function (expr, precedence, flags) {
var result, i, iz;
// F_ALLOW_UNPARATH_NEW becomes false.
result = [this.generateExpression(expr.callee, Precedence.Call, E_TTF)];
result.push('(');
for (i = 0, iz = expr['arguments'].length; i < iz; ++i) {
result.push(this.generateExpression(expr['arguments'][i], Precedence.Assignment, E_TTT));
if (i + 1 < iz) {
result.push(',' + space);
}
}
result.push(')');
if (!(flags & F_ALLOW_CALL)) {
return ['(', result, ')'];
}
return parenthesize(result, Precedence.Call, precedence);
},
NewExpression: function (expr, precedence, flags) {
var result, length, i, iz, itemFlags;
length = expr['arguments'].length;
// F_ALLOW_CALL becomes false.
// F_ALLOW_UNPARATH_NEW may become false.
itemFlags = (flags & F_ALLOW_UNPARATH_NEW && !parentheses && length === 0) ? E_TFT : E_TFF;
result = join(
'new',
this.generateExpression(expr.callee, Precedence.New, itemFlags)
);
if (!(flags & F_ALLOW_UNPARATH_NEW) || parentheses || length > 0) {
result.push('(');
for (i = 0, iz = length; i < iz; ++i) {
result.push(this.generateExpression(expr['arguments'][i], Precedence.Assignment, E_TTT));
if (i + 1 < iz) {
result.push(',' + space);
}
}
result.push(')');
}
return parenthesize(result, Precedence.New, precedence);
},
MemberExpression: function (expr, precedence, flags) {
var result, fragment;
// F_ALLOW_UNPARATH_NEW becomes false.
result = [this.generateExpression(expr.object, Precedence.Call, (flags & F_ALLOW_CALL) ? E_TTF : E_TFF)];
if (expr.computed) {
result.push('[');
result.push(this.generateExpression(expr.property, Precedence.Sequence, flags & F_ALLOW_CALL ? E_TTT : E_TFT));
result.push(']');
} else {
if (expr.object.type === Syntax.Literal && typeof expr.object.value === 'number') {
fragment = toSourceNodeWhenNeeded(result).toString();
// When the following conditions are all true,
// 1. No floating point
// 2. Don't have exponents
// 3. The last character is a decimal digit
// 4. Not hexadecimal OR octal number literal
// we should add a floating point.
if (
fragment.indexOf('.') < 0 &&
!/[eExX]/.test(fragment) &&
esutils.code.isDecimalDigit(fragment.charCodeAt(fragment.length - 1)) &&
!(fragment.length >= 2 && fragment.charCodeAt(0) === 48) // '0'
) {
result.push(' ');
}
}
result.push('.');
result.push(generateIdentifier(expr.property));
}
return parenthesize(result, Precedence.Member, precedence);
},
MetaProperty: function (expr, precedence, flags) {
var result;
result = [];
result.push(typeof expr.meta === "string" ? expr.meta : generateIdentifier(expr.meta));
result.push('.');
result.push(typeof expr.property === "string" ? expr.property : generateIdentifier(expr.property));
return parenthesize(result, Precedence.Member, precedence);
},
UnaryExpression: function (expr, precedence, flags) {
var result, fragment, rightCharCode, leftSource, leftCharCode;
fragment = this.generateExpression(expr.argument, Precedence.Unary, E_TTT);
if (space === '') {
result = join(expr.operator, fragment);
} else {
result = [expr.operator];
if (expr.operator.length > 2) {
// delete, void, typeof
// get `typeof []`, not `typeof[]`
result = join(result, fragment);
} else {
// Prevent inserting spaces between operator and argument if it is unnecessary
// like, `!cond`
leftSource = toSourceNodeWhenNeeded(result).toString();
leftCharCode = leftSource.charCodeAt(leftSource.length - 1);
rightCharCode = fragment.toString().charCodeAt(0);
if (((leftCharCode === 0x2B /* + */ || leftCharCode === 0x2D /* - */) && leftCharCode === rightCharCode) ||
(esutils.code.isIdentifierPartES5(leftCharCode) && esutils.code.isIdentifierPartES5(rightCharCode))) {
result.push(noEmptySpace());
result.push(fragment);
} else {
result.push(fragment);
}
}
}
return parenthesize(result, Precedence.Unary, precedence);
},
YieldExpression: function (expr, precedence, flags) {
var result;
if (expr.delegate) {
result = 'yield*';
} else {
result = 'yield';
}
if (expr.argument) {
result = join(
result,
this.generateExpression(expr.argument, Precedence.Yield, E_TTT)
);
}
return parenthesize(result, Precedence.Yield, precedence);
},
AwaitExpression: function (expr, precedence, flags) {
var result = join(
expr.all ? 'await*' : 'await',
this.generateExpression(expr.argument, Precedence.Await, E_TTT)
);
return parenthesize(result, Precedence.Await, precedence);
},
UpdateExpression: function (expr, precedence, flags) {
if (expr.prefix) {
return parenthesize(
[
expr.operator,
this.generateExpression(expr.argument, Precedence.Unary, E_TTT)
],
Precedence.Unary,
precedence
);
}
return parenthesize(
[
this.generateExpression(expr.argument, Precedence.Postfix, E_TTT),
expr.operator
],
Precedence.Postfix,
precedence
);
},
FunctionExpression: function (expr, precedence, flags) {
var result = [
generateAsyncPrefix(expr, true),
'function'
];
if (expr.id) {
result.push(generateStarSuffix(expr) || noEmptySpace());
result.push(generateIdentifier(expr.id));
} else {
result.push(generateStarSuffix(expr) || space);
}
result.push(this.generateFunctionBody(expr));
return result;
},
ArrayPattern: function (expr, precedence, flags) {
return this.ArrayExpression(expr, precedence, flags, true);
},
ArrayExpression: function (expr, precedence, flags, isPattern) {
var result, multiline, that = this;
if (!expr.elements.length) {
return '[]';
}
multiline = isPattern ? false : expr.elements.length > 1;
result = ['[', multiline ? newline : ''];
withIndent(function (indent) {
var i, iz;
for (i = 0, iz = expr.elements.length; i < iz; ++i) {
if (!expr.elements[i]) {
if (multiline) {
result.push(indent);
}
if (i + 1 === iz) {
result.push(',');
}
} else {
result.push(multiline ? indent : '');
result.push(that.generateExpression(expr.elements[i], Precedence.Assignment, E_TTT));
}
if (i + 1 < iz) {
result.push(',' + (multiline ? newline : space));
}
}
});
if (multiline && !endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(multiline ? base : '');
result.push(']');
return result;
},
RestElement: function(expr, precedence, flags) {
return '...' + this.generatePattern(expr.argument);
},
ClassExpression: function (expr, precedence, flags) {
var result, fragment;
result = ['class'];
if (expr.id) {
result = join(result, this.generateExpression(expr.id, Precedence.Sequence, E_TTT));
}
if (expr.superClass) {
fragment = join('extends', this.generateExpression(expr.superClass, Precedence.Unary, E_TTT));
result = join(result, fragment);
}
result.push(space);
result.push(this.generateStatement(expr.body, S_TFFT));
return result;
},
MethodDefinition: function (expr, precedence, flags) {
var result, fragment;
if (expr['static']) {
result = ['static' + space];
} else {
result = [];
}
if (expr.kind === 'get' || expr.kind === 'set') {
fragment = [
join(expr.kind, this.generatePropertyKey(expr.key, expr.computed)),
this.generateFunctionBody(expr.value)
];
} else {
fragment = [
generateMethodPrefix(expr),
this.generatePropertyKey(expr.key, expr.computed),
this.generateFunctionBody(expr.value)
];
}
return join(result, fragment);
},
Property: function (expr, precedence, flags) {
if (expr.kind === 'get' || expr.kind === 'set') {
return [
expr.kind, noEmptySpace(),
this.generatePropertyKey(expr.key, expr.computed),
this.generateFunctionBody(expr.value)
];
}
if (expr.shorthand) {
if (expr.value.type === "AssignmentPattern") {
return this.AssignmentPattern(expr.value, Precedence.Sequence, E_TTT);
}
return this.generatePropertyKey(expr.key, expr.computed);
}
if (expr.method) {
return [
generateMethodPrefix(expr),
this.generatePropertyKey(expr.key, expr.computed),
this.generateFunctionBody(expr.value)
];
}
return [
this.generatePropertyKey(expr.key, expr.computed),
':' + space,
this.generateExpression(expr.value, Precedence.Assignment, E_TTT)
];
},
ObjectExpression: function (expr, precedence, flags) {
var multiline, result, fragment, that = this;
if (!expr.properties.length) {
return '{}';
}
multiline = expr.properties.length > 1;
withIndent(function () {
fragment = that.generateExpression(expr.properties[0], Precedence.Sequence, E_TTT);
});
if (!multiline) {
// issues 4
// Do not transform from
// dejavu.Class.declare({
// method2: function () {}
// });
// to
// dejavu.Class.declare({method2: function () {
// }});
if (!hasLineTerminator(toSourceNodeWhenNeeded(fragment).toString())) {
return [ '{', space, fragment, space, '}' ];
}
}
withIndent(function (indent) {
var i, iz;
result = [ '{', newline, indent, fragment ];
if (multiline) {
result.push(',' + newline);
for (i = 1, iz = expr.properties.length; i < iz; ++i) {
result.push(indent);
result.push(that.generateExpression(expr.properties[i], Precedence.Sequence, E_TTT));
if (i + 1 < iz) {
result.push(',' + newline);
}
}
}
});
if (!endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(base);
result.push('}');
return result;
},
AssignmentPattern: function(expr, precedence, flags) {
return this.generateAssignment(expr.left, expr.right, '=', precedence, flags);
},
ObjectPattern: function (expr, precedence, flags) {
var result, i, iz, multiline, property, that = this;
if (!expr.properties.length) {
return '{}';
}
multiline = false;
if (expr.properties.length === 1) {
property = expr.properties[0];
if (
property.type === Syntax.Property
&& property.value.type !== Syntax.Identifier
) {
multiline = true;
}
} else {
for (i = 0, iz = expr.properties.length; i < iz; ++i) {
property = expr.properties[i];
if (
property.type === Syntax.Property
&& !property.shorthand
) {
multiline = true;
break;
}
}
}
result = ['{', multiline ? newline : '' ];
withIndent(function (indent) {
var i, iz;
for (i = 0, iz = expr.properties.length; i < iz; ++i) {
result.push(multiline ? indent : '');
result.push(that.generateExpression(expr.properties[i], Precedence.Sequence, E_TTT));
if (i + 1 < iz) {
result.push(',' + (multiline ? newline : space));
}
}
});
if (multiline && !endsWithLineTerminator(toSourceNodeWhenNeeded(result).toString())) {
result.push(newline);
}
result.push(multiline ? base : '');
result.push('}');
return result;
},
ThisExpression: function (expr, precedence, flags) {
return 'this';
},
Super: function (expr, precedence, flags) {
return 'super';
},
Identifier: function (expr, precedence, flags) {
return generateIdentifier(expr);
},
ImportDefaultSpecifier: function (expr, precedence, flags) {
return generateIdentifier(expr.id || expr.local);
},
ImportNamespaceSpecifier: function (expr, precedence, flags) {
var result = ['*'];
var id = expr.id || expr.local;
if (id) {
result.push(space + 'as' + noEmptySpace() + generateIdentifier(id));
}
return result;
},
ImportSpecifier: function (expr, precedence, flags) {
var imported = expr.imported;
var result = [ imported.name ];
var local = expr.local;
if (local && local.name !== imported.name) {
result.push(noEmptySpace() + 'as' + noEmptySpace() + generateIdentifier(local));
}
return result;
},
ExportSpecifier: function (expr, precedence, flags) {
var local = expr.local;
var result = [ local.name ];
var exported = expr.exported;
if (exported && exported.name !== local.name) {
result.push(noEmptySpace() + 'as' + noEmptySpace() + generateIdentifier(exported));
}
return result;
},
Literal: function (expr, precedence, flags) {
var raw;
if (expr.hasOwnProperty('raw') && parse && extra.raw) {
try {
raw = parse(expr.raw).body[0].expression;
if (raw.type === Syntax.Literal) {
if (raw.value === expr.value) {
return expr.raw;
}
}
} catch (e) {
// not use raw property
}
}
if (expr.regex) {
return '/' + expr.regex.pattern + '/' + expr.regex.flags;
}
if (expr.value === null) {
return 'null';
}
if (typeof expr.value === 'string') {
return escapeString(expr.value);
}
if (typeof expr.value === 'number') {
return generateNumber(expr.value);
}
if (typeof expr.value === 'boolean') {
return expr.value ? 'true' : 'false';
}
return generateRegExp(expr.value);
},
GeneratorExpression: function (expr, precedence, flags) {
return this.ComprehensionExpression(expr, precedence, flags);
},
ComprehensionExpression: function (expr, precedence, flags) {
// GeneratorExpression should be parenthesized with (...), ComprehensionExpression with [...]
// Due to https://bugzilla.mozilla.org/show_bug.cgi?id=883468 position of expr.body can differ in Spidermonkey and ES6
var result, i, iz, fragment, that = this;
result = (expr.type === Syntax.GeneratorExpression) ? ['('] : ['['];
if (extra.moz.comprehensionExpressionStartsWithAssignment) {
fragment = this.generateExpression(expr.body, Precedence.Assignment, E_TTT);
result.push(fragment);
}
if (expr.blocks) {
withIndent(function () {
for (i = 0, iz = expr.blocks.length; i < iz; ++i) {
fragment = that.generateExpression(expr.blocks[i], Precedence.Sequence, E_TTT);
if (i > 0 || extra.moz.comprehensionExpressionStartsWithAssignment) {
result = join(result, fragment);
} else {
result.push(fragment);
}
}
});
}
if (expr.filter) {
result = join(result, 'if' + space);
fragment = this.generateExpression(expr.filter, Precedence.Sequence, E_TTT);
result = join(result, [ '(', fragment, ')' ]);
}
if (!extra.moz.comprehensionExpressionStartsWithAssignment) {
fragment = this.generateExpression(expr.body, Precedence.Assignment, E_TTT);
result = join(result, fragment);
}
result.push((expr.type === Syntax.GeneratorExpression) ? ')' : ']');
return result;
},
ComprehensionBlock: function (expr, precedence, flags) {
var fragment;
if (expr.left.type === Syntax.VariableDeclaration) {
fragment = [
expr.left.kind, noEmptySpace(),
this.generateStatement(expr.left.declarations[0], S_FFFF)
];
} else {
fragment = this.generateExpression(expr.left, Precedence.Call, E_TTT);
}
fragment = join(fragment, expr.of ? 'of' : 'in');
fragment = join(fragment, this.generateExpression(expr.right, Precedence.Sequence, E_TTT));
return [ 'for' + space + '(', fragment, ')' ];
},
SpreadElement: function (expr, precedence, flags) {
return [
'...',
this.generateExpression(expr.argument, Precedence.Assignment, E_TTT)
];
},
TaggedTemplateExpression: function (expr, precedence, flags) {
var itemFlags = E_TTF;
if (!(flags & F_ALLOW_CALL)) {
itemFlags = E_TFF;
}
var result = [
this.generateExpression(expr.tag, Precedence.Call, itemFlags),
this.generateExpression(expr.quasi, Precedence.Primary, E_FFT)
];
return parenthesize(result, Precedence.TaggedTemplate, precedence);
},
TemplateElement: function (expr, precedence, flags) {
// Don't use "cooked". Since tagged template can use raw template
// representation. So if we do so, it breaks the script semantics.
return expr.value.raw;
},
TemplateLiteral: function (expr, precedence, flags) {
var result, i, iz;
result = [ '`' ];
for (i = 0, iz = expr.quasis.length; i < iz; ++i) {
result.push(this.generateExpression(expr.quasis[i], Precedence.Primary, E_TTT));
if (i + 1 < iz) {
result.push('${' + space);
result.push(this.generateExpression(expr.expressions[i], Precedence.Sequence, E_TTT));
result.push(space + '}');
}
}
result.push('`');
return result;
},
ModuleSpecifier: function (expr, precedence, flags) {
return this.Literal(expr, precedence, flags);
},
ImportExpression: function(expr, precedence, flag) {
return parenthesize([
'import(',
this.generateExpression(expr.source, Precedence.Assignment, E_TTT),
')'
], Precedence.Call, precedence);
},
};
merge(CodeGenerator.prototype, CodeGenerator.Expression);
CodeGenerator.prototype.generateExpression = function (expr, precedence, flags) {
var result, type;
type = expr.type || Syntax.Property;
if (extra.verbatim && expr.hasOwnProperty(extra.verbatim)) {
return generateVerbatim(expr, precedence);
}
result = this[type](expr, precedence, flags);
if (extra.comment) {
result = addComments(expr, result);
}
return toSourceNodeWhenNeeded(result, expr);
};
CodeGenerator.prototype.generateStatement = function (stmt, flags) {
var result,
fragment;
result = this[stmt.type](stmt, flags);
// Attach comments
if (extra.comment) {
result = addComments(stmt, result);
}
fragment = toSourceNodeWhenNeeded(result).toString();
if (stmt.type === Syntax.Program && !safeConcatenation && newline === '' && fragment.charAt(fragment.length - 1) === '\n') {
result = sourceMap ? toSourceNodeWhenNeeded(result).replaceRight(/\s+$/, '') : fragment.replace(/\s+$/, '');
}
return toSourceNodeWhenNeeded(result, stmt);
};
function generateInternal(node) {
var codegen;
codegen = new CodeGenerator();
if (isStatement(node)) {
return codegen.generateStatement(node, S_TFFF);
}
if (isExpression(node)) {
return codegen.generateExpression(node, Precedence.Sequence, E_TTT);
}
throw new Error('Unknown node type: ' + node.type);
}
function generate(node, options) {
var defaultOptions = getDefaultOptions(), result, pair;
if (options != null) {
// Obsolete options
//
// `options.indent`
// `options.base`
//
// Instead of them, we can use `option.format.indent`.
if (typeof options.indent === 'string') {
defaultOptions.format.indent.style = options.indent;
}
if (typeof options.base === 'number') {
defaultOptions.format.indent.base = options.base;
}
options = updateDeeply(defaultOptions, options);
indent = options.format.indent.style;
if (typeof options.base === 'string') {
base = options.base;
} else {
base = stringRepeat(indent, options.format.indent.base);
}
} else {
options = defaultOptions;
indent = options.format.indent.style;
base = stringRepeat(indent, options.format.indent.base);
}
json = options.format.json;
renumber = options.format.renumber;
hexadecimal = json ? false : options.format.hexadecimal;
quotes = json ? 'double' : options.format.quotes;
escapeless = options.format.escapeless;
newline = options.format.newline;
space = options.format.space;
if (options.format.compact) {
newline = space = indent = base = '';
}
parentheses = options.format.parentheses;
semicolons = options.format.semicolons;
safeConcatenation = options.format.safeConcatenation;
directive = options.directive;
parse = json ? null : options.parse;
sourceMap = options.sourceMap;
sourceCode = options.sourceCode;
preserveBlankLines = options.format.preserveBlankLines && sourceCode !== null;
extra = options;
if (sourceMap) {
if (!exports.browser) {
// We assume environment is node.js
// And prevent from including source-map by browserify
SourceNode = require('source-map').SourceNode;
} else {
SourceNode = global.sourceMap.SourceNode;
}
}
result = generateInternal(node);
if (!sourceMap) {
pair = {code: result.toString(), map: null};
return options.sourceMapWithCode ? pair : pair.code;
}
pair = result.toStringWithSourceMap({
file: options.file,
sourceRoot: options.sourceMapRoot
});
if (options.sourceContent) {
pair.map.setSourceContent(options.sourceMap,
options.sourceContent);
}
if (options.sourceMapWithCode) {
return pair;
}
return pair.map.toString();
}
FORMAT_MINIFY = {
indent: {
style: '',
base: 0
},
renumber: true,
hexadecimal: true,
quotes: 'auto',
escapeless: true,
compact: true,
parentheses: false,
semicolons: false
};
FORMAT_DEFAULTS = getDefaultOptions().format;
exports.version = require('escodegen/package.json').version;
exports.generate = generate;
exports.attachComments = estraverse.attachComments;
exports.Precedence = updateDeeply({}, Precedence);
exports.browser = false;
exports.FORMAT_MINIFY = FORMAT_MINIFY;
exports.FORMAT_DEFAULTS = FORMAT_DEFAULTS;
}());
/* vim: set sw=4 ts=4 et tw=80 : */ | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/escodegen/escodegen.js | escodegen.js |
## Escodegen
[](http://badge.fury.io/js/escodegen)
[](http://travis-ci.org/estools/escodegen)
[](https://david-dm.org/estools/escodegen)
[](https://david-dm.org/estools/escodegen#info=devDependencies)
Escodegen ([escodegen](http://github.com/estools/escodegen)) is an
[ECMAScript](http://www.ecma-international.org/publications/standards/Ecma-262.htm)
(also popularly known as [JavaScript](http://en.wikipedia.org/wiki/JavaScript))
code generator from [Mozilla's Parser API](https://developer.mozilla.org/en/SpiderMonkey/Parser_API)
AST. See the [online generator](https://estools.github.io/escodegen/demo/index.html)
for a demo.
### Install
Escodegen can be used in a web browser:
<script src="escodegen.browser.js"></script>
escodegen.browser.js can be found in tagged revisions on GitHub.
Or in a Node.js application via npm:
npm install escodegen
### Usage
A simple example: the program
escodegen.generate({
type: 'BinaryExpression',
operator: '+',
left: { type: 'Literal', value: 40 },
right: { type: 'Literal', value: 2 }
});
produces the string `'40 + 2'`.
See the [API page](https://github.com/estools/escodegen/wiki/API) for
options. To run the tests, execute `npm test` in the root directory.
### Building browser bundle / minified browser bundle
At first, execute `npm install` to install the all dev dependencies.
After that,
npm run-script build
will generate `escodegen.browser.js`, which can be used in browser environments.
And,
npm run-script build-min
will generate the minified file `escodegen.browser.min.js`.
### License
#### Escodegen
Copyright (C) 2012 [Yusuke Suzuki](http://github.com/Constellation)
(twitter: [@Constellation](http://twitter.com/Constellation)) and other contributors.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL <COPYRIGHT HOLDER> BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/escodegen/README.md | README.md |
export declare const EVENTS: readonly ["xmldecl", "text", "processinginstruction", "doctype", "comment", "opentagstart", "attribute", "opentag", "closetag", "cdata", "error", "end", "ready"];
/**
* Event handler for the
*
* @param text The text data encountered by the parser.
*
*/
export declare type XMLDeclHandler = (decl: XMLDecl) => void;
/**
* Event handler for text data.
*
* @param text The text data encountered by the parser.
*
*/
export declare type TextHandler = (text: string) => void;
/**
* Event handler for processing instructions.
*
* @param data The target and body of the processing instruction.
*/
export declare type PIHandler = (data: {
target: string;
body: string;
}) => void;
/**
* Event handler for doctype.
*
* @param doctype The doctype contents.
*/
export declare type DoctypeHandler = (doctype: string) => void;
/**
* Event handler for comments.
*
* @param comment The comment contents.
*/
export declare type CommentHandler = (comment: string) => void;
/**
* Event handler for the start of an open tag. This is called as soon as we
* have a tag name.
*
* @param tag The tag.
*/
export declare type OpenTagStartHandler<O> = (tag: StartTagForOptions<O>) => void;
export declare type AttributeEventForOptions<O extends SaxesOptions> = O extends {
xmlns: true;
} ? SaxesAttributeNSIncomplete : O extends {
xmlns?: false | undefined;
} ? SaxesAttributePlain : SaxesAttribute;
/**
* Event handler for attributes.
*/
export declare type AttributeHandler<O> = (attribute: AttributeEventForOptions<O>) => void;
/**
* Event handler for an open tag. This is called when the open tag is
* complete. (We've encountered the ">" that ends the open tag.)
*
* @param tag The tag.
*/
export declare type OpenTagHandler<O> = (tag: TagForOptions<O>) => void;
/**
* Event handler for a close tag. Note that for self-closing tags, this is
* called right after ``opentag``.
*
* @param tag The tag.
*/
export declare type CloseTagHandler<O> = (tag: TagForOptions<O>) => void;
/**
* Event handler for a CDATA section. This is called when ending the
* CDATA section.
*
* @param cdata The contents of the CDATA section.
*/
export declare type CDataHandler = (cdata: string) => void;
/**
* Event handler for the stream end. This is called when the stream has been
* closed with ``close`` or by passing ``null`` to ``write``.
*/
export declare type EndHandler = () => void;
/**
* Event handler indicating parser readiness . This is called when the parser
* is ready to parse a new document.
*/
export declare type ReadyHandler = () => void;
/**
* Event handler indicating an error.
*
* @param err The error that occurred.
*/
export declare type ErrorHandler = (err: Error) => void;
export declare type EventName = (typeof EVENTS)[number];
export declare type EventNameToHandler<O, N extends EventName> = {
"xmldecl": XMLDeclHandler;
"text": TextHandler;
"processinginstruction": PIHandler;
"doctype": DoctypeHandler;
"comment": CommentHandler;
"opentagstart": OpenTagStartHandler<O>;
"attribute": AttributeHandler<O>;
"opentag": OpenTagHandler<O>;
"closetag": CloseTagHandler<O>;
"cdata": CDataHandler;
"error": ErrorHandler;
"end": EndHandler;
"ready": ReadyHandler;
}[N];
/**
* This interface defines the structure of attributes when the parser is
* processing namespaces (created with ``xmlns: true``).
*/
export interface SaxesAttributeNS {
/**
* The attribute's name. This is the combination of prefix and local name.
* For instance ``a:b="c"`` would have ``a:b`` for name.
*/
name: string;
/**
* The attribute's prefix. For instance ``a:b="c"`` would have ``"a"`` for
* ``prefix``.
*/
prefix: string;
/**
* The attribute's local name. For instance ``a:b="c"`` would have ``"b"`` for
* ``local``.
*/
local: string;
/** The namespace URI of this attribute. */
uri: string;
/** The attribute's value. */
value: string;
}
/**
* This is an attribute, as recorded by a parser which parses namespaces but
* prior to the URI being resolvable. This is what is passed to the attribute
* event handler.
*/
export declare type SaxesAttributeNSIncomplete = Exclude<SaxesAttributeNS, "uri">;
/**
* This interface defines the structure of attributes when the parser is
* NOT processing namespaces (created with ``xmlns: false``).
*/
export interface SaxesAttributePlain {
/**
* The attribute's name.
*/
name: string;
/** The attribute's value. */
value: string;
}
/**
* A saxes attribute, with or without namespace information.
*/
export declare type SaxesAttribute = SaxesAttributeNS | SaxesAttributePlain;
/**
* This are the fields that MAY be present on a complete tag.
*/
export interface SaxesTag {
/**
* The tag's name. This is the combination of prefix and global name. For
* instance ``<a:b>`` would have ``"a:b"`` for ``name``.
*/
name: string;
/**
* A map of attribute name to attributes. If namespaces are tracked, the
* values in the map are attribute objects. Otherwise, they are strings.
*/
attributes: Record<string, SaxesAttributeNS> | Record<string, string>;
/**
* The namespace bindings in effect.
*/
ns?: Record<string, string>;
/**
* The tag's prefix. For instance ``<a:b>`` would have ``"a"`` for
* ``prefix``. Undefined if we do not track namespaces.
*/
prefix?: string;
/**
* The tag's local name. For instance ``<a:b>`` would
* have ``"b"`` for ``local``. Undefined if we do not track namespaces.
*/
local?: string;
/**
* The namespace URI of this tag. Undefined if we do not track namespaces.
*/
uri?: string;
/** Whether the tag is self-closing (e.g. ``<foo/>``). */
isSelfClosing: boolean;
}
/**
* This type defines the fields that are present on a tag object when
* ``onopentagstart`` is called. This interface is namespace-agnostic.
*/
export declare type SaxesStartTag = Pick<SaxesTag, "name" | "attributes" | "ns">;
/**
* This type defines the fields that are present on a tag object when
* ``onopentagstart`` is called on a parser that does not processes namespaces.
*/
export declare type SaxesStartTagPlain = Pick<SaxesStartTag, "name" | "attributes">;
/**
* This type defines the fields that are present on a tag object when
* ``onopentagstart`` is called on a parser that does process namespaces.
*/
export declare type SaxesStartTagNS = Required<SaxesStartTag>;
/**
* This are the fields that are present on a complete tag produced by a parser
* that does process namespaces.
*/
export declare type SaxesTagNS = Required<SaxesTag> & {
attributes: Record<string, SaxesAttributeNS>;
};
/**
* This are the fields that are present on a complete tag produced by a parser
* that does not process namespaces.
*/
export declare type SaxesTagPlain = Pick<SaxesTag, "name" | "attributes" | "isSelfClosing"> & {
attributes: Record<string, string>;
};
/**
* An XML declaration.
*/
export interface XMLDecl {
/** The version specified by the XML declaration. */
version?: string;
/** The encoding specified by the XML declaration. */
encoding?: string;
/** The value of the standalone parameter */
standalone?: string;
}
/**
* A callback for resolving name prefixes.
*
* @param prefix The prefix to check.
*
* @returns The URI corresponding to the prefix, if any.
*/
export declare type ResolvePrefix = (prefix: string) => string | undefined;
export interface CommonOptions {
/** Whether to accept XML fragments. Unset means ``false``. */
fragment?: boolean;
/** Whether to track positions. Unset means ``true``. */
position?: boolean;
/**
* A file name to use for error reporting. "File name" is a loose concept. You
* could use a URL to some resource, or any descriptive name you like.
*/
fileName?: string;
}
export interface NSOptions {
/** Whether to track namespaces. Unset means ``false``. */
xmlns?: boolean;
/**
* A plain object whose key, value pairs define namespaces known before
* parsing the XML file. It is not legal to pass bindings for the namespaces
* ``"xml"`` or ``"xmlns"``.
*/
additionalNamespaces?: Record<string, string>;
/**
* A function that will be used if the parser cannot resolve a namespace
* prefix on its own.
*/
resolvePrefix?: ResolvePrefix;
}
export interface NSOptionsWithoutNamespaces extends NSOptions {
xmlns?: false;
additionalNamespaces?: undefined;
resolvePrefix?: undefined;
}
export interface NSOptionsWithNamespaces extends NSOptions {
xmlns: true;
}
export interface XMLVersionOptions {
/**
* The default XML version to use. If unspecified, and there is no XML
* encoding declaration, the default version is "1.0".
*/
defaultXMLVersion?: "1.0" | "1.1";
/**
* A flag indicating whether to force the XML version used for parsing to the
* value of ``defaultXMLVersion``. When this flag is ``true``,
* ``defaultXMLVersion`` must be specified. If unspecified, the default value
* of this flag is ``false``.
*/
forceXMLVersion?: boolean;
}
export interface NoForcedXMLVersion extends XMLVersionOptions {
forceXMLVersion?: false;
}
export interface ForcedXMLVersion extends XMLVersionOptions {
forceXMLVersion: true;
defaultXMLVersion: Exclude<XMLVersionOptions["defaultXMLVersion"], undefined>;
}
/**
* The entire set of options supported by saxes.
*/
export declare type SaxesOptions = CommonOptions & NSOptions & XMLVersionOptions;
export declare type TagForOptions<O extends SaxesOptions> = O extends {
xmlns: true;
} ? SaxesTagNS : O extends {
xmlns?: false | undefined;
} ? SaxesTagPlain : SaxesTag;
export declare type StartTagForOptions<O extends SaxesOptions> = O extends {
xmlns: true;
} ? SaxesStartTagNS : O extends {
xmlns?: false | undefined;
} ? SaxesStartTagPlain : SaxesStartTag;
export declare class SaxesParser<O extends SaxesOptions = {}> {
private readonly fragmentOpt;
private readonly xmlnsOpt;
private readonly trackPosition;
private readonly fileName?;
private readonly nameStartCheck;
private readonly nameCheck;
private readonly isName;
private readonly ns;
private openWakaBang;
private text;
private name;
private piTarget;
private entity;
private q;
private tags;
private tag;
private topNS;
private chunk;
private chunkPosition;
private i;
private prevI;
private carriedFromPrevious?;
private forbiddenState;
private attribList;
private state;
private reportedTextBeforeRoot;
private reportedTextAfterRoot;
private closedRoot;
private sawRoot;
private xmlDeclPossible;
private xmlDeclExpects;
private entityReturnState?;
private processAttribs;
private positionAtNewLine;
private doctype;
private getCode;
private isChar;
private pushAttrib;
private _closed;
private currentXMLVersion;
private readonly stateTable;
private xmldeclHandler?;
private textHandler?;
private piHandler?;
private doctypeHandler?;
private commentHandler?;
private openTagStartHandler?;
private openTagHandler?;
private closeTagHandler?;
private cdataHandler?;
private errorHandler?;
private endHandler?;
private readyHandler?;
private attributeHandler?;
/**
* Indicates whether or not the parser is closed. If ``true``, wait for
* the ``ready`` event to write again.
*/
get closed(): boolean;
readonly opt: SaxesOptions;
/**
* The XML declaration for this document.
*/
xmlDecl: XMLDecl;
/**
* The line number of the next character to be read by the parser. This field
* is one-based. (The first line is numbered 1.)
*/
line: number;
/**
* The column number of the next character to be read by the parser. *
* This field is zero-based. (The first column is 0.)
*
* This field counts columns by *Unicode character*. Note that this *can*
* be different from the index of the character in a JavaScript string due
* to how JavaScript handles astral plane characters.
*
* See [[columnIndex]] for a number that corresponds to the JavaScript index.
*/
column: number;
/**
* A map of entity name to expansion.
*/
ENTITIES: Record<string, string>;
/**
* @param opt The parser options.
*/
constructor(opt?: O);
_init(): void;
/**
* The stream position the parser is currently looking at. This field is
* zero-based.
*
* This field is not based on counting Unicode characters but is to be
* interpreted as a plain index into a JavaScript string.
*/
get position(): number;
/**
* The column number of the next character to be read by the parser. *
* This field is zero-based. (The first column in a line is 0.)
*
* This field reports the index at which the next character would be in the
* line if the line were represented as a JavaScript string. Note that this
* *can* be different to a count based on the number of *Unicode characters*
* due to how JavaScript handles astral plane characters.
*
* See [[column]] for a number that corresponds to a count of Unicode
* characters.
*/
get columnIndex(): number;
/**
* Set an event listener on an event. The parser supports one handler per
* event type. If you try to set an event handler over an existing handler,
* the old handler is silently overwritten.
*
* @param name The event to listen to.
*
* @param handler The handler to set.
*/
on<N extends EventName>(name: N, handler: EventNameToHandler<O, N>): void;
/**
* Unset an event handler.
*
* @parma name The event to stop listening to.
*/
off(name: EventName): void;
/**
* Make an error object. The error object will have a message that contains
* the ``fileName`` option passed at the creation of the parser. If position
* tracking was turned on, it will also have line and column number
* information.
*
* @param message The message describing the error to report.
*
* @returns An error object with a properly formatted message.
*/
makeError(message: string): Error;
/**
* Report a parsing error. This method is made public so that client code may
* check for issues that are outside the scope of this project and can report
* errors.
*
* @param message The error to report.
*
* @returns this
*/
fail(message: string): this;
/**
* Write a XML data to the parser.
*
* @param chunk The XML data to write.
*
* @returns this
*/
write(chunk: string | {} | null): this;
/**
* Close the current stream. Perform final well-formedness checks and reset
* the parser tstate.
*
* @returns this
*/
close(): this;
/**
* Get a single code point out of the current chunk. This updates the current
* position if we do position tracking.
*
* This is the algorithm to use for XML 1.0.
*
* @returns The character read.
*/
private getCode10;
/**
* Get a single code point out of the current chunk. This updates the current
* position if we do position tracking.
*
* This is the algorithm to use for XML 1.1.
*
* @returns {number} The character read.
*/
private getCode11;
/**
* Like ``getCode`` but with the return value normalized so that ``NL`` is
* returned for ``NL_LIKE``.
*/
private getCodeNorm;
private unget;
/**
* Capture characters into a buffer until encountering one of a set of
* characters.
*
* @param chars An array of codepoints. Encountering a character in the array
* ends the capture. (``chars`` may safely contain ``NL``.)
*
* @return The character code that made the capture end, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
private captureTo;
/**
* Capture characters into a buffer until encountering a character.
*
* @param char The codepoint that ends the capture. **NOTE ``char`` MAY NOT
* CONTAIN ``NL``.** Passing ``NL`` will result in buggy behavior.
*
* @return ``true`` if we ran into the character. Otherwise, we ran into the
* end of the current chunk.
*/
private captureToChar;
/**
* Capture characters that satisfy ``isNameChar`` into the ``name`` field of
* this parser.
*
* @return The character code that made the test fail, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
private captureNameChars;
/**
* Skip white spaces.
*
* @return The character that ended the skip, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
private skipSpaces;
private setXMLVersion;
private sBegin;
private sBeginWhitespace;
private sDoctype;
private sDoctypeQuote;
private sDTD;
private sDTDQuoted;
private sDTDOpenWaka;
private sDTDOpenWakaBang;
private sDTDComment;
private sDTDCommentEnding;
private sDTDCommentEnded;
private sDTDPI;
private sDTDPIEnding;
private sText;
private sEntity;
private sOpenWaka;
private sOpenWakaBang;
private sComment;
private sCommentEnding;
private sCommentEnded;
private sCData;
private sCDataEnding;
private sCDataEnding2;
private sPIFirstChar;
private sPIRest;
private sPIBody;
private sPIEnding;
private sXMLDeclNameStart;
private sXMLDeclName;
private sXMLDeclEq;
private sXMLDeclValueStart;
private sXMLDeclValue;
private sXMLDeclSeparator;
private sXMLDeclEnding;
private sOpenTag;
private sOpenTagSlash;
private sAttrib;
private sAttribName;
private sAttribNameSawWhite;
private sAttribValue;
private sAttribValueQuoted;
private sAttribValueClosed;
private sAttribValueUnquoted;
private sCloseTag;
private sCloseTagSawWhite;
private handleTextInRoot;
private handleTextOutsideRoot;
private pushAttribNS;
private pushAttribPlain;
/**
* End parsing. This performs final well-formedness checks and resets the
* parser to a clean state.
*
* @returns this
*/
private end;
/**
* Resolve a namespace prefix.
*
* @param prefix The prefix to resolve.
*
* @returns The namespace URI or ``undefined`` if the prefix is not defined.
*/
resolve(prefix: string): string | undefined;
/**
* Parse a qname into its prefix and local name parts.
*
* @param name The name to parse
*
* @returns
*/
private qname;
private processAttribsNS;
private processAttribsPlain;
/**
* Handle a complete open tag. This parser code calls this once it has seen
* the whole tag. This method checks for well-formeness and then emits
* ``onopentag``.
*/
private openTag;
/**
* Handle a complete self-closing tag. This parser code calls this once it has
* seen the whole tag. This method checks for well-formeness and then emits
* ``onopentag`` and ``onclosetag``.
*/
private openSelfClosingTag;
/**
* Handle a complete close tag. This parser code calls this once it has seen
* the whole tag. This method checks for well-formeness and then emits
* ``onclosetag``.
*/
private closeTag;
/**
* Resolves an entity. Makes any necessary well-formedness checks.
*
* @param entity The entity to resolve.
*
* @returns The parsed entity.
*/
private parseEntity;
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/saxes/saxes.d.ts | saxes.d.ts |
# saxes
A sax-style non-validating parser for XML.
Saxes is a fork of [sax](https://github.com/isaacs/sax-js) 1.2.4. All mentions
of sax in this project's documentation are references to sax 1.2.4.
Designed with [node](http://nodejs.org/) in mind, but should work fine in the
browser or other CommonJS implementations.
Saxes does not support Node versions older than 10.
## Notable Differences from Sax.
* Saxes aims to be much stricter than sax with regards to XML
well-formedness. Sax, even in its so-called "strict mode", is not strict. It
silently accepts structures that are not well-formed XML. Projects that need
better compliance with well-formedness constraints cannot use sax as-is.
Consequently, saxes does not support HTML, or pseudo-XML, or bad XML. Saxes
will report well-formedness errors in all these cases but it won't try to
extract data from malformed documents like sax does.
* Saxes is much much faster than sax, mostly because of a substantial redesign
of the internal parsing logic. The speed improvement is not merely due to
removing features that were supported by sax. That helped a bit, but saxes
adds some expensive checks in its aim for conformance with the XML
specification. Redesigning the parsing logic is what accounts for most of the
performance improvement.
* Saxes does not aim to support antiquated platforms. We will not pollute the
source or the default build with support for antiquated platforms. If you want
support for IE 11, you are welcome to produce a PR that adds a *new build*
transpiled to ES5.
* Saxes handles errors differently from sax: it provides a default onerror
handler which throws. You can replace it with your own handler if you want. If
your handler does nothing, there is no `resume` method to call.
* There's no `Stream` API. A revamped API may be introduced later. (It is still
a "streaming parser" in the general sense that you write a character stream to
it.)
* Saxes does not have facilities for limiting the size the data chunks passed to
event handlers. See the FAQ entry for more details.
## Conformance
Saxes supports:
* [XML 1.0 fifth edition](https://www.w3.org/TR/2008/REC-xml-20081126/)
* [XML 1.1 second edition](https://www.w3.org/TR/2006/REC-xml11-20060816/)
* [Namespaces in XML 1.0 (Third Edition)](https://www.w3.org/TR/2009/REC-xml-names-20091208/).
* [Namespaces in XML 1.1 (Second Edition)](https://www.w3.org/TR/2006/REC-xml-names11-20060816/).
## Limitations
This is a non-validating parser so it only verifies whether the document is
well-formed. We do aim to raise errors for all malformed constructs
encountered. However, this parser does not thorougly parse the contents of
DTDs. So most malformedness errors caused by errors **in DTDs** cannot be
reported.
## Regarding `<!DOCTYPE` and `<!ENTITY`
The parser will handle the basic XML entities in text nodes and attribute
values: `& < > ' "`. It's possible to define additional
entities in XML by putting them in the DTD. This parser doesn't do anything with
that. If you want to listen to the `doctype` event, and then fetch the
doctypes, and read the entities and add them to `parser.ENTITIES`, then be my
guest.
## Documentation
The source code contains JSDOC comments. Use them. What follows is a brief
summary of what is available. The final authority is the source code.
**PAY CLOSE ATTENTION TO WHAT IS PUBLIC AND WHAT IS PRIVATE.**
The move to TypeScript makes it so that everything is now formally private,
protected, or public.
If you use anything not public, that's at your own peril.
If there's a mistake in the documentation, raise an issue. If you just assume,
you may assume incorrectly.
## Summary Usage Information
### Example
```javascript
var saxes = require("./lib/saxes"),
parser = new saxes.SaxesParser();
parser.on("error", function (e) {
// an error happened.
});
parser.on("text", function (t) {
// got some text. t is the string of text.
});
parser.on("opentag", function (node) {
// opened a tag. node has "name" and "attributes"
});
parser.on("end", function () {
// parser stream is done, and ready to have more stuff written to it.
});
parser.write('<xml>Hello, <who name="world">world</who>!</xml>').close();
```
### Constructor Arguments
Settings supported:
* `xmlns` - Boolean. If `true`, then namespaces are supported. Default
is `false`.
* `position` - Boolean. If `false`, then don't track line/col/position. Unset is
treated as `true`. Default is unset. Currently, setting this to `false` only
results in a cosmetic change: the errors reported do not contain position
information. sax-js would literally turn off the position-computing logic if
this flag was set to false. The notion was that it would optimize
execution. In saxes at least it turns out that continually testing this flag
causes a cost that offsets the benefits of turning off this logic.
* `fileName` - String. Set a file name for error reporting. This is useful only
when tracking positions. You may leave it unset.
* `fragment` - Boolean. If `true`, parse the XML as an XML fragment. Default is
`false`.
* `additionalNamespaces` - A plain object whose key, value pairs define
namespaces known before parsing the XML file. It is not legal to pass
bindings for the namespaces `"xml"` or `"xmlns"`.
* `defaultXMLVersion` - The default version of the XML specification to use if
the document contains no XML declaration. If the document does contain an XML
declaration, then this setting is ignored. Must be `"1.0"` or `"1.1"`. The
default is `"1.0"`.
* `forceXMLVersion` - Boolean. A flag indicating whether to force the XML
version used for parsing to the value of ``defaultXMLVersion``. When this flag
is ``true``, ``defaultXMLVersion`` must be specified. If unspecified, the
default value of this flag is ``false``.
Example: suppose you are parsing a document that has an XML declaration
specifying XML version 1.1.
If you set ``defaultXMLVersion`` to ``"1.0"`` without setting
``forceXMLVersion`` then the XML declaration will override the value of
``defaultXMLVersion`` and the document will be parsed according to XML 1.1.
If you set ``defaultXMLVersion`` to ``"1.0"`` and set ``forceXMLVersion`` to
``true``, then the XML declaration will be ignored and the document will be
parsed according to XML 1.0.
### Methods
`write` - Write bytes onto the stream. You don't have to pass the whole document
in one `write` call. You can read your source chunk by chunk and call `write`
with each chunk.
`close` - Close the stream. Once closed, no more data may be written until it is
done processing the buffer, which is signaled by the `end` event.
### Properties
The parser has the following properties:
`line`, `column`, `columnIndex`, `position` - Indications of the position in the
XML document where the parser currently is looking. The `columnIndex` property
counts columns as if indexing into a JavaScript string, whereas the `column`
property counts Unicode characters.
`closed` - Boolean indicating whether or not the parser can be written to. If
it's `true`, then wait for the `ready` event to write again.
`opt` - Any options passed into the constructor.
`xmlDecl` - The XML declaration for this document. It contains the fields
`version`, `encoding` and `standalone`. They are all `undefined` before
encountering the XML declaration. If they are undefined after the XML
declaration, the corresponding value was not set by the declaration. There is no
event associated with the XML declaration. In a well-formed document, the XML
declaration may be preceded only by an optional BOM. So by the time any event
generated by the parser happens, the declaration has been processed if present
at all. Otherwise, you have a malformed document, and as stated above, you
cannot rely on the parser data!
### Error Handling
The parser continues to parse even upon encountering errors, and does its best
to continue reporting errors. You should heed all errors reported. After an
error, however, saxes may interpret your document incorrectly. For instance
``<foo a=bc="d"/>`` is invalid XML. Did you mean to have ``<foo a="bc=d"/>`` or
``<foo a="b" c="d"/>`` or some other variation? For the sake of continuing to
provide errors, saxes will continue parsing the document, but the structure it
reports may be incorrect. It is only after the errors are fixed in the document
that saxes can provide a reliable interpretation of the document.
That leaves you with two rules of thumb when using saxes:
* Pay attention to the errors that saxes report. The default `onerror` handler
throws, so by default, you cannot miss errors.
* **ONCE AN ERROR HAS BEEN ENCOUNTERED, STOP RELYING ON THE EVENT HANDLERS OTHER
THAN `onerror`.** As explained above, when saxes runs into a well-formedness
problem, it makes a guess in order to continue reporting more errors. The guess
may be wrong.
### Events
To listen to an event, override `on<eventname>`. The list of supported events
are also in the exported `EVENTS` array.
See the JSDOC comments in the source code for a description of each supported
event.
### Parsing XML Fragments
The XML specification does not define any method by which to parse XML
fragments. However, there are usage scenarios in which it is desirable to parse
fragments. In order to allow this, saxes provides three initialization options.
If you pass the option `fragment: true` to the parser constructor, the parser
will expect an XML fragment. It essentially starts with a parsing state
equivalent to the one it would be in if `parser.write("<foo">)` had been called
right after initialization. In other words, it expects content which is
acceptable inside an element. This also turns off well-formedness checks that
are inappropriate when parsing a fragment.
The option `additionalNamespaces` allows you to define additional prefix-to-URI
bindings known before parsing starts. You would use this over `resolvePrefix` if
you have at the ready a series of namespaces bindings to use.
The option `resolvePrefix` allows you to pass a function which saxes will use if
it is unable to resolve a namespace prefix by itself. You would use this over
`additionalNamespaces` in a context where getting a complete list of defined
namespaces is onerous.
Note that you can use `additionalNamespaces` and `resolvePrefix` together if you
want. `additionalNamespaces` applies before `resolvePrefix`.
The options `additionalNamespaces` and `resolvePrefix` are really meant to be
used for parsing fragments. However, saxes won't prevent you from using them
with `fragment: false`. Note that if you do this, your document may parse
without errors and yet be malformed because the document can refer to namespaces
which are not defined *in* the document.
Of course, `additionalNamespaces` and `resolvePrefix` are used only if `xmlns`
is `true`. If you are parsing a fragment that does not use namespaces, there's
no point in setting these options.
### Performance Tips
* saxes works faster on files that use newlines (``\u000A``) as end of line
markers than files that use other end of line markers (like ``\r`` or
``\r\n``). The XML specification requires that conformant applications behave
as if all characters that are to be treated as end of line characters are
converted to ``\u000A`` prior to parsing. The optimal code path for saxes is a
file in which all end of line characters are already ``\u000A``.
* Don't split Unicode strings you feed to saxes across surrogates. When you
naively split a string in JavaScript, you run the risk of splitting a Unicode
character into two surrogates. e.g. In the following example ``a`` and ``b``
each contain half of a single Unicode character: ``const a = "\u{1F4A9}"[0];
const b = "\u{1F4A9}"[1]`` If you feed such split surrogates to versions of
saxes prior to 4, you'd get errors. Saxes version 4 and over are able to
detect when a chunk of data ends with a surrogate and carry over the surrogate
to the next chunk. However this operation entails slicing and concatenating
strings. If you can feed your data in a way that does not split surrogates,
you should do it. (Obviously, feeding all the data at once with a single write
is fastest.)
* Don't set event handlers you don't need. Saxes has always aimed to avoid doing
work that will just be tossed away but future improvements hope to do this
more aggressively. One way saxes knows whether or not some data is needed is
by checking whether a handler has been set for a specific event.
## FAQ
Q. Why has saxes dropped support for limiting the size of data chunks passed to
event handlers?
A. With sax you could set ``MAX_BUFFER_LENGTH`` to cause the parser to limit the
size of data chunks passed to event handlers. So if you ran into a span of text
above the limit, multiple ``text`` events with smaller data chunks were fired
instead of a single event with a large chunk.
However, that functionality had some problematic characteristics. It had an
arbitrary default value. It was library-wide so all parsers created from a
single instance of the ``sax`` library shared it. This could potentially cause
conflicts among libraries running in the same VM but using sax for different
purposes.
These issues could have been easily fixed, but there were larger issues. The
buffer limit arbitrarily applied to some events but not others. It would split
``text``, ``cdata`` and ``script`` events. However, if a ``comment``,
``doctype``, ``attribute`` or ``processing instruction`` were more than the
limit, the parser would generate an error and you were left picking up the
pieces.
It was not intuitive to use. You'd think setting the limit to 1K would prevent
chunks bigger than 1K to be passed to event handlers. But that was not the
case. A comment in the source code told you that you might go over the limit if
you passed large chunks to ``write``. So if you want a 1K limit, don't pass 64K
chunks to ``write``. Fair enough. You know what limit you want so you can
control the size of the data you pass to ``write``. So you limit the chunks to
``write`` to 1K at a time. Even if you do this, your event handlers may get data
chunks that are 2K in size. Suppose on the previous ``write`` the parser has
just finished processing an open tag, so it is ready for text. Your ``write``
passes 1K of text. You are not above the limit yet, so no event is generated
yet. The next ``write`` passes another 1K of text. It so happens that sax checks
buffer limits only once per ``write``, after the chunk of data has been
processed. Now you've hit the limit and you get a ``text`` event with 2K of
data. So even if you limit your ``write`` calls to the buffer limit you've set,
you may still get events with chunks at twice the buffer size limit you've
specified.
We may consider reinstating an equivalent functionality, provided that it
addresses the issues above and does not cause a huge performance drop for
use-case scenarios that don't need it.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/saxes/README.md | README.md |
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
const ed5 = require("xmlchars/xml/1.0/ed5");
const ed2 = require("xmlchars/xml/1.1/ed2");
const NSed3 = require("xmlchars/xmlns/1.0/ed3");
var isS = ed5.isS;
var isChar10 = ed5.isChar;
var isNameStartChar = ed5.isNameStartChar;
var isNameChar = ed5.isNameChar;
var S_LIST = ed5.S_LIST;
var NAME_RE = ed5.NAME_RE;
var isChar11 = ed2.isChar;
var isNCNameStartChar = NSed3.isNCNameStartChar;
var isNCNameChar = NSed3.isNCNameChar;
var NC_NAME_RE = NSed3.NC_NAME_RE;
const XML_NAMESPACE = "http://www.w3.org/XML/1998/namespace";
const XMLNS_NAMESPACE = "http://www.w3.org/2000/xmlns/";
const rootNS = {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
__proto__: null,
xml: XML_NAMESPACE,
xmlns: XMLNS_NAMESPACE,
};
const XML_ENTITIES = {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
__proto__: null,
amp: "&",
gt: ">",
lt: "<",
quot: "\"",
apos: "'",
};
// EOC: end-of-chunk
const EOC = -1;
const NL_LIKE = -2;
const S_BEGIN = 0; // Initial state.
const S_BEGIN_WHITESPACE = 1; // leading whitespace
const S_DOCTYPE = 2; // <!DOCTYPE
const S_DOCTYPE_QUOTE = 3; // <!DOCTYPE "//blah
const S_DTD = 4; // <!DOCTYPE "//blah" [ ...
const S_DTD_QUOTED = 5; // <!DOCTYPE "//blah" [ "foo
const S_DTD_OPEN_WAKA = 6;
const S_DTD_OPEN_WAKA_BANG = 7;
const S_DTD_COMMENT = 8; // <!--
const S_DTD_COMMENT_ENDING = 9; // <!-- blah -
const S_DTD_COMMENT_ENDED = 10; // <!-- blah --
const S_DTD_PI = 11; // <?
const S_DTD_PI_ENDING = 12; // <?hi "there" ?
const S_TEXT = 13; // general stuff
const S_ENTITY = 14; // & and such
const S_OPEN_WAKA = 15; // <
const S_OPEN_WAKA_BANG = 16; // <!...
const S_COMMENT = 17; // <!--
const S_COMMENT_ENDING = 18; // <!-- blah -
const S_COMMENT_ENDED = 19; // <!-- blah --
const S_CDATA = 20; // <![CDATA[ something
const S_CDATA_ENDING = 21; // ]
const S_CDATA_ENDING_2 = 22; // ]]
const S_PI_FIRST_CHAR = 23; // <?hi, first char
const S_PI_REST = 24; // <?hi, rest of the name
const S_PI_BODY = 25; // <?hi there
const S_PI_ENDING = 26; // <?hi "there" ?
const S_XML_DECL_NAME_START = 27; // <?xml
const S_XML_DECL_NAME = 28; // <?xml foo
const S_XML_DECL_EQ = 29; // <?xml foo=
const S_XML_DECL_VALUE_START = 30; // <?xml foo=
const S_XML_DECL_VALUE = 31; // <?xml foo="bar"
const S_XML_DECL_SEPARATOR = 32; // <?xml foo="bar"
const S_XML_DECL_ENDING = 33; // <?xml ... ?
const S_OPEN_TAG = 34; // <strong
const S_OPEN_TAG_SLASH = 35; // <strong /
const S_ATTRIB = 36; // <a
const S_ATTRIB_NAME = 37; // <a foo
const S_ATTRIB_NAME_SAW_WHITE = 38; // <a foo _
const S_ATTRIB_VALUE = 39; // <a foo=
const S_ATTRIB_VALUE_QUOTED = 40; // <a foo="bar
const S_ATTRIB_VALUE_CLOSED = 41; // <a foo="bar"
const S_ATTRIB_VALUE_UNQUOTED = 42; // <a foo=bar
const S_CLOSE_TAG = 43; // </a
const S_CLOSE_TAG_SAW_WHITE = 44; // </a >
const TAB = 9;
const NL = 0xA;
const CR = 0xD;
const SPACE = 0x20;
const BANG = 0x21;
const DQUOTE = 0x22;
const AMP = 0x26;
const SQUOTE = 0x27;
const MINUS = 0x2D;
const FORWARD_SLASH = 0x2F;
const SEMICOLON = 0x3B;
const LESS = 0x3C;
const EQUAL = 0x3D;
const GREATER = 0x3E;
const QUESTION = 0x3F;
const OPEN_BRACKET = 0x5B;
const CLOSE_BRACKET = 0x5D;
const NEL = 0x85;
const LS = 0x2028; // Line Separator
const isQuote = (c) => c === DQUOTE || c === SQUOTE;
const QUOTES = [DQUOTE, SQUOTE];
const DOCTYPE_TERMINATOR = [...QUOTES, OPEN_BRACKET, GREATER];
const DTD_TERMINATOR = [...QUOTES, LESS, CLOSE_BRACKET];
const XML_DECL_NAME_TERMINATOR = [EQUAL, QUESTION, ...S_LIST];
const ATTRIB_VALUE_UNQUOTED_TERMINATOR = [...S_LIST, GREATER, AMP, LESS];
function nsPairCheck(parser, prefix, uri) {
switch (prefix) {
case "xml":
if (uri !== XML_NAMESPACE) {
parser.fail(`xml prefix must be bound to ${XML_NAMESPACE}.`);
}
break;
case "xmlns":
if (uri !== XMLNS_NAMESPACE) {
parser.fail(`xmlns prefix must be bound to ${XMLNS_NAMESPACE}.`);
}
break;
default:
}
switch (uri) {
case XMLNS_NAMESPACE:
parser.fail(prefix === "" ?
`the default namespace may not be set to ${uri}.` :
`may not assign a prefix (even "xmlns") to the URI \
${XMLNS_NAMESPACE}.`);
break;
case XML_NAMESPACE:
switch (prefix) {
case "xml":
// Assinging the XML namespace to "xml" is fine.
break;
case "":
parser.fail(`the default namespace may not be set to ${uri}.`);
break;
default:
parser.fail("may not assign the xml namespace to another prefix.");
}
break;
default:
}
}
function nsMappingCheck(parser, mapping) {
for (const local of Object.keys(mapping)) {
nsPairCheck(parser, local, mapping[local]);
}
}
const isNCName = (name) => NC_NAME_RE.test(name);
const isName = (name) => NAME_RE.test(name);
const FORBIDDEN_START = 0;
const FORBIDDEN_BRACKET = 1;
const FORBIDDEN_BRACKET_BRACKET = 2;
/**
* The list of supported events.
*/
exports.EVENTS = [
"xmldecl",
"text",
"processinginstruction",
"doctype",
"comment",
"opentagstart",
"attribute",
"opentag",
"closetag",
"cdata",
"error",
"end",
"ready",
];
const EVENT_NAME_TO_HANDLER_NAME = {
xmldecl: "xmldeclHandler",
text: "textHandler",
processinginstruction: "piHandler",
doctype: "doctypeHandler",
comment: "commentHandler",
opentagstart: "openTagStartHandler",
attribute: "attributeHandler",
opentag: "openTagHandler",
closetag: "closeTagHandler",
cdata: "cdataHandler",
error: "errorHandler",
end: "endHandler",
ready: "readyHandler",
};
class SaxesParser {
/**
* @param opt The parser options.
*/
constructor(opt) {
this.opt = opt !== null && opt !== void 0 ? opt : {};
this.fragmentOpt = !!this.opt.fragment;
const xmlnsOpt = this.xmlnsOpt = !!this.opt.xmlns;
this.trackPosition = this.opt.position !== false;
this.fileName = this.opt.fileName;
if (xmlnsOpt) {
// This is the function we use to perform name checks on PIs and entities.
// When namespaces are used, colons are not allowed in PI target names or
// entity names. So the check depends on whether namespaces are used. See:
//
// https://www.w3.org/XML/xml-names-19990114-errata.html
// NE08
//
this.nameStartCheck = isNCNameStartChar;
this.nameCheck = isNCNameChar;
this.isName = isNCName;
// eslint-disable-next-line @typescript-eslint/unbound-method
this.processAttribs = this.processAttribsNS;
// eslint-disable-next-line @typescript-eslint/unbound-method
this.pushAttrib = this.pushAttribNS;
// eslint-disable-next-line @typescript-eslint/no-explicit-any
this.ns = Object.assign({ __proto__: null }, rootNS);
const additional = this.opt.additionalNamespaces;
if (additional != null) {
nsMappingCheck(this, additional);
Object.assign(this.ns, additional);
}
}
else {
this.nameStartCheck = isNameStartChar;
this.nameCheck = isNameChar;
this.isName = isName;
// eslint-disable-next-line @typescript-eslint/unbound-method
this.processAttribs = this.processAttribsPlain;
// eslint-disable-next-line @typescript-eslint/unbound-method
this.pushAttrib = this.pushAttribPlain;
}
//
// The order of the members in this table needs to correspond to the state
// numbers given to the states that correspond to the methods being recorded
// here.
//
this.stateTable = [
/* eslint-disable @typescript-eslint/unbound-method */
this.sBegin,
this.sBeginWhitespace,
this.sDoctype,
this.sDoctypeQuote,
this.sDTD,
this.sDTDQuoted,
this.sDTDOpenWaka,
this.sDTDOpenWakaBang,
this.sDTDComment,
this.sDTDCommentEnding,
this.sDTDCommentEnded,
this.sDTDPI,
this.sDTDPIEnding,
this.sText,
this.sEntity,
this.sOpenWaka,
this.sOpenWakaBang,
this.sComment,
this.sCommentEnding,
this.sCommentEnded,
this.sCData,
this.sCDataEnding,
this.sCDataEnding2,
this.sPIFirstChar,
this.sPIRest,
this.sPIBody,
this.sPIEnding,
this.sXMLDeclNameStart,
this.sXMLDeclName,
this.sXMLDeclEq,
this.sXMLDeclValueStart,
this.sXMLDeclValue,
this.sXMLDeclSeparator,
this.sXMLDeclEnding,
this.sOpenTag,
this.sOpenTagSlash,
this.sAttrib,
this.sAttribName,
this.sAttribNameSawWhite,
this.sAttribValue,
this.sAttribValueQuoted,
this.sAttribValueClosed,
this.sAttribValueUnquoted,
this.sCloseTag,
this.sCloseTagSawWhite,
];
this._init();
}
/**
* Indicates whether or not the parser is closed. If ``true``, wait for
* the ``ready`` event to write again.
*/
get closed() {
return this._closed;
}
_init() {
var _a;
this.openWakaBang = "";
this.text = "";
this.name = "";
this.piTarget = "";
this.entity = "";
this.q = null;
this.tags = [];
this.tag = null;
this.topNS = null;
this.chunk = "";
this.chunkPosition = 0;
this.i = 0;
this.prevI = 0;
this.carriedFromPrevious = undefined;
this.forbiddenState = FORBIDDEN_START;
this.attribList = [];
// The logic is organized so as to minimize the need to check
// this.opt.fragment while parsing.
const { fragmentOpt } = this;
this.state = fragmentOpt ? S_TEXT : S_BEGIN;
// We want these to be all true if we are dealing with a fragment.
this.reportedTextBeforeRoot = this.reportedTextAfterRoot = this.closedRoot =
this.sawRoot = fragmentOpt;
// An XML declaration is intially possible only when parsing whole
// documents.
this.xmlDeclPossible = !fragmentOpt;
this.xmlDeclExpects = ["version"];
this.entityReturnState = undefined;
let { defaultXMLVersion } = this.opt;
if (defaultXMLVersion === undefined) {
if (this.opt.forceXMLVersion === true) {
throw new Error("forceXMLVersion set but defaultXMLVersion is not set");
}
defaultXMLVersion = "1.0";
}
this.setXMLVersion(defaultXMLVersion);
this.positionAtNewLine = 0;
this.doctype = false;
this._closed = false;
this.xmlDecl = {
version: undefined,
encoding: undefined,
standalone: undefined,
};
this.line = 1;
this.column = 0;
this.ENTITIES = Object.create(XML_ENTITIES);
// eslint-disable-next-line no-unused-expressions
(_a = this.readyHandler) === null || _a === void 0 ? void 0 : _a.call(this);
}
/**
* The stream position the parser is currently looking at. This field is
* zero-based.
*
* This field is not based on counting Unicode characters but is to be
* interpreted as a plain index into a JavaScript string.
*/
get position() {
return this.chunkPosition + this.i;
}
/**
* The column number of the next character to be read by the parser. *
* This field is zero-based. (The first column in a line is 0.)
*
* This field reports the index at which the next character would be in the
* line if the line were represented as a JavaScript string. Note that this
* *can* be different to a count based on the number of *Unicode characters*
* due to how JavaScript handles astral plane characters.
*
* See [[column]] for a number that corresponds to a count of Unicode
* characters.
*/
get columnIndex() {
return this.position - this.positionAtNewLine;
}
/**
* Set an event listener on an event. The parser supports one handler per
* event type. If you try to set an event handler over an existing handler,
* the old handler is silently overwritten.
*
* @param name The event to listen to.
*
* @param handler The handler to set.
*/
on(name, handler) {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
this[EVENT_NAME_TO_HANDLER_NAME[name]] = handler;
}
/**
* Unset an event handler.
*
* @parma name The event to stop listening to.
*/
off(name) {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
this[EVENT_NAME_TO_HANDLER_NAME[name]] = undefined;
}
/**
* Make an error object. The error object will have a message that contains
* the ``fileName`` option passed at the creation of the parser. If position
* tracking was turned on, it will also have line and column number
* information.
*
* @param message The message describing the error to report.
*
* @returns An error object with a properly formatted message.
*/
makeError(message) {
var _a;
let msg = (_a = this.fileName) !== null && _a !== void 0 ? _a : "";
if (this.trackPosition) {
if (msg.length > 0) {
msg += ":";
}
msg += `${this.line}:${this.column}`;
}
if (msg.length > 0) {
msg += ": ";
}
return new Error(msg + message);
}
/**
* Report a parsing error. This method is made public so that client code may
* check for issues that are outside the scope of this project and can report
* errors.
*
* @param message The error to report.
*
* @returns this
*/
fail(message) {
const err = this.makeError(message);
const handler = this.errorHandler;
if (handler === undefined) {
throw err;
}
else {
handler(err);
}
return this;
}
/**
* Write a XML data to the parser.
*
* @param chunk The XML data to write.
*
* @returns this
*/
write(chunk) {
if (this.closed) {
return this.fail("cannot write after close; assign an onready handler.");
}
let end = false;
if (chunk === null) {
// We cannot return immediately because carriedFromPrevious may need
// processing.
end = true;
chunk = "";
}
else if (typeof chunk === "object") {
chunk = chunk.toString();
}
// We checked if performing a pre-decomposition of the string into an array
// of single complete characters (``Array.from(chunk)``) would be faster
// than the current repeated calls to ``charCodeAt``. As of August 2018, it
// isn't. (There may be Node-specific code that would perform faster than
// ``Array.from`` but don't want to be dependent on Node.)
if (this.carriedFromPrevious !== undefined) {
// The previous chunk had char we must carry over.
chunk = `${this.carriedFromPrevious}${chunk}`;
this.carriedFromPrevious = undefined;
}
let limit = chunk.length;
const lastCode = chunk.charCodeAt(limit - 1);
if (!end &&
// A trailing CR or surrogate must be carried over to the next
// chunk.
(lastCode === CR || (lastCode >= 0xD800 && lastCode <= 0xDBFF))) {
// The chunk ends with a character that must be carried over. We cannot
// know how to handle it until we get the next chunk or the end of the
// stream. So save it for later.
this.carriedFromPrevious = chunk[limit - 1];
limit--;
chunk = chunk.slice(0, limit);
}
const { stateTable } = this;
this.chunk = chunk;
this.i = 0;
while (this.i < limit) {
// eslint-disable-next-line @typescript-eslint/no-explicit-any
stateTable[this.state].call(this);
}
this.chunkPosition += limit;
return end ? this.end() : this;
}
/**
* Close the current stream. Perform final well-formedness checks and reset
* the parser tstate.
*
* @returns this
*/
close() {
return this.write(null);
}
/**
* Get a single code point out of the current chunk. This updates the current
* position if we do position tracking.
*
* This is the algorithm to use for XML 1.0.
*
* @returns The character read.
*/
getCode10() {
const { chunk, i } = this;
this.prevI = i;
// Yes, we do this instead of doing this.i++. Doing it this way, we do not
// read this.i again, which is a bit faster.
this.i = i + 1;
if (i >= chunk.length) {
return EOC;
}
// Using charCodeAt and handling the surrogates ourselves is faster
// than using codePointAt.
const code = chunk.charCodeAt(i);
this.column++;
if (code < 0xD800) {
if (code >= SPACE || code === TAB) {
return code;
}
switch (code) {
case NL:
this.line++;
this.column = 0;
this.positionAtNewLine = this.position;
return NL;
case CR:
// We may get NaN if we read past the end of the chunk, which is fine.
if (chunk.charCodeAt(i + 1) === NL) {
// A \r\n sequence is converted to \n so we have to skip over the
// next character. We already know it has a size of 1 so ++ is fine
// here.
this.i = i + 2;
}
// Otherwise, a \r is just converted to \n, so we don't have to skip
// ahead.
// In either case, \r becomes \n.
this.line++;
this.column = 0;
this.positionAtNewLine = this.position;
return NL_LIKE;
default:
// If we get here, then code < SPACE and it is not NL CR or TAB.
this.fail("disallowed character.");
return code;
}
}
if (code > 0xDBFF) {
// This is a specialized version of isChar10 that takes into account
// that in this context code > 0xDBFF and code <= 0xFFFF. So it does not
// test cases that don't need testing.
if (!(code >= 0xE000 && code <= 0xFFFD)) {
this.fail("disallowed character.");
}
return code;
}
const final = 0x10000 + ((code - 0xD800) * 0x400) +
(chunk.charCodeAt(i + 1) - 0xDC00);
this.i = i + 2;
// This is a specialized version of isChar10 that takes into account that in
// this context necessarily final >= 0x10000.
if (final > 0x10FFFF) {
this.fail("disallowed character.");
}
return final;
}
/**
* Get a single code point out of the current chunk. This updates the current
* position if we do position tracking.
*
* This is the algorithm to use for XML 1.1.
*
* @returns {number} The character read.
*/
getCode11() {
const { chunk, i } = this;
this.prevI = i;
// Yes, we do this instead of doing this.i++. Doing it this way, we do not
// read this.i again, which is a bit faster.
this.i = i + 1;
if (i >= chunk.length) {
return EOC;
}
// Using charCodeAt and handling the surrogates ourselves is faster
// than using codePointAt.
const code = chunk.charCodeAt(i);
this.column++;
if (code < 0xD800) {
if ((code > 0x1F && code < 0x7F) || (code > 0x9F && code !== LS) ||
code === TAB) {
return code;
}
switch (code) {
case NL: // 0xA
this.line++;
this.column = 0;
this.positionAtNewLine = this.position;
return NL;
case CR: { // 0xD
// We may get NaN if we read past the end of the chunk, which is
// fine.
const next = chunk.charCodeAt(i + 1);
if (next === NL || next === NEL) {
// A CR NL or CR NEL sequence is converted to NL so we have to skip
// over the next character. We already know it has a size of 1.
this.i = i + 2;
}
// Otherwise, a CR is just converted to NL, no skip.
}
/* yes, fall through */
case NEL: // 0x85
case LS: // Ox2028
this.line++;
this.column = 0;
this.positionAtNewLine = this.position;
return NL_LIKE;
default:
this.fail("disallowed character.");
return code;
}
}
if (code > 0xDBFF) {
// This is a specialized version of isCharAndNotRestricted that takes into
// account that in this context code > 0xDBFF and code <= 0xFFFF. So it
// does not test cases that don't need testing.
if (!(code >= 0xE000 && code <= 0xFFFD)) {
this.fail("disallowed character.");
}
return code;
}
const final = 0x10000 + ((code - 0xD800) * 0x400) +
(chunk.charCodeAt(i + 1) - 0xDC00);
this.i = i + 2;
// This is a specialized version of isCharAndNotRestricted that takes into
// account that in this context necessarily final >= 0x10000.
if (final > 0x10FFFF) {
this.fail("disallowed character.");
}
return final;
}
/**
* Like ``getCode`` but with the return value normalized so that ``NL`` is
* returned for ``NL_LIKE``.
*/
getCodeNorm() {
const c = this.getCode();
return c === NL_LIKE ? NL : c;
}
unget() {
this.i = this.prevI;
this.column--;
}
/**
* Capture characters into a buffer until encountering one of a set of
* characters.
*
* @param chars An array of codepoints. Encountering a character in the array
* ends the capture. (``chars`` may safely contain ``NL``.)
*
* @return The character code that made the capture end, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
captureTo(chars) {
let { i: start } = this;
const { chunk } = this;
// eslint-disable-next-line no-constant-condition
while (true) {
const c = this.getCode();
const isNLLike = c === NL_LIKE;
const final = isNLLike ? NL : c;
if (final === EOC || chars.includes(final)) {
this.text += chunk.slice(start, this.prevI);
return final;
}
if (isNLLike) {
this.text += `${chunk.slice(start, this.prevI)}\n`;
start = this.i;
}
}
}
/**
* Capture characters into a buffer until encountering a character.
*
* @param char The codepoint that ends the capture. **NOTE ``char`` MAY NOT
* CONTAIN ``NL``.** Passing ``NL`` will result in buggy behavior.
*
* @return ``true`` if we ran into the character. Otherwise, we ran into the
* end of the current chunk.
*/
captureToChar(char) {
let { i: start } = this;
const { chunk } = this;
// eslint-disable-next-line no-constant-condition
while (true) {
let c = this.getCode();
switch (c) {
case NL_LIKE:
this.text += `${chunk.slice(start, this.prevI)}\n`;
start = this.i;
c = NL;
break;
case EOC:
this.text += chunk.slice(start);
return false;
default:
}
if (c === char) {
this.text += chunk.slice(start, this.prevI);
return true;
}
}
}
/**
* Capture characters that satisfy ``isNameChar`` into the ``name`` field of
* this parser.
*
* @return The character code that made the test fail, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
captureNameChars() {
const { chunk, i: start } = this;
// eslint-disable-next-line no-constant-condition
while (true) {
const c = this.getCode();
if (c === EOC) {
this.name += chunk.slice(start);
return EOC;
}
// NL is not a name char so we don't have to test specifically for it.
if (!isNameChar(c)) {
this.name += chunk.slice(start, this.prevI);
return c === NL_LIKE ? NL : c;
}
}
}
/**
* Skip white spaces.
*
* @return The character that ended the skip, or ``EOC`` if we hit
* the end of the chunk. The return value cannot be NL_LIKE: NL is returned
* instead.
*/
skipSpaces() {
// eslint-disable-next-line no-constant-condition
while (true) {
const c = this.getCodeNorm();
if (c === EOC || !isS(c)) {
return c;
}
}
}
setXMLVersion(version) {
this.currentXMLVersion = version;
/* eslint-disable @typescript-eslint/unbound-method */
if (version === "1.0") {
this.isChar = isChar10;
this.getCode = this.getCode10;
}
else {
this.isChar = isChar11;
this.getCode = this.getCode11;
}
/* eslint-enable @typescript-eslint/unbound-method */
}
// STATE ENGINE METHODS
// This needs to be a state separate from S_BEGIN_WHITESPACE because we want
// to be sure never to come back to this state later.
sBegin() {
// We are essentially peeking at the first character of the chunk. Since
// S_BEGIN can be in effect only when we start working on the first chunk,
// the index at which we must look is necessarily 0. Note also that the
// following test does not depend on decoding surrogates.
// If the initial character is 0xFEFF, ignore it.
if (this.chunk.charCodeAt(0) === 0xFEFF) {
this.i++;
this.column++;
}
this.state = S_BEGIN_WHITESPACE;
}
sBeginWhitespace() {
// We need to know whether we've encountered spaces or not because as soon
// as we run into a space, an XML declaration is no longer possible. Rather
// than slow down skipSpaces even in places where we don't care whether it
// skipped anything or not, we check whether prevI is equal to the value of
// i from before we skip spaces.
const iBefore = this.i;
const c = this.skipSpaces();
if (this.prevI !== iBefore) {
this.xmlDeclPossible = false;
}
switch (c) {
case LESS:
this.state = S_OPEN_WAKA;
// We could naively call closeText but in this state, it is not normal
// to have text be filled with any data.
if (this.text.length !== 0) {
throw new Error("no-empty text at start");
}
break;
case EOC:
break;
default:
this.unget();
this.state = S_TEXT;
this.xmlDeclPossible = false;
}
}
sDoctype() {
var _a;
const c = this.captureTo(DOCTYPE_TERMINATOR);
switch (c) {
case GREATER: {
// eslint-disable-next-line no-unused-expressions
(_a = this.doctypeHandler) === null || _a === void 0 ? void 0 : _a.call(this, this.text);
this.text = "";
this.state = S_TEXT;
this.doctype = true; // just remember that we saw it.
break;
}
case EOC:
break;
default:
this.text += String.fromCodePoint(c);
if (c === OPEN_BRACKET) {
this.state = S_DTD;
}
else if (isQuote(c)) {
this.state = S_DOCTYPE_QUOTE;
this.q = c;
}
}
}
sDoctypeQuote() {
const q = this.q;
if (this.captureToChar(q)) {
this.text += String.fromCodePoint(q);
this.q = null;
this.state = S_DOCTYPE;
}
}
sDTD() {
const c = this.captureTo(DTD_TERMINATOR);
if (c === EOC) {
return;
}
this.text += String.fromCodePoint(c);
if (c === CLOSE_BRACKET) {
this.state = S_DOCTYPE;
}
else if (c === LESS) {
this.state = S_DTD_OPEN_WAKA;
}
else if (isQuote(c)) {
this.state = S_DTD_QUOTED;
this.q = c;
}
}
sDTDQuoted() {
const q = this.q;
if (this.captureToChar(q)) {
this.text += String.fromCodePoint(q);
this.state = S_DTD;
this.q = null;
}
}
sDTDOpenWaka() {
const c = this.getCodeNorm();
this.text += String.fromCodePoint(c);
switch (c) {
case BANG:
this.state = S_DTD_OPEN_WAKA_BANG;
this.openWakaBang = "";
break;
case QUESTION:
this.state = S_DTD_PI;
break;
default:
this.state = S_DTD;
}
}
sDTDOpenWakaBang() {
const char = String.fromCodePoint(this.getCodeNorm());
const owb = this.openWakaBang += char;
this.text += char;
if (owb !== "-") {
this.state = owb === "--" ? S_DTD_COMMENT : S_DTD;
this.openWakaBang = "";
}
}
sDTDComment() {
if (this.captureToChar(MINUS)) {
this.text += "-";
this.state = S_DTD_COMMENT_ENDING;
}
}
sDTDCommentEnding() {
const c = this.getCodeNorm();
this.text += String.fromCodePoint(c);
this.state = c === MINUS ? S_DTD_COMMENT_ENDED : S_DTD_COMMENT;
}
sDTDCommentEnded() {
const c = this.getCodeNorm();
this.text += String.fromCodePoint(c);
if (c === GREATER) {
this.state = S_DTD;
}
else {
this.fail("malformed comment.");
// <!-- blah -- bloo --> will be recorded as
// a comment of " blah -- bloo "
this.state = S_DTD_COMMENT;
}
}
sDTDPI() {
if (this.captureToChar(QUESTION)) {
this.text += "?";
this.state = S_DTD_PI_ENDING;
}
}
sDTDPIEnding() {
const c = this.getCodeNorm();
this.text += String.fromCodePoint(c);
if (c === GREATER) {
this.state = S_DTD;
}
}
sText() {
//
// We did try a version of saxes where the S_TEXT state was split in two
// states: one for text inside the root element, and one for text
// outside. This was avoiding having to test this.tags.length to decide
// what implementation to actually use.
//
// Peformance testing on gigabyte-size files did not show any advantage to
// using the two states solution instead of the current one. Conversely, it
// made the code a bit more complicated elsewhere. For instance, a comment
// can appear before the root element so when a comment ended it was
// necessary to determine whether to return to the S_TEXT state or to the
// new text-outside-root state.
//
if (this.tags.length !== 0) {
this.handleTextInRoot();
}
else {
this.handleTextOutsideRoot();
}
}
sEntity() {
// This is essentially a specialized version of captureToChar(SEMICOLON...)
let { i: start } = this;
const { chunk } = this;
// eslint-disable-next-line no-labels, no-restricted-syntax
loop:
// eslint-disable-next-line no-constant-condition
while (true) {
switch (this.getCode()) {
case NL_LIKE:
this.entity += `${chunk.slice(start, this.prevI)}\n`;
start = this.i;
break;
case SEMICOLON: {
const { entityReturnState } = this;
const entity = this.entity + chunk.slice(start, this.prevI);
this.state = entityReturnState;
let parsed;
if (entity === "") {
this.fail("empty entity name.");
parsed = "&;";
}
else {
parsed = this.parseEntity(entity);
this.entity = "";
}
if (entityReturnState !== S_TEXT || this.textHandler !== undefined) {
this.text += parsed;
}
// eslint-disable-next-line no-labels
break loop;
}
case EOC:
this.entity += chunk.slice(start);
// eslint-disable-next-line no-labels
break loop;
default:
}
}
}
sOpenWaka() {
// Reminder: a state handler is called with at least one character
// available in the current chunk. So the first call to get code inside of
// a state handler cannot return ``EOC``. That's why we don't test
// for it.
const c = this.getCode();
// either a /, ?, !, or text is coming next.
if (isNameStartChar(c)) {
this.state = S_OPEN_TAG;
this.unget();
this.xmlDeclPossible = false;
}
else {
switch (c) {
case FORWARD_SLASH:
this.state = S_CLOSE_TAG;
this.xmlDeclPossible = false;
break;
case BANG:
this.state = S_OPEN_WAKA_BANG;
this.openWakaBang = "";
this.xmlDeclPossible = false;
break;
case QUESTION:
this.state = S_PI_FIRST_CHAR;
break;
default:
this.fail("disallowed character in tag name");
this.state = S_TEXT;
this.xmlDeclPossible = false;
}
}
}
sOpenWakaBang() {
this.openWakaBang += String.fromCodePoint(this.getCodeNorm());
switch (this.openWakaBang) {
case "[CDATA[":
if (!this.sawRoot && !this.reportedTextBeforeRoot) {
this.fail("text data outside of root node.");
this.reportedTextBeforeRoot = true;
}
if (this.closedRoot && !this.reportedTextAfterRoot) {
this.fail("text data outside of root node.");
this.reportedTextAfterRoot = true;
}
this.state = S_CDATA;
this.openWakaBang = "";
break;
case "--":
this.state = S_COMMENT;
this.openWakaBang = "";
break;
case "DOCTYPE":
this.state = S_DOCTYPE;
if (this.doctype || this.sawRoot) {
this.fail("inappropriately located doctype declaration.");
}
this.openWakaBang = "";
break;
default:
// 7 happens to be the maximum length of the string that can possibly
// match one of the cases above.
if (this.openWakaBang.length >= 7) {
this.fail("incorrect syntax.");
}
}
}
sComment() {
if (this.captureToChar(MINUS)) {
this.state = S_COMMENT_ENDING;
}
}
sCommentEnding() {
var _a;
const c = this.getCodeNorm();
if (c === MINUS) {
this.state = S_COMMENT_ENDED;
// eslint-disable-next-line no-unused-expressions
(_a = this.commentHandler) === null || _a === void 0 ? void 0 : _a.call(this, this.text);
this.text = "";
}
else {
this.text += `-${String.fromCodePoint(c)}`;
this.state = S_COMMENT;
}
}
sCommentEnded() {
const c = this.getCodeNorm();
if (c !== GREATER) {
this.fail("malformed comment.");
// <!-- blah -- bloo --> will be recorded as
// a comment of " blah -- bloo "
this.text += `--${String.fromCodePoint(c)}`;
this.state = S_COMMENT;
}
else {
this.state = S_TEXT;
}
}
sCData() {
if (this.captureToChar(CLOSE_BRACKET)) {
this.state = S_CDATA_ENDING;
}
}
sCDataEnding() {
const c = this.getCodeNorm();
if (c === CLOSE_BRACKET) {
this.state = S_CDATA_ENDING_2;
}
else {
this.text += `]${String.fromCodePoint(c)}`;
this.state = S_CDATA;
}
}
sCDataEnding2() {
var _a;
const c = this.getCodeNorm();
switch (c) {
case GREATER: {
// eslint-disable-next-line no-unused-expressions
(_a = this.cdataHandler) === null || _a === void 0 ? void 0 : _a.call(this, this.text);
this.text = "";
this.state = S_TEXT;
break;
}
case CLOSE_BRACKET:
this.text += "]";
break;
default:
this.text += `]]${String.fromCodePoint(c)}`;
this.state = S_CDATA;
}
}
// We need this separate state to check the first character fo the pi target
// with this.nameStartCheck which allows less characters than this.nameCheck.
sPIFirstChar() {
const c = this.getCodeNorm();
// This is first because in the case where the file is well-formed this is
// the branch taken. We optimize for well-formedness.
if (this.nameStartCheck(c)) {
this.piTarget += String.fromCodePoint(c);
this.state = S_PI_REST;
}
else if (c === QUESTION || isS(c)) {
this.fail("processing instruction without a target.");
this.state = c === QUESTION ? S_PI_ENDING : S_PI_BODY;
}
else {
this.fail("disallowed character in processing instruction name.");
this.piTarget += String.fromCodePoint(c);
this.state = S_PI_REST;
}
}
sPIRest() {
// Capture characters into a piTarget while ``this.nameCheck`` run on the
// character read returns true.
const { chunk, i: start } = this;
// eslint-disable-next-line no-constant-condition
while (true) {
const c = this.getCodeNorm();
if (c === EOC) {
this.piTarget += chunk.slice(start);
return;
}
// NL cannot satisfy this.nameCheck so we don't have to test specifically
// for it.
if (!this.nameCheck(c)) {
this.piTarget += chunk.slice(start, this.prevI);
const isQuestion = c === QUESTION;
if (isQuestion || isS(c)) {
if (this.piTarget === "xml") {
if (!this.xmlDeclPossible) {
this.fail("an XML declaration must be at the start of the document.");
}
this.state = isQuestion ? S_XML_DECL_ENDING : S_XML_DECL_NAME_START;
}
else {
this.state = isQuestion ? S_PI_ENDING : S_PI_BODY;
}
}
else {
this.fail("disallowed character in processing instruction name.");
this.piTarget += String.fromCodePoint(c);
}
break;
}
}
}
sPIBody() {
if (this.text.length === 0) {
const c = this.getCodeNorm();
if (c === QUESTION) {
this.state = S_PI_ENDING;
}
else if (!isS(c)) {
this.text = String.fromCodePoint(c);
}
}
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
else if (this.captureToChar(QUESTION)) {
this.state = S_PI_ENDING;
}
}
sPIEnding() {
var _a;
const c = this.getCodeNorm();
if (c === GREATER) {
const { piTarget } = this;
if (piTarget.toLowerCase() === "xml") {
this.fail("the XML declaration must appear at the start of the document.");
}
// eslint-disable-next-line no-unused-expressions
(_a = this.piHandler) === null || _a === void 0 ? void 0 : _a.call(this, {
target: piTarget,
body: this.text,
});
this.piTarget = this.text = "";
this.state = S_TEXT;
}
else if (c === QUESTION) {
// We ran into ?? as part of a processing instruction. We initially took
// the first ? as a sign that the PI was ending, but it is not. So we have
// to add it to the body but we take the new ? as a sign that the PI is
// ending.
this.text += "?";
}
else {
this.text += `?${String.fromCodePoint(c)}`;
this.state = S_PI_BODY;
}
this.xmlDeclPossible = false;
}
sXMLDeclNameStart() {
const c = this.skipSpaces();
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
// It is valid to go to S_XML_DECL_ENDING from this state.
this.state = S_XML_DECL_ENDING;
return;
}
if (c !== EOC) {
this.state = S_XML_DECL_NAME;
this.name = String.fromCodePoint(c);
}
}
sXMLDeclName() {
const c = this.captureTo(XML_DECL_NAME_TERMINATOR);
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
this.state = S_XML_DECL_ENDING;
this.name += this.text;
this.text = "";
this.fail("XML declaration is incomplete.");
return;
}
if (!(isS(c) || c === EQUAL)) {
return;
}
this.name += this.text;
this.text = "";
if (!this.xmlDeclExpects.includes(this.name)) {
switch (this.name.length) {
case 0:
this.fail("did not expect any more name/value pairs.");
break;
case 1:
this.fail(`expected the name ${this.xmlDeclExpects[0]}.`);
break;
default:
this.fail(`expected one of ${this.xmlDeclExpects.join(", ")}`);
}
}
this.state = c === EQUAL ? S_XML_DECL_VALUE_START : S_XML_DECL_EQ;
}
sXMLDeclEq() {
const c = this.getCodeNorm();
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
this.state = S_XML_DECL_ENDING;
this.fail("XML declaration is incomplete.");
return;
}
if (isS(c)) {
return;
}
if (c !== EQUAL) {
this.fail("value required.");
}
this.state = S_XML_DECL_VALUE_START;
}
sXMLDeclValueStart() {
const c = this.getCodeNorm();
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
this.state = S_XML_DECL_ENDING;
this.fail("XML declaration is incomplete.");
return;
}
if (isS(c)) {
return;
}
if (!isQuote(c)) {
this.fail("value must be quoted.");
this.q = SPACE;
}
else {
this.q = c;
}
this.state = S_XML_DECL_VALUE;
}
sXMLDeclValue() {
const c = this.captureTo([this.q, QUESTION]);
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
this.state = S_XML_DECL_ENDING;
this.text = "";
this.fail("XML declaration is incomplete.");
return;
}
if (c === EOC) {
return;
}
const value = this.text;
this.text = "";
switch (this.name) {
case "version": {
this.xmlDeclExpects = ["encoding", "standalone"];
const version = value;
this.xmlDecl.version = version;
// This is the test specified by XML 1.0 but it is fine for XML 1.1.
if (!/^1\.[0-9]+$/.test(version)) {
this.fail("version number must match /^1\\.[0-9]+$/.");
}
// When forceXMLVersion is set, the XML declaration is ignored.
else if (!this.opt.forceXMLVersion) {
this.setXMLVersion(version);
}
break;
}
case "encoding":
if (!/^[A-Za-z][A-Za-z0-9._-]*$/.test(value)) {
this.fail("encoding value must match \
/^[A-Za-z0-9][A-Za-z0-9._-]*$/.");
}
this.xmlDeclExpects = ["standalone"];
this.xmlDecl.encoding = value;
break;
case "standalone":
if (value !== "yes" && value !== "no") {
this.fail("standalone value must match \"yes\" or \"no\".");
}
this.xmlDeclExpects = [];
this.xmlDecl.standalone = value;
break;
default:
// We don't need to raise an error here since we've already raised one
// when checking what name was expected.
}
this.name = "";
this.state = S_XML_DECL_SEPARATOR;
}
sXMLDeclSeparator() {
const c = this.getCodeNorm();
// The question mark character is not valid inside any of the XML
// declaration name/value pairs.
if (c === QUESTION) {
// It is valid to go to S_XML_DECL_ENDING from this state.
this.state = S_XML_DECL_ENDING;
return;
}
if (!isS(c)) {
this.fail("whitespace required.");
this.unget();
}
this.state = S_XML_DECL_NAME_START;
}
sXMLDeclEnding() {
var _a;
const c = this.getCodeNorm();
if (c === GREATER) {
if (this.piTarget !== "xml") {
this.fail("processing instructions are not allowed before root.");
}
else if (this.name !== "version" &&
this.xmlDeclExpects.includes("version")) {
this.fail("XML declaration must contain a version.");
}
// eslint-disable-next-line no-unused-expressions
(_a = this.xmldeclHandler) === null || _a === void 0 ? void 0 : _a.call(this, this.xmlDecl);
this.name = "";
this.piTarget = this.text = "";
this.state = S_TEXT;
}
else {
// We got here because the previous character was a ?, but the question
// mark character is not valid inside any of the XML declaration
// name/value pairs.
this.fail("The character ? is disallowed anywhere in XML declarations.");
}
this.xmlDeclPossible = false;
}
sOpenTag() {
var _a;
const c = this.captureNameChars();
if (c === EOC) {
return;
}
const tag = this.tag = {
name: this.name,
attributes: Object.create(null),
};
this.name = "";
if (this.xmlnsOpt) {
this.topNS = tag.ns = Object.create(null);
}
// eslint-disable-next-line no-unused-expressions
(_a = this.openTagStartHandler) === null || _a === void 0 ? void 0 : _a.call(this, tag);
this.sawRoot = true;
if (!this.fragmentOpt && this.closedRoot) {
this.fail("documents may contain only one root.");
}
switch (c) {
case GREATER:
this.openTag();
break;
case FORWARD_SLASH:
this.state = S_OPEN_TAG_SLASH;
break;
default:
if (!isS(c)) {
this.fail("disallowed character in tag name.");
}
this.state = S_ATTRIB;
}
}
sOpenTagSlash() {
if (this.getCode() === GREATER) {
this.openSelfClosingTag();
}
else {
this.fail("forward-slash in opening tag not followed by >.");
this.state = S_ATTRIB;
}
}
sAttrib() {
const c = this.skipSpaces();
if (c === EOC) {
return;
}
if (isNameStartChar(c)) {
this.unget();
this.state = S_ATTRIB_NAME;
}
else if (c === GREATER) {
this.openTag();
}
else if (c === FORWARD_SLASH) {
this.state = S_OPEN_TAG_SLASH;
}
else {
this.fail("disallowed character in attribute name.");
}
}
sAttribName() {
const c = this.captureNameChars();
if (c === EQUAL) {
this.state = S_ATTRIB_VALUE;
}
else if (isS(c)) {
this.state = S_ATTRIB_NAME_SAW_WHITE;
}
else if (c === GREATER) {
this.fail("attribute without value.");
this.pushAttrib(this.name, this.name);
this.name = this.text = "";
this.openTag();
}
else if (c !== EOC) {
this.fail("disallowed character in attribute name.");
}
}
sAttribNameSawWhite() {
const c = this.skipSpaces();
switch (c) {
case EOC:
return;
case EQUAL:
this.state = S_ATTRIB_VALUE;
break;
default:
this.fail("attribute without value.");
// Should we do this???
// this.tag.attributes[this.name] = "";
this.text = "";
this.name = "";
if (c === GREATER) {
this.openTag();
}
else if (isNameStartChar(c)) {
this.unget();
this.state = S_ATTRIB_NAME;
}
else {
this.fail("disallowed character in attribute name.");
this.state = S_ATTRIB;
}
}
}
sAttribValue() {
const c = this.getCodeNorm();
if (isQuote(c)) {
this.q = c;
this.state = S_ATTRIB_VALUE_QUOTED;
}
else if (!isS(c)) {
this.fail("unquoted attribute value.");
this.state = S_ATTRIB_VALUE_UNQUOTED;
this.unget();
}
}
sAttribValueQuoted() {
// We deliberately do not use captureTo here. The specialized code we use
// here is faster than using captureTo.
const { q, chunk } = this;
let { i: start } = this;
// eslint-disable-next-line no-constant-condition
while (true) {
switch (this.getCode()) {
case q:
this.pushAttrib(this.name, this.text + chunk.slice(start, this.prevI));
this.name = this.text = "";
this.q = null;
this.state = S_ATTRIB_VALUE_CLOSED;
return;
case AMP:
this.text += chunk.slice(start, this.prevI);
this.state = S_ENTITY;
this.entityReturnState = S_ATTRIB_VALUE_QUOTED;
return;
case NL:
case NL_LIKE:
case TAB:
this.text += `${chunk.slice(start, this.prevI)} `;
start = this.i;
break;
case LESS:
this.text += chunk.slice(start, this.prevI);
this.fail("disallowed character.");
return;
case EOC:
this.text += chunk.slice(start);
return;
default:
}
}
}
sAttribValueClosed() {
const c = this.getCodeNorm();
if (isS(c)) {
this.state = S_ATTRIB;
}
else if (c === GREATER) {
this.openTag();
}
else if (c === FORWARD_SLASH) {
this.state = S_OPEN_TAG_SLASH;
}
else if (isNameStartChar(c)) {
this.fail("no whitespace between attributes.");
this.unget();
this.state = S_ATTRIB_NAME;
}
else {
this.fail("disallowed character in attribute name.");
}
}
sAttribValueUnquoted() {
// We don't do anything regarding EOL or space handling for unquoted
// attributes. We already have failed by the time we get here, and the
// contract that saxes upholds states that upon failure, it is not safe to
// rely on the data passed to event handlers (other than
// ``onerror``). Passing "bad" data is not a problem.
const c = this.captureTo(ATTRIB_VALUE_UNQUOTED_TERMINATOR);
switch (c) {
case AMP:
this.state = S_ENTITY;
this.entityReturnState = S_ATTRIB_VALUE_UNQUOTED;
break;
case LESS:
this.fail("disallowed character.");
break;
case EOC:
break;
default:
if (this.text.includes("]]>")) {
this.fail("the string \"]]>\" is disallowed in char data.");
}
this.pushAttrib(this.name, this.text);
this.name = this.text = "";
if (c === GREATER) {
this.openTag();
}
else {
this.state = S_ATTRIB;
}
}
}
sCloseTag() {
const c = this.captureNameChars();
if (c === GREATER) {
this.closeTag();
}
else if (isS(c)) {
this.state = S_CLOSE_TAG_SAW_WHITE;
}
else if (c !== EOC) {
this.fail("disallowed character in closing tag.");
}
}
sCloseTagSawWhite() {
switch (this.skipSpaces()) {
case GREATER:
this.closeTag();
break;
case EOC:
break;
default:
this.fail("disallowed character in closing tag.");
}
}
// END OF STATE ENGINE METHODS
handleTextInRoot() {
// This is essentially a specialized version of captureTo which is optimized
// for performing the ]]> check. A previous version of this code, checked
// ``this.text`` for the presence of ]]>. It simplified the code but was
// very costly when character data contained a lot of entities to be parsed.
//
// Since we are using a specialized loop, we also keep track of the presence
// of ]]> in text data. The sequence ]]> is forbidden to appear as-is.
//
let { i: start, forbiddenState } = this;
const { chunk, textHandler: handler } = this;
// eslint-disable-next-line no-labels, no-restricted-syntax
scanLoop:
// eslint-disable-next-line no-constant-condition
while (true) {
switch (this.getCode()) {
case LESS: {
this.state = S_OPEN_WAKA;
if (handler !== undefined) {
const { text } = this;
const slice = chunk.slice(start, this.prevI);
if (text.length !== 0) {
handler(text + slice);
this.text = "";
}
else if (slice.length !== 0) {
handler(slice);
}
}
forbiddenState = FORBIDDEN_START;
// eslint-disable-next-line no-labels
break scanLoop;
}
case AMP:
this.state = S_ENTITY;
this.entityReturnState = S_TEXT;
if (handler !== undefined) {
this.text += chunk.slice(start, this.prevI);
}
forbiddenState = FORBIDDEN_START;
// eslint-disable-next-line no-labels
break scanLoop;
case CLOSE_BRACKET:
switch (forbiddenState) {
case FORBIDDEN_START:
forbiddenState = FORBIDDEN_BRACKET;
break;
case FORBIDDEN_BRACKET:
forbiddenState = FORBIDDEN_BRACKET_BRACKET;
break;
case FORBIDDEN_BRACKET_BRACKET:
break;
default:
throw new Error("impossible state");
}
break;
case GREATER:
if (forbiddenState === FORBIDDEN_BRACKET_BRACKET) {
this.fail("the string \"]]>\" is disallowed in char data.");
}
forbiddenState = FORBIDDEN_START;
break;
case NL_LIKE:
if (handler !== undefined) {
this.text += `${chunk.slice(start, this.prevI)}\n`;
}
start = this.i;
forbiddenState = FORBIDDEN_START;
break;
case EOC:
if (handler !== undefined) {
this.text += chunk.slice(start);
}
// eslint-disable-next-line no-labels
break scanLoop;
default:
forbiddenState = FORBIDDEN_START;
}
}
this.forbiddenState = forbiddenState;
}
handleTextOutsideRoot() {
// This is essentially a specialized version of captureTo which is optimized
// for a specialized task. We keep track of the presence of non-space
// characters in the text since these are errors when appearing outside the
// document root element.
let { i: start } = this;
const { chunk, textHandler: handler } = this;
let nonSpace = false;
// eslint-disable-next-line no-labels, no-restricted-syntax
outRootLoop:
// eslint-disable-next-line no-constant-condition
while (true) {
const code = this.getCode();
switch (code) {
case LESS: {
this.state = S_OPEN_WAKA;
if (handler !== undefined) {
const { text } = this;
const slice = chunk.slice(start, this.prevI);
if (text.length !== 0) {
handler(text + slice);
this.text = "";
}
else if (slice.length !== 0) {
handler(slice);
}
}
// eslint-disable-next-line no-labels
break outRootLoop;
}
case AMP:
this.state = S_ENTITY;
this.entityReturnState = S_TEXT;
if (handler !== undefined) {
this.text += chunk.slice(start, this.prevI);
}
nonSpace = true;
// eslint-disable-next-line no-labels
break outRootLoop;
case NL_LIKE:
if (handler !== undefined) {
this.text += `${chunk.slice(start, this.prevI)}\n`;
}
start = this.i;
break;
case EOC:
if (handler !== undefined) {
this.text += chunk.slice(start);
}
// eslint-disable-next-line no-labels
break outRootLoop;
default:
if (!isS(code)) {
nonSpace = true;
}
}
}
if (!nonSpace) {
return;
}
// We use the reportedTextBeforeRoot and reportedTextAfterRoot flags
// to avoid reporting errors for every single character that is out of
// place.
if (!this.sawRoot && !this.reportedTextBeforeRoot) {
this.fail("text data outside of root node.");
this.reportedTextBeforeRoot = true;
}
if (this.closedRoot && !this.reportedTextAfterRoot) {
this.fail("text data outside of root node.");
this.reportedTextAfterRoot = true;
}
}
pushAttribNS(name, value) {
var _a;
const { prefix, local } = this.qname(name);
const attr = { name, prefix, local, value };
this.attribList.push(attr);
// eslint-disable-next-line no-unused-expressions
(_a = this.attributeHandler) === null || _a === void 0 ? void 0 : _a.call(this, attr);
if (prefix === "xmlns") {
const trimmed = value.trim();
if (this.currentXMLVersion === "1.0" && trimmed === "") {
this.fail("invalid attempt to undefine prefix in XML 1.0");
}
this.topNS[local] = trimmed;
nsPairCheck(this, local, trimmed);
}
else if (name === "xmlns") {
const trimmed = value.trim();
this.topNS[""] = trimmed;
nsPairCheck(this, "", trimmed);
}
}
pushAttribPlain(name, value) {
var _a;
const attr = { name, value };
this.attribList.push(attr);
// eslint-disable-next-line no-unused-expressions
(_a = this.attributeHandler) === null || _a === void 0 ? void 0 : _a.call(this, attr);
}
/**
* End parsing. This performs final well-formedness checks and resets the
* parser to a clean state.
*
* @returns this
*/
end() {
var _a, _b;
if (!this.sawRoot) {
this.fail("document must contain a root element.");
}
const { tags } = this;
while (tags.length > 0) {
const tag = tags.pop();
this.fail(`unclosed tag: ${tag.name}`);
}
if ((this.state !== S_BEGIN) && (this.state !== S_TEXT)) {
this.fail("unexpected end.");
}
const { text } = this;
if (text.length !== 0) {
// eslint-disable-next-line no-unused-expressions
(_a = this.textHandler) === null || _a === void 0 ? void 0 : _a.call(this, text);
this.text = "";
}
this._closed = true;
// eslint-disable-next-line no-unused-expressions
(_b = this.endHandler) === null || _b === void 0 ? void 0 : _b.call(this);
this._init();
return this;
}
/**
* Resolve a namespace prefix.
*
* @param prefix The prefix to resolve.
*
* @returns The namespace URI or ``undefined`` if the prefix is not defined.
*/
resolve(prefix) {
var _a, _b;
let uri = this.topNS[prefix];
if (uri !== undefined) {
return uri;
}
const { tags } = this;
for (let index = tags.length - 1; index >= 0; index--) {
uri = tags[index].ns[prefix];
if (uri !== undefined) {
return uri;
}
}
uri = this.ns[prefix];
if (uri !== undefined) {
return uri;
}
return (_b = (_a = this.opt).resolvePrefix) === null || _b === void 0 ? void 0 : _b.call(_a, prefix);
}
/**
* Parse a qname into its prefix and local name parts.
*
* @param name The name to parse
*
* @returns
*/
qname(name) {
// This is faster than using name.split(":").
const colon = name.indexOf(":");
if (colon === -1) {
return { prefix: "", local: name };
}
const local = name.slice(colon + 1);
const prefix = name.slice(0, colon);
if (prefix === "" || local === "" || local.includes(":")) {
this.fail(`malformed name: ${name}.`);
}
return { prefix, local };
}
processAttribsNS() {
var _a;
const { attribList } = this;
const tag = this.tag;
{
// add namespace info to tag
const { prefix, local } = this.qname(tag.name);
tag.prefix = prefix;
tag.local = local;
const uri = tag.uri = (_a = this.resolve(prefix)) !== null && _a !== void 0 ? _a : "";
if (prefix !== "") {
if (prefix === "xmlns") {
this.fail("tags may not have \"xmlns\" as prefix.");
}
if (uri === "") {
this.fail(`unbound namespace prefix: ${JSON.stringify(prefix)}.`);
tag.uri = prefix;
}
}
}
if (attribList.length === 0) {
return;
}
const { attributes } = tag;
const seen = new Set();
// Note: do not apply default ns to attributes:
// http://www.w3.org/TR/REC-xml-names/#defaulting
for (const attr of attribList) {
const { name, prefix, local } = attr;
let uri;
let eqname;
if (prefix === "") {
uri = name === "xmlns" ? XMLNS_NAMESPACE : "";
eqname = name;
}
else {
uri = this.resolve(prefix);
// if there's any attributes with an undefined namespace,
// then fail on them now.
if (uri === undefined) {
this.fail(`unbound namespace prefix: ${JSON.stringify(prefix)}.`);
uri = prefix;
}
eqname = `{${uri}}${local}`;
}
if (seen.has(eqname)) {
this.fail(`duplicate attribute: ${eqname}.`);
}
seen.add(eqname);
attr.uri = uri;
attributes[name] = attr;
}
this.attribList = [];
}
processAttribsPlain() {
const { attribList } = this;
// eslint-disable-next-line prefer-destructuring
const attributes = this.tag.attributes;
for (const { name, value } of attribList) {
if (attributes[name] !== undefined) {
this.fail(`duplicate attribute: ${name}.`);
}
attributes[name] = value;
}
this.attribList = [];
}
/**
* Handle a complete open tag. This parser code calls this once it has seen
* the whole tag. This method checks for well-formeness and then emits
* ``onopentag``.
*/
openTag() {
var _a;
this.processAttribs();
const { tags } = this;
const tag = this.tag;
tag.isSelfClosing = false;
// There cannot be any pending text here due to the onopentagstart that was
// necessarily emitted before we get here. So we do not check text.
// eslint-disable-next-line no-unused-expressions
(_a = this.openTagHandler) === null || _a === void 0 ? void 0 : _a.call(this, tag);
tags.push(tag);
this.state = S_TEXT;
this.name = "";
}
/**
* Handle a complete self-closing tag. This parser code calls this once it has
* seen the whole tag. This method checks for well-formeness and then emits
* ``onopentag`` and ``onclosetag``.
*/
openSelfClosingTag() {
var _a, _b, _c;
this.processAttribs();
const { tags } = this;
const tag = this.tag;
tag.isSelfClosing = true;
// There cannot be any pending text here due to the onopentagstart that was
// necessarily emitted before we get here. So we do not check text.
// eslint-disable-next-line no-unused-expressions
(_a = this.openTagHandler) === null || _a === void 0 ? void 0 : _a.call(this, tag);
// eslint-disable-next-line no-unused-expressions
(_b = this.closeTagHandler) === null || _b === void 0 ? void 0 : _b.call(this, tag);
const top = this.tag = (_c = tags[tags.length - 1]) !== null && _c !== void 0 ? _c : null;
if (top === null) {
this.closedRoot = true;
}
this.state = S_TEXT;
this.name = "";
}
/**
* Handle a complete close tag. This parser code calls this once it has seen
* the whole tag. This method checks for well-formeness and then emits
* ``onclosetag``.
*/
closeTag() {
const { tags, name } = this;
// Our state after this will be S_TEXT, no matter what, and we can clear
// tagName now.
this.state = S_TEXT;
this.name = "";
if (name === "") {
this.fail("weird empty close tag.");
this.text += "</>";
return;
}
const handler = this.closeTagHandler;
let l = tags.length;
while (l-- > 0) {
const tag = this.tag = tags.pop();
this.topNS = tag.ns;
// eslint-disable-next-line no-unused-expressions
handler === null || handler === void 0 ? void 0 : handler(tag);
if (tag.name === name) {
break;
}
this.fail("unexpected close tag.");
}
if (l === 0) {
this.closedRoot = true;
}
else if (l < 0) {
this.fail(`unmatched closing tag: ${name}.`);
this.text += `</${name}>`;
}
}
/**
* Resolves an entity. Makes any necessary well-formedness checks.
*
* @param entity The entity to resolve.
*
* @returns The parsed entity.
*/
parseEntity(entity) {
// startsWith would be significantly slower for this test.
// eslint-disable-next-line @typescript-eslint/prefer-string-starts-ends-with
if (entity[0] !== "#") {
const defined = this.ENTITIES[entity];
if (defined !== undefined) {
return defined;
}
this.fail(this.isName(entity) ? "undefined entity." :
"disallowed character in entity name.");
return `&${entity};`;
}
let num = NaN;
if (entity[1] === "x" && /^#x[0-9a-f]+$/i.test(entity)) {
num = parseInt(entity.slice(2), 16);
}
else if (/^#[0-9]+$/.test(entity)) {
num = parseInt(entity.slice(1), 10);
}
// The character reference is required to match the CHAR production.
if (!this.isChar(num)) {
this.fail("malformed character entity.");
return `&${entity};`;
}
return String.fromCodePoint(num);
}
}
exports.SaxesParser = SaxesParser;
//# sourceMappingURL=saxes.js.map | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/saxes/saxes.js | saxes.js |
# prelude.ls [](https://travis-ci.org/gkz/prelude-ls)
is a functionally oriented utility library. It is powerful and flexible. Almost all of its functions are curried. It is written in, and is the recommended base library for, <a href="http://livescript.net">LiveScript</a>.
See **[the prelude.ls site](http://preludels.com)** for examples, a reference, and more.
You can install via npm `npm install prelude-ls`
### Development
`make test` to test
`make build` to build `lib` from `src`
`make build-browser` to build browser versions
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/README.md | README.md |
# 1.1.2
- add `Func.memoize`
- fix `zip-all` and `zip-with-all` corner case (no input)
- build with LiveScript 1.4.0
# 1.1.1
- curry `unique-by`, `minimum-by`
# 1.1.0
- added `List` functions: `maximum-by`, `minimum-by`, `unique-by`
- added `List` functions: `at`, `elem-index`, `elem-indices`, `find-index`, `find-indices`
- added `Str` functions: `capitalize`, `camelize`, `dasherize`
- added `Func` function: `over` - eg. ``same-length = (==) `over` (.length)``
- exported `Str.repeat` through main `prelude` object
- fixed definition of `foldr` and `foldr1`, the new correct definition is backwards incompatible with the old, incorrect one
- fixed issue with `fix`
- improved code coverage
# 1.0.3
- build browser versions
# 1.0.2
- bug fix for `flatten` - slight change with bug fix, flattens arrays only, not array-like objects
# 1.0.1
- bug fixes for `drop-while` and `take-while`
# 1.0.0
* massive update - separated functions into separate modules
* functions do not accept multiple types anymore - use different versions in their respective modules in some cases (eg. `Obj.map`), or use `chars` or `values` in other cases to transform into a list
* objects are no longer transformed into functions, simply use `(obj.)` in LiveScript to do that
* browser version now using browserify - use `prelude = require('prelude-ls')`
* added `compact`, `split`, `flatten`, `difference`, `intersection`, `union`, `count-by`, `group-by`, `chars`, `unchars`, `apply`
* added `lists-to-obj` which takes a list of keys and list of values and zips them up into an object, and the converse `obj-to-lists`
* added `pairs-to-obj` which takes a list of pairs (2 element lists) and creates an object, and the converse `obj-to-pairs`
* removed `cons`, `append` - use the concat operator
* removed `compose` - use the compose operator
* removed `obj-to-func` - use partially applied access (eg. `(obj.)`)
* removed `length` - use `(.length)`
* `sort-by` renamed to `sort-with`
* added new `sort-by`
* removed `compare` - just use the new `sort-by`
* `break-it` renamed `break-list`, (`Str.break-str` for the string version)
* added `Str.repeat` which creates a new string by repeating the input n times
* `unfold` as alias to `unfoldr` is no longer used
* fixed up style and compiled with LiveScript 1.1.1
* use Make instead of Slake
* greatly improved tests
# 0.6.0
* fixed various bugs
* added `fix`, a fixpoint (Y combinator) for anonymous recursive functions
* added `unfoldr` (alias `unfold`)
* calling `replicate` with a string now returns a list of strings
* removed `partial`, just use native partial application in LiveScript using the `_` placeholder, or currying
* added `sort`, `sortBy`, and `compare`
# 0.5.0
* removed `lookup` - use (.prop)
* removed `call` - use (.func arg1, arg2)
* removed `pluck` - use map (.prop), xs
* fixed buys wtih `head` and `last`
* added non-minifed browser version, as `prelude-browser.js`
* renamed `prelude-min.js` to `prelude-browser-min.js`
* renamed `zip` to `zipAll`
* renamed `zipWith` to `zipAllWith`
* added `zip`, a curried zip that takes only two arguments
* added `zipWith`, a curried zipWith that takes only two arguments
# 0.4.0
* added `parition` function
* added `curry` function
* removed `elem` function (use `in`)
* removed `notElem` function (use `not in`)
# 0.3.0
* added `listToObject`
* added `unique`
* added `objToFunc`
* added support for using strings in map and the like
* added support for using objects in map and the like
* added ability to use objects instead of functions in certain cases
* removed `error` (just use throw)
* added `tau` constant
* added `join`
* added `values`
* added `keys`
* added `partial`
* renamed `log` to `ln`
* added alias to `head`: `first`
* added `installPrelude` helper
# 0.2.0
* removed functions that simply warp operators as you can now use operators as functions in LiveScript
* `min/max` are now curried and take only 2 arguments
* added `call`
# 0.1.0
* initial public release
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/CHANGELOG.md | CHANGELOG.md |
var each, map, compact, filter, reject, partition, find, head, first, tail, last, initial, empty, reverse, unique, uniqueBy, fold, foldl, fold1, foldl1, foldr, foldr1, unfoldr, concat, concatMap, flatten, difference, intersection, union, countBy, groupBy, andList, orList, any, all, sort, sortWith, sortBy, sum, product, mean, average, maximum, minimum, maximumBy, minimumBy, scan, scanl, scan1, scanl1, scanr, scanr1, slice, take, drop, splitAt, takeWhile, dropWhile, span, breakList, zip, zipWith, zipAll, zipAllWith, at, elemIndex, elemIndices, findIndex, findIndices, toString$ = {}.toString, slice$ = [].slice;
each = curry$(function(f, xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
f(x);
}
return xs;
});
map = curry$(function(f, xs){
var i$, len$, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
results$.push(f(x));
}
return results$;
});
compact = function(xs){
var i$, len$, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (x) {
results$.push(x);
}
}
return results$;
};
filter = curry$(function(f, xs){
var i$, len$, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (f(x)) {
results$.push(x);
}
}
return results$;
});
reject = curry$(function(f, xs){
var i$, len$, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (!f(x)) {
results$.push(x);
}
}
return results$;
});
partition = curry$(function(f, xs){
var passed, failed, i$, len$, x;
passed = [];
failed = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
(f(x) ? passed : failed).push(x);
}
return [passed, failed];
});
find = curry$(function(f, xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (f(x)) {
return x;
}
}
});
head = first = function(xs){
return xs[0];
};
tail = function(xs){
if (!xs.length) {
return;
}
return xs.slice(1);
};
last = function(xs){
return xs[xs.length - 1];
};
initial = function(xs){
if (!xs.length) {
return;
}
return xs.slice(0, -1);
};
empty = function(xs){
return !xs.length;
};
reverse = function(xs){
return xs.concat().reverse();
};
unique = function(xs){
var result, i$, len$, x;
result = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (!in$(x, result)) {
result.push(x);
}
}
return result;
};
uniqueBy = curry$(function(f, xs){
var seen, i$, len$, x, val, results$ = [];
seen = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
val = f(x);
if (in$(val, seen)) {
continue;
}
seen.push(val);
results$.push(x);
}
return results$;
});
fold = foldl = curry$(function(f, memo, xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
memo = f(memo, x);
}
return memo;
});
fold1 = foldl1 = curry$(function(f, xs){
return fold(f, xs[0], xs.slice(1));
});
foldr = curry$(function(f, memo, xs){
var i$, x;
for (i$ = xs.length - 1; i$ >= 0; --i$) {
x = xs[i$];
memo = f(x, memo);
}
return memo;
});
foldr1 = curry$(function(f, xs){
return foldr(f, xs[xs.length - 1], xs.slice(0, -1));
});
unfoldr = curry$(function(f, b){
var result, x, that;
result = [];
x = b;
while ((that = f(x)) != null) {
result.push(that[0]);
x = that[1];
}
return result;
});
concat = function(xss){
return [].concat.apply([], xss);
};
concatMap = curry$(function(f, xs){
var x;
return [].concat.apply([], (function(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = xs).length; i$ < len$; ++i$) {
x = ref$[i$];
results$.push(f(x));
}
return results$;
}()));
});
flatten = function(xs){
var x;
return [].concat.apply([], (function(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = xs).length; i$ < len$; ++i$) {
x = ref$[i$];
if (toString$.call(x).slice(8, -1) === 'Array') {
results$.push(flatten(x));
} else {
results$.push(x);
}
}
return results$;
}()));
};
difference = function(xs){
var yss, results, i$, len$, x, j$, len1$, ys;
yss = slice$.call(arguments, 1);
results = [];
outer: for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
for (j$ = 0, len1$ = yss.length; j$ < len1$; ++j$) {
ys = yss[j$];
if (in$(x, ys)) {
continue outer;
}
}
results.push(x);
}
return results;
};
intersection = function(xs){
var yss, results, i$, len$, x, j$, len1$, ys;
yss = slice$.call(arguments, 1);
results = [];
outer: for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
for (j$ = 0, len1$ = yss.length; j$ < len1$; ++j$) {
ys = yss[j$];
if (!in$(x, ys)) {
continue outer;
}
}
results.push(x);
}
return results;
};
union = function(){
var xss, results, i$, len$, xs, j$, len1$, x;
xss = slice$.call(arguments);
results = [];
for (i$ = 0, len$ = xss.length; i$ < len$; ++i$) {
xs = xss[i$];
for (j$ = 0, len1$ = xs.length; j$ < len1$; ++j$) {
x = xs[j$];
if (!in$(x, results)) {
results.push(x);
}
}
}
return results;
};
countBy = curry$(function(f, xs){
var results, i$, len$, x, key;
results = {};
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
key = f(x);
if (key in results) {
results[key] += 1;
} else {
results[key] = 1;
}
}
return results;
});
groupBy = curry$(function(f, xs){
var results, i$, len$, x, key;
results = {};
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
key = f(x);
if (key in results) {
results[key].push(x);
} else {
results[key] = [x];
}
}
return results;
});
andList = function(xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (!x) {
return false;
}
}
return true;
};
orList = function(xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (x) {
return true;
}
}
return false;
};
any = curry$(function(f, xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (f(x)) {
return true;
}
}
return false;
});
all = curry$(function(f, xs){
var i$, len$, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
if (!f(x)) {
return false;
}
}
return true;
});
sort = function(xs){
return xs.concat().sort(function(x, y){
if (x > y) {
return 1;
} else if (x < y) {
return -1;
} else {
return 0;
}
});
};
sortWith = curry$(function(f, xs){
return xs.concat().sort(f);
});
sortBy = curry$(function(f, xs){
return xs.concat().sort(function(x, y){
if (f(x) > f(y)) {
return 1;
} else if (f(x) < f(y)) {
return -1;
} else {
return 0;
}
});
});
sum = function(xs){
var result, i$, len$, x;
result = 0;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
result += x;
}
return result;
};
product = function(xs){
var result, i$, len$, x;
result = 1;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
result *= x;
}
return result;
};
mean = average = function(xs){
var sum, i$, len$, x;
sum = 0;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
x = xs[i$];
sum += x;
}
return sum / xs.length;
};
maximum = function(xs){
var max, i$, ref$, len$, x;
max = xs[0];
for (i$ = 0, len$ = (ref$ = xs.slice(1)).length; i$ < len$; ++i$) {
x = ref$[i$];
if (x > max) {
max = x;
}
}
return max;
};
minimum = function(xs){
var min, i$, ref$, len$, x;
min = xs[0];
for (i$ = 0, len$ = (ref$ = xs.slice(1)).length; i$ < len$; ++i$) {
x = ref$[i$];
if (x < min) {
min = x;
}
}
return min;
};
maximumBy = curry$(function(f, xs){
var max, i$, ref$, len$, x;
max = xs[0];
for (i$ = 0, len$ = (ref$ = xs.slice(1)).length; i$ < len$; ++i$) {
x = ref$[i$];
if (f(x) > f(max)) {
max = x;
}
}
return max;
});
minimumBy = curry$(function(f, xs){
var min, i$, ref$, len$, x;
min = xs[0];
for (i$ = 0, len$ = (ref$ = xs.slice(1)).length; i$ < len$; ++i$) {
x = ref$[i$];
if (f(x) < f(min)) {
min = x;
}
}
return min;
});
scan = scanl = curry$(function(f, memo, xs){
var last, x;
last = memo;
return [memo].concat((function(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = xs).length; i$ < len$; ++i$) {
x = ref$[i$];
results$.push(last = f(last, x));
}
return results$;
}()));
});
scan1 = scanl1 = curry$(function(f, xs){
if (!xs.length) {
return;
}
return scan(f, xs[0], xs.slice(1));
});
scanr = curry$(function(f, memo, xs){
xs = xs.concat().reverse();
return scan(f, memo, xs).reverse();
});
scanr1 = curry$(function(f, xs){
if (!xs.length) {
return;
}
xs = xs.concat().reverse();
return scan(f, xs[0], xs.slice(1)).reverse();
});
slice = curry$(function(x, y, xs){
return xs.slice(x, y);
});
take = curry$(function(n, xs){
if (n <= 0) {
return xs.slice(0, 0);
} else {
return xs.slice(0, n);
}
});
drop = curry$(function(n, xs){
if (n <= 0) {
return xs;
} else {
return xs.slice(n);
}
});
splitAt = curry$(function(n, xs){
return [take(n, xs), drop(n, xs)];
});
takeWhile = curry$(function(p, xs){
var len, i;
len = xs.length;
if (!len) {
return xs;
}
i = 0;
while (i < len && p(xs[i])) {
i += 1;
}
return xs.slice(0, i);
});
dropWhile = curry$(function(p, xs){
var len, i;
len = xs.length;
if (!len) {
return xs;
}
i = 0;
while (i < len && p(xs[i])) {
i += 1;
}
return xs.slice(i);
});
span = curry$(function(p, xs){
return [takeWhile(p, xs), dropWhile(p, xs)];
});
breakList = curry$(function(p, xs){
return span(compose$(p, not$), xs);
});
zip = curry$(function(xs, ys){
var result, len, i$, len$, i, x;
result = [];
len = ys.length;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (i === len) {
break;
}
result.push([x, ys[i]]);
}
return result;
});
zipWith = curry$(function(f, xs, ys){
var result, len, i$, len$, i, x;
result = [];
len = ys.length;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (i === len) {
break;
}
result.push(f(x, ys[i]));
}
return result;
});
zipAll = function(){
var xss, minLength, i$, len$, xs, ref$, i, lresult$, j$, results$ = [];
xss = slice$.call(arguments);
minLength = undefined;
for (i$ = 0, len$ = xss.length; i$ < len$; ++i$) {
xs = xss[i$];
minLength <= (ref$ = xs.length) || (minLength = ref$);
}
for (i$ = 0; i$ < minLength; ++i$) {
i = i$;
lresult$ = [];
for (j$ = 0, len$ = xss.length; j$ < len$; ++j$) {
xs = xss[j$];
lresult$.push(xs[i]);
}
results$.push(lresult$);
}
return results$;
};
zipAllWith = function(f){
var xss, minLength, i$, len$, xs, ref$, i, results$ = [];
xss = slice$.call(arguments, 1);
minLength = undefined;
for (i$ = 0, len$ = xss.length; i$ < len$; ++i$) {
xs = xss[i$];
minLength <= (ref$ = xs.length) || (minLength = ref$);
}
for (i$ = 0; i$ < minLength; ++i$) {
i = i$;
results$.push(f.apply(null, (fn$())));
}
return results$;
function fn$(){
var i$, ref$, len$, results$ = [];
for (i$ = 0, len$ = (ref$ = xss).length; i$ < len$; ++i$) {
xs = ref$[i$];
results$.push(xs[i]);
}
return results$;
}
};
at = curry$(function(n, xs){
if (n < 0) {
return xs[xs.length + n];
} else {
return xs[n];
}
});
elemIndex = curry$(function(el, xs){
var i$, len$, i, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (x === el) {
return i;
}
}
});
elemIndices = curry$(function(el, xs){
var i$, len$, i, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (x === el) {
results$.push(i);
}
}
return results$;
});
findIndex = curry$(function(f, xs){
var i$, len$, i, x;
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (f(x)) {
return i;
}
}
});
findIndices = curry$(function(f, xs){
var i$, len$, i, x, results$ = [];
for (i$ = 0, len$ = xs.length; i$ < len$; ++i$) {
i = i$;
x = xs[i$];
if (f(x)) {
results$.push(i);
}
}
return results$;
});
module.exports = {
each: each,
map: map,
filter: filter,
compact: compact,
reject: reject,
partition: partition,
find: find,
head: head,
first: first,
tail: tail,
last: last,
initial: initial,
empty: empty,
reverse: reverse,
difference: difference,
intersection: intersection,
union: union,
countBy: countBy,
groupBy: groupBy,
fold: fold,
fold1: fold1,
foldl: foldl,
foldl1: foldl1,
foldr: foldr,
foldr1: foldr1,
unfoldr: unfoldr,
andList: andList,
orList: orList,
any: any,
all: all,
unique: unique,
uniqueBy: uniqueBy,
sort: sort,
sortWith: sortWith,
sortBy: sortBy,
sum: sum,
product: product,
mean: mean,
average: average,
concat: concat,
concatMap: concatMap,
flatten: flatten,
maximum: maximum,
minimum: minimum,
maximumBy: maximumBy,
minimumBy: minimumBy,
scan: scan,
scan1: scan1,
scanl: scanl,
scanl1: scanl1,
scanr: scanr,
scanr1: scanr1,
slice: slice,
take: take,
drop: drop,
splitAt: splitAt,
takeWhile: takeWhile,
dropWhile: dropWhile,
span: span,
breakList: breakList,
zip: zip,
zipWith: zipWith,
zipAll: zipAll,
zipAllWith: zipAllWith,
at: at,
elemIndex: elemIndex,
elemIndices: elemIndices,
findIndex: findIndex,
findIndices: findIndices
};
function curry$(f, bound){
var context,
_curry = function(args) {
return f.length > 1 ? function(){
var params = args ? args.concat() : [];
context = bound ? context || this : this;
return params.push.apply(params, arguments) <
f.length && arguments.length ?
_curry.call(context, params) : f.apply(context, params);
} : f;
};
return _curry();
}
function in$(x, xs){
var i = -1, l = xs.length >>> 0;
while (++i < l) if (x === xs[i]) return true;
return false;
}
function compose$() {
var functions = arguments;
return function() {
var i, result;
result = functions[0].apply(this, arguments);
for (i = 1; i < functions.length; ++i) {
result = functions[i](result);
}
return result;
};
}
function not$(x){ return !x; } | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/lib/List.js | List.js |
var values, keys, pairsToObj, objToPairs, listsToObj, objToLists, empty, each, map, compact, filter, reject, partition, find;
values = function(object){
var i$, x, results$ = [];
for (i$ in object) {
x = object[i$];
results$.push(x);
}
return results$;
};
keys = function(object){
var x, results$ = [];
for (x in object) {
results$.push(x);
}
return results$;
};
pairsToObj = function(object){
var i$, len$, x, resultObj$ = {};
for (i$ = 0, len$ = object.length; i$ < len$; ++i$) {
x = object[i$];
resultObj$[x[0]] = x[1];
}
return resultObj$;
};
objToPairs = function(object){
var key, value, results$ = [];
for (key in object) {
value = object[key];
results$.push([key, value]);
}
return results$;
};
listsToObj = curry$(function(keys, values){
var i$, len$, i, key, resultObj$ = {};
for (i$ = 0, len$ = keys.length; i$ < len$; ++i$) {
i = i$;
key = keys[i$];
resultObj$[key] = values[i];
}
return resultObj$;
});
objToLists = function(object){
var keys, values, key, value;
keys = [];
values = [];
for (key in object) {
value = object[key];
keys.push(key);
values.push(value);
}
return [keys, values];
};
empty = function(object){
var x;
for (x in object) {
return false;
}
return true;
};
each = curry$(function(f, object){
var i$, x;
for (i$ in object) {
x = object[i$];
f(x);
}
return object;
});
map = curry$(function(f, object){
var k, x, resultObj$ = {};
for (k in object) {
x = object[k];
resultObj$[k] = f(x);
}
return resultObj$;
});
compact = function(object){
var k, x, resultObj$ = {};
for (k in object) {
x = object[k];
if (x) {
resultObj$[k] = x;
}
}
return resultObj$;
};
filter = curry$(function(f, object){
var k, x, resultObj$ = {};
for (k in object) {
x = object[k];
if (f(x)) {
resultObj$[k] = x;
}
}
return resultObj$;
});
reject = curry$(function(f, object){
var k, x, resultObj$ = {};
for (k in object) {
x = object[k];
if (!f(x)) {
resultObj$[k] = x;
}
}
return resultObj$;
});
partition = curry$(function(f, object){
var passed, failed, k, x;
passed = {};
failed = {};
for (k in object) {
x = object[k];
(f(x) ? passed : failed)[k] = x;
}
return [passed, failed];
});
find = curry$(function(f, object){
var i$, x;
for (i$ in object) {
x = object[i$];
if (f(x)) {
return x;
}
}
});
module.exports = {
values: values,
keys: keys,
pairsToObj: pairsToObj,
objToPairs: objToPairs,
listsToObj: listsToObj,
objToLists: objToLists,
empty: empty,
each: each,
map: map,
filter: filter,
compact: compact,
reject: reject,
partition: partition,
find: find
};
function curry$(f, bound){
var context,
_curry = function(args) {
return f.length > 1 ? function(){
var params = args ? args.concat() : [];
context = bound ? context || this : this;
return params.push.apply(params, arguments) <
f.length && arguments.length ?
_curry.call(context, params) : f.apply(context, params);
} : f;
};
return _curry();
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/lib/Obj.js | Obj.js |
var Func, List, Obj, Str, Num, id, isType, replicate, prelude, toString$ = {}.toString;
Func = require('prelude-ls/lib/Func.js');
List = require('prelude-ls/lib/List.js');
Obj = require('prelude-ls/lib/Obj.js');
Str = require('prelude-ls/lib/Str.js');
Num = require('prelude-ls/lib/Num.js');
id = function(x){
return x;
};
isType = curry$(function(type, x){
return toString$.call(x).slice(8, -1) === type;
});
replicate = curry$(function(n, x){
var i$, results$ = [];
for (i$ = 0; i$ < n; ++i$) {
results$.push(x);
}
return results$;
});
Str.empty = List.empty;
Str.slice = List.slice;
Str.take = List.take;
Str.drop = List.drop;
Str.splitAt = List.splitAt;
Str.takeWhile = List.takeWhile;
Str.dropWhile = List.dropWhile;
Str.span = List.span;
Str.breakStr = List.breakList;
prelude = {
Func: Func,
List: List,
Obj: Obj,
Str: Str,
Num: Num,
id: id,
isType: isType,
replicate: replicate
};
prelude.each = List.each;
prelude.map = List.map;
prelude.filter = List.filter;
prelude.compact = List.compact;
prelude.reject = List.reject;
prelude.partition = List.partition;
prelude.find = List.find;
prelude.head = List.head;
prelude.first = List.first;
prelude.tail = List.tail;
prelude.last = List.last;
prelude.initial = List.initial;
prelude.empty = List.empty;
prelude.reverse = List.reverse;
prelude.difference = List.difference;
prelude.intersection = List.intersection;
prelude.union = List.union;
prelude.countBy = List.countBy;
prelude.groupBy = List.groupBy;
prelude.fold = List.fold;
prelude.foldl = List.foldl;
prelude.fold1 = List.fold1;
prelude.foldl1 = List.foldl1;
prelude.foldr = List.foldr;
prelude.foldr1 = List.foldr1;
prelude.unfoldr = List.unfoldr;
prelude.andList = List.andList;
prelude.orList = List.orList;
prelude.any = List.any;
prelude.all = List.all;
prelude.unique = List.unique;
prelude.uniqueBy = List.uniqueBy;
prelude.sort = List.sort;
prelude.sortWith = List.sortWith;
prelude.sortBy = List.sortBy;
prelude.sum = List.sum;
prelude.product = List.product;
prelude.mean = List.mean;
prelude.average = List.average;
prelude.concat = List.concat;
prelude.concatMap = List.concatMap;
prelude.flatten = List.flatten;
prelude.maximum = List.maximum;
prelude.minimum = List.minimum;
prelude.maximumBy = List.maximumBy;
prelude.minimumBy = List.minimumBy;
prelude.scan = List.scan;
prelude.scanl = List.scanl;
prelude.scan1 = List.scan1;
prelude.scanl1 = List.scanl1;
prelude.scanr = List.scanr;
prelude.scanr1 = List.scanr1;
prelude.slice = List.slice;
prelude.take = List.take;
prelude.drop = List.drop;
prelude.splitAt = List.splitAt;
prelude.takeWhile = List.takeWhile;
prelude.dropWhile = List.dropWhile;
prelude.span = List.span;
prelude.breakList = List.breakList;
prelude.zip = List.zip;
prelude.zipWith = List.zipWith;
prelude.zipAll = List.zipAll;
prelude.zipAllWith = List.zipAllWith;
prelude.at = List.at;
prelude.elemIndex = List.elemIndex;
prelude.elemIndices = List.elemIndices;
prelude.findIndex = List.findIndex;
prelude.findIndices = List.findIndices;
prelude.apply = Func.apply;
prelude.curry = Func.curry;
prelude.flip = Func.flip;
prelude.fix = Func.fix;
prelude.over = Func.over;
prelude.split = Str.split;
prelude.join = Str.join;
prelude.lines = Str.lines;
prelude.unlines = Str.unlines;
prelude.words = Str.words;
prelude.unwords = Str.unwords;
prelude.chars = Str.chars;
prelude.unchars = Str.unchars;
prelude.repeat = Str.repeat;
prelude.capitalize = Str.capitalize;
prelude.camelize = Str.camelize;
prelude.dasherize = Str.dasherize;
prelude.values = Obj.values;
prelude.keys = Obj.keys;
prelude.pairsToObj = Obj.pairsToObj;
prelude.objToPairs = Obj.objToPairs;
prelude.listsToObj = Obj.listsToObj;
prelude.objToLists = Obj.objToLists;
prelude.max = Num.max;
prelude.min = Num.min;
prelude.negate = Num.negate;
prelude.abs = Num.abs;
prelude.signum = Num.signum;
prelude.quot = Num.quot;
prelude.rem = Num.rem;
prelude.div = Num.div;
prelude.mod = Num.mod;
prelude.recip = Num.recip;
prelude.pi = Num.pi;
prelude.tau = Num.tau;
prelude.exp = Num.exp;
prelude.sqrt = Num.sqrt;
prelude.ln = Num.ln;
prelude.pow = Num.pow;
prelude.sin = Num.sin;
prelude.tan = Num.tan;
prelude.cos = Num.cos;
prelude.acos = Num.acos;
prelude.asin = Num.asin;
prelude.atan = Num.atan;
prelude.atan2 = Num.atan2;
prelude.truncate = Num.truncate;
prelude.round = Num.round;
prelude.ceiling = Num.ceiling;
prelude.floor = Num.floor;
prelude.isItNaN = Num.isItNaN;
prelude.even = Num.even;
prelude.odd = Num.odd;
prelude.gcd = Num.gcd;
prelude.lcm = Num.lcm;
prelude.VERSION = '1.1.2';
module.exports = prelude;
function curry$(f, bound){
var context,
_curry = function(args) {
return f.length > 1 ? function(){
var params = args ? args.concat() : [];
context = bound ? context || this : this;
return params.push.apply(params, arguments) <
f.length && arguments.length ?
_curry.call(context, params) : f.apply(context, params);
} : f;
};
return _curry();
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/lib/index.js | index.js |
var split, join, lines, unlines, words, unwords, chars, unchars, reverse, repeat, capitalize, camelize, dasherize;
split = curry$(function(sep, str){
return str.split(sep);
});
join = curry$(function(sep, xs){
return xs.join(sep);
});
lines = function(str){
if (!str.length) {
return [];
}
return str.split('\n');
};
unlines = function(it){
return it.join('\n');
};
words = function(str){
if (!str.length) {
return [];
}
return str.split(/[ ]+/);
};
unwords = function(it){
return it.join(' ');
};
chars = function(it){
return it.split('');
};
unchars = function(it){
return it.join('');
};
reverse = function(str){
return str.split('').reverse().join('');
};
repeat = curry$(function(n, str){
var result, i$;
result = '';
for (i$ = 0; i$ < n; ++i$) {
result += str;
}
return result;
});
capitalize = function(str){
return str.charAt(0).toUpperCase() + str.slice(1);
};
camelize = function(it){
return it.replace(/[-_]+(.)?/g, function(arg$, c){
return (c != null ? c : '').toUpperCase();
});
};
dasherize = function(str){
return str.replace(/([^-A-Z])([A-Z]+)/g, function(arg$, lower, upper){
return lower + "-" + (upper.length > 1
? upper
: upper.toLowerCase());
}).replace(/^([A-Z]+)/, function(arg$, upper){
if (upper.length > 1) {
return upper + "-";
} else {
return upper.toLowerCase();
}
});
};
module.exports = {
split: split,
join: join,
lines: lines,
unlines: unlines,
words: words,
unwords: unwords,
chars: chars,
unchars: unchars,
reverse: reverse,
repeat: repeat,
capitalize: capitalize,
camelize: camelize,
dasherize: dasherize
};
function curry$(f, bound){
var context,
_curry = function(args) {
return f.length > 1 ? function(){
var params = args ? args.concat() : [];
context = bound ? context || this : this;
return params.push.apply(params, arguments) <
f.length && arguments.length ?
_curry.call(context, params) : f.apply(context, params);
} : f;
};
return _curry();
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/lib/Str.js | Str.js |
var max, min, negate, abs, signum, quot, rem, div, mod, recip, pi, tau, exp, sqrt, ln, pow, sin, tan, cos, asin, acos, atan, atan2, truncate, round, ceiling, floor, isItNaN, even, odd, gcd, lcm;
max = curry$(function(x$, y$){
return x$ > y$ ? x$ : y$;
});
min = curry$(function(x$, y$){
return x$ < y$ ? x$ : y$;
});
negate = function(x){
return -x;
};
abs = Math.abs;
signum = function(x){
if (x < 0) {
return -1;
} else if (x > 0) {
return 1;
} else {
return 0;
}
};
quot = curry$(function(x, y){
return ~~(x / y);
});
rem = curry$(function(x$, y$){
return x$ % y$;
});
div = curry$(function(x, y){
return Math.floor(x / y);
});
mod = curry$(function(x$, y$){
var ref$;
return (((x$) % (ref$ = y$) + ref$) % ref$);
});
recip = (function(it){
return 1 / it;
});
pi = Math.PI;
tau = pi * 2;
exp = Math.exp;
sqrt = Math.sqrt;
ln = Math.log;
pow = curry$(function(x$, y$){
return Math.pow(x$, y$);
});
sin = Math.sin;
tan = Math.tan;
cos = Math.cos;
asin = Math.asin;
acos = Math.acos;
atan = Math.atan;
atan2 = curry$(function(x, y){
return Math.atan2(x, y);
});
truncate = function(x){
return ~~x;
};
round = Math.round;
ceiling = Math.ceil;
floor = Math.floor;
isItNaN = function(x){
return x !== x;
};
even = function(x){
return x % 2 === 0;
};
odd = function(x){
return x % 2 !== 0;
};
gcd = curry$(function(x, y){
var z;
x = Math.abs(x);
y = Math.abs(y);
while (y !== 0) {
z = x % y;
x = y;
y = z;
}
return x;
});
lcm = curry$(function(x, y){
return Math.abs(Math.floor(x / gcd(x, y) * y));
});
module.exports = {
max: max,
min: min,
negate: negate,
abs: abs,
signum: signum,
quot: quot,
rem: rem,
div: div,
mod: mod,
recip: recip,
pi: pi,
tau: tau,
exp: exp,
sqrt: sqrt,
ln: ln,
pow: pow,
sin: sin,
tan: tan,
cos: cos,
acos: acos,
asin: asin,
atan: atan,
atan2: atan2,
truncate: truncate,
round: round,
ceiling: ceiling,
floor: floor,
isItNaN: isItNaN,
even: even,
odd: odd,
gcd: gcd,
lcm: lcm
};
function curry$(f, bound){
var context,
_curry = function(args) {
return f.length > 1 ? function(){
var params = args ? args.concat() : [];
context = bound ? context || this : this;
return params.push.apply(params, arguments) <
f.length && arguments.length ?
_curry.call(context, params) : f.apply(context, params);
} : f;
};
return _curry();
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/prelude-ls/lib/Num.js | Num.js |
(MIT License)
Copyright (c) 2013 [Ramesh Nair](http://www.hiddentao.com/)
Permission is hereby granted, free of charge, to any person
obtaining a copy of this software and associated documentation
files (the "Software"), to deal in the Software without
restriction, including without limitation the rights to use,
copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the
Software is furnished to do so, subject to the following
conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
OTHER DEALINGS IN THE SOFTWARE.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/fast-levenshtein/LICENSE.md | LICENSE.md |
# fast-levenshtein - Levenshtein algorithm in Javascript
[](http://travis-ci.org/hiddentao/fast-levenshtein)
[](https://badge.fury.io/js/fast-levenshtein)
[](https://www.npmjs.com/package/fast-levenshtein)
[](https://twitter.com/hiddentao)
An efficient Javascript implementation of the [Levenshtein algorithm](http://en.wikipedia.org/wiki/Levenshtein_distance) with locale-specific collator support.
## Features
* Works in node.js and in the browser.
* Better performance than other implementations by not needing to store the whole matrix ([more info](http://www.codeproject.com/Articles/13525/Fast-memory-efficient-Levenshtein-algorithm)).
* Locale-sensitive string comparisions if needed.
* Comprehensive test suite and performance benchmark.
* Small: <1 KB minified and gzipped
## Installation
### node.js
Install using [npm](http://npmjs.org/):
```bash
$ npm install fast-levenshtein
```
### Browser
Using bower:
```bash
$ bower install fast-levenshtein
```
If you are not using any module loader system then the API will then be accessible via the `window.Levenshtein` object.
## Examples
**Default usage**
```javascript
var levenshtein = require('fast-levenshtein');
var distance = levenshtein.get('back', 'book'); // 2
var distance = levenshtein.get('我愛你', '我叫你'); // 1
```
**Locale-sensitive string comparisons**
It supports using [Intl.Collator](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Collator) for locale-sensitive string comparisons:
```javascript
var levenshtein = require('fast-levenshtein');
levenshtein.get('mikailovitch', 'Mikhaïlovitch', { useCollator: true});
// 1
```
## Building and Testing
To build the code and run the tests:
```bash
$ npm install -g grunt-cli
$ npm install
$ npm run build
```
## Performance
_Thanks to [Titus Wormer](https://github.com/wooorm) for [encouraging me](https://github.com/hiddentao/fast-levenshtein/issues/1) to do this._
Benchmarked against other node.js levenshtein distance modules (on Macbook Air 2012, Core i7, 8GB RAM):
```bash
Running suite Implementation comparison [benchmark/speed.js]...
>> levenshtein-edit-distance x 234 ops/sec ±3.02% (73 runs sampled)
>> levenshtein-component x 422 ops/sec ±4.38% (83 runs sampled)
>> levenshtein-deltas x 283 ops/sec ±3.83% (78 runs sampled)
>> natural x 255 ops/sec ±0.76% (88 runs sampled)
>> levenshtein x 180 ops/sec ±3.55% (86 runs sampled)
>> fast-levenshtein x 1,792 ops/sec ±2.72% (95 runs sampled)
Benchmark done.
Fastest test is fast-levenshtein at 4.2x faster than levenshtein-component
```
You can run this benchmark yourself by doing:
```bash
$ npm install
$ npm run build
$ npm run benchmark
```
## Contributing
If you wish to submit a pull request please update and/or create new tests for any changes you make and ensure the grunt build passes.
See [CONTRIBUTING.md](https://github.com/hiddentao/fast-levenshtein/blob/master/CONTRIBUTING.md) for details.
## License
MIT - see [LICENSE.md](https://github.com/hiddentao/fast-levenshtein/blob/master/LICENSE.md)
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/fast-levenshtein/README.md | README.md |
(function() {
'use strict';
var collator;
try {
collator = (typeof Intl !== "undefined" && typeof Intl.Collator !== "undefined") ? Intl.Collator("generic", { sensitivity: "base" }) : null;
} catch (err){
console.log("Collator could not be initialized and wouldn't be used");
}
// arrays to re-use
var prevRow = [],
str2Char = [];
/**
* Based on the algorithm at http://en.wikipedia.org/wiki/Levenshtein_distance.
*/
var Levenshtein = {
/**
* Calculate levenshtein distance of the two strings.
*
* @param str1 String the first string.
* @param str2 String the second string.
* @param [options] Additional options.
* @param [options.useCollator] Use `Intl.Collator` for locale-sensitive string comparison.
* @return Integer the levenshtein distance (0 and above).
*/
get: function(str1, str2, options) {
var useCollator = (options && collator && options.useCollator);
var str1Len = str1.length,
str2Len = str2.length;
// base cases
if (str1Len === 0) return str2Len;
if (str2Len === 0) return str1Len;
// two rows
var curCol, nextCol, i, j, tmp;
// initialise previous row
for (i=0; i<str2Len; ++i) {
prevRow[i] = i;
str2Char[i] = str2.charCodeAt(i);
}
prevRow[str2Len] = str2Len;
var strCmp;
if (useCollator) {
// calculate current row distance from previous row using collator
for (i = 0; i < str1Len; ++i) {
nextCol = i + 1;
for (j = 0; j < str2Len; ++j) {
curCol = nextCol;
// substution
strCmp = 0 === collator.compare(str1.charAt(i), String.fromCharCode(str2Char[j]));
nextCol = prevRow[j] + (strCmp ? 0 : 1);
// insertion
tmp = curCol + 1;
if (nextCol > tmp) {
nextCol = tmp;
}
// deletion
tmp = prevRow[j + 1] + 1;
if (nextCol > tmp) {
nextCol = tmp;
}
// copy current col value into previous (in preparation for next iteration)
prevRow[j] = curCol;
}
// copy last col value into previous (in preparation for next iteration)
prevRow[j] = nextCol;
}
}
else {
// calculate current row distance from previous row without collator
for (i = 0; i < str1Len; ++i) {
nextCol = i + 1;
for (j = 0; j < str2Len; ++j) {
curCol = nextCol;
// substution
strCmp = str1.charCodeAt(i) === str2Char[j];
nextCol = prevRow[j] + (strCmp ? 0 : 1);
// insertion
tmp = curCol + 1;
if (nextCol > tmp) {
nextCol = tmp;
}
// deletion
tmp = prevRow[j + 1] + 1;
if (nextCol > tmp) {
nextCol = tmp;
}
// copy current col value into previous (in preparation for next iteration)
prevRow[j] = curCol;
}
// copy last col value into previous (in preparation for next iteration)
prevRow[j] = nextCol;
}
}
return nextCol;
}
};
// amd
if (typeof define !== "undefined" && define !== null && define.amd) {
define(function() {
return Levenshtein;
});
}
// commonjs
else if (typeof module !== "undefined" && module !== null && typeof exports !== "undefined" && module.exports === exports) {
module.exports = Levenshtein;
}
// web worker
else if (typeof self !== "undefined" && typeof self.postMessage === 'function' && typeof self.importScripts === 'function') {
self.Levenshtein = Levenshtein;
}
// browser main thread
else if (typeof window !== "undefined" && window !== null) {
window.Levenshtein = Levenshtein;
}
}()); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/fast-levenshtein/levenshtein.js | levenshtein.js |
var arrayEach = require('lodash/_arrayEach'),
baseCreate = require('lodash/_baseCreate'),
baseForOwn = require('lodash/_baseForOwn'),
baseIteratee = require('lodash/_baseIteratee'),
getPrototype = require('lodash/_getPrototype'),
isArray = require('lodash/isArray'),
isBuffer = require('lodash/isBuffer'),
isFunction = require('lodash/isFunction'),
isObject = require('lodash/isObject'),
isTypedArray = require('lodash/isTypedArray');
/**
* An alternative to `_.reduce`; this method transforms `object` to a new
* `accumulator` object which is the result of running each of its own
* enumerable string keyed properties thru `iteratee`, with each invocation
* potentially mutating the `accumulator` object. If `accumulator` is not
* provided, a new object with the same `[[Prototype]]` will be used. The
* iteratee is invoked with four arguments: (accumulator, value, key, object).
* Iteratee functions may exit iteration early by explicitly returning `false`.
*
* @static
* @memberOf _
* @since 1.3.0
* @category Object
* @param {Object} object The object to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @param {*} [accumulator] The custom accumulator value.
* @returns {*} Returns the accumulated value.
* @example
*
* _.transform([2, 3, 4], function(result, n) {
* result.push(n *= n);
* return n % 2 == 0;
* }, []);
* // => [4, 9]
*
* _.transform({ 'a': 1, 'b': 2, 'c': 1 }, function(result, value, key) {
* (result[value] || (result[value] = [])).push(key);
* }, {});
* // => { '1': ['a', 'c'], '2': ['b'] }
*/
function transform(object, iteratee, accumulator) {
var isArr = isArray(object),
isArrLike = isArr || isBuffer(object) || isTypedArray(object);
iteratee = baseIteratee(iteratee, 4);
if (accumulator == null) {
var Ctor = object && object.constructor;
if (isArrLike) {
accumulator = isArr ? new Ctor : [];
}
else if (isObject(object)) {
accumulator = isFunction(Ctor) ? baseCreate(getPrototype(object)) : {};
}
else {
accumulator = {};
}
}
(isArrLike ? arrayEach : baseForOwn)(object, function(value, index, object) {
return iteratee(accumulator, value, index, object);
});
return accumulator;
}
module.exports = transform; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/transform.js | transform.js |
var composeArgs = require('lodash/_composeArgs'),
composeArgsRight = require('lodash/_composeArgsRight'),
replaceHolders = require('lodash/_replaceHolders');
/** Used as the internal argument placeholder. */
var PLACEHOLDER = '__lodash_placeholder__';
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_BIND_KEY_FLAG = 2,
WRAP_CURRY_BOUND_FLAG = 4,
WRAP_CURRY_FLAG = 8,
WRAP_ARY_FLAG = 128,
WRAP_REARG_FLAG = 256;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeMin = Math.min;
/**
* Merges the function metadata of `source` into `data`.
*
* Merging metadata reduces the number of wrappers used to invoke a function.
* This is possible because methods like `_.bind`, `_.curry`, and `_.partial`
* may be applied regardless of execution order. Methods like `_.ary` and
* `_.rearg` modify function arguments, making the order in which they are
* executed important, preventing the merging of metadata. However, we make
* an exception for a safe combined case where curried functions have `_.ary`
* and or `_.rearg` applied.
*
* @private
* @param {Array} data The destination metadata.
* @param {Array} source The source metadata.
* @returns {Array} Returns `data`.
*/
function mergeData(data, source) {
var bitmask = data[1],
srcBitmask = source[1],
newBitmask = bitmask | srcBitmask,
isCommon = newBitmask < (WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG | WRAP_ARY_FLAG);
var isCombo =
((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_CURRY_FLAG)) ||
((srcBitmask == WRAP_ARY_FLAG) && (bitmask == WRAP_REARG_FLAG) && (data[7].length <= source[8])) ||
((srcBitmask == (WRAP_ARY_FLAG | WRAP_REARG_FLAG)) && (source[7].length <= source[8]) && (bitmask == WRAP_CURRY_FLAG));
// Exit early if metadata can't be merged.
if (!(isCommon || isCombo)) {
return data;
}
// Use source `thisArg` if available.
if (srcBitmask & WRAP_BIND_FLAG) {
data[2] = source[2];
// Set when currying a bound function.
newBitmask |= bitmask & WRAP_BIND_FLAG ? 0 : WRAP_CURRY_BOUND_FLAG;
}
// Compose partial arguments.
var value = source[3];
if (value) {
var partials = data[3];
data[3] = partials ? composeArgs(partials, value, source[4]) : value;
data[4] = partials ? replaceHolders(data[3], PLACEHOLDER) : source[4];
}
// Compose partial right arguments.
value = source[5];
if (value) {
partials = data[5];
data[5] = partials ? composeArgsRight(partials, value, source[6]) : value;
data[6] = partials ? replaceHolders(data[5], PLACEHOLDER) : source[6];
}
// Use source `argPos` if available.
value = source[7];
if (value) {
data[7] = value;
}
// Use source `ary` if it's smaller.
if (srcBitmask & WRAP_ARY_FLAG) {
data[8] = data[8] == null ? source[8] : nativeMin(data[8], source[8]);
}
// Use source `arity` if one is not provided.
if (data[9] == null) {
data[9] = source[9];
}
// Use source `func` and merge bitmasks.
data[0] = source[0];
data[1] = newBitmask;
return data;
}
module.exports = mergeData; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_mergeData.js | _mergeData.js |
var baseToString = require('lodash/_baseToString'),
castSlice = require('lodash/_castSlice'),
hasUnicode = require('lodash/_hasUnicode'),
isObject = require('lodash/isObject'),
isRegExp = require('lodash/isRegExp'),
stringSize = require('lodash/_stringSize'),
stringToArray = require('lodash/_stringToArray'),
toInteger = require('lodash/toInteger'),
toString = require('lodash/toString');
/** Used as default options for `_.truncate`. */
var DEFAULT_TRUNC_LENGTH = 30,
DEFAULT_TRUNC_OMISSION = '...';
/** Used to match `RegExp` flags from their coerced string values. */
var reFlags = /\w*$/;
/**
* Truncates `string` if it's longer than the given maximum string length.
* The last characters of the truncated string are replaced with the omission
* string which defaults to "...".
*
* @static
* @memberOf _
* @since 4.0.0
* @category String
* @param {string} [string=''] The string to truncate.
* @param {Object} [options={}] The options object.
* @param {number} [options.length=30] The maximum string length.
* @param {string} [options.omission='...'] The string to indicate text is omitted.
* @param {RegExp|string} [options.separator] The separator pattern to truncate to.
* @returns {string} Returns the truncated string.
* @example
*
* _.truncate('hi-diddly-ho there, neighborino');
* // => 'hi-diddly-ho there, neighbo...'
*
* _.truncate('hi-diddly-ho there, neighborino', {
* 'length': 24,
* 'separator': ' '
* });
* // => 'hi-diddly-ho there,...'
*
* _.truncate('hi-diddly-ho there, neighborino', {
* 'length': 24,
* 'separator': /,? +/
* });
* // => 'hi-diddly-ho there...'
*
* _.truncate('hi-diddly-ho there, neighborino', {
* 'omission': ' [...]'
* });
* // => 'hi-diddly-ho there, neig [...]'
*/
function truncate(string, options) {
var length = DEFAULT_TRUNC_LENGTH,
omission = DEFAULT_TRUNC_OMISSION;
if (isObject(options)) {
var separator = 'separator' in options ? options.separator : separator;
length = 'length' in options ? toInteger(options.length) : length;
omission = 'omission' in options ? baseToString(options.omission) : omission;
}
string = toString(string);
var strLength = string.length;
if (hasUnicode(string)) {
var strSymbols = stringToArray(string);
strLength = strSymbols.length;
}
if (length >= strLength) {
return string;
}
var end = length - stringSize(omission);
if (end < 1) {
return omission;
}
var result = strSymbols
? castSlice(strSymbols, 0, end).join('')
: string.slice(0, end);
if (separator === undefined) {
return result + omission;
}
if (strSymbols) {
end += (result.length - end);
}
if (isRegExp(separator)) {
if (string.slice(end).search(separator)) {
var match,
substring = result;
if (!separator.global) {
separator = RegExp(separator.source, toString(reFlags.exec(separator)) + 'g');
}
separator.lastIndex = 0;
while ((match = separator.exec(substring))) {
var newEnd = match.index;
}
result = result.slice(0, newEnd === undefined ? end : newEnd);
}
} else if (string.indexOf(baseToString(separator), end) != end) {
var index = result.lastIndexOf(separator);
if (index > -1) {
result = result.slice(0, index);
}
}
return result + omission;
}
module.exports = truncate; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/truncate.js | truncate.js |
var arrayEach = require('lodash/_arrayEach'),
arrayPush = require('lodash/_arrayPush'),
baseFunctions = require('lodash/_baseFunctions'),
copyArray = require('lodash/_copyArray'),
isFunction = require('lodash/isFunction'),
isObject = require('lodash/isObject'),
keys = require('lodash/keys');
/**
* Adds all own enumerable string keyed function properties of a source
* object to the destination object. If `object` is a function, then methods
* are added to its prototype as well.
*
* **Note:** Use `_.runInContext` to create a pristine `lodash` function to
* avoid conflicts caused by modifying the original.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @param {Function|Object} [object=lodash] The destination object.
* @param {Object} source The object of functions to add.
* @param {Object} [options={}] The options object.
* @param {boolean} [options.chain=true] Specify whether mixins are chainable.
* @returns {Function|Object} Returns `object`.
* @example
*
* function vowels(string) {
* return _.filter(string, function(v) {
* return /[aeiou]/i.test(v);
* });
* }
*
* _.mixin({ 'vowels': vowels });
* _.vowels('fred');
* // => ['e']
*
* _('fred').vowels().value();
* // => ['e']
*
* _.mixin({ 'vowels': vowels }, { 'chain': false });
* _('fred').vowels();
* // => ['e']
*/
function mixin(object, source, options) {
var props = keys(source),
methodNames = baseFunctions(source, props);
var chain = !(isObject(options) && 'chain' in options) || !!options.chain,
isFunc = isFunction(object);
arrayEach(methodNames, function(methodName) {
var func = source[methodName];
object[methodName] = func;
if (isFunc) {
object.prototype[methodName] = function() {
var chainAll = this.__chain__;
if (chain || chainAll) {
var result = object(this.__wrapped__),
actions = result.__actions__ = copyArray(this.__actions__);
actions.push({ 'func': func, 'args': arguments, 'thisArg': object });
result.__chain__ = chainAll;
return result;
}
return func.apply(object, arrayPush([this.value()], arguments));
};
}
});
return object;
}
module.exports = mixin; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/mixin.js | mixin.js |
var LazyWrapper = require('lodash/_LazyWrapper'),
LodashWrapper = require('lodash/_LodashWrapper'),
baseLodash = require('lodash/_baseLodash'),
isArray = require('lodash/isArray'),
isObjectLike = require('lodash/isObjectLike'),
wrapperClone = require('lodash/_wrapperClone');
/** Used for built-in method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Creates a `lodash` object which wraps `value` to enable implicit method
* chain sequences. Methods that operate on and return arrays, collections,
* and functions can be chained together. Methods that retrieve a single value
* or may return a primitive value will automatically end the chain sequence
* and return the unwrapped value. Otherwise, the value must be unwrapped
* with `_#value`.
*
* Explicit chain sequences, which must be unwrapped with `_#value`, may be
* enabled using `_.chain`.
*
* The execution of chained methods is lazy, that is, it's deferred until
* `_#value` is implicitly or explicitly called.
*
* Lazy evaluation allows several methods to support shortcut fusion.
* Shortcut fusion is an optimization to merge iteratee calls; this avoids
* the creation of intermediate arrays and can greatly reduce the number of
* iteratee executions. Sections of a chain sequence qualify for shortcut
* fusion if the section is applied to an array and iteratees accept only
* one argument. The heuristic for whether a section qualifies for shortcut
* fusion is subject to change.
*
* Chaining is supported in custom builds as long as the `_#value` method is
* directly or indirectly included in the build.
*
* In addition to lodash methods, wrappers have `Array` and `String` methods.
*
* The wrapper `Array` methods are:
* `concat`, `join`, `pop`, `push`, `shift`, `sort`, `splice`, and `unshift`
*
* The wrapper `String` methods are:
* `replace` and `split`
*
* The wrapper methods that support shortcut fusion are:
* `at`, `compact`, `drop`, `dropRight`, `dropWhile`, `filter`, `find`,
* `findLast`, `head`, `initial`, `last`, `map`, `reject`, `reverse`, `slice`,
* `tail`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, and `toArray`
*
* The chainable wrapper methods are:
* `after`, `ary`, `assign`, `assignIn`, `assignInWith`, `assignWith`, `at`,
* `before`, `bind`, `bindAll`, `bindKey`, `castArray`, `chain`, `chunk`,
* `commit`, `compact`, `concat`, `conforms`, `constant`, `countBy`, `create`,
* `curry`, `debounce`, `defaults`, `defaultsDeep`, `defer`, `delay`,
* `difference`, `differenceBy`, `differenceWith`, `drop`, `dropRight`,
* `dropRightWhile`, `dropWhile`, `extend`, `extendWith`, `fill`, `filter`,
* `flatMap`, `flatMapDeep`, `flatMapDepth`, `flatten`, `flattenDeep`,
* `flattenDepth`, `flip`, `flow`, `flowRight`, `fromPairs`, `functions`,
* `functionsIn`, `groupBy`, `initial`, `intersection`, `intersectionBy`,
* `intersectionWith`, `invert`, `invertBy`, `invokeMap`, `iteratee`, `keyBy`,
* `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`, `matchesProperty`,
* `memoize`, `merge`, `mergeWith`, `method`, `methodOf`, `mixin`, `negate`,
* `nthArg`, `omit`, `omitBy`, `once`, `orderBy`, `over`, `overArgs`,
* `overEvery`, `overSome`, `partial`, `partialRight`, `partition`, `pick`,
* `pickBy`, `plant`, `property`, `propertyOf`, `pull`, `pullAll`, `pullAllBy`,
* `pullAllWith`, `pullAt`, `push`, `range`, `rangeRight`, `rearg`, `reject`,
* `remove`, `rest`, `reverse`, `sampleSize`, `set`, `setWith`, `shuffle`,
* `slice`, `sort`, `sortBy`, `splice`, `spread`, `tail`, `take`, `takeRight`,
* `takeRightWhile`, `takeWhile`, `tap`, `throttle`, `thru`, `toArray`,
* `toPairs`, `toPairsIn`, `toPath`, `toPlainObject`, `transform`, `unary`,
* `union`, `unionBy`, `unionWith`, `uniq`, `uniqBy`, `uniqWith`, `unset`,
* `unshift`, `unzip`, `unzipWith`, `update`, `updateWith`, `values`,
* `valuesIn`, `without`, `wrap`, `xor`, `xorBy`, `xorWith`, `zip`,
* `zipObject`, `zipObjectDeep`, and `zipWith`
*
* The wrapper methods that are **not** chainable by default are:
* `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clamp`, `clone`,
* `cloneDeep`, `cloneDeepWith`, `cloneWith`, `conformsTo`, `deburr`,
* `defaultTo`, `divide`, `each`, `eachRight`, `endsWith`, `eq`, `escape`,
* `escapeRegExp`, `every`, `find`, `findIndex`, `findKey`, `findLast`,
* `findLastIndex`, `findLastKey`, `first`, `floor`, `forEach`, `forEachRight`,
* `forIn`, `forInRight`, `forOwn`, `forOwnRight`, `get`, `gt`, `gte`, `has`,
* `hasIn`, `head`, `identity`, `includes`, `indexOf`, `inRange`, `invoke`,
* `isArguments`, `isArray`, `isArrayBuffer`, `isArrayLike`, `isArrayLikeObject`,
* `isBoolean`, `isBuffer`, `isDate`, `isElement`, `isEmpty`, `isEqual`,
* `isEqualWith`, `isError`, `isFinite`, `isFunction`, `isInteger`, `isLength`,
* `isMap`, `isMatch`, `isMatchWith`, `isNaN`, `isNative`, `isNil`, `isNull`,
* `isNumber`, `isObject`, `isObjectLike`, `isPlainObject`, `isRegExp`,
* `isSafeInteger`, `isSet`, `isString`, `isUndefined`, `isTypedArray`,
* `isWeakMap`, `isWeakSet`, `join`, `kebabCase`, `last`, `lastIndexOf`,
* `lowerCase`, `lowerFirst`, `lt`, `lte`, `max`, `maxBy`, `mean`, `meanBy`,
* `min`, `minBy`, `multiply`, `noConflict`, `noop`, `now`, `nth`, `pad`,
* `padEnd`, `padStart`, `parseInt`, `pop`, `random`, `reduce`, `reduceRight`,
* `repeat`, `result`, `round`, `runInContext`, `sample`, `shift`, `size`,
* `snakeCase`, `some`, `sortedIndex`, `sortedIndexBy`, `sortedLastIndex`,
* `sortedLastIndexBy`, `startCase`, `startsWith`, `stubArray`, `stubFalse`,
* `stubObject`, `stubString`, `stubTrue`, `subtract`, `sum`, `sumBy`,
* `template`, `times`, `toFinite`, `toInteger`, `toJSON`, `toLength`,
* `toLower`, `toNumber`, `toSafeInteger`, `toString`, `toUpper`, `trim`,
* `trimEnd`, `trimStart`, `truncate`, `unescape`, `uniqueId`, `upperCase`,
* `upperFirst`, `value`, and `words`
*
* @name _
* @constructor
* @category Seq
* @param {*} value The value to wrap in a `lodash` instance.
* @returns {Object} Returns the new `lodash` wrapper instance.
* @example
*
* function square(n) {
* return n * n;
* }
*
* var wrapped = _([1, 2, 3]);
*
* // Returns an unwrapped value.
* wrapped.reduce(_.add);
* // => 6
*
* // Returns a wrapped value.
* var squares = wrapped.map(square);
*
* _.isArray(squares);
* // => false
*
* _.isArray(squares.value());
* // => true
*/
function lodash(value) {
if (isObjectLike(value) && !isArray(value) && !(value instanceof LazyWrapper)) {
if (value instanceof LodashWrapper) {
return value;
}
if (hasOwnProperty.call(value, '__wrapped__')) {
return wrapperClone(value);
}
}
return new LodashWrapper(value);
}
// Ensure wrappers are instances of `baseLodash`.
lodash.prototype = baseLodash.prototype;
lodash.prototype.constructor = lodash;
module.exports = lodash; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/wrapperLodash.js | wrapperLodash.js |
var SetCache = require('lodash/_SetCache'),
arrayIncludes = require('lodash/_arrayIncludes'),
arrayIncludesWith = require('lodash/_arrayIncludesWith'),
arrayMap = require('lodash/_arrayMap'),
baseUnary = require('lodash/_baseUnary'),
cacheHas = require('lodash/_cacheHas');
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeMin = Math.min;
/**
* The base implementation of methods like `_.intersection`, without support
* for iteratee shorthands, that accepts an array of arrays to inspect.
*
* @private
* @param {Array} arrays The arrays to inspect.
* @param {Function} [iteratee] The iteratee invoked per element.
* @param {Function} [comparator] The comparator invoked per element.
* @returns {Array} Returns the new array of shared values.
*/
function baseIntersection(arrays, iteratee, comparator) {
var includes = comparator ? arrayIncludesWith : arrayIncludes,
length = arrays[0].length,
othLength = arrays.length,
othIndex = othLength,
caches = Array(othLength),
maxLength = Infinity,
result = [];
while (othIndex--) {
var array = arrays[othIndex];
if (othIndex && iteratee) {
array = arrayMap(array, baseUnary(iteratee));
}
maxLength = nativeMin(array.length, maxLength);
caches[othIndex] = !comparator && (iteratee || (length >= 120 && array.length >= 120))
? new SetCache(othIndex && array)
: undefined;
}
array = arrays[0];
var index = -1,
seen = caches[0];
outer:
while (++index < length && result.length < maxLength) {
var value = array[index],
computed = iteratee ? iteratee(value) : value;
value = (comparator || value !== 0) ? value : 0;
if (!(seen
? cacheHas(seen, computed)
: includes(result, computed, comparator)
)) {
othIndex = othLength;
while (--othIndex) {
var cache = caches[othIndex];
if (!(cache
? cacheHas(cache, computed)
: includes(arrays[othIndex], computed, comparator))
) {
continue outer;
}
}
if (seen) {
seen.push(computed);
}
result.push(value);
}
}
return result;
}
module.exports = baseIntersection; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseIntersection.js | _baseIntersection.js |
var Symbol = require('lodash/_Symbol'),
Uint8Array = require('lodash/_Uint8Array'),
eq = require('lodash/eq'),
equalArrays = require('lodash/_equalArrays'),
mapToArray = require('lodash/_mapToArray'),
setToArray = require('lodash/_setToArray');
/** Used to compose bitmasks for value comparisons. */
var COMPARE_PARTIAL_FLAG = 1,
COMPARE_UNORDERED_FLAG = 2;
/** `Object#toString` result references. */
var boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
mapTag = '[object Map]',
numberTag = '[object Number]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
symbolTag = '[object Symbol]';
var arrayBufferTag = '[object ArrayBuffer]',
dataViewTag = '[object DataView]';
/** Used to convert symbols to primitives and strings. */
var symbolProto = Symbol ? Symbol.prototype : undefined,
symbolValueOf = symbolProto ? symbolProto.valueOf : undefined;
/**
* A specialized version of `baseIsEqualDeep` for comparing objects of
* the same `toStringTag`.
*
* **Note:** This function only supports comparing values with tags of
* `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {string} tag The `toStringTag` of the objects to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalByTag(object, other, tag, bitmask, customizer, equalFunc, stack) {
switch (tag) {
case dataViewTag:
if ((object.byteLength != other.byteLength) ||
(object.byteOffset != other.byteOffset)) {
return false;
}
object = object.buffer;
other = other.buffer;
case arrayBufferTag:
if ((object.byteLength != other.byteLength) ||
!equalFunc(new Uint8Array(object), new Uint8Array(other))) {
return false;
}
return true;
case boolTag:
case dateTag:
case numberTag:
// Coerce booleans to `1` or `0` and dates to milliseconds.
// Invalid dates are coerced to `NaN`.
return eq(+object, +other);
case errorTag:
return object.name == other.name && object.message == other.message;
case regexpTag:
case stringTag:
// Coerce regexes to strings and treat strings, primitives and objects,
// as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
// for more details.
return object == (other + '');
case mapTag:
var convert = mapToArray;
case setTag:
var isPartial = bitmask & COMPARE_PARTIAL_FLAG;
convert || (convert = setToArray);
if (object.size != other.size && !isPartial) {
return false;
}
// Assume cyclic values are equal.
var stacked = stack.get(object);
if (stacked) {
return stacked == other;
}
bitmask |= COMPARE_UNORDERED_FLAG;
// Recursively compare objects (susceptible to call stack limits).
stack.set(object, other);
var result = equalArrays(convert(object), convert(other), bitmask, customizer, equalFunc, stack);
stack['delete'](object);
return result;
case symbolTag:
if (symbolValueOf) {
return symbolValueOf.call(object) == symbolValueOf.call(other);
}
}
return false;
}
module.exports = equalByTag; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_equalByTag.js | _equalByTag.js |
var isSymbol = require('lodash/isSymbol');
/** Used as references for the maximum length and index of an array. */
var MAX_ARRAY_LENGTH = 4294967295,
MAX_ARRAY_INDEX = MAX_ARRAY_LENGTH - 1;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeFloor = Math.floor,
nativeMin = Math.min;
/**
* The base implementation of `_.sortedIndexBy` and `_.sortedLastIndexBy`
* which invokes `iteratee` for `value` and each element of `array` to compute
* their sort ranking. The iteratee is invoked with one argument; (value).
*
* @private
* @param {Array} array The sorted array to inspect.
* @param {*} value The value to evaluate.
* @param {Function} iteratee The iteratee invoked per element.
* @param {boolean} [retHighest] Specify returning the highest qualified index.
* @returns {number} Returns the index at which `value` should be inserted
* into `array`.
*/
function baseSortedIndexBy(array, value, iteratee, retHighest) {
value = iteratee(value);
var low = 0,
high = array == null ? 0 : array.length,
valIsNaN = value !== value,
valIsNull = value === null,
valIsSymbol = isSymbol(value),
valIsUndefined = value === undefined;
while (low < high) {
var mid = nativeFloor((low + high) / 2),
computed = iteratee(array[mid]),
othIsDefined = computed !== undefined,
othIsNull = computed === null,
othIsReflexive = computed === computed,
othIsSymbol = isSymbol(computed);
if (valIsNaN) {
var setLow = retHighest || othIsReflexive;
} else if (valIsUndefined) {
setLow = othIsReflexive && (retHighest || othIsDefined);
} else if (valIsNull) {
setLow = othIsReflexive && othIsDefined && (retHighest || !othIsNull);
} else if (valIsSymbol) {
setLow = othIsReflexive && othIsDefined && !othIsNull && (retHighest || !othIsSymbol);
} else if (othIsNull || othIsSymbol) {
setLow = false;
} else {
setLow = retHighest ? (computed <= value) : (computed < value);
}
if (setLow) {
low = mid + 1;
} else {
high = mid;
}
}
return nativeMin(high, MAX_ARRAY_INDEX);
}
module.exports = baseSortedIndexBy; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseSortedIndexBy.js | _baseSortedIndexBy.js |
var isLaziable = require('lodash/_isLaziable'),
setData = require('lodash/_setData'),
setWrapToString = require('lodash/_setWrapToString');
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_BIND_KEY_FLAG = 2,
WRAP_CURRY_BOUND_FLAG = 4,
WRAP_CURRY_FLAG = 8,
WRAP_PARTIAL_FLAG = 32,
WRAP_PARTIAL_RIGHT_FLAG = 64;
/**
* Creates a function that wraps `func` to continue currying.
*
* @private
* @param {Function} func The function to wrap.
* @param {number} bitmask The bitmask flags. See `createWrap` for more details.
* @param {Function} wrapFunc The function to create the `func` wrapper.
* @param {*} placeholder The placeholder value.
* @param {*} [thisArg] The `this` binding of `func`.
* @param {Array} [partials] The arguments to prepend to those provided to
* the new function.
* @param {Array} [holders] The `partials` placeholder indexes.
* @param {Array} [argPos] The argument positions of the new function.
* @param {number} [ary] The arity cap of `func`.
* @param {number} [arity] The arity of `func`.
* @returns {Function} Returns the new wrapped function.
*/
function createRecurry(func, bitmask, wrapFunc, placeholder, thisArg, partials, holders, argPos, ary, arity) {
var isCurry = bitmask & WRAP_CURRY_FLAG,
newHolders = isCurry ? holders : undefined,
newHoldersRight = isCurry ? undefined : holders,
newPartials = isCurry ? partials : undefined,
newPartialsRight = isCurry ? undefined : partials;
bitmask |= (isCurry ? WRAP_PARTIAL_FLAG : WRAP_PARTIAL_RIGHT_FLAG);
bitmask &= ~(isCurry ? WRAP_PARTIAL_RIGHT_FLAG : WRAP_PARTIAL_FLAG);
if (!(bitmask & WRAP_CURRY_BOUND_FLAG)) {
bitmask &= ~(WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG);
}
var newData = [
func, bitmask, thisArg, newPartials, newHolders, newPartialsRight,
newHoldersRight, argPos, ary, arity
];
var result = wrapFunc.apply(undefined, newData);
if (isLaziable(func)) {
setData(result, newData);
}
result.placeholder = placeholder;
return setWrapToString(result, func, bitmask);
}
module.exports = createRecurry; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_createRecurry.js | _createRecurry.js |
var baseKeys = require('lodash/_baseKeys'),
getTag = require('lodash/_getTag'),
isArguments = require('lodash/isArguments'),
isArray = require('lodash/isArray'),
isArrayLike = require('lodash/isArrayLike'),
isBuffer = require('lodash/isBuffer'),
isPrototype = require('lodash/_isPrototype'),
isTypedArray = require('lodash/isTypedArray');
/** `Object#toString` result references. */
var mapTag = '[object Map]',
setTag = '[object Set]';
/** Used for built-in method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Checks if `value` is an empty object, collection, map, or set.
*
* Objects are considered empty if they have no own enumerable string keyed
* properties.
*
* Array-like values such as `arguments` objects, arrays, buffers, strings, or
* jQuery-like collections are considered empty if they have a `length` of `0`.
* Similarly, maps and sets are considered empty if they have a `size` of `0`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is empty, else `false`.
* @example
*
* _.isEmpty(null);
* // => true
*
* _.isEmpty(true);
* // => true
*
* _.isEmpty(1);
* // => true
*
* _.isEmpty([1, 2, 3]);
* // => false
*
* _.isEmpty({ 'a': 1 });
* // => false
*/
function isEmpty(value) {
if (value == null) {
return true;
}
if (isArrayLike(value) &&
(isArray(value) || typeof value == 'string' || typeof value.splice == 'function' ||
isBuffer(value) || isTypedArray(value) || isArguments(value))) {
return !value.length;
}
var tag = getTag(value);
if (tag == mapTag || tag == setTag) {
return !value.size;
}
if (isPrototype(value)) {
return !baseKeys(value).length;
}
for (var key in value) {
if (hasOwnProperty.call(value, key)) {
return false;
}
}
return true;
}
module.exports = isEmpty; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/isEmpty.js | isEmpty.js |
;(function(){function n(n){return H(n)&&pn.call(n,"callee")&&!yn.call(n,"callee")}function t(n,t){return n.push.apply(n,t),n}function r(n){return function(t){return null==t?Z:t[n]}}function e(n,t,r,e,u){return u(n,function(n,u,o){r=e?(e=false,n):t(r,n,u,o)}),r}function u(n,t){return j(t,function(t){return n[t]})}function o(n){return n instanceof i?n:new i(n)}function i(n,t){this.__wrapped__=n,this.__actions__=[],this.__chain__=!!t}function c(n,t,r){if(typeof n!="function")throw new TypeError("Expected a function");
return setTimeout(function(){n.apply(Z,r)},t)}function f(n,t){var r=true;return mn(n,function(n,e,u){return r=!!t(n,e,u)}),r}function a(n,t,r){for(var e=-1,u=n.length;++e<u;){var o=n[e],i=t(o);if(null!=i&&(c===Z?i===i:r(i,c)))var c=i,f=o}return f}function l(n,t){var r=[];return mn(n,function(n,e,u){t(n,e,u)&&r.push(n)}),r}function p(n,r,e,u,o){var i=-1,c=n.length;for(e||(e=R),o||(o=[]);++i<c;){var f=n[i];0<r&&e(f)?1<r?p(f,r-1,e,u,o):t(o,f):u||(o[o.length]=f)}return o}function s(n,t){return n&&On(n,t,Dn);
}function h(n,t){return l(t,function(t){return U(n[t])})}function v(n,t){return n>t}function b(n,t,r,e,u){return n===t||(null==n||null==t||!H(n)&&!H(t)?n!==n&&t!==t:y(n,t,r,e,b,u))}function y(n,t,r,e,u,o){var i=Nn(n),c=Nn(t),f=i?"[object Array]":hn.call(n),a=c?"[object Array]":hn.call(t),f="[object Arguments]"==f?"[object Object]":f,a="[object Arguments]"==a?"[object Object]":a,l="[object Object]"==f,c="[object Object]"==a,a=f==a;o||(o=[]);var p=An(o,function(t){return t[0]==n}),s=An(o,function(n){
return n[0]==t});if(p&&s)return p[1]==t;if(o.push([n,t]),o.push([t,n]),a&&!l){if(i)r=T(n,t,r,e,u,o);else n:{switch(f){case"[object Boolean]":case"[object Date]":case"[object Number]":r=J(+n,+t);break n;case"[object Error]":r=n.name==t.name&&n.message==t.message;break n;case"[object RegExp]":case"[object String]":r=n==t+"";break n}r=false}return o.pop(),r}return 1&r||(i=l&&pn.call(n,"__wrapped__"),f=c&&pn.call(t,"__wrapped__"),!i&&!f)?!!a&&(r=B(n,t,r,e,u,o),o.pop(),r):(i=i?n.value():n,f=f?t.value():t,
r=u(i,f,r,e,o),o.pop(),r)}function g(n){return typeof n=="function"?n:null==n?X:(typeof n=="object"?d:r)(n)}function _(n,t){return n<t}function j(n,t){var r=-1,e=M(n)?Array(n.length):[];return mn(n,function(n,u,o){e[++r]=t(n,u,o)}),e}function d(n){var t=_n(n);return function(r){var e=t.length;if(null==r)return!e;for(r=Object(r);e--;){var u=t[e];if(!(u in r&&b(n[u],r[u],3)))return false}return true}}function m(n,t){return n=Object(n),C(t,function(t,r){return r in n&&(t[r]=n[r]),t},{})}function O(n){return xn(I(n,void 0,X),n+"");
}function x(n,t,r){var e=-1,u=n.length;for(0>t&&(t=-t>u?0:u+t),r=r>u?u:r,0>r&&(r+=u),u=t>r?0:r-t>>>0,t>>>=0,r=Array(u);++e<u;)r[e]=n[e+t];return r}function A(n){return x(n,0,n.length)}function E(n,t){var r;return mn(n,function(n,e,u){return r=t(n,e,u),!r}),!!r}function w(n,r){return C(r,function(n,r){return r.func.apply(r.thisArg,t([n],r.args))},n)}function k(n,t,r){var e=!r;r||(r={});for(var u=-1,o=t.length;++u<o;){var i=t[u],c=Z;if(c===Z&&(c=n[i]),e)r[i]=c;else{var f=r,a=f[i];pn.call(f,i)&&J(a,c)&&(c!==Z||i in f)||(f[i]=c);
}}return r}function N(n){return O(function(t,r){var e=-1,u=r.length,o=1<u?r[u-1]:Z,o=3<n.length&&typeof o=="function"?(u--,o):Z;for(t=Object(t);++e<u;){var i=r[e];i&&n(t,i,e,o)}return t})}function F(n){return function(){var t=arguments,r=dn(n.prototype),t=n.apply(r,t);return V(t)?t:r}}function S(n,t,r){function e(){for(var o=-1,i=arguments.length,c=-1,f=r.length,a=Array(f+i),l=this&&this!==on&&this instanceof e?u:n;++c<f;)a[c]=r[c];for(;i--;)a[c++]=arguments[++o];return l.apply(t,a)}if(typeof n!="function")throw new TypeError("Expected a function");
var u=F(n);return e}function T(n,t,r,e,u,o){var i=n.length,c=t.length;if(i!=c&&!(1&r&&c>i))return false;for(var c=-1,f=true,a=2&r?[]:Z;++c<i;){var l=n[c],p=t[c];if(void 0!==Z){f=false;break}if(a){if(!E(t,function(n,t){if(!P(a,t)&&(l===n||u(l,n,r,e,o)))return a.push(t)})){f=false;break}}else if(l!==p&&!u(l,p,r,e,o)){f=false;break}}return f}function B(n,t,r,e,u,o){var i=1&r,c=Dn(n),f=c.length,a=Dn(t).length;if(f!=a&&!i)return false;for(var l=f;l--;){var p=c[l];if(!(i?p in t:pn.call(t,p)))return false}for(a=true;++l<f;){var p=c[l],s=n[p],h=t[p];
if(void 0!==Z||s!==h&&!u(s,h,r,e,o)){a=false;break}i||(i="constructor"==p)}return a&&!i&&(r=n.constructor,e=t.constructor,r!=e&&"constructor"in n&&"constructor"in t&&!(typeof r=="function"&&r instanceof r&&typeof e=="function"&&e instanceof e)&&(a=false)),a}function R(t){return Nn(t)||n(t)}function D(n){var t=[];if(null!=n)for(var r in Object(n))t.push(r);return t}function I(n,t,r){return t=jn(t===Z?n.length-1:t,0),function(){for(var e=arguments,u=-1,o=jn(e.length-t,0),i=Array(o);++u<o;)i[u]=e[t+u];for(u=-1,
o=Array(t+1);++u<t;)o[u]=e[u];return o[t]=r(i),n.apply(this,o)}}function $(n){return(null==n?0:n.length)?p(n,1):[]}function q(n){return n&&n.length?n[0]:Z}function P(n,t,r){var e=null==n?0:n.length;r=typeof r=="number"?0>r?jn(e+r,0):r:0,r=(r||0)-1;for(var u=t===t;++r<e;){var o=n[r];if(u?o===t:o!==o)return r}return-1}function z(n,t){return mn(n,g(t))}function C(n,t,r){return e(n,g(t),r,3>arguments.length,mn)}function G(n,t){var r;if(typeof t!="function")throw new TypeError("Expected a function");return n=Fn(n),
function(){return 0<--n&&(r=t.apply(this,arguments)),1>=n&&(t=Z),r}}function J(n,t){return n===t||n!==n&&t!==t}function M(n){var t;return(t=null!=n)&&(t=n.length,t=typeof t=="number"&&-1<t&&0==t%1&&9007199254740991>=t),t&&!U(n)}function U(n){return!!V(n)&&(n=hn.call(n),"[object Function]"==n||"[object GeneratorFunction]"==n||"[object AsyncFunction]"==n||"[object Proxy]"==n)}function V(n){var t=typeof n;return null!=n&&("object"==t||"function"==t)}function H(n){return null!=n&&typeof n=="object"}function K(n){
return typeof n=="number"||H(n)&&"[object Number]"==hn.call(n)}function L(n){return typeof n=="string"||!Nn(n)&&H(n)&&"[object String]"==hn.call(n)}function Q(n){return typeof n=="string"?n:null==n?"":n+""}function W(n){return null==n?[]:u(n,Dn(n))}function X(n){return n}function Y(n,r,e){var u=Dn(r),o=h(r,u);null!=e||V(r)&&(o.length||!u.length)||(e=r,r=n,n=this,o=h(r,Dn(r)));var i=!(V(e)&&"chain"in e&&!e.chain),c=U(n);return mn(o,function(e){var u=r[e];n[e]=u,c&&(n.prototype[e]=function(){var r=this.__chain__;
if(i||r){var e=n(this.__wrapped__);return(e.__actions__=A(this.__actions__)).push({func:u,args:arguments,thisArg:n}),e.__chain__=r,e}return u.apply(n,t([this.value()],arguments))})}),n}var Z,nn=1/0,tn=/[&<>"']/g,rn=RegExp(tn.source),en=/^(?:0|[1-9]\d*)$/,un=typeof self=="object"&&self&&self.Object===Object&&self,on=typeof global=="object"&&global&&global.Object===Object&&global||un||Function("return this")(),cn=(un=typeof exports=="object"&&exports&&!exports.nodeType&&exports)&&typeof module=="object"&&module&&!module.nodeType&&module,fn=function(n){
return function(t){return null==n?Z:n[t]}}({"&":"&","<":"<",">":">",'"':""","'":"'"}),an=Array.prototype,ln=Object.prototype,pn=ln.hasOwnProperty,sn=0,hn=ln.toString,vn=on._,bn=Object.create,yn=ln.propertyIsEnumerable,gn=on.isFinite,_n=function(n,t){return function(r){return n(t(r))}}(Object.keys,Object),jn=Math.max,dn=function(){function n(){}return function(t){return V(t)?bn?bn(t):(n.prototype=t,t=new n,n.prototype=Z,t):{}}}();i.prototype=dn(o.prototype),i.prototype.constructor=i;
var mn=function(n,t){return function(r,e){if(null==r)return r;if(!M(r))return n(r,e);for(var u=r.length,o=t?u:-1,i=Object(r);(t?o--:++o<u)&&false!==e(i[o],o,i););return r}}(s),On=function(n){return function(t,r,e){var u=-1,o=Object(t);e=e(t);for(var i=e.length;i--;){var c=e[n?i:++u];if(false===r(o[c],c,o))break}return t}}(),xn=X,An=function(n){return function(t,r,e){var u=Object(t);if(!M(t)){var o=g(r);t=Dn(t),r=function(n){return o(u[n],n,u)}}return r=n(t,r,e),-1<r?u[o?t[r]:r]:Z}}(function(n,t,r){var e=null==n?0:n.length;
if(!e)return-1;r=null==r?0:Fn(r),0>r&&(r=jn(e+r,0));n:{for(t=g(t),e=n.length,r+=-1;++r<e;)if(t(n[r],r,n)){n=r;break n}n=-1}return n}),En=O(function(n,t,r){return S(n,t,r)}),wn=O(function(n,t){return c(n,1,t)}),kn=O(function(n,t,r){return c(n,Sn(t)||0,r)}),Nn=Array.isArray,Fn=Number,Sn=Number,Tn=N(function(n,t){k(t,_n(t),n)}),Bn=N(function(n,t){k(t,D(t),n)}),Rn=O(function(n,t){n=Object(n);var r,e=-1,u=t.length,o=2<u?t[2]:Z;if(r=o){r=t[0];var i=t[1];if(V(o)){var c=typeof i;if("number"==c){if(c=M(o))var c=o.length,f=typeof i,c=null==c?9007199254740991:c,c=!!c&&("number"==f||"symbol"!=f&&en.test(i))&&-1<i&&0==i%1&&i<c;
}else c="string"==c&&i in o;r=!!c&&J(o[i],r)}else r=false}for(r&&(u=1);++e<u;)for(o=t[e],r=In(o),i=-1,c=r.length;++i<c;){var f=r[i],a=n[f];(a===Z||J(a,ln[f])&&!pn.call(n,f))&&(n[f]=o[f])}return n}),Dn=_n,In=D,$n=function(n){return xn(I(n,Z,$),n+"")}(function(n,t){return null==n?{}:m(n,t)});o.assignIn=Bn,o.before=G,o.bind=En,o.chain=function(n){return n=o(n),n.__chain__=true,n},o.compact=function(n){return l(n,Boolean)},o.concat=function(){var n=arguments.length;if(!n)return[];for(var r=Array(n-1),e=arguments[0];n--;)r[n-1]=arguments[n];
return t(Nn(e)?A(e):[e],p(r,1))},o.create=function(n,t){var r=dn(n);return null==t?r:Tn(r,t)},o.defaults=Rn,o.defer=wn,o.delay=kn,o.filter=function(n,t){return l(n,g(t))},o.flatten=$,o.flattenDeep=function(n){return(null==n?0:n.length)?p(n,nn):[]},o.iteratee=g,o.keys=Dn,o.map=function(n,t){return j(n,g(t))},o.matches=function(n){return d(Tn({},n))},o.mixin=Y,o.negate=function(n){if(typeof n!="function")throw new TypeError("Expected a function");return function(){return!n.apply(this,arguments)}},o.once=function(n){
return G(2,n)},o.pick=$n,o.slice=function(n,t,r){var e=null==n?0:n.length;return r=r===Z?e:+r,e?x(n,null==t?0:+t,r):[]},o.sortBy=function(n,t){var e=0;return t=g(t),j(j(n,function(n,r,u){return{value:n,index:e++,criteria:t(n,r,u)}}).sort(function(n,t){var r;n:{r=n.criteria;var e=t.criteria;if(r!==e){var u=r!==Z,o=null===r,i=r===r,c=e!==Z,f=null===e,a=e===e;if(!f&&r>e||o&&c&&a||!u&&a||!i){r=1;break n}if(!o&&r<e||f&&u&&i||!c&&i||!a){r=-1;break n}}r=0}return r||n.index-t.index}),r("value"))},o.tap=function(n,t){
return t(n),n},o.thru=function(n,t){return t(n)},o.toArray=function(n){return M(n)?n.length?A(n):[]:W(n)},o.values=W,o.extend=Bn,Y(o,o),o.clone=function(n){return V(n)?Nn(n)?A(n):k(n,_n(n)):n},o.escape=function(n){return(n=Q(n))&&rn.test(n)?n.replace(tn,fn):n},o.every=function(n,t,r){return t=r?Z:t,f(n,g(t))},o.find=An,o.forEach=z,o.has=function(n,t){return null!=n&&pn.call(n,t)},o.head=q,o.identity=X,o.indexOf=P,o.isArguments=n,o.isArray=Nn,o.isBoolean=function(n){return true===n||false===n||H(n)&&"[object Boolean]"==hn.call(n);
},o.isDate=function(n){return H(n)&&"[object Date]"==hn.call(n)},o.isEmpty=function(t){return M(t)&&(Nn(t)||L(t)||U(t.splice)||n(t))?!t.length:!_n(t).length},o.isEqual=function(n,t){return b(n,t)},o.isFinite=function(n){return typeof n=="number"&&gn(n)},o.isFunction=U,o.isNaN=function(n){return K(n)&&n!=+n},o.isNull=function(n){return null===n},o.isNumber=K,o.isObject=V,o.isRegExp=function(n){return H(n)&&"[object RegExp]"==hn.call(n)},o.isString=L,o.isUndefined=function(n){return n===Z},o.last=function(n){
var t=null==n?0:n.length;return t?n[t-1]:Z},o.max=function(n){return n&&n.length?a(n,X,v):Z},o.min=function(n){return n&&n.length?a(n,X,_):Z},o.noConflict=function(){return on._===this&&(on._=vn),this},o.noop=function(){},o.reduce=C,o.result=function(n,t,r){return t=null==n?Z:n[t],t===Z&&(t=r),U(t)?t.call(n):t},o.size=function(n){return null==n?0:(n=M(n)?n:_n(n),n.length)},o.some=function(n,t,r){return t=r?Z:t,E(n,g(t))},o.uniqueId=function(n){var t=++sn;return Q(n)+t},o.each=z,o.first=q,Y(o,function(){
var n={};return s(o,function(t,r){pn.call(o.prototype,r)||(n[r]=t)}),n}(),{chain:false}),o.VERSION="4.17.15",mn("pop join replace reverse split push shift sort splice unshift".split(" "),function(n){var t=(/^(?:replace|split)$/.test(n)?String.prototype:an)[n],r=/^(?:push|sort|unshift)$/.test(n)?"tap":"thru",e=/^(?:pop|join|replace|shift)$/.test(n);o.prototype[n]=function(){var n=arguments;if(e&&!this.__chain__){var u=this.value();return t.apply(Nn(u)?u:[],n)}return this[r](function(r){return t.apply(Nn(r)?r:[],n);
})}}),o.prototype.toJSON=o.prototype.valueOf=o.prototype.value=function(){return w(this.__wrapped__,this.__actions__)},cn&&((cn.exports=o)._=o,un._=o)}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/core.min.js | core.min.js |
var Stack = require('lodash/_Stack'),
equalArrays = require('lodash/_equalArrays'),
equalByTag = require('lodash/_equalByTag'),
equalObjects = require('lodash/_equalObjects'),
getTag = require('lodash/_getTag'),
isArray = require('lodash/isArray'),
isBuffer = require('lodash/isBuffer'),
isTypedArray = require('lodash/isTypedArray');
/** Used to compose bitmasks for value comparisons. */
var COMPARE_PARTIAL_FLAG = 1;
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
objectTag = '[object Object]';
/** Used for built-in method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* A specialized version of `baseIsEqual` for arrays and objects which performs
* deep comparisons and tracks traversed objects enabling objects with circular
* references to be compared.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} [stack] Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function baseIsEqualDeep(object, other, bitmask, customizer, equalFunc, stack) {
var objIsArr = isArray(object),
othIsArr = isArray(other),
objTag = objIsArr ? arrayTag : getTag(object),
othTag = othIsArr ? arrayTag : getTag(other);
objTag = objTag == argsTag ? objectTag : objTag;
othTag = othTag == argsTag ? objectTag : othTag;
var objIsObj = objTag == objectTag,
othIsObj = othTag == objectTag,
isSameTag = objTag == othTag;
if (isSameTag && isBuffer(object)) {
if (!isBuffer(other)) {
return false;
}
objIsArr = true;
objIsObj = false;
}
if (isSameTag && !objIsObj) {
stack || (stack = new Stack);
return (objIsArr || isTypedArray(object))
? equalArrays(object, other, bitmask, customizer, equalFunc, stack)
: equalByTag(object, other, objTag, bitmask, customizer, equalFunc, stack);
}
if (!(bitmask & COMPARE_PARTIAL_FLAG)) {
var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
if (objIsWrapped || othIsWrapped) {
var objUnwrapped = objIsWrapped ? object.value() : object,
othUnwrapped = othIsWrapped ? other.value() : other;
stack || (stack = new Stack);
return equalFunc(objUnwrapped, othUnwrapped, bitmask, customizer, stack);
}
}
if (!isSameTag) {
return false;
}
stack || (stack = new Stack);
return equalObjects(object, other, bitmask, customizer, equalFunc, stack);
}
module.exports = baseIsEqualDeep; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseIsEqualDeep.js | _baseIsEqualDeep.js |
var cloneArrayBuffer = require('lodash/_cloneArrayBuffer'),
cloneDataView = require('lodash/_cloneDataView'),
cloneRegExp = require('lodash/_cloneRegExp'),
cloneSymbol = require('lodash/_cloneSymbol'),
cloneTypedArray = require('lodash/_cloneTypedArray');
/** `Object#toString` result references. */
var boolTag = '[object Boolean]',
dateTag = '[object Date]',
mapTag = '[object Map]',
numberTag = '[object Number]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
symbolTag = '[object Symbol]';
var arrayBufferTag = '[object ArrayBuffer]',
dataViewTag = '[object DataView]',
float32Tag = '[object Float32Array]',
float64Tag = '[object Float64Array]',
int8Tag = '[object Int8Array]',
int16Tag = '[object Int16Array]',
int32Tag = '[object Int32Array]',
uint8Tag = '[object Uint8Array]',
uint8ClampedTag = '[object Uint8ClampedArray]',
uint16Tag = '[object Uint16Array]',
uint32Tag = '[object Uint32Array]';
/**
* Initializes an object clone based on its `toStringTag`.
*
* **Note:** This function only supports cloning values with tags of
* `Boolean`, `Date`, `Error`, `Map`, `Number`, `RegExp`, `Set`, or `String`.
*
* @private
* @param {Object} object The object to clone.
* @param {string} tag The `toStringTag` of the object to clone.
* @param {boolean} [isDeep] Specify a deep clone.
* @returns {Object} Returns the initialized clone.
*/
function initCloneByTag(object, tag, isDeep) {
var Ctor = object.constructor;
switch (tag) {
case arrayBufferTag:
return cloneArrayBuffer(object);
case boolTag:
case dateTag:
return new Ctor(+object);
case dataViewTag:
return cloneDataView(object, isDeep);
case float32Tag: case float64Tag:
case int8Tag: case int16Tag: case int32Tag:
case uint8Tag: case uint8ClampedTag: case uint16Tag: case uint32Tag:
return cloneTypedArray(object, isDeep);
case mapTag:
return new Ctor;
case numberTag:
case stringTag:
return new Ctor(object);
case regexpTag:
return cloneRegExp(object);
case setTag:
return new Ctor;
case symbolTag:
return cloneSymbol(object);
}
}
module.exports = initCloneByTag; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_initCloneByTag.js | _initCloneByTag.js |
var debounce = require('lodash/debounce'),
isObject = require('lodash/isObject');
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/**
* Creates a throttled function that only invokes `func` at most once per
* every `wait` milliseconds. The throttled function comes with a `cancel`
* method to cancel delayed `func` invocations and a `flush` method to
* immediately invoke them. Provide `options` to indicate whether `func`
* should be invoked on the leading and/or trailing edge of the `wait`
* timeout. The `func` is invoked with the last arguments provided to the
* throttled function. Subsequent calls to the throttled function return the
* result of the last `func` invocation.
*
* **Note:** If `leading` and `trailing` options are `true`, `func` is
* invoked on the trailing edge of the timeout only if the throttled function
* is invoked more than once during the `wait` timeout.
*
* If `wait` is `0` and `leading` is `false`, `func` invocation is deferred
* until to the next tick, similar to `setTimeout` with a timeout of `0`.
*
* See [David Corbacho's article](https://css-tricks.com/debouncing-throttling-explained-examples/)
* for details over the differences between `_.throttle` and `_.debounce`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to throttle.
* @param {number} [wait=0] The number of milliseconds to throttle invocations to.
* @param {Object} [options={}] The options object.
* @param {boolean} [options.leading=true]
* Specify invoking on the leading edge of the timeout.
* @param {boolean} [options.trailing=true]
* Specify invoking on the trailing edge of the timeout.
* @returns {Function} Returns the new throttled function.
* @example
*
* // Avoid excessively updating the position while scrolling.
* jQuery(window).on('scroll', _.throttle(updatePosition, 100));
*
* // Invoke `renewToken` when the click event is fired, but not more than once every 5 minutes.
* var throttled = _.throttle(renewToken, 300000, { 'trailing': false });
* jQuery(element).on('click', throttled);
*
* // Cancel the trailing throttled invocation.
* jQuery(window).on('popstate', throttled.cancel);
*/
function throttle(func, wait, options) {
var leading = true,
trailing = true;
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
if (isObject(options)) {
leading = 'leading' in options ? !!options.leading : leading;
trailing = 'trailing' in options ? !!options.trailing : trailing;
}
return debounce(func, wait, {
'leading': leading,
'maxWait': wait,
'trailing': trailing
});
}
module.exports = throttle; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/throttle.js | throttle.js |
module.exports = {
'chunk': require('lodash/chunk'),
'compact': require('lodash/compact'),
'concat': require('lodash/concat'),
'difference': require('lodash/difference'),
'differenceBy': require('lodash/differenceBy'),
'differenceWith': require('lodash/differenceWith'),
'drop': require('lodash/drop'),
'dropRight': require('lodash/dropRight'),
'dropRightWhile': require('lodash/dropRightWhile'),
'dropWhile': require('lodash/dropWhile'),
'fill': require('lodash/fill'),
'findIndex': require('lodash/findIndex'),
'findLastIndex': require('lodash/findLastIndex'),
'first': require('lodash/first'),
'flatten': require('lodash/flatten'),
'flattenDeep': require('lodash/flattenDeep'),
'flattenDepth': require('lodash/flattenDepth'),
'fromPairs': require('lodash/fromPairs'),
'head': require('lodash/head'),
'indexOf': require('lodash/indexOf'),
'initial': require('lodash/initial'),
'intersection': require('lodash/intersection'),
'intersectionBy': require('lodash/intersectionBy'),
'intersectionWith': require('lodash/intersectionWith'),
'join': require('lodash/join'),
'last': require('lodash/last'),
'lastIndexOf': require('lodash/lastIndexOf'),
'nth': require('lodash/nth'),
'pull': require('lodash/pull'),
'pullAll': require('lodash/pullAll'),
'pullAllBy': require('lodash/pullAllBy'),
'pullAllWith': require('lodash/pullAllWith'),
'pullAt': require('lodash/pullAt'),
'remove': require('lodash/remove'),
'reverse': require('lodash/reverse'),
'slice': require('lodash/slice'),
'sortedIndex': require('lodash/sortedIndex'),
'sortedIndexBy': require('lodash/sortedIndexBy'),
'sortedIndexOf': require('lodash/sortedIndexOf'),
'sortedLastIndex': require('lodash/sortedLastIndex'),
'sortedLastIndexBy': require('lodash/sortedLastIndexBy'),
'sortedLastIndexOf': require('lodash/sortedLastIndexOf'),
'sortedUniq': require('lodash/sortedUniq'),
'sortedUniqBy': require('lodash/sortedUniqBy'),
'tail': require('lodash/tail'),
'take': require('lodash/take'),
'takeRight': require('lodash/takeRight'),
'takeRightWhile': require('lodash/takeRightWhile'),
'takeWhile': require('lodash/takeWhile'),
'union': require('lodash/union'),
'unionBy': require('lodash/unionBy'),
'unionWith': require('lodash/unionWith'),
'uniq': require('lodash/uniq'),
'uniqBy': require('lodash/uniqBy'),
'uniqWith': require('lodash/uniqWith'),
'unzip': require('lodash/unzip'),
'unzipWith': require('lodash/unzipWith'),
'without': require('lodash/without'),
'xor': require('lodash/xor'),
'xorBy': require('lodash/xorBy'),
'xorWith': require('lodash/xorWith'),
'zip': require('lodash/zip'),
'zipObject': require('lodash/zipObject'),
'zipObjectDeep': require('lodash/zipObjectDeep'),
'zipWith': require('lodash/zipWith')
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/array.js | array.js |
var Stack = require('lodash/_Stack'),
arrayEach = require('lodash/_arrayEach'),
assignValue = require('lodash/_assignValue'),
baseAssign = require('lodash/_baseAssign'),
baseAssignIn = require('lodash/_baseAssignIn'),
cloneBuffer = require('lodash/_cloneBuffer'),
copyArray = require('lodash/_copyArray'),
copySymbols = require('lodash/_copySymbols'),
copySymbolsIn = require('lodash/_copySymbolsIn'),
getAllKeys = require('lodash/_getAllKeys'),
getAllKeysIn = require('lodash/_getAllKeysIn'),
getTag = require('lodash/_getTag'),
initCloneArray = require('lodash/_initCloneArray'),
initCloneByTag = require('lodash/_initCloneByTag'),
initCloneObject = require('lodash/_initCloneObject'),
isArray = require('lodash/isArray'),
isBuffer = require('lodash/isBuffer'),
isMap = require('lodash/isMap'),
isObject = require('lodash/isObject'),
isSet = require('lodash/isSet'),
keys = require('lodash/keys');
/** Used to compose bitmasks for cloning. */
var CLONE_DEEP_FLAG = 1,
CLONE_FLAT_FLAG = 2,
CLONE_SYMBOLS_FLAG = 4;
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
funcTag = '[object Function]',
genTag = '[object GeneratorFunction]',
mapTag = '[object Map]',
numberTag = '[object Number]',
objectTag = '[object Object]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
symbolTag = '[object Symbol]',
weakMapTag = '[object WeakMap]';
var arrayBufferTag = '[object ArrayBuffer]',
dataViewTag = '[object DataView]',
float32Tag = '[object Float32Array]',
float64Tag = '[object Float64Array]',
int8Tag = '[object Int8Array]',
int16Tag = '[object Int16Array]',
int32Tag = '[object Int32Array]',
uint8Tag = '[object Uint8Array]',
uint8ClampedTag = '[object Uint8ClampedArray]',
uint16Tag = '[object Uint16Array]',
uint32Tag = '[object Uint32Array]';
/** Used to identify `toStringTag` values supported by `_.clone`. */
var cloneableTags = {};
cloneableTags[argsTag] = cloneableTags[arrayTag] =
cloneableTags[arrayBufferTag] = cloneableTags[dataViewTag] =
cloneableTags[boolTag] = cloneableTags[dateTag] =
cloneableTags[float32Tag] = cloneableTags[float64Tag] =
cloneableTags[int8Tag] = cloneableTags[int16Tag] =
cloneableTags[int32Tag] = cloneableTags[mapTag] =
cloneableTags[numberTag] = cloneableTags[objectTag] =
cloneableTags[regexpTag] = cloneableTags[setTag] =
cloneableTags[stringTag] = cloneableTags[symbolTag] =
cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
cloneableTags[errorTag] = cloneableTags[funcTag] =
cloneableTags[weakMapTag] = false;
/**
* The base implementation of `_.clone` and `_.cloneDeep` which tracks
* traversed objects.
*
* @private
* @param {*} value The value to clone.
* @param {boolean} bitmask The bitmask flags.
* 1 - Deep clone
* 2 - Flatten inherited properties
* 4 - Clone symbols
* @param {Function} [customizer] The function to customize cloning.
* @param {string} [key] The key of `value`.
* @param {Object} [object] The parent object of `value`.
* @param {Object} [stack] Tracks traversed objects and their clone counterparts.
* @returns {*} Returns the cloned value.
*/
function baseClone(value, bitmask, customizer, key, object, stack) {
var result,
isDeep = bitmask & CLONE_DEEP_FLAG,
isFlat = bitmask & CLONE_FLAT_FLAG,
isFull = bitmask & CLONE_SYMBOLS_FLAG;
if (customizer) {
result = object ? customizer(value, key, object, stack) : customizer(value);
}
if (result !== undefined) {
return result;
}
if (!isObject(value)) {
return value;
}
var isArr = isArray(value);
if (isArr) {
result = initCloneArray(value);
if (!isDeep) {
return copyArray(value, result);
}
} else {
var tag = getTag(value),
isFunc = tag == funcTag || tag == genTag;
if (isBuffer(value)) {
return cloneBuffer(value, isDeep);
}
if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
result = (isFlat || isFunc) ? {} : initCloneObject(value);
if (!isDeep) {
return isFlat
? copySymbolsIn(value, baseAssignIn(result, value))
: copySymbols(value, baseAssign(result, value));
}
} else {
if (!cloneableTags[tag]) {
return object ? value : {};
}
result = initCloneByTag(value, tag, isDeep);
}
}
// Check for circular references and return its corresponding clone.
stack || (stack = new Stack);
var stacked = stack.get(value);
if (stacked) {
return stacked;
}
stack.set(value, result);
if (isSet(value)) {
value.forEach(function(subValue) {
result.add(baseClone(subValue, bitmask, customizer, subValue, value, stack));
});
} else if (isMap(value)) {
value.forEach(function(subValue, key) {
result.set(key, baseClone(subValue, bitmask, customizer, key, value, stack));
});
}
var keysFunc = isFull
? (isFlat ? getAllKeysIn : getAllKeys)
: (isFlat ? keysIn : keys);
var props = isArr ? undefined : keysFunc(value);
arrayEach(props || value, function(subValue, key) {
if (props) {
key = subValue;
subValue = value[key];
}
// Recursively populate clone (susceptible to call stack limits).
assignValue(result, key, baseClone(subValue, bitmask, customizer, key, value, stack));
});
return result;
}
module.exports = baseClone; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseClone.js | _baseClone.js |
(function(){function n(n,t,r){switch(r.length){case 0:return n.call(t);case 1:return n.call(t,r[0]);case 2:return n.call(t,r[0],r[1]);case 3:return n.call(t,r[0],r[1],r[2])}return n.apply(t,r)}function t(n,t,r,e){for(var u=-1,i=null==n?0:n.length;++u<i;){var o=n[u];t(e,o,r(o),n)}return e}function r(n,t){for(var r=-1,e=null==n?0:n.length;++r<e&&t(n[r],r,n)!==!1;);return n}function e(n,t){for(var r=null==n?0:n.length;r--&&t(n[r],r,n)!==!1;);return n}function u(n,t){for(var r=-1,e=null==n?0:n.length;++r<e;)if(!t(n[r],r,n))return!1;
return!0}function i(n,t){for(var r=-1,e=null==n?0:n.length,u=0,i=[];++r<e;){var o=n[r];t(o,r,n)&&(i[u++]=o)}return i}function o(n,t){return!!(null==n?0:n.length)&&y(n,t,0)>-1}function f(n,t,r){for(var e=-1,u=null==n?0:n.length;++e<u;)if(r(t,n[e]))return!0;return!1}function c(n,t){for(var r=-1,e=null==n?0:n.length,u=Array(e);++r<e;)u[r]=t(n[r],r,n);return u}function a(n,t){for(var r=-1,e=t.length,u=n.length;++r<e;)n[u+r]=t[r];return n}function l(n,t,r,e){var u=-1,i=null==n?0:n.length;for(e&&i&&(r=n[++u]);++u<i;)r=t(r,n[u],u,n);
return r}function s(n,t,r,e){var u=null==n?0:n.length;for(e&&u&&(r=n[--u]);u--;)r=t(r,n[u],u,n);return r}function h(n,t){for(var r=-1,e=null==n?0:n.length;++r<e;)if(t(n[r],r,n))return!0;return!1}function p(n){return n.split("")}function _(n){return n.match(Bt)||[]}function v(n,t,r){var e;return r(n,function(n,r,u){if(t(n,r,u))return e=r,!1}),e}function g(n,t,r,e){for(var u=n.length,i=r+(e?1:-1);e?i--:++i<u;)if(t(n[i],i,n))return i;return-1}function y(n,t,r){return t===t?q(n,t,r):g(n,b,r)}function d(n,t,r,e){
for(var u=r-1,i=n.length;++u<i;)if(e(n[u],t))return u;return-1}function b(n){return n!==n}function w(n,t){var r=null==n?0:n.length;return r?k(n,t)/r:Sn}function m(n){return function(t){return null==t?Y:t[n]}}function x(n){return function(t){return null==n?Y:n[t]}}function j(n,t,r,e,u){return u(n,function(n,u,i){r=e?(e=!1,n):t(r,n,u,i)}),r}function A(n,t){var r=n.length;for(n.sort(t);r--;)n[r]=n[r].value;return n}function k(n,t){for(var r,e=-1,u=n.length;++e<u;){var i=t(n[e]);i!==Y&&(r=r===Y?i:r+i);
}return r}function O(n,t){for(var r=-1,e=Array(n);++r<n;)e[r]=t(r);return e}function I(n,t){return c(t,function(t){return[t,n[t]]})}function R(n){return function(t){return n(t)}}function z(n,t){return c(t,function(t){return n[t]})}function E(n,t){return n.has(t)}function S(n,t){for(var r=-1,e=n.length;++r<e&&y(t,n[r],0)>-1;);return r}function W(n,t){for(var r=n.length;r--&&y(t,n[r],0)>-1;);return r}function L(n,t){for(var r=n.length,e=0;r--;)n[r]===t&&++e;return e}function C(n){return"\\"+Gr[n]}function U(n,t){
return null==n?Y:n[t]}function B(n){return Dr.test(n)}function T(n){return Mr.test(n)}function $(n){for(var t,r=[];!(t=n.next()).done;)r.push(t.value);return r}function D(n){var t=-1,r=Array(n.size);return n.forEach(function(n,e){r[++t]=[e,n]}),r}function M(n,t){return function(r){return n(t(r))}}function F(n,t){for(var r=-1,e=n.length,u=0,i=[];++r<e;){var o=n[r];o!==t&&o!==un||(n[r]=un,i[u++]=r)}return i}function N(n){var t=-1,r=Array(n.size);return n.forEach(function(n){r[++t]=n}),r}function P(n){
var t=-1,r=Array(n.size);return n.forEach(function(n){r[++t]=[n,n]}),r}function q(n,t,r){for(var e=r-1,u=n.length;++e<u;)if(n[e]===t)return e;return-1}function Z(n,t,r){for(var e=r+1;e--;)if(n[e]===t)return e;return e}function K(n){return B(n)?G(n):se(n)}function V(n){return B(n)?H(n):p(n)}function G(n){for(var t=Tr.lastIndex=0;Tr.test(n);)++t;return t}function H(n){return n.match(Tr)||[]}function J(n){return n.match($r)||[]}var Y,Q="4.17.19",X=200,nn="Unsupported core-js use. Try https://npms.io/search?q=ponyfill.",tn="Expected a function",rn="__lodash_hash_undefined__",en=500,un="__lodash_placeholder__",on=1,fn=2,cn=4,an=1,ln=2,sn=1,hn=2,pn=4,_n=8,vn=16,gn=32,yn=64,dn=128,bn=256,wn=512,mn=30,xn="...",jn=800,An=16,kn=1,On=2,In=3,Rn=1/0,zn=9007199254740991,En=1.7976931348623157e308,Sn=NaN,Wn=4294967295,Ln=Wn-1,Cn=Wn>>>1,Un=[["ary",dn],["bind",sn],["bindKey",hn],["curry",_n],["curryRight",vn],["flip",wn],["partial",gn],["partialRight",yn],["rearg",bn]],Bn="[object Arguments]",Tn="[object Array]",$n="[object AsyncFunction]",Dn="[object Boolean]",Mn="[object Date]",Fn="[object DOMException]",Nn="[object Error]",Pn="[object Function]",qn="[object GeneratorFunction]",Zn="[object Map]",Kn="[object Number]",Vn="[object Null]",Gn="[object Object]",Hn="[object Promise]",Jn="[object Proxy]",Yn="[object RegExp]",Qn="[object Set]",Xn="[object String]",nt="[object Symbol]",tt="[object Undefined]",rt="[object WeakMap]",et="[object WeakSet]",ut="[object ArrayBuffer]",it="[object DataView]",ot="[object Float32Array]",ft="[object Float64Array]",ct="[object Int8Array]",at="[object Int16Array]",lt="[object Int32Array]",st="[object Uint8Array]",ht="[object Uint8ClampedArray]",pt="[object Uint16Array]",_t="[object Uint32Array]",vt=/\b__p \+= '';/g,gt=/\b(__p \+=) '' \+/g,yt=/(__e\(.*?\)|\b__t\)) \+\n'';/g,dt=/&(?:amp|lt|gt|quot|#39);/g,bt=/[&<>"']/g,wt=RegExp(dt.source),mt=RegExp(bt.source),xt=/<%-([\s\S]+?)%>/g,jt=/<%([\s\S]+?)%>/g,At=/<%=([\s\S]+?)%>/g,kt=/\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/,Ot=/^\w*$/,It=/[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g,Rt=/[\\^$.*+?()[\]{}|]/g,zt=RegExp(Rt.source),Et=/^\s+|\s+$/g,St=/^\s+/,Wt=/\s+$/,Lt=/\{(?:\n\/\* \[wrapped with .+\] \*\/)?\n?/,Ct=/\{\n\/\* \[wrapped with (.+)\] \*/,Ut=/,? & /,Bt=/[^\x00-\x2f\x3a-\x40\x5b-\x60\x7b-\x7f]+/g,Tt=/\\(\\)?/g,$t=/\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g,Dt=/\w*$/,Mt=/^[-+]0x[0-9a-f]+$/i,Ft=/^0b[01]+$/i,Nt=/^\[object .+?Constructor\]$/,Pt=/^0o[0-7]+$/i,qt=/^(?:0|[1-9]\d*)$/,Zt=/[\xc0-\xd6\xd8-\xf6\xf8-\xff\u0100-\u017f]/g,Kt=/($^)/,Vt=/['\n\r\u2028\u2029\\]/g,Gt="\\ud800-\\udfff",Ht="\\u0300-\\u036f",Jt="\\ufe20-\\ufe2f",Yt="\\u20d0-\\u20ff",Qt=Ht+Jt+Yt,Xt="\\u2700-\\u27bf",nr="a-z\\xdf-\\xf6\\xf8-\\xff",tr="\\xac\\xb1\\xd7\\xf7",rr="\\x00-\\x2f\\x3a-\\x40\\x5b-\\x60\\x7b-\\xbf",er="\\u2000-\\u206f",ur=" \\t\\x0b\\f\\xa0\\ufeff\\n\\r\\u2028\\u2029\\u1680\\u180e\\u2000\\u2001\\u2002\\u2003\\u2004\\u2005\\u2006\\u2007\\u2008\\u2009\\u200a\\u202f\\u205f\\u3000",ir="A-Z\\xc0-\\xd6\\xd8-\\xde",or="\\ufe0e\\ufe0f",fr=tr+rr+er+ur,cr="['\u2019]",ar="["+Gt+"]",lr="["+fr+"]",sr="["+Qt+"]",hr="\\d+",pr="["+Xt+"]",_r="["+nr+"]",vr="[^"+Gt+fr+hr+Xt+nr+ir+"]",gr="\\ud83c[\\udffb-\\udfff]",yr="(?:"+sr+"|"+gr+")",dr="[^"+Gt+"]",br="(?:\\ud83c[\\udde6-\\uddff]){2}",wr="[\\ud800-\\udbff][\\udc00-\\udfff]",mr="["+ir+"]",xr="\\u200d",jr="(?:"+_r+"|"+vr+")",Ar="(?:"+mr+"|"+vr+")",kr="(?:"+cr+"(?:d|ll|m|re|s|t|ve))?",Or="(?:"+cr+"(?:D|LL|M|RE|S|T|VE))?",Ir=yr+"?",Rr="["+or+"]?",zr="(?:"+xr+"(?:"+[dr,br,wr].join("|")+")"+Rr+Ir+")*",Er="\\d*(?:1st|2nd|3rd|(?![123])\\dth)(?=\\b|[A-Z_])",Sr="\\d*(?:1ST|2ND|3RD|(?![123])\\dTH)(?=\\b|[a-z_])",Wr=Rr+Ir+zr,Lr="(?:"+[pr,br,wr].join("|")+")"+Wr,Cr="(?:"+[dr+sr+"?",sr,br,wr,ar].join("|")+")",Ur=RegExp(cr,"g"),Br=RegExp(sr,"g"),Tr=RegExp(gr+"(?="+gr+")|"+Cr+Wr,"g"),$r=RegExp([mr+"?"+_r+"+"+kr+"(?="+[lr,mr,"$"].join("|")+")",Ar+"+"+Or+"(?="+[lr,mr+jr,"$"].join("|")+")",mr+"?"+jr+"+"+kr,mr+"+"+Or,Sr,Er,hr,Lr].join("|"),"g"),Dr=RegExp("["+xr+Gt+Qt+or+"]"),Mr=/[a-z][A-Z]|[A-Z]{2}[a-z]|[0-9][a-zA-Z]|[a-zA-Z][0-9]|[^a-zA-Z0-9 ]/,Fr=["Array","Buffer","DataView","Date","Error","Float32Array","Float64Array","Function","Int8Array","Int16Array","Int32Array","Map","Math","Object","Promise","RegExp","Set","String","Symbol","TypeError","Uint8Array","Uint8ClampedArray","Uint16Array","Uint32Array","WeakMap","_","clearTimeout","isFinite","parseInt","setTimeout"],Nr=-1,Pr={};
Pr[ot]=Pr[ft]=Pr[ct]=Pr[at]=Pr[lt]=Pr[st]=Pr[ht]=Pr[pt]=Pr[_t]=!0,Pr[Bn]=Pr[Tn]=Pr[ut]=Pr[Dn]=Pr[it]=Pr[Mn]=Pr[Nn]=Pr[Pn]=Pr[Zn]=Pr[Kn]=Pr[Gn]=Pr[Yn]=Pr[Qn]=Pr[Xn]=Pr[rt]=!1;var qr={};qr[Bn]=qr[Tn]=qr[ut]=qr[it]=qr[Dn]=qr[Mn]=qr[ot]=qr[ft]=qr[ct]=qr[at]=qr[lt]=qr[Zn]=qr[Kn]=qr[Gn]=qr[Yn]=qr[Qn]=qr[Xn]=qr[nt]=qr[st]=qr[ht]=qr[pt]=qr[_t]=!0,qr[Nn]=qr[Pn]=qr[rt]=!1;var Zr={"\xc0":"A","\xc1":"A","\xc2":"A","\xc3":"A","\xc4":"A","\xc5":"A","\xe0":"a","\xe1":"a","\xe2":"a","\xe3":"a","\xe4":"a","\xe5":"a",
"\xc7":"C","\xe7":"c","\xd0":"D","\xf0":"d","\xc8":"E","\xc9":"E","\xca":"E","\xcb":"E","\xe8":"e","\xe9":"e","\xea":"e","\xeb":"e","\xcc":"I","\xcd":"I","\xce":"I","\xcf":"I","\xec":"i","\xed":"i","\xee":"i","\xef":"i","\xd1":"N","\xf1":"n","\xd2":"O","\xd3":"O","\xd4":"O","\xd5":"O","\xd6":"O","\xd8":"O","\xf2":"o","\xf3":"o","\xf4":"o","\xf5":"o","\xf6":"o","\xf8":"o","\xd9":"U","\xda":"U","\xdb":"U","\xdc":"U","\xf9":"u","\xfa":"u","\xfb":"u","\xfc":"u","\xdd":"Y","\xfd":"y","\xff":"y","\xc6":"Ae",
"\xe6":"ae","\xde":"Th","\xfe":"th","\xdf":"ss","\u0100":"A","\u0102":"A","\u0104":"A","\u0101":"a","\u0103":"a","\u0105":"a","\u0106":"C","\u0108":"C","\u010a":"C","\u010c":"C","\u0107":"c","\u0109":"c","\u010b":"c","\u010d":"c","\u010e":"D","\u0110":"D","\u010f":"d","\u0111":"d","\u0112":"E","\u0114":"E","\u0116":"E","\u0118":"E","\u011a":"E","\u0113":"e","\u0115":"e","\u0117":"e","\u0119":"e","\u011b":"e","\u011c":"G","\u011e":"G","\u0120":"G","\u0122":"G","\u011d":"g","\u011f":"g","\u0121":"g",
"\u0123":"g","\u0124":"H","\u0126":"H","\u0125":"h","\u0127":"h","\u0128":"I","\u012a":"I","\u012c":"I","\u012e":"I","\u0130":"I","\u0129":"i","\u012b":"i","\u012d":"i","\u012f":"i","\u0131":"i","\u0134":"J","\u0135":"j","\u0136":"K","\u0137":"k","\u0138":"k","\u0139":"L","\u013b":"L","\u013d":"L","\u013f":"L","\u0141":"L","\u013a":"l","\u013c":"l","\u013e":"l","\u0140":"l","\u0142":"l","\u0143":"N","\u0145":"N","\u0147":"N","\u014a":"N","\u0144":"n","\u0146":"n","\u0148":"n","\u014b":"n","\u014c":"O",
"\u014e":"O","\u0150":"O","\u014d":"o","\u014f":"o","\u0151":"o","\u0154":"R","\u0156":"R","\u0158":"R","\u0155":"r","\u0157":"r","\u0159":"r","\u015a":"S","\u015c":"S","\u015e":"S","\u0160":"S","\u015b":"s","\u015d":"s","\u015f":"s","\u0161":"s","\u0162":"T","\u0164":"T","\u0166":"T","\u0163":"t","\u0165":"t","\u0167":"t","\u0168":"U","\u016a":"U","\u016c":"U","\u016e":"U","\u0170":"U","\u0172":"U","\u0169":"u","\u016b":"u","\u016d":"u","\u016f":"u","\u0171":"u","\u0173":"u","\u0174":"W","\u0175":"w",
"\u0176":"Y","\u0177":"y","\u0178":"Y","\u0179":"Z","\u017b":"Z","\u017d":"Z","\u017a":"z","\u017c":"z","\u017e":"z","\u0132":"IJ","\u0133":"ij","\u0152":"Oe","\u0153":"oe","\u0149":"'n","\u017f":"s"},Kr={"&":"&","<":"<",">":">",'"':""","'":"'"},Vr={"&":"&","<":"<",">":">",""":'"',"'":"'"},Gr={"\\":"\\","'":"'","\n":"n","\r":"r","\u2028":"u2028","\u2029":"u2029"},Hr=parseFloat,Jr=parseInt,Yr="object"==typeof global&&global&&global.Object===Object&&global,Qr="object"==typeof self&&self&&self.Object===Object&&self,Xr=Yr||Qr||Function("return this")(),ne="object"==typeof exports&&exports&&!exports.nodeType&&exports,te=ne&&"object"==typeof module&&module&&!module.nodeType&&module,re=te&&te.exports===ne,ee=re&&Yr.process,ue=function(){
try{var n=te&&te.require&&te.require("util").types;return n?n:ee&&ee.binding&&ee.binding("util")}catch(n){}}(),ie=ue&&ue.isArrayBuffer,oe=ue&&ue.isDate,fe=ue&&ue.isMap,ce=ue&&ue.isRegExp,ae=ue&&ue.isSet,le=ue&&ue.isTypedArray,se=m("length"),he=x(Zr),pe=x(Kr),_e=x(Vr),ve=function p(x){function q(n){if(oc(n)&&!yh(n)&&!(n instanceof Bt)){if(n instanceof H)return n;if(yl.call(n,"__wrapped__"))return to(n)}return new H(n)}function G(){}function H(n,t){this.__wrapped__=n,this.__actions__=[],this.__chain__=!!t,
this.__index__=0,this.__values__=Y}function Bt(n){this.__wrapped__=n,this.__actions__=[],this.__dir__=1,this.__filtered__=!1,this.__iteratees__=[],this.__takeCount__=Wn,this.__views__=[]}function Gt(){var n=new Bt(this.__wrapped__);return n.__actions__=Uu(this.__actions__),n.__dir__=this.__dir__,n.__filtered__=this.__filtered__,n.__iteratees__=Uu(this.__iteratees__),n.__takeCount__=this.__takeCount__,n.__views__=Uu(this.__views__),n}function Ht(){if(this.__filtered__){var n=new Bt(this);n.__dir__=-1,
n.__filtered__=!0}else n=this.clone(),n.__dir__*=-1;return n}function Jt(){var n=this.__wrapped__.value(),t=this.__dir__,r=yh(n),e=t<0,u=r?n.length:0,i=Ai(0,u,this.__views__),o=i.start,f=i.end,c=f-o,a=e?f:o-1,l=this.__iteratees__,s=l.length,h=0,p=Vl(c,this.__takeCount__);if(!r||!e&&u==c&&p==c)return du(n,this.__actions__);var _=[];n:for(;c--&&h<p;){a+=t;for(var v=-1,g=n[a];++v<s;){var y=l[v],d=y.iteratee,b=y.type,w=d(g);if(b==On)g=w;else if(!w){if(b==kn)continue n;break n}}_[h++]=g}return _}function Yt(n){
var t=-1,r=null==n?0:n.length;for(this.clear();++t<r;){var e=n[t];this.set(e[0],e[1])}}function Qt(){this.__data__=es?es(null):{},this.size=0}function Xt(n){var t=this.has(n)&&delete this.__data__[n];return this.size-=t?1:0,t}function nr(n){var t=this.__data__;if(es){var r=t[n];return r===rn?Y:r}return yl.call(t,n)?t[n]:Y}function tr(n){var t=this.__data__;return es?t[n]!==Y:yl.call(t,n)}function rr(n,t){var r=this.__data__;return this.size+=this.has(n)?0:1,r[n]=es&&t===Y?rn:t,this}function er(n){
var t=-1,r=null==n?0:n.length;for(this.clear();++t<r;){var e=n[t];this.set(e[0],e[1])}}function ur(){this.__data__=[],this.size=0}function ir(n){var t=this.__data__,r=Er(t,n);return!(r<0)&&(r==t.length-1?t.pop():Sl.call(t,r,1),--this.size,!0)}function or(n){var t=this.__data__,r=Er(t,n);return r<0?Y:t[r][1]}function fr(n){return Er(this.__data__,n)>-1}function cr(n,t){var r=this.__data__,e=Er(r,n);return e<0?(++this.size,r.push([n,t])):r[e][1]=t,this}function ar(n){var t=-1,r=null==n?0:n.length;for(this.clear();++t<r;){
var e=n[t];this.set(e[0],e[1])}}function lr(){this.size=0,this.__data__={hash:new Yt,map:new(Xl||er),string:new Yt}}function sr(n){var t=wi(this,n).delete(n);return this.size-=t?1:0,t}function hr(n){return wi(this,n).get(n)}function pr(n){return wi(this,n).has(n)}function _r(n,t){var r=wi(this,n),e=r.size;return r.set(n,t),this.size+=r.size==e?0:1,this}function vr(n){var t=-1,r=null==n?0:n.length;for(this.__data__=new ar;++t<r;)this.add(n[t])}function gr(n){return this.__data__.set(n,rn),this}function yr(n){
return this.__data__.has(n)}function dr(n){this.size=(this.__data__=new er(n)).size}function br(){this.__data__=new er,this.size=0}function wr(n){var t=this.__data__,r=t.delete(n);return this.size=t.size,r}function mr(n){return this.__data__.get(n)}function xr(n){return this.__data__.has(n)}function jr(n,t){var r=this.__data__;if(r instanceof er){var e=r.__data__;if(!Xl||e.length<X-1)return e.push([n,t]),this.size=++r.size,this;r=this.__data__=new ar(e)}return r.set(n,t),this.size=r.size,this}function Ar(n,t){
var r=yh(n),e=!r&&gh(n),u=!r&&!e&&bh(n),i=!r&&!e&&!u&&Ah(n),o=r||e||u||i,f=o?O(n.length,ll):[],c=f.length;for(var a in n)!t&&!yl.call(n,a)||o&&("length"==a||u&&("offset"==a||"parent"==a)||i&&("buffer"==a||"byteLength"==a||"byteOffset"==a)||Wi(a,c))||f.push(a);return f}function kr(n){var t=n.length;return t?n[Xe(0,t-1)]:Y}function Or(n,t){return Yi(Uu(n),$r(t,0,n.length))}function Ir(n){return Yi(Uu(n))}function Rr(n,t,r){(r===Y||Kf(n[t],r))&&(r!==Y||t in n)||Cr(n,t,r)}function zr(n,t,r){var e=n[t];
yl.call(n,t)&&Kf(e,r)&&(r!==Y||t in n)||Cr(n,t,r)}function Er(n,t){for(var r=n.length;r--;)if(Kf(n[r][0],t))return r;return-1}function Sr(n,t,r,e){return vs(n,function(n,u,i){t(e,n,r(n),i)}),e}function Wr(n,t){return n&&Bu(t,Fc(t),n)}function Lr(n,t){return n&&Bu(t,Nc(t),n)}function Cr(n,t,r){"__proto__"==t&&Ul?Ul(n,t,{configurable:!0,enumerable:!0,value:r,writable:!0}):n[t]=r}function Tr(n,t){for(var r=-1,e=t.length,u=el(e),i=null==n;++r<e;)u[r]=i?Y:$c(n,t[r]);return u}function $r(n,t,r){return n===n&&(r!==Y&&(n=n<=r?n:r),
t!==Y&&(n=n>=t?n:t)),n}function Dr(n,t,e,u,i,o){var f,c=t&on,a=t&fn,l=t&cn;if(e&&(f=i?e(n,u,i,o):e(n)),f!==Y)return f;if(!ic(n))return n;var s=yh(n);if(s){if(f=Ii(n),!c)return Uu(n,f)}else{var h=Is(n),p=h==Pn||h==qn;if(bh(n))return ku(n,c);if(h==Gn||h==Bn||p&&!i){if(f=a||p?{}:Ri(n),!c)return a?$u(n,Lr(f,n)):Tu(n,Wr(f,n))}else{if(!qr[h])return i?n:{};f=zi(n,h,c)}}o||(o=new dr);var _=o.get(n);if(_)return _;o.set(n,f),jh(n)?n.forEach(function(r){f.add(Dr(r,t,e,r,n,o))}):mh(n)&&n.forEach(function(r,u){
f.set(u,Dr(r,t,e,u,n,o))});var v=l?a?gi:vi:a?Nc:Fc,g=s?Y:v(n);return r(g||n,function(r,u){g&&(u=r,r=n[u]),zr(f,u,Dr(r,t,e,u,n,o))}),f}function Mr(n){var t=Fc(n);return function(r){return Zr(r,n,t)}}function Zr(n,t,r){var e=r.length;if(null==n)return!e;for(n=cl(n);e--;){var u=r[e],i=t[u],o=n[u];if(o===Y&&!(u in n)||!i(o))return!1}return!0}function Kr(n,t,r){if("function"!=typeof n)throw new sl(tn);return Es(function(){n.apply(Y,r)},t)}function Vr(n,t,r,e){var u=-1,i=o,a=!0,l=n.length,s=[],h=t.length;
if(!l)return s;r&&(t=c(t,R(r))),e?(i=f,a=!1):t.length>=X&&(i=E,a=!1,t=new vr(t));n:for(;++u<l;){var p=n[u],_=null==r?p:r(p);if(p=e||0!==p?p:0,a&&_===_){for(var v=h;v--;)if(t[v]===_)continue n;s.push(p)}else i(t,_,e)||s.push(p)}return s}function Gr(n,t){var r=!0;return vs(n,function(n,e,u){return r=!!t(n,e,u)}),r}function Yr(n,t,r){for(var e=-1,u=n.length;++e<u;){var i=n[e],o=t(i);if(null!=o&&(f===Y?o===o&&!yc(o):r(o,f)))var f=o,c=i}return c}function Qr(n,t,r,e){var u=n.length;for(r=jc(r),r<0&&(r=-r>u?0:u+r),
e=e===Y||e>u?u:jc(e),e<0&&(e+=u),e=r>e?0:Ac(e);r<e;)n[r++]=t;return n}function ne(n,t){var r=[];return vs(n,function(n,e,u){t(n,e,u)&&r.push(n)}),r}function te(n,t,r,e,u){var i=-1,o=n.length;for(r||(r=Si),u||(u=[]);++i<o;){var f=n[i];t>0&&r(f)?t>1?te(f,t-1,r,e,u):a(u,f):e||(u[u.length]=f)}return u}function ee(n,t){return n&&ys(n,t,Fc)}function ue(n,t){return n&&ds(n,t,Fc)}function se(n,t){return i(t,function(t){return rc(n[t])})}function ve(n,t){t=ju(t,n);for(var r=0,e=t.length;null!=n&&r<e;)n=n[Qi(t[r++])];
return r&&r==e?n:Y}function ye(n,t,r){var e=t(n);return yh(n)?e:a(e,r(n))}function de(n){return null==n?n===Y?tt:Vn:Cl&&Cl in cl(n)?ji(n):qi(n)}function be(n,t){return n>t}function we(n,t){return null!=n&&yl.call(n,t)}function me(n,t){return null!=n&&t in cl(n)}function xe(n,t,r){return n>=Vl(t,r)&&n<Kl(t,r)}function je(n,t,r){for(var e=r?f:o,u=n[0].length,i=n.length,a=i,l=el(i),s=1/0,h=[];a--;){var p=n[a];a&&t&&(p=c(p,R(t))),s=Vl(p.length,s),l[a]=!r&&(t||u>=120&&p.length>=120)?new vr(a&&p):Y}p=n[0];
var _=-1,v=l[0];n:for(;++_<u&&h.length<s;){var g=p[_],y=t?t(g):g;if(g=r||0!==g?g:0,!(v?E(v,y):e(h,y,r))){for(a=i;--a;){var d=l[a];if(!(d?E(d,y):e(n[a],y,r)))continue n}v&&v.push(y),h.push(g)}}return h}function Ae(n,t,r,e){return ee(n,function(n,u,i){t(e,r(n),u,i)}),e}function ke(t,r,e){r=ju(r,t),t=Ki(t,r);var u=null==t?t:t[Qi(mo(r))];return null==u?Y:n(u,t,e)}function Oe(n){return oc(n)&&de(n)==Bn}function Ie(n){return oc(n)&&de(n)==ut}function Re(n){return oc(n)&&de(n)==Mn}function ze(n,t,r,e,u){
return n===t||(null==n||null==t||!oc(n)&&!oc(t)?n!==n&&t!==t:Ee(n,t,r,e,ze,u))}function Ee(n,t,r,e,u,i){var o=yh(n),f=yh(t),c=o?Tn:Is(n),a=f?Tn:Is(t);c=c==Bn?Gn:c,a=a==Bn?Gn:a;var l=c==Gn,s=a==Gn,h=c==a;if(h&&bh(n)){if(!bh(t))return!1;o=!0,l=!1}if(h&&!l)return i||(i=new dr),o||Ah(n)?si(n,t,r,e,u,i):hi(n,t,c,r,e,u,i);if(!(r&an)){var p=l&&yl.call(n,"__wrapped__"),_=s&&yl.call(t,"__wrapped__");if(p||_){var v=p?n.value():n,g=_?t.value():t;return i||(i=new dr),u(v,g,r,e,i)}}return!!h&&(i||(i=new dr),pi(n,t,r,e,u,i));
}function Se(n){return oc(n)&&Is(n)==Zn}function We(n,t,r,e){var u=r.length,i=u,o=!e;if(null==n)return!i;for(n=cl(n);u--;){var f=r[u];if(o&&f[2]?f[1]!==n[f[0]]:!(f[0]in n))return!1}for(;++u<i;){f=r[u];var c=f[0],a=n[c],l=f[1];if(o&&f[2]){if(a===Y&&!(c in n))return!1}else{var s=new dr;if(e)var h=e(a,l,c,n,t,s);if(!(h===Y?ze(l,a,an|ln,e,s):h))return!1}}return!0}function Le(n){return!(!ic(n)||Ti(n))&&(rc(n)?jl:Nt).test(Xi(n))}function Ce(n){return oc(n)&&de(n)==Yn}function Ue(n){return oc(n)&&Is(n)==Qn;
}function Be(n){return oc(n)&&uc(n.length)&&!!Pr[de(n)]}function Te(n){return"function"==typeof n?n:null==n?Sa:"object"==typeof n?yh(n)?Pe(n[0],n[1]):Ne(n):Da(n)}function $e(n){if(!$i(n))return Zl(n);var t=[];for(var r in cl(n))yl.call(n,r)&&"constructor"!=r&&t.push(r);return t}function De(n){if(!ic(n))return Pi(n);var t=$i(n),r=[];for(var e in n)("constructor"!=e||!t&&yl.call(n,e))&&r.push(e);return r}function Me(n,t){return n<t}function Fe(n,t){var r=-1,e=Vf(n)?el(n.length):[];return vs(n,function(n,u,i){
e[++r]=t(n,u,i)}),e}function Ne(n){var t=mi(n);return 1==t.length&&t[0][2]?Mi(t[0][0],t[0][1]):function(r){return r===n||We(r,n,t)}}function Pe(n,t){return Ci(n)&&Di(t)?Mi(Qi(n),t):function(r){var e=$c(r,n);return e===Y&&e===t?Mc(r,n):ze(t,e,an|ln)}}function qe(n,t,r,e,u){n!==t&&ys(t,function(i,o){if(u||(u=new dr),ic(i))Ze(n,t,o,r,qe,e,u);else{var f=e?e(Gi(n,o),i,o+"",n,t,u):Y;f===Y&&(f=i),Rr(n,o,f)}},Nc)}function Ze(n,t,r,e,u,i,o){var f=Gi(n,r),c=Gi(t,r),a=o.get(c);if(a)return Rr(n,r,a),Y;var l=i?i(f,c,r+"",n,t,o):Y,s=l===Y;
if(s){var h=yh(c),p=!h&&bh(c),_=!h&&!p&&Ah(c);l=c,h||p||_?yh(f)?l=f:Gf(f)?l=Uu(f):p?(s=!1,l=ku(c,!0)):_?(s=!1,l=Eu(c,!0)):l=[]:_c(c)||gh(c)?(l=f,gh(f)?l=Oc(f):ic(f)&&!rc(f)||(l=Ri(c))):s=!1}s&&(o.set(c,l),u(l,c,e,i,o),o.delete(c)),Rr(n,r,l)}function Ke(n,t){var r=n.length;if(r)return t+=t<0?r:0,Wi(t,r)?n[t]:Y}function Ve(n,t,r){t=t.length?c(t,function(n){return yh(n)?function(t){return ve(t,1===n.length?n[0]:n)}:n}):[Sa];var e=-1;return t=c(t,R(bi())),A(Fe(n,function(n,r,u){return{criteria:c(t,function(t){
return t(n)}),index:++e,value:n}}),function(n,t){return Wu(n,t,r)})}function Ge(n,t){return He(n,t,function(t,r){return Mc(n,r)})}function He(n,t,r){for(var e=-1,u=t.length,i={};++e<u;){var o=t[e],f=ve(n,o);r(f,o)&&iu(i,ju(o,n),f)}return i}function Je(n){return function(t){return ve(t,n)}}function Ye(n,t,r,e){var u=e?d:y,i=-1,o=t.length,f=n;for(n===t&&(t=Uu(t)),r&&(f=c(n,R(r)));++i<o;)for(var a=0,l=t[i],s=r?r(l):l;(a=u(f,s,a,e))>-1;)f!==n&&Sl.call(f,a,1),Sl.call(n,a,1);return n}function Qe(n,t){for(var r=n?t.length:0,e=r-1;r--;){
var u=t[r];if(r==e||u!==i){var i=u;Wi(u)?Sl.call(n,u,1):vu(n,u)}}return n}function Xe(n,t){return n+Ml(Jl()*(t-n+1))}function nu(n,t,r,e){for(var u=-1,i=Kl(Dl((t-n)/(r||1)),0),o=el(i);i--;)o[e?i:++u]=n,n+=r;return o}function tu(n,t){var r="";if(!n||t<1||t>zn)return r;do t%2&&(r+=n),t=Ml(t/2),t&&(n+=n);while(t);return r}function ru(n,t){return Ss(Zi(n,t,Sa),n+"")}function eu(n){return kr(na(n))}function uu(n,t){var r=na(n);return Yi(r,$r(t,0,r.length))}function iu(n,t,r,e){if(!ic(n))return n;t=ju(t,n);
for(var u=-1,i=t.length,o=i-1,f=n;null!=f&&++u<i;){var c=Qi(t[u]),a=r;if("__proto__"===c||"constructor"===c||"prototype"===c)return n;if(u!=o){var l=f[c];a=e?e(l,c,f):Y,a===Y&&(a=ic(l)?l:Wi(t[u+1])?[]:{})}zr(f,c,a),f=f[c]}return n}function ou(n){return Yi(na(n))}function fu(n,t,r){var e=-1,u=n.length;t<0&&(t=-t>u?0:u+t),r=r>u?u:r,r<0&&(r+=u),u=t>r?0:r-t>>>0,t>>>=0;for(var i=el(u);++e<u;)i[e]=n[e+t];return i}function cu(n,t){var r;return vs(n,function(n,e,u){return r=t(n,e,u),!r}),!!r}function au(n,t,r){
var e=0,u=null==n?e:n.length;if("number"==typeof t&&t===t&&u<=Cn){for(;e<u;){var i=e+u>>>1,o=n[i];null!==o&&!yc(o)&&(r?o<=t:o<t)?e=i+1:u=i}return u}return lu(n,t,Sa,r)}function lu(n,t,r,e){var u=0,i=null==n?0:n.length;if(0===i)return 0;t=r(t);for(var o=t!==t,f=null===t,c=yc(t),a=t===Y;u<i;){var l=Ml((u+i)/2),s=r(n[l]),h=s!==Y,p=null===s,_=s===s,v=yc(s);if(o)var g=e||_;else g=a?_&&(e||h):f?_&&h&&(e||!p):c?_&&h&&!p&&(e||!v):!p&&!v&&(e?s<=t:s<t);g?u=l+1:i=l}return Vl(i,Ln)}function su(n,t){for(var r=-1,e=n.length,u=0,i=[];++r<e;){
var o=n[r],f=t?t(o):o;if(!r||!Kf(f,c)){var c=f;i[u++]=0===o?0:o}}return i}function hu(n){return"number"==typeof n?n:yc(n)?Sn:+n}function pu(n){if("string"==typeof n)return n;if(yh(n))return c(n,pu)+"";if(yc(n))return ps?ps.call(n):"";var t=n+"";return"0"==t&&1/n==-Rn?"-0":t}function _u(n,t,r){var e=-1,u=o,i=n.length,c=!0,a=[],l=a;if(r)c=!1,u=f;else if(i>=X){var s=t?null:js(n);if(s)return N(s);c=!1,u=E,l=new vr}else l=t?[]:a;n:for(;++e<i;){var h=n[e],p=t?t(h):h;if(h=r||0!==h?h:0,c&&p===p){for(var _=l.length;_--;)if(l[_]===p)continue n;
t&&l.push(p),a.push(h)}else u(l,p,r)||(l!==a&&l.push(p),a.push(h))}return a}function vu(n,t){return t=ju(t,n),n=Ki(n,t),null==n||delete n[Qi(mo(t))]}function gu(n,t,r,e){return iu(n,t,r(ve(n,t)),e)}function yu(n,t,r,e){for(var u=n.length,i=e?u:-1;(e?i--:++i<u)&&t(n[i],i,n););return r?fu(n,e?0:i,e?i+1:u):fu(n,e?i+1:0,e?u:i)}function du(n,t){var r=n;return r instanceof Bt&&(r=r.value()),l(t,function(n,t){return t.func.apply(t.thisArg,a([n],t.args))},r)}function bu(n,t,r){var e=n.length;if(e<2)return e?_u(n[0]):[];
for(var u=-1,i=el(e);++u<e;)for(var o=n[u],f=-1;++f<e;)f!=u&&(i[u]=Vr(i[u]||o,n[f],t,r));return _u(te(i,1),t,r)}function wu(n,t,r){for(var e=-1,u=n.length,i=t.length,o={};++e<u;){r(o,n[e],e<i?t[e]:Y)}return o}function mu(n){return Gf(n)?n:[]}function xu(n){return"function"==typeof n?n:Sa}function ju(n,t){return yh(n)?n:Ci(n,t)?[n]:Ws(Rc(n))}function Au(n,t,r){var e=n.length;return r=r===Y?e:r,!t&&r>=e?n:fu(n,t,r)}function ku(n,t){if(t)return n.slice();var r=n.length,e=Il?Il(r):new n.constructor(r);
return n.copy(e),e}function Ou(n){var t=new n.constructor(n.byteLength);return new Ol(t).set(new Ol(n)),t}function Iu(n,t){return new n.constructor(t?Ou(n.buffer):n.buffer,n.byteOffset,n.byteLength)}function Ru(n){var t=new n.constructor(n.source,Dt.exec(n));return t.lastIndex=n.lastIndex,t}function zu(n){return hs?cl(hs.call(n)):{}}function Eu(n,t){return new n.constructor(t?Ou(n.buffer):n.buffer,n.byteOffset,n.length)}function Su(n,t){if(n!==t){var r=n!==Y,e=null===n,u=n===n,i=yc(n),o=t!==Y,f=null===t,c=t===t,a=yc(t);
if(!f&&!a&&!i&&n>t||i&&o&&c&&!f&&!a||e&&o&&c||!r&&c||!u)return 1;if(!e&&!i&&!a&&n<t||a&&r&&u&&!e&&!i||f&&r&&u||!o&&u||!c)return-1}return 0}function Wu(n,t,r){for(var e=-1,u=n.criteria,i=t.criteria,o=u.length,f=r.length;++e<o;){var c=Su(u[e],i[e]);if(c){if(e>=f)return c;return c*("desc"==r[e]?-1:1)}}return n.index-t.index}function Lu(n,t,r,e){for(var u=-1,i=n.length,o=r.length,f=-1,c=t.length,a=Kl(i-o,0),l=el(c+a),s=!e;++f<c;)l[f]=t[f];for(;++u<o;)(s||u<i)&&(l[r[u]]=n[u]);for(;a--;)l[f++]=n[u++];return l;
}function Cu(n,t,r,e){for(var u=-1,i=n.length,o=-1,f=r.length,c=-1,a=t.length,l=Kl(i-f,0),s=el(l+a),h=!e;++u<l;)s[u]=n[u];for(var p=u;++c<a;)s[p+c]=t[c];for(;++o<f;)(h||u<i)&&(s[p+r[o]]=n[u++]);return s}function Uu(n,t){var r=-1,e=n.length;for(t||(t=el(e));++r<e;)t[r]=n[r];return t}function Bu(n,t,r,e){var u=!r;r||(r={});for(var i=-1,o=t.length;++i<o;){var f=t[i],c=e?e(r[f],n[f],f,r,n):Y;c===Y&&(c=n[f]),u?Cr(r,f,c):zr(r,f,c)}return r}function Tu(n,t){return Bu(n,ks(n),t)}function $u(n,t){return Bu(n,Os(n),t);
}function Du(n,r){return function(e,u){var i=yh(e)?t:Sr,o=r?r():{};return i(e,n,bi(u,2),o)}}function Mu(n){return ru(function(t,r){var e=-1,u=r.length,i=u>1?r[u-1]:Y,o=u>2?r[2]:Y;for(i=n.length>3&&"function"==typeof i?(u--,i):Y,o&&Li(r[0],r[1],o)&&(i=u<3?Y:i,u=1),t=cl(t);++e<u;){var f=r[e];f&&n(t,f,e,i)}return t})}function Fu(n,t){return function(r,e){if(null==r)return r;if(!Vf(r))return n(r,e);for(var u=r.length,i=t?u:-1,o=cl(r);(t?i--:++i<u)&&e(o[i],i,o)!==!1;);return r}}function Nu(n){return function(t,r,e){
for(var u=-1,i=cl(t),o=e(t),f=o.length;f--;){var c=o[n?f:++u];if(r(i[c],c,i)===!1)break}return t}}function Pu(n,t,r){function e(){return(this&&this!==Xr&&this instanceof e?i:n).apply(u?r:this,arguments)}var u=t&sn,i=Ku(n);return e}function qu(n){return function(t){t=Rc(t);var r=B(t)?V(t):Y,e=r?r[0]:t.charAt(0),u=r?Au(r,1).join(""):t.slice(1);return e[n]()+u}}function Zu(n){return function(t){return l(Oa(oa(t).replace(Ur,"")),n,"")}}function Ku(n){return function(){var t=arguments;switch(t.length){
case 0:return new n;case 1:return new n(t[0]);case 2:return new n(t[0],t[1]);case 3:return new n(t[0],t[1],t[2]);case 4:return new n(t[0],t[1],t[2],t[3]);case 5:return new n(t[0],t[1],t[2],t[3],t[4]);case 6:return new n(t[0],t[1],t[2],t[3],t[4],t[5]);case 7:return new n(t[0],t[1],t[2],t[3],t[4],t[5],t[6])}var r=_s(n.prototype),e=n.apply(r,t);return ic(e)?e:r}}function Vu(t,r,e){function u(){for(var o=arguments.length,f=el(o),c=o,a=di(u);c--;)f[c]=arguments[c];var l=o<3&&f[0]!==a&&f[o-1]!==a?[]:F(f,a);
return o-=l.length,o<e?ui(t,r,Ju,u.placeholder,Y,f,l,Y,Y,e-o):n(this&&this!==Xr&&this instanceof u?i:t,this,f)}var i=Ku(t);return u}function Gu(n){return function(t,r,e){var u=cl(t);if(!Vf(t)){var i=bi(r,3);t=Fc(t),r=function(n){return i(u[n],n,u)}}var o=n(t,r,e);return o>-1?u[i?t[o]:o]:Y}}function Hu(n){return _i(function(t){var r=t.length,e=r,u=H.prototype.thru;for(n&&t.reverse();e--;){var i=t[e];if("function"!=typeof i)throw new sl(tn);if(u&&!o&&"wrapper"==yi(i))var o=new H([],!0)}for(e=o?e:r;++e<r;){
i=t[e];var f=yi(i),c="wrapper"==f?As(i):Y;o=c&&Bi(c[0])&&c[1]==(dn|_n|gn|bn)&&!c[4].length&&1==c[9]?o[yi(c[0])].apply(o,c[3]):1==i.length&&Bi(i)?o[f]():o.thru(i)}return function(){var n=arguments,e=n[0];if(o&&1==n.length&&yh(e))return o.plant(e).value();for(var u=0,i=r?t[u].apply(this,n):e;++u<r;)i=t[u].call(this,i);return i}})}function Ju(n,t,r,e,u,i,o,f,c,a){function l(){for(var y=arguments.length,d=el(y),b=y;b--;)d[b]=arguments[b];if(_)var w=di(l),m=L(d,w);if(e&&(d=Lu(d,e,u,_)),i&&(d=Cu(d,i,o,_)),
y-=m,_&&y<a){return ui(n,t,Ju,l.placeholder,r,d,F(d,w),f,c,a-y)}var x=h?r:this,j=p?x[n]:n;return y=d.length,f?d=Vi(d,f):v&&y>1&&d.reverse(),s&&c<y&&(d.length=c),this&&this!==Xr&&this instanceof l&&(j=g||Ku(j)),j.apply(x,d)}var s=t&dn,h=t&sn,p=t&hn,_=t&(_n|vn),v=t&wn,g=p?Y:Ku(n);return l}function Yu(n,t){return function(r,e){return Ae(r,n,t(e),{})}}function Qu(n,t){return function(r,e){var u;if(r===Y&&e===Y)return t;if(r!==Y&&(u=r),e!==Y){if(u===Y)return e;"string"==typeof r||"string"==typeof e?(r=pu(r),
e=pu(e)):(r=hu(r),e=hu(e)),u=n(r,e)}return u}}function Xu(t){return _i(function(r){return r=c(r,R(bi())),ru(function(e){var u=this;return t(r,function(t){return n(t,u,e)})})})}function ni(n,t){t=t===Y?" ":pu(t);var r=t.length;if(r<2)return r?tu(t,n):t;var e=tu(t,Dl(n/K(t)));return B(t)?Au(V(e),0,n).join(""):e.slice(0,n)}function ti(t,r,e,u){function i(){for(var r=-1,c=arguments.length,a=-1,l=u.length,s=el(l+c),h=this&&this!==Xr&&this instanceof i?f:t;++a<l;)s[a]=u[a];for(;c--;)s[a++]=arguments[++r];
return n(h,o?e:this,s)}var o=r&sn,f=Ku(t);return i}function ri(n){return function(t,r,e){return e&&"number"!=typeof e&&Li(t,r,e)&&(r=e=Y),t=xc(t),r===Y?(r=t,t=0):r=xc(r),e=e===Y?t<r?1:-1:xc(e),nu(t,r,e,n)}}function ei(n){return function(t,r){return"string"==typeof t&&"string"==typeof r||(t=kc(t),r=kc(r)),n(t,r)}}function ui(n,t,r,e,u,i,o,f,c,a){var l=t&_n,s=l?o:Y,h=l?Y:o,p=l?i:Y,_=l?Y:i;t|=l?gn:yn,t&=~(l?yn:gn),t&pn||(t&=~(sn|hn));var v=[n,t,u,p,s,_,h,f,c,a],g=r.apply(Y,v);return Bi(n)&&zs(g,v),g.placeholder=e,
Hi(g,n,t)}function ii(n){var t=fl[n];return function(n,r){if(n=kc(n),r=null==r?0:Vl(jc(r),292),r&&Pl(n)){var e=(Rc(n)+"e").split("e");return e=(Rc(t(e[0]+"e"+(+e[1]+r)))+"e").split("e"),+(e[0]+"e"+(+e[1]-r))}return t(n)}}function oi(n){return function(t){var r=Is(t);return r==Zn?D(t):r==Qn?P(t):I(t,n(t))}}function fi(n,t,r,e,u,i,o,f){var c=t&hn;if(!c&&"function"!=typeof n)throw new sl(tn);var a=e?e.length:0;if(a||(t&=~(gn|yn),e=u=Y),o=o===Y?o:Kl(jc(o),0),f=f===Y?f:jc(f),a-=u?u.length:0,t&yn){var l=e,s=u;
e=u=Y}var h=c?Y:As(n),p=[n,t,r,e,u,l,s,i,o,f];if(h&&Ni(p,h),n=p[0],t=p[1],r=p[2],e=p[3],u=p[4],f=p[9]=p[9]===Y?c?0:n.length:Kl(p[9]-a,0),!f&&t&(_n|vn)&&(t&=~(_n|vn)),t&&t!=sn)_=t==_n||t==vn?Vu(n,t,f):t!=gn&&t!=(sn|gn)||u.length?Ju.apply(Y,p):ti(n,t,r,e);else var _=Pu(n,t,r);return Hi((h?bs:zs)(_,p),n,t)}function ci(n,t,r,e){return n===Y||Kf(n,_l[r])&&!yl.call(e,r)?t:n}function ai(n,t,r,e,u,i){return ic(n)&&ic(t)&&(i.set(t,n),qe(n,t,Y,ai,i),i.delete(t)),n}function li(n){return _c(n)?Y:n}function si(n,t,r,e,u,i){
var o=r&an,f=n.length,c=t.length;if(f!=c&&!(o&&c>f))return!1;var a=i.get(n),l=i.get(t);if(a&&l)return a==t&&l==n;var s=-1,p=!0,_=r&ln?new vr:Y;for(i.set(n,t),i.set(t,n);++s<f;){var v=n[s],g=t[s];if(e)var y=o?e(g,v,s,t,n,i):e(v,g,s,n,t,i);if(y!==Y){if(y)continue;p=!1;break}if(_){if(!h(t,function(n,t){if(!E(_,t)&&(v===n||u(v,n,r,e,i)))return _.push(t)})){p=!1;break}}else if(v!==g&&!u(v,g,r,e,i)){p=!1;break}}return i.delete(n),i.delete(t),p}function hi(n,t,r,e,u,i,o){switch(r){case it:if(n.byteLength!=t.byteLength||n.byteOffset!=t.byteOffset)return!1;
n=n.buffer,t=t.buffer;case ut:return!(n.byteLength!=t.byteLength||!i(new Ol(n),new Ol(t)));case Dn:case Mn:case Kn:return Kf(+n,+t);case Nn:return n.name==t.name&&n.message==t.message;case Yn:case Xn:return n==t+"";case Zn:var f=D;case Qn:var c=e&an;if(f||(f=N),n.size!=t.size&&!c)return!1;var a=o.get(n);if(a)return a==t;e|=ln,o.set(n,t);var l=si(f(n),f(t),e,u,i,o);return o.delete(n),l;case nt:if(hs)return hs.call(n)==hs.call(t)}return!1}function pi(n,t,r,e,u,i){var o=r&an,f=vi(n),c=f.length;if(c!=vi(t).length&&!o)return!1;
for(var a=c;a--;){var l=f[a];if(!(o?l in t:yl.call(t,l)))return!1}var s=i.get(n),h=i.get(t);if(s&&h)return s==t&&h==n;var p=!0;i.set(n,t),i.set(t,n);for(var _=o;++a<c;){l=f[a];var v=n[l],g=t[l];if(e)var y=o?e(g,v,l,t,n,i):e(v,g,l,n,t,i);if(!(y===Y?v===g||u(v,g,r,e,i):y)){p=!1;break}_||(_="constructor"==l)}if(p&&!_){var d=n.constructor,b=t.constructor;d!=b&&"constructor"in n&&"constructor"in t&&!("function"==typeof d&&d instanceof d&&"function"==typeof b&&b instanceof b)&&(p=!1)}return i.delete(n),
i.delete(t),p}function _i(n){return Ss(Zi(n,Y,ho),n+"")}function vi(n){return ye(n,Fc,ks)}function gi(n){return ye(n,Nc,Os)}function yi(n){for(var t=n.name+"",r=is[t],e=yl.call(is,t)?r.length:0;e--;){var u=r[e],i=u.func;if(null==i||i==n)return u.name}return t}function di(n){return(yl.call(q,"placeholder")?q:n).placeholder}function bi(){var n=q.iteratee||Wa;return n=n===Wa?Te:n,arguments.length?n(arguments[0],arguments[1]):n}function wi(n,t){var r=n.__data__;return Ui(t)?r["string"==typeof t?"string":"hash"]:r.map;
}function mi(n){for(var t=Fc(n),r=t.length;r--;){var e=t[r],u=n[e];t[r]=[e,u,Di(u)]}return t}function xi(n,t){var r=U(n,t);return Le(r)?r:Y}function ji(n){var t=yl.call(n,Cl),r=n[Cl];try{n[Cl]=Y;var e=!0}catch(n){}var u=wl.call(n);return e&&(t?n[Cl]=r:delete n[Cl]),u}function Ai(n,t,r){for(var e=-1,u=r.length;++e<u;){var i=r[e],o=i.size;switch(i.type){case"drop":n+=o;break;case"dropRight":t-=o;break;case"take":t=Vl(t,n+o);break;case"takeRight":n=Kl(n,t-o)}}return{start:n,end:t}}function ki(n){var t=n.match(Ct);
return t?t[1].split(Ut):[]}function Oi(n,t,r){t=ju(t,n);for(var e=-1,u=t.length,i=!1;++e<u;){var o=Qi(t[e]);if(!(i=null!=n&&r(n,o)))break;n=n[o]}return i||++e!=u?i:(u=null==n?0:n.length,!!u&&uc(u)&&Wi(o,u)&&(yh(n)||gh(n)))}function Ii(n){var t=n.length,r=new n.constructor(t);return t&&"string"==typeof n[0]&&yl.call(n,"index")&&(r.index=n.index,r.input=n.input),r}function Ri(n){return"function"!=typeof n.constructor||$i(n)?{}:_s(Rl(n))}function zi(n,t,r){var e=n.constructor;switch(t){case ut:return Ou(n);
case Dn:case Mn:return new e(+n);case it:return Iu(n,r);case ot:case ft:case ct:case at:case lt:case st:case ht:case pt:case _t:return Eu(n,r);case Zn:return new e;case Kn:case Xn:return new e(n);case Yn:return Ru(n);case Qn:return new e;case nt:return zu(n)}}function Ei(n,t){var r=t.length;if(!r)return n;var e=r-1;return t[e]=(r>1?"& ":"")+t[e],t=t.join(r>2?", ":" "),n.replace(Lt,"{\n/* [wrapped with "+t+"] */\n")}function Si(n){return yh(n)||gh(n)||!!(Wl&&n&&n[Wl])}function Wi(n,t){var r=typeof n;
return t=null==t?zn:t,!!t&&("number"==r||"symbol"!=r&&qt.test(n))&&n>-1&&n%1==0&&n<t}function Li(n,t,r){if(!ic(r))return!1;var e=typeof t;return!!("number"==e?Vf(r)&&Wi(t,r.length):"string"==e&&t in r)&&Kf(r[t],n)}function Ci(n,t){if(yh(n))return!1;var r=typeof n;return!("number"!=r&&"symbol"!=r&&"boolean"!=r&&null!=n&&!yc(n))||(Ot.test(n)||!kt.test(n)||null!=t&&n in cl(t))}function Ui(n){var t=typeof n;return"string"==t||"number"==t||"symbol"==t||"boolean"==t?"__proto__"!==n:null===n}function Bi(n){
var t=yi(n),r=q[t];if("function"!=typeof r||!(t in Bt.prototype))return!1;if(n===r)return!0;var e=As(r);return!!e&&n===e[0]}function Ti(n){return!!bl&&bl in n}function $i(n){var t=n&&n.constructor;return n===("function"==typeof t&&t.prototype||_l)}function Di(n){return n===n&&!ic(n)}function Mi(n,t){return function(r){return null!=r&&(r[n]===t&&(t!==Y||n in cl(r)))}}function Fi(n){var t=Wf(n,function(n){return r.size===en&&r.clear(),n}),r=t.cache;return t}function Ni(n,t){var r=n[1],e=t[1],u=r|e,i=u<(sn|hn|dn),o=e==dn&&r==_n||e==dn&&r==bn&&n[7].length<=t[8]||e==(dn|bn)&&t[7].length<=t[8]&&r==_n;
if(!i&&!o)return n;e&sn&&(n[2]=t[2],u|=r&sn?0:pn);var f=t[3];if(f){var c=n[3];n[3]=c?Lu(c,f,t[4]):f,n[4]=c?F(n[3],un):t[4]}return f=t[5],f&&(c=n[5],n[5]=c?Cu(c,f,t[6]):f,n[6]=c?F(n[5],un):t[6]),f=t[7],f&&(n[7]=f),e&dn&&(n[8]=null==n[8]?t[8]:Vl(n[8],t[8])),null==n[9]&&(n[9]=t[9]),n[0]=t[0],n[1]=u,n}function Pi(n){var t=[];if(null!=n)for(var r in cl(n))t.push(r);return t}function qi(n){return wl.call(n)}function Zi(t,r,e){return r=Kl(r===Y?t.length-1:r,0),function(){for(var u=arguments,i=-1,o=Kl(u.length-r,0),f=el(o);++i<o;)f[i]=u[r+i];
i=-1;for(var c=el(r+1);++i<r;)c[i]=u[i];return c[r]=e(f),n(t,this,c)}}function Ki(n,t){return t.length<2?n:ve(n,fu(t,0,-1))}function Vi(n,t){for(var r=n.length,e=Vl(t.length,r),u=Uu(n);e--;){var i=t[e];n[e]=Wi(i,r)?u[i]:Y}return n}function Gi(n,t){if(("constructor"!==t||"function"!=typeof n[t])&&"__proto__"!=t)return n[t]}function Hi(n,t,r){var e=t+"";return Ss(n,Ei(e,no(ki(e),r)))}function Ji(n){var t=0,r=0;return function(){var e=Gl(),u=An-(e-r);if(r=e,u>0){if(++t>=jn)return arguments[0]}else t=0;
return n.apply(Y,arguments)}}function Yi(n,t){var r=-1,e=n.length,u=e-1;for(t=t===Y?e:t;++r<t;){var i=Xe(r,u),o=n[i];n[i]=n[r],n[r]=o}return n.length=t,n}function Qi(n){if("string"==typeof n||yc(n))return n;var t=n+"";return"0"==t&&1/n==-Rn?"-0":t}function Xi(n){if(null!=n){try{return gl.call(n)}catch(n){}try{return n+""}catch(n){}}return""}function no(n,t){return r(Un,function(r){var e="_."+r[0];t&r[1]&&!o(n,e)&&n.push(e)}),n.sort()}function to(n){if(n instanceof Bt)return n.clone();var t=new H(n.__wrapped__,n.__chain__);
return t.__actions__=Uu(n.__actions__),t.__index__=n.__index__,t.__values__=n.__values__,t}function ro(n,t,r){t=(r?Li(n,t,r):t===Y)?1:Kl(jc(t),0);var e=null==n?0:n.length;if(!e||t<1)return[];for(var u=0,i=0,o=el(Dl(e/t));u<e;)o[i++]=fu(n,u,u+=t);return o}function eo(n){for(var t=-1,r=null==n?0:n.length,e=0,u=[];++t<r;){var i=n[t];i&&(u[e++]=i)}return u}function uo(){var n=arguments.length;if(!n)return[];for(var t=el(n-1),r=arguments[0],e=n;e--;)t[e-1]=arguments[e];return a(yh(r)?Uu(r):[r],te(t,1));
}function io(n,t,r){var e=null==n?0:n.length;return e?(t=r||t===Y?1:jc(t),fu(n,t<0?0:t,e)):[]}function oo(n,t,r){var e=null==n?0:n.length;return e?(t=r||t===Y?1:jc(t),t=e-t,fu(n,0,t<0?0:t)):[]}function fo(n,t){return n&&n.length?yu(n,bi(t,3),!0,!0):[]}function co(n,t){return n&&n.length?yu(n,bi(t,3),!0):[]}function ao(n,t,r,e){var u=null==n?0:n.length;return u?(r&&"number"!=typeof r&&Li(n,t,r)&&(r=0,e=u),Qr(n,t,r,e)):[]}function lo(n,t,r){var e=null==n?0:n.length;if(!e)return-1;var u=null==r?0:jc(r);
return u<0&&(u=Kl(e+u,0)),g(n,bi(t,3),u)}function so(n,t,r){var e=null==n?0:n.length;if(!e)return-1;var u=e-1;return r!==Y&&(u=jc(r),u=r<0?Kl(e+u,0):Vl(u,e-1)),g(n,bi(t,3),u,!0)}function ho(n){return(null==n?0:n.length)?te(n,1):[]}function po(n){return(null==n?0:n.length)?te(n,Rn):[]}function _o(n,t){return(null==n?0:n.length)?(t=t===Y?1:jc(t),te(n,t)):[]}function vo(n){for(var t=-1,r=null==n?0:n.length,e={};++t<r;){var u=n[t];e[u[0]]=u[1]}return e}function go(n){return n&&n.length?n[0]:Y}function yo(n,t,r){
var e=null==n?0:n.length;if(!e)return-1;var u=null==r?0:jc(r);return u<0&&(u=Kl(e+u,0)),y(n,t,u)}function bo(n){return(null==n?0:n.length)?fu(n,0,-1):[]}function wo(n,t){return null==n?"":ql.call(n,t)}function mo(n){var t=null==n?0:n.length;return t?n[t-1]:Y}function xo(n,t,r){var e=null==n?0:n.length;if(!e)return-1;var u=e;return r!==Y&&(u=jc(r),u=u<0?Kl(e+u,0):Vl(u,e-1)),t===t?Z(n,t,u):g(n,b,u,!0)}function jo(n,t){return n&&n.length?Ke(n,jc(t)):Y}function Ao(n,t){return n&&n.length&&t&&t.length?Ye(n,t):n;
}function ko(n,t,r){return n&&n.length&&t&&t.length?Ye(n,t,bi(r,2)):n}function Oo(n,t,r){return n&&n.length&&t&&t.length?Ye(n,t,Y,r):n}function Io(n,t){var r=[];if(!n||!n.length)return r;var e=-1,u=[],i=n.length;for(t=bi(t,3);++e<i;){var o=n[e];t(o,e,n)&&(r.push(o),u.push(e))}return Qe(n,u),r}function Ro(n){return null==n?n:Yl.call(n)}function zo(n,t,r){var e=null==n?0:n.length;return e?(r&&"number"!=typeof r&&Li(n,t,r)?(t=0,r=e):(t=null==t?0:jc(t),r=r===Y?e:jc(r)),fu(n,t,r)):[]}function Eo(n,t){
return au(n,t)}function So(n,t,r){return lu(n,t,bi(r,2))}function Wo(n,t){var r=null==n?0:n.length;if(r){var e=au(n,t);if(e<r&&Kf(n[e],t))return e}return-1}function Lo(n,t){return au(n,t,!0)}function Co(n,t,r){return lu(n,t,bi(r,2),!0)}function Uo(n,t){if(null==n?0:n.length){var r=au(n,t,!0)-1;if(Kf(n[r],t))return r}return-1}function Bo(n){return n&&n.length?su(n):[]}function To(n,t){return n&&n.length?su(n,bi(t,2)):[]}function $o(n){var t=null==n?0:n.length;return t?fu(n,1,t):[]}function Do(n,t,r){
return n&&n.length?(t=r||t===Y?1:jc(t),fu(n,0,t<0?0:t)):[]}function Mo(n,t,r){var e=null==n?0:n.length;return e?(t=r||t===Y?1:jc(t),t=e-t,fu(n,t<0?0:t,e)):[]}function Fo(n,t){return n&&n.length?yu(n,bi(t,3),!1,!0):[]}function No(n,t){return n&&n.length?yu(n,bi(t,3)):[]}function Po(n){return n&&n.length?_u(n):[]}function qo(n,t){return n&&n.length?_u(n,bi(t,2)):[]}function Zo(n,t){return t="function"==typeof t?t:Y,n&&n.length?_u(n,Y,t):[]}function Ko(n){if(!n||!n.length)return[];var t=0;return n=i(n,function(n){
if(Gf(n))return t=Kl(n.length,t),!0}),O(t,function(t){return c(n,m(t))})}function Vo(t,r){if(!t||!t.length)return[];var e=Ko(t);return null==r?e:c(e,function(t){return n(r,Y,t)})}function Go(n,t){return wu(n||[],t||[],zr)}function Ho(n,t){return wu(n||[],t||[],iu)}function Jo(n){var t=q(n);return t.__chain__=!0,t}function Yo(n,t){return t(n),n}function Qo(n,t){return t(n)}function Xo(){return Jo(this)}function nf(){return new H(this.value(),this.__chain__)}function tf(){this.__values__===Y&&(this.__values__=mc(this.value()));
var n=this.__index__>=this.__values__.length;return{done:n,value:n?Y:this.__values__[this.__index__++]}}function rf(){return this}function ef(n){for(var t,r=this;r instanceof G;){var e=to(r);e.__index__=0,e.__values__=Y,t?u.__wrapped__=e:t=e;var u=e;r=r.__wrapped__}return u.__wrapped__=n,t}function uf(){var n=this.__wrapped__;if(n instanceof Bt){var t=n;return this.__actions__.length&&(t=new Bt(this)),t=t.reverse(),t.__actions__.push({func:Qo,args:[Ro],thisArg:Y}),new H(t,this.__chain__)}return this.thru(Ro);
}function of(){return du(this.__wrapped__,this.__actions__)}function ff(n,t,r){var e=yh(n)?u:Gr;return r&&Li(n,t,r)&&(t=Y),e(n,bi(t,3))}function cf(n,t){return(yh(n)?i:ne)(n,bi(t,3))}function af(n,t){return te(vf(n,t),1)}function lf(n,t){return te(vf(n,t),Rn)}function sf(n,t,r){return r=r===Y?1:jc(r),te(vf(n,t),r)}function hf(n,t){return(yh(n)?r:vs)(n,bi(t,3))}function pf(n,t){return(yh(n)?e:gs)(n,bi(t,3))}function _f(n,t,r,e){n=Vf(n)?n:na(n),r=r&&!e?jc(r):0;var u=n.length;return r<0&&(r=Kl(u+r,0)),
gc(n)?r<=u&&n.indexOf(t,r)>-1:!!u&&y(n,t,r)>-1}function vf(n,t){return(yh(n)?c:Fe)(n,bi(t,3))}function gf(n,t,r,e){return null==n?[]:(yh(t)||(t=null==t?[]:[t]),r=e?Y:r,yh(r)||(r=null==r?[]:[r]),Ve(n,t,r))}function yf(n,t,r){var e=yh(n)?l:j,u=arguments.length<3;return e(n,bi(t,4),r,u,vs)}function df(n,t,r){var e=yh(n)?s:j,u=arguments.length<3;return e(n,bi(t,4),r,u,gs)}function bf(n,t){return(yh(n)?i:ne)(n,Lf(bi(t,3)))}function wf(n){return(yh(n)?kr:eu)(n)}function mf(n,t,r){return t=(r?Li(n,t,r):t===Y)?1:jc(t),
(yh(n)?Or:uu)(n,t)}function xf(n){return(yh(n)?Ir:ou)(n)}function jf(n){if(null==n)return 0;if(Vf(n))return gc(n)?K(n):n.length;var t=Is(n);return t==Zn||t==Qn?n.size:$e(n).length}function Af(n,t,r){var e=yh(n)?h:cu;return r&&Li(n,t,r)&&(t=Y),e(n,bi(t,3))}function kf(n,t){if("function"!=typeof t)throw new sl(tn);return n=jc(n),function(){if(--n<1)return t.apply(this,arguments)}}function Of(n,t,r){return t=r?Y:t,t=n&&null==t?n.length:t,fi(n,dn,Y,Y,Y,Y,t)}function If(n,t){var r;if("function"!=typeof t)throw new sl(tn);
return n=jc(n),function(){return--n>0&&(r=t.apply(this,arguments)),n<=1&&(t=Y),r}}function Rf(n,t,r){t=r?Y:t;var e=fi(n,_n,Y,Y,Y,Y,Y,t);return e.placeholder=Rf.placeholder,e}function zf(n,t,r){t=r?Y:t;var e=fi(n,vn,Y,Y,Y,Y,Y,t);return e.placeholder=zf.placeholder,e}function Ef(n,t,r){function e(t){var r=h,e=p;return h=p=Y,d=t,v=n.apply(e,r)}function u(n){return d=n,g=Es(f,t),b?e(n):v}function i(n){var r=n-y,e=n-d,u=t-r;return w?Vl(u,_-e):u}function o(n){var r=n-y,e=n-d;return y===Y||r>=t||r<0||w&&e>=_;
}function f(){var n=ih();return o(n)?c(n):(g=Es(f,i(n)),Y)}function c(n){return g=Y,m&&h?e(n):(h=p=Y,v)}function a(){g!==Y&&xs(g),d=0,h=y=p=g=Y}function l(){return g===Y?v:c(ih())}function s(){var n=ih(),r=o(n);if(h=arguments,p=this,y=n,r){if(g===Y)return u(y);if(w)return xs(g),g=Es(f,t),e(y)}return g===Y&&(g=Es(f,t)),v}var h,p,_,v,g,y,d=0,b=!1,w=!1,m=!0;if("function"!=typeof n)throw new sl(tn);return t=kc(t)||0,ic(r)&&(b=!!r.leading,w="maxWait"in r,_=w?Kl(kc(r.maxWait)||0,t):_,m="trailing"in r?!!r.trailing:m),
s.cancel=a,s.flush=l,s}function Sf(n){return fi(n,wn)}function Wf(n,t){if("function"!=typeof n||null!=t&&"function"!=typeof t)throw new sl(tn);var r=function(){var e=arguments,u=t?t.apply(this,e):e[0],i=r.cache;if(i.has(u))return i.get(u);var o=n.apply(this,e);return r.cache=i.set(u,o)||i,o};return r.cache=new(Wf.Cache||ar),r}function Lf(n){if("function"!=typeof n)throw new sl(tn);return function(){var t=arguments;switch(t.length){case 0:return!n.call(this);case 1:return!n.call(this,t[0]);case 2:
return!n.call(this,t[0],t[1]);case 3:return!n.call(this,t[0],t[1],t[2])}return!n.apply(this,t)}}function Cf(n){return If(2,n)}function Uf(n,t){if("function"!=typeof n)throw new sl(tn);return t=t===Y?t:jc(t),ru(n,t)}function Bf(t,r){if("function"!=typeof t)throw new sl(tn);return r=null==r?0:Kl(jc(r),0),ru(function(e){var u=e[r],i=Au(e,0,r);return u&&a(i,u),n(t,this,i)})}function Tf(n,t,r){var e=!0,u=!0;if("function"!=typeof n)throw new sl(tn);return ic(r)&&(e="leading"in r?!!r.leading:e,u="trailing"in r?!!r.trailing:u),
Ef(n,t,{leading:e,maxWait:t,trailing:u})}function $f(n){return Of(n,1)}function Df(n,t){return sh(xu(t),n)}function Mf(){if(!arguments.length)return[];var n=arguments[0];return yh(n)?n:[n]}function Ff(n){return Dr(n,cn)}function Nf(n,t){return t="function"==typeof t?t:Y,Dr(n,cn,t)}function Pf(n){return Dr(n,on|cn)}function qf(n,t){return t="function"==typeof t?t:Y,Dr(n,on|cn,t)}function Zf(n,t){return null==t||Zr(n,t,Fc(t))}function Kf(n,t){return n===t||n!==n&&t!==t}function Vf(n){return null!=n&&uc(n.length)&&!rc(n);
}function Gf(n){return oc(n)&&Vf(n)}function Hf(n){return n===!0||n===!1||oc(n)&&de(n)==Dn}function Jf(n){return oc(n)&&1===n.nodeType&&!_c(n)}function Yf(n){if(null==n)return!0;if(Vf(n)&&(yh(n)||"string"==typeof n||"function"==typeof n.splice||bh(n)||Ah(n)||gh(n)))return!n.length;var t=Is(n);if(t==Zn||t==Qn)return!n.size;if($i(n))return!$e(n).length;for(var r in n)if(yl.call(n,r))return!1;return!0}function Qf(n,t){return ze(n,t)}function Xf(n,t,r){r="function"==typeof r?r:Y;var e=r?r(n,t):Y;return e===Y?ze(n,t,Y,r):!!e;
}function nc(n){if(!oc(n))return!1;var t=de(n);return t==Nn||t==Fn||"string"==typeof n.message&&"string"==typeof n.name&&!_c(n)}function tc(n){return"number"==typeof n&&Pl(n)}function rc(n){if(!ic(n))return!1;var t=de(n);return t==Pn||t==qn||t==$n||t==Jn}function ec(n){return"number"==typeof n&&n==jc(n)}function uc(n){return"number"==typeof n&&n>-1&&n%1==0&&n<=zn}function ic(n){var t=typeof n;return null!=n&&("object"==t||"function"==t)}function oc(n){return null!=n&&"object"==typeof n}function fc(n,t){
return n===t||We(n,t,mi(t))}function cc(n,t,r){return r="function"==typeof r?r:Y,We(n,t,mi(t),r)}function ac(n){return pc(n)&&n!=+n}function lc(n){if(Rs(n))throw new il(nn);return Le(n)}function sc(n){return null===n}function hc(n){return null==n}function pc(n){return"number"==typeof n||oc(n)&&de(n)==Kn}function _c(n){if(!oc(n)||de(n)!=Gn)return!1;var t=Rl(n);if(null===t)return!0;var r=yl.call(t,"constructor")&&t.constructor;return"function"==typeof r&&r instanceof r&&gl.call(r)==ml}function vc(n){
return ec(n)&&n>=-zn&&n<=zn}function gc(n){return"string"==typeof n||!yh(n)&&oc(n)&&de(n)==Xn}function yc(n){return"symbol"==typeof n||oc(n)&&de(n)==nt}function dc(n){return n===Y}function bc(n){return oc(n)&&Is(n)==rt}function wc(n){return oc(n)&&de(n)==et}function mc(n){if(!n)return[];if(Vf(n))return gc(n)?V(n):Uu(n);if(Ll&&n[Ll])return $(n[Ll]());var t=Is(n);return(t==Zn?D:t==Qn?N:na)(n)}function xc(n){if(!n)return 0===n?n:0;if(n=kc(n),n===Rn||n===-Rn){return(n<0?-1:1)*En}return n===n?n:0}function jc(n){
var t=xc(n),r=t%1;return t===t?r?t-r:t:0}function Ac(n){return n?$r(jc(n),0,Wn):0}function kc(n){if("number"==typeof n)return n;if(yc(n))return Sn;if(ic(n)){var t="function"==typeof n.valueOf?n.valueOf():n;n=ic(t)?t+"":t}if("string"!=typeof n)return 0===n?n:+n;n=n.replace(Et,"");var r=Ft.test(n);return r||Pt.test(n)?Jr(n.slice(2),r?2:8):Mt.test(n)?Sn:+n}function Oc(n){return Bu(n,Nc(n))}function Ic(n){return n?$r(jc(n),-zn,zn):0===n?n:0}function Rc(n){return null==n?"":pu(n)}function zc(n,t){var r=_s(n);
return null==t?r:Wr(r,t)}function Ec(n,t){return v(n,bi(t,3),ee)}function Sc(n,t){return v(n,bi(t,3),ue)}function Wc(n,t){return null==n?n:ys(n,bi(t,3),Nc)}function Lc(n,t){return null==n?n:ds(n,bi(t,3),Nc)}function Cc(n,t){return n&&ee(n,bi(t,3))}function Uc(n,t){return n&&ue(n,bi(t,3))}function Bc(n){return null==n?[]:se(n,Fc(n))}function Tc(n){return null==n?[]:se(n,Nc(n))}function $c(n,t,r){var e=null==n?Y:ve(n,t);return e===Y?r:e}function Dc(n,t){return null!=n&&Oi(n,t,we)}function Mc(n,t){return null!=n&&Oi(n,t,me);
}function Fc(n){return Vf(n)?Ar(n):$e(n)}function Nc(n){return Vf(n)?Ar(n,!0):De(n)}function Pc(n,t){var r={};return t=bi(t,3),ee(n,function(n,e,u){Cr(r,t(n,e,u),n)}),r}function qc(n,t){var r={};return t=bi(t,3),ee(n,function(n,e,u){Cr(r,e,t(n,e,u))}),r}function Zc(n,t){return Kc(n,Lf(bi(t)))}function Kc(n,t){if(null==n)return{};var r=c(gi(n),function(n){return[n]});return t=bi(t),He(n,r,function(n,r){return t(n,r[0])})}function Vc(n,t,r){t=ju(t,n);var e=-1,u=t.length;for(u||(u=1,n=Y);++e<u;){var i=null==n?Y:n[Qi(t[e])];
i===Y&&(e=u,i=r),n=rc(i)?i.call(n):i}return n}function Gc(n,t,r){return null==n?n:iu(n,t,r)}function Hc(n,t,r,e){return e="function"==typeof e?e:Y,null==n?n:iu(n,t,r,e)}function Jc(n,t,e){var u=yh(n),i=u||bh(n)||Ah(n);if(t=bi(t,4),null==e){var o=n&&n.constructor;e=i?u?new o:[]:ic(n)&&rc(o)?_s(Rl(n)):{}}return(i?r:ee)(n,function(n,r,u){return t(e,n,r,u)}),e}function Yc(n,t){return null==n||vu(n,t)}function Qc(n,t,r){return null==n?n:gu(n,t,xu(r))}function Xc(n,t,r,e){return e="function"==typeof e?e:Y,
null==n?n:gu(n,t,xu(r),e)}function na(n){return null==n?[]:z(n,Fc(n))}function ta(n){return null==n?[]:z(n,Nc(n))}function ra(n,t,r){return r===Y&&(r=t,t=Y),r!==Y&&(r=kc(r),r=r===r?r:0),t!==Y&&(t=kc(t),t=t===t?t:0),$r(kc(n),t,r)}function ea(n,t,r){return t=xc(t),r===Y?(r=t,t=0):r=xc(r),n=kc(n),xe(n,t,r)}function ua(n,t,r){if(r&&"boolean"!=typeof r&&Li(n,t,r)&&(t=r=Y),r===Y&&("boolean"==typeof t?(r=t,t=Y):"boolean"==typeof n&&(r=n,n=Y)),n===Y&&t===Y?(n=0,t=1):(n=xc(n),t===Y?(t=n,n=0):t=xc(t)),n>t){
var e=n;n=t,t=e}if(r||n%1||t%1){var u=Jl();return Vl(n+u*(t-n+Hr("1e-"+((u+"").length-1))),t)}return Xe(n,t)}function ia(n){return Jh(Rc(n).toLowerCase())}function oa(n){return n=Rc(n),n&&n.replace(Zt,he).replace(Br,"")}function fa(n,t,r){n=Rc(n),t=pu(t);var e=n.length;r=r===Y?e:$r(jc(r),0,e);var u=r;return r-=t.length,r>=0&&n.slice(r,u)==t}function ca(n){return n=Rc(n),n&&mt.test(n)?n.replace(bt,pe):n}function aa(n){return n=Rc(n),n&&zt.test(n)?n.replace(Rt,"\\$&"):n}function la(n,t,r){n=Rc(n),t=jc(t);
var e=t?K(n):0;if(!t||e>=t)return n;var u=(t-e)/2;return ni(Ml(u),r)+n+ni(Dl(u),r)}function sa(n,t,r){n=Rc(n),t=jc(t);var e=t?K(n):0;return t&&e<t?n+ni(t-e,r):n}function ha(n,t,r){n=Rc(n),t=jc(t);var e=t?K(n):0;return t&&e<t?ni(t-e,r)+n:n}function pa(n,t,r){return r||null==t?t=0:t&&(t=+t),Hl(Rc(n).replace(St,""),t||0)}function _a(n,t,r){return t=(r?Li(n,t,r):t===Y)?1:jc(t),tu(Rc(n),t)}function va(){var n=arguments,t=Rc(n[0]);return n.length<3?t:t.replace(n[1],n[2])}function ga(n,t,r){return r&&"number"!=typeof r&&Li(n,t,r)&&(t=r=Y),
(r=r===Y?Wn:r>>>0)?(n=Rc(n),n&&("string"==typeof t||null!=t&&!xh(t))&&(t=pu(t),!t&&B(n))?Au(V(n),0,r):n.split(t,r)):[]}function ya(n,t,r){return n=Rc(n),r=null==r?0:$r(jc(r),0,n.length),t=pu(t),n.slice(r,r+t.length)==t}function da(n,t,r){var e=q.templateSettings;r&&Li(n,t,r)&&(t=Y),n=Rc(n),t=zh({},t,e,ci);var u,i,o=zh({},t.imports,e.imports,ci),f=Fc(o),c=z(o,f),a=0,l=t.interpolate||Kt,s="__p += '",h=al((t.escape||Kt).source+"|"+l.source+"|"+(l===At?$t:Kt).source+"|"+(t.evaluate||Kt).source+"|$","g"),p="//# sourceURL="+(yl.call(t,"sourceURL")?(t.sourceURL+"").replace(/\s/g," "):"lodash.templateSources["+ ++Nr+"]")+"\n";
n.replace(h,function(t,r,e,o,f,c){return e||(e=o),s+=n.slice(a,c).replace(Vt,C),r&&(u=!0,s+="' +\n__e("+r+") +\n'"),f&&(i=!0,s+="';\n"+f+";\n__p += '"),e&&(s+="' +\n((__t = ("+e+")) == null ? '' : __t) +\n'"),a=c+t.length,t}),s+="';\n";var _=yl.call(t,"variable")&&t.variable;_||(s="with (obj) {\n"+s+"\n}\n"),s=(i?s.replace(vt,""):s).replace(gt,"$1").replace(yt,"$1;"),s="function("+(_||"obj")+") {\n"+(_?"":"obj || (obj = {});\n")+"var __t, __p = ''"+(u?", __e = _.escape":"")+(i?", __j = Array.prototype.join;\nfunction print() { __p += __j.call(arguments, '') }\n":";\n")+s+"return __p\n}";
var v=Yh(function(){return ol(f,p+"return "+s).apply(Y,c)});if(v.source=s,nc(v))throw v;return v}function ba(n){return Rc(n).toLowerCase()}function wa(n){return Rc(n).toUpperCase()}function ma(n,t,r){if(n=Rc(n),n&&(r||t===Y))return n.replace(Et,"");if(!n||!(t=pu(t)))return n;var e=V(n),u=V(t);return Au(e,S(e,u),W(e,u)+1).join("")}function xa(n,t,r){if(n=Rc(n),n&&(r||t===Y))return n.replace(Wt,"");if(!n||!(t=pu(t)))return n;var e=V(n);return Au(e,0,W(e,V(t))+1).join("")}function ja(n,t,r){if(n=Rc(n),
n&&(r||t===Y))return n.replace(St,"");if(!n||!(t=pu(t)))return n;var e=V(n);return Au(e,S(e,V(t))).join("")}function Aa(n,t){var r=mn,e=xn;if(ic(t)){var u="separator"in t?t.separator:u;r="length"in t?jc(t.length):r,e="omission"in t?pu(t.omission):e}n=Rc(n);var i=n.length;if(B(n)){var o=V(n);i=o.length}if(r>=i)return n;var f=r-K(e);if(f<1)return e;var c=o?Au(o,0,f).join(""):n.slice(0,f);if(u===Y)return c+e;if(o&&(f+=c.length-f),xh(u)){if(n.slice(f).search(u)){var a,l=c;for(u.global||(u=al(u.source,Rc(Dt.exec(u))+"g")),
u.lastIndex=0;a=u.exec(l);)var s=a.index;c=c.slice(0,s===Y?f:s)}}else if(n.indexOf(pu(u),f)!=f){var h=c.lastIndexOf(u);h>-1&&(c=c.slice(0,h))}return c+e}function ka(n){return n=Rc(n),n&&wt.test(n)?n.replace(dt,_e):n}function Oa(n,t,r){return n=Rc(n),t=r?Y:t,t===Y?T(n)?J(n):_(n):n.match(t)||[]}function Ia(t){var r=null==t?0:t.length,e=bi();return t=r?c(t,function(n){if("function"!=typeof n[1])throw new sl(tn);return[e(n[0]),n[1]]}):[],ru(function(e){for(var u=-1;++u<r;){var i=t[u];if(n(i[0],this,e))return n(i[1],this,e);
}})}function Ra(n){return Mr(Dr(n,on))}function za(n){return function(){return n}}function Ea(n,t){return null==n||n!==n?t:n}function Sa(n){return n}function Wa(n){return Te("function"==typeof n?n:Dr(n,on))}function La(n){return Ne(Dr(n,on))}function Ca(n,t){return Pe(n,Dr(t,on))}function Ua(n,t,e){var u=Fc(t),i=se(t,u);null!=e||ic(t)&&(i.length||!u.length)||(e=t,t=n,n=this,i=se(t,Fc(t)));var o=!(ic(e)&&"chain"in e&&!e.chain),f=rc(n);return r(i,function(r){var e=t[r];n[r]=e,f&&(n.prototype[r]=function(){
var t=this.__chain__;if(o||t){var r=n(this.__wrapped__);return(r.__actions__=Uu(this.__actions__)).push({func:e,args:arguments,thisArg:n}),r.__chain__=t,r}return e.apply(n,a([this.value()],arguments))})}),n}function Ba(){return Xr._===this&&(Xr._=xl),this}function Ta(){}function $a(n){return n=jc(n),ru(function(t){return Ke(t,n)})}function Da(n){return Ci(n)?m(Qi(n)):Je(n)}function Ma(n){return function(t){return null==n?Y:ve(n,t)}}function Fa(){return[]}function Na(){return!1}function Pa(){return{};
}function qa(){return""}function Za(){return!0}function Ka(n,t){if(n=jc(n),n<1||n>zn)return[];var r=Wn,e=Vl(n,Wn);t=bi(t),n-=Wn;for(var u=O(e,t);++r<n;)t(r);return u}function Va(n){return yh(n)?c(n,Qi):yc(n)?[n]:Uu(Ws(Rc(n)))}function Ga(n){var t=++dl;return Rc(n)+t}function Ha(n){return n&&n.length?Yr(n,Sa,be):Y}function Ja(n,t){return n&&n.length?Yr(n,bi(t,2),be):Y}function Ya(n){return w(n,Sa)}function Qa(n,t){return w(n,bi(t,2))}function Xa(n){return n&&n.length?Yr(n,Sa,Me):Y}function nl(n,t){
return n&&n.length?Yr(n,bi(t,2),Me):Y}function tl(n){return n&&n.length?k(n,Sa):0}function rl(n,t){return n&&n.length?k(n,bi(t,2)):0}x=null==x?Xr:ge.defaults(Xr.Object(),x,ge.pick(Xr,Fr));var el=x.Array,ul=x.Date,il=x.Error,ol=x.Function,fl=x.Math,cl=x.Object,al=x.RegExp,ll=x.String,sl=x.TypeError,hl=el.prototype,pl=ol.prototype,_l=cl.prototype,vl=x["__core-js_shared__"],gl=pl.toString,yl=_l.hasOwnProperty,dl=0,bl=function(){var n=/[^.]+$/.exec(vl&&vl.keys&&vl.keys.IE_PROTO||"");return n?"Symbol(src)_1."+n:"";
}(),wl=_l.toString,ml=gl.call(cl),xl=Xr._,jl=al("^"+gl.call(yl).replace(Rt,"\\$&").replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g,"$1.*?")+"$"),Al=re?x.Buffer:Y,kl=x.Symbol,Ol=x.Uint8Array,Il=Al?Al.allocUnsafe:Y,Rl=M(cl.getPrototypeOf,cl),zl=cl.create,El=_l.propertyIsEnumerable,Sl=hl.splice,Wl=kl?kl.isConcatSpreadable:Y,Ll=kl?kl.iterator:Y,Cl=kl?kl.toStringTag:Y,Ul=function(){try{var n=xi(cl,"defineProperty");return n({},"",{}),n}catch(n){}}(),Bl=x.clearTimeout!==Xr.clearTimeout&&x.clearTimeout,Tl=ul&&ul.now!==Xr.Date.now&&ul.now,$l=x.setTimeout!==Xr.setTimeout&&x.setTimeout,Dl=fl.ceil,Ml=fl.floor,Fl=cl.getOwnPropertySymbols,Nl=Al?Al.isBuffer:Y,Pl=x.isFinite,ql=hl.join,Zl=M(cl.keys,cl),Kl=fl.max,Vl=fl.min,Gl=ul.now,Hl=x.parseInt,Jl=fl.random,Yl=hl.reverse,Ql=xi(x,"DataView"),Xl=xi(x,"Map"),ns=xi(x,"Promise"),ts=xi(x,"Set"),rs=xi(x,"WeakMap"),es=xi(cl,"create"),us=rs&&new rs,is={},os=Xi(Ql),fs=Xi(Xl),cs=Xi(ns),as=Xi(ts),ls=Xi(rs),ss=kl?kl.prototype:Y,hs=ss?ss.valueOf:Y,ps=ss?ss.toString:Y,_s=function(){
function n(){}return function(t){if(!ic(t))return{};if(zl)return zl(t);n.prototype=t;var r=new n;return n.prototype=Y,r}}();q.templateSettings={escape:xt,evaluate:jt,interpolate:At,variable:"",imports:{_:q}},q.prototype=G.prototype,q.prototype.constructor=q,H.prototype=_s(G.prototype),H.prototype.constructor=H,Bt.prototype=_s(G.prototype),Bt.prototype.constructor=Bt,Yt.prototype.clear=Qt,Yt.prototype.delete=Xt,Yt.prototype.get=nr,Yt.prototype.has=tr,Yt.prototype.set=rr,er.prototype.clear=ur,er.prototype.delete=ir,
er.prototype.get=or,er.prototype.has=fr,er.prototype.set=cr,ar.prototype.clear=lr,ar.prototype.delete=sr,ar.prototype.get=hr,ar.prototype.has=pr,ar.prototype.set=_r,vr.prototype.add=vr.prototype.push=gr,vr.prototype.has=yr,dr.prototype.clear=br,dr.prototype.delete=wr,dr.prototype.get=mr,dr.prototype.has=xr,dr.prototype.set=jr;var vs=Fu(ee),gs=Fu(ue,!0),ys=Nu(),ds=Nu(!0),bs=us?function(n,t){return us.set(n,t),n}:Sa,ws=Ul?function(n,t){return Ul(n,"toString",{configurable:!0,enumerable:!1,value:za(t),
writable:!0})}:Sa,ms=ru,xs=Bl||function(n){return Xr.clearTimeout(n)},js=ts&&1/N(new ts([,-0]))[1]==Rn?function(n){return new ts(n)}:Ta,As=us?function(n){return us.get(n)}:Ta,ks=Fl?function(n){return null==n?[]:(n=cl(n),i(Fl(n),function(t){return El.call(n,t)}))}:Fa,Os=Fl?function(n){for(var t=[];n;)a(t,ks(n)),n=Rl(n);return t}:Fa,Is=de;(Ql&&Is(new Ql(new ArrayBuffer(1)))!=it||Xl&&Is(new Xl)!=Zn||ns&&Is(ns.resolve())!=Hn||ts&&Is(new ts)!=Qn||rs&&Is(new rs)!=rt)&&(Is=function(n){var t=de(n),r=t==Gn?n.constructor:Y,e=r?Xi(r):"";
if(e)switch(e){case os:return it;case fs:return Zn;case cs:return Hn;case as:return Qn;case ls:return rt}return t});var Rs=vl?rc:Na,zs=Ji(bs),Es=$l||function(n,t){return Xr.setTimeout(n,t)},Ss=Ji(ws),Ws=Fi(function(n){var t=[];return 46===n.charCodeAt(0)&&t.push(""),n.replace(It,function(n,r,e,u){t.push(e?u.replace(Tt,"$1"):r||n)}),t}),Ls=ru(function(n,t){return Gf(n)?Vr(n,te(t,1,Gf,!0)):[]}),Cs=ru(function(n,t){var r=mo(t);return Gf(r)&&(r=Y),Gf(n)?Vr(n,te(t,1,Gf,!0),bi(r,2)):[]}),Us=ru(function(n,t){
var r=mo(t);return Gf(r)&&(r=Y),Gf(n)?Vr(n,te(t,1,Gf,!0),Y,r):[]}),Bs=ru(function(n){var t=c(n,mu);return t.length&&t[0]===n[0]?je(t):[]}),Ts=ru(function(n){var t=mo(n),r=c(n,mu);return t===mo(r)?t=Y:r.pop(),r.length&&r[0]===n[0]?je(r,bi(t,2)):[]}),$s=ru(function(n){var t=mo(n),r=c(n,mu);return t="function"==typeof t?t:Y,t&&r.pop(),r.length&&r[0]===n[0]?je(r,Y,t):[]}),Ds=ru(Ao),Ms=_i(function(n,t){var r=null==n?0:n.length,e=Tr(n,t);return Qe(n,c(t,function(n){return Wi(n,r)?+n:n}).sort(Su)),e}),Fs=ru(function(n){
return _u(te(n,1,Gf,!0))}),Ns=ru(function(n){var t=mo(n);return Gf(t)&&(t=Y),_u(te(n,1,Gf,!0),bi(t,2))}),Ps=ru(function(n){var t=mo(n);return t="function"==typeof t?t:Y,_u(te(n,1,Gf,!0),Y,t)}),qs=ru(function(n,t){return Gf(n)?Vr(n,t):[]}),Zs=ru(function(n){return bu(i(n,Gf))}),Ks=ru(function(n){var t=mo(n);return Gf(t)&&(t=Y),bu(i(n,Gf),bi(t,2))}),Vs=ru(function(n){var t=mo(n);return t="function"==typeof t?t:Y,bu(i(n,Gf),Y,t)}),Gs=ru(Ko),Hs=ru(function(n){var t=n.length,r=t>1?n[t-1]:Y;return r="function"==typeof r?(n.pop(),
r):Y,Vo(n,r)}),Js=_i(function(n){var t=n.length,r=t?n[0]:0,e=this.__wrapped__,u=function(t){return Tr(t,n)};return!(t>1||this.__actions__.length)&&e instanceof Bt&&Wi(r)?(e=e.slice(r,+r+(t?1:0)),e.__actions__.push({func:Qo,args:[u],thisArg:Y}),new H(e,this.__chain__).thru(function(n){return t&&!n.length&&n.push(Y),n})):this.thru(u)}),Ys=Du(function(n,t,r){yl.call(n,r)?++n[r]:Cr(n,r,1)}),Qs=Gu(lo),Xs=Gu(so),nh=Du(function(n,t,r){yl.call(n,r)?n[r].push(t):Cr(n,r,[t])}),th=ru(function(t,r,e){var u=-1,i="function"==typeof r,o=Vf(t)?el(t.length):[];
return vs(t,function(t){o[++u]=i?n(r,t,e):ke(t,r,e)}),o}),rh=Du(function(n,t,r){Cr(n,r,t)}),eh=Du(function(n,t,r){n[r?0:1].push(t)},function(){return[[],[]]}),uh=ru(function(n,t){if(null==n)return[];var r=t.length;return r>1&&Li(n,t[0],t[1])?t=[]:r>2&&Li(t[0],t[1],t[2])&&(t=[t[0]]),Ve(n,te(t,1),[])}),ih=Tl||function(){return Xr.Date.now()},oh=ru(function(n,t,r){var e=sn;if(r.length){var u=F(r,di(oh));e|=gn}return fi(n,e,t,r,u)}),fh=ru(function(n,t,r){var e=sn|hn;if(r.length){var u=F(r,di(fh));e|=gn;
}return fi(t,e,n,r,u)}),ch=ru(function(n,t){return Kr(n,1,t)}),ah=ru(function(n,t,r){return Kr(n,kc(t)||0,r)});Wf.Cache=ar;var lh=ms(function(t,r){r=1==r.length&&yh(r[0])?c(r[0],R(bi())):c(te(r,1),R(bi()));var e=r.length;return ru(function(u){for(var i=-1,o=Vl(u.length,e);++i<o;)u[i]=r[i].call(this,u[i]);return n(t,this,u)})}),sh=ru(function(n,t){return fi(n,gn,Y,t,F(t,di(sh)))}),hh=ru(function(n,t){return fi(n,yn,Y,t,F(t,di(hh)))}),ph=_i(function(n,t){return fi(n,bn,Y,Y,Y,t)}),_h=ei(be),vh=ei(function(n,t){
return n>=t}),gh=Oe(function(){return arguments}())?Oe:function(n){return oc(n)&&yl.call(n,"callee")&&!El.call(n,"callee")},yh=el.isArray,dh=ie?R(ie):Ie,bh=Nl||Na,wh=oe?R(oe):Re,mh=fe?R(fe):Se,xh=ce?R(ce):Ce,jh=ae?R(ae):Ue,Ah=le?R(le):Be,kh=ei(Me),Oh=ei(function(n,t){return n<=t}),Ih=Mu(function(n,t){if($i(t)||Vf(t))return Bu(t,Fc(t),n),Y;for(var r in t)yl.call(t,r)&&zr(n,r,t[r])}),Rh=Mu(function(n,t){Bu(t,Nc(t),n)}),zh=Mu(function(n,t,r,e){Bu(t,Nc(t),n,e)}),Eh=Mu(function(n,t,r,e){Bu(t,Fc(t),n,e);
}),Sh=_i(Tr),Wh=ru(function(n,t){n=cl(n);var r=-1,e=t.length,u=e>2?t[2]:Y;for(u&&Li(t[0],t[1],u)&&(e=1);++r<e;)for(var i=t[r],o=Nc(i),f=-1,c=o.length;++f<c;){var a=o[f],l=n[a];(l===Y||Kf(l,_l[a])&&!yl.call(n,a))&&(n[a]=i[a])}return n}),Lh=ru(function(t){return t.push(Y,ai),n($h,Y,t)}),Ch=Yu(function(n,t,r){null!=t&&"function"!=typeof t.toString&&(t=wl.call(t)),n[t]=r},za(Sa)),Uh=Yu(function(n,t,r){null!=t&&"function"!=typeof t.toString&&(t=wl.call(t)),yl.call(n,t)?n[t].push(r):n[t]=[r]},bi),Bh=ru(ke),Th=Mu(function(n,t,r){
qe(n,t,r)}),$h=Mu(function(n,t,r,e){qe(n,t,r,e)}),Dh=_i(function(n,t){var r={};if(null==n)return r;var e=!1;t=c(t,function(t){return t=ju(t,n),e||(e=t.length>1),t}),Bu(n,gi(n),r),e&&(r=Dr(r,on|fn|cn,li));for(var u=t.length;u--;)vu(r,t[u]);return r}),Mh=_i(function(n,t){return null==n?{}:Ge(n,t)}),Fh=oi(Fc),Nh=oi(Nc),Ph=Zu(function(n,t,r){return t=t.toLowerCase(),n+(r?ia(t):t)}),qh=Zu(function(n,t,r){return n+(r?"-":"")+t.toLowerCase()}),Zh=Zu(function(n,t,r){return n+(r?" ":"")+t.toLowerCase()}),Kh=qu("toLowerCase"),Vh=Zu(function(n,t,r){
return n+(r?"_":"")+t.toLowerCase()}),Gh=Zu(function(n,t,r){return n+(r?" ":"")+Jh(t)}),Hh=Zu(function(n,t,r){return n+(r?" ":"")+t.toUpperCase()}),Jh=qu("toUpperCase"),Yh=ru(function(t,r){try{return n(t,Y,r)}catch(n){return nc(n)?n:new il(n)}}),Qh=_i(function(n,t){return r(t,function(t){t=Qi(t),Cr(n,t,oh(n[t],n))}),n}),Xh=Hu(),np=Hu(!0),tp=ru(function(n,t){return function(r){return ke(r,n,t)}}),rp=ru(function(n,t){return function(r){return ke(n,r,t)}}),ep=Xu(c),up=Xu(u),ip=Xu(h),op=ri(),fp=ri(!0),cp=Qu(function(n,t){
return n+t},0),ap=ii("ceil"),lp=Qu(function(n,t){return n/t},1),sp=ii("floor"),hp=Qu(function(n,t){return n*t},1),pp=ii("round"),_p=Qu(function(n,t){return n-t},0);return q.after=kf,q.ary=Of,q.assign=Ih,q.assignIn=Rh,q.assignInWith=zh,q.assignWith=Eh,q.at=Sh,q.before=If,q.bind=oh,q.bindAll=Qh,q.bindKey=fh,q.castArray=Mf,q.chain=Jo,q.chunk=ro,q.compact=eo,q.concat=uo,q.cond=Ia,q.conforms=Ra,q.constant=za,q.countBy=Ys,q.create=zc,q.curry=Rf,q.curryRight=zf,q.debounce=Ef,q.defaults=Wh,q.defaultsDeep=Lh,
q.defer=ch,q.delay=ah,q.difference=Ls,q.differenceBy=Cs,q.differenceWith=Us,q.drop=io,q.dropRight=oo,q.dropRightWhile=fo,q.dropWhile=co,q.fill=ao,q.filter=cf,q.flatMap=af,q.flatMapDeep=lf,q.flatMapDepth=sf,q.flatten=ho,q.flattenDeep=po,q.flattenDepth=_o,q.flip=Sf,q.flow=Xh,q.flowRight=np,q.fromPairs=vo,q.functions=Bc,q.functionsIn=Tc,q.groupBy=nh,q.initial=bo,q.intersection=Bs,q.intersectionBy=Ts,q.intersectionWith=$s,q.invert=Ch,q.invertBy=Uh,q.invokeMap=th,q.iteratee=Wa,q.keyBy=rh,q.keys=Fc,q.keysIn=Nc,
q.map=vf,q.mapKeys=Pc,q.mapValues=qc,q.matches=La,q.matchesProperty=Ca,q.memoize=Wf,q.merge=Th,q.mergeWith=$h,q.method=tp,q.methodOf=rp,q.mixin=Ua,q.negate=Lf,q.nthArg=$a,q.omit=Dh,q.omitBy=Zc,q.once=Cf,q.orderBy=gf,q.over=ep,q.overArgs=lh,q.overEvery=up,q.overSome=ip,q.partial=sh,q.partialRight=hh,q.partition=eh,q.pick=Mh,q.pickBy=Kc,q.property=Da,q.propertyOf=Ma,q.pull=Ds,q.pullAll=Ao,q.pullAllBy=ko,q.pullAllWith=Oo,q.pullAt=Ms,q.range=op,q.rangeRight=fp,q.rearg=ph,q.reject=bf,q.remove=Io,q.rest=Uf,
q.reverse=Ro,q.sampleSize=mf,q.set=Gc,q.setWith=Hc,q.shuffle=xf,q.slice=zo,q.sortBy=uh,q.sortedUniq=Bo,q.sortedUniqBy=To,q.split=ga,q.spread=Bf,q.tail=$o,q.take=Do,q.takeRight=Mo,q.takeRightWhile=Fo,q.takeWhile=No,q.tap=Yo,q.throttle=Tf,q.thru=Qo,q.toArray=mc,q.toPairs=Fh,q.toPairsIn=Nh,q.toPath=Va,q.toPlainObject=Oc,q.transform=Jc,q.unary=$f,q.union=Fs,q.unionBy=Ns,q.unionWith=Ps,q.uniq=Po,q.uniqBy=qo,q.uniqWith=Zo,q.unset=Yc,q.unzip=Ko,q.unzipWith=Vo,q.update=Qc,q.updateWith=Xc,q.values=na,q.valuesIn=ta,
q.without=qs,q.words=Oa,q.wrap=Df,q.xor=Zs,q.xorBy=Ks,q.xorWith=Vs,q.zip=Gs,q.zipObject=Go,q.zipObjectDeep=Ho,q.zipWith=Hs,q.entries=Fh,q.entriesIn=Nh,q.extend=Rh,q.extendWith=zh,Ua(q,q),q.add=cp,q.attempt=Yh,q.camelCase=Ph,q.capitalize=ia,q.ceil=ap,q.clamp=ra,q.clone=Ff,q.cloneDeep=Pf,q.cloneDeepWith=qf,q.cloneWith=Nf,q.conformsTo=Zf,q.deburr=oa,q.defaultTo=Ea,q.divide=lp,q.endsWith=fa,q.eq=Kf,q.escape=ca,q.escapeRegExp=aa,q.every=ff,q.find=Qs,q.findIndex=lo,q.findKey=Ec,q.findLast=Xs,q.findLastIndex=so,
q.findLastKey=Sc,q.floor=sp,q.forEach=hf,q.forEachRight=pf,q.forIn=Wc,q.forInRight=Lc,q.forOwn=Cc,q.forOwnRight=Uc,q.get=$c,q.gt=_h,q.gte=vh,q.has=Dc,q.hasIn=Mc,q.head=go,q.identity=Sa,q.includes=_f,q.indexOf=yo,q.inRange=ea,q.invoke=Bh,q.isArguments=gh,q.isArray=yh,q.isArrayBuffer=dh,q.isArrayLike=Vf,q.isArrayLikeObject=Gf,q.isBoolean=Hf,q.isBuffer=bh,q.isDate=wh,q.isElement=Jf,q.isEmpty=Yf,q.isEqual=Qf,q.isEqualWith=Xf,q.isError=nc,q.isFinite=tc,q.isFunction=rc,q.isInteger=ec,q.isLength=uc,q.isMap=mh,
q.isMatch=fc,q.isMatchWith=cc,q.isNaN=ac,q.isNative=lc,q.isNil=hc,q.isNull=sc,q.isNumber=pc,q.isObject=ic,q.isObjectLike=oc,q.isPlainObject=_c,q.isRegExp=xh,q.isSafeInteger=vc,q.isSet=jh,q.isString=gc,q.isSymbol=yc,q.isTypedArray=Ah,q.isUndefined=dc,q.isWeakMap=bc,q.isWeakSet=wc,q.join=wo,q.kebabCase=qh,q.last=mo,q.lastIndexOf=xo,q.lowerCase=Zh,q.lowerFirst=Kh,q.lt=kh,q.lte=Oh,q.max=Ha,q.maxBy=Ja,q.mean=Ya,q.meanBy=Qa,q.min=Xa,q.minBy=nl,q.stubArray=Fa,q.stubFalse=Na,q.stubObject=Pa,q.stubString=qa,
q.stubTrue=Za,q.multiply=hp,q.nth=jo,q.noConflict=Ba,q.noop=Ta,q.now=ih,q.pad=la,q.padEnd=sa,q.padStart=ha,q.parseInt=pa,q.random=ua,q.reduce=yf,q.reduceRight=df,q.repeat=_a,q.replace=va,q.result=Vc,q.round=pp,q.runInContext=p,q.sample=wf,q.size=jf,q.snakeCase=Vh,q.some=Af,q.sortedIndex=Eo,q.sortedIndexBy=So,q.sortedIndexOf=Wo,q.sortedLastIndex=Lo,q.sortedLastIndexBy=Co,q.sortedLastIndexOf=Uo,q.startCase=Gh,q.startsWith=ya,q.subtract=_p,q.sum=tl,q.sumBy=rl,q.template=da,q.times=Ka,q.toFinite=xc,q.toInteger=jc,
q.toLength=Ac,q.toLower=ba,q.toNumber=kc,q.toSafeInteger=Ic,q.toString=Rc,q.toUpper=wa,q.trim=ma,q.trimEnd=xa,q.trimStart=ja,q.truncate=Aa,q.unescape=ka,q.uniqueId=Ga,q.upperCase=Hh,q.upperFirst=Jh,q.each=hf,q.eachRight=pf,q.first=go,Ua(q,function(){var n={};return ee(q,function(t,r){yl.call(q.prototype,r)||(n[r]=t)}),n}(),{chain:!1}),q.VERSION=Q,r(["bind","bindKey","curry","curryRight","partial","partialRight"],function(n){q[n].placeholder=q}),r(["drop","take"],function(n,t){Bt.prototype[n]=function(r){
r=r===Y?1:Kl(jc(r),0);var e=this.__filtered__&&!t?new Bt(this):this.clone();return e.__filtered__?e.__takeCount__=Vl(r,e.__takeCount__):e.__views__.push({size:Vl(r,Wn),type:n+(e.__dir__<0?"Right":"")}),e},Bt.prototype[n+"Right"]=function(t){return this.reverse()[n](t).reverse()}}),r(["filter","map","takeWhile"],function(n,t){var r=t+1,e=r==kn||r==In;Bt.prototype[n]=function(n){var t=this.clone();return t.__iteratees__.push({iteratee:bi(n,3),type:r}),t.__filtered__=t.__filtered__||e,t}}),r(["head","last"],function(n,t){
var r="take"+(t?"Right":"");Bt.prototype[n]=function(){return this[r](1).value()[0]}}),r(["initial","tail"],function(n,t){var r="drop"+(t?"":"Right");Bt.prototype[n]=function(){return this.__filtered__?new Bt(this):this[r](1)}}),Bt.prototype.compact=function(){return this.filter(Sa)},Bt.prototype.find=function(n){return this.filter(n).head()},Bt.prototype.findLast=function(n){return this.reverse().find(n)},Bt.prototype.invokeMap=ru(function(n,t){return"function"==typeof n?new Bt(this):this.map(function(r){
return ke(r,n,t)})}),Bt.prototype.reject=function(n){return this.filter(Lf(bi(n)))},Bt.prototype.slice=function(n,t){n=jc(n);var r=this;return r.__filtered__&&(n>0||t<0)?new Bt(r):(n<0?r=r.takeRight(-n):n&&(r=r.drop(n)),t!==Y&&(t=jc(t),r=t<0?r.dropRight(-t):r.take(t-n)),r)},Bt.prototype.takeRightWhile=function(n){return this.reverse().takeWhile(n).reverse()},Bt.prototype.toArray=function(){return this.take(Wn)},ee(Bt.prototype,function(n,t){var r=/^(?:filter|find|map|reject)|While$/.test(t),e=/^(?:head|last)$/.test(t),u=q[e?"take"+("last"==t?"Right":""):t],i=e||/^find/.test(t);
u&&(q.prototype[t]=function(){var t=this.__wrapped__,o=e?[1]:arguments,f=t instanceof Bt,c=o[0],l=f||yh(t),s=function(n){var t=u.apply(q,a([n],o));return e&&h?t[0]:t};l&&r&&"function"==typeof c&&1!=c.length&&(f=l=!1);var h=this.__chain__,p=!!this.__actions__.length,_=i&&!h,v=f&&!p;if(!i&&l){t=v?t:new Bt(this);var g=n.apply(t,o);return g.__actions__.push({func:Qo,args:[s],thisArg:Y}),new H(g,h)}return _&&v?n.apply(this,o):(g=this.thru(s),_?e?g.value()[0]:g.value():g)})}),r(["pop","push","shift","sort","splice","unshift"],function(n){
var t=hl[n],r=/^(?:push|sort|unshift)$/.test(n)?"tap":"thru",e=/^(?:pop|shift)$/.test(n);q.prototype[n]=function(){var n=arguments;if(e&&!this.__chain__){var u=this.value();return t.apply(yh(u)?u:[],n)}return this[r](function(r){return t.apply(yh(r)?r:[],n)})}}),ee(Bt.prototype,function(n,t){var r=q[t];if(r){var e=r.name+"";yl.call(is,e)||(is[e]=[]),is[e].push({name:t,func:r})}}),is[Ju(Y,hn).name]=[{name:"wrapper",func:Y}],Bt.prototype.clone=Gt,Bt.prototype.reverse=Ht,Bt.prototype.value=Jt,q.prototype.at=Js,
q.prototype.chain=Xo,q.prototype.commit=nf,q.prototype.next=tf,q.prototype.plant=ef,q.prototype.reverse=uf,q.prototype.toJSON=q.prototype.valueOf=q.prototype.value=of,q.prototype.first=q.prototype.head,Ll&&(q.prototype[Ll]=rf),q},ge=ve();"function"==typeof define&&"object"==typeof define.amd&&define.amd?(Xr._=ge,define(function(){return ge})):te?((te.exports=ge)._=ge,ne._=ge):Xr._=ge}).call(this); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/lodash.min.js | lodash.min.js |
var isObject = require('lodash/isObject'),
now = require('lodash/now'),
toNumber = require('lodash/toNumber');
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeMax = Math.max,
nativeMin = Math.min;
/**
* Creates a debounced function that delays invoking `func` until after `wait`
* milliseconds have elapsed since the last time the debounced function was
* invoked. The debounced function comes with a `cancel` method to cancel
* delayed `func` invocations and a `flush` method to immediately invoke them.
* Provide `options` to indicate whether `func` should be invoked on the
* leading and/or trailing edge of the `wait` timeout. The `func` is invoked
* with the last arguments provided to the debounced function. Subsequent
* calls to the debounced function return the result of the last `func`
* invocation.
*
* **Note:** If `leading` and `trailing` options are `true`, `func` is
* invoked on the trailing edge of the timeout only if the debounced function
* is invoked more than once during the `wait` timeout.
*
* If `wait` is `0` and `leading` is `false`, `func` invocation is deferred
* until to the next tick, similar to `setTimeout` with a timeout of `0`.
*
* See [David Corbacho's article](https://css-tricks.com/debouncing-throttling-explained-examples/)
* for details over the differences between `_.debounce` and `_.throttle`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to debounce.
* @param {number} [wait=0] The number of milliseconds to delay.
* @param {Object} [options={}] The options object.
* @param {boolean} [options.leading=false]
* Specify invoking on the leading edge of the timeout.
* @param {number} [options.maxWait]
* The maximum time `func` is allowed to be delayed before it's invoked.
* @param {boolean} [options.trailing=true]
* Specify invoking on the trailing edge of the timeout.
* @returns {Function} Returns the new debounced function.
* @example
*
* // Avoid costly calculations while the window size is in flux.
* jQuery(window).on('resize', _.debounce(calculateLayout, 150));
*
* // Invoke `sendMail` when clicked, debouncing subsequent calls.
* jQuery(element).on('click', _.debounce(sendMail, 300, {
* 'leading': true,
* 'trailing': false
* }));
*
* // Ensure `batchLog` is invoked once after 1 second of debounced calls.
* var debounced = _.debounce(batchLog, 250, { 'maxWait': 1000 });
* var source = new EventSource('/stream');
* jQuery(source).on('message', debounced);
*
* // Cancel the trailing debounced invocation.
* jQuery(window).on('popstate', debounced.cancel);
*/
function debounce(func, wait, options) {
var lastArgs,
lastThis,
maxWait,
result,
timerId,
lastCallTime,
lastInvokeTime = 0,
leading = false,
maxing = false,
trailing = true;
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
wait = toNumber(wait) || 0;
if (isObject(options)) {
leading = !!options.leading;
maxing = 'maxWait' in options;
maxWait = maxing ? nativeMax(toNumber(options.maxWait) || 0, wait) : maxWait;
trailing = 'trailing' in options ? !!options.trailing : trailing;
}
function invokeFunc(time) {
var args = lastArgs,
thisArg = lastThis;
lastArgs = lastThis = undefined;
lastInvokeTime = time;
result = func.apply(thisArg, args);
return result;
}
function leadingEdge(time) {
// Reset any `maxWait` timer.
lastInvokeTime = time;
// Start the timer for the trailing edge.
timerId = setTimeout(timerExpired, wait);
// Invoke the leading edge.
return leading ? invokeFunc(time) : result;
}
function remainingWait(time) {
var timeSinceLastCall = time - lastCallTime,
timeSinceLastInvoke = time - lastInvokeTime,
timeWaiting = wait - timeSinceLastCall;
return maxing
? nativeMin(timeWaiting, maxWait - timeSinceLastInvoke)
: timeWaiting;
}
function shouldInvoke(time) {
var timeSinceLastCall = time - lastCallTime,
timeSinceLastInvoke = time - lastInvokeTime;
// Either this is the first call, activity has stopped and we're at the
// trailing edge, the system time has gone backwards and we're treating
// it as the trailing edge, or we've hit the `maxWait` limit.
return (lastCallTime === undefined || (timeSinceLastCall >= wait) ||
(timeSinceLastCall < 0) || (maxing && timeSinceLastInvoke >= maxWait));
}
function timerExpired() {
var time = now();
if (shouldInvoke(time)) {
return trailingEdge(time);
}
// Restart the timer.
timerId = setTimeout(timerExpired, remainingWait(time));
}
function trailingEdge(time) {
timerId = undefined;
// Only invoke if we have `lastArgs` which means `func` has been
// debounced at least once.
if (trailing && lastArgs) {
return invokeFunc(time);
}
lastArgs = lastThis = undefined;
return result;
}
function cancel() {
if (timerId !== undefined) {
clearTimeout(timerId);
}
lastInvokeTime = 0;
lastArgs = lastCallTime = lastThis = timerId = undefined;
}
function flush() {
return timerId === undefined ? result : trailingEdge(now());
}
function debounced() {
var time = now(),
isInvoking = shouldInvoke(time);
lastArgs = arguments;
lastThis = this;
lastCallTime = time;
if (isInvoking) {
if (timerId === undefined) {
return leadingEdge(lastCallTime);
}
if (maxing) {
// Handle invocations in a tight loop.
clearTimeout(timerId);
timerId = setTimeout(timerExpired, wait);
return invokeFunc(lastCallTime);
}
}
if (timerId === undefined) {
timerId = setTimeout(timerExpired, wait);
}
return result;
}
debounced.cancel = cancel;
debounced.flush = flush;
return debounced;
}
module.exports = debounce; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/debounce.js | debounce.js |
var baseGetTag = require('lodash/_baseGetTag'),
isLength = require('lodash/isLength'),
isObjectLike = require('lodash/isObjectLike');
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
funcTag = '[object Function]',
mapTag = '[object Map]',
numberTag = '[object Number]',
objectTag = '[object Object]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
weakMapTag = '[object WeakMap]';
var arrayBufferTag = '[object ArrayBuffer]',
dataViewTag = '[object DataView]',
float32Tag = '[object Float32Array]',
float64Tag = '[object Float64Array]',
int8Tag = '[object Int8Array]',
int16Tag = '[object Int16Array]',
int32Tag = '[object Int32Array]',
uint8Tag = '[object Uint8Array]',
uint8ClampedTag = '[object Uint8ClampedArray]',
uint16Tag = '[object Uint16Array]',
uint32Tag = '[object Uint32Array]';
/** Used to identify `toStringTag` values of typed arrays. */
var typedArrayTags = {};
typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
typedArrayTags[uint32Tag] = true;
typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
typedArrayTags[dataViewTag] = typedArrayTags[dateTag] =
typedArrayTags[errorTag] = typedArrayTags[funcTag] =
typedArrayTags[mapTag] = typedArrayTags[numberTag] =
typedArrayTags[objectTag] = typedArrayTags[regexpTag] =
typedArrayTags[setTag] = typedArrayTags[stringTag] =
typedArrayTags[weakMapTag] = false;
/**
* The base implementation of `_.isTypedArray` without Node.js optimizations.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
*/
function baseIsTypedArray(value) {
return isObjectLike(value) &&
isLength(value.length) && !!typedArrayTags[baseGetTag(value)];
}
module.exports = baseIsTypedArray; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseIsTypedArray.js | _baseIsTypedArray.js |
var SetCache = require('lodash/_SetCache'),
arraySome = require('lodash/_arraySome'),
cacheHas = require('lodash/_cacheHas');
/** Used to compose bitmasks for value comparisons. */
var COMPARE_PARTIAL_FLAG = 1,
COMPARE_UNORDERED_FLAG = 2;
/**
* A specialized version of `baseIsEqualDeep` for arrays with support for
* partial deep comparisons.
*
* @private
* @param {Array} array The array to compare.
* @param {Array} other The other array to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `array` and `other` objects.
* @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
*/
function equalArrays(array, other, bitmask, customizer, equalFunc, stack) {
var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
arrLength = array.length,
othLength = other.length;
if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
return false;
}
// Assume cyclic values are equal.
var stacked = stack.get(array);
if (stacked && stack.get(other)) {
return stacked == other;
}
var index = -1,
result = true,
seen = (bitmask & COMPARE_UNORDERED_FLAG) ? new SetCache : undefined;
stack.set(array, other);
stack.set(other, array);
// Ignore non-index properties.
while (++index < arrLength) {
var arrValue = array[index],
othValue = other[index];
if (customizer) {
var compared = isPartial
? customizer(othValue, arrValue, index, other, array, stack)
: customizer(arrValue, othValue, index, array, other, stack);
}
if (compared !== undefined) {
if (compared) {
continue;
}
result = false;
break;
}
// Recursively compare arrays (susceptible to call stack limits).
if (seen) {
if (!arraySome(other, function(othValue, othIndex) {
if (!cacheHas(seen, othIndex) &&
(arrValue === othValue || equalFunc(arrValue, othValue, bitmask, customizer, stack))) {
return seen.push(othIndex);
}
})) {
result = false;
break;
}
} else if (!(
arrValue === othValue ||
equalFunc(arrValue, othValue, bitmask, customizer, stack)
)) {
result = false;
break;
}
}
stack['delete'](array);
stack['delete'](other);
return result;
}
module.exports = equalArrays; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_equalArrays.js | _equalArrays.js |
var baseRandom = require('lodash/_baseRandom'),
isIterateeCall = require('lodash/_isIterateeCall'),
toFinite = require('lodash/toFinite');
/** Built-in method references without a dependency on `root`. */
var freeParseFloat = parseFloat;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeMin = Math.min,
nativeRandom = Math.random;
/**
* Produces a random number between the inclusive `lower` and `upper` bounds.
* If only one argument is provided a number between `0` and the given number
* is returned. If `floating` is `true`, or either `lower` or `upper` are
* floats, a floating-point number is returned instead of an integer.
*
* **Note:** JavaScript follows the IEEE-754 standard for resolving
* floating-point values which can produce unexpected results.
*
* @static
* @memberOf _
* @since 0.7.0
* @category Number
* @param {number} [lower=0] The lower bound.
* @param {number} [upper=1] The upper bound.
* @param {boolean} [floating] Specify returning a floating-point number.
* @returns {number} Returns the random number.
* @example
*
* _.random(0, 5);
* // => an integer between 0 and 5
*
* _.random(5);
* // => also an integer between 0 and 5
*
* _.random(5, true);
* // => a floating-point number between 0 and 5
*
* _.random(1.2, 5.2);
* // => a floating-point number between 1.2 and 5.2
*/
function random(lower, upper, floating) {
if (floating && typeof floating != 'boolean' && isIterateeCall(lower, upper, floating)) {
upper = floating = undefined;
}
if (floating === undefined) {
if (typeof upper == 'boolean') {
floating = upper;
upper = undefined;
}
else if (typeof lower == 'boolean') {
floating = lower;
lower = undefined;
}
}
if (lower === undefined && upper === undefined) {
lower = 0;
upper = 1;
}
else {
lower = toFinite(lower);
if (upper === undefined) {
upper = lower;
lower = 0;
} else {
upper = toFinite(upper);
}
}
if (lower > upper) {
var temp = lower;
lower = upper;
upper = temp;
}
if (floating || lower % 1 || upper % 1) {
var rand = nativeRandom();
return nativeMin(lower + (rand * (upper - lower + freeParseFloat('1e-' + ((rand + '').length - 1)))), upper);
}
return baseRandom(lower, upper);
}
module.exports = random; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/random.js | random.js |
# lodash v4.17.19
The [Lodash](https://lodash.com/) library exported as [Node.js](https://nodejs.org/) modules.
## Installation
Using npm:
```shell
$ npm i -g npm
$ npm i --save lodash
```
In Node.js:
```js
// Load the full build.
var _ = require('lodash');
// Load the core build.
var _ = require('lodash/core');
// Load the FP build for immutable auto-curried iteratee-first data-last methods.
var fp = require('lodash/fp');
// Load method categories.
var array = require('lodash/array');
var object = require('lodash/fp/object');
// Cherry-pick methods for smaller browserify/rollup/webpack bundles.
var at = require('lodash/at');
var curryN = require('lodash/fp/curryN');
```
See the [package source](https://github.com/lodash/lodash/tree/4.17.19-npm) for more details.
**Note:**<br>
Install [n_](https://www.npmjs.com/package/n_) for Lodash use in the Node.js < 6 REPL.
## Support
Tested in Chrome 74-75, Firefox 66-67, IE 11, Edge 18, Safari 11-12, & Node.js 8-12.<br>
Automated [browser](https://saucelabs.com/u/lodash) & [CI](https://travis-ci.org/lodash/lodash/) test runs are available.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/README.md | README.md |
var baseRest = require('lodash/_baseRest'),
createWrap = require('lodash/_createWrap'),
getHolder = require('lodash/_getHolder'),
replaceHolders = require('lodash/_replaceHolders');
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_BIND_KEY_FLAG = 2,
WRAP_PARTIAL_FLAG = 32;
/**
* Creates a function that invokes the method at `object[key]` with `partials`
* prepended to the arguments it receives.
*
* This method differs from `_.bind` by allowing bound functions to reference
* methods that may be redefined or don't yet exist. See
* [Peter Michaux's article](http://peter.michaux.ca/articles/lazy-function-definition-pattern)
* for more details.
*
* The `_.bindKey.placeholder` value, which defaults to `_` in monolithic
* builds, may be used as a placeholder for partially applied arguments.
*
* @static
* @memberOf _
* @since 0.10.0
* @category Function
* @param {Object} object The object to invoke the method on.
* @param {string} key The key of the method.
* @param {...*} [partials] The arguments to be partially applied.
* @returns {Function} Returns the new bound function.
* @example
*
* var object = {
* 'user': 'fred',
* 'greet': function(greeting, punctuation) {
* return greeting + ' ' + this.user + punctuation;
* }
* };
*
* var bound = _.bindKey(object, 'greet', 'hi');
* bound('!');
* // => 'hi fred!'
*
* object.greet = function(greeting, punctuation) {
* return greeting + 'ya ' + this.user + punctuation;
* };
*
* bound('!');
* // => 'hiya fred!'
*
* // Bound with placeholders.
* var bound = _.bindKey(object, 'greet', _, '!');
* bound('hi');
* // => 'hiya fred!'
*/
var bindKey = baseRest(function(object, key, partials) {
var bitmask = WRAP_BIND_FLAG | WRAP_BIND_KEY_FLAG;
if (partials.length) {
var holders = replaceHolders(partials, getHolder(bindKey));
bitmask |= WRAP_PARTIAL_FLAG;
}
return createWrap(key, bitmask, object, partials, holders);
});
// Assign default placeholders.
bindKey.placeholder = {};
module.exports = bindKey; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/bindKey.js | bindKey.js |
var getAllKeys = require('lodash/_getAllKeys');
/** Used to compose bitmasks for value comparisons. */
var COMPARE_PARTIAL_FLAG = 1;
/** Used for built-in method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* A specialized version of `baseIsEqualDeep` for objects with support for
* partial deep comparisons.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalObjects(object, other, bitmask, customizer, equalFunc, stack) {
var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
objProps = getAllKeys(object),
objLength = objProps.length,
othProps = getAllKeys(other),
othLength = othProps.length;
if (objLength != othLength && !isPartial) {
return false;
}
var index = objLength;
while (index--) {
var key = objProps[index];
if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
return false;
}
}
// Assume cyclic values are equal.
var stacked = stack.get(object);
if (stacked && stack.get(other)) {
return stacked == other;
}
var result = true;
stack.set(object, other);
stack.set(other, object);
var skipCtor = isPartial;
while (++index < objLength) {
key = objProps[index];
var objValue = object[key],
othValue = other[key];
if (customizer) {
var compared = isPartial
? customizer(othValue, objValue, key, other, object, stack)
: customizer(objValue, othValue, key, object, other, stack);
}
// Recursively compare objects (susceptible to call stack limits).
if (!(compared === undefined
? (objValue === othValue || equalFunc(objValue, othValue, bitmask, customizer, stack))
: compared
)) {
result = false;
break;
}
skipCtor || (skipCtor = key == 'constructor');
}
if (result && !skipCtor) {
var objCtor = object.constructor,
othCtor = other.constructor;
// Non `Object` object instances with different constructors are not equal.
if (objCtor != othCtor &&
('constructor' in object && 'constructor' in other) &&
!(typeof objCtor == 'function' && objCtor instanceof objCtor &&
typeof othCtor == 'function' && othCtor instanceof othCtor)) {
result = false;
}
}
stack['delete'](object);
stack['delete'](other);
return result;
}
module.exports = equalObjects; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_equalObjects.js | _equalObjects.js |
var composeArgs = require('lodash/_composeArgs'),
composeArgsRight = require('lodash/_composeArgsRight'),
countHolders = require('lodash/_countHolders'),
createCtor = require('lodash/_createCtor'),
createRecurry = require('lodash/_createRecurry'),
getHolder = require('lodash/_getHolder'),
reorder = require('lodash/_reorder'),
replaceHolders = require('lodash/_replaceHolders'),
root = require('lodash/_root');
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_BIND_KEY_FLAG = 2,
WRAP_CURRY_FLAG = 8,
WRAP_CURRY_RIGHT_FLAG = 16,
WRAP_ARY_FLAG = 128,
WRAP_FLIP_FLAG = 512;
/**
* Creates a function that wraps `func` to invoke it with optional `this`
* binding of `thisArg`, partial application, and currying.
*
* @private
* @param {Function|string} func The function or method name to wrap.
* @param {number} bitmask The bitmask flags. See `createWrap` for more details.
* @param {*} [thisArg] The `this` binding of `func`.
* @param {Array} [partials] The arguments to prepend to those provided to
* the new function.
* @param {Array} [holders] The `partials` placeholder indexes.
* @param {Array} [partialsRight] The arguments to append to those provided
* to the new function.
* @param {Array} [holdersRight] The `partialsRight` placeholder indexes.
* @param {Array} [argPos] The argument positions of the new function.
* @param {number} [ary] The arity cap of `func`.
* @param {number} [arity] The arity of `func`.
* @returns {Function} Returns the new wrapped function.
*/
function createHybrid(func, bitmask, thisArg, partials, holders, partialsRight, holdersRight, argPos, ary, arity) {
var isAry = bitmask & WRAP_ARY_FLAG,
isBind = bitmask & WRAP_BIND_FLAG,
isBindKey = bitmask & WRAP_BIND_KEY_FLAG,
isCurried = bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG),
isFlip = bitmask & WRAP_FLIP_FLAG,
Ctor = isBindKey ? undefined : createCtor(func);
function wrapper() {
var length = arguments.length,
args = Array(length),
index = length;
while (index--) {
args[index] = arguments[index];
}
if (isCurried) {
var placeholder = getHolder(wrapper),
holdersCount = countHolders(args, placeholder);
}
if (partials) {
args = composeArgs(args, partials, holders, isCurried);
}
if (partialsRight) {
args = composeArgsRight(args, partialsRight, holdersRight, isCurried);
}
length -= holdersCount;
if (isCurried && length < arity) {
var newHolders = replaceHolders(args, placeholder);
return createRecurry(
func, bitmask, createHybrid, wrapper.placeholder, thisArg,
args, newHolders, argPos, ary, arity - length
);
}
var thisBinding = isBind ? thisArg : this,
fn = isBindKey ? thisBinding[func] : func;
length = args.length;
if (argPos) {
args = reorder(args, argPos);
} else if (isFlip && length > 1) {
args.reverse();
}
if (isAry && ary < length) {
args.length = ary;
}
if (this && this !== root && this instanceof wrapper) {
fn = Ctor || createCtor(fn);
}
return fn.apply(thisBinding, args);
}
return wrapper;
}
module.exports = createHybrid; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_createHybrid.js | _createHybrid.js |
var basePropertyOf = require('lodash/_basePropertyOf');
/** Used to map Latin Unicode letters to basic Latin letters. */
var deburredLetters = {
// Latin-1 Supplement block.
'\xc0': 'A', '\xc1': 'A', '\xc2': 'A', '\xc3': 'A', '\xc4': 'A', '\xc5': 'A',
'\xe0': 'a', '\xe1': 'a', '\xe2': 'a', '\xe3': 'a', '\xe4': 'a', '\xe5': 'a',
'\xc7': 'C', '\xe7': 'c',
'\xd0': 'D', '\xf0': 'd',
'\xc8': 'E', '\xc9': 'E', '\xca': 'E', '\xcb': 'E',
'\xe8': 'e', '\xe9': 'e', '\xea': 'e', '\xeb': 'e',
'\xcc': 'I', '\xcd': 'I', '\xce': 'I', '\xcf': 'I',
'\xec': 'i', '\xed': 'i', '\xee': 'i', '\xef': 'i',
'\xd1': 'N', '\xf1': 'n',
'\xd2': 'O', '\xd3': 'O', '\xd4': 'O', '\xd5': 'O', '\xd6': 'O', '\xd8': 'O',
'\xf2': 'o', '\xf3': 'o', '\xf4': 'o', '\xf5': 'o', '\xf6': 'o', '\xf8': 'o',
'\xd9': 'U', '\xda': 'U', '\xdb': 'U', '\xdc': 'U',
'\xf9': 'u', '\xfa': 'u', '\xfb': 'u', '\xfc': 'u',
'\xdd': 'Y', '\xfd': 'y', '\xff': 'y',
'\xc6': 'Ae', '\xe6': 'ae',
'\xde': 'Th', '\xfe': 'th',
'\xdf': 'ss',
// Latin Extended-A block.
'\u0100': 'A', '\u0102': 'A', '\u0104': 'A',
'\u0101': 'a', '\u0103': 'a', '\u0105': 'a',
'\u0106': 'C', '\u0108': 'C', '\u010a': 'C', '\u010c': 'C',
'\u0107': 'c', '\u0109': 'c', '\u010b': 'c', '\u010d': 'c',
'\u010e': 'D', '\u0110': 'D', '\u010f': 'd', '\u0111': 'd',
'\u0112': 'E', '\u0114': 'E', '\u0116': 'E', '\u0118': 'E', '\u011a': 'E',
'\u0113': 'e', '\u0115': 'e', '\u0117': 'e', '\u0119': 'e', '\u011b': 'e',
'\u011c': 'G', '\u011e': 'G', '\u0120': 'G', '\u0122': 'G',
'\u011d': 'g', '\u011f': 'g', '\u0121': 'g', '\u0123': 'g',
'\u0124': 'H', '\u0126': 'H', '\u0125': 'h', '\u0127': 'h',
'\u0128': 'I', '\u012a': 'I', '\u012c': 'I', '\u012e': 'I', '\u0130': 'I',
'\u0129': 'i', '\u012b': 'i', '\u012d': 'i', '\u012f': 'i', '\u0131': 'i',
'\u0134': 'J', '\u0135': 'j',
'\u0136': 'K', '\u0137': 'k', '\u0138': 'k',
'\u0139': 'L', '\u013b': 'L', '\u013d': 'L', '\u013f': 'L', '\u0141': 'L',
'\u013a': 'l', '\u013c': 'l', '\u013e': 'l', '\u0140': 'l', '\u0142': 'l',
'\u0143': 'N', '\u0145': 'N', '\u0147': 'N', '\u014a': 'N',
'\u0144': 'n', '\u0146': 'n', '\u0148': 'n', '\u014b': 'n',
'\u014c': 'O', '\u014e': 'O', '\u0150': 'O',
'\u014d': 'o', '\u014f': 'o', '\u0151': 'o',
'\u0154': 'R', '\u0156': 'R', '\u0158': 'R',
'\u0155': 'r', '\u0157': 'r', '\u0159': 'r',
'\u015a': 'S', '\u015c': 'S', '\u015e': 'S', '\u0160': 'S',
'\u015b': 's', '\u015d': 's', '\u015f': 's', '\u0161': 's',
'\u0162': 'T', '\u0164': 'T', '\u0166': 'T',
'\u0163': 't', '\u0165': 't', '\u0167': 't',
'\u0168': 'U', '\u016a': 'U', '\u016c': 'U', '\u016e': 'U', '\u0170': 'U', '\u0172': 'U',
'\u0169': 'u', '\u016b': 'u', '\u016d': 'u', '\u016f': 'u', '\u0171': 'u', '\u0173': 'u',
'\u0174': 'W', '\u0175': 'w',
'\u0176': 'Y', '\u0177': 'y', '\u0178': 'Y',
'\u0179': 'Z', '\u017b': 'Z', '\u017d': 'Z',
'\u017a': 'z', '\u017c': 'z', '\u017e': 'z',
'\u0132': 'IJ', '\u0133': 'ij',
'\u0152': 'Oe', '\u0153': 'oe',
'\u0149': "'n", '\u017f': 's'
};
/**
* Used by `_.deburr` to convert Latin-1 Supplement and Latin Extended-A
* letters to basic Latin letters.
*
* @private
* @param {string} letter The matched letter to deburr.
* @returns {string} Returns the deburred letter.
*/
var deburrLetter = basePropertyOf(deburredLetters);
module.exports = deburrLetter; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_deburrLetter.js | _deburrLetter.js |
var assignInWith = require('lodash/assignInWith'),
attempt = require('lodash/attempt'),
baseValues = require('lodash/_baseValues'),
customDefaultsAssignIn = require('lodash/_customDefaultsAssignIn'),
escapeStringChar = require('lodash/_escapeStringChar'),
isError = require('lodash/isError'),
isIterateeCall = require('lodash/_isIterateeCall'),
keys = require('lodash/keys'),
reInterpolate = require('lodash/_reInterpolate'),
templateSettings = require('lodash/templateSettings'),
toString = require('lodash/toString');
/** Used to match empty string literals in compiled template source. */
var reEmptyStringLeading = /\b__p \+= '';/g,
reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
/**
* Used to match
* [ES template delimiters](http://ecma-international.org/ecma-262/7.0/#sec-template-literal-lexical-components).
*/
var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
/** Used to ensure capturing order of template delimiters. */
var reNoMatch = /($^)/;
/** Used to match unescaped characters in compiled string literals. */
var reUnescapedString = /['\n\r\u2028\u2029\\]/g;
/** Used for built-in method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Creates a compiled template function that can interpolate data properties
* in "interpolate" delimiters, HTML-escape interpolated data properties in
* "escape" delimiters, and execute JavaScript in "evaluate" delimiters. Data
* properties may be accessed as free variables in the template. If a setting
* object is given, it takes precedence over `_.templateSettings` values.
*
* **Note:** In the development build `_.template` utilizes
* [sourceURLs](http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl)
* for easier debugging.
*
* For more information on precompiling templates see
* [lodash's custom builds documentation](https://lodash.com/custom-builds).
*
* For more information on Chrome extension sandboxes see
* [Chrome's extensions documentation](https://developer.chrome.com/extensions/sandboxingEval).
*
* @static
* @since 0.1.0
* @memberOf _
* @category String
* @param {string} [string=''] The template string.
* @param {Object} [options={}] The options object.
* @param {RegExp} [options.escape=_.templateSettings.escape]
* The HTML "escape" delimiter.
* @param {RegExp} [options.evaluate=_.templateSettings.evaluate]
* The "evaluate" delimiter.
* @param {Object} [options.imports=_.templateSettings.imports]
* An object to import into the template as free variables.
* @param {RegExp} [options.interpolate=_.templateSettings.interpolate]
* The "interpolate" delimiter.
* @param {string} [options.sourceURL='templateSources[n]']
* The sourceURL of the compiled template.
* @param {string} [options.variable='obj']
* The data object variable name.
* @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
* @returns {Function} Returns the compiled template function.
* @example
*
* // Use the "interpolate" delimiter to create a compiled template.
* var compiled = _.template('hello <%= user %>!');
* compiled({ 'user': 'fred' });
* // => 'hello fred!'
*
* // Use the HTML "escape" delimiter to escape data property values.
* var compiled = _.template('<b><%- value %></b>');
* compiled({ 'value': '<script>' });
* // => '<b><script></b>'
*
* // Use the "evaluate" delimiter to execute JavaScript and generate HTML.
* var compiled = _.template('<% _.forEach(users, function(user) { %><li><%- user %></li><% }); %>');
* compiled({ 'users': ['fred', 'barney'] });
* // => '<li>fred</li><li>barney</li>'
*
* // Use the internal `print` function in "evaluate" delimiters.
* var compiled = _.template('<% print("hello " + user); %>!');
* compiled({ 'user': 'barney' });
* // => 'hello barney!'
*
* // Use the ES template literal delimiter as an "interpolate" delimiter.
* // Disable support by replacing the "interpolate" delimiter.
* var compiled = _.template('hello ${ user }!');
* compiled({ 'user': 'pebbles' });
* // => 'hello pebbles!'
*
* // Use backslashes to treat delimiters as plain text.
* var compiled = _.template('<%= "\\<%- value %\\>" %>');
* compiled({ 'value': 'ignored' });
* // => '<%- value %>'
*
* // Use the `imports` option to import `jQuery` as `jq`.
* var text = '<% jq.each(users, function(user) { %><li><%- user %></li><% }); %>';
* var compiled = _.template(text, { 'imports': { 'jq': jQuery } });
* compiled({ 'users': ['fred', 'barney'] });
* // => '<li>fred</li><li>barney</li>'
*
* // Use the `sourceURL` option to specify a custom sourceURL for the template.
* var compiled = _.template('hello <%= user %>!', { 'sourceURL': '/basic/greeting.jst' });
* compiled(data);
* // => Find the source of "greeting.jst" under the Sources tab or Resources panel of the web inspector.
*
* // Use the `variable` option to ensure a with-statement isn't used in the compiled template.
* var compiled = _.template('hi <%= data.user %>!', { 'variable': 'data' });
* compiled.source;
* // => function(data) {
* // var __t, __p = '';
* // __p += 'hi ' + ((__t = ( data.user )) == null ? '' : __t) + '!';
* // return __p;
* // }
*
* // Use custom template delimiters.
* _.templateSettings.interpolate = /{{([\s\S]+?)}}/g;
* var compiled = _.template('hello {{ user }}!');
* compiled({ 'user': 'mustache' });
* // => 'hello mustache!'
*
* // Use the `source` property to inline compiled templates for meaningful
* // line numbers in error messages and stack traces.
* fs.writeFileSync(path.join(process.cwd(), 'jst.js'), '\
* var JST = {\
* "main": ' + _.template(mainText).source + '\
* };\
* ');
*/
function template(string, options, guard) {
// Based on John Resig's `tmpl` implementation
// (http://ejohn.org/blog/javascript-micro-templating/)
// and Laura Doktorova's doT.js (https://github.com/olado/doT).
var settings = templateSettings.imports._.templateSettings || templateSettings;
if (guard && isIterateeCall(string, options, guard)) {
options = undefined;
}
string = toString(string);
options = assignInWith({}, options, settings, customDefaultsAssignIn);
var imports = assignInWith({}, options.imports, settings.imports, customDefaultsAssignIn),
importsKeys = keys(imports),
importsValues = baseValues(imports, importsKeys);
var isEscaping,
isEvaluating,
index = 0,
interpolate = options.interpolate || reNoMatch,
source = "__p += '";
// Compile the regexp to match each delimiter.
var reDelimiters = RegExp(
(options.escape || reNoMatch).source + '|' +
interpolate.source + '|' +
(interpolate === reInterpolate ? reEsTemplate : reNoMatch).source + '|' +
(options.evaluate || reNoMatch).source + '|$'
, 'g');
// Use a sourceURL for easier debugging.
// The sourceURL gets injected into the source that's eval-ed, so be careful
// with lookup (in case of e.g. prototype pollution), and strip newlines if any.
// A newline wouldn't be a valid sourceURL anyway, and it'd enable code injection.
var sourceURL = hasOwnProperty.call(options, 'sourceURL')
? ('//# sourceURL=' +
(options.sourceURL + '').replace(/[\r\n]/g, ' ') +
'\n')
: '';
string.replace(reDelimiters, function(match, escapeValue, interpolateValue, esTemplateValue, evaluateValue, offset) {
interpolateValue || (interpolateValue = esTemplateValue);
// Escape characters that can't be included in string literals.
source += string.slice(index, offset).replace(reUnescapedString, escapeStringChar);
// Replace delimiters with snippets.
if (escapeValue) {
isEscaping = true;
source += "' +\n__e(" + escapeValue + ") +\n'";
}
if (evaluateValue) {
isEvaluating = true;
source += "';\n" + evaluateValue + ";\n__p += '";
}
if (interpolateValue) {
source += "' +\n((__t = (" + interpolateValue + ")) == null ? '' : __t) +\n'";
}
index = offset + match.length;
// The JS engine embedded in Adobe products needs `match` returned in
// order to produce the correct `offset` value.
return match;
});
source += "';\n";
// If `variable` is not specified wrap a with-statement around the generated
// code to add the data object to the top of the scope chain.
// Like with sourceURL, we take care to not check the option's prototype,
// as this configuration is a code injection vector.
var variable = hasOwnProperty.call(options, 'variable') && options.variable;
if (!variable) {
source = 'with (obj) {\n' + source + '\n}\n';
}
// Cleanup code by stripping empty strings.
source = (isEvaluating ? source.replace(reEmptyStringLeading, '') : source)
.replace(reEmptyStringMiddle, '$1')
.replace(reEmptyStringTrailing, '$1;');
// Frame code as the function body.
source = 'function(' + (variable || 'obj') + ') {\n' +
(variable
? ''
: 'obj || (obj = {});\n'
) +
"var __t, __p = ''" +
(isEscaping
? ', __e = _.escape'
: ''
) +
(isEvaluating
? ', __j = Array.prototype.join;\n' +
"function print() { __p += __j.call(arguments, '') }\n"
: ';\n'
) +
source +
'return __p\n}';
var result = attempt(function() {
return Function(importsKeys, sourceURL + 'return ' + source)
.apply(undefined, importsValues);
});
// Provide the compiled function's source by its `toString` method or
// the `source` property as a convenience for inlining compiled templates.
result.source = source;
if (isError(result)) {
throw result;
}
return result;
}
module.exports = template; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/template.js | template.js |
var assignMergeValue = require('lodash/_assignMergeValue'),
cloneBuffer = require('lodash/_cloneBuffer'),
cloneTypedArray = require('lodash/_cloneTypedArray'),
copyArray = require('lodash/_copyArray'),
initCloneObject = require('lodash/_initCloneObject'),
isArguments = require('lodash/isArguments'),
isArray = require('lodash/isArray'),
isArrayLikeObject = require('lodash/isArrayLikeObject'),
isBuffer = require('lodash/isBuffer'),
isFunction = require('lodash/isFunction'),
isObject = require('lodash/isObject'),
isPlainObject = require('lodash/isPlainObject'),
isTypedArray = require('lodash/isTypedArray'),
safeGet = require('lodash/_safeGet'),
toPlainObject = require('lodash/toPlainObject');
/**
* A specialized version of `baseMerge` for arrays and objects which performs
* deep merges and tracks traversed objects enabling objects with circular
* references to be merged.
*
* @private
* @param {Object} object The destination object.
* @param {Object} source The source object.
* @param {string} key The key of the value to merge.
* @param {number} srcIndex The index of `source`.
* @param {Function} mergeFunc The function to merge values.
* @param {Function} [customizer] The function to customize assigned values.
* @param {Object} [stack] Tracks traversed source values and their merged
* counterparts.
*/
function baseMergeDeep(object, source, key, srcIndex, mergeFunc, customizer, stack) {
var objValue = safeGet(object, key),
srcValue = safeGet(source, key),
stacked = stack.get(srcValue);
if (stacked) {
assignMergeValue(object, key, stacked);
return;
}
var newValue = customizer
? customizer(objValue, srcValue, (key + ''), object, source, stack)
: undefined;
var isCommon = newValue === undefined;
if (isCommon) {
var isArr = isArray(srcValue),
isBuff = !isArr && isBuffer(srcValue),
isTyped = !isArr && !isBuff && isTypedArray(srcValue);
newValue = srcValue;
if (isArr || isBuff || isTyped) {
if (isArray(objValue)) {
newValue = objValue;
}
else if (isArrayLikeObject(objValue)) {
newValue = copyArray(objValue);
}
else if (isBuff) {
isCommon = false;
newValue = cloneBuffer(srcValue, true);
}
else if (isTyped) {
isCommon = false;
newValue = cloneTypedArray(srcValue, true);
}
else {
newValue = [];
}
}
else if (isPlainObject(srcValue) || isArguments(srcValue)) {
newValue = objValue;
if (isArguments(objValue)) {
newValue = toPlainObject(objValue);
}
else if (!isObject(objValue) || isFunction(objValue)) {
newValue = initCloneObject(srcValue);
}
}
else {
isCommon = false;
}
}
if (isCommon) {
// Recursively merge objects and arrays (susceptible to call stack limits).
stack.set(srcValue, newValue);
mergeFunc(newValue, srcValue, srcIndex, customizer, stack);
stack['delete'](srcValue);
}
assignMergeValue(object, key, newValue);
}
module.exports = baseMergeDeep; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_baseMergeDeep.js | _baseMergeDeep.js |
var MapCache = require('lodash/_MapCache');
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/**
* Creates a function that memoizes the result of `func`. If `resolver` is
* provided, it determines the cache key for storing the result based on the
* arguments provided to the memoized function. By default, the first argument
* provided to the memoized function is used as the map cache key. The `func`
* is invoked with the `this` binding of the memoized function.
*
* **Note:** The cache is exposed as the `cache` property on the memoized
* function. Its creation may be customized by replacing the `_.memoize.Cache`
* constructor with one whose instances implement the
* [`Map`](http://ecma-international.org/ecma-262/7.0/#sec-properties-of-the-map-prototype-object)
* method interface of `clear`, `delete`, `get`, `has`, and `set`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to have its output memoized.
* @param {Function} [resolver] The function to resolve the cache key.
* @returns {Function} Returns the new memoized function.
* @example
*
* var object = { 'a': 1, 'b': 2 };
* var other = { 'c': 3, 'd': 4 };
*
* var values = _.memoize(_.values);
* values(object);
* // => [1, 2]
*
* values(other);
* // => [3, 4]
*
* object.a = 2;
* values(object);
* // => [1, 2]
*
* // Modify the result cache.
* values.cache.set(object, ['a', 'b']);
* values(object);
* // => ['a', 'b']
*
* // Replace `_.memoize.Cache`.
* _.memoize.Cache = WeakMap;
*/
function memoize(func, resolver) {
if (typeof func != 'function' || (resolver != null && typeof resolver != 'function')) {
throw new TypeError(FUNC_ERROR_TEXT);
}
var memoized = function() {
var args = arguments,
key = resolver ? resolver.apply(this, args) : args[0],
cache = memoized.cache;
if (cache.has(key)) {
return cache.get(key);
}
var result = func.apply(this, args);
memoized.cache = cache.set(key, result) || cache;
return result;
};
memoized.cache = new (memoize.Cache || MapCache);
return memoized;
}
// Expose `MapCache`.
memoize.Cache = MapCache;
module.exports = memoize; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/memoize.js | memoize.js |
module.exports = {
'castArray': require('lodash/castArray'),
'clone': require('lodash/clone'),
'cloneDeep': require('lodash/cloneDeep'),
'cloneDeepWith': require('lodash/cloneDeepWith'),
'cloneWith': require('lodash/cloneWith'),
'conformsTo': require('lodash/conformsTo'),
'eq': require('lodash/eq'),
'gt': require('lodash/gt'),
'gte': require('lodash/gte'),
'isArguments': require('lodash/isArguments'),
'isArray': require('lodash/isArray'),
'isArrayBuffer': require('lodash/isArrayBuffer'),
'isArrayLike': require('lodash/isArrayLike'),
'isArrayLikeObject': require('lodash/isArrayLikeObject'),
'isBoolean': require('lodash/isBoolean'),
'isBuffer': require('lodash/isBuffer'),
'isDate': require('lodash/isDate'),
'isElement': require('lodash/isElement'),
'isEmpty': require('lodash/isEmpty'),
'isEqual': require('lodash/isEqual'),
'isEqualWith': require('lodash/isEqualWith'),
'isError': require('lodash/isError'),
'isFinite': require('lodash/isFinite'),
'isFunction': require('lodash/isFunction'),
'isInteger': require('lodash/isInteger'),
'isLength': require('lodash/isLength'),
'isMap': require('lodash/isMap'),
'isMatch': require('lodash/isMatch'),
'isMatchWith': require('lodash/isMatchWith'),
'isNaN': require('lodash/isNaN'),
'isNative': require('lodash/isNative'),
'isNil': require('lodash/isNil'),
'isNull': require('lodash/isNull'),
'isNumber': require('lodash/isNumber'),
'isObject': require('lodash/isObject'),
'isObjectLike': require('lodash/isObjectLike'),
'isPlainObject': require('lodash/isPlainObject'),
'isRegExp': require('lodash/isRegExp'),
'isSafeInteger': require('lodash/isSafeInteger'),
'isSet': require('lodash/isSet'),
'isString': require('lodash/isString'),
'isSymbol': require('lodash/isSymbol'),
'isTypedArray': require('lodash/isTypedArray'),
'isUndefined': require('lodash/isUndefined'),
'isWeakMap': require('lodash/isWeakMap'),
'isWeakSet': require('lodash/isWeakSet'),
'lt': require('lodash/lt'),
'lte': require('lodash/lte'),
'toArray': require('lodash/toArray'),
'toFinite': require('lodash/toFinite'),
'toInteger': require('lodash/toInteger'),
'toLength': require('lodash/toLength'),
'toNumber': require('lodash/toNumber'),
'toPlainObject': require('lodash/toPlainObject'),
'toSafeInteger': require('lodash/toSafeInteger'),
'toString': require('lodash/toString')
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/lang.js | lang.js |
var rsAstralRange = '\\ud800-\\udfff',
rsComboMarksRange = '\\u0300-\\u036f',
reComboHalfMarksRange = '\\ufe20-\\ufe2f',
rsComboSymbolsRange = '\\u20d0-\\u20ff',
rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,
rsDingbatRange = '\\u2700-\\u27bf',
rsLowerRange = 'a-z\\xdf-\\xf6\\xf8-\\xff',
rsMathOpRange = '\\xac\\xb1\\xd7\\xf7',
rsNonCharRange = '\\x00-\\x2f\\x3a-\\x40\\x5b-\\x60\\x7b-\\xbf',
rsPunctuationRange = '\\u2000-\\u206f',
rsSpaceRange = ' \\t\\x0b\\f\\xa0\\ufeff\\n\\r\\u2028\\u2029\\u1680\\u180e\\u2000\\u2001\\u2002\\u2003\\u2004\\u2005\\u2006\\u2007\\u2008\\u2009\\u200a\\u202f\\u205f\\u3000',
rsUpperRange = 'A-Z\\xc0-\\xd6\\xd8-\\xde',
rsVarRange = '\\ufe0e\\ufe0f',
rsBreakRange = rsMathOpRange + rsNonCharRange + rsPunctuationRange + rsSpaceRange;
/** Used to compose unicode capture groups. */
var rsApos = "['\u2019]",
rsBreak = '[' + rsBreakRange + ']',
rsCombo = '[' + rsComboRange + ']',
rsDigits = '\\d+',
rsDingbat = '[' + rsDingbatRange + ']',
rsLower = '[' + rsLowerRange + ']',
rsMisc = '[^' + rsAstralRange + rsBreakRange + rsDigits + rsDingbatRange + rsLowerRange + rsUpperRange + ']',
rsFitz = '\\ud83c[\\udffb-\\udfff]',
rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',
rsNonAstral = '[^' + rsAstralRange + ']',
rsRegional = '(?:\\ud83c[\\udde6-\\uddff]){2}',
rsSurrPair = '[\\ud800-\\udbff][\\udc00-\\udfff]',
rsUpper = '[' + rsUpperRange + ']',
rsZWJ = '\\u200d';
/** Used to compose unicode regexes. */
var rsMiscLower = '(?:' + rsLower + '|' + rsMisc + ')',
rsMiscUpper = '(?:' + rsUpper + '|' + rsMisc + ')',
rsOptContrLower = '(?:' + rsApos + '(?:d|ll|m|re|s|t|ve))?',
rsOptContrUpper = '(?:' + rsApos + '(?:D|LL|M|RE|S|T|VE))?',
reOptMod = rsModifier + '?',
rsOptVar = '[' + rsVarRange + ']?',
rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',
rsOrdLower = '\\d*(?:1st|2nd|3rd|(?![123])\\dth)(?=\\b|[A-Z_])',
rsOrdUpper = '\\d*(?:1ST|2ND|3RD|(?![123])\\dTH)(?=\\b|[a-z_])',
rsSeq = rsOptVar + reOptMod + rsOptJoin,
rsEmoji = '(?:' + [rsDingbat, rsRegional, rsSurrPair].join('|') + ')' + rsSeq;
/** Used to match complex or compound words. */
var reUnicodeWord = RegExp([
rsUpper + '?' + rsLower + '+' + rsOptContrLower + '(?=' + [rsBreak, rsUpper, '$'].join('|') + ')',
rsMiscUpper + '+' + rsOptContrUpper + '(?=' + [rsBreak, rsUpper + rsMiscLower, '$'].join('|') + ')',
rsUpper + '?' + rsMiscLower + '+' + rsOptContrLower,
rsUpper + '+' + rsOptContrUpper,
rsOrdUpper,
rsOrdLower,
rsDigits,
rsEmoji
].join('|'), 'g');
/**
* Splits a Unicode `string` into an array of its words.
*
* @private
* @param {string} The string to inspect.
* @returns {Array} Returns the words of `string`.
*/
function unicodeWords(string) {
return string.match(reUnicodeWord) || [];
}
module.exports = unicodeWords; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_unicodeWords.js | _unicodeWords.js |
;(function() {
/** Used as a safe reference for `undefined` in pre-ES5 environments. */
var undefined;
/** Used as the semantic version number. */
var VERSION = '4.17.15';
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/** Used to compose bitmasks for value comparisons. */
var COMPARE_PARTIAL_FLAG = 1,
COMPARE_UNORDERED_FLAG = 2;
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_PARTIAL_FLAG = 32;
/** Used as references for various `Number` constants. */
var INFINITY = 1 / 0,
MAX_SAFE_INTEGER = 9007199254740991;
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
asyncTag = '[object AsyncFunction]',
boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
funcTag = '[object Function]',
genTag = '[object GeneratorFunction]',
numberTag = '[object Number]',
objectTag = '[object Object]',
proxyTag = '[object Proxy]',
regexpTag = '[object RegExp]',
stringTag = '[object String]';
/** Used to match HTML entities and HTML characters. */
var reUnescapedHtml = /[&<>"']/g,
reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
/** Used to detect unsigned integer values. */
var reIsUint = /^(?:0|[1-9]\d*)$/;
/** Used to map characters to HTML entities. */
var htmlEscapes = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
};
/** Detect free variable `global` from Node.js. */
var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
/** Detect free variable `self`. */
var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
/** Used as a reference to the global object. */
var root = freeGlobal || freeSelf || Function('return this')();
/** Detect free variable `exports`. */
var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
/** Detect free variable `module`. */
var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
/*--------------------------------------------------------------------------*/
/**
* Appends the elements of `values` to `array`.
*
* @private
* @param {Array} array The array to modify.
* @param {Array} values The values to append.
* @returns {Array} Returns `array`.
*/
function arrayPush(array, values) {
array.push.apply(array, values);
return array;
}
/**
* The base implementation of `_.findIndex` and `_.findLastIndex` without
* support for iteratee shorthands.
*
* @private
* @param {Array} array The array to inspect.
* @param {Function} predicate The function invoked per iteration.
* @param {number} fromIndex The index to search from.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {number} Returns the index of the matched value, else `-1`.
*/
function baseFindIndex(array, predicate, fromIndex, fromRight) {
var length = array.length,
index = fromIndex + (fromRight ? 1 : -1);
while ((fromRight ? index-- : ++index < length)) {
if (predicate(array[index], index, array)) {
return index;
}
}
return -1;
}
/**
* The base implementation of `_.property` without support for deep paths.
*
* @private
* @param {string} key The key of the property to get.
* @returns {Function} Returns the new accessor function.
*/
function baseProperty(key) {
return function(object) {
return object == null ? undefined : object[key];
};
}
/**
* The base implementation of `_.propertyOf` without support for deep paths.
*
* @private
* @param {Object} object The object to query.
* @returns {Function} Returns the new accessor function.
*/
function basePropertyOf(object) {
return function(key) {
return object == null ? undefined : object[key];
};
}
/**
* The base implementation of `_.reduce` and `_.reduceRight`, without support
* for iteratee shorthands, which iterates over `collection` using `eachFunc`.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {*} accumulator The initial value.
* @param {boolean} initAccum Specify using the first or last element of
* `collection` as the initial value.
* @param {Function} eachFunc The function to iterate over `collection`.
* @returns {*} Returns the accumulated value.
*/
function baseReduce(collection, iteratee, accumulator, initAccum, eachFunc) {
eachFunc(collection, function(value, index, collection) {
accumulator = initAccum
? (initAccum = false, value)
: iteratee(accumulator, value, index, collection);
});
return accumulator;
}
/**
* The base implementation of `_.values` and `_.valuesIn` which creates an
* array of `object` property values corresponding to the property names
* of `props`.
*
* @private
* @param {Object} object The object to query.
* @param {Array} props The property names to get values for.
* @returns {Object} Returns the array of property values.
*/
function baseValues(object, props) {
return baseMap(props, function(key) {
return object[key];
});
}
/**
* Used by `_.escape` to convert characters to HTML entities.
*
* @private
* @param {string} chr The matched character to escape.
* @returns {string} Returns the escaped character.
*/
var escapeHtmlChar = basePropertyOf(htmlEscapes);
/**
* Creates a unary function that invokes `func` with its argument transformed.
*
* @private
* @param {Function} func The function to wrap.
* @param {Function} transform The argument transform.
* @returns {Function} Returns the new function.
*/
function overArg(func, transform) {
return function(arg) {
return func(transform(arg));
};
}
/*--------------------------------------------------------------------------*/
/** Used for built-in method references. */
var arrayProto = Array.prototype,
objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/** Used to generate unique IDs. */
var idCounter = 0;
/**
* Used to resolve the
* [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
* of values.
*/
var nativeObjectToString = objectProto.toString;
/** Used to restore the original `_` reference in `_.noConflict`. */
var oldDash = root._;
/** Built-in value references. */
var objectCreate = Object.create,
propertyIsEnumerable = objectProto.propertyIsEnumerable;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeIsFinite = root.isFinite,
nativeKeys = overArg(Object.keys, Object),
nativeMax = Math.max;
/*------------------------------------------------------------------------*/
/**
* Creates a `lodash` object which wraps `value` to enable implicit method
* chain sequences. Methods that operate on and return arrays, collections,
* and functions can be chained together. Methods that retrieve a single value
* or may return a primitive value will automatically end the chain sequence
* and return the unwrapped value. Otherwise, the value must be unwrapped
* with `_#value`.
*
* Explicit chain sequences, which must be unwrapped with `_#value`, may be
* enabled using `_.chain`.
*
* The execution of chained methods is lazy, that is, it's deferred until
* `_#value` is implicitly or explicitly called.
*
* Lazy evaluation allows several methods to support shortcut fusion.
* Shortcut fusion is an optimization to merge iteratee calls; this avoids
* the creation of intermediate arrays and can greatly reduce the number of
* iteratee executions. Sections of a chain sequence qualify for shortcut
* fusion if the section is applied to an array and iteratees accept only
* one argument. The heuristic for whether a section qualifies for shortcut
* fusion is subject to change.
*
* Chaining is supported in custom builds as long as the `_#value` method is
* directly or indirectly included in the build.
*
* In addition to lodash methods, wrappers have `Array` and `String` methods.
*
* The wrapper `Array` methods are:
* `concat`, `join`, `pop`, `push`, `shift`, `sort`, `splice`, and `unshift`
*
* The wrapper `String` methods are:
* `replace` and `split`
*
* The wrapper methods that support shortcut fusion are:
* `at`, `compact`, `drop`, `dropRight`, `dropWhile`, `filter`, `find`,
* `findLast`, `head`, `initial`, `last`, `map`, `reject`, `reverse`, `slice`,
* `tail`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, and `toArray`
*
* The chainable wrapper methods are:
* `after`, `ary`, `assign`, `assignIn`, `assignInWith`, `assignWith`, `at`,
* `before`, `bind`, `bindAll`, `bindKey`, `castArray`, `chain`, `chunk`,
* `commit`, `compact`, `concat`, `conforms`, `constant`, `countBy`, `create`,
* `curry`, `debounce`, `defaults`, `defaultsDeep`, `defer`, `delay`,
* `difference`, `differenceBy`, `differenceWith`, `drop`, `dropRight`,
* `dropRightWhile`, `dropWhile`, `extend`, `extendWith`, `fill`, `filter`,
* `flatMap`, `flatMapDeep`, `flatMapDepth`, `flatten`, `flattenDeep`,
* `flattenDepth`, `flip`, `flow`, `flowRight`, `fromPairs`, `functions`,
* `functionsIn`, `groupBy`, `initial`, `intersection`, `intersectionBy`,
* `intersectionWith`, `invert`, `invertBy`, `invokeMap`, `iteratee`, `keyBy`,
* `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`, `matchesProperty`,
* `memoize`, `merge`, `mergeWith`, `method`, `methodOf`, `mixin`, `negate`,
* `nthArg`, `omit`, `omitBy`, `once`, `orderBy`, `over`, `overArgs`,
* `overEvery`, `overSome`, `partial`, `partialRight`, `partition`, `pick`,
* `pickBy`, `plant`, `property`, `propertyOf`, `pull`, `pullAll`, `pullAllBy`,
* `pullAllWith`, `pullAt`, `push`, `range`, `rangeRight`, `rearg`, `reject`,
* `remove`, `rest`, `reverse`, `sampleSize`, `set`, `setWith`, `shuffle`,
* `slice`, `sort`, `sortBy`, `splice`, `spread`, `tail`, `take`, `takeRight`,
* `takeRightWhile`, `takeWhile`, `tap`, `throttle`, `thru`, `toArray`,
* `toPairs`, `toPairsIn`, `toPath`, `toPlainObject`, `transform`, `unary`,
* `union`, `unionBy`, `unionWith`, `uniq`, `uniqBy`, `uniqWith`, `unset`,
* `unshift`, `unzip`, `unzipWith`, `update`, `updateWith`, `values`,
* `valuesIn`, `without`, `wrap`, `xor`, `xorBy`, `xorWith`, `zip`,
* `zipObject`, `zipObjectDeep`, and `zipWith`
*
* The wrapper methods that are **not** chainable by default are:
* `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clamp`, `clone`,
* `cloneDeep`, `cloneDeepWith`, `cloneWith`, `conformsTo`, `deburr`,
* `defaultTo`, `divide`, `each`, `eachRight`, `endsWith`, `eq`, `escape`,
* `escapeRegExp`, `every`, `find`, `findIndex`, `findKey`, `findLast`,
* `findLastIndex`, `findLastKey`, `first`, `floor`, `forEach`, `forEachRight`,
* `forIn`, `forInRight`, `forOwn`, `forOwnRight`, `get`, `gt`, `gte`, `has`,
* `hasIn`, `head`, `identity`, `includes`, `indexOf`, `inRange`, `invoke`,
* `isArguments`, `isArray`, `isArrayBuffer`, `isArrayLike`, `isArrayLikeObject`,
* `isBoolean`, `isBuffer`, `isDate`, `isElement`, `isEmpty`, `isEqual`,
* `isEqualWith`, `isError`, `isFinite`, `isFunction`, `isInteger`, `isLength`,
* `isMap`, `isMatch`, `isMatchWith`, `isNaN`, `isNative`, `isNil`, `isNull`,
* `isNumber`, `isObject`, `isObjectLike`, `isPlainObject`, `isRegExp`,
* `isSafeInteger`, `isSet`, `isString`, `isUndefined`, `isTypedArray`,
* `isWeakMap`, `isWeakSet`, `join`, `kebabCase`, `last`, `lastIndexOf`,
* `lowerCase`, `lowerFirst`, `lt`, `lte`, `max`, `maxBy`, `mean`, `meanBy`,
* `min`, `minBy`, `multiply`, `noConflict`, `noop`, `now`, `nth`, `pad`,
* `padEnd`, `padStart`, `parseInt`, `pop`, `random`, `reduce`, `reduceRight`,
* `repeat`, `result`, `round`, `runInContext`, `sample`, `shift`, `size`,
* `snakeCase`, `some`, `sortedIndex`, `sortedIndexBy`, `sortedLastIndex`,
* `sortedLastIndexBy`, `startCase`, `startsWith`, `stubArray`, `stubFalse`,
* `stubObject`, `stubString`, `stubTrue`, `subtract`, `sum`, `sumBy`,
* `template`, `times`, `toFinite`, `toInteger`, `toJSON`, `toLength`,
* `toLower`, `toNumber`, `toSafeInteger`, `toString`, `toUpper`, `trim`,
* `trimEnd`, `trimStart`, `truncate`, `unescape`, `uniqueId`, `upperCase`,
* `upperFirst`, `value`, and `words`
*
* @name _
* @constructor
* @category Seq
* @param {*} value The value to wrap in a `lodash` instance.
* @returns {Object} Returns the new `lodash` wrapper instance.
* @example
*
* function square(n) {
* return n * n;
* }
*
* var wrapped = _([1, 2, 3]);
*
* // Returns an unwrapped value.
* wrapped.reduce(_.add);
* // => 6
*
* // Returns a wrapped value.
* var squares = wrapped.map(square);
*
* _.isArray(squares);
* // => false
*
* _.isArray(squares.value());
* // => true
*/
function lodash(value) {
return value instanceof LodashWrapper
? value
: new LodashWrapper(value);
}
/**
* The base implementation of `_.create` without support for assigning
* properties to the created object.
*
* @private
* @param {Object} proto The object to inherit from.
* @returns {Object} Returns the new object.
*/
var baseCreate = (function() {
function object() {}
return function(proto) {
if (!isObject(proto)) {
return {};
}
if (objectCreate) {
return objectCreate(proto);
}
object.prototype = proto;
var result = new object;
object.prototype = undefined;
return result;
};
}());
/**
* The base constructor for creating `lodash` wrapper objects.
*
* @private
* @param {*} value The value to wrap.
* @param {boolean} [chainAll] Enable explicit method chain sequences.
*/
function LodashWrapper(value, chainAll) {
this.__wrapped__ = value;
this.__actions__ = [];
this.__chain__ = !!chainAll;
}
LodashWrapper.prototype = baseCreate(lodash.prototype);
LodashWrapper.prototype.constructor = LodashWrapper;
/*------------------------------------------------------------------------*/
/**
* Assigns `value` to `key` of `object` if the existing value is not equivalent
* using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
* for equality comparisons.
*
* @private
* @param {Object} object The object to modify.
* @param {string} key The key of the property to assign.
* @param {*} value The value to assign.
*/
function assignValue(object, key, value) {
var objValue = object[key];
if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
(value === undefined && !(key in object))) {
baseAssignValue(object, key, value);
}
}
/**
* The base implementation of `assignValue` and `assignMergeValue` without
* value checks.
*
* @private
* @param {Object} object The object to modify.
* @param {string} key The key of the property to assign.
* @param {*} value The value to assign.
*/
function baseAssignValue(object, key, value) {
object[key] = value;
}
/**
* The base implementation of `_.delay` and `_.defer` which accepts `args`
* to provide to `func`.
*
* @private
* @param {Function} func The function to delay.
* @param {number} wait The number of milliseconds to delay invocation.
* @param {Array} args The arguments to provide to `func`.
* @returns {number|Object} Returns the timer id or timeout object.
*/
function baseDelay(func, wait, args) {
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
return setTimeout(function() { func.apply(undefined, args); }, wait);
}
/**
* The base implementation of `_.forEach` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array|Object} Returns `collection`.
*/
var baseEach = createBaseEach(baseForOwn);
/**
* The base implementation of `_.every` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if all elements pass the predicate check,
* else `false`
*/
function baseEvery(collection, predicate) {
var result = true;
baseEach(collection, function(value, index, collection) {
result = !!predicate(value, index, collection);
return result;
});
return result;
}
/**
* The base implementation of methods like `_.max` and `_.min` which accepts a
* `comparator` to determine the extremum value.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} iteratee The iteratee invoked per iteration.
* @param {Function} comparator The comparator used to compare values.
* @returns {*} Returns the extremum value.
*/
function baseExtremum(array, iteratee, comparator) {
var index = -1,
length = array.length;
while (++index < length) {
var value = array[index],
current = iteratee(value);
if (current != null && (computed === undefined
? (current === current && !false)
: comparator(current, computed)
)) {
var computed = current,
result = value;
}
}
return result;
}
/**
* The base implementation of `_.filter` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {Array} Returns the new filtered array.
*/
function baseFilter(collection, predicate) {
var result = [];
baseEach(collection, function(value, index, collection) {
if (predicate(value, index, collection)) {
result.push(value);
}
});
return result;
}
/**
* The base implementation of `_.flatten` with support for restricting flattening.
*
* @private
* @param {Array} array The array to flatten.
* @param {number} depth The maximum recursion depth.
* @param {boolean} [predicate=isFlattenable] The function invoked per iteration.
* @param {boolean} [isStrict] Restrict to values that pass `predicate` checks.
* @param {Array} [result=[]] The initial result value.
* @returns {Array} Returns the new flattened array.
*/
function baseFlatten(array, depth, predicate, isStrict, result) {
var index = -1,
length = array.length;
predicate || (predicate = isFlattenable);
result || (result = []);
while (++index < length) {
var value = array[index];
if (depth > 0 && predicate(value)) {
if (depth > 1) {
// Recursively flatten arrays (susceptible to call stack limits).
baseFlatten(value, depth - 1, predicate, isStrict, result);
} else {
arrayPush(result, value);
}
} else if (!isStrict) {
result[result.length] = value;
}
}
return result;
}
/**
* The base implementation of `baseForOwn` which iterates over `object`
* properties returned by `keysFunc` and invokes `iteratee` for each property.
* Iteratee functions may exit iteration early by explicitly returning `false`.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {Function} keysFunc The function to get the keys of `object`.
* @returns {Object} Returns `object`.
*/
var baseFor = createBaseFor();
/**
* The base implementation of `_.forOwn` without support for iteratee shorthands.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Object} Returns `object`.
*/
function baseForOwn(object, iteratee) {
return object && baseFor(object, iteratee, keys);
}
/**
* The base implementation of `_.functions` which creates an array of
* `object` function property names filtered from `props`.
*
* @private
* @param {Object} object The object to inspect.
* @param {Array} props The property names to filter.
* @returns {Array} Returns the function names.
*/
function baseFunctions(object, props) {
return baseFilter(props, function(key) {
return isFunction(object[key]);
});
}
/**
* The base implementation of `getTag` without fallbacks for buggy environments.
*
* @private
* @param {*} value The value to query.
* @returns {string} Returns the `toStringTag`.
*/
function baseGetTag(value) {
return objectToString(value);
}
/**
* The base implementation of `_.gt` which doesn't coerce arguments.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {boolean} Returns `true` if `value` is greater than `other`,
* else `false`.
*/
function baseGt(value, other) {
return value > other;
}
/**
* The base implementation of `_.isArguments`.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an `arguments` object,
*/
var baseIsArguments = noop;
/**
* The base implementation of `_.isDate` without Node.js optimizations.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a date object, else `false`.
*/
function baseIsDate(value) {
return isObjectLike(value) && baseGetTag(value) == dateTag;
}
/**
* The base implementation of `_.isEqual` which supports partial comparisons
* and tracks traversed objects.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @param {boolean} bitmask The bitmask flags.
* 1 - Unordered comparison
* 2 - Partial comparison
* @param {Function} [customizer] The function to customize comparisons.
* @param {Object} [stack] Tracks traversed `value` and `other` objects.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
*/
function baseIsEqual(value, other, bitmask, customizer, stack) {
if (value === other) {
return true;
}
if (value == null || other == null || (!isObjectLike(value) && !isObjectLike(other))) {
return value !== value && other !== other;
}
return baseIsEqualDeep(value, other, bitmask, customizer, baseIsEqual, stack);
}
/**
* A specialized version of `baseIsEqual` for arrays and objects which performs
* deep comparisons and tracks traversed objects enabling objects with circular
* references to be compared.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} [stack] Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function baseIsEqualDeep(object, other, bitmask, customizer, equalFunc, stack) {
var objIsArr = isArray(object),
othIsArr = isArray(other),
objTag = objIsArr ? arrayTag : baseGetTag(object),
othTag = othIsArr ? arrayTag : baseGetTag(other);
objTag = objTag == argsTag ? objectTag : objTag;
othTag = othTag == argsTag ? objectTag : othTag;
var objIsObj = objTag == objectTag,
othIsObj = othTag == objectTag,
isSameTag = objTag == othTag;
stack || (stack = []);
var objStack = find(stack, function(entry) {
return entry[0] == object;
});
var othStack = find(stack, function(entry) {
return entry[0] == other;
});
if (objStack && othStack) {
return objStack[1] == other;
}
stack.push([object, other]);
stack.push([other, object]);
if (isSameTag && !objIsObj) {
var result = (objIsArr)
? equalArrays(object, other, bitmask, customizer, equalFunc, stack)
: equalByTag(object, other, objTag, bitmask, customizer, equalFunc, stack);
stack.pop();
return result;
}
if (!(bitmask & COMPARE_PARTIAL_FLAG)) {
var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
if (objIsWrapped || othIsWrapped) {
var objUnwrapped = objIsWrapped ? object.value() : object,
othUnwrapped = othIsWrapped ? other.value() : other;
var result = equalFunc(objUnwrapped, othUnwrapped, bitmask, customizer, stack);
stack.pop();
return result;
}
}
if (!isSameTag) {
return false;
}
var result = equalObjects(object, other, bitmask, customizer, equalFunc, stack);
stack.pop();
return result;
}
/**
* The base implementation of `_.isRegExp` without Node.js optimizations.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
*/
function baseIsRegExp(value) {
return isObjectLike(value) && baseGetTag(value) == regexpTag;
}
/**
* The base implementation of `_.iteratee`.
*
* @private
* @param {*} [value=_.identity] The value to convert to an iteratee.
* @returns {Function} Returns the iteratee.
*/
function baseIteratee(func) {
if (typeof func == 'function') {
return func;
}
if (func == null) {
return identity;
}
return (typeof func == 'object' ? baseMatches : baseProperty)(func);
}
/**
* The base implementation of `_.lt` which doesn't coerce arguments.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {boolean} Returns `true` if `value` is less than `other`,
* else `false`.
*/
function baseLt(value, other) {
return value < other;
}
/**
* The base implementation of `_.map` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
*/
function baseMap(collection, iteratee) {
var index = -1,
result = isArrayLike(collection) ? Array(collection.length) : [];
baseEach(collection, function(value, key, collection) {
result[++index] = iteratee(value, key, collection);
});
return result;
}
/**
* The base implementation of `_.matches` which doesn't clone `source`.
*
* @private
* @param {Object} source The object of property values to match.
* @returns {Function} Returns the new spec function.
*/
function baseMatches(source) {
var props = nativeKeys(source);
return function(object) {
var length = props.length;
if (object == null) {
return !length;
}
object = Object(object);
while (length--) {
var key = props[length];
if (!(key in object &&
baseIsEqual(source[key], object[key], COMPARE_PARTIAL_FLAG | COMPARE_UNORDERED_FLAG)
)) {
return false;
}
}
return true;
};
}
/**
* The base implementation of `_.pick` without support for individual
* property identifiers.
*
* @private
* @param {Object} object The source object.
* @param {string[]} paths The property paths to pick.
* @returns {Object} Returns the new object.
*/
function basePick(object, props) {
object = Object(object);
return reduce(props, function(result, key) {
if (key in object) {
result[key] = object[key];
}
return result;
}, {});
}
/**
* The base implementation of `_.rest` which doesn't validate or coerce arguments.
*
* @private
* @param {Function} func The function to apply a rest parameter to.
* @param {number} [start=func.length-1] The start position of the rest parameter.
* @returns {Function} Returns the new function.
*/
function baseRest(func, start) {
return setToString(overRest(func, start, identity), func + '');
}
/**
* The base implementation of `_.slice` without an iteratee call guard.
*
* @private
* @param {Array} array The array to slice.
* @param {number} [start=0] The start position.
* @param {number} [end=array.length] The end position.
* @returns {Array} Returns the slice of `array`.
*/
function baseSlice(array, start, end) {
var index = -1,
length = array.length;
if (start < 0) {
start = -start > length ? 0 : (length + start);
}
end = end > length ? length : end;
if (end < 0) {
end += length;
}
length = start > end ? 0 : ((end - start) >>> 0);
start >>>= 0;
var result = Array(length);
while (++index < length) {
result[index] = array[index + start];
}
return result;
}
/**
* Copies the values of `source` to `array`.
*
* @private
* @param {Array} source The array to copy values from.
* @param {Array} [array=[]] The array to copy values to.
* @returns {Array} Returns `array`.
*/
function copyArray(source) {
return baseSlice(source, 0, source.length);
}
/**
* The base implementation of `_.some` without support for iteratee shorthands.
*
* @private
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
*/
function baseSome(collection, predicate) {
var result;
baseEach(collection, function(value, index, collection) {
result = predicate(value, index, collection);
return !result;
});
return !!result;
}
/**
* The base implementation of `wrapperValue` which returns the result of
* performing a sequence of actions on the unwrapped `value`, where each
* successive action is supplied the return value of the previous.
*
* @private
* @param {*} value The unwrapped value.
* @param {Array} actions Actions to perform to resolve the unwrapped value.
* @returns {*} Returns the resolved value.
*/
function baseWrapperValue(value, actions) {
var result = value;
return reduce(actions, function(result, action) {
return action.func.apply(action.thisArg, arrayPush([result], action.args));
}, result);
}
/**
* Compares values to sort them in ascending order.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {number} Returns the sort order indicator for `value`.
*/
function compareAscending(value, other) {
if (value !== other) {
var valIsDefined = value !== undefined,
valIsNull = value === null,
valIsReflexive = value === value,
valIsSymbol = false;
var othIsDefined = other !== undefined,
othIsNull = other === null,
othIsReflexive = other === other,
othIsSymbol = false;
if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) ||
(valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) ||
(valIsNull && othIsDefined && othIsReflexive) ||
(!valIsDefined && othIsReflexive) ||
!valIsReflexive) {
return 1;
}
if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) ||
(othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) ||
(othIsNull && valIsDefined && valIsReflexive) ||
(!othIsDefined && valIsReflexive) ||
!othIsReflexive) {
return -1;
}
}
return 0;
}
/**
* Copies properties of `source` to `object`.
*
* @private
* @param {Object} source The object to copy properties from.
* @param {Array} props The property identifiers to copy.
* @param {Object} [object={}] The object to copy properties to.
* @param {Function} [customizer] The function to customize copied values.
* @returns {Object} Returns `object`.
*/
function copyObject(source, props, object, customizer) {
var isNew = !object;
object || (object = {});
var index = -1,
length = props.length;
while (++index < length) {
var key = props[index];
var newValue = customizer
? customizer(object[key], source[key], key, object, source)
: undefined;
if (newValue === undefined) {
newValue = source[key];
}
if (isNew) {
baseAssignValue(object, key, newValue);
} else {
assignValue(object, key, newValue);
}
}
return object;
}
/**
* Creates a function like `_.assign`.
*
* @private
* @param {Function} assigner The function to assign values.
* @returns {Function} Returns the new assigner function.
*/
function createAssigner(assigner) {
return baseRest(function(object, sources) {
var index = -1,
length = sources.length,
customizer = length > 1 ? sources[length - 1] : undefined;
customizer = (assigner.length > 3 && typeof customizer == 'function')
? (length--, customizer)
: undefined;
object = Object(object);
while (++index < length) {
var source = sources[index];
if (source) {
assigner(object, source, index, customizer);
}
}
return object;
});
}
/**
* Creates a `baseEach` or `baseEachRight` function.
*
* @private
* @param {Function} eachFunc The function to iterate over a collection.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseEach(eachFunc, fromRight) {
return function(collection, iteratee) {
if (collection == null) {
return collection;
}
if (!isArrayLike(collection)) {
return eachFunc(collection, iteratee);
}
var length = collection.length,
index = fromRight ? length : -1,
iterable = Object(collection);
while ((fromRight ? index-- : ++index < length)) {
if (iteratee(iterable[index], index, iterable) === false) {
break;
}
}
return collection;
};
}
/**
* Creates a base function for methods like `_.forIn` and `_.forOwn`.
*
* @private
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseFor(fromRight) {
return function(object, iteratee, keysFunc) {
var index = -1,
iterable = Object(object),
props = keysFunc(object),
length = props.length;
while (length--) {
var key = props[fromRight ? length : ++index];
if (iteratee(iterable[key], key, iterable) === false) {
break;
}
}
return object;
};
}
/**
* Creates a function that produces an instance of `Ctor` regardless of
* whether it was invoked as part of a `new` expression or by `call` or `apply`.
*
* @private
* @param {Function} Ctor The constructor to wrap.
* @returns {Function} Returns the new wrapped function.
*/
function createCtor(Ctor) {
return function() {
// Use a `switch` statement to work with class constructors. See
// http://ecma-international.org/ecma-262/7.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
// for more details.
var args = arguments;
var thisBinding = baseCreate(Ctor.prototype),
result = Ctor.apply(thisBinding, args);
// Mimic the constructor's `return` behavior.
// See https://es5.github.io/#x13.2.2 for more details.
return isObject(result) ? result : thisBinding;
};
}
/**
* Creates a `_.find` or `_.findLast` function.
*
* @private
* @param {Function} findIndexFunc The function to find the collection index.
* @returns {Function} Returns the new find function.
*/
function createFind(findIndexFunc) {
return function(collection, predicate, fromIndex) {
var iterable = Object(collection);
if (!isArrayLike(collection)) {
var iteratee = baseIteratee(predicate, 3);
collection = keys(collection);
predicate = function(key) { return iteratee(iterable[key], key, iterable); };
}
var index = findIndexFunc(collection, predicate, fromIndex);
return index > -1 ? iterable[iteratee ? collection[index] : index] : undefined;
};
}
/**
* Creates a function that wraps `func` to invoke it with the `this` binding
* of `thisArg` and `partials` prepended to the arguments it receives.
*
* @private
* @param {Function} func The function to wrap.
* @param {number} bitmask The bitmask flags. See `createWrap` for more details.
* @param {*} thisArg The `this` binding of `func`.
* @param {Array} partials The arguments to prepend to those provided to
* the new function.
* @returns {Function} Returns the new wrapped function.
*/
function createPartial(func, bitmask, thisArg, partials) {
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
var isBind = bitmask & WRAP_BIND_FLAG,
Ctor = createCtor(func);
function wrapper() {
var argsIndex = -1,
argsLength = arguments.length,
leftIndex = -1,
leftLength = partials.length,
args = Array(leftLength + argsLength),
fn = (this && this !== root && this instanceof wrapper) ? Ctor : func;
while (++leftIndex < leftLength) {
args[leftIndex] = partials[leftIndex];
}
while (argsLength--) {
args[leftIndex++] = arguments[++argsIndex];
}
return fn.apply(isBind ? thisArg : this, args);
}
return wrapper;
}
/**
* A specialized version of `baseIsEqualDeep` for arrays with support for
* partial deep comparisons.
*
* @private
* @param {Array} array The array to compare.
* @param {Array} other The other array to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `array` and `other` objects.
* @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
*/
function equalArrays(array, other, bitmask, customizer, equalFunc, stack) {
var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
arrLength = array.length,
othLength = other.length;
if (arrLength != othLength && !(isPartial && othLength > arrLength)) {
return false;
}
var index = -1,
result = true,
seen = (bitmask & COMPARE_UNORDERED_FLAG) ? [] : undefined;
// Ignore non-index properties.
while (++index < arrLength) {
var arrValue = array[index],
othValue = other[index];
var compared;
if (compared !== undefined) {
if (compared) {
continue;
}
result = false;
break;
}
// Recursively compare arrays (susceptible to call stack limits).
if (seen) {
if (!baseSome(other, function(othValue, othIndex) {
if (!indexOf(seen, othIndex) &&
(arrValue === othValue || equalFunc(arrValue, othValue, bitmask, customizer, stack))) {
return seen.push(othIndex);
}
})) {
result = false;
break;
}
} else if (!(
arrValue === othValue ||
equalFunc(arrValue, othValue, bitmask, customizer, stack)
)) {
result = false;
break;
}
}
return result;
}
/**
* A specialized version of `baseIsEqualDeep` for comparing objects of
* the same `toStringTag`.
*
* **Note:** This function only supports comparing values with tags of
* `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {string} tag The `toStringTag` of the objects to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalByTag(object, other, tag, bitmask, customizer, equalFunc, stack) {
switch (tag) {
case boolTag:
case dateTag:
case numberTag:
// Coerce booleans to `1` or `0` and dates to milliseconds.
// Invalid dates are coerced to `NaN`.
return eq(+object, +other);
case errorTag:
return object.name == other.name && object.message == other.message;
case regexpTag:
case stringTag:
// Coerce regexes to strings and treat strings, primitives and objects,
// as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring
// for more details.
return object == (other + '');
}
return false;
}
/**
* A specialized version of `baseIsEqualDeep` for objects with support for
* partial deep comparisons.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {number} bitmask The bitmask flags. See `baseIsEqual` for more details.
* @param {Function} customizer The function to customize comparisons.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Object} stack Tracks traversed `object` and `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalObjects(object, other, bitmask, customizer, equalFunc, stack) {
var isPartial = bitmask & COMPARE_PARTIAL_FLAG,
objProps = keys(object),
objLength = objProps.length,
othProps = keys(other),
othLength = othProps.length;
if (objLength != othLength && !isPartial) {
return false;
}
var index = objLength;
while (index--) {
var key = objProps[index];
if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) {
return false;
}
}
var result = true;
var skipCtor = isPartial;
while (++index < objLength) {
key = objProps[index];
var objValue = object[key],
othValue = other[key];
var compared;
// Recursively compare objects (susceptible to call stack limits).
if (!(compared === undefined
? (objValue === othValue || equalFunc(objValue, othValue, bitmask, customizer, stack))
: compared
)) {
result = false;
break;
}
skipCtor || (skipCtor = key == 'constructor');
}
if (result && !skipCtor) {
var objCtor = object.constructor,
othCtor = other.constructor;
// Non `Object` object instances with different constructors are not equal.
if (objCtor != othCtor &&
('constructor' in object && 'constructor' in other) &&
!(typeof objCtor == 'function' && objCtor instanceof objCtor &&
typeof othCtor == 'function' && othCtor instanceof othCtor)) {
result = false;
}
}
return result;
}
/**
* A specialized version of `baseRest` which flattens the rest array.
*
* @private
* @param {Function} func The function to apply a rest parameter to.
* @returns {Function} Returns the new function.
*/
function flatRest(func) {
return setToString(overRest(func, undefined, flatten), func + '');
}
/**
* Checks if `value` is a flattenable `arguments` object or array.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is flattenable, else `false`.
*/
function isFlattenable(value) {
return isArray(value) || isArguments(value);
}
/**
* Checks if `value` is a valid array-like index.
*
* @private
* @param {*} value The value to check.
* @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
* @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
*/
function isIndex(value, length) {
var type = typeof value;
length = length == null ? MAX_SAFE_INTEGER : length;
return !!length &&
(type == 'number' ||
(type != 'symbol' && reIsUint.test(value))) &&
(value > -1 && value % 1 == 0 && value < length);
}
/**
* Checks if the given arguments are from an iteratee call.
*
* @private
* @param {*} value The potential iteratee value argument.
* @param {*} index The potential iteratee index or key argument.
* @param {*} object The potential iteratee object argument.
* @returns {boolean} Returns `true` if the arguments are from an iteratee call,
* else `false`.
*/
function isIterateeCall(value, index, object) {
if (!isObject(object)) {
return false;
}
var type = typeof index;
if (type == 'number'
? (isArrayLike(object) && isIndex(index, object.length))
: (type == 'string' && index in object)
) {
return eq(object[index], value);
}
return false;
}
/**
* This function is like
* [`Object.keys`](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
* except that it includes inherited enumerable properties.
*
* @private
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
*/
function nativeKeysIn(object) {
var result = [];
if (object != null) {
for (var key in Object(object)) {
result.push(key);
}
}
return result;
}
/**
* Converts `value` to a string using `Object.prototype.toString`.
*
* @private
* @param {*} value The value to convert.
* @returns {string} Returns the converted string.
*/
function objectToString(value) {
return nativeObjectToString.call(value);
}
/**
* A specialized version of `baseRest` which transforms the rest array.
*
* @private
* @param {Function} func The function to apply a rest parameter to.
* @param {number} [start=func.length-1] The start position of the rest parameter.
* @param {Function} transform The rest array transform.
* @returns {Function} Returns the new function.
*/
function overRest(func, start, transform) {
start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
return function() {
var args = arguments,
index = -1,
length = nativeMax(args.length - start, 0),
array = Array(length);
while (++index < length) {
array[index] = args[start + index];
}
index = -1;
var otherArgs = Array(start + 1);
while (++index < start) {
otherArgs[index] = args[index];
}
otherArgs[start] = transform(array);
return func.apply(this, otherArgs);
};
}
/**
* Sets the `toString` method of `func` to return `string`.
*
* @private
* @param {Function} func The function to modify.
* @param {Function} string The `toString` result.
* @returns {Function} Returns `func`.
*/
var setToString = identity;
/*------------------------------------------------------------------------*/
/**
* Creates an array with all falsey values removed. The values `false`, `null`,
* `0`, `""`, `undefined`, and `NaN` are falsey.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Array
* @param {Array} array The array to compact.
* @returns {Array} Returns the new array of filtered values.
* @example
*
* _.compact([0, 1, false, 2, '', 3]);
* // => [1, 2, 3]
*/
function compact(array) {
return baseFilter(array, Boolean);
}
/**
* Creates a new array concatenating `array` with any additional arrays
* and/or values.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Array
* @param {Array} array The array to concatenate.
* @param {...*} [values] The values to concatenate.
* @returns {Array} Returns the new concatenated array.
* @example
*
* var array = [1];
* var other = _.concat(array, 2, [3], [[4]]);
*
* console.log(other);
* // => [1, 2, 3, [4]]
*
* console.log(array);
* // => [1]
*/
function concat() {
var length = arguments.length;
if (!length) {
return [];
}
var args = Array(length - 1),
array = arguments[0],
index = length;
while (index--) {
args[index - 1] = arguments[index];
}
return arrayPush(isArray(array) ? copyArray(array) : [array], baseFlatten(args, 1));
}
/**
* This method is like `_.find` except that it returns the index of the first
* element `predicate` returns truthy for instead of the element itself.
*
* @static
* @memberOf _
* @since 1.1.0
* @category Array
* @param {Array} array The array to inspect.
* @param {Function} [predicate=_.identity] The function invoked per iteration.
* @param {number} [fromIndex=0] The index to search from.
* @returns {number} Returns the index of the found element, else `-1`.
* @example
*
* var users = [
* { 'user': 'barney', 'active': false },
* { 'user': 'fred', 'active': false },
* { 'user': 'pebbles', 'active': true }
* ];
*
* _.findIndex(users, function(o) { return o.user == 'barney'; });
* // => 0
*
* // The `_.matches` iteratee shorthand.
* _.findIndex(users, { 'user': 'fred', 'active': false });
* // => 1
*
* // The `_.matchesProperty` iteratee shorthand.
* _.findIndex(users, ['active', false]);
* // => 0
*
* // The `_.property` iteratee shorthand.
* _.findIndex(users, 'active');
* // => 2
*/
function findIndex(array, predicate, fromIndex) {
var length = array == null ? 0 : array.length;
if (!length) {
return -1;
}
var index = fromIndex == null ? 0 : toInteger(fromIndex);
if (index < 0) {
index = nativeMax(length + index, 0);
}
return baseFindIndex(array, baseIteratee(predicate, 3), index);
}
/**
* Flattens `array` a single level deep.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Array
* @param {Array} array The array to flatten.
* @returns {Array} Returns the new flattened array.
* @example
*
* _.flatten([1, [2, [3, [4]], 5]]);
* // => [1, 2, [3, [4]], 5]
*/
function flatten(array) {
var length = array == null ? 0 : array.length;
return length ? baseFlatten(array, 1) : [];
}
/**
* Recursively flattens `array`.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Array
* @param {Array} array The array to flatten.
* @returns {Array} Returns the new flattened array.
* @example
*
* _.flattenDeep([1, [2, [3, [4]], 5]]);
* // => [1, 2, 3, 4, 5]
*/
function flattenDeep(array) {
var length = array == null ? 0 : array.length;
return length ? baseFlatten(array, INFINITY) : [];
}
/**
* Gets the first element of `array`.
*
* @static
* @memberOf _
* @since 0.1.0
* @alias first
* @category Array
* @param {Array} array The array to query.
* @returns {*} Returns the first element of `array`.
* @example
*
* _.head([1, 2, 3]);
* // => 1
*
* _.head([]);
* // => undefined
*/
function head(array) {
return (array && array.length) ? array[0] : undefined;
}
/**
* Gets the index at which the first occurrence of `value` is found in `array`
* using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
* for equality comparisons. If `fromIndex` is negative, it's used as the
* offset from the end of `array`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Array
* @param {Array} array The array to inspect.
* @param {*} value The value to search for.
* @param {number} [fromIndex=0] The index to search from.
* @returns {number} Returns the index of the matched value, else `-1`.
* @example
*
* _.indexOf([1, 2, 1, 2], 2);
* // => 1
*
* // Search from the `fromIndex`.
* _.indexOf([1, 2, 1, 2], 2, 2);
* // => 3
*/
function indexOf(array, value, fromIndex) {
var length = array == null ? 0 : array.length;
if (typeof fromIndex == 'number') {
fromIndex = fromIndex < 0 ? nativeMax(length + fromIndex, 0) : fromIndex;
} else {
fromIndex = 0;
}
var index = (fromIndex || 0) - 1,
isReflexive = value === value;
while (++index < length) {
var other = array[index];
if ((isReflexive ? other === value : other !== other)) {
return index;
}
}
return -1;
}
/**
* Gets the last element of `array`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Array
* @param {Array} array The array to query.
* @returns {*} Returns the last element of `array`.
* @example
*
* _.last([1, 2, 3]);
* // => 3
*/
function last(array) {
var length = array == null ? 0 : array.length;
return length ? array[length - 1] : undefined;
}
/**
* Creates a slice of `array` from `start` up to, but not including, `end`.
*
* **Note:** This method is used instead of
* [`Array#slice`](https://mdn.io/Array/slice) to ensure dense arrays are
* returned.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Array
* @param {Array} array The array to slice.
* @param {number} [start=0] The start position.
* @param {number} [end=array.length] The end position.
* @returns {Array} Returns the slice of `array`.
*/
function slice(array, start, end) {
var length = array == null ? 0 : array.length;
start = start == null ? 0 : +start;
end = end === undefined ? length : +end;
return length ? baseSlice(array, start, end) : [];
}
/*------------------------------------------------------------------------*/
/**
* Creates a `lodash` wrapper instance that wraps `value` with explicit method
* chain sequences enabled. The result of such sequences must be unwrapped
* with `_#value`.
*
* @static
* @memberOf _
* @since 1.3.0
* @category Seq
* @param {*} value The value to wrap.
* @returns {Object} Returns the new `lodash` wrapper instance.
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36 },
* { 'user': 'fred', 'age': 40 },
* { 'user': 'pebbles', 'age': 1 }
* ];
*
* var youngest = _
* .chain(users)
* .sortBy('age')
* .map(function(o) {
* return o.user + ' is ' + o.age;
* })
* .head()
* .value();
* // => 'pebbles is 1'
*/
function chain(value) {
var result = lodash(value);
result.__chain__ = true;
return result;
}
/**
* This method invokes `interceptor` and returns `value`. The interceptor
* is invoked with one argument; (value). The purpose of this method is to
* "tap into" a method chain sequence in order to modify intermediate results.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Seq
* @param {*} value The value to provide to `interceptor`.
* @param {Function} interceptor The function to invoke.
* @returns {*} Returns `value`.
* @example
*
* _([1, 2, 3])
* .tap(function(array) {
* // Mutate input array.
* array.pop();
* })
* .reverse()
* .value();
* // => [2, 1]
*/
function tap(value, interceptor) {
interceptor(value);
return value;
}
/**
* This method is like `_.tap` except that it returns the result of `interceptor`.
* The purpose of this method is to "pass thru" values replacing intermediate
* results in a method chain sequence.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Seq
* @param {*} value The value to provide to `interceptor`.
* @param {Function} interceptor The function to invoke.
* @returns {*} Returns the result of `interceptor`.
* @example
*
* _(' abc ')
* .chain()
* .trim()
* .thru(function(value) {
* return [value];
* })
* .value();
* // => ['abc']
*/
function thru(value, interceptor) {
return interceptor(value);
}
/**
* Creates a `lodash` wrapper instance with explicit method chain sequences enabled.
*
* @name chain
* @memberOf _
* @since 0.1.0
* @category Seq
* @returns {Object} Returns the new `lodash` wrapper instance.
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36 },
* { 'user': 'fred', 'age': 40 }
* ];
*
* // A sequence without explicit chaining.
* _(users).head();
* // => { 'user': 'barney', 'age': 36 }
*
* // A sequence with explicit chaining.
* _(users)
* .chain()
* .head()
* .pick('user')
* .value();
* // => { 'user': 'barney' }
*/
function wrapperChain() {
return chain(this);
}
/**
* Executes the chain sequence to resolve the unwrapped value.
*
* @name value
* @memberOf _
* @since 0.1.0
* @alias toJSON, valueOf
* @category Seq
* @returns {*} Returns the resolved unwrapped value.
* @example
*
* _([1, 2, 3]).value();
* // => [1, 2, 3]
*/
function wrapperValue() {
return baseWrapperValue(this.__wrapped__, this.__actions__);
}
/*------------------------------------------------------------------------*/
/**
* Checks if `predicate` returns truthy for **all** elements of `collection`.
* Iteration is stopped once `predicate` returns falsey. The predicate is
* invoked with three arguments: (value, index|key, collection).
*
* **Note:** This method returns `true` for
* [empty collections](https://en.wikipedia.org/wiki/Empty_set) because
* [everything is true](https://en.wikipedia.org/wiki/Vacuous_truth) of
* elements of empty collections.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [predicate=_.identity] The function invoked per iteration.
* @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
* @returns {boolean} Returns `true` if all elements pass the predicate check,
* else `false`.
* @example
*
* _.every([true, 1, null, 'yes'], Boolean);
* // => false
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': false },
* { 'user': 'fred', 'age': 40, 'active': false }
* ];
*
* // The `_.matches` iteratee shorthand.
* _.every(users, { 'user': 'barney', 'active': false });
* // => false
*
* // The `_.matchesProperty` iteratee shorthand.
* _.every(users, ['active', false]);
* // => true
*
* // The `_.property` iteratee shorthand.
* _.every(users, 'active');
* // => false
*/
function every(collection, predicate, guard) {
predicate = guard ? undefined : predicate;
return baseEvery(collection, baseIteratee(predicate));
}
/**
* Iterates over elements of `collection`, returning an array of all elements
* `predicate` returns truthy for. The predicate is invoked with three
* arguments: (value, index|key, collection).
*
* **Note:** Unlike `_.remove`, this method returns a new array.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [predicate=_.identity] The function invoked per iteration.
* @returns {Array} Returns the new filtered array.
* @see _.reject
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': true },
* { 'user': 'fred', 'age': 40, 'active': false }
* ];
*
* _.filter(users, function(o) { return !o.active; });
* // => objects for ['fred']
*
* // The `_.matches` iteratee shorthand.
* _.filter(users, { 'age': 36, 'active': true });
* // => objects for ['barney']
*
* // The `_.matchesProperty` iteratee shorthand.
* _.filter(users, ['active', false]);
* // => objects for ['fred']
*
* // The `_.property` iteratee shorthand.
* _.filter(users, 'active');
* // => objects for ['barney']
*/
function filter(collection, predicate) {
return baseFilter(collection, baseIteratee(predicate));
}
/**
* Iterates over elements of `collection`, returning the first element
* `predicate` returns truthy for. The predicate is invoked with three
* arguments: (value, index|key, collection).
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to inspect.
* @param {Function} [predicate=_.identity] The function invoked per iteration.
* @param {number} [fromIndex=0] The index to search from.
* @returns {*} Returns the matched element, else `undefined`.
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': true },
* { 'user': 'fred', 'age': 40, 'active': false },
* { 'user': 'pebbles', 'age': 1, 'active': true }
* ];
*
* _.find(users, function(o) { return o.age < 40; });
* // => object for 'barney'
*
* // The `_.matches` iteratee shorthand.
* _.find(users, { 'age': 1, 'active': true });
* // => object for 'pebbles'
*
* // The `_.matchesProperty` iteratee shorthand.
* _.find(users, ['active', false]);
* // => object for 'fred'
*
* // The `_.property` iteratee shorthand.
* _.find(users, 'active');
* // => object for 'barney'
*/
var find = createFind(findIndex);
/**
* Iterates over elements of `collection` and invokes `iteratee` for each element.
* The iteratee is invoked with three arguments: (value, index|key, collection).
* Iteratee functions may exit iteration early by explicitly returning `false`.
*
* **Note:** As with other "Collections" methods, objects with a "length"
* property are iterated like arrays. To avoid this behavior use `_.forIn`
* or `_.forOwn` for object iteration.
*
* @static
* @memberOf _
* @since 0.1.0
* @alias each
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @returns {Array|Object} Returns `collection`.
* @see _.forEachRight
* @example
*
* _.forEach([1, 2], function(value) {
* console.log(value);
* });
* // => Logs `1` then `2`.
*
* _.forEach({ 'a': 1, 'b': 2 }, function(value, key) {
* console.log(key);
* });
* // => Logs 'a' then 'b' (iteration order is not guaranteed).
*/
function forEach(collection, iteratee) {
return baseEach(collection, baseIteratee(iteratee));
}
/**
* Creates an array of values by running each element in `collection` thru
* `iteratee`. The iteratee is invoked with three arguments:
* (value, index|key, collection).
*
* Many lodash methods are guarded to work as iteratees for methods like
* `_.every`, `_.filter`, `_.map`, `_.mapValues`, `_.reject`, and `_.some`.
*
* The guarded methods are:
* `ary`, `chunk`, `curry`, `curryRight`, `drop`, `dropRight`, `every`,
* `fill`, `invert`, `parseInt`, `random`, `range`, `rangeRight`, `repeat`,
* `sampleSize`, `slice`, `some`, `sortBy`, `split`, `take`, `takeRight`,
* `template`, `trim`, `trimEnd`, `trimStart`, and `words`
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
* @example
*
* function square(n) {
* return n * n;
* }
*
* _.map([4, 8], square);
* // => [16, 64]
*
* _.map({ 'a': 4, 'b': 8 }, square);
* // => [16, 64] (iteration order is not guaranteed)
*
* var users = [
* { 'user': 'barney' },
* { 'user': 'fred' }
* ];
*
* // The `_.property` iteratee shorthand.
* _.map(users, 'user');
* // => ['barney', 'fred']
*/
function map(collection, iteratee) {
return baseMap(collection, baseIteratee(iteratee));
}
/**
* Reduces `collection` to a value which is the accumulated result of running
* each element in `collection` thru `iteratee`, where each successive
* invocation is supplied the return value of the previous. If `accumulator`
* is not given, the first element of `collection` is used as the initial
* value. The iteratee is invoked with four arguments:
* (accumulator, value, index|key, collection).
*
* Many lodash methods are guarded to work as iteratees for methods like
* `_.reduce`, `_.reduceRight`, and `_.transform`.
*
* The guarded methods are:
* `assign`, `defaults`, `defaultsDeep`, `includes`, `merge`, `orderBy`,
* and `sortBy`
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @param {*} [accumulator] The initial value.
* @returns {*} Returns the accumulated value.
* @see _.reduceRight
* @example
*
* _.reduce([1, 2], function(sum, n) {
* return sum + n;
* }, 0);
* // => 3
*
* _.reduce({ 'a': 1, 'b': 2, 'c': 1 }, function(result, value, key) {
* (result[value] || (result[value] = [])).push(key);
* return result;
* }, {});
* // => { '1': ['a', 'c'], '2': ['b'] } (iteration order is not guaranteed)
*/
function reduce(collection, iteratee, accumulator) {
return baseReduce(collection, baseIteratee(iteratee), accumulator, arguments.length < 3, baseEach);
}
/**
* Gets the size of `collection` by returning its length for array-like
* values or the number of own enumerable string keyed properties for objects.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object|string} collection The collection to inspect.
* @returns {number} Returns the collection size.
* @example
*
* _.size([1, 2, 3]);
* // => 3
*
* _.size({ 'a': 1, 'b': 2 });
* // => 2
*
* _.size('pebbles');
* // => 7
*/
function size(collection) {
if (collection == null) {
return 0;
}
collection = isArrayLike(collection) ? collection : nativeKeys(collection);
return collection.length;
}
/**
* Checks if `predicate` returns truthy for **any** element of `collection`.
* Iteration is stopped once `predicate` returns truthy. The predicate is
* invoked with three arguments: (value, index|key, collection).
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [predicate=_.identity] The function invoked per iteration.
* @param- {Object} [guard] Enables use as an iteratee for methods like `_.map`.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
* @example
*
* _.some([null, 0, 'yes', false], Boolean);
* // => true
*
* var users = [
* { 'user': 'barney', 'active': true },
* { 'user': 'fred', 'active': false }
* ];
*
* // The `_.matches` iteratee shorthand.
* _.some(users, { 'user': 'barney', 'active': false });
* // => false
*
* // The `_.matchesProperty` iteratee shorthand.
* _.some(users, ['active', false]);
* // => true
*
* // The `_.property` iteratee shorthand.
* _.some(users, 'active');
* // => true
*/
function some(collection, predicate, guard) {
predicate = guard ? undefined : predicate;
return baseSome(collection, baseIteratee(predicate));
}
/**
* Creates an array of elements, sorted in ascending order by the results of
* running each element in a collection thru each iteratee. This method
* performs a stable sort, that is, it preserves the original sort order of
* equal elements. The iteratees are invoked with one argument: (value).
*
* @static
* @memberOf _
* @since 0.1.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {...(Function|Function[])} [iteratees=[_.identity]]
* The iteratees to sort by.
* @returns {Array} Returns the new sorted array.
* @example
*
* var users = [
* { 'user': 'fred', 'age': 48 },
* { 'user': 'barney', 'age': 36 },
* { 'user': 'fred', 'age': 40 },
* { 'user': 'barney', 'age': 34 }
* ];
*
* _.sortBy(users, [function(o) { return o.user; }]);
* // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]]
*
* _.sortBy(users, ['user', 'age']);
* // => objects for [['barney', 34], ['barney', 36], ['fred', 40], ['fred', 48]]
*/
function sortBy(collection, iteratee) {
var index = 0;
iteratee = baseIteratee(iteratee);
return baseMap(baseMap(collection, function(value, key, collection) {
return { 'value': value, 'index': index++, 'criteria': iteratee(value, key, collection) };
}).sort(function(object, other) {
return compareAscending(object.criteria, other.criteria) || (object.index - other.index);
}), baseProperty('value'));
}
/*------------------------------------------------------------------------*/
/**
* Creates a function that invokes `func`, with the `this` binding and arguments
* of the created function, while it's called less than `n` times. Subsequent
* calls to the created function return the result of the last `func` invocation.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Function
* @param {number} n The number of calls at which `func` is no longer invoked.
* @param {Function} func The function to restrict.
* @returns {Function} Returns the new restricted function.
* @example
*
* jQuery(element).on('click', _.before(5, addContactToList));
* // => Allows adding up to 4 contacts to the list.
*/
function before(n, func) {
var result;
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
n = toInteger(n);
return function() {
if (--n > 0) {
result = func.apply(this, arguments);
}
if (n <= 1) {
func = undefined;
}
return result;
};
}
/**
* Creates a function that invokes `func` with the `this` binding of `thisArg`
* and `partials` prepended to the arguments it receives.
*
* The `_.bind.placeholder` value, which defaults to `_` in monolithic builds,
* may be used as a placeholder for partially applied arguments.
*
* **Note:** Unlike native `Function#bind`, this method doesn't set the "length"
* property of bound functions.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to bind.
* @param {*} thisArg The `this` binding of `func`.
* @param {...*} [partials] The arguments to be partially applied.
* @returns {Function} Returns the new bound function.
* @example
*
* function greet(greeting, punctuation) {
* return greeting + ' ' + this.user + punctuation;
* }
*
* var object = { 'user': 'fred' };
*
* var bound = _.bind(greet, object, 'hi');
* bound('!');
* // => 'hi fred!'
*
* // Bound with placeholders.
* var bound = _.bind(greet, object, _, '!');
* bound('hi');
* // => 'hi fred!'
*/
var bind = baseRest(function(func, thisArg, partials) {
return createPartial(func, WRAP_BIND_FLAG | WRAP_PARTIAL_FLAG, thisArg, partials);
});
/**
* Defers invoking the `func` until the current call stack has cleared. Any
* additional arguments are provided to `func` when it's invoked.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to defer.
* @param {...*} [args] The arguments to invoke `func` with.
* @returns {number} Returns the timer id.
* @example
*
* _.defer(function(text) {
* console.log(text);
* }, 'deferred');
* // => Logs 'deferred' after one millisecond.
*/
var defer = baseRest(function(func, args) {
return baseDelay(func, 1, args);
});
/**
* Invokes `func` after `wait` milliseconds. Any additional arguments are
* provided to `func` when it's invoked.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to delay.
* @param {number} wait The number of milliseconds to delay invocation.
* @param {...*} [args] The arguments to invoke `func` with.
* @returns {number} Returns the timer id.
* @example
*
* _.delay(function(text) {
* console.log(text);
* }, 1000, 'later');
* // => Logs 'later' after one second.
*/
var delay = baseRest(function(func, wait, args) {
return baseDelay(func, toNumber(wait) || 0, args);
});
/**
* Creates a function that negates the result of the predicate `func`. The
* `func` predicate is invoked with the `this` binding and arguments of the
* created function.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Function
* @param {Function} predicate The predicate to negate.
* @returns {Function} Returns the new negated function.
* @example
*
* function isEven(n) {
* return n % 2 == 0;
* }
*
* _.filter([1, 2, 3, 4, 5, 6], _.negate(isEven));
* // => [1, 3, 5]
*/
function negate(predicate) {
if (typeof predicate != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
return function() {
var args = arguments;
return !predicate.apply(this, args);
};
}
/**
* Creates a function that is restricted to invoking `func` once. Repeat calls
* to the function return the value of the first invocation. The `func` is
* invoked with the `this` binding and arguments of the created function.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Function
* @param {Function} func The function to restrict.
* @returns {Function} Returns the new restricted function.
* @example
*
* var initialize = _.once(createApplication);
* initialize();
* initialize();
* // => `createApplication` is invoked once
*/
function once(func) {
return before(2, func);
}
/*------------------------------------------------------------------------*/
/**
* Creates a shallow clone of `value`.
*
* **Note:** This method is loosely based on the
* [structured clone algorithm](https://mdn.io/Structured_clone_algorithm)
* and supports cloning arrays, array buffers, booleans, date objects, maps,
* numbers, `Object` objects, regexes, sets, strings, symbols, and typed
* arrays. The own enumerable properties of `arguments` objects are cloned
* as plain objects. An empty object is returned for uncloneable values such
* as error objects, functions, DOM nodes, and WeakMaps.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to clone.
* @returns {*} Returns the cloned value.
* @see _.cloneDeep
* @example
*
* var objects = [{ 'a': 1 }, { 'b': 2 }];
*
* var shallow = _.clone(objects);
* console.log(shallow[0] === objects[0]);
* // => true
*/
function clone(value) {
if (!isObject(value)) {
return value;
}
return isArray(value) ? copyArray(value) : copyObject(value, nativeKeys(value));
}
/**
* Performs a
* [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
* comparison between two values to determine if they are equivalent.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
* @example
*
* var object = { 'a': 1 };
* var other = { 'a': 1 };
*
* _.eq(object, object);
* // => true
*
* _.eq(object, other);
* // => false
*
* _.eq('a', 'a');
* // => true
*
* _.eq('a', Object('a'));
* // => false
*
* _.eq(NaN, NaN);
* // => true
*/
function eq(value, other) {
return value === other || (value !== value && other !== other);
}
/**
* Checks if `value` is likely an `arguments` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an `arguments` object,
* else `false`.
* @example
*
* _.isArguments(function() { return arguments; }());
* // => true
*
* _.isArguments([1, 2, 3]);
* // => false
*/
var isArguments = baseIsArguments(function() { return arguments; }()) ? baseIsArguments : function(value) {
return isObjectLike(value) && hasOwnProperty.call(value, 'callee') &&
!propertyIsEnumerable.call(value, 'callee');
};
/**
* Checks if `value` is classified as an `Array` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an array, else `false`.
* @example
*
* _.isArray([1, 2, 3]);
* // => true
*
* _.isArray(document.body.children);
* // => false
*
* _.isArray('abc');
* // => false
*
* _.isArray(_.noop);
* // => false
*/
var isArray = Array.isArray;
/**
* Checks if `value` is array-like. A value is considered array-like if it's
* not a function and has a `value.length` that's an integer greater than or
* equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is array-like, else `false`.
* @example
*
* _.isArrayLike([1, 2, 3]);
* // => true
*
* _.isArrayLike(document.body.children);
* // => true
*
* _.isArrayLike('abc');
* // => true
*
* _.isArrayLike(_.noop);
* // => false
*/
function isArrayLike(value) {
return value != null && isLength(value.length) && !isFunction(value);
}
/**
* Checks if `value` is classified as a boolean primitive or object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a boolean, else `false`.
* @example
*
* _.isBoolean(false);
* // => true
*
* _.isBoolean(null);
* // => false
*/
function isBoolean(value) {
return value === true || value === false ||
(isObjectLike(value) && baseGetTag(value) == boolTag);
}
/**
* Checks if `value` is classified as a `Date` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a date object, else `false`.
* @example
*
* _.isDate(new Date);
* // => true
*
* _.isDate('Mon April 23 2012');
* // => false
*/
var isDate = baseIsDate;
/**
* Checks if `value` is an empty object, collection, map, or set.
*
* Objects are considered empty if they have no own enumerable string keyed
* properties.
*
* Array-like values such as `arguments` objects, arrays, buffers, strings, or
* jQuery-like collections are considered empty if they have a `length` of `0`.
* Similarly, maps and sets are considered empty if they have a `size` of `0`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is empty, else `false`.
* @example
*
* _.isEmpty(null);
* // => true
*
* _.isEmpty(true);
* // => true
*
* _.isEmpty(1);
* // => true
*
* _.isEmpty([1, 2, 3]);
* // => false
*
* _.isEmpty({ 'a': 1 });
* // => false
*/
function isEmpty(value) {
if (isArrayLike(value) &&
(isArray(value) || isString(value) ||
isFunction(value.splice) || isArguments(value))) {
return !value.length;
}
return !nativeKeys(value).length;
}
/**
* Performs a deep comparison between two values to determine if they are
* equivalent.
*
* **Note:** This method supports comparing arrays, array buffers, booleans,
* date objects, error objects, maps, numbers, `Object` objects, regexes,
* sets, strings, symbols, and typed arrays. `Object` objects are compared
* by their own, not inherited, enumerable properties. Functions and DOM
* nodes are compared by strict equality, i.e. `===`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
* @example
*
* var object = { 'a': 1 };
* var other = { 'a': 1 };
*
* _.isEqual(object, other);
* // => true
*
* object === other;
* // => false
*/
function isEqual(value, other) {
return baseIsEqual(value, other);
}
/**
* Checks if `value` is a finite primitive number.
*
* **Note:** This method is based on
* [`Number.isFinite`](https://mdn.io/Number/isFinite).
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a finite number, else `false`.
* @example
*
* _.isFinite(3);
* // => true
*
* _.isFinite(Number.MIN_VALUE);
* // => true
*
* _.isFinite(Infinity);
* // => false
*
* _.isFinite('3');
* // => false
*/
function isFinite(value) {
return typeof value == 'number' && nativeIsFinite(value);
}
/**
* Checks if `value` is classified as a `Function` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a function, else `false`.
* @example
*
* _.isFunction(_);
* // => true
*
* _.isFunction(/abc/);
* // => false
*/
function isFunction(value) {
if (!isObject(value)) {
return false;
}
// The use of `Object#toString` avoids issues with the `typeof` operator
// in Safari 9 which returns 'object' for typed arrays and other constructors.
var tag = baseGetTag(value);
return tag == funcTag || tag == genTag || tag == asyncTag || tag == proxyTag;
}
/**
* Checks if `value` is a valid array-like length.
*
* **Note:** This method is loosely based on
* [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
* @example
*
* _.isLength(3);
* // => true
*
* _.isLength(Number.MIN_VALUE);
* // => false
*
* _.isLength(Infinity);
* // => false
*
* _.isLength('3');
* // => false
*/
function isLength(value) {
return typeof value == 'number' &&
value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
}
/**
* Checks if `value` is the
* [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
* of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an object, else `false`.
* @example
*
* _.isObject({});
* // => true
*
* _.isObject([1, 2, 3]);
* // => true
*
* _.isObject(_.noop);
* // => true
*
* _.isObject(null);
* // => false
*/
function isObject(value) {
var type = typeof value;
return value != null && (type == 'object' || type == 'function');
}
/**
* Checks if `value` is object-like. A value is object-like if it's not `null`
* and has a `typeof` result of "object".
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is object-like, else `false`.
* @example
*
* _.isObjectLike({});
* // => true
*
* _.isObjectLike([1, 2, 3]);
* // => true
*
* _.isObjectLike(_.noop);
* // => false
*
* _.isObjectLike(null);
* // => false
*/
function isObjectLike(value) {
return value != null && typeof value == 'object';
}
/**
* Checks if `value` is `NaN`.
*
* **Note:** This method is based on
* [`Number.isNaN`](https://mdn.io/Number/isNaN) and is not the same as
* global [`isNaN`](https://mdn.io/isNaN) which returns `true` for
* `undefined` and other non-number values.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
* @example
*
* _.isNaN(NaN);
* // => true
*
* _.isNaN(new Number(NaN));
* // => true
*
* isNaN(undefined);
* // => true
*
* _.isNaN(undefined);
* // => false
*/
function isNaN(value) {
// An `NaN` primitive is the only value that is not equal to itself.
// Perform the `toStringTag` check first to avoid errors with some
// ActiveX objects in IE.
return isNumber(value) && value != +value;
}
/**
* Checks if `value` is `null`.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is `null`, else `false`.
* @example
*
* _.isNull(null);
* // => true
*
* _.isNull(void 0);
* // => false
*/
function isNull(value) {
return value === null;
}
/**
* Checks if `value` is classified as a `Number` primitive or object.
*
* **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are
* classified as numbers, use the `_.isFinite` method.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a number, else `false`.
* @example
*
* _.isNumber(3);
* // => true
*
* _.isNumber(Number.MIN_VALUE);
* // => true
*
* _.isNumber(Infinity);
* // => true
*
* _.isNumber('3');
* // => false
*/
function isNumber(value) {
return typeof value == 'number' ||
(isObjectLike(value) && baseGetTag(value) == numberTag);
}
/**
* Checks if `value` is classified as a `RegExp` object.
*
* @static
* @memberOf _
* @since 0.1.0
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a regexp, else `false`.
* @example
*
* _.isRegExp(/abc/);
* // => true
*
* _.isRegExp('/abc/');
* // => false
*/
var isRegExp = baseIsRegExp;
/**
* Checks if `value` is classified as a `String` primitive or object.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a string, else `false`.
* @example
*
* _.isString('abc');
* // => true
*
* _.isString(1);
* // => false
*/
function isString(value) {
return typeof value == 'string' ||
(!isArray(value) && isObjectLike(value) && baseGetTag(value) == stringTag);
}
/**
* Checks if `value` is `undefined`.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is `undefined`, else `false`.
* @example
*
* _.isUndefined(void 0);
* // => true
*
* _.isUndefined(null);
* // => false
*/
function isUndefined(value) {
return value === undefined;
}
/**
* Converts `value` to an array.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Lang
* @param {*} value The value to convert.
* @returns {Array} Returns the converted array.
* @example
*
* _.toArray({ 'a': 1, 'b': 2 });
* // => [1, 2]
*
* _.toArray('abc');
* // => ['a', 'b', 'c']
*
* _.toArray(1);
* // => []
*
* _.toArray(null);
* // => []
*/
function toArray(value) {
if (!isArrayLike(value)) {
return values(value);
}
return value.length ? copyArray(value) : [];
}
/**
* Converts `value` to an integer.
*
* **Note:** This method is loosely based on
* [`ToInteger`](http://www.ecma-international.org/ecma-262/7.0/#sec-tointeger).
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to convert.
* @returns {number} Returns the converted integer.
* @example
*
* _.toInteger(3.2);
* // => 3
*
* _.toInteger(Number.MIN_VALUE);
* // => 0
*
* _.toInteger(Infinity);
* // => 1.7976931348623157e+308
*
* _.toInteger('3.2');
* // => 3
*/
var toInteger = Number;
/**
* Converts `value` to a number.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to process.
* @returns {number} Returns the number.
* @example
*
* _.toNumber(3.2);
* // => 3.2
*
* _.toNumber(Number.MIN_VALUE);
* // => 5e-324
*
* _.toNumber(Infinity);
* // => Infinity
*
* _.toNumber('3.2');
* // => 3.2
*/
var toNumber = Number;
/**
* Converts `value` to a string. An empty string is returned for `null`
* and `undefined` values. The sign of `-0` is preserved.
*
* @static
* @memberOf _
* @since 4.0.0
* @category Lang
* @param {*} value The value to convert.
* @returns {string} Returns the converted string.
* @example
*
* _.toString(null);
* // => ''
*
* _.toString(-0);
* // => '-0'
*
* _.toString([1, 2, 3]);
* // => '1,2,3'
*/
function toString(value) {
if (typeof value == 'string') {
return value;
}
return value == null ? '' : (value + '');
}
/*------------------------------------------------------------------------*/
/**
* Assigns own enumerable string keyed properties of source objects to the
* destination object. Source objects are applied from left to right.
* Subsequent sources overwrite property assignments of previous sources.
*
* **Note:** This method mutates `object` and is loosely based on
* [`Object.assign`](https://mdn.io/Object/assign).
*
* @static
* @memberOf _
* @since 0.10.0
* @category Object
* @param {Object} object The destination object.
* @param {...Object} [sources] The source objects.
* @returns {Object} Returns `object`.
* @see _.assignIn
* @example
*
* function Foo() {
* this.a = 1;
* }
*
* function Bar() {
* this.c = 3;
* }
*
* Foo.prototype.b = 2;
* Bar.prototype.d = 4;
*
* _.assign({ 'a': 0 }, new Foo, new Bar);
* // => { 'a': 1, 'c': 3 }
*/
var assign = createAssigner(function(object, source) {
copyObject(source, nativeKeys(source), object);
});
/**
* This method is like `_.assign` except that it iterates over own and
* inherited source properties.
*
* **Note:** This method mutates `object`.
*
* @static
* @memberOf _
* @since 4.0.0
* @alias extend
* @category Object
* @param {Object} object The destination object.
* @param {...Object} [sources] The source objects.
* @returns {Object} Returns `object`.
* @see _.assign
* @example
*
* function Foo() {
* this.a = 1;
* }
*
* function Bar() {
* this.c = 3;
* }
*
* Foo.prototype.b = 2;
* Bar.prototype.d = 4;
*
* _.assignIn({ 'a': 0 }, new Foo, new Bar);
* // => { 'a': 1, 'b': 2, 'c': 3, 'd': 4 }
*/
var assignIn = createAssigner(function(object, source) {
copyObject(source, nativeKeysIn(source), object);
});
/**
* Creates an object that inherits from the `prototype` object. If a
* `properties` object is given, its own enumerable string keyed properties
* are assigned to the created object.
*
* @static
* @memberOf _
* @since 2.3.0
* @category Object
* @param {Object} prototype The object to inherit from.
* @param {Object} [properties] The properties to assign to the object.
* @returns {Object} Returns the new object.
* @example
*
* function Shape() {
* this.x = 0;
* this.y = 0;
* }
*
* function Circle() {
* Shape.call(this);
* }
*
* Circle.prototype = _.create(Shape.prototype, {
* 'constructor': Circle
* });
*
* var circle = new Circle;
* circle instanceof Circle;
* // => true
*
* circle instanceof Shape;
* // => true
*/
function create(prototype, properties) {
var result = baseCreate(prototype);
return properties == null ? result : assign(result, properties);
}
/**
* Assigns own and inherited enumerable string keyed properties of source
* objects to the destination object for all destination properties that
* resolve to `undefined`. Source objects are applied from left to right.
* Once a property is set, additional values of the same property are ignored.
*
* **Note:** This method mutates `object`.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The destination object.
* @param {...Object} [sources] The source objects.
* @returns {Object} Returns `object`.
* @see _.defaultsDeep
* @example
*
* _.defaults({ 'a': 1 }, { 'b': 2 }, { 'a': 3 });
* // => { 'a': 1, 'b': 2 }
*/
var defaults = baseRest(function(object, sources) {
object = Object(object);
var index = -1;
var length = sources.length;
var guard = length > 2 ? sources[2] : undefined;
if (guard && isIterateeCall(sources[0], sources[1], guard)) {
length = 1;
}
while (++index < length) {
var source = sources[index];
var props = keysIn(source);
var propsIndex = -1;
var propsLength = props.length;
while (++propsIndex < propsLength) {
var key = props[propsIndex];
var value = object[key];
if (value === undefined ||
(eq(value, objectProto[key]) && !hasOwnProperty.call(object, key))) {
object[key] = source[key];
}
}
}
return object;
});
/**
* Checks if `path` is a direct property of `object`.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @param {Array|string} path The path to check.
* @returns {boolean} Returns `true` if `path` exists, else `false`.
* @example
*
* var object = { 'a': { 'b': 2 } };
* var other = _.create({ 'a': _.create({ 'b': 2 }) });
*
* _.has(object, 'a');
* // => true
*
* _.has(object, 'a.b');
* // => true
*
* _.has(object, ['a', 'b']);
* // => true
*
* _.has(other, 'a');
* // => false
*/
function has(object, path) {
return object != null && hasOwnProperty.call(object, path);
}
/**
* Creates an array of the own enumerable property names of `object`.
*
* **Note:** Non-object values are coerced to objects. See the
* [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
* for more details.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.keys(new Foo);
* // => ['a', 'b'] (iteration order is not guaranteed)
*
* _.keys('hi');
* // => ['0', '1']
*/
var keys = nativeKeys;
/**
* Creates an array of the own and inherited enumerable property names of `object`.
*
* **Note:** Non-object values are coerced to objects.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.keysIn(new Foo);
* // => ['a', 'b', 'c'] (iteration order is not guaranteed)
*/
var keysIn = nativeKeysIn;
/**
* Creates an object composed of the picked `object` properties.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The source object.
* @param {...(string|string[])} [paths] The property paths to pick.
* @returns {Object} Returns the new object.
* @example
*
* var object = { 'a': 1, 'b': '2', 'c': 3 };
*
* _.pick(object, ['a', 'c']);
* // => { 'a': 1, 'c': 3 }
*/
var pick = flatRest(function(object, paths) {
return object == null ? {} : basePick(object, paths);
});
/**
* This method is like `_.get` except that if the resolved value is a
* function it's invoked with the `this` binding of its parent object and
* its result is returned.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @param {Array|string} path The path of the property to resolve.
* @param {*} [defaultValue] The value returned for `undefined` resolved values.
* @returns {*} Returns the resolved value.
* @example
*
* var object = { 'a': [{ 'b': { 'c1': 3, 'c2': _.constant(4) } }] };
*
* _.result(object, 'a[0].b.c1');
* // => 3
*
* _.result(object, 'a[0].b.c2');
* // => 4
*
* _.result(object, 'a[0].b.c3', 'default');
* // => 'default'
*
* _.result(object, 'a[0].b.c3', _.constant('default'));
* // => 'default'
*/
function result(object, path, defaultValue) {
var value = object == null ? undefined : object[path];
if (value === undefined) {
value = defaultValue;
}
return isFunction(value) ? value.call(object) : value;
}
/**
* Creates an array of the own enumerable string keyed property values of `object`.
*
* **Note:** Non-object values are coerced to objects.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property values.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.values(new Foo);
* // => [1, 2] (iteration order is not guaranteed)
*
* _.values('hi');
* // => ['h', 'i']
*/
function values(object) {
return object == null ? [] : baseValues(object, keys(object));
}
/*------------------------------------------------------------------------*/
/**
* Converts the characters "&", "<", ">", '"', and "'" in `string` to their
* corresponding HTML entities.
*
* **Note:** No other characters are escaped. To escape additional
* characters use a third-party library like [_he_](https://mths.be/he).
*
* Though the ">" character is escaped for symmetry, characters like
* ">" and "/" don't need escaping in HTML and have no special meaning
* unless they're part of a tag or unquoted attribute value. See
* [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
* (under "semi-related fun fact") for more details.
*
* When working with HTML you should always
* [quote attribute values](http://wonko.com/post/html-escaping) to reduce
* XSS vectors.
*
* @static
* @since 0.1.0
* @memberOf _
* @category String
* @param {string} [string=''] The string to escape.
* @returns {string} Returns the escaped string.
* @example
*
* _.escape('fred, barney, & pebbles');
* // => 'fred, barney, & pebbles'
*/
function escape(string) {
string = toString(string);
return (string && reHasUnescapedHtml.test(string))
? string.replace(reUnescapedHtml, escapeHtmlChar)
: string;
}
/*------------------------------------------------------------------------*/
/**
* This method returns the first argument it receives.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @param {*} value Any value.
* @returns {*} Returns `value`.
* @example
*
* var object = { 'a': 1 };
*
* console.log(_.identity(object) === object);
* // => true
*/
function identity(value) {
return value;
}
/**
* Creates a function that invokes `func` with the arguments of the created
* function. If `func` is a property name, the created function returns the
* property value for a given element. If `func` is an array or object, the
* created function returns `true` for elements that contain the equivalent
* source properties, otherwise it returns `false`.
*
* @static
* @since 4.0.0
* @memberOf _
* @category Util
* @param {*} [func=_.identity] The value to convert to a callback.
* @returns {Function} Returns the callback.
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': true },
* { 'user': 'fred', 'age': 40, 'active': false }
* ];
*
* // The `_.matches` iteratee shorthand.
* _.filter(users, _.iteratee({ 'user': 'barney', 'active': true }));
* // => [{ 'user': 'barney', 'age': 36, 'active': true }]
*
* // The `_.matchesProperty` iteratee shorthand.
* _.filter(users, _.iteratee(['user', 'fred']));
* // => [{ 'user': 'fred', 'age': 40 }]
*
* // The `_.property` iteratee shorthand.
* _.map(users, _.iteratee('user'));
* // => ['barney', 'fred']
*
* // Create custom iteratee shorthands.
* _.iteratee = _.wrap(_.iteratee, function(iteratee, func) {
* return !_.isRegExp(func) ? iteratee(func) : function(string) {
* return func.test(string);
* };
* });
*
* _.filter(['abc', 'def'], /ef/);
* // => ['def']
*/
var iteratee = baseIteratee;
/**
* Creates a function that performs a partial deep comparison between a given
* object and `source`, returning `true` if the given object has equivalent
* property values, else `false`.
*
* **Note:** The created function is equivalent to `_.isMatch` with `source`
* partially applied.
*
* Partial comparisons will match empty array and empty object `source`
* values against any array or object value, respectively. See `_.isEqual`
* for a list of supported value comparisons.
*
* @static
* @memberOf _
* @since 3.0.0
* @category Util
* @param {Object} source The object of property values to match.
* @returns {Function} Returns the new spec function.
* @example
*
* var objects = [
* { 'a': 1, 'b': 2, 'c': 3 },
* { 'a': 4, 'b': 5, 'c': 6 }
* ];
*
* _.filter(objects, _.matches({ 'a': 4, 'c': 6 }));
* // => [{ 'a': 4, 'b': 5, 'c': 6 }]
*/
function matches(source) {
return baseMatches(assign({}, source));
}
/**
* Adds all own enumerable string keyed function properties of a source
* object to the destination object. If `object` is a function, then methods
* are added to its prototype as well.
*
* **Note:** Use `_.runInContext` to create a pristine `lodash` function to
* avoid conflicts caused by modifying the original.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @param {Function|Object} [object=lodash] The destination object.
* @param {Object} source The object of functions to add.
* @param {Object} [options={}] The options object.
* @param {boolean} [options.chain=true] Specify whether mixins are chainable.
* @returns {Function|Object} Returns `object`.
* @example
*
* function vowels(string) {
* return _.filter(string, function(v) {
* return /[aeiou]/i.test(v);
* });
* }
*
* _.mixin({ 'vowels': vowels });
* _.vowels('fred');
* // => ['e']
*
* _('fred').vowels().value();
* // => ['e']
*
* _.mixin({ 'vowels': vowels }, { 'chain': false });
* _('fred').vowels();
* // => ['e']
*/
function mixin(object, source, options) {
var props = keys(source),
methodNames = baseFunctions(source, props);
if (options == null &&
!(isObject(source) && (methodNames.length || !props.length))) {
options = source;
source = object;
object = this;
methodNames = baseFunctions(source, keys(source));
}
var chain = !(isObject(options) && 'chain' in options) || !!options.chain,
isFunc = isFunction(object);
baseEach(methodNames, function(methodName) {
var func = source[methodName];
object[methodName] = func;
if (isFunc) {
object.prototype[methodName] = function() {
var chainAll = this.__chain__;
if (chain || chainAll) {
var result = object(this.__wrapped__),
actions = result.__actions__ = copyArray(this.__actions__);
actions.push({ 'func': func, 'args': arguments, 'thisArg': object });
result.__chain__ = chainAll;
return result;
}
return func.apply(object, arrayPush([this.value()], arguments));
};
}
});
return object;
}
/**
* Reverts the `_` variable to its previous value and returns a reference to
* the `lodash` function.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @returns {Function} Returns the `lodash` function.
* @example
*
* var lodash = _.noConflict();
*/
function noConflict() {
if (root._ === this) {
root._ = oldDash;
}
return this;
}
/**
* This method returns `undefined`.
*
* @static
* @memberOf _
* @since 2.3.0
* @category Util
* @example
*
* _.times(2, _.noop);
* // => [undefined, undefined]
*/
function noop() {
// No operation performed.
}
/**
* Generates a unique ID. If `prefix` is given, the ID is appended to it.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Util
* @param {string} [prefix=''] The value to prefix the ID with.
* @returns {string} Returns the unique ID.
* @example
*
* _.uniqueId('contact_');
* // => 'contact_104'
*
* _.uniqueId();
* // => '105'
*/
function uniqueId(prefix) {
var id = ++idCounter;
return toString(prefix) + id;
}
/*------------------------------------------------------------------------*/
/**
* Computes the maximum value of `array`. If `array` is empty or falsey,
* `undefined` is returned.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Math
* @param {Array} array The array to iterate over.
* @returns {*} Returns the maximum value.
* @example
*
* _.max([4, 2, 8, 6]);
* // => 8
*
* _.max([]);
* // => undefined
*/
function max(array) {
return (array && array.length)
? baseExtremum(array, identity, baseGt)
: undefined;
}
/**
* Computes the minimum value of `array`. If `array` is empty or falsey,
* `undefined` is returned.
*
* @static
* @since 0.1.0
* @memberOf _
* @category Math
* @param {Array} array The array to iterate over.
* @returns {*} Returns the minimum value.
* @example
*
* _.min([4, 2, 8, 6]);
* // => 2
*
* _.min([]);
* // => undefined
*/
function min(array) {
return (array && array.length)
? baseExtremum(array, identity, baseLt)
: undefined;
}
/*------------------------------------------------------------------------*/
// Add methods that return wrapped values in chain sequences.
lodash.assignIn = assignIn;
lodash.before = before;
lodash.bind = bind;
lodash.chain = chain;
lodash.compact = compact;
lodash.concat = concat;
lodash.create = create;
lodash.defaults = defaults;
lodash.defer = defer;
lodash.delay = delay;
lodash.filter = filter;
lodash.flatten = flatten;
lodash.flattenDeep = flattenDeep;
lodash.iteratee = iteratee;
lodash.keys = keys;
lodash.map = map;
lodash.matches = matches;
lodash.mixin = mixin;
lodash.negate = negate;
lodash.once = once;
lodash.pick = pick;
lodash.slice = slice;
lodash.sortBy = sortBy;
lodash.tap = tap;
lodash.thru = thru;
lodash.toArray = toArray;
lodash.values = values;
// Add aliases.
lodash.extend = assignIn;
// Add methods to `lodash.prototype`.
mixin(lodash, lodash);
/*------------------------------------------------------------------------*/
// Add methods that return unwrapped values in chain sequences.
lodash.clone = clone;
lodash.escape = escape;
lodash.every = every;
lodash.find = find;
lodash.forEach = forEach;
lodash.has = has;
lodash.head = head;
lodash.identity = identity;
lodash.indexOf = indexOf;
lodash.isArguments = isArguments;
lodash.isArray = isArray;
lodash.isBoolean = isBoolean;
lodash.isDate = isDate;
lodash.isEmpty = isEmpty;
lodash.isEqual = isEqual;
lodash.isFinite = isFinite;
lodash.isFunction = isFunction;
lodash.isNaN = isNaN;
lodash.isNull = isNull;
lodash.isNumber = isNumber;
lodash.isObject = isObject;
lodash.isRegExp = isRegExp;
lodash.isString = isString;
lodash.isUndefined = isUndefined;
lodash.last = last;
lodash.max = max;
lodash.min = min;
lodash.noConflict = noConflict;
lodash.noop = noop;
lodash.reduce = reduce;
lodash.result = result;
lodash.size = size;
lodash.some = some;
lodash.uniqueId = uniqueId;
// Add aliases.
lodash.each = forEach;
lodash.first = head;
mixin(lodash, (function() {
var source = {};
baseForOwn(lodash, function(func, methodName) {
if (!hasOwnProperty.call(lodash.prototype, methodName)) {
source[methodName] = func;
}
});
return source;
}()), { 'chain': false });
/*------------------------------------------------------------------------*/
/**
* The semantic version number.
*
* @static
* @memberOf _
* @type {string}
*/
lodash.VERSION = VERSION;
// Add `Array` methods to `lodash.prototype`.
baseEach(['pop', 'join', 'replace', 'reverse', 'split', 'push', 'shift', 'sort', 'splice', 'unshift'], function(methodName) {
var func = (/^(?:replace|split)$/.test(methodName) ? String.prototype : arrayProto)[methodName],
chainName = /^(?:push|sort|unshift)$/.test(methodName) ? 'tap' : 'thru',
retUnwrapped = /^(?:pop|join|replace|shift)$/.test(methodName);
lodash.prototype[methodName] = function() {
var args = arguments;
if (retUnwrapped && !this.__chain__) {
var value = this.value();
return func.apply(isArray(value) ? value : [], args);
}
return this[chainName](function(value) {
return func.apply(isArray(value) ? value : [], args);
});
};
});
// Add chain sequence methods to the `lodash` wrapper.
lodash.prototype.toJSON = lodash.prototype.valueOf = lodash.prototype.value = wrapperValue;
/*--------------------------------------------------------------------------*/
if (freeModule) {
// Export for Node.js.
(freeModule.exports = lodash)._ = lodash;
// Export for CommonJS support.
freeExports._ = lodash;
}
}.call(this)); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/core.js | core.js |
var LodashWrapper = require('lodash/_LodashWrapper'),
flatRest = require('lodash/_flatRest'),
getData = require('lodash/_getData'),
getFuncName = require('lodash/_getFuncName'),
isArray = require('lodash/isArray'),
isLaziable = require('lodash/_isLaziable');
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/** Used to compose bitmasks for function metadata. */
var WRAP_CURRY_FLAG = 8,
WRAP_PARTIAL_FLAG = 32,
WRAP_ARY_FLAG = 128,
WRAP_REARG_FLAG = 256;
/**
* Creates a `_.flow` or `_.flowRight` function.
*
* @private
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new flow function.
*/
function createFlow(fromRight) {
return flatRest(function(funcs) {
var length = funcs.length,
index = length,
prereq = LodashWrapper.prototype.thru;
if (fromRight) {
funcs.reverse();
}
while (index--) {
var func = funcs[index];
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
if (prereq && !wrapper && getFuncName(func) == 'wrapper') {
var wrapper = new LodashWrapper([], true);
}
}
index = wrapper ? index : length;
while (++index < length) {
func = funcs[index];
var funcName = getFuncName(func),
data = funcName == 'wrapper' ? getData(func) : undefined;
if (data && isLaziable(data[0]) &&
data[1] == (WRAP_ARY_FLAG | WRAP_CURRY_FLAG | WRAP_PARTIAL_FLAG | WRAP_REARG_FLAG) &&
!data[4].length && data[9] == 1
) {
wrapper = wrapper[getFuncName(data[0])].apply(wrapper, data[3]);
} else {
wrapper = (func.length == 1 && isLaziable(func))
? wrapper[funcName]()
: wrapper.thru(func);
}
}
return function() {
var args = arguments,
value = args[0];
if (wrapper && args.length == 1 && isArray(value)) {
return wrapper.plant(value).value();
}
var index = 0,
result = length ? funcs[index].apply(this, args) : value;
while (++index < length) {
result = funcs[index].call(this, result);
}
return result;
};
});
}
module.exports = createFlow; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_createFlow.js | _createFlow.js |
var baseSetData = require('lodash/_baseSetData'),
createBind = require('lodash/_createBind'),
createCurry = require('lodash/_createCurry'),
createHybrid = require('lodash/_createHybrid'),
createPartial = require('lodash/_createPartial'),
getData = require('lodash/_getData'),
mergeData = require('lodash/_mergeData'),
setData = require('lodash/_setData'),
setWrapToString = require('lodash/_setWrapToString'),
toInteger = require('lodash/toInteger');
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
/** Used to compose bitmasks for function metadata. */
var WRAP_BIND_FLAG = 1,
WRAP_BIND_KEY_FLAG = 2,
WRAP_CURRY_FLAG = 8,
WRAP_CURRY_RIGHT_FLAG = 16,
WRAP_PARTIAL_FLAG = 32,
WRAP_PARTIAL_RIGHT_FLAG = 64;
/* Built-in method references for those with the same name as other `lodash` methods. */
var nativeMax = Math.max;
/**
* Creates a function that either curries or invokes `func` with optional
* `this` binding and partially applied arguments.
*
* @private
* @param {Function|string} func The function or method name to wrap.
* @param {number} bitmask The bitmask flags.
* 1 - `_.bind`
* 2 - `_.bindKey`
* 4 - `_.curry` or `_.curryRight` of a bound function
* 8 - `_.curry`
* 16 - `_.curryRight`
* 32 - `_.partial`
* 64 - `_.partialRight`
* 128 - `_.rearg`
* 256 - `_.ary`
* 512 - `_.flip`
* @param {*} [thisArg] The `this` binding of `func`.
* @param {Array} [partials] The arguments to be partially applied.
* @param {Array} [holders] The `partials` placeholder indexes.
* @param {Array} [argPos] The argument positions of the new function.
* @param {number} [ary] The arity cap of `func`.
* @param {number} [arity] The arity of `func`.
* @returns {Function} Returns the new wrapped function.
*/
function createWrap(func, bitmask, thisArg, partials, holders, argPos, ary, arity) {
var isBindKey = bitmask & WRAP_BIND_KEY_FLAG;
if (!isBindKey && typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
var length = partials ? partials.length : 0;
if (!length) {
bitmask &= ~(WRAP_PARTIAL_FLAG | WRAP_PARTIAL_RIGHT_FLAG);
partials = holders = undefined;
}
ary = ary === undefined ? ary : nativeMax(toInteger(ary), 0);
arity = arity === undefined ? arity : toInteger(arity);
length -= holders ? holders.length : 0;
if (bitmask & WRAP_PARTIAL_RIGHT_FLAG) {
var partialsRight = partials,
holdersRight = holders;
partials = holders = undefined;
}
var data = isBindKey ? undefined : getData(func);
var newData = [
func, bitmask, thisArg, partials, holders, partialsRight, holdersRight,
argPos, ary, arity
];
if (data) {
mergeData(newData, data);
}
func = newData[0];
bitmask = newData[1];
thisArg = newData[2];
partials = newData[3];
holders = newData[4];
arity = newData[9] = newData[9] === undefined
? (isBindKey ? 0 : func.length)
: nativeMax(newData[9] - length, 0);
if (!arity && bitmask & (WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG)) {
bitmask &= ~(WRAP_CURRY_FLAG | WRAP_CURRY_RIGHT_FLAG);
}
if (!bitmask || bitmask == WRAP_BIND_FLAG) {
var result = createBind(func, bitmask, thisArg);
} else if (bitmask == WRAP_CURRY_FLAG || bitmask == WRAP_CURRY_RIGHT_FLAG) {
result = createCurry(func, bitmask, arity);
} else if ((bitmask == WRAP_PARTIAL_FLAG || bitmask == (WRAP_BIND_FLAG | WRAP_PARTIAL_FLAG)) && !holders.length) {
result = createPartial(func, bitmask, thisArg, partials);
} else {
result = createHybrid.apply(undefined, newData);
}
var setter = data ? baseSetData : setData;
return setWrapToString(setter(result, newData), func, bitmask);
}
module.exports = createWrap; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/_createWrap.js | _createWrap.js |
var mapping = require('lodash/fp/_mapping'),
fallbackHolder = require('lodash/fp/placeholder');
/** Built-in value reference. */
var push = Array.prototype.push;
/**
* Creates a function, with an arity of `n`, that invokes `func` with the
* arguments it receives.
*
* @private
* @param {Function} func The function to wrap.
* @param {number} n The arity of the new function.
* @returns {Function} Returns the new function.
*/
function baseArity(func, n) {
return n == 2
? function(a, b) { return func.apply(undefined, arguments); }
: function(a) { return func.apply(undefined, arguments); };
}
/**
* Creates a function that invokes `func`, with up to `n` arguments, ignoring
* any additional arguments.
*
* @private
* @param {Function} func The function to cap arguments for.
* @param {number} n The arity cap.
* @returns {Function} Returns the new function.
*/
function baseAry(func, n) {
return n == 2
? function(a, b) { return func(a, b); }
: function(a) { return func(a); };
}
/**
* Creates a clone of `array`.
*
* @private
* @param {Array} array The array to clone.
* @returns {Array} Returns the cloned array.
*/
function cloneArray(array) {
var length = array ? array.length : 0,
result = Array(length);
while (length--) {
result[length] = array[length];
}
return result;
}
/**
* Creates a function that clones a given object using the assignment `func`.
*
* @private
* @param {Function} func The assignment function.
* @returns {Function} Returns the new cloner function.
*/
function createCloner(func) {
return function(object) {
return func({}, object);
};
}
/**
* A specialized version of `_.spread` which flattens the spread array into
* the arguments of the invoked `func`.
*
* @private
* @param {Function} func The function to spread arguments over.
* @param {number} start The start position of the spread.
* @returns {Function} Returns the new function.
*/
function flatSpread(func, start) {
return function() {
var length = arguments.length,
lastIndex = length - 1,
args = Array(length);
while (length--) {
args[length] = arguments[length];
}
var array = args[start],
otherArgs = args.slice(0, start);
if (array) {
push.apply(otherArgs, array);
}
if (start != lastIndex) {
push.apply(otherArgs, args.slice(start + 1));
}
return func.apply(this, otherArgs);
};
}
/**
* Creates a function that wraps `func` and uses `cloner` to clone the first
* argument it receives.
*
* @private
* @param {Function} func The function to wrap.
* @param {Function} cloner The function to clone arguments.
* @returns {Function} Returns the new immutable function.
*/
function wrapImmutable(func, cloner) {
return function() {
var length = arguments.length;
if (!length) {
return;
}
var args = Array(length);
while (length--) {
args[length] = arguments[length];
}
var result = args[0] = cloner.apply(undefined, args);
func.apply(undefined, args);
return result;
};
}
/**
* The base implementation of `convert` which accepts a `util` object of methods
* required to perform conversions.
*
* @param {Object} util The util object.
* @param {string} name The name of the function to convert.
* @param {Function} func The function to convert.
* @param {Object} [options] The options object.
* @param {boolean} [options.cap=true] Specify capping iteratee arguments.
* @param {boolean} [options.curry=true] Specify currying.
* @param {boolean} [options.fixed=true] Specify fixed arity.
* @param {boolean} [options.immutable=true] Specify immutable operations.
* @param {boolean} [options.rearg=true] Specify rearranging arguments.
* @returns {Function|Object} Returns the converted function or object.
*/
function baseConvert(util, name, func, options) {
var isLib = typeof name == 'function',
isObj = name === Object(name);
if (isObj) {
options = func;
func = name;
name = undefined;
}
if (func == null) {
throw new TypeError;
}
options || (options = {});
var config = {
'cap': 'cap' in options ? options.cap : true,
'curry': 'curry' in options ? options.curry : true,
'fixed': 'fixed' in options ? options.fixed : true,
'immutable': 'immutable' in options ? options.immutable : true,
'rearg': 'rearg' in options ? options.rearg : true
};
var defaultHolder = isLib ? func : fallbackHolder,
forceCurry = ('curry' in options) && options.curry,
forceFixed = ('fixed' in options) && options.fixed,
forceRearg = ('rearg' in options) && options.rearg,
pristine = isLib ? func.runInContext() : undefined;
var helpers = isLib ? func : {
'ary': util.ary,
'assign': util.assign,
'clone': util.clone,
'curry': util.curry,
'forEach': util.forEach,
'isArray': util.isArray,
'isError': util.isError,
'isFunction': util.isFunction,
'isWeakMap': util.isWeakMap,
'iteratee': util.iteratee,
'keys': util.keys,
'rearg': util.rearg,
'toInteger': util.toInteger,
'toPath': util.toPath
};
var ary = helpers.ary,
assign = helpers.assign,
clone = helpers.clone,
curry = helpers.curry,
each = helpers.forEach,
isArray = helpers.isArray,
isError = helpers.isError,
isFunction = helpers.isFunction,
isWeakMap = helpers.isWeakMap,
keys = helpers.keys,
rearg = helpers.rearg,
toInteger = helpers.toInteger,
toPath = helpers.toPath;
var aryMethodKeys = keys(mapping.aryMethod);
var wrappers = {
'castArray': function(castArray) {
return function() {
var value = arguments[0];
return isArray(value)
? castArray(cloneArray(value))
: castArray.apply(undefined, arguments);
};
},
'iteratee': function(iteratee) {
return function() {
var func = arguments[0],
arity = arguments[1],
result = iteratee(func, arity),
length = result.length;
if (config.cap && typeof arity == 'number') {
arity = arity > 2 ? (arity - 2) : 1;
return (length && length <= arity) ? result : baseAry(result, arity);
}
return result;
};
},
'mixin': function(mixin) {
return function(source) {
var func = this;
if (!isFunction(func)) {
return mixin(func, Object(source));
}
var pairs = [];
each(keys(source), function(key) {
if (isFunction(source[key])) {
pairs.push([key, func.prototype[key]]);
}
});
mixin(func, Object(source));
each(pairs, function(pair) {
var value = pair[1];
if (isFunction(value)) {
func.prototype[pair[0]] = value;
} else {
delete func.prototype[pair[0]];
}
});
return func;
};
},
'nthArg': function(nthArg) {
return function(n) {
var arity = n < 0 ? 1 : (toInteger(n) + 1);
return curry(nthArg(n), arity);
};
},
'rearg': function(rearg) {
return function(func, indexes) {
var arity = indexes ? indexes.length : 0;
return curry(rearg(func, indexes), arity);
};
},
'runInContext': function(runInContext) {
return function(context) {
return baseConvert(util, runInContext(context), options);
};
}
};
/*--------------------------------------------------------------------------*/
/**
* Casts `func` to a function with an arity capped iteratee if needed.
*
* @private
* @param {string} name The name of the function to inspect.
* @param {Function} func The function to inspect.
* @returns {Function} Returns the cast function.
*/
function castCap(name, func) {
if (config.cap) {
var indexes = mapping.iterateeRearg[name];
if (indexes) {
return iterateeRearg(func, indexes);
}
var n = !isLib && mapping.iterateeAry[name];
if (n) {
return iterateeAry(func, n);
}
}
return func;
}
/**
* Casts `func` to a curried function if needed.
*
* @private
* @param {string} name The name of the function to inspect.
* @param {Function} func The function to inspect.
* @param {number} n The arity of `func`.
* @returns {Function} Returns the cast function.
*/
function castCurry(name, func, n) {
return (forceCurry || (config.curry && n > 1))
? curry(func, n)
: func;
}
/**
* Casts `func` to a fixed arity function if needed.
*
* @private
* @param {string} name The name of the function to inspect.
* @param {Function} func The function to inspect.
* @param {number} n The arity cap.
* @returns {Function} Returns the cast function.
*/
function castFixed(name, func, n) {
if (config.fixed && (forceFixed || !mapping.skipFixed[name])) {
var data = mapping.methodSpread[name],
start = data && data.start;
return start === undefined ? ary(func, n) : flatSpread(func, start);
}
return func;
}
/**
* Casts `func` to an rearged function if needed.
*
* @private
* @param {string} name The name of the function to inspect.
* @param {Function} func The function to inspect.
* @param {number} n The arity of `func`.
* @returns {Function} Returns the cast function.
*/
function castRearg(name, func, n) {
return (config.rearg && n > 1 && (forceRearg || !mapping.skipRearg[name]))
? rearg(func, mapping.methodRearg[name] || mapping.aryRearg[n])
: func;
}
/**
* Creates a clone of `object` by `path`.
*
* @private
* @param {Object} object The object to clone.
* @param {Array|string} path The path to clone by.
* @returns {Object} Returns the cloned object.
*/
function cloneByPath(object, path) {
path = toPath(path);
var index = -1,
length = path.length,
lastIndex = length - 1,
result = clone(Object(object)),
nested = result;
while (nested != null && ++index < length) {
var key = path[index],
value = nested[key];
if (value != null &&
!(isFunction(value) || isError(value) || isWeakMap(value))) {
nested[key] = clone(index == lastIndex ? value : Object(value));
}
nested = nested[key];
}
return result;
}
/**
* Converts `lodash` to an immutable auto-curried iteratee-first data-last
* version with conversion `options` applied.
*
* @param {Object} [options] The options object. See `baseConvert` for more details.
* @returns {Function} Returns the converted `lodash`.
*/
function convertLib(options) {
return _.runInContext.convert(options)(undefined);
}
/**
* Create a converter function for `func` of `name`.
*
* @param {string} name The name of the function to convert.
* @param {Function} func The function to convert.
* @returns {Function} Returns the new converter function.
*/
function createConverter(name, func) {
var realName = mapping.aliasToReal[name] || name,
methodName = mapping.remap[realName] || realName,
oldOptions = options;
return function(options) {
var newUtil = isLib ? pristine : helpers,
newFunc = isLib ? pristine[methodName] : func,
newOptions = assign(assign({}, oldOptions), options);
return baseConvert(newUtil, realName, newFunc, newOptions);
};
}
/**
* Creates a function that wraps `func` to invoke its iteratee, with up to `n`
* arguments, ignoring any additional arguments.
*
* @private
* @param {Function} func The function to cap iteratee arguments for.
* @param {number} n The arity cap.
* @returns {Function} Returns the new function.
*/
function iterateeAry(func, n) {
return overArg(func, function(func) {
return typeof func == 'function' ? baseAry(func, n) : func;
});
}
/**
* Creates a function that wraps `func` to invoke its iteratee with arguments
* arranged according to the specified `indexes` where the argument value at
* the first index is provided as the first argument, the argument value at
* the second index is provided as the second argument, and so on.
*
* @private
* @param {Function} func The function to rearrange iteratee arguments for.
* @param {number[]} indexes The arranged argument indexes.
* @returns {Function} Returns the new function.
*/
function iterateeRearg(func, indexes) {
return overArg(func, function(func) {
var n = indexes.length;
return baseArity(rearg(baseAry(func, n), indexes), n);
});
}
/**
* Creates a function that invokes `func` with its first argument transformed.
*
* @private
* @param {Function} func The function to wrap.
* @param {Function} transform The argument transform.
* @returns {Function} Returns the new function.
*/
function overArg(func, transform) {
return function() {
var length = arguments.length;
if (!length) {
return func();
}
var args = Array(length);
while (length--) {
args[length] = arguments[length];
}
var index = config.rearg ? 0 : (length - 1);
args[index] = transform(args[index]);
return func.apply(undefined, args);
};
}
/**
* Creates a function that wraps `func` and applys the conversions
* rules by `name`.
*
* @private
* @param {string} name The name of the function to wrap.
* @param {Function} func The function to wrap.
* @returns {Function} Returns the converted function.
*/
function wrap(name, func, placeholder) {
var result,
realName = mapping.aliasToReal[name] || name,
wrapped = func,
wrapper = wrappers[realName];
if (wrapper) {
wrapped = wrapper(func);
}
else if (config.immutable) {
if (mapping.mutate.array[realName]) {
wrapped = wrapImmutable(func, cloneArray);
}
else if (mapping.mutate.object[realName]) {
wrapped = wrapImmutable(func, createCloner(func));
}
else if (mapping.mutate.set[realName]) {
wrapped = wrapImmutable(func, cloneByPath);
}
}
each(aryMethodKeys, function(aryKey) {
each(mapping.aryMethod[aryKey], function(otherName) {
if (realName == otherName) {
var data = mapping.methodSpread[realName],
afterRearg = data && data.afterRearg;
result = afterRearg
? castFixed(realName, castRearg(realName, wrapped, aryKey), aryKey)
: castRearg(realName, castFixed(realName, wrapped, aryKey), aryKey);
result = castCap(realName, result);
result = castCurry(realName, result, aryKey);
return false;
}
});
return !result;
});
result || (result = wrapped);
if (result == func) {
result = forceCurry ? curry(result, 1) : function() {
return func.apply(this, arguments);
};
}
result.convert = createConverter(realName, func);
result.placeholder = func.placeholder = placeholder;
return result;
}
/*--------------------------------------------------------------------------*/
if (!isObj) {
return wrap(name, func, defaultHolder);
}
var _ = func;
// Convert methods by ary cap.
var pairs = [];
each(aryMethodKeys, function(aryKey) {
each(mapping.aryMethod[aryKey], function(key) {
var func = _[mapping.remap[key] || key];
if (func) {
pairs.push([key, wrap(key, func, _)]);
}
});
});
// Convert remaining methods.
each(keys(_), function(key) {
var func = _[key];
if (typeof func == 'function') {
var length = pairs.length;
while (length--) {
if (pairs[length][0] == key) {
return;
}
}
func.convert = createConverter(key, func);
pairs.push([key, func]);
}
});
// Assign to `_` leaving `_.prototype` unchanged to allow chaining.
each(pairs, function(pair) {
_[pair[0]] = pair[1];
});
_.convert = convertLib;
_.placeholder = _;
// Assign aliases.
each(keys(_), function(key) {
each(mapping.realToAlias[key] || [], function(alias) {
_[alias] = _[key];
});
});
return _;
}
module.exports = baseConvert; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/fp/_baseConvert.js | _baseConvert.js |
exports.aliasToReal = {
// Lodash aliases.
'each': 'forEach',
'eachRight': 'forEachRight',
'entries': 'toPairs',
'entriesIn': 'toPairsIn',
'extend': 'assignIn',
'extendAll': 'assignInAll',
'extendAllWith': 'assignInAllWith',
'extendWith': 'assignInWith',
'first': 'head',
// Methods that are curried variants of others.
'conforms': 'conformsTo',
'matches': 'isMatch',
'property': 'get',
// Ramda aliases.
'__': 'placeholder',
'F': 'stubFalse',
'T': 'stubTrue',
'all': 'every',
'allPass': 'overEvery',
'always': 'constant',
'any': 'some',
'anyPass': 'overSome',
'apply': 'spread',
'assoc': 'set',
'assocPath': 'set',
'complement': 'negate',
'compose': 'flowRight',
'contains': 'includes',
'dissoc': 'unset',
'dissocPath': 'unset',
'dropLast': 'dropRight',
'dropLastWhile': 'dropRightWhile',
'equals': 'isEqual',
'identical': 'eq',
'indexBy': 'keyBy',
'init': 'initial',
'invertObj': 'invert',
'juxt': 'over',
'omitAll': 'omit',
'nAry': 'ary',
'path': 'get',
'pathEq': 'matchesProperty',
'pathOr': 'getOr',
'paths': 'at',
'pickAll': 'pick',
'pipe': 'flow',
'pluck': 'map',
'prop': 'get',
'propEq': 'matchesProperty',
'propOr': 'getOr',
'props': 'at',
'symmetricDifference': 'xor',
'symmetricDifferenceBy': 'xorBy',
'symmetricDifferenceWith': 'xorWith',
'takeLast': 'takeRight',
'takeLastWhile': 'takeRightWhile',
'unapply': 'rest',
'unnest': 'flatten',
'useWith': 'overArgs',
'where': 'conformsTo',
'whereEq': 'isMatch',
'zipObj': 'zipObject'
};
/** Used to map ary to method names. */
exports.aryMethod = {
'1': [
'assignAll', 'assignInAll', 'attempt', 'castArray', 'ceil', 'create',
'curry', 'curryRight', 'defaultsAll', 'defaultsDeepAll', 'floor', 'flow',
'flowRight', 'fromPairs', 'invert', 'iteratee', 'memoize', 'method', 'mergeAll',
'methodOf', 'mixin', 'nthArg', 'over', 'overEvery', 'overSome','rest', 'reverse',
'round', 'runInContext', 'spread', 'template', 'trim', 'trimEnd', 'trimStart',
'uniqueId', 'words', 'zipAll'
],
'2': [
'add', 'after', 'ary', 'assign', 'assignAllWith', 'assignIn', 'assignInAllWith',
'at', 'before', 'bind', 'bindAll', 'bindKey', 'chunk', 'cloneDeepWith',
'cloneWith', 'concat', 'conformsTo', 'countBy', 'curryN', 'curryRightN',
'debounce', 'defaults', 'defaultsDeep', 'defaultTo', 'delay', 'difference',
'divide', 'drop', 'dropRight', 'dropRightWhile', 'dropWhile', 'endsWith', 'eq',
'every', 'filter', 'find', 'findIndex', 'findKey', 'findLast', 'findLastIndex',
'findLastKey', 'flatMap', 'flatMapDeep', 'flattenDepth', 'forEach',
'forEachRight', 'forIn', 'forInRight', 'forOwn', 'forOwnRight', 'get',
'groupBy', 'gt', 'gte', 'has', 'hasIn', 'includes', 'indexOf', 'intersection',
'invertBy', 'invoke', 'invokeMap', 'isEqual', 'isMatch', 'join', 'keyBy',
'lastIndexOf', 'lt', 'lte', 'map', 'mapKeys', 'mapValues', 'matchesProperty',
'maxBy', 'meanBy', 'merge', 'mergeAllWith', 'minBy', 'multiply', 'nth', 'omit',
'omitBy', 'overArgs', 'pad', 'padEnd', 'padStart', 'parseInt', 'partial',
'partialRight', 'partition', 'pick', 'pickBy', 'propertyOf', 'pull', 'pullAll',
'pullAt', 'random', 'range', 'rangeRight', 'rearg', 'reject', 'remove',
'repeat', 'restFrom', 'result', 'sampleSize', 'some', 'sortBy', 'sortedIndex',
'sortedIndexOf', 'sortedLastIndex', 'sortedLastIndexOf', 'sortedUniqBy',
'split', 'spreadFrom', 'startsWith', 'subtract', 'sumBy', 'take', 'takeRight',
'takeRightWhile', 'takeWhile', 'tap', 'throttle', 'thru', 'times', 'trimChars',
'trimCharsEnd', 'trimCharsStart', 'truncate', 'union', 'uniqBy', 'uniqWith',
'unset', 'unzipWith', 'without', 'wrap', 'xor', 'zip', 'zipObject',
'zipObjectDeep'
],
'3': [
'assignInWith', 'assignWith', 'clamp', 'differenceBy', 'differenceWith',
'findFrom', 'findIndexFrom', 'findLastFrom', 'findLastIndexFrom', 'getOr',
'includesFrom', 'indexOfFrom', 'inRange', 'intersectionBy', 'intersectionWith',
'invokeArgs', 'invokeArgsMap', 'isEqualWith', 'isMatchWith', 'flatMapDepth',
'lastIndexOfFrom', 'mergeWith', 'orderBy', 'padChars', 'padCharsEnd',
'padCharsStart', 'pullAllBy', 'pullAllWith', 'rangeStep', 'rangeStepRight',
'reduce', 'reduceRight', 'replace', 'set', 'slice', 'sortedIndexBy',
'sortedLastIndexBy', 'transform', 'unionBy', 'unionWith', 'update', 'xorBy',
'xorWith', 'zipWith'
],
'4': [
'fill', 'setWith', 'updateWith'
]
};
/** Used to map ary to rearg configs. */
exports.aryRearg = {
'2': [1, 0],
'3': [2, 0, 1],
'4': [3, 2, 0, 1]
};
/** Used to map method names to their iteratee ary. */
exports.iterateeAry = {
'dropRightWhile': 1,
'dropWhile': 1,
'every': 1,
'filter': 1,
'find': 1,
'findFrom': 1,
'findIndex': 1,
'findIndexFrom': 1,
'findKey': 1,
'findLast': 1,
'findLastFrom': 1,
'findLastIndex': 1,
'findLastIndexFrom': 1,
'findLastKey': 1,
'flatMap': 1,
'flatMapDeep': 1,
'flatMapDepth': 1,
'forEach': 1,
'forEachRight': 1,
'forIn': 1,
'forInRight': 1,
'forOwn': 1,
'forOwnRight': 1,
'map': 1,
'mapKeys': 1,
'mapValues': 1,
'partition': 1,
'reduce': 2,
'reduceRight': 2,
'reject': 1,
'remove': 1,
'some': 1,
'takeRightWhile': 1,
'takeWhile': 1,
'times': 1,
'transform': 2
};
/** Used to map method names to iteratee rearg configs. */
exports.iterateeRearg = {
'mapKeys': [1],
'reduceRight': [1, 0]
};
/** Used to map method names to rearg configs. */
exports.methodRearg = {
'assignInAllWith': [1, 0],
'assignInWith': [1, 2, 0],
'assignAllWith': [1, 0],
'assignWith': [1, 2, 0],
'differenceBy': [1, 2, 0],
'differenceWith': [1, 2, 0],
'getOr': [2, 1, 0],
'intersectionBy': [1, 2, 0],
'intersectionWith': [1, 2, 0],
'isEqualWith': [1, 2, 0],
'isMatchWith': [2, 1, 0],
'mergeAllWith': [1, 0],
'mergeWith': [1, 2, 0],
'padChars': [2, 1, 0],
'padCharsEnd': [2, 1, 0],
'padCharsStart': [2, 1, 0],
'pullAllBy': [2, 1, 0],
'pullAllWith': [2, 1, 0],
'rangeStep': [1, 2, 0],
'rangeStepRight': [1, 2, 0],
'setWith': [3, 1, 2, 0],
'sortedIndexBy': [2, 1, 0],
'sortedLastIndexBy': [2, 1, 0],
'unionBy': [1, 2, 0],
'unionWith': [1, 2, 0],
'updateWith': [3, 1, 2, 0],
'xorBy': [1, 2, 0],
'xorWith': [1, 2, 0],
'zipWith': [1, 2, 0]
};
/** Used to map method names to spread configs. */
exports.methodSpread = {
'assignAll': { 'start': 0 },
'assignAllWith': { 'start': 0 },
'assignInAll': { 'start': 0 },
'assignInAllWith': { 'start': 0 },
'defaultsAll': { 'start': 0 },
'defaultsDeepAll': { 'start': 0 },
'invokeArgs': { 'start': 2 },
'invokeArgsMap': { 'start': 2 },
'mergeAll': { 'start': 0 },
'mergeAllWith': { 'start': 0 },
'partial': { 'start': 1 },
'partialRight': { 'start': 1 },
'without': { 'start': 1 },
'zipAll': { 'start': 0 }
};
/** Used to identify methods which mutate arrays or objects. */
exports.mutate = {
'array': {
'fill': true,
'pull': true,
'pullAll': true,
'pullAllBy': true,
'pullAllWith': true,
'pullAt': true,
'remove': true,
'reverse': true
},
'object': {
'assign': true,
'assignAll': true,
'assignAllWith': true,
'assignIn': true,
'assignInAll': true,
'assignInAllWith': true,
'assignInWith': true,
'assignWith': true,
'defaults': true,
'defaultsAll': true,
'defaultsDeep': true,
'defaultsDeepAll': true,
'merge': true,
'mergeAll': true,
'mergeAllWith': true,
'mergeWith': true,
},
'set': {
'set': true,
'setWith': true,
'unset': true,
'update': true,
'updateWith': true
}
};
/** Used to map real names to their aliases. */
exports.realToAlias = (function() {
var hasOwnProperty = Object.prototype.hasOwnProperty,
object = exports.aliasToReal,
result = {};
for (var key in object) {
var value = object[key];
if (hasOwnProperty.call(result, value)) {
result[value].push(key);
} else {
result[value] = [key];
}
}
return result;
}());
/** Used to map method names to other names. */
exports.remap = {
'assignAll': 'assign',
'assignAllWith': 'assignWith',
'assignInAll': 'assignIn',
'assignInAllWith': 'assignInWith',
'curryN': 'curry',
'curryRightN': 'curryRight',
'defaultsAll': 'defaults',
'defaultsDeepAll': 'defaultsDeep',
'findFrom': 'find',
'findIndexFrom': 'findIndex',
'findLastFrom': 'findLast',
'findLastIndexFrom': 'findLastIndex',
'getOr': 'get',
'includesFrom': 'includes',
'indexOfFrom': 'indexOf',
'invokeArgs': 'invoke',
'invokeArgsMap': 'invokeMap',
'lastIndexOfFrom': 'lastIndexOf',
'mergeAll': 'merge',
'mergeAllWith': 'mergeWith',
'padChars': 'pad',
'padCharsEnd': 'padEnd',
'padCharsStart': 'padStart',
'propertyOf': 'get',
'rangeStep': 'range',
'rangeStepRight': 'rangeRight',
'restFrom': 'rest',
'spreadFrom': 'spread',
'trimChars': 'trim',
'trimCharsEnd': 'trimEnd',
'trimCharsStart': 'trimStart',
'zipAll': 'zip'
};
/** Used to track methods that skip fixing their arity. */
exports.skipFixed = {
'castArray': true,
'flow': true,
'flowRight': true,
'iteratee': true,
'mixin': true,
'rearg': true,
'runInContext': true
};
/** Used to track methods that skip rearranging arguments. */
exports.skipRearg = {
'add': true,
'assign': true,
'assignIn': true,
'bind': true,
'bindKey': true,
'concat': true,
'difference': true,
'divide': true,
'eq': true,
'gt': true,
'gte': true,
'isEqual': true,
'lt': true,
'lte': true,
'matchesProperty': true,
'merge': true,
'multiply': true,
'overArgs': true,
'partial': true,
'partialRight': true,
'propertyOf': true,
'random': true,
'range': true,
'rangeRight': true,
'subtract': true,
'zip': true,
'zipObject': true,
'zipObjectDeep': true
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/lodash/fp/_mapping.js | _mapping.js |
Port of the OpenBSD `bcrypt_pbkdf` function to pure Javascript. `npm`-ified
version of [Devi Mandiri's port](https://github.com/devi/tmp/blob/master/js/bcrypt_pbkdf.js),
with some minor performance improvements. The code is copied verbatim (and
un-styled) from Devi's work.
This product includes software developed by Niels Provos.
## API
### `bcrypt_pbkdf.pbkdf(pass, passlen, salt, saltlen, key, keylen, rounds)`
Derive a cryptographic key of arbitrary length from a given password and salt,
using the OpenBSD `bcrypt_pbkdf` function. This is a combination of Blowfish and
SHA-512.
See [this article](http://www.tedunangst.com/flak/post/bcrypt-pbkdf) for
further information.
Parameters:
* `pass`, a Uint8Array of length `passlen`
* `passlen`, an integer Number
* `salt`, a Uint8Array of length `saltlen`
* `saltlen`, an integer Number
* `key`, a Uint8Array of length `keylen`, will be filled with output
* `keylen`, an integer Number
* `rounds`, an integer Number, number of rounds of the PBKDF to run
### `bcrypt_pbkdf.hash(sha2pass, sha2salt, out)`
Calculate a Blowfish hash, given SHA2-512 output of a password and salt. Used as
part of the inner round function in the PBKDF.
Parameters:
* `sha2pass`, a Uint8Array of length 64
* `sha2salt`, a Uint8Array of length 64
* `out`, a Uint8Array of length 32, will be filled with output
## License
This source form is a 1:1 port from the OpenBSD `blowfish.c` and `bcrypt_pbkdf.c`.
As a result, it retains the original copyright and license. The two files are
under slightly different (but compatible) licenses, and are here combined in
one file. For each of the full license texts see `LICENSE`.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/bcrypt-pbkdf/README.md | README.md |
# Contributing
This repository uses [cr.joyent.us](https://cr.joyent.us) (Gerrit) for new
changes. Anyone can submit changes. To get started, see the [cr.joyent.us user
guide](https://github.com/joyent/joyent-gerrit/blob/master/docs/user/README.md).
This repo does not use GitHub pull requests.
See the [Joyent Engineering
Guidelines](https://github.com/joyent/eng/blob/master/docs/index.md) for general
best practices expected in this repository.
If you're changing something non-trivial or user-facing, you may want to submit
an issue first.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/bcrypt-pbkdf/CONTRIBUTING.md | CONTRIBUTING.md |
'use strict';
var crypto_hash_sha512 = require('tweetnacl').lowlevel.crypto_hash;
/*
* This file is a 1:1 port from the OpenBSD blowfish.c and bcrypt_pbkdf.c. As a
* result, it retains the original copyright and license. The two files are
* under slightly different (but compatible) licenses, and are here combined in
* one file.
*
* Credit for the actual porting work goes to:
* Devi Mandiri <[email protected]>
*/
/*
* The Blowfish portions are under the following license:
*
* Blowfish block cipher for OpenBSD
* Copyright 1997 Niels Provos <[email protected]>
* All rights reserved.
*
* Implementation advice by David Mazieres <[email protected]>.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/*
* The bcrypt_pbkdf portions are under the following license:
*
* Copyright (c) 2013 Ted Unangst <[email protected]>
*
* Permission to use, copy, modify, and distribute this software for any
* purpose with or without fee is hereby granted, provided that the above
* copyright notice and this permission notice appear in all copies.
*
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
* ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
* OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
*/
/*
* Performance improvements (Javascript-specific):
*
* Copyright 2016, Joyent Inc
* Author: Alex Wilson <[email protected]>
*
* Permission to use, copy, modify, and distribute this software for any
* purpose with or without fee is hereby granted, provided that the above
* copyright notice and this permission notice appear in all copies.
*
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
* ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
* OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
*/
// Ported from OpenBSD bcrypt_pbkdf.c v1.9
var BLF_J = 0;
var Blowfish = function() {
this.S = [
new Uint32Array([
0xd1310ba6, 0x98dfb5ac, 0x2ffd72db, 0xd01adfb7,
0xb8e1afed, 0x6a267e96, 0xba7c9045, 0xf12c7f99,
0x24a19947, 0xb3916cf7, 0x0801f2e2, 0x858efc16,
0x636920d8, 0x71574e69, 0xa458fea3, 0xf4933d7e,
0x0d95748f, 0x728eb658, 0x718bcd58, 0x82154aee,
0x7b54a41d, 0xc25a59b5, 0x9c30d539, 0x2af26013,
0xc5d1b023, 0x286085f0, 0xca417918, 0xb8db38ef,
0x8e79dcb0, 0x603a180e, 0x6c9e0e8b, 0xb01e8a3e,
0xd71577c1, 0xbd314b27, 0x78af2fda, 0x55605c60,
0xe65525f3, 0xaa55ab94, 0x57489862, 0x63e81440,
0x55ca396a, 0x2aab10b6, 0xb4cc5c34, 0x1141e8ce,
0xa15486af, 0x7c72e993, 0xb3ee1411, 0x636fbc2a,
0x2ba9c55d, 0x741831f6, 0xce5c3e16, 0x9b87931e,
0xafd6ba33, 0x6c24cf5c, 0x7a325381, 0x28958677,
0x3b8f4898, 0x6b4bb9af, 0xc4bfe81b, 0x66282193,
0x61d809cc, 0xfb21a991, 0x487cac60, 0x5dec8032,
0xef845d5d, 0xe98575b1, 0xdc262302, 0xeb651b88,
0x23893e81, 0xd396acc5, 0x0f6d6ff3, 0x83f44239,
0x2e0b4482, 0xa4842004, 0x69c8f04a, 0x9e1f9b5e,
0x21c66842, 0xf6e96c9a, 0x670c9c61, 0xabd388f0,
0x6a51a0d2, 0xd8542f68, 0x960fa728, 0xab5133a3,
0x6eef0b6c, 0x137a3be4, 0xba3bf050, 0x7efb2a98,
0xa1f1651d, 0x39af0176, 0x66ca593e, 0x82430e88,
0x8cee8619, 0x456f9fb4, 0x7d84a5c3, 0x3b8b5ebe,
0xe06f75d8, 0x85c12073, 0x401a449f, 0x56c16aa6,
0x4ed3aa62, 0x363f7706, 0x1bfedf72, 0x429b023d,
0x37d0d724, 0xd00a1248, 0xdb0fead3, 0x49f1c09b,
0x075372c9, 0x80991b7b, 0x25d479d8, 0xf6e8def7,
0xe3fe501a, 0xb6794c3b, 0x976ce0bd, 0x04c006ba,
0xc1a94fb6, 0x409f60c4, 0x5e5c9ec2, 0x196a2463,
0x68fb6faf, 0x3e6c53b5, 0x1339b2eb, 0x3b52ec6f,
0x6dfc511f, 0x9b30952c, 0xcc814544, 0xaf5ebd09,
0xbee3d004, 0xde334afd, 0x660f2807, 0x192e4bb3,
0xc0cba857, 0x45c8740f, 0xd20b5f39, 0xb9d3fbdb,
0x5579c0bd, 0x1a60320a, 0xd6a100c6, 0x402c7279,
0x679f25fe, 0xfb1fa3cc, 0x8ea5e9f8, 0xdb3222f8,
0x3c7516df, 0xfd616b15, 0x2f501ec8, 0xad0552ab,
0x323db5fa, 0xfd238760, 0x53317b48, 0x3e00df82,
0x9e5c57bb, 0xca6f8ca0, 0x1a87562e, 0xdf1769db,
0xd542a8f6, 0x287effc3, 0xac6732c6, 0x8c4f5573,
0x695b27b0, 0xbbca58c8, 0xe1ffa35d, 0xb8f011a0,
0x10fa3d98, 0xfd2183b8, 0x4afcb56c, 0x2dd1d35b,
0x9a53e479, 0xb6f84565, 0xd28e49bc, 0x4bfb9790,
0xe1ddf2da, 0xa4cb7e33, 0x62fb1341, 0xcee4c6e8,
0xef20cada, 0x36774c01, 0xd07e9efe, 0x2bf11fb4,
0x95dbda4d, 0xae909198, 0xeaad8e71, 0x6b93d5a0,
0xd08ed1d0, 0xafc725e0, 0x8e3c5b2f, 0x8e7594b7,
0x8ff6e2fb, 0xf2122b64, 0x8888b812, 0x900df01c,
0x4fad5ea0, 0x688fc31c, 0xd1cff191, 0xb3a8c1ad,
0x2f2f2218, 0xbe0e1777, 0xea752dfe, 0x8b021fa1,
0xe5a0cc0f, 0xb56f74e8, 0x18acf3d6, 0xce89e299,
0xb4a84fe0, 0xfd13e0b7, 0x7cc43b81, 0xd2ada8d9,
0x165fa266, 0x80957705, 0x93cc7314, 0x211a1477,
0xe6ad2065, 0x77b5fa86, 0xc75442f5, 0xfb9d35cf,
0xebcdaf0c, 0x7b3e89a0, 0xd6411bd3, 0xae1e7e49,
0x00250e2d, 0x2071b35e, 0x226800bb, 0x57b8e0af,
0x2464369b, 0xf009b91e, 0x5563911d, 0x59dfa6aa,
0x78c14389, 0xd95a537f, 0x207d5ba2, 0x02e5b9c5,
0x83260376, 0x6295cfa9, 0x11c81968, 0x4e734a41,
0xb3472dca, 0x7b14a94a, 0x1b510052, 0x9a532915,
0xd60f573f, 0xbc9bc6e4, 0x2b60a476, 0x81e67400,
0x08ba6fb5, 0x571be91f, 0xf296ec6b, 0x2a0dd915,
0xb6636521, 0xe7b9f9b6, 0xff34052e, 0xc5855664,
0x53b02d5d, 0xa99f8fa1, 0x08ba4799, 0x6e85076a]),
new Uint32Array([
0x4b7a70e9, 0xb5b32944, 0xdb75092e, 0xc4192623,
0xad6ea6b0, 0x49a7df7d, 0x9cee60b8, 0x8fedb266,
0xecaa8c71, 0x699a17ff, 0x5664526c, 0xc2b19ee1,
0x193602a5, 0x75094c29, 0xa0591340, 0xe4183a3e,
0x3f54989a, 0x5b429d65, 0x6b8fe4d6, 0x99f73fd6,
0xa1d29c07, 0xefe830f5, 0x4d2d38e6, 0xf0255dc1,
0x4cdd2086, 0x8470eb26, 0x6382e9c6, 0x021ecc5e,
0x09686b3f, 0x3ebaefc9, 0x3c971814, 0x6b6a70a1,
0x687f3584, 0x52a0e286, 0xb79c5305, 0xaa500737,
0x3e07841c, 0x7fdeae5c, 0x8e7d44ec, 0x5716f2b8,
0xb03ada37, 0xf0500c0d, 0xf01c1f04, 0x0200b3ff,
0xae0cf51a, 0x3cb574b2, 0x25837a58, 0xdc0921bd,
0xd19113f9, 0x7ca92ff6, 0x94324773, 0x22f54701,
0x3ae5e581, 0x37c2dadc, 0xc8b57634, 0x9af3dda7,
0xa9446146, 0x0fd0030e, 0xecc8c73e, 0xa4751e41,
0xe238cd99, 0x3bea0e2f, 0x3280bba1, 0x183eb331,
0x4e548b38, 0x4f6db908, 0x6f420d03, 0xf60a04bf,
0x2cb81290, 0x24977c79, 0x5679b072, 0xbcaf89af,
0xde9a771f, 0xd9930810, 0xb38bae12, 0xdccf3f2e,
0x5512721f, 0x2e6b7124, 0x501adde6, 0x9f84cd87,
0x7a584718, 0x7408da17, 0xbc9f9abc, 0xe94b7d8c,
0xec7aec3a, 0xdb851dfa, 0x63094366, 0xc464c3d2,
0xef1c1847, 0x3215d908, 0xdd433b37, 0x24c2ba16,
0x12a14d43, 0x2a65c451, 0x50940002, 0x133ae4dd,
0x71dff89e, 0x10314e55, 0x81ac77d6, 0x5f11199b,
0x043556f1, 0xd7a3c76b, 0x3c11183b, 0x5924a509,
0xf28fe6ed, 0x97f1fbfa, 0x9ebabf2c, 0x1e153c6e,
0x86e34570, 0xeae96fb1, 0x860e5e0a, 0x5a3e2ab3,
0x771fe71c, 0x4e3d06fa, 0x2965dcb9, 0x99e71d0f,
0x803e89d6, 0x5266c825, 0x2e4cc978, 0x9c10b36a,
0xc6150eba, 0x94e2ea78, 0xa5fc3c53, 0x1e0a2df4,
0xf2f74ea7, 0x361d2b3d, 0x1939260f, 0x19c27960,
0x5223a708, 0xf71312b6, 0xebadfe6e, 0xeac31f66,
0xe3bc4595, 0xa67bc883, 0xb17f37d1, 0x018cff28,
0xc332ddef, 0xbe6c5aa5, 0x65582185, 0x68ab9802,
0xeecea50f, 0xdb2f953b, 0x2aef7dad, 0x5b6e2f84,
0x1521b628, 0x29076170, 0xecdd4775, 0x619f1510,
0x13cca830, 0xeb61bd96, 0x0334fe1e, 0xaa0363cf,
0xb5735c90, 0x4c70a239, 0xd59e9e0b, 0xcbaade14,
0xeecc86bc, 0x60622ca7, 0x9cab5cab, 0xb2f3846e,
0x648b1eaf, 0x19bdf0ca, 0xa02369b9, 0x655abb50,
0x40685a32, 0x3c2ab4b3, 0x319ee9d5, 0xc021b8f7,
0x9b540b19, 0x875fa099, 0x95f7997e, 0x623d7da8,
0xf837889a, 0x97e32d77, 0x11ed935f, 0x16681281,
0x0e358829, 0xc7e61fd6, 0x96dedfa1, 0x7858ba99,
0x57f584a5, 0x1b227263, 0x9b83c3ff, 0x1ac24696,
0xcdb30aeb, 0x532e3054, 0x8fd948e4, 0x6dbc3128,
0x58ebf2ef, 0x34c6ffea, 0xfe28ed61, 0xee7c3c73,
0x5d4a14d9, 0xe864b7e3, 0x42105d14, 0x203e13e0,
0x45eee2b6, 0xa3aaabea, 0xdb6c4f15, 0xfacb4fd0,
0xc742f442, 0xef6abbb5, 0x654f3b1d, 0x41cd2105,
0xd81e799e, 0x86854dc7, 0xe44b476a, 0x3d816250,
0xcf62a1f2, 0x5b8d2646, 0xfc8883a0, 0xc1c7b6a3,
0x7f1524c3, 0x69cb7492, 0x47848a0b, 0x5692b285,
0x095bbf00, 0xad19489d, 0x1462b174, 0x23820e00,
0x58428d2a, 0x0c55f5ea, 0x1dadf43e, 0x233f7061,
0x3372f092, 0x8d937e41, 0xd65fecf1, 0x6c223bdb,
0x7cde3759, 0xcbee7460, 0x4085f2a7, 0xce77326e,
0xa6078084, 0x19f8509e, 0xe8efd855, 0x61d99735,
0xa969a7aa, 0xc50c06c2, 0x5a04abfc, 0x800bcadc,
0x9e447a2e, 0xc3453484, 0xfdd56705, 0x0e1e9ec9,
0xdb73dbd3, 0x105588cd, 0x675fda79, 0xe3674340,
0xc5c43465, 0x713e38d8, 0x3d28f89e, 0xf16dff20,
0x153e21e7, 0x8fb03d4a, 0xe6e39f2b, 0xdb83adf7]),
new Uint32Array([
0xe93d5a68, 0x948140f7, 0xf64c261c, 0x94692934,
0x411520f7, 0x7602d4f7, 0xbcf46b2e, 0xd4a20068,
0xd4082471, 0x3320f46a, 0x43b7d4b7, 0x500061af,
0x1e39f62e, 0x97244546, 0x14214f74, 0xbf8b8840,
0x4d95fc1d, 0x96b591af, 0x70f4ddd3, 0x66a02f45,
0xbfbc09ec, 0x03bd9785, 0x7fac6dd0, 0x31cb8504,
0x96eb27b3, 0x55fd3941, 0xda2547e6, 0xabca0a9a,
0x28507825, 0x530429f4, 0x0a2c86da, 0xe9b66dfb,
0x68dc1462, 0xd7486900, 0x680ec0a4, 0x27a18dee,
0x4f3ffea2, 0xe887ad8c, 0xb58ce006, 0x7af4d6b6,
0xaace1e7c, 0xd3375fec, 0xce78a399, 0x406b2a42,
0x20fe9e35, 0xd9f385b9, 0xee39d7ab, 0x3b124e8b,
0x1dc9faf7, 0x4b6d1856, 0x26a36631, 0xeae397b2,
0x3a6efa74, 0xdd5b4332, 0x6841e7f7, 0xca7820fb,
0xfb0af54e, 0xd8feb397, 0x454056ac, 0xba489527,
0x55533a3a, 0x20838d87, 0xfe6ba9b7, 0xd096954b,
0x55a867bc, 0xa1159a58, 0xcca92963, 0x99e1db33,
0xa62a4a56, 0x3f3125f9, 0x5ef47e1c, 0x9029317c,
0xfdf8e802, 0x04272f70, 0x80bb155c, 0x05282ce3,
0x95c11548, 0xe4c66d22, 0x48c1133f, 0xc70f86dc,
0x07f9c9ee, 0x41041f0f, 0x404779a4, 0x5d886e17,
0x325f51eb, 0xd59bc0d1, 0xf2bcc18f, 0x41113564,
0x257b7834, 0x602a9c60, 0xdff8e8a3, 0x1f636c1b,
0x0e12b4c2, 0x02e1329e, 0xaf664fd1, 0xcad18115,
0x6b2395e0, 0x333e92e1, 0x3b240b62, 0xeebeb922,
0x85b2a20e, 0xe6ba0d99, 0xde720c8c, 0x2da2f728,
0xd0127845, 0x95b794fd, 0x647d0862, 0xe7ccf5f0,
0x5449a36f, 0x877d48fa, 0xc39dfd27, 0xf33e8d1e,
0x0a476341, 0x992eff74, 0x3a6f6eab, 0xf4f8fd37,
0xa812dc60, 0xa1ebddf8, 0x991be14c, 0xdb6e6b0d,
0xc67b5510, 0x6d672c37, 0x2765d43b, 0xdcd0e804,
0xf1290dc7, 0xcc00ffa3, 0xb5390f92, 0x690fed0b,
0x667b9ffb, 0xcedb7d9c, 0xa091cf0b, 0xd9155ea3,
0xbb132f88, 0x515bad24, 0x7b9479bf, 0x763bd6eb,
0x37392eb3, 0xcc115979, 0x8026e297, 0xf42e312d,
0x6842ada7, 0xc66a2b3b, 0x12754ccc, 0x782ef11c,
0x6a124237, 0xb79251e7, 0x06a1bbe6, 0x4bfb6350,
0x1a6b1018, 0x11caedfa, 0x3d25bdd8, 0xe2e1c3c9,
0x44421659, 0x0a121386, 0xd90cec6e, 0xd5abea2a,
0x64af674e, 0xda86a85f, 0xbebfe988, 0x64e4c3fe,
0x9dbc8057, 0xf0f7c086, 0x60787bf8, 0x6003604d,
0xd1fd8346, 0xf6381fb0, 0x7745ae04, 0xd736fccc,
0x83426b33, 0xf01eab71, 0xb0804187, 0x3c005e5f,
0x77a057be, 0xbde8ae24, 0x55464299, 0xbf582e61,
0x4e58f48f, 0xf2ddfda2, 0xf474ef38, 0x8789bdc2,
0x5366f9c3, 0xc8b38e74, 0xb475f255, 0x46fcd9b9,
0x7aeb2661, 0x8b1ddf84, 0x846a0e79, 0x915f95e2,
0x466e598e, 0x20b45770, 0x8cd55591, 0xc902de4c,
0xb90bace1, 0xbb8205d0, 0x11a86248, 0x7574a99e,
0xb77f19b6, 0xe0a9dc09, 0x662d09a1, 0xc4324633,
0xe85a1f02, 0x09f0be8c, 0x4a99a025, 0x1d6efe10,
0x1ab93d1d, 0x0ba5a4df, 0xa186f20f, 0x2868f169,
0xdcb7da83, 0x573906fe, 0xa1e2ce9b, 0x4fcd7f52,
0x50115e01, 0xa70683fa, 0xa002b5c4, 0x0de6d027,
0x9af88c27, 0x773f8641, 0xc3604c06, 0x61a806b5,
0xf0177a28, 0xc0f586e0, 0x006058aa, 0x30dc7d62,
0x11e69ed7, 0x2338ea63, 0x53c2dd94, 0xc2c21634,
0xbbcbee56, 0x90bcb6de, 0xebfc7da1, 0xce591d76,
0x6f05e409, 0x4b7c0188, 0x39720a3d, 0x7c927c24,
0x86e3725f, 0x724d9db9, 0x1ac15bb4, 0xd39eb8fc,
0xed545578, 0x08fca5b5, 0xd83d7cd3, 0x4dad0fc4,
0x1e50ef5e, 0xb161e6f8, 0xa28514d9, 0x6c51133c,
0x6fd5c7e7, 0x56e14ec4, 0x362abfce, 0xddc6c837,
0xd79a3234, 0x92638212, 0x670efa8e, 0x406000e0]),
new Uint32Array([
0x3a39ce37, 0xd3faf5cf, 0xabc27737, 0x5ac52d1b,
0x5cb0679e, 0x4fa33742, 0xd3822740, 0x99bc9bbe,
0xd5118e9d, 0xbf0f7315, 0xd62d1c7e, 0xc700c47b,
0xb78c1b6b, 0x21a19045, 0xb26eb1be, 0x6a366eb4,
0x5748ab2f, 0xbc946e79, 0xc6a376d2, 0x6549c2c8,
0x530ff8ee, 0x468dde7d, 0xd5730a1d, 0x4cd04dc6,
0x2939bbdb, 0xa9ba4650, 0xac9526e8, 0xbe5ee304,
0xa1fad5f0, 0x6a2d519a, 0x63ef8ce2, 0x9a86ee22,
0xc089c2b8, 0x43242ef6, 0xa51e03aa, 0x9cf2d0a4,
0x83c061ba, 0x9be96a4d, 0x8fe51550, 0xba645bd6,
0x2826a2f9, 0xa73a3ae1, 0x4ba99586, 0xef5562e9,
0xc72fefd3, 0xf752f7da, 0x3f046f69, 0x77fa0a59,
0x80e4a915, 0x87b08601, 0x9b09e6ad, 0x3b3ee593,
0xe990fd5a, 0x9e34d797, 0x2cf0b7d9, 0x022b8b51,
0x96d5ac3a, 0x017da67d, 0xd1cf3ed6, 0x7c7d2d28,
0x1f9f25cf, 0xadf2b89b, 0x5ad6b472, 0x5a88f54c,
0xe029ac71, 0xe019a5e6, 0x47b0acfd, 0xed93fa9b,
0xe8d3c48d, 0x283b57cc, 0xf8d56629, 0x79132e28,
0x785f0191, 0xed756055, 0xf7960e44, 0xe3d35e8c,
0x15056dd4, 0x88f46dba, 0x03a16125, 0x0564f0bd,
0xc3eb9e15, 0x3c9057a2, 0x97271aec, 0xa93a072a,
0x1b3f6d9b, 0x1e6321f5, 0xf59c66fb, 0x26dcf319,
0x7533d928, 0xb155fdf5, 0x03563482, 0x8aba3cbb,
0x28517711, 0xc20ad9f8, 0xabcc5167, 0xccad925f,
0x4de81751, 0x3830dc8e, 0x379d5862, 0x9320f991,
0xea7a90c2, 0xfb3e7bce, 0x5121ce64, 0x774fbe32,
0xa8b6e37e, 0xc3293d46, 0x48de5369, 0x6413e680,
0xa2ae0810, 0xdd6db224, 0x69852dfd, 0x09072166,
0xb39a460a, 0x6445c0dd, 0x586cdecf, 0x1c20c8ae,
0x5bbef7dd, 0x1b588d40, 0xccd2017f, 0x6bb4e3bb,
0xdda26a7e, 0x3a59ff45, 0x3e350a44, 0xbcb4cdd5,
0x72eacea8, 0xfa6484bb, 0x8d6612ae, 0xbf3c6f47,
0xd29be463, 0x542f5d9e, 0xaec2771b, 0xf64e6370,
0x740e0d8d, 0xe75b1357, 0xf8721671, 0xaf537d5d,
0x4040cb08, 0x4eb4e2cc, 0x34d2466a, 0x0115af84,
0xe1b00428, 0x95983a1d, 0x06b89fb4, 0xce6ea048,
0x6f3f3b82, 0x3520ab82, 0x011a1d4b, 0x277227f8,
0x611560b1, 0xe7933fdc, 0xbb3a792b, 0x344525bd,
0xa08839e1, 0x51ce794b, 0x2f32c9b7, 0xa01fbac9,
0xe01cc87e, 0xbcc7d1f6, 0xcf0111c3, 0xa1e8aac7,
0x1a908749, 0xd44fbd9a, 0xd0dadecb, 0xd50ada38,
0x0339c32a, 0xc6913667, 0x8df9317c, 0xe0b12b4f,
0xf79e59b7, 0x43f5bb3a, 0xf2d519ff, 0x27d9459c,
0xbf97222c, 0x15e6fc2a, 0x0f91fc71, 0x9b941525,
0xfae59361, 0xceb69ceb, 0xc2a86459, 0x12baa8d1,
0xb6c1075e, 0xe3056a0c, 0x10d25065, 0xcb03a442,
0xe0ec6e0e, 0x1698db3b, 0x4c98a0be, 0x3278e964,
0x9f1f9532, 0xe0d392df, 0xd3a0342b, 0x8971f21e,
0x1b0a7441, 0x4ba3348c, 0xc5be7120, 0xc37632d8,
0xdf359f8d, 0x9b992f2e, 0xe60b6f47, 0x0fe3f11d,
0xe54cda54, 0x1edad891, 0xce6279cf, 0xcd3e7e6f,
0x1618b166, 0xfd2c1d05, 0x848fd2c5, 0xf6fb2299,
0xf523f357, 0xa6327623, 0x93a83531, 0x56cccd02,
0xacf08162, 0x5a75ebb5, 0x6e163697, 0x88d273cc,
0xde966292, 0x81b949d0, 0x4c50901b, 0x71c65614,
0xe6c6c7bd, 0x327a140a, 0x45e1d006, 0xc3f27b9a,
0xc9aa53fd, 0x62a80f00, 0xbb25bfe2, 0x35bdd2f6,
0x71126905, 0xb2040222, 0xb6cbcf7c, 0xcd769c2b,
0x53113ec0, 0x1640e3d3, 0x38abbd60, 0x2547adf0,
0xba38209c, 0xf746ce76, 0x77afa1c5, 0x20756060,
0x85cbfe4e, 0x8ae88dd8, 0x7aaaf9b0, 0x4cf9aa7e,
0x1948c25c, 0x02fb8a8c, 0x01c36ae4, 0xd6ebe1f9,
0x90d4f869, 0xa65cdea0, 0x3f09252d, 0xc208e69f,
0xb74e6132, 0xce77e25b, 0x578fdfe3, 0x3ac372e6])
];
this.P = new Uint32Array([
0x243f6a88, 0x85a308d3, 0x13198a2e, 0x03707344,
0xa4093822, 0x299f31d0, 0x082efa98, 0xec4e6c89,
0x452821e6, 0x38d01377, 0xbe5466cf, 0x34e90c6c,
0xc0ac29b7, 0xc97c50dd, 0x3f84d5b5, 0xb5470917,
0x9216d5d9, 0x8979fb1b]);
};
function F(S, x8, i) {
return (((S[0][x8[i+3]] +
S[1][x8[i+2]]) ^
S[2][x8[i+1]]) +
S[3][x8[i]]);
};
Blowfish.prototype.encipher = function(x, x8) {
if (x8 === undefined) {
x8 = new Uint8Array(x.buffer);
if (x.byteOffset !== 0)
x8 = x8.subarray(x.byteOffset);
}
x[0] ^= this.P[0];
for (var i = 1; i < 16; i += 2) {
x[1] ^= F(this.S, x8, 0) ^ this.P[i];
x[0] ^= F(this.S, x8, 4) ^ this.P[i+1];
}
var t = x[0];
x[0] = x[1] ^ this.P[17];
x[1] = t;
};
Blowfish.prototype.decipher = function(x) {
var x8 = new Uint8Array(x.buffer);
if (x.byteOffset !== 0)
x8 = x8.subarray(x.byteOffset);
x[0] ^= this.P[17];
for (var i = 16; i > 0; i -= 2) {
x[1] ^= F(this.S, x8, 0) ^ this.P[i];
x[0] ^= F(this.S, x8, 4) ^ this.P[i-1];
}
var t = x[0];
x[0] = x[1] ^ this.P[0];
x[1] = t;
};
function stream2word(data, databytes){
var i, temp = 0;
for (i = 0; i < 4; i++, BLF_J++) {
if (BLF_J >= databytes) BLF_J = 0;
temp = (temp << 8) | data[BLF_J];
}
return temp;
};
Blowfish.prototype.expand0state = function(key, keybytes) {
var d = new Uint32Array(2), i, k;
var d8 = new Uint8Array(d.buffer);
for (i = 0, BLF_J = 0; i < 18; i++) {
this.P[i] ^= stream2word(key, keybytes);
}
BLF_J = 0;
for (i = 0; i < 18; i += 2) {
this.encipher(d, d8);
this.P[i] = d[0];
this.P[i+1] = d[1];
}
for (i = 0; i < 4; i++) {
for (k = 0; k < 256; k += 2) {
this.encipher(d, d8);
this.S[i][k] = d[0];
this.S[i][k+1] = d[1];
}
}
};
Blowfish.prototype.expandstate = function(data, databytes, key, keybytes) {
var d = new Uint32Array(2), i, k;
for (i = 0, BLF_J = 0; i < 18; i++) {
this.P[i] ^= stream2word(key, keybytes);
}
for (i = 0, BLF_J = 0; i < 18; i += 2) {
d[0] ^= stream2word(data, databytes);
d[1] ^= stream2word(data, databytes);
this.encipher(d);
this.P[i] = d[0];
this.P[i+1] = d[1];
}
for (i = 0; i < 4; i++) {
for (k = 0; k < 256; k += 2) {
d[0] ^= stream2word(data, databytes);
d[1] ^= stream2word(data, databytes);
this.encipher(d);
this.S[i][k] = d[0];
this.S[i][k+1] = d[1];
}
}
BLF_J = 0;
};
Blowfish.prototype.enc = function(data, blocks) {
for (var i = 0; i < blocks; i++) {
this.encipher(data.subarray(i*2));
}
};
Blowfish.prototype.dec = function(data, blocks) {
for (var i = 0; i < blocks; i++) {
this.decipher(data.subarray(i*2));
}
};
var BCRYPT_BLOCKS = 8,
BCRYPT_HASHSIZE = 32;
function bcrypt_hash(sha2pass, sha2salt, out) {
var state = new Blowfish(),
cdata = new Uint32Array(BCRYPT_BLOCKS), i,
ciphertext = new Uint8Array([79,120,121,99,104,114,111,109,97,116,105,
99,66,108,111,119,102,105,115,104,83,119,97,116,68,121,110,97,109,
105,116,101]); //"OxychromaticBlowfishSwatDynamite"
state.expandstate(sha2salt, 64, sha2pass, 64);
for (i = 0; i < 64; i++) {
state.expand0state(sha2salt, 64);
state.expand0state(sha2pass, 64);
}
for (i = 0; i < BCRYPT_BLOCKS; i++)
cdata[i] = stream2word(ciphertext, ciphertext.byteLength);
for (i = 0; i < 64; i++)
state.enc(cdata, cdata.byteLength / 8);
for (i = 0; i < BCRYPT_BLOCKS; i++) {
out[4*i+3] = cdata[i] >>> 24;
out[4*i+2] = cdata[i] >>> 16;
out[4*i+1] = cdata[i] >>> 8;
out[4*i+0] = cdata[i];
}
};
function bcrypt_pbkdf(pass, passlen, salt, saltlen, key, keylen, rounds) {
var sha2pass = new Uint8Array(64),
sha2salt = new Uint8Array(64),
out = new Uint8Array(BCRYPT_HASHSIZE),
tmpout = new Uint8Array(BCRYPT_HASHSIZE),
countsalt = new Uint8Array(saltlen+4),
i, j, amt, stride, dest, count,
origkeylen = keylen;
if (rounds < 1)
return -1;
if (passlen === 0 || saltlen === 0 || keylen === 0 ||
keylen > (out.byteLength * out.byteLength) || saltlen > (1<<20))
return -1;
stride = Math.floor((keylen + out.byteLength - 1) / out.byteLength);
amt = Math.floor((keylen + stride - 1) / stride);
for (i = 0; i < saltlen; i++)
countsalt[i] = salt[i];
crypto_hash_sha512(sha2pass, pass, passlen);
for (count = 1; keylen > 0; count++) {
countsalt[saltlen+0] = count >>> 24;
countsalt[saltlen+1] = count >>> 16;
countsalt[saltlen+2] = count >>> 8;
countsalt[saltlen+3] = count;
crypto_hash_sha512(sha2salt, countsalt, saltlen + 4);
bcrypt_hash(sha2pass, sha2salt, tmpout);
for (i = out.byteLength; i--;)
out[i] = tmpout[i];
for (i = 1; i < rounds; i++) {
crypto_hash_sha512(sha2salt, tmpout, tmpout.byteLength);
bcrypt_hash(sha2pass, sha2salt, tmpout);
for (j = 0; j < out.byteLength; j++)
out[j] ^= tmpout[j];
}
amt = Math.min(amt, keylen);
for (i = 0; i < amt; i++) {
dest = i * stride + (count - 1);
if (dest >= origkeylen)
break;
key[dest] = out[i];
}
keylen -= i;
}
return 0;
};
module.exports = {
BLOCKS: BCRYPT_BLOCKS,
HASHSIZE: BCRYPT_HASHSIZE,
hash: bcrypt_hash,
pbkdf: bcrypt_pbkdf
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/bcrypt-pbkdf/index.js | index.js |
# Acorn
A tiny, fast JavaScript parser written in JavaScript.
## Community
Acorn is open source software released under an
[MIT license](https://github.com/acornjs/acorn/blob/master/acorn/LICENSE).
You are welcome to
[report bugs](https://github.com/acornjs/acorn/issues) or create pull
requests on [github](https://github.com/acornjs/acorn). For questions
and discussion, please use the
[Tern discussion forum](https://discuss.ternjs.net).
## Installation
The easiest way to install acorn is from [`npm`](https://www.npmjs.com/):
```sh
npm install acorn
```
Alternately, you can download the source and build acorn yourself:
```sh
git clone https://github.com/acornjs/acorn.git
cd acorn
npm install
```
## Interface
**parse**`(input, options)` is the main interface to the library. The
`input` parameter is a string, `options` can be undefined or an object
setting some of the options listed below. The return value will be an
abstract syntax tree object as specified by the [ESTree
spec](https://github.com/estree/estree).
```javascript
let acorn = require("acorn");
console.log(acorn.parse("1 + 1"));
```
When encountering a syntax error, the parser will raise a
`SyntaxError` object with a meaningful message. The error object will
have a `pos` property that indicates the string offset at which the
error occurred, and a `loc` object that contains a `{line, column}`
object referring to that same position.
Options can be provided by passing a second argument, which should be
an object containing any of these fields:
- **ecmaVersion**: Indicates the ECMAScript version to parse. Must be
either 3, 5, 6 (2015), 7 (2016), 8 (2017), 9 (2018), 10 (2019) or 11
(2020, partial support). This influences support for strict mode,
the set of reserved words, and support for new syntax features.
Default is 10.
**NOTE**: Only 'stage 4' (finalized) ECMAScript features are being
implemented by Acorn. Other proposed new features can be implemented
through plugins.
- **sourceType**: Indicate the mode the code should be parsed in. Can be
either `"script"` or `"module"`. This influences global strict mode
and parsing of `import` and `export` declarations.
**NOTE**: If set to `"module"`, then static `import` / `export` syntax
will be valid, even if `ecmaVersion` is less than 6.
- **onInsertedSemicolon**: If given a callback, that callback will be
called whenever a missing semicolon is inserted by the parser. The
callback will be given the character offset of the point where the
semicolon is inserted as argument, and if `locations` is on, also a
`{line, column}` object representing this position.
- **onTrailingComma**: Like `onInsertedSemicolon`, but for trailing
commas.
- **allowReserved**: If `false`, using a reserved word will generate
an error. Defaults to `true` for `ecmaVersion` 3, `false` for higher
versions. When given the value `"never"`, reserved words and
keywords can also not be used as property names (as in Internet
Explorer's old parser).
- **allowReturnOutsideFunction**: By default, a return statement at
the top level raises an error. Set this to `true` to accept such
code.
- **allowImportExportEverywhere**: By default, `import` and `export`
declarations can only appear at a program's top level. Setting this
option to `true` allows them anywhere where a statement is allowed.
- **allowAwaitOutsideFunction**: By default, `await` expressions can
only appear inside `async` functions. Setting this option to
`true` allows to have top-level `await` expressions. They are
still not allowed in non-`async` functions, though.
- **allowHashBang**: When this is enabled (off by default), if the
code starts with the characters `#!` (as in a shellscript), the
first line will be treated as a comment.
- **locations**: When `true`, each node has a `loc` object attached
with `start` and `end` subobjects, each of which contains the
one-based line and zero-based column numbers in `{line, column}`
form. Default is `false`.
- **onToken**: If a function is passed for this option, each found
token will be passed in same format as tokens returned from
`tokenizer().getToken()`.
If array is passed, each found token is pushed to it.
Note that you are not allowed to call the parser from the
callback—that will corrupt its internal state.
- **onComment**: If a function is passed for this option, whenever a
comment is encountered the function will be called with the
following parameters:
- `block`: `true` if the comment is a block comment, false if it
is a line comment.
- `text`: The content of the comment.
- `start`: Character offset of the start of the comment.
- `end`: Character offset of the end of the comment.
When the `locations` options is on, the `{line, column}` locations
of the comment’s start and end are passed as two additional
parameters.
If array is passed for this option, each found comment is pushed
to it as object in Esprima format:
```javascript
{
"type": "Line" | "Block",
"value": "comment text",
"start": Number,
"end": Number,
// If `locations` option is on:
"loc": {
"start": {line: Number, column: Number}
"end": {line: Number, column: Number}
},
// If `ranges` option is on:
"range": [Number, Number]
}
```
Note that you are not allowed to call the parser from the
callback—that will corrupt its internal state.
- **ranges**: Nodes have their start and end characters offsets
recorded in `start` and `end` properties (directly on the node,
rather than the `loc` object, which holds line/column data. To also
add a
[semi-standardized](https://bugzilla.mozilla.org/show_bug.cgi?id=745678)
`range` property holding a `[start, end]` array with the same
numbers, set the `ranges` option to `true`.
- **program**: It is possible to parse multiple files into a single
AST by passing the tree produced by parsing the first file as the
`program` option in subsequent parses. This will add the toplevel
forms of the parsed file to the "Program" (top) node of an existing
parse tree.
- **sourceFile**: When the `locations` option is `true`, you can pass
this option to add a `source` attribute in every node’s `loc`
object. Note that the contents of this option are not examined or
processed in any way; you are free to use whatever format you
choose.
- **directSourceFile**: Like `sourceFile`, but a `sourceFile` property
will be added (regardless of the `location` option) directly to the
nodes, rather than the `loc` object.
- **preserveParens**: If this option is `true`, parenthesized expressions
are represented by (non-standard) `ParenthesizedExpression` nodes
that have a single `expression` property containing the expression
inside parentheses.
**parseExpressionAt**`(input, offset, options)` will parse a single
expression in a string, and return its AST. It will not complain if
there is more of the string left after the expression.
**tokenizer**`(input, options)` returns an object with a `getToken`
method that can be called repeatedly to get the next token, a `{start,
end, type, value}` object (with added `loc` property when the
`locations` option is enabled and `range` property when the `ranges`
option is enabled). When the token's type is `tokTypes.eof`, you
should stop calling the method, since it will keep returning that same
token forever.
In ES6 environment, returned result can be used as any other
protocol-compliant iterable:
```javascript
for (let token of acorn.tokenizer(str)) {
// iterate over the tokens
}
// transform code to array of tokens:
var tokens = [...acorn.tokenizer(str)];
```
**tokTypes** holds an object mapping names to the token type objects
that end up in the `type` properties of tokens.
**getLineInfo**`(input, offset)` can be used to get a `{line,
column}` object for a given program string and offset.
### The `Parser` class
Instances of the **`Parser`** class contain all the state and logic
that drives a parse. It has static methods `parse`,
`parseExpressionAt`, and `tokenizer` that match the top-level
functions by the same name.
When extending the parser with plugins, you need to call these methods
on the extended version of the class. To extend a parser with plugins,
you can use its static `extend` method.
```javascript
var acorn = require("acorn");
var jsx = require("acorn-jsx");
var JSXParser = acorn.Parser.extend(jsx());
JSXParser.parse("foo(<bar/>)");
```
The `extend` method takes any number of plugin values, and returns a
new `Parser` class that includes the extra parser logic provided by
the plugins.
## Command line interface
The `bin/acorn` utility can be used to parse a file from the command
line. It accepts as arguments its input file and the following
options:
- `--ecma3|--ecma5|--ecma6|--ecma7|--ecma8|--ecma9|--ecma10`: Sets the ECMAScript version
to parse. Default is version 9.
- `--module`: Sets the parsing mode to `"module"`. Is set to `"script"` otherwise.
- `--locations`: Attaches a "loc" object to each node with "start" and
"end" subobjects, each of which contains the one-based line and
zero-based column numbers in `{line, column}` form.
- `--allow-hash-bang`: If the code starts with the characters #! (as
in a shellscript), the first line will be treated as a comment.
- `--compact`: No whitespace is used in the AST output.
- `--silent`: Do not output the AST, just return the exit status.
- `--help`: Print the usage information and quit.
The utility spits out the syntax tree as JSON data.
## Existing plugins
- [`acorn-jsx`](https://github.com/RReverser/acorn-jsx): Parse [Facebook JSX syntax extensions](https://github.com/facebook/jsx)
Plugins for ECMAScript proposals:
- [`acorn-stage3`](https://github.com/acornjs/acorn-stage3): Parse most stage 3 proposals, bundling:
- [`acorn-class-fields`](https://github.com/acornjs/acorn-class-fields): Parse [class fields proposal](https://github.com/tc39/proposal-class-fields)
- [`acorn-import-meta`](https://github.com/acornjs/acorn-import-meta): Parse [import.meta proposal](https://github.com/tc39/proposal-import-meta)
- [`acorn-numeric-separator`](https://github.com/acornjs/acorn-numeric-separator): Parse [numeric separator proposal](https://github.com/tc39/proposal-numeric-separator)
- [`acorn-private-methods`](https://github.com/acornjs/acorn-private-methods): parse [private methods, getters and setters proposal](https://github.com/tc39/proposal-private-methods)n
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/acorn/README.md | README.md |
## 7.3.1 (2020-06-11)
### Bug fixes
Make the string in the `version` export match the actual library version.
## 7.3.0 (2020-06-11)
### Bug fixes
Fix a bug that caused parsing of object patterns with a property named `set` that had a default value to fail.
### New features
Add support for optional chaining (`?.`).
## 7.2.0 (2020-05-09)
### Bug fixes
Fix precedence issue in parsing of async arrow functions.
### New features
Add support for nullish coalescing.
Add support for `import.meta`.
Support `export * as ...` syntax.
Upgrade to Unicode 13.
## 6.4.1 (2020-03-09)
### Bug fixes
More carefully check for valid UTF16 surrogate pairs in regexp validator.
## 7.1.1 (2020-03-01)
### Bug fixes
Treat `\8` and `\9` as invalid escapes in template strings.
Allow unicode escapes in property names that are keywords.
Don't error on an exponential operator expression as argument to `await`.
More carefully check for valid UTF16 surrogate pairs in regexp validator.
## 7.1.0 (2019-09-24)
### Bug fixes
Disallow trailing object literal commas when ecmaVersion is less than 5.
### New features
Add a static `acorn` property to the `Parser` class that contains the entire module interface, to allow plugins to access the instance of the library that they are acting on.
## 7.0.0 (2019-08-13)
### Breaking changes
Changes the node format for dynamic imports to use the `ImportExpression` node type, as defined in [ESTree](https://github.com/estree/estree/blob/master/es2020.md#importexpression).
Makes 10 (ES2019) the default value for the `ecmaVersion` option.
## 6.3.0 (2019-08-12)
### New features
`sourceType: "module"` can now be used even when `ecmaVersion` is less than 6, to parse module-style code that otherwise conforms to an older standard.
## 6.2.1 (2019-07-21)
### Bug fixes
Fix bug causing Acorn to treat some characters as identifier characters that shouldn't be treated as such.
Fix issue where setting the `allowReserved` option to `"never"` allowed reserved words in some circumstances.
## 6.2.0 (2019-07-04)
### Bug fixes
Improve valid assignment checking in `for`/`in` and `for`/`of` loops.
Disallow binding `let` in patterns.
### New features
Support bigint syntax with `ecmaVersion` >= 11.
Support dynamic `import` syntax with `ecmaVersion` >= 11.
Upgrade to Unicode version 12.
## 6.1.1 (2019-02-27)
### Bug fixes
Fix bug that caused parsing default exports of with names to fail.
## 6.1.0 (2019-02-08)
### Bug fixes
Fix scope checking when redefining a `var` as a lexical binding.
### New features
Split up `parseSubscripts` to use an internal `parseSubscript` method to make it easier to extend with plugins.
## 6.0.7 (2019-02-04)
### Bug fixes
Check that exported bindings are defined.
Don't treat `\u180e` as a whitespace character.
Check for duplicate parameter names in methods.
Don't allow shorthand properties when they are generators or async methods.
Forbid binding `await` in async arrow function's parameter list.
## 6.0.6 (2019-01-30)
### Bug fixes
The content of class declarations and expressions is now always parsed in strict mode.
Don't allow `let` or `const` to bind the variable name `let`.
Treat class declarations as lexical.
Don't allow a generator function declaration as the sole body of an `if` or `else`.
Ignore `"use strict"` when after an empty statement.
Allow string line continuations with special line terminator characters.
Treat `for` bodies as part of the `for` scope when checking for conflicting bindings.
Fix bug with parsing `yield` in a `for` loop initializer.
Implement special cases around scope checking for functions.
## 6.0.5 (2019-01-02)
### Bug fixes
Fix TypeScript type for `Parser.extend` and add `allowAwaitOutsideFunction` to options type.
Don't treat `let` as a keyword when the next token is `{` on the next line.
Fix bug that broke checking for parentheses around an object pattern in a destructuring assignment when `preserveParens` was on.
## 6.0.4 (2018-11-05)
### Bug fixes
Further improvements to tokenizing regular expressions in corner cases.
## 6.0.3 (2018-11-04)
### Bug fixes
Fix bug in tokenizing an expression-less return followed by a function followed by a regular expression.
Remove stray symlink in the package tarball.
## 6.0.2 (2018-09-26)
### Bug fixes
Fix bug where default expressions could fail to parse inside an object destructuring assignment expression.
## 6.0.1 (2018-09-14)
### Bug fixes
Fix wrong value in `version` export.
## 6.0.0 (2018-09-14)
### Bug fixes
Better handle variable-redefinition checks for catch bindings and functions directly under if statements.
Forbid `new.target` in top-level arrow functions.
Fix issue with parsing a regexp after `yield` in some contexts.
### New features
The package now comes with TypeScript definitions.
### Breaking changes
The default value of the `ecmaVersion` option is now 9 (2018).
Plugins work differently, and will have to be rewritten to work with this version.
The loose parser and walker have been moved into separate packages (`acorn-loose` and `acorn-walk`).
## 5.7.3 (2018-09-10)
### Bug fixes
Fix failure to tokenize regexps after expressions like `x.of`.
Better error message for unterminated template literals.
## 5.7.2 (2018-08-24)
### Bug fixes
Properly handle `allowAwaitOutsideFunction` in for statements.
Treat function declarations at the top level of modules like let bindings.
Don't allow async function declarations as the only statement under a label.
## 5.7.0 (2018-06-15)
### New features
Upgraded to Unicode 11.
## 5.6.0 (2018-05-31)
### New features
Allow U+2028 and U+2029 in string when ECMAVersion >= 10.
Allow binding-less catch statements when ECMAVersion >= 10.
Add `allowAwaitOutsideFunction` option for parsing top-level `await`.
## 5.5.3 (2018-03-08)
### Bug fixes
A _second_ republish of the code in 5.5.1, this time with yarn, to hopefully get valid timestamps.
## 5.5.2 (2018-03-08)
### Bug fixes
A republish of the code in 5.5.1 in an attempt to solve an issue with the file timestamps in the npm package being 0.
## 5.5.1 (2018-03-06)
### Bug fixes
Fix misleading error message for octal escapes in template strings.
## 5.5.0 (2018-02-27)
### New features
The identifier character categorization is now based on Unicode version 10.
Acorn will now validate the content of regular expressions, including new ES9 features.
## 5.4.0 (2018-02-01)
### Bug fixes
Disallow duplicate or escaped flags on regular expressions.
Disallow octal escapes in strings in strict mode.
### New features
Add support for async iteration.
Add support for object spread and rest.
## 5.3.0 (2017-12-28)
### Bug fixes
Fix parsing of floating point literals with leading zeroes in loose mode.
Allow duplicate property names in object patterns.
Don't allow static class methods named `prototype`.
Disallow async functions directly under `if` or `else`.
Parse right-hand-side of `for`/`of` as an assignment expression.
Stricter parsing of `for`/`in`.
Don't allow unicode escapes in contextual keywords.
### New features
Parsing class members was factored into smaller methods to allow plugins to hook into it.
## 5.2.1 (2017-10-30)
### Bug fixes
Fix a token context corruption bug.
## 5.2.0 (2017-10-30)
### Bug fixes
Fix token context tracking for `class` and `function` in property-name position.
Make sure `%*` isn't parsed as a valid operator.
Allow shorthand properties `get` and `set` to be followed by default values.
Disallow `super` when not in callee or object position.
### New features
Support [`directive` property](https://github.com/estree/estree/compare/b3de58c9997504d6fba04b72f76e6dd1619ee4eb...1da8e603237144f44710360f8feb7a9977e905e0) on directive expression statements.
## 5.1.2 (2017-09-04)
### Bug fixes
Disable parsing of legacy HTML-style comments in modules.
Fix parsing of async methods whose names are keywords.
## 5.1.1 (2017-07-06)
### Bug fixes
Fix problem with disambiguating regexp and division after a class.
## 5.1.0 (2017-07-05)
### Bug fixes
Fix tokenizing of regexps in an object-desctructuring `for`/`of` loop and after `yield`.
Parse zero-prefixed numbers with non-octal digits as decimal.
Allow object/array patterns in rest parameters.
Don't error when `yield` is used as a property name.
Allow `async` as a shorthand object property.
### New features
Implement the [template literal revision proposal](https://github.com/tc39/proposal-template-literal-revision) for ES9.
## 5.0.3 (2017-04-01)
### Bug fixes
Fix spurious duplicate variable definition errors for named functions.
## 5.0.2 (2017-03-30)
### Bug fixes
A binary operator after a parenthesized arrow expression is no longer incorrectly treated as an error.
## 5.0.0 (2017-03-28)
### Bug fixes
Raise an error for duplicated lexical bindings.
Fix spurious error when an assignement expression occurred after a spread expression.
Accept regular expressions after `of` (in `for`/`of`), `yield` (in a generator), and braced arrow functions.
Allow labels in front or `var` declarations, even in strict mode.
### Breaking changes
Parse declarations following `export default` as declaration nodes, not expressions. This means that class and function declarations nodes can now have `null` as their `id`.
## 4.0.11 (2017-02-07)
### Bug fixes
Allow all forms of member expressions to be parenthesized as lvalue.
## 4.0.10 (2017-02-07)
### Bug fixes
Don't expect semicolons after default-exported functions or classes, even when they are expressions.
Check for use of `'use strict'` directives in non-simple parameter functions, even when already in strict mode.
## 4.0.9 (2017-02-06)
### Bug fixes
Fix incorrect error raised for parenthesized simple assignment targets, so that `(x) = 1` parses again.
## 4.0.8 (2017-02-03)
### Bug fixes
Solve spurious parenthesized pattern errors by temporarily erring on the side of accepting programs that our delayed errors don't handle correctly yet.
## 4.0.7 (2017-02-02)
### Bug fixes
Accept invalidly rejected code like `(x).y = 2` again.
Don't raise an error when a function _inside_ strict code has a non-simple parameter list.
## 4.0.6 (2017-02-02)
### Bug fixes
Fix exponential behavior (manifesting itself as a complete hang for even relatively small source files) introduced by the new 'use strict' check.
## 4.0.5 (2017-02-02)
### Bug fixes
Disallow parenthesized pattern expressions.
Allow keywords as export names.
Don't allow the `async` keyword to be parenthesized.
Properly raise an error when a keyword contains a character escape.
Allow `"use strict"` to appear after other string literal expressions.
Disallow labeled declarations.
## 4.0.4 (2016-12-19)
### Bug fixes
Fix crash when `export` was followed by a keyword that can't be
exported.
## 4.0.3 (2016-08-16)
### Bug fixes
Allow regular function declarations inside single-statement `if` branches in loose mode. Forbid them entirely in strict mode.
Properly parse properties named `async` in ES2017 mode.
Fix bug where reserved words were broken in ES2017 mode.
## 4.0.2 (2016-08-11)
### Bug fixes
Don't ignore period or 'e' characters after octal numbers.
Fix broken parsing for call expressions in default parameter values of arrow functions.
## 4.0.1 (2016-08-08)
### Bug fixes
Fix false positives in duplicated export name errors.
## 4.0.0 (2016-08-07)
### Breaking changes
The default `ecmaVersion` option value is now 7.
A number of internal method signatures changed, so plugins might need to be updated.
### Bug fixes
The parser now raises errors on duplicated export names.
`arguments` and `eval` can now be used in shorthand properties.
Duplicate parameter names in non-simple argument lists now always produce an error.
### New features
The `ecmaVersion` option now also accepts year-style version numbers
(2015, etc).
Support for `async`/`await` syntax when `ecmaVersion` is >= 8.
Support for trailing commas in call expressions when `ecmaVersion` is >= 8.
## 3.3.0 (2016-07-25)
### Bug fixes
Fix bug in tokenizing of regexp operator after a function declaration.
Fix parser crash when parsing an array pattern with a hole.
### New features
Implement check against complex argument lists in functions that enable strict mode in ES7.
## 3.2.0 (2016-06-07)
### Bug fixes
Improve handling of lack of unicode regexp support in host
environment.
Properly reject shorthand properties whose name is a keyword.
### New features
Visitors created with `visit.make` now have their base as _prototype_, rather than copying properties into a fresh object.
## 3.1.0 (2016-04-18)
### Bug fixes
Properly tokenize the division operator directly after a function expression.
Allow trailing comma in destructuring arrays.
## 3.0.4 (2016-02-25)
### Fixes
Allow update expressions as left-hand-side of the ES7 exponential operator.
## 3.0.2 (2016-02-10)
### Fixes
Fix bug that accidentally made `undefined` a reserved word when parsing ES7.
## 3.0.0 (2016-02-10)
### Breaking changes
The default value of the `ecmaVersion` option is now 6 (used to be 5).
Support for comprehension syntax (which was dropped from the draft spec) has been removed.
### Fixes
`let` and `yield` are now “contextual keywords”, meaning you can mostly use them as identifiers in ES5 non-strict code.
A parenthesized class or function expression after `export default` is now parsed correctly.
### New features
When `ecmaVersion` is set to 7, Acorn will parse the exponentiation operator (`**`).
The identifier character ranges are now based on Unicode 8.0.0.
Plugins can now override the `raiseRecoverable` method to override the way non-critical errors are handled.
## 2.7.0 (2016-01-04)
### Fixes
Stop allowing rest parameters in setters.
Disallow `y` rexexp flag in ES5.
Disallow `\00` and `\000` escapes in strict mode.
Raise an error when an import name is a reserved word.
## 2.6.2 (2015-11-10)
### Fixes
Don't crash when no options object is passed.
## 2.6.0 (2015-11-09)
### Fixes
Add `await` as a reserved word in module sources.
Disallow `yield` in a parameter default value for a generator.
Forbid using a comma after a rest pattern in an array destructuring.
### New features
Support parsing stdin in command-line tool.
## 2.5.0 (2015-10-27)
### Fixes
Fix tokenizer support in the command-line tool.
Stop allowing `new.target` outside of functions.
Remove legacy `guard` and `guardedHandler` properties from try nodes.
Stop allowing multiple `__proto__` properties on an object literal in strict mode.
Don't allow rest parameters to be non-identifier patterns.
Check for duplicate paramter names in arrow functions.
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/acorn/CHANGELOG.md | CHANGELOG.md |
export as namespace acorn
export = acorn
declare namespace acorn {
function parse(input: string, options?: Options): Node
function parseExpressionAt(input: string, pos?: number, options?: Options): Node
function tokenizer(input: string, options?: Options): {
getToken(): Token
[Symbol.iterator](): Iterator<Token>
}
interface Options {
ecmaVersion?: 3 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 2015 | 2016 | 2017 | 2018 | 2019 | 2020
sourceType?: 'script' | 'module'
onInsertedSemicolon?: (lastTokEnd: number, lastTokEndLoc?: Position) => void
onTrailingComma?: (lastTokEnd: number, lastTokEndLoc?: Position) => void
allowReserved?: boolean | 'never'
allowReturnOutsideFunction?: boolean
allowImportExportEverywhere?: boolean
allowAwaitOutsideFunction?: boolean
allowHashBang?: boolean
locations?: boolean
onToken?: ((token: Token) => any) | Token[]
onComment?: ((
isBlock: boolean, text: string, start: number, end: number, startLoc?: Position,
endLoc?: Position
) => void) | Comment[]
ranges?: boolean
program?: Node
sourceFile?: string
directSourceFile?: string
preserveParens?: boolean
}
class Parser {
constructor(options: Options, input: string, startPos?: number)
parse(this: Parser): Node
static parse(this: typeof Parser, input: string, options?: Options): Node
static parseExpressionAt(this: typeof Parser, input: string, pos: number, options?: Options): Node
static tokenizer(this: typeof Parser, input: string, options?: Options): {
getToken(): Token
[Symbol.iterator](): Iterator<Token>
}
static extend(this: typeof Parser, ...plugins: ((BaseParser: typeof Parser) => typeof Parser)[]): typeof Parser
}
interface Position { line: number; column: number; offset: number }
const defaultOptions: Options
function getLineInfo(input: string, offset: number): Position
class SourceLocation {
start: Position
end: Position
source?: string | null
constructor(p: Parser, start: Position, end: Position)
}
class Node {
type: string
start: number
end: number
loc?: SourceLocation
sourceFile?: string
range?: [number, number]
constructor(parser: Parser, pos: number, loc?: SourceLocation)
}
class TokenType {
label: string
keyword: string
beforeExpr: boolean
startsExpr: boolean
isLoop: boolean
isAssign: boolean
prefix: boolean
postfix: boolean
binop: number
updateContext?: (prevType: TokenType) => void
constructor(label: string, conf?: any)
}
const tokTypes: {
num: TokenType
regexp: TokenType
string: TokenType
name: TokenType
eof: TokenType
bracketL: TokenType
bracketR: TokenType
braceL: TokenType
braceR: TokenType
parenL: TokenType
parenR: TokenType
comma: TokenType
semi: TokenType
colon: TokenType
dot: TokenType
question: TokenType
arrow: TokenType
template: TokenType
ellipsis: TokenType
backQuote: TokenType
dollarBraceL: TokenType
eq: TokenType
assign: TokenType
incDec: TokenType
prefix: TokenType
logicalOR: TokenType
logicalAND: TokenType
bitwiseOR: TokenType
bitwiseXOR: TokenType
bitwiseAND: TokenType
equality: TokenType
relational: TokenType
bitShift: TokenType
plusMin: TokenType
modulo: TokenType
star: TokenType
slash: TokenType
starstar: TokenType
_break: TokenType
_case: TokenType
_catch: TokenType
_continue: TokenType
_debugger: TokenType
_default: TokenType
_do: TokenType
_else: TokenType
_finally: TokenType
_for: TokenType
_function: TokenType
_if: TokenType
_return: TokenType
_switch: TokenType
_throw: TokenType
_try: TokenType
_var: TokenType
_const: TokenType
_while: TokenType
_with: TokenType
_new: TokenType
_this: TokenType
_super: TokenType
_class: TokenType
_extends: TokenType
_export: TokenType
_import: TokenType
_null: TokenType
_true: TokenType
_false: TokenType
_in: TokenType
_instanceof: TokenType
_typeof: TokenType
_void: TokenType
_delete: TokenType
}
class TokContext {
constructor(token: string, isExpr: boolean, preserveSpace: boolean, override?: (p: Parser) => void)
}
const tokContexts: {
b_stat: TokContext
b_expr: TokContext
b_tmpl: TokContext
p_stat: TokContext
p_expr: TokContext
q_tmpl: TokContext
f_expr: TokContext
}
function isIdentifierStart(code: number, astral?: boolean): boolean
function isIdentifierChar(code: number, astral?: boolean): boolean
interface AbstractToken {
}
interface Comment extends AbstractToken {
type: string
value: string
start: number
end: number
loc?: SourceLocation
range?: [number, number]
}
class Token {
type: TokenType
value: any
start: number
end: number
loc?: SourceLocation
range?: [number, number]
constructor(p: Parser)
}
function isNewLine(code: number): boolean
const lineBreak: RegExp
const lineBreakG: RegExp
const version: string
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/acorn/dist/acorn.d.ts | acorn.d.ts |
'use strict';
var path = require('path');
var fs = require('fs');
var acorn = require('acorn');
var infile, forceFile, silent = false, compact = false, tokenize = false;
var options = {};
function help(status) {
var print = (status === 0) ? console.log : console.error;
print("usage: " + path.basename(process.argv[1]) + " [--ecma3|--ecma5|--ecma6|--ecma7|--ecma8|--ecma9|...|--ecma2015|--ecma2016|--ecma2017|--ecma2018|...]");
print(" [--tokenize] [--locations] [---allow-hash-bang] [--compact] [--silent] [--module] [--help] [--] [infile]");
process.exit(status);
}
for (var i = 2; i < process.argv.length; ++i) {
var arg = process.argv[i];
if ((arg === "-" || arg[0] !== "-") && !infile) { infile = arg; }
else if (arg === "--" && !infile && i + 2 === process.argv.length) { forceFile = infile = process.argv[++i]; }
else if (arg === "--locations") { options.locations = true; }
else if (arg === "--allow-hash-bang") { options.allowHashBang = true; }
else if (arg === "--silent") { silent = true; }
else if (arg === "--compact") { compact = true; }
else if (arg === "--help") { help(0); }
else if (arg === "--tokenize") { tokenize = true; }
else if (arg === "--module") { options.sourceType = "module"; }
else {
var match = arg.match(/^--ecma(\d+)$/);
if (match)
{ options.ecmaVersion = +match[1]; }
else
{ help(1); }
}
}
function run(code) {
var result;
try {
if (!tokenize) {
result = acorn.parse(code, options);
} else {
result = [];
var tokenizer = acorn.tokenizer(code, options), token;
do {
token = tokenizer.getToken();
result.push(token);
} while (token.type !== acorn.tokTypes.eof)
}
} catch (e) {
console.error(infile && infile !== "-" ? e.message.replace(/\(\d+:\d+\)$/, function (m) { return m.slice(0, 1) + infile + " " + m.slice(1); }) : e.message);
process.exit(1);
}
if (!silent) { console.log(JSON.stringify(result, null, compact ? null : 2)); }
}
if (forceFile || infile && infile !== "-") {
run(fs.readFileSync(infile, "utf8"));
} else {
var code = "";
process.stdin.resume();
process.stdin.on("data", function (chunk) { return code += chunk; });
process.stdin.on("end", function () { return run(code); });
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/acorn/dist/bin.js | bin.js |
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) :
typeof define === 'function' && define.amd ? define(['exports'], factory) :
(global = global || self, factory(global.acorn = {}));
}(this, (function (exports) { 'use strict';
// Reserved word lists for various dialects of the language
var reservedWords = {
3: "abstract boolean byte char class double enum export extends final float goto implements import int interface long native package private protected public short static super synchronized throws transient volatile",
5: "class enum extends super const export import",
6: "enum",
strict: "implements interface let package private protected public static yield",
strictBind: "eval arguments"
};
// And the keywords
var ecma5AndLessKeywords = "break case catch continue debugger default do else finally for function if return switch throw try var while with null true false instanceof typeof void delete new in this";
var keywords = {
5: ecma5AndLessKeywords,
"5module": ecma5AndLessKeywords + " export import",
6: ecma5AndLessKeywords + " const class extends export import super"
};
var keywordRelationalOperator = /^in(stanceof)?$/;
// ## Character categories
// Big ugly regular expressions that match characters in the
// whitespace, identifier, and identifier-start categories. These
// are only applied when a character is found to actually have a
// code point above 128.
// Generated by `bin/generate-identifier-regex.js`.
var nonASCIIidentifierStartChars = "\xaa\xb5\xba\xc0-\xd6\xd8-\xf6\xf8-\u02c1\u02c6-\u02d1\u02e0-\u02e4\u02ec\u02ee\u0370-\u0374\u0376\u0377\u037a-\u037d\u037f\u0386\u0388-\u038a\u038c\u038e-\u03a1\u03a3-\u03f5\u03f7-\u0481\u048a-\u052f\u0531-\u0556\u0559\u0560-\u0588\u05d0-\u05ea\u05ef-\u05f2\u0620-\u064a\u066e\u066f\u0671-\u06d3\u06d5\u06e5\u06e6\u06ee\u06ef\u06fa-\u06fc\u06ff\u0710\u0712-\u072f\u074d-\u07a5\u07b1\u07ca-\u07ea\u07f4\u07f5\u07fa\u0800-\u0815\u081a\u0824\u0828\u0840-\u0858\u0860-\u086a\u08a0-\u08b4\u08b6-\u08c7\u0904-\u0939\u093d\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098c\u098f\u0990\u0993-\u09a8\u09aa-\u09b0\u09b2\u09b6-\u09b9\u09bd\u09ce\u09dc\u09dd\u09df-\u09e1\u09f0\u09f1\u09fc\u0a05-\u0a0a\u0a0f\u0a10\u0a13-\u0a28\u0a2a-\u0a30\u0a32\u0a33\u0a35\u0a36\u0a38\u0a39\u0a59-\u0a5c\u0a5e\u0a72-\u0a74\u0a85-\u0a8d\u0a8f-\u0a91\u0a93-\u0aa8\u0aaa-\u0ab0\u0ab2\u0ab3\u0ab5-\u0ab9\u0abd\u0ad0\u0ae0\u0ae1\u0af9\u0b05-\u0b0c\u0b0f\u0b10\u0b13-\u0b28\u0b2a-\u0b30\u0b32\u0b33\u0b35-\u0b39\u0b3d\u0b5c\u0b5d\u0b5f-\u0b61\u0b71\u0b83\u0b85-\u0b8a\u0b8e-\u0b90\u0b92-\u0b95\u0b99\u0b9a\u0b9c\u0b9e\u0b9f\u0ba3\u0ba4\u0ba8-\u0baa\u0bae-\u0bb9\u0bd0\u0c05-\u0c0c\u0c0e-\u0c10\u0c12-\u0c28\u0c2a-\u0c39\u0c3d\u0c58-\u0c5a\u0c60\u0c61\u0c80\u0c85-\u0c8c\u0c8e-\u0c90\u0c92-\u0ca8\u0caa-\u0cb3\u0cb5-\u0cb9\u0cbd\u0cde\u0ce0\u0ce1\u0cf1\u0cf2\u0d04-\u0d0c\u0d0e-\u0d10\u0d12-\u0d3a\u0d3d\u0d4e\u0d54-\u0d56\u0d5f-\u0d61\u0d7a-\u0d7f\u0d85-\u0d96\u0d9a-\u0db1\u0db3-\u0dbb\u0dbd\u0dc0-\u0dc6\u0e01-\u0e30\u0e32\u0e33\u0e40-\u0e46\u0e81\u0e82\u0e84\u0e86-\u0e8a\u0e8c-\u0ea3\u0ea5\u0ea7-\u0eb0\u0eb2\u0eb3\u0ebd\u0ec0-\u0ec4\u0ec6\u0edc-\u0edf\u0f00\u0f40-\u0f47\u0f49-\u0f6c\u0f88-\u0f8c\u1000-\u102a\u103f\u1050-\u1055\u105a-\u105d\u1061\u1065\u1066\u106e-\u1070\u1075-\u1081\u108e\u10a0-\u10c5\u10c7\u10cd\u10d0-\u10fa\u10fc-\u1248\u124a-\u124d\u1250-\u1256\u1258\u125a-\u125d\u1260-\u1288\u128a-\u128d\u1290-\u12b0\u12b2-\u12b5\u12b8-\u12be\u12c0\u12c2-\u12c5\u12c8-\u12d6\u12d8-\u1310\u1312-\u1315\u1318-\u135a\u1380-\u138f\u13a0-\u13f5\u13f8-\u13fd\u1401-\u166c\u166f-\u167f\u1681-\u169a\u16a0-\u16ea\u16ee-\u16f8\u1700-\u170c\u170e-\u1711\u1720-\u1731\u1740-\u1751\u1760-\u176c\u176e-\u1770\u1780-\u17b3\u17d7\u17dc\u1820-\u1878\u1880-\u18a8\u18aa\u18b0-\u18f5\u1900-\u191e\u1950-\u196d\u1970-\u1974\u1980-\u19ab\u19b0-\u19c9\u1a00-\u1a16\u1a20-\u1a54\u1aa7\u1b05-\u1b33\u1b45-\u1b4b\u1b83-\u1ba0\u1bae\u1baf\u1bba-\u1be5\u1c00-\u1c23\u1c4d-\u1c4f\u1c5a-\u1c7d\u1c80-\u1c88\u1c90-\u1cba\u1cbd-\u1cbf\u1ce9-\u1cec\u1cee-\u1cf3\u1cf5\u1cf6\u1cfa\u1d00-\u1dbf\u1e00-\u1f15\u1f18-\u1f1d\u1f20-\u1f45\u1f48-\u1f4d\u1f50-\u1f57\u1f59\u1f5b\u1f5d\u1f5f-\u1f7d\u1f80-\u1fb4\u1fb6-\u1fbc\u1fbe\u1fc2-\u1fc4\u1fc6-\u1fcc\u1fd0-\u1fd3\u1fd6-\u1fdb\u1fe0-\u1fec\u1ff2-\u1ff4\u1ff6-\u1ffc\u2071\u207f\u2090-\u209c\u2102\u2107\u210a-\u2113\u2115\u2118-\u211d\u2124\u2126\u2128\u212a-\u2139\u213c-\u213f\u2145-\u2149\u214e\u2160-\u2188\u2c00-\u2c2e\u2c30-\u2c5e\u2c60-\u2ce4\u2ceb-\u2cee\u2cf2\u2cf3\u2d00-\u2d25\u2d27\u2d2d\u2d30-\u2d67\u2d6f\u2d80-\u2d96\u2da0-\u2da6\u2da8-\u2dae\u2db0-\u2db6\u2db8-\u2dbe\u2dc0-\u2dc6\u2dc8-\u2dce\u2dd0-\u2dd6\u2dd8-\u2dde\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303c\u3041-\u3096\u309b-\u309f\u30a1-\u30fa\u30fc-\u30ff\u3105-\u312f\u3131-\u318e\u31a0-\u31bf\u31f0-\u31ff\u3400-\u4dbf\u4e00-\u9ffc\ua000-\ua48c\ua4d0-\ua4fd\ua500-\ua60c\ua610-\ua61f\ua62a\ua62b\ua640-\ua66e\ua67f-\ua69d\ua6a0-\ua6ef\ua717-\ua71f\ua722-\ua788\ua78b-\ua7bf\ua7c2-\ua7ca\ua7f5-\ua801\ua803-\ua805\ua807-\ua80a\ua80c-\ua822\ua840-\ua873\ua882-\ua8b3\ua8f2-\ua8f7\ua8fb\ua8fd\ua8fe\ua90a-\ua925\ua930-\ua946\ua960-\ua97c\ua984-\ua9b2\ua9cf\ua9e0-\ua9e4\ua9e6-\ua9ef\ua9fa-\ua9fe\uaa00-\uaa28\uaa40-\uaa42\uaa44-\uaa4b\uaa60-\uaa76\uaa7a\uaa7e-\uaaaf\uaab1\uaab5\uaab6\uaab9-\uaabd\uaac0\uaac2\uaadb-\uaadd\uaae0-\uaaea\uaaf2-\uaaf4\uab01-\uab06\uab09-\uab0e\uab11-\uab16\uab20-\uab26\uab28-\uab2e\uab30-\uab5a\uab5c-\uab69\uab70-\uabe2\uac00-\ud7a3\ud7b0-\ud7c6\ud7cb-\ud7fb\uf900-\ufa6d\ufa70-\ufad9\ufb00-\ufb06\ufb13-\ufb17\ufb1d\ufb1f-\ufb28\ufb2a-\ufb36\ufb38-\ufb3c\ufb3e\ufb40\ufb41\ufb43\ufb44\ufb46-\ufbb1\ufbd3-\ufd3d\ufd50-\ufd8f\ufd92-\ufdc7\ufdf0-\ufdfb\ufe70-\ufe74\ufe76-\ufefc\uff21-\uff3a\uff41-\uff5a\uff66-\uffbe\uffc2-\uffc7\uffca-\uffcf\uffd2-\uffd7\uffda-\uffdc";
var nonASCIIidentifierChars = "\u200c\u200d\xb7\u0300-\u036f\u0387\u0483-\u0487\u0591-\u05bd\u05bf\u05c1\u05c2\u05c4\u05c5\u05c7\u0610-\u061a\u064b-\u0669\u0670\u06d6-\u06dc\u06df-\u06e4\u06e7\u06e8\u06ea-\u06ed\u06f0-\u06f9\u0711\u0730-\u074a\u07a6-\u07b0\u07c0-\u07c9\u07eb-\u07f3\u07fd\u0816-\u0819\u081b-\u0823\u0825-\u0827\u0829-\u082d\u0859-\u085b\u08d3-\u08e1\u08e3-\u0903\u093a-\u093c\u093e-\u094f\u0951-\u0957\u0962\u0963\u0966-\u096f\u0981-\u0983\u09bc\u09be-\u09c4\u09c7\u09c8\u09cb-\u09cd\u09d7\u09e2\u09e3\u09e6-\u09ef\u09fe\u0a01-\u0a03\u0a3c\u0a3e-\u0a42\u0a47\u0a48\u0a4b-\u0a4d\u0a51\u0a66-\u0a71\u0a75\u0a81-\u0a83\u0abc\u0abe-\u0ac5\u0ac7-\u0ac9\u0acb-\u0acd\u0ae2\u0ae3\u0ae6-\u0aef\u0afa-\u0aff\u0b01-\u0b03\u0b3c\u0b3e-\u0b44\u0b47\u0b48\u0b4b-\u0b4d\u0b55-\u0b57\u0b62\u0b63\u0b66-\u0b6f\u0b82\u0bbe-\u0bc2\u0bc6-\u0bc8\u0bca-\u0bcd\u0bd7\u0be6-\u0bef\u0c00-\u0c04\u0c3e-\u0c44\u0c46-\u0c48\u0c4a-\u0c4d\u0c55\u0c56\u0c62\u0c63\u0c66-\u0c6f\u0c81-\u0c83\u0cbc\u0cbe-\u0cc4\u0cc6-\u0cc8\u0cca-\u0ccd\u0cd5\u0cd6\u0ce2\u0ce3\u0ce6-\u0cef\u0d00-\u0d03\u0d3b\u0d3c\u0d3e-\u0d44\u0d46-\u0d48\u0d4a-\u0d4d\u0d57\u0d62\u0d63\u0d66-\u0d6f\u0d81-\u0d83\u0dca\u0dcf-\u0dd4\u0dd6\u0dd8-\u0ddf\u0de6-\u0def\u0df2\u0df3\u0e31\u0e34-\u0e3a\u0e47-\u0e4e\u0e50-\u0e59\u0eb1\u0eb4-\u0ebc\u0ec8-\u0ecd\u0ed0-\u0ed9\u0f18\u0f19\u0f20-\u0f29\u0f35\u0f37\u0f39\u0f3e\u0f3f\u0f71-\u0f84\u0f86\u0f87\u0f8d-\u0f97\u0f99-\u0fbc\u0fc6\u102b-\u103e\u1040-\u1049\u1056-\u1059\u105e-\u1060\u1062-\u1064\u1067-\u106d\u1071-\u1074\u1082-\u108d\u108f-\u109d\u135d-\u135f\u1369-\u1371\u1712-\u1714\u1732-\u1734\u1752\u1753\u1772\u1773\u17b4-\u17d3\u17dd\u17e0-\u17e9\u180b-\u180d\u1810-\u1819\u18a9\u1920-\u192b\u1930-\u193b\u1946-\u194f\u19d0-\u19da\u1a17-\u1a1b\u1a55-\u1a5e\u1a60-\u1a7c\u1a7f-\u1a89\u1a90-\u1a99\u1ab0-\u1abd\u1abf\u1ac0\u1b00-\u1b04\u1b34-\u1b44\u1b50-\u1b59\u1b6b-\u1b73\u1b80-\u1b82\u1ba1-\u1bad\u1bb0-\u1bb9\u1be6-\u1bf3\u1c24-\u1c37\u1c40-\u1c49\u1c50-\u1c59\u1cd0-\u1cd2\u1cd4-\u1ce8\u1ced\u1cf4\u1cf7-\u1cf9\u1dc0-\u1df9\u1dfb-\u1dff\u203f\u2040\u2054\u20d0-\u20dc\u20e1\u20e5-\u20f0\u2cef-\u2cf1\u2d7f\u2de0-\u2dff\u302a-\u302f\u3099\u309a\ua620-\ua629\ua66f\ua674-\ua67d\ua69e\ua69f\ua6f0\ua6f1\ua802\ua806\ua80b\ua823-\ua827\ua82c\ua880\ua881\ua8b4-\ua8c5\ua8d0-\ua8d9\ua8e0-\ua8f1\ua8ff-\ua909\ua926-\ua92d\ua947-\ua953\ua980-\ua983\ua9b3-\ua9c0\ua9d0-\ua9d9\ua9e5\ua9f0-\ua9f9\uaa29-\uaa36\uaa43\uaa4c\uaa4d\uaa50-\uaa59\uaa7b-\uaa7d\uaab0\uaab2-\uaab4\uaab7\uaab8\uaabe\uaabf\uaac1\uaaeb-\uaaef\uaaf5\uaaf6\uabe3-\uabea\uabec\uabed\uabf0-\uabf9\ufb1e\ufe00-\ufe0f\ufe20-\ufe2f\ufe33\ufe34\ufe4d-\ufe4f\uff10-\uff19\uff3f";
var nonASCIIidentifierStart = new RegExp("[" + nonASCIIidentifierStartChars + "]");
var nonASCIIidentifier = new RegExp("[" + nonASCIIidentifierStartChars + nonASCIIidentifierChars + "]");
nonASCIIidentifierStartChars = nonASCIIidentifierChars = null;
// These are a run-length and offset encoded representation of the
// >0xffff code points that are a valid part of identifiers. The
// offset starts at 0x10000, and each pair of numbers represents an
// offset to the next range, and then a size of the range. They were
// generated by bin/generate-identifier-regex.js
// eslint-disable-next-line comma-spacing
var astralIdentifierStartCodes = [0,11,2,25,2,18,2,1,2,14,3,13,35,122,70,52,268,28,4,48,48,31,14,29,6,37,11,29,3,35,5,7,2,4,43,157,19,35,5,35,5,39,9,51,157,310,10,21,11,7,153,5,3,0,2,43,2,1,4,0,3,22,11,22,10,30,66,18,2,1,11,21,11,25,71,55,7,1,65,0,16,3,2,2,2,28,43,28,4,28,36,7,2,27,28,53,11,21,11,18,14,17,111,72,56,50,14,50,14,35,349,41,7,1,79,28,11,0,9,21,107,20,28,22,13,52,76,44,33,24,27,35,30,0,3,0,9,34,4,0,13,47,15,3,22,0,2,0,36,17,2,24,85,6,2,0,2,3,2,14,2,9,8,46,39,7,3,1,3,21,2,6,2,1,2,4,4,0,19,0,13,4,159,52,19,3,21,2,31,47,21,1,2,0,185,46,42,3,37,47,21,0,60,42,14,0,72,26,230,43,117,63,32,7,3,0,3,7,2,1,2,23,16,0,2,0,95,7,3,38,17,0,2,0,29,0,11,39,8,0,22,0,12,45,20,0,35,56,264,8,2,36,18,0,50,29,113,6,2,1,2,37,22,0,26,5,2,1,2,31,15,0,328,18,190,0,80,921,103,110,18,195,2749,1070,4050,582,8634,568,8,30,114,29,19,47,17,3,32,20,6,18,689,63,129,74,6,0,67,12,65,1,2,0,29,6135,9,1237,43,8,8952,286,50,2,18,3,9,395,2309,106,6,12,4,8,8,9,5991,84,2,70,2,1,3,0,3,1,3,3,2,11,2,0,2,6,2,64,2,3,3,7,2,6,2,27,2,3,2,4,2,0,4,6,2,339,3,24,2,24,2,30,2,24,2,30,2,24,2,30,2,24,2,30,2,24,2,7,2357,44,11,6,17,0,370,43,1301,196,60,67,8,0,1205,3,2,26,2,1,2,0,3,0,2,9,2,3,2,0,2,0,7,0,5,0,2,0,2,0,2,2,2,1,2,0,3,0,2,0,2,0,2,0,2,0,2,1,2,0,3,3,2,6,2,3,2,3,2,0,2,9,2,16,6,2,2,4,2,16,4421,42717,35,4148,12,221,3,5761,15,7472,3104,541,1507,4938];
// eslint-disable-next-line comma-spacing
var astralIdentifierCodes = [509,0,227,0,150,4,294,9,1368,2,2,1,6,3,41,2,5,0,166,1,574,3,9,9,370,1,154,10,176,2,54,14,32,9,16,3,46,10,54,9,7,2,37,13,2,9,6,1,45,0,13,2,49,13,9,3,2,11,83,11,7,0,161,11,6,9,7,3,56,1,2,6,3,1,3,2,10,0,11,1,3,6,4,4,193,17,10,9,5,0,82,19,13,9,214,6,3,8,28,1,83,16,16,9,82,12,9,9,84,14,5,9,243,14,166,9,71,5,2,1,3,3,2,0,2,1,13,9,120,6,3,6,4,0,29,9,41,6,2,3,9,0,10,10,47,15,406,7,2,7,17,9,57,21,2,13,123,5,4,0,2,1,2,6,2,0,9,9,49,4,2,1,2,4,9,9,330,3,19306,9,135,4,60,6,26,9,1014,0,2,54,8,3,82,0,12,1,19628,1,5319,4,4,5,9,7,3,6,31,3,149,2,1418,49,513,54,5,49,9,0,15,0,23,4,2,14,1361,6,2,16,3,6,2,1,2,4,262,6,10,9,419,13,1495,6,110,6,6,9,4759,9,787719,239];
// This has a complexity linear to the value of the code. The
// assumption is that looking up astral identifier characters is
// rare.
function isInAstralSet(code, set) {
var pos = 0x10000;
for (var i = 0; i < set.length; i += 2) {
pos += set[i];
if (pos > code) { return false }
pos += set[i + 1];
if (pos >= code) { return true }
}
}
// Test whether a given character code starts an identifier.
function isIdentifierStart(code, astral) {
if (code < 65) { return code === 36 }
if (code < 91) { return true }
if (code < 97) { return code === 95 }
if (code < 123) { return true }
if (code <= 0xffff) { return code >= 0xaa && nonASCIIidentifierStart.test(String.fromCharCode(code)) }
if (astral === false) { return false }
return isInAstralSet(code, astralIdentifierStartCodes)
}
// Test whether a given character is part of an identifier.
function isIdentifierChar(code, astral) {
if (code < 48) { return code === 36 }
if (code < 58) { return true }
if (code < 65) { return false }
if (code < 91) { return true }
if (code < 97) { return code === 95 }
if (code < 123) { return true }
if (code <= 0xffff) { return code >= 0xaa && nonASCIIidentifier.test(String.fromCharCode(code)) }
if (astral === false) { return false }
return isInAstralSet(code, astralIdentifierStartCodes) || isInAstralSet(code, astralIdentifierCodes)
}
// ## Token types
// The assignment of fine-grained, information-carrying type objects
// allows the tokenizer to store the information it has about a
// token in a way that is very cheap for the parser to look up.
// All token type variables start with an underscore, to make them
// easy to recognize.
// The `beforeExpr` property is used to disambiguate between regular
// expressions and divisions. It is set on all token types that can
// be followed by an expression (thus, a slash after them would be a
// regular expression).
//
// The `startsExpr` property is used to check if the token ends a
// `yield` expression. It is set on all token types that either can
// directly start an expression (like a quotation mark) or can
// continue an expression (like the body of a string).
//
// `isLoop` marks a keyword as starting a loop, which is important
// to know when parsing a label, in order to allow or disallow
// continue jumps to that label.
var TokenType = function TokenType(label, conf) {
if ( conf === void 0 ) conf = {};
this.label = label;
this.keyword = conf.keyword;
this.beforeExpr = !!conf.beforeExpr;
this.startsExpr = !!conf.startsExpr;
this.isLoop = !!conf.isLoop;
this.isAssign = !!conf.isAssign;
this.prefix = !!conf.prefix;
this.postfix = !!conf.postfix;
this.binop = conf.binop || null;
this.updateContext = null;
};
function binop(name, prec) {
return new TokenType(name, {beforeExpr: true, binop: prec})
}
var beforeExpr = {beforeExpr: true}, startsExpr = {startsExpr: true};
// Map keyword names to token types.
var keywords$1 = {};
// Succinct definitions of keyword token types
function kw(name, options) {
if ( options === void 0 ) options = {};
options.keyword = name;
return keywords$1[name] = new TokenType(name, options)
}
var types = {
num: new TokenType("num", startsExpr),
regexp: new TokenType("regexp", startsExpr),
string: new TokenType("string", startsExpr),
name: new TokenType("name", startsExpr),
eof: new TokenType("eof"),
// Punctuation token types.
bracketL: new TokenType("[", {beforeExpr: true, startsExpr: true}),
bracketR: new TokenType("]"),
braceL: new TokenType("{", {beforeExpr: true, startsExpr: true}),
braceR: new TokenType("}"),
parenL: new TokenType("(", {beforeExpr: true, startsExpr: true}),
parenR: new TokenType(")"),
comma: new TokenType(",", beforeExpr),
semi: new TokenType(";", beforeExpr),
colon: new TokenType(":", beforeExpr),
dot: new TokenType("."),
question: new TokenType("?", beforeExpr),
questionDot: new TokenType("?."),
arrow: new TokenType("=>", beforeExpr),
template: new TokenType("template"),
invalidTemplate: new TokenType("invalidTemplate"),
ellipsis: new TokenType("...", beforeExpr),
backQuote: new TokenType("`", startsExpr),
dollarBraceL: new TokenType("${", {beforeExpr: true, startsExpr: true}),
// Operators. These carry several kinds of properties to help the
// parser use them properly (the presence of these properties is
// what categorizes them as operators).
//
// `binop`, when present, specifies that this operator is a binary
// operator, and will refer to its precedence.
//
// `prefix` and `postfix` mark the operator as a prefix or postfix
// unary operator.
//
// `isAssign` marks all of `=`, `+=`, `-=` etcetera, which act as
// binary operators with a very low precedence, that should result
// in AssignmentExpression nodes.
eq: new TokenType("=", {beforeExpr: true, isAssign: true}),
assign: new TokenType("_=", {beforeExpr: true, isAssign: true}),
incDec: new TokenType("++/--", {prefix: true, postfix: true, startsExpr: true}),
prefix: new TokenType("!/~", {beforeExpr: true, prefix: true, startsExpr: true}),
logicalOR: binop("||", 1),
logicalAND: binop("&&", 2),
bitwiseOR: binop("|", 3),
bitwiseXOR: binop("^", 4),
bitwiseAND: binop("&", 5),
equality: binop("==/!=/===/!==", 6),
relational: binop("</>/<=/>=", 7),
bitShift: binop("<</>>/>>>", 8),
plusMin: new TokenType("+/-", {beforeExpr: true, binop: 9, prefix: true, startsExpr: true}),
modulo: binop("%", 10),
star: binop("*", 10),
slash: binop("/", 10),
starstar: new TokenType("**", {beforeExpr: true}),
coalesce: binop("??", 1),
// Keyword token types.
_break: kw("break"),
_case: kw("case", beforeExpr),
_catch: kw("catch"),
_continue: kw("continue"),
_debugger: kw("debugger"),
_default: kw("default", beforeExpr),
_do: kw("do", {isLoop: true, beforeExpr: true}),
_else: kw("else", beforeExpr),
_finally: kw("finally"),
_for: kw("for", {isLoop: true}),
_function: kw("function", startsExpr),
_if: kw("if"),
_return: kw("return", beforeExpr),
_switch: kw("switch"),
_throw: kw("throw", beforeExpr),
_try: kw("try"),
_var: kw("var"),
_const: kw("const"),
_while: kw("while", {isLoop: true}),
_with: kw("with"),
_new: kw("new", {beforeExpr: true, startsExpr: true}),
_this: kw("this", startsExpr),
_super: kw("super", startsExpr),
_class: kw("class", startsExpr),
_extends: kw("extends", beforeExpr),
_export: kw("export"),
_import: kw("import", startsExpr),
_null: kw("null", startsExpr),
_true: kw("true", startsExpr),
_false: kw("false", startsExpr),
_in: kw("in", {beforeExpr: true, binop: 7}),
_instanceof: kw("instanceof", {beforeExpr: true, binop: 7}),
_typeof: kw("typeof", {beforeExpr: true, prefix: true, startsExpr: true}),
_void: kw("void", {beforeExpr: true, prefix: true, startsExpr: true}),
_delete: kw("delete", {beforeExpr: true, prefix: true, startsExpr: true})
};
// Matches a whole line break (where CRLF is considered a single
// line break). Used to count lines.
var lineBreak = /\r\n?|\n|\u2028|\u2029/;
var lineBreakG = new RegExp(lineBreak.source, "g");
function isNewLine(code, ecma2019String) {
return code === 10 || code === 13 || (!ecma2019String && (code === 0x2028 || code === 0x2029))
}
var nonASCIIwhitespace = /[\u1680\u2000-\u200a\u202f\u205f\u3000\ufeff]/;
var skipWhiteSpace = /(?:\s|\/\/.*|\/\*[^]*?\*\/)*/g;
var ref = Object.prototype;
var hasOwnProperty = ref.hasOwnProperty;
var toString = ref.toString;
// Checks if an object has a property.
function has(obj, propName) {
return hasOwnProperty.call(obj, propName)
}
var isArray = Array.isArray || (function (obj) { return (
toString.call(obj) === "[object Array]"
); });
function wordsRegexp(words) {
return new RegExp("^(?:" + words.replace(/ /g, "|") + ")$")
}
// These are used when `options.locations` is on, for the
// `startLoc` and `endLoc` properties.
var Position = function Position(line, col) {
this.line = line;
this.column = col;
};
Position.prototype.offset = function offset (n) {
return new Position(this.line, this.column + n)
};
var SourceLocation = function SourceLocation(p, start, end) {
this.start = start;
this.end = end;
if (p.sourceFile !== null) { this.source = p.sourceFile; }
};
// The `getLineInfo` function is mostly useful when the
// `locations` option is off (for performance reasons) and you
// want to find the line/column position for a given character
// offset. `input` should be the code string that the offset refers
// into.
function getLineInfo(input, offset) {
for (var line = 1, cur = 0;;) {
lineBreakG.lastIndex = cur;
var match = lineBreakG.exec(input);
if (match && match.index < offset) {
++line;
cur = match.index + match[0].length;
} else {
return new Position(line, offset - cur)
}
}
}
// A second optional argument can be given to further configure
// the parser process. These options are recognized:
var defaultOptions = {
// `ecmaVersion` indicates the ECMAScript version to parse. Must be
// either 3, 5, 6 (2015), 7 (2016), 8 (2017), 9 (2018), or 10
// (2019). This influences support for strict mode, the set of
// reserved words, and support for new syntax features. The default
// is 10.
ecmaVersion: 10,
// `sourceType` indicates the mode the code should be parsed in.
// Can be either `"script"` or `"module"`. This influences global
// strict mode and parsing of `import` and `export` declarations.
sourceType: "script",
// `onInsertedSemicolon` can be a callback that will be called
// when a semicolon is automatically inserted. It will be passed
// the position of the comma as an offset, and if `locations` is
// enabled, it is given the location as a `{line, column}` object
// as second argument.
onInsertedSemicolon: null,
// `onTrailingComma` is similar to `onInsertedSemicolon`, but for
// trailing commas.
onTrailingComma: null,
// By default, reserved words are only enforced if ecmaVersion >= 5.
// Set `allowReserved` to a boolean value to explicitly turn this on
// an off. When this option has the value "never", reserved words
// and keywords can also not be used as property names.
allowReserved: null,
// When enabled, a return at the top level is not considered an
// error.
allowReturnOutsideFunction: false,
// When enabled, import/export statements are not constrained to
// appearing at the top of the program.
allowImportExportEverywhere: false,
// When enabled, await identifiers are allowed to appear at the top-level scope,
// but they are still not allowed in non-async functions.
allowAwaitOutsideFunction: false,
// When enabled, hashbang directive in the beginning of file
// is allowed and treated as a line comment.
allowHashBang: false,
// When `locations` is on, `loc` properties holding objects with
// `start` and `end` properties in `{line, column}` form (with
// line being 1-based and column 0-based) will be attached to the
// nodes.
locations: false,
// A function can be passed as `onToken` option, which will
// cause Acorn to call that function with object in the same
// format as tokens returned from `tokenizer().getToken()`. Note
// that you are not allowed to call the parser from the
// callback—that will corrupt its internal state.
onToken: null,
// A function can be passed as `onComment` option, which will
// cause Acorn to call that function with `(block, text, start,
// end)` parameters whenever a comment is skipped. `block` is a
// boolean indicating whether this is a block (`/* */`) comment,
// `text` is the content of the comment, and `start` and `end` are
// character offsets that denote the start and end of the comment.
// When the `locations` option is on, two more parameters are
// passed, the full `{line, column}` locations of the start and
// end of the comments. Note that you are not allowed to call the
// parser from the callback—that will corrupt its internal state.
onComment: null,
// Nodes have their start and end characters offsets recorded in
// `start` and `end` properties (directly on the node, rather than
// the `loc` object, which holds line/column data. To also add a
// [semi-standardized][range] `range` property holding a `[start,
// end]` array with the same numbers, set the `ranges` option to
// `true`.
//
// [range]: https://bugzilla.mozilla.org/show_bug.cgi?id=745678
ranges: false,
// It is possible to parse multiple files into a single AST by
// passing the tree produced by parsing the first file as
// `program` option in subsequent parses. This will add the
// toplevel forms of the parsed file to the `Program` (top) node
// of an existing parse tree.
program: null,
// When `locations` is on, you can pass this to record the source
// file in every node's `loc` object.
sourceFile: null,
// This value, if given, is stored in every node, whether
// `locations` is on or off.
directSourceFile: null,
// When enabled, parenthesized expressions are represented by
// (non-standard) ParenthesizedExpression nodes
preserveParens: false
};
// Interpret and default an options object
function getOptions(opts) {
var options = {};
for (var opt in defaultOptions)
{ options[opt] = opts && has(opts, opt) ? opts[opt] : defaultOptions[opt]; }
if (options.ecmaVersion >= 2015)
{ options.ecmaVersion -= 2009; }
if (options.allowReserved == null)
{ options.allowReserved = options.ecmaVersion < 5; }
if (isArray(options.onToken)) {
var tokens = options.onToken;
options.onToken = function (token) { return tokens.push(token); };
}
if (isArray(options.onComment))
{ options.onComment = pushComment(options, options.onComment); }
return options
}
function pushComment(options, array) {
return function(block, text, start, end, startLoc, endLoc) {
var comment = {
type: block ? "Block" : "Line",
value: text,
start: start,
end: end
};
if (options.locations)
{ comment.loc = new SourceLocation(this, startLoc, endLoc); }
if (options.ranges)
{ comment.range = [start, end]; }
array.push(comment);
}
}
// Each scope gets a bitset that may contain these flags
var
SCOPE_TOP = 1,
SCOPE_FUNCTION = 2,
SCOPE_VAR = SCOPE_TOP | SCOPE_FUNCTION,
SCOPE_ASYNC = 4,
SCOPE_GENERATOR = 8,
SCOPE_ARROW = 16,
SCOPE_SIMPLE_CATCH = 32,
SCOPE_SUPER = 64,
SCOPE_DIRECT_SUPER = 128;
function functionFlags(async, generator) {
return SCOPE_FUNCTION | (async ? SCOPE_ASYNC : 0) | (generator ? SCOPE_GENERATOR : 0)
}
// Used in checkLVal and declareName to determine the type of a binding
var
BIND_NONE = 0, // Not a binding
BIND_VAR = 1, // Var-style binding
BIND_LEXICAL = 2, // Let- or const-style binding
BIND_FUNCTION = 3, // Function declaration
BIND_SIMPLE_CATCH = 4, // Simple (identifier pattern) catch binding
BIND_OUTSIDE = 5; // Special case for function names as bound inside the function
var Parser = function Parser(options, input, startPos) {
this.options = options = getOptions(options);
this.sourceFile = options.sourceFile;
this.keywords = wordsRegexp(keywords[options.ecmaVersion >= 6 ? 6 : options.sourceType === "module" ? "5module" : 5]);
var reserved = "";
if (options.allowReserved !== true) {
for (var v = options.ecmaVersion;; v--)
{ if (reserved = reservedWords[v]) { break } }
if (options.sourceType === "module") { reserved += " await"; }
}
this.reservedWords = wordsRegexp(reserved);
var reservedStrict = (reserved ? reserved + " " : "") + reservedWords.strict;
this.reservedWordsStrict = wordsRegexp(reservedStrict);
this.reservedWordsStrictBind = wordsRegexp(reservedStrict + " " + reservedWords.strictBind);
this.input = String(input);
// Used to signal to callers of `readWord1` whether the word
// contained any escape sequences. This is needed because words with
// escape sequences must not be interpreted as keywords.
this.containsEsc = false;
// Set up token state
// The current position of the tokenizer in the input.
if (startPos) {
this.pos = startPos;
this.lineStart = this.input.lastIndexOf("\n", startPos - 1) + 1;
this.curLine = this.input.slice(0, this.lineStart).split(lineBreak).length;
} else {
this.pos = this.lineStart = 0;
this.curLine = 1;
}
// Properties of the current token:
// Its type
this.type = types.eof;
// For tokens that include more information than their type, the value
this.value = null;
// Its start and end offset
this.start = this.end = this.pos;
// And, if locations are used, the {line, column} object
// corresponding to those offsets
this.startLoc = this.endLoc = this.curPosition();
// Position information for the previous token
this.lastTokEndLoc = this.lastTokStartLoc = null;
this.lastTokStart = this.lastTokEnd = this.pos;
// The context stack is used to superficially track syntactic
// context to predict whether a regular expression is allowed in a
// given position.
this.context = this.initialContext();
this.exprAllowed = true;
// Figure out if it's a module code.
this.inModule = options.sourceType === "module";
this.strict = this.inModule || this.strictDirective(this.pos);
// Used to signify the start of a potential arrow function
this.potentialArrowAt = -1;
// Positions to delayed-check that yield/await does not exist in default parameters.
this.yieldPos = this.awaitPos = this.awaitIdentPos = 0;
// Labels in scope.
this.labels = [];
// Thus-far undefined exports.
this.undefinedExports = {};
// If enabled, skip leading hashbang line.
if (this.pos === 0 && options.allowHashBang && this.input.slice(0, 2) === "#!")
{ this.skipLineComment(2); }
// Scope tracking for duplicate variable names (see scope.js)
this.scopeStack = [];
this.enterScope(SCOPE_TOP);
// For RegExp validation
this.regexpState = null;
};
var prototypeAccessors = { inFunction: { configurable: true },inGenerator: { configurable: true },inAsync: { configurable: true },allowSuper: { configurable: true },allowDirectSuper: { configurable: true },treatFunctionsAsVar: { configurable: true } };
Parser.prototype.parse = function parse () {
var node = this.options.program || this.startNode();
this.nextToken();
return this.parseTopLevel(node)
};
prototypeAccessors.inFunction.get = function () { return (this.currentVarScope().flags & SCOPE_FUNCTION) > 0 };
prototypeAccessors.inGenerator.get = function () { return (this.currentVarScope().flags & SCOPE_GENERATOR) > 0 };
prototypeAccessors.inAsync.get = function () { return (this.currentVarScope().flags & SCOPE_ASYNC) > 0 };
prototypeAccessors.allowSuper.get = function () { return (this.currentThisScope().flags & SCOPE_SUPER) > 0 };
prototypeAccessors.allowDirectSuper.get = function () { return (this.currentThisScope().flags & SCOPE_DIRECT_SUPER) > 0 };
prototypeAccessors.treatFunctionsAsVar.get = function () { return this.treatFunctionsAsVarInScope(this.currentScope()) };
// Switch to a getter for 7.0.0.
Parser.prototype.inNonArrowFunction = function inNonArrowFunction () { return (this.currentThisScope().flags & SCOPE_FUNCTION) > 0 };
Parser.extend = function extend () {
var plugins = [], len = arguments.length;
while ( len-- ) plugins[ len ] = arguments[ len ];
var cls = this;
for (var i = 0; i < plugins.length; i++) { cls = plugins[i](cls); }
return cls
};
Parser.parse = function parse (input, options) {
return new this(options, input).parse()
};
Parser.parseExpressionAt = function parseExpressionAt (input, pos, options) {
var parser = new this(options, input, pos);
parser.nextToken();
return parser.parseExpression()
};
Parser.tokenizer = function tokenizer (input, options) {
return new this(options, input)
};
Object.defineProperties( Parser.prototype, prototypeAccessors );
var pp = Parser.prototype;
// ## Parser utilities
var literal = /^(?:'((?:\\.|[^'])*?)'|"((?:\\.|[^"])*?)")/;
pp.strictDirective = function(start) {
for (;;) {
// Try to find string literal.
skipWhiteSpace.lastIndex = start;
start += skipWhiteSpace.exec(this.input)[0].length;
var match = literal.exec(this.input.slice(start));
if (!match) { return false }
if ((match[1] || match[2]) === "use strict") {
skipWhiteSpace.lastIndex = start + match[0].length;
var spaceAfter = skipWhiteSpace.exec(this.input), end = spaceAfter.index + spaceAfter[0].length;
var next = this.input.charAt(end);
return next === ";" || next === "}" ||
(lineBreak.test(spaceAfter[0]) &&
!(/[(`.[+\-/*%<>=,?^&]/.test(next) || next === "!" && this.input.charAt(end + 1) === "="))
}
start += match[0].length;
// Skip semicolon, if any.
skipWhiteSpace.lastIndex = start;
start += skipWhiteSpace.exec(this.input)[0].length;
if (this.input[start] === ";")
{ start++; }
}
};
// Predicate that tests whether the next token is of the given
// type, and if yes, consumes it as a side effect.
pp.eat = function(type) {
if (this.type === type) {
this.next();
return true
} else {
return false
}
};
// Tests whether parsed token is a contextual keyword.
pp.isContextual = function(name) {
return this.type === types.name && this.value === name && !this.containsEsc
};
// Consumes contextual keyword if possible.
pp.eatContextual = function(name) {
if (!this.isContextual(name)) { return false }
this.next();
return true
};
// Asserts that following token is given contextual keyword.
pp.expectContextual = function(name) {
if (!this.eatContextual(name)) { this.unexpected(); }
};
// Test whether a semicolon can be inserted at the current position.
pp.canInsertSemicolon = function() {
return this.type === types.eof ||
this.type === types.braceR ||
lineBreak.test(this.input.slice(this.lastTokEnd, this.start))
};
pp.insertSemicolon = function() {
if (this.canInsertSemicolon()) {
if (this.options.onInsertedSemicolon)
{ this.options.onInsertedSemicolon(this.lastTokEnd, this.lastTokEndLoc); }
return true
}
};
// Consume a semicolon, or, failing that, see if we are allowed to
// pretend that there is a semicolon at this position.
pp.semicolon = function() {
if (!this.eat(types.semi) && !this.insertSemicolon()) { this.unexpected(); }
};
pp.afterTrailingComma = function(tokType, notNext) {
if (this.type === tokType) {
if (this.options.onTrailingComma)
{ this.options.onTrailingComma(this.lastTokStart, this.lastTokStartLoc); }
if (!notNext)
{ this.next(); }
return true
}
};
// Expect a token of a given type. If found, consume it, otherwise,
// raise an unexpected token error.
pp.expect = function(type) {
this.eat(type) || this.unexpected();
};
// Raise an unexpected token error.
pp.unexpected = function(pos) {
this.raise(pos != null ? pos : this.start, "Unexpected token");
};
function DestructuringErrors() {
this.shorthandAssign =
this.trailingComma =
this.parenthesizedAssign =
this.parenthesizedBind =
this.doubleProto =
-1;
}
pp.checkPatternErrors = function(refDestructuringErrors, isAssign) {
if (!refDestructuringErrors) { return }
if (refDestructuringErrors.trailingComma > -1)
{ this.raiseRecoverable(refDestructuringErrors.trailingComma, "Comma is not permitted after the rest element"); }
var parens = isAssign ? refDestructuringErrors.parenthesizedAssign : refDestructuringErrors.parenthesizedBind;
if (parens > -1) { this.raiseRecoverable(parens, "Parenthesized pattern"); }
};
pp.checkExpressionErrors = function(refDestructuringErrors, andThrow) {
if (!refDestructuringErrors) { return false }
var shorthandAssign = refDestructuringErrors.shorthandAssign;
var doubleProto = refDestructuringErrors.doubleProto;
if (!andThrow) { return shorthandAssign >= 0 || doubleProto >= 0 }
if (shorthandAssign >= 0)
{ this.raise(shorthandAssign, "Shorthand property assignments are valid only in destructuring patterns"); }
if (doubleProto >= 0)
{ this.raiseRecoverable(doubleProto, "Redefinition of __proto__ property"); }
};
pp.checkYieldAwaitInDefaultParams = function() {
if (this.yieldPos && (!this.awaitPos || this.yieldPos < this.awaitPos))
{ this.raise(this.yieldPos, "Yield expression cannot be a default value"); }
if (this.awaitPos)
{ this.raise(this.awaitPos, "Await expression cannot be a default value"); }
};
pp.isSimpleAssignTarget = function(expr) {
if (expr.type === "ParenthesizedExpression")
{ return this.isSimpleAssignTarget(expr.expression) }
return expr.type === "Identifier" || expr.type === "MemberExpression"
};
var pp$1 = Parser.prototype;
// ### Statement parsing
// Parse a program. Initializes the parser, reads any number of
// statements, and wraps them in a Program node. Optionally takes a
// `program` argument. If present, the statements will be appended
// to its body instead of creating a new node.
pp$1.parseTopLevel = function(node) {
var exports = {};
if (!node.body) { node.body = []; }
while (this.type !== types.eof) {
var stmt = this.parseStatement(null, true, exports);
node.body.push(stmt);
}
if (this.inModule)
{ for (var i = 0, list = Object.keys(this.undefinedExports); i < list.length; i += 1)
{
var name = list[i];
this.raiseRecoverable(this.undefinedExports[name].start, ("Export '" + name + "' is not defined"));
} }
this.adaptDirectivePrologue(node.body);
this.next();
node.sourceType = this.options.sourceType;
return this.finishNode(node, "Program")
};
var loopLabel = {kind: "loop"}, switchLabel = {kind: "switch"};
pp$1.isLet = function(context) {
if (this.options.ecmaVersion < 6 || !this.isContextual("let")) { return false }
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length, nextCh = this.input.charCodeAt(next);
// For ambiguous cases, determine if a LexicalDeclaration (or only a
// Statement) is allowed here. If context is not empty then only a Statement
// is allowed. However, `let [` is an explicit negative lookahead for
// ExpressionStatement, so special-case it first.
if (nextCh === 91) { return true } // '['
if (context) { return false }
if (nextCh === 123) { return true } // '{'
if (isIdentifierStart(nextCh, true)) {
var pos = next + 1;
while (isIdentifierChar(this.input.charCodeAt(pos), true)) { ++pos; }
var ident = this.input.slice(next, pos);
if (!keywordRelationalOperator.test(ident)) { return true }
}
return false
};
// check 'async [no LineTerminator here] function'
// - 'async /*foo*/ function' is OK.
// - 'async /*\n*/ function' is invalid.
pp$1.isAsyncFunction = function() {
if (this.options.ecmaVersion < 8 || !this.isContextual("async"))
{ return false }
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length;
return !lineBreak.test(this.input.slice(this.pos, next)) &&
this.input.slice(next, next + 8) === "function" &&
(next + 8 === this.input.length || !isIdentifierChar(this.input.charAt(next + 8)))
};
// Parse a single statement.
//
// If expecting a statement and finding a slash operator, parse a
// regular expression literal. This is to handle cases like
// `if (foo) /blah/.exec(foo)`, where looking at the previous token
// does not help.
pp$1.parseStatement = function(context, topLevel, exports) {
var starttype = this.type, node = this.startNode(), kind;
if (this.isLet(context)) {
starttype = types._var;
kind = "let";
}
// Most types of statements are recognized by the keyword they
// start with. Many are trivial to parse, some require a bit of
// complexity.
switch (starttype) {
case types._break: case types._continue: return this.parseBreakContinueStatement(node, starttype.keyword)
case types._debugger: return this.parseDebuggerStatement(node)
case types._do: return this.parseDoStatement(node)
case types._for: return this.parseForStatement(node)
case types._function:
// Function as sole body of either an if statement or a labeled statement
// works, but not when it is part of a labeled statement that is the sole
// body of an if statement.
if ((context && (this.strict || context !== "if" && context !== "label")) && this.options.ecmaVersion >= 6) { this.unexpected(); }
return this.parseFunctionStatement(node, false, !context)
case types._class:
if (context) { this.unexpected(); }
return this.parseClass(node, true)
case types._if: return this.parseIfStatement(node)
case types._return: return this.parseReturnStatement(node)
case types._switch: return this.parseSwitchStatement(node)
case types._throw: return this.parseThrowStatement(node)
case types._try: return this.parseTryStatement(node)
case types._const: case types._var:
kind = kind || this.value;
if (context && kind !== "var") { this.unexpected(); }
return this.parseVarStatement(node, kind)
case types._while: return this.parseWhileStatement(node)
case types._with: return this.parseWithStatement(node)
case types.braceL: return this.parseBlock(true, node)
case types.semi: return this.parseEmptyStatement(node)
case types._export:
case types._import:
if (this.options.ecmaVersion > 10 && starttype === types._import) {
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length, nextCh = this.input.charCodeAt(next);
if (nextCh === 40 || nextCh === 46) // '(' or '.'
{ return this.parseExpressionStatement(node, this.parseExpression()) }
}
if (!this.options.allowImportExportEverywhere) {
if (!topLevel)
{ this.raise(this.start, "'import' and 'export' may only appear at the top level"); }
if (!this.inModule)
{ this.raise(this.start, "'import' and 'export' may appear only with 'sourceType: module'"); }
}
return starttype === types._import ? this.parseImport(node) : this.parseExport(node, exports)
// If the statement does not start with a statement keyword or a
// brace, it's an ExpressionStatement or LabeledStatement. We
// simply start parsing an expression, and afterwards, if the
// next token is a colon and the expression was a simple
// Identifier node, we switch to interpreting it as a label.
default:
if (this.isAsyncFunction()) {
if (context) { this.unexpected(); }
this.next();
return this.parseFunctionStatement(node, true, !context)
}
var maybeName = this.value, expr = this.parseExpression();
if (starttype === types.name && expr.type === "Identifier" && this.eat(types.colon))
{ return this.parseLabeledStatement(node, maybeName, expr, context) }
else { return this.parseExpressionStatement(node, expr) }
}
};
pp$1.parseBreakContinueStatement = function(node, keyword) {
var isBreak = keyword === "break";
this.next();
if (this.eat(types.semi) || this.insertSemicolon()) { node.label = null; }
else if (this.type !== types.name) { this.unexpected(); }
else {
node.label = this.parseIdent();
this.semicolon();
}
// Verify that there is an actual destination to break or
// continue to.
var i = 0;
for (; i < this.labels.length; ++i) {
var lab = this.labels[i];
if (node.label == null || lab.name === node.label.name) {
if (lab.kind != null && (isBreak || lab.kind === "loop")) { break }
if (node.label && isBreak) { break }
}
}
if (i === this.labels.length) { this.raise(node.start, "Unsyntactic " + keyword); }
return this.finishNode(node, isBreak ? "BreakStatement" : "ContinueStatement")
};
pp$1.parseDebuggerStatement = function(node) {
this.next();
this.semicolon();
return this.finishNode(node, "DebuggerStatement")
};
pp$1.parseDoStatement = function(node) {
this.next();
this.labels.push(loopLabel);
node.body = this.parseStatement("do");
this.labels.pop();
this.expect(types._while);
node.test = this.parseParenExpression();
if (this.options.ecmaVersion >= 6)
{ this.eat(types.semi); }
else
{ this.semicolon(); }
return this.finishNode(node, "DoWhileStatement")
};
// Disambiguating between a `for` and a `for`/`in` or `for`/`of`
// loop is non-trivial. Basically, we have to parse the init `var`
// statement or expression, disallowing the `in` operator (see
// the second parameter to `parseExpression`), and then check
// whether the next token is `in` or `of`. When there is no init
// part (semicolon immediately after the opening parenthesis), it
// is a regular `for` loop.
pp$1.parseForStatement = function(node) {
this.next();
var awaitAt = (this.options.ecmaVersion >= 9 && (this.inAsync || (!this.inFunction && this.options.allowAwaitOutsideFunction)) && this.eatContextual("await")) ? this.lastTokStart : -1;
this.labels.push(loopLabel);
this.enterScope(0);
this.expect(types.parenL);
if (this.type === types.semi) {
if (awaitAt > -1) { this.unexpected(awaitAt); }
return this.parseFor(node, null)
}
var isLet = this.isLet();
if (this.type === types._var || this.type === types._const || isLet) {
var init$1 = this.startNode(), kind = isLet ? "let" : this.value;
this.next();
this.parseVar(init$1, true, kind);
this.finishNode(init$1, "VariableDeclaration");
if ((this.type === types._in || (this.options.ecmaVersion >= 6 && this.isContextual("of"))) && init$1.declarations.length === 1) {
if (this.options.ecmaVersion >= 9) {
if (this.type === types._in) {
if (awaitAt > -1) { this.unexpected(awaitAt); }
} else { node.await = awaitAt > -1; }
}
return this.parseForIn(node, init$1)
}
if (awaitAt > -1) { this.unexpected(awaitAt); }
return this.parseFor(node, init$1)
}
var refDestructuringErrors = new DestructuringErrors;
var init = this.parseExpression(true, refDestructuringErrors);
if (this.type === types._in || (this.options.ecmaVersion >= 6 && this.isContextual("of"))) {
if (this.options.ecmaVersion >= 9) {
if (this.type === types._in) {
if (awaitAt > -1) { this.unexpected(awaitAt); }
} else { node.await = awaitAt > -1; }
}
this.toAssignable(init, false, refDestructuringErrors);
this.checkLVal(init);
return this.parseForIn(node, init)
} else {
this.checkExpressionErrors(refDestructuringErrors, true);
}
if (awaitAt > -1) { this.unexpected(awaitAt); }
return this.parseFor(node, init)
};
pp$1.parseFunctionStatement = function(node, isAsync, declarationPosition) {
this.next();
return this.parseFunction(node, FUNC_STATEMENT | (declarationPosition ? 0 : FUNC_HANGING_STATEMENT), false, isAsync)
};
pp$1.parseIfStatement = function(node) {
this.next();
node.test = this.parseParenExpression();
// allow function declarations in branches, but only in non-strict mode
node.consequent = this.parseStatement("if");
node.alternate = this.eat(types._else) ? this.parseStatement("if") : null;
return this.finishNode(node, "IfStatement")
};
pp$1.parseReturnStatement = function(node) {
if (!this.inFunction && !this.options.allowReturnOutsideFunction)
{ this.raise(this.start, "'return' outside of function"); }
this.next();
// In `return` (and `break`/`continue`), the keywords with
// optional arguments, we eagerly look for a semicolon or the
// possibility to insert one.
if (this.eat(types.semi) || this.insertSemicolon()) { node.argument = null; }
else { node.argument = this.parseExpression(); this.semicolon(); }
return this.finishNode(node, "ReturnStatement")
};
pp$1.parseSwitchStatement = function(node) {
this.next();
node.discriminant = this.parseParenExpression();
node.cases = [];
this.expect(types.braceL);
this.labels.push(switchLabel);
this.enterScope(0);
// Statements under must be grouped (by label) in SwitchCase
// nodes. `cur` is used to keep the node that we are currently
// adding statements to.
var cur;
for (var sawDefault = false; this.type !== types.braceR;) {
if (this.type === types._case || this.type === types._default) {
var isCase = this.type === types._case;
if (cur) { this.finishNode(cur, "SwitchCase"); }
node.cases.push(cur = this.startNode());
cur.consequent = [];
this.next();
if (isCase) {
cur.test = this.parseExpression();
} else {
if (sawDefault) { this.raiseRecoverable(this.lastTokStart, "Multiple default clauses"); }
sawDefault = true;
cur.test = null;
}
this.expect(types.colon);
} else {
if (!cur) { this.unexpected(); }
cur.consequent.push(this.parseStatement(null));
}
}
this.exitScope();
if (cur) { this.finishNode(cur, "SwitchCase"); }
this.next(); // Closing brace
this.labels.pop();
return this.finishNode(node, "SwitchStatement")
};
pp$1.parseThrowStatement = function(node) {
this.next();
if (lineBreak.test(this.input.slice(this.lastTokEnd, this.start)))
{ this.raise(this.lastTokEnd, "Illegal newline after throw"); }
node.argument = this.parseExpression();
this.semicolon();
return this.finishNode(node, "ThrowStatement")
};
// Reused empty array added for node fields that are always empty.
var empty = [];
pp$1.parseTryStatement = function(node) {
this.next();
node.block = this.parseBlock();
node.handler = null;
if (this.type === types._catch) {
var clause = this.startNode();
this.next();
if (this.eat(types.parenL)) {
clause.param = this.parseBindingAtom();
var simple = clause.param.type === "Identifier";
this.enterScope(simple ? SCOPE_SIMPLE_CATCH : 0);
this.checkLVal(clause.param, simple ? BIND_SIMPLE_CATCH : BIND_LEXICAL);
this.expect(types.parenR);
} else {
if (this.options.ecmaVersion < 10) { this.unexpected(); }
clause.param = null;
this.enterScope(0);
}
clause.body = this.parseBlock(false);
this.exitScope();
node.handler = this.finishNode(clause, "CatchClause");
}
node.finalizer = this.eat(types._finally) ? this.parseBlock() : null;
if (!node.handler && !node.finalizer)
{ this.raise(node.start, "Missing catch or finally clause"); }
return this.finishNode(node, "TryStatement")
};
pp$1.parseVarStatement = function(node, kind) {
this.next();
this.parseVar(node, false, kind);
this.semicolon();
return this.finishNode(node, "VariableDeclaration")
};
pp$1.parseWhileStatement = function(node) {
this.next();
node.test = this.parseParenExpression();
this.labels.push(loopLabel);
node.body = this.parseStatement("while");
this.labels.pop();
return this.finishNode(node, "WhileStatement")
};
pp$1.parseWithStatement = function(node) {
if (this.strict) { this.raise(this.start, "'with' in strict mode"); }
this.next();
node.object = this.parseParenExpression();
node.body = this.parseStatement("with");
return this.finishNode(node, "WithStatement")
};
pp$1.parseEmptyStatement = function(node) {
this.next();
return this.finishNode(node, "EmptyStatement")
};
pp$1.parseLabeledStatement = function(node, maybeName, expr, context) {
for (var i$1 = 0, list = this.labels; i$1 < list.length; i$1 += 1)
{
var label = list[i$1];
if (label.name === maybeName)
{ this.raise(expr.start, "Label '" + maybeName + "' is already declared");
} }
var kind = this.type.isLoop ? "loop" : this.type === types._switch ? "switch" : null;
for (var i = this.labels.length - 1; i >= 0; i--) {
var label$1 = this.labels[i];
if (label$1.statementStart === node.start) {
// Update information about previous labels on this node
label$1.statementStart = this.start;
label$1.kind = kind;
} else { break }
}
this.labels.push({name: maybeName, kind: kind, statementStart: this.start});
node.body = this.parseStatement(context ? context.indexOf("label") === -1 ? context + "label" : context : "label");
this.labels.pop();
node.label = expr;
return this.finishNode(node, "LabeledStatement")
};
pp$1.parseExpressionStatement = function(node, expr) {
node.expression = expr;
this.semicolon();
return this.finishNode(node, "ExpressionStatement")
};
// Parse a semicolon-enclosed block of statements, handling `"use
// strict"` declarations when `allowStrict` is true (used for
// function bodies).
pp$1.parseBlock = function(createNewLexicalScope, node, exitStrict) {
if ( createNewLexicalScope === void 0 ) createNewLexicalScope = true;
if ( node === void 0 ) node = this.startNode();
node.body = [];
this.expect(types.braceL);
if (createNewLexicalScope) { this.enterScope(0); }
while (this.type !== types.braceR) {
var stmt = this.parseStatement(null);
node.body.push(stmt);
}
if (exitStrict) { this.strict = false; }
this.next();
if (createNewLexicalScope) { this.exitScope(); }
return this.finishNode(node, "BlockStatement")
};
// Parse a regular `for` loop. The disambiguation code in
// `parseStatement` will already have parsed the init statement or
// expression.
pp$1.parseFor = function(node, init) {
node.init = init;
this.expect(types.semi);
node.test = this.type === types.semi ? null : this.parseExpression();
this.expect(types.semi);
node.update = this.type === types.parenR ? null : this.parseExpression();
this.expect(types.parenR);
node.body = this.parseStatement("for");
this.exitScope();
this.labels.pop();
return this.finishNode(node, "ForStatement")
};
// Parse a `for`/`in` and `for`/`of` loop, which are almost
// same from parser's perspective.
pp$1.parseForIn = function(node, init) {
var isForIn = this.type === types._in;
this.next();
if (
init.type === "VariableDeclaration" &&
init.declarations[0].init != null &&
(
!isForIn ||
this.options.ecmaVersion < 8 ||
this.strict ||
init.kind !== "var" ||
init.declarations[0].id.type !== "Identifier"
)
) {
this.raise(
init.start,
((isForIn ? "for-in" : "for-of") + " loop variable declaration may not have an initializer")
);
} else if (init.type === "AssignmentPattern") {
this.raise(init.start, "Invalid left-hand side in for-loop");
}
node.left = init;
node.right = isForIn ? this.parseExpression() : this.parseMaybeAssign();
this.expect(types.parenR);
node.body = this.parseStatement("for");
this.exitScope();
this.labels.pop();
return this.finishNode(node, isForIn ? "ForInStatement" : "ForOfStatement")
};
// Parse a list of variable declarations.
pp$1.parseVar = function(node, isFor, kind) {
node.declarations = [];
node.kind = kind;
for (;;) {
var decl = this.startNode();
this.parseVarId(decl, kind);
if (this.eat(types.eq)) {
decl.init = this.parseMaybeAssign(isFor);
} else if (kind === "const" && !(this.type === types._in || (this.options.ecmaVersion >= 6 && this.isContextual("of")))) {
this.unexpected();
} else if (decl.id.type !== "Identifier" && !(isFor && (this.type === types._in || this.isContextual("of")))) {
this.raise(this.lastTokEnd, "Complex binding patterns require an initialization value");
} else {
decl.init = null;
}
node.declarations.push(this.finishNode(decl, "VariableDeclarator"));
if (!this.eat(types.comma)) { break }
}
return node
};
pp$1.parseVarId = function(decl, kind) {
decl.id = this.parseBindingAtom();
this.checkLVal(decl.id, kind === "var" ? BIND_VAR : BIND_LEXICAL, false);
};
var FUNC_STATEMENT = 1, FUNC_HANGING_STATEMENT = 2, FUNC_NULLABLE_ID = 4;
// Parse a function declaration or literal (depending on the
// `statement & FUNC_STATEMENT`).
// Remove `allowExpressionBody` for 7.0.0, as it is only called with false
pp$1.parseFunction = function(node, statement, allowExpressionBody, isAsync) {
this.initFunction(node);
if (this.options.ecmaVersion >= 9 || this.options.ecmaVersion >= 6 && !isAsync) {
if (this.type === types.star && (statement & FUNC_HANGING_STATEMENT))
{ this.unexpected(); }
node.generator = this.eat(types.star);
}
if (this.options.ecmaVersion >= 8)
{ node.async = !!isAsync; }
if (statement & FUNC_STATEMENT) {
node.id = (statement & FUNC_NULLABLE_ID) && this.type !== types.name ? null : this.parseIdent();
if (node.id && !(statement & FUNC_HANGING_STATEMENT))
// If it is a regular function declaration in sloppy mode, then it is
// subject to Annex B semantics (BIND_FUNCTION). Otherwise, the binding
// mode depends on properties of the current scope (see
// treatFunctionsAsVar).
{ this.checkLVal(node.id, (this.strict || node.generator || node.async) ? this.treatFunctionsAsVar ? BIND_VAR : BIND_LEXICAL : BIND_FUNCTION); }
}
var oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
this.enterScope(functionFlags(node.async, node.generator));
if (!(statement & FUNC_STATEMENT))
{ node.id = this.type === types.name ? this.parseIdent() : null; }
this.parseFunctionParams(node);
this.parseFunctionBody(node, allowExpressionBody, false);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, (statement & FUNC_STATEMENT) ? "FunctionDeclaration" : "FunctionExpression")
};
pp$1.parseFunctionParams = function(node) {
this.expect(types.parenL);
node.params = this.parseBindingList(types.parenR, false, this.options.ecmaVersion >= 8);
this.checkYieldAwaitInDefaultParams();
};
// Parse a class declaration or literal (depending on the
// `isStatement` parameter).
pp$1.parseClass = function(node, isStatement) {
this.next();
// ecma-262 14.6 Class Definitions
// A class definition is always strict mode code.
var oldStrict = this.strict;
this.strict = true;
this.parseClassId(node, isStatement);
this.parseClassSuper(node);
var classBody = this.startNode();
var hadConstructor = false;
classBody.body = [];
this.expect(types.braceL);
while (this.type !== types.braceR) {
var element = this.parseClassElement(node.superClass !== null);
if (element) {
classBody.body.push(element);
if (element.type === "MethodDefinition" && element.kind === "constructor") {
if (hadConstructor) { this.raise(element.start, "Duplicate constructor in the same class"); }
hadConstructor = true;
}
}
}
this.strict = oldStrict;
this.next();
node.body = this.finishNode(classBody, "ClassBody");
return this.finishNode(node, isStatement ? "ClassDeclaration" : "ClassExpression")
};
pp$1.parseClassElement = function(constructorAllowsSuper) {
var this$1 = this;
if (this.eat(types.semi)) { return null }
var method = this.startNode();
var tryContextual = function (k, noLineBreak) {
if ( noLineBreak === void 0 ) noLineBreak = false;
var start = this$1.start, startLoc = this$1.startLoc;
if (!this$1.eatContextual(k)) { return false }
if (this$1.type !== types.parenL && (!noLineBreak || !this$1.canInsertSemicolon())) { return true }
if (method.key) { this$1.unexpected(); }
method.computed = false;
method.key = this$1.startNodeAt(start, startLoc);
method.key.name = k;
this$1.finishNode(method.key, "Identifier");
return false
};
method.kind = "method";
method.static = tryContextual("static");
var isGenerator = this.eat(types.star);
var isAsync = false;
if (!isGenerator) {
if (this.options.ecmaVersion >= 8 && tryContextual("async", true)) {
isAsync = true;
isGenerator = this.options.ecmaVersion >= 9 && this.eat(types.star);
} else if (tryContextual("get")) {
method.kind = "get";
} else if (tryContextual("set")) {
method.kind = "set";
}
}
if (!method.key) { this.parsePropertyName(method); }
var key = method.key;
var allowsDirectSuper = false;
if (!method.computed && !method.static && (key.type === "Identifier" && key.name === "constructor" ||
key.type === "Literal" && key.value === "constructor")) {
if (method.kind !== "method") { this.raise(key.start, "Constructor can't have get/set modifier"); }
if (isGenerator) { this.raise(key.start, "Constructor can't be a generator"); }
if (isAsync) { this.raise(key.start, "Constructor can't be an async method"); }
method.kind = "constructor";
allowsDirectSuper = constructorAllowsSuper;
} else if (method.static && key.type === "Identifier" && key.name === "prototype") {
this.raise(key.start, "Classes may not have a static property named prototype");
}
this.parseClassMethod(method, isGenerator, isAsync, allowsDirectSuper);
if (method.kind === "get" && method.value.params.length !== 0)
{ this.raiseRecoverable(method.value.start, "getter should have no params"); }
if (method.kind === "set" && method.value.params.length !== 1)
{ this.raiseRecoverable(method.value.start, "setter should have exactly one param"); }
if (method.kind === "set" && method.value.params[0].type === "RestElement")
{ this.raiseRecoverable(method.value.params[0].start, "Setter cannot use rest params"); }
return method
};
pp$1.parseClassMethod = function(method, isGenerator, isAsync, allowsDirectSuper) {
method.value = this.parseMethod(isGenerator, isAsync, allowsDirectSuper);
return this.finishNode(method, "MethodDefinition")
};
pp$1.parseClassId = function(node, isStatement) {
if (this.type === types.name) {
node.id = this.parseIdent();
if (isStatement)
{ this.checkLVal(node.id, BIND_LEXICAL, false); }
} else {
if (isStatement === true)
{ this.unexpected(); }
node.id = null;
}
};
pp$1.parseClassSuper = function(node) {
node.superClass = this.eat(types._extends) ? this.parseExprSubscripts() : null;
};
// Parses module export declaration.
pp$1.parseExport = function(node, exports) {
this.next();
// export * from '...'
if (this.eat(types.star)) {
if (this.options.ecmaVersion >= 11) {
if (this.eatContextual("as")) {
node.exported = this.parseIdent(true);
this.checkExport(exports, node.exported.name, this.lastTokStart);
} else {
node.exported = null;
}
}
this.expectContextual("from");
if (this.type !== types.string) { this.unexpected(); }
node.source = this.parseExprAtom();
this.semicolon();
return this.finishNode(node, "ExportAllDeclaration")
}
if (this.eat(types._default)) { // export default ...
this.checkExport(exports, "default", this.lastTokStart);
var isAsync;
if (this.type === types._function || (isAsync = this.isAsyncFunction())) {
var fNode = this.startNode();
this.next();
if (isAsync) { this.next(); }
node.declaration = this.parseFunction(fNode, FUNC_STATEMENT | FUNC_NULLABLE_ID, false, isAsync);
} else if (this.type === types._class) {
var cNode = this.startNode();
node.declaration = this.parseClass(cNode, "nullableID");
} else {
node.declaration = this.parseMaybeAssign();
this.semicolon();
}
return this.finishNode(node, "ExportDefaultDeclaration")
}
// export var|const|let|function|class ...
if (this.shouldParseExportStatement()) {
node.declaration = this.parseStatement(null);
if (node.declaration.type === "VariableDeclaration")
{ this.checkVariableExport(exports, node.declaration.declarations); }
else
{ this.checkExport(exports, node.declaration.id.name, node.declaration.id.start); }
node.specifiers = [];
node.source = null;
} else { // export { x, y as z } [from '...']
node.declaration = null;
node.specifiers = this.parseExportSpecifiers(exports);
if (this.eatContextual("from")) {
if (this.type !== types.string) { this.unexpected(); }
node.source = this.parseExprAtom();
} else {
for (var i = 0, list = node.specifiers; i < list.length; i += 1) {
// check for keywords used as local names
var spec = list[i];
this.checkUnreserved(spec.local);
// check if export is defined
this.checkLocalExport(spec.local);
}
node.source = null;
}
this.semicolon();
}
return this.finishNode(node, "ExportNamedDeclaration")
};
pp$1.checkExport = function(exports, name, pos) {
if (!exports) { return }
if (has(exports, name))
{ this.raiseRecoverable(pos, "Duplicate export '" + name + "'"); }
exports[name] = true;
};
pp$1.checkPatternExport = function(exports, pat) {
var type = pat.type;
if (type === "Identifier")
{ this.checkExport(exports, pat.name, pat.start); }
else if (type === "ObjectPattern")
{ for (var i = 0, list = pat.properties; i < list.length; i += 1)
{
var prop = list[i];
this.checkPatternExport(exports, prop);
} }
else if (type === "ArrayPattern")
{ for (var i$1 = 0, list$1 = pat.elements; i$1 < list$1.length; i$1 += 1) {
var elt = list$1[i$1];
if (elt) { this.checkPatternExport(exports, elt); }
} }
else if (type === "Property")
{ this.checkPatternExport(exports, pat.value); }
else if (type === "AssignmentPattern")
{ this.checkPatternExport(exports, pat.left); }
else if (type === "RestElement")
{ this.checkPatternExport(exports, pat.argument); }
else if (type === "ParenthesizedExpression")
{ this.checkPatternExport(exports, pat.expression); }
};
pp$1.checkVariableExport = function(exports, decls) {
if (!exports) { return }
for (var i = 0, list = decls; i < list.length; i += 1)
{
var decl = list[i];
this.checkPatternExport(exports, decl.id);
}
};
pp$1.shouldParseExportStatement = function() {
return this.type.keyword === "var" ||
this.type.keyword === "const" ||
this.type.keyword === "class" ||
this.type.keyword === "function" ||
this.isLet() ||
this.isAsyncFunction()
};
// Parses a comma-separated list of module exports.
pp$1.parseExportSpecifiers = function(exports) {
var nodes = [], first = true;
// export { x, y as z } [from '...']
this.expect(types.braceL);
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.afterTrailingComma(types.braceR)) { break }
} else { first = false; }
var node = this.startNode();
node.local = this.parseIdent(true);
node.exported = this.eatContextual("as") ? this.parseIdent(true) : node.local;
this.checkExport(exports, node.exported.name, node.exported.start);
nodes.push(this.finishNode(node, "ExportSpecifier"));
}
return nodes
};
// Parses import declaration.
pp$1.parseImport = function(node) {
this.next();
// import '...'
if (this.type === types.string) {
node.specifiers = empty;
node.source = this.parseExprAtom();
} else {
node.specifiers = this.parseImportSpecifiers();
this.expectContextual("from");
node.source = this.type === types.string ? this.parseExprAtom() : this.unexpected();
}
this.semicolon();
return this.finishNode(node, "ImportDeclaration")
};
// Parses a comma-separated list of module imports.
pp$1.parseImportSpecifiers = function() {
var nodes = [], first = true;
if (this.type === types.name) {
// import defaultObj, { x, y as z } from '...'
var node = this.startNode();
node.local = this.parseIdent();
this.checkLVal(node.local, BIND_LEXICAL);
nodes.push(this.finishNode(node, "ImportDefaultSpecifier"));
if (!this.eat(types.comma)) { return nodes }
}
if (this.type === types.star) {
var node$1 = this.startNode();
this.next();
this.expectContextual("as");
node$1.local = this.parseIdent();
this.checkLVal(node$1.local, BIND_LEXICAL);
nodes.push(this.finishNode(node$1, "ImportNamespaceSpecifier"));
return nodes
}
this.expect(types.braceL);
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.afterTrailingComma(types.braceR)) { break }
} else { first = false; }
var node$2 = this.startNode();
node$2.imported = this.parseIdent(true);
if (this.eatContextual("as")) {
node$2.local = this.parseIdent();
} else {
this.checkUnreserved(node$2.imported);
node$2.local = node$2.imported;
}
this.checkLVal(node$2.local, BIND_LEXICAL);
nodes.push(this.finishNode(node$2, "ImportSpecifier"));
}
return nodes
};
// Set `ExpressionStatement#directive` property for directive prologues.
pp$1.adaptDirectivePrologue = function(statements) {
for (var i = 0; i < statements.length && this.isDirectiveCandidate(statements[i]); ++i) {
statements[i].directive = statements[i].expression.raw.slice(1, -1);
}
};
pp$1.isDirectiveCandidate = function(statement) {
return (
statement.type === "ExpressionStatement" &&
statement.expression.type === "Literal" &&
typeof statement.expression.value === "string" &&
// Reject parenthesized strings.
(this.input[statement.start] === "\"" || this.input[statement.start] === "'")
)
};
var pp$2 = Parser.prototype;
// Convert existing expression atom to assignable pattern
// if possible.
pp$2.toAssignable = function(node, isBinding, refDestructuringErrors) {
if (this.options.ecmaVersion >= 6 && node) {
switch (node.type) {
case "Identifier":
if (this.inAsync && node.name === "await")
{ this.raise(node.start, "Cannot use 'await' as identifier inside an async function"); }
break
case "ObjectPattern":
case "ArrayPattern":
case "RestElement":
break
case "ObjectExpression":
node.type = "ObjectPattern";
if (refDestructuringErrors) { this.checkPatternErrors(refDestructuringErrors, true); }
for (var i = 0, list = node.properties; i < list.length; i += 1) {
var prop = list[i];
this.toAssignable(prop, isBinding);
// Early error:
// AssignmentRestProperty[Yield, Await] :
// `...` DestructuringAssignmentTarget[Yield, Await]
//
// It is a Syntax Error if |DestructuringAssignmentTarget| is an |ArrayLiteral| or an |ObjectLiteral|.
if (
prop.type === "RestElement" &&
(prop.argument.type === "ArrayPattern" || prop.argument.type === "ObjectPattern")
) {
this.raise(prop.argument.start, "Unexpected token");
}
}
break
case "Property":
// AssignmentProperty has type === "Property"
if (node.kind !== "init") { this.raise(node.key.start, "Object pattern can't contain getter or setter"); }
this.toAssignable(node.value, isBinding);
break
case "ArrayExpression":
node.type = "ArrayPattern";
if (refDestructuringErrors) { this.checkPatternErrors(refDestructuringErrors, true); }
this.toAssignableList(node.elements, isBinding);
break
case "SpreadElement":
node.type = "RestElement";
this.toAssignable(node.argument, isBinding);
if (node.argument.type === "AssignmentPattern")
{ this.raise(node.argument.start, "Rest elements cannot have a default value"); }
break
case "AssignmentExpression":
if (node.operator !== "=") { this.raise(node.left.end, "Only '=' operator can be used for specifying default value."); }
node.type = "AssignmentPattern";
delete node.operator;
this.toAssignable(node.left, isBinding);
// falls through to AssignmentPattern
case "AssignmentPattern":
break
case "ParenthesizedExpression":
this.toAssignable(node.expression, isBinding, refDestructuringErrors);
break
case "ChainExpression":
this.raiseRecoverable(node.start, "Optional chaining cannot appear in left-hand side");
break
case "MemberExpression":
if (!isBinding) { break }
default:
this.raise(node.start, "Assigning to rvalue");
}
} else if (refDestructuringErrors) { this.checkPatternErrors(refDestructuringErrors, true); }
return node
};
// Convert list of expression atoms to binding list.
pp$2.toAssignableList = function(exprList, isBinding) {
var end = exprList.length;
for (var i = 0; i < end; i++) {
var elt = exprList[i];
if (elt) { this.toAssignable(elt, isBinding); }
}
if (end) {
var last = exprList[end - 1];
if (this.options.ecmaVersion === 6 && isBinding && last && last.type === "RestElement" && last.argument.type !== "Identifier")
{ this.unexpected(last.argument.start); }
}
return exprList
};
// Parses spread element.
pp$2.parseSpread = function(refDestructuringErrors) {
var node = this.startNode();
this.next();
node.argument = this.parseMaybeAssign(false, refDestructuringErrors);
return this.finishNode(node, "SpreadElement")
};
pp$2.parseRestBinding = function() {
var node = this.startNode();
this.next();
// RestElement inside of a function parameter must be an identifier
if (this.options.ecmaVersion === 6 && this.type !== types.name)
{ this.unexpected(); }
node.argument = this.parseBindingAtom();
return this.finishNode(node, "RestElement")
};
// Parses lvalue (assignable) atom.
pp$2.parseBindingAtom = function() {
if (this.options.ecmaVersion >= 6) {
switch (this.type) {
case types.bracketL:
var node = this.startNode();
this.next();
node.elements = this.parseBindingList(types.bracketR, true, true);
return this.finishNode(node, "ArrayPattern")
case types.braceL:
return this.parseObj(true)
}
}
return this.parseIdent()
};
pp$2.parseBindingList = function(close, allowEmpty, allowTrailingComma) {
var elts = [], first = true;
while (!this.eat(close)) {
if (first) { first = false; }
else { this.expect(types.comma); }
if (allowEmpty && this.type === types.comma) {
elts.push(null);
} else if (allowTrailingComma && this.afterTrailingComma(close)) {
break
} else if (this.type === types.ellipsis) {
var rest = this.parseRestBinding();
this.parseBindingListItem(rest);
elts.push(rest);
if (this.type === types.comma) { this.raise(this.start, "Comma is not permitted after the rest element"); }
this.expect(close);
break
} else {
var elem = this.parseMaybeDefault(this.start, this.startLoc);
this.parseBindingListItem(elem);
elts.push(elem);
}
}
return elts
};
pp$2.parseBindingListItem = function(param) {
return param
};
// Parses assignment pattern around given atom if possible.
pp$2.parseMaybeDefault = function(startPos, startLoc, left) {
left = left || this.parseBindingAtom();
if (this.options.ecmaVersion < 6 || !this.eat(types.eq)) { return left }
var node = this.startNodeAt(startPos, startLoc);
node.left = left;
node.right = this.parseMaybeAssign();
return this.finishNode(node, "AssignmentPattern")
};
// Verify that a node is an lval — something that can be assigned
// to.
// bindingType can be either:
// 'var' indicating that the lval creates a 'var' binding
// 'let' indicating that the lval creates a lexical ('let' or 'const') binding
// 'none' indicating that the binding should be checked for illegal identifiers, but not for duplicate references
pp$2.checkLVal = function(expr, bindingType, checkClashes) {
if ( bindingType === void 0 ) bindingType = BIND_NONE;
switch (expr.type) {
case "Identifier":
if (bindingType === BIND_LEXICAL && expr.name === "let")
{ this.raiseRecoverable(expr.start, "let is disallowed as a lexically bound name"); }
if (this.strict && this.reservedWordsStrictBind.test(expr.name))
{ this.raiseRecoverable(expr.start, (bindingType ? "Binding " : "Assigning to ") + expr.name + " in strict mode"); }
if (checkClashes) {
if (has(checkClashes, expr.name))
{ this.raiseRecoverable(expr.start, "Argument name clash"); }
checkClashes[expr.name] = true;
}
if (bindingType !== BIND_NONE && bindingType !== BIND_OUTSIDE) { this.declareName(expr.name, bindingType, expr.start); }
break
case "ChainExpression":
this.raiseRecoverable(expr.start, "Optional chaining cannot appear in left-hand side");
break
case "MemberExpression":
if (bindingType) { this.raiseRecoverable(expr.start, "Binding member expression"); }
break
case "ObjectPattern":
for (var i = 0, list = expr.properties; i < list.length; i += 1)
{
var prop = list[i];
this.checkLVal(prop, bindingType, checkClashes);
}
break
case "Property":
// AssignmentProperty has type === "Property"
this.checkLVal(expr.value, bindingType, checkClashes);
break
case "ArrayPattern":
for (var i$1 = 0, list$1 = expr.elements; i$1 < list$1.length; i$1 += 1) {
var elem = list$1[i$1];
if (elem) { this.checkLVal(elem, bindingType, checkClashes); }
}
break
case "AssignmentPattern":
this.checkLVal(expr.left, bindingType, checkClashes);
break
case "RestElement":
this.checkLVal(expr.argument, bindingType, checkClashes);
break
case "ParenthesizedExpression":
this.checkLVal(expr.expression, bindingType, checkClashes);
break
default:
this.raise(expr.start, (bindingType ? "Binding" : "Assigning to") + " rvalue");
}
};
// A recursive descent parser operates by defining functions for all
var pp$3 = Parser.prototype;
// Check if property name clashes with already added.
// Object/class getters and setters are not allowed to clash —
// either with each other or with an init property — and in
// strict mode, init properties are also not allowed to be repeated.
pp$3.checkPropClash = function(prop, propHash, refDestructuringErrors) {
if (this.options.ecmaVersion >= 9 && prop.type === "SpreadElement")
{ return }
if (this.options.ecmaVersion >= 6 && (prop.computed || prop.method || prop.shorthand))
{ return }
var key = prop.key;
var name;
switch (key.type) {
case "Identifier": name = key.name; break
case "Literal": name = String(key.value); break
default: return
}
var kind = prop.kind;
if (this.options.ecmaVersion >= 6) {
if (name === "__proto__" && kind === "init") {
if (propHash.proto) {
if (refDestructuringErrors) {
if (refDestructuringErrors.doubleProto < 0)
{ refDestructuringErrors.doubleProto = key.start; }
// Backwards-compat kludge. Can be removed in version 6.0
} else { this.raiseRecoverable(key.start, "Redefinition of __proto__ property"); }
}
propHash.proto = true;
}
return
}
name = "$" + name;
var other = propHash[name];
if (other) {
var redefinition;
if (kind === "init") {
redefinition = this.strict && other.init || other.get || other.set;
} else {
redefinition = other.init || other[kind];
}
if (redefinition)
{ this.raiseRecoverable(key.start, "Redefinition of property"); }
} else {
other = propHash[name] = {
init: false,
get: false,
set: false
};
}
other[kind] = true;
};
// ### Expression parsing
// These nest, from the most general expression type at the top to
// 'atomic', nondivisible expression types at the bottom. Most of
// the functions will simply let the function(s) below them parse,
// and, *if* the syntactic construct they handle is present, wrap
// the AST node that the inner parser gave them in another node.
// Parse a full expression. The optional arguments are used to
// forbid the `in` operator (in for loops initalization expressions)
// and provide reference for storing '=' operator inside shorthand
// property assignment in contexts where both object expression
// and object pattern might appear (so it's possible to raise
// delayed syntax error at correct position).
pp$3.parseExpression = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseMaybeAssign(noIn, refDestructuringErrors);
if (this.type === types.comma) {
var node = this.startNodeAt(startPos, startLoc);
node.expressions = [expr];
while (this.eat(types.comma)) { node.expressions.push(this.parseMaybeAssign(noIn, refDestructuringErrors)); }
return this.finishNode(node, "SequenceExpression")
}
return expr
};
// Parse an assignment expression. This includes applications of
// operators like `+=`.
pp$3.parseMaybeAssign = function(noIn, refDestructuringErrors, afterLeftParse) {
if (this.isContextual("yield")) {
if (this.inGenerator) { return this.parseYield(noIn) }
// The tokenizer will assume an expression is allowed after
// `yield`, but this isn't that kind of yield
else { this.exprAllowed = false; }
}
var ownDestructuringErrors = false, oldParenAssign = -1, oldTrailingComma = -1;
if (refDestructuringErrors) {
oldParenAssign = refDestructuringErrors.parenthesizedAssign;
oldTrailingComma = refDestructuringErrors.trailingComma;
refDestructuringErrors.parenthesizedAssign = refDestructuringErrors.trailingComma = -1;
} else {
refDestructuringErrors = new DestructuringErrors;
ownDestructuringErrors = true;
}
var startPos = this.start, startLoc = this.startLoc;
if (this.type === types.parenL || this.type === types.name)
{ this.potentialArrowAt = this.start; }
var left = this.parseMaybeConditional(noIn, refDestructuringErrors);
if (afterLeftParse) { left = afterLeftParse.call(this, left, startPos, startLoc); }
if (this.type.isAssign) {
var node = this.startNodeAt(startPos, startLoc);
node.operator = this.value;
node.left = this.type === types.eq ? this.toAssignable(left, false, refDestructuringErrors) : left;
if (!ownDestructuringErrors) {
refDestructuringErrors.parenthesizedAssign = refDestructuringErrors.trailingComma = refDestructuringErrors.doubleProto = -1;
}
if (refDestructuringErrors.shorthandAssign >= node.left.start)
{ refDestructuringErrors.shorthandAssign = -1; } // reset because shorthand default was used correctly
this.checkLVal(left);
this.next();
node.right = this.parseMaybeAssign(noIn);
return this.finishNode(node, "AssignmentExpression")
} else {
if (ownDestructuringErrors) { this.checkExpressionErrors(refDestructuringErrors, true); }
}
if (oldParenAssign > -1) { refDestructuringErrors.parenthesizedAssign = oldParenAssign; }
if (oldTrailingComma > -1) { refDestructuringErrors.trailingComma = oldTrailingComma; }
return left
};
// Parse a ternary conditional (`?:`) operator.
pp$3.parseMaybeConditional = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseExprOps(noIn, refDestructuringErrors);
if (this.checkExpressionErrors(refDestructuringErrors)) { return expr }
if (this.eat(types.question)) {
var node = this.startNodeAt(startPos, startLoc);
node.test = expr;
node.consequent = this.parseMaybeAssign();
this.expect(types.colon);
node.alternate = this.parseMaybeAssign(noIn);
return this.finishNode(node, "ConditionalExpression")
}
return expr
};
// Start the precedence parser.
pp$3.parseExprOps = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseMaybeUnary(refDestructuringErrors, false);
if (this.checkExpressionErrors(refDestructuringErrors)) { return expr }
return expr.start === startPos && expr.type === "ArrowFunctionExpression" ? expr : this.parseExprOp(expr, startPos, startLoc, -1, noIn)
};
// Parse binary operators with the operator precedence parsing
// algorithm. `left` is the left-hand side of the operator.
// `minPrec` provides context that allows the function to stop and
// defer further parser to one of its callers when it encounters an
// operator that has a lower precedence than the set it is parsing.
pp$3.parseExprOp = function(left, leftStartPos, leftStartLoc, minPrec, noIn) {
var prec = this.type.binop;
if (prec != null && (!noIn || this.type !== types._in)) {
if (prec > minPrec) {
var logical = this.type === types.logicalOR || this.type === types.logicalAND;
var coalesce = this.type === types.coalesce;
if (coalesce) {
// Handle the precedence of `tt.coalesce` as equal to the range of logical expressions.
// In other words, `node.right` shouldn't contain logical expressions in order to check the mixed error.
prec = types.logicalAND.binop;
}
var op = this.value;
this.next();
var startPos = this.start, startLoc = this.startLoc;
var right = this.parseExprOp(this.parseMaybeUnary(null, false), startPos, startLoc, prec, noIn);
var node = this.buildBinary(leftStartPos, leftStartLoc, left, right, op, logical || coalesce);
if ((logical && this.type === types.coalesce) || (coalesce && (this.type === types.logicalOR || this.type === types.logicalAND))) {
this.raiseRecoverable(this.start, "Logical expressions and coalesce expressions cannot be mixed. Wrap either by parentheses");
}
return this.parseExprOp(node, leftStartPos, leftStartLoc, minPrec, noIn)
}
}
return left
};
pp$3.buildBinary = function(startPos, startLoc, left, right, op, logical) {
var node = this.startNodeAt(startPos, startLoc);
node.left = left;
node.operator = op;
node.right = right;
return this.finishNode(node, logical ? "LogicalExpression" : "BinaryExpression")
};
// Parse unary operators, both prefix and postfix.
pp$3.parseMaybeUnary = function(refDestructuringErrors, sawUnary) {
var startPos = this.start, startLoc = this.startLoc, expr;
if (this.isContextual("await") && (this.inAsync || (!this.inFunction && this.options.allowAwaitOutsideFunction))) {
expr = this.parseAwait();
sawUnary = true;
} else if (this.type.prefix) {
var node = this.startNode(), update = this.type === types.incDec;
node.operator = this.value;
node.prefix = true;
this.next();
node.argument = this.parseMaybeUnary(null, true);
this.checkExpressionErrors(refDestructuringErrors, true);
if (update) { this.checkLVal(node.argument); }
else if (this.strict && node.operator === "delete" &&
node.argument.type === "Identifier")
{ this.raiseRecoverable(node.start, "Deleting local variable in strict mode"); }
else { sawUnary = true; }
expr = this.finishNode(node, update ? "UpdateExpression" : "UnaryExpression");
} else {
expr = this.parseExprSubscripts(refDestructuringErrors);
if (this.checkExpressionErrors(refDestructuringErrors)) { return expr }
while (this.type.postfix && !this.canInsertSemicolon()) {
var node$1 = this.startNodeAt(startPos, startLoc);
node$1.operator = this.value;
node$1.prefix = false;
node$1.argument = expr;
this.checkLVal(expr);
this.next();
expr = this.finishNode(node$1, "UpdateExpression");
}
}
if (!sawUnary && this.eat(types.starstar))
{ return this.buildBinary(startPos, startLoc, expr, this.parseMaybeUnary(null, false), "**", false) }
else
{ return expr }
};
// Parse call, dot, and `[]`-subscript expressions.
pp$3.parseExprSubscripts = function(refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseExprAtom(refDestructuringErrors);
if (expr.type === "ArrowFunctionExpression" && this.input.slice(this.lastTokStart, this.lastTokEnd) !== ")")
{ return expr }
var result = this.parseSubscripts(expr, startPos, startLoc);
if (refDestructuringErrors && result.type === "MemberExpression") {
if (refDestructuringErrors.parenthesizedAssign >= result.start) { refDestructuringErrors.parenthesizedAssign = -1; }
if (refDestructuringErrors.parenthesizedBind >= result.start) { refDestructuringErrors.parenthesizedBind = -1; }
}
return result
};
pp$3.parseSubscripts = function(base, startPos, startLoc, noCalls) {
var maybeAsyncArrow = this.options.ecmaVersion >= 8 && base.type === "Identifier" && base.name === "async" &&
this.lastTokEnd === base.end && !this.canInsertSemicolon() && base.end - base.start === 5 &&
this.potentialArrowAt === base.start;
var optionalChained = false;
while (true) {
var element = this.parseSubscript(base, startPos, startLoc, noCalls, maybeAsyncArrow, optionalChained);
if (element.optional) { optionalChained = true; }
if (element === base || element.type === "ArrowFunctionExpression") {
if (optionalChained) {
var chainNode = this.startNodeAt(startPos, startLoc);
chainNode.expression = element;
element = this.finishNode(chainNode, "ChainExpression");
}
return element
}
base = element;
}
};
pp$3.parseSubscript = function(base, startPos, startLoc, noCalls, maybeAsyncArrow, optionalChained) {
var optionalSupported = this.options.ecmaVersion >= 11;
var optional = optionalSupported && this.eat(types.questionDot);
if (noCalls && optional) { this.raise(this.lastTokStart, "Optional chaining cannot appear in the callee of new expressions"); }
var computed = this.eat(types.bracketL);
if (computed || (optional && this.type !== types.parenL && this.type !== types.backQuote) || this.eat(types.dot)) {
var node = this.startNodeAt(startPos, startLoc);
node.object = base;
node.property = computed ? this.parseExpression() : this.parseIdent(this.options.allowReserved !== "never");
node.computed = !!computed;
if (computed) { this.expect(types.bracketR); }
if (optionalSupported) {
node.optional = optional;
}
base = this.finishNode(node, "MemberExpression");
} else if (!noCalls && this.eat(types.parenL)) {
var refDestructuringErrors = new DestructuringErrors, oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
var exprList = this.parseExprList(types.parenR, this.options.ecmaVersion >= 8, false, refDestructuringErrors);
if (maybeAsyncArrow && !optional && !this.canInsertSemicolon() && this.eat(types.arrow)) {
this.checkPatternErrors(refDestructuringErrors, false);
this.checkYieldAwaitInDefaultParams();
if (this.awaitIdentPos > 0)
{ this.raise(this.awaitIdentPos, "Cannot use 'await' as identifier inside an async function"); }
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), exprList, true)
}
this.checkExpressionErrors(refDestructuringErrors, true);
this.yieldPos = oldYieldPos || this.yieldPos;
this.awaitPos = oldAwaitPos || this.awaitPos;
this.awaitIdentPos = oldAwaitIdentPos || this.awaitIdentPos;
var node$1 = this.startNodeAt(startPos, startLoc);
node$1.callee = base;
node$1.arguments = exprList;
if (optionalSupported) {
node$1.optional = optional;
}
base = this.finishNode(node$1, "CallExpression");
} else if (this.type === types.backQuote) {
if (optional || optionalChained) {
this.raise(this.start, "Optional chaining cannot appear in the tag of tagged template expressions");
}
var node$2 = this.startNodeAt(startPos, startLoc);
node$2.tag = base;
node$2.quasi = this.parseTemplate({isTagged: true});
base = this.finishNode(node$2, "TaggedTemplateExpression");
}
return base
};
// Parse an atomic expression — either a single token that is an
// expression, an expression started by a keyword like `function` or
// `new`, or an expression wrapped in punctuation like `()`, `[]`,
// or `{}`.
pp$3.parseExprAtom = function(refDestructuringErrors) {
// If a division operator appears in an expression position, the
// tokenizer got confused, and we force it to read a regexp instead.
if (this.type === types.slash) { this.readRegexp(); }
var node, canBeArrow = this.potentialArrowAt === this.start;
switch (this.type) {
case types._super:
if (!this.allowSuper)
{ this.raise(this.start, "'super' keyword outside a method"); }
node = this.startNode();
this.next();
if (this.type === types.parenL && !this.allowDirectSuper)
{ this.raise(node.start, "super() call outside constructor of a subclass"); }
// The `super` keyword can appear at below:
// SuperProperty:
// super [ Expression ]
// super . IdentifierName
// SuperCall:
// super ( Arguments )
if (this.type !== types.dot && this.type !== types.bracketL && this.type !== types.parenL)
{ this.unexpected(); }
return this.finishNode(node, "Super")
case types._this:
node = this.startNode();
this.next();
return this.finishNode(node, "ThisExpression")
case types.name:
var startPos = this.start, startLoc = this.startLoc, containsEsc = this.containsEsc;
var id = this.parseIdent(false);
if (this.options.ecmaVersion >= 8 && !containsEsc && id.name === "async" && !this.canInsertSemicolon() && this.eat(types._function))
{ return this.parseFunction(this.startNodeAt(startPos, startLoc), 0, false, true) }
if (canBeArrow && !this.canInsertSemicolon()) {
if (this.eat(types.arrow))
{ return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), [id], false) }
if (this.options.ecmaVersion >= 8 && id.name === "async" && this.type === types.name && !containsEsc) {
id = this.parseIdent(false);
if (this.canInsertSemicolon() || !this.eat(types.arrow))
{ this.unexpected(); }
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), [id], true)
}
}
return id
case types.regexp:
var value = this.value;
node = this.parseLiteral(value.value);
node.regex = {pattern: value.pattern, flags: value.flags};
return node
case types.num: case types.string:
return this.parseLiteral(this.value)
case types._null: case types._true: case types._false:
node = this.startNode();
node.value = this.type === types._null ? null : this.type === types._true;
node.raw = this.type.keyword;
this.next();
return this.finishNode(node, "Literal")
case types.parenL:
var start = this.start, expr = this.parseParenAndDistinguishExpression(canBeArrow);
if (refDestructuringErrors) {
if (refDestructuringErrors.parenthesizedAssign < 0 && !this.isSimpleAssignTarget(expr))
{ refDestructuringErrors.parenthesizedAssign = start; }
if (refDestructuringErrors.parenthesizedBind < 0)
{ refDestructuringErrors.parenthesizedBind = start; }
}
return expr
case types.bracketL:
node = this.startNode();
this.next();
node.elements = this.parseExprList(types.bracketR, true, true, refDestructuringErrors);
return this.finishNode(node, "ArrayExpression")
case types.braceL:
return this.parseObj(false, refDestructuringErrors)
case types._function:
node = this.startNode();
this.next();
return this.parseFunction(node, 0)
case types._class:
return this.parseClass(this.startNode(), false)
case types._new:
return this.parseNew()
case types.backQuote:
return this.parseTemplate()
case types._import:
if (this.options.ecmaVersion >= 11) {
return this.parseExprImport()
} else {
return this.unexpected()
}
default:
this.unexpected();
}
};
pp$3.parseExprImport = function() {
var node = this.startNode();
// Consume `import` as an identifier for `import.meta`.
// Because `this.parseIdent(true)` doesn't check escape sequences, it needs the check of `this.containsEsc`.
if (this.containsEsc) { this.raiseRecoverable(this.start, "Escape sequence in keyword import"); }
var meta = this.parseIdent(true);
switch (this.type) {
case types.parenL:
return this.parseDynamicImport(node)
case types.dot:
node.meta = meta;
return this.parseImportMeta(node)
default:
this.unexpected();
}
};
pp$3.parseDynamicImport = function(node) {
this.next(); // skip `(`
// Parse node.source.
node.source = this.parseMaybeAssign();
// Verify ending.
if (!this.eat(types.parenR)) {
var errorPos = this.start;
if (this.eat(types.comma) && this.eat(types.parenR)) {
this.raiseRecoverable(errorPos, "Trailing comma is not allowed in import()");
} else {
this.unexpected(errorPos);
}
}
return this.finishNode(node, "ImportExpression")
};
pp$3.parseImportMeta = function(node) {
this.next(); // skip `.`
var containsEsc = this.containsEsc;
node.property = this.parseIdent(true);
if (node.property.name !== "meta")
{ this.raiseRecoverable(node.property.start, "The only valid meta property for import is 'import.meta'"); }
if (containsEsc)
{ this.raiseRecoverable(node.start, "'import.meta' must not contain escaped characters"); }
if (this.options.sourceType !== "module")
{ this.raiseRecoverable(node.start, "Cannot use 'import.meta' outside a module"); }
return this.finishNode(node, "MetaProperty")
};
pp$3.parseLiteral = function(value) {
var node = this.startNode();
node.value = value;
node.raw = this.input.slice(this.start, this.end);
if (node.raw.charCodeAt(node.raw.length - 1) === 110) { node.bigint = node.raw.slice(0, -1); }
this.next();
return this.finishNode(node, "Literal")
};
pp$3.parseParenExpression = function() {
this.expect(types.parenL);
var val = this.parseExpression();
this.expect(types.parenR);
return val
};
pp$3.parseParenAndDistinguishExpression = function(canBeArrow) {
var startPos = this.start, startLoc = this.startLoc, val, allowTrailingComma = this.options.ecmaVersion >= 8;
if (this.options.ecmaVersion >= 6) {
this.next();
var innerStartPos = this.start, innerStartLoc = this.startLoc;
var exprList = [], first = true, lastIsComma = false;
var refDestructuringErrors = new DestructuringErrors, oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, spreadStart;
this.yieldPos = 0;
this.awaitPos = 0;
// Do not save awaitIdentPos to allow checking awaits nested in parameters
while (this.type !== types.parenR) {
first ? first = false : this.expect(types.comma);
if (allowTrailingComma && this.afterTrailingComma(types.parenR, true)) {
lastIsComma = true;
break
} else if (this.type === types.ellipsis) {
spreadStart = this.start;
exprList.push(this.parseParenItem(this.parseRestBinding()));
if (this.type === types.comma) { this.raise(this.start, "Comma is not permitted after the rest element"); }
break
} else {
exprList.push(this.parseMaybeAssign(false, refDestructuringErrors, this.parseParenItem));
}
}
var innerEndPos = this.start, innerEndLoc = this.startLoc;
this.expect(types.parenR);
if (canBeArrow && !this.canInsertSemicolon() && this.eat(types.arrow)) {
this.checkPatternErrors(refDestructuringErrors, false);
this.checkYieldAwaitInDefaultParams();
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
return this.parseParenArrowList(startPos, startLoc, exprList)
}
if (!exprList.length || lastIsComma) { this.unexpected(this.lastTokStart); }
if (spreadStart) { this.unexpected(spreadStart); }
this.checkExpressionErrors(refDestructuringErrors, true);
this.yieldPos = oldYieldPos || this.yieldPos;
this.awaitPos = oldAwaitPos || this.awaitPos;
if (exprList.length > 1) {
val = this.startNodeAt(innerStartPos, innerStartLoc);
val.expressions = exprList;
this.finishNodeAt(val, "SequenceExpression", innerEndPos, innerEndLoc);
} else {
val = exprList[0];
}
} else {
val = this.parseParenExpression();
}
if (this.options.preserveParens) {
var par = this.startNodeAt(startPos, startLoc);
par.expression = val;
return this.finishNode(par, "ParenthesizedExpression")
} else {
return val
}
};
pp$3.parseParenItem = function(item) {
return item
};
pp$3.parseParenArrowList = function(startPos, startLoc, exprList) {
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), exprList)
};
// New's precedence is slightly tricky. It must allow its argument to
// be a `[]` or dot subscript expression, but not a call — at least,
// not without wrapping it in parentheses. Thus, it uses the noCalls
// argument to parseSubscripts to prevent it from consuming the
// argument list.
var empty$1 = [];
pp$3.parseNew = function() {
if (this.containsEsc) { this.raiseRecoverable(this.start, "Escape sequence in keyword new"); }
var node = this.startNode();
var meta = this.parseIdent(true);
if (this.options.ecmaVersion >= 6 && this.eat(types.dot)) {
node.meta = meta;
var containsEsc = this.containsEsc;
node.property = this.parseIdent(true);
if (node.property.name !== "target")
{ this.raiseRecoverable(node.property.start, "The only valid meta property for new is 'new.target'"); }
if (containsEsc)
{ this.raiseRecoverable(node.start, "'new.target' must not contain escaped characters"); }
if (!this.inNonArrowFunction())
{ this.raiseRecoverable(node.start, "'new.target' can only be used in functions"); }
return this.finishNode(node, "MetaProperty")
}
var startPos = this.start, startLoc = this.startLoc, isImport = this.type === types._import;
node.callee = this.parseSubscripts(this.parseExprAtom(), startPos, startLoc, true);
if (isImport && node.callee.type === "ImportExpression") {
this.raise(startPos, "Cannot use new with import()");
}
if (this.eat(types.parenL)) { node.arguments = this.parseExprList(types.parenR, this.options.ecmaVersion >= 8, false); }
else { node.arguments = empty$1; }
return this.finishNode(node, "NewExpression")
};
// Parse template expression.
pp$3.parseTemplateElement = function(ref) {
var isTagged = ref.isTagged;
var elem = this.startNode();
if (this.type === types.invalidTemplate) {
if (!isTagged) {
this.raiseRecoverable(this.start, "Bad escape sequence in untagged template literal");
}
elem.value = {
raw: this.value,
cooked: null
};
} else {
elem.value = {
raw: this.input.slice(this.start, this.end).replace(/\r\n?/g, "\n"),
cooked: this.value
};
}
this.next();
elem.tail = this.type === types.backQuote;
return this.finishNode(elem, "TemplateElement")
};
pp$3.parseTemplate = function(ref) {
if ( ref === void 0 ) ref = {};
var isTagged = ref.isTagged; if ( isTagged === void 0 ) isTagged = false;
var node = this.startNode();
this.next();
node.expressions = [];
var curElt = this.parseTemplateElement({isTagged: isTagged});
node.quasis = [curElt];
while (!curElt.tail) {
if (this.type === types.eof) { this.raise(this.pos, "Unterminated template literal"); }
this.expect(types.dollarBraceL);
node.expressions.push(this.parseExpression());
this.expect(types.braceR);
node.quasis.push(curElt = this.parseTemplateElement({isTagged: isTagged}));
}
this.next();
return this.finishNode(node, "TemplateLiteral")
};
pp$3.isAsyncProp = function(prop) {
return !prop.computed && prop.key.type === "Identifier" && prop.key.name === "async" &&
(this.type === types.name || this.type === types.num || this.type === types.string || this.type === types.bracketL || this.type.keyword || (this.options.ecmaVersion >= 9 && this.type === types.star)) &&
!lineBreak.test(this.input.slice(this.lastTokEnd, this.start))
};
// Parse an object literal or binding pattern.
pp$3.parseObj = function(isPattern, refDestructuringErrors) {
var node = this.startNode(), first = true, propHash = {};
node.properties = [];
this.next();
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.options.ecmaVersion >= 5 && this.afterTrailingComma(types.braceR)) { break }
} else { first = false; }
var prop = this.parseProperty(isPattern, refDestructuringErrors);
if (!isPattern) { this.checkPropClash(prop, propHash, refDestructuringErrors); }
node.properties.push(prop);
}
return this.finishNode(node, isPattern ? "ObjectPattern" : "ObjectExpression")
};
pp$3.parseProperty = function(isPattern, refDestructuringErrors) {
var prop = this.startNode(), isGenerator, isAsync, startPos, startLoc;
if (this.options.ecmaVersion >= 9 && this.eat(types.ellipsis)) {
if (isPattern) {
prop.argument = this.parseIdent(false);
if (this.type === types.comma) {
this.raise(this.start, "Comma is not permitted after the rest element");
}
return this.finishNode(prop, "RestElement")
}
// To disallow parenthesized identifier via `this.toAssignable()`.
if (this.type === types.parenL && refDestructuringErrors) {
if (refDestructuringErrors.parenthesizedAssign < 0) {
refDestructuringErrors.parenthesizedAssign = this.start;
}
if (refDestructuringErrors.parenthesizedBind < 0) {
refDestructuringErrors.parenthesizedBind = this.start;
}
}
// Parse argument.
prop.argument = this.parseMaybeAssign(false, refDestructuringErrors);
// To disallow trailing comma via `this.toAssignable()`.
if (this.type === types.comma && refDestructuringErrors && refDestructuringErrors.trailingComma < 0) {
refDestructuringErrors.trailingComma = this.start;
}
// Finish
return this.finishNode(prop, "SpreadElement")
}
if (this.options.ecmaVersion >= 6) {
prop.method = false;
prop.shorthand = false;
if (isPattern || refDestructuringErrors) {
startPos = this.start;
startLoc = this.startLoc;
}
if (!isPattern)
{ isGenerator = this.eat(types.star); }
}
var containsEsc = this.containsEsc;
this.parsePropertyName(prop);
if (!isPattern && !containsEsc && this.options.ecmaVersion >= 8 && !isGenerator && this.isAsyncProp(prop)) {
isAsync = true;
isGenerator = this.options.ecmaVersion >= 9 && this.eat(types.star);
this.parsePropertyName(prop, refDestructuringErrors);
} else {
isAsync = false;
}
this.parsePropertyValue(prop, isPattern, isGenerator, isAsync, startPos, startLoc, refDestructuringErrors, containsEsc);
return this.finishNode(prop, "Property")
};
pp$3.parsePropertyValue = function(prop, isPattern, isGenerator, isAsync, startPos, startLoc, refDestructuringErrors, containsEsc) {
if ((isGenerator || isAsync) && this.type === types.colon)
{ this.unexpected(); }
if (this.eat(types.colon)) {
prop.value = isPattern ? this.parseMaybeDefault(this.start, this.startLoc) : this.parseMaybeAssign(false, refDestructuringErrors);
prop.kind = "init";
} else if (this.options.ecmaVersion >= 6 && this.type === types.parenL) {
if (isPattern) { this.unexpected(); }
prop.kind = "init";
prop.method = true;
prop.value = this.parseMethod(isGenerator, isAsync);
} else if (!isPattern && !containsEsc &&
this.options.ecmaVersion >= 5 && !prop.computed && prop.key.type === "Identifier" &&
(prop.key.name === "get" || prop.key.name === "set") &&
(this.type !== types.comma && this.type !== types.braceR && this.type !== types.eq)) {
if (isGenerator || isAsync) { this.unexpected(); }
prop.kind = prop.key.name;
this.parsePropertyName(prop);
prop.value = this.parseMethod(false);
var paramCount = prop.kind === "get" ? 0 : 1;
if (prop.value.params.length !== paramCount) {
var start = prop.value.start;
if (prop.kind === "get")
{ this.raiseRecoverable(start, "getter should have no params"); }
else
{ this.raiseRecoverable(start, "setter should have exactly one param"); }
} else {
if (prop.kind === "set" && prop.value.params[0].type === "RestElement")
{ this.raiseRecoverable(prop.value.params[0].start, "Setter cannot use rest params"); }
}
} else if (this.options.ecmaVersion >= 6 && !prop.computed && prop.key.type === "Identifier") {
if (isGenerator || isAsync) { this.unexpected(); }
this.checkUnreserved(prop.key);
if (prop.key.name === "await" && !this.awaitIdentPos)
{ this.awaitIdentPos = startPos; }
prop.kind = "init";
if (isPattern) {
prop.value = this.parseMaybeDefault(startPos, startLoc, prop.key);
} else if (this.type === types.eq && refDestructuringErrors) {
if (refDestructuringErrors.shorthandAssign < 0)
{ refDestructuringErrors.shorthandAssign = this.start; }
prop.value = this.parseMaybeDefault(startPos, startLoc, prop.key);
} else {
prop.value = prop.key;
}
prop.shorthand = true;
} else { this.unexpected(); }
};
pp$3.parsePropertyName = function(prop) {
if (this.options.ecmaVersion >= 6) {
if (this.eat(types.bracketL)) {
prop.computed = true;
prop.key = this.parseMaybeAssign();
this.expect(types.bracketR);
return prop.key
} else {
prop.computed = false;
}
}
return prop.key = this.type === types.num || this.type === types.string ? this.parseExprAtom() : this.parseIdent(this.options.allowReserved !== "never")
};
// Initialize empty function node.
pp$3.initFunction = function(node) {
node.id = null;
if (this.options.ecmaVersion >= 6) { node.generator = node.expression = false; }
if (this.options.ecmaVersion >= 8) { node.async = false; }
};
// Parse object or class method.
pp$3.parseMethod = function(isGenerator, isAsync, allowDirectSuper) {
var node = this.startNode(), oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.initFunction(node);
if (this.options.ecmaVersion >= 6)
{ node.generator = isGenerator; }
if (this.options.ecmaVersion >= 8)
{ node.async = !!isAsync; }
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
this.enterScope(functionFlags(isAsync, node.generator) | SCOPE_SUPER | (allowDirectSuper ? SCOPE_DIRECT_SUPER : 0));
this.expect(types.parenL);
node.params = this.parseBindingList(types.parenR, false, this.options.ecmaVersion >= 8);
this.checkYieldAwaitInDefaultParams();
this.parseFunctionBody(node, false, true);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, "FunctionExpression")
};
// Parse arrow function expression with given parameters.
pp$3.parseArrowExpression = function(node, params, isAsync) {
var oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.enterScope(functionFlags(isAsync, false) | SCOPE_ARROW);
this.initFunction(node);
if (this.options.ecmaVersion >= 8) { node.async = !!isAsync; }
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
node.params = this.toAssignableList(params, true);
this.parseFunctionBody(node, true, false);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, "ArrowFunctionExpression")
};
// Parse function body and check parameters.
pp$3.parseFunctionBody = function(node, isArrowFunction, isMethod) {
var isExpression = isArrowFunction && this.type !== types.braceL;
var oldStrict = this.strict, useStrict = false;
if (isExpression) {
node.body = this.parseMaybeAssign();
node.expression = true;
this.checkParams(node, false);
} else {
var nonSimple = this.options.ecmaVersion >= 7 && !this.isSimpleParamList(node.params);
if (!oldStrict || nonSimple) {
useStrict = this.strictDirective(this.end);
// If this is a strict mode function, verify that argument names
// are not repeated, and it does not try to bind the words `eval`
// or `arguments`.
if (useStrict && nonSimple)
{ this.raiseRecoverable(node.start, "Illegal 'use strict' directive in function with non-simple parameter list"); }
}
// Start a new scope with regard to labels and the `inFunction`
// flag (restore them to their old value afterwards).
var oldLabels = this.labels;
this.labels = [];
if (useStrict) { this.strict = true; }
// Add the params to varDeclaredNames to ensure that an error is thrown
// if a let/const declaration in the function clashes with one of the params.
this.checkParams(node, !oldStrict && !useStrict && !isArrowFunction && !isMethod && this.isSimpleParamList(node.params));
// Ensure the function name isn't a forbidden identifier in strict mode, e.g. 'eval'
if (this.strict && node.id) { this.checkLVal(node.id, BIND_OUTSIDE); }
node.body = this.parseBlock(false, undefined, useStrict && !oldStrict);
node.expression = false;
this.adaptDirectivePrologue(node.body.body);
this.labels = oldLabels;
}
this.exitScope();
};
pp$3.isSimpleParamList = function(params) {
for (var i = 0, list = params; i < list.length; i += 1)
{
var param = list[i];
if (param.type !== "Identifier") { return false
} }
return true
};
// Checks function params for various disallowed patterns such as using "eval"
// or "arguments" and duplicate parameters.
pp$3.checkParams = function(node, allowDuplicates) {
var nameHash = {};
for (var i = 0, list = node.params; i < list.length; i += 1)
{
var param = list[i];
this.checkLVal(param, BIND_VAR, allowDuplicates ? null : nameHash);
}
};
// Parses a comma-separated list of expressions, and returns them as
// an array. `close` is the token type that ends the list, and
// `allowEmpty` can be turned on to allow subsequent commas with
// nothing in between them to be parsed as `null` (which is needed
// for array literals).
pp$3.parseExprList = function(close, allowTrailingComma, allowEmpty, refDestructuringErrors) {
var elts = [], first = true;
while (!this.eat(close)) {
if (!first) {
this.expect(types.comma);
if (allowTrailingComma && this.afterTrailingComma(close)) { break }
} else { first = false; }
var elt = (void 0);
if (allowEmpty && this.type === types.comma)
{ elt = null; }
else if (this.type === types.ellipsis) {
elt = this.parseSpread(refDestructuringErrors);
if (refDestructuringErrors && this.type === types.comma && refDestructuringErrors.trailingComma < 0)
{ refDestructuringErrors.trailingComma = this.start; }
} else {
elt = this.parseMaybeAssign(false, refDestructuringErrors);
}
elts.push(elt);
}
return elts
};
pp$3.checkUnreserved = function(ref) {
var start = ref.start;
var end = ref.end;
var name = ref.name;
if (this.inGenerator && name === "yield")
{ this.raiseRecoverable(start, "Cannot use 'yield' as identifier inside a generator"); }
if (this.inAsync && name === "await")
{ this.raiseRecoverable(start, "Cannot use 'await' as identifier inside an async function"); }
if (this.keywords.test(name))
{ this.raise(start, ("Unexpected keyword '" + name + "'")); }
if (this.options.ecmaVersion < 6 &&
this.input.slice(start, end).indexOf("\\") !== -1) { return }
var re = this.strict ? this.reservedWordsStrict : this.reservedWords;
if (re.test(name)) {
if (!this.inAsync && name === "await")
{ this.raiseRecoverable(start, "Cannot use keyword 'await' outside an async function"); }
this.raiseRecoverable(start, ("The keyword '" + name + "' is reserved"));
}
};
// Parse the next token as an identifier. If `liberal` is true (used
// when parsing properties), it will also convert keywords into
// identifiers.
pp$3.parseIdent = function(liberal, isBinding) {
var node = this.startNode();
if (this.type === types.name) {
node.name = this.value;
} else if (this.type.keyword) {
node.name = this.type.keyword;
// To fix https://github.com/acornjs/acorn/issues/575
// `class` and `function` keywords push new context into this.context.
// But there is no chance to pop the context if the keyword is consumed as an identifier such as a property name.
// If the previous token is a dot, this does not apply because the context-managing code already ignored the keyword
if ((node.name === "class" || node.name === "function") &&
(this.lastTokEnd !== this.lastTokStart + 1 || this.input.charCodeAt(this.lastTokStart) !== 46)) {
this.context.pop();
}
} else {
this.unexpected();
}
this.next(!!liberal);
this.finishNode(node, "Identifier");
if (!liberal) {
this.checkUnreserved(node);
if (node.name === "await" && !this.awaitIdentPos)
{ this.awaitIdentPos = node.start; }
}
return node
};
// Parses yield expression inside generator.
pp$3.parseYield = function(noIn) {
if (!this.yieldPos) { this.yieldPos = this.start; }
var node = this.startNode();
this.next();
if (this.type === types.semi || this.canInsertSemicolon() || (this.type !== types.star && !this.type.startsExpr)) {
node.delegate = false;
node.argument = null;
} else {
node.delegate = this.eat(types.star);
node.argument = this.parseMaybeAssign(noIn);
}
return this.finishNode(node, "YieldExpression")
};
pp$3.parseAwait = function() {
if (!this.awaitPos) { this.awaitPos = this.start; }
var node = this.startNode();
this.next();
node.argument = this.parseMaybeUnary(null, false);
return this.finishNode(node, "AwaitExpression")
};
var pp$4 = Parser.prototype;
// This function is used to raise exceptions on parse errors. It
// takes an offset integer (into the current `input`) to indicate
// the location of the error, attaches the position to the end
// of the error message, and then raises a `SyntaxError` with that
// message.
pp$4.raise = function(pos, message) {
var loc = getLineInfo(this.input, pos);
message += " (" + loc.line + ":" + loc.column + ")";
var err = new SyntaxError(message);
err.pos = pos; err.loc = loc; err.raisedAt = this.pos;
throw err
};
pp$4.raiseRecoverable = pp$4.raise;
pp$4.curPosition = function() {
if (this.options.locations) {
return new Position(this.curLine, this.pos - this.lineStart)
}
};
var pp$5 = Parser.prototype;
var Scope = function Scope(flags) {
this.flags = flags;
// A list of var-declared names in the current lexical scope
this.var = [];
// A list of lexically-declared names in the current lexical scope
this.lexical = [];
// A list of lexically-declared FunctionDeclaration names in the current lexical scope
this.functions = [];
};
// The functions in this module keep track of declared variables in the current scope in order to detect duplicate variable names.
pp$5.enterScope = function(flags) {
this.scopeStack.push(new Scope(flags));
};
pp$5.exitScope = function() {
this.scopeStack.pop();
};
// The spec says:
// > At the top level of a function, or script, function declarations are
// > treated like var declarations rather than like lexical declarations.
pp$5.treatFunctionsAsVarInScope = function(scope) {
return (scope.flags & SCOPE_FUNCTION) || !this.inModule && (scope.flags & SCOPE_TOP)
};
pp$5.declareName = function(name, bindingType, pos) {
var redeclared = false;
if (bindingType === BIND_LEXICAL) {
var scope = this.currentScope();
redeclared = scope.lexical.indexOf(name) > -1 || scope.functions.indexOf(name) > -1 || scope.var.indexOf(name) > -1;
scope.lexical.push(name);
if (this.inModule && (scope.flags & SCOPE_TOP))
{ delete this.undefinedExports[name]; }
} else if (bindingType === BIND_SIMPLE_CATCH) {
var scope$1 = this.currentScope();
scope$1.lexical.push(name);
} else if (bindingType === BIND_FUNCTION) {
var scope$2 = this.currentScope();
if (this.treatFunctionsAsVar)
{ redeclared = scope$2.lexical.indexOf(name) > -1; }
else
{ redeclared = scope$2.lexical.indexOf(name) > -1 || scope$2.var.indexOf(name) > -1; }
scope$2.functions.push(name);
} else {
for (var i = this.scopeStack.length - 1; i >= 0; --i) {
var scope$3 = this.scopeStack[i];
if (scope$3.lexical.indexOf(name) > -1 && !((scope$3.flags & SCOPE_SIMPLE_CATCH) && scope$3.lexical[0] === name) ||
!this.treatFunctionsAsVarInScope(scope$3) && scope$3.functions.indexOf(name) > -1) {
redeclared = true;
break
}
scope$3.var.push(name);
if (this.inModule && (scope$3.flags & SCOPE_TOP))
{ delete this.undefinedExports[name]; }
if (scope$3.flags & SCOPE_VAR) { break }
}
}
if (redeclared) { this.raiseRecoverable(pos, ("Identifier '" + name + "' has already been declared")); }
};
pp$5.checkLocalExport = function(id) {
// scope.functions must be empty as Module code is always strict.
if (this.scopeStack[0].lexical.indexOf(id.name) === -1 &&
this.scopeStack[0].var.indexOf(id.name) === -1) {
this.undefinedExports[id.name] = id;
}
};
pp$5.currentScope = function() {
return this.scopeStack[this.scopeStack.length - 1]
};
pp$5.currentVarScope = function() {
for (var i = this.scopeStack.length - 1;; i--) {
var scope = this.scopeStack[i];
if (scope.flags & SCOPE_VAR) { return scope }
}
};
// Could be useful for `this`, `new.target`, `super()`, `super.property`, and `super[property]`.
pp$5.currentThisScope = function() {
for (var i = this.scopeStack.length - 1;; i--) {
var scope = this.scopeStack[i];
if (scope.flags & SCOPE_VAR && !(scope.flags & SCOPE_ARROW)) { return scope }
}
};
var Node = function Node(parser, pos, loc) {
this.type = "";
this.start = pos;
this.end = 0;
if (parser.options.locations)
{ this.loc = new SourceLocation(parser, loc); }
if (parser.options.directSourceFile)
{ this.sourceFile = parser.options.directSourceFile; }
if (parser.options.ranges)
{ this.range = [pos, 0]; }
};
// Start an AST node, attaching a start offset.
var pp$6 = Parser.prototype;
pp$6.startNode = function() {
return new Node(this, this.start, this.startLoc)
};
pp$6.startNodeAt = function(pos, loc) {
return new Node(this, pos, loc)
};
// Finish an AST node, adding `type` and `end` properties.
function finishNodeAt(node, type, pos, loc) {
node.type = type;
node.end = pos;
if (this.options.locations)
{ node.loc.end = loc; }
if (this.options.ranges)
{ node.range[1] = pos; }
return node
}
pp$6.finishNode = function(node, type) {
return finishNodeAt.call(this, node, type, this.lastTokEnd, this.lastTokEndLoc)
};
// Finish node at given position
pp$6.finishNodeAt = function(node, type, pos, loc) {
return finishNodeAt.call(this, node, type, pos, loc)
};
// The algorithm used to determine whether a regexp can appear at a
var TokContext = function TokContext(token, isExpr, preserveSpace, override, generator) {
this.token = token;
this.isExpr = !!isExpr;
this.preserveSpace = !!preserveSpace;
this.override = override;
this.generator = !!generator;
};
var types$1 = {
b_stat: new TokContext("{", false),
b_expr: new TokContext("{", true),
b_tmpl: new TokContext("${", false),
p_stat: new TokContext("(", false),
p_expr: new TokContext("(", true),
q_tmpl: new TokContext("`", true, true, function (p) { return p.tryReadTemplateToken(); }),
f_stat: new TokContext("function", false),
f_expr: new TokContext("function", true),
f_expr_gen: new TokContext("function", true, false, null, true),
f_gen: new TokContext("function", false, false, null, true)
};
var pp$7 = Parser.prototype;
pp$7.initialContext = function() {
return [types$1.b_stat]
};
pp$7.braceIsBlock = function(prevType) {
var parent = this.curContext();
if (parent === types$1.f_expr || parent === types$1.f_stat)
{ return true }
if (prevType === types.colon && (parent === types$1.b_stat || parent === types$1.b_expr))
{ return !parent.isExpr }
// The check for `tt.name && exprAllowed` detects whether we are
// after a `yield` or `of` construct. See the `updateContext` for
// `tt.name`.
if (prevType === types._return || prevType === types.name && this.exprAllowed)
{ return lineBreak.test(this.input.slice(this.lastTokEnd, this.start)) }
if (prevType === types._else || prevType === types.semi || prevType === types.eof || prevType === types.parenR || prevType === types.arrow)
{ return true }
if (prevType === types.braceL)
{ return parent === types$1.b_stat }
if (prevType === types._var || prevType === types._const || prevType === types.name)
{ return false }
return !this.exprAllowed
};
pp$7.inGeneratorContext = function() {
for (var i = this.context.length - 1; i >= 1; i--) {
var context = this.context[i];
if (context.token === "function")
{ return context.generator }
}
return false
};
pp$7.updateContext = function(prevType) {
var update, type = this.type;
if (type.keyword && prevType === types.dot)
{ this.exprAllowed = false; }
else if (update = type.updateContext)
{ update.call(this, prevType); }
else
{ this.exprAllowed = type.beforeExpr; }
};
// Token-specific context update code
types.parenR.updateContext = types.braceR.updateContext = function() {
if (this.context.length === 1) {
this.exprAllowed = true;
return
}
var out = this.context.pop();
if (out === types$1.b_stat && this.curContext().token === "function") {
out = this.context.pop();
}
this.exprAllowed = !out.isExpr;
};
types.braceL.updateContext = function(prevType) {
this.context.push(this.braceIsBlock(prevType) ? types$1.b_stat : types$1.b_expr);
this.exprAllowed = true;
};
types.dollarBraceL.updateContext = function() {
this.context.push(types$1.b_tmpl);
this.exprAllowed = true;
};
types.parenL.updateContext = function(prevType) {
var statementParens = prevType === types._if || prevType === types._for || prevType === types._with || prevType === types._while;
this.context.push(statementParens ? types$1.p_stat : types$1.p_expr);
this.exprAllowed = true;
};
types.incDec.updateContext = function() {
// tokExprAllowed stays unchanged
};
types._function.updateContext = types._class.updateContext = function(prevType) {
if (prevType.beforeExpr && prevType !== types.semi && prevType !== types._else &&
!(prevType === types._return && lineBreak.test(this.input.slice(this.lastTokEnd, this.start))) &&
!((prevType === types.colon || prevType === types.braceL) && this.curContext() === types$1.b_stat))
{ this.context.push(types$1.f_expr); }
else
{ this.context.push(types$1.f_stat); }
this.exprAllowed = false;
};
types.backQuote.updateContext = function() {
if (this.curContext() === types$1.q_tmpl)
{ this.context.pop(); }
else
{ this.context.push(types$1.q_tmpl); }
this.exprAllowed = false;
};
types.star.updateContext = function(prevType) {
if (prevType === types._function) {
var index = this.context.length - 1;
if (this.context[index] === types$1.f_expr)
{ this.context[index] = types$1.f_expr_gen; }
else
{ this.context[index] = types$1.f_gen; }
}
this.exprAllowed = true;
};
types.name.updateContext = function(prevType) {
var allowed = false;
if (this.options.ecmaVersion >= 6 && prevType !== types.dot) {
if (this.value === "of" && !this.exprAllowed ||
this.value === "yield" && this.inGeneratorContext())
{ allowed = true; }
}
this.exprAllowed = allowed;
};
// This file contains Unicode properties extracted from the ECMAScript
// specification. The lists are extracted like so:
// $$('#table-binary-unicode-properties > figure > table > tbody > tr > td:nth-child(1) code').map(el => el.innerText)
// #table-binary-unicode-properties
var ecma9BinaryProperties = "ASCII ASCII_Hex_Digit AHex Alphabetic Alpha Any Assigned Bidi_Control Bidi_C Bidi_Mirrored Bidi_M Case_Ignorable CI Cased Changes_When_Casefolded CWCF Changes_When_Casemapped CWCM Changes_When_Lowercased CWL Changes_When_NFKC_Casefolded CWKCF Changes_When_Titlecased CWT Changes_When_Uppercased CWU Dash Default_Ignorable_Code_Point DI Deprecated Dep Diacritic Dia Emoji Emoji_Component Emoji_Modifier Emoji_Modifier_Base Emoji_Presentation Extender Ext Grapheme_Base Gr_Base Grapheme_Extend Gr_Ext Hex_Digit Hex IDS_Binary_Operator IDSB IDS_Trinary_Operator IDST ID_Continue IDC ID_Start IDS Ideographic Ideo Join_Control Join_C Logical_Order_Exception LOE Lowercase Lower Math Noncharacter_Code_Point NChar Pattern_Syntax Pat_Syn Pattern_White_Space Pat_WS Quotation_Mark QMark Radical Regional_Indicator RI Sentence_Terminal STerm Soft_Dotted SD Terminal_Punctuation Term Unified_Ideograph UIdeo Uppercase Upper Variation_Selector VS White_Space space XID_Continue XIDC XID_Start XIDS";
var ecma10BinaryProperties = ecma9BinaryProperties + " Extended_Pictographic";
var ecma11BinaryProperties = ecma10BinaryProperties;
var unicodeBinaryProperties = {
9: ecma9BinaryProperties,
10: ecma10BinaryProperties,
11: ecma11BinaryProperties
};
// #table-unicode-general-category-values
var unicodeGeneralCategoryValues = "Cased_Letter LC Close_Punctuation Pe Connector_Punctuation Pc Control Cc cntrl Currency_Symbol Sc Dash_Punctuation Pd Decimal_Number Nd digit Enclosing_Mark Me Final_Punctuation Pf Format Cf Initial_Punctuation Pi Letter L Letter_Number Nl Line_Separator Zl Lowercase_Letter Ll Mark M Combining_Mark Math_Symbol Sm Modifier_Letter Lm Modifier_Symbol Sk Nonspacing_Mark Mn Number N Open_Punctuation Ps Other C Other_Letter Lo Other_Number No Other_Punctuation Po Other_Symbol So Paragraph_Separator Zp Private_Use Co Punctuation P punct Separator Z Space_Separator Zs Spacing_Mark Mc Surrogate Cs Symbol S Titlecase_Letter Lt Unassigned Cn Uppercase_Letter Lu";
// #table-unicode-script-values
var ecma9ScriptValues = "Adlam Adlm Ahom Ahom Anatolian_Hieroglyphs Hluw Arabic Arab Armenian Armn Avestan Avst Balinese Bali Bamum Bamu Bassa_Vah Bass Batak Batk Bengali Beng Bhaiksuki Bhks Bopomofo Bopo Brahmi Brah Braille Brai Buginese Bugi Buhid Buhd Canadian_Aboriginal Cans Carian Cari Caucasian_Albanian Aghb Chakma Cakm Cham Cham Cherokee Cher Common Zyyy Coptic Copt Qaac Cuneiform Xsux Cypriot Cprt Cyrillic Cyrl Deseret Dsrt Devanagari Deva Duployan Dupl Egyptian_Hieroglyphs Egyp Elbasan Elba Ethiopic Ethi Georgian Geor Glagolitic Glag Gothic Goth Grantha Gran Greek Grek Gujarati Gujr Gurmukhi Guru Han Hani Hangul Hang Hanunoo Hano Hatran Hatr Hebrew Hebr Hiragana Hira Imperial_Aramaic Armi Inherited Zinh Qaai Inscriptional_Pahlavi Phli Inscriptional_Parthian Prti Javanese Java Kaithi Kthi Kannada Knda Katakana Kana Kayah_Li Kali Kharoshthi Khar Khmer Khmr Khojki Khoj Khudawadi Sind Lao Laoo Latin Latn Lepcha Lepc Limbu Limb Linear_A Lina Linear_B Linb Lisu Lisu Lycian Lyci Lydian Lydi Mahajani Mahj Malayalam Mlym Mandaic Mand Manichaean Mani Marchen Marc Masaram_Gondi Gonm Meetei_Mayek Mtei Mende_Kikakui Mend Meroitic_Cursive Merc Meroitic_Hieroglyphs Mero Miao Plrd Modi Modi Mongolian Mong Mro Mroo Multani Mult Myanmar Mymr Nabataean Nbat New_Tai_Lue Talu Newa Newa Nko Nkoo Nushu Nshu Ogham Ogam Ol_Chiki Olck Old_Hungarian Hung Old_Italic Ital Old_North_Arabian Narb Old_Permic Perm Old_Persian Xpeo Old_South_Arabian Sarb Old_Turkic Orkh Oriya Orya Osage Osge Osmanya Osma Pahawh_Hmong Hmng Palmyrene Palm Pau_Cin_Hau Pauc Phags_Pa Phag Phoenician Phnx Psalter_Pahlavi Phlp Rejang Rjng Runic Runr Samaritan Samr Saurashtra Saur Sharada Shrd Shavian Shaw Siddham Sidd SignWriting Sgnw Sinhala Sinh Sora_Sompeng Sora Soyombo Soyo Sundanese Sund Syloti_Nagri Sylo Syriac Syrc Tagalog Tglg Tagbanwa Tagb Tai_Le Tale Tai_Tham Lana Tai_Viet Tavt Takri Takr Tamil Taml Tangut Tang Telugu Telu Thaana Thaa Thai Thai Tibetan Tibt Tifinagh Tfng Tirhuta Tirh Ugaritic Ugar Vai Vaii Warang_Citi Wara Yi Yiii Zanabazar_Square Zanb";
var ecma10ScriptValues = ecma9ScriptValues + " Dogra Dogr Gunjala_Gondi Gong Hanifi_Rohingya Rohg Makasar Maka Medefaidrin Medf Old_Sogdian Sogo Sogdian Sogd";
var ecma11ScriptValues = ecma10ScriptValues + " Elymaic Elym Nandinagari Nand Nyiakeng_Puachue_Hmong Hmnp Wancho Wcho";
var unicodeScriptValues = {
9: ecma9ScriptValues,
10: ecma10ScriptValues,
11: ecma11ScriptValues
};
var data = {};
function buildUnicodeData(ecmaVersion) {
var d = data[ecmaVersion] = {
binary: wordsRegexp(unicodeBinaryProperties[ecmaVersion] + " " + unicodeGeneralCategoryValues),
nonBinary: {
General_Category: wordsRegexp(unicodeGeneralCategoryValues),
Script: wordsRegexp(unicodeScriptValues[ecmaVersion])
}
};
d.nonBinary.Script_Extensions = d.nonBinary.Script;
d.nonBinary.gc = d.nonBinary.General_Category;
d.nonBinary.sc = d.nonBinary.Script;
d.nonBinary.scx = d.nonBinary.Script_Extensions;
}
buildUnicodeData(9);
buildUnicodeData(10);
buildUnicodeData(11);
var pp$8 = Parser.prototype;
var RegExpValidationState = function RegExpValidationState(parser) {
this.parser = parser;
this.validFlags = "gim" + (parser.options.ecmaVersion >= 6 ? "uy" : "") + (parser.options.ecmaVersion >= 9 ? "s" : "");
this.unicodeProperties = data[parser.options.ecmaVersion >= 11 ? 11 : parser.options.ecmaVersion];
this.source = "";
this.flags = "";
this.start = 0;
this.switchU = false;
this.switchN = false;
this.pos = 0;
this.lastIntValue = 0;
this.lastStringValue = "";
this.lastAssertionIsQuantifiable = false;
this.numCapturingParens = 0;
this.maxBackReference = 0;
this.groupNames = [];
this.backReferenceNames = [];
};
RegExpValidationState.prototype.reset = function reset (start, pattern, flags) {
var unicode = flags.indexOf("u") !== -1;
this.start = start | 0;
this.source = pattern + "";
this.flags = flags;
this.switchU = unicode && this.parser.options.ecmaVersion >= 6;
this.switchN = unicode && this.parser.options.ecmaVersion >= 9;
};
RegExpValidationState.prototype.raise = function raise (message) {
this.parser.raiseRecoverable(this.start, ("Invalid regular expression: /" + (this.source) + "/: " + message));
};
// If u flag is given, this returns the code point at the index (it combines a surrogate pair).
// Otherwise, this returns the code unit of the index (can be a part of a surrogate pair).
RegExpValidationState.prototype.at = function at (i, forceU) {
if ( forceU === void 0 ) forceU = false;
var s = this.source;
var l = s.length;
if (i >= l) {
return -1
}
var c = s.charCodeAt(i);
if (!(forceU || this.switchU) || c <= 0xD7FF || c >= 0xE000 || i + 1 >= l) {
return c
}
var next = s.charCodeAt(i + 1);
return next >= 0xDC00 && next <= 0xDFFF ? (c << 10) + next - 0x35FDC00 : c
};
RegExpValidationState.prototype.nextIndex = function nextIndex (i, forceU) {
if ( forceU === void 0 ) forceU = false;
var s = this.source;
var l = s.length;
if (i >= l) {
return l
}
var c = s.charCodeAt(i), next;
if (!(forceU || this.switchU) || c <= 0xD7FF || c >= 0xE000 || i + 1 >= l ||
(next = s.charCodeAt(i + 1)) < 0xDC00 || next > 0xDFFF) {
return i + 1
}
return i + 2
};
RegExpValidationState.prototype.current = function current (forceU) {
if ( forceU === void 0 ) forceU = false;
return this.at(this.pos, forceU)
};
RegExpValidationState.prototype.lookahead = function lookahead (forceU) {
if ( forceU === void 0 ) forceU = false;
return this.at(this.nextIndex(this.pos, forceU), forceU)
};
RegExpValidationState.prototype.advance = function advance (forceU) {
if ( forceU === void 0 ) forceU = false;
this.pos = this.nextIndex(this.pos, forceU);
};
RegExpValidationState.prototype.eat = function eat (ch, forceU) {
if ( forceU === void 0 ) forceU = false;
if (this.current(forceU) === ch) {
this.advance(forceU);
return true
}
return false
};
function codePointToString(ch) {
if (ch <= 0xFFFF) { return String.fromCharCode(ch) }
ch -= 0x10000;
return String.fromCharCode((ch >> 10) + 0xD800, (ch & 0x03FF) + 0xDC00)
}
/**
* Validate the flags part of a given RegExpLiteral.
*
* @param {RegExpValidationState} state The state to validate RegExp.
* @returns {void}
*/
pp$8.validateRegExpFlags = function(state) {
var validFlags = state.validFlags;
var flags = state.flags;
for (var i = 0; i < flags.length; i++) {
var flag = flags.charAt(i);
if (validFlags.indexOf(flag) === -1) {
this.raise(state.start, "Invalid regular expression flag");
}
if (flags.indexOf(flag, i + 1) > -1) {
this.raise(state.start, "Duplicate regular expression flag");
}
}
};
/**
* Validate the pattern part of a given RegExpLiteral.
*
* @param {RegExpValidationState} state The state to validate RegExp.
* @returns {void}
*/
pp$8.validateRegExpPattern = function(state) {
this.regexp_pattern(state);
// The goal symbol for the parse is |Pattern[~U, ~N]|. If the result of
// parsing contains a |GroupName|, reparse with the goal symbol
// |Pattern[~U, +N]| and use this result instead. Throw a *SyntaxError*
// exception if _P_ did not conform to the grammar, if any elements of _P_
// were not matched by the parse, or if any Early Error conditions exist.
if (!state.switchN && this.options.ecmaVersion >= 9 && state.groupNames.length > 0) {
state.switchN = true;
this.regexp_pattern(state);
}
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-Pattern
pp$8.regexp_pattern = function(state) {
state.pos = 0;
state.lastIntValue = 0;
state.lastStringValue = "";
state.lastAssertionIsQuantifiable = false;
state.numCapturingParens = 0;
state.maxBackReference = 0;
state.groupNames.length = 0;
state.backReferenceNames.length = 0;
this.regexp_disjunction(state);
if (state.pos !== state.source.length) {
// Make the same messages as V8.
if (state.eat(0x29 /* ) */)) {
state.raise("Unmatched ')'");
}
if (state.eat(0x5D /* ] */) || state.eat(0x7D /* } */)) {
state.raise("Lone quantifier brackets");
}
}
if (state.maxBackReference > state.numCapturingParens) {
state.raise("Invalid escape");
}
for (var i = 0, list = state.backReferenceNames; i < list.length; i += 1) {
var name = list[i];
if (state.groupNames.indexOf(name) === -1) {
state.raise("Invalid named capture referenced");
}
}
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-Disjunction
pp$8.regexp_disjunction = function(state) {
this.regexp_alternative(state);
while (state.eat(0x7C /* | */)) {
this.regexp_alternative(state);
}
// Make the same message as V8.
if (this.regexp_eatQuantifier(state, true)) {
state.raise("Nothing to repeat");
}
if (state.eat(0x7B /* { */)) {
state.raise("Lone quantifier brackets");
}
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-Alternative
pp$8.regexp_alternative = function(state) {
while (state.pos < state.source.length && this.regexp_eatTerm(state))
{ }
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-Term
pp$8.regexp_eatTerm = function(state) {
if (this.regexp_eatAssertion(state)) {
// Handle `QuantifiableAssertion Quantifier` alternative.
// `state.lastAssertionIsQuantifiable` is true if the last eaten Assertion
// is a QuantifiableAssertion.
if (state.lastAssertionIsQuantifiable && this.regexp_eatQuantifier(state)) {
// Make the same message as V8.
if (state.switchU) {
state.raise("Invalid quantifier");
}
}
return true
}
if (state.switchU ? this.regexp_eatAtom(state) : this.regexp_eatExtendedAtom(state)) {
this.regexp_eatQuantifier(state);
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-Assertion
pp$8.regexp_eatAssertion = function(state) {
var start = state.pos;
state.lastAssertionIsQuantifiable = false;
// ^, $
if (state.eat(0x5E /* ^ */) || state.eat(0x24 /* $ */)) {
return true
}
// \b \B
if (state.eat(0x5C /* \ */)) {
if (state.eat(0x42 /* B */) || state.eat(0x62 /* b */)) {
return true
}
state.pos = start;
}
// Lookahead / Lookbehind
if (state.eat(0x28 /* ( */) && state.eat(0x3F /* ? */)) {
var lookbehind = false;
if (this.options.ecmaVersion >= 9) {
lookbehind = state.eat(0x3C /* < */);
}
if (state.eat(0x3D /* = */) || state.eat(0x21 /* ! */)) {
this.regexp_disjunction(state);
if (!state.eat(0x29 /* ) */)) {
state.raise("Unterminated group");
}
state.lastAssertionIsQuantifiable = !lookbehind;
return true
}
}
state.pos = start;
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-Quantifier
pp$8.regexp_eatQuantifier = function(state, noError) {
if ( noError === void 0 ) noError = false;
if (this.regexp_eatQuantifierPrefix(state, noError)) {
state.eat(0x3F /* ? */);
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-QuantifierPrefix
pp$8.regexp_eatQuantifierPrefix = function(state, noError) {
return (
state.eat(0x2A /* * */) ||
state.eat(0x2B /* + */) ||
state.eat(0x3F /* ? */) ||
this.regexp_eatBracedQuantifier(state, noError)
)
};
pp$8.regexp_eatBracedQuantifier = function(state, noError) {
var start = state.pos;
if (state.eat(0x7B /* { */)) {
var min = 0, max = -1;
if (this.regexp_eatDecimalDigits(state)) {
min = state.lastIntValue;
if (state.eat(0x2C /* , */) && this.regexp_eatDecimalDigits(state)) {
max = state.lastIntValue;
}
if (state.eat(0x7D /* } */)) {
// SyntaxError in https://www.ecma-international.org/ecma-262/8.0/#sec-term
if (max !== -1 && max < min && !noError) {
state.raise("numbers out of order in {} quantifier");
}
return true
}
}
if (state.switchU && !noError) {
state.raise("Incomplete quantifier");
}
state.pos = start;
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-Atom
pp$8.regexp_eatAtom = function(state) {
return (
this.regexp_eatPatternCharacters(state) ||
state.eat(0x2E /* . */) ||
this.regexp_eatReverseSolidusAtomEscape(state) ||
this.regexp_eatCharacterClass(state) ||
this.regexp_eatUncapturingGroup(state) ||
this.regexp_eatCapturingGroup(state)
)
};
pp$8.regexp_eatReverseSolidusAtomEscape = function(state) {
var start = state.pos;
if (state.eat(0x5C /* \ */)) {
if (this.regexp_eatAtomEscape(state)) {
return true
}
state.pos = start;
}
return false
};
pp$8.regexp_eatUncapturingGroup = function(state) {
var start = state.pos;
if (state.eat(0x28 /* ( */)) {
if (state.eat(0x3F /* ? */) && state.eat(0x3A /* : */)) {
this.regexp_disjunction(state);
if (state.eat(0x29 /* ) */)) {
return true
}
state.raise("Unterminated group");
}
state.pos = start;
}
return false
};
pp$8.regexp_eatCapturingGroup = function(state) {
if (state.eat(0x28 /* ( */)) {
if (this.options.ecmaVersion >= 9) {
this.regexp_groupSpecifier(state);
} else if (state.current() === 0x3F /* ? */) {
state.raise("Invalid group");
}
this.regexp_disjunction(state);
if (state.eat(0x29 /* ) */)) {
state.numCapturingParens += 1;
return true
}
state.raise("Unterminated group");
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-ExtendedAtom
pp$8.regexp_eatExtendedAtom = function(state) {
return (
state.eat(0x2E /* . */) ||
this.regexp_eatReverseSolidusAtomEscape(state) ||
this.regexp_eatCharacterClass(state) ||
this.regexp_eatUncapturingGroup(state) ||
this.regexp_eatCapturingGroup(state) ||
this.regexp_eatInvalidBracedQuantifier(state) ||
this.regexp_eatExtendedPatternCharacter(state)
)
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-InvalidBracedQuantifier
pp$8.regexp_eatInvalidBracedQuantifier = function(state) {
if (this.regexp_eatBracedQuantifier(state, true)) {
state.raise("Nothing to repeat");
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-SyntaxCharacter
pp$8.regexp_eatSyntaxCharacter = function(state) {
var ch = state.current();
if (isSyntaxCharacter(ch)) {
state.lastIntValue = ch;
state.advance();
return true
}
return false
};
function isSyntaxCharacter(ch) {
return (
ch === 0x24 /* $ */ ||
ch >= 0x28 /* ( */ && ch <= 0x2B /* + */ ||
ch === 0x2E /* . */ ||
ch === 0x3F /* ? */ ||
ch >= 0x5B /* [ */ && ch <= 0x5E /* ^ */ ||
ch >= 0x7B /* { */ && ch <= 0x7D /* } */
)
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-PatternCharacter
// But eat eager.
pp$8.regexp_eatPatternCharacters = function(state) {
var start = state.pos;
var ch = 0;
while ((ch = state.current()) !== -1 && !isSyntaxCharacter(ch)) {
state.advance();
}
return state.pos !== start
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-ExtendedPatternCharacter
pp$8.regexp_eatExtendedPatternCharacter = function(state) {
var ch = state.current();
if (
ch !== -1 &&
ch !== 0x24 /* $ */ &&
!(ch >= 0x28 /* ( */ && ch <= 0x2B /* + */) &&
ch !== 0x2E /* . */ &&
ch !== 0x3F /* ? */ &&
ch !== 0x5B /* [ */ &&
ch !== 0x5E /* ^ */ &&
ch !== 0x7C /* | */
) {
state.advance();
return true
}
return false
};
// GroupSpecifier ::
// [empty]
// `?` GroupName
pp$8.regexp_groupSpecifier = function(state) {
if (state.eat(0x3F /* ? */)) {
if (this.regexp_eatGroupName(state)) {
if (state.groupNames.indexOf(state.lastStringValue) !== -1) {
state.raise("Duplicate capture group name");
}
state.groupNames.push(state.lastStringValue);
return
}
state.raise("Invalid group");
}
};
// GroupName ::
// `<` RegExpIdentifierName `>`
// Note: this updates `state.lastStringValue` property with the eaten name.
pp$8.regexp_eatGroupName = function(state) {
state.lastStringValue = "";
if (state.eat(0x3C /* < */)) {
if (this.regexp_eatRegExpIdentifierName(state) && state.eat(0x3E /* > */)) {
return true
}
state.raise("Invalid capture group name");
}
return false
};
// RegExpIdentifierName ::
// RegExpIdentifierStart
// RegExpIdentifierName RegExpIdentifierPart
// Note: this updates `state.lastStringValue` property with the eaten name.
pp$8.regexp_eatRegExpIdentifierName = function(state) {
state.lastStringValue = "";
if (this.regexp_eatRegExpIdentifierStart(state)) {
state.lastStringValue += codePointToString(state.lastIntValue);
while (this.regexp_eatRegExpIdentifierPart(state)) {
state.lastStringValue += codePointToString(state.lastIntValue);
}
return true
}
return false
};
// RegExpIdentifierStart ::
// UnicodeIDStart
// `$`
// `_`
// `\` RegExpUnicodeEscapeSequence[+U]
pp$8.regexp_eatRegExpIdentifierStart = function(state) {
var start = state.pos;
var forceU = this.options.ecmaVersion >= 11;
var ch = state.current(forceU);
state.advance(forceU);
if (ch === 0x5C /* \ */ && this.regexp_eatRegExpUnicodeEscapeSequence(state, forceU)) {
ch = state.lastIntValue;
}
if (isRegExpIdentifierStart(ch)) {
state.lastIntValue = ch;
return true
}
state.pos = start;
return false
};
function isRegExpIdentifierStart(ch) {
return isIdentifierStart(ch, true) || ch === 0x24 /* $ */ || ch === 0x5F /* _ */
}
// RegExpIdentifierPart ::
// UnicodeIDContinue
// `$`
// `_`
// `\` RegExpUnicodeEscapeSequence[+U]
// <ZWNJ>
// <ZWJ>
pp$8.regexp_eatRegExpIdentifierPart = function(state) {
var start = state.pos;
var forceU = this.options.ecmaVersion >= 11;
var ch = state.current(forceU);
state.advance(forceU);
if (ch === 0x5C /* \ */ && this.regexp_eatRegExpUnicodeEscapeSequence(state, forceU)) {
ch = state.lastIntValue;
}
if (isRegExpIdentifierPart(ch)) {
state.lastIntValue = ch;
return true
}
state.pos = start;
return false
};
function isRegExpIdentifierPart(ch) {
return isIdentifierChar(ch, true) || ch === 0x24 /* $ */ || ch === 0x5F /* _ */ || ch === 0x200C /* <ZWNJ> */ || ch === 0x200D /* <ZWJ> */
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-AtomEscape
pp$8.regexp_eatAtomEscape = function(state) {
if (
this.regexp_eatBackReference(state) ||
this.regexp_eatCharacterClassEscape(state) ||
this.regexp_eatCharacterEscape(state) ||
(state.switchN && this.regexp_eatKGroupName(state))
) {
return true
}
if (state.switchU) {
// Make the same message as V8.
if (state.current() === 0x63 /* c */) {
state.raise("Invalid unicode escape");
}
state.raise("Invalid escape");
}
return false
};
pp$8.regexp_eatBackReference = function(state) {
var start = state.pos;
if (this.regexp_eatDecimalEscape(state)) {
var n = state.lastIntValue;
if (state.switchU) {
// For SyntaxError in https://www.ecma-international.org/ecma-262/8.0/#sec-atomescape
if (n > state.maxBackReference) {
state.maxBackReference = n;
}
return true
}
if (n <= state.numCapturingParens) {
return true
}
state.pos = start;
}
return false
};
pp$8.regexp_eatKGroupName = function(state) {
if (state.eat(0x6B /* k */)) {
if (this.regexp_eatGroupName(state)) {
state.backReferenceNames.push(state.lastStringValue);
return true
}
state.raise("Invalid named reference");
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-CharacterEscape
pp$8.regexp_eatCharacterEscape = function(state) {
return (
this.regexp_eatControlEscape(state) ||
this.regexp_eatCControlLetter(state) ||
this.regexp_eatZero(state) ||
this.regexp_eatHexEscapeSequence(state) ||
this.regexp_eatRegExpUnicodeEscapeSequence(state, false) ||
(!state.switchU && this.regexp_eatLegacyOctalEscapeSequence(state)) ||
this.regexp_eatIdentityEscape(state)
)
};
pp$8.regexp_eatCControlLetter = function(state) {
var start = state.pos;
if (state.eat(0x63 /* c */)) {
if (this.regexp_eatControlLetter(state)) {
return true
}
state.pos = start;
}
return false
};
pp$8.regexp_eatZero = function(state) {
if (state.current() === 0x30 /* 0 */ && !isDecimalDigit(state.lookahead())) {
state.lastIntValue = 0;
state.advance();
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-ControlEscape
pp$8.regexp_eatControlEscape = function(state) {
var ch = state.current();
if (ch === 0x74 /* t */) {
state.lastIntValue = 0x09; /* \t */
state.advance();
return true
}
if (ch === 0x6E /* n */) {
state.lastIntValue = 0x0A; /* \n */
state.advance();
return true
}
if (ch === 0x76 /* v */) {
state.lastIntValue = 0x0B; /* \v */
state.advance();
return true
}
if (ch === 0x66 /* f */) {
state.lastIntValue = 0x0C; /* \f */
state.advance();
return true
}
if (ch === 0x72 /* r */) {
state.lastIntValue = 0x0D; /* \r */
state.advance();
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-ControlLetter
pp$8.regexp_eatControlLetter = function(state) {
var ch = state.current();
if (isControlLetter(ch)) {
state.lastIntValue = ch % 0x20;
state.advance();
return true
}
return false
};
function isControlLetter(ch) {
return (
(ch >= 0x41 /* A */ && ch <= 0x5A /* Z */) ||
(ch >= 0x61 /* a */ && ch <= 0x7A /* z */)
)
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-RegExpUnicodeEscapeSequence
pp$8.regexp_eatRegExpUnicodeEscapeSequence = function(state, forceU) {
if ( forceU === void 0 ) forceU = false;
var start = state.pos;
var switchU = forceU || state.switchU;
if (state.eat(0x75 /* u */)) {
if (this.regexp_eatFixedHexDigits(state, 4)) {
var lead = state.lastIntValue;
if (switchU && lead >= 0xD800 && lead <= 0xDBFF) {
var leadSurrogateEnd = state.pos;
if (state.eat(0x5C /* \ */) && state.eat(0x75 /* u */) && this.regexp_eatFixedHexDigits(state, 4)) {
var trail = state.lastIntValue;
if (trail >= 0xDC00 && trail <= 0xDFFF) {
state.lastIntValue = (lead - 0xD800) * 0x400 + (trail - 0xDC00) + 0x10000;
return true
}
}
state.pos = leadSurrogateEnd;
state.lastIntValue = lead;
}
return true
}
if (
switchU &&
state.eat(0x7B /* { */) &&
this.regexp_eatHexDigits(state) &&
state.eat(0x7D /* } */) &&
isValidUnicode(state.lastIntValue)
) {
return true
}
if (switchU) {
state.raise("Invalid unicode escape");
}
state.pos = start;
}
return false
};
function isValidUnicode(ch) {
return ch >= 0 && ch <= 0x10FFFF
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-IdentityEscape
pp$8.regexp_eatIdentityEscape = function(state) {
if (state.switchU) {
if (this.regexp_eatSyntaxCharacter(state)) {
return true
}
if (state.eat(0x2F /* / */)) {
state.lastIntValue = 0x2F; /* / */
return true
}
return false
}
var ch = state.current();
if (ch !== 0x63 /* c */ && (!state.switchN || ch !== 0x6B /* k */)) {
state.lastIntValue = ch;
state.advance();
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-DecimalEscape
pp$8.regexp_eatDecimalEscape = function(state) {
state.lastIntValue = 0;
var ch = state.current();
if (ch >= 0x31 /* 1 */ && ch <= 0x39 /* 9 */) {
do {
state.lastIntValue = 10 * state.lastIntValue + (ch - 0x30 /* 0 */);
state.advance();
} while ((ch = state.current()) >= 0x30 /* 0 */ && ch <= 0x39 /* 9 */)
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-CharacterClassEscape
pp$8.regexp_eatCharacterClassEscape = function(state) {
var ch = state.current();
if (isCharacterClassEscape(ch)) {
state.lastIntValue = -1;
state.advance();
return true
}
if (
state.switchU &&
this.options.ecmaVersion >= 9 &&
(ch === 0x50 /* P */ || ch === 0x70 /* p */)
) {
state.lastIntValue = -1;
state.advance();
if (
state.eat(0x7B /* { */) &&
this.regexp_eatUnicodePropertyValueExpression(state) &&
state.eat(0x7D /* } */)
) {
return true
}
state.raise("Invalid property name");
}
return false
};
function isCharacterClassEscape(ch) {
return (
ch === 0x64 /* d */ ||
ch === 0x44 /* D */ ||
ch === 0x73 /* s */ ||
ch === 0x53 /* S */ ||
ch === 0x77 /* w */ ||
ch === 0x57 /* W */
)
}
// UnicodePropertyValueExpression ::
// UnicodePropertyName `=` UnicodePropertyValue
// LoneUnicodePropertyNameOrValue
pp$8.regexp_eatUnicodePropertyValueExpression = function(state) {
var start = state.pos;
// UnicodePropertyName `=` UnicodePropertyValue
if (this.regexp_eatUnicodePropertyName(state) && state.eat(0x3D /* = */)) {
var name = state.lastStringValue;
if (this.regexp_eatUnicodePropertyValue(state)) {
var value = state.lastStringValue;
this.regexp_validateUnicodePropertyNameAndValue(state, name, value);
return true
}
}
state.pos = start;
// LoneUnicodePropertyNameOrValue
if (this.regexp_eatLoneUnicodePropertyNameOrValue(state)) {
var nameOrValue = state.lastStringValue;
this.regexp_validateUnicodePropertyNameOrValue(state, nameOrValue);
return true
}
return false
};
pp$8.regexp_validateUnicodePropertyNameAndValue = function(state, name, value) {
if (!has(state.unicodeProperties.nonBinary, name))
{ state.raise("Invalid property name"); }
if (!state.unicodeProperties.nonBinary[name].test(value))
{ state.raise("Invalid property value"); }
};
pp$8.regexp_validateUnicodePropertyNameOrValue = function(state, nameOrValue) {
if (!state.unicodeProperties.binary.test(nameOrValue))
{ state.raise("Invalid property name"); }
};
// UnicodePropertyName ::
// UnicodePropertyNameCharacters
pp$8.regexp_eatUnicodePropertyName = function(state) {
var ch = 0;
state.lastStringValue = "";
while (isUnicodePropertyNameCharacter(ch = state.current())) {
state.lastStringValue += codePointToString(ch);
state.advance();
}
return state.lastStringValue !== ""
};
function isUnicodePropertyNameCharacter(ch) {
return isControlLetter(ch) || ch === 0x5F /* _ */
}
// UnicodePropertyValue ::
// UnicodePropertyValueCharacters
pp$8.regexp_eatUnicodePropertyValue = function(state) {
var ch = 0;
state.lastStringValue = "";
while (isUnicodePropertyValueCharacter(ch = state.current())) {
state.lastStringValue += codePointToString(ch);
state.advance();
}
return state.lastStringValue !== ""
};
function isUnicodePropertyValueCharacter(ch) {
return isUnicodePropertyNameCharacter(ch) || isDecimalDigit(ch)
}
// LoneUnicodePropertyNameOrValue ::
// UnicodePropertyValueCharacters
pp$8.regexp_eatLoneUnicodePropertyNameOrValue = function(state) {
return this.regexp_eatUnicodePropertyValue(state)
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-CharacterClass
pp$8.regexp_eatCharacterClass = function(state) {
if (state.eat(0x5B /* [ */)) {
state.eat(0x5E /* ^ */);
this.regexp_classRanges(state);
if (state.eat(0x5D /* ] */)) {
return true
}
// Unreachable since it threw "unterminated regular expression" error before.
state.raise("Unterminated character class");
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-ClassRanges
// https://www.ecma-international.org/ecma-262/8.0/#prod-NonemptyClassRanges
// https://www.ecma-international.org/ecma-262/8.0/#prod-NonemptyClassRangesNoDash
pp$8.regexp_classRanges = function(state) {
while (this.regexp_eatClassAtom(state)) {
var left = state.lastIntValue;
if (state.eat(0x2D /* - */) && this.regexp_eatClassAtom(state)) {
var right = state.lastIntValue;
if (state.switchU && (left === -1 || right === -1)) {
state.raise("Invalid character class");
}
if (left !== -1 && right !== -1 && left > right) {
state.raise("Range out of order in character class");
}
}
}
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-ClassAtom
// https://www.ecma-international.org/ecma-262/8.0/#prod-ClassAtomNoDash
pp$8.regexp_eatClassAtom = function(state) {
var start = state.pos;
if (state.eat(0x5C /* \ */)) {
if (this.regexp_eatClassEscape(state)) {
return true
}
if (state.switchU) {
// Make the same message as V8.
var ch$1 = state.current();
if (ch$1 === 0x63 /* c */ || isOctalDigit(ch$1)) {
state.raise("Invalid class escape");
}
state.raise("Invalid escape");
}
state.pos = start;
}
var ch = state.current();
if (ch !== 0x5D /* ] */) {
state.lastIntValue = ch;
state.advance();
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-ClassEscape
pp$8.regexp_eatClassEscape = function(state) {
var start = state.pos;
if (state.eat(0x62 /* b */)) {
state.lastIntValue = 0x08; /* <BS> */
return true
}
if (state.switchU && state.eat(0x2D /* - */)) {
state.lastIntValue = 0x2D; /* - */
return true
}
if (!state.switchU && state.eat(0x63 /* c */)) {
if (this.regexp_eatClassControlLetter(state)) {
return true
}
state.pos = start;
}
return (
this.regexp_eatCharacterClassEscape(state) ||
this.regexp_eatCharacterEscape(state)
)
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-ClassControlLetter
pp$8.regexp_eatClassControlLetter = function(state) {
var ch = state.current();
if (isDecimalDigit(ch) || ch === 0x5F /* _ */) {
state.lastIntValue = ch % 0x20;
state.advance();
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-HexEscapeSequence
pp$8.regexp_eatHexEscapeSequence = function(state) {
var start = state.pos;
if (state.eat(0x78 /* x */)) {
if (this.regexp_eatFixedHexDigits(state, 2)) {
return true
}
if (state.switchU) {
state.raise("Invalid escape");
}
state.pos = start;
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-DecimalDigits
pp$8.regexp_eatDecimalDigits = function(state) {
var start = state.pos;
var ch = 0;
state.lastIntValue = 0;
while (isDecimalDigit(ch = state.current())) {
state.lastIntValue = 10 * state.lastIntValue + (ch - 0x30 /* 0 */);
state.advance();
}
return state.pos !== start
};
function isDecimalDigit(ch) {
return ch >= 0x30 /* 0 */ && ch <= 0x39 /* 9 */
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-HexDigits
pp$8.regexp_eatHexDigits = function(state) {
var start = state.pos;
var ch = 0;
state.lastIntValue = 0;
while (isHexDigit(ch = state.current())) {
state.lastIntValue = 16 * state.lastIntValue + hexToInt(ch);
state.advance();
}
return state.pos !== start
};
function isHexDigit(ch) {
return (
(ch >= 0x30 /* 0 */ && ch <= 0x39 /* 9 */) ||
(ch >= 0x41 /* A */ && ch <= 0x46 /* F */) ||
(ch >= 0x61 /* a */ && ch <= 0x66 /* f */)
)
}
function hexToInt(ch) {
if (ch >= 0x41 /* A */ && ch <= 0x46 /* F */) {
return 10 + (ch - 0x41 /* A */)
}
if (ch >= 0x61 /* a */ && ch <= 0x66 /* f */) {
return 10 + (ch - 0x61 /* a */)
}
return ch - 0x30 /* 0 */
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-annexB-LegacyOctalEscapeSequence
// Allows only 0-377(octal) i.e. 0-255(decimal).
pp$8.regexp_eatLegacyOctalEscapeSequence = function(state) {
if (this.regexp_eatOctalDigit(state)) {
var n1 = state.lastIntValue;
if (this.regexp_eatOctalDigit(state)) {
var n2 = state.lastIntValue;
if (n1 <= 3 && this.regexp_eatOctalDigit(state)) {
state.lastIntValue = n1 * 64 + n2 * 8 + state.lastIntValue;
} else {
state.lastIntValue = n1 * 8 + n2;
}
} else {
state.lastIntValue = n1;
}
return true
}
return false
};
// https://www.ecma-international.org/ecma-262/8.0/#prod-OctalDigit
pp$8.regexp_eatOctalDigit = function(state) {
var ch = state.current();
if (isOctalDigit(ch)) {
state.lastIntValue = ch - 0x30; /* 0 */
state.advance();
return true
}
state.lastIntValue = 0;
return false
};
function isOctalDigit(ch) {
return ch >= 0x30 /* 0 */ && ch <= 0x37 /* 7 */
}
// https://www.ecma-international.org/ecma-262/8.0/#prod-Hex4Digits
// https://www.ecma-international.org/ecma-262/8.0/#prod-HexDigit
// And HexDigit HexDigit in https://www.ecma-international.org/ecma-262/8.0/#prod-HexEscapeSequence
pp$8.regexp_eatFixedHexDigits = function(state, length) {
var start = state.pos;
state.lastIntValue = 0;
for (var i = 0; i < length; ++i) {
var ch = state.current();
if (!isHexDigit(ch)) {
state.pos = start;
return false
}
state.lastIntValue = 16 * state.lastIntValue + hexToInt(ch);
state.advance();
}
return true
};
// Object type used to represent tokens. Note that normally, tokens
// simply exist as properties on the parser object. This is only
// used for the onToken callback and the external tokenizer.
var Token = function Token(p) {
this.type = p.type;
this.value = p.value;
this.start = p.start;
this.end = p.end;
if (p.options.locations)
{ this.loc = new SourceLocation(p, p.startLoc, p.endLoc); }
if (p.options.ranges)
{ this.range = [p.start, p.end]; }
};
// ## Tokenizer
var pp$9 = Parser.prototype;
// Move to the next token
pp$9.next = function(ignoreEscapeSequenceInKeyword) {
if (!ignoreEscapeSequenceInKeyword && this.type.keyword && this.containsEsc)
{ this.raiseRecoverable(this.start, "Escape sequence in keyword " + this.type.keyword); }
if (this.options.onToken)
{ this.options.onToken(new Token(this)); }
this.lastTokEnd = this.end;
this.lastTokStart = this.start;
this.lastTokEndLoc = this.endLoc;
this.lastTokStartLoc = this.startLoc;
this.nextToken();
};
pp$9.getToken = function() {
this.next();
return new Token(this)
};
// If we're in an ES6 environment, make parsers iterable
if (typeof Symbol !== "undefined")
{ pp$9[Symbol.iterator] = function() {
var this$1 = this;
return {
next: function () {
var token = this$1.getToken();
return {
done: token.type === types.eof,
value: token
}
}
}
}; }
// Toggle strict mode. Re-reads the next number or string to please
// pedantic tests (`"use strict"; 010;` should fail).
pp$9.curContext = function() {
return this.context[this.context.length - 1]
};
// Read a single token, updating the parser object's token-related
// properties.
pp$9.nextToken = function() {
var curContext = this.curContext();
if (!curContext || !curContext.preserveSpace) { this.skipSpace(); }
this.start = this.pos;
if (this.options.locations) { this.startLoc = this.curPosition(); }
if (this.pos >= this.input.length) { return this.finishToken(types.eof) }
if (curContext.override) { return curContext.override(this) }
else { this.readToken(this.fullCharCodeAtPos()); }
};
pp$9.readToken = function(code) {
// Identifier or keyword. '\uXXXX' sequences are allowed in
// identifiers, so '\' also dispatches to that.
if (isIdentifierStart(code, this.options.ecmaVersion >= 6) || code === 92 /* '\' */)
{ return this.readWord() }
return this.getTokenFromCode(code)
};
pp$9.fullCharCodeAtPos = function() {
var code = this.input.charCodeAt(this.pos);
if (code <= 0xd7ff || code >= 0xe000) { return code }
var next = this.input.charCodeAt(this.pos + 1);
return (code << 10) + next - 0x35fdc00
};
pp$9.skipBlockComment = function() {
var startLoc = this.options.onComment && this.curPosition();
var start = this.pos, end = this.input.indexOf("*/", this.pos += 2);
if (end === -1) { this.raise(this.pos - 2, "Unterminated comment"); }
this.pos = end + 2;
if (this.options.locations) {
lineBreakG.lastIndex = start;
var match;
while ((match = lineBreakG.exec(this.input)) && match.index < this.pos) {
++this.curLine;
this.lineStart = match.index + match[0].length;
}
}
if (this.options.onComment)
{ this.options.onComment(true, this.input.slice(start + 2, end), start, this.pos,
startLoc, this.curPosition()); }
};
pp$9.skipLineComment = function(startSkip) {
var start = this.pos;
var startLoc = this.options.onComment && this.curPosition();
var ch = this.input.charCodeAt(this.pos += startSkip);
while (this.pos < this.input.length && !isNewLine(ch)) {
ch = this.input.charCodeAt(++this.pos);
}
if (this.options.onComment)
{ this.options.onComment(false, this.input.slice(start + startSkip, this.pos), start, this.pos,
startLoc, this.curPosition()); }
};
// Called at the start of the parse and after every token. Skips
// whitespace and comments, and.
pp$9.skipSpace = function() {
loop: while (this.pos < this.input.length) {
var ch = this.input.charCodeAt(this.pos);
switch (ch) {
case 32: case 160: // ' '
++this.pos;
break
case 13:
if (this.input.charCodeAt(this.pos + 1) === 10) {
++this.pos;
}
case 10: case 8232: case 8233:
++this.pos;
if (this.options.locations) {
++this.curLine;
this.lineStart = this.pos;
}
break
case 47: // '/'
switch (this.input.charCodeAt(this.pos + 1)) {
case 42: // '*'
this.skipBlockComment();
break
case 47:
this.skipLineComment(2);
break
default:
break loop
}
break
default:
if (ch > 8 && ch < 14 || ch >= 5760 && nonASCIIwhitespace.test(String.fromCharCode(ch))) {
++this.pos;
} else {
break loop
}
}
}
};
// Called at the end of every token. Sets `end`, `val`, and
// maintains `context` and `exprAllowed`, and skips the space after
// the token, so that the next one's `start` will point at the
// right position.
pp$9.finishToken = function(type, val) {
this.end = this.pos;
if (this.options.locations) { this.endLoc = this.curPosition(); }
var prevType = this.type;
this.type = type;
this.value = val;
this.updateContext(prevType);
};
// ### Token reading
// This is the function that is called to fetch the next token. It
// is somewhat obscure, because it works in character codes rather
// than characters, and because operator parsing has been inlined
// into it.
//
// All in the name of speed.
//
pp$9.readToken_dot = function() {
var next = this.input.charCodeAt(this.pos + 1);
if (next >= 48 && next <= 57) { return this.readNumber(true) }
var next2 = this.input.charCodeAt(this.pos + 2);
if (this.options.ecmaVersion >= 6 && next === 46 && next2 === 46) { // 46 = dot '.'
this.pos += 3;
return this.finishToken(types.ellipsis)
} else {
++this.pos;
return this.finishToken(types.dot)
}
};
pp$9.readToken_slash = function() { // '/'
var next = this.input.charCodeAt(this.pos + 1);
if (this.exprAllowed) { ++this.pos; return this.readRegexp() }
if (next === 61) { return this.finishOp(types.assign, 2) }
return this.finishOp(types.slash, 1)
};
pp$9.readToken_mult_modulo_exp = function(code) { // '%*'
var next = this.input.charCodeAt(this.pos + 1);
var size = 1;
var tokentype = code === 42 ? types.star : types.modulo;
// exponentiation operator ** and **=
if (this.options.ecmaVersion >= 7 && code === 42 && next === 42) {
++size;
tokentype = types.starstar;
next = this.input.charCodeAt(this.pos + 2);
}
if (next === 61) { return this.finishOp(types.assign, size + 1) }
return this.finishOp(tokentype, size)
};
pp$9.readToken_pipe_amp = function(code) { // '|&'
var next = this.input.charCodeAt(this.pos + 1);
if (next === code) { return this.finishOp(code === 124 ? types.logicalOR : types.logicalAND, 2) }
if (next === 61) { return this.finishOp(types.assign, 2) }
return this.finishOp(code === 124 ? types.bitwiseOR : types.bitwiseAND, 1)
};
pp$9.readToken_caret = function() { // '^'
var next = this.input.charCodeAt(this.pos + 1);
if (next === 61) { return this.finishOp(types.assign, 2) }
return this.finishOp(types.bitwiseXOR, 1)
};
pp$9.readToken_plus_min = function(code) { // '+-'
var next = this.input.charCodeAt(this.pos + 1);
if (next === code) {
if (next === 45 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 62 &&
(this.lastTokEnd === 0 || lineBreak.test(this.input.slice(this.lastTokEnd, this.pos)))) {
// A `-->` line comment
this.skipLineComment(3);
this.skipSpace();
return this.nextToken()
}
return this.finishOp(types.incDec, 2)
}
if (next === 61) { return this.finishOp(types.assign, 2) }
return this.finishOp(types.plusMin, 1)
};
pp$9.readToken_lt_gt = function(code) { // '<>'
var next = this.input.charCodeAt(this.pos + 1);
var size = 1;
if (next === code) {
size = code === 62 && this.input.charCodeAt(this.pos + 2) === 62 ? 3 : 2;
if (this.input.charCodeAt(this.pos + size) === 61) { return this.finishOp(types.assign, size + 1) }
return this.finishOp(types.bitShift, size)
}
if (next === 33 && code === 60 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 45 &&
this.input.charCodeAt(this.pos + 3) === 45) {
// `<!--`, an XML-style comment that should be interpreted as a line comment
this.skipLineComment(4);
this.skipSpace();
return this.nextToken()
}
if (next === 61) { size = 2; }
return this.finishOp(types.relational, size)
};
pp$9.readToken_eq_excl = function(code) { // '=!'
var next = this.input.charCodeAt(this.pos + 1);
if (next === 61) { return this.finishOp(types.equality, this.input.charCodeAt(this.pos + 2) === 61 ? 3 : 2) }
if (code === 61 && next === 62 && this.options.ecmaVersion >= 6) { // '=>'
this.pos += 2;
return this.finishToken(types.arrow)
}
return this.finishOp(code === 61 ? types.eq : types.prefix, 1)
};
pp$9.readToken_question = function() { // '?'
if (this.options.ecmaVersion >= 11) {
var next = this.input.charCodeAt(this.pos + 1);
if (next === 46) {
var next2 = this.input.charCodeAt(this.pos + 2);
if (next2 < 48 || next2 > 57) { return this.finishOp(types.questionDot, 2) }
}
if (next === 63) { return this.finishOp(types.coalesce, 2) }
}
return this.finishOp(types.question, 1)
};
pp$9.getTokenFromCode = function(code) {
switch (code) {
// The interpretation of a dot depends on whether it is followed
// by a digit or another two dots.
case 46: // '.'
return this.readToken_dot()
// Punctuation tokens.
case 40: ++this.pos; return this.finishToken(types.parenL)
case 41: ++this.pos; return this.finishToken(types.parenR)
case 59: ++this.pos; return this.finishToken(types.semi)
case 44: ++this.pos; return this.finishToken(types.comma)
case 91: ++this.pos; return this.finishToken(types.bracketL)
case 93: ++this.pos; return this.finishToken(types.bracketR)
case 123: ++this.pos; return this.finishToken(types.braceL)
case 125: ++this.pos; return this.finishToken(types.braceR)
case 58: ++this.pos; return this.finishToken(types.colon)
case 96: // '`'
if (this.options.ecmaVersion < 6) { break }
++this.pos;
return this.finishToken(types.backQuote)
case 48: // '0'
var next = this.input.charCodeAt(this.pos + 1);
if (next === 120 || next === 88) { return this.readRadixNumber(16) } // '0x', '0X' - hex number
if (this.options.ecmaVersion >= 6) {
if (next === 111 || next === 79) { return this.readRadixNumber(8) } // '0o', '0O' - octal number
if (next === 98 || next === 66) { return this.readRadixNumber(2) } // '0b', '0B' - binary number
}
// Anything else beginning with a digit is an integer, octal
// number, or float.
case 49: case 50: case 51: case 52: case 53: case 54: case 55: case 56: case 57: // 1-9
return this.readNumber(false)
// Quotes produce strings.
case 34: case 39: // '"', "'"
return this.readString(code)
// Operators are parsed inline in tiny state machines. '=' (61) is
// often referred to. `finishOp` simply skips the amount of
// characters it is given as second argument, and returns a token
// of the type given by its first argument.
case 47: // '/'
return this.readToken_slash()
case 37: case 42: // '%*'
return this.readToken_mult_modulo_exp(code)
case 124: case 38: // '|&'
return this.readToken_pipe_amp(code)
case 94: // '^'
return this.readToken_caret()
case 43: case 45: // '+-'
return this.readToken_plus_min(code)
case 60: case 62: // '<>'
return this.readToken_lt_gt(code)
case 61: case 33: // '=!'
return this.readToken_eq_excl(code)
case 63: // '?'
return this.readToken_question()
case 126: // '~'
return this.finishOp(types.prefix, 1)
}
this.raise(this.pos, "Unexpected character '" + codePointToString$1(code) + "'");
};
pp$9.finishOp = function(type, size) {
var str = this.input.slice(this.pos, this.pos + size);
this.pos += size;
return this.finishToken(type, str)
};
pp$9.readRegexp = function() {
var escaped, inClass, start = this.pos;
for (;;) {
if (this.pos >= this.input.length) { this.raise(start, "Unterminated regular expression"); }
var ch = this.input.charAt(this.pos);
if (lineBreak.test(ch)) { this.raise(start, "Unterminated regular expression"); }
if (!escaped) {
if (ch === "[") { inClass = true; }
else if (ch === "]" && inClass) { inClass = false; }
else if (ch === "/" && !inClass) { break }
escaped = ch === "\\";
} else { escaped = false; }
++this.pos;
}
var pattern = this.input.slice(start, this.pos);
++this.pos;
var flagsStart = this.pos;
var flags = this.readWord1();
if (this.containsEsc) { this.unexpected(flagsStart); }
// Validate pattern
var state = this.regexpState || (this.regexpState = new RegExpValidationState(this));
state.reset(start, pattern, flags);
this.validateRegExpFlags(state);
this.validateRegExpPattern(state);
// Create Literal#value property value.
var value = null;
try {
value = new RegExp(pattern, flags);
} catch (e) {
// ESTree requires null if it failed to instantiate RegExp object.
// https://github.com/estree/estree/blob/a27003adf4fd7bfad44de9cef372a2eacd527b1c/es5.md#regexpliteral
}
return this.finishToken(types.regexp, {pattern: pattern, flags: flags, value: value})
};
// Read an integer in the given radix. Return null if zero digits
// were read, the integer value otherwise. When `len` is given, this
// will return `null` unless the integer has exactly `len` digits.
pp$9.readInt = function(radix, len) {
var start = this.pos, total = 0;
for (var i = 0, e = len == null ? Infinity : len; i < e; ++i) {
var code = this.input.charCodeAt(this.pos), val = (void 0);
if (code >= 97) { val = code - 97 + 10; } // a
else if (code >= 65) { val = code - 65 + 10; } // A
else if (code >= 48 && code <= 57) { val = code - 48; } // 0-9
else { val = Infinity; }
if (val >= radix) { break }
++this.pos;
total = total * radix + val;
}
if (this.pos === start || len != null && this.pos - start !== len) { return null }
return total
};
pp$9.readRadixNumber = function(radix) {
var start = this.pos;
this.pos += 2; // 0x
var val = this.readInt(radix);
if (val == null) { this.raise(this.start + 2, "Expected number in radix " + radix); }
if (this.options.ecmaVersion >= 11 && this.input.charCodeAt(this.pos) === 110) {
val = typeof BigInt !== "undefined" ? BigInt(this.input.slice(start, this.pos)) : null;
++this.pos;
} else if (isIdentifierStart(this.fullCharCodeAtPos())) { this.raise(this.pos, "Identifier directly after number"); }
return this.finishToken(types.num, val)
};
// Read an integer, octal integer, or floating-point number.
pp$9.readNumber = function(startsWithDot) {
var start = this.pos;
if (!startsWithDot && this.readInt(10) === null) { this.raise(start, "Invalid number"); }
var octal = this.pos - start >= 2 && this.input.charCodeAt(start) === 48;
if (octal && this.strict) { this.raise(start, "Invalid number"); }
var next = this.input.charCodeAt(this.pos);
if (!octal && !startsWithDot && this.options.ecmaVersion >= 11 && next === 110) {
var str$1 = this.input.slice(start, this.pos);
var val$1 = typeof BigInt !== "undefined" ? BigInt(str$1) : null;
++this.pos;
if (isIdentifierStart(this.fullCharCodeAtPos())) { this.raise(this.pos, "Identifier directly after number"); }
return this.finishToken(types.num, val$1)
}
if (octal && /[89]/.test(this.input.slice(start, this.pos))) { octal = false; }
if (next === 46 && !octal) { // '.'
++this.pos;
this.readInt(10);
next = this.input.charCodeAt(this.pos);
}
if ((next === 69 || next === 101) && !octal) { // 'eE'
next = this.input.charCodeAt(++this.pos);
if (next === 43 || next === 45) { ++this.pos; } // '+-'
if (this.readInt(10) === null) { this.raise(start, "Invalid number"); }
}
if (isIdentifierStart(this.fullCharCodeAtPos())) { this.raise(this.pos, "Identifier directly after number"); }
var str = this.input.slice(start, this.pos);
var val = octal ? parseInt(str, 8) : parseFloat(str);
return this.finishToken(types.num, val)
};
// Read a string value, interpreting backslash-escapes.
pp$9.readCodePoint = function() {
var ch = this.input.charCodeAt(this.pos), code;
if (ch === 123) { // '{'
if (this.options.ecmaVersion < 6) { this.unexpected(); }
var codePos = ++this.pos;
code = this.readHexChar(this.input.indexOf("}", this.pos) - this.pos);
++this.pos;
if (code > 0x10FFFF) { this.invalidStringToken(codePos, "Code point out of bounds"); }
} else {
code = this.readHexChar(4);
}
return code
};
function codePointToString$1(code) {
// UTF-16 Decoding
if (code <= 0xFFFF) { return String.fromCharCode(code) }
code -= 0x10000;
return String.fromCharCode((code >> 10) + 0xD800, (code & 1023) + 0xDC00)
}
pp$9.readString = function(quote) {
var out = "", chunkStart = ++this.pos;
for (;;) {
if (this.pos >= this.input.length) { this.raise(this.start, "Unterminated string constant"); }
var ch = this.input.charCodeAt(this.pos);
if (ch === quote) { break }
if (ch === 92) { // '\'
out += this.input.slice(chunkStart, this.pos);
out += this.readEscapedChar(false);
chunkStart = this.pos;
} else {
if (isNewLine(ch, this.options.ecmaVersion >= 10)) { this.raise(this.start, "Unterminated string constant"); }
++this.pos;
}
}
out += this.input.slice(chunkStart, this.pos++);
return this.finishToken(types.string, out)
};
// Reads template string tokens.
var INVALID_TEMPLATE_ESCAPE_ERROR = {};
pp$9.tryReadTemplateToken = function() {
this.inTemplateElement = true;
try {
this.readTmplToken();
} catch (err) {
if (err === INVALID_TEMPLATE_ESCAPE_ERROR) {
this.readInvalidTemplateToken();
} else {
throw err
}
}
this.inTemplateElement = false;
};
pp$9.invalidStringToken = function(position, message) {
if (this.inTemplateElement && this.options.ecmaVersion >= 9) {
throw INVALID_TEMPLATE_ESCAPE_ERROR
} else {
this.raise(position, message);
}
};
pp$9.readTmplToken = function() {
var out = "", chunkStart = this.pos;
for (;;) {
if (this.pos >= this.input.length) { this.raise(this.start, "Unterminated template"); }
var ch = this.input.charCodeAt(this.pos);
if (ch === 96 || ch === 36 && this.input.charCodeAt(this.pos + 1) === 123) { // '`', '${'
if (this.pos === this.start && (this.type === types.template || this.type === types.invalidTemplate)) {
if (ch === 36) {
this.pos += 2;
return this.finishToken(types.dollarBraceL)
} else {
++this.pos;
return this.finishToken(types.backQuote)
}
}
out += this.input.slice(chunkStart, this.pos);
return this.finishToken(types.template, out)
}
if (ch === 92) { // '\'
out += this.input.slice(chunkStart, this.pos);
out += this.readEscapedChar(true);
chunkStart = this.pos;
} else if (isNewLine(ch)) {
out += this.input.slice(chunkStart, this.pos);
++this.pos;
switch (ch) {
case 13:
if (this.input.charCodeAt(this.pos) === 10) { ++this.pos; }
case 10:
out += "\n";
break
default:
out += String.fromCharCode(ch);
break
}
if (this.options.locations) {
++this.curLine;
this.lineStart = this.pos;
}
chunkStart = this.pos;
} else {
++this.pos;
}
}
};
// Reads a template token to search for the end, without validating any escape sequences
pp$9.readInvalidTemplateToken = function() {
for (; this.pos < this.input.length; this.pos++) {
switch (this.input[this.pos]) {
case "\\":
++this.pos;
break
case "$":
if (this.input[this.pos + 1] !== "{") {
break
}
// falls through
case "`":
return this.finishToken(types.invalidTemplate, this.input.slice(this.start, this.pos))
// no default
}
}
this.raise(this.start, "Unterminated template");
};
// Used to read escaped characters
pp$9.readEscapedChar = function(inTemplate) {
var ch = this.input.charCodeAt(++this.pos);
++this.pos;
switch (ch) {
case 110: return "\n" // 'n' -> '\n'
case 114: return "\r" // 'r' -> '\r'
case 120: return String.fromCharCode(this.readHexChar(2)) // 'x'
case 117: return codePointToString$1(this.readCodePoint()) // 'u'
case 116: return "\t" // 't' -> '\t'
case 98: return "\b" // 'b' -> '\b'
case 118: return "\u000b" // 'v' -> '\u000b'
case 102: return "\f" // 'f' -> '\f'
case 13: if (this.input.charCodeAt(this.pos) === 10) { ++this.pos; } // '\r\n'
case 10: // ' \n'
if (this.options.locations) { this.lineStart = this.pos; ++this.curLine; }
return ""
case 56:
case 57:
if (inTemplate) {
var codePos = this.pos - 1;
this.invalidStringToken(
codePos,
"Invalid escape sequence in template string"
);
return null
}
default:
if (ch >= 48 && ch <= 55) {
var octalStr = this.input.substr(this.pos - 1, 3).match(/^[0-7]+/)[0];
var octal = parseInt(octalStr, 8);
if (octal > 255) {
octalStr = octalStr.slice(0, -1);
octal = parseInt(octalStr, 8);
}
this.pos += octalStr.length - 1;
ch = this.input.charCodeAt(this.pos);
if ((octalStr !== "0" || ch === 56 || ch === 57) && (this.strict || inTemplate)) {
this.invalidStringToken(
this.pos - 1 - octalStr.length,
inTemplate
? "Octal literal in template string"
: "Octal literal in strict mode"
);
}
return String.fromCharCode(octal)
}
if (isNewLine(ch)) {
// Unicode new line characters after \ get removed from output in both
// template literals and strings
return ""
}
return String.fromCharCode(ch)
}
};
// Used to read character escape sequences ('\x', '\u', '\U').
pp$9.readHexChar = function(len) {
var codePos = this.pos;
var n = this.readInt(16, len);
if (n === null) { this.invalidStringToken(codePos, "Bad character escape sequence"); }
return n
};
// Read an identifier, and return it as a string. Sets `this.containsEsc`
// to whether the word contained a '\u' escape.
//
// Incrementally adds only escaped chars, adding other chunks as-is
// as a micro-optimization.
pp$9.readWord1 = function() {
this.containsEsc = false;
var word = "", first = true, chunkStart = this.pos;
var astral = this.options.ecmaVersion >= 6;
while (this.pos < this.input.length) {
var ch = this.fullCharCodeAtPos();
if (isIdentifierChar(ch, astral)) {
this.pos += ch <= 0xffff ? 1 : 2;
} else if (ch === 92) { // "\"
this.containsEsc = true;
word += this.input.slice(chunkStart, this.pos);
var escStart = this.pos;
if (this.input.charCodeAt(++this.pos) !== 117) // "u"
{ this.invalidStringToken(this.pos, "Expecting Unicode escape sequence \\uXXXX"); }
++this.pos;
var esc = this.readCodePoint();
if (!(first ? isIdentifierStart : isIdentifierChar)(esc, astral))
{ this.invalidStringToken(escStart, "Invalid Unicode escape"); }
word += codePointToString$1(esc);
chunkStart = this.pos;
} else {
break
}
first = false;
}
return word + this.input.slice(chunkStart, this.pos)
};
// Read an identifier or keyword token. Will check for reserved
// words when necessary.
pp$9.readWord = function() {
var word = this.readWord1();
var type = types.name;
if (this.keywords.test(word)) {
type = keywords$1[word];
}
return this.finishToken(type, word)
};
// Acorn is a tiny, fast JavaScript parser written in JavaScript.
var version = "7.3.1";
Parser.acorn = {
Parser: Parser,
version: version,
defaultOptions: defaultOptions,
Position: Position,
SourceLocation: SourceLocation,
getLineInfo: getLineInfo,
Node: Node,
TokenType: TokenType,
tokTypes: types,
keywordTypes: keywords$1,
TokContext: TokContext,
tokContexts: types$1,
isIdentifierChar: isIdentifierChar,
isIdentifierStart: isIdentifierStart,
Token: Token,
isNewLine: isNewLine,
lineBreak: lineBreak,
lineBreakG: lineBreakG,
nonASCIIwhitespace: nonASCIIwhitespace
};
// The main exported interface (under `self.acorn` when in the
// browser) is a `parse` function that takes a code string and
// returns an abstract syntax tree as specified by [Mozilla parser
// API][api].
//
// [api]: https://developer.mozilla.org/en-US/docs/SpiderMonkey/Parser_API
function parse(input, options) {
return Parser.parse(input, options)
}
// This function tries to parse a single expression at a given
// offset in a string. Useful for parsing mixed-language formats
// that embed JavaScript expressions.
function parseExpressionAt(input, pos, options) {
return Parser.parseExpressionAt(input, pos, options)
}
// Acorn is organized as a tokenizer and a recursive-descent parser.
// The `tokenizer` export provides an interface to the tokenizer.
function tokenizer(input, options) {
return Parser.tokenizer(input, options)
}
exports.Node = Node;
exports.Parser = Parser;
exports.Position = Position;
exports.SourceLocation = SourceLocation;
exports.TokContext = TokContext;
exports.Token = Token;
exports.TokenType = TokenType;
exports.defaultOptions = defaultOptions;
exports.getLineInfo = getLineInfo;
exports.isIdentifierChar = isIdentifierChar;
exports.isIdentifierStart = isIdentifierStart;
exports.isNewLine = isNewLine;
exports.keywordTypes = keywords$1;
exports.lineBreak = lineBreak;
exports.lineBreakG = lineBreakG;
exports.nonASCIIwhitespace = nonASCIIwhitespace;
exports.parse = parse;
exports.parseExpressionAt = parseExpressionAt;
exports.tokContexts = types$1;
exports.tokTypes = types;
exports.tokenizer = tokenizer;
exports.version = version;
Object.defineProperty(exports, '__esModule', { value: true });
}))); | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/acorn/dist/acorn.js | acorn.js |
sshpk
=========
Parse, convert, fingerprint and use SSH keys (both public and private) in pure
node -- no `ssh-keygen` or other external dependencies.
Supports RSA, DSA, ECDSA (nistp-\*) and ED25519 key types, in PEM (PKCS#1,
PKCS#8) and OpenSSH formats.
This library has been extracted from
[`node-http-signature`](https://github.com/joyent/node-http-signature)
(work by [Mark Cavage](https://github.com/mcavage) and
[Dave Eddy](https://github.com/bahamas10)) and
[`node-ssh-fingerprint`](https://github.com/bahamas10/node-ssh-fingerprint)
(work by Dave Eddy), with additions (including ECDSA support) by
[Alex Wilson](https://github.com/arekinath).
Install
-------
```
npm install sshpk
```
Examples
--------
```js
var sshpk = require('sshpk');
var fs = require('fs');
/* Read in an OpenSSH-format public key */
var keyPub = fs.readFileSync('id_rsa.pub');
var key = sshpk.parseKey(keyPub, 'ssh');
/* Get metadata about the key */
console.log('type => %s', key.type);
console.log('size => %d bits', key.size);
console.log('comment => %s', key.comment);
/* Compute key fingerprints, in new OpenSSH (>6.7) format, and old MD5 */
console.log('fingerprint => %s', key.fingerprint().toString());
console.log('old-style fingerprint => %s', key.fingerprint('md5').toString());
```
Example output:
```
type => rsa
size => 2048 bits
comment => [email protected]
fingerprint => SHA256:PYC9kPVC6J873CSIbfp0LwYeczP/W4ffObNCuDJ1u5w
old-style fingerprint => a0:c8:ad:6c:32:9a:32:fa:59:cc:a9:8c:0a:0d:6e:bd
```
More examples: converting between formats:
```js
/* Read in a PEM public key */
var keyPem = fs.readFileSync('id_rsa.pem');
var key = sshpk.parseKey(keyPem, 'pem');
/* Convert to PEM PKCS#8 public key format */
var pemBuf = key.toBuffer('pkcs8');
/* Convert to SSH public key format (and return as a string) */
var sshKey = key.toString('ssh');
```
Signing and verifying:
```js
/* Read in an OpenSSH/PEM *private* key */
var keyPriv = fs.readFileSync('id_ecdsa');
var key = sshpk.parsePrivateKey(keyPriv, 'pem');
var data = 'some data';
/* Sign some data with the key */
var s = key.createSign('sha1');
s.update(data);
var signature = s.sign();
/* Now load the public key (could also use just key.toPublic()) */
var keyPub = fs.readFileSync('id_ecdsa.pub');
key = sshpk.parseKey(keyPub, 'ssh');
/* Make a crypto.Verifier with this key */
var v = key.createVerify('sha1');
v.update(data);
var valid = v.verify(signature);
/* => true! */
```
Matching fingerprints with keys:
```js
var fp = sshpk.parseFingerprint('SHA256:PYC9kPVC6J873CSIbfp0LwYeczP/W4ffObNCuDJ1u5w');
var keys = [sshpk.parseKey(...), sshpk.parseKey(...), ...];
keys.forEach(function (key) {
if (fp.matches(key))
console.log('found it!');
});
```
Usage
-----
## Public keys
### `parseKey(data[, format = 'auto'[, options]])`
Parses a key from a given data format and returns a new `Key` object.
Parameters
- `data` -- Either a Buffer or String, containing the key
- `format` -- String name of format to use, valid options are:
- `auto`: choose automatically from all below
- `pem`: supports both PKCS#1 and PKCS#8
- `ssh`: standard OpenSSH format,
- `pkcs1`, `pkcs8`: variants of `pem`
- `rfc4253`: raw OpenSSH wire format
- `openssh`: new post-OpenSSH 6.5 internal format, produced by
`ssh-keygen -o`
- `dnssec`: `.key` file format output by `dnssec-keygen` etc
- `putty`: the PuTTY `.ppk` file format (supports truncated variant without
all the lines from `Private-Lines:` onwards)
- `options` -- Optional Object, extra options, with keys:
- `filename` -- Optional String, name for the key being parsed
(eg. the filename that was opened). Used to generate
Error messages
- `passphrase` -- Optional String, encryption passphrase used to decrypt an
encrypted PEM file
### `Key.isKey(obj)`
Returns `true` if the given object is a valid `Key` object created by a version
of `sshpk` compatible with this one.
Parameters
- `obj` -- Object to identify
### `Key#type`
String, the type of key. Valid options are `rsa`, `dsa`, `ecdsa`.
### `Key#size`
Integer, "size" of the key in bits. For RSA/DSA this is the size of the modulus;
for ECDSA this is the bit size of the curve in use.
### `Key#comment`
Optional string, a key comment used by some formats (eg the `ssh` format).
### `Key#curve`
Only present if `this.type === 'ecdsa'`, string containing the name of the
named curve used with this key. Possible values include `nistp256`, `nistp384`
and `nistp521`.
### `Key#toBuffer([format = 'ssh'])`
Convert the key into a given data format and return the serialized key as
a Buffer.
Parameters
- `format` -- String name of format to use, for valid options see `parseKey()`
### `Key#toString([format = 'ssh])`
Same as `this.toBuffer(format).toString()`.
### `Key#fingerprint([algorithm = 'sha256'[, hashType = 'ssh']])`
Creates a new `Fingerprint` object representing this Key's fingerprint.
Parameters
- `algorithm` -- String name of hash algorithm to use, valid options are `md5`,
`sha1`, `sha256`, `sha384`, `sha512`
- `hashType` -- String name of fingerprint hash type to use, valid options are
`ssh` (the type of fingerprint used by OpenSSH, e.g. in
`ssh-keygen`), `spki` (used by HPKP, some OpenSSL applications)
### `Key#createVerify([hashAlgorithm])`
Creates a `crypto.Verifier` specialized to use this Key (and the correct public
key algorithm to match it). The returned Verifier has the same API as a regular
one, except that the `verify()` function takes only the target signature as an
argument.
Parameters
- `hashAlgorithm` -- optional String name of hash algorithm to use, any
supported by OpenSSL are valid, usually including
`sha1`, `sha256`.
`v.verify(signature[, format])` Parameters
- `signature` -- either a Signature object, or a Buffer or String
- `format` -- optional String, name of format to interpret given String with.
Not valid if `signature` is a Signature or Buffer.
### `Key#createDiffieHellman()`
### `Key#createDH()`
Creates a Diffie-Hellman key exchange object initialized with this key and all
necessary parameters. This has the same API as a `crypto.DiffieHellman`
instance, except that functions take `Key` and `PrivateKey` objects as
arguments, and return them where indicated for.
This is only valid for keys belonging to a cryptosystem that supports DHE
or a close analogue (i.e. `dsa`, `ecdsa` and `curve25519` keys). An attempt
to call this function on other keys will yield an `Error`.
## Private keys
### `parsePrivateKey(data[, format = 'auto'[, options]])`
Parses a private key from a given data format and returns a new
`PrivateKey` object.
Parameters
- `data` -- Either a Buffer or String, containing the key
- `format` -- String name of format to use, valid options are:
- `auto`: choose automatically from all below
- `pem`: supports both PKCS#1 and PKCS#8
- `ssh`, `openssh`: new post-OpenSSH 6.5 internal format, produced by
`ssh-keygen -o`
- `pkcs1`, `pkcs8`: variants of `pem`
- `rfc4253`: raw OpenSSH wire format
- `dnssec`: `.private` format output by `dnssec-keygen` etc.
- `options` -- Optional Object, extra options, with keys:
- `filename` -- Optional String, name for the key being parsed
(eg. the filename that was opened). Used to generate
Error messages
- `passphrase` -- Optional String, encryption passphrase used to decrypt an
encrypted PEM file
### `generatePrivateKey(type[, options])`
Generates a new private key of a certain key type, from random data.
Parameters
- `type` -- String, type of key to generate. Currently supported are `'ecdsa'`
and `'ed25519'`
- `options` -- optional Object, with keys:
- `curve` -- optional String, for `'ecdsa'` keys, specifies the curve to use.
If ECDSA is specified and this option is not given, defaults to
using `'nistp256'`.
### `PrivateKey.isPrivateKey(obj)`
Returns `true` if the given object is a valid `PrivateKey` object created by a
version of `sshpk` compatible with this one.
Parameters
- `obj` -- Object to identify
### `PrivateKey#type`
String, the type of key. Valid options are `rsa`, `dsa`, `ecdsa`.
### `PrivateKey#size`
Integer, "size" of the key in bits. For RSA/DSA this is the size of the modulus;
for ECDSA this is the bit size of the curve in use.
### `PrivateKey#curve`
Only present if `this.type === 'ecdsa'`, string containing the name of the
named curve used with this key. Possible values include `nistp256`, `nistp384`
and `nistp521`.
### `PrivateKey#toBuffer([format = 'pkcs1'])`
Convert the key into a given data format and return the serialized key as
a Buffer.
Parameters
- `format` -- String name of format to use, valid options are listed under
`parsePrivateKey`. Note that ED25519 keys default to `openssh`
format instead (as they have no `pkcs1` representation).
### `PrivateKey#toString([format = 'pkcs1'])`
Same as `this.toBuffer(format).toString()`.
### `PrivateKey#toPublic()`
Extract just the public part of this private key, and return it as a `Key`
object.
### `PrivateKey#fingerprint([algorithm = 'sha256'])`
Same as `this.toPublic().fingerprint()`.
### `PrivateKey#createVerify([hashAlgorithm])`
Same as `this.toPublic().createVerify()`.
### `PrivateKey#createSign([hashAlgorithm])`
Creates a `crypto.Sign` specialized to use this PrivateKey (and the correct
key algorithm to match it). The returned Signer has the same API as a regular
one, except that the `sign()` function takes no arguments, and returns a
`Signature` object.
Parameters
- `hashAlgorithm` -- optional String name of hash algorithm to use, any
supported by OpenSSL are valid, usually including
`sha1`, `sha256`.
`v.sign()` Parameters
- none
### `PrivateKey#derive(newType)`
Derives a related key of type `newType` from this key. Currently this is
only supported to change between `ed25519` and `curve25519` keys which are
stored with the same private key (but usually distinct public keys in order
to avoid degenerate keys that lead to a weak Diffie-Hellman exchange).
Parameters
- `newType` -- String, type of key to derive, either `ed25519` or `curve25519`
## Fingerprints
### `parseFingerprint(fingerprint[, options])`
Pre-parses a fingerprint, creating a `Fingerprint` object that can be used to
quickly locate a key by using the `Fingerprint#matches` function.
Parameters
- `fingerprint` -- String, the fingerprint value, in any supported format
- `options` -- Optional Object, with properties:
- `algorithms` -- Array of strings, names of hash algorithms to limit
support to. If `fingerprint` uses a hash algorithm not on
this list, throws `InvalidAlgorithmError`.
- `hashType` -- String, the type of hash the fingerprint uses, either `ssh`
or `spki` (normally auto-detected based on the format, but
can be overridden)
- `type` -- String, the entity this fingerprint identifies, either `key` or
`certificate`
### `Fingerprint.isFingerprint(obj)`
Returns `true` if the given object is a valid `Fingerprint` object created by a
version of `sshpk` compatible with this one.
Parameters
- `obj` -- Object to identify
### `Fingerprint#toString([format])`
Returns a fingerprint as a string, in the given format.
Parameters
- `format` -- Optional String, format to use, valid options are `hex` and
`base64`. If this `Fingerprint` uses the `md5` algorithm, the
default format is `hex`. Otherwise, the default is `base64`.
### `Fingerprint#matches(keyOrCertificate)`
Verifies whether or not this `Fingerprint` matches a given `Key` or
`Certificate`. This function uses double-hashing to avoid leaking timing
information. Returns a boolean.
Note that a `Key`-type Fingerprint will always return `false` if asked to match
a `Certificate` and vice versa.
Parameters
- `keyOrCertificate` -- a `Key` object or `Certificate` object, the entity to
match this fingerprint against
## Signatures
### `parseSignature(signature, algorithm, format)`
Parses a signature in a given format, creating a `Signature` object. Useful
for converting between the SSH and ASN.1 (PKCS/OpenSSL) signature formats, and
also returned as output from `PrivateKey#createSign().sign()`.
A Signature object can also be passed to a verifier produced by
`Key#createVerify()` and it will automatically be converted internally into the
correct format for verification.
Parameters
- `signature` -- a Buffer (binary) or String (base64), data of the actual
signature in the given format
- `algorithm` -- a String, name of the algorithm to be used, possible values
are `rsa`, `dsa`, `ecdsa`
- `format` -- a String, either `asn1` or `ssh`
### `Signature.isSignature(obj)`
Returns `true` if the given object is a valid `Signature` object created by a
version of `sshpk` compatible with this one.
Parameters
- `obj` -- Object to identify
### `Signature#toBuffer([format = 'asn1'])`
Converts a Signature to the given format and returns it as a Buffer.
Parameters
- `format` -- a String, either `asn1` or `ssh`
### `Signature#toString([format = 'asn1'])`
Same as `this.toBuffer(format).toString('base64')`.
## Certificates
`sshpk` includes basic support for parsing certificates in X.509 (PEM) format
and the OpenSSH certificate format. This feature is intended to be used mainly
to access basic metadata about certificates, extract public keys from them, and
also to generate simple self-signed certificates from an existing key.
Notably, there is no implementation of CA chain-of-trust verification, and only
very minimal support for key usage restrictions. Please do the security world
a favour, and DO NOT use this code for certificate verification in the
traditional X.509 CA chain style.
### `parseCertificate(data, format)`
Parameters
- `data` -- a Buffer or String
- `format` -- a String, format to use, one of `'openssh'`, `'pem'` (X.509 in a
PEM wrapper), or `'x509'` (raw DER encoded)
### `createSelfSignedCertificate(subject, privateKey[, options])`
Parameters
- `subject` -- an Identity, the subject of the certificate
- `privateKey` -- a PrivateKey, the key of the subject: will be used both to be
placed in the certificate and also to sign it (since this is
a self-signed certificate)
- `options` -- optional Object, with keys:
- `lifetime` -- optional Number, lifetime of the certificate from now in
seconds
- `validFrom`, `validUntil` -- optional Dates, beginning and end of
certificate validity period. If given
`lifetime` will be ignored
- `serial` -- optional Buffer, the serial number of the certificate
- `purposes` -- optional Array of String, X.509 key usage restrictions
### `createCertificate(subject, key, issuer, issuerKey[, options])`
Parameters
- `subject` -- an Identity, the subject of the certificate
- `key` -- a Key, the public key of the subject
- `issuer` -- an Identity, the issuer of the certificate who will sign it
- `issuerKey` -- a PrivateKey, the issuer's private key for signing
- `options` -- optional Object, with keys:
- `lifetime` -- optional Number, lifetime of the certificate from now in
seconds
- `validFrom`, `validUntil` -- optional Dates, beginning and end of
certificate validity period. If given
`lifetime` will be ignored
- `serial` -- optional Buffer, the serial number of the certificate
- `purposes` -- optional Array of String, X.509 key usage restrictions
### `Certificate#subjects`
Array of `Identity` instances describing the subject of this certificate.
### `Certificate#issuer`
The `Identity` of the Certificate's issuer (signer).
### `Certificate#subjectKey`
The public key of the subject of the certificate, as a `Key` instance.
### `Certificate#issuerKey`
The public key of the signing issuer of this certificate, as a `Key` instance.
May be `undefined` if the issuer's key is unknown (e.g. on an X509 certificate).
### `Certificate#serial`
The serial number of the certificate. As this is normally a 64-bit or wider
integer, it is returned as a Buffer.
### `Certificate#purposes`
Array of Strings indicating the X.509 key usage purposes that this certificate
is valid for. The possible strings at the moment are:
* `'signature'` -- key can be used for digital signatures
* `'identity'` -- key can be used to attest about the identity of the signer
(X.509 calls this `nonRepudiation`)
* `'codeSigning'` -- key can be used to sign executable code
* `'keyEncryption'` -- key can be used to encrypt other keys
* `'encryption'` -- key can be used to encrypt data (only applies for RSA)
* `'keyAgreement'` -- key can be used for key exchange protocols such as
Diffie-Hellman
* `'ca'` -- key can be used to sign other certificates (is a Certificate
Authority)
* `'crl'` -- key can be used to sign Certificate Revocation Lists (CRLs)
### `Certificate#getExtension(nameOrOid)`
Retrieves information about a certificate extension, if present, or returns
`undefined` if not. The string argument `nameOrOid` should be either the OID
(for X509 extensions) or the name (for OpenSSH extensions) of the extension
to retrieve.
The object returned will have the following properties:
* `format` -- String, set to either `'x509'` or `'openssh'`
* `name` or `oid` -- String, only one set based on value of `format`
* `data` -- Buffer, the raw data inside the extension
### `Certificate#getExtensions()`
Returns an Array of all present certificate extensions, in the same manner and
format as `getExtension()`.
### `Certificate#isExpired([when])`
Tests whether the Certificate is currently expired (i.e. the `validFrom` and
`validUntil` dates specify a range of time that does not include the current
time).
Parameters
- `when` -- optional Date, if specified, tests whether the Certificate was or
will be expired at the specified time instead of now
Returns a Boolean.
### `Certificate#isSignedByKey(key)`
Tests whether the Certificate was validly signed by the given (public) Key.
Parameters
- `key` -- a Key instance
Returns a Boolean.
### `Certificate#isSignedBy(certificate)`
Tests whether this Certificate was validly signed by the subject of the given
certificate. Also tests that the issuer Identity of this Certificate and the
subject Identity of the other Certificate are equivalent.
Parameters
- `certificate` -- another Certificate instance
Returns a Boolean.
### `Certificate#fingerprint([hashAlgo])`
Returns the X509-style fingerprint of the entire certificate (as a Fingerprint
instance). This matches what a web-browser or similar would display as the
certificate fingerprint and should not be confused with the fingerprint of the
subject's public key.
Parameters
- `hashAlgo` -- an optional String, any hash function name
### `Certificate#toBuffer([format])`
Serializes the Certificate to a Buffer and returns it.
Parameters
- `format` -- an optional String, output format, one of `'openssh'`, `'pem'` or
`'x509'`. Defaults to `'x509'`.
Returns a Buffer.
### `Certificate#toString([format])`
- `format` -- an optional String, output format, one of `'openssh'`, `'pem'` or
`'x509'`. Defaults to `'pem'`.
Returns a String.
## Certificate identities
### `identityForHost(hostname)`
Constructs a host-type Identity for a given hostname.
Parameters
- `hostname` -- the fully qualified DNS name of the host
Returns an Identity instance.
### `identityForUser(uid)`
Constructs a user-type Identity for a given UID.
Parameters
- `uid` -- a String, user identifier (login name)
Returns an Identity instance.
### `identityForEmail(email)`
Constructs an email-type Identity for a given email address.
Parameters
- `email` -- a String, email address
Returns an Identity instance.
### `identityFromDN(dn)`
Parses an LDAP-style DN string (e.g. `'CN=foo, C=US'`) and turns it into an
Identity instance.
Parameters
- `dn` -- a String
Returns an Identity instance.
### `identityFromArray(arr)`
Constructs an Identity from an array of DN components (see `Identity#toArray()`
for the format).
Parameters
- `arr` -- an Array of Objects, DN components with `name` and `value`
Returns an Identity instance.
Supported attributes in DNs:
| Attribute name | OID |
| -------------- | --- |
| `cn` | `2.5.4.3` |
| `o` | `2.5.4.10` |
| `ou` | `2.5.4.11` |
| `l` | `2.5.4.7` |
| `s` | `2.5.4.8` |
| `c` | `2.5.4.6` |
| `sn` | `2.5.4.4` |
| `postalCode` | `2.5.4.17` |
| `serialNumber` | `2.5.4.5` |
| `street` | `2.5.4.9` |
| `x500UniqueIdentifier` | `2.5.4.45` |
| `role` | `2.5.4.72` |
| `telephoneNumber` | `2.5.4.20` |
| `description` | `2.5.4.13` |
| `dc` | `0.9.2342.19200300.100.1.25` |
| `uid` | `0.9.2342.19200300.100.1.1` |
| `mail` | `0.9.2342.19200300.100.1.3` |
| `title` | `2.5.4.12` |
| `gn` | `2.5.4.42` |
| `initials` | `2.5.4.43` |
| `pseudonym` | `2.5.4.65` |
### `Identity#toString()`
Returns the identity as an LDAP-style DN string.
e.g. `'CN=foo, O=bar corp, C=us'`
### `Identity#type`
The type of identity. One of `'host'`, `'user'`, `'email'` or `'unknown'`
### `Identity#hostname`
### `Identity#uid`
### `Identity#email`
Set when `type` is `'host'`, `'user'`, or `'email'`, respectively. Strings.
### `Identity#cn`
The value of the first `CN=` in the DN, if any. It's probably better to use
the `#get()` method instead of this property.
### `Identity#get(name[, asArray])`
Returns the value of a named attribute in the Identity DN. If there is no
attribute of the given name, returns `undefined`. If multiple components
of the DN contain an attribute of this name, an exception is thrown unless
the `asArray` argument is given as `true` -- then they will be returned as
an Array in the same order they appear in the DN.
Parameters
- `name` -- a String
- `asArray` -- an optional Boolean
### `Identity#toArray()`
Returns the Identity as an Array of DN component objects. This looks like:
```js
[ {
"name": "cn",
"value": "Joe Bloggs"
},
{
"name": "o",
"value": "Organisation Ltd"
} ]
```
Each object has a `name` and a `value` property. The returned objects may be
safely modified.
Errors
------
### `InvalidAlgorithmError`
The specified algorithm is not valid, either because it is not supported, or
because it was not included on a list of allowed algorithms.
Thrown by `Fingerprint.parse`, `Key#fingerprint`.
Properties
- `algorithm` -- the algorithm that could not be validated
### `FingerprintFormatError`
The fingerprint string given could not be parsed as a supported fingerprint
format, or the specified fingerprint format is invalid.
Thrown by `Fingerprint.parse`, `Fingerprint#toString`.
Properties
- `fingerprint` -- if caused by a fingerprint, the string value given
- `format` -- if caused by an invalid format specification, the string value given
### `KeyParseError`
The key data given could not be parsed as a valid key.
Properties
- `keyName` -- `filename` that was given to `parseKey`
- `format` -- the `format` that was trying to parse the key (see `parseKey`)
- `innerErr` -- the inner Error thrown by the format parser
### `KeyEncryptedError`
The key is encrypted with a symmetric key (ie, it is password protected). The
parsing operation would succeed if it was given the `passphrase` option.
Properties
- `keyName` -- `filename` that was given to `parseKey`
- `format` -- the `format` that was trying to parse the key (currently can only
be `"pem"`)
### `CertificateParseError`
The certificate data given could not be parsed as a valid certificate.
Properties
- `certName` -- `filename` that was given to `parseCertificate`
- `format` -- the `format` that was trying to parse the key
(see `parseCertificate`)
- `innerErr` -- the inner Error thrown by the format parser
Friends of sshpk
----------------
* [`sshpk-agent`](https://github.com/arekinath/node-sshpk-agent) is a library
for speaking the `ssh-agent` protocol from node.js, which uses `sshpk`
| zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/README.md | README.md |
module.exports = Identity;
var assert = require('assert-plus');
var algs = require('sshpk/lib/algs');
var crypto = require('crypto');
var Fingerprint = require('sshpk/lib/fingerprint');
var Signature = require('sshpk/lib/signature');
var errs = require('sshpk/lib/errors');
var util = require('util');
var utils = require('sshpk/lib/utils');
var asn1 = require('asn1');
var Buffer = require('safer-buffer').Buffer;
/*JSSTYLED*/
var DNS_NAME_RE = /^([*]|[a-z0-9][a-z0-9\-]{0,62})(?:\.([*]|[a-z0-9][a-z0-9\-]{0,62}))*$/i;
var oids = {};
oids.cn = '2.5.4.3';
oids.o = '2.5.4.10';
oids.ou = '2.5.4.11';
oids.l = '2.5.4.7';
oids.s = '2.5.4.8';
oids.c = '2.5.4.6';
oids.sn = '2.5.4.4';
oids.postalCode = '2.5.4.17';
oids.serialNumber = '2.5.4.5';
oids.street = '2.5.4.9';
oids.x500UniqueIdentifier = '2.5.4.45';
oids.role = '2.5.4.72';
oids.telephoneNumber = '2.5.4.20';
oids.description = '2.5.4.13';
oids.dc = '0.9.2342.19200300.100.1.25';
oids.uid = '0.9.2342.19200300.100.1.1';
oids.mail = '0.9.2342.19200300.100.1.3';
oids.title = '2.5.4.12';
oids.gn = '2.5.4.42';
oids.initials = '2.5.4.43';
oids.pseudonym = '2.5.4.65';
oids.emailAddress = '1.2.840.113549.1.9.1';
var unoids = {};
Object.keys(oids).forEach(function (k) {
unoids[oids[k]] = k;
});
function Identity(opts) {
var self = this;
assert.object(opts, 'options');
assert.arrayOfObject(opts.components, 'options.components');
this.components = opts.components;
this.componentLookup = {};
this.components.forEach(function (c) {
if (c.name && !c.oid)
c.oid = oids[c.name];
if (c.oid && !c.name)
c.name = unoids[c.oid];
if (self.componentLookup[c.name] === undefined)
self.componentLookup[c.name] = [];
self.componentLookup[c.name].push(c);
});
if (this.componentLookup.cn && this.componentLookup.cn.length > 0) {
this.cn = this.componentLookup.cn[0].value;
}
assert.optionalString(opts.type, 'options.type');
if (opts.type === undefined) {
if (this.components.length === 1 &&
this.componentLookup.cn &&
this.componentLookup.cn.length === 1 &&
this.componentLookup.cn[0].value.match(DNS_NAME_RE)) {
this.type = 'host';
this.hostname = this.componentLookup.cn[0].value;
} else if (this.componentLookup.dc &&
this.components.length === this.componentLookup.dc.length) {
this.type = 'host';
this.hostname = this.componentLookup.dc.map(
function (c) {
return (c.value);
}).join('.');
} else if (this.componentLookup.uid &&
this.components.length ===
this.componentLookup.uid.length) {
this.type = 'user';
this.uid = this.componentLookup.uid[0].value;
} else if (this.componentLookup.cn &&
this.componentLookup.cn.length === 1 &&
this.componentLookup.cn[0].value.match(DNS_NAME_RE)) {
this.type = 'host';
this.hostname = this.componentLookup.cn[0].value;
} else if (this.componentLookup.uid &&
this.componentLookup.uid.length === 1) {
this.type = 'user';
this.uid = this.componentLookup.uid[0].value;
} else if (this.componentLookup.mail &&
this.componentLookup.mail.length === 1) {
this.type = 'email';
this.email = this.componentLookup.mail[0].value;
} else if (this.componentLookup.cn &&
this.componentLookup.cn.length === 1) {
this.type = 'user';
this.uid = this.componentLookup.cn[0].value;
} else {
this.type = 'unknown';
}
} else {
this.type = opts.type;
if (this.type === 'host')
this.hostname = opts.hostname;
else if (this.type === 'user')
this.uid = opts.uid;
else if (this.type === 'email')
this.email = opts.email;
else
throw (new Error('Unknown type ' + this.type));
}
}
Identity.prototype.toString = function () {
return (this.components.map(function (c) {
var n = c.name.toUpperCase();
/*JSSTYLED*/
n = n.replace(/=/g, '\\=');
var v = c.value;
/*JSSTYLED*/
v = v.replace(/,/g, '\\,');
return (n + '=' + v);
}).join(', '));
};
Identity.prototype.get = function (name, asArray) {
assert.string(name, 'name');
var arr = this.componentLookup[name];
if (arr === undefined || arr.length === 0)
return (undefined);
if (!asArray && arr.length > 1)
throw (new Error('Multiple values for attribute ' + name));
if (!asArray)
return (arr[0].value);
return (arr.map(function (c) {
return (c.value);
}));
};
Identity.prototype.toArray = function (idx) {
return (this.components.map(function (c) {
return ({
name: c.name,
value: c.value
});
}));
};
/*
* These are from X.680 -- PrintableString allowed chars are in section 37.4
* table 8. Spec for IA5Strings is "1,6 + SPACE + DEL" where 1 refers to
* ISO IR #001 (standard ASCII control characters) and 6 refers to ISO IR #006
* (the basic ASCII character set).
*/
/* JSSTYLED */
var NOT_PRINTABLE = /[^a-zA-Z0-9 '(),+.\/:=?-]/;
/* JSSTYLED */
var NOT_IA5 = /[^\x00-\x7f]/;
Identity.prototype.toAsn1 = function (der, tag) {
der.startSequence(tag);
this.components.forEach(function (c) {
der.startSequence(asn1.Ber.Constructor | asn1.Ber.Set);
der.startSequence();
der.writeOID(c.oid);
/*
* If we fit in a PrintableString, use that. Otherwise use an
* IA5String or UTF8String.
*
* If this identity was parsed from a DN, use the ASN.1 types
* from the original representation (otherwise this might not
* be a full match for the original in some validators).
*/
if (c.asn1type === asn1.Ber.Utf8String ||
c.value.match(NOT_IA5)) {
var v = Buffer.from(c.value, 'utf8');
der.writeBuffer(v, asn1.Ber.Utf8String);
} else if (c.asn1type === asn1.Ber.IA5String ||
c.value.match(NOT_PRINTABLE)) {
der.writeString(c.value, asn1.Ber.IA5String);
} else {
var type = asn1.Ber.PrintableString;
if (c.asn1type !== undefined)
type = c.asn1type;
der.writeString(c.value, type);
}
der.endSequence();
der.endSequence();
});
der.endSequence();
};
function globMatch(a, b) {
if (a === '**' || b === '**')
return (true);
var aParts = a.split('.');
var bParts = b.split('.');
if (aParts.length !== bParts.length)
return (false);
for (var i = 0; i < aParts.length; ++i) {
if (aParts[i] === '*' || bParts[i] === '*')
continue;
if (aParts[i] !== bParts[i])
return (false);
}
return (true);
}
Identity.prototype.equals = function (other) {
if (!Identity.isIdentity(other, [1, 0]))
return (false);
if (other.components.length !== this.components.length)
return (false);
for (var i = 0; i < this.components.length; ++i) {
if (this.components[i].oid !== other.components[i].oid)
return (false);
if (!globMatch(this.components[i].value,
other.components[i].value)) {
return (false);
}
}
return (true);
};
Identity.forHost = function (hostname) {
assert.string(hostname, 'hostname');
return (new Identity({
type: 'host',
hostname: hostname,
components: [ { name: 'cn', value: hostname } ]
}));
};
Identity.forUser = function (uid) {
assert.string(uid, 'uid');
return (new Identity({
type: 'user',
uid: uid,
components: [ { name: 'uid', value: uid } ]
}));
};
Identity.forEmail = function (email) {
assert.string(email, 'email');
return (new Identity({
type: 'email',
email: email,
components: [ { name: 'mail', value: email } ]
}));
};
Identity.parseDN = function (dn) {
assert.string(dn, 'dn');
var parts = [''];
var idx = 0;
var rem = dn;
while (rem.length > 0) {
var m;
/*JSSTYLED*/
if ((m = /^,/.exec(rem)) !== null) {
parts[++idx] = '';
rem = rem.slice(m[0].length);
/*JSSTYLED*/
} else if ((m = /^\\,/.exec(rem)) !== null) {
parts[idx] += ',';
rem = rem.slice(m[0].length);
/*JSSTYLED*/
} else if ((m = /^\\./.exec(rem)) !== null) {
parts[idx] += m[0];
rem = rem.slice(m[0].length);
/*JSSTYLED*/
} else if ((m = /^[^\\,]+/.exec(rem)) !== null) {
parts[idx] += m[0];
rem = rem.slice(m[0].length);
} else {
throw (new Error('Failed to parse DN'));
}
}
var cmps = parts.map(function (c) {
c = c.trim();
var eqPos = c.indexOf('=');
while (eqPos > 0 && c.charAt(eqPos - 1) === '\\')
eqPos = c.indexOf('=', eqPos + 1);
if (eqPos === -1) {
throw (new Error('Failed to parse DN'));
}
/*JSSTYLED*/
var name = c.slice(0, eqPos).toLowerCase().replace(/\\=/g, '=');
var value = c.slice(eqPos + 1);
return ({ name: name, value: value });
});
return (new Identity({ components: cmps }));
};
Identity.fromArray = function (components) {
assert.arrayOfObject(components, 'components');
components.forEach(function (cmp) {
assert.object(cmp, 'component');
assert.string(cmp.name, 'component.name');
if (!Buffer.isBuffer(cmp.value) &&
!(typeof (cmp.value) === 'string')) {
throw (new Error('Invalid component value'));
}
});
return (new Identity({ components: components }));
};
Identity.parseAsn1 = function (der, top) {
var components = [];
der.readSequence(top);
var end = der.offset + der.length;
while (der.offset < end) {
der.readSequence(asn1.Ber.Constructor | asn1.Ber.Set);
var after = der.offset + der.length;
der.readSequence();
var oid = der.readOID();
var type = der.peek();
var value;
switch (type) {
case asn1.Ber.PrintableString:
case asn1.Ber.IA5String:
case asn1.Ber.OctetString:
case asn1.Ber.T61String:
value = der.readString(type);
break;
case asn1.Ber.Utf8String:
value = der.readString(type, true);
value = value.toString('utf8');
break;
case asn1.Ber.CharacterString:
case asn1.Ber.BMPString:
value = der.readString(type, true);
value = value.toString('utf16le');
break;
default:
throw (new Error('Unknown asn1 type ' + type));
}
components.push({ oid: oid, asn1type: type, value: value });
der._offset = after;
}
der._offset = end;
return (new Identity({
components: components
}));
};
Identity.isIdentity = function (obj, ver) {
return (utils.isCompatible(obj, Identity, ver));
};
/*
* API versions for Identity:
* [1,0] -- initial ver
*/
Identity.prototype._sshpkApiVersion = [1, 0];
Identity._oldVersionDetect = function (obj) {
return ([1, 0]);
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/identity.js | identity.js |
module.exports = Fingerprint;
var assert = require('assert-plus');
var Buffer = require('safer-buffer').Buffer;
var algs = require('sshpk/lib/algs');
var crypto = require('crypto');
var errs = require('sshpk/lib/errors');
var Key = require('sshpk/lib/key');
var PrivateKey = require('sshpk/lib/private-key');
var Certificate = require('sshpk/lib/certificate');
var utils = require('sshpk/lib/utils');
var FingerprintFormatError = errs.FingerprintFormatError;
var InvalidAlgorithmError = errs.InvalidAlgorithmError;
function Fingerprint(opts) {
assert.object(opts, 'options');
assert.string(opts.type, 'options.type');
assert.buffer(opts.hash, 'options.hash');
assert.string(opts.algorithm, 'options.algorithm');
this.algorithm = opts.algorithm.toLowerCase();
if (algs.hashAlgs[this.algorithm] !== true)
throw (new InvalidAlgorithmError(this.algorithm));
this.hash = opts.hash;
this.type = opts.type;
this.hashType = opts.hashType;
}
Fingerprint.prototype.toString = function (format) {
if (format === undefined) {
if (this.algorithm === 'md5' || this.hashType === 'spki')
format = 'hex';
else
format = 'base64';
}
assert.string(format);
switch (format) {
case 'hex':
if (this.hashType === 'spki')
return (this.hash.toString('hex'));
return (addColons(this.hash.toString('hex')));
case 'base64':
if (this.hashType === 'spki')
return (this.hash.toString('base64'));
return (sshBase64Format(this.algorithm,
this.hash.toString('base64')));
default:
throw (new FingerprintFormatError(undefined, format));
}
};
Fingerprint.prototype.matches = function (other) {
assert.object(other, 'key or certificate');
if (this.type === 'key' && this.hashType !== 'ssh') {
utils.assertCompatible(other, Key, [1, 7], 'key with spki');
if (PrivateKey.isPrivateKey(other)) {
utils.assertCompatible(other, PrivateKey, [1, 6],
'privatekey with spki support');
}
} else if (this.type === 'key') {
utils.assertCompatible(other, Key, [1, 0], 'key');
} else {
utils.assertCompatible(other, Certificate, [1, 0],
'certificate');
}
var theirHash = other.hash(this.algorithm, this.hashType);
var theirHash2 = crypto.createHash(this.algorithm).
update(theirHash).digest('base64');
if (this.hash2 === undefined)
this.hash2 = crypto.createHash(this.algorithm).
update(this.hash).digest('base64');
return (this.hash2 === theirHash2);
};
/*JSSTYLED*/
var base64RE = /^[A-Za-z0-9+\/=]+$/;
/*JSSTYLED*/
var hexRE = /^[a-fA-F0-9]+$/;
Fingerprint.parse = function (fp, options) {
assert.string(fp, 'fingerprint');
var alg, hash, enAlgs;
if (Array.isArray(options)) {
enAlgs = options;
options = {};
}
assert.optionalObject(options, 'options');
if (options === undefined)
options = {};
if (options.enAlgs !== undefined)
enAlgs = options.enAlgs;
if (options.algorithms !== undefined)
enAlgs = options.algorithms;
assert.optionalArrayOfString(enAlgs, 'algorithms');
var hashType = 'ssh';
if (options.hashType !== undefined)
hashType = options.hashType;
assert.string(hashType, 'options.hashType');
var parts = fp.split(':');
if (parts.length == 2) {
alg = parts[0].toLowerCase();
if (!base64RE.test(parts[1]))
throw (new FingerprintFormatError(fp));
try {
hash = Buffer.from(parts[1], 'base64');
} catch (e) {
throw (new FingerprintFormatError(fp));
}
} else if (parts.length > 2) {
alg = 'md5';
if (parts[0].toLowerCase() === 'md5')
parts = parts.slice(1);
parts = parts.map(function (p) {
while (p.length < 2)
p = '0' + p;
if (p.length > 2)
throw (new FingerprintFormatError(fp));
return (p);
});
parts = parts.join('');
if (!hexRE.test(parts) || parts.length % 2 !== 0)
throw (new FingerprintFormatError(fp));
try {
hash = Buffer.from(parts, 'hex');
} catch (e) {
throw (new FingerprintFormatError(fp));
}
} else {
if (hexRE.test(fp)) {
hash = Buffer.from(fp, 'hex');
} else if (base64RE.test(fp)) {
hash = Buffer.from(fp, 'base64');
} else {
throw (new FingerprintFormatError(fp));
}
switch (hash.length) {
case 32:
alg = 'sha256';
break;
case 16:
alg = 'md5';
break;
case 20:
alg = 'sha1';
break;
case 64:
alg = 'sha512';
break;
default:
throw (new FingerprintFormatError(fp));
}
/* Plain hex/base64: guess it's probably SPKI unless told. */
if (options.hashType === undefined)
hashType = 'spki';
}
if (alg === undefined)
throw (new FingerprintFormatError(fp));
if (algs.hashAlgs[alg] === undefined)
throw (new InvalidAlgorithmError(alg));
if (enAlgs !== undefined) {
enAlgs = enAlgs.map(function (a) { return a.toLowerCase(); });
if (enAlgs.indexOf(alg) === -1)
throw (new InvalidAlgorithmError(alg));
}
return (new Fingerprint({
algorithm: alg,
hash: hash,
type: options.type || 'key',
hashType: hashType
}));
};
function addColons(s) {
/*JSSTYLED*/
return (s.replace(/(.{2})(?=.)/g, '$1:'));
}
function base64Strip(s) {
/*JSSTYLED*/
return (s.replace(/=*$/, ''));
}
function sshBase64Format(alg, h) {
return (alg.toUpperCase() + ':' + base64Strip(h));
}
Fingerprint.isFingerprint = function (obj, ver) {
return (utils.isCompatible(obj, Fingerprint, ver));
};
/*
* API versions for Fingerprint:
* [1,0] -- initial ver
* [1,1] -- first tagged ver
* [1,2] -- hashType and spki support
*/
Fingerprint.prototype._sshpkApiVersion = [1, 2];
Fingerprint._oldVersionDetect = function (obj) {
assert.func(obj.toString);
assert.func(obj.matches);
return ([1, 0]);
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/fingerprint.js | fingerprint.js |
var assert = require('assert-plus');
var util = require('util');
function FingerprintFormatError(fp, format) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, FingerprintFormatError);
this.name = 'FingerprintFormatError';
this.fingerprint = fp;
this.format = format;
this.message = 'Fingerprint format is not supported, or is invalid: ';
if (fp !== undefined)
this.message += ' fingerprint = ' + fp;
if (format !== undefined)
this.message += ' format = ' + format;
}
util.inherits(FingerprintFormatError, Error);
function InvalidAlgorithmError(alg) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, InvalidAlgorithmError);
this.name = 'InvalidAlgorithmError';
this.algorithm = alg;
this.message = 'Algorithm "' + alg + '" is not supported';
}
util.inherits(InvalidAlgorithmError, Error);
function KeyParseError(name, format, innerErr) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, KeyParseError);
this.name = 'KeyParseError';
this.format = format;
this.keyName = name;
this.innerErr = innerErr;
this.message = 'Failed to parse ' + name + ' as a valid ' + format +
' format key: ' + innerErr.message;
}
util.inherits(KeyParseError, Error);
function SignatureParseError(type, format, innerErr) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, SignatureParseError);
this.name = 'SignatureParseError';
this.type = type;
this.format = format;
this.innerErr = innerErr;
this.message = 'Failed to parse the given data as a ' + type +
' signature in ' + format + ' format: ' + innerErr.message;
}
util.inherits(SignatureParseError, Error);
function CertificateParseError(name, format, innerErr) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, CertificateParseError);
this.name = 'CertificateParseError';
this.format = format;
this.certName = name;
this.innerErr = innerErr;
this.message = 'Failed to parse ' + name + ' as a valid ' + format +
' format certificate: ' + innerErr.message;
}
util.inherits(CertificateParseError, Error);
function KeyEncryptedError(name, format) {
if (Error.captureStackTrace)
Error.captureStackTrace(this, KeyEncryptedError);
this.name = 'KeyEncryptedError';
this.format = format;
this.keyName = name;
this.message = 'The ' + format + ' format key ' + name + ' is ' +
'encrypted (password-protected), and no passphrase was ' +
'provided in `options`';
}
util.inherits(KeyEncryptedError, Error);
module.exports = {
FingerprintFormatError: FingerprintFormatError,
InvalidAlgorithmError: InvalidAlgorithmError,
KeyParseError: KeyParseError,
SignatureParseError: SignatureParseError,
KeyEncryptedError: KeyEncryptedError,
CertificateParseError: CertificateParseError
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/errors.js | errors.js |
var Buffer = require('safer-buffer').Buffer;
var algInfo = {
'dsa': {
parts: ['p', 'q', 'g', 'y'],
sizePart: 'p'
},
'rsa': {
parts: ['e', 'n'],
sizePart: 'n'
},
'ecdsa': {
parts: ['curve', 'Q'],
sizePart: 'Q'
},
'ed25519': {
parts: ['A'],
sizePart: 'A'
}
};
algInfo['curve25519'] = algInfo['ed25519'];
var algPrivInfo = {
'dsa': {
parts: ['p', 'q', 'g', 'y', 'x']
},
'rsa': {
parts: ['n', 'e', 'd', 'iqmp', 'p', 'q']
},
'ecdsa': {
parts: ['curve', 'Q', 'd']
},
'ed25519': {
parts: ['A', 'k']
}
};
algPrivInfo['curve25519'] = algPrivInfo['ed25519'];
var hashAlgs = {
'md5': true,
'sha1': true,
'sha256': true,
'sha384': true,
'sha512': true
};
/*
* Taken from
* http://csrc.nist.gov/groups/ST/toolkit/documents/dss/NISTReCur.pdf
*/
var curves = {
'nistp256': {
size: 256,
pkcs8oid: '1.2.840.10045.3.1.7',
p: Buffer.from(('00' +
'ffffffff 00000001 00000000 00000000' +
'00000000 ffffffff ffffffff ffffffff').
replace(/ /g, ''), 'hex'),
a: Buffer.from(('00' +
'FFFFFFFF 00000001 00000000 00000000' +
'00000000 FFFFFFFF FFFFFFFF FFFFFFFC').
replace(/ /g, ''), 'hex'),
b: Buffer.from((
'5ac635d8 aa3a93e7 b3ebbd55 769886bc' +
'651d06b0 cc53b0f6 3bce3c3e 27d2604b').
replace(/ /g, ''), 'hex'),
s: Buffer.from(('00' +
'c49d3608 86e70493 6a6678e1 139d26b7' +
'819f7e90').
replace(/ /g, ''), 'hex'),
n: Buffer.from(('00' +
'ffffffff 00000000 ffffffff ffffffff' +
'bce6faad a7179e84 f3b9cac2 fc632551').
replace(/ /g, ''), 'hex'),
G: Buffer.from(('04' +
'6b17d1f2 e12c4247 f8bce6e5 63a440f2' +
'77037d81 2deb33a0 f4a13945 d898c296' +
'4fe342e2 fe1a7f9b 8ee7eb4a 7c0f9e16' +
'2bce3357 6b315ece cbb64068 37bf51f5').
replace(/ /g, ''), 'hex')
},
'nistp384': {
size: 384,
pkcs8oid: '1.3.132.0.34',
p: Buffer.from(('00' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff ffffffff fffffffe' +
'ffffffff 00000000 00000000 ffffffff').
replace(/ /g, ''), 'hex'),
a: Buffer.from(('00' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFF' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFE' +
'FFFFFFFF 00000000 00000000 FFFFFFFC').
replace(/ /g, ''), 'hex'),
b: Buffer.from((
'b3312fa7 e23ee7e4 988e056b e3f82d19' +
'181d9c6e fe814112 0314088f 5013875a' +
'c656398d 8a2ed19d 2a85c8ed d3ec2aef').
replace(/ /g, ''), 'hex'),
s: Buffer.from(('00' +
'a335926a a319a27a 1d00896a 6773a482' +
'7acdac73').
replace(/ /g, ''), 'hex'),
n: Buffer.from(('00' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff c7634d81 f4372ddf' +
'581a0db2 48b0a77a ecec196a ccc52973').
replace(/ /g, ''), 'hex'),
G: Buffer.from(('04' +
'aa87ca22 be8b0537 8eb1c71e f320ad74' +
'6e1d3b62 8ba79b98 59f741e0 82542a38' +
'5502f25d bf55296c 3a545e38 72760ab7' +
'3617de4a 96262c6f 5d9e98bf 9292dc29' +
'f8f41dbd 289a147c e9da3113 b5f0b8c0' +
'0a60b1ce 1d7e819d 7a431d7c 90ea0e5f').
replace(/ /g, ''), 'hex')
},
'nistp521': {
size: 521,
pkcs8oid: '1.3.132.0.35',
p: Buffer.from((
'01ffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffff').replace(/ /g, ''), 'hex'),
a: Buffer.from(('01FF' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFF' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFF' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFF' +
'FFFFFFFF FFFFFFFF FFFFFFFF FFFFFFFC').
replace(/ /g, ''), 'hex'),
b: Buffer.from(('51' +
'953eb961 8e1c9a1f 929a21a0 b68540ee' +
'a2da725b 99b315f3 b8b48991 8ef109e1' +
'56193951 ec7e937b 1652c0bd 3bb1bf07' +
'3573df88 3d2c34f1 ef451fd4 6b503f00').
replace(/ /g, ''), 'hex'),
s: Buffer.from(('00' +
'd09e8800 291cb853 96cc6717 393284aa' +
'a0da64ba').replace(/ /g, ''), 'hex'),
n: Buffer.from(('01ff' +
'ffffffff ffffffff ffffffff ffffffff' +
'ffffffff ffffffff ffffffff fffffffa' +
'51868783 bf2f966b 7fcc0148 f709a5d0' +
'3bb5c9b8 899c47ae bb6fb71e 91386409').
replace(/ /g, ''), 'hex'),
G: Buffer.from(('04' +
'00c6 858e06b7 0404e9cd 9e3ecb66 2395b442' +
'9c648139 053fb521 f828af60 6b4d3dba' +
'a14b5e77 efe75928 fe1dc127 a2ffa8de' +
'3348b3c1 856a429b f97e7e31 c2e5bd66' +
'0118 39296a78 9a3bc004 5c8a5fb4 2c7d1bd9' +
'98f54449 579b4468 17afbd17 273e662c' +
'97ee7299 5ef42640 c550b901 3fad0761' +
'353c7086 a272c240 88be9476 9fd16650').
replace(/ /g, ''), 'hex')
}
};
module.exports = {
info: algInfo,
privInfo: algPrivInfo,
hashAlgs: hashAlgs,
curves: curves
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/algs.js | algs.js |
module.exports = {
bufferSplit: bufferSplit,
addRSAMissing: addRSAMissing,
calculateDSAPublic: calculateDSAPublic,
calculateED25519Public: calculateED25519Public,
calculateX25519Public: calculateX25519Public,
mpNormalize: mpNormalize,
mpDenormalize: mpDenormalize,
ecNormalize: ecNormalize,
countZeros: countZeros,
assertCompatible: assertCompatible,
isCompatible: isCompatible,
opensslKeyDeriv: opensslKeyDeriv,
opensshCipherInfo: opensshCipherInfo,
publicFromPrivateECDSA: publicFromPrivateECDSA,
zeroPadToLength: zeroPadToLength,
writeBitString: writeBitString,
readBitString: readBitString,
pbkdf2: pbkdf2
};
var assert = require('assert-plus');
var Buffer = require('safer-buffer').Buffer;
var PrivateKey = require('sshpk/lib/private-key');
var Key = require('sshpk/lib/key');
var crypto = require('crypto');
var algs = require('sshpk/lib/algs');
var asn1 = require('asn1');
var ec = require('ecc-jsbn/lib/ec');
var jsbn = require('jsbn').BigInteger;
var nacl = require('tweetnacl');
var MAX_CLASS_DEPTH = 3;
function isCompatible(obj, klass, needVer) {
if (obj === null || typeof (obj) !== 'object')
return (false);
if (needVer === undefined)
needVer = klass.prototype._sshpkApiVersion;
if (obj instanceof klass &&
klass.prototype._sshpkApiVersion[0] == needVer[0])
return (true);
var proto = Object.getPrototypeOf(obj);
var depth = 0;
while (proto.constructor.name !== klass.name) {
proto = Object.getPrototypeOf(proto);
if (!proto || ++depth > MAX_CLASS_DEPTH)
return (false);
}
if (proto.constructor.name !== klass.name)
return (false);
var ver = proto._sshpkApiVersion;
if (ver === undefined)
ver = klass._oldVersionDetect(obj);
if (ver[0] != needVer[0] || ver[1] < needVer[1])
return (false);
return (true);
}
function assertCompatible(obj, klass, needVer, name) {
if (name === undefined)
name = 'object';
assert.ok(obj, name + ' must not be null');
assert.object(obj, name + ' must be an object');
if (needVer === undefined)
needVer = klass.prototype._sshpkApiVersion;
if (obj instanceof klass &&
klass.prototype._sshpkApiVersion[0] == needVer[0])
return;
var proto = Object.getPrototypeOf(obj);
var depth = 0;
while (proto.constructor.name !== klass.name) {
proto = Object.getPrototypeOf(proto);
assert.ok(proto && ++depth <= MAX_CLASS_DEPTH,
name + ' must be a ' + klass.name + ' instance');
}
assert.strictEqual(proto.constructor.name, klass.name,
name + ' must be a ' + klass.name + ' instance');
var ver = proto._sshpkApiVersion;
if (ver === undefined)
ver = klass._oldVersionDetect(obj);
assert.ok(ver[0] == needVer[0] && ver[1] >= needVer[1],
name + ' must be compatible with ' + klass.name + ' klass ' +
'version ' + needVer[0] + '.' + needVer[1]);
}
var CIPHER_LEN = {
'des-ede3-cbc': { key: 24, iv: 8 },
'aes-128-cbc': { key: 16, iv: 16 },
'aes-256-cbc': { key: 32, iv: 16 }
};
var PKCS5_SALT_LEN = 8;
function opensslKeyDeriv(cipher, salt, passphrase, count) {
assert.buffer(salt, 'salt');
assert.buffer(passphrase, 'passphrase');
assert.number(count, 'iteration count');
var clen = CIPHER_LEN[cipher];
assert.object(clen, 'supported cipher');
salt = salt.slice(0, PKCS5_SALT_LEN);
var D, D_prev, bufs;
var material = Buffer.alloc(0);
while (material.length < clen.key + clen.iv) {
bufs = [];
if (D_prev)
bufs.push(D_prev);
bufs.push(passphrase);
bufs.push(salt);
D = Buffer.concat(bufs);
for (var j = 0; j < count; ++j)
D = crypto.createHash('md5').update(D).digest();
material = Buffer.concat([material, D]);
D_prev = D;
}
return ({
key: material.slice(0, clen.key),
iv: material.slice(clen.key, clen.key + clen.iv)
});
}
/* See: RFC2898 */
function pbkdf2(hashAlg, salt, iterations, size, passphrase) {
var hkey = Buffer.alloc(salt.length + 4);
salt.copy(hkey);
var gen = 0, ts = [];
var i = 1;
while (gen < size) {
var t = T(i++);
gen += t.length;
ts.push(t);
}
return (Buffer.concat(ts).slice(0, size));
function T(I) {
hkey.writeUInt32BE(I, hkey.length - 4);
var hmac = crypto.createHmac(hashAlg, passphrase);
hmac.update(hkey);
var Ti = hmac.digest();
var Uc = Ti;
var c = 1;
while (c++ < iterations) {
hmac = crypto.createHmac(hashAlg, passphrase);
hmac.update(Uc);
Uc = hmac.digest();
for (var x = 0; x < Ti.length; ++x)
Ti[x] ^= Uc[x];
}
return (Ti);
}
}
/* Count leading zero bits on a buffer */
function countZeros(buf) {
var o = 0, obit = 8;
while (o < buf.length) {
var mask = (1 << obit);
if ((buf[o] & mask) === mask)
break;
obit--;
if (obit < 0) {
o++;
obit = 8;
}
}
return (o*8 + (8 - obit) - 1);
}
function bufferSplit(buf, chr) {
assert.buffer(buf);
assert.string(chr);
var parts = [];
var lastPart = 0;
var matches = 0;
for (var i = 0; i < buf.length; ++i) {
if (buf[i] === chr.charCodeAt(matches))
++matches;
else if (buf[i] === chr.charCodeAt(0))
matches = 1;
else
matches = 0;
if (matches >= chr.length) {
var newPart = i + 1;
parts.push(buf.slice(lastPart, newPart - matches));
lastPart = newPart;
matches = 0;
}
}
if (lastPart <= buf.length)
parts.push(buf.slice(lastPart, buf.length));
return (parts);
}
function ecNormalize(buf, addZero) {
assert.buffer(buf);
if (buf[0] === 0x00 && buf[1] === 0x04) {
if (addZero)
return (buf);
return (buf.slice(1));
} else if (buf[0] === 0x04) {
if (!addZero)
return (buf);
} else {
while (buf[0] === 0x00)
buf = buf.slice(1);
if (buf[0] === 0x02 || buf[0] === 0x03)
throw (new Error('Compressed elliptic curve points ' +
'are not supported'));
if (buf[0] !== 0x04)
throw (new Error('Not a valid elliptic curve point'));
if (!addZero)
return (buf);
}
var b = Buffer.alloc(buf.length + 1);
b[0] = 0x0;
buf.copy(b, 1);
return (b);
}
function readBitString(der, tag) {
if (tag === undefined)
tag = asn1.Ber.BitString;
var buf = der.readString(tag, true);
assert.strictEqual(buf[0], 0x00, 'bit strings with unused bits are ' +
'not supported (0x' + buf[0].toString(16) + ')');
return (buf.slice(1));
}
function writeBitString(der, buf, tag) {
if (tag === undefined)
tag = asn1.Ber.BitString;
var b = Buffer.alloc(buf.length + 1);
b[0] = 0x00;
buf.copy(b, 1);
der.writeBuffer(b, tag);
}
function mpNormalize(buf) {
assert.buffer(buf);
while (buf.length > 1 && buf[0] === 0x00 && (buf[1] & 0x80) === 0x00)
buf = buf.slice(1);
if ((buf[0] & 0x80) === 0x80) {
var b = Buffer.alloc(buf.length + 1);
b[0] = 0x00;
buf.copy(b, 1);
buf = b;
}
return (buf);
}
function mpDenormalize(buf) {
assert.buffer(buf);
while (buf.length > 1 && buf[0] === 0x00)
buf = buf.slice(1);
return (buf);
}
function zeroPadToLength(buf, len) {
assert.buffer(buf);
assert.number(len);
while (buf.length > len) {
assert.equal(buf[0], 0x00);
buf = buf.slice(1);
}
while (buf.length < len) {
var b = Buffer.alloc(buf.length + 1);
b[0] = 0x00;
buf.copy(b, 1);
buf = b;
}
return (buf);
}
function bigintToMpBuf(bigint) {
var buf = Buffer.from(bigint.toByteArray());
buf = mpNormalize(buf);
return (buf);
}
function calculateDSAPublic(g, p, x) {
assert.buffer(g);
assert.buffer(p);
assert.buffer(x);
g = new jsbn(g);
p = new jsbn(p);
x = new jsbn(x);
var y = g.modPow(x, p);
var ybuf = bigintToMpBuf(y);
return (ybuf);
}
function calculateED25519Public(k) {
assert.buffer(k);
var kp = nacl.sign.keyPair.fromSeed(new Uint8Array(k));
return (Buffer.from(kp.publicKey));
}
function calculateX25519Public(k) {
assert.buffer(k);
var kp = nacl.box.keyPair.fromSeed(new Uint8Array(k));
return (Buffer.from(kp.publicKey));
}
function addRSAMissing(key) {
assert.object(key);
assertCompatible(key, PrivateKey, [1, 1]);
var d = new jsbn(key.part.d.data);
var buf;
if (!key.part.dmodp) {
var p = new jsbn(key.part.p.data);
var dmodp = d.mod(p.subtract(1));
buf = bigintToMpBuf(dmodp);
key.part.dmodp = {name: 'dmodp', data: buf};
key.parts.push(key.part.dmodp);
}
if (!key.part.dmodq) {
var q = new jsbn(key.part.q.data);
var dmodq = d.mod(q.subtract(1));
buf = bigintToMpBuf(dmodq);
key.part.dmodq = {name: 'dmodq', data: buf};
key.parts.push(key.part.dmodq);
}
}
function publicFromPrivateECDSA(curveName, priv) {
assert.string(curveName, 'curveName');
assert.buffer(priv);
var params = algs.curves[curveName];
var p = new jsbn(params.p);
var a = new jsbn(params.a);
var b = new jsbn(params.b);
var curve = new ec.ECCurveFp(p, a, b);
var G = curve.decodePointHex(params.G.toString('hex'));
var d = new jsbn(mpNormalize(priv));
var pub = G.multiply(d);
pub = Buffer.from(curve.encodePointHex(pub), 'hex');
var parts = [];
parts.push({name: 'curve', data: Buffer.from(curveName)});
parts.push({name: 'Q', data: pub});
var key = new Key({type: 'ecdsa', curve: curve, parts: parts});
return (key);
}
function opensshCipherInfo(cipher) {
var inf = {};
switch (cipher) {
case '3des-cbc':
inf.keySize = 24;
inf.blockSize = 8;
inf.opensslName = 'des-ede3-cbc';
break;
case 'blowfish-cbc':
inf.keySize = 16;
inf.blockSize = 8;
inf.opensslName = 'bf-cbc';
break;
case 'aes128-cbc':
case 'aes128-ctr':
case '[email protected]':
inf.keySize = 16;
inf.blockSize = 16;
inf.opensslName = 'aes-128-' + cipher.slice(7, 10);
break;
case 'aes192-cbc':
case 'aes192-ctr':
case '[email protected]':
inf.keySize = 24;
inf.blockSize = 16;
inf.opensslName = 'aes-192-' + cipher.slice(7, 10);
break;
case 'aes256-cbc':
case 'aes256-ctr':
case '[email protected]':
inf.keySize = 32;
inf.blockSize = 16;
inf.opensslName = 'aes-256-' + cipher.slice(7, 10);
break;
default:
throw (new Error(
'Unsupported openssl cipher "' + cipher + '"'));
}
return (inf);
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/utils.js | utils.js |
module.exports = Signature;
var assert = require('assert-plus');
var Buffer = require('safer-buffer').Buffer;
var algs = require('sshpk/lib/algs');
var crypto = require('crypto');
var errs = require('sshpk/lib/errors');
var utils = require('sshpk/lib/utils');
var asn1 = require('asn1');
var SSHBuffer = require('sshpk/lib/ssh-buffer');
var InvalidAlgorithmError = errs.InvalidAlgorithmError;
var SignatureParseError = errs.SignatureParseError;
function Signature(opts) {
assert.object(opts, 'options');
assert.arrayOfObject(opts.parts, 'options.parts');
assert.string(opts.type, 'options.type');
var partLookup = {};
for (var i = 0; i < opts.parts.length; ++i) {
var part = opts.parts[i];
partLookup[part.name] = part;
}
this.type = opts.type;
this.hashAlgorithm = opts.hashAlgo;
this.curve = opts.curve;
this.parts = opts.parts;
this.part = partLookup;
}
Signature.prototype.toBuffer = function (format) {
if (format === undefined)
format = 'asn1';
assert.string(format, 'format');
var buf;
var stype = 'ssh-' + this.type;
switch (this.type) {
case 'rsa':
switch (this.hashAlgorithm) {
case 'sha256':
stype = 'rsa-sha2-256';
break;
case 'sha512':
stype = 'rsa-sha2-512';
break;
case 'sha1':
case undefined:
break;
default:
throw (new Error('SSH signature ' +
'format does not support hash ' +
'algorithm ' + this.hashAlgorithm));
}
if (format === 'ssh') {
buf = new SSHBuffer({});
buf.writeString(stype);
buf.writePart(this.part.sig);
return (buf.toBuffer());
} else {
return (this.part.sig.data);
}
break;
case 'ed25519':
if (format === 'ssh') {
buf = new SSHBuffer({});
buf.writeString(stype);
buf.writePart(this.part.sig);
return (buf.toBuffer());
} else {
return (this.part.sig.data);
}
break;
case 'dsa':
case 'ecdsa':
var r, s;
if (format === 'asn1') {
var der = new asn1.BerWriter();
der.startSequence();
r = utils.mpNormalize(this.part.r.data);
s = utils.mpNormalize(this.part.s.data);
der.writeBuffer(r, asn1.Ber.Integer);
der.writeBuffer(s, asn1.Ber.Integer);
der.endSequence();
return (der.buffer);
} else if (format === 'ssh' && this.type === 'dsa') {
buf = new SSHBuffer({});
buf.writeString('ssh-dss');
r = this.part.r.data;
if (r.length > 20 && r[0] === 0x00)
r = r.slice(1);
s = this.part.s.data;
if (s.length > 20 && s[0] === 0x00)
s = s.slice(1);
if ((this.hashAlgorithm &&
this.hashAlgorithm !== 'sha1') ||
r.length + s.length !== 40) {
throw (new Error('OpenSSH only supports ' +
'DSA signatures with SHA1 hash'));
}
buf.writeBuffer(Buffer.concat([r, s]));
return (buf.toBuffer());
} else if (format === 'ssh' && this.type === 'ecdsa') {
var inner = new SSHBuffer({});
r = this.part.r.data;
inner.writeBuffer(r);
inner.writePart(this.part.s);
buf = new SSHBuffer({});
/* XXX: find a more proper way to do this? */
var curve;
if (r[0] === 0x00)
r = r.slice(1);
var sz = r.length * 8;
if (sz === 256)
curve = 'nistp256';
else if (sz === 384)
curve = 'nistp384';
else if (sz === 528)
curve = 'nistp521';
buf.writeString('ecdsa-sha2-' + curve);
buf.writeBuffer(inner.toBuffer());
return (buf.toBuffer());
}
throw (new Error('Invalid signature format'));
default:
throw (new Error('Invalid signature data'));
}
};
Signature.prototype.toString = function (format) {
assert.optionalString(format, 'format');
return (this.toBuffer(format).toString('base64'));
};
Signature.parse = function (data, type, format) {
if (typeof (data) === 'string')
data = Buffer.from(data, 'base64');
assert.buffer(data, 'data');
assert.string(format, 'format');
assert.string(type, 'type');
var opts = {};
opts.type = type.toLowerCase();
opts.parts = [];
try {
assert.ok(data.length > 0, 'signature must not be empty');
switch (opts.type) {
case 'rsa':
return (parseOneNum(data, type, format, opts));
case 'ed25519':
return (parseOneNum(data, type, format, opts));
case 'dsa':
case 'ecdsa':
if (format === 'asn1')
return (parseDSAasn1(data, type, format, opts));
else if (opts.type === 'dsa')
return (parseDSA(data, type, format, opts));
else
return (parseECDSA(data, type, format, opts));
default:
throw (new InvalidAlgorithmError(type));
}
} catch (e) {
if (e instanceof InvalidAlgorithmError)
throw (e);
throw (new SignatureParseError(type, format, e));
}
};
function parseOneNum(data, type, format, opts) {
if (format === 'ssh') {
try {
var buf = new SSHBuffer({buffer: data});
var head = buf.readString();
} catch (e) {
/* fall through */
}
if (buf !== undefined) {
var msg = 'SSH signature does not match expected ' +
'type (expected ' + type + ', got ' + head + ')';
switch (head) {
case 'ssh-rsa':
assert.strictEqual(type, 'rsa', msg);
opts.hashAlgo = 'sha1';
break;
case 'rsa-sha2-256':
assert.strictEqual(type, 'rsa', msg);
opts.hashAlgo = 'sha256';
break;
case 'rsa-sha2-512':
assert.strictEqual(type, 'rsa', msg);
opts.hashAlgo = 'sha512';
break;
case 'ssh-ed25519':
assert.strictEqual(type, 'ed25519', msg);
opts.hashAlgo = 'sha512';
break;
default:
throw (new Error('Unknown SSH signature ' +
'type: ' + head));
}
var sig = buf.readPart();
assert.ok(buf.atEnd(), 'extra trailing bytes');
sig.name = 'sig';
opts.parts.push(sig);
return (new Signature(opts));
}
}
opts.parts.push({name: 'sig', data: data});
return (new Signature(opts));
}
function parseDSAasn1(data, type, format, opts) {
var der = new asn1.BerReader(data);
der.readSequence();
var r = der.readString(asn1.Ber.Integer, true);
var s = der.readString(asn1.Ber.Integer, true);
opts.parts.push({name: 'r', data: utils.mpNormalize(r)});
opts.parts.push({name: 's', data: utils.mpNormalize(s)});
return (new Signature(opts));
}
function parseDSA(data, type, format, opts) {
if (data.length != 40) {
var buf = new SSHBuffer({buffer: data});
var d = buf.readBuffer();
if (d.toString('ascii') === 'ssh-dss')
d = buf.readBuffer();
assert.ok(buf.atEnd(), 'extra trailing bytes');
assert.strictEqual(d.length, 40, 'invalid inner length');
data = d;
}
opts.parts.push({name: 'r', data: data.slice(0, 20)});
opts.parts.push({name: 's', data: data.slice(20, 40)});
return (new Signature(opts));
}
function parseECDSA(data, type, format, opts) {
var buf = new SSHBuffer({buffer: data});
var r, s;
var inner = buf.readBuffer();
var stype = inner.toString('ascii');
if (stype.slice(0, 6) === 'ecdsa-') {
var parts = stype.split('-');
assert.strictEqual(parts[0], 'ecdsa');
assert.strictEqual(parts[1], 'sha2');
opts.curve = parts[2];
switch (opts.curve) {
case 'nistp256':
opts.hashAlgo = 'sha256';
break;
case 'nistp384':
opts.hashAlgo = 'sha384';
break;
case 'nistp521':
opts.hashAlgo = 'sha512';
break;
default:
throw (new Error('Unsupported ECDSA curve: ' +
opts.curve));
}
inner = buf.readBuffer();
assert.ok(buf.atEnd(), 'extra trailing bytes on outer');
buf = new SSHBuffer({buffer: inner});
r = buf.readPart();
} else {
r = {data: inner};
}
s = buf.readPart();
assert.ok(buf.atEnd(), 'extra trailing bytes');
r.name = 'r';
s.name = 's';
opts.parts.push(r);
opts.parts.push(s);
return (new Signature(opts));
}
Signature.isSignature = function (obj, ver) {
return (utils.isCompatible(obj, Signature, ver));
};
/*
* API versions for Signature:
* [1,0] -- initial ver
* [2,0] -- support for rsa in full ssh format, compat with sshpk-agent
* hashAlgorithm property
* [2,1] -- first tagged version
*/
Signature.prototype._sshpkApiVersion = [2, 1];
Signature._oldVersionDetect = function (obj) {
assert.func(obj.toBuffer);
if (obj.hasOwnProperty('hashAlgorithm'))
return ([2, 0]);
return ([1, 0]);
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/signature.js | signature.js |
module.exports = {
DiffieHellman: DiffieHellman,
generateECDSA: generateECDSA,
generateED25519: generateED25519
};
var assert = require('assert-plus');
var crypto = require('crypto');
var Buffer = require('safer-buffer').Buffer;
var algs = require('sshpk/lib/algs');
var utils = require('sshpk/lib/utils');
var nacl = require('tweetnacl');
var Key = require('sshpk/lib/key');
var PrivateKey = require('sshpk/lib/private-key');
var CRYPTO_HAVE_ECDH = (crypto.createECDH !== undefined);
var ecdh = require('ecc-jsbn');
var ec = require('ecc-jsbn/lib/ec');
var jsbn = require('jsbn').BigInteger;
function DiffieHellman(key) {
utils.assertCompatible(key, Key, [1, 4], 'key');
this._isPriv = PrivateKey.isPrivateKey(key, [1, 3]);
this._algo = key.type;
this._curve = key.curve;
this._key = key;
if (key.type === 'dsa') {
if (!CRYPTO_HAVE_ECDH) {
throw (new Error('Due to bugs in the node 0.10 ' +
'crypto API, node 0.12.x or later is required ' +
'to use DH'));
}
this._dh = crypto.createDiffieHellman(
key.part.p.data, undefined,
key.part.g.data, undefined);
this._p = key.part.p;
this._g = key.part.g;
if (this._isPriv)
this._dh.setPrivateKey(key.part.x.data);
this._dh.setPublicKey(key.part.y.data);
} else if (key.type === 'ecdsa') {
if (!CRYPTO_HAVE_ECDH) {
this._ecParams = new X9ECParameters(this._curve);
if (this._isPriv) {
this._priv = new ECPrivate(
this._ecParams, key.part.d.data);
}
return;
}
var curve = {
'nistp256': 'prime256v1',
'nistp384': 'secp384r1',
'nistp521': 'secp521r1'
}[key.curve];
this._dh = crypto.createECDH(curve);
if (typeof (this._dh) !== 'object' ||
typeof (this._dh.setPrivateKey) !== 'function') {
CRYPTO_HAVE_ECDH = false;
DiffieHellman.call(this, key);
return;
}
if (this._isPriv)
this._dh.setPrivateKey(key.part.d.data);
this._dh.setPublicKey(key.part.Q.data);
} else if (key.type === 'curve25519') {
if (this._isPriv) {
utils.assertCompatible(key, PrivateKey, [1, 5], 'key');
this._priv = key.part.k.data;
}
} else {
throw (new Error('DH not supported for ' + key.type + ' keys'));
}
}
DiffieHellman.prototype.getPublicKey = function () {
if (this._isPriv)
return (this._key.toPublic());
return (this._key);
};
DiffieHellman.prototype.getPrivateKey = function () {
if (this._isPriv)
return (this._key);
else
return (undefined);
};
DiffieHellman.prototype.getKey = DiffieHellman.prototype.getPrivateKey;
DiffieHellman.prototype._keyCheck = function (pk, isPub) {
assert.object(pk, 'key');
if (!isPub)
utils.assertCompatible(pk, PrivateKey, [1, 3], 'key');
utils.assertCompatible(pk, Key, [1, 4], 'key');
if (pk.type !== this._algo) {
throw (new Error('A ' + pk.type + ' key cannot be used in ' +
this._algo + ' Diffie-Hellman'));
}
if (pk.curve !== this._curve) {
throw (new Error('A key from the ' + pk.curve + ' curve ' +
'cannot be used with a ' + this._curve +
' Diffie-Hellman'));
}
if (pk.type === 'dsa') {
assert.deepEqual(pk.part.p, this._p,
'DSA key prime does not match');
assert.deepEqual(pk.part.g, this._g,
'DSA key generator does not match');
}
};
DiffieHellman.prototype.setKey = function (pk) {
this._keyCheck(pk);
if (pk.type === 'dsa') {
this._dh.setPrivateKey(pk.part.x.data);
this._dh.setPublicKey(pk.part.y.data);
} else if (pk.type === 'ecdsa') {
if (CRYPTO_HAVE_ECDH) {
this._dh.setPrivateKey(pk.part.d.data);
this._dh.setPublicKey(pk.part.Q.data);
} else {
this._priv = new ECPrivate(
this._ecParams, pk.part.d.data);
}
} else if (pk.type === 'curve25519') {
var k = pk.part.k;
if (!pk.part.k)
k = pk.part.r;
this._priv = k.data;
if (this._priv[0] === 0x00)
this._priv = this._priv.slice(1);
this._priv = this._priv.slice(0, 32);
}
this._key = pk;
this._isPriv = true;
};
DiffieHellman.prototype.setPrivateKey = DiffieHellman.prototype.setKey;
DiffieHellman.prototype.computeSecret = function (otherpk) {
this._keyCheck(otherpk, true);
if (!this._isPriv)
throw (new Error('DH exchange has not been initialized with ' +
'a private key yet'));
var pub;
if (this._algo === 'dsa') {
return (this._dh.computeSecret(
otherpk.part.y.data));
} else if (this._algo === 'ecdsa') {
if (CRYPTO_HAVE_ECDH) {
return (this._dh.computeSecret(
otherpk.part.Q.data));
} else {
pub = new ECPublic(
this._ecParams, otherpk.part.Q.data);
return (this._priv.deriveSharedSecret(pub));
}
} else if (this._algo === 'curve25519') {
pub = otherpk.part.A.data;
while (pub[0] === 0x00 && pub.length > 32)
pub = pub.slice(1);
var priv = this._priv;
assert.strictEqual(pub.length, 32);
assert.strictEqual(priv.length, 32);
var secret = nacl.box.before(new Uint8Array(pub),
new Uint8Array(priv));
return (Buffer.from(secret));
}
throw (new Error('Invalid algorithm: ' + this._algo));
};
DiffieHellman.prototype.generateKey = function () {
var parts = [];
var priv, pub;
if (this._algo === 'dsa') {
this._dh.generateKeys();
parts.push({name: 'p', data: this._p.data});
parts.push({name: 'q', data: this._key.part.q.data});
parts.push({name: 'g', data: this._g.data});
parts.push({name: 'y', data: this._dh.getPublicKey()});
parts.push({name: 'x', data: this._dh.getPrivateKey()});
this._key = new PrivateKey({
type: 'dsa',
parts: parts
});
this._isPriv = true;
return (this._key);
} else if (this._algo === 'ecdsa') {
if (CRYPTO_HAVE_ECDH) {
this._dh.generateKeys();
parts.push({name: 'curve',
data: Buffer.from(this._curve)});
parts.push({name: 'Q', data: this._dh.getPublicKey()});
parts.push({name: 'd', data: this._dh.getPrivateKey()});
this._key = new PrivateKey({
type: 'ecdsa',
curve: this._curve,
parts: parts
});
this._isPriv = true;
return (this._key);
} else {
var n = this._ecParams.getN();
var r = new jsbn(crypto.randomBytes(n.bitLength()));
var n1 = n.subtract(jsbn.ONE);
priv = r.mod(n1).add(jsbn.ONE);
pub = this._ecParams.getG().multiply(priv);
priv = Buffer.from(priv.toByteArray());
pub = Buffer.from(this._ecParams.getCurve().
encodePointHex(pub), 'hex');
this._priv = new ECPrivate(this._ecParams, priv);
parts.push({name: 'curve',
data: Buffer.from(this._curve)});
parts.push({name: 'Q', data: pub});
parts.push({name: 'd', data: priv});
this._key = new PrivateKey({
type: 'ecdsa',
curve: this._curve,
parts: parts
});
this._isPriv = true;
return (this._key);
}
} else if (this._algo === 'curve25519') {
var pair = nacl.box.keyPair();
priv = Buffer.from(pair.secretKey);
pub = Buffer.from(pair.publicKey);
priv = Buffer.concat([priv, pub]);
assert.strictEqual(priv.length, 64);
assert.strictEqual(pub.length, 32);
parts.push({name: 'A', data: pub});
parts.push({name: 'k', data: priv});
this._key = new PrivateKey({
type: 'curve25519',
parts: parts
});
this._isPriv = true;
return (this._key);
}
throw (new Error('Invalid algorithm: ' + this._algo));
};
DiffieHellman.prototype.generateKeys = DiffieHellman.prototype.generateKey;
/* These are helpers for using ecc-jsbn (for node 0.10 compatibility). */
function X9ECParameters(name) {
var params = algs.curves[name];
assert.object(params);
var p = new jsbn(params.p);
var a = new jsbn(params.a);
var b = new jsbn(params.b);
var n = new jsbn(params.n);
var h = jsbn.ONE;
var curve = new ec.ECCurveFp(p, a, b);
var G = curve.decodePointHex(params.G.toString('hex'));
this.curve = curve;
this.g = G;
this.n = n;
this.h = h;
}
X9ECParameters.prototype.getCurve = function () { return (this.curve); };
X9ECParameters.prototype.getG = function () { return (this.g); };
X9ECParameters.prototype.getN = function () { return (this.n); };
X9ECParameters.prototype.getH = function () { return (this.h); };
function ECPublic(params, buffer) {
this._params = params;
if (buffer[0] === 0x00)
buffer = buffer.slice(1);
this._pub = params.getCurve().decodePointHex(buffer.toString('hex'));
}
function ECPrivate(params, buffer) {
this._params = params;
this._priv = new jsbn(utils.mpNormalize(buffer));
}
ECPrivate.prototype.deriveSharedSecret = function (pubKey) {
assert.ok(pubKey instanceof ECPublic);
var S = pubKey._pub.multiply(this._priv);
return (Buffer.from(S.getX().toBigInteger().toByteArray()));
};
function generateED25519() {
var pair = nacl.sign.keyPair();
var priv = Buffer.from(pair.secretKey);
var pub = Buffer.from(pair.publicKey);
assert.strictEqual(priv.length, 64);
assert.strictEqual(pub.length, 32);
var parts = [];
parts.push({name: 'A', data: pub});
parts.push({name: 'k', data: priv.slice(0, 32)});
var key = new PrivateKey({
type: 'ed25519',
parts: parts
});
return (key);
}
/* Generates a new ECDSA private key on a given curve. */
function generateECDSA(curve) {
var parts = [];
var key;
if (CRYPTO_HAVE_ECDH) {
/*
* Node crypto doesn't expose key generation directly, but the
* ECDH instances can generate keys. It turns out this just
* calls into the OpenSSL generic key generator, and we can
* read its output happily without doing an actual DH. So we
* use that here.
*/
var osCurve = {
'nistp256': 'prime256v1',
'nistp384': 'secp384r1',
'nistp521': 'secp521r1'
}[curve];
var dh = crypto.createECDH(osCurve);
dh.generateKeys();
parts.push({name: 'curve',
data: Buffer.from(curve)});
parts.push({name: 'Q', data: dh.getPublicKey()});
parts.push({name: 'd', data: dh.getPrivateKey()});
key = new PrivateKey({
type: 'ecdsa',
curve: curve,
parts: parts
});
return (key);
} else {
var ecParams = new X9ECParameters(curve);
/* This algorithm taken from FIPS PUB 186-4 (section B.4.1) */
var n = ecParams.getN();
/*
* The crypto.randomBytes() function can only give us whole
* bytes, so taking a nod from X9.62, we round up.
*/
var cByteLen = Math.ceil((n.bitLength() + 64) / 8);
var c = new jsbn(crypto.randomBytes(cByteLen));
var n1 = n.subtract(jsbn.ONE);
var priv = c.mod(n1).add(jsbn.ONE);
var pub = ecParams.getG().multiply(priv);
priv = Buffer.from(priv.toByteArray());
pub = Buffer.from(ecParams.getCurve().
encodePointHex(pub), 'hex');
parts.push({name: 'curve', data: Buffer.from(curve)});
parts.push({name: 'Q', data: pub});
parts.push({name: 'd', data: priv});
key = new PrivateKey({
type: 'ecdsa',
curve: curve,
parts: parts
});
return (key);
}
} | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/dhe.js | dhe.js |
module.exports = {
Verifier: Verifier,
Signer: Signer
};
var nacl = require('tweetnacl');
var stream = require('stream');
var util = require('util');
var assert = require('assert-plus');
var Buffer = require('safer-buffer').Buffer;
var Signature = require('sshpk/lib/signature');
function Verifier(key, hashAlgo) {
if (hashAlgo.toLowerCase() !== 'sha512')
throw (new Error('ED25519 only supports the use of ' +
'SHA-512 hashes'));
this.key = key;
this.chunks = [];
stream.Writable.call(this, {});
}
util.inherits(Verifier, stream.Writable);
Verifier.prototype._write = function (chunk, enc, cb) {
this.chunks.push(chunk);
cb();
};
Verifier.prototype.update = function (chunk) {
if (typeof (chunk) === 'string')
chunk = Buffer.from(chunk, 'binary');
this.chunks.push(chunk);
};
Verifier.prototype.verify = function (signature, fmt) {
var sig;
if (Signature.isSignature(signature, [2, 0])) {
if (signature.type !== 'ed25519')
return (false);
sig = signature.toBuffer('raw');
} else if (typeof (signature) === 'string') {
sig = Buffer.from(signature, 'base64');
} else if (Signature.isSignature(signature, [1, 0])) {
throw (new Error('signature was created by too old ' +
'a version of sshpk and cannot be verified'));
}
assert.buffer(sig);
return (nacl.sign.detached.verify(
new Uint8Array(Buffer.concat(this.chunks)),
new Uint8Array(sig),
new Uint8Array(this.key.part.A.data)));
};
function Signer(key, hashAlgo) {
if (hashAlgo.toLowerCase() !== 'sha512')
throw (new Error('ED25519 only supports the use of ' +
'SHA-512 hashes'));
this.key = key;
this.chunks = [];
stream.Writable.call(this, {});
}
util.inherits(Signer, stream.Writable);
Signer.prototype._write = function (chunk, enc, cb) {
this.chunks.push(chunk);
cb();
};
Signer.prototype.update = function (chunk) {
if (typeof (chunk) === 'string')
chunk = Buffer.from(chunk, 'binary');
this.chunks.push(chunk);
};
Signer.prototype.sign = function () {
var sig = nacl.sign.detached(
new Uint8Array(Buffer.concat(this.chunks)),
new Uint8Array(Buffer.concat([
this.key.part.k.data, this.key.part.A.data])));
var sigBuf = Buffer.from(sig);
var sigObj = Signature.parse(sigBuf, 'ed25519', 'raw');
sigObj.hashAlgorithm = 'sha512';
return (sigObj);
}; | zhihu-crawler | /zhihu_crawler-0.0.2.tar.gz/zhihu_crawler-0.0.2/zhihu_crawler/common/node_modules/sshpk/lib/ed-compat.js | ed-compat.js |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.