instance_id
stringlengths 12
53
| patch
stringlengths 264
37.7k
| repo
stringlengths 8
49
| base_commit
stringlengths 40
40
| hints_text
stringclasses 114
values | test_patch
stringlengths 224
315k
| problem_statement
stringlengths 40
10.4k
| version
stringclasses 1
value | FAIL_TO_PASS
sequencelengths 1
4.94k
| PASS_TO_PASS
sequencelengths 0
6.23k
| created_at
stringlengths 25
25
| __index_level_0__
int64 4.13k
6.41k
|
---|---|---|---|---|---|---|---|---|---|---|---|
networkx__networkx-2713 | diff --git a/networkx/algorithms/community/quality.py b/networkx/algorithms/community/quality.py
index 7de690af7..e04ff260d 100644
--- a/networkx/algorithms/community/quality.py
+++ b/networkx/algorithms/community/quality.py
@@ -114,7 +114,10 @@ def inter_community_edges(G, partition):
# for block in partition))
# return sum(1 for u, v in G.edges() if aff[u] != aff[v])
#
- return nx.quotient_graph(G, partition, create_using=nx.MultiGraph()).size()
+ if G.is_directed():
+ return nx.quotient_graph(G, partition, create_using=nx.MultiDiGraph()).size()
+ else:
+ return nx.quotient_graph(G, partition, create_using=nx.MultiGraph()).size()
def inter_community_non_edges(G, partition):
diff --git a/networkx/algorithms/simple_paths.py b/networkx/algorithms/simple_paths.py
index 763fa24d7..a2ef79671 100644
--- a/networkx/algorithms/simple_paths.py
+++ b/networkx/algorithms/simple_paths.py
@@ -333,7 +333,6 @@ def shortest_simple_paths(G, source, target, weight=None):
for path in listA:
if path[:i] == root:
ignore_edges.add((path[i - 1], path[i]))
- ignore_nodes.add(root[-1])
try:
length, spur = shortest_path_func(G, root[-1], target,
ignore_nodes=ignore_nodes,
@@ -343,6 +342,7 @@ def shortest_simple_paths(G, source, target, weight=None):
listB.push(root_length + length, path)
except nx.NetworkXNoPath:
pass
+ ignore_nodes.add(root[-1])
if listB:
path = listB.pop()
@@ -447,6 +447,8 @@ def _bidirectional_pred_succ(G, source, target, ignore_nodes=None, ignore_edges=
succ is a dictionary of successors from w to the target.
"""
# does BFS from both source and target and meets in the middle
+ if ignore_nodes and (source in ignore_nodes or target in ignore_nodes):
+ raise nx.NetworkXNoPath("No path between %s and %s." % (source, target))
if target == source:
return ({target: None}, {source: None}, source)
@@ -605,6 +607,8 @@ def _bidirectional_dijkstra(G, source, target, weight='weight',
shortest_path
shortest_path_length
"""
+ if ignore_nodes and (source in ignore_nodes or target in ignore_nodes):
+ raise nx.NetworkXNoPath("No path between %s and %s." % (source, target))
if source == target:
return (0, [source])
| networkx/networkx | 9f6c9cd6a561d41192bc29f14fd9bc16bcaad919 | diff --git a/networkx/algorithms/community/tests/test_quality.py b/networkx/algorithms/community/tests/test_quality.py
index 0c5b94c5a..79ce7e7f6 100644
--- a/networkx/algorithms/community/tests/test_quality.py
+++ b/networkx/algorithms/community/tests/test_quality.py
@@ -12,6 +12,7 @@ module.
"""
from __future__ import division
+from nose.tools import assert_equal
from nose.tools import assert_almost_equal
import networkx as nx
@@ -19,6 +20,7 @@ from networkx import barbell_graph
from networkx.algorithms.community import coverage
from networkx.algorithms.community import modularity
from networkx.algorithms.community import performance
+from networkx.algorithms.community.quality import inter_community_edges
class TestPerformance(object):
@@ -61,3 +63,17 @@ def test_modularity():
assert_almost_equal(-16 / (14 ** 2), modularity(G, C))
C = [{0, 1, 2}, {3, 4, 5}]
assert_almost_equal((35 * 2) / (14 ** 2), modularity(G, C))
+
+
+def test_inter_community_edges_with_digraphs():
+ G = nx.complete_graph(2, create_using = nx.DiGraph())
+ partition = [{0}, {1}]
+ assert_equal(inter_community_edges(G, partition), 2)
+
+ G = nx.complete_graph(10, create_using = nx.DiGraph())
+ partition = [{0}, {1, 2}, {3, 4, 5}, {6, 7, 8, 9}]
+ assert_equal(inter_community_edges(G, partition), 70)
+
+ G = nx.cycle_graph(4, create_using = nx.DiGraph())
+ partition = [{0, 1}, {2, 3}]
+ assert_equal(inter_community_edges(G, partition), 2)
diff --git a/networkx/algorithms/tests/test_simple_paths.py b/networkx/algorithms/tests/test_simple_paths.py
index e29255c32..4c701e487 100644
--- a/networkx/algorithms/tests/test_simple_paths.py
+++ b/networkx/algorithms/tests/test_simple_paths.py
@@ -220,6 +220,40 @@ def test_directed_weighted_shortest_simple_path():
cost = this_cost
+def test_weighted_shortest_simple_path_issue2427():
+ G = nx.Graph()
+ G.add_edge('IN', 'OUT', weight = 2)
+ G.add_edge('IN', 'A', weight = 1)
+ G.add_edge('IN', 'B', weight = 2)
+ G.add_edge('B', 'OUT', weight = 2)
+ assert_equal(list(nx.shortest_simple_paths(G, 'IN', 'OUT', weight = "weight")),
+ [['IN', 'OUT'], ['IN', 'B', 'OUT']])
+ G = nx.Graph()
+ G.add_edge('IN', 'OUT', weight = 10)
+ G.add_edge('IN', 'A', weight = 1)
+ G.add_edge('IN', 'B', weight = 1)
+ G.add_edge('B', 'OUT', weight = 1)
+ assert_equal(list(nx.shortest_simple_paths(G, 'IN', 'OUT', weight = "weight")),
+ [['IN', 'B', 'OUT'], ['IN', 'OUT']])
+
+
+def test_directed_weighted_shortest_simple_path_issue2427():
+ G = nx.DiGraph()
+ G.add_edge('IN', 'OUT', weight = 2)
+ G.add_edge('IN', 'A', weight = 1)
+ G.add_edge('IN', 'B', weight = 2)
+ G.add_edge('B', 'OUT', weight = 2)
+ assert_equal(list(nx.shortest_simple_paths(G, 'IN', 'OUT', weight = "weight")),
+ [['IN', 'OUT'], ['IN', 'B', 'OUT']])
+ G = nx.DiGraph()
+ G.add_edge('IN', 'OUT', weight = 10)
+ G.add_edge('IN', 'A', weight = 1)
+ G.add_edge('IN', 'B', weight = 1)
+ G.add_edge('B', 'OUT', weight = 1)
+ assert_equal(list(nx.shortest_simple_paths(G, 'IN', 'OUT', weight = "weight")),
+ [['IN', 'B', 'OUT'], ['IN', 'OUT']])
+
+
def test_weight_name():
G = nx.cycle_graph(7)
nx.set_edge_attributes(G, 1, 'weight')
@@ -303,6 +337,38 @@ def test_bidirectional_shortest_path_restricted_directed_cycle():
)
+def test_bidirectional_shortest_path_ignore():
+ G = nx.Graph()
+ nx.add_path(G, [1, 2])
+ nx.add_path(G, [1, 3])
+ nx.add_path(G, [1, 4])
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_shortest_path,
+ G,
+ 1, 2,
+ ignore_nodes=[1],
+ )
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_shortest_path,
+ G,
+ 1, 2,
+ ignore_nodes=[2],
+ )
+ G = nx.Graph()
+ nx.add_path(G, [1, 3])
+ nx.add_path(G, [1, 4])
+ nx.add_path(G, [3, 2])
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_shortest_path,
+ G,
+ 1, 2,
+ ignore_nodes=[1, 2],
+ )
+
+
def validate_path(G, s, t, soln_len, path):
assert_equal(path[0], s)
assert_equal(path[-1], t)
@@ -362,3 +428,30 @@ def test_bidirectional_dijkstra_no_path():
nx.add_path(G, [1, 2, 3])
nx.add_path(G, [4, 5, 6])
path = _bidirectional_dijkstra(G, 1, 6)
+
+
+def test_bidirectional_dijkstra_ignore():
+ G = nx.Graph()
+ nx.add_path(G, [1, 2, 10])
+ nx.add_path(G, [1, 3, 10])
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_dijkstra,
+ G,
+ 1, 2,
+ ignore_nodes=[1],
+ )
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_dijkstra,
+ G,
+ 1, 2,
+ ignore_nodes=[2],
+ )
+ assert_raises(
+ nx.NetworkXNoPath,
+ _bidirectional_dijkstra,
+ G,
+ 1, 2,
+ ignore_nodes=[1, 2],
+ )
| inter_community_non_edges ignore directionality
Hi,
I think the function:
nx.algorithms.community.quality.inter_community_non_edges()
does not work properly for directed graph. It always return the non-edge of a undirected graph, basically halving the number of edges. This mean that the performance function (nx.algorithms.community.performance) will never by higher than 50% for a directed graph.
I'm using version '2.0.dev_20170801111157', python 3.5.1
Best,
Nicolas | 0.0 | [
"networkx/algorithms/community/tests/test_quality.py::TestPerformance::test_bad_partition",
"networkx/algorithms/community/tests/test_quality.py::TestPerformance::test_good_partition",
"networkx/algorithms/community/tests/test_quality.py::TestCoverage::test_bad_partition",
"networkx/algorithms/community/tests/test_quality.py::TestCoverage::test_good_partition",
"networkx/algorithms/community/tests/test_quality.py::test_modularity",
"networkx/algorithms/community/tests/test_quality.py::test_inter_community_edges_with_digraphs",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_empty_list",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_trivial_path",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_trivial_nonpath",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_simple_path",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_non_simple_path",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_cycle",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_missing_node",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_directed_path",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_directed_non_path",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_directed_cycle",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_multigraph",
"networkx/algorithms/tests/test_simple_paths.py::TestIsSimplePath::test_multidigraph",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths_cutoff",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths_multigraph",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths_multigraph_with_cutoff",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths_directed",
"networkx/algorithms/tests/test_simple_paths.py::test_all_simple_paths_empty",
"networkx/algorithms/tests/test_simple_paths.py::test_hamiltonian_path",
"networkx/algorithms/tests/test_simple_paths.py::test_cutoff_zero",
"networkx/algorithms/tests/test_simple_paths.py::test_source_missing",
"networkx/algorithms/tests/test_simple_paths.py::test_target_missing",
"networkx/algorithms/tests/test_simple_paths.py::test_shortest_simple_paths",
"networkx/algorithms/tests/test_simple_paths.py::test_shortest_simple_paths_directed",
"networkx/algorithms/tests/test_simple_paths.py::test_Greg_Bernstein",
"networkx/algorithms/tests/test_simple_paths.py::test_weighted_shortest_simple_path",
"networkx/algorithms/tests/test_simple_paths.py::test_directed_weighted_shortest_simple_path",
"networkx/algorithms/tests/test_simple_paths.py::test_weighted_shortest_simple_path_issue2427",
"networkx/algorithms/tests/test_simple_paths.py::test_directed_weighted_shortest_simple_path_issue2427",
"networkx/algorithms/tests/test_simple_paths.py::test_weight_name",
"networkx/algorithms/tests/test_simple_paths.py::test_ssp_source_missing",
"networkx/algorithms/tests/test_simple_paths.py::test_ssp_target_missing",
"networkx/algorithms/tests/test_simple_paths.py::test_ssp_multigraph",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_shortest_path_restricted_cycle",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_shortest_path_restricted_wheel",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_shortest_path_restricted_directed_cycle",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_shortest_path_ignore",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_dijksta_restricted",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_dijkstra_no_path",
"networkx/algorithms/tests/test_simple_paths.py::test_bidirectional_dijkstra_ignore"
] | [] | 2017-10-15 17:09:15+00:00 | 4,131 |
|
networkx__networkx-3822 | diff --git a/networkx/generators/random_graphs.py b/networkx/generators/random_graphs.py
index e4f2c569d..745f64e4d 100644
--- a/networkx/generators/random_graphs.py
+++ b/networkx/generators/random_graphs.py
@@ -1000,6 +1000,11 @@ def random_lobster(n, p1, p2, seed=None):
leaf nodes. A caterpillar is a tree that reduces to a path graph
when pruning all leaf nodes; setting `p2` to zero produces a caterpillar.
+ This implementation iterates on the probabilities `p1` and `p2` to add
+ edges at levels 1 and 2, respectively. Graphs are therefore constructed
+ iteratively with uniform randomness at each level rather than being selected
+ uniformly at random from the set of all possible lobsters.
+
Parameters
----------
n : int
@@ -1011,19 +1016,29 @@ def random_lobster(n, p1, p2, seed=None):
seed : integer, random_state, or None (default)
Indicator of random number generation state.
See :ref:`Randomness<randomness>`.
+
+ Raises
+ ------
+ NetworkXError
+ If `p1` or `p2` parameters are >= 1 because the while loops would never finish.
"""
+ p1, p2 = abs(p1), abs(p2)
+ if any([p >= 1 for p in [p1, p2]]):
+ raise nx.NetworkXError("Probability values for `p1` and `p2` must both be < 1.")
+
# a necessary ingredient in any self-respecting graph library
llen = int(2 * seed.random() * n + 0.5)
L = path_graph(llen)
# build caterpillar: add edges to path graph with probability p1
current_node = llen - 1
for n in range(llen):
- if seed.random() < p1: # add fuzzy caterpillar parts
+ while seed.random() < p1: # add fuzzy caterpillar parts
current_node += 1
L.add_edge(n, current_node)
- if seed.random() < p2: # add crunchy lobster bits
+ cat_node = current_node
+ while seed.random() < p2: # add crunchy lobster bits
current_node += 1
- L.add_edge(current_node - 1, current_node)
+ L.add_edge(cat_node, current_node)
return L # voila, un lobster!
| networkx/networkx | a4d024c54f06d17d2f9ab26595a0b20ed6858f5c | diff --git a/networkx/generators/tests/test_random_graphs.py b/networkx/generators/tests/test_random_graphs.py
index f958dceab..8f2d68415 100644
--- a/networkx/generators/tests/test_random_graphs.py
+++ b/networkx/generators/tests/test_random_graphs.py
@@ -91,7 +91,38 @@ class TestGeneratorsRandom:
constructor = [(10, 20, 0.8), (20, 40, 0.8)]
G = random_shell_graph(constructor, seed)
+ def is_caterpillar(g):
+ """
+ A tree is a caterpillar iff all nodes of degree >=3 are surrounded
+ by at most two nodes of degree two or greater.
+ ref: http://mathworld.wolfram.com/CaterpillarGraph.html
+ """
+ deg_over_3 = [n for n in g if g.degree(n) >= 3]
+ for n in deg_over_3:
+ nbh_deg_over_2 = [nbh for nbh in g.neighbors(n) if g.degree(nbh) >= 2]
+ if not len(nbh_deg_over_2) <= 2:
+ return False
+ return True
+
+ def is_lobster(g):
+ """
+ A tree is a lobster if it has the property that the removal of leaf
+ nodes leaves a caterpillar graph (Gallian 2007)
+ ref: http://mathworld.wolfram.com/LobsterGraph.html
+ """
+ non_leafs = [n for n in g if g.degree(n) > 1]
+ return is_caterpillar(g.subgraph(non_leafs))
+
G = random_lobster(10, 0.1, 0.5, seed)
+ assert max([G.degree(n) for n in G.nodes()]) > 3
+ assert is_lobster(G)
+ pytest.raises(NetworkXError, random_lobster, 10, 0.1, 1, seed)
+ pytest.raises(NetworkXError, random_lobster, 10, 1, 1, seed)
+ pytest.raises(NetworkXError, random_lobster, 10, 1, 0.5, seed)
+
+ # docstring says this should be a caterpillar
+ G = random_lobster(10, 0.1, 0.0, seed)
+ assert is_caterpillar(G)
# difficult to find seed that requires few tries
seq = random_powerlaw_tree_sequence(10, 3, seed=14, tries=1)
| Wrong random_lobster implementation?
Hi, it seems that [networkx.random_lobster's implementation logic](https://github.com/networkx/networkx/blob/4e9771f04192e94a5cbdd71249a983d124a56593/networkx/generators/random_graphs.py#L1009) is not aligned with the common definition as given in [wolfram mathworld](http://mathworld.wolfram.com/LobsterGraph.html) or [Wikipedia](https://en.wikipedia.org/wiki/List_of_graphs#Lobster).
For example, it can not produce the simplest lobster graph examples such as . | 0.0 | [
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_random_graph"
] | [
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_dual_barabasi_albert",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_extended_barabasi_albert",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_random_zero_regular_graph",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_gnp",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_gnm",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_watts_strogatz_big_k",
"networkx/generators/tests/test_random_graphs.py::TestGeneratorsRandom::test_random_kernel_graph"
] | 2020-02-16 23:49:40+00:00 | 4,132 |
|
networkx__networkx-3848 | diff --git a/networkx/algorithms/connectivity/cuts.py b/networkx/algorithms/connectivity/cuts.py
index 846cd4729..dd59e3db9 100644
--- a/networkx/algorithms/connectivity/cuts.py
+++ b/networkx/algorithms/connectivity/cuts.py
@@ -281,7 +281,7 @@ def minimum_st_node_cut(G, s, t, flow_func=None, auxiliary=None, residual=None):
if mapping is None:
raise nx.NetworkXError('Invalid auxiliary digraph.')
if G.has_edge(s, t) or G.has_edge(t, s):
- return []
+ return {}
kwargs = dict(flow_func=flow_func, residual=residual, auxiliary=H)
# The edge cut in the auxiliary digraph corresponds to the node cut in the
| networkx/networkx | 3f4f9c3379a5d70fc58852154aab7b1051ff96d6 | diff --git a/networkx/algorithms/connectivity/tests/test_cuts.py b/networkx/algorithms/connectivity/tests/test_cuts.py
index b98fbfa5e..257797a6f 100644
--- a/networkx/algorithms/connectivity/tests/test_cuts.py
+++ b/networkx/algorithms/connectivity/tests/test_cuts.py
@@ -268,7 +268,7 @@ def tests_minimum_st_node_cut():
G.add_nodes_from([0, 1, 2, 3, 7, 8, 11, 12])
G.add_edges_from([(7, 11), (1, 11), (1, 12), (12, 8), (0, 1)])
nodelist = minimum_st_node_cut(G, 7, 11)
- assert(nodelist == [])
+ assert(nodelist == {})
def test_invalid_auxiliary():
| `minimum_st_node_cut` returns empty list instead of set for adjacent nodes
https://github.com/networkx/networkx/blob/3f4f9c3379a5d70fc58852154aab7b1051ff96d6/networkx/algorithms/connectivity/cuts.py#L284
Should read `return {}`. Was questioning my sanity for a bit there 😉 | 0.0 | [
"networkx/algorithms/connectivity/tests/test_cuts.py::tests_minimum_st_node_cut"
] | [
"networkx/algorithms/connectivity/tests/test_cuts.py::test_articulation_points",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_brandes_erlebach_book",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_white_harary_paper",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_petersen_cutset",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_octahedral_cutset",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_icosahedral_cutset",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_node_cutset_exception",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_node_cutset_random_graphs",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_edge_cutset_random_graphs",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_empty_graphs",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_unbounded",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_missing_source",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_missing_target",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_not_weakly_connected",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_not_connected",
"networkx/algorithms/connectivity/tests/test_cuts.py::tests_min_cut_complete",
"networkx/algorithms/connectivity/tests/test_cuts.py::tests_min_cut_complete_directed",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_invalid_auxiliary",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_interface_only_source",
"networkx/algorithms/connectivity/tests/test_cuts.py::test_interface_only_target"
] | 2020-03-04 08:11:36+00:00 | 4,133 |
|
networkx__networkx-4066 | diff --git a/networkx/relabel.py b/networkx/relabel.py
index 737b5af3d..26f50d241 100644
--- a/networkx/relabel.py
+++ b/networkx/relabel.py
@@ -13,7 +13,7 @@ def relabel_nodes(G, mapping, copy=True):
mapping : dictionary
A dictionary with the old labels as keys and new labels as values.
- A partial mapping is allowed.
+ A partial mapping is allowed. Mapping 2 nodes to a single node is allowed.
copy : bool (optional, default=True)
If True return a copy, or if False relabel the nodes in place.
@@ -64,6 +64,27 @@ def relabel_nodes(G, mapping, copy=True):
>>> list(H)
[0, 1, 4]
+ In a multigraph, relabeling two or more nodes to the same new node
+ will retain all edges, but may change the edge keys in the process:
+
+ >>> G = nx.MultiGraph()
+ >>> G.add_edge(0, 1, value="a") # returns the key for this edge
+ 0
+ >>> G.add_edge(0, 2, value="b")
+ 0
+ >>> G.add_edge(0, 3, value="c")
+ 0
+ >>> mapping = {1: 4, 2: 4, 3: 4}
+ >>> H = nx.relabel_nodes(G, mapping, copy=True)
+ >>> print(H[0])
+ {4: {0: {'value': 'a'}, 1: {'value': 'b'}, 2: {'value': 'c'}}}
+
+ This works for in-place relabeling too:
+
+ >>> G = nx.relabel_nodes(G, mapping, copy=False)
+ >>> print(G[0])
+ {4: {0: {'value': 'a'}, 1: {'value': 'b'}, 2: {'value': 'c'}}}
+
Notes
-----
Only the nodes specified in the mapping will be relabeled.
@@ -77,6 +98,13 @@ def relabel_nodes(G, mapping, copy=True):
graph is not possible in-place and an exception is raised.
In that case, use copy=True.
+ If a relabel operation on a multigraph would cause two or more
+ edges to have the same source, target and key, the second edge must
+ be assigned a new key to retain all edges. The new key is set
+ to the lowest non-negative integer not already used as a key
+ for edges between these two nodes. Note that this means non-numeric
+ keys may be replaced by numeric keys.
+
See Also
--------
convert_node_labels_to_integers
@@ -136,6 +164,15 @@ def _relabel_inplace(G, mapping):
(new if old == source else source, new, key, data)
for (source, _, key, data) in G.in_edges(old, data=True, keys=True)
]
+ # Ensure new edges won't overwrite existing ones
+ seen = set()
+ for i, (source, target, key, data) in enumerate(new_edges):
+ if (target in G[source] and key in G[source][target]):
+ new_key = 0 if not isinstance(key, (int, float)) else key
+ while (new_key in G[source][target] or (target, new_key) in seen):
+ new_key += 1
+ new_edges[i] = (source, target, new_key, data)
+ seen.add((target, new_key))
else:
new_edges = [
(new, new if old == target else target, data)
@@ -156,10 +193,25 @@ def _relabel_copy(G, mapping):
H.add_nodes_from(mapping.get(n, n) for n in G)
H._node.update((mapping.get(n, n), d.copy()) for n, d in G.nodes.items())
if G.is_multigraph():
- H.add_edges_from(
+ new_edges = [
(mapping.get(n1, n1), mapping.get(n2, n2), k, d.copy())
for (n1, n2, k, d) in G.edges(keys=True, data=True)
- )
+ ]
+
+ # check for conflicting edge-keys
+ undirected = not G.is_directed()
+ seen_edges = set()
+ for i, (source, target, key, data) in enumerate(new_edges):
+ while (source, target, key) in seen_edges:
+ if not isinstance(key, (int, float)):
+ key = 0
+ key += 1
+ seen_edges.add((source, target, key))
+ if undirected:
+ seen_edges.add((target, source, key))
+ new_edges[i] = (source, target, key, data)
+
+ H.add_edges_from(new_edges)
else:
H.add_edges_from(
(mapping.get(n1, n1), mapping.get(n2, n2), d.copy())
| networkx/networkx | 5638e1ff3d01e21c7d950615a699eb1f99987b8d | diff --git a/networkx/tests/test_relabel.py b/networkx/tests/test_relabel.py
index 9b8ad2998..909904bc9 100644
--- a/networkx/tests/test_relabel.py
+++ b/networkx/tests/test_relabel.py
@@ -183,3 +183,109 @@ class TestRelabel:
G = nx.MultiDiGraph([(1, 1)])
G = nx.relabel_nodes(G, {1: 0}, copy=False)
assert_nodes_equal(G.nodes(), [0])
+
+# def test_relabel_multidigraph_inout_inplace(self):
+# pass
+ def test_relabel_multidigraph_inout_merge_nodes(self):
+ for MG in (nx.MultiGraph, nx.MultiDiGraph):
+ for cc in (True, False):
+ G = MG([(0, 4), (1, 4), (4, 2), (4, 3)])
+ G[0][4][0]["value"] = "a"
+ G[1][4][0]["value"] = "b"
+ G[4][2][0]["value"] = "c"
+ G[4][3][0]["value"] = "d"
+ G.add_edge(0, 4, key="x", value="e")
+ G.add_edge(4, 3, key="x", value="f")
+ mapping = {0: 9, 1: 9, 2: 9, 3: 9}
+ H = nx.relabel_nodes(G, mapping, copy=cc)
+ # No ordering on keys enforced
+ assert {"value": "a"} in H[9][4].values()
+ assert {"value": "b"} in H[9][4].values()
+ assert {"value": "c"} in H[4][9].values()
+ assert len(H[4][9]) == 3 if G.is_directed() else 6
+ assert {"value": "d"} in H[4][9].values()
+ assert {"value": "e"} in H[9][4].values()
+ assert {"value": "f"} in H[4][9].values()
+ assert len(H[9][4]) == 3 if G.is_directed() else 6
+
+ def test_relabel_multigraph_merge_inplace(self):
+ G = nx.MultiGraph([(0, 1), (0, 2), (0, 3), (0, 1), (0, 2), (0, 3)])
+ G[0][1][0]["value"] = "a"
+ G[0][2][0]["value"] = "b"
+ G[0][3][0]["value"] = "c"
+ mapping = {1: 4, 2: 4, 3: 4}
+ nx.relabel_nodes(G, mapping, copy=False)
+ # No ordering on keys enforced
+ assert {"value": "a"} in G[0][4].values()
+ assert {"value": "b"} in G[0][4].values()
+ assert {"value": "c"} in G[0][4].values()
+
+ def test_relabel_multidigraph_merge_inplace(self):
+ G = nx.MultiDiGraph([(0, 1), (0, 2), (0, 3)])
+ G[0][1][0]["value"] = "a"
+ G[0][2][0]["value"] = "b"
+ G[0][3][0]["value"] = "c"
+ mapping = {1: 4, 2: 4, 3: 4}
+ nx.relabel_nodes(G, mapping, copy=False)
+ # No ordering on keys enforced
+ assert {"value": "a"} in G[0][4].values()
+ assert {"value": "b"} in G[0][4].values()
+ assert {"value": "c"} in G[0][4].values()
+
+ def test_relabel_multidigraph_inout_copy(self):
+ G = nx.MultiDiGraph([(0, 4), (1, 4), (4, 2), (4, 3)])
+ G[0][4][0]["value"] = "a"
+ G[1][4][0]["value"] = "b"
+ G[4][2][0]["value"] = "c"
+ G[4][3][0]["value"] = "d"
+ G.add_edge(0, 4, key="x", value="e")
+ G.add_edge(4, 3, key="x", value="f")
+ mapping = {0: 9, 1: 9, 2: 9, 3: 9}
+ H = nx.relabel_nodes(G, mapping, copy=True)
+ # No ordering on keys enforced
+ assert {"value": "a"} in H[9][4].values()
+ assert {"value": "b"} in H[9][4].values()
+ assert {"value": "c"} in H[4][9].values()
+ assert len(H[4][9]) == 3
+ assert {"value": "d"} in H[4][9].values()
+ assert {"value": "e"} in H[9][4].values()
+ assert {"value": "f"} in H[4][9].values()
+ assert len(H[9][4]) == 3
+
+ def test_relabel_multigraph_merge_copy(self):
+ G = nx.MultiGraph([(0, 1), (0, 2), (0, 3)])
+ G[0][1][0]["value"] = "a"
+ G[0][2][0]["value"] = "b"
+ G[0][3][0]["value"] = "c"
+ mapping = {1: 4, 2: 4, 3: 4}
+ H = nx.relabel_nodes(G, mapping, copy=True)
+ assert {"value": "a"} in H[0][4].values()
+ assert {"value": "b"} in H[0][4].values()
+ assert {"value": "c"} in H[0][4].values()
+
+ def test_relabel_multidigraph_merge_copy(self):
+ G = nx.MultiDiGraph([(0, 1), (0, 2), (0, 3)])
+ G[0][1][0]["value"] = "a"
+ G[0][2][0]["value"] = "b"
+ G[0][3][0]["value"] = "c"
+ mapping = {1: 4, 2: 4, 3: 4}
+ H = nx.relabel_nodes(G, mapping, copy=True)
+ assert {"value": "a"} in H[0][4].values()
+ assert {"value": "b"} in H[0][4].values()
+ assert {"value": "c"} in H[0][4].values()
+
+ def test_relabel_multigraph_nonnumeric_key(self):
+ for MG in (nx.MultiGraph, nx.MultiDiGraph):
+ for cc in (True, False):
+ G = nx.MultiGraph()
+ G.add_edge(0, 1, key="I", value="a")
+ G.add_edge(0, 2, key="II", value="b")
+ G.add_edge(0, 3, key="II", value="c")
+ mapping = {1: 4, 2: 4, 3: 4}
+ nx.relabel_nodes(G, mapping, copy=False)
+ assert {"value": "a"} in G[0][4].values()
+ assert {"value": "b"} in G[0][4].values()
+ assert {"value": "c"} in G[0][4].values()
+ assert 0 in G[0][4]
+ assert "I" in G[0][4]
+ assert "II" in G[0][4]
| relabel_nodes on MultiGraphs does not preserve both edges when two nodes are replaced by one
When the graph contains edges (0,1) and (0,2), and I relabel both 1 and 2 to 3, I expected two edges from (0,3) but only one node is preserved.
Multi*Graph supports parallel edges between nodes and I expected it to preserve both edges when "merging" the two old nodes together.
Tested on both networkx 2.4 and the latest version on master.
```python
import networkx as nx
G = nx.MultiDiGraph([(0,1),(0,2)])
G[0][1][0]["value"] = "a"
G[0][2][0]["value"] = "b"
print(G[0])
# Output:
# {1: {0: {'value': 'a'}}, 2: {0: {'value': 'b'}}}
mapping = {1:3, 2:3}
nx.relabel_nodes(G, mapping, copy=False)
print(G[0])
# Output:
# {3: {0: {'value': 'b'}}}
# Expected:
# {3: {0: {'value': 'a'}, 1: {'value': 'b'}}}
``` | 0.0 | [
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_inout_merge_nodes",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_merge_inplace",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_merge_inplace",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_inout_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_merge_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_merge_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_nonnumeric_key"
] | [
"networkx/tests/test_relabel.py::TestRelabel::test_convert_node_labels_to_integers",
"networkx/tests/test_relabel.py::TestRelabel::test_convert_to_integers2",
"networkx/tests/test_relabel.py::TestRelabel::test_convert_to_integers_raise",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_function",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_graph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_orderedgraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_digraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_multigraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_multidigraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_isolated_nodes_to_same",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_missing",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_copy_name",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_toposort",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_selfloop"
] | 2020-07-11 12:56:42+00:00 | 4,134 |
|
networkx__networkx-4125 | diff --git a/networkx/readwrite/edgelist.py b/networkx/readwrite/edgelist.py
index 72afe1a7c..5183071d1 100644
--- a/networkx/readwrite/edgelist.py
+++ b/networkx/readwrite/edgelist.py
@@ -270,7 +270,11 @@ def parse_edgelist(
elif data is True:
# no edge types specified
try: # try to evaluate as dictionary
- edgedata = dict(literal_eval(" ".join(d)))
+ if delimiter == ",":
+ edgedata_str = ",".join(d)
+ else:
+ edgedata_str = " ".join(d)
+ edgedata = dict(literal_eval(edgedata_str.strip()))
except BaseException as e:
raise TypeError(
f"Failed to convert edge data ({d}) " f"to dictionary."
| networkx/networkx | ab7429c62806f1242c36fb81c1b1d801b4cca7a3 | diff --git a/networkx/readwrite/tests/test_edgelist.py b/networkx/readwrite/tests/test_edgelist.py
index 2e1a2424f..31f214566 100644
--- a/networkx/readwrite/tests/test_edgelist.py
+++ b/networkx/readwrite/tests/test_edgelist.py
@@ -100,6 +100,48 @@ class TestEdgelist:
G.edges(data=True), [(1, 2, {"weight": 2.0}), (2, 3, {"weight": 3.0})]
)
+ def test_read_edgelist_5(self):
+ s = b"""\
+# comment line
+1 2 {'weight':2.0, 'color':'green'}
+# comment line
+2 3 {'weight':3.0, 'color':'red'}
+"""
+ bytesIO = io.BytesIO(s)
+ G = nx.read_edgelist(bytesIO, nodetype=int, data=False)
+ assert_edges_equal(G.edges(), [(1, 2), (2, 3)])
+
+ bytesIO = io.BytesIO(s)
+ G = nx.read_edgelist(bytesIO, nodetype=int, data=True)
+ assert_edges_equal(
+ G.edges(data=True),
+ [
+ (1, 2, {"weight": 2.0, "color": "green"}),
+ (2, 3, {"weight": 3.0, "color": "red"}),
+ ],
+ )
+
+ def test_read_edgelist_6(self):
+ s = b"""\
+# comment line
+1, 2, {'weight':2.0, 'color':'green'}
+# comment line
+2, 3, {'weight':3.0, 'color':'red'}
+"""
+ bytesIO = io.BytesIO(s)
+ G = nx.read_edgelist(bytesIO, nodetype=int, data=False, delimiter=",")
+ assert_edges_equal(G.edges(), [(1, 2), (2, 3)])
+
+ bytesIO = io.BytesIO(s)
+ G = nx.read_edgelist(bytesIO, nodetype=int, data=True, delimiter=",")
+ assert_edges_equal(
+ G.edges(data=True),
+ [
+ (1, 2, {"weight": 2.0, "color": "green"}),
+ (2, 3, {"weight": 3.0, "color": "red"}),
+ ],
+ )
+
def test_write_edgelist_1(self):
fh = io.BytesIO()
G = nx.OrderedGraph()
| Parse edgelist bug
When calling `parse_edgelist()` using comma delimiters, it will fail to parse correctly fro edges with multiple attributes.
Ex. of bad edge:
`1,2,{'test':1, 'test_other':2}`
`parse_edgelist()` will recognize the comma in the braces as delimiter and try to split them up. | 0.0 | [
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_6"
] | [
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_1",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_2",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_3",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_4",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_read_edgelist_5",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_write_edgelist_1",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_write_edgelist_2",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_write_edgelist_3",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_write_edgelist_4",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_unicode",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_latin1_issue",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_latin1",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_edgelist_graph",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_edgelist_digraph",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_edgelist_integers",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_edgelist_multigraph",
"networkx/readwrite/tests/test_edgelist.py::TestEdgelist::test_edgelist_multidigraph"
] | 2020-08-03 00:13:47+00:00 | 4,135 |
|
networkx__networkx-4246 | diff --git a/networkx/algorithms/centrality/betweenness.py b/networkx/algorithms/centrality/betweenness.py
index 0829d9b0b..6907f25b1 100644
--- a/networkx/algorithms/centrality/betweenness.py
+++ b/networkx/algorithms/centrality/betweenness.py
@@ -101,20 +101,20 @@ def betweenness_centrality(
.. [1] Ulrik Brandes:
A Faster Algorithm for Betweenness Centrality.
Journal of Mathematical Sociology 25(2):163-177, 2001.
- http://www.inf.uni-konstanz.de/algo/publications/b-fabc-01.pdf
+ https://doi.org/10.1080/0022250X.2001.9990249
.. [2] Ulrik Brandes:
On Variants of Shortest-Path Betweenness
Centrality and their Generic Computation.
Social Networks 30(2):136-145, 2008.
- http://www.inf.uni-konstanz.de/algo/publications/b-vspbc-08.pdf
+ https://doi.org/10.1016/j.socnet.2007.11.001
.. [3] Ulrik Brandes and Christian Pich:
Centrality Estimation in Large Networks.
International Journal of Bifurcation and Chaos 17(7):2303-2318, 2007.
- http://www.inf.uni-konstanz.de/algo/publications/bp-celn-06.pdf
+ https://dx.doi.org/10.1142/S0218127407018403
.. [4] Linton C. Freeman:
A set of measures of centrality based on betweenness.
Sociometry 40: 35–41, 1977
- http://moreno.ss.uci.edu/23.pdf
+ https://doi.org/10.2307/3033543
"""
betweenness = dict.fromkeys(G, 0.0) # b[v]=0 for v in G
if k is None:
diff --git a/networkx/algorithms/euler.py b/networkx/algorithms/euler.py
index 6f8283f27..52042580a 100644
--- a/networkx/algorithms/euler.py
+++ b/networkx/algorithms/euler.py
@@ -224,7 +224,7 @@ def has_eulerian_path(G):
- at most one vertex has in_degree - out_degree = 1,
- every other vertex has equal in_degree and out_degree,
- and all of its vertices with nonzero degree belong to a
- - single connected component of the underlying undirected graph.
+ single connected component of the underlying undirected graph.
An undirected graph has an Eulerian path iff:
- exactly zero or two vertices have odd degree,
@@ -246,16 +246,28 @@ def has_eulerian_path(G):
eulerian_path
"""
if G.is_directed():
+ # Remove isolated nodes (if any) without altering the input graph
+ nodes_remove = [v for v in G if G.in_degree[v] == 0 and G.out_degree[v] == 0]
+ if nodes_remove:
+ G = G.copy()
+ G.remove_nodes_from(nodes_remove)
+
ins = G.in_degree
outs = G.out_degree
- semibalanced_ins = sum(ins(v) - outs(v) == 1 for v in G)
- semibalanced_outs = sum(outs(v) - ins(v) == 1 for v in G)
+ unbalanced_ins = 0
+ unbalanced_outs = 0
+ for v in G:
+ if ins[v] - outs[v] == 1:
+ unbalanced_ins += 1
+ elif outs[v] - ins[v] == 1:
+ unbalanced_outs += 1
+ elif ins[v] != outs[v]:
+ return False
+
return (
- semibalanced_ins <= 1
- and semibalanced_outs <= 1
- and sum(G.in_degree(v) != G.out_degree(v) for v in G) <= 2
- and nx.is_weakly_connected(G)
+ unbalanced_ins <= 1 and unbalanced_outs <= 1 and nx.is_weakly_connected(G)
)
+
else:
return sum(d % 2 == 1 for v, d in G.degree()) in (0, 2) and nx.is_connected(G)
diff --git a/networkx/algorithms/minors.py b/networkx/algorithms/minors.py
index 336fe254f..7958bceca 100644
--- a/networkx/algorithms/minors.py
+++ b/networkx/algorithms/minors.py
@@ -445,37 +445,36 @@ def contracted_nodes(G, u, v, self_loops=True, copy=True):
not be the same as the edge keys for the old edges. This is
natural because edge keys are unique only within each pair of nodes.
+ This function is also available as `identified_nodes`.
+
Examples
--------
Contracting two nonadjacent nodes of the cycle graph on four nodes `C_4`
yields the path graph (ignoring parallel edges)::
- >>> G = nx.cycle_graph(4)
- >>> M = nx.contracted_nodes(G, 1, 3)
- >>> P3 = nx.path_graph(3)
- >>> nx.is_isomorphic(M, P3)
- True
-
- >>> G = nx.MultiGraph(P3)
- >>> M = nx.contracted_nodes(G, 0, 2)
- >>> M.edges
- MultiEdgeView([(0, 1, 0), (0, 1, 1)])
-
- >>> G = nx.Graph([(1, 2), (2, 2)])
- >>> H = nx.contracted_nodes(G, 1, 2, self_loops=False)
- >>> list(H.nodes())
- [1]
- >>> list(H.edges())
- [(1, 1)]
-
- See also
+ >>> G = nx.cycle_graph(4)
+ >>> M = nx.contracted_nodes(G, 1, 3)
+ >>> P3 = nx.path_graph(3)
+ >>> nx.is_isomorphic(M, P3)
+ True
+
+ >>> G = nx.MultiGraph(P3)
+ >>> M = nx.contracted_nodes(G, 0, 2)
+ >>> M.edges
+ MultiEdgeView([(0, 1, 0), (0, 1, 1)])
+
+ >>> G = nx.Graph([(1, 2), (2, 2)])
+ >>> H = nx.contracted_nodes(G, 1, 2, self_loops=False)
+ >>> list(H.nodes())
+ [1]
+ >>> list(H.edges())
+ [(1, 1)]
+
+ See Also
--------
contracted_edge
quotient_graph
- Notes
- -----
- This function is also available as `identified_nodes`.
"""
# Copying has significant overhead and can be disabled if needed
if copy:
diff --git a/networkx/classes/function.py b/networkx/classes/function.py
index d17e22f60..700cac158 100644
--- a/networkx/classes/function.py
+++ b/networkx/classes/function.py
@@ -576,23 +576,14 @@ def info(G, n=None):
"""
if n is None:
- n_nodes = G.number_of_nodes()
- n_edges = G.number_of_edges()
- return "".join(
- [
- type(G).__name__,
- f" named '{G.name}'" if G.name else "",
- f" with {n_nodes} nodes and {n_edges} edges",
- ]
- )
- else:
- if n not in G:
- raise nx.NetworkXError(f"node {n} not in graph")
- info = "" # append this all to a string
- info += f"Node {n} has the following properties:\n"
- info += f"Degree: {G.degree(n)}\n"
- info += "Neighbors: "
- info += " ".join(str(nbr) for nbr in G.neighbors(n))
+ return str(G)
+ if n not in G:
+ raise nx.NetworkXError(f"node {n} not in graph")
+ info = "" # append this all to a string
+ info += f"Node {n} has the following properties:\n"
+ info += f"Degree: {G.degree(n)}\n"
+ info += "Neighbors: "
+ info += " ".join(str(nbr) for nbr in G.neighbors(n))
return info
diff --git a/networkx/classes/graph.py b/networkx/classes/graph.py
index ae34047bc..8629ee808 100644
--- a/networkx/classes/graph.py
+++ b/networkx/classes/graph.py
@@ -370,20 +370,31 @@ class Graph:
self.graph["name"] = s
def __str__(self):
- """Returns the graph name.
+ """Returns a short summary of the graph.
Returns
-------
- name : string
- The name of the graph.
+ info : string
+ Graph information as provided by `nx.info`
Examples
--------
>>> G = nx.Graph(name="foo")
>>> str(G)
- 'foo'
+ "Graph named 'foo' with 0 nodes and 0 edges"
+
+ >>> G = nx.path_graph(3)
+ >>> str(G)
+ 'Graph with 3 nodes and 2 edges'
+
"""
- return self.name
+ return "".join(
+ [
+ type(self).__name__,
+ f" named '{self.name}'" if self.name else "",
+ f" with {self.number_of_nodes()} nodes and {self.number_of_edges()} edges",
+ ]
+ )
def __iter__(self):
"""Iterate over the nodes. Use: 'for n in G'.
| networkx/networkx | 2f0c56ffdc923c5fce04935447772ba9f68b69f9 | diff --git a/networkx/algorithms/tests/test_euler.py b/networkx/algorithms/tests/test_euler.py
index f136ab0ff..4a0905bc8 100644
--- a/networkx/algorithms/tests/test_euler.py
+++ b/networkx/algorithms/tests/test_euler.py
@@ -134,6 +134,22 @@ class TestHasEulerianPath:
G = nx.path_graph(6, create_using=nx.DiGraph)
assert nx.has_eulerian_path(G)
+ def test_has_eulerian_path_directed_graph(self):
+ # Test directed graphs and returns False
+ G = nx.DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (0, 2)])
+ assert not nx.has_eulerian_path(G)
+
+ def test_has_eulerian_path_isolated_node(self):
+ # Test directed graphs without isolated node returns True
+ G = nx.DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 0)])
+ assert nx.has_eulerian_path(G)
+
+ # Test directed graphs with isolated node returns True
+ G.add_node(3)
+ assert nx.has_eulerian_path(G)
+
class TestFindPathStart:
def testfind_path_start(self):
diff --git a/networkx/classes/tests/historical_tests.py b/networkx/classes/tests/historical_tests.py
index 8f53c4c53..c19396add 100644
--- a/networkx/classes/tests/historical_tests.py
+++ b/networkx/classes/tests/historical_tests.py
@@ -21,7 +21,6 @@ class HistoricalTests:
def test_name(self):
G = self.G(name="test")
- assert str(G) == "test"
assert G.name == "test"
H = self.G()
assert H.name == ""
diff --git a/networkx/classes/tests/test_graph.py b/networkx/classes/tests/test_graph.py
index 03902b092..e90f99510 100644
--- a/networkx/classes/tests/test_graph.py
+++ b/networkx/classes/tests/test_graph.py
@@ -182,9 +182,18 @@ class BaseAttrGraphTester(BaseGraphTester):
G = self.Graph(name="")
assert G.name == ""
G = self.Graph(name="test")
- assert G.__str__() == "test"
assert G.name == "test"
+ def test_str_unnamed(self):
+ G = self.Graph()
+ G.add_edges_from([(1, 2), (2, 3)])
+ assert str(G) == f"{type(G).__name__} with 3 nodes and 2 edges"
+
+ def test_str_named(self):
+ G = self.Graph(name="foo")
+ G.add_edges_from([(1, 2), (2, 3)])
+ assert str(G) == f"{type(G).__name__} named 'foo' with 3 nodes and 2 edges"
+
def test_graph_chain(self):
G = self.Graph([(0, 1), (1, 2)])
DG = G.to_directed(as_view=True)
| Issue with networkx.has_eulerian_path()
This issue adds to #3976
There appears to be a problem with the `has_eulerian_path` function. It returns the wrong answer on this example:
```
test_graph = nx.DiGraph()
test_graph.add_edges_from([(0, 1), (1,2), (0,2)])
print(nx.has_eulerian_path(test_graph))
print(list(nx.eulerian_path(test_graph)))
```
Output:
```
True
[(0, 0), (0, 1), (1, 2)]
```
This directed graph does not have a Eulerian path, and, adding to #3976, the path returned by `eulerian_path` is not an actual Eulerian path, since it (1) does not include the edge `(0,2)` and (2) includes a self-loop `(0,0)` that is not in the graph.
My hunch is that the problem is in this line: https://github.com/networkx/networkx/blob/3351206a3ce5b3a39bb2fc451e93ef545b96c95b/networkx/algorithms/euler.py#L256
I think changing that `2` to be `semibalanced_ins+semibalanced_outs` may do the trick, since the definition is
>"...every _other_ vertex has equal in\_degree and out\_degree..."
Going to see about fixing and adding this test. Appreciate any clues, ideas or tips.
| 0.0 | [
"networkx/algorithms/tests/test_euler.py::TestHasEulerianPath::test_has_eulerian_path_directed_graph",
"networkx/algorithms/tests/test_euler.py::TestHasEulerianPath::test_has_eulerian_path_isolated_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_str_unnamed",
"networkx/classes/tests/test_graph.py::TestGraph::test_str_named"
] | [
"networkx/algorithms/tests/test_euler.py::TestIsEulerian::test_is_eulerian",
"networkx/algorithms/tests/test_euler.py::TestIsEulerian::test_is_eulerian2",
"networkx/algorithms/tests/test_euler.py::TestEulerianCircuit::test_eulerian_circuit_cycle",
"networkx/algorithms/tests/test_euler.py::TestEulerianCircuit::test_eulerian_circuit_digraph",
"networkx/algorithms/tests/test_euler.py::TestEulerianCircuit::test_multigraph",
"networkx/algorithms/tests/test_euler.py::TestEulerianCircuit::test_multigraph_with_keys",
"networkx/algorithms/tests/test_euler.py::TestEulerianCircuit::test_not_eulerian",
"networkx/algorithms/tests/test_euler.py::TestIsSemiEulerian::test_is_semieulerian",
"networkx/algorithms/tests/test_euler.py::TestHasEulerianPath::test_has_eulerian_path_cyclic",
"networkx/algorithms/tests/test_euler.py::TestHasEulerianPath::test_has_eulerian_path_non_cyclic",
"networkx/algorithms/tests/test_euler.py::TestFindPathStart::testfind_path_start",
"networkx/algorithms/tests/test_euler.py::TestEulerianPath::test_eulerian_path",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_disconnected",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_null_graph",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_null_multigraph",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_on_empty_graph",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_on_eulerian",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_on_eulerian_multigraph",
"networkx/algorithms/tests/test_euler.py::TestEulerize::test_on_complete_graph",
"networkx/classes/tests/test_graph.py::TestGraph::test_contains",
"networkx/classes/tests/test_graph.py::TestGraph::test_order",
"networkx/classes/tests/test_graph.py::TestGraph::test_nodes",
"networkx/classes/tests/test_graph.py::TestGraph::test_has_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_has_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_neighbors",
"networkx/classes/tests/test_graph.py::TestGraph::test_memory_leak",
"networkx/classes/tests/test_graph.py::TestGraph::test_edges",
"networkx/classes/tests/test_graph.py::TestGraph::test_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_size",
"networkx/classes/tests/test_graph.py::TestGraph::test_nbunch_iter",
"networkx/classes/tests/test_graph.py::TestGraph::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloop_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloops",
"networkx/classes/tests/test_graph.py::TestGraph::test_weighted_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_name",
"networkx/classes/tests/test_graph.py::TestGraph::test_graph_chain",
"networkx/classes/tests/test_graph.py::TestGraph::test_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_class_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_fresh_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_graph_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_node_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_node_attr2",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_lookup",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr2",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr3",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr4",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_undirected",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_directed",
"networkx/classes/tests/test_graph.py::TestGraph::test_subgraph",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloops_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_pickle",
"networkx/classes/tests/test_graph.py::TestGraph::test_data_input",
"networkx/classes/tests/test_graph.py::TestGraph::test_adjacency",
"networkx/classes/tests/test_graph.py::TestGraph::test_getitem",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_nodes_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_nodes_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_edges_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_edges_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_clear",
"networkx/classes/tests/test_graph.py::TestGraph::test_clear_edges",
"networkx/classes/tests/test_graph.py::TestGraph::test_edges_data",
"networkx/classes/tests/test_graph.py::TestGraph::test_get_edge_data",
"networkx/classes/tests/test_graph.py::TestGraph::test_update",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_correct_nodes",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_correct_edges",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_add_node",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_remove_node",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_node_attr_dict",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_edge_attr_dict",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_graph_attr_dict"
] | 2020-10-08 20:32:04+00:00 | 4,136 |
|
networkx__networkx-4461 | diff --git a/doc/reference/algorithms/approximation.rst b/doc/reference/algorithms/approximation.rst
index 5b207d4fb..fe3dc53e0 100644
--- a/doc/reference/algorithms/approximation.rst
+++ b/doc/reference/algorithms/approximation.rst
@@ -107,3 +107,13 @@ Vertex Cover
:toctree: generated/
min_weighted_vertex_cover
+
+
+Max Cut
+-------
+.. automodule:: networkx.algorithms.approximation.maxcut
+.. autosummary::
+ :toctree: generated/
+
+ randomized_partitioning
+ one_exchange
diff --git a/networkx/algorithms/approximation/__init__.py b/networkx/algorithms/approximation/__init__.py
index 99e8340b4..631a2c353 100644
--- a/networkx/algorithms/approximation/__init__.py
+++ b/networkx/algorithms/approximation/__init__.py
@@ -20,3 +20,4 @@ from networkx.algorithms.approximation.ramsey import *
from networkx.algorithms.approximation.steinertree import *
from networkx.algorithms.approximation.vertex_cover import *
from networkx.algorithms.approximation.treewidth import *
+from networkx.algorithms.approximation.maxcut import *
diff --git a/networkx/algorithms/approximation/maxcut.py b/networkx/algorithms/approximation/maxcut.py
new file mode 100644
index 000000000..47e5c70ca
--- /dev/null
+++ b/networkx/algorithms/approximation/maxcut.py
@@ -0,0 +1,111 @@
+import networkx as nx
+from networkx.utils.decorators import py_random_state
+
+__all__ = ["randomized_partitioning", "one_exchange"]
+
+
[email protected]_implemented_for("directed", "multigraph")
+@py_random_state(1)
+def randomized_partitioning(G, seed=None, p=0.5, weight=None):
+ """Compute a random partitioning of the graph nodes and its cut value.
+
+ A partitioning is calculated by observing each node
+ and deciding to add it to the partition with probability `p`,
+ returning a random cut and its corresponding value (the
+ sum of weights of edges connecting different partitions).
+
+ Parameters
+ ----------
+ G : NetworkX graph
+
+ seed : integer, random_state, or None (default)
+ Indicator of random number generation state.
+ See :ref:`Randomness<randomness>`.
+
+ p : scalar
+ Probability for each node to be part of the first partition.
+ Should be in [0,1]
+
+ weight : object
+ Edge attribute key to use as weight. If not specified, edges
+ have weight one.
+
+ Returns
+ -------
+ cut_size : scalar
+ Value of the minimum cut.
+
+ partition : pair of node sets
+ A partitioning of the nodes that defines a minimum cut.
+ """
+ cut = {node for node in G.nodes() if seed.random() < p}
+ cut_size = nx.algorithms.cut_size(G, cut, weight=weight)
+ partition = (cut, G.nodes - cut)
+ return cut_size, partition
+
+
+def _swap_node_partition(cut, node):
+ return cut - {node} if node in cut else cut.union({node})
+
+
[email protected]_implemented_for("directed", "multigraph")
+@py_random_state(2)
+def one_exchange(G, initial_cut=None, seed=None, weight=None):
+ """Compute a partitioning of the graphs nodes and the corresponding cut value.
+
+ Use a greedy one exchange strategy to find a locally maximal cut
+ and its value, it works by finding the best node (one that gives
+ the highest gain to the cut value) to add to the current cut
+ and repeats this process until no improvement can be made.
+
+ Parameters
+ ----------
+ G : networkx Graph
+ Graph to find a maximum cut for.
+
+ initial_cut : set
+ Cut to use as a starting point. If not supplied the algorithm
+ starts with an empty cut.
+
+ seed : integer, random_state, or None (default)
+ Indicator of random number generation state.
+ See :ref:`Randomness<randomness>`.
+
+ weight : object
+ Edge attribute key to use as weight. If not specified, edges
+ have weight one.
+
+ Returns
+ -------
+ cut_value : scalar
+ Value of the maximum cut.
+
+ partition : pair of node sets
+ A partitioning of the nodes that defines a maximum cut.
+ """
+ if initial_cut is None:
+ initial_cut = set()
+ cut = set(initial_cut)
+ current_cut_size = nx.algorithms.cut_size(G, cut, weight=weight)
+ while True:
+ nodes = list(G.nodes())
+ # Shuffling the nodes ensures random tie-breaks in the following call to max
+ seed.shuffle(nodes)
+ best_node_to_swap = max(
+ nodes,
+ key=lambda v: nx.algorithms.cut_size(
+ G, _swap_node_partition(cut, v), weight=weight
+ ),
+ default=None,
+ )
+ potential_cut = _swap_node_partition(cut, best_node_to_swap)
+ potential_cut_size = nx.algorithms.cut_size(G, potential_cut, weight=weight)
+
+ if potential_cut_size > current_cut_size:
+ cut = potential_cut
+ current_cut_size = potential_cut_size
+ else:
+ break
+
+ partition = (cut, G.nodes - cut)
+ return current_cut_size, partition
diff --git a/networkx/generators/classic.py b/networkx/generators/classic.py
index 9968d20f7..b89aca3dc 100644
--- a/networkx/generators/classic.py
+++ b/networkx/generators/classic.py
@@ -188,7 +188,7 @@ def barbell_graph(m1, m2, create_using=None):
return G
-def binomial_tree(n):
+def binomial_tree(n, create_using=None):
"""Returns the Binomial Tree of order n.
The binomial tree of order 0 consists of a single node. A binomial tree of order k
@@ -200,16 +200,21 @@ def binomial_tree(n):
n : int
Order of the binomial tree.
+ create_using : NetworkX graph constructor, optional (default=nx.Graph)
+ Graph type to create. If graph instance, then cleared before populated.
+
Returns
-------
G : NetworkX graph
A binomial tree of $2^n$ nodes and $2^n - 1$ edges.
"""
- G = nx.empty_graph(1)
+ G = nx.empty_graph(1, create_using)
+
N = 1
for i in range(n):
- edges = [(u + N, v + N) for (u, v) in G.edges]
+ # Use G.edges() to ensure 2-tuples. G.edges is 3-tuple for MultiGraph
+ edges = [(u + N, v + N) for (u, v) in G.edges()]
G.add_edges_from(edges)
G.add_edge(0, N)
N *= 2
| networkx/networkx | f542e5fb92d12ec42570d14df867d144a9e8ba4f | diff --git a/networkx/algorithms/approximation/tests/test_maxcut.py b/networkx/algorithms/approximation/tests/test_maxcut.py
new file mode 100644
index 000000000..897d25594
--- /dev/null
+++ b/networkx/algorithms/approximation/tests/test_maxcut.py
@@ -0,0 +1,82 @@
+import random
+
+import networkx as nx
+from networkx.algorithms.approximation import maxcut
+
+
+def _is_valid_cut(G, set1, set2):
+ union = set1.union(set2)
+ assert union == set(G.nodes)
+ assert len(set1) + len(set2) == G.number_of_nodes()
+
+
+def _cut_is_locally_optimal(G, cut_size, set1):
+ # test if cut can be locally improved
+ for i, node in enumerate(set1):
+ cut_size_without_node = nx.algorithms.cut_size(
+ G, set1 - {node}, weight="weight"
+ )
+ assert cut_size_without_node <= cut_size
+
+
+def test_random_partitioning():
+ G = nx.complete_graph(5)
+ _, (set1, set2) = maxcut.randomized_partitioning(G, seed=5)
+ _is_valid_cut(G, set1, set2)
+
+
+def test_random_partitioning_all_to_one():
+ G = nx.complete_graph(5)
+ _, (set1, set2) = maxcut.randomized_partitioning(G, p=1)
+ _is_valid_cut(G, set1, set2)
+ assert len(set1) == G.number_of_nodes()
+ assert len(set2) == 0
+
+
+def test_one_exchange_basic():
+ G = nx.complete_graph(5)
+ random.seed(5)
+ for (u, v, w) in G.edges(data=True):
+ w["weight"] = random.randrange(-100, 100, 1) / 10
+
+ initial_cut = set(random.sample(G.nodes(), k=5))
+ cut_size, (set1, set2) = maxcut.one_exchange(
+ G, initial_cut, weight="weight", seed=5
+ )
+
+ _is_valid_cut(G, set1, set2)
+ _cut_is_locally_optimal(G, cut_size, set1)
+
+
+def test_one_exchange_optimal():
+ # Greedy one exchange should find the optimal solution for this graph (14)
+ G = nx.Graph()
+ G.add_edge(1, 2, weight=3)
+ G.add_edge(1, 3, weight=3)
+ G.add_edge(1, 4, weight=3)
+ G.add_edge(1, 5, weight=3)
+ G.add_edge(2, 3, weight=5)
+
+ cut_size, (set1, set2) = maxcut.one_exchange(G, weight="weight", seed=5)
+
+ _is_valid_cut(G, set1, set2)
+ _cut_is_locally_optimal(G, cut_size, set1)
+ # check global optimality
+ assert cut_size == 14
+
+
+def test_negative_weights():
+ G = nx.complete_graph(5)
+ random.seed(5)
+ for (u, v, w) in G.edges(data=True):
+ w["weight"] = -1 * random.random()
+
+ initial_cut = set(random.sample(G.nodes(), k=5))
+ cut_size, (set1, set2) = maxcut.one_exchange(G, initial_cut, weight="weight")
+
+ # make sure it is a valid cut
+ _is_valid_cut(G, set1, set2)
+ # check local optimality
+ _cut_is_locally_optimal(G, cut_size, set1)
+ # test that all nodes are in the same partition
+ assert len(set1) == len(G.nodes) or len(set2) == len(G.nodes)
diff --git a/networkx/generators/tests/test_classic.py b/networkx/generators/tests/test_classic.py
index c98eb8f5a..3ec36f0f9 100644
--- a/networkx/generators/tests/test_classic.py
+++ b/networkx/generators/tests/test_classic.py
@@ -138,10 +138,12 @@ class TestGeneratorClassic:
assert_edges_equal(mb.edges(), b.edges())
def test_binomial_tree(self):
- for n in range(0, 4):
- b = nx.binomial_tree(n)
- assert nx.number_of_nodes(b) == 2 ** n
- assert nx.number_of_edges(b) == (2 ** n - 1)
+ graphs = (None, nx.Graph, nx.DiGraph, nx.MultiGraph, nx.MultiDiGraph)
+ for create_using in graphs:
+ for n in range(0, 4):
+ b = nx.binomial_tree(n, create_using)
+ assert nx.number_of_nodes(b) == 2 ** n
+ assert nx.number_of_edges(b) == (2 ** n - 1)
def test_complete_graph(self):
# complete_graph(m) is a connected graph with
| Allow binomial_tree() to create directed graphs (via create_using parameter)
`binomial_tree() ` doesn't seem to allow the `create_using` parameter to get passed and as a result, one can't create a directed binomial graph.
This seem like a inadvertent omission and easy to fix. I will put in a PR to address this soon. | 0.0 | [
"networkx/algorithms/approximation/tests/test_maxcut.py::test_random_partitioning",
"networkx/algorithms/approximation/tests/test_maxcut.py::test_random_partitioning_all_to_one",
"networkx/algorithms/approximation/tests/test_maxcut.py::test_one_exchange_basic",
"networkx/algorithms/approximation/tests/test_maxcut.py::test_one_exchange_optimal",
"networkx/algorithms/approximation/tests/test_maxcut.py::test_negative_weights",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_balanced_tree",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_balanced_tree_star",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_balanced_tree_path",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_full_rary_tree",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_full_rary_tree_balanced",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_full_rary_tree_path",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_full_rary_tree_empty",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_full_rary_tree_3_20",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_barbell_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_binomial_tree",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_digraph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_circular_ladder_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_circulant_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_cycle_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_dorogovtsev_goltsev_mendes_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_create_using",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_empty_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_ladder_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_lollipop_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_null_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_path_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_star_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_trivial_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_turan_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_wheel_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_0_partite_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_1_partite_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_2_partite_graph",
"networkx/generators/tests/test_classic.py::TestGeneratorClassic::test_complete_multipartite_graph"
] | [] | 2020-12-14 02:51:50+00:00 | 4,137 |
|
networkx__networkx-4667 | diff --git a/networkx/algorithms/bipartite/matching.py b/networkx/algorithms/bipartite/matching.py
index 279e01604..2059d6bdf 100644
--- a/networkx/algorithms/bipartite/matching.py
+++ b/networkx/algorithms/bipartite/matching.py
@@ -346,14 +346,14 @@ def _is_connected_by_alternating_path(G, v, matched_edges, unmatched_edges, targ
will continue only through edges *not* in the given matching.
"""
- if along_matched:
- edges = itertools.cycle([matched_edges, unmatched_edges])
- else:
- edges = itertools.cycle([unmatched_edges, matched_edges])
visited = set()
- stack = [(u, iter(G[u]), next(edges))]
+ # Follow matched edges when depth is even,
+ # and follow unmatched edges when depth is odd.
+ initial_depth = 0 if along_matched else 1
+ stack = [(u, iter(G[u]), initial_depth)]
while stack:
- parent, children, valid_edges = stack[-1]
+ parent, children, depth = stack[-1]
+ valid_edges = matched_edges if depth % 2 else unmatched_edges
try:
child = next(children)
if child not in visited:
@@ -361,7 +361,7 @@ def _is_connected_by_alternating_path(G, v, matched_edges, unmatched_edges, targ
if child in targets:
return True
visited.add(child)
- stack.append((child, iter(G[child]), next(edges)))
+ stack.append((child, iter(G[child]), depth + 1))
except StopIteration:
stack.pop()
return False
diff --git a/networkx/algorithms/tournament.py b/networkx/algorithms/tournament.py
index 2205f5556..cedd2bc06 100644
--- a/networkx/algorithms/tournament.py
+++ b/networkx/algorithms/tournament.py
@@ -276,7 +276,6 @@ def is_reachable(G, s, t):
tournaments."
*Electronic Colloquium on Computational Complexity*. 2001.
<http://eccc.hpi-web.de/report/2001/092/>
-
"""
def two_neighborhood(G, v):
| networkx/networkx | e03144e0780898fed4afa976fff86f2710aa5d40 | diff --git a/networkx/algorithms/bipartite/tests/test_matching.py b/networkx/algorithms/bipartite/tests/test_matching.py
index d4fcd9802..3c6cc35fd 100644
--- a/networkx/algorithms/bipartite/tests/test_matching.py
+++ b/networkx/algorithms/bipartite/tests/test_matching.py
@@ -180,6 +180,16 @@ class TestMatching:
for u, v in G.edges():
assert u in vertex_cover or v in vertex_cover
+ def test_vertex_cover_issue_3306(self):
+ G = nx.Graph()
+ edges = [(0, 2), (1, 0), (1, 1), (1, 2), (2, 2)]
+ G.add_edges_from([((i, "L"), (j, "R")) for i, j in edges])
+
+ matching = maximum_matching(G)
+ vertex_cover = to_vertex_cover(G, matching)
+ for u, v in G.edges():
+ assert u in vertex_cover or v in vertex_cover
+
def test_unorderable_nodes(self):
a = object()
b = object()
diff --git a/networkx/algorithms/tests/test_tournament.py b/networkx/algorithms/tests/test_tournament.py
index 8de6f7c3e..42a31f9be 100644
--- a/networkx/algorithms/tests/test_tournament.py
+++ b/networkx/algorithms/tests/test_tournament.py
@@ -1,132 +1,157 @@
"""Unit tests for the :mod:`networkx.algorithms.tournament` module."""
from itertools import combinations
-
-
+import pytest
from networkx import DiGraph
from networkx.algorithms.tournament import is_reachable
from networkx.algorithms.tournament import is_strongly_connected
from networkx.algorithms.tournament import is_tournament
from networkx.algorithms.tournament import random_tournament
from networkx.algorithms.tournament import hamiltonian_path
+from networkx.algorithms.tournament import score_sequence
+from networkx.algorithms.tournament import tournament_matrix
+from networkx.algorithms.tournament import index_satisfying
+
+
+def test_condition_not_satisfied():
+ condition = lambda x: x > 0
+ iter_in = [0]
+ assert index_satisfying(iter_in, condition) == 1
+
+
+def test_empty_iterable():
+ condition = lambda x: x > 0
+ with pytest.raises(ValueError):
+ index_satisfying([], condition)
-class TestIsTournament:
- """Unit tests for the :func:`networkx.tournament.is_tournament`
- function.
+def test_is_tournament():
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+ assert is_tournament(G)
+
+
+def test_self_loops():
+ """A tournament must have no self-loops."""
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+ G.add_edge(0, 0)
+ assert not is_tournament(G)
+
+
+def test_missing_edges():
+ """A tournament must not have any pair of nodes without at least
+ one edge joining the pair.
"""
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3)])
+ assert not is_tournament(G)
+
- def test_is_tournament(self):
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+def test_bidirectional_edges():
+ """A tournament must not have any pair of nodes with greater
+ than one edge joining the pair.
+
+ """
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+ G.add_edge(1, 0)
+ assert not is_tournament(G)
+
+
+def test_graph_is_tournament():
+ for _ in range(10):
+ G = random_tournament(5)
assert is_tournament(G)
- def test_self_loops(self):
- """A tournament must have no self-loops."""
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
- G.add_edge(0, 0)
- assert not is_tournament(G)
- def test_missing_edges(self):
- """A tournament must not have any pair of nodes without at least
- one edge joining the pair.
+def test_graph_is_tournament_seed():
+ for _ in range(10):
+ G = random_tournament(5, seed=1)
+ assert is_tournament(G)
- """
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3)])
- assert not is_tournament(G)
- def test_bidirectional_edges(self):
- """A tournament must not have any pair of nodes with greater
- than one edge joining the pair.
+def test_graph_is_tournament_one_node():
+ G = random_tournament(1)
+ assert is_tournament(G)
- """
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
- G.add_edge(1, 0)
- assert not is_tournament(G)
+def test_graph_is_tournament_zero_node():
+ G = random_tournament(0)
+ assert is_tournament(G)
-class TestRandomTournament:
- """Unit tests for the :func:`networkx.tournament.random_tournament`
- function.
- """
+def test_hamiltonian_empty_graph():
+ path = hamiltonian_path(DiGraph())
+ assert len(path) == 0
- def test_graph_is_tournament(self):
- for n in range(10):
- G = random_tournament(5)
- assert is_tournament(G)
- def test_graph_is_tournament_seed(self):
- for n in range(10):
- G = random_tournament(5, seed=1)
- assert is_tournament(G)
+def test_path_is_hamiltonian():
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+ path = hamiltonian_path(G)
+ assert len(path) == 4
+ assert all(v in G[u] for u, v in zip(path, path[1:]))
-class TestHamiltonianPath:
- """Unit tests for the :func:`networkx.tournament.hamiltonian_path`
- function.
+def test_hamiltonian_cycle():
+ """Tests that :func:`networkx.tournament.hamiltonian_path`
+ returns a Hamiltonian cycle when provided a strongly connected
+ tournament.
"""
+ G = DiGraph()
+ G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
+ path = hamiltonian_path(G)
+ assert len(path) == 4
+ assert all(v in G[u] for u, v in zip(path, path[1:]))
+ assert path[0] in G[path[-1]]
- def test_path_is_hamiltonian(self):
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
- path = hamiltonian_path(G)
- assert len(path) == 4
- assert all(v in G[u] for u, v in zip(path, path[1:]))
- def test_hamiltonian_cycle(self):
- """Tests that :func:`networkx.tournament.hamiltonian_path`
- returns a Hamiltonian cycle when provided a strongly connected
- tournament.
+def test_score_sequence_edge():
+ G = DiGraph([(0, 1)])
+ assert score_sequence(G) == [0, 1]
- """
- G = DiGraph()
- G.add_edges_from([(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (0, 2)])
- path = hamiltonian_path(G)
- assert len(path) == 4
- assert all(v in G[u] for u, v in zip(path, path[1:]))
- assert path[0] in G[path[-1]]
+def test_score_sequence_triangle():
+ G = DiGraph([(0, 1), (1, 2), (2, 0)])
+ assert score_sequence(G) == [1, 1, 1]
-class TestReachability:
- """Unit tests for the :func:`networkx.tournament.is_reachable`
- function.
- """
+def test_tournament_matrix():
+ np = pytest.importorskip("numpy")
+ npt = pytest.importorskip("numpy.testing")
+ G = DiGraph([(0, 1)])
+ m = tournament_matrix(G)
+ npt.assert_array_equal(m.todense(), np.array([[0, 1], [-1, 0]]))
- def test_reachable_pair(self):
- """Tests for a reachable pair of nodes."""
- G = DiGraph([(0, 1), (1, 2), (2, 0)])
- assert is_reachable(G, 0, 2)
- def test_same_node_is_reachable(self):
- """Tests that a node is always reachable from itself."""
- # G is an arbitrary tournament on ten nodes.
- G = DiGraph(sorted(p) for p in combinations(range(10), 2))
- assert all(is_reachable(G, v, v) for v in G)
+def test_reachable_pair():
+ """Tests for a reachable pair of nodes."""
+ G = DiGraph([(0, 1), (1, 2), (2, 0)])
+ assert is_reachable(G, 0, 2)
- def test_unreachable_pair(self):
- """Tests for an unreachable pair of nodes."""
- G = DiGraph([(0, 1), (0, 2), (1, 2)])
- assert not is_reachable(G, 1, 0)
+def test_same_node_is_reachable():
+ """Tests that a node is always reachable from it."""
+ # G is an arbitrary tournament on ten nodes.
+ G = DiGraph(sorted(p) for p in combinations(range(10), 2))
+ assert all(is_reachable(G, v, v) for v in G)
-class TestStronglyConnected:
- """Unit tests for the
- :func:`networkx.tournament.is_strongly_connected` function.
- """
+def test_unreachable_pair():
+ """Tests for an unreachable pair of nodes."""
+ G = DiGraph([(0, 1), (0, 2), (1, 2)])
+ assert not is_reachable(G, 1, 0)
+
+
+def test_is_strongly_connected():
+ """Tests for a strongly connected tournament."""
+ G = DiGraph([(0, 1), (1, 2), (2, 0)])
+ assert is_strongly_connected(G)
- def test_is_strongly_connected(self):
- """Tests for a strongly connected tournament."""
- G = DiGraph([(0, 1), (1, 2), (2, 0)])
- assert is_strongly_connected(G)
- def test_not_strongly_connected(self):
- """Tests for a tournament that is not strongly connected."""
- G = DiGraph([(0, 1), (0, 2), (1, 2)])
- assert not is_strongly_connected(G)
+def test_not_strongly_connected():
+ """Tests for a tournament that is not strongly connected."""
+ G = DiGraph([(0, 1), (0, 2), (1, 2)])
+ assert not is_strongly_connected(G)
| hopcroft_karp_matching / to_vertex_cover bug
`to_vertex_cover` must give the **minimum vertex cover** as documented. Wasn't it fixed in #2384 ?
The following code gives random results:
```python
import networkx as nx
nodesU = ['r1','r2','r3','r4','r5','r6','r7','r8']
nodesV = ['c1','c2','c3','c4','c5','c6','c7','c8']
edges = [
('r1','c2'),('r1','c4'),('r1','c5'),('r1','c8'),
('r2','c1'),('r2','c2'),('r2','c3'),('r2','c6'),('r2','c7'),
('r3','c4'),('r3','c5'),
('r4','c2'),('r4','c8'),
('r5','c2'),('r5','c8'),
('r6','c1'),('r6','c2'),('r6','c3'),('r6','c5'),('r6','c6'),('r6','c7'),
('r7','c2'),('r7','c8'),
('r8','c2'),('r8','c4')]
G = nx.Graph()
G.add_nodes_from(nodesU, bipartite=0)
G.add_nodes_from(nodesV, bipartite=1)
G.add_edges_from(edges)
M = nx.bipartite.hopcroft_karp_matching(G, top_nodes = nodesU)
print('matchings M = ', M)
print('|M| = ', len(M))
K = nx.bipartite.to_vertex_cover(G, M, top_nodes = nodesU)
print('minimum cover K = ', K)
print('|K| = ', len(K))
```
Here are the results of multiple runs:
```
matchings M = {'c1': 'r6', 'r7': 'c2', 'r5': 'c8', 'r1': 'c5', 'r8': 'c4', 'r6': 'c1', 'c5': 'r1', 'c8': 'r5', 'r2': 'c7', 'c7': 'r2', 'c2': 'r7', 'c4': 'r8'}
|M| = 12
minimum cover K = {'c1', 'c5', 'c7', 'c2', 'c8', 'c4'}
|K| = 6
matchings M = {'c6': 'r6', 'r6': 'c6', 'r1': 'c4', 'r4': 'c2', 'c3': 'r2', 'r5': 'c8', 'c5': 'r3', 'r3': 'c5', 'c8': 'r5', 'r2': 'c3', 'c4': 'r1', 'c2': 'r4'}
|M| = 12
minimum cover K = {'c6', 'r6', 'c4', 'c5', 'c2', 'c3', 'c8', 'r2'}
|K| = 8
matchings M = {'c8': 'r7', 'r7': 'c8', 'c1': 'r2', 'r3': 'c4', 'r6': 'c7', 'c5': 'r1', 'c2': 'r4', 'c7': 'r6', 'r4': 'c2', 'r2': 'c1', 'c4': 'r3', 'r1': 'c5'}
|M| = 12
minimum cover K = {'r2', 'c8', 'c4', 'c2', 'c5'}
|K| = 5
matchings M = {'r3': 'c4', 'r6': 'c1', 'r1': 'c5', 'c2': 'r7', 'r7': 'c2', 'c8': 'r5', 'r2': 'c7', 'c5': 'r1', 'r5': 'c8', 'c1': 'r6', 'c7': 'r2', 'c4': 'r3'}
|M| = 12
minimum cover K = {'c8', 'c5', 'c7', 'r6', 'c2', 'c4'}
|K| = 6
```
I'm using networkx 2.2 on debian/python3 and windows miniconda3.
The graph is from: [http://tryalgo.org/en/matching/2016/08/05/konig/](http://tryalgo.org/en/matching/2016/08/05/konig/)
Also why `hopcroft_karp_matching` returns matchings and opposite edges ? | 0.0 | [
"networkx/algorithms/bipartite/tests/test_matching.py::TestMatching::test_vertex_cover_issue_3306"
] | [
"networkx/algorithms/bipartite/tests/test_matching.py::TestMatching::test_issue_2127",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMatching::test_vertex_cover_issue_2384",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMatching::test_unorderable_nodes",
"networkx/algorithms/bipartite/tests/test_matching.py::test_eppstein_matching",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_incomplete_graph",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_with_no_full_matching",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_square",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_smaller_left",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_smaller_top_nodes_right",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_smaller_right",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_negative_weights",
"networkx/algorithms/bipartite/tests/test_matching.py::TestMinimumWeightFullMatching::test_minimum_weight_full_matching_different_weight_key",
"networkx/algorithms/tests/test_tournament.py::test_condition_not_satisfied",
"networkx/algorithms/tests/test_tournament.py::test_empty_iterable",
"networkx/algorithms/tests/test_tournament.py::test_is_tournament",
"networkx/algorithms/tests/test_tournament.py::test_self_loops",
"networkx/algorithms/tests/test_tournament.py::test_missing_edges",
"networkx/algorithms/tests/test_tournament.py::test_bidirectional_edges",
"networkx/algorithms/tests/test_tournament.py::test_graph_is_tournament",
"networkx/algorithms/tests/test_tournament.py::test_graph_is_tournament_seed",
"networkx/algorithms/tests/test_tournament.py::test_graph_is_tournament_one_node",
"networkx/algorithms/tests/test_tournament.py::test_graph_is_tournament_zero_node",
"networkx/algorithms/tests/test_tournament.py::test_hamiltonian_empty_graph",
"networkx/algorithms/tests/test_tournament.py::test_path_is_hamiltonian",
"networkx/algorithms/tests/test_tournament.py::test_hamiltonian_cycle",
"networkx/algorithms/tests/test_tournament.py::test_score_sequence_edge",
"networkx/algorithms/tests/test_tournament.py::test_score_sequence_triangle",
"networkx/algorithms/tests/test_tournament.py::test_tournament_matrix",
"networkx/algorithms/tests/test_tournament.py::test_reachable_pair",
"networkx/algorithms/tests/test_tournament.py::test_same_node_is_reachable",
"networkx/algorithms/tests/test_tournament.py::test_unreachable_pair",
"networkx/algorithms/tests/test_tournament.py::test_is_strongly_connected",
"networkx/algorithms/tests/test_tournament.py::test_not_strongly_connected"
] | 2021-03-11 07:17:35+00:00 | 4,138 |
|
networkx__networkx-4753 | diff --git a/networkx/utils/misc.py b/networkx/utils/misc.py
index 4caffbe18..bd58a4ed6 100644
--- a/networkx/utils/misc.py
+++ b/networkx/utils/misc.py
@@ -19,6 +19,30 @@ import uuid
from itertools import tee, chain
import networkx as nx
+__all__ = [
+ "is_string_like",
+ "iterable",
+ "empty_generator",
+ "flatten",
+ "make_list_of_ints",
+ "is_list_of_ints",
+ "make_str",
+ "generate_unique_node",
+ "default_opener",
+ "dict_to_numpy_array",
+ "dict_to_numpy_array1",
+ "dict_to_numpy_array2",
+ "is_iterator",
+ "arbitrary_element",
+ "consume",
+ "pairwise",
+ "groups",
+ "to_tuple",
+ "create_random_state",
+ "create_py_random_state",
+ "PythonRandomInterface",
+]
+
# some cookbook stuff
# used in deciding whether something is a bunch of nodes, edges, etc.
diff --git a/networkx/utils/random_sequence.py b/networkx/utils/random_sequence.py
index 7bd68c798..ad9b34a3c 100644
--- a/networkx/utils/random_sequence.py
+++ b/networkx/utils/random_sequence.py
@@ -7,6 +7,16 @@ import networkx as nx
from networkx.utils import py_random_state
+__all__ = [
+ "powerlaw_sequence",
+ "zipf_rv",
+ "cumulative_distribution",
+ "discrete_sequence",
+ "random_weighted_sample",
+ "weighted_choice",
+]
+
+
# The same helpers for choosing random sequences from distributions
# uses Python's random module
# https://docs.python.org/3/library/random.html
| networkx/networkx | 63f550fef6712d5ea3ae09bbc341d696d65e55e3 | diff --git a/networkx/utils/tests/test__init.py b/networkx/utils/tests/test__init.py
new file mode 100644
index 000000000..ecbcce36d
--- /dev/null
+++ b/networkx/utils/tests/test__init.py
@@ -0,0 +1,11 @@
+import pytest
+
+
+def test_utils_namespace():
+ """Ensure objects are not unintentionally exposed in utils namespace."""
+ with pytest.raises(ImportError):
+ from networkx.utils import nx
+ with pytest.raises(ImportError):
+ from networkx.utils import sys
+ with pytest.raises(ImportError):
+ from networkx.utils import defaultdict, deque
| `networkx.utils.misc` missing `__all__`
The `misc` module in the `utils` package doesn't define an `__all__`. As a consequence, some non-networkx functionality including e.g. objects from `collections` and builtin modules are incorrectly exposed in the `networkx.utils` namespace, including networkx itself:
```python
from networkx.utils import nx # yikes
``` | 0.0 | [
"networkx/utils/tests/test__init.py::test_utils_namespace"
] | [] | 2021-04-23 18:53:02+00:00 | 4,139 |
|
networkx__networkx-5287 | diff --git a/networkx/algorithms/community/louvain.py b/networkx/algorithms/community/louvain.py
index ba7153521..9ee9f51c4 100644
--- a/networkx/algorithms/community/louvain.py
+++ b/networkx/algorithms/community/louvain.py
@@ -185,6 +185,7 @@ def louvain_partitions(
)
if new_mod - mod <= threshold:
return
+ mod = new_mod
graph = _gen_graph(graph, inner_partition)
partition, inner_partition, improvement = _one_level(
graph, m, partition, resolution, is_directed, seed
diff --git a/networkx/readwrite/json_graph/tree.py b/networkx/readwrite/json_graph/tree.py
index 2de2ca9b6..615907eeb 100644
--- a/networkx/readwrite/json_graph/tree.py
+++ b/networkx/readwrite/json_graph/tree.py
@@ -75,6 +75,8 @@ def tree_data(G, root, attrs=None, ident="id", children="children"):
raise TypeError("G is not a tree.")
if not G.is_directed():
raise TypeError("G is not directed.")
+ if not nx.is_weakly_connected(G):
+ raise TypeError("G is not weakly connected.")
# NOTE: to be removed in 3.0
if attrs is not None:
| networkx/networkx | 0cc70051fa0a979b1f1eab4af5b6587a6ebf8334 | diff --git a/networkx/algorithms/community/tests/test_louvain.py b/networkx/algorithms/community/tests/test_louvain.py
index 81bde05b6..8f623fab1 100644
--- a/networkx/algorithms/community/tests/test_louvain.py
+++ b/networkx/algorithms/community/tests/test_louvain.py
@@ -110,3 +110,15 @@ def test_resolution():
partition3 = louvain_communities(G, resolution=2, seed=12)
assert len(partition1) <= len(partition2) <= len(partition3)
+
+
+def test_threshold():
+ G = nx.LFR_benchmark_graph(
+ 250, 3, 1.5, 0.009, average_degree=5, min_community=20, seed=10
+ )
+ partition1 = louvain_communities(G, threshold=0.2, seed=2)
+ partition2 = louvain_communities(G, seed=2)
+ mod1 = modularity(G, partition1)
+ mod2 = modularity(G, partition2)
+
+ assert mod1 < mod2
diff --git a/networkx/readwrite/json_graph/tests/test_tree.py b/networkx/readwrite/json_graph/tests/test_tree.py
index bfbf18163..848edd0b1 100644
--- a/networkx/readwrite/json_graph/tests/test_tree.py
+++ b/networkx/readwrite/json_graph/tests/test_tree.py
@@ -35,6 +35,11 @@ def test_exceptions():
with pytest.raises(TypeError, match="is not directed."):
G = nx.path_graph(3)
tree_data(G, 0)
+ with pytest.raises(TypeError, match="is not weakly connected."):
+ G = nx.path_graph(3, create_using=nx.DiGraph)
+ G.add_edge(2, 0)
+ G.add_node(3)
+ tree_data(G, 0)
with pytest.raises(nx.NetworkXError, match="must be different."):
G = nx.MultiDiGraph()
G.add_node(0)
| `json_graph.tree_data` can cause maximum recursion depth error.
<!-- If you have a general question about NetworkX, please use the discussions tab to create a new discussion -->
<!--- Provide a general summary of the issue in the Title above -->
### Current Behavior
<!--- Tell us what happens instead of the expected behavior -->
Currently the algorithm compares the `n_nodes` with `n_edges` to check if `G` is a tree. https://github.com/networkx/networkx/blob/0cc70051fa0a979b1f1eab4af5b6587a6ebf8334/networkx/readwrite/json_graph/tree.py#L74-L75
This check can be bypassed with specific inputs and cause a recursion error.
### Expected Behavior
<!--- Tell us what should happen -->
The code should check whether there are cycles with `root` as the source and raise an exception.
Another possible fix would be to check if the graph is not weakly connected.
### Steps to Reproduce
<!--- Provide a minimal example that reproduces the bug -->
```Python3
>>> import networkx as nx
>>> G = nx.DiGraph([(1, 2), (2, 3), (3, 1)])
>>> G.add_node(4)
>>> data = nx.json_graph.tree_data(G, 1)
RecursionError: maximum recursion depth exceeded
```
### Environment
<!--- Please provide details about your local environment -->
Python version: 3.8.10
NetworkX version: 2.7rc1.dev0
| 0.0 | [
"networkx/algorithms/community/tests/test_louvain.py::test_threshold",
"networkx/readwrite/json_graph/tests/test_tree.py::test_exceptions"
] | [
"networkx/algorithms/community/tests/test_louvain.py::test_modularity_increase",
"networkx/algorithms/community/tests/test_louvain.py::test_valid_partition",
"networkx/algorithms/community/tests/test_louvain.py::test_partition",
"networkx/algorithms/community/tests/test_louvain.py::test_none_weight_param",
"networkx/algorithms/community/tests/test_louvain.py::test_quality",
"networkx/algorithms/community/tests/test_louvain.py::test_multigraph",
"networkx/algorithms/community/tests/test_louvain.py::test_resolution",
"networkx/readwrite/json_graph/tests/test_tree.py::test_graph",
"networkx/readwrite/json_graph/tests/test_tree.py::test_graph_attributes",
"networkx/readwrite/json_graph/tests/test_tree.py::test_attrs_deprecation"
] | 2022-01-28 11:20:36+00:00 | 4,140 |
|
networkx__networkx-5394 | diff --git a/doc/developer/deprecations.rst b/doc/developer/deprecations.rst
index 77d814532..a155ab765 100644
--- a/doc/developer/deprecations.rst
+++ b/doc/developer/deprecations.rst
@@ -114,3 +114,4 @@ Version 3.0
* In ``algorithms/distance_measures.py`` remove ``extrema_bounding``.
* In ``utils/misc.py`` remove ``dict_to_numpy_array1`` and ``dict_to_numpy_array2``.
* In ``utils/misc.py`` remove ``to_tuple``.
+* In ``algorithms/matching.py``, remove parameter ``maxcardinality`` from ``min_weight_matching``.
diff --git a/doc/release/release_dev.rst b/doc/release/release_dev.rst
index 53b5562bb..dc6240670 100644
--- a/doc/release/release_dev.rst
+++ b/doc/release/release_dev.rst
@@ -46,6 +46,10 @@ Improvements
API Changes
-----------
+- [`#5394 <https://github.com/networkx/networkx/pull/5394>`_]
+ The function ``min_weight_matching`` no longer acts upon the parameter ``maxcardinality``
+ because setting it to False would result in the min_weight_matching being no edges
+ at all. The only resonable option is True. The parameter will be removed completely in v3.0.
Deprecations
------------
diff --git a/networkx/algorithms/matching.py b/networkx/algorithms/matching.py
index 2a57e9a13..fda0b42cd 100644
--- a/networkx/algorithms/matching.py
+++ b/networkx/algorithms/matching.py
@@ -227,28 +227,49 @@ def is_perfect_matching(G, matching):
@not_implemented_for("multigraph")
@not_implemented_for("directed")
-def min_weight_matching(G, maxcardinality=False, weight="weight"):
+def min_weight_matching(G, maxcardinality=None, weight="weight"):
"""Computing a minimum-weight maximal matching of G.
- Use reciprocal edge weights with the maximum-weight algorithm.
+ Use the maximum-weight algorithm with edge weights subtracted
+ from the maximum weight of all edges.
A matching is a subset of edges in which no node occurs more than once.
The weight of a matching is the sum of the weights of its edges.
A maximal matching cannot add more edges and still be a matching.
The cardinality of a matching is the number of matched edges.
- This method replaces the weights with their reciprocal and
- then runs :func:`max_weight_matching`.
- Read the documentation of max_weight_matching for more information.
+ This method replaces the edge weights with 1 plus the maximum edge weight
+ minus the original edge weight.
+
+ new_weight = (max_weight + 1) - edge_weight
+
+ then runs :func:`max_weight_matching` with the new weights.
+ The max weight matching with these new weights corresponds
+ to the min weight matching using the original weights.
+ Adding 1 to the max edge weight keeps all edge weights positive
+ and as integers if they started as integers.
+
+ You might worry that adding 1 to each weight would make the algorithm
+ favor matchings with more edges. But we use the parameter
+ `maxcardinality=True` in `max_weight_matching` to ensure that the
+ number of edges in the competing matchings are the same and thus
+ the optimum does not change due to changes in the number of edges.
+
+ Read the documentation of `max_weight_matching` for more information.
Parameters
----------
G : NetworkX graph
Undirected graph
- maxcardinality: bool, optional (default=False)
- If maxcardinality is True, compute the maximum-cardinality matching
- with minimum weight among all maximum-cardinality matchings.
+ maxcardinality: bool
+ .. deprecated:: 2.8
+ The `maxcardinality` parameter will be removed in v3.0.
+ It doesn't make sense to set it to False when looking for
+ a min weight matching because then we just return no edges.
+
+ If maxcardinality is True, compute the maximum-cardinality matching
+ with minimum weight among all maximum-cardinality matchings.
weight: string, optional (default='weight')
Edge data key corresponding to the edge weight.
@@ -258,15 +279,25 @@ def min_weight_matching(G, maxcardinality=False, weight="weight"):
-------
matching : set
A minimal weight matching of the graph.
+
+ See Also
+ --------
+ max_weight_matching
"""
+ if maxcardinality not in (True, None):
+ raise nx.NetworkXError(
+ "The argument maxcardinality does not make sense "
+ "in the context of minimum weight matchings."
+ "It is deprecated and will be removed in v3.0."
+ )
if len(G.edges) == 0:
- return max_weight_matching(G, maxcardinality, weight)
+ return max_weight_matching(G, maxcardinality=True, weight=weight)
G_edges = G.edges(data=weight, default=1)
- min_weight = min(w for _, _, w in G_edges)
+ max_weight = 1 + max(w for _, _, w in G_edges)
InvG = nx.Graph()
- edges = ((u, v, 1 / (1 + w - min_weight)) for u, v, w in G_edges)
+ edges = ((u, v, max_weight - w) for u, v, w in G_edges)
InvG.add_weighted_edges_from(edges, weight=weight)
- return max_weight_matching(InvG, maxcardinality, weight)
+ return max_weight_matching(InvG, maxcardinality=True, weight=weight)
@not_implemented_for("multigraph")
| networkx/networkx | c39511522b272bf65b6a415169cd48d1bf3ff5c1 | diff --git a/networkx/algorithms/tests/test_matching.py b/networkx/algorithms/tests/test_matching.py
index ed436aada..1dcc69dc6 100644
--- a/networkx/algorithms/tests/test_matching.py
+++ b/networkx/algorithms/tests/test_matching.py
@@ -19,15 +19,13 @@ class TestMaxWeightMatching:
assert nx.max_weight_matching(G) == set()
assert nx.min_weight_matching(G) == set()
- def test_trivial2(self):
- """Self loop"""
+ def test_selfloop(self):
G = nx.Graph()
G.add_edge(0, 0, weight=100)
assert nx.max_weight_matching(G) == set()
assert nx.min_weight_matching(G) == set()
- def test_trivial3(self):
- """Single edge"""
+ def test_single_edge(self):
G = nx.Graph()
G.add_edge(0, 1)
assert edges_equal(
@@ -37,8 +35,7 @@ class TestMaxWeightMatching:
nx.min_weight_matching(G), matching_dict_to_set({0: 1, 1: 0})
)
- def test_trivial4(self):
- """Small graph"""
+ def test_two_path(self):
G = nx.Graph()
G.add_edge("one", "two", weight=10)
G.add_edge("two", "three", weight=11)
@@ -51,8 +48,7 @@ class TestMaxWeightMatching:
matching_dict_to_set({"one": "two", "two": "one"}),
)
- def test_trivial5(self):
- """Path"""
+ def test_path(self):
G = nx.Graph()
G.add_edge(1, 2, weight=5)
G.add_edge(2, 3, weight=11)
@@ -70,8 +66,20 @@ class TestMaxWeightMatching:
nx.min_weight_matching(G, 1), matching_dict_to_set({1: 2, 3: 4})
)
- def test_trivial6(self):
- """Small graph with arbitrary weight attribute"""
+ def test_square(self):
+ G = nx.Graph()
+ G.add_edge(1, 4, weight=2)
+ G.add_edge(2, 3, weight=2)
+ G.add_edge(1, 2, weight=1)
+ G.add_edge(3, 4, weight=4)
+ assert edges_equal(
+ nx.max_weight_matching(G), matching_dict_to_set({1: 2, 3: 4})
+ )
+ assert edges_equal(
+ nx.min_weight_matching(G), matching_dict_to_set({1: 4, 2: 3})
+ )
+
+ def test_edge_attribute_name(self):
G = nx.Graph()
G.add_edge("one", "two", weight=10, abcd=11)
G.add_edge("two", "three", weight=11, abcd=10)
@@ -85,7 +93,6 @@ class TestMaxWeightMatching:
)
def test_floating_point_weights(self):
- """Floating point weights"""
G = nx.Graph()
G.add_edge(1, 2, weight=math.pi)
G.add_edge(2, 3, weight=math.exp(1))
@@ -99,7 +106,6 @@ class TestMaxWeightMatching:
)
def test_negative_weights(self):
- """Negative weights"""
G = nx.Graph()
G.add_edge(1, 2, weight=2)
G.add_edge(1, 3, weight=-2)
| min_weight_matching gives incorrect results
<!-- If you have a general question about NetworkX, please use the discussions tab to create a new discussion -->
<!--- Provide a general summary of the issue in the Title above -->
Consider the following graph:
```python
G = nx.Graph()
G.add_edge(1, 4, weight=2)
G.add_edge(2, 3, weight=2)
G.add_edge(1, 2, weight=1)
G.add_edge(3, 4, weight=4)
nx.min_weight_matching(G)
```
### Current Behavior
<!--- Tell us what happens instead of the expected behavior -->
The program returns `{(2, 1), (4, 3)}`
### Expected Behavior
<!--- Tell us what should happen -->
There are two maximal matchings in the graph, (1,2)(3,4) with weight 1+4=5, and (1,4)(2,3) with weight 2+2=4. The minimum weight maximal matching should be (1,4)(2,3) which has weight 4.
### Steps to Reproduce
<!--- Provide a minimal example that reproduces the bug -->
Run the code provided
### Environment
<!--- Please provide details about your local environment -->
Python version: 3.9
NetworkX version: 2.7.1
### Additional context
<!--- Add any other context about the problem here, screenshots, etc. -->
From the documentation and the code, `min_weight_matching` is simply taking the reciprocal of the weights and uses `max_weight_matching`. This logic seems to be faulty in this example: 1/1+1/4>1/2+1/2. | 0.0 | [
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_square"
] | [
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_trivial1",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_selfloop",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_single_edge",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_two_path",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_path",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_edge_attribute_name",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_floating_point_weights",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_negative_weights",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_s_blossom",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_s_t_blossom",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nested_s_blossom",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nested_s_blossom_relabel",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nested_s_blossom_expand",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_s_blossom_relabel_expand",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nested_s_blossom_relabel_expand",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nasty_blossom1",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nasty_blossom2",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nasty_blossom_least_slack",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nasty_blossom_augmenting",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_nasty_blossom_expand_recursively",
"networkx/algorithms/tests/test_matching.py::TestMaxWeightMatching::test_wrong_graph_type",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_dict",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_empty_matching",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_single_edge",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_edge_order",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_valid_matching",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_invalid_input",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_selfloops",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_invalid_matching",
"networkx/algorithms/tests/test_matching.py::TestIsMatching::test_invalid_edge",
"networkx/algorithms/tests/test_matching.py::TestIsMaximalMatching::test_dict",
"networkx/algorithms/tests/test_matching.py::TestIsMaximalMatching::test_valid",
"networkx/algorithms/tests/test_matching.py::TestIsMaximalMatching::test_not_matching",
"networkx/algorithms/tests/test_matching.py::TestIsMaximalMatching::test_not_maximal",
"networkx/algorithms/tests/test_matching.py::TestIsPerfectMatching::test_dict",
"networkx/algorithms/tests/test_matching.py::TestIsPerfectMatching::test_valid",
"networkx/algorithms/tests/test_matching.py::TestIsPerfectMatching::test_valid_not_path",
"networkx/algorithms/tests/test_matching.py::TestIsPerfectMatching::test_not_matching",
"networkx/algorithms/tests/test_matching.py::TestIsPerfectMatching::test_maximal_but_not_perfect",
"networkx/algorithms/tests/test_matching.py::TestMaximalMatching::test_valid_matching",
"networkx/algorithms/tests/test_matching.py::TestMaximalMatching::test_single_edge_matching",
"networkx/algorithms/tests/test_matching.py::TestMaximalMatching::test_self_loops",
"networkx/algorithms/tests/test_matching.py::TestMaximalMatching::test_ordering",
"networkx/algorithms/tests/test_matching.py::TestMaximalMatching::test_wrong_graph_type"
] | 2022-03-15 22:32:49+00:00 | 4,141 |
|
networkx__networkx-5523 | diff --git a/networkx/algorithms/planarity.py b/networkx/algorithms/planarity.py
index 4d1441efc..8f4b29096 100644
--- a/networkx/algorithms/planarity.py
+++ b/networkx/algorithms/planarity.py
@@ -24,6 +24,18 @@ def check_planarity(G, counterexample=False):
If the graph is planar `certificate` is a PlanarEmbedding
otherwise it is a Kuratowski subgraph.
+ Examples
+ --------
+ >>> G = nx.Graph([(0, 1), (0, 2)])
+ >>> is_planar, P = nx.check_planarity(G)
+ >>> print(is_planar)
+ True
+
+ When `G` is planar, a `PlanarEmbedding` instance is returned:
+
+ >>> P.get_data()
+ {0: [1, 2], 1: [0], 2: [0]}
+
Notes
-----
A (combinatorial) embedding consists of cyclic orderings of the incident
@@ -716,6 +728,8 @@ class PlanarEmbedding(nx.DiGraph):
The planar embedding is given by a `combinatorial embedding
<https://en.wikipedia.org/wiki/Graph_embedding#Combinatorial_embedding>`_.
+ .. note:: `check_planarity` is the preferred way to check if a graph is planar.
+
**Neighbor ordering:**
In comparison to a usual graph structure, the embedding also stores the
@@ -761,6 +775,13 @@ class PlanarEmbedding(nx.DiGraph):
For a half-edge (u, v) that is orientated such that u is below v then the
face that belongs to (u, v) is to the right of this half-edge.
+ See Also
+ --------
+ is _planar :
+ Preferred way to check if an existing graph is planar.
+ check_planarity :
+ A convenient way to create a `PlanarEmbedding`. If not planar, it returns a subgraph that shows this.
+
Examples
--------
diff --git a/networkx/algorithms/similarity.py b/networkx/algorithms/similarity.py
index d5f463536..2447a9dce 100644
--- a/networkx/algorithms/similarity.py
+++ b/networkx/algorithms/similarity.py
@@ -745,18 +745,30 @@ def optimize_edit_paths(
N = len(pending_h)
# assert Ce.C.shape == (M + N, M + N)
- g_ind = [
- i
- for i in range(M)
- if pending_g[i][:2] == (u, u)
- or any(pending_g[i][:2] in ((p, u), (u, p)) for p, q in matched_uv)
- ]
- h_ind = [
- j
- for j in range(N)
- if pending_h[j][:2] == (v, v)
- or any(pending_h[j][:2] in ((q, v), (v, q)) for p, q in matched_uv)
- ]
+ # only attempt to match edges after one node match has been made
+ # this will stop self-edges on the first node being automatically deleted
+ # even when a substitution is the better option
+ if matched_uv:
+ g_ind = [
+ i
+ for i in range(M)
+ if pending_g[i][:2] == (u, u)
+ or any(
+ pending_g[i][:2] in ((p, u), (u, p), (p, p)) for p, q in matched_uv
+ )
+ ]
+ h_ind = [
+ j
+ for j in range(N)
+ if pending_h[j][:2] == (v, v)
+ or any(
+ pending_h[j][:2] in ((q, v), (v, q), (q, q)) for p, q in matched_uv
+ )
+ ]
+ else:
+ g_ind = []
+ h_ind = []
+
m = len(g_ind)
n = len(h_ind)
@@ -782,9 +794,9 @@ def optimize_edit_paths(
for p, q in matched_uv
):
continue
- if g == (u, u):
+ if g == (u, u) or any(g == (p, p) for p, q in matched_uv):
continue
- if h == (v, v):
+ if h == (v, v) or any(h == (q, q) for p, q in matched_uv):
continue
C[k, l] = inf
diff --git a/networkx/algorithms/tree/recognition.py b/networkx/algorithms/tree/recognition.py
index 5fbff544e..52da959b6 100644
--- a/networkx/algorithms/tree/recognition.py
+++ b/networkx/algorithms/tree/recognition.py
@@ -157,6 +157,21 @@ def is_forest(G):
b : bool
A boolean that is True if `G` is a forest.
+ Raises
+ ------
+ NetworkXPointlessConcept
+ If `G` is empty.
+
+ Examples
+ --------
+ >>> G = nx.Graph()
+ >>> G.add_edges_from([(1, 2), (1, 3), (2, 4), (2, 5)])
+ >>> nx.is_forest(G)
+ True
+ >>> G.add_edge(4, 1)
+ >>> nx.is_forest(G)
+ False
+
Notes
-----
In another convention, a directed forest is known as a *polyforest* and
@@ -198,6 +213,21 @@ def is_tree(G):
b : bool
A boolean that is True if `G` is a tree.
+ Raises
+ ------
+ NetworkXPointlessConcept
+ If `G` is empty.
+
+ Examples
+ --------
+ >>> G = nx.Graph()
+ >>> G.add_edges_from([(1, 2), (1, 3), (2, 4), (2, 5)])
+ >>> nx.is_tree(G) # n-1 edges
+ True
+ >>> G.add_edge(3, 4)
+ >>> nx.is_tree(G) # n edges
+ False
+
Notes
-----
In another convention, a directed tree is known as a *polytree* and then
diff --git a/networkx/utils/decorators.py b/networkx/utils/decorators.py
index e8e4bff55..4e065c50b 100644
--- a/networkx/utils/decorators.py
+++ b/networkx/utils/decorators.py
@@ -464,6 +464,9 @@ class argmap:
function constructs an object (like a file handle) that requires
post-processing (like closing).
+ Note: try_finally decorators cannot be used to decorate generator
+ functions.
+
Examples
--------
Most of these examples use `@argmap(...)` to apply the decorator to
@@ -606,6 +609,38 @@ class argmap:
# this code doesn't need to worry about closing the file
print(file.read())
+ Decorators with try_finally = True cannot be used with generator functions,
+ because the `finally` block is evaluated before the generator is exhausted::
+
+ @argmap(open_file, "file", try_finally=True)
+ def file_to_lines(file):
+ for line in file.readlines():
+ yield line
+
+ is equivalent to::
+
+ def file_to_lines_wrapped(file):
+ for line in file.readlines():
+ yield line
+
+ def file_to_lines_wrapper(file):
+ try:
+ file = open_file(file)
+ return file_to_lines_wrapped(file)
+ finally:
+ file.close()
+
+ which behaves similarly to::
+
+ def file_to_lines_whoops(file):
+ file = open_file(file)
+ file.close()
+ for line in file.readlines():
+ yield line
+
+ because the `finally` block of `file_to_lines_wrapper` is executed before
+ the caller has a chance to exhaust the iterator.
+
Notes
-----
An object of this class is callable and intended to be used when
@@ -805,15 +840,8 @@ class argmap:
argmap._lazy_compile
"""
- if inspect.isgeneratorfunction(f):
-
- def func(*args, __wrapper=None, **kwargs):
- yield from argmap._lazy_compile(__wrapper)(*args, **kwargs)
-
- else:
-
- def func(*args, __wrapper=None, **kwargs):
- return argmap._lazy_compile(__wrapper)(*args, **kwargs)
+ def func(*args, __wrapper=None, **kwargs):
+ return argmap._lazy_compile(__wrapper)(*args, **kwargs)
# standard function-wrapping stuff
func.__name__ = f.__name__
@@ -843,6 +871,14 @@ class argmap:
# this is used to variously call self.assemble and self.compile
func.__argmap__ = self
+ if hasattr(f, "__argmap__"):
+ func.__is_generator = f.__is_generator
+ else:
+ func.__is_generator = inspect.isgeneratorfunction(f)
+
+ if self._finally and func.__is_generator:
+ raise nx.NetworkXError("argmap cannot decorate generators with try_finally")
+
return func
__count = 0
@@ -1162,12 +1198,7 @@ class argmap:
fname = cls._name(f)
def_sig = f'def {fname}({", ".join(def_sig)}):'
- if inspect.isgeneratorfunction(f):
- _return = "yield from"
- else:
- _return = "return"
-
- call_sig = f"{_return} {{}}({', '.join(call_sig)})"
+ call_sig = f"return {{}}({', '.join(call_sig)})"
return cls.Signature(fname, sig, def_sig, call_sig, names, npos, args, kwargs)
| networkx/networkx | b79768389070c5533a5ae21afce15dd06cd2cff0 | diff --git a/networkx/algorithms/tests/test_similarity.py b/networkx/algorithms/tests/test_similarity.py
index c4fd17f12..9b620dece 100644
--- a/networkx/algorithms/tests/test_similarity.py
+++ b/networkx/algorithms/tests/test_similarity.py
@@ -894,3 +894,27 @@ class TestSimilarity:
assert expected_paths == list(paths)
assert expected_map == index_map
+
+ def test_symmetry_with_custom_matching(self):
+ print("G2 is edge (a,b) and G3 is edge (a,a)")
+ print("but node order for G2 is (a,b) while for G3 it is (b,a)")
+
+ a, b = "A", "B"
+ G2 = nx.Graph()
+ G2.add_nodes_from((a, b))
+ G2.add_edges_from([(a, b)])
+ G3 = nx.Graph()
+ G3.add_nodes_from((b, a))
+ G3.add_edges_from([(a, a)])
+ for G in (G2, G3):
+ for n in G:
+ G.nodes[n]["attr"] = n
+ for e in G.edges:
+ G.edges[e]["attr"] = e
+ match = lambda x, y: x == y
+
+ print("Starting G2 to G3 GED calculation")
+ assert nx.graph_edit_distance(G2, G3, node_match=match, edge_match=match) == 1
+
+ print("Starting G3 to G2 GED calculation")
+ assert nx.graph_edit_distance(G3, G2, node_match=match, edge_match=match) == 1
diff --git a/networkx/utils/tests/test_decorators.py b/networkx/utils/tests/test_decorators.py
index eee48fd48..3be29e549 100644
--- a/networkx/utils/tests/test_decorators.py
+++ b/networkx/utils/tests/test_decorators.py
@@ -380,29 +380,25 @@ class TestArgmap:
# context exits are called in reverse
assert container == ["c", "b", "a"]
- def test_contextmanager_iterator(self):
+ def test_tryfinally_generator(self):
container = []
- def contextmanager(x):
- nonlocal container
- return x, lambda: container.append(x)
+ def singleton(x):
+ return (x,)
- @argmap(contextmanager, 0, 1, 2, try_finally=True)
+ with pytest.raises(nx.NetworkXError):
+
+ @argmap(singleton, 0, 1, 2, try_finally=True)
+ def foo(x, y, z):
+ yield from (x, y, z)
+
+ @argmap(singleton, 0, 1, 2)
def foo(x, y, z):
- yield from (x, y, z)
+ return x + y + z
q = foo("a", "b", "c")
- assert next(q) == "a"
- assert container == []
- assert next(q) == "b"
- assert container == []
- assert next(q) == "c"
- assert container == []
- with pytest.raises(StopIteration):
- next(q)
- # context exits are called in reverse
- assert container == ["c", "b", "a"]
+ assert q == ("a", "b", "c")
def test_actual_vararg(self):
@argmap(lambda x: -x, 4)
@@ -485,7 +481,25 @@ finally:
pass#"""
)
- def nop(self):
- print(foo.__argmap__.assemble(foo.__wrapped__))
- argmap._lazy_compile(foo)
- print(foo._code)
+ def test_immediate_raise(self):
+ @not_implemented_for("directed")
+ def yield_nodes(G):
+ yield from G
+
+ G = nx.Graph([(1, 2)])
+ D = nx.DiGraph()
+
+ # test first call (argmap is compiled and executed)
+ with pytest.raises(nx.NetworkXNotImplemented):
+ node_iter = yield_nodes(D)
+
+ # test second call (argmap is only executed)
+ with pytest.raises(nx.NetworkXNotImplemented):
+ node_iter = yield_nodes(D)
+
+ # ensure that generators still make generators
+ node_iter = yield_nodes(G)
+ next(node_iter)
+ next(node_iter)
+ with pytest.raises(StopIteration):
+ next(node_iter)
| Update documentation for planar embedding
Let's update the documentation to make it clear that the `check_planarity` function is the primary interface for the planar embedding tools. Also, the class `PlanarEmbedding` is tricky to make sure it maintains the planar data structure. People not familiar with those ideas should definitely start with the `check_planarity` function.
See discussion in #5079 | 0.0 | [
"networkx/utils/tests/test_decorators.py::TestArgmap::test_tryfinally_generator",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_immediate_raise"
] | [
"networkx/utils/tests/test_decorators.py::test_not_implemented_decorator",
"networkx/utils/tests/test_decorators.py::test_not_implemented_decorator_key",
"networkx/utils/tests/test_decorators.py::test_not_implemented_decorator_raise",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg0_str",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg0_fobj",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg0_pathlib",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg1_str",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg1_fobj",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg2default_str",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg2default_fobj",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg2default_fobj_path_none",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_arg4default_fobj",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_kwarg_str",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_kwarg_fobj",
"networkx/utils/tests/test_decorators.py::TestOpenFileDecorator::test_writer_kwarg_path_none",
"networkx/utils/tests/test_decorators.py::test_preserve_random_state",
"networkx/utils/tests/test_decorators.py::test_random_state_string_arg_index",
"networkx/utils/tests/test_decorators.py::test_py_random_state_string_arg_index",
"networkx/utils/tests/test_decorators.py::test_random_state_invalid_arg_index",
"networkx/utils/tests/test_decorators.py::test_py_random_state_invalid_arg_index",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_trivial_function",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_trivial_iterator",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_contextmanager",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_actual_vararg",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_signature_destroying_intermediate_decorator",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_actual_kwarg",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_nested_tuple",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_flatten",
"networkx/utils/tests/test_decorators.py::TestArgmap::test_indent"
] | 2022-04-12 17:38:58+00:00 | 4,142 |
|
networkx__networkx-5550 | diff --git a/doc/reference/algorithms/planarity.rst b/doc/reference/algorithms/planarity.rst
index cad00dc8e..03ded5fa8 100644
--- a/doc/reference/algorithms/planarity.rst
+++ b/doc/reference/algorithms/planarity.rst
@@ -7,5 +7,5 @@ Planarity
:toctree: generated/
check_planarity
-.. autoclass:: PlanarEmbedding
- :members:
\ No newline at end of file
+ is_planar
+ PlanarEmbedding
diff --git a/networkx/algorithms/bridges.py b/networkx/algorithms/bridges.py
index 959a67fec..eda4a4f6d 100644
--- a/networkx/algorithms/bridges.py
+++ b/networkx/algorithms/bridges.py
@@ -35,6 +35,9 @@ def bridges(G, root=None):
NodeNotFound
If `root` is not in the graph `G`.
+ NetworkXNotImplemented
+ If `G` is a directed graph.
+
Examples
--------
The barbell graph with parameter zero has a single bridge:
@@ -99,6 +102,9 @@ def has_bridges(G, root=None):
NodeNotFound
If `root` is not in the graph `G`.
+ NetworkXNotImplemented
+ If `G` is a directed graph.
+
Examples
--------
The barbell graph with parameter zero has a single bridge::
@@ -158,6 +164,11 @@ def local_bridges(G, with_span=True, weight=None):
The local bridges as an edge 2-tuple of nodes `(u, v)` or
as a 3-tuple `(u, v, span)` when `with_span is True`.
+ Raises
+ ------
+ NetworkXNotImplemented
+ If `G` is a directed graph or multigraph.
+
Examples
--------
A cycle graph has every edge a local bridge with span N-1.
diff --git a/networkx/algorithms/community/modularity_max.py b/networkx/algorithms/community/modularity_max.py
index 264a0dc07..ca1cfb9e2 100644
--- a/networkx/algorithms/community/modularity_max.py
+++ b/networkx/algorithms/community/modularity_max.py
@@ -326,6 +326,8 @@ def greedy_modularity_communities(
raise ValueError(f"best_n must be between 1 and {len(G)}. Got {best_n}.")
if best_n < cutoff:
raise ValueError(f"Must have best_n >= cutoff. Got {best_n} < {cutoff}")
+ if best_n == 1:
+ return [set(G)]
else:
best_n = G.number_of_nodes()
if n_communities is not None:
@@ -351,7 +353,19 @@ def greedy_modularity_communities(
# continue merging communities until one of the breaking criteria is satisfied
while len(communities) > cutoff:
- dq = next(community_gen)
+ try:
+ dq = next(community_gen)
+ # StopIteration occurs when communities are the connected components
+ except StopIteration:
+ communities = sorted(communities, key=len, reverse=True)
+ # if best_n requires more merging, merge big sets for highest modularity
+ while len(communities) > best_n:
+ comm1, comm2, *rest = communities
+ communities = [comm1 ^ comm2]
+ communities.extend(rest)
+ return communities
+
+ # keep going unless max_mod is reached or best_n says to merge more
if dq < 0 and len(communities) <= best_n:
break
communities = next(community_gen)
diff --git a/networkx/algorithms/covering.py b/networkx/algorithms/covering.py
index 174044270..b2aeb82b5 100644
--- a/networkx/algorithms/covering.py
+++ b/networkx/algorithms/covering.py
@@ -12,36 +12,42 @@ __all__ = ["min_edge_cover", "is_edge_cover"]
@not_implemented_for("directed")
@not_implemented_for("multigraph")
def min_edge_cover(G, matching_algorithm=None):
- """Returns a set of edges which constitutes
- the minimum edge cover of the graph.
+ """Returns the min cardinality edge cover of the graph as a set of edges.
A smallest edge cover can be found in polynomial time by finding
a maximum matching and extending it greedily so that all nodes
- are covered.
+ are covered. This function follows that process. A maximum matching
+ algorithm can be specified for the first step of the algorithm.
+ The resulting set may return a set with one 2-tuple for each edge,
+ (the usual case) or with both 2-tuples `(u, v)` and `(v, u)` for
+ each edge. The latter is only done when a bipartite matching algorithm
+ is specified as `matching_algorithm`.
Parameters
----------
G : NetworkX graph
- An undirected bipartite graph.
+ An undirected graph.
matching_algorithm : function
- A function that returns a maximum cardinality matching in a
- given bipartite graph. The function must take one input, the
- graph ``G``, and return a dictionary mapping each node to its
- mate. If not specified,
+ A function that returns a maximum cardinality matching for `G`.
+ The function must take one input, the graph `G`, and return
+ either a set of edges (with only one direction for the pair of nodes)
+ or a dictionary mapping each node to its mate. If not specified,
+ :func:`~networkx.algorithms.matching.max_weight_matching` is used.
+ Common bipartite matching functions include
:func:`~networkx.algorithms.bipartite.matching.hopcroft_karp_matching`
- will be used. Other possibilities include
- :func:`~networkx.algorithms.bipartite.matching.eppstein_matching`,
- or matching algorithms in the
- :mod:`networkx.algorithms.matching` module.
+ or
+ :func:`~networkx.algorithms.bipartite.matching.eppstein_matching`.
Returns
-------
min_cover : set
- It contains all the edges of minimum edge cover
- in form of tuples. It contains both the edges `(u, v)` and `(v, u)`
- for given nodes `u` and `v` among the edges of minimum edge cover.
+ A set of the edges in a minimum edge cover in the form of tuples.
+ It contains only one of the equivalent 2-tuples `(u, v)` and `(v, u)`
+ for each edge. If a bipartite method is used to compute the matching,
+ the returned set contains both the 2-tuples `(u, v)` and `(v, u)`
+ for each edge of a minimum edge cover.
Notes
-----
@@ -53,9 +59,13 @@ def min_edge_cover(G, matching_algorithm=None):
is bounded by the worst-case running time of the function
``matching_algorithm``.
- Minimum edge cover for bipartite graph can also be found using the
- function present in :mod:`networkx.algorithms.bipartite.covering`
+ Minimum edge cover for `G` can also be found using the `min_edge_covering`
+ function in :mod:`networkx.algorithms.bipartite.covering` which is
+ simply this function with a default matching algorithm of
+ :func:`~networkx.algorithms.bipartite.matching.hopcraft_karp_matching`
"""
+ if len(G) == 0:
+ return set()
if nx.number_of_isolates(G) > 0:
# ``min_cover`` does not exist as there is an isolated node
raise nx.NetworkXException(
@@ -66,11 +76,12 @@ def min_edge_cover(G, matching_algorithm=None):
maximum_matching = matching_algorithm(G)
# ``min_cover`` is superset of ``maximum_matching``
try:
- min_cover = set(
- maximum_matching.items()
- ) # bipartite matching case returns dict
+ # bipartite matching algs return dict so convert if needed
+ min_cover = set(maximum_matching.items())
+ bipartite_cover = True
except AttributeError:
min_cover = maximum_matching
+ bipartite_cover = False
# iterate for uncovered nodes
uncovered_nodes = set(G) - {v for u, v in min_cover} - {u for u, v in min_cover}
for v in uncovered_nodes:
@@ -82,7 +93,8 @@ def min_edge_cover(G, matching_algorithm=None):
# multigraph.)
u = arbitrary_element(G[v])
min_cover.add((u, v))
- min_cover.add((v, u))
+ if bipartite_cover:
+ min_cover.add((v, u))
return min_cover
diff --git a/networkx/algorithms/planarity.py b/networkx/algorithms/planarity.py
index 4d1441efc..bc6ff2f0f 100644
--- a/networkx/algorithms/planarity.py
+++ b/networkx/algorithms/planarity.py
@@ -1,7 +1,39 @@
from collections import defaultdict
import networkx as nx
-__all__ = ["check_planarity", "PlanarEmbedding"]
+__all__ = ["check_planarity", "is_planar", "PlanarEmbedding"]
+
+
+def is_planar(G):
+ """Returns True if and only if `G` is planar.
+
+ A graph is *planar* iff it can be drawn in a plane without
+ any edge intersections.
+
+ Parameters
+ ----------
+ G : NetworkX graph
+
+ Returns
+ -------
+ bool
+ Whether the graph is planar.
+
+ Examples
+ --------
+ >>> G = nx.Graph([(0, 1), (0, 2)])
+ >>> nx.is_planar(G)
+ True
+ >>> nx.is_planar(nx.complete_graph(5))
+ False
+
+ See Also
+ --------
+ check_planarity :
+ Check if graph is planar *and* return a `PlanarEmbedding` instance if True.
+ """
+
+ return check_planarity(G, counterexample=False)[0]
def check_planarity(G, counterexample=False):
@@ -24,6 +56,18 @@ def check_planarity(G, counterexample=False):
If the graph is planar `certificate` is a PlanarEmbedding
otherwise it is a Kuratowski subgraph.
+ Examples
+ --------
+ >>> G = nx.Graph([(0, 1), (0, 2)])
+ >>> is_planar, P = nx.check_planarity(G)
+ >>> print(is_planar)
+ True
+
+ When `G` is planar, a `PlanarEmbedding` instance is returned:
+
+ >>> P.get_data()
+ {0: [1, 2], 1: [0], 2: [0]}
+
Notes
-----
A (combinatorial) embedding consists of cyclic orderings of the incident
@@ -37,6 +81,11 @@ def check_planarity(G, counterexample=False):
A counterexample is only generated if the corresponding parameter is set,
because the complexity of the counterexample generation is higher.
+ See also
+ --------
+ is_planar :
+ Check for planarity without creating a `PlanarEmbedding` or counterexample.
+
References
----------
.. [1] Ulrik Brandes:
@@ -716,6 +765,8 @@ class PlanarEmbedding(nx.DiGraph):
The planar embedding is given by a `combinatorial embedding
<https://en.wikipedia.org/wiki/Graph_embedding#Combinatorial_embedding>`_.
+ .. note:: `check_planarity` is the preferred way to check if a graph is planar.
+
**Neighbor ordering:**
In comparison to a usual graph structure, the embedding also stores the
@@ -761,6 +812,15 @@ class PlanarEmbedding(nx.DiGraph):
For a half-edge (u, v) that is orientated such that u is below v then the
face that belongs to (u, v) is to the right of this half-edge.
+ See Also
+ --------
+ is_planar :
+ Preferred way to check if an existing graph is planar.
+
+ check_planarity :
+ A convenient way to create a `PlanarEmbedding`. If not planar,
+ it returns a subgraph that shows this.
+
Examples
--------
diff --git a/networkx/algorithms/tournament.py b/networkx/algorithms/tournament.py
index bace64bae..a002e022e 100644
--- a/networkx/algorithms/tournament.py
+++ b/networkx/algorithms/tournament.py
@@ -82,6 +82,13 @@ def is_tournament(G):
bool
Whether the given graph is a tournament graph.
+ Examples
+ --------
+ >>> from networkx.algorithms import tournament
+ >>> G = nx.DiGraph([(0, 1), (1, 2), (2, 0)])
+ >>> tournament.is_tournament(G)
+ True
+
Notes
-----
Some definitions require a self-loop on each node, but that is not
@@ -114,6 +121,13 @@ def hamiltonian_path(G):
path : list
A list of nodes which form a Hamiltonian path in `G`.
+ Examples
+ --------
+ >>> from networkx.algorithms import tournament
+ >>> G = nx.DiGraph([(0, 1), (0, 2), (0, 3), (1, 2), (1, 3), (2, 3)])
+ >>> tournament.hamiltonian_path(G)
+ [0, 1, 2, 3]
+
Notes
-----
This is a recursive implementation with an asymptotic running time
@@ -185,6 +199,13 @@ def score_sequence(G):
list
A sorted list of the out-degrees of the nodes of `G`.
+ Examples
+ --------
+ >>> from networkx.algorithms import tournament
+ >>> G = nx.DiGraph([(1, 0), (1, 3), (0, 2), (0, 3), (2, 1), (3, 2)])
+ >>> tournament.score_sequence(G)
+ [1, 1, 2, 2]
+
"""
return sorted(d for v, d in G.out_degree())
@@ -260,6 +281,15 @@ def is_reachable(G, s, t):
bool
Whether there is a path from `s` to `t` in `G`.
+ Examples
+ --------
+ >>> from networkx.algorithms import tournament
+ >>> G = nx.DiGraph([(1, 0), (1, 3), (1, 2), (2, 3), (2, 0), (3, 0)])
+ >>> tournament.is_reachable(G, 1, 3)
+ True
+ >>> tournament.is_reachable(G, 3, 2)
+ False
+
Notes
-----
Although this function is more theoretically efficient than the
@@ -331,6 +361,16 @@ def is_strongly_connected(G):
bool
Whether the tournament is strongly connected.
+ Examples
+ --------
+ >>> from networkx.algorithms import tournament
+ >>> G = nx.DiGraph([(0, 1), (0, 2), (0, 3), (1, 2), (1, 3), (2, 3), (3, 0)])
+ >>> tournament.is_strongly_connected(G)
+ True
+ >>> G.remove_edge(1, 3)
+ >>> tournament.is_strongly_connected(G)
+ False
+
Notes
-----
Although this function is more theoretically efficient than the
diff --git a/networkx/algorithms/tree/recognition.py b/networkx/algorithms/tree/recognition.py
index 52da959b6..88b2abe8b 100644
--- a/networkx/algorithms/tree/recognition.py
+++ b/networkx/algorithms/tree/recognition.py
@@ -96,6 +96,16 @@ def is_arborescence(G):
b : bool
A boolean that is True if `G` is an arborescence.
+ Examples
+ --------
+ >>> G = nx.DiGraph([(0, 1), (0, 2), (2, 3), (3, 4)])
+ >>> nx.is_arborescence(G)
+ True
+ >>> G.remove_edge(0, 1)
+ >>> G.add_edge(1, 2) # maximum in-degree is 2
+ >>> nx.is_arborescence(G)
+ False
+
Notes
-----
In another convention, an arborescence is known as a *tree*.
@@ -125,6 +135,16 @@ def is_branching(G):
b : bool
A boolean that is True if `G` is a branching.
+ Examples
+ --------
+ >>> G = nx.DiGraph([(0, 1), (1, 2), (2, 3), (3, 4)])
+ >>> nx.is_branching(G)
+ True
+ >>> G.remove_edge(2, 3)
+ >>> G.add_edge(3, 1) # maximum in-degree is 2
+ >>> nx.is_branching(G)
+ False
+
Notes
-----
In another convention, a branching is also known as a *forest*.
| networkx/networkx | 1e5f0bde4cf4cbe4b65bf6e7be775a49556dcf23 | diff --git a/networkx/algorithms/community/tests/test_modularity_max.py b/networkx/algorithms/community/tests/test_modularity_max.py
index 784e4a97b..acdb19d64 100644
--- a/networkx/algorithms/community/tests/test_modularity_max.py
+++ b/networkx/algorithms/community/tests/test_modularity_max.py
@@ -44,6 +44,17 @@ def test_modularity_communities_categorical_labels(func):
assert set(func(G)) == expected
+def test_greedy_modularity_communities_components():
+ # Test for gh-5530
+ G = nx.Graph([(0, 1), (2, 3), (4, 5), (5, 6)])
+ # usual case with 3 components
+ assert greedy_modularity_communities(G) == [{4, 5, 6}, {0, 1}, {2, 3}]
+ # best_n can make the algorithm continue even when modularity goes down
+ assert greedy_modularity_communities(G, best_n=3) == [{4, 5, 6}, {0, 1}, {2, 3}]
+ assert greedy_modularity_communities(G, best_n=2) == [{0, 1, 4, 5, 6}, {2, 3}]
+ assert greedy_modularity_communities(G, best_n=1) == [{0, 1, 2, 3, 4, 5, 6}]
+
+
def test_greedy_modularity_communities_relabeled():
# Test for gh-4966
G = nx.balanced_tree(2, 2)
@@ -306,7 +317,7 @@ def test_cutoff_parameter():
def test_best_n():
G = nx.barbell_graph(5, 3)
- # Same result as without enforcing n_communities:
+ # Same result as without enforcing cutoff:
best_n = 3
expected = [frozenset(range(5)), frozenset(range(8, 13)), frozenset(range(5, 8))]
assert greedy_modularity_communities(G, best_n=best_n) == expected
diff --git a/networkx/algorithms/tests/test_covering.py b/networkx/algorithms/tests/test_covering.py
index 78487b734..40971963e 100644
--- a/networkx/algorithms/tests/test_covering.py
+++ b/networkx/algorithms/tests/test_covering.py
@@ -14,28 +14,44 @@ class TestMinEdgeCover:
assert nx.min_edge_cover(G) == {(0, 0)}
def test_graph_single_edge(self):
- G = nx.Graph()
- G.add_edge(0, 1)
+ G = nx.Graph([(0, 1)])
assert nx.min_edge_cover(G) in ({(0, 1)}, {(1, 0)})
+ def test_graph_two_edge_path(self):
+ G = nx.path_graph(3)
+ min_cover = nx.min_edge_cover(G)
+ assert len(min_cover) == 2
+ for u, v in G.edges:
+ assert (u, v) in min_cover or (v, u) in min_cover
+
def test_bipartite_explicit(self):
G = nx.Graph()
G.add_nodes_from([1, 2, 3, 4], bipartite=0)
G.add_nodes_from(["a", "b", "c"], bipartite=1)
G.add_edges_from([(1, "a"), (1, "b"), (2, "b"), (2, "c"), (3, "c"), (4, "a")])
+ # Use bipartite method by prescribing the algorithm
min_cover = nx.min_edge_cover(
G, nx.algorithms.bipartite.matching.eppstein_matching
)
- min_cover2 = nx.min_edge_cover(G)
assert nx.is_edge_cover(G, min_cover)
assert len(min_cover) == 8
+ # Use the default method which is not specialized for bipartite
+ min_cover2 = nx.min_edge_cover(G)
+ assert nx.is_edge_cover(G, min_cover2)
+ assert len(min_cover2) == 4
- def test_complete_graph(self):
+ def test_complete_graph_even(self):
G = nx.complete_graph(10)
min_cover = nx.min_edge_cover(G)
assert nx.is_edge_cover(G, min_cover)
assert len(min_cover) == 5
+ def test_complete_graph_odd(self):
+ G = nx.complete_graph(11)
+ min_cover = nx.min_edge_cover(G)
+ assert nx.is_edge_cover(G, min_cover)
+ assert len(min_cover) == 6
+
class TestIsEdgeCover:
"""Tests for :func:`networkx.algorithms.is_edge_cover`"""
| greedy_modularity_communities raises StopIteration for unconnected (?) graph
<!--- Provide a general summary of the issue in the Title above -->
After an update of `networkx` from 2.6.3 to 2.8, one of my tests suddenly failed with
```
Traceback (most recent call last):
File "/usr/lib/python3.10/runpy.py", line 196, in _run_module_as_main
return _run_code(code, main_globals, None,
File "/usr/lib/python3.10/runpy.py", line 86, in _run_code
exec(code, run_globals)
File "/home/gereon/Develop/lexedata/src/lexedata/report/nonconcatenative_morphemes.py", line 159, in <module>
networkx.algorithms.community.greedy_modularity_communities(graph),
File "/home/gereon/.local/etc/lexedata/lib/python3.10/site-packages/networkx/algorithms/community/modularity_max.py", line 354, in greedy_modularity_communities
dq = next(community_gen)
StopIteration
```
It turns out that the internals of `greedy_modularity_communities` have changed, so the function doesn't work any more with unconnected graphs.
### Current Behavior
<!--- Tell us what happens instead of the expected behavior -->
`networkx.algorithms.community.greedy_modularity_communities` fails with undocumented error message for unconnected graphs, or graphs without edges
### Expected Behavior
<!--- Tell us what should happen -->
- Either document the requirement to have a connected graph with at least one edge and throw `ValueError` instead of `StopIteration` when it is not fulfilled, or run the graph through `nx.connected_components()` first.
- If the graph has only one node and no edges, return that node.
### Steps to Reproduce
<!--- Provide a minimal example that reproduces the bug -->
```
>>> G = nx.Graph()
>>> G.add_edges_from([(0,1),(2,3)])
>>> greedy_modularity_communities(G)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/gereon/.local/etc/lexedata/lib/python3.10/site-packages/networkx/algorithms/community/modularity_max.py", line 354, in greedy_modularity_communities
dq = next(community_gen)
StopIteration
```
```
>>> G = nx.Graph()
>>> G.add_nodes_from([1])
>>> greedy_modularity_communities(G)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/gereon/.local/etc/lexedata/lib/python3.10/site-packages/networkx/algorithms/community/modularity_max.py", line 350, in greedy_modularity_communities
communities = next(community_gen)
File "/home/gereon/.local/etc/lexedata/lib/python3.10/site-packages/networkx/algorithms/community/modularity_max.py", line 79, in _greedy_modularity_communities_generator
q0 = 1 / m
ZeroDivisionError: division by zero
```
### Environment
<!--- Please provide details about your local environment -->
Python version: 3.10.2
NetworkX version: 2.8 | 0.0 | [
"networkx/algorithms/community/tests/test_modularity_max.py::test_greedy_modularity_communities_components",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_graph_two_edge_path",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_bipartite_explicit",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_complete_graph_odd"
] | [
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities[greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities[naive_greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_categorical_labels[greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_categorical_labels[naive_greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greedy_modularity_communities_relabeled",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greedy_modularity_communities_directed",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_weighted[greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_weighted[naive_greedy_modularity_communities]",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_floating_point",
"networkx/algorithms/community/tests/test_modularity_max.py::test_modularity_communities_directed_weighted",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greedy_modularity_communities_multigraph",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greedy_modularity_communities_multigraph_weighted",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greed_modularity_communities_multidigraph",
"networkx/algorithms/community/tests/test_modularity_max.py::test_greed_modularity_communities_multidigraph_weighted",
"networkx/algorithms/community/tests/test_modularity_max.py::test_resolution_parameter_impact",
"networkx/algorithms/community/tests/test_modularity_max.py::test_cutoff_parameter",
"networkx/algorithms/community/tests/test_modularity_max.py::test_best_n",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_empty_graph",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_graph_with_loop",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_graph_single_edge",
"networkx/algorithms/tests/test_covering.py::TestMinEdgeCover::test_complete_graph_even",
"networkx/algorithms/tests/test_covering.py::TestIsEdgeCover::test_empty_graph",
"networkx/algorithms/tests/test_covering.py::TestIsEdgeCover::test_graph_with_loop",
"networkx/algorithms/tests/test_covering.py::TestIsEdgeCover::test_graph_single_edge"
] | 2022-04-17 20:42:01+00:00 | 4,143 |
|
networkx__networkx-5894 | diff --git a/networkx/classes/graph.py b/networkx/classes/graph.py
index ebbc8b535..47d5f81d9 100644
--- a/networkx/classes/graph.py
+++ b/networkx/classes/graph.py
@@ -41,6 +41,28 @@ class _CachedPropertyResetterAdj:
del od["adj"]
+class _CachedPropertyResetterNode:
+ """Data Descriptor class for _node that resets ``nodes`` cached_property when needed
+
+ This assumes that the ``cached_property`` ``G.node`` should be reset whenever
+ ``G._node`` is set to a new value.
+
+ This object sits on a class and ensures that any instance of that
+ class clears its cached property "nodes" whenever the underlying
+ instance attribute "_node" is set to a new object. It only affects
+ the set process of the obj._adj attribute. All get/del operations
+ act as they normally would.
+
+ For info on Data Descriptors see: https://docs.python.org/3/howto/descriptor.html
+ """
+
+ def __set__(self, obj, value):
+ od = obj.__dict__
+ od["_node"] = value
+ if "nodes" in od:
+ del od["nodes"]
+
+
class Graph:
"""
Base class for undirected graphs.
@@ -282,6 +304,7 @@ class Graph:
"""
_adj = _CachedPropertyResetterAdj()
+ _node = _CachedPropertyResetterNode()
node_dict_factory = dict
node_attr_dict_factory = dict
| networkx/networkx | 98060487ad192918cfc2415fc0b5c309ff2d3565 | diff --git a/networkx/algorithms/shortest_paths/unweighted.py b/networkx/algorithms/shortest_paths/unweighted.py
index 5363b24dc..9d1dff5b2 100644
--- a/networkx/algorithms/shortest_paths/unweighted.py
+++ b/networkx/algorithms/shortest_paths/unweighted.py
@@ -460,8 +460,7 @@ def all_pairs_shortest_path(G, cutoff=None):
def predecessor(G, source, target=None, cutoff=None, return_seen=None):
- """Returns dict of predecessors for the path from source to all nodes in G
-
+ """Returns dict of predecessors for the path from source to all nodes in G.
Parameters
----------
@@ -477,12 +476,23 @@ def predecessor(G, source, target=None, cutoff=None, return_seen=None):
cutoff : integer, optional
Depth to stop the search. Only paths of length <= cutoff are returned.
+ return_seen : bool, optional (default=None)
+ Whether to return a dictionary, keyed by node, of the level (number of
+ hops) to reach the node (as seen during breadth-first-search).
Returns
-------
pred : dictionary
Dictionary, keyed by node, of predecessors in the shortest path.
+
+ (pred, seen): tuple of dictionaries
+ If `return_seen` argument is set to `True`, then a tuple of dictionaries
+ is returned. The first element is the dictionary, keyed by node, of
+ predecessors in the shortest path. The second element is the dictionary,
+ keyed by node, of the level (number of hops) to reach the node (as seen
+ during breadth-first-search).
+
Examples
--------
>>> G = nx.path_graph(4)
@@ -490,6 +500,9 @@ def predecessor(G, source, target=None, cutoff=None, return_seen=None):
[0, 1, 2, 3]
>>> nx.predecessor(G, 0)
{0: [], 1: [0], 2: [1], 3: [2]}
+ >>> nx.predecessor(G, 0, return_seen=True)
+ ({0: [], 1: [0], 2: [1], 3: [2]}, {0: 0, 1: 1, 2: 2, 3: 3})
+
"""
if source not in G:
diff --git a/networkx/classes/tests/test_graph.py b/networkx/classes/tests/test_graph.py
index ebaa04bbd..2adb159bf 100644
--- a/networkx/classes/tests/test_graph.py
+++ b/networkx/classes/tests/test_graph.py
@@ -178,6 +178,11 @@ class BaseGraphTester:
G._adj = {}
assert id(G.adj) != id(old_adj)
+ old_nodes = G.nodes
+ assert id(G.nodes) == id(old_nodes)
+ G._node = {}
+ assert id(G.nodes) != id(old_nodes)
+
def test_attributes_cached(self):
G = self.K3.copy()
assert id(G.nodes) == id(G.nodes)
| Critical NetworkX 2.8.X bug with mutable cached_properties
### Current Behavior
The `nodes()` method of a Graph is decorated with `@cached_property`.<br>
This leads to the assumption that a Graph's `nodes()` method should return a static value.<br>
This assumption is incorrect.
Notably, the `@cached_property` decorator completely breaks `Graph.subgraph()`.<br>
Trace:
Graph.subgraph -> graphviews.subgraph_view -> [this line](https://github.com/networkx/networkx/blob/ead0e65bda59862e329f2e6f1da47919c6b07ca9/networkx/classes/graphviews.py#L149) prevents [this line](https://github.com/networkx/networkx/blob/ead0e65bda59862e329f2e6f1da47919c6b07ca9/networkx/classes/graphviews.py#L152) from functioning correctly
Subgraphs are shown as containing the wrong nodes as a result, until `del G.nodes` is manually run.<br>
This breaks things.
### Expected Behavior
`@cached_property` decorators should not break functionality.
### Steps to Reproduce
- Initialize a Graph, `G`
- Populate `G` with node objects that are complex enough that the following inequality assertion will pass: `newG = nx.freeze(G.__class__()); newG._graph = G; newG.graph = G.graph; assert(newG.nodes()!=G.nodes)`.
- Pick a subset of `G.nodes`, call this `subG_nodes`
- `subgraph = G.subgraph(subG_nodes)`
- In NetworkX 2.7, the nodes of `subgraph` will be a subset of the nodes of `G`. In NetworkX 2.8, the nodes of `subgraph` and the nodes of `G` will be a fully disjoint set.
### Environment
<!--- Please provide details about your local environment -->
Python version: 3.9.10
NetworkX version: 2.8.5 vs 2.7.0
| 0.0 | [
"networkx/classes/tests/test_graph.py::TestGraph::test_cache_reset"
] | [
"networkx/classes/tests/test_graph.py::TestGraph::test_contains",
"networkx/classes/tests/test_graph.py::TestGraph::test_order",
"networkx/classes/tests/test_graph.py::TestGraph::test_nodes",
"networkx/classes/tests/test_graph.py::TestGraph::test_none_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_has_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_has_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_neighbors",
"networkx/classes/tests/test_graph.py::TestGraph::test_memory_leak",
"networkx/classes/tests/test_graph.py::TestGraph::test_edges",
"networkx/classes/tests/test_graph.py::TestGraph::test_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_size",
"networkx/classes/tests/test_graph.py::TestGraph::test_nbunch_iter",
"networkx/classes/tests/test_graph.py::TestGraph::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloop_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloops",
"networkx/classes/tests/test_graph.py::TestGraph::test_attributes_cached",
"networkx/classes/tests/test_graph.py::TestGraph::test_weighted_degree",
"networkx/classes/tests/test_graph.py::TestGraph::test_name",
"networkx/classes/tests/test_graph.py::TestGraph::test_str_unnamed",
"networkx/classes/tests/test_graph.py::TestGraph::test_str_named",
"networkx/classes/tests/test_graph.py::TestGraph::test_graph_chain",
"networkx/classes/tests/test_graph.py::TestGraph::test_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_class_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_fresh_copy",
"networkx/classes/tests/test_graph.py::TestGraph::test_graph_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_node_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_node_attr2",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_lookup",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr2",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr3",
"networkx/classes/tests/test_graph.py::TestGraph::test_edge_attr4",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_undirected",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_directed_as_view",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_undirected_as_view",
"networkx/classes/tests/test_graph.py::TestGraph::test_directed_class",
"networkx/classes/tests/test_graph.py::TestGraph::test_to_directed",
"networkx/classes/tests/test_graph.py::TestGraph::test_subgraph",
"networkx/classes/tests/test_graph.py::TestGraph::test_selfloops_attr",
"networkx/classes/tests/test_graph.py::TestGraph::test_pickle",
"networkx/classes/tests/test_graph.py::TestGraph::test_data_input",
"networkx/classes/tests/test_graph.py::TestGraph::test_adjacency",
"networkx/classes/tests/test_graph.py::TestGraph::test_getitem",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_nodes_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_node",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_nodes_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_add_edges_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_edge",
"networkx/classes/tests/test_graph.py::TestGraph::test_remove_edges_from",
"networkx/classes/tests/test_graph.py::TestGraph::test_clear",
"networkx/classes/tests/test_graph.py::TestGraph::test_clear_edges",
"networkx/classes/tests/test_graph.py::TestGraph::test_edges_data",
"networkx/classes/tests/test_graph.py::TestGraph::test_get_edge_data",
"networkx/classes/tests/test_graph.py::TestGraph::test_update",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_correct_nodes",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_correct_edges",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_add_node",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_remove_node",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_node_attr_dict",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_edge_attr_dict",
"networkx/classes/tests/test_graph.py::TestEdgeSubgraph::test_graph_attr_dict"
] | 2022-07-25 14:18:48+00:00 | 4,144 |
|
networkx__networkx-5903 | diff --git a/networkx/relabel.py b/networkx/relabel.py
index 35e71536a..65297d573 100644
--- a/networkx/relabel.py
+++ b/networkx/relabel.py
@@ -114,9 +114,13 @@ def relabel_nodes(G, mapping, copy=True):
--------
convert_node_labels_to_integers
"""
- # you can pass a function f(old_label)->new_label
+ # you can pass a function f(old_label) -> new_label
+ # or a class e.g. str(old_label) -> new_label
# but we'll just make a dictionary here regardless
- if not hasattr(mapping, "__getitem__"):
+ # To allow classes, we check if __getitem__ is a bound method using __self__
+ if not (
+ hasattr(mapping, "__getitem__") and hasattr(mapping.__getitem__, "__self__")
+ ):
m = {n: mapping(n) for n in G}
else:
m = mapping
| networkx/networkx | 28f78cfa9a386620ee1179582fda1db5ffc59f84 | diff --git a/networkx/tests/test_relabel.py b/networkx/tests/test_relabel.py
index 46b74482b..9a9db76eb 100644
--- a/networkx/tests/test_relabel.py
+++ b/networkx/tests/test_relabel.py
@@ -106,6 +106,12 @@ class TestRelabel:
H = nx.relabel_nodes(G, mapping)
assert nodes_equal(H.nodes(), [65, 66, 67, 68])
+ def test_relabel_nodes_classes(self):
+ G = nx.empty_graph()
+ G.add_edges_from([(0, 1), (0, 2), (1, 2), (2, 3)])
+ H = nx.relabel_nodes(G, str)
+ assert nodes_equal(H.nodes, ["0", "1", "2", "3"])
+
def test_relabel_nodes_graph(self):
G = nx.Graph([("A", "B"), ("A", "C"), ("B", "C"), ("C", "D")])
mapping = {"A": "aardvark", "B": "bear", "C": "cat", "D": "dog"}
| `relabel_nodes` does not work for callables with `__getitem__`
<!-- If you have a general question about NetworkX, please use the discussions tab to create a new discussion -->
<!--- Provide a general summary of the issue in the Title above -->
`relabel_nodes` accepts mappings in the form of functions. It differentiates function from mapping by testing`not hasattr(mapping, "__getitem__")`. This test is ambiguous for callables that do possess '__getitem__`, e.g. `str`.
Would it be better to test `isinstance(mapping, typing.Mapping)` and document accordingly?
### Current Behavior
<!--- Tell us what happens instead of the expected behavior -->
```python
import networkx as nx
G = nx.DiGraph()
G.add_nodes_from([1, 2])
# works
# nx.relabel_nodes(G, lambda x: str(x))
# error
nx.relabel_nodes(G, str)
```
```
Traceback (most recent call last):
File "...\networkx_2_8_4_relabel_callable.py", line 10, in <module>
nx.relabel_nodes(G, str)
File "...\lib\site-packages\networkx\relabel.py", line 121, in relabel_nodes
return _relabel_copy(G, m)
File "...\lib\site-packages\networkx\relabel.py", line 193, in _relabel_copy
H.add_nodes_from(mapping.get(n, n) for n in G)
File "...\lib\site-packages\networkx\classes\digraph.py", line 519, in add_nodes_from
for n in nodes_for_adding:
File "...\lib\site-packages\networkx\relabel.py", line 193, in <genexpr>
H.add_nodes_from(mapping.get(n, n) for n in G)
AttributeError: type object 'str' has no attribute 'get'
```
### Expected Behavior
<!--- Tell us what should happen -->
`relabel_nodes` works for callables.
### Steps to Reproduce
<!--- Provide a minimal example that reproduces the bug -->
See current behavior.
### Environment
<!--- Please provide details about your local environment -->
Python version: 3.9
NetworkX version: 2.8.5
### Additional context
<!--- Add any other context about the problem here, screenshots, etc. -->
| 0.0 | [
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_classes"
] | [
"networkx/tests/test_relabel.py::TestRelabel::test_convert_node_labels_to_integers",
"networkx/tests/test_relabel.py::TestRelabel::test_convert_to_integers2",
"networkx/tests/test_relabel.py::TestRelabel::test_convert_to_integers_raise",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_function",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_graph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_orderedgraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_digraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_multigraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_multidigraph",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_isolated_nodes_to_same",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_nodes_missing",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_copy_name",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_toposort",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_selfloop",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_inout_merge_nodes",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_merge_inplace",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_merge_inplace",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_inout_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_merge_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multidigraph_merge_copy",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_multigraph_nonnumeric_key",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_circular",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_preserve_node_order_full_mapping_with_copy_true",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_preserve_node_order_full_mapping_with_copy_false",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_preserve_node_order_partial_mapping_with_copy_true",
"networkx/tests/test_relabel.py::TestRelabel::test_relabel_preserve_node_order_partial_mapping_with_copy_false"
] | 2022-07-29 02:02:29+00:00 | 4,145 |
|
networkx__networkx-5988 | diff --git a/networkx/algorithms/dag.py b/networkx/algorithms/dag.py
index 826b87ff6..d5e2735b1 100644
--- a/networkx/algorithms/dag.py
+++ b/networkx/algorithms/dag.py
@@ -1006,7 +1006,15 @@ def dag_longest_path(G, weight="weight", default_weight=1, topo_order=None):
dist = {} # stores {v : (length, u)}
for v in topo_order:
us = [
- (dist[u][0] + data.get(weight, default_weight), u)
+ (
+ dist[u][0]
+ + (
+ max(data.values(), key=lambda x: x.get(weight, default_weight))
+ if G.is_multigraph()
+ else data
+ ).get(weight, default_weight),
+ u,
+ )
for u, data in G.pred[v].items()
]
@@ -1068,8 +1076,13 @@ def dag_longest_path_length(G, weight="weight", default_weight=1):
"""
path = nx.dag_longest_path(G, weight, default_weight)
path_length = 0
- for (u, v) in pairwise(path):
- path_length += G[u][v].get(weight, default_weight)
+ if G.is_multigraph():
+ for u, v in pairwise(path):
+ i = max(G[u][v], key=lambda x: G[u][v][x].get(weight, default_weight))
+ path_length += G[u][v][i].get(weight, default_weight)
+ else:
+ for (u, v) in pairwise(path):
+ path_length += G[u][v].get(weight, default_weight)
return path_length
| networkx/networkx | 1ce75f0f3604abd0551fa9baf20c65c3747fb328 | diff --git a/networkx/algorithms/tests/test_dag.py b/networkx/algorithms/tests/test_dag.py
index 56f16c4f5..7ad6a77fe 100644
--- a/networkx/algorithms/tests/test_dag.py
+++ b/networkx/algorithms/tests/test_dag.py
@@ -60,6 +60,31 @@ class TestDagLongestPath:
# this will raise NotImplementedError when nodes need to be ordered
nx.dag_longest_path(G)
+ def test_multigraph_unweighted(self):
+ edges = [(1, 2), (2, 3), (2, 3), (3, 4), (4, 5), (1, 3), (1, 5), (3, 5)]
+ G = nx.MultiDiGraph(edges)
+ assert nx.dag_longest_path(G) == [1, 2, 3, 4, 5]
+
+ def test_multigraph_weighted(self):
+ G = nx.MultiDiGraph()
+ edges = [
+ (1, 2, 2),
+ (2, 3, 2),
+ (1, 3, 1),
+ (1, 3, 5),
+ (1, 3, 2),
+ ]
+ G.add_weighted_edges_from(edges)
+ assert nx.dag_longest_path(G) == [1, 3]
+
+ def test_multigraph_weighted_default_weight(self):
+ G = nx.MultiDiGraph([(1, 2), (2, 3)]) # Unweighted edges
+ G.add_weighted_edges_from([(1, 3, 1), (1, 3, 5), (1, 3, 2)])
+
+ # Default value for default weight is 1
+ assert nx.dag_longest_path(G) == [1, 3]
+ assert nx.dag_longest_path(G, default_weight=3) == [1, 2, 3]
+
class TestDagLongestPathLength:
"""Unit tests for computing the length of a longest path in a
@@ -91,6 +116,23 @@ class TestDagLongestPathLength:
G.add_weighted_edges_from(edges)
assert nx.dag_longest_path_length(G) == 5
+ def test_multigraph_unweighted(self):
+ edges = [(1, 2), (2, 3), (2, 3), (3, 4), (4, 5), (1, 3), (1, 5), (3, 5)]
+ G = nx.MultiDiGraph(edges)
+ assert nx.dag_longest_path_length(G) == 4
+
+ def test_multigraph_weighted(self):
+ G = nx.MultiDiGraph()
+ edges = [
+ (1, 2, 2),
+ (2, 3, 2),
+ (1, 3, 1),
+ (1, 3, 5),
+ (1, 3, 2),
+ ]
+ G.add_weighted_edges_from(edges)
+ assert nx.dag_longest_path_length(G) == 5
+
class TestDAG:
@classmethod
| Weighted MultiDiGraphs never use weights in dag_longest_path and dag_longest_path_length
### Current Behavior
Given any MultiDiGraph, using dag_longest_path will always evaluate using the default_weight keyword argument.
This is because dag_longest_path uses `G.pred[v].items()` to grab the data dictionary, but the data dictionary for a MultiDiGraph is embedded inside of another dictionary with the edge number as a key. When dag_longest_path calls `data.get(weight, default_weight)` on this, it will always raise a KeyError on weight and use default_weight instead.
dag_longest_path_length also calls dict.get() on the wrong dictionary, making it return bad results for weighted MultiDiGraphs even if dag_longest_path returns the correct path.
### Expected Behavior
A MultiDiGraph should either evaluate correctly using weights or raise a NotImplementedError.
### Steps to Reproduce
```
MDG = nx.MultiDiGraph([("A", "B", {"cost": 5}), ("B", "C", {"cost": 10}), ("A", "C", {"cost": 40})])
print(nx.dag_longest_path(MDG, weight="cost"), nx.dag_longest_path_length(MDG, weight="cost"))
# prints ['A', 'B', 'C'] 2
# should be ['A', 'C'] 40
```
Incorrect paths are especially noticeable when a weight key is given, but default_weight is set to 0, as it will always return a list containing only the starting node.
### Environment
Python version: 3.10
NetworkX version: 2.8.5
### Additional context
I have a fix for this issue ready if you would like the functions to support MultiDiGraphs. | 0.0 | [
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_multigraph_weighted",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_multigraph_weighted_default_weight",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPathLength::test_multigraph_weighted"
] | [
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_empty",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_unweighted1",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_unweighted2",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_weighted",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_undirected_not_implemented",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_unorderable_nodes",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPath::test_multigraph_unweighted",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPathLength::test_unweighted",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPathLength::test_undirected_not_implemented",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPathLength::test_weighted",
"networkx/algorithms/tests/test_dag.py::TestDagLongestPathLength::test_multigraph_unweighted",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort1",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_is_directed_acyclic_graph",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort2",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort3",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort4",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort5",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_topological_sort6",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_1",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_2",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_3",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_4",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_multigraph_1",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_all_topological_sorts_multigraph_2",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_ancestors",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_descendants",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_transitive_closure",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_reflexive_transitive_closure",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_transitive_closure_dag",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_transitive_reduction",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_antichains",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_lexicographical_topological_sort",
"networkx/algorithms/tests/test_dag.py::TestDAG::test_lexicographical_topological_sort2",
"networkx/algorithms/tests/test_dag.py::test_topological_generations",
"networkx/algorithms/tests/test_dag.py::test_topological_generations_empty",
"networkx/algorithms/tests/test_dag.py::test_topological_generations_cycle",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_cycle",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_cycle2",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_cycle3",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_cycle4",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_selfloop",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_raise",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_bipartite",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_rary_tree",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_disconnected",
"networkx/algorithms/tests/test_dag.py::test_is_aperiodic_disconnected2",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_single_root",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_multiple_roots",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_already_arborescence",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_already_branching",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_not_acyclic",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_undirected",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_multigraph",
"networkx/algorithms/tests/test_dag.py::TestDagToBranching::test_multidigraph",
"networkx/algorithms/tests/test_dag.py::test_ancestors_descendants_undirected",
"networkx/algorithms/tests/test_dag.py::test_compute_v_structures_raise",
"networkx/algorithms/tests/test_dag.py::test_compute_v_structures"
] | 2022-09-17 21:14:45+00:00 | 4,146 |
|
networkx__networkx-6098 | diff --git a/examples/drawing/plot_eigenvalues.py b/examples/drawing/plot_eigenvalues.py
index b0df67ae9..67322cfd1 100644
--- a/examples/drawing/plot_eigenvalues.py
+++ b/examples/drawing/plot_eigenvalues.py
@@ -14,7 +14,7 @@ m = 5000 # 5000 edges
G = nx.gnm_random_graph(n, m, seed=5040) # Seed for reproducibility
L = nx.normalized_laplacian_matrix(G)
-e = numpy.linalg.eigvals(L.A)
+e = numpy.linalg.eigvals(L.toarray())
print("Largest eigenvalue:", max(e))
print("Smallest eigenvalue:", min(e))
plt.hist(e, bins=100) # histogram with 100 bins
diff --git a/networkx/algorithms/flow/maxflow.py b/networkx/algorithms/flow/maxflow.py
index 8d2fb8f03..b0098d0a0 100644
--- a/networkx/algorithms/flow/maxflow.py
+++ b/networkx/algorithms/flow/maxflow.py
@@ -12,14 +12,6 @@ from .utils import build_flow_dict
# Define the default flow function for computing maximum flow.
default_flow_func = preflow_push
-# Functions that don't support cutoff for minimum cut computations.
-flow_funcs = [
- boykov_kolmogorov,
- dinitz,
- edmonds_karp,
- preflow_push,
- shortest_augmenting_path,
-]
__all__ = ["maximum_flow", "maximum_flow_value", "minimum_cut", "minimum_cut_value"]
@@ -452,7 +444,7 @@ def minimum_cut(flowG, _s, _t, capacity="capacity", flow_func=None, **kwargs):
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
@@ -603,7 +595,7 @@ def minimum_cut_value(flowG, _s, _t, capacity="capacity", flow_func=None, **kwar
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
diff --git a/networkx/drawing/nx_pylab.py b/networkx/drawing/nx_pylab.py
index 09e5ed3a9..674c28a45 100644
--- a/networkx/drawing/nx_pylab.py
+++ b/networkx/drawing/nx_pylab.py
@@ -650,6 +650,42 @@ def draw_networkx_edges(
# undirected graphs (for performance reasons) and use FancyArrowPatches
# for directed graphs.
# The `arrows` keyword can be used to override the default behavior
+ use_linecollection = not G.is_directed()
+ if arrows in (True, False):
+ use_linecollection = not arrows
+
+ # Some kwargs only apply to FancyArrowPatches. Warn users when they use
+ # non-default values for these kwargs when LineCollection is being used
+ # instead of silently ignoring the specified option
+ if use_linecollection and any(
+ [
+ arrowstyle is not None,
+ arrowsize != 10,
+ connectionstyle != "arc3",
+ min_source_margin != 0,
+ min_target_margin != 0,
+ ]
+ ):
+ import warnings
+
+ msg = (
+ "\n\nThe {0} keyword argument is not applicable when drawing edges\n"
+ "with LineCollection.\n\n"
+ "To make this warning go away, either specify `arrows=True` to\n"
+ "force FancyArrowPatches or use the default value for {0}.\n"
+ "Note that using FancyArrowPatches may be slow for large graphs.\n"
+ )
+ if arrowstyle is not None:
+ msg = msg.format("arrowstyle")
+ if arrowsize != 10:
+ msg = msg.format("arrowsize")
+ if connectionstyle != "arc3":
+ msg = msg.format("connectionstyle")
+ if min_source_margin != 0:
+ msg = msg.format("min_source_margin")
+ if min_target_margin != 0:
+ msg = msg.format("min_target_margin")
+ warnings.warn(msg, category=UserWarning, stacklevel=2)
if arrowstyle == None:
if G.is_directed():
@@ -657,10 +693,6 @@ def draw_networkx_edges(
else:
arrowstyle = "-"
- use_linecollection = not G.is_directed()
- if arrows in (True, False):
- use_linecollection = not arrows
-
if ax is None:
ax = plt.gca()
diff --git a/networkx/readwrite/gml.py b/networkx/readwrite/gml.py
index da5ccca58..758c0c73b 100644
--- a/networkx/readwrite/gml.py
+++ b/networkx/readwrite/gml.py
@@ -363,6 +363,11 @@ def parse_gml_lines(lines, label, destringizer):
value = destringizer(value)
except ValueError:
pass
+ # Special handling for empty lists and tuples
+ if value == "()":
+ value = tuple()
+ if value == "[]":
+ value = list()
curr_token = next(tokens)
elif category == Pattern.DICT_START:
curr_token, value = parse_dict(curr_token)
@@ -728,12 +733,9 @@ def generate_gml(G, stringizer=None):
for key, value in value.items():
yield from stringize(key, value, (), next_indent)
yield indent + "]"
- elif (
- isinstance(value, (list, tuple))
- and key != "label"
- and value
- and not in_list
- ):
+ elif isinstance(value, (list, tuple)) and key != "label" and not in_list:
+ if len(value) == 0:
+ yield indent + key + " " + f'"{value!r}"'
if len(value) == 1:
yield indent + key + " " + f'"{LIST_START_VALUE}"'
for val in value:
| networkx/networkx | bcf607cf7ce4009ca37786b2fcd84e548f1833f5 | diff --git a/networkx/algorithms/approximation/tests/test_dominating_set.py b/networkx/algorithms/approximation/tests/test_dominating_set.py
index 892ce34e2..6b90d85ec 100644
--- a/networkx/algorithms/approximation/tests/test_dominating_set.py
+++ b/networkx/algorithms/approximation/tests/test_dominating_set.py
@@ -1,3 +1,5 @@
+import pytest
+
import networkx as nx
from networkx.algorithms.approximation import (
min_edge_dominating_set,
@@ -37,6 +39,11 @@ class TestMinWeightDominatingSet:
G = nx.relabel_nodes(G, {0: 9, 9: 0})
assert min_weighted_dominating_set(G) == {9}
+ def test_null_graph(self):
+ """Tests that the unique dominating set for the null graph is an empty set"""
+ G = nx.Graph()
+ assert min_weighted_dominating_set(G) == set()
+
def test_min_edge_dominating_set(self):
graph = nx.path_graph(5)
dom_set = min_edge_dominating_set(graph)
@@ -65,3 +72,7 @@ class TestMinWeightDominatingSet:
for dom_edge in dom_set:
found |= u == dom_edge[0] or u == dom_edge[1]
assert found, "Non adjacent edge found!"
+
+ graph = nx.Graph() # empty Networkx graph
+ with pytest.raises(ValueError, match="Expected non-empty NetworkX graph!"):
+ min_edge_dominating_set(graph)
diff --git a/networkx/algorithms/flow/tests/test_maxflow.py b/networkx/algorithms/flow/tests/test_maxflow.py
index 36bc5ec7c..d4f9052ce 100644
--- a/networkx/algorithms/flow/tests/test_maxflow.py
+++ b/networkx/algorithms/flow/tests/test_maxflow.py
@@ -20,6 +20,7 @@ flow_funcs = {
preflow_push,
shortest_augmenting_path,
}
+
max_min_funcs = {nx.maximum_flow, nx.minimum_cut}
flow_value_funcs = {nx.maximum_flow_value, nx.minimum_cut_value}
interface_funcs = max_min_funcs & flow_value_funcs
@@ -427,25 +428,24 @@ class TestMaxFlowMinCutInterface:
def test_minimum_cut_no_cutoff(self):
G = self.G
- for flow_func in flow_funcs:
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut_value,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut_value,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
def test_kwargs(self):
G = self.H
@@ -539,11 +539,20 @@ class TestCutoff:
assert k <= R.graph["flow_value"] <= (2 * k)
R = edmonds_karp(G, "s", "t", cutoff=k)
assert k <= R.graph["flow_value"] <= (2 * k)
+ R = dinitz(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
+ R = boykov_kolmogorov(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
def test_complete_graph_cutoff(self):
G = nx.complete_graph(5)
nx.set_edge_attributes(G, {(u, v): 1 for u, v in G.edges()}, "capacity")
- for flow_func in [shortest_augmenting_path, edmonds_karp]:
+ for flow_func in [
+ shortest_augmenting_path,
+ edmonds_karp,
+ dinitz,
+ boykov_kolmogorov,
+ ]:
for cutoff in [3, 2, 1]:
result = nx.maximum_flow_value(
G, 0, 4, flow_func=flow_func, cutoff=cutoff
diff --git a/networkx/algorithms/tests/test_richclub.py b/networkx/algorithms/tests/test_richclub.py
index 1ed42d459..5638ddbf0 100644
--- a/networkx/algorithms/tests/test_richclub.py
+++ b/networkx/algorithms/tests/test_richclub.py
@@ -80,6 +80,17 @@ def test_rich_club_exception2():
nx.rich_club_coefficient(G)
+def test_rich_club_selfloop():
+ G = nx.Graph() # or DiGraph, MultiGraph, MultiDiGraph, etc
+ G.add_edge(1, 1) # self loop
+ G.add_edge(1, 2)
+ with pytest.raises(
+ Exception,
+ match="rich_club_coefficient is not implemented for " "graphs with self loops.",
+ ):
+ nx.rich_club_coefficient(G)
+
+
# def test_richclub2_normalized():
# T = nx.balanced_tree(2,10)
# rcNorm = nx.richclub.rich_club_coefficient(T,Q=2)
diff --git a/networkx/classes/tests/test_multigraph.py b/networkx/classes/tests/test_multigraph.py
index 0584f5620..07f7f5db6 100644
--- a/networkx/classes/tests/test_multigraph.py
+++ b/networkx/classes/tests/test_multigraph.py
@@ -303,6 +303,9 @@ class TestMultiGraph(BaseMultiGraphTester, _TestGraph):
G = self.Graph()
G.add_edge(*(0, 1))
assert G.adj == {0: {1: {0: {}}}, 1: {0: {0: {}}}}
+ G = self.Graph()
+ with pytest.raises(ValueError):
+ G.add_edge(None, "anything")
def test_add_edge_conflicting_key(self):
G = self.Graph()
diff --git a/networkx/drawing/tests/test_pylab.py b/networkx/drawing/tests/test_pylab.py
index f642dcc47..cef2702df 100644
--- a/networkx/drawing/tests/test_pylab.py
+++ b/networkx/drawing/tests/test_pylab.py
@@ -1,6 +1,7 @@
"""Unit tests for matplotlib drawing functions."""
import itertools
import os
+import warnings
import pytest
@@ -396,6 +397,7 @@ def test_labels_and_colors():
G,
pos,
edgelist=[(4, 5), (5, 6), (6, 7), (7, 4)],
+ arrows=True,
min_source_margin=0.5,
min_target_margin=0.75,
width=8,
@@ -752,3 +754,38 @@ def test_draw_networkx_edges_undirected_selfloop_colors():
for fap, clr, slp in zip(ax.patches, edge_colors[-3:], sl_points):
assert fap.get_path().contains_point(slp)
assert mpl.colors.same_color(fap.get_edgecolor(), clr)
+ plt.delaxes(ax)
+
+
[email protected](
+ "fap_only_kwarg", # Non-default values for kwargs that only apply to FAPs
+ (
+ {"arrowstyle": "-"},
+ {"arrowsize": 20},
+ {"connectionstyle": "arc3,rad=0.2"},
+ {"min_source_margin": 10},
+ {"min_target_margin": 10},
+ ),
+)
+def test_user_warnings_for_unused_edge_drawing_kwargs(fap_only_kwarg):
+ """Users should get a warning when they specify a non-default value for
+ one of the kwargs that applies only to edges drawn with FancyArrowPatches,
+ but FancyArrowPatches aren't being used under the hood."""
+ G = nx.path_graph(3)
+ pos = {n: (n, n) for n in G}
+ fig, ax = plt.subplots()
+ # By default, an undirected graph will use LineCollection to represent
+ # the edges
+ kwarg_name = list(fap_only_kwarg.keys())[0]
+ with pytest.warns(
+ UserWarning, match=f"\n\nThe {kwarg_name} keyword argument is not applicable"
+ ):
+ nx.draw_networkx_edges(G, pos, ax=ax, **fap_only_kwarg)
+ # FancyArrowPatches are always used when `arrows=True` is specified.
+ # Check that warnings are *not* raised in this case
+ with warnings.catch_warnings():
+ # Escalate warnings -> errors so tests fail if warnings are raised
+ warnings.simplefilter("error")
+ nx.draw_networkx_edges(G, pos, ax=ax, arrows=True, **fap_only_kwarg)
+
+ plt.delaxes(ax)
diff --git a/networkx/readwrite/tests/test_gml.py b/networkx/readwrite/tests/test_gml.py
index 6e6ce0f3a..68b0da6f8 100644
--- a/networkx/readwrite/tests/test_gml.py
+++ b/networkx/readwrite/tests/test_gml.py
@@ -571,10 +571,6 @@ graph
G = nx.Graph()
G.graph["data"] = frozenset([1, 2, 3])
assert_generate_error(G, stringizer=literal_stringizer)
- G = nx.Graph()
- G.graph["data"] = []
- assert_generate_error(G)
- assert_generate_error(G, stringizer=len)
def test_label_kwarg(self):
G = nx.parse_gml(self.simple_data, label="id")
@@ -712,3 +708,23 @@ class TestPropertyLists:
f.seek(0)
graph = nx.read_gml(f)
assert graph.nodes(data=True)["n1"] == {"properties": ["element"]}
+
+
[email protected]("coll", (list(), tuple()))
+def test_stringize_empty_list_tuple(coll):
+ G = nx.path_graph(2)
+ G.nodes[0]["test"] = coll # test serializing an empty collection
+ f = io.BytesIO()
+ nx.write_gml(G, f) # Smoke test - should not raise
+ f.seek(0)
+ H = nx.read_gml(f)
+ assert H.nodes["0"]["test"] == coll # Check empty list round-trips properly
+ # Check full round-tripping. Note that nodes are loaded as strings by
+ # default, so there needs to be some remapping prior to comparison
+ H = nx.relabel_nodes(H, {"0": 0, "1": 1})
+ assert nx.utils.graphs_equal(G, H)
+ # Same as above, but use destringizer for node remapping. Should have no
+ # effect on node attr
+ f.seek(0)
+ H = nx.read_gml(f, destringizer=int)
+ assert nx.utils.graphs_equal(G, H)
| `connectionstyle` argument of `nx.draw_networkx_edges()` does not work properly for multigraphs and undirected graphs
`connectionstyle` argument of `nx.draw_networkx_edges()` does not work properly for MultiGraphs and Undirected graphs. Consider the following example:
```
G=nx.DiGraph([(1,2),(3,1),(3,2)])
positions = {1:(0,0),2:(1,-2), 3:(2,0)}
nx.draw_networkx_nodes(G, pos=positions, node_size = 500)
nx.draw_networkx_edges(G, pos=positions, arrowstyle="-", connectionstyle="arc3,rad=0.3");
```
Output is the following:
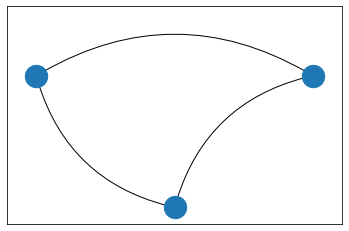
The outputs of the same code snippet when G is created as a multigraph and an undirected graph (`G=nx.MultiGraph([(1,2),(3,1),(3,2)])` and `G=nx.Graph([(1,2),(3,1),(3,2)])`) as follows:
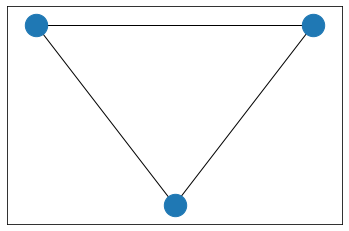
| 0.0 | [
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll0]",
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll1]"
] | [
"networkx/algorithms/approximation/tests/test_dominating_set.py::TestMinWeightDominatingSet::test_min_weighted_dominating_set",
"networkx/algorithms/approximation/tests/test_dominating_set.py::TestMinWeightDominatingSet::test_star_graph",
"networkx/algorithms/approximation/tests/test_dominating_set.py::TestMinWeightDominatingSet::test_null_graph",
"networkx/algorithms/approximation/tests/test_dominating_set.py::TestMinWeightDominatingSet::test_min_edge_dominating_set",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph3",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph4",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_wikipedia_dinitz_example",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_optional_capacity",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_path",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph5",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_disconnected",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_not_in_graph",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_coincide",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_multigraphs_raise",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxFlowMinCutInterface::test_flow_func_not_callable",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_global_relabel_freq",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_makes_enough_space",
"networkx/algorithms/flow/tests/test_maxflow.py::test_shortest_augmenting_path_two_phase",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_cutoff",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_complete_graph_cutoff",
"networkx/algorithms/tests/test_richclub.py::test_richclub",
"networkx/algorithms/tests/test_richclub.py::test_richclub_seed",
"networkx/algorithms/tests/test_richclub.py::test_richclub_normalized",
"networkx/algorithms/tests/test_richclub.py::test_richclub2",
"networkx/algorithms/tests/test_richclub.py::test_richclub3",
"networkx/algorithms/tests/test_richclub.py::test_richclub4",
"networkx/algorithms/tests/test_richclub.py::test_richclub_exception",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_exception2",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_selfloop",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_contains",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_order",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nodes",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_none_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_has_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_neighbors",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_memory_leak",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_size",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nbunch_iter",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloop_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_cache_reset",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_attributes_cached",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_weighted_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_name",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_str_unnamed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_str_named",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_graph_chain",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_class_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_fresh_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_graph_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_node_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_node_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr3",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_directed_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_undirected_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_directed_class",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_subgraph",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloops_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_pickle",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_clear",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_clear_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edges_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_update",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_has_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_get_edge_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_adjacency",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_undirected",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_directed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_number_of_edges_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_lookup",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr4",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_data_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_data_multigraph_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod0-True-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod1-False-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod2-False-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod3-False-edges3]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod4-False-edges4]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod0-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod1-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod2-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_getitem",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edge_conflicting_key",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_multigraph_add_edges_from_four_tuple_misordered",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_multiedge",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_correct_nodes",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_correct_edges",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_node_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_edge_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_graph_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_contains",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_order",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nodes",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_none_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_has_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_neighbors",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_memory_leak",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_size",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nbunch_iter",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloop_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_cache_reset",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_attributes_cached",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_weighted_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_name",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_str_unnamed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_str_named",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_graph_chain",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_class_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_fresh_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_graph_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_node_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_node_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr3",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_directed_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_undirected_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_directed_class",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_subgraph",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloops_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_pickle",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_clear",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_clear_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edges_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_update",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_has_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_get_edge_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_adjacency",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_undirected",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_directed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_number_of_edges_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_lookup",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr4",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_data_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_data_multigraph_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod0-True-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod1-False-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod2-False-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod3-False-edges3]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod4-False-edges4]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod0-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod1-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod2-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_getitem",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edge_conflicting_key",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_multigraph_add_edges_from_four_tuple_misordered",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_multiedge",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml_cytoscape_bug",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_read_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_labels_are_strings",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_relabel_duplicate",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_tuplelabels",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_quotes",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_unicode_node",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_special_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_name",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_graph_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_data_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_escape_unescape",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_exceptions",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_label_kwarg",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_outofrange_integers",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_multi_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_one_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_list_property",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_single_element_list_property"
] | 2022-10-18 22:48:20+00:00 | 4,147 |
|
networkx__networkx-6101 | diff --git a/examples/drawing/plot_eigenvalues.py b/examples/drawing/plot_eigenvalues.py
index b0df67ae9..67322cfd1 100644
--- a/examples/drawing/plot_eigenvalues.py
+++ b/examples/drawing/plot_eigenvalues.py
@@ -14,7 +14,7 @@ m = 5000 # 5000 edges
G = nx.gnm_random_graph(n, m, seed=5040) # Seed for reproducibility
L = nx.normalized_laplacian_matrix(G)
-e = numpy.linalg.eigvals(L.A)
+e = numpy.linalg.eigvals(L.toarray())
print("Largest eigenvalue:", max(e))
print("Smallest eigenvalue:", min(e))
plt.hist(e, bins=100) # histogram with 100 bins
diff --git a/networkx/algorithms/flow/maxflow.py b/networkx/algorithms/flow/maxflow.py
index 8d2fb8f03..b0098d0a0 100644
--- a/networkx/algorithms/flow/maxflow.py
+++ b/networkx/algorithms/flow/maxflow.py
@@ -12,14 +12,6 @@ from .utils import build_flow_dict
# Define the default flow function for computing maximum flow.
default_flow_func = preflow_push
-# Functions that don't support cutoff for minimum cut computations.
-flow_funcs = [
- boykov_kolmogorov,
- dinitz,
- edmonds_karp,
- preflow_push,
- shortest_augmenting_path,
-]
__all__ = ["maximum_flow", "maximum_flow_value", "minimum_cut", "minimum_cut_value"]
@@ -452,7 +444,7 @@ def minimum_cut(flowG, _s, _t, capacity="capacity", flow_func=None, **kwargs):
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
@@ -603,7 +595,7 @@ def minimum_cut_value(flowG, _s, _t, capacity="capacity", flow_func=None, **kwar
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
diff --git a/networkx/readwrite/gml.py b/networkx/readwrite/gml.py
index da5ccca58..758c0c73b 100644
--- a/networkx/readwrite/gml.py
+++ b/networkx/readwrite/gml.py
@@ -363,6 +363,11 @@ def parse_gml_lines(lines, label, destringizer):
value = destringizer(value)
except ValueError:
pass
+ # Special handling for empty lists and tuples
+ if value == "()":
+ value = tuple()
+ if value == "[]":
+ value = list()
curr_token = next(tokens)
elif category == Pattern.DICT_START:
curr_token, value = parse_dict(curr_token)
@@ -728,12 +733,9 @@ def generate_gml(G, stringizer=None):
for key, value in value.items():
yield from stringize(key, value, (), next_indent)
yield indent + "]"
- elif (
- isinstance(value, (list, tuple))
- and key != "label"
- and value
- and not in_list
- ):
+ elif isinstance(value, (list, tuple)) and key != "label" and not in_list:
+ if len(value) == 0:
+ yield indent + key + " " + f'"{value!r}"'
if len(value) == 1:
yield indent + key + " " + f'"{LIST_START_VALUE}"'
for val in value:
| networkx/networkx | bcf607cf7ce4009ca37786b2fcd84e548f1833f5 | diff --git a/networkx/algorithms/flow/tests/test_maxflow.py b/networkx/algorithms/flow/tests/test_maxflow.py
index 36bc5ec7c..d4f9052ce 100644
--- a/networkx/algorithms/flow/tests/test_maxflow.py
+++ b/networkx/algorithms/flow/tests/test_maxflow.py
@@ -20,6 +20,7 @@ flow_funcs = {
preflow_push,
shortest_augmenting_path,
}
+
max_min_funcs = {nx.maximum_flow, nx.minimum_cut}
flow_value_funcs = {nx.maximum_flow_value, nx.minimum_cut_value}
interface_funcs = max_min_funcs & flow_value_funcs
@@ -427,25 +428,24 @@ class TestMaxFlowMinCutInterface:
def test_minimum_cut_no_cutoff(self):
G = self.G
- for flow_func in flow_funcs:
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut_value,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut_value,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
def test_kwargs(self):
G = self.H
@@ -539,11 +539,20 @@ class TestCutoff:
assert k <= R.graph["flow_value"] <= (2 * k)
R = edmonds_karp(G, "s", "t", cutoff=k)
assert k <= R.graph["flow_value"] <= (2 * k)
+ R = dinitz(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
+ R = boykov_kolmogorov(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
def test_complete_graph_cutoff(self):
G = nx.complete_graph(5)
nx.set_edge_attributes(G, {(u, v): 1 for u, v in G.edges()}, "capacity")
- for flow_func in [shortest_augmenting_path, edmonds_karp]:
+ for flow_func in [
+ shortest_augmenting_path,
+ edmonds_karp,
+ dinitz,
+ boykov_kolmogorov,
+ ]:
for cutoff in [3, 2, 1]:
result = nx.maximum_flow_value(
G, 0, 4, flow_func=flow_func, cutoff=cutoff
diff --git a/networkx/algorithms/tests/test_richclub.py b/networkx/algorithms/tests/test_richclub.py
index 1ed42d459..5638ddbf0 100644
--- a/networkx/algorithms/tests/test_richclub.py
+++ b/networkx/algorithms/tests/test_richclub.py
@@ -80,6 +80,17 @@ def test_rich_club_exception2():
nx.rich_club_coefficient(G)
+def test_rich_club_selfloop():
+ G = nx.Graph() # or DiGraph, MultiGraph, MultiDiGraph, etc
+ G.add_edge(1, 1) # self loop
+ G.add_edge(1, 2)
+ with pytest.raises(
+ Exception,
+ match="rich_club_coefficient is not implemented for " "graphs with self loops.",
+ ):
+ nx.rich_club_coefficient(G)
+
+
# def test_richclub2_normalized():
# T = nx.balanced_tree(2,10)
# rcNorm = nx.richclub.rich_club_coefficient(T,Q=2)
diff --git a/networkx/classes/tests/test_multigraph.py b/networkx/classes/tests/test_multigraph.py
index 0584f5620..07f7f5db6 100644
--- a/networkx/classes/tests/test_multigraph.py
+++ b/networkx/classes/tests/test_multigraph.py
@@ -303,6 +303,9 @@ class TestMultiGraph(BaseMultiGraphTester, _TestGraph):
G = self.Graph()
G.add_edge(*(0, 1))
assert G.adj == {0: {1: {0: {}}}, 1: {0: {0: {}}}}
+ G = self.Graph()
+ with pytest.raises(ValueError):
+ G.add_edge(None, "anything")
def test_add_edge_conflicting_key(self):
G = self.Graph()
diff --git a/networkx/readwrite/tests/test_gml.py b/networkx/readwrite/tests/test_gml.py
index 6e6ce0f3a..68b0da6f8 100644
--- a/networkx/readwrite/tests/test_gml.py
+++ b/networkx/readwrite/tests/test_gml.py
@@ -571,10 +571,6 @@ graph
G = nx.Graph()
G.graph["data"] = frozenset([1, 2, 3])
assert_generate_error(G, stringizer=literal_stringizer)
- G = nx.Graph()
- G.graph["data"] = []
- assert_generate_error(G)
- assert_generate_error(G, stringizer=len)
def test_label_kwarg(self):
G = nx.parse_gml(self.simple_data, label="id")
@@ -712,3 +708,23 @@ class TestPropertyLists:
f.seek(0)
graph = nx.read_gml(f)
assert graph.nodes(data=True)["n1"] == {"properties": ["element"]}
+
+
[email protected]("coll", (list(), tuple()))
+def test_stringize_empty_list_tuple(coll):
+ G = nx.path_graph(2)
+ G.nodes[0]["test"] = coll # test serializing an empty collection
+ f = io.BytesIO()
+ nx.write_gml(G, f) # Smoke test - should not raise
+ f.seek(0)
+ H = nx.read_gml(f)
+ assert H.nodes["0"]["test"] == coll # Check empty list round-trips properly
+ # Check full round-tripping. Note that nodes are loaded as strings by
+ # default, so there needs to be some remapping prior to comparison
+ H = nx.relabel_nodes(H, {"0": 0, "1": 1})
+ assert nx.utils.graphs_equal(G, H)
+ # Same as above, but use destringizer for node remapping. Should have no
+ # effect on node attr
+ f.seek(0)
+ H = nx.read_gml(f, destringizer=int)
+ assert nx.utils.graphs_equal(G, H)
| Improve test coverage for multigraph class
I discovered there are still some red spots in this link https://app.codecov.io/gh/networkx/networkx/blob/main/networkx/classes/multigraph.py and will like to work on it.
The tests will be added in https://github.com/networkx/networkx/blob/main/networkx/classes/tests/test_multigraph.py
Current Behavior
We don't test all the paths the code can take us.
Expected Behavior
We should be testing everything so there aren't any surprises.
Steps to Reproduce
Visit https://app.codecov.io/gh/networkx/networkx/blob/main//networkx/classes/multigraph.py
| 0.0 | [
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll0]",
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll1]"
] | [
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph3",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph4",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_wikipedia_dinitz_example",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_optional_capacity",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_path",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph5",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_disconnected",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_not_in_graph",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_coincide",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_multigraphs_raise",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxFlowMinCutInterface::test_flow_func_not_callable",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_global_relabel_freq",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_makes_enough_space",
"networkx/algorithms/flow/tests/test_maxflow.py::test_shortest_augmenting_path_two_phase",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_cutoff",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_complete_graph_cutoff",
"networkx/algorithms/tests/test_richclub.py::test_richclub",
"networkx/algorithms/tests/test_richclub.py::test_richclub_seed",
"networkx/algorithms/tests/test_richclub.py::test_richclub_normalized",
"networkx/algorithms/tests/test_richclub.py::test_richclub2",
"networkx/algorithms/tests/test_richclub.py::test_richclub3",
"networkx/algorithms/tests/test_richclub.py::test_richclub4",
"networkx/algorithms/tests/test_richclub.py::test_richclub_exception",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_exception2",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_selfloop",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_contains",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_order",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nodes",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_none_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_has_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_neighbors",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_memory_leak",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_size",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nbunch_iter",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloop_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_cache_reset",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_attributes_cached",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_weighted_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_name",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_str_unnamed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_str_named",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_graph_chain",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_class_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_fresh_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_graph_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_node_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_node_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr3",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_directed_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_undirected_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_directed_class",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_subgraph",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_selfloops_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_pickle",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_clear",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_clear_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edges_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_update",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_has_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_get_edge_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_adjacency",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_undirected",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_to_directed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_number_of_edges_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_lookup",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_edge_attr4",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_data_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_data_multigraph_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod0-True-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod1-False-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod2-False-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod3-False-edges3]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input[dod4-False-edges4]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod0-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod1-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_mgi_none[dod2-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_non_multigraph_input_raise[dod2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_getitem",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edge_conflicting_key",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_add_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_multigraph_add_edges_from_four_tuple_misordered",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraph::test_remove_multiedge",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_correct_nodes",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_correct_edges",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_node_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_edge_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestEdgeSubgraph::test_graph_attr_dict",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_contains",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_order",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nodes",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_none_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_has_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_neighbors",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_memory_leak",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_size",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nbunch_iter",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_nbunch_iter_node_format_raise",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloop_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_cache_reset",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_attributes_cached",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_weighted_degree",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_name",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_str_unnamed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_str_named",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_graph_chain",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_class_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_fresh_copy",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_graph_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_node_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_node_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr2",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr3",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_directed_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_undirected_as_view",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_directed_class",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_subgraph",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_selfloops_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_pickle",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_nodes_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_clear",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_clear_edges",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edges_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_update",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_has_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_get_edge_data",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_adjacency",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_undirected",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_to_directed",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_number_of_edges_selfloops",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_lookup",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_edge_attr4",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_data_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_data_multigraph_input",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod0-True-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod1-False-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod2-False-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod3-False-edges3]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input[dod4-False-edges4]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod0-edges0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod1-edges1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_mgi_none[dod2-edges2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod0]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod1]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_non_multigraph_input_raise[dod2]",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_getitem",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_node",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edge_conflicting_key",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_add_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_multigraph_add_edges_from_four_tuple_misordered",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_edge",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_edges_from",
"networkx/classes/tests/test_multigraph.py::TestMultiGraphSubclass::test_remove_multiedge",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml_cytoscape_bug",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_read_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_labels_are_strings",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_relabel_duplicate",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_tuplelabels",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_quotes",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_unicode_node",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_special_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_name",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_graph_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_data_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_escape_unescape",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_exceptions",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_label_kwarg",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_outofrange_integers",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_multi_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_one_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_list_property",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_single_element_list_property"
] | 2022-10-19 11:49:52+00:00 | 4,148 |
|
networkx__networkx-6104 | diff --git a/examples/drawing/plot_eigenvalues.py b/examples/drawing/plot_eigenvalues.py
index b0df67ae9..67322cfd1 100644
--- a/examples/drawing/plot_eigenvalues.py
+++ b/examples/drawing/plot_eigenvalues.py
@@ -14,7 +14,7 @@ m = 5000 # 5000 edges
G = nx.gnm_random_graph(n, m, seed=5040) # Seed for reproducibility
L = nx.normalized_laplacian_matrix(G)
-e = numpy.linalg.eigvals(L.A)
+e = numpy.linalg.eigvals(L.toarray())
print("Largest eigenvalue:", max(e))
print("Smallest eigenvalue:", min(e))
plt.hist(e, bins=100) # histogram with 100 bins
diff --git a/networkx/algorithms/flow/maxflow.py b/networkx/algorithms/flow/maxflow.py
index 8d2fb8f03..b0098d0a0 100644
--- a/networkx/algorithms/flow/maxflow.py
+++ b/networkx/algorithms/flow/maxflow.py
@@ -12,14 +12,6 @@ from .utils import build_flow_dict
# Define the default flow function for computing maximum flow.
default_flow_func = preflow_push
-# Functions that don't support cutoff for minimum cut computations.
-flow_funcs = [
- boykov_kolmogorov,
- dinitz,
- edmonds_karp,
- preflow_push,
- shortest_augmenting_path,
-]
__all__ = ["maximum_flow", "maximum_flow_value", "minimum_cut", "minimum_cut_value"]
@@ -452,7 +444,7 @@ def minimum_cut(flowG, _s, _t, capacity="capacity", flow_func=None, **kwargs):
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
@@ -603,7 +595,7 @@ def minimum_cut_value(flowG, _s, _t, capacity="capacity", flow_func=None, **kwar
if not callable(flow_func):
raise nx.NetworkXError("flow_func has to be callable.")
- if kwargs.get("cutoff") is not None and flow_func in flow_funcs:
+ if kwargs.get("cutoff") is not None and flow_func is preflow_push:
raise nx.NetworkXError("cutoff should not be specified.")
R = flow_func(flowG, _s, _t, capacity=capacity, value_only=True, **kwargs)
diff --git a/networkx/readwrite/gml.py b/networkx/readwrite/gml.py
index da5ccca58..758c0c73b 100644
--- a/networkx/readwrite/gml.py
+++ b/networkx/readwrite/gml.py
@@ -363,6 +363,11 @@ def parse_gml_lines(lines, label, destringizer):
value = destringizer(value)
except ValueError:
pass
+ # Special handling for empty lists and tuples
+ if value == "()":
+ value = tuple()
+ if value == "[]":
+ value = list()
curr_token = next(tokens)
elif category == Pattern.DICT_START:
curr_token, value = parse_dict(curr_token)
@@ -728,12 +733,9 @@ def generate_gml(G, stringizer=None):
for key, value in value.items():
yield from stringize(key, value, (), next_indent)
yield indent + "]"
- elif (
- isinstance(value, (list, tuple))
- and key != "label"
- and value
- and not in_list
- ):
+ elif isinstance(value, (list, tuple)) and key != "label" and not in_list:
+ if len(value) == 0:
+ yield indent + key + " " + f'"{value!r}"'
if len(value) == 1:
yield indent + key + " " + f'"{LIST_START_VALUE}"'
for val in value:
| networkx/networkx | bcf607cf7ce4009ca37786b2fcd84e548f1833f5 | diff --git a/networkx/algorithms/flow/tests/test_maxflow.py b/networkx/algorithms/flow/tests/test_maxflow.py
index 36bc5ec7c..d4f9052ce 100644
--- a/networkx/algorithms/flow/tests/test_maxflow.py
+++ b/networkx/algorithms/flow/tests/test_maxflow.py
@@ -20,6 +20,7 @@ flow_funcs = {
preflow_push,
shortest_augmenting_path,
}
+
max_min_funcs = {nx.maximum_flow, nx.minimum_cut}
flow_value_funcs = {nx.maximum_flow_value, nx.minimum_cut_value}
interface_funcs = max_min_funcs & flow_value_funcs
@@ -427,25 +428,24 @@ class TestMaxFlowMinCutInterface:
def test_minimum_cut_no_cutoff(self):
G = self.G
- for flow_func in flow_funcs:
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
- pytest.raises(
- nx.NetworkXError,
- nx.minimum_cut_value,
- G,
- "x",
- "y",
- flow_func=flow_func,
- cutoff=1.0,
- )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
+ pytest.raises(
+ nx.NetworkXError,
+ nx.minimum_cut_value,
+ G,
+ "x",
+ "y",
+ flow_func=preflow_push,
+ cutoff=1.0,
+ )
def test_kwargs(self):
G = self.H
@@ -539,11 +539,20 @@ class TestCutoff:
assert k <= R.graph["flow_value"] <= (2 * k)
R = edmonds_karp(G, "s", "t", cutoff=k)
assert k <= R.graph["flow_value"] <= (2 * k)
+ R = dinitz(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
+ R = boykov_kolmogorov(G, "s", "t", cutoff=k)
+ assert k <= R.graph["flow_value"] <= (2 * k)
def test_complete_graph_cutoff(self):
G = nx.complete_graph(5)
nx.set_edge_attributes(G, {(u, v): 1 for u, v in G.edges()}, "capacity")
- for flow_func in [shortest_augmenting_path, edmonds_karp]:
+ for flow_func in [
+ shortest_augmenting_path,
+ edmonds_karp,
+ dinitz,
+ boykov_kolmogorov,
+ ]:
for cutoff in [3, 2, 1]:
result = nx.maximum_flow_value(
G, 0, 4, flow_func=flow_func, cutoff=cutoff
diff --git a/networkx/algorithms/tests/test_richclub.py b/networkx/algorithms/tests/test_richclub.py
index 1ed42d459..5638ddbf0 100644
--- a/networkx/algorithms/tests/test_richclub.py
+++ b/networkx/algorithms/tests/test_richclub.py
@@ -80,6 +80,17 @@ def test_rich_club_exception2():
nx.rich_club_coefficient(G)
+def test_rich_club_selfloop():
+ G = nx.Graph() # or DiGraph, MultiGraph, MultiDiGraph, etc
+ G.add_edge(1, 1) # self loop
+ G.add_edge(1, 2)
+ with pytest.raises(
+ Exception,
+ match="rich_club_coefficient is not implemented for " "graphs with self loops.",
+ ):
+ nx.rich_club_coefficient(G)
+
+
# def test_richclub2_normalized():
# T = nx.balanced_tree(2,10)
# rcNorm = nx.richclub.rich_club_coefficient(T,Q=2)
diff --git a/networkx/readwrite/tests/test_gml.py b/networkx/readwrite/tests/test_gml.py
index 6e6ce0f3a..68b0da6f8 100644
--- a/networkx/readwrite/tests/test_gml.py
+++ b/networkx/readwrite/tests/test_gml.py
@@ -571,10 +571,6 @@ graph
G = nx.Graph()
G.graph["data"] = frozenset([1, 2, 3])
assert_generate_error(G, stringizer=literal_stringizer)
- G = nx.Graph()
- G.graph["data"] = []
- assert_generate_error(G)
- assert_generate_error(G, stringizer=len)
def test_label_kwarg(self):
G = nx.parse_gml(self.simple_data, label="id")
@@ -712,3 +708,23 @@ class TestPropertyLists:
f.seek(0)
graph = nx.read_gml(f)
assert graph.nodes(data=True)["n1"] == {"properties": ["element"]}
+
+
[email protected]("coll", (list(), tuple()))
+def test_stringize_empty_list_tuple(coll):
+ G = nx.path_graph(2)
+ G.nodes[0]["test"] = coll # test serializing an empty collection
+ f = io.BytesIO()
+ nx.write_gml(G, f) # Smoke test - should not raise
+ f.seek(0)
+ H = nx.read_gml(f)
+ assert H.nodes["0"]["test"] == coll # Check empty list round-trips properly
+ # Check full round-tripping. Note that nodes are loaded as strings by
+ # default, so there needs to be some remapping prior to comparison
+ H = nx.relabel_nodes(H, {"0": 0, "1": 1})
+ assert nx.utils.graphs_equal(G, H)
+ # Same as above, but use destringizer for node remapping. Should have no
+ # effect on node attr
+ f.seek(0)
+ H = nx.read_gml(f, destringizer=int)
+ assert nx.utils.graphs_equal(G, H)
| boykov_kolmogorov and dinitz algorithms allow cutoff but are not tested
I noticed in *test_maxflow.py* that cutoff tests don't include boykov_kolmogorov and dinitz algorithms. I'm already working on this! | 0.0 | [
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll0]",
"networkx/readwrite/tests/test_gml.py::test_stringize_empty_list_tuple[coll1]"
] | [
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph1",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph2",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph3",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph4",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_wikipedia_dinitz_example",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_optional_capacity",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph_infcap_path",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_graph_infcap_edges",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_digraph5",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_disconnected",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_not_in_graph",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_source_target_coincide",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxflowMinCutCommon::test_multigraphs_raise",
"networkx/algorithms/flow/tests/test_maxflow.py::TestMaxFlowMinCutInterface::test_flow_func_not_callable",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_global_relabel_freq",
"networkx/algorithms/flow/tests/test_maxflow.py::test_preflow_push_makes_enough_space",
"networkx/algorithms/flow/tests/test_maxflow.py::test_shortest_augmenting_path_two_phase",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_cutoff",
"networkx/algorithms/flow/tests/test_maxflow.py::TestCutoff::test_complete_graph_cutoff",
"networkx/algorithms/tests/test_richclub.py::test_richclub",
"networkx/algorithms/tests/test_richclub.py::test_richclub_seed",
"networkx/algorithms/tests/test_richclub.py::test_richclub_normalized",
"networkx/algorithms/tests/test_richclub.py::test_richclub2",
"networkx/algorithms/tests/test_richclub.py::test_richclub3",
"networkx/algorithms/tests/test_richclub.py::test_richclub4",
"networkx/algorithms/tests/test_richclub.py::test_richclub_exception",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_exception2",
"networkx/algorithms/tests/test_richclub.py::test_rich_club_selfloop",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml_cytoscape_bug",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_parse_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_read_gml",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_labels_are_strings",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_relabel_duplicate",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_tuplelabels",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_quotes",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_unicode_node",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_special_float_label",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_name",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_graph_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_data_types",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_escape_unescape",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_exceptions",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_label_kwarg",
"networkx/readwrite/tests/test_gml.py::TestGraph::test_outofrange_integers",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_multi_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_writing_graph_with_one_element_property_list",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_list_property",
"networkx/readwrite/tests/test_gml.py::TestPropertyLists::test_reading_graph_with_single_element_list_property"
] | 2022-10-19 14:04:07+00:00 | 4,149 |
|
networkx__networkx-6149 | diff --git a/networkx/algorithms/swap.py b/networkx/algorithms/swap.py
index 9b2f06db6..c02a4b3ca 100644
--- a/networkx/algorithms/swap.py
+++ b/networkx/algorithms/swap.py
@@ -46,7 +46,7 @@ def directed_edge_swap(G, *, nswap=1, max_tries=100, seed=None):
NetworkXError
If `G` is not directed, or
If nswap > max_tries, or
- If there are fewer than 4 nodes in `G`
+ If there are fewer than 4 nodes or 3 edges in `G`.
NetworkXAlgorithmError
If the number of swap attempts exceeds `max_tries` before `nswap` swaps are made
@@ -70,7 +70,9 @@ def directed_edge_swap(G, *, nswap=1, max_tries=100, seed=None):
if nswap > max_tries:
raise nx.NetworkXError("Number of swaps > number of tries allowed.")
if len(G) < 4:
- raise nx.NetworkXError("Graph has less than four nodes.")
+ raise nx.NetworkXError("DiGraph has fewer than four nodes.")
+ if len(G.edges) < 3:
+ raise nx.NetworkXError("DiGraph has fewer than 3 edges")
# Instead of choosing uniformly at random from a generated edge list,
# this algorithm chooses nonuniformly from the set of nodes with
@@ -161,6 +163,15 @@ def double_edge_swap(G, nswap=1, max_tries=100, seed=None):
G : graph
The graph after double edge swaps.
+ Raises
+ ------
+ NetworkXError
+ If `G` is directed, or
+ If `nswap` > `max_tries`, or
+ If there are fewer than 4 nodes or 2 edges in `G`.
+ NetworkXAlgorithmError
+ If the number of swap attempts exceeds `max_tries` before `nswap` swaps are made
+
Notes
-----
Does not enforce any connectivity constraints.
@@ -174,7 +185,9 @@ def double_edge_swap(G, nswap=1, max_tries=100, seed=None):
if nswap > max_tries:
raise nx.NetworkXError("Number of swaps > number of tries allowed.")
if len(G) < 4:
- raise nx.NetworkXError("Graph has less than four nodes.")
+ raise nx.NetworkXError("Graph has fewer than four nodes.")
+ if len(G.edges) < 2:
+ raise nx.NetworkXError("Graph has fewer than 2 edges")
# Instead of choosing uniformly at random from a generated edge list,
# this algorithm chooses nonuniformly from the set of nodes with
# probability weighted by degree.
@@ -285,7 +298,7 @@ def connected_double_edge_swap(G, nswap=1, _window_threshold=3, seed=None):
if not nx.is_connected(G):
raise nx.NetworkXError("Graph not connected")
if len(G) < 4:
- raise nx.NetworkXError("Graph has less than four nodes.")
+ raise nx.NetworkXError("Graph has fewer than four nodes.")
n = 0
swapcount = 0
deg = G.degree()
| networkx/networkx | 9374d1ab1cc732a1a86ef1ed2438bc51e834f20d | diff --git a/networkx/algorithms/tests/test_swap.py b/networkx/algorithms/tests/test_swap.py
index 2bc25df23..49dd5f8e8 100644
--- a/networkx/algorithms/tests/test_swap.py
+++ b/networkx/algorithms/tests/test_swap.py
@@ -19,9 +19,6 @@ def test_edge_cases_directed_edge_swap():
"Maximum number of swap attempts \\(11\\) exceeded "
"before desired swaps achieved \\(\\d\\)."
)
- graph = nx.DiGraph([(0, 1), (2, 3)])
- with pytest.raises(nx.NetworkXAlgorithmError, match=e):
- nx.directed_edge_swap(graph, nswap=4, max_tries=10, seed=1)
graph = nx.DiGraph([(0, 0), (0, 1), (1, 0), (2, 3), (3, 2)])
with pytest.raises(nx.NetworkXAlgorithmError, match=e):
nx.directed_edge_swap(graph, nswap=1, max_tries=10, seed=1)
@@ -138,3 +135,22 @@ def test_degree_seq_c4():
degrees = sorted(d for n, d in G.degree())
G = nx.double_edge_swap(G, 1, 100)
assert degrees == sorted(d for n, d in G.degree())
+
+
+def test_fewer_than_4_nodes():
+ G = nx.DiGraph()
+ G.add_nodes_from([0, 1, 2])
+ with pytest.raises(nx.NetworkXError, match=".*fewer than four nodes."):
+ nx.directed_edge_swap(G)
+
+
+def test_less_than_3_edges():
+ G = nx.DiGraph([(0, 1), (1, 2)])
+ G.add_nodes_from([3, 4])
+ with pytest.raises(nx.NetworkXError, match=".*fewer than 3 edges"):
+ nx.directed_edge_swap(G)
+
+ G = nx.Graph()
+ G.add_nodes_from([0, 1, 2, 3])
+ with pytest.raises(nx.NetworkXError, match=".*fewer than 2 edges"):
+ nx.double_edge_swap(G)
| directed_edge_swap: ZeroDivisionError when passing a digraph without edges
I was working on improving test coverage for `swap.py` and tested `directed_edge_swap` with a digraph without edges. That resulted in a ZeroDivisionError from `nx.utils.cumulative_distribution`.
### Steps to Reproduce
```python
from networkx as nx
G = nx.DiGraph()
G.add_nodes_from([0, 2, 4, 3])
G = nx.directed_edge_swap(G, nswap=1, max_tries=500, seed=1)
```
### Current Behavior
ZeroDivisionError is raised because in `nx.utils.cumulative_distribution` there's a division by the sum of the input distribution that is zero. The distribution that `nx.utils.cumulative_distribution` is called with in this example is the degrees of a digraph without edges (all degrees are zero). This is the error log.
```
Traceback (most recent call last):
File "test.py", line 12, in <module>
G = nx.directed_edge_swap(G, nswap=1, max_tries=500, seed=1)
File "/home/paula/Outreachy/networkX/networkx/networkx/utils/decorators.py", line 766, in func
return argmap._lazy_compile(__wrapper)(*args, **kwargs)
File "<class 'networkx.utils.decorators.argmap'> compilation 21", line 5, in argmap_directed_edge_swap_17
File "/home/paula/Outreachy/networkX/networkx/networkx/algorithms/swap.py", line 81, in directed_edge_swap
cdf = nx.utils.cumulative_distribution(degrees) # cdf of degree
File "/home/paula/Outreachy/networkX/networkx/networkx/utils/random_sequence.py", line 103, in cumulative_distribution
cdf.append(cdf[i] + distribution[i] / psum)
ZeroDivisionError: division by zero
```
### Expected Behavior
I think the best is to raise an exception in `directed_edge_swap` with a message that exposes this situation. Also, this should be added to the [doc entry](https://output.circle-artifacts.com/output/job/ab098af6-f76c-4bf9-a732-5c243aca96da/artifacts/0/doc/build/html/reference/algorithms/generated/networkx.algorithms.swap.directed_edge_swap.html#directed-edge-swap).
### Environment
Python version: 3.8
NetworkX version: 2.8.7
### Further Discoveries
Something else that should be added is that if the digraph has less than 3 edges then is impossible to swap edges. In that case, the current behavior is that the max number of tries is reached. In my opinion, this case should raise an exception with an appropriate message. Also, this could be mentioned in the [doc entry](https://output.circle-artifacts.com/output/job/ab098af6-f76c-4bf9-a732-5c243aca96da/artifacts/0/doc/build/html/reference/algorithms/generated/networkx.algorithms.swap.directed_edge_swap.html#directed-edge-swap).
I will work on these changes and will look closely at the other functions in `swap.py`.
Also, I think it will be good to review the functions that use `nx.utils.cumulative_distribution` to check for this error. I can do that too.
| 0.0 | [
"networkx/algorithms/tests/test_swap.py::test_fewer_than_4_nodes",
"networkx/algorithms/tests/test_swap.py::test_less_than_3_edges"
] | [
"networkx/algorithms/tests/test_swap.py::test_directed_edge_swap",
"networkx/algorithms/tests/test_swap.py::test_edge_cases_directed_edge_swap",
"networkx/algorithms/tests/test_swap.py::test_double_edge_swap",
"networkx/algorithms/tests/test_swap.py::test_double_edge_swap_seed",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap_low_window_threshold",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap_star",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap_star_low_window_threshold",
"networkx/algorithms/tests/test_swap.py::test_directed_edge_swap_small",
"networkx/algorithms/tests/test_swap.py::test_directed_edge_swap_tries",
"networkx/algorithms/tests/test_swap.py::test_directed_exception_undirected",
"networkx/algorithms/tests/test_swap.py::test_directed_edge_max_tries",
"networkx/algorithms/tests/test_swap.py::test_double_edge_swap_small",
"networkx/algorithms/tests/test_swap.py::test_double_edge_swap_tries",
"networkx/algorithms/tests/test_swap.py::test_double_edge_directed",
"networkx/algorithms/tests/test_swap.py::test_double_edge_max_tries",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap_small",
"networkx/algorithms/tests/test_swap.py::test_connected_double_edge_swap_not_connected",
"networkx/algorithms/tests/test_swap.py::test_degree_seq_c4"
] | 2022-10-29 17:06:53+00:00 | 4,150 |
|
networkx__networkx-6240 | diff --git a/networkx/algorithms/traversal/depth_first_search.py b/networkx/algorithms/traversal/depth_first_search.py
index 0ccca4ff9..c250787bf 100644
--- a/networkx/algorithms/traversal/depth_first_search.py
+++ b/networkx/algorithms/traversal/depth_first_search.py
@@ -364,12 +364,15 @@ def dfs_labeled_edges(G, source=None, depth_limit=None):
edges: generator
A generator of triples of the form (*u*, *v*, *d*), where (*u*,
*v*) is the edge being explored in the depth-first search and *d*
- is one of the strings 'forward', 'nontree', or 'reverse'. A
- 'forward' edge is one in which *u* has been visited but *v* has
+ is one of the strings 'forward', 'nontree', 'reverse', or 'reverse-depth_limit'.
+ A 'forward' edge is one in which *u* has been visited but *v* has
not. A 'nontree' edge is one in which both *u* and *v* have been
visited but the edge is not in the DFS tree. A 'reverse' edge is
- on in which both *u* and *v* have been visited and the edge is in
- the DFS tree.
+ one in which both *u* and *v* have been visited and the edge is in
+ the DFS tree. When the `depth_limit` is reached via a 'forward' edge,
+ a 'reverse' edge is immediately generated rather than the subtree
+ being explored. To indicate this flavor of 'reverse' edge, the string
+ yielded is 'reverse-depth_limit'.
Examples
--------
@@ -436,6 +439,8 @@ def dfs_labeled_edges(G, source=None, depth_limit=None):
visited.add(child)
if depth_now > 1:
stack.append((child, depth_now - 1, iter(G[child])))
+ else:
+ yield parent, child, "reverse-depth_limit"
except StopIteration:
stack.pop()
if stack:
| networkx/networkx | d82815dba6c8ddce19cd49f700298dc82a58f066 | diff --git a/networkx/algorithms/traversal/tests/test_dfs.py b/networkx/algorithms/traversal/tests/test_dfs.py
index 765280954..0eb698b0f 100644
--- a/networkx/algorithms/traversal/tests/test_dfs.py
+++ b/networkx/algorithms/traversal/tests/test_dfs.py
@@ -59,11 +59,43 @@ class TestDFS:
edges = list(nx.dfs_labeled_edges(self.G, source=0))
forward = [(u, v) for (u, v, d) in edges if d == "forward"]
assert forward == [(0, 0), (0, 1), (1, 2), (2, 4), (1, 3)]
+ assert edges == [
+ (0, 0, "forward"),
+ (0, 1, "forward"),
+ (1, 0, "nontree"),
+ (1, 2, "forward"),
+ (2, 1, "nontree"),
+ (2, 4, "forward"),
+ (4, 2, "nontree"),
+ (4, 0, "nontree"),
+ (2, 4, "reverse"),
+ (1, 2, "reverse"),
+ (1, 3, "forward"),
+ (3, 1, "nontree"),
+ (3, 0, "nontree"),
+ (1, 3, "reverse"),
+ (0, 1, "reverse"),
+ (0, 3, "nontree"),
+ (0, 4, "nontree"),
+ (0, 0, "reverse"),
+ ]
def test_dfs_labeled_disconnected_edges(self):
edges = list(nx.dfs_labeled_edges(self.D))
forward = [(u, v) for (u, v, d) in edges if d == "forward"]
assert forward == [(0, 0), (0, 1), (2, 2), (2, 3)]
+ assert edges == [
+ (0, 0, "forward"),
+ (0, 1, "forward"),
+ (1, 0, "nontree"),
+ (0, 1, "reverse"),
+ (0, 0, "reverse"),
+ (2, 2, "forward"),
+ (2, 3, "forward"),
+ (3, 2, "nontree"),
+ (2, 3, "reverse"),
+ (2, 2, "reverse"),
+ ]
def test_dfs_tree_isolates(self):
G = nx.Graph()
@@ -141,12 +173,79 @@ class TestDepthLimitedSearch:
edges = nx.dfs_edges(self.G, source=9, depth_limit=4)
assert list(edges) == [(9, 8), (8, 7), (7, 2), (2, 1), (2, 3), (9, 10)]
- def test_dls_labeled_edges(self):
+ def test_dls_labeled_edges_depth_1(self):
edges = list(nx.dfs_labeled_edges(self.G, source=5, depth_limit=1))
forward = [(u, v) for (u, v, d) in edges if d == "forward"]
assert forward == [(5, 5), (5, 4), (5, 6)]
+ # Note: reverse-depth_limit edge types were not reported before gh-6240
+ assert edges == [
+ (5, 5, "forward"),
+ (5, 4, "forward"),
+ (5, 4, "reverse-depth_limit"),
+ (5, 6, "forward"),
+ (5, 6, "reverse-depth_limit"),
+ (5, 5, "reverse"),
+ ]
- def test_dls_labeled_disconnected_edges(self):
+ def test_dls_labeled_edges_depth_2(self):
edges = list(nx.dfs_labeled_edges(self.G, source=6, depth_limit=2))
forward = [(u, v) for (u, v, d) in edges if d == "forward"]
assert forward == [(6, 6), (6, 5), (5, 4)]
+ assert edges == [
+ (6, 6, "forward"),
+ (6, 5, "forward"),
+ (5, 4, "forward"),
+ (5, 4, "reverse-depth_limit"),
+ (5, 6, "nontree"),
+ (6, 5, "reverse"),
+ (6, 6, "reverse"),
+ ]
+
+ def test_dls_labeled_disconnected_edges(self):
+ edges = list(nx.dfs_labeled_edges(self.D, depth_limit=1))
+ assert edges == [
+ (0, 0, "forward"),
+ (0, 1, "forward"),
+ (0, 1, "reverse-depth_limit"),
+ (0, 0, "reverse"),
+ (2, 2, "forward"),
+ (2, 3, "forward"),
+ (2, 3, "reverse-depth_limit"),
+ (2, 7, "forward"),
+ (2, 7, "reverse-depth_limit"),
+ (2, 2, "reverse"),
+ (8, 8, "forward"),
+ (8, 7, "nontree"),
+ (8, 9, "forward"),
+ (8, 9, "reverse-depth_limit"),
+ (8, 8, "reverse"),
+ (10, 10, "forward"),
+ (10, 9, "nontree"),
+ (10, 10, "reverse"),
+ ]
+ # large depth_limit has no impact
+ edges = list(nx.dfs_labeled_edges(self.D, depth_limit=19))
+ assert edges == [
+ (0, 0, "forward"),
+ (0, 1, "forward"),
+ (1, 0, "nontree"),
+ (0, 1, "reverse"),
+ (0, 0, "reverse"),
+ (2, 2, "forward"),
+ (2, 3, "forward"),
+ (3, 2, "nontree"),
+ (2, 3, "reverse"),
+ (2, 7, "forward"),
+ (7, 2, "nontree"),
+ (7, 8, "forward"),
+ (8, 7, "nontree"),
+ (8, 9, "forward"),
+ (9, 8, "nontree"),
+ (9, 10, "forward"),
+ (10, 9, "nontree"),
+ (9, 10, "reverse"),
+ (8, 9, "reverse"),
+ (7, 8, "reverse"),
+ (2, 7, "reverse"),
+ (2, 2, "reverse"),
+ ]
| nx.dfs_labeled_edges does not return last visited edge if depth_limit is specified
`nx.dfs_labeled_edges` does not return last (deepest) visited edge when traversing backwards if **depth_limit** is specified.
### Current Behavior
```
graph = nx.path_graph(5, nx.DiGraph)
list(nx.dfs_labeled_edges(graph, source=0))
[(0, 0, 'forward'),
(0, 1, 'forward'),
(1, 2, 'forward'),
(2, 3, 'forward'),
(3, 4, 'forward'),
(3, 4, 'reverse'),
(2, 3, 'reverse'),
(1, 2, 'reverse'),
(0, 1, 'reverse'),
(0, 0, 'reverse')]
list(nx.dfs_labeled_edges(graph, source=0, depth_limit=4))
[(0, 0, 'forward'),
(0, 1, 'forward'),
(1, 2, 'forward'),
(2, 3, 'forward'),
(3, 4, 'forward'),
(2, 3, 'reverse'),
(1, 2, 'reverse'),
(0, 1, 'reverse'),
(0, 0, 'reverse')]
```
Note that edge `(3, 4, 'reverse')` is missing.
### Expected Behavior
```
graph = nx.path_graph(5, nx.DiGraph)
list(nx.dfs_labeled_edges(graph, source=0, depth_limit=4))
[(0, 0, 'forward'),
(0, 1, 'forward'),
(1, 2, 'forward'),
(2, 3, 'forward'),
(3, 4, 'forward'),
(3, 4, 'reverse'),
(2, 3, 'reverse'),
(1, 2, 'reverse'),
(0, 1, 'reverse'),
(0, 0, 'reverse')]
```
### Environment
Python version: 3.10
NetworkX version: 2.8.8 and 3.0rc1
| 0.0 | [
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_labeled_edges_depth_1",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_labeled_edges_depth_2",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_labeled_disconnected_edges"
] | [
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_preorder_nodes",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_postorder_nodes",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_successor",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_predecessor",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_dfs_tree",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_dfs_edges",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_dfs_labeled_edges",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_dfs_labeled_disconnected_edges",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDFS::test_dfs_tree_isolates",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_preorder_nodes",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_postorder_nodes",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_successor",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_predecessor",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_tree",
"networkx/algorithms/traversal/tests/test_dfs.py::TestDepthLimitedSearch::test_dls_edges"
] | 2022-11-27 09:07:26+00:00 | 4,151 |
|
networkx__networkx-6908 | diff --git a/doc/conf.py b/doc/conf.py
index ec7c640cf..f2eac1f60 100644
--- a/doc/conf.py
+++ b/doc/conf.py
@@ -1,3 +1,4 @@
+import os
from datetime import date
from sphinx_gallery.sorting import ExplicitOrder, FileNameSortKey
from warnings import filterwarnings
@@ -81,12 +82,15 @@ exclude_patterns = ["release/release_template.rst"]
project = "NetworkX"
copyright = f"2004-{date.today().year}, NetworkX Developers"
+# Used in networkx.utils.backends for cleaner rendering of functions.
+# We need to set this before we import networkx.
+os.environ["_NETWORKX_BUILDING_DOCS_"] = "True"
+import networkx
+
# The default replacements for |version| and |release|, also used in various
# other places throughout the built documents.
#
# The short X.Y version.
-import networkx
-
version = networkx.__version__
# The full version, including dev info
release = networkx.__version__.replace("_", "")
diff --git a/doc/developer/deprecations.rst b/doc/developer/deprecations.rst
index 7da13d5d4..c6339b550 100644
--- a/doc/developer/deprecations.rst
+++ b/doc/developer/deprecations.rst
@@ -68,6 +68,8 @@ Version 3.2
* Remove pydot functionality ``drawing/nx_pydot.py``, if pydot is still not being maintained. See #5723
* In ``readwrite/json_graph/node_link.py`` remove the ``attrs` keyword code
and docstring in ``node_link_data`` and ``node_link_graph``. Also the associated tests.
+* Remove renamed function ``join()`` in ``algorithms/tree/operations.py`` and
+ in ``doc/reference/algorithms/trees.rst``
Version 3.3
~~~~~~~~~~~
diff --git a/doc/reference/algorithms/tree.rst b/doc/reference/algorithms/tree.rst
index 9f14fda7c..4c7c3e6f5 100644
--- a/doc/reference/algorithms/tree.rst
+++ b/doc/reference/algorithms/tree.rst
@@ -49,6 +49,7 @@ Operations
.. autosummary::
:toctree: generated/
+ join_trees
join
Spanning Trees
diff --git a/networkx/algorithms/minors/contraction.py b/networkx/algorithms/minors/contraction.py
index ff4df958d..1b4da3522 100644
--- a/networkx/algorithms/minors/contraction.py
+++ b/networkx/algorithms/minors/contraction.py
@@ -497,6 +497,18 @@ def contracted_nodes(G, u, v, self_loops=True, copy=True):
>>> list(H.edges())
[(1, 1)]
+ In a ``MultiDiGraph`` with a self loop, the in and out edges will
+ be treated separately as edges, so while contracting a node which
+ has a self loop the contraction will add multiple edges:
+
+ >>> G = nx.MultiDiGraph([(1, 2), (2, 2)])
+ >>> H = nx.contracted_nodes(G, 1, 2)
+ >>> list(H.edges()) # edge 1->2, 2->2, 2<-2 from the original Graph G
+ [(1, 1), (1, 1), (1, 1)]
+ >>> H = nx.contracted_nodes(G, 1, 2, self_loops=False)
+ >>> list(H.edges()) # edge 2->2, 2<-2 from the original Graph G
+ [(1, 1), (1, 1)]
+
See Also
--------
contracted_edge
diff --git a/networkx/algorithms/polynomials.py b/networkx/algorithms/polynomials.py
index 54d00fa57..57ecf0d09 100644
--- a/networkx/algorithms/polynomials.py
+++ b/networkx/algorithms/polynomials.py
@@ -250,10 +250,10 @@ def chromatic_polynomial(G):
cases are listed in [2]_.
The chromatic polynomial is a specialization of the Tutte polynomial; in
- particular, `X_G(x) = `T_G(x, 0)` [6]_.
+ particular, ``X_G(x) = T_G(x, 0)`` [6]_.
The chromatic polynomial may take negative arguments, though evaluations
- may not have chromatic interpretations. For instance, `X_G(-1)` enumerates
+ may not have chromatic interpretations. For instance, ``X_G(-1)`` enumerates
the acyclic orientations of `G` [7]_.
References
diff --git a/networkx/algorithms/tree/operations.py b/networkx/algorithms/tree/operations.py
index 169a2e2be..3682110ce 100644
--- a/networkx/algorithms/tree/operations.py
+++ b/networkx/algorithms/tree/operations.py
@@ -4,13 +4,42 @@ from itertools import accumulate, chain
import networkx as nx
-__all__ = ["join"]
+__all__ = ["join", "join_trees"]
def join(rooted_trees, label_attribute=None):
- """Returns a new rooted tree with a root node joined with the roots
+ """A deprecated name for `join_trees`
+
+ Returns a new rooted tree with a root node joined with the roots
of each of the given rooted trees.
+ .. deprecated:: 3.2
+
+ `join` is deprecated in NetworkX v3.2 and will be removed in v3.4.
+ It has been renamed join_trees with the same syntax/interface.
+
+ """
+ import warnings
+
+ warnings.warn(
+ "The function `join` is deprecated and is renamed `join_trees`.\n"
+ "The ``join`` function itself will be removed in v3.4",
+ DeprecationWarning,
+ stacklevel=2,
+ )
+
+ return join_trees(rooted_trees, label_attribute=label_attribute)
+
+
+def join_trees(rooted_trees, label_attribute=None):
+ """Returns a new rooted tree made by joining `rooted_trees`
+
+ Constructs a new tree by joining each tree in `rooted_trees`.
+ A new root node is added and connected to each of the roots
+ of the input trees. While copying the nodes from the trees,
+ relabeling to integers occurs and the old name stored as an
+ attribute of the new node in the returned graph.
+
Parameters
----------
rooted_trees : list
@@ -35,6 +64,10 @@ def join(rooted_trees, label_attribute=None):
Notes
-----
+ Trees are stored in NetworkX as NetworkX Graphs. There is no specific
+ enforcement of the fact that these are trees. Testing for each tree
+ can be done using :func:`networkx.is_tree`.
+
Graph, edge, and node attributes are propagated from the given
rooted trees to the created tree. If there are any overlapping graph
attributes, those from later trees will overwrite those from earlier
diff --git a/networkx/utils/backends.py b/networkx/utils/backends.py
index c7871c77a..ae7b030a7 100644
--- a/networkx/utils/backends.py
+++ b/networkx/utils/backends.py
@@ -817,3 +817,19 @@ class _dispatch:
def _restore_dispatch(name):
return _registered_algorithms[name]
+
+
+if os.environ.get("_NETWORKX_BUILDING_DOCS_"):
+ # When building docs with Sphinx, use the original function with the
+ # dispatched __doc__, b/c Sphinx renders normal Python functions better.
+ # This doesn't show e.g. `*, backend=None, **backend_kwargs` in the
+ # signatures, which is probably okay. It does allow the docstring to be
+ # updated based on the installed backends.
+ _orig_dispatch = _dispatch
+
+ def _dispatch(func=None, **kwargs): # type: ignore[no-redef]
+ if func is None:
+ return partial(_dispatch, **kwargs)
+ dispatched_func = _orig_dispatch(func, **kwargs)
+ func.__doc__ = dispatched_func.__doc__
+ return func
| networkx/networkx | 88097f7d7f798ec49eb868691dde77cf791a67ec | diff --git a/networkx/algorithms/tree/tests/test_operations.py b/networkx/algorithms/tree/tests/test_operations.py
index 989691199..d85d40c4d 100644
--- a/networkx/algorithms/tree/tests/test_operations.py
+++ b/networkx/algorithms/tree/tests/test_operations.py
@@ -1,7 +1,3 @@
-"""Unit tests for the :mod:`networkx.algorithms.tree.operations` module.
-
-"""
-
from itertools import chain
import networkx as nx
@@ -16,36 +12,29 @@ def _check_label_attribute(input_trees, res_tree, label_attribute="_old"):
return res_attr_set == input_label_set
-class TestJoin:
- """Unit tests for the :func:`networkx.tree.join` function."""
-
- def test_empty_sequence(self):
- """Tests that joining the empty sequence results in the tree
- with one node.
-
- """
- T = nx.join([])
- assert len(T) == 1
- assert T.number_of_edges() == 0
-
- def test_single(self):
- """Tests that joining just one tree yields a tree with one more
- node.
-
- """
- T = nx.empty_graph(1)
- trees = [(T, 0)]
- actual = nx.join(trees)
- expected = nx.path_graph(2)
- assert nodes_equal(list(expected), list(actual))
- assert edges_equal(list(expected.edges()), list(actual.edges()))
- assert _check_label_attribute(trees, actual)
-
- def test_basic(self):
- """Tests for joining multiple subtrees at a root node."""
- trees = [(nx.full_rary_tree(2, 2**2 - 1), 0) for i in range(2)]
- label_attribute = "old_values"
- actual = nx.join(trees, label_attribute)
- expected = nx.full_rary_tree(2, 2**3 - 1)
- assert nx.is_isomorphic(actual, expected)
- assert _check_label_attribute(trees, actual, label_attribute)
+def test_empty_sequence():
+ """Joining the empty sequence results in the tree with one node."""
+ T = nx.join_trees([])
+ assert len(T) == 1
+ assert T.number_of_edges() == 0
+
+
+def test_single():
+ """Joining just one tree yields a tree with one more node."""
+ T = nx.empty_graph(1)
+ trees = [(T, 0)]
+ actual = nx.join_trees(trees)
+ expected = nx.path_graph(2)
+ assert nodes_equal(list(expected), list(actual))
+ assert edges_equal(list(expected.edges()), list(actual.edges()))
+ assert _check_label_attribute(trees, actual)
+
+
+def test_basic():
+ """Joining multiple subtrees at a root node."""
+ trees = [(nx.full_rary_tree(2, 2**2 - 1), 0) for i in range(2)]
+ label_attribute = "old_values"
+ actual = nx.join_trees(trees, label_attribute)
+ expected = nx.full_rary_tree(2, 2**3 - 1)
+ assert nx.is_isomorphic(actual, expected)
+ assert _check_label_attribute(trees, actual, label_attribute)
| Rename nx.join to something which gives more context about operations on trees
While reviewing #6503 [@dschult noted](https://github.com/networkx/networkx/pull/6503#issuecomment-1707200999) that the current function name `nx.join` doesn't give enough information to the user. The function is used to "join" trees.
This will require a deprecation cycle. | 0.0 | [
"networkx/algorithms/tree/tests/test_operations.py::test_empty_sequence",
"networkx/algorithms/tree/tests/test_operations.py::test_single",
"networkx/algorithms/tree/tests/test_operations.py::test_basic"
] | [] | 2023-09-06 20:15:05+00:00 | 4,152 |
|
networkx__networkx-6937 | diff --git a/CONTRIBUTING.rst b/CONTRIBUTING.rst
index 2ab68ed29..e92e9373a 100644
--- a/CONTRIBUTING.rst
+++ b/CONTRIBUTING.rst
@@ -403,7 +403,7 @@ Then create a baseline image to compare against later::
$ pytest -k test_barbell --mpl-generate-path=networkx/drawing/tests/baseline
-.. note: In order to keep the size of the repository from becoming too large, we
+.. note:: In order to keep the size of the repository from becoming too large, we
prefer to limit the size and number of baseline images we include.
And test::
@@ -413,27 +413,7 @@ And test::
Documentation
-------------
-Building the documentation locally requires that the additional dependencies
-specified in ``requirements/doc.txt`` be installed in your development
-environment.
-
-The documentation is built with ``sphinx``. To build the documentation locally,
-navigate to the ``doc/`` directory and::
-
- make html
-
-This will generate both the reference documentation as well as the example
-gallery. If you want to build the documentation *without* building the
-gallery examples use::
-
- make html-noplot
-
-The build products are stored in ``doc/build/`` and can be viewed directly.
-For example, to view the built html, open ``build/html/index.html``
-in your preferred web browser.
-
-.. note: ``sphinx`` supports many other output formats. Type ``make`` without
- any arguments to see all the built-in options.
+.. include:: ../README.rst
Adding examples
~~~~~~~~~~~~~~~
diff --git a/doc/README.md b/doc/README.rst
similarity index 57%
rename from doc/README.md
rename to doc/README.rst
index c806ffca2..09a0f8278 100644
--- a/doc/README.md
+++ b/doc/README.rst
@@ -1,5 +1,3 @@
-# Building docs
-
We use Sphinx for generating the API and reference documentation.
Pre-built versions can be found at
@@ -8,33 +6,37 @@ Pre-built versions can be found at
for both the stable and the latest (i.e., development) releases.
-## Instructions
+Instructions
+~~~~~~~~~~~~
After installing NetworkX and its dependencies, install the Python
packages needed to build the documentation by entering the root
-directory and executing:
+directory and executing::
pip install -r requirements/doc.txt
Building the example gallery additionally requires the dependencies
-listed in `requirements/extra.txt` and `requirements/example.txt`:
+listed in ``requirements/extra.txt`` and ``requirements/example.txt``::
pip install -r requirements/extra.txt
pip install -r requirements/example.txt
-To build the HTML documentation, enter `doc/` and execute:
+To build the HTML documentation, enter ``doc/`` and execute::
make html
-This will generate a `build/html` subdirectory containing the built
-documentation. If the dependencies in `extra.txt` and `example.txt`
+This will generate a ``build/html`` subdirectory containing the built
+documentation. If the dependencies in ``extra.txt`` and ``example.txt``
are **not** installed, build the HTML documentation without generating
-figures by using:
+figures by using::
make html-noplot
-To build the PDF documentation, enter:
+To build the PDF documentation, enter::
make latexpdf
You will need to have LaTeX installed for this.
+
+.. note:: ``sphinx`` supports many other output formats. Type ``make`` without
+ any arguments to see all the built-in options.
diff --git a/doc/developer/deprecations.rst b/doc/developer/deprecations.rst
index c6339b550..5dd39577c 100644
--- a/doc/developer/deprecations.rst
+++ b/doc/developer/deprecations.rst
@@ -66,8 +66,6 @@ Version 3.2
* In ``generators/directed.py`` remove the ``create_using`` keyword argument
for the ``scale_free_graph`` function.
* Remove pydot functionality ``drawing/nx_pydot.py``, if pydot is still not being maintained. See #5723
-* In ``readwrite/json_graph/node_link.py`` remove the ``attrs` keyword code
- and docstring in ``node_link_data`` and ``node_link_graph``. Also the associated tests.
* Remove renamed function ``join()`` in ``algorithms/tree/operations.py`` and
in ``doc/reference/algorithms/trees.rst``
diff --git a/networkx/algorithms/graphical.py b/networkx/algorithms/graphical.py
index da27e5f1f..20e9ced75 100644
--- a/networkx/algorithms/graphical.py
+++ b/networkx/algorithms/graphical.py
@@ -104,6 +104,13 @@ def is_valid_degree_sequence_havel_hakimi(deg_sequence):
valid : bool
True if deg_sequence is graphical and False if not.
+ Examples
+ --------
+ >>> G = nx.Graph([(1, 2), (1, 3), (2, 3), (3, 4), (4, 2), (5, 1), (5, 4)])
+ >>> sequence = (d for _, d in G.degree())
+ >>> nx.is_valid_degree_sequence_havel_hakimi(sequence)
+ True
+
Notes
-----
The ZZ condition says that for the sequence d if
@@ -177,6 +184,13 @@ def is_valid_degree_sequence_erdos_gallai(deg_sequence):
valid : bool
True if deg_sequence is graphical and False if not.
+ Examples
+ --------
+ >>> G = nx.Graph([(1, 2), (1, 3), (2, 3), (3, 4), (4, 2), (5, 1), (5, 4)])
+ >>> sequence = (d for _, d in G.degree())
+ >>> nx.is_valid_degree_sequence_erdos_gallai(sequence)
+ True
+
Notes
-----
@@ -252,6 +266,13 @@ def is_multigraphical(sequence):
valid : bool
True if deg_sequence is a multigraphic degree sequence and False if not.
+ Examples
+ --------
+ >>> G = nx.MultiGraph([(1, 2), (1, 3), (2, 3), (3, 4), (4, 2), (5, 1), (5, 4)])
+ >>> sequence = (d for _, d in G.degree())
+ >>> nx.is_multigraphical(sequence)
+ True
+
Notes
-----
The worst-case run time is $O(n)$ where $n$ is the length of the sequence.
@@ -292,6 +313,13 @@ def is_pseudographical(sequence):
valid : bool
True if the sequence is a pseudographic degree sequence and False if not.
+ Examples
+ --------
+ >>> G = nx.Graph([(1, 2), (1, 3), (2, 3), (3, 4), (4, 2), (5, 1), (5, 4)])
+ >>> sequence = (d for _, d in G.degree())
+ >>> nx.is_pseudographical(sequence)
+ True
+
Notes
-----
The worst-case run time is $O(n)$ where n is the length of the sequence.
@@ -326,6 +354,14 @@ def is_digraphical(in_sequence, out_sequence):
valid : bool
True if in and out-sequences are digraphic False if not.
+ Examples
+ --------
+ >>> G = nx.DiGraph([(1, 2), (1, 3), (2, 3), (3, 4), (4, 2), (5, 1), (5, 4)])
+ >>> in_seq = (d for n, d in G.in_degree())
+ >>> out_seq = (d for n, d in G.out_degree())
+ >>> nx.is_digraphical(in_seq, out_seq)
+ True
+
Notes
-----
This algorithm is from Kleitman and Wang [1]_.
diff --git a/networkx/classes/reportviews.py b/networkx/classes/reportviews.py
index 4503566e7..59a16243f 100644
--- a/networkx/classes/reportviews.py
+++ b/networkx/classes/reportviews.py
@@ -757,7 +757,7 @@ class OutEdgeDataView:
def __setstate__(self, state):
self.__init__(**state)
- def __init__(self, viewer, nbunch=None, data=False, default=None):
+ def __init__(self, viewer, nbunch=None, data=False, *, default=None):
self._viewer = viewer
adjdict = self._adjdict = viewer._adjdict
if nbunch is None:
@@ -902,7 +902,7 @@ class OutMultiEdgeDataView(OutEdgeDataView):
def __setstate__(self, state):
self.__init__(**state)
- def __init__(self, viewer, nbunch=None, data=False, keys=False, default=None):
+ def __init__(self, viewer, nbunch=None, data=False, *, default=None, keys=False):
self._viewer = viewer
adjdict = self._adjdict = viewer._adjdict
self.keys = keys
@@ -1085,10 +1085,10 @@ class OutEdgeView(Set, Mapping):
return self._adjdict[u][v]
# EdgeDataView methods
- def __call__(self, nbunch=None, data=False, default=None):
+ def __call__(self, nbunch=None, data=False, *, default=None):
if nbunch is None and data is False:
return self
- return self.dataview(self, nbunch, data, default)
+ return self.dataview(self, nbunch, data, default=default)
def data(self, data=True, default=None, nbunch=None):
"""
@@ -1166,7 +1166,7 @@ class OutEdgeView(Set, Mapping):
"""
if nbunch is None and data is False:
return self
- return self.dataview(self, nbunch, data, default)
+ return self.dataview(self, nbunch, data, default=default)
# String Methods
def __str__(self):
@@ -1352,15 +1352,15 @@ class OutMultiEdgeView(OutEdgeView):
u, v, k = e
return self._adjdict[u][v][k]
- def __call__(self, nbunch=None, data=False, keys=False, default=None):
+ def __call__(self, nbunch=None, data=False, *, default=None, keys=False):
if nbunch is None and data is False and keys is True:
return self
- return self.dataview(self, nbunch, data, keys, default)
+ return self.dataview(self, nbunch, data, default=default, keys=keys)
- def data(self, data=True, keys=False, default=None, nbunch=None):
+ def data(self, data=True, default=None, nbunch=None, keys=False):
if nbunch is None and data is False and keys is True:
return self
- return self.dataview(self, nbunch, data, keys, default)
+ return self.dataview(self, nbunch, data, default=default, keys=keys)
class MultiEdgeView(OutMultiEdgeView):
diff --git a/networkx/generators/atlas.py b/networkx/generators/atlas.py
index a5c7f492c..6f4388d2b 100644
--- a/networkx/generators/atlas.py
+++ b/networkx/generators/atlas.py
@@ -2,6 +2,7 @@
Generators for the small graph atlas.
"""
import gzip
+import importlib.resources
import os
import os.path
from itertools import islice
@@ -16,9 +17,6 @@ __all__ = ["graph_atlas", "graph_atlas_g"]
#: including) this number.
NUM_GRAPHS = 1253
-#: The absolute path representing the directory containing this file.
-THIS_DIR = os.path.dirname(os.path.abspath(__file__))
-
#: The path to the data file containing the graph edge lists.
#:
#: This is the absolute path of the gzipped text file containing the
@@ -51,7 +49,9 @@ THIS_DIR = os.path.dirname(os.path.abspath(__file__))
#: f.write(bytes(f'NODES {len(G)}\n', encoding='utf-8'))
#: write_edgelist(G, f, data=False)
#:
-ATLAS_FILE = os.path.join(THIS_DIR, "atlas.dat.gz")
+
+# Path to the atlas file
+ATLAS_FILE = importlib.resources.files("networkx.generators") / "atlas.dat.gz"
def _generate_graphs():
diff --git a/networkx/readwrite/json_graph/node_link.py b/networkx/readwrite/json_graph/node_link.py
index 65bcf4d63..98979c301 100644
--- a/networkx/readwrite/json_graph/node_link.py
+++ b/networkx/readwrite/json_graph/node_link.py
@@ -33,7 +33,6 @@ def _to_tuple(x):
def node_link_data(
G,
- attrs=None,
*,
source="source",
target="target",
@@ -47,29 +46,6 @@ def node_link_data(
Parameters
----------
G : NetworkX graph
-
- attrs : dict
- A dictionary that contains five keys 'source', 'target', 'name',
- 'key' and 'link'. The corresponding values provide the attribute
- names for storing NetworkX-internal graph data. The values should
- be unique. Default value::
-
- dict(source='source', target='target', name='id',
- key='key', link='links')
-
- If some user-defined graph data use these attribute names as data keys,
- they may be silently dropped.
-
- .. deprecated:: 2.8.6
-
- The `attrs` keyword argument will be replaced with `source`, `target`, `name`,
- `key` and `link`. in networkx 3.2
-
- If the `attrs` keyword and the new keywords are both used in a single function call (not recommended)
- the `attrs` keyword argument will take precedence.
-
- The values of the keywords must be unique.
-
source : string
A string that provides the 'source' attribute name for storing NetworkX-internal graph data.
target : string
@@ -134,31 +110,6 @@ def node_link_data(
--------
node_link_graph, adjacency_data, tree_data
"""
- # ------ TODO: Remove between the lines after signature change is complete ----- #
- if attrs is not None:
- import warnings
-
- msg = (
- "\n\nThe `attrs` keyword argument of node_link_data is deprecated\n"
- "and will be removed in networkx 3.2. It is replaced with explicit\n"
- "keyword arguments: `source`, `target`, `name`, `key` and `link`.\n"
- "To make this warning go away, and ensure usage is forward\n"
- "compatible, replace `attrs` with the keywords. "
- "For example:\n\n"
- " >>> node_link_data(G, attrs={'target': 'foo', 'name': 'bar'})\n\n"
- "should instead be written as\n\n"
- " >>> node_link_data(G, target='foo', name='bar')\n\n"
- "in networkx 3.2.\n"
- "The default values of the keywords will not change.\n"
- )
- warnings.warn(msg, DeprecationWarning, stacklevel=2)
-
- source = attrs.get("source", "source")
- target = attrs.get("target", "target")
- name = attrs.get("name", "name")
- key = attrs.get("key", "key")
- link = attrs.get("link", "links")
- # -------------------------------------------------- #
multigraph = G.is_multigraph()
# Allow 'key' to be omitted from attrs if the graph is not a multigraph.
@@ -185,7 +136,6 @@ def node_link_graph(
data,
directed=False,
multigraph=True,
- attrs=None,
*,
source="source",
target="target",
@@ -207,24 +157,6 @@ def node_link_graph(
multigraph : bool
If True, and multigraph not specified in data, return a multigraph.
- attrs : dict
- A dictionary that contains five keys 'source', 'target', 'name',
- 'key' and 'link'. The corresponding values provide the attribute
- names for storing NetworkX-internal graph data. Default value:
-
- dict(source='source', target='target', name='id',
- key='key', link='links')
-
- .. deprecated:: 2.8.6
-
- The `attrs` keyword argument will be replaced with the individual keywords: `source`, `target`, `name`,
- `key` and `link`. in networkx 3.2.
-
- If the `attrs` keyword and the new keywords are both used in a single function call (not recommended)
- the `attrs` keyword argument will take precedence.
-
- The values of the keywords must be unique.
-
source : string
A string that provides the 'source' attribute name for storing NetworkX-internal graph data.
target : string
@@ -277,31 +209,6 @@ def node_link_graph(
--------
node_link_data, adjacency_data, tree_data
"""
- # ------ TODO: Remove between the lines after signature change is complete ----- #
- if attrs is not None:
- import warnings
-
- msg = (
- "\n\nThe `attrs` keyword argument of node_link_graph is deprecated\n"
- "and will be removed in networkx 3.2. It is replaced with explicit\n"
- "keyword arguments: `source`, `target`, `name`, `key` and `link`.\n"
- "To make this warning go away, and ensure usage is forward\n"
- "compatible, replace `attrs` with the keywords. "
- "For example:\n\n"
- " >>> node_link_graph(data, attrs={'target': 'foo', 'name': 'bar'})\n\n"
- "should instead be written as\n\n"
- " >>> node_link_graph(data, target='foo', name='bar')\n\n"
- "in networkx 3.2.\n"
- "The default values of the keywords will not change.\n"
- )
- warnings.warn(msg, DeprecationWarning, stacklevel=2)
-
- source = attrs.get("source", "source")
- target = attrs.get("target", "target")
- name = attrs.get("name", "name")
- key = attrs.get("key", "key")
- link = attrs.get("link", "links")
- # -------------------------------------------------- #
multigraph = data.get("multigraph", multigraph)
directed = data.get("directed", directed)
if multigraph:
| networkx/networkx | 0eb2f98ce29fd84da4907cd9c7dd33e2d561410e | diff --git a/networkx/algorithms/flow/tests/test_maxflow_large_graph.py b/networkx/algorithms/flow/tests/test_maxflow_large_graph.py
index fd36c1153..7b4859c8a 100644
--- a/networkx/algorithms/flow/tests/test_maxflow_large_graph.py
+++ b/networkx/algorithms/flow/tests/test_maxflow_large_graph.py
@@ -2,6 +2,7 @@
"""
import bz2
+import importlib.resources
import os
import pickle
@@ -48,8 +49,11 @@ def gen_pyramid(N):
def read_graph(name):
- dirname = os.path.dirname(__file__)
- fname = os.path.join(dirname, name + ".gpickle.bz2")
+ fname = (
+ importlib.resources.files("networkx.algorithms.flow.tests")
+ / f"{name}.gpickle.bz2"
+ )
+
with bz2.BZ2File(fname, "rb") as f:
G = pickle.load(f)
return G
diff --git a/networkx/algorithms/flow/tests/test_mincost.py b/networkx/algorithms/flow/tests/test_mincost.py
index 65603d3c1..5b1794b15 100644
--- a/networkx/algorithms/flow/tests/test_mincost.py
+++ b/networkx/algorithms/flow/tests/test_mincost.py
@@ -1,4 +1,5 @@
import bz2
+import importlib.resources
import os
import pickle
@@ -461,7 +462,10 @@ class TestMinCostFlow:
# pytest.raises(nx.NetworkXUnfeasible, nx.capacity_scaling, G)
def test_large(self):
- fname = os.path.join(os.path.dirname(__file__), "netgen-2.gpickle.bz2")
+ fname = (
+ importlib.resources.files("networkx.algorithms.flow.tests")
+ / "netgen-2.gpickle.bz2"
+ )
with bz2.BZ2File(fname, "rb") as f:
G = pickle.load(f)
flowCost, flowDict = nx.network_simplex(G)
diff --git a/networkx/algorithms/flow/tests/test_networksimplex.py b/networkx/algorithms/flow/tests/test_networksimplex.py
index 40c4bf080..5b3b5f6dd 100644
--- a/networkx/algorithms/flow/tests/test_networksimplex.py
+++ b/networkx/algorithms/flow/tests/test_networksimplex.py
@@ -1,4 +1,5 @@
import bz2
+import importlib.resources
import os
import pickle
@@ -141,7 +142,11 @@ def test_google_or_tools_example2():
def test_large():
- fname = os.path.join(os.path.dirname(__file__), "netgen-2.gpickle.bz2")
+ fname = (
+ importlib.resources.files("networkx.algorithms.flow.tests")
+ / "netgen-2.gpickle.bz2"
+ )
+
with bz2.BZ2File(fname, "rb") as f:
G = pickle.load(f)
flowCost, flowDict = nx.network_simplex(G)
diff --git a/networkx/algorithms/isomorphism/tests/test_isomorphvf2.py b/networkx/algorithms/isomorphism/tests/test_isomorphvf2.py
index 31f670a32..f658bcae4 100644
--- a/networkx/algorithms/isomorphism/tests/test_isomorphvf2.py
+++ b/networkx/algorithms/isomorphism/tests/test_isomorphvf2.py
@@ -2,6 +2,7 @@
Tests for VF2 isomorphism algorithm.
"""
+import importlib.resources
import os
import random
import struct
@@ -118,18 +119,18 @@ class TestVF2GraphDB:
return graph
def test_graph(self):
- head, tail = os.path.split(__file__)
- g1 = self.create_graph(os.path.join(head, "iso_r01_s80.A99"))
- g2 = self.create_graph(os.path.join(head, "iso_r01_s80.B99"))
+ head = importlib.resources.files("networkx.algorithms.isomorphism.tests")
+ g1 = self.create_graph(head / "iso_r01_s80.A99")
+ g2 = self.create_graph(head / "iso_r01_s80.B99")
gm = iso.GraphMatcher(g1, g2)
assert gm.is_isomorphic()
def test_subgraph(self):
# A is the subgraph
# B is the full graph
- head, tail = os.path.split(__file__)
- subgraph = self.create_graph(os.path.join(head, "si2_b06_m200.A99"))
- graph = self.create_graph(os.path.join(head, "si2_b06_m200.B99"))
+ head = importlib.resources.files("networkx.algorithms.isomorphism.tests")
+ subgraph = self.create_graph(head / "si2_b06_m200.A99")
+ graph = self.create_graph(head / "si2_b06_m200.B99")
gm = iso.GraphMatcher(graph, subgraph)
assert gm.subgraph_is_isomorphic()
# Just testing some cases
diff --git a/networkx/classes/tests/test_reportviews.py b/networkx/classes/tests/test_reportviews.py
index 48148c240..a68d6eb82 100644
--- a/networkx/classes/tests/test_reportviews.py
+++ b/networkx/classes/tests/test_reportviews.py
@@ -872,8 +872,12 @@ class TestMultiEdgeView(TestEdgeView):
assert len(e) == 3
elist = sorted([(i, i + 1, 0) for i in range(8)] + [(1, 2, 3)])
assert sorted(ev) == elist
- # test order of arguments:graph, nbunch, data, keys, default
- ev = evr((1, 2), "foo", True, 1)
+ # test that the keyword arguments are passed correctly
+ ev = evr((1, 2), "foo", keys=True, default=1)
+ with pytest.raises(TypeError):
+ evr((1, 2), "foo", True, 1)
+ with pytest.raises(TypeError):
+ evr((1, 2), "foo", True, default=1)
for e in ev:
if set(e[:2]) == {1, 2}:
assert e[2] in {0, 3}
diff --git a/networkx/conftest.py b/networkx/conftest.py
index a2b63a3b2..682a91a5d 100644
--- a/networkx/conftest.py
+++ b/networkx/conftest.py
@@ -101,11 +101,6 @@ def set_warnings():
warnings.filterwarnings(
"ignore", category=DeprecationWarning, message="nx.nx_pydot"
)
- warnings.filterwarnings(
- "ignore",
- category=DeprecationWarning,
- message="\n\nThe `attrs` keyword argument of node_link",
- )
warnings.filterwarnings(
"ignore",
category=DeprecationWarning,
diff --git a/networkx/readwrite/json_graph/tests/test_node_link.py b/networkx/readwrite/json_graph/tests/test_node_link.py
index 54078c471..a432666b6 100644
--- a/networkx/readwrite/json_graph/tests/test_node_link.py
+++ b/networkx/readwrite/json_graph/tests/test_node_link.py
@@ -6,31 +6,6 @@ import networkx as nx
from networkx.readwrite.json_graph import node_link_data, node_link_graph
-# TODO: To be removed when signature change complete
-def test_attrs_deprecation(recwarn):
- G = nx.path_graph(3)
-
- # No warnings when `attrs` kwarg not used
- data = node_link_data(G)
- H = node_link_graph(data)
- assert len(recwarn) == 0
-
- # Future warning raised with `attrs` kwarg
- attrs = {
- "source": "source",
- "target": "target",
- "name": "id",
- "key": "key",
- "link": "links",
- }
- data = node_link_data(G, attrs=attrs)
- assert len(recwarn) == 1
-
- recwarn.clear()
- H = node_link_graph(data, attrs=attrs)
- assert len(recwarn) == 1
-
-
class TestNodeLink:
# TODO: To be removed when signature change complete
def test_custom_attrs_dep(self):
@@ -48,9 +23,7 @@ class TestNodeLink:
"link": "c_links",
}
- H = node_link_graph(
- node_link_data(G, attrs=attrs), multigraph=False, attrs=attrs
- )
+ H = node_link_graph(node_link_data(G, **attrs), multigraph=False, **attrs)
assert nx.is_isomorphic(G, H)
assert H.graph["foo"] == "bar"
assert H.nodes[1]["color"] == "red"
@@ -63,20 +36,16 @@ class TestNodeLink:
"source": "c_source",
"target": "c_target",
}
- H = node_link_graph(
- node_link_data(G, attrs=attrs), multigraph=False, attrs=attrs
- )
+ H = node_link_graph(node_link_data(G, **attrs), multigraph=False, **attrs)
assert nx.is_isomorphic(G, H)
assert H.graph["foo"] == "bar"
assert H.nodes[1]["color"] == "red"
assert H[1][2]["width"] == 7
- # TODO: To be removed when signature change complete
def test_exception_dep(self):
with pytest.raises(nx.NetworkXError):
G = nx.MultiDiGraph()
- attrs = {"name": "node", "source": "node", "target": "node", "key": "node"}
- node_link_data(G, attrs)
+ node_link_data(G, name="node", source="node", target="node", key="node")
def test_graph(self):
G = nx.path_graph(4)
| Unify documentation README with contributor guide
It was pointed out in #6766 that we have a README in the `doc/` folder that describes how to build the documentation. I think the go-to reference for this information is the contributor guide, and these two documents are not necessarily in sync. However, contributors have also indicated that having a README in the doc folder is nice. Therefore I would propose the following:
1. Reformat `doc/README.md` to .rst, then
2. `.. include` `doc/README` in the `Documentation` section of `CONTRIBUTING.rst`
This should check all boxes: it preserves the README in the `doc/` folder, de-duplicates the source of info (preventing divergence of the README from the contributor guide), and guarantees that the info from the README shows up in the html version of the contributor guide. | 0.0 | [
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_iterkeys",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_iterkeys",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_iterkeys"
] | [
"networkx/algorithms/flow/tests/test_maxflow_large_graph.py::TestMaxflowLargeGraph::test_complete_graph",
"networkx/algorithms/flow/tests/test_maxflow_large_graph.py::TestMaxflowLargeGraph::test_pyramid",
"networkx/algorithms/flow/tests/test_maxflow_large_graph.py::TestMaxflowLargeGraph::test_gl1",
"networkx/algorithms/flow/tests/test_maxflow_large_graph.py::TestMaxflowLargeGraph::test_wlm3",
"networkx/algorithms/flow/tests/test_maxflow_large_graph.py::TestMaxflowLargeGraph::test_preflow_push_global_relabel",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_simple_digraph",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_negcycle_infcap",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_sum_demands_not_zero",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_no_flow_satisfying_demands",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_transshipment",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_max_flow_min_cost",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_digraph1",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_digraph2",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_digraph3",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_zero_capacity_edges",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_digon",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_deadend",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_infinite_capacity_neg_digon",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_finite_capacity_neg_digon",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_multidigraph",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_negative_selfloops",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_bone_shaped",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_exceptions",
"networkx/algorithms/flow/tests/test_mincost.py::TestMinCostFlow::test_large",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_infinite_demand_raise",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_neg_infinite_demand_raise",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_infinite_weight_raise",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_nonzero_net_demand_raise",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_negative_capacity_raise",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_no_flow_satisfying_demands",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_sum_demands_not_zero",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_google_or_tools_example",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_google_or_tools_example2",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_large",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_simple_digraph",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_negcycle_infcap",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_transshipment",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_digraph1",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_zero_capacity_edges",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_digon",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_deadend",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_infinite_capacity_neg_digon",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_multidigraph",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_negative_selfloops",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_bone_shaped",
"networkx/algorithms/flow/tests/test_networksimplex.py::test_graphs_type_exceptions",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestWikipediaExample::test_graph",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestWikipediaExample::test_subgraph",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestWikipediaExample::test_subgraph_mono",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestVF2GraphDB::test_graph",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestVF2GraphDB::test_subgraph",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::TestAtlas::test_graph_atlas",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_multiedge",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_selfloop",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_selfloop_mono",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_isomorphism_iter1",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_monomorphism_iter1",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_isomorphism_iter2",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_multiple",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_noncomparable_nodes",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_monomorphism_edge_match",
"networkx/algorithms/isomorphism/tests/test_isomorphvf2.py::test_isomorphvf2pp_multidigraphs",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestNodeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_viewtype",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestNodeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::test_nodedataview_unhashable",
"networkx/classes/tests/test_reportviews.py::TestNodeViewSetOps::test_len",
"networkx/classes/tests/test_reportviews.py::TestNodeViewSetOps::test_and",
"networkx/classes/tests/test_reportviews.py::TestNodeViewSetOps::test_or",
"networkx/classes/tests/test_reportviews.py::TestNodeViewSetOps::test_xor",
"networkx/classes/tests/test_reportviews.py::TestNodeViewSetOps::test_sub",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewSetOps::test_len",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewSetOps::test_and",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewSetOps::test_or",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewSetOps::test_xor",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewSetOps::test_sub",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewDefaultSetOps::test_len",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewDefaultSetOps::test_and",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewDefaultSetOps::test_or",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewDefaultSetOps::test_xor",
"networkx/classes/tests/test_reportviews.py::TestNodeDataViewDefaultSetOps::test_sub",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_iterdata",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeDataView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestMultiEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutMultiEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_contains",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_call",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_data",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_or",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_sub",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_xor",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_and",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInMultiEdgeView::test_contains_with_nbunch",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestDiDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestOutDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestInDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestMultiDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestDiMultiDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestOutMultiDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_pickle",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_iter",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_len",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_str",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_repr",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_nbunch",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_getitem",
"networkx/classes/tests/test_reportviews.py::TestInMultiDegreeView::test_weight",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[NodeView-list(G.nodes]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[NodeDataView-list(G.nodes.data]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[EdgeView-list(G.edges]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[InEdgeView-list(G.in_edges]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[OutEdgeView-list(G.edges]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[MultiEdgeView-list(G.edges]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[InMultiEdgeView-list(G.in_edges]",
"networkx/classes/tests/test_reportviews.py::test_slicing_reportviews[OutMultiEdgeView-list(G.edges]",
"networkx/classes/tests/test_reportviews.py::test_cache_dict_get_set_state[Graph]",
"networkx/classes/tests/test_reportviews.py::test_cache_dict_get_set_state[DiGraph]",
"networkx/classes/tests/test_reportviews.py::test_cache_dict_get_set_state[MultiGraph]",
"networkx/classes/tests/test_reportviews.py::test_cache_dict_get_set_state[MultiDiGraph]",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_custom_attrs_dep",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_exception_dep",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_graph",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_graph_attributes",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_digraph",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_multigraph",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_graph_with_tuple_nodes",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_unicode_keys",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_exception",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_string_ids",
"networkx/readwrite/json_graph/tests/test_node_link.py::TestNodeLink::test_custom_attrs"
] | 2023-09-21 21:09:12+00:00 | 4,153 |
|
networkx__networkx-6957 | diff --git a/.circleci/config.yml b/.circleci/config.yml
index a1e6ef043..f01de2033 100644
--- a/.circleci/config.yml
+++ b/.circleci/config.yml
@@ -38,6 +38,10 @@ jobs:
pip install -r requirements/extra.txt
pip install -r requirements/example.txt
pip install -r requirements/doc.txt
+ # Install trusted backends, but not their dependencies.
+ # We only need to use the "networkx.plugin_info" entry-point.
+ # This is the nightly wheel for nx-cugraph.
+ pip install nx-cugraph-cu11 --extra-index-url https://rapidsdev:[email protected]/simple --no-deps
pip list
- save_cache:
@@ -49,7 +53,7 @@ jobs:
name: Install
command: |
source venv/bin/activate
- pip install -e .
+ pip install -e . --no-deps
- run:
name: Build docs
diff --git a/.github/workflows/deploy-docs.yml b/.github/workflows/deploy-docs.yml
index edd211f6f..92862bce9 100644
--- a/.github/workflows/deploy-docs.yml
+++ b/.github/workflows/deploy-docs.yml
@@ -32,6 +32,10 @@ jobs:
pip install -r requirements/example.txt
pip install -U -r requirements/doc.txt
pip install .
+ # Install trusted backends, but not their dependencies.
+ # We only need to use the "networkx.plugin_info" entry-point.
+ # This is the nightly wheel for nx-cugraph.
+ pip install nx-cugraph-cu11 --extra-index-url https://rapidsdev:[email protected]/simple --no-deps
pip list
# To set up a cross-repository deploy key:
diff --git a/doc/conf.py b/doc/conf.py
index b32f8d7a7..4161a4ee0 100644
--- a/doc/conf.py
+++ b/doc/conf.py
@@ -247,3 +247,48 @@ numpydoc_show_class_members = False
def setup(app):
app.add_css_file("custom.css")
app.add_js_file("copybutton.js")
+
+
+# Monkeypatch numpydoc to show "Backends" section
+from numpydoc.docscrape import NumpyDocString
+
+orig_setitem = NumpyDocString.__setitem__
+
+
+def new_setitem(self, key, val):
+ if key != "Backends":
+ orig_setitem(self, key, val)
+ return
+ # Update how we show backend information in the online docs.
+ # Start by creating an "admonition" section to make it stand out.
+ newval = [".. admonition:: Additional backends implement this function", ""]
+ for line in val:
+ if line and not line.startswith(" "):
+ # This line must identify a backend; let's try to add a link
+ backend, *rest = line.split(" ")
+ url = networkx.utils.backends.plugin_info.get(backend, {}).get("url")
+ if url:
+ line = f"`{backend} <{url}>`_ " + " ".join(rest)
+ newval.append(f" {line}")
+ self._parsed_data[key] = newval
+
+
+NumpyDocString.__setitem__ = new_setitem
+
+from numpydoc.docscrape_sphinx import SphinxDocString
+
+orig_str = SphinxDocString.__str__
+
+
+def new_str(self, indent=0, func_role="obj"):
+ rv = orig_str(self, indent=indent, func_role=func_role)
+ if "Backends" in self:
+ lines = self._str_section("Backends")
+ # Remove "Backends" as a section and add a divider instead
+ lines[0] = "----"
+ lines = self._str_indent(lines, indent)
+ rv += "\n".join(lines)
+ return rv
+
+
+SphinxDocString.__str__ = new_str
diff --git a/doc/developer/deprecations.rst b/doc/developer/deprecations.rst
index 266fb4055..e20426196 100644
--- a/doc/developer/deprecations.rst
+++ b/doc/developer/deprecations.rst
@@ -61,3 +61,5 @@ Version 3.4
* Remove ``normalized`` kwarg from ``algorithms.s_metric``
* Remove renamed function ``join()`` in ``algorithms/tree/operations.py`` and
in ``doc/reference/algorithms/trees.rst``
+* Remove ``strongly_connected_components_recursive`` from
+ ``algorithms/components/strongly_connected.py``
diff --git a/doc/release/release_dev.rst b/doc/release/release_dev.rst
index 519ffe407..152516a7b 100644
--- a/doc/release/release_dev.rst
+++ b/doc/release/release_dev.rst
@@ -68,6 +68,9 @@ Deprecations
- [`#6841 <https://github.com/networkx/pull/6841>`_]
Deprecate the ``normalized`` keyword of the ``s_metric`` function. Ignore
value of ``normalized`` for future compatibility.
+- [`#6957 <https://github.com/networkx/pull/6957>`_]
+ Deprecate ``strongly_connected_components_recursive`` function in favor of
+ the non-recursive implmentation ``strongly_connected_components``.
Merged PRs
----------
diff --git a/networkx/algorithms/components/strongly_connected.py b/networkx/algorithms/components/strongly_connected.py
index 3486d4034..5bf5b9947 100644
--- a/networkx/algorithms/components/strongly_connected.py
+++ b/networkx/algorithms/components/strongly_connected.py
@@ -178,6 +178,11 @@ def kosaraju_strongly_connected_components(G, source=None):
def strongly_connected_components_recursive(G):
"""Generate nodes in strongly connected components of graph.
+ .. deprecated:: 3.2
+
+ This function is deprecated and will be removed in a future version of
+ NetworkX. Use `strongly_connected_components` instead.
+
Recursive version of algorithm.
Parameters
@@ -236,35 +241,18 @@ def strongly_connected_components_recursive(G):
Information Processing Letters 49(1): 9-14, (1994)..
"""
+ import warnings
+
+ warnings.warn(
+ (
+ "\n\nstrongly_connected_components_recursive is deprecated and will be\n"
+ "removed in the future. Use strongly_connected_components instead."
+ ),
+ category=DeprecationWarning,
+ stacklevel=2,
+ )
- def visit(v, cnt):
- root[v] = cnt
- visited[v] = cnt
- cnt += 1
- stack.append(v)
- for w in G[v]:
- if w not in visited:
- yield from visit(w, cnt)
- if w not in component:
- root[v] = min(root[v], root[w])
- if root[v] == visited[v]:
- component[v] = root[v]
- tmpc = {v} # hold nodes in this component
- while stack[-1] != v:
- w = stack.pop()
- component[w] = root[v]
- tmpc.add(w)
- stack.remove(v)
- yield tmpc
-
- visited = {}
- component = {}
- root = {}
- cnt = 0
- stack = []
- for source in G:
- if source not in visited:
- yield from visit(source, cnt)
+ yield from strongly_connected_components(G)
@not_implemented_for("undirected")
diff --git a/networkx/utils/backends.py b/networkx/utils/backends.py
index ae7b030a7..0ce1a9e00 100644
--- a/networkx/utils/backends.py
+++ b/networkx/utils/backends.py
@@ -96,11 +96,14 @@ from ..exception import NetworkXNotImplemented
__all__ = ["_dispatch"]
-def _get_plugins():
+def _get_plugins(group, *, load_and_call=False):
if sys.version_info < (3, 10):
- items = entry_points()["networkx.plugins"]
+ eps = entry_points()
+ if group not in eps:
+ return {}
+ items = eps[group]
else:
- items = entry_points(group="networkx.plugins")
+ items = entry_points(group=group)
rv = {}
for ep in items:
if ep.name in rv:
@@ -109,14 +112,24 @@ def _get_plugins():
RuntimeWarning,
stacklevel=2,
)
+ elif load_and_call:
+ try:
+ rv[ep.name] = ep.load()()
+ except Exception as exc:
+ warnings.warn(
+ f"Error encountered when loading info for plugin {ep.name}: {exc}",
+ RuntimeWarning,
+ stacklevel=2,
+ )
else:
rv[ep.name] = ep
# nx-loopback plugin is only available when testing (added in conftest.py)
- del rv["nx-loopback"]
+ rv.pop("nx-loopback", None)
return rv
-plugins = _get_plugins()
+plugins = _get_plugins("networkx.plugins")
+plugin_info = _get_plugins("networkx.plugin_info", load_and_call=True)
_registered_algorithms = {}
@@ -234,7 +247,7 @@ class _dispatch:
# standard function-wrapping stuff
# __annotations__ not used
self.__name__ = func.__name__
- self.__doc__ = func.__doc__
+ # self.__doc__ = func.__doc__ # __doc__ handled as cached property
self.__defaults__ = func.__defaults__
# We "magically" add `backend=` keyword argument to allow backend to be specified
if func.__kwdefaults__:
@@ -246,6 +259,10 @@ class _dispatch:
self.__dict__.update(func.__dict__)
self.__wrapped__ = func
+ # Supplement docstring with backend info; compute and cache when needed
+ self._orig_doc = func.__doc__
+ self._cached_doc = None
+
self.orig_func = func
self.name = name
self.edge_attrs = edge_attrs
@@ -306,6 +323,16 @@ class _dispatch:
# Compute and cache the signature on-demand
self._sig = None
+ # Load and cache backends on-demand
+ self._backends = {}
+
+ # Which backends implement this function?
+ self.backends = {
+ backend
+ for backend, info in plugin_info.items()
+ if "functions" in info and name in info["functions"]
+ }
+
if name in _registered_algorithms:
raise KeyError(
f"Algorithm already exists in dispatch registry: {name}"
@@ -313,6 +340,18 @@ class _dispatch:
_registered_algorithms[name] = self
return self
+ @property
+ def __doc__(self):
+ if (rv := self._cached_doc) is not None:
+ return rv
+ rv = self._cached_doc = self._make_doc()
+ return rv
+
+ @__doc__.setter
+ def __doc__(self, val):
+ self._orig_doc = val
+ self._cached_doc = None
+
@property
def __signature__(self):
if self._sig is None:
@@ -462,7 +501,7 @@ class _dispatch:
f"{self.name}() has networkx and {plugin_name} graphs, but NetworkX is not "
f"configured to automatically convert graphs from networkx to {plugin_name}."
)
- backend = plugins[plugin_name].load()
+ backend = self._load_backend(plugin_name)
if hasattr(backend, self.name):
if "networkx" in plugin_names:
# We need to convert networkx graphs to backend graphs
@@ -494,9 +533,15 @@ class _dispatch:
# Default: run with networkx on networkx inputs
return self.orig_func(*args, **kwargs)
+ def _load_backend(self, plugin_name):
+ if plugin_name in self._backends:
+ return self._backends[plugin_name]
+ rv = self._backends[plugin_name] = plugins[plugin_name].load()
+ return rv
+
def _can_backend_run(self, plugin_name, /, *args, **kwargs):
"""Can the specified backend run this algorithms with these arguments?"""
- backend = plugins[plugin_name].load()
+ backend = self._load_backend(plugin_name)
return hasattr(backend, self.name) and (
not hasattr(backend, "can_run") or backend.can_run(self.name, args, kwargs)
)
@@ -645,7 +690,7 @@ class _dispatch:
# It should be safe to assume that we either have networkx graphs or backend graphs.
# Future work: allow conversions between backends.
- backend = plugins[plugin_name].load()
+ backend = self._load_backend(plugin_name)
for gname in self.graphs:
if gname in self.list_graphs:
bound.arguments[gname] = [
@@ -704,7 +749,7 @@ class _dispatch:
def _convert_and_call(self, plugin_name, args, kwargs, *, fallback_to_nx=False):
"""Call this dispatchable function with a backend, converting graphs if necessary."""
- backend = plugins[plugin_name].load()
+ backend = self._load_backend(plugin_name)
if not self._can_backend_run(plugin_name, *args, **kwargs):
if fallback_to_nx:
return self.orig_func(*args, **kwargs)
@@ -729,7 +774,7 @@ class _dispatch:
self, plugin_name, args, kwargs, *, fallback_to_nx=False
):
"""Call this dispatchable function with a backend; for use with testing."""
- backend = plugins[plugin_name].load()
+ backend = self._load_backend(plugin_name)
if not self._can_backend_run(plugin_name, *args, **kwargs):
if fallback_to_nx:
return self.orig_func(*args, **kwargs)
@@ -807,6 +852,49 @@ class _dispatch:
return backend.convert_to_nx(result, name=self.name)
+ def _make_doc(self):
+ if not self.backends:
+ return self._orig_doc
+ lines = [
+ "Backends",
+ "--------",
+ ]
+ for backend in sorted(self.backends):
+ info = plugin_info[backend]
+ if "short_summary" in info:
+ lines.append(f"{backend} : {info['short_summary']}")
+ else:
+ lines.append(backend)
+ if "functions" not in info or self.name not in info["functions"]:
+ lines.append("")
+ continue
+
+ func_info = info["functions"][self.name]
+ if "extra_docstring" in func_info:
+ lines.extend(
+ f" {line}" if line else line
+ for line in func_info["extra_docstring"].split("\n")
+ )
+ add_gap = True
+ else:
+ add_gap = False
+ if "extra_parameters" in func_info:
+ if add_gap:
+ lines.append("")
+ lines.append(" Extra parameters:")
+ extra_parameters = func_info["extra_parameters"]
+ for param in sorted(extra_parameters):
+ lines.append(f" {param}")
+ if desc := extra_parameters[param]:
+ lines.append(f" {desc}")
+ lines.append("")
+ else:
+ lines.append("")
+
+ lines.pop() # Remove last empty line
+ to_add = "\n ".join(lines)
+ return f"{self._orig_doc.rstrip()}\n\n {to_add}"
+
def __reduce__(self):
"""Allow this object to be serialized with pickle.
| networkx/networkx | ba11717e40ac4466f322f1c448eb70a5914c8b6e | diff --git a/networkx/algorithms/components/tests/test_strongly_connected.py b/networkx/algorithms/components/tests/test_strongly_connected.py
index ab4e89395..f1c773026 100644
--- a/networkx/algorithms/components/tests/test_strongly_connected.py
+++ b/networkx/algorithms/components/tests/test_strongly_connected.py
@@ -63,7 +63,8 @@ class TestStronglyConnected:
def test_tarjan_recursive(self):
scc = nx.strongly_connected_components_recursive
for G, C in self.gc:
- assert {frozenset(g) for g in scc(G)} == C
+ with pytest.deprecated_call():
+ assert {frozenset(g) for g in scc(G)} == C
def test_kosaraju(self):
scc = nx.kosaraju_strongly_connected_components
@@ -168,7 +169,8 @@ class TestStronglyConnected:
G = nx.DiGraph()
assert list(nx.strongly_connected_components(G)) == []
assert list(nx.kosaraju_strongly_connected_components(G)) == []
- assert list(nx.strongly_connected_components_recursive(G)) == []
+ with pytest.deprecated_call():
+ assert list(nx.strongly_connected_components_recursive(G)) == []
assert len(nx.condensation(G)) == 0
pytest.raises(
nx.NetworkXPointlessConcept, nx.is_strongly_connected, nx.DiGraph()
@@ -181,7 +183,8 @@ class TestStronglyConnected:
with pytest.raises(NetworkXNotImplemented):
next(nx.kosaraju_strongly_connected_components(G))
with pytest.raises(NetworkXNotImplemented):
- next(nx.strongly_connected_components_recursive(G))
+ with pytest.deprecated_call():
+ next(nx.strongly_connected_components_recursive(G))
pytest.raises(NetworkXNotImplemented, nx.is_strongly_connected, G)
pytest.raises(
nx.NetworkXPointlessConcept, nx.is_strongly_connected, nx.DiGraph()
@@ -191,7 +194,6 @@ class TestStronglyConnected:
strong_cc_methods = (
nx.strongly_connected_components,
nx.kosaraju_strongly_connected_components,
- nx.strongly_connected_components_recursive,
)
@pytest.mark.parametrize("get_components", strong_cc_methods)
diff --git a/networkx/conftest.py b/networkx/conftest.py
index 0cad73f21..1702bdce5 100644
--- a/networkx/conftest.py
+++ b/networkx/conftest.py
@@ -117,6 +117,11 @@ def set_warnings():
warnings.filterwarnings(
"ignore", category=DeprecationWarning, message="function `join` is deprecated"
)
+ warnings.filterwarnings(
+ "ignore",
+ category=DeprecationWarning,
+ message="\n\nstrongly_connected_components_recursive",
+ )
@pytest.fixture(autouse=True)
| Discrepancy in 'strongly_connected_components_recursive' for Detecting SCCs
Hey NetworkX team! 👋
### Description:
While attempting to identify strongly connected components (SCCs) of a graph using the `strongly_connected_components`, `strongly_connected_components_recursive`, and `kosaraju_strongly_connected_components` methods provided by `networkx`, inconsistent SCCs were observed. Notably, the `strongly_connected_components_recursive` method identified node `8` as a separate SCC, while the other two methods included node `8` in a larger SCC.\
For context, I've minimized my original, much larger graph to a smaller version to isolate and highlight the issue.
### Observed Behavior:
- Using `strongly_connected_components`: [{0, 2, 3, 5, 6, 7, 8, 10}, {1}, {4}, {9}]
- Using `strongly_connected_components_recursive`: [{8}, {0, 2, 3, 5, 6, 7, 10}, {1}, {4}, {9}]
- Using `kosaraju_strongly_connected_components`: [{1}, {0, 2, 3, 5, 6, 7, 8, 10}, {9}, {4}]
### Expected Behavior:
All three methods should produce consistent SCCs. Node `8` should be part of the larger SCC `{0, 2, 3, 5, 6, 7, 8, 10}` based on the provided edges.
### Steps to Reproduce:
1. Create a directed graph with the provided nodes and edges.
2. Compute SCCs using the `strongly_connected_components`, `strongly_connected_components_recursive`, and `kosaraju_strongly_connected_components` methods.
### Environment:
Python version: 3.8.10
NetworkX version: 3.1
```python
import networkx as nx
from matplotlib import pyplot as plt
class Graph:
def __init__(self):
self.graph = nx.DiGraph()
def add_nodes(self, nodes):
for node in nodes:
self.graph.add_node(node)
def add_edges(self, edges):
for edge in edges:
self.graph.add_edge(edge[0], edge[1])
def compute_scc(self):
"""Compute the result using three functions and print them"""
result1 = list(nx.strongly_connected_components(self.graph))
result2 = list(nx.strongly_connected_components_recursive(self.graph))
result3 = list(nx.kosaraju_strongly_connected_components(self.graph))
print("Using strongly_connected_components:", result1)
print("Using strongly_connected_components_recursive:", result2)
print("Using kosaraju_strongly_connected_components:", result3)
if __name__ == "__main__":
nodes = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
edges = [
(0, 2), (0, 8), (0, 10), (2, 3), (3, 10),
(3, 0), (4, 6), (4, 1), (5, 6), (5, 7),
(6, 2), (6, 5), (7, 10), (8, 7), (9, 8),
(10, 6)
]
graph_instance = Graph()
graph_instance.add_nodes(nodes)
graph_instance.add_edges(edges)
graph_instance.compute_scc()
| 0.0 | [
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_tarjan_recursive",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_null_graph"
] | [
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_tarjan",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_kosaraju",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_number_strongly_connected_components",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_is_strongly_connected",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_contract_scc1",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_contract_scc_isolate",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_contract_scc_edge",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_condensation_mapping_and_members",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_connected_mutability[strongly_connected_components]",
"networkx/algorithms/components/tests/test_strongly_connected.py::TestStronglyConnected::test_connected_mutability[kosaraju_strongly_connected_components]"
] | 2023-09-26 06:55:22+00:00 | 4,154 |
|
networkx__networkx-7024 | diff --git a/networkx/algorithms/clique.py b/networkx/algorithms/clique.py
index b725eabb4..7fd7e8166 100644
--- a/networkx/algorithms/clique.py
+++ b/networkx/algorithms/clique.py
@@ -18,11 +18,8 @@ __all__ = [
"find_cliques_recursive",
"make_max_clique_graph",
"make_clique_bipartite",
- "graph_clique_number",
- "graph_number_of_cliques",
"node_clique_number",
"number_of_cliques",
- "cliques_containing_node",
"enumerate_all_cliques",
"max_weight_clique",
]
@@ -511,106 +508,6 @@ def make_clique_bipartite(G, fpos=None, create_using=None, name=None):
return B
-def graph_clique_number(G, cliques=None):
- """Returns the clique number of the graph.
-
- The *clique number* of a graph is the size of the largest clique in
- the graph.
-
- .. deprecated:: 3.0
-
- graph_clique_number is deprecated in NetworkX 3.0 and will be removed
- in v3.2. The graph clique number can be computed directly with::
-
- max(len(c) for c in nx.find_cliques(G))
-
-
- Parameters
- ----------
- G : NetworkX graph
- An undirected graph.
-
- cliques : list
- A list of cliques, each of which is itself a list of nodes. If
- not specified, the list of all cliques will be computed, as by
- :func:`find_cliques`.
-
- Returns
- -------
- int
- The size of the largest clique in `G`.
-
- Notes
- -----
- You should provide `cliques` if you have already computed the list
- of maximal cliques, in order to avoid an exponential time search for
- maximal cliques.
-
- """
- import warnings
-
- warnings.warn(
- (
- "\n\ngraph_clique_number is deprecated and will be removed.\n"
- "Use: ``max(len(c) for c in nx.find_cliques(G))`` instead."
- ),
- DeprecationWarning,
- stacklevel=2,
- )
- if len(G.nodes) < 1:
- return 0
- if cliques is None:
- cliques = find_cliques(G)
- return max([len(c) for c in cliques] or [1])
-
-
-def graph_number_of_cliques(G, cliques=None):
- """Returns the number of maximal cliques in the graph.
-
- .. deprecated:: 3.0
-
- graph_number_of_cliques is deprecated and will be removed in v3.2.
- The number of maximal cliques can be computed directly with::
-
- sum(1 for _ in nx.find_cliques(G))
-
- Parameters
- ----------
- G : NetworkX graph
- An undirected graph.
-
- cliques : list
- A list of cliques, each of which is itself a list of nodes. If
- not specified, the list of all cliques will be computed, as by
- :func:`find_cliques`.
-
- Returns
- -------
- int
- The number of maximal cliques in `G`.
-
- Notes
- -----
- You should provide `cliques` if you have already computed the list
- of maximal cliques, in order to avoid an exponential time search for
- maximal cliques.
-
- """
- import warnings
-
- warnings.warn(
- (
- "\n\ngraph_number_of_cliques is deprecated and will be removed.\n"
- "Use: ``sum(1 for _ in nx.find_cliques(G))`` instead."
- ),
- DeprecationWarning,
- stacklevel=2,
- )
- if cliques is None:
- cliques = list(find_cliques(G))
- return len(cliques)
-
-
@nx._dispatch
def node_clique_number(G, nodes=None, cliques=None, separate_nodes=False):
"""Returns the size of the largest maximal clique containing each given node.
@@ -678,29 +575,9 @@ def node_clique_number(G, nodes=None, cliques=None, separate_nodes=False):
def number_of_cliques(G, nodes=None, cliques=None):
"""Returns the number of maximal cliques for each node.
- .. deprecated:: 3.0
-
- number_of_cliques is deprecated and will be removed in v3.2.
- Use the result of `find_cliques` directly to compute the number of
- cliques containing each node::
-
- {n: sum(1 for c in nx.find_cliques(G) if n in c) for n in G}
-
Returns a single or list depending on input nodes.
Optional list of cliques can be input if already computed.
"""
- import warnings
-
- warnings.warn(
- (
- "\n\nnumber_of_cliques is deprecated and will be removed.\n"
- "Use the result of find_cliques directly to compute the number\n"
- "of cliques containing each node:\n\n"
- " {n: sum(1 for c in nx.find_cliques(G) if n in c) for n in G}\n\n"
- ),
- DeprecationWarning,
- stacklevel=2,
- )
if cliques is None:
cliques = list(find_cliques(G))
@@ -718,49 +595,6 @@ def number_of_cliques(G, nodes=None, cliques=None):
return numcliq
-def cliques_containing_node(G, nodes=None, cliques=None):
- """Returns a list of cliques containing the given node.
-
- .. deprecated:: 3.0
-
- cliques_containing_node is deprecated and will be removed in 3.2.
- Use the result of `find_cliques` directly to compute the cliques that
- contain each node::
-
- {n: [c for c in nx.find_cliques(G) if n in c] for n in G}
-
- Returns a single list or list of lists depending on input nodes.
- Optional list of cliques can be input if already computed.
- """
- import warnings
-
- warnings.warn(
- (
- "\n\ncliques_containing_node is deprecated and will be removed.\n"
- "Use the result of find_cliques directly to compute maximal cliques\n"
- "containing each node:\n\n"
- " {n: [c for c in nx.find_cliques(G) if n in c] for n in G}\n\n"
- ),
- DeprecationWarning,
- stacklevel=2,
- )
- if cliques is None:
- cliques = list(find_cliques(G))
-
- if nodes is None:
- nodes = list(G.nodes()) # none, get entire graph
-
- if not isinstance(nodes, list): # check for a list
- v = nodes
- # assume it is a single value
- vcliques = [c for c in cliques if v in c]
- else:
- vcliques = {}
- for v in nodes:
- vcliques[v] = [c for c in cliques if v in c]
- return vcliques
-
-
class MaxWeightClique:
"""A class for the maximum weight clique algorithm.
diff --git a/networkx/algorithms/connectivity/edge_augmentation.py b/networkx/algorithms/connectivity/edge_augmentation.py
index ed9bd7c33..c1215509e 100644
--- a/networkx/algorithms/connectivity/edge_augmentation.py
+++ b/networkx/algorithms/connectivity/edge_augmentation.py
@@ -68,7 +68,7 @@ def is_k_edge_connected(G, k):
if k == 1:
return nx.is_connected(G)
elif k == 2:
- return not nx.has_bridges(G)
+ return nx.is_connected(G) and not nx.has_bridges(G)
else:
return nx.edge_connectivity(G, cutoff=k) >= k
| networkx/networkx | 3b95b3b39e4bb57831e9611b820054218af15a71 | diff --git a/networkx/algorithms/connectivity/tests/test_edge_augmentation.py b/networkx/algorithms/connectivity/tests/test_edge_augmentation.py
index 37ee29740..e1d92d996 100644
--- a/networkx/algorithms/connectivity/tests/test_edge_augmentation.py
+++ b/networkx/algorithms/connectivity/tests/test_edge_augmentation.py
@@ -75,6 +75,11 @@ def test_is_k_edge_connected():
assert is_k_edge_connected(G, k=3)
assert is_k_edge_connected(G, k=4)
+ G = nx.compose(nx.complete_graph([0, 1, 2]), nx.complete_graph([3, 4, 5]))
+ assert not is_k_edge_connected(G, k=1)
+ assert not is_k_edge_connected(G, k=2)
+ assert not is_k_edge_connected(G, k=3)
+
def test_is_k_edge_connected_exceptions():
pytest.raises(
diff --git a/networkx/algorithms/tests/test_clique.py b/networkx/algorithms/tests/test_clique.py
index 1bcbd4fe1..3bee21098 100644
--- a/networkx/algorithms/tests/test_clique.py
+++ b/networkx/algorithms/tests/test_clique.py
@@ -67,98 +67,66 @@ class TestCliques:
with pytest.raises(ValueError):
list(nx.find_cliques_recursive(self.G, [2, 6, 4, 1]))
- def test_clique_number(self):
- G = self.G
- with pytest.deprecated_call():
- assert nx.graph_clique_number(G) == 4
- with pytest.deprecated_call():
- assert nx.graph_clique_number(G, cliques=self.cl) == 4
-
- def test_clique_number2(self):
- G = nx.Graph()
- G.add_nodes_from([1, 2, 3])
- with pytest.deprecated_call():
- assert nx.graph_clique_number(G) == 1
-
- def test_clique_number3(self):
- G = nx.Graph()
- with pytest.deprecated_call():
- assert nx.graph_clique_number(G) == 0
-
def test_number_of_cliques(self):
G = self.G
- with pytest.deprecated_call():
- assert nx.graph_number_of_cliques(G) == 5
- with pytest.deprecated_call():
- assert nx.graph_number_of_cliques(G, cliques=self.cl) == 5
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, 1) == 1
- with pytest.deprecated_call():
- assert list(nx.number_of_cliques(G, [1]).values()) == [1]
- with pytest.deprecated_call():
- assert list(nx.number_of_cliques(G, [1, 2]).values()) == [1, 2]
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, [1, 2]) == {1: 1, 2: 2}
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, 2) == 2
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G) == {
- 1: 1,
- 2: 2,
- 3: 1,
- 4: 2,
- 5: 1,
- 6: 2,
- 7: 1,
- 8: 1,
- 9: 1,
- 10: 1,
- 11: 1,
- }
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, nodes=list(G)) == {
- 1: 1,
- 2: 2,
- 3: 1,
- 4: 2,
- 5: 1,
- 6: 2,
- 7: 1,
- 8: 1,
- 9: 1,
- 10: 1,
- 11: 1,
- }
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, nodes=[2, 3, 4]) == {2: 2, 3: 1, 4: 2}
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, cliques=self.cl) == {
- 1: 1,
- 2: 2,
- 3: 1,
- 4: 2,
- 5: 1,
- 6: 2,
- 7: 1,
- 8: 1,
- 9: 1,
- 10: 1,
- 11: 1,
- }
- with pytest.deprecated_call():
- assert nx.number_of_cliques(G, list(G), cliques=self.cl) == {
- 1: 1,
- 2: 2,
- 3: 1,
- 4: 2,
- 5: 1,
- 6: 2,
- 7: 1,
- 8: 1,
- 9: 1,
- 10: 1,
- 11: 1,
- }
+ assert nx.number_of_cliques(G, 1) == 1
+ assert list(nx.number_of_cliques(G, [1]).values()) == [1]
+ assert list(nx.number_of_cliques(G, [1, 2]).values()) == [1, 2]
+ assert nx.number_of_cliques(G, [1, 2]) == {1: 1, 2: 2}
+ assert nx.number_of_cliques(G, 2) == 2
+ assert nx.number_of_cliques(G) == {
+ 1: 1,
+ 2: 2,
+ 3: 1,
+ 4: 2,
+ 5: 1,
+ 6: 2,
+ 7: 1,
+ 8: 1,
+ 9: 1,
+ 10: 1,
+ 11: 1,
+ }
+ assert nx.number_of_cliques(G, nodes=list(G)) == {
+ 1: 1,
+ 2: 2,
+ 3: 1,
+ 4: 2,
+ 5: 1,
+ 6: 2,
+ 7: 1,
+ 8: 1,
+ 9: 1,
+ 10: 1,
+ 11: 1,
+ }
+ assert nx.number_of_cliques(G, nodes=[2, 3, 4]) == {2: 2, 3: 1, 4: 2}
+ assert nx.number_of_cliques(G, cliques=self.cl) == {
+ 1: 1,
+ 2: 2,
+ 3: 1,
+ 4: 2,
+ 5: 1,
+ 6: 2,
+ 7: 1,
+ 8: 1,
+ 9: 1,
+ 10: 1,
+ 11: 1,
+ }
+ assert nx.number_of_cliques(G, list(G), cliques=self.cl) == {
+ 1: 1,
+ 2: 2,
+ 3: 1,
+ 4: 2,
+ 5: 1,
+ 6: 2,
+ 7: 1,
+ 8: 1,
+ 9: 1,
+ 10: 1,
+ 11: 1,
+ }
def test_node_clique_number(self):
G = self.G
@@ -196,34 +164,6 @@ class TestCliques:
assert nx.node_clique_number(G, [1, 2], cliques=self.cl) == {1: 4, 2: 4}
assert nx.node_clique_number(G, 1, cliques=self.cl) == 4
- def test_cliques_containing_node(self):
- G = self.G
- with pytest.deprecated_call():
- assert nx.cliques_containing_node(G, 1) == [[2, 6, 1, 3]]
- with pytest.deprecated_call():
- assert list(nx.cliques_containing_node(G, [1]).values()) == [[[2, 6, 1, 3]]]
- with pytest.deprecated_call():
- assert [
- sorted(c) for c in list(nx.cliques_containing_node(G, [1, 2]).values())
- ] == [[[2, 6, 1, 3]], [[2, 6, 1, 3], [2, 6, 4]]]
- with pytest.deprecated_call():
- result = nx.cliques_containing_node(G, [1, 2])
- for k, v in result.items():
- result[k] = sorted(v)
- assert result == {1: [[2, 6, 1, 3]], 2: [[2, 6, 1, 3], [2, 6, 4]]}
- with pytest.deprecated_call():
- assert nx.cliques_containing_node(G, 1) == [[2, 6, 1, 3]]
- expected = [{2, 6, 1, 3}, {2, 6, 4}]
- with pytest.deprecated_call():
- answer = [set(c) for c in nx.cliques_containing_node(G, 2)]
- assert answer in (expected, list(reversed(expected)))
-
- with pytest.deprecated_call():
- answer = [set(c) for c in nx.cliques_containing_node(G, 2, cliques=self.cl)]
- assert answer in (expected, list(reversed(expected)))
- with pytest.deprecated_call():
- assert len(nx.cliques_containing_node(G)) == 11
-
def test_make_clique_bipartite(self):
G = self.G
B = nx.make_clique_bipartite(G)
| ```is_k_edge_connected``` incorrectly returns True for k=2 with multi-component graphs without bridges
### Current Behavior
The implementation of ```is_k_edge_connected``` currently defers to ```is_connected``` for k=1, to ```has_bridges``` for k=2, and runs the full check for k>2.
As a result, this returns True for a multi-component graph without bridges.
### Expected Behavior
Since the graph has multiple components, it is not connected in the first place and ```is_k_edge_connected``` should return False for all (positive) values of k.
### Steps to Reproduce
```
import networkx as nx
G = nx.compose(nx.complete_graph([0, 1, 2]), nx.complete_graph([3, 4, 5]))
nx.connectivity.is_k_edge_connected(G, 2)
```
This returns True but should return False.
### Environment
Python version: 3.11.2
NetworkX version: 3.1
### Additional context
As defined in the NetworkX documentation, a bridge is an edge whose removal increases the number of connected components. It appears to me, then, that a graph not having bridges is a necessary-but-not-sufficient condition for a graph being 2-edge-connected.
| 0.0 | [
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_is_k_edge_connected"
] | [
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_weight_key",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_is_locally_k_edge_connected_exceptions",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_is_k_edge_connected_exceptions",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_is_locally_k_edge_connected",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_null_graph",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_cliques",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_clique_and_node",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_point_graph",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_edgeless_graph",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_invalid_k",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_unfeasible",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_tarjan",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_configuration",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_shell",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_karate",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_star",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_barbell",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_bridge",
"networkx/algorithms/connectivity/tests/test_edge_augmentation.py::test_gnp_augmentation",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_find_cliques1",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_selfloops",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_find_cliques2",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_find_cliques3",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_number_of_cliques",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_node_clique_number",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_make_clique_bipartite",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_make_max_clique_graph",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_directed",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_find_cliques_trivial",
"networkx/algorithms/tests/test_clique.py::TestCliques::test_make_max_clique_graph_create_using",
"networkx/algorithms/tests/test_clique.py::TestEnumerateAllCliques::test_paper_figure_4"
] | 2023-10-17 16:13:05+00:00 | 4,155 |
|
neurostuff__PyMARE-114 | diff --git a/.github/workflows/python-publish.yml b/.github/workflows/python-publish.yml
index 88d6b34..bee4ac8 100644
--- a/.github/workflows/python-publish.yml
+++ b/.github/workflows/python-publish.yml
@@ -17,7 +17,7 @@ jobs:
- name: Set up Python
uses: actions/setup-python@v2
with:
- python-version: '3.7'
+ python-version: '3.8'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
diff --git a/pymare/__init__.py b/pymare/__init__.py
index e91cc9b..6f112e9 100644
--- a/pymare/__init__.py
+++ b/pymare/__init__.py
@@ -1,4 +1,7 @@
"""PyMARE: Python Meta-Analysis & Regression Engine."""
+import sys
+import warnings
+
from .core import Dataset, meta_regression
from .effectsize import OneSampleEffectSizeConverter, TwoSampleEffectSizeConverter
@@ -13,3 +16,34 @@ from . import _version
__version__ = _version.get_versions()["version"]
del _version
+
+
+def _py367_deprecation_warning():
+ """Deprecation warnings message.
+
+ Notes
+ -----
+ Adapted from NiMARE.
+ """
+ py36_warning = (
+ "Python 3.6 and 3.7 support is deprecated and will be removed in release 0.0.5 of PyMARE. "
+ "Consider switching to Python 3.8, 3.9."
+ )
+ warnings.filterwarnings("once", message=py36_warning)
+ warnings.warn(message=py36_warning, category=FutureWarning, stacklevel=3)
+
+
+def _python_deprecation_warnings():
+ """Raise deprecation warnings.
+
+ Notes
+ -----
+ Adapted from NiMARE.
+ """
+ if sys.version_info.major == 3 and (
+ sys.version_info.minor == 6 or sys.version_info.minor == 7
+ ):
+ _py367_deprecation_warning()
+
+
+_python_deprecation_warnings()
diff --git a/setup.cfg b/setup.cfg
index a71d2e7..17959f6 100644
--- a/setup.cfg
+++ b/setup.cfg
@@ -97,5 +97,7 @@ max-line-length = 99
exclude=*build/,_version.py
putty-ignore =
*/__init__.py : +F401
+per-file-ignores =
+ */__init__.py:D401
ignore = E203,E402,E722,W503
docstring-convention = numpy
| neurostuff/PyMARE | 001f5d62f003782b3ccba3fff69ea8f57fa32340 | diff --git a/.github/workflows/testing.yml b/.github/workflows/testing.yml
index 555c1b5..050df84 100644
--- a/.github/workflows/testing.yml
+++ b/.github/workflows/testing.yml
@@ -40,6 +40,14 @@ jobs:
matrix:
os: ["ubuntu-latest", "macos-latest"]
python-version: ["3.6", "3.7", "3.8", "3.9"]
+ include:
+ # ubuntu-20.04 is used only to test python 3.6
+ - os: "ubuntu-20.04"
+ python-version: "3.6"
+ exclude:
+ # ubuntu-latest does not support python 3.6
+ - os: "ubuntu-latest"
+ python-version: "3.6"
name: ${{ matrix.os }} with Python ${{ matrix.python-version }}
defaults:
run:
@@ -73,7 +81,7 @@ jobs:
- name: Set up Python environment
uses: actions/setup-python@v2
with:
- python-version: "3.7"
+ python-version: "3.8"
- name: "Install the package"
shell: bash {0}
| Deprecate Python 3.6 and 3.7
Since we are deprecating Python 3.6 and 3.7 in NiMARE, I thought we could add deprecation warnings here as well. @tsalo | 0.0 | [
"pymare/tests/test_effectsize_base.py::test_compute_measure"
] | [
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data0-directed-expected0]",
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data1-undirected-expected1]",
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data2-concordant-expected2]",
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data3-directed-expected3]",
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data4-undirected-expected4]",
"pymare/tests/test_combination_tests.py::test_combination_test[StoufferCombinationTest-data5-concordant-expected5]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data6-directed-expected6]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data7-undirected-expected7]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data8-concordant-expected8]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data9-directed-expected9]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data10-undirected-expected10]",
"pymare/tests/test_combination_tests.py::test_combination_test[FisherCombinationTest-data11-concordant-expected11]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data0-directed-expected0]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data1-undirected-expected1]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data2-concordant-expected2]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data3-directed-expected3]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data4-undirected-expected4]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[StoufferCombinationTest-data5-concordant-expected5]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data6-directed-expected6]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data7-undirected-expected7]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data8-concordant-expected8]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data9-directed-expected9]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data10-undirected-expected10]",
"pymare/tests/test_combination_tests.py::test_combination_test_from_dataset[FisherCombinationTest-data11-concordant-expected11]",
"pymare/tests/test_core.py::test_dataset_init",
"pymare/tests/test_core.py::test_dataset_init_2D",
"pymare/tests/test_core.py::test_dataset_init_from_df",
"pymare/tests/test_core.py::test_meta_regression_1",
"pymare/tests/test_core.py::test_meta_regression_2",
"pymare/tests/test_datasets.py::test_michael2013",
"pymare/tests/test_effectsize_base.py::test_EffectSizeConverter_smoke_test",
"pymare/tests/test_effectsize_base.py::test_esc_implicit_dtype_conversion",
"pymare/tests/test_effectsize_base.py::test_EffectSizeConverter_from_df",
"pymare/tests/test_effectsize_base.py::test_EffectSizeConverter_to_dataset",
"pymare/tests/test_effectsize_base.py::test_2d_array_conversion",
"pymare/tests/test_effectsize_base.py::test_convert_r_and_n_to_rz",
"pymare/tests/test_effectsize_base.py::test_convert_r_to_itself",
"pymare/tests/test_effectsize_expressions.py::test_Expression_init",
"pymare/tests/test_effectsize_expressions.py::test_select_expressions",
"pymare/tests/test_estimators.py::test_weighted_least_squares_estimator",
"pymare/tests/test_estimators.py::test_dersimonian_laird_estimator",
"pymare/tests/test_estimators.py::test_2d_DL_estimator",
"pymare/tests/test_estimators.py::test_hedges_estimator",
"pymare/tests/test_estimators.py::test_2d_hedges",
"pymare/tests/test_estimators.py::test_variance_based_maximum_likelihood_estimator",
"pymare/tests/test_estimators.py::test_variance_based_restricted_maximum_likelihood_estimator",
"pymare/tests/test_estimators.py::test_sample_size_based_maximum_likelihood_estimator",
"pymare/tests/test_estimators.py::test_sample_size_based_restricted_maximum_likelihood_estimator",
"pymare/tests/test_estimators.py::test_2d_looping",
"pymare/tests/test_estimators.py::test_2d_loop_warning",
"pymare/tests/test_results.py::test_meta_regression_results_from_arrays",
"pymare/tests/test_results.py::test_combination_test_results_from_arrays",
"pymare/tests/test_results.py::test_meta_regression_results_init_1d",
"pymare/tests/test_results.py::test_meta_regression_results_init_2d",
"pymare/tests/test_results.py::test_mrr_fe_se",
"pymare/tests/test_results.py::test_mrr_get_fe_stats",
"pymare/tests/test_results.py::test_mrr_get_re_stats",
"pymare/tests/test_results.py::test_mrr_get_heterogeneity_stats",
"pymare/tests/test_results.py::test_mrr_to_df",
"pymare/tests/test_results.py::test_estimator_summary",
"pymare/tests/test_results.py::test_exact_perm_test_2d_no_mods",
"pymare/tests/test_results.py::test_exact_perm_test_1d_no_mods",
"pymare/tests/test_results.py::test_approx_perm_test_with_n_based_estimator",
"pymare/tests/test_results.py::test_stouffers_perm_test_exact",
"pymare/tests/test_results.py::test_stouffers_perm_test_approx",
"pymare/tests/test_stats.py::test_q_gen",
"pymare/tests/test_stats.py::test_q_profile",
"pymare/tests/test_stats.py::test_var_to_ci",
"pymare/tests/test_utils.py::test_get_resource_path",
"pymare/tests/test_utils.py::test_check_inputs_shape"
] | 2023-01-25 16:52:55+00:00 | 4,156 |
|
newrelic__newrelic-lambda-cli-110 | diff --git a/README.md b/README.md
index f386693..99329ca 100644
--- a/README.md
+++ b/README.md
@@ -154,6 +154,7 @@ newrelic-lambda layers install \
| `--exclude` or `-e` | No | A function name to exclude while installing layers. Can provide multiple `--exclude` arguments. Only checked when `all`, `installed` and `not-installed` are used. See `newrelic-lambda functions list` for function names. |
| `--layer-arn` or `-l` | No | Specify a specific layer version ARN to use. This is auto detected by default. |
| `--upgrade` or `-u` | No | Permit upgrade to the latest layer version for this region and runtime. |
+| `--enable-extension` or `-x` | No | Enable the [New Relic Lambda Extension](https://github.com/newrelic/newrelic-lambda-extension). |
| `--aws-profile` or `-p` | No | The AWS profile to use for this command. Can also use `AWS_PROFILE`. Will also check `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY` environment variables if not using AWS CLI. |
| `--aws-region` or `-r` | No | The AWS region this function is located. Can use `AWS_DEFAULT_REGION` environment variable. Defaults to AWS session region. |
diff --git a/newrelic_lambda_cli/cli/layers.py b/newrelic_lambda_cli/cli/layers.py
index 9807f0e..7a8ab3f 100644
--- a/newrelic_lambda_cli/cli/layers.py
+++ b/newrelic_lambda_cli/cli/layers.py
@@ -63,6 +63,12 @@ def register(group):
help="Permit upgrade of function layers to new version.",
is_flag=True,
)
[email protected](
+ "--enable-extension",
+ "-x",
+ help="Enable the New Relic Lambda Extension",
+ is_flag=True,
+)
@click.pass_context
def install(
ctx,
@@ -74,6 +80,7 @@ def install(
excludes,
layer_arn,
upgrade,
+ enable_extension,
):
"""Install New Relic AWS Lambda Layers"""
session = boto3.Session(profile_name=aws_profile, region_name=aws_region)
@@ -92,6 +99,7 @@ def install(
layer_arn,
nr_account_id,
upgrade,
+ enable_extension,
ctx.obj["VERBOSE"],
)
for function in functions
diff --git a/newrelic_lambda_cli/layers.py b/newrelic_lambda_cli/layers.py
index ce3d47a..9215a24 100644
--- a/newrelic_lambda_cli/layers.py
+++ b/newrelic_lambda_cli/layers.py
@@ -19,7 +19,9 @@ def index(region, runtime):
return layers_response.get("Layers", [])
-def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
+def _add_new_relic(
+ config, region, layer_arn, account_id, allow_upgrade, enable_extension
+):
runtime = config["Configuration"]["Runtime"]
if runtime not in utils.RUNTIME_CONFIG:
failure(
@@ -36,7 +38,7 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
"upgrade or reinstall to latest layer version."
% config["Configuration"]["FunctionArn"]
)
- return False
+ return True
existing_layers = [
layer["Arn"]
@@ -96,10 +98,23 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
if handler != runtime_handler:
update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_HANDLER"] = handler
+ if enable_extension:
+ update_kwargs["Environment"]["Variables"][
+ "NEW_RELIC_LAMBDA_EXTENSION_ENABLED"
+ ] = "true"
+
return update_kwargs
-def install(session, function_arn, layer_arn, account_id, allow_upgrade, verbose):
+def install(
+ session,
+ function_arn,
+ layer_arn,
+ account_id,
+ allow_upgrade,
+ enable_extension,
+ verbose,
+):
client = session.client("lambda")
config = get_function(session, function_arn)
if not config:
@@ -108,7 +123,9 @@ def install(session, function_arn, layer_arn, account_id, allow_upgrade, verbose
region = session.region_name
- update_kwargs = _add_new_relic(config, region, layer_arn, account_id, allow_upgrade)
+ update_kwargs = _add_new_relic(
+ config, region, layer_arn, account_id, allow_upgrade, enable_extension
+ )
if not update_kwargs:
return False
| newrelic/newrelic-lambda-cli | 8e5399c6072a052c0df445ccb06559f5e2df5b3d | diff --git a/tests/test_layers.py b/tests/test_layers.py
index 971702f..90ee31f 100644
--- a/tests/test_layers.py
+++ b/tests/test_layers.py
@@ -5,7 +5,7 @@ def test_add_new_relic(mock_function_config):
config = mock_function_config("python3.7")
assert config["Configuration"]["Handler"] == "original_handler"
- update_kwargs = _add_new_relic(config, "us-east-1", None, "12345", False)
+ update_kwargs = _add_new_relic(config, "us-east-1", None, "12345", False, True)
assert update_kwargs["FunctionName"] == config["Configuration"]["FunctionArn"]
assert update_kwargs["Handler"] == "newrelic_lambda_wrapper.handler"
assert update_kwargs["Environment"]["Variables"]["NEW_RELIC_ACCOUNT_ID"] == "12345"
@@ -13,6 +13,10 @@ def test_add_new_relic(mock_function_config):
update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_HANDLER"]
== config["Configuration"]["Handler"]
)
+ assert (
+ update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_EXTENSION_ENABLED"]
+ == "true"
+ )
def test_remove_new_relic(mock_function_config):
| [layers install] Add `NEW_RELIC_LAMBDA_EXTENSION_ENABLED=true` environment variable
[NOTE]: # ( ^^ Provide a general summary of the request in the title above. ^^ )
## Summary
[NOTE]: # ( Provide a brief overview of what the new feature is all about. )
New Relic Lambda Layer required `NEW_RELIC_LAMBDA_EXTENSION_ENABLED=true` environment variable. We have to add this variable manually because `laryers install` command doesn't add it.
https://docs.newrelic.co.jp/docs/serverless-function-monitoring/aws-lambda-monitoring/enable-lambda-monitoring/enable-serverless-monitoring-using-lambda-layer
## Desired Behaviour
[NOTE]: # ( Tell us how the new feature should work. Be specific. )
[TIP]: # ( Do NOT give us access or passwords to your New Relic account or API keys! )
The `layers install` command will add `NEW_RELIC_LAMBDA_EXTENSION_ENABLED=true`.
## Possible Solution
[NOTE]: # ( Not required. Suggest how to implement the addition or change. )
How about add env var [here](https://github.com/newrelic/newrelic-lambda-cli/blob/7eb3075186dc2df9246676ea388e8b6542aa40c0/newrelic_lambda_cli/layers.py#L95-L99
)?
## Additional context
[TIP]: # ( Why does this feature matter to you? What unique circumstances do you have? )
| 0.0 | [
"tests/test_layers.py::test_add_new_relic"
] | [
"tests/test_layers.py::test_remove_new_relic"
] | 2020-10-15 18:50:09+00:00 | 4,157 |
|
newrelic__newrelic-lambda-cli-111 | diff --git a/README.md b/README.md
index f386693..47ba380 100644
--- a/README.md
+++ b/README.md
@@ -26,18 +26,26 @@ A CLI to install the New Relic AWS Lambda integration and layers.
* Installs and configures a New Relic AWS Lambda layer onto your AWS Lambda functions
* Automatically selects the correct New Relic layer for your function's runtime and region
* Wraps your AWS Lambda functions without requiring a code change
-* Supports Node.js and Python AWS Lambda runtimes
+* Supports Go, Java, .NET, Node.js and Python AWS Lambda runtimes
* Easily uninstall the AWS Lambda layer with a single command
## Runtimes Supported
+* dotnetcore3.1
+* java8.al2
+* java11
* nodejs10.x
* nodejs12.x
+* provided
+* provided.al2
* python2.7
* python3.6
* python3.7
* python3.8
+**Note:** Automatic handler wrapping is only supported for Node.js and Python. For other runtimes,
+manual function wrapping is required using the runtime specific New Relic agent.
+
## Requirements
* Python >= 3.3
@@ -154,6 +162,7 @@ newrelic-lambda layers install \
| `--exclude` or `-e` | No | A function name to exclude while installing layers. Can provide multiple `--exclude` arguments. Only checked when `all`, `installed` and `not-installed` are used. See `newrelic-lambda functions list` for function names. |
| `--layer-arn` or `-l` | No | Specify a specific layer version ARN to use. This is auto detected by default. |
| `--upgrade` or `-u` | No | Permit upgrade to the latest layer version for this region and runtime. |
+| `--enable-extension` or `-x` | No | Enable the [New Relic Lambda Extension](https://github.com/newrelic/newrelic-lambda-extension). |
| `--aws-profile` or `-p` | No | The AWS profile to use for this command. Can also use `AWS_PROFILE`. Will also check `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY` environment variables if not using AWS CLI. |
| `--aws-region` or `-r` | No | The AWS region this function is located. Can use `AWS_DEFAULT_REGION` environment variable. Defaults to AWS session region. |
diff --git a/newrelic_lambda_cli/cli/layers.py b/newrelic_lambda_cli/cli/layers.py
index 9807f0e..7a8ab3f 100644
--- a/newrelic_lambda_cli/cli/layers.py
+++ b/newrelic_lambda_cli/cli/layers.py
@@ -63,6 +63,12 @@ def register(group):
help="Permit upgrade of function layers to new version.",
is_flag=True,
)
[email protected](
+ "--enable-extension",
+ "-x",
+ help="Enable the New Relic Lambda Extension",
+ is_flag=True,
+)
@click.pass_context
def install(
ctx,
@@ -74,6 +80,7 @@ def install(
excludes,
layer_arn,
upgrade,
+ enable_extension,
):
"""Install New Relic AWS Lambda Layers"""
session = boto3.Session(profile_name=aws_profile, region_name=aws_region)
@@ -92,6 +99,7 @@ def install(
layer_arn,
nr_account_id,
upgrade,
+ enable_extension,
ctx.obj["VERBOSE"],
)
for function in functions
diff --git a/newrelic_lambda_cli/layers.py b/newrelic_lambda_cli/layers.py
index ce3d47a..55c2d1f 100644
--- a/newrelic_lambda_cli/layers.py
+++ b/newrelic_lambda_cli/layers.py
@@ -19,7 +19,9 @@ def index(region, runtime):
return layers_response.get("Layers", [])
-def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
+def _add_new_relic(
+ config, region, layer_arn, account_id, allow_upgrade, enable_extension
+):
runtime = config["Configuration"]["Runtime"]
if runtime not in utils.RUNTIME_CONFIG:
failure(
@@ -30,13 +32,20 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
handler = config["Configuration"]["Handler"]
runtime_handler = utils.RUNTIME_CONFIG.get(runtime, {}).get("Handler")
- if not allow_upgrade and handler == runtime_handler:
+
+ existing_newrelic_layer = [
+ layer["Arn"]
+ for layer in config["Configuration"].get("Layers", [])
+ if layer["Arn"].startswith(utils.get_arn_prefix(region))
+ ]
+
+ if not allow_upgrade and existing_newrelic_layer:
success(
"Already installed on function '%s'. Pass --upgrade (or -u) to allow "
"upgrade or reinstall to latest layer version."
% config["Configuration"]["FunctionArn"]
)
- return False
+ return True
existing_layers = [
layer["Arn"]
@@ -45,6 +54,7 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
]
new_relic_layers = []
+
if layer_arn:
new_relic_layers = [layer_arn]
else:
@@ -80,7 +90,6 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
update_kwargs = {
"FunctionName": config["Configuration"]["FunctionArn"],
- "Handler": runtime_handler,
"Environment": {
"Variables": config["Configuration"]
.get("Environment", {})
@@ -89,17 +98,34 @@ def _add_new_relic(config, region, layer_arn, account_id, allow_upgrade):
"Layers": new_relic_layers + existing_layers,
}
+ # Only used by Python and Node.js runtimes
+ if runtime_handler:
+ update_kwargs["Handler"] = runtime_handler
+
# Update the account id
update_kwargs["Environment"]["Variables"]["NEW_RELIC_ACCOUNT_ID"] = str(account_id)
# Update the NEW_RELIC_LAMBDA_HANDLER envvars only when it's a new install.
- if handler != runtime_handler:
+ if runtime_handler and handler != runtime_handler:
update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_HANDLER"] = handler
+ if enable_extension:
+ update_kwargs["Environment"]["Variables"][
+ "NEW_RELIC_LAMBDA_EXTENSION_ENABLED"
+ ] = "true"
+
return update_kwargs
-def install(session, function_arn, layer_arn, account_id, allow_upgrade, verbose):
+def install(
+ session,
+ function_arn,
+ layer_arn,
+ account_id,
+ allow_upgrade,
+ enable_extension,
+ verbose,
+):
client = session.client("lambda")
config = get_function(session, function_arn)
if not config:
@@ -108,7 +134,9 @@ def install(session, function_arn, layer_arn, account_id, allow_upgrade, verbose
region = session.region_name
- update_kwargs = _add_new_relic(config, region, layer_arn, account_id, allow_upgrade)
+ update_kwargs = _add_new_relic(
+ config, region, layer_arn, account_id, allow_upgrade, enable_extension
+ )
if not update_kwargs:
return False
diff --git a/newrelic_lambda_cli/utils.py b/newrelic_lambda_cli/utils.py
index 547e915..4b9977c 100644
--- a/newrelic_lambda_cli/utils.py
+++ b/newrelic_lambda_cli/utils.py
@@ -8,8 +8,13 @@ from click.exceptions import Exit
NEW_RELIC_ARN_PREFIX_TEMPLATE = "arn:aws:lambda:%s:451483290750"
RUNTIME_CONFIG = {
+ "dotnetcore3.1": {},
+ "java11": {},
+ "java8.al2": {},
"nodejs10.x": {"Handler": "newrelic-lambda-wrapper.handler"},
"nodejs12.x": {"Handler": "newrelic-lambda-wrapper.handler"},
+ "provided": {},
+ "provided.al2": {},
"python2.7": {"Handler": "newrelic_lambda_wrapper.handler"},
"python3.6": {"Handler": "newrelic_lambda_wrapper.handler"},
"python3.7": {"Handler": "newrelic_lambda_wrapper.handler"},
| newrelic/newrelic-lambda-cli | 8e5399c6072a052c0df445ccb06559f5e2df5b3d | diff --git a/tests/test_layers.py b/tests/test_layers.py
index 971702f..90ee31f 100644
--- a/tests/test_layers.py
+++ b/tests/test_layers.py
@@ -5,7 +5,7 @@ def test_add_new_relic(mock_function_config):
config = mock_function_config("python3.7")
assert config["Configuration"]["Handler"] == "original_handler"
- update_kwargs = _add_new_relic(config, "us-east-1", None, "12345", False)
+ update_kwargs = _add_new_relic(config, "us-east-1", None, "12345", False, True)
assert update_kwargs["FunctionName"] == config["Configuration"]["FunctionArn"]
assert update_kwargs["Handler"] == "newrelic_lambda_wrapper.handler"
assert update_kwargs["Environment"]["Variables"]["NEW_RELIC_ACCOUNT_ID"] == "12345"
@@ -13,6 +13,10 @@ def test_add_new_relic(mock_function_config):
update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_HANDLER"]
== config["Configuration"]["Handler"]
)
+ assert (
+ update_kwargs["Environment"]["Variables"]["NEW_RELIC_LAMBDA_EXTENSION_ENABLED"]
+ == "true"
+ )
def test_remove_new_relic(mock_function_config):
| [layers install] Support installing layer for .NET Core and Java runtime.
[NOTE]: # ( ^^ Provide a general summary of the request in the title above. ^^ )
## Summary
Now New Relic Lambda extension can support .NET Core, Java and Go in addition to node.js and Python.
https://github.com/newrelic/newrelic-lambda-extension/tree/main/examples
It's good to support these runtimes.
[NOTE]: # ( Provide a brief overview of what the new feature is all about. )
## Desired Behaviour
When the runtime is specified as one of `dotnetcore3.1`, `java8.al2`, `java11`, layers install command will add `NewRelicLambdaExtension` layer.
The layer endpoint returns an available layer.
https://ap-southeast-1.layers.newrelic-external.com/get-layers?CompatibleRuntime=dotnetcore3.1
It seems when code is written in Go, we need another approach since we should use `provided` runtime.
[NOTE]: # ( Tell us how the new feature should work. Be specific. )
[TIP]: # ( Do NOT give us access or passwords to your New Relic account or API keys! )
## Possible Solution
[NOTE]: # ( Not required. Suggest how to implement the addition or change. )
How about adding the following key-value pairs to the [RUNTIME_CONFIG](https://github.com/newrelic/newrelic-lambda-cli/blob/v0.2.6/newrelic_lambda_cli/utils.py#L10-L17)
```
"dotnetcore3.1": {"Handler": "NewRelicExampleDotnet::NewRelicExampleDotnet.Function::FunctionHandler"},
"java8.al2": {"Handler": "com.newrelic.lambda.example.App::handleRequest"},
"java11": {"Handler": "com.newrelic.lambda.example.App::handleRequest"},
```
## Additional context
[TIP]: # ( Why does this feature matter to you? What unique circumstances do you have? )
| 0.0 | [
"tests/test_layers.py::test_add_new_relic"
] | [
"tests/test_layers.py::test_remove_new_relic"
] | 2020-10-16 14:18:04+00:00 | 4,158 |
|
nficano__humps-194 | diff --git a/humps/main.py b/humps/main.py
index 9c58ac8..5ea96e7 100644
--- a/humps/main.py
+++ b/humps/main.py
@@ -21,8 +21,8 @@ if is_py3: # pragma: no cover
ACRONYM_RE = re.compile(r"([A-Z]+)$|([A-Z]+)(?=[A-Z0-9])")
PASCAL_RE = re.compile(r"([^\-_\s]+)")
-SPLIT_RE = re.compile(r"([\-_\s]*[A-Z]+[^A-Z\-_\s]+[\-_\s]*)")
-UNDERSCORE_RE = re.compile(r"(?<=[^\-_\s])[\-_\s]+([^\-_\s])")
+SPLIT_RE = re.compile(r"([\-_\s]*[A-Z]+[^A-Z\-_\s]*[\-_\s]*)")
+UNDERSCORE_RE = re.compile(r"([^\-_\s])[\-_\s]+([^\-_\s])")
def pascalize(str_or_iter):
| nficano/humps | ee698d9d2154518cdb36dd5d69b9fc41145902a9 | diff --git a/tests/test_decamelize.py b/tests/test_decamelize.py
index 2d0e128..7d2092a 100644
--- a/tests/test_decamelize.py
+++ b/tests/test_decamelize.py
@@ -27,6 +27,10 @@ import humps
("_itemID", "_item_id"),
# Fixed issue #4. 2021-05-01
("memMB", "mem_mb"),
+ # Fixed issue #127. 2021-09-13
+ ("sizeX", "size_x"),
+ # Fixed issue #168. 2021-09-13
+ ("aB", "a_b"),
],
)
def test_decamelize(input_str, expected_output):
diff --git a/tests/test_separate_words.py b/tests/test_separate_words.py
index e8a8ec0..ca9aa16 100644
--- a/tests/test_separate_words.py
+++ b/tests/test_separate_words.py
@@ -30,6 +30,10 @@ from humps.main import separate_words
# Fixes issue #128
("whatever_hi", "whatever_hi"),
("whatever_10", "whatever_10"),
+ # Fixes issue #127
+ ("sizeX", "size_X"),
+ # Fixes issue #168
+ ("aB", "a_B"),
],
)
def test_separate_words(input_str, expected_output):
| sizeX decamelized as sizex
**Describe the bug**
String 'sizeX' is correctly identified as camelCase but is converted to snake_case as 'sizex'.
**To Reproduce**
```python
>>> humps.is_camelcase("sizeX")
True
>>> humps.decamelize("sizeX")
'sizex'
>>> humps.decamelize("camelCaseTest")
'camel_case_test'
```
**Expected behavior**
```python
>>> humps.decamelize("sizeX")
'size_x'
```
**Desktop (please complete the following information):**
- OS: Ubuntu 18.04, Python 3.8
- Version 1.6.1 | 0.0 | [
"tests/test_decamelize.py::test_decamelize[sizeX-size_x]",
"tests/test_decamelize.py::test_decamelize[aB-a_b]",
"tests/test_separate_words.py::test_separate_words[sizeX-size_X]",
"tests/test_separate_words.py::test_separate_words[aB-a_B]"
] | [
"tests/test_decamelize.py::test_decamelize[symbol-symbol]",
"tests/test_decamelize.py::test_decamelize[lastPrice-last_price]",
"tests/test_decamelize.py::test_decamelize[changePct-change_pct]",
"tests/test_decamelize.py::test_decamelize[impliedVolatility-implied_volatility]",
"tests/test_decamelize.py::test_decamelize[_symbol-_symbol]",
"tests/test_decamelize.py::test_decamelize[changePct_-change_pct_]",
"tests/test_decamelize.py::test_decamelize[_lastPrice__-_last_price__]",
"tests/test_decamelize.py::test_decamelize[__impliedVolatility_-__implied_volatility_]",
"tests/test_decamelize.py::test_decamelize[API-API]",
"tests/test_decamelize.py::test_decamelize[_API_-_API_]",
"tests/test_decamelize.py::test_decamelize[__API__-__API__]",
"tests/test_decamelize.py::test_decamelize[APIResponse-api_response]",
"tests/test_decamelize.py::test_decamelize[_APIResponse_-_api_response_]",
"tests/test_decamelize.py::test_decamelize[__APIResponse__-__api_response__]",
"tests/test_decamelize.py::test_decamelize[_itemID-_item_id]",
"tests/test_decamelize.py::test_decamelize[memMB-mem_mb]",
"tests/test_decamelize.py::test_decamelize_dict_list",
"tests/test_separate_words.py::test_separate_words[HelloWorld-Hello_World]",
"tests/test_separate_words.py::test_separate_words[_HelloWorld-_Hello_World]",
"tests/test_separate_words.py::test_separate_words[__HelloWorld-__Hello_World]",
"tests/test_separate_words.py::test_separate_words[HelloWorld_-Hello_World_]",
"tests/test_separate_words.py::test_separate_words[HelloWorld__-Hello_World__]",
"tests/test_separate_words.py::test_separate_words[helloWorld-hello_World]",
"tests/test_separate_words.py::test_separate_words[_helloWorld-_hello_World]",
"tests/test_separate_words.py::test_separate_words[__helloWorld-__hello_World]",
"tests/test_separate_words.py::test_separate_words[helloWorld_-hello_World_]",
"tests/test_separate_words.py::test_separate_words[helloWorld__-hello_World__]",
"tests/test_separate_words.py::test_separate_words[hello_world-hello_world]",
"tests/test_separate_words.py::test_separate_words[_hello_world-_hello_world]",
"tests/test_separate_words.py::test_separate_words[__hello_world-__hello_world]",
"tests/test_separate_words.py::test_separate_words[hello_world_-hello_world_]",
"tests/test_separate_words.py::test_separate_words[hello_world__-hello_world__]",
"tests/test_separate_words.py::test_separate_words[whatever_hi-whatever_hi]",
"tests/test_separate_words.py::test_separate_words[whatever_10-whatever_10]"
] | 2021-09-13 19:22:01+00:00 | 4,159 |
|
nginxinc__crossplane-23 | diff --git a/crossplane/analyzer.py b/crossplane/analyzer.py
index f5a5ffb..ec89dab 100644
--- a/crossplane/analyzer.py
+++ b/crossplane/analyzer.py
@@ -1920,7 +1920,7 @@ def analyze(fname, stmt, term, ctx=()):
reason = '"%s" directive is not allowed here' % directive
raise NgxParserDirectiveContextError(reason, fname, line)
- valid_flag = lambda x: x in ('on', 'off')
+ valid_flag = lambda x: x.lower() in ('on', 'off')
# do this in reverse because we only throw errors at the end if no masks
# are valid, and typically the first bit mask is what the parser expects
@@ -1942,6 +1942,8 @@ def analyze(fname, stmt, term, ctx=()):
(mask & NGX_CONF_1MORE and n_args >= 1) or
(mask & NGX_CONF_2MORE and n_args >= 2)):
return
+ elif mask & NGX_CONF_FLAG and n_args == 1 and not valid_flag(args[0]):
+ reason = 'invalid value "%s" in "%%s" directive, it must be "on" or "off"' % args[0]
else:
reason = 'invalid number of arguments in "%s" directive'
| nginxinc/crossplane | 8709d938119f967ce938dd5163b233ce5439d30d | diff --git a/tests/test_analyze.py b/tests/test_analyze.py
index ccb4091..8c36815 100644
--- a/tests/test_analyze.py
+++ b/tests/test_analyze.py
@@ -31,3 +31,30 @@ def test_state_directive():
raise Exception("bad context for 'state' passed: " + repr(ctx))
except crossplane.errors.NgxParserDirectiveContextError:
continue
+
+
+def test_flag_directive_args():
+ fname = '/path/to/nginx.conf'
+ ctx = ('events',)
+
+ # an NGINX_CONF_FLAG directive
+ stmt = {
+ 'directive': 'accept_mutex',
+ 'line': 2 # this is arbitrary
+ }
+
+ good_args = [['on'], ['off'], ['On'], ['Off'], ['ON'], ['OFF']]
+
+ for args in good_args:
+ stmt['args'] = args
+ crossplane.analyzer.analyze(fname, stmt, term=';', ctx=ctx)
+
+ bad_args = [['1'], ['0'], ['true'], ['okay'], ['']]
+
+ for args in bad_args:
+ stmt['args'] = args
+ try:
+ crossplane.analyzer.analyze(fname, stmt, term=';', ctx=ctx)
+ raise Exception('bad args for flag directive: ' + repr(args))
+ except crossplane.errors.NgxParserDirectiveArgumentsError as e:
+ assert e.strerror.endswith('it must be "on" or "off"')
| NGINX_CONF_FLAG directives should support uppercase ON or OFF as args
`crossplane parse` throws an error for this config but `nginx` does not:
```nginx
events {
accept_mutex OFF;
}
``` | 0.0 | [
"tests/test_analyze.py::test_flag_directive_args"
] | [
"tests/test_analyze.py::test_state_directive"
] | 2018-01-18 01:31:29+00:00 | 4,160 |
|
nhoad__flake8-unused-arguments-25 | diff --git a/README.md b/README.md
index dd58c30..5f892ea 100644
--- a/README.md
+++ b/README.md
@@ -10,6 +10,7 @@ This package adds the following warnings:
Configuration options also exist:
- `unused-arguments-ignore-abstract-functions` - don't show warnings for abstract functions.
- `unused-arguments-ignore-overload-functions` - don't show warnings for overload functions.
+ - `unused-arguments-ignore-override-functions` - don't show warnings for overridden functions.
- `unused-arguments-ignore-stub-functions` - don't show warnings for empty functions.
- `unused-arguments-ignore-variadic-names` - don't show warnings for unused *args and **kwargs.
- `unused-arguments-ignore-lambdas` - don't show warnings for all lambdas.
diff --git a/flake8_unused_arguments.py b/flake8_unused_arguments.py
index 88074c7..518bce1 100644
--- a/flake8_unused_arguments.py
+++ b/flake8_unused_arguments.py
@@ -16,6 +16,7 @@ class Plugin:
ignore_abstract = False
ignore_overload = False
+ ignore_override = False
ignore_stubs = False
ignore_variadic_names = False
ignore_lambdas = False
@@ -45,6 +46,15 @@ class Plugin:
help="If provided, then unused arguments for functions decorated with overload will be ignored.",
)
+ option_manager.add_option(
+ "--unused-arguments-ignore-override-functions",
+ action="store_true",
+ parse_from_config=True,
+ default=cls.ignore_override,
+ dest="unused_arguments_ignore_override_functions",
+ help="If provided, then unused arguments for functions decorated with override will be ignored.",
+ )
+
option_manager.add_option(
"--unused-arguments-ignore-stub-functions",
action="store_true",
@@ -100,6 +110,7 @@ class Plugin:
def parse_options(cls, options: optparse.Values) -> None:
cls.ignore_abstract = options.unused_arguments_ignore_abstract_functions
cls.ignore_overload = options.unused_arguments_ignore_overload_functions
+ cls.ignore_override = options.unused_arguments_ignore_override_functions
cls.ignore_stubs = options.unused_arguments_ignore_stub_functions
cls.ignore_variadic_names = options.unused_arguments_ignore_variadic_names
cls.ignore_lambdas = options.unused_arguments_ignore_lambdas
@@ -117,6 +128,10 @@ class Plugin:
if self.ignore_overload and "overload" in decorator_names:
continue
+ # ignore overridden functions
+ if self.ignore_override and "override" in decorator_names:
+ continue
+
# ignore abstractmethods, it's not a surprise when they're empty
if self.ignore_abstract and "abstractmethod" in decorator_names:
continue
| nhoad/flake8-unused-arguments | 8f713ff998dfe78a5a1b468559d8e28fd5e86346 | diff --git a/test_unused_arguments.py b/test_unused_arguments.py
index 9fa6446..caf5d62 100644
--- a/test_unused_arguments.py
+++ b/test_unused_arguments.py
@@ -196,6 +196,24 @@ def test_is_stub_function(function, expected_result):
),
(
"""
+ @override
+ def foo(a):
+ pass
+ """,
+ {"ignore_override": False},
+ [(3, 8, "U100 Unused argument 'a'", "unused argument")],
+ ),
+ (
+ """
+ @override
+ def foo(a):
+ pass
+ """,
+ {"ignore_override": True},
+ [],
+ ),
+ (
+ """
def foo(a):
pass
""",
| (Feature) Ignore overridden methods in subclasses
It would be great if there were a way to ignore inherited arguments in overridden methods in a subclass. For example, suppose we have the following code:
```py
class BaseClass:
def execute(self, context):
...
class MyClass(BaseClass):
def execute(self, context):
# Implementation doesn't use `context`
```
`BaseClass` is defined in a third-party library and I have no control over it. Currently, `context` is invoked by the third-party code as a keyword argument, which means that I can't name the argument `_context` because it'd trigger an exception.
Of course, I can use `noqa: U100` on that line to suppress the error. It's tedious if you have a lot of these, but it's not a blocker. However, there's one case where we don't want to do that, when we add arguments in a compatible way:
```py
class MyClass2(BaseClass):
def execute(self, context, some_flag=False):
# Implementation doesn't use `context`
...
```
Here, we don't care if the implementation doesn't use arguments defined in the superclass because we need to preserve the signature. _However_, we should still care about arguments that _we_ define that aren't being used.
I'll be the first to admit this is non-trivial. The first implementation I can think of off the top of my head would require you to traverse the MRO and find the method in its parents, then compare call signatures. Proper caching will mitigate the performance impact on large codebases, but it'll still be a pain. | 0.0 | [
"test_unused_arguments.py::test_integration[\\n"
] | [
"test_unused_arguments.py::test_get_argument_names[def",
"test_unused_arguments.py::test_get_argument_names[async",
"test_unused_arguments.py::test_get_argument_names[\\n",
"test_unused_arguments.py::test_get_argument_names[l",
"test_unused_arguments.py::test_get_unused_arguments[def",
"test_unused_arguments.py::test_get_unused_arguments[\\n",
"test_unused_arguments.py::test_get_unused_arguments[l",
"test_unused_arguments.py::test_get_decorator_names[\\n",
"test_unused_arguments.py::test_get_decorator_names[lambda",
"test_unused_arguments.py::test_is_stub_function[def",
"test_unused_arguments.py::test_is_stub_function[lambda:",
"test_unused_arguments.py::test_is_stub_function[\\n",
"test_unused_arguments.py::test_integration[foo",
"test_unused_arguments.py::test_check_version",
"test_unused_arguments.py::test_function_finder[False-expected0]",
"test_unused_arguments.py::test_function_finder[True-expected1]",
"test_unused_arguments.py::test_is_dunder_method[def",
"test_unused_arguments.py::test_is_dunder_method[async",
"test_unused_arguments.py::test_is_dunder_method[lambda:"
] | 2023-02-02 15:40:33+00:00 | 4,161 |
|
nickstenning__honcho-171 | diff --git a/honcho/export/templates/upstart/process.conf b/honcho/export/templates/upstart/process.conf
index 8a05378..fe6b451 100644
--- a/honcho/export/templates/upstart/process.conf
+++ b/honcho/export/templates/upstart/process.conf
@@ -2,8 +2,7 @@ start on starting {{ group_name }}
stop on stopping {{ group_name }}
respawn
-exec su - {{ user }} -s {{ shell }} -c 'cd {{ app_root }};
-{%- for k, v in process.env.items() -%}
- export {{ k }}={{ v | shellquote }};
-{%- endfor -%}
-exec {{ process.cmd }} >> {{ log }}/{{ process.name|dashrepl }}.log 2>&1'
+{% for k, v in process.env.items() -%}
+env {{ k }}={{ v | shellquote }}
+{% endfor %}
+exec su - {{ user }} -m -s {{ shell }} -c 'cd {{ app_root }}; exec {{ process.cmd }} >> {{ log }}/{{ process.name|dashrepl }}.log 2>&1'
diff --git a/honcho/manager.py b/honcho/manager.py
index 218f2b4..ff31a8e 100644
--- a/honcho/manager.py
+++ b/honcho/manager.py
@@ -53,7 +53,7 @@ class Manager(object):
self._terminating = False
- def add_process(self, name, cmd, quiet=False, env=None):
+ def add_process(self, name, cmd, quiet=False, env=None, cwd=None):
"""
Add a process to this manager instance. The process will not be started
until #loop() is called.
@@ -63,7 +63,8 @@ class Manager(object):
name=name,
quiet=quiet,
colour=next(self._colours),
- env=env)
+ env=env,
+ cwd=cwd)
self._processes[name] = {}
self._processes[name]['obj'] = proc
diff --git a/honcho/process.py b/honcho/process.py
index 669c8ef..c211af1 100644
--- a/honcho/process.py
+++ b/honcho/process.py
@@ -21,12 +21,14 @@ class Process(object):
name=None,
colour=None,
quiet=False,
- env=None):
+ env=None,
+ cwd=None):
self.cmd = cmd
self.colour = colour
self.quiet = quiet
self.name = name
self.env = os.environ.copy() if env is None else env
+ self.cwd = cwd
# This is a honcho.environ.Env object, to allow for stubbing of
# external calls, not the operating system environment.
@@ -36,7 +38,7 @@ class Process(object):
def run(self, events=None, ignore_signals=False):
self._events = events
- self._child = self._child_ctor(self.cmd, env=self.env)
+ self._child = self._child_ctor(self.cmd, env=self.env, cwd=self.cwd)
self._send_message({'pid': self._child.pid}, type='start')
# Don't pay attention to SIGINT/SIGTERM. The process itself is
| nickstenning/honcho | 824775779ddf30606e7514b4639e81a2d6f25393 | diff --git a/tests/integration/test_export.py b/tests/integration/test_export.py
index bbb2397..09543b7 100644
--- a/tests/integration/test_export.py
+++ b/tests/integration/test_export.py
@@ -37,3 +37,37 @@ def test_export_upstart(testenv):
'trunk-web-1.conf'):
expected = testenv.path('elephant', filename)
assert os.path.exists(expected)
+
+
[email protected]('testenv', [{
+ 'Procfile': "web: python web.py",
+ '.env': """
+NORMAL=ok
+SQ_SPACES='sqspace sqspace'
+DQ_SPACES="dqspace dqspace"
+SQ="it's got single quotes"
+DQ='it has "double" quotes'
+EXCL='an exclamation mark!'
+SQ_DOLLAR='costs $UNINTERPOLATED amount'
+DQ_DOLLAR="costs $UNINTERPOLATED amount"
+"""
+}], indirect=True)
+def test_export_upstart_environment(testenv):
+ ret, out, err = testenv.run_honcho([
+ 'export',
+ 'upstart',
+ testenv.path('test'),
+ '-a', 'envvars',
+ ])
+
+ assert ret == 0
+
+ lines = open(testenv.path('test', 'envvars-web-1.conf')).readlines()
+ assert 'env NORMAL=ok\n' in lines
+ assert "env SQ_SPACES='sqspace sqspace'\n" in lines
+ assert "env DQ_SPACES='dqspace dqspace'\n" in lines
+ assert "env SQ='it'\"'\"'s got single quotes'\n" in lines
+ assert "env DQ='it has \"double\" quotes'\n" in lines
+ assert "env EXCL='an exclamation mark!'\n" in lines
+ assert "env SQ_DOLLAR='costs $UNINTERPOLATED amount'\n" in lines
+ assert "env DQ_DOLLAR='costs $UNINTERPOLATED amount'\n" in lines
diff --git a/tests/test_manager.py b/tests/test_manager.py
index abee53c..ae028c6 100644
--- a/tests/test_manager.py
+++ b/tests/test_manager.py
@@ -59,12 +59,13 @@ class FakeEnv(object):
class FakeProcess(object):
- def __init__(self, cmd, name=None, colour=None, quiet=None, env=None):
+ def __init__(self, cmd, name=None, colour=None, quiet=None, env=None, cwd=None):
self.cmd = cmd
self.name = name
self.colour = colour
self.quiet = quiet
self.env = env
+ self.cwd = cwd
self._events = None
self._options = {}
@@ -229,6 +230,10 @@ class TestManager(object):
with pytest.raises(AssertionError):
self.m.add_process('foo', 'another command')
+ def test_add_process_sets_cwd(self):
+ proc = self.m.add_process('foo', 'ruby server.rb', cwd='foo-dir')
+ assert proc.cwd == 'foo-dir'
+
def test_loop_with_empty_manager_returns_immediately(self):
self.m.loop()
diff --git a/tests/test_process.py b/tests/test_process.py
index 59e1538..9002212 100644
--- a/tests/test_process.py
+++ b/tests/test_process.py
@@ -184,3 +184,9 @@ class TestProcess(object):
proc.run(self.q)
msg = self.q.find_message({'returncode': 42})
assert msg.type == 'stop'
+
+ def test_cwd_passed_along(self):
+ proc = Process('echo 123', cwd='fake-dir')
+ proc._child_ctor = FakePopen
+ proc.run(self.q)
+ assert proc._child.kwargs['cwd'] == 'fake-dir'
| Exported upstart configuration silently fails with quoted variables
At or near d8be8f4a8 (version 0.5.0), quoted variables in exported upstart scripts became invalid and fail silently. Previously, quoted `.env` variables with spaces generated upstart configurations with correctly-nested double and single quotes. This is an example generated by 84a1f7d (also version 0.5.0):
```
VAR="foo bar"
```
```
start on starting things-stuff
stop on stopping things-stuff
respawn
exec su - migurski -s /bin/sh -c 'cd /home/migurski/things; export PORT=5000; export VAR="foo bar"; python stuff.py >> /var/log/things/stuff-1.log 2>&1'
```
Starting at d8be8f4a8 and still in 0.6.6, the exported configuration from the configuration above began producing this invalid and failing upstart configuration, due to the single quotes:
```
start on starting things-stuff
stop on stopping things-stuff
respawn
exec su - migurski -s /bin/sh -c 'cd /home/migurski/things;export VAR='foo bar';export PORT=5000;python stuff.py >> /var/log/things/stuff-1.log 2>&1'
```
Here are my Procfile and python script for testing:
```
stuff: python stuff.py
```
```python
from sys import stderr
from os import environ
from time import sleep
while True:
print >> stderr, repr(environ['VAR'])
sleep(5)
``` | 0.0 | [
"tests/test_manager.py::TestManager::test_add_process_sets_cwd",
"tests/test_process.py::TestProcess::test_cwd_passed_along"
] | [
"tests/test_manager.py::TestManager::test_init_sets_default_printer_width",
"tests/test_manager.py::TestManager::test_add_process_updates_printer_width",
"tests/test_manager.py::TestManager::test_add_process_sets_name",
"tests/test_manager.py::TestManager::test_add_process_sets_cmd",
"tests/test_manager.py::TestManager::test_add_process_sets_colour",
"tests/test_manager.py::TestManager::test_add_process_sets_unique_colours",
"tests/test_manager.py::TestManager::test_add_process_sets_quiet",
"tests/test_manager.py::TestManager::test_add_process_name_must_be_unique",
"tests/test_manager.py::TestManager::test_loop_with_empty_manager_returns_immediately",
"tests/test_manager.py::TestManager::test_loop_calls_process_run",
"tests/test_manager.py::TestManager::test_printer_receives_messages_in_correct_order",
"tests/test_manager.py::TestManager::test_printer_receives_lines_multi_process",
"tests/test_manager.py::TestManager::test_returncode_set_by_first_exiting_process",
"tests/test_manager.py::TestManager::test_printer_receives_lines_after_stop",
"tests/test_process.py::TestProcess::test_ctor_cmd",
"tests/test_process.py::TestProcess::test_ctor_name",
"tests/test_process.py::TestProcess::test_ctor_colour",
"tests/test_process.py::TestProcess::test_ctor_quiet",
"tests/test_process.py::TestProcess::test_output_receives_start_with_pid",
"tests/test_process.py::TestProcess::test_message_contains_name",
"tests/test_process.py::TestProcess::test_message_contains_time",
"tests/test_process.py::TestProcess::test_message_contains_colour",
"tests/test_process.py::TestProcess::test_output_receives_lines",
"tests/test_process.py::TestProcess::test_output_receives_lines_invalid_utf8",
"tests/test_process.py::TestProcess::test_output_does_not_receive_lines_when_quiet",
"tests/test_process.py::TestProcess::test_output_receives_stop",
"tests/test_process.py::TestProcess::test_output_receives_stop_with_returncode"
] | 2016-03-28 14:30:24+00:00 | 4,162 |
|
nickstenning__honcho-174 | diff --git a/honcho/command.py b/honcho/command.py
index 3796a39..9a7323d 100644
--- a/honcho/command.py
+++ b/honcho/command.py
@@ -39,6 +39,7 @@ def _add_common_args(parser, with_defaults=False):
help='procfile directory (default: .)')
parser.add_argument('-f', '--procfile',
metavar='FILE',
+ default=suppress,
help='procfile path (default: Procfile)')
parser.add_argument('-v', '--version',
action='version',
| nickstenning/honcho | 5af4cf1d98926fa63eae711e6a89f8e2ef5d8539 | diff --git a/tests/integration/test_start.py b/tests/integration/test_start.py
index 2388bda..73c8693 100644
--- a/tests/integration/test_start.py
+++ b/tests/integration/test_start.py
@@ -53,6 +53,39 @@ def test_start_env_procfile(testenv):
assert 'mongoose' in out
[email protected]('testenv', [{
+ 'Procfile': 'foo: {0} test.py'.format(python_bin),
+ 'Procfile.dev': 'bar: {0} test_dev.py'.format(python_bin),
+ 'test.py': script,
+ 'test_dev.py': textwrap.dedent("""
+ from __future__ import print_function
+ print("mongoose")
+ """)
+}], indirect=True)
+def test_start_procfile_after_command(testenv):
+ # Regression test for #173: Ensure that -f argument can be provided after
+ # command
+ ret, out, err = testenv.run_honcho(['start', '-f', 'Procfile.dev'])
+
+ assert 'mongoose' in out
+
+
[email protected]('testenv', [{
+ 'Procfile': 'foo: {0} test.py'.format(python_bin),
+ 'Procfile.dev': 'bar: {0} test_dev.py'.format(python_bin),
+ 'test.py': script,
+ 'test_dev.py': textwrap.dedent("""
+ from __future__ import print_function
+ print("mongoose")
+ """)
+}], indirect=True)
+def test_start_procfile_before_command(testenv):
+ # Test case for #173: Ensure that -f argument can be provided before command
+ ret, out, err = testenv.run_honcho(['-f', 'Procfile.dev', 'start'])
+
+ assert 'mongoose' in out
+
+
@pytest.mark.parametrize('testenv', [{
'Procfile': 'foo: {0} test.py'.format(python_bin),
'test.py': 'import sys; sys.exit(42)',
| -f argument before command is suppressed
System information: Mac OS X, 10.11.1
Honcho version: 0.7.0
With the latest release of honcho (0.7.0), I've noticed that the -f argument is processed properly **after** a command, but not **before** a command. -e and -d arguments don't exhibit this order-dependent behavior.
Examples:
```
$ honcho -f my_procfile check
2016-04-12 23:49:22 [45546] [ERROR] Procfile does not exist or is not a file
$ honcho check -f my_procfile
2016-04-12 23:49:24 [45548] [INFO] Valid procfile detected (postgres, rabbit, redis, flower)
``` | 0.0 | [
"tests/integration/test_start.py::test_start_procfile_before_command[testenv0]"
] | [
"tests/integration/test_start.py::test_start[testenv0]",
"tests/integration/test_start.py::test_start_env[testenv0]",
"tests/integration/test_start.py::test_start_env_procfile[testenv0]",
"tests/integration/test_start.py::test_start_procfile_after_command[testenv0]",
"tests/integration/test_start.py::test_start_returncode[testenv0]"
] | 2016-04-13 06:21:38+00:00 | 4,163 |
|
nickstenning__honcho-218 | diff --git a/doc/using_procfiles.rst b/doc/using_procfiles.rst
index 02cd95b..d11dc91 100644
--- a/doc/using_procfiles.rst
+++ b/doc/using_procfiles.rst
@@ -18,10 +18,10 @@ source tree that contains zero or more lines of the form::
<process type>: <command>
-The ``process type`` is a string which may contain alphanumerics and underscores
-(``[A-Za-z0-9_]+``), and uniquely identifies one type of process which can be
-run to form your application. For example: ``web``, ``worker``, or
-``my_process_123``.
+The ``process type`` is a string which may contain alphanumerics as well as
+underscores and dashes (``[A-Za-z0-9_-]+``), and uniquely identifies one type
+of process which can be run to form your application. For example: ``web``,
+``worker``, or ``my_process_123``.
``command`` is a shell commandline which will be executed to spawn a process of
the specified type.
diff --git a/honcho/environ.py b/honcho/environ.py
index 646d950..09448f3 100644
--- a/honcho/environ.py
+++ b/honcho/environ.py
@@ -13,7 +13,7 @@ if compat.ON_WINDOWS:
import ctypes
-PROCFILE_LINE = re.compile(r'^([A-Za-z0-9_]+):\s*(.+)$')
+PROCFILE_LINE = re.compile(r'^([A-Za-z0-9_-]+):\s*(.+)$')
class Env(object):
| nickstenning/honcho | 5abd2a2864cf4f46a10c3cfc4f4dfe55144cbd5b | diff --git a/tests/test_environ.py b/tests/test_environ.py
index c7260a4..a9720d7 100644
--- a/tests/test_environ.py
+++ b/tests/test_environ.py
@@ -155,10 +155,17 @@ def test_environ_parse(content, commands):
[
# Invalid characters
"""
- -foo: command
+ +foo: command
""",
{}
],
+ [
+ # Valid -/_ characters
+ """
+ -foo_bar: command
+ """,
+ {'-foo_bar': 'command'}
+ ],
[
# Shell metacharacters
"""
| Dashes in process types
I believe that process types, according to this [Heroku page](https://devcenter.heroku.com/articles/procfile), must be alphanumeric.
If I create a Procfile with the following content
```
process-type-with-dashes: python -c 'print("hello")'
```
and then run `honcho start`, honcho fails silently.
Also, when I run `honcho check`, it says it is a valid Procfile.
Perhaps it would be helpful if:
- `honcho start` would complain if there is an invalid Procfile
- `honcho check` complains if the process types have dashes
I am using honcho v1.0.1 with python 3.7.0 on macOS.
Thank you for all of your work, I wish I had time to create a PR for this, but I figured creating an issue was better than nothing. | 0.0 | [
"tests/test_environ.py::test_parse_procfile[\\n"
] | [
"tests/test_environ.py::test_environ_parse[\\n",
"tests/test_environ.py::test_parse_procfile_ordered",
"tests/test_environ.py::TestProcfile::test_has_no_processes_after_init",
"tests/test_environ.py::TestProcfile::test_add_process",
"tests/test_environ.py::TestProcfile::test_add_process_ensures_unique_name",
"tests/test_environ.py::test_expand_processes_name",
"tests/test_environ.py::test_expand_processes_name_multiple",
"tests/test_environ.py::test_expand_processes_name_concurrency",
"tests/test_environ.py::test_expand_processes_name_concurrency_multiple",
"tests/test_environ.py::test_expand_processes_command",
"tests/test_environ.py::test_expand_processes_port_not_defaulted",
"tests/test_environ.py::test_expand_processes_port",
"tests/test_environ.py::test_expand_processes_port_multiple",
"tests/test_environ.py::test_expand_processes_port_from_env",
"tests/test_environ.py::test_expand_processes_port_from_env_coerced_to_number",
"tests/test_environ.py::test_expand_processes_port_from_env_overrides",
"tests/test_environ.py::test_expand_processes_port_concurrency",
"tests/test_environ.py::test_expand_processes_quiet",
"tests/test_environ.py::test_expand_processes_quiet_multiple",
"tests/test_environ.py::test_expand_processes_env",
"tests/test_environ.py::test_expand_processes_env_multiple",
"tests/test_environ.py::test_set_env_process_name"
] | 2020-03-07 00:24:54+00:00 | 4,164 |
|
nickw444__nessclient-29 | diff --git a/nessclient/event.py b/nessclient/event.py
index d4aab6c..2877a70 100644
--- a/nessclient/event.py
+++ b/nessclient/event.py
@@ -96,11 +96,13 @@ class SystemStatusEvent(BaseEvent):
@classmethod
def decode(cls, packet: Packet) -> 'SystemStatusEvent':
- data = bytearray.fromhex(packet.data)
+ event_type = int(packet.data[0:2], 16)
+ zone = int(packet.data[2:4])
+ area = int(packet.data[4:6], 16)
return SystemStatusEvent(
- type=SystemStatusEvent.EventType(data[0]),
- zone=data[1],
- area=data[2],
+ type=SystemStatusEvent.EventType(event_type),
+ zone=zone,
+ area=area,
timestamp=packet.timestamp,
address=packet.address,
)
| nickw444/nessclient | 3c59b0d2f49984bab822f995e57c3e05bef4880e | diff --git a/nessclient_tests/test_event.py b/nessclient_tests/test_event.py
index a8a1d1b..85c904e 100644
--- a/nessclient_tests/test_event.py
+++ b/nessclient_tests/test_event.py
@@ -127,6 +127,20 @@ class SystemStatusEventTestCase(unittest.TestCase):
self.assertEqual(event.type,
SystemStatusEvent.EventType.SEALED)
+ def test_zone_unsealed_with_zone_15(self):
+ pkt = make_packet(CommandType.SYSTEM_STATUS, '001500')
+ event = SystemStatusEvent.decode(pkt)
+ self.assertEqual(event.area, 0)
+ self.assertEqual(event.zone, 15)
+ self.assertEqual(event.type, SystemStatusEvent.EventType.UNSEALED)
+
+ def test_zone_unsealed_with_zone_16(self):
+ pkt = make_packet(CommandType.SYSTEM_STATUS, '001600')
+ event = SystemStatusEvent.decode(pkt)
+ self.assertEqual(event.area, 0)
+ self.assertEqual(event.zone, 16)
+ self.assertEqual(event.type, SystemStatusEvent.EventType.UNSEALED)
+
def make_packet(command: CommandType, data: str):
return Packet(address=0, command=command,
diff --git a/nessclient_tests/test_packet.py b/nessclient_tests/test_packet.py
index 3464909..9ef6bd3 100644
--- a/nessclient_tests/test_packet.py
+++ b/nessclient_tests/test_packet.py
@@ -131,3 +131,14 @@ class PacketTestCase(unittest.TestCase):
self.assertEqual(pkt.data, '000700')
self.assertEqual(pkt.timestamp, datetime.datetime(
year=2019, month=2, day=25, hour=18, minute=0, second=0))
+
+ def test_decode_zone_16(self):
+ pkt = Packet.decode('8700036100160019022823032274')
+ self.assertEqual(pkt.start, 0x87)
+ self.assertEqual(pkt.address, 0x00)
+ self.assertEqual(pkt.length, 3)
+ self.assertEqual(pkt.seq, 0x00)
+ self.assertEqual(pkt.command, CommandType.SYSTEM_STATUS)
+ self.assertEqual(pkt.data, '001600')
+ self.assertEqual(pkt.timestamp, datetime.datetime(
+ year=2019, month=2, day=28, hour=23, minute=3, second=22))
| Zones 15 and 16 cause Nessclient 0.9.13 to loose connection
Hi Nick,
I'm not too sure about the best way to get in touch with you regarding the issue I'm having with the current build of nessclient.
With P199E options 1, 2, 3 and 6 enabled I get the following error when triggering Zones 15 and 16. I only have zones 1-4, 15 and 16 connected to my panel at the moment.
I'm pretty sure the issue is in the decode function of the event class as it's converting decimal 15/16 to hex 21/22 and saving that as the zone.
Unfortunately i'm not yet confident enough in python (or github) to create a pull request to fix this but I think you need to change
`zone=data[1],` on line 102 of event.py to
`zone=int(data[1], 16),`
The debug log for outputs 15 and 16 is copied below.
### Zone 16:
```
DEBUG:nessclient.client:Decoding data: '8700036100160019022823032274'
DEBUG:nessclient.packet:Decoding bytes: '8700036100160019022823032274'
<SystemStatusEvent {'address': 0, 'timestamp': datetime.datetime(2019, 2, 28, 23, 3, 22), 'type': <EventType.UNSEALED: 0>, 'zone': 22, 'area': 0}>
ERROR:asyncio:Task exception was never retrieved
future: <Task finished coro=<Client.keepalive() done, defined at /usr/local/lib/python3.7/site-packages/nessclient/client.py:145> exception=IndexError('list index out of range')>
Traceback (most recent call last):
File "/usr/local/lib/python3.7/site-packages/nessclient/client.py", line 148, in keepalive
self._update_loop(),
File "/usr/local/lib/python3.7/site-packages/nessclient/client.py", line 131, in _recv_loop
self.alarm.handle_event(event)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 50, in handle_event
self._handle_system_status_event(event)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 81, in _handle_system_status_event
return self._update_zone(event.zone, True)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 113, in _update_zone
zone = self.zones[zone_id - 1]
IndexError: list index out of range
```
### Zone 15
```
DEBUG:nessclient.client:Decoding data: '8700036100150019022823094849'
DEBUG:nessclient.packet:Decoding bytes: '8700036100150019022823094849'
<SystemStatusEvent {'address': 0, 'timestamp': datetime.datetime(2019, 2, 28, 23, 9, 48), 'type': <EventType.UNSEALED: 0>, 'zone': 21, 'area': 0}>
ERROR:asyncio:Task exception was never retrieved
future: <Task finished coro=<Client.keepalive() done, defined at /usr/local/lib/python3.7/site-packages/nessclient/client.py:145> exception=IndexError('list index out of range')>
Traceback (most recent call last):
File "/usr/local/lib/python3.7/site-packages/nessclient/client.py", line 148, in keepalive
self._update_loop(),
File "/usr/local/lib/python3.7/site-packages/nessclient/client.py", line 131, in _recv_loop
self.alarm.handle_event(event)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 50, in handle_event
self._handle_system_status_event(event)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 81, in _handle_system_status_event
return self._update_zone(event.zone, True)
File "/usr/local/lib/python3.7/site-packages/nessclient/alarm.py", line 113, in _update_zone
zone = self.zones[zone_id - 1]
IndexError: list index out of range
```
Regards,
Anthony
| 0.0 | [
"nessclient_tests/test_event.py::SystemStatusEventTestCase::test_zone_unsealed_with_zone_15",
"nessclient_tests/test_event.py::SystemStatusEventTestCase::test_zone_unsealed_with_zone_16"
] | [
"nessclient_tests/test_event.py::UtilsTestCase::test_pack_unsigned_short_data_enum",
"nessclient_tests/test_event.py::ArmingUpdateTestCase::test_area1_armed",
"nessclient_tests/test_event.py::ArmingUpdateTestCase::test_encode",
"nessclient_tests/test_event.py::ZoneUpdateTestCase::test_encode",
"nessclient_tests/test_event.py::ZoneUpdateTestCase::test_zone_in_alarm_with_zones",
"nessclient_tests/test_event.py::ZoneUpdateTestCase::test_zone_in_delay_no_zones",
"nessclient_tests/test_event.py::ZoneUpdateTestCase::test_zone_in_delay_with_zones",
"nessclient_tests/test_event.py::ViewStateUpdateTestCase::test_normal_state",
"nessclient_tests/test_event.py::OutputsUpdateTestCase::test_panic_outputs",
"nessclient_tests/test_event.py::MiscellaneousAlarmsUpdateTestCase::test_misc_alarms_install_end",
"nessclient_tests/test_event.py::MiscellaneousAlarmsUpdateTestCase::test_misc_alarms_multi",
"nessclient_tests/test_event.py::MiscellaneousAlarmsUpdateTestCase::test_misc_alarms_panic",
"nessclient_tests/test_event.py::SystemStatusEventTestCase::test_exit_delay_end",
"nessclient_tests/test_event.py::SystemStatusEventTestCase::test_zone_sealed",
"nessclient_tests/test_packet.py::PacketTestCase::test_bad_timestamp",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_encode_identity",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_status_update_response",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_with_address",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_with_address_and_time",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_without_address",
"nessclient_tests/test_packet.py::PacketTestCase::test_decode_zone_16",
"nessclient_tests/test_packet.py::PacketTestCase::test_encode_cecode2",
"nessclient_tests/test_packet.py::PacketTestCase::test_encode_decode1",
"nessclient_tests/test_packet.py::PacketTestCase::test_system_status_packet_decode",
"nessclient_tests/test_packet.py::PacketTestCase::test_user_interface_packet_decode"
] | 2019-02-28 20:56:13+00:00 | 4,165 |
|
niedakh__pqdm-56 | diff --git a/pqdm/_base.py b/pqdm/_base.py
index fe85482..3c119f5 100644
--- a/pqdm/_base.py
+++ b/pqdm/_base.py
@@ -1,11 +1,12 @@
import copy
from concurrent.futures import Executor, as_completed
-from typing import Any, Callable, Iterable
+from typing import Any, Callable, Iterable, Union
from tqdm import tqdm as tqdm_cli
from tqdm.notebook import tqdm as tqdm_notebook
+from typing_extensions import Literal
-from pqdm.constants import ArgumentPassing
+from pqdm.constants import ArgumentPassing, ExceptionBehaviour
from pqdm.utils import _inside_jupyter, _divide_kwargs
TQDM = tqdm_notebook if _inside_jupyter() else tqdm_cli
@@ -26,6 +27,7 @@ def _parallel_process(
n_jobs: int,
executor: Executor,
argument_type: str = 'direct',
+ exception_behaviour: Union[Literal['ignore'], Literal['immediate'], Literal['deferred']] = 'ignore',
**kwargs
):
executor_opts, tqdm_opts = _divide_kwargs(kwargs, executor)
@@ -69,10 +71,19 @@ def _parallel_process(
collecting_opts['total'] = len(futures)
results = []
+ exceptions = []
for i, future in TQDM(enumerate(futures), **collecting_opts):
try:
results.append(future.result())
except Exception as e:
- results.append(e)
+ if exception_behaviour == ExceptionBehaviour.IMMEDIATE:
+ raise e
+ if exception_behaviour == ExceptionBehaviour.IGNORE:
+ results.append(e)
+ if exception_behaviour == ExceptionBehaviour.DEFERRED:
+ exceptions.append(e)
+
+ if exceptions:
+ raise Exception(*exceptions)
return results
diff --git a/pqdm/constants.py b/pqdm/constants.py
index 7fc76ca..5728586 100644
--- a/pqdm/constants.py
+++ b/pqdm/constants.py
@@ -4,3 +4,9 @@ from typing import NamedTuple
class ArgumentPassing(NamedTuple):
AS_ARGS = 'args'
AS_KWARGS = 'kwargs'
+
+
+class ExceptionBehaviour(NamedTuple):
+ IGNORE = 'ignore'
+ IMMEDIATE = 'immediate'
+ DEFERRED = 'deferred'
diff --git a/pqdm/processes.py b/pqdm/processes.py
index 5a69502..fc026da 100644
--- a/pqdm/processes.py
+++ b/pqdm/processes.py
@@ -13,6 +13,7 @@ def pqdm(
n_jobs: int,
argument_type: Optional[Union[Literal['kwargs'], Literal['args']]] = None,
bounded: bool = False,
+ exception_behaviour: Union[Literal['ignore'], Literal['immediate'], Literal['deferred']] = 'ignore',
**kwargs
):
return _parallel_process(
@@ -21,5 +22,6 @@ def pqdm(
argument_type=argument_type,
n_jobs=n_jobs,
executor=BoundedProcessPoolExecutor if bounded else ProcessPoolExecutor,
+ exception_behaviour=exception_behaviour,
**kwargs
)
diff --git a/pqdm/threads.py b/pqdm/threads.py
index b9c5b8b..7957974 100644
--- a/pqdm/threads.py
+++ b/pqdm/threads.py
@@ -13,6 +13,7 @@ def pqdm(
n_jobs: int,
argument_type: Optional[Union[Literal['kwargs'], Literal['args']]] = None,
bounded: bool = False,
+ exception_behaviour: Union[Literal['ignore'], Literal['immediate'], Literal['deferred']] = 'ignore',
**kwargs
):
return _parallel_process(
@@ -21,5 +22,6 @@ def pqdm(
argument_type=argument_type,
n_jobs=n_jobs,
executor=BoundedThreadPoolExecutor if bounded else ThreadPoolExecutor,
+ exception_behaviour=exception_behaviour,
**kwargs
)
| niedakh/pqdm | 40cf767613ff87e15eab8eb0aa810909b333d20e | diff --git a/tests/test_pqdm.py b/tests/test_pqdm.py
index 8ebcca8..e98293a 100644
--- a/tests/test_pqdm.py
+++ b/tests/test_pqdm.py
@@ -9,6 +9,15 @@ from pqdm.processes import pqdm as pqdm_processes
from pqdm.threads import pqdm as pqdm_threads
+run_for_threads_and_processes = pytest.mark.parametrize("pqdm_method", [pqdm_threads, pqdm_processes], ids=["threads", "processes"])
+
+
+class ExceptionWithValueEquality(Exception):
+ """ Value equality is required for comparisons when processes are involved. """
+ def __eq__(self, other):
+ return type(self) is type(other) and self.args == other.args
+
+
def multiply_args(a, b):
return a * b
@@ -17,6 +26,13 @@ def multiply_list(x):
return x[0] * x[1]
+def raises_exceptions(obj):
+ if isinstance(obj, BaseException):
+ raise obj
+ else:
+ return obj
+
+
RESULT = [1 * 2, 2 * 3, 3 * 4, 4 * 5]
TEST_DATA = [
@@ -51,42 +67,52 @@ TEST_DATA = [
]
-
[email protected]("function, input_list, kwargs", TEST_DATA)
-def test_pqdm_threads_work_with_argument_types(function, input_list, kwargs):
- result = pqdm_threads(input_list, function, **kwargs)
- assert result == RESULT
+TEST_DATA_WITH_EXCEPTIONS = [
+ ExceptionWithValueEquality(1),
+ "SomeObjectWithValueEquality",
+ ExceptionWithValueEquality(2),
+]
+@run_for_threads_and_processes
@pytest.mark.parametrize("function, input_list, kwargs", TEST_DATA)
-def test_pqdm_processes_work_with_argument_types(function, input_list, kwargs):
- result = pqdm_processes(input_list, function, **kwargs)
+def test_argument_types(pqdm_method, function, input_list, kwargs):
+ result = pqdm_method(input_list, function, **kwargs)
assert result == RESULT
+@run_for_threads_and_processes
@pytest.mark.parametrize("function, input_list, kwargs", TEST_DATA)
-def test_pqdm_processes_pushes_argument_to_tqdm(function, input_list, kwargs):
+def test_pqdm_pushes_argument_to_tqdm(pqdm_method, function, input_list, kwargs):
output = io.StringIO("")
kwargs['desc'] = 'Testing'
kwargs['file'] = output
- result = pqdm_processes(input_list, function, **kwargs)
+ result = pqdm_method(input_list, function, **kwargs)
text = output.getvalue()
assert 'Testing:' in text
assert result == RESULT
[email protected]("function, input_list, kwargs", TEST_DATA)
-def test_pqdm_threads_pushes_argument_to_tqdm(function, input_list, kwargs):
- output = io.StringIO("")
+@run_for_threads_and_processes
+def test_exceptions_ignored(pqdm_method):
+ results = pqdm_method(TEST_DATA_WITH_EXCEPTIONS, raises_exceptions, n_jobs=2, exception_behaviour='ignore')
+ assert results == TEST_DATA_WITH_EXCEPTIONS
- kwargs['desc'] = 'Testing'
- kwargs['file'] = output
- result = pqdm_threads(input_list, function, **kwargs)
+@run_for_threads_and_processes
+def test_exceptions_immediately(pqdm_method):
+ with pytest.raises(Exception) as exc:
+ pqdm_method(TEST_DATA_WITH_EXCEPTIONS, raises_exceptions, n_jobs=2, exception_behaviour='immediate')
- text = output.getvalue()
- assert 'Testing:' in text
- assert result == RESULT
+ assert exc.value == TEST_DATA_WITH_EXCEPTIONS[0]
+
+
+@run_for_threads_and_processes
+def test_exceptions_deferred(pqdm_method):
+ with pytest.raises(Exception) as exc:
+ pqdm_method(TEST_DATA_WITH_EXCEPTIONS, raises_exceptions, n_jobs=2, exception_behaviour='deferred')
+
+ assert exc.value.args == (TEST_DATA_WITH_EXCEPTIONS[0], TEST_DATA_WITH_EXCEPTIONS[2])
| Is it possible that pqdm passes over error messages
* Parallel TQDM version: 0.1.0
* Python version: 3.6
* Operating System: linux cluster
### Description
I wanted:
Read chunk of a big file
process and save chunk to a new files
Run this in parallel with pqdm.threads
### What I Did
```
def process_chunck(genome):
D=pd.read_hdf(input_tmp_file,where=f"Genome == {genome}")
out= process ...
out.to_parquet(output_file)
from pqdm.threads import pqdm
pqdm(all_genomes, process_chunck, n_jobs=threads)
```
Now there was a bug in my function `process_chunk` which was not raised.
What can I do to do better error handling with pqdm?
| 0.0 | [
"tests/test_pqdm.py::test_exceptions_ignored[threads]",
"tests/test_pqdm.py::test_exceptions_ignored[processes]",
"tests/test_pqdm.py::test_exceptions_immediately[threads]",
"tests/test_pqdm.py::test_exceptions_immediately[processes]",
"tests/test_pqdm.py::test_exceptions_deferred[threads]",
"tests/test_pqdm.py::test_exceptions_deferred[processes]"
] | [
"tests/test_pqdm.py::test_argument_types[multiply_args-input_list0-kwargs0-threads]",
"tests/test_pqdm.py::test_argument_types[multiply_args-input_list0-kwargs0-processes]",
"tests/test_pqdm.py::test_argument_types[multiply_args-input_list1-kwargs1-threads]",
"tests/test_pqdm.py::test_argument_types[multiply_args-input_list1-kwargs1-processes]",
"tests/test_pqdm.py::test_argument_types[multiply_list-input_list2-kwargs2-threads]",
"tests/test_pqdm.py::test_argument_types[multiply_list-input_list2-kwargs2-processes]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_args-input_list0-kwargs0-threads]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_args-input_list0-kwargs0-processes]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_args-input_list1-kwargs1-threads]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_args-input_list1-kwargs1-processes]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_list-input_list2-kwargs2-threads]",
"tests/test_pqdm.py::test_pqdm_pushes_argument_to_tqdm[multiply_list-input_list2-kwargs2-processes]"
] | 2020-09-29 17:31:11+00:00 | 4,166 |
|
nielstron__quantulum3-211 | diff --git a/quantulum3/_lang/en_US/parser.py b/quantulum3/_lang/en_US/parser.py
index 6f5ebe8..c2caad4 100644
--- a/quantulum3/_lang/en_US/parser.py
+++ b/quantulum3/_lang/en_US/parser.py
@@ -57,6 +57,7 @@ def clean_surface(surface, span):
###############################################################################
def split_spellout_sequence(text, span):
+ negatives = reg.negatives(lang)
units = reg.units(lang)
tens = reg.tens(lang)
scales = reg.scales(lang)
@@ -78,19 +79,22 @@ def split_spellout_sequence(text, span):
)
# if should start a new seqquence
# split on:
+ # a minus word (minus ten)
# unit -> unit (one two three)
# unit -> tens (five twenty)
# tens -> tens (twenty thirty)
# same scale starts (hundred and one hundred and two)
should_split = False
- if prev_word_rank == 1 and rank in [1, 2]:
+ if word in negatives:
+ should_split = True
+ elif prev_word_rank == 1 and rank in [1, 2]:
should_split = True
elif prev_word_rank == 2 and rank == 2:
should_split = True
elif rank >= 3 and rank == prev_scale:
should_split = True
prev_scale = rank
- if should_split:
+ if should_split and last_word_end > 0:
# yield up to here
adjust = 0
if prev_word.lower() in [
@@ -141,6 +145,7 @@ def extract_spellout_values(text):
):
continue
try:
+ is_negative = False
surface, span = clean_surface(seq, span)
if not surface:
continue
@@ -160,6 +165,9 @@ def extract_spellout_values(text):
except ValueError:
match = re.search(reg.numberwords_regex(), word)
scale, increment = reg.numberwords(lang)[match.group(0)]
+ if scale < 0: # negative, must be the first word in the sequence
+ is_negative = True
+ continue
if (
scale > 0
and increment == 0
@@ -178,11 +186,14 @@ def extract_spellout_values(text):
if scale > 100 or word == "and":
result += curr
curr = 0.0
+ value = result + curr
+ if is_negative:
+ value = -value
values.append(
{
"old_surface": surface,
"old_span": span,
- "new_surface": str(result + curr),
+ "new_surface": str(value),
}
)
except (KeyError, AttributeError):
diff --git a/quantulum3/_lang/en_US/regex.py b/quantulum3/_lang/en_US/regex.py
index 2bb8506..f2d995f 100644
--- a/quantulum3/_lang/en_US/regex.py
+++ b/quantulum3/_lang/en_US/regex.py
@@ -64,6 +64,8 @@ MULTIPLICATION_OPERATORS = {" times "}
DIVISION_OPERATORS = {" per ", " a "}
+NEGATIVES = {"minus", "negative"}
+
GROUPING_OPERATORS = {",", " "}
DECIMAL_OPERATORS = {"."}
diff --git a/quantulum3/_lang/en_US/speak.py b/quantulum3/_lang/en_US/speak.py
index de40fca..68236c7 100644
--- a/quantulum3/_lang/en_US/speak.py
+++ b/quantulum3/_lang/en_US/speak.py
@@ -37,6 +37,10 @@ def unit_to_spoken(unit, count=1):
# derived unit
denominator_dimensions = [i for i in unit.dimensions if i["power"] > 0]
denominator_string = parser.name_from_dimensions(denominator_dimensions, lang)
- plural_denominator_string = load.pluralize(denominator_string)
+ if denominator_string:
+ plural_denominator_string = load.pluralize(denominator_string)
+ else:
+ # in case the denominator is empty, its plural is, too
+ plural_denominator_string = denominator_string
unit_string = unit.name.replace(denominator_string, plural_denominator_string)
return unit_string
diff --git a/quantulum3/regex.py b/quantulum3/regex.py
index 42b02fd..750eaf7 100644
--- a/quantulum3/regex.py
+++ b/quantulum3/regex.py
@@ -46,6 +46,10 @@ def powers(lang="en_US"):
return _get_regex(lang).POWERS
+def negatives(lang="en_US"):
+ return _get_regex(lang).NEGATIVES
+
+
def exponents_regex(lang="en_US"):
return _get_regex(lang).EXPONENTS_REGEX
@@ -75,6 +79,8 @@ def numberwords(lang="en_US"):
numwords.update(miscnum(lang))
+ for word in negatives(lang):
+ numwords[word] = (-1, 0)
for idx, word in enumerate(units(lang)):
numwords[word] = (1, idx)
for idx, word in enumerate(tens(lang)):
| nielstron/quantulum3 | 06774a10370220a08dc4ccd27ceda50536e7312b | diff --git a/quantulum3/_lang/en_US/tests/extract_spellout_values.py b/quantulum3/_lang/en_US/tests/extract_spellout_values.py
index 8833396..84dd698 100644
--- a/quantulum3/_lang/en_US/tests/extract_spellout_values.py
+++ b/quantulum3/_lang/en_US/tests/extract_spellout_values.py
@@ -35,6 +35,14 @@ TEST_CASES = [
("hundred and five hundred and six", ["105.0", "106.0"]), # this is ambiguous..
("hundred and five twenty two", ["105.0", "22.0"]),
("hundred and five twenty two million", ["105.0", "22000000.0"]),
+ ## negatives
+ ("minus ten", ["-10.0"]),
+ ("minus a million and a half", ["-1000000.5"]),
+ ("negative million and a half", ["-1000000.5"]),
+ ## negative splitting
+ ("minus twenty five and thirty six", ["-25.0", "36.0"]),
+ ("twenty five and minus thirty six", ["25.0", "-36.0"]),
+ ("negative twenty five and minus thirty six", ["-25.0", "-36.0"]),
]
diff --git a/quantulum3/tests/test_hypothesis.py b/quantulum3/tests/test_hypothesis.py
index 5cc0f66..c7d25b4 100644
--- a/quantulum3/tests/test_hypothesis.py
+++ b/quantulum3/tests/test_hypothesis.py
@@ -6,7 +6,7 @@
import unittest
-from hypothesis import given, settings
+from hypothesis import example, given, settings
from hypothesis import strategies as st
from .. import parser as p
@@ -30,6 +30,7 @@ class TestNoErrors(unittest.TestCase):
p.extract_spellout_values(s, lang=lang)
@given(st.text(), multilang_strategy)
+ @example("0/B", "en_US")
@settings(deadline=None)
def test_inline_parse_and_expand(self, s, lang):
# Just assert that this does not throw any exceptions
| negative spelled out numbers are not recognized as negative
**Describe the bug**
```python
from quantulum3 import parser
parser.parse("minus ten")
# returns 10 should return -10.
```
| 0.0 | [
"quantulum3/_lang/en_US/tests/extract_spellout_values.py::ExtractSpellout::test_spellout_values",
"quantulum3/tests/test_hypothesis.py::TestNoErrors::test_inline_parse_and_expand"
] | [
"quantulum3/tests/test_hypothesis.py::TestNoErrors::test_clean_text",
"quantulum3/tests/test_hypothesis.py::TestNoErrors::test_extract_spellout_values",
"quantulum3/tests/test_hypothesis.py::TestNoErrors::test_parse"
] | 2023-01-07 14:04:21+00:00 | 4,167 |
|
niftycode__imessage_reader-12 | diff --git a/imessage_reader/create_sqlite.py b/imessage_reader/create_sqlite.py
index 2c2aedc..1bf6d24 100644
--- a/imessage_reader/create_sqlite.py
+++ b/imessage_reader/create_sqlite.py
@@ -5,7 +5,7 @@
Create a SQLite3 database containing iMessage data (user id, text, date, service)
Python 3.8+
Date created: April 30th, 2021
-Date modified: August 7th, 2021
+Date modified: August 28th, 2021
"""
import sqlite3
@@ -41,11 +41,12 @@ class CreateDatabase:
message TEXT,
date TEXT,
service TEXT,
- destination_caller_id TEXT)''')
+ destination_caller_id TEXT,
+ is_from_me TEXT)''')
for data in self.imessage_data:
- cur.execute('''INSERT INTO Messages (user_id, message, date, service, destination_caller_id)
- VALUES(?, ?, ?, ?, ?)''', (data.user_id, data.text, data.date, data.service, data.account))
+ cur.execute('''INSERT INTO Messages (user_id, message, date, service, destination_caller_id, is_from_me)
+ VALUES(?, ?, ?, ?, ?, ?)''', (data.user_id, data.text, data.date, data.service, data.account, data.is_from_me))
conn.commit()
cur.close()
diff --git a/imessage_reader/fetch_data.py b/imessage_reader/fetch_data.py
index 30501e1..6e6f0f2 100644
--- a/imessage_reader/fetch_data.py
+++ b/imessage_reader/fetch_data.py
@@ -7,7 +7,7 @@ Python 3.8+
Author: niftycode
Modified by: thecircleisround
Date created: October 8th, 2020
-Date modified: August 6th, 2021
+Date modified: August 28th, 2021
"""
import sys
@@ -28,6 +28,7 @@ class MessageData:
date: str
service: str
account: str
+ is_from_me: str
def __str__(self):
"""
@@ -37,7 +38,8 @@ class MessageData:
f"message: {self.text}\n" \
f"date: {self.date}\n" \
f"service: {self.service}\n" \
- f"destination caller id: {self.account}\n"
+ f"destination caller id: {self.account}\n "\
+ f"is_from_me: {self.is_from_me}\n"
# noinspection PyMethodMayBeStatic
@@ -55,7 +57,8 @@ class FetchData:
"datetime((date / 1000000000) + 978307200, 'unixepoch', 'localtime')," \
"handle.id, " \
"handle.service, " \
- "message.destination_caller_id " \
+ "message.destination_caller_id, " \
+ "message.is_from_me "\
"FROM message " \
"JOIN handle on message.handle_id=handle.ROWID"
@@ -77,7 +80,7 @@ class FetchData:
data = []
for row in rval:
- data.append(MessageData(row[2], row[0], row[1], row[3], row[4]))
+ data.append(MessageData(row[2], row[0], row[1], row[3], row[4], row[5]))
return data
@@ -137,6 +140,8 @@ class FetchData:
dates = []
service = []
account = []
+ is_from_me = []
+
for data in fetched_data:
users.append(data.user_id)
@@ -144,7 +149,8 @@ class FetchData:
dates.append(data.date)
service.append(data.service)
account.append(data.account)
+ is_from_me.append(data.is_from_me)
- data = list(zip(users, messages, dates, service, account))
+ data = list(zip(users, messages, dates, service, account, is_from_me))
return data
diff --git a/imessage_reader/write_excel.py b/imessage_reader/write_excel.py
index 47412f9..3f88a6d 100644
--- a/imessage_reader/write_excel.py
+++ b/imessage_reader/write_excel.py
@@ -6,7 +6,7 @@
Write Excel file containing iMessage data (user id, text, date, service, account)
Python 3.8+
Date created: October 1st, 2020
-Date modified: August 7th, 2021
+Date modified: August 28th, 2021
"""
from datetime import datetime
@@ -40,6 +40,7 @@ class ExelWriter:
dates = []
services = []
accounts = []
+ is_from_me = []
for data in self.imessage_data:
users.append(data.user_id)
@@ -47,6 +48,7 @@ class ExelWriter:
dates.append(data.date)
services.append(data.service)
accounts.append(data.account)
+ is_from_me.append(data.is_from_me)
# Call openpyxl.Workbook() to create a new blank Excel workbook
workbook = openpyxl.Workbook()
@@ -75,6 +77,9 @@ class ExelWriter:
sheet['E1'] = 'Destination Caller ID'
sheet['E1'].font = bold16font
+ sheet['F1'] = 'Is From Me'
+ sheet['F1'].font = bold16font
+
# Write users to 1st column
users_row = 2
for user in users:
@@ -105,6 +110,12 @@ class ExelWriter:
sheet.cell(row=account_row, column=5).value = account
account_row += 1
+ # Write is_from_me to 6th column
+ is_from_me_row = 2
+ for from_me in is_from_me:
+ sheet.cell(row=is_from_me_row, column=6).value = from_me
+ is_from_me_row += 1
+
# Save the workbook (excel file)
try:
workbook.save(self.file_path + f'iMessage-Data_{datetime.now().strftime("%Y-%m-%d")}.xlsx')
| niftycode/imessage_reader | 3a972bebf1c16e02e12322d3e81a7ea60400d5a2 | diff --git a/tests/test_create_sqlite.py b/tests/test_create_sqlite.py
index a0a3f35..673a51c 100644
--- a/tests/test_create_sqlite.py
+++ b/tests/test_create_sqlite.py
@@ -18,7 +18,8 @@ def message_data_one_row():
text='Hello Max!',
date='2021-04-11 17:02:34',
service='iMessage',
- account='+01 555 17172')]
+ account='+01 555 17172',
+ is_from_me = 1)]
return message_data_list
diff --git a/tests/test_message_data.py b/tests/test_message_data.py
index fe8acf8..5b99916 100644
--- a/tests/test_message_data.py
+++ b/tests/test_message_data.py
@@ -14,7 +14,8 @@ def message_data_one_row():
'Hello!',
'2020-10-27 17:19:20',
'SMS',
- '+01 555 17172')
+ '+01 555 17172',
+ 1)
@pytest.fixture(scope='function')
@@ -31,17 +32,19 @@ def initialize_db(tmpdir):
text TEXT UNIQUE,
date TEXT UNIQUE,
service TEXT UNIQUE,
- account TEXT UNIQUE
+ account TEXT UNIQUE,
+ is_from_me INTEGER
);
''')
- cur.execute('''INSERT OR IGNORE INTO message(user_id, text, date, service, account)
- VALUES ( ?, ?, ?, ?, ?)''',
+ cur.execute('''INSERT OR IGNORE INTO message(user_id, text, date, service, account, is_from_me)
+ VALUES ( ?, ?, ?, ?, ?, ?)''',
('[email protected]',
'Hello Kendra!',
'2020-10-27 17:19:20',
'iMessage',
- '+01 555 17172'))
+ '+01 555 17172',
+ 1))
conn.commit()
@@ -54,7 +57,7 @@ def test_message_data(message_data_one_row):
def test_db_data(initialize_db):
- sql_command = 'SELECT user_id, text, date, service, account from message'
+ sql_command = 'SELECT user_id, text, date, service, account, is_from_me from message'
rval = common.fetch_db_data(initialize_db, sql_command)
assert(isinstance(rval, list))
assert(isinstance(rval[0][0], str))
@@ -62,3 +65,4 @@ def test_db_data(initialize_db):
assert (isinstance(rval[0][2], str))
assert (isinstance(rval[0][3], str))
assert (isinstance(rval[0][4], str))
+ assert (isinstance(rval[0][5], int))
diff --git a/tests/test_write_excel.py b/tests/test_write_excel.py
index 8ba4092..aaa6816 100644
--- a/tests/test_write_excel.py
+++ b/tests/test_write_excel.py
@@ -19,7 +19,8 @@ def message_data_one_row():
text='Hello!',
date='2020-10-27 17:19:20',
service='SMS',
- account='+01 555 17172')]
+ account='+01 555 17172',
+ is_from_me=1)]
return message_data_list
| Add ability to filter sent/received messages
Awesome app! Thanks for putting it together. I've been able to access both the excel and sqlite output it creates to look at my messages. My only problem (right now) is that I can't figure out a way to tell if I sent or received the message. I can filter by `sender` to isolate conversations to an individual text thread but without any context it's hard to tell if I sent the message or received it.
You might be able to use the `is_from_me` column on the `message` table in the original database to add a flag or some logic on it. I'm going to keep looking into a little myself but thanks again for putting this together! | 0.0 | [
"tests/test_create_sqlite.py::test_create_sqlite",
"tests/test_message_data.py::test_message_data",
"tests/test_write_excel.py::test_write_excel"
] | [
"tests/test_message_data.py::test_db_data"
] | 2021-08-28 17:04:02+00:00 | 4,168 |
|
nilearn__nilearn-2678 | diff --git a/.github/ISSUE_TEMPLATE/bug_report.md b/.github/ISSUE_TEMPLATE/bug_report.md
index 89db404bd..f62478c2d 100644
--- a/.github/ISSUE_TEMPLATE/bug_report.md
+++ b/.github/ISSUE_TEMPLATE/bug_report.md
@@ -6,21 +6,20 @@ labels: 'Bug'
assignees: ''
---
+<!--Provide a brief description of the bug.-->
-<!--
-Include this information:
--------------------------
-What version of Nilearn are you using?
-What were you trying to do?
-What did you expect will happen?
-What actually happened?
+<!--Please fill in the following information, to the best of your ability.-->
+Nilearn version:
-List the steps you performed that revealed the bug to you.
-Include any code samples. Enclose them in triple back-ticks (```)
-Like this:
+### Expected behavior
+
+
+### Actual behavior
+
+
+### Steps and code to reproduce bug
+
+```python
```
-<code>
-```
--->
diff --git a/.github/ISSUE_TEMPLATE/documentation.md b/.github/ISSUE_TEMPLATE/documentation.md
index 5e8bd7e6f..97c126845 100644
--- a/.github/ISSUE_TEMPLATE/documentation.md
+++ b/.github/ISSUE_TEMPLATE/documentation.md
@@ -5,8 +5,7 @@ title: ''
labels: Documentation
assignees: ''
---
-
-
-#### Proposed documentation enhancement
<!--Describe your proposed enhancement in detail.-->
+<!--List any pages that would be impacted by the enhancement.-->
+### Affected pages
diff --git a/.github/ISSUE_TEMPLATE/feature_request.md b/.github/ISSUE_TEMPLATE/feature_request.md
index bd5fbf55f..3e98ff321 100644
--- a/.github/ISSUE_TEMPLATE/feature_request.md
+++ b/.github/ISSUE_TEMPLATE/feature_request.md
@@ -6,12 +6,15 @@ labels: 'Enhancement'
assignees: ''
---
+<!--Provide a brief description what you would like changes and why.-->
-<!--
-Include the following:
-------------------------
-What would you like changed/added and why?
-What would be the benefit? Does the change make something easier to use?
-Clarifies something?
-If it is a new feature, what is the benefit?
--->
+
+<!--Please fill in the following information, to the best of your ability.-->
+### Benefits to the change
+
+
+### Pseudocode for the new behavior, if applicable
+
+```python
+
+```
diff --git a/doc/whats_new.rst b/doc/whats_new.rst
index 18cc7ae5f..08a50a88c 100644
--- a/doc/whats_new.rst
+++ b/doc/whats_new.rst
@@ -18,6 +18,9 @@ NEW
Fixes
-----
+- Fix altered, non-zero baseline in design matrices where multiple events in the same condition
+ end at the same time (https://github.com/nilearn/nilearn/issues/2674).
+
- Fix testing issues on ARM machine.
Enhancements
diff --git a/nilearn/surface/__init__.py b/nilearn/surface/__init__.py
index d367acbd4..4dfdc2ea0 100644
--- a/nilearn/surface/__init__.py
+++ b/nilearn/surface/__init__.py
@@ -3,7 +3,10 @@ Functions for surface manipulation.
"""
from .surface import (vol_to_surf, load_surf_data,
- load_surf_mesh, check_mesh_and_data, Mesh)
+ load_surf_mesh, load_surface,
+ check_mesh_and_data, check_surface,
+ Mesh, Surface)
__all__ = ['vol_to_surf', 'load_surf_data', 'load_surf_mesh',
- 'check_mesh_and_data', 'Mesh']
+ 'load_surface', 'check_surface', 'check_mesh_and_data',
+ 'Mesh', 'Surface']
diff --git a/nilearn/surface/surface.py b/nilearn/surface/surface.py
index 9f7cda88e..f799c0dce 100644
--- a/nilearn/surface/surface.py
+++ b/nilearn/surface/surface.py
@@ -33,6 +33,8 @@ from nilearn.image import get_data
# Create a namedtuple object for meshes
Mesh = namedtuple("mesh", ["coordinates", "faces"])
+# Create a namedtuple object for surfaces
+Surface = namedtuple("surface", ["mesh", "data"])
def _uniform_ball_cloud(n_points=20, dim=3, n_monte_carlo=50000):
"""Get points uniformly spaced in the unit ball."""
@@ -895,6 +897,55 @@ def load_surf_mesh(surf_mesh):
return mesh
+def load_surface(surface):
+ """Loads a surface.
+
+ Parameters
+ ----------
+ surface : Surface-like (see description)
+ The surface to be loaded.
+ A surface can be:
+ - a nilearn.surface.Surface
+ - a sequence (mesh, data) where:
+ - mesh can be:
+ - a nilearn.surface.Mesh
+ - a path to .gii or .gii.gz etc.
+ - a sequence of two numpy arrays,
+ the first containing vertex coordinates
+ and the second containing triangles.
+ - data can be:
+ - a path to .gii or .gii.gz etc.
+ - a numpy array with shape (n_vertices,)
+ or (n_time_points, n_vertices)
+
+ Returns
+ --------
+ surface : Surface
+ With the fields "mesh" (Mesh object) and "data" (numpy.ndarray).
+
+ """
+ # Handle the case where we received a Surface
+ # object with mesh and data attributes
+ if hasattr(surface, "mesh") and hasattr(surface, "data"):
+ mesh = load_surf_mesh(surface.mesh)
+ data = load_surf_data(surface.data)
+ # Handle the case where we received a sequence
+ # (mesh, data)
+ elif isinstance(surface, (list, tuple, np.ndarray)):
+ if len(surface) == 2:
+ mesh = load_surf_mesh(surface[0])
+ data = load_surf_data(surface[1])
+ else:
+ raise ValueError("`load_surface` accepts iterables "
+ "of length 2 to define a surface. "
+ "You provided a {} of length {}.".format(
+ type(surface), len(surface)))
+ else:
+ raise ValueError("Wrong parameter `surface` in `load_surface`. "
+ "Please refer to the documentation for more information.")
+ return Surface(mesh, data)
+
+
def _check_mesh(mesh):
"""Check that mesh data is either a str, or a dict with sufficient
entries.
@@ -918,7 +969,32 @@ def _check_mesh(mesh):
def check_mesh_and_data(mesh, data):
- """Load surface mesh and data, check that they have compatible shapes."""
+ """Load surface mesh and data, check that they have compatible shapes.
+
+ Parameters
+ ----------
+ mesh : str or numpy.ndarray or Mesh
+ Either a file containing surface mesh geometry (valid formats
+ are .gii .gii.gz or Freesurfer specific files such as .orig, .pial,
+ .sphere, .white, .inflated) or two Numpy arrays organized in a list,
+ tuple or a namedtuple with the fields "coordinates" and "faces", or a
+ Mesh object with "coordinates" and "faces" attributes.
+
+ data : str or numpy.ndarray
+ Either a file containing surface data (valid format are .gii,
+ .gii.gz, .mgz, .nii, .nii.gz, or Freesurfer specific files such as
+ .thickness, .curv, .sulc, .annot, .label), lists of 1D data files are
+ returned as 2D arrays, or a Numpy array containing surface data.
+
+ Returns
+ -------
+ mesh : Mesh
+ Checked mesh.
+
+ data : numpy.ndarray
+ Checked data.
+
+ """
mesh = load_surf_mesh(mesh)
data = load_surf_data(data)
# Check that mesh coordinates has a number of nodes
@@ -936,3 +1012,38 @@ def check_mesh_and_data(mesh, data):
"Maximum face index is {} while coordinates array has length {}.".format(
mesh.faces.max(), len(mesh.coordinates)))
return mesh, data
+
+
+def check_surface(surface):
+ """Load a surface as a Surface object.
+ This function will make sure that the surfaces's
+ mesh and data have compatible shapes.
+
+ Parameters
+ ----------
+ surface : Surface-like (see description)
+ The surface to be loaded.
+ A surface can be:
+ - a nilearn.surface.Surface
+ - a sequence (mesh, data) where:
+ - mesh can be:
+ - a nilearn.surface.Mesh
+ - a path to .gii or .gii.gz etc.
+ - a sequence of two numpy arrays,
+ the first containing vertex coordinates
+ and the second containing triangles.
+ - data can be:
+ - a path to .gii or .gii.gz etc.
+ - a numpy array with shape (n_vertices,)
+ or (n_time_points, n_vertices)
+
+ Returns
+ -------
+ surface : Surface
+ Checked surface object.
+
+ """
+ surface = load_surface(surface)
+ mesh, data = check_mesh_and_data(surface.mesh,
+ surface.data)
+ return Surface(mesh, data)
| nilearn/nilearn | 6f6fa805904ed23436d35ed6d571a0c45fe5378a | diff --git a/nilearn/datasets/tests/test_neurovault.py b/nilearn/datasets/tests/test_neurovault.py
index 74cccbbe3..53c907a8e 100644
--- a/nilearn/datasets/tests/test_neurovault.py
+++ b/nilearn/datasets/tests/test_neurovault.py
@@ -431,7 +431,7 @@ def test_result_filter():
filter_0 = neurovault.ResultFilter(query_terms={'a': 0},
callable_filter=lambda d: len(d) < 5,
b=1)
- assert np.unicode(filter_0) == u'ResultFilter'
+ assert str(filter_0) == 'ResultFilter'
assert filter_0['a'] == 0
assert filter_0({'a': 0, 'b': 1, 'c': 2})
assert not filter_0({'a': 0, 'b': 1, 'c': 2, 'd': 3, 'e': 4})
diff --git a/nilearn/decomposition/tests/test_canica.py b/nilearn/decomposition/tests/test_canica.py
index ce2b58fa6..07c5e59a2 100644
--- a/nilearn/decomposition/tests/test_canica.py
+++ b/nilearn/decomposition/tests/test_canica.py
@@ -158,8 +158,13 @@ def test_percentile_range():
canica = CanICA(n_components=edge_case, threshold=float(edge_case))
with warnings.catch_warnings(record=True) as warning:
canica.fit(data)
- assert len(warning) == 1 # ensure single warning
- assert "critical threshold" in str(warning[-1].message)
+ # Filter out deprecation warnings
+ not_deprecation_warning = [not issubclass(w.category,
+ DeprecationWarning)
+ for w in warning]
+ assert sum(not_deprecation_warning) == 1 # ensure single warning
+ idx_critical_warning = not_deprecation_warning.index(True)
+ assert "critical threshold" in str(warning[idx_critical_warning].message)
def test_masker_attributes_with_fit():
diff --git a/nilearn/input_data/tests/test_nifti_maps_masker.py b/nilearn/input_data/tests/test_nifti_maps_masker.py
index 34dd02765..cb142c9f8 100644
--- a/nilearn/input_data/tests/test_nifti_maps_masker.py
+++ b/nilearn/input_data/tests/test_nifti_maps_masker.py
@@ -132,7 +132,8 @@ def test_nifti_maps_masker_with_nans():
# nans
maps_data = get_data(maps_img)
- mask_data = get_data(mask_img)
+ mask_data = np.array(get_data(mask_img),
+ dtype=np.float64)
maps_data[:, 9, 9] = np.nan
maps_data[:, 5, 5] = np.inf
diff --git a/nilearn/surface/tests/test_surface.py b/nilearn/surface/tests/test_surface.py
index 1c5879bc2..8d0c15d7f 100644
--- a/nilearn/surface/tests/test_surface.py
+++ b/nilearn/surface/tests/test_surface.py
@@ -4,6 +4,7 @@ import os
import tempfile
import warnings
+from collections import namedtuple
from distutils.version import LooseVersion
import nibabel as nb
@@ -20,7 +21,7 @@ from nilearn import datasets
from nilearn import image
from nilearn.image import resampling
from nilearn.image.tests.test_resampling import rotation
-from nilearn.surface import Mesh
+from nilearn.surface import Mesh, Surface
from nilearn.surface import surface
from nilearn.surface import load_surf_data, load_surf_mesh, vol_to_surf
from nilearn.surface.surface import (_gifti_img_to_mesh,
@@ -47,6 +48,23 @@ class MeshLikeObject(object):
def faces(self):
return self._faces
+class SurfaceLikeObject(object):
+ """Class with attributes mesh and
+ data to be used for testing purposes.
+ """
+ def __init__(self, mesh, data):
+ self._mesh = mesh
+ self._data = data
+ @classmethod
+ def fromarrays(cls, coordinates, faces, data):
+ return cls(MeshLikeObject(coordinates, faces), data)
+ @property
+ def mesh(self):
+ return self._mesh
+ @property
+ def data(self):
+ return self._data
+
def test_load_surf_data_array():
# test loading and squeezing data from numpy array
@@ -182,6 +200,39 @@ def test_load_surf_mesh():
assert_array_equal(mesh_like.faces, loaded_mesh.faces)
+def test_load_surface():
+ coords, faces = generate_surf()
+ mesh = Mesh(coords, faces)
+ data = mesh[0][:,0]
+ surf = Surface(mesh, data)
+ surf_like_obj = SurfaceLikeObject(mesh, data)
+ # Load the surface from:
+ # - Surface-like objects having the right attributes
+ # - a list of length 2 (mesh, data)
+ for loadings in [surf,
+ surf_like_obj,
+ [mesh, data]]:
+ s = surface.load_surface(loadings)
+ assert_array_equal(s.data, data)
+ assert_array_equal(s.data, surf.data)
+ assert_array_equal(s.mesh.coordinates, coords)
+ assert_array_equal(s.mesh.coordinates, surf.mesh.coordinates)
+ assert_array_equal(s.mesh.faces, surf.mesh.faces)
+ # Giving an iterable of length other than 2 will raise an error
+ # Length 3
+ with pytest.raises(ValueError,
+ match="`load_surface` accepts iterables of length 2"):
+ s = surface.load_surface([coords, faces, data])
+ # Length 1
+ with pytest.raises(ValueError,
+ match="`load_surface` accepts iterables of length 2"):
+ s = surface.load_surface([coords])
+ # Giving other objects will raise an error
+ with pytest.raises(ValueError,
+ match="Wrong parameter `surface` in `load_surface`"):
+ s = surface.load_surface("foo")
+
+
def test_load_surf_mesh_list():
# test if correct list is returned
mesh = generate_surf()
@@ -661,9 +712,41 @@ def test_check_mesh_and_data():
wrong_faces = rng.randint(coords.shape[0] + 1, size=(30, 3))
wrong_mesh = Mesh(coords, wrong_faces)
# Check that check_mesh_and_data raises an error with the resulting wrong mesh
- with pytest.raises(ValueError, match="Mismatch between the indices of faces and the number of nodes."):
+ with pytest.raises(ValueError,
+ match="Mismatch between the indices of faces and the number of nodes."):
surface.check_mesh_and_data(wrong_mesh, data)
# Alter the data and check that an error is raised
data = mesh[0][::2, 0]
- with pytest.raises(ValueError, match="Mismatch between number of nodes in mesh"):
+ with pytest.raises(ValueError,
+ match="Mismatch between number of nodes in mesh"):
surface.check_mesh_and_data(mesh, data)
+
+
+def test_check_surface():
+ coords, faces = generate_surf()
+ mesh = Mesh(coords, faces)
+ data = mesh[0][:,0]
+ surf = Surface(mesh, data)
+ s = surface.check_surface(surf)
+ assert_array_equal(s.data, data)
+ assert_array_equal(s.data, surf.data)
+ assert_array_equal(s.mesh.coordinates, coords)
+ assert_array_equal(s.mesh.coordinates, mesh.coordinates)
+ assert_array_equal(s.mesh.faces, faces)
+ assert_array_equal(s.mesh.faces, mesh.faces)
+ # Generate faces such that max index is larger than
+ # the length of coordinates array.
+ rng = np.random.RandomState(42)
+ wrong_faces = rng.randint(coords.shape[0] + 1, size=(30, 3))
+ wrong_mesh = Mesh(coords, wrong_faces)
+ wrong_surface = Surface(wrong_mesh, data)
+ # Check that check_mesh_and_data raises an error with the resulting wrong mesh
+ with pytest.raises(ValueError,
+ match="Mismatch between the indices of faces and the number of nodes."):
+ surface.check_surface(wrong_surface)
+ # Alter the data and check that an error is raised
+ wrong_data = mesh[0][::2, 0]
+ wrong_surface = Surface(mesh, wrong_data)
+ with pytest.raises(ValueError,
+ match="Mismatch between number of nodes in mesh"):
+ surface.check_surface(wrong_surface)
| Improve Issue Templates
As discussed in last meeting:
TODO:
- [x] Fix labels in current issue templates which are not added automatically
- [ ] Improve existing templates
- [x] Add new templates? (Documentation Improvement, Question, others...?) | 0.0 | [
"nilearn/datasets/tests/test_neurovault.py::test_remove_none_strings",
"nilearn/datasets/tests/test_neurovault.py::test_append_filters_to_query",
"nilearn/datasets/tests/test_neurovault.py::test_get_batch",
"nilearn/datasets/tests/test_neurovault.py::test_scroll_server_results",
"nilearn/datasets/tests/test_neurovault.py::test_is_null",
"nilearn/datasets/tests/test_neurovault.py::test_not_null",
"nilearn/datasets/tests/test_neurovault.py::test_not_equal",
"nilearn/datasets/tests/test_neurovault.py::test_order_comp",
"nilearn/datasets/tests/test_neurovault.py::test_is_in",
"nilearn/datasets/tests/test_neurovault.py::test_not_in",
"nilearn/datasets/tests/test_neurovault.py::test_contains",
"nilearn/datasets/tests/test_neurovault.py::test_not_contains",
"nilearn/datasets/tests/test_neurovault.py::test_pattern",
"nilearn/datasets/tests/test_neurovault.py::test_result_filter",
"nilearn/datasets/tests/test_neurovault.py::test_result_filter_combinations",
"nilearn/datasets/tests/test_neurovault.py::test_simple_download",
"nilearn/datasets/tests/test_neurovault.py::test_neurosynth_words_vectorized",
"nilearn/datasets/tests/test_neurovault.py::test_write_read_metadata",
"nilearn/datasets/tests/test_neurovault.py::test_add_absolute_paths",
"nilearn/datasets/tests/test_neurovault.py::test_json_add_collection_dir",
"nilearn/datasets/tests/test_neurovault.py::test_json_add_im_files_paths",
"nilearn/datasets/tests/test_neurovault.py::test_split_terms",
"nilearn/datasets/tests/test_neurovault.py::test_move_unknown_terms_to_local_filter",
"nilearn/datasets/tests/test_neurovault.py::test_move_col_id",
"nilearn/datasets/tests/test_neurovault.py::test_download_image_terms",
"nilearn/datasets/tests/test_neurovault.py::test_download_image",
"nilearn/datasets/tests/test_neurovault.py::test_fetch_neurovault",
"nilearn/datasets/tests/test_neurovault.py::test_fetch_neurovault_errors",
"nilearn/datasets/tests/test_neurovault.py::test_fetch_neurovault_ids",
"nilearn/decomposition/tests/test_canica.py::test_threshold_bound",
"nilearn/surface/tests/test_surface.py::test_load_surf_data_array",
"nilearn/surface/tests/test_surface.py::test_load_surf_data_gii_gz",
"nilearn/surface/tests/test_surface.py::test_load_surf_data_file_freesurfer",
"nilearn/surface/tests/test_surface.py::test_load_surf_data_file_error",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh",
"nilearn/surface/tests/test_surface.py::test_load_surface",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh_list",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh_file_gii_gz",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh_file_freesurfer",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh_file_error",
"nilearn/surface/tests/test_surface.py::test_load_surf_mesh_file_glob",
"nilearn/surface/tests/test_surface.py::test_flat_mesh[xy0]",
"nilearn/surface/tests/test_surface.py::test_flat_mesh[xy1]",
"nilearn/surface/tests/test_surface.py::test_flat_mesh[xy2]",
"nilearn/surface/tests/test_surface.py::test_vertex_outer_normals",
"nilearn/surface/tests/test_surface.py::test_load_uniform_ball_cloud",
"nilearn/surface/tests/test_surface.py::test_sample_locations",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[None-depth0]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[None-depth1]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[None-depth2]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[None-depth3]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[10-depth0]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[10-depth1]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[10-depth2]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_depth[10-depth3]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[None-1]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[None-7]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[depth2-8]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[depth3-8]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[depth4-8]",
"nilearn/surface/tests/test_surface.py::test_sample_locations_between_surfaces[depth5-8]",
"nilearn/surface/tests/test_surface.py::test_masked_indices",
"nilearn/surface/tests/test_surface.py::test_projection_matrix",
"nilearn/surface/tests/test_surface.py::test_sampling_affine",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-True-auto]",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-True-line]",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-True-ball]",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-False-auto]",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-False-line]",
"nilearn/surface/tests/test_surface.py::test_sampling[linear-False-ball]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-True-auto]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-True-line]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-True-ball]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-False-auto]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-False-line]",
"nilearn/surface/tests/test_surface.py::test_sampling[nearest-False-ball]",
"nilearn/surface/tests/test_surface.py::test_sampling_between_surfaces[linear]",
"nilearn/surface/tests/test_surface.py::test_sampling_between_surfaces[nearest]",
"nilearn/surface/tests/test_surface.py::test_choose_kind",
"nilearn/surface/tests/test_surface.py::test_check_mesh",
"nilearn/surface/tests/test_surface.py::test_check_mesh_and_data",
"nilearn/surface/tests/test_surface.py::test_check_surface"
] | [] | 2021-02-01 18:45:49+00:00 | 4,169 |
|
nilearn__nilearn-3003 | diff --git a/doc/whats_new.rst b/doc/whats_new.rst
index a3db610d8..38aae48a7 100644
--- a/doc/whats_new.rst
+++ b/doc/whats_new.rst
@@ -18,6 +18,12 @@ Enhancements
When `two_sided` is `False`, only values greater than or equal to the threshold
are retained.
+- :func:`nilearn.signal.clean` raises a warning when the user sets
+ parameters `detrend` and `standardize_confound` to False.
+ The user is suggested to set one of
+ those options to `True`, or standardize/demean the confounds before using the
+ function.
+
Changes
-------
diff --git a/nilearn/_utils/data_gen.py b/nilearn/_utils/data_gen.py
index a8855459a..9f70959a2 100644
--- a/nilearn/_utils/data_gen.py
+++ b/nilearn/_utils/data_gen.py
@@ -12,7 +12,6 @@ from scipy import ndimage
from sklearn.utils import check_random_state
import scipy.linalg
-import nibabel
from nibabel import Nifti1Image
@@ -143,7 +142,7 @@ def generate_maps(shape, n_regions, overlap=0, border=1,
mask[border:-border, border:-border, border:-border] = 1
ts = generate_regions_ts(mask.sum(), n_regions, overlap=overlap,
rand_gen=rand_gen, window=window)
- mask_img = nibabel.Nifti1Image(mask, affine)
+ mask_img = Nifti1Image(mask, affine)
return masking.unmask(ts, mask_img), mask_img
@@ -191,7 +190,7 @@ def generate_labeled_regions(shape, n_regions, rand_gen=None, labels=None,
row[row > 0] = n
data = np.zeros(shape, dtype=dtype)
data[np.ones(shape, dtype=bool)] = regions.sum(axis=0).T
- return nibabel.Nifti1Image(data, affine)
+ return Nifti1Image(data, affine)
def generate_labeled_regions_large(shape, n_regions, rand_gen=None,
@@ -206,7 +205,7 @@ def generate_labeled_regions_large(shape, n_regions, rand_gen=None,
data = rand_gen.randint(n_regions + 1, size=shape)
if len(np.unique(data)) != n_regions + 1:
raise ValueError("Some labels are missing. Maybe shape is too small.")
- return nibabel.Nifti1Image(data, affine)
+ return Nifti1Image(data, affine)
def generate_fake_fmri(shape=(10, 11, 12), length=17, kind="noise",
@@ -293,8 +292,8 @@ def generate_fake_fmri(shape=(10, 11, 12), length=17, kind="noise",
shift[2]:shift[2] + width[2]] = 1
if n_blocks is None:
- return (nibabel.Nifti1Image(fmri, affine),
- nibabel.Nifti1Image(mask, affine))
+ return (Nifti1Image(fmri, affine),
+ Nifti1Image(mask, affine))
block_size = 3 if block_size is None else block_size
flat_fmri = fmri[mask.astype(bool)]
@@ -330,8 +329,8 @@ def generate_fake_fmri(shape=(10, 11, 12), length=17, kind="noise",
else target.astype(np.float64)
fmri = np.zeros(fmri.shape)
fmri[mask.astype(bool)] = flat_fmri
- return (nibabel.Nifti1Image(fmri, affine),
- nibabel.Nifti1Image(mask, affine), target)
+ return (Nifti1Image(fmri, affine),
+ Nifti1Image(mask, affine), target)
def generate_fake_fmri_data_and_design(shapes, rk=3, affine=np.eye(4)):
diff --git a/nilearn/plotting/js_plotting_utils.py b/nilearn/plotting/js_plotting_utils.py
index 252ace1e7..9618d59a4 100644
--- a/nilearn/plotting/js_plotting_utils.py
+++ b/nilearn/plotting/js_plotting_utils.py
@@ -14,7 +14,7 @@ from matplotlib import cm as mpl_cm
# included here for backward compatibility
from nilearn.plotting.html_document import (
- HTMLDocument, set_max_img_views_before_warning,) # noqa
+ HTMLDocument, set_max_img_views_before_warning,) # noqa: F401
from .._utils.extmath import fast_abs_percentile
from .._utils.param_validation import check_threshold
from .. import surface
diff --git a/nilearn/reporting/glm_reporter.py b/nilearn/reporting/glm_reporter.py
index 50ea2e291..914b4351a 100644
--- a/nilearn/reporting/glm_reporter.py
+++ b/nilearn/reporting/glm_reporter.py
@@ -620,11 +620,11 @@ def _mask_to_svg(mask_img, bg_img):
"""
if mask_img:
- mask_plot = plot_roi(roi_img=mask_img, # noqa: F841
- bg_img=bg_img,
- display_mode='z',
- cmap='Set1',
- )
+ plot_roi(roi_img=mask_img,
+ bg_img=bg_img,
+ display_mode='z',
+ cmap='Set1',
+ )
mask_plot_svg = _plot_to_svg(plt.gcf())
# prevents sphinx-gallery & jupyter from scraping & inserting plots
plt.close()
@@ -899,7 +899,7 @@ def _stat_map_to_svg(stat_img,
raise ValueError('Invalid plot type provided. Acceptable options are'
"'slice' or 'glass'.")
with pd.option_context('display.precision', 2):
- stat_map_plot = _add_params_to_plot(table_details, stat_map_plot)
+ _add_params_to_plot(table_details, stat_map_plot)
fig = plt.gcf()
stat_map_svg = _plot_to_svg(fig)
# prevents sphinx-gallery & jupyter from scraping & inserting plots
@@ -933,10 +933,10 @@ def _add_params_to_plot(table_details, stat_map_plot):
wrap=True,
)
fig = list(stat_map_plot.axes.values())[0].ax.figure
- fig = _resize_plot_inches(plot=fig,
- width_change=.2,
- height_change=1,
- )
+ _resize_plot_inches(plot=fig,
+ width_change=.2,
+ height_change=1,
+ )
if stat_map_plot._black_bg:
suptitle_text.set_color('w')
return stat_map_plot
diff --git a/nilearn/signal.py b/nilearn/signal.py
index d1732b847..5809be638 100644
--- a/nilearn/signal.py
+++ b/nilearn/signal.py
@@ -529,6 +529,10 @@ def clean(signals, runs=None, detrend=True, standardize='zscore',
--------
nilearn.image.clean_img
"""
+ # Raise warning for some parameter combinations when confounds present
+ if confounds is not None:
+ _check_signal_parameters(detrend, standardize_confounds)
+
# Read confounds and signals
signals, runs, confounds = _sanitize_inputs(
signals, runs, confounds, sample_mask, ensure_finite
@@ -824,3 +828,15 @@ def _sanitize_signals(signals, ensure_finite):
if mask.any():
signals[mask] = 0
return _ensure_float(signals)
+
+
+def _check_signal_parameters(detrend, standardize_confounds):
+ """Raise warning if the combination is illogical"""
+ if not detrend and not standardize_confounds:
+ warnings.warn("When confounds are provided, one must perform detrend "
+ "and/or standarize confounds. You provided detrend={0}, "
+ "standardize_confounds={1}. If confounds were not "
+ "standardized or demeaned before passing to signal.clean"
+ " signal will not be correctly cleaned. ".format(
+ detrend, standardize_confounds)
+ )
diff --git a/nilearn/surface/surface.py b/nilearn/surface/surface.py
index 319a78fe1..c2c7261ca 100644
--- a/nilearn/surface/surface.py
+++ b/nilearn/surface/surface.py
@@ -3,10 +3,9 @@ Functions for surface manipulation.
"""
import os
import warnings
-import collections
import gzip
from distutils.version import LooseVersion
-from collections import namedtuple
+from collections import (namedtuple, Mapping)
import numpy as np
@@ -959,7 +958,7 @@ def _check_mesh(mesh):
"""
if isinstance(mesh, str):
return datasets.fetch_surf_fsaverage(mesh)
- if not isinstance(mesh, collections.Mapping):
+ if not isinstance(mesh, Mapping):
raise TypeError("The mesh should be a str or a dictionary, "
"you provided: {}.".format(type(mesh).__name__))
missing = {'pial_left', 'pial_right', 'sulc_left', 'sulc_right',
| nilearn/nilearn | 8ea37cdc13e8c953c4bbc396be3510bbc484299e | diff --git a/nilearn/tests/test_signal.py b/nilearn/tests/test_signal.py
index 76eed877d..1453c4c38 100644
--- a/nilearn/tests/test_signal.py
+++ b/nilearn/tests/test_signal.py
@@ -505,13 +505,17 @@ def test_clean_confounds():
# Test without standardizing that constant parts of confounds are
# accounted for
- np.testing.assert_almost_equal(nisignal.clean(np.ones((20, 2)),
- standardize=False,
- confounds=np.ones(20),
- standardize_confounds=False,
- detrend=False,
- ).mean(),
- np.zeros((20, 2)))
+ # passing standardize_confounds=False, detrend=False should raise warning
+ warning_message = r"must perform detrend and/or standarize confounds"
+ with pytest.warns(UserWarning, match=warning_message):
+ np.testing.assert_almost_equal(
+ nisignal.clean(np.ones((20, 2)),
+ standardize=False,
+ confounds=np.ones(20),
+ standardize_confounds=False,
+ detrend=False,
+ ).mean(),
+ np.zeros((20, 2)))
# Test to check that confounders effects are effectively removed from
# the signals when having a detrending and filtering operation together.
| Prevent user passing `standardize_confounds=False` with confound variables to `signal.clean`
<!--Provide a brief description what you would like changes and why.-->
While working on the test in #2946, I noticed there's no mechanism in place to prevent user from passing some illogical options, such as `standardize_confounds=False, detrend=False, standardize=False` and `standardize_confounds=False, detrend=False` when passing confound variables. When confound variables are present, they should always be demeaned or standardised.
<!--Please fill in the following information, to the best of your ability.-->
### Benefits to the change
Reduce the chance of mistake for users who decided not to use the default parameters.
### Pseudocode for the new behaviour, if applicable
I have thought of several ways:
1. Raise error when bad parameter combinations are passed to the function
2. Raise warning and fall back to default options
3. Warn the user without changing the provided parameters (proposed by @NicolasGensollen)
Here's a list of option combinations that should not be allowed in `signal.clean`.
|`standardize_confounds`|`detrend`|`standardize`|
|--|--|--|
|False|False|zscore|
|False|False|psc|
|False|False|False| | 0.0 | [
"nilearn/tests/test_signal.py::test_clean_confounds"
] | [
"nilearn/tests/test_signal.py::test_butterworth",
"nilearn/tests/test_signal.py::test_standardize",
"nilearn/tests/test_signal.py::test_detrend",
"nilearn/tests/test_signal.py::test_mean_of_squares",
"nilearn/tests/test_signal.py::test_row_sum_of_squares",
"nilearn/tests/test_signal.py::test_clean_detrending",
"nilearn/tests/test_signal.py::test_clean_t_r",
"nilearn/tests/test_signal.py::test_clean_frequencies",
"nilearn/tests/test_signal.py::test_clean_runs",
"nilearn/tests/test_signal.py::test_clean_frequencies_using_power_spectrum_density",
"nilearn/tests/test_signal.py::test_clean_finite_no_inplace_mod",
"nilearn/tests/test_signal.py::test_high_variance_confounds",
"nilearn/tests/test_signal.py::test_clean_psc",
"nilearn/tests/test_signal.py::test_clean_zscore",
"nilearn/tests/test_signal.py::test_cosine_filter",
"nilearn/tests/test_signal.py::test_sample_mask"
] | 2021-10-01 16:42:05+00:00 | 4,170 |
|
nilearn__nilearn-3353 | diff --git a/nilearn/datasets/atlas.py b/nilearn/datasets/atlas.py
index 3841234e7..4cb32d34a 100644
--- a/nilearn/datasets/atlas.py
+++ b/nilearn/datasets/atlas.py
@@ -12,7 +12,6 @@ import shutil
import nibabel as nb
import numpy as np
import pandas as pd
-from numpy.lib import recfunctions
import re
from sklearn.utils import Bunch
@@ -36,7 +35,8 @@ def fetch_atlas_difumo(dimension=64, resolution_mm=2, data_dir=None,
Dictionaries of Functional Modes, or “DiFuMo”, can serve as
:term:`probabilistic atlases<Probabilistic atlas>` to extract
- functional signals with different dimensionalities (64, 128, 256, 512, and 1024).
+ functional signals with different dimensionalities (64, 128,
+ 256, 512, and 1024).
These modes are optimized to represent well raw :term:`BOLD` timeseries,
over a with range of experimental conditions.
See :footcite:`Dadi2020`.
@@ -139,13 +139,12 @@ def fetch_atlas_difumo(dimension=64, resolution_mm=2, data_dir=None,
fdescr = _get_dataset_descr(dataset_name)
- params = dict(description=fdescr, maps=files_[1], labels=labels)
-
- return Bunch(**params)
+ return Bunch(description=fdescr, maps=files_[1], labels=labels)
@fill_doc
-def fetch_atlas_craddock_2012(data_dir=None, url=None, resume=True, verbose=1):
+def fetch_atlas_craddock_2012(data_dir=None, url=None, resume=True,
+ verbose=1, homogeneity=None, grp_mean=True):
"""Download and return file names for the Craddock 2012 parcellation.
This function returns a :term:`probabilistic atlas<Probabilistic atlas>`.
@@ -163,6 +162,11 @@ def fetch_atlas_craddock_2012(data_dir=None, url=None, resume=True, verbose=1):
%(url)s
%(resume)s
%(verbose)s
+ homogeneity: :obj:`str`, optional
+ The choice of the homogeneity ('spatial' or 'temporal' or 'random')
+ grp_mean: :obj:`bool`, optional
+ The choice of the parcellation (with group_mean or without)
+ Default=True.
Returns
-------
@@ -181,6 +185,14 @@ def fetch_atlas_craddock_2012(data_dir=None, url=None, resume=True, verbose=1):
parcellation obtained with random clustering.
- 'description': :obj:`str`, general description of the dataset.
+ Warns
+ -----
+ FutureWarning
+ If an homogeneity input is provided, the current behavior
+ (returning multiple maps) is deprecated.
+ Starting in version 0.13, one map will be returned depending on
+ the homogeneity value.
+
References
----------
.. footbibliography::
@@ -192,25 +204,45 @@ def fetch_atlas_craddock_2012(data_dir=None, url=None, resume=True, verbose=1):
opts = {'uncompress': True}
dataset_name = "craddock_2012"
+
keys = ("scorr_mean", "tcorr_mean",
"scorr_2level", "tcorr_2level",
"random")
filenames = [
- ("scorr05_mean_all.nii.gz", url, opts),
- ("tcorr05_mean_all.nii.gz", url, opts),
- ("scorr05_2level_all.nii.gz", url, opts),
- ("tcorr05_2level_all.nii.gz", url, opts),
- ("random_all.nii.gz", url, opts)
+ ("scorr05_mean_all.nii.gz", url, opts),
+ ("tcorr05_mean_all.nii.gz", url, opts),
+ ("scorr05_2level_all.nii.gz", url, opts),
+ ("tcorr05_2level_all.nii.gz", url, opts),
+ ("random_all.nii.gz", url, opts)
]
data_dir = _get_dataset_dir(dataset_name, data_dir=data_dir,
verbose=verbose)
+
sub_files = _fetch_files(data_dir, filenames, resume=resume,
verbose=verbose)
fdescr = _get_dataset_descr(dataset_name)
- params = dict([('description', fdescr)] + list(zip(keys, sub_files)))
+ if homogeneity:
+ if homogeneity in ['spatial', 'temporal']:
+ if grp_mean:
+ filename = [(homogeneity[0] + "corr05_mean_all.nii.gz",
+ url, opts)]
+ else:
+ filename = [(homogeneity[0]
+ + "corr05_2level_all.nii.gz", url, opts)]
+ else:
+ filename = [("random_all.nii.gz", url, opts)]
+ data = _fetch_files(data_dir, filename, resume=resume, verbose=verbose)
+ params = dict(map=data[0], description=fdescr)
+ else:
+ params = dict([('description', fdescr)] + list(zip(keys, sub_files)))
+ warnings.warn(category=FutureWarning,
+ message="The default behavior of the function will "
+ "be deprecated and replaced in release 0.13 "
+ "to use the new parameters homogeneity "
+ "and grp_mean.")
return Bunch(**params)
@@ -531,11 +563,12 @@ def fetch_atlas_juelich(atlas_name, data_dir=None,
if is_probabilistic and symmetric_split:
raise ValueError("Region splitting not supported for probabilistic "
"atlases")
- atlas_img, atlas_filename, names, _ = _get_atlas_data_and_labels("Juelich",
- atlas_name,
- data_dir=data_dir,
- resume=resume,
- verbose=verbose)
+ atlas_img, atlas_filename, names, _ = \
+ _get_atlas_data_and_labels("Juelich",
+ atlas_name,
+ data_dir=data_dir,
+ resume=resume,
+ verbose=verbose)
atlas_niimg = check_niimg(atlas_img)
atlas_data = get_data(atlas_niimg)
@@ -811,8 +844,9 @@ def fetch_coords_power_2011(legacy_format=True):
@fill_doc
-def fetch_atlas_smith_2009(data_dir=None, mirror='origin', url=None,
- resume=True, verbose=1):
+def fetch_atlas_smith_2009(data_dir=None, url=None, resume=True,
+ verbose=1, mirror='origin', dimension=None,
+ resting=True):
"""Download and load the Smith :term:`ICA` and BrainMap
:term:`Probabilistic atlas` (2009).
@@ -821,13 +855,19 @@ def fetch_atlas_smith_2009(data_dir=None, mirror='origin', url=None,
Parameters
----------
%(data_dir)s
+ %(url)s
+ %(resume)s
+ %(verbose)s
mirror : :obj:`str`, optional
By default, the dataset is downloaded from the original website of the
atlas. Specifying "nitrc" will force download from a mirror, with
potentially higher bandwidth. Default='origin'.
- %(url)s
- %(resume)s
- %(verbose)s
+ dimension: :obj:`int`, optional
+ Number of dimensions in the dictionary. Valid resolutions
+ available are {10, 20, 70}.
+ resting: :obj:`bool`, optional
+ Either to fetch the resting-:term:`fMRI` or BrainMap components
+ Default=True.
Returns
-------
@@ -856,6 +896,14 @@ def fetch_atlas_smith_2009(data_dir=None, mirror='origin', url=None,
The shape of the image is ``(91, 109, 91, 70)``.
- 'description': :obj:`str`, description of the atlas.
+ Warns
+ -----
+ FutureWarning
+ If a dimension input is provided, the current behavior
+ (returning multiple maps) is deprecated.
+ Starting in version 0.13, one map will be returned depending on
+ the dimension value.
+
References
----------
.. footbibliography::
@@ -871,42 +919,55 @@ def fetch_atlas_smith_2009(data_dir=None, mirror='origin', url=None,
url = "http://www.fmrib.ox.ac.uk/datasets/brainmap+rsns/"
elif mirror == 'nitrc':
url = [
- 'https://www.nitrc.org/frs/download.php/7730/',
- 'https://www.nitrc.org/frs/download.php/7729/',
- 'https://www.nitrc.org/frs/download.php/7731/',
- 'https://www.nitrc.org/frs/download.php/7726/',
- 'https://www.nitrc.org/frs/download.php/7728/',
- 'https://www.nitrc.org/frs/download.php/7727/',
+ 'https://www.nitrc.org/frs/download.php/7730/',
+ 'https://www.nitrc.org/frs/download.php/7729/',
+ 'https://www.nitrc.org/frs/download.php/7731/',
+ 'https://www.nitrc.org/frs/download.php/7726/',
+ 'https://www.nitrc.org/frs/download.php/7728/',
+ 'https://www.nitrc.org/frs/download.php/7727/',
]
else:
raise ValueError('Unknown mirror "%s". Mirror must be "origin" '
- 'or "nitrc"' % str(mirror))
+ 'or "nitrc"' % str(mirror))
- files = [
- 'rsn20.nii.gz',
- 'PNAS_Smith09_rsn10.nii.gz',
- 'rsn70.nii.gz',
- 'bm20.nii.gz',
- 'PNAS_Smith09_bm10.nii.gz',
- 'bm70.nii.gz'
- ]
+ files = {
+ 'rsn20': 'rsn20.nii.gz',
+ 'rsn10': 'PNAS_Smith09_rsn10.nii.gz',
+ 'rsn70': 'rsn70.nii.gz',
+ 'bm20': 'bm20.nii.gz',
+ 'bm10': 'PNAS_Smith09_bm10.nii.gz',
+ 'bm70': 'bm70.nii.gz'
+ }
if isinstance(url, str):
url = [url] * len(files)
- files = [(f, u + f, {}) for f, u in zip(files, url)]
-
dataset_name = 'smith_2009'
data_dir = _get_dataset_dir(dataset_name, data_dir=data_dir,
verbose=verbose)
- files_ = _fetch_files(data_dir, files, resume=resume,
- verbose=verbose)
fdescr = _get_dataset_descr(dataset_name)
- keys = ['rsn20', 'rsn10', 'rsn70', 'bm20', 'bm10', 'bm70']
- params = dict(zip(keys, files_))
- params['description'] = fdescr
+ if dimension:
+ key = f"{'rsn' if resting else 'bm'}{dimension}"
+ key_index = list(files).index(key)
+
+ file = [(files[key], url[key_index] + files[key], {})]
+ data = _fetch_files(data_dir, file, resume=resume,
+ verbose=verbose)
+ params = Bunch(map=data[0], description=fdescr)
+ else:
+ keys = list(files.keys())
+ files = [(f, u + f, {}) for f, u in zip(files.values(), url)]
+ files_ = _fetch_files(data_dir, files, resume=resume,
+ verbose=verbose)
+ params = dict(zip(keys, files_))
+ params['description'] = fdescr
+ warnings.warn(category=FutureWarning,
+ message="The default behavior of the function will "
+ "be deprecated and replaced in release 0.13 "
+ "to use the new parameters dimension and "
+ "resting.")
return Bunch(**params)
@@ -996,7 +1057,7 @@ def fetch_atlas_yeo_2011(data_dir=None, url=None, resume=True, verbose=1):
for f in basenames]
data_dir = _get_dataset_dir(dataset_name, data_dir=data_dir,
- verbose=verbose)
+ verbose=verbose)
sub_files = _fetch_files(data_dir, filenames, resume=resume,
verbose=verbose)
@@ -1139,8 +1200,9 @@ def fetch_atlas_aal(version='SPM12', data_dir=None, url=None, resume=True,
@fill_doc
-def fetch_atlas_basc_multiscale_2015(version='sym', data_dir=None, url=None,
- resume=True, verbose=1):
+def fetch_atlas_basc_multiscale_2015(data_dir=None, url=None, resume=True,
+ verbose=1, resolution=None,
+ version='sym'):
"""Downloads and loads multiscale functional brain parcellations.
This :term:`Deterministic atlas` includes group brain parcellations
@@ -1148,11 +1210,11 @@ def fetch_atlas_basc_multiscale_2015(version='sym', data_dir=None, url=None,
:term:`functional magnetic resonance images<fMRI>` from about 200 young
healthy subjects.
- Multiple scales (number of networks) are available, among
+ Multiple resolutions (number of networks) are available, among
7, 12, 20, 36, 64, 122, 197, 325, 444. The brain parcellations
have been generated using a method called bootstrap analysis of
stable clusters called as BASC :footcite:`Bellec2010`,
- and the scales have been selected using a data-driven method
+ and the resolutions have been selected using a data-driven method
called MSTEPS :footcite:`Bellec2013`.
Note that two versions of the template are available, 'sym' or 'asym'.
@@ -1169,14 +1231,17 @@ def fetch_atlas_basc_multiscale_2015(version='sym', data_dir=None, url=None,
Parameters
----------
- version : {'sym', 'asym'}, optional
- Available versions are 'sym' or 'asym'. By default all scales of
- brain parcellations of version 'sym' will be returned.
- Default='sym'.
%(data_dir)s
%(url)s
%(resume)s
%(verbose)s
+ resolution: :ob:`int`, optional
+ Number of networks in the dictionary. Valid resolutions
+ available are {7, 12, 20, 36, 64, 122, 197, 325, 444}
+ version : {'sym', 'asym'}, optional
+ Available versions are 'sym' or 'asym'. By default all scales of
+ brain parcellations of version 'sym' will be returned.
+ Default='sym'.
Returns
-------
@@ -1190,6 +1255,14 @@ def fetch_atlas_basc_multiscale_2015(version='sym', data_dir=None, url=None,
values from 0 to the selected number of networks (scale).
- "description": :obj:`str`, details about the data release.
+ Warns
+ -----
+ FutureWarning
+ If a resolution input is provided, the current behavior
+ (returning multiple maps) is deprecated.
+ Starting in version 0.13, one map will be returned depending on
+ the resolution value.
+
References
----------
.. footbibliography::
@@ -1206,32 +1279,47 @@ def fetch_atlas_basc_multiscale_2015(version='sym', data_dir=None, url=None,
'does not exist. Please choose one among them %s.' %
(version, str(versions)))
- keys = ['scale007', 'scale012', 'scale020', 'scale036', 'scale064',
- 'scale122', 'scale197', 'scale325', 'scale444']
-
if version == 'sym':
url = "https://ndownloader.figshare.com/files/1861819"
elif version == 'asym':
url = "https://ndownloader.figshare.com/files/1861820"
opts = {'uncompress': True}
+ keys = ['scale007', 'scale012', 'scale020', 'scale036', 'scale064',
+ 'scale122', 'scale197', 'scale325', 'scale444']
+
dataset_name = "basc_multiscale_2015"
data_dir = _get_dataset_dir(dataset_name, data_dir=data_dir,
verbose=verbose)
folder_name = 'template_cambridge_basc_multiscale_nii_' + version
- basenames = ['template_cambridge_basc_multiscale_' + version +
- '_' + key + '.nii.gz' for key in keys]
-
- filenames = [(os.path.join(folder_name, basename), url, opts)
- for basename in basenames]
- data = _fetch_files(data_dir, filenames, resume=resume, verbose=verbose)
+ fdescr = _get_dataset_descr(dataset_name)
- descr = _get_dataset_descr(dataset_name)
+ if resolution:
+ basename = 'template_cambridge_basc_multiscale_' + version + \
+ f'_scale{resolution:03}' + '.nii.gz'
- params = dict(zip(keys, data))
- params['description'] = descr
+ filename = [(os.path.join(folder_name, basename), url, opts)]
+ data = _fetch_files(data_dir, filename, resume=resume, verbose=verbose)
+ params = Bunch(map=data[0], description=fdescr)
+ else:
+ basenames = ['template_cambridge_basc_multiscale_' + version +
+ '_' + key + '.nii.gz' for key in keys]
+ filenames = [(os.path.join(folder_name, basename), url, opts)
+ for basename in basenames]
+ data = _fetch_files(data_dir, filenames, resume=resume,
+ verbose=verbose)
+
+ descr = _get_dataset_descr(dataset_name)
+
+ params = dict(zip(keys, data))
+ params['description'] = descr
+ warnings.warn(category=FutureWarning,
+ message="The default behavior of the function will "
+ "be deprecated and replaced in release 0.13 "
+ "to use the new parameters resolution and "
+ "and version.")
return Bunch(**params)
@@ -1548,7 +1636,7 @@ def fetch_atlas_surf_destrieux(data_dir=None, url=None,
annot_left = nb.freesurfer.read_annot(annots[0])
annot_right = nb.freesurfer.read_annot(annots[1])
- return Bunch(labels=annot_left[2], map_left=annot_left[0],
+ return Bunch(labels=annot_left[2], map_left=annot_left[0],
map_right=annot_right[0], description=fdescr)
@@ -1727,8 +1815,8 @@ def fetch_atlas_pauli_2017(version='prob', data_dir=None, verbose=1):
url_maps = 'https://osf.io/5mqfx/download'
filename = 'pauli_2017_det.nii.gz'
else:
- raise NotImplementedError('{} is no valid version for '.format(version) + \
- 'the Pauli atlas')
+ raise NotImplementedError(f'{version} is no valid version for '
+ + 'the Pauli atlas')
url_labels = 'https://osf.io/6qrcb/download'
dataset_name = 'pauli_2017'
@@ -1738,10 +1826,10 @@ def fetch_atlas_pauli_2017(version='prob', data_dir=None, verbose=1):
files = [(filename,
url_maps,
- {'move':filename}),
+ {'move': filename}),
('labels.txt',
url_labels,
- {'move':'labels.txt'})]
+ {'move': 'labels.txt'})]
atlas_file, labels = _fetch_files(data_dir, files)
labels = np.loadtxt(labels, dtype=str)[:, 1].tolist()
@@ -1849,7 +1937,8 @@ def fetch_atlas_schaefer_2018(n_rois=400, yeo_networks=7, resolution_mm=1,
"options: {}".format(n_rois, valid_n_rois))
if yeo_networks not in valid_yeo_networks:
raise ValueError("Requested yeo_networks={} not available. Valid "
- "options: {}".format(yeo_networks,valid_yeo_networks))
+ "options: {}".format(yeo_networks,
+ valid_yeo_networks))
if resolution_mm not in valid_resolution_mm:
raise ValueError("Requested resolution_mm={} not available. Valid "
"options: {}".format(resolution_mm,
| nilearn/nilearn | e1e40d82f4ebbbe560a66f29c872635901a59bc4 | diff --git a/doc/changes/latest.rst b/doc/changes/latest.rst
index 1116285f6..44c8470f7 100644
--- a/doc/changes/latest.rst
+++ b/doc/changes/latest.rst
@@ -16,6 +16,7 @@ Enhancements
Changes
-------
+- The behavior of :func:`~nilearn.datasets.fetch_atlas_craddock_2012`, :func:`~nilearn.datasets.fetch_atlas_smith_2009` and :func:`~nilearn.datasets.fetch_atlas_basc_multiscale_2015` is updated with their new parameters to return one map along with a deprecation cycle (:gh:`3353` by `Ahmad Chamma`_).
0.10.1rc1
diff --git a/nilearn/datasets/tests/test_atlas.py b/nilearn/datasets/tests/test_atlas.py
index 648e37b3f..d09d98d57 100644
--- a/nilearn/datasets/tests/test_atlas.py
+++ b/nilearn/datasets/tests/test_atlas.py
@@ -263,6 +263,25 @@ def test_fetch_atlas_craddock_2012(tmp_path, request_mocker):
local_archive = Path(
__file__).parent / "data" / "craddock_2011_parcellations.tar.gz"
request_mocker.url_mapping["*craddock*"] = local_archive
+ bunch = atlas.fetch_atlas_craddock_2012(data_dir=tmp_path,
+ verbose=0, homogeneity='spatial')
+ bunch_rand = atlas.fetch_atlas_craddock_2012(data_dir=tmp_path,
+ verbose=0,
+ homogeneity='random')
+ bunch_no_mean = atlas.fetch_atlas_craddock_2012(data_dir=tmp_path,
+ verbose=0,
+ grp_mean=False,
+ homogeneity='spatial')
+ assert request_mocker.url_count == 1
+ assert bunch['map'] == str(tmp_path / 'craddock_2012'
+ / 'scorr05_mean_all.nii.gz')
+ assert bunch_rand['map'] == str(tmp_path / 'craddock_2012'
+ / 'random_all.nii.gz')
+ assert bunch_no_mean['map'] == str(tmp_path / 'craddock_2012'
+ / 'scorr05_2level_all.nii.gz')
+ assert bunch.description != ''
+
+ # Old code
bunch = atlas.fetch_atlas_craddock_2012(data_dir=tmp_path,
verbose=0)
@@ -283,6 +302,13 @@ def test_fetch_atlas_craddock_2012(tmp_path, request_mocker):
def test_fetch_atlas_smith_2009(tmp_path, request_mocker):
+ bunch = atlas.fetch_atlas_smith_2009(data_dir=tmp_path, verbose=0,
+ dimension=20)
+ assert bunch['map'] == str(tmp_path / 'smith_2009' / 'rsn20.nii.gz')
+ assert len(bunch.keys()) == 2
+ assert bunch.description != ''
+
+ # Old code
bunch = atlas.fetch_atlas_smith_2009(data_dir=tmp_path, verbose=0)
keys = ("rsn20", "rsn10", "rsn70",
@@ -412,7 +438,8 @@ def test_fetch_atlas_difumo(tmp_path, request_mocker):
"CSF": ["" for _ in range(dim)]}
)
root = Path("{0}".format(dim))
- archive = {root / "labels_{0}_dictionary.csv".format(dim): labels.to_csv(index=False),
+ archive = {root / "labels_{0}_dictionary.csv".format(dim):
+ labels.to_csv(index=False),
root / "2mm" / "maps.nii.gz": "",
root / "3mm" / "maps.nii.gz": ""}
request_mocker.url_mapping[url] = dict_to_archive(archive, "zip")
@@ -481,6 +508,40 @@ def test_fetch_atlas_aal_version_error(tmp_path, request_mocker):
def test_fetch_atlas_basc_multiscale_2015(tmp_path, request_mocker):
+ # default version='sym',
+ data_sym = atlas.fetch_atlas_basc_multiscale_2015(data_dir=tmp_path,
+ verbose=0, resolution=7)
+ # version='asym'
+ data_asym = atlas.fetch_atlas_basc_multiscale_2015(version='asym',
+ verbose=0,
+ data_dir=tmp_path,
+ resolution=7)
+
+ dataset_name = 'basc_multiscale_2015'
+ name_sym = 'template_cambridge_basc_multiscale_nii_sym'
+ basename_sym = 'template_cambridge_basc_multiscale_sym_scale007.nii.gz'
+
+ assert data_sym['map'] == str(tmp_path / dataset_name / name_sym
+ / basename_sym)
+
+ name_asym = 'template_cambridge_basc_multiscale_nii_asym'
+ basename_asym = 'template_cambridge_basc_multiscale_asym_scale007.nii.gz'
+ assert data_asym['map'] == str(tmp_path / dataset_name / name_asym
+ / basename_asym)
+
+ assert len(data_sym) == 2
+ with pytest.raises(
+ ValueError,
+ match='The version of Brain parcellations requested "aym"'):
+ atlas.fetch_atlas_basc_multiscale_2015(version="aym",
+ data_dir=tmp_path,
+ verbose=0)
+
+ assert request_mocker.url_count == 2
+ assert data_sym.description != ''
+ assert data_asym.description != ''
+
+ # Old code
# default version='sym',
data_sym = atlas.fetch_atlas_basc_multiscale_2015(data_dir=tmp_path,
verbose=0)
@@ -556,9 +617,9 @@ def test_fetch_atlas_surf_destrieux(tmp_path, request_mocker, verbose=0):
# Create mock annots
for hemi in ('left', 'right'):
nibabel.freesurfer.write_annot(
- os.path.join(data_dir,
- '%s.aparc.a2009s.annot' % hemi),
- np.arange(4), np.zeros((4, 5)), 5 * ['a'],)
+ os.path.join(data_dir,
+ '%s.aparc.a2009s.annot' % hemi),
+ np.arange(4), np.zeros((4, 5)), 5 * ['a'],)
bunch = atlas.fetch_atlas_surf_destrieux(data_dir=tmp_path, verbose=0)
# Our mock annots have 4 labels
| Ensure `fetch_atlas_*` functions returns one atlas map only
<!--Provide a brief description what you would like changes and why.-->
Some of the possibly older multiscale atlas fetcher is slightly inconsistent with how the later implemented ones
For example, `nilearn.dataset.fetch_atlas_basc_multiscale_2015` would return all resolutions:
https://github.com/nilearn/nilearn/blob/2fd6665664f27dfbf1f10aeeba660adc9b560361/nilearn/datasets/atlas.py#L965-L972
Craddock returns all atlas construction methods
https://github.com/nilearn/nilearn/blob/1607b52458c28953a87bbe6f42448b7b4e30a72f/nilearn/datasets/atlas.py#L142-L148
For some latter implemented ones - Difumo would require resolution to be specified:
https://github.com/nilearn/nilearn/blob/1607b52458c28953a87bbe6f42448b7b4e30a72f/nilearn/datasets/atlas.py#L46-L58
Julich needs atlas name:
https://github.com/nilearn/nilearn/blob/1607b52458c28953a87bbe6f42448b7b4e30a72f/nilearn/datasets/atlas.py#L363-L370
<!--Please fill in the following information, to the best of your ability.-->
### Benefits to the change
Improve consistency. Ensuring `fetch_atlas_*` functions only return one atlas map would be more intuitive.
I hope the atlas path will be under the label `maps`, so it's consistent amongst atlas fetchers.
### Pseudocode for the new behavior, if applicable
Using `nilearn.dataset.fetch_atlas_basc_multiscale_2015` as an example:
```python
>> parcellations = fetch_atlas_basc_multiscale_2015(resolution=None) # old behaviour
>> parcellations.keys()
dict_keys(['scale007', 'scale012', 'scale020', 'scale036', 'scale064', 'scale122', 'scale197', 'scale325', 'scale444', 'description'])
>>parcellations.scale007
'/path/to/nilearn_data/basc_multiscale_2015/template_cambridge_basc_multiscale_nii_sym/template_cambridge_basc_multiscale_sym_scale007.nii.gz'
>> parcellation = fetch_atlas_basc_multiscale_2015(resolution=7)
>> parcellation.keys()
dict_keys(['maps', 'description'])
>> parcellations.map
'/path/to/nilearn_data/basc_multiscale_2015/template_cambridge_basc_multiscale_nii_sym/template_cambridge_basc_multiscale_sym_scale007.nii.gz'
```
Are there any other older atlas fetcher that I am missing here?
Happy to do the PR! | 0.0 | [
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_craddock_2012",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_smith_2009",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_basc_multiscale_2015"
] | [
"nilearn/datasets/tests/test_atlas.py::test_get_dataset_dir",
"nilearn/datasets/tests/test_atlas.py::test_downloader",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_source",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl_errors[HarvardOxford-cortl-prob-1mm]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl_errors[Juelich-prob-1mm]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[HarvardOxford--Cortical-cort-prob-1mm-False-False]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[HarvardOxford--Subcortical-sub-maxprob-thr0-1mm-False-True]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[HarvardOxford--Cortical-Lateralized-cortl-maxprob-thr0-1mm-True-True]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[Juelich--prob-1mm-False-False]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[Juelich--maxprob-thr0-1mm-False-False]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_fsl[Juelich--maxprob-thr0-1mm-False-True]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_coords_power_2011",
"nilearn/datasets/tests/test_atlas.py::test_fetch_coords_seitzman_2018",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_destrieux_2009[True]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_destrieux_2009[False]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_msdl",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_yeo_2011",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_difumo",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_aal[SPM5-zip-uploads]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_aal[SPM8-zip-uploads]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_aal[SPM12-gztar-AAL_files]",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_aal_version_error",
"nilearn/datasets/tests/test_atlas.py::test_fetch_coords_dosenbach_2010",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_allen_2011",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_surf_destrieux",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_talairach",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_pauli_2017",
"nilearn/datasets/tests/test_atlas.py::test_fetch_atlas_schaefer_2018"
] | 2022-08-31 11:18:48+00:00 | 4,171 |
|
nilearn__nilearn-3439 | diff --git a/nilearn/_utils/glm.py b/nilearn/_utils/glm.py
index 591bd6f54..79da26cd3 100644
--- a/nilearn/_utils/glm.py
+++ b/nilearn/_utils/glm.py
@@ -341,11 +341,24 @@ def _check_run_sample_masks(n_runs, sample_masks):
raise TypeError(
f"sample_mask has an unhandled type: {sample_masks.__class__}"
)
- if not isinstance(sample_masks, (list, tuple)):
+
+ if isinstance(sample_masks, np.ndarray):
sample_masks = (sample_masks, )
- if len(sample_masks) != n_runs:
+
+ checked_sample_masks = [_convert_bool2index(sm) for sm in sample_masks]
+
+ if len(checked_sample_masks) != n_runs:
raise ValueError(
- f"Number of sample_mask ({len(sample_masks)}) not matching "
- f"number of runs ({n_runs})."
+ f"Number of sample_mask ({len(checked_sample_masks)}) not "
+ f"matching number of runs ({n_runs})."
)
- return sample_masks
+ return checked_sample_masks
+
+
+def _convert_bool2index(sample_mask):
+ """Convert boolean to index. """
+ check_boolean = [type(i) is bool or type(i) is np.bool_
+ for i in sample_mask]
+ if all(check_boolean):
+ sample_mask = np.where(sample_mask)[0]
+ return sample_mask
diff --git a/nilearn/datasets/struct.py b/nilearn/datasets/struct.py
index 05a2602a9..89c3b1a09 100644
--- a/nilearn/datasets/struct.py
+++ b/nilearn/datasets/struct.py
@@ -37,10 +37,6 @@ _LEGACY_FORMAT_MSG = (
"instead of recarrays."
)
-# workaround for
-# https://github.com/nilearn/nilearn/pull/2738#issuecomment-869018842
-_MNI_RES_WARNING_ALREADY_SHOWN = False
-
@fill_doc
def fetch_icbm152_2009(data_dir=None, url=None, resume=True, verbose=1):
@@ -172,7 +168,7 @@ def load_mni152_template(resolution=None):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template is re-sampled with the
specified resolution.
@@ -200,14 +196,7 @@ def load_mni152_template(resolution=None):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning,
- stacklevel=2)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
brain_template = check_niimg(MNI152_FILE_PATH)
@@ -237,7 +226,7 @@ def load_mni152_gm_template(resolution=None):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template is re-sampled with the
specified resolution.
@@ -256,13 +245,7 @@ def load_mni152_gm_template(resolution=None):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
gm_template = check_niimg(GM_MNI152_FILE_PATH)
@@ -292,7 +275,7 @@ def load_mni152_wm_template(resolution=None):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template is re-sampled with the
specified resolution.
@@ -311,13 +294,7 @@ def load_mni152_wm_template(resolution=None):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
wm_template = check_niimg(WM_MNI152_FILE_PATH)
@@ -346,7 +323,7 @@ def load_mni152_brain_mask(resolution=None, threshold=0.2):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template loaded is first
re-sampled with the specified resolution.
@@ -372,13 +349,7 @@ def load_mni152_brain_mask(resolution=None, threshold=0.2):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
# Load MNI template
target_img = load_mni152_template(resolution=resolution)
@@ -397,7 +368,7 @@ def load_mni152_gm_mask(resolution=None, threshold=0.2, n_iter=2):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template loaded is first
re-sampled with the specified resolution.
@@ -425,13 +396,7 @@ def load_mni152_gm_mask(resolution=None, threshold=0.2, n_iter=2):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
# Load MNI template
gm_target = load_mni152_gm_template(resolution=resolution)
@@ -455,7 +420,7 @@ def load_mni152_wm_mask(resolution=None, threshold=0.2, n_iter=2):
Parameters
----------
- resolution: int, optional, Default = 2
+ resolution: int, optional, Default = 1
If resolution is different from 1, the template loaded is first
re-sampled with the specified resolution.
@@ -483,13 +448,7 @@ def load_mni152_wm_mask(resolution=None, threshold=0.2, n_iter=2):
"""
- global _MNI_RES_WARNING_ALREADY_SHOWN
- if resolution is None:
- if not _MNI_RES_WARNING_ALREADY_SHOWN:
- warnings.warn("Default resolution of the MNI template will change "
- "from 2mm to 1mm in version 0.10.0", FutureWarning)
- _MNI_RES_WARNING_ALREADY_SHOWN = True
- resolution = 2
+ resolution = resolution or 1
# Load MNI template
wm_target = load_mni152_wm_template(resolution=resolution)
diff --git a/nilearn/image/image.py b/nilearn/image/image.py
index 0bbcfd0b8..257f16a17 100644
--- a/nilearn/image/image.py
+++ b/nilearn/image/image.py
@@ -631,24 +631,24 @@ def index_img(imgs, index):
>>> joint_mni_image = concat_imgs([datasets.load_mni152_template(),
... datasets.load_mni152_template()])
>>> print(joint_mni_image.shape)
- (99, 117, 95, 2)
+ (197, 233, 189, 2)
We can now select one slice from the last dimension of this 4D-image::
>>> single_mni_image = index_img(joint_mni_image, 1)
>>> print(single_mni_image.shape)
- (99, 117, 95)
+ (197, 233, 189)
We can also select multiple frames using the `slice` constructor::
>>> five_mni_images = concat_imgs([datasets.load_mni152_template()] * 5)
>>> print(five_mni_images.shape)
- (99, 117, 95, 5)
+ (197, 233, 189, 5)
>>> first_three_images = index_img(five_mni_images,
... slice(0, 3))
>>> print(first_three_images.shape)
- (99, 117, 95, 3)
+ (197, 233, 189, 3)
"""
imgs = check_niimg_4d(imgs)
diff --git a/nilearn/image/resampling.py b/nilearn/image/resampling.py
index 679a6c11a..d4d77d5ef 100644
--- a/nilearn/image/resampling.py
+++ b/nilearn/image/resampling.py
@@ -131,7 +131,7 @@ def coord_transform(x, y, z, affine):
>>> niimg = datasets.load_mni152_template()
>>> # Find the MNI coordinates of the voxel (50, 50, 50)
>>> image.coord_transform(50, 50, 50, niimg.affine)
- (2.0, -34.0, 28.0)
+ (-48.0, -84.0, -22.0)
"""
squeeze = (not hasattr(x, '__iter__'))
diff --git a/nilearn/signal.py b/nilearn/signal.py
index 9991b3c30..661725409 100644
--- a/nilearn/signal.py
+++ b/nilearn/signal.py
@@ -528,11 +528,14 @@ def clean(signals, runs=None, detrend=True, standardize='zscore',
signal, as if all were in the same array.
Default is None.
- sample_mask : None, :class:`numpy.ndarray`, :obj:`list`,\
- :obj:`tuple`, or :obj:`list` of
- shape: (number of scans - number of volumes removed, )
+ sample_mask : None, Any type compatible with numpy-array indexing, \
+ or :obj:`list` of
+ shape: (number of scans - number of volumes removed, ) for explicit \
+ index, or (number of scans, ) for binary mask
Masks the niimgs along time/fourth dimension to perform scrubbing
(remove volumes with high motion) and/or non-steady-state volumes.
+ When passing binary mask with boolean values, ``True`` refers to
+ volumes kept, and ``False`` for volumes removed.
This masking step is applied before signal cleaning. When supplying run
information, sample_mask must be a list containing sets of indexes for
each run.
| nilearn/nilearn | d75d3ce6caa9bfa04cf6e7c23c5d919e011ad005 | diff --git a/doc/changes/latest.rst b/doc/changes/latest.rst
index 1650fd8a9..42c302d53 100644
--- a/doc/changes/latest.rst
+++ b/doc/changes/latest.rst
@@ -28,6 +28,7 @@ Enhancements
- :func:`~signal.clean` imputes scrubbed volumes (defined through ``sample_masks``) with cubic spline function before applying butterworth filter (:gh:`3385` by `Hao-Ting Wang`_).
- As part of making the User Guide more user-friendly, the introduction was reworked (:gh:`3380` by `Alexis Thual`_)
- Added instructions for maintainers to make sure LaTeX dependencies are installed before building and deploying the stable docs (:gh:`3426` by `Yasmin Mzayek`_).
+- Parameter ``sample_masks`` in :func:`~signal.clean` and masker functions accept binary mask (:gh:`3439` by `Hao-Ting Wang`_).
Changes
-------
@@ -49,3 +50,4 @@ Changes
* Joblib -- v1.0.0
(:gh:`3440` by `Yasmin Mzayek`_).
+- In release ``0.10.0`` the default resolution for loaded MNI152 templates will be 1mm instead of 2mm (:gh:`3433` by `Yasmin Mzayek`_).
diff --git a/nilearn/datasets/tests/test_struct.py b/nilearn/datasets/tests/test_struct.py
index 7159a4e9d..0afca3853 100644
--- a/nilearn/datasets/tests/test_struct.py
+++ b/nilearn/datasets/tests/test_struct.py
@@ -148,8 +148,8 @@ def test_fetch_oasis_vbm(tmp_path, request_mocker, legacy_format):
def test_load_mni152_template():
# All subjects
- template_nii_1mm = struct.load_mni152_template(resolution=1)
- template_nii_2mm = struct.load_mni152_template()
+ template_nii_1mm = struct.load_mni152_template()
+ template_nii_2mm = struct.load_mni152_template(resolution=2)
assert template_nii_1mm.shape == (197, 233, 189)
assert template_nii_2mm.shape == (99, 117, 95)
assert template_nii_1mm.header.get_zooms() == (1.0, 1.0, 1.0)
@@ -158,8 +158,8 @@ def test_load_mni152_template():
def test_load_mni152_gm_template():
# All subjects
- gm_template_nii_1mm = struct.load_mni152_gm_template(resolution=1)
- gm_template_nii_2mm = struct.load_mni152_gm_template()
+ gm_template_nii_1mm = struct.load_mni152_gm_template()
+ gm_template_nii_2mm = struct.load_mni152_gm_template(resolution=2)
assert gm_template_nii_1mm.shape == (197, 233, 189)
assert gm_template_nii_2mm.shape == (99, 117, 95)
assert gm_template_nii_1mm.header.get_zooms() == (1.0, 1.0, 1.0)
@@ -168,8 +168,8 @@ def test_load_mni152_gm_template():
def test_load_mni152_wm_template():
# All subjects
- wm_template_nii_1mm = struct.load_mni152_wm_template(resolution=1)
- wm_template_nii_2mm = struct.load_mni152_wm_template()
+ wm_template_nii_1mm = struct.load_mni152_wm_template()
+ wm_template_nii_2mm = struct.load_mni152_wm_template(resolution=2)
assert wm_template_nii_1mm.shape == (197, 233, 189)
assert wm_template_nii_2mm.shape == (99, 117, 95)
assert wm_template_nii_1mm.header.get_zooms() == (1.0, 1.0, 1.0)
@@ -177,8 +177,8 @@ def test_load_mni152_wm_template():
def test_load_mni152_brain_mask():
- brain_mask_1mm = struct.load_mni152_brain_mask(resolution=1)
- brain_mask_2mm = struct.load_mni152_brain_mask()
+ brain_mask_1mm = struct.load_mni152_brain_mask()
+ brain_mask_2mm = struct.load_mni152_brain_mask(resolution=2)
assert isinstance(brain_mask_1mm, nibabel.Nifti1Image)
assert isinstance(brain_mask_2mm, nibabel.Nifti1Image)
# standard MNI template shape
@@ -187,8 +187,8 @@ def test_load_mni152_brain_mask():
def test_load_mni152_gm_mask():
- gm_mask_1mm = struct.load_mni152_gm_mask(resolution=1)
- gm_mask_2mm = struct.load_mni152_gm_mask()
+ gm_mask_1mm = struct.load_mni152_gm_mask()
+ gm_mask_2mm = struct.load_mni152_gm_mask(resolution=2)
assert isinstance(gm_mask_1mm, nibabel.Nifti1Image)
assert isinstance(gm_mask_2mm, nibabel.Nifti1Image)
# standard MNI template shape
@@ -197,8 +197,8 @@ def test_load_mni152_gm_mask():
def test_load_mni152_wm_mask():
- wm_mask_1mm = struct.load_mni152_wm_mask(resolution=1)
- wm_mask_2mm = struct.load_mni152_wm_mask()
+ wm_mask_1mm = struct.load_mni152_wm_mask()
+ wm_mask_2mm = struct.load_mni152_wm_mask(resolution=2)
assert isinstance(wm_mask_1mm, nibabel.Nifti1Image)
assert isinstance(wm_mask_2mm, nibabel.Nifti1Image)
# standard MNI template shape
@@ -206,26 +206,6 @@ def test_load_mni152_wm_mask():
assert wm_mask_2mm.shape == (99, 117, 95)
[email protected]("part", ["_brain", "_gm", "_wm"])
[email protected]("kind", ["template", "mask"])
-def test_mni152_resolution_warnings(part, kind):
- struct._MNI_RES_WARNING_ALREADY_SHOWN = False
- if kind == "template" and part == "_brain":
- part = ""
- loader = getattr(struct, f"load_mni152{part}_{kind}")
- try:
- loader.cache_clear()
- except AttributeError:
- pass
- with warnings.catch_warnings(record=True) as w:
- loader(resolution=1)
- assert len(w) == 0
- with warnings.catch_warnings(record=True) as w:
- loader()
- loader()
- assert len(w) == 1
-
-
def test_fetch_icbm152_brain_gm_mask(tmp_path, request_mocker):
dataset = struct.fetch_icbm152_2009(data_dir=str(tmp_path), verbose=0)
struct.load_mni152_template().to_filename(dataset.gm)
diff --git a/nilearn/tests/test_signal.py b/nilearn/tests/test_signal.py
index de0e1fdd6..9dfd365e3 100644
--- a/nilearn/tests/test_signal.py
+++ b/nilearn/tests/test_signal.py
@@ -125,21 +125,6 @@ def test_butterworth():
# single timeseries
data = rng.standard_normal(size=n_samples)
data_original = data.copy()
- '''
- May be only on py3.5:
- Bug in scipy 1.1.0 generates an unavoidable FutureWarning.
- (More info: https://github.com/scipy/scipy/issues/9086)
- The number of warnings generated is overwhelming TravisCI's log limit,
- causing it to fail tests.
- This hack prevents that and will be removed in future.
- '''
- buggy_scipy = (
- _compare_version(scipy.__version__, '<', '1.2')
- and _compare_version(scipy.__version__, '>', '1.0')
- )
- if buggy_scipy:
- warnings.simplefilter('ignore')
- ''' END HACK '''
out_single = nisignal.butterworth(data, sampling,
low_pass=low_pass, high_pass=high_pass,
copy=True)
@@ -785,13 +770,20 @@ def test_sample_mask():
sample_mask = np.arange(signals.shape[0])
scrub_index = [2, 3, 6, 7, 8, 30, 31, 32]
sample_mask = np.delete(sample_mask, scrub_index)
+ sample_mask_binary = np.full(signals.shape[0], True)
+ sample_mask_binary[scrub_index] = False
scrub_clean = clean(signals, confounds=confounds, sample_mask=sample_mask)
assert scrub_clean.shape[0] == sample_mask.shape[0]
+ # test the binary mask
+ scrub_clean_bin = clean(signals, confounds=confounds,
+ sample_mask=sample_mask_binary)
+ np.testing.assert_equal(scrub_clean_bin, scrub_clean)
+
# list of sample_mask for each run
runs = np.ones(signals.shape[0])
- runs[0:signals.shape[0] // 2] = 0
+ runs[:signals.shape[0] // 2] = 0
sample_mask_sep = [np.arange(20), np.arange(20)]
scrub_index = [[6, 7, 8], [10, 11, 12]]
sample_mask_sep = [np.delete(sm, si)
@@ -800,6 +792,15 @@ def test_sample_mask():
sample_mask=sample_mask_sep, runs=runs)
assert scrub_sep_mask.shape[0] == signals.shape[0] - 6
+ # test for binary mask per run
+ sample_mask_sep_binary = [np.full(signals.shape[0] // 2, True),
+ np.full(signals.shape[0] // 2, True)]
+ sample_mask_sep_binary[0][scrub_index[0]] = False
+ sample_mask_sep_binary[1][scrub_index[1]] = False
+ scrub_sep_mask = clean(signals, confounds=confounds,
+ sample_mask=sample_mask_sep_binary, runs=runs)
+ assert scrub_sep_mask.shape[0] == signals.shape[0] - 6
+
# 1D sample mask with runs labels
with pytest.raises(ValueError,
match=r'Number of sample_mask \(\d\) not matching'):
| Pass binary mask to `sample_masks` instead of indices
> Just wondering: wouldn't it be more handy to give a binary vector with length `n_scans` for `sample_mask` ?
_Originally posted by @bthirion in https://github.com/nilearn/nilearn/pull/3193#discussion_r838897961_
I did some archaeology and found out why I implemented index, rather than binary vector. I think it was for staying consistent to the OG `sample_mask`:
>I had a look at the relevant code of `sample_mask` application for resolving #2777. Currently, the timeseries censoring is done in the `base_masker` module:
https://github.com/nilearn/nilearn/blob/4cdd8fe8aad085d21e5d8367ad5b94d8efbe5151/nilearn/input_data/base_masker.py#L71-L73
_Originally posted by @htwangtw in https://github.com/nilearn/nilearn/issues/1012#issuecomment-847064092_
And the old doc of `sample_mask`:
https://github.com/nilearn/nilearn/blob/4cdd8fe8aad085d21e5d8367ad5b94d8efbe5151/nilearn/input_data/nifti_masker.py#L172-L177
Here's all the back ground info I can find. We can change it to binary vector as we no longer use the `index_img` function, and is using `signal.clean` to handle things. Let me know how you think we should move forward, given these info.
cc @pbellec as I know you raised the same question before. | 0.0 | [
"nilearn/datasets/tests/test_struct.py::test_load_mni152_template",
"nilearn/datasets/tests/test_struct.py::test_load_mni152_gm_template",
"nilearn/datasets/tests/test_struct.py::test_load_mni152_wm_template",
"nilearn/datasets/tests/test_struct.py::test_load_mni152_brain_mask",
"nilearn/datasets/tests/test_struct.py::test_load_mni152_gm_mask",
"nilearn/datasets/tests/test_struct.py::test_load_mni152_wm_mask",
"nilearn/tests/test_signal.py::test_sample_mask"
] | [
"nilearn/datasets/tests/test_struct.py::test_get_dataset_dir",
"nilearn/datasets/tests/test_struct.py::test_fetch_icbm152_2009",
"nilearn/datasets/tests/test_struct.py::test_fetch_oasis_vbm[True]",
"nilearn/datasets/tests/test_struct.py::test_fetch_oasis_vbm[False]",
"nilearn/datasets/tests/test_struct.py::test_fetch_icbm152_brain_gm_mask",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage3]",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage4]",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage5]",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage6]",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage7]",
"nilearn/datasets/tests/test_struct.py::test_fetch_surf_fsaverage[fsaverage]",
"nilearn/tests/test_signal.py::test_butterworth",
"nilearn/tests/test_signal.py::test_standardize",
"nilearn/tests/test_signal.py::test_detrend",
"nilearn/tests/test_signal.py::test_mean_of_squares",
"nilearn/tests/test_signal.py::test_row_sum_of_squares",
"nilearn/tests/test_signal.py::test_clean_detrending",
"nilearn/tests/test_signal.py::test_clean_t_r",
"nilearn/tests/test_signal.py::test_clean_frequencies",
"nilearn/tests/test_signal.py::test_clean_runs",
"nilearn/tests/test_signal.py::test_clean_confounds",
"nilearn/tests/test_signal.py::test_clean_frequencies_using_power_spectrum_density",
"nilearn/tests/test_signal.py::test_clean_finite_no_inplace_mod",
"nilearn/tests/test_signal.py::test_high_variance_confounds",
"nilearn/tests/test_signal.py::test_clean_psc",
"nilearn/tests/test_signal.py::test_clean_zscore",
"nilearn/tests/test_signal.py::test_create_cosine_drift_terms",
"nilearn/tests/test_signal.py::test_handle_scrubbed_volumes"
] | 2022-12-01 19:25:22+00:00 | 4,172 |
|
nilearn__nilearn-3474 | diff --git a/nilearn/signal.py b/nilearn/signal.py
index 5f5b85c6a..0de6716fc 100644
--- a/nilearn/signal.py
+++ b/nilearn/signal.py
@@ -37,11 +37,14 @@ def _standardize(signals, detrend=False, standardize='zscore'):
If detrending of timeseries is requested.
Default=False.
- standardize : {'zscore', 'psc', True, False}, optional
+ standardize : {'zscore_sample', 'zscore', 'psc', True, False}, optional
Strategy to standardize the signal:
+ - 'zscore_sample': The signal is z-scored. Timeseries are shifted
+ to zero mean and scaled to unit variance. Uses sample std.
- 'zscore': The signal is z-scored. Timeseries are shifted
- to zero mean and scaled to unit variance.
+ to zero mean and scaled to unit variance. Uses population std
+ by calling default :obj:`numpy.std` with N - ``ddof=0``.
- 'psc': Timeseries are shifted to zero mean value and scaled
to percent signal change (as compared to original mean signal).
- True: The signal is z-scored (same as option `zscore`).
@@ -55,7 +58,7 @@ def _standardize(signals, detrend=False, standardize='zscore'):
std_signals : :class:`numpy.ndarray`
Copy of signals, standardized.
"""
- if standardize not in [True, False, 'psc', 'zscore']:
+ if standardize not in [True, False, 'psc', 'zscore', 'zscore_sample']:
raise ValueError('{} is no valid standardize strategy.'
.format(standardize))
@@ -70,13 +73,36 @@ def _standardize(signals, detrend=False, standardize='zscore'):
'would lead to zero values. Skipping.')
return signals
+ elif (standardize == 'zscore_sample'):
+ if not detrend:
+ # remove mean if not already detrended
+ signals = signals - signals.mean(axis=0)
+
+ std = signals.std(axis=0, ddof=1)
+ # avoid numerical problems
+ std[std < np.finfo(np.float64).eps] = 1.
+ signals /= std
+
elif (standardize == 'zscore') or (standardize is True):
+ std_strategy_default = (
+ "The default strategy for standardize is currently 'zscore' "
+ "which incorrectly uses population std to calculate sample "
+ "zscores. The new strategy 'zscore_sample' corrects this "
+ "behavior by using the sample std. In release 0.13, the "
+ "default strategy will be replaced by the new strategy and "
+ "the 'zscore' option will be removed. Please use "
+ "'zscore_sample' instead."
+ )
+ warnings.warn(category=FutureWarning,
+ message=std_strategy_default,
+ stacklevel=3)
if not detrend:
# remove mean if not already detrended
signals = signals - signals.mean(axis=0)
std = signals.std(axis=0)
- std[std < np.finfo(np.float64).eps] = 1. # avoid numerical problems
+ # avoid numerical problems
+ std[std < np.finfo(np.float64).eps] = 1.
signals /= std
elif standardize == 'psc':
@@ -609,11 +635,14 @@ def clean(signals, runs=None, detrend=True, standardize='zscore',
%(high_pass)s
%(detrend)s
- standardize : {'zscore', 'psc', False}, optional
+ standardize : {'zscore_sample', 'zscore', 'psc', True, False}, optional
Strategy to standardize the signal:
+ - 'zscore_sample': The signal is z-scored. Timeseries are shifted
+ to zero mean and scaled to unit variance. Uses sample std.
- 'zscore': The signal is z-scored. Timeseries are shifted
- to zero mean and scaled to unit variance.
+ to zero mean and scaled to unit variance. Uses population std
+ by calling default :obj:`numpy.std` with N - ``ddof=0``.
- 'psc': Timeseries are shifted to zero mean value and scaled
to percent signal change (as compared to original mean signal).
- True: The signal is z-scored (same as option `zscore`).
| nilearn/nilearn | 1d99bbd6ade1c82a0e66787005efda3b5805d0fc | diff --git a/doc/changes/latest.rst b/doc/changes/latest.rst
index c33b0a80b..1eeb02a23 100644
--- a/doc/changes/latest.rst
+++ b/doc/changes/latest.rst
@@ -17,9 +17,11 @@ Fixes
- :func:`~nilearn.interfaces.fmriprep.load_confounds` can support searching preprocessed data in native space. (:gh:`3531` by `Hao-Ting Wang`_)
+- Add correct "zscore_sample" strategy to ``signal._standardize`` which will replace the default "zscore" strategy in release 0.13 (:gh:`3474` by `Yasmin Mzayek`_).
Enhancements
------------
+
- Updated example :ref:`sphx_glr_auto_examples_02_decoding_plot_haxby_frem.py` to include section on plotting a confusion matrix from a decoder family object (:gh:`3483` by `Michelle Wang`_).
- Surface plotting methods no longer automatically rescale background maps, which, among other things, allows to use curvature sign as a background map (:gh:`3173` by `Alexis Thual`_).
diff --git a/nilearn/tests/test_signal.py b/nilearn/tests/test_signal.py
index 3149326e2..d8274981e 100644
--- a/nilearn/tests/test_signal.py
+++ b/nilearn/tests/test_signal.py
@@ -251,6 +251,14 @@ def test_standardize():
a = rng.random_sample((n_samples, n_features))
a += np.linspace(0, 2., n_features)
+ # Test raise error when strategy is not valid option
+ with pytest.raises(ValueError, match="no valid standardize strategy"):
+ nisignal._standardize(a, standardize="foo")
+
+ # test warning for strategy that will be removed
+ with pytest.warns(FutureWarning, match="default strategy for standardize"):
+ nisignal._standardize(a, standardize="zscore")
+
# transpose array to fit _standardize input.
# Without trend removal
b = nisignal._standardize(a, standardize='zscore')
@@ -258,16 +266,32 @@ def test_standardize():
np.testing.assert_almost_equal(stds, np.ones(n_features))
np.testing.assert_almost_equal(b.sum(axis=0), np.zeros(n_features))
+ # Repeating test above but for new correct strategy
+ b = nisignal._standardize(a, standardize='zscore_sample')
+ stds = np.std(b)
+ np.testing.assert_almost_equal(stds, np.ones(n_features), decimal=1)
+ np.testing.assert_almost_equal(b.sum(axis=0), np.zeros(n_features))
+
# With trend removal
a = np.atleast_2d(np.linspace(0, 2., n_features)).T
b = nisignal._standardize(a, detrend=True, standardize=False)
np.testing.assert_almost_equal(b, np.zeros(b.shape))
+ b = nisignal._standardize(a, detrend=True, standardize="zscore_sample")
+ np.testing.assert_almost_equal(b, np.zeros(b.shape))
+
length_1_signal = np.atleast_2d(np.linspace(0, 2., n_features))
np.testing.assert_array_equal(length_1_signal,
nisignal._standardize(length_1_signal,
standardize='zscore'))
+ # Repeating test above but for new correct strategy
+ length_1_signal = np.atleast_2d(np.linspace(0, 2., n_features))
+ np.testing.assert_array_equal(
+ length_1_signal,
+ nisignal._standardize(length_1_signal, standardize="zscore_sample")
+ )
+
def test_detrend():
"""Test custom detrend implementation."""
@@ -827,9 +851,18 @@ def test_clean_zscore():
length=n_samples)
signals += rng.standard_normal(size=(1, n_features))
- cleaned_signals = clean(signals, standardize='zscore')
+ cleaned_signals_ = clean(signals, standardize='zscore')
+ np.testing.assert_almost_equal(cleaned_signals_.mean(0), 0)
+ np.testing.assert_almost_equal(cleaned_signals_.std(0), 1)
+
+ # Repeating test above but for new correct strategy
+ cleaned_signals = clean(signals, standardize='zscore_sample')
np.testing.assert_almost_equal(cleaned_signals.mean(0), 0)
- np.testing.assert_almost_equal(cleaned_signals.std(0), 1)
+ np.testing.assert_almost_equal(cleaned_signals.std(0), 1, decimal=3)
+
+ # Show outcome from two zscore strategies is not equal
+ with pytest.raises(AssertionError):
+ np.testing.assert_array_equal(cleaned_signals_, cleaned_signals)
def test_create_cosine_drift_terms():
| signal clean should not use population standard deviation
Nilearn version: 0.9.2
In signal.clean, the calculation simply uses numpy.std, this method by default uses N / ddf=0 for calculation, indicating population std. For fMRI studies, especially when std are calculated along time axis, the data is usually a sample of the population. The method should use sample std instead.
### Expected behavior
Given dataframe
1 4 7
2 5 8
3 6 9
z-scored sample should be:
(anp - anp.mean(axis=0))/anp.std(axis=0, ddof=1)
array([[-1., -1., -1.],
[ 0., 0., 0.],
[ 1., 1., 1.]])
### Actual behavior
nilearn.signal.clean(anp)
array([[-1.2247448, -1.2247448, -1.2247448],
[ 0. , 0. , 0. ],
[ 1.2247448, 1.2247448, 1.2247448]], dtype=float32)
### Steps and code to reproduce bug
I think it would be beneficial if we change the default behavior.
However, given the scope of this change, I think it's worth adding the ddof as an option instead of changing the default action. | 0.0 | [
"nilearn/tests/test_signal.py::test_standardize",
"nilearn/tests/test_signal.py::test_clean_zscore"
] | [
"nilearn/tests/test_signal.py::test_butterworth",
"nilearn/tests/test_signal.py::test_detrend",
"nilearn/tests/test_signal.py::test_mean_of_squares",
"nilearn/tests/test_signal.py::test_row_sum_of_squares",
"nilearn/tests/test_signal.py::test_clean_detrending",
"nilearn/tests/test_signal.py::test_clean_t_r",
"nilearn/tests/test_signal.py::test_clean_kwargs",
"nilearn/tests/test_signal.py::test_clean_frequencies",
"nilearn/tests/test_signal.py::test_clean_runs",
"nilearn/tests/test_signal.py::test_clean_confounds",
"nilearn/tests/test_signal.py::test_clean_frequencies_using_power_spectrum_density",
"nilearn/tests/test_signal.py::test_clean_finite_no_inplace_mod",
"nilearn/tests/test_signal.py::test_high_variance_confounds",
"nilearn/tests/test_signal.py::test_clean_psc",
"nilearn/tests/test_signal.py::test_create_cosine_drift_terms",
"nilearn/tests/test_signal.py::test_sample_mask",
"nilearn/tests/test_signal.py::test_handle_scrubbed_volumes"
] | 2023-01-27 10:07:38+00:00 | 4,173 |
|
nilearn__nilearn-3733 | diff --git a/.pre-commit-config.yaml b/.pre-commit-config.yaml
index 93461cdd0..3f3893823 100644
--- a/.pre-commit-config.yaml
+++ b/.pre-commit-config.yaml
@@ -98,7 +98,7 @@ repos:
- id: flynt
- repo: https://github.com/asottile/pyupgrade
- rev: v3.3.2
+ rev: v3.4.0
hooks:
- id: pyupgrade
args: [--py36-plus]
diff --git a/nilearn/decoding/decoder.py b/nilearn/decoding/decoder.py
index 0767b5f72..63be82ade 100644
--- a/nilearn/decoding/decoder.py
+++ b/nilearn/decoding/decoder.py
@@ -304,10 +304,18 @@ def _parallel_fit(
X_test = selector.transform(X_test)
# If there is no parameter grid, then we use a suitable grid (by default)
- param_grid = _check_param_grid(estimator, X_train, y_train, param_grid)
+ param_grid = ParameterGrid(
+ _check_param_grid(estimator, X_train, y_train, param_grid)
+ )
+
+ # collect all parameter names from the grid
+ all_params = set()
+ for params in param_grid:
+ all_params.update(params.keys())
+
best_score = None
- for param in ParameterGrid(param_grid):
- estimator = clone(estimator).set_params(**param)
+ for params in param_grid:
+ estimator = clone(estimator).set_params(**params)
estimator.fit(X_train, y_train)
if is_classification:
@@ -332,8 +340,13 @@ def _parallel_fit(
dummy_output = estimator.constant_
if isinstance(estimator, (RidgeCV, RidgeClassifierCV)):
- param["best_alpha"] = estimator.alpha_
- best_param = param
+ params["best_alpha"] = estimator.alpha_
+ best_params = params
+
+ # fill in any missing param from param_grid
+ for param in all_params:
+ if param not in best_params:
+ best_params[param] = getattr(estimator, param)
if best_coef is not None:
if do_screening:
@@ -346,7 +359,7 @@ def _parallel_fit(
class_index,
best_coef,
best_intercept,
- best_param,
+ best_params,
best_score,
dummy_output,
)
| nilearn/nilearn | 3edbbb88c61477c6115c2b812c4b3f74345a56cf | diff --git a/doc/changes/latest.rst b/doc/changes/latest.rst
index bb4253eb1..2150f15d0 100644
--- a/doc/changes/latest.rst
+++ b/doc/changes/latest.rst
@@ -11,6 +11,8 @@ NEW
Fixes
-----
+- :bdg-dark:`Code` Fix bug where the `cv_params_` attribute of fitter Decoder objects sometimes had missing entries if `grid_param` is a sequence of dicts with different keys (:gh:`3733` by `Michelle Wang`_).
+
Enhancements
------------
diff --git a/nilearn/decoding/tests/test_decoder.py b/nilearn/decoding/tests/test_decoder.py
index b97b4d0fb..c8dd1c728 100644
--- a/nilearn/decoding/tests/test_decoder.py
+++ b/nilearn/decoding/tests/test_decoder.py
@@ -441,6 +441,31 @@ def test_parallel_fit_builtin_cv(
assert isinstance(best_param[fitted_param_name], (float, int))
+def test_decoder_param_grid_sequence(binary_classification_data):
+ X, y, _ = binary_classification_data
+ n_cv_folds = 10
+ param_grid = [
+ {
+ "penalty": ["l2"],
+ "C": [100, 1000],
+ "random_state": [42], # fix the seed for consistent behaviour
+ },
+ {
+ "penalty": ["l1"],
+ "dual": [False], # "dual" is not in the first dict
+ "C": [100, 10],
+ "random_state": [42], # fix the seed for consistent behaviour
+ },
+ ]
+
+ model = Decoder(param_grid=param_grid, cv=n_cv_folds)
+ model.fit(X, y)
+
+ for best_params in model.cv_params_.values():
+ for param_list in best_params.values():
+ assert len(param_list) == n_cv_folds
+
+
def test_decoder_binary_classification_with_masker_object(
binary_classification_data,
):
| [BUG]: missing values in `Decoder.cv_params_` when `param_grid` is a list of dicts with different keys
If `param_grid` is a list of dicts and the dicts have different keys, the fitted Decoder's `cv_params_` output can contain lists that have less elements than the number of CV folds. For example, if `param_grid` is
```python
param_grid = [
{
"penalty": ["l2"],
"C": [100, 1000],
},
{
"penalty": ["l1"],
"dual": [False], # "dual" is not in the first dict
"C": [100, 1000],
},
]
```
Then it is possible that `decoder.cv_params_["shoe"]` has something like
```python
{
'C': [1000, 1000, 100, 100, 1000],
'dual': [False, False, False], # less than 5, not aligned with the other hyperparameter values
'penalty': ['l1', 'l2', 'l1', 'l1', 'l2'],
}
```
_Originally posted by @michellewang in https://github.com/nilearn/nilearn/pull/3626#discussion_r1172699079_
| 0.0 | [
"nilearn/decoding/tests/test_decoder.py::test_decoder_param_grid_sequence"
] | [
"nilearn/decoding/tests/test_decoder.py::test_check_param_grid_regression[regressor0-param0]",
"nilearn/decoding/tests/test_decoder.py::test_check_param_grid_regression[regressor1-C]",
"nilearn/decoding/tests/test_decoder.py::test_check_param_grid_classification[classifier0-C]",
"nilearn/decoding/tests/test_decoder.py::test_check_param_grid_classification[classifier1-C]",
"nilearn/decoding/tests/test_decoder.py::test_check_param_grid_classification[classifier2-param2]",
"nilearn/decoding/tests/test_decoder.py::test_non_supported_estimator_error[log_l1]",
"nilearn/decoding/tests/test_decoder.py::test_non_supported_estimator_error[estimator1]",
"nilearn/decoding/tests/test_decoder.py::test_check_parameter_grid_is_empty",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid0]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid1]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid2]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid3]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid4]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid5]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid6]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid7]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid8]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid9]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid[param_grid10]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid_warning[param_grid0-True]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid_warning[param_grid1-False]",
"nilearn/decoding/tests/test_decoder.py::test_wrap_param_grid_is_none",
"nilearn/decoding/tests/test_decoder.py::test_check_inputs_length[DecoderRegressor]",
"nilearn/decoding/tests/test_decoder.py::test_check_inputs_length[Decoder]",
"nilearn/decoding/tests/test_decoder.py::test_check_inputs_length[FREMRegressor]",
"nilearn/decoding/tests/test_decoder.py::test_check_inputs_length[FREMClassifier]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[svc]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[svc_l2]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[svc_l1]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[logistic]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[logistic_l1]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[logistic_l2]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[ridge]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[ridge_classifier]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[ridge_regressor]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[svr]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[dummy_classifier]",
"nilearn/decoding/tests/test_decoder.py::test_check_supported_estimator[dummy_regressor]",
"nilearn/decoding/tests/test_decoder.py::test_check_unsupported_estimator[ridgo]",
"nilearn/decoding/tests/test_decoder.py::test_check_unsupported_estimator[svb]",
"nilearn/decoding/tests/test_decoder.py::test_parallel_fit",
"nilearn/decoding/tests/test_decoder.py::test_parallel_fit_builtin_cv[estimator0-alphas-best_alpha-False-param_values0]",
"nilearn/decoding/tests/test_decoder.py::test_parallel_fit_builtin_cv[estimator0-alphas-best_alpha-False-param_values1]",
"nilearn/decoding/tests/test_decoder.py::test_parallel_fit_builtin_cv[estimator1-alphas-best_alpha-True-param_values0]",
"nilearn/decoding/tests/test_decoder.py::test_parallel_fit_builtin_cv[estimator1-alphas-best_alpha-True-param_values1]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_with_logistic_model",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_screening[100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_screening[20]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_screening[None]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_clustering[100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_clustering[99]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_cross_validation[cv0]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_binary_classification_cross_validation[cv1]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier_with_callable",
"nilearn/decoding/tests/test_decoder.py::test_decoder_error_model_not_fitted",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier_strategy_prior",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier_strategy_most_frequent",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier_roc_scoring",
"nilearn/decoding/tests/test_decoder.py::test_decoder_error_not_implemented",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_classifier_default_scoring",
"nilearn/decoding/tests/test_decoder.py::test_decoder_classification_string_label",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[ridge-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[ridge-20]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[ridge-1]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[ridge-None]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[svr-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[svr-20]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[svr-1]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_screening[svr-None]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_clustering[ridge-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_clustering[ridge-99]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_clustering[svr-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_regression_clustering[svr-99]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_regression",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_regression_default_scoring_metric_is_r2",
"nilearn/decoding/tests/test_decoder.py::test_decoder_dummy_regression_other_strategy",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_masker",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_masker_dummy_classifier",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_screening[100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_screening[20]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_screening[None]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_clustering[svc_l2-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_clustering[svc_l2-99]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_clustering[svc_l1-100]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_clustering[svc_l1-99]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_cross_validation[cv0]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_cross_validation[cv1]",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_apply_mask_shape",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_classification_apply_mask_attributes",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_error_incorrect_cv",
"nilearn/decoding/tests/test_decoder.py::test_decoder_multiclass_warnings"
] | 2023-05-03 15:25:06+00:00 | 4,174 |
|
nilearn__nilearn-3742 | diff --git a/.pre-commit-config.yaml b/.pre-commit-config.yaml
index ee5f62336..cc165dd6e 100644
--- a/.pre-commit-config.yaml
+++ b/.pre-commit-config.yaml
@@ -38,7 +38,6 @@ files: |
exclude: |
(?x)^(
- doc/changes/(names|latest).rst
| doc/glm/first_level_model\\.rst
| introduction\\.rst
| maintenance\\.rst
diff --git a/nilearn/_utils/data_gen.py b/nilearn/_utils/data_gen.py
index e88b09dd3..003d0f5eb 100644
--- a/nilearn/_utils/data_gen.py
+++ b/nilearn/_utils/data_gen.py
@@ -1399,6 +1399,11 @@ def _write_bids_derivative_func(
shape = [n_voxels, n_voxels, n_voxels, n_time_points]
+ entities_to_include = [
+ *_bids_entities()["raw"],
+ *_bids_entities()["derivatives"]
+ ]
+
for space in ("MNI", "T1w"):
for desc in ("preproc", "fmriprep"):
# Only space 'T1w' include both descriptions.
@@ -1408,10 +1413,6 @@ def _write_bids_derivative_func(
fields["entities"]["space"] = space
fields["entities"]["desc"] = desc
- entities_to_include = [
- *_bids_entities()["raw"], *_bids_entities()["derivatives"]
- ]
-
bold_path = func_path / _create_bids_filename(
fields=fields, entities_to_include=entities_to_include
)
diff --git a/nilearn/glm/first_level/first_level.py b/nilearn/glm/first_level/first_level.py
index c40dd40f1..bda0bd566 100644
--- a/nilearn/glm/first_level/first_level.py
+++ b/nilearn/glm/first_level/first_level.py
@@ -1334,16 +1334,9 @@ def _get_confounds(
List of fullpath to the confounds.tsv files
"""
- supported_filters = (
- _bids_entities()["raw"]
- + _bids_entities()["derivatives"]
- )
- # confounds use a desc-confounds,
- # so we must remove desc if it was passed as a filter
- supported_filters.remove("desc")
filters = _make_bids_files_filter(
task_label=task_label,
- supported_filters=supported_filters,
+ supported_filters=_bids_entities()["raw"],
extra_filter=img_filters,
verbose=verbose,
)
diff --git a/pyproject.toml b/pyproject.toml
index 28f52010f..81d6d398f 100644
--- a/pyproject.toml
+++ b/pyproject.toml
@@ -181,5 +181,5 @@ doctest_optionflags = "NORMALIZE_WHITESPACE ELLIPSIS"
junit_family = "xunit2"
[tool.codespell]
-skip = "./.git,plotly-gl3d-latest.min.js,jquery.min.js,localizer_behavioural.tsv,.mypy_cache,env,venv"
+skip = "./.git,plotly-gl3d-latest.min.js,jquery.min.js,localizer_behavioural.tsv,.mypy_cache,env,venv,./doc/auto_examples"
ignore-words = ".github/codespell_ignore_words.txt"
| nilearn/nilearn | 44933309ecd09add795ce1c3ea5edaab4294da87 | diff --git a/doc/changes/latest.rst b/doc/changes/latest.rst
index 66430988a..5c9e7f486 100644
--- a/doc/changes/latest.rst
+++ b/doc/changes/latest.rst
@@ -11,6 +11,7 @@ NEW
Fixes
-----
+- Fix bug in :func:`~glm.first_level.first_level_from_bids` that returned no confound files if the corresponding bold files contained derivatives BIDS entities (:gh:`3742` by `Rémi Gau`_).
- :bdg-dark:`Code` Fix bug where the `cv_params_` attribute of fitter Decoder objects sometimes had missing entries if `grid_param` is a sequence of dicts with different keys (:gh:`3733` by `Michelle Wang`_).
Enhancements
diff --git a/nilearn/glm/tests/test_first_level.py b/nilearn/glm/tests/test_first_level.py
index 26eaaece7..8b7f9dd95 100644
--- a/nilearn/glm/tests/test_first_level.py
+++ b/nilearn/glm/tests/test_first_level.py
@@ -1112,10 +1112,11 @@ def test_first_level_from_bids(tmp_path,
slice_time_ref=None,
)
- assert len(models) == n_sub
- assert len(models) == len(m_imgs)
- assert len(models) == len(m_events)
- assert len(models) == len(m_confounds)
+ _check_output_first_level_from_bids(n_sub,
+ models,
+ m_imgs,
+ m_events,
+ m_confounds)
n_imgs_expected = n_ses * n_runs[task_index]
@@ -1144,10 +1145,11 @@ def test_first_level_from_bids_select_one_run_per_session(bids_dataset):
slice_time_ref=None,
)
- assert len(models) == n_sub
- assert len(models) == len(m_imgs)
- assert len(models) == len(m_events)
- assert len(models) == len(m_confounds)
+ _check_output_first_level_from_bids(n_sub,
+ models,
+ m_imgs,
+ m_events,
+ m_confounds)
n_imgs_expected = n_ses
assert len(m_imgs[0]) == n_imgs_expected
@@ -1165,10 +1167,11 @@ def test_first_level_from_bids_select_all_runs_of_one_session(bids_dataset):
slice_time_ref=None,
)
- assert len(models) == n_sub
- assert len(models) == len(m_imgs)
- assert len(models) == len(m_events)
- assert len(models) == len(m_confounds)
+ _check_output_first_level_from_bids(n_sub,
+ models,
+ m_imgs,
+ m_events,
+ m_confounds)
n_imgs_expected = n_runs[0]
assert len(m_imgs[0]) == n_imgs_expected
@@ -1217,13 +1220,36 @@ def test_first_level_from_bids_several_labels_per_entity(tmp_path, entity):
img_filters=[("desc", "preproc"), (entity, "A")],
slice_time_ref=None,
)
+
+ _check_output_first_level_from_bids(n_sub,
+ models,
+ m_imgs,
+ m_events,
+ m_confounds)
+ n_imgs_expected = n_ses * n_runs[0]
+ assert len(m_imgs[0]) == n_imgs_expected
+
+
+def _check_output_first_level_from_bids(n_sub,
+ models,
+ m_imgs,
+ m_events,
+ m_confounds):
assert len(models) == n_sub
+ assert all(isinstance(model, FirstLevelModel) for model in models)
assert len(models) == len(m_imgs)
+ for imgs in m_imgs:
+ assert isinstance(imgs, list)
+ assert all(Path(img_).exists() for img_ in imgs)
assert len(models) == len(m_events)
+ for events in m_events:
+ assert isinstance(events, list)
+ assert all(isinstance(event_, pd.DataFrame) for event_ in events)
assert len(models) == len(m_confounds)
-
- n_imgs_expected = n_ses * n_runs[0]
- assert len(m_imgs[0]) == n_imgs_expected
+ for confounds in m_confounds:
+ assert isinstance(confounds, list)
+ assert all(isinstance(confound_, pd.DataFrame)
+ for confound_ in confounds)
def test_first_level_from_bids_with_subject_labels(bids_dataset):
diff --git a/nilearn/plotting/tests/test_matrix_plotting.py b/nilearn/plotting/tests/test_matrix_plotting.py
index 54c2158f3..d289fe534 100644
--- a/nilearn/plotting/tests/test_matrix_plotting.py
+++ b/nilearn/plotting/tests/test_matrix_plotting.py
@@ -96,7 +96,7 @@ def test_sanitize_reorder_error(reorder):
else:
param_to_print.append(str(item))
with pytest.raises(ValueError,
- match=("Parameter reorder needs to be one of:\n"
+ match=("Parameter reorder needs to be one of:\\n"
f"{', '.join(param_to_print)}.")):
_sanitize_reorder(reorder)
| [BUG] first_level_from_bids confused by "res" filter when looking for confounds.tsv
### Is there an existing issue for this?
- [X] I have searched the existing issues
### Operating system
- [X] Linux
- [ ] Mac
- [ ] Windows
### Operating system version
CentOS Linux release 7.6.1810
### Python version
- [ ] 3.11
- [X] 3.10
- [ ] 3.9
- [ ] 3.8
- [ ] 3.7
### nilearn version
0.10.0
### Expected behavior
When running the command below one can expect the model_confounds to be found in a bids-valid directory.
(models,
models_run_imgs,
models_events,
models_confounds,) = first_level_from_bids(data_dir, task_label, derivatives_folder=derivatives_folder,space_label=space_label,img_filters=[("desc", "preproc"),("res","2")], smoothing_fwhm=6, slice_time_ref=0.481, n_jobs=-1, signal_scaling=(0,1))
### Current behavior & error messages
This is what I got:
models_confounds were al None.
What I did to fix it was simply add an extra line in /nilearn/glm/first_level/first_level.py for removing "res" from the filters:
supported_filters.remove("desc")
**supported_filters.remove("res")**
filters = _make_bids_files_filter(
task_label=task_label,
supported_filters=supported_filters,
extra_filter=img_filters,
verbose=verbose,
)
confounds = get_bids_files(
derivatives_path,
modality_folder="func",
file_tag="desc-confounds*",
file_type="tsv",
sub_label=sub_label,
filters=filters,
)
### Steps and code to reproduce bug
```python
# Paste your code here
```
| 0.0 | [
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[res]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[den]"
] | [
"nilearn/glm/tests/test_first_level.py::test_scaling",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_repetition_time_warnings",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_repetition_time_errors[not",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_repetition_time_errors[-1-ValueError-positive]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_slice_timing_ref_warnings",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_slice_timing_ref_errors[not",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_set_slice_timing_ref_errors[2-ValueError-between",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_get_metadata_from_derivatives",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_get_RepetitionTime_from_derivatives",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_get_StartTime_from_derivatives",
"nilearn/glm/tests/test_first_level.py::test_first_level_with_scaling",
"nilearn/glm/tests/test_first_level.py::test_first_level_with_no_signal_scaling",
"nilearn/glm/tests/test_first_level.py::test_first_level_residuals",
"nilearn/glm/tests/test_first_level.py::test_get_voxelwise_attributes_should_return_as_many_as_design_matrices[shapes0]",
"nilearn/glm/tests/test_first_level.py::test_get_voxelwise_attributes_should_return_as_many_as_design_matrices[shapes1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_predictions_r_square",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-0-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-0-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-0-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-1-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-1-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-1-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-2-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-2-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-0-2-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-0-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-0-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-0-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-1-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-1-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-1-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-2-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-2-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[MNI-1-2-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-0-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-0-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-0-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-1-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-1-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-1-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-2-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-2-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-0-2-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-0-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-0-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-0-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-1-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-1-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-1-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-2-n_runs0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-2-n_runs1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids[T1w-1-2-n_runs2]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_select_one_run_per_session",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_select_all_runs_of_one_session",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_smoke_test_for_verbose_argument[0]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_smoke_test_for_verbose_argument[1]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[acq]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[ce]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[dir]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[rec]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_several_labels_per_entity[echo]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_with_subject_labels",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_no_duplicate_sub_labels",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_input_dataset_path",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_task_label[42-TypeError]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_task_label[$$$-ValueError]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_sub_labels[42-TypeError-must",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_sub_labels[sub_labels1-TypeError-must",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_sub_labels[sub_labels2-TypeError-must",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_space_label[42-TypeError]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_space_label[$$$-ValueError]",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_img_filter[foo-TypeError-'img_filters'",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_img_filter[img_filters1-TypeError-Filters",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_img_filter[img_filters2-ValueError-bids",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_validation_img_filter[img_filters3-ValueError-is",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_too_many_bold_files",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_with_missing_events",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_no_tr",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_no_bold_file",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_with_one_events_missing",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_one_confound_missing",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_all_confounds_missing",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_no_derivatives",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_no_session",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_mismatch_run_index",
"nilearn/glm/tests/test_first_level.py::test_first_level_from_bids_deprecated_slice_time_default",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes_error[foo-bar]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes_error[1-2]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes_error[fig2-axes2]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes[fig0-None-True]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes[fig1-None-True]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes[None-None-True]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_figure_and_axes[None-axes3-False]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_labels",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri[full]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri[lower]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri[diag]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri_error[None]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri_error[foo]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_tri_error[2]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder[False]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder[True]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder[complete]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder[single]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder[average]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder_error[None]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder_error[foo]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_sanitize_reorder_error[2]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_errors[matrix0-lab0-False]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_errors[matrix1-None-True]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_errors[matrix2-lab2-",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_with_labels_and_different_tri[full]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_with_labels_and_different_tri[lower]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_with_labels_and_different_tri[diag]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_labels[lab0]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_labels[lab1]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_labels[None]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_set_title[foo]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_set_title[foo",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_set_title[",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_set_title[None]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_grid[full]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_grid[lower]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_grid[diag]",
"nilearn/plotting/tests/test_matrix_plotting.py::test_matrix_plotting_reorder",
"nilearn/plotting/tests/test_matrix_plotting.py::test_show_design_matrix",
"nilearn/plotting/tests/test_matrix_plotting.py::test_show_event_plot",
"nilearn/plotting/tests/test_matrix_plotting.py::test_show_contrast_matrix"
] | 2023-06-01 14:16:53+00:00 | 4,175 |
|
nion-software__niondata-20 | diff --git a/nion/data/Core.py b/nion/data/Core.py
index ca5f2c4..43e17b0 100755
--- a/nion/data/Core.py
+++ b/nion/data/Core.py
@@ -386,7 +386,7 @@ def function_register_template(image_xdata: DataAndMetadata.DataAndMetadata, tem
ccorr_xdata = function_match_template(image_xdata, template_xdata)
error, ccoeff, max_pos = TemplateMatching.find_ccorr_max(ccorr_xdata.data)
if not error:
- return ccoeff, max_pos
+ return ccoeff, tuple(max_pos[i] - image_xdata.data_shape[i] * 0.5 for i in range(len(image_xdata.data_shape)))
def function_shift(src: DataAndMetadata.DataAndMetadata, shift: typing.Tuple[float, ...], *, order: int = 1) -> DataAndMetadata.DataAndMetadata:
| nion-software/niondata | 422c897b7a2295ad907b7beaef73dcae5a2ca520 | diff --git a/nion/data/test/Core_test.py b/nion/data/test/Core_test.py
index 54ef2a1..054d822 100755
--- a/nion/data/test/Core_test.py
+++ b/nion/data/test/Core_test.py
@@ -932,7 +932,7 @@ class TestCore(unittest.TestCase):
template_xdata = DataAndMetadata.new_data_and_metadata(data[40:60])
ccoeff, max_pos = Core.function_register_template(image_xdata, template_xdata)
self.assertEqual(len(max_pos), 1)
- self.assertAlmostEqual(max_pos[0], 50, places=1)
+ self.assertAlmostEqual(max_pos[0], 0, places=1)
self.assertAlmostEqual(ccoeff, 1.0, places=1)
def test_register_template_for_2d_data(self):
@@ -941,7 +941,7 @@ class TestCore(unittest.TestCase):
template_xdata = DataAndMetadata.new_data_and_metadata(data[40:60, 15:20])
ccoeff, max_pos = Core.function_register_template(image_xdata, template_xdata)
self.assertEqual(len(max_pos), 2)
- self.assertTrue(numpy.allclose(max_pos, (50, 17), atol=0.1))
+ self.assertTrue(numpy.allclose(max_pos, (0, -33), atol=0.1))
self.assertAlmostEqual(ccoeff, 1.0, places=1)
def test_sequence_join(self):
| Should register_template return offset relative to center pixel?
```
quality, offset = xd.register_template(img, tmpl_img)
offset = Geometry.FloatPoint(y=img.data_shape[0] * 0.5 - offset[0], x=img.data_shape[1] * 0.5 - offset[1])
```
| 0.0 | [
"nion/data/test/Core_test.py::TestCore::test_register_template_for_1d_data",
"nion/data/test/Core_test.py::TestCore::test_register_template_for_2d_data"
] | [
"nion/data/test/Core_test.py::TestCore::test_ability_to_take_1d_slice_with_newaxis",
"nion/data/test/Core_test.py::TestCore::test_align_with_bounds_works_on_1d_data",
"nion/data/test/Core_test.py::TestCore::test_align_with_bounds_works_on_2d_data",
"nion/data/test/Core_test.py::TestCore::test_align_works_on_1d_data",
"nion/data/test/Core_test.py::TestCore::test_align_works_on_2d_data",
"nion/data/test/Core_test.py::TestCore::test_align_works_on_nx1_data",
"nion/data/test/Core_test.py::TestCore::test_auto_correlation_keeps_calibration",
"nion/data/test/Core_test.py::TestCore::test_concatenate_calibrations",
"nion/data/test/Core_test.py::TestCore::test_concatenate_propagates_data_descriptor",
"nion/data/test/Core_test.py::TestCore::test_create_display_from_rgba_sequence_should_work",
"nion/data/test/Core_test.py::TestCore::test_create_rgba_image_from_uint16",
"nion/data/test/Core_test.py::TestCore::test_crop_out_of_bounds_produces_proper_size_data",
"nion/data/test/Core_test.py::TestCore::test_crop_rotated_produces_proper_size_data",
"nion/data/test/Core_test.py::TestCore::test_cross_correlation_keeps_calibration",
"nion/data/test/Core_test.py::TestCore::test_display_data_2d_not_a_view",
"nion/data/test/Core_test.py::TestCore::test_display_rgba_with_1d_rgba",
"nion/data/test/Core_test.py::TestCore::test_fft_1d_forward_and_back_is_consistent",
"nion/data/test/Core_test.py::TestCore::test_fft_1d_rms_is_same_as_original",
"nion/data/test/Core_test.py::TestCore::test_fft_forward_and_back_is_consistent",
"nion/data/test/Core_test.py::TestCore::test_fft_rms_is_same_as_original",
"nion/data/test/Core_test.py::TestCore::test_fft_works_on_rgba_data",
"nion/data/test/Core_test.py::TestCore::test_fourier_filter_gives_sensible_units_when_source_has_no_units",
"nion/data/test/Core_test.py::TestCore::test_fourier_filter_gives_sensible_units_when_source_has_units",
"nion/data/test/Core_test.py::TestCore::test_line_profile_uses_integer_coordinates",
"nion/data/test/Core_test.py::TestCore::test_line_profile_width_adjusts_intensity_calibration",
"nion/data/test/Core_test.py::TestCore::test_line_profile_width_computation_does_not_affect_source_intensity",
"nion/data/test/Core_test.py::TestCore::test_match_template_for_1d_data",
"nion/data/test/Core_test.py::TestCore::test_match_template_for_2d_data",
"nion/data/test/Core_test.py::TestCore::test_mean_over_two_axes_returns_correct_calibrations",
"nion/data/test/Core_test.py::TestCore::test_pick_grabs_datum_index_from_3d",
"nion/data/test/Core_test.py::TestCore::test_pick_grabs_datum_index_from_4d",
"nion/data/test/Core_test.py::TestCore::test_pick_grabs_datum_index_from_sequence_of_3d",
"nion/data/test/Core_test.py::TestCore::test_pick_grabs_datum_index_from_sequence_of_4d",
"nion/data/test/Core_test.py::TestCore::test_resize_works_to_make_one_dimension_larger_and_one_smaller",
"nion/data/test/Core_test.py::TestCore::test_resize_works_to_make_one_dimension_larger_and_one_smaller_with_odd_dimensions",
"nion/data/test/Core_test.py::TestCore::test_rgb_slice_of_sequence_works",
"nion/data/test/Core_test.py::TestCore::test_sequence_join",
"nion/data/test/Core_test.py::TestCore::test_sequence_split",
"nion/data/test/Core_test.py::TestCore::test_shift_nx1_data_produces_nx1_data",
"nion/data/test/Core_test.py::TestCore::test_slice_of_2d_works",
"nion/data/test/Core_test.py::TestCore::test_slice_of_sequence_works",
"nion/data/test/Core_test.py::TestCore::test_slice_sum_grabs_signal_index",
"nion/data/test/Core_test.py::TestCore::test_slice_sum_works_on_2d_data",
"nion/data/test/Core_test.py::TestCore::test_squeeze_removes_collection_dimension",
"nion/data/test/Core_test.py::TestCore::test_squeeze_removes_datum_dimension",
"nion/data/test/Core_test.py::TestCore::test_squeeze_removes_sequence_dimension",
"nion/data/test/Core_test.py::TestCore::test_sum_over_rgb_produces_correct_data",
"nion/data/test/Core_test.py::TestCore::test_sum_over_two_axes_returns_correct_calibrations",
"nion/data/test/Core_test.py::TestCore::test_sum_over_two_axes_returns_correct_shape"
] | 2020-05-05 08:29:06+00:00 | 4,176 |
|
nion-software__nionutils-19 | diff --git a/nion/utils/Converter.py b/nion/utils/Converter.py
index 8bfacb2..178a127 100644
--- a/nion/utils/Converter.py
+++ b/nion/utils/Converter.py
@@ -43,7 +43,7 @@ class IntegerToStringConverter(ConverterLike[int, str]):
def convert_back(self, formatted_value: typing.Optional[str]) -> typing.Optional[int]:
""" Convert string to value using standard int conversion """
- formatted_value = re.sub("[^0-9]", "", formatted_value) if self.__fuzzy and formatted_value else None
+ formatted_value = re.sub("[+-](?!\d)|(?<=\.)\w*|[^-+0-9]", "", formatted_value) if self.__fuzzy and formatted_value else None
if formatted_value:
return int(formatted_value)
else:
| nion-software/nionutils | c4b09457ab9433dde6f224279fe8f35265c6c041 | diff --git a/nion/utils/test/Converter_test.py b/nion/utils/test/Converter_test.py
index 2fcbf1b..65b8717 100644
--- a/nion/utils/test/Converter_test.py
+++ b/nion/utils/test/Converter_test.py
@@ -23,6 +23,12 @@ class TestConverter(unittest.TestCase):
self.assertAlmostEqual(converter.convert_back(converter.convert(-100)) or 0.0, -100)
self.assertAlmostEqual(converter.convert_back(converter.convert(100)) or 0.0, 100)
+ def test_integer_to_string_converter(self) -> None:
+ converter = Converter.IntegerToStringConverter()
+ self.assertEqual(converter.convert_back("-1"), -1)
+ self.assertEqual(converter.convert_back("2.45653"), 2)
+ self.assertEqual(converter.convert_back("-adcv-2.15sa56aas"), -2)
+ self.assertEqual(converter.convert_back("xx4."), 4)
if __name__ == '__main__':
| Regex in IntegerToStringConverter not handling negative numbers
https://github.com/nion-software/nionutils/blob/c4b09457ab9433dde6f224279fe8f35265c6c041/nion/utils/Converter.py#L46
This regex is not sufficient to properly handle negative numbers. It will also fail to convert floating point numbers. See the following examples:
```
from nion.utils import Converter
conv = Converter.IntegerToStringConverter()
conv.convert_back("-1")
>>> 1
conv.convert_back("1.243")
>>> 1243
| 0.0 | [
"nion/utils/test/Converter_test.py::TestConverter::test_integer_to_string_converter"
] | [
"nion/utils/test/Converter_test.py::TestConverter::test_float_to_scaled_integer_with_negative_min"
] | 2022-05-13 12:15:05+00:00 | 4,177 |
|
nipy__heudiconv-304 | diff --git a/README.md b/README.md
index adfb4ba..1b45439 100644
--- a/README.md
+++ b/README.md
@@ -76,6 +76,8 @@ dicomstack.
- YouTube:
- ["Heudiconv Example"](https://www.youtube.com/watch?v=O1kZAuR7E00) by [James Kent](https://github.com/jdkent)
+- Blog post:
+ - ["BIDS Tutorial Series: HeuDiConv Walkthrough"](http://reproducibility.stanford.edu/bids-tutorial-series-part-2a/) by the [Stanford Center for Reproducible Neuroscience](http://reproducibility.stanford.edu/)
## How it works (in some more detail)
diff --git a/heudiconv/cli/run.py b/heudiconv/cli/run.py
index 0197912..0d984fc 100644
--- a/heudiconv/cli/run.py
+++ b/heudiconv/cli/run.py
@@ -1,3 +1,5 @@
+#!/usr/bin/env python
+
import os
import os.path as op
from argparse import ArgumentParser
@@ -215,12 +217,11 @@ def get_parser():
parser.add_argument('--dcmconfig', default=None,
help='JSON file for additional dcm2niix configuration')
submission = parser.add_argument_group('Conversion submission options')
- submission.add_argument('-q', '--queue', default=None,
- help='select batch system to submit jobs to instead'
- ' of running the conversion serially')
- submission.add_argument('--sbargs', dest='sbatch_args', default=None,
- help='Additional sbatch arguments if running with '
- 'queue arg')
+ submission.add_argument('-q', '--queue', choices=("SLURM", None),
+ default=None,
+ help='batch system to submit jobs in parallel')
+ submission.add_argument('--queue-args', dest='queue_args', default=None,
+ help='Additional queue arguments')
return parser
@@ -281,27 +282,28 @@ def process_args(args):
continue
if args.queue:
- if seqinfo and not dicoms:
- # flatten them all and provide into batching, which again
- # would group them... heh
- dicoms = sum(seqinfo.values(), [])
- raise NotImplementedError(
- "we already grouped them so need to add a switch to avoid "
- "any grouping, so no outdir prefix doubled etc")
-
- progname = op.abspath(inspect.getfile(inspect.currentframe()))
-
- queue_conversion(progname,
+ # if seqinfo and not dicoms:
+ # # flatten them all and provide into batching, which again
+ # # would group them... heh
+ # dicoms = sum(seqinfo.values(), [])
+ # raise NotImplementedError(
+ # "we already grouped them so need to add a switch to avoid "
+ # "any grouping, so no outdir prefix doubled etc")
+
+ pyscript = op.abspath(inspect.getfile(inspect.currentframe()))
+
+ studyid = sid
+ if session:
+ studyid += "-%s" % session
+ if locator:
+ studyid += "-%s" % locator
+ # remove any separators
+ studyid = studyid.replace(op.sep, '_')
+
+ queue_conversion(pyscript,
args.queue,
- study_outdir,
- heuristic.filename,
- dicoms,
- sid,
- args.anon_cmd,
- args.converter,
- session,
- args.with_prov,
- args.bids)
+ studyid,
+ args.queue_args)
continue
anon_sid = anonymize_sid(sid, args.anon_cmd) if args.anon_cmd else None
diff --git a/heudiconv/dicoms.py b/heudiconv/dicoms.py
index 1206894..b94013f 100644
--- a/heudiconv/dicoms.py
+++ b/heudiconv/dicoms.py
@@ -4,7 +4,6 @@ import os.path as op
import logging
from collections import OrderedDict
import tarfile
-from nibabel.nicom import csareader
from heudiconv.external.pydicom import dcm
from .utils import SeqInfo, load_json, set_readonly
diff --git a/heudiconv/parser.py b/heudiconv/parser.py
index 2ed0c04..8ceba64 100644
--- a/heudiconv/parser.py
+++ b/heudiconv/parser.py
@@ -186,7 +186,7 @@ def get_study_sessions(dicom_dir_template, files_opt, heuristic, outdir,
"`infotoids` to heuristic file or "
"provide `--subjects` option")
lgr.warn("Heuristic is missing an `infotoids` method, assigning "
- "empty method and using provided subject id %s."
+ "empty method and using provided subject id %s. "
"Provide `session` and `locator` fields for best results."
, sid)
def infotoids(seqinfos, outdir):
diff --git a/heudiconv/queue.py b/heudiconv/queue.py
index 89c912f..ba8ad66 100644
--- a/heudiconv/queue.py
+++ b/heudiconv/queue.py
@@ -1,35 +1,62 @@
+import subprocess
+import sys
import os
-import os.path as op
import logging
lgr = logging.getLogger(__name__)
-# start with SLURM but extend past that #TODO
-def queue_conversion(progname, queue, outdir, heuristic, dicoms, sid,
- anon_cmd, converter, session,with_prov, bids):
-
- # Rework this...
- convertcmd = ' '.join(['python', progname,
- '-o', outdir,
- '-f', heuristic,
- '-s', sid,
- '--anon-cmd', anon_cmd,
- '-c', converter])
- if session:
- convertcmd += " --ses '%s'" % session
- if with_prov:
- convertcmd += " --with-prov"
- if bids:
- convertcmd += " --bids"
- if dicoms:
- convertcmd += " --files"
- convertcmd += [" '%s'" % f for f in dicoms]
-
- script_file = 'dicom-%s.sh' % sid
- with open(script_file, 'wt') as fp:
- fp.writelines(['#!/bin/bash\n', convertcmd])
- outcmd = 'sbatch -J dicom-%s -p %s -N1 -c2 --mem=20G %s' \
- % (sid, queue, script_file)
-
- os.system(outcmd)
+def queue_conversion(pyscript, queue, studyid, queue_args=None):
+ """
+ Write out conversion arguments to file and submit to a job scheduler.
+ Parses `sys.argv` for heudiconv arguments.
+
+ Parameters
+ ----------
+ pyscript: file
+ path to `heudiconv` script
+ queue: string
+ batch scheduler to use
+ studyid: string
+ identifier for conversion
+ queue_args: string (optional)
+ additional queue arguments for job submission
+
+ Returns
+ -------
+ proc: int
+ Queue submission exit code
+ """
+
+ SUPPORTED_QUEUES = {'SLURM': 'sbatch'}
+ if queue not in SUPPORTED_QUEUES:
+ raise NotImplementedError("Queuing with %s is not supported", queue)
+
+ args = sys.argv[1:]
+ # search args for queue flag
+ for i, arg in enumerate(args):
+ if arg in ["-q", "--queue"]:
+ break
+ if i == len(args) - 1:
+ raise RuntimeError(
+ "Queue flag not found (must be provided as a command-line arg)"
+ )
+ # remove queue flag and value
+ del args[i:i+2]
+
+ # make arguments executable again
+ args.insert(0, pyscript)
+ pypath = sys.executable or "python"
+ args.insert(0, pypath)
+ convertcmd = " ".join(args)
+
+ # will overwrite across subjects
+ queue_file = os.path.abspath('heudiconv-%s.sh' % queue)
+ with open(queue_file, 'wt') as fp:
+ fp.writelines(['#!/bin/bash\n', convertcmd, '\n'])
+
+ cmd = [SUPPORTED_QUEUES[queue], queue_file]
+ if queue_args:
+ cmd.insert(1, queue_args)
+ proc = subprocess.call(cmd)
+ return proc
| nipy/heudiconv | 4e142b4d5332d3a65f06f460b6d99bd9543eea82 | diff --git a/heudiconv/tests/test_queue.py b/heudiconv/tests/test_queue.py
new file mode 100644
index 0000000..a90dd9b
--- /dev/null
+++ b/heudiconv/tests/test_queue.py
@@ -0,0 +1,46 @@
+import os
+import sys
+import subprocess
+
+from heudiconv.cli.run import main as runner
+from .utils import TESTS_DATA_PATH
+import pytest
+from nipype.utils.filemanip import which
+
[email protected](which("sbatch"), reason="skip a real slurm call")
[email protected](
+ 'invocation', [
+ "--files %s/01-fmap_acq-3mm" % TESTS_DATA_PATH, # our new way with automated groupping
+ "-d %s/{subject}/* -s 01-fmap_acq-3mm" % TESTS_DATA_PATH # "old" way specifying subject
+ ])
+def test_queue_no_slurm(tmpdir, invocation):
+ tmpdir.chdir()
+ hargs = invocation.split(" ")
+ hargs.extend(["-f", "reproin", "-b", "--minmeta", "--queue", "SLURM"])
+
+ # simulate command-line call
+ _sys_args = sys.argv
+ sys.argv = ['heudiconv'] + hargs
+
+ try:
+ with pytest.raises(OSError):
+ runner(hargs)
+ # should have generated a slurm submission script
+ slurm_cmd_file = (tmpdir / 'heudiconv-SLURM.sh').strpath
+ assert slurm_cmd_file
+ # check contents and ensure args match
+ with open(slurm_cmd_file) as fp:
+ lines = fp.readlines()
+ assert lines[0] == "#!/bin/bash\n"
+ cmd = lines[1]
+
+ # check that all flags we gave still being called
+ for arg in hargs:
+ # except --queue <queue>
+ if arg in ['--queue', 'SLURM']:
+ assert arg not in cmd
+ else:
+ assert arg in cmd
+ finally:
+ # revert before breaking something
+ sys.argv = _sys_args
| Issues w/ slurm
Greetings,
heudiconv version 0.5.3.
I am trying to run a basic heudiconv on one subject for testing w/ slurm (running on two nodes, partition name = heudiconv) to create my heuristic.
1) Command as follows:
heudiconv -d /srv/lab/fmri/mft/dicom4bids/data/{subject}*/* -f /srv/lab/fmri/mft/dicom4bids/data/convertall.py -s sub01 -q heudiconv -o /srv/lab/fmri/mft/dicom4bids/data/output/
This yields:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
Traceback (most recent call last):
File "/usr/local/anaconda3/envs/fmri/bin/heudiconv", line 11, in <module>
sys.exit(main())
File "/usr/local/anaconda3/envs/fmri/lib/python3.5/site-packages/heudiconv/cli/run.py", line 125, in main
process_args(args)
File "/usr/local/anaconda3/envs/fmri/lib/python3.5/site-packages/heudiconv/cli/run.py", line 296, in process_args
study_outdir,
UnboundLocalError: local variable 'study_outdir' referenced before assignment
2) Now, adding "-l unknown" to the above command executes, but does not create a .heudiconv folder:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
WARNING: Skipping unknown locator dataset
3) Curiously, removing the -q flag and the -l flag executes the command properly and creates .heudiconv under /output:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
INFO: PROCESSING STARTS: {'session': None, 'outdir': '/srv/lab/fmri/mft/dicom4bids/data/output/', 'subject': 'sub01'}
INFO: Processing 4976 dicoms
INFO: Analyzing 4976 dicoms
INFO: Generated sequence info with 10 entries
INFO: PROCESSING DONE: {'session': None, 'outdir': '/srv/lab/fmri/mft/dicom4bids/data/output/', 'subject': 'sub01'}
4) Anyone else experienced these issues? How come that adding -q breaks the locator inference? I still believe that a working scheduler integration for heudiconv will be extremely valuable w/ increasing data sets and I am happy to contribute. Perhaps a starting point would be to clarify what the -l flag actually requires. In the help menu for -l, it says: study path under outdir. If provided, it overloads the value provided by the heuristic. If --datalad is enabled, every directory within locator becomes a super-dataset thus establishing a hierarchy. Setting to "unknown" will skip that dataset.
Thanks in advance!
| 0.0 | [
"heudiconv/tests/test_queue.py::test_queue_no_slurm[--files",
"heudiconv/tests/test_queue.py::test_queue_no_slurm[-d"
] | [] | 2019-02-05 18:30:31+00:00 | 4,178 |
|
nipy__heudiconv-306 | diff --git a/heudiconv/dicoms.py b/heudiconv/dicoms.py
index b94013f..13a200b 100644
--- a/heudiconv/dicoms.py
+++ b/heudiconv/dicoms.py
@@ -353,7 +353,7 @@ def compress_dicoms(dicom_list, out_prefix, tempdirs, overwrite):
return outtar
-def embed_nifti(dcmfiles, niftifile, infofile, bids_info, force, min_meta):
+def embed_nifti(dcmfiles, niftifile, infofile, bids_info, min_meta):
"""
If `niftifile` doesn't exist, it gets created out of the `dcmfiles` stack,
@@ -370,7 +370,6 @@ def embed_nifti(dcmfiles, niftifile, infofile, bids_info, force, min_meta):
niftifile
infofile
bids_info
- force
min_meta
Returns
@@ -387,10 +386,11 @@ def embed_nifti(dcmfiles, niftifile, infofile, bids_info, force, min_meta):
if not min_meta:
import dcmstack as ds
- stack = ds.parse_and_stack(dcmfiles, force=force).values()
+ stack = ds.parse_and_stack(dcmfiles, force=True).values()
if len(stack) > 1:
raise ValueError('Found multiple series')
- stack = stack[0]
+ # may be odict now - iter to be safe
+ stack = next(iter(stack))
#Create the nifti image using the data array
if not op.exists(niftifile):
@@ -458,7 +458,7 @@ def embed_metadata_from_dicoms(bids, item_dicoms, outname, outname_bids,
item_dicoms = list(map(op.abspath, item_dicoms))
embedfunc = Node(Function(input_names=['dcmfiles', 'niftifile', 'infofile',
- 'bids_info', 'force', 'min_meta'],
+ 'bids_info', 'min_meta'],
output_names=['outfile', 'meta'],
function=embed_nifti),
name='embedder')
@@ -466,13 +466,10 @@ def embed_metadata_from_dicoms(bids, item_dicoms, outname, outname_bids,
embedfunc.inputs.niftifile = op.abspath(outname)
embedfunc.inputs.infofile = op.abspath(scaninfo)
embedfunc.inputs.min_meta = min_meta
- if bids:
- embedfunc.inputs.bids_info = load_json(op.abspath(outname_bids))
- else:
- embedfunc.inputs.bids_info = None
- embedfunc.inputs.force = True
+ embedfunc.inputs.bids_info = load_json(op.abspath(outname_bids)) if bids else None
embedfunc.base_dir = tmpdir
cwd = os.getcwd()
+
lgr.debug("Embedding into %s based on dicoms[0]=%s for nifti %s",
scaninfo, item_dicoms[0], outname)
try:
| nipy/heudiconv | b85e5e18d6e15b36fcfa59f606eac5f3660a1143 | diff --git a/heudiconv/tests/test_dicoms.py b/heudiconv/tests/test_dicoms.py
index 536d9d4..de8e9a1 100644
--- a/heudiconv/tests/test_dicoms.py
+++ b/heudiconv/tests/test_dicoms.py
@@ -1,10 +1,11 @@
import os.path as op
+import json
import pytest
from heudiconv.external.pydicom import dcm
from heudiconv.cli.run import main as runner
-from heudiconv.dicoms import parse_private_csa_header
+from heudiconv.dicoms import parse_private_csa_header, embed_nifti
from .utils import TESTS_DATA_PATH
# Public: Private DICOM tags
@@ -23,3 +24,37 @@ def test_private_csa_header(tmpdir):
assert parse_private_csa_header(dcm_data, pub, priv) != ''
# and quickly run heudiconv with no conversion
runner(['--files', dcm_file, '-c' 'none', '-f', 'reproin'])
+
+
+def test_nifti_embed(tmpdir):
+ """Test dcmstack's additional fields"""
+ tmpdir.chdir()
+ # set up testing files
+ dcmfiles = [op.join(TESTS_DATA_PATH, 'axasc35.dcm')]
+ infofile = 'infofile.json'
+
+ # 1) nifti does not exist
+ out = embed_nifti(dcmfiles, 'nifti.nii', 'infofile.json', None, False)
+ # string -> json
+ out = json.loads(out)
+ # should have created nifti file
+ assert op.exists('nifti.nii')
+
+ # 2) nifti exists
+ nifti, info = embed_nifti(dcmfiles, 'nifti.nii', 'infofile.json', None, False)
+ assert op.exists(nifti)
+ assert op.exists(info)
+ with open(info) as fp:
+ out2 = json.load(fp)
+
+ assert out == out2
+
+ # 3) with existing metadata
+ bids = {"existing": "data"}
+ nifti, info = embed_nifti(dcmfiles, 'nifti.nii', 'infofile.json', bids, False)
+ with open(info) as fp:
+ out3 = json.load(fp)
+
+ assert out3["existing"]
+ del out3["existing"]
+ assert out3 == out2 == out
| ERROR: Embedding failed: 'odict_values' object does not support indexing & BIDS validator
Dear Heudiconv's experts,
I have run heudiconv docker image on FDG PET data with the following command line et execution message:
```
docker run --rm -it -v /Users/mattvan83/fMRIprep/COMAJ:/data:ro -v /Users/mattvan83/fMRIprep/BIDS:/output nipy/heudiconv:latest -d /data/{subject}/*/* -s s001 -f /data/IMAP_AGEWELL_bids.py -b -o /output
/src/heudiconv/heudiconv/dicoms.py:7: UserWarning: The DICOM readers are highly experimental, unstable, and only work for Siemens time-series at the moment
Please use with caution. We would be grateful for your help in improving them
from nibabel.nicom import csareader
INFO: Running heudiconv version 0.5.2-dev
INFO: Need to process 1 study sessions
INFO: PROCESSING STARTS: {'subject': 's001', 'outdir': '/output/', 'session': None}
INFO: Processing 47 dicoms
INFO: Analyzing 47 dicoms
INFO: Generated sequence info with 1 entries
INFO: Doing conversion using dcm2niix
INFO: Converting /output/sub-s001/pet/sub-s001_task-rest_acq-FDG_rec-AC_run-01_pet (47 DICOMs) -> /output/sub-s001/pet . Converter: dcm2niix . Output types: ('nii.gz',)
181230-10:03:23,191 nipype.workflow INFO:
[Node] Setting-up "convert" in "/tmp/dcm2niixadifah8v/convert".
INFO: [Node] Setting-up "convert" in "/tmp/dcm2niixadifah8v/convert".
181230-10:03:23,603 nipype.workflow INFO:
[Node] Running "convert" ("nipype.interfaces.dcm2nii.Dcm2niix"), a CommandLine Interface with command:
dcm2niix -b y -z y -x n -t n -m n -f pet -o . -s n -v n /tmp/dcm2niixadifah8v/convert
INFO: [Node] Running "convert" ("nipype.interfaces.dcm2nii.Dcm2niix"), a CommandLine Interface with command:
dcm2niix -b y -z y -x n -t n -m n -f pet -o . -s n -v n /tmp/dcm2niixadifah8v/convert
181230-10:03:24,486 nipype.interface INFO:
stdout 2018-12-30T10:03:24.485856:Chris Rorden's dcm2niiX version v1.0.20180622 GCC6.3.0 (64-bit Linux)
INFO: stdout 2018-12-30T10:03:24.485856:Chris Rorden's dcm2niiX version v1.0.20180622 GCC6.3.0 (64-bit Linux)
181230-10:03:24,486 nipype.interface INFO:
stdout 2018-12-30T10:03:24.485856:Found 47 DICOM file(s)
INFO: stdout 2018-12-30T10:03:24.485856:Found 47 DICOM file(s)
181230-10:03:24,486 nipype.interface INFO:
stdout 2018-12-30T10:03:24.485856:Convert 47 DICOM as ./pet (256x256x47x1)
INFO: stdout 2018-12-30T10:03:24.485856:Convert 47 DICOM as ./pet (256x256x47x1)
181230-10:03:24,832 nipype.interface INFO:
stdout 2018-12-30T10:03:24.832191:compress: "/usr/bin/pigz" -n -f -6 "./pet.nii"
INFO: stdout 2018-12-30T10:03:24.832191:compress: "/usr/bin/pigz" -n -f -6 "./pet.nii"
181230-10:03:24,832 nipype.interface INFO:
stdout 2018-12-30T10:03:24.832191:Conversion required 1.112542 seconds (0.126743 for core code).
INFO: stdout 2018-12-30T10:03:24.832191:Conversion required 1.112542 seconds (0.126743 for core code).
181230-10:03:24,898 nipype.workflow INFO:
[Node] Finished "convert".
INFO: [Node] Finished "convert".
181230-10:03:25,70 nipype.workflow INFO:
[Node] Setting-up "embedder" in "/tmp/embedmetaf18vnj85/embedder".
INFO: [Node] Setting-up "embedder" in "/tmp/embedmetaf18vnj85/embedder".
181230-10:03:25,227 nipype.workflow INFO:
[Node] Running "embedder" ("nipype.interfaces.utility.wrappers.Function")
INFO: [Node] Running "embedder" ("nipype.interfaces.utility.wrappers.Function")
181230-10:03:26,887 nipype.workflow WARNING:
[Node] Error on "embedder" (/tmp/embedmetaf18vnj85/embedder)
WARNING: [Node] Error on "embedder" (/tmp/embedmetaf18vnj85/embedder)
ERROR: Embedding failed: 'odict_values' object does not support indexing
INFO: Post-treating /output/sub-s001/pet/sub-s001_task-rest_acq-FDG_rec-AC_run-01_pet.json file
INFO: Populating template files under /output/
INFO: PROCESSING DONE: {'subject': 's001', 'outdir': '/output/', 'session': None}
```
It seems that there is an error of embedder following the message above:
```
181230-10:03:26,887 nipype.workflow WARNING:
[Node] Error on "embedder" (/tmp/embedmetaf18vnj85/embedder)
WARNING: [Node] Error on "embedder" (/tmp/embedmetaf18vnj85/embedder)
ERROR: Embedding failed: 'odict_values' object does not support indexing
```
What is the impact of this error ?
Moreover, it seems that my BIDS PET data are in wrong format according BIDS validator:
```
Error 1: [Code 61] QUICK_VALIDATION_FAILED
Quick validation failed - the general folder structure does not resemble a BIDS dataset. Have you chosen the right folder (with "sub-*/" subfolders)? Check for structural/naming issues and presence of at least one subject.
```
I have used the following heuristics:
```
import os
def create_key(template, outtype=('nii.gz'), annotation_classes=None):
if template is None or not template:
raise ValueError('Template must be a valid format string')
return (template, outtype, annotation_classes)
def infotodict(seqinfo):
"""Heuristic evaluator for determining which runs belong where
allowed template fields - follow python string module:
item: index within category
subject: participant id
seqitem: run number during scanning
subindex: sub index within group
"""
pet = create_key('sub-{subject}/pet/sub-{subject}_task-rest_acq-{acq}_rec-{rec}_run-{item:02d}_pet')
info = {pet:[]}
for idx, s in enumerate(seqinfo):
if (s.dim3 == 47) and (s.TR == -1) and ('CERVEAU 3D SALENGRO' in s.protocol_name):
rectype = s.series_description.split('_')[-1]
info[pet].append({'item': s.series_id, 'acq': 'FDG', 'rec': rectype}) # append if multiple series meet criteria
# You can even put checks in place for your protocol
msg = []
if len(info[pet]) != 1: msg.append('Missing correct number of pet runs')
if msg:
raise ValueError('\n'.join(msg))
return info
```
Thanks in advance for any help.
Best,
Matt | 0.0 | [
"heudiconv/tests/test_dicoms.py::test_nifti_embed"
] | [
"heudiconv/tests/test_dicoms.py::test_private_csa_header"
] | 2019-02-06 19:29:37+00:00 | 4,179 |
|
nipy__heudiconv-328 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index 40cd074..42283e6 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -11,7 +11,7 @@ import csv
from random import sample
from glob import glob
-from heudiconv.external.pydicom import dcm
+from .external.pydicom import dcm
from .parser import find_files
from .utils import (
diff --git a/heudiconv/cli/run.py b/heudiconv/cli/run.py
index 0d984fc..f51d167 100644
--- a/heudiconv/cli/run.py
+++ b/heudiconv/cli/run.py
@@ -142,7 +142,7 @@ def get_parser():
group.add_argument('--files', nargs='*',
help='Files (tarballs, dicoms) or directories '
'containing files to process. Cannot be provided if '
- 'using --dicom_dir_template or --subjects')
+ 'using --dicom_dir_template.')
parser.add_argument('-s', '--subjects', dest='subjs', type=str, nargs='*',
help='list of subjects - required for dicom template. '
'If not provided, DICOMS would first be "sorted" and '
@@ -173,8 +173,6 @@ def get_parser():
'single argument and return a single anonymized ID. '
'Also see --conv-outdir')
parser.add_argument('-f', '--heuristic', dest='heuristic',
- # some commands might not need heuristic
- # required=True,
help='Name of a known heuristic or path to the Python'
'script containing heuristic')
parser.add_argument('-p', '--with-prov', action='store_true',
@@ -221,7 +219,9 @@ def get_parser():
default=None,
help='batch system to submit jobs in parallel')
submission.add_argument('--queue-args', dest='queue_args', default=None,
- help='Additional queue arguments')
+ help='Additional queue arguments passed as '
+ 'single string of Argument=Value pairs space '
+ 'separated.')
return parser
@@ -246,6 +246,13 @@ def process_args(args):
if not args.heuristic:
raise RuntimeError("No heuristic specified - add to arguments and rerun")
+ if args.queue:
+ lgr.info("Queuing %s conversion", args.queue)
+ iterarg, iterables = ("files", len(args.files)) if args.files else \
+ ("subjects", len(args.subjs))
+ queue_conversion(args.queue, iterarg, iterables, args.queue_args)
+ sys.exit(0)
+
heuristic = load_heuristic(args.heuristic)
study_sessions = get_study_sessions(args.dicom_dir_template, args.files,
@@ -281,31 +288,6 @@ def process_args(args):
lgr.warning("Skipping unknown locator dataset")
continue
- if args.queue:
- # if seqinfo and not dicoms:
- # # flatten them all and provide into batching, which again
- # # would group them... heh
- # dicoms = sum(seqinfo.values(), [])
- # raise NotImplementedError(
- # "we already grouped them so need to add a switch to avoid "
- # "any grouping, so no outdir prefix doubled etc")
-
- pyscript = op.abspath(inspect.getfile(inspect.currentframe()))
-
- studyid = sid
- if session:
- studyid += "-%s" % session
- if locator:
- studyid += "-%s" % locator
- # remove any separators
- studyid = studyid.replace(op.sep, '_')
-
- queue_conversion(pyscript,
- args.queue,
- studyid,
- args.queue_args)
- continue
-
anon_sid = anonymize_sid(sid, args.anon_cmd) if args.anon_cmd else None
if args.anon_cmd:
lgr.info('Anonymized {} to {}'.format(sid, anon_sid))
diff --git a/heudiconv/dicoms.py b/heudiconv/dicoms.py
index 13a200b..9ef9b9c 100644
--- a/heudiconv/dicoms.py
+++ b/heudiconv/dicoms.py
@@ -4,8 +4,8 @@ import os.path as op
import logging
from collections import OrderedDict
import tarfile
-from heudiconv.external.pydicom import dcm
+from .external.pydicom import dcm
from .utils import SeqInfo, load_json, set_readonly
lgr = logging.getLogger(__name__)
@@ -55,10 +55,10 @@ def group_dicoms_into_seqinfos(files, file_filter, dcmfilter, grouping):
lgr.info('Filtering out {0} dicoms based on their filename'.format(
nfl_before-nfl_after))
for fidx, filename in enumerate(files):
- from heudiconv.external.dcmstack import ds
+ import nibabel.nicom.dicomwrappers as dw
# TODO after getting a regression test check if the same behavior
# with stop_before_pixels=True
- mw = ds.wrapper_from_data(dcm.read_file(filename, force=True))
+ mw = dw.wrapper_from_data(dcm.read_file(filename, force=True))
for sig in ('iop', 'ICE_Dims', 'SequenceName'):
try:
@@ -385,7 +385,7 @@ def embed_nifti(dcmfiles, niftifile, infofile, bids_info, min_meta):
import re
if not min_meta:
- import dcmstack as ds
+ from heudiconv.external.dcmstack import ds
stack = ds.parse_and_stack(dcmfiles, force=True).values()
if len(stack) > 1:
raise ValueError('Found multiple series')
diff --git a/heudiconv/external/dcmstack.py b/heudiconv/external/dcmstack.py
index 80ebc33..4e00eb2 100644
--- a/heudiconv/external/dcmstack.py
+++ b/heudiconv/external/dcmstack.py
@@ -7,7 +7,7 @@ from .pydicom import dcm # to assure that we have it one way or another
try:
import dcmstack as ds
except ImportError as e:
- from heudiconv import lgr
+ from .. import lgr
# looks different between py2 and 3 so we go for very rudimentary matching
e_str = str(e)
# there were changes from how
diff --git a/heudiconv/queue.py b/heudiconv/queue.py
index ba8ad66..8e091ca 100644
--- a/heudiconv/queue.py
+++ b/heudiconv/queue.py
@@ -1,62 +1,112 @@
import subprocess
import sys
import os
-
import logging
+from .utils import which
+
lgr = logging.getLogger(__name__)
-def queue_conversion(pyscript, queue, studyid, queue_args=None):
- """
- Write out conversion arguments to file and submit to a job scheduler.
- Parses `sys.argv` for heudiconv arguments.
-
- Parameters
- ----------
- pyscript: file
- path to `heudiconv` script
- queue: string
- batch scheduler to use
- studyid: string
- identifier for conversion
- queue_args: string (optional)
- additional queue arguments for job submission
-
- Returns
- -------
- proc: int
- Queue submission exit code
- """
-
- SUPPORTED_QUEUES = {'SLURM': 'sbatch'}
- if queue not in SUPPORTED_QUEUES:
- raise NotImplementedError("Queuing with %s is not supported", queue)
-
- args = sys.argv[1:]
- # search args for queue flag
- for i, arg in enumerate(args):
- if arg in ["-q", "--queue"]:
- break
- if i == len(args) - 1:
- raise RuntimeError(
- "Queue flag not found (must be provided as a command-line arg)"
- )
- # remove queue flag and value
- del args[i:i+2]
-
- # make arguments executable again
- args.insert(0, pyscript)
- pypath = sys.executable or "python"
- args.insert(0, pypath)
+def queue_conversion(queue, iterarg, iterables, queue_args=None):
+ """
+ Write out conversion arguments to file and submit to a job scheduler.
+ Parses `sys.argv` for heudiconv arguments.
+
+ Parameters
+ ----------
+ queue: string
+ Batch scheduler to use
+ iterarg: str
+ Multi-argument to index (`subjects` OR `files`)
+ iterables: int
+ Number of `iterarg` arguments
+ queue_args: string (optional)
+ Additional queue arguments for job submission
+
+ """
+
+ SUPPORTED_QUEUES = {'SLURM': 'sbatch'}
+ if queue not in SUPPORTED_QUEUES:
+ raise NotImplementedError("Queuing with %s is not supported", queue)
+
+ for i in range(iterables):
+ args = clean_args(sys.argv[1:], iterarg, i)
+ # make arguments executable
+ heudiconv_exec = which("heudiconv") or "heudiconv"
+ args.insert(0, heudiconv_exec)
convertcmd = " ".join(args)
# will overwrite across subjects
queue_file = os.path.abspath('heudiconv-%s.sh' % queue)
with open(queue_file, 'wt') as fp:
- fp.writelines(['#!/bin/bash\n', convertcmd, '\n'])
+ fp.write("#!/bin/bash\n")
+ if queue_args:
+ for qarg in queue_args.split():
+ fp.write("#SBATCH %s\n" % qarg)
+ fp.write(convertcmd + "\n")
cmd = [SUPPORTED_QUEUES[queue], queue_file]
- if queue_args:
- cmd.insert(1, queue_args)
proc = subprocess.call(cmd)
- return proc
+ lgr.info("Submitted %d jobs", iterables)
+
+def clean_args(hargs, iterarg, iteridx):
+ """
+ Filters arguments for batch submission.
+
+ Parameters
+ ----------
+ hargs: list
+ Command-line arguments
+ iterarg: str
+ Multi-argument to index (`subjects` OR `files`)
+ iteridx: int
+ `iterarg` index to submit
+
+ Returns
+ -------
+ cmdargs : list
+ Filtered arguments for batch submission
+
+ Example
+ --------
+ >>> from heudiconv.queue import clean_args
+ >>> cmd = ['heudiconv', '-d', '/some/{subject}/path',
+ ... '-q', 'SLURM',
+ ... '-s', 'sub-1', 'sub-2', 'sub-3', 'sub-4']
+ >>> clean_args(cmd, 'subjects', 0)
+ ['heudiconv', '-d', '/some/{subject}/path', '-s', 'sub-1']
+ """
+
+ if iterarg == "subjects":
+ iterarg = ['-s', '--subjects']
+ elif iterarg == "files":
+ iterarg = ['--files']
+ else:
+ raise ValueError("Cannot index %s" % iterarg)
+
+ # remove these or cause an infinite loop
+ queue_args = ['-q', '--queue', '--queue-args']
+
+ # control variables for multi-argument parsing
+ is_iterarg = False
+ itercount = 0
+
+ indicies = []
+ cmdargs = hargs[:]
+
+ for i, arg in enumerate(hargs):
+ if arg.startswith('-') and is_iterarg:
+ # moving on to another argument
+ is_iterarg = False
+ if is_iterarg:
+ if iteridx != itercount:
+ indicies.append(i)
+ itercount += 1
+ if arg in iterarg:
+ is_iterarg = True
+ if arg in queue_args:
+ indicies.extend([i, i+1])
+
+ for j in sorted(indicies, reverse=True):
+ del cmdargs[j]
+ return cmdargs
diff --git a/heudiconv/utils.py b/heudiconv/utils.py
index a4cc1c9..847dfaf 100644
--- a/heudiconv/utils.py
+++ b/heudiconv/utils.py
@@ -12,6 +12,9 @@ import os.path as op
from pathlib import Path
from collections import namedtuple
from glob import glob
+from subprocess import check_output
+
+from nipype.utils.filemanip import which
import logging
lgr = logging.getLogger(__name__)
@@ -103,18 +106,17 @@ def docstring_parameter(*sub):
def anonymize_sid(sid, anon_sid_cmd):
- import sys
- from subprocess import check_output
-
+
cmd = [anon_sid_cmd, sid]
shell_return = check_output(cmd)
- ### Handle subprocess returning a bytes literal string to a python3 interpreter
- if all([sys.version_info[0] > 2, isinstance(shell_return, bytes), isinstance(sid, str)]):
+ if all([sys.version_info[0] > 2,
+ isinstance(shell_return, bytes),
+ isinstance(sid, str)]):
anon_sid = shell_return.decode()
else:
anon_sid = shell_return
-
+
return anon_sid.strip()
| nipy/heudiconv | 3467341dbf50bfa813f915b3e639d62105cb9ee0 | diff --git a/heudiconv/tests/test_queue.py b/heudiconv/tests/test_queue.py
index a90dd9b..8d80448 100644
--- a/heudiconv/tests/test_queue.py
+++ b/heudiconv/tests/test_queue.py
@@ -3,9 +3,9 @@ import sys
import subprocess
from heudiconv.cli.run import main as runner
+from heudiconv.queue import clean_args, which
from .utils import TESTS_DATA_PATH
import pytest
-from nipype.utils.filemanip import which
@pytest.mark.skipif(which("sbatch"), reason="skip a real slurm call")
@pytest.mark.parametrize(
@@ -23,7 +23,7 @@ def test_queue_no_slurm(tmpdir, invocation):
sys.argv = ['heudiconv'] + hargs
try:
- with pytest.raises(OSError):
+ with pytest.raises(OSError): # SLURM should not be installed
runner(hargs)
# should have generated a slurm submission script
slurm_cmd_file = (tmpdir / 'heudiconv-SLURM.sh').strpath
@@ -44,3 +44,50 @@ def test_queue_no_slurm(tmpdir, invocation):
finally:
# revert before breaking something
sys.argv = _sys_args
+
+def test_argument_filtering(tmpdir):
+ cmd_files = [
+ 'heudiconv',
+ '--files',
+ '/fake/path/to/files',
+ '/another/fake/path',
+ '-f',
+ 'convertall',
+ '-q',
+ 'SLURM',
+ '--queue-args',
+ '--cpus-per-task=4 --contiguous --time=10'
+ ]
+ filtered = [
+ 'heudiconv',
+ '--files',
+ '/another/fake/path',
+ '-f',
+ 'convertall',
+ ]
+ assert clean_args(cmd_files, 'files', 1) == filtered
+
+ cmd_subjects = [
+ 'heudiconv',
+ '-d',
+ '/some/{subject}/path',
+ '--queue',
+ 'SLURM',
+ '--subjects',
+ 'sub1',
+ 'sub2',
+ 'sub3',
+ 'sub4',
+ '-f',
+ 'convertall'
+ ]
+ filtered = [
+ 'heudiconv',
+ '-d',
+ '/some/{subject}/path',
+ '--subjects',
+ 'sub3',
+ '-f',
+ 'convertall'
+ ]
+ assert clean_args(cmd_subjects, 'subjects', 2) == filtered
| Issues w/ slurm
Greetings,
heudiconv version 0.5.3.
I am trying to run a basic heudiconv on one subject for testing w/ slurm (running on two nodes, partition name = heudiconv) to create my heuristic.
1) Command as follows:
heudiconv -d /srv/lab/fmri/mft/dicom4bids/data/{subject}*/* -f /srv/lab/fmri/mft/dicom4bids/data/convertall.py -s sub01 -q heudiconv -o /srv/lab/fmri/mft/dicom4bids/data/output/
This yields:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
Traceback (most recent call last):
File "/usr/local/anaconda3/envs/fmri/bin/heudiconv", line 11, in <module>
sys.exit(main())
File "/usr/local/anaconda3/envs/fmri/lib/python3.5/site-packages/heudiconv/cli/run.py", line 125, in main
process_args(args)
File "/usr/local/anaconda3/envs/fmri/lib/python3.5/site-packages/heudiconv/cli/run.py", line 296, in process_args
study_outdir,
UnboundLocalError: local variable 'study_outdir' referenced before assignment
2) Now, adding "-l unknown" to the above command executes, but does not create a .heudiconv folder:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
WARNING: Skipping unknown locator dataset
3) Curiously, removing the -q flag and the -l flag executes the command properly and creates .heudiconv under /output:
INFO: Running heudiconv version 0.5.3
INFO: Need to process 1 study sessions
INFO: PROCESSING STARTS: {'session': None, 'outdir': '/srv/lab/fmri/mft/dicom4bids/data/output/', 'subject': 'sub01'}
INFO: Processing 4976 dicoms
INFO: Analyzing 4976 dicoms
INFO: Generated sequence info with 10 entries
INFO: PROCESSING DONE: {'session': None, 'outdir': '/srv/lab/fmri/mft/dicom4bids/data/output/', 'subject': 'sub01'}
4) Anyone else experienced these issues? How come that adding -q breaks the locator inference? I still believe that a working scheduler integration for heudiconv will be extremely valuable w/ increasing data sets and I am happy to contribute. Perhaps a starting point would be to clarify what the -l flag actually requires. In the help menu for -l, it says: study path under outdir. If provided, it overloads the value provided by the heuristic. If --datalad is enabled, every directory within locator becomes a super-dataset thus establishing a hierarchy. Setting to "unknown" will skip that dataset.
Thanks in advance!
| 0.0 | [
"heudiconv/tests/test_queue.py::test_queue_no_slurm[--files",
"heudiconv/tests/test_queue.py::test_queue_no_slurm[-d",
"heudiconv/tests/test_queue.py::test_argument_filtering"
] | [] | 2019-04-01 19:39:38+00:00 | 4,180 |
|
nipy__heudiconv-342 | diff --git a/heudiconv/convert.py b/heudiconv/convert.py
index c115039..7acfdf7 100644
--- a/heudiconv/convert.py
+++ b/heudiconv/convert.py
@@ -572,7 +572,7 @@ def save_converted_files(res, item_dicoms, bids, outtype, prefix, outname_bids,
echo_number = fileinfo.get('EchoNumber', 1)
- supported_multiecho = ['_bold', '_epi', '_sbref', '_T1w']
+ supported_multiecho = ['_bold', '_epi', '_sbref', '_T1w', '_PDT2']
# Now, decide where to insert it.
# Insert it **before** the following string(s), whichever appears first.
for imgtype in supported_multiecho:
diff --git a/heudiconv/heuristics/reproin.py b/heudiconv/heuristics/reproin.py
index 7de451d..4829941 100644
--- a/heudiconv/heuristics/reproin.py
+++ b/heudiconv/heuristics/reproin.py
@@ -28,10 +28,11 @@ per each session.
Sequence names on the scanner must follow this specification to avoid manual
conversion/handling:
- [PREFIX:]<seqtype[-label]>[_ses-<SESID>][_task-<TASKID>][_acq-<ACQLABEL>][_run-<RUNID>][_dir-<DIR>][<more BIDS>][__<custom>]
+ [PREFIX:][WIP ]<seqtype[-label]>[_ses-<SESID>][_task-<TASKID>][_acq-<ACQLABEL>][_run-<RUNID>][_dir-<DIR>][<more BIDS>][__<custom>]
where
[PREFIX:] - leading capital letters followed by : are stripped/ignored
+ [WIP ] - prefix is stripped/ignored (added by Philips for patch sequences)
<...> - value to be entered
[...] - optional -- might be nearly mandatory for some modalities (e.g.,
run for functional) and very optional for others
@@ -104,6 +105,16 @@ __<custom> (optional)
Although we still support "-" and "+" used within SESID and TASKID, their use is
not recommended, thus not listed here
+
+## Scanner specifics
+
+We perform following actions regardless of the type of scanner, but applied
+generally to accommodate limitations imposed by different manufacturers/models:
+
+### Philips
+
+- We replace all ( with { and ) with } to be able e.g. to specify session {date}
+- "WIP " prefix unconditionally added by the scanner is stripped
"""
import os
@@ -426,16 +437,15 @@ def ls(study_session, seqinfo):
# So we just need subdir and file_suffix!
def infotodict(seqinfo):
"""Heuristic evaluator for determining which runs belong where
-
- allowed template fields - follow python string module:
-
+
+ allowed template fields - follow python string module:
+
item: index within category
subject: participant id
seqitem: run number during scanning
subindex: sub index within group
session: scan index for longitudinal acq
"""
-
seqinfo = fix_seqinfo(seqinfo)
lgr.info("Processing %d seqinfo entries", len(seqinfo))
and_dicom = ('dicom', 'nii.gz')
@@ -841,6 +851,7 @@ def parse_series_spec(series_spec):
# https://github.com/ReproNim/reproin/issues/14
# where PU: prefix is added by the scanner
series_spec = re.sub("^[A-Z]*:", "", series_spec)
+ series_spec = re.sub("^WIP ", "", series_spec) # remove Philips WIP prefix
# Remove possible suffix we don't care about after __
series_spec = series_spec.split('__', 1)[0]
@@ -888,7 +899,9 @@ def parse_series_spec(series_spec):
# sanitize values, which must not have _ and - is undesirable ATM as well
# TODO: BIDSv2.0 -- allows "-" so replace with it instead
- value = str(value).replace('_', 'X').replace('-', 'X')
+ value = str(value) \
+ .replace('_', 'X').replace('-', 'X') \
+ .replace('(', '{').replace(')', '}') # for Philips
if key in ['ses', 'run', 'task', 'acq']:
# those we care about explicitly
diff --git a/heudiconv/utils.py b/heudiconv/utils.py
index 847dfaf..abea820 100644
--- a/heudiconv/utils.py
+++ b/heudiconv/utils.py
@@ -251,7 +251,17 @@ def treat_infofile(filename):
j = json.load(f)
j_slim = slim_down_info(j)
- j_pretty = json_dumps_pretty(j_slim, indent=2, sort_keys=True)
+ dumps_kw = dict(indent=2, sort_keys=True)
+ try:
+ j_pretty = json_dumps_pretty(j_slim, **dumps_kw)
+ except AssertionError as exc:
+ lgr.warning(
+ "Prettyfication of .json failed (%s). "
+ "Original .json will be kept as is. Please share (if you could) "
+ "that file (%s) with HeuDiConv developers"
+ % (str(exc), filename)
+ )
+ j_pretty = json.dumps(j_slim, **dumps_kw)
set_readonly(filename, False)
with open(filename, 'wt') as fp:
| nipy/heudiconv | 8cdf06da2798aa6f75a218780146e1cbca30fe97 | diff --git a/heudiconv/heuristics/test_reproin.py b/heudiconv/heuristics/test_reproin.py
index 2517b29..0a63dc1 100644
--- a/heudiconv/heuristics/test_reproin.py
+++ b/heudiconv/heuristics/test_reproin.py
@@ -166,6 +166,7 @@ def test_parse_series_spec():
assert \
pdpn(" PREFIX:bids_func_ses+_task-boo_run+ ") == \
pdpn("PREFIX:bids_func_ses+_task-boo_run+") == \
+ pdpn("WIP func_ses+_task-boo_run+") == \
pdpn("bids_func_ses+_run+_task-boo") == \
{
'seqtype': 'func',
@@ -202,3 +203,9 @@ def test_parse_series_spec():
'acq': 'MPRAGE',
'seqtype_label': 'T1w'
}
+
+ # Check for currently used {date}, which should also should get adjusted
+ # from (date) since Philips does not allow for {}
+ assert pdpn("func_ses-{date}") == \
+ pdpn("func_ses-(date)") == \
+ {'seqtype': 'func', 'session': '{date}'}
\ No newline at end of file
| AssertionError line 239 in Utils.py
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
```
Traceback (most recent call last):
File "/home/ggilmore/.local/bin/heudiconv", line 11, in <module>
load_entry_point('heudiconv==0.5.4.dev1', 'console_scripts', 'heudiconv')()
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/cli/run.py", line 127, in main
process_args(args)
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/cli/run.py", line 341, in process_args
dcmconfig=args.dcmconfig,)
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/convert.py", line 199, in prep_conversion
dcmconfig=dcmconfig,)
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/convert.py", line 318, in convert
treat_infofile(scaninfo)
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/utils.py", line 252, in treat_infofile
j_pretty = json_dumps_pretty(j_slim, indent=2, sort_keys=True)
File "/home/ggilmore/.local/lib/python2.7/site-packages/heudiconv/utils.py", line 239, in json_dumps_pretty
"Values differed when they should have not. "\
AssertionError: Values differed when they should have not. Report to the heudiconv developers
```
This works for all my scan sequences except I get this assertion failure on my CT study series. Could this be an issue with the CT sequence?
### Platform details:
Choose one:
- [x ] Local environment
<!-- If selected, please provide OS and python version -->
Ubuntu 16.04, python 2.7
- [ ] Container
<!-- If selected, please provide container name and tag"-->
- Heudiconv version:
<!-- To check: run heudiconv with just the --version flag -->
version 0.5.4.dev1 | 0.0 | [
"heudiconv/heuristics/test_reproin.py::test_parse_series_spec"
] | [
"heudiconv/heuristics/test_reproin.py::test_get_dups_marked",
"heudiconv/heuristics/test_reproin.py::test_filter_files",
"heudiconv/heuristics/test_reproin.py::test_md5sum",
"heudiconv/heuristics/test_reproin.py::test_fix_canceled_runs",
"heudiconv/heuristics/test_reproin.py::test_fix_dbic_protocol",
"heudiconv/heuristics/test_reproin.py::test_sanitize_str",
"heudiconv/heuristics/test_reproin.py::test_fixupsubjectid"
] | 2019-05-13 22:02:00+00:00 | 4,181 |
|
nipy__heudiconv-373 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index 1bbfcf6..9569278 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -240,6 +240,27 @@ def add_participant_record(studydir, subject, age, sex):
known_subjects = {l.split('\t')[0] for l in f.readlines()}
if participant_id in known_subjects:
return
+ else:
+ # Populate particpants.json (an optional file to describe column names in
+ # participant.tsv). This auto generation will make BIDS-validator happy.
+ participants_json = op.join(studydir, 'participants.json')
+ if not op.lexists(participants_json):
+ save_json(participants_json,
+ OrderedDict([
+ ("participant_id", OrderedDict([
+ ("Description", "Participant identifier")])),
+ ("age", OrderedDict([
+ ("Description", "Age in years (TODO - verify) as in the initial"
+ " session, might not be correct for other sessions")])),
+ ("sex", OrderedDict([
+ ("Description", "self-rated by participant, M for male/F for "
+ "female (TODO: verify)")])),
+ ("group", OrderedDict([
+ ("Description", "(TODO: adjust - by default everyone is in "
+ "control group)")])),
+ ]),
+ sort_keys=False,
+ indent=2)
# Add a new participant
with open(participants_tsv, 'a') as f:
f.write(
@@ -311,7 +332,8 @@ def save_scans_key(item, bids_files):
def add_rows_to_scans_keys_file(fn, newrows):
"""
- Add new rows to file fn for scans key filename
+ Add new rows to file fn for scans key filename and generate accompanying json
+ descriptor to make BIDS validator happy.
Parameters
----------
@@ -334,6 +356,25 @@ def add_rows_to_scans_keys_file(fn, newrows):
os.unlink(fn)
else:
fnames2info = newrows
+ # Populate _scans.json (an optional file to describe column names in
+ # _scans.tsv). This auto generation will make BIDS-validator happy.
+ scans_json = '.'.join(fn.split('.')[:-1] + ['json'])
+ if not op.lexists(scans_json):
+ save_json(scans_json,
+ OrderedDict([
+ ("filename", OrderedDict([
+ ("Description", "Name of the nifti file")])),
+ ("acq_time", OrderedDict([
+ ("LongName", "Acquisition time"),
+ ("Description", "Acquisition time of the particular scan")])),
+ ("operator", OrderedDict([
+ ("Description", "Name of the operator")])),
+ ("randstr", OrderedDict([
+ ("LongName", "Random string"),
+ ("Description", "md5 hash of UIDs")])),
+ ]),
+ sort_keys=False,
+ indent=2)
header = ['filename', 'acq_time', 'operator', 'randstr']
# prepare all the data rows
diff --git a/heudiconv/convert.py b/heudiconv/convert.py
index 5a5db05..1c16bb0 100644
--- a/heudiconv/convert.py
+++ b/heudiconv/convert.py
@@ -580,7 +580,7 @@ def save_converted_files(res, item_dicoms, bids_options, outtype, prefix, outnam
else:
echo_number = echo_times.index(fileinfo['EchoTime']) + 1
- supported_multiecho = ['_bold', '_epi', '_sbref', '_T1w', '_PDT2']
+ supported_multiecho = ['_bold', '_phase', '_epi', '_sbref', '_T1w', '_PDT2']
# Now, decide where to insert it.
# Insert it **before** the following string(s), whichever appears first.
for imgtype in supported_multiecho:
diff --git a/heudiconv/info.py b/heudiconv/info.py
index b970b3b..feeae46 100644
--- a/heudiconv/info.py
+++ b/heudiconv/info.py
@@ -8,6 +8,19 @@ __longdesc__ = """Convert DICOM dirs based on heuristic info - HeuDiConv
uses the dcmstack package and dcm2niix tool to convert DICOM directories or
tarballs into collections of NIfTI files following pre-defined heuristic(s)."""
+CLASSIFIERS = [
+ 'Environment :: Console',
+ 'Intended Audience :: Science/Research',
+ 'License :: OSI Approved :: Apache Software License',
+ 'Programming Language :: Python :: 2.7',
+ 'Programming Language :: Python :: 3.5',
+ 'Programming Language :: Python :: 3.6',
+ 'Programming Language :: Python :: 3.7',
+ 'Topic :: Scientific/Engineering'
+]
+
+PYTHON_REQUIRES = ">=2.7,!=3.0.*,!=3.1.*,!=3.2.*,!=3.3.*,!=3.4.*"
+
REQUIRES = [
'nibabel',
'pydicom',
diff --git a/heudiconv/utils.py b/heudiconv/utils.py
index abea820..2453e9d 100644
--- a/heudiconv/utils.py
+++ b/heudiconv/utils.py
@@ -19,6 +19,11 @@ from nipype.utils.filemanip import which
import logging
lgr = logging.getLogger(__name__)
+if sys.version_info[0] > 2:
+ from json.decoder import JSONDecodeError
+else:
+ JSONDecodeError = ValueError
+
seqinfo_fields = [
'total_files_till_now', # 0
@@ -172,10 +177,15 @@ def load_json(filename):
-------
data : dict
"""
- with open(filename, 'r') as fp:
- data = json.load(fp)
- return data
+ try:
+ with open(filename, 'r') as fp:
+ data = json.load(fp)
+ except JSONDecodeError:
+ lgr.error("{fname} is not a valid json file".format(fname=filename))
+ raise
+ return data
+
def assure_no_file_exists(path):
"""Check if file or symlink (git-annex?) exists, and if so -- remove"""
diff --git a/setup.py b/setup.py
index 6017447..c3a804a 100755
--- a/setup.py
+++ b/setup.py
@@ -47,11 +47,13 @@ def main():
description=ldict['__description__'],
long_description=ldict['__longdesc__'],
license=ldict['__license__'],
+ classifiers=ldict['CLASSIFIERS'],
packages=heudiconv_pkgs,
entry_points={'console_scripts': [
'heudiconv=heudiconv.cli.run:main',
'heudiconv_monitor=heudiconv.cli.monitor:main',
]},
+ python_requires=ldict['PYTHON_REQUIRES'],
install_requires=ldict['REQUIRES'],
extras_require=ldict['EXTRA_REQUIRES'],
package_data={
| nipy/heudiconv | d31d19d6904d59ca407f5899e405f6de4ba7d00f | diff --git a/heudiconv/tests/test_heuristics.py b/heudiconv/tests/test_heuristics.py
index c9485c6..f36bbb4 100644
--- a/heudiconv/tests/test_heuristics.py
+++ b/heudiconv/tests/test_heuristics.py
@@ -165,7 +165,13 @@ def test_notop(tmpdir, bidsoptions):
runner(args)
assert op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4'))
- for fname in ['CHANGES', 'dataset_description.json', 'participants.tsv', 'README']:
+ for fname in [
+ 'CHANGES',
+ 'dataset_description.json',
+ 'participants.tsv',
+ 'README',
+ 'participants.json'
+ ]:
if 'notop' in bidsoptions:
assert not op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4', fname))
else:
diff --git a/heudiconv/tests/test_main.py b/heudiconv/tests/test_main.py
index 7dcd32e..f4b7700 100644
--- a/heudiconv/tests/test_main.py
+++ b/heudiconv/tests/test_main.py
@@ -22,6 +22,7 @@ from mock import patch
from os.path import join as opj
from six.moves import StringIO
import stat
+import os.path as op
@patch('sys.stdout', new_callable=StringIO)
@@ -205,6 +206,7 @@ def test_add_rows_to_scans_keys_file(tmpdir):
assert dates == sorted(dates)
_check_rows(fn, rows)
+ assert op.exists(opj(tmpdir.strpath, 'file.json'))
# add a new one
extra_rows = {
'a_new_file.nii.gz': ['2016adsfasd23', '', 'fasadfasdf'],
diff --git a/heudiconv/tests/test_utils.py b/heudiconv/tests/test_utils.py
index 47faec4..ad84673 100644
--- a/heudiconv/tests/test_utils.py
+++ b/heudiconv/tests/test_utils.py
@@ -1,10 +1,16 @@
+import json
import os
import os.path as op
+
from heudiconv.utils import (
get_known_heuristics_with_descriptions,
get_heuristic_description,
load_heuristic,
- json_dumps_pretty)
+ json_dumps_pretty,
+ load_json,
+ create_tree,
+ save_json,
+ JSONDecodeError)
import pytest
from .utils import HEURISTICS_PATH
@@ -58,4 +64,24 @@ def test_json_dumps_pretty():
'Mar 3 2017 10:46:13 by eja'
# just the date which reveals the issue
# tstr = 'Mar 3 2017 10:46:13 by eja'
- assert pretty({'WipMemBlock': tstr}) == '{\n "WipMemBlock": "%s"\n}' % tstr
\ No newline at end of file
+ assert pretty({'WipMemBlock': tstr}) == '{\n "WipMemBlock": "%s"\n}' % tstr
+
+
+def test_load_json(tmp_path, caplog):
+ # test invalid json
+ ifname = 'invalid.json'
+ invalid_json_file = str(tmp_path / ifname)
+ create_tree(str(tmp_path), {ifname: u"I'm Jason Bourne"})
+
+ with pytest.raises(JSONDecodeError):
+ load_json(str(invalid_json_file))
+
+ assert ifname in caplog.text
+
+ # test valid json
+ vcontent = {"secret": "spy"}
+ vfname = "valid.json"
+ valid_json_file = str(tmp_path / vfname)
+ save_json(valid_json_file, vcontent)
+
+ assert load_json(valid_json_file) == vcontent
| Support for complex multi-echo fMRI
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
Functional phase data (from magnitude+phase reconstruction of fMRI data) is not currently supported as a multi-echo data type. I know that there are plans for a more robust form of support for multi-volume sequences in #346, but in the meantime I think that `_phase` needs to be added to the [list of supported multi-echo data types](https://github.com/nipy/heudiconv/blob/5cfb6f2835f76b8c9d6f19c45e8be0759535900f/heudiconv/convert.py#L583).
### Platform details:
Choose one:
- [X] Local environment: OSX Mojave, Python 3.6.2
<!-- If selected, please provide OS and python version -->
- [ ] Container
<!-- If selected, please provide container name and tag"-->
- Heudiconv version: latest, as of 5cfb6f2
<!-- To check: run heudiconv with just the --version flag --> | 0.0 | [
"heudiconv/tests/test_heuristics.py::test_smoke_convertall",
"heudiconv/tests/test_heuristics.py::test_ls",
"heudiconv/tests/test_main.py::test_main_help",
"heudiconv/tests/test_main.py::test_main_version",
"heudiconv/tests/test_main.py::test_create_file_if_missing",
"heudiconv/tests/test_main.py::test_populate_bids_templates",
"heudiconv/tests/test_main.py::test_add_participant_record",
"heudiconv/tests/test_main.py::test_get_formatted_scans_key_row",
"heudiconv/tests/test_main.py::test_add_rows_to_scans_keys_file",
"heudiconv/tests/test_main.py::test__find_subj_ses",
"heudiconv/tests/test_main.py::test_make_readonly",
"heudiconv/tests/test_utils.py::test_get_known_heuristics_with_descriptions",
"heudiconv/tests/test_utils.py::test_get_heuristic_description",
"heudiconv/tests/test_utils.py::test_load_heuristic",
"heudiconv/tests/test_utils.py::test_json_dumps_pretty",
"heudiconv/tests/test_utils.py::test_load_json"
] | [] | 2019-09-18 16:13:35+00:00 | 4,182 |
|
nipy__heudiconv-376 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index 1bbfcf6..9569278 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -240,6 +240,27 @@ def add_participant_record(studydir, subject, age, sex):
known_subjects = {l.split('\t')[0] for l in f.readlines()}
if participant_id in known_subjects:
return
+ else:
+ # Populate particpants.json (an optional file to describe column names in
+ # participant.tsv). This auto generation will make BIDS-validator happy.
+ participants_json = op.join(studydir, 'participants.json')
+ if not op.lexists(participants_json):
+ save_json(participants_json,
+ OrderedDict([
+ ("participant_id", OrderedDict([
+ ("Description", "Participant identifier")])),
+ ("age", OrderedDict([
+ ("Description", "Age in years (TODO - verify) as in the initial"
+ " session, might not be correct for other sessions")])),
+ ("sex", OrderedDict([
+ ("Description", "self-rated by participant, M for male/F for "
+ "female (TODO: verify)")])),
+ ("group", OrderedDict([
+ ("Description", "(TODO: adjust - by default everyone is in "
+ "control group)")])),
+ ]),
+ sort_keys=False,
+ indent=2)
# Add a new participant
with open(participants_tsv, 'a') as f:
f.write(
@@ -311,7 +332,8 @@ def save_scans_key(item, bids_files):
def add_rows_to_scans_keys_file(fn, newrows):
"""
- Add new rows to file fn for scans key filename
+ Add new rows to file fn for scans key filename and generate accompanying json
+ descriptor to make BIDS validator happy.
Parameters
----------
@@ -334,6 +356,25 @@ def add_rows_to_scans_keys_file(fn, newrows):
os.unlink(fn)
else:
fnames2info = newrows
+ # Populate _scans.json (an optional file to describe column names in
+ # _scans.tsv). This auto generation will make BIDS-validator happy.
+ scans_json = '.'.join(fn.split('.')[:-1] + ['json'])
+ if not op.lexists(scans_json):
+ save_json(scans_json,
+ OrderedDict([
+ ("filename", OrderedDict([
+ ("Description", "Name of the nifti file")])),
+ ("acq_time", OrderedDict([
+ ("LongName", "Acquisition time"),
+ ("Description", "Acquisition time of the particular scan")])),
+ ("operator", OrderedDict([
+ ("Description", "Name of the operator")])),
+ ("randstr", OrderedDict([
+ ("LongName", "Random string"),
+ ("Description", "md5 hash of UIDs")])),
+ ]),
+ sort_keys=False,
+ indent=2)
header = ['filename', 'acq_time', 'operator', 'randstr']
# prepare all the data rows
diff --git a/heudiconv/cli/run.py b/heudiconv/cli/run.py
index cad83bf..ab69f10 100644
--- a/heudiconv/cli/run.py
+++ b/heudiconv/cli/run.py
@@ -16,7 +16,7 @@ import inspect
import logging
lgr = logging.getLogger(__name__)
-INIT_MSG = "Running {packname} version {version}".format
+INIT_MSG = "Running {packname} version {version} latest {latest}".format
def is_interactive():
@@ -245,8 +245,16 @@ def process_args(args):
outdir = op.abspath(args.outdir)
+ import etelemetry
+ try:
+ latest = etelemetry.get_project("nipy/heudiconv")
+ except Exception as e:
+ lgr.warning("Could not check for version updates: ", e)
+ latest = {"version": 'Unknown'}
+
lgr.info(INIT_MSG(packname=__packagename__,
- version=__version__))
+ version=__version__,
+ latest=latest["version"]))
if args.command:
process_extra_commands(outdir, args)
diff --git a/heudiconv/info.py b/heudiconv/info.py
index 8c6b67c..feeae46 100644
--- a/heudiconv/info.py
+++ b/heudiconv/info.py
@@ -8,6 +8,19 @@ __longdesc__ = """Convert DICOM dirs based on heuristic info - HeuDiConv
uses the dcmstack package and dcm2niix tool to convert DICOM directories or
tarballs into collections of NIfTI files following pre-defined heuristic(s)."""
+CLASSIFIERS = [
+ 'Environment :: Console',
+ 'Intended Audience :: Science/Research',
+ 'License :: OSI Approved :: Apache Software License',
+ 'Programming Language :: Python :: 2.7',
+ 'Programming Language :: Python :: 3.5',
+ 'Programming Language :: Python :: 3.6',
+ 'Programming Language :: Python :: 3.7',
+ 'Topic :: Scientific/Engineering'
+]
+
+PYTHON_REQUIRES = ">=2.7,!=3.0.*,!=3.1.*,!=3.2.*,!=3.3.*,!=3.4.*"
+
REQUIRES = [
'nibabel',
'pydicom',
@@ -15,6 +28,7 @@ REQUIRES = [
'nipype >=1.0.0,!=1.2.1,!=1.2.2; python_version == "2.7"',
'pathlib',
'dcmstack>=0.7',
+ 'etelemetry',
]
TESTS_REQUIRES = [
diff --git a/setup.py b/setup.py
index 6017447..c3a804a 100755
--- a/setup.py
+++ b/setup.py
@@ -47,11 +47,13 @@ def main():
description=ldict['__description__'],
long_description=ldict['__longdesc__'],
license=ldict['__license__'],
+ classifiers=ldict['CLASSIFIERS'],
packages=heudiconv_pkgs,
entry_points={'console_scripts': [
'heudiconv=heudiconv.cli.run:main',
'heudiconv_monitor=heudiconv.cli.monitor:main',
]},
+ python_requires=ldict['PYTHON_REQUIRES'],
install_requires=ldict['REQUIRES'],
extras_require=ldict['EXTRA_REQUIRES'],
package_data={
| nipy/heudiconv | fe0f2c89b96f6ee7f63d44ed3b19f004ba977c56 | diff --git a/heudiconv/tests/test_heuristics.py b/heudiconv/tests/test_heuristics.py
index c9485c6..f36bbb4 100644
--- a/heudiconv/tests/test_heuristics.py
+++ b/heudiconv/tests/test_heuristics.py
@@ -165,7 +165,13 @@ def test_notop(tmpdir, bidsoptions):
runner(args)
assert op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4'))
- for fname in ['CHANGES', 'dataset_description.json', 'participants.tsv', 'README']:
+ for fname in [
+ 'CHANGES',
+ 'dataset_description.json',
+ 'participants.tsv',
+ 'README',
+ 'participants.json'
+ ]:
if 'notop' in bidsoptions:
assert not op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4', fname))
else:
diff --git a/heudiconv/tests/test_main.py b/heudiconv/tests/test_main.py
index 7dcd32e..f4b7700 100644
--- a/heudiconv/tests/test_main.py
+++ b/heudiconv/tests/test_main.py
@@ -22,6 +22,7 @@ from mock import patch
from os.path import join as opj
from six.moves import StringIO
import stat
+import os.path as op
@patch('sys.stdout', new_callable=StringIO)
@@ -205,6 +206,7 @@ def test_add_rows_to_scans_keys_file(tmpdir):
assert dates == sorted(dates)
_check_rows(fn, rows)
+ assert op.exists(opj(tmpdir.strpath, 'file.json'))
# add a new one
extra_rows = {
'a_new_file.nii.gz': ['2016adsfasd23', '', 'fasadfasdf'],
| Generate participants.json to accompany .tsv where fields are described
ATM we are generating `participants.tsv` with columns age, sex, group. bids-validator then whines
```
./participants.tsv
Evidence: Columns: age, sex, group not defined, please define in: /participants.json
```
We should then create/define something like
```json
{
"participant_id": {
"Description": "participant id"
},
"age": {
"Description": "Age in years (TODO - verify) as in the initial session, might not be correct for other sessions"
},
"sex": {
"Description": "self-rated by participant, M for male/F for female (TODO: verify)"
},
"group": {
"Description": "(TODO: adjust - by default everyone is in control group)"
}
}
``` | 0.0 | [
"heudiconv/tests/test_main.py::test_add_rows_to_scans_keys_file"
] | [
"heudiconv/tests/test_heuristics.py::test_smoke_convertall",
"heudiconv/tests/test_heuristics.py::test_ls",
"heudiconv/tests/test_main.py::test_main_help",
"heudiconv/tests/test_main.py::test_main_version",
"heudiconv/tests/test_main.py::test_create_file_if_missing",
"heudiconv/tests/test_main.py::test_populate_bids_templates",
"heudiconv/tests/test_main.py::test_add_participant_record",
"heudiconv/tests/test_main.py::test_get_formatted_scans_key_row",
"heudiconv/tests/test_main.py::test__find_subj_ses",
"heudiconv/tests/test_main.py::test_make_readonly"
] | 2019-09-20 04:25:31+00:00 | 4,183 |
|
nipy__heudiconv-379 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index 1bbfcf6..9569278 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -240,6 +240,27 @@ def add_participant_record(studydir, subject, age, sex):
known_subjects = {l.split('\t')[0] for l in f.readlines()}
if participant_id in known_subjects:
return
+ else:
+ # Populate particpants.json (an optional file to describe column names in
+ # participant.tsv). This auto generation will make BIDS-validator happy.
+ participants_json = op.join(studydir, 'participants.json')
+ if not op.lexists(participants_json):
+ save_json(participants_json,
+ OrderedDict([
+ ("participant_id", OrderedDict([
+ ("Description", "Participant identifier")])),
+ ("age", OrderedDict([
+ ("Description", "Age in years (TODO - verify) as in the initial"
+ " session, might not be correct for other sessions")])),
+ ("sex", OrderedDict([
+ ("Description", "self-rated by participant, M for male/F for "
+ "female (TODO: verify)")])),
+ ("group", OrderedDict([
+ ("Description", "(TODO: adjust - by default everyone is in "
+ "control group)")])),
+ ]),
+ sort_keys=False,
+ indent=2)
# Add a new participant
with open(participants_tsv, 'a') as f:
f.write(
@@ -311,7 +332,8 @@ def save_scans_key(item, bids_files):
def add_rows_to_scans_keys_file(fn, newrows):
"""
- Add new rows to file fn for scans key filename
+ Add new rows to file fn for scans key filename and generate accompanying json
+ descriptor to make BIDS validator happy.
Parameters
----------
@@ -334,6 +356,25 @@ def add_rows_to_scans_keys_file(fn, newrows):
os.unlink(fn)
else:
fnames2info = newrows
+ # Populate _scans.json (an optional file to describe column names in
+ # _scans.tsv). This auto generation will make BIDS-validator happy.
+ scans_json = '.'.join(fn.split('.')[:-1] + ['json'])
+ if not op.lexists(scans_json):
+ save_json(scans_json,
+ OrderedDict([
+ ("filename", OrderedDict([
+ ("Description", "Name of the nifti file")])),
+ ("acq_time", OrderedDict([
+ ("LongName", "Acquisition time"),
+ ("Description", "Acquisition time of the particular scan")])),
+ ("operator", OrderedDict([
+ ("Description", "Name of the operator")])),
+ ("randstr", OrderedDict([
+ ("LongName", "Random string"),
+ ("Description", "md5 hash of UIDs")])),
+ ]),
+ sort_keys=False,
+ indent=2)
header = ['filename', 'acq_time', 'operator', 'randstr']
# prepare all the data rows
diff --git a/heudiconv/info.py b/heudiconv/info.py
index b970b3b..feeae46 100644
--- a/heudiconv/info.py
+++ b/heudiconv/info.py
@@ -8,6 +8,19 @@ __longdesc__ = """Convert DICOM dirs based on heuristic info - HeuDiConv
uses the dcmstack package and dcm2niix tool to convert DICOM directories or
tarballs into collections of NIfTI files following pre-defined heuristic(s)."""
+CLASSIFIERS = [
+ 'Environment :: Console',
+ 'Intended Audience :: Science/Research',
+ 'License :: OSI Approved :: Apache Software License',
+ 'Programming Language :: Python :: 2.7',
+ 'Programming Language :: Python :: 3.5',
+ 'Programming Language :: Python :: 3.6',
+ 'Programming Language :: Python :: 3.7',
+ 'Topic :: Scientific/Engineering'
+]
+
+PYTHON_REQUIRES = ">=2.7,!=3.0.*,!=3.1.*,!=3.2.*,!=3.3.*,!=3.4.*"
+
REQUIRES = [
'nibabel',
'pydicom',
diff --git a/heudiconv/utils.py b/heudiconv/utils.py
index abea820..2453e9d 100644
--- a/heudiconv/utils.py
+++ b/heudiconv/utils.py
@@ -19,6 +19,11 @@ from nipype.utils.filemanip import which
import logging
lgr = logging.getLogger(__name__)
+if sys.version_info[0] > 2:
+ from json.decoder import JSONDecodeError
+else:
+ JSONDecodeError = ValueError
+
seqinfo_fields = [
'total_files_till_now', # 0
@@ -172,10 +177,15 @@ def load_json(filename):
-------
data : dict
"""
- with open(filename, 'r') as fp:
- data = json.load(fp)
- return data
+ try:
+ with open(filename, 'r') as fp:
+ data = json.load(fp)
+ except JSONDecodeError:
+ lgr.error("{fname} is not a valid json file".format(fname=filename))
+ raise
+ return data
+
def assure_no_file_exists(path):
"""Check if file or symlink (git-annex?) exists, and if so -- remove"""
diff --git a/setup.py b/setup.py
index 6017447..c3a804a 100755
--- a/setup.py
+++ b/setup.py
@@ -47,11 +47,13 @@ def main():
description=ldict['__description__'],
long_description=ldict['__longdesc__'],
license=ldict['__license__'],
+ classifiers=ldict['CLASSIFIERS'],
packages=heudiconv_pkgs,
entry_points={'console_scripts': [
'heudiconv=heudiconv.cli.run:main',
'heudiconv_monitor=heudiconv.cli.monitor:main',
]},
+ python_requires=ldict['PYTHON_REQUIRES'],
install_requires=ldict['REQUIRES'],
extras_require=ldict['EXTRA_REQUIRES'],
package_data={
| nipy/heudiconv | d31d19d6904d59ca407f5899e405f6de4ba7d00f | diff --git a/heudiconv/tests/test_heuristics.py b/heudiconv/tests/test_heuristics.py
index c9485c6..f36bbb4 100644
--- a/heudiconv/tests/test_heuristics.py
+++ b/heudiconv/tests/test_heuristics.py
@@ -165,7 +165,13 @@ def test_notop(tmpdir, bidsoptions):
runner(args)
assert op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4'))
- for fname in ['CHANGES', 'dataset_description.json', 'participants.tsv', 'README']:
+ for fname in [
+ 'CHANGES',
+ 'dataset_description.json',
+ 'participants.tsv',
+ 'README',
+ 'participants.json'
+ ]:
if 'notop' in bidsoptions:
assert not op.exists(pjoin(tmppath, 'Halchenko/Yarik/950_bids_test4', fname))
else:
diff --git a/heudiconv/tests/test_main.py b/heudiconv/tests/test_main.py
index 7dcd32e..f4b7700 100644
--- a/heudiconv/tests/test_main.py
+++ b/heudiconv/tests/test_main.py
@@ -22,6 +22,7 @@ from mock import patch
from os.path import join as opj
from six.moves import StringIO
import stat
+import os.path as op
@patch('sys.stdout', new_callable=StringIO)
@@ -205,6 +206,7 @@ def test_add_rows_to_scans_keys_file(tmpdir):
assert dates == sorted(dates)
_check_rows(fn, rows)
+ assert op.exists(opj(tmpdir.strpath, 'file.json'))
# add a new one
extra_rows = {
'a_new_file.nii.gz': ['2016adsfasd23', '', 'fasadfasdf'],
diff --git a/heudiconv/tests/test_utils.py b/heudiconv/tests/test_utils.py
index 47faec4..ad84673 100644
--- a/heudiconv/tests/test_utils.py
+++ b/heudiconv/tests/test_utils.py
@@ -1,10 +1,16 @@
+import json
import os
import os.path as op
+
from heudiconv.utils import (
get_known_heuristics_with_descriptions,
get_heuristic_description,
load_heuristic,
- json_dumps_pretty)
+ json_dumps_pretty,
+ load_json,
+ create_tree,
+ save_json,
+ JSONDecodeError)
import pytest
from .utils import HEURISTICS_PATH
@@ -58,4 +64,24 @@ def test_json_dumps_pretty():
'Mar 3 2017 10:46:13 by eja'
# just the date which reveals the issue
# tstr = 'Mar 3 2017 10:46:13 by eja'
- assert pretty({'WipMemBlock': tstr}) == '{\n "WipMemBlock": "%s"\n}' % tstr
\ No newline at end of file
+ assert pretty({'WipMemBlock': tstr}) == '{\n "WipMemBlock": "%s"\n}' % tstr
+
+
+def test_load_json(tmp_path, caplog):
+ # test invalid json
+ ifname = 'invalid.json'
+ invalid_json_file = str(tmp_path / ifname)
+ create_tree(str(tmp_path), {ifname: u"I'm Jason Bourne"})
+
+ with pytest.raises(JSONDecodeError):
+ load_json(str(invalid_json_file))
+
+ assert ifname in caplog.text
+
+ # test valid json
+ vcontent = {"secret": "spy"}
+ vfname = "valid.json"
+ valid_json_file = str(tmp_path / vfname)
+ save_json(valid_json_file, vcontent)
+
+ assert load_json(valid_json_file) == vcontent
| enhance explicitness about what json files heudiconv fails to read
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
I was helping a user troubleshoot a problem with heudiconv, as it was tripping up on an invalid json file, but the user only thought heudiconv was touching files in the subject directory inside the bids directory, and not looking in other places inside the bids directory (which it does).
A clue that could have gotten this user on track faster would be to print which file was the invalid json file.
To help, the `load_json` function could be changed to something like:
```
try:
with open(filename, 'r') as fp:
data = json.load(fp)
except json.JSONDecodeError:
print("{fname} is not a valid json file".format(fname=filename)
raise
```
Thoughts on this?
If positive I can open a pull request.
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
### Platform details:
Choose one:
- [ ] Local environment
<!-- If selected, please provide OS and python version -->
- [x] Container
<!-- If selected, please provide container name and tag"-->
- Heudiconv version: 0.5.4
<!-- To check: run heudiconv with just the --version flag -->
| 0.0 | [
"heudiconv/tests/test_heuristics.py::test_smoke_convertall",
"heudiconv/tests/test_heuristics.py::test_ls",
"heudiconv/tests/test_main.py::test_main_help",
"heudiconv/tests/test_main.py::test_main_version",
"heudiconv/tests/test_main.py::test_create_file_if_missing",
"heudiconv/tests/test_main.py::test_populate_bids_templates",
"heudiconv/tests/test_main.py::test_add_participant_record",
"heudiconv/tests/test_main.py::test_get_formatted_scans_key_row",
"heudiconv/tests/test_main.py::test_add_rows_to_scans_keys_file",
"heudiconv/tests/test_main.py::test__find_subj_ses",
"heudiconv/tests/test_main.py::test_make_readonly",
"heudiconv/tests/test_utils.py::test_get_known_heuristics_with_descriptions",
"heudiconv/tests/test_utils.py::test_get_heuristic_description",
"heudiconv/tests/test_utils.py::test_load_heuristic",
"heudiconv/tests/test_utils.py::test_json_dumps_pretty",
"heudiconv/tests/test_utils.py::test_load_json"
] | [] | 2019-10-03 14:21:10+00:00 | 4,184 |
|
nipy__heudiconv-407 | diff --git a/heudiconv/cli/run.py b/heudiconv/cli/run.py
index ed17aad..516e163 100644
--- a/heudiconv/cli/run.py
+++ b/heudiconv/cli/run.py
@@ -248,11 +248,11 @@ def process_args(args):
outdir = op.abspath(args.outdir)
- import etelemetry
try:
+ import etelemetry
latest = etelemetry.get_project("nipy/heudiconv")
except Exception as e:
- lgr.warning("Could not check for version updates: ", e)
+ lgr.warning("Could not check for version updates: %s", str(e))
latest = {"version": 'Unknown'}
lgr.info(INIT_MSG(packname=__packagename__,
diff --git a/heudiconv/dicoms.py b/heudiconv/dicoms.py
index 8249aad..7c8c450 100644
--- a/heudiconv/dicoms.py
+++ b/heudiconv/dicoms.py
@@ -58,7 +58,9 @@ def group_dicoms_into_seqinfos(files, file_filter, dcmfilter, grouping):
import nibabel.nicom.dicomwrappers as dw
# TODO after getting a regression test check if the same behavior
# with stop_before_pixels=True
- mw = dw.wrapper_from_data(dcm.read_file(filename, force=True))
+ mw = dw.wrapper_from_data(
+ dcm.read_file(filename, stop_before_pixels=True, force=True)
+ )
for sig in ('iop', 'ICE_Dims', 'SequenceName'):
try:
| nipy/heudiconv | 536056875c111b67574e6dcf86f29c3d454d7435 | diff --git a/heudiconv/tests/test_dicoms.py b/heudiconv/tests/test_dicoms.py
index de8e9a1..4786cae 100644
--- a/heudiconv/tests/test_dicoms.py
+++ b/heudiconv/tests/test_dicoms.py
@@ -15,7 +15,7 @@ DICOM_FIELDS_TO_TEST = {
def test_private_csa_header(tmpdir):
dcm_file = op.join(TESTS_DATA_PATH, 'axasc35.dcm')
- dcm_data = dcm.read_file(dcm_file)
+ dcm_data = dcm.read_file(dcm_file, stop_before_pixels=True)
for pub, priv in DICOM_FIELDS_TO_TEST.items():
# ensure missing public tag
with pytest.raises(AttributeError):
diff --git a/heudiconv/tests/test_main.py b/heudiconv/tests/test_main.py
index f4b7700..2ee2d95 100644
--- a/heudiconv/tests/test_main.py
+++ b/heudiconv/tests/test_main.py
@@ -1,6 +1,9 @@
# TODO: break this up by modules
-from heudiconv.cli.run import main as runner
+from heudiconv.cli.run import (
+ main as runner,
+ process_args,
+)
from heudiconv import __version__
from heudiconv.utils import (create_file_if_missing,
set_readonly,
@@ -271,3 +274,16 @@ def test_cache(tmpdir):
assert (cachedir / 'dicominfo.tsv').exists()
assert (cachedir / 'S01.auto.txt').exists()
assert (cachedir / 'S01.edit.txt').exists()
+
+
+def test_no_etelemetry():
+ # smoke test at large - just verifying that no crash if no etelemetry
+ class args:
+ outdir = '/dev/null'
+ command = 'ls'
+ heuristic = 'reproin'
+ files = [] # Nothing to list
+
+ # must not fail if etelemetry no found
+ with patch.dict('sys.modules', {'etelemetry': None}):
+ process_args(args)
| something (etelemetry?) causes logger to puke a traceback upon startup
```shell
bids@rolando:~$ heudiconv -o /tmp/cmrr -l '' --files .... -f cmrr-test.py
Traceback (most recent call last):
File "/usr/lib/python2.7/logging/__init__.py", line 868, in emit
msg = self.format(record)
File "/usr/lib/python2.7/logging/__init__.py", line 741, in format
return fmt.format(record)
File "/usr/lib/python2.7/logging/__init__.py", line 465, in format
record.message = record.getMessage()
File "/usr/lib/python2.7/logging/__init__.py", line 329, in getMessage
msg = msg % self.args
TypeError: not all arguments converted during string formatting
Logged from file run.py, line 255
INFO: Running heudiconv version 0.6.0 latest Unknown
...
``` | 0.0 | [
"heudiconv/tests/test_main.py::test_no_etelemetry"
] | [
"heudiconv/tests/test_dicoms.py::test_private_csa_header",
"heudiconv/tests/test_dicoms.py::test_nifti_embed",
"heudiconv/tests/test_main.py::test_main_help",
"heudiconv/tests/test_main.py::test_main_version",
"heudiconv/tests/test_main.py::test_create_file_if_missing",
"heudiconv/tests/test_main.py::test_populate_bids_templates",
"heudiconv/tests/test_main.py::test_add_participant_record",
"heudiconv/tests/test_main.py::test_get_formatted_scans_key_row",
"heudiconv/tests/test_main.py::test_add_rows_to_scans_keys_file",
"heudiconv/tests/test_main.py::test__find_subj_ses",
"heudiconv/tests/test_main.py::test_make_readonly"
] | 2019-12-24 00:42:32+00:00 | 4,185 |
|
nipy__heudiconv-424 | diff --git a/CHANGELOG.md b/CHANGELOG.md
index 5d4b1e2..32e72bb 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -360,3 +360,11 @@ TODO Summary
[#434]: https://github.com/nipy/heudiconv/issues/434
[#436]: https://github.com/nipy/heudiconv/issues/436
[#437]: https://github.com/nipy/heudiconv/issues/437
+[#425]: https://github.com/nipy/heudiconv/issues/425
+[#420]: https://github.com/nipy/heudiconv/issues/420
+[#425]: https://github.com/nipy/heudiconv/issues/425
+[#430]: https://github.com/nipy/heudiconv/issues/430
+[#432]: https://github.com/nipy/heudiconv/issues/432
+[#434]: https://github.com/nipy/heudiconv/issues/434
+[#436]: https://github.com/nipy/heudiconv/issues/436
+[#437]: https://github.com/nipy/heudiconv/issues/437
diff --git a/docs/installation.rst b/docs/installation.rst
index 86668d3..0bb2380 100644
--- a/docs/installation.rst
+++ b/docs/installation.rst
@@ -7,23 +7,23 @@ Installation
Local
=====
-Released versions of HeuDiConv are available on `PyPI <https://pypi.org/project/heudiconv/>`_
-and `conda <https://github.com/conda-forge/heudiconv-feedstock#installing-heudiconv>`_.
+Released versions of HeuDiConv are available on `PyPI <https://pypi.org/project/heudiconv/>`_
+and `conda <https://github.com/conda-forge/heudiconv-feedstock#installing-heudiconv>`_.
If installing through ``PyPI``, eg::
pip install heudiconv[all]
-Manual installation of `dcm2niix <https://github.com/rordenlab/dcm2niix#install>`_
+Manual installation of `dcm2niix <https://github.com/rordenlab/dcm2niix#install>`_
is required.
-On Debian-based systems we recommend using `NeuroDebian <http://neuro.debian.net>`_
+On Debian-based systems we recommend using `NeuroDebian <http://neuro.debian.net>`_
which provides the `heudiconv package <http://neuro.debian.net/pkgs/heudiconv.html>`_.
Docker
======
-If `Docker <https://docs.docker.com/install/>`_ is available on your system, you
-can visit `our page on Docker Hub <https://hub.docker.com/r/nipy/heudiconv/tags>`_
+If `Docker <https://docs.docker.com/install/>`_ is available on your system, you
+can visit `our page on Docker Hub <https://hub.docker.com/r/nipy/heudiconv/tags>`_
to view available releases. To pull the latest release, run::
$ docker pull nipy/heudiconv:0.8.0
@@ -31,8 +31,8 @@ to view available releases. To pull the latest release, run::
Singularity
===========
-If `Singularity <https://www.sylabs.io/singularity/>`_ is available on your system,
-you can use it to pull and convert our Docker images! For example, to pull and
+If `Singularity <https://www.sylabs.io/singularity/>`_ is available on your system,
+you can use it to pull and convert our Docker images! For example, to pull and
build the latest release, you can run::
$ singularity pull docker://nipy/heudiconv:0.8.0
diff --git a/heudiconv/convert.py b/heudiconv/convert.py
index 7a0ea36..8e2c3c6 100644
--- a/heudiconv/convert.py
+++ b/heudiconv/convert.py
@@ -82,7 +82,7 @@ def conversion_info(subject, outdir, info, filegroup, ses):
def prep_conversion(sid, dicoms, outdir, heuristic, converter, anon_sid,
- anon_outdir, with_prov, ses, bids_options, seqinfo,
+ anon_outdir, with_prov, ses, bids_options, seqinfo,
min_meta, overwrite, dcmconfig, grouping):
if dicoms:
lgr.info("Processing %d dicoms", len(dicoms))
@@ -233,6 +233,157 @@ def prep_conversion(sid, dicoms, outdir, heuristic, converter, anon_sid,
getattr(heuristic, 'DEFAULT_FIELDS', {}))
+def update_complex_name(metadata, filename, suffix):
+ """
+ Insert `_rec-<magnitude|phase>` entity into filename if data are from a
+ sequence with magnitude/phase part.
+
+ Parameters
+ ----------
+ metadata : dict
+ Scan metadata dictionary from BIDS sidecar file.
+ filename : str
+ Incoming filename
+ suffix : str
+ An index used for cases where a single scan produces multiple files,
+ but the differences between those files are unknown.
+
+ Returns
+ -------
+ filename : str
+ Updated filename with rec entity added in appropriate position.
+ """
+ # Some scans separate magnitude/phase differently
+ unsupported_types = ['_bold', '_phase',
+ '_magnitude', '_magnitude1', '_magnitude2',
+ '_phasediff', '_phase1', '_phase2']
+ if any(ut in filename for ut in unsupported_types):
+ return filename
+
+ # Check to see if it is magnitude or phase part:
+ if 'M' in metadata.get('ImageType'):
+ mag_or_phase = 'magnitude'
+ elif 'P' in metadata.get('ImageType'):
+ mag_or_phase = 'phase'
+ else:
+ mag_or_phase = suffix
+
+ # Determine scan suffix
+ filetype = '_' + filename.split('_')[-1]
+
+ # Insert rec label
+ if not ('_rec-%s' % mag_or_phase) in filename:
+ # If "_rec-" is specified, prepend the 'mag_or_phase' value.
+ if '_rec-' in filename:
+ raise BIDSError(
+ "Reconstruction label for images will be automatically set, "
+ "remove from heuristic"
+ )
+
+ # Insert it **before** the following string(s), whichever appears first.
+ for label in ['_dir', '_run', '_mod', '_echo', '_recording', '_proc', '_space', filetype]:
+ if (label == filetype) or (label in filename):
+ filename = filename.replace(
+ label, "_rec-%s%s" % (mag_or_phase, label)
+ )
+ break
+
+ return filename
+
+
+def update_multiecho_name(metadata, filename, echo_times):
+ """
+ Insert `_echo-<num>` entity into filename if data are from a multi-echo
+ sequence.
+
+ Parameters
+ ----------
+ metadata : dict
+ Scan metadata dictionary from BIDS sidecar file.
+ filename : str
+ Incoming filename
+ echo_times : list
+ List of all echo times from scan. Used to determine the echo *number*
+ (i.e., index) if field is missing from metadata.
+
+ Returns
+ -------
+ filename : str
+ Updated filename with echo entity added, if appropriate.
+ """
+ # Field maps separate echoes differently
+ unsupported_types = [
+ '_magnitude', '_magnitude1', '_magnitude2',
+ '_phasediff', '_phase1', '_phase2', '_fieldmap'
+ ]
+ if any(ut in filename for ut in unsupported_types):
+ return filename
+
+ # Get the EchoNumber from json file info. If not present, use EchoTime
+ if 'EchoNumber' in metadata.keys():
+ echo_number = metadata['EchoNumber']
+ else:
+ echo_number = echo_times.index(metadata['EchoTime']) + 1
+
+ # Determine scan suffix
+ filetype = '_' + filename.split('_')[-1]
+
+ # Insert it **before** the following string(s), whichever appears first.
+ for label in ['_recording', '_proc', '_space', filetype]:
+ if (label == filetype) or (label in filename):
+ filename = filename.replace(
+ label, "_echo-%s%s" % (echo_number, label)
+ )
+ break
+
+ return filename
+
+
+def update_uncombined_name(metadata, filename, channel_names):
+ """
+ Insert `_ch-<num>` entity into filename if data are from a sequence
+ with "save uncombined".
+
+ Parameters
+ ----------
+ metadata : dict
+ Scan metadata dictionary from BIDS sidecar file.
+ filename : str
+ Incoming filename
+ channel_names : list
+ List of all channel names from scan. Used to determine the channel
+ *number* (i.e., index) if field is missing from metadata.
+
+ Returns
+ -------
+ filename : str
+ Updated filename with ch entity added, if appropriate.
+ """
+ # In case any scan types separate channels differently
+ unsupported_types = []
+ if any(ut in filename for ut in unsupported_types):
+ return filename
+
+ # Determine the channel number
+ channel_number = ''.join([c for c in metadata['CoilString'] if c.isdigit()])
+ if not channel_number:
+ channel_number = channel_names.index(metadata['CoilString']) + 1
+ channel_number = str(channel_number).zfill(2)
+
+ # Determine scan suffix
+ filetype = '_' + filename.split('_')[-1]
+
+ # Insert it **before** the following string(s), whichever appears first.
+ # Choosing to put channel near the end since it's not in the specification yet.
+ for label in ['_recording', '_proc', '_space', filetype]:
+ if (label == filetype) or (label in filename):
+ filename = filename.replace(
+ label, "_ch-%s%s" % (channel_number, label)
+ )
+ break
+ return filename
+
+
def convert(items, converter, scaninfo_suffix, custom_callable, with_prov,
bids_options, outdir, min_meta, overwrite, symlink=True, prov_file=None,
dcmconfig=None):
@@ -534,14 +685,17 @@ def save_converted_files(res, item_dicoms, bids_options, outtype, prefix, outnam
# series. To do that, the most straightforward way is to read the
# echo times for all bids_files and see if they are all the same or not.
- # Check for varying echo times
- echo_times = sorted(list(set(
- b.get('EchoTime', nan)
- for b in bids_metas
- if b
- )))
-
- is_multiecho = len(echo_times) > 1
+ # Collect some metadata across all images
+ echo_times, channel_names, image_types = set(), set(), set()
+ for metadata in bids_metas:
+ if not metadata:
+ continue
+ echo_times.add(metadata.get('EchoTime', nan))
+ channel_names.add(metadata.get('CoilString', nan))
+ image_types.update(metadata.get('ImageType', [nan]))
+ is_multiecho = len(set(filter(bool, echo_times))) > 1 # Check for varying echo times
+ is_uncombined = len(set(filter(bool, channel_names))) > 1 # Check for uncombined data
+ is_complex = 'M' in image_types and 'P' in image_types # Determine if data are complex (magnitude + phase)
### Loop through the bids_files, set the output name and save files
for fl, suffix, bids_file, bids_meta in zip(res_files, suffixes, bids_files, bids_metas):
@@ -552,65 +706,22 @@ def save_converted_files(res, item_dicoms, bids_options, outtype, prefix, outnam
# and we don't want to modify it for all the bids_files):
this_prefix_basename = prefix_basename
- # _sbref sequences reconstructing magnitude and phase generate
- # two NIfTI files IN THE SAME SERIES, so we cannot just add
- # the suffix, if we want to be bids compliant:
- if bids_meta and this_prefix_basename.endswith('_sbref') \
- and len(suffixes) > len(echo_times):
- if len(suffixes) != len(echo_times)*2:
- lgr.warning(
- "Got %d suffixes for %d echo times, which isn't "
- "multiple of two as if it was magnitude + phase pairs",
- len(suffixes), len(echo_times)
+ # Update name for certain criteria
+ if bids_file:
+ if is_multiecho:
+ this_prefix_basename = update_multiecho_name(
+ bids_meta, this_prefix_basename, echo_times
+ )
+
+ if is_complex:
+ this_prefix_basename = update_complex_name(
+ bids_meta, this_prefix_basename, suffix
+ )
+
+ if is_uncombined:
+ this_prefix_basename = update_uncombined_name(
+ bids_meta, this_prefix_basename, channel_names
)
- # Check to see if it is magnitude or phase reconstruction:
- if 'M' in bids_meta.get('ImageType'):
- mag_or_phase = 'magnitude'
- elif 'P' in bids_meta.get('ImageType'):
- mag_or_phase = 'phase'
- else:
- mag_or_phase = suffix
-
- # Insert reconstruction label
- if not ("_rec-%s" % mag_or_phase) in this_prefix_basename:
-
- # If "_rec-" is specified, prepend the 'mag_or_phase' value.
- if ('_rec-' in this_prefix_basename):
- raise BIDSError(
- "Reconstruction label for multi-echo single-band"
- " reference images will be automatically set, remove"
- " from heuristic"
- )
-
- # If not, insert "_rec-" + 'mag_or_phase' into the prefix_basename
- # **before** "_run", "_echo" or "_sbref", whichever appears first:
- for label in ['_run', '_echo', '_sbref']:
- if (label in this_prefix_basename):
- this_prefix_basename = this_prefix_basename.replace(
- label, "_rec-%s%s" % (mag_or_phase, label)
- )
- break
-
- # Now check if this run is multi-echo
- # (Note: it can be _sbref and multiecho, so don't use "elif"):
- # For multi-echo sequences, we have to specify the echo number in
- # the file name:
- if bids_meta and is_multiecho:
- # Get the EchoNumber from json file info. If not present, use EchoTime
- if 'EchoNumber' in bids_meta:
- echo_number = bids_meta['EchoNumber']
- else:
- echo_number = echo_times.index(bids_meta['EchoTime']) + 1
-
- supported_multiecho = ['_bold', '_phase', '_epi', '_sbref', '_T1w', '_PDT2']
- # Now, decide where to insert it.
- # Insert it **before** the following string(s), whichever appears first.
- for imgtype in supported_multiecho:
- if (imgtype in this_prefix_basename):
- this_prefix_basename = this_prefix_basename.replace(
- imgtype, "_echo-%d%s" % (echo_number, imgtype)
- )
- break
# Fallback option:
# If we have failed to modify this_prefix_basename, because it didn't fall
| nipy/heudiconv | 75f2850f7f07a95ff5875aba1dfd76c09e4be201 | diff --git a/heudiconv/tests/test_convert.py b/heudiconv/tests/test_convert.py
new file mode 100644
index 0000000..7593cb0
--- /dev/null
+++ b/heudiconv/tests/test_convert.py
@@ -0,0 +1,78 @@
+"""Test functions in heudiconv.convert module.
+"""
+import pytest
+
+from heudiconv.convert import (update_complex_name,
+ update_multiecho_name,
+ update_uncombined_name)
+from heudiconv.bids import BIDSError
+
+
+def test_update_complex_name():
+ """Unit testing for heudiconv.convert.update_complex_name(), which updates
+ filenames with the rec field if appropriate.
+ """
+ # Standard name update
+ fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
+ metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'P', 'MB', 'TE3', 'ND', 'MOSAIC']}
+ suffix = 3
+ out_fn_true = 'sub-X_ses-Y_task-Z_rec-phase_run-01_sbref'
+ out_fn_test = update_complex_name(metadata, fn, suffix)
+ assert out_fn_test == out_fn_true
+ # Catch an unsupported type and *do not* update
+ fn = 'sub-X_ses-Y_task-Z_run-01_phase'
+ out_fn_test = update_complex_name(metadata, fn, suffix)
+ assert out_fn_test == fn
+ # Data type is missing from metadata so use suffix
+ fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
+ metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'MB', 'TE3', 'ND', 'MOSAIC']}
+ out_fn_true = 'sub-X_ses-Y_task-Z_rec-3_run-01_sbref'
+ out_fn_test = update_complex_name(metadata, fn, suffix)
+ assert out_fn_test == out_fn_true
+ # Catch existing field with value that *does not match* metadata
+ # and raise Exception
+ fn = 'sub-X_ses-Y_task-Z_rec-magnitude_run-01_sbref'
+ metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'P', 'MB', 'TE3', 'ND', 'MOSAIC']}
+ suffix = 3
+ with pytest.raises(BIDSError):
+ assert update_complex_name(metadata, fn, suffix)
+
+
+def test_update_multiecho_name():
+ """Unit testing for heudiconv.convert.update_multiecho_name(), which updates
+ filenames with the echo field if appropriate.
+ """
+ # Standard name update
+ fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ metadata = {'EchoTime': 0.01,
+ 'EchoNumber': 1}
+ echo_times = [0.01, 0.02, 0.03]
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_echo-1_bold'
+ out_fn_test = update_multiecho_name(metadata, fn, echo_times)
+ assert out_fn_test == out_fn_true
+ # EchoNumber field is missing from metadata, so use echo_times
+ metadata = {'EchoTime': 0.01}
+ out_fn_test = update_multiecho_name(metadata, fn, echo_times)
+ assert out_fn_test == out_fn_true
+ # Catch an unsupported type and *do not* update
+ fn = 'sub-X_ses-Y_task-Z_run-01_phasediff'
+ out_fn_test = update_multiecho_name(metadata, fn, echo_times)
+ assert out_fn_test == fn
+
+
+def test_update_uncombined_name():
+ """Unit testing for heudiconv.convert.update_uncombined_name(), which updates
+ filenames with the ch field if appropriate.
+ """
+ # Standard name update
+ fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ metadata = {'CoilString': 'H1'}
+ channel_names = ['H1', 'H2', 'H3', 'HEA;HEP']
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-01_bold'
+ out_fn_test = update_uncombined_name(metadata, fn, channel_names)
+ assert out_fn_test == out_fn_true
+ # CoilString field has no number in it
+ metadata = {'CoilString': 'HEA;HEP'}
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-04_bold'
+ out_fn_test = update_uncombined_name(metadata, fn, channel_names)
+ assert out_fn_test == out_fn_true
| Converting uncombined (channel-level) data
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
In order to support channel-level data, I was thinking that [convert.save_converted_files](https://github.com/nipy/heudiconv/blob/fdea77ce6263d3f92e51dd8a24ac506e7a2fce4e/heudiconv/convert.py#L462) should include a check for the `CoilString` metadata field and should insert a `coil` or `channel` entity into the filenames before copying them to the output directory.
It might be worth it to move the multi-echo, complex (currently only for SBRefs), and uncombined data renaming methods into distinct functions as well.
### Platform details:
Choose one:
- [ ] Local environment
<!-- If selected, please provide OS and python version -->
- [ ] Container
<!-- If selected, please provide container name and tag"-->
- Heudiconv version:
<!-- To check: run heudiconv with just the --version flag -->
| 0.0 | [
"heudiconv/tests/test_convert.py::test_update_complex_name",
"heudiconv/tests/test_convert.py::test_update_multiecho_name",
"heudiconv/tests/test_convert.py::test_update_uncombined_name"
] | [] | 2020-02-24 14:34:45+00:00 | 4,186 |
|
nipy__heudiconv-461 | diff --git a/heudiconv/convert.py b/heudiconv/convert.py
index a145732..2cbdf05 100644
--- a/heudiconv/convert.py
+++ b/heudiconv/convert.py
@@ -2,9 +2,7 @@ import filelock
import os
import os.path as op
import logging
-from math import nan
import shutil
-import sys
import random
import re
@@ -239,7 +237,7 @@ def prep_conversion(sid, dicoms, outdir, heuristic, converter, anon_sid,
getattr(heuristic, 'DEFAULT_FIELDS', {}))
-def update_complex_name(metadata, filename, suffix):
+def update_complex_name(metadata, filename):
"""
Insert `_part-<mag|phase>` entity into filename if data are from a
sequence with magnitude/phase part.
@@ -250,14 +248,11 @@ def update_complex_name(metadata, filename, suffix):
Scan metadata dictionary from BIDS sidecar file.
filename : str
Incoming filename
- suffix : str
- An index used for cases where a single scan produces multiple files,
- but the differences between those files are unknown.
Returns
-------
filename : str
- Updated filename with rec entity added in appropriate position.
+ Updated filename with part entity added in appropriate position.
"""
# Some scans separate magnitude/phase differently
# A small note: _phase is deprecated, but this may add part-mag to
@@ -275,12 +270,12 @@ def update_complex_name(metadata, filename, suffix):
elif 'P' in metadata.get('ImageType'):
mag_or_phase = 'phase'
else:
- mag_or_phase = suffix
+ raise RuntimeError("Data type could not be inferred from the metadata.")
# Determine scan suffix
filetype = '_' + filename.split('_')[-1]
- # Insert rec label
+ # Insert part label
if not ('_part-%s' % mag_or_phase) in filename:
# If "_part-" is specified, prepend the 'mag_or_phase' value.
if '_part-' in filename:
@@ -290,7 +285,21 @@ def update_complex_name(metadata, filename, suffix):
)
# Insert it **before** the following string(s), whichever appears first.
- for label in ['_recording', '_proc', '_space', filetype]:
+ # https://bids-specification.readthedocs.io/en/stable/99-appendices/09-entities.html
+ entities_after_part = [
+ "_proc",
+ "_hemi",
+ "_space",
+ "_split",
+ "_recording",
+ "_chunk",
+ "_res",
+ "_den",
+ "_label",
+ "_desc",
+ filetype,
+ ]
+ for label in entities_after_part:
if (label == filetype) or (label in filename):
filename = filename.replace(
label, "_part-%s%s" % (mag_or_phase, label)
@@ -320,7 +329,8 @@ def update_multiecho_name(metadata, filename, echo_times):
filename : str
Updated filename with echo entity added, if appropriate.
"""
- # Field maps separate echoes differently
+ # Field maps separate echoes differently, so do not attempt to update any filenames with these
+ # suffixes
unsupported_types = [
'_magnitude', '_magnitude1', '_magnitude2',
'_phasediff', '_phase1', '_phase2', '_fieldmap'
@@ -328,18 +338,43 @@ def update_multiecho_name(metadata, filename, echo_times):
if any(ut in filename for ut in unsupported_types):
return filename
- # Get the EchoNumber from json file info. If not present, use EchoTime
+ if not isinstance(echo_times, list):
+ raise TypeError(f'Argument "echo_times" must be a list, not a {type(echo_times)}')
+
+ # Get the EchoNumber from json file info. If not present, use EchoTime.
if 'EchoNumber' in metadata.keys():
echo_number = metadata['EchoNumber']
- else:
+ elif 'EchoTime' in metadata.keys():
echo_number = echo_times.index(metadata['EchoTime']) + 1
+ else:
+ raise KeyError(
+ 'Either "EchoNumber" or "EchoTime" must be in metadata keys. '
+ f'Keys detected: {metadata.keys()}'
+ )
# Determine scan suffix
filetype = '_' + filename.split('_')[-1]
# Insert it **before** the following string(s), whichever appears first.
- # https://bids-specification.readthedocs.io/en/stable/99-appendices/04-entity-table.html
- for label in ['_flip', '_inv', '_mt', '_part', '_recording', '_proc', '_space', filetype]:
+ # https://bids-specification.readthedocs.io/en/stable/99-appendices/09-entities.html
+ entities_after_echo = [
+ "_flip",
+ "_inv",
+ "_mt",
+ "_part",
+ "_proc",
+ "_hemi",
+ "_space",
+ "_split",
+ "_recording",
+ "_chunk",
+ "_res",
+ "_den",
+ "_label",
+ "_desc",
+ filetype,
+ ]
+ for label in entities_after_echo:
if (label == filetype) or (label in filename):
filename = filename.replace(
label, "_echo-%s%s" % (echo_number, label)
@@ -374,6 +409,9 @@ def update_uncombined_name(metadata, filename, channel_names):
if any(ut in filename for ut in unsupported_types):
return filename
+ if not isinstance(channel_names, list):
+ raise TypeError(f'Argument "channel_names" must be a list, not a {type(channel_names)}')
+
# Determine the channel number
channel_number = ''.join([c for c in metadata['CoilString'] if c.isdigit()])
if not channel_number:
@@ -385,7 +423,21 @@ def update_uncombined_name(metadata, filename, channel_names):
# Insert it **before** the following string(s), whichever appears first.
# Choosing to put channel near the end since it's not in the specification yet.
- for label in ['_recording', '_proc', '_space', filetype]:
+ # See https://bids-specification.readthedocs.io/en/stable/99-appendices/09-entities.html
+ entities_after_ch = [
+ "_proc",
+ "_hemi",
+ "_space",
+ "_split",
+ "_recording",
+ "_chunk",
+ "_res",
+ "_den",
+ "_label",
+ "_desc",
+ filetype,
+ ]
+ for label in entities_after_ch:
if (label == filetype) or (label in filename):
filename = filename.replace(
label, "_ch-%s%s" % (channel_number, label)
@@ -731,12 +783,17 @@ def save_converted_files(res, item_dicoms, bids_options, outtype, prefix, outnam
for metadata in bids_metas:
if not metadata:
continue
- echo_times.add(metadata.get('EchoTime', nan))
- channel_names.add(metadata.get('CoilString', nan))
- image_types.update(metadata.get('ImageType', [nan]))
+
+ # If the field is not available, fill that entry in the set with a False.
+ echo_times.add(metadata.get('EchoTime', False))
+ channel_names.add(metadata.get('CoilString', False))
+ image_types.update(metadata.get('ImageType', [False]))
+
is_multiecho = len(set(filter(bool, echo_times))) > 1 # Check for varying echo times
is_uncombined = len(set(filter(bool, channel_names))) > 1 # Check for uncombined data
is_complex = 'M' in image_types and 'P' in image_types # Determine if data are complex (magnitude + phase)
+ echo_times = sorted(echo_times) # also converts to list
+ channel_names = sorted(channel_names) # also converts to list
### Loop through the bids_files, set the output name and save files
for fl, suffix, bids_file, bids_meta in zip(res_files, suffixes, bids_files, bids_metas):
@@ -756,7 +813,7 @@ def save_converted_files(res, item_dicoms, bids_options, outtype, prefix, outnam
if is_complex:
this_prefix_basename = update_complex_name(
- bids_meta, this_prefix_basename, suffix
+ bids_meta, this_prefix_basename
)
if is_uncombined:
| nipy/heudiconv | 3f9a504270f83d00bb483c8f52ae0c228fc7d808 | diff --git a/heudiconv/tests/test_convert.py b/heudiconv/tests/test_convert.py
index 6a29895..f8316ee 100644
--- a/heudiconv/tests/test_convert.py
+++ b/heudiconv/tests/test_convert.py
@@ -4,15 +4,16 @@ import os.path as op
from glob import glob
import pytest
-from .utils import TESTS_DATA_PATH
-
-from heudiconv.convert import (update_complex_name,
- update_multiecho_name,
- update_uncombined_name,
- DW_IMAGE_IN_FMAP_FOLDER_WARNING,
- )
from heudiconv.bids import BIDSError
from heudiconv.cli.run import main as runner
+from heudiconv.convert import (
+ DW_IMAGE_IN_FMAP_FOLDER_WARNING,
+ update_complex_name,
+ update_multiecho_name,
+ update_uncombined_name,
+)
+
+from .utils import TESTS_DATA_PATH
def test_update_complex_name():
@@ -20,29 +21,36 @@ def test_update_complex_name():
filenames with the part field if appropriate.
"""
# Standard name update
- fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'P', 'MB', 'TE3', 'ND', 'MOSAIC']}
- suffix = 3
out_fn_true = 'sub-X_ses-Y_task-Z_run-01_part-phase_sbref'
- out_fn_test = update_complex_name(metadata, fn, suffix)
+ out_fn_test = update_complex_name(metadata, base_fn)
assert out_fn_test == out_fn_true
+
# Catch an unsupported type and *do not* update
- fn = 'sub-X_ses-Y_task-Z_run-01_phase'
- out_fn_test = update_complex_name(metadata, fn, suffix)
- assert out_fn_test == fn
- # Data type is missing from metadata so use suffix
- fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_phase'
+ out_fn_test = update_complex_name(metadata, base_fn)
+ assert out_fn_test == base_fn
+
+ # Data type is missing from metadata so raise a RuntimeError
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_sbref'
metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'MB', 'TE3', 'ND', 'MOSAIC']}
- out_fn_true = 'sub-X_ses-Y_task-Z_run-01_part-3_sbref'
- out_fn_test = update_complex_name(metadata, fn, suffix)
- assert out_fn_test == out_fn_true
- # Catch existing field with value that *does not match* metadata
- # and raise Exception
- fn = 'sub-X_ses-Y_task-Z_run-01_part-mag_sbref'
+ with pytest.raises(RuntimeError):
+ update_complex_name(metadata, base_fn)
+
+ # Catch existing field with value (part is already in the filename)
+ # that *does not match* metadata and raise Exception
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_part-mag_sbref'
metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'P', 'MB', 'TE3', 'ND', 'MOSAIC']}
- suffix = 3
with pytest.raises(BIDSError):
- assert update_complex_name(metadata, fn, suffix)
+ update_complex_name(metadata, base_fn)
+
+ # Catch existing field with value (part is already in the filename)
+ # that *does match* metadata and do not update
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_part-phase_sbref'
+ metadata = {'ImageType': ['ORIGINAL', 'PRIMARY', 'P', 'MB', 'TE3', 'ND', 'MOSAIC']}
+ out_fn_test = update_complex_name(metadata, base_fn)
+ assert out_fn_test == base_fn
def test_update_multiecho_name():
@@ -50,21 +58,43 @@ def test_update_multiecho_name():
filenames with the echo field if appropriate.
"""
# Standard name update
- fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
metadata = {'EchoTime': 0.01,
'EchoNumber': 1}
echo_times = [0.01, 0.02, 0.03]
out_fn_true = 'sub-X_ses-Y_task-Z_run-01_echo-1_bold'
- out_fn_test = update_multiecho_name(metadata, fn, echo_times)
+ out_fn_test = update_multiecho_name(metadata, base_fn, echo_times)
assert out_fn_test == out_fn_true
+
# EchoNumber field is missing from metadata, so use echo_times
metadata = {'EchoTime': 0.01}
- out_fn_test = update_multiecho_name(metadata, fn, echo_times)
+ out_fn_test = update_multiecho_name(metadata, base_fn, echo_times)
assert out_fn_test == out_fn_true
+
# Catch an unsupported type and *do not* update
- fn = 'sub-X_ses-Y_task-Z_run-01_phasediff'
- out_fn_test = update_multiecho_name(metadata, fn, echo_times)
- assert out_fn_test == fn
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_phasediff'
+ out_fn_test = update_multiecho_name(metadata, base_fn, echo_times)
+ assert out_fn_test == base_fn
+
+ # EchoTime is missing, but use EchoNumber (which is the first thing it checks)
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_echo-1_bold'
+ metadata = {'EchoNumber': 1}
+ echo_times = [False, 0.02, 0.03]
+ out_fn_test = update_multiecho_name(metadata, base_fn, echo_times)
+ assert out_fn_test == out_fn_true
+
+ # Both EchoTime and EchoNumber are missing, which raises a KeyError
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ metadata = {}
+ echo_times = [False, 0.02, 0.03]
+ with pytest.raises(KeyError):
+ update_multiecho_name(metadata, base_fn, echo_times)
+
+ # Providing echo times as something other than a list should raise a TypeError
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ with pytest.raises(TypeError):
+ update_multiecho_name(metadata, base_fn, set(echo_times))
def test_update_uncombined_name():
@@ -72,18 +102,39 @@ def test_update_uncombined_name():
filenames with the ch field if appropriate.
"""
# Standard name update
- fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
metadata = {'CoilString': 'H1'}
channel_names = ['H1', 'H2', 'H3', 'HEA;HEP']
out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-01_bold'
- out_fn_test = update_uncombined_name(metadata, fn, channel_names)
+ out_fn_test = update_uncombined_name(metadata, base_fn, channel_names)
assert out_fn_test == out_fn_true
- # CoilString field has no number in it
+
+ # CoilString field has no number in it, so we index the channel_names list
metadata = {'CoilString': 'HEA;HEP'}
out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-04_bold'
- out_fn_test = update_uncombined_name(metadata, fn, channel_names)
+ out_fn_test = update_uncombined_name(metadata, base_fn, channel_names)
assert out_fn_test == out_fn_true
+ # Extract the number from the CoilString and use that
+ channel_names = ['H1', 'B1', 'H3', 'HEA;HEP']
+ metadata = {'CoilString': 'H1'}
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-01_bold'
+ out_fn_test = update_uncombined_name(metadata, base_fn, channel_names)
+ assert out_fn_test == out_fn_true
+
+ # NOTE: Extracting the number does not protect against multiple coils with the same number
+ # (but, say, different letters)
+ # Note that this is still "ch-01"
+ metadata = {'CoilString': 'B1'}
+ out_fn_true = 'sub-X_ses-Y_task-Z_run-01_ch-01_bold'
+ out_fn_test = update_uncombined_name(metadata, base_fn, channel_names)
+ assert out_fn_test == out_fn_true
+
+ # Providing echo times as something other than a list should raise a TypeError
+ base_fn = 'sub-X_ses-Y_task-Z_run-01_bold'
+ with pytest.raises(TypeError):
+ update_uncombined_name(metadata, base_fn, set(channel_names))
+
def test_b0dwi_for_fmap(tmpdir, caplog):
"""Make sure we raise a warning when .bvec and .bval files
| Multi-echo and uncombined name updaters assume lists instead of sets
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
When converting multi-echo data without an EchoNumber metadata field, `heudiconv.convert.update_multiecho_name()` attempts to index `echo_times`. The problem is that this parameter is a `set`, rather than a `list`, so doing this raises an error. The same should apply to `heudiconv.convert.update_uncombined_name()`.
Here is the traceback:
```python
Traceback (most recent call last):
File "/opt/miniconda-latest/bin/heudiconv", line 33, in <module>
sys.exit(load_entry_point('heudiconv', 'console_scripts', 'heudiconv')())
File "/home/code/heudiconv/heudiconv/cli/run.py", line 24, in main
workflow(**kwargs)
File "/home/code/heudiconv/heudiconv/main.py", line 344, in workflow
grouping=grouping,)
File "/home/code/heudiconv/heudiconv/convert.py", line 211, in prep_conversion
dcmconfig=dcmconfig,)
File "/home/code/heudiconv/heudiconv/convert.py", line 459, in convert
overwrite=overwrite)
File "/home/code/heudiconv/heudiconv/convert.py", line 713, in save_converted_files
bids_meta, this_prefix_basename, echo_times
File "/home/code/heudiconv/heudiconv/convert.py", line 326, in update_multiecho_name
echo_number = echo_times.index(metadata['EchoTime']) + 1
AttributeError: 'set' object has no attribute 'index'
```
This wasn't caught by the tests for these functions because I mistakenly used lists as the arguments in the tests.
EDIT: Introduced in #424, so at least it hasn't affected any releases.
### Platform details:
Choose one:
- [ ] Local environment
<!-- If selected, please provide OS and python version -->
- [X] Container: Docker using current master (as of f4c2dd2).
<!-- If selected, please provide container name and tag"-->
- Heudiconv version: Current master (as of f4c2dd2).
<!-- To check: run heudiconv with just the --version flag -->
| 0.0 | [
"heudiconv/tests/test_convert.py::test_update_complex_name",
"heudiconv/tests/test_convert.py::test_update_multiecho_name",
"heudiconv/tests/test_convert.py::test_update_uncombined_name"
] | [] | 2020-06-26 21:12:27+00:00 | 4,187 |
|
nipy__heudiconv-500 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index e07c347..fc630aa 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -47,6 +47,36 @@ class BIDSError(Exception):
BIDS_VERSION = "1.4.1"
+def maybe_na(val):
+ """Return 'n/a' if non-None value represented as str is not empty
+
+ Primarily for the consistent use of lower case 'n/a' so 'N/A' and 'NA'
+ are also treated as 'n/a'
+ """
+ if val is not None:
+ val = str(val)
+ val = val.strip()
+ return 'n/a' if (not val or val in ('N/A', 'NA')) else val
+
+
+def treat_age(age):
+ """Age might encounter 'Y' suffix or be a float"""
+ age = str(age)
+ if age.endswith('M'):
+ age = age.rstrip('M')
+ age = float(age) / 12
+ age = ('%.2f' if age != int(age) else '%d') % age
+ else:
+ age = age.rstrip('Y')
+ if age:
+ # strip all leading 0s but allow to scan a newborn (age 0Y)
+ age = '0' if not age.lstrip('0') else age.lstrip('0')
+ if age.startswith('.'):
+ # we had float point value, let's prepend 0
+ age = '0' + age
+ return age
+
+
def populate_bids_templates(path, defaults={}):
"""Premake BIDS text files with templates"""
@@ -278,12 +308,13 @@ def add_participant_record(studydir, subject, age, sex):
"control group)")])),
]),
sort_keys=False)
+
# Add a new participant
with open(participants_tsv, 'a') as f:
f.write(
'\t'.join(map(str, [participant_id,
- age.lstrip('0').rstrip('Y') if age else 'N/A',
- sex if sex else 'n/a',
+ maybe_na(treat_age(age)),
+ maybe_na(sex),
'control'])) + '\n')
| nipy/heudiconv | 6b80704e297fff859504a5df455a7f7cac88aa81 | diff --git a/heudiconv/tests/test_bids.py b/heudiconv/tests/test_bids.py
new file mode 100644
index 0000000..a980e35
--- /dev/null
+++ b/heudiconv/tests/test_bids.py
@@ -0,0 +1,26 @@
+"""Test functions in heudiconv.bids module.
+"""
+
+from heudiconv.bids import (
+ maybe_na,
+ treat_age,
+)
+
+
+def test_maybe_na():
+ for na in '', ' ', None, 'n/a', 'N/A', 'NA':
+ assert maybe_na(na) == 'n/a'
+ for notna in 0, 1, False, True, 'value':
+ assert maybe_na(notna) == str(notna)
+
+
+def test_treat_age():
+ assert treat_age(0) == '0'
+ assert treat_age('0') == '0'
+ assert treat_age('0000') == '0'
+ assert treat_age('0000Y') == '0'
+ assert treat_age('000.1Y') == '0.1'
+ assert treat_age('1M') == '0.08'
+ assert treat_age('12M') == '1'
+ assert treat_age('0000.1') == '0.1'
+ assert treat_age(0000.1) == '0.1'
\ No newline at end of file
| Participants.tsv will null values
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->
In participants.tsv age and sex have null values (empty cells) instead of a 'n/a', which causes bids validation to fail.
I'm not sure these N/A replacements catch all the edge cases:
https://github.com/nipy/heudiconv/blob/master/heudiconv/bids.py#L285
I would've expected age to be fixed by this commit:
https://github.com/nipy/heudiconv/commit/7a7ef5c5f9852ef2b479aafcd5e09e462a08193c#diff-9f5ed69cb0b31e87bd735166c54cbd41b50710d545dc0e48d4d42f21269271c2
But I believe the n/a replacement for sex has been around awhile, and is in the version I'm using (0.9.0), but sex is still missing from participants.tsv.
ex:
```
participant_id age sex group
sub-111 control
```
Yarik, you can checkout the UIC sub-travhuman data for an example.
I sorted this out on my own with pandas and numpy:
```
import pandas as pd
import numpy as np
participants_df = pd.read_csv('participants.tsv', sep='\t')
participants_df = participants_df.replace(np.nan, 'n/a')
participants_df.to_csv('participants.tsv', sep="\t")
```
but it's probably worth doing within heudiconv.
### Platform details:
Choose one:
- [ ] Local environment
<!-- If selected, please provide OS and python version -->
- [x] Container
<!-- If selected, please provide container name and tag"-->
Docker container: nipy/heudiconv:0.9.0
- Heudiconv version: 0.9.0
<!-- To check: run heudiconv with just the --version flag -->
Thanks,
Joshua | 0.0 | [
"heudiconv/tests/test_bids.py::test_maybe_na",
"heudiconv/tests/test_bids.py::test_treat_age"
] | [] | 2021-03-26 17:37:58+00:00 | 4,188 |
|
nipy__heudiconv-523 | diff --git a/heudiconv/bids.py b/heudiconv/bids.py
index 714e4a3..fa5d260 100644
--- a/heudiconv/bids.py
+++ b/heudiconv/bids.py
@@ -157,7 +157,7 @@ def populate_aggregated_jsons(path):
# TODO: if we are to fix it, then old ones (without _acq) should be
# removed first
task = re.sub('.*_(task-[^_\.]*(_acq-[^_\.]*)?)_.*', r'\1', fpath)
- json_ = load_json(fpath)
+ json_ = load_json(fpath, retry=100)
if task not in tasks:
tasks[task] = json_
else:
@@ -212,7 +212,7 @@ def populate_aggregated_jsons(path):
"CogAtlasID": "http://www.cognitiveatlas.org/task/id/TODO",
}
if op.lexists(task_file):
- j = load_json(task_file)
+ j = load_json(task_file, retry=100)
# Retain possibly modified placeholder fields
for f in placeholders:
if f in j:
diff --git a/heudiconv/utils.py b/heudiconv/utils.py
index 3dc402f..eb2756b 100644
--- a/heudiconv/utils.py
+++ b/heudiconv/utils.py
@@ -14,6 +14,7 @@ from collections import namedtuple
from glob import glob
from subprocess import check_output
from datetime import datetime
+from time import sleep
from nipype.utils.filemanip import which
@@ -147,24 +148,40 @@ def write_config(outfile, info):
fp.writelines(PrettyPrinter().pformat(info))
-def load_json(filename):
+def load_json(filename, retry=0):
"""Load data from a json file
Parameters
----------
filename : str
Filename to load data from.
+ retry: int, optional
+ Number of times to retry opening/loading the file in case of
+ failure. Code will sleep for 0.1 seconds between retries.
+ Could be used in code which is not sensitive to order effects
+ (e.g. like populating bids templates where the last one to
+ do it, would make sure it would be the correct/final state).
Returns
-------
data : dict
"""
- try:
- with open(filename, 'r') as fp:
- data = json.load(fp)
- except JSONDecodeError:
- lgr.error("{fname} is not a valid json file".format(fname=filename))
- raise
+ assert retry >= 0
+ for i in range(retry + 1): # >= 10 sec wait
+ try:
+ try:
+ with open(filename, 'r') as fp:
+ data = json.load(fp)
+ break
+ except JSONDecodeError:
+ lgr.error("{fname} is not a valid json file".format(fname=filename))
+ raise
+ except (JSONDecodeError, FileNotFoundError) as exc:
+ if i >= retry:
+ raise
+ lgr.warning("Caught %s. Will retry again", exc)
+ sleep(0.1)
+ continue
return data
| nipy/heudiconv | 21d5104b0a79ac32ff8c01aa1dde3ef5b115cff2 | diff --git a/heudiconv/tests/test_utils.py b/heudiconv/tests/test_utils.py
index b8c8c16..f43a09b 100644
--- a/heudiconv/tests/test_utils.py
+++ b/heudiconv/tests/test_utils.py
@@ -2,6 +2,8 @@ import json
import os
import os.path as op
+import mock
+
from heudiconv.utils import (
get_known_heuristics_with_descriptions,
get_heuristic_description,
@@ -77,6 +79,13 @@ def test_load_json(tmpdir, caplog):
with pytest.raises(JSONDecodeError):
load_json(str(invalid_json_file))
+ # and even if we ask to retry a few times -- should be the same
+ with pytest.raises(JSONDecodeError):
+ load_json(str(invalid_json_file), retry=3)
+
+ with pytest.raises(FileNotFoundError):
+ load_json("absent123not.there", retry=3)
+
assert ifname in caplog.text
# test valid json
@@ -87,6 +96,23 @@ def test_load_json(tmpdir, caplog):
assert load_json(valid_json_file) == vcontent
+ calls = [0]
+ json_load = json.load
+
+ def json_load_patched(fp):
+ calls[0] += 1
+ if calls[0] == 1:
+ # just reuse bad file
+ load_json(str(invalid_json_file))
+ elif calls[0] == 2:
+ raise FileNotFoundError()
+ else:
+ return json_load(fp)
+
+ with mock.patch.object(json, 'load', json_load_patched):
+ assert load_json(valid_json_file, retry=3) == vcontent
+
+
def test_get_datetime():
"""
| FileNotFoundError: [Errno 2] No such file or directory: '_task-rest_bold.json'
<!-- DO NOT DELETE THIS!
This template is used to facilitate issue resolution.
All text in <!-> tags will not be displayed.
-->
### Summary
<!-- If you are having conversion troubles, please share as much
relevant information as possible. This includes, but is not limited to:
- log of conversion
- heuristic
-->I try to implement bids conversion with heudiconv on our local HPC using a singularity container ( heudiconv-0.9.0). As I will work with a large cohort (>2000 subjects) I am currently implementing a parallelization across nodes via SLURM and within each node with GNU parallel with a test dataset (5 times the same subject). In doing so, a test run fails with the following error.
```
`local:5/0/100%/0.0s 0: Traceback (most recent call last):
0: File "/opt/miniconda-latest/bin/heudiconv", line 33, in <module>
0: sys.exit(load_entry_point('heudiconv', 'console_scripts', 'heudiconv')())
0: File "/src/heudiconv/heudiconv/cli/run.py", line 24, in main
0: workflow(**kwargs)
0: File "/src/heudiconv/heudiconv/main.py", line 351, in workflow
0: grouping=grouping,)
0: File "/src/heudiconv/heudiconv/convert.py", line 238, in prep_conversion
0: getattr(heuristic, 'DEFAULT_FIELDS', {}))
0: File "/src/heudiconv/heudiconv/bids.py", line 94, in populate_bids_templates
0: populate_aggregated_jsons(path)
0: File "/src/heudiconv/heudiconv/bids.py", line 126, in populate_aggregated_jsons
0: json_ = load_json(fpath)
0: File "/src/heudiconv/heudiconv/utils.py", line 177, in load_json
0: with open(filename, 'r') as fp:
0: FileNotFoundError: [Errno 2] No such file or directory: '/bids/sub-ewgenia001/ses-1/func/sub-ewgenia001_ses-1_task-rest_bold.json'`
```
Interestingly this affects only 4 of 5 subjects with the remaining one (seemingly always the first subject completing) without issues. All this sounds a little bit like race-condition as discussed in #362. However, as far as I understand a fix has been implemented and I am working with datalad creating a ephemeral clone of the dataset on a scratch partition for each subject before applying heudiconv to it (scripts below). Maybe I am misunderstanding something but shouldn't the latter somehow address the race condition with the processes writing to a separate/cloned top-level file?
Any input would be highly appreciated. Happy to provide further details.
[heudiconv_heuristic.txt](https://github.com/nipy/heudiconv/files/6776972/heudiconv_heuristic.txt)
[pipelines_parallelization.txt](https://github.com/nipy/heudiconv/files/6776973/pipelines_parallelization.txt) (batch script parallelizing pipelines_processing across subjects with GNU parallel)
[pipelines_processing.txt](https://github.com/nipy/heudiconv/files/6776974/pipelines_processing.txt)
### Platform details:
Choose one:
- [ x] Container (heudiconv 0.9.0)
<!-- If selected, please provide container name and tag"--> | 0.0 | [
"heudiconv/tests/test_utils.py::test_load_json"
] | [
"heudiconv/tests/test_utils.py::test_get_known_heuristics_with_descriptions",
"heudiconv/tests/test_utils.py::test_get_heuristic_description",
"heudiconv/tests/test_utils.py::test_load_heuristic",
"heudiconv/tests/test_utils.py::test_json_dumps_pretty",
"heudiconv/tests/test_utils.py::test_get_datetime"
] | 2021-09-13 19:14:52+00:00 | 4,189 |
|
nipy__nipype-2065 | diff --git a/README.rst b/README.rst
index aa41f34d6..5064198dd 100644
--- a/README.rst
+++ b/README.rst
@@ -33,7 +33,7 @@ NIPYPE: Neuroimaging in Python: Pipelines and Interfaces
.. image:: https://img.shields.io/badge/gitter-join%20chat%20%E2%86%92-brightgreen.svg?style=flat
:target: http://gitter.im/nipy/nipype
:alt: Chat
-
+
.. image:: https://zenodo.org/badge/DOI/10.5281/zenodo.581704.svg
:target: https://doi.org/10.5281/zenodo.581704
diff --git a/nipype/interfaces/mipav/developer.py b/nipype/interfaces/mipav/developer.py
index ac42f7c5a..141a7de1c 100644
--- a/nipype/interfaces/mipav/developer.py
+++ b/nipype/interfaces/mipav/developer.py
@@ -722,10 +722,10 @@ class JistIntensityMp2rageMaskingInputSpec(CommandLineInputSpec):
inSkip = traits.Enum("true", "false", desc="Skip zero values", argstr="--inSkip %s")
inMasking = traits.Enum("binary", "proba", desc="Whether to use a binary threshold or a weighted average based on the probability.", argstr="--inMasking %s")
xPrefExt = traits.Enum("nrrd", desc="Output File Type", argstr="--xPrefExt %s")
- outSignal = traits.Either(traits.Bool, File(), hash_files=False, desc="Signal Proba Image", argstr="--outSignal %s")
- outSignal2 = traits.Either(traits.Bool, File(), hash_files=False, desc="Signal Mask Image", argstr="--outSignal2 %s")
- outMasked = traits.Either(traits.Bool, File(), hash_files=False, desc="Masked T1 Map Image", argstr="--outMasked %s")
- outMasked2 = traits.Either(traits.Bool, File(), hash_files=False, desc="Masked Iso Image", argstr="--outMasked2 %s")
+ outSignal = traits.Either(traits.Bool, File(), hash_files=False, desc="Signal Proba Image", argstr="--outSignal_Proba %s")
+ outSignal2 = traits.Either(traits.Bool, File(), hash_files=False, desc="Signal Mask Image", argstr="--outSignal_Mask %s")
+ outMasked = traits.Either(traits.Bool, File(), hash_files=False, desc="Masked T1 Map Image", argstr="--outMasked_T1_Map %s")
+ outMasked2 = traits.Either(traits.Bool, File(), hash_files=False, desc="Masked Iso Image", argstr="--outMasked_T1weighted %s")
null = traits.Str(desc="Execution Time", argstr="--null %s")
xDefaultMem = traits.Int(desc="Set default maximum heap size", argstr="-xDefaultMem %d")
xMaxProcess = traits.Int(1, desc="Set default maximum number of processes.", argstr="-xMaxProcess %d", usedefault=True)
| nipy/nipype | a63c52d97df65d316a5c97a40dd9c7e5a63d237c | diff --git a/nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py b/nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py
index 95700af1b..0fd3ed52e 100644
--- a/nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py
+++ b/nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py
@@ -26,16 +26,16 @@ def test_JistIntensityMp2rageMasking_inputs():
),
null=dict(argstr='--null %s',
),
- outMasked=dict(argstr='--outMasked %s',
+ outMasked=dict(argstr='--outMasked_T1_Map %s',
hash_files=False,
),
- outMasked2=dict(argstr='--outMasked2 %s',
+ outMasked2=dict(argstr='--outMasked_T1weighted %s',
hash_files=False,
),
- outSignal=dict(argstr='--outSignal %s',
+ outSignal=dict(argstr='--outSignal_Proba %s',
hash_files=False,
),
- outSignal2=dict(argstr='--outSignal2 %s',
+ outSignal2=dict(argstr='--outSignal_Mask %s',
hash_files=False,
),
terminal_output=dict(nohash=True,
| JistIntensityMp2rageMasking interface incompatible with current CBS Tools release
For the command underlying the interface mipav.JistIntensityMp2rageMasking the parameter names have changed in the current CBS Tools release, which makes the interface fail. I meant to do a PR but then saw the mipav/developer.py file is autogenerated and should not be be edited.
These would be the required changes:
https://github.com/nipy/nipype/compare/master...juhuntenburg:fix/cbstools_params
| 0.0 | [
"nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py::test_JistIntensityMp2rageMasking_inputs"
] | [
"nipype/interfaces/mipav/tests/test_auto_JistIntensityMp2rageMasking.py::test_JistIntensityMp2rageMasking_outputs"
] | 2017-06-05 17:17:48+00:00 | 4,190 |
|
nipy__nipype-2085 | diff --git a/.mailmap b/.mailmap
index af5a39bd6..f7d32274f 100644
--- a/.mailmap
+++ b/.mailmap
@@ -83,6 +83,7 @@ Michael Waskom <[email protected]> Michael Waskom <[email protected]>
Michael Waskom <[email protected]> Michael Waskom <[email protected]>
Michael Waskom <[email protected]> mwaskom <[email protected]>
Michael Waskom <[email protected]> mwaskom <[email protected]>
+Michael Waskom <[email protected]> mwaskom <[email protected]>
Oscar Esteban <[email protected]> Oscar Esteban <code@oscaresteban>
Oscar Esteban <[email protected]> oesteban <[email protected]>
Russell Poldrack <[email protected]> Russ Poldrack <[email protected]>
diff --git a/nipype/interfaces/afni/__init__.py b/nipype/interfaces/afni/__init__.py
index 60076eefc..6fb20abf4 100644
--- a/nipype/interfaces/afni/__init__.py
+++ b/nipype/interfaces/afni/__init__.py
@@ -17,7 +17,7 @@ from .preprocess import (Allineate, Automask, AutoTcorrelate,
Seg, SkullStrip, TCorr1D, TCorrMap, TCorrelate,
TShift, Volreg, Warp, QwarpPlusMinus)
from .svm import (SVMTest, SVMTrain)
-from .utils import (AFNItoNIFTI, Autobox, BrickStat, Calc, Copy,
+from .utils import (AFNItoNIFTI, Autobox, BrickStat, Calc, Copy, Edge3,
Eval, FWHMx,
MaskTool, Merge, Notes, Refit, Resample, TCat, TStat, To3D,
Unifize, ZCutUp, GCOR,)
diff --git a/nipype/interfaces/afni/utils.py b/nipype/interfaces/afni/utils.py
index 0e6455496..8500f998d 100644
--- a/nipype/interfaces/afni/utils.py
+++ b/nipype/interfaces/afni/utils.py
@@ -394,6 +394,99 @@ class Copy(AFNICommand):
output_spec = AFNICommandOutputSpec
+class Edge3InputSpec(AFNICommandInputSpec):
+ in_file = File(
+ desc='input file to 3dedge3',
+ argstr='-input %s',
+ position=0,
+ mandatory=True,
+ exists=True,
+ copyfile=False)
+ out_file = File(
+ desc='output image file name',
+ position=-1,
+ argstr='-prefix %s')
+ datum = traits.Enum(
+ 'byte','short','float',
+ argstr='-datum %s',
+ desc='specify data type for output. Valid types are \'byte\', '
+ '\'short\' and \'float\'.')
+ fscale = traits.Bool(
+ desc='Force scaling of the output to the maximum integer range.',
+ argstr='-fscale',
+ xor=['gscale', 'nscale', 'scale_floats'])
+ gscale = traits.Bool(
+ desc='Same as \'-fscale\', but also forces each output sub-brick to '
+ 'to get the same scaling factor.',
+ argstr='-gscale',
+ xor=['fscale', 'nscale', 'scale_floats'])
+ nscale = traits.Bool(
+ desc='Don\'t do any scaling on output to byte or short datasets.',
+ argstr='-nscale',
+ xor=['fscale', 'gscale', 'scale_floats'])
+ scale_floats = traits.Float(
+ desc='Multiply input by VAL, but only if the input datum is '
+ 'float. This is needed when the input dataset '
+ 'has a small range, like 0 to 2.0 for instance. '
+ 'With such a range, very few edges are detected due to '
+ 'what I suspect to be truncation problems. '
+ 'Multiplying such a dataset by 10000 fixes the problem '
+ 'and the scaling is undone at the output.',
+ argstr='-scale_floats %f',
+ xor=['fscale', 'gscale', 'nscale'])
+ verbose = traits.Bool(
+ desc='Print out some information along the way.',
+ argstr='-verbose')
+
+
+class Edge3(AFNICommand):
+ """Does 3D Edge detection using the library 3DEdge
+ by Gregoire Malandain ([email protected]).
+
+ For complete details, see the `3dedge3 Documentation.
+ <https://afni.nimh.nih.gov/pub/dist/doc/program_help/3dedge3.html>`_
+
+ references_ = [{'entry': BibTeX('@article{Deriche1987,'
+ 'author={R. Deriche},'
+ 'title={Optimal edge detection using recursive filtering},'
+ 'journal={International Journal of Computer Vision},'
+ 'volume={2},',
+ 'pages={167-187},'
+ 'year={1987},'
+ '}'),
+ 'tags': ['method'],
+ },
+ {'entry': BibTeX('@article{MongaDericheMalandainCocquerez1991,'
+ 'author={O. Monga, R. Deriche, G. Malandain, J.P. Cocquerez},'
+ 'title={Recursive filtering and edge tracking: two primary tools for 3D edge detection},'
+ 'journal={Image and vision computing},'
+ 'volume={9},',
+ 'pages={203-214},'
+ 'year={1991},'
+ '}'),
+ 'tags': ['method'],
+ },
+ ]
+
+ Examples
+ ========
+
+ >>> from nipype.interfaces import afni
+ >>> edge3 = afni.Edge3()
+ >>> edge3.inputs.in_file = 'functional.nii'
+ >>> edge3.inputs.out_file = 'edges.nii'
+ >>> edge3.inputs.datum = 'byte'
+ >>> edge3.cmdline # doctest: +ALLOW_UNICODE
+ '3dedge3 -input functional.nii -datum byte -prefix edges.nii'
+ >>> res = edge3.run() # doctest: +SKIP
+
+ """
+
+ _cmd = '3dedge3'
+ input_spec = Edge3InputSpec
+ output_spec = AFNICommandOutputSpec
+
+
class EvalInputSpec(AFNICommandInputSpec):
in_file_a = File(
desc='input file to 1deval',
diff --git a/nipype/interfaces/base.py b/nipype/interfaces/base.py
index 8d7c53cde..2f8b1bf0e 100644
--- a/nipype/interfaces/base.py
+++ b/nipype/interfaces/base.py
@@ -1381,6 +1381,35 @@ def _get_ram_mb(pid, pyfunc=False):
return mem_mb
+def _canonicalize_env(env):
+ """Windows requires that environment be dicts with bytes as keys and values
+ This function converts any unicode entries for Windows only, returning the
+ dictionary untouched in other environments.
+
+ Parameters
+ ----------
+ env : dict
+ environment dictionary with unicode or bytes keys and values
+
+ Returns
+ -------
+ env : dict
+ Windows: environment dictionary with bytes keys and values
+ Other: untouched input ``env``
+ """
+ if os.name != 'nt':
+ return env
+
+ out_env = {}
+ for key, val in env:
+ if not isinstance(key, bytes):
+ key = key.encode('utf-8')
+ if not isinstance(val, bytes):
+ val = key.encode('utf-8')
+ out_env[key] = val
+ return out_env
+
+
# Get max resources used for process
def get_max_resources_used(pid, mem_mb, num_threads, pyfunc=False):
"""Function to get the RAM and threads usage of a process
@@ -1435,6 +1464,8 @@ def run_command(runtime, output=None, timeout=0.01, redirect_x=False):
raise RuntimeError('Xvfb was not found, X redirection aborted')
cmdline = 'xvfb-run -a ' + cmdline
+ env = _canonicalize_env(runtime.environ)
+
default_encoding = locale.getdefaultlocale()[1]
if default_encoding is None:
default_encoding = 'UTF-8'
@@ -1449,14 +1480,14 @@ def run_command(runtime, output=None, timeout=0.01, redirect_x=False):
stderr=stderr,
shell=True,
cwd=runtime.cwd,
- env=runtime.environ)
+ env=env)
else:
proc = subprocess.Popen(cmdline,
stdout=PIPE,
stderr=PIPE,
shell=True,
cwd=runtime.cwd,
- env=runtime.environ)
+ env=env)
result = {}
errfile = os.path.join(runtime.cwd, 'stderr.nipype')
outfile = os.path.join(runtime.cwd, 'stdout.nipype')
diff --git a/nipype/interfaces/fsl/epi.py b/nipype/interfaces/fsl/epi.py
index 1f4a7ded1..38c65efee 100644
--- a/nipype/interfaces/fsl/epi.py
+++ b/nipype/interfaces/fsl/epi.py
@@ -143,6 +143,9 @@ class TOPUPInputSpec(FSLCommandInputSpec):
out_warp_prefix = traits.Str("warpfield", argstr='--dfout=%s', hash_files=False,
desc='prefix for the warpfield images (in mm)',
usedefault=True)
+ out_mat_prefix = traits.Str("xfm", argstr='--rbmout=%s', hash_files=False,
+ desc='prefix for the realignment matrices',
+ usedefault=True)
out_jac_prefix = traits.Str("jac", argstr='--jacout=%s',
hash_files=False,
desc='prefix for the warpfield images',
@@ -221,6 +224,7 @@ class TOPUPOutputSpec(TraitedSpec):
out_field = File(desc='name of image file with field (Hz)')
out_warps = traits.List(File(exists=True), desc='warpfield images')
out_jacs = traits.List(File(exists=True), desc='Jacobian images')
+ out_mats = traits.List(File(exists=True), desc='realignment matrices')
out_corrected = File(desc='name of 4D image file with unwarped images')
out_logfile = File(desc='name of log-file')
@@ -247,7 +251,7 @@ class TOPUP(FSLCommand):
'topup --config=b02b0.cnf --datain=topup_encoding.txt \
--imain=b0_b0rev.nii --out=b0_b0rev_base --iout=b0_b0rev_corrected.nii.gz \
--fout=b0_b0rev_field.nii.gz --jacout=jac --logout=b0_b0rev_topup.log \
---dfout=warpfield'
+--rbmout=xfm --dfout=warpfield'
>>> res = topup.run() # doctest: +SKIP
"""
@@ -289,6 +293,9 @@ class TOPUP(FSLCommand):
outputs['out_jacs'] = [
fmt(prefix=self.inputs.out_jac_prefix, i=i, ext=ext)
for i in range(1, n_vols + 1)]
+ outputs['out_mats'] = [
+ fmt(prefix=self.inputs.out_mat_prefix, i=i, ext=".mat")
+ for i in range(1, n_vols + 1)]
if isdefined(self.inputs.encoding_direction):
outputs['out_enc_file'] = self._get_encfilename()
| nipy/nipype | 50402604f068de9d8f99b402e533fc01e387dd8e | diff --git a/nipype/interfaces/afni/tests/test_auto_Edge3.py b/nipype/interfaces/afni/tests/test_auto_Edge3.py
new file mode 100644
index 000000000..51a4dc865
--- /dev/null
+++ b/nipype/interfaces/afni/tests/test_auto_Edge3.py
@@ -0,0 +1,57 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from __future__ import unicode_literals
+from ..utils import Edge3
+
+
+def test_Edge3_inputs():
+ input_map = dict(args=dict(argstr='%s',
+ ),
+ datum=dict(argstr='-datum %s',
+ ),
+ environ=dict(nohash=True,
+ usedefault=True,
+ ),
+ fscale=dict(argstr='-fscale',
+ xor=['gscale', 'nscale', 'scale_floats'],
+ ),
+ gscale=dict(argstr='-gscale',
+ xor=['fscale', 'nscale', 'scale_floats'],
+ ),
+ ignore_exception=dict(nohash=True,
+ usedefault=True,
+ ),
+ in_file=dict(argstr='-input %s',
+ copyfile=False,
+ mandatory=True,
+ position=0,
+ ),
+ nscale=dict(argstr='-nscale',
+ xor=['fscale', 'gscale', 'scale_floats'],
+ ),
+ out_file=dict(argstr='-prefix %s',
+ position=-1,
+ ),
+ outputtype=dict(),
+ scale_floats=dict(argstr='-scale_floats %f',
+ xor=['fscale', 'gscale', 'nscale'],
+ ),
+ terminal_output=dict(nohash=True,
+ ),
+ verbose=dict(argstr='-verbose',
+ ),
+ )
+ inputs = Edge3.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+
+
+def test_Edge3_outputs():
+ output_map = dict(out_file=dict(),
+ )
+ outputs = Edge3.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
diff --git a/nipype/interfaces/fsl/tests/test_auto_TOPUP.py b/nipype/interfaces/fsl/tests/test_auto_TOPUP.py
index 28083c6dc..88f11a77d 100644
--- a/nipype/interfaces/fsl/tests/test_auto_TOPUP.py
+++ b/nipype/interfaces/fsl/tests/test_auto_TOPUP.py
@@ -64,6 +64,10 @@ def test_TOPUP_inputs():
name_source=['in_file'],
name_template='%s_topup.log',
),
+ out_mat_prefix=dict(argstr='--rbmout=%s',
+ hash_files=False,
+ usedefault=True,
+ ),
out_warp_prefix=dict(argstr='--dfout=%s',
hash_files=False,
usedefault=True,
| Issues of interfacing with PETPVC modules
Hi @effigies @alexsavio, I have run it in a Node and the content of the file report.rst as following:
Node: petpvc
============
Hierarchy : pvc
Exec ID : pvc
Original Inputs
---------------
* alpha : <undefined>
* args : <undefined>
* debug : False
* environ : {}
* fwhm_x : 2.0
* fwhm_y : 2.0
* fwhm_z : 2.0
* ignore_exception : False
* in_file : C:\Users\135173\Desktop\pvc_test\PET_nii\x_WB_CTAC_Body.nii.gz
* mask_file : C:\Users\135173\Desktop\pvc_test\mask_nill\skin_mask.nii.gz
* n_deconv : <undefined>
* n_iter : <undefined>
* out_file : C:\Users\135173\Desktop\pvc_test\pet_pvc_rbv.nii.gz
* pvc : RBV
* stop_crit : <undefined>
* terminal_output : stream
Thanks.
| 0.0 | [
"nipype/interfaces/afni/tests/test_auto_Edge3.py::test_Edge3_inputs",
"nipype/interfaces/afni/tests/test_auto_Edge3.py::test_Edge3_outputs",
"nipype/interfaces/fsl/tests/test_auto_TOPUP.py::test_TOPUP_inputs",
"nipype/interfaces/fsl/tests/test_auto_TOPUP.py::test_TOPUP_outputs"
] | [] | 2017-06-21 17:05:10+00:00 | 4,191 |
|
nipy__nipype-2238 | diff --git a/nipype/interfaces/base.py b/nipype/interfaces/base.py
index 79812d4b1..f8e845d94 100644
--- a/nipype/interfaces/base.py
+++ b/nipype/interfaces/base.py
@@ -38,7 +38,7 @@ from .. import config, logging, LooseVersion, __version__
from ..utils.provenance import write_provenance
from ..utils.misc import is_container, trim, str2bool
from ..utils.filemanip import (md5, hash_infile, FileNotFoundError, hash_timestamp,
- split_filename, to_str)
+ split_filename, to_str, read_stream)
from .traits_extension import (
traits, Undefined, TraitDictObject, TraitListObject, TraitError, isdefined,
File, Directory, DictStrStr, has_metadata, ImageFile)
@@ -1268,9 +1268,7 @@ class Stream(object):
self._buf = ''
self._rows = []
self._lastidx = 0
- self.default_encoding = locale.getdefaultlocale()[1]
- if self.default_encoding is None:
- self.default_encoding = 'UTF-8'
+ self.default_encoding = locale.getdefaultlocale()[1] or 'UTF-8'
def fileno(self):
"Pass-through for file descriptor."
@@ -1349,10 +1347,6 @@ def run_command(runtime, output=None, timeout=0.01):
cmdline = runtime.cmdline
env = _canonicalize_env(runtime.environ)
- default_encoding = locale.getdefaultlocale()[1]
- if default_encoding is None:
- default_encoding = 'UTF-8'
-
errfile = None
outfile = None
stdout = sp.PIPE
@@ -1420,19 +1414,22 @@ def run_command(runtime, output=None, timeout=0.01):
if output == 'allatonce':
stdout, stderr = proc.communicate()
- result['stdout'] = stdout.decode(default_encoding).split('\n')
- result['stderr'] = stderr.decode(default_encoding).split('\n')
+ result['stdout'] = read_stream(stdout, logger=iflogger)
+ result['stderr'] = read_stream(stderr, logger=iflogger)
elif output.startswith('file'):
proc.wait()
if outfile is not None:
stdout.flush()
- result['stdout'] = [line.decode(default_encoding).strip()
- for line in open(outfile, 'rb').readlines()]
+ with open(outfile, 'rb') as ofh:
+ stdoutstr = ofh.read()
+ result['stdout'] = read_stream(stdoutstr, logger=iflogger)
+
if errfile is not None:
stderr.flush()
- result['stderr'] = [line.decode(default_encoding).strip()
- for line in open(errfile, 'rb').readlines()]
+ with open(errfile, 'rb') as efh:
+ stderrstr = efh.read()
+ result['stderr'] = read_stream(stderrstr, logger=iflogger)
if output == 'file':
result['merged'] = result['stdout']
diff --git a/nipype/interfaces/freesurfer/preprocess.py b/nipype/interfaces/freesurfer/preprocess.py
index 0138062e5..5f39f1cc9 100644
--- a/nipype/interfaces/freesurfer/preprocess.py
+++ b/nipype/interfaces/freesurfer/preprocess.py
@@ -2387,8 +2387,8 @@ class ConcatenateLTAInputSpec(FSTraitedSpec):
File(exists=True), 'identity.nofile', argstr='%s', position=-2,
mandatory=True, desc='maps dst1(src2) to dst2')
out_file = File(
- 'concat.lta', usedefault=True, position=-1, argstr='%s',
- hash_files=False,
+ position=-1, argstr='%s', hash_files=False, name_source=['in_lta1'],
+ name_template='%s_concat', keep_extension=True,
desc='the combined LTA maps: src1 to dst2 = LTA2*LTA1')
# Inversion and transform type
@@ -2434,7 +2434,7 @@ class ConcatenateLTA(FSCommand):
>>> conc_lta.inputs.in_lta1 = 'lta1.lta'
>>> conc_lta.inputs.in_lta2 = 'lta2.lta'
>>> conc_lta.cmdline # doctest: +ALLOW_UNICODE
- 'mri_concatenate_lta lta1.lta lta2.lta concat.lta'
+ 'mri_concatenate_lta lta1.lta lta2.lta lta1_concat.lta'
You can use 'identity.nofile' as the filename for in_lta2, e.g.:
@@ -2459,8 +2459,3 @@ class ConcatenateLTA(FSCommand):
if name == 'out_type':
value = {'VOX2VOX': 0, 'RAS2RAS': 1}[value]
return super(ConcatenateLTA, self)._format_arg(name, spec, value)
-
- def _list_outputs(self):
- outputs = self.output_spec().get()
- outputs['out_file'] = os.path.abspath(self.inputs.out_file)
- return outputs
diff --git a/nipype/utils/filemanip.py b/nipype/utils/filemanip.py
index e321a597a..72cb1fc15 100644
--- a/nipype/utils/filemanip.py
+++ b/nipype/utils/filemanip.py
@@ -5,16 +5,13 @@
"""
from __future__ import print_function, division, unicode_literals, absolute_import
-from builtins import str, bytes, open
-
-from future import standard_library
-standard_library.install_aliases()
import sys
import pickle
import subprocess
import gzip
import hashlib
+import locale
from hashlib import md5
import os
import re
@@ -23,10 +20,15 @@ import posixpath
import simplejson as json
import numpy as np
+from builtins import str, bytes, open
+
from .. import logging, config
from .misc import is_container
from ..interfaces.traits_extension import isdefined
+from future import standard_library
+standard_library.install_aliases()
+
fmlogger = logging.getLogger('utils')
@@ -596,6 +598,26 @@ def crash2txt(filename, record):
fp.write(''.join(record['traceback']))
+def read_stream(stream, logger=None, encoding=None):
+ """
+ Robustly reads a stream, sending a warning to a logger
+ if some decoding error was raised.
+
+ >>> read_stream(bytearray([65, 0xc7, 65, 10, 66])) # doctest: +ELLIPSIS +ALLOW_UNICODE
+ ['A...A', 'B']
+
+
+ """
+ default_encoding = encoding or locale.getdefaultlocale()[1] or 'UTF-8'
+ logger = logger or fmlogger
+ try:
+ out = stream.decode(default_encoding)
+ except UnicodeDecodeError as err:
+ out = stream.decode(default_encoding, errors='replace')
+ logger.warning('Error decoding string: %s', err)
+ return out.splitlines()
+
+
def savepkl(filename, record):
if filename.endswith('pklz'):
pkl_file = gzip.open(filename, 'wb')
| nipy/nipype | 66411f4edbc03a43a56e49ee791652cc7d824572 | diff --git a/nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py b/nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py
index 1f957d35f..2357c8709 100644
--- a/nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py
+++ b/nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py
@@ -28,8 +28,10 @@ def test_ConcatenateLTA_inputs():
),
out_file=dict(argstr='%s',
hash_files=False,
+ keep_extension=True,
+ name_source=['in_lta1'],
+ name_template='%s_concat',
position=-1,
- usedefault=True,
),
out_type=dict(argstr='-out_type %d',
),
| UnicodeDecodeError: 'utf-8' codec can't decode in run_command
Running FMRIPREP using singularity.
```
Node: _autorecon30
Working directory: /scratch/03763/oesteban/fmriprep-phase2/work/ds000172/sub-control01/fmriprep_wf/single_subject_control01_wf/anat_preproc_wf/surface_recon_wf/autorecon_resume_wf/autorecon3/mapflow/_autorecon
30
Node inputs:
T1_files = <undefined>
T2_file = <undefined>
args = <undefined>
big_ventricles = <undefined>
brainstem = <undefined>
directive = autorecon3
environ = {}
expert = <undefined>
flags = <undefined>
hemi = lh
hippocampal_subfields_T1 = <undefined>
hippocampal_subfields_T2 = <undefined>
hires = <undefined>
ignore_exception = False
mprage = <undefined>
mri_aparc2aseg = <undefined>
mri_ca_label = <undefined>
mri_ca_normalize = <undefined>
mri_ca_register = <undefined>
mri_edit_wm_with_aseg = <undefined>
mri_em_register = <undefined>
mri_fill = <undefined>
mri_mask = <undefined>
mri_normalize = <undefined>
mri_pretess = <undefined>
mri_remove_neck = <undefined>
mri_segment = <undefined>
mri_segstats = <undefined>
mri_tessellate = <undefined>
mri_watershed = <undefined>
mris_anatomical_stats = <undefined>
mris_ca_label = <undefined>
mris_fix_topology = <undefined>
mris_inflate = <undefined>
mris_make_surfaces = <undefined>
mris_register = <undefined>
mris_smooth = <undefined>
mris_sphere = <undefined>
mris_surf2vol = <undefined>
mrisp_paint = <undefined>
openmp = 8
parallel = <undefined>
subject_id = sub-control01
subjects_dir = /work/03843/crn_plab/stampede2/fmriprep-phase2/derivatives/ds000172/freesurfer
talairach = <undefined>
terminal_output = <undefined>
use_T2 = False
xopts = <undefined>
Traceback (most recent call last):
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/plugins/multiproc.py", line 51, in run_node
result['result'] = node.run(updatehash=updatehash)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/engine/nodes.py", line 407, in run
self._run_interface()
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/engine/nodes.py", line 517, in _run_interface
self._result = self._run_command(execute)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/engine/nodes.py", line 650, in _run_command
result = self._interface.run()
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/interfaces/base.py", line 1088, in run
runtime = self._run_interface(runtime)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/interfaces/base.py", line 1654, in _run_interface
runtime = run_command(runtime, output=self.terminal_output)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/interfaces/base.py", line 1423, in run_command
result['stdout'] = stdout.decode(default_encoding).split('\n')
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xc7 in position 9789: invalid continuation byte
``` | 0.0 | [
"nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py::test_ConcatenateLTA_inputs"
] | [
"nipype/interfaces/freesurfer/tests/test_auto_ConcatenateLTA.py::test_ConcatenateLTA_outputs"
] | 2017-10-19 16:42:19+00:00 | 4,192 |
|
nipy__nipype-2248 | diff --git a/.travis.yml b/.travis.yml
index a7630ca91..681d3dd76 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -34,10 +34,10 @@ before_install:
hash -r &&
conda config --set always_yes yes --set changeps1 no &&
conda update -q conda &&
- conda install python=${TRAVIS_PYTHON_VERSION} &&
conda config --add channels conda-forge &&
- conda install -y nipype icu &&
- rm -r ${CONDA_HOME}/lib/python${TRAVIS_PYTHON_VERSION}/site-packages/nipype*;
+ conda install python=${TRAVIS_PYTHON_VERSION} &&
+ conda install -y icu &&
+ pip install -r requirements.txt &&
pushd $HOME;
git clone https://github.com/INCF/pybids.git;
cd pybids;
diff --git a/nipype/interfaces/afni/__init__.py b/nipype/interfaces/afni/__init__.py
index bebcdab4b..6a1e7df76 100644
--- a/nipype/interfaces/afni/__init__.py
+++ b/nipype/interfaces/afni/__init__.py
@@ -22,7 +22,7 @@ from .svm import (SVMTest, SVMTrain)
from .utils import (ABoverlap, AFNItoNIFTI, Autobox, Axialize, BrickStat,
Bucket, Calc, Cat, CatMatvec, CenterMass, Copy, Dot,
Edge3, Eval, FWHMx, MaskTool, Merge, Notes, NwarpApply,
- OneDToolPy,
+ NwarpCat, OneDToolPy,
Refit, Resample, TCat, TCatSubBrick, TStat, To3D, Unifize,
Undump, ZCutUp, GCOR,
Zcat, Zeropad)
diff --git a/nipype/interfaces/afni/utils.py b/nipype/interfaces/afni/utils.py
index f7281542a..824557878 100644
--- a/nipype/interfaces/afni/utils.py
+++ b/nipype/interfaces/afni/utils.py
@@ -1588,6 +1588,123 @@ class NwarpApply(AFNICommandBase):
input_spec = NwarpApplyInputSpec
output_spec = AFNICommandOutputSpec
+
+class NwarpCatInputSpec(AFNICommandInputSpec):
+ in_files = traits.List(
+ traits.Either(
+ traits.File(),
+ traits.Tuple(traits.Enum('IDENT', 'INV', 'SQRT', 'SQRTINV'),
+ traits.File())),
+ descr="list of tuples of 3D warps and associated functions",
+ mandatory=True,
+ argstr="%s",
+ position=-1)
+ space = traits.String(
+ desc='string to attach to the output dataset as its atlas space '
+ 'marker.',
+ argstr='-space %s')
+ inv_warp = traits.Bool(
+ desc='invert the final warp before output',
+ argstr='-iwarp')
+ interp = traits.Enum(
+ 'linear', 'quintic', 'wsinc5',
+ desc='specify a different interpolation method than might '
+ 'be used for the warp',
+ argstr='-interp %s',
+ default='wsinc5')
+ expad = traits.Int(
+ desc='Pad the nonlinear warps by the given number of voxels voxels in '
+ 'all directions. The warp displacements are extended by linear '
+ 'extrapolation from the faces of the input grid..',
+ argstr='-expad %d')
+ out_file = File(
+ name_template='%s_NwarpCat',
+ desc='output image file name',
+ argstr='-prefix %s',
+ name_source='in_files')
+ verb = traits.Bool(
+ desc='be verbose',
+ argstr='-verb')
+
+
+class NwarpCat(AFNICommand):
+ """Catenates (composes) 3D warps defined on a grid, OR via a matrix.
+
+ .. note::
+
+ * All transformations are from DICOM xyz (in mm) to DICOM xyz.
+
+ * Matrix warps are in files that end in '.1D' or in '.txt'. A matrix
+ warp file should have 12 numbers in it, as output (for example), by
+ '3dAllineate -1Dmatrix_save'.
+
+ * Nonlinear warps are in dataset files (AFNI .HEAD/.BRIK or NIfTI .nii)
+ with 3 sub-bricks giving the DICOM order xyz grid displacements in mm.
+
+ * If all the input warps are matrices, then the output is a matrix
+ and will be written to the file 'prefix.aff12.1D'.
+ Unless the prefix already contains the string '.1D', in which case
+ the filename is just the prefix.
+
+ * If 'prefix' is just 'stdout', then the output matrix is written
+ to standard output.
+ In any of these cases, the output format is 12 numbers in one row.
+
+ * If any of the input warps are datasets, they must all be defined on
+ the same 3D grid!
+ And of course, then the output will be a dataset on the same grid.
+ However, you can expand the grid using the '-expad' option.
+
+ * The order of operations in the final (output) warp is, for the
+ case of 3 input warps:
+
+ OUTPUT(x) = warp3( warp2( warp1(x) ) )
+
+ That is, warp1 is applied first, then warp2, et cetera.
+ The 3D x coordinates are taken from each grid location in the
+ first dataset defined on a grid.
+
+ For complete details, see the `3dNwarpCat Documentation.
+ <https://afni.nimh.nih.gov/pub/dist/doc/program_help/3dNwarpCat.html>`_
+
+ Examples
+ ========
+
+ >>> from nipype.interfaces import afni
+ >>> nwarpcat = afni.NwarpCat()
+ >>> nwarpcat.inputs.in_files = ['Q25_warp+tlrc.HEAD', ('IDENT', 'structural.nii')]
+ >>> nwarpcat.inputs.out_file = 'Fred_total_WARP'
+ >>> nwarpcat.cmdline # doctest: +ALLOW_UNICODE
+ "3dNwarpCat -prefix Fred_total_WARP Q25_warp+tlrc.HEAD 'IDENT(structural.nii)'"
+ >>> res = nwarpcat.run() # doctest: +SKIP
+
+ """
+ _cmd = '3dNwarpCat'
+ input_spec = NwarpCatInputSpec
+ output_spec = AFNICommandOutputSpec
+
+ def _format_arg(self, name, spec, value):
+ if name == 'in_files':
+ return spec.argstr % (' '.join(["'" + v[0] + "(" + v[1] + ")'"
+ if isinstance(v, tuple) else v
+ for v in value]))
+ return super(NwarpCat, self)._format_arg(name, spec, value)
+
+ def _gen_filename(self, name):
+ if name == 'out_file':
+ return self._gen_fname(self.inputs.in_files[0][0],
+ suffix='_NwarpCat')
+
+ def _list_outputs(self):
+ outputs = self.output_spec().get()
+ if isdefined(self.inputs.out_file):
+ outputs['out_file'] = os.path.abspath(self.inputs.out_file)
+ else:
+ outputs['out_file'] = os.path.abspath(self._gen_fname(
+ self.inputs.in_files[0], suffix='_NwarpCat+tlrc', ext='.HEAD'))
+ return outputs
+
+
class OneDToolPyInputSpec(AFNIPythonCommandInputSpec):
in_file = File(
desc='input file to OneDTool',
diff --git a/nipype/interfaces/bids_utils.py b/nipype/interfaces/bids_utils.py
index 0bbc89509..0259a8035 100644
--- a/nipype/interfaces/bids_utils.py
+++ b/nipype/interfaces/bids_utils.py
@@ -6,15 +6,15 @@ available interfaces are:
BIDSDataGrabber: Query data from BIDS dataset using pybids grabbids.
-Change directory to provide relative paths for doctests
->>> import os
->>> import bids
->>> filepath = os.path.realpath(os.path.dirname(bids.__file__))
->>> datadir = os.path.realpath(os.path.join(filepath, 'grabbids/tests/data/'))
->>> os.chdir(datadir)
+ Change directory to provide relative paths for doctests
+ >>> import os
+ >>> filepath = os.path.dirname( os.path.realpath( __file__ ) )
+ >>> datadir = os.path.realpath(os.path.join(filepath, '../testing/data'))
+ >>> os.chdir(datadir)
"""
from os.path import join, dirname
+import json
from .. import logging
from .base import (traits,
DynamicTraitedSpec,
@@ -24,13 +24,11 @@ from .base import (traits,
Str,
Undefined)
+have_pybids = True
try:
from bids import grabbids as gb
- import json
except ImportError:
have_pybids = False
-else:
- have_pybids = True
LOGGER = logging.getLogger('workflows')
@@ -56,9 +54,6 @@ class BIDSDataGrabber(BaseInterface):
Examples
--------
- >>> from nipype.interfaces.bids_utils import BIDSDataGrabber
- >>> from os.path import basename
-
By default, the BIDSDataGrabber fetches anatomical and functional images
from a project, and makes BIDS entities (e.g. subject) available for
filtering outputs.
@@ -66,12 +61,7 @@ class BIDSDataGrabber(BaseInterface):
>>> bg = BIDSDataGrabber()
>>> bg.inputs.base_dir = 'ds005/'
>>> bg.inputs.subject = '01'
- >>> results = bg.run()
- >>> basename(results.outputs.anat[0]) # doctest: +ALLOW_UNICODE
- 'sub-01_T1w.nii.gz'
-
- >>> basename(results.outputs.func[0]) # doctest: +ALLOW_UNICODE
- 'sub-01_task-mixedgamblestask_run-01_bold.nii.gz'
+ >>> results = bg.run() # doctest: +SKIP
Dynamically created, user-defined output fields can also be defined to
@@ -83,9 +73,7 @@ class BIDSDataGrabber(BaseInterface):
>>> bg.inputs.base_dir = 'ds005/'
>>> bg.inputs.subject = '01'
>>> bg.inputs.output_query['dwi'] = dict(modality='dwi')
- >>> results = bg.run()
- >>> basename(results.outputs.dwi[0]) # doctest: +ALLOW_UNICODE
- 'sub-01_dwi.nii.gz'
+ >>> results = bg.run() # doctest: +SKIP
"""
input_spec = BIDSDataGrabberInputSpec
@@ -104,32 +92,32 @@ class BIDSDataGrabber(BaseInterface):
If no matching items, returns Undefined.
"""
super(BIDSDataGrabber, self).__init__(**kwargs)
- if not have_pybids:
- raise ImportError(
- "The BIDSEventsGrabber interface requires pybids."
- " Please make sure it is installed.")
if not isdefined(self.inputs.output_query):
self.inputs.output_query = {"func": {"modality": "func"},
"anat": {"modality": "anat"}}
- # If infields is None, use all BIDS entities
- if infields is None:
+ # If infields is empty, use all BIDS entities
+ if not infields is None and have_pybids:
bids_config = join(dirname(gb.__file__), 'config', 'bids.json')
bids_config = json.load(open(bids_config, 'r'))
infields = [i['name'] for i in bids_config['entities']]
- self._infields = infields
+ self._infields = infields or []
# used for mandatory inputs check
undefined_traits = {}
- for key in infields:
+ for key in self._infields:
self.inputs.add_trait(key, traits.Any)
undefined_traits[key] = kwargs[key] if key in kwargs else Undefined
self.inputs.trait_set(trait_change_notify=False, **undefined_traits)
def _run_interface(self, runtime):
+ if not have_pybids:
+ raise ImportError(
+ "The BIDSEventsGrabber interface requires pybids."
+ " Please make sure it is installed.")
return runtime
def _list_outputs(self):
diff --git a/nipype/interfaces/io.py b/nipype/interfaces/io.py
index f2e3fcd94..4d3220b04 100644
--- a/nipype/interfaces/io.py
+++ b/nipype/interfaces/io.py
@@ -1188,15 +1188,18 @@ class SelectFilesInputSpec(DynamicTraitedSpec, BaseInterfaceInputSpec):
base_directory = Directory(exists=True,
desc="Root path common to templates.")
sort_filelist = traits.Bool(True, usedefault=True,
- desc="When matching mutliple files, return them in sorted order.")
+ desc="When matching mutliple files, return them"
+ " in sorted order.")
raise_on_empty = traits.Bool(True, usedefault=True,
- desc="Raise an exception if a template pattern matches no files.")
+ desc="Raise an exception if a template pattern "
+ "matches no files.")
force_lists = traits.Either(traits.Bool(), traits.List(Str()),
default=False, usedefault=True,
- desc=("Whether to return outputs as a list even when only one file "
- "matches the template. Either a boolean that applies to all "
- "output fields or a list of output field names to coerce to "
- " a list"))
+ desc=("Whether to return outputs as a list even"
+ " when only one file matches the template. "
+ "Either a boolean that applies to all output "
+ "fields or a list of output field names to "
+ "coerce to a list"))
class SelectFiles(IOBase):
@@ -1296,6 +1299,8 @@ class SelectFiles(IOBase):
for field, template in list(self._templates.items()):
+ find_dirs = template[-1] == os.sep
+
# Build the full template path
if isdefined(self.inputs.base_directory):
template = op.abspath(op.join(
@@ -1303,6 +1308,10 @@ class SelectFiles(IOBase):
else:
template = op.abspath(template)
+ # re-add separator if searching exclusively for directories
+ if find_dirs:
+ template += os.sep
+
# Fill in the template and glob for files
filled_template = template.format(**info)
filelist = glob.glob(filled_template)
diff --git a/nipype/testing/data/ds005/filler.txt b/nipype/testing/data/ds005/filler.txt
new file mode 100644
index 000000000..e69de29bb
diff --git a/nipype/utils/filemanip.py b/nipype/utils/filemanip.py
index 72cb1fc15..e8a9ea22b 100644
--- a/nipype/utils/filemanip.py
+++ b/nipype/utils/filemanip.py
@@ -646,3 +646,20 @@ def write_rst_dict(info, prefix=''):
for key, value in sorted(info.items()):
out.append('{}* {} : {}'.format(prefix, key, str(value)))
return '\n'.join(out) + '\n\n'
+
+
+def dist_is_editable(dist):
+ """Is distribution an editable install?
+
+ Parameters
+ ----------
+ dist : string
+ Package name
+
+ # Borrowed from `pip`'s' API
+ """
+ for path_item in sys.path:
+ egg_link = os.path.join(path_item, dist + '.egg-link')
+ if os.path.isfile(egg_link):
+ return True
+ return False
| nipy/nipype | 1a7401328baf4aca0f163ca18d3a88b913412eb3 | diff --git a/nipype/interfaces/afni/tests/test_auto_NwarpCat.py b/nipype/interfaces/afni/tests/test_auto_NwarpCat.py
new file mode 100644
index 000000000..6e5077e64
--- /dev/null
+++ b/nipype/interfaces/afni/tests/test_auto_NwarpCat.py
@@ -0,0 +1,56 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from __future__ import unicode_literals
+from ..utils import NwarpCat
+
+
+def test_NwarpCat_inputs():
+ input_map = dict(args=dict(argstr='%s',
+ ),
+ environ=dict(nohash=True,
+ usedefault=True,
+ ),
+ expad=dict(argstr='-expad %d',
+ ),
+ ignore_exception=dict(nohash=True,
+ usedefault=True,
+ ),
+ in_files=dict(argstr='%s',
+ descr='list of tuples of 3D warps and associated functions',
+ mandatory=True,
+ position=-1,
+ ),
+ interp=dict(argstr='-interp %s',
+ ),
+ inv_warp=dict(argstr='-iwarp',
+ ),
+ num_threads=dict(nohash=True,
+ usedefault=True,
+ ),
+ out_file=dict(argstr='-prefix %s',
+ name_source='in_files',
+ name_template='%s_NwarpCat',
+ ),
+ outputtype=dict(),
+ space=dict(argstr='-space %s',
+ ),
+ terminal_output=dict(deprecated='1.0.0',
+ nohash=True,
+ ),
+ verb=dict(argstr='-verb',
+ ),
+ )
+ inputs = NwarpCat.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+
+
+def test_NwarpCat_outputs():
+ output_map = dict(out_file=dict(),
+ )
+ outputs = NwarpCat.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
diff --git a/nipype/interfaces/tests/test_bids.py b/nipype/interfaces/tests/test_bids.py
new file mode 100644
index 000000000..aa5bc6c35
--- /dev/null
+++ b/nipype/interfaces/tests/test_bids.py
@@ -0,0 +1,50 @@
+import os
+import json
+import sys
+
+import pytest
+from nipype.interfaces.bids_utils import BIDSDataGrabber
+from nipype.utils.filemanip import dist_is_editable
+
+have_pybids = True
+try:
+ import bids
+ from bids import grabbids as gb
+ filepath = os.path.realpath(os.path.dirname(bids.__file__))
+ datadir = os.path.realpath(os.path.join(filepath, 'grabbids/tests/data/'))
+except ImportError:
+ have_pybids = False
+
+
+# There are three reasons these tests will be skipped:
[email protected](not have_pybids,
+ reason="Pybids is not installed")
[email protected](sys.version_info < (3, 0),
+ reason="Pybids no longer supports Python 2")
[email protected](not dist_is_editable('pybids'),
+ reason="Pybids is not installed in editable mode")
+def test_bids_grabber(tmpdir):
+ tmpdir.chdir()
+ bg = BIDSDataGrabber()
+ bg.inputs.base_dir = os.path.join(datadir, 'ds005')
+ bg.inputs.subject = '01'
+ results = bg.run()
+ assert os.path.basename(results.outputs.anat[0]) == 'sub-01_T1w.nii.gz'
+ assert os.path.basename(results.outputs.func[0]) == (
+ 'sub-01_task-mixedgamblestask_run-01_bold.nii.gz')
+
+
[email protected](not have_pybids,
+ reason="Pybids is not installed")
[email protected](sys.version_info < (3, 0),
+ reason="Pybids no longer supports Python 2")
[email protected](not dist_is_editable('pybids'),
+ reason="Pybids is not installed in editable mode")
+def test_bids_fields(tmpdir):
+ tmpdir.chdir()
+ bg = BIDSDataGrabber(infields = ['subject'], outfields = ['dwi'])
+ bg.inputs.base_dir = os.path.join(datadir, 'ds005')
+ bg.inputs.subject = '01'
+ bg.inputs.output_query['dwi'] = dict(modality='dwi')
+ results = bg.run()
+ assert os.path.basename(results.outputs.dwi[0]) == 'sub-01_dwi.nii.gz'
diff --git a/nipype/interfaces/tests/test_resource_monitor.py b/nipype/interfaces/tests/test_resource_monitor.py
index 660f11455..8374ba7ac 100644
--- a/nipype/interfaces/tests/test_resource_monitor.py
+++ b/nipype/interfaces/tests/test_resource_monitor.py
@@ -45,6 +45,7 @@ class UseResources(CommandLine):
_always_run = True
[email protected](reason="inconsistent readings")
@pytest.mark.skipif(os.getenv('CI_SKIP_TEST', False), reason='disabled in CI tests')
@pytest.mark.parametrize("mem_gb,n_procs", [(0.5, 3), (2.2, 8), (0.8, 4), (1.5, 1)])
def test_cmdline_profiling(tmpdir, mem_gb, n_procs):
| Testing the install: "UNEXPECTED EXCEPTION: ImportError("No module named 'bids'",)"
### Summary
While testing the installation with `python -c "import nipype; nipype.test()" ` I got the following error
```
==================================================================================================================================== FAILURES =====================================================================================================================================
_____________________________________________________________________________________________________________________ [doctest] nipype.interfaces.bids_utils ______________________________________________________________________________________________________________________
UNEXPECTED EXCEPTION: ImportError("No module named 'bids'",)
Traceback (most recent call last):
File "/home/athanell/anaconda3/envs/SAH/lib/python3.5/doctest.py", line 1321, in __run
compileflags, 1), test.globs)
File "<doctest nipype.interfaces.bids_utils[1]>", line 1, in <module>
ImportError: No module named 'bids'
/home/athanell/.local/lib/python3.5/site-packages/nipype/interfaces/bids_utils.py:11: UnexpectedException
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! Interrupted: stopping after 1 failures !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
================================================================================================================ 1 failed, 143 passed, 5 skipped in 29.53 seconds =================================================================================================================
```
Is this something that will hinder the usage of nipype and should be fixed? If yes how should I go about it?
Thank you
### Platform details:
```
{'nibabel_version': '2.1.0', 'sys_executable': '/home/athanell/anaconda3/envs/SAH/bin/python', 'scipy_version': '0.19.1', 'networkx_version': '2.0', 'sys_platform': 'linux', 'pkg_path': '/home/athanell/.local/lib/python3.5/site-packages/nipype', 'commit_source': 'installation', 'nipype_version': '1.0.0-dev', 'numpy_version': '1.13.3', 'sys_version': '3.5.4 |Anaconda, Inc.| (default, Oct 5 2017, 08:00:22) \n[GCC 7.2.0]', 'commit_hash': 'e4e95f3', 'traits_version': '4.6.0'}
1.0.0-dev
```
| 0.0 | [
"nipype/interfaces/afni/tests/test_auto_NwarpCat.py::test_NwarpCat_inputs",
"nipype/interfaces/afni/tests/test_auto_NwarpCat.py::test_NwarpCat_outputs"
] | [] | 2017-10-23 22:15:33+00:00 | 4,193 |
|
nipy__nipype-2301 | diff --git a/CHANGES b/CHANGES
index 0845eee28..0480ad874 100644
--- a/CHANGES
+++ b/CHANGES
@@ -7,6 +7,7 @@ Upcoming release
###### [Full changelog](https://github.com/nipy/nipype/milestone/13)
+* FIX: MultiProc mishandling crashes (https://github.com/nipy/nipype/pull/2301)
* MAINT: Revise use of `subprocess.Popen` (https://github.com/nipy/nipype/pull/2289)
* ENH: Memorize version checks (https://github.com/nipy/nipype/pull/2274, https://github.com/nipy/nipype/pull/2295)
diff --git a/nipype/interfaces/ants/registration.py b/nipype/interfaces/ants/registration.py
index 6d82a2e9f..785205952 100644
--- a/nipype/interfaces/ants/registration.py
+++ b/nipype/interfaces/ants/registration.py
@@ -1,5 +1,6 @@
# -*- coding: utf-8 -*-
-"""The ants module provides basic functions for interfacing with ants functions.
+"""The ants module provides basic functions for interfacing with ants
+ functions.
Change directory to provide relative paths for doctests
>>> import os
@@ -7,7 +8,8 @@
>>> datadir = os.path.realpath(os.path.join(filepath, '../../testing/data'))
>>> os.chdir(datadir)
"""
-from __future__ import print_function, division, unicode_literals, absolute_import
+from __future__ import (print_function, division, unicode_literals,
+ absolute_import)
from builtins import range, str
import os
@@ -20,17 +22,19 @@ class ANTSInputSpec(ANTSCommandInputSpec):
dimension = traits.Enum(3, 2, argstr='%d', usedefault=False,
position=1, desc='image dimension (2 or 3)')
fixed_image = InputMultiPath(File(exists=True), mandatory=True,
- desc=('image to which the moving image is warped'))
+ desc=('image to which the moving image is '
+ 'warped'))
moving_image = InputMultiPath(File(exists=True), argstr='%s',
mandatory=True,
- desc=('image to apply transformation to (generally a coregistered '
+ desc=('image to apply transformation to '
+ '(generally a coregistered'
'functional)'))
# Not all metrics are appropriate for all modalities. Also, not all metrics
-# are efficeint or appropriate at all resolution levels, Some metrics perform
-# well for gross global registraiton, but do poorly for small changes (i.e.
-# Mattes), and some metrics do well for small changes but don't work well for
-# gross level changes (i.e. 'CC').
+# are efficeint or appropriate at all resolution levels, Some metrics
+# perform well for gross global registraiton, but do poorly for small
+# changes (i.e. Mattes), and some metrics do well for small changes but
+# don't work well for gross level changes (i.e. 'CC').
#
# This is a two stage registration. in the first stage
# [ 'Mattes', .................]
@@ -49,10 +53,18 @@ class ANTSInputSpec(ANTSCommandInputSpec):
metric = traits.List(traits.Enum('CC', 'MI', 'SMI', 'PR', 'SSD',
'MSQ', 'PSE'), mandatory=True, desc='')
- metric_weight = traits.List(traits.Float(), requires=['metric'], desc='')
- radius = traits.List(traits.Int(), requires=['metric'], desc='')
+ metric_weight = traits.List(traits.Float(), value=[1.0], usedefault=True,
+ requires=['metric'], mandatory=True,
+ desc='the metric weight(s) for each stage. '
+ 'The weights must sum to 1 per stage.')
- output_transform_prefix = Str('out', usedefault=True, argstr='--output-naming %s',
+ radius = traits.List(traits.Int(), requires=['metric'], mandatory=True,
+ desc='radius of the region (i.e. number of layers'
+ ' around a voxel point)'
+ ' that is used for computing cross correlation')
+
+ output_transform_prefix = Str('out', usedefault=True,
+ argstr='--output-naming %s',
mandatory=True, desc='')
transformation_model = traits.Enum('Diff', 'Elast', 'Exp', 'Greedy Exp',
'SyN', argstr='%s', mandatory=True,
diff --git a/nipype/pipeline/plugins/multiproc.py b/nipype/pipeline/plugins/multiproc.py
index 16bfb51a0..b26d02951 100644
--- a/nipype/pipeline/plugins/multiproc.py
+++ b/nipype/pipeline/plugins/multiproc.py
@@ -238,8 +238,10 @@ class MultiProcPlugin(DistributedPluginBase):
num_subnodes = self.procs[jobid].num_subnodes()
except Exception:
traceback = format_exception(*sys.exc_info())
- self._report_crash(self.procs[jobid], traceback=traceback)
- self._clean_queue(jobid, graph)
+ self._clean_queue(
+ jobid, graph,
+ result={'result': None, 'traceback': traceback}
+ )
self.proc_pending[jobid] = False
continue
if num_subnodes > 1:
@@ -275,10 +277,13 @@ class MultiProcPlugin(DistributedPluginBase):
logger.debug('Running node %s on master thread',
self.procs[jobid])
try:
- self.procs[jobid].run()
+ self.procs[jobid].run(updatehash=updatehash)
except Exception:
traceback = format_exception(*sys.exc_info())
- self._report_crash(self.procs[jobid], traceback=traceback)
+ self._clean_queue(
+ jobid, graph,
+ result={'result': None, 'traceback': traceback}
+ )
# Release resources
self._task_finished_cb(jobid)
| nipy/nipype | 10a0a418a4762915cbf9a6e7bf38e3428edc8e94 | diff --git a/nipype/interfaces/ants/tests/test_registration.py b/nipype/interfaces/ants/tests/test_registration.py
new file mode 100644
index 000000000..745b825c6
--- /dev/null
+++ b/nipype/interfaces/ants/tests/test_registration.py
@@ -0,0 +1,22 @@
+# emacs: -*- mode: python; py-indent-offset: 4; indent-tabs-mode: nil -*-
+# vi: set ft=python sts=4 ts=4 sw=4 et:
+from __future__ import unicode_literals
+from nipype.interfaces.ants import registration
+import os
+import pytest
+
+
+def test_ants_mand(tmpdir):
+ tmpdir.chdir()
+ filepath = os.path.dirname(os.path.realpath(__file__))
+ datadir = os.path.realpath(os.path.join(filepath, '../../../testing/data'))
+
+ ants = registration.ANTS()
+ ants.inputs.transformation_model = "SyN"
+ ants.inputs.moving_image = [os.path.join(datadir, 'resting.nii')]
+ ants.inputs.fixed_image = [os.path.join(datadir, 'T1.nii')]
+ ants.inputs.metric = ['MI']
+
+ with pytest.raises(ValueError) as er:
+ ants.run()
+ assert "ANTS requires a value for input 'radius'" in str(er.value)
| [BUG] MultiProc crashes are not correctly handled
### Summary
Since the latest refactor, MultiProc calls `self._report_crash` with a `traceback` argument and such function is not defined:
```
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/engine/workflows.py", line 591, in run
runner.run(execgraph, updatehash=updatehash, config=self.config)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/plugins/base.py", line 182, in run
self._send_procs_to_workers(updatehash=updatehash, graph=graph)
File "/usr/local/miniconda/lib/python3.6/site-packages/niworkflows/nipype/pipeline/plugins/multiproc.py", line 250, in _send_procs_to_workers
self._report_crash(self.procs[jobid], traceback=traceback)
TypeError: _report_crash() got an unexpected keyword argument 'traceback'
```
I'll propose a fix today. | 0.0 | [
"nipype/interfaces/ants/tests/test_registration.py::test_ants_mand"
] | [] | 2017-11-22 19:21:36+00:00 | 4,194 |
|
nipy__nipype-2349 | diff --git a/nipype/interfaces/io.py b/nipype/interfaces/io.py
index fc3617036..6bb9a943f 100644
--- a/nipype/interfaces/io.py
+++ b/nipype/interfaces/io.py
@@ -1555,16 +1555,12 @@ class DataFinder(IOBase):
class FSSourceInputSpec(BaseInterfaceInputSpec):
- subjects_dir = Directory(
- mandatory=True, desc='Freesurfer subjects directory.')
- subject_id = Str(
- mandatory=True, desc='Subject name for whom to retrieve data')
- hemi = traits.Enum(
- 'both',
- 'lh',
- 'rh',
- usedefault=True,
- desc='Selects hemisphere specific outputs')
+ subjects_dir = Directory(exists=True, mandatory=True,
+ desc='Freesurfer subjects directory.')
+ subject_id = Str(mandatory=True,
+ desc='Subject name for whom to retrieve data')
+ hemi = traits.Enum('both', 'lh', 'rh', usedefault=True,
+ desc='Selects hemisphere specific outputs')
class FSSourceOutputSpec(TraitedSpec):
| nipy/nipype | 045b28ef9056fac1107bc4f0707859d043f3bfd1 | diff --git a/nipype/interfaces/tests/test_io.py b/nipype/interfaces/tests/test_io.py
index a2103eadf..76fc9e257 100644
--- a/nipype/interfaces/tests/test_io.py
+++ b/nipype/interfaces/tests/test_io.py
@@ -16,7 +16,7 @@ from collections import namedtuple
import pytest
import nipype
import nipype.interfaces.io as nio
-from nipype.interfaces.base import Undefined
+from nipype.interfaces.base import Undefined, TraitError
# Check for boto
noboto = False
@@ -498,6 +498,12 @@ def test_freesurfersource():
assert fss.inputs.subjects_dir == Undefined
+def test_freesurfersource_incorrectdir():
+ fss = nio.FreeSurferSource()
+ with pytest.raises(TraitError) as err:
+ fss.inputs.subjects_dir = 'path/to/no/existing/directory'
+
+
def test_jsonsink_input():
ds = nio.JSONFileSink()
| FreeSurferSource doesn't check subjects_dir
### Summary
`FreeSurferSource` doesn't check `subjects_dir` is an existing path.
### How to replicate the behavior
```
from nipype.interfaces.io import FreeSurferSource
fs = FreeSurferSource()
fs.inputs.subjects_dir = 'path/to/no/existing/directory'
fs.inputs.subject_id = 'sub-01'
res = fs.run()
```
### Actual behavior
Doesn't give an error.
### Expected behavior
Should check if directory exists (and possibly if there are files for `subject_id` ?).
| 0.0 | [
"nipype/interfaces/tests/test_io.py::test_freesurfersource_incorrectdir"
] | [
"nipype/interfaces/tests/test_io.py::test_datagrabber",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args0-inputs_att0-expected0]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args1-inputs_att1-expected1]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args2-inputs_att2-expected2]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args3-inputs_att3-expected3]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args4-inputs_att4-expected4]",
"nipype/interfaces/tests/test_io.py::test_selectfiles_valueerror",
"nipype/interfaces/tests/test_io.py::test_datagrabber_order",
"nipype/interfaces/tests/test_io.py::test_datasink",
"nipype/interfaces/tests/test_io.py::test_datasink_localcopy",
"nipype/interfaces/tests/test_io.py::test_datasink_substitutions",
"nipype/interfaces/tests/test_io.py::test_datasink_copydir_1",
"nipype/interfaces/tests/test_io.py::test_datasink_copydir_2",
"nipype/interfaces/tests/test_io.py::test_datafinder_depth",
"nipype/interfaces/tests/test_io.py::test_datafinder_unpack",
"nipype/interfaces/tests/test_io.py::test_freesurfersource",
"nipype/interfaces/tests/test_io.py::test_jsonsink_input",
"nipype/interfaces/tests/test_io.py::test_jsonsink[inputs_attributes0]",
"nipype/interfaces/tests/test_io.py::test_jsonsink[inputs_attributes1]"
] | 2017-12-29 08:57:36+00:00 | 4,195 |
|
nipy__nipype-2363 | diff --git a/CHANGES b/CHANGES
index fa1716688..8dcca2ba6 100644
--- a/CHANGES
+++ b/CHANGES
@@ -1,6 +1,7 @@
Upcoming release (0.14.1)
=========================
+* FIX: Errors parsing ``$DISPLAY`` (https://github.com/nipy/nipype/pull/2363)
* FIX: MultiProc starting workers at dubious wd (https://github.com/nipy/nipype/pull/2368)
* REF+FIX: Move BIDSDataGrabber to `interfaces.io` + fix correct default behavior (https://github.com/nipy/nipype/pull/2336)
* ENH: Add AFNI interface for 3dConvertDset (https://github.com/nipy/nipype/pull/2337)
diff --git a/nipype/utils/config.py b/nipype/utils/config.py
index 15264b9ed..30b826d23 100644
--- a/nipype/utils/config.py
+++ b/nipype/utils/config.py
@@ -312,11 +312,11 @@ class NipypeConfig(object):
def _mock():
pass
- # Store a fake Xvfb object
- ndisp = int(sysdisplay.split(':')[-1])
+ # Store a fake Xvfb object. Format - <host>:<display>[.<screen>]
+ ndisp = sysdisplay.split(':')[-1].split('.')[0]
Xvfb = namedtuple('Xvfb', ['new_display', 'stop'])
- self._display = Xvfb(ndisp, _mock)
- return sysdisplay
+ self._display = Xvfb(int(ndisp), _mock)
+ return self.get_display()
else:
if 'darwin' in sys.platform:
raise RuntimeError(
@@ -343,8 +343,7 @@ class NipypeConfig(object):
if not hasattr(self._display, 'new_display'):
setattr(self._display, 'new_display',
self._display.vdisplay_num)
-
- return ':%d' % self._display.new_display
+ return self.get_display()
def stop_display(self):
"""Closes the display if started"""
| nipy/nipype | f3b09125eceec7b90ad801176892aab06e012f8c | diff --git a/nipype/utils/tests/test_config.py b/nipype/utils/tests/test_config.py
index 9c322128e..65ada4c64 100644
--- a/nipype/utils/tests/test_config.py
+++ b/nipype/utils/tests/test_config.py
@@ -28,6 +28,17 @@ xvfbpatch_old.Xvfb.return_value = MagicMock(
spec=['vdisplay_num', 'start', 'stop'], vdisplay_num=2010)
[email protected]('dispvar', [':12', 'localhost:12', 'localhost:12.1'])
+def test_display_parse(monkeypatch, dispvar):
+ """Check that when $DISPLAY is defined, the display is correctly parsed"""
+ config._display = None
+ config._config.remove_option('execution', 'display_variable')
+ monkeypatch.setenv('DISPLAY', dispvar)
+ assert config.get_display() == ':12'
+ # Test that it was correctly cached
+ assert config.get_display() == ':12'
+
+
@pytest.mark.parametrize('dispnum', range(5))
def test_display_config(monkeypatch, dispnum):
"""Check that the display_variable option is used ($DISPLAY not set)"""
@@ -46,7 +57,7 @@ def test_display_system(monkeypatch, dispnum):
config._display = None
config._config.remove_option('execution', 'display_variable')
dispstr = ':%d' % dispnum
- monkeypatch.setitem(os.environ, 'DISPLAY', dispstr)
+ monkeypatch.setenv('DISPLAY', dispstr)
assert config.get_display() == dispstr
# Test that it was correctly cached
assert config.get_display() == dispstr
@@ -58,7 +69,7 @@ def test_display_config_and_system(monkeypatch):
config._display = None
dispstr = ':10'
config.set('execution', 'display_variable', dispstr)
- monkeypatch.setitem(os.environ, 'DISPLAY', ':0')
+ monkeypatch.setenv('DISPLAY', ':0')
assert config.get_display() == dispstr
# Test that it was correctly cached
assert config.get_display() == dispstr
@@ -72,10 +83,17 @@ def test_display_noconfig_nosystem_patched(monkeypatch):
config._config.remove_option('execution', 'display_variable')
monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ":2010"
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
+
def test_display_empty_patched(monkeypatch):
"""
@@ -85,12 +103,18 @@ def test_display_empty_patched(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ':2010'
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_noconfig_nosystem_patched_oldxvfbwrapper(monkeypatch):
"""
@@ -102,10 +126,16 @@ def test_display_noconfig_nosystem_patched_oldxvfbwrapper(monkeypatch):
config._config.remove_option('execution', 'display_variable')
monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch_old)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ":2010"
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_empty_patched_oldxvfbwrapper(monkeypatch):
"""
@@ -115,12 +145,18 @@ def test_display_empty_patched_oldxvfbwrapper(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch_old)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ':2010'
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_noconfig_nosystem_notinstalled(monkeypatch):
"""
@@ -130,7 +166,7 @@ def test_display_noconfig_nosystem_notinstalled(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
+ monkeypatch.delenv('DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', None)
with pytest.raises(RuntimeError):
config.get_display()
@@ -144,13 +180,14 @@ def test_display_empty_notinstalled(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', None)
with pytest.raises(RuntimeError):
config.get_display()
@pytest.mark.skipif(not has_Xvfb, reason='xvfbwrapper not installed')
[email protected]('darwin' in sys.platform, reason='macosx requires root for Xvfb')
def test_display_noconfig_nosystem_installed(monkeypatch):
"""
Check that actually uses xvfbwrapper when installed (not mocked)
@@ -159,7 +196,7 @@ def test_display_noconfig_nosystem_installed(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
+ monkeypatch.delenv('DISPLAY', raising=False)
newdisp = config.get_display()
assert int(newdisp.split(':')[-1]) > 1000
# Test that it was correctly cached
@@ -167,6 +204,7 @@ def test_display_noconfig_nosystem_installed(monkeypatch):
@pytest.mark.skipif(not has_Xvfb, reason='xvfbwrapper not installed')
[email protected]('darwin' in sys.platform, reason='macosx requires root for Xvfb')
def test_display_empty_installed(monkeypatch):
"""
Check that actually uses xvfbwrapper when installed (not mocked)
@@ -175,7 +213,7 @@ def test_display_empty_installed(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
newdisp = config.get_display()
assert int(newdisp.split(':')[-1]) > 1000
# Test that it was correctly cached
@@ -191,7 +229,7 @@ def test_display_empty_macosx(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', '')
+ monkeypatch.delenv('DISPLAY', '')
monkeypatch.setattr(sys, 'platform', 'darwin')
with pytest.raises(RuntimeError):
| issue with MIPAV module
Dear all,
I've tried to run the example on MP2RAGE-skullstripping http://nipype.readthedocs.io/en/latest/users/examples/smri_cbs_skullstripping.html with nipype 0.14.0 and CBStools version 3.0. I use a conda environment based on python 2.7.
Here is the error message:
Traceback:
Traceback (most recent call last):
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/pipeline/plugins/linear.py", line 43, in run
node.run(updatehash=updatehash)
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/pipeline/engine/nodes.py", line 407, in run
self._run_interface()
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/pipeline/engine/nodes.py", line 517, in _run_interface
self._result = self._run_command(execute)
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/pipeline/engine/nodes.py", line 650, in _run_command
result = self._interface.run()
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/interfaces/base.py", line 1063, in run
env['DISPLAY'] = config.get_display()
File "/home/raid1/fbeyer/local/miniconda2/envs/nip14/lib/python2.7/site-packages/nipype/utils/config.py", line 286, in get_display
ndisp = int(sysdisplay.split(':')[-1])
ValueError: invalid literal for int() with base 10: '0.0'
When running the MIPAV command separately it works fine.
Do you have an idea what could be the reason for this?
Best
Frauke | 0.0 | [
"nipype/utils/tests/test_config.py::test_display_parse[localhost:12]",
"nipype/utils/tests/test_config.py::test_display_parse[localhost:12.1]"
] | [
"nipype/utils/tests/test_config.py::test_display_parse[:12]",
"nipype/utils/tests/test_config.py::test_display_config[0]",
"nipype/utils/tests/test_config.py::test_display_config[1]",
"nipype/utils/tests/test_config.py::test_display_config[2]",
"nipype/utils/tests/test_config.py::test_display_config[3]",
"nipype/utils/tests/test_config.py::test_display_config[4]",
"nipype/utils/tests/test_config.py::test_display_system[0]",
"nipype/utils/tests/test_config.py::test_display_system[1]",
"nipype/utils/tests/test_config.py::test_display_system[2]",
"nipype/utils/tests/test_config.py::test_display_system[3]",
"nipype/utils/tests/test_config.py::test_display_system[4]",
"nipype/utils/tests/test_config.py::test_display_config_and_system",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_patched",
"nipype/utils/tests/test_config.py::test_display_empty_patched",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_patched_oldxvfbwrapper",
"nipype/utils/tests/test_config.py::test_display_empty_patched_oldxvfbwrapper",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_notinstalled",
"nipype/utils/tests/test_config.py::test_display_empty_notinstalled",
"nipype/utils/tests/test_config.py::test_display_empty_macosx",
"nipype/utils/tests/test_config.py::test_cwd_cached"
] | 2018-01-09 22:24:45+00:00 | 4,196 |
|
nipy__nipype-2375 | diff --git a/CHANGES b/CHANGES
index fa1716688..8dcca2ba6 100644
--- a/CHANGES
+++ b/CHANGES
@@ -1,6 +1,7 @@
Upcoming release (0.14.1)
=========================
+* FIX: Errors parsing ``$DISPLAY`` (https://github.com/nipy/nipype/pull/2363)
* FIX: MultiProc starting workers at dubious wd (https://github.com/nipy/nipype/pull/2368)
* REF+FIX: Move BIDSDataGrabber to `interfaces.io` + fix correct default behavior (https://github.com/nipy/nipype/pull/2336)
* ENH: Add AFNI interface for 3dConvertDset (https://github.com/nipy/nipype/pull/2337)
diff --git a/nipype/interfaces/spm/preprocess.py b/nipype/interfaces/spm/preprocess.py
index 7dd47ef46..47c9fd77a 100644
--- a/nipype/interfaces/spm/preprocess.py
+++ b/nipype/interfaces/spm/preprocess.py
@@ -125,8 +125,7 @@ class SliceTiming(SPMCommand):
class RealignInputSpec(SPMCommandInputSpec):
in_files = InputMultiPath(
- traits.Either(
- traits.List(ImageFileSPM(exists=True)), ImageFileSPM(exists=True)),
+ ImageFileSPM(exists=True),
field='data',
mandatory=True,
copyfile=True,
diff --git a/nipype/utils/config.py b/nipype/utils/config.py
index 15264b9ed..30b826d23 100644
--- a/nipype/utils/config.py
+++ b/nipype/utils/config.py
@@ -312,11 +312,11 @@ class NipypeConfig(object):
def _mock():
pass
- # Store a fake Xvfb object
- ndisp = int(sysdisplay.split(':')[-1])
+ # Store a fake Xvfb object. Format - <host>:<display>[.<screen>]
+ ndisp = sysdisplay.split(':')[-1].split('.')[0]
Xvfb = namedtuple('Xvfb', ['new_display', 'stop'])
- self._display = Xvfb(ndisp, _mock)
- return sysdisplay
+ self._display = Xvfb(int(ndisp), _mock)
+ return self.get_display()
else:
if 'darwin' in sys.platform:
raise RuntimeError(
@@ -343,8 +343,7 @@ class NipypeConfig(object):
if not hasattr(self._display, 'new_display'):
setattr(self._display, 'new_display',
self._display.vdisplay_num)
-
- return ':%d' % self._display.new_display
+ return self.get_display()
def stop_display(self):
"""Closes the display if started"""
| nipy/nipype | f3b09125eceec7b90ad801176892aab06e012f8c | diff --git a/nipype/utils/tests/test_config.py b/nipype/utils/tests/test_config.py
index 9c322128e..65ada4c64 100644
--- a/nipype/utils/tests/test_config.py
+++ b/nipype/utils/tests/test_config.py
@@ -28,6 +28,17 @@ xvfbpatch_old.Xvfb.return_value = MagicMock(
spec=['vdisplay_num', 'start', 'stop'], vdisplay_num=2010)
[email protected]('dispvar', [':12', 'localhost:12', 'localhost:12.1'])
+def test_display_parse(monkeypatch, dispvar):
+ """Check that when $DISPLAY is defined, the display is correctly parsed"""
+ config._display = None
+ config._config.remove_option('execution', 'display_variable')
+ monkeypatch.setenv('DISPLAY', dispvar)
+ assert config.get_display() == ':12'
+ # Test that it was correctly cached
+ assert config.get_display() == ':12'
+
+
@pytest.mark.parametrize('dispnum', range(5))
def test_display_config(monkeypatch, dispnum):
"""Check that the display_variable option is used ($DISPLAY not set)"""
@@ -46,7 +57,7 @@ def test_display_system(monkeypatch, dispnum):
config._display = None
config._config.remove_option('execution', 'display_variable')
dispstr = ':%d' % dispnum
- monkeypatch.setitem(os.environ, 'DISPLAY', dispstr)
+ monkeypatch.setenv('DISPLAY', dispstr)
assert config.get_display() == dispstr
# Test that it was correctly cached
assert config.get_display() == dispstr
@@ -58,7 +69,7 @@ def test_display_config_and_system(monkeypatch):
config._display = None
dispstr = ':10'
config.set('execution', 'display_variable', dispstr)
- monkeypatch.setitem(os.environ, 'DISPLAY', ':0')
+ monkeypatch.setenv('DISPLAY', ':0')
assert config.get_display() == dispstr
# Test that it was correctly cached
assert config.get_display() == dispstr
@@ -72,10 +83,17 @@ def test_display_noconfig_nosystem_patched(monkeypatch):
config._config.remove_option('execution', 'display_variable')
monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ":2010"
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
+
def test_display_empty_patched(monkeypatch):
"""
@@ -85,12 +103,18 @@ def test_display_empty_patched(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ':2010'
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_noconfig_nosystem_patched_oldxvfbwrapper(monkeypatch):
"""
@@ -102,10 +126,16 @@ def test_display_noconfig_nosystem_patched_oldxvfbwrapper(monkeypatch):
config._config.remove_option('execution', 'display_variable')
monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch_old)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ":2010"
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_empty_patched_oldxvfbwrapper(monkeypatch):
"""
@@ -115,12 +145,18 @@ def test_display_empty_patched_oldxvfbwrapper(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', xvfbpatch_old)
+ monkeypatch.setattr(sys, 'platform', value='linux')
assert config.get_display() == ':2010'
# Test that it was correctly cached
assert config.get_display() == ':2010'
+ # Check that raises in Mac
+ config._display = None
+ monkeypatch.setattr(sys, 'platform', value='darwin')
+ with pytest.raises(RuntimeError):
+ config.get_display()
def test_display_noconfig_nosystem_notinstalled(monkeypatch):
"""
@@ -130,7 +166,7 @@ def test_display_noconfig_nosystem_notinstalled(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
+ monkeypatch.delenv('DISPLAY', raising=False)
monkeypatch.setitem(sys.modules, 'xvfbwrapper', None)
with pytest.raises(RuntimeError):
config.get_display()
@@ -144,13 +180,14 @@ def test_display_empty_notinstalled(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
monkeypatch.setitem(sys.modules, 'xvfbwrapper', None)
with pytest.raises(RuntimeError):
config.get_display()
@pytest.mark.skipif(not has_Xvfb, reason='xvfbwrapper not installed')
[email protected]('darwin' in sys.platform, reason='macosx requires root for Xvfb')
def test_display_noconfig_nosystem_installed(monkeypatch):
"""
Check that actually uses xvfbwrapper when installed (not mocked)
@@ -159,7 +196,7 @@ def test_display_noconfig_nosystem_installed(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', raising=False)
+ monkeypatch.delenv('DISPLAY', raising=False)
newdisp = config.get_display()
assert int(newdisp.split(':')[-1]) > 1000
# Test that it was correctly cached
@@ -167,6 +204,7 @@ def test_display_noconfig_nosystem_installed(monkeypatch):
@pytest.mark.skipif(not has_Xvfb, reason='xvfbwrapper not installed')
[email protected]('darwin' in sys.platform, reason='macosx requires root for Xvfb')
def test_display_empty_installed(monkeypatch):
"""
Check that actually uses xvfbwrapper when installed (not mocked)
@@ -175,7 +213,7 @@ def test_display_empty_installed(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.setitem(os.environ, 'DISPLAY', '')
+ monkeypatch.setenv('DISPLAY', '')
newdisp = config.get_display()
assert int(newdisp.split(':')[-1]) > 1000
# Test that it was correctly cached
@@ -191,7 +229,7 @@ def test_display_empty_macosx(monkeypatch):
config._display = None
if config.has_option('execution', 'display_variable'):
config._config.remove_option('execution', 'display_variable')
- monkeypatch.delitem(os.environ, 'DISPLAY', '')
+ monkeypatch.delenv('DISPLAY', '')
monkeypatch.setattr(sys, 'platform', 'darwin')
with pytest.raises(RuntimeError):
| different error message from SPM interaces
### Summary
#1949 introduced `ImageFileSPM` that gives a nice error message, when input file is `nii.gz`, but `Realign` gives still the old error message " the path '/data/ds000114/sub-03/func/sub-03_task-fingerfootlips_bold.nii.gz' does not exist."
### How to replicate the behavior
Compare these two codes:
```
from nipype.interfaces.spm import Smooth
smooth = Smooth(in_files='/existing/file.nii.gz')
smooth.run()
```
and
```
from nipype.interfaces.spm import Realign
realign = Realign(in_files='/existing/file.nii.gz')
realign.run()
```
### Actual behavior
The first code gives a nice error:
`TraitError: /existing/file.nii.gz is not included in allowed types: .img, .nii, .hdr`
the second gives:
`TraitError: Each element of the 'in_files' trait of a RealignInputSpec instance must be a list of items which are an existing file name or an existing file name, but a value of '/existing/file.nii.gz' <class 'str'> was specified.`
### Expected behavior
Both examples should give the same error.
| 0.0 | [
"nipype/utils/tests/test_config.py::test_display_parse[localhost:12]",
"nipype/utils/tests/test_config.py::test_display_parse[localhost:12.1]"
] | [
"nipype/utils/tests/test_config.py::test_display_parse[:12]",
"nipype/utils/tests/test_config.py::test_display_config[0]",
"nipype/utils/tests/test_config.py::test_display_config[1]",
"nipype/utils/tests/test_config.py::test_display_config[2]",
"nipype/utils/tests/test_config.py::test_display_config[3]",
"nipype/utils/tests/test_config.py::test_display_config[4]",
"nipype/utils/tests/test_config.py::test_display_system[0]",
"nipype/utils/tests/test_config.py::test_display_system[1]",
"nipype/utils/tests/test_config.py::test_display_system[2]",
"nipype/utils/tests/test_config.py::test_display_system[3]",
"nipype/utils/tests/test_config.py::test_display_system[4]",
"nipype/utils/tests/test_config.py::test_display_config_and_system",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_patched",
"nipype/utils/tests/test_config.py::test_display_empty_patched",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_patched_oldxvfbwrapper",
"nipype/utils/tests/test_config.py::test_display_empty_patched_oldxvfbwrapper",
"nipype/utils/tests/test_config.py::test_display_noconfig_nosystem_notinstalled",
"nipype/utils/tests/test_config.py::test_display_empty_notinstalled",
"nipype/utils/tests/test_config.py::test_display_empty_macosx",
"nipype/utils/tests/test_config.py::test_cwd_cached"
] | 2018-01-16 21:55:55+00:00 | 4,197 |
|
nipy__nipype-2409 | diff --git a/nipype/algorithms/icc.py b/nipype/algorithms/icc.py
index 133fa7974..5d5ec1c39 100644
--- a/nipype/algorithms/icc.py
+++ b/nipype/algorithms/icc.py
@@ -80,7 +80,6 @@ class ICC(BaseInterface):
def _list_outputs(self):
outputs = self._outputs().get()
outputs['icc_map'] = os.path.abspath('icc_map.nii')
- outputs['sessions_F_map'] = os.path.abspath('sessions_F_map.nii')
outputs['session_var_map'] = os.path.abspath('session_var_map.nii')
outputs['subject_var_map'] = os.path.abspath('subject_var_map.nii')
return outputs
diff --git a/nipype/interfaces/afni/utils.py b/nipype/interfaces/afni/utils.py
index 188ba158c..8acdf29f9 100644
--- a/nipype/interfaces/afni/utils.py
+++ b/nipype/interfaces/afni/utils.py
@@ -1434,7 +1434,7 @@ class MergeInputSpec(AFNICommandInputSpec):
name_template='%s_merge',
desc='output image file name',
argstr='-prefix %s',
- name_source='in_file')
+ name_source='in_files')
doall = traits.Bool(
desc='apply options to all sub-bricks in dataset', argstr='-doall')
blurfwhm = traits.Int(
@@ -2635,9 +2635,10 @@ class ZcatInputSpec(AFNICommandInputSpec):
mandatory=True,
copyfile=False)
out_file = File(
- name_template='zcat',
+ name_template='%s_zcat',
desc='output dataset prefix name (default \'zcat\')',
- argstr='-prefix %s')
+ argstr='-prefix %s',
+ name_source='in_files')
datum = traits.Enum(
'byte',
'short',
diff --git a/nipype/interfaces/base/traits_extension.py b/nipype/interfaces/base/traits_extension.py
index 5b3e9f94d..6dfef8ebf 100644
--- a/nipype/interfaces/base/traits_extension.py
+++ b/nipype/interfaces/base/traits_extension.py
@@ -217,6 +217,16 @@ class ImageFile(File):
super(ImageFile, self).__init__(value, filter, auto_set, entries,
exists, **metadata)
+ def info(self):
+ existing = 'n existing' if self.exists else ''
+ comma = ',' if self.exists and not self.allow_compressed else ''
+ uncompressed = ' uncompressed' if not self.allow_compressed else ''
+ with_ext = ' (valid extensions: [{}])'.format(
+ ', '.join(self.grab_exts())) if self.types else ''
+ return 'a{existing}{comma}{uncompressed} file{with_ext}'.format(
+ existing=existing, comma=comma, uncompressed=uncompressed,
+ with_ext=with_ext)
+
def grab_exts(self):
# TODO: file type validation
exts = []
@@ -243,11 +253,11 @@ class ImageFile(File):
"""
validated_value = super(ImageFile, self).validate(object, name, value)
if validated_value and self.types:
- self._exts = self.grab_exts()
- if not any(validated_value.endswith(x) for x in self._exts):
+ _exts = self.grab_exts()
+ if not any(validated_value.endswith(x) for x in _exts):
raise TraitError(
args="{} is not included in allowed types: {}".format(
- validated_value, ', '.join(self._exts)))
+ validated_value, ', '.join(_exts)))
return validated_value
@@ -322,15 +332,11 @@ class MultiPath(traits.List):
newvalue = value
+ inner_trait = self.inner_traits()[0]
if not isinstance(value, list) \
- or (self.inner_traits() and
- isinstance(self.inner_traits()[0].trait_type,
- traits.List) and not
- isinstance(self.inner_traits()[0].trait_type,
- InputMultiPath) and
- isinstance(value, list) and
- value and not
- isinstance(value[0], list)):
+ or (isinstance(inner_trait.trait_type, traits.List) and
+ not isinstance(inner_trait.trait_type, InputMultiPath) and
+ not isinstance(value[0], list)):
newvalue = [value]
value = super(MultiPath, self).validate(object, name, newvalue)
diff --git a/nipype/interfaces/spm/preprocess.py b/nipype/interfaces/spm/preprocess.py
index 47c9fd77a..c08cc4686 100644
--- a/nipype/interfaces/spm/preprocess.py
+++ b/nipype/interfaces/spm/preprocess.py
@@ -125,7 +125,8 @@ class SliceTiming(SPMCommand):
class RealignInputSpec(SPMCommandInputSpec):
in_files = InputMultiPath(
- ImageFileSPM(exists=True),
+ traits.Either(ImageFileSPM(exists=True),
+ traits.List(ImageFileSPM(exists=True))),
field='data',
mandatory=True,
copyfile=True,
diff --git a/nipype/pipeline/plugins/base.py b/nipype/pipeline/plugins/base.py
index d7b7d6417..ff84937bc 100644
--- a/nipype/pipeline/plugins/base.py
+++ b/nipype/pipeline/plugins/base.py
@@ -7,13 +7,13 @@ from __future__ import (print_function, division, unicode_literals,
absolute_import)
from builtins import range, object, open
+import sys
from copy import deepcopy
from glob import glob
import os
import shutil
-import sys
from time import sleep, time
-from traceback import format_exc
+from traceback import format_exception
import numpy as np
import scipy.sparse as ssp
@@ -159,7 +159,7 @@ class DistributedPluginBase(PluginBase):
graph,
result={
'result': None,
- 'traceback': format_exc()
+ 'traceback': '\n'.join(format_exception(*sys.exc_info()))
}))
else:
if result:
@@ -244,7 +244,7 @@ class DistributedPluginBase(PluginBase):
mapnodesubids = self.procs[jobid].get_subnodes()
numnodes = len(mapnodesubids)
logger.debug('Adding %d jobs for mapnode %s', numnodes,
- self.procs[jobid]._id)
+ self.procs[jobid])
for i in range(numnodes):
self.mapnodesubids[self.depidx.shape[0] + i] = jobid
self.procs.extend(mapnodesubids)
@@ -274,7 +274,7 @@ class DistributedPluginBase(PluginBase):
slots = None
else:
slots = max(0, self.max_jobs - num_jobs)
- logger.debug('Slots available: %s' % slots)
+ logger.debug('Slots available: %s', slots)
if (num_jobs >= self.max_jobs) or (slots == 0):
break
@@ -303,14 +303,14 @@ class DistributedPluginBase(PluginBase):
self.proc_done[jobid] = True
self.proc_pending[jobid] = True
# Send job to task manager and add to pending tasks
- logger.info('Submitting: %s ID: %d' %
- (self.procs[jobid]._id, jobid))
+ logger.info('Submitting: %s ID: %d',
+ self.procs[jobid], jobid)
if self._status_callback:
self._status_callback(self.procs[jobid], 'start')
if not self._local_hash_check(jobid, graph):
if self.procs[jobid].run_without_submitting:
- logger.debug('Running node %s on master thread' %
+ logger.debug('Running node %s on master thread',
self.procs[jobid])
try:
self.procs[jobid].run()
@@ -327,8 +327,8 @@ class DistributedPluginBase(PluginBase):
self.proc_pending[jobid] = False
else:
self.pending_tasks.insert(0, (tid, jobid))
- logger.info('Finished submitting: %s ID: %d' %
- (self.procs[jobid]._id, jobid))
+ logger.info('Finished submitting: %s ID: %d',
+ self.procs[jobid], jobid)
else:
break
@@ -337,22 +337,38 @@ class DistributedPluginBase(PluginBase):
self.procs[jobid].config['execution']['local_hash_check']):
return False
- cached, updated = self.procs[jobid].is_cached()
+ try:
+ cached, updated = self.procs[jobid].is_cached()
+ except Exception:
+ logger.warning(
+ 'Error while checking node hash, forcing re-run. '
+ 'Although this error may not prevent the workflow from running, '
+ 'it could indicate a major problem. Please report a new issue '
+ 'at https://github.com/nipy/nipype/issues adding the following '
+ 'information:\n\n\tNode: %s\n\tInterface: %s.%s\n\tTraceback:\n%s',
+ self.procs[jobid],
+ self.procs[jobid].interface.__module__,
+ self.procs[jobid].interface.__class__.__name__,
+ '\n'.join(format_exception(*sys.exc_info()))
+ )
+ return False
+
logger.debug('Checking hash "%s" locally: cached=%s, updated=%s.',
- self.procs[jobid].fullname, cached, updated)
+ self.procs[jobid], cached, updated)
overwrite = self.procs[jobid].overwrite
- always_run = self.procs[jobid]._interface.always_run
+ always_run = self.procs[jobid].interface.always_run
if cached and updated and (overwrite is False or
overwrite is None and not always_run):
logger.debug('Skipping cached node %s with ID %s.',
- self.procs[jobid]._id, jobid)
+ self.procs[jobid], jobid)
try:
self._task_finished_cb(jobid, cached=True)
self._remove_node_dirs()
except Exception:
- logger.debug('Error skipping cached node %s (%s).',
- self.procs[jobid]._id, jobid)
+ logger.debug('Error skipping cached node %s (%s).\n\n%s',
+ self.procs[jobid], jobid,
+ '\n'.join(format_exception(*sys.exc_info())))
self._clean_queue(jobid, graph)
self.proc_pending[jobid] = False
return True
@@ -364,7 +380,7 @@ class DistributedPluginBase(PluginBase):
This is called when a job is completed.
"""
logger.info('[Job %d] %s (%s).', jobid, 'Cached'
- if cached else 'Completed', self.procs[jobid].fullname)
+ if cached else 'Completed', self.procs[jobid])
if self._status_callback:
self._status_callback(self.procs[jobid], 'end')
# Update job and worker queues
@@ -481,7 +497,7 @@ class SGELikeBatchManagerBase(DistributedPluginBase):
taskid, timeout, node_dir))
raise IOError(error_message)
except IOError as e:
- result_data['traceback'] = format_exc()
+ result_data['traceback'] = '\n'.join(format_exception(*sys.exc_info()))
else:
results_file = glob(os.path.join(node_dir, 'result_*.pklz'))[0]
result_data = loadpkl(results_file)
| nipy/nipype | db72d88aa16cd03446148162a85921f825515f9b | diff --git a/nipype/interfaces/afni/tests/test_auto_Merge.py b/nipype/interfaces/afni/tests/test_auto_Merge.py
index 02c8f9ca2..7136d04df 100644
--- a/nipype/interfaces/afni/tests/test_auto_Merge.py
+++ b/nipype/interfaces/afni/tests/test_auto_Merge.py
@@ -32,7 +32,7 @@ def test_Merge_inputs():
),
out_file=dict(
argstr='-prefix %s',
- name_source='in_file',
+ name_source='in_files',
name_template='%s_merge',
),
outputtype=dict(),
diff --git a/nipype/interfaces/afni/tests/test_auto_Zcat.py b/nipype/interfaces/afni/tests/test_auto_Zcat.py
index d15deb91f..4a631c6ad 100644
--- a/nipype/interfaces/afni/tests/test_auto_Zcat.py
+++ b/nipype/interfaces/afni/tests/test_auto_Zcat.py
@@ -36,7 +36,8 @@ def test_Zcat_inputs():
),
out_file=dict(
argstr='-prefix %s',
- name_template='zcat',
+ name_source='in_files',
+ name_template='%s_zcat',
),
outputtype=dict(),
terminal_output=dict(
| With NiPype Memory with version 0.12.1 and >=0.13.0
Hi Nipype team,
### Summary
Trying to understand what is causing this difference between versions 0.12.1 and >=0.13.0. Is this with Memory or Realign. I am suspecting that this is the problem. But, I am sure I am missing some important details on this ?
We are experiencing this in package pypreprocess which is reliant on Nipype. See full traceback in https://github.com/neurospin/pypreprocess/issues/288
### Actual behavior
```python
Nipype version:0.13.0
Traceback (most recent call last):
File "report_nipype.py", line 11, in <module>
print(realign)
File "/home/kamalakar/miniconda2/envs/pypreprocess0_13/lib/python2.7/site-packages/nipype/caching/memory.py", line 98, in __repr__
self.base_dir)
ValueError: Single '}' encountered in format string
```
### Expected behavior
```python
Nipype version:0.12.1
PipeFunc(nipype.interfaces.spm.preprocess.Realign, base_dir=/tmp/nipype_mem)
```
### How to replicate the behavior
```python
import nipype
print("Nipype version:{0}".format(nipype.__version__))
import nipype.interfaces.spm as spm
from nipype.caching import Memory
mem = Memory(base_dir='/tmp/')
realign = mem.cache(spm.Realign)
print(realign)
```
Thanks a lot for your time.
| 0.0 | [
"nipype/interfaces/afni/tests/test_auto_Merge.py::test_Merge_inputs",
"nipype/interfaces/afni/tests/test_auto_Zcat.py::test_Zcat_inputs"
] | [
"nipype/interfaces/afni/tests/test_auto_Merge.py::test_Merge_outputs",
"nipype/interfaces/afni/tests/test_auto_Zcat.py::test_Zcat_outputs"
] | 2018-01-26 15:25:08+00:00 | 4,198 |
|
nipy__nipype-2429 | diff --git a/doc/_templates/gse.html b/doc/_templates/gse.html
index 1f0f0557d..25f13feb1 100644
--- a/doc/_templates/gse.html
+++ b/doc/_templates/gse.html
@@ -24,20 +24,17 @@
</style>
<div class="sidebarblock">
- <div id="cse-search-form">Loading</div>
-
- <script src="http://www.google.com/jsapi" type="text/javascript"></script>
- <script type="text/javascript">
- google.load('search', '1', {language : 'en'});
- google.setOnLoadCallback(function() {
- var customSearchControl = new google.search.CustomSearchControl(
- '010960497803984932957:u8pmqf7fdoq');
-
- customSearchControl.setResultSetSize(google.search.Search.FILTERED_CSE_RESULTSET);
- var options = new google.search.DrawOptions();
- options.enableSearchboxOnly("{{pathto('searchresults')}}");
- customSearchControl.draw('cse-search-form', options);
- }, true);
+ <script>
+ (function() {
+ var cx = '010960497803984932957:u8pmqf7fdoq';
+ var gcse = document.createElement('script');
+ gcse.type = 'text/javascript';
+ gcse.async = true;
+ gcse.src = 'https://cse.google.com/cse.js?cx=' + cx;
+ var s = document.getElementsByTagName('script')[0];
+ s.parentNode.insertBefore(gcse, s);
+ })();
</script>
+ <gcse:search></gcse:search>
</div>
{% endblock %}
diff --git a/doc/conf.py b/doc/conf.py
index 9891454e6..144c36f0d 100644
--- a/doc/conf.py
+++ b/doc/conf.py
@@ -73,7 +73,7 @@ master_doc = 'index'
# General information about the project.
project = u'nipype'
-copyright = u'2009-17, Neuroimaging in Python team'
+copyright = u'2009-18, Neuroimaging in Python team'
# The version info for the project you're documenting, acts as replacement for
# |version| and |release|, also used in various other places throughout the
diff --git a/doc/searchresults.rst b/doc/searchresults.rst
index bef389467..d79eaebfb 100644
--- a/doc/searchresults.rst
+++ b/doc/searchresults.rst
@@ -6,168 +6,17 @@ Search results
.. raw:: html
- <div id="cse" style="width: 100%;">Loading</div>
- <script src="http://www.google.com/jsapi" type="text/javascript"></script>
- <script type="text/javascript">
- function parseQueryFromUrl () {
- var queryParamName = "q";
- var search = window.location.search.substr(1);
- var parts = search.split('&');
- for (var i = 0; i < parts.length; i++) {
- var keyvaluepair = parts[i].split('=');
- if (decodeURIComponent(keyvaluepair[0]) == queryParamName) {
- return decodeURIComponent(keyvaluepair[1].replace(/\+/g, ' '));
- }
- }
- return '';
- }
- google.load('search', '1', {language : 'en', style : google.loader.themes.MINIMALIST});
- google.setOnLoadCallback(function() {
- var customSearchControl = new google.search.CustomSearchControl(
- '010960497803984932957:u8pmqf7fdoq');
-
- customSearchControl.setResultSetSize(google.search.Search.FILTERED_CSE_RESULTSET);
- customSearchControl.draw('cse');
- var queryFromUrl = parseQueryFromUrl();
- if (queryFromUrl) {
- customSearchControl.execute(queryFromUrl);
- }
- }, true);
- </script>
- <link rel="stylesheet" href="http://www.google.com/cse/style/look/default.css" type="text/css" /> <style type="text/css">
- .gsc-control-cse {
- font-family: Arial, sans-serif;
- border-color: #FFFFFF;
- background-color: #FFFFFF;
- }
- input.gsc-input {
- border-color: #BCCDF0;
- }
- input.gsc-search-button {
- border-color: #666666;
- background-color: #CECECE;
- }
- .gsc-tabHeader.gsc-tabhInactive {
- border-color: #E9E9E9;
- background-color: #E9E9E9;
- }
- .gsc-tabHeader.gsc-tabhActive {
- border-top-color: #FF9900;
- border-left-color: #E9E9E9;
- border-right-color: #E9E9E9;
- background-color: #FFFFFF;
- }
- .gsc-tabsArea {
- border-color: #E9E9E9;
- }
- .gsc-webResult.gsc-result,
- .gsc-results .gsc-imageResult {
- border-color: #FFFFFF;
- background-color: #FFFFFF;
- }
- .gsc-webResult.gsc-result:hover,
- .gsc-imageResult:hover {
- border-color: #FFFFFF;
- background-color: #FFFFFF;
- }
- .gs-webResult.gs-result a.gs-title:link,
- .gs-webResult.gs-result a.gs-title:link b,
- .gs-imageResult a.gs-title:link,
- .gs-imageResult a.gs-title:link b {
- color: #0000CC;
- }
- .gs-webResult.gs-result a.gs-title:visited,
- .gs-webResult.gs-result a.gs-title:visited b,
- .gs-imageResult a.gs-title:visited,
- .gs-imageResult a.gs-title:visited b {
- color: #0000CC;
- }
- .gs-webResult.gs-result a.gs-title:hover,
- .gs-webResult.gs-result a.gs-title:hover b,
- .gs-imageResult a.gs-title:hover,
- .gs-imageResult a.gs-title:hover b {
- color: #0000CC;
- }
- .gs-webResult.gs-result a.gs-title:active,
- .gs-webResult.gs-result a.gs-title:active b,
- .gs-imageResult a.gs-title:active,
- .gs-imageResult a.gs-title:active b {
- color: #0000CC;
- }
- .gsc-cursor-page {
- color: #0000CC;
- }
- a.gsc-trailing-more-results:link {
- color: #0000CC;
- }
- .gs-webResult .gs-snippet,
- .gs-imageResult .gs-snippet,
- .gs-fileFormatType {
- color: #000000;
- }
- .gs-webResult div.gs-visibleUrl,
- .gs-imageResult div.gs-visibleUrl {
- color: #008000;
- }
- .gs-webResult div.gs-visibleUrl-short {
- color: #008000;
- }
- .gs-webResult div.gs-visibleUrl-short {
- display: none;
- }
- .gs-webResult div.gs-visibleUrl-long {
- display: block;
- }
- .gsc-cursor-box {
- border-color: #FFFFFF;
- }
- .gsc-results .gsc-cursor-box .gsc-cursor-page {
- border-color: #E9E9E9;
- background-color: #FFFFFF;
- color: #0000CC;
- }
- .gsc-results .gsc-cursor-box .gsc-cursor-current-page {
- border-color: #FF9900;
- background-color: #FFFFFF;
- color: #0000CC;
- }
- .gs-promotion {
- border-color: #336699;
- background-color: #FFFFFF;
- }
- .gs-promotion a.gs-title:link,
- .gs-promotion a.gs-title:link *,
- .gs-promotion .gs-snippet a:link {
- color: #0000CC;
- }
- .gs-promotion a.gs-title:visited,
- .gs-promotion a.gs-title:visited *,
- .gs-promotion .gs-snippet a:visited {
- color: #0000CC;
- }
- .gs-promotion a.gs-title:hover,
- .gs-promotion a.gs-title:hover *,
- .gs-promotion .gs-snippet a:hover {
- color: #0000CC;
- }
- .gs-promotion a.gs-title:active,
- .gs-promotion a.gs-title:active *,
- .gs-promotion .gs-snippet a:active {
- color: #0000CC;
- }
- .gs-promotion .gs-snippet,
- .gs-promotion .gs-title .gs-promotion-title-right,
- .gs-promotion .gs-title .gs-promotion-title-right * {
- color: #000000;
- }
- .gs-promotion .gs-visibleUrl,
- .gs-promotion .gs-visibleUrl-short {
- color: #008000;
- }
- /* Manually added - the layout goes wrong without this */
- .gsc-tabsArea,
- .gsc-webResult:after,
- .gsc-resultsHeader {
- clear: none;
- }
- </style>
+ <div id="cse" style="width: 100%;">Loading
+ <script>
+ (function() {
+ var cx = '010960497803984932957:u8pmqf7fdoq';
+ var gcse = document.createElement('script');
+ gcse.type = 'text/javascript';
+ gcse.async = true;
+ gcse.src = 'https://cse.google.com/cse.js?cx=' + cx;
+ var s = document.getElementsByTagName('script')[0];
+ s.parentNode.insertBefore(gcse, s);
+ })();
+ </script>
+ <gcse:search></gcse:search>
+ </div>
diff --git a/nipype/interfaces/mrtrix3/preprocess.py b/nipype/interfaces/mrtrix3/preprocess.py
index ca5996bea..740513194 100644
--- a/nipype/interfaces/mrtrix3/preprocess.py
+++ b/nipype/interfaces/mrtrix3/preprocess.py
@@ -27,7 +27,7 @@ class ResponseSDInputSpec(MRTrix3BaseInputSpec):
'tournier',
'tax',
argstr='%s',
- position=-6,
+ position=1,
mandatory=True,
desc='response estimation algorithm (multi-tissue)')
in_file = File(
@@ -74,7 +74,7 @@ class ResponseSD(MRTrix3Base):
>>> resp.inputs.algorithm = 'tournier'
>>> resp.inputs.grad_fsl = ('bvecs', 'bvals')
>>> resp.cmdline # doctest: +ELLIPSIS
- 'dwi2response -fslgrad bvecs bvals tournier dwi.mif wm.txt'
+ 'dwi2response tournier -fslgrad bvecs bvals dwi.mif wm.txt'
>>> resp.run() # doctest: +SKIP
"""
| nipy/nipype | 168dfee8862e3a635d8fd6f0f4469ad0950e55b9 | diff --git a/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py b/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
index 29a89f097..01104d2d2 100644
--- a/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
+++ b/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
@@ -8,7 +8,7 @@ def test_ResponseSD_inputs():
algorithm=dict(
argstr='%s',
mandatory=True,
- position=-6,
+ position=1,
),
args=dict(argstr='%s', ),
bval_scale=dict(argstr='-bvalue_scaling %s', ),
| Mrtrix3 `dwi2response` - bad algorithm argument position
### Summary
Th Mrtrix3 `dwi2response` CL wrapper generates the following runtime error:
```shell
dwi2response:
mrinfo: [ERROR] no diffusion encoding information found in image "<DWI_FILE>"
dwi2response: [ERROR] Script requires diffusion gradient table: either in image header, or using -grad / -fslgrad option
```
It turns out that the command generated by `nipype` does not respect (my version of) the Mrtrix3 CL format.
### Actual behavior
Generated command (not runnable):
```shell
dwi2response -fslgrad <BVEC_FILE> <BVAL_FILE> -mask <MASK_FILE> tournier <WM_FILE>
```
### Expected behavior
Runnable command:
```shell
dwi2response tournier -fslgrad <BVEC_FILE> <BVAL_FILE> -mask <MASK_FILE> <WM_FILE>
```
### Environment
- `MRtrix 3.0_RC2-117-gf098f097 dwi2response bin version: 3.0_RC2-117-gf098f097`
- `Python 2.7.12`
- `nipype v1.0.0`
### Quick and dirty solution
I'm really not sure how clean it is, but it worked for me; in the `ResponseSDInputSpec` class, I changed `position=-6` to `position=1` in the `algorithm` traits.
| 0.0 | [
"nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py::test_ResponseSD_inputs"
] | [
"nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py::test_ResponseSD_outputs"
] | 2018-02-07 15:08:46+00:00 | 4,199 |
|
nipy__nipype-2432 | diff --git a/.travis.yml b/.travis.yml
index 08d923467..1e2ed0c3d 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -1,55 +1,64 @@
-cache:
- apt: true
+dist: trusty
+sudo: required
language: python
+# our build matrix
python:
- 2.7
- 3.4
- 3.5
- 3.6
+
env:
- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler" CI_SKIP_TEST=1
- INSTALL_DEB_DEPENDECIES=false NIPYPE_EXTRAS="doc,tests,fmri,profiler" CI_SKIP_TEST=1
- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler,duecredit" CI_SKIP_TEST=1
- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler" PIP_FLAGS="--pre" CI_SKIP_TEST=1
+
+addons:
+ apt:
+ packages:
+ - xvfb
+ - fusefat
+ - graphviz
+
+cache:
+ directories:
+ - ${HOME}/.cache
+
before_install:
-- function apt_inst {
- if $INSTALL_DEB_DEPENDECIES; then sudo rm -rf /dev/shm; fi &&
- if $INSTALL_DEB_DEPENDECIES; then sudo ln -s /run/shm /dev/shm; fi &&
- bash <(wget -q -O- http://neuro.debian.net/_files/neurodebian-travis.sh) &&
- sudo apt-get -y update &&
- sudo apt-get -y install xvfb fusefat graphviz &&
- if $INSTALL_DEB_DEPENDECIES; then travis_retry sudo apt-get install -y -qq
- fsl afni elastix fsl-atlases; fi &&
- if $INSTALL_DEB_DEPENDECIES; then
+- if $INSTALL_DEB_DEPENDECIES; then sudo rm -rf /dev/shm; sudo ln -s /run/shm /dev/shm; fi
+- travis_retry bash <(wget -q -O- http://neuro.debian.net/_files/neurodebian-travis.sh);
+- if $INSTALL_DEB_DEPENDECIES; then
+ travis_retry sudo apt-get -y update &&
+ travis_retry sudo apt-get install -y -qq fsl afni elastix fsl-atlases;
+ fi;
+- if $INSTALL_DEB_DEPENDECIES; then
source /etc/fsl/fsl.sh;
source /etc/afni/afni.sh;
- export FSLOUTPUTTYPE=NIFTI_GZ; fi }
-- function conda_inst {
- export CONDA_HOME=$HOME/conda &&
- wget https://repo.continuum.io/miniconda/Miniconda${TRAVIS_PYTHON_VERSION:0:1}-latest-Linux-x86_64.sh
- -O /home/travis/.cache/conda.sh &&
- bash /home/travis/.cache/conda.sh -b -p ${CONDA_HOME} &&
- export PATH=${CONDA_HOME}/bin:$PATH &&
- hash -r &&
- conda config --set always_yes yes --set changeps1 no &&
- conda update -q conda &&
- conda config --add channels conda-forge &&
- conda install python=${TRAVIS_PYTHON_VERSION} &&
- conda install -y icu &&
- pip install -r requirements.txt &&
- pushd $HOME;
- git clone https://github.com/INCF/pybids.git;
- cd pybids;
- pip install -e .;
- popd; }
-# Add install of vtk and mayavi to test mesh (disabled): conda install -y vtk mayavi
-- travis_retry apt_inst
-- travis_retry conda_inst
+ export FSLOUTPUTTYPE=NIFTI_GZ;
+ fi;
+
+# handle python operations separately to reduce timeouts
+- wget https://repo.continuum.io/miniconda/Miniconda${TRAVIS_PYTHON_VERSION:0:1}-latest-Linux-x86_64.sh
+ -O /home/travis/.cache/conda.sh
+- bash ${HOME}/.cache/conda.sh -b -p ${HOME}/conda
+- export PATH=${HOME}/conda/bin:$PATH
+- hash -r
+- conda config --set always_yes yes --set changeps1 no
+- travis_retry conda update -q conda
+- conda config --add channels conda-forge
+- travis_retry conda install -y python=${TRAVIS_PYTHON_VERSION} icu
+- travis_retry pip install -r requirements.txt
+- travis_retry git clone https://github.com/INCF/pybids.git ${HOME}/pybids &&
+ pip install -e ${HOME}/pybids
+
install:
- travis_retry pip install $PIP_FLAGS -e .[$NIPYPE_EXTRAS]
+
script:
- py.test -v --doctest-modules nipype
+
deploy:
provider: pypi
user: satra
diff --git a/nipype/interfaces/c3.py b/nipype/interfaces/c3.py
index 3a3284b32..f4778e7d9 100644
--- a/nipype/interfaces/c3.py
+++ b/nipype/interfaces/c3.py
@@ -10,9 +10,16 @@
"""
from __future__ import (print_function, division, unicode_literals,
absolute_import)
+import os
+from glob import glob
from .base import (CommandLineInputSpec, traits, TraitedSpec, File,
- SEMLikeCommandLine)
+ SEMLikeCommandLine, InputMultiPath, OutputMultiPath,
+ CommandLine, isdefined)
+from ..utils.filemanip import split_filename
+from .. import logging
+
+iflogger = logging.getLogger("interface")
class C3dAffineToolInputSpec(CommandLineInputSpec):
@@ -52,3 +59,150 @@ class C3dAffineTool(SEMLikeCommandLine):
_cmd = 'c3d_affine_tool'
_outputs_filenames = {'itk_transform': 'affine.txt'}
+
+
+class C3dInputSpec(CommandLineInputSpec):
+ in_file = InputMultiPath(
+ File(),
+ position=1,
+ argstr="%s",
+ mandatory=True,
+ desc="Input file (wildcard and multiple are supported).")
+ out_file = File(
+ exists=False,
+ argstr="-o %s",
+ position=-1,
+ xor=["out_files"],
+ desc="Output file of last image on the stack.")
+ out_files = InputMultiPath(
+ File(),
+ argstr="-oo %s",
+ xor=["out_file"],
+ position=-1,
+ desc=("Write all images on the convert3d stack as multiple files."
+ " Supports both list of output files or a pattern for the output"
+ " filenames (using %d substituion)."))
+ pix_type = traits.Enum(
+ "float", "char", "uchar", "short", "ushort", "int", "uint", "double",
+ argstr="-type %s",
+ desc=("Specifies the pixel type for the output image. By default,"
+ " images are written in floating point (float) format"))
+ scale = traits.Either(
+ traits.Int(), traits.Float(),
+ argstr="-scale %s",
+ desc=("Multiplies the intensity of each voxel in the last image on the"
+ " stack by the given factor."))
+ shift = traits.Either(
+ traits.Int(), traits.Float(),
+ argstr="-shift %s",
+ desc='Adds the given constant to every voxel.')
+ interp = traits.Enum(
+ "Linear", "NearestNeighbor", "Cubic", "Sinc", "Gaussian",
+ argstr="-interpolation %s",
+ desc=("Specifies the interpolation used with -resample and other"
+ " commands. Default is Linear."))
+ resample = traits.Str(
+ argstr="-resample %s",
+ desc=("Resamples the image, keeping the bounding box the same, but"
+ " changing the number of voxels in the image. The dimensions can be"
+ " specified as a percentage, for example to double the number of voxels"
+ " in each direction. The -interpolation flag affects how sampling is"
+ " performed."))
+ smooth = traits.Str(
+ argstr="-smooth %s",
+ desc=("Applies Gaussian smoothing to the image. The parameter vector"
+ " specifies the standard deviation of the Gaussian kernel."))
+ multicomp_split = traits.Bool(
+ False,
+ usedefault=True,
+ argstr="-mcr",
+ position=0,
+ desc="Enable reading of multi-component images.")
+ is_4d = traits.Bool(
+ False,
+ usedefault=True,
+ desc=("Changes command to support 4D file operations (default is"
+ " false)."))
+
+
+class C3dOutputSpec(TraitedSpec):
+ out_files = OutputMultiPath(File(exists=False))
+
+
+class C3d(CommandLine):
+ """
+ Convert3d is a command-line tool for converting 3D (or 4D) images between
+ common file formats. The tool also includes a growing list of commands for
+ image manipulation, such as thresholding and resampling. The tool can also
+ be used to obtain information about image files. More information on
+ Convert3d can be found at:
+ https://sourceforge.net/p/c3d/git/ci/master/tree/doc/c3d.md
+
+
+ Example
+ =======
+
+ >>> from nipype.interfaces.c3 import C3d
+ >>> c3 = C3d()
+ >>> c3.inputs.in_file = "T1.nii"
+ >>> c3.inputs.pix_type = "short"
+ >>> c3.inputs.out_file = "T1.img"
+ >>> c3.cmdline
+ 'c3d T1.nii -type short -o T1.img'
+ >>> c3.inputs.is_4d = True
+ >>> c3.inputs.in_file = "epi.nii"
+ >>> c3.inputs.out_file = "epi.img"
+ >>> c3.cmdline
+ 'c4d epi.nii -type short -o epi.img'
+ """
+ input_spec = C3dInputSpec
+ output_spec = C3dOutputSpec
+
+ _cmd = "c3d"
+
+ def __init__(self, **inputs):
+ super(C3d, self).__init__(**inputs)
+ self.inputs.on_trait_change(self._is_4d, "is_4d")
+ if self.inputs.is_4d:
+ self._is_4d()
+
+ def _is_4d(self):
+ self._cmd = "c4d" if self.inputs.is_4d else "c3d"
+
+ def _run_interface(self, runtime):
+ cmd = self._cmd
+ if (not isdefined(self.inputs.out_file)
+ and not isdefined(self.inputs.out_files)):
+ # Convert3d does not want to override file, by default
+ # so we define a new output file
+ self._gen_outfile()
+ runtime = super(C3d, self)._run_interface(runtime)
+ self._cmd = cmd
+ return runtime
+
+ def _gen_outfile(self):
+ # if many infiles, raise exception
+ if (len(self.inputs.in_file) > 1) or ("*" in self.inputs.in_file[0]):
+ raise AttributeError("Multiple in_files found - specify either"
+ " `out_file` or `out_files`.")
+ _, fn, ext = split_filename(self.inputs.in_file[0])
+ self.inputs.out_file = fn + "_generated" + ext
+ # if generated file will overwrite, raise error
+ if os.path.exists(os.path.abspath(self.inputs.out_file)):
+ raise IOError("File already found - to overwrite, use `out_file`.")
+ iflogger.info("Generating `out_file`.")
+
+ def _list_outputs(self):
+ outputs = self.output_spec().get()
+ if isdefined(self.inputs.out_file):
+ outputs["out_files"] = os.path.abspath(self.inputs.out_file)
+ if isdefined(self.inputs.out_files):
+ if len(self.inputs.out_files) == 1:
+ _out_files = glob(os.path.abspath(self.inputs.out_files[0]))
+ else:
+ _out_files = [os.path.abspath(f) for f in self.inputs.out_files
+ if os.path.exists(os.path.abspath(f))]
+ outputs["out_files"] = _out_files
+
+ return outputs
+
diff --git a/nipype/interfaces/mrtrix3/preprocess.py b/nipype/interfaces/mrtrix3/preprocess.py
index ca5996bea..740513194 100644
--- a/nipype/interfaces/mrtrix3/preprocess.py
+++ b/nipype/interfaces/mrtrix3/preprocess.py
@@ -27,7 +27,7 @@ class ResponseSDInputSpec(MRTrix3BaseInputSpec):
'tournier',
'tax',
argstr='%s',
- position=-6,
+ position=1,
mandatory=True,
desc='response estimation algorithm (multi-tissue)')
in_file = File(
@@ -74,7 +74,7 @@ class ResponseSD(MRTrix3Base):
>>> resp.inputs.algorithm = 'tournier'
>>> resp.inputs.grad_fsl = ('bvecs', 'bvals')
>>> resp.cmdline # doctest: +ELLIPSIS
- 'dwi2response -fslgrad bvecs bvals tournier dwi.mif wm.txt'
+ 'dwi2response tournier -fslgrad bvecs bvals dwi.mif wm.txt'
>>> resp.run() # doctest: +SKIP
"""
diff --git a/nipype/pipeline/engine/base.py b/nipype/pipeline/engine/base.py
index 51449632b..0883023f6 100644
--- a/nipype/pipeline/engine/base.py
+++ b/nipype/pipeline/engine/base.py
@@ -84,9 +84,12 @@ class EngineBase(object):
A clone of node or workflow must have a new name
"""
if name == self.name:
- raise ValueError('Cloning requires a new name, "%s" is in use.' % name)
+ raise ValueError('Cloning requires a new name, "%s" is '
+ 'in use.' % name)
clone = deepcopy(self)
clone.name = name
+ if hasattr(clone, '_id'):
+ clone._id = name
return clone
def _check_outputs(self, parameter):
| nipy/nipype | d7a8085d9230c4f43489ba93742ea1f6401f3ede | diff --git a/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py b/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
index 29a89f097..01104d2d2 100644
--- a/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
+++ b/nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py
@@ -8,7 +8,7 @@ def test_ResponseSD_inputs():
algorithm=dict(
argstr='%s',
mandatory=True,
- position=-6,
+ position=1,
),
args=dict(argstr='%s', ),
bval_scale=dict(argstr='-bvalue_scaling %s', ),
diff --git a/nipype/interfaces/tests/test_auto_C3d.py b/nipype/interfaces/tests/test_auto_C3d.py
new file mode 100644
index 000000000..18300e2af
--- /dev/null
+++ b/nipype/interfaces/tests/test_auto_C3d.py
@@ -0,0 +1,61 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from __future__ import unicode_literals
+from ..c3 import C3d
+
+
+def test_C3d_inputs():
+ input_map = dict(
+ args=dict(argstr='%s', ),
+ environ=dict(
+ nohash=True,
+ usedefault=True,
+ ),
+ ignore_exception=dict(
+ deprecated='1.0.0',
+ nohash=True,
+ usedefault=True,
+ ),
+ in_file=dict(
+ argstr='%s',
+ mandatory=True,
+ position=1,
+ ),
+ interp=dict(argstr='-interpolation %s', ),
+ is_4d=dict(usedefault=True, ),
+ multicomp_split=dict(
+ argstr='-mcr',
+ position=0,
+ usedefault=True,
+ ),
+ out_file=dict(
+ argstr='-o %s',
+ position=-1,
+ xor=['out_files'],
+ ),
+ out_files=dict(
+ argstr='-oo %s',
+ position=-1,
+ xor=['out_file'],
+ ),
+ pix_type=dict(argstr='-type %s', ),
+ resample=dict(argstr='-resample %s', ),
+ scale=dict(argstr='-scale %s', ),
+ shift=dict(argstr='-shift %s', ),
+ smooth=dict(argstr='-smooth %s', ),
+ terminal_output=dict(
+ deprecated='1.0.0',
+ nohash=True,
+ ),
+ )
+ inputs = C3d.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+def test_C3d_outputs():
+ output_map = dict(out_files=dict(), )
+ outputs = C3d.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
diff --git a/nipype/pipeline/engine/tests/test_base.py b/nipype/pipeline/engine/tests/test_base.py
index 54356fd6c..fd87aa687 100644
--- a/nipype/pipeline/engine/tests/test_base.py
+++ b/nipype/pipeline/engine/tests/test_base.py
@@ -6,6 +6,8 @@ from __future__ import print_function, unicode_literals
import pytest
from ..base import EngineBase
from ....interfaces import base as nib
+from ....interfaces import utility as niu
+from ... import engine as pe
class InputSpec(nib.TraitedSpec):
@@ -64,3 +66,24 @@ def test_clone():
with pytest.raises(ValueError):
base.clone('nodename')
+
+def test_clone_node_iterables(tmpdir):
+ tmpdir.chdir()
+
+ def addstr(string):
+ return ('%s + 2' % string)
+
+ subject_list = ['sub-001', 'sub-002']
+ inputnode = pe.Node(niu.IdentityInterface(fields=['subject']),
+ name='inputnode')
+ inputnode.iterables = [('subject', subject_list)]
+
+ node_1 = pe.Node(niu.Function(input_names='string',
+ output_names='string',
+ function=addstr), name='node_1')
+ node_2 = node_1.clone('node_2')
+
+ workflow = pe.Workflow(name='iter_clone_wf')
+ workflow.connect([(inputnode, node_1, [('subject', 'string')]),
+ (node_1, node_2, [('string', 'string')])])
+ workflow.run()
| workflow with iterables and cloned nodes fail when expanding iterables
### Summary
When running a workflow which includes a cloned node and iterables the workflow will fail when expanding the iterables because the id of the cloned node will be the same as the original one.
### Actual behavior
Will result in an error:
Traceback (most recent call last):
File "<ipython-input-55-177d6eaeef2c>", line 27, in <module>
workflow.run()
File "/data/eaxfjord/anaconda2/lib/python2.7/site-packages/nipype/pipeline/engine/workflows.py", line 592, in run
execgraph = generate_expanded_graph(deepcopy(flatgraph))
File "/data/eaxfjord/anaconda2/lib/python2.7/site-packages/nipype/pipeline/engine/utils.py", line 1042, in generate_expanded_graph
iterable_prefix, inode.synchronize)
File "/data/eaxfjord/anaconda2/lib/python2.7/site-packages/nipype/pipeline/engine/utils.py", line 733, in _merge_graphs
raise Exception(("Execution graph does not have a unique set of node "
Exception: Execution graph does not have a unique set of node names. Please rerun the workflow
### Expected behavior
Will execute normally without the errors.
### How to replicate the behavior
The following workflow will produce the error.
### Script/Workflow details
```python
from nipype.interfaces import utility as niu
from nipype.pipeline import engine as pe
def addstr(string):
string = ('%s+2' % string )
return string
subject_list = ['sub-001', 'sub-002']
inputnode = pe.Node(niu.IdentityInterface(fields = ['subject']),
name = 'inputnode')
inputnode.iterables = [('subject', subject_list)]
node_1 = pe.Node(niu.Function(input_names='string',
output_names= 'string',
function = addstr),name='node_1')
node_2 = node_1.clone('node_2')
workflow = pe.Workflow(name='my_workflow')
workflow.connect([(inputnode, node_1, [('subject','string')]),
(node_1, node_2, [('string','string')])])
workflow.run()
```
### Platform details:
/data/eaxfjord/anaconda2/lib/python2.7/site-packages/h5py/__init__.py:34: FutureWarning: Conversion of the second argument of issubdtype from `float` to `np.floating` is deprecated. In future, it will be treated as `np.float64 == np.dtype(float).type`.
from ._conv import register_converters as _register_converters
{'nibabel_version': '2.2.1', 'sys_executable': '/data/eaxfjord/anaconda2/bin/python', 'networkx_version': '2.1', 'numpy_version': '1.14.0', 'sys_platform': 'linux2', 'sys_version': '2.7.13 |Anaconda custom (64-bit)| (default, Dec 20 2016, 23:09:15) \n[GCC 4.4.7 20120313 (Red Hat 4.4.7-1)]', 'commit_source': 'installation', 'commit_hash': '0a5948a0', 'pkg_path': '/data/eaxfjord/anaconda2/lib/python2.7/site-packages/nipype', 'nipype_version': '1.0.0', 'traits_version': '4.6.0', 'scipy_version': '1.0.0'}
1.0.0
### Execution environment
- My python environment outside container
| 0.0 | [
"nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py::test_ResponseSD_inputs",
"nipype/interfaces/mrtrix3/tests/test_auto_ResponseSD.py::test_ResponseSD_outputs",
"nipype/interfaces/tests/test_auto_C3d.py::test_C3d_inputs",
"nipype/interfaces/tests/test_auto_C3d.py::test_C3d_outputs",
"nipype/pipeline/engine/tests/test_base.py::test_create[valid1]",
"nipype/pipeline/engine/tests/test_base.py::test_create[valid_node]",
"nipype/pipeline/engine/tests/test_base.py::test_create[valid-node]",
"nipype/pipeline/engine/tests/test_base.py::test_create[ValidNode0]",
"nipype/pipeline/engine/tests/test_base.py::test_create_invalid[invalid*1]",
"nipype/pipeline/engine/tests/test_base.py::test_create_invalid[invalid.1]",
"nipype/pipeline/engine/tests/test_base.py::test_create_invalid[invalid@]",
"nipype/pipeline/engine/tests/test_base.py::test_create_invalid[in/valid]",
"nipype/pipeline/engine/tests/test_base.py::test_create_invalid[None]",
"nipype/pipeline/engine/tests/test_base.py::test_hierarchy",
"nipype/pipeline/engine/tests/test_base.py::test_clone",
"nipype/pipeline/engine/tests/test_base.py::test_clone_node_iterables"
] | [] | 2018-02-08 16:41:43+00:00 | 4,200 |
|
nipy__nipype-2471 | diff --git a/.zenodo.json b/.zenodo.json
index 1058e3b15..29e7f047a 100644
--- a/.zenodo.json
+++ b/.zenodo.json
@@ -558,7 +558,7 @@
"name": "Flandin, Guillaume"
},
{
- "affiliation": "Stereotaxy Core, Brain & Spine Institute",
+ "affiliation": "University College London",
"name": "P\u00e9rez-Garc\u00eda, Fernando",
"orcid": "0000-0001-9090-3024"
},
diff --git a/nipype/interfaces/niftyreg/regutils.py b/nipype/interfaces/niftyreg/regutils.py
index 7c3ed28ea..0910b7d65 100644
--- a/nipype/interfaces/niftyreg/regutils.py
+++ b/nipype/interfaces/niftyreg/regutils.py
@@ -122,7 +122,7 @@ warpfield.nii -res im2_res.nii.gz'
# Need this overload to properly constraint the interpolation type input
def _format_arg(self, name, spec, value):
if name == 'inter_val':
- inter_val = {'NN': 0, 'LIN': 1, 'CUB': 3, 'SINC': 5}
+ inter_val = {'NN': 0, 'LIN': 1, 'CUB': 3, 'SINC': 4}
return spec.argstr % inter_val[value]
else:
return super(RegResample, self)._format_arg(name, spec, value)
@@ -295,6 +295,15 @@ class RegToolsInputSpec(NiftyRegCommandInputSpec):
desc=desc,
argstr='-smoG %f %f %f')
+ # Interpolation type
+ inter_val = traits.Enum(
+ 'NN',
+ 'LIN',
+ 'CUB',
+ 'SINC',
+ desc='Interpolation order to use to warp the floating image',
+ argstr='-interp %d')
+
class RegToolsOutputSpec(TraitedSpec):
""" Output Spec for RegTools. """
@@ -326,6 +335,14 @@ class RegTools(NiftyRegCommand):
output_spec = RegToolsOutputSpec
_suffix = '_tools'
+ # Need this overload to properly constraint the interpolation type input
+ def _format_arg(self, name, spec, value):
+ if name == 'inter_val':
+ inter_val = {'NN': 0, 'LIN': 1, 'CUB': 3, 'SINC': 4}
+ return spec.argstr % inter_val[value]
+ else:
+ return super(RegTools, self)._format_arg(name, spec, value)
+
class RegAverageInputSpec(NiftyRegCommandInputSpec):
""" Input Spec for RegAverage. """
| nipy/nipype | 6ca791d9c5ec0efb9f56cc9e44758d6e53ffb800 | diff --git a/nipype/interfaces/niftyreg/tests/test_auto_RegTools.py b/nipype/interfaces/niftyreg/tests/test_auto_RegTools.py
index 97ea5e6c9..f0f66083c 100644
--- a/nipype/interfaces/niftyreg/tests/test_auto_RegTools.py
+++ b/nipype/interfaces/niftyreg/tests/test_auto_RegTools.py
@@ -24,6 +24,7 @@ def test_RegTools_inputs():
argstr='-in %s',
mandatory=True,
),
+ inter_val=dict(argstr='-interp %d', ),
iso_flag=dict(argstr='-iso', ),
mask_file=dict(argstr='-nan %s', ),
mul_val=dict(argstr='-mul %s', ),
| NiftyReg's RegTools is missing interpolation order argument | 0.0 | [
"nipype/interfaces/niftyreg/tests/test_auto_RegTools.py::test_RegTools_inputs"
] | [
"nipype/interfaces/niftyreg/tests/test_auto_RegTools.py::test_RegTools_outputs"
] | 2018-02-28 18:35:59+00:00 | 4,201 |
|
nipy__nipype-2479 | diff --git a/.travis.yml b/.travis.yml
index d57a4205d..fa5c199a8 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -12,7 +12,7 @@ python:
env:
- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler" CI_SKIP_TEST=1
- INSTALL_DEB_DEPENDECIES=false NIPYPE_EXTRAS="doc,tests,fmri,profiler" CI_SKIP_TEST=1
-- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler,duecredit" CI_SKIP_TEST=1
+- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler,duecredit,ssh" CI_SKIP_TEST=1
- INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,fmri,profiler" PIP_FLAGS="--pre" CI_SKIP_TEST=1
addons:
diff --git a/docker/generate_dockerfiles.sh b/docker/generate_dockerfiles.sh
index 2f0ed5eaa..e1f21b313 100755
--- a/docker/generate_dockerfiles.sh
+++ b/docker/generate_dockerfiles.sh
@@ -103,7 +103,7 @@ function generate_main_dockerfile() {
--arg PYTHON_VERSION_MAJOR=3 PYTHON_VERSION_MINOR=6 BUILD_DATE VCS_REF VERSION \
--miniconda env_name=neuro \
conda_install='python=${PYTHON_VERSION_MAJOR}.${PYTHON_VERSION_MINOR}
- icu=58.1 libxml2 libxslt matplotlib mkl numpy
+ icu=58.1 libxml2 libxslt matplotlib mkl numpy paramiko
pandas psutil scikit-learn scipy traits=4.6.0' \
pip_opts="-e" \
pip_install="/src/nipype[all]" \
diff --git a/nipype/info.py b/nipype/info.py
index 7cee71d6b..6e704cbc2 100644
--- a/nipype/info.py
+++ b/nipype/info.py
@@ -163,7 +163,8 @@ EXTRA_REQUIRES = {
'profiler': ['psutil>=5.0'],
'duecredit': ['duecredit'],
'xvfbwrapper': ['xvfbwrapper'],
- 'pybids': ['pybids']
+ 'pybids': ['pybids'],
+ 'ssh': ['paramiko'],
# 'mesh': ['mayavi'] # Enable when it works
}
diff --git a/nipype/interfaces/io.py b/nipype/interfaces/io.py
index c4fdd7521..ee8b1a28c 100644
--- a/nipype/interfaces/io.py
+++ b/nipype/interfaces/io.py
@@ -31,6 +31,7 @@ import os.path as op
import shutil
import subprocess
import re
+import copy
import tempfile
from os.path import join, dirname
from warnings import warn
@@ -38,7 +39,9 @@ from warnings import warn
import sqlite3
from .. import config, logging
-from ..utils.filemanip import copyfile, list_to_filename, filename_to_list
+from ..utils.filemanip import (
+ copyfile, list_to_filename, filename_to_list,
+ get_related_files, related_filetype_sets)
from ..utils.misc import human_order_sorted, str2bool
from .base import (
TraitedSpec, traits, Str, File, Directory, BaseInterface, InputMultiPath,
@@ -2412,6 +2415,65 @@ class SSHDataGrabber(DataGrabber):
and self.inputs.template[-1] != '$'):
self.inputs.template += '$'
+ def _get_files_over_ssh(self, template):
+ """Get the files matching template over an SSH connection."""
+ # Connect over SSH
+ client = self._get_ssh_client()
+ sftp = client.open_sftp()
+ sftp.chdir(self.inputs.base_directory)
+
+ # Get all files in the dir, and filter for desired files
+ template_dir = os.path.dirname(template)
+ template_base = os.path.basename(template)
+ every_file_in_dir = sftp.listdir(template_dir)
+ if self.inputs.template_expression == 'fnmatch':
+ outfiles = fnmatch.filter(every_file_in_dir, template_base)
+ elif self.inputs.template_expression == 'regexp':
+ regexp = re.compile(template_base)
+ outfiles = list(filter(regexp.match, every_file_in_dir))
+ else:
+ raise ValueError('template_expression value invalid')
+
+ if len(outfiles) == 0:
+ # no files
+ msg = 'Output template: %s returned no files' % template
+ if self.inputs.raise_on_empty:
+ raise IOError(msg)
+ else:
+ warn(msg)
+
+ # return value
+ outfiles = None
+
+ else:
+ # found files, sort and save to outputs
+ if self.inputs.sort_filelist:
+ outfiles = human_order_sorted(outfiles)
+
+ # actually download the files, if desired
+ if self.inputs.download_files:
+ files_to_download = copy.copy(outfiles) # make sure new list!
+
+ # check to see if there are any related files to download
+ for file_to_download in files_to_download:
+ related_to_current = get_related_files(
+ file_to_download, include_this_file=False)
+ existing_related_not_downloading = [
+ f for f in related_to_current
+ if f in every_file_in_dir and f not in files_to_download]
+ files_to_download.extend(existing_related_not_downloading)
+
+ for f in files_to_download:
+ try:
+ sftp.get(os.path.join(template_dir, f), f)
+ except IOError:
+ iflogger.info('remote file %s not found' % f)
+
+ # return value
+ outfiles = list_to_filename(outfiles)
+
+ return outfiles
+
def _list_outputs(self):
try:
paramiko
@@ -2439,32 +2501,10 @@ class SSHDataGrabber(DataGrabber):
isdefined(self.inputs.field_template) and \
key in self.inputs.field_template:
template = self.inputs.field_template[key]
+
if not args:
- client = self._get_ssh_client()
- sftp = client.open_sftp()
- sftp.chdir(self.inputs.base_directory)
- filelist = sftp.listdir()
- if self.inputs.template_expression == 'fnmatch':
- filelist = fnmatch.filter(filelist, template)
- elif self.inputs.template_expression == 'regexp':
- regexp = re.compile(template)
- filelist = list(filter(regexp.match, filelist))
- else:
- raise ValueError('template_expression value invalid')
- if len(filelist) == 0:
- msg = 'Output key: %s Template: %s returned no files' % (
- key, template)
- if self.inputs.raise_on_empty:
- raise IOError(msg)
- else:
- warn(msg)
- else:
- if self.inputs.sort_filelist:
- filelist = human_order_sorted(filelist)
- outputs[key] = list_to_filename(filelist)
- if self.inputs.download_files:
- for f in filelist:
- sftp.get(f, f)
+ outputs[key] = self._get_files_over_ssh(template)
+
for argnum, arglist in enumerate(args):
maxlen = 1
for arg in arglist:
@@ -2498,44 +2538,18 @@ class SSHDataGrabber(DataGrabber):
e.message +
": Template %s failed to convert with args %s"
% (template, str(tuple(argtuple))))
- client = self._get_ssh_client()
- sftp = client.open_sftp()
- sftp.chdir(self.inputs.base_directory)
- filledtemplate_dir = os.path.dirname(filledtemplate)
- filledtemplate_base = os.path.basename(filledtemplate)
- filelist = sftp.listdir(filledtemplate_dir)
- if self.inputs.template_expression == 'fnmatch':
- outfiles = fnmatch.filter(filelist,
- filledtemplate_base)
- elif self.inputs.template_expression == 'regexp':
- regexp = re.compile(filledtemplate_base)
- outfiles = list(filter(regexp.match, filelist))
- else:
- raise ValueError('template_expression value invalid')
- if len(outfiles) == 0:
- msg = 'Output key: %s Template: %s returned no files' % (
- key, filledtemplate)
- if self.inputs.raise_on_empty:
- raise IOError(msg)
- else:
- warn(msg)
- outputs[key].append(None)
- else:
- if self.inputs.sort_filelist:
- outfiles = human_order_sorted(outfiles)
- outputs[key].append(list_to_filename(outfiles))
- if self.inputs.download_files:
- for f in outfiles:
- try:
- sftp.get(
- os.path.join(filledtemplate_dir, f), f)
- except IOError:
- iflogger.info('remote file %s not found',
- f)
+
+ outputs[key].append(self._get_files_over_ssh(filledtemplate))
+
+ # disclude where there was any invalid matches
if any([val is None for val in outputs[key]]):
outputs[key] = []
+
+ # no outputs is None, not empty list
if len(outputs[key]) == 0:
outputs[key] = None
+
+ # one output is the item, not a list
elif len(outputs[key]) == 1:
outputs[key] = outputs[key][0]
diff --git a/nipype/pipeline/engine/utils.py b/nipype/pipeline/engine/utils.py
index 2b6bb6ed3..301a35844 100644
--- a/nipype/pipeline/engine/utils.py
+++ b/nipype/pipeline/engine/utils.py
@@ -1050,7 +1050,17 @@ def generate_expanded_graph(graph_in):
expansions = defaultdict(list)
for node in graph_in.nodes():
for src_id in list(old_edge_dict.keys()):
- if node.itername.startswith(src_id):
+ # Drop the original JoinNodes; only concerned with
+ # generated Nodes
+ if hasattr(node, 'joinfield'):
+ continue
+ # Patterns:
+ # - src_id : Non-iterable node
+ # - src_id.[a-z]\d+ : IdentityInterface w/ iterables
+ # - src_id.[a-z]I.[a-z]\d+ : Non-IdentityInterface w/ iterables
+ # - src_idJ\d+ : JoinNode(IdentityInterface)
+ if re.match(src_id + r'((\.[a-z](I\.[a-z])?|J)\d+)?$',
+ node.itername):
expansions[src_id].append(node)
for in_id, in_nodes in list(expansions.items()):
logger.debug("The join node %s input %s was expanded"
| nipy/nipype | e6792158568a51f0e6cdef77c6ca12ab6266a7dd | diff --git a/nipype/interfaces/tests/test_io.py b/nipype/interfaces/tests/test_io.py
index 6aafb5b6b..1eea2b31f 100644
--- a/nipype/interfaces/tests/test_io.py
+++ b/nipype/interfaces/tests/test_io.py
@@ -5,6 +5,7 @@ from __future__ import print_function, unicode_literals
from builtins import str, zip, range, open
from future import standard_library
import os
+import copy
import simplejson
import glob
import shutil
@@ -37,6 +38,32 @@ try:
except ImportError:
noboto3 = True
+# Check for paramiko
+try:
+ import paramiko
+ no_paramiko = False
+
+ # Check for localhost SSH Server
+ # FIXME: Tests requiring this are never run on CI
+ try:
+ proxy = None
+ client = paramiko.SSHClient()
+ client.load_system_host_keys()
+ client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
+ client.connect('127.0.0.1', username=os.getenv('USER'), sock=proxy,
+ timeout=10)
+
+ no_local_ssh = False
+
+ except (paramiko.SSHException,
+ paramiko.ssh_exception.NoValidConnectionsError,
+ OSError):
+ no_local_ssh = True
+
+except ImportError:
+ no_paramiko = True
+ no_local_ssh = True
+
# Check for fakes3
standard_library.install_aliases()
from subprocess import check_call, CalledProcessError
@@ -316,7 +343,7 @@ def test_datasink_to_s3(dummy_input, tmpdir):
aws_access_key_id='mykey',
aws_secret_access_key='mysecret',
service_name='s3',
- endpoint_url='http://localhost:4567',
+ endpoint_url='http://127.0.0.1:4567',
use_ssl=False)
resource.meta.client.meta.events.unregister('before-sign.s3', fix_s3_host)
@@ -611,3 +638,52 @@ def test_bids_infields_outfields(tmpdir):
bg = nio.BIDSDataGrabber()
for outfield in ['anat', 'func']:
assert outfield in bg._outputs().traits()
+
+
[email protected](no_paramiko, reason="paramiko library is not available")
[email protected](no_local_ssh, reason="SSH Server is not running")
+def test_SSHDataGrabber(tmpdir):
+ """Test SSHDataGrabber by connecting to localhost and collecting some data.
+ """
+ old_cwd = tmpdir.chdir()
+
+ source_dir = tmpdir.mkdir('source')
+ source_hdr = source_dir.join('somedata.hdr')
+ source_dat = source_dir.join('somedata.img')
+ source_hdr.ensure() # create
+ source_dat.ensure() # create
+
+ # ssh client that connects to localhost, current user, regardless of
+ # ~/.ssh/config
+ def _mock_get_ssh_client(self):
+ proxy = None
+ client = paramiko.SSHClient()
+ client.load_system_host_keys()
+ client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
+ client.connect('127.0.0.1', username=os.getenv('USER'), sock=proxy,
+ timeout=10)
+ return client
+ MockSSHDataGrabber = copy.copy(nio.SSHDataGrabber)
+ MockSSHDataGrabber._get_ssh_client = _mock_get_ssh_client
+
+ # grabber to get files from source_dir matching test.hdr
+ ssh_grabber = MockSSHDataGrabber(infields=['test'],
+ outfields=['test_file'])
+ ssh_grabber.inputs.base_directory = str(source_dir)
+ ssh_grabber.inputs.hostname = '127.0.0.1'
+ ssh_grabber.inputs.field_template = dict(test_file='%s.hdr')
+ ssh_grabber.inputs.template = ''
+ ssh_grabber.inputs.template_args = dict(test_file=[['test']])
+ ssh_grabber.inputs.test = 'somedata'
+ ssh_grabber.inputs.sort_filelist = True
+
+ runtime = ssh_grabber.run()
+
+ # did we successfully get the header?
+ assert runtime.outputs.test_file == str(tmpdir.join(source_hdr.basename))
+ # did we successfully get the data?
+ assert (tmpdir.join(source_hdr.basename) # header file
+ .new(ext='.img') # data file
+ .check(file=True, exists=True)) # exists?
+
+ old_cwd.chdir()
diff --git a/nipype/pipeline/engine/tests/test_join.py b/nipype/pipeline/engine/tests/test_join.py
index 436d29d9e..54ff15048 100644
--- a/nipype/pipeline/engine/tests/test_join.py
+++ b/nipype/pipeline/engine/tests/test_join.py
@@ -7,11 +7,9 @@ from __future__ import (print_function, division, unicode_literals,
absolute_import)
from builtins import open
-import os
-
from ... import engine as pe
from ....interfaces import base as nib
-from ....interfaces.utility import IdentityInterface
+from ....interfaces.utility import IdentityInterface, Function, Merge
from ....interfaces.base import traits, File
@@ -612,3 +610,20 @@ def test_nested_workflow_join(tmpdir):
# there should be six nodes in total
assert len(result.nodes()) == 6, \
"The number of expanded nodes is incorrect."
+
+
+def test_name_prefix_join(tmpdir):
+ tmpdir.chdir()
+
+ def sq(x):
+ return x ** 2
+
+ wf = pe.Workflow('wf', base_dir=tmpdir.strpath)
+ square = pe.Node(Function(function=sq), name='square')
+ square.iterables = [('x', [1, 2])]
+ square_join = pe.JoinNode(Merge(1, ravel_inputs=True),
+ name='square_join',
+ joinsource='square',
+ joinfield=['in1'])
+ wf.connect(square, 'out', square_join, "in1")
+ wf.run()
| Issue with node name that starts with another node's name
I think the [line ~801 in util.py](https://github.com/nipy/nipype/edit/master/nipype/pipeline/engine/utils.py#L801) should be something like this:
for node in graph_in.nodes():
for src_id, edge_data in list(old_edge_dict.items()):
if node.itername.startswith(src_id + '.'): # <-- add '.' to src_id
expansions[src_id].append(node)
For example, if the node "input" feeds into "input_files", the "input_files" can be included if you just test for node.itername.startswith(src_id). This change would prevent "input_files" from being included.
Edit: removed last part of my comment. | 0.0 | [
"nipype/pipeline/engine/tests/test_join.py::test_name_prefix_join"
] | [
"nipype/interfaces/tests/test_io.py::test_datagrabber",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args0-inputs_att0-expected0]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args1-inputs_att1-expected1]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args2-inputs_att2-expected2]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args3-inputs_att3-expected3]",
"nipype/interfaces/tests/test_io.py::test_selectfiles[SF_args4-inputs_att4-expected4]",
"nipype/interfaces/tests/test_io.py::test_selectfiles_valueerror",
"nipype/interfaces/tests/test_io.py::test_datagrabber_order",
"nipype/interfaces/tests/test_io.py::test_datasink",
"nipype/interfaces/tests/test_io.py::test_datasink_localcopy",
"nipype/interfaces/tests/test_io.py::test_datasink_substitutions",
"nipype/interfaces/tests/test_io.py::test_datasink_copydir_1",
"nipype/interfaces/tests/test_io.py::test_datasink_copydir_2",
"nipype/interfaces/tests/test_io.py::test_datafinder_depth",
"nipype/interfaces/tests/test_io.py::test_datafinder_unpack",
"nipype/interfaces/tests/test_io.py::test_freesurfersource",
"nipype/interfaces/tests/test_io.py::test_freesurfersource_incorrectdir",
"nipype/interfaces/tests/test_io.py::test_jsonsink_input",
"nipype/interfaces/tests/test_io.py::test_jsonsink[inputs_attributes0]",
"nipype/interfaces/tests/test_io.py::test_jsonsink[inputs_attributes1]",
"nipype/pipeline/engine/tests/test_join.py::test_join_expansion",
"nipype/pipeline/engine/tests/test_join.py::test_node_joinsource",
"nipype/pipeline/engine/tests/test_join.py::test_set_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_unique_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_multiple_join_nodes",
"nipype/pipeline/engine/tests/test_join.py::test_identity_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_multifield_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_synchronize_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_itersource_join_source_node",
"nipype/pipeline/engine/tests/test_join.py::test_itersource_two_join_nodes",
"nipype/pipeline/engine/tests/test_join.py::test_set_join_node_file_input",
"nipype/pipeline/engine/tests/test_join.py::test_nested_workflow_join"
] | 2018-03-01 22:23:30+00:00 | 4,202 |
|
nipy__nipype-2490 | diff --git a/nipype/utils/nipype2boutiques.py b/nipype/utils/nipype2boutiques.py
index 9f228f5c5..21ecbc0ee 100644
--- a/nipype/utils/nipype2boutiques.py
+++ b/nipype/utils/nipype2boutiques.py
@@ -2,7 +2,7 @@
from __future__ import (print_function, division, unicode_literals,
absolute_import)
-from builtins import str, open
+from builtins import str, open, bytes
# This tool exports a Nipype interface in the Boutiques (https://github.com/boutiques) JSON format.
# Boutiques tools can be imported in CBRAIN (https://github.com/aces/cbrain) among other platforms.
#
@@ -40,10 +40,12 @@ def generate_boutiques_descriptor(
raise Exception("Undefined module.")
# Retrieves Nipype interface
- if isinstance(module, str):
+ if isinstance(module, (str, bytes)):
import_module(module)
module_name = str(module)
module = sys.modules[module]
+ else:
+ module_name = str(module.__name__)
interface = getattr(module, interface_name)()
inputs = interface.input_spec()
@@ -249,7 +251,7 @@ def create_tempfile():
Creates a temp file and returns its name.
'''
fileTemp = tempfile.NamedTemporaryFile(delete=False)
- fileTemp.write("hello")
+ fileTemp.write(b"hello")
fileTemp.close()
return fileTemp.name
@@ -283,6 +285,8 @@ def must_generate_value(name, type, ignored_template_inputs, spec_info, spec,
# Best guess to detect string restrictions...
if "' or '" in spec_info:
return False
+ if spec.default or spec.default_value():
+ return False
if not ignored_template_inputs:
return True
return not (name in ignored_template_inputs)
| nipy/nipype | 88dbce1ce5439440bcc14c9aa46666c40f642152 | diff --git a/nipype/utils/tests/test_nipype2boutiques.py b/nipype/utils/tests/test_nipype2boutiques.py
new file mode 100644
index 000000000..f1d0c46ee
--- /dev/null
+++ b/nipype/utils/tests/test_nipype2boutiques.py
@@ -0,0 +1,17 @@
+# -*- coding: utf-8 -*-
+# emacs: -*- mode: python; py-indent-offset: 4; indent-tabs-mode: nil -*-
+# vi: set ft=python sts=4 ts=4 sw=4 et:
+from future import standard_library
+standard_library.install_aliases()
+
+from ..nipype2boutiques import generate_boutiques_descriptor
+
+
+def test_generate():
+ generate_boutiques_descriptor(module='nipype.interfaces.ants.registration',
+ interface_name='ANTS',
+ ignored_template_inputs=(),
+ docker_image=None,
+ docker_index=None,
+ verbose=False,
+ ignore_template_numbers=False)
| UnboundLocalError: local variable 'module_name' referenced before assignment
### Summary
Discovered for myself `nipypecli` and decided to give it a try while composing cmdline invocation just following the errors it was spitting out at me and stopping when error didn't give a hint what I could have specified incorrectly:
```
$> nipypecli convert boutiques -m nipype.interfaces.ants.registration -i ANTS -o test
Traceback (most recent call last):
File "/usr/bin/nipypecli", line 11, in <module>
load_entry_point('nipype==1.0.1', 'console_scripts', 'nipypecli')()
File "/usr/lib/python2.7/dist-packages/click/core.py", line 722, in __call__
return self.main(*args, **kwargs)
File "/usr/lib/python2.7/dist-packages/click/core.py", line 697, in main
rv = self.invoke(ctx)
File "/usr/lib/python2.7/dist-packages/click/core.py", line 1066, in invoke
return _process_result(sub_ctx.command.invoke(sub_ctx))
File "/usr/lib/python2.7/dist-packages/click/core.py", line 1066, in invoke
return _process_result(sub_ctx.command.invoke(sub_ctx))
File "/usr/lib/python2.7/dist-packages/click/core.py", line 895, in invoke
return ctx.invoke(self.callback, **ctx.params)
File "/usr/lib/python2.7/dist-packages/click/core.py", line 535, in invoke
return callback(*args, **kwargs)
File "/usr/lib/python2.7/dist-packages/nipype/scripts/cli.py", line 254, in boutiques
verbose, ignore_template_numbers)
File "/usr/lib/python2.7/dist-packages/nipype/utils/nipype2boutiques.py", line 56, in generate_boutiques_descriptor
'command-line'] = "nipype_cmd " + module_name + " " + interface_name + " "
UnboundLocalError: local variable 'module_name' referenced before assignment
```
| 0.0 | [
"nipype/utils/tests/test_nipype2boutiques.py::test_generate"
] | [] | 2018-03-07 21:23:46+00:00 | 4,203 |
|
nipy__nipype-2503 | diff --git a/doc/users/index.rst b/doc/users/index.rst
index d509191f0..a66d8d7a1 100644
--- a/doc/users/index.rst
+++ b/doc/users/index.rst
@@ -12,9 +12,12 @@
install
neurodocker
-
caching_tutorial
+.. toctree::
+ Nipype tutorials <https://miykael.github.io/nipype_tutorial/>
+ Porcupine graphical interface <https://timvanmourik.github.io/Porcupine/getting-started/>
+
.. toctree::
:maxdepth: 1
diff --git a/nipype/interfaces/afni/__init__.py b/nipype/interfaces/afni/__init__.py
index b28e5961f..925011a19 100644
--- a/nipype/interfaces/afni/__init__.py
+++ b/nipype/interfaces/afni/__init__.py
@@ -18,7 +18,7 @@ from .svm import (SVMTest, SVMTrain)
from .utils import (
ABoverlap, AFNItoNIFTI, Autobox, Axialize, BrickStat, Bucket, Calc, Cat,
CatMatvec, CenterMass, ConvertDset, Copy, Dot, Edge3, Eval, FWHMx,
- MaskTool, Merge, Notes, NwarpApply, NwarpCat, OneDToolPy, Refit, Resample,
- TCat, TCatSubBrick, TStat, To3D, Unifize, Undump, ZCutUp, GCOR, Zcat,
- Zeropad)
+ MaskTool, Merge, Notes, NwarpApply, NwarpAdjust, NwarpCat, OneDToolPy,
+ Refit, Resample, TCat, TCatSubBrick, TStat, To3D, Unifize, Undump, ZCutUp,
+ GCOR, Zcat, Zeropad)
from .model import (Deconvolve, Remlfit, Synthesize)
diff --git a/nipype/interfaces/afni/utils.py b/nipype/interfaces/afni/utils.py
index 6cc187a97..9f306c7b8 100644
--- a/nipype/interfaces/afni/utils.py
+++ b/nipype/interfaces/afni/utils.py
@@ -1511,6 +1511,81 @@ class Notes(CommandLine):
return outputs
+class NwarpAdjustInputSpec(AFNICommandInputSpec):
+ warps = InputMultiPath(
+ File(exists=True),
+ minlen=5,
+ mandatory=True,
+ argstr='-nwarp %s',
+ desc='List of input 3D warp datasets')
+ in_files = InputMultiPath(
+ File(exists=True),
+ minlen=5,
+ argstr='-source %s',
+ desc='List of input 3D datasets to be warped by the adjusted warp '
+ 'datasets. There must be exactly as many of these datasets as '
+ 'there are input warps.')
+ out_file = File(
+ desc='Output mean dataset, only needed if in_files are also given. '
+ 'The output dataset will be on the common grid shared by the '
+ 'source datasets.',
+ argstr='-prefix %s',
+ name_source='in_files',
+ name_template='%s_NwarpAdjust',
+ keep_extension=True,
+ requires=['in_files'])
+
+
+class NwarpAdjust(AFNICommandBase):
+ """This program takes as input a bunch of 3D warps, averages them,
+ and computes the inverse of this average warp. It then composes
+ each input warp with this inverse average to 'adjust' the set of
+ warps. Optionally, it can also read in a set of 1-brick datasets
+ corresponding to the input warps, and warp each of them, and average
+ those.
+
+ For complete details, see the `3dNwarpAdjust Documentation.
+ <https://afni.nimh.nih.gov/pub/dist/doc/program_help/3dNwarpAdjust.html>`_
+
+ Examples
+ ========
+
+ >>> from nipype.interfaces import afni
+ >>> adjust = afni.NwarpAdjust()
+ >>> adjust.inputs.warps = ['func2anat_InverseWarp.nii.gz', 'func2anat_InverseWarp.nii.gz', 'func2anat_InverseWarp.nii.gz', 'func2anat_InverseWarp.nii.gz', 'func2anat_InverseWarp.nii.gz']
+ >>> adjust.cmdline
+ '3dNwarpAdjust -nwarp func2anat_InverseWarp.nii.gz func2anat_InverseWarp.nii.gz func2anat_InverseWarp.nii.gz func2anat_InverseWarp.nii.gz func2anat_InverseWarp.nii.gz'
+ >>> res = adjust.run() # doctest: +SKIP
+
+ """
+ _cmd = '3dNwarpAdjust'
+ input_spec = NwarpAdjustInputSpec
+ output_spec = AFNICommandOutputSpec
+
+ def _parse_inputs(self, skip=None):
+ if not self.inputs.in_files:
+ if skip is None:
+ skip = []
+ skip += ['out_file']
+ return super(NwarpAdjust, self)._parse_inputs(skip=skip)
+
+ def _list_outputs(self):
+ outputs = self.output_spec().get()
+
+ if self.inputs.in_files:
+ if self.inputs.out_file:
+ outputs['out_file'] = os.path.abspath(self.inputs.out_file)
+ else:
+ basename = os.path.basename(self.inputs.in_files[0])
+ basename_noext, ext = op.splitext(basename)
+ if '.gz' in ext:
+ basename_noext, ext2 = op.splitext(basename_noext)
+ ext = ext2 + ext
+ outputs['out_file'] = os.path.abspath(
+ basename_noext + '_NwarpAdjust' + ext)
+ return outputs
+
+
class NwarpApplyInputSpec(CommandLineInputSpec):
in_file = traits.Either(
File(exists=True),
| nipy/nipype | 27b33ef128f761cc7166205fc42ef7c04a8d4e4f | diff --git a/nipype/interfaces/afni/tests/test_auto_NwarpAdjust.py b/nipype/interfaces/afni/tests/test_auto_NwarpAdjust.py
new file mode 100644
index 000000000..cf427dd41
--- /dev/null
+++ b/nipype/interfaces/afni/tests/test_auto_NwarpAdjust.py
@@ -0,0 +1,51 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from __future__ import unicode_literals
+from ..utils import NwarpAdjust
+
+
+def test_NwarpAdjust_inputs():
+ input_map = dict(
+ args=dict(argstr='%s', ),
+ environ=dict(
+ nohash=True,
+ usedefault=True,
+ ),
+ ignore_exception=dict(
+ deprecated='1.0.0',
+ nohash=True,
+ usedefault=True,
+ ),
+ in_files=dict(argstr='-source %s', ),
+ num_threads=dict(
+ nohash=True,
+ usedefault=True,
+ ),
+ out_file=dict(
+ argstr='-prefix %s',
+ keep_extension=True,
+ name_source='in_files',
+ name_template='%s_NwarpAdjust',
+ requires=['in_files'],
+ ),
+ outputtype=dict(),
+ terminal_output=dict(
+ deprecated='1.0.0',
+ nohash=True,
+ ),
+ warps=dict(
+ argstr='-nwarp %s',
+ mandatory=True,
+ ),
+ )
+ inputs = NwarpAdjust.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+def test_NwarpAdjust_outputs():
+ output_map = dict(out_file=dict(), )
+ outputs = NwarpAdjust.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
| add porcupine and nipype tutorial pointers in the user section of the documentation
porcupine: https://timvanmourik.github.io/Porcupine/examples/
tutorial: https://miykael.github.io/nipype_tutorial/
to both quickstart and to user docs. | 0.0 | [
"nipype/interfaces/afni/tests/test_auto_NwarpAdjust.py::test_NwarpAdjust_outputs",
"nipype/interfaces/afni/tests/test_auto_NwarpAdjust.py::test_NwarpAdjust_inputs"
] | [] | 2018-03-22 16:30:05+00:00 | 4,204 |
|
nipy__nipype-2597 | diff --git a/nipype/pipeline/engine/utils.py b/nipype/pipeline/engine/utils.py
index 08d357ff6..0a59aac26 100644
--- a/nipype/pipeline/engine/utils.py
+++ b/nipype/pipeline/engine/utils.py
@@ -1054,12 +1054,14 @@ def generate_expanded_graph(graph_in):
for src_id in list(old_edge_dict.keys()):
# Drop the original JoinNodes; only concerned with
# generated Nodes
- if hasattr(node, 'joinfield'):
+ if hasattr(node, 'joinfield') and node.itername == src_id:
continue
# Patterns:
# - src_id : Non-iterable node
- # - src_id.[a-z]\d+ : IdentityInterface w/ iterables
- # - src_id.[a-z]I.[a-z]\d+ : Non-IdentityInterface w/ iterables
+ # - src_id.[a-z]\d+ :
+ # IdentityInterface w/ iterables or nested JoinNode
+ # - src_id.[a-z]I.[a-z]\d+ :
+ # Non-IdentityInterface w/ iterables
# - src_idJ\d+ : JoinNode(IdentityInterface)
if re.match(src_id + r'((\.[a-z](I\.[a-z])?|J)\d+)?$',
node.itername):
| nipy/nipype | 9eaa2a32c8cb3569633a79d6f7968270453f9aed | diff --git a/nipype/pipeline/engine/tests/test_join.py b/nipype/pipeline/engine/tests/test_join.py
index 54ff15048..77fc0f2fd 100644
--- a/nipype/pipeline/engine/tests/test_join.py
+++ b/nipype/pipeline/engine/tests/test_join.py
@@ -627,3 +627,35 @@ def test_name_prefix_join(tmpdir):
joinfield=['in1'])
wf.connect(square, 'out', square_join, "in1")
wf.run()
+
+
+def test_join_nestediters(tmpdir):
+ tmpdir.chdir()
+
+ def exponent(x, p):
+ return x ** p
+
+ wf = pe.Workflow('wf', base_dir=tmpdir.strpath)
+
+ xs = pe.Node(IdentityInterface(['x']),
+ iterables=[('x', [1, 2])],
+ name='xs')
+ ps = pe.Node(IdentityInterface(['p']),
+ iterables=[('p', [3, 4])],
+ name='ps')
+ exp = pe.Node(Function(function=exponent), name='exp')
+ exp_joinx = pe.JoinNode(Merge(1, ravel_inputs=True),
+ name='exp_joinx',
+ joinsource='xs',
+ joinfield=['in1'])
+ exp_joinp = pe.JoinNode(Merge(1, ravel_inputs=True),
+ name='exp_joinp',
+ joinsource='ps',
+ joinfield=['in1'])
+ wf.connect([
+ (xs, exp, [('x', 'x')]),
+ (ps, exp, [('p', 'p')]),
+ (exp, exp_joinx, [('out', 'in1')]),
+ (exp_joinx, exp_joinp, [('out', 'in1')])])
+
+ wf.run()
| PR #2479 has broken my package
### Summary
PR #2479 has broken my package (https://pypi.org/project/arcana/)
I am not quite sure what the rationale behind the changes are so it is difficult to know how to debug or whether there is something I can change in my package.
### Actual behavior
Workflow exits with error
```
File "/Users/tclose/git/ni/arcana/test/mwe/nipype_pr2479/test.py", line 71, in <module>
study.data('out')
File "/Users/tclose/git/ni/arcana/arcana/study/base.py", line 325, in data
visit_ids=visit_ids)
File "/Users/tclose/git/ni/arcana/arcana/runner/base.py", line 37, in run
return workflow.run(plugin=self._plugin)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/workflows.py", line 595, in run
runner.run(execgraph, updatehash=updatehash, config=self.config)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/plugins/linear.py", line 44, in run
node.run(updatehash=updatehash)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 480, in run
result = self._run_interface(execute=True)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 564, in _run_interface
return self._run_command(execute)
File "/Users/tclose/git/ni/arcana/arcana/node.py", line 59, in _run_command
result = self.nipype_cls._run_command(self, *args, **kwargs)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 888, in _run_command
self._collate_join_field_inputs()
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 898, in _collate_join_field_inputs
val = self._collate_input_value(field)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 928, in _collate_input_value
for idx in range(self._next_slot_index)
File "/Users/tclose/git/ni/nipype/nipype/pipeline/engine/nodes.py", line 947, in _slot_value
field, index, e))
AttributeError: The join node pipeline1.pipeline1_subject_session_outputs does not have a slot field subject_session_pairsJ1 to hold the subject_session_pairs value at index 0: 'DynamicTraitedSpec' object has no attribute 'subject_session_pairsJ1'
```
### Expected behavior
The workflow runs without error
### How to replicate the behavior
See script below
### Script/Workflow details
I have tried to come up with a MWE that doesn't use my package but it was proving difficult. However, you can now install my package with pip
`pip install arcana`
and run the following
```
import os.path
import shutil
from nipype import config
config.enable_debug_mode()
import nipype # @IgnorePep8
from nipype.interfaces.utility import IdentityInterface # @IgnorePep8
from arcana.dataset import DatasetMatch, DatasetSpec # @IgnorePep8
from arcana.data_format import text_format # @IgnorePep8
from arcana.study.base import Study, StudyMetaClass # @IgnorePep8
from arcana.archive.local import LocalArchive # @IgnorePep8
from arcana.runner import LinearRunner # @IgnorePep8
BASE_ARCHIVE_DIR = os.path.join(os.path.dirname(__file__), 'archives')
BASE_WORK_DIR = os.path.join(os.path.dirname(__file__), 'work')
print(nipype.get_info())
print(nipype.__version__)
class TestStudy(Study):
__metaclass__ = StudyMetaClass
add_data_specs = [
DatasetSpec('in', text_format),
DatasetSpec('out', text_format, 'pipeline')]
def pipeline(self, **kwargs):
pipeline = self.create_pipeline(
name='pipeline1',
inputs=[DatasetSpec('in', text_format)],
outputs=[DatasetSpec('out', text_format)],
desc="A dummy pipeline used to test 'run_pipeline' method",
version=1,
citations=[],
**kwargs)
ident = pipeline.create_node(IdentityInterface(['a']),
name="ident")
# Connect inputs
pipeline.connect_input('in', ident, 'a')
# Connect outputs
pipeline.connect_output('out', ident, 'a')
return pipeline
# Create archives
shutil.rmtree(BASE_ARCHIVE_DIR, ignore_errors=True)
shutil.rmtree(BASE_WORK_DIR, ignore_errors=True)
os.makedirs(BASE_ARCHIVE_DIR)
for sess in (['ARCHIVE1', 'SUBJECT', 'VISIT'],
['ARCHIVE2', 'SUBJECT1', 'VISIT1'],
['ARCHIVE2', 'SUBJECT1', 'VISIT2'],
['ARCHIVE2', 'SUBJECT2', 'VISIT1'],
['ARCHIVE2', 'SUBJECT2', 'VISIT2']):
sess_dir = os.path.join(*([BASE_ARCHIVE_DIR] + sess))
os.makedirs(sess_dir)
with open(os.path.join(sess_dir, 'in.txt'), 'w') as f:
f.write('in')
archive1_path = os.path.join(BASE_ARCHIVE_DIR, 'ARCHIVE1')
archive2_path = os.path.join(BASE_ARCHIVE_DIR, 'ARCHIVE2')
work1_path = os.path.join(BASE_WORK_DIR, 'WORK1')
work2_path = os.path.join(BASE_WORK_DIR, 'WORK2')
# Attempt to run with archive with 2 subjects and 2 visits
study = TestStudy('two',
LocalArchive(archive2_path),
LinearRunner(work2_path),
inputs=[DatasetMatch('in', text_format, 'in')])
# Fails here
study2.data('out')
print("Ran study 2")
#
study1 = TestStudy('one',
LocalArchive(archive1_path),
LinearRunner(work1_path),
inputs=[DatasetMatch('in', text_format, 'in')])
study1.data('out')
print("Ran study 1")
```
to reproduce the error
### Platform details:
{'nibabel_version': '2.2.1', 'sys_executable': '/usr/local/opt/python@2/bin/python2.7', 'networkx_version': '1.9', 'numpy_version': '1.14.3', 'sys_platform': 'darwin', 'sys_version': '2.7.15 (default, May 1 2018, 16:44:08) \n[GCC 4.2.1 Compatible Apple LLVM 9.1.0 (clang-902.0.39.1)]', 'commit_source': 'repository', 'commit_hash': '5a96ea54a', 'pkg_path': '/Users/tclose/git/ni/nipype/nipype', 'nipype_version': '1.0.4-dev+g5a96ea54a', 'traits_version': '4.6.0', 'scipy_version': '1.1.0'}
1.0.4-dev+g5a96ea54a
(problem arose in 1.0.1)
### Execution environment
My Homebrew python 2 environment outside container
| 0.0 | [
"nipype/pipeline/engine/tests/test_join.py::test_join_nestediters"
] | [
"nipype/pipeline/engine/tests/test_join.py::test_node_joinsource",
"nipype/pipeline/engine/tests/test_join.py::test_set_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_join_expansion",
"nipype/pipeline/engine/tests/test_join.py::test_identity_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_unique_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_itersource_join_source_node",
"nipype/pipeline/engine/tests/test_join.py::test_multiple_join_nodes",
"nipype/pipeline/engine/tests/test_join.py::test_nested_workflow_join",
"nipype/pipeline/engine/tests/test_join.py::test_multifield_join_node",
"nipype/pipeline/engine/tests/test_join.py::test_set_join_node_file_input",
"nipype/pipeline/engine/tests/test_join.py::test_name_prefix_join",
"nipype/pipeline/engine/tests/test_join.py::test_itersource_two_join_nodes",
"nipype/pipeline/engine/tests/test_join.py::test_synchronize_join_node"
] | 2018-05-25 13:56:19+00:00 | 4,205 |
|
nipy__nipype-2628 | diff --git a/nipype/interfaces/fsl/dti.py b/nipype/interfaces/fsl/dti.py
index f318b5cb1..d8812cec6 100644
--- a/nipype/interfaces/fsl/dti.py
+++ b/nipype/interfaces/fsl/dti.py
@@ -171,7 +171,7 @@ class FSLXCommandInputSpec(FSLCommandInputSpec):
low=0,
value=0,
usedefault=True,
- argstr='--burninnoard=%d',
+ argstr='--burnin_noard=%d',
desc=('num of burnin jumps before the ard is'
' imposed'))
sample_every = traits.Range(
@@ -424,7 +424,7 @@ class BEDPOSTX5(FSLXCommand):
>>> bedp = fsl.BEDPOSTX5(bvecs='bvecs', bvals='bvals', dwi='diffusion.nii',
... mask='mask.nii', n_fibres=1)
>>> bedp.cmdline
- 'bedpostx bedpostx -b 0 --burninnoard=0 --forcedir -n 1 -j 5000 \
+ 'bedpostx bedpostx -b 0 --burnin_noard=0 --forcedir -n 1 -j 5000 \
-s 1 --updateproposalevery=40'
"""
| nipy/nipype | 7ea4f1bd3e99a276ea99423a2534a68556e036c7 | diff --git a/nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py b/nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py
index b105405c7..1ac5db111 100644
--- a/nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py
+++ b/nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py
@@ -15,7 +15,7 @@ def test_BEDPOSTX5_inputs():
usedefault=True,
),
burn_in_no_ard=dict(
- argstr='--burninnoard=%d',
+ argstr='--burnin_noard=%d',
usedefault=True,
),
bvals=dict(mandatory=True, ),
diff --git a/nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py b/nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py
index b215f70bc..fd85eee3b 100644
--- a/nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py
+++ b/nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py
@@ -15,7 +15,7 @@ def test_FSLXCommand_inputs():
usedefault=True,
),
burn_in_no_ard=dict(
- argstr='--burninnoard=%d',
+ argstr='--burnin_noard=%d',
usedefault=True,
),
bvals=dict(
diff --git a/nipype/interfaces/fsl/tests/test_auto_XFibres5.py b/nipype/interfaces/fsl/tests/test_auto_XFibres5.py
index 25da78450..d72bb3bb4 100644
--- a/nipype/interfaces/fsl/tests/test_auto_XFibres5.py
+++ b/nipype/interfaces/fsl/tests/test_auto_XFibres5.py
@@ -15,7 +15,7 @@ def test_XFibres5_inputs():
usedefault=True,
),
burn_in_no_ard=dict(
- argstr='--burninnoard=%d',
+ argstr='--burnin_noard=%d',
usedefault=True,
),
bvals=dict(
| Wrong argstr for fsl:bedpostx
### Summary
File fsl/dti.py, Line 174:
An argument "burninnoard" for bedpostx is set. This argument does not exist, bedpostx crashes.
The correct argument is "burnin_noard".
### Solution
Please change
argstr='--burninnoard=%d',
to
argstr='--burnin_noard=%d', | 0.0 | [
"nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py::test_BEDPOSTX5_inputs",
"nipype/interfaces/fsl/tests/test_auto_XFibres5.py::test_XFibres5_inputs",
"nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py::test_FSLXCommand_inputs"
] | [
"nipype/interfaces/fsl/tests/test_auto_FSLXCommand.py::test_FSLXCommand_outputs",
"nipype/interfaces/fsl/tests/test_auto_XFibres5.py::test_XFibres5_outputs",
"nipype/interfaces/fsl/tests/test_auto_BEDPOSTX5.py::test_BEDPOSTX5_outputs"
] | 2018-07-01 13:55:18+00:00 | 4,206 |
|
nipy__nipype-2754 | diff --git a/nipype/info.py b/nipype/info.py
index 5efb4282b..936c6b63e 100644
--- a/nipype/info.py
+++ b/nipype/info.py
@@ -108,7 +108,7 @@ DATEUTIL_MIN_VERSION = '2.2'
PYTEST_MIN_VERSION = '3.0'
FUTURE_MIN_VERSION = '0.16.0'
SIMPLEJSON_MIN_VERSION = '3.8.0'
-PROV_VERSION = '1.5.0'
+PROV_VERSION = '1.5.2'
CLICK_MIN_VERSION = '6.6.0'
PYDOT_MIN_VERSION = '1.2.3'
@@ -139,7 +139,8 @@ REQUIRES = [
'traits>=%s' % TRAITS_MIN_VERSION,
'future>=%s' % FUTURE_MIN_VERSION,
'simplejson>=%s' % SIMPLEJSON_MIN_VERSION,
- 'prov==%s' % PROV_VERSION,
+ 'prov>=%s' % PROV_VERSION,
+ 'neurdflib',
'click>=%s' % CLICK_MIN_VERSION,
'funcsigs',
'pytest>=%s' % PYTEST_MIN_VERSION,
diff --git a/nipype/interfaces/meshfix.py b/nipype/interfaces/meshfix.py
index 4b9db519a..02a0db403 100644
--- a/nipype/interfaces/meshfix.py
+++ b/nipype/interfaces/meshfix.py
@@ -38,10 +38,10 @@ class MeshFixInputSpec(CommandLineInputSpec):
dont_clean = traits.Bool(argstr='--no-clean', desc="Don't Clean")
save_as_stl = traits.Bool(
- xor=['save_as_vmrl', 'save_as_freesurfer_mesh'],
+ xor=['save_as_vrml', 'save_as_freesurfer_mesh'],
argstr='--stl',
desc="Result is saved in stereolithographic format (.stl)")
- save_as_vmrl = traits.Bool(
+ save_as_vrml = traits.Bool(
argstr='--wrl',
xor=['save_as_stl', 'save_as_freesurfer_mesh'],
desc="Result is saved in VRML1.0 format (.wrl)")
@@ -210,7 +210,7 @@ class MeshFix(CommandLine):
if self.inputs.save_as_stl or self.inputs.output_type == 'stl':
self.inputs.output_type = 'stl'
self.inputs.save_as_stl = True
- if self.inputs.save_as_vmrl or self.inputs.output_type == 'vmrl':
- self.inputs.output_type = 'vmrl'
- self.inputs.save_as_vmrl = True
+ if self.inputs.save_as_vrml or self.inputs.output_type == 'vrml':
+ self.inputs.output_type = 'vrml'
+ self.inputs.save_as_vrml = True
return name + '_fixed.' + self.inputs.output_type
diff --git a/nipype/pipeline/plugins/legacymultiproc.py b/nipype/pipeline/plugins/legacymultiproc.py
index d93e6e77d..dd88a5505 100644
--- a/nipype/pipeline/plugins/legacymultiproc.py
+++ b/nipype/pipeline/plugins/legacymultiproc.py
@@ -11,7 +11,7 @@ from __future__ import (print_function, division, unicode_literals,
# Import packages
import os
-from multiprocessing import Process, Pool, cpu_count, pool
+from multiprocessing import Pool, cpu_count, pool
from traceback import format_exception
import sys
from logging import INFO
@@ -74,23 +74,23 @@ def run_node(node, updatehash, taskid):
return result
-class NonDaemonProcess(Process):
- """A non-daemon process to support internal multiprocessing.
- """
-
- def _get_daemon(self):
- return False
-
- def _set_daemon(self, value):
- pass
-
- daemon = property(_get_daemon, _set_daemon)
-
-
class NonDaemonPool(pool.Pool):
"""A process pool with non-daemon processes.
"""
- Process = NonDaemonProcess
+ def Process(self, *args, **kwds):
+ proc = super(NonDaemonPool, self).Process(*args, **kwds)
+
+ class NonDaemonProcess(proc.__class__):
+ """Monkey-patch process to ensure it is never daemonized"""
+ @property
+ def daemon(self):
+ return False
+
+ @daemon.setter
+ def daemon(self, val):
+ pass
+ proc.__class__ = NonDaemonProcess
+ return proc
class LegacyMultiProcPlugin(DistributedPluginBase):
diff --git a/requirements.txt b/requirements.txt
index 5ef00ec98..1d1a4d1f9 100644
--- a/requirements.txt
+++ b/requirements.txt
@@ -6,7 +6,8 @@ python-dateutil>=2.2
nibabel>=2.1.0
future>=0.16.0
simplejson>=3.8.0
-prov==1.5.0
+prov>=1.5.2
+neurdflib
click>=6.6.0
funcsigs
configparser
| nipy/nipype | 39675df33fadf4459e46b0dcdb78425b853dd22d | diff --git a/nipype/interfaces/tests/test_auto_MeshFix.py b/nipype/interfaces/tests/test_auto_MeshFix.py
index 3cc1541d6..9f50145ba 100644
--- a/nipype/interfaces/tests/test_auto_MeshFix.py
+++ b/nipype/interfaces/tests/test_auto_MeshFix.py
@@ -67,9 +67,9 @@ def test_MeshFix_inputs():
),
save_as_stl=dict(
argstr='--stl',
- xor=['save_as_vmrl', 'save_as_freesurfer_mesh'],
+ xor=['save_as_vrml', 'save_as_freesurfer_mesh'],
),
- save_as_vmrl=dict(
+ save_as_vrml=dict(
argstr='--wrl',
xor=['save_as_stl', 'save_as_freesurfer_mesh'],
),
| Apparent multiprocessing API change in Python 3.7
### Summary
There's a Python 3.7 failure that just began exhibiting on Travis.
```Python
[gw0] [ 99%] FAILED pipeline/plugins/test/test_legacymultiproc_nondaemon.py::test_run_multiproc_nondaemon_true
...
=================================== FAILURES ===================================
______________________ test_run_multiproc_nondaemon_true _______________________
[gw0] linux -- Python 3.7.1 /home/travis/virtualenv/python3.7.1/bin/python
def test_run_multiproc_nondaemon_true():
# with nondaemon_flag = True, the execution should succeed
> result = run_multiproc_nondaemon_with_flag(True)
/home/travis/build/nipy/nipype/nipype/pipeline/plugins/tests/test_legacymultiproc_nondaemon.py:165:
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
/home/travis/build/nipy/nipype/nipype/pipeline/plugins/tests/test_legacymultiproc_nondaemon.py:132: in run_multiproc_nondaemon_with_flag
'non_daemon': nondaemon_flag
/home/travis/build/nipy/nipype/nipype/pipeline/engine/workflows.py:579: in run
runner = plugin_mod(plugin_args=plugin_args)
/home/travis/build/nipy/nipype/nipype/pipeline/plugins/legacymultiproc.py:162: in __init__
initargs=(self._cwd,)
/opt/python/3.7.1/lib/python3.7/multiprocessing/pool.py:177: in __init__
self._repopulate_pool()
/opt/python/3.7.1/lib/python3.7/multiprocessing/pool.py:238: in _repopulate_pool
self._wrap_exception)
/opt/python/3.7.1/lib/python3.7/multiprocessing/pool.py:252: in _repopulate_pool_static
wrap_exception)
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
self = <[AttributeError("'NonDaemonProcess' object has no attribute '_closed'") raised in repr()] NonDaemonProcess object at 0x7fa0ad3d24a8>
group = <multiprocessing.context.ForkContext object at 0x7fa0d7aeda90>
target = <function worker at 0x7fa0af430840>, name = None
args = (<multiprocessing.queues.SimpleQueue object at 0x7fa0ad2afcf8>, <multiprocessing.queues.SimpleQueue object at 0x7fa0ad10d6a0>, <built-in function chdir>, ('/tmp/test_engine_y5eb4eh0',), 10, True)
kwargs = {}
def __init__(self, group=None, target=None, name=None, args=(), kwargs={},
*, daemon=None):
> assert group is None, 'group argument must be None for now'
E AssertionError: group argument must be None for now
/opt/python/3.7.1/lib/python3.7/multiprocessing/process.py:74: AssertionError
```
### Platform details:
```
Python: 3.7
INSTALL_DEB_DEPENDECIES=true NIPYPE_EXTRAS="doc,tests,nipy,profiler" CI_SKIP_TEST=1
```
### Execution environment
Travis
| 0.0 | [
"nipype/interfaces/tests/test_auto_MeshFix.py::test_MeshFix_inputs"
] | [
"nipype/interfaces/tests/test_auto_MeshFix.py::test_MeshFix_outputs"
] | 2018-10-25 16:53:11+00:00 | 4,207 |
|
nipy__nipype-3248 | diff --git a/.zenodo.json b/.zenodo.json
index cb9ffacc3..a8ae4c70e 100644
--- a/.zenodo.json
+++ b/.zenodo.json
@@ -697,6 +697,10 @@
"affiliation": "Sagol School of Neuroscience, Tel Aviv University",
"name": "Baratz, Zvi"
},
+ {
+ "affiliation": "Sagol School of Neuroscience, Tel Aviv University",
+ "name": "Ben-Zvi, Gal"
+ },
{
"name": "Matsubara, K"
},
diff --git a/nipype/interfaces/mrtrix3/preprocess.py b/nipype/interfaces/mrtrix3/preprocess.py
index 9384ef43c..aa3347c7f 100644
--- a/nipype/interfaces/mrtrix3/preprocess.py
+++ b/nipype/interfaces/mrtrix3/preprocess.py
@@ -181,15 +181,17 @@ class DWIBiasCorrectInputSpec(MRTrix3BaseInputSpec):
)
in_mask = File(argstr="-mask %s", desc="input mask image for bias field estimation")
use_ants = traits.Bool(
- argstr="-ants",
+ argstr="ants",
mandatory=True,
desc="use ANTS N4 to estimate the inhomogeneity field",
+ position=0,
xor=["use_fsl"],
)
use_fsl = traits.Bool(
- argstr="-fsl",
+ argstr="fsl",
mandatory=True,
desc="use FSL FAST to estimate the inhomogeneity field",
+ position=0,
xor=["use_ants"],
)
bias = File(argstr="-bias %s", desc="bias field")
@@ -224,14 +226,20 @@ class DWIBiasCorrect(MRTrix3Base):
>>> bias_correct.inputs.in_file = 'dwi.mif'
>>> bias_correct.inputs.use_ants = True
>>> bias_correct.cmdline
- 'dwibiascorrect -ants dwi.mif dwi_biascorr.mif'
+ 'dwibiascorrect ants dwi.mif dwi_biascorr.mif'
>>> bias_correct.run() # doctest: +SKIP
"""
_cmd = "dwibiascorrect"
input_spec = DWIBiasCorrectInputSpec
output_spec = DWIBiasCorrectOutputSpec
-
+ def _format_arg(self, name, trait_spec, value):
+ if name in ("use_ants", "use_fsl"):
+ ver = self.version
+ # Changed in version 3.0, after release candidates
+ if ver is not None and (ver[0] < "3" or ver.startswith("3.0_RC")):
+ return f"-{trait_spec.argstr}"
+ return super()._format_arg(name, trait_spec, value)
class ResponseSDInputSpec(MRTrix3BaseInputSpec):
algorithm = traits.Enum(
| nipy/nipype | 3976524934c6742af65a44ac45c3fa092347f70b | diff --git a/nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py b/nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py
index f67dd5466..0028748ab 100644
--- a/nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py
+++ b/nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py
@@ -58,13 +58,15 @@ def test_DWIBiasCorrect_inputs():
position=-1,
),
use_ants=dict(
- argstr="-ants",
+ argstr="ants",
mandatory=True,
+ position=0,
xor=["use_fsl"],
),
use_fsl=dict(
- argstr="-fsl",
+ argstr="fsl",
mandatory=True,
+ position=0,
xor=["use_ants"],
),
)
| Mrtrix3's dwibiascorrect interface crashes due to "-"
Interface crashes because of "-" before algorithm specification.
```python
RuntimeError: Command:
```
```bash
dwibiascorrect -mask <mask_file> -ants <in_file> <out_file>
Standard output:
Standard error:
Error: argument algorithm: invalid choice: <in_file> (choose from 'ants', 'fsl')
Usage: dwibiascorrect algorithm [ options ] ...
(Run dwibiascorrect -help for more information)
Return code: 1
```
The expected behavior would be to execute the following command:
```bash
dwibiascorrect ants [options] <in_file> <out_file>
```
To replicate the behavior, simply execute:
```python
import nipype.interface.mrtrix3 as mrt
bc = mrt.DWIBiasCorrect()
bc.inputs.in_file = <in_file>
bc.inputs.out_file = <out_file>
bc.inputs.use_ants = True
bc.run()
```
Running on a 64 bit Linux machine (Ubuntu)
```
{'commit_hash': '72945ef34',
'commit_source': 'installation',
'networkx_version': '2.4',
'nibabel_version': '3.0.0',
'nipype_version': '1.5.0',
'numpy_version': '1.19.0',
'pkg_path': '/home/groot/Projects/labbing/venv/lib/python3.7/site-packages/nipype',
'scipy_version': '1.5.0',
'sys_executable': '/home/groot/Projects/labbing/venv/bin/python',
'sys_platform': 'linux',
'sys_version': '3.7.8 (default, Jun 29 2020, 04:26:04) \n[GCC 9.3.0]',
'traits_version': '6.1.1'}
```
- My python environment outside container
| 0.0 | [
"nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py::test_DWIBiasCorrect_inputs"
] | [
"nipype/interfaces/mrtrix3/tests/test_auto_DWIBiasCorrect.py::test_DWIBiasCorrect_outputs"
] | 2020-09-10 16:14:52+00:00 | 4,208 |
|
nipy__nipype-3278 | diff --git a/.zenodo.json b/.zenodo.json
index 6672d5bde..e525f1432 100644
--- a/.zenodo.json
+++ b/.zenodo.json
@@ -788,6 +788,11 @@
{
"name": "Tambini, Arielle"
},
+ {
+ "affiliation": "Weill Cornell Medicine",
+ "name": "Xie, Xihe",
+ "orcid": "0000-0001-6595-2473"
+ },
{
"affiliation": "Max Planck Institute for Human Cognitive and Brain Sciences, Leipzig, Germany.",
"name": "Mihai, Paul Glad",
diff --git a/nipype/interfaces/mrtrix3/__init__.py b/nipype/interfaces/mrtrix3/__init__.py
index f60e83731..53af56ef6 100644
--- a/nipype/interfaces/mrtrix3/__init__.py
+++ b/nipype/interfaces/mrtrix3/__init__.py
@@ -18,6 +18,7 @@ from .preprocess import (
ResponseSD,
ACTPrepareFSL,
ReplaceFSwithFIRST,
+ DWIPreproc,
DWIDenoise,
MRDeGibbs,
DWIBiasCorrect,
diff --git a/nipype/interfaces/mrtrix3/preprocess.py b/nipype/interfaces/mrtrix3/preprocess.py
index aa3347c7f..ef67365f0 100644
--- a/nipype/interfaces/mrtrix3/preprocess.py
+++ b/nipype/interfaces/mrtrix3/preprocess.py
@@ -233,6 +233,7 @@ class DWIBiasCorrect(MRTrix3Base):
_cmd = "dwibiascorrect"
input_spec = DWIBiasCorrectInputSpec
output_spec = DWIBiasCorrectOutputSpec
+
def _format_arg(self, name, trait_spec, value):
if name in ("use_ants", "use_fsl"):
ver = self.version
@@ -241,6 +242,139 @@ class DWIBiasCorrect(MRTrix3Base):
return f"-{trait_spec.argstr}"
return super()._format_arg(name, trait_spec, value)
+
+class DWIPreprocInputSpec(MRTrix3BaseInputSpec):
+ in_file = File(
+ exists=True, argstr="%s", position=0, mandatory=True, desc="input DWI image"
+ )
+ out_file = File(
+ "preproc.mif",
+ argstr="%s",
+ mandatory=True,
+ position=1,
+ usedefault=True,
+ desc="output file after preprocessing",
+ )
+ rpe_options = traits.Enum(
+ "none",
+ "pair",
+ "all",
+ "header",
+ argstr="-rpe_%s",
+ position=2,
+ mandatory=True,
+ desc='Specify acquisition phase-encoding design. "none" for no reversed phase-encoding image, "all" for all DWIs have opposing phase-encoding acquisition, "pair" for using a pair of b0 volumes for inhomogeneity field estimation only, and "header" for phase-encoding information can be found in the image header(s)',
+ )
+ pe_dir = traits.Str(
+ argstr="-pe_dir %s",
+ mandatory=True,
+ desc="Specify the phase encoding direction of the input series, can be a signed axis number (e.g. -0, 1, +2), an axis designator (e.g. RL, PA, IS), or NIfTI axis codes (e.g. i-, j, k)",
+ )
+ ro_time = traits.Float(
+ argstr="-readout_time %f",
+ desc="Total readout time of input series (in seconds)",
+ )
+ in_epi = File(
+ exists=True,
+ argstr="-se_epi %s",
+ desc="Provide an additional image series consisting of spin-echo EPI images, which is to be used exclusively by topup for estimating the inhomogeneity field (i.e. it will not form part of the output image series)",
+ )
+ align_seepi = traits.Bool(
+ argstr="-align_seepi",
+ desc="Achieve alignment between the SE-EPI images used for inhomogeneity field estimation, and the DWIs",
+ )
+ eddy_options = traits.Str(
+ argstr='-eddy_options "%s"',
+ desc="Manually provide additional command-line options to the eddy command",
+ )
+ topup_options = traits.Str(
+ argstr='-topup_options "%s"',
+ desc="Manually provide additional command-line options to the topup command",
+ )
+ export_grad_mrtrix = traits.Bool(
+ argstr="-export_grad_mrtrix", desc="export new gradient files in mrtrix format"
+ )
+ export_grad_fsl = traits.Bool(
+ argstr="-export_grad_fsl", desc="export gradient files in FSL format"
+ )
+ out_grad_mrtrix = File(
+ "grad.b",
+ argstr="%s",
+ usedefault=True,
+ requires=["export_grad_mrtrix"],
+ desc="name of new gradient file",
+ )
+ out_grad_fsl = traits.Tuple(
+ File("grad.bvecs", usedefault=True, desc="bvecs"),
+ File("grad.bvals", usedefault=True, desc="bvals"),
+ argstr="%s, %s",
+ requires=["export_grad_fsl"],
+ desc="Output (bvecs, bvals) gradients FSL format",
+ )
+
+
+class DWIPreprocOutputSpec(TraitedSpec):
+ out_file = File(argstr="%s", desc="output preprocessed image series")
+ out_grad_mrtrix = File(
+ "grad.b",
+ argstr="%s",
+ usedefault=True,
+ desc="preprocessed gradient file in mrtrix3 format",
+ )
+ out_fsl_bvec = File(
+ "grad.bvecs",
+ argstr="%s",
+ usedefault=True,
+ desc="exported fsl gradient bvec file",
+ )
+ out_fsl_bval = File(
+ "grad.bvals",
+ argstr="%s",
+ usedefault=True,
+ desc="exported fsl gradient bval file",
+ )
+
+
+class DWIPreproc(MRTrix3Base):
+ """
+ Perform diffusion image pre-processing using FSL's eddy tool; including inhomogeneity distortion correction using FSL's topup tool if possible
+
+ For more information, see
+ <https://mrtrix.readthedocs.io/en/latest/reference/commands/dwifslpreproc.html>
+
+ Example
+ -------
+
+ >>> import nipype.interfaces.mrtrix3 as mrt
+ >>> preproc = mrt.DWIPreproc()
+ >>> preproc.inputs.in_file = 'dwi.mif'
+ >>> preproc.inputs.rpe_options = 'none'
+ >>> preproc.inputs.out_file = "preproc.mif"
+ >>> preproc.inputs.eddy_options = '--slm=linear --repol' # linear second level model and replace outliers
+ >>> preproc.inputs.export_grad_mrtrix = True # export final gradient table in MRtrix format
+ >>> preproc.inputs.ro_time = 0.165240 # 'TotalReadoutTime' in BIDS JSON metadata files
+ >>> preproc.inputs.pe_dir = 'j' # 'PhaseEncodingDirection' in BIDS JSON metadata files
+ >>> preproc.cmdline
+ 'dwifslpreproc dwi.mif preproc.mif -rpe_none -eddy_options "--slm=linear --repol" -export_grad_mrtrix grad.b -pe_dir j -readout_time 0.165240'
+ >>> preproc.run() # doctest: +SKIP
+ """
+
+ _cmd = "dwifslpreproc"
+ input_spec = DWIPreprocInputSpec
+ output_spec = DWIPreprocOutputSpec
+
+ def _list_outputs(self):
+ outputs = self.output_spec().get()
+ outputs["out_file"] = op.abspath(self.inputs.out_file)
+ if self.inputs.export_grad_mrtrix:
+ outputs["out_grad_mrtrix"] = op.abspath(self.inputs.out_grad_mrtrix)
+ if self.inputs.export_grad_fsl:
+ outputs["out_fsl_bvec"] = op.abspath(self.inputs.out_grad_fsl[0])
+ outputs["out_fsl_bval"] = op.abspath(self.inputs.out_grad_fsl[1])
+
+ return outputs
+
+
class ResponseSDInputSpec(MRTrix3BaseInputSpec):
algorithm = traits.Enum(
"msmt_5tt",
| nipy/nipype | 54f50294064c081f866b10085f6e6486e3fe2620 | diff --git a/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py b/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py
new file mode 100644
index 000000000..76fae6548
--- /dev/null
+++ b/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py
@@ -0,0 +1,54 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from ..preprocess import DWIPreproc
+
+
+def test_DWIPreproc_inputs():
+ input_map = dict(
+ align_seepi=dict(argstr="-align_seepi"),
+ args=dict(argstr="%s"),
+ bval_scale=dict(argstr="-bvalue_scaling %s"),
+ eddy_options=dict(argstr='-eddy_options "%s"'),
+ environ=dict(nohash=True, usedefault=True),
+ export_grad_fsl=dict(argstr="-export_grad_fsl"),
+ export_grad_mrtrix=dict(argstr="-export_grad_mrtrix"),
+ grad_file=dict(argstr="-grad %s", extensions=None, xor=["grad_fsl"]),
+ grad_fsl=dict(argstr="-fslgrad %s %s", xor=["grad_file"]),
+ in_bval=dict(extensions=None),
+ in_bvec=dict(argstr="-fslgrad %s %s", extensions=None),
+ in_epi=dict(argstr="-se_epi %s", extensions=None),
+ in_file=dict(argstr="%s", extensions=None, mandatory=True, position=0),
+ nthreads=dict(argstr="-nthreads %d", nohash=True),
+ out_file=dict(
+ argstr="%s", extensions=None, mandatory=True, position=1, usedefault=True
+ ),
+ out_grad_fsl=dict(argstr="%s, %s", requires=["export_grad_fsl"]),
+ out_grad_mrtrix=dict(
+ argstr="%s",
+ extensions=None,
+ requires=["export_grad_mrtrix"],
+ usedefault=True,
+ ),
+ pe_dir=dict(argstr="-pe_dir %s", mandatory=True),
+ ro_time=dict(argstr="-readout_time %f"),
+ rpe_options=dict(argstr="-rpe_%s", mandatory=True, position=2),
+ topup_options=dict(argstr='-topup_options "%s"'),
+ )
+ inputs = DWIPreproc.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+
+
+def test_DWIPreproc_outputs():
+ output_map = dict(
+ out_file=dict(argstr="%s", extensions=None),
+ out_fsl_bval=dict(argstr="%s", extensions=None, usedefault=True),
+ out_fsl_bvec=dict(argstr="%s", extensions=None, usedefault=True),
+ out_grad_mrtrix=dict(argstr="%s", extensions=None, usedefault=True),
+ )
+ outputs = DWIPreproc.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
| Add `dwifslpreproc` mrtrix3 interface
### Summary
Add the new mrtrix3 `dwifslpreproc` command as an interface. See https://mrtrix.readthedocs.io/en/latest/reference/commands/dwifslpreproc.html
### Actual behavior
no `dwipreproc` or `dwifslpreproc` interface in current release
### Expected behavior
Interface exists under mrtrix3's preprocess module
I'm currently working on a pull request... There are a few other functionalities from mrtrix3 that needs to be updated as interfaces, thinking of submitting this as a hackathon project for the upcoming brainhack global...
| 0.0 | [
"nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py::test_DWIPreproc_inputs",
"nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py::test_DWIPreproc_outputs"
] | [] | 2020-11-30 18:12:57+00:00 | 4,209 |
|
nipy__nipype-3309 | diff --git a/nipype/interfaces/afni/__init__.py b/nipype/interfaces/afni/__init__.py
index d5f2bb436..3629090ac 100644
--- a/nipype/interfaces/afni/__init__.py
+++ b/nipype/interfaces/afni/__init__.py
@@ -29,6 +29,7 @@ from .preprocess import (
LFCD,
Maskave,
Means,
+ NetCorr,
OutlierCount,
QualityIndex,
ROIStats,
diff --git a/nipype/interfaces/afni/preprocess.py b/nipype/interfaces/afni/preprocess.py
index 1d53aac98..ada49fc9a 100644
--- a/nipype/interfaces/afni/preprocess.py
+++ b/nipype/interfaces/afni/preprocess.py
@@ -2556,6 +2556,186 @@ class TCorrMap(AFNICommand):
return super(TCorrMap, self)._format_arg(name, trait_spec, value)
+class NetCorrInputSpec(AFNICommandInputSpec):
+ in_file = File(
+ desc="input time series file (4D data set)",
+ exists=True,
+ argstr="-inset %s",
+ mandatory=True)
+ in_rois = File(
+ desc="input set of ROIs, each labelled with distinct integers",
+ exists=True,
+ argstr="-in_rois %s",
+ mandatory=True)
+ mask = File(
+ desc="can include a whole brain mask within which to "
+ "calculate correlation. Otherwise, data should be "
+ "masked already",
+ exists=True,
+ argstr="-mask %s")
+ weight_ts = File(
+ desc="input a 1D file WTS of weights that will be applied "
+ "multiplicatively to each ROI's average time series. "
+ "WTS can be a column- or row-file of values, but it "
+ "must have the same length as the input time series "
+ "volume. "
+ "If the initial average time series was A[n] for "
+ "n=0,..,(N-1) time points, then applying a set of "
+ "weights W[n] of the same length from WTS would "
+ "produce a new time series: B[n] = A[n] * W[n]",
+ exists=True,
+ argstr="-weight_ts %s")
+ fish_z = traits.Bool(
+ desc="switch to also output a matrix of Fisher Z-transform "
+ "values for the corr coefs (r): "
+ "Z = atanh(r) , "
+ "(with Z=4 being output along matrix diagonals where "
+ "r=1, as the r-to-Z conversion is ceilinged at "
+ "Z = atanh(r=0.999329) = 4, which is still *quite* a "
+ "high Pearson-r value",
+ argstr="-fish_z")
+ part_corr = traits.Bool(
+ desc="output the partial correlation matrix",
+ argstr="-part_corr")
+ ts_out = traits.Bool(
+ desc="switch to output the mean time series of the ROIs that "
+ "have been used to generate the correlation matrices. "
+ "Output filenames mirror those of the correlation "
+ "matrix files, with a '.netts' postfix",
+ argstr="-ts_out")
+ ts_label = traits.Bool(
+ desc="additional switch when using '-ts_out'. Using this "
+ "option will insert the integer ROI label at the start "
+ "of each line of the *.netts file created. Thus, for "
+ "a time series of length N, each line will have N+1 "
+ "numbers, where the first is the integer ROI label "
+ "and the subsequent N are scientific notation values",
+ argstr="-ts_label")
+ ts_indiv = traits.Bool(
+ desc="switch to create a directory for each network that "
+ "contains the average time series for each ROI in "
+ "individual files (each file has one line). "
+ "The directories are labelled PREFIX_000_INDIV/, "
+ "PREFIX_001_INDIV/, etc. (one per network). Within each "
+ "directory, the files are labelled ROI_001.netts, "
+ "ROI_002.netts, etc., with the numbers given by the "
+ "actual ROI integer labels",
+ argstr="-ts_indiv")
+ ts_wb_corr = traits.Bool(
+ desc="switch to create a set of whole brain correlation maps. "
+ "Performs whole brain correlation for each "
+ "ROI's average time series; this will automatically "
+ "create a directory for each network that contains the "
+ "set of whole brain correlation maps (Pearson 'r's). "
+ "The directories are labelled as above for '-ts_indiv' "
+ "Within each directory, the files are labelled "
+ "WB_CORR_ROI_001+orig, WB_CORR_ROI_002+orig, etc., with "
+ "the numbers given by the actual ROI integer labels",
+ argstr="-ts_wb_corr")
+ ts_wb_Z = traits.Bool(
+ desc="same as above in '-ts_wb_corr', except that the maps "
+ "have been Fisher transformed to Z-scores the relation: "
+ "Z=atanh(r). "
+ "To avoid infinities in the transform, Pearson values "
+ "are effectively capped at |r| = 0.999329 (where |Z| = 4.0). "
+ "Files are labelled WB_Z_ROI_001+orig, etc",
+ argstr="-ts_wb_Z")
+ ts_wb_strlabel = traits.Bool(
+ desc="by default, '-ts_wb_{corr,Z}' output files are named "
+ "using the int number of a given ROI, such as: "
+ "WB_Z_ROI_001+orig. "
+ "With this option, one can replace the int (such as '001') "
+ "with the string label (such as 'L-thalamus') "
+ "*if* one has a labeltable attached to the file",
+ argstr="-ts_wb_strlabel")
+ nifti = traits.Bool(
+ desc="output any correlation map files as NIFTI files "
+ "(default is BRIK/HEAD). Only useful if using "
+ "'-ts_wb_corr' and/or '-ts_wb_Z'",
+ argstr="-nifti")
+ output_mask_nonnull = traits.Bool(
+ desc="internally, this program checks for where there are "
+ "nonnull time series, because we don't like those, in "
+ "general. With this flag, the user can output the "
+ "determined mask of non-null time series.",
+ argstr="-output_mask_nonnull")
+ push_thru_many_zeros = traits.Bool(
+ desc="by default, this program will grind to a halt and "
+ "refuse to calculate if any ROI contains >10 percent "
+ "of voxels with null times series (i.e., each point is "
+ "0), as of April, 2017. This is because it seems most "
+ "likely that hidden badness is responsible. However, "
+ "if the user still wants to carry on the calculation "
+ "anyways, then this option will allow one to push on "
+ "through. However, if any ROI *only* has null time "
+ "series, then the program will not calculate and the "
+ "user will really, really, really need to address their masking",
+ argstr="-push_thru_many_zeros")
+ ignore_LT = traits.Bool(
+ desc="switch to ignore any label table labels in the "
+ "'-in_rois' file, if there are any labels attached",
+ argstr="-ignore_LT")
+ out_file = File(
+ desc="output file name part",
+ name_template="%s_netcorr",
+ argstr="-prefix %s",
+ position=1,
+ name_source="in_file",
+ )
+
+class NetCorrOutputSpec(TraitedSpec):
+ out_corr_matrix = File(desc="output correlation matrix between ROIs written to a text file with .netcc suffix")
+ out_corr_maps = traits.List(File(), desc="output correlation maps in Pearson and/or Z-scores")
+
+class NetCorr(AFNICommand):
+ """Calculate correlation matrix of a set of ROIs (using mean time series of
+ each). Several networks may be analyzed simultaneously, one per brick.
+
+ For complete details, see the `3dNetCorr Documentation
+ <https://afni.nimh.nih.gov/pub/dist/doc/program_help/3dNetCorr.html>`_.
+
+ Examples
+ --------
+ >>> from nipype.interfaces import afni
+ >>> ncorr = afni.NetCorr()
+ >>> ncorr.inputs.in_file = 'functional.nii'
+ >>> ncorr.inputs.mask = 'mask.nii'
+ >>> ncorr.inputs.in_rois = 'maps.nii'
+ >>> ncorr.inputs.ts_wb_corr = True
+ >>> ncorr.inputs.ts_wb_Z = True
+ >>> ncorr.inputs.fish_z = True
+ >>> ncorr.inputs.out_file = 'sub0.tp1.ncorr'
+ >>> ncorr.cmdline
+ '3dNetCorr -prefix sub0.tp1.ncorr -fish_z -inset functional.nii -in_rois maps.nii -mask mask.nii -ts_wb_Z -ts_wb_corr'
+ >>> res = ncorr.run() # doctest: +SKIP
+
+ """
+
+ _cmd = "3dNetCorr"
+ input_spec = NetCorrInputSpec
+ output_spec = NetCorrOutputSpec
+
+ def _list_outputs(self):
+ import glob
+
+ outputs = self.output_spec().get()
+
+ if not isdefined(self.inputs.out_file):
+ prefix = self._gen_fname(self.inputs.in_file, suffix="_netcorr")
+ else:
+ prefix = self.inputs.out_file
+
+ # All outputs should be in the same directory as the prefix
+ odir = os.path.dirname(os.path.abspath(prefix))
+ outputs["out_corr_matrix"] = glob.glob(os.path.join(odir, "*.netcc"))[0]
+
+ if isdefined(self.inputs.ts_wb_corr) or isdefined(self.inputs.ts_Z_corr):
+ corrdir = os.path.join(odir, prefix + "_000_INDIV")
+ outputs["out_corr_maps"] = glob.glob(os.path.join(corrdir, "*.nii.gz"))
+
+ return outputs
+
+
class TCorrelateInputSpec(AFNICommandInputSpec):
xset = File(
desc="input xset",
diff --git a/nipype/interfaces/fsl/model.py b/nipype/interfaces/fsl/model.py
index e06377cb4..d7484c0f9 100644
--- a/nipype/interfaces/fsl/model.py
+++ b/nipype/interfaces/fsl/model.py
@@ -1886,7 +1886,7 @@ class SmoothEstimateInputSpec(FSLCommandInputSpec):
class SmoothEstimateOutputSpec(TraitedSpec):
dlh = traits.Float(desc="smoothness estimate sqrt(det(Lambda))")
volume = traits.Int(desc="number of voxels in mask")
- resels = traits.Float(desc="number of resels")
+ resels = traits.Float(desc="volume of resel, in voxels, defined as FWHM_x * FWHM_y * FWHM_z")
class SmoothEstimate(FSLCommand):
diff --git a/nipype/interfaces/fsl/preprocess.py b/nipype/interfaces/fsl/preprocess.py
index f2fbe8c07..54a41be03 100644
--- a/nipype/interfaces/fsl/preprocess.py
+++ b/nipype/interfaces/fsl/preprocess.py
@@ -35,6 +35,7 @@ class BETInputSpec(FSLCommandInputSpec):
argstr="%s",
position=0,
mandatory=True,
+ copyfile=False,
)
out_file = File(
desc="name of output skull stripped image",
@@ -164,15 +165,27 @@ class BET(FSLCommand):
self.raise_exception(runtime)
return runtime
+ def _format_arg(self, name, spec, value):
+ formatted = super(BET, self)._format_arg(name, spec, value)
+ if name == "in_file":
+ # Convert to relative path to prevent BET failure
+ # with long paths.
+ return op.relpath(formatted, start=os.getcwd())
+ return formatted
+
def _gen_outfilename(self):
out_file = self.inputs.out_file
+ # Generate default output filename if non specified.
if not isdefined(out_file) and isdefined(self.inputs.in_file):
out_file = self._gen_fname(self.inputs.in_file, suffix="_brain")
- return os.path.abspath(out_file)
+ # Convert to relative path to prevent BET failure
+ # with long paths.
+ return op.relpath(out_file, start=os.getcwd())
+ return out_file
def _list_outputs(self):
outputs = self.output_spec().get()
- outputs["out_file"] = self._gen_outfilename()
+ outputs["out_file"] = os.path.abspath(self._gen_outfilename())
basename = os.path.basename(outputs["out_file"])
cwd = os.path.dirname(outputs["out_file"])
@@ -1309,10 +1322,7 @@ class FNIRT(FSLCommand):
if key == "out_intensitymap_file" and isdefined(outputs[key]):
basename = FNIRT.intensitymap_file_basename(outputs[key])
- outputs[key] = [
- outputs[key],
- "%s.txt" % basename,
- ]
+ outputs[key] = [outputs[key], "%s.txt" % basename]
return outputs
def _format_arg(self, name, spec, value):
| nipy/nipype | 47fe00b387e205f3f884691a208ef7f20df4119f | diff --git a/nipype/interfaces/afni/tests/test_auto_NetCorr.py b/nipype/interfaces/afni/tests/test_auto_NetCorr.py
new file mode 100644
index 000000000..e613dc13e
--- /dev/null
+++ b/nipype/interfaces/afni/tests/test_auto_NetCorr.py
@@ -0,0 +1,99 @@
+# AUTO-GENERATED by tools/checkspecs.py - DO NOT EDIT
+from ..preprocess import NetCorr
+
+
+def test_NetCorr_inputs():
+ input_map = dict(
+ args=dict(
+ argstr="%s",
+ ),
+ environ=dict(
+ nohash=True,
+ usedefault=True,
+ ),
+ fish_z=dict(
+ argstr="-fish_z",
+ ),
+ ignore_LT=dict(
+ argstr="-ignore_LT",
+ ),
+ in_file=dict(
+ argstr="-inset %s",
+ extensions=None,
+ mandatory=True,
+ ),
+ in_rois=dict(
+ argstr="-in_rois %s",
+ extensions=None,
+ mandatory=True,
+ ),
+ mask=dict(
+ argstr="-mask %s",
+ extensions=None,
+ ),
+ nifti=dict(
+ argstr="-nifti",
+ ),
+ num_threads=dict(
+ nohash=True,
+ usedefault=True,
+ ),
+ out_file=dict(
+ argstr="-prefix %s",
+ extensions=None,
+ name_source="in_file",
+ name_template="%s_netcorr",
+ position=1,
+ ),
+ output_mask_nonnull=dict(
+ argstr="-output_mask_nonnull",
+ ),
+ outputtype=dict(),
+ part_corr=dict(
+ argstr="-part_corr",
+ ),
+ push_thru_many_zeros=dict(
+ argstr="-push_thru_many_zeros",
+ ),
+ ts_indiv=dict(
+ argstr="-ts_indiv",
+ ),
+ ts_label=dict(
+ argstr="-ts_label",
+ ),
+ ts_out=dict(
+ argstr="-ts_out",
+ ),
+ ts_wb_Z=dict(
+ argstr="-ts_wb_Z",
+ ),
+ ts_wb_corr=dict(
+ argstr="-ts_wb_corr",
+ ),
+ ts_wb_strlabel=dict(
+ argstr="-ts_wb_strlabel",
+ ),
+ weight_ts=dict(
+ argstr="-weight_ts %s",
+ extensions=None,
+ ),
+ )
+ inputs = NetCorr.input_spec()
+
+ for key, metadata in list(input_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(inputs.traits()[key], metakey) == value
+
+
+def test_NetCorr_outputs():
+ output_map = dict(
+ out_corr_maps=dict(),
+ out_corr_matrix=dict(
+ extensions=None,
+ ),
+ )
+ outputs = NetCorr.output_spec()
+
+ for key, metadata in list(output_map.items()):
+ for metakey, value in list(metadata.items()):
+ assert getattr(outputs.traits()[key], metakey) == value
diff --git a/nipype/interfaces/fsl/tests/test_preprocess.py b/nipype/interfaces/fsl/tests/test_preprocess.py
index 438f3f0ec..6b1b6cb61 100644
--- a/nipype/interfaces/fsl/tests/test_preprocess.py
+++ b/nipype/interfaces/fsl/tests/test_preprocess.py
@@ -31,6 +31,9 @@ def setup_infile(tmpdir):
@pytest.mark.skipif(no_fsl(), reason="fsl is not installed")
def test_bet(setup_infile):
tmp_infile, tp_dir = setup_infile
+ # BET converts the in_file path to be relative to prevent
+ # failure with long paths.
+ tmp_infile = os.path.relpath(tmp_infile, start=os.getcwd())
better = fsl.BET()
assert better.cmd == "bet"
@@ -41,8 +44,7 @@ def test_bet(setup_infile):
# Test generated outfile name
better.inputs.in_file = tmp_infile
outfile = fsl_name(better, "foo_brain")
- outpath = os.path.join(os.getcwd(), outfile)
- realcmd = "bet %s %s" % (tmp_infile, outpath)
+ realcmd = "bet %s %s" % (tmp_infile, outfile)
assert better.cmdline == realcmd
# Test specified outfile name
outfile = fsl_name(better, "/newdata/bar")
@@ -79,12 +81,11 @@ def test_bet(setup_infile):
# test each of our arguments
better = fsl.BET()
outfile = fsl_name(better, "foo_brain")
- outpath = os.path.join(os.getcwd(), outfile)
for name, settings in list(opt_map.items()):
better = fsl.BET(**{name: settings[1]})
# Add mandatory input
better.inputs.in_file = tmp_infile
- realcmd = " ".join([better.cmd, tmp_infile, outpath, settings[0]])
+ realcmd = " ".join([better.cmd, tmp_infile, outfile, settings[0]])
assert better.cmdline == realcmd
| FSL (+6.0) BET chokes with long filenames
Would adding the copyfile metadata just fix this, @satra?
I think I've seen this issue reported before, but I can't seem to find it. Feel free to link and close this if it is a duplicate | 0.0 | [
"nipype/interfaces/afni/tests/test_auto_NetCorr.py::test_NetCorr_inputs",
"nipype/interfaces/afni/tests/test_auto_NetCorr.py::test_NetCorr_outputs"
] | [] | 2021-03-04 06:44:05+00:00 | 4,210 |
|
nipy__nipype-3325 | diff --git a/nipype/interfaces/fsl/model.py b/nipype/interfaces/fsl/model.py
index 8c269caac..15fb36f6c 100644
--- a/nipype/interfaces/fsl/model.py
+++ b/nipype/interfaces/fsl/model.py
@@ -2245,7 +2245,7 @@ class RandomiseInputSpec(FSLCommandInputSpec):
desc=("carry out Threshold-Free Cluster Enhancement with 2D " "optimisation"),
argstr="--T2",
)
- f_only = traits.Bool(desc="calculate f-statistics only", argstr="--f_only")
+ f_only = traits.Bool(desc="calculate f-statistics only", argstr="--fonly")
raw_stats_imgs = traits.Bool(
desc="output raw ( unpermuted ) statistic images", argstr="-R"
)
| nipy/nipype | 6c060304f380c46b2f05c5afdc7171dbbdfadc58 | diff --git a/nipype/interfaces/fsl/tests/test_auto_Randomise.py b/nipype/interfaces/fsl/tests/test_auto_Randomise.py
index cf816c56d..9b0b74bf2 100644
--- a/nipype/interfaces/fsl/tests/test_auto_Randomise.py
+++ b/nipype/interfaces/fsl/tests/test_auto_Randomise.py
@@ -37,7 +37,7 @@ def test_Randomise_inputs():
argstr="-S %.2f",
),
f_only=dict(
- argstr="--f_only",
+ argstr="--fonly",
),
fcon=dict(
argstr="-f %s",
| fsl randomise f_only flag contains typo, can't work
### Summary
when calling randomise with f_only=True, nipypes interfaces appends --f_only instead of --fonly. This is incorrect and prevents the call from working.
The offending line is [here](https://github.com/nipy/nipype/blob/master/nipype/interfaces/fsl/model.py#L2250), where the last bit should be `argstr="--fonly"`
### Actual behavior
appending --fonly to the randomise call.
### Expected behavior
--f_only is appended, breaking the call.
### Script/Workflow details
```
fsl_randomise = mem.cache(fsl.Randomise)
randomise_results = fsl_randomise(
in_file=`fourdimage.nii`,
mask=`mask.nii`,
num_perm=10000,
f_only=True,
**kwargs
)
```
### Platform details:
```
{'commit_hash': 'c5ce0e2c9',
'commit_source': 'installation',
'networkx_version': '2.5',
'nibabel_version': '3.2.1',
'nipype_version': '1.4.2',
'numpy_version': '1.20.1',
'pkg_path': '/usr/local/miniconda/lib/python3.7/site-packages/nipype',
'scipy_version': '1.6.1',
'sys_executable': '/usr/local/miniconda/bin/python',
'sys_platform': 'linux',
'sys_version': '3.7.4 (default, Aug 13 2019, 20:35:49) \n[GCC 7.3.0]',
'traits_version': '6.2.0'}
``` | 0.0 | [
"nipype/interfaces/fsl/tests/test_auto_Randomise.py::test_Randomise_inputs"
] | [
"nipype/interfaces/fsl/tests/test_auto_Randomise.py::test_Randomise_outputs"
] | 2021-04-09 16:06:42+00:00 | 4,211 |
|
nipy__nipype-3470 | diff --git a/.zenodo.json b/.zenodo.json
index f715cad42..4e8b3022a 100644
--- a/.zenodo.json
+++ b/.zenodo.json
@@ -892,11 +892,7 @@
"orcid": "0000-0002-5312-6729"
}
],
- "keywords": [
- "neuroimaging",
- "workflow",
- "pipeline"
- ],
+ "keywords": ["neuroimaging", "workflow", "pipeline"],
"license": "Apache-2.0",
"upload_type": "software"
}
diff --git a/doc/interfaces.rst b/doc/interfaces.rst
index bad49381c..7b74d00d4 100644
--- a/doc/interfaces.rst
+++ b/doc/interfaces.rst
@@ -8,7 +8,7 @@ Interfaces and Workflows
:Release: |version|
:Date: |today|
-Previous versions: `1.8.2 <http://nipype.readthedocs.io/en/1.8.2/>`_ `1.8.1 <http://nipype.readthedocs.io/en/1.8.1/>`_
+Previous versions: `1.8.3 <http://nipype.readthedocs.io/en/1.8.3/>`_ `1.8.2 <http://nipype.readthedocs.io/en/1.8.2/>`_
Workflows
---------
diff --git a/nipype/info.py b/nipype/info.py
index b4f8373a1..4de8f9ff0 100644
--- a/nipype/info.py
+++ b/nipype/info.py
@@ -5,7 +5,7 @@ docs. In setup.py in particular, we exec this file, so it cannot import nipy
# nipype version information
# Remove .dev0 for release
-__version__ = "1.8.3"
+__version__ = "1.8.4.dev0"
def get_nipype_gitversion():
diff --git a/nipype/interfaces/mrtrix3/preprocess.py b/nipype/interfaces/mrtrix3/preprocess.py
index 928833aaf..be87930a3 100644
--- a/nipype/interfaces/mrtrix3/preprocess.py
+++ b/nipype/interfaces/mrtrix3/preprocess.py
@@ -5,21 +5,26 @@
import os.path as op
from ..base import (
- CommandLineInputSpec,
CommandLine,
- traits,
- TraitedSpec,
+ CommandLineInputSpec,
+ Directory,
File,
- isdefined,
- Undefined,
InputMultiObject,
+ TraitedSpec,
+ Undefined,
+ isdefined,
+ traits,
)
-from .base import MRTrix3BaseInputSpec, MRTrix3Base
+from .base import MRTrix3Base, MRTrix3BaseInputSpec
class DWIDenoiseInputSpec(MRTrix3BaseInputSpec):
in_file = File(
- exists=True, argstr="%s", position=-2, mandatory=True, desc="input DWI image"
+ exists=True,
+ argstr="%s",
+ position=-2,
+ mandatory=True,
+ desc="input DWI image",
)
mask = File(exists=True, argstr="-mask %s", position=1, desc="mask image")
extent = traits.Tuple(
@@ -88,7 +93,11 @@ class DWIDenoise(MRTrix3Base):
class MRDeGibbsInputSpec(MRTrix3BaseInputSpec):
in_file = File(
- exists=True, argstr="%s", position=-2, mandatory=True, desc="input DWI image"
+ exists=True,
+ argstr="%s",
+ position=-2,
+ mandatory=True,
+ desc="input DWI image",
)
axes = traits.ListInt(
default_value=[0, 1],
@@ -177,7 +186,11 @@ class MRDeGibbs(MRTrix3Base):
class DWIBiasCorrectInputSpec(MRTrix3BaseInputSpec):
in_file = File(
- exists=True, argstr="%s", position=-2, mandatory=True, desc="input DWI image"
+ exists=True,
+ argstr="%s",
+ position=-2,
+ mandatory=True,
+ desc="input DWI image",
)
in_mask = File(argstr="-mask %s", desc="input mask image for bias field estimation")
use_ants = traits.Bool(
@@ -252,7 +265,11 @@ class DWIBiasCorrect(MRTrix3Base):
class DWIPreprocInputSpec(MRTrix3BaseInputSpec):
in_file = File(
- exists=True, argstr="%s", position=0, mandatory=True, desc="input DWI image"
+ exists=True,
+ argstr="%s",
+ position=0,
+ mandatory=True,
+ desc="input DWI image",
)
out_file = File(
"preproc.mif",
@@ -274,7 +291,6 @@ class DWIPreprocInputSpec(MRTrix3BaseInputSpec):
)
pe_dir = traits.Str(
argstr="-pe_dir %s",
- mandatory=True,
desc="Specify the phase encoding direction of the input series, can be a signed axis number (e.g. -0, 1, +2), an axis designator (e.g. RL, PA, IS), or NIfTI axis codes (e.g. i-, j, k)",
)
ro_time = traits.Float(
@@ -290,33 +306,49 @@ class DWIPreprocInputSpec(MRTrix3BaseInputSpec):
argstr="-align_seepi",
desc="Achieve alignment between the SE-EPI images used for inhomogeneity field estimation, and the DWIs",
)
- eddy_options = traits.Str(
- argstr='-eddy_options "%s"',
- desc="Manually provide additional command-line options to the eddy command",
+ json_import = File(
+ exists=True,
+ argstr="-json_import %s",
+ desc="Import image header information from an associated JSON file (may be necessary to determine phase encoding information)",
)
topup_options = traits.Str(
argstr='-topup_options "%s"',
desc="Manually provide additional command-line options to the topup command",
)
- export_grad_mrtrix = traits.Bool(
- argstr="-export_grad_mrtrix", desc="export new gradient files in mrtrix format"
+ eddy_options = traits.Str(
+ argstr='-eddy_options "%s"',
+ desc="Manually provide additional command-line options to the eddy command",
+ )
+ eddy_mask = File(
+ exists=True,
+ argstr="-eddy_mask %s",
+ desc="Provide a processing mask to use for eddy, instead of having dwifslpreproc generate one internally using dwi2mask",
+ )
+ eddy_slspec = File(
+ exists=True,
+ argstr="-eddy_slspec %s",
+ desc="Provide a file containing slice groupings for eddy's slice-to-volume registration",
+ )
+ eddyqc_text = Directory(
+ exists=False,
+ argstr="-eddyqc_text %s",
+ desc="Copy the various text-based statistical outputs generated by eddy, and the output of eddy_qc (if installed), into an output directory",
)
- export_grad_fsl = traits.Bool(
- argstr="-export_grad_fsl", desc="export gradient files in FSL format"
+ eddyqc_all = Directory(
+ exists=False,
+ argstr="-eddyqc_all %s",
+ desc="Copy ALL outputs generated by eddy (including images), and the output of eddy_qc (if installed), into an output directory",
)
out_grad_mrtrix = File(
"grad.b",
- argstr="%s",
- usedefault=True,
- requires=["export_grad_mrtrix"],
- desc="name of new gradient file",
+ argstr="-export_grad_mrtrix %s",
+ desc="export new gradient files in mrtrix format",
)
out_grad_fsl = traits.Tuple(
- File("grad.bvecs", usedefault=True, desc="bvecs"),
- File("grad.bvals", usedefault=True, desc="bvals"),
- argstr="%s, %s",
- requires=["export_grad_fsl"],
- desc="Output (bvecs, bvals) gradients FSL format",
+ File("grad.bvecs", desc="bvecs"),
+ File("grad.bvals", desc="bvals"),
+ argstr="-export_grad_fsl %s, %s",
+ desc="export gradient files in FSL format",
)
@@ -358,7 +390,7 @@ class DWIPreproc(MRTrix3Base):
>>> preproc.inputs.rpe_options = 'none'
>>> preproc.inputs.out_file = "preproc.mif"
>>> preproc.inputs.eddy_options = '--slm=linear --repol' # linear second level model and replace outliers
- >>> preproc.inputs.export_grad_mrtrix = True # export final gradient table in MRtrix format
+ >>> preproc.inputs.out_grad_mrtrix = "grad.b" # export final gradient table in MRtrix format
>>> preproc.inputs.ro_time = 0.165240 # 'TotalReadoutTime' in BIDS JSON metadata files
>>> preproc.inputs.pe_dir = 'j' # 'PhaseEncodingDirection' in BIDS JSON metadata files
>>> preproc.cmdline
@@ -394,7 +426,11 @@ class ResponseSDInputSpec(MRTrix3BaseInputSpec):
desc="response estimation algorithm (multi-tissue)",
)
in_file = File(
- exists=True, argstr="%s", position=-5, mandatory=True, desc="input DWI image"
+ exists=True,
+ argstr="%s",
+ position=-5,
+ mandatory=True,
+ desc="input DWI image",
)
mtt_file = File(argstr="%s", position=-4, desc="input 5tt image")
wm_file = File(
@@ -518,10 +554,17 @@ class ReplaceFSwithFIRSTInputSpec(CommandLineInputSpec):
desc="input anatomical image",
)
in_t1w = File(
- exists=True, argstr="%s", mandatory=True, position=-3, desc="input T1 image"
+ exists=True,
+ argstr="%s",
+ mandatory=True,
+ position=-3,
+ desc="input T1 image",
)
in_config = File(
- exists=True, argstr="%s", position=-2, desc="connectome configuration file"
+ exists=True,
+ argstr="%s",
+ position=-2,
+ desc="connectome configuration file",
)
out_file = File(
| nipy/nipype | 3b505327f412a2c4215d0763f932d0eac6e3a11b | diff --git a/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py b/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py
index bc53d67b4..7c0231bd7 100644
--- a/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py
+++ b/nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py
@@ -13,19 +13,27 @@ def test_DWIPreproc_inputs():
bval_scale=dict(
argstr="-bvalue_scaling %s",
),
+ eddy_mask=dict(
+ argstr="-eddy_mask %s",
+ extensions=None,
+ ),
eddy_options=dict(
argstr='-eddy_options "%s"',
),
+ eddy_slspec=dict(
+ argstr="-eddy_slspec %s",
+ extensions=None,
+ ),
+ eddyqc_all=dict(
+ argstr="-eddyqc_all %s",
+ ),
+ eddyqc_text=dict(
+ argstr="-eddyqc_text %s",
+ ),
environ=dict(
nohash=True,
usedefault=True,
),
- export_grad_fsl=dict(
- argstr="-export_grad_fsl",
- ),
- export_grad_mrtrix=dict(
- argstr="-export_grad_mrtrix",
- ),
grad_file=dict(
argstr="-grad %s",
extensions=None,
@@ -52,6 +60,10 @@ def test_DWIPreproc_inputs():
mandatory=True,
position=0,
),
+ json_import=dict(
+ argstr="-json_import %s",
+ extensions=None,
+ ),
nthreads=dict(
argstr="-nthreads %d",
nohash=True,
@@ -71,18 +83,14 @@ def test_DWIPreproc_inputs():
usedefault=True,
),
out_grad_fsl=dict(
- argstr="%s, %s",
- requires=["export_grad_fsl"],
+ argstr="-export_grad_fsl %s, %s",
),
out_grad_mrtrix=dict(
- argstr="%s",
+ argstr="-export_grad_mrtrix %s",
extensions=None,
- requires=["export_grad_mrtrix"],
- usedefault=True,
),
pe_dir=dict(
argstr="-pe_dir %s",
- mandatory=True,
),
ro_time=dict(
argstr="-readout_time %f",
| Mrtrix3's DWIPreproc force inputs that are not mandatory
### Summary
The interface to Mrtrix3's dwifslpreproc forces some inputs that are not really necessary according to [the original CLI on which it relies](https://mrtrix.readthedocs.io/en/dev/reference/commands/dwifslpreproc.html).
### Actual behavior
The interface requires inputs to be set for out_grad_mrtrix, pe_dir, etc.
### Expected behavior
Ideally, it would change the necessary inputs (that are different and depends on the way you use this CLI).
A simpler solution would be to simply reduce the mandatory inputs to those that truly are.
python -c "import nipype; from pprint import pprint; pprint(nipype.get_info())"
-->
```
{'commit_hash': 'd77fc3c',
'commit_source': 'installation',
'networkx_version': '2.6.3',
'nibabel_version': '3.2.1',
'nipype_version': '1.8.0',
'numpy_version': '1.20.0',
'pkg_path': '/home/groot/Projects/PhD/venv/lib/python3.9/site-packages/nipype',
'scipy_version': '1.7.3',
'sys_executable': '/home/groot/Projects/PhD/venv/bin/python',
'sys_platform': 'linux',
'sys_version': '3.9.12 (main, Mar 24 2022, 16:20:11) \n[GCC 9.4.0]',
'traits_version': '6.3.2'}
```
### Execution environment
- My python environment outside container
| 0.0 | [
"nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py::test_DWIPreproc_inputs"
] | [
"nipype/interfaces/mrtrix3/tests/test_auto_DWIPreproc.py::test_DWIPreproc_outputs"
] | 2022-05-11 20:10:09+00:00 | 4,212 |
|
nipype__pydra-211 | diff --git a/pydra/engine/core.py b/pydra/engine/core.py
index 5e3e8ef..e630588 100644
--- a/pydra/engine/core.py
+++ b/pydra/engine/core.py
@@ -381,7 +381,7 @@ class TaskBase:
orig_inputs = attr.asdict(self.inputs)
map_copyfiles = copyfile_input(self.inputs, self.output_dir)
modified_inputs = template_update(self.inputs, map_copyfiles)
- if modified_inputs is not None:
+ if modified_inputs:
self.inputs = attr.evolve(self.inputs, **modified_inputs)
self.audit.start_audit(odir)
result = Result(output=None, runtime=None, errored=False)
diff --git a/pydra/engine/helpers_file.py b/pydra/engine/helpers_file.py
index 7c2098b..2afac73 100644
--- a/pydra/engine/helpers_file.py
+++ b/pydra/engine/helpers_file.py
@@ -505,7 +505,7 @@ def template_update(inputs, map_copyfiles=None):
f"output_file_template metadata for "
"{fld.name} should be a string"
)
- return {k: v for k, v in dict_.items() if getattr(inputs, k) != v}
+ return {k: v for k, v in dict_.items() if getattr(inputs, k) is not v}
def is_local_file(f):
diff --git a/pydra/engine/task.py b/pydra/engine/task.py
index 4872445..aa7fff5 100644
--- a/pydra/engine/task.py
+++ b/pydra/engine/task.py
@@ -176,15 +176,20 @@ class FunctionTask(TaskBase):
)
else:
if not isinstance(return_info, tuple):
- return_info = (return_info,)
- output_spec = SpecInfo(
- name="Output",
- fields=[
- ("out{}".format(n + 1), t)
- for n, t in enumerate(return_info)
- ],
- bases=(BaseSpec,),
- )
+ output_spec = SpecInfo(
+ name="Output",
+ fields=[("out", return_info)],
+ bases=(BaseSpec,),
+ )
+ else:
+ output_spec = SpecInfo(
+ name="Output",
+ fields=[
+ ("out{}".format(n + 1), t)
+ for n, t in enumerate(return_info)
+ ],
+ bases=(BaseSpec,),
+ )
elif "return" in func.__annotations__:
raise NotImplementedError("Branch not implemented")
self.output_spec = output_spec
| nipype/pydra | 73ac9828d1e0a81720907cf2d518cafea34afba9 | diff --git a/pydra/engine/tests/test_task.py b/pydra/engine/tests/test_task.py
index 2d6a15e..e624a39 100644
--- a/pydra/engine/tests/test_task.py
+++ b/pydra/engine/tests/test_task.py
@@ -27,6 +27,17 @@ def test_output():
assert res.output.out == 5
+def test_numpy():
+ """ checking if mark.task works for numpy functions"""
+ np = pytest.importorskip("numpy")
+ fft = mark.annotate({"a": np.ndarray, "return": float})(np.fft.fft)
+ fft = mark.task(fft)()
+ arr = np.array([[1, 10], [2, 20]])
+ fft.inputs.a = arr
+ res = fft()
+ assert np.allclose(np.fft.fft(arr), res.output.out)
+
+
@pytest.mark.xfail(reason="cp.dumps(func) depends on the system/setup, TODO!!")
def test_checksum():
nn = funaddtwo(a=3)
@@ -38,7 +49,9 @@ def test_checksum():
def test_annotated_func():
@mark.task
- def testfunc(a: int, b: float = 0.1) -> ty.NamedTuple("Output", [("out1", float)]):
+ def testfunc(
+ a: int, b: float = 0.1
+ ) -> ty.NamedTuple("Output", [("out_out", float)]):
return a + b
funky = testfunc(a=1)
@@ -48,14 +61,14 @@ def test_annotated_func():
assert getattr(funky.inputs, "a") == 1
assert getattr(funky.inputs, "b") == 0.1
assert getattr(funky.inputs, "_func") is not None
- assert set(funky.output_names) == set(["out1"])
+ assert set(funky.output_names) == set(["out_out"])
# assert funky.inputs.hash == '17772c3aec9540a8dd3e187eecd2301a09c9a25c6e371ddd86e31e3a1ecfeefa'
assert funky.__class__.__name__ + "_" + funky.inputs.hash == funky.checksum
result = funky()
assert hasattr(result, "output")
- assert hasattr(result.output, "out1")
- assert result.output.out1 == 1.1
+ assert hasattr(result.output, "out_out")
+ assert result.output.out_out == 1.1
assert os.path.exists(funky.cache_dir / funky.checksum / "_result.pklz")
funky.result() # should not recompute
@@ -64,7 +77,7 @@ def test_annotated_func():
assert funky.result() is None
funky()
result = funky.result()
- assert result.output.out1 == 2.1
+ assert result.output.out_out == 2.1
help = funky.help(returnhelp=True)
assert help == [
@@ -74,7 +87,7 @@ def test_annotated_func():
"- b: float (default: 0.1)",
"- _func: str",
"Output Parameters:",
- "- out1: float",
+ "- out_out: float",
]
@@ -150,13 +163,13 @@ def test_halfannotated_func():
assert getattr(funky.inputs, "a") == 10
assert getattr(funky.inputs, "b") == 20
assert getattr(funky.inputs, "_func") is not None
- assert set(funky.output_names) == set(["out1"])
+ assert set(funky.output_names) == set(["out"])
assert funky.__class__.__name__ + "_" + funky.inputs.hash == funky.checksum
result = funky()
assert hasattr(result, "output")
- assert hasattr(result.output, "out1")
- assert result.output.out1 == 30
+ assert hasattr(result.output, "out")
+ assert result.output.out == 30
assert os.path.exists(funky.cache_dir / funky.checksum / "_result.pklz")
@@ -165,7 +178,7 @@ def test_halfannotated_func():
assert funky.result() is None
funky()
result = funky.result()
- assert result.output.out1 == 31
+ assert result.output.out == 31
help = funky.help(returnhelp=True)
assert help == [
@@ -175,7 +188,7 @@ def test_halfannotated_func():
"- b: _empty",
"- _func: str",
"Output Parameters:",
- "- out1: int",
+ "- out: int",
]
diff --git a/pydra/mark/tests/test_functions.py b/pydra/mark/tests/test_functions.py
index ae963eb..31c764e 100644
--- a/pydra/mark/tests/test_functions.py
+++ b/pydra/mark/tests/test_functions.py
@@ -87,6 +87,16 @@ def test_return_annotated_task():
assert res.output.squared == 4.0
+def test_return_halfannotated_annotated_task():
+ @task
+ @annotate({"in_val": float, "return": float})
+ def square(in_val):
+ return in_val ** 2
+
+ res = square(in_val=2.0)()
+ assert res.output.out == 4.0
+
+
def test_return_annotated_task_multiple_output():
@task
@annotate({"in_val": float, "return": {"squared": float, "cubed": float}})
| template_update does not take type of value into account
the `template_update` function cannot simply check for equality of objects.
https://github.com/nipype/pydra/blob/master/pydra/engine/helpers_file.py#L508
<summary>
Code
<details>
```
import numpy as np
fft = pydra.mark.annotate({'a': np.ndarray, 'return': float})(np.fft.fft)
fft = pydra.mark.task(fft)()
foo(a=np.ones((100)))
```
</details>
</summary>
<summary>
Error
<details>
```
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-34-af82eb0ea503> in <module>
2 fft = pydra.mark.annotate({'a': np.ndarray, 'return': float})(np.fft.fft)
3 fft = pydra.mark.task(fft)()
----> 4 foo(a=np.ones((100)))
~/pydra/engine/core.py in __call__(self, submitter, plugin, rerun, **kwargs)
354 "TODO: linear workflow execution - assign submitter or plugin for now"
355 )
--> 356 res = self._run(rerun=rerun, **kwargs)
357 return res
358
~/pydra/engine/core.py in _run(self, rerun, **kwargs)
378 orig_inputs = attr.asdict(self.inputs)
379 map_copyfiles = copyfile_input(self.inputs, self.output_dir)
--> 380 modified_inputs = template_update(self.inputs, map_copyfiles)
381 if modified_inputs is not None:
382 self.inputs = attr.evolve(self.inputs, **modified_inputs)
~/pydra/engine/helpers_file.py in template_update(inputs, map_copyfiles)
506 "{fld.name} should be a string"
507 )
--> 508 return {k: v for k, v in dict_.items() if getattr(inputs, k) != v}
509
510
~/pydra/engine/helpers_file.py in <dictcomp>(.0)
506 "{fld.name} should be a string"
507 )
--> 508 return {k: v for k, v in dict_.items() if getattr(inputs, k) != v}
509
510
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()```
</details>
</summary>
| 0.0 | [
"pydra/engine/tests/test_task.py::test_numpy",
"pydra/engine/tests/test_task.py::test_halfannotated_func",
"pydra/mark/tests/test_functions.py::test_return_halfannotated_annotated_task"
] | [
"pydra/engine/tests/test_task.py::test_output",
"pydra/engine/tests/test_task.py::test_annotated_func",
"pydra/engine/tests/test_task.py::test_annotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_annotated_func_multreturn_exception",
"pydra/engine/tests/test_task.py::test_halfannotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_notannotated_func",
"pydra/engine/tests/test_task.py::test_notannotated_func_returnlist",
"pydra/engine/tests/test_task.py::test_halfannotated_func_multrun_returnlist",
"pydra/engine/tests/test_task.py::test_notannotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_exception_func",
"pydra/engine/tests/test_task.py::test_audit_prov",
"pydra/engine/tests/test_task.py::test_audit_all",
"pydra/engine/tests/test_task.py::test_shell_cmd",
"pydra/engine/tests/test_task.py::test_container_cmds",
"pydra/engine/tests/test_task.py::test_docker_cmd",
"pydra/engine/tests/test_task.py::test_singularity_cmd",
"pydra/engine/tests/test_task.py::test_functask_callable",
"pydra/engine/tests/test_task.py::test_taskhooks",
"pydra/mark/tests/test_functions.py::test_task_equivalence",
"pydra/mark/tests/test_functions.py::test_annotation_equivalence",
"pydra/mark/tests/test_functions.py::test_annotation_override",
"pydra/mark/tests/test_functions.py::test_invalid_annotation",
"pydra/mark/tests/test_functions.py::test_annotated_task",
"pydra/mark/tests/test_functions.py::test_return_annotated_task",
"pydra/mark/tests/test_functions.py::test_return_annotated_task_multiple_output",
"pydra/mark/tests/test_functions.py::test_return_halfannotated_task_multiple_output"
] | 2020-03-04 19:12:21+00:00 | 4,213 |
|
nipype__pydra-222 | diff --git a/pydra/engine/helpers.py b/pydra/engine/helpers.py
index 1a441f1..3cd9c65 100644
--- a/pydra/engine/helpers.py
+++ b/pydra/engine/helpers.py
@@ -10,7 +10,7 @@ from hashlib import sha256
import subprocess as sp
from .specs import Runtime, File, attr_fields
-from .helpers_file import is_existing_file, hash_file, copyfile, is_existing_file
+from .helpers_file import hash_file, copyfile, is_existing_file
def ensure_list(obj, tuple2list=False):
@@ -444,12 +444,14 @@ def hash_function(obj):
def hash_value(value, tp=None, metadata=None):
"""calculating hash or returning values recursively"""
+ if metadata is None:
+ metadata = {}
if isinstance(value, (tuple, list)):
return [hash_value(el, tp, metadata) for el in value]
elif isinstance(value, dict):
dict_hash = {k: hash_value(v, tp, metadata) for (k, v) in value.items()}
# returning a sorted object
- return sorted(dict_hash.items(), key=lambda x: x[0])
+ return [list(el) for el in sorted(dict_hash.items(), key=lambda x: x[0])]
else: # not a container
if (
(tp is File or "pydra.engine.specs.File" in str(tp))
@@ -457,8 +459,6 @@ def hash_value(value, tp=None, metadata=None):
and "container_path" not in metadata
):
return hash_file(value)
- elif isinstance(value, tuple):
- return list(value)
else:
return value
| nipype/pydra | 349d954add42e4297ce127c2fa61f49346cc19a1 | diff --git a/pydra/engine/tests/test_helpers.py b/pydra/engine/tests/test_helpers.py
index 862f6b1..5043735 100644
--- a/pydra/engine/tests/test_helpers.py
+++ b/pydra/engine/tests/test_helpers.py
@@ -4,17 +4,18 @@ import pytest
import cloudpickle as cp
from .utils import multiply
-from .. import helpers
+from ..helpers import hash_value, hash_function, save, create_pyscript
from .. import helpers_file
+from ..specs import File
def test_save(tmpdir):
outdir = Path(tmpdir)
with pytest.raises(ValueError):
- helpers.save(tmpdir)
+ save(tmpdir)
foo = multiply(name="mult", x=1, y=2)
# save task
- helpers.save(outdir, task=foo)
+ save(outdir, task=foo)
del foo
# load saved task
task_pkl = outdir / "_task.pklz"
@@ -24,7 +25,7 @@ def test_save(tmpdir):
# execute task and save result
res = foo()
assert res.output.out == 2
- helpers.save(outdir, result=res)
+ save(outdir, result=res)
del res
# load saved result
res_pkl = outdir / "_result.pklz"
@@ -35,10 +36,10 @@ def test_save(tmpdir):
def test_create_pyscript(tmpdir):
outdir = Path(tmpdir)
with pytest.raises(Exception):
- helpers.create_pyscript(outdir, "checksum")
+ create_pyscript(outdir, "checksum")
foo = multiply(name="mult", x=1, y=2)
- helpers.save(outdir, task=foo)
- pyscript = helpers.create_pyscript(outdir, foo.checksum)
+ save(outdir, task=foo)
+ pyscript = create_pyscript(outdir, foo.checksum)
assert pyscript.exists()
@@ -50,3 +51,76 @@ def test_hash_file(tmpdir):
helpers_file.hash_file(outdir / "test.file")
== "9f86d081884c7d659a2feaa0c55ad015a3bf4f1b2b0b822cd15d6c15b0f00a08"
)
+
+
+def test_hashfun_float():
+ import math
+
+ pi_50 = 3.14159265358979323846264338327950288419716939937510
+ pi_15 = 3.141592653589793
+ pi_10 = 3.1415926536
+ # comparing for x that have the same x.as_integer_ratio()
+ assert (
+ math.pi.as_integer_ratio()
+ == pi_50.as_integer_ratio()
+ == pi_15.as_integer_ratio()
+ )
+ assert hash_function(math.pi) == hash_function(pi_15) == hash_function(pi_50)
+ # comparing for x that have different x.as_integer_ratio()
+ assert math.pi.as_integer_ratio() != pi_10.as_integer_ratio()
+ assert hash_function(math.pi) != hash_function(pi_10)
+
+
+def test_hash_value_dict():
+ dict1 = {"a": 10, "b": 5}
+ dict2 = {"b": 5, "a": 10}
+ assert (
+ hash_value(dict1)
+ == hash_value(dict2)
+ == [["a", hash_value(10)], ["b", hash_value(5)]]
+ == [["a", 10], ["b", 5]]
+ )
+
+
+def test_hash_value_list_tpl():
+ lst = [2, 5.6, "ala"]
+ tpl = (2, 5.6, "ala")
+ assert hash_value(lst) == [hash_value(2), hash_value(5.6), hash_value("ala")] == lst
+ assert hash_value(lst) == hash_value(tpl)
+
+
+def test_hash_value_list_dict():
+ lst = [2, {"a": "ala", "b": 1}]
+ hash_value(lst)
+ assert (
+ hash_value(lst)
+ == [hash_value(2), hash_value([["a", "ala"], ["b", 1]])]
+ == [2, [["a", "ala"], ["b", 1]]]
+ )
+
+
+def test_hash_value_files(tmpdir):
+ file_1 = tmpdir.join("file_1.txt")
+ file_2 = tmpdir.join("file_2.txt")
+ with open(file_1, "w") as f:
+ f.write("hello")
+ with open(file_2, "w") as f:
+ f.write("hello")
+
+ assert hash_value(file_1, tp=File) == hash_value(file_2, tp=File)
+ assert hash_value(file_1, tp=str) != hash_value(file_2, tp=str)
+ assert hash_value(file_1) != hash_value(file_2)
+
+
+def test_hash_value_files_list(tmpdir):
+ file_1 = tmpdir.join("file_1.txt")
+ file_2 = tmpdir.join("file_2.txt")
+ with open(file_1, "w") as f:
+ f.write("hello")
+ with open(file_2, "w") as f:
+ f.write("hi")
+
+ assert hash_value([file_1, file_2], tp=File) == [
+ hash_value(file_1, tp=File),
+ hash_value(file_2, tp=File),
+ ]
diff --git a/pydra/engine/tests/test_specs.py b/pydra/engine/tests/test_specs.py
index 67ea41e..da98ff8 100644
--- a/pydra/engine/tests/test_specs.py
+++ b/pydra/engine/tests/test_specs.py
@@ -289,7 +289,7 @@ def test_input_file_hash_4(tmpdir):
# checking specific hash value
hash1 = inputs(in_file=[{"file": file, "int": 3}]).hash
- assert hash1 == "0148961a66106e15e55de3ab7e8c49e6227b6db7cdc614dcbae20406feb2548a"
+ assert hash1 == "e0555e78a40a02611674b0f48da97cdd28eee7e9885ecc17392b560c14826f06"
# the same file, but int field changes
hash1a = inputs(in_file=[{"file": file, "int": 5}]).hash
| review what should be included checksum
for example I'm removing bindings and input that have output_file_template | 0.0 | [
"pydra/engine/tests/test_helpers.py::test_hash_value_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_files",
"pydra/engine/tests/test_helpers.py::test_hash_value_files_list",
"pydra/engine/tests/test_specs.py::test_input_file_hash_4"
] | [
"pydra/engine/tests/test_helpers.py::test_save",
"pydra/engine/tests/test_helpers.py::test_create_pyscript",
"pydra/engine/tests/test_helpers.py::test_hash_file",
"pydra/engine/tests/test_helpers.py::test_hashfun_float",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_tpl",
"pydra/engine/tests/test_specs.py::test_basespec",
"pydra/engine/tests/test_specs.py::test_runtime",
"pydra/engine/tests/test_specs.py::test_result",
"pydra/engine/tests/test_specs.py::test_shellspec",
"pydra/engine/tests/test_specs.py::test_container",
"pydra/engine/tests/test_specs.py::test_docker",
"pydra/engine/tests/test_specs.py::test_singularity",
"pydra/engine/tests/test_specs.py::test_lazy_inp",
"pydra/engine/tests/test_specs.py::test_lazy_out",
"pydra/engine/tests/test_specs.py::test_laxy_errorattr",
"pydra/engine/tests/test_specs.py::test_lazy_getvale",
"pydra/engine/tests/test_specs.py::test_input_file_hash_1",
"pydra/engine/tests/test_specs.py::test_input_file_hash_2",
"pydra/engine/tests/test_specs.py::test_input_file_hash_2a",
"pydra/engine/tests/test_specs.py::test_input_file_hash_3"
] | 2020-04-02 01:31:19+00:00 | 4,214 |
|
nipype__pydra-224 | diff --git a/pydra/engine/helpers.py b/pydra/engine/helpers.py
index 1a441f1..3cd9c65 100644
--- a/pydra/engine/helpers.py
+++ b/pydra/engine/helpers.py
@@ -10,7 +10,7 @@ from hashlib import sha256
import subprocess as sp
from .specs import Runtime, File, attr_fields
-from .helpers_file import is_existing_file, hash_file, copyfile, is_existing_file
+from .helpers_file import hash_file, copyfile, is_existing_file
def ensure_list(obj, tuple2list=False):
@@ -444,12 +444,14 @@ def hash_function(obj):
def hash_value(value, tp=None, metadata=None):
"""calculating hash or returning values recursively"""
+ if metadata is None:
+ metadata = {}
if isinstance(value, (tuple, list)):
return [hash_value(el, tp, metadata) for el in value]
elif isinstance(value, dict):
dict_hash = {k: hash_value(v, tp, metadata) for (k, v) in value.items()}
# returning a sorted object
- return sorted(dict_hash.items(), key=lambda x: x[0])
+ return [list(el) for el in sorted(dict_hash.items(), key=lambda x: x[0])]
else: # not a container
if (
(tp is File or "pydra.engine.specs.File" in str(tp))
@@ -457,8 +459,6 @@ def hash_value(value, tp=None, metadata=None):
and "container_path" not in metadata
):
return hash_file(value)
- elif isinstance(value, tuple):
- return list(value)
else:
return value
diff --git a/pydra/engine/helpers_state.py b/pydra/engine/helpers_state.py
index 1be966a..6aad048 100644
--- a/pydra/engine/helpers_state.py
+++ b/pydra/engine/helpers_state.py
@@ -10,6 +10,16 @@ from .helpers import ensure_list
logger = logging.getLogger("pydra")
+class PydraStateError(Exception):
+ """Custom error for Pydra State"""
+
+ def __init__(self, value):
+ self.value = value
+
+ def __str__(self):
+ return "{}".format(self.value)
+
+
def splitter2rpn(splitter, other_states=None, state_fields=True):
"""
Translate user-provided splitter into *reverse polish notation*.
@@ -49,7 +59,7 @@ def _ordering(
if type(el[0]) is str and el[0].startswith("_"):
node_nm = el[0][1:]
if node_nm not in other_states and state_fields:
- raise Exception(
+ raise PydraStateError(
"can't ask for splitter from {}, other nodes that are connected: {}".format(
node_nm, other_states.keys()
)
@@ -64,7 +74,7 @@ def _ordering(
if type(el[1]) is str and el[1].startswith("_"):
node_nm = el[1][1:]
if node_nm not in other_states and state_fields:
- raise Exception(
+ raise PydraStateError(
"can't ask for splitter from {}, other nodes that are connected: {}".format(
node_nm, other_states.keys()
)
@@ -87,7 +97,7 @@ def _ordering(
if type(el[0]) is str and el[0].startswith("_"):
node_nm = el[0][1:]
if node_nm not in other_states and state_fields:
- raise Exception(
+ raise PydraStateError(
"can't ask for splitter from {}, other nodes that are connected: {}".format(
node_nm, other_states.keys()
)
@@ -102,7 +112,7 @@ def _ordering(
if type(el[1]) is str and el[1].startswith("_"):
node_nm = el[1][1:]
if node_nm not in other_states and state_fields:
- raise Exception(
+ raise PydraStateError(
"can't ask for splitter from {}, other nodes that are connected: {}".format(
node_nm, other_states.keys()
)
@@ -125,7 +135,7 @@ def _ordering(
if el.startswith("_"):
node_nm = el[1:]
if node_nm not in other_states and state_fields:
- raise Exception(
+ raise PydraStateError(
"can't ask for splitter from {}, other nodes that are connected: {}".format(
node_nm, other_states.keys()
)
@@ -156,7 +166,7 @@ def _ordering(
state_fields=state_fields,
)
else:
- raise Exception("splitter has to be a string, a tuple or a list")
+ raise PydraStateError("splitter has to be a string, a tuple or a list")
if i > 0:
output_splitter.append(current_sign)
@@ -702,7 +712,7 @@ def _single_op_splits_groups(op_single, combiner, inner_inputs, groups):
return [], {}, [], combiner
else:
# TODO: probably not needed, should be already check by st.combiner_validation
- raise Exception(
+ raise PydraStateError(
"all fields from the combiner have to be in splitter_rpn: {}, but combiner: {} is set".format(
[op_single], combiner
)
@@ -733,7 +743,7 @@ def combine_final_groups(combiner, groups, groups_stack, keys):
elif gr in grs_removed:
pass
else:
- raise Exception(
+ raise PydraStateError(
"input {} not ready to combine, you have to combine {} "
"first".format(comb, groups_stack[-1])
)
diff --git a/pydra/engine/state.py b/pydra/engine/state.py
index 92df1be..40c8111 100644
--- a/pydra/engine/state.py
+++ b/pydra/engine/state.py
@@ -111,7 +111,9 @@ class State:
@splitter.setter
def splitter(self, splitter):
if splitter and not isinstance(splitter, (str, tuple, list)):
- raise Exception("splitter has to be a string, a tuple or a list")
+ raise hlpst.PydraStateError(
+ "splitter has to be a string, a tuple or a list"
+ )
if splitter:
self._splitter = hlpst.add_name_splitter(splitter, self.name)
else:
@@ -209,7 +211,7 @@ class State:
def combiner(self, combiner):
if combiner:
if not isinstance(combiner, (str, list)):
- raise Exception("combiner has to be a string or a list")
+ raise hlpst.PydraStateError("combiner has to be a string or a list")
self._combiner = hlpst.add_name_combiner(ensure_list(combiner), self.name)
else:
self._combiner = []
@@ -251,11 +253,13 @@ class State:
def other_states(self, other_states):
if other_states:
if not isinstance(other_states, dict):
- raise Exception("other states has to be a dictionary")
+ raise hlpst.PydraStateError("other states has to be a dictionary")
else:
for key, val in other_states.items():
if not val:
- raise Exception(f"connection from node {key} is empty")
+ raise hlpst.PydraStateError(
+ f"connection from node {key} is empty"
+ )
self._other_states = other_states
else:
self._other_states = {}
@@ -360,7 +364,9 @@ class State:
):
return "[Left, Right]" # Left and Right parts separated in outer scalar
else:
- raise Exception("Left and Right splitters are mixed - splitter invalid")
+ raise hlpst.PydraStateError(
+ "Left and Right splitters are mixed - splitter invalid"
+ )
def set_input_groups(self, state_fields=True):
"""Evaluate groups, especially the final groups that address the combiner."""
@@ -409,7 +415,7 @@ class State:
):
last_gr = last_gr - 1
if left_nm[1:] not in self.other_states:
- raise Exception(
+ raise hlpst.PydraStateError(
f"can't ask for splitter from {left_nm[1:]}, other nodes that are connected: {self.other_states}"
)
st = self.other_states[left_nm[1:]][0]
@@ -434,7 +440,7 @@ class State:
)
self.keys_final += keys_f_st # st.keys_final
if not hasattr(st, "group_for_inputs_final"):
- raise Exception("previous state has to run first")
+ raise hlpst.PydraStateError("previous state has to run first")
group_for_inputs = group_for_inputs_f_st
groups_stack = groups_stack_f_st
self.left_combiner_all += combiner_all_st
@@ -487,7 +493,7 @@ class State:
or spl.startswith("_")
or spl.split(".")[0] == self.name
):
- raise Exception(
+ raise hlpst.PydraStateError(
"can't include {} in the splitter, consider using _{}".format(
spl, spl.split(".")[0]
)
@@ -497,9 +503,11 @@ class State:
""" validating if the combiner is correct (after all states are connected)"""
if self.combiner:
if not self.splitter:
- raise Exception("splitter has to be set before setting combiner")
+ raise hlpst.PydraStateError(
+ "splitter has to be set before setting combiner"
+ )
if set(self._combiner) - set(self.splitter_rpn):
- raise Exception("all combiners have to be in the splitter")
+ raise hlpst.PydraStateError("all combiners have to be in the splitter")
def prepare_states(self, inputs, cont_dim=None):
"""
| nipype/pydra | 349d954add42e4297ce127c2fa61f49346cc19a1 | diff --git a/pydra/engine/tests/test_helpers.py b/pydra/engine/tests/test_helpers.py
index 862f6b1..5043735 100644
--- a/pydra/engine/tests/test_helpers.py
+++ b/pydra/engine/tests/test_helpers.py
@@ -4,17 +4,18 @@ import pytest
import cloudpickle as cp
from .utils import multiply
-from .. import helpers
+from ..helpers import hash_value, hash_function, save, create_pyscript
from .. import helpers_file
+from ..specs import File
def test_save(tmpdir):
outdir = Path(tmpdir)
with pytest.raises(ValueError):
- helpers.save(tmpdir)
+ save(tmpdir)
foo = multiply(name="mult", x=1, y=2)
# save task
- helpers.save(outdir, task=foo)
+ save(outdir, task=foo)
del foo
# load saved task
task_pkl = outdir / "_task.pklz"
@@ -24,7 +25,7 @@ def test_save(tmpdir):
# execute task and save result
res = foo()
assert res.output.out == 2
- helpers.save(outdir, result=res)
+ save(outdir, result=res)
del res
# load saved result
res_pkl = outdir / "_result.pklz"
@@ -35,10 +36,10 @@ def test_save(tmpdir):
def test_create_pyscript(tmpdir):
outdir = Path(tmpdir)
with pytest.raises(Exception):
- helpers.create_pyscript(outdir, "checksum")
+ create_pyscript(outdir, "checksum")
foo = multiply(name="mult", x=1, y=2)
- helpers.save(outdir, task=foo)
- pyscript = helpers.create_pyscript(outdir, foo.checksum)
+ save(outdir, task=foo)
+ pyscript = create_pyscript(outdir, foo.checksum)
assert pyscript.exists()
@@ -50,3 +51,76 @@ def test_hash_file(tmpdir):
helpers_file.hash_file(outdir / "test.file")
== "9f86d081884c7d659a2feaa0c55ad015a3bf4f1b2b0b822cd15d6c15b0f00a08"
)
+
+
+def test_hashfun_float():
+ import math
+
+ pi_50 = 3.14159265358979323846264338327950288419716939937510
+ pi_15 = 3.141592653589793
+ pi_10 = 3.1415926536
+ # comparing for x that have the same x.as_integer_ratio()
+ assert (
+ math.pi.as_integer_ratio()
+ == pi_50.as_integer_ratio()
+ == pi_15.as_integer_ratio()
+ )
+ assert hash_function(math.pi) == hash_function(pi_15) == hash_function(pi_50)
+ # comparing for x that have different x.as_integer_ratio()
+ assert math.pi.as_integer_ratio() != pi_10.as_integer_ratio()
+ assert hash_function(math.pi) != hash_function(pi_10)
+
+
+def test_hash_value_dict():
+ dict1 = {"a": 10, "b": 5}
+ dict2 = {"b": 5, "a": 10}
+ assert (
+ hash_value(dict1)
+ == hash_value(dict2)
+ == [["a", hash_value(10)], ["b", hash_value(5)]]
+ == [["a", 10], ["b", 5]]
+ )
+
+
+def test_hash_value_list_tpl():
+ lst = [2, 5.6, "ala"]
+ tpl = (2, 5.6, "ala")
+ assert hash_value(lst) == [hash_value(2), hash_value(5.6), hash_value("ala")] == lst
+ assert hash_value(lst) == hash_value(tpl)
+
+
+def test_hash_value_list_dict():
+ lst = [2, {"a": "ala", "b": 1}]
+ hash_value(lst)
+ assert (
+ hash_value(lst)
+ == [hash_value(2), hash_value([["a", "ala"], ["b", 1]])]
+ == [2, [["a", "ala"], ["b", 1]]]
+ )
+
+
+def test_hash_value_files(tmpdir):
+ file_1 = tmpdir.join("file_1.txt")
+ file_2 = tmpdir.join("file_2.txt")
+ with open(file_1, "w") as f:
+ f.write("hello")
+ with open(file_2, "w") as f:
+ f.write("hello")
+
+ assert hash_value(file_1, tp=File) == hash_value(file_2, tp=File)
+ assert hash_value(file_1, tp=str) != hash_value(file_2, tp=str)
+ assert hash_value(file_1) != hash_value(file_2)
+
+
+def test_hash_value_files_list(tmpdir):
+ file_1 = tmpdir.join("file_1.txt")
+ file_2 = tmpdir.join("file_2.txt")
+ with open(file_1, "w") as f:
+ f.write("hello")
+ with open(file_2, "w") as f:
+ f.write("hi")
+
+ assert hash_value([file_1, file_2], tp=File) == [
+ hash_value(file_1, tp=File),
+ hash_value(file_2, tp=File),
+ ]
diff --git a/pydra/engine/tests/test_specs.py b/pydra/engine/tests/test_specs.py
index 67ea41e..da98ff8 100644
--- a/pydra/engine/tests/test_specs.py
+++ b/pydra/engine/tests/test_specs.py
@@ -289,7 +289,7 @@ def test_input_file_hash_4(tmpdir):
# checking specific hash value
hash1 = inputs(in_file=[{"file": file, "int": 3}]).hash
- assert hash1 == "0148961a66106e15e55de3ab7e8c49e6227b6db7cdc614dcbae20406feb2548a"
+ assert hash1 == "e0555e78a40a02611674b0f48da97cdd28eee7e9885ecc17392b560c14826f06"
# the same file, but int field changes
hash1a = inputs(in_file=[{"file": file, "int": 5}]).hash
diff --git a/pydra/engine/tests/test_state.py b/pydra/engine/tests/test_state.py
index 5d451cd..b3c46d2 100644
--- a/pydra/engine/tests/test_state.py
+++ b/pydra/engine/tests/test_state.py
@@ -1,6 +1,7 @@
import pytest
from ..state import State
+from ..helpers_state import PydraStateError
@pytest.mark.parametrize(
@@ -95,27 +96,27 @@ def test_state_1(
def test_state_2_err():
- with pytest.raises(Exception) as exinfo:
+ with pytest.raises(PydraStateError) as exinfo:
st = State("NA", splitter={"a"})
assert "splitter has to be a string, a tuple or a list" == str(exinfo.value)
def test_state_3_err():
- with pytest.raises(Exception) as exinfo:
+ with pytest.raises(PydraStateError) as exinfo:
st = State("NA", splitter=["a", "b"], combiner=("a", "b"))
assert "combiner has to be a string or a list" == str(exinfo.value)
def test_state_4_err():
st = State("NA", splitter="a", combiner=["a", "b"])
- with pytest.raises(Exception) as exinfo:
+ with pytest.raises(PydraStateError) as exinfo:
st.combiner_validation()
assert "all combiners have to be in the splitter" in str(exinfo.value)
def test_state_5_err():
st = State("NA", combiner="a")
- with pytest.raises(Exception) as exinfo:
+ with pytest.raises(PydraStateError) as exinfo:
st.combiner_validation()
assert "splitter has to be set before" in str(exinfo.value)
@@ -166,7 +167,7 @@ def test_state_connect_1b_exception():
"""can't provide explicitly NA.a (should be _NA)"""
st1 = State(name="NA", splitter="a", other_states={})
st2 = State(name="NB", splitter="NA.a")
- with pytest.raises(Exception) as excinfo:
+ with pytest.raises(PydraStateError) as excinfo:
st2.splitter_validation()
assert "consider using _NA" in str(excinfo.value)
@@ -175,7 +176,7 @@ def test_state_connect_1b_exception():
def test_state_connect_1c_exception(splitter2, other_states2):
"""can't ask for splitter from node that is not connected"""
st1 = State(name="NA", splitter="a")
- with pytest.raises(Exception) as excinfo:
+ with pytest.raises(PydraStateError) as excinfo:
st2 = State(name="NB", splitter=splitter2, other_states=other_states2)
st2.splitter_validation()
@@ -691,7 +692,7 @@ def test_state_connect_innerspl_1a():
def test_state_connect_innerspl_1b():
"""incorrect splitter - Right & Left parts in scalar splitter"""
- with pytest.raises(Exception):
+ with pytest.raises(PydraStateError):
st1 = State(name="NA", splitter="a")
st2 = State(name="NB", splitter=("_NA", "b"), other_states={"NA": (st1, "b")})
@@ -1718,7 +1719,7 @@ def test_connect_splitters(
],
)
def test_connect_splitters_exception_1(splitter, other_states):
- with pytest.raises(Exception) as excinfo:
+ with pytest.raises(PydraStateError) as excinfo:
st = State(name="CN", splitter=splitter, other_states=other_states)
assert "Left and Right splitters are mixed" in str(excinfo.value)
@@ -1729,13 +1730,13 @@ def test_connect_splitters_exception_2():
splitter="_NB",
other_states={"NA": (State(name="NA", splitter="a"), "b")},
)
- with pytest.raises(Exception) as excinfo:
+ with pytest.raises(PydraStateError) as excinfo:
st.set_input_groups()
assert "can't ask for splitter from NB" in str(excinfo.value)
def test_connect_splitters_exception_3():
- with pytest.raises(Exception) as excinfo:
+ with pytest.raises(PydraStateError) as excinfo:
st = State(
name="CN",
splitter="_NB",
| add pydra-specific exceptions
Once the API is more stable, I can add specific error objects like `NodeError` and so on. | 0.0 | [
"pydra/engine/tests/test_helpers.py::test_save",
"pydra/engine/tests/test_helpers.py::test_create_pyscript",
"pydra/engine/tests/test_helpers.py::test_hash_file",
"pydra/engine/tests/test_helpers.py::test_hashfun_float",
"pydra/engine/tests/test_helpers.py::test_hash_value_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_tpl",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_files",
"pydra/engine/tests/test_helpers.py::test_hash_value_files_list",
"pydra/engine/tests/test_specs.py::test_basespec",
"pydra/engine/tests/test_specs.py::test_runtime",
"pydra/engine/tests/test_specs.py::test_result",
"pydra/engine/tests/test_specs.py::test_shellspec",
"pydra/engine/tests/test_specs.py::test_container",
"pydra/engine/tests/test_specs.py::test_docker",
"pydra/engine/tests/test_specs.py::test_singularity",
"pydra/engine/tests/test_specs.py::test_lazy_inp",
"pydra/engine/tests/test_specs.py::test_lazy_out",
"pydra/engine/tests/test_specs.py::test_laxy_errorattr",
"pydra/engine/tests/test_specs.py::test_lazy_getvale",
"pydra/engine/tests/test_specs.py::test_input_file_hash_1",
"pydra/engine/tests/test_specs.py::test_input_file_hash_2",
"pydra/engine/tests/test_specs.py::test_input_file_hash_2a",
"pydra/engine/tests/test_specs.py::test_input_file_hash_3",
"pydra/engine/tests/test_specs.py::test_input_file_hash_4",
"pydra/engine/tests/test_state.py::test_state_1[inputs0-a-1-states_ind0-states_val0-group_for_inputs0-groups_stack0]",
"pydra/engine/tests/test_state.py::test_state_1[inputs1-splitter1-1-states_ind1-states_val1-group_for_inputs1-groups_stack1]",
"pydra/engine/tests/test_state.py::test_state_1[inputs2-splitter2-2-states_ind2-states_val2-group_for_inputs2-groups_stack2]",
"pydra/engine/tests/test_state.py::test_state_1[inputs3-a-1-states_ind3-states_val3-group_for_inputs3-groups_stack3]",
"pydra/engine/tests/test_state.py::test_state_1[inputs4-splitter4-2-states_ind4-states_val4-group_for_inputs4-groups_stack4]",
"pydra/engine/tests/test_state.py::test_state_2_err",
"pydra/engine/tests/test_state.py::test_state_3_err",
"pydra/engine/tests/test_state.py::test_state_4_err",
"pydra/engine/tests/test_state.py::test_state_5_err",
"pydra/engine/tests/test_state.py::test_state_connect_1",
"pydra/engine/tests/test_state.py::test_state_connect_1a",
"pydra/engine/tests/test_state.py::test_state_connect_1b_exception",
"pydra/engine/tests/test_state.py::test_state_connect_1c_exception[_NA-other_states20]",
"pydra/engine/tests/test_state.py::test_state_connect_1c_exception[_N-other_states21]",
"pydra/engine/tests/test_state.py::test_state_connect_2",
"pydra/engine/tests/test_state.py::test_state_connect_2a",
"pydra/engine/tests/test_state.py::test_state_connect_2b",
"pydra/engine/tests/test_state.py::test_state_connect_3",
"pydra/engine/tests/test_state.py::test_state_connect_3a",
"pydra/engine/tests/test_state.py::test_state_connect_3b",
"pydra/engine/tests/test_state.py::test_state_connect_4",
"pydra/engine/tests/test_state.py::test_state_connect_5",
"pydra/engine/tests/test_state.py::test_state_connect_6",
"pydra/engine/tests/test_state.py::test_state_connect_6a",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_1",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_1a",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_1b",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_2",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_2a",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_3",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_4",
"pydra/engine/tests/test_state.py::test_state_combine_1",
"pydra/engine/tests/test_state.py::test_state_connect_combine_1",
"pydra/engine/tests/test_state.py::test_state_connect_combine_2",
"pydra/engine/tests/test_state.py::test_state_connect_combine_3",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_combine_1",
"pydra/engine/tests/test_state.py::test_state_connect_innerspl_combine_2",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_1",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_2",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_3",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_4",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_5",
"pydra/engine/tests/test_state.py::test_state_connect_combine_left_6",
"pydra/engine/tests/test_state.py::test_connect_splitters[None-other_states0-_NA-_NA-None]",
"pydra/engine/tests/test_state.py::test_connect_splitters[b-other_states1-expected_splitter1-_NA-CN.b]",
"pydra/engine/tests/test_state.py::test_connect_splitters[splitter2-other_states2-expected_splitter2-_NA-expected_right2]",
"pydra/engine/tests/test_state.py::test_connect_splitters[None-other_states3-expected_splitter3-expected_left3-None]",
"pydra/engine/tests/test_state.py::test_connect_splitters[b-other_states4-expected_splitter4-expected_left4-CN.b]",
"pydra/engine/tests/test_state.py::test_connect_splitters[splitter5-other_states5-expected_splitter5-expected_left5-CN.b]",
"pydra/engine/tests/test_state.py::test_connect_splitters_exception_1[splitter0-other_states0]",
"pydra/engine/tests/test_state.py::test_connect_splitters_exception_1[splitter1-other_states1]",
"pydra/engine/tests/test_state.py::test_connect_splitters_exception_1[splitter2-other_states2]",
"pydra/engine/tests/test_state.py::test_connect_splitters_exception_2",
"pydra/engine/tests/test_state.py::test_connect_splitters_exception_3"
] | [] | 2020-04-02 01:52:06+00:00 | 4,215 |
|
nipype__pydra-236 | diff --git a/pydra/engine/helpers.py b/pydra/engine/helpers.py
index 2762111..e10335e 100644
--- a/pydra/engine/helpers.py
+++ b/pydra/engine/helpers.py
@@ -513,3 +513,31 @@ def output_from_inputfields(output_spec, inputs):
(field_name, attr.ib(type=File, metadata={"value": value}))
)
return output_spec
+
+
+def get_available_cpus():
+ """
+ Return the number of CPUs available to the current process or, if that is not
+ available, the total number of CPUs on the system.
+
+ Returns
+ -------
+ n_proc : :obj:`int`
+ The number of available CPUs.
+ """
+ # Will not work on some systems or if psutil is not installed.
+ # See https://psutil.readthedocs.io/en/latest/#psutil.Process.cpu_affinity
+ try:
+ import psutil
+
+ return len(psutil.Process().cpu_affinity())
+ except (AttributeError, ImportError, NotImplementedError):
+ pass
+
+ # Not available on all systems, including macOS.
+ # See https://docs.python.org/3/library/os.html#os.sched_getaffinity
+ if hasattr(os, "sched_getaffinity"):
+ return len(os.sched_getaffinity(0))
+
+ # Last resort
+ return os.cpu_count()
diff --git a/pydra/engine/workers.py b/pydra/engine/workers.py
index ff418b8..615ec52 100644
--- a/pydra/engine/workers.py
+++ b/pydra/engine/workers.py
@@ -7,7 +7,7 @@ from pathlib import Path
import concurrent.futures as cf
-from .helpers import create_pyscript, read_and_display_async, save
+from .helpers import create_pyscript, get_available_cpus, read_and_display_async, save
import logging
@@ -164,7 +164,7 @@ class ConcurrentFuturesWorker(Worker):
def __init__(self, n_procs=None):
"""Initialize Worker."""
super(ConcurrentFuturesWorker, self).__init__()
- self.n_procs = n_procs
+ self.n_procs = get_available_cpus() if n_procs is None else n_procs
# added cpu_count to verify, remove once confident and let PPE handle
self.pool = cf.ProcessPoolExecutor(self.n_procs)
# self.loop = asyncio.get_event_loop()
| nipype/pydra | c9c967e5fa161e897e4b7f3ffb8bdb16868b8e5b | diff --git a/pydra/engine/tests/test_helpers.py b/pydra/engine/tests/test_helpers.py
index 97a30fb..b0e298d 100644
--- a/pydra/engine/tests/test_helpers.py
+++ b/pydra/engine/tests/test_helpers.py
@@ -1,10 +1,18 @@
+import os
from pathlib import Path
+import platform
import pytest
import cloudpickle as cp
from .utils import multiply
-from ..helpers import hash_value, hash_function, save, create_pyscript
+from ..helpers import (
+ hash_value,
+ hash_function,
+ get_available_cpus,
+ save,
+ create_pyscript,
+)
from .. import helpers_file
from ..specs import File, Directory
@@ -155,3 +163,22 @@ def test_hash_value_nested(tmpdir):
[file_1, [file_2, file_3]], tp=File
)
assert hash_value(tmpdir, tp=Directory) == helpers_file.hash_dir(tmpdir)
+
+
+def test_get_available_cpus():
+ assert get_available_cpus() > 0
+ try:
+ import psutil
+
+ has_psutil = True
+ except ImportError:
+ has_psutil = False
+
+ if hasattr(os, "sched_getaffinity"):
+ assert get_available_cpus() == len(os.sched_getaffinity(0))
+
+ if has_psutil and platform.system().lower() != "darwin":
+ assert get_available_cpus() == len(psutil.Process().cpu_affinity())
+
+ if platform.system().lower() == "darwin":
+ assert get_available_cpus() == os.cpu_count()
| Change default `n_procs` arg in `ConcurrentFuturesWorker`
What would you like changed/added and why?
- Change the `n_procs` default to the number of CPUs the current process has access to, not the number of CPUs on the machine.
What would be the benefit? Does the change make something easier to use?
- The benefit would be that the CPUs are not oversubscribed.
By default, `n_procs` is set to the number of processors on the machine (that is the default behavior of [concurrent.futures.ProcessPoolExecutor](https://docs.python.org/3/library/concurrent.futures.html#concurrent.futures.ProcessPoolExecutor), but this could oversubscribe the CPUs. Instead, if `n_procs` is `None`, I propose that `n_procs` should be translated to `len(os.sched_getaffinity(0))`, which returns the number of _usable_ CPUs ([documentation](https://docs.python.org/3.8/library/os.html#os.cpu_count)). This would help with things like SLURM, where jobs can be started with access to a subset of CPUs on a machine. | 0.0 | [
"pydra/engine/tests/test_helpers.py::test_save",
"pydra/engine/tests/test_helpers.py::test_create_pyscript",
"pydra/engine/tests/test_helpers.py::test_hash_file",
"pydra/engine/tests/test_helpers.py::test_hashfun_float",
"pydra/engine/tests/test_helpers.py::test_hash_value_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_tpl",
"pydra/engine/tests/test_helpers.py::test_hash_value_list_dict",
"pydra/engine/tests/test_helpers.py::test_hash_value_files",
"pydra/engine/tests/test_helpers.py::test_hash_value_files_list",
"pydra/engine/tests/test_helpers.py::test_hash_value_dir",
"pydra/engine/tests/test_helpers.py::test_hash_value_nested",
"pydra/engine/tests/test_helpers.py::test_get_available_cpus"
] | [] | 2020-04-19 17:22:06+00:00 | 4,216 |
|
nipype__pydra-261 | diff --git a/.azure-pipelines/windows.yml b/.azure-pipelines/windows.yml
index f2907a5..6365a53 100644
--- a/.azure-pipelines/windows.yml
+++ b/.azure-pipelines/windows.yml
@@ -35,4 +35,9 @@ jobs:
- script: |
pytest -vs -n auto --cov pydra --cov-config .coveragerc --cov-report xml:cov.xml --doctest-modules pydra
displayName: 'Pytest tests'
-
+ - script: |
+ python -m pip install codecov
+ codecov --file cov.xml
+ displayName: 'Upload To Codecov'
+ env:
+ CODECOV_TOKEN: $(CODECOV_TOKEN)
diff --git a/README.md b/README.md
index 44f2bda..17b5c8c 100644
--- a/README.md
+++ b/README.md
@@ -18,6 +18,7 @@ Feature list:
and Dask (this is an experimental implementation with limited testing)
[[API Documentation](https://nipype.github.io/pydra/)]
+[[PyCon 2020 Poster](https://docs.google.com/presentation/d/10tS2I34rS0G9qz6v29qVd77OUydjP_FdBklrgAGmYSw/edit?usp=sharing)]
### Tutorial
This tutorial will walk you through the main concepts of Pydra!
diff --git a/pydra/engine/task.py b/pydra/engine/task.py
index 8cb088a..d8aaa60 100644
--- a/pydra/engine/task.py
+++ b/pydra/engine/task.py
@@ -199,19 +199,21 @@ class FunctionTask(TaskBase):
del inputs["_func"]
self.output_ = None
output = cp.loads(self.inputs._func)(**inputs)
- if output is not None:
- output_names = [el[0] for el in self.output_spec.fields]
- self.output_ = {}
- if len(output_names) > 1:
- if len(output_names) == len(output):
+ output_names = [el[0] for el in self.output_spec.fields]
+ if output is None:
+ self.output_ = dict((nm, None) for nm in output_names)
+ else:
+ if len(output_names) == 1:
+ # if only one element in the fields, everything should be returned together
+ self.output_ = {output_names[0]: output}
+ else:
+ if isinstance(output, tuple) and len(output_names) == len(output):
self.output_ = dict(zip(output_names, output))
else:
raise Exception(
f"expected {len(self.output_spec.fields)} elements, "
- f"but {len(output)} were returned"
+ f"but {output} were returned"
)
- else: # if only one element in the fields, everything should be returned together
- self.output_[output_names[0]] = output
class ShellCommandTask(TaskBase):
| nipype/pydra | 0067eeee42a8db39a80653e3821056c6ad87ead2 | diff --git a/pydra/engine/tests/test_task.py b/pydra/engine/tests/test_task.py
index f7f4182..eaa21f9 100644
--- a/pydra/engine/tests/test_task.py
+++ b/pydra/engine/tests/test_task.py
@@ -309,6 +309,31 @@ def test_exception_func():
assert pytest.raises(Exception, bad_funk)
+def test_result_none_1():
+ """ checking if None is properly returned as the result"""
+
+ @mark.task
+ def fun_none(x):
+ return None
+
+ task = fun_none(name="none", x=3)
+ res = task()
+ assert res.output.out is None
+
+
+def test_result_none_2():
+ """ checking if None is properly set for all outputs """
+
+ @mark.task
+ def fun_none(x) -> (ty.Any, ty.Any):
+ return None
+
+ task = fun_none(name="none", x=3)
+ res = task()
+ assert res.output.out1 is None
+ assert res.output.out2 is None
+
+
def test_audit_prov(tmpdir):
@mark.task
def testfunc(a: int, b: float = 0.1) -> ty.NamedTuple("Output", [("out", float)]):
| Task crashes when function returns None
in both cases the named outputs should be set to `None`.
```
import pydra
import typing as ty
import os
@pydra.mark.task
@pydra.mark.annotate({"return": {"b": ty.Any}})
def test_multiout(val, val2):
return None
if __name__ == "__main__":
cache_dir = os.path.join(os.getcwd(), 'cache3')
task = test_multiout(name="mo2",
val=[0, 1, 2],
val2=[4, 5, 6],
cache_dir=cache_dir)
with pydra.Submitter(plugin="cf", n_procs=2) as sub:
sub(runnable=task)
results = task.result()
print(results)
```
this should also be checked for multiple outputs.
```
@pydra.mark.task
@pydra.mark.annotate({"return": {"a": ty.Any, "b": ty.Any}})
def test_multiout(val, val2):
return None
``` | 0.0 | [
"pydra/engine/tests/test_task.py::test_result_none_1",
"pydra/engine/tests/test_task.py::test_result_none_2"
] | [
"pydra/engine/tests/test_task.py::test_output",
"pydra/engine/tests/test_task.py::test_numpy",
"pydra/engine/tests/test_task.py::test_annotated_func",
"pydra/engine/tests/test_task.py::test_annotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_annotated_func_multreturn_exception",
"pydra/engine/tests/test_task.py::test_halfannotated_func",
"pydra/engine/tests/test_task.py::test_halfannotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_notannotated_func",
"pydra/engine/tests/test_task.py::test_notannotated_func_returnlist",
"pydra/engine/tests/test_task.py::test_halfannotated_func_multrun_returnlist",
"pydra/engine/tests/test_task.py::test_notannotated_func_multreturn",
"pydra/engine/tests/test_task.py::test_exception_func",
"pydra/engine/tests/test_task.py::test_audit_prov",
"pydra/engine/tests/test_task.py::test_audit_all",
"pydra/engine/tests/test_task.py::test_shell_cmd",
"pydra/engine/tests/test_task.py::test_container_cmds",
"pydra/engine/tests/test_task.py::test_docker_cmd",
"pydra/engine/tests/test_task.py::test_singularity_cmd",
"pydra/engine/tests/test_task.py::test_functask_callable",
"pydra/engine/tests/test_task.py::test_taskhooks"
] | 2020-05-17 05:34:08+00:00 | 4,217 |
|
nipype__pydra-344 | diff --git a/pydra/engine/core.py b/pydra/engine/core.py
index b04a50e..90bd3d3 100644
--- a/pydra/engine/core.py
+++ b/pydra/engine/core.py
@@ -34,7 +34,6 @@ from .helpers import (
ensure_list,
record_error,
hash_function,
- output_from_inputfields,
)
from .helpers_file import copyfile_input, template_update
from .graph import DiGraph
@@ -312,7 +311,7 @@ class TaskBase:
@property
def output_names(self):
"""Get the names of the outputs generated by the task."""
- return output_from_inputfields(self.output_spec, self.inputs, names_only=True)
+ return [f.name for f in attr.fields(make_klass(self.output_spec))]
@property
def can_resume(self):
@@ -411,7 +410,7 @@ class TaskBase:
try:
self.audit.monitor()
self._run_task()
- result.output = self._collect_outputs()
+ result.output = self._collect_outputs(output_dir=odir)
except Exception as e:
record_error(self.output_dir, e)
result.errored = True
@@ -429,12 +428,11 @@ class TaskBase:
self.hooks.post_run(self, result)
return result
- def _collect_outputs(self):
+ def _collect_outputs(self, output_dir):
run_output = self.output_
- self.output_spec = output_from_inputfields(self.output_spec, self.inputs)
output_klass = make_klass(self.output_spec)
output = output_klass(**{f.name: None for f in attr.fields(output_klass)})
- other_output = output.collect_additional_outputs(self.inputs, self.output_dir)
+ other_output = output.collect_additional_outputs(self.inputs, output_dir)
return attr.evolve(output, **run_output, **other_output)
def split(self, splitter, overwrite=False, **kwargs):
@@ -996,7 +994,22 @@ class Workflow(TaskBase):
)
self._connections += new_connections
- fields = [(name, ty.Any) for name, _ in self._connections]
+ fields = []
+ for con in self._connections:
+ wf_out_nm, lf = con
+ task_nm, task_out_nm = lf.name, lf.field
+ if task_out_nm == "all_":
+ help_string = f"all outputs from {task_nm}"
+ fields.append((wf_out_nm, dict, {"help_string": help_string}))
+ else:
+ # getting information about the output field from the task output_spec
+ # providing proper type and some help string
+ task_output_spec = getattr(self, task_nm).output_spec
+ out_fld = attr.fields_dict(make_klass(task_output_spec))[task_out_nm]
+ help_string = (
+ f"{out_fld.metadata.get('help_string', '')} (from {task_nm})"
+ )
+ fields.append((wf_out_nm, out_fld.type, {"help_string": help_string}))
self.output_spec = SpecInfo(name="Output", fields=fields, bases=(BaseSpec,))
logger.info("Added %s to %s", self.output_spec, self)
diff --git a/pydra/engine/helpers.py b/pydra/engine/helpers.py
index c1a30bf..975ef8e 100644
--- a/pydra/engine/helpers.py
+++ b/pydra/engine/helpers.py
@@ -155,8 +155,14 @@ def copyfile_workflow(wf_path, result):
""" if file in the wf results, the file will be copied to the workflow directory"""
for field in attr_fields(result.output):
value = getattr(result.output, field.name)
- new_value = _copyfile_single_value(wf_path=wf_path, value=value)
- if new_value != value:
+ # if the field is a path or it can contain a path _copyfile_single_value is run
+ # to move all files and directories to the workflow directory
+ if field.type in [File, Directory, MultiOutputObj] or type(value) in [
+ list,
+ tuple,
+ dict,
+ ]:
+ new_value = _copyfile_single_value(wf_path=wf_path, value=value)
setattr(result.output, field.name, new_value)
return result
@@ -672,7 +678,7 @@ def hash_value(value, tp=None, metadata=None):
return value
-def output_from_inputfields(output_spec, inputs, names_only=False):
+def output_from_inputfields(output_spec, input_spec):
"""
Collect values from output from input fields.
If names_only is False, the output_spec is updated,
@@ -682,30 +688,26 @@ def output_from_inputfields(output_spec, inputs, names_only=False):
----------
output_spec :
TODO
- inputs :
+ input_spec :
TODO
"""
current_output_spec_names = [f.name for f in attr.fields(make_klass(output_spec))]
new_fields = []
- for fld in attr_fields(inputs):
+ for fld in attr.fields(make_klass(input_spec)):
if "output_file_template" in fld.metadata:
- value = getattr(inputs, fld.name)
if "output_field_name" in fld.metadata:
field_name = fld.metadata["output_field_name"]
else:
field_name = fld.name
# not adding if the field already in teh output_spec
if field_name not in current_output_spec_names:
+ # TODO: should probably remove some of the keys
new_fields.append(
- (field_name, attr.ib(type=File, metadata={"value": value}))
+ (field_name, attr.ib(type=File, metadata=fld.metadata))
)
- if names_only:
- new_names = [el[0] for el in new_fields]
- return current_output_spec_names + new_names
- else:
- output_spec.fields += new_fields
- return output_spec
+ output_spec.fields += new_fields
+ return output_spec
def get_available_cpus():
diff --git a/pydra/engine/task.py b/pydra/engine/task.py
index 3a5f0d7..f86c917 100644
--- a/pydra/engine/task.py
+++ b/pydra/engine/task.py
@@ -57,7 +57,13 @@ from .specs import (
SingularitySpec,
attr_fields,
)
-from .helpers import ensure_list, execute, position_adjustment, argstr_formatting
+from .helpers import (
+ ensure_list,
+ execute,
+ position_adjustment,
+ argstr_formatting,
+ output_from_inputfields,
+)
from .helpers_file import template_update, is_local_file
@@ -276,6 +282,7 @@ class ShellCommandTask(TaskBase):
output_spec = SpecInfo(name="Output", fields=[], bases=(ShellOutSpec,))
self.output_spec = output_spec
+ self.output_spec = output_from_inputfields(self.output_spec, self.input_spec)
super().__init__(
name=name,
| nipype/pydra | 79573fde5a43a5cdff714b819f2b49185d8f85c9 | diff --git a/pydra/engine/tests/test_numpy_examples.py b/pydra/engine/tests/test_numpy_examples.py
index 55884ac..35b8972 100644
--- a/pydra/engine/tests/test_numpy_examples.py
+++ b/pydra/engine/tests/test_numpy_examples.py
@@ -19,6 +19,22 @@ def arrayout(val):
def test_multiout(plugin):
""" testing a simple function that returns a numpy array"""
+ wf = Workflow("wf", input_spec=["val"], val=2)
+ wf.add(arrayout(name="mo", val=wf.lzin.val))
+
+ wf.set_output([("array", wf.mo.lzout.b)])
+
+ with Submitter(plugin=plugin, n_procs=2) as sub:
+ sub(runnable=wf)
+
+ results = wf.result(return_inputs=True)
+
+ assert results[0] == {"wf.val": 2}
+ assert np.array_equal(results[1].output.array, np.array([2, 2]))
+
+
+def test_multiout_st(plugin):
+ """ testing a simple function that returns a numpy array, adding splitter"""
wf = Workflow("wf", input_spec=["val"], val=[0, 1, 2])
wf.add(arrayout(name="mo", val=wf.lzin.val))
wf.mo.split("val").combine("val")
diff --git a/pydra/engine/tests/utils.py b/pydra/engine/tests/utils.py
index ad08788..b2fbdab 100644
--- a/pydra/engine/tests/utils.py
+++ b/pydra/engine/tests/utils.py
@@ -164,7 +164,7 @@ def fun_dict(d):
@mark.task
-def fun_write_file(filename: ty.Union[str, File, Path], text="hello"):
+def fun_write_file(filename: ty.Union[str, File, Path], text="hello") -> File:
with open(filename, "w") as f:
f.write(text)
return Path(filename).absolute()
| More issues with numpy arrays
Still having errors with comparing arrays, now in `copyfile_workflow`. It's somewhat confusing that `value` can be the actual output or the path to output file.
Error:
```python
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-2-418998bcfc4f> in <module>
27
28 with pydra.Submitter(plugin="cf") as sub:
---> 29 sub(wf2)
30
31 wf2.result(return_inputs=True)
~/Desktop/nlo/pydra/pydra/engine/submitter.py in __call__(self, runnable, cache_locations, rerun)
57 self.loop.run_until_complete(self.submit_workflow(runnable, rerun=rerun))
58 else:
---> 59 self.loop.run_until_complete(self.submit(runnable, wait=True, rerun=rerun))
60 if is_workflow(runnable):
61 # resetting all connections with LazyFields
~/anaconda3/envs/pydra/lib/python3.7/asyncio/base_events.py in run_until_complete(self, future)
581 raise RuntimeError('Event loop stopped before Future completed.')
582
--> 583 return future.result()
584
585 def stop(self):
~/Desktop/nlo/pydra/pydra/engine/submitter.py in submit(self, runnable, wait, rerun)
122 # run coroutines concurrently and wait for execution
123 # wait until all states complete or error
--> 124 await asyncio.gather(*futures)
125 return
126 # pass along futures to be awaited independently
~/Desktop/nlo/pydra/pydra/engine/submitter.py in submit_workflow(self, workflow, rerun)
69 await self.worker.run_el(workflow, rerun=rerun)
70 else:
---> 71 await workflow._run(self, rerun=rerun)
72
73 async def submit(self, runnable, wait=False, rerun=False):
~/Desktop/nlo/pydra/pydra/engine/core.py in _run(self, submitter, rerun, **kwargs)
907 self.hooks.post_run_task(self, result)
908 self.audit.finalize_audit(result=result)
--> 909 save(odir, result=result, task=self)
910 os.chdir(cwd)
911 self.hooks.post_run(self, result)
~/Desktop/nlo/pydra/pydra/engine/helpers.py in save(task_path, result, task)
115 if Path(task_path).name.startswith("Workflow"):
116 # copy files to the workflow directory
--> 117 result = copyfile_workflow(wf_path=task_path, result=result)
118 with (task_path / "_result.pklz").open("wb") as fp:
119 cp.dump(result, fp)
~/Desktop/nlo/pydra/pydra/engine/helpers.py in copyfile_workflow(wf_path, result)
128 value = getattr(result.output, field.name)
129 new_value = _copyfile_single_value(wf_path=wf_path, value=value)
--> 130 if new_value != value:
131 setattr(result.output, field.name, new_value)
132 return result
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
```
To replicate:
```python
import pydra
import typing as ty
@pydra.mark.task
@pydra.mark.annotate({"return": {'scores': ty.Any}})
def calc_metric(score):
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.model_selection import cross_val_score
X, y = datasets.load_digits(n_class=10, return_X_y=True)
X_tr, X_tt, y_tr, y_tt = train_test_split(X, y)
clf = SVC()
clf.fit(X_tr, y_tr)
cv_scores = cross_val_score(clf, X_tt, y_tt, scoring=f'{score}_macro')
return(cv_scores)
# workflow
wf2 = pydra.Workflow(name="svm",
input_spec=['score'], score=['precision', 'recall'])
wf2.split('score')
wf2.add(calc_metric(name='calc_metric', score=wf2.lzin.score))
wf2.set_output([("scores", wf2.calc_metric.lzout.scores)])
with pydra.Submitter(plugin="cf") as sub:
sub(wf2)
wf2.result(return_inputs=True)
``` | 0.0 | [
"pydra/engine/tests/test_numpy_examples.py::test_multiout[cf]"
] | [
"pydra/engine/tests/test_numpy_examples.py::test_multiout_st[cf]"
] | 2020-09-12 16:23:10+00:00 | 4,218 |
|
nipype__pydra-614 | diff --git a/.pre-commit-config.yaml b/.pre-commit-config.yaml
index 37543f0..d4499fe 100644
--- a/.pre-commit-config.yaml
+++ b/.pre-commit-config.yaml
@@ -9,7 +9,7 @@ repos:
- id: check-yaml
- id: check-added-large-files
- repo: https://github.com/psf/black
- rev: 22.12.0
+ rev: 23.1.0
hooks:
- id: black
- repo: https://github.com/codespell-project/codespell
diff --git a/pydra/__init__.py b/pydra/__init__.py
index cb6823d..0416c9b 100644
--- a/pydra/__init__.py
+++ b/pydra/__init__.py
@@ -23,7 +23,6 @@ from . import mark
def check_latest_version():
-
import etelemetry
return etelemetry.check_available_version("nipype/pydra", __version__, lgr=logger)
diff --git a/pydra/engine/core.py b/pydra/engine/core.py
index e0ccd75..31127ca 100644
--- a/pydra/engine/core.py
+++ b/pydra/engine/core.py
@@ -696,7 +696,7 @@ class TaskBase:
def _combined_output(self, return_inputs=False):
combined_results = []
- for (gr, ind_l) in self.state.final_combined_ind_mapping.items():
+ for gr, ind_l in self.state.final_combined_ind_mapping.items():
combined_results_gr = []
for ind in ind_l:
result = load_result(self.checksum_states(ind), self.cache_locations)
@@ -1253,7 +1253,7 @@ class Workflow(TaskBase):
for task in self.graph.nodes:
self.create_connections(task, detailed=True)
# adding wf outputs
- for (wf_out, lf) in self._connections:
+ for wf_out, lf in self._connections:
self.graph.add_edges_description((self.name, wf_out, lf.name, lf.field))
dotfile = self.graph.create_dotfile_detailed(outdir=outdir, name=name)
else:
diff --git a/pydra/engine/graph.py b/pydra/engine/graph.py
index 01de5cc..7b2724e 100644
--- a/pydra/engine/graph.py
+++ b/pydra/engine/graph.py
@@ -85,7 +85,7 @@ class DiGraph:
"""Add edges to the graph (nodes should be already set)."""
if edges:
edges = ensure_list(edges)
- for (nd_out, nd_in) in edges:
+ for nd_out, nd_in in edges:
if nd_out not in self.nodes or nd_in not in self.nodes:
raise Exception(
f"edge {(nd_out, nd_in)} can't be added to the graph"
@@ -131,7 +131,7 @@ class DiGraph:
self.predecessors[nd.name] = []
self.successors[nd.name] = []
- for (nd_out, nd_in) in self.edges:
+ for nd_out, nd_in in self.edges:
self.predecessors[nd_in.name].append(nd_out)
self.successors[nd_out.name].append(nd_in)
@@ -148,7 +148,7 @@ class DiGraph:
def add_edges(self, new_edges):
"""Add new edges and sort the new graph."""
self.edges = self._edges + ensure_list(new_edges)
- for (nd_out, nd_in) in ensure_list(new_edges):
+ for nd_out, nd_in in ensure_list(new_edges):
self.predecessors[nd_in.name].append(nd_out)
self.successors[nd_out.name].append(nd_in)
if self._sorted_nodes is not None:
diff --git a/pydra/engine/helpers_state.py b/pydra/engine/helpers_state.py
index d4e527b..4eb9248 100644
--- a/pydra/engine/helpers_state.py
+++ b/pydra/engine/helpers_state.py
@@ -237,7 +237,7 @@ def remove_inp_from_splitter_rpn(splitter_rpn, inputs_to_remove):
stack_inp = []
stack_sgn = []
from_last_sign = []
- for (ii, el) in enumerate(splitter_rpn_copy):
+ for ii, el in enumerate(splitter_rpn_copy):
# element is a sign
if el == "." or el == "*":
stack_sgn.append((ii, el))
@@ -589,7 +589,7 @@ def combine_final_groups(combiner, groups, groups_stack, keys):
gr_final = list(gr_final)
map_gr_nr = {nr: i for (i, nr) in enumerate(sorted(gr_final))}
groups_final_map = {}
- for (inp, gr) in groups_final.items():
+ for inp, gr in groups_final.items():
if isinstance(gr, int):
groups_final_map[inp] = map_gr_nr[gr]
elif isinstance(gr, list):
diff --git a/pydra/engine/task.py b/pydra/engine/task.py
index 135af50..06f5133 100644
--- a/pydra/engine/task.py
+++ b/pydra/engine/task.py
@@ -371,7 +371,7 @@ class ShellCommandTask(TaskBase):
return value
def _command_shelltask_executable(self, field):
- """Returining position and value for executable ShellTask input"""
+ """Returning position and value for executable ShellTask input"""
pos = 0 # executable should be the first el. of the command
value = self._field_value(field)
if value is None:
@@ -379,7 +379,7 @@ class ShellCommandTask(TaskBase):
return pos, ensure_list(value, tuple2list=True)
def _command_shelltask_args(self, field):
- """Returining position and value for args ShellTask input"""
+ """Returning position and value for args ShellTask input"""
pos = -1 # assuming that args is the last el. of the command
value = self._field_value(field, check_file=True)
if value is None:
@@ -396,7 +396,7 @@ class ShellCommandTask(TaskBase):
argstr = field.metadata.get("argstr", None)
formatter = field.metadata.get("formatter", None)
if argstr is None and formatter is None:
- # assuming that input that has no arstr is not used in the command,
+ # assuming that input that has no argstr is not used in the command,
# or a formatter is not provided too.
return None
pos = field.metadata.get("position", None)
@@ -429,7 +429,7 @@ class ShellCommandTask(TaskBase):
cmd_add = []
# formatter that creates a custom command argument
- # it can thake the value of the filed, all inputs, or the value of other fields.
+ # it can take the value of the field, all inputs, or the value of other fields.
if "formatter" in field.metadata:
call_args = inspect.getfullargspec(field.metadata["formatter"])
call_args_val = {}
@@ -453,12 +453,16 @@ class ShellCommandTask(TaskBase):
cmd_add += split_cmd(cmd_el_str)
elif field.type is bool:
# if value is simply True the original argstr is used,
- # if False, nothing is added to the command
+ # if False, nothing is added to the command.
if value is True:
cmd_add.append(argstr)
else:
sep = field.metadata.get("sep", " ")
- if argstr.endswith("...") and isinstance(value, list):
+ if (
+ argstr.endswith("...")
+ and isinstance(value, ty.Iterable)
+ and not isinstance(value, (str, bytes))
+ ):
argstr = argstr.replace("...", "")
# if argstr has a more complex form, with "{input_field}"
if "{" in argstr and "}" in argstr:
@@ -474,7 +478,9 @@ class ShellCommandTask(TaskBase):
else:
# in case there are ... when input is not a list
argstr = argstr.replace("...", "")
- if isinstance(value, list):
+ if isinstance(value, ty.Iterable) and not isinstance(
+ value, (str, bytes)
+ ):
cmd_el_str = sep.join([str(val) for val in value])
value = cmd_el_str
# if argstr has a more complex form, with "{input_field}"
@@ -505,10 +511,10 @@ class ShellCommandTask(TaskBase):
command_args = self.container_args + self.command_args
else:
command_args = self.command_args
- # Skip the executable, which can be a multi-part command, e.g. 'docker run'.
+ # Skip the executable, which can be a multipart command, e.g. 'docker run'.
cmdline = command_args[0]
for arg in command_args[1:]:
- # If there are spaces in the arg and it is not enclosed by matching
+ # If there are spaces in the arg, and it is not enclosed by matching
# quotes, add quotes to escape the space. Not sure if this should
# be expanded to include other special characters apart from spaces
if " " in arg:
@@ -600,7 +606,7 @@ class ContainerTask(ShellCommandTask):
def _field_value(self, field, check_file=False):
"""
Checking value of the specific field, if value is not set, None is returned.
- If check_file is True, checking if field is a a local file
+ If check_file is True, checking if field is a local file
and settings bindings if needed.
"""
value = super()._field_value(field)
@@ -654,7 +660,6 @@ class ContainerTask(ShellCommandTask):
or "pydra.engine.specs.File" in str(fld.type)
or "pydra.engine.specs.Directory" in str(fld.type)
):
-
if fld.name == "image":
continue
file = Path(getattr(self.inputs, fld.name))
@@ -855,12 +860,12 @@ def split_cmd(cmd: str):
str
the command line string split into process args
"""
- # Check whether running on posix or windows system
+ # Check whether running on posix or Windows system
on_posix = platform.system() != "Windows"
args = shlex.split(cmd, posix=on_posix)
cmd_args = []
for arg in args:
- match = re.match("('|\")(.*)\\1$", arg)
+ match = re.match("(['\"])(.*)\\1$", arg)
if match:
cmd_args.append(match.group(2))
else:
diff --git a/pydra/engine/workers.py b/pydra/engine/workers.py
index 54b8129..152a868 100644
--- a/pydra/engine/workers.py
+++ b/pydra/engine/workers.py
@@ -758,7 +758,6 @@ class SGEWorker(DistributedWorker):
self.output_by_jobid[jobid] = (rc, stdout, stderr)
for task_pkl, ind, rerun in tasks_to_run:
-
self.jobid_by_task_uid[Path(task_pkl).parent.name] = jobid
if error_file:
@@ -813,7 +812,6 @@ class SGEWorker(DistributedWorker):
await asyncio.sleep(self.poll_delay)
async def _poll_job(self, jobid, cache_dir):
-
cmd = (f"qstat", "-j", jobid)
logger.debug(f"Polling job {jobid}")
rc, stdout, stderr = await read_and_display_async(*cmd, hide_display=True)
| nipype/pydra | a9a116d886fd9263ade5fcf4a3fb2a73d1676064 | diff --git a/pydra/engine/tests/test_shelltask.py b/pydra/engine/tests/test_shelltask.py
index 79f1a8c..f77dd06 100644
--- a/pydra/engine/tests/test_shelltask.py
+++ b/pydra/engine/tests/test_shelltask.py
@@ -1674,6 +1674,40 @@ def test_shell_cmd_inputspec_12(tmpdir, plugin, results_function):
assert shelly.output_dir == res.output.file_copy.parent
+def test_shell_cmd_inputspec_with_iterable():
+ """Test formatting of argstr with different iterable types."""
+
+ input_spec = SpecInfo(
+ name="Input",
+ fields=[
+ (
+ "iterable_1",
+ ty.Iterable[int],
+ {
+ "help_string": "iterable input 1",
+ "argstr": "--in1",
+ },
+ ),
+ (
+ "iterable_2",
+ ty.Iterable[str],
+ {
+ "help_string": "iterable input 2",
+ "argstr": "--in2...",
+ },
+ ),
+ ],
+ bases=(ShellSpec,),
+ )
+
+ task = ShellCommandTask(name="test", input_spec=input_spec, executable="test")
+
+ for iterable_type in (list, tuple):
+ task.inputs.iterable_1 = iterable_type(range(3))
+ task.inputs.iterable_2 = iterable_type(["bar", "foo"])
+ assert task.cmdline == "test --in1 0 1 2 --in2 bar --in2 foo"
+
+
@pytest.mark.parametrize("results_function", [result_no_submitter, result_submitter])
def test_shell_cmd_inputspec_copyfile_1(plugin, results_function, tmpdir):
"""shelltask changes a file in place,
@@ -3251,6 +3285,7 @@ def test_shell_cmd_outputspec_8d(tmpdir, plugin, results_function):
"""
customised output_spec, adding Directory to the output named by input spec
"""
+
# For /tmp/some_dict/test this function returns "/test"
def get_lowest_directory(directory_path):
return str(directory_path).replace(str(Path(directory_path).parents[0]), "")
@@ -4395,7 +4430,8 @@ def test_shell_cmd_non_existing_outputs_1(tmpdir):
def test_shell_cmd_non_existing_outputs_2(tmpdir):
"""Checking that non existing output files do not return a phantom path,
- but return NOTHING instead. This test has one existing and one non existing output file."""
+ but return NOTHING instead. This test has one existing and one non existing output file.
+ """
input_spec = SpecInfo(
name="Input",
fields=[
@@ -4460,7 +4496,8 @@ def test_shell_cmd_non_existing_outputs_2(tmpdir):
def test_shell_cmd_non_existing_outputs_3(tmpdir):
"""Checking that non existing output files do not return a phantom path,
- but return NOTHING instead. This test has an existing mandatory output and another non existing output file."""
+ but return NOTHING instead. This test has an existing mandatory output and another non existing output file.
+ """
input_spec = SpecInfo(
name="Input",
fields=[
@@ -4886,7 +4923,6 @@ def test_shellspec_formatter_splitter_2(tmpdir):
@no_win
def test_shellcommand_error_msg(tmpdir):
-
script_path = Path(tmpdir) / "script.sh"
with open(script_path, "w") as f:
| Proper way to format a tuple parameter
I have got an interface which expects a parameter `foo` as a triple with the following format `--foo 1 2 3`
I reached for the following definition as a first attempt:
```python
input_spec = pydra.specs.SpecInfo(
name="Input",
fields=[
(
"foo",
ty.Tuple[int, int, int],
{
"help_string": "foo triple",
"argstr": "--foo {foo}",
},
),
],
bases=(pydra.specs.ShellSpec,),
```
Which does not work since it produces `--foo (1, 2, 3)`.
If I use `--foo...` and instantiate the interface with a list instead of tuple then I get `--foo 1 --foo 2 --foo 3`.
If I use `formatter` instead of `argstr` with `"formatter": lambda field: f"--foo {' '.join(field)}"`, I get an error because formatter seem to be called with each element of the list instead of the entire list (`TypeError: sequence item 0: expected str instance, int found`).
I am probably missing another option, hence this support request 🙏 | 0.0 | [
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_with_iterable"
] | [
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_1_strip[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_1_strip[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_2b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_3[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_4[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_5[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_6[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_7[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_1[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_2[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_2[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3c_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3c[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_3c[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4c_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_4d_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_5_nosubm[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_5_nosubm[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_5a_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_6[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_6[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_6a_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_6b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_6b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7c[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_7c[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_8[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_8[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_8a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_8a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9b[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9b[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9c[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9c[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9d[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_9d[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_10[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_10[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_10_err",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputsspec_11",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_12[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_12[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_1a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_1a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_typeval_1",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_typeval_2",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_1a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_1a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_2[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_2[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_3[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_state_3[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_state_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_copyfile_state_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_2[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_2a[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_3[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_3a[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_state_1[cf]",
"pydra/engine/tests/test_shelltask.py::test_wf_shell_cmd_ndst_1[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_1a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_1a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_1b_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_2[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_2[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_2a_exception[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_3[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_3[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_4[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_4[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_4a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_4a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_5[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_5[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_5a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_5a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_5b_error",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_6[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_6[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_6a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_7[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_7[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_7a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_7a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8a[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8a[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8b_error",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8c[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8c[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8d[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_8d[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_state_outputspec_1[cf-result_no_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_state_outputspec_1[cf-result_submitter]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_outputspec_wf_1[cf]",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_1",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_1a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_2",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_2a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_3",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_3a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_4",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_4a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_5",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_5a",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_5b",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_inputspec_outputspec_6_except",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_1",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_2",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_3",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_4",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_multi_1",
"pydra/engine/tests/test_shelltask.py::test_shell_cmd_non_existing_outputs_multi_2",
"pydra/engine/tests/test_shelltask.py::test_shellspec_formatter_1",
"pydra/engine/tests/test_shelltask.py::test_shellspec_formatter_splitter_2",
"pydra/engine/tests/test_shelltask.py::test_shellcommand_error_msg"
] | 2022-12-31 16:44:03+00:00 | 4,219 |
|
nirum__tableprint-17 | diff --git a/Makefile b/Makefile
index 6ad5c19..6fa34eb 100644
--- a/Makefile
+++ b/Makefile
@@ -9,7 +9,7 @@ upload:
twine upload dist/*
test2:
- python2 /Users/nirum/anaconda/bin/nosetests --logging-level=INFO
+ python2 -m nose --logging-level=INFO
test:
nosetests -v --with-coverage --cover-package=tableprint --logging-level=INFO
diff --git a/tableprint/metadata.py b/tableprint/metadata.py
index 2b1f034..c67d4f8 100644
--- a/tableprint/metadata.py
+++ b/tableprint/metadata.py
@@ -1,7 +1,7 @@
# -*- coding: utf-8 -*-
# Version info
-__version__ = '0.8.0'
+__version__ = '0.8.1'
__license__ = 'MIT'
# Project description(s)
diff --git a/tableprint/printer.py b/tableprint/printer.py
index eb2b4bf..45f85b8 100644
--- a/tableprint/printer.py
+++ b/tableprint/printer.py
@@ -22,13 +22,16 @@ from .utils import ansi_len, format_line, parse_width
__all__ = ('table', 'header', 'row', 'hrule', 'top', 'bottom', 'banner', 'dataframe', 'TableContext')
+# Defaults
STYLE = 'round'
WIDTH = 11
FMT = '5g'
+ALIGN = 'right'
+ALIGNMENTS = {"left": "<", "right": ">", "center": "^"}
class TableContext:
- def __init__(self, headers, width=WIDTH, style=STYLE, add_hr=True, out=sys.stdout):
+ def __init__(self, headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True, out=sys.stdout):
"""Context manager for table printing
Parameters
@@ -39,6 +42,9 @@ class TableContext:
width : int or array_like, optional
The width of each column in the table (Default: 11)
+ align : string
+ The alignment to use ('left', 'center', or 'right'). (Default: 'right')
+
style : string or tuple, optional
A formatting style. (Default: 'round')
@@ -52,9 +58,9 @@ class TableContext:
t.row(np.random.randn(3))
"""
self.out = out
- self.config = {'width': width, 'style': style}
+ self.config = {'width': width, 'style': style, 'align': align}
self.headers = header(headers, add_hr=add_hr, **self.config)
- self.bottom = bottom(len(headers), **self.config)
+ self.bottom = bottom(len(headers), width=width, style=style)
def __call__(self, data):
self.out.write(row(data, **self.config) + '\n')
@@ -70,7 +76,7 @@ class TableContext:
self.out.flush()
-def table(data, headers=None, format_spec=FMT, width=WIDTH, style=STYLE, out=sys.stdout):
+def table(data, headers=None, format_spec=FMT, width=WIDTH, align=ALIGN, style=STYLE, out=sys.stdout):
"""Print a table with the given data
Parameters
@@ -87,22 +93,26 @@ def table(data, headers=None, format_spec=FMT, width=WIDTH, style=STYLE, out=sys
width : int or array_like, optional
The width of each column in the table (Default: 11)
+ align : string
+ The alignment to use ('left', 'center', or 'right'). (Default: 'right')
+
style : string or tuple, optional
A formatting style. (Default: 'fancy_grid')
out : writer, optional
A file handle or object that has write() and flush() methods (Default: sys.stdout)
"""
+ # Number of columns in the table.
ncols = len(data[0]) if headers is None else len(headers)
tablestyle = STYLES[style]
widths = parse_width(width, ncols)
# Initialize with a hr or the header
tablestr = [hrule(ncols, widths, tablestyle.top)] \
- if headers is None else [header(headers, widths, style)]
+ if headers is None else [header(headers, width=widths, align=align, style=style)]
# parse each row
- tablestr += [row(d, widths, format_spec, style) for d in data]
+ tablestr += [row(d, widths, format_spec, align, style) for d in data]
# only add the final border if there was data in the table
if len(data) > 0:
@@ -113,7 +123,7 @@ def table(data, headers=None, format_spec=FMT, width=WIDTH, style=STYLE, out=sys
out.flush()
-def header(headers, width=WIDTH, style=STYLE, add_hr=True):
+def header(headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True):
"""Returns a formatted row of column header strings
Parameters
@@ -134,9 +144,10 @@ def header(headers, width=WIDTH, style=STYLE, add_hr=True):
"""
tablestyle = STYLES[style]
widths = parse_width(width, len(headers))
+ alignment = ALIGNMENTS[align]
# string formatter
- data = map(lambda x: ('{:^%d}' % (x[0] + ansi_len(x[1]))).format(x[1]), zip(widths, headers))
+ data = map(lambda x: ('{:%s%d}' % (alignment, x[0] + ansi_len(x[1]))).format(x[1]), zip(widths, headers))
# build the formatted str
headerstr = format_line(data, tablestyle.row)
@@ -149,7 +160,7 @@ def header(headers, width=WIDTH, style=STYLE, add_hr=True):
return headerstr
-def row(values, width=WIDTH, format_spec=FMT, style=STYLE):
+def row(values, width=WIDTH, format_spec=FMT, align=ALIGN, style=STYLE):
"""Returns a formatted row of data
Parameters
@@ -163,6 +174,9 @@ def row(values, width=WIDTH, format_spec=FMT, style=STYLE):
format_spec : string
The precision format string used to format numbers in the values array (Default: '5g')
+ align : string
+ The alignment to use ('left', 'center', or 'right'). (Default: 'right')
+
style : namedtuple, optional
A line formatting style
@@ -187,10 +201,10 @@ def row(values, width=WIDTH, format_spec=FMT, style=STYLE):
width, datum, prec = val
if isinstance(datum, string_types):
- return ('{:>%i}' % (width + ansi_len(datum))).format(datum)
+ return ('{:%s%i}' % (ALIGNMENTS[align], width + ansi_len(datum))).format(datum)
elif isinstance(datum, Number):
- return ('{:>%i.%s}' % (width, prec)).format(datum)
+ return ('{:%s%i.%s}' % (ALIGNMENTS[align], width, prec)).format(datum)
else:
raise ValueError('Elements in the values array must be strings, ints, or floats')
@@ -255,7 +269,7 @@ def banner(message, width=30, style='banner', out=sys.stdout):
out : writer
An object that has write() and flush() methods (Default: sys.stdout)
"""
- out.write(header([message], max(width, len(message)), style) + '\n')
+ out.write(header([message], width=max(width, len(message)), style=style) + '\n')
out.flush()
| nirum/tableprint | 0e658c09c0fd2e77c52b5403e4326093ac5fdff5 | diff --git a/tests/test_io.py b/tests/test_io.py
index c866986..d07a075 100644
--- a/tests/test_io.py
+++ b/tests/test_io.py
@@ -11,18 +11,18 @@ def test_context():
with TableContext('ABC', style='round', width=5, out=output) as t:
t([1, 2, 3])
t([4, 5, 6])
- assert output.getvalue() == '╭───────┬───────┬───────╮\n│ A │ B │ C │\n├───────┼───────┼───────┤\n│ 1 │ 2 │ 3 │\n│ 4 │ 5 │ 6 │\n╰───────┴───────┴───────╯\n'
+ assert output.getvalue() == '╭───────┬───────┬───────╮\n│ A │ B │ C │\n├───────┼───────┼───────┤\n│ 1 │ 2 │ 3 │\n│ 4 │ 5 │ 6 │\n╰───────┴───────┴───────╯\n'
def test_table():
"""Tests the table function"""
output = StringIO()
table([[1, 2, 3], [4, 5, 6]], 'ABC', style='round', width=5, out=output)
- assert output.getvalue() == '╭───────┬───────┬───────╮\n│ A │ B │ C │\n├───────┼───────┼───────┤\n│ 1 │ 2 │ 3 │\n│ 4 │ 5 │ 6 │\n╰───────┴───────┴───────╯\n'
+ assert output.getvalue() == '╭───────┬───────┬───────╮\n│ A │ B │ C │\n├───────┼───────┼───────┤\n│ 1 │ 2 │ 3 │\n│ 4 │ 5 │ 6 │\n╰───────┴───────┴───────╯\n'
output = StringIO()
table(["bar"], "foo", style='grid', width=3, out=output)
- assert output.getvalue() == '+---+---+---+\n| f | o | o |\n+---+---+---+\n| b| a| r|\n+---+---+---+\n'
+ assert output.getvalue() == '+---+---+---+\n| f| o| o|\n+---+---+---+\n| b| a| r|\n+---+---+---+\n'
def test_frame():
@@ -30,7 +30,7 @@ def test_frame():
df = pd.DataFrame({'a': [1,], 'b': [2,], 'c': [3,]})
output = StringIO()
dataframe(df, width=4, style='fancy_grid', out=output)
- assert output.getvalue() == '╒════╤════╤════╕\n│ a │ b │ c │\n╞════╪════╪════╡\n│ 1│ 2│ 3│\n╘════╧════╧════╛\n'
+ assert output.getvalue() == '╒════╤════╤════╕\n│ a│ b│ c│\n╞════╪════╪════╡\n│ 1│ 2│ 3│\n╘════╧════╧════╛\n'
def test_banner():
| Feature request: Horizontal alignment
This is a simple, lightweight, and awesome package and exactly what I needed to print some database data in my CLI app. Any chance you could add two parameters for horizontal alignment that takes a string for the alignment of the cell data, such as "left", "center", or "right"? Something like header_align and row_align?? As it is right now I have to pad all the strings to be the same length as the longest one otherwise the table is difficult to read.
Thanks so much! | 0.0 | [
"tests/test_io.py::test_context",
"tests/test_io.py::test_table",
"tests/test_io.py::test_frame"
] | [
"tests/test_io.py::test_banner",
"tests/test_io.py::test_hrule"
] | 2019-02-19 01:07:56+00:00 | 4,220 |
|
nirum__tableprint-19 | diff --git a/README.md b/README.md
index a7d8fba..4aac2fe 100644
--- a/README.md
+++ b/README.md
@@ -57,7 +57,7 @@ with tp.TableContext("ABC") as t:
Hosted at Read The Docs: [tableprint.readthedocs.org](http://tableprint.readthedocs.org)
## 📦 Dependencies
-- Python 3.6, 3.5, 3.4, or 2.7
+- Python 3.5+ or 2.7
- [future](https://pypi.org/project/future/)
- [six](https://pypi.org/project/six/)
@@ -67,6 +67,7 @@ Thanks to: [@nowox](https://github.com/nowox), [@nicktimko](https://github.com/n
## 🛠 Changelog
| Version | Release Date | Description |
| ---: | :---: | :--- |
+| 0.9.0 | May 16 2020 | Adds support for automatically determining the table's width.
| 0.8.0 | Oct 24 2017 | Improves support for international languages, removes numpy dependency
| 0.7.0 | May 26 2017 | Adds a TableContext context manager for easy creation of dynamic tables (tables that update periodically). Adds the ability to pass a list or tuple of widths to specify different widths for different columns
| 0.6.9 | May 25 2017 | Splitting the tableprint.py module into a pacakge with multiple files
diff --git a/tableprint/__init__.py b/tableprint/__init__.py
index 9ad1910..cd7fae9 100644
--- a/tableprint/__init__.py
+++ b/tableprint/__init__.py
@@ -1,7 +1,5 @@
# -*- coding: utf-8 -*-
-"""
-Tableprint
-"""
+"""Tableprint."""
from .metadata import __author__, __version__
from .printer import *
from .style import *
diff --git a/tableprint/metadata.py b/tableprint/metadata.py
index 6567af6..5505964 100644
--- a/tableprint/metadata.py
+++ b/tableprint/metadata.py
@@ -1,7 +1,7 @@
# -*- coding: utf-8 -*-
# Version info
-__version__ = '0.8.2'
+__version__ = '0.9.0'
__license__ = 'MIT'
# Project description(s)
diff --git a/tableprint/printer.py b/tableprint/printer.py
index 45f85b8..ac8d3ab 100644
--- a/tableprint/printer.py
+++ b/tableprint/printer.py
@@ -12,45 +12,50 @@ Usage
"""
from __future__ import print_function, unicode_literals
-import sys
+from itertools import chain, starmap
from numbers import Number
+import sys
from six import string_types
from .style import LineStyle, STYLES
-from .utils import ansi_len, format_line, parse_width
+from .utils import ansi_len, format_line, parse_width, max_width
__all__ = ('table', 'header', 'row', 'hrule', 'top', 'bottom', 'banner', 'dataframe', 'TableContext')
# Defaults
STYLE = 'round'
-WIDTH = 11
FMT = '5g'
ALIGN = 'right'
ALIGNMENTS = {"left": "<", "right": ">", "center": "^"}
class TableContext:
- def __init__(self, headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True, out=sys.stdout):
+ def __init__(self, headers, width=11, align=ALIGN, style=STYLE, add_hr=True, out=sys.stdout):
"""Context manager for table printing
Parameters
----------
- headers : array_like
+ headers: array_like
A list of N strings consisting of the header of each of the N columns
- width : int or array_like, optional
+ width: int or array_like, optional
The width of each column in the table (Default: 11)
- align : string
+ align: str
The alignment to use ('left', 'center', or 'right'). (Default: 'right')
- style : string or tuple, optional
+ style: str or tuple, optional
A formatting style. (Default: 'round')
- add_hr : boolean, optional
+ add_hr: boolean, optional
Whether or not to add a horizontal rule (hr) after the headers
+ out: IO writer, optional
+ Object used to manage IO (displaying the table). Must have a write() method
+ that takes a string argument, and a flush() method. See sys.stdout for an
+ example. (Default: 'sys.stdout')
+
Usage
-----
>>> with TableContext("ABC") as t:
@@ -76,32 +81,41 @@ class TableContext:
self.out.flush()
-def table(data, headers=None, format_spec=FMT, width=WIDTH, align=ALIGN, style=STYLE, out=sys.stdout):
+def table(data, headers=None, format_spec=FMT, width=None, align=ALIGN, style=STYLE, out=sys.stdout):
"""Print a table with the given data
Parameters
----------
- data : array_like
+ data: array_like
An (m x n) array containing the data to print (m rows of n columns)
- headers : list, optional
+ headers: list, optional
A list of n strings consisting of the header of each of the n columns (Default: None)
- format_spec : string, optional
+ format_spec: string, optional
Format specification for formatting numbers (Default: '5g')
- width : int or array_like, optional
- The width of each column in the table (Default: 11)
+ width: int or None or array_like, optional
+ The width of each column in the table. If None, tries to estimate an appropriate width
+ based on the length of the data in the table. (Default: None)
- align : string
+ align: string
The alignment to use ('left', 'center', or 'right'). (Default: 'right')
- style : string or tuple, optional
+ style: string or tuple, optional
A formatting style. (Default: 'fancy_grid')
- out : writer, optional
- A file handle or object that has write() and flush() methods (Default: sys.stdout)
+ out: IO writer, optional
+ File handle or object used to manage IO (displaying the table). Must have a write()
+ method that takes a string argument, and a flush() method. See sys.stdout for an
+ example. (Default: 'sys.stdout')
"""
+ # Auto-width.
+ if width is None:
+ max_header_width = 0 if headers is None else max_width(headers, FMT)
+ max_data_width = max_width(chain(*data), format_spec)
+ width = max(max_header_width, max_data_width)
+
# Number of columns in the table.
ncols = len(data[0]) if headers is None else len(headers)
tablestyle = STYLES[style]
@@ -123,18 +137,18 @@ def table(data, headers=None, format_spec=FMT, width=WIDTH, align=ALIGN, style=S
out.flush()
-def header(headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True):
+def header(headers, width=None, align=ALIGN, style=STYLE, add_hr=True):
"""Returns a formatted row of column header strings
Parameters
----------
- headers : list of strings
+ headers: list of strings
A list of n strings, the column headers
- width : int
- The width of each column (Default: 11)
+ width: int
+ The width of each column. If None, automatically determines the width. (Default: None)
- style : string or tuple, optional
+ style: string or tuple, optional
A formatting style (see STYLES)
Returns
@@ -142,6 +156,9 @@ def header(headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True):
headerstr : string
A string consisting of the full header row to print
"""
+ if width is None:
+ width = max_width(headers, FMT)
+
tablestyle = STYLES[style]
widths = parse_width(width, len(headers))
alignment = ALIGNMENTS[align]
@@ -160,31 +177,34 @@ def header(headers, width=WIDTH, align=ALIGN, style=STYLE, add_hr=True):
return headerstr
-def row(values, width=WIDTH, format_spec=FMT, align=ALIGN, style=STYLE):
+def row(values, width=None, format_spec=FMT, align=ALIGN, style=STYLE):
"""Returns a formatted row of data
Parameters
----------
- values : array_like
+ values: array_like
An iterable array of data (numbers or strings), each value is printed in a separate column
- width : int
- The width of each column (Default: 11)
+ width: int
+ The width of each column. If None, automatically determines the width. (Default: None)
- format_spec : string
+ format_spec: string
The precision format string used to format numbers in the values array (Default: '5g')
- align : string
+ align: string
The alignment to use ('left', 'center', or 'right'). (Default: 'right')
- style : namedtuple, optional
+ style: namedtuple, optional
A line formatting style
Returns
-------
- rowstr : string
+ rowstr: string
A string consisting of the full row of data to print
"""
+ if width is None:
+ width = max_width(values, format_spec)
+
tablestyle = STYLES[style]
widths = parse_width(width, len(values))
@@ -195,39 +215,34 @@ def row(values, width=WIDTH, format_spec=FMT, align=ALIGN, style=STYLE):
format_spec = [format_spec] * len(list(values))
# mapping function for string formatting
- def mapdata(val):
-
- # unpack
- width, datum, prec = val
-
+ def mapdata(width, datum, prec):
+ """Formats an individual piece of data."""
if isinstance(datum, string_types):
return ('{:%s%i}' % (ALIGNMENTS[align], width + ansi_len(datum))).format(datum)
-
elif isinstance(datum, Number):
return ('{:%s%i.%s}' % (ALIGNMENTS[align], width, prec)).format(datum)
-
else:
raise ValueError('Elements in the values array must be strings, ints, or floats')
# string formatter
- data = map(mapdata, zip(widths, values, format_spec))
+ data = starmap(mapdata, zip(widths, values, format_spec))
# build the row string
return format_line(data, tablestyle.row)
-def hrule(n=1, width=WIDTH, linestyle=LineStyle('', '─', '─', '')):
+def hrule(n=1, width=11, linestyle=LineStyle('', '─', '─', '')):
"""Returns a formatted string used as a border between table rows
Parameters
----------
- n : int
+ n: int
The number of columns in the table
- width : int
+ width: int
The width of each column (Default: 11)
- linestyle : tuple
+ linestyle: tuple
A LineStyle namedtuple containing the characters for (begin, hr, sep, end).
(Default: ('', '─', '─', ''))
@@ -242,12 +257,12 @@ def hrule(n=1, width=WIDTH, linestyle=LineStyle('', '─', '─', '')):
return linestyle.begin + hrstr + linestyle.end
-def top(n, width=WIDTH, style=STYLE):
+def top(n, width=11, style=STYLE):
"""Prints the top row of a table"""
return hrule(n, width, linestyle=STYLES[style].top)
-def bottom(n, width=WIDTH, style=STYLE):
+def bottom(n, width=11, style=STYLE):
"""Prints the top row of a table"""
return hrule(n, width, linestyle=STYLES[style].bottom)
@@ -257,16 +272,16 @@ def banner(message, width=30, style='banner', out=sys.stdout):
Parameters
----------
- message : string
+ message: string
The message to print in the banner
- width : int
+ width: int
The minimum width of the banner (Default: 30)
- style : string
+ style: string
A line formatting style (Default: 'banner')
- out : writer
+ out: writer
An object that has write() and flush() methods (Default: sys.stdout)
"""
out.write(header([message], width=max(width, len(message)), style=style) + '\n')
@@ -278,7 +293,7 @@ def dataframe(df, **kwargs):
Parameters
----------
- df : DataFrame
+ df: DataFrame
A pandas DataFrame with the table to print
"""
table(df.values, list(df.columns), **kwargs)
diff --git a/tableprint/utils.py b/tableprint/utils.py
index b4ed756..5f50636 100644
--- a/tableprint/utils.py
+++ b/tableprint/utils.py
@@ -1,12 +1,16 @@
# -*- coding: utf-8 -*-
-"""
-Tableprint utilities
-"""
+"""Tableprint utilities."""
+
from __future__ import print_function, unicode_literals
-from wcwidth import wcswidth
+
+from functools import reduce
import math
import re
+from numbers import Number
+from six import string_types
+from wcwidth import wcswidth
+
__all__ = ('humantime',)
@@ -78,7 +82,7 @@ def format_line(data, linestyle):
def parse_width(width, n):
- """Parses an int or array of widths
+ """Parses an int or array of widths.
Parameters
----------
@@ -89,7 +93,22 @@ def parse_width(width, n):
widths = [width] * n
else:
- assert len(width) == n, "Widths and data do not match"
+ assert len(width) == n, "Widths and data do not match."
widths = width
return widths
+
+
+def max_width(data, format_spec):
+ """Computes the maximum formatted width of an iterable of data."""
+
+ def compute_width(d):
+ """Computes the formatted width of single element."""
+ if isinstance(d, string_types):
+ return len(d)
+ if isinstance(d, Number):
+ return len(('{:0.%s}' % format_spec).format(d))
+ else:
+ raise ValueError('Elements in the values array must be strings, ints, or floats')
+
+ return reduce(max, map(compute_width, data))
| nirum/tableprint | 37e8db5d7ae74213b49a17987572094860abd786 | diff --git a/tests/test_functions.py b/tests/test_functions.py
index 7b24178..694929d 100644
--- a/tests/test_functions.py
+++ b/tests/test_functions.py
@@ -5,7 +5,7 @@ import pytest
def test_borders():
- """Tests printing of the top and bottom borders"""
+ """Tests printing of the top and bottom borders."""
# top
assert top(5, width=2, style='round') == '╭────┬────┬────┬────┬────╮'
@@ -17,7 +17,7 @@ def test_borders():
def test_row():
- """Tests printing of a single row of data"""
+ """Tests printing of a single row of data."""
# valid
assert row("abc", width=3, style='round') == '│ a │ b │ c │'
diff --git a/tests/test_utils.py b/tests/test_utils.py
index 92e3cc9..20d84ac 100644
--- a/tests/test_utils.py
+++ b/tests/test_utils.py
@@ -1,11 +1,26 @@
# -*- coding: utf-8 -*-
from __future__ import unicode_literals
+import numpy as np
from tableprint import humantime, LineStyle
-from tableprint.utils import format_line
+from tableprint.utils import format_line, max_width
import pytest
+def test_max_width():
+ """Tests the auto width feature"""
+
+ # Max width for strings.
+ assert max_width(['a', 'b', 'c'], None) == 1
+ assert max_width(['a', 'b', 'foo'], None) == 3
+
+ # Max width for numbers.
+ assert max_width([1, 2, 3], '0f') == 1
+ assert max_width([1, 2, 3], '2f') == 4
+ assert max_width([np.pi, np.pi], '3f') == 5
+
+
def test_format_line():
+ """Tests line formatting"""
# using ASCII
assert format_line(['foo', 'bar'], LineStyle('(', '_', '+', ')')) == '(foo+bar)'
@@ -19,6 +34,7 @@ def test_format_line():
def test_humantime():
+ """Tests the humantime utility"""
# test numeric input
assert humantime(1e6) == u'1 weeks, 4 days, 13 hours, 46 min., 40 s'
| Auto-width
Love tableprint!
Since everything is monospaced, it should be easy to set the width on each column automatically based on the longest string value of each column (and its header). Could you please implement this? It would save precious horizontal space and fit more columns. | 0.0 | [
"tests/test_functions.py::test_borders",
"tests/test_functions.py::test_row",
"tests/test_utils.py::test_max_width",
"tests/test_utils.py::test_format_line",
"tests/test_utils.py::test_humantime"
] | [] | 2020-05-16 18:52:04+00:00 | 4,221 |
|
nithinmurali__pygsheets-272 | diff --git a/.gitignore b/.gitignore
index 9bc512f..3556492 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,22 +1,28 @@
+# Python
*.pyc
-.DS_Store
-.coverage
-
+build/
+dist/
venv/
-.idea/
.cache/
.pytest_cache/
-build/
-dist/
-# docs/
+pygsheets.egg-info/
+.pytest_cache/
+
+# File types
*.json
*.csv
+
+# Tests
test/*.json
test/usages
test/data/*.json
test/manual_test1.py
-pygsheets.egg-info/
+# Other
+.idea/
+.DS_Store
+.python-version
+.coverage
diff --git a/pygsheets/authorization.py b/pygsheets/authorization.py
index 6c1b37f..0d4420b 100644
--- a/pygsheets/authorization.py
+++ b/pygsheets/authorization.py
@@ -59,7 +59,6 @@ _SCOPES = ('https://www.googleapis.com/auth/spreadsheets', 'https://www.googleap
_deprecated_keyword_mapping = {
'outh_file': 'client_secret',
'outh_creds_store': 'credentials_directory',
- 'outh_nonlocal': 'non_local_authorization',
'service_file': 'service_account_file',
'credentials': 'custom_credentials'
}
@@ -86,13 +85,15 @@ def authorize(client_secret='client_secret.json',
:param kwargs: Parameters to be handed into the client constructor.
:returns: :class:`Client`
"""
- v = vars()
+
for key in kwargs:
if key in ['outh_file', 'outh_creds_store', 'service_file', 'credentials']:
warnings.warn('The argument {} is deprecated. Use {} instead.'.format(key, _deprecated_keyword_mapping[key])
, category=DeprecationWarning)
- v[_deprecated_keyword_mapping[key]] = kwargs[key]
- del kwargs[key]
+ client_secret = kwargs.get('outh_file', client_secret)
+ service_account_file = kwargs.get('service_file', service_account_file)
+ credentials_directory = kwargs.get('outh_creds_store', credentials_directory)
+ custom_credentials = kwargs.get('credentials', custom_credentials)
if custom_credentials is not None:
credentials = custom_credentials
| nithinmurali/pygsheets | 136c295143f6365bdbca70328a876b1cc66baab3 | diff --git a/test/authorization_tests.py b/test/authorization_tests.py
index eee5b06..16389cb 100644
--- a/test/authorization_tests.py
+++ b/test/authorization_tests.py
@@ -26,4 +26,8 @@ class TestAuthorization(object):
self.sheet = c.create('test_sheet')
self.sheet.share('[email protected]')
- self.sheet.delete()
\ No newline at end of file
+ self.sheet.delete()
+
+ def test_deprecated_kwargs_removal(self):
+ c = pygsheets.authorize(service_file=self.base_path + '/pygsheettest_service_account.json')
+ assert isinstance(c, Client)
\ No newline at end of file
| RuntimeError: dictionary changed size during iteration
https://github.com/nithinmurali/pygsheets/blob/0e2468fe9a1957b19f1cebe3667c601c32942bbd/pygsheets/authorization.py#L94
You can't delete keys from a dictionary you are currently iterating. | 0.0 | [
"test/authorization_tests.py::TestAuthorization::test_deprecated_kwargs_removal"
] | [
"test/authorization_tests.py::TestAuthorization::test_service_account_authorization",
"test/authorization_tests.py::TestAuthorization::test_user_credentials_loading"
] | 2018-08-23 06:30:01+00:00 | 4,222 |
|
nithinmurali__pygsheets-572 | diff --git a/pygsheets/authorization.py b/pygsheets/authorization.py
index 3da1580..7c921c3 100644
--- a/pygsheets/authorization.py
+++ b/pygsheets/authorization.py
@@ -5,6 +5,7 @@ import warnings
from google.oauth2 import service_account
from google.oauth2.credentials import Credentials
+from google.auth.exceptions import RefreshError
from google_auth_oauthlib.flow import Flow, InstalledAppFlow
from google.auth.transport.requests import Request
@@ -16,6 +17,22 @@ except NameError:
pass
+def _get_initial_user_authentication_credentials(client_secret_file, local, scopes):
+ if local:
+ flow = InstalledAppFlow.from_client_secrets_file(client_secret_file, scopes)
+ credentials = flow.run_local_server()
+ else:
+ flow = Flow.from_client_secrets_file(client_secret_file, scopes=scopes,
+ redirect_uri='urn:ietf:wg:oauth:2.0:oob')
+ auth_url, _ = flow.authorization_url(prompt='consent')
+
+ print('Please go to this URL and finish the authentication flow: {}'.format(auth_url))
+ code = input('Enter the authorization code: ')
+ flow.fetch_token(code=code)
+ credentials = flow.credentials
+ return credentials
+
+
def _get_user_authentication_credentials(client_secret_file, scopes, credential_directory=None, local=False):
"""Returns user credentials."""
if credential_directory is None:
@@ -37,20 +54,13 @@ def _get_user_authentication_credentials(client_secret_file, scopes, credential_
if credentials:
if credentials.expired and credentials.refresh_token:
- credentials.refresh(Request())
+ try:
+ credentials.refresh(Request())
+ except RefreshError as exc:
+ print(f'Refresh token is obsolete {exc}. Executing the initial flow')
+ credentials = _get_initial_user_authentication_credentials(client_secret_file, local, scopes)
else:
- if local:
- flow = InstalledAppFlow.from_client_secrets_file(client_secret_file, scopes)
- credentials = flow.run_local_server()
- else:
- flow = Flow.from_client_secrets_file(client_secret_file, scopes=scopes,
- redirect_uri='urn:ietf:wg:oauth:2.0:oob')
- auth_url, _ = flow.authorization_url(prompt='consent')
-
- print('Please go to this URL and finish the authentication flow: {}'.format(auth_url))
- code = input('Enter the authorization code: ')
- flow.fetch_token(code=code)
- credentials = flow.credentials
+ credentials = _get_initial_user_authentication_credentials(client_secret_file, local, scopes)
# Save the credentials for the next run
credentials_as_dict = {
| nithinmurali/pygsheets | 265f16caff33a7ee6eb0d648ec740d0e3eccf445 | diff --git a/tests/authorization_test.py b/tests/authorization_test.py
index d2abca3..8b5073d 100644
--- a/tests/authorization_test.py
+++ b/tests/authorization_test.py
@@ -1,8 +1,12 @@
+from google.auth.exceptions import RefreshError
+from google.oauth2.credentials import Credentials
import os
import sys
+from unittest.mock import patch
sys.path.append(os.path.dirname(os.path.dirname(os.path.abspath(__file__))))
import pygsheets
+from pygsheets.authorization import _SCOPES
from pygsheets.client import Client
from googleapiclient.http import HttpError
@@ -38,3 +42,35 @@ class TestAuthorization(object):
c = pygsheets.authorize(service_file=self.base_path + '/pygsheettest_service_account.json', retries=3)
assert isinstance(c, Client)
assert c.sheet.retries == 3
+
+ def test_should_reload_client_secret_on_refresh_error(self):
+ # First connection
+ initial_c = pygsheets.authorize(
+ client_secret=self.base_path + "/client_secret.json",
+ credentials_directory=self.base_path,
+ )
+ credentials_filepath = self.base_path + "/sheets.googleapis.com-python.json"
+ assert os.path.exists(credentials_filepath)
+
+ # After a while, the refresh token is not working and raises RefreshError
+ refresh_c = None
+ with patch(
+ "pygsheets.authorization._get_initial_user_authentication_credentials"
+ ) as mock_initial_credentials:
+ real_credentials = Credentials.from_authorized_user_file(
+ credentials_filepath, scopes=_SCOPES
+ )
+ mock_initial_credentials.return_value = real_credentials
+
+ with patch("pygsheets.authorization.Credentials") as mock_credentials:
+ mock_credentials.from_authorized_user_file.return_value.refresh.side_effect = RefreshError(
+ "Error using refresh token"
+ )
+ mock_initial_credentials
+ refresh_c = pygsheets.authorize(
+ client_secret=self.base_path + "/client_secret.json",
+ credentials_directory=self.base_path,
+ )
+
+ mock_initial_credentials.assert_called_once()
+ assert isinstance(refresh_c, Client)
| Token has been expired or revoked
Hello! How can I disable frequent refresh of the Token?
Quite often, you have to renew the token for the errors “Token has been expired or revoked.” | 0.0 | [
"tests/authorization_test.py::TestAuthorization::test_should_reload_client_secret_on_refresh_error"
] | [
"tests/authorization_test.py::TestAuthorization::test_service_account_authorization",
"tests/authorization_test.py::TestAuthorization::test_user_credentials_loading",
"tests/authorization_test.py::TestAuthorization::test_deprecated_kwargs_removal",
"tests/authorization_test.py::TestAuthorization::test_kwargs_passed_to_client"
] | 2023-02-08 18:25:00+00:00 | 4,223 |
|
nokia__moler-191 | diff --git a/moler/cmd/unix/scp.py b/moler/cmd/unix/scp.py
index 8033ef50..4db8aa82 100644
--- a/moler/cmd/unix/scp.py
+++ b/moler/cmd/unix/scp.py
@@ -2,6 +2,7 @@
"""
SCP command module.
"""
+
from moler.cmd.unix.genericunix import GenericUnixCommand
from moler.exceptions import CommandFailure
from moler.exceptions import ParsingDone
@@ -15,7 +16,7 @@ __email__ = '[email protected], [email protected], michal.erns
class Scp(GenericUnixCommand):
def __init__(self, connection, source, dest, password="", options="", prompt=None, newline_chars=None,
- known_hosts_on_failure='keygen', encrypt_password=True, runner=None):
+ known_hosts_on_failure='keygen', encrypt_password=True, runner=None, repeat_password=True):
"""
Represents Unix command scp.
@@ -28,6 +29,7 @@ class Scp(GenericUnixCommand):
:param known_hosts_on_failure: "rm" or "keygen" how to deal with error. If empty then scp fails.
:param encrypt_password: If True then * will be in logs when password is sent, otherwise plain text
:param runner: Runner to run command
+ :param repeat_password: If True then repeat last password if no more provided. If False then exception is set.
"""
super(Scp, self).__init__(connection=connection, prompt=prompt, newline_chars=newline_chars, runner=runner)
self.source = source
@@ -44,6 +46,8 @@ class Scp(GenericUnixCommand):
self._passwords = [password]
else:
self._passwords = list(password) # copy of list of passwords to modify
+ self.repeat_password = repeat_password
+ self._last_password = ""
def build_command_string(self):
"""
@@ -63,8 +67,8 @@ class Scp(GenericUnixCommand):
Put your parsing code here.
:param line: Line to process, can be only part of line. New line chars are removed from line.
- :param is_full_line: True if line had new line chars, False otherwise
- :return: Nothing
+ :param is_full_line: True if line had new line chars, False otherwise.
+ :return: None.
"""
try:
self._know_hosts_verification(line)
@@ -79,35 +83,38 @@ class Scp(GenericUnixCommand):
self._sent_password = False # Clear flag for multi passwords connections
return super(Scp, self).on_new_line(line, is_full_line)
- _re_parse_success = re.compile(r'^(?P<FILENAME>\S+)\s+.*\d+\%.*')
+ _re_parse_success = re.compile(r'^(?P<FILENAME>\S+)\s+.*\d+%.*')
def _parse_success(self, line):
"""
Parses line if success.
:param line: Line from device.
- :return: Nothing but raises ParsingDone if matches success
+ :return: None.
+ :raises ParsingDone: if matches success.
"""
if self._regex_helper.search_compiled(Scp._re_parse_success, line):
if 'FILE_NAMES' not in self.current_ret.keys():
self.current_ret['FILE_NAMES'] = list()
self.current_ret['FILE_NAMES'].append(self._regex_helper.group('FILENAME'))
- raise ParsingDone
+ raise ParsingDone()
_re_parse_failed = re.compile(
- r'(?P<FAILED>cannot access|Could not|no such|denied|not a regular file|Is a directory|No route to host|lost connection)')
+ r'cannot access|Could not|no such|denied|not a regular file|Is a directory|No route to host|"'
+ r'lost connection|Not a directory', re.IGNORECASE)
def _parse_failed(self, line):
"""
Parses line if failed.
:param line: Line from device.
- :return: Nothing but raises ParsingDone if matches fail
+ :return: None.
+ :raises ParsingDone: if matches fail.
"""
if self._regex_helper.search_compiled(Scp._re_parse_failed, line):
self.set_exception(CommandFailure(self, "Command failed in line >>{}<<.".format(line)))
- raise ParsingDone
+ raise ParsingDone()
_re_parse_permission_denied = re.compile(
r'Permission denied, please try again|Permission denied \(publickey,password\)')
@@ -117,14 +124,20 @@ class Scp(GenericUnixCommand):
Sends password if necessary.
:param line: Line from device.
- :return: Nothing but raises ParsingDone if password was sent.
+ :return: None.
+ :raises ParsingDone: if password was sent.
"""
if (not self._sent_password) and self._is_password_requested(line):
try:
pwd = self._passwords.pop(0)
+ self._last_password = pwd
self.connection.sendline(pwd, encrypt=self.encrypt_password)
except IndexError:
- self.set_exception(CommandFailure(self, "Password was requested but no more passwords provided."))
+ if self.repeat_password:
+ self.connection.sendline(self._last_password, encrypt=self.encrypt_password)
+ else:
+ self.set_exception(CommandFailure(self, "Password was requested but no more passwords provided."))
+ self.break_cmd()
self._sent_password = True
raise ParsingDone()
@@ -135,7 +148,7 @@ class Scp(GenericUnixCommand):
Parses line if password is requested.
:param line: Line from device.
- :return: Match object if matches, otherwise None
+ :return: Match object if matches, otherwise None.
"""
return self._regex_helper.search_compiled(Scp._re_password, line)
@@ -144,20 +157,22 @@ class Scp(GenericUnixCommand):
Sends yes to device if needed.
:param line: Line from device.
- :return: Nothing
+ :return: None.
+ :raises ParsingDone: if line handled by this method.
"""
if (not self._sent_continue_connecting) and self._parse_continue_connecting(line):
self.connection.sendline('yes')
self._sent_continue_connecting = True
+ raise ParsingDone()
- _re_continue_connecting = re.compile(r'\(yes\/no\)|\'yes\'\sor\s\'no\'')
+ _re_continue_connecting = re.compile(r'\(yes/no\)|\'yes\'\sor\s\'no\'')
def _parse_continue_connecting(self, line):
"""
Parses continue connecting.
:param line: Line from device.
- :return: Match object if matches, None otherwise
+ :return: Match object if matches, None otherwise.
"""
return self._regex_helper.search_compiled(Scp._re_continue_connecting, line)
@@ -167,11 +182,14 @@ class Scp(GenericUnixCommand):
"""
Parses hosts file.
- :param line: Line from device
- :return: Nothing
+ :param line: Line from device.
+ :return: None.
+ :raises ParsingDone: if line handled by this method.
+
"""
if (self.known_hosts_on_failure is not None) and self._regex_helper.search_compiled(Scp._re_host_key, line):
self._hosts_file = self._regex_helper.group("PATH")
+ raise ParsingDone()
_re_id_dsa = re.compile("id_dsa:", re.IGNORECASE)
@@ -181,22 +199,25 @@ class Scp(GenericUnixCommand):
"""
Parses host key verification.
- :param line: Line from device
- :return: Nothing
+ :param line: Line from device.
+ :return: None.
+ :raises ParsingDone: if line handled by this method.
"""
if self._regex_helper.search_compiled(Scp._re_id_dsa, line):
self.connection.sendline("")
+ raise ParsingDone()
elif self._regex_helper.search_compiled(Scp._re_host_key_verification_failure, line):
if self._hosts_file:
- self.handle_failed_host_key_verification()
+ self._handle_failed_host_key_verification()
else:
self.set_exception(CommandFailure(self, "Command failed in line >>{}<<.".format(line)))
+ raise ParsingDone()
- def handle_failed_host_key_verification(self):
+ def _handle_failed_host_key_verification(self):
"""
Handles failed host key verification.
- :return: Nothing
+ :return: None.
"""
if "rm" == self.known_hosts_on_failure:
self.connection.sendline("\nrm -f " + self._hosts_file)
| nokia/moler | c0ca25a34f537640c26534e2c05e1955f241fcf5 | diff --git a/test/cmd/unix/test_cmd_scp.py b/test/cmd/unix/test_cmd_scp.py
index 053a62b1..9e168beb 100644
--- a/test/cmd/unix/test_cmd_scp.py
+++ b/test/cmd/unix/test_cmd_scp.py
@@ -77,6 +77,18 @@ ute@debdev:~/Desktop$"""
scp_cmd()
+def test_scp_raise_exception_failure_not_a_directory(buffer_connection):
+ command_output = """
+ute@debdev:~/Desktop$ scp test ute@localhost:/home/ute
+Not a directory
+ute@debdev:~/Desktop$"""
+ buffer_connection.remote_inject_response([command_output])
+ scp_cmd = Scp(connection=buffer_connection.moler_connection, source="test", dest="ute@localhost:/home/ute",
+ known_hosts_on_failure="")
+ with pytest.raises(CommandFailure):
+ scp_cmd()
+
+
def test_scp_raise_exception_ldap_password(buffer_connection):
command_output = """
ute@debdev:~/Desktop$ scp test.txt ute@localhost:/home/ute
@@ -86,7 +98,7 @@ test.txt 100% 104
ute@debdev:~/Desktop$"""
buffer_connection.remote_inject_response([command_output])
scp_cmd = Scp(connection=buffer_connection.moler_connection, source="test.txt", dest="ute@localhost:/home/ute",
- known_hosts_on_failure="", password="pass")
+ known_hosts_on_failure="", password="pass", repeat_password=False)
with pytest.raises(CommandFailure):
scp_cmd()
| Update failure patters in scp command
Not a directory | 0.0 | [
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_failure_not_a_directory",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_ldap_password"
] | [
"test/cmd/unix/test_cmd_scp.py::test_scp_returns_proper_command_string",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_failure",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_failure_key_verification_no_key_file",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_failure_key_verification_no_known_hosts_on_failure",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_failure_key_verification_permission_denied",
"test/cmd/unix/test_cmd_scp.py::test_scp_raise_exception_ldap_password_coppied"
] | 2019-08-08 08:18:14+00:00 | 4,224 |
|
nolanbconaway__binoculars-4 | diff --git a/lib/binoculars/__init__.py b/lib/binoculars/__init__.py
index ab04d17..7a4885d 100644
--- a/lib/binoculars/__init__.py
+++ b/lib/binoculars/__init__.py
@@ -7,7 +7,7 @@ from scipy import stats
def binomial_jeffreys_interval(p: float, n: int, tail: str, z: float = 1.96):
"""Use compute a jeffrey's interval via beta distirbution CDF."""
- alpha = stats.norm.sf(z)
+ alpha = stats.norm.sf(z) * 2
a = n * p + 0.5
b = n - n * p + 0.5
if tail == "lower":
@@ -41,6 +41,18 @@ def binomial_normal_interval(p: float, n: int, tail: str, z: float = 1.96):
raise ValueError("Invalid tail! Choose from: lower, upper")
+def binomial_clopper_pearson_interval(p: float, n: int, tail: str, z: float = 1.96):
+ """Return the clopper-pearson interval for a proportion."""
+ alpha = stats.norm.sf(z) * 2
+ k = p * n
+ if tail == "lower":
+ return stats.beta.ppf(alpha / 2, k, n - k + 1)
+ elif tail == "upper":
+ return stats.beta.ppf(1 - alpha / 2, k + 1, n - k)
+ else:
+ raise ValueError("Invalid tail! Choose from: lower, upper")
+
+
def binomial_confidence(
p: float, n: int, tail: str = None, z: float = 1.96, method="jeffrey"
) -> Union[float, Tuple[float]]:
@@ -53,13 +65,14 @@ def binomial_confidence(
n : int
The n parameter of the binomial for the distributionon,
tail : str
- Tail of the CI to return, either lower or upper. If not provided, this function returns
- a tuple of (lower, upper). if provided, it returns a float value.
+ Tail of the CI to return, either lower or upper. If not provided, this
+ function returns a tuple of (lower, upper). if provided, it returns a float
+ value.
z : float
Optional Z critical value. Default 1.96 for 95%.
method : str
- Optional approximation method. By default this uses Jeffrey's interval. Options:
- jeffrey, wilson, normal.
+ Optional approximation method. By default this uses Jeffrey's interval.
+ Options: jeffrey, wilson, normal, clopper-pearson.
Returns
A tuple of (lower, upper) confidence interval values, or a single value.
@@ -69,10 +82,13 @@ def binomial_confidence(
"jeffrey": binomial_jeffreys_interval,
"wilson": binomial_wilson_interval,
"normal": binomial_normal_interval,
+ "clopper-pearson": binomial_clopper_pearson_interval,
}[method]
except KeyError:
- raise ValueError("Invalid method! Choose from: jeffrey, wilson, normal")
+ raise ValueError(
+ "Invalid method! Choose from: jeffrey, wilson, normal, clopper-pearson"
+ )
if tail is not None:
return func(p=p, n=n, z=z, tail=tail)
diff --git a/readme.md b/readme.md
index 2f37517..76827e6 100644
--- a/readme.md
+++ b/readme.md
@@ -14,6 +14,7 @@ Presently, the package implements:
- [The Normal Approximation](https://en.wikipedia.org/wiki/Binomial_proportion_confidence_interval#Normal_approximation_interval)
- [The Wilson Interval](https://en.wikipedia.org/wiki/Binomial_proportion_confidence_interval#Wilson_score_interval) (no continuity correction)
- [Jeffrey's interval](https://en.wikipedia.org/wiki/Binomial_proportion_confidence_interval#Jeffreys_interval) (via scipy.stats.beta)
+- [Clopper-Pearson interval](https://en.wikipedia.org/wiki/Binomial_proportion_confidence_interval#Clopper%E2%80%93Pearson_interval) (also via scipy.stats.beta)
If you haven't spent a lot of time thinking about which interval _you_ should use (and why would you want to?), I suggest using the Wilson interval or Jeffrey's interval. Jeffrey's interval is returned by default by the `binomial_confidence` function in this package.
diff --git a/setup.py b/setup.py
index 070b04b..359819e 100644
--- a/setup.py
+++ b/setup.py
@@ -15,7 +15,7 @@ LONG_DESCRIPTION = (THIS_DIRECTORY / "readme.md").read_text()
setup(
name="binoculars",
- version="0.1.2",
+ version="0.1.3",
description="Various calculations for binomial confidence intervals.",
long_description=LONG_DESCRIPTION,
long_description_content_type="text/markdown",
@@ -35,5 +35,4 @@ setup(
extras_require=dict(
test=["black==20.8b1", "pytest==6.2.1", "pytest-cov==2.10.1", "codecov==2.1.11"]
),
- package_data={"shabadoo": ["version"]},
)
| nolanbconaway/binoculars | fa6efdd5b2b671668a2be50ea647fc06c156dcaa | diff --git a/.github/workflows/test_on_push.yml b/.github/workflows/test_on_push.yml
index e3e403f..3569dce 100644
--- a/.github/workflows/test_on_push.yml
+++ b/.github/workflows/test_on_push.yml
@@ -7,7 +7,7 @@ jobs:
runs-on: ubuntu-latest
strategy:
matrix:
- python-version: [3.6, 3.7, 3.8]
+ python-version: [3.6, 3.7, 3.8, 3.9]
steps:
- uses: actions/checkout@master
diff --git a/test_binoculars.py b/test_binoculars.py
index 3300fac..efe4166 100644
--- a/test_binoculars.py
+++ b/test_binoculars.py
@@ -3,8 +3,10 @@ import pytest
import binoculars
+METHODS = ["jeffrey", "wilson", "normal", "clopper-pearson"]
[email protected]("method", ["jeffrey", "wilson", "normal"])
+
[email protected]("method", METHODS)
@pytest.mark.parametrize("n", [5, 1e2, 1e3, 1e5, 1e8])
@pytest.mark.parametrize("p", [0.01, 0.5, 0.99])
def test_lower_less_upper(method, n, p):
@@ -21,7 +23,7 @@ def test_lower_less_upper(method, n, p):
assert u == binoculars.binomial_confidence(p, n, method=method, tail="upper")
[email protected]("method", ["jeffrey", "wilson", "normal"])
[email protected]("method", METHODS)
@pytest.mark.parametrize("lower_n, greater_n", [(2, 3), (10, 20), (100, 200)])
def test_more_certain_with_n(method, lower_n, greater_n):
"""Test that certainty diminishes with greater N."""
@@ -32,7 +34,7 @@ def test_more_certain_with_n(method, lower_n, greater_n):
assert lower_u > greater_u
[email protected]("method", ["jeffrey", "wilson", "normal"])
[email protected]("method", METHODS)
@pytest.mark.parametrize("lower_z, greater_z", [(1, 1.01), (1.96, 2.58)])
def test_z_certainty(method, lower_z, greater_z):
"""Test that the interval tightens with lower Z"""
@@ -45,7 +47,7 @@ def test_z_certainty(method, lower_z, greater_z):
assert lower_u < greater_u
[email protected]("method", ["jeffrey", "wilson", "normal"])
[email protected]("method", METHODS)
def test_invalid_tail_error(method):
with pytest.raises(ValueError):
binoculars.binomial_confidence(0.1, 10, tail="NOPE", method=method)
| Cloper Pearson?
For completeness and elegance it would be interesting to also have this method:
https://en.wikipedia.org/wiki/Binomial_proportion_confidence_interval#Clopper%E2%80%93Pearson_interval
which, if I understood correctly is the most conservative.
What do you think? | 0.0 | [
"test_binoculars.py::test_lower_less_upper[0.01-5-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.01-100.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.01-1000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.01-100000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.01-100000000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.5-5-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.5-100.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.5-1000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.5-100000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.5-100000000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.99-5-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.99-100.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.99-1000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.99-100000.0-clopper-pearson]",
"test_binoculars.py::test_lower_less_upper[0.99-100000000.0-clopper-pearson]",
"test_binoculars.py::test_more_certain_with_n[2-3-clopper-pearson]",
"test_binoculars.py::test_more_certain_with_n[10-20-clopper-pearson]",
"test_binoculars.py::test_more_certain_with_n[100-200-clopper-pearson]",
"test_binoculars.py::test_z_certainty[1-1.01-clopper-pearson]",
"test_binoculars.py::test_z_certainty[1.96-2.58-clopper-pearson]"
] | [
"test_binoculars.py::test_lower_less_upper[0.01-5-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.01-5-wilson]",
"test_binoculars.py::test_lower_less_upper[0.01-5-normal]",
"test_binoculars.py::test_lower_less_upper[0.01-100.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.01-100.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.01-100.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.01-1000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.01-1000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.01-1000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.01-100000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.01-100000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.01-100000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.01-100000000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.01-100000000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.01-100000000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.5-5-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.5-5-wilson]",
"test_binoculars.py::test_lower_less_upper[0.5-5-normal]",
"test_binoculars.py::test_lower_less_upper[0.5-100.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.5-100.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.5-100.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.5-1000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.5-1000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.5-1000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.5-100000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.5-100000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.5-100000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.5-100000000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.5-100000000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.5-100000000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.99-5-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.99-5-wilson]",
"test_binoculars.py::test_lower_less_upper[0.99-5-normal]",
"test_binoculars.py::test_lower_less_upper[0.99-100.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.99-100.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.99-100.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.99-1000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.99-1000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.99-1000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.99-100000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.99-100000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.99-100000.0-normal]",
"test_binoculars.py::test_lower_less_upper[0.99-100000000.0-jeffrey]",
"test_binoculars.py::test_lower_less_upper[0.99-100000000.0-wilson]",
"test_binoculars.py::test_lower_less_upper[0.99-100000000.0-normal]",
"test_binoculars.py::test_more_certain_with_n[2-3-jeffrey]",
"test_binoculars.py::test_more_certain_with_n[2-3-wilson]",
"test_binoculars.py::test_more_certain_with_n[2-3-normal]",
"test_binoculars.py::test_more_certain_with_n[10-20-jeffrey]",
"test_binoculars.py::test_more_certain_with_n[10-20-wilson]",
"test_binoculars.py::test_more_certain_with_n[10-20-normal]",
"test_binoculars.py::test_more_certain_with_n[100-200-jeffrey]",
"test_binoculars.py::test_more_certain_with_n[100-200-wilson]",
"test_binoculars.py::test_more_certain_with_n[100-200-normal]",
"test_binoculars.py::test_z_certainty[1-1.01-jeffrey]",
"test_binoculars.py::test_z_certainty[1-1.01-wilson]",
"test_binoculars.py::test_z_certainty[1-1.01-normal]",
"test_binoculars.py::test_z_certainty[1.96-2.58-jeffrey]",
"test_binoculars.py::test_z_certainty[1.96-2.58-wilson]",
"test_binoculars.py::test_z_certainty[1.96-2.58-normal]",
"test_binoculars.py::test_invalid_tail_error[jeffrey]",
"test_binoculars.py::test_invalid_tail_error[wilson]",
"test_binoculars.py::test_invalid_tail_error[normal]",
"test_binoculars.py::test_invalid_tail_error[clopper-pearson]",
"test_binoculars.py::test_invalid_method_error"
] | 2021-07-03 14:11:51+00:00 | 4,225 |
|
nolar__kopf-618 | diff --git a/docs/handlers.rst b/docs/handlers.rst
index 5703ccb..4e7dcaa 100644
--- a/docs/handlers.rst
+++ b/docs/handlers.rst
@@ -235,24 +235,25 @@ partials (`functools.partial`), or the inner functions in the closures:
- item1
- item2
-Sub-handlers can be implemented either imperatively::
+Sub-handlers can be implemented either imperatively
+(where it requires :doc:`asynchronous handlers <async>` and ``async/await``)::
import functools
import kopf
@kopf.on.create('zalando.org', 'v1', 'kopfexamples')
- def create_fn(spec, **_):
+ async def create_fn(spec, **_):
fns = {}
for item in spec.get('items', []):
fns[item] = functools.partial(handle_item, item=item)
- kopf.execute(fns)
+ await kopf.execute(fns=fns)
def handle_item(item, *, spec, **_):
pass
-Or decoratively::
+Or declaratively with decorators::
import kopf
@@ -261,9 +262,8 @@ Or decoratively::
for item in spec.get('items', []):
- @kopf.on.this(id=item)
+ @kopf.subhandler(id=item)
def handle_item(item=item, **_):
-
pass
Both of these ways are equivalent.
diff --git a/docs/idempotence.rst b/docs/idempotence.rst
index 22d396d..325e50b 100644
--- a/docs/idempotence.rst
+++ b/docs/idempotence.rst
@@ -4,8 +4,8 @@ Idempotence
Kopf provides tools to make the handlers idempotent.
-`kopf.register` function and `kopf.on.this` decorator allow to schedule
-arbitrary sub-handlers for the execution in the current cycle.
+The `kopf.register` function and the `kopf.subhandler` decorator allow
+to schedule arbitrary sub-handlers for the execution in the current cycle.
`kopf.execute` coroutine executes arbitrary sub-handlers
directly in the place of invocation, and returns when all they have succeeded.
diff --git a/examples/07-subhandlers/example.py b/examples/07-subhandlers/example.py
index 3314372..2d6d7d8 100644
--- a/examples/07-subhandlers/example.py
+++ b/examples/07-subhandlers/example.py
@@ -6,6 +6,6 @@ def create_fn(spec, **kwargs):
for item in spec.get('items', []):
- @kopf.on.this(id=item)
+ @kopf.subhandler(id=item)
async def create_item_fn(item=item, **kwargs):
print(f"=== Handling creation for {item}. ===")
diff --git a/kopf/__init__.py b/kopf/__init__.py
index 1e6c1a8..ead0560 100644
--- a/kopf/__init__.py
+++ b/kopf/__init__.py
@@ -31,12 +31,11 @@ from kopf.engines.posting import (
exception,
)
from kopf.on import (
+ subhandler,
+ register,
daemon,
timer,
)
-from kopf.on import (
- register,
-)
from kopf.reactor import (
lifecycles, # as a separate name on the public namespace
)
diff --git a/kopf/on.py b/kopf/on.py
index 8604e12..4472237 100644
--- a/kopf/on.py
+++ b/kopf/on.py
@@ -418,9 +418,7 @@ def timer( # lgtm[py/similar-function]
return decorator
-# TODO: find a better name: `@kopf.on.this` is confusing and does not fully
-# TODO: match with the `@kopf.on.{cause}` pattern, where cause is create/update/delete.
-def this( # lgtm[py/similar-function]
+def subhandler( # lgtm[py/similar-function]
*,
id: Optional[str] = None,
errors: Optional[handlers.ErrorsMode] = None,
@@ -434,7 +432,7 @@ def this( # lgtm[py/similar-function]
when: Optional[callbacks.WhenFilterFn] = None,
) -> ResourceChangingDecorator:
"""
- ``@kopf.on.this()`` decorator for the dynamically generated sub-handlers.
+ ``@kopf.subhandler()`` decorator for the dynamically generated sub-handlers.
Can be used only inside of the handler function.
It is efficiently a syntax sugar to look like all other handlers::
@@ -444,9 +442,8 @@ def this( # lgtm[py/similar-function]
for task in spec.get('tasks', []):
- @kopf.on.this(id=f'task_{task}')
+ @kopf.subhandler(id=f'task_{task}')
def create_task(*, spec, task=task, **kwargs):
-
pass
In this example, having spec.tasks set to ``[abc, def]``, this will create
@@ -517,11 +514,11 @@ def register( # lgtm[py/similar-function]
def create_it(spec, **kwargs):
for task in spec.get('tasks', []):
- @kopf.on.this(id=task)
+ @kopf.subhandler(id=task)
def create_single_task(task=task, **_):
pass
"""
- decorator = this(
+ decorator = subhandler(
id=id, registry=registry,
errors=errors, timeout=timeout, retries=retries, backoff=backoff, cooldown=cooldown,
labels=labels, annotations=annotations, when=when,
@@ -529,6 +526,10 @@ def register( # lgtm[py/similar-function]
return decorator(fn)
+# DEPRECATED: for backward compatibility, the original name of @kopf.on.this() is kept.
+this = subhandler
+
+
def _warn_deprecated_signatures(
fn: Callable[..., Any],
) -> None:
diff --git a/kopf/reactor/handling.py b/kopf/reactor/handling.py
index 2c5fb86..c814c26 100644
--- a/kopf/reactor/handling.py
+++ b/kopf/reactor/handling.py
@@ -50,7 +50,7 @@ class HandlerChildrenRetry(TemporaryError):
# The task-local context; propagated down the stack instead of multiple kwargs.
-# Used in `@kopf.on.this` and `kopf.execute()` to add/get the sub-handlers.
+# Used in `@kopf.subhandler` and `kopf.execute()` to add/get the sub-handlers.
sublifecycle_var: ContextVar[Optional[lifecycles.LifeCycleFn]] = ContextVar('sublifecycle_var')
subregistry_var: ContextVar[registries.ResourceChangingRegistry] = ContextVar('subregistry_var')
subsettings_var: ContextVar[configuration.OperatorSettings] = ContextVar('subsettings_var')
@@ -77,7 +77,7 @@ async def execute(
If no explicit functions or handlers or registry are passed,
the sub-handlers of the current handler are assumed, as accumulated
- in the per-handler registry with ``@kopf.on.this``.
+ in the per-handler registry with ``@kopf.subhandler``.
If the call to this method for the sub-handlers is not done explicitly
in the handler, it is done implicitly after the handler is exited.
@@ -137,7 +137,7 @@ async def execute(
elif subexecuted_var.get():
return
- # If no explicit args were passed, implicitly use the accumulated handlers from `@kopf.on.this`.
+ # If no explicit args were passed, use the accumulated handlers from `@kopf.subhandler`.
else:
subexecuted_var.set(True)
subregistry = subregistry_var.get()
@@ -336,7 +336,7 @@ async def invoke_handler(
Ensure the global context for this asyncio task is set to the handler and
its cause -- for proper population of the sub-handlers via the decorators
- (see `@kopf.on.this`).
+ (see `@kopf.subhandler`).
"""
# For the field-handlers, the old/new/diff values must match the field, not the whole object.
@@ -349,7 +349,7 @@ async def invoke_handler(
diff = diffs.reduce(cause.diff, handler.field)
cause = causation.enrich_cause(cause=cause, old=old, new=new, diff=diff)
- # Store the context of the current resource-object-event-handler, to be used in `@kopf.on.this`,
+ # Store the context of the current handler, to be used in `@kopf.subhandler`,
# and maybe other places, and consumed in the recursive `execute()` calls for the children.
# This replaces the multiple kwargs passing through the whole call stack (easy to forget).
with invocation.context([
| nolar/kopf | 1e517d07c9cd6b590ed9dfbea8f1a670b298c144 | diff --git a/tests/registries/test_decorators.py b/tests/registries/test_decorators.py
index bc824ac..fd110bb 100644
--- a/tests/registries/test_decorators.py
+++ b/tests/registries/test_decorators.py
@@ -425,7 +425,7 @@ def test_subhandler_fails_with_no_parent_handler():
# Check the actual behaviour of the decorator.
with pytest.raises(LookupError):
- @kopf.on.this()
+ @kopf.subhandler()
def fn(**_):
pass
@@ -437,7 +437,7 @@ def test_subhandler_declaratively(parent_handler, cause_factory):
subregistry_var.set(registry)
with context([(handler_var, parent_handler)]):
- @kopf.on.this()
+ @kopf.subhandler()
def fn(**_):
pass
diff --git a/tests/registries/test_subhandlers_ids.py b/tests/registries/test_subhandlers_ids.py
index 92a4c82..7a4ec4a 100644
--- a/tests/registries/test_subhandlers_ids.py
+++ b/tests/registries/test_subhandlers_ids.py
@@ -15,7 +15,7 @@ def test_with_no_parent(
registry = resource_registry_cls()
with context([(handler_var, None)]):
- kopf.on.this(registry=registry)(child_fn)
+ kopf.subhandler(registry=registry)(child_fn)
handlers = registry.get_handlers(cause)
assert len(handlers) == 1
@@ -30,7 +30,7 @@ def test_with_parent(
registry = resource_registry_cls()
with context([(handler_var, parent_handler)]):
- kopf.on.this(registry=registry)(child_fn)
+ kopf.subhandler(registry=registry)(child_fn)
handlers = registry.get_handlers(cause)
assert len(handlers) == 1
| Add more readable @kopf.subhandler instead of @kopf.on.this/kopf.register
## Background
A long time ago, when Kopf was only prototyped, sub-handlers were implemented via `@kopf.on.this()` decorator. The logic was so that if we all other handlers as `@kopf.on.{something}`, sub-handler should be also declared to be triggered "on" something, and keep sub-handlers declarative. That naming turned to be highly confusing, and so a more imperative way was introduced: `kopf.register()` function (not a decorator). And also even more imperative way: `await kopf.execute()` function.
Much later, daemons and timers were added as `@kopf.daemon` and `@kopf.timer`, because they could not be expressed with the "on-something" expression (the timers could be "on-schedule", but the daemons definitely could not be named that way).
And so, the "on-something" semantics was already violated.
## Proposal
It makes no sense to keep it as `@kopf.on.this` anymore. Why not make it `@kopf.subhandler`, and return the good old declarative way of declaring the sub-handlers — in addition to the existing imperative ways?
## Example
```python
import kopf
@kopf.on.creation('kopfexamples')
def create_fn(name, **_):
for i in range(5):
@kopf.subhandler(id=str(i))
def sub_fn(i=i, **_):
print(f"{name}: {i}")
```
Looks more readable than `@kopf.on.this()`.
## Side-changes
Also, consider `@kopf.sub()` as a shortcut.
Also, consider adding the on-creation/on-deletion aliases for the existing on-create/on-delete decorators — for English readability.
However, the aliases go against Python Zen:
```
There should be one-- and preferably only one --obvious way to do it.
``` | 0.0 | [
"tests/registries/test_decorators.py::test_subhandler_fails_with_no_parent_handler",
"tests/registries/test_decorators.py::test_subhandler_declaratively",
"tests/registries/test_subhandlers_ids.py::test_with_no_parent[resource-watching-registry]",
"tests/registries/test_subhandlers_ids.py::test_with_no_parent[resource-changing-registry]",
"tests/registries/test_subhandlers_ids.py::test_with_no_parent[simple-registry]",
"tests/registries/test_subhandlers_ids.py::test_with_parent[resource-watching-registry]",
"tests/registries/test_subhandlers_ids.py::test_with_parent[resource-changing-registry]",
"tests/registries/test_subhandlers_ids.py::test_with_parent[simple-registry]"
] | [
"tests/registries/test_decorators.py::test_on_startup_minimal",
"tests/registries/test_decorators.py::test_on_cleanup_minimal",
"tests/registries/test_decorators.py::test_on_probe_minimal",
"tests/registries/test_decorators.py::test_on_resume_minimal[create]",
"tests/registries/test_decorators.py::test_on_resume_minimal[update]",
"tests/registries/test_decorators.py::test_on_resume_minimal[delete]",
"tests/registries/test_decorators.py::test_on_resume_minimal[resume]",
"tests/registries/test_decorators.py::test_on_create_minimal",
"tests/registries/test_decorators.py::test_on_update_minimal",
"tests/registries/test_decorators.py::test_on_delete_minimal",
"tests/registries/test_decorators.py::test_on_field_minimal",
"tests/registries/test_decorators.py::test_on_field_fails_without_field",
"tests/registries/test_decorators.py::test_on_startup_with_all_kwargs",
"tests/registries/test_decorators.py::test_on_cleanup_with_all_kwargs",
"tests/registries/test_decorators.py::test_on_probe_with_all_kwargs",
"tests/registries/test_decorators.py::test_on_resume_with_all_kwargs[create]",
"tests/registries/test_decorators.py::test_on_resume_with_all_kwargs[update]",
"tests/registries/test_decorators.py::test_on_resume_with_all_kwargs[delete]",
"tests/registries/test_decorators.py::test_on_resume_with_all_kwargs[resume]",
"tests/registries/test_decorators.py::test_on_create_with_all_kwargs",
"tests/registries/test_decorators.py::test_on_update_with_all_kwargs",
"tests/registries/test_decorators.py::test_on_delete_with_all_kwargs[optional]",
"tests/registries/test_decorators.py::test_on_delete_with_all_kwargs[mandatory]",
"tests/registries/test_decorators.py::test_on_field_with_all_kwargs",
"tests/registries/test_decorators.py::test_subhandler_imperatively",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[event-kwargs0]",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[resume-kwargs1]",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[create-kwargs2]",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[update-kwargs3]",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[delete-kwargs4]",
"tests/registries/test_decorators.py::test_labels_filter_with_nones[field-kwargs5]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[event-kwargs0]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[resume-kwargs1]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[create-kwargs2]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[update-kwargs3]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[delete-kwargs4]",
"tests/registries/test_decorators.py::test_annotations_filter_with_nones[field-kwargs5]"
] | 2020-12-15 18:56:14+00:00 | 4,226 |
|
nolar__kopf-650 | diff --git a/kopf/reactor/registries.py b/kopf/reactor/registries.py
index 87dcff3..d42f4a7 100644
--- a/kopf/reactor/registries.py
+++ b/kopf/reactor/registries.py
@@ -340,13 +340,13 @@ def prematch(
) -> bool:
# Kwargs are lazily evaluated on the first _actual_ use, and shared for all filters since then.
kwargs: MutableMapping[str, Any] = {}
- return all([
- _matches_resource(handler, cause.resource),
- _matches_labels(handler, cause, kwargs),
- _matches_annotations(handler, cause, kwargs),
- _matches_field_values(handler, cause, kwargs),
- _matches_filter_callback(handler, cause, kwargs),
- ])
+ return (
+ _matches_resource(handler, cause.resource) and
+ _matches_labels(handler, cause, kwargs) and
+ _matches_annotations(handler, cause, kwargs) and
+ _matches_field_values(handler, cause, kwargs) and
+ _matches_filter_callback(handler, cause, kwargs) # the callback comes in the end!
+ )
def match(
@@ -355,14 +355,14 @@ def match(
) -> bool:
# Kwargs are lazily evaluated on the first _actual_ use, and shared for all filters since then.
kwargs: MutableMapping[str, Any] = {}
- return all([
- _matches_resource(handler, cause.resource),
- _matches_labels(handler, cause, kwargs),
- _matches_annotations(handler, cause, kwargs),
- _matches_field_values(handler, cause, kwargs),
- _matches_field_changes(handler, cause, kwargs),
- _matches_filter_callback(handler, cause, kwargs),
- ])
+ return (
+ _matches_resource(handler, cause.resource) and
+ _matches_labels(handler, cause, kwargs) and
+ _matches_annotations(handler, cause, kwargs) and
+ _matches_field_values(handler, cause, kwargs) and
+ _matches_field_changes(handler, cause, kwargs) and
+ _matches_filter_callback(handler, cause, kwargs) # the callback comes in the end!
+ )
def _matches_resource(
| nolar/kopf | 81bdef3b6933b911ec9d2eb791c377ba95b64581 | diff --git a/tests/registries/test_matching_of_callbacks.py b/tests/registries/test_matching_of_callbacks.py
new file mode 100644
index 0000000..5d1e02d
--- /dev/null
+++ b/tests/registries/test_matching_of_callbacks.py
@@ -0,0 +1,75 @@
+import dataclasses
+from unittest.mock import Mock
+
+import pytest
+
+from kopf.reactor.causation import ResourceWatchingCause
+from kopf.reactor.registries import match, prematch
+from kopf.structs.bodies import Body
+from kopf.structs.dicts import parse_field
+from kopf.structs.handlers import ResourceWatchingHandler
+from kopf.structs.references import Resource
+
+
+# Used in the tests. Must be global-scoped, or its qualname will be affected.
+def some_fn(x=None):
+ pass
+
+
[email protected]()
+def callback():
+ mock = Mock()
+ mock.return_value = True
+ return mock
+
+
[email protected](params=['annotations', 'labels', 'value', 'when'])
+def handler(request, callback, selector):
+ handler = ResourceWatchingHandler(
+ selector=selector,
+ annotations={'known': 'value'},
+ labels={'known': 'value'},
+ field=parse_field('spec.field'),
+ value='value',
+ when=None,
+ fn=some_fn, id='a', errors=None, timeout=None, retries=None, backoff=None, # irrelevant
+ )
+ if request.param in ['annotations', 'labels']:
+ handler = dataclasses.replace(handler, **{request.param: {'known': callback}})
+ else:
+ handler = dataclasses.replace(handler, **{request.param: callback})
+ return handler
+
+
[email protected]()
+def cause(cause_factory, callback):
+ return cause_factory(
+ cls=ResourceWatchingCause,
+ body=Body(dict(
+ metadata=dict(
+ labels={'known': 'value'},
+ annotations={'known': 'value'},
+ ),
+ spec=dict(
+ field='value',
+ ),
+ )))
+
+
[email protected]('match_fn', [match, prematch])
+def test_callback_is_called_with_matching_resource(
+ match_fn, callback, handler, cause,
+):
+ result = match_fn(handler=handler, cause=cause)
+ assert result
+ assert callback.called
+
+
[email protected]('match_fn', [match, prematch])
+def test_callback_is_not_called_with_mismatching_resource(
+ match_fn, callback, handler, cause,
+):
+ cause = dataclasses.replace(cause, resource=Resource(group='x', version='y', plural='z'))
+ result = match_fn(handler=handler, cause=cause)
+ assert not result
+ assert not callback.called
| Error when starting up kopf
## Long story short
Kopf starts up with error when using a handler with a callback & a core resource handler.
## Description
Not sure if this is a bug or just a poorly written callback but it doesn't seem to make sense why the callback is being called when handling core resources.
When I start kopf with the below handlers errors are logged by kopf on startup. However if I remove the `@kopf.on.create('apps', 'v1', 'deployments')` handler or change the callback to use `dict.get()` method then everything starts up correctly.
```python
import kopf
def is_good_enough(spec, **_):
return spec['field'] in spec['items']
@kopf.on.create('company.com', 'v1', 'testresources', when=is_good_enough)
def my_create(spec, **_):
pass
@kopf.on.create('apps', 'v1', 'deployments')
def create(spec, **kwargs):
pass
```
</details>
<details><summary>The exact command to reproduce the issue</summary>
```bash
kopf run handler.py --standalone
```
</details>
<details><summary>The full output of the command that failed</summary>
```
2021-01-19 16:43:29,972] kopf.reactor.activit [INFO ] Initial authentication has been initiated.
[2021-01-19 16:43:30,129] kopf.activities.auth [INFO ] Activity 'login_via_client' succeeded.
[2021-01-19 16:43:30,129] kopf.reactor.activit [INFO ] Initial authentication has finished.
[2021-01-19 16:43:30,359] kopf.objects [ERROR ] [kube-system/coredns] Throttling for 1 seconds due to an unexpected error:
Traceback (most recent call last):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/effects.py", line 200, in throttled
yield should_run
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/processing.py", line 79, in process_resource_event
delays, matched = await process_resource_causes(
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/processing.py", line 167, in process_resource_causes
not registry._resource_changing.prematch(cause=resource_changing_cause)):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 211, in prematch
if prematch(handler=handler, cause=cause):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 348, in prematch
_matches_filter_callback(handler, cause, kwargs),
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 501, in _matches_filter_callback
return handler.when(**kwargs)
File "/Users/etilley/Documents/kubernetes/kopf/handler-testing/handler.py", line 11, in is_good_enough
return spec['field'] in spec['items']
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/structs/dicts.py", line 249, in __getitem__
return resolve(self._src, self._path + (item,))
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/structs/dicts.py", line 83, in resolve
result = result[key]
KeyError: 'field'
[2021-01-19 16:43:30,368] kopf.objects [ERROR ] [local-path-storage/local-path-provisioner] Throttling for 1 seconds due to an unexpected error:
Traceback (most recent call last):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/effects.py", line 200, in throttled
yield should_run
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/processing.py", line 79, in process_resource_event
delays, matched = await process_resource_causes(
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/processing.py", line 167, in process_resource_causes
not registry._resource_changing.prematch(cause=resource_changing_cause)):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 211, in prematch
if prematch(handler=handler, cause=cause):
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 348, in prematch
_matches_filter_callback(handler, cause, kwargs),
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/reactor/registries.py", line 501, in _matches_filter_callback
return handler.when(**kwargs)
File "/Users/etilley/Documents/kubernetes/kopf/handler-testing/handler.py", line 11, in is_good_enough
return spec['field'] in spec['items']
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/structs/dicts.py", line 249, in __getitem__
return resolve(self._src, self._path + (item,))
File "/Users/etilley/.pyenv/versions/3.8.0/lib/python3.8/site-packages/kopf/structs/dicts.py", line 83, in resolve
result = result[key]
KeyError: 'field'
[2021-01-19 16:43:31,361] kopf.objects [INFO ] [kube-system/coredns] Throttling is over. Switching back to normal operations.
[2021-01-19 16:43:31,369] kopf.objects [INFO ] [local-path-storage/local-path-provisioner] Throttling is over. Switching back to normal operations.
```
</details>
## Environment
<!-- The following commands can help:
`kopf --version` or `pip show kopf`
`kubectl version`
`python --version`
-->
* Kopf version: 1.29.0rc2
* Kubernetes version: v1.19.1
* Python version: 3.8.0
* OS/platform: mac OS
```
Package Version
------------------ ---------
aiohttp 3.6.2
aiojobs 0.2.2
astroid 2.3.3
async-timeout 3.0.1
attrs 19.3.0
boto3 1.16.30
botocore 1.19.30
cachetools 3.1.1
certifi 2019.9.11
cffi 1.14.3
chardet 3.0.4
Click 7.0
cryptography 3.1.1
deepdiff 4.2.0
dictdiffer 0.8.1
elasticsearch 7.5.1
elasticsearch-dsl 7.1.0
google-auth 1.7.1
idna 2.8
iniconfig 1.1.1
iso8601 0.1.12
isort 4.3.21
javaobj-py3 0.4.1
Jinja2 2.10.3
jmespath 0.10.0
jsondiff 1.2.0
kopf 1.29.0rc2
kubernetes 10.0.1
kubetest 0.8.1
lazy-object-proxy 1.4.3
MarkupSafe 1.1.1
mccabe 0.6.1
multidict 4.6.1
oauthlib 3.1.0
ordered-set 3.1.1
packaging 20.4
pip 19.2.3
pluggy 0.13.1
prometheus-client 0.7.1
py 1.9.0
pyasn1 0.4.8
pyasn1-modules 0.2.7
pycparser 2.20
pycryptodomex 3.9.8
pyjks 20.0.0
pykube-ng 19.10.0
pylint 2.4.4
pymongo 3.9.0
pyOpenSSL 19.1.0
pyparsing 2.4.7
pytest 6.1.2
python-dateutil 2.8.1
python-json-logger 2.0.0
PyYAML 5.1.2
rabbitmq-admin 0.2
requests 2.22.0
requests-oauthlib 1.3.0
rope 0.18.0
rsa 4.0
s3transfer 0.3.3
setuptools 41.2.0
six 1.13.0
toml 0.10.2
twofish 0.3.0
typing-extensions 3.7.4.1
urllib3 1.25.7
websocket-client 0.56.0
wrapt 1.11.2
yarl 1.3.0
```
| 0.0 | [
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[annotations-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[annotations-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[annotations-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[annotations-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[labels-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[labels-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[labels-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[labels-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[value-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[value-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[value-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[value-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[when-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[when-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[when-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_not_called_with_mismatching_resource[when-cluster-prematch]"
] | [
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[annotations-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[annotations-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[annotations-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[annotations-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[labels-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[labels-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[labels-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[labels-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[value-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[value-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[value-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[value-cluster-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[when-namespaced-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[when-namespaced-prematch]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[when-cluster-match]",
"tests/registries/test_matching_of_callbacks.py::test_callback_is_called_with_matching_resource[when-cluster-prematch]"
] | 2021-01-22 17:22:08+00:00 | 4,227 |
|
nolar__kopf-675 | diff --git a/kopf/reactor/daemons.py b/kopf/reactor/daemons.py
index f083e6a..240330e 100644
--- a/kopf/reactor/daemons.py
+++ b/kopf/reactor/daemons.py
@@ -25,6 +25,8 @@ import time
import warnings
from typing import Collection, List, Mapping, MutableMapping, Sequence
+import aiojobs
+
from kopf.engines import loggers
from kopf.reactor import causation, effects, handling, lifecycles
from kopf.storage import states
@@ -209,31 +211,71 @@ async def daemon_killer(
*,
settings: configuration.OperatorSettings,
memories: containers.ResourceMemories,
+ freeze_checker: primitives.ToggleSet,
) -> None:
"""
- An operator's root task to kill the daemons on the operator's shutdown.
- """
+ An operator's root task to kill the daemons on the operator's demand.
+
+ The "demand" comes in two cases: when the operator is exiting (gracefully
+ or not), and when the operator is pausing because of peering. In that case,
+ all watch-streams are disconnected, and all daemons/timers should stop.
+
+ When pausing, the daemons/timers are stopped via their regular stopping
+ procedure: with graceful or forced termination, backoffs, timeouts.
+
+ .. warning::
+
+ Each daemon will be respawned on the next K8s watch-event strictly
+ after the previous daemon is fully stopped.
+ There are never 2 instances of the same daemon running in parallel.
- # Sleep forever, or until cancelled, which happens when the operator begins its shutdown.
+ In normal cases (enough time is given to stop), this is usually done
+ by the post-freeze re-listing event. In rare cases when the unfreeze
+ happens faster than the daemon is stopped (highly unlikely to happen),
+ that event can be missed because the daemon is being stopped yet,
+ so the respawn can happen with a significant delay.
+
+ This issue is considered low-priority & auxiliary, so as the peering
+ itself. It can be fixed later. Workaround: make daemons to exit fast.
+ """
+ # Unlimited job pool size —- the same as if we would be managing the tasks directly.
+ # Unlimited timeout in `close()` -- since we have our own per-daemon timeout management.
+ scheduler: aiojobs.Scheduler = await aiojobs.create_scheduler(limit=None, close_timeout=99999)
try:
- await asyncio.Event().wait()
+ while True:
+
+ # Stay here while the operator is running normally, until it is frozen.
+ await freeze_checker.wait_for(True)
+
+ # The stopping tasks are "fire-and-forget" -- we do not get (or care of) the result.
+ # The daemons remain resumable, since they exit not on their own accord.
+ for memory in memories.iter_all_memories():
+ for daemon in memory.running_daemons.values():
+ await scheduler.spawn(stop_daemon(
+ settings=settings,
+ daemon=daemon,
+ reason=primitives.DaemonStoppingReason.OPERATOR_PAUSING))
+
+ # Stay here while the operator is frozen, until it is resumed.
+ # The fresh stream of watch-events will spawn new daemons naturally.
+ await freeze_checker.wait_for(False)
# Terminate all running daemons when the operator exits (and this task is cancelled).
finally:
- tasks = [
- aiotasks.create_task(
- name=f"stop daemon {daemon.handler.id}",
- coro=stop_daemon(daemon=daemon, settings=settings))
- for memory in memories.iter_all_memories()
- for daemon in memory.running_daemons.values()
- ]
- await aiotasks.wait(tasks)
+ for memory in memories.iter_all_memories():
+ for daemon in memory.running_daemons.values():
+ await scheduler.spawn(stop_daemon(
+ settings=settings,
+ daemon=daemon,
+ reason=primitives.DaemonStoppingReason.OPERATOR_EXITING))
+ await scheduler.close()
async def stop_daemon(
*,
settings: configuration.OperatorSettings,
daemon: containers.Daemon,
+ reason: primitives.DaemonStoppingReason,
) -> None:
"""
Stop a single daemon.
@@ -255,7 +297,7 @@ async def stop_daemon(
raise RuntimeError(f"Unsupported daemon handler: {handler!r}")
# Whatever happens with other flags & logs & timings, this flag must be surely set.
- daemon.stopper.set(reason=primitives.DaemonStoppingReason.OPERATOR_EXITING)
+ daemon.stopper.set(reason=reason)
await _wait_for_instant_exit(settings=settings, daemon=daemon)
if daemon.task.done():
@@ -336,7 +378,7 @@ async def _runner(
finally:
# Prevent future re-spawns for those exited on their own, for no reason.
- # Only the filter-mismatching daemons can be re-spawned on future events.
+ # Only the filter-mismatching or peering-frozen daemons can be re-spawned.
if stopper.reason == primitives.DaemonStoppingReason.NONE:
memory.forever_stopped.add(handler.id)
diff --git a/kopf/reactor/orchestration.py b/kopf/reactor/orchestration.py
index ec0b426..3271581 100644
--- a/kopf/reactor/orchestration.py
+++ b/kopf/reactor/orchestration.py
@@ -87,8 +87,8 @@ async def ochestrator(
settings: configuration.OperatorSettings,
identity: peering.Identity,
insights: references.Insights,
+ freeze_checker: primitives.ToggleSet,
) -> None:
- freeze_checker = primitives.ToggleSet()
freeze_blocker = await freeze_checker.make_toggle(name='peering CRD is absent')
ensemble = Ensemble(freeze_blocker=freeze_blocker, freeze_checker=freeze_checker)
try:
diff --git a/kopf/reactor/running.py b/kopf/reactor/running.py
index e66ea48..3c0b33f 100644
--- a/kopf/reactor/running.py
+++ b/kopf/reactor/running.py
@@ -177,6 +177,7 @@ async def spawn_tasks(
event_queue: posting.K8sEventQueue = asyncio.Queue()
signal_flag: aiotasks.Future = asyncio.Future()
started_flag: asyncio.Event = asyncio.Event()
+ freeze_checker = primitives.ToggleSet()
tasks: MutableSequence[aiotasks.Task] = []
# Map kwargs into the settings object.
@@ -223,7 +224,8 @@ async def spawn_tasks(
name="daemon killer", flag=started_flag, logger=logger,
coro=daemons.daemon_killer(
settings=settings,
- memories=memories)))
+ memories=memories,
+ freeze_checker=freeze_checker)))
# Keeping the credentials fresh and valid via the authentication handlers on demand.
tasks.append(aiotasks.create_guarded_task(
@@ -281,6 +283,7 @@ async def spawn_tasks(
settings=settings,
insights=insights,
identity=identity,
+ freeze_checker=freeze_checker,
processor=functools.partial(processing.process_resource_event,
lifecycle=lifecycle,
registry=registry,
diff --git a/kopf/structs/primitives.py b/kopf/structs/primitives.py
index 9980aba..d0d7476 100644
--- a/kopf/structs/primitives.py
+++ b/kopf/structs/primitives.py
@@ -230,6 +230,7 @@ class DaemonStoppingReason(enum.Flag):
DONE = enum.auto() # whatever the reason and the status, the asyncio task has exited.
FILTERS_MISMATCH = enum.auto() # the resource does not match the filters anymore.
RESOURCE_DELETED = enum.auto() # the resource was deleted, the asyncio task is still awaited.
+ OPERATOR_PAUSING = enum.auto() # the operator is pausing, the asyncio task is still awaited.
OPERATOR_EXITING = enum.auto() # the operator is exiting, the asyncio task is still awaited.
DAEMON_SIGNALLED = enum.auto() # the stopper flag was set, the asyncio task is still awaited.
DAEMON_CANCELLED = enum.auto() # the asyncio task was cancelled, the thread can be running.
| nolar/kopf | 9d5f8da8ea5a8073e3bb1291e24f2a56afd44315 | diff --git a/tests/handling/daemons/conftest.py b/tests/handling/daemons/conftest.py
index e2636ea..22b1812 100644
--- a/tests/handling/daemons/conftest.py
+++ b/tests/handling/daemons/conftest.py
@@ -6,10 +6,12 @@ import freezegun
import pytest
import kopf
+from kopf.reactor.daemons import daemon_killer
from kopf.reactor.processing import process_resource_event
from kopf.structs.bodies import RawBody
from kopf.structs.containers import ResourceMemories
from kopf.structs.memos import Memo
+from kopf.structs.primitives import ToggleSet
class DaemonDummy:
@@ -76,6 +78,31 @@ def simulate_cycle(k8s_mocked, registry, settings, resource, memories, mocker):
return _simulate_cycle
[email protected]()
+async def freeze_checker():
+ return ToggleSet()
+
+
[email protected]()
+async def freeze_toggle(freeze_checker: ToggleSet):
+ return await freeze_checker.make_toggle(name="freeze_toggle fixture")
+
+
[email protected]()
+async def background_daemon_killer(settings, memories, freeze_checker):
+ """
+ Run the daemon killer in the background.
+ """
+ task = asyncio.create_task(daemon_killer(
+ settings=settings, memories=memories, freeze_checker=freeze_checker))
+ yield
+ task.cancel()
+ try:
+ await task
+ except asyncio.CancelledError:
+ pass
+
+
@pytest.fixture()
def frozen_time():
"""
diff --git a/tests/handling/daemons/test_daemon_termination.py b/tests/handling/daemons/test_daemon_termination.py
index 2d89d22..3ec39de 100644
--- a/tests/handling/daemons/test_daemon_termination.py
+++ b/tests/handling/daemons/test_daemon_termination.py
@@ -18,7 +18,7 @@ async def test_daemon_exits_gracefully_and_instantly_via_stopper(
dummy.steps['called'].set()
await kwargs['stopped'].wait()
- # 0th cycle:tTrigger spawning and wait until ready. Assume the finalizers are already added.
+ # 0th cycle: trigger spawning and wait until ready. Assume the finalizers are already added.
finalizer = settings.persistence.finalizer
event_object = {'metadata': {'finalizers': [finalizer]}}
await simulate_cycle(event_object)
@@ -39,6 +39,41 @@ async def test_daemon_exits_gracefully_and_instantly_via_stopper(
assert k8s_mocked.patch_obj.call_args_list[0][1]['patch']['metadata']['finalizers'] == []
[email protected]('background_daemon_killer')
+async def test_daemon_exits_gracefully_and_instantly_via_peering_freeze(
+ settings, memories, resource, dummy, simulate_cycle, freeze_toggle,
+ caplog, assert_logs, k8s_mocked, frozen_time, mocker, timer):
+ caplog.set_level(logging.DEBUG)
+
+ # A daemon-under-test.
+ @kopf.daemon(*resource, id='fn')
+ async def fn(**kwargs):
+ dummy.kwargs = kwargs
+ dummy.steps['called'].set()
+ await kwargs['stopped'].wait()
+
+ # 0th cycle: trigger spawning and wait until ready. Assume the finalizers are already added.
+ finalizer = settings.persistence.finalizer
+ event_object = {'metadata': {'finalizers': [finalizer]}}
+ await simulate_cycle(event_object)
+ await dummy.steps['called'].wait()
+
+ # 1st stage: trigger termination due to peering freeze.
+ mocker.resetall()
+ await freeze_toggle.turn_to(True)
+
+ # Check that the daemon has exited near-instantly, with no delays.
+ with timer:
+ await dummy.wait_for_daemon_done()
+ assert timer.seconds < 0.01 # near-instantly
+
+ # There is no way to test for re-spawning here: it is done by watch-events,
+ # which are tested by the peering freezes elsewhere (test_daemon_spawning.py).
+ # We only test that it is capable for respawning (not forever-stopped):
+ memory = await memories.recall(event_object)
+ assert not memory.forever_stopped
+
+
async def test_daemon_exits_instantly_via_cancellation_with_backoff(
settings, resource, dummy, simulate_cycle,
caplog, assert_logs, k8s_mocked, frozen_time, mocker):
| timers continue when freezing operations
## Long story short
Thanks for creating kopf. I am currently developing an operator that updates Egress NetworkPolicy resources to sync its `spec.egress[*].to[*].ipBlock.cidr` keys with A records for DNS hostnames.
This operator used timers (looking into daemons to follow DNS TTL). I run an operator in k8s with cluster peering. For development I start a local instance with --dev.
I see the operator in k8s detects the development instance:
```
Freezing operations in favour of [<Peer rtoma@devsystem/20210208145159/nz5: priority=666, lifetime=60, lastseen='2021-02-08T14:52:01.160123'>].
```
The development instance's log show:
```
Resuming operations after the freeze. Conflicting operators with the same priority are gone.
```
Still both operator instances show `Timer 'NAME' succeeded` messages. I searched the docs, but could not find anything.
## Description
<!-- Please provide as much information as possible. Lack of information may result in a delayed response. As a guideline, use the following placeholders, and add yours as needed. -->
<details><summary>The code snippet to reproduce the issue</summary>
```python
import kopf
```
</details>
<details><summary>The exact command to reproduce the issue</summary>
```bash
kopf run ...
```
</details>
<details><summary>The full output of the command that failed</summary>
```
```
</details>
## Environment
<!-- The following commands can help:
`kopf --version` or `pip show kopf`
`kubectl version`
`python --version`
-->
* Kopf version: 1.29.1
* Kubernetes version: 1.19.5
* Python version: 3.9.1
* OS/platform: k8s on pi4, local on arm mbp
<details><summary>Python packages installed</summary>
<!-- use `pip freeze --all` -->
```
```
</details>
| 0.0 | [
"tests/handling/daemons/test_daemon_termination.py::test_daemon_is_abandoned_due_to_cancellation_timeout_reached[namespaced]"
] | [
"tests/handling/daemons/test_daemon_termination.py::test_daemon_exits_gracefully_and_instantly_via_stopper[namespaced]",
"tests/handling/daemons/test_daemon_termination.py::test_daemon_exits_gracefully_and_instantly_via_stopper[cluster]",
"tests/handling/daemons/test_daemon_termination.py::test_daemon_exits_instantly_via_cancellation_with_backoff[namespaced]",
"tests/handling/daemons/test_daemon_termination.py::test_daemon_exits_instantly_via_cancellation_with_backoff[cluster]",
"tests/handling/daemons/test_daemon_termination.py::test_daemon_exits_slowly_via_cancellation_with_backoff[namespaced]",
"tests/handling/daemons/test_daemon_termination.py::test_daemon_is_abandoned_due_to_cancellation_timeout_reached[cluster]"
] | 2021-02-08 23:54:30+00:00 | 4,228 |
|
nolar__kopf-726 | diff --git a/kopf/toolkits/hierarchies.py b/kopf/toolkits/hierarchies.py
index 578b8ec..2c54a73 100644
--- a/kopf/toolkits/hierarchies.py
+++ b/kopf/toolkits/hierarchies.py
@@ -2,6 +2,7 @@
All the functions to properly build the object hierarchies.
"""
import collections.abc
+import enum
import warnings
from typing import Any, Iterable, Iterator, Mapping, MutableMapping, Optional, Union, cast
@@ -13,6 +14,10 @@ K8sObject = Union[MutableMapping[Any, Any], thirdparty.PykubeObject, thirdparty.
K8sObjects = Union[K8sObject, Iterable[K8sObject]]
+class _UNSET(enum.Enum):
+ token = enum.auto()
+
+
def append_owner_reference(
objs: K8sObjects,
owner: Optional[bodies.Body] = None,
@@ -82,7 +87,7 @@ def remove_owner_reference(
def label(
objs: K8sObjects,
- labels: Optional[Mapping[str, Union[None, str]]] = None,
+ labels: Union[Mapping[str, Union[None, str]], _UNSET] = _UNSET.token,
*,
forced: bool = False,
nested: Optional[Union[str, Iterable[dicts.FieldSpec]]] = None,
@@ -97,9 +102,11 @@ def label(
forced = force
# Try to use the current object being handled if possible.
- if labels is None:
+ if isinstance(labels, _UNSET):
real_owner = _guess_owner(None)
labels = real_owner.get('metadata', {}).get('labels', {})
+ if isinstance(labels, _UNSET):
+ raise RuntimeError("Impossible error: labels are not resolved.") # for type-checking
# Set labels based on the explicitly specified or guessed ones.
for obj in cast(Iterator[K8sObject], dicts.walk(objs, nested=nested)):
@@ -124,7 +131,7 @@ def label(
def harmonize_naming(
objs: K8sObjects,
- name: Optional[str] = None,
+ name: Union[None, str, _UNSET] = _UNSET.token,
*,
forced: bool = False,
strict: bool = False,
@@ -145,9 +152,11 @@ def harmonize_naming(
"""
# Try to use the current object being handled if possible.
- if name is None:
+ if isinstance(name, _UNSET):
real_owner = _guess_owner(None)
name = real_owner.get('metadata', {}).get('name', None)
+ if isinstance(name, _UNSET):
+ raise RuntimeError("Impossible error: the name is not resolved.") # for type-checking
if name is None:
raise LookupError("Name must be set explicitly: couldn't find it automatically.")
@@ -184,7 +193,7 @@ def harmonize_naming(
def adjust_namespace(
objs: K8sObjects,
- namespace: Optional[str] = None,
+ namespace: Union[None, str, _UNSET] = _UNSET.token,
*,
forced: bool = False,
) -> None:
@@ -198,11 +207,11 @@ def adjust_namespace(
"""
# Try to use the current object being handled if possible.
- if namespace is None:
+ if isinstance(namespace, _UNSET):
real_owner = _guess_owner(None)
namespace = real_owner.get('metadata', {}).get('namespace', None)
- if namespace is None:
- raise LookupError("Namespace must be set explicitly: couldn't find it automatically.")
+ if isinstance(namespace, _UNSET):
+ raise RuntimeError("Impossible error: the namespace is not resolved.") # for type-checking
# Set namespace based on the explicitly specified or guessed namespace.
for obj in cast(Iterator[K8sObject], dicts.walk(objs)):
| nolar/kopf | e90ceaa9faeb000ea1ede52067b4389dd5609c92 | diff --git a/tests/hierarchies/test_contextual_owner.py b/tests/hierarchies/test_contextual_owner.py
index 0acc456..1138a28 100644
--- a/tests/hierarchies/test_contextual_owner.py
+++ b/tests/hierarchies/test_contextual_owner.py
@@ -108,10 +108,11 @@ def test_when_empty_for_name_harmonization(owner):
def test_when_empty_for_namespace_adjustment(owner):
+ # An absent namespace means a cluster-scoped resource -- a valid case.
+ obj = {}
owner._replace_with({})
- with pytest.raises(LookupError) as e:
- kopf.adjust_namespace([])
- assert 'Namespace must be set explicitly' in str(e.value)
+ kopf.adjust_namespace(obj)
+ assert obj['metadata']['namespace'] is None
def test_when_empty_for_adopting(owner):
| Regression in kopf.adopt() in 1.30.
## Long story short
The ``kopf.adopt()`` function now only appears to work where parent and child resources are both namespaced. This is not the only combination that is possible, and so code that previous relied on other possibilities now fails.
## Description
For owner references, it is believed the following working combinations work.
* Parent is namespaced, child is namespaced.
* Parent is cluster scoped (non namespaced), child is cluster scoped.
* Parent is cluster scoped, child is namespaced.
Note that the following are believed not to be possible.
* Parent is namespaced, child is namespaced but child is in a different namespace to the parent.
* Parent is namespaced, child is cluster scoped.
Prior to 1.30, all three of the above working combinations were supported. In 1.30, only the first now works.
If you attempt to have the others, you trigger the check:
* https://github.com/nolar/kopf/blob/e71dc607f31ccd0697895e83c3b795c3f685b3d2/kopf/toolkits/hierarchies.py#L204
and get an exception saying that parent needs to be namespaced.
## Environment
* Kopf version: 1.30.2
* Kubernetes version: 1.19
* Python version: 3.7
* OS/platform: Fedora
| 0.0 | [
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_namespace_adjustment[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_namespace_adjustment[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_namespace_adjustment[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_namespace_adjustment[event-watching-cause-cluster]"
] | [
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_owner_references_appending",
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_owner_references_removal",
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_name_harmonization",
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_namespace_adjustment",
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_labelling",
"tests/hierarchies/test_contextual_owner.py::test_when_unset_for_adopting",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_name_harmonization[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_name_harmonization[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_name_harmonization[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_name_harmonization[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_adopting[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_adopting[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_adopting[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_empty_for_adopting[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_name_harmonization[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_name_harmonization[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_name_harmonization[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_name_harmonization[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_namespace_adjustment[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_namespace_adjustment[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_namespace_adjustment[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_namespace_adjustment[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_appending[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_appending[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_appending[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_appending[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_removal[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_removal[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_removal[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_owner_references_removal[event-watching-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_labelling[state-changing-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_labelling[state-changing-cause-cluster]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_labelling[event-watching-cause-namespaced]",
"tests/hierarchies/test_contextual_owner.py::test_when_set_for_labelling[event-watching-cause-cluster]"
] | 2021-03-29 20:16:36+00:00 | 4,229 |
|
nolar__kopf-741 | diff --git a/docs/resources.rst b/docs/resources.rst
index 283cc34..5cf94fe 100644
--- a/docs/resources.rst
+++ b/docs/resources.rst
@@ -194,3 +194,15 @@ if there are some accidental overlaps in the specifications.
Keep the short forms only for prototyping and experimentation mode,
and for ad-hoc operators with custom resources (not reusable and running
in controlled clusters where no other similar resources can be defined).
+
+.. warning::
+
+ Some API groups are served by API extensions: e.g. ``metrics.k8s.io``.
+ If the extension's deployment/service/pods are down, such a group will
+ not be scannable (failing with "HTTP 503 Service Unavailable")
+ and will block scanning the whole cluster if resources are specified
+ with no group name (e.g. ``('pods')`` instead of ``('v1', 'pods')``).
+
+ To avoid scanning the whole cluster and all (even unused) API groups,
+ it is recommended to specify at least the group names for all resources,
+ especially in reusable and publicly distributed operators.
diff --git a/kopf/engines/peering.py b/kopf/engines/peering.py
index 257af61..4787c1d 100644
--- a/kopf/engines/peering.py
+++ b/kopf/engines/peering.py
@@ -265,13 +265,13 @@ def detect_own_id(*, manual: bool) -> Identity:
return Identity(f'{user}@{host}' if manual else f'{user}@{host}/{now}/{rnd}')
-def guess_selector(settings: configuration.OperatorSettings) -> Optional[references.Selector]:
+def guess_selectors(settings: configuration.OperatorSettings) -> Iterable[references.Selector]:
if settings.peering.standalone:
- return None
+ return []
elif settings.peering.clusterwide:
- return references.CLUSTER_PEERINGS
+ return [references.CLUSTER_PEERINGS_K, references.CLUSTER_PEERINGS_Z]
elif settings.peering.namespaced:
- return references.NAMESPACED_PEERINGS
+ return [references.NAMESPACED_PEERINGS_K, references.NAMESPACED_PEERINGS_Z]
else:
raise TypeError("Unidentified peering mode (none of standalone/cluster/namespaced).")
@@ -289,10 +289,10 @@ async def touch_command(
insights.ready_resources.wait(),
})
- selector = guess_selector(settings=settings)
- resource = insights.backbone.get(selector) if selector else None
- if resource is None:
- raise RuntimeError(f"Cannot find the peering resource {selector}.")
+ selectors = guess_selectors(settings=settings)
+ resources = [insights.backbone[s] for s in selectors if s in insights.backbone]
+ if not resources:
+ raise RuntimeError(f"Cannot find the peering resource for {selectors}.")
await aiotasks.wait({
aiotasks.create_guarded_task(
@@ -305,4 +305,5 @@ async def touch_command(
lifetime=lifetime),
)
for namespace in insights.namespaces
+ for resource in resources
})
diff --git a/kopf/reactor/orchestration.py b/kopf/reactor/orchestration.py
index 6b624fa..9906400 100644
--- a/kopf/reactor/orchestration.py
+++ b/kopf/reactor/orchestration.py
@@ -127,9 +127,8 @@ async def adjust_tasks(
identity: peering.Identity,
ensemble: Ensemble,
) -> None:
- peering_selector = peering.guess_selector(settings=settings)
- peering_resource = insights.backbone.get(peering_selector) if peering_selector else None
- peering_resources = {peering_resource} if peering_resource is not None else set()
+ peering_selectors = peering.guess_selectors(settings=settings)
+ peering_resources = {insights.backbone[s] for s in peering_selectors if s in insights.backbone}
# Pause or resume all streams if the peering CRDs are absent but required.
# Ignore the CRD absence in auto-detection mode: pause only when (and if) the CRDs are added.
diff --git a/kopf/structs/references.py b/kopf/structs/references.py
index bbf3c74..26534e2 100644
--- a/kopf/structs/references.py
+++ b/kopf/structs/references.py
@@ -389,14 +389,16 @@ class Selector:
# Some predefined API endpoints that we use in the framework itself (not exposed to the operators).
# Note: the CRDs are versionless: we do not look into its ``spec`` stanza, we only watch for
# the fact of changes, so the schema does not matter, any cluster-preferred API version would work.
-# Note: the peering resources are either zalando.org/v1 or kopf.dev/v1; both cannot co-exist because
-# they would share the names, so K8s will not let this. It is done for domain name transitioning.
+# Note: the peering resources are usually either zalando.org/v1 or kopf.dev/v1; if both co-exist,
+# then both will be served (for keepalives and pausing). It is done for domain name transitioning.
CRDS = Selector('apiextensions.k8s.io', 'customresourcedefinitions')
EVENTS = Selector('v1', 'events')
EVENTS_K8S = Selector('events.k8s.io', 'events') # only for exclusion from EVERYTHING
NAMESPACES = Selector('v1', 'namespaces')
-CLUSTER_PEERINGS = Selector('clusterkopfpeerings')
-NAMESPACED_PEERINGS = Selector('kopfpeerings')
+CLUSTER_PEERINGS_K = Selector('kopf.dev/v1', 'clusterkopfpeerings')
+CLUSTER_PEERINGS_Z = Selector('zalando.org/v1', 'clusterkopfpeerings')
+NAMESPACED_PEERINGS_K = Selector('kopf.dev/v1', 'kopfpeerings')
+NAMESPACED_PEERINGS_Z = Selector('zalando.org/v1', 'kopfpeerings')
MUTATING_WEBHOOK = Selector('admissionregistration.k8s.io', 'mutatingwebhookconfigurations')
VALIDATING_WEBHOOK = Selector('admissionregistration.k8s.io', 'validatingwebhookconfigurations')
@@ -426,8 +428,9 @@ class Backbone(Mapping[Selector, Resource]):
self._revised = asyncio.Condition()
self.selectors = [
NAMESPACES, EVENTS, CRDS,
- CLUSTER_PEERINGS, NAMESPACED_PEERINGS,
MUTATING_WEBHOOK, VALIDATING_WEBHOOK,
+ CLUSTER_PEERINGS_K, NAMESPACED_PEERINGS_K,
+ CLUSTER_PEERINGS_Z, NAMESPACED_PEERINGS_Z,
]
def __len__(self) -> int:
| nolar/kopf | 7c86ca29ea8de4cdd42ff16872a17c98cbfa684a | diff --git a/tests/peering/test_resource_guessing.py b/tests/peering/test_resource_guessing.py
index 7f3c4e1..65212f1 100644
--- a/tests/peering/test_resource_guessing.py
+++ b/tests/peering/test_resource_guessing.py
@@ -1,20 +1,21 @@
import pytest
-from kopf.engines.peering import guess_selector
-from kopf.structs.references import CLUSTER_PEERINGS, NAMESPACED_PEERINGS
+from kopf.engines.peering import guess_selectors
+from kopf.structs.references import CLUSTER_PEERINGS_K, CLUSTER_PEERINGS_Z, \
+ NAMESPACED_PEERINGS_K, NAMESPACED_PEERINGS_Z
[email protected]('namespaced, expected_resource', [
- (False, CLUSTER_PEERINGS),
- (True, NAMESPACED_PEERINGS),
[email protected]('namespaced, expected_selectors', [
+ (False, [CLUSTER_PEERINGS_K, CLUSTER_PEERINGS_Z]),
+ (True, [NAMESPACED_PEERINGS_K, NAMESPACED_PEERINGS_Z]),
])
@pytest.mark.parametrize('mandatory', [False, True])
-def test_resource_when_not_standalone(settings, namespaced, mandatory, expected_resource):
+def test_resource_when_not_standalone(settings, namespaced, mandatory, expected_selectors):
settings.peering.standalone = False
settings.peering.namespaced = namespaced
settings.peering.mandatory = mandatory
- selector = guess_selector(settings=settings)
- assert selector == expected_resource
+ selectors = guess_selectors(settings=settings)
+ assert selectors == expected_selectors
@pytest.mark.parametrize('namespaced', [False, True])
@@ -23,5 +24,5 @@ def test_resource_when_standalone(settings, namespaced, mandatory):
settings.peering.standalone = True
settings.peering.namespaced = namespaced
settings.peering.mandatory = mandatory
- selector = guess_selector(settings=settings)
- assert selector is None
+ selectors = guess_selectors(settings=settings)
+ assert not selectors
diff --git a/tests/references/test_backbone.py b/tests/references/test_backbone.py
index 9087c07..2f858aa 100644
--- a/tests/references/test_backbone.py
+++ b/tests/references/test_backbone.py
@@ -3,12 +3,15 @@ import asyncio
import async_timeout
import pytest
-from kopf.structs.references import CLUSTER_PEERINGS, CRDS, EVENTS, NAMESPACED_PEERINGS, \
+from kopf.structs.references import CLUSTER_PEERINGS_K, CLUSTER_PEERINGS_Z, CRDS, EVENTS, \
+ NAMESPACED_PEERINGS_K, NAMESPACED_PEERINGS_Z, \
NAMESPACES, Backbone, Resource, Selector
@pytest.mark.parametrize('selector', [
- CRDS, EVENTS, NAMESPACES, CLUSTER_PEERINGS, NAMESPACED_PEERINGS,
+ CRDS, EVENTS, NAMESPACES,
+ CLUSTER_PEERINGS_K, NAMESPACED_PEERINGS_K,
+ CLUSTER_PEERINGS_Z, NAMESPACED_PEERINGS_Z,
])
def test_empty_backbone(selector: Selector):
backbone = Backbone()
@@ -24,10 +27,10 @@ def test_empty_backbone(selector: Selector):
(CRDS, Resource('apiextensions.k8s.io', 'vX', 'customresourcedefinitions')),
(EVENTS, Resource('', 'v1', 'events')),
(NAMESPACES, Resource('', 'v1', 'namespaces')),
- (CLUSTER_PEERINGS, Resource('kopf.dev', 'v1', 'clusterkopfpeerings')),
- (NAMESPACED_PEERINGS, Resource('kopf.dev', 'v1', 'kopfpeerings')),
- (CLUSTER_PEERINGS, Resource('zalando.org', 'v1', 'clusterkopfpeerings')),
- (NAMESPACED_PEERINGS, Resource('zalando.org', 'v1', 'kopfpeerings')),
+ (CLUSTER_PEERINGS_K, Resource('kopf.dev', 'v1', 'clusterkopfpeerings')),
+ (NAMESPACED_PEERINGS_K, Resource('kopf.dev', 'v1', 'kopfpeerings')),
+ (CLUSTER_PEERINGS_Z, Resource('zalando.org', 'v1', 'clusterkopfpeerings')),
+ (NAMESPACED_PEERINGS_Z, Resource('zalando.org', 'v1', 'kopfpeerings')),
])
async def test_refill_populates_the_resources(selector: Selector, resource: Resource):
backbone = Backbone()
| Group_filter in scan_resources is always to None
## Long story short
In my operator I'm scaling down all deployments in the cluster. By doing that it's scaling down also metrics-server. If the operator resume it tries to contact the /apis/metrics.k8s.io/v1beta1 endpoint at the startup and fail because metrics-server is down. This raise an APIError and stop the process.
## Description
Even if I explicitly define in my handlers the groups and version of the resources I want to handle, the operator always tries to fetch all available resources in the cluster.
This is because of in `kopf/structs/references.py` Line 398-399
```python
CLUSTER_PEERINGS = Selector('clusterkopfpeerings')
NAMESPACED_PEERINGS = Selector('kopfpeerings')
```
The `group` value isn't set.
Due to that in `/kopf/reactor/observation` the return of the test at line 103 is always `None`
```python
group_filter = None if None in groups else {group for group in groups if group is not None}
```
This is rending metrics-server as a mandatory component to run kopf in a cluster.
## Environment
<!-- The following commands can help:
`kopf --version` or `pip show kopf`
`kubectl version`
`python --version`
-->
* Kopf version: 1.30.3
* Kubernetes version: 1.18
* Python version: 3.7.9
| 0.0 | [
"tests/peering/test_resource_guessing.py::test_resource_when_not_standalone[False-False-expected_selectors0]",
"tests/peering/test_resource_guessing.py::test_resource_when_not_standalone[False-True-expected_selectors1]",
"tests/peering/test_resource_guessing.py::test_resource_when_not_standalone[True-False-expected_selectors0]",
"tests/peering/test_resource_guessing.py::test_resource_when_not_standalone[True-True-expected_selectors1]",
"tests/peering/test_resource_guessing.py::test_resource_when_standalone[False-False]",
"tests/peering/test_resource_guessing.py::test_resource_when_standalone[False-True]",
"tests/peering/test_resource_guessing.py::test_resource_when_standalone[True-False]",
"tests/peering/test_resource_guessing.py::test_resource_when_standalone[True-True]",
"tests/references/test_backbone.py::test_empty_backbone[selector0]",
"tests/references/test_backbone.py::test_empty_backbone[selector1]",
"tests/references/test_backbone.py::test_empty_backbone[selector2]",
"tests/references/test_backbone.py::test_empty_backbone[selector3]",
"tests/references/test_backbone.py::test_empty_backbone[selector4]",
"tests/references/test_backbone.py::test_empty_backbone[selector5]",
"tests/references/test_backbone.py::test_empty_backbone[selector6]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector0-resource0]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector1-resource1]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector2-resource2]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector3-resource3]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector4-resource4]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector5-resource5]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector6-resource6]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector7-resource7]",
"tests/references/test_backbone.py::test_refill_populates_the_resources[selector8-resource8]",
"tests/references/test_backbone.py::test_refill_is_cumulative_ie_does_not_reset",
"tests/references/test_backbone.py::test_waiting_for_absent_resources_never_ends",
"tests/references/test_backbone.py::test_waiting_for_preexisting_resources_ends_instantly",
"tests/references/test_backbone.py::test_waiting_for_delayed_resources_ends_once_delivered"
] | [] | 2021-04-25 21:11:57+00:00 | 4,230 |
Subsets and Splits