text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.inspector;
import javax.swing.tree.DefaultMutableTreeNode;
public class TreeUtils {
public static DiagnosticsNode maybeGetDiagnostic(DefaultMutableTreeNode treeNode) {
if (treeNode == null) {
return null;
}
final Object userObject = treeNode.getUserObject();
return (userObject instanceof DiagnosticsNode) ? (DiagnosticsNode)userObject : null;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/inspector/TreeUtils.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/inspector/TreeUtils.java",
"repo_id": "flutter-intellij",
"token_count": 176
} | 486 |
<?xml version="1.0" encoding="UTF-8"?>
<form xmlns="http://www.intellij.com/uidesigner/form/" version="1" bind-to-class="io.flutter.module.settings.FlutterCreateParams">
<grid id="27dc6" binding="mainPanel" layout-manager="GridLayoutManager" row-count="2" column-count="3" same-size-horizontally="false" same-size-vertically="false" hgap="-1" vgap="-1">
<margin top="0" left="0" bottom="0" right="0"/>
<constraints>
<xy x="20" y="20" width="500" height="400"/>
</constraints>
<properties/>
<border type="none"/>
<children>
<vspacer id="f2cc0">
<constraints>
<grid row="1" column="0" row-span="1" col-span="1" vsize-policy="6" hsize-policy="1" anchor="0" fill="2" indent="0" use-parent-layout="false"/>
</constraints>
</vspacer>
<component id="ef6df" class="javax.swing.JLabel" binding="infoLabel">
<constraints>
<grid row="0" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<horizontalAlignment value="4"/>
<icon value="icons/general/information.png"/>
<text value=""/>
<toolTipText value="Offline mode auto-selected."/>
</properties>
</component>
<grid id="d7bac" layout-manager="GridLayoutManager" row-count="1" column-count="1" same-size-horizontally="false" same-size-vertically="false" hgap="-1" vgap="-1">
<margin top="0" left="0" bottom="0" right="3"/>
<constraints>
<grid row="0" column="2" row-span="1" col-span="1" vsize-policy="3" hsize-policy="3" anchor="0" fill="3" indent="0" use-parent-layout="false"/>
</constraints>
<properties/>
<border type="none"/>
<children>
<component id="681d2" class="javax.swing.JCheckBox" binding="createProjectOfflineCheckBox" default-binding="true">
<constraints>
<grid row="0" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="1" anchor="4" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<actionCommand value="Create project offline"/>
<text value="Create project &offline" noi18n="true"/>
<toolTipText value="Run the flutter create command in "offline" mode."/>
</properties>
</component>
</children>
</grid>
</children>
</grid>
</form>
| flutter-intellij/flutter-idea/src/io/flutter/module/settings/FlutterCreateParams.form/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/module/settings/FlutterCreateParams.form",
"repo_id": "flutter-intellij",
"token_count": 1113
} | 487 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.preview;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import com.intellij.icons.AllIcons;
import com.intellij.ide.CommonActionsManager;
import com.intellij.ide.DefaultTreeExpander;
import com.intellij.ide.TreeExpander;
import com.intellij.openapi.actionSystem.*;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.components.PersistentStateComponent;
import com.intellij.openapi.components.Storage;
import com.intellij.openapi.editor.Caret;
import com.intellij.openapi.editor.Editor;
import com.intellij.openapi.editor.event.CaretEvent;
import com.intellij.openapi.editor.event.CaretListener;
import com.intellij.openapi.fileEditor.*;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.ui.SimpleToolWindowPanel;
import com.intellij.openapi.ui.Splitter;
import com.intellij.openapi.util.io.FileUtil;
import com.intellij.openapi.util.text.StringUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.openapi.wm.ToolWindow;
import com.intellij.openapi.wm.ex.ToolWindowEx;
import com.intellij.ui.*;
import com.intellij.ui.content.Content;
import com.intellij.ui.content.ContentFactory;
import com.intellij.ui.content.ContentManager;
import com.intellij.ui.speedSearch.SpeedSearchUtil;
import com.intellij.ui.treeStructure.Tree;
import com.intellij.util.messages.MessageBusConnection;
import io.flutter.FlutterInitializer;
import io.flutter.FlutterUtils;
import io.flutter.dart.FlutterDartAnalysisServer;
import io.flutter.dart.FlutterOutlineListener;
import io.flutter.editor.PropertyEditorPanel;
import io.flutter.inspector.InspectorGroupManagerService;
import io.flutter.settings.FlutterSettings;
import io.flutter.utils.CustomIconMaker;
import io.flutter.utils.EventStream;
import org.dartlang.analysis.server.protocol.Element;
import org.dartlang.analysis.server.protocol.FlutterOutline;
import org.dartlang.analysis.server.protocol.FlutterOutlineAttribute;
import org.dartlang.analysis.server.protocol.FlutterOutlineKind;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.swing.*;
import javax.swing.event.TreeSelectionEvent;
import javax.swing.event.TreeSelectionListener;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.DefaultTreeModel;
import javax.swing.tree.TreeNode;
import javax.swing.tree.TreePath;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.List;
import java.util.*;
@com.intellij.openapi.components.State(
name = "FlutterPreviewView",
storages = {@Storage("$WORKSPACE_FILE$")}
)
public class PreviewView implements PersistentStateComponent<PreviewViewState> {
public static final String TOOL_WINDOW_ID = "Flutter Outline";
@NotNull
private final PreviewViewState state = new PreviewViewState();
@NotNull
private final Project project;
@NotNull
private final FlutterDartAnalysisServer flutterAnalysisServer;
private final InspectorGroupManagerService inspectorGroupManagerService;
private SimpleToolWindowPanel windowPanel;
private boolean isSettingSplitterProportion = false;
private Splitter splitter;
private Splitter propertyEditSplitter;
private JScrollPane scrollPane;
private OutlineTree tree;
private @Nullable PreviewArea previewArea;
private final Set<FlutterOutline> outlinesWithWidgets = Sets.newHashSet();
private final Map<FlutterOutline, DefaultMutableTreeNode> outlineToNodeMap = Maps.newHashMap();
private final EventStream<VirtualFile> currentFile;
private String currentFilePath;
FileEditor currentFileEditor;
private Editor currentEditor;
private FlutterOutline currentOutline;
private final EventStream<List<FlutterOutline>> activeOutlines;
private final WidgetEditToolbar widgetEditToolbar;
private final FlutterOutlineListener outlineListener = new FlutterOutlineListener() {
@Override
public void outlineUpdated(@NotNull String filePath, @NotNull FlutterOutline outline, @Nullable String instrumentedCode) {
if (Objects.equals(currentFilePath, filePath)) {
ApplicationManager.getApplication().invokeLater(() -> updateOutline(outline));
}
}
};
private final CaretListener caretListener = new CaretListener() {
@Override
public void caretPositionChanged(CaretEvent e) {
final Caret caret = e.getCaret();
if (caret != null) {
ApplicationManager.getApplication().invokeLater(() -> applyEditorSelectionToTree(caret));
}
}
@Override
public void caretAdded(@NotNull CaretEvent e) {
}
@Override
public void caretRemoved(@NotNull CaretEvent e) {
}
};
private final TreeSelectionListener treeSelectionListener = this::handleTreeSelectionEvent;
private PropertyEditorPanel propertyEditPanel;
private DefaultActionGroup propertyEditToolbarGroup;
public PreviewView(@NotNull Project project) {
this.project = project;
currentFile = new EventStream<>();
activeOutlines = new EventStream<>(ImmutableList.of());
flutterAnalysisServer = FlutterDartAnalysisServer.getInstance(project);
inspectorGroupManagerService = InspectorGroupManagerService.getInstance(project);
// Show preview for the file selected when the view is being opened.
final VirtualFile[] selectedFiles = FileEditorManager.getInstance(project).getSelectedFiles();
if (selectedFiles.length != 0) {
setSelectedFile(selectedFiles[0]);
}
final FileEditor[] selectedEditors = FileEditorManager.getInstance(project).getSelectedEditors();
if (selectedEditors.length != 0) {
setSelectedEditor(selectedEditors[0]);
}
// Listen for selecting files.
final MessageBusConnection bus = project.getMessageBus().connect(project);
bus.subscribe(FileEditorManagerListener.FILE_EDITOR_MANAGER, new FileEditorManagerListener() {
@Override
public void selectionChanged(@NotNull FileEditorManagerEvent event) {
setSelectedFile(event.getNewFile());
setSelectedEditor(event.getNewEditor());
}
});
widgetEditToolbar = new WidgetEditToolbar(
FlutterSettings.getInstance().isEnableHotUi(),
activeOutlines,
currentFile,
project,
flutterAnalysisServer
);
}
@NotNull
@Override
public PreviewViewState getState() {
return this.state;
}
@Override
public void loadState(@NotNull PreviewViewState state) {
this.state.copyFrom(state);
}
public void initToolWindow(@NotNull ToolWindow toolWindow) {
final ContentFactory contentFactory = ContentFactory.getInstance();
final ContentManager contentManager = toolWindow.getContentManager();
final Content content = contentFactory.createContent(null, null, false);
content.setCloseable(false);
windowPanel = new OutlineComponent(this);
content.setComponent(windowPanel);
windowPanel.setToolbar(widgetEditToolbar.getToolbar().getComponent());
final DefaultMutableTreeNode rootNode = new DefaultMutableTreeNode();
tree = new OutlineTree(rootNode);
tree.setCellRenderer(new OutlineTreeCellRenderer());
tree.expandAll();
initTreePopup();
// Add collapse all, expand all, and show only widgets buttons.
if (toolWindow instanceof ToolWindowEx) {
final ToolWindowEx toolWindowEx = (ToolWindowEx)toolWindow;
final CommonActionsManager actions = CommonActionsManager.getInstance();
final TreeExpander expander = new DefaultTreeExpander(tree);
final AnAction expandAllAction = actions.createExpandAllAction(expander, tree);
expandAllAction.getTemplatePresentation().setIcon(AllIcons.Actions.Expandall);
final AnAction collapseAllAction = actions.createCollapseAllAction(expander, tree);
collapseAllAction.getTemplatePresentation().setIcon(AllIcons.Actions.Collapseall);
final ShowOnlyWidgetsAction showOnlyWidgetsAction = new ShowOnlyWidgetsAction();
toolWindowEx.setTitleActions(Arrays.asList(expandAllAction, collapseAllAction, showOnlyWidgetsAction));
}
new TreeSpeedSearch(tree) {
@Override
protected String getElementText(Object element) {
final TreePath path = (TreePath)element;
final DefaultMutableTreeNode node = (DefaultMutableTreeNode)path.getLastPathComponent();
final Object object = node.getUserObject();
if (object instanceof OutlineObject) {
return ((OutlineObject)object).getSpeedSearchString();
}
return null;
}
};
tree.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
if (e.getClickCount() > 1) {
final TreePath selectionPath = tree.getSelectionPath();
if (selectionPath != null) {
selectPath(selectionPath, true);
}
}
}
});
tree.addTreeSelectionListener(treeSelectionListener);
scrollPane = ScrollPaneFactory.createScrollPane(tree);
content.setPreferredFocusableComponent(tree);
contentManager.addContent(content);
contentManager.setSelectedContent(content);
splitter = new Splitter(true);
setSplitterProportion(getState().getSplitterProportion());
getState().addListener(e -> {
final float newProportion = getState().getSplitterProportion();
if (splitter.getProportion() != newProportion) {
setSplitterProportion(newProportion);
}
});
//noinspection Convert2Lambda
splitter.addPropertyChangeListener("proportion", new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
if (!isSettingSplitterProportion) {
getState().setSplitterProportion(splitter.getProportion());
}
}
});
scrollPane.setMinimumSize(new Dimension(1, 1));
splitter.setFirstComponent(scrollPane);
windowPanel.setContent(splitter);
}
private void initTreePopup() {
tree.addMouseListener(new PopupHandler() {
public void invokePopup(Component comp, int x, int y) {
// Ensure that at least one Widget item is selected.
final List<FlutterOutline> selectedOutlines = getOutlinesSelectedInTree();
if (selectedOutlines.isEmpty()) {
return;
}
for (FlutterOutline outline : selectedOutlines) {
if (outline.getDartElement() != null) {
return;
}
}
widgetEditToolbar.createPopupMenu(comp, x, y);
}
});
}
private OutlineOffsetConverter getOutlineOffsetConverter() {
return new OutlineOffsetConverter(project, currentFile.getValue());
}
private void handleTreeSelectionEvent(TreeSelectionEvent e) {
final TreePath selectionPath = e.getNewLeadSelectionPath();
if (selectionPath != null) {
ApplicationManager.getApplication().invokeLater(() -> selectPath(selectionPath, false));
}
activeOutlines.setValue(getOutlinesSelectedInTree());
}
private void selectPath(TreePath selectionPath, boolean focusEditor) {
final FlutterOutline outline = getOutlineOfPath(selectionPath);
jumpToOutlineInEditor(outline, focusEditor);
}
private void jumpToOutlineInEditor(FlutterOutline outline, boolean focusEditor) {
if (outline == null) {
return;
}
final int offset = outline.getDartElement() != null ? outline.getDartElement().getLocation().getOffset() : outline.getOffset();
final int editorOffset = getOutlineOffsetConverter().getConvertedFileOffset(offset);
sendAnalyticEvent("jumpToSource");
if (currentFile != null) {
currentEditor.getCaretModel().removeCaretListener(caretListener);
try {
new OpenFileDescriptor(project, currentFile.getValue(), editorOffset).navigate(focusEditor);
}
finally {
currentEditor.getCaretModel().addCaretListener(caretListener);
}
}
// TODO(jacobr): refactor the previewArea to listen on the stream of
// selected outlines instead.
if (previewArea != null) {
previewArea.select(ImmutableList.of(outline), currentEditor);
}
}
// TODO: Add parent relationship info to FlutterOutline instead of this O(n^2) traversal.
private Element getElementParentFor(@Nullable Element element) {
if (element == null) {
return null;
}
for (FlutterOutline outline : outlineToNodeMap.keySet()) {
final List<FlutterOutline> children = outline.getChildren();
if (children != null) {
for (FlutterOutline child : children) {
if (child.getDartElement() == element) {
return outline.getDartElement();
}
}
}
}
return null;
}
private DefaultTreeModel getTreeModel() {
return (DefaultTreeModel)tree.getModel();
}
private DefaultMutableTreeNode getRootNode() {
return (DefaultMutableTreeNode)getTreeModel().getRoot();
}
private void updateOutline(@NotNull FlutterOutline outline) {
currentOutline = outline;
final DefaultMutableTreeNode rootNode = getRootNode();
rootNode.removeAllChildren();
outlinesWithWidgets.clear();
outlineToNodeMap.clear();
if (outline.getChildren() != null) {
computeOutlinesWithWidgets(outline);
updateOutlineImpl(rootNode, outline.getChildren());
}
getTreeModel().reload(rootNode);
tree.expandAll();
if (currentEditor != null) {
final Caret caret = currentEditor.getCaretModel().getPrimaryCaret();
applyEditorSelectionToTree(caret);
}
if (FlutterSettings.getInstance().isEnableHotUi() && propertyEditPanel == null) {
propertyEditSplitter = new Splitter(false, 0.75f);
propertyEditPanel = new PropertyEditorPanel(inspectorGroupManagerService, project, flutterAnalysisServer, false, false, project);
propertyEditPanel.initalize(null, activeOutlines, currentFile);
propertyEditToolbarGroup = new DefaultActionGroup();
final ActionToolbar windowToolbar = ActionManager.getInstance().createActionToolbar("PropertyArea", propertyEditToolbarGroup, true);
final SimpleToolWindowPanel window = new SimpleToolWindowPanel(true, true);
window.setToolbar(windowToolbar.getComponent());
final JScrollPane propertyScrollPane = ScrollPaneFactory.createScrollPane(propertyEditPanel);
window.setContent(propertyScrollPane);
propertyEditSplitter.setFirstComponent(window.getComponent());
final InspectorGroupManagerService.Client inspectorStateServiceClient = new InspectorGroupManagerService.Client(project) {
@Override
public void onInspectorAvailabilityChanged() {
super.onInspectorAvailabilityChanged();
// Only show the screen mirror if there is a running device and
// the inspector supports the neccessary apis.
if (getInspectorService() != null && getInspectorService().isHotUiScreenMirrorSupported()) {
// Wait to create the preview area until it is needed.
if (previewArea == null) {
previewArea = new PreviewArea(project, outlinesWithWidgets, project);
}
propertyEditSplitter.setSecondComponent(previewArea.getComponent());
}
else {
propertyEditSplitter.setSecondComponent(null);
}
}
};
inspectorGroupManagerService.addListener(inspectorStateServiceClient, project);
splitter.setSecondComponent(propertyEditSplitter);
}
// TODO(jacobr): this is the wrong spot.
if (propertyEditToolbarGroup != null) {
final TitleAction propertyTitleAction = new TitleAction("Properties");
propertyEditToolbarGroup.removeAll();
propertyEditToolbarGroup.add(propertyTitleAction);
}
}
private boolean computeOutlinesWithWidgets(FlutterOutline outline) {
boolean hasWidget = false;
if (outline.getDartElement() == null) {
outlinesWithWidgets.add(outline);
hasWidget = true;
}
final List<FlutterOutline> children = outline.getChildren();
if (children != null) {
for (final FlutterOutline child : children) {
if (computeOutlinesWithWidgets(child)) {
outlinesWithWidgets.add(outline);
hasWidget = true;
}
}
}
return hasWidget;
}
private void updateOutlineImpl(@NotNull DefaultMutableTreeNode parent, @NotNull List<FlutterOutline> outlines) {
int index = 0;
for (final FlutterOutline outline : outlines) {
if (FlutterSettings.getInstance().isShowOnlyWidgets() && !outlinesWithWidgets.contains(outline)) {
continue;
}
final OutlineObject object = new OutlineObject(outline);
final DefaultMutableTreeNode node = new DefaultMutableTreeNode(object);
outlineToNodeMap.put(outline, node);
getTreeModel().insertNodeInto(node, parent, index++);
if (outline.getChildren() != null) {
updateOutlineImpl(node, outline.getChildren());
}
}
}
@NotNull
private List<FlutterOutline> getOutlinesSelectedInTree() {
final List<FlutterOutline> selectedOutlines = new ArrayList<>();
final DefaultMutableTreeNode[] selectedNodes = tree.getSelectedNodes(DefaultMutableTreeNode.class, null);
for (DefaultMutableTreeNode selectedNode : selectedNodes) {
final FlutterOutline outline = getOutlineOfNode(selectedNode);
selectedOutlines.add(outline);
}
return selectedOutlines;
}
static private FlutterOutline getOutlineOfNode(DefaultMutableTreeNode node) {
final OutlineObject object = (OutlineObject)node.getUserObject();
return object.outline;
}
@Nullable
private FlutterOutline getOutlineOfPath(@Nullable TreePath path) {
if (path == null) {
return null;
}
final DefaultMutableTreeNode node = (DefaultMutableTreeNode)path.getLastPathComponent();
return getOutlineOfNode(node);
}
private void addOutlinesCoveredByRange(List<FlutterOutline> covered, int start, int end, @Nullable FlutterOutline outline) {
if (outline == null) {
return;
}
final OutlineOffsetConverter converter = getOutlineOffsetConverter();
final int outlineStart = converter.getConvertedOutlineOffset(outline);
final int outlineEnd = converter.getConvertedOutlineEnd(outline);
// The outline ends before, or starts after the selection.
if (outlineEnd < start || outlineStart > end) {
return;
}
// The outline is covered by the selection.
if (outlineStart >= start && outlineEnd <= end) {
covered.add(outline);
return;
}
// The outline covers the selection.
if (outlineStart <= start && end <= outlineEnd) {
if (outline.getChildren() != null) {
for (FlutterOutline child : outline.getChildren()) {
addOutlinesCoveredByRange(covered, start, end, child);
}
}
}
}
private void setSelectedFile(VirtualFile newFile) {
if (currentFile.getValue() != null) {
flutterAnalysisServer.removeOutlineListener(currentFilePath, outlineListener);
currentFile.setValue(null);
currentFilePath = null;
}
// If not a Dart file, ignore it.
if (newFile != null && !FlutterUtils.isDartFile(newFile)) {
newFile = null;
}
// Show the toolbar if the new file is a Dart file, or hide otherwise.
if (windowPanel != null) {
if (newFile != null) {
windowPanel.setToolbar(widgetEditToolbar.getToolbar().getComponent());
}
else if (windowPanel.isToolbarVisible()) {
windowPanel.setToolbar(null);
}
}
// If the tree is already created, clear it now, until the outline for the new file is received.
if (tree != null) {
final DefaultMutableTreeNode rootNode = getRootNode();
rootNode.removeAllChildren();
getTreeModel().reload(rootNode);
}
// Subscribe for the outline for the new file.
if (newFile != null) {
currentFile.setValue(newFile);
currentFilePath = FileUtil.toSystemDependentName(newFile.getPath());
flutterAnalysisServer.addOutlineListener(currentFilePath, outlineListener);
}
}
private void setSelectedEditor(FileEditor newEditor) {
if (currentEditor != null) {
currentEditor.getCaretModel().removeCaretListener(caretListener);
}
if (newEditor instanceof TextEditor) {
currentFileEditor = newEditor;
currentEditor = ((TextEditor)newEditor).getEditor();
currentEditor.getCaretModel().addCaretListener(caretListener);
}
}
private void applyEditorSelectionToTree(Caret caret) {
final List<FlutterOutline> selectedOutlines = new ArrayList<>();
// Try to find outlines covered by the selection.
addOutlinesCoveredByRange(selectedOutlines, caret.getSelectionStart(), caret.getSelectionEnd(), currentOutline);
// If no covered outlines, try to find the outline under the caret.
if (selectedOutlines.isEmpty()) {
final FlutterOutline outline = getOutlineOffsetConverter().findOutlineAtOffset(currentOutline, caret.getOffset());
if (outline != null) {
selectedOutlines.add(outline);
}
}
activeOutlines.setValue(selectedOutlines);
applyOutlinesSelectionToTree(selectedOutlines);
// TODO(jacobr): refactor the previewArea to listen on the stream of
// selected outlines instead.
if (previewArea != null) {
previewArea.select(selectedOutlines, currentEditor);
}
}
private void applyOutlinesSelectionToTree(List<FlutterOutline> outlines) {
final List<TreePath> selectedPaths = new ArrayList<>();
TreeNode[] lastNodePath = null;
TreePath lastTreePath = null;
for (FlutterOutline outline : outlines) {
final DefaultMutableTreeNode selectedNode = outlineToNodeMap.get(outline);
if (selectedNode != null) {
lastNodePath = selectedNode.getPath();
lastTreePath = new TreePath(lastNodePath);
selectedPaths.add(lastTreePath);
}
}
if (lastNodePath != null) {
// Ensure that all parent nodes are expected.
tree.scrollPathToVisible(lastTreePath);
// Ensure that the top-level declaration (class) is on the top of the tree.
if (lastNodePath.length >= 2) {
scrollTreeToNodeOnTop(lastNodePath[1]);
}
// Ensure that the selected node is still visible, even if the top-level declaration is long.
tree.scrollPathToVisible(lastTreePath);
}
// Now actually select the node.
tree.removeTreeSelectionListener(treeSelectionListener);
tree.setSelectionPaths(selectedPaths.toArray(new TreePath[0]));
tree.addTreeSelectionListener(treeSelectionListener);
// JTree attempts to show as much of the node as possible, so scrolls horizontally.
// But we actually need to see the whole hierarchy, so we scroll back to zero.
scrollPane.getHorizontalScrollBar().setValue(0);
}
private void scrollTreeToNodeOnTop(TreeNode node) {
if (node instanceof DefaultMutableTreeNode) {
final DefaultMutableTreeNode defaultNode = (DefaultMutableTreeNode)node;
final Rectangle bounds = tree.getPathBounds(new TreePath(defaultNode.getPath()));
// Set the height to the visible tree height to force the node to top.
if (bounds != null) {
bounds.height = tree.getVisibleRect().height;
tree.scrollRectToVisible(bounds);
}
}
}
private void setSplitterProportion(float value) {
isSettingSplitterProportion = true;
try {
splitter.setProportion(value);
}
finally {
isSettingSplitterProportion = false;
}
doLayoutRecursively(splitter);
}
// TODO(jacobr): is this method really useful? This is from re-applying the
// PreviewArea but it doesn't really seem that useful.
private static void doLayoutRecursively(Component component) {
if (component != null) {
component.doLayout();
if (component instanceof Container) {
final Container container = (Container)component;
for (Component child : container.getComponents()) {
doLayoutRecursively(child);
}
}
}
}
private void sendAnalyticEvent(@NotNull String name) {
FlutterInitializer.getAnalytics().sendEvent("preview", name);
}
private class ShowOnlyWidgetsAction extends AnAction implements Toggleable, RightAlignedToolbarAction {
ShowOnlyWidgetsAction() {
super("Show Only Widgets", "Show only widgets", AllIcons.General.Filter);
}
@Override
public void actionPerformed(@NotNull AnActionEvent e) {
final FlutterSettings flutterSettings = FlutterSettings.getInstance();
flutterSettings.setShowOnlyWidgets(!flutterSettings.isShowOnlyWidgets());
if (currentOutline != null) {
updateOutline(currentOutline);
}
}
@Override
public void update(AnActionEvent e) {
final Presentation presentation = e.getPresentation();
presentation.putClientProperty(SELECTED_PROPERTY, FlutterSettings.getInstance().isShowOnlyWidgets());
presentation.setEnabled(currentOutline != null);
}
}
}
/**
* We subclass {@link SimpleToolWindowPanel} to implement "getData" and return {@link PlatformDataKeys#FILE_EDITOR},
* so that Undo/Redo actions work.
*/
class OutlineComponent extends SimpleToolWindowPanel {
private final PreviewView myView;
OutlineComponent(PreviewView view) {
super(true, true);
myView = view;
}
@Override
public Object getData(@NotNull String dataId) {
if (PlatformDataKeys.FILE_EDITOR.is(dataId)) {
return myView.currentFileEditor;
}
return super.getData(dataId);
}
}
class OutlineTree extends Tree {
OutlineTree(DefaultMutableTreeNode model) {
super(model);
setRootVisible(false);
setToggleClickCount(0);
}
void expandAll() {
for (int row = 0; row < getRowCount(); row++) {
expandRow(row);
}
}
}
class OutlineObject {
private static final CustomIconMaker iconMaker = new CustomIconMaker();
private static final Map<Icon, LayeredIcon> flutterDecoratedIcons = new HashMap<>();
final FlutterOutline outline;
private Icon icon;
OutlineObject(FlutterOutline outline) {
this.outline = outline;
}
Icon getIcon() {
if (outline.getKind().equals(FlutterOutlineKind.DART_ELEMENT)) {
return null;
}
if (icon == null) {
final String className = outline.getClassName();
if (icon == null) {
icon = iconMaker.fromWidgetName(className);
}
}
return icon;
}
/**
* Return the string that is suitable for speed search. It has every name part separated so that we search only inside individual name
* parts, but not in their accidential concatenation.
*/
@NotNull
String getSpeedSearchString() {
final StringBuilder builder = new StringBuilder();
final Element dartElement = outline.getDartElement();
if (dartElement != null) {
builder.append(dartElement.getName());
}
else {
builder.append(outline.getClassName());
}
if (outline.getVariableName() != null) {
builder.append('|');
builder.append(outline.getVariableName());
}
final List<FlutterOutlineAttribute> attributes = outline.getAttributes();
if (attributes != null) {
for (FlutterOutlineAttribute attribute : attributes) {
builder.append(attribute.getName());
builder.append(':');
builder.append(attribute.getLabel());
}
}
return builder.toString();
}
}
class OutlineTreeCellRenderer extends ColoredTreeCellRenderer {
private JTree tree;
private boolean selected;
public void customizeCellRenderer(
@NotNull final JTree tree,
final Object value,
final boolean selected,
final boolean expanded,
final boolean leaf,
final int row,
final boolean hasFocus
) {
final Object userObject = ((DefaultMutableTreeNode)value).getUserObject();
if (!(userObject instanceof OutlineObject)) {
return;
}
final OutlineObject node = (OutlineObject)userObject;
final FlutterOutline outline = node.outline;
this.tree = tree;
this.selected = selected;
// Render a Dart element.
final Element dartElement = outline.getDartElement();
if (dartElement != null) {
final Icon icon = DartElementPresentationUtil.getIcon(dartElement);
setIcon(icon);
final boolean renderInBold = hasWidgetChild(outline) && ModelUtils.isBuildMethod(dartElement);
DartElementPresentationUtil.renderElement(dartElement, this, renderInBold);
return;
}
final Icon icon = node.getIcon();
if (icon != null) {
setIcon(icon);
}
// Render the widget class.
appendSearch(outline.getClassName(), SimpleTextAttributes.REGULAR_ATTRIBUTES);
// Render the variable.
if (outline.getVariableName() != null) {
append(" ");
appendSearch(outline.getVariableName(), SimpleTextAttributes.GRAYED_ATTRIBUTES);
}
// Render a generic label.
if (outline.getKind().equals(FlutterOutlineKind.GENERIC) && outline.getLabel() != null) {
append(" ");
final String label = outline.getLabel();
appendSearch(label, SimpleTextAttributes.GRAYED_ATTRIBUTES);
}
// Append all attributes.
final List<FlutterOutlineAttribute> attributes = outline.getAttributes();
if (attributes != null) {
if (attributes.size() == 1 && isAttributeElidable(attributes.get(0).getName())) {
final FlutterOutlineAttribute attribute = attributes.get(0);
append(" ");
appendSearch(attribute.getLabel(), SimpleTextAttributes.GRAYED_ATTRIBUTES);
}
else {
for (int i = 0; i < attributes.size(); i++) {
final FlutterOutlineAttribute attribute = attributes.get(i);
if (i > 0) {
append(",");
}
append(" ");
if (!StringUtil.equals("data", attribute.getName())) {
appendSearch(attribute.getName(), SimpleTextAttributes.GRAYED_ATTRIBUTES);
append(": ", SimpleTextAttributes.GRAYED_ATTRIBUTES);
}
appendSearch(attribute.getLabel(), SimpleTextAttributes.GRAYED_ATTRIBUTES);
}
}
}
}
private boolean hasWidgetChild(FlutterOutline outline) {
if (outline.getChildren() == null) {
return false;
}
for (FlutterOutline child : outline.getChildren()) {
if (child.getDartElement() == null) {
return true;
}
}
return false;
}
void appendSearch(@NotNull String text, @NotNull SimpleTextAttributes attributes) {
SpeedSearchUtil.appendFragmentsForSpeedSearch(tree, text, attributes, selected, this);
}
private static boolean isAttributeElidable(String name) {
return StringUtil.equals("text", name) || StringUtil.equals("icon", name);
}
}
/**
* Delegate to the given action, but do not render the action's icon.
*/
class TextOnlyActionWrapper extends AnAction {
private final AnAction action;
public TextOnlyActionWrapper(AnAction action) {
super(action.getTemplatePresentation().getText());
this.action = action;
}
@Override
public void actionPerformed(@NotNull AnActionEvent event) {
action.actionPerformed(event);
}
}
| flutter-intellij/flutter-idea/src/io/flutter/preview/PreviewView.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/preview/PreviewView.java",
"repo_id": "flutter-intellij",
"token_count": 10844
} | 488 |
/*
* Copyright 2016 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run;
import com.intellij.execution.configuration.EnvironmentVariablesTextFieldWithBrowseButton;
import com.intellij.openapi.options.ConfigurationException;
import com.intellij.openapi.options.SettingsEditor;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.ui.TextFieldWithBrowseButton;
import com.intellij.openapi.util.io.FileUtil;
import com.intellij.openapi.util.text.StringUtil;
import org.jetbrains.annotations.NotNull;
import javax.swing.*;
import static com.jetbrains.lang.dart.ide.runner.server.ui.DartCommandLineConfigurationEditorForm.initDartFileTextWithBrowse;
public class FlutterConfigurationEditorForm extends SettingsEditor<SdkRunConfig> {
private JPanel myMainPanel;
private JLabel myDartFileLabel;
private TextFieldWithBrowseButton myFileField;
private com.intellij.ui.components.fields.ExpandableTextField myAdditionalArgumentsField;
private JTextField myBuildFlavorField;
private com.intellij.ui.components.fields.ExpandableTextField myAttachArgsField;
private EnvironmentVariablesTextFieldWithBrowseButton myEnvironmentVariables;
private JLabel myEnvvarLabel;
public FlutterConfigurationEditorForm(final Project project) {
initDartFileTextWithBrowse(project, myFileField);
}
@Override
protected void resetEditorFrom(@NotNull final SdkRunConfig config) {
final SdkFields fields = config.getFields();
myFileField.setText(FileUtil.toSystemDependentName(StringUtil.notNullize(fields.getFilePath())));
myBuildFlavorField.setText(fields.getBuildFlavor());
myAdditionalArgumentsField.setText(fields.getAdditionalArgs());
myAttachArgsField.setText(fields.getAttachArgs());
myEnvironmentVariables.setEnvs(fields.getEnvs());
}
@Override
protected void applyEditorTo(@NotNull final SdkRunConfig config) throws ConfigurationException {
final SdkFields fields = new SdkFields();
fields.setFilePath(StringUtil.nullize(FileUtil.toSystemIndependentName(myFileField.getText().trim()), true));
fields.setBuildFlavor(StringUtil.nullize(myBuildFlavorField.getText().trim()));
fields.setAdditionalArgs(StringUtil.nullize(myAdditionalArgumentsField.getText().trim()));
fields.setAttachArgs(StringUtil.nullize(myAttachArgsField.getText().trim()));
fields.setEnvs(myEnvironmentVariables.getEnvs());
config.setFields(fields);
}
@NotNull
@Override
protected JComponent createEditor() {
return myMainPanel;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/FlutterConfigurationEditorForm.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/FlutterConfigurationEditorForm.java",
"repo_id": "flutter-intellij",
"token_count": 823
} | 489 |
/*
* Copyright (C) 2018 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.flutter.run;
import com.intellij.execution.ExecutionException;
import com.intellij.execution.Executor;
import com.intellij.execution.configurations.GeneralCommandLine;
import com.intellij.execution.configurations.RunConfiguration;
import com.intellij.execution.configurations.RuntimeConfigurationError;
import com.intellij.execution.runners.ExecutionEnvironment;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.module.ModuleUtilCore;
import com.intellij.openapi.options.SettingsEditor;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.util.Disposer;
import com.intellij.openapi.util.text.StringUtil;
import com.intellij.psi.PsiElement;
import com.intellij.refactoring.listeners.RefactoringElementListener;
import com.jetbrains.lang.dart.sdk.DartConfigurable;
import com.jetbrains.lang.dart.sdk.DartSdk;
import io.flutter.FlutterBundle;
import io.flutter.dart.DartPlugin;
import io.flutter.pub.PubRoot;
import io.flutter.run.common.RunMode;
import io.flutter.run.daemon.FlutterApp;
import io.flutter.sdk.FlutterSdkManager;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public class SdkAttachConfig extends SdkRunConfig {
public PubRoot pubRoot;
public SdkAttachConfig(SdkRunConfig config) {
//noinspection ConstantConditions
super(config.getProject(), config.getFactory(), config.getName());
setFields(config.getFields()); // TODO(messick): Delete if not needed.
}
@Nullable
@Override
public RefactoringElementListener getRefactoringElementListener(PsiElement element) {
return null;
}
@NotNull
@Override
public SettingsEditor<? extends RunConfiguration> getConfigurationEditor() {
throw new IllegalStateException("Attach configurations are not editable");
}
@NotNull
@Override
public LaunchState getState(@NotNull Executor executor, @NotNull ExecutionEnvironment env) throws ExecutionException {
try {
checkRunnable(env.getProject());
}
catch (RuntimeConfigurationError e) {
throw new ExecutionException(e);
}
final SdkFields launchFields = getFields();
final MainFile mainFile = MainFile.verify(launchFields.getFilePath(), env.getProject()).get();
final Project project = env.getProject();
final RunMode mode = RunMode.fromEnv(env);
final Module module = ModuleUtilCore.findModuleForFile(mainFile.getFile(), env.getProject());
final LaunchState.CreateAppCallback createAppCallback = (@Nullable FlutterDevice device) -> {
if (device == null) return null;
final GeneralCommandLine command = getCommand(env, device);
final FlutterApp app = FlutterApp.start(env, project, module, mode, device, command,
StringUtil.capitalize(mode.mode()) + "App",
"StopApp");
// Stop the app if the Flutter SDK changes.
final FlutterSdkManager.Listener sdkListener = new FlutterSdkManager.Listener() {
@Override
public void flutterSdkRemoved() {
app.shutdownAsync();
}
};
FlutterSdkManager.getInstance(project).addListener(sdkListener);
Disposer.register(app, () -> FlutterSdkManager.getInstance(project).removeListener(sdkListener));
return app;
};
final LaunchState launcher = new AttachState(env, mainFile.getAppDir(), mainFile.getFile(), this, createAppCallback);
addConsoleFilters(launcher, env, mainFile, module);
return launcher;
}
@NotNull
@Override
public GeneralCommandLine getCommand(@NotNull ExecutionEnvironment env, @NotNull FlutterDevice device) throws ExecutionException {
return getFields().createFlutterSdkAttachCommand(env.getProject(), FlutterLaunchMode.fromEnv(env), device);
}
private void checkRunnable(@NotNull Project project) throws RuntimeConfigurationError {
final DartSdk sdk = DartPlugin.getDartSdk(project);
if (sdk == null) {
throw new RuntimeConfigurationError(FlutterBundle.message("dart.sdk.is.not.configured"),
() -> DartConfigurable.openDartSettings(project));
}
final MainFile.Result main = MainFile.verify(getFields().getFilePath(), project);
if (!main.canLaunch()) {
throw new RuntimeConfigurationError(main.getError());
}
if (PubRoot.forDirectory(main.get().getAppDir()) == null) {
throw new RuntimeConfigurationError("Entrypoint isn't within a Flutter pub root");
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/SdkAttachConfig.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/SdkAttachConfig.java",
"repo_id": "flutter-intellij",
"token_count": 1721
} | 490 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.bazelTest;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSyntaxException;
import com.intellij.execution.ExecutionException;
import com.intellij.execution.ExecutionResult;
import com.intellij.execution.configurations.RunProfile;
import com.intellij.execution.configurations.RunProfileState;
import com.intellij.execution.executors.DefaultDebugExecutor;
import com.intellij.execution.executors.DefaultRunExecutor;
import com.intellij.execution.process.*;
import com.intellij.execution.runners.ExecutionEnvironment;
import com.intellij.execution.runners.GenericProgramRunner;
import com.intellij.execution.ui.RunContentDescriptor;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.roots.ContentEntry;
import com.intellij.openapi.roots.ModuleRootManager;
import com.intellij.openapi.util.Computable;
import com.intellij.openapi.util.Key;
import com.intellij.openapi.vfs.LocalFileSystem;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.xdebugger.XDebugProcess;
import com.intellij.xdebugger.XDebugProcessStarter;
import com.intellij.xdebugger.XDebugSession;
import com.intellij.xdebugger.XDebuggerManager;
import com.jetbrains.lang.dart.sdk.DartSdkLibUtil;
import com.jetbrains.lang.dart.util.DartUrlResolver;
import io.flutter.FlutterMessages;
import io.flutter.FlutterUtils;
import io.flutter.ObservatoryConnector;
import io.flutter.bazel.Workspace;
import io.flutter.bazel.WorkspaceCache;
import io.flutter.run.FlutterPositionMapper;
import io.flutter.run.common.CommonTestConfigUtils;
import io.flutter.run.test.FlutterTestRunner;
import io.flutter.settings.FlutterSettings;
import io.flutter.utils.JsonUtils;
import io.flutter.utils.StdoutJsonParser;
import io.flutter.utils.UrlUtils;
import org.apache.commons.lang3.StringUtils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
/**
* The Bazel version of the {@link FlutterTestRunner}. Runs a Bazel Flutter test configuration in the debugger.
*/
public class BazelTestRunner extends GenericProgramRunner {
private static final Logger LOG = Logger.getInstance(BazelTestRunner.class);
@NotNull
@Override
public String getRunnerId() {
return "FlutterBazelTestRunner";
}
/**
* Only allow this runner to execute for the Run and Debug executors.
*/
@Override
public boolean canRun(@NotNull String executorId, @NotNull RunProfile profile) {
return (DefaultRunExecutor.EXECUTOR_ID.equals(executorId) || DefaultDebugExecutor.EXECUTOR_ID.equals(executorId)) &&
profile instanceof BazelTestConfig;
}
@Nullable
@Override
protected RunContentDescriptor doExecute(@NotNull RunProfileState state, @NotNull ExecutionEnvironment env)
throws ExecutionException {
return runInDebugger((BazelTestLaunchState)state, env);
}
protected RunContentDescriptor runInDebugger(@NotNull BazelTestLaunchState launcher, @NotNull ExecutionEnvironment env)
throws ExecutionException {
// Start process and create console.
final ExecutionResult executionResult = launcher.execute(env.getExecutor(), this);
final Connector connector = new Connector(executionResult.getProcessHandler(), env.getProject());
// Set up source file mapping.
final DartUrlResolver resolver = DartUrlResolver.getInstance(env.getProject(), launcher.getTestFile());
final FlutterPositionMapper.Analyzer analyzer = FlutterPositionMapper.Analyzer.create(env.getProject(), launcher.getTestFile());
final BazelPositionMapper mapper =
new BazelPositionMapper(env.getProject(), env.getProject().getBaseDir()/*this is different, incorrect?*/, resolver, analyzer,
connector);
// Create the debug session.
final XDebuggerManager manager = XDebuggerManager.getInstance(env.getProject());
final XDebugSession session = manager.startSession(env, new XDebugProcessStarter() {
@Override
@NotNull
public XDebugProcess start(@NotNull final XDebugSession session) {
return new BazelTestDebugProcess(env, session, executionResult, resolver, connector, mapper);
}
});
return session.getRunContentDescriptor();
}
/**
* Provides observatory URI, as received from the test process.
*/
private static final class Connector implements ObservatoryConnector {
private final StdoutJsonParser stdoutParser = new StdoutJsonParser();
private final ProcessListener listener;
private String observatoryUri;
private String runfilesDir;
private String workspaceDirName;
private static final String STARTED_PROCESS = "test.startedProcess";
private static final String OBSERVATORY_URI_KEY = "vmServiceUri";
private static final String RUNFILES_DIR_KEY = "runfilesDir";
private static final String WORKSPACE_DIR_NAME_KEY = "workspaceDirName";
public Connector(ProcessHandler handler, Project project) {
Workspace workspace = WorkspaceCache.getInstance(project).get();
assert(workspace != null);
String configWarningPrefix = workspace.getConfigWarningPrefix();
listener = new ProcessAdapter() {
@Override
public void onTextAvailable(@NotNull ProcessEvent event, @NotNull Key outputType) {
final String text = event.getText();
if (configWarningPrefix != null && text.startsWith(configWarningPrefix)) {
FlutterMessages.showWarning(
"Configuration warning",
UrlUtils.generateHtmlFragmentWithHrefTags(text.substring(configWarningPrefix.length())),
null
);
}
if (!outputType.equals(ProcessOutputTypes.STDOUT)) {
return;
}
if (FlutterSettings.getInstance().isVerboseLogging()) {
LOG.info("[<-- " + text.trim() + "]");
}
stdoutParser.appendOutput(text);
for (String line : stdoutParser.getAvailableLines()) {
if (line.startsWith("[{")) {
line = line.trim();
final String json = line.substring(1, line.length() - 1);
dispatchJson(json);
}
}
}
@Override
public void processWillTerminate(@NotNull ProcessEvent event, boolean willBeDestroyed) {
handler.removeProcessListener(listener);
}
};
handler.addProcessListener(listener);
}
@Nullable
@Override
public String getWebSocketUrl() {
if (observatoryUri == null || !observatoryUri.startsWith("http:")) {
return null;
}
return CommonTestConfigUtils.convertHttpServiceProtocolToWs(observatoryUri);
}
@Nullable
@Override
public String getBrowserUrl() {
return observatoryUri;
}
@Nullable
public String getRunfilesDir() {
return runfilesDir;
}
@Nullable
public String getWorkspaceDirName() {
return workspaceDirName;
}
@Nullable
@Override
public String getRemoteBaseUrl() {
return null;
}
@Override
public void onDebuggerPaused(@NotNull Runnable resume) {
}
@Override
public void onDebuggerResumed() {
}
private void dispatchJson(String json) {
final JsonObject obj;
try {
final JsonElement elem = JsonUtils.parseString(json);
obj = elem.getAsJsonObject();
}
catch (JsonSyntaxException e) {
FlutterUtils.warn(LOG, "Unable to parse JSON from Flutter test", e);
return;
}
final JsonPrimitive primId = obj.getAsJsonPrimitive("id");
if (primId != null) {
// Not an event.
LOG.info("Ignored JSON from Flutter test: " + json);
return;
}
final JsonPrimitive primEvent = obj.getAsJsonPrimitive("event");
if (primEvent == null) {
FlutterUtils.warn(LOG, "Missing event field in JSON from Flutter test: " + obj);
return;
}
final String eventName = primEvent.getAsString();
if (eventName == null) {
FlutterUtils.warn(LOG, "Unexpected event field in JSON from Flutter test: " + obj);
return;
}
final JsonObject params = obj.getAsJsonObject("params");
if (params == null) {
FlutterUtils.warn(LOG, "Missing parameters in event from Flutter test: " + obj);
return;
}
if (eventName.equals(STARTED_PROCESS)) {
final JsonPrimitive primUri = params.getAsJsonPrimitive(OBSERVATORY_URI_KEY);
if (primUri != null) {
observatoryUri = primUri.getAsString();
}
final JsonPrimitive primRunfilesDir = params.getAsJsonPrimitive(RUNFILES_DIR_KEY);
if (primRunfilesDir != null) {
runfilesDir = primRunfilesDir.getAsString();
}
final JsonPrimitive primWorkspaceDirName = params.getAsJsonPrimitive(WORKSPACE_DIR_NAME_KEY);
if (primWorkspaceDirName != null) {
workspaceDirName = primWorkspaceDirName.getAsString();
}
}
}
}
private static final class BazelPositionMapper extends FlutterPositionMapper {
@NotNull final Connector connector;
public BazelPositionMapper(@NotNull final Project project,
@NotNull final VirtualFile sourceRoot,
@NotNull final DartUrlResolver resolver,
@Nullable final Analyzer analyzer,
@NotNull final Connector connector) {
super(project, sourceRoot, resolver, analyzer);
this.connector = connector;
}
/**
* Attempt to find a local Dart file corresponding to a script in Observatory.
*/
@Nullable
@Override
protected VirtualFile findLocalFile(@NotNull final String uri) {
// Get the workspace directory name provided by the test harness.
final String workspaceDirName = connector.getWorkspaceDirName();
// Verify the returned workspace directory name, we weren't passed a workspace name or if the valid workspace name does not start the
// uri then return the super invocation of this method. This prevents the unknown URI type from being passed to the analysis server.
if (StringUtils.isEmpty(workspaceDirName) || !uri.startsWith(workspaceDirName + ":/")) return super.findLocalFile(uri);
final String pathFromWorkspace = uri.substring(workspaceDirName.length() + 1);
// For each root in each module, look for a bazel workspace path, if found attempt to compute the VirtualFile, return when found.
return ApplicationManager.getApplication().runReadAction((Computable<VirtualFile>)() -> {
for (Module module : DartSdkLibUtil.getModulesWithDartSdkEnabled(getProject())) {
for (ContentEntry contentEntry : ModuleRootManager.getInstance(module).getContentEntries()) {
final VirtualFile includedRoot = contentEntry.getFile();
if (includedRoot == null) continue;
final String includedRootPath = includedRoot.getPath();
final int workspaceOffset = includedRootPath.indexOf(workspaceDirName);
if (workspaceOffset == -1) continue;
final String pathToWorkspace = includedRootPath.substring(0, workspaceOffset + workspaceDirName.length());
final VirtualFile virtualFile = LocalFileSystem.getInstance().findFileByPath(pathToWorkspace + pathFromWorkspace);
if (virtualFile != null) {
return virtualFile;
}
}
}
return super.findLocalFile(uri);
});
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/bazelTest/BazelTestRunner.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/bazelTest/BazelTestRunner.java",
"repo_id": "flutter-intellij",
"token_count": 4394
} | 491 |
/*
* Copyright 2021 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.coverage;
import com.intellij.coverage.BaseCoverageSuite;
import com.intellij.coverage.CoverageEngine;
import com.intellij.coverage.CoverageFileProvider;
import com.intellij.coverage.CoverageRunner;
import com.intellij.openapi.project.Project;
import org.jetbrains.annotations.NotNull;
public class FlutterCoverageSuite extends BaseCoverageSuite {
@NotNull final private FlutterCoverageEngine coverageEngine;
public FlutterCoverageSuite(@NotNull FlutterCoverageEngine coverageEngine) {
this.coverageEngine = coverageEngine;
}
public FlutterCoverageSuite(CoverageRunner runner,
String name,
CoverageFileProvider coverageDataFileProvider,
Project project,
@NotNull FlutterCoverageEngine coverageEngine
) {
super(name, coverageDataFileProvider, System.currentTimeMillis(), false, false,
false, runner, project);
this.coverageEngine = coverageEngine;
}
@Override
public @NotNull CoverageEngine getCoverageEngine() {
return coverageEngine;
}
@Override
public void deleteCachedCoverageData() {
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/coverage/FlutterCoverageSuite.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/coverage/FlutterCoverageSuite.java",
"repo_id": "flutter-intellij",
"token_count": 489
} | 492 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.sdk;
import com.google.common.collect.ImmutableList;
import com.intellij.execution.ExecutionException;
import com.intellij.execution.configurations.GeneralCommandLine;
import com.intellij.execution.process.*;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.util.io.FileUtil;
import com.intellij.openapi.vfs.VirtualFile;
import io.flutter.FlutterBundle;
import io.flutter.FlutterInitializer;
import io.flutter.FlutterMessages;
import io.flutter.android.IntelliJAndroidSdk;
import io.flutter.console.FlutterConsoles;
import io.flutter.dart.DartPlugin;
import io.flutter.utils.MostlySilentColoredProcessHandler;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.nio.charset.StandardCharsets;
import java.util.*;
import java.util.function.Consumer;
/**
* A Flutter command to run, with its arguments.
*/
public class FlutterCommand {
private static final Logger LOG = Logger.getInstance(FlutterCommand.class);
private static final Set<Type> pubRelatedCommands = new HashSet<>(
Arrays.asList(Type.PUB_GET, Type.PUB_UPGRADE, Type.PUB_OUTDATED, Type.UPGRADE));
@NotNull
protected final FlutterSdk sdk;
@Nullable
protected final VirtualFile workDir;
@NotNull
private final Type type;
@NotNull
protected final List<String> args;
/**
* @see FlutterSdk for methods to create specific commands.
*/
FlutterCommand(@NotNull FlutterSdk sdk, @Nullable VirtualFile workDir, @NotNull Type type, String... args) {
this.sdk = sdk;
this.workDir = workDir;
this.type = type;
this.args = ImmutableList.copyOf(args);
}
/**
* Returns a displayable version of the command that will be run.
*/
public String getDisplayCommand() {
final List<String> words = new ArrayList<>();
words.add("flutter");
words.addAll(type.subCommand);
words.addAll(args);
return String.join(" ", words);
}
protected boolean isPubRelatedCommand() {
return pubRelatedCommands.contains(type);
}
/**
* Starts running the command, without showing its output in a console.
* <p>
* If unable to start (for example, if a command is already running), returns null.
*/
public Process start(@Nullable Consumer<ProcessOutput> onDone, @Nullable ProcessListener processListener) {
// TODO(skybrian) add Project parameter if it turns out later that we need to set ANDROID_HOME.
final ColoredProcessHandler handler = startProcessOrShowError(null);
if (handler == null) {
return null;
}
if (processListener != null) {
handler.addProcessListener(processListener);
}
// Capture all process output if requested.
if (onDone != null) {
final CapturingProcessAdapter listener = new CapturingProcessAdapter() {
@Override
public void processTerminated(@NotNull ProcessEvent event) {
super.processTerminated(event);
onDone.accept(getOutput());
}
};
handler.addProcessListener(listener);
}
// Transition to "running" state.
handler.startNotify();
return handler.getProcess();
}
/**
* Starts running the command, showing its output in a non-module console.
* <p>
* Shows the output in a tab in the tool window that's not associated
* with a particular module. Returns the process handler.
* <p>
* If unable to start (for example, if a command is already running), returns null.
*/
public ColoredProcessHandler startInConsole(@NotNull Project project) {
final ColoredProcessHandler handler = startProcessOrShowError(project);
if (handler != null) {
FlutterConsoles.displayProcessLater(handler, project, null, handler::startNotify);
}
return handler;
}
/**
* Starts running the command, showing its output in a module console.
* <p>
* Shows the output in the tool window's tab corresponding to the passed-in module.
* Returns the process.
* <p>
* If unable to start (for example, if a command is already running), returns null.
*/
public Process startInModuleConsole(@NotNull Module module, @Nullable Runnable onDone, @Nullable ProcessListener processListener) {
final ColoredProcessHandler handler = startProcessOrShowError(module.getProject());
if (handler == null) {
return null;
}
if (processListener != null) {
handler.addProcessListener(processListener);
}
handler.addProcessListener(new ProcessAdapter() {
@Override
public void processTerminated(@NotNull ProcessEvent event) {
if (onDone != null) {
onDone.run();
}
}
});
FlutterConsoles.displayProcessLater(handler, module.getProject(), module, handler::startNotify);
return handler.getProcess();
}
@Override
public String toString() {
return "FlutterCommand(" + getDisplayCommand() + ")";
}
/**
* Starts a process that runs a flutter command, unless one is already running.
* <p>
* Returns the handler if successfully started.
*/
@Nullable
public ColoredProcessHandler startProcess(boolean sendAnalytics) {
try {
final GeneralCommandLine commandLine = createGeneralCommandLine(null);
LOG.info(commandLine.toString());
final ColoredProcessHandler handler = new ColoredProcessHandler(commandLine);
if (sendAnalytics) {
type.sendAnalyticsEvent();
}
return handler;
}
catch (ExecutionException e) {
FlutterMessages.showError(
type.title,
FlutterBundle.message("flutter.command.exception.message", e.getMessage()),
null);
return null;
}
}
/**
* Starts a process that runs a flutter command, unless one is already running.
* <p>
* If a project is supplied, it will be used to determine the ANDROID_HOME variable for the subprocess.
* <p>
* Returns the handler if successfully started.
*/
@NotNull
public FlutterCommandStartResult startProcess(@Nullable Project project) {
if (isPubRelatedCommand()) {
DartPlugin.setPubActionInProgress(true);
}
final ColoredProcessHandler handler;
try {
final GeneralCommandLine commandLine = createGeneralCommandLine(project);
LOG.info(commandLine.toString());
handler = new MostlySilentColoredProcessHandler(commandLine);
handler.addProcessListener(new ProcessAdapter() {
@Override
public void processTerminated(@NotNull final ProcessEvent event) {
if (isPubRelatedCommand()) {
DartPlugin.setPubActionInProgress(false);
}
}
});
type.sendAnalyticsEvent();
return new FlutterCommandStartResult(handler);
}
catch (ExecutionException e) {
if (isPubRelatedCommand()) {
DartPlugin.setPubActionInProgress(false);
}
return new FlutterCommandStartResult(e);
}
}
/**
* Starts a process that runs a flutter command, unless one is already running.
* <p>
* If a project is supplied, it will be used to determine the ANDROID_HOME variable for the subprocess.
* <p>
* Returns the handler if successfully started, or null if there was a problem.
*/
@Nullable
public ColoredProcessHandler startProcessOrShowError(@Nullable Project project) {
final FlutterCommandStartResult result = startProcess(project);
if (result.status == FlutterCommandStartResultStatus.EXCEPTION && result.exception != null) {
FlutterMessages.showError(
type.title,
FlutterBundle.message("flutter.command.exception.message", result.exception.getMessage()),
project
);
}
return result.processHandler;
}
/**
* Creates the command line to run.
* <p>
* If a project is supplied, it will be used to determine the ANDROID_HOME variable for the subprocess.
*/
@NotNull
public GeneralCommandLine createGeneralCommandLine(@Nullable Project project) {
final GeneralCommandLine line = new GeneralCommandLine();
line.setCharset(StandardCharsets.UTF_8);
line.withEnvironment(FlutterSdkUtil.FLUTTER_HOST_ENV, (new FlutterSdkUtil()).getFlutterHostEnvValue());
final String androidHome = IntelliJAndroidSdk.chooseAndroidHome(project, false);
if (androidHome != null) {
line.withEnvironment("ANDROID_HOME", androidHome);
}
line.setExePath(FileUtil.toSystemDependentName(sdk.getHomePath() + "/bin/" + FlutterSdkUtil.flutterScriptName()));
if (workDir != null) {
line.setWorkDirectory(workDir.getPath());
}
if (!isDoctorCommand()) {
line.addParameter("--no-color");
}
line.addParameters(type.subCommand);
line.addParameters(args);
return line;
}
private boolean isDoctorCommand() {
return type == Type.DOCTOR;
}
enum Type {
ATTACH("Flutter attach", "attach"),
BUILD("Flutter build", "build"),
CHANNEL("Flutter channel", "channel"),
CLEAN("Flutter clean", "clean"),
CONFIG("Flutter config", "config"),
CREATE("Flutter create", "create"),
DOCTOR("Flutter doctor", "doctor", "--verbose"),
PUB_GET("Flutter pub get", "pub", "get"),
PUB_UPGRADE("Flutter pub upgrade", "pub", "upgrade"),
PUB_OUTDATED("Flutter pub outdated", "pub", "outdated"),
PUB("Flutter pub", "pub"),
RUN("Flutter run", "run"),
UPGRADE("Flutter upgrade", "upgrade"),
VERSION("Flutter version", "--version"),
TEST("Flutter test", "test");
final public String title;
final ImmutableList<String> subCommand;
Type(String title, String... subCommand) {
this.title = title;
this.subCommand = ImmutableList.copyOf(subCommand);
}
void sendAnalyticsEvent() {
final String action = String.join("_", subCommand).replaceAll("-", "");
FlutterInitializer.getAnalytics().sendEvent("flutter", action);
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/sdk/FlutterCommand.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/sdk/FlutterCommand.java",
"repo_id": "flutter-intellij",
"token_count": 3443
} | 493 |
/*
* Copyright 2016 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.settings;
import com.google.common.annotations.VisibleForTesting;
import com.intellij.ide.util.PropertiesComponent;
import com.intellij.openapi.components.ServiceManager;
import com.intellij.openapi.util.registry.Registry;
import com.intellij.util.EventDispatcher;
import com.jetbrains.lang.dart.analyzer.DartClosingLabelManager;
import io.flutter.analytics.Analytics;
import java.util.EventListener;
public class FlutterSettings {
private static final String reloadOnSaveKey = "io.flutter.reloadOnSave";
private static final String openInspectorOnAppLaunchKey = "io.flutter.openInspectorOnAppLaunch";
private static final String perserveLogsDuringHotReloadAndRestartKey = "io.flutter.persereLogsDuringHotReloadAndRestart";
private static final String verboseLoggingKey = "io.flutter.verboseLogging";
private static final String formatCodeOnSaveKey = "io.flutter.formatCodeOnSave";
private static final String organizeImportsOnSaveKey = "io.flutter.organizeImportsOnSave";
private static final String showOnlyWidgetsKey = "io.flutter.showOnlyWidgets";
private static final String syncAndroidLibrariesKey = "io.flutter.syncAndroidLibraries";
private static final String showStructuredErrorsKey = "io.flutter.showStructuredErrors";
private static final String includeAllStackTracesKey = "io.flutter.includeAllStackTraces";
private static final String showBuildMethodGuidesKey = "io.flutter.editor.showBuildMethodGuides";
private static final String enableHotUiKey = "io.flutter.editor.enableHotUi";
private static final String enableBazelHotRestartKey = "io.flutter.editor.enableBazelHotRestart";
private static final String showBazelHotRestartWarningKey = "io.flutter.showBazelHotRestartWarning";
private static final String enableJcefBrowserKey = "io.flutter.enableJcefBrowser";
private static final String fontPackagesKey = "io.flutter.fontPackages";
private static final String allowTestsInSourcesRootKey = "io.flutter.allowTestsInSources";
private static final String showBazelIosRunNotificationKey = "io.flutter.hideBazelIosRunNotification";
// TODO(helin24): This is to change the embedded browser setting back to true only once for Big Sur users. If we
// switch to enabling the embedded browser for everyone, then delete this key.
private static final String changeBigSurToTrueKey = "io.flutter.setBigSurToTrueKey2";
/**
* Registry key to suggest all run configurations instead of just one.
* <p>
* Useful for {@link io.flutter.run.bazelTest.FlutterBazelTestConfigurationType} to show both watch and regular configurations
* in the left-hand gutter.
*/
private static final String suggestAllRunConfigurationsFromContextKey = "suggest.all.run.configurations.from.context";
private static FlutterSettings testInstance;
/**
* This is only used for testing.
*/
@VisibleForTesting
public static void setInstance(FlutterSettings instance) {
testInstance = instance;
}
public static FlutterSettings getInstance() {
if (testInstance != null) {
return testInstance;
}
return ServiceManager.getService(FlutterSettings.class);
}
protected static PropertiesComponent getPropertiesComponent() {
return PropertiesComponent.getInstance();
}
public interface Listener extends EventListener {
void settingsChanged();
}
private final EventDispatcher<Listener> dispatcher = EventDispatcher.create(Listener.class);
public FlutterSettings() {
}
public void sendSettingsToAnalytics(Analytics analytics) {
// Send data on the number of experimental features enabled by users.
analytics.sendEvent("settings", "ping");
if (isReloadOnSave()) {
analytics.sendEvent("settings", afterLastPeriod(reloadOnSaveKey));
}
if (isOpenInspectorOnAppLaunch()) {
analytics.sendEvent("settings", afterLastPeriod(openInspectorOnAppLaunchKey));
}
if (isPerserveLogsDuringHotReloadAndRestart()) {
analytics.sendEvent("settings", afterLastPeriod(perserveLogsDuringHotReloadAndRestartKey));
}
if (isFormatCodeOnSave()) {
analytics.sendEvent("settings", afterLastPeriod(formatCodeOnSaveKey));
if (isOrganizeImportsOnSave()) {
analytics.sendEvent("settings", afterLastPeriod(organizeImportsOnSaveKey));
}
}
if (isShowOnlyWidgets()) {
analytics.sendEvent("settings", afterLastPeriod(showOnlyWidgetsKey));
}
if (isSyncingAndroidLibraries()) {
analytics.sendEvent("settings", afterLastPeriod(syncAndroidLibrariesKey));
}
if (isShowBuildMethodGuides()) {
analytics.sendEvent("settings", afterLastPeriod(showBuildMethodGuidesKey));
}
if (isShowStructuredErrors()) {
analytics.sendEvent("settings", afterLastPeriod(showStructuredErrorsKey));
if (isIncludeAllStackTraces()) {
analytics.sendEvent("settings", afterLastPeriod(includeAllStackTracesKey));
}
}
if (showAllRunConfigurationsInContext()) {
analytics.sendEvent("settings", "showAllRunConfigurations");
}
if (isEnableHotUi()) {
analytics.sendEvent("settings", afterLastPeriod(enableHotUiKey));
}
if (isShowBazelHotRestartWarning()) {
analytics.sendEvent("settings", afterLastPeriod(showBazelHotRestartWarningKey));
}
if (isChangeBigSurToTrue()) {
analytics.sendEvent("settings", afterLastPeriod(changeBigSurToTrueKey));
}
if (isAllowTestsInSourcesRoot()) {
analytics.sendEvent("settings", afterLastPeriod(allowTestsInSourcesRootKey));
}
if (!getFontPackages().isEmpty()) {
analytics.sendEvent("settings", afterLastPeriod(fontPackagesKey));
}
if (isShowBazelIosRunNotification()) {
analytics.sendEvent("settings", afterLastPeriod(showBazelIosRunNotificationKey));
}
}
public void addListener(Listener listener) {
dispatcher.addListener(listener);
}
public void removeListener(Listener listener) {
dispatcher.removeListener(listener);
}
public boolean isReloadOnSave() {
return getPropertiesComponent().getBoolean(reloadOnSaveKey, true);
}
public void setReloadOnSave(boolean value) {
getPropertiesComponent().setValue(reloadOnSaveKey, value, true);
fireEvent();
}
public boolean isFormatCodeOnSave() {
return getPropertiesComponent().getBoolean(formatCodeOnSaveKey, false);
}
public void setFormatCodeOnSave(boolean value) {
getPropertiesComponent().setValue(formatCodeOnSaveKey, value, false);
fireEvent();
}
public boolean isOrganizeImportsOnSave() {
return getPropertiesComponent().getBoolean(organizeImportsOnSaveKey, false);
}
public void setOrganizeImportsOnSave(boolean value) {
getPropertiesComponent().setValue(organizeImportsOnSaveKey, value, false);
fireEvent();
}
public boolean isShowOnlyWidgets() {
return getPropertiesComponent().getBoolean(showOnlyWidgetsKey, false);
}
public void setShowOnlyWidgets(boolean value) {
getPropertiesComponent().setValue(showOnlyWidgetsKey, value, false);
fireEvent();
}
public boolean isSyncingAndroidLibraries() {
return getPropertiesComponent().getBoolean(syncAndroidLibrariesKey, false);
}
public void setSyncingAndroidLibraries(boolean value) {
getPropertiesComponent().setValue(syncAndroidLibrariesKey, value, false);
fireEvent();
}
public boolean isShowStructuredErrors() {
return getPropertiesComponent().getBoolean(showStructuredErrorsKey, true);
}
public void setShowStructuredErrors(boolean value) {
getPropertiesComponent().setValue(showStructuredErrorsKey, value, true);
fireEvent();
}
public boolean isIncludeAllStackTraces() {
return getPropertiesComponent().getBoolean(includeAllStackTracesKey, true);
}
public void setIncludeAllStackTraces(boolean value) {
getPropertiesComponent().setValue(includeAllStackTracesKey, value, true);
fireEvent();
}
public boolean isOpenInspectorOnAppLaunch() {
return getPropertiesComponent().getBoolean(openInspectorOnAppLaunchKey, false);
}
public void setOpenInspectorOnAppLaunch(boolean value) {
getPropertiesComponent().setValue(openInspectorOnAppLaunchKey, value, false);
fireEvent();
}
public boolean isPerserveLogsDuringHotReloadAndRestart() {
return getPropertiesComponent().getBoolean(perserveLogsDuringHotReloadAndRestartKey, false);
}
public void setPerserveLogsDuringHotReloadAndRestart(boolean value) {
getPropertiesComponent().setValue(perserveLogsDuringHotReloadAndRestartKey, value, false);
fireEvent();
}
/**
* Tells IntelliJ to show all run configurations possible when the user clicks on the left-hand green arrow to run a test.
* <p>
* Useful for {@link io.flutter.run.bazelTest.FlutterBazelTestConfigurationType} to show both watch and regular configurations
* in the left-hand gutter.
*/
public boolean showAllRunConfigurationsInContext() {
return Registry.is(suggestAllRunConfigurationsFromContextKey, false);
}
public void setShowAllRunConfigurationsInContext(boolean value) {
Registry.get(suggestAllRunConfigurationsFromContextKey).setValue(value);
fireEvent();
}
public boolean isEnableJcefBrowser() {
return getPropertiesComponent().getBoolean(enableJcefBrowserKey, false);
}
public void setEnableJcefBrowser(boolean value) {
getPropertiesComponent().setValue(enableJcefBrowserKey, value, false);
fireEvent();
}
public boolean isVerboseLogging() {
return getPropertiesComponent().getBoolean(verboseLoggingKey, false);
}
public void setVerboseLogging(boolean value) {
getPropertiesComponent().setValue(verboseLoggingKey, value, false);
fireEvent();
}
public boolean isShowBuildMethodGuides() {
return getPropertiesComponent().getBoolean(showBuildMethodGuidesKey, true);
}
public void setShowBuildMethodGuides(boolean value) {
getPropertiesComponent().setValue(showBuildMethodGuidesKey, value, true);
fireEvent();
}
public boolean isShowClosingLabels() {
return DartClosingLabelManager.getInstance().getShowClosingLabels();
}
public void setShowClosingLabels(boolean value) {
DartClosingLabelManager.getInstance().setShowClosingLabels(value);
}
public String getFontPackages() {
return getPropertiesComponent().getValue(fontPackagesKey, "");
}
public void setFontPackages(String value) {
getPropertiesComponent().setValue(fontPackagesKey, value == null ? "" : value);
}
protected void fireEvent() {
dispatcher.getMulticaster().settingsChanged();
}
private static String afterLastPeriod(String str) {
final int index = str.lastIndexOf('.');
return index == -1 ? str : str.substring(index + 1);
}
public boolean isEnableHotUi() {
return getPropertiesComponent().getBoolean(enableHotUiKey, false);
}
public void setEnableHotUi(boolean value) {
getPropertiesComponent().setValue(enableHotUiKey, value, false);
fireEvent();
}
public boolean isEnableHotUiInCodeEditor() {
// We leave this setting off for now to avoid possible performance and
// usability issues rendering previews directly in the code editor.
return false;
}
public boolean isEnableBazelHotRestart() {
return getPropertiesComponent().getBoolean(enableBazelHotRestartKey, false);
}
public void setEnableBazelHotRestart(boolean value) {
getPropertiesComponent().setValue(enableBazelHotRestartKey, value, false);
fireEvent();
}
public boolean isShowBazelHotRestartWarning() {
return getPropertiesComponent().getBoolean(showBazelHotRestartWarningKey, true);
}
public void setShowBazelHotRestartWarning(boolean value) {
getPropertiesComponent().setValue(showBazelHotRestartWarningKey, value, true);
fireEvent();
}
public boolean isChangeBigSurToTrue() {
return getPropertiesComponent().getBoolean(changeBigSurToTrueKey, true);
}
public void setChangeBigSurToTrue(boolean value) {
getPropertiesComponent().setValue(changeBigSurToTrueKey, value, true);
fireEvent();
}
public boolean isAllowTestsInSourcesRoot() {
return getPropertiesComponent().getBoolean(allowTestsInSourcesRootKey, false);
}
public void setAllowTestsInSourcesRoot(boolean value) {
getPropertiesComponent().setValue(allowTestsInSourcesRootKey, value, false);
fireEvent();
}
public boolean isShowBazelIosRunNotification() {
return getPropertiesComponent().getBoolean(showBazelIosRunNotificationKey, true);
}
public void setShowBazelIosRunNotification(boolean value) {
getPropertiesComponent().setValue(showBazelIosRunNotificationKey, value, true);
fireEvent();
}
}
| flutter-intellij/flutter-idea/src/io/flutter/settings/FlutterSettings.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/settings/FlutterSettings.java",
"repo_id": "flutter-intellij",
"token_count": 4007
} | 494 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils;
import org.jdom.Element;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.HashMap;
import java.util.Map;
/**
* Utilities for reading and writing IntelliJ run configurations to and from the disk.
*/
public class ElementIO {
public static void addOption(@NotNull Element element, @NotNull String name, @Nullable String value) {
if (value == null) return;
final Element child = new Element("option");
child.setAttribute("name", name);
child.setAttribute("value", value);
element.addContent(child);
}
public static Map<String, String> readOptions(Element element) {
final Map<String, String> result = new HashMap<>();
for (Element child : element.getChildren()) {
if ("option".equals(child.getName())) {
final String name = child.getAttributeValue("name");
final String value = child.getAttributeValue("value");
if (name != null && value != null) {
result.put(name, value);
}
}
}
return result;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/utils/ElementIO.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/utils/ElementIO.java",
"repo_id": "flutter-intellij",
"token_count": 412
} | 495 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils;
import org.jetbrains.annotations.NotNull;
import java.util.ArrayList;
import java.util.List;
public class ThreadUtil {
public static ThreadGroup getRootThreadGroup() {
ThreadGroup threadGroup = Thread.currentThread().getThreadGroup();
ThreadGroup parentThreadGroup;
while ((parentThreadGroup = threadGroup.getParent()) != null) {
threadGroup = parentThreadGroup;
}
return threadGroup;
}
/**
* Returns all active threads of the current group.
*/
public static List<Thread> getAllThreads() {
final ThreadGroup rootThreadGroup = getRootThreadGroup();
Thread[] threads = new Thread[rootThreadGroup.activeCount()];
while (rootThreadGroup.enumerate(threads, true) == threads.length) {
threads = new Thread[threads.length * 2];
}
return getThreadList(threads);
}
/**
* Returns all active threads of the current group.
*/
public static List<Thread> getCurrentGroupThreads() {
Thread[] threads = new Thread[Thread.activeCount()];
while (Thread.enumerate(threads) == threads.length) {
threads = new Thread[threads.length * 2];
}
return getThreadList(threads);
}
/**
* Return the list of thread in the given array, which ends with null.
*/
@NotNull
private static List<Thread> getThreadList(Thread[] threads) {
final List<Thread> result = new ArrayList<>(threads.length);
for (Thread thread : threads) {
if (thread == null) {
break;
}
result.add(thread);
}
return result;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/utils/ThreadUtil.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/utils/ThreadUtil.java",
"repo_id": "flutter-intellij",
"token_count": 567
} | 496 |
/*
* Copyright 2019 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils.math;
import java.util.Arrays;
/**
* 4D column vector.
* <p>
* This class is ported from the Vector4 class in the Dart vector_math
* package. The code is ported as is without concern for making the code
* consistent with Java api conventions to keep the code consistent with
* the Dart code to simplify using Transform Matrixes returned by Flutter.
*/
@SuppressWarnings("Duplicates")
class Vector4 implements Vector {
final double[] _v4storage;
/**
* Construct a new vector with the specified values.
*/
Vector4(double x, double y, double z, double w) {
_v4storage = new double[]{x, y, z, w};
}
/**
* Zero vector.
*/
Vector4() {
_v4storage = new double[4];
}
/**
* Constructs Vector4 with given double[] as [.getStorage()].
*/
Vector4(double[] values) {
_v4storage = values;
}
/**
* Set the values of [result] to the minimum of [a] and [b] for each line.
*/
public static void min(Vector4 a, Vector4 b, Vector4 result) {
result.setX(Math.min(a.getX(), b.getX()));
result.setY(Math.min(a.getY(), b.getY()));
result.setZ(Math.min(a.getZ(), b.getZ()));
result.setW(Math.min(a.getW(), b.getW()));
}
/**
* Set the values of [result] to the maximum of [a] and [b] for each line.
*/
public static void max(Vector4 a, Vector4 b, Vector4 result) {
result.setX(Math.max(a.getX(), b.getX()));
result.setY(Math.max(a.getY(), b.getY()));
result.setZ(Math.max(a.getZ(), b.getZ()));
result.setW(Math.max(a.getW(), b.getW()));
}
/*
* Interpolate between [min] and [max] with the amount of [a] using a linear
* interpolation and store the values in [result].
*/
public static void mix(Vector4 min, Vector4 max, double a, Vector4 result) {
result.setX(min.getX() + a * (max.getX() - min.getX()));
result.setY(min.getY() + a * (max.getY() - min.getY()));
result.setZ(min.getZ() + a * (max.getZ() - min.getZ()));
result.setW(min.getW() + a * (max.getW() - min.getW()));
}
/**
* Constructs the identity vector.
*/
public static Vector4 identity() {
final Vector4 ret = new Vector4();
ret.setIdentity();
return ret;
}
/**
* Splat [value] into all lanes of the vector.
*/
public static Vector4 all(double value) {
final Vector4 ret = new Vector4();
ret.splat(value);
return ret;
}
/**
* Copy of [other].
*/
public static Vector4 copy(Vector4 other) {
final Vector4 ret = new Vector4();
ret.setFrom(other);
return ret;
}
/**
* The components of the vector.
*/
@Override()
public double[] getStorage() {
return _v4storage;
}
/**
* Set the values of the vector.
*/
public void setValues(double x_, double y_, double z_, double w_) {
_v4storage[3] = w_;
_v4storage[2] = z_;
_v4storage[1] = y_;
_v4storage[0] = x_;
}
/**
* Zero the vector.
*/
public void setZero() {
_v4storage[0] = 0.0;
_v4storage[1] = 0.0;
_v4storage[2] = 0.0;
_v4storage[3] = 0.0;
}
/**
* Set to the identity vector.
*/
public void setIdentity() {
_v4storage[0] = 0.0;
_v4storage[1] = 0.0;
_v4storage[2] = 0.0;
_v4storage[3] = 1.0;
}
/**
* Set the values by copying them from [other].
*/
public void setFrom(Vector4 other) {
final double[] otherStorage = other._v4storage;
_v4storage[3] = otherStorage[3];
_v4storage[2] = otherStorage[2];
_v4storage[1] = otherStorage[1];
_v4storage[0] = otherStorage[0];
}
/**
* Splat [arg] into all lanes of the vector.
*/
public void splat(double arg) {
_v4storage[3] = arg;
_v4storage[2] = arg;
_v4storage[1] = arg;
_v4storage[0] = arg;
}
/**
* Returns a printable string
*/
@Override()
public String toString() {
return "" + _v4storage[0] + "," + _v4storage[1] + "," + _v4storage[2] + "," + _v4storage[3];
}
/**
* Check if two vectors are the same.
*/
@Override()
public boolean equals(Object o) {
if (!(o instanceof Vector4)) {
return false;
}
final Vector4 other = (Vector4)o;
return (_v4storage[0] == other._v4storage[0]) &&
(_v4storage[1] == other._v4storage[1]) &&
(_v4storage[2] == other._v4storage[2]) &&
(_v4storage[3] == other._v4storage[3]);
}
@Override()
public int hashCode() {
return Arrays.hashCode(_v4storage);
}
/**
* Negate.
*/
public Vector4 operatorNegate() {
final Vector4 ret = clone();
ret.negate();
return ret;
}
/**
* Subtract two vectors.
*/
public Vector4 operatorSub(Vector4 other) {
final Vector4 ret = clone();
ret.sub(other);
return ret;
}
/**
* Add two vectors.
*/
public Vector4 operatorAdd(Vector4 other) {
final Vector4 ret = clone();
ret.add(other);
return ret;
}
/**
* Scale.
*/
public Vector4 operatorDiv(double scale) {
final Vector4 ret = clone();
ret.scale(1.0 / scale);
return ret;
}
/**
* Scale.
*/
public Vector4 operatorScale(double scale) {
final Vector4 ret = clone();
ret.scale(scale);
return ret;
}
/**
* Length.
*/
public double getLength() {
return Math.sqrt(getLength2());
}
/**
* Set the length of the vector. A negative [value] will change the vectors
* orientation and a [value] of zero will set the vector to zero.
*/
public void setLength(double value) {
if (value == 0.0) {
setZero();
}
else {
double l = getLength();
if (l == 0.0) {
return;
}
l = value / l;
_v4storage[0] *= l;
_v4storage[1] *= l;
_v4storage[2] *= l;
_v4storage[3] *= l;
}
}
/**
* Length squared.
*/
public double getLength2() {
double sum;
sum = (_v4storage[0] * _v4storage[0]);
sum += (_v4storage[1] * _v4storage[1]);
sum += (_v4storage[2] * _v4storage[2]);
sum += (_v4storage[3] * _v4storage[3]);
return sum;
}
/**
* Normalizes [this].
*/
public double normalize() {
final double l = getLength();
if (l == 0.0) {
return 0.0;
}
final double d = 1.0 / l;
_v4storage[0] *= d;
_v4storage[1] *= d;
_v4storage[2] *= d;
_v4storage[3] *= d;
return l;
}
/**
* Normalizes copy of [this].
*/
public Vector4 normalized() {
final Vector4 ret = clone();
ret.normalize();
return ret;
}
/**
* Normalize vector into [out].
*/
public Vector4 normalizeInto(Vector4 out) {
out.setFrom(this);
out.normalize();
return out;
}
/**
* Distance from [this] to [arg]
*/
public double distanceTo(Vector4 arg) {
return Math.sqrt(distanceToSquared(arg));
}
/**
* Squared distance from [this] to [arg]
*/
public double distanceToSquared(Vector4 arg) {
final double[] argStorage = arg._v4storage;
final double dx = _v4storage[0] - argStorage[0];
final double dy = _v4storage[1] - argStorage[1];
final double dz = _v4storage[2] - argStorage[2];
final double dw = _v4storage[3] - argStorage[3];
return dx * dx + dy * dy + dz * dz + dw * dw;
}
/**
* Inner product.
*/
public double dot(Vector4 other) {
final double[] otherStorage = other._v4storage;
double sum;
sum = _v4storage[0] * otherStorage[0];
sum += _v4storage[1] * otherStorage[1];
sum += _v4storage[2] * otherStorage[2];
sum += _v4storage[3] * otherStorage[3];
return sum;
}
/**
* Multiplies [this] by [arg].
*/
public void applyMatrix4(Matrix4 arg) {
final double v1 = _v4storage[0];
final double v2 = _v4storage[1];
final double v3 = _v4storage[2];
final double v4 = _v4storage[3];
final double[] argStorage = arg.getStorage();
_v4storage[0] = argStorage[0] * v1 +
argStorage[4] * v2 +
argStorage[8] * v3 +
argStorage[12] * v4;
_v4storage[1] = argStorage[1] * v1 +
argStorage[5] * v2 +
argStorage[9] * v3 +
argStorage[13] * v4;
_v4storage[2] = argStorage[2] * v1 +
argStorage[6] * v2 +
argStorage[10] * v3 +
argStorage[14] * v4;
_v4storage[3] = argStorage[3] * v1 +
argStorage[7] * v2 +
argStorage[11] * v3 +
argStorage[15] * v4;
}
/**
* Relative error between [this] and [correct]
*/
double relativeError(Vector4 correct) {
final double correct_norm = correct.getLength();
final double diff_norm = (this.operatorSub(correct)).getLength();
return diff_norm / correct_norm;
}
/**
* Absolute error between [this] and [correct]
*/
double absoluteError(Vector4 correct) {
return (this.operatorSub(correct)).getLength();
}
/**
* True if any component is infinite.
*/
public boolean isInfinite() {
boolean is_infinite;
is_infinite = Double.isInfinite(_v4storage[0]);
is_infinite = is_infinite || Double.isInfinite(_v4storage[1]);
is_infinite = is_infinite || Double.isInfinite(_v4storage[2]);
is_infinite = is_infinite || Double.isInfinite(_v4storage[3]);
return is_infinite;
}
/**
* True if any component is NaN.
*/
public boolean isNaN() {
boolean is_nan;
is_nan = Double.isNaN(_v4storage[0]);
is_nan = is_nan || Double.isNaN(_v4storage[1]);
is_nan = is_nan || Double.isNaN(_v4storage[2]);
is_nan = is_nan || Double.isNaN(_v4storage[3]);
return is_nan;
}
public void add(Vector4 arg) {
final double[] argStorage = arg._v4storage;
_v4storage[0] = _v4storage[0] + argStorage[0];
_v4storage[1] = _v4storage[1] + argStorage[1];
_v4storage[2] = _v4storage[2] + argStorage[2];
_v4storage[3] = _v4storage[3] + argStorage[3];
}
/**
* Add [arg] scaled by [factor] to [this].
*/
public void addScaled(Vector4 arg, double factor) {
final double[] argStorage = arg._v4storage;
_v4storage[0] = _v4storage[0] + argStorage[0] * factor;
_v4storage[1] = _v4storage[1] + argStorage[1] * factor;
_v4storage[2] = _v4storage[2] + argStorage[2] * factor;
_v4storage[3] = _v4storage[3] + argStorage[3] * factor;
}
/**
* Subtract [arg] from [this].
*/
public void sub(Vector4 arg) {
final double[] argStorage = arg._v4storage;
_v4storage[0] = _v4storage[0] - argStorage[0];
_v4storage[1] = _v4storage[1] - argStorage[1];
_v4storage[2] = _v4storage[2] - argStorage[2];
_v4storage[3] = _v4storage[3] - argStorage[3];
}
/**
* Multiply [this] by [arg].
*/
public void multiply(Vector4 arg) {
final double[] argStorage = arg._v4storage;
_v4storage[0] = _v4storage[0] * argStorage[0];
_v4storage[1] = _v4storage[1] * argStorage[1];
_v4storage[2] = _v4storage[2] * argStorage[2];
_v4storage[3] = _v4storage[3] * argStorage[3];
}
/**
* Divide [this] by [arg].
*/
public void div(Vector4 arg) {
final double[] argStorage = arg._v4storage;
_v4storage[0] = _v4storage[0] / argStorage[0];
_v4storage[1] = _v4storage[1] / argStorage[1];
_v4storage[2] = _v4storage[2] / argStorage[2];
_v4storage[3] = _v4storage[3] / argStorage[3];
}
/**
* Scale [this] by [arg].
*/
public void scale(double arg) {
_v4storage[0] = _v4storage[0] * arg;
_v4storage[1] = _v4storage[1] * arg;
_v4storage[2] = _v4storage[2] * arg;
_v4storage[3] = _v4storage[3] * arg;
}
/**
* Create a copy of [this] scaled by [arg].
*/
public Vector4 scaled(double arg) {
final Vector4 ret = clone();
ret.scale(arg);
return ret;
}
/**
* Negate [this].
*/
public void negate() {
_v4storage[0] = -_v4storage[0];
_v4storage[1] = -_v4storage[1];
_v4storage[2] = -_v4storage[2];
_v4storage[3] = -_v4storage[3];
}
/**
* Set [this] to the absolute.
*/
public void absolute() {
_v4storage[3] = Math.abs(_v4storage[3]);
_v4storage[2] = Math.abs(_v4storage[2]);
_v4storage[1] = Math.abs(_v4storage[1]);
_v4storage[0] = Math.abs(_v4storage[0]);
}
/**
* Clamp each entry n in [this] in the range [min[n]]-[max[n]].
*/
public void clamp(Vector4 min, Vector4 max) {
final double[] minStorage = min.getStorage();
final double[] maxStorage = max.getStorage();
_v4storage[0] = VectorUtil.clamp(
_v4storage[0], minStorage[0], maxStorage[0]);
_v4storage[1] = VectorUtil.clamp(
_v4storage[1], minStorage[1], maxStorage[1]);
_v4storage[2] = VectorUtil.clamp(
_v4storage[2], minStorage[2], maxStorage[2]);
_v4storage[3] = VectorUtil.clamp(
_v4storage[3], minStorage[3], maxStorage[3]);
}
/**
* Clamp entries in [this] in the range [min]-[max].
*/
public void clampScalar(double min, double max) {
_v4storage[0] = VectorUtil.clamp(_v4storage[0], min, max);
_v4storage[1] = VectorUtil.clamp(_v4storage[1], min, max);
_v4storage[2] = VectorUtil.clamp(_v4storage[2], min, max);
_v4storage[3] = VectorUtil.clamp(_v4storage[3], min, max);
}
/**
* Floor entries in [this].
*/
public void floor() {
_v4storage[0] = Math.floor(_v4storage[0]);
_v4storage[1] = Math.floor(_v4storage[1]);
_v4storage[2] = Math.floor(_v4storage[2]);
_v4storage[3] = Math.floor(_v4storage[3]);
}
/**
* Ceil entries in [this].
*/
public void ceil() {
_v4storage[0] = Math.ceil(_v4storage[0]);
_v4storage[1] = Math.ceil(_v4storage[1]);
_v4storage[2] = Math.ceil(_v4storage[2]);
_v4storage[3] = Math.ceil(_v4storage[3]);
}
/**
* Round entries in [this].
*/
public void round() {
_v4storage[0] = Math.round(_v4storage[0]);
_v4storage[1] = Math.round(_v4storage[1]);
_v4storage[2] = Math.round(_v4storage[2]);
_v4storage[3] = Math.round(_v4storage[3]);
}
/**
* Round entries in [this] towards zero.
*/
public void roundToZero() {
_v4storage[0] = _v4storage[0] < 0.0
? Math.ceil(_v4storage[0])
: Math.floor(_v4storage[0]);
_v4storage[1] = _v4storage[1] < 0.0
? Math.ceil(_v4storage[1])
: Math.floor(_v4storage[1]);
_v4storage[2] = _v4storage[2] < 0.0
? Math.ceil(_v4storage[2])
: Math.floor(_v4storage[2]);
_v4storage[3] = _v4storage[3] < 0.0
? Math.ceil(_v4storage[3])
: Math.floor(_v4storage[3]);
}
/**
* Create a copy of [this].
*/
@SuppressWarnings("MethodDoesntCallSuperMethod")
@Override
public Vector4 clone() {
return Vector4.copy(this);
}
/**
* Copy [this]
*/
public Vector4 copyInto(Vector4 arg) {
final double[] argStorage = arg._v4storage;
argStorage[0] = _v4storage[0];
argStorage[1] = _v4storage[1];
argStorage[2] = _v4storage[2];
argStorage[3] = _v4storage[3];
return arg;
}
public double getX() {
return _v4storage[0];
}
public void setX(double arg) {
_v4storage[0] = arg;
}
public double getY() {
return _v4storage[1];
}
public void setY(double arg) {
_v4storage[1] = arg;
}
public double getZ() {
return _v4storage[2];
}
public void setZ(double arg) {
_v4storage[2] = arg;
}
public double getW() {
return _v4storage[3];
}
public void setW(double arg) {
_v4storage[3] = arg;
}
} | flutter-intellij/flutter-idea/src/io/flutter/utils/math/Vector4.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/utils/math/Vector4.java",
"repo_id": "flutter-intellij",
"token_count": 6801
} | 497 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.view;
import com.intellij.ui.JBColor;
import com.intellij.ui.LoadingNode;
import com.intellij.util.ui.JBUI;
import com.intellij.util.ui.UIUtil;
import com.intellij.util.ui.tree.WideSelectionTreeUI;
import org.jetbrains.annotations.NotNull;
import javax.swing.*;
import javax.swing.tree.TreeCellRenderer;
import java.awt.*;
public abstract class InspectorColoredTreeCellRenderer extends MultiIconSimpleColoredComponent implements TreeCellRenderer {
/**
* Defines whether the tree has focus or not
*/
private boolean myFocused;
private boolean myFocusedCalculated;
protected JTree myTree;
private boolean myOpaque = true;
@Override
public final Component getTreeCellRendererComponent(JTree tree,
Object value,
boolean selected,
boolean expanded,
boolean leaf,
int row,
boolean hasFocus) {
myTree = tree;
clear();
myFocusedCalculated = false;
// We paint background if and only if tree path is selected and tree has focus.
// If path is selected and tree is not focused then we just paint focused border.
if (UIUtil.isFullRowSelectionLAF()) {
setBackground(selected ? UIUtil.getTreeSelectionBackground() : null);
}
else if (WideSelectionTreeUI.isWideSelection(tree)) {
setPaintFocusBorder(false);
if (selected) {
setBackground(hasFocus ? UIUtil.getTreeSelectionBackground() : UIUtil.getTreeUnfocusedSelectionBackground());
}
}
else if (selected) {
setPaintFocusBorder(true);
if (isFocused()) {
setBackground(UIUtil.getTreeSelectionBackground());
}
else {
setBackground(null);
}
}
else {
setBackground(null);
}
if (value instanceof LoadingNode) {
setForeground(JBColor.GRAY);
}
else {
setForeground(tree.getForeground());
setIcon(null);
}
super.setOpaque(myOpaque || selected && hasFocus || selected && isFocused()); // draw selection background even for non-opaque tree
if (tree.getUI() instanceof InspectorTreeUI && UIUtil.isUnderAquaBasedLookAndFeel()) {
setMyBorder(null);
setIpad(JBUI.insets(0, 2));
}
customizeCellRenderer(tree, value, selected, expanded, leaf, row, hasFocus);
return this;
}
public JTree getTree() {
return myTree;
}
protected final boolean isFocused() {
if (!myFocusedCalculated) {
myFocused = calcFocusedState();
myFocusedCalculated = true;
}
return myFocused;
}
private boolean calcFocusedState() {
return myTree.hasFocus();
}
@Override
public void setOpaque(boolean isOpaque) {
myOpaque = isOpaque;
super.setOpaque(isOpaque);
}
public InspectorTreeUI getUI() {
return (InspectorTreeUI)myTree.getUI();
}
@Override
public Font getFont() {
final Font font = super.getFont();
// Cell renderers could have no parent and no explicit set font.
// Take tree font in this case.
if (font != null) {
return font;
}
final JTree tree = getTree();
return tree != null ? tree.getFont() : null;
}
/**
* This method is invoked only for customization of component.
* All component attributes are cleared when this method is being invoked.
*/
public abstract void customizeCellRenderer(@NotNull JTree tree,
Object value,
boolean selected,
boolean expanded,
boolean leaf,
int row,
boolean hasFocus);
}
| flutter-intellij/flutter-idea/src/io/flutter/view/InspectorColoredTreeCellRenderer.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/view/InspectorColoredTreeCellRenderer.java",
"repo_id": "flutter-intellij",
"token_count": 1833
} | 498 |
/*
* Copyright 2020 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.vmService;
import com.google.gson.JsonObject;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.diagnostic.Logger;
import io.flutter.NotificationManager;
import io.flutter.utils.EventStream;
import io.flutter.utils.StreamSubscription;
import org.dartlang.vm.service.VmService;
import org.dartlang.vm.service.consumer.ServiceExtensionConsumer;
import org.dartlang.vm.service.element.RPCError;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
public class DisplayRefreshRateManager {
private static final Logger LOG = Logger.getInstance(DisplayRefreshRateManager.class);
private static final String INVALID_DISPLAY_REFRESH_RATE = "INVALID_DISPLAY_REFRESH_RATE";
public static final double defaultRefreshRate = 60.0;
private final VMServiceManager vmServiceManager;
private final VmService vmService;
private final EventStream<Double> displayRefreshRateStream;
DisplayRefreshRateManager(VMServiceManager vmServiceManager, VmService vmService) {
this.vmServiceManager = vmServiceManager;
this.vmService = vmService;
displayRefreshRateStream = new EventStream<>();
}
public void queryRefreshRate() {
// This needs to happen on the UI thread.
//noinspection CodeBlock2Expr
ApplicationManager.getApplication().invokeLater(() -> {
getDisplayRefreshRate().thenAcceptAsync(displayRefreshRateStream::setValue);
});
}
public int getTargetMicrosPerFrame() {
Double fps = getCurrentDisplayRefreshRateRaw();
if (fps == null) {
fps = defaultRefreshRate;
}
return (int)Math.round((Math.floor(1000000.0f / fps)));
}
/**
* Returns a StreamSubscription providing the queried display refresh rate.
* <p>
* The current value of the subscription can be null occasionally during initial application startup and for a brief time when doing a
* hot restart.
*/
public StreamSubscription<Double> getCurrentDisplayRefreshRate(Consumer<Double> onValue, boolean onUIThread) {
return displayRefreshRateStream.listen(onValue, onUIThread);
}
/**
* Return the current display refresh rate, if any.
* <p>
* Note that this may not be immediately populated at app startup for Flutter apps. In that case, this will return
* the default value (defaultRefreshRate). Clients that wish to be notified when the refresh rate is discovered
* should prefer the StreamSubscription variant of this method (getCurrentDisplayRefreshRate()).
*/
public Double getCurrentDisplayRefreshRateRaw() {
synchronized (displayRefreshRateStream) {
Double fps = displayRefreshRateStream.getValue();
if (fps == null) {
fps = defaultRefreshRate;
}
return fps;
}
}
private CompletableFuture<Double> getDisplayRefreshRate() {
final CompletableFuture<Double> displayRefreshRate = new CompletableFuture<>();
vmServiceManager.getFlutterViewId().whenComplete((String id, Throwable throwable) -> {
if (throwable != null) {
// We often see "java.lang.RuntimeException: Method not found" from here; perhaps a race condition?
LOG.warn(throwable.getMessage());
// Fail gracefully by returning the default.
displayRefreshRate.complete(defaultRefreshRate);
}
else {
invokeGetDisplayRefreshRate(id).whenComplete((Double refreshRate, Throwable t) -> {
if (t != null) {
LOG.warn(t.getMessage());
// Fail gracefully by returning the default.
displayRefreshRate.complete(defaultRefreshRate);
}
else {
displayRefreshRate.complete(refreshRate);
}
});
}
});
return displayRefreshRate;
}
private CompletableFuture<Double> invokeGetDisplayRefreshRate(String flutterViewId) {
final CompletableFuture<Double> ret = new CompletableFuture<>();
final JsonObject params = new JsonObject();
params.addProperty("viewId", flutterViewId);
vmService.callServiceExtension(
vmServiceManager.getCurrentFlutterIsolateRaw().getId(), ServiceExtensions.displayRefreshRate, params,
new ServiceExtensionConsumer() {
@Override
public void onError(RPCError error) {
ret.completeExceptionally(new RuntimeException(error.getMessage()));
}
@Override
public void received(JsonObject object) {
final String fpsField = "fps";
if (object == null || !object.has(fpsField)) {
ret.complete(null);
}
else {
final double fps = object.get(fpsField).getAsDouble();
// Defend against invalid refresh rate for Flutter Desktop devices (0.0).
if (invalidFps(fps)) {
NotificationManager.showWarning(
"Flutter device frame rate invalid",
"Device returned a target frame rate of " + fps + " FPS." + " Using 60 FPS instead.",
INVALID_DISPLAY_REFRESH_RATE,
true
);
ret.complete(defaultRefreshRate);
}
else {
ret.complete(fps);
}
}
}
}
);
return ret;
}
private boolean invalidFps(double fps) {
// 24 FPS is the lowest frame rate that can be considered smooth.
return fps < 24.0;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/vmService/DisplayRefreshRateManager.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/vmService/DisplayRefreshRateManager.java",
"repo_id": "flutter-intellij",
"token_count": 1988
} | 499 |
package io.flutter.vmService.frame;
import com.intellij.icons.AllIcons;
import com.intellij.openapi.util.text.StringUtil;
import com.intellij.testFramework.LightVirtualFile;
import com.intellij.ui.ColoredTextContainer;
import com.intellij.ui.SimpleTextAttributes;
import com.intellij.util.SmartList;
import com.intellij.xdebugger.XSourcePosition;
import com.intellij.xdebugger.evaluation.XDebuggerEvaluator;
import com.intellij.xdebugger.frame.XCompositeNode;
import com.intellij.xdebugger.frame.XStackFrame;
import com.intellij.xdebugger.frame.XValueChildrenList;
import io.flutter.vmService.DartVmServiceDebugProcess;
import org.dartlang.vm.service.consumer.GetObjectConsumer;
import org.dartlang.vm.service.element.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.List;
public class DartVmServiceStackFrame extends XStackFrame {
@NotNull private final DartVmServiceDebugProcess myDebugProcess;
@NotNull private final String myIsolateId;
@NotNull private final Frame myVmFrame;
@Nullable private final InstanceRef myException;
@Nullable private final XSourcePosition mySourcePosition;
@Nullable private final List<Frame> myVmFrames;
private boolean myIsDroppableFrame;
public DartVmServiceStackFrame(@NotNull final DartVmServiceDebugProcess debugProcess,
@NotNull final String isolateId,
@NotNull final Frame vmFrame,
@Nullable List<Frame> vmFrames,
@Nullable final InstanceRef exception) {
myDebugProcess = debugProcess;
myIsolateId = isolateId;
myVmFrame = vmFrame;
myVmFrames = vmFrames;
myException = exception;
if (vmFrame.getLocation() == null) {
mySourcePosition = null;
}
else {
mySourcePosition = debugProcess.getSourcePosition(isolateId, vmFrame.getLocation().getScript(), vmFrame.getLocation().getTokenPos());
}
}
@NotNull
public String getIsolateId() {
return myIsolateId;
}
@Nullable
@Override
public XSourcePosition getSourcePosition() {
return mySourcePosition;
}
public int getFrameIndex() {
return myVmFrames == null ? 0 : myVmFrames.indexOf(myVmFrame);
}
public void setIsDroppableFrame(boolean value) {
myIsDroppableFrame = value;
}
private boolean isLastFrame() {
if (myVmFrames == null) {
return true;
}
return getFrameIndex() == (myVmFrames.size() - 1);
}
@Override
public void customizePresentation(@NotNull final ColoredTextContainer component) {
final String unoptimizedPrefix = "[Unoptimized] ";
String name = StringUtil.trimEnd(myVmFrame.getCode().getName(), "="); // trim setter postfix
name = StringUtil.trimStart(name, unoptimizedPrefix);
final boolean causal = myVmFrame.getKind() == FrameKind.AsyncCausal;
component.append(name, causal ? SimpleTextAttributes.REGULAR_ITALIC_ATTRIBUTES : SimpleTextAttributes.REGULAR_ATTRIBUTES);
if (mySourcePosition != null) {
final String text = " (" + mySourcePosition.getFile().getName() + ":" + (mySourcePosition.getLine() + 1) + ")";
component.append(text, SimpleTextAttributes.GRAY_ATTRIBUTES);
}
component.setIcon(AllIcons.Debugger.Frame);
}
@NotNull
@Override
public Object getEqualityObject() {
return myVmFrame.getLocation().getScript().getId() + ":" + myVmFrame.getCode().getId();
}
@Override
public void computeChildren(@NotNull final XCompositeNode node) {
if (myException != null) {
final DartVmServiceValue exception = new DartVmServiceValue(myDebugProcess, myIsolateId, "exception", myException, null, null, true);
node.addChildren(XValueChildrenList.singleton(exception), false);
}
final ElementList<BoundVariable> vars = myVmFrame.getVars();
if (vars == null) {
node.addChildren(XValueChildrenList.EMPTY, true);
return;
}
BoundVariable thisVar = null;
for (BoundVariable var : vars) {
if ("this".equals(var.getName())) {
// in some cases "this" var is not the first one in the list, no idea why
thisVar = var;
break;
}
}
addStaticFieldsIfPresentAndThenAllVars(node, thisVar, vars);
}
private void addStaticFieldsIfPresentAndThenAllVars(@NotNull final XCompositeNode node,
@Nullable final BoundVariable thisVar,
@NotNull final ElementList<BoundVariable> vars) {
if (thisVar == null) {
addVars(node, vars);
return;
}
final Object thisVarValue = thisVar.getValue();
if (!(thisVarValue instanceof InstanceRef)) {
addVars(node, vars);
return;
}
// InstanceRef
final ClassRef classRef = ((InstanceRef)thisVarValue).getClassRef();
myDebugProcess.getVmServiceWrapper().getObject(myIsolateId, classRef.getId(), new GetObjectConsumer() {
@Override
public void received(Obj classObj) {
final SmartList<FieldRef> staticFields = new SmartList<>();
for (FieldRef fieldRef : ((ClassObj)classObj).getFields()) {
if (fieldRef.isStatic()) {
staticFields.add(fieldRef);
}
}
if (!staticFields.isEmpty()) {
final XValueChildrenList list = new XValueChildrenList();
list.addTopGroup(new DartStaticFieldsGroup(myDebugProcess, myIsolateId, ((ClassObj)classObj).getName(), staticFields));
node.addChildren(list, false);
}
addVars(node, vars);
}
@Override
public void received(Sentinel sentinel) {
node.setErrorMessage(sentinel.getValueAsString());
}
@Override
public void onError(RPCError error) {
node.setErrorMessage(error.getMessage());
}
});
}
private void addVars(@NotNull final XCompositeNode node, @NotNull final ElementList<BoundVariable> vars) {
final XValueChildrenList childrenList = new XValueChildrenList(vars.size());
for (BoundVariable var : vars) {
final Object value = var.getValue();
if (value instanceof InstanceRef) {
final InstanceRef instanceRef = (InstanceRef)value;
final DartVmServiceValue.LocalVarSourceLocation varLocation =
"this".equals(var.getName())
? null
: new DartVmServiceValue.LocalVarSourceLocation(myVmFrame.getLocation().getScript(), var.getDeclarationTokenPos());
childrenList.add(new DartVmServiceValue(myDebugProcess, myIsolateId, var.getName(), instanceRef, varLocation, null, false));
}
}
node.addChildren(childrenList, true);
}
@Nullable
@Override
public XDebuggerEvaluator getEvaluator() {
return new DartVmServiceEvaluatorInFrame(myDebugProcess, myIsolateId, myVmFrame);
}
public boolean isInDartSdkPatchFile() {
return mySourcePosition != null && (mySourcePosition.getFile() instanceof LightVirtualFile);
}
public boolean canDrop() {
return myIsDroppableFrame && !isLastFrame();
}
public void dropFrame() {
myDebugProcess.dropFrame(this);
}
}
| flutter-intellij/flutter-idea/src/io/flutter/vmService/frame/DartVmServiceStackFrame.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/vmService/frame/DartVmServiceStackFrame.java",
"repo_id": "flutter-intellij",
"token_count": 2722
} | 500 |
// Copyright 2000-2021 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license that can be found in the LICENSE file.
package org.jetbrains.android.facet;
import com.android.SdkConstants;
import com.android.tools.idea.gradle.project.Info;
import com.intellij.facet.FacetType;
import com.intellij.framework.detection.DetectedFrameworkDescription;
import com.intellij.framework.detection.FacetBasedFrameworkDetector;
import com.intellij.framework.detection.FileContentPattern;
import com.intellij.framework.detection.FrameworkDetectionContext;
import com.intellij.ide.highlighter.XmlFileType;
import com.intellij.notification.Notification;
import com.intellij.notification.NotificationGroupManager;
import com.intellij.notification.NotificationListener;
import com.intellij.notification.NotificationType;
import com.intellij.openapi.fileTypes.FileType;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.options.ShowSettingsUtil;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.util.Pair;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.patterns.ElementPattern;
import com.intellij.util.indexing.FileContent;
import org.jetbrains.android.util.AndroidBundle;
import org.jetbrains.annotations.NotNull;
import javax.swing.event.HyperlinkEvent;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
public class AndroidFrameworkDetector extends FacetBasedFrameworkDetector<AndroidFacet, AndroidFacetConfiguration> {
public AndroidFrameworkDetector() {
super("android");
}
@Override
public List<? extends DetectedFrameworkDescription> detect(@NotNull Collection<? extends VirtualFile> newFiles,
@NotNull FrameworkDetectionContext context) {
Project project = context.getProject();
if (project != null) {
Info gradleProjectInfo = Info.getInstance(project);
// See https://code.google.com/p/android/issues/detail?id=203384
// Since this method is invoked before sync, 'isBuildWithGradle' may return false even for Gradle projects. If that happens, we fall
// back to checking that a project has a suitable Gradle file at its toplevel.
if (gradleProjectInfo.isBuildWithGradle() || gradleProjectInfo.hasTopLevelGradleFile()) {
return Collections.emptyList();
}
}
return super.detect(newFiles, context);
}
private static boolean getFirstAsBoolean(@NotNull Pair<String, VirtualFile> pair) {
return Boolean.parseBoolean(pair.getFirst());
}
@NotNull
public static Notification showDexOptionNotification(@NotNull Module module, @NotNull String propertyName) {
Project project = module.getProject();
Notification notification = NotificationGroupManager.getInstance().getNotificationGroup("Android Module Importing").createNotification(
AndroidBundle.message("android.facet.importing.title", module.getName()),
"'" + propertyName +
"' property is detected in " + SdkConstants.FN_PROJECT_PROPERTIES +
" file.<br>You may enable related option in <a href='configure'>Settings | Compiler | Android DX</a>",
NotificationType.INFORMATION).setListener(new NotificationListener.Adapter() {
@Override
protected void hyperlinkActivated(@NotNull Notification notification, @NotNull HyperlinkEvent event) {
notification.expire();
ShowSettingsUtil.getInstance().showSettingsDialog(
project, AndroidBundle.message("android.dex.compiler.configurable.display.name"));
}
});
notification.notify(project);
return notification;
}
@NotNull
@Override
public FacetType<AndroidFacet, AndroidFacetConfiguration> getFacetType() {
return AndroidFacet.getFacetType();
}
@Override
@NotNull
public FileType getFileType() {
return XmlFileType.INSTANCE;
}
@Override
@NotNull
public ElementPattern<FileContent> createSuitableFilePattern() {
return FileContentPattern.fileContent().withName(SdkConstants.FN_ANDROID_MANIFEST_XML);
}
}
| flutter-intellij/flutter-idea/src/org/jetbrains/android/facet/AndroidFrameworkDetector.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/org/jetbrains/android/facet/AndroidFrameworkDetector.java",
"repo_id": "flutter-intellij",
"token_count": 1330
} | 501 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.testing;
import com.intellij.openapi.Disposable;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.application.PathManager;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.roots.impl.libraries.ApplicationLibraryTable;
import com.intellij.openapi.roots.libraries.Library;
import com.intellij.openapi.util.Disposer;
import com.intellij.openapi.util.io.FileUtil;
import com.intellij.openapi.vfs.newvfs.impl.VfsRootAccess;
import com.intellij.util.PathUtil;
import io.flutter.dart.DartPlugin;
import io.flutter.sdk.FlutterSdk;
import io.flutter.sdk.FlutterSdkUtil;
import org.jetbrains.annotations.NotNull;
import org.junit.Assert;
import java.io.File;
import java.util.Collections;
public class FlutterTestUtils {
public static final String BASE_TEST_DATA_PATH = findTestDataPath();
public static final String SDK_HOME_PATH = BASE_TEST_DATA_PATH + "/sdk";
public static void configureFlutterSdk(@NotNull final Module module, @NotNull final Disposable disposable, final boolean realSdk) {
final String sdkHome;
if (realSdk) {
sdkHome = System.getProperty("flutter.sdk");
if (sdkHome == null) {
Assert.fail("To run tests that use the flutter tools you need to add '-Dflutter.sdk=[real SDK home]' to the VM Options field of " +
"the corresponding JUnit run configuration (Run | Edit Configurations)");
}
if (!FlutterSdkUtil.isFlutterSdkHome(sdkHome)) {
Assert.fail("Incorrect path to the Flutter SDK (" + sdkHome + ") is set as '-Dflutter.sdk' VM option of " +
"the corresponding JUnit run configuration (Run | Edit Configurations)");
}
VfsRootAccess.allowRootAccess(disposable, sdkHome);
}
else {
sdkHome = SDK_HOME_PATH;
}
ApplicationManager.getApplication().runWriteAction(() -> {
FlutterSdkUtil.setFlutterSdkPath(module.getProject(), sdkHome);
DartPlugin.enableDartSdk(module);
});
Disposer.register(disposable, () -> ApplicationManager.getApplication().runWriteAction(() -> {
if (!module.isDisposed()) {
DartPlugin.disableDartSdk(Collections.singletonList(module));
}
final ApplicationLibraryTable libraryTable = ApplicationLibraryTable.getApplicationTable();
final Library library = libraryTable.getLibraryByName(FlutterSdk.FLUTTER_SDK_GLOBAL_LIB_NAME);
if (library != null) {
libraryTable.removeLibrary(library);
}
}));
}
private static String findTestDataPath() {
if (new File(PathManager.getHomePath() + "/contrib").isDirectory()) {
// started from IntelliJ IDEA Ultimate project
return FileUtil.toSystemIndependentName(PathManager.getHomePath() + "/contrib/flutter-intellij/testData");
}
final File f = new File("testData");
if (f.isDirectory()) {
// started from flutter plugin project
return FileUtil.toSystemIndependentName(f.getAbsolutePath());
}
final String parentPath = PathUtil.getParentPath(PathManager.getHomePath());
if (new File(parentPath + "/intellij-plugins").isDirectory()) {
// started from IntelliJ IDEA Community Edition + flutter plugin project
return FileUtil.toSystemIndependentName(parentPath + "/intellij-plugins/flutter-intellij/testData");
}
if (new File(parentPath + "/contrib").isDirectory()) {
// started from IntelliJ IDEA Community + flutter plugin project
return FileUtil.toSystemIndependentName(parentPath + "/contrib/flutter-intellij/testData");
}
return "";
}
}
| flutter-intellij/flutter-idea/testSrc/integration/io/flutter/testing/FlutterTestUtils.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/integration/io/flutter/testing/FlutterTestUtils.java",
"repo_id": "flutter-intellij",
"token_count": 1335
} | 502 |
package io.flutter.devtools;
import io.flutter.bazel.WorkspaceCache;
import io.flutter.sdk.FlutterSdkUtil;
import io.flutter.sdk.FlutterSdkVersion;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
public class DevToolsUrlTest {
@Test
public void testGetUrlString() {
final String devtoolsHost = "127.0.0.1";
final int devtoolsPort = 9100;
final String serviceProtocolUri = "http://127.0.0.1:50224/WTFTYus3IPU=/";
final String page = "timeline";
final FlutterSdkUtil mockSdkUtil = mock(FlutterSdkUtil.class);
when(mockSdkUtil.getFlutterHostEnvValue()).thenReturn("IntelliJ-IDEA");
final WorkspaceCache notBazelWorkspaceCache = mock(WorkspaceCache.class);
when(notBazelWorkspaceCache.isBazel()).thenReturn(false);
final WorkspaceCache bazelWorkspaceCache = mock(WorkspaceCache.class);
when(bazelWorkspaceCache.isBazel()).thenReturn(true);
FlutterSdkVersion newVersion = new FlutterSdkVersion("3.3.0");
assertEquals(
"http://127.0.0.1:9100/timeline?ide=IntelliJ-IDEA&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil)).getUrlString()
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=IntelliJ-IDEA&embed=true&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, true, null, null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil)).getUrlString()
);
assertEquals(
"http://127.0.0.1:9100/?ide=IntelliJ-IDEA",
(new DevToolsUrl(devtoolsHost, devtoolsPort, null, null, false, null, null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil).getUrlString())
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=IntelliJ-IDEA&backgroundColor=ffffff&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, "ffffff", null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil).getUrlString())
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=IntelliJ-IDEA&backgroundColor=ffffff&fontSize=12.0&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, "ffffff", 12.0f, newVersion, notBazelWorkspaceCache, null, mockSdkUtil).getUrlString())
);
when(mockSdkUtil.getFlutterHostEnvValue()).thenReturn("Android-Studio");
assertEquals(
"http://127.0.0.1:9100/timeline?ide=Android-Studio&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil).getUrlString())
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=Android-Studio&backgroundColor=3c3f41&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, "3c3f41", null, newVersion, notBazelWorkspaceCache, null, mockSdkUtil).getUrlString())
);
FlutterSdkVersion oldVersion = new FlutterSdkVersion("3.0.0");
assertEquals(
"http://127.0.0.1:9100/#/?ide=Android-Studio&page=timeline&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, oldVersion, notBazelWorkspaceCache, null, mockSdkUtil)).getUrlString()
);
assertEquals(
"http://127.0.0.1:9100/#/?ide=Android-Studio&page=timeline&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, null, notBazelWorkspaceCache, null, mockSdkUtil)).getUrlString()
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=Android-Studio&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, null, bazelWorkspaceCache, null, mockSdkUtil)).getUrlString()
);
assertEquals(
"http://127.0.0.1:9100/timeline?ide=Android-Studio&ideFeature=toolWindow&uri=http%3A%2F%2F127.0.0.1%3A50224%2FWTFTYus3IPU%3D%2F",
(new DevToolsUrl(devtoolsHost, devtoolsPort, serviceProtocolUri, page, false, null, null, null, bazelWorkspaceCache, DevToolsIdeFeature.TOOL_WINDOW, mockSdkUtil)).getUrlString()
);
}
} | flutter-intellij/flutter-idea/testSrc/unit/io/flutter/devtools/DevToolsUrlTest.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/devtools/DevToolsUrlTest.java",
"repo_id": "flutter-intellij",
"token_count": 2005
} | 503 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.daemon;
import com.google.common.base.Joiner;
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import org.junit.Before;
import org.junit.Test;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import static io.flutter.testing.JsonTesting.curly;
import static org.junit.Assert.assertEquals;
/**
* Verifies that we can read events sent using the Flutter daemon protocol.
*/
public class DaemonEventTest {
private List<String> log;
private DaemonEvent.Listener listener;
@Before
public void setUp() {
log = new ArrayList<>();
listener = new DaemonEvent.Listener() {
// daemon domain
@Override
public void onDaemonLogMessage(DaemonEvent.DaemonLogMessage event) {
logEvent(event, event.level, event.message, event.stackTrace);
}
@Override
public void onDaemonShowMessage(DaemonEvent.DaemonShowMessage event) {
logEvent(event, event.level, event.title, event.message);
}
// app domain
@Override
public void onAppStarting(DaemonEvent.AppStarting event) {
logEvent(event, event.appId, event.deviceId, event.directory, event.launchMode);
}
@Override
public void onAppDebugPort(DaemonEvent.AppDebugPort event) {
logEvent(event, event.appId, event.wsUri, event.baseUri);
}
@Override
public void onAppStarted(DaemonEvent.AppStarted event) {
logEvent(event, event.appId);
}
@Override
public void onAppLog(DaemonEvent.AppLog event) {
logEvent(event, event.appId, event.log, event.error);
}
@Override
public void onAppProgressStarting(DaemonEvent.AppProgress event) {
logEvent(event, "starting", event.appId, event.id, event.getType(), event.message);
}
@Override
public void onAppProgressFinished(DaemonEvent.AppProgress event) {
logEvent(event, "finished", event.appId, event.id, event.getType(), event.message);
}
@Override
public void onAppStopped(DaemonEvent.AppStopped event) {
if (event.error != null) {
logEvent(event, event.appId, event.error);
}
else {
logEvent(event, event.appId);
}
}
// device domain
@Override
public void onDeviceAdded(DaemonEvent.DeviceAdded event) {
logEvent(event, event.id, event.name, event.platform, event.category);
}
@Override
public void onDeviceRemoved(DaemonEvent.DeviceRemoved event) {
logEvent(event, event.id, event.name, event.platform);
}
};
}
@Test
public void shouldIgnoreUnknownEvent() {
send("unknown.message", curly());
checkLog();
}
// daemon domain
@Test
public void canReceiveLogMessage() {
send("daemon.logMessage", curly("level:\"spam\"", "message:\"Make money fast\"", "stackTrace:\"Las Vegas\""));
checkLog("DaemonLogMessage: spam, Make money fast, Las Vegas");
}
@Test
public void canReceiveShowMessage() {
send("daemon.showMessage", curly("level:\"info\"", "title:\"Spam\"", "message:\"Make money fast\""));
checkLog("DaemonShowMessage: info, Spam, Make money fast");
}
// app domain
@Test
public void canReceiveAppStarting() {
send("app.start", curly("appId:42", "deviceId:456", "directory:somedir", "launchMode:run"));
checkLog("AppStarting: 42, 456, somedir, run");
}
@Test
public void canReceiveDebugPort() {
// The port parameter is deprecated; should ignore it.
send("app.debugPort", curly("appId:42", "port:456", "wsUri:\"example.com\"", "baseUri:\"belongto:us\""));
checkLog("AppDebugPort: 42, example.com, belongto:us");
}
@Test
public void canReceiveAppStarted() {
send("app.started", curly("appId:42"));
checkLog("AppStarted: 42");
}
@Test
public void canReceiveAppLog() {
send("app.log", curly("appId:42", "log:\"Oh no!\"", "error:true"));
checkLog("AppLog: 42, Oh no!, true");
}
@Test
public void canReceiveProgressStarting() {
send("app.progress", curly("appId:42", "id:opaque", "progressId:very.hot", "message:\"Please wait\""));
checkLog("AppProgress: starting, 42, opaque, very.hot, Please wait");
}
@Test
public void canReceiveProgressFinished() {
send("app.progress", curly("appId:42", "id:opaque", "progressId:very.hot", "message:\"All done!\"", "finished:true"));
checkLog("AppProgress: finished, 42, opaque, very.hot, All done!");
}
@Test
public void canReceiveAppStopped() {
send("app.stop", curly("appId:42"));
checkLog("AppStopped: 42");
}
@Test
public void canReceiveAppStoppedWithError() {
send("app.stop", curly("appId:42", "error:\"foobar\""));
checkLog("AppStopped: 42, foobar");
}
// device domain
@Test
public void canReceiveDeviceAdded() {
send("device.added", curly("id:9000", "name:\"Banana Jr\"", "platform:\"feet\"", "category:\"mobile\""));
checkLog("DeviceAdded: 9000, Banana Jr, feet, mobile");
}
@Test
public void canReceiveDeviceRemoved() {
send("device.removed", curly("id:9000", "name:\"Banana Jr\"", "platform:\"feet\""));
checkLog("DeviceRemoved: 9000, Banana Jr, feet");
}
private void send(String eventName, String params) {
DaemonEvent.dispatch(
GSON.fromJson(curly("event:\"" + eventName + "\"", "params:" + params),
JsonObject.class), listener);
}
private void logEvent(DaemonEvent event, Object... items) {
log.add(event.getClass().getSimpleName() + ": " + Joiner.on(", ").join(items));
}
private void checkLog(String... expectedEntries) {
assertEquals("log entries are different", Arrays.asList(expectedEntries), log);
log.clear();
}
private static final Gson GSON = new Gson();
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/run/daemon/DaemonEventTest.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/run/daemon/DaemonEventTest.java",
"repo_id": "flutter-intellij",
"token_count": 2222
} | 504 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils;
import com.google.common.collect.ImmutableSet;
import com.intellij.openapi.vfs.VirtualFile;
import io.flutter.testing.TestDir;
import org.junit.Rule;
import org.junit.Test;
import java.util.concurrent.atomic.AtomicInteger;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotEquals;
/**
* Verifies that we can watch files and directories.
*/
public class FileWatchTest {
@Rule
public final TestDir tmp = new TestDir();
@Test
public void shouldFireEvents() throws Exception {
final VirtualFile dir = tmp.ensureDir("abc");
final AtomicInteger eventCount = new AtomicInteger();
final FileWatch fileWatch = FileWatch.subscribe(dir, ImmutableSet.of("child"), eventCount::incrementAndGet);
assertEquals(0, eventCount.get());
// create
tmp.writeFile("abc/child", "");
final int count;
// The number of events fired is an implementation detail of the VFS. We just need at least one.
assertNotEquals(0, count = eventCount.get());
// modify
tmp.writeFile("abc/child", "hello");
assertEquals(count + 1, eventCount.get());
// delete
tmp.deleteFile("abc/child");
assertEquals(count + 2, eventCount.get());
}
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/utils/FileWatchTest.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/utils/FileWatchTest.java",
"repo_id": "flutter-intellij",
"token_count": 452
} | 505 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service;
import org.dartlang.vm.service.element.Event;
/**
* Interface used by {@link VmService} to notify others of VM events.
*/
public interface VmServiceListener {
void connectionOpened();
/**
* Called when a VM event has been received.
*
* @param streamId the stream identifier (e.g. {@link VmService#DEBUG_STREAM_ID}
* @param event the event
*/
void received(String streamId, Event event);
void connectionClosed();
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/VmServiceListener.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/VmServiceListener.java",
"repo_id": "flutter-intellij",
"token_count": 306
} | 506 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonPrimitive;
/**
* A {@link BoundField} represents a field bound to a particular value in an {@link Instance}.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class BoundField extends Element {
public BoundField(JsonObject json) {
super(json);
}
/**
* @return one of <code>String</code> or <code>int</code>
*/
public Object getName() {
final JsonElement elem = json.get("name");
if (elem != null && elem.isJsonPrimitive()) {
final JsonPrimitive p = (JsonPrimitive) elem;
if (p.isString()) return p.getAsString();
if (p.isNumber()) return p.getAsInt();
}
return null;
}
/**
* @return one of <code>InstanceRef</code> or <code>Sentinel</code>
*/
public InstanceRef getValue() {
final JsonElement elem = json.get("value");
if (elem == null || !elem.isJsonObject()) {
return null;
}
final JsonObject child = elem.getAsJsonObject();
final String type = child.get("type").getAsString();
if ("Sentinel".equals(type)) {
return null;
}
return new InstanceRef(child);
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/BoundField.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/BoundField.java",
"repo_id": "flutter-intellij",
"token_count": 648
} | 507 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonArray;
import com.google.gson.JsonObject;
/**
* See getCpuSamples and CpuSample.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class CpuSamples extends Response {
public CpuSamples(JsonObject json) {
super(json);
}
/**
* A list of functions seen in the relevant samples. These references can be looked up using the
* indices provided in a `CpuSample` `stack` to determine which function was on the stack.
*/
public ElementList<ProfileFunction> getFunctions() {
return new ElementList<ProfileFunction>(json.get("functions").getAsJsonArray()) {
@Override
protected ProfileFunction basicGet(JsonArray array, int index) {
return new ProfileFunction(array.get(index).getAsJsonObject());
}
};
}
/**
* The maximum possible stack depth for samples.
*/
public int getMaxStackDepth() {
return getAsInt("maxStackDepth");
}
/**
* The process ID for the VM.
*/
public int getPid() {
return getAsInt("pid");
}
/**
* The number of samples returned.
*/
public int getSampleCount() {
return getAsInt("sampleCount");
}
/**
* The sampling rate for the profiler in microseconds.
*/
public int getSamplePeriod() {
return getAsInt("samplePeriod");
}
/**
* A list of samples collected in the range `[timeOriginMicros, timeOriginMicros +
* timeExtentMicros]`
*/
public ElementList<CpuSample> getSamples() {
return new ElementList<CpuSample>(json.get("samples").getAsJsonArray()) {
@Override
protected CpuSample basicGet(JsonArray array, int index) {
return new CpuSample(array.get(index).getAsJsonObject());
}
};
}
/**
* The duration of time covered by the returned samples.
*/
public int getTimeExtentMicros() {
return getAsInt("timeExtentMicros");
}
/**
* The start of the period of time in which the returned samples were collected.
*/
public int getTimeOriginMicros() {
return getAsInt("timeOriginMicros");
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/CpuSamples.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/CpuSamples.java",
"repo_id": "flutter-intellij",
"token_count": 896
} | 508 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonObject;
/**
* {@link LibraryRef} is a reference to a {@link Library}.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class LibraryRef extends ObjRef {
public LibraryRef(JsonObject json) {
super(json);
}
/**
* The name of this library.
*/
public String getName() {
return getAsString("name");
}
/**
* The uri of this library.
*/
public String getUri() {
return getAsString("uri");
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/LibraryRef.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/LibraryRef.java",
"repo_id": "flutter-intellij",
"token_count": 367
} | 509 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonObject;
import java.util.List;
/**
* The {@link SourceReportRange} class represents a range of executable code (function, method,
* constructor, etc) in the running program. It is part of a SourceReport.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class SourceReportRange extends Element {
public SourceReportRange(JsonObject json) {
super(json);
}
/**
* Branch coverage information for this range. Provided only when the BranchCoverage report has
* been requested and the range has been compiled.
*
* Can return <code>null</code>.
*/
public SourceReportCoverage getBranchCoverage() {
JsonObject obj = (JsonObject) json.get("branchCoverage");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new SourceReportCoverage(obj);
}
/**
* Has this range been compiled by the Dart VM?
*/
public boolean getCompiled() {
return getAsBoolean("compiled");
}
/**
* Code coverage information for this range. Provided only when the Coverage report has been
* requested and the range has been compiled.
*
* Can return <code>null</code>.
*/
public SourceReportCoverage getCoverage() {
JsonObject obj = (JsonObject) json.get("coverage");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new SourceReportCoverage(obj);
}
/**
* The token position at which this range ends. Inclusive.
*/
public int getEndPos() {
return getAsInt("endPos");
}
/**
* The error while attempting to compile this range, if this report was generated with
* forceCompile=true.
*
* Can return <code>null</code>.
*/
public ErrorRef getError() {
JsonObject obj = (JsonObject) json.get("error");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new ErrorRef(obj);
}
/**
* Possible breakpoint information for this range, represented as a sorted list of token
* positions (or line numbers if reportLines was enabled). Provided only when the when the
* PossibleBreakpoint report has been requested and the range has been compiled.
*
* Can return <code>null</code>.
*/
public List<Integer> getPossibleBreakpoints() {
return getListInt("possibleBreakpoints");
}
/**
* An index into the script table of the SourceReport, indicating which script contains this
* range of code.
*/
public int getScriptIndex() {
return getAsInt("scriptIndex");
}
/**
* The token position at which this range begins.
*/
public int getStartPos() {
return getAsInt("startPos");
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/SourceReportRange.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/SourceReportRange.java",
"repo_id": "flutter-intellij",
"token_count": 1234
} | 510 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.startup.StartupActivity;
import io.flutter.android.AndroidModuleLibraryManager;
//import io.flutter.project.FlutterProjectCreator;
import io.flutter.settings.FlutterSettings;
import io.flutter.utils.AddToAppUtils;
import io.flutter.utils.AndroidUtils;
import io.flutter.utils.GradleUtils;
import org.jetbrains.annotations.NotNull;
public class FlutterStudioStartupActivity implements StartupActivity {
@Override
public void runActivity(@NotNull Project project) {
if (AndroidUtils.isAndroidProject(project)) {
GradleUtils.addGradleListeners(project);
}
if (!AddToAppUtils.initializeAndDetectFlutter(project)) {
return;
}
// Unset this flag for all projects, mainly to ease the upgrade from 3.0.1 to 3.1.
// TODO(messick) Delete once 3.0.x has 0 7DA's.
//FlutterProjectCreator.disableUserConfig(project);
// Monitor Android dependencies.
if (FlutterSettings.getInstance().isSyncingAndroidLibraries() ||
System.getProperty("flutter.android.library.sync", null) != null) {
// TODO(messick): Remove the flag once this sync mechanism is stable.
AndroidModuleLibraryManager.startWatching(project);
}
}
}
| flutter-intellij/flutter-studio/src/io/flutter/FlutterStudioStartupActivity.java/0 | {
"file_path": "flutter-intellij/flutter-studio/src/io/flutter/FlutterStudioStartupActivity.java",
"repo_id": "flutter-intellij",
"token_count": 471
} | 511 |
/*
* Copyright 2020 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils;
import com.android.tools.idea.gradle.dsl.model.BuildModelContext;
import com.android.tools.idea.gradle.project.model.GradleModuleModel;
import com.android.tools.idea.gradle.util.GradleProjectSystemUtil;
import com.android.tools.idea.projectsystem.AndroidProjectRootUtil;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.project.ProjectUtil;
import com.intellij.openapi.vfs.VirtualFile;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.jetbrains.annotations.SystemIndependent;
// Copied from GradleModelSource.ResolvedConfigurationFileLocationProviderImpl
// This file must be ignored in pre-4.1 builds.
public class AndroidLocationProvider implements BuildModelContext.ResolvedConfigurationFileLocationProvider {
@Nullable
@Override
public VirtualFile getGradleBuildFile(@NotNull Module module) {
GradleModuleModel moduleModel = GradleProjectSystemUtil.getGradleModuleModel(module);
if (moduleModel != null) {
return moduleModel.getBuildFile();
}
return null;
}
@Nullable
@Override
public @SystemIndependent String getGradleProjectRootPath(@NotNull Module module) {
return AndroidProjectRootUtil.getModuleDirPath(module);
}
@Nullable
@Override
public @SystemIndependent String getGradleProjectRootPath(@NotNull Project project) {
VirtualFile projectDir = ProjectUtil.guessProjectDir(project);
if (projectDir == null) return null;
return projectDir.getPath();
}
}
| flutter-intellij/flutter-studio/src/io/flutter/utils/AndroidLocationProvider.java/0 | {
"file_path": "flutter-intellij/flutter-studio/src/io/flutter/utils/AndroidLocationProvider.java",
"repo_id": "flutter-intellij",
"token_count": 526
} | 512 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package com.android.tools.idea.tests.gui.framework.fixture.newProjectWizard;
import static com.google.common.collect.Lists.newArrayList;
import com.android.tools.adtui.ASGallery;
import com.android.tools.idea.tests.gui.framework.GuiTests;
import com.android.tools.idea.tests.gui.framework.fixture.wizard.AbstractWizardFixture;
import com.android.tools.idea.tests.gui.framework.matcher.Matchers;
import com.intellij.openapi.progress.ProgressManager;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.project.ProjectManager;
import io.flutter.module.FlutterProjectType;
import java.util.List;
import javax.swing.JDialog;
import javax.swing.JRootPane;
import org.fest.swing.core.Robot;
import org.fest.swing.fixture.JListFixture;
import org.fest.swing.timing.Wait;
import org.jetbrains.annotations.NotNull;
// Adapted from com.android.tools.idea.tests.gui.framework.fixture.newProjectWizard.NewProjectWizardFixture
@SuppressWarnings("UnusedReturnValue")
public class NewFlutterProjectWizardFixture extends AbstractWizardFixture<NewFlutterProjectWizardFixture> {
private NewFlutterProjectWizardFixture(@NotNull Robot robot, @NotNull JDialog target) {
super(NewFlutterProjectWizardFixture.class, robot, target);
}
@NotNull
public static NewFlutterProjectWizardFixture find(@NotNull Robot robot) {
JDialog dialog = GuiTests.waitUntilShowing(robot, Matchers.byTitle(JDialog.class, "Create New Flutter Project"));
return new NewFlutterProjectWizardFixture(robot, dialog);
}
public NewFlutterProjectWizardFixture chooseProjectType(@NotNull String activity) {
JListFixture listFixture = new JListFixture(robot(), robot().finder().findByType(target(), ASGallery.class));
listFixture.replaceCellReader((jList, index) -> String.valueOf(jList.getModel().getElementAt(index)));
listFixture.clickItem(activity);
return this;
}
@NotNull
public FlutterProjectStepFixture<NewFlutterProjectWizardFixture> getFlutterProjectStep(@NotNull FlutterProjectType type) {
String projectType;
switch (type) {
case APP:
projectType = "application";
break;
case MODULE:
projectType = "module";
break;
case PACKAGE:
projectType = "package";
break;
case PLUGIN:
projectType = "plugin";
break;
default:
throw new IllegalArgumentException();
}
JRootPane rootPane = findStepWithTitle("Configure the new Flutter " + projectType);
return new FlutterProjectStepFixture<>(this, rootPane);
}
@NotNull
public FlutterSettingsStepFixture getFlutterSettingsStep() {
JRootPane rootPane = findStepWithTitle("Set the package name");
return new FlutterSettingsStepFixture<>(this, rootPane);
}
@NotNull
public NewFlutterProjectWizardFixture clickFinish() {
List<Project> previouslyOpenProjects = newArrayList(ProjectManager.getInstance().getOpenProjects());
super.clickFinish(Wait.seconds(30));
List<Project> newOpenProjects = newArrayList();
Wait.seconds(5).expecting("Project to be created")
.until(() -> {
newOpenProjects.addAll(newArrayList(ProjectManager.getInstance().getOpenProjects()));
newOpenProjects.removeAll(previouslyOpenProjects);
return !newOpenProjects.isEmpty();
});
// Wait for 'flutter create' to finish
Wait.seconds(30).expecting("Modal Progress Indicator to finish")
.until(() -> {
robot().waitForIdle();
return !ProgressManager.getInstance().hasModalProgressIndicator();
});
return myself();
}
}
| flutter-intellij/flutter-studio/testSrc/com/android/tools/idea/tests/gui/framework/fixture/newProjectWizard/NewFlutterProjectWizardFixture.java/0 | {
"file_path": "flutter-intellij/flutter-studio/testSrc/com/android/tools/idea/tests/gui/framework/fixture/newProjectWizard/NewFlutterProjectWizardFixture.java",
"repo_id": "flutter-intellij",
"token_count": 1290
} | 513 |
name: flutter_intellij
version: 0.1.0
environment:
sdk: '>=3.0.0-0.0.dev <4.0.0'
dependencies:
args: any
path: any
dev_dependencies:
grinder: ^0.9.0
http: ^1.1.2
lints: ^3.0.0
meta: any
| flutter-intellij/pubspec.yaml/0 | {
"file_path": "flutter-intellij/pubspec.yaml",
"repo_id": "flutter-intellij",
"token_count": 106
} | 514 |
# Generated file - do not edit.
# suppress inspection "UnusedProperty" for whole file
activeBlue=ff007aff
activeBlue.darkColor=ff0a84ff
activeBlue.darkElevatedColor=ff0a84ff
activeBlue.darkHighContrastColor=ff409cff
activeBlue.darkHighContrastElevatedColor=ff409cff
activeBlue.elevatedColor=ff007aff
activeBlue.highContrastColor=ff0040dd
activeBlue.highContrastElevatedColor=ff0040dd
activeGreen=ff34c759
activeGreen.darkColor=ff30d158
activeGreen.darkElevatedColor=ff30d158
activeGreen.darkHighContrastColor=ff30db5b
activeGreen.darkHighContrastElevatedColor=ff30db5b
activeGreen.elevatedColor=ff34c759
activeGreen.highContrastColor=ff248a3d
activeGreen.highContrastElevatedColor=ff248a3d
activeOrange=ffff9500
activeOrange.darkColor=ffff9f0a
activeOrange.darkElevatedColor=ffff9f0a
activeOrange.darkHighContrastColor=ffffb340
activeOrange.darkHighContrastElevatedColor=ffffb340
activeOrange.elevatedColor=ffff9500
activeOrange.highContrastColor=ffc93400
activeOrange.highContrastElevatedColor=ffc93400
black=ff000000
darkBackgroundGray=ff171717
destructiveRed=ffff3b30
destructiveRed.darkColor=ffff453a
destructiveRed.darkElevatedColor=ffff453a
destructiveRed.darkHighContrastColor=ffff6961
destructiveRed.darkHighContrastElevatedColor=ffff6961
destructiveRed.elevatedColor=ffff3b30
destructiveRed.highContrastColor=ffd70015
destructiveRed.highContrastElevatedColor=ffd70015
extraLightBackgroundGray=ffefeff4
inactiveGray=ff999999
inactiveGray.darkColor=ff757575
inactiveGray.darkElevatedColor=ff757575
inactiveGray.darkHighContrastColor=ff757575
inactiveGray.darkHighContrastElevatedColor=ff757575
inactiveGray.elevatedColor=ff999999
inactiveGray.highContrastColor=ff999999
inactiveGray.highContrastElevatedColor=ff999999
label=ff000000
label.darkColor=ffffffff
label.darkElevatedColor=ffffffff
label.darkHighContrastColor=ffffffff
label.darkHighContrastElevatedColor=ffffffff
label.elevatedColor=ff000000
label.highContrastColor=ff000000
label.highContrastElevatedColor=ff000000
lightBackgroundGray=ffe5e5ea
link=ff007aff
link.darkColor=ff0984ff
link.darkElevatedColor=ff0984ff
link.darkHighContrastColor=ff0984ff
link.darkHighContrastElevatedColor=ff0984ff
link.elevatedColor=ff007aff
link.highContrastColor=ff007aff
link.highContrastElevatedColor=ff007aff
opaqueSeparator=ffc6c6c8
opaqueSeparator.darkColor=ff38383a
opaqueSeparator.darkElevatedColor=ff38383a
opaqueSeparator.darkHighContrastColor=ff38383a
opaqueSeparator.darkHighContrastElevatedColor=ff38383a
opaqueSeparator.elevatedColor=ffc6c6c8
opaqueSeparator.highContrastColor=ffc6c6c8
opaqueSeparator.highContrastElevatedColor=ffc6c6c8
placeholderText=4c3c3c43
placeholderText.darkColor=4cebebf5
placeholderText.darkElevatedColor=4cebebf5
placeholderText.darkHighContrastColor=60ebebf5
placeholderText.darkHighContrastElevatedColor=60ebebf5
placeholderText.elevatedColor=4c3c3c43
placeholderText.highContrastColor=603c3c43
placeholderText.highContrastElevatedColor=603c3c43
quaternaryLabel=2d3c3c43
quaternaryLabel.darkColor=28ebebf5
quaternaryLabel.darkElevatedColor=28ebebf5
quaternaryLabel.darkHighContrastColor=3debebf5
quaternaryLabel.darkHighContrastElevatedColor=3debebf5
quaternaryLabel.elevatedColor=2d3c3c43
quaternaryLabel.highContrastColor=423c3c43
quaternaryLabel.highContrastElevatedColor=423c3c43
quaternarySystemFill=14747480
quaternarySystemFill.darkColor=2d767680
quaternarySystemFill.darkElevatedColor=2d767680
quaternarySystemFill.darkHighContrastColor=42767680
quaternarySystemFill.darkHighContrastElevatedColor=42767680
quaternarySystemFill.elevatedColor=14747480
quaternarySystemFill.highContrastColor=28747480
quaternarySystemFill.highContrastElevatedColor=28747480
secondaryLabel=993c3c43
secondaryLabel.darkColor=99ebebf5
secondaryLabel.darkElevatedColor=99ebebf5
secondaryLabel.darkHighContrastColor=adebebf5
secondaryLabel.darkHighContrastElevatedColor=adebebf5
secondaryLabel.elevatedColor=993c3c43
secondaryLabel.highContrastColor=ad3c3c43
secondaryLabel.highContrastElevatedColor=ad3c3c43
secondarySystemBackground=fff2f2f7
secondarySystemBackground.darkColor=ff1c1c1e
secondarySystemBackground.darkElevatedColor=ff2c2c2e
secondarySystemBackground.darkHighContrastColor=ff242426
secondarySystemBackground.darkHighContrastElevatedColor=ff363638
secondarySystemBackground.elevatedColor=fff2f2f7
secondarySystemBackground.highContrastColor=ffebebf0
secondarySystemBackground.highContrastElevatedColor=ffebebf0
secondarySystemFill=28787880
secondarySystemFill.darkColor=51787880
secondarySystemFill.darkElevatedColor=51787880
secondarySystemFill.darkHighContrastColor=66787880
secondarySystemFill.darkHighContrastElevatedColor=66787880
secondarySystemFill.elevatedColor=28787880
secondarySystemFill.highContrastColor=3d787880
secondarySystemFill.highContrastElevatedColor=3d787880
secondarySystemGroupedBackground=ffffffff
secondarySystemGroupedBackground.darkColor=ff1c1c1e
secondarySystemGroupedBackground.darkElevatedColor=ff2c2c2e
secondarySystemGroupedBackground.darkHighContrastColor=ff242426
secondarySystemGroupedBackground.darkHighContrastElevatedColor=ff363638
secondarySystemGroupedBackground.elevatedColor=ffffffff
secondarySystemGroupedBackground.highContrastColor=ffffffff
secondarySystemGroupedBackground.highContrastElevatedColor=ffffffff
separator=493c3c43
separator.darkColor=99545458
separator.darkElevatedColor=99545458
separator.darkHighContrastColor=ad545458
separator.darkHighContrastElevatedColor=ad545458
separator.elevatedColor=493c3c43
separator.highContrastColor=5e3c3c43
separator.highContrastElevatedColor=5e3c3c43
systemBackground=ffffffff
systemBackground.darkColor=ff000000
systemBackground.darkElevatedColor=ff1c1c1e
systemBackground.darkHighContrastColor=ff000000
systemBackground.darkHighContrastElevatedColor=ff242426
systemBackground.elevatedColor=ffffffff
systemBackground.highContrastColor=ffffffff
systemBackground.highContrastElevatedColor=ffffffff
systemBlue=ff007aff
systemBlue.darkColor=ff0a84ff
systemBlue.darkElevatedColor=ff0a84ff
systemBlue.darkHighContrastColor=ff409cff
systemBlue.darkHighContrastElevatedColor=ff409cff
systemBlue.elevatedColor=ff007aff
systemBlue.highContrastColor=ff0040dd
systemBlue.highContrastElevatedColor=ff0040dd
systemFill=33787880
systemFill.darkColor=5b787880
systemFill.darkElevatedColor=5b787880
systemFill.darkHighContrastColor=70787880
systemFill.darkHighContrastElevatedColor=70787880
systemFill.elevatedColor=33787880
systemFill.highContrastColor=47787880
systemFill.highContrastElevatedColor=47787880
systemGreen=ff34c759
systemGreen.darkColor=ff30d158
systemGreen.darkElevatedColor=ff30d158
systemGreen.darkHighContrastColor=ff30db5b
systemGreen.darkHighContrastElevatedColor=ff30db5b
systemGreen.elevatedColor=ff34c759
systemGreen.highContrastColor=ff248a3d
systemGreen.highContrastElevatedColor=ff248a3d
systemGrey=ff8e8e93
systemGrey.darkColor=ff8e8e93
systemGrey.darkElevatedColor=ff8e8e93
systemGrey.darkHighContrastColor=ffaeaeb2
systemGrey.darkHighContrastElevatedColor=ffaeaeb2
systemGrey.elevatedColor=ff8e8e93
systemGrey.highContrastColor=ff6c6c70
systemGrey.highContrastElevatedColor=ff6c6c70
systemGrey2=ffaeaeb2
systemGrey2.darkColor=ff636366
systemGrey2.darkElevatedColor=ff636366
systemGrey2.darkHighContrastColor=ff7c7c80
systemGrey2.darkHighContrastElevatedColor=ff7c7c80
systemGrey2.elevatedColor=ffaeaeb2
systemGrey2.highContrastColor=ff8e8e93
systemGrey2.highContrastElevatedColor=ff8e8e93
systemGrey3=ffc7c7cc
systemGrey3.darkColor=ff48484a
systemGrey3.darkElevatedColor=ff48484a
systemGrey3.darkHighContrastColor=ff545456
systemGrey3.darkHighContrastElevatedColor=ff545456
systemGrey3.elevatedColor=ffc7c7cc
systemGrey3.highContrastColor=ffaeaeb2
systemGrey3.highContrastElevatedColor=ffaeaeb2
systemGrey4=ffd1d1d6
systemGrey4.darkColor=ff3a3a3c
systemGrey4.darkElevatedColor=ff3a3a3c
systemGrey4.darkHighContrastColor=ff444446
systemGrey4.darkHighContrastElevatedColor=ff444446
systemGrey4.elevatedColor=ffd1d1d6
systemGrey4.highContrastColor=ffbcbcc0
systemGrey4.highContrastElevatedColor=ffbcbcc0
systemGrey5=ffe5e5ea
systemGrey5.darkColor=ff2c2c2e
systemGrey5.darkElevatedColor=ff2c2c2e
systemGrey5.darkHighContrastColor=ff363638
systemGrey5.darkHighContrastElevatedColor=ff363638
systemGrey5.elevatedColor=ffe5e5ea
systemGrey5.highContrastColor=ffd8d8dc
systemGrey5.highContrastElevatedColor=ffd8d8dc
systemGrey6=fff2f2f7
systemGrey6.darkColor=ff1c1c1e
systemGrey6.darkElevatedColor=ff1c1c1e
systemGrey6.darkHighContrastColor=ff242426
systemGrey6.darkHighContrastElevatedColor=ff242426
systemGrey6.elevatedColor=fff2f2f7
systemGrey6.highContrastColor=ffebebf0
systemGrey6.highContrastElevatedColor=ffebebf0
systemGroupedBackground=fff2f2f7
systemGroupedBackground.darkColor=ff000000
systemGroupedBackground.darkElevatedColor=ff1c1c1e
systemGroupedBackground.darkHighContrastColor=ff000000
systemGroupedBackground.darkHighContrastElevatedColor=ff242426
systemGroupedBackground.elevatedColor=fff2f2f7
systemGroupedBackground.highContrastColor=ffebebf0
systemGroupedBackground.highContrastElevatedColor=ffebebf0
systemIndigo=ff5856d6
systemIndigo.darkColor=ff5e5ce6
systemIndigo.darkElevatedColor=ff5e5ce6
systemIndigo.darkHighContrastColor=ff7d7aff
systemIndigo.darkHighContrastElevatedColor=ff7d7aff
systemIndigo.elevatedColor=ff5856d6
systemIndigo.highContrastColor=ff3634a3
systemIndigo.highContrastElevatedColor=ff3634a3
systemOrange=ffff9500
systemOrange.darkColor=ffff9f0a
systemOrange.darkElevatedColor=ffff9f0a
systemOrange.darkHighContrastColor=ffffb340
systemOrange.darkHighContrastElevatedColor=ffffb340
systemOrange.elevatedColor=ffff9500
systemOrange.highContrastColor=ffc93400
systemOrange.highContrastElevatedColor=ffc93400
systemPink=ffff2d55
systemPink.darkColor=ffff375f
systemPink.darkElevatedColor=ffff375f
systemPink.darkHighContrastColor=ffff6482
systemPink.darkHighContrastElevatedColor=ffff6482
systemPink.elevatedColor=ffff2d55
systemPink.highContrastColor=ffd30f45
systemPink.highContrastElevatedColor=ffd30f45
systemPurple=ffaf52de
systemPurple.darkColor=ffbf5af2
systemPurple.darkElevatedColor=ffbf5af2
systemPurple.darkHighContrastColor=ffda8fff
systemPurple.darkHighContrastElevatedColor=ffda8fff
systemPurple.elevatedColor=ffaf52de
systemPurple.highContrastColor=ff8944ab
systemPurple.highContrastElevatedColor=ff8944ab
systemRed=ffff3b30
systemRed.darkColor=ffff453a
systemRed.darkElevatedColor=ffff453a
systemRed.darkHighContrastColor=ffff6961
systemRed.darkHighContrastElevatedColor=ffff6961
systemRed.elevatedColor=ffff3b30
systemRed.highContrastColor=ffd70015
systemRed.highContrastElevatedColor=ffd70015
systemTeal=ff5ac8fa
systemTeal.darkColor=ff64d2ff
systemTeal.darkElevatedColor=ff64d2ff
systemTeal.darkHighContrastColor=ff70d7ff
systemTeal.darkHighContrastElevatedColor=ff70d7ff
systemTeal.elevatedColor=ff5ac8fa
systemTeal.highContrastColor=ff0071a4
systemTeal.highContrastElevatedColor=ff0071a4
systemYellow=ffffcc00
systemYellow.darkColor=ffffd60a
systemYellow.darkElevatedColor=ffffd60a
systemYellow.darkHighContrastColor=ffffd426
systemYellow.darkHighContrastElevatedColor=ffffd426
systemYellow.elevatedColor=ffffcc00
systemYellow.highContrastColor=ffa05a00
systemYellow.highContrastElevatedColor=ffa05a00
tertiaryLabel=4c3c3c43
tertiaryLabel.darkColor=4cebebf5
tertiaryLabel.darkElevatedColor=4cebebf5
tertiaryLabel.darkHighContrastColor=60ebebf5
tertiaryLabel.darkHighContrastElevatedColor=60ebebf5
tertiaryLabel.elevatedColor=4c3c3c43
tertiaryLabel.highContrastColor=603c3c43
tertiaryLabel.highContrastElevatedColor=603c3c43
tertiarySystemBackground=ffffffff
tertiarySystemBackground.darkColor=ff2c2c2e
tertiarySystemBackground.darkElevatedColor=ff3a3a3c
tertiarySystemBackground.darkHighContrastColor=ff363638
tertiarySystemBackground.darkHighContrastElevatedColor=ff444446
tertiarySystemBackground.elevatedColor=ffffffff
tertiarySystemBackground.highContrastColor=ffffffff
tertiarySystemBackground.highContrastElevatedColor=ffffffff
tertiarySystemFill=1e767680
tertiarySystemFill.darkColor=3d767680
tertiarySystemFill.darkElevatedColor=3d767680
tertiarySystemFill.darkHighContrastColor=51767680
tertiarySystemFill.darkHighContrastElevatedColor=51767680
tertiarySystemFill.elevatedColor=1e767680
tertiarySystemFill.highContrastColor=33767680
tertiarySystemFill.highContrastElevatedColor=33767680
tertiarySystemGroupedBackground=fff2f2f7
tertiarySystemGroupedBackground.darkColor=ff2c2c2e
tertiarySystemGroupedBackground.darkElevatedColor=ff3a3a3c
tertiarySystemGroupedBackground.darkHighContrastColor=ff363638
tertiarySystemGroupedBackground.darkHighContrastElevatedColor=ff444446
tertiarySystemGroupedBackground.elevatedColor=fff2f2f7
tertiarySystemGroupedBackground.highContrastColor=ffebebf0
tertiarySystemGroupedBackground.highContrastElevatedColor=ffebebf0
white=ffffffff
| flutter-intellij/resources/flutter/colors/cupertino.properties/0 | {
"file_path": "flutter-intellij/resources/flutter/colors/cupertino.properties",
"repo_id": "flutter-intellij",
"token_count": 4501
} | 515 |
fonts:
- icons: FontAwesomeIcons
- family: FontAwesomeBrands
fonts:
- asset: fonts/fa-brands-400.ttf
class: IconDataBrands
- family: FontAwesomeRegular
fonts:
- asset: fonts/fa-icon-400.ttf
class: IconDataRegular
- family: FontAwesomeSolid
fonts:
- asset: fonts/fa-solid-900.ttf
class: IconDataSolid
| flutter-intellij/resources/iconAssetMaps/FontAwesomeIcons/asset_map.yaml/0 | {
"file_path": "flutter-intellij/resources/iconAssetMaps/FontAwesomeIcons/asset_map.yaml",
"repo_id": "flutter-intellij",
"token_count": 154
} | 516 |
# Changelog
## 0.0.1
- Initial version
| flutter-intellij/tool/plugin/CHANGELOG.md/0 | {
"file_path": "flutter-intellij/tool/plugin/CHANGELOG.md",
"repo_id": "flutter-intellij",
"token_count": 18
} | 517 |
e2e09d9fba1187f8d6aafaa34d4172f56f1ffb72
| flutter/bin/internal/openssl.version/0 | {
"file_path": "flutter/bin/internal/openssl.version",
"repo_id": "flutter",
"token_count": 29
} | 518 |
package com.example.a11y_assessments
import io.flutter.embedding.android.FlutterActivity
class MainActivity : FlutterActivity()
| flutter/dev/a11y_assessments/android/app/src/main/kotlin/com/example/a11y_assessments/MainActivity.kt/0 | {
"file_path": "flutter/dev/a11y_assessments/android/app/src/main/kotlin/com/example/a11y_assessments/MainActivity.kt",
"repo_id": "flutter",
"token_count": 39
} | 519 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'use_cases.dart';
class SliderUseCase extends UseCase {
@override
String get name => 'Slider';
@override
String get route => '/slider';
@override
Widget build(BuildContext context) => const MainWidget();
}
class MainWidget extends StatefulWidget {
const MainWidget({super.key});
@override
State<MainWidget> createState() => MainWidgetState();
}
class MainWidgetState extends State<MainWidget> {
double currentSliderValue = 20;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text('Slider'),
),
body: Center(
child: Slider(
value: currentSliderValue,
max: 100,
divisions: 5,
label: currentSliderValue.round().toString(),
onChanged: (double value) {
setState(() {
currentSliderValue = value;
});
},
),
),
);
}
}
| flutter/dev/a11y_assessments/lib/use_cases/slider.dart/0 | {
"file_path": "flutter/dev/a11y_assessments/lib/use_cases/slider.dart",
"repo_id": "flutter",
"token_count": 475
} | 520 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:a11y_assessments/use_cases/slider.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'test_utils.dart';
void main() {
testWidgets('slider can run', (WidgetTester tester) async {
await pumpsUseCase(tester, SliderUseCase());
expect(find.byType(Slider), findsOneWidget);
await tester.tapAt(tester.getCenter(find.byType(Slider)));
await tester.pumpAndSettle();
final MainWidgetState state = tester.state<MainWidgetState>(find.byType(MainWidget));
expect(state.currentSliderValue, 60);
});
}
| flutter/dev/a11y_assessments/test/slider_test.dart/0 | {
"file_path": "flutter/dev/a11y_assessments/test/slider_test.dart",
"repo_id": "flutter",
"token_count": 256
} | 521 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This test is a use case of flutter/flutter#60796
// the test should be run as:
// flutter drive -t test/using_array.dart --driver test_driver/scrolling_test_e2e_test.dart
import 'package:complex_layout/main.dart' as app;
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
/// Generates the [PointerEvent] to simulate a drag operation from
/// `center - totalMove/2` to `center + totalMove/2`.
Iterable<PointerEvent> dragInputEvents(
final Duration epoch,
final Offset center, {
final Offset totalMove = const Offset(0, -400),
final Duration totalTime = const Duration(milliseconds: 2000),
final double frequency = 90,
}) sync* {
final Offset startLocation = center - totalMove / 2;
// The issue is about 120Hz input on 90Hz refresh rate device.
// We test 90Hz input on 60Hz device here, which shows similar pattern.
final int moveEventCount = totalTime.inMicroseconds * frequency ~/ const Duration(seconds: 1).inMicroseconds;
final Offset movePerEvent = totalMove / moveEventCount.toDouble();
yield PointerAddedEvent(
timeStamp: epoch,
position: startLocation,
);
yield PointerDownEvent(
timeStamp: epoch,
position: startLocation,
pointer: 1,
);
for (int t = 0; t < moveEventCount + 1; t++) {
final Offset position = startLocation + movePerEvent * t.toDouble();
yield PointerMoveEvent(
timeStamp: epoch + totalTime * t ~/ moveEventCount,
position: position,
delta: movePerEvent,
pointer: 1,
);
}
final Offset position = startLocation + totalMove;
yield PointerUpEvent(
timeStamp: epoch + totalTime,
position: position,
pointer: 1,
);
}
enum TestScenario {
resampleOn90Hz,
resampleOn59Hz,
resampleOff90Hz,
resampleOff59Hz,
}
class ResampleFlagVariant extends TestVariant<TestScenario> {
ResampleFlagVariant(this.binding);
final IntegrationTestWidgetsFlutterBinding binding;
@override
final Set<TestScenario> values = Set<TestScenario>.from(TestScenario.values);
late TestScenario currentValue;
bool get resample {
return switch (currentValue) {
TestScenario.resampleOn90Hz || TestScenario.resampleOn59Hz => true,
TestScenario.resampleOff90Hz || TestScenario.resampleOff59Hz => false,
};
}
double get frequency {
return switch (currentValue) {
TestScenario.resampleOn90Hz || TestScenario.resampleOff90Hz => 90.0,
TestScenario.resampleOn59Hz || TestScenario.resampleOff59Hz => 59.0,
};
}
Map<String, dynamic>? result;
@override
String describeValue(TestScenario value) {
return switch (value) {
TestScenario.resampleOn90Hz => 'resample on with 90Hz input',
TestScenario.resampleOn59Hz => 'resample on with 59Hz input',
TestScenario.resampleOff90Hz => 'resample off with 90Hz input',
TestScenario.resampleOff59Hz => 'resample off with 59Hz input',
};
}
@override
Future<bool> setUp(TestScenario value) async {
currentValue = value;
final bool original = binding.resamplingEnabled;
binding.resamplingEnabled = resample;
return original;
}
@override
Future<void> tearDown(TestScenario value, bool memento) async {
binding.resamplingEnabled = memento;
binding.reportData![describeValue(value)] = result;
}
}
Future<void> main() async {
final WidgetsBinding widgetsBinding = IntegrationTestWidgetsFlutterBinding.ensureInitialized();
assert(widgetsBinding is IntegrationTestWidgetsFlutterBinding);
final IntegrationTestWidgetsFlutterBinding binding = widgetsBinding as IntegrationTestWidgetsFlutterBinding;
binding.framePolicy = LiveTestWidgetsFlutterBindingFramePolicy.benchmarkLive;
binding.reportData ??= <String, dynamic>{};
final ResampleFlagVariant variant = ResampleFlagVariant(binding);
testWidgets('Smoothness test', (WidgetTester tester) async {
app.main();
await tester.pumpAndSettle();
final Finder scrollerFinder = find.byKey(const ValueKey<String>('complex-scroll'));
final ListView scroller = tester.widget<ListView>(scrollerFinder);
final ScrollController? controller = scroller.controller;
final List<int> frameTimestamp = <int>[];
final List<double> scrollOffset = <double>[];
final List<Duration> delays = <Duration>[];
binding.addPersistentFrameCallback((Duration timeStamp) {
if (controller?.hasClients ?? false) {
// This if is necessary because by the end of the test the widget tree
// is destroyed.
frameTimestamp.add(timeStamp.inMicroseconds);
scrollOffset.add(controller!.offset);
}
});
Duration now() => binding.currentSystemFrameTimeStamp;
Future<void> scroll() async {
// Extra 50ms to avoid timeouts.
final Duration startTime = const Duration(milliseconds: 500) + now();
for (final PointerEvent event in dragInputEvents(
startTime,
tester.getCenter(scrollerFinder),
frequency: variant.frequency,
)) {
await tester.binding.delayed(event.timeStamp - now());
// This now measures how accurate the above delayed is.
final Duration delay = now() - event.timeStamp;
if (delays.length < frameTimestamp.length) {
while (delays.length < frameTimestamp.length - 1) {
delays.add(Duration.zero);
}
delays.add(delay);
} else if (delays.last < delay) {
delays.last = delay;
}
tester.binding.handlePointerEventForSource(event, source: TestBindingEventSource.test);
}
}
for (int n = 0; n < 5; n++) {
await scroll();
}
variant.result = scrollSummary(scrollOffset, delays, frameTimestamp);
await tester.pumpAndSettle();
scrollOffset.clear();
delays.clear();
await tester.idle();
}, semanticsEnabled: false, variant: variant);
}
/// Calculates the smoothness measure from `scrollOffset` and `delays` list.
///
/// Smoothness (`abs_jerk`) is measured by the absolute value of the discrete
/// 2nd derivative of the scroll offset.
///
/// It was experimented that jerk (3rd derivative of the position) is a good
/// measure the smoothness.
/// Here we are using 2nd derivative instead because the input is completely
/// linear and the expected acceleration should be strictly zero.
/// Observed acceleration is jumping from positive to negative within
/// adjacent frames, meaning mathematically the discrete 3-rd derivative
/// (`f[3] - 3*f[2] + 3*f[1] - f[0]`) is not a good approximation of jerk
/// (continuous 3-rd derivative), while discrete 2nd
/// derivative (`f[2] - 2*f[1] + f[0]`) on the other hand is a better measure
/// of how the scrolling deviate away from linear, and given the acceleration
/// should average to zero within two frames, it's also a good approximation
/// for jerk in terms of physics.
/// We use abs rather than square because square (2-norm) amplifies the
/// effect of the data point that's relatively large, but in this metric
/// we prefer smaller data point to have similar effect.
/// This is also why we count the number of data that's larger than a
/// threshold (and the result is tested not sensitive to this threshold),
/// which is effectively a 0-norm.
///
/// Frames that are too slow to build (longer than 40ms) or with input delay
/// longer than 16ms (1/60Hz) is filtered out to separate the janky due to slow
/// response.
///
/// The returned map has keys:
/// `average_abs_jerk`: average for the overall smoothness. The smaller this
/// number the more smooth the scrolling is.
/// `janky_count`: number of frames with `abs_jerk` larger than 0.5. The frames
/// that take longer than the frame budget to build are ignored, so increase of
/// this number itself may not represent a regression.
/// `dropped_frame_count`: number of frames that are built longer than 40ms and
/// are not used for smoothness measurement.
/// `frame_timestamp`: the list of the timestamp for each frame, in the time
/// order.
/// `scroll_offset`: the scroll offset for each frame. Its length is the same as
/// `frame_timestamp`.
/// `input_delay`: the list of maximum delay time of the input simulation during
/// a frame. Its length is the same as `frame_timestamp`
Map<String, dynamic> scrollSummary(
List<double> scrollOffset,
List<Duration> delays,
List<int> frameTimestamp,
) {
double jankyCount = 0;
double absJerkAvg = 0;
int lostFrame = 0;
for (int i = 1; i < scrollOffset.length-1; i += 1) {
if (frameTimestamp[i+1] - frameTimestamp[i-1] > 40E3 ||
(i >= delays.length || delays[i] > const Duration(milliseconds: 16))) {
// filter data points from slow frame building or input simulation artifact
lostFrame += 1;
continue;
}
//
final double absJerk = (scrollOffset[i-1] + scrollOffset[i+1] - 2*scrollOffset[i]).abs();
absJerkAvg += absJerk;
if (absJerk > 0.5) {
jankyCount += 1;
}
}
// expect(lostFrame < 0.1 * frameTimestamp.length, true);
absJerkAvg /= frameTimestamp.length - lostFrame;
return <String, dynamic>{
'janky_count': jankyCount,
'average_abs_jerk': absJerkAvg,
'dropped_frame_count': lostFrame,
'frame_timestamp': List<int>.from(frameTimestamp),
'scroll_offset': List<double>.from(scrollOffset),
'input_delay': delays.map<int>((Duration data) => data.inMicroseconds).toList(),
};
}
| flutter/dev/benchmarks/complex_layout/test/measure_scroll_smoothness.dart/0 | {
"file_path": "flutter/dev/benchmarks/complex_layout/test/measure_scroll_smoothness.dart",
"repo_id": "flutter",
"token_count": 3165
} | 522 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class CullOpacityPage extends StatefulWidget {
const CullOpacityPage({super.key});
@override
State<StatefulWidget> createState() => _CullOpacityPageState();
}
class _CullOpacityPageState extends State<CullOpacityPage> with SingleTickerProviderStateMixin {
late Animation<double> _offsetY;
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(vsync: this, duration: const Duration(seconds: 2));
// Animations are typically implemented using the AnimatedBuilder widget.
// This code uses a manual listener for historical reasons and will remain
// in order to preserve compatibility with the history of measurements for
// this benchmark.
_offsetY = Tween<double>(begin: 0, end: -1000.0).animate(_controller)..addListener(() {
setState(() {});
});
_controller.repeat();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Stack(children: List<Widget>.generate(50, (int i) => Positioned(
left: 0,
top: (200 * i).toDouble() + _offsetY.value,
child: Opacity(
opacity: 0.5,
child: RepaintBoundary(
child: Container(
// Slightly change width to invalidate raster cache.
width: 1000 - (_offsetY.value / 100),
height: 100, color: Colors.red,
),
),
),
)));
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/cull_opacity.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/cull_opacity.dart",
"repo_id": "flutter",
"token_count": 587
} | 523 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/material.dart';
class PostBackdropFilterPage extends StatefulWidget {
const PostBackdropFilterPage({super.key});
@override
State<PostBackdropFilterPage> createState() => _PostBackdropFilterPageState();
}
class _PostBackdropFilterPageState extends State<PostBackdropFilterPage> with TickerProviderStateMixin {
bool _includeBackdropFilter = false;
late AnimationController animation;
@override
void initState() {
super.initState();
animation = AnimationController(vsync: this, duration: const Duration(seconds: 1));
}
@override
void dispose() {
animation.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
Widget getConditionalBackdrop() {
if (_includeBackdropFilter) {
return Column(
children: <Widget>[
const SizedBox(height: 20),
ClipRect(
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: 10.0, sigmaY: 10.0),
child: const Text('BackdropFilter'),
),
),
const SizedBox(height: 20),
],
);
} else {
return const SizedBox(height: 20);
}
}
return Scaffold(
backgroundColor: Colors.grey,
body: Stack(
children: <Widget>[
Text('0' * 10000, style: const TextStyle(color: Colors.yellow)),
Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: RepaintBoundary(
child: Center(
child: AnimatedBuilder(
animation: animation,
builder: (BuildContext c, Widget? w) {
final int val = (animation.value * 255).round();
return Container(
width: 50,
height: 50,
color: Color.fromARGB(255, val, val, val));
}),
)),
),
getConditionalBackdrop(),
RepaintBoundary(
child: ColoredBox(
color: Colors.white,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Include BackdropFilter:'),
Checkbox(
key: const Key('bdf-checkbox'), // this key is used by the driver test
value: _includeBackdropFilter,
onChanged: (bool? v) => setState(() { _includeBackdropFilter = v ?? false; }),
),
MaterialButton(
key: const Key('bdf-animate'), // this key is used by the driver test
child: const Text('Animate'),
onPressed: () => setState(() { animation.repeat(); }),
),
],
),
),
),
],
),
],
),
);
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/post_backdrop_filter.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/post_backdrop_filter.dart",
"repo_id": "flutter",
"token_count": 1748
} | 524 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'recorder.dart';
import 'test_data.dart';
/// The height of each row.
const double kRowHeight = 20.0;
/// Number of rows.
const int kRows = 100;
/// Number of columns.
const int kColumns = 10;
/// The amount the picture is scrolled on every iteration of the benchmark.
const double kScrollDelta = 2.0;
/// Draws one complex picture, then moves a clip around it simulating scrolling
/// large static content.
///
/// This benchmark measures how efficient we are at taking advantage of the
/// static picture when all that changes is the clip.
///
/// See also:
///
/// * `bench_text_out_of_picture_bounds.dart`, which measures a volatile
/// picture with a static clip.
/// * https://github.com/flutter/flutter/issues/42987, which this benchmark is
/// based on.
class BenchDynamicClipOnStaticPicture extends SceneBuilderRecorder {
BenchDynamicClipOnStaticPicture() : super(name: benchmarkName) {
// If the scrollable extent is too small, the benchmark may end up
// scrolling the picture out of the clip area entirely, resulting in
// bogus metric values.
const double maxScrollExtent = kDefaultTotalSampleCount * kScrollDelta;
const double pictureHeight = kRows * kRowHeight;
if (maxScrollExtent > pictureHeight) {
throw Exception(
'Bad combination of constant values kRowHeight, kRows, and '
'kScrollData. With these numbers there is risk that the picture '
'will scroll out of the clip entirely. To fix the issue reduce '
'kScrollDelta, or increase either kRows or kRowHeight.'
);
}
// Create one static picture, then never change it again.
const Color black = Color.fromARGB(255, 0, 0, 0);
final PictureRecorder pictureRecorder = PictureRecorder();
final Canvas canvas = Canvas(pictureRecorder);
viewSize = view.physicalSize / view.devicePixelRatio;
clipSize = Size(
viewSize.width / 2,
viewSize.height / 5,
);
final double cellWidth = viewSize.width / kColumns;
final List<Paragraph> paragraphs = generateLaidOutParagraphs(
paragraphCount: 500,
minWordCountPerParagraph: 3,
maxWordCountPerParagraph: 3,
widthConstraint: cellWidth,
color: black,
);
int paragraphCounter = 0;
double yOffset = 0.0;
for (int row = 0; row < kRows; row += 1) {
for (int column = 0; column < kColumns; column += 1) {
final double left = cellWidth * column;
canvas.save();
canvas.clipRect(Rect.fromLTWH(
left,
yOffset,
cellWidth,
20.0,
));
canvas.drawParagraph(
paragraphs[paragraphCounter % paragraphs.length],
Offset(left, yOffset),
);
canvas.restore();
paragraphCounter += 1;
}
yOffset += kRowHeight;
}
picture = pictureRecorder.endRecording();
}
static const String benchmarkName = 'dynamic_clip_on_static_picture';
late Size viewSize;
late Size clipSize;
late Picture picture;
double pictureVerticalOffset = 0.0;
@override
void onDrawFrame(SceneBuilder sceneBuilder) {
// Render the exact same picture, but offset it as if it's being scrolled.
// This will move the clip along the Y axis in picture's local coordinates
// causing a repaint. If we're not efficient at managing clips and/or
// repaints this will jank (see https://github.com/flutter/flutter/issues/42987).
final Rect clip = Rect.fromLTWH(0.0, 0.0, clipSize.width, clipSize.height);
sceneBuilder.pushClipRect(clip);
sceneBuilder.pushOffset(0.0, pictureVerticalOffset);
sceneBuilder.addPicture(Offset.zero, picture);
sceneBuilder.pop();
sceneBuilder.pop();
pictureVerticalOffset -= kScrollDelta;
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/web/bench_dynamic_clip_on_static_picture.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/web/bench_dynamic_clip_on_static_picture.dart",
"repo_id": "flutter",
"token_count": 1344
} | 525 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'recorder.dart';
/// Creates a [Wrap] inside a ListView.
///
/// Tests large number of DOM nodes since image breaks up large canvas.
class BenchWrapBoxScroll extends WidgetRecorder {
BenchWrapBoxScroll() : super(name: benchmarkName);
static const String benchmarkName = 'bench_wrapbox_scroll';
@override
Widget createWidget() {
return MaterialApp(
theme: ThemeData(
primarySwatch: Colors.blue,
),
title: 'WrapBox Scroll Benchmark',
home: const Scaffold(body: MyHomePage()),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
late ScrollController scrollController;
int block = 0;
static const Duration stepDuration = Duration(milliseconds: 500);
static const double stepDistance = 400;
@override
void initState() {
super.initState();
scrollController = ScrollController();
// Without the timer the animation doesn't begin.
Timer.run(() async {
while (block < 25) {
await scrollController.animateTo((block % 5) * stepDistance,
duration: stepDuration, curve: Curves.easeInOut);
block++;
}
});
}
@override
void dispose() {
scrollController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return ListView(
controller: scrollController,
children: <Widget>[
Wrap(
children: <Widget>[
for (int i = 0; i < 30; i++)
FractionallySizedBox(
widthFactor: 0.2,
child: ProductPreview(i)), //need case1
for (int i = 0; i < 30; i++) ProductPreview(i), //need case2
],
),
]);
}
}
class ProductPreview extends StatelessWidget {
const ProductPreview(this.previewIndex, {super.key});
final int previewIndex;
@override
Widget build(BuildContext context) {
return GestureDetector(
behavior: HitTestBehavior.translucent,
onTap: () => print('tap'),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Container(
margin: const EdgeInsets.all(23),
padding: const EdgeInsets.all(18),
decoration: const BoxDecoration(
color: Color(0xfff9f9f9),
shape: BoxShape.circle,
),
child: Image.network(
'assets/assets/Icon-192.png',
width: 100,
height: 100,
),
),
const Text(
'title',
),
const SizedBox(
height: 14,
),
Wrap(
alignment: WrapAlignment.center,
children: <Widget>[
ProductOption(
optionText: '$previewIndex: option1',
),
ProductOption(
optionText: '$previewIndex: option2',
),
ProductOption(
optionText: '$previewIndex: option3',
),
ProductOption(
optionText: '$previewIndex: option4',
),
ProductOption(
optionText: '$previewIndex: option5',
),
],
),
],
),
);
}
}
class ProductOption extends StatelessWidget {
const ProductOption({
super.key,
required this.optionText,
});
final String optionText;
@override
Widget build(BuildContext context) {
return Container(
constraints: const BoxConstraints(minWidth: 56),
margin: const EdgeInsets.all(2),
padding: const EdgeInsets.symmetric(horizontal: 11, vertical: 5),
decoration: BoxDecoration(
border: Border.all(
color: const Color(0xffebebeb),
),
borderRadius: const BorderRadius.all(Radius.circular(15)),
),
child: Text(
optionText,
maxLines: 1,
textAlign: TextAlign.center,
overflow: TextOverflow.ellipsis,
),
);
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/web/bench_wrapbox_scroll.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/web/bench_wrapbox_scroll.dart",
"repo_id": "flutter",
"token_count": 1958
} | 526 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:macrobenchmarks/common.dart';
import 'util.dart';
void main() {
macroPerfTest(
'picture_cache_perf',
kPictureCacheRouteName,
pageDelay: const Duration(seconds: 1),
driverOps: (FlutterDriver driver) async {
final SerializableFinder tabBarView = find.byValueKey('tabbar_view');
Future<void> scrollOnce(double offset) async {
// Technically it's not scrolling but moving
await driver.scroll(tabBarView, offset, 0.0, const Duration(milliseconds: 300));
await Future<void>.delayed(const Duration(milliseconds: 500));
}
for (int i = 0; i < 3; i += 1) {
await scrollOnce(-300.0);
await scrollOnce(-300.0);
await scrollOnce(300.0);
await scrollOnce(300.0);
}
},
);
}
| flutter/dev/benchmarks/macrobenchmarks/test_driver/picture_cache_perf_test.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/test_driver/picture_cache_perf_test.dart",
"repo_id": "flutter",
"token_count": 377
} | 527 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/animation.dart';
import '../common.dart';
const int _kNumIters = 10000;
void _testCurve(Curve curve, {required String name, required String description, required BenchmarkResultPrinter printer}) {
final Stopwatch watch = Stopwatch();
print('$description benchmark...');
watch.start();
for (int i = 0; i < _kNumIters; i += 1) {
final double t = i / _kNumIters.toDouble();
curve.transform(t);
}
watch.stop();
printer.addResult(
description: description,
value: watch.elapsedMicroseconds / _kNumIters,
unit: 'µs per iteration',
name: name,
);
}
void main() {
assert(false, "Don't run benchmarks in debug mode! Use 'flutter run --release'.");
final BenchmarkResultPrinter printer = BenchmarkResultPrinter();
_testCurve(
const Cubic(0.0, 0.25, 0.5, 1.0),
name: 'cubic_animation_transform_iteration',
description: 'Cubic animation transform',
printer: printer,
);
final CatmullRomCurve catmullRomCurve = CatmullRomCurve(const <Offset>[
Offset(0.09, 0.99),
Offset(0.21, 0.01),
Offset(0.28, 0.99),
Offset(0.38, -0.00),
Offset(0.43, 0.99),
Offset(0.54, -0.01),
Offset(0.59, 0.98),
Offset(0.70, 0.04),
Offset(0.78, 0.98),
Offset(0.88, -0.00),
]);
_testCurve(
catmullRomCurve,
name: 'catmullrom_transform_iteration',
description: 'CatmullRomCurve animation transform',
printer: printer,
);
printer.printToStdout();
}
| flutter/dev/benchmarks/microbenchmarks/lib/geometry/curves_bench.dart/0 | {
"file_path": "flutter/dev/benchmarks/microbenchmarks/lib/geometry/curves_bench.dart",
"repo_id": "flutter",
"token_count": 630
} | 528 |
# Project-wide Gradle settings.
# IDE (e.g. Android Studio) users:
# Gradle settings configured through the IDE *will override*
# any settings specified in this file.
# For more details on how to configure your build environment visit
# http://www.gradle.org/docs/current/userguide/build_environment.html
# Specifies the JVM arguments used for the daemon process.
# The setting is particularly useful for tweaking memory settings.
org.gradle.jvmargs=-Xmx4G -XX:MaxMetaspaceSize=2G -XX:+HeapDumpOnOutOfMemoryError -Dfile.encoding=UTF-8
# When configured, Gradle will run in incubating parallel mode.
# This option should only be used with decoupled projects. More details, visit
# http://www.gradle.org/docs/current/userguide/multi_project_builds.html#sec:decoupled_projects
# org.gradle.parallel=true
# AndroidX package structure to make it clearer which packages are bundled with the
# Android operating system, and which are packaged with your app"s APK
# https://developer.android.com/topic/libraries/support-library/androidx-rn
android.useAndroidX=true
# Automatically convert third-party libraries to use AndroidX
android.enableJetifier=true
# Kotlin code style for this project: "official" or "obsolete":
kotlin.code.style=official
| flutter/dev/benchmarks/multiple_flutters/android/gradle.properties/0 | {
"file_path": "flutter/dev/benchmarks/multiple_flutters/android/gradle.properties",
"repo_id": "flutter",
"token_count": 346
} | 529 |
# platform_views_layout
## Scrolling benchmark
To run the scrolling benchmark on a device:
```
flutter drive --profile test_driver/scroll_perf.dart
```
Results should be in the file `build/platform_views_scroll_perf.timeline_summary.json`.
More detailed logs should be in `build/platform_views_scroll_perf.timeline.json`.
## Startup benchmark
To measure startup time on a device:
```
flutter run --profile --trace-startup
```
The results should be in the logs.
Additional results should be in the file `build/start_up_info.json`.
| flutter/dev/benchmarks/platform_views_layout/README.md/0 | {
"file_path": "flutter/dev/benchmarks/platform_views_layout/README.md",
"repo_id": "flutter",
"token_count": 163
} | 530 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
class AndroidPlatformView extends StatelessWidget {
/// Creates a platform view for Android, which is rendered as a
/// native view.
const AndroidPlatformView({
super.key,
required this.viewType,
});
/// The unique identifier for the view type to be embedded by this widget.
///
/// A PlatformViewFactory for this type must have been registered.
final String viewType;
@override
Widget build(BuildContext context) {
return PlatformViewLink(
viewType: viewType,
surfaceFactory:
(BuildContext context, PlatformViewController controller) {
return AndroidViewSurface(
controller: controller as AndroidViewController,
gestureRecognizers: const <Factory<OneSequenceGestureRecognizer>>{},
hitTestBehavior: PlatformViewHitTestBehavior.opaque,
);
},
onCreatePlatformView: (PlatformViewCreationParams params) {
return PlatformViewsService.initExpensiveAndroidView(
id: params.id,
viewType: viewType,
layoutDirection: TextDirection.ltr,
creationParamsCodec: const StandardMessageCodec(),
)
..addOnPlatformViewCreatedListener(params.onPlatformViewCreated)
..create();
},
);
}
}
| flutter/dev/benchmarks/platform_views_layout_hybrid_composition/lib/android_platform_view.dart/0 | {
"file_path": "flutter/dev/benchmarks/platform_views_layout_hybrid_composition/lib/android_platform_view.dart",
"repo_id": "flutter",
"token_count": 563
} | 531 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'stock_arrow.dart';
import 'stock_data.dart';
typedef StockRowActionCallback = void Function(Stock stock);
class StockRow extends StatelessWidget {
StockRow({
required this.stock,
this.onPressed,
this.onDoubleTap,
this.onLongPressed,
}) : super(key: ObjectKey(stock));
final Stock stock;
final StockRowActionCallback? onPressed;
final StockRowActionCallback? onDoubleTap;
final StockRowActionCallback? onLongPressed;
static const double kHeight = 79.0;
GestureTapCallback? _getHandler(StockRowActionCallback? callback) {
return callback == null ? null : () => callback(stock);
}
@override
Widget build(BuildContext context) {
final String lastSale = '\$${stock.lastSale.toStringAsFixed(2)}';
String changeInPrice = '${stock.percentChange.toStringAsFixed(2)}%';
if (stock.percentChange > 0) {
changeInPrice = '+$changeInPrice';
}
return InkWell(
key: ValueKey<String>(stock.symbol),
onTap: _getHandler(onPressed),
onDoubleTap: _getHandler(onDoubleTap),
onLongPress: _getHandler(onLongPressed),
child: Container(
padding: const EdgeInsets.fromLTRB(16.0, 16.0, 16.0, 20.0),
decoration: BoxDecoration(
border: Border(
bottom: BorderSide(color: Theme.of(context).dividerColor)
)
),
child: Row(
children: <Widget>[
Container(
margin: const EdgeInsets.only(right: 5.0),
child: Hero(
tag: stock,
child: StockArrow(percentChange: stock.percentChange),
),
),
Expanded(
child: Row(
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: DefaultTextStyle.of(context).style.textBaseline,
children: <Widget>[
Expanded(
flex: 2,
child: Text(
stock.symbol
),
),
Expanded(
child: Text(
lastSale,
textAlign: TextAlign.right,
),
),
Expanded(
child: Text(
changeInPrice,
textAlign: TextAlign.right,
),
),
],
),
),
],
),
),
);
}
}
| flutter/dev/benchmarks/test_apps/stocks/lib/stock_row.dart/0 | {
"file_path": "flutter/dev/benchmarks/test_apps/stocks/lib/stock_row.dart",
"repo_id": "flutter",
"token_count": 1350
} | 532 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:core' hide print;
import 'dart:io' hide exit;
import 'dart:typed_data';
import 'package:analyzer/dart/analysis/features.dart';
import 'package:analyzer/dart/analysis/results.dart';
import 'package:analyzer/dart/analysis/utilities.dart';
import 'package:analyzer/dart/ast/ast.dart';
import 'package:analyzer/dart/ast/visitor.dart';
import 'package:crypto/crypto.dart';
import 'package:meta/meta.dart';
import 'package:path/path.dart' as path;
import 'allowlist.dart';
import 'custom_rules/analyze.dart';
import 'custom_rules/avoid_future_catcherror.dart';
import 'custom_rules/no_double_clamp.dart';
import 'custom_rules/no_stop_watches.dart';
import 'run_command.dart';
import 'utils.dart';
final String flutterRoot = path.dirname(path.dirname(path.dirname(path.fromUri(Platform.script))));
final String flutter = path.join(flutterRoot, 'bin', Platform.isWindows ? 'flutter.bat' : 'flutter');
final String flutterPackages = path.join(flutterRoot, 'packages');
final String flutterExamples = path.join(flutterRoot, 'examples');
/// The path to the `dart` executable; set at the top of `main`
late final String dart;
/// The path to the `pub` executable; set at the top of `main`
late final String pub;
/// When you call this, you can pass additional arguments to pass custom
/// arguments to flutter analyze. For example, you might want to call this
/// script with the parameter --dart-sdk to use custom dart sdk.
///
/// For example:
/// bin/cache/dart-sdk/bin/dart dev/bots/analyze.dart --dart-sdk=/tmp/dart-sdk
Future<void> main(List<String> arguments) async {
final String dartSdk = path.join(
Directory.current.absolute.path,
_getDartSdkFromArguments(arguments) ?? path.join(flutterRoot, 'bin', 'cache', 'dart-sdk'),
);
dart = path.join(dartSdk, 'bin', Platform.isWindows ? 'dart.exe' : 'dart');
pub = path.join(dartSdk, 'bin', Platform.isWindows ? 'pub.bat' : 'pub');
printProgress('STARTING ANALYSIS');
await run(arguments);
if (hasError) {
reportErrorsAndExit('${bold}Analysis failed.$reset');
}
reportSuccessAndExit('${bold}Analysis successful.$reset');
}
/// Scans [arguments] for an argument of the form `--dart-sdk` or
/// `--dart-sdk=...` and returns the configured SDK, if any.
String? _getDartSdkFromArguments(List<String> arguments) {
String? result;
for (int i = 0; i < arguments.length; i += 1) {
if (arguments[i] == '--dart-sdk') {
if (result != null) {
foundError(<String>['The --dart-sdk argument must not be used more than once.']);
return null;
}
if (i + 1 < arguments.length) {
result = arguments[i + 1];
} else {
foundError(<String>['--dart-sdk must be followed by a path.']);
return null;
}
}
if (arguments[i].startsWith('--dart-sdk=')) {
if (result != null) {
foundError(<String>['The --dart-sdk argument must not be used more than once.']);
return null;
}
result = arguments[i].substring('--dart-sdk='.length);
}
}
return result;
}
Future<void> run(List<String> arguments) async {
bool assertsEnabled = false;
assert(() { assertsEnabled = true; return true; }());
if (!assertsEnabled) {
foundError(<String>['The analyze.dart script must be run with --enable-asserts.']);
}
printProgress('TargetPlatform tool/framework consistency');
await verifyTargetPlatform(flutterRoot);
printProgress('All tool test files end in _test.dart...');
await verifyToolTestsEndInTestDart(flutterRoot);
printProgress('No sync*/async*');
await verifyNoSyncAsyncStar(flutterPackages);
await verifyNoSyncAsyncStar(flutterExamples, minimumMatches: 200);
printProgress('No runtimeType in toString...');
await verifyNoRuntimeTypeInToString(flutterRoot);
printProgress('Debug mode instead of checked mode...');
await verifyNoCheckedMode(flutterRoot);
printProgress('Links for creating GitHub issues');
await verifyIssueLinks(flutterRoot);
printProgress('Unexpected binaries...');
await verifyNoBinaries(flutterRoot);
printProgress('Trailing spaces...');
await verifyNoTrailingSpaces(flutterRoot); // assumes no unexpected binaries, so should be after verifyNoBinaries
printProgress('Spaces after flow control statements...');
await verifySpacesAfterFlowControlStatements(flutterRoot);
printProgress('Deprecations...');
await verifyDeprecations(flutterRoot);
printProgress('Goldens...');
await verifyGoldenTags(flutterPackages);
printProgress('Skip test comments...');
await verifySkipTestComments(flutterRoot);
printProgress('Licenses...');
await verifyNoMissingLicense(flutterRoot);
printProgress('Test imports...');
await verifyNoTestImports(flutterRoot);
printProgress('Bad imports (framework)...');
await verifyNoBadImportsInFlutter(flutterRoot);
printProgress('Bad imports (tools)...');
await verifyNoBadImportsInFlutterTools(flutterRoot);
printProgress('Internationalization...');
await verifyInternationalizations(flutterRoot, dart);
printProgress('Integration test timeouts...');
await verifyIntegrationTestTimeouts(flutterRoot);
printProgress('null initialized debug fields...');
await verifyNullInitializedDebugExpensiveFields(flutterRoot);
printProgress('Taboo words...');
await verifyTabooDocumentation(flutterRoot);
// Ensure that all package dependencies are in sync.
printProgress('Package dependencies...');
await runCommand(flutter, <String>['update-packages', '--verify-only'],
workingDirectory: flutterRoot,
);
/// Ensure that no new dependencies have been accidentally
/// added to core packages.
printProgress('Package Allowlist...');
await _checkConsumerDependencies();
// Analyze all the Dart code in the repo.
printProgress('Dart analysis...');
final CommandResult dartAnalyzeResult = await _runFlutterAnalyze(flutterRoot, options: <String>[
'--flutter-repo',
...arguments,
]);
if (dartAnalyzeResult.exitCode == 0) {
// Only run the private lints when the code is free of type errors. The
// lints are easier to write when they can assume, for example, there is no
// inheritance cycles.
final List<AnalyzeRule> rules = <AnalyzeRule>[noDoubleClamp, noStopwatches];
final String ruleNames = rules.map((AnalyzeRule rule) => '\n * $rule').join();
printProgress('Analyzing code in the framework with the following rules:$ruleNames');
await analyzeWithRules(flutterRoot, rules,
includePaths: <String>['packages/flutter/lib'],
excludePaths: <String>['packages/flutter/lib/fix_data'],
);
final List<AnalyzeRule> testRules = <AnalyzeRule>[noStopwatches];
final String testRuleNames = testRules.map((AnalyzeRule rule) => '\n * $rule').join();
printProgress('Analyzing code in the test folder with the following rules:$testRuleNames');
await analyzeWithRules(flutterRoot, testRules,
includePaths: <String>['packages/flutter/test'],
);
final List<AnalyzeRule> toolRules = <AnalyzeRule>[AvoidFutureCatchError()];
final String toolRuleNames = toolRules.map((AnalyzeRule rule) => '\n * $rule').join();
printProgress('Analyzing code in the tool with the following rules:$toolRuleNames');
await analyzeToolWithRules(flutterRoot, toolRules);
} else {
printProgress('Skipped performing further analysis in the framework because "flutter analyze" finished with a non-zero exit code.');
}
printProgress('Executable allowlist...');
await _checkForNewExecutables();
// Try with the --watch analyzer, to make sure it returns success also.
// The --benchmark argument exits after one run.
// We specify a failureMessage so that the actual output is muted in the case where _runFlutterAnalyze above already failed.
printProgress('Dart analysis (with --watch)...');
await _runFlutterAnalyze(flutterRoot, failureMessage: 'Dart analyzer failed when --watch was used.', options: <String>[
'--flutter-repo',
'--watch',
'--benchmark',
...arguments,
]);
// Analyze the code in `{@tool snippet}` sections in the repo.
printProgress('Snippet code...');
await runCommand(dart,
<String>['--enable-asserts', path.join(flutterRoot, 'dev', 'bots', 'analyze_snippet_code.dart'), '--verbose'],
workingDirectory: flutterRoot,
);
// Make sure that all of the existing samples are linked from at least one API doc comment.
printProgress('Code sample link validation...');
await runCommand(dart,
<String>['--enable-asserts', path.join(flutterRoot, 'dev', 'bots', 'check_code_samples.dart')],
workingDirectory: flutterRoot,
);
// Try analysis against a big version of the gallery; generate into a temporary directory.
printProgress('Dart analysis (mega gallery)...');
final Directory outDir = Directory.systemTemp.createTempSync('flutter_mega_gallery.');
try {
await runCommand(dart,
<String>[
path.join(flutterRoot, 'dev', 'tools', 'mega_gallery.dart'),
'--out',
outDir.path,
],
workingDirectory: flutterRoot,
);
await _runFlutterAnalyze(outDir.path, failureMessage: 'Dart analyzer failed on mega_gallery benchmark.', options: <String>[
'--watch',
'--benchmark',
...arguments,
]);
} finally {
outDir.deleteSync(recursive: true);
}
// Ensure gen_default links the correct files
printProgress('Correct file names in gen_defaults.dart...');
await verifyTokenTemplatesUpdateCorrectFiles(flutterRoot);
// Ensure integration test files are up-to-date with the app template.
printProgress('Up to date integration test template files...');
await verifyIntegrationTestTemplateFiles(flutterRoot);
}
// TESTS
FeatureSet _parsingFeatureSet() => FeatureSet.latestLanguageVersion();
_Line _getLine(ParseStringResult parseResult, int offset) {
final int lineNumber = parseResult.lineInfo.getLocation(offset).lineNumber;
final String content = parseResult.content.substring(
parseResult.lineInfo.getOffsetOfLine(lineNumber - 1),
parseResult.lineInfo.getOffsetOfLine(lineNumber) - 1,
);
return _Line(lineNumber, content);
}
Future<void> verifyTargetPlatform(String workingDirectory) async {
final File framework = File('$workingDirectory/packages/flutter/lib/src/foundation/platform.dart');
final Set<String> frameworkPlatforms = <String>{};
List<String> lines = framework.readAsLinesSync();
int index = 0;
while (true) {
if (index >= lines.length) {
foundError(<String>['${framework.path}: Can no longer find TargetPlatform enum.']);
return;
}
if (lines[index].startsWith('enum TargetPlatform {')) {
index += 1;
break;
}
index += 1;
}
while (true) {
if (index >= lines.length) {
foundError(<String>['${framework.path}: Could not find end of TargetPlatform enum.']);
return;
}
String line = lines[index].trim();
final int comment = line.indexOf('//');
if (comment >= 0) {
line = line.substring(0, comment);
}
if (line == '}') {
break;
}
if (line.isNotEmpty) {
if (line.endsWith(',')) {
frameworkPlatforms.add(line.substring(0, line.length - 1));
} else {
foundError(<String>['${framework.path}:$index: unparseable line when looking for TargetPlatform values']);
}
}
index += 1;
}
final File tool = File('$workingDirectory/packages/flutter_tools/lib/src/resident_runner.dart');
final Set<String> toolPlatforms = <String>{};
lines = tool.readAsLinesSync();
index = 0;
while (true) {
if (index >= lines.length) {
foundError(<String>['${tool.path}: Can no longer find nextPlatform logic.']);
return;
}
if (lines[index].trim().startsWith('const List<String> platforms = <String>[')) {
index += 1;
break;
}
index += 1;
}
while (true) {
if (index >= lines.length) {
foundError(<String>['${tool.path}: Could not find end of nextPlatform logic.']);
return;
}
final String line = lines[index].trim();
if (line.startsWith("'") && line.endsWith("',")) {
toolPlatforms.add(line.substring(1, line.length - 2));
} else if (line == '];') {
break;
} else {
foundError(<String>['${tool.path}:$index: unparseable line when looking for nextPlatform values']);
}
index += 1;
}
final Set<String> frameworkExtra = frameworkPlatforms.difference(toolPlatforms);
if (frameworkExtra.isNotEmpty) {
foundError(<String>['TargetPlatform has some extra values not found in the tool: ${frameworkExtra.join(", ")}']);
}
final Set<String> toolExtra = toolPlatforms.difference(frameworkPlatforms);
if (toolExtra.isNotEmpty) {
foundError(<String>['The nextPlatform logic in the tool has some extra values not found in TargetPlatform: ${toolExtra.join(", ")}']);
}
}
/// Verify Token Templates are mapped to correct file names while generating
/// M3 defaults in /dev/tools/gen_defaults/bin/gen_defaults.dart.
Future<void> verifyTokenTemplatesUpdateCorrectFiles(String workingDirectory) async {
final List<String> errors = <String>[];
String getMaterialDirPath(List<String> lines) {
final String line = lines.firstWhere((String line) => line.contains('String materialLib'));
final String relativePath = line.substring(line.indexOf("'") + 1, line.lastIndexOf("'"));
return path.join(workingDirectory, relativePath);
}
String getFileName(String line) {
const String materialLibString = r"'$materialLib/";
final String leftClamp = line.substring(line.indexOf(materialLibString) + materialLibString.length);
return leftClamp.substring(0, leftClamp.indexOf("'"));
}
final String genDefaultsBinDir = '$workingDirectory/dev/tools/gen_defaults/bin';
final File file = File(path.join(genDefaultsBinDir, 'gen_defaults.dart'));
final List<String> lines = file.readAsLinesSync();
final String materialDirPath = getMaterialDirPath(lines);
bool atLeastOneTargetLineExists = false;
for (final String line in lines) {
if (line.contains('updateFile();')) {
atLeastOneTargetLineExists = true;
final String fileName = getFileName(line);
final String filePath = path.join(materialDirPath, fileName);
final File file = File(filePath);
if (!file.existsSync()) {
errors.add('file $filePath does not exist.');
}
}
}
assert(atLeastOneTargetLineExists, 'No lines exist that this test expects to '
'verify. Check if the target file is correct or remove this test');
// Fail if any errors
if (errors.isNotEmpty) {
final String s = errors.length > 1 ? 's' : '';
final String itThem = errors.length > 1 ? 'them' : 'it';
foundError(<String>[
...errors,
'${bold}Please correct the file name$s or remove $itThem from /dev/tools/gen_defaults/bin/gen_defaults.dart$reset',
]);
}
}
/// Verify tool test files end in `_test.dart`.
///
/// The test runner will only recognize files ending in `_test.dart` as tests to
/// be run: https://github.com/dart-lang/test/tree/master/pkgs/test#running-tests
Future<void> verifyToolTestsEndInTestDart(String workingDirectory) async {
final String toolsTestPath = path.join(
workingDirectory,
'packages',
'flutter_tools',
'test',
);
final List<String> violations = <String>[];
// detect files that contains calls to test(), testUsingContext(), and testWithoutContext()
final RegExp callsTestFunctionPattern = RegExp(r'(test\(.*\)|testUsingContext\(.*\)|testWithoutContext\(.*\))');
await for (final File file in _allFiles(toolsTestPath, 'dart', minimumMatches: 300)) {
final bool isValidTestFile = file.path.endsWith('_test.dart');
if (isValidTestFile) {
continue;
}
final bool isTestData = file.path.contains(r'test_data');
if (isTestData) {
continue;
}
final bool isInTestShard = file.path.contains(r'.shard/');
if (!isInTestShard) {
continue;
}
final bool callsTestFunction = file.readAsStringSync().contains(callsTestFunctionPattern);
if (!callsTestFunction) {
continue;
}
violations.add(file.path);
}
if (violations.isNotEmpty) {
foundError(<String>[
'${bold}Found flutter_tools tests that do not end in `_test.dart`; these will not be run by the test runner$reset',
...violations,
]);
}
}
Future<void> verifyNoSyncAsyncStar(String workingDirectory, {int minimumMatches = 2000 }) async {
final RegExp syncPattern = RegExp(r'\s*?a?sync\*\s*?{');
final RegExp ignorePattern = RegExp(r'^\s*?// The following uses a?sync\* because:? ');
final RegExp commentPattern = RegExp(r'^\s*?//');
final List<String> errors = <String>[];
await for (final File file in _allFiles(workingDirectory, 'dart', minimumMatches: minimumMatches)) {
if (file.path.contains('test')) {
continue;
}
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index += 1) {
final String line = lines[index];
if (line.startsWith(commentPattern)) {
continue;
}
if (line.contains(syncPattern)) {
int lookBehindIndex = index - 1;
bool hasExplanation = false;
while (lookBehindIndex >= 0 && lines[lookBehindIndex].startsWith(commentPattern)) {
if (lines[lookBehindIndex].startsWith(ignorePattern)) {
hasExplanation = true;
break;
}
lookBehindIndex -= 1;
}
if (!hasExplanation) {
errors.add('${file.path}:$index: sync*/async* without an explanation.');
}
}
}
}
if (errors.isNotEmpty) {
foundError(<String>[
'${bold}Do not use sync*/async* methods. See https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#avoid-syncasync for details.$reset',
...errors,
]);
}
}
final RegExp _findGoldenTestPattern = RegExp(r'matchesGoldenFile\(');
final RegExp _findGoldenDefinitionPattern = RegExp(r'matchesGoldenFile\(Object');
final RegExp _leadingComment = RegExp(r'//');
final RegExp _goldenTagPattern1 = RegExp(r'@Tags\(');
final RegExp _goldenTagPattern2 = RegExp(r"'reduced-test-set'");
/// Only golden file tests in the flutter package are subject to reduced testing,
/// for example, invocations in flutter_test to validate comparator
/// functionality do not require tagging.
const String _ignoreGoldenTag = '// flutter_ignore: golden_tag (see analyze.dart)';
const String _ignoreGoldenTagForFile = '// flutter_ignore_for_file: golden_tag (see analyze.dart)';
Future<void> verifyGoldenTags(String workingDirectory, { int minimumMatches = 2000 }) async {
final List<String> errors = <String>[];
await for (final File file in _allFiles(workingDirectory, 'dart', minimumMatches: minimumMatches)) {
bool needsTag = false;
bool hasTagNotation = false;
bool hasReducedTag = false;
bool ignoreForFile = false;
final List<String> lines = file.readAsLinesSync();
for (final String line in lines) {
if (line.contains(_goldenTagPattern1)) {
hasTagNotation = true;
}
if (line.contains(_goldenTagPattern2)) {
hasReducedTag = true;
}
if (line.contains(_findGoldenTestPattern)
&& !line.contains(_findGoldenDefinitionPattern)
&& !line.contains(_leadingComment)
&& !line.contains(_ignoreGoldenTag)) {
needsTag = true;
}
if (line.contains(_ignoreGoldenTagForFile)) {
ignoreForFile = true;
}
// If the file is being ignored or a reduced test tag is already accounted
// for, skip parsing the rest of the lines for golden file tests.
if (ignoreForFile || (hasTagNotation && hasReducedTag)) {
break;
}
}
// If a reduced test tag is already accounted for, move on to the next file.
if (ignoreForFile || (hasTagNotation && hasReducedTag)) {
continue;
}
// If there are golden file tests, ensure they are tagged for all reduced
// test environments.
if (needsTag) {
if (!hasTagNotation) {
errors.add('${file.path}: Files containing golden tests must be tagged using '
"@Tags(<String>['reduced-test-set']) at the top of the file before import statements.");
} else if (!hasReducedTag) {
errors.add('${file.path}: Files containing golden tests must be tagged with '
"'reduced-test-set'.");
}
}
}
if (errors.isNotEmpty) {
foundError(<String>[
...errors,
'${bold}See: https://github.com/flutter/flutter/wiki/Writing-a-golden-file-test-for-package:flutter$reset',
]);
}
}
final RegExp _findDeprecationPattern = RegExp(r'@[Dd]eprecated');
final RegExp _deprecationStartPattern = RegExp(r'^(?<indent> *)@Deprecated\($'); // flutter_ignore: deprecation_syntax (see analyze.dart)
final RegExp _deprecationMessagePattern = RegExp(r"^ *'(?<message>.+) '$");
final RegExp _deprecationVersionPattern = RegExp(r"^ *'This feature was deprecated after v(?<major>\d+)\.(?<minor>\d+)\.(?<patch>\d+)(?<build>-\d+\.\d+\.pre)?\.',?$");
final RegExp _deprecationEndPattern = RegExp(r'^ *\)$');
/// Some deprecation notices are special, for example they're used to annotate members that
/// will never go away and were never allowed but which we are trying to show messages for.
/// (One example would be a library that intentionally conflicts with a member in another
/// library to indicate that it is incompatible with that other library. Another would be
/// the regexp just above...)
const String _ignoreDeprecation = ' // flutter_ignore: deprecation_syntax (see analyze.dart)';
/// Some deprecation notices are exempt for historical reasons. They must have an issue listed.
final RegExp _legacyDeprecation = RegExp(r' // flutter_ignore: deprecation_syntax, https://github.com/flutter/flutter/issues/\d+$');
Future<void> verifyDeprecations(String workingDirectory, { int minimumMatches = 2000 }) async {
final List<String> errors = <String>[];
await for (final File file in _allFiles(workingDirectory, 'dart', minimumMatches: minimumMatches)) {
int lineNumber = 0;
final List<String> lines = file.readAsLinesSync();
final List<int> linesWithDeprecations = <int>[];
for (final String line in lines) {
if (line.contains(_findDeprecationPattern) &&
!line.endsWith(_ignoreDeprecation) &&
!line.contains(_legacyDeprecation)) {
linesWithDeprecations.add(lineNumber);
}
lineNumber += 1;
}
for (int lineNumber in linesWithDeprecations) {
try {
final RegExpMatch? startMatch = _deprecationStartPattern.firstMatch(lines[lineNumber]);
if (startMatch == null) {
throw 'Deprecation notice does not match required pattern.';
}
final String indent = startMatch.namedGroup('indent')!;
lineNumber += 1;
if (lineNumber >= lines.length) {
throw 'Incomplete deprecation notice.';
}
RegExpMatch? versionMatch;
String? message;
do {
final RegExpMatch? messageMatch = _deprecationMessagePattern.firstMatch(lines[lineNumber]);
if (messageMatch == null) {
String possibleReason = '';
if (lines[lineNumber].trimLeft().startsWith('"')) {
possibleReason = ' You might have used double quotes (") for the string instead of single quotes (\').';
} else if (!lines[lineNumber].contains("'")) {
possibleReason = ' It might be missing the line saying "This feature was deprecated after...".';
} else if (!lines[lineNumber].trimRight().endsWith(" '")) {
if (lines[lineNumber].contains('This feature was deprecated')) {
possibleReason = ' There might not be an explanatory message.';
} else {
possibleReason = ' There might be a missing space character at the end of the line.';
}
}
throw 'Deprecation notice does not match required pattern.$possibleReason';
}
if (!lines[lineNumber].startsWith("$indent '")) {
throw 'Unexpected deprecation notice indent.';
}
if (message == null) {
message = messageMatch.namedGroup('message');
final String firstChar = String.fromCharCode(message!.runes.first);
if (firstChar.toUpperCase() != firstChar) {
throw 'Deprecation notice should be a grammatically correct sentence and start with a capital letter; see style guide: https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo';
}
} else {
message += messageMatch.namedGroup('message')!;
}
lineNumber += 1;
if (lineNumber >= lines.length) {
throw 'Incomplete deprecation notice.';
}
versionMatch = _deprecationVersionPattern.firstMatch(lines[lineNumber]);
} while (versionMatch == null);
final int major = int.parse(versionMatch.namedGroup('major')!);
final int minor = int.parse(versionMatch.namedGroup('minor')!);
final int patch = int.parse(versionMatch.namedGroup('patch')!);
final bool hasBuild = versionMatch.namedGroup('build') != null;
// There was a beta release that was mistakenly labeled 3.1.0 without a build.
final bool specialBeta = major == 3 && minor == 1 && patch == 0;
if (!specialBeta && (major > 1 || (major == 1 && minor >= 20))) {
if (!hasBuild) {
throw 'Deprecation notice does not accurately indicate a beta branch version number; please see https://flutter.dev/docs/development/tools/sdk/releases to find the latest beta build version number.';
}
}
if (!message.endsWith('.') && !message.endsWith('!') && !message.endsWith('?')) {
throw 'Deprecation notice should be a grammatically correct sentence and end with a period; notice appears to be "$message".';
}
if (!lines[lineNumber].startsWith("$indent '")) {
throw 'Unexpected deprecation notice indent.';
}
lineNumber += 1;
if (lineNumber >= lines.length) {
throw 'Incomplete deprecation notice.';
}
if (!lines[lineNumber].contains(_deprecationEndPattern)) {
throw 'End of deprecation notice does not match required pattern.';
}
if (!lines[lineNumber].startsWith('$indent)')) {
throw 'Unexpected deprecation notice indent.';
}
} catch (error) {
errors.add('${file.path}:${lineNumber + 1}: $error');
}
}
}
// Fail if any errors
if (errors.isNotEmpty) {
foundError(<String>[
...errors,
'${bold}See: https://github.com/flutter/flutter/wiki/Tree-hygiene#handling-breaking-changes$reset',
]);
}
}
String _generateLicense(String prefix) {
return '${prefix}Copyright 2014 The Flutter Authors. All rights reserved.\n'
'${prefix}Use of this source code is governed by a BSD-style license that can be\n'
'${prefix}found in the LICENSE file.';
}
Future<void> verifyNoMissingLicense(String workingDirectory, { bool checkMinimums = true }) async {
final int? overrideMinimumMatches = checkMinimums ? null : 0;
await _verifyNoMissingLicenseForExtension(workingDirectory, 'dart', overrideMinimumMatches ?? 2000, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'java', overrideMinimumMatches ?? 39, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'h', overrideMinimumMatches ?? 30, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'm', overrideMinimumMatches ?? 30, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'cc', overrideMinimumMatches ?? 10, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'cpp', overrideMinimumMatches ?? 0, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'swift', overrideMinimumMatches ?? 10, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'gradle', overrideMinimumMatches ?? 80, _generateLicense('// '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'gn', overrideMinimumMatches ?? 0, _generateLicense('# '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'sh', overrideMinimumMatches ?? 1, _generateLicense('# '), header: r'#!/usr/bin/env bash\n',);
await _verifyNoMissingLicenseForExtension(workingDirectory, 'bat', overrideMinimumMatches ?? 1, _generateLicense('REM '), header: r'@ECHO off\n');
await _verifyNoMissingLicenseForExtension(workingDirectory, 'ps1', overrideMinimumMatches ?? 1, _generateLicense('# '));
await _verifyNoMissingLicenseForExtension(workingDirectory, 'html', overrideMinimumMatches ?? 1, '<!-- ${_generateLicense('')} -->', trailingBlank: false, header: r'<!DOCTYPE HTML>\n');
await _verifyNoMissingLicenseForExtension(workingDirectory, 'xml', overrideMinimumMatches ?? 1, '<!-- ${_generateLicense('')} -->', header: r'(<\?xml version="1.0" encoding="utf-8"\?>\n)?');
await _verifyNoMissingLicenseForExtension(workingDirectory, 'frag', overrideMinimumMatches ?? 1, _generateLicense('// '), header: r'#version 320 es(\n)+');
}
Future<void> _verifyNoMissingLicenseForExtension(
String workingDirectory,
String extension,
int minimumMatches,
String license, {
bool trailingBlank = true,
// The "header" is a regular expression matching the header that comes before
// the license in some files.
String header = '',
}) async {
assert(!license.endsWith('\n'));
final String licensePattern = RegExp.escape('$license\n${trailingBlank ? '\n' : ''}');
final List<String> errors = <String>[];
await for (final File file in _allFiles(workingDirectory, extension, minimumMatches: minimumMatches)) {
final String contents = file.readAsStringSync().replaceAll('\r\n', '\n');
if (contents.isEmpty) {
continue; // let's not go down the /bin/true rabbit hole
}
if (!contents.startsWith(RegExp(header + licensePattern))) {
errors.add(file.path);
}
}
// Fail if any errors
if (errors.isNotEmpty) {
final String fileDoes = errors.length == 1 ? 'file does' : '${errors.length} files do';
foundError(<String>[
'${bold}The following $fileDoes not have the right license header for $extension files:$reset',
...errors.map<String>((String error) => ' $error'),
'The expected license header is:',
if (header.isNotEmpty) 'A header matching the regular expression "$header",',
if (header.isNotEmpty) 'followed by the following license text:',
license,
if (trailingBlank) '...followed by a blank line.',
]);
}
}
class _Line {
_Line(this.line, this.content);
final int line;
final String content;
}
Iterable<_Line> _getTestSkips(File file) {
final ParseStringResult parseResult = parseFile(
featureSet: _parsingFeatureSet(),
path: file.absolute.path,
);
final _TestSkipLinesVisitor<CompilationUnit> visitor = _TestSkipLinesVisitor<CompilationUnit>(parseResult);
visitor.visitCompilationUnit(parseResult.unit);
return visitor.skips;
}
class _TestSkipLinesVisitor<T> extends RecursiveAstVisitor<T> {
_TestSkipLinesVisitor(this.parseResult) : skips = <_Line>{};
final ParseStringResult parseResult;
final Set<_Line> skips;
static bool isTestMethod(String name) {
return name.startsWith('test') || name == 'group' || name == 'expect';
}
@override
T? visitMethodInvocation(MethodInvocation node) {
if (isTestMethod(node.methodName.toString())) {
for (final Expression argument in node.argumentList.arguments) {
if (argument is NamedExpression && argument.name.label.name == 'skip') {
skips.add(_getLine(parseResult, argument.beginToken.charOffset));
}
}
}
return super.visitMethodInvocation(node);
}
}
final RegExp _skipTestCommentPattern = RegExp(r'//(.*)$');
const Pattern _skipTestIntentionalPattern = '[intended]';
final Pattern _skipTestTrackingBugPattern = RegExp(r'https+?://github.com/.*/issues/\d+');
Future<void> verifySkipTestComments(String workingDirectory) async {
final List<String> errors = <String>[];
final Stream<File> testFiles =_allFiles(workingDirectory, 'dart', minimumMatches: 1500)
.where((File f) => f.path.endsWith('_test.dart'));
await for (final File file in testFiles) {
for (final _Line skip in _getTestSkips(file)) {
final Match? match = _skipTestCommentPattern.firstMatch(skip.content);
final String? skipComment = match?.group(1);
if (skipComment == null ||
!(skipComment.contains(_skipTestIntentionalPattern) ||
skipComment.contains(_skipTestTrackingBugPattern))) {
errors.add('${file.path}:${skip.line}: skip test without a justification comment.');
}
}
}
// Fail if any errors
if (errors.isNotEmpty) {
foundError(<String>[
...errors,
'\n${bold}See: https://github.com/flutter/flutter/wiki/Tree-hygiene#skipped-tests$reset',
]);
}
}
final RegExp _testImportPattern = RegExp(r'''import (['"])([^'"]+_test\.dart)\1''');
const Set<String> _exemptTestImports = <String>{
'package:flutter_test/flutter_test.dart',
'hit_test.dart',
'package:test_api/src/backend/live_test.dart',
'package:integration_test/integration_test.dart',
};
Future<void> verifyNoTestImports(String workingDirectory) async {
final List<String> errors = <String>[];
assert("// foo\nimport 'binding_test.dart' as binding;\n'".contains(_testImportPattern));
final List<File> dartFiles = await _allFiles(path.join(workingDirectory, 'packages'), 'dart', minimumMatches: 1500).toList();
for (final File file in dartFiles) {
for (final String line in file.readAsLinesSync()) {
final Match? match = _testImportPattern.firstMatch(line);
if (match != null && !_exemptTestImports.contains(match.group(2))) {
errors.add(file.path);
}
}
}
// Fail if any errors
if (errors.isNotEmpty) {
final String s = errors.length == 1 ? '' : 's';
foundError(<String>[
'${bold}The following file$s import a test directly. Test utilities should be in their own file.$reset',
...errors,
]);
}
}
Future<void> verifyNoBadImportsInFlutter(String workingDirectory) async {
final List<String> errors = <String>[];
final String libPath = path.join(workingDirectory, 'packages', 'flutter', 'lib');
final String srcPath = path.join(workingDirectory, 'packages', 'flutter', 'lib', 'src');
// Verify there's one libPath/*.dart for each srcPath/*/.
final List<String> packages = Directory(libPath).listSync()
.where((FileSystemEntity entity) => entity is File && path.extension(entity.path) == '.dart')
.map<String>((FileSystemEntity entity) => path.basenameWithoutExtension(entity.path))
.toList()..sort();
final List<String> directories = Directory(srcPath).listSync()
.whereType<Directory>()
.map<String>((Directory entity) => path.basename(entity.path))
.toList()..sort();
if (!_listEquals<String>(packages, directories)) {
errors.add(<String>[
'flutter/lib/*.dart does not match flutter/lib/src/*/:',
'These are the exported packages:',
...packages.map<String>((String path) => ' lib/$path.dart'),
'These are the directories:',
...directories.map<String>((String path) => ' lib/src/$path/'),
].join('\n'));
}
// Verify that the imports are well-ordered.
final Map<String, Set<String>> dependencyMap = <String, Set<String>>{};
for (final String directory in directories) {
dependencyMap[directory] = await _findFlutterDependencies(path.join(srcPath, directory), errors, checkForMeta: directory != 'foundation');
}
assert(dependencyMap['material']!.contains('widgets') &&
dependencyMap['widgets']!.contains('rendering') &&
dependencyMap['rendering']!.contains('painting')); // to make sure we're convinced _findFlutterDependencies is finding some
for (final String package in dependencyMap.keys) {
if (dependencyMap[package]!.contains(package)) {
errors.add(
'One of the files in the $yellow$package$reset package imports that package recursively.'
);
}
}
for (final String key in dependencyMap.keys) {
for (final String dependency in dependencyMap[key]!) {
if (dependencyMap[dependency] != null) {
continue;
}
// Sanity check before performing _deepSearch, to ensure there's no rogue
// dependencies.
final String validFilenames = dependencyMap.keys.map((String name) => '$name.dart').join(', ');
errors.add(
'$key imported package:flutter/$dependency.dart '
'which is not one of the valid exports { $validFilenames }.\n'
'Consider changing $dependency.dart to one of them.'
);
}
}
for (final String package in dependencyMap.keys) {
final List<String>? loop = _deepSearch<String>(dependencyMap, package);
if (loop != null) {
errors.add('${yellow}Dependency loop:$reset ${loop.join(' depends on ')}');
}
}
// Fail if any errors
if (errors.isNotEmpty) {
foundError(<String>[
if (errors.length == 1)
'${bold}An error was detected when looking at import dependencies within the Flutter package:$reset'
else
'${bold}Multiple errors were detected when looking at import dependencies within the Flutter package:$reset',
...errors,
]);
}
}
Future<void> verifyNoBadImportsInFlutterTools(String workingDirectory) async {
final List<String> errors = <String>[];
final List<File> files = await _allFiles(path.join(workingDirectory, 'packages', 'flutter_tools', 'lib'), 'dart', minimumMatches: 200).toList();
for (final File file in files) {
if (file.readAsStringSync().contains('package:flutter_tools/')) {
errors.add('$yellow${file.path}$reset imports flutter_tools.');
}
}
// Fail if any errors
if (errors.isNotEmpty) {
foundError(<String>[
if (errors.length == 1)
'${bold}An error was detected when looking at import dependencies within the flutter_tools package:$reset'
else
'${bold}Multiple errors were detected when looking at import dependencies within the flutter_tools package:$reset',
...errors.map((String paragraph) => '$paragraph\n'),
]);
}
}
Future<void> verifyIntegrationTestTimeouts(String workingDirectory) async {
final List<String> errors = <String>[];
final String dev = path.join(workingDirectory, 'dev');
final List<File> files = await _allFiles(dev, 'dart', minimumMatches: 1)
.where((File file) => file.path.contains('test_driver') && (file.path.endsWith('_test.dart') || file.path.endsWith('util.dart')))
.toList();
for (final File file in files) {
final String contents = file.readAsStringSync();
final int testCount = ' test('.allMatches(contents).length;
final int timeoutNoneCount = 'timeout: Timeout.none'.allMatches(contents).length;
if (testCount != timeoutNoneCount) {
errors.add('$yellow${file.path}$reset has at least $testCount test(s) but only $timeoutNoneCount `Timeout.none`(s).');
}
}
if (errors.isNotEmpty) {
foundError(<String>[
if (errors.length == 1)
'${bold}An error was detected when looking at integration test timeouts:$reset'
else
'${bold}Multiple errors were detected when looking at integration test timeouts:$reset',
...errors.map((String paragraph) => '$paragraph\n'),
]);
}
}
Future<void> verifyInternationalizations(String workingDirectory, String dartExecutable) async {
final EvalResult materialGenResult = await _evalCommand(
dartExecutable,
<String>[
path.join('dev', 'tools', 'localization', 'bin', 'gen_localizations.dart'),
'--material',
'--remove-undefined',
],
workingDirectory: workingDirectory,
);
final EvalResult cupertinoGenResult = await _evalCommand(
dartExecutable,
<String>[
path.join('dev', 'tools', 'localization', 'bin', 'gen_localizations.dart'),
'--cupertino',
'--remove-undefined',
],
workingDirectory: workingDirectory,
);
final String materialLocalizationsFile = path.join(workingDirectory, 'packages', 'flutter_localizations', 'lib', 'src', 'l10n', 'generated_material_localizations.dart');
final String cupertinoLocalizationsFile = path.join(workingDirectory, 'packages', 'flutter_localizations', 'lib', 'src', 'l10n', 'generated_cupertino_localizations.dart');
final String expectedMaterialResult = await File(materialLocalizationsFile).readAsString();
final String expectedCupertinoResult = await File(cupertinoLocalizationsFile).readAsString();
if (materialGenResult.stdout.trim() != expectedMaterialResult.trim()) {
foundError(<String>[
'<<<<<<< $materialLocalizationsFile',
expectedMaterialResult.trim(),
'=======',
materialGenResult.stdout.trim(),
'>>>>>>> gen_localizations',
'The contents of $materialLocalizationsFile are different from that produced by gen_localizations.',
'',
'Did you forget to run gen_localizations.dart after updating a .arb file?',
]);
}
if (cupertinoGenResult.stdout.trim() != expectedCupertinoResult.trim()) {
foundError(<String>[
'<<<<<<< $cupertinoLocalizationsFile',
expectedCupertinoResult.trim(),
'=======',
cupertinoGenResult.stdout.trim(),
'>>>>>>> gen_localizations',
'The contents of $cupertinoLocalizationsFile are different from that produced by gen_localizations.',
'',
'Did you forget to run gen_localizations.dart after updating a .arb file?',
]);
}
}
/// Verifies that all instances of "checked mode" have been migrated to "debug mode".
Future<void> verifyNoCheckedMode(String workingDirectory) async {
final String flutterPackages = path.join(workingDirectory, 'packages');
final List<File> files = await _allFiles(flutterPackages, 'dart', minimumMatches: 400)
.where((File file) => path.extension(file.path) == '.dart')
.toList();
final List<String> problems = <String>[];
for (final File file in files) {
int lineCount = 0;
for (final String line in file.readAsLinesSync()) {
if (line.toLowerCase().contains('checked mode')) {
problems.add('${file.path}:$lineCount uses deprecated "checked mode" instead of "debug mode".');
}
lineCount += 1;
}
}
if (problems.isNotEmpty) {
foundError(problems);
}
}
Future<void> verifyNoRuntimeTypeInToString(String workingDirectory) async {
final String flutterLib = path.join(workingDirectory, 'packages', 'flutter', 'lib');
final Set<String> excludedFiles = <String>{
path.join(flutterLib, 'src', 'foundation', 'object.dart'), // Calls this from within an assert.
};
final List<File> files = await _allFiles(flutterLib, 'dart', minimumMatches: 400)
.where((File file) => !excludedFiles.contains(file.path))
.toList();
final RegExp toStringRegExp = RegExp(r'^\s+String\s+to(.+?)?String(.+?)?\(\)\s+(\{|=>)');
final List<String> problems = <String>[];
for (final File file in files) {
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index++) {
if (toStringRegExp.hasMatch(lines[index])) {
final int sourceLine = index + 1;
bool checkForRuntimeType(String line) {
if (line.contains(r'$runtimeType') || line.contains('runtimeType.toString()')) {
problems.add('${file.path}:$sourceLine}: toString calls runtimeType.toString');
return true;
}
return false;
}
if (checkForRuntimeType(lines[index])) {
continue;
}
if (lines[index].contains('=>')) {
while (!lines[index].contains(';')) {
index++;
assert(index < lines.length, 'Source file $file has unterminated toString method.');
if (checkForRuntimeType(lines[index])) {
break;
}
}
} else {
int openBraceCount = '{'.allMatches(lines[index]).length - '}'.allMatches(lines[index]).length;
while (!lines[index].contains('}') && openBraceCount > 0) {
index++;
assert(index < lines.length, 'Source file $file has unbalanced braces in a toString method.');
if (checkForRuntimeType(lines[index])) {
break;
}
openBraceCount += '{'.allMatches(lines[index]).length;
openBraceCount -= '}'.allMatches(lines[index]).length;
}
}
}
}
}
if (problems.isNotEmpty) {
foundError(problems);
}
}
Future<void> verifyNoTrailingSpaces(String workingDirectory, { int minimumMatches = 4000 }) async {
final List<File> files = await _allFiles(workingDirectory, null, minimumMatches: minimumMatches)
.where((File file) => path.basename(file.path) != 'serviceaccount.enc')
.where((File file) => path.basename(file.path) != 'Ahem.ttf')
.where((File file) => path.extension(file.path) != '.snapshot')
.where((File file) => path.extension(file.path) != '.png')
.where((File file) => path.extension(file.path) != '.jpg')
.where((File file) => path.extension(file.path) != '.ico')
.where((File file) => path.extension(file.path) != '.jar')
.where((File file) => path.extension(file.path) != '.swp')
.toList();
final List<String> problems = <String>[];
for (final File file in files) {
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index += 1) {
if (lines[index].endsWith(' ')) {
problems.add('${file.path}:${index + 1}: trailing U+0020 space character');
} else if (lines[index].endsWith('\t')) {
problems.add('${file.path}:${index + 1}: trailing U+0009 tab character');
}
}
if (lines.isNotEmpty && lines.last == '') {
problems.add('${file.path}:${lines.length}: trailing blank line');
}
}
if (problems.isNotEmpty) {
foundError(problems);
}
}
final RegExp _flowControlStatementWithoutSpace = RegExp(r'(^|[ \t])(if|switch|for|do|while|catch)\(', multiLine: true);
Future<void> verifySpacesAfterFlowControlStatements(String workingDirectory, { int minimumMatches = 4000 }) async {
const Set<String> extensions = <String>{
'.dart',
'.java',
'.js',
'.kt',
'.swift',
'.c',
'.cc',
'.cpp',
'.h',
'.m',
};
final List<File> files = await _allFiles(workingDirectory, null, minimumMatches: minimumMatches)
.where((File file) => extensions.contains(path.extension(file.path)))
.toList();
final List<String> problems = <String>[];
for (final File file in files) {
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index += 1) {
if (lines[index].contains(_flowControlStatementWithoutSpace)) {
problems.add('${file.path}:${index + 1}: no space after flow control statement');
}
}
}
if (problems.isNotEmpty) {
foundError(problems);
}
}
String _bullets(String value) => ' * $value';
Future<void> verifyIssueLinks(String workingDirectory) async {
const String issueLinkPrefix = 'https://github.com/flutter/flutter/issues/new';
const Set<String> stops = <String>{ '\n', ' ', "'", '"', r'\', ')', '>' };
assert(!stops.contains('.')); // instead of "visit https://foo." say "visit: https://", it copy-pastes better
const String kGiveTemplates =
'Prefer to provide a link either to $issueLinkPrefix/choose (the list of issue '
'templates) or to a specific template directly ($issueLinkPrefix?template=...).\n';
final Set<String> templateNames =
Directory(path.join(workingDirectory, '.github', 'ISSUE_TEMPLATE'))
.listSync()
.whereType<File>()
.where((File file) => path.extension(file.path) == '.md' || path.extension(file.path) == '.yml')
.map<String>((File file) => path.basename(file.path))
.toSet();
final String kTemplates = 'The available templates are:\n${templateNames.map(_bullets).join("\n")}';
final List<String> problems = <String>[];
final Set<String> suggestions = <String>{};
final List<File> files = await _gitFiles(workingDirectory);
for (final File file in files) {
if (path.basename(file.path).endsWith('_test.dart') || path.basename(file.path) == 'analyze.dart') {
continue; // Skip tests, they're not public-facing.
}
final Uint8List bytes = file.readAsBytesSync();
// We allow invalid UTF-8 here so that binaries don't trip us up.
// There's a separate test in this file that verifies that all text
// files are actually valid UTF-8 (see verifyNoBinaries below).
final String contents = utf8.decode(bytes, allowMalformed: true);
int start = 0;
while ((start = contents.indexOf(issueLinkPrefix, start)) >= 0) {
int end = start + issueLinkPrefix.length;
while (end < contents.length && !stops.contains(contents[end])) {
end += 1;
}
final String url = contents.substring(start, end);
if (url == issueLinkPrefix) {
if (file.path != path.join(workingDirectory, 'dev', 'bots', 'analyze.dart')) {
problems.add('${file.path} contains a direct link to $issueLinkPrefix.');
suggestions.add(kGiveTemplates);
suggestions.add(kTemplates);
}
} else if (url.startsWith('$issueLinkPrefix?')) {
final Uri parsedUrl = Uri.parse(url);
final List<String>? templates = parsedUrl.queryParametersAll['template'];
if (templates == null) {
problems.add('${file.path} contains $url, which has no "template" argument specified.');
suggestions.add(kGiveTemplates);
suggestions.add(kTemplates);
} else if (templates.length != 1) {
problems.add('${file.path} contains $url, which has ${templates.length} templates specified.');
suggestions.add(kGiveTemplates);
suggestions.add(kTemplates);
} else if (!templateNames.contains(templates.single)) {
problems.add('${file.path} contains $url, which specifies a non-existent template ("${templates.single}").');
suggestions.add(kTemplates);
} else if (parsedUrl.queryParametersAll.keys.length > 1) {
problems.add('${file.path} contains $url, which the analyze.dart script is not sure how to handle.');
suggestions.add('Update analyze.dart to handle the URLs above, or change them to the expected pattern.');
}
} else if (url != '$issueLinkPrefix/choose') {
problems.add('${file.path} contains $url, which the analyze.dart script is not sure how to handle.');
suggestions.add('Update analyze.dart to handle the URLs above, or change them to the expected pattern.');
}
start = end;
}
}
assert(problems.isEmpty == suggestions.isEmpty);
if (problems.isNotEmpty) {
foundError(<String>[
...problems,
...suggestions,
]);
}
}
@immutable
class Hash256 {
const Hash256(this.a, this.b, this.c, this.d);
factory Hash256.fromDigest(Digest digest) {
assert(digest.bytes.length == 32);
return Hash256(
digest.bytes[ 0] << 56 |
digest.bytes[ 1] << 48 |
digest.bytes[ 2] << 40 |
digest.bytes[ 3] << 32 |
digest.bytes[ 4] << 24 |
digest.bytes[ 5] << 16 |
digest.bytes[ 6] << 8 |
digest.bytes[ 7] << 0,
digest.bytes[ 8] << 56 |
digest.bytes[ 9] << 48 |
digest.bytes[10] << 40 |
digest.bytes[11] << 32 |
digest.bytes[12] << 24 |
digest.bytes[13] << 16 |
digest.bytes[14] << 8 |
digest.bytes[15] << 0,
digest.bytes[16] << 56 |
digest.bytes[17] << 48 |
digest.bytes[18] << 40 |
digest.bytes[19] << 32 |
digest.bytes[20] << 24 |
digest.bytes[21] << 16 |
digest.bytes[22] << 8 |
digest.bytes[23] << 0,
digest.bytes[24] << 56 |
digest.bytes[25] << 48 |
digest.bytes[26] << 40 |
digest.bytes[27] << 32 |
digest.bytes[28] << 24 |
digest.bytes[29] << 16 |
digest.bytes[30] << 8 |
digest.bytes[31] << 0,
);
}
final int a;
final int b;
final int c;
final int d;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is Hash256
&& other.a == a
&& other.b == b
&& other.c == c
&& other.d == d;
}
@override
int get hashCode => Object.hash(a, b, c, d);
}
// DO NOT ADD ANY ENTRIES TO THIS LIST.
// We have a policy of not checking in binaries into this repository.
// If you are adding/changing template images, use the flutter_template_images
// package and a .img.tmpl placeholder instead.
// If you have other binaries to add, please consult Hixie for advice.
final Set<Hash256> _legacyBinaries = <Hash256>{
// DEFAULT ICON IMAGES
// packages/flutter_tools/templates/app/android.tmpl/app/src/main/res/mipmap-hdpi/ic_launcher.png
// packages/flutter_tools/templates/module/android/host_app_common/app.tmpl/src/main/res/mipmap-hdpi/ic_launcher.png
// (also used by many examples)
const Hash256(0x6A7C8F0D703E3682, 0x108F9662F8133022, 0x36240D3F8F638BB3, 0x91E32BFB96055FEF),
// packages/flutter_tools/templates/app/android.tmpl/app/src/main/res/mipmap-mdpi/ic_launcher.png
// (also used by many examples)
const Hash256(0xC7C0C0189145E4E3, 0x2A401C61C9BDC615, 0x754B0264E7AFAE24, 0xE834BB81049EAF81),
// packages/flutter_tools/templates/app/android.tmpl/app/src/main/res/mipmap-xhdpi/ic_launcher.png
// (also used by many examples)
const Hash256(0xE14AA40904929BF3, 0x13FDED22CF7E7FFC, 0xBF1D1AAC4263B5EF, 0x1BE8BFCE650397AA),
// packages/flutter_tools/templates/app/android.tmpl/app/src/main/res/mipmap-xxhdpi/ic_launcher.png
// (also used by many examples)
const Hash256(0x4D470BF22D5C17D8, 0x4EDC5F82516D1BA8, 0xA1C09559CD761CEF, 0xB792F86D9F52B540),
// packages/flutter_tools/templates/app/android.tmpl/app/src/main/res/mipmap-xxxhdpi/ic_launcher.png
// (also used by many examples)
const Hash256(0x3C34E1F298D0C9EA, 0x3455D46DB6B7759C, 0x8211A49E9EC6E44B, 0x635FC5C87DFB4180),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by a few examples)
const Hash256(0x7770183009E91411, 0x2DE7D8EF1D235A6A, 0x30C5834424858E0D, 0x2F8253F6B8D31926),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x5925DAB509451F9E, 0xCBB12CE8A625F9D4, 0xC104718EE20CAFF8, 0xB1B51032D1CD8946),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0xC4D9A284C12301D0, 0xF50E248EC53ED51A, 0x19A10147B774B233, 0x08399250B0D44C55),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0xBF97F9D3233F33E1, 0x389B09F7B8ADD537, 0x41300CB834D6C7A5, 0xCA32CBED363A4FB2),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x285442F69A06B45D, 0x9D79DF80321815B5, 0x46473548A37B7881, 0x9B68959C7B8ED237),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x2AB64AF8AC727EA9, 0x9C6AB9EAFF847F46, 0xFBF2A9A0A78A0ABC, 0xBF3180F3851645B4),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x9DCA09F4E5ED5684, 0xD3C4DFF41F4E8B7C, 0xB864B438172D72BE, 0x069315FA362930F9),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0xD5AD04DE321EF37C, 0xACC5A7B960AFCCE7, 0x1BDCB96FA020C482, 0x49C1545DD1A0F497),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x809ABFE75C440770, 0xC13C4E2E46D09603, 0xC22053E9D4E0E227, 0x5DCB9C1DCFBB2C75),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x3DB08CB79E7B01B9, 0xE81F956E3A0AE101, 0x48D0FAFDE3EA7AA7, 0x0048DF905AA52CFD),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x23C13D463F5DCA5C, 0x1F14A14934003601, 0xC29F1218FD461016, 0xD8A22CEF579A665F),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0x6DB7726530D71D3F, 0x52CB59793EB69131, 0x3BAA04796E129E1E, 0x043C0A58A1BFFD2F),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/AppIcon.appiconset/[email protected]
// (also used by many examples)
const Hash256(0xCEE565F5E6211656, 0x9B64980B209FD5CA, 0x4B3D3739011F5343, 0x250B33A1A2C6EB65),
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/LaunchImage.imageset/LaunchImage.png
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/LaunchImage.imageset/[email protected]
// packages/flutter_tools/templates/app/ios.tmpl/Runner/Assets.xcassets/LaunchImage.imageset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/LaunchImage.imageset/LaunchImage.png
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/LaunchImage.imageset/[email protected]
// packages/flutter_tools/templates/module/ios/host_app_ephemeral/Runner.tmpl/Assets.xcassets/LaunchImage.imageset/[email protected]
// (also used by many examples)
const Hash256(0x93AE7D494FAD0FB3, 0x0CBF3AE746A39C4B, 0xC7A0F8BBF87FBB58, 0x7A3F3C01F3C5CE20),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_1024.png
// (also used by a few examples)
const Hash256(0xB18BEBAAD1AD6724, 0xE48BCDF699BA3927, 0xDF3F258FEBE646A3, 0xAB5C62767C6BAB40),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_128.png
// (also used by a few examples)
const Hash256(0xF90D839A289ECADB, 0xF2B0B3400DA43EB8, 0x08B84908335AE4A0, 0x07457C4D5A56A57C),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_16.png
// (also used by a few examples)
const Hash256(0x592C2ABF84ADB2D3, 0x91AED8B634D3233E, 0x2C65369F06018DCD, 0x8A4B27BA755EDCBE),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_256.png
// (also used by a few examples)
const Hash256(0x75D9A0C034113CA8, 0xA1EC11C24B81F208, 0x6630A5A5C65C7D26, 0xA5DC03A1C0A4478C),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_32.png
// (also used by a few examples)
const Hash256(0xA896E65745557732, 0xC72BD4EE3A10782F, 0xE2AA95590B5AF659, 0x869E5808DB9C01C1),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_512.png
// (also used by a few examples)
const Hash256(0x3A69A8A1AAC5D9A8, 0x374492AF4B6D07A4, 0xCE637659EB24A784, 0x9C4DFB261D75C6A3),
// packages/flutter_tools/templates/app/macos.tmpl/Runner/Assets.xcassets/AppIcon.appiconset/app_icon_64.png
// (also used by a few examples)
const Hash256(0xD29D4E0AF9256DC9, 0x2D0A8F8810608A5E, 0x64A132AD8B397CA2, 0xC4DDC0B1C26A68C3),
// packages/flutter_tools/templates/app/web/icons/Icon-192.png.copy.tmpl
// dev/integration_tests/flutter_gallery/web/icons/Icon-192.png
const Hash256(0x3DCE99077602F704, 0x21C1C6B2A240BC9B, 0x83D64D86681D45F2, 0x154143310C980BE3),
// packages/flutter_tools/templates/app/web/icons/Icon-512.png.copy.tmpl
// dev/integration_tests/flutter_gallery/web/icons/Icon-512.png
const Hash256(0xBACCB205AE45f0B4, 0x21BE1657259B4943, 0xAC40C95094AB877F, 0x3BCBE12CD544DCBE),
// packages/flutter_tools/templates/app/web/favicon.png.copy.tmpl
// dev/integration_tests/flutter_gallery/web/favicon.png
const Hash256(0x7AB2525F4B86B65D, 0x3E4C70358A17E5A1, 0xAAF6F437f99CBCC0, 0x46DAD73d59BB9015),
// GALLERY ICONS
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-hdpi/ic_background.png
const Hash256(0x03CFDE53C249475C, 0x277E8B8E90AC8A13, 0xE5FC13C358A94CCB, 0x67CA866C9862A0DD),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-hdpi/ic_foreground.png
const Hash256(0x86A83E23A505EFCC, 0x39C358B699EDE12F, 0xC088EE516A1D0C73, 0xF3B5D74DDAD164B1),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-hdpi/ic_launcher.png
const Hash256(0xD813B1A77320355E, 0xB68C485CD47D0F0F, 0x3C7E1910DCD46F08, 0x60A6401B8DC13647),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xhdpi/ic_background.png
const Hash256(0x35AFA76BD5D6053F, 0xEE927436C78A8794, 0xA8BA5F5D9FC9653B, 0xE5B96567BB7215ED),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xhdpi/ic_foreground.png
const Hash256(0x263CE9B4F1F69B43, 0xEBB08AE9FE8F80E7, 0x95647A59EF2C040B, 0xA8AEB246861A7DFF),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xhdpi/ic_launcher.png
const Hash256(0x5E1A93C3653BAAFF, 0x1AAC6BCEB8DCBC2F, 0x2AE7D68ECB07E507, 0xCB1FA8354B28313A),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxhdpi/ic_background.png
const Hash256(0xA5C77499151DDEC6, 0xDB40D0AC7321FD74, 0x0646C0C0F786743F, 0x8F3C3C408CAC5E8C),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxhdpi/ic_foreground.png
const Hash256(0x33DE450980A2A16B, 0x1982AC7CDC1E7B01, 0x919E07E0289C2139, 0x65F85BCED8895FEF),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxhdpi/ic_launcher.png
const Hash256(0xC3B8577F4A89BA03, 0x830944FB06C3566B, 0x4C99140A2CA52958, 0x089BFDC3079C59B7),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxxhdpi/ic_background.png
const Hash256(0xDEBC241D6F9C5767, 0x8980FDD46FA7ED0C, 0x5B8ACD26BCC5E1BC, 0x473C89B432D467AD),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxxhdpi/ic_foreground.png
const Hash256(0xBEFE5F7E82BF8B64, 0x148D869E3742004B, 0xF821A9F5A1BCDC00, 0x357D246DCC659DC2),
// dev/integration_tests/flutter_gallery/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.png
const Hash256(0xC385404341FF9EDD, 0x30FBE76F0EC99155, 0x8EA4F4AFE8CC0C60, 0x1CA3EDEF177E1DA8),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-1024.png
const Hash256(0x6BE5751A29F57A80, 0x36A4B31CC542C749, 0x984E49B22BD65CAA, 0x75AE8B2440848719),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-120.png
const Hash256(0x9972A2264BFA8F8D, 0x964AFE799EADC1FA, 0x2247FB31097F994A, 0x1495DC32DF071793),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-152.png
const Hash256(0x4C7CC9B09BEEDA24, 0x45F57D6967753910, 0x57D68E1A6B883D2C, 0x8C52701A74F1400F),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-167.png
const Hash256(0x66DACAC1CFE4D349, 0xDBE994CB9125FFD7, 0x2D795CFC9CF9F739, 0xEDBB06CE25082E9C),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-180.png
const Hash256(0x5188621015EBC327, 0xC9EF63AD76E60ECE, 0xE82BDC3E4ABF09E2, 0xEE0139FA7C0A2BE5),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-20.png
const Hash256(0x27D2752D04EE9A6B, 0x78410E208F74A6CD, 0xC90D9E03B73B8C60, 0xD05F7D623E790487),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-29.png
const Hash256(0xBB20556B2826CF85, 0xD5BAC73AA69C2AC3, 0x8E71DAD64F15B855, 0xB30CB73E0AF89307),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-40.png
const Hash256(0x623820FA45CDB0AC, 0x808403E34AD6A53E, 0xA3E9FDAE83EE0931, 0xB020A3A4EF2CDDE7),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-58.png
const Hash256(0xC6D631D1E107215E, 0xD4A58FEC5F3AA4B5, 0x0AE9724E07114C0C, 0x453E5D87C2CAD3B3),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-60.png
const Hash256(0x4B6F58D1EB8723C6, 0xE717A0D09FEC8806, 0x90C6D1EF4F71836E, 0x618672827979B1A2),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-76.png
const Hash256(0x0A1744CC7634D508, 0xE85DD793331F0C8A, 0x0B7C6DDFE0975D8F, 0x29E91C905BBB1BED),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-80.png
const Hash256(0x24032FBD1E6519D6, 0x0BA93C0D5C189554, 0xF50EAE23756518A2, 0x3FABACF4BD5DAF08),
// dev/integration_tests/flutter_gallery/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-87.png
const Hash256(0xC17BAE6DF6BB234A, 0xE0AF4BEB0B805F12, 0x14E74EB7AA9A30F1, 0x5763689165DA7DDF),
// STOCKS ICONS
// dev/benchmarks/test_apps/stocks/android/app/src/main/res/mipmap-hdpi/ic_launcher.png
const Hash256(0x74052AB5241D4418, 0x7085180608BC3114, 0xD12493C50CD8BBC7, 0x56DED186C37ACE84),
// dev/benchmarks/test_apps/stocks/android/app/src/main/res/mipmap-mdpi/ic_launcher.png
const Hash256(0xE37947332E3491CB, 0x82920EE86A086FEA, 0xE1E0A70B3700A7DA, 0xDCAFBDD8F40E2E19),
// dev/benchmarks/test_apps/stocks/android/app/src/main/res/mipmap-xhdpi/ic_launcher.png
const Hash256(0xE608CDFC0C8579FB, 0xE38873BAAF7BC944, 0x9C9D2EE3685A4FAE, 0x671EF0C8BC41D17C),
// dev/benchmarks/test_apps/stocks/android/app/src/main/res/mipmap-xxhdpi/ic_launcher.png
const Hash256(0xBD53D86977DF9C54, 0xF605743C5ABA114C, 0x9D51D1A8BB917E1A, 0x14CAA26C335CAEBD),
// dev/benchmarks/test_apps/stocks/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.png
const Hash256(0x64E4D02262C4F3D0, 0xBB4FDC21CD0A816C, 0x4CD2A0194E00FB0F, 0x1C3AE4142FAC0D15),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0x5BA3283A76918FC0, 0xEE127D0F22D7A0B6, 0xDF03DAED61669427, 0x93D89DDD87A08117),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xCD7F26ED31DEA42A, 0x535D155EC6261499, 0x34E6738255FDB2C4, 0xBD8D4BDDE9A99B05),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-76.png
const Hash256(0x3FA1225FC9A96A7E, 0xCD071BC42881AB0E, 0x7747EB72FFB72459, 0xA37971BBAD27EE24),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xCD867001ACD7BBDB, 0x25CDFD452AE89FA2, 0x8C2DC980CAF55F48, 0x0B16C246CFB389BC),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0x848E9736E5C4915A, 0x7945BCF6B32FD56B, 0x1F1E7CDDD914352E, 0xC9681D38EF2A70DA),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-Notification.png
const Hash256(0x654BA7D6C4E05CA0, 0x7799878884EF8F11, 0xA383E1F24CEF5568, 0x3C47604A966983C8),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-Small-40.png
const Hash256(0x743056FE7D83FE42, 0xA2990825B6AD0415, 0x1AF73D0D43B227AA, 0x07EBEA9B767381D9),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xA7E1570812D119CF, 0xEF4B602EF28DD0A4, 0x100D066E66F5B9B9, 0x881765DC9303343B),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xB4102839A1E41671, 0x62DACBDEFA471953, 0xB1EE89A0AB7594BE, 0x1D9AC1E67DC2B2CE),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/Icon-Small.png
const Hash256(0x70AC6571B593A967, 0xF1CBAEC9BC02D02D, 0x93AD766D8290ADE6, 0x840139BF9F219019),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0x5D87A78386DA2C43, 0xDDA8FEF2CA51438C, 0xE5A276FE28C6CF0A, 0xEBE89085B56665B6),
// dev/benchmarks/test_apps/stocks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0x4D9F5E81F668DA44, 0xB20A77F8BF7BA2E1, 0xF384533B5AD58F07, 0xB3A2F93F8635CD96),
// LEGACY ICONS
// dev/benchmarks/complex_layout/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/benchmarks/microbenchmarks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// examples/flutter_view/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// (not really sure where this came from, or why neither the template nor most examples use them)
const Hash256(0x6E645DC9ED913AAD, 0xB50ED29EEB16830D, 0xB32CA12F39121DB9, 0xB7BC1449DDDBF8B8),
// dev/benchmarks/macrobenchmarks/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/integration_tests/codegen/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/integration_tests/ios_add2app/ios_add2app/Assets.xcassets/AppIcon.appiconset/[email protected]
// dev/integration_tests/release_smoke_test/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xDEFAC77E08EC71EC, 0xA04CCA3C95D1FC33, 0xB9F26E1CB15CB051, 0x47DEFC79CDD7C158),
// examples/flutter_view/ios/Runner/ic_add.png
// examples/platform_view/ios/Runner/ic_add.png
const Hash256(0x3CCE7450334675E2, 0xE3AABCA20B028993, 0x127BE82FE0EB3DFF, 0x8B027B3BAF052F2F),
// examples/image_list/images/coast.jpg
const Hash256(0xDA957FD30C51B8D2, 0x7D74C2C918692DC4, 0xD3C5C99BB00F0D6B, 0x5EBB30395A6EDE82),
// examples/image_list/ios/Runner/Assets.xcassets/AppIcon.appiconset/[email protected]
const Hash256(0xB5792CA06F48A431, 0xD4379ABA2160BD5D, 0xE92339FC64C6A0D3, 0x417AA359634CD905),
// TEST ASSETS
// dev/benchmarks/macrobenchmarks/assets/999x1000.png
const Hash256(0x553E9C36DFF3E610, 0x6A608BDE822A0019, 0xDE4F1769B6FBDB97, 0xBC3C20E26B839F59),
// dev/bots/test/analyze-test-input/root/packages/foo/serviceaccount.enc
const Hash256(0xA8100AE6AA1940D0, 0xB663BB31CD466142, 0xEBBDBD5187131B92, 0xD93818987832EB89),
// dev/automated_tests/icon/test.png
const Hash256(0xE214B4A0FEEEC6FA, 0x8E7AA8CC9BFBEC40, 0xBCDAC2F2DEBC950F, 0x75AF8EBF02BCE459),
// dev/integration_tests/android_splash_screens/splash_screen_kitchen_sink/android/app/src/main/res/drawable-land-xxhdpi/flutter_splash_screen.png
// dev/integration_tests/android_splash_screens/splash_screen_kitchen_sink/android/app/src/main/res/mipmap-land-xxhdpi/flutter_splash_screen.png
const Hash256(0x2D4F8D7A3DFEF9D3, 0xA0C66938E169AB58, 0x8C6BBBBD1973E34E, 0x03C428416D010182),
// dev/integration_tests/android_splash_screens/splash_screen_kitchen_sink/android/app/src/main/res/drawable-xxhdpi/flutter_splash_screen.png
// dev/integration_tests/android_splash_screens/splash_screen_kitchen_sink/android/app/src/main/res/mipmap-xxhdpi/flutter_splash_screen.png
const Hash256(0xCD46C01BAFA3B243, 0xA6AA1645EEDDE481, 0x143AC8ABAB1A0996, 0x22CAA9D41F74649A),
// dev/integration_tests/flutter_driver_screenshot_test/assets/red_square.png
const Hash256(0x40054377E1E084F4, 0x4F4410CE8F44C210, 0xABA945DFC55ED0EF, 0x23BDF9469E32F8D3),
// dev/integration_tests/flutter_driver_screenshot_test/test_driver/goldens/red_square_image/iPhone7,2.png
const Hash256(0x7F9D27C7BC418284, 0x01214E21CA886B2F, 0x40D9DA2B31AE7754, 0x71D68375F9C8A824),
// examples/flutter_view/assets/flutter-mark-square-64.png
// examples/platform_view/assets/flutter-mark-square-64.png
const Hash256(0xF416B0D8AC552EC8, 0x819D1F492D1AB5E6, 0xD4F20CF45DB47C22, 0x7BB431FEFB5B67B2),
// packages/flutter_tools/test/data/intellij/plugins/Dart/lib/Dart.jar
const Hash256(0x576E489D788A13DB, 0xBF40E4A39A3DAB37, 0x15CCF0002032E79C, 0xD260C69B29E06646),
// packages/flutter_tools/test/data/intellij/plugins/flutter-intellij.jar
const Hash256(0x4C67221E25626CB2, 0x3F94E1F49D34E4CF, 0x3A9787A514924FC5, 0x9EF1E143E5BC5690),
// MISCELLANEOUS
// dev/bots/serviceaccount.enc
const Hash256(0x1F19ADB4D80AFE8C, 0xE61899BA776B1A8D, 0xCA398C75F5F7050D, 0xFB0E72D7FBBBA69B),
// dev/docs/favicon.ico
const Hash256(0x67368CA1733E933A, 0xCA3BC56EF0695012, 0xE862C371AD4412F0, 0x3EC396039C609965),
// dev/snippets/assets/code_sample.png
const Hash256(0xAB2211A47BDA001D, 0x173A52FD9C75EBC7, 0xE158942FFA8243AD, 0x2A148871990D4297),
// dev/snippets/assets/code_snippet.png
const Hash256(0xDEC70574DA46DFBB, 0xFA657A771F3E1FBD, 0xB265CFC6B2AA5FE3, 0x93BA4F325D1520BA),
// packages/flutter_tools/static/Ahem.ttf
const Hash256(0x63D2ABD0041C3E3B, 0x4B52AD8D382353B5, 0x3C51C6785E76CE56, 0xED9DACAD2D2E31C4),
};
Future<void> verifyNoBinaries(String workingDirectory, { Set<Hash256>? legacyBinaries }) async {
// Please do not add anything to the _legacyBinaries set above.
// We have a policy of not checking in binaries into this repository.
// If you are adding/changing template images, use the flutter_template_images
// package and a .img.tmpl placeholder instead.
// If you have other binaries to add, please consult Hixie for advice.
assert(
_legacyBinaries
.expand<int>((Hash256 hash) => <int>[hash.a, hash.b, hash.c, hash.d])
.reduce((int value, int element) => value ^ element) == 0x606B51C908B40BFA // Please do not modify this line.
);
legacyBinaries ??= _legacyBinaries;
if (!Platform.isWindows) { // TODO(ianh): Port this to Windows
final List<File> files = await _gitFiles(workingDirectory);
final List<String> problems = <String>[];
for (final File file in files) {
final Uint8List bytes = file.readAsBytesSync();
try {
utf8.decode(bytes);
} on FormatException catch (error) {
final Digest digest = sha256.convert(bytes);
if (!legacyBinaries.contains(Hash256.fromDigest(digest))) {
problems.add('${file.path}:${error.offset}: file is not valid UTF-8');
}
}
}
if (problems.isNotEmpty) {
foundError(<String>[
...problems,
'All files in this repository must be UTF-8. In particular, images and other binaries',
'must not be checked into this repository. This is because we are very sensitive to the',
'size of the repository as it is distributed to all our developers. If you have a binary',
'to which you need access, you should consider how to fetch it from another repository;',
'for example, the "assets-for-api-docs" repository is used for images in API docs.',
'To add assets to flutter_tools templates, see the instructions in the wiki:',
'https://github.com/flutter/flutter/wiki/Managing-template-image-assets',
]);
}
}
}
// UTILITY FUNCTIONS
bool _listEquals<T>(List<T> a, List<T> b) {
if (a.length != b.length) {
return false;
}
for (int index = 0; index < a.length; index += 1) {
if (a[index] != b[index]) {
return false;
}
}
return true;
}
Future<List<File>> _gitFiles(String workingDirectory, {bool runSilently = true}) async {
final EvalResult evalResult = await _evalCommand(
'git', <String>['ls-files', '-z'],
workingDirectory: workingDirectory,
runSilently: runSilently,
);
if (evalResult.exitCode != 0) {
foundError(<String>[
'git ls-files failed with exit code ${evalResult.exitCode}',
'${bold}stdout:$reset',
evalResult.stdout,
'${bold}stderr:$reset',
evalResult.stderr,
]);
}
final List<String> filenames = evalResult
.stdout
.split('\x00');
assert(filenames.last.isEmpty); // git ls-files gives a trailing blank 0x00
filenames.removeLast();
return filenames
.map<File>((String filename) => File(path.join(workingDirectory, filename)))
.toList();
}
Stream<File> _allFiles(String workingDirectory, String? extension, { required int minimumMatches }) async* {
final Set<String> gitFileNamesSet = <String>{};
gitFileNamesSet.addAll((await _gitFiles(workingDirectory)).map((File f) => path.canonicalize(f.absolute.path)));
assert(extension == null || !extension.startsWith('.'), 'Extension argument should not start with a period.');
final Set<FileSystemEntity> pending = <FileSystemEntity>{ Directory(workingDirectory) };
int matches = 0;
while (pending.isNotEmpty) {
final FileSystemEntity entity = pending.first;
pending.remove(entity);
if (path.extension(entity.path) == '.tmpl') {
continue;
}
if (entity is File) {
if (!gitFileNamesSet.contains(path.canonicalize(entity.absolute.path))) {
continue;
}
if (_isGeneratedPluginRegistrant(entity)) {
continue;
}
if (path.basename(entity.path) == 'flutter_export_environment.sh') {
continue;
}
if (path.basename(entity.path) == 'gradlew.bat') {
continue;
}
if (path.basename(entity.path) == '.DS_Store') {
continue;
}
if (extension == null || path.extension(entity.path) == '.$extension') {
matches += 1;
yield entity;
}
} else if (entity is Directory) {
if (File(path.join(entity.path, '.dartignore')).existsSync()) {
continue;
}
if (path.basename(entity.path) == '.git') {
continue;
}
if (path.basename(entity.path) == '.idea') {
continue;
}
if (path.basename(entity.path) == '.gradle') {
continue;
}
if (path.basename(entity.path) == '.dart_tool') {
continue;
}
if (path.basename(entity.path) == '.idea') {
continue;
}
if (path.basename(entity.path) == 'build') {
continue;
}
pending.addAll(entity.listSync());
}
}
assert(matches >= minimumMatches, 'Expected to find at least $minimumMatches files with extension ".$extension" in "$workingDirectory", but only found $matches.');
}
class EvalResult {
EvalResult({
required this.stdout,
required this.stderr,
this.exitCode = 0,
});
final String stdout;
final String stderr;
final int exitCode;
}
// TODO(ianh): Refactor this to reuse the code in run_command.dart
Future<EvalResult> _evalCommand(String executable, List<String> arguments, {
required String workingDirectory,
Map<String, String>? environment,
bool allowNonZeroExit = false,
bool runSilently = false,
}) async {
final String commandDescription = '${path.relative(executable, from: workingDirectory)} ${arguments.join(' ')}';
final String relativeWorkingDir = path.relative(workingDirectory);
if (!runSilently) {
print('RUNNING: cd $cyan$relativeWorkingDir$reset; $green$commandDescription$reset');
}
final Stopwatch time = Stopwatch()..start();
final Process process = await Process.start(executable, arguments,
workingDirectory: workingDirectory,
environment: environment,
);
final Future<List<List<int>>> savedStdout = process.stdout.toList();
final Future<List<List<int>>> savedStderr = process.stderr.toList();
final int exitCode = await process.exitCode;
final EvalResult result = EvalResult(
stdout: utf8.decode((await savedStdout).expand<int>((List<int> ints) => ints).toList()),
stderr: utf8.decode((await savedStderr).expand<int>((List<int> ints) => ints).toList()),
exitCode: exitCode,
);
if (!runSilently) {
print('ELAPSED TIME: $bold${prettyPrintDuration(time.elapsed)}$reset for $commandDescription in $relativeWorkingDir');
}
if (exitCode != 0 && !allowNonZeroExit) {
foundError(<String>[
result.stderr,
'${bold}ERROR:$red Last command exited with $exitCode.$reset',
'${bold}Command:$red $commandDescription$reset',
'${bold}Relative working directory:$red $relativeWorkingDir$reset',
]);
}
return result;
}
Future<void> _checkConsumerDependencies() async {
const List<String> kCorePackages = <String>[
'flutter',
'flutter_test',
'flutter_driver',
'flutter_localizations',
'integration_test',
'fuchsia_remote_debug_protocol',
];
final Set<String> dependencies = <String>{};
// Parse the output of pub deps --json to determine all of the
// current packages used by the core set of flutter packages.
for (final String package in kCorePackages) {
final ProcessResult result = await Process.run(flutter, <String>[
'pub',
'deps',
'--json',
'--directory=${path.join(flutterRoot, 'packages', package)}',
]);
if (result.exitCode != 0) {
foundError(<String>[
result.stdout.toString(),
result.stderr.toString(),
]);
return;
}
final Map<String, Object?> rawJson = json.decode(result.stdout as String) as Map<String, Object?>;
final Map<String, Map<String, Object?>> dependencyTree = <String, Map<String, Object?>>{
for (final Map<String, Object?> package in (rawJson['packages']! as List<Object?>).cast<Map<String, Object?>>())
package['name']! as String : package,
};
final List<Map<String, Object?>> workset = <Map<String, Object?>>[];
workset.add(dependencyTree[package]!);
while (workset.isNotEmpty) {
final Map<String, Object?> currentPackage = workset.removeLast();
if (currentPackage['kind'] == 'dev') {
continue;
}
dependencies.add(currentPackage['name']! as String);
final List<String> currentDependencies = (currentPackage['dependencies']! as List<Object?>).cast<String>();
for (final String dependency in currentDependencies) {
// Don't add dependencies we've already seen or we will get stuck
// forever if there are any circular references.
// TODO(dantup): Consider failing gracefully with the names of the
// packages once the cycle between test_api and matcher is resolved.
// https://github.com/dart-lang/test/issues/1979
if (!dependencies.contains(dependency)) {
workset.add(dependencyTree[dependency]!);
}
}
}
}
final Set<String> removed = kCorePackageAllowList.difference(dependencies);
final Set<String> added = dependencies.difference(kCorePackageAllowList);
String plural(int n, String s, String p) => n == 1 ? s : p;
if (added.isNotEmpty) {
foundError(<String>[
'The transitive closure of package dependencies contains ${plural(added.length, "a non-allowlisted package", "non-allowlisted packages")}:',
' ${added.join(', ')}',
'We strongly desire to keep the number of dependencies to a minimum and',
'therefore would much prefer not to add new dependencies.',
'See dev/bots/allowlist.dart for instructions on how to update the package',
'allowlist if you nonetheless believe this is a necessary addition.',
]);
}
if (removed.isNotEmpty) {
foundError(<String>[
'Excellent news! ${plural(removed.length, "A package dependency has been removed!", "Multiple package dependencies have been removed!")}',
' ${removed.join(', ')}',
'To make sure we do not accidentally add ${plural(removed.length, "this dependency", "these dependencies")} back in the future,',
'please remove ${plural(removed.length, "this", "these")} packages from the allow-list in dev/bots/allowlist.dart.',
'Thanks!',
]);
}
}
const String _kDebugOnlyAnnotation = '@_debugOnly';
final RegExp _nullInitializedField = RegExp(r'kDebugMode \? [\w<> ,{}()]+ : null;');
Future<void> verifyNullInitializedDebugExpensiveFields(String workingDirectory, {int minimumMatches = 400}) async {
final String flutterLib = path.join(workingDirectory, 'packages', 'flutter', 'lib');
final List<File> files = await _allFiles(flutterLib, 'dart', minimumMatches: minimumMatches)
.toList();
final List<String> errors = <String>[];
for (final File file in files) {
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index += 1) {
final String line = lines[index];
if (!line.contains(_kDebugOnlyAnnotation)) {
continue;
}
final String nextLine = lines[index + 1];
if (_nullInitializedField.firstMatch(nextLine) == null) {
errors.add('${file.path}:$index');
}
}
}
if (errors.isNotEmpty) {
foundError(<String>[
'${bold}ERROR: ${red}fields annotated with @_debugOnly must null initialize.$reset',
'to ensure both the field and initializer are removed from profile/release mode.',
'These fields should be written as:\n',
'field = kDebugMode ? <DebugValue> : null;\n',
'Errors were found in the following files:',
...errors,
]);
}
}
final RegExp tabooPattern = RegExp(r'^ *///.*\b(simply|note:|note that)\b', caseSensitive: false);
Future<void> verifyTabooDocumentation(String workingDirectory, { int minimumMatches = 100 }) async {
final List<String> errors = <String>[];
await for (final File file in _allFiles(workingDirectory, 'dart', minimumMatches: minimumMatches)) {
final List<String> lines = file.readAsLinesSync();
for (int index = 0; index < lines.length; index += 1) {
final String line = lines[index];
final Match? match = tabooPattern.firstMatch(line);
if (match != null) {
errors.add('${file.path}:${index + 1}: Found use of the taboo word "${match.group(1)}" in documentation string.');
}
}
}
if (errors.isNotEmpty) {
foundError(<String>[
'${bold}Avoid the word "simply" in documentation. See https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#use-the-passive-voice-recommend-do-not-require-never-say-things-are-simple for details.$reset',
'${bold}In many cases these words can be omitted without loss of generality; in other cases it may require a bit of rewording to avoid implying that the task is simple.$reset',
'${bold}Similarly, avoid using "note:" or the phrase "note that". See https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#avoid-empty-prose for details.$reset',
...errors,
]);
}
}
const List<String> _kIgnoreList = <String>[
'Runner.rc.tmpl',
'flutter_window.cpp',
];
final String _kIntegrationTestsRelativePath = path.join('dev', 'integration_tests');
final String _kTemplateRelativePath = path.join('packages', 'flutter_tools', 'templates', 'app_shared', 'windows.tmpl', 'runner');
final String _kWindowsRunnerSubPath = path.join('windows', 'runner');
const String _kProjectNameKey = '{{projectName}}';
const String _kTmplExt = '.tmpl';
final String _kLicensePath = path.join('dev', 'conductor', 'core', 'lib', 'src', 'proto', 'license_header.txt');
String _getFlutterLicense(String flutterRoot) {
return '${File(path.join(flutterRoot, _kLicensePath)).readAsLinesSync().join("\n")}\n\n';
}
String _removeLicenseIfPresent(String fileContents, String license) {
if (fileContents.startsWith(license)) {
return fileContents.substring(license.length);
}
return fileContents;
}
Future<void> verifyIntegrationTestTemplateFiles(String flutterRoot) async {
final List<String> errors = <String>[];
final String license = _getFlutterLicense(flutterRoot);
final String integrationTestsPath = path.join(flutterRoot, _kIntegrationTestsRelativePath);
final String templatePath = path.join(flutterRoot, _kTemplateRelativePath);
final Iterable<Directory>subDirs = Directory(integrationTestsPath).listSync().toList().whereType<Directory>();
for (final Directory testPath in subDirs) {
final String projectName = path.basename(testPath.path);
final String runnerPath = path.join(testPath.path, _kWindowsRunnerSubPath);
final Directory runner = Directory(runnerPath);
if (!runner.existsSync()) {
continue;
}
final Iterable<File> files = Directory(templatePath).listSync().toList().whereType<File>();
for (final File templateFile in files) {
final String fileName = path.basename(templateFile.path);
if (_kIgnoreList.contains(fileName)) {
continue;
}
String templateFileContents = templateFile.readAsLinesSync().join('\n');
String appFilePath = path.join(runnerPath, fileName);
if (fileName.endsWith(_kTmplExt)) {
appFilePath = appFilePath.substring(0, appFilePath.length - _kTmplExt.length); // Remove '.tmpl' from app file path
templateFileContents = templateFileContents.replaceAll(_kProjectNameKey, projectName); // Substitute template project name
}
String appFileContents = File(appFilePath).readAsLinesSync().join('\n');
appFileContents = _removeLicenseIfPresent(appFileContents, license);
if (appFileContents != templateFileContents) {
int indexOfDifference;
for (indexOfDifference = 0; indexOfDifference < appFileContents.length; indexOfDifference++) {
if (indexOfDifference >= templateFileContents.length || templateFileContents.codeUnitAt(indexOfDifference) != appFileContents.codeUnitAt(indexOfDifference)) {
break;
}
}
final String error = '''
Error: file $fileName mismatched for integration test $testPath
Verify the integration test has been migrated to the latest app template.
=====$appFilePath======
$appFileContents
=====${templateFile.path}======
$templateFileContents
==========
Diff at character #$indexOfDifference
''';
errors.add(error);
}
}
}
if (errors.isNotEmpty) {
foundError(errors);
}
}
Future<CommandResult> _runFlutterAnalyze(String workingDirectory, {
List<String> options = const <String>[],
String? failureMessage,
}) async {
return runCommand(
flutter,
<String>['analyze', ...options],
workingDirectory: workingDirectory,
failureMessage: failureMessage,
);
}
// These files legitimately require executable permissions
const Set<String> kExecutableAllowlist = <String>{
'bin/dart',
'bin/flutter',
'bin/internal/update_dart_sdk.sh',
'dev/bots/accept_android_sdk_licenses.sh',
'dev/bots/codelabs_build_test.sh',
'dev/bots/docs.sh',
'dev/conductor/bin/conductor',
'dev/conductor/bin/packages_autoroller',
'dev/conductor/core/lib/src/proto/compile_proto.sh',
'dev/customer_testing/ci.sh',
'dev/integration_tests/flutter_gallery/tool/run_instrumentation_test.sh',
'dev/integration_tests/ios_add2app_life_cycle/build_and_test.sh',
'dev/integration_tests/deferred_components_test/download_assets.sh',
'dev/integration_tests/deferred_components_test/run_release_test.sh',
'dev/tools/gen_keycodes/bin/gen_keycodes',
'dev/tools/repackage_gradle_wrapper.sh',
'packages/flutter_tools/bin/macos_assemble.sh',
'packages/flutter_tools/bin/tool_backend.sh',
'packages/flutter_tools/bin/xcode_backend.sh',
};
Future<void> _checkForNewExecutables() async {
// 0b001001001
const int executableBitMask = 0x49;
final List<File> files = await _gitFiles(flutterRoot);
final List<String> errors = <String>[];
for (final File file in files) {
final String relativePath = path.relative(
file.path,
from: flutterRoot,
);
final FileStat stat = file.statSync();
final bool isExecutable = stat.mode & executableBitMask != 0x0;
if (isExecutable && !kExecutableAllowlist.contains(relativePath)) {
errors.add('$relativePath is executable: ${(stat.mode & 0x1FF).toRadixString(2)}');
}
}
if (errors.isNotEmpty) {
throw Exception(
'${errors.join('\n')}\n'
'found ${errors.length} unexpected executable file'
'${errors.length == 1 ? '' : 's'}! If this was intended, you '
'must add this file to kExecutableAllowlist in dev/bots/analyze.dart',
);
}
}
final RegExp _importPattern = RegExp(r'''^\s*import (['"])package:flutter/([^.]+)\.dart\1''');
final RegExp _importMetaPattern = RegExp(r'''^\s*import (['"])package:meta/meta\.dart\1''');
Future<Set<String>> _findFlutterDependencies(String srcPath, List<String> errors, { bool checkForMeta = false }) async {
return _allFiles(srcPath, 'dart', minimumMatches: 1)
.map<Set<String>>((File file) {
final Set<String> result = <String>{};
for (final String line in file.readAsLinesSync()) {
Match? match = _importPattern.firstMatch(line);
if (match != null) {
result.add(match.group(2)!);
}
if (checkForMeta) {
match = _importMetaPattern.firstMatch(line);
if (match != null) {
errors.add(
'${file.path}\nThis package imports the ${yellow}meta$reset package.\n'
'You should instead import the "foundation.dart" library.'
);
}
}
}
return result;
})
.reduce((Set<String>? value, Set<String> element) {
value ??= <String>{};
value.addAll(element);
return value;
});
}
List<T>? _deepSearch<T>(Map<T, Set<T>> map, T start, [ Set<T>? seen ]) {
if (map[start] == null) {
return null; // We catch these separately.
}
for (final T key in map[start]!) {
if (key == start) {
continue; // we catch these separately
}
if (seen != null && seen.contains(key)) {
return <T>[start, key];
}
final List<T>? result = _deepSearch<T>(
map,
key,
<T>{
if (seen == null) start else ...seen,
key,
},
);
if (result != null) {
result.insert(0, start);
// Only report the shortest chains.
// For example a->b->a, rather than c->a->b->a.
// Since we visit every node, we know the shortest chains are those
// that start and end on the loop.
if (result.first == result.last) {
return result;
}
}
}
return null;
}
bool _isGeneratedPluginRegistrant(File file) {
final String filename = path.basename(file.path);
return !file.path.contains('.pub-cache')
&& (filename == 'GeneratedPluginRegistrant.java' ||
filename == 'GeneratedPluginRegistrant.swift' ||
filename == 'GeneratedPluginRegistrant.h' ||
filename == 'GeneratedPluginRegistrant.m' ||
filename == 'generated_plugin_registrant.dart' ||
filename == 'generated_plugin_registrant.h' ||
filename == 'generated_plugin_registrant.cc');
}
| flutter/dev/bots/analyze.dart/0 | {
"file_path": "flutter/dev/bots/analyze.dart",
"repo_id": "flutter",
"token_count": 37559
} | 533 |
// THIS FILE HAS NO LICENSE.
// IT IS PART OF A TEST FOR MISSING LICENSES.
| flutter/dev/bots/test/analyze-test-input/root/packages/foo/foo.dart/0 | {
"file_path": "flutter/dev/bots/test/analyze-test-input/root/packages/foo/foo.dart",
"repo_id": "flutter",
"token_count": 24
} | 534 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io' show ProcessResult;
import 'dart:typed_data';
import 'package:file/file.dart';
import 'package:file/memory.dart';
import 'package:path/path.dart' as path;
import 'package:platform/platform.dart' show FakePlatform, Platform;
import '../../../packages/flutter_tools/test/src/fake_process_manager.dart';
import '../prepare_package/archive_creator.dart';
import '../prepare_package/archive_publisher.dart';
import '../prepare_package/common.dart';
import '../prepare_package/process_runner.dart';
import 'common.dart';
void main() {
const String testRef = 'deadbeefdeadbeefdeadbeefdeadbeefdeadbeef';
test('Throws on missing executable', () async {
// Uses a *real* process manager, since we want to know what happens if
// it can't find an executable.
final ProcessRunner processRunner = ProcessRunner(subprocessOutput: false);
expect(
expectAsync1((List<String> commandLine) async {
return processRunner.runProcess(commandLine);
})(<String>['this_executable_better_not_exist_2857632534321']),
throwsA(isA<PreparePackageException>()));
await expectLater(
() => processRunner.runProcess(<String>['this_executable_better_not_exist_2857632534321']),
throwsA(isA<PreparePackageException>().having(
(PreparePackageException error) => error.message,
'message',
contains('ProcessException: Failed to find "this_executable_better_not_exist_2857632534321" in the search path'),
)),
);
});
for (final String platformName in <String>[Platform.macOS, Platform.linux, Platform.windows]) {
final FakePlatform platform = FakePlatform(
operatingSystem: platformName,
environment: <String, String>{
'DEPOT_TOOLS': platformName == Platform.windows ? path.join('D:', 'depot_tools'): '/depot_tools',
},
);
group('ProcessRunner for $platform', () {
test('Returns stdout', () async {
final FakeProcessManager fakeProcessManager = FakeProcessManager.list(<FakeCommand>[
const FakeCommand(
command: <String>['echo', 'test',],
stdout: 'output',
stderr: 'error',
),
]);
final ProcessRunner processRunner = ProcessRunner(
subprocessOutput: false, platform: platform, processManager: fakeProcessManager);
final String output = await processRunner.runProcess(<String>['echo', 'test']);
expect(output, equals('output'));
});
test('Throws on process failure', () async {
final FakeProcessManager fakeProcessManager = FakeProcessManager.list(<FakeCommand>[
const FakeCommand(
command: <String>['echo', 'test',],
stdout: 'output',
stderr: 'error',
exitCode: -1,
),
]);
final ProcessRunner processRunner = ProcessRunner(
subprocessOutput: false, platform: platform, processManager: fakeProcessManager);
expect(
expectAsync1((List<String> commandLine) async {
return processRunner.runProcess(commandLine);
})(<String>['echo', 'test']),
throwsA(isA<PreparePackageException>()));
});
});
group('ArchiveCreator for $platformName', () {
late ArchiveCreator creator;
late Directory tempDir;
Directory flutterDir;
Directory cacheDir;
late FakeProcessManager processManager;
late FileSystem fs;
final List<List<String>> args = <List<String>>[];
final List<Map<Symbol, dynamic>> namedArgs = <Map<Symbol, dynamic>>[];
late String flutter;
late String dart;
Future<Uint8List> fakeHttpReader(Uri url, {Map<String, String>? headers}) {
return Future<Uint8List>.value(Uint8List(0));
}
setUp(() async {
processManager = FakeProcessManager.list(<FakeCommand>[]);
args.clear();
namedArgs.clear();
fs = MemoryFileSystem.test();
tempDir = fs.systemTempDirectory;
flutterDir = fs.directory(path.join(tempDir.path, 'flutter'));
flutterDir.createSync(recursive: true);
cacheDir = fs.directory(path.join(flutterDir.path, 'bin', 'cache'));
cacheDir.createSync(recursive: true);
creator = ArchiveCreator(
tempDir,
tempDir,
testRef,
Branch.beta,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
httpReader: fakeHttpReader,
);
flutter = path.join(creator.flutterRoot.absolute.path,
'bin', 'flutter');
dart = path.join(creator.flutterRoot.absolute.path,
'bin', 'cache', 'dart-sdk', 'bin', 'dart');
});
tearDown(() async {
tryToDelete(tempDir);
});
test('sets PUB_CACHE properly', () async {
final String createBase = path.join(tempDir.absolute.path, 'create_');
final String archiveName = path.join(tempDir.absolute.path,
'flutter_${platformName}_v1.2.3-beta${platform.isLinux ? '.tar.xz' : '.zip'}');
processManager.addCommands(convertResults(<String, List<ProcessResult>?>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter': null,
'git reset --hard $testRef': null,
'git remote set-url origin https://github.com/flutter/flutter.git': null,
'git gc --prune=now --aggressive': null,
'git describe --tags --exact-match $testRef': <ProcessResult>[ProcessResult(0, 0, 'v1.2.3', '')],
'$flutter --version --machine': <ProcessResult>[
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
],
'$dart --version': <ProcessResult>[
ProcessResult(0, 0, 'Dart SDK version: 2.17.0-63.0.beta (beta) (Wed Jan 26 03:48:52 2022 -0800) on "${platformName}_x64"', ''),
],
if (platform.isWindows) '7za x ${path.join(tempDir.path, 'mingit.zip')}': null,
'$flutter doctor': null,
'$flutter update-packages': null,
'$flutter precache': null,
'$flutter ide-config': null,
'$flutter create --template=app ${createBase}app': null,
'$flutter create --template=package ${createBase}package': null,
'$flutter create --template=plugin ${createBase}plugin': null,
'$flutter pub cache list': <ProcessResult>[ProcessResult(0,0,'{"packages":{}}','')],
'git clean -f -x -- **/.packages': null,
'git clean -f -x -- **/.dart_tool/': null,
if (platform.isMacOS) 'codesign -vvvv --check-notarization ${path.join(tempDir.path, 'flutter', 'bin', 'cache', 'dart-sdk', 'bin', 'dart')}': null,
if (platform.isWindows) 'attrib -h .git': null,
if (platform.isWindows) '7za a -tzip -mx=9 $archiveName flutter': null
else if (platform.isMacOS) 'zip -r -9 --symlinks $archiveName flutter': null
else if (platform.isLinux) 'tar cJf $archiveName --verbose flutter': null,
}));
await creator.initializeRepo();
await creator.createArchive();
});
test('calls the right commands for archive output', () async {
final String createBase = path.join(tempDir.absolute.path, 'create_');
final String archiveName = path.join(tempDir.absolute.path,
'flutter_${platformName}_v1.2.3-beta${platform.isLinux ? '.tar.xz' : '.zip'}');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter': null,
'git reset --hard $testRef': null,
'git remote set-url origin https://github.com/flutter/flutter.git': null,
'git gc --prune=now --aggressive': null,
'git describe --tags --exact-match $testRef': <ProcessResult>[ProcessResult(0, 0, 'v1.2.3', '')],
'$flutter --version --machine': <ProcessResult>[
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
],
'$dart --version': <ProcessResult>[
ProcessResult(0, 0, 'Dart SDK version: 2.17.0-63.0.beta (beta) (Wed Jan 26 03:48:52 2022 -0800) on "${platformName}_x64"', ''),
],
if (platform.isWindows) '7za x ${path.join(tempDir.path, 'mingit.zip')}': null,
'$flutter doctor': null,
'$flutter update-packages': null,
'$flutter precache': null,
'$flutter ide-config': null,
'$flutter create --template=app ${createBase}app': null,
'$flutter create --template=package ${createBase}package': null,
'$flutter create --template=plugin ${createBase}plugin': null,
'$flutter pub cache list': <ProcessResult>[ProcessResult(0,0,'{"packages":{}}','')],
'git clean -f -x -- **/.packages': null,
'git clean -f -x -- **/.dart_tool/': null,
if (platform.isMacOS) 'codesign -vvvv --check-notarization ${path.join(tempDir.path, 'flutter', 'bin', 'cache', 'dart-sdk', 'bin', 'dart')}': null,
if (platform.isWindows) 'attrib -h .git': null,
if (platform.isWindows) '7za a -tzip -mx=9 $archiveName flutter': null
else if (platform.isMacOS) 'zip -r -9 --symlinks $archiveName flutter': null
else if (platform.isLinux) 'tar cJf $archiveName --verbose flutter': null,
};
processManager.addCommands(convertResults(calls));
creator = ArchiveCreator(
tempDir,
tempDir,
testRef,
Branch.beta,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
httpReader: fakeHttpReader,
);
await creator.initializeRepo();
await creator.createArchive();
});
test('adds the arch name to the archive for non-x64', () async {
final String createBase = path.join(tempDir.absolute.path, 'create_');
final String archiveName = path.join(tempDir.absolute.path,
'flutter_${platformName}_arm64_v1.2.3-beta${platform.isLinux ? '.tar.xz' : '.zip'}');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter': null,
'git reset --hard $testRef': null,
'git remote set-url origin https://github.com/flutter/flutter.git': null,
'git gc --prune=now --aggressive': null,
'git describe --tags --exact-match $testRef': <ProcessResult>[ProcessResult(0, 0, 'v1.2.3', '')],
'$flutter --version --machine': <ProcessResult>[
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
],
'$dart --version': <ProcessResult>[
ProcessResult(0, 0, 'Dart SDK version: 2.17.0-63.0.beta (beta) (Wed Jan 26 03:48:52 2022 -0800) on "${platformName}_arm64"', ''),
],
if (platform.isWindows) '7za x ${path.join(tempDir.path, 'mingit.zip')}': null,
'$flutter doctor': null,
'$flutter update-packages': null,
'$flutter precache': null,
'$flutter ide-config': null,
'$flutter create --template=app ${createBase}app': null,
'$flutter create --template=package ${createBase}package': null,
'$flutter create --template=plugin ${createBase}plugin': null,
'$flutter pub cache list': <ProcessResult>[ProcessResult(0,0,'{"packages":{}}','')],
'git clean -f -x -- **/.packages': null,
'git clean -f -x -- **/.dart_tool/': null,
if (platform.isMacOS) 'codesign -vvvv --check-notarization ${path.join(tempDir.path, 'flutter', 'bin', 'cache', 'dart-sdk', 'bin', 'dart')}': null,
if (platform.isWindows) 'attrib -h .git': null,
if (platform.isWindows) '7za a -tzip -mx=9 $archiveName flutter': null
else if (platform.isMacOS) 'zip -r -9 --symlinks $archiveName flutter': null
else if (platform.isLinux) 'tar cJf $archiveName --verbose flutter': null,
};
processManager.addCommands(convertResults(calls));
creator = ArchiveCreator(
tempDir,
tempDir,
testRef,
Branch.beta,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
httpReader: fakeHttpReader,
);
await creator.initializeRepo();
await creator.createArchive();
});
test('throws when a command errors out', () async {
final Map<String, List<ProcessResult>> calls = <String, List<ProcessResult>>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter':
<ProcessResult>[ProcessResult(0, 0, 'output1', '')],
'git reset --hard $testRef': <ProcessResult>[ProcessResult(0, -1, 'output2', '')],
};
processManager.addCommands(convertResults(calls));
expect(expectAsync0(creator.initializeRepo), throwsA(isA<PreparePackageException>()));
});
test('non-strict mode calls the right commands', () async {
final String createBase = path.join(tempDir.absolute.path, 'create_');
final String archiveName = path.join(tempDir.absolute.path,
'flutter_${platformName}_v1.2.3-beta${platform.isLinux ? '.tar.xz' : '.zip'}');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter': null,
'git reset --hard $testRef': null,
'git remote set-url origin https://github.com/flutter/flutter.git': null,
'git gc --prune=now --aggressive': null,
'git describe --tags --abbrev=0 $testRef': <ProcessResult>[ProcessResult(0, 0, 'v1.2.3', '')],
'$flutter --version --machine': <ProcessResult>[
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
],
'$dart --version': <ProcessResult>[
ProcessResult(0, 0, 'Dart SDK version: 2.17.0-63.0.beta (beta) (Wed Jan 26 03:48:52 2022 -0800) on "${platformName}_x64"', ''),
],
if (platform.isWindows) '7za x ${path.join(tempDir.path, 'mingit.zip')}': null,
'$flutter doctor': null,
'$flutter update-packages': null,
'$flutter precache': null,
'$flutter ide-config': null,
'$flutter create --template=app ${createBase}app': null,
'$flutter create --template=package ${createBase}package': null,
'$flutter create --template=plugin ${createBase}plugin': null,
'$flutter pub cache list': <ProcessResult>[ProcessResult(0,0,'{"packages":{}}','')],
'git clean -f -x -- **/.packages': null,
'git clean -f -x -- **/.dart_tool/': null,
if (platform.isWindows) 'attrib -h .git': null,
if (platform.isWindows) '7za a -tzip -mx=9 $archiveName flutter': null
else if (platform.isMacOS) 'zip -r -9 --symlinks $archiveName flutter': null
else if (platform.isLinux) 'tar cJf $archiveName --verbose flutter': null,
};
processManager.addCommands(convertResults(calls));
creator = ArchiveCreator(
tempDir,
tempDir,
testRef,
Branch.beta,
fs: fs,
strict: false,
processManager: processManager,
subprocessOutput: false,
platform: platform,
httpReader: fakeHttpReader,
);
await creator.initializeRepo();
await creator.createArchive();
});
test('fails if binary is not codesigned', () async {
final String createBase = path.join(tempDir.absolute.path, 'create_');
final String archiveName = path.join(tempDir.absolute.path,
'flutter_${platformName}_v1.2.3-beta${platform.isLinux ? '.tar.xz' : '.zip'}');
final ProcessResult codesignFailure = ProcessResult(1, 1, '', 'code object is not signed at all');
final String binPath = path.join(tempDir.path, 'flutter', 'bin', 'cache', 'dart-sdk', 'bin', 'dart');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
'git clone -b beta https://flutter.googlesource.com/mirrors/flutter': null,
'git reset --hard $testRef': null,
'git remote set-url origin https://github.com/flutter/flutter.git': null,
'git gc --prune=now --aggressive': null,
'git describe --tags --exact-match $testRef': <ProcessResult>[ProcessResult(0, 0, 'v1.2.3', '')],
'$flutter --version --machine': <ProcessResult>[
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
ProcessResult(0, 0, '{"dartSdkVersion": "3.2.1"}', ''),
],
'$dart --version': <ProcessResult>[
ProcessResult(0, 0, 'Dart SDK version: 2.17.0-63.0.beta (beta) (Wed Jan 26 03:48:52 2022 -0800) on "${platformName}_x64"', ''),
],
if (platform.isWindows) '7za x ${path.join(tempDir.path, 'mingit.zip')}': null,
'$flutter doctor': null,
'$flutter update-packages': null,
'$flutter precache': null,
'$flutter ide-config': null,
'$flutter create --template=app ${createBase}app': null,
'$flutter create --template=package ${createBase}package': null,
'$flutter create --template=plugin ${createBase}plugin': null,
'$flutter pub cache list': <ProcessResult>[ProcessResult(0,0,'{"packages":{}}','')],
'git clean -f -x -- **/.packages': null,
'git clean -f -x -- **/.dart_tool/': null,
if (platform.isMacOS) 'codesign -vvvv --check-notarization $binPath': <ProcessResult>[codesignFailure],
if (platform.isWindows) 'attrib -h .git': null,
if (platform.isWindows) '7za a -tzip -mx=9 $archiveName flutter': null
else if (platform.isMacOS) 'zip -r -9 --symlinks $archiveName flutter': null
else if (platform.isLinux) 'tar cJf $archiveName flutter': null,
};
processManager.addCommands(convertResults(calls));
creator = ArchiveCreator(
tempDir,
tempDir,
testRef,
Branch.beta,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
httpReader: fakeHttpReader,
);
await creator.initializeRepo();
await expectLater(
() => creator.createArchive(),
throwsA(isA<PreparePackageException>().having(
(PreparePackageException exception) => exception.message,
'message',
contains('The binary $binPath was not codesigned!'),
)),
);
}, skip: !platform.isMacOS); // [intended] codesign is only available on macOS
});
group('ArchivePublisher for $platformName', () {
late FakeProcessManager processManager;
late Directory tempDir;
late FileSystem fs;
final String gsutilCall = platform.isWindows
? 'python3 ${path.join("D:", "depot_tools", "gsutil.py")}'
: 'python3 ${path.join("/", "depot_tools", "gsutil.py")}';
final String releasesName = 'releases_$platformName.json';
final String archiveName = platform.isLinux ? 'archive.tar.xz' : 'archive.zip';
final String archiveMime = platform.isLinux ? 'application/x-gtar' : 'application/zip';
final String gsArchivePath = 'gs://flutter_infra_release/releases/stable/$platformName/$archiveName';
setUp(() async {
fs = MemoryFileSystem.test(style: platform.isWindows ? FileSystemStyle.windows : FileSystemStyle.posix);
processManager = FakeProcessManager.list(<FakeCommand>[]);
tempDir = fs.systemTempDirectory.createTempSync('flutter_prepage_package_test.');
});
tearDown(() async {
tryToDelete(tempDir);
});
test('calls the right processes', () async {
final String archivePath = path.join(tempDir.absolute.path, archiveName);
final String jsonPath = path.join(tempDir.absolute.path, releasesName);
final String gsJsonPath = 'gs://flutter_infra_release/releases/$releasesName';
final String releasesJson = '''
{
"base_url": "https://storage.googleapis.com/flutter_infra_release/releases",
"current_release": {
"beta": "3ea4d06340a97a1e9d7cae97567c64e0569dcaa2",
"beta": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"
},
"releases": [
{
"hash": "5a58b36e36b8d7aace89d3950e6deb307956a6a0",
"channel": "beta",
"version": "v0.2.3",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.3-beta.zip",
"sha256": "4fe85a822093e81cb5a66c7fc263f68de39b5797b294191b6d75e7afcc86aff8",
"dart_sdk_arch": "x64"
},
{
"hash": "b9bd51cc36b706215915711e580851901faebb40",
"channel": "beta",
"version": "v0.2.2",
"release_date": "2018-03-16T18:48:13.375013Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.2-beta.zip",
"sha256": "6073331168cdb37a4637a5dc073d6a7ef4e466321effa2c529fa27d2253a4d4b",
"dart_sdk_arch": "x64"
},
{
"hash": "$testRef",
"channel": "stable",
"version": "v0.0.0",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "stable/$platformName/flutter_${platformName}_v0.0.0-beta.zip",
"sha256": "5dd34873b3a3e214a32fd30c2c319a0f46e608afb72f0d450b2d621a6d02aebd",
"dart_sdk_arch": "x64"
}
]
}
''';
fs.file(jsonPath).writeAsStringSync(releasesJson);
fs.file(archivePath).writeAsStringSync('archive contents');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
// This process fails because the file does NOT already exist
'$gsutilCall -- cp $gsJsonPath $jsonPath': null,
'$gsutilCall -- stat $gsArchivePath': <ProcessResult>[ProcessResult(0, 1, '', '')],
'$gsutilCall -- rm $gsArchivePath': null,
'$gsutilCall -- -h Content-Type:$archiveMime cp $archivePath $gsArchivePath': null,
'$gsutilCall -- rm $gsJsonPath': null,
'$gsutilCall -- -h Content-Type:application/json -h Cache-Control:max-age=60 cp $jsonPath $gsJsonPath': null,
};
processManager.addCommands(convertResults(calls));
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
outputFile.createSync();
assert(tempDir.existsSync());
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'x64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
assert(tempDir.existsSync());
await publisher.generateLocalMetadata();
await publisher.publishArchive();
final File releaseFile = fs.file(jsonPath);
expect(releaseFile.existsSync(), isTrue);
final String contents = releaseFile.readAsStringSync();
// Make sure new data is added.
expect(contents, contains('"hash": "$testRef"'));
expect(contents, contains('"channel": "stable"'));
expect(contents, contains('"archive": "stable/$platformName/$archiveName"'));
expect(contents, contains('"sha256": "f69f4865f861193a91d1c5544a894167a7137b788d10bac8edbf5d095f45cb4d"'));
// Make sure existing entries are preserved.
expect(contents, contains('"hash": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"'));
expect(contents, contains('"hash": "b9bd51cc36b706215915711e580851901faebb40"'));
expect(contents, contains('"channel": "beta"'));
expect(contents, contains('"channel": "beta"'));
// Make sure old matching entries are removed.
expect(contents, isNot(contains('v0.0.0')));
final Map<String, dynamic> jsonData = json.decode(contents) as Map<String, dynamic>;
final List<dynamic> releases = jsonData['releases'] as List<dynamic>;
expect(releases.length, equals(3));
// Make sure the new entry is first (and hopefully it takes less than a
// minute to go from publishArchive above to this line!).
expect(
DateTime.now().difference(DateTime.parse((releases[0] as Map<String, dynamic>)['release_date'] as String)),
lessThan(const Duration(minutes: 1)),
);
const JsonEncoder encoder = JsonEncoder.withIndent(' ');
expect(contents, equals(encoder.convert(jsonData)));
});
test('contains Dart SDK version info', () async {
final String archivePath = path.join(tempDir.absolute.path, archiveName);
final String jsonPath = path.join(tempDir.absolute.path, releasesName);
final String gsJsonPath = 'gs://flutter_infra_release/releases/$releasesName';
final String releasesJson = '''
{
"base_url": "https://storage.googleapis.com/flutter_infra_release/releases",
"current_release": {
"beta": "3ea4d06340a97a1e9d7cae97567c64e0569dcaa2",
"beta": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"
},
"releases": [
{
"hash": "5a58b36e36b8d7aace89d3950e6deb307956a6a0",
"channel": "beta",
"version": "v0.2.3",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.3-beta.zip",
"sha256": "4fe85a822093e81cb5a66c7fc263f68de39b5797b294191b6d75e7afcc86aff8"
},
{
"hash": "b9bd51cc36b706215915711e580851901faebb40",
"channel": "beta",
"version": "v0.2.2",
"release_date": "2018-03-16T18:48:13.375013Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.2-beta.zip",
"sha256": "6073331168cdb37a4637a5dc073d6a7ef4e466321effa2c529fa27d2253a4d4b"
},
{
"hash": "$testRef",
"channel": "stable",
"version": "v0.0.0",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "stable/$platformName/flutter_${platformName}_v0.0.0-beta.zip",
"sha256": "5dd34873b3a3e214a32fd30c2c319a0f46e608afb72f0d450b2d621a6d02aebd"
}
]
}
''';
fs.file(jsonPath).writeAsStringSync(releasesJson);
fs.file(archivePath).writeAsStringSync('archive contents');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
// This process fails because the file does NOT already exist
'$gsutilCall -- cp $gsJsonPath $jsonPath': null,
'$gsutilCall -- stat $gsArchivePath': <ProcessResult>[ProcessResult(0, 1, '', '')],
'$gsutilCall -- rm $gsArchivePath': null,
'$gsutilCall -- -h Content-Type:$archiveMime cp $archivePath $gsArchivePath': null,
'$gsutilCall -- rm $gsJsonPath': null,
'$gsutilCall -- -h Content-Type:application/json -h Cache-Control:max-age=60 cp $jsonPath $gsJsonPath': null,
};
processManager.addCommands(convertResults(calls));
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
outputFile.createSync();
assert(tempDir.existsSync());
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'x64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
assert(tempDir.existsSync());
await publisher.generateLocalMetadata();
await publisher.publishArchive();
final File releaseFile = fs.file(jsonPath);
expect(releaseFile.existsSync(), isTrue);
final String contents = releaseFile.readAsStringSync();
expect(contents, contains('"dart_sdk_version": "3.2.1"'));
expect(contents, contains('"dart_sdk_arch": "x64"'));
});
test('Supports multiple architectures', () async {
final String archivePath = path.join(tempDir.absolute.path, archiveName);
final String jsonPath = path.join(tempDir.absolute.path, releasesName);
final String gsJsonPath = 'gs://flutter_infra_release/releases/$releasesName';
final String releasesJson = '''
{
"base_url": "https://storage.googleapis.com/flutter_infra_release/releases",
"current_release": {
"beta": "3ea4d06340a97a1e9d7cae97567c64e0569dcaa2",
"beta": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"
},
"releases": [
{
"hash": "deadbeefdeadbeefdeadbeefdeadbeefdeadbeef",
"channel": "stable",
"version": "v1.2.3",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.3-beta.zip",
"sha256": "4fe85a822093e81cb5a66c7fc263f68de39b5797b294191b6d75e7afcc86aff8",
"dart_sdk_arch": "x64"
}
]
}
''';
fs.file(jsonPath).writeAsStringSync(releasesJson);
fs.file(archivePath).writeAsStringSync('archive contents');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
// This process fails because the file does NOT already exist
'$gsutilCall -- cp $gsJsonPath $jsonPath': null,
'$gsutilCall -- stat $gsArchivePath': <ProcessResult>[ProcessResult(0, 1, '', '')],
'$gsutilCall -- rm $gsArchivePath': null,
'$gsutilCall -- -h Content-Type:$archiveMime cp $archivePath $gsArchivePath': null,
'$gsutilCall -- rm $gsJsonPath': null,
'$gsutilCall -- -h Content-Type:application/json -h Cache-Control:max-age=60 cp $jsonPath $gsJsonPath': null,
};
processManager.addCommands(convertResults(calls));
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
outputFile.createSync();
assert(tempDir.existsSync());
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'arm64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
assert(tempDir.existsSync());
await publisher.generateLocalMetadata();
await publisher.publishArchive();
final File releaseFile = fs.file(jsonPath);
expect(releaseFile.existsSync(), isTrue);
final String contents = releaseFile.readAsStringSync();
final Map<String, dynamic> releases = jsonDecode(contents) as Map<String, dynamic>;
expect((releases['releases'] as List<dynamic>).length, equals(2));
});
test('updates base_url from old bucket to new bucket', () async {
final String archivePath = path.join(tempDir.absolute.path, archiveName);
final String jsonPath = path.join(tempDir.absolute.path, releasesName);
final String gsJsonPath = 'gs://flutter_infra_release/releases/$releasesName';
final String releasesJson = '''
{
"base_url": "https://storage.googleapis.com/flutter_infra_release/releases",
"current_release": {
"beta": "3ea4d06340a97a1e9d7cae97567c64e0569dcaa2",
"beta": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"
},
"releases": [
{
"hash": "5a58b36e36b8d7aace89d3950e6deb307956a6a0",
"channel": "beta",
"version": "v0.2.3",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.3-beta.zip",
"sha256": "4fe85a822093e81cb5a66c7fc263f68de39b5797b294191b6d75e7afcc86aff8"
},
{
"hash": "b9bd51cc36b706215915711e580851901faebb40",
"channel": "beta",
"version": "v0.2.2",
"release_date": "2018-03-16T18:48:13.375013Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.2-beta.zip",
"sha256": "6073331168cdb37a4637a5dc073d6a7ef4e466321effa2c529fa27d2253a4d4b"
},
{
"hash": "$testRef",
"channel": "stable",
"version": "v0.0.0",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "stable/$platformName/flutter_${platformName}_v0.0.0-beta.zip",
"sha256": "5dd34873b3a3e214a32fd30c2c319a0f46e608afb72f0d450b2d621a6d02aebd"
}
]
}
''';
fs.file(jsonPath).writeAsStringSync(releasesJson);
fs.file(archivePath).writeAsStringSync('archive contents');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
// This process fails because the file does NOT already exist
'$gsutilCall -- cp $gsJsonPath $jsonPath': null,
'$gsutilCall -- stat $gsArchivePath': <ProcessResult>[ProcessResult(0, 1, '', '')],
'$gsutilCall -- rm $gsArchivePath': null,
'$gsutilCall -- -h Content-Type:$archiveMime cp $archivePath $gsArchivePath': null,
'$gsutilCall -- rm $gsJsonPath': null,
'$gsutilCall -- -h Content-Type:application/json -h Cache-Control:max-age=60 cp $jsonPath $gsJsonPath': null,
};
processManager.addCommands(convertResults(calls));
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
outputFile.createSync();
assert(tempDir.existsSync());
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'x64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
assert(tempDir.existsSync());
await publisher.generateLocalMetadata();
await publisher.publishArchive();
final File releaseFile = fs.file(jsonPath);
expect(releaseFile.existsSync(), isTrue);
final String contents = releaseFile.readAsStringSync();
final Map<String, dynamic> jsonData = json.decode(contents) as Map<String, dynamic>;
expect(jsonData['base_url'], 'https://storage.googleapis.com/flutter_infra_release/releases');
});
test('publishArchive throws if forceUpload is false and artifact already exists on cloud storage', () async {
final String archiveName = platform.isLinux ? 'archive.tar.xz' : 'archive.zip';
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'x64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
final Map<String, List<ProcessResult>> calls = <String, List<ProcessResult>>{
// This process returns 0 because file already exists
'$gsutilCall -- stat $gsArchivePath': <ProcessResult>[ProcessResult(0, 0, '', '')],
};
processManager.addCommands(convertResults(calls));
expect(() async => publisher.publishArchive(), throwsException);
});
test('publishArchive does not throw if forceUpload is true and artifact already exists on cloud storage', () async {
final String archiveName = platform.isLinux ? 'archive.tar.xz' : 'archive.zip';
final File outputFile = fs.file(path.join(tempDir.absolute.path, archiveName));
final ArchivePublisher publisher = ArchivePublisher(
tempDir,
testRef,
Branch.stable,
<String, String>{
'frameworkVersionFromGit': 'v1.2.3',
'dartSdkVersion': '3.2.1',
'dartTargetArch': 'x64',
},
outputFile,
false,
fs: fs,
processManager: processManager,
subprocessOutput: false,
platform: platform,
);
final String archivePath = path.join(tempDir.absolute.path, archiveName);
final String jsonPath = path.join(tempDir.absolute.path, releasesName);
final String gsJsonPath = 'gs://flutter_infra_release/releases/$releasesName';
final String releasesJson = '''
{
"base_url": "https://storage.googleapis.com/flutter_infra_release/releases",
"current_release": {
"beta": "3ea4d06340a97a1e9d7cae97567c64e0569dcaa2",
"beta": "5a58b36e36b8d7aace89d3950e6deb307956a6a0"
},
"releases": [
{
"hash": "5a58b36e36b8d7aace89d3950e6deb307956a6a0",
"channel": "beta",
"version": "v0.2.3",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.3-beta.zip",
"sha256": "4fe85a822093e81cb5a66c7fc263f68de39b5797b294191b6d75e7afcc86aff8"
},
{
"hash": "b9bd51cc36b706215915711e580851901faebb40",
"channel": "beta",
"version": "v0.2.2",
"release_date": "2018-03-16T18:48:13.375013Z",
"archive": "beta/$platformName/flutter_${platformName}_v0.2.2-beta.zip",
"sha256": "6073331168cdb37a4637a5dc073d6a7ef4e466321effa2c529fa27d2253a4d4b"
},
{
"hash": "$testRef",
"channel": "stable",
"version": "v0.0.0",
"release_date": "2018-03-20T01:47:02.851729Z",
"archive": "stable/$platformName/flutter_${platformName}_v0.0.0-beta.zip",
"sha256": "5dd34873b3a3e214a32fd30c2c319a0f46e608afb72f0d450b2d621a6d02aebd"
}
]
}
''';
fs.file(jsonPath).writeAsStringSync(releasesJson);
fs.file(archivePath).writeAsStringSync('archive contents');
final Map<String, List<ProcessResult>?> calls = <String, List<ProcessResult>?>{
'$gsutilCall -- cp $gsJsonPath $jsonPath': null,
'$gsutilCall -- rm $gsArchivePath': null,
'$gsutilCall -- -h Content-Type:$archiveMime cp $archivePath $gsArchivePath': null,
'$gsutilCall -- rm $gsJsonPath': null,
'$gsutilCall -- -h Content-Type:application/json -h Cache-Control:max-age=60 cp $jsonPath $gsJsonPath': null,
};
processManager.addCommands(convertResults(calls));
assert(tempDir.existsSync());
await publisher.generateLocalMetadata();
await publisher.publishArchive(true);
});
});
}
}
List<FakeCommand> convertResults(Map<String, List<ProcessResult>?> results) {
final List<FakeCommand> commands = <FakeCommand>[];
for (final String key in results.keys) {
final List<ProcessResult>? candidates = results[key];
final List<String> args = key.split(' ');
if (candidates == null) {
commands.add(FakeCommand(
command: args,
));
} else {
for (final ProcessResult result in candidates) {
commands.add(FakeCommand(
command: args,
exitCode: result.exitCode,
stderr: result.stderr.toString(),
stdout: result.stdout.toString(),
));
}
}
}
return commands;
}
| flutter/dev/bots/test/prepare_package_test.dart/0 | {
"file_path": "flutter/dev/bots/test/prepare_package_test.dart",
"repo_id": "flutter",
"token_count": 17734
} | 535 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:conductor_core/src/proto/conductor_state.pb.dart' as pb;
import 'package:conductor_core/src/state.dart';
import 'package:file/file.dart';
import 'package:file/memory.dart';
import './common.dart';
void main() {
test('writeStateToFile() pretty-prints JSON with 2 spaces', () {
final MemoryFileSystem fileSystem = MemoryFileSystem.test();
final File stateFile = fileSystem.file('/path/to/statefile.json')
..createSync(recursive: true);
const String candidateBranch = 'flutter-2.3-candidate.0';
final pb.ConductorState state = pb.ConductorState.create()
..releaseChannel = 'stable'
..releaseVersion = '2.3.4'
..engine = (pb.Repository.create()
..candidateBranch = candidateBranch
..upstream = (pb.Remote.create()
..name = 'upstream'
..url = '[email protected]:flutter/engine.git'
)
)
..framework = (pb.Repository.create()
..candidateBranch = candidateBranch
..upstream = (pb.Remote.create()
..name = 'upstream'
..url = '[email protected]:flutter/flutter.git'
)
);
writeStateToFile(
stateFile,
state,
<String>['[status] hello world'],
);
final String serializedState = stateFile.readAsStringSync();
const String expectedString = '''
{
"releaseChannel": "stable",
"releaseVersion": "2.3.4",
"engine": {
"candidateBranch": "flutter-2.3-candidate.0",
"upstream": {
"name": "upstream",
"url": "[email protected]:flutter/engine.git"
}
},
"framework": {
"candidateBranch": "flutter-2.3-candidate.0",
"upstream": {
"name": "upstream",
"url": "[email protected]:flutter/flutter.git"
}
},
"logs": [
"[status] hello world"
]
}''';
expect(serializedState, expectedString);
});
}
| flutter/dev/conductor/core/test/state_test.dart/0 | {
"file_path": "flutter/dev/conductor/core/test/state_test.dart",
"repo_id": "flutter",
"token_count": 813
} | 536 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'dart:typed_data';
import 'package:collection/collection.dart';
import 'package:flutter_devicelab/framework/devices.dart';
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/task_result.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:flutter_devicelab/tasks/integration_tests.dart';
import 'package:path/path.dart' as path;
import 'package:standard_message_codec/standard_message_codec.dart';
Future<void> main() async {
deviceOperatingSystem = DeviceOperatingSystem.ios;
await task(() async {
await createFlavorsTest().call();
await createIntegrationTestFlavorsTest().call();
// test install and uninstall of flavors app
final String projectDir = '${flutterDirectory.path}/dev/integration_tests/flavors';
final TaskResult installTestsResult = await inDirectory(
projectDir,
() async {
final List<TaskResult> testResults = <TaskResult>[
await _testInstallDebugPaidFlavor(projectDir),
await _testInstallBogusFlavor(),
];
final TaskResult? firstInstallFailure = testResults
.firstWhereOrNull((TaskResult element) => element.failed);
return firstInstallFailure ?? TaskResult.success(null);
},
);
return installTestsResult;
});
}
Future<TaskResult> _testInstallDebugPaidFlavor(String projectDir) async {
await evalFlutter(
'install',
options: <String>['--flavor', 'paid'],
);
final Uint8List assetManifestFileData = File(
path.join(
projectDir,
'build',
'ios',
'iphoneos',
'Paid App.app',
'Frameworks',
'App.framework',
'flutter_assets',
'AssetManifest.bin',
),
).readAsBytesSync();
final Map<Object?, Object?> assetManifest = const StandardMessageCodec()
.decodeMessage(ByteData.sublistView(assetManifestFileData)) as Map<Object?, Object?>;
if (assetManifest.containsKey('assets/free/free.txt')) {
return TaskResult.failure('Expected the asset "assets/free/free.txt", which '
' was declared with a flavor of "free" to not be included in the asset bundle '
' because the --flavor was set to "paid".');
}
if (!assetManifest.containsKey('assets/paid/paid.txt')) {
return TaskResult.failure('Expected the asset "assets/paid/paid.txt", which '
' was declared with a flavor of "paid" to be included in the asset bundle '
' because the --flavor was set to "paid".');
}
await flutter(
'install',
options: <String>['--flavor', 'paid', '--uninstall-only'],
);
return TaskResult.success(null);
}
Future<TaskResult> _testInstallBogusFlavor() async {
final StringBuffer stderr = StringBuffer();
await evalFlutter(
'install',
canFail: true,
stderr: stderr,
options: <String>['--flavor', 'bogus'],
);
final String stderrString = stderr.toString();
if (!stderrString.contains('The Xcode project defines schemes: free, paid')) {
print(stderrString);
return TaskResult.failure('Should not succeed with bogus flavor');
}
return TaskResult.success(null);
}
| flutter/dev/devicelab/bin/tasks/flavors_test_ios.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/flavors_test_ios.dart",
"repo_id": "flutter",
"token_count": 1178
} | 537 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_devicelab/framework/devices.dart';
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:flutter_devicelab/tasks/perf_tests.dart';
Future<void> main() async {
deviceOperatingSystem = DeviceOperatingSystem.ios;
await task(PerfTest(
'${flutterDirectory.path}/dev/benchmarks/macrobenchmarks',
'test_driver/large_image_changer.dart',
'large_image_changer',
// This benchmark doesn't care about frame times, frame times will be heavily
// impacted by IO time for loading the image initially.
benchmarkScoreKeys: <String>[
'average_cpu_usage',
'average_gpu_usage',
'average_memory_usage',
'90th_percentile_memory_usage',
'99th_percentile_memory_usage',
'new_gen_gc_count',
'old_gen_gc_count',
],
).run);
}
| flutter/dev/devicelab/bin/tasks/large_image_changer_perf_ios.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/large_image_changer_perf_ios.dart",
"repo_id": "flutter",
"token_count": 373
} | 538 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/ios.dart';
import 'package:flutter_devicelab/framework/task_result.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:path/path.dart' as path;
Future<void> main() async {
await task(() async {
final String projectDirectory = '${flutterDirectory.path}/dev/integration_tests/flutter_gallery';
await inDirectory(projectDirectory, () async {
section('Build gallery app');
await flutter(
'build',
options: <String>[
'macos',
'-v',
'--debug',
],
);
});
section('Run platform unit tests');
if (!await runXcodeTests(
platformDirectory: path.join(projectDirectory, 'macos'),
destination: 'platform=macOS',
testName: 'native_ui_tests_macos',
skipCodesign: true,
)) {
return TaskResult.failure('Platform unit tests failed');
}
return TaskResult.success(null);
});
}
| flutter/dev/devicelab/bin/tasks/native_ui_tests_macos.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/native_ui_tests_macos.dart",
"repo_id": "flutter",
"token_count": 447
} | 539 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io';
import 'package:collection/collection.dart';
import 'package:metrics_center/metrics_center.dart';
/// Authenticate and connect to gcloud storage.
///
/// It supports both token and credential authentications.
Future<FlutterDestination> connectFlutterDestination() async {
const String kTokenPath = 'TOKEN_PATH';
const String kGcpProject = 'GCP_PROJECT';
final Map<String, String> env = Platform.environment;
final bool isTesting = env['IS_TESTING'] == 'true';
if (env.containsKey(kTokenPath) && env.containsKey(kGcpProject)) {
return FlutterDestination.makeFromAccessToken(
File(env[kTokenPath]!).readAsStringSync(),
env[kGcpProject]!,
isTesting: isTesting,
);
}
return FlutterDestination.makeFromCredentialsJson(
jsonDecode(env['BENCHMARK_GCP_CREDENTIALS']!) as Map<String, dynamic>,
isTesting: isTesting,
);
}
/// Parse results and append additional benchmark tags into Metric Points.
///
/// An example of `resultsJson`:
/// {
/// "CommitBranch": "master",
/// "CommitSha": "abc",
/// "BuilderName": "test",
/// "ResultData": {
/// "average_frame_build_time_millis": 0.4550425531914895,
/// "90th_percentile_frame_build_time_millis": 0.473
/// },
/// "BenchmarkScoreKeys": [
/// "average_frame_build_time_millis",
/// "90th_percentile_frame_build_time_millis"
/// ]
/// }
///
/// An example of `benchmarkTags`:
/// {
/// "arch": "intel",
/// "device_type": "Moto G Play",
/// "device_version": "android-25",
/// "host_type": "linux",
/// "host_version": "debian-10.11"
/// }
List<MetricPoint> parse(Map<String, dynamic> resultsJson, Map<String, dynamic> benchmarkTags, String taskName) {
print('Results to upload to skia perf: $resultsJson');
print('Benchmark tags to upload to skia perf: $benchmarkTags');
final List<String> scoreKeys =
(resultsJson['BenchmarkScoreKeys'] as List<dynamic>?)?.cast<String>() ?? const <String>[];
final Map<String, dynamic> resultData =
resultsJson['ResultData'] as Map<String, dynamic>? ?? const <String, dynamic>{};
final String gitBranch = (resultsJson['CommitBranch'] as String).trim();
final String gitSha = (resultsJson['CommitSha'] as String).trim();
final List<MetricPoint> metricPoints = <MetricPoint>[];
for (final String scoreKey in scoreKeys) {
Map<String, String> tags = <String, String>{
kGithubRepoKey: kFlutterFrameworkRepo,
kGitRevisionKey: gitSha,
'branch': gitBranch,
kNameKey: taskName,
kSubResultKey: scoreKey,
};
// Append additional benchmark tags, which will surface in Skia Perf dashboards.
tags = mergeMaps<String, String>(
tags, benchmarkTags.map((String key, dynamic value) => MapEntry<String, String>(key, value.toString())));
metricPoints.add(
MetricPoint(
(resultData[scoreKey] as num).toDouble(),
tags,
),
);
}
return metricPoints;
}
/// Upload metrics to GCS bucket used by Skia Perf.
///
/// Skia Perf picks up all available files under the folder, and
/// is robust to duplicate entries.
///
/// Files will be named based on `taskName`, such as
/// `complex_layout_scroll_perf__timeline_summary_values.json`.
/// If no `taskName` is specified, data will be saved to
/// `default_values.json`.
Future<void> upload(
FlutterDestination metricsDestination,
List<MetricPoint> metricPoints,
int commitTimeSinceEpoch,
String taskName,
) async {
await metricsDestination.update(
metricPoints,
DateTime.fromMillisecondsSinceEpoch(
commitTimeSinceEpoch,
isUtc: true,
),
taskName,
);
}
/// Upload JSON results to skia perf.
///
/// Flutter infrastructure's workflow is:
/// 1. Run DeviceLab test, writing results to a known path
/// 2. Request service account token from luci auth (valid for at least 3 minutes)
/// 3. Upload results from (1) to skia perf.
Future<void> uploadToSkiaPerf(String? resultsPath, String? commitTime, String? taskName, String? benchmarkTags) async {
int commitTimeSinceEpoch;
if (resultsPath == null) {
return;
}
if (commitTime != null) {
commitTimeSinceEpoch = 1000 * int.parse(commitTime);
} else {
commitTimeSinceEpoch = DateTime.now().millisecondsSinceEpoch;
}
taskName = taskName ?? 'default';
final Map<String, dynamic> benchmarkTagsMap = jsonDecode(benchmarkTags ?? '{}') as Map<String, dynamic>;
final File resultFile = File(resultsPath);
Map<String, dynamic> resultsJson = <String, dynamic>{};
resultsJson = json.decode(await resultFile.readAsString()) as Map<String, dynamic>;
final List<MetricPoint> metricPoints = parse(resultsJson, benchmarkTagsMap, taskName);
final FlutterDestination metricsDestination = await connectFlutterDestination();
await upload(
metricsDestination,
metricPoints,
commitTimeSinceEpoch,
metricFileName(taskName, benchmarkTagsMap),
);
}
/// Create metric file name based on `taskName`, `arch`, `host type`, and `device type`.
///
/// Same `taskName` may run on different platforms. Considering host/device tags to
/// use different metric file names.
///
/// This affects only the metric file name which contains metric data, and does not affect
/// real host/device tags.
///
/// For example:
/// Old file name: `backdrop_filter_perf__timeline_summary`
/// New file name: `backdrop_filter_perf__timeline_summary_intel_linux_motoG4`
String metricFileName(
String taskName,
Map<String, dynamic> benchmarkTagsMap,
) {
final StringBuffer fileName = StringBuffer(taskName);
if (benchmarkTagsMap.containsKey('arch')) {
fileName
..write('_')
..write(_fileNameFormat(benchmarkTagsMap['arch'] as String));
}
if (benchmarkTagsMap.containsKey('host_type')) {
fileName
..write('_')
..write(_fileNameFormat(benchmarkTagsMap['host_type'] as String));
}
if (benchmarkTagsMap.containsKey('device_type')) {
fileName
..write('_')
..write(_fileNameFormat(benchmarkTagsMap['device_type'] as String));
}
return fileName.toString();
}
/// Format `fileName` removing non letter and number characters.
String _fileNameFormat(String fileName) {
return fileName.replaceAll(RegExp('[^a-zA-Z0-9]'), '');
}
| flutter/dev/devicelab/lib/framework/metrics_center.dart/0 | {
"file_path": "flutter/dev/devicelab/lib/framework/metrics_center.dart",
"repo_id": "flutter",
"token_count": 2210
} | 540 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io';
import 'dart:math' as math;
import '../framework/devices.dart';
import '../framework/framework.dart';
import '../framework/task_result.dart';
import '../framework/utils.dart';
import 'build_test_task.dart';
final Directory galleryDirectory = dir('${flutterDirectory.path}/dev/integration_tests/flutter_gallery');
/// Temp function during gallery tests transition to build+test model.
///
/// https://github.com/flutter/flutter/issues/103542
TaskFunction createGalleryTransitionBuildTest(List<String> args, {bool semanticsEnabled = false}) {
return GalleryTransitionBuildTest(args, semanticsEnabled: semanticsEnabled).call;
}
TaskFunction createGalleryTransitionTest({bool semanticsEnabled = false}) {
return GalleryTransitionTest(semanticsEnabled: semanticsEnabled).call;
}
TaskFunction createGalleryTransitionE2EBuildTest(
List<String> args, {
bool semanticsEnabled = false,
bool? enableImpeller,
}) {
return GalleryTransitionBuildTest(
args,
testFile: semanticsEnabled ? 'transitions_perf_e2e_with_semantics' : 'transitions_perf_e2e',
needFullTimeline: false,
timelineSummaryFile: 'e2e_perf_summary',
transitionDurationFile: null,
timelineTraceFile: null,
driverFile: 'transitions_perf_e2e_test',
enableImpeller: enableImpeller,
).call;
}
TaskFunction createGalleryTransitionE2ETest({
bool semanticsEnabled = false,
bool? enableImpeller,
}) {
return GalleryTransitionTest(
testFile: semanticsEnabled
? 'transitions_perf_e2e_with_semantics'
: 'transitions_perf_e2e',
needFullTimeline: false,
timelineSummaryFile: 'e2e_perf_summary',
transitionDurationFile: null,
timelineTraceFile: null,
driverFile: 'transitions_perf_e2e_test',
enableImpeller: enableImpeller,
).call;
}
TaskFunction createGalleryTransitionHybridBuildTest(
List<String> args, {
bool semanticsEnabled = false,
}) {
return GalleryTransitionBuildTest(
args,
semanticsEnabled: semanticsEnabled,
driverFile: semanticsEnabled ? 'transitions_perf_hybrid_with_semantics_test' : 'transitions_perf_hybrid_test',
).call;
}
TaskFunction createGalleryTransitionHybridTest({bool semanticsEnabled = false}) {
return GalleryTransitionTest(
semanticsEnabled: semanticsEnabled,
driverFile: semanticsEnabled
? 'transitions_perf_hybrid_with_semantics_test'
: 'transitions_perf_hybrid_test',
).call;
}
class GalleryTransitionTest {
GalleryTransitionTest({
this.semanticsEnabled = false,
this.testFile = 'transitions_perf',
this.needFullTimeline = true,
this.timelineSummaryFile = 'transitions.timeline_summary',
this.timelineTraceFile = 'transitions.timeline',
this.transitionDurationFile = 'transition_durations.timeline',
this.driverFile,
this.measureCpuGpu = true,
this.measureMemory = true,
this.enableImpeller,
});
final bool semanticsEnabled;
final bool needFullTimeline;
final bool measureCpuGpu;
final bool measureMemory;
final bool? enableImpeller;
final String testFile;
final String timelineSummaryFile;
final String? timelineTraceFile;
final String? transitionDurationFile;
final String? driverFile;
Future<TaskResult> call() async {
final Device device = await devices.workingDevice;
await device.unlock();
final String deviceId = device.deviceId;
final Directory galleryDirectory = dir('${flutterDirectory.path}/dev/integration_tests/flutter_gallery');
await inDirectory<void>(galleryDirectory, () async {
String? applicationBinaryPath;
if (deviceOperatingSystem == DeviceOperatingSystem.android) {
section('BUILDING APPLICATION');
await flutter(
'build',
options: <String>[
'apk',
'--no-android-gradle-daemon',
'--profile',
'-t',
'test_driver/$testFile.dart',
'--target-platform',
'android-arm,android-arm64',
],
);
applicationBinaryPath = 'build/app/outputs/flutter-apk/app-profile.apk';
}
final String testDriver = driverFile ?? (semanticsEnabled
? '${testFile}_with_semantics_test'
: '${testFile}_test');
section('DRIVE START');
await flutter('drive', options: <String>[
'--no-dds',
'--profile',
if (enableImpeller != null && enableImpeller!) '--enable-impeller',
if (enableImpeller != null && !enableImpeller!) '--no-enable-impeller',
if (needFullTimeline)
'--trace-startup',
if (applicationBinaryPath != null)
'--use-application-binary=$applicationBinaryPath'
else
...<String>[
'-t',
'test_driver/$testFile.dart',
],
'--driver',
'test_driver/$testDriver.dart',
'-d',
deviceId,
'-v',
'--verbose-system-logs'
]);
});
final String testOutputDirectory = Platform.environment['FLUTTER_TEST_OUTPUTS_DIR'] ?? '${galleryDirectory.path}/build';
final Map<String, dynamic> summary = json.decode(
file('$testOutputDirectory/$timelineSummaryFile.json').readAsStringSync(),
) as Map<String, dynamic>;
if (transitionDurationFile != null) {
final Map<String, dynamic> original = json.decode(
file('$testOutputDirectory/$transitionDurationFile.json').readAsStringSync(),
) as Map<String, dynamic>;
final Map<String, List<int>> transitions = <String, List<int>>{};
for (final String key in original.keys) {
transitions[key] = List<int>.from(original[key] as List<dynamic>);
}
summary['transitions'] = transitions;
summary['missed_transition_count'] = _countMissedTransitions(transitions);
}
final bool isAndroid = deviceOperatingSystem == DeviceOperatingSystem.android;
return TaskResult.success(summary,
detailFiles: <String>[
if (transitionDurationFile != null)
'$testOutputDirectory/$transitionDurationFile.json',
if (timelineTraceFile != null)
'$testOutputDirectory/$timelineTraceFile.json',
],
benchmarkScoreKeys: <String>[
if (transitionDurationFile != null)
'missed_transition_count',
'average_frame_build_time_millis',
'worst_frame_build_time_millis',
'90th_percentile_frame_build_time_millis',
'99th_percentile_frame_build_time_millis',
'average_frame_rasterizer_time_millis',
'stddev_frame_rasterizer_time_millis',
'worst_frame_rasterizer_time_millis',
'90th_percentile_frame_rasterizer_time_millis',
'99th_percentile_frame_rasterizer_time_millis',
'average_layer_cache_count',
'90th_percentile_layer_cache_count',
'99th_percentile_layer_cache_count',
'worst_layer_cache_count',
'average_layer_cache_memory',
'90th_percentile_layer_cache_memory',
'99th_percentile_layer_cache_memory',
'worst_layer_cache_memory',
'average_picture_cache_count',
'90th_percentile_picture_cache_count',
'99th_percentile_picture_cache_count',
'worst_picture_cache_count',
'average_picture_cache_memory',
'90th_percentile_picture_cache_memory',
'99th_percentile_picture_cache_memory',
'worst_picture_cache_memory',
if (measureCpuGpu && !isAndroid) ...<String>[
// See https://github.com/flutter/flutter/issues/68888
if (summary['average_cpu_usage'] != null) 'average_cpu_usage',
if (summary['average_gpu_usage'] != null) 'average_gpu_usage',
],
if (measureMemory && !isAndroid) ...<String>[
// See https://github.com/flutter/flutter/issues/68888
if (summary['average_memory_usage'] != null) 'average_memory_usage',
if (summary['90th_percentile_memory_usage'] != null) '90th_percentile_memory_usage',
if (summary['99th_percentile_memory_usage'] != null) '99th_percentile_memory_usage',
],
],
);
}
}
class GalleryTransitionBuildTest extends BuildTestTask {
GalleryTransitionBuildTest(
super.args, {
this.semanticsEnabled = false,
this.testFile = 'transitions_perf',
this.needFullTimeline = true,
this.timelineSummaryFile = 'transitions.timeline_summary',
this.timelineTraceFile = 'transitions.timeline',
this.transitionDurationFile = 'transition_durations.timeline',
this.driverFile,
this.measureCpuGpu = true,
this.measureMemory = true,
this.enableImpeller,
}) : super(workingDirectory: galleryDirectory);
final bool semanticsEnabled;
final bool needFullTimeline;
final bool measureCpuGpu;
final bool measureMemory;
final bool? enableImpeller;
final String testFile;
final String timelineSummaryFile;
final String? timelineTraceFile;
final String? transitionDurationFile;
final String? driverFile;
final String testOutputDirectory = Platform.environment['FLUTTER_TEST_OUTPUTS_DIR'] ?? '${galleryDirectory.path}/build';
@override
void copyArtifacts() {
if (applicationBinaryPath == null) {
return;
}
if (deviceOperatingSystem == DeviceOperatingSystem.android) {
copy(
file('${galleryDirectory.path}/build/app/outputs/flutter-apk/app-profile.apk'),
Directory(applicationBinaryPath!),
);
} else if (deviceOperatingSystem == DeviceOperatingSystem.ios) {
recursiveCopy(
Directory('${galleryDirectory.path}/build/ios/iphoneos'),
Directory(applicationBinaryPath!),
);
}
}
@override
List<String> getBuildArgs(DeviceOperatingSystem deviceOperatingSystem) {
if (deviceOperatingSystem == DeviceOperatingSystem.android) {
return <String>[
'apk',
'--no-android-gradle-daemon',
'--profile',
'-t',
'test_driver/$testFile.dart',
'--target-platform',
'android-arm,android-arm64',
];
} else if (deviceOperatingSystem == DeviceOperatingSystem.ios) {
return <String>[
'ios',
'--codesign',
'--profile',
'-t',
'test_driver/$testFile.dart',
];
}
throw Exception('$deviceOperatingSystem has no build configuration');
}
@override
List<String> getTestArgs(DeviceOperatingSystem deviceOperatingSystem, String deviceId) {
final String testDriver = driverFile ?? (semanticsEnabled ? '${testFile}_with_semantics_test' : '${testFile}_test');
return <String>[
'--no-dds',
'--profile',
if (enableImpeller != null && enableImpeller!) '--enable-impeller',
if (enableImpeller != null && !enableImpeller!) '--no-enable-impeller',
if (needFullTimeline) '--trace-startup',
'-t',
'test_driver/$testFile.dart',
if (applicationBinaryPath != null) '--use-application-binary=${getApplicationBinaryPath()}',
'--driver',
'test_driver/$testDriver.dart',
'-d',
deviceId,
];
}
@override
Future<TaskResult> parseTaskResult() async {
final Map<String, dynamic> summary = json.decode(
file('$testOutputDirectory/$timelineSummaryFile.json').readAsStringSync(),
) as Map<String, dynamic>;
if (transitionDurationFile != null) {
final Map<String, dynamic> original = json.decode(
file('$testOutputDirectory/$transitionDurationFile.json').readAsStringSync(),
) as Map<String, dynamic>;
final Map<String, List<int>> transitions = <String, List<int>>{};
for (final String key in original.keys) {
transitions[key] = List<int>.from(original[key] as List<dynamic>);
}
summary['transitions'] = transitions;
summary['missed_transition_count'] = _countMissedTransitions(transitions);
}
final bool isAndroid = deviceOperatingSystem == DeviceOperatingSystem.android;
return TaskResult.success(
summary,
detailFiles: <String>[
if (transitionDurationFile != null) '$testOutputDirectory/$transitionDurationFile.json',
if (timelineTraceFile != null) '$testOutputDirectory/$timelineTraceFile.json',
],
benchmarkScoreKeys: <String>[
if (transitionDurationFile != null) 'missed_transition_count',
'average_frame_build_time_millis',
'worst_frame_build_time_millis',
'90th_percentile_frame_build_time_millis',
'99th_percentile_frame_build_time_millis',
'average_frame_rasterizer_time_millis',
'worst_frame_rasterizer_time_millis',
'90th_percentile_frame_rasterizer_time_millis',
'99th_percentile_frame_rasterizer_time_millis',
'average_layer_cache_count',
'90th_percentile_layer_cache_count',
'99th_percentile_layer_cache_count',
'worst_layer_cache_count',
'average_layer_cache_memory',
'90th_percentile_layer_cache_memory',
'99th_percentile_layer_cache_memory',
'worst_layer_cache_memory',
'average_picture_cache_count',
'90th_percentile_picture_cache_count',
'99th_percentile_picture_cache_count',
'worst_picture_cache_count',
'average_picture_cache_memory',
'90th_percentile_picture_cache_memory',
'99th_percentile_picture_cache_memory',
'worst_picture_cache_memory',
if (measureCpuGpu && !isAndroid) ...<String>[
// See https://github.com/flutter/flutter/issues/68888
if (summary['average_cpu_usage'] != null) 'average_cpu_usage',
if (summary['average_gpu_usage'] != null) 'average_gpu_usage',
],
if (measureMemory && !isAndroid) ...<String>[
// See https://github.com/flutter/flutter/issues/68888
if (summary['average_memory_usage'] != null) 'average_memory_usage',
if (summary['90th_percentile_memory_usage'] != null) '90th_percentile_memory_usage',
if (summary['99th_percentile_memory_usage'] != null) '99th_percentile_memory_usage',
],
],
);
}
@override
String getApplicationBinaryPath() {
if (deviceOperatingSystem == DeviceOperatingSystem.android) {
return '$applicationBinaryPath/app-profile.apk';
} else if (deviceOperatingSystem == DeviceOperatingSystem.ios) {
return '$applicationBinaryPath/Flutter Gallery.app';
} else {
return applicationBinaryPath!;
}
}
}
int _countMissedTransitions(Map<String, List<int>> transitions) {
const int kTransitionBudget = 100000; // µs
int count = 0;
transitions.forEach((String demoName, List<int> durations) {
final int longestDuration = durations.reduce(math.max);
if (longestDuration > kTransitionBudget) {
print('$demoName missed transition time budget ($longestDuration µs > $kTransitionBudget µs)');
count++;
}
});
return count;
}
| flutter/dev/devicelab/lib/tasks/gallery.dart/0 | {
"file_path": "flutter/dev/devicelab/lib/tasks/gallery.dart",
"repo_id": "flutter",
"token_count": 5820
} | 541 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/test.dart';
export 'package:test/test.dart' hide isInstanceOf;
/// A matcher that compares the type of the actual value to the type argument T.
TypeMatcher<T> isInstanceOf<T>() => isA<T>();
| flutter/dev/devicelab/test/common.dart/0 | {
"file_path": "flutter/dev/devicelab/test/common.dart",
"repo_id": "flutter",
"token_count": 113
} | 542 |
<!-- Google Tag Manager (noscript) -->
<noscript><iframe src="https://www.googletagmanager.com/ns.html?id=GTM-ND4LWWZ"
height="0" width="0" style="display:none;visibility:hidden"></iframe></noscript>
<!-- End Google Tag Manager (noscript) -->
| flutter/dev/docs/analytics-footer.html/0 | {
"file_path": "flutter/dev/docs/analytics-footer.html",
"repo_id": "flutter",
"token_count": 83
} | 543 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The name of the route containing the text field tests.
const String textFieldRoute = 'textField';
/// The string supplied to the [ValueKey] for the normal text field.
const String normalTextFieldKeyValue = 'textFieldNormal';
/// The string supplied to the [ValueKey] for the password text field.
const String passwordTextFieldKeyValue = 'passwordField';
/// The string supplied to the [ValueKey] for the page navigation back button.
const String backButtonKeyValue = 'back';
| flutter/dev/integration_tests/android_semantics_testing/lib/src/tests/text_field_constants.dart/0 | {
"file_path": "flutter/dev/integration_tests/android_semantics_testing/lib/src/tests/text_field_constants.dart",
"repo_id": "flutter",
"token_count": 159
} | 544 |
# Integration test for touch events on embedded Android views
This test verifies that the synthesized motion events that get to embedded
Android view are equal to the motion events that originally hit the FlutterView.
The test app's Android code listens to MotionEvents that get to FlutterView and
to an embedded Android view and sends them over a platform channel to the Dart
code where the events are matched.
This is what the app looks like:
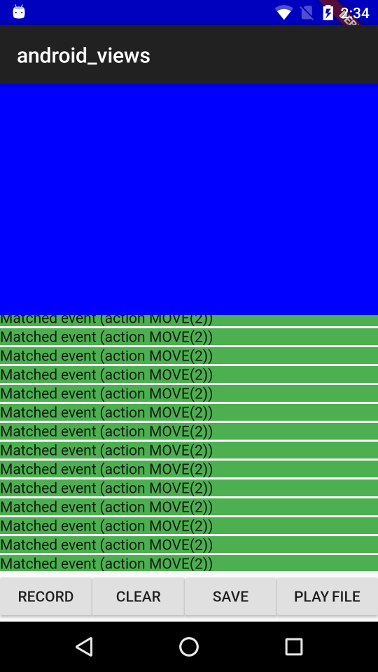
The blue part is the embedded Android view because it is positioned at the top
left corner, the coordinate systems for FlutterView and for the embedded view's
virtual display has the same origin (this makes the MotionEvent comparison
easier as we don't need to translate the coordinates).
The app includes the following control buttons:
* RECORD - Start listening for MotionEvents for 3 seconds, matched/unmatched events are
displayed in the listview as they arrive.
* CLEAR - Clears the events that were recorded so far.
* SAVE - Saves the events that hit FlutterView to a file.
* PLAY FILE - Send a list of events from a bundled asset file to FlutterView.
A recorded touch events sequence is bundled as an asset in the
assets_for_android_view package which lives in the goldens repository.
When running this test with `flutter drive` the record touch sequences is
replayed and the test asserts that the events that got to FlutterView are
equivalent to the ones that got to the embedded view.
| flutter/dev/integration_tests/android_views/README.md/0 | {
"file_path": "flutter/dev/integration_tests/android_views/README.md",
"repo_id": "flutter",
"token_count": 378
} | 545 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_driver/driver_extension.dart';
import 'motion_events_page.dart';
import 'page.dart';
import 'wm_integrations.dart';
final List<PageWidget> _allPages = <PageWidget>[
const MotionEventsPage(),
const WindowManagerIntegrationsPage(),
];
void main() {
enableFlutterDriverExtension(handler: driverDataHandler.handleMessage);
runApp(const MaterialApp(home: Home()));
}
class Home extends StatelessWidget {
const Home({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView(
children: _allPages.map((PageWidget p) => _buildPageListTile(context, p)).toList(),
),
);
}
Widget _buildPageListTile(BuildContext context, PageWidget page) {
return ListTile(
title: Text(page.title),
key: page.tileKey,
onTap: () { _pushPage(context, page); },
);
}
void _pushPage(BuildContext context, PageWidget page) {
Navigator.of(context).push(MaterialPageRoute<void>(
builder: (_) => Scaffold(
body: page,
)));
}
}
| flutter/dev/integration_tests/android_views/lib/main.dart/0 | {
"file_path": "flutter/dev/integration_tests/android_views/lib/main.dart",
"repo_id": "flutter",
"token_count": 454
} | 546 |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>CFBundleDevelopmentRegion</key>
<string>$(DEVELOPMENT_LANGUAGE)</string>
<key>CFBundleDisplayName</key>
<string>Channels</string>
<key>CFBundleExecutable</key>
<string>$(EXECUTABLE_NAME)</string>
<key>CFBundleIdentifier</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleInfoDictionaryVersion</key>
<string>6.0</string>
<key>CFBundleName</key>
<string>channels</string>
<key>CFBundlePackageType</key>
<string>APPL</string>
<key>CFBundleShortVersionString</key>
<string>$(FLUTTER_BUILD_NAME)</string>
<key>CFBundleSignature</key>
<string>????</string>
<key>CFBundleVersion</key>
<string>$(FLUTTER_BUILD_NUMBER)</string>
<key>LSRequiresIPhoneOS</key>
<true/>
<key>UILaunchStoryboardName</key>
<string>LaunchScreen</string>
<key>UIMainStoryboardFile</key>
<string>Main</string>
<key>UISupportedInterfaceOrientations</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>UISupportedInterfaceOrientations~ipad</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationPortraitUpsideDown</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>CADisableMinimumFrameDurationOnPhone</key>
<true/>
<key>UIApplicationSupportsIndirectInputEvents</key>
<true/>
</dict>
</plist>
| flutter/dev/integration_tests/channels/ios/Runner/Info.plist/0 | {
"file_path": "flutter/dev/integration_tests/channels/ios/Runner/Info.plist",
"repo_id": "flutter",
"token_count": 646
} | 547 |
# This is a Gradle generated file for dependency locking.
# Manual edits can break the build and are not advised.
# This file is expected to be part of source control.
com.android.tools.build:aapt2:4.1.3-6503028
| flutter/dev/integration_tests/deferred_components_test/android/app/gradle/dependency-locks/_internal_aapt2_binary.lockfile/0 | {
"file_path": "flutter/dev/integration_tests/deferred_components_test/android/app/gradle/dependency-locks/_internal_aapt2_binary.lockfile",
"repo_id": "flutter",
"token_count": 61
} | 548 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
include ':app', ':component1'
def localPropertiesFile = new File(rootProject.projectDir, "local.properties")
def properties = new Properties()
assert localPropertiesFile.exists()
localPropertiesFile.withReader("UTF-8") { reader -> properties.load(reader) }
def flutterSdkPath = properties.getProperty("flutter.sdk")
assert flutterSdkPath != null, "flutter.sdk not set in local.properties"
apply from: "$flutterSdkPath/packages/flutter_tools/gradle/app_plugin_loader.gradle"
| flutter/dev/integration_tests/deferred_components_test/android/settings.gradle/0 | {
"file_path": "flutter/dev/integration_tests/deferred_components_test/android/settings.gradle",
"repo_id": "flutter",
"token_count": 189
} | 549 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class _ContactCategory extends StatelessWidget {
const _ContactCategory({ this.icon, this.children });
final IconData? icon;
final List<Widget>? children;
@override
Widget build(BuildContext context) {
final ThemeData themeData = Theme.of(context);
return Container(
padding: const EdgeInsets.symmetric(vertical: 16.0),
decoration: BoxDecoration(
border: Border(bottom: BorderSide(color: themeData.dividerColor))
),
child: DefaultTextStyle(
style: Theme.of(context).textTheme.titleMedium!,
child: SafeArea(
top: false,
bottom: false,
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Container(
padding: const EdgeInsets.symmetric(vertical: 24.0),
width: 72.0,
child: Icon(icon, color: themeData.primaryColor),
),
Expanded(child: Column(children: children!)),
],
),
),
),
);
}
}
class _ContactItem extends StatelessWidget {
const _ContactItem({ this.icon, required this.lines, this.tooltip, this.onPressed })
: assert(lines.length > 1);
final IconData? icon;
final List<String> lines;
final String? tooltip;
final VoidCallback? onPressed;
@override
Widget build(BuildContext context) {
final ThemeData themeData = Theme.of(context);
return MergeSemantics(
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 16.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Expanded(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
...lines.sublist(0, lines.length - 1).map<Widget>((String line) => Text(line)),
Text(lines.last, style: themeData.textTheme.bodySmall),
],
),
),
if (icon != null)
SizedBox(
width: 72.0,
child: IconButton(
icon: Icon(icon),
color: themeData.primaryColor,
onPressed: onPressed,
),
),
],
),
),
);
}
}
class ContactsDemo extends StatefulWidget {
const ContactsDemo({super.key});
static const String routeName = '/contacts';
@override
ContactsDemoState createState() => ContactsDemoState();
}
enum AppBarBehavior { normal, pinned, floating, snapping }
class ContactsDemoState extends State<ContactsDemo> {
final double _appBarHeight = 256.0;
AppBarBehavior _appBarBehavior = AppBarBehavior.pinned;
@override
Widget build(BuildContext context) {
return Theme(
data: ThemeData(
useMaterial3: false,
brightness: Brightness.light,
primarySwatch: Colors.indigo,
platform: Theme.of(context).platform,
),
child: Scaffold(
body: CustomScrollView(
slivers: <Widget>[
SliverAppBar(
expandedHeight: _appBarHeight,
pinned: _appBarBehavior == AppBarBehavior.pinned,
floating: _appBarBehavior == AppBarBehavior.floating || _appBarBehavior == AppBarBehavior.snapping,
snap: _appBarBehavior == AppBarBehavior.snapping,
actions: <Widget>[
IconButton(
icon: const Icon(Icons.create),
tooltip: 'Edit',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text("Editing isn't supported in this screen."),
));
},
),
PopupMenuButton<AppBarBehavior>(
onSelected: (AppBarBehavior value) {
setState(() {
_appBarBehavior = value;
});
},
itemBuilder: (BuildContext context) => <PopupMenuItem<AppBarBehavior>>[
const PopupMenuItem<AppBarBehavior>(
value: AppBarBehavior.normal,
child: Text('App bar scrolls away'),
),
const PopupMenuItem<AppBarBehavior>(
value: AppBarBehavior.pinned,
child: Text('App bar stays put'),
),
const PopupMenuItem<AppBarBehavior>(
value: AppBarBehavior.floating,
child: Text('App bar floats'),
),
const PopupMenuItem<AppBarBehavior>(
value: AppBarBehavior.snapping,
child: Text('App bar snaps'),
),
],
),
],
flexibleSpace: FlexibleSpaceBar(
title: const Text('Ali Connors'),
background: Stack(
fit: StackFit.expand,
children: <Widget>[
Image.asset(
'people/ali_landscape.png',
package: 'flutter_gallery_assets',
fit: BoxFit.cover,
height: _appBarHeight,
),
// This gradient ensures that the toolbar icons are distinct
// against the background image.
const DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: Alignment(0, .35),
colors: <Color>[Color(0xC0000000), Color(0x00000000)],
),
),
),
],
),
),
),
SliverList(
delegate: SliverChildListDelegate(<Widget>[
AnnotatedRegion<SystemUiOverlayStyle>(
value: SystemUiOverlayStyle.dark,
child: _ContactCategory(
icon: Icons.call,
children: <Widget>[
_ContactItem(
icon: Icons.message,
tooltip: 'Send message',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Pretend that this opened your SMS application.'),
));
},
lines: const <String>[
'(650) 555-1234',
'Mobile',
],
),
_ContactItem(
icon: Icons.message,
tooltip: 'Send message',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('A messaging app appears.'),
));
},
lines: const <String>[
'(323) 555-6789',
'Work',
],
),
_ContactItem(
icon: Icons.message,
tooltip: 'Send message',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Imagine if you will, a messaging application.'),
));
},
lines: const <String>[
'(650) 555-6789',
'Home',
],
),
],
),
),
_ContactCategory(
icon: Icons.contact_mail,
children: <Widget>[
_ContactItem(
icon: Icons.email,
tooltip: 'Send personal e-mail',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Here, your e-mail application would open.'),
));
},
lines: const <String>[
'[email protected]',
'Personal',
],
),
_ContactItem(
icon: Icons.email,
tooltip: 'Send work e-mail',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Summon your favorite e-mail application here.'),
));
},
lines: const <String>[
'[email protected]',
'Work',
],
),
],
),
_ContactCategory(
icon: Icons.location_on,
children: <Widget>[
_ContactItem(
icon: Icons.map,
tooltip: 'Open map',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('This would show a map of San Francisco.'),
));
},
lines: const <String>[
'2000 Main Street',
'San Francisco, CA',
'Home',
],
),
_ContactItem(
icon: Icons.map,
tooltip: 'Open map',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('This would show a map of Mountain View.'),
));
},
lines: const <String>[
'1600 Amphitheater Parkway',
'Mountain View, CA',
'Work',
],
),
_ContactItem(
icon: Icons.map,
tooltip: 'Open map',
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('This would also show a map, if this was not a demo.'),
));
},
lines: const <String>[
'126 Severyns Ave',
'Mountain View, CA',
'Jet Travel',
],
),
],
),
_ContactCategory(
icon: Icons.today,
children: <Widget>[
_ContactItem(
lines: const <String>[
'Birthday',
'January 9th, 1989',
],
),
_ContactItem(
lines: const <String>[
'Wedding anniversary',
'June 21st, 2014',
],
),
_ContactItem(
lines: const <String>[
'First day in office',
'January 20th, 2015',
],
),
_ContactItem(
lines: const <String>[
'Last day in office',
'August 9th, 2018',
],
),
],
),
]),
),
],
),
),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/contacts_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/contacts_demo.dart",
"repo_id": "flutter",
"token_count": 7621
} | 550 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery/demo.dart';
enum BannerDemoAction {
reset,
showMultipleActions,
showLeading,
}
class BannerDemo extends StatefulWidget {
const BannerDemo({ super.key });
static const String routeName = '/material/banner';
@override
State<BannerDemo> createState() => _BannerDemoState();
}
class _BannerDemoState extends State<BannerDemo> {
static const int _numItems = 20;
bool _displayBanner = true;
bool _showMultipleActions = true;
bool _showLeading = true;
void handleDemoAction(BannerDemoAction action) {
setState(() {
switch (action) {
case BannerDemoAction.reset:
_displayBanner = true;
_showMultipleActions = true;
_showLeading = true;
case BannerDemoAction.showMultipleActions:
_showMultipleActions = !_showMultipleActions;
case BannerDemoAction.showLeading:
_showLeading = !_showLeading;
}
});
}
@override
Widget build(BuildContext context) {
final Widget banner = MaterialBanner(
content: const Text('Your password was updated on your other device. Please sign in again.'),
leading: _showLeading ? const CircleAvatar(child: Icon(Icons.access_alarm)) : null,
actions: <Widget>[
TextButton(
child: const Text('SIGN IN'),
onPressed: () {
setState(() {
_displayBanner = false;
});
},
),
if (_showMultipleActions)
TextButton(
child: const Text('DISMISS'),
onPressed: () {
setState(() {
_displayBanner = false;
});
},
),
],
);
return Scaffold(
appBar: AppBar(
title: const Text('Banner'),
actions: <Widget>[
MaterialDemoDocumentationButton(BannerDemo.routeName),
PopupMenuButton<BannerDemoAction>(
onSelected: handleDemoAction,
itemBuilder: (BuildContext context) => <PopupMenuEntry<BannerDemoAction>>[
const PopupMenuItem<BannerDemoAction>(
value: BannerDemoAction.reset,
child: Text('Reset the banner'),
),
const PopupMenuDivider(),
CheckedPopupMenuItem<BannerDemoAction>(
value: BannerDemoAction.showMultipleActions,
checked: _showMultipleActions,
child: const Text('Multiple actions'),
),
CheckedPopupMenuItem<BannerDemoAction>(
value: BannerDemoAction.showLeading,
checked: _showLeading,
child: const Text('Leading icon'),
),
],
),
],
),
body: ListView.builder(itemCount: _displayBanner ? _numItems + 1 : _numItems, itemBuilder: (BuildContext context, int index) {
if (index == 0 && _displayBanner) {
return banner;
}
return ListTile(title: Text('Item ${_displayBanner ? index : index + 1}'),);
}),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/material/banner_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/material/banner_demo.dart",
"repo_id": "flutter",
"token_count": 1466
} | 551 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:collection/collection.dart' show lowerBound;
import 'package:flutter/material.dart';
import 'package:flutter/semantics.dart';
import '../../gallery/demo.dart';
enum LeaveBehindDemoAction {
reset,
horizontalSwipe,
leftSwipe,
rightSwipe,
confirmDismiss,
}
class LeaveBehindItem implements Comparable<LeaveBehindItem> {
LeaveBehindItem({ this.index, this.name, this.subject, this.body });
LeaveBehindItem.from(LeaveBehindItem item)
: index = item.index, name = item.name, subject = item.subject, body = item.body;
final int? index;
final String? name;
final String? subject;
final String? body;
@override
int compareTo(LeaveBehindItem other) => index!.compareTo(other.index!);
}
class LeaveBehindDemo extends StatefulWidget {
const LeaveBehindDemo({ super.key });
static const String routeName = '/material/leave-behind';
@override
LeaveBehindDemoState createState() => LeaveBehindDemoState();
}
class LeaveBehindDemoState extends State<LeaveBehindDemo> {
DismissDirection _dismissDirection = DismissDirection.horizontal;
bool _confirmDismiss = true;
late List<LeaveBehindItem> leaveBehindItems;
void initListItems() {
leaveBehindItems = List<LeaveBehindItem>.generate(16, (int index) {
return LeaveBehindItem(
index: index,
name: 'Item $index Sender',
subject: 'Subject: $index',
body: "[$index] first line of the message's body...",
);
});
}
@override
void initState() {
super.initState();
initListItems();
}
void handleDemoAction(LeaveBehindDemoAction action) {
setState(() {
switch (action) {
case LeaveBehindDemoAction.reset:
initListItems();
case LeaveBehindDemoAction.horizontalSwipe:
_dismissDirection = DismissDirection.horizontal;
case LeaveBehindDemoAction.leftSwipe:
_dismissDirection = DismissDirection.endToStart;
case LeaveBehindDemoAction.rightSwipe:
_dismissDirection = DismissDirection.startToEnd;
case LeaveBehindDemoAction.confirmDismiss:
_confirmDismiss = !_confirmDismiss;
}
});
}
void handleUndo(LeaveBehindItem item) {
final int insertionIndex = lowerBound(leaveBehindItems, item);
setState(() {
leaveBehindItems.insert(insertionIndex, item);
});
}
void _handleArchive(LeaveBehindItem item) {
setState(() {
leaveBehindItems.remove(item);
});
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('You archived item ${item.index}'),
action: SnackBarAction(
label: 'UNDO',
onPressed: () { handleUndo(item); },
),
));
}
void _handleDelete(LeaveBehindItem item) {
setState(() {
leaveBehindItems.remove(item);
});
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('You deleted item ${item.index}'),
action: SnackBarAction(
label: 'UNDO',
onPressed: () { handleUndo(item); },
),
));
}
@override
Widget build(BuildContext context) {
Widget body;
if (leaveBehindItems.isEmpty) {
body = Center(
child: ElevatedButton(
onPressed: () => handleDemoAction(LeaveBehindDemoAction.reset),
child: const Text('Reset the list'),
),
);
} else {
body = Scrollbar(
child: ListView(
primary: true,
children: leaveBehindItems.map<Widget>((LeaveBehindItem item) {
return _LeaveBehindListItem(
confirmDismiss: _confirmDismiss,
item: item,
onArchive: _handleArchive,
onDelete: _handleDelete,
dismissDirection: _dismissDirection,
);
}).toList(),
),
);
}
return Scaffold(
appBar: AppBar(
title: const Text('Swipe to dismiss'),
actions: <Widget>[
MaterialDemoDocumentationButton(LeaveBehindDemo.routeName),
PopupMenuButton<LeaveBehindDemoAction>(
onSelected: handleDemoAction,
itemBuilder: (BuildContext context) => <PopupMenuEntry<LeaveBehindDemoAction>>[
const PopupMenuItem<LeaveBehindDemoAction>(
value: LeaveBehindDemoAction.reset,
child: Text('Reset the list'),
),
const PopupMenuDivider(),
CheckedPopupMenuItem<LeaveBehindDemoAction>(
value: LeaveBehindDemoAction.horizontalSwipe,
checked: _dismissDirection == DismissDirection.horizontal,
child: const Text('Horizontal swipe'),
),
CheckedPopupMenuItem<LeaveBehindDemoAction>(
value: LeaveBehindDemoAction.leftSwipe,
checked: _dismissDirection == DismissDirection.endToStart,
child: const Text('Only swipe left'),
),
CheckedPopupMenuItem<LeaveBehindDemoAction>(
value: LeaveBehindDemoAction.rightSwipe,
checked: _dismissDirection == DismissDirection.startToEnd,
child: const Text('Only swipe right'),
),
CheckedPopupMenuItem<LeaveBehindDemoAction>(
value: LeaveBehindDemoAction.confirmDismiss,
checked: _confirmDismiss,
child: const Text('Confirm dismiss'),
),
],
),
],
),
body: body,
);
}
}
class _LeaveBehindListItem extends StatelessWidget {
const _LeaveBehindListItem({
required this.item,
required this.onArchive,
required this.onDelete,
required this.dismissDirection,
required this.confirmDismiss,
});
final LeaveBehindItem item;
final DismissDirection dismissDirection;
final void Function(LeaveBehindItem) onArchive;
final void Function(LeaveBehindItem) onDelete;
final bool confirmDismiss;
void _handleArchive() {
onArchive(item);
}
void _handleDelete() {
onDelete(item);
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
return Semantics(
customSemanticsActions: <CustomSemanticsAction, VoidCallback>{
const CustomSemanticsAction(label: 'Archive'): _handleArchive,
const CustomSemanticsAction(label: 'Delete'): _handleDelete,
},
child: Dismissible(
key: ObjectKey(item),
direction: dismissDirection,
onDismissed: (DismissDirection direction) {
if (direction == DismissDirection.endToStart) {
_handleArchive();
} else {
_handleDelete();
}
},
confirmDismiss: !confirmDismiss ? null : (DismissDirection dismissDirection) async {
switch (dismissDirection) {
case DismissDirection.endToStart:
return await _showConfirmationDialog(context, 'archive') ?? false;
case DismissDirection.startToEnd:
return await _showConfirmationDialog(context, 'delete') ?? false;
case DismissDirection.horizontal:
case DismissDirection.vertical:
case DismissDirection.up:
case DismissDirection.down:
case DismissDirection.none:
assert(false);
}
return false;
},
background: ColoredBox(
color: theme.primaryColor,
child: const Center(
child: ListTile(
leading: Icon(Icons.delete, color: Colors.white, size: 36.0),
),
),
),
secondaryBackground: ColoredBox(
color: theme.primaryColor,
child: const Center(
child: ListTile(
trailing: Icon(Icons.archive, color: Colors.white, size: 36.0),
),
),
),
child: Container(
decoration: BoxDecoration(
color: theme.canvasColor,
border: Border(bottom: BorderSide(color: theme.dividerColor)),
),
child: ListTile(
title: Text(item.name!),
subtitle: Text('${item.subject}\n${item.body}'),
isThreeLine: true,
),
),
),
);
}
Future<bool?> _showConfirmationDialog(BuildContext context, String action) {
return showDialog<bool>(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('Do you want to $action this item?'),
actions: <Widget>[
TextButton(
child: const Text('Yes'),
onPressed: () {
Navigator.pop(context, true); // showDialog() returns true
},
),
TextButton(
child: const Text('No'),
onPressed: () {
Navigator.pop(context, false); // showDialog() returns false
},
),
],
);
},
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/material/leave_behind_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/material/leave_behind_demo.dart",
"repo_id": "flutter",
"token_count": 4000
} | 552 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery/demo.dart';
const String _explanatoryText =
"When the Scaffold's floating action button changes, the new button fades and "
'turns into view. In this demo, changing tabs can cause the app to be rebuilt '
'with a FloatingActionButton that the Scaffold distinguishes from the others '
'by its key.';
class _Page {
_Page({ this.label, this.colors, this.icon });
final String? label;
final MaterialColor? colors;
final IconData? icon;
Color get labelColor => colors != null ? colors!.shade300 : Colors.grey.shade300;
bool get fabDefined => colors != null && icon != null;
Color get fabColor => colors!.shade400;
Icon get fabIcon => Icon(icon);
Key get fabKey => ValueKey<Color>(fabColor);
}
final List<_Page> _allPages = <_Page>[
_Page(label: 'Pink', colors: Colors.pink, icon: Icons.add),
_Page(label: 'Eco', colors: Colors.green, icon: Icons.create),
_Page(label: 'No'),
_Page(label: 'Teal', colors: Colors.teal, icon: Icons.add),
_Page(label: 'Red', colors: Colors.red, icon: Icons.create),
];
class TabsFabDemo extends StatefulWidget {
const TabsFabDemo({super.key});
static const String routeName = '/material/tabs-fab';
@override
State<TabsFabDemo> createState() => _TabsFabDemoState();
}
class _TabsFabDemoState extends State<TabsFabDemo> with SingleTickerProviderStateMixin {
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
TabController? _controller;
late _Page _selectedPage;
bool _extendedButtons = false;
@override
void initState() {
super.initState();
_controller = TabController(vsync: this, length: _allPages.length);
_controller!.addListener(_handleTabSelection);
_selectedPage = _allPages[0];
}
@override
void dispose() {
_controller!.dispose();
super.dispose();
}
void _handleTabSelection() {
setState(() {
_selectedPage = _allPages[_controller!.index];
});
}
void _showExplanatoryText() {
_scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return Container(
decoration: BoxDecoration(
border: Border(top: BorderSide(color: Theme.of(context).dividerColor))
),
child: Padding(
padding: const EdgeInsets.all(32.0),
child: Text(_explanatoryText, style: Theme.of(context).textTheme.titleMedium),
),
);
});
}
Widget buildTabView(_Page page) {
return Builder(
builder: (BuildContext context) {
return Container(
key: ValueKey<String?>(page.label),
padding: const EdgeInsets.fromLTRB(48.0, 48.0, 48.0, 96.0),
child: Card(
child: Center(
child: Text(page.label!,
style: TextStyle(
color: page.labelColor,
fontSize: 32.0,
),
textAlign: TextAlign.center,
),
),
),
);
}
);
}
Widget? buildFloatingActionButton(_Page page) {
if (!page.fabDefined) {
return null;
}
if (_extendedButtons) {
return FloatingActionButton.extended(
key: ValueKey<Key>(page.fabKey),
tooltip: 'Show explanation',
backgroundColor: page.fabColor,
icon: page.fabIcon,
label: Text(page.label!.toUpperCase()),
onPressed: _showExplanatoryText,
);
}
return FloatingActionButton(
key: page.fabKey,
tooltip: 'Show explanation',
backgroundColor: page.fabColor,
onPressed: _showExplanatoryText,
child: page.fabIcon,
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: const Text('FAB per tab'),
bottom: TabBar(
controller: _controller,
tabs: _allPages.map<Widget>((_Page page) => Tab(text: page.label!.toUpperCase())).toList(),
),
actions: <Widget>[
MaterialDemoDocumentationButton(TabsFabDemo.routeName),
IconButton(
icon: const Icon(Icons.sentiment_very_satisfied, semanticLabel: 'Toggle extended buttons'),
onPressed: () {
setState(() {
_extendedButtons = !_extendedButtons;
});
},
),
],
),
floatingActionButton: buildFloatingActionButton(_selectedPage),
body: TabBarView(
controller: _controller,
children: _allPages.map<Widget>(buildTabView).toList(),
),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/material/tabs_fab_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/material/tabs_fab_demo.dart",
"repo_id": "flutter",
"token_count": 1948
} | 553 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/material.dart';
class CutCornersBorder extends OutlineInputBorder {
const CutCornersBorder({
super.borderSide = BorderSide.none,
super.borderRadius = const BorderRadius.all(Radius.circular(2.0)),
this.cut = 7.0,
super.gapPadding = 2.0,
});
@override
CutCornersBorder copyWith({
BorderSide? borderSide,
BorderRadius? borderRadius,
double? gapPadding,
double? cut,
}) {
return CutCornersBorder(
borderSide: borderSide ?? this.borderSide,
borderRadius: borderRadius ?? this.borderRadius,
gapPadding: gapPadding ?? this.gapPadding,
cut: cut ?? this.cut,
);
}
final double cut;
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is CutCornersBorder) {
final CutCornersBorder outline = a;
return CutCornersBorder(
borderRadius: BorderRadius.lerp(outline.borderRadius, borderRadius, t)!,
borderSide: BorderSide.lerp(outline.borderSide, borderSide, t),
cut: cut,
gapPadding: outline.gapPadding,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is CutCornersBorder) {
final CutCornersBorder outline = b;
return CutCornersBorder(
borderRadius: BorderRadius.lerp(borderRadius, outline.borderRadius, t)!,
borderSide: BorderSide.lerp(borderSide, outline.borderSide, t),
cut: cut,
gapPadding: outline.gapPadding,
);
}
return super.lerpTo(b, t);
}
Path _notchedCornerPath(Rect center, [double start = 0.0, double? extent = 0.0]) {
final Path path = Path();
if (start > 0.0 || extent! > 0.0) {
path.relativeMoveTo(extent! + start, center.top);
_notchedSidesAndBottom(center, path);
path..lineTo(center.left + cut, center.top)..lineTo(start, center.top);
} else {
path.moveTo(center.left + cut, center.top);
_notchedSidesAndBottom(center, path);
path.lineTo(center.left + cut, center.top);
}
return path;
}
Path _notchedSidesAndBottom(Rect center, Path path) {
return path
..lineTo(center.right - cut, center.top)
..lineTo(center.right, center.top + cut)
..lineTo(center.right, center.top + center.height - cut)
..lineTo(center.right - cut, center.top + center.height)
..lineTo(center.left + cut, center.top + center.height)
..lineTo(center.left, center.top + center.height - cut)
..lineTo(center.left, center.top + cut);
}
@override
void paint(
Canvas canvas,
Rect rect, {
double? gapStart,
double gapExtent = 0.0,
double gapPercentage = 0.0,
TextDirection? textDirection,
}) {
assert(gapPercentage >= 0.0 && gapPercentage <= 1.0);
final Paint paint = borderSide.toPaint();
final RRect outer = borderRadius.toRRect(rect);
if (gapStart == null || gapExtent <= 0.0 || gapPercentage == 0.0) {
canvas.drawPath(_notchedCornerPath(outer.middleRect), paint);
} else {
final double? extent = lerpDouble(0.0, gapExtent + gapPadding * 2.0, gapPercentage);
switch (textDirection) {
case TextDirection.rtl: {
final Path path = _notchedCornerPath(outer.middleRect, gapStart + gapPadding - extent!, extent);
canvas.drawPath(path, paint);
break;
}
case TextDirection.ltr: {
final Path path = _notchedCornerPath(outer.middleRect, gapStart - gapPadding, extent);
canvas.drawPath(path, paint);
break;
}
case null:
break;
}
}
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/shrine/supplemental/cut_corners_border.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/shrine/supplemental/cut_corners_border.dart",
"repo_id": "flutter",
"token_count": 1547
} | 554 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher.dart';
import 'demos.dart';
import 'example_code_parser.dart';
import 'syntax_highlighter.dart';
@immutable
class ComponentDemoTabData {
const ComponentDemoTabData({
this.demoWidget,
this.exampleCodeTag,
this.description,
this.tabName,
this.documentationUrl,
});
final Widget? demoWidget;
final String? exampleCodeTag;
final String? description;
final String? tabName;
final String? documentationUrl;
@override
bool operator==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ComponentDemoTabData
&& other.tabName == tabName
&& other.description == description
&& other.documentationUrl == documentationUrl;
}
@override
int get hashCode => Object.hash(tabName, description, documentationUrl);
}
class TabbedComponentDemoScaffold extends StatefulWidget {
const TabbedComponentDemoScaffold({
super.key,
this.title,
this.demos,
this.actions,
this.isScrollable = true,
this.showExampleCodeAction = true,
});
final List<ComponentDemoTabData>? demos;
final String? title;
final List<Widget>? actions;
final bool isScrollable;
final bool showExampleCodeAction;
@override
State<TabbedComponentDemoScaffold> createState() => _TabbedComponentDemoScaffoldState();
}
class _TabbedComponentDemoScaffoldState extends State<TabbedComponentDemoScaffold> {
void _showExampleCode(BuildContext context) {
final String? tag = widget.demos![DefaultTabController.of(context).index].exampleCodeTag;
if (tag != null) {
Navigator.push(context, MaterialPageRoute<FullScreenCodeDialog>(
builder: (BuildContext context) => FullScreenCodeDialog(exampleCodeTag: tag)
));
}
}
Future<void> _showApiDocumentation(BuildContext context) async {
final String? url = widget.demos![DefaultTabController.of(context).index].documentationUrl;
if (url == null) {
return;
}
final Uri uri = Uri.parse(url);
if (await canLaunchUrl(uri)) {
await launchUrl(uri);
} else if (context.mounted) {
showDialog<void>(
context: context,
builder: (BuildContext context) {
return SimpleDialog(
title: const Text("Couldn't display URL:"),
children: <Widget>[
Padding(
padding: const EdgeInsets.symmetric(horizontal: 16.0),
child: Text(url),
),
],
);
},
);
}
}
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: widget.demos!.length,
child: Scaffold(
appBar: AppBar(
title: Text(widget.title!),
actions: <Widget>[
...?widget.actions,
Builder(
builder: (BuildContext context) {
return IconButton(
icon: const Icon(Icons.library_books, semanticLabel: 'Show documentation'),
onPressed: () => _showApiDocumentation(context),
);
},
),
if (widget.showExampleCodeAction)
Builder(
builder: (BuildContext context) {
return IconButton(
icon: const Icon(Icons.code),
tooltip: 'Show example code',
onPressed: () => _showExampleCode(context),
);
},
),
],
bottom: TabBar(
isScrollable: widget.isScrollable,
tabs: widget.demos!.map<Widget>((ComponentDemoTabData data) => Tab(text: data.tabName)).toList(),
),
),
body: TabBarView(
children: widget.demos!.map<Widget>((ComponentDemoTabData demo) {
return SafeArea(
top: false,
bottom: false,
child: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.all(16.0),
child: Text(demo.description!,
style: Theme.of(context).textTheme.titleMedium,
),
),
Expanded(child: demo.demoWidget!),
],
),
);
}).toList(),
),
),
);
}
}
class FullScreenCodeDialog extends StatefulWidget {
const FullScreenCodeDialog({ super.key, this.exampleCodeTag });
final String? exampleCodeTag;
@override
FullScreenCodeDialogState createState() => FullScreenCodeDialogState();
}
class FullScreenCodeDialogState extends State<FullScreenCodeDialog> {
String? _exampleCode;
@override
void didChangeDependencies() {
getExampleCode(widget.exampleCodeTag, DefaultAssetBundle.of(context)).then((String? code) {
if (mounted) {
setState(() {
_exampleCode = code ?? 'Example code not found';
});
}
});
super.didChangeDependencies();
}
@override
Widget build(BuildContext context) {
final SyntaxHighlighterStyle style = Theme.of(context).brightness == Brightness.dark
? SyntaxHighlighterStyle.darkThemeStyle
: SyntaxHighlighterStyle.lightThemeStyle;
Widget body;
if (_exampleCode == null) {
body = const Center(
child: CircularProgressIndicator(),
);
} else {
body = SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: RichText(
text: TextSpan(
style: const TextStyle(fontFamily: 'monospace', fontSize: 10.0),
children: <TextSpan>[
DartSyntaxHighlighter(style).format(_exampleCode),
],
),
),
),
);
}
return Scaffold(
appBar: AppBar(
leading: IconButton(
icon: const Icon(
Icons.clear,
semanticLabel: 'Close',
),
onPressed: () { Navigator.pop(context); },
),
title: const Text('Example code'),
),
body: body,
);
}
}
class MaterialDemoDocumentationButton extends StatelessWidget {
MaterialDemoDocumentationButton(String routeName, { super.key })
: documentationUrl = kDemoDocumentationUrl[routeName],
assert(
kDemoDocumentationUrl[routeName] != null,
'A documentation URL was not specified for demo route $routeName in kAllGalleryDemos',
);
final String? documentationUrl;
@override
Widget build(BuildContext context) {
return IconButton(
icon: const Icon(Icons.library_books),
tooltip: 'API documentation',
onPressed: () => launchUrl(Uri.parse(documentationUrl!), mode: LaunchMode.inAppWebView),
);
}
}
class CupertinoDemoDocumentationButton extends StatelessWidget {
CupertinoDemoDocumentationButton(String routeName, { super.key })
: documentationUrl = kDemoDocumentationUrl[routeName],
assert(
kDemoDocumentationUrl[routeName] != null,
'A documentation URL was not specified for demo route $routeName in kAllGalleryDemos',
);
final String? documentationUrl;
@override
Widget build(BuildContext context) {
return CupertinoButton(
padding: EdgeInsets.zero,
child: Semantics(
label: 'API documentation',
child: const Icon(CupertinoIcons.book),
),
onPressed: () => launchUrl(Uri.parse(documentationUrl!), mode: LaunchMode.inAppWebView),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/gallery/demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/gallery/demo.dart",
"repo_id": "flutter",
"token_count": 3308
} | 555 |
name: flutter_gallery
environment:
sdk: '>=3.2.0-0 <4.0.0'
dependencies:
flutter:
sdk: flutter
collection: 1.18.0
device_info: 2.0.3
intl: 0.19.0
connectivity: 3.0.6
string_scanner: 1.2.0
url_launcher: 6.2.5
cupertino_icons: 1.0.6
video_player: 2.8.3
scoped_model: 2.0.0
shrine_images: 2.0.2
# Also update dev/benchmarks/complex_layout/pubspec.yaml
# and dev/benchmarks/macrobenchmarks/pubspec.yaml
flutter_gallery_assets: 1.0.2
characters: 1.3.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
clock: 1.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
connectivity_for_web: 0.4.0+1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
connectivity_macos: 0.2.1+2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
connectivity_platform_interface: 2.0.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
csslib: 1.0.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
device_info_platform_interface: 2.0.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
html: 0.15.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
material_color_utilities: 0.8.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
meta: 1.12.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
path: 1.9.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
plugin_platform_interface: 2.1.8 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
source_span: 1.10.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
term_glyph: 1.2.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_android: 6.3.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_ios: 6.2.5 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_linux: 3.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_macos: 3.1.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_platform_interface: 2.3.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_web: 2.3.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
url_launcher_windows: 3.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
vector_math: 2.1.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
video_player_android: 2.4.12 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
video_player_avfoundation: 2.5.6 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
video_player_platform_interface: 6.2.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
video_player_web: 2.3.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
web: 0.5.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
dev_dependencies:
flutter_test:
sdk: flutter
flutter_driver:
sdk: flutter
flutter_goldens:
sdk: flutter
test: 1.25.2
integration_test:
sdk: flutter
_fe_analyzer_shared: 67.0.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
analyzer: 6.4.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
args: 2.4.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
async: 2.11.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
boolean_selector: 2.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
convert: 3.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
coverage: 1.7.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
crypto: 3.0.3 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
fake_async: 1.3.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
file: 7.0.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
frontend_server_client: 3.2.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
glob: 2.1.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
http_multi_server: 3.2.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
http_parser: 4.0.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
io: 1.0.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
js: 0.7.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
leak_tracker: 10.0.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
leak_tracker_flutter_testing: 3.0.3 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
leak_tracker_testing: 3.0.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
logging: 1.2.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
matcher: 0.12.16+1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
mime: 1.0.5 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
node_preamble: 2.0.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
package_config: 2.1.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
platform: 3.1.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
pool: 1.5.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
process: 5.0.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
pub_semver: 2.1.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
shelf: 1.4.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
shelf_packages_handler: 3.0.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
shelf_static: 1.1.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
shelf_web_socket: 1.0.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
source_map_stack_trace: 2.1.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
source_maps: 0.10.12 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
stack_trace: 1.11.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
stream_channel: 2.1.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
sync_http: 0.3.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
test_api: 0.7.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
test_core: 0.6.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
typed_data: 1.3.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
vm_service: 14.2.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
watcher: 1.1.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
web_socket_channel: 2.4.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
webdriver: 3.0.3 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
webkit_inspection_protocol: 1.2.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
yaml: 3.1.2 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
flutter:
uses-material-design: true
assets:
- lib/gallery/example_code.dart
- packages/flutter_gallery_assets/people/ali_landscape.png
- packages/flutter_gallery_assets/monochrome/red-square-1024x1024.png
- packages/flutter_gallery_assets/logos/flutter_white/logo.png
- packages/flutter_gallery_assets/logos/fortnightly/fortnightly_logo.png
- packages/flutter_gallery_assets/videos/bee.mp4
- packages/flutter_gallery_assets/videos/butterfly.mp4
- packages/flutter_gallery_assets/animated_images/animated_flutter_lgtm.gif
- packages/flutter_gallery_assets/animated_images/animated_flutter_stickers.webp
- packages/flutter_gallery_assets/food/butternut_squash_soup.png
- packages/flutter_gallery_assets/food/cherry_pie.png
- packages/flutter_gallery_assets/food/chopped_beet_leaves.png
- packages/flutter_gallery_assets/food/fruits.png
- packages/flutter_gallery_assets/food/pesto_pasta.png
- packages/flutter_gallery_assets/food/roasted_chicken.png
- packages/flutter_gallery_assets/food/spanakopita.png
- packages/flutter_gallery_assets/food/spinach_onion_salad.png
- packages/flutter_gallery_assets/food/icons/fish.png
- packages/flutter_gallery_assets/food/icons/healthy.png
- packages/flutter_gallery_assets/food/icons/main.png
- packages/flutter_gallery_assets/food/icons/meat.png
- packages/flutter_gallery_assets/food/icons/quick.png
- packages/flutter_gallery_assets/food/icons/spicy.png
- packages/flutter_gallery_assets/food/icons/veggie.png
- packages/flutter_gallery_assets/logos/pesto/logo_small.png
- packages/flutter_gallery_assets/places/india_chennai_flower_market.png
- packages/flutter_gallery_assets/places/india_thanjavur_market.png
- packages/flutter_gallery_assets/places/india_tanjore_bronze_works.png
- packages/flutter_gallery_assets/places/india_tanjore_market_merchant.png
- packages/flutter_gallery_assets/places/india_tanjore_thanjavur_temple.png
- packages/flutter_gallery_assets/places/india_pondicherry_salt_farm.png
- packages/flutter_gallery_assets/places/india_chennai_highway.png
- packages/flutter_gallery_assets/places/india_chettinad_silk_maker.png
- packages/flutter_gallery_assets/places/india_tanjore_thanjavur_temple_carvings.png
- packages/flutter_gallery_assets/places/india_chettinad_produce.png
- packages/flutter_gallery_assets/places/india_tanjore_market_technology.png
- packages/flutter_gallery_assets/places/india_pondicherry_beach.png
- packages/flutter_gallery_assets/places/india_pondicherry_fisherman.png
- packages/flutter_gallery_assets/products/backpack.png
- packages/flutter_gallery_assets/products/belt.png
- packages/flutter_gallery_assets/products/cup.png
- packages/flutter_gallery_assets/products/deskset.png
- packages/flutter_gallery_assets/products/dress.png
- packages/flutter_gallery_assets/products/earrings.png
- packages/flutter_gallery_assets/products/flatwear.png
- packages/flutter_gallery_assets/products/hat.png
- packages/flutter_gallery_assets/products/jacket.png
- packages/flutter_gallery_assets/products/jumper.png
- packages/flutter_gallery_assets/products/kitchen_quattro.png
- packages/flutter_gallery_assets/products/napkins.png
- packages/flutter_gallery_assets/products/planters.png
- packages/flutter_gallery_assets/products/platter.png
- packages/flutter_gallery_assets/products/scarf.png
- packages/flutter_gallery_assets/products/shirt.png
- packages/flutter_gallery_assets/products/sunnies.png
- packages/flutter_gallery_assets/products/sweater.png
- packages/flutter_gallery_assets/products/sweats.png
- packages/flutter_gallery_assets/products/table.png
- packages/flutter_gallery_assets/products/teaset.png
- packages/flutter_gallery_assets/products/top.png
- packages/flutter_gallery_assets/people/square/ali.png
- packages/flutter_gallery_assets/people/square/peter.png
- packages/flutter_gallery_assets/people/square/sandra.png
- packages/flutter_gallery_assets/people/square/stella.png
- packages/flutter_gallery_assets/people/square/trevor.png
- packages/shrine_images/diamond.png
- packages/shrine_images/slanted_menu.png
- packages/shrine_images/0-0.jpg
- packages/shrine_images/1-0.jpg
- packages/shrine_images/2-0.jpg
- packages/shrine_images/3-0.jpg
- packages/shrine_images/4-0.jpg
- packages/shrine_images/5-0.jpg
- packages/shrine_images/6-0.jpg
- packages/shrine_images/7-0.jpg
- packages/shrine_images/8-0.jpg
- packages/shrine_images/9-0.jpg
- packages/shrine_images/10-0.jpg
- packages/shrine_images/11-0.jpg
- packages/shrine_images/12-0.jpg
- packages/shrine_images/13-0.jpg
- packages/shrine_images/14-0.jpg
- packages/shrine_images/15-0.jpg
- packages/shrine_images/16-0.jpg
- packages/shrine_images/17-0.jpg
- packages/shrine_images/18-0.jpg
- packages/shrine_images/19-0.jpg
- packages/shrine_images/20-0.jpg
- packages/shrine_images/21-0.jpg
- packages/shrine_images/22-0.jpg
- packages/shrine_images/23-0.jpg
- packages/shrine_images/24-0.jpg
- packages/shrine_images/25-0.jpg
- packages/shrine_images/26-0.jpg
- packages/shrine_images/27-0.jpg
- packages/shrine_images/28-0.jpg
- packages/shrine_images/29-0.jpg
- packages/shrine_images/30-0.jpg
- packages/shrine_images/31-0.jpg
- packages/shrine_images/32-0.jpg
- packages/shrine_images/33-0.jpg
- packages/shrine_images/34-0.jpg
- packages/shrine_images/35-0.jpg
- packages/shrine_images/36-0.jpg
- packages/shrine_images/37-0.jpg
fonts:
- family: Raleway
fonts:
- asset: packages/flutter_gallery_assets/fonts/raleway/Raleway-Regular.ttf
- asset: packages/flutter_gallery_assets/fonts/raleway/Raleway-Medium.ttf
weight: 500
- asset: packages/flutter_gallery_assets/fonts/raleway/Raleway-SemiBold.ttf
weight: 600
- family: AbrilFatface
fonts:
- asset: packages/flutter_gallery_assets/fonts/abrilfatface/AbrilFatface-Regular.ttf
- family: GalleryIcons
fonts:
- asset: packages/flutter_gallery_assets/fonts/private/gallery_icons/GalleryIcons.ttf
- family: GoogleSans
fonts:
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-BoldItalic.ttf
weight: 700
style: italic
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-Bold.ttf
weight: 700
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-Italic.ttf
weight: 400
style: italic
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-MediumItalic.ttf
weight: 500
style: italic
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-Medium.ttf
weight: 500
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSans-Regular.ttf
weight: 400
- family: GoogleSansDisplay
fonts:
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-BoldItalic.ttf
weight: 700
style: italic
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-Bold.ttf
weight: 700
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-Italic.ttf
weight: 400
style: italic
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-MediumItalic.ttf
style: italic
weight: 500
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-Medium.ttf
weight: 500
- asset: packages/flutter_gallery_assets/fonts/private/googlesans/GoogleSansDisplay-Regular.ttf
weight: 400
- family: LibreFranklin
fonts:
- asset: packages/flutter_gallery_assets/fonts/librefranklin/LibreFranklin-Bold.ttf
- asset: packages/flutter_gallery_assets/fonts/librefranklin/LibreFranklin-Light.ttf
- asset: packages/flutter_gallery_assets/fonts/librefranklin/LibreFranklin-Medium.ttf
- asset: packages/flutter_gallery_assets/fonts/librefranklin/LibreFranklin-Regular.ttf
- family: Merriweather
fonts:
- asset: packages/flutter_gallery_assets/fonts/merriweather/Merriweather-BlackItalic.ttf
- asset: packages/flutter_gallery_assets/fonts/merriweather/Merriweather-Italic.ttf
- asset: packages/flutter_gallery_assets/fonts/merriweather/Merriweather-Regular.ttf
- asset: packages/flutter_gallery_assets/fonts/merriweather/Merriweather-Light.ttf
# PUBSPEC CHECKSUM: aa94
| flutter/dev/integration_tests/flutter_gallery/pubspec.yaml/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/pubspec.yaml",
"repo_id": "flutter",
"token_count": 6538
} | 556 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_gallery/gallery/app.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final TestWidgetsFlutterBinding binding = TestWidgetsFlutterBinding.ensureInitialized();
if (binding is LiveTestWidgetsFlutterBinding) {
binding.framePolicy = LiveTestWidgetsFlutterBindingFramePolicy.fullyLive;
}
// Regression test for https://github.com/flutter/flutter/pull/5168
testWidgets('Pesto appbar heroics', (WidgetTester tester) async {
await tester.pumpWidget(
// The bug only manifests itself when the screen's orientation is portrait
const Center(
child: SizedBox(
width: 450.0,
height: 800.0,
child: GalleryApp(testMode: true),
),
)
);
await tester.pump(); // see https://github.com/flutter/flutter/issues/1865
await tester.pump(); // triggers a frame
await tester.tap(find.text('Studies'));
await tester.pumpAndSettle();
await tester.tap(find.text('Pesto'));
await tester.pumpAndSettle();
await tester.tap(find.text('Roasted Chicken'));
await tester.pumpAndSettle();
await tester.drag(find.text('Roasted Chicken'), const Offset(0.0, -300.0));
await tester.pumpAndSettle();
Navigator.pop(find.byType(Scaffold).evaluate().single);
await tester.pumpAndSettle();
});
testWidgets('Pesto can be scrolled all the way down', (WidgetTester tester) async {
await tester.pumpWidget(const GalleryApp(testMode: true));
await tester.pump(); // see https://github.com/flutter/flutter/issues/1865
await tester.pump(); // triggers a frame
await tester.tap(find.text('Studies'));
await tester.pumpAndSettle();
await tester.tap(find.text('Pesto'));
await tester.pumpAndSettle();
await tester.fling(find.text('Roasted Chicken'), const Offset(0.0, -200.0), 10000.0);
await tester.pumpAndSettle(); // start and finish fling
expect(find.text('Spanakopita'), findsOneWidget);
});
}
| flutter/dev/integration_tests/flutter_gallery/test/pesto_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/test/pesto_test.dart",
"repo_id": "flutter",
"token_count": 804
} | 557 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert' show JsonEncoder, json;
import 'package:file/file.dart';
import 'package:file/local.dart';
import 'package:flutter_driver/flutter_driver.dart';
import 'package:flutter_gallery/demo_lists.dart';
import 'package:path/path.dart' as path;
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
const FileSystem _fs = LocalFileSystem();
/// The demos we don't run as part of the integration test.
///
/// Demo names are formatted as 'DEMO_NAME@DEMO_CATEGORY' (see
/// `demo_lists.dart` for more examples).
const List<String> kSkippedDemos = <String>[
// This demo is flaky on CI due to hitting the network.
// See: https://github.com/flutter/flutter/issues/100497
'Video@Media',
];
// All of the gallery demos, identified as "title@category".
//
// These names are reported by the test app, see _handleMessages()
// in transitions_perf.dart.
List<String> _allDemos = <String>[];
/// Extracts event data from [events] recorded by timeline, validates it, turns
/// it into a histogram, and saves to a JSON file.
Future<void> saveDurationsHistogram(List<Map<String, dynamic>> events, String outputPath) async {
final Map<String, List<int>> durations = <String, List<int>>{};
Map<String, dynamic>? startEvent;
int? frameStart;
// Save the duration of the first frame after each 'Start Transition' event.
for (final Map<String, dynamic> event in events) {
final String eventName = event['name'] as String;
if (eventName == 'Start Transition') {
assert(startEvent == null);
startEvent = event;
} else if (startEvent != null && eventName == 'Frame') {
final String phase = event['ph'] as String;
final int timestamp = event['ts'] as int;
if (phase == 'B' || phase == 'b') {
assert(frameStart == null);
frameStart = timestamp;
} else {
assert(phase == 'E' || phase == 'e');
final String routeName = (startEvent['args'] as Map<String, dynamic>)['to'] as String;
durations[routeName] ??= <int>[];
durations[routeName]!.add(timestamp - frameStart!);
startEvent = null;
frameStart = null;
}
}
}
// Verify that the durations data is valid.
if (durations.keys.isEmpty) {
throw 'no "Start Transition" timeline events found';
}
final Map<String, int> unexpectedValueCounts = <String, int>{};
durations.forEach((String routeName, List<int> values) {
if (values.length != 2) {
unexpectedValueCounts[routeName] = values.length;
}
});
if (unexpectedValueCounts.isNotEmpty) {
final StringBuffer error = StringBuffer('Some routes recorded wrong number of values (expected 2 values/route):\n\n');
// When run with --trace-startup, the VM stores trace events in an endless buffer instead of a ring buffer.
error.write('You must add the --trace-startup parameter to run the test. \n\n');
unexpectedValueCounts.forEach((String routeName, int count) {
error.writeln(' - $routeName recorded $count values.');
});
error.writeln('\nFull event sequence:');
final Iterator<Map<String, dynamic>> eventIter = events.iterator;
String lastEventName = '';
String lastRouteName = '';
while (eventIter.moveNext()) {
final String eventName = eventIter.current['name'] as String;
if (!<String>['Start Transition', 'Frame'].contains(eventName)) {
continue;
}
final String routeName = eventName == 'Start Transition'
? (eventIter.current['args'] as Map<String, dynamic>)['to'] as String
: '';
if (eventName == lastEventName && routeName == lastRouteName) {
error.write('.');
} else {
error.write('\n - $eventName $routeName .');
}
lastEventName = eventName;
lastRouteName = routeName;
}
throw error;
}
// Save the durations Map to a file.
final File file = await _fs.file(outputPath).create(recursive: true);
await file.writeAsString(const JsonEncoder.withIndent(' ').convert(durations));
}
/// Scrolls each demo menu item into view, launches it, then returns to the
/// home screen twice.
Future<void> runDemos(List<String> demos, FlutterDriver driver) async {
final SerializableFinder demoList = find.byValueKey('GalleryDemoList');
String? currentDemoCategory;
for (final String demo in demos) {
if (kSkippedDemos.contains(demo)) {
continue;
}
final String demoName = demo.substring(0, demo.indexOf('@'));
final String demoCategory = demo.substring(demo.indexOf('@') + 1);
print('> $demo');
final SerializableFinder demoCategoryItem = find.text(demoCategory);
if (currentDemoCategory == null) {
await driver.scrollIntoView(demoCategoryItem);
await driver.tap(demoCategoryItem);
} else if (currentDemoCategory != demoCategory) {
await driver.tap(find.byTooltip('Back'));
await driver.scrollIntoView(demoCategoryItem);
await driver.tap(demoCategoryItem);
// Scroll back to the top
await driver.scroll(demoList, 0.0, 10000.0, const Duration(milliseconds: 100));
}
currentDemoCategory = demoCategory;
final SerializableFinder demoItem = find.text(demoName);
await driver.scrollUntilVisible(demoList, demoItem,
dyScroll: -48.0,
alignment: 0.5,
);
for (int i = 0; i < 2; i += 1) {
await driver.tap(demoItem); // Launch the demo
if (kUnsynchronizedDemos.contains(demo)) {
await driver.runUnsynchronized<void>(() async {
await driver.tap(find.pageBack());
});
} else {
await driver.tap(find.pageBack());
}
}
print('< Success');
}
// Return to the home screen
await driver.tap(find.byTooltip('Back'));
}
void main([List<String> args = const <String>[]]) {
final bool withSemantics = args.contains('--with_semantics');
final bool hybrid = args.contains('--hybrid');
group('flutter gallery transitions', () {
late FlutterDriver driver;
setUpAll(() async {
driver = await FlutterDriver.connect();
// Wait for the first frame to be rasterized.
await driver.waitUntilFirstFrameRasterized();
if (withSemantics) {
print('Enabling semantics...');
await driver.setSemantics(true);
}
// See _handleMessages() in transitions_perf.dart.
_allDemos = List<String>.from(json.decode(await driver.requestData('demoNames')) as List<dynamic>);
if (_allDemos.isEmpty) {
throw 'no demo names found';
}
});
tearDownAll(() async {
await driver.close();
});
test('find.bySemanticsLabel', () async {
// Assert that we can use semantics related finders in profile mode.
final int id = await driver.getSemanticsId(find.bySemanticsLabel('Material'));
expect(id, greaterThan(-1));
},
skip: !withSemantics, // [intended] test only makes sense when semantics are turned on.
timeout: Timeout.none,
);
test('all demos', () async {
// Collect timeline data for just a limited set of demos to avoid OOMs.
final Timeline timeline = await driver.traceAction(
() async {
if (hybrid) {
await driver.requestData('profileDemos');
} else {
await runDemos(kProfiledDemos, driver);
}
},
streams: const <TimelineStream>[
TimelineStream.dart,
TimelineStream.embedder,
TimelineStream.gc,
],
retainPriorEvents: true,
);
// Save the duration (in microseconds) of the first timeline Frame event
// that follows a 'Start Transition' event. The Gallery app adds a
// 'Start Transition' event when a demo is launched (see GalleryItem).
final TimelineSummary summary = TimelineSummary.summarize(timeline);
await summary.writeTimelineToFile('transitions', pretty: true);
final String histogramPath = path.join(testOutputsDirectory, 'transition_durations.timeline.json');
await saveDurationsHistogram(
List<Map<String, dynamic>>.from(timeline.json['traceEvents'] as List<dynamic>),
histogramPath);
// Execute the remaining tests.
if (hybrid) {
await driver.requestData('restDemos');
} else {
final Set<String> unprofiledDemos = Set<String>.from(_allDemos)..removeAll(kProfiledDemos);
await runDemos(unprofiledDemos.toList(), driver);
}
}, timeout: Timeout.none);
});
}
| flutter/dev/integration_tests/flutter_gallery/test_driver/transitions_perf_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/test_driver/transitions_perf_test.dart",
"repo_id": "flutter",
"token_count": 3125
} | 558 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "TextFieldFactory.h"
@interface PlatformTextField: NSObject<FlutterPlatformView>
@property (strong, nonatomic) UITextField *textField;
@end
@implementation PlatformTextField
- (instancetype)init
{
self = [super init];
if (self) {
_textField = [[UITextField alloc] init];
_textField.text = @"Platform Text Field";
}
return self;
}
- (UIView *)view {
return self.textField;
}
@end
@implementation TextFieldFactory
- (NSObject<FlutterPlatformView> *)createWithFrame:(CGRect)frame viewIdentifier:(int64_t)viewId arguments:(id)args {
return [[PlatformTextField alloc] init];
}
@end
| flutter/dev/integration_tests/ios_platform_view_tests/ios/Runner/TextFieldFactory.m/0 | {
"file_path": "flutter/dev/integration_tests/ios_platform_view_tests/ios/Runner/TextFieldFactory.m",
"repo_id": "flutter",
"token_count": 256
} | 559 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery_localizations.dart';
import 'material_demo_types.dart';
class ChipDemo extends StatelessWidget {
const ChipDemo({
super.key,
required this.type,
});
final ChipDemoType type;
String _title(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
switch (type) {
case ChipDemoType.action:
return localizations.demoActionChipTitle;
case ChipDemoType.choice:
return localizations.demoChoiceChipTitle;
case ChipDemoType.filter:
return localizations.demoFilterChipTitle;
case ChipDemoType.input:
return localizations.demoInputChipTitle;
}
}
@override
Widget build(BuildContext context) {
Widget? buttons;
switch (type) {
case ChipDemoType.action:
buttons = _ActionChipDemo();
case ChipDemoType.choice:
buttons = _ChoiceChipDemo();
case ChipDemoType.filter:
buttons = _FilterChipDemo();
case ChipDemoType.input:
buttons = _InputChipDemo();
}
return Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: Text(_title(context)),
),
body: buttons,
);
}
}
// BEGIN chipDemoAction
class _ActionChipDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: ActionChip(
onPressed: () {},
avatar: const Icon(
Icons.brightness_5,
color: Colors.black54,
),
label: Text(GalleryLocalizations.of(context)!.chipTurnOnLights),
),
);
}
}
// END
// BEGIN chipDemoChoice
class _ChoiceChipDemo extends StatefulWidget {
@override
_ChoiceChipDemoState createState() => _ChoiceChipDemoState();
}
class _ChoiceChipDemoState extends State<_ChoiceChipDemo>
with RestorationMixin {
final RestorableIntN _indexSelected = RestorableIntN(null);
@override
String get restorationId => 'choice_chip_demo';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_indexSelected, 'choice_chip');
}
@override
void dispose() {
_indexSelected.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Wrap(
children: <Widget>[
ChoiceChip(
label: Text(localizations.chipSmall),
selected: _indexSelected.value == 0,
onSelected: (bool value) {
setState(() {
_indexSelected.value = value ? 0 : -1;
});
},
),
const SizedBox(width: 8),
ChoiceChip(
label: Text(localizations.chipMedium),
selected: _indexSelected.value == 1,
onSelected: (bool value) {
setState(() {
_indexSelected.value = value ? 1 : -1;
});
},
),
const SizedBox(width: 8),
ChoiceChip(
label: Text(localizations.chipLarge),
selected: _indexSelected.value == 2,
onSelected: (bool value) {
setState(() {
_indexSelected.value = value ? 2 : -1;
});
},
),
],
),
const SizedBox(height: 12),
// Disabled chips
Wrap(
children: <Widget>[
ChoiceChip(
label: Text(localizations.chipSmall),
selected: _indexSelected.value == 0,
),
const SizedBox(width: 8),
ChoiceChip(
label: Text(localizations.chipMedium),
selected: _indexSelected.value == 1,
),
const SizedBox(width: 8),
ChoiceChip(
label: Text(localizations.chipLarge),
selected: _indexSelected.value == 2,
),
],
),
],
),
);
}
}
// END
// BEGIN chipDemoFilter
class _FilterChipDemo extends StatefulWidget {
@override
_FilterChipDemoState createState() => _FilterChipDemoState();
}
class _FilterChipDemoState extends State<_FilterChipDemo>
with RestorationMixin {
final RestorableBool isSelectedElevator = RestorableBool(false);
final RestorableBool isSelectedWasher = RestorableBool(false);
final RestorableBool isSelectedFireplace = RestorableBool(false);
@override
String get restorationId => 'filter_chip_demo';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(isSelectedElevator, 'selected_elevator');
registerForRestoration(isSelectedWasher, 'selected_washer');
registerForRestoration(isSelectedFireplace, 'selected_fireplace');
}
@override
void dispose() {
isSelectedElevator.dispose();
isSelectedWasher.dispose();
isSelectedFireplace.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Wrap(
spacing: 8.0,
children: <Widget>[
FilterChip(
label: Text(localizations.chipElevator),
selected: isSelectedElevator.value,
onSelected: (bool value) {
setState(() {
isSelectedElevator.value = !isSelectedElevator.value;
});
},
),
FilterChip(
label: Text(localizations.chipWasher),
selected: isSelectedWasher.value,
onSelected: (bool value) {
setState(() {
isSelectedWasher.value = !isSelectedWasher.value;
});
},
),
FilterChip(
label: Text(localizations.chipFireplace),
selected: isSelectedFireplace.value,
onSelected: (bool value) {
setState(() {
isSelectedFireplace.value = !isSelectedFireplace.value;
});
},
),
],
),
const SizedBox(height: 12),
// Disabled chips
Wrap(
spacing: 8.0,
children: <Widget>[
FilterChip(
label: Text(localizations.chipElevator),
selected: isSelectedElevator.value,
onSelected: null,
),
FilterChip(
label: Text(localizations.chipWasher),
selected: isSelectedWasher.value,
onSelected: null,
),
FilterChip(
label: Text(localizations.chipFireplace),
selected: isSelectedFireplace.value,
onSelected: null,
),
],
),
],
),
);
}
}
// END
// BEGIN chipDemoInput
class _InputChipDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
InputChip(
onPressed: () {},
onDeleted: () {},
avatar: const Icon(
Icons.directions_bike,
size: 20,
color: Colors.black54,
),
deleteIconColor: Colors.black54,
label: Text(GalleryLocalizations.of(context)!.chipBiking),
),
const SizedBox(height: 12),
// Disabled chip
InputChip(
avatar: const Icon(
Icons.directions_bike,
size: 20,
color: Colors.black54,
),
deleteIconColor: Colors.black54,
label: Text(GalleryLocalizations.of(context)!.chipBiking),
),
],
),
);
}
}
// END
| flutter/dev/integration_tests/new_gallery/lib/demos/material/chip_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/demos/material/chip_demo.dart",
"repo_id": "flutter",
"token_count": 4196
} | 560 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery_localizations.dart';
import 'material_demo_types.dart';
class TabsDemo extends StatelessWidget {
const TabsDemo({super.key, required this.type});
final TabsDemoType type;
@override
Widget build(BuildContext context) {
Widget tabs;
switch (type) {
case TabsDemoType.scrollable:
tabs = _TabsScrollableDemo();
case TabsDemoType.nonScrollable:
tabs = _TabsNonScrollableDemo();
}
return tabs;
}
}
// BEGIN tabsScrollableDemo
class _TabsScrollableDemo extends StatefulWidget {
@override
__TabsScrollableDemoState createState() => __TabsScrollableDemoState();
}
class __TabsScrollableDemoState extends State<_TabsScrollableDemo>
with SingleTickerProviderStateMixin, RestorationMixin {
TabController? _tabController;
final RestorableInt tabIndex = RestorableInt(0);
@override
String get restorationId => 'tab_scrollable_demo';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(tabIndex, 'tab_index');
_tabController!.index = tabIndex.value;
}
@override
void initState() {
_tabController = TabController(
length: 12,
vsync: this,
);
_tabController!.addListener(() {
// When the tab controller's value is updated, make sure to update the
// tab index value, which is state restorable.
setState(() {
tabIndex.value = _tabController!.index;
});
});
super.initState();
}
@override
void dispose() {
_tabController!.dispose();
tabIndex.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final List<String> tabs = <String>[
localizations.colorsRed,
localizations.colorsOrange,
localizations.colorsGreen,
localizations.colorsBlue,
localizations.colorsIndigo,
localizations.colorsPurple,
localizations.colorsRed,
localizations.colorsOrange,
localizations.colorsGreen,
localizations.colorsBlue,
localizations.colorsIndigo,
localizations.colorsPurple,
];
return Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: Text(localizations.demoTabsScrollingTitle),
bottom: TabBar(
controller: _tabController,
isScrollable: true,
tabs: <Widget>[
for (final String tab in tabs) Tab(text: tab),
],
),
),
body: TabBarView(
controller: _tabController,
children: <Widget>[
for (final String tab in tabs)
Center(
child: Text(tab),
),
],
),
);
}
}
// END
// BEGIN tabsNonScrollableDemo
class _TabsNonScrollableDemo extends StatefulWidget {
@override
__TabsNonScrollableDemoState createState() => __TabsNonScrollableDemoState();
}
class __TabsNonScrollableDemoState extends State<_TabsNonScrollableDemo>
with SingleTickerProviderStateMixin, RestorationMixin {
late TabController _tabController;
final RestorableInt tabIndex = RestorableInt(0);
@override
String get restorationId => 'tab_non_scrollable_demo';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(tabIndex, 'tab_index');
_tabController.index = tabIndex.value;
}
@override
void initState() {
super.initState();
_tabController = TabController(
length: 3,
vsync: this,
);
_tabController.addListener(() {
// When the tab controller's value is updated, make sure to update the
// tab index value, which is state restorable.
setState(() {
tabIndex.value = _tabController.index;
});
});
}
@override
void dispose() {
_tabController.dispose();
tabIndex.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final List<String> tabs = <String>[
localizations.colorsRed,
localizations.colorsOrange,
localizations.colorsGreen,
];
return Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: Text(
localizations.demoTabsNonScrollingTitle,
),
bottom: TabBar(
controller: _tabController,
tabs: <Widget>[
for (final String tab in tabs) Tab(text: tab),
],
),
),
body: TabBarView(
controller: _tabController,
children: <Widget>[
for (final String tab in tabs)
Center(
child: Text(tab),
),
],
),
);
}
}
// END
| flutter/dev/integration_tests/new_gallery/lib/demos/material/tabs_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/demos/material/tabs_demo.dart",
"repo_id": "flutter",
"token_count": 1971
} | 561 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:dual_screen/dual_screen.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:google_fonts/google_fonts.dart';
import 'package:url_launcher/url_launcher_string.dart';
import '../codeviewer/code_displayer.dart';
import '../codeviewer/code_style.dart';
import '../constants.dart';
import '../data/demos.dart';
import '../data/gallery_options.dart';
import '../feature_discovery/feature_discovery.dart';
import '../gallery_localizations.dart';
import '../layout/adaptive.dart';
import '../themes/gallery_theme_data.dart';
import '../themes/material_demo_theme_data.dart';
import 'splash.dart';
enum _DemoState {
normal,
options,
info,
code,
fullscreen,
}
class DemoPage extends StatefulWidget {
const DemoPage({
super.key,
required this.slug,
});
static const String baseRoute = '/demo';
final String? slug;
@override
State<DemoPage> createState() => _DemoPageState();
}
class _DemoPageState extends State<DemoPage> {
late Map<String?, GalleryDemo> slugToDemoMap;
@override
void didChangeDependencies() {
super.didChangeDependencies();
// To make sure that we do not rebuild the map for every update to the demo
// page, we save it in a variable. The cost of running `slugToDemo` is
// still only close to constant, as it's just iterating over all of the
// demos.
slugToDemoMap = Demos.asSlugToDemoMap(context);
}
@override
Widget build(BuildContext context) {
if (widget.slug == null || !slugToDemoMap.containsKey(widget.slug)) {
// Return to root if invalid slug.
Navigator.of(context).pop();
}
return ScaffoldMessenger(
child: GalleryDemoPage(
restorationId: widget.slug!,
demo: slugToDemoMap[widget.slug]!,
));
}
}
class GalleryDemoPage extends StatefulWidget {
const GalleryDemoPage({
super.key,
required this.restorationId,
required this.demo,
});
final String restorationId;
final GalleryDemo demo;
@override
State<GalleryDemoPage> createState() => _GalleryDemoPageState();
}
class _GalleryDemoPageState extends State<GalleryDemoPage>
with RestorationMixin, TickerProviderStateMixin {
final RestorableInt _demoStateIndex = RestorableInt(_DemoState.normal.index);
final RestorableInt _configIndex = RestorableInt(0);
bool? _isDesktop;
late AnimationController _codeBackgroundColorController;
@override
String get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_demoStateIndex, 'demo_state');
registerForRestoration(_configIndex, 'configuration_index');
}
GalleryDemoConfiguration get _currentConfig {
return widget.demo.configurations[_configIndex.value];
}
bool get _hasOptions => widget.demo.configurations.length > 1;
@override
void initState() {
super.initState();
_codeBackgroundColorController = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 300),
);
}
@override
void dispose() {
_demoStateIndex.dispose();
_configIndex.dispose();
_codeBackgroundColorController.dispose();
super.dispose();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_isDesktop ??= isDisplayDesktop(context);
}
/// Sets state and updates the background color for code.
void setStateAndUpdate(VoidCallback callback) {
setState(() {
callback();
if (_demoStateIndex.value == _DemoState.code.index) {
_codeBackgroundColorController.forward();
} else {
_codeBackgroundColorController.reverse();
}
});
}
void _handleTap(_DemoState newState) {
final int newStateIndex = newState.index;
// Do not allow normal state for desktop.
if (_demoStateIndex.value == newStateIndex && isDisplayDesktop(context)) {
if (_demoStateIndex.value == _DemoState.fullscreen.index) {
setStateAndUpdate(() {
_demoStateIndex.value =
_hasOptions ? _DemoState.options.index : _DemoState.info.index;
});
}
return;
}
setStateAndUpdate(() {
_demoStateIndex.value = _demoStateIndex.value == newStateIndex
? _DemoState.normal.index
: newStateIndex;
});
}
Future<void> _showDocumentation(BuildContext context) async {
final String url = _currentConfig.documentationUrl;
if (await canLaunchUrlString(url)) {
await launchUrlString(url);
} else if (context.mounted) {
await showDialog<void>(
context: context,
builder: (BuildContext context) {
return SimpleDialog(
title: Text(GalleryLocalizations.of(context)!.demoInvalidURL),
children: <Widget>[
Padding(
padding: const EdgeInsets.symmetric(horizontal: 16),
child: Text(url),
),
],
);
},
);
}
}
void _resolveState(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
final bool isFoldable = isDisplayFoldable(context);
if (_DemoState.values[_demoStateIndex.value] == _DemoState.fullscreen &&
!isDesktop) {
// Do not allow fullscreen state for mobile.
_demoStateIndex.value = _DemoState.normal.index;
} else if (_DemoState.values[_demoStateIndex.value] == _DemoState.normal &&
(isDesktop || isFoldable)) {
// Do not allow normal state for desktop.
_demoStateIndex.value =
_hasOptions ? _DemoState.options.index : _DemoState.info.index;
} else if (isDesktop != _isDesktop) {
_isDesktop = isDesktop;
// When going from desktop to mobile, return to normal state.
if (!isDesktop) {
_demoStateIndex.value = _DemoState.normal.index;
}
}
}
@override
Widget build(BuildContext context) {
final bool isFoldable = isDisplayFoldable(context);
final bool isDesktop = isDisplayDesktop(context);
_resolveState(context);
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final Color iconColor = colorScheme.onSurface;
final Color selectedIconColor = colorScheme.primary;
final double appBarPadding = isDesktop ? 20.0 : 0.0;
final _DemoState currentDemoState = _DemoState.values[_demoStateIndex.value];
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final GalleryOptions options = GalleryOptions.of(context);
final AppBar appBar = AppBar(
systemOverlayStyle: options.resolvedSystemUiOverlayStyle(),
backgroundColor: Colors.transparent,
leading: Padding(
padding: EdgeInsetsDirectional.only(start: appBarPadding),
child: IconButton(
key: const ValueKey<String>('Back'),
icon: const BackButtonIcon(),
tooltip: MaterialLocalizations.of(context).backButtonTooltip,
onPressed: () {
Navigator.maybePop(context);
},
),
),
actions: <Widget>[
if (_hasOptions)
IconButton(
icon: FeatureDiscovery(
title: localizations.demoOptionsFeatureTitle,
description: localizations.demoOptionsFeatureDescription,
showOverlay: !isDisplayDesktop(context) && !options.isTestMode,
color: colorScheme.primary,
onTap: () => _handleTap(_DemoState.options),
child: Icon(
Icons.tune,
color: currentDemoState == _DemoState.options
? selectedIconColor
: iconColor,
),
),
tooltip: localizations.demoOptionsTooltip,
onPressed: () => _handleTap(_DemoState.options),
),
IconButton(
icon: const Icon(Icons.info),
tooltip: localizations.demoInfoTooltip,
color: currentDemoState == _DemoState.info
? selectedIconColor
: iconColor,
onPressed: () => _handleTap(_DemoState.info),
),
IconButton(
icon: const Icon(Icons.code),
tooltip: localizations.demoCodeTooltip,
color: currentDemoState == _DemoState.code
? selectedIconColor
: iconColor,
onPressed: () => _handleTap(_DemoState.code),
),
IconButton(
icon: const Icon(Icons.library_books),
tooltip: localizations.demoDocumentationTooltip,
color: iconColor,
onPressed: () => _showDocumentation(context),
),
if (isDesktop)
IconButton(
icon: const Icon(Icons.fullscreen),
tooltip: localizations.demoFullscreenTooltip,
color: currentDemoState == _DemoState.fullscreen
? selectedIconColor
: iconColor,
onPressed: () => _handleTap(_DemoState.fullscreen),
),
SizedBox(width: appBarPadding),
],
);
final MediaQueryData mediaQuery = MediaQuery.of(context);
final double bottomSafeArea = mediaQuery.padding.bottom;
final double contentHeight = mediaQuery.size.height -
mediaQuery.padding.top -
mediaQuery.padding.bottom -
appBar.preferredSize.height;
final double maxSectionHeight = isDesktop ? contentHeight : contentHeight - 64;
final double horizontalPadding = isDesktop ? mediaQuery.size.width * 0.12 : 0.0;
const double maxSectionWidth = 420.0;
Widget section;
switch (currentDemoState) {
case _DemoState.options:
section = _DemoSectionOptions(
maxHeight: maxSectionHeight,
maxWidth: maxSectionWidth,
configurations: widget.demo.configurations,
configIndex: _configIndex.value,
onConfigChanged: (int index) {
setStateAndUpdate(() {
_configIndex.value = index;
if (!isDesktop) {
_demoStateIndex.value = _DemoState.normal.index;
}
});
},
);
case _DemoState.info:
section = _DemoSectionInfo(
maxHeight: maxSectionHeight,
maxWidth: maxSectionWidth,
title: _currentConfig.title,
description: _currentConfig.description,
);
case _DemoState.code:
final TextStyle codeTheme = GoogleFonts.robotoMono(
fontSize: 12 * options.textScaleFactor(context),
);
section = CodeStyle(
baseStyle: codeTheme.copyWith(color: const Color(0xFFFAFBFB)),
numberStyle: codeTheme.copyWith(color: const Color(0xFFBD93F9)),
commentStyle: codeTheme.copyWith(color: const Color(0xFF808080)),
keywordStyle: codeTheme.copyWith(color: const Color(0xFF1CDEC9)),
stringStyle: codeTheme.copyWith(color: const Color(0xFFFFA65C)),
punctuationStyle: codeTheme.copyWith(color: const Color(0xFF8BE9FD)),
classStyle: codeTheme.copyWith(color: const Color(0xFFD65BAD)),
constantStyle: codeTheme.copyWith(color: const Color(0xFFFF8383)),
child: _DemoSectionCode(
maxHeight: maxSectionHeight,
codeWidget: CodeDisplayPage(
_currentConfig.code,
),
),
);
case _DemoState.normal:
case _DemoState.fullscreen:
section = Container();
}
Widget body;
Widget demoContent = ScaffoldMessenger(
child: DemoWrapper(
height: contentHeight,
buildRoute: _currentConfig.buildRoute,
),
);
if (isDesktop) {
final bool isFullScreen = currentDemoState == _DemoState.fullscreen;
final Widget sectionAndDemo = Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
if (!isFullScreen) Expanded(child: section),
SizedBox(width: !isFullScreen ? 48.0 : 0),
Expanded(child: demoContent),
],
);
body = SafeArea(
child: Padding(
padding: const EdgeInsets.only(top: 56),
child: sectionAndDemo,
),
);
} else if (isFoldable) {
body = Padding(
padding: const EdgeInsets.only(top: 12.0),
child: TwoPane(
startPane: demoContent,
endPane: section,
),
);
} else {
section = AnimatedSize(
duration: const Duration(milliseconds: 200),
alignment: Alignment.topCenter,
curve: Curves.easeIn,
child: section,
);
final bool isDemoNormal = currentDemoState == _DemoState.normal;
// Add a tap gesture to collapse the currently opened section.
demoContent = Semantics(
label:
'${GalleryLocalizations.of(context)!.demo}, ${widget.demo.title}',
child: MouseRegion(
cursor: isDemoNormal ? MouseCursor.defer : SystemMouseCursors.click,
child: GestureDetector(
onTap: isDemoNormal
? null
: () {
setStateAndUpdate(() {
_demoStateIndex.value = _DemoState.normal.index;
});
},
child: Semantics(
excludeSemantics: !isDemoNormal,
child: demoContent,
),
),
),
);
body = SafeArea(
bottom: false,
child: ListView(
// Use a non-scrollable ListView to enable animation of shifting the
// demo offscreen.
physics: const NeverScrollableScrollPhysics(),
children: <Widget>[
section,
demoContent,
// Fake the safe area to ensure the animation looks correct.
SizedBox(height: bottomSafeArea),
],
),
);
}
Widget page;
if (isDesktop || isFoldable) {
page = AnimatedBuilder(
animation: _codeBackgroundColorController,
builder: (BuildContext context, Widget? child) {
Brightness themeBrightness;
switch (GalleryOptions.of(context).themeMode) {
case ThemeMode.system:
themeBrightness = MediaQuery.of(context).platformBrightness;
case ThemeMode.light:
themeBrightness = Brightness.light;
case ThemeMode.dark:
themeBrightness = Brightness.dark;
}
Widget contents = Container(
padding: EdgeInsets.symmetric(horizontal: horizontalPadding),
child: ApplyTextOptions(
child: Scaffold(
appBar: appBar,
body: body,
backgroundColor: Colors.transparent,
),
),
);
if (themeBrightness == Brightness.light) {
// If it is currently in light mode, add a
// dark background for code.
final Widget codeBackground = SafeArea(
child: Container(
padding: const EdgeInsets.only(top: 56),
child: Container(
color: ColorTween(
begin: Colors.transparent,
end: GalleryThemeData.darkThemeData.canvasColor,
).animate(_codeBackgroundColorController).value,
),
),
);
contents = Stack(
children: <Widget>[
codeBackground,
contents,
],
);
}
return ColoredBox(
color: colorScheme.background,
child: contents,
);
});
} else {
page = ColoredBox(
color: colorScheme.background,
child: ApplyTextOptions(
child: Scaffold(
appBar: appBar,
body: body,
resizeToAvoidBottomInset: false,
),
),
);
}
// Add the splash page functionality for desktop.
if (isDesktop) {
page = MediaQuery.removePadding(
removeTop: true,
context: context,
child: SplashPage(
child: page,
),
);
}
return FeatureDiscoveryController(page);
}
}
class _DemoSectionOptions extends StatelessWidget {
const _DemoSectionOptions({
required this.maxHeight,
required this.maxWidth,
required this.configurations,
required this.configIndex,
required this.onConfigChanged,
});
final double maxHeight;
final double maxWidth;
final List<GalleryDemoConfiguration> configurations;
final int configIndex;
final ValueChanged<int> onConfigChanged;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final ColorScheme colorScheme = Theme.of(context).colorScheme;
return Align(
alignment: AlignmentDirectional.topStart,
child: Container(
constraints: BoxConstraints(maxHeight: maxHeight, maxWidth: maxWidth),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Padding(
padding: const EdgeInsetsDirectional.only(
start: 24,
top: 12,
end: 24,
),
child: Text(
GalleryLocalizations.of(context)!.demoOptionsTooltip,
style: textTheme.headlineMedium!.apply(
color: colorScheme.onSurface,
fontSizeDelta:
isDisplayDesktop(context) ? desktopDisplay1FontDelta : 0,
),
),
),
Divider(
thickness: 1,
height: 16,
color: colorScheme.onSurface,
),
Flexible(
child: ListView(
shrinkWrap: true,
children: <Widget>[
for (final GalleryDemoConfiguration configuration in configurations)
_DemoSectionOptionsItem(
title: configuration.title,
isSelected: configuration == configurations[configIndex],
onTap: () {
onConfigChanged(configurations.indexOf(configuration));
},
),
],
),
),
const SizedBox(height: 12),
],
),
),
);
}
}
class _DemoSectionOptionsItem extends StatelessWidget {
const _DemoSectionOptionsItem({
required this.title,
required this.isSelected,
this.onTap,
});
final String title;
final bool isSelected;
final GestureTapCallback? onTap;
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
return Material(
color: isSelected ? colorScheme.surface : null,
child: InkWell(
onTap: onTap,
child: Container(
constraints: const BoxConstraints(minWidth: double.infinity),
padding: const EdgeInsets.symmetric(horizontal: 24, vertical: 8),
child: Text(
title,
style: Theme.of(context).textTheme.bodyMedium!.apply(
color:
isSelected ? colorScheme.primary : colorScheme.onSurface,
),
),
),
),
);
}
}
class _DemoSectionInfo extends StatelessWidget {
const _DemoSectionInfo({
required this.maxHeight,
required this.maxWidth,
required this.title,
required this.description,
});
final double maxHeight;
final double maxWidth;
final String title;
final String description;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final ColorScheme colorScheme = Theme.of(context).colorScheme;
return Align(
alignment: AlignmentDirectional.topStart,
child: Container(
padding: const EdgeInsetsDirectional.only(
start: 24,
top: 12,
end: 24,
bottom: 32,
),
constraints: BoxConstraints(maxHeight: maxHeight, maxWidth: maxWidth),
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
SelectableText(
title,
style: textTheme.headlineMedium!.apply(
color: colorScheme.onSurface,
fontSizeDelta:
isDisplayDesktop(context) ? desktopDisplay1FontDelta : 0,
),
),
const SizedBox(height: 12),
SelectableText(
description,
style: textTheme.bodyMedium!.apply(
color: colorScheme.onSurface,
),
),
],
),
),
),
);
}
}
class DemoWrapper extends StatelessWidget {
const DemoWrapper({
super.key,
required this.height,
required this.buildRoute,
});
final double height;
final WidgetBuilder buildRoute;
@override
Widget build(BuildContext context) {
return Container(
padding: const EdgeInsets.only(left: 16, right: 16, bottom: 16),
height: height,
child: ClipRRect(
clipBehavior: Clip.antiAliasWithSaveLayer,
borderRadius: const BorderRadius.vertical(
top: Radius.circular(10.0),
bottom: Radius.circular(2.0),
),
child: Theme(
data: MaterialDemoThemeData.themeData.copyWith(
platform: GalleryOptions.of(context).platform,
),
child: CupertinoTheme(
data: const CupertinoThemeData()
.copyWith(brightness: Brightness.light),
child: ApplyTextOptions(
child: Builder(builder: buildRoute),
),
),
),
),
);
}
}
class _DemoSectionCode extends StatelessWidget {
const _DemoSectionCode({
this.maxHeight,
this.codeWidget,
});
final double? maxHeight;
final Widget? codeWidget;
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
return Theme(
data: GalleryThemeData.darkThemeData,
child: Padding(
padding: const EdgeInsets.only(bottom: 16),
child: Container(
color: isDesktop ? null : GalleryThemeData.darkThemeData.canvasColor,
padding: const EdgeInsets.symmetric(horizontal: 16),
height: maxHeight,
child: codeWidget,
),
),
);
}
}
class CodeDisplayPage extends StatelessWidget {
const CodeDisplayPage(this.code, {super.key});
final CodeDisplayer code;
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
final TextSpan richTextCode = code(context);
final String plainTextCode = richTextCode.toPlainText();
void showSnackBarOnCopySuccess(dynamic result) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(
GalleryLocalizations.of(context)!
.demoCodeViewerCopiedToClipboardMessage,
),
),
);
}
void showSnackBarOnCopyFailure(Object exception) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(
GalleryLocalizations.of(context)!
.demoCodeViewerFailedToCopyToClipboardMessage(exception),
),
),
);
}
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Padding(
padding: isDesktop
? const EdgeInsets.only(bottom: 8)
: const EdgeInsets.symmetric(vertical: 8),
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.white.withOpacity(0.15),
padding: const EdgeInsets.symmetric(horizontal: 8),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(4)),
),
),
onPressed: () async {
await Clipboard.setData(ClipboardData(text: plainTextCode))
.then(showSnackBarOnCopySuccess)
.catchError(showSnackBarOnCopyFailure);
},
child: Text(
GalleryLocalizations.of(context)!.demoCodeViewerCopyAll,
style: Theme.of(context).textTheme.labelLarge!.copyWith(
color: Colors.white,
fontWeight: FontWeight.w500,
),
),
),
),
Expanded(
child: SingleChildScrollView(
child: Container(
padding: const EdgeInsets.symmetric(vertical: 8),
child: SelectableText.rich(
richTextCode,
textDirection: TextDirection.ltr,
),
),
),
),
],
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/pages/demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/pages/demo.dart",
"repo_id": "flutter",
"token_count": 11435
} | 562 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../layout/adaptive.dart';
import '../../layout/highlight_focus.dart';
import '../../layout/image_placeholder.dart';
import 'model/destination.dart';
// Width and height for thumbnail images.
const double mobileThumbnailSize = 60.0;
class DestinationCard extends StatelessWidget {
const DestinationCard({
super.key,
required this.destination,
});
final Destination destination;
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
final TextTheme textTheme = Theme.of(context).textTheme;
final Widget card = isDesktop
? Padding(
padding: const EdgeInsets.only(bottom: 40),
child: Semantics(
container: true,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(4)),
child: _DestinationImage(destination: destination),
),
Padding(
padding: const EdgeInsets.only(top: 20, bottom: 10),
child: SelectableText(
destination.destination,
style: textTheme.titleMedium,
),
),
SelectableText(
destination.subtitle(context),
semanticsLabel: destination.subtitleSemantics(context),
style: textTheme.titleSmall,
),
],
),
),
)
: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ListTile(
contentPadding: const EdgeInsetsDirectional.only(end: 8),
leading: ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(4)),
child: SizedBox(
width: mobileThumbnailSize,
height: mobileThumbnailSize,
child: _DestinationImage(destination: destination),
),
),
title: SelectableText(destination.destination,
style: textTheme.titleMedium),
subtitle: SelectableText(
destination.subtitle(context),
semanticsLabel: destination.subtitleSemantics(context),
style: textTheme.titleSmall,
),
),
const Divider(thickness: 1),
],
);
return HighlightFocus(
debugLabel: 'DestinationCard: ${destination.destination}',
highlightColor: Colors.red.withOpacity(0.1),
onPressed: () {},
child: card,
);
}
}
class _DestinationImage extends StatelessWidget {
const _DestinationImage({
required this.destination,
});
final Destination destination;
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
return Semantics(
label: destination.assetSemanticLabel,
child: ExcludeSemantics(
child: FadeInImagePlaceholder(
image: AssetImage(
destination.assetName,
package: 'flutter_gallery_assets',
),
fit: BoxFit.cover,
width: isDesktop ? null : mobileThumbnailSize,
height: isDesktop ? null : mobileThumbnailSize,
placeholder: LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
return Container(
color: Colors.black.withOpacity(0.1),
width: constraints.maxWidth,
height: constraints.maxWidth / destination.imageAspectRatio,
);
}),
),
),
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/crane/item_cards.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/crane/item_cards.dart",
"repo_id": "flutter",
"token_count": 1904
} | 563 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:animations/animations.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import '../../data/gallery_options.dart';
import '../../gallery_localizations.dart';
import '../../layout/adaptive.dart';
import '../../layout/text_scale.dart';
import 'charts/line_chart.dart';
import 'charts/pie_chart.dart';
import 'charts/vertical_fraction_bar.dart';
import 'colors.dart';
import 'data.dart';
import 'formatters.dart';
class FinancialEntityView extends StatelessWidget {
const FinancialEntityView({
super.key,
required this.heroLabel,
required this.heroAmount,
required this.wholeAmount,
required this.segments,
required this.financialEntityCards,
}) : assert(segments.length == financialEntityCards.length);
/// The amounts to assign each item.
final List<RallyPieChartSegment> segments;
final String heroLabel;
final double heroAmount;
final double wholeAmount;
final List<FinancialEntityCategoryView> financialEntityCards;
@override
Widget build(BuildContext context) {
final double maxWidth = pieChartMaxSize + (cappedTextScale(context) - 1.0) * 100.0;
return LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
return Column(
children: <Widget>[
ConstrainedBox(
constraints: BoxConstraints(
// We decrease the max height to ensure the [RallyPieChart] does
// not take up the full height when it is smaller than
// [kPieChartMaxSize].
maxHeight: math.min(
constraints.biggest.shortestSide * 0.9,
maxWidth,
),
),
child: RallyPieChart(
heroLabel: heroLabel,
heroAmount: heroAmount,
wholeAmount: wholeAmount,
segments: segments,
),
),
const SizedBox(height: 24),
Container(
height: 1,
constraints: BoxConstraints(maxWidth: maxWidth),
color: RallyColors.inputBackground,
),
Container(
constraints: BoxConstraints(maxWidth: maxWidth),
color: RallyColors.cardBackground,
child: Column(
children: financialEntityCards,
),
),
],
);
});
}
}
/// A reusable widget to show balance information of a single entity as a card.
class FinancialEntityCategoryView extends StatelessWidget {
const FinancialEntityCategoryView({
super.key,
required this.indicatorColor,
required this.indicatorFraction,
required this.title,
required this.subtitle,
required this.semanticsLabel,
required this.amount,
required this.suffix,
});
final Color indicatorColor;
final double indicatorFraction;
final String title;
final String subtitle;
final String semanticsLabel;
final String amount;
final Widget suffix;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Semantics.fromProperties(
properties: SemanticsProperties(
button: true,
enabled: true,
label: semanticsLabel,
),
excludeSemantics: true,
// TODO(x): State restoration of FinancialEntityCategoryDetailsPage on mobile is blocked because OpenContainer does not support restorablePush, https://github.com/flutter/gallery/issues/570.
child: OpenContainer(
transitionDuration: const Duration(milliseconds: 350),
openBuilder: (BuildContext context, void Function() openContainer) =>
FinancialEntityCategoryDetailsPage(),
openColor: RallyColors.primaryBackground,
closedColor: RallyColors.primaryBackground,
closedElevation: 0,
closedBuilder: (BuildContext context, void Function() openContainer) {
return TextButton(
style: TextButton.styleFrom(foregroundColor: Colors.black),
onPressed: openContainer,
child: Column(
children: <Widget>[
Container(
padding:
const EdgeInsets.symmetric(vertical: 16, horizontal: 8),
child: Row(
children: <Widget>[
Container(
alignment: Alignment.center,
height: 32 + 60 * (cappedTextScale(context) - 1),
padding: const EdgeInsets.symmetric(horizontal: 12),
child: VerticalFractionBar(
color: indicatorColor,
fraction: indicatorFraction,
),
),
Expanded(
child: Wrap(
alignment: WrapAlignment.spaceBetween,
crossAxisAlignment: WrapCrossAlignment.center,
children: <Widget>[
Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
title,
style: textTheme.bodyMedium!
.copyWith(fontSize: 16),
),
Text(
subtitle,
style: textTheme.bodyMedium!
.copyWith(color: RallyColors.gray60),
),
],
),
Text(
amount,
style: textTheme.bodyLarge!.copyWith(
fontSize: 20,
color: RallyColors.gray,
),
),
],
),
),
Container(
constraints: const BoxConstraints(minWidth: 32),
padding: const EdgeInsetsDirectional.only(start: 12),
child: suffix,
),
],
),
),
const Divider(
height: 1,
indent: 16,
endIndent: 16,
color: RallyColors.dividerColor,
),
],
),
);
},
),
);
}
}
/// Data model for [FinancialEntityCategoryView].
class FinancialEntityCategoryModel {
const FinancialEntityCategoryModel(
this.indicatorColor,
this.indicatorFraction,
this.title,
this.subtitle,
this.usdAmount,
this.suffix,
);
final Color indicatorColor;
final double indicatorFraction;
final String title;
final String subtitle;
final double usdAmount;
final Widget suffix;
}
FinancialEntityCategoryView buildFinancialEntityFromAccountData(
AccountData model,
int accountDataIndex,
BuildContext context,
) {
final String amount = usdWithSignFormat(context).format(model.primaryAmount);
final String shortAccountNumber = model.accountNumber.substring(6);
return FinancialEntityCategoryView(
suffix: const Icon(Icons.chevron_right, color: Colors.grey),
title: model.name,
subtitle: '• • • • • • $shortAccountNumber',
semanticsLabel: GalleryLocalizations.of(context)!.rallyAccountAmount(
model.name,
shortAccountNumber,
amount,
),
indicatorColor: RallyColors.accountColor(accountDataIndex),
indicatorFraction: 1,
amount: amount,
);
}
FinancialEntityCategoryView buildFinancialEntityFromBillData(
BillData model,
int billDataIndex,
BuildContext context,
) {
final String amount = usdWithSignFormat(context).format(model.primaryAmount);
return FinancialEntityCategoryView(
suffix: const Icon(Icons.chevron_right, color: Colors.grey),
title: model.name,
subtitle: model.dueDate,
semanticsLabel: GalleryLocalizations.of(context)!.rallyBillAmount(
model.name,
model.dueDate,
amount,
),
indicatorColor: RallyColors.billColor(billDataIndex),
indicatorFraction: 1,
amount: amount,
);
}
FinancialEntityCategoryView buildFinancialEntityFromBudgetData(
BudgetData model,
int budgetDataIndex,
BuildContext context,
) {
final String amountUsed = usdWithSignFormat(context).format(model.amountUsed);
final String primaryAmount = usdWithSignFormat(context).format(model.primaryAmount);
final String amount =
usdWithSignFormat(context).format(model.primaryAmount - model.amountUsed);
return FinancialEntityCategoryView(
suffix: Text(
GalleryLocalizations.of(context)!.rallyFinanceLeft,
style: Theme.of(context)
.textTheme
.bodyMedium!
.copyWith(color: RallyColors.gray60, fontSize: 10),
),
title: model.name,
subtitle: '$amountUsed / $primaryAmount',
semanticsLabel: GalleryLocalizations.of(context)!.rallyBudgetAmount(
model.name,
model.amountUsed,
model.primaryAmount,
amount,
),
indicatorColor: RallyColors.budgetColor(budgetDataIndex),
indicatorFraction: model.amountUsed / model.primaryAmount,
amount: amount,
);
}
List<FinancialEntityCategoryView> buildAccountDataListViews(
List<AccountData> items,
BuildContext context,
) {
return List<FinancialEntityCategoryView>.generate(
items.length,
(int i) => buildFinancialEntityFromAccountData(items[i], i, context),
);
}
List<FinancialEntityCategoryView> buildBillDataListViews(
List<BillData> items,
BuildContext context,
) {
return List<FinancialEntityCategoryView>.generate(
items.length,
(int i) => buildFinancialEntityFromBillData(items[i], i, context),
);
}
List<FinancialEntityCategoryView> buildBudgetDataListViews(
List<BudgetData> items,
BuildContext context,
) {
return <FinancialEntityCategoryView>[
for (int i = 0; i < items.length; i++)
buildFinancialEntityFromBudgetData(items[i], i, context)
];
}
class FinancialEntityCategoryDetailsPage extends StatelessWidget {
FinancialEntityCategoryDetailsPage({super.key});
final List<DetailedEventData> items =
DummyDataService.getDetailedEventItems();
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
return ApplyTextOptions(
child: Scaffold(
appBar: AppBar(
elevation: 0,
centerTitle: true,
title: Text(
GalleryLocalizations.of(context)!.rallyAccountDataChecking,
style:
Theme.of(context).textTheme.bodyMedium!.copyWith(fontSize: 18),
),
),
body: Column(
children: <Widget>[
SizedBox(
height: 200,
width: double.infinity,
child: RallyLineChart(events: items),
),
Expanded(
child: Padding(
padding: isDesktop ? const EdgeInsets.all(40) : EdgeInsets.zero,
child: ListView(
shrinkWrap: true,
children: <Widget>[
for (final DetailedEventData detailedEventData in items)
_DetailedEventCard(
title: detailedEventData.title,
date: detailedEventData.date,
amount: detailedEventData.amount,
),
],
),
),
),
],
),
),
);
}
}
class _DetailedEventCard extends StatelessWidget {
const _DetailedEventCard({
required this.title,
required this.date,
required this.amount,
});
final String title;
final DateTime date;
final double amount;
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
return TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.black,
padding: const EdgeInsets.symmetric(horizontal: 16),
),
onPressed: () {},
child: Column(
children: <Widget>[
Container(
padding: const EdgeInsets.symmetric(vertical: 16),
width: double.infinity,
child: isDesktop
? Row(
children: <Widget>[
Expanded(
child: _EventTitle(title: title),
),
_EventDate(date: date),
Expanded(
child: Align(
alignment: AlignmentDirectional.centerEnd,
child: _EventAmount(amount: amount),
),
),
],
)
: Wrap(
alignment: WrapAlignment.spaceBetween,
children: <Widget>[
Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
_EventTitle(title: title),
_EventDate(date: date),
],
),
_EventAmount(amount: amount),
],
),
),
SizedBox(
height: 1,
child: Container(
color: RallyColors.dividerColor,
),
),
],
),
);
}
}
class _EventAmount extends StatelessWidget {
const _EventAmount({required this.amount});
final double amount;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Text(
usdWithSignFormat(context).format(amount),
style: textTheme.bodyLarge!.copyWith(
fontSize: 20,
color: RallyColors.gray,
),
);
}
}
class _EventDate extends StatelessWidget {
const _EventDate({required this.date});
final DateTime date;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Text(
shortDateFormat(context).format(date),
semanticsLabel: longDateFormat(context).format(date),
style: textTheme.bodyMedium!.copyWith(color: RallyColors.gray60),
);
}
}
class _EventTitle extends StatelessWidget {
const _EventTitle({required this.title});
final String title;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Text(
title,
style: textTheme.bodyMedium!.copyWith(fontSize: 16),
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/rally/finance.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/rally/finance.dart",
"repo_id": "flutter",
"token_count": 7062
} | 564 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:animations/animations.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import '../../layout/adaptive.dart';
import 'colors.dart';
import 'mail_view_page.dart';
import 'model/email_model.dart';
import 'model/email_store.dart';
import 'profile_avatar.dart';
const String _assetsPackage = 'flutter_gallery_assets';
const String _iconAssetLocation = 'reply/icons';
class MailPreviewCard extends StatelessWidget {
const MailPreviewCard({
super.key,
required this.id,
required this.email,
required this.onDelete,
required this.onStar,
required this.isStarred,
required this.onStarredMailbox,
});
final int id;
final Email email;
final VoidCallback onDelete;
final VoidCallback onStar;
final bool isStarred;
final bool onStarredMailbox;
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
// TODO(x): State restoration of mail view page is blocked because OpenContainer does not support restorablePush, https://github.com/flutter/gallery/issues/570.
return OpenContainer(
openBuilder: (BuildContext context, void Function() closedContainer) {
return MailViewPage(id: id, email: email);
},
openColor: theme.cardColor,
closedShape: const RoundedRectangleBorder(
),
closedElevation: 0,
closedColor: theme.cardColor,
closedBuilder: (BuildContext context, void Function() openContainer) {
final bool isDesktop = isDisplayDesktop(context);
final ColorScheme colorScheme = theme.colorScheme;
final _MailPreview mailPreview = _MailPreview(
id: id,
email: email,
onTap: openContainer,
onStar: onStar,
onDelete: onDelete,
);
if (isDesktop) {
return mailPreview;
} else {
return Dismissible(
key: ObjectKey(email),
dismissThresholds: const <DismissDirection, double>{
DismissDirection.startToEnd: 0.8,
DismissDirection.endToStart: 0.4,
},
onDismissed: (DismissDirection direction) {
switch (direction) {
case DismissDirection.endToStart:
if (onStarredMailbox) {
onStar();
}
case DismissDirection.startToEnd:
onDelete();
case DismissDirection.vertical:
case DismissDirection.horizontal:
case DismissDirection.up:
case DismissDirection.down:
case DismissDirection.none:
break;
}
},
background: _DismissibleContainer(
icon: 'twotone_delete',
backgroundColor: colorScheme.primary,
iconColor: ReplyColors.blue50,
alignment: Alignment.centerLeft,
padding: const EdgeInsetsDirectional.only(start: 20),
),
confirmDismiss: (DismissDirection direction) async {
if (direction == DismissDirection.endToStart) {
if (onStarredMailbox) {
return true;
}
onStar();
return false;
} else {
return true;
}
},
secondaryBackground: _DismissibleContainer(
icon: 'twotone_star',
backgroundColor: isStarred
? colorScheme.secondary
: theme.scaffoldBackgroundColor,
iconColor: isStarred
? colorScheme.onSecondary
: colorScheme.onBackground,
alignment: Alignment.centerRight,
padding: const EdgeInsetsDirectional.only(end: 20),
),
child: mailPreview,
);
}
},
);
}
}
class _DismissibleContainer extends StatelessWidget {
const _DismissibleContainer({
required this.icon,
required this.backgroundColor,
required this.iconColor,
required this.alignment,
required this.padding,
});
final String icon;
final Color backgroundColor;
final Color iconColor;
final Alignment alignment;
final EdgeInsetsDirectional padding;
@override
Widget build(BuildContext context) {
return AnimatedContainer(
alignment: alignment,
curve: Easing.legacy,
color: backgroundColor,
duration: kThemeAnimationDuration,
padding: padding,
child: Material(
color: Colors.transparent,
child: ImageIcon(
AssetImage(
'reply/icons/$icon.png',
package: 'flutter_gallery_assets',
),
size: 36,
color: iconColor,
),
),
);
}
}
class _MailPreview extends StatelessWidget {
const _MailPreview({
required this.id,
required this.email,
required this.onTap,
this.onStar,
this.onDelete,
});
final int id;
final Email email;
final VoidCallback onTap;
final VoidCallback? onStar;
final VoidCallback? onDelete;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final EmailStore emailStore = Provider.of<EmailStore>(
context,
listen: false,
);
return InkWell(
onTap: () {
Provider.of<EmailStore>(
context,
listen: false,
).selectedEmailId = id;
onTap();
},
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return ConstrainedBox(
constraints: BoxConstraints(maxHeight: constraints.maxHeight),
child: Padding(
padding: const EdgeInsets.all(20),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Text(
'${email.sender} - ${email.time}',
style: textTheme.bodySmall,
),
const SizedBox(height: 4),
Text(email.subject, style: textTheme.headlineSmall),
const SizedBox(height: 16),
],
),
),
_MailPreviewActionBar(
avatar: email.avatar,
isStarred: emailStore.isEmailStarred(email.id),
onStar: onStar,
onDelete: onDelete,
),
],
),
Padding(
padding: const EdgeInsetsDirectional.only(
end: 20,
),
child: Text(
email.message,
overflow: TextOverflow.ellipsis,
maxLines: 1,
style: textTheme.bodyMedium,
),
),
if (email.containsPictures) ...<Widget>[
const Flexible(
child: Column(
children: <Widget>[
SizedBox(height: 20),
_PicturePreview(),
],
),
),
],
],
),
),
);
},
),
);
}
}
class _PicturePreview extends StatelessWidget {
const _PicturePreview();
bool _shouldShrinkImage() {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.android:
return true;
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return false;
}
}
@override
Widget build(BuildContext context) {
return SizedBox(
height: 96,
child: ListView.builder(
itemCount: 4,
scrollDirection: Axis.horizontal,
itemBuilder: (BuildContext context, int index) {
return Padding(
padding: const EdgeInsetsDirectional.only(end: 4),
child: Image.asset(
'reply/attachments/paris_${index + 1}.jpg',
gaplessPlayback: true,
package: 'flutter_gallery_assets',
cacheWidth: _shouldShrinkImage() ? 200 : null,
),
);
},
),
);
}
}
class _MailPreviewActionBar extends StatelessWidget {
const _MailPreviewActionBar({
required this.avatar,
required this.isStarred,
this.onStar,
this.onDelete,
});
final String avatar;
final bool isStarred;
final VoidCallback? onStar;
final VoidCallback? onDelete;
@override
Widget build(BuildContext context) {
final bool isDark = Theme.of(context).brightness == Brightness.dark;
final Color color = isDark ? ReplyColors.white50 : ReplyColors.blue600;
final bool isDesktop = isDisplayDesktop(context);
final Color starredIconColor =
isStarred ? Theme.of(context).colorScheme.secondary : color;
return Row(
children: <Widget>[
if (isDesktop) ...<Widget>[
IconButton(
icon: ImageIcon(
const AssetImage(
'$_iconAssetLocation/twotone_star.png',
package: _assetsPackage,
),
color: starredIconColor,
),
onPressed: onStar,
),
IconButton(
icon: ImageIcon(
const AssetImage(
'$_iconAssetLocation/twotone_delete.png',
package: _assetsPackage,
),
color: color,
),
onPressed: onDelete,
),
IconButton(
icon: Icon(
Icons.more_vert,
color: color,
),
onPressed: () {},
),
const SizedBox(width: 12),
],
ProfileAvatar(avatar: avatar),
],
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/reply/mail_card_preview.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/reply/mail_card_preview.dart",
"repo_id": "flutter",
"token_count": 5402
} | 565 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:scoped_model/scoped_model.dart';
import 'product.dart';
import 'products_repository.dart';
double _salesTaxRate = 0.06;
double _shippingCostPerItem = 7;
class AppStateModel extends Model {
// All the available products.
List<Product> _availableProducts = <Product>[];
// The currently selected category of products.
Category _selectedCategory = categoryAll;
// The IDs and quantities of products currently in the cart.
final Map<int, int> _productsInCart = <int, int>{};
Map<int, int> get productsInCart => Map<int, int>.from(_productsInCart);
// Total number of items in the cart.
int get totalCartQuantity => _productsInCart.values.fold(0, (int v, int e) => v + e);
Category get selectedCategory => _selectedCategory;
// Totaled prices of the items in the cart.
double get subtotalCost {
return _productsInCart.keys
.map((int id) => _availableProducts[id].price * _productsInCart[id]!)
.fold(0.0, (double sum, int e) => sum + e);
}
// Total shipping cost for the items in the cart.
double get shippingCost {
return _shippingCostPerItem *
_productsInCart.values.fold(0.0, (num sum, int e) => sum + e);
}
// Sales tax for the items in the cart
double get tax => subtotalCost * _salesTaxRate;
// Total cost to order everything in the cart.
double get totalCost => subtotalCost + shippingCost + tax;
// Returns a copy of the list of available products, filtered by category.
List<Product> getProducts() {
if (_selectedCategory == categoryAll) {
return List<Product>.from(_availableProducts);
} else {
return _availableProducts
.where((Product p) => p.category == _selectedCategory)
.toList();
}
}
// Adds a product to the cart.
void addProductToCart(int productId) {
if (!_productsInCart.containsKey(productId)) {
_productsInCart[productId] = 1;
} else {
_productsInCart[productId] = _productsInCart[productId]! + 1;
}
notifyListeners();
}
// Adds products to the cart by a certain amount.
// quantity must be non-null positive value.
void addMultipleProductsToCart(int productId, int quantity) {
assert(quantity > 0);
if (!_productsInCart.containsKey(productId)) {
_productsInCart[productId] = quantity;
} else {
_productsInCart[productId] = _productsInCart[productId]! + quantity;
}
notifyListeners();
}
// Removes an item from the cart.
void removeItemFromCart(int productId) {
if (_productsInCart.containsKey(productId)) {
if (_productsInCart[productId] == 1) {
_productsInCart.remove(productId);
} else {
_productsInCart[productId] = _productsInCart[productId]! - 1;
}
}
notifyListeners();
}
// Returns the Product instance matching the provided id.
Product getProductById(int id) {
return _availableProducts.firstWhere((Product p) => p.id == id);
}
// Removes everything from the cart.
void clearCart() {
_productsInCart.clear();
notifyListeners();
}
// Loads the list of available products from the repo.
void loadProducts() {
_availableProducts = ProductsRepository.loadProducts(categoryAll);
notifyListeners();
}
void setCategory(Category newCategory) {
_selectedCategory = newCategory;
notifyListeners();
}
@override
String toString() {
return 'AppStateModel(totalCost: $totalCost)';
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/shrine/model/app_state_model.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/shrine/model/app_state_model.dart",
"repo_id": "flutter",
"token_count": 1198
} | 566 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../data/gallery_options.dart';
import '../../gallery_localizations.dart';
import 'home.dart';
import 'routes.dart' as routes;
const Color _primaryColor = Color(0xFF6200EE);
class StarterApp extends StatelessWidget {
const StarterApp({super.key});
static const String defaultRoute = routes.defaultRoute;
@override
Widget build(BuildContext context) {
return MaterialApp(
restorationScopeId: 'starter_app',
title: GalleryLocalizations.of(context)!.starterAppTitle,
debugShowCheckedModeBanner: false,
localizationsDelegates: GalleryLocalizations.localizationsDelegates,
supportedLocales: GalleryLocalizations.supportedLocales,
locale: GalleryOptions.of(context).locale,
initialRoute: StarterApp.defaultRoute,
routes: <String, WidgetBuilder>{
StarterApp.defaultRoute: (BuildContext context) => const _Home(),
},
theme: ThemeData(
highlightColor: Colors.transparent,
colorScheme: const ColorScheme(
primary: _primaryColor,
primaryContainer: Color(0xFF3700B3),
secondary: Color(0xFF03DAC6),
secondaryContainer: Color(0xFF018786),
background: Colors.white,
surface: Colors.white,
onBackground: Colors.black,
error: Color(0xFFB00020),
onError: Colors.white,
onPrimary: Colors.white,
onSecondary: Colors.black,
onSurface: Colors.black,
brightness: Brightness.light,
),
dividerTheme: const DividerThemeData(
thickness: 1,
color: Color(0xFFE5E5E5),
),
platform: GalleryOptions.of(context).platform,
),
);
}
}
class _Home extends StatelessWidget {
const _Home();
@override
Widget build(BuildContext context) {
return const ApplyTextOptions(
child: HomePage(),
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/starter/app.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/starter/app.dart",
"repo_id": "flutter",
"token_count": 804
} | 567 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_driver/driver_extension.dart';
/// This application displays text passed through a --dart-define.
void main() {
enableFlutterDriverExtension();
runApp(
const Center(
child: Text(
String.fromEnvironment('test.valueA') + String.fromEnvironment('test.valueB'),
textDirection: TextDirection.ltr,
),
),
);
}
| flutter/dev/integration_tests/ui/lib/defines.dart/0 | {
"file_path": "flutter/dev/integration_tests/ui/lib/defines.dart",
"repo_id": "flutter",
"token_count": 191
} | 568 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:html' as html;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'package:meta/dart2js.dart';
// Tests that the framework prints stack traces in all build modes.
//
// Regression test for https://github.com/flutter/flutter/issues/68616.
//
// See also `dev/integration_tests/web/lib/stack_trace.dart` that tests the
// framework's ability to parse stack traces in all build modes.
Future<void> main() async {
final StringBuffer errorMessage = StringBuffer();
debugPrint = (String? message, { int? wrapWidth }) {
errorMessage.writeln(message);
};
runApp(const ThrowingWidget());
// Let the framework flush error messages.
await Future<void>.delayed(Duration.zero);
final StringBuffer output = StringBuffer();
if (_errorMessageFormattedCorrectly(errorMessage.toString())) {
output.writeln('--- TEST SUCCEEDED ---');
} else {
output.writeln('--- UNEXPECTED ERROR MESSAGE FORMAT ---');
output.writeln(errorMessage);
output.writeln('--- TEST FAILED ---');
}
print(output);
html.HttpRequest.request(
'/test-result',
method: 'POST',
sendData: '$output',
);
}
bool _errorMessageFormattedCorrectly(String errorMessage) {
if (!errorMessage.contains('Test error message')) {
return false;
}
// In release mode symbols are minified. No sense testing the contents of the stack trace.
if (kReleaseMode) {
return true;
}
const List<String> expectedFunctions = <String>[
'topLevelFunction',
'secondLevelFunction',
'thirdLevelFunction',
];
return expectedFunctions.every(errorMessage.contains);
}
class ThrowingWidget extends StatefulWidget {
const ThrowingWidget({super.key});
@override
State<ThrowingWidget> createState() => _ThrowingWidgetState();
}
class _ThrowingWidgetState extends State<ThrowingWidget> {
@override
void initState() {
super.initState();
topLevelFunction();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
@noInline
void topLevelFunction() {
secondLevelFunction();
}
@noInline
void secondLevelFunction() {
thirdLevelFunction();
}
@noInline
void thirdLevelFunction() {
throw Exception('Test error message');
}
| flutter/dev/integration_tests/web/lib/framework_stack_trace.dart/0 | {
"file_path": "flutter/dev/integration_tests/web/lib/framework_stack_trace.dart",
"repo_id": "flutter",
"token_count": 751
} | 569 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
MyAppState createState() => MyAppState();
}
class MyAppState extends State<MyApp> {
String kMessage = 'ABC';
@override
Widget build(BuildContext context) {
// cause cast error.
print(kMessage as int);
return const Text('Hello');
}
}
| flutter/dev/integration_tests/web_e2e_tests/lib/profile_diagnostics_main.dart/0 | {
"file_path": "flutter/dev/integration_tests/web_e2e_tests/lib/profile_diagnostics_main.dart",
"repo_id": "flutter",
"token_count": 185
} | 570 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/scheduler.dart';
int seed = 0;
void main() {
runApp(MaterialApp(
title: 'Text tester',
home: const Home(),
routes: <String, WidgetBuilder>{
'underlines': (BuildContext context) => const Underlines(),
'fallback': (BuildContext context) => const Fallback(),
'bidi': (BuildContext context) => const Bidi(),
'fuzzer': (BuildContext context) => Fuzzer(seed: seed),
'zalgo': (BuildContext context) => Zalgo(seed: seed),
'painting': (BuildContext context) => Painting(seed: seed),
},
));
}
class Home extends StatefulWidget {
const Home({ super.key });
@override
State<Home> createState() => _HomeState();
}
class _HomeState extends State<Home> {
@override
Widget build(BuildContext context) {
return Material(
child: Column(
children: <Widget>[
Expanded(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.white,
backgroundColor: Colors.red.shade800,
),
onPressed: () { Navigator.pushNamed(context, 'underlines'); },
child: const Text('Test Underlines'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.white,
backgroundColor: Colors.orange.shade700,
),
onPressed: () { Navigator.pushNamed(context, 'fallback'); },
child: const Text('Test Font Fallback'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.black,
backgroundColor: Colors.yellow.shade700,
),
onPressed: () { Navigator.pushNamed(context, 'bidi'); },
child: const Text('Test Bidi Formatting'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.black,
backgroundColor: Colors.green.shade400,
),
onPressed: () { Navigator.pushNamed(context, 'fuzzer'); },
child: const Text('TextSpan Fuzzer'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.white,
backgroundColor: Colors.blue.shade400,
),
onPressed: () { Navigator.pushNamed(context, 'zalgo'); },
child: const Text('Diacritics Fuzzer'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.black,
backgroundColor: Colors.purple.shade200,
),
onPressed: () { Navigator.pushNamed(context, 'painting'); },
child: const Text('Painting Fuzzer'),
),
],
),
),
Padding(
padding: const EdgeInsets.symmetric(horizontal: 20.0),
child: Slider(
max: 1024.0,
value: seed.toDouble(),
label: '$seed',
divisions: 1025,
onChanged: (double value) {
setState(() {
seed = value.round();
});
},
),
),
Padding(
padding: const EdgeInsets.only(bottom: 10.0),
child: Text('Random seed for fuzzers: $seed'),
),
],
),
);
}
}
class Fuzzer extends StatefulWidget {
const Fuzzer({ super.key, required this.seed });
final int seed;
@override
State<Fuzzer> createState() => _FuzzerState();
}
class _FuzzerState extends State<Fuzzer> with SingleTickerProviderStateMixin {
TextSpan _textSpan = const TextSpan(text: 'Welcome to the Flutter text fuzzer.');
late final Ticker _ticker = createTicker(_updateTextSpan)..start();
late final math.Random _random = math.Random(widget.seed); // providing a seed is important for reproducibility;
@override
void initState() {
super.initState();
_updateTextSpan(null);
}
@override
void dispose() {
_ticker.dispose();
super.dispose();
}
void _updateTextSpan(Duration? duration) {
setState(() {
_textSpan = _fiddleWith(_textSpan);
});
}
TextSpan _fiddleWith(TextSpan node) {
return TextSpan(
text: _fiddleWithText(node.text),
style: _fiddleWithStyle(node.style),
children: _fiddleWithChildren(node.children?.map((InlineSpan child) => _fiddleWith(child as TextSpan)).toList() ?? <TextSpan>[]),
);
}
String? _fiddleWithText(String? text) {
if (_random.nextInt(10) > 0) {
return text;
}
return _createRandomText();
}
TextStyle? _fiddleWithStyle(TextStyle? style) {
if (style == null) {
switch (_random.nextInt(20)) {
case 0:
return const TextStyle();
case 1:
style = const TextStyle(); // is mutated below
default:
return null;
}
}
if (_random.nextInt(200) == 0) {
return null;
}
return TextStyle(
color: _fiddleWithColor(style.color),
decoration: _fiddleWithDecoration(style.decoration),
decorationColor: _fiddleWithColor(style.decorationColor),
decorationStyle: _fiddleWithDecorationStyle(style.decorationStyle),
fontWeight: _fiddleWithFontWeight(style.fontWeight),
fontStyle: _fiddleWithFontStyle(style.fontStyle),
// TODO(ianh): Check textBaseline once we support that
fontFamily: _fiddleWithFontFamily(style.fontFamily),
fontSize: _fiddleWithDouble(style.fontSize, 14.0, 100.0),
letterSpacing: _fiddleWithDouble(style.letterSpacing, 0.0, 30.0),
wordSpacing: _fiddleWithDouble(style.wordSpacing, 0.0, 30.0),
height: _fiddleWithDouble(style.height, 1.0, 1.9),
);
}
Color? _fiddleWithColor(Color? value) {
switch (_random.nextInt(10)) {
case 0:
if (value == null) {
return pickFromList<MaterialColor>(_random, Colors.primaries)[(_random.nextInt(9) + 1) * 100];
}
switch (_random.nextInt(4)) {
case 0:
return value.withAlpha(value.alpha + _random.nextInt(10) - 5);
case 1:
return value.withRed(value.red + _random.nextInt(10) - 5);
case 2:
return value.withGreen(value.green + _random.nextInt(10) - 5);
case 3:
return value.withBlue(value.blue + _random.nextInt(10) - 5);
}
case 1:
return null;
}
return value;
}
TextDecoration? _fiddleWithDecoration(TextDecoration? value) {
if (_random.nextInt(10) > 0) {
return value;
}
switch (_random.nextInt(100)) {
case 10:
return TextDecoration.underline;
case 11:
return TextDecoration.underline;
case 12:
return TextDecoration.underline;
case 13:
return TextDecoration.underline;
case 20:
return TextDecoration.lineThrough;
case 30:
return TextDecoration.overline;
case 90:
return TextDecoration.combine(<TextDecoration>[TextDecoration.underline, TextDecoration.lineThrough]);
case 91:
return TextDecoration.combine(<TextDecoration>[TextDecoration.underline, TextDecoration.overline]);
case 92:
return TextDecoration.combine(<TextDecoration>[TextDecoration.lineThrough, TextDecoration.overline]);
case 93:
return TextDecoration.combine(<TextDecoration>[TextDecoration.underline, TextDecoration.lineThrough, TextDecoration.overline]);
}
return null;
}
TextDecorationStyle? _fiddleWithDecorationStyle(TextDecorationStyle? value) {
switch (_random.nextInt(10)) {
case 0:
return null;
case 1:
return pickFromList<TextDecorationStyle>(_random, TextDecorationStyle.values);
}
return value;
}
FontWeight? _fiddleWithFontWeight(FontWeight? value) {
switch (_random.nextInt(10)) {
case 0:
return null;
case 1:
return pickFromList<FontWeight>(_random, FontWeight.values);
}
return value;
}
FontStyle? _fiddleWithFontStyle(FontStyle? value) {
switch (_random.nextInt(10)) {
case 0:
return null;
case 1:
return pickFromList<FontStyle>(_random, FontStyle.values);
}
return value;
}
String? _fiddleWithFontFamily(String? value) {
switch (_random.nextInt(10)) {
case 0:
return null;
case 1:
return 'sans-serif';
case 2:
return 'sans-serif-condensed';
case 3:
return 'serif';
case 4:
return 'monospace';
case 5:
return 'serif-monospace';
case 6:
return 'casual';
case 7:
return 'cursive';
case 8:
return 'sans-serif-smallcaps';
}
return value;
}
double? _fiddleWithDouble(double? value, double defaultValue, double max) {
switch (_random.nextInt(10)) {
case 0:
if (value == null) {
return math.min(defaultValue * (0.95 + _random.nextDouble() * 0.1), max);
}
return math.min(value * (0.51 + _random.nextDouble()), max);
case 1:
return null;
}
return value;
}
List<TextSpan>? _fiddleWithChildren(List<TextSpan> children) {
switch (_random.nextInt(100)) {
case 0:
case 1:
case 2:
case 3:
case 4:
children.insert(_random.nextInt(children.length + 1), _createRandomTextSpan());
case 10:
children = children.reversed.toList();
case 20:
if (children.isEmpty) {
break;
}
if (_random.nextInt(10) > 0) {
break;
}
final int index = _random.nextInt(children.length);
if (depthOf(children[index]) < 3) {
children.removeAt(index);
}
}
if (children.isEmpty && _random.nextBool()) {
return null;
}
return children;
}
int depthOf(TextSpan node) {
if (node.children == null || (node.children?.isEmpty ?? false)) {
return 0;
}
int result = 0;
for (final TextSpan child in node.children!.cast<TextSpan>()) {
result = math.max(result, depthOf(child));
}
return result;
}
TextSpan _createRandomTextSpan() {
return TextSpan(
text: _createRandomText(),
);
}
String? _createRandomText() {
switch (_random.nextInt(90)) {
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
return 'ABC';
case 7:
case 8:
case 9:
case 10:
return 'أمثولة'; // "Example" or "lesson" in Arabic
case 11:
case 12:
case 13:
case 14:
return 'אבג'; // Hebrew ABC
case 15:
return '';
case 16:
return ' ';
case 17:
return '\n';
case 18:
return '\t';
case 19:
return '\r';
case 20:
return Unicode.RLE;
case 21:
return Unicode.LRE;
case 22:
return Unicode.LRO;
case 23:
return Unicode.RLO;
case 24:
case 25:
case 26:
case 27:
return Unicode.PDF;
case 28:
return Unicode.LRM;
case 29:
return Unicode.RLM;
case 30:
return Unicode.RLI;
case 31:
return Unicode.LRI;
case 32:
return Unicode.FSI;
case 33:
case 34:
case 35:
return Unicode.PDI;
case 36:
case 37:
case 38:
case 39:
case 40:
return ' Hello ';
case 41:
case 42:
case 43:
case 44:
case 45:
return ' World ';
case 46:
return 'Flutter';
case 47:
return 'Fuzz';
case 48:
return 'Test';
case 49:
return '𠜎𠜱𠝹𠱓𠱸𠲖𠳏𠳕𠴕𠵼𠵿𠸎𠸏𠹷𠺝𠺢𠻗𠻹𠻺𠼭𠼮𠽌𠾴𠾼𠿪𡁜𡁯𡁵𡁶𡁻𡃁𡃉𡇙𢃇𢞵𢫕𢭃𢯊𢱑𢱕𢳂𢴈𢵌𢵧𢺳𣲷𤓓𤶸𤷪𥄫𦉘𦟌𦧲𦧺𧨾𨅝𨈇𨋢𨳊𨳍𨳒𩶘'; // http://www.i18nguy.com/unicode/supplementary-test.html
case 50: // any random character
return String.fromCharCode(_random.nextInt(0x10FFFF + 1));
case 51:
return '\u00DF'; // SS
case 52:
return '\u2002'; // En space
case 53:
return '\u2003'; // Em space
case 54:
return '\u200B'; // zero-width space
case 55:
return '\u00A0'; // non-breaking space
case 56:
return '\u00FF'; // y-diaresis
case 57:
return '\u0178'; // Y-diaresis
case 58:
return '\u2060'; // Word Joiner
case 59: // random BMP character
case 60: // random BMP character
case 61: // random BMP character
case 62: // random BMP character
case 63: // random BMP character
return String.fromCharCode(_random.nextInt(0xFFFF));
case 64: // random emoji
case 65: // random emoji
return String.fromCharCode(0x1F000 + _random.nextInt(0x9FF));
case 66:
final String value = zalgo(_random, _random.nextInt(4) + 2);
return 'Z{$value}Z';
case 67:
return 'Οὐχὶ ταὐτὰ παρίσταταί μοι γιγνώσκειν';
case 68:
return 'გთხოვთ ახლავე გაიაროთ რეგისტრაცია';
case 69:
return 'Зарегистрируйтесь сейчас';
case 70:
return 'แผ่นดินฮั่นเสื่อมโทรมแสนสังเวช';
case 71:
return 'ᚻᛖ ᚳᚹᚫᚦ ᚦᚫᛏ ᚻᛖ ᛒᚢᛞᛖ ᚩᚾ ᚦᚫᛗ ᛚᚪᚾᛞᛖ ᚾᚩᚱᚦᚹᛖᚪᚱᛞᚢᛗ ᚹᛁᚦ ᚦᚪ ᚹᛖᛥᚫ';
case 72:
return '⡌⠁⠧⠑ ⠼⠁⠒ ⡍⠜⠇⠑⠹⠰⠎ ⡣⠕⠌';
case 73:
return 'コンニチハ';
case 74:
case 75:
case 76:
case 77:
case 78:
case 79:
case 80:
case 81:
case 82:
final StringBuffer buffer = StringBuffer();
final int targetLength = _random.nextInt(8) + 1;
for (int index = 0; index < targetLength; index += 1) {
if (_random.nextInt(20) > 0) {
buffer.writeCharCode(randomCharacter(_random));
} else {
buffer.write(zalgo(_random, _random.nextInt(2) + 1, includeSpacingCombiningMarks: true));
}
}
return buffer.toString();
}
return null;
}
@override
Widget build(BuildContext context) {
return ColoredBox(
color: Colors.black,
child: Column(
children: <Widget>[
Expanded(
child: SingleChildScrollView(
child: SafeArea(
child: Padding(
padding: const EdgeInsets.all(10.0),
child: RichText(text: _textSpan),
),
),
),
),
Material(
child: SwitchListTile(
title: const Text('Enable Fuzzer'),
value: _ticker.isActive,
onChanged: (bool value) {
setState(() {
if (value) {
_ticker.start();
} else {
_ticker.stop();
debugPrint(_textSpan.toStringDeep());
}
});
},
),
),
],
),
);
}
}
class Underlines extends StatefulWidget {
const Underlines({ super.key });
@override
State<Underlines> createState() => _UnderlinesState();
}
class _UnderlinesState extends State<Underlines> {
String _text = 'i';
final TextStyle _style = TextStyle(
inherit: false,
color: Colors.yellow.shade200,
fontSize: 48.0,
fontFamily: 'sans-serif',
decorationColor: Colors.yellow.shade500,
);
Widget _wrap(TextDecorationStyle? style) {
return Align(
alignment: Alignment.centerLeft,
heightFactor: 1.0,
child: Container(
decoration: const BoxDecoration(color: Color(0xFF333333), border: Border(right: BorderSide(color: Colors.white, width: 0.0))),
child: Text(_text, style: style != null ? _style.copyWith(decoration: TextDecoration.underline, decorationStyle: style) : _style),
),
);
}
@override
Widget build(BuildContext context) {
final Size size = MediaQuery.of(context).size;
return ColoredBox(
color: Colors.black,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
child: SingleChildScrollView(
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: size.width * 0.1,
vertical: size.height * 0.1,
),
child: ListBody(
children: <Widget>[
_wrap(null),
for (final TextDecorationStyle style in TextDecorationStyle.values) _wrap(style),
],
),
),
),
),
Material(
child: Container(
alignment: AlignmentDirectional.centerEnd,
padding: const EdgeInsets.all(8),
child: OverflowBar(
spacing: 8,
children: <Widget>[
TextButton(
onPressed: () {
setState(() {
_text += 'i';
});
},
style: TextButton.styleFrom(
backgroundColor: Colors.yellow,
),
child: const Text('ADD i'),
),
TextButton(
onPressed: () {
setState(() {
_text += 'w';
});
},
style: TextButton.styleFrom(
backgroundColor: Colors.yellow,
),
child: const Text('ADD w'),
),
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.white,
backgroundColor: Colors.red,
),
onPressed: _text == '' ? null : () {
setState(() {
_text = _text.substring(0, _text.length - 1);
});
},
child: const Text('REMOVE'),
),
],
),
),
),
],
),
);
}
}
class Fallback extends StatefulWidget {
const Fallback({ super.key });
@override
State<Fallback> createState() => _FallbackState();
}
class _FallbackState extends State<Fallback> {
static const String multiScript = 'A1!aÀàĀāƁƀḂⱠꜲꬰəͲἀἏЀЖԠꙐꙮՁخࡔࠇܦআਉઐଘஇఘಧൺඣᭆᯔᮯ᳇ꠈᜅᩌꪈ༇ꥄꡙꫤ᧰៘꧁꧂ᜰᨏᯤᢆᣭᗗꗃⵞ𐒎߷ጩꬤ𖠺‡₩℻Ⅷ↹⋇⏳ⓖ╋▒◛⚧⑆שׁ🅕㊼龜ポ䷤🂡';
static const List<String> androidFonts = <String>[
'sans-serif',
'sans-serif-condensed',
'serif',
'monospace',
'serif-monospace',
'casual',
'cursive',
'sans-serif-smallcaps',
];
static const TextStyle style = TextStyle(
inherit: false,
color: Colors.white,
);
double _fontSize = 3.0;
@override
Widget build(BuildContext context) {
final Size size = MediaQuery.of(context).size;
return ColoredBox(
color: Colors.black,
child: Column(
children: <Widget>[
Expanded(
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: SingleChildScrollView(
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: size.width * 0.1,
vertical: size.height * 0.1,
),
child: IntrinsicWidth(
child: ListBody(
children: <Widget>[
for (final String font in androidFonts)
Text(
multiScript,
style: style.copyWith(
fontFamily: font,
fontSize: math.exp(_fontSize),
),
),
],
),
),
),
),
),
),
Material(
child: Padding(
padding: const EdgeInsets.only(left: 20.0, right: 20.0, bottom: 20.0),
child: Row(
crossAxisAlignment: CrossAxisAlignment.end,
children: <Widget>[
const Padding(
padding: EdgeInsets.only(bottom: 10.0),
child: Text('Font size'),
),
Expanded(
child: Slider(
min: 2.0,
max: 5.0,
value: _fontSize,
label: '${math.exp(_fontSize).round()}',
onChanged: (double value) {
setState(() {
_fontSize = value;
});
},
),
),
],
),
),
),
],
),
);
}
}
class Bidi extends StatefulWidget {
const Bidi({ super.key });
@override
State<Bidi> createState() => _BidiState();
}
class _BidiState extends State<Bidi> {
@override
Widget build(BuildContext context) {
return ColoredBox(
color: Colors.black,
child: ListView(
padding: const EdgeInsets.symmetric(vertical: 40.0, horizontal: 20.0),
children: <Widget>[
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(text: 'abc', style: TextStyle(fontWeight: FontWeight.w100, fontSize: 40.0, color: Colors.blue.shade100)),
TextSpan(text: 'ghi', style: TextStyle(fontWeight: FontWeight.w400, fontSize: 40.0, color: Colors.blue.shade500)),
TextSpan(text: 'mno', style: TextStyle(fontWeight: FontWeight.w900, fontSize: 40.0, color: Colors.blue.shade900)),
TextSpan(text: 'LKJ', style: TextStyle(fontWeight: FontWeight.w500, fontSize: 40.0, color: Colors.blue.shade700)),
TextSpan(text: 'FED', style: TextStyle(fontWeight: FontWeight.w300, fontSize: 40.0, color: Colors.blue.shade300)),
],
),
textAlign: TextAlign.center,
textDirection: TextDirection.ltr,
),
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(text: '${Unicode.LRO}abc', style: TextStyle(fontWeight: FontWeight.w100, fontSize: 40.0, color: Colors.blue.shade100)),
TextSpan(text: '${Unicode.RLO}DEF', style: TextStyle(fontWeight: FontWeight.w300, fontSize: 40.0, color: Colors.blue.shade300)),
TextSpan(text: '${Unicode.LRO}ghi', style: TextStyle(fontWeight: FontWeight.w400, fontSize: 40.0, color: Colors.blue.shade500)),
TextSpan(text: '${Unicode.RLO}JKL', style: TextStyle(fontWeight: FontWeight.w500, fontSize: 40.0, color: Colors.blue.shade700)),
TextSpan(text: '${Unicode.LRO}mno', style: TextStyle(fontWeight: FontWeight.w900, fontSize: 40.0, color: Colors.blue.shade900)),
],
),
textAlign: TextAlign.center,
textDirection: TextDirection.ltr,
),
const SizedBox(height: 40.0),
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(text: '${Unicode.LRO}abc${Unicode.RLO}D', style: TextStyle(fontWeight: FontWeight.w100, fontSize: 40.0, color: Colors.orange.shade100)),
TextSpan(text: 'EF${Unicode.LRO}gh', style: TextStyle(fontWeight: FontWeight.w500, fontSize: 50.0, color: Colors.orange.shade500)),
TextSpan(text: 'i${Unicode.RLO}JKL${Unicode.LRO}mno', style: TextStyle(fontWeight: FontWeight.w900, fontSize: 60.0, color: Colors.orange.shade900)),
],
),
textAlign: TextAlign.center,
textDirection: TextDirection.ltr,
),
RichText(
text: TextSpan(
children: <TextSpan>[
TextSpan(text: 'abc', style: TextStyle(fontWeight: FontWeight.w100, fontSize: 40.0, color: Colors.orange.shade100)),
TextSpan(text: 'gh', style: TextStyle(fontWeight: FontWeight.w500, fontSize: 50.0, color: Colors.orange.shade500)),
TextSpan(text: 'imno', style: TextStyle(fontWeight: FontWeight.w900, fontSize: 60.0, color: Colors.orange.shade900)),
TextSpan(text: 'LKJ', style: TextStyle(fontWeight: FontWeight.w900, fontSize: 60.0, color: Colors.orange.shade900)),
TextSpan(text: 'FE', style: TextStyle(fontWeight: FontWeight.w500, fontSize: 50.0, color: Colors.orange.shade500)),
TextSpan(text: 'D', style: TextStyle(fontWeight: FontWeight.w100, fontSize: 40.0, color: Colors.orange.shade100)),
],
),
textAlign: TextAlign.center,
textDirection: TextDirection.ltr,
),
const SizedBox(height: 40.0),
const Text('The pairs of lines above should match exactly.', textAlign: TextAlign.center, style: TextStyle(inherit: false, fontSize: 14.0, color: Colors.white)),
],
),
);
}
}
class Zalgo extends StatefulWidget {
const Zalgo({ super.key, required this.seed });
final int seed;
@override
State<Zalgo> createState() => _ZalgoState();
}
class _ZalgoState extends State<Zalgo> with SingleTickerProviderStateMixin {
String? _text;
late final Ticker _ticker = createTicker(_update)..start();
math.Random _random = math.Random();
@override
void initState() {
super.initState();
_random = math.Random(widget.seed); // providing a seed is important for reproducibility;
_update(null);
}
@override
void dispose() {
_ticker.dispose();
super.dispose();
}
bool _allowSpacing = false;
bool _varyBase = false;
void _update(Duration? duration) {
setState(() {
_text = zalgo(
_random,
6 + _random.nextInt(10),
includeSpacingCombiningMarks: _allowSpacing,
base: _varyBase ? null : 'O',
);
});
}
@override
Widget build(BuildContext context) {
return ColoredBox(
color: Colors.black,
child: Column(
children: <Widget>[
Expanded(
child: Center(
child: RichText(
text: TextSpan(
text: _text,
style: TextStyle(
inherit: false,
fontSize: 96.0,
color: Colors.red.shade200,
),
),
),
),
),
Material(
child: Column(
children: <Widget>[
SwitchListTile(
title: const Text('Enable Fuzzer'),
value: _ticker.isActive,
onChanged: (bool value) {
setState(() {
if (value) {
_ticker.start();
} else {
_ticker.stop();
}
});
},
),
SwitchListTile(
title: const Text('Allow spacing combining marks'),
value: _allowSpacing,
onChanged: (bool value) {
setState(() {
_allowSpacing = value;
_random = math.Random(widget.seed); // reset for reproducibility
});
},
),
SwitchListTile(
title: const Text('Vary base character'),
value: _varyBase,
onChanged: (bool value) {
setState(() {
_varyBase = value;
_random = math.Random(widget.seed); // reset for reproducibility
});
},
),
],
),
),
],
),
);
}
}
class Painting extends StatefulWidget {
const Painting({ super.key, required this.seed });
final int seed;
@override
State<Painting> createState() => _PaintingState();
}
class _PaintingState extends State<Painting> with SingleTickerProviderStateMixin {
String? _text;
late final Ticker _ticker = createTicker(_update)..start();
math.Random _random = math.Random();
@override
void initState() {
super.initState();
_random = math.Random(widget.seed); // providing a seed is important for reproducibility;
_update(null);
}
@override
void dispose() {
_ticker.dispose();
super.dispose();
}
final GlobalKey intrinsicKey = GlobalKey();
final GlobalKey controlKey = GlobalKey();
bool _ellipsize = false;
void _update(Duration? duration) {
setState(() {
final StringBuffer buffer = StringBuffer();
final int targetLength = _random.nextInt(20) + (_ellipsize ? MediaQuery.of(context).size.width.round() : 1);
for (int index = 0; index < targetLength; index += 1) {
if (_random.nextInt(5) > 0) {
buffer.writeCharCode(randomCharacter(_random));
} else {
buffer.write(zalgo(_random, _random.nextInt(2) + 1, includeSpacingCombiningMarks: true));
}
}
_text = buffer.toString();
});
SchedulerBinding.instance.addPostFrameCallback((Duration duration) {
if (mounted && intrinsicKey.currentContext?.size?.height != controlKey.currentContext?.size?.height) {
debugPrint('Found some text that unexpectedly renders at different heights.');
debugPrint('Text: $_text');
debugPrint(_text?.runes.map<String>((int index) {
final String hexa = index.toRadixString(16).padLeft(4, '0');
return 'U+$hexa';
}).join(' '));
setState(() {
_ticker.stop();
});
}
});
}
@override
Widget build(BuildContext context) {
final Size size = MediaQuery.of(context).size;
return ColoredBox(
color: Colors.black,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
child: Padding(
padding: EdgeInsets.only(top: size.height * 0.1),
child: Stack(
alignment: Alignment.center,
children: <Widget>[
Positioned(
top: 0.0,
left: 0.0,
right: 0.0,
child: Align(
alignment: Alignment.topCenter,
child: IntrinsicWidth( // to test shrink-wrap vs rendering
child: RichText(
key: intrinsicKey,
textAlign: TextAlign.center,
overflow: _ellipsize ? TextOverflow.ellipsis : TextOverflow.clip,
text: TextSpan(
text: _text,
style: const TextStyle(
inherit: false,
fontSize: 28.0,
color: Colors.red,
),
),
),
),
),
),
Positioned(
top: 0.0,
left: 0.0,
right: 0.0,
child: RichText(
key: controlKey,
textAlign: TextAlign.center,
overflow: _ellipsize ? TextOverflow.ellipsis : TextOverflow.clip,
text: TextSpan(
text: _text,
style: const TextStyle(
inherit: false,
fontSize: 28.0,
color: Colors.white,
),
),
),
),
],
),
),
),
Material(
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
SwitchListTile(
title: const Text('Enable Fuzzer'),
value: _ticker.isActive,
onChanged: (bool value) {
setState(() {
if (value) {
_ticker.start();
} else {
_ticker.stop();
}
});
},
),
SwitchListTile(
title: const Text('Enable Ellipses'),
value: _ellipsize,
onChanged: (bool value) {
setState(() {
_ellipsize = value;
_random = math.Random(widget.seed); // reset for reproducibility
if (!_ticker.isActive) {
_update(null);
}
});
},
),
const ListTile(
title: Text('There should be no red visible.'),
),
Container(
alignment: AlignmentDirectional.centerEnd,
padding: const EdgeInsets.all(8),
child: OverflowBar(
spacing: 8,
children: <Widget>[
TextButton(
onPressed: _ticker.isActive ? null : () => _update(null),
child: const Text('ITERATE'),
),
TextButton(
onPressed: _ticker.isActive ? null : () {
print('The currently visible text is: $_text');
print(_text?.runes.map<String>((int value) => 'U+${value.toRadixString(16).padLeft(4, '0').toUpperCase()}').join(' '));
},
child: const Text('DUMP TEXT TO LOGS'),
),
],
),
),
],
),
),
],
),
);
}
}
String zalgo(math.Random random, int targetLength, { bool includeSpacingCombiningMarks = false, String? base }) {
// The following three tables are derived from UnicodeData.txt:
// http://unicode.org/Public/UNIDATA/UnicodeData.txt
// There are three groups, character classes Mc, Me, and Mn.
const List<int> enclosingCombiningMarks = <int>[ // Me
0x00488, 0x00489, 0x01ABE, 0x020DD, 0x020DE, 0x020DF, 0x020E0,
0x020E2, 0x020E3, 0x020E4, 0x0A670, 0x0A671, 0x0A672,
];
const List<int> nonspacingCombiningMarks = <int>[ // Mn
0x00300, 0x00301, 0x00302, 0x00303, 0x00304, 0x00305, 0x00306,
0x00307, 0x00308, 0x00309, 0x0030A, 0x0030B, 0x0030C, 0x0030D,
0x0030E, 0x0030F, 0x00310, 0x00311, 0x00312, 0x00313, 0x00314,
0x00315, 0x00316, 0x00317, 0x00318, 0x00319, 0x0031A, 0x0031B,
0x0031C, 0x0031D, 0x0031E, 0x0031F, 0x00320, 0x00321, 0x00322,
0x00323, 0x00324, 0x00325, 0x00326, 0x00327, 0x00328, 0x00329,
0x0032A, 0x0032B, 0x0032C, 0x0032D, 0x0032E, 0x0032F, 0x00330,
0x00331, 0x00332, 0x00333, 0x00334, 0x00335, 0x00336, 0x00337,
0x00338, 0x00339, 0x0033A, 0x0033B, 0x0033C, 0x0033D, 0x0033E,
0x0033F, 0x00340, 0x00341, 0x00342, 0x00343, 0x00344, 0x00345,
0x00346, 0x00347, 0x00348, 0x00349, 0x0034A, 0x0034B, 0x0034C,
0x0034D, 0x0034E, 0x0034F, 0x00350, 0x00351, 0x00352, 0x00353,
0x00354, 0x00355, 0x00356, 0x00357, 0x00358, 0x00359, 0x0035A,
0x0035B, 0x0035C, 0x0035D, 0x0035E, 0x0035F, 0x00360, 0x00361,
0x00362, 0x00363, 0x00364, 0x00365, 0x00366, 0x00367, 0x00368,
0x00369, 0x0036A, 0x0036B, 0x0036C, 0x0036D, 0x0036E, 0x0036F,
0x00483, 0x00484, 0x00485, 0x00486, 0x00487, 0x00591, 0x00592,
0x00593, 0x00594, 0x00595, 0x00596, 0x00597, 0x00598, 0x00599,
0x0059A, 0x0059B, 0x0059C, 0x0059D, 0x0059E, 0x0059F, 0x005A0,
0x005A1, 0x005A2, 0x005A3, 0x005A4, 0x005A5, 0x005A6, 0x005A7,
0x005A8, 0x005A9, 0x005AA, 0x005AB, 0x005AC, 0x005AD, 0x005AE,
0x005AF, 0x005B0, 0x005B1, 0x005B2, 0x005B3, 0x005B4, 0x005B5,
0x005B6, 0x005B7, 0x005B8, 0x005B9, 0x005BA, 0x005BB, 0x005BC,
0x005BD, 0x005BF, 0x005C1, 0x005C2, 0x005C4, 0x005C5, 0x005C7,
0x00610, 0x00611, 0x00612, 0x00613, 0x00614, 0x00615, 0x00616,
0x00617, 0x00618, 0x00619, 0x0061A, 0x0064B, 0x0064C, 0x0064D,
0x0064E, 0x0064F, 0x00650, 0x00651, 0x00652, 0x00653, 0x00654,
0x00655, 0x00656, 0x00657, 0x00658, 0x00659, 0x0065A, 0x0065B,
0x0065C, 0x0065D, 0x0065E, 0x0065F, 0x00670, 0x006D6, 0x006D7,
0x006D8, 0x006D9, 0x006DA, 0x006DB, 0x006DC, 0x006DF, 0x006E0,
0x006E1, 0x006E2, 0x006E3, 0x006E4, 0x006E7, 0x006E8, 0x006EA,
0x006EB, 0x006EC, 0x006ED, 0x00711, 0x00730, 0x00731, 0x00732,
0x00733, 0x00734, 0x00735, 0x00736, 0x00737, 0x00738, 0x00739,
0x0073A, 0x0073B, 0x0073C, 0x0073D, 0x0073E, 0x0073F, 0x00740,
0x00741, 0x00742, 0x00743, 0x00744, 0x00745, 0x00746, 0x00747,
0x00748, 0x00749, 0x0074A, 0x007A6, 0x007A7, 0x007A8, 0x007A9,
0x007AA, 0x007AB, 0x007AC, 0x007AD, 0x007AE, 0x007AF, 0x007B0,
0x007EB, 0x007EC, 0x007ED, 0x007EE, 0x007EF, 0x007F0, 0x007F1,
0x007F2, 0x007F3, 0x00816, 0x00817, 0x00818, 0x00819, 0x0081B,
0x0081C, 0x0081D, 0x0081E, 0x0081F, 0x00820, 0x00821, 0x00822,
0x00823, 0x00825, 0x00826, 0x00827, 0x00829, 0x0082A, 0x0082B,
0x0082C, 0x0082D, 0x00859, 0x0085A, 0x0085B, 0x008D4, 0x008D5,
0x008D6, 0x008D7, 0x008D8, 0x008D9, 0x008DA, 0x008DB, 0x008DC,
0x008DD, 0x008DE, 0x008DF, 0x008E0, 0x008E1, 0x008E3, 0x008E4,
0x008E5, 0x008E6, 0x008E7, 0x008E8, 0x008E9, 0x008EA, 0x008EB,
0x008EC, 0x008ED, 0x008EE, 0x008EF, 0x008F0, 0x008F1, 0x008F2,
0x008F3, 0x008F4, 0x008F5, 0x008F6, 0x008F7, 0x008F8, 0x008F9,
0x008FA, 0x008FB, 0x008FC, 0x008FD, 0x008FE, 0x008FF, 0x00900,
0x00901, 0x00902, 0x0093A, 0x0093C, 0x00941, 0x00942, 0x00943,
0x00944, 0x00945, 0x00946, 0x00947, 0x00948, 0x0094D, 0x00951,
0x00952, 0x00953, 0x00954, 0x00955, 0x00956, 0x00957, 0x00962,
0x00963, 0x00981, 0x009BC, 0x009C1, 0x009C2, 0x009C3, 0x009C4,
0x009CD, 0x009E2, 0x009E3, 0x00A01, 0x00A02, 0x00A3C, 0x00A41,
0x00A42, 0x00A47, 0x00A48, 0x00A4B, 0x00A4C, 0x00A4D, 0x00A51,
0x00A70, 0x00A71, 0x00A75, 0x00A81, 0x00A82, 0x00ABC, 0x00AC1,
0x00AC2, 0x00AC3, 0x00AC4, 0x00AC5, 0x00AC7, 0x00AC8, 0x00ACD,
0x00AE2, 0x00AE3, 0x00AFA, 0x00AFB, 0x00AFC, 0x00AFD, 0x00AFE,
0x00AFF, 0x00B01, 0x00B3C, 0x00B3F, 0x00B41, 0x00B42, 0x00B43,
0x00B44, 0x00B4D, 0x00B56, 0x00B62, 0x00B63, 0x00B82, 0x00BC0,
0x00BCD, 0x00C00, 0x00C3E, 0x00C3F, 0x00C40, 0x00C46, 0x00C47,
0x00C48, 0x00C4A, 0x00C4B, 0x00C4C, 0x00C4D, 0x00C55, 0x00C56,
0x00C62, 0x00C63, 0x00C81, 0x00CBC, 0x00CBF, 0x00CC6, 0x00CCC,
0x00CCD, 0x00CE2, 0x00CE3, 0x00D00, 0x00D01, 0x00D3B, 0x00D3C,
0x00D41, 0x00D42, 0x00D43, 0x00D44, 0x00D4D, 0x00D62, 0x00D63,
0x00DCA, 0x00DD2, 0x00DD3, 0x00DD4, 0x00DD6, 0x00E31, 0x00E34,
0x00E35, 0x00E36, 0x00E37, 0x00E38, 0x00E39, 0x00E3A, 0x00E47,
0x00E48, 0x00E49, 0x00E4A, 0x00E4B, 0x00E4C, 0x00E4D, 0x00E4E,
0x00EB1, 0x00EB4, 0x00EB5, 0x00EB6, 0x00EB7, 0x00EB8, 0x00EB9,
0x00EBB, 0x00EBC, 0x00EC8, 0x00EC9, 0x00ECA, 0x00ECB, 0x00ECC,
0x00ECD, 0x00F18, 0x00F19, 0x00F35, 0x00F37, 0x00F39, 0x00F71,
0x00F72, 0x00F73, 0x00F74, 0x00F75, 0x00F76, 0x00F77, 0x00F78,
0x00F79, 0x00F7A, 0x00F7B, 0x00F7C, 0x00F7D, 0x00F7E, 0x00F80,
0x00F81, 0x00F82, 0x00F83, 0x00F84, 0x00F86, 0x00F87, 0x00F8D,
0x00F8E, 0x00F8F, 0x00F90, 0x00F91, 0x00F92, 0x00F93, 0x00F94,
0x00F95, 0x00F96, 0x00F97, 0x00F99, 0x00F9A, 0x00F9B, 0x00F9C,
0x00F9D, 0x00F9E, 0x00F9F, 0x00FA0, 0x00FA1, 0x00FA2, 0x00FA3,
0x00FA4, 0x00FA5, 0x00FA6, 0x00FA7, 0x00FA8, 0x00FA9, 0x00FAA,
0x00FAB, 0x00FAC, 0x00FAD, 0x00FAE, 0x00FAF, 0x00FB0, 0x00FB1,
0x00FB2, 0x00FB3, 0x00FB4, 0x00FB5, 0x00FB6, 0x00FB7, 0x00FB8,
0x00FB9, 0x00FBA, 0x00FBB, 0x00FBC, 0x00FC6, 0x0102D, 0x0102E,
0x0102F, 0x01030, 0x01032, 0x01033, 0x01034, 0x01035, 0x01036,
0x01037, 0x01039, 0x0103A, 0x0103D, 0x0103E, 0x01058, 0x01059,
0x0105E, 0x0105F, 0x01060, 0x01071, 0x01072, 0x01073, 0x01074,
0x01082, 0x01085, 0x01086, 0x0108D, 0x0109D, 0x0135D, 0x0135E,
0x0135F, 0x01712, 0x01713, 0x01714, 0x01732, 0x01733, 0x01734,
0x01752, 0x01753, 0x01772, 0x01773, 0x017B4, 0x017B5, 0x017B7,
0x017B8, 0x017B9, 0x017BA, 0x017BB, 0x017BC, 0x017BD, 0x017C6,
0x017C9, 0x017CA, 0x017CB, 0x017CC, 0x017CD, 0x017CE, 0x017CF,
0x017D0, 0x017D1, 0x017D2, 0x017D3, 0x017DD, 0x0180B, 0x0180C,
0x0180D, 0x01885, 0x01886, 0x018A9, 0x01920, 0x01921, 0x01922,
0x01927, 0x01928, 0x01932, 0x01939, 0x0193A, 0x0193B, 0x01A17,
0x01A18, 0x01A1B, 0x01A56, 0x01A58, 0x01A59, 0x01A5A, 0x01A5B,
0x01A5C, 0x01A5D, 0x01A5E, 0x01A60, 0x01A62, 0x01A65, 0x01A66,
0x01A67, 0x01A68, 0x01A69, 0x01A6A, 0x01A6B, 0x01A6C, 0x01A73,
0x01A74, 0x01A75, 0x01A76, 0x01A77, 0x01A78, 0x01A79, 0x01A7A,
0x01A7B, 0x01A7C, 0x01A7F, 0x01AB0, 0x01AB1, 0x01AB2, 0x01AB3,
0x01AB4, 0x01AB5, 0x01AB6, 0x01AB7, 0x01AB8, 0x01AB9, 0x01ABA,
0x01ABB, 0x01ABC, 0x01ABD, 0x01B00, 0x01B01, 0x01B02, 0x01B03,
0x01B34, 0x01B36, 0x01B37, 0x01B38, 0x01B39, 0x01B3A, 0x01B3C,
0x01B42, 0x01B6B, 0x01B6C, 0x01B6D, 0x01B6E, 0x01B6F, 0x01B70,
0x01B71, 0x01B72, 0x01B73, 0x01B80, 0x01B81, 0x01BA2, 0x01BA3,
0x01BA4, 0x01BA5, 0x01BA8, 0x01BA9, 0x01BAB, 0x01BAC, 0x01BAD,
0x01BE6, 0x01BE8, 0x01BE9, 0x01BED, 0x01BEF, 0x01BF0, 0x01BF1,
0x01C2C, 0x01C2D, 0x01C2E, 0x01C2F, 0x01C30, 0x01C31, 0x01C32,
0x01C33, 0x01C36, 0x01C37, 0x01CD0, 0x01CD1, 0x01CD2, 0x01CD4,
0x01CD5, 0x01CD6, 0x01CD7, 0x01CD8, 0x01CD9, 0x01CDA, 0x01CDB,
0x01CDC, 0x01CDD, 0x01CDE, 0x01CDF, 0x01CE0, 0x01CE2, 0x01CE3,
0x01CE4, 0x01CE5, 0x01CE6, 0x01CE7, 0x01CE8, 0x01CED, 0x01CF4,
0x01CF8, 0x01CF9, 0x01DC0, 0x01DC1, 0x01DC2, 0x01DC3, 0x01DC4,
0x01DC5, 0x01DC6, 0x01DC7, 0x01DC8, 0x01DC9, 0x01DCA, 0x01DCB,
0x01DCC, 0x01DCD, 0x01DCE, 0x01DCF, 0x01DD0, 0x01DD1, 0x01DD2,
0x01DD3, 0x01DD4, 0x01DD5, 0x01DD6, 0x01DD7, 0x01DD8, 0x01DD9,
0x01DDA, 0x01DDB, 0x01DDC, 0x01DDD, 0x01DDE, 0x01DDF, 0x01DE0,
0x01DE1, 0x01DE2, 0x01DE3, 0x01DE4, 0x01DE5, 0x01DE6, 0x01DE7,
0x01DE8, 0x01DE9, 0x01DEA, 0x01DEB, 0x01DEC, 0x01DED, 0x01DEE,
0x01DEF, 0x01DF0, 0x01DF1, 0x01DF2, 0x01DF3, 0x01DF4, 0x01DF5,
0x01DF6, 0x01DF7, 0x01DF8, 0x01DF9, 0x01DFB, 0x01DFC, 0x01DFD,
0x01DFE, 0x01DFF, 0x020D0, 0x020D1, 0x020D2, 0x020D3, 0x020D4,
0x020D5, 0x020D6, 0x020D7, 0x020D8, 0x020D9, 0x020DA, 0x020DB,
0x020DC, 0x020E1, 0x020E5, 0x020E6, 0x020E7, 0x020E8, 0x020E9,
0x020EA, 0x020EB, 0x020EC, 0x020ED, 0x020EE, 0x020EF, 0x020F0,
0x02CEF, 0x02CF0, 0x02CF1, 0x02D7F, 0x02DE0, 0x02DE1, 0x02DE2,
0x02DE3, 0x02DE4, 0x02DE5, 0x02DE6, 0x02DE7, 0x02DE8, 0x02DE9,
0x02DEA, 0x02DEB, 0x02DEC, 0x02DED, 0x02DEE, 0x02DEF, 0x02DF0,
0x02DF1, 0x02DF2, 0x02DF3, 0x02DF4, 0x02DF5, 0x02DF6, 0x02DF7,
0x02DF8, 0x02DF9, 0x02DFA, 0x02DFB, 0x02DFC, 0x02DFD, 0x02DFE,
0x02DFF, 0x0302A, 0x0302B, 0x0302C, 0x0302D, 0x03099, 0x0309A,
0x0A66F, 0x0A674, 0x0A675, 0x0A676, 0x0A677, 0x0A678, 0x0A679,
0x0A67A, 0x0A67B, 0x0A67C, 0x0A67D, 0x0A69E, 0x0A69F, 0x0A6F0,
0x0A6F1, 0x0A802, 0x0A806, 0x0A80B, 0x0A825, 0x0A826, 0x0A8C4,
0x0A8C5, 0x0A8E0, 0x0A8E1, 0x0A8E2, 0x0A8E3, 0x0A8E4, 0x0A8E5,
0x0A8E6, 0x0A8E7, 0x0A8E8, 0x0A8E9, 0x0A8EA, 0x0A8EB, 0x0A8EC,
0x0A8ED, 0x0A8EE, 0x0A8EF, 0x0A8F0, 0x0A8F1, 0x0A926, 0x0A927,
0x0A928, 0x0A929, 0x0A92A, 0x0A92B, 0x0A92C, 0x0A92D, 0x0A947,
0x0A948, 0x0A949, 0x0A94A, 0x0A94B, 0x0A94C, 0x0A94D, 0x0A94E,
0x0A94F, 0x0A950, 0x0A951, 0x0A980, 0x0A981, 0x0A982, 0x0A9B3,
0x0A9B6, 0x0A9B7, 0x0A9B8, 0x0A9B9, 0x0A9BC, 0x0A9E5, 0x0AA29,
0x0AA2A, 0x0AA2B, 0x0AA2C, 0x0AA2D, 0x0AA2E, 0x0AA31, 0x0AA32,
0x0AA35, 0x0AA36, 0x0AA43, 0x0AA4C, 0x0AA7C, 0x0AAB0, 0x0AAB2,
0x0AAB3, 0x0AAB4, 0x0AAB7, 0x0AAB8, 0x0AABE, 0x0AABF, 0x0AAC1,
0x0AAEC, 0x0AAED, 0x0AAF6, 0x0ABE5, 0x0ABE8, 0x0ABED, 0x0FB1E,
0x0FE00, 0x0FE01, 0x0FE02, 0x0FE03, 0x0FE04, 0x0FE05, 0x0FE06,
0x0FE07, 0x0FE08, 0x0FE09, 0x0FE0A, 0x0FE0B, 0x0FE0C, 0x0FE0D,
0x0FE0E, 0x0FE0F, 0x0FE20, 0x0FE21, 0x0FE22, 0x0FE23, 0x0FE24,
0x0FE25, 0x0FE26, 0x0FE27, 0x0FE28, 0x0FE29, 0x0FE2A, 0x0FE2B,
0x0FE2C, 0x0FE2D, 0x0FE2E, 0x0FE2F, 0x101FD, 0x102E0, 0x10376,
0x10377, 0x10378, 0x10379, 0x1037A, 0x10A01, 0x10A02, 0x10A03,
0x10A05, 0x10A06, 0x10A0C, 0x10A0D, 0x10A0E, 0x10A0F, 0x10A38,
0x10A39, 0x10A3A, 0x10A3F, 0x10AE5, 0x10AE6, 0x11001, 0x11038,
0x11039, 0x1103A, 0x1103B, 0x1103C, 0x1103D, 0x1103E, 0x1103F,
0x11040, 0x11041, 0x11042, 0x11043, 0x11044, 0x11045, 0x11046,
0x1107F, 0x11080, 0x11081, 0x110B3, 0x110B4, 0x110B5, 0x110B6,
0x110B9, 0x110BA, 0x11100, 0x11101, 0x11102, 0x11127, 0x11128,
0x11129, 0x1112A, 0x1112B, 0x1112D, 0x1112E, 0x1112F, 0x11130,
0x11131, 0x11132, 0x11133, 0x11134, 0x11173, 0x11180, 0x11181,
0x111B6, 0x111B7, 0x111B8, 0x111B9, 0x111BA, 0x111BB, 0x111BC,
0x111BD, 0x111BE, 0x111CA, 0x111CB, 0x111CC, 0x1122F, 0x11230,
0x11231, 0x11234, 0x11236, 0x11237, 0x1123E, 0x112DF, 0x112E3,
0x112E4, 0x112E5, 0x112E6, 0x112E7, 0x112E8, 0x112E9, 0x112EA,
0x11300, 0x11301, 0x1133C, 0x11340, 0x11366, 0x11367, 0x11368,
0x11369, 0x1136A, 0x1136B, 0x1136C, 0x11370, 0x11371, 0x11372,
0x11373, 0x11374, 0x11438, 0x11439, 0x1143A, 0x1143B, 0x1143C,
0x1143D, 0x1143E, 0x1143F, 0x11442, 0x11443, 0x11444, 0x11446,
0x114B3, 0x114B4, 0x114B5, 0x114B6, 0x114B7, 0x114B8, 0x114BA,
0x114BF, 0x114C0, 0x114C2, 0x114C3, 0x115B2, 0x115B3, 0x115B4,
0x115B5, 0x115BC, 0x115BD, 0x115BF, 0x115C0, 0x115DC, 0x115DD,
0x11633, 0x11634, 0x11635, 0x11636, 0x11637, 0x11638, 0x11639,
0x1163A, 0x1163D, 0x1163F, 0x11640, 0x116AB, 0x116AD, 0x116B0,
0x116B1, 0x116B2, 0x116B3, 0x116B4, 0x116B5, 0x116B7, 0x1171D,
0x1171E, 0x1171F, 0x11722, 0x11723, 0x11724, 0x11725, 0x11727,
0x11728, 0x11729, 0x1172A, 0x1172B, 0x11A01, 0x11A02, 0x11A03,
0x11A04, 0x11A05, 0x11A06, 0x11A09, 0x11A0A, 0x11A33, 0x11A34,
0x11A35, 0x11A36, 0x11A37, 0x11A38, 0x11A3B, 0x11A3C, 0x11A3D,
0x11A3E, 0x11A47, 0x11A51, 0x11A52, 0x11A53, 0x11A54, 0x11A55,
0x11A56, 0x11A59, 0x11A5A, 0x11A5B, 0x11A8A, 0x11A8B, 0x11A8C,
0x11A8D, 0x11A8E, 0x11A8F, 0x11A90, 0x11A91, 0x11A92, 0x11A93,
0x11A94, 0x11A95, 0x11A96, 0x11A98, 0x11A99, 0x11C30, 0x11C31,
0x11C32, 0x11C33, 0x11C34, 0x11C35, 0x11C36, 0x11C38, 0x11C39,
0x11C3A, 0x11C3B, 0x11C3C, 0x11C3D, 0x11C3F, 0x11C92, 0x11C93,
0x11C94, 0x11C95, 0x11C96, 0x11C97, 0x11C98, 0x11C99, 0x11C9A,
0x11C9B, 0x11C9C, 0x11C9D, 0x11C9E, 0x11C9F, 0x11CA0, 0x11CA1,
0x11CA2, 0x11CA3, 0x11CA4, 0x11CA5, 0x11CA6, 0x11CA7, 0x11CAA,
0x11CAB, 0x11CAC, 0x11CAD, 0x11CAE, 0x11CAF, 0x11CB0, 0x11CB2,
0x11CB3, 0x11CB5, 0x11CB6, 0x11D31, 0x11D32, 0x11D33, 0x11D34,
0x11D35, 0x11D36, 0x11D3A, 0x11D3C, 0x11D3D, 0x11D3F, 0x11D40,
0x11D41, 0x11D42, 0x11D43, 0x11D44, 0x11D45, 0x11D47, 0x16AF0,
0x16AF1, 0x16AF2, 0x16AF3, 0x16AF4, 0x16B30, 0x16B31, 0x16B32,
0x16B33, 0x16B34, 0x16B35, 0x16B36, 0x16F8F, 0x16F90, 0x16F91,
0x16F92, 0x1BC9D, 0x1BC9E, 0x1D167, 0x1D168, 0x1D169, 0x1D17B,
0x1D17C, 0x1D17D, 0x1D17E, 0x1D17F, 0x1D180, 0x1D181, 0x1D182,
0x1D185, 0x1D186, 0x1D187, 0x1D188, 0x1D189, 0x1D18A, 0x1D18B,
0x1D1AA, 0x1D1AB, 0x1D1AC, 0x1D1AD, 0x1D242, 0x1D243, 0x1D244,
0x1DA00, 0x1DA01, 0x1DA02, 0x1DA03, 0x1DA04, 0x1DA05, 0x1DA06,
0x1DA07, 0x1DA08, 0x1DA09, 0x1DA0A, 0x1DA0B, 0x1DA0C, 0x1DA0D,
0x1DA0E, 0x1DA0F, 0x1DA10, 0x1DA11, 0x1DA12, 0x1DA13, 0x1DA14,
0x1DA15, 0x1DA16, 0x1DA17, 0x1DA18, 0x1DA19, 0x1DA1A, 0x1DA1B,
0x1DA1C, 0x1DA1D, 0x1DA1E, 0x1DA1F, 0x1DA20, 0x1DA21, 0x1DA22,
0x1DA23, 0x1DA24, 0x1DA25, 0x1DA26, 0x1DA27, 0x1DA28, 0x1DA29,
0x1DA2A, 0x1DA2B, 0x1DA2C, 0x1DA2D, 0x1DA2E, 0x1DA2F, 0x1DA30,
0x1DA31, 0x1DA32, 0x1DA33, 0x1DA34, 0x1DA35, 0x1DA36, 0x1DA3B,
0x1DA3C, 0x1DA3D, 0x1DA3E, 0x1DA3F, 0x1DA40, 0x1DA41, 0x1DA42,
0x1DA43, 0x1DA44, 0x1DA45, 0x1DA46, 0x1DA47, 0x1DA48, 0x1DA49,
0x1DA4A, 0x1DA4B, 0x1DA4C, 0x1DA4D, 0x1DA4E, 0x1DA4F, 0x1DA50,
0x1DA51, 0x1DA52, 0x1DA53, 0x1DA54, 0x1DA55, 0x1DA56, 0x1DA57,
0x1DA58, 0x1DA59, 0x1DA5A, 0x1DA5B, 0x1DA5C, 0x1DA5D, 0x1DA5E,
0x1DA5F, 0x1DA60, 0x1DA61, 0x1DA62, 0x1DA63, 0x1DA64, 0x1DA65,
0x1DA66, 0x1DA67, 0x1DA68, 0x1DA69, 0x1DA6A, 0x1DA6B, 0x1DA6C,
0x1DA75, 0x1DA84, 0x1DA9B, 0x1DA9C, 0x1DA9D, 0x1DA9E, 0x1DA9F,
0x1DAA1, 0x1DAA2, 0x1DAA3, 0x1DAA4, 0x1DAA5, 0x1DAA6, 0x1DAA7,
0x1DAA8, 0x1DAA9, 0x1DAAA, 0x1DAAB, 0x1DAAC, 0x1DAAD, 0x1DAAE,
0x1DAAF, 0x1E000, 0x1E001, 0x1E002, 0x1E003, 0x1E004, 0x1E005,
0x1E006, 0x1E008, 0x1E009, 0x1E00A, 0x1E00B, 0x1E00C, 0x1E00D,
0x1E00E, 0x1E00F, 0x1E010, 0x1E011, 0x1E012, 0x1E013, 0x1E014,
0x1E015, 0x1E016, 0x1E017, 0x1E018, 0x1E01B, 0x1E01C, 0x1E01D,
0x1E01E, 0x1E01F, 0x1E020, 0x1E021, 0x1E023, 0x1E024, 0x1E026,
0x1E027, 0x1E028, 0x1E029, 0x1E02A, 0x1E8D0, 0x1E8D1, 0x1E8D2,
0x1E8D3, 0x1E8D4, 0x1E8D5, 0x1E8D6, 0x1E944, 0x1E945, 0x1E946,
0x1E947, 0x1E948, 0x1E949, 0x1E94A, 0xE0100, 0xE0101, 0xE0102,
0xE0103, 0xE0104, 0xE0105, 0xE0106, 0xE0107, 0xE0108, 0xE0109,
0xE010A, 0xE010B, 0xE010C, 0xE010D, 0xE010E, 0xE010F, 0xE0110,
0xE0111, 0xE0112, 0xE0113, 0xE0114, 0xE0115, 0xE0116, 0xE0117,
0xE0118, 0xE0119, 0xE011A, 0xE011B, 0xE011C, 0xE011D, 0xE011E,
0xE011F, 0xE0120, 0xE0121, 0xE0122, 0xE0123, 0xE0124, 0xE0125,
0xE0126, 0xE0127, 0xE0128, 0xE0129, 0xE012A, 0xE012B, 0xE012C,
0xE012D, 0xE012E, 0xE012F, 0xE0130, 0xE0131, 0xE0132, 0xE0133,
0xE0134, 0xE0135, 0xE0136, 0xE0137, 0xE0138, 0xE0139, 0xE013A,
0xE013B, 0xE013C, 0xE013D, 0xE013E, 0xE013F, 0xE0140, 0xE0141,
0xE0142, 0xE0143, 0xE0144, 0xE0145, 0xE0146, 0xE0147, 0xE0148,
0xE0149, 0xE014A, 0xE014B, 0xE014C, 0xE014D, 0xE014E, 0xE014F,
0xE0150, 0xE0151, 0xE0152, 0xE0153, 0xE0154, 0xE0155, 0xE0156,
0xE0157, 0xE0158, 0xE0159, 0xE015A, 0xE015B, 0xE015C, 0xE015D,
0xE015E, 0xE015F, 0xE0160, 0xE0161, 0xE0162, 0xE0163, 0xE0164,
0xE0165, 0xE0166, 0xE0167, 0xE0168, 0xE0169, 0xE016A, 0xE016B,
0xE016C, 0xE016D, 0xE016E, 0xE016F, 0xE0170, 0xE0171, 0xE0172,
0xE0173, 0xE0174, 0xE0175, 0xE0176, 0xE0177, 0xE0178, 0xE0179,
0xE017A, 0xE017B, 0xE017C, 0xE017D, 0xE017E, 0xE017F, 0xE0180,
0xE0181, 0xE0182, 0xE0183, 0xE0184, 0xE0185, 0xE0186, 0xE0187,
0xE0188, 0xE0189, 0xE018A, 0xE018B, 0xE018C, 0xE018D, 0xE018E,
0xE018F, 0xE0190, 0xE0191, 0xE0192, 0xE0193, 0xE0194, 0xE0195,
0xE0196, 0xE0197, 0xE0198, 0xE0199, 0xE019A, 0xE019B, 0xE019C,
0xE019D, 0xE019E, 0xE019F, 0xE01A0, 0xE01A1, 0xE01A2, 0xE01A3,
0xE01A4, 0xE01A5, 0xE01A6, 0xE01A7, 0xE01A8, 0xE01A9, 0xE01AA,
0xE01AB, 0xE01AC, 0xE01AD, 0xE01AE, 0xE01AF, 0xE01B0, 0xE01B1,
0xE01B2, 0xE01B3, 0xE01B4, 0xE01B5, 0xE01B6, 0xE01B7, 0xE01B8,
0xE01B9, 0xE01BA, 0xE01BB, 0xE01BC, 0xE01BD, 0xE01BE, 0xE01BF,
0xE01C0, 0xE01C1, 0xE01C2, 0xE01C3, 0xE01C4, 0xE01C5, 0xE01C6,
0xE01C7, 0xE01C8, 0xE01C9, 0xE01CA, 0xE01CB, 0xE01CC, 0xE01CD,
0xE01CE, 0xE01CF, 0xE01D0, 0xE01D1, 0xE01D2, 0xE01D3, 0xE01D4,
0xE01D5, 0xE01D6, 0xE01D7, 0xE01D8, 0xE01D9, 0xE01DA, 0xE01DB,
0xE01DC, 0xE01DD, 0xE01DE, 0xE01DF, 0xE01E0, 0xE01E1, 0xE01E2,
0xE01E3, 0xE01E4, 0xE01E5, 0xE01E6, 0xE01E7, 0xE01E8, 0xE01E9,
0xE01EA, 0xE01EB, 0xE01EC, 0xE01ED, 0xE01EE, 0xE01EF,
];
const List<int> spacingCombiningMarks = <int>[ // Mc
0x00903, 0x0093B, 0x0093E, 0x0093F, 0x00940, 0x00949, 0x0094A,
0x0094B, 0x0094C, 0x0094E, 0x0094F, 0x00982, 0x00983, 0x009BE,
0x009BF, 0x009C0, 0x009C7, 0x009C8, 0x009CB, 0x009CC, 0x009D7,
0x00A03, 0x00A3E, 0x00A3F, 0x00A40, 0x00A83, 0x00ABE, 0x00ABF,
0x00AC0, 0x00AC9, 0x00ACB, 0x00ACC, 0x00B02, 0x00B03, 0x00B3E,
0x00B40, 0x00B47, 0x00B48, 0x00B4B, 0x00B4C, 0x00B57, 0x00BBE,
0x00BBF, 0x00BC1, 0x00BC2, 0x00BC6, 0x00BC7, 0x00BC8, 0x00BCA,
0x00BCB, 0x00BCC, 0x00BD7, 0x00C01, 0x00C02, 0x00C03, 0x00C41,
0x00C42, 0x00C43, 0x00C44, 0x00C82, 0x00C83, 0x00CBE, 0x00CC0,
0x00CC1, 0x00CC2, 0x00CC3, 0x00CC4, 0x00CC7, 0x00CC8, 0x00CCA,
0x00CCB, 0x00CD5, 0x00CD6, 0x00D02, 0x00D03, 0x00D3E, 0x00D3F,
0x00D40, 0x00D46, 0x00D47, 0x00D48, 0x00D4A, 0x00D4B, 0x00D4C,
0x00D57, 0x00D82, 0x00D83, 0x00DCF, 0x00DD0, 0x00DD1, 0x00DD8,
0x00DD9, 0x00DDA, 0x00DDB, 0x00DDC, 0x00DDD, 0x00DDE, 0x00DDF,
0x00DF2, 0x00DF3, 0x00F3E, 0x00F3F, 0x00F7F, 0x0102B, 0x0102C,
0x01031, 0x01038, 0x0103B, 0x0103C, 0x01056, 0x01057, 0x01062,
0x01063, 0x01064, 0x01067, 0x01068, 0x01069, 0x0106A, 0x0106B,
0x0106C, 0x0106D, 0x01083, 0x01084, 0x01087, 0x01088, 0x01089,
0x0108A, 0x0108B, 0x0108C, 0x0108F, 0x0109A, 0x0109B, 0x0109C,
0x017B6, 0x017BE, 0x017BF, 0x017C0, 0x017C1, 0x017C2, 0x017C3,
0x017C4, 0x017C5, 0x017C7, 0x017C8, 0x01923, 0x01924, 0x01925,
0x01926, 0x01929, 0x0192A, 0x0192B, 0x01930, 0x01931, 0x01933,
0x01934, 0x01935, 0x01936, 0x01937, 0x01938, 0x01A19, 0x01A1A,
0x01A55, 0x01A57, 0x01A61, 0x01A63, 0x01A64, 0x01A6D, 0x01A6E,
0x01A6F, 0x01A70, 0x01A71, 0x01A72, 0x01B04, 0x01B35, 0x01B3B,
0x01B3D, 0x01B3E, 0x01B3F, 0x01B40, 0x01B41, 0x01B43, 0x01B44,
0x01B82, 0x01BA1, 0x01BA6, 0x01BA7, 0x01BAA, 0x01BE7, 0x01BEA,
0x01BEB, 0x01BEC, 0x01BEE, 0x01BF2, 0x01BF3, 0x01C24, 0x01C25,
0x01C26, 0x01C27, 0x01C28, 0x01C29, 0x01C2A, 0x01C2B, 0x01C34,
0x01C35, 0x01CE1, 0x01CF2, 0x01CF3, 0x01CF7, 0x0302E, 0x0302F,
0x0A823, 0x0A824, 0x0A827, 0x0A880, 0x0A881, 0x0A8B4, 0x0A8B5,
0x0A8B6, 0x0A8B7, 0x0A8B8, 0x0A8B9, 0x0A8BA, 0x0A8BB, 0x0A8BC,
0x0A8BD, 0x0A8BE, 0x0A8BF, 0x0A8C0, 0x0A8C1, 0x0A8C2, 0x0A8C3,
0x0A952, 0x0A953, 0x0A983, 0x0A9B4, 0x0A9B5, 0x0A9BA, 0x0A9BB,
0x0A9BD, 0x0A9BE, 0x0A9BF, 0x0A9C0, 0x0AA2F, 0x0AA30, 0x0AA33,
0x0AA34, 0x0AA4D, 0x0AA7B, 0x0AA7D, 0x0AAEB, 0x0AAEE, 0x0AAEF,
0x0AAF5, 0x0ABE3, 0x0ABE4, 0x0ABE6, 0x0ABE7, 0x0ABE9, 0x0ABEA,
0x0ABEC, 0x11000, 0x11002, 0x11082, 0x110B0, 0x110B1, 0x110B2,
0x110B7, 0x110B8, 0x1112C, 0x11182, 0x111B3, 0x111B4, 0x111B5,
0x111BF, 0x111C0, 0x1122C, 0x1122D, 0x1122E, 0x11232, 0x11233,
0x11235, 0x112E0, 0x112E1, 0x112E2, 0x11302, 0x11303, 0x1133E,
0x1133F, 0x11341, 0x11342, 0x11343, 0x11344, 0x11347, 0x11348,
0x1134B, 0x1134C, 0x1134D, 0x11357, 0x11362, 0x11363, 0x11435,
0x11436, 0x11437, 0x11440, 0x11441, 0x11445, 0x114B0, 0x114B1,
0x114B2, 0x114B9, 0x114BB, 0x114BC, 0x114BD, 0x114BE, 0x114C1,
0x115AF, 0x115B0, 0x115B1, 0x115B8, 0x115B9, 0x115BA, 0x115BB,
0x115BE, 0x11630, 0x11631, 0x11632, 0x1163B, 0x1163C, 0x1163E,
0x116AC, 0x116AE, 0x116AF, 0x116B6, 0x11720, 0x11721, 0x11726,
0x11A07, 0x11A08, 0x11A39, 0x11A57, 0x11A58, 0x11A97, 0x11C2F,
0x11C3E, 0x11CA9, 0x11CB1, 0x11CB4, 0x16F51, 0x16F52, 0x16F53,
0x16F54, 0x16F55, 0x16F56, 0x16F57, 0x16F58, 0x16F59, 0x16F5A,
0x16F5B, 0x16F5C, 0x16F5D, 0x16F5E, 0x16F5F, 0x16F60, 0x16F61,
0x16F62, 0x16F63, 0x16F64, 0x16F65, 0x16F66, 0x16F67, 0x16F68,
0x16F69, 0x16F6A, 0x16F6B, 0x16F6C, 0x16F6D, 0x16F6E, 0x16F6F,
0x16F70, 0x16F71, 0x16F72, 0x16F73, 0x16F74, 0x16F75, 0x16F76,
0x16F77, 0x16F78, 0x16F79, 0x16F7A, 0x16F7B, 0x16F7C, 0x16F7D,
0x16F7E, 0x1D165, 0x1D166, 0x1D16D, 0x1D16E, 0x1D16F, 0x1D170,
0x1D171, 0x1D172,
];
final Set<int> these = <int>{};
int combiningCount = enclosingCombiningMarks.length + nonspacingCombiningMarks.length;
if (includeSpacingCombiningMarks) {
combiningCount += spacingCombiningMarks.length;
}
for (int count = 0; count < targetLength; count += 1) {
int characterCode = random.nextInt(combiningCount);
if (characterCode < enclosingCombiningMarks.length) {
these.add(enclosingCombiningMarks[characterCode]);
} else {
characterCode -= enclosingCombiningMarks.length;
if (characterCode < nonspacingCombiningMarks.length) {
these.add(nonspacingCombiningMarks[characterCode]);
} else {
characterCode -= nonspacingCombiningMarks.length;
these.add(spacingCombiningMarks[characterCode]);
}
}
}
base ??= String.fromCharCode(randomCharacter(random));
final List<int> characters = these.toList();
return base + String.fromCharCodes(characters);
}
T pickFromList<T>(math.Random random, List<T> list) {
return list[random.nextInt(list.length)];
}
class Range {
const Range(this.start, this.end);
final int start;
final int end;
}
int randomCharacter(math.Random random) {
// all ranges of non-control, non-combining characters
const List<Range> characterRanges = <Range>[
Range(0x00020, 0x0007e),
Range(0x000a0, 0x000ac),
Range(0x000ae, 0x002ff),
Range(0x00370, 0x00377),
Range(0x0037a, 0x0037f),
Range(0x00384, 0x0038a),
Range(0x0038c, 0x0038c),
Range(0x0038e, 0x003a1),
Range(0x003a3, 0x00482),
Range(0x0048a, 0x0052f),
Range(0x00531, 0x00556),
Range(0x00559, 0x0055f),
Range(0x00561, 0x00587),
Range(0x00589, 0x0058a),
Range(0x0058d, 0x0058f),
Range(0x005be, 0x005be),
Range(0x005c0, 0x005c0),
Range(0x005c3, 0x005c3),
Range(0x005c6, 0x005c6),
Range(0x005d0, 0x005ea),
Range(0x005f0, 0x005f4),
Range(0x00606, 0x0060f),
Range(0x0061b, 0x0061b),
Range(0x0061e, 0x0064a),
Range(0x00660, 0x0066f),
Range(0x00671, 0x006d5),
Range(0x006de, 0x006de),
Range(0x006e5, 0x006e6),
Range(0x006e9, 0x006e9),
Range(0x006ee, 0x0070d),
Range(0x00710, 0x00710),
Range(0x00712, 0x0072f),
Range(0x0074d, 0x007a5),
Range(0x007b1, 0x007b1),
Range(0x007c0, 0x007ea),
Range(0x007f4, 0x007fa),
Range(0x00800, 0x00815),
Range(0x0081a, 0x0081a),
Range(0x00824, 0x00824),
Range(0x00828, 0x00828),
Range(0x00830, 0x0083e),
Range(0x00840, 0x00858),
Range(0x0085e, 0x0085e),
Range(0x00860, 0x0086a),
Range(0x008a0, 0x008b4),
Range(0x008b6, 0x008bd),
Range(0x00904, 0x00939),
Range(0x0093d, 0x0093d),
Range(0x00950, 0x00950),
Range(0x00958, 0x00961),
Range(0x00964, 0x00980),
Range(0x00985, 0x0098c),
Range(0x0098f, 0x00990),
Range(0x00993, 0x009a8),
Range(0x009aa, 0x009b0),
Range(0x009b2, 0x009b2),
Range(0x009b6, 0x009b9),
Range(0x009bd, 0x009bd),
Range(0x009ce, 0x009ce),
Range(0x009dc, 0x009dd),
Range(0x009df, 0x009e1),
Range(0x009e6, 0x009fd),
Range(0x00a05, 0x00a0a),
Range(0x00a0f, 0x00a10),
Range(0x00a13, 0x00a28),
Range(0x00a2a, 0x00a30),
Range(0x00a32, 0x00a33),
Range(0x00a35, 0x00a36),
Range(0x00a38, 0x00a39),
Range(0x00a59, 0x00a5c),
Range(0x00a5e, 0x00a5e),
Range(0x00a66, 0x00a6f),
Range(0x00a72, 0x00a74),
Range(0x00a85, 0x00a8d),
Range(0x00a8f, 0x00a91),
Range(0x00a93, 0x00aa8),
Range(0x00aaa, 0x00ab0),
Range(0x00ab2, 0x00ab3),
Range(0x00ab5, 0x00ab9),
Range(0x00abd, 0x00abd),
Range(0x00ad0, 0x00ad0),
Range(0x00ae0, 0x00ae1),
Range(0x00ae6, 0x00af1),
Range(0x00af9, 0x00af9),
Range(0x00b05, 0x00b0c),
Range(0x00b0f, 0x00b10),
Range(0x00b13, 0x00b28),
Range(0x00b2a, 0x00b30),
Range(0x00b32, 0x00b33),
Range(0x00b35, 0x00b39),
Range(0x00b3d, 0x00b3d),
Range(0x00b5c, 0x00b5d),
Range(0x00b5f, 0x00b61),
Range(0x00b66, 0x00b77),
Range(0x00b83, 0x00b83),
Range(0x00b85, 0x00b8a),
Range(0x00b8e, 0x00b90),
Range(0x00b92, 0x00b95),
Range(0x00b99, 0x00b9a),
Range(0x00b9c, 0x00b9c),
Range(0x00b9e, 0x00b9f),
Range(0x00ba3, 0x00ba4),
Range(0x00ba8, 0x00baa),
Range(0x00bae, 0x00bb9),
Range(0x00bd0, 0x00bd0),
Range(0x00be6, 0x00bfa),
Range(0x00c05, 0x00c0c),
Range(0x00c0e, 0x00c10),
Range(0x00c12, 0x00c28),
Range(0x00c2a, 0x00c39),
Range(0x00c3d, 0x00c3d),
Range(0x00c58, 0x00c5a),
Range(0x00c60, 0x00c61),
Range(0x00c66, 0x00c6f),
Range(0x00c78, 0x00c80),
Range(0x00c85, 0x00c8c),
Range(0x00c8e, 0x00c90),
Range(0x00c92, 0x00ca8),
Range(0x00caa, 0x00cb3),
Range(0x00cb5, 0x00cb9),
Range(0x00cbd, 0x00cbd),
Range(0x00cde, 0x00cde),
Range(0x00ce0, 0x00ce1),
Range(0x00ce6, 0x00cef),
Range(0x00cf1, 0x00cf2),
Range(0x00d05, 0x00d0c),
Range(0x00d0e, 0x00d10),
Range(0x00d12, 0x00d3a),
Range(0x00d3d, 0x00d3d),
Range(0x00d4e, 0x00d4f),
Range(0x00d54, 0x00d56),
Range(0x00d58, 0x00d61),
Range(0x00d66, 0x00d7f),
Range(0x00d85, 0x00d96),
Range(0x00d9a, 0x00db1),
Range(0x00db3, 0x00dbb),
Range(0x00dbd, 0x00dbd),
Range(0x00dc0, 0x00dc6),
Range(0x00de6, 0x00def),
Range(0x00df4, 0x00df4),
Range(0x00e01, 0x00e30),
Range(0x00e32, 0x00e33),
Range(0x00e3f, 0x00e46),
Range(0x00e4f, 0x00e5b),
Range(0x00e81, 0x00e82),
Range(0x00e84, 0x00e84),
Range(0x00e87, 0x00e88),
Range(0x00e8a, 0x00e8a),
Range(0x00e8d, 0x00e8d),
Range(0x00e94, 0x00e97),
Range(0x00e99, 0x00e9f),
Range(0x00ea1, 0x00ea3),
Range(0x00ea5, 0x00ea5),
Range(0x00ea7, 0x00ea7),
Range(0x00eaa, 0x00eab),
Range(0x00ead, 0x00eb0),
Range(0x00eb2, 0x00eb3),
Range(0x00ebd, 0x00ebd),
Range(0x00ec0, 0x00ec4),
Range(0x00ec6, 0x00ec6),
Range(0x00ed0, 0x00ed9),
Range(0x00edc, 0x00edf),
Range(0x00f00, 0x00f17),
Range(0x00f1a, 0x00f34),
Range(0x00f36, 0x00f36),
Range(0x00f38, 0x00f38),
Range(0x00f3a, 0x00f3d),
Range(0x00f40, 0x00f47),
Range(0x00f49, 0x00f6c),
Range(0x00f85, 0x00f85),
Range(0x00f88, 0x00f8c),
Range(0x00fbe, 0x00fc5),
Range(0x00fc7, 0x00fcc),
Range(0x00fce, 0x00fda),
Range(0x01000, 0x0102a),
Range(0x0103f, 0x01055),
Range(0x0105a, 0x0105d),
Range(0x01061, 0x01061),
Range(0x01065, 0x01066),
Range(0x0106e, 0x01070),
Range(0x01075, 0x01081),
Range(0x0108e, 0x0108e),
Range(0x01090, 0x01099),
Range(0x0109e, 0x010c5),
Range(0x010c7, 0x010c7),
Range(0x010cd, 0x010cd),
Range(0x010d0, 0x01248),
Range(0x0124a, 0x0124d),
Range(0x01250, 0x01256),
Range(0x01258, 0x01258),
Range(0x0125a, 0x0125d),
Range(0x01260, 0x01288),
Range(0x0128a, 0x0128d),
Range(0x01290, 0x012b0),
Range(0x012b2, 0x012b5),
Range(0x012b8, 0x012be),
Range(0x012c0, 0x012c0),
Range(0x012c2, 0x012c5),
Range(0x012c8, 0x012d6),
Range(0x012d8, 0x01310),
Range(0x01312, 0x01315),
Range(0x01318, 0x0135a),
Range(0x01360, 0x0137c),
Range(0x01380, 0x01399),
Range(0x013a0, 0x013f5),
Range(0x013f8, 0x013fd),
Range(0x01400, 0x0169c),
Range(0x016a0, 0x016f8),
Range(0x01700, 0x0170c),
Range(0x0170e, 0x01711),
Range(0x01720, 0x01731),
Range(0x01735, 0x01736),
Range(0x01740, 0x01751),
Range(0x01760, 0x0176c),
Range(0x0176e, 0x01770),
Range(0x01780, 0x017b3),
Range(0x017d4, 0x017dc),
Range(0x017e0, 0x017e9),
Range(0x017f0, 0x017f9),
Range(0x01800, 0x0180a),
Range(0x01810, 0x01819),
Range(0x01820, 0x01877),
Range(0x01880, 0x01884),
Range(0x01887, 0x018a8),
Range(0x018aa, 0x018aa),
Range(0x018b0, 0x018f5),
Range(0x01900, 0x0191e),
Range(0x01940, 0x01940),
Range(0x01944, 0x0196d),
Range(0x01970, 0x01974),
Range(0x01980, 0x019ab),
Range(0x019b0, 0x019c9),
Range(0x019d0, 0x019da),
Range(0x019de, 0x01a16),
Range(0x01a1e, 0x01a54),
Range(0x01a80, 0x01a89),
Range(0x01a90, 0x01a99),
Range(0x01aa0, 0x01aad),
Range(0x01b05, 0x01b33),
Range(0x01b45, 0x01b4b),
Range(0x01b50, 0x01b6a),
Range(0x01b74, 0x01b7c),
Range(0x01b83, 0x01ba0),
Range(0x01bae, 0x01be5),
Range(0x01bfc, 0x01c23),
Range(0x01c3b, 0x01c49),
Range(0x01c4d, 0x01c88),
Range(0x01cc0, 0x01cc7),
Range(0x01cd3, 0x01cd3),
Range(0x01ce9, 0x01cec),
Range(0x01cee, 0x01cf1),
Range(0x01cf5, 0x01cf6),
Range(0x01d00, 0x01dbf),
Range(0x01e00, 0x01f15),
Range(0x01f18, 0x01f1d),
Range(0x01f20, 0x01f45),
Range(0x01f48, 0x01f4d),
Range(0x01f50, 0x01f57),
Range(0x01f59, 0x01f59),
Range(0x01f5b, 0x01f5b),
Range(0x01f5d, 0x01f5d),
Range(0x01f5f, 0x01f7d),
Range(0x01f80, 0x01fb4),
Range(0x01fb6, 0x01fc4),
Range(0x01fc6, 0x01fd3),
Range(0x01fd6, 0x01fdb),
Range(0x01fdd, 0x01fef),
Range(0x01ff2, 0x01ff4),
Range(0x01ff6, 0x01ffe),
Range(0x02000, 0x0200a),
Range(0x02010, 0x02029),
Range(0x0202f, 0x0205f),
Range(0x02070, 0x02071),
Range(0x02074, 0x0208e),
Range(0x02090, 0x0209c),
Range(0x020a0, 0x020bf),
Range(0x02100, 0x0218b),
Range(0x02190, 0x02426),
Range(0x02440, 0x0244a),
Range(0x02460, 0x02b73),
Range(0x02b76, 0x02b95),
Range(0x02b98, 0x02bb9),
Range(0x02bbd, 0x02bc8),
Range(0x02bca, 0x02bd2),
Range(0x02bec, 0x02bef),
Range(0x02c00, 0x02c2e),
Range(0x02c30, 0x02c5e),
Range(0x02c60, 0x02cee),
Range(0x02cf2, 0x02cf3),
Range(0x02cf9, 0x02d25),
Range(0x02d27, 0x02d27),
Range(0x02d2d, 0x02d2d),
Range(0x02d30, 0x02d67),
Range(0x02d6f, 0x02d70),
Range(0x02d80, 0x02d96),
Range(0x02da0, 0x02da6),
Range(0x02da8, 0x02dae),
Range(0x02db0, 0x02db6),
Range(0x02db8, 0x02dbe),
Range(0x02dc0, 0x02dc6),
Range(0x02dc8, 0x02dce),
Range(0x02dd0, 0x02dd6),
Range(0x02dd8, 0x02dde),
Range(0x02e00, 0x02e49),
Range(0x02e80, 0x02e99),
Range(0x02e9b, 0x02ef3),
Range(0x02f00, 0x02fd5),
Range(0x02ff0, 0x02ffb),
Range(0x03000, 0x03029),
Range(0x03030, 0x0303f),
Range(0x03041, 0x03096),
Range(0x0309b, 0x030ff),
Range(0x03105, 0x0312e),
Range(0x03131, 0x0318e),
Range(0x03190, 0x031ba),
Range(0x031c0, 0x031e3),
Range(0x031f0, 0x0321e),
Range(0x03220, 0x032fe),
Range(0x03300, 0x04db5),
Range(0x04dc0, 0x09fea),
Range(0x0a000, 0x0a48c),
Range(0x0a490, 0x0a4c6),
Range(0x0a4d0, 0x0a62b),
Range(0x0a640, 0x0a66e),
Range(0x0a673, 0x0a673),
Range(0x0a67e, 0x0a69d),
Range(0x0a6a0, 0x0a6ef),
Range(0x0a6f2, 0x0a6f7),
Range(0x0a700, 0x0a7ae),
Range(0x0a7b0, 0x0a7b7),
Range(0x0a7f7, 0x0a801),
Range(0x0a803, 0x0a805),
Range(0x0a807, 0x0a80a),
Range(0x0a80c, 0x0a822),
Range(0x0a828, 0x0a82b),
Range(0x0a830, 0x0a839),
Range(0x0a840, 0x0a877),
Range(0x0a882, 0x0a8b3),
Range(0x0a8ce, 0x0a8d9),
Range(0x0a8f2, 0x0a8fd),
Range(0x0a900, 0x0a925),
Range(0x0a92e, 0x0a946),
Range(0x0a95f, 0x0a97c),
Range(0x0a984, 0x0a9b2),
Range(0x0a9c1, 0x0a9cd),
Range(0x0a9cf, 0x0a9d9),
Range(0x0a9de, 0x0a9e4),
Range(0x0a9e6, 0x0a9fe),
Range(0x0aa00, 0x0aa28),
Range(0x0aa40, 0x0aa42),
Range(0x0aa44, 0x0aa4b),
Range(0x0aa50, 0x0aa59),
Range(0x0aa5c, 0x0aa7a),
Range(0x0aa7e, 0x0aaaf),
Range(0x0aab1, 0x0aab1),
Range(0x0aab5, 0x0aab6),
Range(0x0aab9, 0x0aabd),
Range(0x0aac0, 0x0aac0),
Range(0x0aac2, 0x0aac2),
Range(0x0aadb, 0x0aaea),
Range(0x0aaf0, 0x0aaf4),
Range(0x0ab01, 0x0ab06),
Range(0x0ab09, 0x0ab0e),
Range(0x0ab11, 0x0ab16),
Range(0x0ab20, 0x0ab26),
Range(0x0ab28, 0x0ab2e),
Range(0x0ab30, 0x0ab65),
Range(0x0ab70, 0x0abe2),
Range(0x0abeb, 0x0abeb),
Range(0x0abf0, 0x0abf9),
Range(0x0ac00, 0x0d7a3),
Range(0x0d7b0, 0x0d7c6),
Range(0x0d7cb, 0x0d7fb),
Range(0x0f900, 0x0fa6d),
Range(0x0fa70, 0x0fad9),
Range(0x0fb00, 0x0fb06),
Range(0x0fb13, 0x0fb17),
Range(0x0fb1d, 0x0fb1d),
Range(0x0fb1f, 0x0fb36),
Range(0x0fb38, 0x0fb3c),
Range(0x0fb3e, 0x0fb3e),
Range(0x0fb40, 0x0fb41),
Range(0x0fb43, 0x0fb44),
Range(0x0fb46, 0x0fbc1),
Range(0x0fbd3, 0x0fd3f),
Range(0x0fd50, 0x0fd8f),
Range(0x0fd92, 0x0fdc7),
Range(0x0fdf0, 0x0fdfd),
Range(0x0fe10, 0x0fe19),
Range(0x0fe30, 0x0fe52),
Range(0x0fe54, 0x0fe66),
Range(0x0fe68, 0x0fe6b),
Range(0x0fe70, 0x0fe74),
Range(0x0fe76, 0x0fefc),
Range(0x0ff01, 0x0ffbe),
Range(0x0ffc2, 0x0ffc7),
Range(0x0ffca, 0x0ffcf),
Range(0x0ffd2, 0x0ffd7),
Range(0x0ffda, 0x0ffdc),
Range(0x0ffe0, 0x0ffe6),
Range(0x0ffe8, 0x0ffee),
Range(0x0fffc, 0x0fffd),
Range(0x10000, 0x1000b),
Range(0x1000d, 0x10026),
Range(0x10028, 0x1003a),
Range(0x1003c, 0x1003d),
Range(0x1003f, 0x1004d),
Range(0x10050, 0x1005d),
Range(0x10080, 0x100fa),
Range(0x10100, 0x10102),
Range(0x10107, 0x10133),
Range(0x10137, 0x1018e),
Range(0x10190, 0x1019b),
Range(0x101a0, 0x101a0),
Range(0x101d0, 0x101fc),
Range(0x10280, 0x1029c),
Range(0x102a0, 0x102d0),
Range(0x102e1, 0x102fb),
Range(0x10300, 0x10323),
Range(0x1032d, 0x1034a),
Range(0x10350, 0x10375),
Range(0x10380, 0x1039d),
Range(0x1039f, 0x103c3),
Range(0x103c8, 0x103d5),
Range(0x10400, 0x1049d),
Range(0x104a0, 0x104a9),
Range(0x104b0, 0x104d3),
Range(0x104d8, 0x104fb),
Range(0x10500, 0x10527),
Range(0x10530, 0x10563),
Range(0x1056f, 0x1056f),
Range(0x10600, 0x10736),
Range(0x10740, 0x10755),
Range(0x10760, 0x10767),
Range(0x10800, 0x10805),
Range(0x10808, 0x10808),
Range(0x1080a, 0x10835),
Range(0x10837, 0x10838),
Range(0x1083c, 0x1083c),
Range(0x1083f, 0x10855),
Range(0x10857, 0x1089e),
Range(0x108a7, 0x108af),
Range(0x108e0, 0x108f2),
Range(0x108f4, 0x108f5),
Range(0x108fb, 0x1091b),
Range(0x1091f, 0x10939),
Range(0x1093f, 0x1093f),
Range(0x10980, 0x109b7),
Range(0x109bc, 0x109cf),
Range(0x109d2, 0x10a00),
Range(0x10a10, 0x10a13),
Range(0x10a15, 0x10a17),
Range(0x10a19, 0x10a33),
Range(0x10a40, 0x10a47),
Range(0x10a50, 0x10a58),
Range(0x10a60, 0x10a9f),
Range(0x10ac0, 0x10ae4),
Range(0x10aeb, 0x10af6),
Range(0x10b00, 0x10b35),
Range(0x10b39, 0x10b55),
Range(0x10b58, 0x10b72),
Range(0x10b78, 0x10b91),
Range(0x10b99, 0x10b9c),
Range(0x10ba9, 0x10baf),
Range(0x10c00, 0x10c48),
Range(0x10c80, 0x10cb2),
Range(0x10cc0, 0x10cf2),
Range(0x10cfa, 0x10cff),
Range(0x10e60, 0x10e7e),
Range(0x11003, 0x11037),
Range(0x11047, 0x1104d),
Range(0x11052, 0x1106f),
Range(0x11083, 0x110af),
Range(0x110bb, 0x110bc),
Range(0x110be, 0x110c1),
Range(0x110d0, 0x110e8),
Range(0x110f0, 0x110f9),
Range(0x11103, 0x11126),
Range(0x11136, 0x11143),
Range(0x11150, 0x11172),
Range(0x11174, 0x11176),
Range(0x11183, 0x111b2),
Range(0x111c1, 0x111c9),
Range(0x111cd, 0x111cd),
Range(0x111d0, 0x111df),
Range(0x111e1, 0x111f4),
Range(0x11200, 0x11211),
Range(0x11213, 0x1122b),
Range(0x11238, 0x1123d),
Range(0x11280, 0x11286),
Range(0x11288, 0x11288),
Range(0x1128a, 0x1128d),
Range(0x1128f, 0x1129d),
Range(0x1129f, 0x112a9),
Range(0x112b0, 0x112de),
Range(0x112f0, 0x112f9),
Range(0x11305, 0x1130c),
Range(0x1130f, 0x11310),
Range(0x11313, 0x11328),
Range(0x1132a, 0x11330),
Range(0x11332, 0x11333),
Range(0x11335, 0x11339),
Range(0x1133d, 0x1133d),
Range(0x11350, 0x11350),
Range(0x1135d, 0x11361),
Range(0x11400, 0x11434),
Range(0x11447, 0x11459),
Range(0x1145b, 0x1145b),
Range(0x1145d, 0x1145d),
Range(0x11480, 0x114af),
Range(0x114c4, 0x114c7),
Range(0x114d0, 0x114d9),
Range(0x11580, 0x115ae),
Range(0x115c1, 0x115db),
Range(0x11600, 0x1162f),
Range(0x11641, 0x11644),
Range(0x11650, 0x11659),
Range(0x11660, 0x1166c),
Range(0x11680, 0x116aa),
Range(0x116c0, 0x116c9),
Range(0x11700, 0x11719),
Range(0x11730, 0x1173f),
Range(0x118a0, 0x118f2),
Range(0x118ff, 0x118ff),
Range(0x11a00, 0x11a00),
Range(0x11a0b, 0x11a32),
Range(0x11a3a, 0x11a3a),
Range(0x11a3f, 0x11a46),
Range(0x11a50, 0x11a50),
Range(0x11a5c, 0x11a83),
Range(0x11a86, 0x11a89),
Range(0x11a9a, 0x11a9c),
Range(0x11a9e, 0x11aa2),
Range(0x11ac0, 0x11af8),
Range(0x11c00, 0x11c08),
Range(0x11c0a, 0x11c2e),
Range(0x11c40, 0x11c45),
Range(0x11c50, 0x11c6c),
Range(0x11c70, 0x11c8f),
Range(0x11d00, 0x11d06),
Range(0x11d08, 0x11d09),
Range(0x11d0b, 0x11d30),
Range(0x11d46, 0x11d46),
Range(0x11d50, 0x11d59),
Range(0x12000, 0x12399),
Range(0x12400, 0x1246e),
Range(0x12470, 0x12474),
Range(0x12480, 0x12543),
Range(0x13000, 0x1342e),
Range(0x14400, 0x14646),
Range(0x16800, 0x16a38),
Range(0x16a40, 0x16a5e),
Range(0x16a60, 0x16a69),
Range(0x16a6e, 0x16a6f),
Range(0x16ad0, 0x16aed),
Range(0x16af5, 0x16af5),
Range(0x16b00, 0x16b2f),
Range(0x16b37, 0x16b45),
Range(0x16b50, 0x16b59),
Range(0x16b5b, 0x16b61),
Range(0x16b63, 0x16b77),
Range(0x16b7d, 0x16b8f),
Range(0x16f00, 0x16f44),
Range(0x16f50, 0x16f50),
Range(0x16f93, 0x16f9f),
Range(0x16fe0, 0x16fe1),
Range(0x17000, 0x187ec),
Range(0x18800, 0x18af2),
Range(0x1b000, 0x1b11e),
Range(0x1b170, 0x1b2fb),
Range(0x1bc00, 0x1bc6a),
Range(0x1bc70, 0x1bc7c),
Range(0x1bc80, 0x1bc88),
Range(0x1bc90, 0x1bc99),
Range(0x1bc9c, 0x1bc9c),
Range(0x1bc9f, 0x1bc9f),
Range(0x1d000, 0x1d0f5),
Range(0x1d100, 0x1d126),
Range(0x1d129, 0x1d164),
Range(0x1d16a, 0x1d16c),
Range(0x1d183, 0x1d184),
Range(0x1d18c, 0x1d1a9),
Range(0x1d1ae, 0x1d1e8),
Range(0x1d200, 0x1d241),
Range(0x1d245, 0x1d245),
Range(0x1d300, 0x1d356),
Range(0x1d360, 0x1d371),
Range(0x1d400, 0x1d454),
Range(0x1d456, 0x1d49c),
Range(0x1d49e, 0x1d49f),
Range(0x1d4a2, 0x1d4a2),
Range(0x1d4a5, 0x1d4a6),
Range(0x1d4a9, 0x1d4ac),
Range(0x1d4ae, 0x1d4b9),
Range(0x1d4bb, 0x1d4bb),
Range(0x1d4bd, 0x1d4c3),
Range(0x1d4c5, 0x1d505),
Range(0x1d507, 0x1d50a),
Range(0x1d50d, 0x1d514),
Range(0x1d516, 0x1d51c),
Range(0x1d51e, 0x1d539),
Range(0x1d53b, 0x1d53e),
Range(0x1d540, 0x1d544),
Range(0x1d546, 0x1d546),
Range(0x1d54a, 0x1d550),
Range(0x1d552, 0x1d6a5),
Range(0x1d6a8, 0x1d7cb),
Range(0x1d7ce, 0x1d9ff),
Range(0x1da37, 0x1da3a),
Range(0x1da6d, 0x1da74),
Range(0x1da76, 0x1da83),
Range(0x1da85, 0x1da8b),
Range(0x1e800, 0x1e8c4),
Range(0x1e8c7, 0x1e8cf),
Range(0x1e900, 0x1e943),
Range(0x1e950, 0x1e959),
Range(0x1e95e, 0x1e95f),
Range(0x1ee00, 0x1ee03),
Range(0x1ee05, 0x1ee1f),
Range(0x1ee21, 0x1ee22),
Range(0x1ee24, 0x1ee24),
Range(0x1ee27, 0x1ee27),
Range(0x1ee29, 0x1ee32),
Range(0x1ee34, 0x1ee37),
Range(0x1ee39, 0x1ee39),
Range(0x1ee3b, 0x1ee3b),
Range(0x1ee42, 0x1ee42),
Range(0x1ee47, 0x1ee47),
Range(0x1ee49, 0x1ee49),
Range(0x1ee4b, 0x1ee4b),
Range(0x1ee4d, 0x1ee4f),
Range(0x1ee51, 0x1ee52),
Range(0x1ee54, 0x1ee54),
Range(0x1ee57, 0x1ee57),
Range(0x1ee59, 0x1ee59),
Range(0x1ee5b, 0x1ee5b),
Range(0x1ee5d, 0x1ee5d),
Range(0x1ee5f, 0x1ee5f),
Range(0x1ee61, 0x1ee62),
Range(0x1ee64, 0x1ee64),
Range(0x1ee67, 0x1ee6a),
Range(0x1ee6c, 0x1ee72),
Range(0x1ee74, 0x1ee77),
Range(0x1ee79, 0x1ee7c),
Range(0x1ee7e, 0x1ee7e),
Range(0x1ee80, 0x1ee89),
Range(0x1ee8b, 0x1ee9b),
Range(0x1eea1, 0x1eea3),
Range(0x1eea5, 0x1eea9),
Range(0x1eeab, 0x1eebb),
Range(0x1eef0, 0x1eef1),
Range(0x1f000, 0x1f02b),
Range(0x1f030, 0x1f093),
Range(0x1f0a0, 0x1f0ae),
Range(0x1f0b1, 0x1f0bf),
Range(0x1f0c1, 0x1f0cf),
Range(0x1f0d1, 0x1f0f5),
Range(0x1f100, 0x1f10c),
Range(0x1f110, 0x1f12e),
Range(0x1f130, 0x1f16b),
Range(0x1f170, 0x1f1ac),
Range(0x1f1e6, 0x1f202),
Range(0x1f210, 0x1f23b),
Range(0x1f240, 0x1f248),
Range(0x1f250, 0x1f251),
Range(0x1f260, 0x1f265),
Range(0x1f300, 0x1f6d4),
Range(0x1f6e0, 0x1f6ec),
Range(0x1f6f0, 0x1f6f8),
Range(0x1f700, 0x1f773),
Range(0x1f780, 0x1f7d4),
Range(0x1f800, 0x1f80b),
Range(0x1f810, 0x1f847),
Range(0x1f850, 0x1f859),
Range(0x1f860, 0x1f887),
Range(0x1f890, 0x1f8ad),
Range(0x1f900, 0x1f90b),
Range(0x1f910, 0x1f93e),
Range(0x1f940, 0x1f94c),
Range(0x1f950, 0x1f96b),
Range(0x1f980, 0x1f997),
Range(0x1f9c0, 0x1f9c0),
Range(0x1f9d0, 0x1f9e6),
Range(0x20000, 0x2a6d6),
Range(0x2a700, 0x2b734),
Range(0x2b740, 0x2b81d),
Range(0x2b820, 0x2cea1),
Range(0x2ceb0, 0x2ebe0),
Range(0x2f800, 0x2fa1d),
];
final Range range = pickFromList<Range>(random, characterRanges);
if (range.start == range.end) {
return range.start;
}
return range.start + random.nextInt(range.end - range.start);
}
| flutter/dev/manual_tests/lib/text.dart/0 | {
"file_path": "flutter/dev/manual_tests/lib/text.dart",
"repo_id": "flutter",
"token_count": 46392
} | 571 |
{
"version": "v0_206",
"md.comp.badge.color": "error",
"md.comp.badge.large.color": "error",
"md.comp.badge.large.label-text.color": "onError",
"md.comp.badge.large.label-text.text-style": "labelSmall",
"md.comp.badge.large.shape": "md.sys.shape.corner.full",
"md.comp.badge.large.size": 16.0,
"md.comp.badge.shape": "md.sys.shape.corner.full",
"md.comp.badge.size": 6.0
}
| flutter/dev/tools/gen_defaults/data/badge.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/badge.json",
"repo_id": "flutter",
"token_count": 176
} | 572 |
{
"version": "v0_206",
"md.comp.suggestion-chip.container.height": 32.0,
"md.comp.suggestion-chip.container.shape": "md.sys.shape.corner.small",
"md.comp.suggestion-chip.disabled.label-text.color": "onSurface",
"md.comp.suggestion-chip.disabled.label-text.opacity": 0.38,
"md.comp.suggestion-chip.dragged.container.elevation": "md.sys.elevation.level4",
"md.comp.suggestion-chip.dragged.label-text.color": "onSurfaceVariant",
"md.comp.suggestion-chip.dragged.state-layer.color": "onSurfaceVariant",
"md.comp.suggestion-chip.dragged.state-layer.opacity": "md.sys.state.dragged.state-layer-opacity",
"md.comp.suggestion-chip.elevated.container.color": "surfaceContainerLow",
"md.comp.suggestion-chip.elevated.container.elevation": "md.sys.elevation.level1",
"md.comp.suggestion-chip.elevated.container.shadow-color": "shadow",
"md.comp.suggestion-chip.elevated.disabled.container.color": "onSurface",
"md.comp.suggestion-chip.elevated.disabled.container.elevation": "md.sys.elevation.level0",
"md.comp.suggestion-chip.elevated.disabled.container.opacity": 0.12,
"md.comp.suggestion-chip.elevated.focus.container.elevation": "md.sys.elevation.level1",
"md.comp.suggestion-chip.elevated.hover.container.elevation": "md.sys.elevation.level2",
"md.comp.suggestion-chip.elevated.pressed.container.elevation": "md.sys.elevation.level1",
"md.comp.suggestion-chip.flat.container.elevation": "md.sys.elevation.level0",
"md.comp.suggestion-chip.flat.disabled.outline.color": "onSurface",
"md.comp.suggestion-chip.flat.disabled.outline.opacity": 0.12,
"md.comp.suggestion-chip.flat.focus.outline.color": "onSurfaceVariant",
"md.comp.suggestion-chip.flat.outline.color": "outline",
"md.comp.suggestion-chip.flat.outline.width": 1.0,
"md.comp.suggestion-chip.focus.indicator.color": "secondary",
"md.comp.suggestion-chip.focus.indicator.outline.offset": "md.sys.state.focus-indicator.outer-offset",
"md.comp.suggestion-chip.focus.indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.suggestion-chip.focus.label-text.color": "onSurfaceVariant",
"md.comp.suggestion-chip.focus.state-layer.color": "onSurfaceVariant",
"md.comp.suggestion-chip.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.suggestion-chip.hover.label-text.color": "onSurfaceVariant",
"md.comp.suggestion-chip.hover.state-layer.color": "onSurfaceVariant",
"md.comp.suggestion-chip.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.suggestion-chip.label-text.color": "onSurfaceVariant",
"md.comp.suggestion-chip.label-text.text-style": "labelLarge",
"md.comp.suggestion-chip.pressed.label-text.color": "onSurfaceVariant",
"md.comp.suggestion-chip.pressed.state-layer.color": "onSurfaceVariant",
"md.comp.suggestion-chip.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity",
"md.comp.suggestion-chip.with-leading-icon.disabled.leading-icon.color": "onSurface",
"md.comp.suggestion-chip.with-leading-icon.disabled.leading-icon.opacity": 0.38,
"md.comp.suggestion-chip.with-leading-icon.dragged.leading-icon.color": "primary",
"md.comp.suggestion-chip.with-leading-icon.focus.leading-icon.color": "primary",
"md.comp.suggestion-chip.with-leading-icon.hover.leading-icon.color": "primary",
"md.comp.suggestion-chip.with-leading-icon.leading-icon.color": "primary",
"md.comp.suggestion-chip.with-leading-icon.leading-icon.size": 18.0,
"md.comp.suggestion-chip.with-leading-icon.pressed.leading-icon.color": "primary"
}
| flutter/dev/tools/gen_defaults/data/chip_suggestion.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/chip_suggestion.json",
"repo_id": "flutter",
"token_count": 1334
} | 573 |
{
"version": "v0_206",
"md.comp.filled-tonal-icon-button.container.color": "secondaryContainer",
"md.comp.filled-tonal-icon-button.container.height": 40.0,
"md.comp.filled-tonal-icon-button.container.shape": "md.sys.shape.corner.full",
"md.comp.filled-tonal-icon-button.container.width": 40.0,
"md.comp.filled-tonal-icon-button.disabled.container.color": "onSurface",
"md.comp.filled-tonal-icon-button.disabled.container.opacity": 0.12,
"md.comp.filled-tonal-icon-button.disabled.icon.color": "onSurface",
"md.comp.filled-tonal-icon-button.disabled.icon.opacity": 0.38,
"md.comp.filled-tonal-icon-button.focus.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.focus.indicator.color": "secondary",
"md.comp.filled-tonal-icon-button.focus.indicator.outline.offset": "md.sys.state.focus-indicator.outer-offset",
"md.comp.filled-tonal-icon-button.focus.indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.filled-tonal-icon-button.focus.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.filled-tonal-icon-button.hover.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.hover.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.filled-tonal-icon-button.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.icon.size": 24.0,
"md.comp.filled-tonal-icon-button.pressed.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.pressed.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity",
"md.comp.filled-tonal-icon-button.selected.container.color": "secondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.focus.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.focus.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.hover.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.hover.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.pressed.icon.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.selected.pressed.state-layer.color": "onSecondaryContainer",
"md.comp.filled-tonal-icon-button.toggle.unselected.focus.icon.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.focus.state-layer.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.hover.icon.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.hover.state-layer.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.icon.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.pressed.icon.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.toggle.unselected.pressed.state-layer.color": "onSurfaceVariant",
"md.comp.filled-tonal-icon-button.unselected.container.color": "surfaceContainerHighest"
}
| flutter/dev/tools/gen_defaults/data/icon_button_filled_tonal.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/icon_button_filled_tonal.json",
"repo_id": "flutter",
"token_count": 1208
} | 574 |
{
"version": "v0_206",
"md.comp.outlined-segmented-button.container.height": 40.0,
"md.comp.outlined-segmented-button.disabled.icon.color": "onSurface",
"md.comp.outlined-segmented-button.disabled.icon.opacity": 0.38,
"md.comp.outlined-segmented-button.disabled.label-text.color": "onSurface",
"md.comp.outlined-segmented-button.disabled.label-text.opacity": 0.38,
"md.comp.outlined-segmented-button.disabled.outline.color": "onSurface",
"md.comp.outlined-segmented-button.disabled.outline.opacity": 0.12,
"md.comp.outlined-segmented-button.focus.indicator.color": "secondary",
"md.comp.outlined-segmented-button.focus.indicator.outline.offset": "md.sys.state.focus-indicator.outer-offset",
"md.comp.outlined-segmented-button.focus.indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.outlined-segmented-button.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.outlined-segmented-button.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.outlined-segmented-button.label-text.text-style": "labelLarge",
"md.comp.outlined-segmented-button.outline.color": "outline",
"md.comp.outlined-segmented-button.outline.width": 1.0,
"md.comp.outlined-segmented-button.pressed.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.outlined-segmented-button.selected.container.color": "secondaryContainer",
"md.comp.outlined-segmented-button.selected.focus.icon.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.focus.label-text.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.focus.state-layer.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.hover.icon.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.hover.label-text.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.hover.state-layer.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.label-text.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.pressed.icon.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.pressed.label-text.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.pressed.state-layer.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.selected.with-icon.icon.color": "onSecondaryContainer",
"md.comp.outlined-segmented-button.shape": "md.sys.shape.corner.full",
"md.comp.outlined-segmented-button.unselected.focus.icon.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.focus.label-text.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.focus.state-layer.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.hover.icon.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.hover.label-text.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.hover.state-layer.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.label-text.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.pressed.icon.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.pressed.label-text.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.pressed.state-layer.color": "onSurface",
"md.comp.outlined-segmented-button.unselected.with-icon.icon.color": "onSurface",
"md.comp.outlined-segmented-button.with-icon.icon.size": 18.0
}
| flutter/dev/tools/gen_defaults/data/segmented_button_outlined.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/segmented_button_outlined.json",
"repo_id": "flutter",
"token_count": 1288
} | 575 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class ActionChipTemplate extends TokenTemplate {
const ActionChipTemplate(super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
super.textThemePrefix = '_textTheme.'
});
static const String tokenGroup = 'md.comp.assist-chip';
static const String flatVariant = '.flat';
static const String elevatedVariant = '.elevated';
@override
String generate() => '''
class _${blockName}DefaultsM3 extends ChipThemeData {
_${blockName}DefaultsM3(this.context, this.isEnabled, this._chipVariant)
: super(
shape: ${shape("$tokenGroup.container")},
showCheckmark: true,
);
final BuildContext context;
final bool isEnabled;
final _ChipVariant _chipVariant;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
double? get elevation => _chipVariant == _ChipVariant.flat
? ${elevation("$tokenGroup$flatVariant.container")}
: isEnabled ? ${elevation("$tokenGroup$elevatedVariant.container")} : ${elevation("$tokenGroup$elevatedVariant.disabled.container")};
@override
double? get pressElevation => ${elevation("$tokenGroup$elevatedVariant.pressed.container")};
@override
TextStyle? get labelStyle => ${textStyle("$tokenGroup.label-text")}?.copyWith(
color: isEnabled
? ${color("$tokenGroup.label-text.color")}
: ${color("$tokenGroup.disabled.label-text.color")},
);
@override
MaterialStateProperty<Color?>? get color =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.disabled.container")}
: ${componentColor("$tokenGroup$elevatedVariant.disabled.container")};
}
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.container")}
: ${componentColor("$tokenGroup$elevatedVariant.container")};
});
@override
Color? get shadowColor => _chipVariant == _ChipVariant.flat
? ${colorOrTransparent("$tokenGroup$flatVariant.container.shadow-color")}
: ${colorOrTransparent("$tokenGroup$elevatedVariant.container.shadow-color")};
@override
Color? get surfaceTintColor => ${colorOrTransparent("$tokenGroup.container.surface-tint-layer.color")};
@override
Color? get checkmarkColor => null;
@override
Color? get deleteIconColor => null;
@override
BorderSide? get side => _chipVariant == _ChipVariant.flat
? isEnabled
? ${border('$tokenGroup$flatVariant.outline')}
: ${border('$tokenGroup$flatVariant.disabled.outline')}
: const BorderSide(color: Colors.transparent);
@override
IconThemeData? get iconTheme => IconThemeData(
color: isEnabled
? ${color("$tokenGroup.with-icon.icon.color")}
: ${color("$tokenGroup.with-icon.disabled.icon.color")},
size: ${getToken("$tokenGroup.with-icon.icon.size")},
);
@override
EdgeInsetsGeometry? get padding => const EdgeInsets.all(8.0);
/// The label padding of the chip scales with the font size specified in the
/// [labelStyle], and the system font size settings that scale font sizes
/// globally.
///
/// The chip at effective font size 14.0 starts with 8px on each side and as
/// the font size scales up to closer to 28.0, the label padding is linearly
/// interpolated from 8px to 4px. Once the label has a font size of 2 or
/// higher, label padding remains 4px.
@override
EdgeInsetsGeometry? get labelPadding {
final double fontSize = labelStyle?.fontSize ?? 14.0;
final double fontSizeRatio = MediaQuery.textScalerOf(context).scale(fontSize) / 14.0;
return EdgeInsets.lerp(
const EdgeInsets.symmetric(horizontal: 8.0),
const EdgeInsets.symmetric(horizontal: 4.0),
clampDouble(fontSizeRatio - 1.0, 0.0, 1.0),
)!;
}
}
''';
}
| flutter/dev/tools/gen_defaults/lib/action_chip_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/action_chip_template.dart",
"repo_id": "flutter",
"token_count": 1415
} | 576 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class FABTemplate extends TokenTemplate {
const FABTemplate(super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
super.textThemePrefix = '_textTheme.',
});
@override
String generate() => '''
class _${blockName}DefaultsM3 extends FloatingActionButtonThemeData {
_${blockName}DefaultsM3(this.context, this.type, this.hasChild)
: super(
elevation: ${elevation("md.comp.fab.primary.container")},
focusElevation: ${elevation("md.comp.fab.primary.focus.container")},
hoverElevation: ${elevation("md.comp.fab.primary.hover.container")},
highlightElevation: ${elevation("md.comp.fab.primary.pressed.container")},
enableFeedback: true,
sizeConstraints: const BoxConstraints.tightFor(
width: ${getToken("md.comp.fab.primary.container.width")},
height: ${getToken("md.comp.fab.primary.container.height")},
),
smallSizeConstraints: const BoxConstraints.tightFor(
width: ${getToken("md.comp.fab.primary.small.container.width")},
height: ${getToken("md.comp.fab.primary.small.container.height")},
),
largeSizeConstraints: const BoxConstraints.tightFor(
width: ${getToken("md.comp.fab.primary.large.container.width")},
height: ${getToken("md.comp.fab.primary.large.container.height")},
),
extendedSizeConstraints: const BoxConstraints.tightFor(
height: ${getToken("md.comp.extended-fab.primary.container.height")},
),
extendedIconLabelSpacing: 8.0,
);
final BuildContext context;
final _FloatingActionButtonType type;
final bool hasChild;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
bool get _isExtended => type == _FloatingActionButtonType.extended;
@override Color? get foregroundColor => ${componentColor("md.comp.fab.primary.icon")};
@override Color? get backgroundColor => ${componentColor("md.comp.fab.primary.container")};
@override Color? get splashColor => ${componentColor("md.comp.fab.primary.pressed.state-layer")};
@override Color? get focusColor => ${componentColor("md.comp.fab.primary.focus.state-layer")};
@override Color? get hoverColor => ${componentColor("md.comp.fab.primary.hover.state-layer")};
@override
ShapeBorder? get shape {
switch (type) {
case _FloatingActionButtonType.regular:
return ${shape("md.comp.fab.primary.container")};
case _FloatingActionButtonType.small:
return ${shape("md.comp.fab.primary.small.container")};
case _FloatingActionButtonType.large:
return ${shape("md.comp.fab.primary.large.container")};
case _FloatingActionButtonType.extended:
return ${shape("md.comp.extended-fab.primary.container")};
}
}
@override
double? get iconSize {
switch (type) {
case _FloatingActionButtonType.regular: return ${getToken("md.comp.fab.primary.icon.size")};
case _FloatingActionButtonType.small: return ${getToken("md.comp.fab.primary.small.icon.size")};
case _FloatingActionButtonType.large: return ${getToken("md.comp.fab.primary.large.icon.size")};
case _FloatingActionButtonType.extended: return ${getToken("md.comp.extended-fab.primary.icon.size")};
}
}
@override EdgeInsetsGeometry? get extendedPadding => EdgeInsetsDirectional.only(start: hasChild && _isExtended ? 16.0 : 20.0, end: 20.0);
@override TextStyle? get extendedTextStyle => ${textStyle("md.comp.extended-fab.primary.label-text")};
}
''';
}
| flutter/dev/tools/gen_defaults/lib/fab_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/fab_template.dart",
"repo_id": "flutter",
"token_count": 1367
} | 577 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class SegmentedButtonTemplate extends TokenTemplate {
const SegmentedButtonTemplate(this.tokenGroup, super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
});
final String tokenGroup;
String _layerOpacity(String layerToken) {
if (tokenAvailable(layerToken)) {
final String layerValue = getToken(layerToken) as String;
if (tokenAvailable(layerValue)) {
final String? opacityValue = opacity(layerValue);
if (opacityValue != null) {
return '.withOpacity($opacityValue)';
}
}
}
return '';
}
String _stateColor(String componentToken, String type, String state) {
final String baseColor = color('$componentToken.$type.$state.state-layer.color', '');
if (baseColor.isEmpty) {
return 'null';
}
final String opacity = _layerOpacity('$componentToken.$state.state-layer.opacity');
return '$baseColor$opacity';
}
@override
String generate() => '''
class _${blockName}DefaultsM3 extends SegmentedButtonThemeData {
_${blockName}DefaultsM3(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override ButtonStyle? get style {
return ButtonStyle(
textStyle: MaterialStatePropertyAll<TextStyle?>(${textStyle('$tokenGroup.label-text')}),
backgroundColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return ${componentColor('$tokenGroup.disabled')};
}
if (states.contains(MaterialState.selected)) {
return ${componentColor('$tokenGroup.selected.container')};
}
return ${componentColor('$tokenGroup.unselected.container')};
}),
foregroundColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return ${componentColor('$tokenGroup.disabled.label-text')};
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return ${componentColor('$tokenGroup.selected.pressed.label-text')};
}
if (states.contains(MaterialState.hovered)) {
return ${componentColor('$tokenGroup.selected.hover.label-text')};
}
if (states.contains(MaterialState.focused)) {
return ${componentColor('$tokenGroup.selected.focus.label-text')};
}
return ${componentColor('$tokenGroup.selected.label-text')};
} else {
if (states.contains(MaterialState.pressed)) {
return ${componentColor('$tokenGroup.unselected.pressed.label-text')};
}
if (states.contains(MaterialState.hovered)) {
return ${componentColor('$tokenGroup.unselected.hover.label-text')};
}
if (states.contains(MaterialState.focused)) {
return ${componentColor('$tokenGroup.unselected.focus.label-text')};
}
return ${componentColor('$tokenGroup.unselected.label-text')};
}
}),
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return ${_stateColor(tokenGroup, 'selected', 'pressed')};
}
if (states.contains(MaterialState.hovered)) {
return ${_stateColor(tokenGroup, 'selected', 'hover')};
}
if (states.contains(MaterialState.focused)) {
return ${_stateColor(tokenGroup, 'selected', 'focus')};
}
} else {
if (states.contains(MaterialState.pressed)) {
return ${_stateColor(tokenGroup, 'unselected', 'pressed')};
}
if (states.contains(MaterialState.hovered)) {
return ${_stateColor(tokenGroup, 'unselected', 'hover')};
}
if (states.contains(MaterialState.focused)) {
return ${_stateColor(tokenGroup, 'unselected', 'focus')};
}
}
return null;
}),
surfaceTintColor: const MaterialStatePropertyAll<Color>(Colors.transparent),
elevation: const MaterialStatePropertyAll<double>(0),
iconSize: const MaterialStatePropertyAll<double?>(${getToken('$tokenGroup.with-icon.icon.size')}),
side: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return ${border("$tokenGroup.disabled.outline")};
}
return ${border("$tokenGroup.outline")};
}),
shape: const MaterialStatePropertyAll<OutlinedBorder>(${shape(tokenGroup, '')}),
minimumSize: const MaterialStatePropertyAll<Size?>(Size.fromHeight(${getToken('$tokenGroup.container.height')})),
);
}
@override
Widget? get selectedIcon => const Icon(Icons.check);
static MaterialStateProperty<Color?> resolveStateColor(Color? unselectedColor, Color? selectedColor){
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return selectedColor?.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return selectedColor?.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return selectedColor?.withOpacity(0.1);
}
} else {
if (states.contains(MaterialState.pressed)) {
return unselectedColor?.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return unselectedColor?.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return unselectedColor?.withOpacity(0.1);
}
}
return Colors.transparent;
});
}
}
''';
}
| flutter/dev/tools/gen_defaults/lib/segmented_button_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/segmented_button_template.dart",
"repo_id": "flutter",
"token_count": 2433
} | 578 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io' hide Platform;
import 'package:args/args.dart';
import 'package:gen_keycodes/android_code_gen.dart';
import 'package:gen_keycodes/base_code_gen.dart';
import 'package:gen_keycodes/gtk_code_gen.dart';
import 'package:gen_keycodes/ios_code_gen.dart';
import 'package:gen_keycodes/keyboard_keys_code_gen.dart';
import 'package:gen_keycodes/keyboard_maps_code_gen.dart';
import 'package:gen_keycodes/logical_key_data.dart';
import 'package:gen_keycodes/macos_code_gen.dart';
import 'package:gen_keycodes/physical_key_data.dart';
import 'package:gen_keycodes/testing_key_codes_cc_gen.dart';
import 'package:gen_keycodes/testing_key_codes_java_gen.dart';
import 'package:gen_keycodes/utils.dart';
import 'package:gen_keycodes/web_code_gen.dart';
import 'package:gen_keycodes/windows_code_gen.dart';
import 'package:http/http.dart' as http;
import 'package:path/path.dart' as path;
/// Get contents of the file that contains the physical key mapping in Chromium
/// source.
Future<String> getChromiumCodes() async {
final Uri keyCodesUri = Uri.parse('https://chromium.googlesource.com/codesearch/chromium/src/+/refs/heads/master/ui/events/keycodes/dom/dom_code_data.inc?format=TEXT');
return utf8.decode(base64.decode(await http.read(keyCodesUri)));
}
/// Get contents of the file that contains the logical key mapping in Chromium
/// source.
Future<String> getChromiumKeys() async {
final Uri keyCodesUri = Uri.parse('https://chromium.googlesource.com/codesearch/chromium/src/+/refs/heads/master/ui/events/keycodes/dom/dom_key_data.inc?format=TEXT');
return utf8.decode(base64.decode(await http.read(keyCodesUri)));
}
/// Get contents of the file that contains the key codes in Android source.
Future<String> getAndroidKeyCodes() async {
final Uri keyCodesUri = Uri.parse('https://android.googlesource.com/platform/frameworks/native/+/master/include/android/keycodes.h?format=TEXT');
return utf8.decode(base64.decode(await http.read(keyCodesUri)));
}
Future<String> getWindowsKeyCodes() async {
final Uri keyCodesUri = Uri.parse('https://raw.githubusercontent.com/tpn/winsdk-10/master/Include/10.0.10240.0/um/WinUser.h');
return http.read(keyCodesUri);
}
/// Get contents of the file that contains the scan codes in Android source.
/// Yes, this is just the generic keyboard layout file for base Android distro
/// This is because there isn't any facility in Android to get the keyboard
/// layout, so we're using this to match scan codes with symbol names for most
/// common keyboards. Other than some special keyboards and game pads, this
/// should be OK.
Future<String> getAndroidScanCodes() async {
final Uri scanCodesUri = Uri.parse('https://android.googlesource.com/platform/frameworks/base/+/master/data/keyboards/Generic.kl?format=TEXT');
return utf8.decode(base64.decode(await http.read(scanCodesUri)));
}
Future<String> getGlfwKeyCodes() async {
final Uri keyCodesUri = Uri.parse('https://raw.githubusercontent.com/glfw/glfw/master/include/GLFW/glfw3.h');
return http.read(keyCodesUri);
}
Future<String> getGtkKeyCodes() async {
final Uri keyCodesUri = Uri.parse('https://gitlab.gnome.org/GNOME/gtk/-/raw/gtk-3-24/gdk/gdkkeysyms.h');
return http.read(keyCodesUri);
}
String readDataFile(String fileName) {
return File(path.join(dataRoot, fileName)).readAsStringSync();
}
bool _assertsEnabled() {
bool enabledAsserts = false;
assert(() {
enabledAsserts = true;
return true;
}());
return enabledAsserts;
}
Future<void> generate(String name, String outDir, BaseCodeGenerator generator) {
final File codeFile = File(outDir);
if (!codeFile.existsSync()) {
codeFile.createSync(recursive: true);
}
print('Writing ${name.padRight(15)}${codeFile.absolute}');
return codeFile.writeAsString(generator.generate());
}
Future<void> main(List<String> rawArguments) async {
if (!_assertsEnabled()) {
print('The gen_keycodes script must be run with --enable-asserts.');
return;
}
final ArgParser argParser = ArgParser();
argParser.addOption(
'engine-root',
defaultsTo: path.join(flutterRoot.path, '..', 'engine', 'src', 'flutter'),
help: 'The path to the root of the flutter/engine repository. This is used '
'to place the generated engine mapping files. If --engine-root is not '
r'specified, it will default to $flutterRoot/../engine/src/flutter, '
'assuming the engine gclient folder is placed at the same folder as '
'the flutter/flutter repository.',
);
argParser.addOption(
'physical-data',
defaultsTo: path.join(dataRoot, 'physical_key_data.g.json'),
help: 'The path to where the physical key data file should be written when '
'collected, and read from when generating output code. If --physical-data is '
'not specified, the output will be written to/read from the current '
"directory. If the output directory doesn't exist, it, and the path to "
'it, will be created.',
);
argParser.addOption(
'logical-data',
defaultsTo: path.join(dataRoot, 'logical_key_data.g.json'),
help: 'The path to where the logical key data file should be written when '
'collected, and read from when generating output code. If --logical-data is '
'not specified, the output will be written to/read from the current '
"directory. If the output directory doesn't exist, it, and the path to "
'it, will be created.',
);
argParser.addOption(
'code',
defaultsTo: path.join(flutterRoot.path, 'packages', 'flutter', 'lib', 'src', 'services', 'keyboard_key.g.dart'),
help: 'The path to where the output "keyboard_key.g.dart" file should be '
'written. If --code is not specified, the output will be written to the '
'correct directory in the flutter tree. If the output directory does not '
'exist, it, and the path to it, will be created.',
);
argParser.addOption(
'maps',
defaultsTo: path.join(flutterRoot.path, 'packages', 'flutter', 'lib', 'src', 'services', 'keyboard_maps.g.dart'),
help: 'The path to where the output "keyboard_maps.g.dart" file should be '
'written. If --maps is not specified, the output will be written to the '
'correct directory in the flutter tree. If the output directory does not '
'exist, it, and the path to it, will be created.',
);
argParser.addFlag(
'collect',
negatable: false,
help: 'If this flag is set, then collect and parse header files from '
'Chromium and Android instead of reading pre-parsed data from '
'"physical_key_data.g.json" and "logical_key_data.g.json", and then '
'update these files with the fresh data.',
);
argParser.addFlag(
'help',
negatable: false,
help: 'Print help for this command.',
);
final ArgResults parsedArguments = argParser.parse(rawArguments);
if (parsedArguments['help'] as bool) {
print(argParser.usage);
exit(0);
}
PlatformCodeGenerator.engineRoot = parsedArguments['engine-root'] as String;
PhysicalKeyData physicalData;
LogicalKeyData logicalData;
if (parsedArguments['collect'] as bool) {
// Physical
final String baseHidCodes = await getChromiumCodes();
final String supplementalHidCodes = readDataFile('supplemental_hid_codes.inc');
final String androidScanCodes = await getAndroidScanCodes();
final String androidToDomKey = readDataFile('android_key_name_to_name.json');
physicalData = PhysicalKeyData(
<String>[baseHidCodes, supplementalHidCodes].join('\n'),
androidScanCodes,
androidToDomKey,
);
// Logical
final String gtkKeyCodes = await getGtkKeyCodes();
final String webLogicalKeys = await getChromiumKeys();
final String supplementalKeyData = readDataFile('supplemental_key_data.inc');
final String gtkToDomKey = readDataFile('gtk_logical_name_mapping.json');
final String windowsKeyCodes = await getWindowsKeyCodes();
final String windowsToDomKey = readDataFile('windows_logical_to_window_vk.json');
final String macosLogicalToPhysical = readDataFile('macos_logical_to_physical.json');
final String iosLogicalToPhysical = readDataFile('ios_logical_to_physical.json');
final String androidKeyCodes = await getAndroidKeyCodes();
final String glfwKeyCodes = await getGlfwKeyCodes();
final String glfwToDomKey = readDataFile('glfw_key_name_to_name.json');
logicalData = LogicalKeyData(
<String>[webLogicalKeys, supplementalKeyData].join('\n'),
gtkKeyCodes,
gtkToDomKey,
windowsKeyCodes,
windowsToDomKey,
androidKeyCodes,
androidToDomKey,
macosLogicalToPhysical,
iosLogicalToPhysical,
glfwKeyCodes,
glfwToDomKey,
physicalData,
);
// Write data files
const JsonEncoder encoder = JsonEncoder.withIndent(' ');
final String physicalJson = encoder.convert(physicalData.toJson());
File(parsedArguments['physical-data'] as String).writeAsStringSync('$physicalJson\n');
final String logicalJson = encoder.convert(logicalData.toJson());
File(parsedArguments['logical-data'] as String).writeAsStringSync('$logicalJson\n');
} else {
physicalData = PhysicalKeyData.fromJson(json.decode(await File(parsedArguments['physical-data'] as String).readAsString()) as Map<String, dynamic>);
logicalData = LogicalKeyData.fromJson(json.decode(await File(parsedArguments['logical-data'] as String).readAsString()) as Map<String, dynamic>);
}
final Map<String, bool> layoutGoals = parseMapOfBool(readDataFile('layout_goals.json'));
await generate('key codes',
parsedArguments['code'] as String,
KeyboardKeysCodeGenerator(physicalData, logicalData));
await generate('key maps',
parsedArguments['maps'] as String,
KeyboardMapsCodeGenerator(physicalData, logicalData));
await generate('engine utils',
path.join(PlatformCodeGenerator.engineRoot,
'shell', 'platform', 'embedder', 'test_utils', 'key_codes.g.h'),
KeyCodesCcGenerator(physicalData, logicalData));
await generate('android utils',
path.join(PlatformCodeGenerator.engineRoot, 'shell', 'platform',
path.join('android', 'test', 'io', 'flutter', 'util', 'KeyCodes.java')),
KeyCodesJavaGenerator(physicalData, logicalData));
final Map<String, PlatformCodeGenerator> platforms = <String, PlatformCodeGenerator>{
'android': AndroidCodeGenerator(
physicalData,
logicalData,
),
'macos': MacOSCodeGenerator(
physicalData,
logicalData,
layoutGoals,
),
'ios': IOSCodeGenerator(
physicalData,
logicalData,
),
'windows': WindowsCodeGenerator(
physicalData,
logicalData,
readDataFile('windows_scancode_logical_map.json')
),
'linux': GtkCodeGenerator(
physicalData,
logicalData,
readDataFile('gtk_modifier_bit_mapping.json'),
readDataFile('gtk_lock_bit_mapping.json'),
layoutGoals,
),
'web': WebCodeGenerator(
physicalData,
logicalData,
readDataFile('web_logical_location_mapping.json'),
),
};
await Future.wait(platforms.entries.map((MapEntry<String, PlatformCodeGenerator> entry) {
final String platform = entry.key;
final PlatformCodeGenerator codeGenerator = entry.value;
return generate('$platform map',
codeGenerator.outputPath(platform),
codeGenerator);
}));
}
| flutter/dev/tools/gen_keycodes/bin/gen_keycodes.dart/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/bin/gen_keycodes.dart",
"repo_id": "flutter",
"token_count": 4112
} | 579 |
package io.flutter.util;
// DO NOT EDIT -- DO NOT EDIT -- DO NOT EDIT
// This file is generated by
// flutter/flutter:dev/tools/gen_keycodes/bin/gen_keycodes.dart and should not
// be edited directly.
//
// Edit the template
// flutter/flutter:dev/tools/gen_keycodes/data/key_codes_java.tmpl
// instead.
//
// See flutter/flutter:dev/tools/gen_keycodes/README.md for more information.
/**
* This class contains keyboard constants to be used in unit tests. They should not be used in
* production code.
*/
public class KeyCodes {
@@@PHYSICAL_KEY_DEFINITIONS@@@
@@@LOGICAL_KEY_DEFINITIONS@@@
}
| flutter/dev/tools/gen_keycodes/data/key_codes_java.tmpl/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/key_codes_java.tmpl",
"repo_id": "flutter",
"token_count": 200
} | 580 |
{
"ControlRight": "ControlRight",
"AltRight": "AltRight",
"Numpad1": "Numpad1",
"Numpad2": "Numpad2",
"Numpad3": "Numpad3",
"Numpad4": "Numpad4",
"Numpad5": "Numpad5",
"Numpad6": "Numpad6",
"Numpad7": "Numpad7",
"Numpad8": "Numpad8",
"Numpad9": "Numpad9",
"Numpad0": "Numpad0",
"NumpadAdd": "NumpadAdd",
"NumpadDecimal": "NumpadDecimal",
"NumpadDivide": "NumpadDivide",
"NumpadEqual": "NumpadEqual",
"NumpadMultiply": "NumpadMultiply",
"NumpadSubtract": "NumpadSubtract"
}
| flutter/dev/tools/gen_keycodes/data/windows_scancode_logical_map.json/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/windows_scancode_logical_map.json",
"repo_id": "flutter",
"token_count": 262
} | 581 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:path/path.dart' as path;
import 'base_code_gen.dart';
import 'constants.dart';
import 'logical_key_data.dart';
import 'physical_key_data.dart';
import 'utils.dart';
/// Generates the key mapping for Windows, based on the information in the key
/// data structure given to it.
class WindowsCodeGenerator extends PlatformCodeGenerator {
WindowsCodeGenerator(
super.keyData,
super.logicalData,
String scancodeToLogical,
) : _scancodeToLogical = parseMapOfString(scancodeToLogical);
/// This generates the map of Windows scan codes to physical keys.
String get _windowsScanCodeMap {
final OutputLines<int> lines = OutputLines<int>('Windows scancode map');
for (final PhysicalKeyEntry entry in keyData.entries) {
if (entry.windowsScanCode != null) {
lines.add(entry.windowsScanCode!,
' {${toHex(entry.windowsScanCode)}, ${toHex(entry.usbHidCode)}}, // ${entry.constantName}');
}
}
return lines.sortedJoin().trimRight();
}
/// This generates the map of Windows key codes to logical keys.
String get _windowsLogicalKeyCodeMap {
final OutputLines<int> lines = OutputLines<int>('Windows logical map');
for (final LogicalKeyEntry entry in logicalData.entries) {
zipStrict(entry.windowsValues, entry.windowsNames,
(int windowsValue, String windowsName) {
lines.add(windowsValue,
' {${toHex(windowsValue)}, ${toHex(entry.value, digits: 11)}}, '
'// $windowsName -> ${entry.constantName}');
},
);
}
return lines.sortedJoin().trimRight();
}
/// This generates the map from scan code to logical keys.
///
/// Normally logical keys should only be derived from key codes, but since some
/// key codes are either 0 or ambiguous (multiple keys using the same key
/// code), these keys are resolved by scan codes.
String get _scanCodeToLogicalMap {
final OutputLines<int> lines = OutputLines<int>('Windows scancode to logical map');
_scancodeToLogical.forEach((String scanCodeName, String logicalName) {
final PhysicalKeyEntry physicalEntry = keyData.entryByName(scanCodeName);
final LogicalKeyEntry logicalEntry = logicalData.entryByName(logicalName);
lines.add(physicalEntry.windowsScanCode!,
' {${toHex(physicalEntry.windowsScanCode)}, ${toHex(logicalEntry.value, digits: 11)}}, '
'// ${physicalEntry.constantName} -> ${logicalEntry.constantName}');
});
return lines.sortedJoin().trimRight();
}
final Map<String, String> _scancodeToLogical;
/// This generates the mask values for the part of a key code that defines its plane.
String get _maskConstants {
final StringBuffer buffer = StringBuffer();
const List<MaskConstant> maskConstants = <MaskConstant>[
kValueMask,
kUnicodePlane,
kWindowsPlane,
];
for (final MaskConstant constant in maskConstants) {
buffer.writeln('const uint64_t KeyboardKeyEmbedderHandler::${constant.lowerCamelName} = ${toHex(constant.value, digits: 11)};');
}
return buffer.toString().trimRight();
}
@override
String get templatePath => path.join(dataRoot, 'windows_flutter_key_map_cc.tmpl');
@override
String outputPath(String platform) => path.join(PlatformCodeGenerator.engineRoot,
'shell', 'platform', 'windows', 'flutter_key_map.g.cc');
@override
Map<String, String> mappings() {
return <String, String>{
'WINDOWS_SCAN_CODE_MAP': _windowsScanCodeMap,
'WINDOWS_SCAN_CODE_TO_LOGICAL_MAP': _scanCodeToLogicalMap,
'WINDOWS_KEY_CODE_MAP': _windowsLogicalKeyCodeMap,
'MASK_CONSTANTS': _maskConstants,
};
}
}
| flutter/dev/tools/gen_keycodes/lib/windows_code_gen.dart/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/lib/windows_code_gen.dart",
"repo_id": "flutter",
"token_count": 1371
} | 582 |
#!/usr/bin/env bash
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
set -e
# Update this URL to get another version of the Gradle wrapper.
# If the AOSP folks have changed the layout of their templates, you may also need to update the
# script below to grab the right files...
WRAPPER_SRC_URL="https://android.googlesource.com/platform/tools/base/+archive/0b5c1398d1d04ac245a310de98825cb7b3278e2a/templates.tgz"
case "$(uname -s)" in
Darwin)
SHASUM="shasum"
;;
*)
SHASUM="sha1sum"
;;
esac
function follow_links() {
cd -P "${1%/*}"
file="$PWD/${1##*/}"
while [ -h "$file" ]; do
# On Mac OS, readlink -f doesn't work.
cd -P "${file%/*}"
file="$(readlink "$file")"
cd -P "${file%/*}"
file="$PWD/${file##*/}"
done
echo "$PWD/${file##*/}"
}
# Convert a filesystem path to a format usable by Dart's URI parser.
function path_uri() {
# Reduce multiple leading slashes to a single slash.
echo "$1" | sed -E -e "s,^/+,/,"
}
PROG_NAME="$(path_uri "$(follow_links "$BASH_SOURCE")")"
BIN_DIR="$(cd "${PROG_NAME%/*}" ; pwd -P)"
FLUTTER_ROOT="$(cd "${BIN_DIR}/../.." ; pwd -P)"
WRAPPER_VERSION_PATH="$FLUTTER_ROOT/bin/internal/gradle_wrapper.version"
WRAPPER_TEMP_DIR="$FLUTTER_ROOT/bin/cache/gradle-wrapper-temp"
echo "Downloading gradle wrapper..."
rm -rf "$WRAPPER_TEMP_DIR"
mkdir "$WRAPPER_TEMP_DIR"
curl --continue-at - --location --output "$WRAPPER_TEMP_DIR/templates.tgz" "$WRAPPER_SRC_URL" 2>&1
echo
echo "Repackaging files..."
mkdir "$WRAPPER_TEMP_DIR/unpack"
tar xzf "$WRAPPER_TEMP_DIR/templates.tgz" -C "$WRAPPER_TEMP_DIR/unpack" gradle NOTICE
mkdir "$WRAPPER_TEMP_DIR/repack"
mv "$WRAPPER_TEMP_DIR/unpack/gradle/wrapper"/* "$WRAPPER_TEMP_DIR/repack/"
mv "$WRAPPER_TEMP_DIR/unpack/NOTICE" "$WRAPPER_TEMP_DIR/repack/"
pushd "$WRAPPER_TEMP_DIR/repack" > /dev/null
STAMP=`for h in $(find . -type f); do $SHASUM $h; done | $SHASUM | cut -d' ' -f1`
echo "Packaged files:"
tar cvzf ../gradle-wrapper.tgz *
popd > /dev/null
echo
echo "Uploading repackaged gradle wrapper..."
echo "Content hash: $STAMP"
gsutil.py cp -n "$WRAPPER_TEMP_DIR/gradle-wrapper.tgz" "gs://flutter_infra_release/gradle-wrapper/$STAMP/gradle-wrapper.tgz"
echo "flutter_infra_release/gradle-wrapper/$STAMP/gradle-wrapper.tgz" > "$WRAPPER_VERSION_PATH"
rm -rf "$WRAPPER_TEMP_DIR"
echo
echo "All done. Updated bin/internal/gradle_wrapper.version, don't forget to commit!"
| flutter/dev/tools/repackage_gradle_wrapper.sh/0 | {
"file_path": "flutter/dev/tools/repackage_gradle_wrapper.sh",
"repo_id": "flutter",
"token_count": 1024
} | 583 |
<svg id="svg_build_00" xmlns="http://www.w3.org/2000/svg" width="48px" height="48px" >
</svg>
| flutter/dev/tools/vitool/test_assets/empty_svg_1_48x48.svg/0 | {
"file_path": "flutter/dev/tools/vitool/test_assets/empty_svg_1_48x48.svg",
"repo_id": "flutter",
"token_count": 46
} | 584 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:developer' as developer;
import 'package:flutter_test/flutter_test.dart';
import 'package:vm_service/vm_service.dart';
import 'package:vm_service/vm_service_io.dart';
// This test ensures that the engine starts the VM with the timeline streams
// for "Dart", "Embedder", and "GC" recorded from startup.
void main() {
late VmService vmService;
setUpAll(() async {
final developer.ServiceProtocolInfo info = await developer.Service.getInfo();
if (info.serverUri == null) {
fail('This test _must_ be run with --enable-vmservice.');
}
vmService = await vmServiceConnectUri(
'ws://localhost:${info.serverUri!.port}${info.serverUri!.path}ws',
);
});
tearDownAll(() async {
await vmService.dispose();
});
test('Image cache tracing', () async {
final TimelineFlags flags = await vmService.getVMTimelineFlags();
expect(flags.recordedStreams, containsAll(<String>[
'Dart',
'Embedder',
'GC',
]));
}, skip: isBrowser); // [intended] uses dart:isolate and io.
}
| flutter/dev/tracing_tests/test/default_streams_test.dart/0 | {
"file_path": "flutter/dev/tracing_tests/test/default_streams_test.dart",
"repo_id": "flutter",
"token_count": 417
} | 585 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.