text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoNavigationBar].
void main() => runApp(const NavBarApp());
class NavBarApp extends StatelessWidget {
const NavBarApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: NavBarExample(),
);
}
}
class NavBarExample extends StatefulWidget {
const NavBarExample({super.key});
@override
State<NavBarExample> createState() => _NavBarExampleState();
}
class _NavBarExampleState extends State<NavBarExample> {
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
// Try removing opacity to observe the lack of a blur effect and of sliding content.
backgroundColor: CupertinoColors.systemGrey.withOpacity(0.5),
middle: const Text('CupertinoNavigationBar Sample'),
),
child: Column(
children: <Widget>[
Container(height: 50, color: CupertinoColors.systemRed),
Container(height: 50, color: CupertinoColors.systemGreen),
Container(height: 50, color: CupertinoColors.systemBlue),
Container(height: 50, color: CupertinoColors.systemYellow),
],
),
);
}
}
| flutter/examples/api/lib/cupertino/nav_bar/cupertino_navigation_bar.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/nav_bar/cupertino_navigation_bar.0.dart",
"repo_id": "flutter",
"token_count": 526
} | 586 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoSwitch].
void main() => runApp(const CupertinoSwitchApp());
class CupertinoSwitchApp extends StatelessWidget {
const CupertinoSwitchApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: CupertinoSwitchExample(),
);
}
}
class CupertinoSwitchExample extends StatefulWidget {
const CupertinoSwitchExample({super.key});
@override
State<CupertinoSwitchExample> createState() => _CupertinoSwitchExampleState();
}
class _CupertinoSwitchExampleState extends State<CupertinoSwitchExample> {
bool switchValue = true;
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoSwitch Sample'),
),
child: Center(
child: CupertinoSwitch(
// This bool value toggles the switch.
value: switchValue,
activeColor: CupertinoColors.activeBlue,
onChanged: (bool? value) {
// This is called when the user toggles the switch.
setState(() {
switchValue = value ?? false;
});
},
),
),
);
}
}
| flutter/examples/api/lib/cupertino/switch/cupertino_switch.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/switch/cupertino_switch.0.dart",
"repo_id": "flutter",
"token_count": 552
} | 587 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AppBar].
List<String> titles = <String>[
'Cloud',
'Beach',
'Sunny',
];
void main() => runApp(const AppBarApp());
class AppBarApp extends StatelessWidget {
const AppBarApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(colorSchemeSeed: const Color(0xff6750a4), useMaterial3: true),
home: const AppBarExample(),
);
}
}
class AppBarExample extends StatelessWidget {
const AppBarExample({super.key});
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final Color oddItemColor = colorScheme.primary.withOpacity(0.05);
final Color evenItemColor = colorScheme.primary.withOpacity(0.15);
const int tabsCount = 3;
return DefaultTabController(
initialIndex: 1,
length: tabsCount,
child: Scaffold(
appBar: AppBar(
title: const Text('AppBar Sample'),
// This check specifies which nested Scrollable's scroll notification
// should be listened to.
//
// When `ThemeData.useMaterial3` is true and scroll view has
// scrolled underneath the app bar, this updates the app bar
// background color and elevation.
//
// This sets `notification.depth == 1` to listen to the scroll
// notification from the nested `ListView.builder`.
notificationPredicate: (ScrollNotification notification) {
return notification.depth == 1;
},
// The elevation value of the app bar when scroll view has
// scrolled underneath the app bar.
scrolledUnderElevation: 4.0,
shadowColor: Theme.of(context).shadowColor,
bottom: TabBar(
tabs: <Widget>[
Tab(
icon: const Icon(Icons.cloud_outlined),
text: titles[0],
),
Tab(
icon: const Icon(Icons.beach_access_sharp),
text: titles[1],
),
Tab(
icon: const Icon(Icons.brightness_5_sharp),
text: titles[2],
),
],
),
),
body: TabBarView(
children: <Widget>[
ListView.builder(
itemCount: 25,
itemBuilder: (BuildContext context, int index) {
return ListTile(
tileColor: index.isOdd ? oddItemColor : evenItemColor,
title: Text('${titles[0]} $index'),
);
},
),
ListView.builder(
itemCount: 25,
itemBuilder: (BuildContext context, int index) {
return ListTile(
tileColor: index.isOdd ? oddItemColor : evenItemColor,
title: Text('${titles[1]} $index'),
);
},
),
ListView.builder(
itemCount: 25,
itemBuilder: (BuildContext context, int index) {
return ListTile(
tileColor: index.isOdd ? oddItemColor : evenItemColor,
title: Text('${titles[2]} $index'),
);
},
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/app_bar/app_bar.3.dart/0 | {
"file_path": "flutter/examples/api/lib/material/app_bar/app_bar.3.dart",
"repo_id": "flutter",
"token_count": 1673
} | 588 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [BottomNavigationBar].
void main() => runApp(const BottomNavigationBarExampleApp());
class BottomNavigationBarExampleApp extends StatelessWidget {
const BottomNavigationBarExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: BottomNavigationBarExample(),
);
}
}
class BottomNavigationBarExample extends StatefulWidget {
const BottomNavigationBarExample({super.key});
@override
State<BottomNavigationBarExample> createState() => _BottomNavigationBarExampleState();
}
class _BottomNavigationBarExampleState extends State<BottomNavigationBarExample> {
int _selectedIndex = 0;
final ScrollController _homeController = ScrollController();
Widget _listViewBody() {
return ListView.separated(
controller: _homeController,
itemBuilder: (BuildContext context, int index) {
return Center(
child: Text(
'Item $index',
),
);
},
separatorBuilder: (BuildContext context, int index) => const Divider(
thickness: 1,
),
itemCount: 50);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('BottomNavigationBar Sample'),
),
body: _listViewBody(),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.open_in_new_rounded),
label: 'Open Dialog',
),
],
currentIndex: _selectedIndex,
selectedItemColor: Colors.amber[800],
onTap: (int index) {
switch (index) {
case 0:
// only scroll to top when current index is selected.
if (_selectedIndex == index) {
_homeController.animateTo(
0.0,
duration: const Duration(milliseconds: 500),
curve: Curves.easeOut,
);
}
case 1:
showModal(context);
}
setState(
() {
_selectedIndex = index;
},
);
},
),
);
}
void showModal(BuildContext context) {
showDialog<void>(
context: context,
builder: (BuildContext context) => AlertDialog(
content: const Text('Example Dialog'),
actions: <TextButton>[
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Close'),
)
],
),
);
}
}
| flutter/examples/api/lib/material/bottom_navigation_bar/bottom_navigation_bar.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/bottom_navigation_bar/bottom_navigation_bar.2.dart",
"repo_id": "flutter",
"token_count": 1324
} | 589 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [DeletableChipAttributes.onDeleted].
void main() => runApp(const OnDeletedExampleApp());
class OnDeletedExampleApp extends StatelessWidget {
const OnDeletedExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('DeletableChipAttributes.onDeleted Sample')),
body: const Center(
child: OnDeletedExample(),
),
),
);
}
}
class Actor {
const Actor(this.name, this.initials);
final String name;
final String initials;
}
class CastList extends StatefulWidget {
const CastList({super.key});
@override
State createState() => CastListState();
}
class CastListState extends State<CastList> {
final List<Actor> _cast = <Actor>[
const Actor('Aaron Burr', 'AB'),
const Actor('Alexander Hamilton', 'AH'),
const Actor('Eliza Hamilton', 'EH'),
const Actor('James Madison', 'JM'),
];
Iterable<Widget> get actorWidgets {
return _cast.map((Actor actor) {
return Padding(
padding: const EdgeInsets.all(4.0),
child: Chip(
avatar: CircleAvatar(child: Text(actor.initials)),
label: Text(actor.name),
onDeleted: () {
setState(() {
_cast.removeWhere((Actor entry) {
return entry.name == actor.name;
});
});
},
),
);
});
}
@override
Widget build(BuildContext context) {
return Wrap(
children: actorWidgets.toList(),
);
}
}
class OnDeletedExample extends StatefulWidget {
const OnDeletedExample({super.key});
@override
State<OnDeletedExample> createState() => _OnDeletedExampleState();
}
class _OnDeletedExampleState extends State<OnDeletedExample> {
@override
Widget build(BuildContext context) {
return const CastList();
}
}
| flutter/examples/api/lib/material/chip/deletable_chip_attributes.on_deleted.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/chip/deletable_chip_attributes.on_deleted.0.dart",
"repo_id": "flutter",
"token_count": 798
} | 590 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ElevatedButton].
void main() => runApp(const ElevatedButtonExampleApp());
class ElevatedButtonExampleApp extends StatelessWidget {
const ElevatedButtonExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ElevatedButton Sample')),
body: const ElevatedButtonExample(),
),
);
}
}
class ElevatedButtonExample extends StatefulWidget {
const ElevatedButtonExample({super.key});
@override
State<ElevatedButtonExample> createState() => _ElevatedButtonExampleState();
}
class _ElevatedButtonExampleState extends State<ElevatedButtonExample> {
@override
Widget build(BuildContext context) {
final ButtonStyle style = ElevatedButton.styleFrom(textStyle: const TextStyle(fontSize: 20));
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
style: style,
onPressed: null,
child: const Text('Disabled'),
),
const SizedBox(height: 30),
ElevatedButton(
style: style,
onPressed: () {},
child: const Text('Enabled'),
),
],
),
);
}
}
| flutter/examples/api/lib/material/elevated_button/elevated_button.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/elevated_button/elevated_button.0.dart",
"repo_id": "flutter",
"token_count": 576
} | 591 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [IconButton] with toggle feature.
void main() {
runApp(const IconButtonToggleApp());
}
class IconButtonToggleApp extends StatelessWidget {
const IconButtonToggleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
colorSchemeSeed: const Color(0xff6750a4),
useMaterial3: true,
),
title: 'Icon Button Types',
home: const Scaffold(
body: DemoIconToggleButtons(),
),
);
}
}
class DemoIconToggleButtons extends StatefulWidget {
const DemoIconToggleButtons({super.key});
@override
State<DemoIconToggleButtons> createState() => _DemoIconToggleButtonsState();
}
class _DemoIconToggleButtonsState extends State<DemoIconToggleButtons> {
bool standardSelected = false;
bool filledSelected = false;
bool tonalSelected = false;
bool outlinedSelected = false;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IconButton(
isSelected: standardSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: () {
setState(() {
standardSelected = !standardSelected;
});
},
),
const SizedBox(width: 10),
IconButton(
isSelected: standardSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: null,
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IconButton.filled(
isSelected: filledSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: () {
setState(() {
filledSelected = !filledSelected;
});
},
),
const SizedBox(width: 10),
IconButton.filled(
isSelected: filledSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: null,
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IconButton.filledTonal(
isSelected: tonalSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: () {
setState(() {
tonalSelected = !tonalSelected;
});
},
),
const SizedBox(width: 10),
IconButton.filledTonal(
isSelected: tonalSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: null,
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IconButton.outlined(
isSelected: outlinedSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: () {
setState(() {
outlinedSelected = !outlinedSelected;
});
},
),
const SizedBox(width: 10),
IconButton.outlined(
isSelected: outlinedSelected,
icon: const Icon(Icons.settings_outlined),
selectedIcon: const Icon(Icons.settings),
onPressed: null,
),
],
),
],
);
}
}
| flutter/examples/api/lib/material/icon_button/icon_button.3.dart/0 | {
"file_path": "flutter/examples/api/lib/material/icon_button/icon_button.3.dart",
"repo_id": "flutter",
"token_count": 2131
} | 592 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InputDecorator].
void main() => runApp(const PrefixIconExampleApp());
class PrefixIconExampleApp extends StatelessWidget {
const PrefixIconExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(body: InputDecoratorExample()),
);
}
}
class InputDecoratorExample extends StatelessWidget {
const InputDecoratorExample({super.key});
@override
Widget build(BuildContext context) {
return const TextField(
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'Enter name',
prefixIcon: Align(
widthFactor: 1.0,
heightFactor: 1.0,
child: Icon(
Icons.person,
),
),
),
);
}
}
| flutter/examples/api/lib/material/input_decorator/input_decoration.prefix_icon.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/input_decorator/input_decoration.prefix_icon.0.dart",
"repo_id": "flutter",
"token_count": 393
} | 593 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [CheckboxMenuButton].
void main() => runApp(const MenuApp());
class MyCheckboxMenu extends StatefulWidget {
const MyCheckboxMenu({super.key, required this.message});
final String message;
@override
State<MyCheckboxMenu> createState() => _MyCheckboxMenuState();
}
class _MyCheckboxMenuState extends State<MyCheckboxMenu> {
final FocusNode _buttonFocusNode = FocusNode(debugLabel: 'Menu Button');
static const SingleActivator _showShortcut = SingleActivator(LogicalKeyboardKey.keyS, control: true);
bool _showingMessage = false;
@override
void dispose() {
_buttonFocusNode.dispose();
super.dispose();
}
void _setMessageVisibility(bool visible) {
setState(() {
_showingMessage = visible;
});
}
@override
Widget build(BuildContext context) {
return CallbackShortcuts(
bindings: <ShortcutActivator, VoidCallback>{
_showShortcut: () {
_setMessageVisibility(!_showingMessage);
},
},
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
MenuAnchor(
childFocusNode: _buttonFocusNode,
menuChildren: <Widget>[
CheckboxMenuButton(
value: _showingMessage,
onChanged: (bool? value) {
_setMessageVisibility(value!);
},
child: const Text('Show Message'),
),
],
builder: (BuildContext context, MenuController controller, Widget? child) {
return TextButton(
focusNode: _buttonFocusNode,
onPressed: () {
if (controller.isOpen) {
controller.close();
} else {
controller.open();
}
},
child: const Text('OPEN MENU'),
);
},
),
Expanded(
child: Container(
alignment: Alignment.center,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
_showingMessage ? widget.message : '',
style: Theme.of(context).textTheme.headlineSmall,
),
),
],
),
),
),
],
),
);
}
}
class MenuApp extends StatelessWidget {
const MenuApp({super.key});
static const String kMessage = '"Talk less. Smile more." - A. Burr';
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(body: SafeArea(child: MyCheckboxMenu(message: kMessage))),
);
}
}
| flutter/examples/api/lib/material/menu_anchor/checkbox_menu_button.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/menu_anchor/checkbox_menu_button.0.dart",
"repo_id": "flutter",
"token_count": 1472
} | 594 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [PageTransitionsTheme].
void main() => runApp(const PageTransitionsThemeApp());
class PageTransitionsThemeApp extends StatelessWidget {
const PageTransitionsThemeApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
useMaterial3: true,
pageTransitionsTheme: const PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
TargetPlatform.android: ZoomPageTransitionsBuilder(
allowSnapshotting: false,
),
},
),
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.blueGrey,
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.of(context).push(
MaterialPageRoute<SecondPage>(
builder: (BuildContext context) => const SecondPage(),
),
);
},
child: const Text('To SecondPage'),
),
),
);
}
}
class SecondPage extends StatelessWidget {
const SecondPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.purple[200],
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text('Back to HomePage'),
),
),
);
}
}
| flutter/examples/api/lib/material/page_transitions_theme/page_transitions_theme.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/page_transitions_theme/page_transitions_theme.1.dart",
"repo_id": "flutter",
"token_count": 739
} | 595 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RadioListTile].
void main() => runApp(const RadioListTileApp());
class RadioListTileApp extends StatelessWidget {
const RadioListTileApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const RadioListTileExample(),
);
}
}
enum Groceries { pickles, tomato, lettuce }
class RadioListTileExample extends StatefulWidget {
const RadioListTileExample({super.key});
@override
State<RadioListTileExample> createState() => _RadioListTileExampleState();
}
class _RadioListTileExampleState extends State<RadioListTileExample> {
Groceries? _groceryItem = Groceries.pickles;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('RadioListTile Sample')),
body: Column(
children: <Widget>[
RadioListTile<Groceries>(
value: Groceries.pickles,
groupValue: _groceryItem,
onChanged: (Groceries? value) {
setState(() {
_groceryItem = value;
});
},
title: const Text('Pickles'),
subtitle: const Text('Supporting text'),
),
RadioListTile<Groceries>(
value: Groceries.tomato,
groupValue: _groceryItem,
onChanged: (Groceries? value) {
setState(() {
_groceryItem = value;
});
},
title: const Text('Tomato'),
subtitle: const Text(
'Longer supporting text to demonstrate how the text wraps and the radio is centered vertically with the text.'),
),
RadioListTile<Groceries>(
value: Groceries.lettuce,
groupValue: _groceryItem,
onChanged: (Groceries? value) {
setState(() {
_groceryItem = value;
});
},
title: const Text('Lettuce'),
subtitle: const Text(
"Longer supporting text to demonstrate how the text wraps and how setting 'RadioListTile.isThreeLine = true' aligns the radio to the top vertically with the text."),
isThreeLine: true,
),
],
),
);
}
}
| flutter/examples/api/lib/material/radio_list_tile/radio_list_tile.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/radio_list_tile/radio_list_tile.1.dart",
"repo_id": "flutter",
"token_count": 1092
} | 596 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SearchBar].
void main() => runApp(const SearchBarApp());
class SearchBarApp extends StatefulWidget {
const SearchBarApp({super.key});
@override
State<SearchBarApp> createState() => _SearchBarAppState();
}
class _SearchBarAppState extends State<SearchBarApp> {
bool isDark = false;
@override
Widget build(BuildContext context) {
final ThemeData themeData = ThemeData(useMaterial3: true, brightness: isDark ? Brightness.dark : Brightness.light);
return MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(title: const Text('Search Bar Sample')),
body: Padding(
padding: const EdgeInsets.all(8.0),
child: SearchAnchor(
builder: (BuildContext context, SearchController controller) {
return SearchBar(
controller: controller,
padding: const MaterialStatePropertyAll<EdgeInsets>(EdgeInsets.symmetric(horizontal: 16.0)),
onTap: () { controller.openView(); },
onChanged: (_) { controller.openView(); },
leading: const Icon(Icons.search),
trailing: <Widget>[
Tooltip(
message: 'Change brightness mode',
child: IconButton(
isSelected: isDark,
onPressed: () {
setState(() {
isDark = !isDark;
});
},
icon: const Icon(Icons.wb_sunny_outlined),
selectedIcon: const Icon(Icons.brightness_2_outlined),
),
)
],
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return List<ListTile>.generate(5, (int index) {
final String item = 'item $index';
return ListTile(
title: Text(item),
onTap: () {
setState(() {
controller.closeView(item);
});
},
);
});
}),
),
),
);
}
}
| flutter/examples/api/lib/material/search_anchor/search_bar.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/search_anchor/search_bar.0.dart",
"repo_id": "flutter",
"token_count": 1232
} | 597 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Switch].
void main() => runApp(const SwitchApp());
class SwitchApp extends StatelessWidget {
const SwitchApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('Switch Sample')),
body: const Center(
child: SwitchExample(),
),
),
);
}
}
class SwitchExample extends StatefulWidget {
const SwitchExample({super.key});
@override
State<SwitchExample> createState() => _SwitchExampleState();
}
class _SwitchExampleState extends State<SwitchExample> {
bool light = true;
@override
Widget build(BuildContext context) {
return Switch(
// This bool value toggles the switch.
value: light,
activeColor: Colors.red,
onChanged: (bool value) {
// This is called when the user toggles the switch.
setState(() {
light = value;
});
},
);
}
}
| flutter/examples/api/lib/material/switch/switch.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/switch/switch.0.dart",
"repo_id": "flutter",
"token_count": 441
} | 598 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TextField].
void main() => runApp(const TextFieldExampleApp());
class TextFieldExampleApp extends StatelessWidget {
const TextFieldExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: TextFieldExample(),
);
}
}
class TextFieldExample extends StatefulWidget {
const TextFieldExample({super.key});
@override
State<TextFieldExample> createState() => _TextFieldExampleState();
}
class _TextFieldExampleState extends State<TextFieldExample> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: TextField(
controller: _controller,
onSubmitted: (String value) async {
await showDialog<void>(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: const Text('Thanks!'),
content: Text('You typed "$value", which has length ${value.characters.length}.'),
actions: <Widget>[
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('OK'),
),
],
);
},
);
},
),
),
);
}
}
| flutter/examples/api/lib/material/text_field/text_field.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/text_field/text_field.1.dart",
"repo_id": "flutter",
"token_count": 801
} | 599 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Examples for [LinearBorder] and [LinearBorderEdge].
void main() {
runApp(const ExampleApp());
}
class ExampleApp extends StatelessWidget {
const ExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.light(useMaterial3: true),
home: const Directionality(
// TRY THIS: Switch to TextDirection.rtl to see how the borders change.
textDirection: TextDirection.ltr,
child: Home(),
),
);
}
}
class SampleCard extends StatelessWidget {
const SampleCard({super.key, required this.title, required this.subtitle, required this.children});
final String title;
final String subtitle;
final List<Widget> children;
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final TextTheme textTheme = theme.textTheme;
final ColorScheme colorScheme = theme.colorScheme;
return Card(
child: Padding(
padding: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(title, style: textTheme.titleMedium),
Text(subtitle, style: textTheme.bodyMedium!.copyWith(color: colorScheme.secondary)),
const SizedBox(height: 16),
Row(
children: List<Widget>.generate(children.length * 2 - 1, (int index) {
return index.isEven ? children[index ~/ 2] : const SizedBox(width: 16);
}),
),
],
),
),
);
}
}
class Home extends StatefulWidget {
const Home({super.key});
@override
State<Home> createState() => _HomeState();
}
class _HomeState extends State<Home> {
final LinearBorder shape0 = LinearBorder.top();
final LinearBorder shape1 = LinearBorder.top(size: 0);
late LinearBorder shape = shape0;
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final BorderSide primarySide0 = BorderSide(width: 0, color: colorScheme.inversePrimary); // hairline
final BorderSide primarySide2 = BorderSide(width: 2, color: colorScheme.onPrimaryContainer);
final BorderSide primarySide3 = BorderSide(width: 3, color: colorScheme.inversePrimary);
return Scaffold(
body: SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.all(16),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
// Demonstrates using LinearBorder.bottom() to define
// an underline border for the standard button types.
// The underline's color and width is defined by the ButtonStyle's
// side parameter. The side can also be specified as a
// LinearBorder parameter and if both are specified then the
// ButtonStyle's side is used. This set up makes it possible
// for a button theme to specify the shape and for individual
// buttons to specify the shape border's color and width.
SampleCard(
title: 'LinearBorder.bottom()',
subtitle: 'Standard button widgets',
children: <Widget>[
TextButton(
style: TextButton.styleFrom(
side: primarySide3,
shape: LinearBorder.bottom(),
),
onPressed: () {},
child: const Text('Text'),
),
OutlinedButton(
style: OutlinedButton.styleFrom(
side: primarySide3,
shape: LinearBorder.bottom(),
),
onPressed: () {},
child: const Text('Outlined'),
),
ElevatedButton(
style: ElevatedButton.styleFrom(
side: primarySide3,
shape: LinearBorder.bottom(),
),
onPressed: () {},
child: const Text('Elevated'),
),
],
),
const SizedBox(height: 32),
// Demonstrates creating LinearBorders with a single edge
// by using the convenience constructors like LinearBorder.start().
// The edges are drawn with a BorderSide with width:0, which
// means that a "hairline" line is stroked. Wider borders are
// drawn with filled rectangles.
SampleCard(
title: 'LinearBorder',
subtitle: 'Convenience constructors',
children: <Widget>[
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: LinearBorder.start(),
),
onPressed: () {},
child: const Text('Start()'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: LinearBorder.end(),
),
onPressed: () {},
child: const Text('End()'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: LinearBorder.top(),
),
onPressed: () {},
child: const Text('Top()'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: LinearBorder.bottom(),
),
onPressed: () {},
child: const Text('Bottom()'),
),
],
),
const SizedBox(height: 32),
// Demonstrates creating LinearBorders with a single edge
// that's smaller than the button's bounding box. The size
// parameter specifies a percentage of the available space
// and alignment is -1 for start-alignment, 0 for centered,
// and 1 for end-alignment.
SampleCard(
title: 'LinearBorder',
subtitle: 'Size and alignment parameters',
children: <Widget>[
TextButton(
style: TextButton.styleFrom(
side: primarySide2,
shape: LinearBorder.bottom(
size: 0.5,
),
),
onPressed: () {},
child: const Text('Center'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide2,
shape: LinearBorder.bottom(
size: 0.75,
alignment: -1,
),
),
onPressed: () {},
child: const Text('Start'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide2,
shape: LinearBorder.bottom(
size: 0.75,
alignment: 1,
),
),
onPressed: () {},
child: const Text('End'),
),
],
),
const SizedBox(height: 32),
// Demonstrates creating LinearBorders with more than one edge.
// In these cases the default constructor is used and each edge
// is defined with one LinearBorderEdge object.
SampleCard(
title: 'LinearBorder',
subtitle: 'LinearBorderEdge parameters',
children: <Widget>[
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: const LinearBorder(
top: LinearBorderEdge(),
bottom: LinearBorderEdge(),
),
),
onPressed: () {},
child: const Text('Horizontal'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: const LinearBorder(
start: LinearBorderEdge(),
end: LinearBorderEdge(),
),
),
onPressed: () {},
child: const Text('Vertical'),
),
TextButton(
style: TextButton.styleFrom(
side: primarySide0,
shape: const LinearBorder(
start: LinearBorderEdge(),
bottom: LinearBorderEdge(),
),
),
onPressed: () {},
child: const Text('Corner'),
),
],
),
const SizedBox(height: 32),
// Demonstrates that changing properties of LinearBorders
// causes them to animate to their new configuration.
SampleCard(
title: 'Interpolation',
subtitle: 'LinearBorder.top() => LinearBorder.top(size: 0)',
children: <Widget>[
IconButton(
icon: const Icon(Icons.play_arrow),
onPressed: () {
setState(() {
shape = shape == shape0 ? shape1 : shape0;
});
},
),
TextButton(
style: TextButton.styleFrom(
side: primarySide3,
shape: shape,
),
onPressed: () {},
child: const Text('Press Play'),
),
TextButton(
style: ButtonStyle(
side: MaterialStateProperty.resolveWith<BorderSide?>((Set<MaterialState> states) {
return states.contains(MaterialState.hovered) ? primarySide3 : null;
}),
shape: MaterialStateProperty.resolveWith<OutlinedBorder>((Set<MaterialState> states) {
return states.contains(MaterialState.hovered) ? shape0 : shape1;
}),
),
onPressed: () {},
child: const Text('Hover'),
),
],
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/painting/linear_border/linear_border.0.dart/0 | {
"file_path": "flutter/examples/api/lib/painting/linear_border/linear_border.0.dart",
"repo_id": "flutter",
"token_count": 6223
} | 600 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [TextInputControl].
void main() => runApp(const TextInputControlExampleApp());
class TextInputControlExampleApp extends StatelessWidget {
const TextInputControlExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: TextInputControlExample(),
);
}
}
class TextInputControlExample extends StatefulWidget {
const TextInputControlExample({super.key});
@override
MyStatefulWidgetState createState() => MyStatefulWidgetState();
}
class MyStatefulWidgetState extends State<TextInputControlExample> {
final TextEditingController _controller = TextEditingController();
final FocusNode _focusNode = FocusNode();
@override
void dispose() {
super.dispose();
_controller.dispose();
_focusNode.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: TextField(
autofocus: true,
controller: _controller,
focusNode: _focusNode,
decoration: InputDecoration(
suffix: IconButton(
icon: const Icon(Icons.clear),
tooltip: 'Clear and unfocus',
onPressed: () {
_controller.clear();
_focusNode.unfocus();
},
),
),
),
),
bottomSheet: const MyVirtualKeyboard(),
);
}
}
class MyVirtualKeyboard extends StatefulWidget {
const MyVirtualKeyboard({super.key});
@override
MyVirtualKeyboardState createState() => MyVirtualKeyboardState();
}
class MyVirtualKeyboardState extends State<MyVirtualKeyboard> {
final MyTextInputControl _inputControl = MyTextInputControl();
@override
void initState() {
super.initState();
_inputControl.register();
}
@override
void dispose() {
super.dispose();
_inputControl.unregister();
}
void _handleKeyPress(String key) {
_inputControl.processUserInput(key);
}
@override
Widget build(BuildContext context) {
return ValueListenableBuilder<bool>(
valueListenable: _inputControl.visible,
builder: (_, bool visible, __) {
return Visibility(
visible: visible,
child: FocusScope(
canRequestFocus: false,
child: TextFieldTapRegion(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
for (final String key in <String>['A', 'B', 'C'])
ElevatedButton(
child: Text(key),
onPressed: () => _handleKeyPress(key),
),
],
),
),
),
);
},
);
}
}
class MyTextInputControl with TextInputControl {
TextEditingValue _editingState = TextEditingValue.empty;
final ValueNotifier<bool> _visible = ValueNotifier<bool>(false);
/// The input control's visibility state for updating the visual presentation.
ValueListenable<bool> get visible => _visible;
/// Register the input control.
void register() => TextInput.setInputControl(this);
/// Restore the original platform input control.
void unregister() => TextInput.restorePlatformInputControl();
@override
void show() => _visible.value = true;
@override
void hide() => _visible.value = false;
@override
void setEditingState(TextEditingValue value) => _editingState = value;
/// Process user input.
///
/// Updates the internal editing state by inserting the input text,
/// and by replacing the current selection if any.
void processUserInput(String input) {
_editingState = _editingState.copyWith(
text: _insertText(input),
selection: _replaceSelection(input),
);
// Request the attached client to update accordingly.
TextInput.updateEditingValue(_editingState);
}
String _insertText(String input) {
final String text = _editingState.text;
final TextSelection selection = _editingState.selection;
return text.replaceRange(selection.start, selection.end, input);
}
TextSelection _replaceSelection(String input) {
final TextSelection selection = _editingState.selection;
return TextSelection.collapsed(offset: selection.start + input.length);
}
}
| flutter/examples/api/lib/services/text_input/text_input_control.0.dart/0 | {
"file_path": "flutter/examples/api/lib/services/text_input/text_input_control.0.dart",
"repo_id": "flutter",
"token_count": 1697
} | 601 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverAnimatedGrid].
void main() => runApp(const SliverAnimatedGridSample());
class SliverAnimatedGridSample extends StatefulWidget {
const SliverAnimatedGridSample({super.key});
@override
State<SliverAnimatedGridSample> createState() => _SliverAnimatedGridSampleState();
}
class _SliverAnimatedGridSampleState extends State<SliverAnimatedGridSample> {
final GlobalKey<SliverAnimatedGridState> _listKey = GlobalKey<SliverAnimatedGridState>();
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
final GlobalKey<ScaffoldMessengerState> _scaffoldMessengerKey = GlobalKey<ScaffoldMessengerState>();
late ListModel<int> _list;
int? _selectedItem;
late int _nextItem; // The next item inserted when the user presses the '+' button.
@override
void initState() {
super.initState();
_list = ListModel<int>(
listKey: _listKey,
initialItems: <int>[0, 1, 2, 3, 4, 5],
removedItemBuilder: _buildRemovedItem,
);
_nextItem = 6;
}
// Used to build list items that haven't been removed.
Widget _buildItem(BuildContext context, int index, Animation<double> animation) {
return CardItem(
animation: animation,
item: _list[index],
selected: _selectedItem == _list[index],
onTap: () {
setState(() {
_selectedItem = _selectedItem == _list[index] ? null : _list[index];
});
},
);
}
// Used to build an item after it has been removed from the list. This
// method is needed because a removed item remains visible until its
// animation has completed (even though it's gone as far this ListModel is
// concerned). The widget will be used by the
// [AnimatedGridState.removeItem] method's
// [AnimatedGridRemovedItemBuilder] parameter.
Widget _buildRemovedItem(int item, BuildContext context, Animation<double> animation) {
return CardItem(
animation: animation,
removing: true,
item: item,
);
}
// Insert the "next item" into the list model.
void _insert() {
final int index = _selectedItem == null ? _list.length : _list.indexOf(_selectedItem!);
_list.insert(index, _nextItem++);
}
// Remove the selected item from the list model.
void _remove() {
if (_selectedItem != null) {
_list.removeAt(_list.indexOf(_selectedItem!));
} else {
_list.removeAt(_list.length - 1);
}
setState(() {
_selectedItem = null;
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
scaffoldMessengerKey: _scaffoldMessengerKey,
debugShowCheckedModeBanner: false,
home: Scaffold(
key: _scaffoldKey,
body: CustomScrollView(
slivers: <Widget>[
SliverAppBar(
title: const Text(
'SliverAnimatedGrid',
style: TextStyle(fontSize: 30),
),
expandedHeight: 60,
centerTitle: true,
backgroundColor: Colors.amber[900],
leading: IconButton(
icon: const Icon(Icons.remove_circle),
onPressed: _remove,
tooltip: 'Remove the selected item, or the last item if none selected.',
iconSize: 32,
),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.add_circle),
onPressed: _insert,
tooltip: 'Insert a new item.',
iconSize: 32,
),
],
),
SliverAnimatedGrid(
key: _listKey,
initialItemCount: _list.length,
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 100.0,
mainAxisSpacing: 10.0,
crossAxisSpacing: 10.0,
),
itemBuilder: _buildItem,
),
],
),
),
);
}
}
typedef RemovedItemBuilder = Widget Function(int item, BuildContext context, Animation<double> animation);
// Keeps a Dart [List] in sync with an [AnimatedGrid].
//
// The [insert] and [removeAt] methods apply to both the internal list and
// the animated list that belongs to [listKey].
//
// This class only exposes as much of the Dart List API as is needed by the
// sample app. More list methods are easily added, however methods that
// mutate the list must make the same changes to the animated list in terms
// of [AnimatedGridState.insertItem] and [AnimatedGrid.removeItem].
class ListModel<E> {
ListModel({
required this.listKey,
required this.removedItemBuilder,
Iterable<E>? initialItems,
}) : _items = List<E>.from(initialItems ?? <E>[]);
final GlobalKey<SliverAnimatedGridState> listKey;
final RemovedItemBuilder removedItemBuilder;
final List<E> _items;
SliverAnimatedGridState get _animatedGrid => listKey.currentState!;
void insert(int index, E item) {
_items.insert(index, item);
_animatedGrid.insertItem(index);
}
E removeAt(int index) {
final E removedItem = _items.removeAt(index);
if (removedItem != null) {
_animatedGrid.removeItem(
index,
(BuildContext context, Animation<double> animation) => removedItemBuilder(index, context, animation),
);
}
return removedItem;
}
int get length => _items.length;
E operator [](int index) => _items[index];
int indexOf(E item) => _items.indexOf(item);
}
// Displays its integer item as 'Item N' on a Card whose color is based on
// the item's value.
//
// The card turns gray when [selected] is true. This widget's height
// is based on the [animation] parameter. It varies as the animation value
// transitions from 0.0 to 1.0.
class CardItem extends StatelessWidget {
const CardItem({
super.key,
this.onTap,
this.selected = false,
this.removing = false,
required this.animation,
required this.item,
}) : assert(item >= 0);
final Animation<double> animation;
final VoidCallback? onTap;
final int item;
final bool selected;
final bool removing;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.only(
left: 2.0,
right: 2.0,
top: 2.0,
),
child: ScaleTransition(
scale: CurvedAnimation(parent: animation, curve: removing ? Curves.easeInOut : Curves.bounceOut),
child: GestureDetector(
onTap: onTap,
child: SizedBox(
height: 80.0,
child: Card(
color: selected ? Colors.black12 : Colors.primaries[item % Colors.primaries.length],
child: Center(
child: Text(
(item + 1).toString(),
style: Theme.of(context).textTheme.headlineMedium,
),
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/animated_grid/sliver_animated_grid.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/animated_grid/sliver_animated_grid.0.dart",
"repo_id": "flutter",
"token_count": 2875
} | 602 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AspectRatio].
void main() => runApp(const AspectRatioApp());
class AspectRatioApp extends StatelessWidget {
const AspectRatioApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('AspectRatio Sample')),
body: const AspectRatioExample(),
),
);
}
}
class AspectRatioExample extends StatelessWidget {
const AspectRatioExample({super.key});
@override
Widget build(BuildContext context) {
return Container(
color: Colors.blue,
alignment: Alignment.center,
width: double.infinity,
height: 100.0,
child: AspectRatio(
aspectRatio: 16 / 9,
child: Container(
color: Colors.green,
),
),
);
}
}
| flutter/examples/api/lib/widgets/basic/aspect_ratio.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/aspect_ratio.0.dart",
"repo_id": "flutter",
"token_count": 389
} | 603 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MouseRegion.onExit].
void main() => runApp(const MouseRegionApp());
class MouseRegionApp extends StatelessWidget {
const MouseRegionApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('MouseRegion.onExit Sample')),
body: const Center(
child: MouseRegionExample(),
),
),
);
}
}
// A region that hides its content one second after being hovered.
class MyTimedButton extends StatefulWidget {
const MyTimedButton({super.key, required this.onEnterButton, required this.onExitButton});
final VoidCallback onEnterButton;
final VoidCallback onExitButton;
@override
State<MyTimedButton> createState() => _MyTimedButton();
}
class _MyTimedButton extends State<MyTimedButton> {
bool regionIsHidden = false;
bool hovered = false;
Future<void> startCountdown() async {
await Future<void>.delayed(const Duration(seconds: 1));
hideButton();
}
void hideButton() {
setState(() {
regionIsHidden = true;
});
// This statement is necessary.
if (hovered) {
widget.onExitButton();
}
}
@override
Widget build(BuildContext context) {
return SizedBox(
width: 100,
height: 100,
child: MouseRegion(
child: regionIsHidden
? null
: MouseRegion(
onEnter: (_) {
widget.onEnterButton();
setState(() {
hovered = true;
});
startCountdown();
},
onExit: (_) {
setState(() {
hovered = false;
});
widget.onExitButton();
},
child: Container(color: Colors.red),
),
),
);
}
}
class MouseRegionExample extends StatefulWidget {
const MouseRegionExample({super.key});
@override
State<MouseRegionExample> createState() => _MouseRegionExampleState();
}
class _MouseRegionExampleState extends State<MouseRegionExample> {
Key key = UniqueKey();
bool hovering = false;
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
ElevatedButton(
onPressed: () {
setState(() {
key = UniqueKey();
});
},
child: const Text('Refresh'),
),
if (hovering) const Text('Hovering'),
if (!hovering) const Text('Not hovering'),
MyTimedButton(
key: key,
onEnterButton: () {
setState(() {
hovering = true;
});
},
onExitButton: () {
setState(() {
hovering = false;
});
},
),
],
);
}
}
| flutter/examples/api/lib/widgets/basic/mouse_region.on_exit.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/mouse_region.on_exit.1.dart",
"repo_id": "flutter",
"token_count": 1377
} | 604 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Focus].
void main() => runApp(const FocusExampleApp());
class FocusExampleApp extends StatelessWidget {
const FocusExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: FocusExample(),
);
}
}
class FocusableText extends StatelessWidget {
const FocusableText(
this.data, {
super.key,
required this.autofocus,
});
/// The string to display as the text for this widget.
final String data;
/// Whether or not to focus this widget initially if nothing else is focused.
final bool autofocus;
@override
Widget build(BuildContext context) {
return Focus(
autofocus: autofocus,
child: Builder(builder: (BuildContext context) {
// The contents of this Builder are being made focusable. It is inside
// of a Builder because the builder provides the correct context
// variable for Focus.of() to be able to find the Focus widget that is
// the Builder's parent. Without the builder, the context variable used
// would be the one given the FocusableText build function, and that
// would start looking for a Focus widget ancestor of the FocusableText
// instead of finding the one inside of its build function.
return Container(
padding: const EdgeInsets.all(8.0),
// Change the color based on whether or not this Container has focus.
color: Focus.of(context).hasPrimaryFocus ? Colors.black12 : null,
child: Text(data),
);
}),
);
}
}
class FocusExample extends StatelessWidget {
const FocusExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView.builder(
itemBuilder: (BuildContext context, int index) => FocusableText(
'Item $index',
autofocus: index == 0,
),
itemCount: 50,
),
);
}
}
| flutter/examples/api/lib/widgets/focus_scope/focus.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/focus_scope/focus.1.dart",
"repo_id": "flutter",
"token_count": 735
} | 605 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Image.frameBuilder].
void main() => runApp(const FrameBuilderExampleApp());
class FrameBuilderExampleApp extends StatelessWidget {
const FrameBuilderExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: FrameBuilderExample(),
);
}
}
class FrameBuilderExample extends StatelessWidget {
const FrameBuilderExample({super.key});
@override
@override
Widget build(BuildContext context) {
return DecoratedBox(
decoration: BoxDecoration(
color: Colors.white,
border: Border.all(),
borderRadius: BorderRadius.circular(20),
),
child: Image.network(
'https://flutter.github.io/assets-for-api-docs/assets/widgets/puffin.jpg',
frameBuilder: (BuildContext context, Widget child, int? frame, bool wasSynchronouslyLoaded) {
if (wasSynchronouslyLoaded) {
return child;
}
return AnimatedOpacity(
opacity: frame == null ? 0 : 1,
duration: const Duration(seconds: 1),
curve: Curves.easeOut,
child: child,
);
},
),
);
}
}
| flutter/examples/api/lib/widgets/image/image.frame_builder.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/image/image.frame_builder.0.dart",
"repo_id": "flutter",
"token_count": 535
} | 606 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [LayoutBuilder].
void main() => runApp(const LayoutBuilderExampleApp());
class LayoutBuilderExampleApp extends StatelessWidget {
const LayoutBuilderExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: LayoutBuilderExample(),
);
}
}
class LayoutBuilderExample extends StatelessWidget {
const LayoutBuilderExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('LayoutBuilder Example')),
body: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
if (constraints.maxWidth > 600) {
return _buildWideContainers();
} else {
return _buildNormalContainer();
}
},
),
);
}
Widget _buildNormalContainer() {
return Center(
child: Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
);
}
Widget _buildWideContainers() {
return Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
Container(
height: 100.0,
width: 100.0,
color: Colors.yellow,
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/layout_builder/layout_builder.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/layout_builder/layout_builder.0.dart",
"repo_id": "flutter",
"token_count": 691
} | 607 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [NestedScrollViewState].
void main() => runApp(const NestedScrollViewStateExampleApp());
class NestedScrollViewStateExampleApp extends StatelessWidget {
const NestedScrollViewStateExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: NestedScrollViewStateExample(),
);
}
}
final GlobalKey<NestedScrollViewState> globalKey = GlobalKey();
class NestedScrollViewStateExample extends StatelessWidget {
const NestedScrollViewStateExample({super.key});
@override
@override
Widget build(BuildContext context) {
return NestedScrollView(
key: globalKey,
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
return const <Widget>[
SliverAppBar(
title: Text('NestedScrollViewState Demo!'),
),
];
},
body: const CustomScrollView(
// Body slivers go here!
),
);
}
ScrollController get innerController {
return globalKey.currentState!.innerController;
}
}
| flutter/examples/api/lib/widgets/nested_scroll_view/nested_scroll_view_state.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/nested_scroll_view/nested_scroll_view_state.0.dart",
"repo_id": "flutter",
"token_count": 435
} | 608 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [showGeneralDialog].
void main() => runApp(const GeneralDialogApp());
class GeneralDialogApp extends StatelessWidget {
const GeneralDialogApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
restorationScopeId: 'app',
home: GeneralDialogExample(),
);
}
}
class GeneralDialogExample extends StatelessWidget {
const GeneralDialogExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: OutlinedButton(
onPressed: () {
/// This shows an alert dialog.
Navigator.of(context).restorablePush(_dialogBuilder);
},
child: const Text('Open Dialog'),
),
),
);
}
@pragma('vm:entry-point')
static Route<Object?> _dialogBuilder(BuildContext context, Object? arguments) {
return RawDialogRoute<void>(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) {
return const AlertDialog(title: Text('Alert!'));
},
);
}
}
| flutter/examples/api/lib/widgets/routes/show_general_dialog.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/routes/show_general_dialog.0.dart",
"repo_id": "flutter",
"token_count": 494
} | 609 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [CallbackShortcuts].
void main() => runApp(const CallbackShortcutsApp());
class CallbackShortcutsApp extends StatelessWidget {
const CallbackShortcutsApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('CallbackShortcuts Sample')),
body: const Center(
child: CallbackShortcutsExample(),
),
),
);
}
}
class CallbackShortcutsExample extends StatefulWidget {
const CallbackShortcutsExample({super.key});
@override
State<CallbackShortcutsExample> createState() => _CallbackShortcutsExampleState();
}
class _CallbackShortcutsExampleState extends State<CallbackShortcutsExample> {
int count = 0;
@override
Widget build(BuildContext context) {
return CallbackShortcuts(
bindings: <ShortcutActivator, VoidCallback>{
const SingleActivator(LogicalKeyboardKey.arrowUp): () {
setState(() => count = count + 1);
},
const SingleActivator(LogicalKeyboardKey.arrowDown): () {
setState(() => count = count - 1);
},
},
child: Focus(
autofocus: true,
child: Column(
children: <Widget>[
const Text('Press the up arrow key to add to the counter'),
const Text('Press the down arrow key to subtract from the counter'),
Text('count: $count'),
],
),
),
);
}
}
| flutter/examples/api/lib/widgets/shortcuts/callback_shortcuts.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/shortcuts/callback_shortcuts.0.dart",
"repo_id": "flutter",
"token_count": 637
} | 610 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverFillRemaining].
void main() => runApp(const SliverFillRemainingExampleApp());
class SliverFillRemainingExampleApp extends StatelessWidget {
const SliverFillRemainingExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('SliverFillRemaining Sample')),
body: const SliverFillRemainingExample(),
),
);
}
}
class SliverFillRemainingExample extends StatelessWidget {
const SliverFillRemainingExample({super.key});
@override
Widget build(BuildContext context) {
return CustomScrollView(
// The ScrollPhysics are overridden here to illustrate the functionality
// of fillOverscroll on all devices this sample may be run on.
// fillOverscroll only changes the behavior of your layout when applied to
// Scrollables that allow for overscroll. BouncingScrollPhysics are one
// example, which are provided by default on the iOS platform.
// BouncingScrollPhysics is combined with AlwaysScrollableScrollPhysics to
// allow for the overscroll, regardless of the depth of the scrollable.
physics: const BouncingScrollPhysics(parent: AlwaysScrollableScrollPhysics()),
slivers: <Widget>[
SliverToBoxAdapter(
child: Container(
color: Colors.tealAccent[700],
height: 150.0,
),
),
SliverFillRemaining(
hasScrollBody: false,
// Switch for different overscroll behavior in your layout. If your
// ScrollPhysics do not allow for overscroll, setting fillOverscroll
// to true will have no effect.
fillOverscroll: true,
child: Container(
color: Colors.teal[100],
child: Align(
alignment: Alignment.bottomCenter,
child: Padding(
padding: const EdgeInsets.all(16.0),
child: ElevatedButton(
onPressed: () {
/* Place your onPressed code here! */
},
child: const Text('Bottom Pinned Button!'),
),
),
),
),
),
],
);
}
}
| flutter/examples/api/lib/widgets/sliver_fill/sliver_fill_remaining.3.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/sliver_fill/sliver_fill_remaining.3.dart",
"repo_id": "flutter",
"token_count": 988
} | 611 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'package:flutter/material.dart';
/// Flutter code sample for [MatrixTransition].
void main() => runApp(const MatrixTransitionExampleApp());
class MatrixTransitionExampleApp extends StatelessWidget {
const MatrixTransitionExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MatrixTransitionExample(),
);
}
}
class MatrixTransitionExample extends StatefulWidget {
const MatrixTransitionExample({super.key});
@override
State<MatrixTransitionExample> createState() => _MatrixTransitionExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _MatrixTransitionExampleState extends State<MatrixTransitionExample> with TickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat();
_animation = CurvedAnimation(
parent: _controller,
curve: Curves.linear,
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: MatrixTransition(
animation: _animation,
child: const Padding(
padding: EdgeInsets.all(8.0),
child: FlutterLogo(size: 150.0),
),
onTransform: (double value) {
return Matrix4.identity()
..setEntry(3, 2, 0.004)
..rotateY(pi * 2.0 * value);
},
),
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/matrix_transition.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/matrix_transition.0.dart",
"repo_id": "flutter",
"token_count": 701
} | 612 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/route/show_cupertino_dialog.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tap on button displays cupertino dialog', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CupertinoDialogApp(),
);
final Finder dialogTitle = find.text('Title');
expect(dialogTitle, findsNothing);
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(dialogTitle, findsOneWidget);
await tester.tap(find.text('Yes'));
await tester.pumpAndSettle();
expect(dialogTitle, findsNothing);
});
}
| flutter/examples/api/test/cupertino/route/show_cupertino_dialog.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/route/show_cupertino_dialog.0_test.dart",
"repo_id": "flutter",
"token_count": 299
} | 613 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/autocomplete/autocomplete.3.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('can search and find options after waiting for fake network delay and debounce delay', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'a');
await tester.pump(example.fakeAPIDuration);
// No results yet, need to also wait for the debounce duration.
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.pump(example.debounceDuration);
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsOneWidget);
expect(find.text('chameleon'), findsOneWidget);
await tester.enterText(find.byType(TextFormField), 'aa');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
});
testWidgets('debounce is reset each time a character is entered', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
await tester.enterText(find.byType(TextFormField), 'c');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'ch');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'cha');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'cham');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
// Despite the total elapsed time being greater than debounceDuration +
// fakeAPIDuration, the search has not yet completed, because the debounce
// was reset each time text input happened.
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'chame');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsOneWidget);
});
testWidgets('multiple pending requests', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
await tester.enterText(find.byType(TextFormField), 'a');
// Wait until the debounce duration has expired, but the request is still
// pending.
await tester.pump(example.debounceDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'aa');
// Wait until the first request has completed.
await tester.pump(example.fakeAPIDuration - example.debounceDuration);
// The results from the first request are thrown away since the query has
// changed.
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
// Wait until the second request has completed.
await tester.pump(example.fakeAPIDuration);
// The results of the second request are reflected.
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
});
}
| flutter/examples/api/test/material/autocomplete/autocomplete.3_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/autocomplete/autocomplete.3_test.dart",
"repo_id": "flutter",
"token_count": 1508
} | 614 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/checkbox_list_tile/custom_labeled_checkbox.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tapping LabeledCheckbox toggles the checkbox', (WidgetTester tester) async {
await tester.pumpWidget(
const example.LabeledCheckboxApp(),
);
// Checkbox is initially unchecked.
Checkbox checkbox = tester.widget(find.byType(Checkbox));
expect(checkbox.value, isFalse);
// Tap the LabeledCheckBoxApp to toggle the checkbox.
await tester.tap(find.byType(example.LabeledCheckbox));
await tester.pumpAndSettle();
// Checkbox is now checked.
checkbox = tester.widget(find.byType(Checkbox));
expect(checkbox.value, isTrue);
});
}
| flutter/examples/api/test/material/checkbox_list_tile/custom_labeled_checkbox.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/checkbox_list_tile/custom_labeled_checkbox.1_test.dart",
"repo_id": "flutter",
"token_count": 328
} | 615 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/elevated_button/elevated_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ElevatedButton Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ElevatedButtonExampleApp(),
);
expect(find.widgetWithText(AppBar, 'ElevatedButton Sample'), findsOneWidget);
final Finder disabledButton = find.widgetWithText(ElevatedButton, 'Disabled');
expect(disabledButton, findsOneWidget);
expect(tester.widget<ElevatedButton>(disabledButton).onPressed.runtimeType, Null);
final Finder enabledButton = find.widgetWithText(ElevatedButton, 'Enabled');
expect(enabledButton, findsOneWidget);
expect(tester.widget<ElevatedButton>(enabledButton).onPressed.runtimeType, VoidCallback);
});
}
| flutter/examples/api/test/material/elevated_button/elevated_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/elevated_button/elevated_button.0_test.dart",
"repo_id": "flutter",
"token_count": 331
} | 616 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/input_chip/input_chip.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ChipApp(),
);
expect(find.byType(InputChip), findsNWidgets(3));
await tester.tap(find.byIcon(Icons.clear).at(0));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsNWidgets(2));
await tester.tap(find.byIcon(Icons.clear).at(0));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsNWidgets(1));
await tester.tap(find.byIcon(Icons.clear).at(0));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsNWidgets(0));
await tester.tap(find.text('Reset'));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsNWidgets(3));
});
}
| flutter/examples/api/test/material/input_chip/input_chip.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/input_chip/input_chip.0_test.dart",
"repo_id": "flutter",
"token_count": 412
} | 617 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/material/menu_anchor/menu_accelerator_label.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can open menu', (WidgetTester tester) async {
Finder findMenu(String label) {
return find
.ancestor(
of: find.text(label, findRichText: true),
matching: find.byType(FocusScope),
)
.first;
}
await tester.pumpWidget(const example.MenuAcceleratorApp());
await tester.sendKeyDownEvent(LogicalKeyboardKey.altLeft);
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.keyF, character: 'f');
await tester.pumpAndSettle();
await tester.pump();
expect(find.text('About', findRichText: true), findsOneWidget);
expect(tester.getRect(findMenu('About')).left, equals(4.0));
expect(tester.getRect(findMenu('About')).top, equals(48.0));
expect(tester.getRect(findMenu('About')).right, closeTo(98.5, 0.1));
expect(tester.getRect(findMenu('About')).bottom, equals(208.0));
expect(find.text('Save', findRichText: true), findsOneWidget);
expect(find.text('Quit', findRichText: true), findsOneWidget);
expect(find.text('Magnify', findRichText: true), findsNothing);
expect(find.text('Minify', findRichText: true), findsNothing);
// Open the About dialog.
await tester.sendKeyEvent(LogicalKeyboardKey.keyA, character: 'a');
await tester.sendKeyUpEvent(LogicalKeyboardKey.altLeft);
await tester.pumpAndSettle();
expect(find.text('Save', findRichText: true), findsNothing);
expect(find.text('Quit', findRichText: true), findsNothing);
expect(find.text('Magnify', findRichText: true), findsNothing);
expect(find.text('Minify', findRichText: true), findsNothing);
expect(find.text('Close'), findsOneWidget);
await tester.tap(find.text('Close'));
await tester.pumpAndSettle();
expect(find.text('Close'), findsNothing);
});
testWidgets('MenuBar is wrapped in a SafeArea', (WidgetTester tester) async {
const double safeAreaPadding = 100.0;
await tester.pumpWidget(
const MediaQuery(
data: MediaQueryData(
padding: EdgeInsets.symmetric(vertical: safeAreaPadding),
),
child: example.MenuAcceleratorApp(),
),
);
expect(tester.getTopLeft(find.byType(MenuBar)), const Offset(0.0, safeAreaPadding));
});
}
| flutter/examples/api/test/material/menu_anchor/menu_accelerator_label.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/menu_anchor/menu_accelerator_label.0_test.dart",
"repo_id": "flutter",
"token_count": 991
} | 618 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/popup_menu/popup_menu.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can open popup menu', (WidgetTester tester) async {
const String menuItem = 'Item 1';
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.PopupMenuApp(),
),
),
);
expect(find.text(menuItem), findsNothing);
// Open popup menu.
await tester.tap(find.byIcon(Icons.adaptive.more));
await tester.pumpAndSettle();
expect(find.text(menuItem), findsOneWidget);
// Close popup menu.
await tester.tapAt(const Offset(1, 1));
await tester.pumpAndSettle();
expect(find.text(menuItem), findsNothing);
});
}
| flutter/examples/api/test/material/popup_menu/popup_menu.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/popup_menu/popup_menu.0_test.dart",
"repo_id": "flutter",
"token_count": 364
} | 619 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/reorderable_list/reorderable_list_view.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Dragged item color is updated', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ReorderableApp(),
);
final ThemeData theme = Theme.of(tester.element(find.byType(MaterialApp)));
// Dragged item is wrapped in a Material widget with correct color.
final TestGesture drag = await tester.startGesture(tester.getCenter(find.text('Item 1')));
await tester.pump(kLongPressTimeout + kPressTimeout);
await tester.pumpAndSettle();
final Material material = tester.widget<Material>(find.ancestor(
of: find.text('Item 1'),
matching: find.byType(Material),
));
expect(material.color, theme.colorScheme.secondary);
// Ends the drag gesture.
await drag.moveTo(tester.getCenter(find.text('Item 4')));
await drag.up();
await tester.pumpAndSettle();
});
}
| flutter/examples/api/test/material/reorderable_list/reorderable_list_view.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/reorderable_list/reorderable_list_view.1_test.dart",
"repo_id": "flutter",
"token_count": 435
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/stepper/stepper.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Stepper Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.StepperExampleApp(),
);
expect(find.widgetWithText(AppBar, 'Stepper Sample'), findsOneWidget);
expect(find.text('Step 1 title').hitTestable(), findsOneWidget);
expect(find.text('Step 2 title').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 1').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 2').hitTestable(), findsNothing);
final Stepper stepper = tester.widget<Stepper>(find.byType(Stepper));
// current: 0 & clicks cancel
stepper.onStepCancel?.call();
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 2').hitTestable(), findsNothing);
// current: 0 & clicks 0th step
stepper.onStepTapped?.call(0);
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 2').hitTestable(), findsNothing);
// current: 0 & clicks continue
stepper.onStepContinue?.call();
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsNothing);
expect(find.text('Content for Step 2').hitTestable(), findsOneWidget);
// current: 1 & clicks 1st step
stepper.onStepTapped?.call(1);
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsNothing);
expect(find.text('Content for Step 2').hitTestable(), findsOneWidget);
// current: 1 & clicks continue
stepper.onStepContinue?.call();
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsNothing);
expect(find.text('Content for Step 2').hitTestable(), findsOneWidget);
// current: 1 & clicks cancel
stepper.onStepCancel?.call();
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 2').hitTestable(), findsNothing);
// current: 0 & clicks 1st step
stepper.onStepTapped?.call(1);
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsNothing);
expect(find.text('Content for Step 2').hitTestable(), findsOneWidget);
// current: 1 & clicks 0th step
stepper.onStepTapped?.call(0);
await tester.pumpAndSettle();
expect(find.text('Content for Step 1').hitTestable(), findsOneWidget);
expect(find.text('Content for Step 2').hitTestable(), findsNothing);
});
}
| flutter/examples/api/test/material/stepper/stepper.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/stepper/stepper.0_test.dart",
"repo_id": "flutter",
"token_count": 998
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/theme_data/theme_data.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ThemeData basics', (WidgetTester tester) async {
await tester.pumpWidget(const example.ThemeDataExampleApp());
final ColorScheme colorScheme = ColorScheme.fromSeed(
seedColor: Colors.indigo,
);
final Material fabMaterial = tester.widget<Material>(
find.descendant(of: find.byType(FloatingActionButton), matching: find.byType(Material)),
);
expect(fabMaterial.color, colorScheme.tertiary);
final RichText iconRichText = tester.widget<RichText>(
find.descendant(of: find.byIcon(Icons.add), matching: find.byType(RichText)),
);
expect(iconRichText.text.style!.color, colorScheme.onTertiary);
expect(find.text('8 Points'), isNotNull);
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
expect(find.text('9 Points'), isNotNull);
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
expect(find.text('10 Points'), isNotNull);
});
}
| flutter/examples/api/test/material/theme_data/theme_data.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/theme_data/theme_data.0_test.dart",
"repo_id": "flutter",
"token_count": 467
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/sample_templates/cupertino.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
// This is an example of a test for API example code.
//
// It only tests that the example is presenting what it is supposed to, but you
// should also test the basic functionality of the example to make sure that it
// functions as expected.
void main() {
testWidgets('Example app has a placeholder', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SampleApp(),
);
expect(find.byType(Placeholder), findsOneWidget);
});
}
| flutter/examples/api/test/sample_templates/cupertino.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/sample_templates/cupertino.0_test.dart",
"repo_id": "flutter",
"token_count": 242
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/clip_rrect.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ClipRRect fits to its child', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ClipRRectApp(),
);
final Finder clipRRectFinder = find.byType(ClipRRect);
final Finder logoFinder = find.byType(FlutterLogo);
expect(clipRRectFinder, findsOneWidget);
expect(logoFinder, findsOneWidget);
final Rect clipRect = tester.getRect(clipRRectFinder);
final Rect containerRect = tester.getRect(logoFinder);
expect(clipRect, equals(containerRect));
});
}
| flutter/examples/api/test/widgets/basic/clip_rrect.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/clip_rrect.1_test.dart",
"repo_id": "flutter",
"token_count": 300
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_api_samples/widgets/binding/widget_binding_observer.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('App tracks lifecycle states', (WidgetTester tester) async {
Future<void> setAppLifeCycleState(AppLifecycleState state) async {
final ByteData? message =
const StringCodec().encodeMessage(state.toString());
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/lifecycle', message, (_) {});
}
await tester.pumpWidget(
const example.WidgetBindingObserverExampleApp(),
);
expect(find.text('There are no AppLifecycleStates to show.'), findsOneWidget);
await setAppLifeCycleState(AppLifecycleState.resumed);
await tester.pumpAndSettle();
expect(find.text('state is: AppLifecycleState.resumed'), findsOneWidget);
await setAppLifeCycleState(AppLifecycleState.inactive);
await tester.pumpAndSettle();
expect(find.text('state is: AppLifecycleState.inactive'), findsOneWidget);
await setAppLifeCycleState(AppLifecycleState.paused);
await tester.pumpAndSettle();
// Can't look for paused text here because rendering is paused.
await setAppLifeCycleState(AppLifecycleState.inactive);
await tester.pumpAndSettle();
expect(find.text('state is: AppLifecycleState.inactive'), findsNWidgets(2));
await setAppLifeCycleState(AppLifecycleState.resumed);
await tester.pumpAndSettle();
expect(find.text('state is: AppLifecycleState.resumed'), findsNWidgets(2));
});
}
| flutter/examples/api/test/widgets/binding/widget_binding_observer.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/binding/widget_binding_observer.0_test.dart",
"repo_id": "flutter",
"token_count": 628
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_api_samples/widgets/navigator_pop_handler/navigator_pop_handler.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
import '../navigator_utils.dart';
void main() {
testWidgets("System back gesture operates on current tab's nested Navigator", (WidgetTester tester) async {
await tester.pumpWidget(
const example.NavigatorPopHandlerApp(),
);
expect(find.text('Bottom nav - tab Home Tab - route _TabPage.home'), findsOneWidget);
// Go to the next route in this tab.
await tester.tap(find.text('Go to another route in this nested Navigator'));
await tester.pumpAndSettle();
expect(find.text('Bottom nav - tab Home Tab - route _TabPage.one'), findsOneWidget);
// Go to another tab.
await tester.tap(find.text('Go to One'));
await tester.pumpAndSettle();
expect(find.text('Bottom nav - tab Tab One - route _TabPage.home'), findsOneWidget);
// Return to the home tab. The navigation state is preserved.
await tester.tap(find.text('Go to Home'));
await tester.pumpAndSettle();
expect(find.text('Bottom nav - tab Home Tab - route _TabPage.one'), findsOneWidget);
// A back pops the navigation stack of the current tab's nested Navigator.
await simulateSystemBack();
await tester.pumpAndSettle();
expect(find.text('Bottom nav - tab Home Tab - route _TabPage.home'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/navigator_pop_handler/navigator_pop_handler.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/navigator_pop_handler/navigator_pop_handler.1_test.dart",
"repo_id": "flutter",
"token_count": 511
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/scrollbar/raw_scrollbar.desktop.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can hide default scrollbar on desktop', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ScrollbarApp(),
);
// Two from left list view where scroll configuration is not set.
// One from right list view where scroll configuration is set.
expect(find.byType(Scrollbar), findsNWidgets(3));
});
}
| flutter/examples/api/test/widgets/scrollbar/raw_scrollbar.desktop.0.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/scrollbar/raw_scrollbar.desktop.0.dart",
"repo_id": "flutter",
"token_count": 226
} | 627 |
## To run the Hello World demo:
```
flutter run
```
## To run the Hello World demo showing Arabic:
```
flutter run lib/arabic.dart
```
| flutter/examples/hello_world/README.md/0 | {
"file_path": "flutter/examples/hello_world/README.md",
"repo_id": "flutter",
"token_count": 48
} | 628 |
#include "Generated.xcconfig"
| flutter/examples/hello_world/ios/Flutter/Release.xcconfig/0 | {
"file_path": "flutter/examples/hello_world/ios/Flutter/Release.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter_driver/flutter_driver.dart';
import 'package:test/test.dart';
import 'package:webdriver/async_io.dart';
// TODO(web): Migrate this test to a normal integration_test with a WidgetTester.
/// The following test is used as a simple smoke test for verifying Flutter
/// Framework and Flutter Web Engine integration.
void main() {
group('Hello World App', () {
final SerializableFinder titleFinder = find.byValueKey('title');
late FlutterDriver driver;
// Connect to the Flutter driver before running any tests.
setUpAll(() async {
driver = await FlutterDriver.connect();
});
// Close the connection to the driver after the tests have completed.
tearDownAll(() async {
driver.close();
});
test('title is correct', () async {
expect(await driver.getText(titleFinder), 'Hello, world!');
});
test('enable accessibility', () async {
await driver.setSemantics(true);
// TODO(ianh): this delay violates our style guide. We should instead wait for a triggering event.
await Future<void>.delayed(const Duration(seconds: 2));
final WebElement? fltSemantics = await driver.webDriver.execute(
'return document.querySelector("flt-semantics")',
<dynamic>[],
) as WebElement?;
expect(fltSemantics, isNotNull);
});
});
}
| flutter/examples/hello_world/test_driver/smoke_web_engine_test.dart/0 | {
"file_path": "flutter/examples/hello_world/test_driver/smoke_web_engine_test.dart",
"repo_id": "flutter",
"token_count": 504
} | 630 |
# Image List
An example that sets up a local HTTP server for serving one
image then creates a single flutter widget with five copies of requested
image and prints how long the loading took.
This is used in [$FH/flutter/devicelab/bin/tasks/image_list_reported_duration.dart] test.
| flutter/examples/image_list/README.md/0 | {
"file_path": "flutter/examples/image_list/README.md",
"repo_id": "flutter",
"token_count": 78
} | 631 |
# Examples of Flutter's layered architecture
This directory contains several self-contained examples that illustrate
Flutter's layered architecture.
* [*raw/*](raw/) These examples show how to program against the lowest layer of
the system. They manually receive input packets and construct composited
scenes.
* [*rendering/*](rendering/) These examples use Flutter's render tree to
structure your app using a retained tree of visual objects. These objects
coordinate to determine their size and position on screen and to handle
events.
* [*widgets/*](widgets/) These examples use Flutter's widgets to build more
elaborate apps using a reactive framework.
* [*services/*](services/) These examples use services available in Flutter to
interact with the host platform.
To run each example, specify the demo file on the `flutter run`
command line, for example:
```
flutter run raw/spinning_square.dart
flutter run rendering/spinning_square.dart
flutter run widgets/spinning_square.dart
```
| flutter/examples/layers/README.md/0 | {
"file_path": "flutter/examples/layers/README.md",
"repo_id": "flutter",
"token_count": 249
} | 632 |
#include "Generated.xcconfig"
| flutter/examples/layers/ios/Flutter/Debug.xcconfig/0 | {
"file_path": "flutter/examples/layers/ios/Flutter/Debug.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/rendering.dart';
const double kTwoPi = 2 * math.pi;
class SectorConstraints extends Constraints {
const SectorConstraints({
this.minDeltaRadius = 0.0,
this.maxDeltaRadius = double.infinity,
this.minDeltaTheta = 0.0,
this.maxDeltaTheta = kTwoPi,
}) : assert(maxDeltaRadius >= minDeltaRadius),
assert(maxDeltaTheta >= minDeltaTheta);
const SectorConstraints.tight({ double deltaRadius = 0.0, double deltaTheta = 0.0 })
: minDeltaRadius = deltaRadius,
maxDeltaRadius = deltaRadius,
minDeltaTheta = deltaTheta,
maxDeltaTheta = deltaTheta;
final double minDeltaRadius;
final double maxDeltaRadius;
final double minDeltaTheta;
final double maxDeltaTheta;
double constrainDeltaRadius(double deltaRadius) {
return deltaRadius.clamp(minDeltaRadius, maxDeltaRadius);
}
double constrainDeltaTheta(double deltaTheta) {
return deltaTheta.clamp(minDeltaTheta, maxDeltaTheta);
}
@override
bool get isTight => minDeltaTheta >= maxDeltaTheta && minDeltaTheta >= maxDeltaTheta;
@override
bool get isNormalized => minDeltaRadius <= maxDeltaRadius && minDeltaTheta <= maxDeltaTheta;
@override
bool debugAssertIsValid({
bool isAppliedConstraint = false,
InformationCollector? informationCollector,
}) {
assert(isNormalized);
return isNormalized;
}
}
class SectorDimensions {
const SectorDimensions({ this.deltaRadius = 0.0, this.deltaTheta = 0.0 });
factory SectorDimensions.withConstraints(
SectorConstraints constraints, {
double deltaRadius = 0.0,
double deltaTheta = 0.0,
}) {
return SectorDimensions(
deltaRadius: constraints.constrainDeltaRadius(deltaRadius),
deltaTheta: constraints.constrainDeltaTheta(deltaTheta),
);
}
final double deltaRadius;
final double deltaTheta;
}
class SectorParentData extends ParentData {
double radius = 0.0;
double theta = 0.0;
}
/// Base class for [RenderObject]s that live in a polar coordinate space.
///
/// In a polar coordinate system each point on a plane is determined by a
/// distance from a reference point ("radius") and an angle from a reference
/// direction ("theta").
///
/// See also:
///
/// * <https://en.wikipedia.org/wiki/Polar_coordinate_system>, which defines
/// the polar coordinate space.
/// * [RenderBox], which is the base class for [RenderObject]s that live in a
/// Cartesian coordinate space.
abstract class RenderSector extends RenderObject {
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SectorParentData) {
child.parentData = SectorParentData();
}
}
// RenderSectors always use SectorParentData subclasses, as they need to be
// able to read their position information for painting and hit testing.
@override
SectorParentData? get parentData => super.parentData as SectorParentData?;
SectorDimensions getIntrinsicDimensions(SectorConstraints constraints, double radius) {
return SectorDimensions.withConstraints(constraints);
}
@override
SectorConstraints get constraints => super.constraints as SectorConstraints;
@override
void debugAssertDoesMeetConstraints() {
assert(deltaRadius < double.infinity);
assert(deltaTheta < double.infinity);
assert(constraints.minDeltaRadius <= deltaRadius);
assert(deltaRadius <= math.max(constraints.minDeltaRadius, constraints.maxDeltaRadius));
assert(constraints.minDeltaTheta <= deltaTheta);
assert(deltaTheta <= math.max(constraints.minDeltaTheta, constraints.maxDeltaTheta));
}
@override
void performResize() {
// default behavior for subclasses that have sizedByParent = true
deltaRadius = constraints.constrainDeltaRadius(0.0);
deltaTheta = constraints.constrainDeltaTheta(0.0);
}
@override
void performLayout() {
// descendants have to either override performLayout() to set both
// the dimensions and lay out children, or, set sizedByParent to
// true so that performResize()'s logic above does its thing.
assert(sizedByParent);
}
@override
Rect get paintBounds => Rect.fromLTWH(0.0, 0.0, 2.0 * deltaRadius, 2.0 * deltaRadius);
@override
Rect get semanticBounds => Rect.fromLTWH(-deltaRadius, -deltaRadius, 2.0 * deltaRadius, 2.0 * deltaRadius);
bool hitTest(SectorHitTestResult result, { required double radius, required double theta }) {
if (radius < parentData!.radius || radius >= parentData!.radius + deltaRadius ||
theta < parentData!.theta || theta >= parentData!.theta + deltaTheta) {
return false;
}
hitTestChildren(result, radius: radius, theta: theta);
result.add(SectorHitTestEntry(this, radius: radius, theta: theta));
return true;
}
void hitTestChildren(SectorHitTestResult result, { required double radius, required double theta }) { }
late double deltaRadius;
late double deltaTheta;
}
abstract class RenderDecoratedSector extends RenderSector {
RenderDecoratedSector(BoxDecoration? decoration) : _decoration = decoration;
BoxDecoration? _decoration;
BoxDecoration? get decoration => _decoration;
set decoration(BoxDecoration? value) {
if (value == _decoration) {
return;
}
_decoration = value;
markNeedsPaint();
}
// offset must point to the center of the circle
@override
void paint(PaintingContext context, Offset offset) {
assert(parentData is SectorParentData);
if (_decoration == null) {
return;
}
if (_decoration!.color != null) {
final Canvas canvas = context.canvas;
final Paint paint = Paint()..color = _decoration!.color!;
final Path path = Path();
final double outerRadius = parentData!.radius + deltaRadius;
final Rect outerBounds = Rect.fromLTRB(offset.dx-outerRadius, offset.dy-outerRadius, offset.dx+outerRadius, offset.dy+outerRadius);
path.arcTo(outerBounds, parentData!.theta, deltaTheta, true);
final double innerRadius = parentData!.radius;
final Rect innerBounds = Rect.fromLTRB(offset.dx-innerRadius, offset.dy-innerRadius, offset.dx+innerRadius, offset.dy+innerRadius);
path.arcTo(innerBounds, parentData!.theta + deltaTheta, -deltaTheta, false);
path.close();
canvas.drawPath(path, paint);
}
}
}
class SectorChildListParentData extends SectorParentData with ContainerParentDataMixin<RenderSector> { }
class RenderSectorWithChildren extends RenderDecoratedSector with ContainerRenderObjectMixin<RenderSector, SectorChildListParentData> {
RenderSectorWithChildren(super.decoration);
@override
void hitTestChildren(SectorHitTestResult result, { required double radius, required double theta }) {
RenderSector? child = lastChild;
while (child != null) {
if (child.hitTest(result, radius: radius, theta: theta)) {
return;
}
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.previousSibling;
}
}
@override
void visitChildren(RenderObjectVisitor visitor) {
RenderSector? child = lastChild;
while (child != null) {
visitor(child);
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.previousSibling;
}
}
}
class RenderSectorRing extends RenderSectorWithChildren {
// lays out RenderSector children in a ring
RenderSectorRing({
BoxDecoration? decoration,
double deltaRadius = double.infinity,
double padding = 0.0,
}) : _padding = padding,
assert(deltaRadius >= 0.0),
_desiredDeltaRadius = deltaRadius,
super(decoration);
double _desiredDeltaRadius;
double get desiredDeltaRadius => _desiredDeltaRadius;
set desiredDeltaRadius(double value) {
assert(value >= 0);
if (_desiredDeltaRadius != value) {
_desiredDeltaRadius = value;
markNeedsLayout();
}
}
double _padding;
double get padding => _padding;
set padding(double value) {
// TODO(ianh): avoid code duplication
if (_padding != value) {
_padding = value;
markNeedsLayout();
}
}
@override
void setupParentData(RenderObject child) {
// TODO(ianh): avoid code duplication
if (child.parentData is! SectorChildListParentData) {
child.parentData = SectorChildListParentData();
}
}
@override
SectorDimensions getIntrinsicDimensions(SectorConstraints constraints, double radius) {
final double outerDeltaRadius = constraints.constrainDeltaRadius(desiredDeltaRadius);
final double innerDeltaRadius = math.max(0.0, outerDeltaRadius - padding * 2.0);
final double childRadius = radius + padding;
final double paddingTheta = math.atan(padding / (radius + outerDeltaRadius));
double innerTheta = paddingTheta; // increments with each child
double remainingDeltaTheta = math.max(0.0, constraints.maxDeltaTheta - (innerTheta + paddingTheta));
RenderSector? child = firstChild;
while (child != null) {
final SectorConstraints innerConstraints = SectorConstraints(
maxDeltaRadius: innerDeltaRadius,
maxDeltaTheta: remainingDeltaTheta,
);
final SectorDimensions childDimensions = child.getIntrinsicDimensions(innerConstraints, childRadius);
innerTheta += childDimensions.deltaTheta;
remainingDeltaTheta -= childDimensions.deltaTheta;
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
if (child != null) {
innerTheta += paddingTheta;
remainingDeltaTheta -= paddingTheta;
}
}
return SectorDimensions.withConstraints(
constraints,
deltaRadius: outerDeltaRadius,
deltaTheta: innerTheta,
);
}
@override
void performLayout() {
assert(parentData is SectorParentData);
deltaRadius = constraints.constrainDeltaRadius(desiredDeltaRadius);
assert(deltaRadius < double.infinity);
final double innerDeltaRadius = deltaRadius - padding * 2.0;
final double childRadius = parentData!.radius + padding;
final double paddingTheta = math.atan(padding / (parentData!.radius + deltaRadius));
double innerTheta = paddingTheta; // increments with each child
double remainingDeltaTheta = constraints.maxDeltaTheta - (innerTheta + paddingTheta);
RenderSector? child = firstChild;
while (child != null) {
final SectorConstraints innerConstraints = SectorConstraints(
maxDeltaRadius: innerDeltaRadius,
maxDeltaTheta: remainingDeltaTheta,
);
assert(child.parentData is SectorParentData);
child.parentData!.theta = innerTheta;
child.parentData!.radius = childRadius;
child.layout(innerConstraints, parentUsesSize: true);
innerTheta += child.deltaTheta;
remainingDeltaTheta -= child.deltaTheta;
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
if (child != null) {
innerTheta += paddingTheta;
remainingDeltaTheta -= paddingTheta;
}
}
deltaTheta = innerTheta;
}
// offset must point to the center of our circle
// each sector then knows how to paint itself at its location
@override
void paint(PaintingContext context, Offset offset) {
// TODO(ianh): avoid code duplication
super.paint(context, offset);
RenderSector? child = firstChild;
while (child != null) {
context.paintChild(child, offset);
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
}
}
}
class RenderSectorSlice extends RenderSectorWithChildren {
// lays out RenderSector children in a stack
RenderSectorSlice({
BoxDecoration? decoration,
double deltaTheta = kTwoPi,
double padding = 0.0,
}) : _padding = padding, _desiredDeltaTheta = deltaTheta, super(decoration);
double _desiredDeltaTheta;
double get desiredDeltaTheta => _desiredDeltaTheta;
set desiredDeltaTheta(double value) {
if (_desiredDeltaTheta != value) {
_desiredDeltaTheta = value;
markNeedsLayout();
}
}
double _padding;
double get padding => _padding;
set padding(double value) {
// TODO(ianh): avoid code duplication
if (_padding != value) {
_padding = value;
markNeedsLayout();
}
}
@override
void setupParentData(RenderObject child) {
// TODO(ianh): avoid code duplication
if (child.parentData is! SectorChildListParentData) {
child.parentData = SectorChildListParentData();
}
}
@override
SectorDimensions getIntrinsicDimensions(SectorConstraints constraints, double radius) {
assert(parentData is SectorParentData);
final double paddingTheta = math.atan(padding / parentData!.radius);
final double outerDeltaTheta = constraints.constrainDeltaTheta(desiredDeltaTheta);
final double innerDeltaTheta = outerDeltaTheta - paddingTheta * 2.0;
double childRadius = parentData!.radius + padding;
double remainingDeltaRadius = constraints.maxDeltaRadius - (padding * 2.0);
RenderSector? child = firstChild;
while (child != null) {
final SectorConstraints innerConstraints = SectorConstraints(
maxDeltaRadius: remainingDeltaRadius,
maxDeltaTheta: innerDeltaTheta,
);
final SectorDimensions childDimensions = child.getIntrinsicDimensions(innerConstraints, childRadius);
childRadius += childDimensions.deltaRadius;
remainingDeltaRadius -= childDimensions.deltaRadius;
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
childRadius += padding;
remainingDeltaRadius -= padding;
}
return SectorDimensions.withConstraints(
constraints,
deltaRadius: childRadius - parentData!.radius,
deltaTheta: outerDeltaTheta,
);
}
@override
void performLayout() {
assert(parentData is SectorParentData);
deltaTheta = constraints.constrainDeltaTheta(desiredDeltaTheta);
assert(deltaTheta <= kTwoPi);
final double paddingTheta = math.atan(padding / parentData!.radius);
final double innerTheta = parentData!.theta + paddingTheta;
final double innerDeltaTheta = deltaTheta - paddingTheta * 2.0;
double childRadius = parentData!.radius + padding;
double remainingDeltaRadius = constraints.maxDeltaRadius - (padding * 2.0);
RenderSector? child = firstChild;
while (child != null) {
final SectorConstraints innerConstraints = SectorConstraints(
maxDeltaRadius: remainingDeltaRadius,
maxDeltaTheta: innerDeltaTheta,
);
child.parentData!.theta = innerTheta;
child.parentData!.radius = childRadius;
child.layout(innerConstraints, parentUsesSize: true);
childRadius += child.deltaRadius;
remainingDeltaRadius -= child.deltaRadius;
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
childRadius += padding;
remainingDeltaRadius -= padding;
}
deltaRadius = childRadius - parentData!.radius;
}
// offset must point to the center of our circle
// each sector then knows how to paint itself at its location
@override
void paint(PaintingContext context, Offset offset) {
// TODO(ianh): avoid code duplication
super.paint(context, offset);
RenderSector? child = firstChild;
while (child != null) {
assert(child.parentData is SectorChildListParentData);
context.paintChild(child, offset);
final SectorChildListParentData childParentData = child.parentData! as SectorChildListParentData;
child = childParentData.nextSibling;
}
}
}
class RenderBoxToRenderSectorAdapter extends RenderBox with RenderObjectWithChildMixin<RenderSector> {
RenderBoxToRenderSectorAdapter({ double innerRadius = 0.0, RenderSector? child })
: _innerRadius = innerRadius {
this.child = child;
}
double _innerRadius;
double get innerRadius => _innerRadius;
set innerRadius(double value) {
_innerRadius = value;
markNeedsLayout();
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SectorParentData) {
child.parentData = SectorParentData();
}
}
@override
double computeMinIntrinsicWidth(double height) {
if (child == null) {
return 0.0;
}
return getIntrinsicDimensions(height: height).width;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child == null) {
return 0.0;
}
return getIntrinsicDimensions(height: height).width;
}
@override
double computeMinIntrinsicHeight(double width) {
if (child == null) {
return 0.0;
}
return getIntrinsicDimensions(width: width).height;
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child == null) {
return 0.0;
}
return getIntrinsicDimensions(width: width).height;
}
Size getIntrinsicDimensions({
double width = double.infinity,
double height = double.infinity,
}) {
assert(child is RenderSector);
assert(child!.parentData is SectorParentData);
if (!width.isFinite && !height.isFinite) {
return Size.zero;
}
final double maxChildDeltaRadius = math.max(0.0, math.min(width, height) / 2.0 - innerRadius);
final SectorDimensions childDimensions = child!.getIntrinsicDimensions(SectorConstraints(maxDeltaRadius: maxChildDeltaRadius), innerRadius);
final double dimension = (innerRadius + childDimensions.deltaRadius) * 2.0;
return Size.square(dimension);
}
@override
void performLayout() {
if (child == null || (!constraints.hasBoundedWidth && !constraints.hasBoundedHeight)) {
size = constraints.constrain(Size.zero);
child?.layout(SectorConstraints(maxDeltaRadius: innerRadius), parentUsesSize: true);
return;
}
assert(child is RenderSector);
assert(child!.parentData is SectorParentData);
final double maxChildDeltaRadius = math.min(constraints.maxWidth, constraints.maxHeight) / 2.0 - innerRadius;
child!.parentData!.radius = innerRadius;
child!.parentData!.theta = 0.0;
child!.layout(SectorConstraints(maxDeltaRadius: maxChildDeltaRadius), parentUsesSize: true);
final double dimension = (innerRadius + child!.deltaRadius) * 2.0;
size = constraints.constrain(Size(dimension, dimension));
}
@override
void paint(PaintingContext context, Offset offset) {
super.paint(context, offset);
if (child != null) {
final Rect bounds = offset & size;
// we move the offset to the center of the circle for the RenderSectors
context.paintChild(child!, bounds.center);
}
}
@override
bool hitTest(BoxHitTestResult result, { required Offset position }) {
if (child == null) {
return false;
}
double x = position.dx;
double y = position.dy;
// translate to our origin
x -= size.width / 2.0;
y -= size.height / 2.0;
// convert to radius/theta
final double radius = math.sqrt(x * x + y * y);
final double theta = (math.atan2(x, -y) - math.pi / 2.0) % kTwoPi;
if (radius < innerRadius) {
return false;
}
if (radius >= innerRadius + child!.deltaRadius) {
return false;
}
if (theta > child!.deltaTheta) {
return false;
}
child!.hitTest(SectorHitTestResult.wrap(result), radius: radius, theta: theta);
result.add(BoxHitTestEntry(this, position));
return true;
}
}
class RenderSolidColor extends RenderDecoratedSector {
RenderSolidColor(
this.backgroundColor, {
this.desiredDeltaRadius = double.infinity,
this.desiredDeltaTheta = kTwoPi,
}) : super(BoxDecoration(color: backgroundColor));
double desiredDeltaRadius;
double desiredDeltaTheta;
final Color backgroundColor;
@override
SectorDimensions getIntrinsicDimensions(SectorConstraints constraints, double radius) {
return SectorDimensions.withConstraints(constraints, deltaTheta: desiredDeltaTheta);
}
@override
void performLayout() {
deltaRadius = constraints.constrainDeltaRadius(desiredDeltaRadius);
deltaTheta = constraints.constrainDeltaTheta(desiredDeltaTheta);
}
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
if (event is PointerDownEvent) {
decoration = const BoxDecoration(color: Color(0xFFFF0000));
} else if (event is PointerUpEvent) {
decoration = BoxDecoration(color: backgroundColor);
}
}
}
/// The result of performing a hit test on [RenderSector]s.
class SectorHitTestResult extends HitTestResult {
/// Creates an empty hit test result for hit testing on [RenderSector].
SectorHitTestResult() : super();
/// Wraps `result` to create a [HitTestResult] that implements the
/// [SectorHitTestResult] protocol for hit testing on [RenderSector]s.
///
/// This method is used by [RenderObject]s that adapt between the
/// [RenderSector]-world and the non-[RenderSector]-world to convert a (subtype of)
/// [HitTestResult] to a [SectorHitTestResult] for hit testing on [RenderSector]s.
///
/// The [HitTestEntry]s added to the returned [SectorHitTestResult] are also
/// added to the wrapped `result` (both share the same underlying data
/// structure to store [HitTestEntry]s).
///
/// See also:
///
/// * [HitTestResult.wrap], which turns a [SectorHitTestResult] back into a
/// generic [HitTestResult].
SectorHitTestResult.wrap(super.result) : super.wrap();
// TODO(goderbauer): Add convenience methods to transform hit test positions
// once we have RenderSector implementations that move the origin of their
// children (e.g. RenderSectorTransform analogs to RenderTransform).
}
/// A hit test entry used by [RenderSector].
class SectorHitTestEntry extends HitTestEntry {
/// Creates a box hit test entry.
SectorHitTestEntry(RenderSector super.target, { required this.radius, required this.theta });
@override
RenderSector get target => super.target as RenderSector;
/// The radius component of the hit test position in the local coordinates of
/// [target].
final double radius;
/// The theta component of the hit test position in the local coordinates of
/// [target].
final double theta;
}
| flutter/examples/layers/rendering/src/sector_layout.dart/0 | {
"file_path": "flutter/examples/layers/rendering/src/sector_layout.dart",
"repo_id": "flutter",
"token_count": 7647
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class _GesturePainter extends CustomPainter {
const _GesturePainter({
required this.zoom,
required this.offset,
required this.swatch,
required this.forward,
required this.scaleEnabled,
required this.tapEnabled,
required this.doubleTapEnabled,
required this.longPressEnabled,
});
final double zoom;
final Offset offset;
final MaterialColor swatch;
final bool forward;
final bool scaleEnabled;
final bool tapEnabled;
final bool doubleTapEnabled;
final bool longPressEnabled;
@override
void paint(Canvas canvas, Size size) {
final Offset center = size.center(Offset.zero) * zoom + offset;
final double radius = size.width / 2.0 * zoom;
final Gradient gradient = RadialGradient(
colors: forward
? <Color>[swatch.shade50, swatch.shade900]
: <Color>[swatch.shade900, swatch.shade50],
);
final Paint paint = Paint()
..shader = gradient.createShader(Rect.fromCircle(
center: center,
radius: radius,
));
canvas.drawCircle(center, radius, paint);
}
@override
bool shouldRepaint(_GesturePainter oldPainter) {
return oldPainter.zoom != zoom
|| oldPainter.offset != offset
|| oldPainter.swatch != swatch
|| oldPainter.forward != forward
|| oldPainter.scaleEnabled != scaleEnabled
|| oldPainter.tapEnabled != tapEnabled
|| oldPainter.doubleTapEnabled != doubleTapEnabled
|| oldPainter.longPressEnabled != longPressEnabled;
}
}
class GestureDemo extends StatefulWidget {
const GestureDemo({super.key});
@override
GestureDemoState createState() => GestureDemoState();
}
class GestureDemoState extends State<GestureDemo> {
late Offset _startingFocalPoint;
late Offset _previousOffset;
Offset _offset = Offset.zero;
late double _previousZoom;
double _zoom = 1.0;
static const List<MaterialColor> kSwatches = <MaterialColor>[
Colors.red,
Colors.pink,
Colors.purple,
Colors.deepPurple,
Colors.indigo,
Colors.blue,
Colors.lightBlue,
Colors.cyan,
Colors.green,
Colors.lightGreen,
Colors.lime,
Colors.yellow,
Colors.amber,
Colors.orange,
Colors.deepOrange,
Colors.brown,
Colors.grey,
Colors.blueGrey,
];
int _swatchIndex = 0;
MaterialColor _swatch = kSwatches.first;
MaterialColor get swatch => _swatch;
bool _forward = true;
bool _scaleEnabled = true;
bool _tapEnabled = true;
bool _doubleTapEnabled = true;
bool _longPressEnabled = true;
void _handleScaleStart(ScaleStartDetails details) {
setState(() {
_startingFocalPoint = details.focalPoint;
_previousOffset = _offset;
_previousZoom = _zoom;
});
}
void _handleScaleUpdate(ScaleUpdateDetails details) {
setState(() {
_zoom = _previousZoom * details.scale;
// Ensure that item under the focal point stays in the same place despite zooming
final Offset normalizedOffset = (_startingFocalPoint - _previousOffset) / _previousZoom;
_offset = details.focalPoint - normalizedOffset * _zoom;
});
}
void _handleScaleReset() {
setState(() {
_zoom = 1.0;
_offset = Offset.zero;
});
}
void _handleColorChange() {
setState(() {
_swatchIndex += 1;
if (_swatchIndex == kSwatches.length) {
_swatchIndex = 0;
}
_swatch = kSwatches[_swatchIndex];
});
}
void _handleDirectionChange() {
setState(() {
_forward = !_forward;
});
}
@override
Widget build(BuildContext context) {
return Stack(
fit: StackFit.expand,
children: <Widget>[
GestureDetector(
onScaleStart: _scaleEnabled ? _handleScaleStart : null,
onScaleUpdate: _scaleEnabled ? _handleScaleUpdate : null,
onTap: _tapEnabled ? _handleColorChange : null,
onDoubleTap: _doubleTapEnabled ? _handleScaleReset : null,
onLongPress: _longPressEnabled ? _handleDirectionChange : null,
child: CustomPaint(
painter: _GesturePainter(
zoom: _zoom,
offset: _offset,
swatch: swatch,
forward: _forward,
scaleEnabled: _scaleEnabled,
tapEnabled: _tapEnabled,
doubleTapEnabled: _doubleTapEnabled,
longPressEnabled: _longPressEnabled,
),
),
),
Positioned(
bottom: 0.0,
left: 0.0,
child: Card(
child: Container(
padding: const EdgeInsets.all(4.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Row(
children: <Widget>[
Checkbox(
value: _scaleEnabled,
onChanged: (bool? value) { setState(() { _scaleEnabled = value!; }); },
),
const Text('Scale'),
],
),
Row(
children: <Widget>[
Checkbox(
value: _tapEnabled,
onChanged: (bool? value) { setState(() { _tapEnabled = value!; }); },
),
const Text('Tap'),
],
),
Row(
children: <Widget>[
Checkbox(
value: _doubleTapEnabled,
onChanged: (bool? value) { setState(() { _doubleTapEnabled = value!; }); },
),
const Text('Double Tap'),
],
),
Row(
children: <Widget>[
Checkbox(
value: _longPressEnabled,
onChanged: (bool? value) { setState(() { _longPressEnabled = value!; }); },
),
const Text('Long Press'),
],
),
],
),
),
),
),
],
);
}
}
void main() {
runApp(MaterialApp(
theme: ThemeData.dark(),
home: Scaffold(
appBar: AppBar(title: const Text('Gestures Demo')),
body: const GestureDemo(),
),
));
}
| flutter/examples/layers/widgets/gestures.dart/0 | {
"file_path": "flutter/examples/layers/widgets/gestures.dart",
"repo_id": "flutter",
"token_count": 3091
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "AppDelegate.h"
#import "GeneratedPluginRegistrant.h"
#import "PlatformViewController.h"
@implementation AppDelegate {
FlutterResult _flutterResult;
}
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[GeneratedPluginRegistrant registerWithRegistry:self];
FlutterViewController* controller =
(FlutterViewController*)self.window.rootViewController;
FlutterMethodChannel* channel =
[FlutterMethodChannel methodChannelWithName:@"samples.flutter.io/platform_view"
binaryMessenger:controller];
[channel setMethodCallHandler:^(FlutterMethodCall* call, FlutterResult result) {
if ([@"switchView" isEqualToString:call.method]) {
_flutterResult = result;
PlatformViewController* platformViewController =
[controller.storyboard instantiateViewControllerWithIdentifier:@"PlatformView"];
platformViewController.counter = ((NSNumber*)call.arguments).intValue;
platformViewController.delegate = self;
UINavigationController* navigationController =
[[UINavigationController alloc] initWithRootViewController:platformViewController];
navigationController.navigationBar.topItem.title = @"Platform View";
[controller presentViewController:navigationController animated:NO completion:nil];
} else {
result(FlutterMethodNotImplemented);
}
}];
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
- (void)didUpdateCounter:(int)counter {
_flutterResult([NSNumber numberWithInt:counter]);
}
@end
| flutter/examples/platform_view/ios/Runner/AppDelegate.m/0 | {
"file_path": "flutter/examples/platform_view/ios/Runner/AppDelegate.m",
"repo_id": "flutter",
"token_count": 546
} | 636 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/platform_view/macos/Flutter/Flutter-Release.xcconfig/0 | {
"file_path": "flutter/examples/platform_view/macos/Flutter/Flutter-Release.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 637 |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.Cocoa.XIB" version="3.0" toolsVersion="21208.1" targetRuntime="MacOSX.Cocoa" propertyAccessControl="none" useAutolayout="YES" customObjectInstantitationMethod="direct">
<dependencies>
<deployment identifier="macosx"/>
<plugIn identifier="com.apple.InterfaceBuilder.CocoaPlugin" version="21208.1"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<objects>
<customObject id="-2" userLabel="File's Owner" customClass="PlatformViewController" customModule="platform_view" customModuleProvider="target">
<connections>
<outlet property="label" destination="Eug-C1-PRv" id="wbw-1a-uP2"/>
<outlet property="view" destination="Hz6-mo-xeY" id="0bl-1N-x8E"/>
</connections>
</customObject>
<customObject id="-1" userLabel="First Responder" customClass="FirstResponder"/>
<customObject id="-3" userLabel="Application" customClass="NSObject"/>
<customView wantsLayer="YES" id="Hz6-mo-xeY" userLabel="Platform View">
<rect key="frame" x="0.0" y="0.0" width="587" height="356"/>
<autoresizingMask key="autoresizingMask" flexibleMaxX="YES" flexibleMinY="YES"/>
<subviews>
<stackView distribution="fill" orientation="vertical" alignment="leading" spacing="0.0" horizontalStackHuggingPriority="249.99998474121094" verticalStackHuggingPriority="249.99998474121094" detachesHiddenViews="YES" translatesAutoresizingMaskIntoConstraints="NO" id="asX-Cr-4ZL">
<rect key="frame" x="0.0" y="0.0" width="587" height="356"/>
<subviews>
<customView translatesAutoresizingMaskIntoConstraints="NO" id="LRL-SV-BW7" userLabel="Top">
<rect key="frame" x="0.0" y="70" width="587" height="286"/>
<subviews>
<textField horizontalHuggingPriority="251" verticalHuggingPriority="750" translatesAutoresizingMaskIntoConstraints="NO" id="Eug-C1-PRv">
<rect key="frame" x="193" y="133" width="202" height="21"/>
<textFieldCell key="cell" lineBreakMode="clipping" title="Button tapped X time(s)." id="e4z-H9-rSi">
<font key="font" metaFont="system" size="18"/>
<color key="textColor" name="labelColor" catalog="System" colorSpace="catalog"/>
<color key="backgroundColor" name="textBackgroundColor" catalog="System" colorSpace="catalog"/>
</textFieldCell>
</textField>
<button verticalHuggingPriority="750" translatesAutoresizingMaskIntoConstraints="NO" id="Jn3-XC-Bej">
<rect key="frame" x="204" y="90" width="179" height="32"/>
<buttonCell key="cell" type="push" title="Continue in Flutter View" bezelStyle="rounded" alignment="center" borderStyle="border" imageScaling="proportionallyDown" inset="2" id="1Sq-bc-nHD">
<behavior key="behavior" pushIn="YES" lightByBackground="YES" lightByGray="YES"/>
<font key="font" metaFont="system"/>
</buttonCell>
<connections>
<action selector="pop:" target="-2" id="KiK-HY-d8D"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstItem="Jn3-XC-Bej" firstAttribute="centerX" secondItem="Eug-C1-PRv" secondAttribute="centerX" id="0Wb-kD-KeP"/>
<constraint firstItem="Jn3-XC-Bej" firstAttribute="top" secondItem="Eug-C1-PRv" secondAttribute="bottom" constant="16" id="55w-Yj-iSV"/>
<constraint firstItem="Eug-C1-PRv" firstAttribute="centerX" secondItem="LRL-SV-BW7" secondAttribute="centerX" id="E6g-Q5-V9j"/>
<constraint firstItem="Eug-C1-PRv" firstAttribute="centerY" secondItem="LRL-SV-BW7" secondAttribute="centerY" id="FPs-Lq-4vX"/>
</constraints>
</customView>
<customView translatesAutoresizingMaskIntoConstraints="NO" id="ocC-s8-csF" userLabel="Bottom">
<rect key="frame" x="0.0" y="0.0" width="587" height="70"/>
<subviews>
<textField horizontalHuggingPriority="251" verticalHuggingPriority="750" translatesAutoresizingMaskIntoConstraints="NO" id="ibp-9J-zlZ">
<rect key="frame" x="18" y="12" width="100" height="35"/>
<textFieldCell key="cell" lineBreakMode="clipping" title="macOS" id="gQ0-QK-wuE">
<font key="font" metaFont="system" size="30"/>
<color key="textColor" name="labelColor" catalog="System" colorSpace="catalog"/>
<color key="backgroundColor" name="textBackgroundColor" catalog="System" colorSpace="catalog"/>
</textFieldCell>
</textField>
<button verticalHuggingPriority="750" misplaced="YES" translatesAutoresizingMaskIntoConstraints="NO" id="p1C-yr-atP">
<rect key="frame" x="540" y="13" width="34" height="32"/>
<buttonCell key="cell" type="push" title="+" bezelStyle="rounded" alignment="center" borderStyle="border" imageScaling="proportionallyDown" inset="2" id="Zhj-im-lGH">
<behavior key="behavior" pushIn="YES" lightByBackground="YES" lightByGray="YES"/>
<font key="font" metaFont="system"/>
</buttonCell>
<connections>
<action selector="increment:" target="-2" id="t7P-hc-bBq"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstAttribute="bottom" secondItem="p1C-yr-atP" secondAttribute="bottom" constant="20" symbolic="YES" id="O6I-0q-C2q"/>
<constraint firstItem="ibp-9J-zlZ" firstAttribute="leading" secondItem="ocC-s8-csF" secondAttribute="leading" constant="20" symbolic="YES" id="RaJ-kB-iMn"/>
<constraint firstAttribute="bottom" secondItem="ibp-9J-zlZ" secondAttribute="bottom" constant="12" id="bHW-eU-Qry"/>
<constraint firstAttribute="height" constant="70" id="ipW-qa-zLH"/>
<constraint firstAttribute="trailing" secondItem="p1C-yr-atP" secondAttribute="trailing" constant="20" symbolic="YES" id="rQt-7N-1IH"/>
</constraints>
</customView>
</subviews>
<visibilityPriorities>
<integer value="1000"/>
<integer value="1000"/>
</visibilityPriorities>
<customSpacing>
<real value="3.4028234663852886e+38"/>
<real value="3.4028234663852886e+38"/>
</customSpacing>
</stackView>
</subviews>
<constraints>
<constraint firstAttribute="trailing" secondItem="asX-Cr-4ZL" secondAttribute="trailing" id="2mi-qA-Xg7"/>
<constraint firstAttribute="bottom" secondItem="asX-Cr-4ZL" secondAttribute="bottom" id="4yQ-Dc-p85"/>
<constraint firstItem="asX-Cr-4ZL" firstAttribute="top" secondItem="Hz6-mo-xeY" secondAttribute="top" id="QUl-ar-P9E"/>
<constraint firstItem="asX-Cr-4ZL" firstAttribute="leading" secondItem="Hz6-mo-xeY" secondAttribute="leading" id="o34-Uf-9SV"/>
</constraints>
<point key="canvasLocation" x="138.5" y="96"/>
</customView>
</objects>
</document>
| flutter/examples/platform_view/macos/Runner/PlatformViewController.xib/0 | {
"file_path": "flutter/examples/platform_view/macos/Runner/PlatformViewController.xib",
"repo_id": "flutter",
"token_count": 4625
} | 638 |
# Take our settings from the repo's main analysis_options.yaml file, and include
# additional rules that are specific to production code.
include: ../analysis_options.yaml
linter:
rules:
- public_member_api_docs # see https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#documentation-dartdocs-javadocs-etc
- no_runtimeType_toString # use objectRuntimeType from package:foundation
| flutter/packages/analysis_options.yaml/0 | {
"file_path": "flutter/packages/analysis_options.yaml",
"repo_id": "flutter",
"token_count": 127
} | 639 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are from the Widgets library. *
# For fixes to
# * Actions: fix_actions.yaml
# * BuildContext: fix_build_context.yaml
# * Element: fix_element.yaml
# * ListWheelScrollView: fix_list_wheel_scroll_view.yaml
version: 1
transforms:
# Changes made in TBD
- title: "Migrate to focusNode.enclosingScope!"
date: 2023-11-29
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
getter: 'focusScopeNode'
inClass: 'NavigatorState'
changes:
- kind: 'rename'
newName: 'focusNode.enclosingScope!'
# Changes made in https://github.com/flutter/flutter/pull/138509
- title: "Migrate to 'PlatformMenuBar.child'"
date: 2023-11-15
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'body'
inClass: 'PlatformMenuBar'
changes:
- kind: 'rename'
newName: 'child'
- title: "Migrate PlatformMenuBar(body:) to PlatformMenuBar(child:)"
date: 2023-11-15
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'PlatformMenuBar'
changes:
- kind: 'renameParameter'
oldName: 'body'
newName: 'child'
# Changes made in https://github.com/flutter/flutter/pull/123352
- title: "Migrate to 'rootElement'"
date: 2023-03-13
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'renderViewElement'
inClass: 'WidgetsBinding'
changes:
- kind: 'rename'
newName: 'rootElement'
# Changes made in https://github.com/flutter/flutter/pull/122555
- title: "Migrate to 'decorationClipBehavior'"
date: 2023-03-13
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'clipBehavior'
inClass: 'ScrollableDetails'
changes:
- kind: 'rename'
newName: 'decorationClipBehavior'
# Changes made in https://github.com/flutter/flutter/pull/122555
- title: "Migrate to 'decorationClipBehavior'"
date: 2023-03-13
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'ScrollableDetails'
changes:
- kind: 'renameParameter'
oldName: 'clipBehavior'
newName: 'decorationClipBehavior'
# Changes made in https://github.com/flutter/flutter/pull/119647
- title: "Migrate to 'fromView'"
date: 2022-10-28
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: 'fromWindow'
inClass: 'MediaQueryData'
changes:
- kind: 'rename'
newName: 'fromView'
# Changes made in https://github.com/flutter/flutter/pull/119186 and https://github.com/flutter/flutter/pull/81067
- title: "Remove 'vsync'"
date: 2023-01-30
element:
uris: ['widgets.dart', 'material.dart', 'cupertino.dart']
constructor: ''
inClass: 'AnimatedSize'
changes:
- kind: 'removeParameter'
name: 'vsync'
# Changes made in https://github.com/flutter/flutter/pull/114459
- title: "Migrate to 'boldTextOf'"
date: 2022-10-28
element:
uris: ['widgets.dart', 'material.dart', 'cupertino.dart']
method: 'boldTextOverride'
inClass: 'MediaQuery'
changes:
- kind: 'rename'
newName: 'boldTextOf'
# Changes made in https://github.com/flutter/flutter/pull/87839
- title: "Migrate to 'disallowIndicator'"
date: 2021-08-06
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'disallowGlow'
inClass: 'OverscrollIndicatorNotification'
changes:
- kind: 'rename'
newName: 'disallowIndicator'
# Changes made in https://github.com/flutter/flutter/pull/66305
- title: "Migrate to 'clipBehavior'"
date: 2020-09-22
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'overflow'
inClass: 'Stack'
changes:
- kind: 'rename'
newName: 'clipBehavior'
# Changes made in https://github.com/flutter/flutter/pull/66305
- title: "Migrate to 'clipBehavior'"
date: 2020-09-22
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'Stack'
oneOf:
- if: "overflow == 'Overflow.clip'"
changes:
- kind: 'addParameter'
index: 0
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "overflow == 'Overflow.clip'"
- kind: 'removeParameter'
name: 'overflow'
- if: "overflow == 'Overflow.visible'"
changes:
- kind: 'addParameter'
index: 0
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "overflow == 'Overflow.visible'"
- kind: 'removeParameter'
name: 'overflow'
variables:
overflow:
kind: 'fragment'
value: 'arguments[overflow]'
# Changes made in https://github.com/flutter/flutter/pull/45941
- title: "Rename to 'deferFirstFrame'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'deferFirstFrameReport'
inClass: 'WidgetsBinding'
changes:
- kind: 'rename'
newName: 'deferFirstFrame'
# Changes made in https://github.com/flutter/flutter/pull/45941
- title: "Rename to 'allowFirstFrame'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'allowFirstFrameReport'
inClass: 'WidgetsBinding'
changes:
- kind: 'rename'
newName: 'allowFirstFrame'
# Changes made in https://github.com/flutter/flutter/pull/44189
- title: "Rename to 'dependOnInheritedElement'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'inheritFromElement'
inClass: 'StatefulElement'
changes:
- kind: 'rename'
newName: 'dependOnInheritedElement'
# Changes made in https://github.com/flutter/flutter/pull/61648
- title: "Migrate to 'autovalidateMode'"
date: 2020-09-02
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'autovalidate'
inClass: 'Form'
changes:
- kind: 'rename'
newName: 'autovalidateMode'
# Changes made in https://github.com/flutter/flutter/pull/61648
- title: "Migrate to 'autovalidateMode'"
date: 2020-09-02
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'Form'
oneOf:
- if: "autovalidate == 'true'"
changes:
- kind: 'addParameter'
index: 0
name: 'autovalidateMode'
style: optional_named
argumentValue:
expression: 'AutovalidateMode.always'
requiredIf: "autovalidate == 'true'"
- kind: 'removeParameter'
name: 'autovalidate'
- if: "autovalidate == 'false'"
changes:
- kind: 'addParameter'
index: 0
name: 'autovalidateMode'
style: optional_named
argumentValue:
expression: 'AutovalidateMode.disabled'
requiredIf: "autovalidate == 'false'"
- kind: 'removeParameter'
name: 'autovalidate'
variables:
autovalidate:
kind: 'fragment'
value: 'arguments[autovalidate]'
# Changes made in https://github.com/flutter/flutter/pull/61648
- title: "Migrate to 'autovalidateMode'"
date: 2020-09-02
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'autovalidate'
inClass: 'FormField'
changes:
- kind: 'rename'
newName: 'autovalidateMode'
# Changes made in https://github.com/flutter/flutter/pull/61648
- title: "Migrate to 'autovalidateMode'"
date: 2020-09-02
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'FormField'
oneOf:
- if: "autovalidate == 'true'"
changes:
- kind: 'addParameter'
index: 0
name: 'autovalidateMode'
style: optional_named
argumentValue:
expression: 'AutovalidateMode.always'
requiredIf: "autovalidate == 'true'"
- kind: 'removeParameter'
name: 'autovalidate'
- if: "autovalidate == 'false'"
changes:
- kind: 'addParameter'
index: 0
name: 'autovalidateMode'
style: optional_named
argumentValue:
expression: 'AutovalidateMode.disabled'
requiredIf: "autovalidate == 'false'"
- kind: 'removeParameter'
name: 'autovalidate'
variables:
autovalidate:
kind: 'fragment'
value: 'arguments[autovalidate]'
# Changes made in https://github.com/flutter/flutter/pull/70726.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'Navigator'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68736.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'MediaQuery'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68925.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'SliverAnimatedList'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68925.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'AnimatedList'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68921.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'Shortcuts'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68917.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'Focus'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68917.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'FocusTraversalGroup'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68917.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'FocusTraversalOrder'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68911.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'localeOf'
inClass: 'Localizations'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeLocaleOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68910.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'of'
inClass: 'Router'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeOf'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/59127
- title: "Migrate to 'label'"
date: 2020-07-09
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'title'
inClass: 'BottomNavigationBarItem'
changes:
- kind: 'rename'
newName: 'label'
# Changes made in https://github.com/flutter/flutter/pull/59127
- title: "Migrate to 'label'"
date: 2020-07-09
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'BottomNavigationBarItem'
changes:
- kind: 'renameParameter'
oldName: 'title'
newName: 'label'
# Changes made in https://github.com/flutter/flutter/pull/79160
- title: "Migrate to 'dragAnchorStrategy'"
date: 2021-04-05
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'dragAnchor'
inClass: 'Draggable'
changes:
- kind: 'rename'
newName: 'dragAnchorStrategy'
# Changes made in https://github.com/flutter/flutter/pull/79160
- title: "Migrate to 'dragAnchorStrategy'"
date: 2021-04-05
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'Draggable'
oneOf:
- if: "dragAnchor == 'DragAnchor.child'"
changes:
- kind: 'addParameter'
index: 9
name: 'dragAnchorStrategy'
style: optional_named
argumentValue:
expression: 'childDragAnchorStrategy'
requiredIf: "dragAnchor == 'DragAnchor.child'"
- kind: 'removeParameter'
name: 'dragAnchor'
- if: "dragAnchor == 'DragAnchor.pointer'"
changes:
- kind: 'addParameter'
index: 9
name: 'dragAnchorStrategy'
style: optional_named
argumentValue:
expression: 'pointerDragAnchorStrategy'
requiredIf: "dragAnchor == 'DragAnchor.pointer'"
- kind: 'removeParameter'
name: 'dragAnchor'
variables:
dragAnchor:
kind: 'fragment'
value: 'arguments[dragAnchor]'
# Changes made in https://github.com/flutter/flutter/pull/79160
- title: "Migrate to 'dragAnchorStrategy'"
date: 2021-04-05
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'dragAnchor'
inClass: 'LongPressDraggable'
changes:
- kind: 'rename'
newName: 'dragAnchorStrategy'
# Changes made in https://github.com/flutter/flutter/pull/79160
- title: "Migrate to 'dragAnchorStrategy'"
date: 2021-04-05
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'LongPressDraggable'
oneOf:
- if: "dragAnchor == 'DragAnchor.child'"
changes:
- kind: 'addParameter'
index: 9
name: 'dragAnchorStrategy'
style: optional_named
argumentValue:
expression: 'childDragAnchorStrategy'
requiredIf: "dragAnchor == 'DragAnchor.child'"
- kind: 'removeParameter'
name: 'dragAnchor'
- if: "dragAnchor == 'DragAnchor.pointer'"
changes:
- kind: 'addParameter'
index: 9
name: 'dragAnchorStrategy'
style: optional_named
argumentValue:
expression: 'pointerDragAnchorStrategy'
requiredIf: "dragAnchor == 'DragAnchor.pointer'"
- kind: 'removeParameter'
name: 'dragAnchor'
variables:
dragAnchor:
kind: 'fragment'
value: 'arguments[dragAnchor]'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'RenderObjectToWidgetElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'RenderObjectToWidgetElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'RenderObjectToWidgetElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'SliverMultiBoxAdaptorElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'SliverMultiBoxAdaptorElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'SliverMultiBoxAdaptorElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'SingleChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'SingleChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'SingleChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'MultiChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'MultiChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'MultiChildRenderObjectElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'ListWheelElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'ListWheelElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'ListWheelElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'removeRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'removeChildRenderObject'
inClass: 'LeafRenderObjectElement'
changes:
- kind: 'rename'
newName: 'removeRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'moveRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'moveChildRenderObject'
inClass: 'LeafRenderObjectElement'
changes:
- kind: 'rename'
newName: 'moveRenderObjectChild'
# Changes made in https://github.com/flutter/flutter/pull/64254
- title: "Migrate to 'insertRenderObjectChild'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'insertChildRenderObject'
inClass: 'LeafRenderObjectElement'
changes:
- kind: 'rename'
newName: 'insertRenderObjectChild'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'clipToSize'
inClass: 'ListWheelViewport'
changes:
- kind: 'rename'
newName: 'clipBehavior'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'ListWheelViewport'
oneOf:
- if: "clipToSize == 'true'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "clipToSize == 'true'"
- kind: 'removeParameter'
name: 'clipToSize'
- if: "clipToSize == 'false'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "clipToSize == 'false'"
- kind: 'removeParameter'
name: 'clipToSize'
variables:
clipToSize:
kind: 'fragment'
value: 'arguments[clipToSize]'
# Changes made in https://github.com/flutter/flutter/pull/96957
- title: "Migrate to 'thumbVisibility'"
date: 2022-01-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'isAlwaysShown'
inClass: 'RawScrollbar'
changes:
- kind: 'rename'
newName: 'thumbVisibility'
# Changes made in https://github.com/flutter/flutter/pull/96957
- title: "Migrate to 'thumbVisibility'"
date: 2022-01-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'RawScrollbar'
changes:
- kind: 'renameParameter'
oldName: 'isAlwaysShown'
newName: 'thumbVisibility'
# Changes made in https://github.com/flutter/flutter/pull/100381
- title: "Migrate 'TextSelectionOverlay.fadeDuration' to SelectionOverlay.fadeDuration"
date: 2022-03-18
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'fadeDuration'
inClass: 'TextSelectionOverlay'
changes:
- kind: 'replacedBy'
newElement:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'fadeDuration'
inClass: 'SelectionOverlay'
# Changes made in https://github.com/flutter/flutter/pull/78588
- title: "Migrate to 'buildOverscrollIndicator'"
date: 2021-03-18
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'buildViewportChrome'
inClass: 'ScrollBehavior'
changes:
- kind: 'rename'
newName: 'buildOverscrollIndicator'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_widgets/fix_widgets.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_widgets/fix_widgets.yaml",
"repo_id": "flutter",
"token_count": 13469
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show Color, Rect, Size;
import 'package:flutter/foundation.dart';
import 'animations.dart';
export 'dart:ui' show Color, Rect, Size;
export 'animation.dart' show Animation;
export 'curves.dart' show Curve;
// Examples can assume:
// late Animation<Offset> _animation;
// late AnimationController _controller;
/// A typedef used by [Animatable.fromCallback] to create an [Animatable]
/// from a callback.
typedef AnimatableCallback<T> = T Function(double value);
/// An object that can produce a value of type `T` given an [Animation<double>]
/// as input.
///
/// Typically, the values of the input animation are nominally in the range 0.0
/// to 1.0. In principle, however, any value could be provided.
///
/// The main subclass of [Animatable] is [Tween].
abstract class Animatable<T> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const Animatable();
/// Create a new [Animatable] from the provided [callback].
///
/// See also:
///
/// * [Animation.drive], which provides an example for how this can be
/// used.
const factory Animatable.fromCallback(AnimatableCallback<T> callback) = _CallbackAnimatable<T>;
/// Returns the value of the object at point `t`.
///
/// The value of `t` is nominally a fraction in the range 0.0 to 1.0, though
/// in practice it may extend outside this range.
///
/// See also:
///
/// * [evaluate], which is a shorthand for applying [transform] to the value
/// of an [Animation].
/// * [Curve.transform], a similar method for easing curves.
T transform(double t);
/// The current value of this object for the given [Animation].
///
/// This function is implemented by deferring to [transform]. Subclasses that
/// want to provide custom behavior should override [transform], not
/// [evaluate].
///
/// See also:
///
/// * [transform], which is similar but takes a `t` value directly instead of
/// an [Animation].
/// * [animate], which creates an [Animation] out of this object, continually
/// applying [evaluate].
T evaluate(Animation<double> animation) => transform(animation.value);
/// Returns a new [Animation] that is driven by the given animation but that
/// takes on values determined by this object.
///
/// Essentially this returns an [Animation] that automatically applies the
/// [evaluate] method to the parent's value.
///
/// See also:
///
/// * [AnimationController.drive], which does the same thing from the
/// opposite starting point.
Animation<T> animate(Animation<double> parent) {
return _AnimatedEvaluation<T>(parent, this);
}
/// Returns a new [Animatable] whose value is determined by first evaluating
/// the given parent and then evaluating this object.
///
/// This allows [Tween]s to be chained before obtaining an [Animation].
Animatable<T> chain(Animatable<double> parent) {
return _ChainedEvaluation<T>(parent, this);
}
}
// A concrete subclass of `Animatable` used by `Animatable.fromCallback`.
class _CallbackAnimatable<T> extends Animatable<T> {
const _CallbackAnimatable(this._callback);
final AnimatableCallback<T> _callback;
@override
T transform(double t) {
return _callback(t);
}
}
class _AnimatedEvaluation<T> extends Animation<T> with AnimationWithParentMixin<double> {
_AnimatedEvaluation(this.parent, this._evaluatable);
@override
final Animation<double> parent;
final Animatable<T> _evaluatable;
@override
T get value => _evaluatable.evaluate(parent);
@override
String toString() {
return '$parent\u27A9$_evaluatable\u27A9$value';
}
@override
String toStringDetails() {
return '${super.toStringDetails()} $_evaluatable';
}
}
class _ChainedEvaluation<T> extends Animatable<T> {
_ChainedEvaluation(this._parent, this._evaluatable);
final Animatable<double> _parent;
final Animatable<T> _evaluatable;
@override
T transform(double t) {
return _evaluatable.transform(_parent.transform(t));
}
@override
String toString() {
return '$_parent\u27A9$_evaluatable';
}
}
/// A linear interpolation between a beginning and ending value.
///
/// [Tween] is useful if you want to interpolate across a range.
///
/// To use a [Tween] object with an animation, call the [Tween] object's
/// [animate] method and pass it the [Animation] object that you want to
/// modify.
///
/// You can chain [Tween] objects together using the [chain] method, so that a
/// single [Animation] object is configured by multiple [Tween] objects called
/// in succession. This is different than calling the [animate] method twice,
/// which results in two separate [Animation] objects, each configured with a
/// single [Tween].
///
/// {@tool snippet}
///
/// Suppose `_controller` is an [AnimationController], and we want to create an
/// [Animation<Offset>] that is controlled by that controller, and save it in
/// `_animation`. Here are two possible ways of expressing this:
///
/// ```dart
/// _animation = _controller.drive(
/// Tween<Offset>(
/// begin: const Offset(100.0, 50.0),
/// end: const Offset(200.0, 300.0),
/// ),
/// );
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// ```dart
/// _animation = Tween<Offset>(
/// begin: const Offset(100.0, 50.0),
/// end: const Offset(200.0, 300.0),
/// ).animate(_controller);
/// ```
/// {@end-tool}
///
/// In both cases, the `_animation` variable holds an object that, over the
/// lifetime of the `_controller`'s animation, returns a value
/// (`_animation.value`) that depicts a point along the line between the two
/// offsets above. If we used a [MaterialPointArcTween] instead of a
/// [Tween<Offset>] in the code above, the points would follow a pleasing curve
/// instead of a straight line, with no other changes necessary.
///
/// ## Performance optimizations
///
/// Tweens are mutable; specifically, their [begin] and [end] values can be
/// changed at runtime. An object created with [Animation.drive] using a [Tween]
/// will immediately honor changes to that underlying [Tween] (though the
/// listeners will only be triggered if the [Animation] is actively animating).
/// This can be used to change an animation on the fly without having to
/// recreate all the objects in the chain from the [AnimationController] to the
/// final [Tween].
///
/// If a [Tween]'s values are never changed, however, a further optimization can
/// be applied: the object can be stored in a `static final` variable, so that
/// the exact same instance is used whenever the [Tween] is needed. This is
/// preferable to creating an identical [Tween] afresh each time a [State.build]
/// method is called, for example.
///
/// ## Types with special considerations
///
/// Classes with [lerp] static methods typically have corresponding dedicated
/// [Tween] subclasses that call that method. For example, [ColorTween] uses
/// [Color.lerp] to implement the [ColorTween.lerp] method.
///
/// Types that define `+` and `-` operators to combine values (`T + T → T` and
/// `T - T → T`) and an `*` operator to scale by multiplying with a double (`T *
/// double → T`) can be directly used with `Tween<T>`.
///
/// This does not extend to any type with `+`, `-`, and `*` operators. In
/// particular, [int] does not satisfy this precise contract (`int * double`
/// actually returns [num], not [int]). There are therefore two specific classes
/// that can be used to interpolate integers:
///
/// * [IntTween], which is an approximation of a linear interpolation (using
/// [double.round]).
/// * [StepTween], which uses [double.floor] to ensure that the result is
/// never greater than it would be using if a `Tween<double>`.
///
/// The relevant operators on [Size] also don't fulfill this contract, so
/// [SizeTween] uses [Size.lerp].
///
/// In addition, some of the types that _do_ have suitable `+`, `-`, and `*`
/// operators still have dedicated [Tween] subclasses that perform the
/// interpolation in a more specialized manner. One such class is
/// [MaterialPointArcTween], which is mentioned above. The [AlignmentTween], and
/// [AlignmentGeometryTween], and [FractionalOffsetTween] are another group of
/// [Tween]s that use dedicated `lerp` methods instead of merely relying on the
/// operators (in particular, this allows them to handle null values in a more
/// useful manner).
///
/// ## Nullability
///
/// The [begin] and [end] fields are nullable; a [Tween] does not have to
/// have non-null values specified when it is created.
///
/// If `T` is nullable, then [lerp] and [transform] may return null.
/// This is typically seen in the case where [begin] is null and `t`
/// is 0.0, or [end] is null and `t` is 1.0, or both are null (at any
/// `t` value).
///
/// If `T` is not nullable, then [begin] and [end] must both be set to
/// non-null values before using [lerp] or [transform], otherwise they
/// will throw.
///
/// ## Implementing a Tween
///
/// To specialize this class for a new type, the subclass should implement
/// the [lerp] method (and a constructor). The other methods of this class
/// are all defined in terms of [lerp].
class Tween<T extends Object?> extends Animatable<T> {
/// Creates a tween.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
Tween({
this.begin,
this.end,
});
/// The value this variable has at the beginning of the animation.
///
/// See the constructor for details about whether this property may be null
/// (it varies from subclass to subclass).
T? begin;
/// The value this variable has at the end of the animation.
///
/// See the constructor for details about whether this property may be null
/// (it varies from subclass to subclass).
T? end;
/// Returns the value this variable has at the given animation clock value.
///
/// The default implementation of this method uses the `+`, `-`, and `*`
/// operators on `T`. The [begin] and [end] properties must therefore be
/// non-null by the time this method is called.
///
/// In general, however, it is possible for this to return null, especially
/// when `t`=0.0 and [begin] is null, or `t`=1.0 and [end] is null.
@protected
T lerp(double t) {
assert(begin != null);
assert(end != null);
assert(() {
// Assertions that attempt to catch common cases of tweening types
// that do not conform to the Tween requirements.
dynamic result;
try {
// ignore: avoid_dynamic_calls
result = (begin as dynamic) + ((end as dynamic) - (begin as dynamic)) * t;
result as T;
return true;
} on NoSuchMethodError {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot lerp between "$begin" and "$end".'),
ErrorDescription(
'The type ${begin.runtimeType} might not fully implement `+`, `-`, and/or `*`. '
'See "Types with special considerations" at https://api.flutter.dev/flutter/animation/Tween-class.html '
'for more information.',
),
if (begin is Color || end is Color)
ErrorHint('To lerp colors, consider ColorTween instead.')
else if (begin is Rect || end is Rect)
ErrorHint('To lerp rects, consider RectTween instead.')
else
ErrorHint(
'There may be a dedicated "${begin.runtimeType}Tween" for this type, '
'or you may need to create one.',
),
]);
} on TypeError {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot lerp between "$begin" and "$end".'),
ErrorDescription(
'The type ${begin.runtimeType} returned a ${result.runtimeType} after '
'multiplication with a double value. '
'See "Types with special considerations" at https://api.flutter.dev/flutter/animation/Tween-class.html '
'for more information.',
),
if (begin is int || end is int)
ErrorHint('To lerp int values, consider IntTween or StepTween instead.')
else
ErrorHint(
'There may be a dedicated "${begin.runtimeType}Tween" for this type, '
'or you may need to create one.',
),
]);
}
}());
// ignore: avoid_dynamic_calls
return (begin as dynamic) + ((end as dynamic) - (begin as dynamic)) * t as T;
}
/// Returns the interpolated value for the current value of the given animation.
///
/// This method returns `begin` and `end` when the animation values are 0.0 or
/// 1.0, respectively.
///
/// This function is implemented by deferring to [lerp]. Subclasses that want
/// to provide custom behavior should override [lerp], not [transform] (nor
/// [evaluate]).
///
/// See the constructor for details about whether the [begin] and [end]
/// properties may be null when this is called. It varies from subclass to
/// subclass.
@override
T transform(double t) {
if (t == 0.0) {
return begin as T;
}
if (t == 1.0) {
return end as T;
}
return lerp(t);
}
@override
String toString() => '${objectRuntimeType(this, 'Animatable')}($begin \u2192 $end)';
}
/// A [Tween] that evaluates its [parent] in reverse.
class ReverseTween<T extends Object?> extends Tween<T> {
/// Construct a [Tween] that evaluates its [parent] in reverse.
ReverseTween(this.parent)
: super(begin: parent.end, end: parent.begin);
/// This tween's value is the same as the parent's value evaluated in reverse.
///
/// This tween's [begin] is the parent's [end] and its [end] is the parent's
/// [begin]. The [lerp] method returns `parent.lerp(1.0 - t)` and its
/// [evaluate] method is similar.
final Tween<T> parent;
@override
T lerp(double t) => parent.lerp(1.0 - t);
}
/// An interpolation between two colors.
///
/// This class specializes the interpolation of [Tween<Color>] to use
/// [Color.lerp].
///
/// The values can be null, representing no color (which is distinct to
/// transparent black, as represented by [Colors.transparent]).
///
/// See [Tween] for a discussion on how to use interpolation objects.
class ColorTween extends Tween<Color?> {
/// Creates a [Color] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as transparent.
///
/// We recommend that you do not pass [Colors.transparent] as [begin]
/// or [end] if you want the effect of fading in or out of transparent.
/// Instead prefer null. [Colors.transparent] refers to black transparent and
/// thus will fade out of or into black which is likely unwanted.
ColorTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
Color? lerp(double t) => Color.lerp(begin, end, t);
}
/// An interpolation between two sizes.
///
/// This class specializes the interpolation of [Tween<Size>] to use
/// [Size.lerp].
///
/// The values can be null, representing [Size.zero].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class SizeTween extends Tween<Size?> {
/// Creates a [Size] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as an empty size.
SizeTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
Size? lerp(double t) => Size.lerp(begin, end, t);
}
/// An interpolation between two rectangles.
///
/// This class specializes the interpolation of [Tween<Rect>] to use
/// [Rect.lerp].
///
/// The values can be null, representing a zero-sized rectangle at the
/// origin ([Rect.zero]).
///
/// See [Tween] for a discussion on how to use interpolation objects.
class RectTween extends Tween<Rect?> {
/// Creates a [Rect] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as an empty rect at the top left corner.
RectTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
Rect? lerp(double t) => Rect.lerp(begin, end, t);
}
/// An interpolation between two integers that rounds.
///
/// This class specializes the interpolation of [Tween<int>] to be
/// appropriate for integers by interpolating between the given begin
/// and end values and then rounding the result to the nearest
/// integer.
///
/// This is the closest approximation to a linear tween that is possible with an
/// integer. Compare to [StepTween] and [Tween<double>].
///
/// The [begin] and [end] values must be set to non-null values before
/// calling [lerp] or [transform].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class IntTween extends Tween<int> {
/// Creates an int tween.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
IntTween({ super.begin, super.end });
// The inherited lerp() function doesn't work with ints because it multiplies
// the begin and end types by a double, and int * double returns a double.
@override
int lerp(double t) => (begin! + (end! - begin!) * t).round();
}
/// An interpolation between two integers that floors.
///
/// This class specializes the interpolation of [Tween<int>] to be
/// appropriate for integers by interpolating between the given begin
/// and end values and then using [double.floor] to return the current
/// integer component, dropping the fractional component.
///
/// This results in a value that is never greater than the equivalent
/// value from a linear double interpolation. Compare to [IntTween].
///
/// The [begin] and [end] values must be set to non-null values before
/// calling [lerp] or [transform].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class StepTween extends Tween<int> {
/// Creates an [int] tween that floors.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
StepTween({ super.begin, super.end });
// The inherited lerp() function doesn't work with ints because it multiplies
// the begin and end types by a double, and int * double returns a double.
@override
int lerp(double t) => (begin! + (end! - begin!) * t).floor();
}
/// A tween with a constant value.
class ConstantTween<T> extends Tween<T> {
/// Create a tween whose [begin] and [end] values equal [value].
ConstantTween(T value) : super(begin: value, end: value);
/// This tween doesn't interpolate, it always returns the same value.
@override
T lerp(double t) => begin as T;
@override
String toString() => '${objectRuntimeType(this, 'ConstantTween')}(value: $begin)';
}
/// Transforms the value of the given animation by the given curve.
///
/// This class differs from [CurvedAnimation] in that [CurvedAnimation] applies
/// a curve to an existing [Animation] object whereas [CurveTween] can be
/// chained with another [Tween] prior to receiving the underlying [Animation].
/// ([CurvedAnimation] also has the additional ability of having different
/// curves when the animation is going forward vs when it is going backward,
/// which can be useful in some scenarios.)
///
/// {@tool snippet}
///
/// The following code snippet shows how you can apply a curve to a linear
/// animation produced by an [AnimationController] `controller`:
///
/// ```dart
/// final Animation<double> animation = _controller.drive(
/// CurveTween(curve: Curves.ease),
/// );
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CurvedAnimation], for an alternative way of expressing the sample above.
/// * [AnimationController], for examples of creating and disposing of an
/// [AnimationController].
class CurveTween extends Animatable<double> {
/// Creates a curve tween.
CurveTween({ required this.curve });
/// The curve to use when transforming the value of the animation.
Curve curve;
@override
double transform(double t) {
if (t == 0.0 || t == 1.0) {
assert(curve.transform(t).round() == t);
return t;
}
return curve.transform(t);
}
@override
String toString() => '${objectRuntimeType(this, 'CurveTween')}(curve: $curve)';
}
| flutter/packages/flutter/lib/src/animation/tween.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/animation/tween.dart",
"repo_id": "flutter",
"token_count": 6382
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/widgets.dart';
import 'button.dart';
import 'colors.dart';
import 'text_selection_toolbar_button.dart';
import 'theme.dart';
// These values were measured from a screenshot of the native context menu on
// macOS 13.2 on a Macbook Pro.
const TextStyle _kToolbarButtonFontStyle = TextStyle(
inherit: false,
fontSize: 14.0,
letterSpacing: -0.15,
fontWeight: FontWeight.w400,
);
// This value was measured from a screenshot of the native context menu on
// macOS 13.2 on a Macbook Pro.
const EdgeInsets _kToolbarButtonPadding = EdgeInsets.fromLTRB(
8.0,
2.0,
8.0,
5.0,
);
/// A button in the style of the Mac context menu buttons.
class CupertinoDesktopTextSelectionToolbarButton extends StatefulWidget {
/// Creates an instance of CupertinoDesktopTextSelectionToolbarButton.
const CupertinoDesktopTextSelectionToolbarButton({
super.key,
required this.onPressed,
required Widget this.child,
}) : buttonItem = null,
text = null;
/// Create an instance of [CupertinoDesktopTextSelectionToolbarButton] whose child is
/// a [Text] widget styled like the default Mac context menu button.
const CupertinoDesktopTextSelectionToolbarButton.text({
super.key,
required this.onPressed,
required this.text,
}) : buttonItem = null,
child = null;
/// Create an instance of [CupertinoDesktopTextSelectionToolbarButton] from
/// the given [ContextMenuButtonItem].
CupertinoDesktopTextSelectionToolbarButton.buttonItem({
super.key,
required ContextMenuButtonItem this.buttonItem,
}) : onPressed = buttonItem.onPressed,
text = null,
child = null;
/// {@macro flutter.cupertino.CupertinoTextSelectionToolbarButton.onPressed}
final VoidCallback? onPressed;
/// {@macro flutter.cupertino.CupertinoTextSelectionToolbarButton.child}
final Widget? child;
/// {@macro flutter.cupertino.CupertinoTextSelectionToolbarButton.onPressed}
final ContextMenuButtonItem? buttonItem;
/// {@macro flutter.cupertino.CupertinoTextSelectionToolbarButton.text}
final String? text;
@override
State<CupertinoDesktopTextSelectionToolbarButton> createState() =>
_CupertinoDesktopTextSelectionToolbarButtonState();
}
class _CupertinoDesktopTextSelectionToolbarButtonState
extends State<CupertinoDesktopTextSelectionToolbarButton> {
bool _isHovered = false;
void _onEnter(PointerEnterEvent event) {
setState(() {
_isHovered = true;
});
}
void _onExit(PointerExitEvent event) {
setState(() {
_isHovered = false;
});
}
@override
Widget build(BuildContext context) {
final Widget child = widget.child ??
Text(
widget.text ??
CupertinoTextSelectionToolbarButton.getButtonLabel(
context,
widget.buttonItem!,
),
overflow: TextOverflow.ellipsis,
style: _kToolbarButtonFontStyle.copyWith(
color: _isHovered
? CupertinoTheme.of(context).primaryContrastingColor
: const CupertinoDynamicColor.withBrightness(
color: CupertinoColors.black,
darkColor: CupertinoColors.white,
).resolveFrom(context),
),
);
return SizedBox(
width: double.infinity,
child: MouseRegion(
onEnter: _onEnter,
onExit: _onExit,
child: CupertinoButton(
alignment: Alignment.centerLeft,
borderRadius: const BorderRadius.all(Radius.circular(4.0)),
color: _isHovered ? CupertinoTheme.of(context).primaryColor : null,
minSize: 0.0,
onPressed: widget.onPressed,
padding: _kToolbarButtonPadding,
pressedOpacity: 0.7,
child: child,
),
),
);
}
}
| flutter/packages/flutter/lib/src/cupertino/desktop_text_selection_toolbar_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/desktop_text_selection_toolbar_button.dart",
"repo_id": "flutter",
"token_count": 1555
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'dart:ui' show ImageFilter, lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'interface_level.dart';
import 'localizations.dart';
const double _kBackGestureWidth = 20.0;
const double _kMinFlingVelocity = 1.0; // Screen widths per second.
// An eyeballed value for the maximum time it takes for a page to animate forward
// if the user releases a page mid swipe.
const int _kMaxDroppedSwipePageForwardAnimationTime = 800; // Milliseconds.
// The maximum time for a page to get reset to it's original position if the
// user releases a page mid swipe.
const int _kMaxPageBackAnimationTime = 300; // Milliseconds.
/// Barrier color used for a barrier visible during transitions for Cupertino
/// page routes.
///
/// This barrier color is only used for full-screen page routes with
/// `fullscreenDialog: false`.
///
/// By default, `fullscreenDialog` Cupertino route transitions have no
/// `barrierColor`, and [CupertinoDialogRoute]s and [CupertinoModalPopupRoute]s
/// have a `barrierColor` defined by [kCupertinoModalBarrierColor].
///
/// A relatively rigorous eyeball estimation.
const Color _kCupertinoPageTransitionBarrierColor = Color(0x18000000);
/// Barrier color for a Cupertino modal barrier.
///
/// Extracted from https://developer.apple.com/design/resources/.
const Color kCupertinoModalBarrierColor = CupertinoDynamicColor.withBrightness(
color: Color(0x33000000),
darkColor: Color(0x7A000000),
);
// The duration of the transition used when a modal popup is shown.
const Duration _kModalPopupTransitionDuration = Duration(milliseconds: 335);
// Offset from offscreen to the right to fully on screen.
final Animatable<Offset> _kRightMiddleTween = Tween<Offset>(
begin: const Offset(1.0, 0.0),
end: Offset.zero,
);
// Offset from fully on screen to 1/3 offscreen to the left.
final Animatable<Offset> _kMiddleLeftTween = Tween<Offset>(
begin: Offset.zero,
end: const Offset(-1.0/3.0, 0.0),
);
// Offset from offscreen below to fully on screen.
final Animatable<Offset> _kBottomUpTween = Tween<Offset>(
begin: const Offset(0.0, 1.0),
end: Offset.zero,
);
/// A mixin that replaces the entire screen with an iOS transition for a
/// [PageRoute].
///
/// {@template flutter.cupertino.cupertinoRouteTransitionMixin}
/// The page slides in from the right and exits in reverse. The page also shifts
/// to the left in parallax when another page enters to cover it.
///
/// The page slides in from the bottom and exits in reverse with no parallax
/// effect for fullscreen dialogs.
/// {@endtemplate}
///
/// See also:
///
/// * [MaterialRouteTransitionMixin], which is a mixin that provides
/// platform-appropriate transitions for a [PageRoute].
/// * [CupertinoPageRoute], which is a [PageRoute] that leverages this mixin.
mixin CupertinoRouteTransitionMixin<T> on PageRoute<T> {
/// Builds the primary contents of the route.
@protected
Widget buildContent(BuildContext context);
/// {@template flutter.cupertino.CupertinoRouteTransitionMixin.title}
/// A title string for this route.
///
/// Used to auto-populate [CupertinoNavigationBar] and
/// [CupertinoSliverNavigationBar]'s `middle`/`largeTitle` widgets when
/// one is not manually supplied.
/// {@endtemplate}
String? get title;
ValueNotifier<String?>? _previousTitle;
/// The title string of the previous [CupertinoPageRoute].
///
/// The [ValueListenable]'s value is readable after the route is installed
/// onto a [Navigator]. The [ValueListenable] will also notify its listeners
/// if the value changes (such as by replacing the previous route).
///
/// The [ValueListenable] itself will be null before the route is installed.
/// Its content value will be null if the previous route has no title or
/// is not a [CupertinoPageRoute].
///
/// See also:
///
/// * [ValueListenableBuilder], which can be used to listen and rebuild
/// widgets based on a ValueListenable.
ValueListenable<String?> get previousTitle {
assert(
_previousTitle != null,
'Cannot read the previousTitle for a route that has not yet been installed',
);
return _previousTitle!;
}
@override
void dispose() {
_previousTitle?.dispose();
super.dispose();
}
@override
void didChangePrevious(Route<dynamic>? previousRoute) {
final String? previousTitleString = previousRoute is CupertinoRouteTransitionMixin
? previousRoute.title
: null;
if (_previousTitle == null) {
_previousTitle = ValueNotifier<String?>(previousTitleString);
} else {
_previousTitle!.value = previousTitleString;
}
super.didChangePrevious(previousRoute);
}
@override
// A relatively rigorous eyeball estimation.
Duration get transitionDuration => const Duration(milliseconds: 500);
@override
Color? get barrierColor => fullscreenDialog ? null : _kCupertinoPageTransitionBarrierColor;
@override
String? get barrierLabel => null;
@override
bool canTransitionTo(TransitionRoute<dynamic> nextRoute) {
// Don't perform outgoing animation if the next route is a fullscreen dialog.
return nextRoute is CupertinoRouteTransitionMixin && !nextRoute.fullscreenDialog;
}
/// True if an iOS-style back swipe pop gesture is currently underway for [route].
///
/// This just checks the route's [NavigatorState.userGestureInProgress].
///
/// See also:
///
/// * [popGestureEnabled], which returns true if a user-triggered pop gesture
/// would be allowed.
static bool isPopGestureInProgress(PageRoute<dynamic> route) {
return route.navigator!.userGestureInProgress;
}
/// True if an iOS-style back swipe pop gesture is currently underway for this route.
///
/// See also:
///
/// * [isPopGestureInProgress], which returns true if a Cupertino pop gesture
/// is currently underway for specific route.
/// * [popGestureEnabled], which returns true if a user-triggered pop gesture
/// would be allowed.
bool get popGestureInProgress => isPopGestureInProgress(this);
/// Whether a pop gesture can be started by the user.
///
/// Returns true if the user can edge-swipe to a previous route.
///
/// Returns false once [isPopGestureInProgress] is true, but
/// [isPopGestureInProgress] can only become true if [popGestureEnabled] was
/// true first.
///
/// This should only be used between frames, not during build.
bool get popGestureEnabled => _isPopGestureEnabled(this);
static bool _isPopGestureEnabled<T>(PageRoute<T> route) {
// If there's nothing to go back to, then obviously we don't support
// the back gesture.
if (route.isFirst) {
return false;
}
// If the route wouldn't actually pop if we popped it, then the gesture
// would be really confusing (or would skip internal routes), so disallow it.
if (route.willHandlePopInternally) {
return false;
}
// If attempts to dismiss this route might be vetoed such as in a page
// with forms, then do not allow the user to dismiss the route with a swipe.
if (route.hasScopedWillPopCallback
|| route.popDisposition == RoutePopDisposition.doNotPop) {
return false;
}
// Fullscreen dialogs aren't dismissible by back swipe.
if (route.fullscreenDialog) {
return false;
}
// If we're in an animation already, we cannot be manually swiped.
if (route.animation!.status != AnimationStatus.completed) {
return false;
}
// If we're being popped into, we also cannot be swiped until the pop above
// it completes. This translates to our secondary animation being
// dismissed.
if (route.secondaryAnimation!.status != AnimationStatus.dismissed) {
return false;
}
// If we're in a gesture already, we cannot start another.
if (isPopGestureInProgress(route)) {
return false;
}
// Looks like a back gesture would be welcome!
return true;
}
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
final Widget child = buildContent(context);
return Semantics(
scopesRoute: true,
explicitChildNodes: true,
child: child,
);
}
// Called by _CupertinoBackGestureDetector when a pop ("back") drag start
// gesture is detected. The returned controller handles all of the subsequent
// drag events.
static _CupertinoBackGestureController<T> _startPopGesture<T>(PageRoute<T> route) {
assert(_isPopGestureEnabled(route));
return _CupertinoBackGestureController<T>(
navigator: route.navigator!,
getIsCurrent: () => route.isCurrent,
getIsActive: () => route.isActive,
controller: route.controller!, // protected access
);
}
/// Returns a [CupertinoFullscreenDialogTransition] if [route] is a full
/// screen dialog, otherwise a [CupertinoPageTransition] is returned.
///
/// Used by [CupertinoPageRoute.buildTransitions].
///
/// This method can be applied to any [PageRoute], not just
/// [CupertinoPageRoute]. It's typically used to provide a Cupertino style
/// horizontal transition for material widgets when the target platform
/// is [TargetPlatform.iOS].
///
/// See also:
///
/// * [CupertinoPageTransitionsBuilder], which uses this method to define a
/// [PageTransitionsBuilder] for the [PageTransitionsTheme].
static Widget buildPageTransitions<T>(
PageRoute<T> route,
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
// Check if the route has an animation that's currently participating
// in a back swipe gesture.
//
// In the middle of a back gesture drag, let the transition be linear to
// match finger motions.
final bool linearTransition = isPopGestureInProgress(route);
if (route.fullscreenDialog) {
return CupertinoFullscreenDialogTransition(
primaryRouteAnimation: animation,
secondaryRouteAnimation: secondaryAnimation,
linearTransition: linearTransition,
child: child,
);
} else {
return CupertinoPageTransition(
primaryRouteAnimation: animation,
secondaryRouteAnimation: secondaryAnimation,
linearTransition: linearTransition,
child: _CupertinoBackGestureDetector<T>(
enabledCallback: () => _isPopGestureEnabled<T>(route),
onStartPopGesture: () => _startPopGesture<T>(route),
getIsCurrent: () => route.isCurrent,
getIsActive: () => route.isActive,
child: child,
),
);
}
}
@override
Widget buildTransitions(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) {
return buildPageTransitions<T>(this, context, animation, secondaryAnimation, child);
}
}
/// A modal route that replaces the entire screen with an iOS transition.
///
/// {@macro flutter.cupertino.cupertinoRouteTransitionMixin}
///
/// By default, when a modal route is replaced by another, the previous route
/// remains in memory. To free all the resources when this is not necessary, set
/// [maintainState] to false.
///
/// The type `T` specifies the return type of the route which can be supplied as
/// the route is popped from the stack via [Navigator.pop] when an optional
/// `result` can be provided.
///
/// If `barrierDismissible` is true, then pressing the escape key on the keyboard
/// will cause the current route to be popped with null as the value.
///
/// See also:
///
/// * [CupertinoRouteTransitionMixin], for a mixin that provides iOS transition
/// for this modal route.
/// * [MaterialPageRoute], for an adaptive [PageRoute] that uses a
/// platform-appropriate transition.
/// * [CupertinoPageScaffold], for applications that have one page with a fixed
/// navigation bar on top.
/// * [CupertinoTabScaffold], for applications that have a tab bar at the
/// bottom with multiple pages.
/// * [CupertinoPage], for a [Page] version of this class.
class CupertinoPageRoute<T> extends PageRoute<T> with CupertinoRouteTransitionMixin<T> {
/// Creates a page route for use in an iOS designed app.
///
/// The [builder], [maintainState], and [fullscreenDialog] arguments must not
/// be null.
CupertinoPageRoute({
required this.builder,
this.title,
super.settings,
this.maintainState = true,
super.fullscreenDialog,
super.allowSnapshotting = true,
super.barrierDismissible = false,
}) {
assert(opaque);
}
/// Builds the primary contents of the route.
final WidgetBuilder builder;
@override
Widget buildContent(BuildContext context) => builder(context);
@override
final String? title;
@override
final bool maintainState;
@override
String get debugLabel => '${super.debugLabel}(${settings.name})';
}
// A page-based version of CupertinoPageRoute.
//
// This route uses the builder from the page to build its content. This ensures
// the content is up to date after page updates.
class _PageBasedCupertinoPageRoute<T> extends PageRoute<T> with CupertinoRouteTransitionMixin<T> {
_PageBasedCupertinoPageRoute({
required CupertinoPage<T> page,
super.allowSnapshotting = true,
}) : super(settings: page) {
assert(opaque);
}
CupertinoPage<T> get _page => settings as CupertinoPage<T>;
@override
Widget buildContent(BuildContext context) => _page.child;
@override
String? get title => _page.title;
@override
bool get maintainState => _page.maintainState;
@override
bool get fullscreenDialog => _page.fullscreenDialog;
@override
String get debugLabel => '${super.debugLabel}(${_page.name})';
}
/// A page that creates a cupertino style [PageRoute].
///
/// {@macro flutter.cupertino.cupertinoRouteTransitionMixin}
///
/// By default, when a created modal route is replaced by another, the previous
/// route remains in memory. To free all the resources when this is not
/// necessary, set [maintainState] to false.
///
/// The type `T` specifies the return type of the route which can be supplied as
/// the route is popped from the stack via [Navigator.transitionDelegate] by
/// providing the optional `result` argument to the
/// [RouteTransitionRecord.markForPop] in the [TransitionDelegate.resolve].
///
/// See also:
///
/// * [CupertinoPageRoute], for a [PageRoute] version of this class.
class CupertinoPage<T> extends Page<T> {
/// Creates a cupertino page.
const CupertinoPage({
required this.child,
this.maintainState = true,
this.title,
this.fullscreenDialog = false,
this.allowSnapshotting = true,
super.key,
super.name,
super.arguments,
super.restorationId,
});
/// The content to be shown in the [Route] created by this page.
final Widget child;
/// {@macro flutter.cupertino.CupertinoRouteTransitionMixin.title}
final String? title;
/// {@macro flutter.widgets.ModalRoute.maintainState}
final bool maintainState;
/// {@macro flutter.widgets.PageRoute.fullscreenDialog}
final bool fullscreenDialog;
/// {@macro flutter.widgets.TransitionRoute.allowSnapshotting}
final bool allowSnapshotting;
@override
Route<T> createRoute(BuildContext context) {
return _PageBasedCupertinoPageRoute<T>(page: this, allowSnapshotting: allowSnapshotting);
}
}
/// Provides an iOS-style page transition animation.
///
/// The page slides in from the right and exits in reverse. It also shifts to the left in
/// a parallax motion when another page enters to cover it.
class CupertinoPageTransition extends StatelessWidget {
/// Creates an iOS-style page transition.
///
/// * `primaryRouteAnimation` is a linear route animation from 0.0 to 1.0
/// when this screen is being pushed.
/// * `secondaryRouteAnimation` is a linear route animation from 0.0 to 1.0
/// when another screen is being pushed on top of this one.
/// * `linearTransition` is whether to perform the transitions linearly.
/// Used to precisely track back gesture drags.
CupertinoPageTransition({
super.key,
required Animation<double> primaryRouteAnimation,
required Animation<double> secondaryRouteAnimation,
required this.child,
required bool linearTransition,
}) : _primaryPositionAnimation =
(linearTransition
? primaryRouteAnimation
: CurvedAnimation(
parent: primaryRouteAnimation,
curve: Curves.fastEaseInToSlowEaseOut,
reverseCurve: Curves.fastEaseInToSlowEaseOut.flipped,
)
).drive(_kRightMiddleTween),
_secondaryPositionAnimation =
(linearTransition
? secondaryRouteAnimation
: CurvedAnimation(
parent: secondaryRouteAnimation,
curve: Curves.linearToEaseOut,
reverseCurve: Curves.easeInToLinear,
)
).drive(_kMiddleLeftTween),
_primaryShadowAnimation =
(linearTransition
? primaryRouteAnimation
: CurvedAnimation(
parent: primaryRouteAnimation,
curve: Curves.linearToEaseOut,
)
).drive(_CupertinoEdgeShadowDecoration.kTween);
// When this page is coming in to cover another page.
final Animation<Offset> _primaryPositionAnimation;
// When this page is becoming covered by another page.
final Animation<Offset> _secondaryPositionAnimation;
final Animation<Decoration> _primaryShadowAnimation;
/// The widget below this widget in the tree.
final Widget child;
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
final TextDirection textDirection = Directionality.of(context);
return SlideTransition(
position: _secondaryPositionAnimation,
textDirection: textDirection,
transformHitTests: false,
child: SlideTransition(
position: _primaryPositionAnimation,
textDirection: textDirection,
child: DecoratedBoxTransition(
decoration: _primaryShadowAnimation,
child: child,
),
),
);
}
}
/// An iOS-style transition used for summoning fullscreen dialogs.
///
/// For example, used when creating a new calendar event by bringing in the next
/// screen from the bottom.
class CupertinoFullscreenDialogTransition extends StatelessWidget {
/// Creates an iOS-style transition used for summoning fullscreen dialogs.
///
/// * `primaryRouteAnimation` is a linear route animation from 0.0 to 1.0
/// when this screen is being pushed.
/// * `secondaryRouteAnimation` is a linear route animation from 0.0 to 1.0
/// when another screen is being pushed on top of this one.
/// * `linearTransition` is whether to perform the secondary transition linearly.
/// Used to precisely track back gesture drags.
CupertinoFullscreenDialogTransition({
super.key,
required Animation<double> primaryRouteAnimation,
required Animation<double> secondaryRouteAnimation,
required this.child,
required bool linearTransition,
}) : _positionAnimation = CurvedAnimation(
parent: primaryRouteAnimation,
curve: Curves.linearToEaseOut,
// The curve must be flipped so that the reverse animation doesn't play
// an ease-in curve, which iOS does not use.
reverseCurve: Curves.linearToEaseOut.flipped,
).drive(_kBottomUpTween),
_secondaryPositionAnimation =
(linearTransition
? secondaryRouteAnimation
: CurvedAnimation(
parent: secondaryRouteAnimation,
curve: Curves.linearToEaseOut,
reverseCurve: Curves.easeInToLinear,
)
).drive(_kMiddleLeftTween);
final Animation<Offset> _positionAnimation;
// When this page is becoming covered by another page.
final Animation<Offset> _secondaryPositionAnimation;
/// The widget below this widget in the tree.
final Widget child;
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
final TextDirection textDirection = Directionality.of(context);
return SlideTransition(
position: _secondaryPositionAnimation,
textDirection: textDirection,
transformHitTests: false,
child: SlideTransition(
position: _positionAnimation,
child: child,
),
);
}
}
/// This is the widget side of [_CupertinoBackGestureController].
///
/// This widget provides a gesture recognizer which, when it determines the
/// route can be closed with a back gesture, creates the controller and
/// feeds it the input from the gesture recognizer.
///
/// The gesture data is converted from absolute coordinates to logical
/// coordinates by this widget.
///
/// The type `T` specifies the return type of the route with which this gesture
/// detector is associated.
class _CupertinoBackGestureDetector<T> extends StatefulWidget {
const _CupertinoBackGestureDetector({
super.key,
required this.enabledCallback,
required this.onStartPopGesture,
required this.child,
required this.getIsActive,
required this.getIsCurrent,
});
final Widget child;
final ValueGetter<bool> enabledCallback;
final ValueGetter<_CupertinoBackGestureController<T>> onStartPopGesture;
final ValueGetter<bool> getIsActive;
final ValueGetter<bool> getIsCurrent;
@override
_CupertinoBackGestureDetectorState<T> createState() => _CupertinoBackGestureDetectorState<T>();
}
class _CupertinoBackGestureDetectorState<T> extends State<_CupertinoBackGestureDetector<T>> {
_CupertinoBackGestureController<T>? _backGestureController;
late HorizontalDragGestureRecognizer _recognizer;
@override
void initState() {
super.initState();
_recognizer = HorizontalDragGestureRecognizer(debugOwner: this)
..onStart = _handleDragStart
..onUpdate = _handleDragUpdate
..onEnd = _handleDragEnd
..onCancel = _handleDragCancel;
}
@override
void dispose() {
_recognizer.dispose();
// If this is disposed during a drag, call navigator.didStopUserGesture.
if (_backGestureController != null) {
WidgetsBinding.instance.addPostFrameCallback((_) {
if (_backGestureController?.navigator.mounted ?? false) {
_backGestureController?.navigator.didStopUserGesture();
}
_backGestureController = null;
});
}
super.dispose();
}
void _handleDragStart(DragStartDetails details) {
assert(mounted);
assert(_backGestureController == null);
_backGestureController = widget.onStartPopGesture();
}
void _handleDragUpdate(DragUpdateDetails details) {
assert(mounted);
assert(_backGestureController != null);
_backGestureController!.dragUpdate(_convertToLogical(details.primaryDelta! / context.size!.width));
}
void _handleDragEnd(DragEndDetails details) {
assert(mounted);
assert(_backGestureController != null);
_backGestureController!.dragEnd(_convertToLogical(details.velocity.pixelsPerSecond.dx / context.size!.width));
_backGestureController = null;
}
void _handleDragCancel() {
assert(mounted);
// This can be called even if start is not called, paired with the "down" event
// that we don't consider here.
_backGestureController?.dragEnd(0.0);
_backGestureController = null;
}
void _handlePointerDown(PointerDownEvent event) {
if (widget.enabledCallback()) {
_recognizer.addPointer(event);
}
}
double _convertToLogical(double value) {
return switch (Directionality.of(context)) {
TextDirection.rtl => -value,
TextDirection.ltr => value,
};
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
// For devices with notches, the drag area needs to be larger on the side
// that has the notch.
double dragAreaWidth = Directionality.of(context) == TextDirection.ltr ?
MediaQuery.paddingOf(context).left :
MediaQuery.paddingOf(context).right;
dragAreaWidth = max(dragAreaWidth, _kBackGestureWidth);
return Stack(
fit: StackFit.passthrough,
children: <Widget>[
widget.child,
PositionedDirectional(
start: 0.0,
width: dragAreaWidth,
top: 0.0,
bottom: 0.0,
child: Listener(
onPointerDown: _handlePointerDown,
behavior: HitTestBehavior.translucent,
),
),
],
);
}
}
/// A controller for an iOS-style back gesture.
///
/// This is created by a [CupertinoPageRoute] in response from a gesture caught
/// by a [_CupertinoBackGestureDetector] widget, which then also feeds it input
/// from the gesture. It controls the animation controller owned by the route,
/// based on the input provided by the gesture detector.
///
/// This class works entirely in logical coordinates (0.0 is new page dismissed,
/// 1.0 is new page on top).
///
/// The type `T` specifies the return type of the route with which this gesture
/// detector controller is associated.
class _CupertinoBackGestureController<T> {
/// Creates a controller for an iOS-style back gesture.
_CupertinoBackGestureController({
required this.navigator,
required this.controller,
required this.getIsActive,
required this.getIsCurrent,
}) {
navigator.didStartUserGesture();
}
final AnimationController controller;
final NavigatorState navigator;
final ValueGetter<bool> getIsActive;
final ValueGetter<bool> getIsCurrent;
/// The drag gesture has changed by [fractionalDelta]. The total range of the
/// drag should be 0.0 to 1.0.
void dragUpdate(double delta) {
controller.value -= delta;
}
/// The drag gesture has ended with a horizontal motion of
/// [fractionalVelocity] as a fraction of screen width per second.
void dragEnd(double velocity) {
// Fling in the appropriate direction.
//
// This curve has been determined through rigorously eyeballing native iOS
// animations.
const Curve animationCurve = Curves.fastLinearToSlowEaseIn;
final bool isCurrent = getIsCurrent();
final bool animateForward;
if (!isCurrent) {
// If the page has already been navigated away from, then the animation
// direction depends on whether or not it's still in the navigation stack,
// regardless of velocity or drag position. For example, if a route is
// being slowly dragged back by just a few pixels, but then a programmatic
// pop occurs, the route should still be animated off the screen.
// See https://github.com/flutter/flutter/issues/141268.
animateForward = getIsActive();
} else if (velocity.abs() >= _kMinFlingVelocity) {
// If the user releases the page before mid screen with sufficient velocity,
// or after mid screen, we should animate the page out. Otherwise, the page
// should be animated back in.
animateForward = velocity <= 0;
} else {
animateForward = controller.value > 0.5;
}
if (animateForward) {
// The closer the panel is to dismissing, the shorter the animation is.
// We want to cap the animation time, but we want to use a linear curve
// to determine it.
final int droppedPageForwardAnimationTime = min(
lerpDouble(_kMaxDroppedSwipePageForwardAnimationTime, 0, controller.value)!.floor(),
_kMaxPageBackAnimationTime,
);
controller.animateTo(1.0, duration: Duration(milliseconds: droppedPageForwardAnimationTime), curve: animationCurve);
} else {
if (isCurrent) {
// This route is destined to pop at this point. Reuse navigator's pop.
navigator.pop();
}
// The popping may have finished inline if already at the target destination.
if (controller.isAnimating) {
// Otherwise, use a custom popping animation duration and curve.
final int droppedPageBackAnimationTime = lerpDouble(0, _kMaxDroppedSwipePageForwardAnimationTime, controller.value)!.floor();
controller.animateBack(0.0, duration: Duration(milliseconds: droppedPageBackAnimationTime), curve: animationCurve);
}
}
if (controller.isAnimating) {
// Keep the userGestureInProgress in true state so we don't change the
// curve of the page transition mid-flight since CupertinoPageTransition
// depends on userGestureInProgress.
late AnimationStatusListener animationStatusCallback;
animationStatusCallback = (AnimationStatus status) {
navigator.didStopUserGesture();
controller.removeStatusListener(animationStatusCallback);
};
controller.addStatusListener(animationStatusCallback);
} else {
navigator.didStopUserGesture();
}
}
}
// A custom [Decoration] used to paint an extra shadow on the start edge of the
// box it's decorating. It's like a [BoxDecoration] with only a gradient except
// it paints on the start side of the box instead of behind the box.
class _CupertinoEdgeShadowDecoration extends Decoration {
const _CupertinoEdgeShadowDecoration._([this._colors]);
static DecorationTween kTween = DecorationTween(
begin: const _CupertinoEdgeShadowDecoration._(), // No decoration initially.
end: const _CupertinoEdgeShadowDecoration._(
// Eyeballed gradient used to mimic a drop shadow on the start side only.
<Color>[
Color(0x04000000),
Color(0x00000000),
],
),
);
// Colors used to paint a gradient at the start edge of the box it is
// decorating.
//
// The first color in the list is used at the start of the gradient, which
// is located at the start edge of the decorated box.
//
// If this is null, no shadow is drawn.
//
// The list must have at least two colors in it (otherwise it would not be a
// gradient).
final List<Color>? _colors;
// Linearly interpolate between two edge shadow decorations decorations.
//
// The `t` argument represents position on the timeline, with 0.0 meaning
// that the interpolation has not started, returning `a` (or something
// equivalent to `a`), 1.0 meaning that the interpolation has finished,
// returning `b` (or something equivalent to `b`), and values in between
// meaning that the interpolation is at the relevant point on the timeline
// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
// 1.0, so negative values and values greater than 1.0 are valid (and can
// easily be generated by curves such as [Curves.elasticInOut]).
//
// Values for `t` are usually obtained from an [Animation<double>], such as
// an [AnimationController].
//
// See also:
//
// * [Decoration.lerp].
static _CupertinoEdgeShadowDecoration? lerp(
_CupertinoEdgeShadowDecoration? a,
_CupertinoEdgeShadowDecoration? b,
double t,
) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!._colors == null ? b : _CupertinoEdgeShadowDecoration._(b._colors!.map<Color>((Color color) => Color.lerp(null, color, t)!).toList());
}
if (b == null) {
return a._colors == null ? a : _CupertinoEdgeShadowDecoration._(a._colors.map<Color>((Color color) => Color.lerp(null, color, 1.0 - t)!).toList());
}
assert(b._colors != null || a._colors != null);
// If it ever becomes necessary, we could allow decorations with different
// length' here, similarly to how it is handled in [LinearGradient.lerp].
assert(b._colors == null || a._colors == null || a._colors.length == b._colors.length);
return _CupertinoEdgeShadowDecoration._(
<Color>[
for (int i = 0; i < b._colors!.length; i += 1)
Color.lerp(a._colors?[i], b._colors[i], t)!,
],
);
}
@override
_CupertinoEdgeShadowDecoration lerpFrom(Decoration? a, double t) {
if (a is _CupertinoEdgeShadowDecoration) {
return _CupertinoEdgeShadowDecoration.lerp(a, this, t)!;
}
return _CupertinoEdgeShadowDecoration.lerp(null, this, t)!;
}
@override
_CupertinoEdgeShadowDecoration lerpTo(Decoration? b, double t) {
if (b is _CupertinoEdgeShadowDecoration) {
return _CupertinoEdgeShadowDecoration.lerp(this, b, t)!;
}
return _CupertinoEdgeShadowDecoration.lerp(this, null, t)!;
}
@override
_CupertinoEdgeShadowPainter createBoxPainter([ VoidCallback? onChanged ]) {
return _CupertinoEdgeShadowPainter(this, onChanged);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _CupertinoEdgeShadowDecoration
&& other._colors == _colors;
}
@override
int get hashCode => _colors.hashCode;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IterableProperty<Color>('colors', _colors));
}
}
/// A [BoxPainter] used to draw the page transition shadow using gradients.
class _CupertinoEdgeShadowPainter extends BoxPainter {
_CupertinoEdgeShadowPainter(
this._decoration,
super.onChanged,
) : assert(_decoration._colors == null || _decoration._colors.length > 1);
final _CupertinoEdgeShadowDecoration _decoration;
@override
void paint(Canvas canvas, Offset offset, ImageConfiguration configuration) {
final List<Color>? colors = _decoration._colors;
if (colors == null) {
return;
}
// The following code simulates drawing a [LinearGradient] configured as
// follows:
//
// LinearGradient(
// begin: AlignmentDirectional(0.90, 0.0), // Spans 5% of the page.
// colors: _decoration._colors,
// )
//
// A performance evaluation on Feb 8, 2021 showed, that drawing the gradient
// manually as implemented below is more performant than relying on
// [LinearGradient.createShader] because compiling that shader takes a long
// time. On an iPhone XR, the implementation below reduced the worst frame
// time for a cupertino page transition of a newly installed app from ~95ms
// down to ~30ms, mainly because there's no longer a need to compile a
// shader for the LinearGradient.
//
// The implementation below divides the width of the shadow into multiple
// bands of equal width, one for each color interval defined by
// `_decoration._colors`. Band x is filled with a gradient going from
// `_decoration._colors[x]` to `_decoration._colors[x + 1]` by drawing a
// bunch of 1px wide rects. The rects change their color by lerping between
// the two colors that define the interval of the band.
// Shadow spans 5% of the page.
final double shadowWidth = 0.05 * configuration.size!.width;
final double shadowHeight = configuration.size!.height;
final double bandWidth = shadowWidth / (colors.length - 1);
final TextDirection? textDirection = configuration.textDirection;
assert(textDirection != null);
final (double shadowDirection, double start) = switch (textDirection!) {
TextDirection.rtl => (1, offset.dx + configuration.size!.width),
TextDirection.ltr => (-1, offset.dx),
};
int bandColorIndex = 0;
for (int dx = 0; dx < shadowWidth; dx += 1) {
if (dx ~/ bandWidth != bandColorIndex) {
bandColorIndex += 1;
}
final Paint paint = Paint()
..color = Color.lerp(colors[bandColorIndex], colors[bandColorIndex + 1], (dx % bandWidth) / bandWidth)!;
final double x = start + shadowDirection * dx;
canvas.drawRect(Rect.fromLTWH(x - 1.0, offset.dy, 1.0, shadowHeight), paint);
}
}
}
/// A route that shows a modal iOS-style popup that slides up from the
/// bottom of the screen.
///
/// Such a popup is an alternative to a menu or a dialog and prevents the user
/// from interacting with the rest of the app.
///
/// It is used internally by [showCupertinoModalPopup] or can be directly pushed
/// onto the [Navigator] stack to enable state restoration. See
/// [showCupertinoModalPopup] for a state restoration app example.
///
/// The `barrierColor` argument determines the [Color] of the barrier underneath
/// the popup. When unspecified, the barrier color defaults to a light opacity
/// black scrim based on iOS's dialog screens. To correctly have iOS resolve
/// to the appropriate modal colors, pass in
/// `CupertinoDynamicColor.resolve(kCupertinoModalBarrierColor, context)`.
///
/// The `barrierDismissible` argument determines whether clicking outside the
/// popup results in dismissal. It is `true` by default.
///
/// The `semanticsDismissible` argument is used to determine whether the
/// semantics of the modal barrier are included in the semantics tree.
///
/// The `routeSettings` argument is used to provide [RouteSettings] to the
/// created Route.
///
/// {@macro flutter.widgets.RawDialogRoute}
///
/// See also:
///
/// * [DisplayFeatureSubScreen], which documents the specifics of how
/// [DisplayFeature]s can split the screen into sub-screens.
/// * [CupertinoActionSheet], which is the widget usually returned by the
/// `builder` argument.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/views/action-sheets/>
class CupertinoModalPopupRoute<T> extends PopupRoute<T> {
/// A route that shows a modal iOS-style popup that slides up from the
/// bottom of the screen.
CupertinoModalPopupRoute({
required this.builder,
this.barrierLabel = 'Dismiss',
this.barrierColor = kCupertinoModalBarrierColor,
bool barrierDismissible = true,
bool semanticsDismissible = false,
super.filter,
super.settings,
this.anchorPoint,
}) : _barrierDismissible = barrierDismissible,
_semanticsDismissible = semanticsDismissible;
/// A builder that builds the widget tree for the [CupertinoModalPopupRoute].
///
/// The [builder] argument typically builds a [CupertinoActionSheet] widget.
///
/// Content below the widget is dimmed with a [ModalBarrier]. The widget built
/// by the [builder] does not share a context with the route it was originally
/// built from. Use a [StatefulBuilder] or a custom [StatefulWidget] if the
/// widget needs to update dynamically.
final WidgetBuilder builder;
final bool _barrierDismissible;
final bool _semanticsDismissible;
@override
final String barrierLabel;
@override
final Color? barrierColor;
@override
bool get barrierDismissible => _barrierDismissible;
@override
bool get semanticsDismissible => _semanticsDismissible;
@override
Duration get transitionDuration => _kModalPopupTransitionDuration;
Animation<double>? _animation;
late Tween<Offset> _offsetTween;
/// {@macro flutter.widgets.DisplayFeatureSubScreen.anchorPoint}
final Offset? anchorPoint;
@override
Animation<double> createAnimation() {
assert(_animation == null);
_animation = CurvedAnimation(
parent: super.createAnimation(),
// These curves were initially measured from native iOS horizontal page
// route animations and seemed to be a good match here as well.
curve: Curves.linearToEaseOut,
reverseCurve: Curves.linearToEaseOut.flipped,
);
_offsetTween = Tween<Offset>(
begin: const Offset(0.0, 1.0),
end: Offset.zero,
);
return _animation!;
}
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
return CupertinoUserInterfaceLevel(
data: CupertinoUserInterfaceLevelData.elevated,
child: DisplayFeatureSubScreen(
anchorPoint: anchorPoint,
child: Builder(builder: builder),
),
);
}
@override
Widget buildTransitions(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) {
return Align(
alignment: Alignment.bottomCenter,
child: FractionalTranslation(
translation: _offsetTween.evaluate(_animation!),
child: child,
),
);
}
}
/// Shows a modal iOS-style popup that slides up from the bottom of the screen.
///
/// Such a popup is an alternative to a menu or a dialog and prevents the user
/// from interacting with the rest of the app.
///
/// The `context` argument is used to look up the [Navigator] for the popup.
/// It is only used when the method is called. Its corresponding widget can be
/// safely removed from the tree before the popup is closed.
///
/// The `barrierColor` argument determines the [Color] of the barrier underneath
/// the popup. When unspecified, the barrier color defaults to a light opacity
/// black scrim based on iOS's dialog screens.
///
/// The `barrierDismissible` argument determines whether clicking outside the
/// popup results in dismissal. It is `true` by default.
///
/// The `useRootNavigator` argument is used to determine whether to push the
/// popup to the [Navigator] furthest from or nearest to the given `context`. It
/// is `true` by default.
///
/// The `semanticsDismissible` argument is used to determine whether the
/// semantics of the modal barrier are included in the semantics tree.
///
/// The `routeSettings` argument is used to provide [RouteSettings] to the
/// created Route.
///
/// The `builder` argument typically builds a [CupertinoActionSheet] widget.
/// Content below the widget is dimmed with a [ModalBarrier]. The widget built
/// by the `builder` does not share a context with the location that
/// [showCupertinoModalPopup] is originally called from. Use a
/// [StatefulBuilder] or a custom [StatefulWidget] if the widget needs to
/// update dynamically.
///
/// {@macro flutter.widgets.RawDialogRoute}
///
/// Returns a `Future` that resolves to the value that was passed to
/// [Navigator.pop] when the popup was closed.
///
/// ### State Restoration in Modals
///
/// Using this method will not enable state restoration for the modal. In order
/// to enable state restoration for a modal, use [Navigator.restorablePush]
/// or [Navigator.restorablePushNamed] with [CupertinoModalPopupRoute].
///
/// For more information about state restoration, see [RestorationManager].
///
/// {@tool dartpad}
/// This sample demonstrates how to create a restorable Cupertino modal route.
/// This is accomplished by enabling state restoration by specifying
/// [CupertinoApp.restorationScopeId] and using [Navigator.restorablePush] to
/// push [CupertinoModalPopupRoute] when the [CupertinoButton] is tapped.
///
/// {@macro flutter.widgets.RestorationManager}
///
/// ** See code in examples/api/lib/cupertino/route/show_cupertino_modal_popup.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [DisplayFeatureSubScreen], which documents the specifics of how
/// [DisplayFeature]s can split the screen into sub-screens.
/// * [CupertinoActionSheet], which is the widget usually returned by the
/// `builder` argument to [showCupertinoModalPopup].
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/views/action-sheets/>
Future<T?> showCupertinoModalPopup<T>({
required BuildContext context,
required WidgetBuilder builder,
ImageFilter? filter,
Color barrierColor = kCupertinoModalBarrierColor,
bool barrierDismissible = true,
bool useRootNavigator = true,
bool semanticsDismissible = false,
RouteSettings? routeSettings,
Offset? anchorPoint,
}) {
return Navigator.of(context, rootNavigator: useRootNavigator).push(
CupertinoModalPopupRoute<T>(
builder: builder,
filter: filter,
barrierColor: CupertinoDynamicColor.resolve(barrierColor, context),
barrierDismissible: barrierDismissible,
semanticsDismissible: semanticsDismissible,
settings: routeSettings,
anchorPoint: anchorPoint,
),
);
}
// The curve and initial scale values were mostly eyeballed from iOS, however
// they reuse the same animation curve that was modeled after native page
// transitions.
final Animatable<double> _dialogScaleTween = Tween<double>(begin: 1.3, end: 1.0)
.chain(CurveTween(curve: Curves.linearToEaseOut));
Widget _buildCupertinoDialogTransitions(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) {
final CurvedAnimation fadeAnimation = CurvedAnimation(
parent: animation,
curve: Curves.easeInOut,
);
if (animation.status == AnimationStatus.reverse) {
return FadeTransition(
opacity: fadeAnimation,
child: child,
);
}
return FadeTransition(
opacity: fadeAnimation,
child: ScaleTransition(
scale: animation.drive(_dialogScaleTween),
child: child,
),
);
}
/// Displays an iOS-style dialog above the current contents of the app, with
/// iOS-style entrance and exit animations, modal barrier color, and modal
/// barrier behavior (by default, the dialog is not dismissible with a tap on
/// the barrier).
///
/// This function takes a `builder` which typically builds a [CupertinoAlertDialog]
/// widget. Content below the dialog is dimmed with a [ModalBarrier]. The widget
/// returned by the `builder` does not share a context with the location that
/// [showCupertinoDialog] is originally called from. Use a [StatefulBuilder] or
/// a custom [StatefulWidget] if the dialog needs to update dynamically.
///
/// The `context` argument is used to look up the [Navigator] for the dialog.
/// It is only used when the method is called. Its corresponding widget can
/// be safely removed from the tree before the dialog is closed.
///
/// The `useRootNavigator` argument is used to determine whether to push the
/// dialog to the [Navigator] furthest from or nearest to the given `context`.
/// By default, `useRootNavigator` is `true` and the dialog route created by
/// this method is pushed to the root navigator.
///
/// {@macro flutter.widgets.RawDialogRoute}
///
/// If the application has multiple [Navigator] objects, it may be necessary to
/// call `Navigator.of(context, rootNavigator: true).pop(result)` to close the
/// dialog rather than just `Navigator.pop(context, result)`.
///
/// Returns a [Future] that resolves to the value (if any) that was passed to
/// [Navigator.pop] when the dialog was closed.
///
/// ### State Restoration in Dialogs
///
/// Using this method will not enable state restoration for the dialog. In order
/// to enable state restoration for a dialog, use [Navigator.restorablePush]
/// or [Navigator.restorablePushNamed] with [CupertinoDialogRoute].
///
/// For more information about state restoration, see [RestorationManager].
///
/// {@tool dartpad}
/// This sample demonstrates how to create a restorable Cupertino dialog. This is
/// accomplished by enabling state restoration by specifying
/// [CupertinoApp.restorationScopeId] and using [Navigator.restorablePush] to
/// push [CupertinoDialogRoute] when the [CupertinoButton] is tapped.
///
/// {@macro flutter.widgets.RestorationManager}
///
/// ** See code in examples/api/lib/cupertino/route/show_cupertino_dialog.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoAlertDialog], an iOS-style alert dialog.
/// * [showDialog], which displays a Material-style dialog.
/// * [showGeneralDialog], which allows for customization of the dialog popup.
/// * [DisplayFeatureSubScreen], which documents the specifics of how
/// [DisplayFeature]s can split the screen into sub-screens.
/// * <https://developer.apple.com/design/human-interface-guidelines/alerts/>
Future<T?> showCupertinoDialog<T>({
required BuildContext context,
required WidgetBuilder builder,
String? barrierLabel,
bool useRootNavigator = true,
bool barrierDismissible = false,
RouteSettings? routeSettings,
Offset? anchorPoint,
}) {
return Navigator.of(context, rootNavigator: useRootNavigator).push<T>(CupertinoDialogRoute<T>(
builder: builder,
context: context,
barrierDismissible: barrierDismissible,
barrierLabel: barrierLabel,
barrierColor: CupertinoDynamicColor.resolve(kCupertinoModalBarrierColor, context),
settings: routeSettings,
anchorPoint: anchorPoint,
));
}
/// A dialog route that shows an iOS-style dialog.
///
/// It is used internally by [showCupertinoDialog] or can be directly pushed
/// onto the [Navigator] stack to enable state restoration. See
/// [showCupertinoDialog] for a state restoration app example.
///
/// This function takes a `builder` which typically builds a [Dialog] widget.
/// Content below the dialog is dimmed with a [ModalBarrier]. The widget
/// returned by the `builder` does not share a context with the location that
/// `showDialog` is originally called from. Use a [StatefulBuilder] or a
/// custom [StatefulWidget] if the dialog needs to update dynamically.
///
/// The `context` argument is used to look up
/// [CupertinoLocalizations.modalBarrierDismissLabel], which provides the
/// modal with a localized accessibility label that will be used for the
/// modal's barrier. However, a custom `barrierLabel` can be passed in as well.
///
/// The `barrierDismissible` argument is used to indicate whether tapping on the
/// barrier will dismiss the dialog. It is `true` by default and cannot be `null`.
///
/// The `barrierColor` argument is used to specify the color of the modal
/// barrier that darkens everything below the dialog. If `null`, then
/// [CupertinoDynamicColor.resolve] is used to compute the modal color.
///
/// The `settings` argument define the settings for this route. See
/// [RouteSettings] for details.
///
/// {@macro flutter.widgets.RawDialogRoute}
///
/// See also:
///
/// * [showCupertinoDialog], which is a way to display
/// an iOS-style dialog.
/// * [showGeneralDialog], which allows for customization of the dialog popup.
/// * [showDialog], which displays a Material dialog.
/// * [DisplayFeatureSubScreen], which documents the specifics of how
/// [DisplayFeature]s can split the screen into sub-screens.
class CupertinoDialogRoute<T> extends RawDialogRoute<T> {
/// A dialog route that shows an iOS-style dialog.
CupertinoDialogRoute({
required WidgetBuilder builder,
required BuildContext context,
super.barrierDismissible,
Color? barrierColor,
String? barrierLabel,
// This transition duration was eyeballed comparing with iOS
super.transitionDuration = const Duration(milliseconds: 250),
super.transitionBuilder = _buildCupertinoDialogTransitions,
super.settings,
super.anchorPoint,
}) : super(
pageBuilder: (BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
return builder(context);
},
barrierLabel: barrierLabel ?? CupertinoLocalizations.of(context).modalBarrierDismissLabel,
barrierColor: barrierColor ?? CupertinoDynamicColor.resolve(kCupertinoModalBarrierColor, context),
);
}
| flutter/packages/flutter/lib/src/cupertino/route.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/route.dart",
"repo_id": "flutter",
"token_count": 16353
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'icon_theme_data.dart';
import 'text_theme.dart';
export 'package:flutter/foundation.dart' show Brightness;
// Values derived from https://developer.apple.com/design/resources/.
const _CupertinoThemeDefaults _kDefaultTheme = _CupertinoThemeDefaults(
null,
CupertinoColors.systemBlue,
CupertinoColors.systemBackground,
CupertinoDynamicColor.withBrightness(
color: Color(0xF0F9F9F9),
darkColor: Color(0xF01D1D1D),
// Values extracted from navigation bar. For toolbar or tabbar the dark color is 0xF0161616.
),
CupertinoColors.systemBackground,
false,
_CupertinoTextThemeDefaults(CupertinoColors.label, CupertinoColors.inactiveGray),
);
/// Applies a visual styling theme to descendant Cupertino widgets.
///
/// Affects the color and text styles of Cupertino widgets whose styling
/// are not overridden when constructing the respective widgets instances.
///
/// Descendant widgets can retrieve the current [CupertinoThemeData] by calling
/// [CupertinoTheme.of]. An [InheritedWidget] dependency is created when
/// an ancestor [CupertinoThemeData] is retrieved via [CupertinoTheme.of].
///
/// The [CupertinoTheme] widget implies an [IconTheme] widget, whose
/// [IconTheme.data] has the same color as [CupertinoThemeData.primaryColor]
///
/// See also:
///
/// * [CupertinoThemeData], specifies the theme's visual styling.
/// * [CupertinoApp], which will automatically add a [CupertinoTheme] based on the
/// value of [CupertinoApp.theme].
/// * [Theme], a Material theme which will automatically add a [CupertinoTheme]
/// with a [CupertinoThemeData] derived from the Material [ThemeData].
class CupertinoTheme extends StatelessWidget {
/// Creates a [CupertinoTheme] to change descendant Cupertino widgets' styling.
const CupertinoTheme({
super.key,
required this.data,
required this.child,
});
/// The [CupertinoThemeData] styling for this theme.
final CupertinoThemeData data;
/// Retrieves the [CupertinoThemeData] from the closest ancestor [CupertinoTheme]
/// widget, or a default [CupertinoThemeData] if no [CupertinoTheme] ancestor
/// exists.
///
/// Resolves all the colors defined in that [CupertinoThemeData] against the
/// given [BuildContext] on a best-effort basis.
static CupertinoThemeData of(BuildContext context) {
final _InheritedCupertinoTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<_InheritedCupertinoTheme>();
return (inheritedTheme?.theme.data ?? const CupertinoThemeData()).resolveFrom(context);
}
/// Retrieves the [Brightness] to use for descendant Cupertino widgets, based
/// on the value of [CupertinoThemeData.brightness] in the given [context].
///
/// If no [CupertinoTheme] can be found in the given [context], or its `brightness`
/// is null, it will fall back to [MediaQueryData.platformBrightness].
///
/// Throws an exception if no valid [CupertinoTheme] or [MediaQuery] widgets
/// exist in the ancestry tree.
///
/// See also:
///
/// * [maybeBrightnessOf], which returns null if no valid [CupertinoTheme] or
/// [MediaQuery] exists, instead of throwing.
/// * [CupertinoThemeData.brightness], the property takes precedence over
/// [MediaQueryData.platformBrightness] for descendant Cupertino widgets.
static Brightness brightnessOf(BuildContext context) {
final _InheritedCupertinoTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<_InheritedCupertinoTheme>();
return inheritedTheme?.theme.data.brightness ?? MediaQuery.platformBrightnessOf(context);
}
/// Retrieves the [Brightness] to use for descendant Cupertino widgets, based
/// on the value of [CupertinoThemeData.brightness] in the given [context].
///
/// If no [CupertinoTheme] can be found in the given [context], it will fall
/// back to [MediaQueryData.platformBrightness].
///
/// Returns null if no valid [CupertinoTheme] or [MediaQuery] widgets exist in
/// the ancestry tree.
///
/// See also:
///
/// * [CupertinoThemeData.brightness], the property takes precedence over
/// [MediaQueryData.platformBrightness] for descendant Cupertino widgets.
/// * [brightnessOf], which throws if no valid [CupertinoTheme] or
/// [MediaQuery] exists, instead of returning null.
static Brightness? maybeBrightnessOf(BuildContext context) {
final _InheritedCupertinoTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<_InheritedCupertinoTheme>();
return inheritedTheme?.theme.data.brightness ?? MediaQuery.maybePlatformBrightnessOf(context);
}
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
Widget build(BuildContext context) {
return _InheritedCupertinoTheme(
theme: this,
child: IconTheme(
data: CupertinoIconThemeData(color: data.primaryColor),
child: child,
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
data.debugFillProperties(properties);
}
}
class _InheritedCupertinoTheme extends InheritedTheme {
const _InheritedCupertinoTheme({
required this.theme,
required super.child,
});
final CupertinoTheme theme;
@override
Widget wrap(BuildContext context, Widget child) {
return CupertinoTheme(data: theme.data, child: child);
}
@override
bool updateShouldNotify(_InheritedCupertinoTheme old) => theme.data != old.theme.data;
}
/// Styling specifications for a [CupertinoTheme].
///
/// All constructor parameters can be null, in which case a
/// [CupertinoColors.activeBlue] based default iOS theme styling is used.
///
/// Parameters can also be partially specified, in which case some parameters
/// will cascade down to other dependent parameters to create a cohesive
/// visual effect. For instance, if a [primaryColor] is specified, it would
/// cascade down to affect some fonts in [textTheme] if [textTheme] is not
/// specified.
///
/// See also:
///
/// * [CupertinoTheme], in which this [CupertinoThemeData] is inserted.
/// * [ThemeData], a Material equivalent that also configures Cupertino
/// styling via a [CupertinoThemeData] subclass [MaterialBasedCupertinoThemeData].
@immutable
class CupertinoThemeData extends NoDefaultCupertinoThemeData with Diagnosticable {
/// Creates a [CupertinoTheme] styling specification.
///
/// Unspecified parameters default to a reasonable iOS default style.
const CupertinoThemeData({
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
}) : this.raw(
brightness,
primaryColor,
primaryContrastingColor,
textTheme,
barBackgroundColor,
scaffoldBackgroundColor,
applyThemeToAll,
);
/// Same as the default constructor but with positional arguments to avoid
/// forgetting any and to specify all arguments.
///
/// Used by subclasses to get the superclass's defaulting behaviors.
@protected
const CupertinoThemeData.raw(
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
) : this._rawWithDefaults(
brightness,
primaryColor,
primaryContrastingColor,
textTheme,
barBackgroundColor,
scaffoldBackgroundColor,
applyThemeToAll,
_kDefaultTheme,
);
const CupertinoThemeData._rawWithDefaults(
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
this._defaults,
) : super(
brightness: brightness,
primaryColor: primaryColor,
primaryContrastingColor: primaryContrastingColor,
textTheme: textTheme,
barBackgroundColor: barBackgroundColor,
scaffoldBackgroundColor: scaffoldBackgroundColor,
applyThemeToAll: applyThemeToAll,
);
final _CupertinoThemeDefaults _defaults;
@override
Color get primaryColor => super.primaryColor ?? _defaults.primaryColor;
@override
Color get primaryContrastingColor => super.primaryContrastingColor ?? _defaults.primaryContrastingColor;
@override
CupertinoTextThemeData get textTheme {
return super.textTheme ?? _defaults.textThemeDefaults.createDefaults(primaryColor: primaryColor);
}
@override
Color get barBackgroundColor => super.barBackgroundColor ?? _defaults.barBackgroundColor;
@override
Color get scaffoldBackgroundColor => super.scaffoldBackgroundColor ?? _defaults.scaffoldBackgroundColor;
@override
bool get applyThemeToAll => super.applyThemeToAll ?? _defaults.applyThemeToAll;
@override
NoDefaultCupertinoThemeData noDefault() {
return NoDefaultCupertinoThemeData(
brightness: super.brightness,
primaryColor: super.primaryColor,
primaryContrastingColor: super.primaryContrastingColor,
textTheme: super.textTheme,
barBackgroundColor: super.barBackgroundColor,
scaffoldBackgroundColor: super.scaffoldBackgroundColor,
applyThemeToAll: super.applyThemeToAll,
);
}
@override
CupertinoThemeData resolveFrom(BuildContext context) {
Color? convertColor(Color? color) => CupertinoDynamicColor.maybeResolve(color, context);
return CupertinoThemeData._rawWithDefaults(
brightness,
convertColor(super.primaryColor),
convertColor(super.primaryContrastingColor),
super.textTheme?.resolveFrom(context),
convertColor(super.barBackgroundColor),
convertColor(super.scaffoldBackgroundColor),
applyThemeToAll,
_defaults.resolveFrom(context, super.textTheme == null),
);
}
@override
CupertinoThemeData copyWith({
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
}) {
return CupertinoThemeData._rawWithDefaults(
brightness ?? super.brightness,
primaryColor ?? super.primaryColor,
primaryContrastingColor ?? super.primaryContrastingColor,
textTheme ?? super.textTheme,
barBackgroundColor ?? super.barBackgroundColor,
scaffoldBackgroundColor ?? super.scaffoldBackgroundColor,
applyThemeToAll ?? super.applyThemeToAll,
_defaults,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
const CupertinoThemeData defaultData = CupertinoThemeData();
properties.add(EnumProperty<Brightness>('brightness', brightness, defaultValue: null));
properties.add(createCupertinoColorProperty('primaryColor', primaryColor, defaultValue: defaultData.primaryColor));
properties.add(createCupertinoColorProperty('primaryContrastingColor', primaryContrastingColor, defaultValue: defaultData.primaryContrastingColor));
properties.add(createCupertinoColorProperty('barBackgroundColor', barBackgroundColor, defaultValue: defaultData.barBackgroundColor));
properties.add(createCupertinoColorProperty('scaffoldBackgroundColor', scaffoldBackgroundColor, defaultValue: defaultData.scaffoldBackgroundColor));
properties.add(DiagnosticsProperty<bool>('applyThemeToAll', applyThemeToAll, defaultValue: defaultData.applyThemeToAll));
textTheme.debugFillProperties(properties);
}
@override
bool operator == (Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is CupertinoThemeData
&& other.brightness == brightness
&& other.primaryColor == primaryColor
&& other.primaryContrastingColor == primaryContrastingColor
&& other.textTheme == textTheme
&& other.barBackgroundColor == barBackgroundColor
&& other.scaffoldBackgroundColor == scaffoldBackgroundColor
&& other.applyThemeToAll == applyThemeToAll;
}
@override
int get hashCode => Object.hash(
brightness,
primaryColor,
primaryContrastingColor,
textTheme,
barBackgroundColor,
scaffoldBackgroundColor,
applyThemeToAll,
);
}
/// Styling specifications for a cupertino theme without default values for
/// unspecified properties.
///
/// Unlike [CupertinoThemeData] instances of this class do not return default
/// values for properties that have been left unspecified in the constructor.
/// Instead, unspecified properties will return null. This is used by
/// Material's [ThemeData.cupertinoOverrideTheme].
///
/// See also:
///
/// * [CupertinoThemeData], which uses reasonable default values for
/// unspecified theme properties.
class NoDefaultCupertinoThemeData {
/// Creates a [NoDefaultCupertinoThemeData] styling specification.
///
/// Unspecified properties default to null.
const NoDefaultCupertinoThemeData({
this.brightness,
this.primaryColor,
this.primaryContrastingColor,
this.textTheme,
this.barBackgroundColor,
this.scaffoldBackgroundColor,
this.applyThemeToAll,
});
/// The brightness override for Cupertino descendants.
///
/// Defaults to null. If a non-null [Brightness] is specified, the value will
/// take precedence over the ambient [MediaQueryData.platformBrightness], when
/// determining the brightness of descendant Cupertino widgets.
///
/// If coming from a Material [Theme] and unspecified, [brightness] will be
/// derived from the Material [ThemeData]'s [brightness].
///
/// See also:
///
/// * [MaterialBasedCupertinoThemeData], a [CupertinoThemeData] that defers
/// [brightness] to its Material [Theme] parent if it's unspecified.
///
/// * [CupertinoTheme.brightnessOf], a method used to retrieve the overall
/// [Brightness] from a [BuildContext], for Cupertino widgets.
final Brightness? brightness;
/// A color used on interactive elements of the theme.
///
/// This color is generally used on text and icons in buttons and tappable
/// elements. Defaults to [CupertinoColors.activeBlue].
///
/// If coming from a Material [Theme] and unspecified, [primaryColor] will be
/// derived from the Material [ThemeData]'s `colorScheme.primary`. However, in
/// iOS styling, the [primaryColor] is more sparsely used than in Material
/// Design where the [primaryColor] can appear on non-interactive surfaces like
/// the [AppBar] background, [TextField] borders etc.
///
/// See also:
///
/// * [MaterialBasedCupertinoThemeData], a [CupertinoThemeData] that defers
/// [primaryColor] to its Material [Theme] parent if it's unspecified.
final Color? primaryColor;
/// A color that must be easy to see when rendered on a [primaryColor] background.
///
/// For example, this color is used for a [CupertinoButton]'s text and icons
/// when the button's background is [primaryColor].
///
/// If coming from a Material [Theme] and unspecified, [primaryContrastingColor]
/// will be derived from the Material [ThemeData]'s `colorScheme.onPrimary`.
///
/// See also:
///
/// * [MaterialBasedCupertinoThemeData], a [CupertinoThemeData] that defers
/// [primaryContrastingColor] to its Material [Theme] parent if it's unspecified.
final Color? primaryContrastingColor;
/// Text styles used by Cupertino widgets.
///
/// Derived from [primaryColor] if unspecified.
final CupertinoTextThemeData? textTheme;
/// Background color of the top nav bar and bottom tab bar.
///
/// Defaults to a light gray in light mode, or a dark translucent gray color in
/// dark mode.
final Color? barBackgroundColor;
/// Background color of the scaffold.
///
/// Defaults to [CupertinoColors.systemBackground].
final Color? scaffoldBackgroundColor;
/// Flag to apply this theme to all descendant Cupertino widgets.
///
/// Certain Cupertino widgets previously didn't use theming, matching past
/// versions of iOS. For example, [CupertinoSwitch]s always used
/// [CupertinoColors.systemGreen] when active.
///
/// Today, however, these widgets can indeed be themed on iOS. Moreover on
/// macOS, the accent color is reflected in these widgets. Turning this flag
/// on ensures that descendant Cupertino widgets will be themed accordingly.
///
/// This flag currently applies to the following widgets:
/// - [CupertinoSwitch] & [Switch.adaptive]
///
/// Defaults to false.
final bool? applyThemeToAll;
/// Returns an instance of the theme data whose property getters only return
/// the construction time specifications with no derived values.
///
/// Used in Material themes to let unspecified properties fallback to Material
/// theme properties instead of iOS defaults.
NoDefaultCupertinoThemeData noDefault() => this;
/// Returns a new theme data with all its colors resolved against the
/// given [BuildContext].
///
/// Called by [CupertinoTheme.of] to resolve colors defined in the retrieved
/// [CupertinoThemeData].
@protected
NoDefaultCupertinoThemeData resolveFrom(BuildContext context) {
Color? convertColor(Color? color) => CupertinoDynamicColor.maybeResolve(color, context);
return NoDefaultCupertinoThemeData(
brightness: brightness,
primaryColor: convertColor(primaryColor),
primaryContrastingColor: convertColor(primaryContrastingColor),
textTheme: textTheme?.resolveFrom(context),
barBackgroundColor: convertColor(barBackgroundColor),
scaffoldBackgroundColor: convertColor(scaffoldBackgroundColor),
applyThemeToAll: applyThemeToAll,
);
}
/// Creates a copy of the theme data with specified attributes overridden.
///
/// Only the current instance's specified attributes are copied instead of
/// derived values. For instance, if the current [textTheme] is implied from
/// the current [primaryColor] because it was not specified, copying with a
/// different [primaryColor] will also change the copy's implied [textTheme].
NoDefaultCupertinoThemeData copyWith({
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor ,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
}) {
return NoDefaultCupertinoThemeData(
brightness: brightness ?? this.brightness,
primaryColor: primaryColor ?? this.primaryColor,
primaryContrastingColor: primaryContrastingColor ?? this.primaryContrastingColor,
textTheme: textTheme ?? this.textTheme,
barBackgroundColor: barBackgroundColor ?? this.barBackgroundColor,
scaffoldBackgroundColor: scaffoldBackgroundColor ?? this.scaffoldBackgroundColor,
applyThemeToAll: applyThemeToAll ?? this.applyThemeToAll,
);
}
}
@immutable
class _CupertinoThemeDefaults {
const _CupertinoThemeDefaults(
this.brightness,
this.primaryColor,
this.primaryContrastingColor,
this.barBackgroundColor,
this.scaffoldBackgroundColor,
this.applyThemeToAll,
this.textThemeDefaults,
);
final Brightness? brightness;
final Color primaryColor;
final Color primaryContrastingColor;
final Color barBackgroundColor;
final Color scaffoldBackgroundColor;
final bool applyThemeToAll;
final _CupertinoTextThemeDefaults textThemeDefaults;
_CupertinoThemeDefaults resolveFrom(BuildContext context, bool resolveTextTheme) {
Color convertColor(Color color) => CupertinoDynamicColor.resolve(color, context);
return _CupertinoThemeDefaults(
brightness,
convertColor(primaryColor),
convertColor(primaryContrastingColor),
convertColor(barBackgroundColor),
convertColor(scaffoldBackgroundColor),
applyThemeToAll,
resolveTextTheme ? textThemeDefaults.resolveFrom(context) : textThemeDefaults,
);
}
}
@immutable
class _CupertinoTextThemeDefaults {
const _CupertinoTextThemeDefaults(
this.labelColor,
this.inactiveGray,
);
final Color labelColor;
final Color inactiveGray;
_CupertinoTextThemeDefaults resolveFrom(BuildContext context) {
return _CupertinoTextThemeDefaults(
CupertinoDynamicColor.resolve(labelColor, context),
CupertinoDynamicColor.resolve(inactiveGray, context),
);
}
CupertinoTextThemeData createDefaults({ required Color primaryColor }) {
return _DefaultCupertinoTextThemeData(
primaryColor: primaryColor,
labelColor: labelColor,
inactiveGray: inactiveGray,
);
}
}
// CupertinoTextThemeData with no text styles explicitly specified.
// The implementation of this class may need to be updated when any of the default
// text styles changes.
class _DefaultCupertinoTextThemeData extends CupertinoTextThemeData {
const _DefaultCupertinoTextThemeData({
required this.labelColor,
required this.inactiveGray,
required super.primaryColor,
});
final Color labelColor;
final Color inactiveGray;
@override
TextStyle get textStyle => super.textStyle.copyWith(color: labelColor);
@override
TextStyle get tabLabelTextStyle => super.tabLabelTextStyle.copyWith(color: inactiveGray);
@override
TextStyle get navTitleTextStyle => super.navTitleTextStyle.copyWith(color: labelColor);
@override
TextStyle get navLargeTitleTextStyle => super.navLargeTitleTextStyle.copyWith(color: labelColor);
@override
TextStyle get pickerTextStyle => super.pickerTextStyle.copyWith(color: labelColor);
@override
TextStyle get dateTimePickerTextStyle => super.dateTimePickerTextStyle.copyWith(color: labelColor);
}
| flutter/packages/flutter/lib/src/cupertino/theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/theme.dart",
"repo_id": "flutter",
"token_count": 6671
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:meta/meta.dart';
import 'basic_types.dart';
import 'constants.dart';
import 'diagnostics.dart';
import 'print.dart';
import 'stack_frame.dart';
export 'basic_types.dart' show IterableFilter;
export 'diagnostics.dart' show DiagnosticLevel, DiagnosticPropertiesBuilder, DiagnosticsNode, DiagnosticsTreeStyle;
export 'stack_frame.dart' show StackFrame;
// Examples can assume:
// late String runtimeType;
// late bool draconisAlive;
// late bool draconisAmulet;
// late Diagnosticable draconis;
// void methodThatMayThrow() { }
// class Trace implements StackTrace { late StackTrace vmTrace; }
// class Chain implements StackTrace { Trace toTrace() => Trace(); }
/// Signature for [FlutterError.onError] handler.
typedef FlutterExceptionHandler = void Function(FlutterErrorDetails details);
/// Signature for [DiagnosticPropertiesBuilder] transformer.
typedef DiagnosticPropertiesTransformer = Iterable<DiagnosticsNode> Function(Iterable<DiagnosticsNode> properties);
/// Signature for [FlutterErrorDetails.informationCollector] callback
/// and other callbacks that collect information describing an error.
typedef InformationCollector = Iterable<DiagnosticsNode> Function();
/// Signature for a function that demangles [StackTrace] objects into a format
/// that can be parsed by [StackFrame].
///
/// See also:
///
/// * [FlutterError.demangleStackTrace], which shows an example implementation.
typedef StackTraceDemangler = StackTrace Function(StackTrace details);
/// Partial information from a stack frame for stack filtering purposes.
///
/// See also:
///
/// * [RepetitiveStackFrameFilter], which uses this class to compare against [StackFrame]s.
@immutable
class PartialStackFrame {
/// Creates a new [PartialStackFrame] instance.
const PartialStackFrame({
required this.package,
required this.className,
required this.method,
});
/// An `<asynchronous suspension>` line in a stack trace.
static const PartialStackFrame asynchronousSuspension = PartialStackFrame(
package: '',
className: '',
method: 'asynchronous suspension',
);
/// The package to match, e.g. `package:flutter/src/foundation/assertions.dart`,
/// or `dart:ui/window.dart`.
final Pattern package;
/// The class name for the method.
///
/// On web, this is ignored, since class names are not available.
///
/// On all platforms, top level methods should use the empty string.
final String className;
/// The method name for this frame line.
///
/// On web, private methods are wrapped with `[]`.
final String method;
/// Tests whether the [StackFrame] matches the information in this
/// [PartialStackFrame].
bool matches(StackFrame stackFrame) {
final String stackFramePackage = '${stackFrame.packageScheme}:${stackFrame.package}/${stackFrame.packagePath}';
// Ideally this wouldn't be necessary.
// TODO(dnfield): https://github.com/dart-lang/sdk/issues/40117
if (kIsWeb) {
return package.allMatches(stackFramePackage).isNotEmpty
&& stackFrame.method == (method.startsWith('_') ? '[$method]' : method);
}
return package.allMatches(stackFramePackage).isNotEmpty
&& stackFrame.method == method
&& stackFrame.className == className;
}
}
/// A class that filters stack frames for additional filtering on
/// [FlutterError.defaultStackFilter].
abstract class StackFilter {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const StackFilter();
/// Filters the list of [StackFrame]s by updating corresponding indices in
/// `reasons`.
///
/// To elide a frame or number of frames, set the string.
void filter(List<StackFrame> stackFrames, List<String?> reasons);
}
/// A [StackFilter] that filters based on repeating lists of
/// [PartialStackFrame]s.
///
/// See also:
///
/// * [FlutterError.addDefaultStackFilter], a method to register additional
/// stack filters for [FlutterError.defaultStackFilter].
/// * [StackFrame], a class that can help with parsing stack frames.
/// * [PartialStackFrame], a class that helps match partial method information
/// to a stack frame.
class RepetitiveStackFrameFilter extends StackFilter {
/// Creates a new RepetitiveStackFrameFilter. All parameters are required and must not be
/// null.
const RepetitiveStackFrameFilter({
required this.frames,
required this.replacement,
});
/// The shape of this repetitive stack pattern.
final List<PartialStackFrame> frames;
/// The number of frames in this pattern.
int get numFrames => frames.length;
/// The string to replace the frames with.
///
/// If the same replacement string is used multiple times in a row, the
/// [FlutterError.defaultStackFilter] will insert a repeat count after this
/// line rather than repeating it.
final String replacement;
List<String> get _replacements => List<String>.filled(numFrames, replacement);
@override
void filter(List<StackFrame> stackFrames, List<String?> reasons) {
for (int index = 0; index < stackFrames.length - numFrames; index += 1) {
if (_matchesFrames(stackFrames.skip(index).take(numFrames).toList())) {
reasons.setRange(index, index + numFrames, _replacements);
index += numFrames - 1;
}
}
}
bool _matchesFrames(List<StackFrame> stackFrames) {
if (stackFrames.length < numFrames) {
return false;
}
for (int index = 0; index < stackFrames.length; index++) {
if (!frames[index].matches(stackFrames[index])) {
return false;
}
}
return true;
}
}
abstract class _ErrorDiagnostic extends DiagnosticsProperty<List<Object>> {
/// This constructor provides a reliable hook for a kernel transformer to find
/// error messages that need to be rewritten to include object references for
/// interactive display of errors.
_ErrorDiagnostic(
String message, {
DiagnosticsTreeStyle style = DiagnosticsTreeStyle.flat,
DiagnosticLevel level = DiagnosticLevel.info,
}) : super(
null,
<Object>[message],
showName: false,
showSeparator: false,
defaultValue: null,
style: style,
level: level,
);
/// In debug builds, a kernel transformer rewrites calls to the default
/// constructors for [ErrorSummary], [ErrorDescription], and [ErrorHint] to use
/// this constructor.
//
// ```dart
// _ErrorDiagnostic('Element $element must be $color')
// ```
// Desugars to:
// ```dart
// _ErrorDiagnostic.fromParts(<Object>['Element ', element, ' must be ', color])
// ```
//
// Slightly more complex case:
// ```dart
// _ErrorDiagnostic('Element ${element.runtimeType} must be $color')
// ```
// Desugars to:
//```dart
// _ErrorDiagnostic.fromParts(<Object>[
// 'Element ',
// DiagnosticsProperty(null, element, description: element.runtimeType?.toString()),
// ' must be ',
// color,
// ])
// ```
_ErrorDiagnostic._fromParts(
List<Object> messageParts, {
DiagnosticsTreeStyle style = DiagnosticsTreeStyle.flat,
DiagnosticLevel level = DiagnosticLevel.info,
}) : super(
null,
messageParts,
showName: false,
showSeparator: false,
defaultValue: null,
style: style,
level: level,
);
@override
String toString({
TextTreeConfiguration? parentConfiguration,
DiagnosticLevel minLevel = DiagnosticLevel.info,
}) {
return valueToString(parentConfiguration: parentConfiguration);
}
@override
List<Object> get value => super.value!;
@override
String valueToString({ TextTreeConfiguration? parentConfiguration }) {
return value.join();
}
}
/// An explanation of the problem and its cause, any information that may help
/// track down the problem, background information, etc.
///
/// Use [ErrorDescription] for any part of an error message where neither
/// [ErrorSummary] or [ErrorHint] is appropriate.
///
/// In debug builds, values interpolated into the `message` are
/// expanded and placed into [value], which is of type [List<Object>].
/// This allows IDEs to examine values interpolated into error messages.
///
/// See also:
///
/// * [ErrorSummary], which provides a short (one line) description of the
/// problem that was detected.
/// * [ErrorHint], which provides specific, non-obvious advice that may be
/// applicable.
/// * [ErrorSpacer], which renders as a blank line.
/// * [FlutterError], which is the most common place to use an
/// [ErrorDescription].
class ErrorDescription extends _ErrorDiagnostic {
/// A lint enforces that this constructor can only be called with a string
/// literal to match the limitations of the Dart Kernel transformer that
/// optionally extracts out objects referenced using string interpolation in
/// the message passed in.
///
/// The message will display with the same text regardless of whether the
/// kernel transformer is used. The kernel transformer is required so that
/// debugging tools can provide interactive displays of objects described by
/// the error.
ErrorDescription(super.message) : super(level: DiagnosticLevel.info);
/// Calls to the default constructor may be rewritten to use this constructor
/// in debug mode using a kernel transformer.
// ignore: unused_element
ErrorDescription._fromParts(super.messageParts) : super._fromParts(level: DiagnosticLevel.info);
}
/// A short (one line) description of the problem that was detected.
///
/// Error summaries from the same source location should have little variance,
/// so that they can be recognized as related. For example, they shouldn't
/// include hash codes.
///
/// A [FlutterError] must start with an [ErrorSummary] and may not contain
/// multiple summaries.
///
/// In debug builds, values interpolated into the `message` are
/// expanded and placed into [value], which is of type [List<Object>].
/// This allows IDEs to examine values interpolated into error messages.
///
/// See also:
///
/// * [ErrorDescription], which provides an explanation of the problem and its
/// cause, any information that may help track down the problem, background
/// information, etc.
/// * [ErrorHint], which provides specific, non-obvious advice that may be
/// applicable.
/// * [FlutterError], which is the most common place to use an [ErrorSummary].
class ErrorSummary extends _ErrorDiagnostic {
/// A lint enforces that this constructor can only be called with a string
/// literal to match the limitations of the Dart Kernel transformer that
/// optionally extracts out objects referenced using string interpolation in
/// the message passed in.
///
/// The message will display with the same text regardless of whether the
/// kernel transformer is used. The kernel transformer is required so that
/// debugging tools can provide interactive displays of objects described by
/// the error.
ErrorSummary(super.message) : super(level: DiagnosticLevel.summary);
/// Calls to the default constructor may be rewritten to use this constructor
/// in debug mode using a kernel transformer.
// ignore: unused_element
ErrorSummary._fromParts(super.messageParts) : super._fromParts(level: DiagnosticLevel.summary);
}
/// An [ErrorHint] provides specific, non-obvious advice that may be applicable.
///
/// If your message provides obvious advice that is always applicable, it is an
/// [ErrorDescription] not a hint.
///
/// In debug builds, values interpolated into the `message` are
/// expanded and placed into [value], which is of type [List<Object>].
/// This allows IDEs to examine values interpolated into error messages.
///
/// See also:
///
/// * [ErrorSummary], which provides a short (one line) description of the
/// problem that was detected.
/// * [ErrorDescription], which provides an explanation of the problem and its
/// cause, any information that may help track down the problem, background
/// information, etc.
/// * [ErrorSpacer], which renders as a blank line.
/// * [FlutterError], which is the most common place to use an [ErrorHint].
class ErrorHint extends _ErrorDiagnostic {
/// A lint enforces that this constructor can only be called with a string
/// literal to match the limitations of the Dart Kernel transformer that
/// optionally extracts out objects referenced using string interpolation in
/// the message passed in.
///
/// The message will display with the same text regardless of whether the
/// kernel transformer is used. The kernel transformer is required so that
/// debugging tools can provide interactive displays of objects described by
/// the error.
ErrorHint(super.message) : super(level:DiagnosticLevel.hint);
/// Calls to the default constructor may be rewritten to use this constructor
/// in debug mode using a kernel transformer.
// ignore: unused_element
ErrorHint._fromParts(super.messageParts) : super._fromParts(level:DiagnosticLevel.hint);
}
/// An [ErrorSpacer] creates an empty [DiagnosticsNode], that can be used to
/// tune the spacing between other [DiagnosticsNode] objects.
class ErrorSpacer extends DiagnosticsProperty<void> {
/// Creates an empty space to insert into a list of [DiagnosticsNode] objects
/// typically within a [FlutterError] object.
ErrorSpacer() : super(
'',
null,
description: '',
showName: false,
);
}
/// Class for information provided to [FlutterExceptionHandler] callbacks.
///
/// {@tool snippet}
/// This is an example of using [FlutterErrorDetails] when calling
/// [FlutterError.reportError].
///
/// ```dart
/// void main() {
/// try {
/// // Try to do something!
/// } catch (error) {
/// // Catch & report error.
/// FlutterError.reportError(FlutterErrorDetails(
/// exception: error,
/// library: 'Flutter test framework',
/// context: ErrorSummary('while running async test code'),
/// ));
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [FlutterError.onError], which is called whenever the Flutter framework
/// catches an error.
class FlutterErrorDetails with Diagnosticable {
/// Creates a [FlutterErrorDetails] object with the given arguments setting
/// the object's properties.
///
/// The framework calls this constructor when catching an exception that will
/// subsequently be reported using [FlutterError.onError].
const FlutterErrorDetails({
required this.exception,
this.stack,
this.library = 'Flutter framework',
this.context,
this.stackFilter,
this.informationCollector,
this.silent = false,
});
/// Creates a copy of the error details but with the given fields replaced
/// with new values.
FlutterErrorDetails copyWith({
DiagnosticsNode? context,
Object? exception,
InformationCollector? informationCollector,
String? library,
bool? silent,
StackTrace? stack,
IterableFilter<String>? stackFilter,
}) {
return FlutterErrorDetails(
context: context ?? this.context,
exception: exception ?? this.exception,
informationCollector: informationCollector ?? this.informationCollector,
library: library ?? this.library,
silent: silent ?? this.silent,
stack: stack ?? this.stack,
stackFilter: stackFilter ?? this.stackFilter,
);
}
/// Transformers to transform [DiagnosticsNode] in [DiagnosticPropertiesBuilder]
/// into a more descriptive form.
///
/// There are layers that attach certain [DiagnosticsNode] into
/// [FlutterErrorDetails] that require knowledge from other layers to parse.
/// To correctly interpret those [DiagnosticsNode], register transformers in
/// the layers that possess the knowledge.
///
/// See also:
///
/// * [WidgetsBinding.initInstances], which registers its transformer.
static final List<DiagnosticPropertiesTransformer> propertiesTransformers =
<DiagnosticPropertiesTransformer>[];
/// The exception. Often this will be an [AssertionError], maybe specifically
/// a [FlutterError]. However, this could be any value at all.
final Object exception;
/// The stack trace from where the [exception] was thrown (as opposed to where
/// it was caught).
///
/// StackTrace objects are opaque except for their [toString] function.
///
/// If this field is not null, then the [stackFilter] callback, if any, will
/// be called with the result of calling [toString] on this object and
/// splitting that result on line breaks. If there's no [stackFilter]
/// callback, then [FlutterError.defaultStackFilter] is used instead. That
/// function expects the stack to be in the format used by
/// [StackTrace.toString].
final StackTrace? stack;
/// A human-readable brief name describing the library that caught the error
/// message. This is used by the default error handler in the header dumped to
/// the console.
final String? library;
/// A [DiagnosticsNode] that provides a human-readable description of where
/// the error was caught (as opposed to where it was thrown).
///
/// The node, e.g. an [ErrorDescription], should be in a form that will make
/// sense in English when following the word "thrown", as in "thrown while
/// obtaining the image from the network" (for the context "while obtaining
/// the image from the network").
///
/// {@tool snippet}
/// This is an example of using and [ErrorDescription] as the
/// [FlutterErrorDetails.context] when calling [FlutterError.reportError].
///
/// ```dart
/// void maybeDoSomething() {
/// try {
/// // Try to do something!
/// } catch (error) {
/// // Catch & report error.
/// FlutterError.reportError(FlutterErrorDetails(
/// exception: error,
/// library: 'Flutter test framework',
/// context: ErrorDescription('while dispatching notifications for $runtimeType'),
/// ));
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ErrorDescription], which provides an explanation of the problem and
/// its cause, any information that may help track down the problem,
/// background information, etc.
/// * [ErrorSummary], which provides a short (one line) description of the
/// problem that was detected.
/// * [ErrorHint], which provides specific, non-obvious advice that may be
/// applicable.
/// * [FlutterError], which is the most common place to use
/// [FlutterErrorDetails].
final DiagnosticsNode? context;
/// A callback which filters the [stack] trace. Receives an iterable of
/// strings representing the frames encoded in the way that
/// [StackTrace.toString()] provides. Should return an iterable of lines to
/// output for the stack.
///
/// If this is not provided, then [FlutterError.dumpErrorToConsole] will use
/// [FlutterError.defaultStackFilter] instead.
///
/// If the [FlutterError.defaultStackFilter] behavior is desired, then the
/// callback should manually call that function. That function expects the
/// incoming list to be in the [StackTrace.toString()] format. The output of
/// that function, however, does not always follow this format.
///
/// This won't be called if [stack] is null.
final IterableFilter<String>? stackFilter;
/// A callback which will provide information that could help with debugging
/// the problem.
///
/// Information collector callbacks can be expensive, so the generated
/// information should be cached by the caller, rather than the callback being
/// called multiple times.
///
/// The callback is expected to return an iterable of [DiagnosticsNode] objects,
/// typically implemented using `sync*` and `yield`.
///
/// {@tool snippet}
/// In this example, the information collector returns two pieces of information,
/// one broadly-applicable statement regarding how the error happened, and one
/// giving a specific piece of information that may be useful in some cases but
/// may also be irrelevant most of the time (an argument to the method).
///
/// ```dart
/// void climbElevator(int pid) {
/// try {
/// // ...
/// } catch (error, stack) {
/// FlutterError.reportError(FlutterErrorDetails(
/// exception: error,
/// stack: stack,
/// informationCollector: () => <DiagnosticsNode>[
/// ErrorDescription('This happened while climbing the space elevator.'),
/// ErrorHint('The process ID is: $pid'),
/// ],
/// ));
/// }
/// }
/// ```
/// {@end-tool}
///
/// The following classes may be of particular use:
///
/// * [ErrorDescription], for information that is broadly applicable to the
/// situation being described.
/// * [ErrorHint], for specific information that may not always be applicable
/// but can be helpful in certain situations.
/// * [DiagnosticsStackTrace], for reporting stack traces.
/// * [ErrorSpacer], for adding spaces (a blank line) between other items.
///
/// For objects that implement [Diagnosticable] one may consider providing
/// additional information by yielding the output of the object's
/// [Diagnosticable.toDiagnosticsNode] method.
final InformationCollector? informationCollector;
/// Whether this error should be ignored by the default error reporting
/// behavior in release mode.
///
/// If this is false, the default, then the default error handler will always
/// dump this error to the console.
///
/// If this is true, then the default error handler would only dump this error
/// to the console in debug mode. In release mode, the error is ignored.
///
/// This is used by certain exception handlers that catch errors that could be
/// triggered by environmental conditions (as opposed to logic errors). For
/// example, the HTTP library sets this flag so as to not report every 404
/// error to the console on end-user devices, while still allowing a custom
/// error handler to see the errors even in release builds.
final bool silent;
/// Converts the [exception] to a string.
///
/// This applies some additional logic to make [AssertionError] exceptions
/// prettier, to handle exceptions that stringify to empty strings, to handle
/// objects that don't inherit from [Exception] or [Error], and so forth.
String exceptionAsString() {
String? longMessage;
if (exception is AssertionError) {
// Regular _AssertionErrors thrown by assert() put the message last, after
// some code snippets. This leads to ugly messages. To avoid this, we move
// the assertion message up to before the code snippets, separated by a
// newline, if we recognize that format is being used.
final Object? message = (exception as AssertionError).message;
final String fullMessage = exception.toString();
if (message is String && message != fullMessage) {
if (fullMessage.length > message.length) {
final int position = fullMessage.lastIndexOf(message);
if (position == fullMessage.length - message.length &&
position > 2 &&
fullMessage.substring(position - 2, position) == ': ') {
// Add a linebreak so that the filename at the start of the
// assertion message is always on its own line.
String body = fullMessage.substring(0, position - 2);
final int splitPoint = body.indexOf(' Failed assertion:');
if (splitPoint >= 0) {
body = '${body.substring(0, splitPoint)}\n${body.substring(splitPoint + 1)}';
}
longMessage = '${message.trimRight()}\n$body';
}
}
}
longMessage ??= fullMessage;
} else if (exception is String) {
longMessage = exception as String;
} else if (exception is Error || exception is Exception) {
longMessage = exception.toString();
} else {
longMessage = ' $exception';
}
longMessage = longMessage.trimRight();
if (longMessage.isEmpty) {
longMessage = ' <no message available>';
}
return longMessage;
}
Diagnosticable? _exceptionToDiagnosticable() {
final Object exception = this.exception;
if (exception is FlutterError) {
return exception;
}
if (exception is AssertionError && exception.message is FlutterError) {
return exception.message! as FlutterError;
}
return null;
}
/// Returns a short (one line) description of the problem that was detected.
///
/// If the exception contains an [ErrorSummary] that summary is used,
/// otherwise the summary is inferred from the string representation of the
/// exception.
///
/// In release mode, this always returns a [DiagnosticsNode.message] with a
/// formatted version of the exception.
DiagnosticsNode get summary {
String formatException() => exceptionAsString().split('\n')[0].trimLeft();
if (kReleaseMode) {
return DiagnosticsNode.message(formatException());
}
final Diagnosticable? diagnosticable = _exceptionToDiagnosticable();
DiagnosticsNode? summary;
if (diagnosticable != null) {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
debugFillProperties(builder);
summary = builder.properties.cast<DiagnosticsNode?>().firstWhere((DiagnosticsNode? node) => node!.level == DiagnosticLevel.summary, orElse: () => null);
}
return summary ?? ErrorSummary(formatException());
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final DiagnosticsNode verb = ErrorDescription('thrown${ context != null ? ErrorDescription(" $context") : ""}');
final Diagnosticable? diagnosticable = _exceptionToDiagnosticable();
if (exception is num) {
properties.add(ErrorDescription('The number $exception was $verb.'));
} else {
final DiagnosticsNode errorName;
if (exception is AssertionError) {
errorName = ErrorDescription('assertion');
} else if (exception is String) {
errorName = ErrorDescription('message');
} else if (exception is Error || exception is Exception) {
errorName = ErrorDescription('${exception.runtimeType}');
} else {
errorName = ErrorDescription('${exception.runtimeType} object');
}
properties.add(ErrorDescription('The following $errorName was $verb:'));
if (diagnosticable != null) {
diagnosticable.debugFillProperties(properties);
} else {
// Many exception classes put their type at the head of their message.
// This is redundant with the way we display exceptions, so attempt to
// strip out that header when we see it.
final String prefix = '${exception.runtimeType}: ';
String message = exceptionAsString();
if (message.startsWith(prefix)) {
message = message.substring(prefix.length);
}
properties.add(ErrorSummary(message));
}
}
if (stack != null) {
if (exception is AssertionError && diagnosticable == null) {
// After popping off any dart: stack frames, are there at least two more
// stack frames coming from package flutter?
//
// If not: Error is in user code (user violated assertion in framework).
// If so: Error is in Framework. We either need an assertion higher up
// in the stack, or we've violated our own assertions.
final List<StackFrame> stackFrames = StackFrame.fromStackTrace(FlutterError.demangleStackTrace(stack!))
.skipWhile((StackFrame frame) => frame.packageScheme == 'dart')
.toList();
final bool ourFault = stackFrames.length >= 2
&& stackFrames[0].package == 'flutter'
&& stackFrames[1].package == 'flutter';
if (ourFault) {
properties.add(ErrorSpacer());
properties.add(ErrorHint(
'Either the assertion indicates an error in the framework itself, or we should '
'provide substantially more information in this error message to help you determine '
'and fix the underlying cause.\n'
'In either case, please report this assertion by filing a bug on GitHub:\n'
' https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
));
}
}
properties.add(ErrorSpacer());
properties.add(DiagnosticsStackTrace('When the exception was thrown, this was the stack', stack, stackFilter: stackFilter));
}
if (informationCollector != null) {
properties.add(ErrorSpacer());
informationCollector!().forEach(properties.add);
}
}
@override
String toStringShort() {
return library != null ? 'Exception caught by $library' : 'Exception caught';
}
@override
String toString({DiagnosticLevel minLevel = DiagnosticLevel.info}) {
return toDiagnosticsNode(style: DiagnosticsTreeStyle.error).toStringDeep(minLevel: minLevel);
}
@override
DiagnosticsNode toDiagnosticsNode({ String? name, DiagnosticsTreeStyle? style }) {
return _FlutterErrorDetailsNode(
name: name,
value: this,
style: style,
);
}
}
/// Error class used to report Flutter-specific assertion failures and
/// contract violations.
///
/// See also:
///
/// * <https://flutter.dev/docs/testing/errors>, more information about error
/// handling in Flutter.
class FlutterError extends Error with DiagnosticableTreeMixin implements AssertionError {
/// Create an error message from a string.
///
/// The message may have newlines in it. The first line should be a terse
/// description of the error, e.g. "Incorrect GlobalKey usage" or "setState()
/// or markNeedsBuild() called during build". Subsequent lines should contain
/// substantial additional information, ideally sufficient to develop a
/// correct solution to the problem.
///
/// In some cases, when a [FlutterError] is reported to the user, only the first
/// line is included. For example, Flutter will typically only fully report
/// the first exception at runtime, displaying only the first line of
/// subsequent errors.
///
/// All sentences in the error should be correctly punctuated (i.e.,
/// do end the error message with a period).
///
/// This constructor defers to the [FlutterError.fromParts] constructor.
/// The first line is wrapped in an implied [ErrorSummary], and subsequent
/// lines are wrapped in implied [ErrorDescription]s. Consider using the
/// [FlutterError.fromParts] constructor to provide more detail, e.g.
/// using [ErrorHint]s or other [DiagnosticsNode]s.
factory FlutterError(String message) {
final List<String> lines = message.split('\n');
return FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(lines.first),
...lines.skip(1).map<DiagnosticsNode>((String line) => ErrorDescription(line)),
]);
}
/// Create an error message from a list of [DiagnosticsNode]s.
///
/// By convention, there should be exactly one [ErrorSummary] in the list,
/// and it should be the first entry.
///
/// Other entries are typically [ErrorDescription]s (for material that is
/// always applicable for this error) and [ErrorHint]s (for material that may
/// be sometimes useful, but may not always apply). Other [DiagnosticsNode]
/// subclasses, such as [DiagnosticsStackTrace], may
/// also be used.
///
/// When using an [ErrorSummary], [ErrorDescription]s, and [ErrorHint]s, in
/// debug builds, values interpolated into the `message` arguments of those
/// classes' constructors are expanded and placed into the
/// [DiagnosticsProperty.value] property of those objects (which is of type
/// [List<Object>]). This allows IDEs to examine values interpolated into
/// error messages.
///
/// Alternatively, to include a specific [Diagnosticable] object into the
/// error message and have the object describe itself in detail (see
/// [DiagnosticsNode.toStringDeep]), consider calling
/// [Diagnosticable.toDiagnosticsNode] on that object and using that as one of
/// the values passed to this constructor.
///
/// {@tool snippet}
/// In this example, an error is thrown in debug mode if certain conditions
/// are not met. The error message includes a description of an object that
/// implements the [Diagnosticable] interface, `draconis`.
///
/// ```dart
/// void controlDraconis() {
/// assert(() {
/// if (!draconisAlive || !draconisAmulet) {
/// throw FlutterError.fromParts(<DiagnosticsNode>[
/// ErrorSummary('Cannot control Draconis in current state.'),
/// ErrorDescription('Draconis can only be controlled while alive and while the amulet is wielded.'),
/// if (!draconisAlive)
/// ErrorHint('Draconis is currently not alive.'),
/// if (!draconisAmulet)
/// ErrorHint('The Amulet of Draconis is currently not wielded.'),
/// draconis.toDiagnosticsNode(name: 'Draconis'),
/// ]);
/// }
/// return true;
/// }());
/// // ...
/// }
/// ```
/// {@end-tool}
FlutterError.fromParts(this.diagnostics) : assert(diagnostics.isNotEmpty, FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Empty FlutterError')])) {
assert(
diagnostics.first.level == DiagnosticLevel.summary,
FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('FlutterError is missing a summary.'),
ErrorDescription(
'All FlutterError objects should start with a short (one line) '
'summary description of the problem that was detected.',
),
DiagnosticsProperty<FlutterError>('Malformed', this, expandableValue: true, showSeparator: false, style: DiagnosticsTreeStyle.whitespace),
ErrorDescription(
'\nThis error should still help you solve your problem, '
'however please also report this malformed error in the '
'framework by filing a bug on GitHub:\n'
' https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]),
);
assert(() {
final Iterable<DiagnosticsNode> summaries = diagnostics.where((DiagnosticsNode node) => node.level == DiagnosticLevel.summary);
if (summaries.length > 1) {
final List<DiagnosticsNode> message = <DiagnosticsNode>[
ErrorSummary('FlutterError contained multiple error summaries.'),
ErrorDescription(
'All FlutterError objects should have only a single short '
'(one line) summary description of the problem that was '
'detected.',
),
DiagnosticsProperty<FlutterError>('Malformed', this, expandableValue: true, showSeparator: false, style: DiagnosticsTreeStyle.whitespace),
ErrorDescription('\nThe malformed error has ${summaries.length} summaries.'),
];
int i = 1;
for (final DiagnosticsNode summary in summaries) {
message.add(DiagnosticsProperty<DiagnosticsNode>('Summary $i', summary, expandableValue : true));
i += 1;
}
message.add(ErrorDescription(
'\nThis error should still help you solve your problem, '
'however please also report this malformed error in the '
'framework by filing a bug on GitHub:\n'
' https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
));
throw FlutterError.fromParts(message);
}
return true;
}());
}
/// The information associated with this error, in structured form.
///
/// The first node is typically an [ErrorSummary] giving a short description
/// of the problem, suitable for an index of errors, a log, etc.
///
/// Subsequent nodes should give information specific to this error. Typically
/// these will be [ErrorDescription]s or [ErrorHint]s, but they could be other
/// objects also. For instance, an error relating to a timer could include a
/// stack trace of when the timer was scheduled using the
/// [DiagnosticsStackTrace] class.
final List<DiagnosticsNode> diagnostics;
/// The message associated with this error.
///
/// This is generated by serializing the [diagnostics].
@override
String get message => toString();
/// Called whenever the Flutter framework catches an error.
///
/// The default behavior is to call [presentError].
///
/// You can set this to your own function to override this default behavior.
/// For example, you could report all errors to your server. Consider calling
/// [presentError] from your custom error handler in order to see the logs in
/// the console as well.
///
/// If the error handler throws an exception, it will not be caught by the
/// Flutter framework.
///
/// Set this to null to silently catch and ignore errors. This is not
/// recommended.
///
/// Do not call [onError] directly, instead, call [reportError], which
/// forwards to [onError] if it is not null.
///
/// See also:
///
/// * <https://flutter.dev/docs/testing/errors>, more information about error
/// handling in Flutter.
static FlutterExceptionHandler? onError = presentError;
/// Called by the Flutter framework before attempting to parse a [StackTrace].
///
/// Some [StackTrace] implementations have a different [toString] format from
/// what the framework expects, like ones from `package:stack_trace`. To make
/// sure we can still parse and filter mangled [StackTrace]s, the framework
/// first calls this function to demangle them.
///
/// This should be set in any environment that could propagate an unusual
/// stack trace to the framework. Otherwise, the default behavior is to assume
/// all stack traces are in a format usually generated by Dart.
///
/// The following example demangles `package:stack_trace` traces by converting
/// them into VM traces, which the framework is able to parse:
///
/// ```dart
/// FlutterError.demangleStackTrace = (StackTrace stack) {
/// // Trace and Chain are classes in package:stack_trace
/// if (stack is Trace) {
/// return stack.vmTrace;
/// }
/// if (stack is Chain) {
/// return stack.toTrace().vmTrace;
/// }
/// return stack;
/// };
/// ```
static StackTraceDemangler demangleStackTrace = _defaultStackTraceDemangler;
static StackTrace _defaultStackTraceDemangler(StackTrace stackTrace) => stackTrace;
/// Called whenever the Flutter framework wants to present an error to the
/// users.
///
/// The default behavior is to call [dumpErrorToConsole].
///
/// Plugins can override how an error is to be presented to the user. For
/// example, the structured errors service extension sets its own method when
/// the extension is enabled. If you want to change how Flutter responds to an
/// error, use [onError] instead.
static FlutterExceptionHandler presentError = dumpErrorToConsole;
static int _errorCount = 0;
/// Resets the count of errors used by [dumpErrorToConsole] to decide whether
/// to show a complete error message or an abbreviated one.
///
/// After this is called, the next error message will be shown in full.
static void resetErrorCount() {
_errorCount = 0;
}
/// The width to which [dumpErrorToConsole] will wrap lines.
///
/// This can be used to ensure strings will not exceed the length at which
/// they will wrap, e.g. when placing ASCII art diagrams in messages.
static const int wrapWidth = 100;
/// Prints the given exception details to the console.
///
/// The first time this is called, it dumps a very verbose message to the
/// console using [debugPrint].
///
/// Subsequent calls only dump the first line of the exception, unless
/// `forceReport` is set to true (in which case it dumps the verbose message).
///
/// Call [resetErrorCount] to cause this method to go back to acting as if it
/// had not been called before (so the next message is verbose again).
///
/// The default behavior for the [onError] handler is to call this function.
static void dumpErrorToConsole(FlutterErrorDetails details, { bool forceReport = false }) {
bool isInDebugMode = false;
assert(() {
// In debug mode, we ignore the "silent" flag.
isInDebugMode = true;
return true;
}());
final bool reportError = isInDebugMode || !details.silent;
if (!reportError && !forceReport) {
return;
}
if (_errorCount == 0 || forceReport) {
// Diagnostics is only available in debug mode. In profile and release modes fallback to plain print.
if (isInDebugMode) {
debugPrint(
TextTreeRenderer(
wrapWidthProperties: wrapWidth,
maxDescendentsTruncatableNode: 5,
).render(details.toDiagnosticsNode(style: DiagnosticsTreeStyle.error)).trimRight(),
);
} else {
debugPrintStack(
stackTrace: details.stack,
label: details.exception.toString(),
maxFrames: 100,
);
}
} else {
debugPrint('Another exception was thrown: ${details.summary}');
}
_errorCount += 1;
}
static final List<StackFilter> _stackFilters = <StackFilter>[];
/// Adds a stack filtering function to [defaultStackFilter].
///
/// For example, the framework adds common patterns of element building to
/// elide tree-walking patterns in the stack trace.
///
/// Added filters are checked in order of addition. The first matching filter
/// wins, and subsequent filters will not be checked.
static void addDefaultStackFilter(StackFilter filter) {
_stackFilters.add(filter);
}
/// Converts a stack to a string that is more readable by omitting stack
/// frames that correspond to Dart internals.
///
/// This is the default filter used by [dumpErrorToConsole] if the
/// [FlutterErrorDetails] object has no [FlutterErrorDetails.stackFilter]
/// callback.
///
/// This function expects its input to be in the format used by
/// [StackTrace.toString()]. The output of this function is similar to that
/// format but the frame numbers will not be consecutive (frames are elided)
/// and the final line may be prose rather than a stack frame.
static Iterable<String> defaultStackFilter(Iterable<String> frames) {
final Map<String, int> removedPackagesAndClasses = <String, int>{
'dart:async-patch': 0,
'dart:async': 0,
'package:stack_trace': 0,
'class _AssertionError': 0,
'class _FakeAsync': 0,
'class _FrameCallbackEntry': 0,
'class _Timer': 0,
'class _RawReceivePortImpl': 0,
};
int skipped = 0;
final List<StackFrame> parsedFrames = StackFrame.fromStackString(frames.join('\n'));
for (int index = 0; index < parsedFrames.length; index += 1) {
final StackFrame frame = parsedFrames[index];
final String className = 'class ${frame.className}';
final String package = '${frame.packageScheme}:${frame.package}';
if (removedPackagesAndClasses.containsKey(className)) {
skipped += 1;
removedPackagesAndClasses.update(className, (int value) => value + 1);
parsedFrames.removeAt(index);
index -= 1;
} else if (removedPackagesAndClasses.containsKey(package)) {
skipped += 1;
removedPackagesAndClasses.update(package, (int value) => value + 1);
parsedFrames.removeAt(index);
index -= 1;
}
}
final List<String?> reasons = List<String?>.filled(parsedFrames.length, null);
for (final StackFilter filter in _stackFilters) {
filter.filter(parsedFrames, reasons);
}
final List<String> result = <String>[];
// Collapse duplicated reasons.
for (int index = 0; index < parsedFrames.length; index += 1) {
final int start = index;
while (index < reasons.length - 1 && reasons[index] != null && reasons[index + 1] == reasons[index]) {
index++;
}
String suffix = '';
if (reasons[index] != null) {
if (index != start) {
suffix = ' (${index - start + 2} frames)';
} else {
suffix = ' (1 frame)';
}
}
final String resultLine = '${reasons[index] ?? parsedFrames[index].source}$suffix';
result.add(resultLine);
}
// Only include packages we actually elided from.
final List<String> where = <String>[
for (final MapEntry<String, int> entry in removedPackagesAndClasses.entries)
if (entry.value > 0)
entry.key,
]..sort();
if (skipped == 1) {
result.add('(elided one frame from ${where.single})');
} else if (skipped > 1) {
if (where.length > 1) {
where[where.length - 1] = 'and ${where.last}';
}
if (where.length > 2) {
result.add('(elided $skipped frames from ${where.join(", ")})');
} else {
result.add('(elided $skipped frames from ${where.join(" ")})');
}
}
return result;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
diagnostics.forEach(properties.add);
}
@override
String toStringShort() => 'FlutterError';
@override
String toString({DiagnosticLevel minLevel = DiagnosticLevel.info}) {
if (kReleaseMode) {
final Iterable<_ErrorDiagnostic> errors = diagnostics.whereType<_ErrorDiagnostic>();
return errors.isNotEmpty ? errors.first.valueToString() : toStringShort();
}
// Avoid wrapping lines.
final TextTreeRenderer renderer = TextTreeRenderer(wrapWidth: 4000000000);
return diagnostics.map((DiagnosticsNode node) => renderer.render(node).trimRight()).join('\n');
}
/// Calls [onError] with the given details, unless it is null.
///
/// {@tool snippet}
/// When calling this from a `catch` block consider annotating the method
/// containing the `catch` block with
/// `@pragma('vm:notify-debugger-on-exception')` to allow an attached debugger
/// to treat the exception as unhandled. This means instead of executing the
/// `catch` block, the debugger can break at the original source location from
/// which the exception was thrown.
///
/// ```dart
/// @pragma('vm:notify-debugger-on-exception')
/// void doSomething() {
/// try {
/// methodThatMayThrow();
/// } catch (exception, stack) {
/// FlutterError.reportError(FlutterErrorDetails(
/// exception: exception,
/// stack: stack,
/// library: 'example library',
/// context: ErrorDescription('while doing something'),
/// ));
/// }
/// }
/// ```
/// {@end-tool}
static void reportError(FlutterErrorDetails details) {
onError?.call(details);
}
}
/// Dump the stack to the console using [debugPrint] and
/// [FlutterError.defaultStackFilter].
///
/// If the `stackTrace` parameter is null, the [StackTrace.current] is used to
/// obtain the stack.
///
/// The `maxFrames` argument can be given to limit the stack to the given number
/// of lines before filtering is applied. By default, all stack lines are
/// included.
///
/// The `label` argument, if present, will be printed before the stack.
void debugPrintStack({StackTrace? stackTrace, String? label, int? maxFrames}) {
if (label != null) {
debugPrint(label);
}
if (stackTrace == null) {
stackTrace = StackTrace.current;
} else {
stackTrace = FlutterError.demangleStackTrace(stackTrace);
}
Iterable<String> lines = stackTrace.toString().trimRight().split('\n');
if (kIsWeb && lines.isNotEmpty) {
// Remove extra call to StackTrace.current for web platform.
// TODO(ferhat): remove when https://github.com/flutter/flutter/issues/37635
// is addressed.
lines = lines.skipWhile((String line) {
return line.contains('StackTrace.current') ||
line.contains('dart-sdk/lib/_internal') ||
line.contains('dart:sdk_internal');
});
}
if (maxFrames != null) {
lines = lines.take(maxFrames);
}
debugPrint(FlutterError.defaultStackFilter(lines).join('\n'));
}
/// Diagnostic with a [StackTrace] [value] suitable for displaying stack traces
/// as part of a [FlutterError] object.
class DiagnosticsStackTrace extends DiagnosticsBlock {
/// Creates a diagnostic for a stack trace.
///
/// [name] describes a name the stack trace is given, e.g.
/// `When the exception was thrown, this was the stack`.
/// [stackFilter] provides an optional filter to use to filter which frames
/// are included. If no filter is specified, [FlutterError.defaultStackFilter]
/// is used.
/// [showSeparator] indicates whether to include a ':' after the [name].
DiagnosticsStackTrace(
String name,
StackTrace? stack, {
IterableFilter<String>? stackFilter,
super.showSeparator,
}) : super(
name: name,
value: stack,
properties: _applyStackFilter(stack, stackFilter),
style: DiagnosticsTreeStyle.flat,
allowTruncate: true,
);
/// Creates a diagnostic describing a single frame from a StackTrace.
DiagnosticsStackTrace.singleFrame(
String name, {
required String frame,
super.showSeparator,
}) : super(
name: name,
properties: <DiagnosticsNode>[_createStackFrame(frame)],
style: DiagnosticsTreeStyle.whitespace,
);
static List<DiagnosticsNode> _applyStackFilter(
StackTrace? stack,
IterableFilter<String>? stackFilter,
) {
if (stack == null) {
return <DiagnosticsNode>[];
}
final IterableFilter<String> filter = stackFilter ?? FlutterError.defaultStackFilter;
final Iterable<String> frames = filter('${FlutterError.demangleStackTrace(stack)}'.trimRight().split('\n'));
return frames.map<DiagnosticsNode>(_createStackFrame).toList();
}
static DiagnosticsNode _createStackFrame(String frame) {
return DiagnosticsNode.message(frame, allowWrap: false);
}
@override
bool get allowTruncate => false;
}
class _FlutterErrorDetailsNode extends DiagnosticableNode<FlutterErrorDetails> {
_FlutterErrorDetailsNode({
super.name,
required super.value,
required super.style,
});
@override
DiagnosticPropertiesBuilder? get builder {
final DiagnosticPropertiesBuilder? builder = super.builder;
if (builder == null) {
return null;
}
Iterable<DiagnosticsNode> properties = builder.properties;
for (final DiagnosticPropertiesTransformer transformer in FlutterErrorDetails.propertiesTransformers) {
properties = transformer(properties);
}
return DiagnosticPropertiesBuilder.fromProperties(properties.toList());
}
}
| flutter/packages/flutter/lib/src/foundation/assertions.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/assertions.dart",
"repo_id": "flutter",
"token_count": 15963
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Framework code should use this method in favor of calling `toString` on
/// [Object.runtimeType].
///
/// Calling `toString` on a runtime type is a non-trivial operation that can
/// negatively impact performance. If asserts are enabled, this method will
/// return `object.runtimeType.toString()`; otherwise, it will return the
/// [optimizedValue], which must be a simple constant string.
String objectRuntimeType(Object? object, String optimizedValue) {
assert(() {
optimizedValue = object.runtimeType.toString();
return true;
}());
return optimizedValue;
}
| flutter/packages/flutter/lib/src/foundation/object.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/object.dart",
"repo_id": "flutter",
"token_count": 194
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'drag_details.dart';
export 'drag_details.dart' show DragEndDetails, DragUpdateDetails;
/// Interface for objects that receive updates about drags.
///
/// This interface is used in various ways. For example,
/// [MultiDragGestureRecognizer] uses it to update its clients when it
/// recognizes a gesture. Similarly, the scrolling infrastructure in the widgets
/// library uses it to notify the [DragScrollActivity] when the user drags the
/// scrollable.
abstract class Drag {
/// The pointer has moved.
void update(DragUpdateDetails details) { }
/// The pointer is no longer in contact with the screen.
///
/// The velocity at which the pointer was moving when it stopped contacting
/// the screen is available in the `details`.
void end(DragEndDetails details) { }
/// The input from the pointer is no longer directed towards this receiver.
///
/// For example, the user might have been interrupted by a system-modal dialog
/// in the middle of the drag.
void cancel() { }
}
| flutter/packages/flutter/lib/src/gestures/drag.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/drag.dart",
"repo_id": "flutter",
"token_count": 299
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'constants.dart';
import 'events.dart';
import 'recognizer.dart';
import 'velocity_tracker.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
export 'events.dart' show PointerDownEvent, PointerEvent, PointerPanZoomStartEvent;
export 'recognizer.dart' show DragStartBehavior;
export 'velocity_tracker.dart' show Velocity;
/// The default conversion factor when treating mouse scrolling as scaling.
///
/// The value was arbitrarily chosen to feel natural for most mousewheels on
/// all supported platforms.
const double kDefaultMouseScrollToScaleFactor = 200;
/// The default conversion factor when treating trackpad scrolling as scaling.
///
/// This factor matches the default [kDefaultMouseScrollToScaleFactor] of 200 to
/// feel natural for most trackpads, and the convention that scrolling up means
/// zooming in.
const Offset kDefaultTrackpadScrollToScaleFactor = Offset(0, -1/kDefaultMouseScrollToScaleFactor);
/// The possible states of a [ScaleGestureRecognizer].
enum _ScaleState {
/// The recognizer is ready to start recognizing a gesture.
ready,
/// The sequence of pointer events seen thus far is consistent with a scale
/// gesture but the gesture has not been accepted definitively.
possible,
/// The sequence of pointer events seen thus far has been accepted
/// definitively as a scale gesture.
accepted,
/// The sequence of pointer events seen thus far has been accepted
/// definitively as a scale gesture and the pointers established a focal point
/// and initial scale.
started,
}
class _PointerPanZoomData {
_PointerPanZoomData.fromStartEvent(
this.parent,
PointerPanZoomStartEvent event
) : _position = event.position,
_pan = Offset.zero,
_scale = 1,
_rotation = 0;
_PointerPanZoomData.fromUpdateEvent(
this.parent,
PointerPanZoomUpdateEvent event
) : _position = event.position,
_pan = event.pan,
_scale = event.scale,
_rotation = event.rotation;
final ScaleGestureRecognizer parent;
final Offset _position;
final Offset _pan;
final double _scale;
final double _rotation;
Offset get focalPoint {
if (parent.trackpadScrollCausesScale) {
return _position;
}
return _position + _pan;
}
double get scale {
if (parent.trackpadScrollCausesScale) {
return _scale * math.exp(
(_pan.dx * parent.trackpadScrollToScaleFactor.dx) +
(_pan.dy * parent.trackpadScrollToScaleFactor.dy)
);
}
return _scale;
}
double get rotation => _rotation;
@override
String toString() => '_PointerPanZoomData(parent: $parent, _position: $_position, _pan: $_pan, _scale: $_scale, _rotation: $_rotation)';
}
/// Details for [GestureScaleStartCallback].
class ScaleStartDetails {
/// Creates details for [GestureScaleStartCallback].
ScaleStartDetails({
this.focalPoint = Offset.zero,
Offset? localFocalPoint,
this.pointerCount = 0,
this.sourceTimeStamp,
}) : localFocalPoint = localFocalPoint ?? focalPoint;
/// The initial focal point of the pointers in contact with the screen.
///
/// Reported in global coordinates.
///
/// See also:
///
/// * [localFocalPoint], which is the same value reported in local
/// coordinates.
final Offset focalPoint;
/// The initial focal point of the pointers in contact with the screen.
///
/// Reported in local coordinates. Defaults to [focalPoint] if not set in the
/// constructor.
///
/// See also:
///
/// * [focalPoint], which is the same value reported in global
/// coordinates.
final Offset localFocalPoint;
/// The number of pointers being tracked by the gesture recognizer.
///
/// Typically this is the number of fingers being used to pan the widget using the gesture
/// recognizer.
final int pointerCount;
/// Recorded timestamp of the source pointer event that triggered the scale
/// event.
///
/// Could be null if triggered from proxied events such as accessibility.
final Duration? sourceTimeStamp;
@override
String toString() => 'ScaleStartDetails(focalPoint: $focalPoint, localFocalPoint: $localFocalPoint, pointersCount: $pointerCount)';
}
/// Details for [GestureScaleUpdateCallback].
class ScaleUpdateDetails {
/// Creates details for [GestureScaleUpdateCallback].
///
/// The [scale], [horizontalScale], and [verticalScale] arguments must be
/// greater than or equal to zero.
ScaleUpdateDetails({
this.focalPoint = Offset.zero,
Offset? localFocalPoint,
this.scale = 1.0,
this.horizontalScale = 1.0,
this.verticalScale = 1.0,
this.rotation = 0.0,
this.pointerCount = 0,
this.focalPointDelta = Offset.zero,
this.sourceTimeStamp,
}) : assert(scale >= 0.0),
assert(horizontalScale >= 0.0),
assert(verticalScale >= 0.0),
localFocalPoint = localFocalPoint ?? focalPoint;
/// The amount the gesture's focal point has moved in the coordinate space of
/// the event receiver since the previous update.
///
/// Defaults to zero if not specified in the constructor.
final Offset focalPointDelta;
/// The focal point of the pointers in contact with the screen.
///
/// Reported in global coordinates.
///
/// See also:
///
/// * [localFocalPoint], which is the same value reported in local
/// coordinates.
final Offset focalPoint;
/// The focal point of the pointers in contact with the screen.
///
/// Reported in local coordinates. Defaults to [focalPoint] if not set in the
/// constructor.
///
/// See also:
///
/// * [focalPoint], which is the same value reported in global
/// coordinates.
final Offset localFocalPoint;
/// The scale implied by the average distance between the pointers in contact
/// with the screen.
///
/// This value must be greater than or equal to zero.
///
/// See also:
///
/// * [horizontalScale], which is the scale along the horizontal axis.
/// * [verticalScale], which is the scale along the vertical axis.
final double scale;
/// The scale implied by the average distance along the horizontal axis
/// between the pointers in contact with the screen.
///
/// This value must be greater than or equal to zero.
///
/// See also:
///
/// * [scale], which is the general scale implied by the pointers.
/// * [verticalScale], which is the scale along the vertical axis.
final double horizontalScale;
/// The scale implied by the average distance along the vertical axis
/// between the pointers in contact with the screen.
///
/// This value must be greater than or equal to zero.
///
/// See also:
///
/// * [scale], which is the general scale implied by the pointers.
/// * [horizontalScale], which is the scale along the horizontal axis.
final double verticalScale;
/// The angle implied by the first two pointers to enter in contact with
/// the screen.
///
/// Expressed in radians.
final double rotation;
/// The number of pointers being tracked by the gesture recognizer.
///
/// Typically this is the number of fingers being used to pan the widget using the gesture
/// recognizer. Due to platform limitations, trackpad gestures count as two fingers
/// even if more than two fingers are used.
final int pointerCount;
/// Recorded timestamp of the source pointer event that triggered the scale
/// event.
///
/// Could be null if triggered from proxied events such as accessibility.
final Duration? sourceTimeStamp;
@override
String toString() => 'ScaleUpdateDetails('
'focalPoint: $focalPoint,'
' localFocalPoint: $localFocalPoint,'
' scale: $scale,'
' horizontalScale: $horizontalScale,'
' verticalScale: $verticalScale,'
' rotation: $rotation,'
' pointerCount: $pointerCount,'
' focalPointDelta: $focalPointDelta,'
' sourceTimeStamp: $sourceTimeStamp)';
}
/// Details for [GestureScaleEndCallback].
class ScaleEndDetails {
/// Creates details for [GestureScaleEndCallback].
ScaleEndDetails({ this.velocity = Velocity.zero, this.scaleVelocity = 0, this.pointerCount = 0 });
/// The velocity of the last pointer to be lifted off of the screen.
final Velocity velocity;
/// The final velocity of the scale factor reported by the gesture.
final double scaleVelocity;
/// The number of pointers being tracked by the gesture recognizer.
///
/// Typically this is the number of fingers being used to pan the widget using the gesture
/// recognizer.
final int pointerCount;
@override
String toString() => 'ScaleEndDetails(velocity: $velocity, scaleVelocity: $scaleVelocity, pointerCount: $pointerCount)';
}
/// Signature for when the pointers in contact with the screen have established
/// a focal point and initial scale of 1.0.
typedef GestureScaleStartCallback = void Function(ScaleStartDetails details);
/// Signature for when the pointers in contact with the screen have indicated a
/// new focal point and/or scale.
typedef GestureScaleUpdateCallback = void Function(ScaleUpdateDetails details);
/// Signature for when the pointers are no longer in contact with the screen.
typedef GestureScaleEndCallback = void Function(ScaleEndDetails details);
bool _isFlingGesture(Velocity velocity) {
final double speedSquared = velocity.pixelsPerSecond.distanceSquared;
return speedSquared > kMinFlingVelocity * kMinFlingVelocity;
}
/// Defines a line between two pointers on screen.
///
/// [_LineBetweenPointers] is an abstraction of a line between two pointers in
/// contact with the screen. Used to track the rotation of a scale gesture.
class _LineBetweenPointers {
/// Creates a [_LineBetweenPointers]. None of the [pointerStartLocation], [pointerStartId]
/// [pointerEndLocation] and [pointerEndId] must be null. [pointerStartId] and [pointerEndId]
/// should be different.
_LineBetweenPointers({
this.pointerStartLocation = Offset.zero,
this.pointerStartId = 0,
this.pointerEndLocation = Offset.zero,
this.pointerEndId = 1,
}) : assert(pointerStartId != pointerEndId);
// The location and the id of the pointer that marks the start of the line.
final Offset pointerStartLocation;
final int pointerStartId;
// The location and the id of the pointer that marks the end of the line.
final Offset pointerEndLocation;
final int pointerEndId;
}
/// Recognizes a scale gesture.
///
/// [ScaleGestureRecognizer] tracks the pointers in contact with the screen and
/// calculates their focal point, indicated scale, and rotation. When a focal
/// point is established, the recognizer calls [onStart]. As the focal point,
/// scale, and rotation change, the recognizer calls [onUpdate]. When the
/// pointers are no longer in contact with the screen, the recognizer calls
/// [onEnd].
class ScaleGestureRecognizer extends OneSequenceGestureRecognizer {
/// Create a gesture recognizer for interactions intended for scaling content.
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
ScaleGestureRecognizer({
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
this.dragStartBehavior = DragStartBehavior.down,
this.trackpadScrollCausesScale = false,
this.trackpadScrollToScaleFactor = kDefaultTrackpadScrollToScaleFactor,
});
/// Determines what point is used as the starting point in all calculations
/// involving this gesture.
///
/// When set to [DragStartBehavior.down], the scale is calculated starting
/// from the position where the pointer first contacted the screen.
///
/// When set to [DragStartBehavior.start], the scale is calculated starting
/// from the position where the scale gesture began. The scale gesture may
/// begin after the time that the pointer first contacted the screen if there
/// are multiple listeners competing for the gesture. In that case, the
/// gesture arena waits to determine whether or not the gesture is a scale
/// gesture before giving the gesture to this GestureRecognizer. This happens
/// in the case of nested GestureDetectors, for example.
///
/// Defaults to [DragStartBehavior.down].
///
/// See also:
///
/// * https://flutter.dev/docs/development/ui/advanced/gestures#gesture-disambiguation,
/// which provides more information about the gesture arena.
DragStartBehavior dragStartBehavior;
/// The pointers in contact with the screen have established a focal point and
/// initial scale of 1.0.
///
/// This won't be called until the gesture arena has determined that this
/// GestureRecognizer has won the gesture.
///
/// See also:
///
/// * https://flutter.dev/docs/development/ui/advanced/gestures#gesture-disambiguation,
/// which provides more information about the gesture arena.
GestureScaleStartCallback? onStart;
/// The pointers in contact with the screen have indicated a new focal point
/// and/or scale.
GestureScaleUpdateCallback? onUpdate;
/// The pointers are no longer in contact with the screen.
GestureScaleEndCallback? onEnd;
_ScaleState _state = _ScaleState.ready;
Matrix4? _lastTransform;
/// {@template flutter.gestures.scale.trackpadScrollCausesScale}
/// Whether scrolling up/down on a trackpad should cause scaling instead of
/// panning.
///
/// Defaults to false.
/// {@endtemplate}
bool trackpadScrollCausesScale;
/// {@template flutter.gestures.scale.trackpadScrollToScaleFactor}
/// A factor to control the direction and magnitude of scale when converting
/// trackpad scrolling.
///
/// Incoming trackpad pan offsets will be divided by this factor to get scale
/// values. Increasing this offset will reduce the amount of scaling caused by
/// a fixed amount of trackpad scrolling.
///
/// Defaults to [kDefaultTrackpadScrollToScaleFactor].
/// {@endtemplate}
Offset trackpadScrollToScaleFactor;
/// The number of pointers being tracked by the gesture recognizer.
///
/// Typically this is the number of fingers being used to pan the widget using the gesture
/// recognizer.
int get pointerCount {
// PointerPanZoom protocol doesn't contain the exact number of pointers
// used on the trackpad, as it isn't exposed by all platforms. However, it
// will always be at least two.
return (2 * _pointerPanZooms.length) + _pointerQueue.length;
}
late Offset _initialFocalPoint;
Offset? _currentFocalPoint;
late double _initialSpan;
late double _currentSpan;
late double _initialHorizontalSpan;
late double _currentHorizontalSpan;
late double _initialVerticalSpan;
late double _currentVerticalSpan;
late Offset _localFocalPoint;
_LineBetweenPointers? _initialLine;
_LineBetweenPointers? _currentLine;
final Map<int, Offset> _pointerLocations = <int, Offset>{};
final List<int> _pointerQueue = <int>[]; // A queue to sort pointers in order of entrance
final Map<int, VelocityTracker> _velocityTrackers = <int, VelocityTracker>{};
VelocityTracker? _scaleVelocityTracker;
late Offset _delta;
final Map<int, _PointerPanZoomData> _pointerPanZooms = <int, _PointerPanZoomData>{};
double _initialPanZoomScaleFactor = 1;
double _initialPanZoomRotationFactor = 0;
Duration? _initialEventTimestamp;
double get _pointerScaleFactor => _initialSpan > 0.0 ? _currentSpan / _initialSpan : 1.0;
double get _pointerHorizontalScaleFactor => _initialHorizontalSpan > 0.0 ? _currentHorizontalSpan / _initialHorizontalSpan : 1.0;
double get _pointerVerticalScaleFactor => _initialVerticalSpan > 0.0 ? _currentVerticalSpan / _initialVerticalSpan : 1.0;
double get _scaleFactor {
double scale = _pointerScaleFactor;
for (final _PointerPanZoomData p in _pointerPanZooms.values) {
scale *= p.scale / _initialPanZoomScaleFactor;
}
return scale;
}
double get _horizontalScaleFactor {
double scale = _pointerHorizontalScaleFactor;
for (final _PointerPanZoomData p in _pointerPanZooms.values) {
scale *= p.scale / _initialPanZoomScaleFactor;
}
return scale;
}
double get _verticalScaleFactor {
double scale = _pointerVerticalScaleFactor;
for (final _PointerPanZoomData p in _pointerPanZooms.values) {
scale *= p.scale / _initialPanZoomScaleFactor;
}
return scale;
}
double _computeRotationFactor() {
double factor = 0.0;
if (_initialLine != null && _currentLine != null) {
final double fx = _initialLine!.pointerStartLocation.dx;
final double fy = _initialLine!.pointerStartLocation.dy;
final double sx = _initialLine!.pointerEndLocation.dx;
final double sy = _initialLine!.pointerEndLocation.dy;
final double nfx = _currentLine!.pointerStartLocation.dx;
final double nfy = _currentLine!.pointerStartLocation.dy;
final double nsx = _currentLine!.pointerEndLocation.dx;
final double nsy = _currentLine!.pointerEndLocation.dy;
final double angle1 = math.atan2(fy - sy, fx - sx);
final double angle2 = math.atan2(nfy - nsy, nfx - nsx);
factor = angle2 - angle1;
}
for (final _PointerPanZoomData p in _pointerPanZooms.values) {
factor += p.rotation;
}
factor -= _initialPanZoomRotationFactor;
return factor;
}
@override
void addAllowedPointer(PointerDownEvent event) {
super.addAllowedPointer(event);
_velocityTrackers[event.pointer] = VelocityTracker.withKind(event.kind);
_initialEventTimestamp = event.timeStamp;
if (_state == _ScaleState.ready) {
_state = _ScaleState.possible;
_initialSpan = 0.0;
_currentSpan = 0.0;
_initialHorizontalSpan = 0.0;
_currentHorizontalSpan = 0.0;
_initialVerticalSpan = 0.0;
_currentVerticalSpan = 0.0;
}
}
@override
bool isPointerPanZoomAllowed(PointerPanZoomStartEvent event) => true;
@override
void addAllowedPointerPanZoom(PointerPanZoomStartEvent event) {
super.addAllowedPointerPanZoom(event);
startTrackingPointer(event.pointer, event.transform);
_velocityTrackers[event.pointer] = VelocityTracker.withKind(event.kind);
_initialEventTimestamp = event.timeStamp;
if (_state == _ScaleState.ready) {
_state = _ScaleState.possible;
_initialPanZoomScaleFactor = 1.0;
_initialPanZoomRotationFactor = 0.0;
}
}
@override
void handleEvent(PointerEvent event) {
assert(_state != _ScaleState.ready);
bool didChangeConfiguration = false;
bool shouldStartIfAccepted = false;
if (event is PointerMoveEvent) {
final VelocityTracker tracker = _velocityTrackers[event.pointer]!;
if (!event.synthesized) {
tracker.addPosition(event.timeStamp, event.position);
}
_pointerLocations[event.pointer] = event.position;
shouldStartIfAccepted = true;
_lastTransform = event.transform;
} else if (event is PointerDownEvent) {
_pointerLocations[event.pointer] = event.position;
_pointerQueue.add(event.pointer);
didChangeConfiguration = true;
shouldStartIfAccepted = true;
_lastTransform = event.transform;
} else if (event is PointerUpEvent || event is PointerCancelEvent) {
_pointerLocations.remove(event.pointer);
_pointerQueue.remove(event.pointer);
didChangeConfiguration = true;
_lastTransform = event.transform;
} else if (event is PointerPanZoomStartEvent) {
assert(_pointerPanZooms[event.pointer] == null);
_pointerPanZooms[event.pointer] = _PointerPanZoomData.fromStartEvent(this, event);
didChangeConfiguration = true;
shouldStartIfAccepted = true;
_lastTransform = event.transform;
} else if (event is PointerPanZoomUpdateEvent) {
assert(_pointerPanZooms[event.pointer] != null);
if (!event.synthesized && !trackpadScrollCausesScale) {
_velocityTrackers[event.pointer]!.addPosition(event.timeStamp, event.pan);
}
_pointerPanZooms[event.pointer] = _PointerPanZoomData.fromUpdateEvent(this, event);
_lastTransform = event.transform;
shouldStartIfAccepted = true;
} else if (event is PointerPanZoomEndEvent) {
assert(_pointerPanZooms[event.pointer] != null);
_pointerPanZooms.remove(event.pointer);
didChangeConfiguration = true;
}
_updateLines();
_update();
if (!didChangeConfiguration || _reconfigure(event.pointer)) {
_advanceStateMachine(shouldStartIfAccepted, event);
}
stopTrackingIfPointerNoLongerDown(event);
}
void _update() {
final Offset? previousFocalPoint = _currentFocalPoint;
// Compute the focal point
Offset focalPoint = Offset.zero;
for (final int pointer in _pointerLocations.keys) {
focalPoint += _pointerLocations[pointer]!;
}
for (final _PointerPanZoomData p in _pointerPanZooms.values) {
focalPoint += p.focalPoint;
}
_currentFocalPoint = focalPoint / math.max(1, _pointerLocations.length + _pointerPanZooms.length).toDouble();
if (previousFocalPoint == null) {
_localFocalPoint = PointerEvent.transformPosition(
_lastTransform,
_currentFocalPoint!,
);
_delta = Offset.zero;
} else {
final Offset localPreviousFocalPoint = _localFocalPoint;
_localFocalPoint = PointerEvent.transformPosition(
_lastTransform,
_currentFocalPoint!,
);
_delta = _localFocalPoint - localPreviousFocalPoint;
}
final int count = _pointerLocations.keys.length;
Offset pointerFocalPoint = Offset.zero;
for (final int pointer in _pointerLocations.keys) {
pointerFocalPoint += _pointerLocations[pointer]!;
}
if (count > 0) {
pointerFocalPoint = pointerFocalPoint / count.toDouble();
}
// Span is the average deviation from focal point. Horizontal and vertical
// spans are the average deviations from the focal point's horizontal and
// vertical coordinates, respectively.
double totalDeviation = 0.0;
double totalHorizontalDeviation = 0.0;
double totalVerticalDeviation = 0.0;
for (final int pointer in _pointerLocations.keys) {
totalDeviation += (pointerFocalPoint - _pointerLocations[pointer]!).distance;
totalHorizontalDeviation += (pointerFocalPoint.dx - _pointerLocations[pointer]!.dx).abs();
totalVerticalDeviation += (pointerFocalPoint.dy - _pointerLocations[pointer]!.dy).abs();
}
_currentSpan = count > 0 ? totalDeviation / count : 0.0;
_currentHorizontalSpan = count > 0 ? totalHorizontalDeviation / count : 0.0;
_currentVerticalSpan = count > 0 ? totalVerticalDeviation / count : 0.0;
}
/// Updates [_initialLine] and [_currentLine] accordingly to the situation of
/// the registered pointers.
void _updateLines() {
final int count = _pointerLocations.keys.length;
assert(_pointerQueue.length >= count);
/// In case of just one pointer registered, reconfigure [_initialLine]
if (count < 2) {
_initialLine = _currentLine;
} else if (_initialLine != null &&
_initialLine!.pointerStartId == _pointerQueue[0] &&
_initialLine!.pointerEndId == _pointerQueue[1]) {
/// Rotation updated, set the [_currentLine]
_currentLine = _LineBetweenPointers(
pointerStartId: _pointerQueue[0],
pointerStartLocation: _pointerLocations[_pointerQueue[0]]!,
pointerEndId: _pointerQueue[1],
pointerEndLocation: _pointerLocations[_pointerQueue[1]]!,
);
} else {
/// A new rotation process is on the way, set the [_initialLine]
_initialLine = _LineBetweenPointers(
pointerStartId: _pointerQueue[0],
pointerStartLocation: _pointerLocations[_pointerQueue[0]]!,
pointerEndId: _pointerQueue[1],
pointerEndLocation: _pointerLocations[_pointerQueue[1]]!,
);
_currentLine = _initialLine;
}
}
bool _reconfigure(int pointer) {
_initialFocalPoint = _currentFocalPoint!;
_initialSpan = _currentSpan;
_initialLine = _currentLine;
_initialHorizontalSpan = _currentHorizontalSpan;
_initialVerticalSpan = _currentVerticalSpan;
if (_pointerPanZooms.isEmpty) {
_initialPanZoomScaleFactor = 1.0;
_initialPanZoomRotationFactor = 0.0;
} else {
_initialPanZoomScaleFactor = _scaleFactor / _pointerScaleFactor;
_initialPanZoomRotationFactor = _pointerPanZooms.values.map((_PointerPanZoomData x) => x.rotation).reduce((double a, double b) => a + b);
}
if (_state == _ScaleState.started) {
if (onEnd != null) {
final VelocityTracker tracker = _velocityTrackers[pointer]!;
Velocity velocity = tracker.getVelocity();
if (_isFlingGesture(velocity)) {
final Offset pixelsPerSecond = velocity.pixelsPerSecond;
if (pixelsPerSecond.distanceSquared > kMaxFlingVelocity * kMaxFlingVelocity) {
velocity = Velocity(pixelsPerSecond: (pixelsPerSecond / pixelsPerSecond.distance) * kMaxFlingVelocity);
}
invokeCallback<void>('onEnd', () => onEnd!(ScaleEndDetails(velocity: velocity, scaleVelocity: _scaleVelocityTracker?.getVelocity().pixelsPerSecond.dx ?? -1, pointerCount: pointerCount)));
} else {
invokeCallback<void>('onEnd', () => onEnd!(ScaleEndDetails(scaleVelocity: _scaleVelocityTracker?.getVelocity().pixelsPerSecond.dx ?? -1, pointerCount: pointerCount)));
}
}
_state = _ScaleState.accepted;
_scaleVelocityTracker = VelocityTracker.withKind(PointerDeviceKind.touch); // arbitrary PointerDeviceKind
return false;
}
_scaleVelocityTracker = VelocityTracker.withKind(PointerDeviceKind.touch); // arbitrary PointerDeviceKind
return true;
}
void _advanceStateMachine(bool shouldStartIfAccepted, PointerEvent event) {
if (_state == _ScaleState.ready) {
_state = _ScaleState.possible;
}
if (_state == _ScaleState.possible) {
final double spanDelta = (_currentSpan - _initialSpan).abs();
final double focalPointDelta = (_currentFocalPoint! - _initialFocalPoint).distance;
if (spanDelta > computeScaleSlop(event.kind) || focalPointDelta > computePanSlop(event.kind, gestureSettings) || math.max(_scaleFactor / _pointerScaleFactor, _pointerScaleFactor / _scaleFactor) > 1.05) {
resolve(GestureDisposition.accepted);
}
} else if (_state.index >= _ScaleState.accepted.index) {
resolve(GestureDisposition.accepted);
}
if (_state == _ScaleState.accepted && shouldStartIfAccepted) {
_initialEventTimestamp = event.timeStamp;
_state = _ScaleState.started;
_dispatchOnStartCallbackIfNeeded();
}
if (_state == _ScaleState.started) {
_scaleVelocityTracker?.addPosition(event.timeStamp, Offset(_scaleFactor, 0));
if (onUpdate != null) {
invokeCallback<void>('onUpdate', () {
onUpdate!(ScaleUpdateDetails(
scale: _scaleFactor,
horizontalScale: _horizontalScaleFactor,
verticalScale: _verticalScaleFactor,
focalPoint: _currentFocalPoint!,
localFocalPoint: _localFocalPoint,
rotation: _computeRotationFactor(),
pointerCount: pointerCount,
focalPointDelta: _delta,
sourceTimeStamp: event.timeStamp
));
});
}
}
}
void _dispatchOnStartCallbackIfNeeded() {
assert(_state == _ScaleState.started);
if (onStart != null) {
invokeCallback<void>('onStart', () {
onStart!(ScaleStartDetails(
focalPoint: _currentFocalPoint!,
localFocalPoint: _localFocalPoint,
pointerCount: pointerCount,
sourceTimeStamp: _initialEventTimestamp,
));
});
}
_initialEventTimestamp = null;
}
@override
void acceptGesture(int pointer) {
if (_state == _ScaleState.possible) {
_state = _ScaleState.started;
_dispatchOnStartCallbackIfNeeded();
if (dragStartBehavior == DragStartBehavior.start) {
_initialFocalPoint = _currentFocalPoint!;
_initialSpan = _currentSpan;
_initialLine = _currentLine;
_initialHorizontalSpan = _currentHorizontalSpan;
_initialVerticalSpan = _currentVerticalSpan;
if (_pointerPanZooms.isEmpty) {
_initialPanZoomScaleFactor = 1.0;
_initialPanZoomRotationFactor = 0.0;
} else {
_initialPanZoomScaleFactor = _scaleFactor / _pointerScaleFactor;
_initialPanZoomRotationFactor = _pointerPanZooms.values.map((_PointerPanZoomData x) => x.rotation).reduce((double a, double b) => a + b);
}
}
}
}
@override
void rejectGesture(int pointer) {
_pointerPanZooms.remove(pointer);
_pointerLocations.remove(pointer);
_pointerQueue.remove(pointer);
stopTrackingPointer(pointer);
}
@override
void didStopTrackingLastPointer(int pointer) {
switch (_state) {
case _ScaleState.possible:
resolve(GestureDisposition.rejected);
case _ScaleState.ready:
assert(false); // We should have not seen a pointer yet
case _ScaleState.accepted:
break;
case _ScaleState.started:
assert(false); // We should be in the accepted state when user is done
}
_state = _ScaleState.ready;
}
@override
void dispose() {
_velocityTrackers.clear();
super.dispose();
}
@override
String get debugDescription => 'scale';
}
| flutter/packages/flutter/lib/src/gestures/scale.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/scale.dart",
"repo_id": "flutter",
"token_count": 9739
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$ellipsis_search = _AnimatedIconData(
Size(96.0, 96.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(57.62369742449878, 56.510753652468935),
Offset(57.50386046799878, 56.38647829016894),
Offset(57.38402351149878, 56.26220292786894),
Offset(57.26418655499878, 56.13792756556894),
Offset(57.144349598398776, 56.01365220316894),
Offset(57.02451264189878, 55.88937684086894),
Offset(56.90467568539878, 55.76510147856894),
Offset(56.78483872889878, 55.64082611626894),
Offset(56.66500177229878, 55.516550753968936),
Offset(56.54516481579878, 55.39227539166894),
Offset(56.42532785929878, 55.26800002926894),
Offset(56.30549090279878, 55.143724666968936),
Offset(56.18565394629878, 55.01944930466894),
Offset(56.06581698969878, 54.895173942368935),
Offset(55.94598003319878, 54.77089858006894),
Offset(55.89372930109525, 54.63379537519879),
Offset(55.99467401074788, 54.46761572737509),
Offset(56.09561872053984, 54.30143607948509),
Offset(56.19656343029247, 54.13525643166141),
Offset(56.297508139945094, 53.969076783837714),
Offset(56.398452849697726, 53.80289713591403),
Offset(56.49939755945036, 53.63671748809034),
Offset(56.297960835674125, 53.25914221256317),
Offset(55.78881270857159, 52.69340023656423),
Offset(56.006714995568615, 52.88005225271881),
Offset(56.182356492271566, 53.03050427381422),
Offset(56.32344115337003, 53.15135539844181),
Offset(56.43452394733299, 53.246507348148306),
Offset(56.52071628832435, 53.320338487990085),
Offset(56.5859271296418, 53.37619717205798),
Offset(56.63368824673307, 53.417108669232874),
Offset(56.66699682004896, 53.44564032188472),
Offset(56.68847407317482, 53.46403743305161),
Offset(56.70040259814858, 53.474255238779065),
Offset(56.70477862272339, 53.478003679517734),
Offset(56.70335264548873, 53.47678220759329),
Offset(56.69766279856795, 53.471908365221054),
Offset(56.689065722138324, 53.46454423118308),
Offset(56.678761173675056, 53.45571750075846),
Offset(56.667817449874256, 53.44634326178278),
Offset(56.657150392580974, 53.43720601142315),
Offset(56.64761589840118, 53.429038898603),
Offset(56.639944407998925, 53.42246760846087),
Offset(56.63479944561546, 53.418060506474255),
Offset(56.632765532511705, 53.416318285285435),
Offset(56.63275314854877, 53.416307677358),
Offset(56.63275314854877, 53.416307677358),
Offset(56.63275314854877, 53.416307677358),
Offset(56.63275314854877, 53.416307677358),
],
),
_PathCubicTo(
<Offset>[
Offset(59.11722254046772, 54.78101039685442),
Offset(58.99738558396772, 54.65673503455442),
Offset(58.877548627467725, 54.532459672254426),
Offset(58.75771167096772, 54.40818430995442),
Offset(58.63787471436772, 54.28390894755442),
Offset(58.518037757867724, 54.159633585254426),
Offset(58.39820080136772, 54.03535822295442),
Offset(58.27836384486772, 53.911082860654425),
Offset(58.15852688826772, 53.78680749835442),
Offset(58.03868993176772, 53.66253213605442),
Offset(57.918852975267725, 53.538256773654425),
Offset(57.79901601876772, 53.41398141135442),
Offset(57.67917906226772, 53.28970604905442),
Offset(57.559342105667724, 53.16543068675442),
Offset(57.43950514916772, 53.04115532445442),
Offset(57.485490128564514, 52.790279302768965),
Offset(57.907338162449705, 52.252441786684095),
Offset(58.32918619648207, 51.71460427052383),
Offset(58.75103423046726, 51.176766754438965),
Offset(59.17288226435245, 50.63892923835408),
Offset(59.59473029833764, 50.101091722169215),
Offset(60.01657833232283, 49.56325420608434),
Offset(60.01932238869081, 48.94920461646341),
Offset(59.563472601893324, 48.32173455588634),
Offset(59.82481548777557, 48.45807534383447),
Offset(60.035472549991965, 48.56797368582478),
Offset(60.204683589459826, 48.656249922511655),
Offset(60.33791164477868, 48.72575408359739),
Offset(60.441287132232546, 48.77968436513692),
Offset(60.51949828685408, 48.820486587135186),
Offset(60.57678097234404, 48.850370570581376),
Offset(60.61672988153154, 48.871211642026296),
Offset(60.64248880031156, 48.88464989297123),
Offset(60.65679537406957, 48.892113534204164),
Offset(60.66204379484951, 48.89485159926458),
Offset(60.66033353741787, 48.89395936971321),
Offset(60.65350937308716, 48.890399250068874),
Offset(60.643198399323495, 48.885020085900294),
Offset(60.630839555044176, 48.87857256175406),
Offset(60.617714110608446, 48.87172510757334),
Offset(60.60492048840596, 48.86505076277184),
Offset(60.593485215014766, 48.85908505921234),
Offset(60.58428435040535, 48.85428503136227),
Offset(60.57811369779046, 48.85106584428997),
Offset(60.575674307513765, 48.84979323114302),
Offset(60.57565945470678, 48.849785482535665),
Offset(60.57565945470678, 48.849785482535665),
Offset(60.57565945470678, 48.849785482535665),
Offset(60.57565945470678, 48.849785482535665),
],
<Offset>[
Offset(60.01637695941208, 52.53311272229554),
Offset(59.896540002912076, 52.408837359995545),
Offset(59.77670304641208, 52.28456199769555),
Offset(59.65686608991208, 52.160286635395536),
Offset(59.537029133312075, 52.03601127299554),
Offset(59.41719217681208, 51.91173591069554),
Offset(59.29735522031208, 51.78746054839554),
Offset(59.177518263812075, 51.663185186095546),
Offset(59.05768130721208, 51.538909823795535),
Offset(58.937844350712076, 51.41463446149554),
Offset(58.81800739421208, 51.29035909909554),
Offset(58.69817043771208, 51.16608373679554),
Offset(58.57833348121208, 51.041808374495545),
Offset(58.45849652461208, 50.917533012195534),
Offset(58.33865956811208, 50.79325764989554),
Offset(58.44378588577579, 50.39452751777087),
Offset(59.058828957998486, 49.37369990758731),
Offset(59.673872030373076, 48.35287229731656),
Offset(60.28891510269577, 47.332044687133006),
Offset(60.90395817491846, 46.31121707694944),
Offset(61.51900124724116, 45.290389466665886),
Offset(62.13404431956385, 44.26956185648232),
Offset(62.259712355426515, 43.348200728562944),
Offset(61.83595003585357, 42.64051158499319),
Offset(62.12344568343471, 42.711470130505994),
Offset(62.35518334223874, 42.76866670842857),
Offset(62.54132744639319, 42.81461006443314),
Offset(62.68788772451427, 42.85078349611087),
Offset(62.80160806784497, 42.87885150418633),
Offset(62.88764586631069, 42.90008701536577),
Offset(62.95066086939573, 42.91564013069819),
Offset(62.9946074933042, 42.92648686364521),
Offset(63.02294412474014, 42.933480799617264),
Offset(63.038682367138975, 42.93736525073486),
Offset(63.04445600098332, 42.9387902763426),
Offset(63.042574596918385, 42.938325915587605),
Offset(63.03506753378623, 42.936473051777625),
Offset(63.02372473381152, 42.93367346647948),
Offset(63.010129131515164, 42.93031785336868),
Offset(62.99569021485936, 42.92675409672298),
Offset(62.981616326115095, 42.92328043483138),
Offset(62.969036717078595, 42.92017558518285),
Offset(62.958915115642, 42.91767741130702),
Offset(62.952126961590935, 42.916001985810205),
Offset(62.94944345985745, 42.91533965446192),
Offset(62.94942712071989, 42.915335621700216),
Offset(62.94942712071989, 42.915335621700216),
Offset(62.94942712071989, 42.915335621700216),
Offset(62.94942712071989, 42.915335621700216),
],
<Offset>[
Offset(60.01637695941208, 50.07184435071208),
Offset(59.896540002912076, 49.94756898841208),
Offset(59.77670304641208, 49.82329362611208),
Offset(59.65686608991208, 49.69901826381208),
Offset(59.537029133312075, 49.57474290141208),
Offset(59.41719217681208, 49.45046753911208),
Offset(59.29735522031208, 49.32619217681208),
Offset(59.177518263812075, 49.20191681451208),
Offset(59.05768130721208, 49.07764145221208),
Offset(58.937844350712076, 48.95336608991208),
Offset(58.81800739421208, 48.82909072751208),
Offset(58.69817043771208, 48.70481536521208),
Offset(58.57833348121208, 48.58054000291208),
Offset(58.45849652461208, 48.456264640612076),
Offset(58.33865956811208, 48.33198927831208),
Offset(58.44378588577579, 47.77137070715779),
Offset(59.058828957998486, 46.22170752948048),
Offset(59.673872030373076, 44.67204435170307),
Offset(60.28891510269577, 43.12238117402577),
Offset(60.90395817491846, 41.57271799634846),
Offset(61.51900124724116, 40.023054818571154),
Offset(62.13404431956385, 38.47339164089385),
Offset(62.259712355426515, 37.21554892931651),
Offset(61.83595003585357, 36.42002629848357),
Offset(62.12344568343471, 36.4193965118447),
Offset(62.35518334223874, 36.41888886839874),
Offset(62.54132744639319, 36.41848110198319),
Offset(62.68788772451427, 36.41816004771427),
Offset(62.80160806784497, 36.41791093245497),
Offset(62.88764586631069, 36.4177224584507),
Offset(62.95066086939573, 36.417584418075734),
Offset(62.9946074933042, 36.4174881488042),
Offset(63.02294412474014, 36.41742607471014),
Offset(63.038682367138975, 36.41739159858898),
Offset(63.04445600098332, 36.417378950893315),
Offset(63.042574596918385, 36.41738307228838),
Offset(63.03506753378623, 36.41739951722624),
Offset(63.02372473381152, 36.417424364711515),
Offset(63.010129131515164, 36.417454147175164),
Offset(62.99569021485936, 36.41748577699936),
Offset(62.981616326115095, 36.41751660719509),
Offset(62.969036717078595, 36.4175441640286),
Offset(62.958915115642, 36.41756633636199),
Offset(62.952126961590935, 36.41758120646094),
Offset(62.94944345985745, 36.41758708492745),
Offset(62.94942712071989, 36.41758712071989),
Offset(62.94942712071989, 36.41758712071989),
Offset(62.94942712071989, 36.41758712071989),
Offset(62.94942712071989, 36.41758712071989),
],
),
_PathCubicTo(
<Offset>[
Offset(60.01637695941208, 44.60068993176772),
Offset(59.896540002912076, 44.47641456946772),
Offset(59.77670304641208, 44.352139207167724),
Offset(59.65686608991208, 44.22786384486772),
Offset(59.537029133312075, 44.10358848246772),
Offset(59.41719217681208, 43.979313120167724),
Offset(59.29735522031208, 43.85503775786772),
Offset(59.177518263812075, 43.73076239556772),
Offset(59.05768130721208, 43.60648703326772),
Offset(58.937844350712076, 43.48221167096772),
Offset(58.81800739421208, 43.35793630856772),
Offset(58.69817043771208, 43.23366094626772),
Offset(58.57833348121208, 43.10938558396772),
Offset(58.45849652461208, 42.98511022166772),
Offset(58.33865956811208, 42.86083485936772),
Offset(58.44378588577579, 41.94035441423051),
Offset(59.058828957998486, 39.2151424482157),
Offset(59.673872030373076, 36.48993048207206),
Offset(60.28891510269577, 33.76471851605726),
Offset(60.90395817491846, 31.039506550042443),
Offset(61.51900124724116, 28.314294583927637),
Offset(62.13404431956385, 25.58908261791283),
Offset(62.259712355426515, 23.5832748633608),
Offset(61.83595003585357, 22.59250713958332),
Offset(62.12344568343471, 22.432743621605564),
Offset(62.35518334223874, 22.303965242071957),
Offset(62.54132744639319, 22.20052354762982),
Offset(62.68788772451427, 22.11907888637867),
Offset(62.80160806784497, 22.05588362666254),
Offset(62.88764586631069, 22.00807176767408),
Offset(62.95066086939573, 21.973053851184037),
Offset(62.9946074933042, 21.948632378031544),
Offset(63.02294412474014, 21.932885496421555),
Offset(63.038682367138975, 21.92413963541956),
Offset(63.04445600098332, 21.920931183179505),
Offset(63.042574596918385, 21.92197669372786),
Offset(63.03506753378623, 21.926148425807156),
Offset(63.02372473381152, 21.93245170602349),
Offset(63.010129131515164, 21.940006885624168),
Offset(62.99569021485936, 21.94803070142844),
Offset(62.981616326115095, 21.955851668445952),
Offset(62.969036717078595, 21.962842252864764),
Offset(62.958915115642, 21.968466903765343),
Offset(62.952126961590935, 21.972239132600446),
Offset(62.94944345985745, 21.973730374923758),
Offset(62.94942712071989, 21.97373945470677),
Offset(62.94942712071989, 21.97373945470677),
Offset(62.94942712071989, 21.97373945470677),
Offset(62.94942712071989, 21.97373945470677),
],
<Offset>[
Offset(55.58154300555442, 40.16584435071208),
Offset(55.46170604905442, 40.04156898841208),
Offset(55.341869092554425, 39.917293626112084),
Offset(55.22203213605442, 39.79301826381208),
Offset(55.10219517945442, 39.66874290141208),
Offset(54.982358222954424, 39.544467539112084),
Offset(54.86252126645442, 39.42019217681208),
Offset(54.74268430995442, 39.29591681451208),
Offset(54.62284735335442, 39.17164145221208),
Offset(54.50301039685442, 39.04736608991208),
Offset(54.383173440354426, 38.92309072751208),
Offset(54.263336483854424, 38.79881536521208),
Offset(54.14349952735442, 38.67454000291208),
Offset(54.023662570754425, 38.55026464061208),
Offset(53.90382561425442, 38.42598927831208),
Offset(53.717253409954964, 37.21380954643979),
Offset(53.3794146437701, 33.53571324376249),
Offset(53.04157587771383, 29.857616940933074),
Offset(52.70373711162896, 26.17952063825577),
Offset(52.36589834544409, 22.50142433557846),
Offset(52.028059579359216, 18.823328032801157),
Offset(51.69022081327434, 15.145231730123854),
Offset(51.209599844133415, 12.533133381006508),
Offset(50.627574843376344, 11.384102561113572),
Offset(50.786079126264475, 11.095347340254705),
Offset(50.91384249150479, 10.86259439455874),
Offset(51.01646887208166, 10.675634757573192),
Offset(51.09727159719739, 10.528432370914274),
Offset(51.159968760166926, 10.414213797064974),
Offset(51.207403772355185, 10.327799050590698),
Offset(51.24214568222138, 10.264507966755737),
Offset(51.266374668526296, 10.220368804304208),
Offset(51.281997435281234, 10.191908024680146),
Offset(51.29067436255417, 10.176100830038976),
Offset(51.29385752619458, 10.170301900803324),
Offset(51.29282025622321, 10.172191547658386),
Offset(51.288681405188875, 10.179731500666236),
Offset(51.282427806600296, 10.19112399561152),
Offset(51.27493217593407, 10.204779162835159),
Offset(51.26697160279334, 10.219281339139357),
Offset(51.25921227961184, 10.233416888275087),
Offset(51.25227679406234, 10.246051610978604),
Offset(51.24669647592227, 10.256217557081996),
Offset(51.242953979299976, 10.263035451330936),
Offset(51.24147449075302, 10.265730709997449),
Offset(51.241465482535666, 10.265747120719887),
Offset(51.241465482535666, 10.265747120719887),
Offset(51.241465482535666, 10.265747120719887),
Offset(51.241465482535666, 10.265747120719887),
],
<Offset>[
Offset(50.11037695941208, 40.16584435071208),
Offset(49.99054000291208, 40.04156898841208),
Offset(49.87070304641208, 39.917293626112084),
Offset(49.75086608991208, 39.79301826381208),
Offset(49.631029133312076, 39.66874290141208),
Offset(49.51119217681208, 39.544467539112084),
Offset(49.39135522031208, 39.42019217681208),
Offset(49.27151826381208, 39.29591681451208),
Offset(49.15168130721208, 39.17164145221208),
Offset(49.03184435071208, 39.04736608991208),
Offset(48.91200739421208, 38.92309072751208),
Offset(48.79217043771208, 38.79881536521208),
Offset(48.67233348121208, 38.67454000291208),
Offset(48.55249652461208, 38.55026464061208),
Offset(48.43265956811208, 38.42598927831208),
Offset(47.886224725057794, 37.21380954643979),
Offset(46.37283467228049, 33.53571324376249),
Offset(44.859444619603074, 29.857616940933074),
Offset(43.346054566925766, 26.17952063825577),
Offset(41.83266451414846, 22.50142433557846),
Offset(40.31927446147115, 18.823328032801157),
Offset(38.80588440879385, 15.145231730123854),
Offset(37.57729680711651, 12.533133381006508),
Offset(36.80002629848357, 11.384102561113572),
Offset(36.799396511844705, 11.095347340254705),
Offset(36.79888886839874, 10.86259439455874),
Offset(36.798481101983185, 10.675634757573192),
Offset(36.798160047714276, 10.528432370914274),
Offset(36.79791093245497, 10.414213797064974),
Offset(36.79772245845069, 10.327799050590698),
Offset(36.79758441807573, 10.264507966755737),
Offset(36.797488148804206, 10.220368804304208),
Offset(36.797426074710145, 10.191908024680146),
Offset(36.79739159858897, 10.176100830038976),
Offset(36.79737895089332, 10.170301900803324),
Offset(36.79738307228838, 10.172191547658386),
Offset(36.79739951722624, 10.179731500666236),
Offset(36.79742436471152, 10.19112399561152),
Offset(36.79745414717516, 10.204779162835159),
Offset(36.79748577699936, 10.219281339139357),
Offset(36.79751660719509, 10.233416888275087),
Offset(36.7975441640286, 10.246051610978604),
Offset(36.797566336361996, 10.256217557081996),
Offset(36.79758120646093, 10.263035451330936),
Offset(36.79758708492745, 10.265730709997449),
Offset(36.79758712071989, 10.265747120719887),
Offset(36.79758712071989, 10.265747120719887),
Offset(36.79758712071989, 10.265747120719887),
Offset(36.79758712071989, 10.265747120719887),
],
),
_PathCubicTo(
<Offset>[
Offset(44.63922254046772, 40.16584435071208),
Offset(44.51938558396772, 40.04156898841208),
Offset(44.39954862746772, 39.917293626112084),
Offset(44.27971167096772, 39.79301826381208),
Offset(44.15987471436772, 39.66874290141208),
Offset(44.04003775786772, 39.544467539112084),
Offset(43.92020080136772, 39.42019217681208),
Offset(43.80036384486772, 39.29591681451208),
Offset(43.68052688826772, 39.17164145221208),
Offset(43.56068993176772, 39.04736608991208),
Offset(43.440852975267724, 38.92309072751208),
Offset(43.32101601876772, 38.79881536521208),
Offset(43.20117906226772, 38.67454000291208),
Offset(43.08134210566772, 38.55026464061208),
Offset(42.96150514916772, 38.42598927831208),
Offset(42.05520843213051, 37.21380954643979),
Offset(39.36626959101571, 33.53571324376249),
Offset(36.677330749972064, 29.857616940933074),
Offset(33.988391908957254, 26.17952063825577),
Offset(31.299453067842446, 22.50142433557846),
Offset(28.610514226827636, 18.823328032801157),
Offset(25.92157538581283, 15.145231730123854),
Offset(23.945022741160805, 12.533133381006508),
Offset(22.97250713958332, 11.384102561113572),
Offset(22.812743621605566, 11.095347340254705),
Offset(22.68396524207196, 10.86259439455874),
Offset(22.580523547629824, 10.675634757573192),
Offset(22.499078886378673, 10.528432370914274),
Offset(22.43588362666254, 10.414213797064974),
Offset(22.38807176767408, 10.327799050590698),
Offset(22.35305385118404, 10.264507966755737),
Offset(22.328632378031546, 10.220368804304208),
Offset(22.312885496421558, 10.191908024680146),
Offset(22.304139635419563, 10.176100830038976),
Offset(22.300931183179507, 10.170301900803324),
Offset(22.301976693727863, 10.172191547658386),
Offset(22.30614842580716, 10.179731500666236),
Offset(22.31245170602349, 10.19112399561152),
Offset(22.32000688562417, 10.204779162835159),
Offset(22.328030701428442, 10.219281339139357),
Offset(22.335851668445954, 10.233416888275087),
Offset(22.342842252864767, 10.246051610978604),
Offset(22.348466903765345, 10.256217557081996),
Offset(22.35223913260045, 10.263035451330936),
Offset(22.35373037492376, 10.265730709997449),
Offset(22.353739454706773, 10.265747120719887),
Offset(22.353739454706773, 10.265747120719887),
Offset(22.353739454706773, 10.265747120719887),
Offset(22.353739454706773, 10.265747120719887),
],
<Offset>[
Offset(40.20437695941208, 44.60068993176772),
Offset(40.08454000291208, 44.47641456946772),
Offset(39.96470304641208, 44.352139207167724),
Offset(39.84486608991208, 44.22786384486772),
Offset(39.72502913331208, 44.10358848246772),
Offset(39.60519217681208, 43.979313120167724),
Offset(39.48535522031208, 43.85503775786772),
Offset(39.36551826381208, 43.73076239556772),
Offset(39.24568130721208, 43.60648703326772),
Offset(39.12584435071208, 43.48221167096772),
Offset(39.006007394212084, 43.35793630856772),
Offset(38.88617043771208, 43.23366094626772),
Offset(38.76633348121208, 43.10938558396772),
Offset(38.64649652461208, 42.98511022166772),
Offset(38.52665956811208, 42.86083485936772),
Offset(37.32866356433979, 41.94035441423051),
Offset(33.686840386562494, 39.2151424482157),
Offset(30.045017208833077, 36.48993048207207),
Offset(26.403194031155767, 33.764718516057265),
Offset(22.761370853378462, 31.03950655004245),
Offset(19.119547675701156, 28.314294583927644),
Offset(15.477724498023854, 25.589082617912837),
Offset(12.894881258806514, 23.583274863360806),
Offset(11.764102561113575, 22.592507139583326),
Offset(11.475347340254707, 22.43274362160557),
Offset(11.242594394558743, 22.303965242071964),
Offset(11.055634757573195, 22.200523547629828),
Offset(10.908432370914277, 22.119078886378677),
Offset(10.794213797064977, 22.055883626662546),
Offset(10.707799050590701, 22.008071767674085),
Offset(10.64450796675574, 21.973053851184044),
Offset(10.60036880430421, 21.94863237803155),
Offset(10.571908024680148, 21.932885496421562),
Offset(10.556100830038979, 21.924139635419568),
Offset(10.550301900803326, 21.920931183179516),
Offset(10.552191547658389, 21.921976693727867),
Offset(10.559731500666238, 21.926148425807163),
Offset(10.571123995611522, 21.932451706023496),
Offset(10.584779162835162, 21.94000688562418),
Offset(10.59928133913936, 21.948030701428447),
Offset(10.61341688827509, 21.95585166844596),
Offset(10.626051610978607, 21.96284225286477),
Offset(10.636217557081999, 21.96846690376535),
Offset(10.643035451330938, 21.972239132600453),
Offset(10.645730709997451, 21.973730374923765),
Offset(10.64574712071989, 21.973739454706777),
Offset(10.64574712071989, 21.973739454706777),
Offset(10.64574712071989, 21.973739454706777),
Offset(10.64574712071989, 21.973739454706777),
],
<Offset>[
Offset(40.20437695941208, 50.07184435071208),
Offset(40.08454000291208, 49.94756898841208),
Offset(39.96470304641208, 49.82329362611208),
Offset(39.84486608991208, 49.69901826381208),
Offset(39.72502913331208, 49.57474290141208),
Offset(39.60519217681208, 49.45046753911208),
Offset(39.48535522031208, 49.32619217681208),
Offset(39.36551826381208, 49.20191681451208),
Offset(39.24568130721208, 49.07764145221208),
Offset(39.12584435071208, 48.95336608991208),
Offset(39.006007394212084, 48.82909072751208),
Offset(38.88617043771208, 48.70481536521208),
Offset(38.76633348121208, 48.58054000291208),
Offset(38.64649652461208, 48.456264640612076),
Offset(38.52665956811208, 48.33198927831208),
Offset(37.32866356433979, 47.77137070715779),
Offset(33.686840386562494, 46.22170752948048),
Offset(30.045017208833077, 44.67204435170307),
Offset(26.403194031155767, 43.122381174025776),
Offset(22.761370853378462, 41.57271799634846),
Offset(19.119547675701156, 40.023054818571154),
Offset(15.477724498023854, 38.47339164089385),
Offset(12.894881258806514, 37.21554892931651),
Offset(11.764102561113575, 36.42002629848357),
Offset(11.475347340254707, 36.41939651184471),
Offset(11.242594394558743, 36.41888886839874),
Offset(11.055634757573195, 36.41848110198319),
Offset(10.908432370914277, 36.418160047714274),
Offset(10.794213797064977, 36.41791093245497),
Offset(10.707799050590701, 36.4177224584507),
Offset(10.64450796675574, 36.417584418075734),
Offset(10.60036880430421, 36.4174881488042),
Offset(10.571908024680148, 36.41742607471014),
Offset(10.556100830038979, 36.41739159858898),
Offset(10.550301900803326, 36.41737895089332),
Offset(10.552191547658389, 36.41738307228839),
Offset(10.559731500666238, 36.41739951722624),
Offset(10.571123995611522, 36.41742436471152),
Offset(10.584779162835162, 36.417454147175164),
Offset(10.59928133913936, 36.41748577699936),
Offset(10.61341688827509, 36.4175166071951),
Offset(10.626051610978607, 36.4175441640286),
Offset(10.636217557081999, 36.417566336362),
Offset(10.643035451330938, 36.41758120646094),
Offset(10.645730709997451, 36.41758708492745),
Offset(10.64574712071989, 36.41758712071989),
Offset(10.64574712071989, 36.41758712071989),
Offset(10.64574712071989, 36.41758712071989),
Offset(10.64574712071989, 36.41758712071989),
],
),
_PathCubicTo(
<Offset>[
Offset(40.20437695941208, 55.54301039685442),
Offset(40.08454000291208, 55.41873503455442),
Offset(39.96470304641208, 55.294459672254426),
Offset(39.84486608991208, 55.17018430995442),
Offset(39.72502913331208, 55.04590894755442),
Offset(39.60519217681208, 54.921633585254426),
Offset(39.48535522031208, 54.79735822295442),
Offset(39.36551826381208, 54.673082860654425),
Offset(39.24568130721208, 54.54880749835442),
Offset(39.12584435071208, 54.424532136054424),
Offset(39.006007394212084, 54.300256773654425),
Offset(38.88617043771208, 54.17598141135442),
Offset(38.76633348121208, 54.051706049054424),
Offset(38.64649652461208, 53.92743068675442),
Offset(38.52665956811208, 53.80315532445442),
Offset(37.32866356433979, 53.60239939205497),
Offset(33.686840386562494, 53.2282875009701),
Offset(30.045017208833077, 52.854175609813836),
Offset(26.403194031155767, 52.480063718728964),
Offset(22.761370853378462, 52.105951827644084),
Offset(19.119547675701156, 51.73183993645922),
Offset(15.477724498023854, 51.357728045374344),
Offset(12.894881258806514, 50.847851966333415),
Offset(11.764102561113575, 50.24757484337634),
Offset(11.475347340254707, 50.40607912626448),
Offset(11.242594394558743, 50.53384249150479),
Offset(11.055634757573195, 50.636468872081664),
Offset(10.908432370914277, 50.717271597197396),
Offset(10.794213797064977, 50.77996876016692),
Offset(10.707799050590701, 50.82740377235519),
Offset(10.64450796675574, 50.86214568222138),
Offset(10.60036880430421, 50.8863746685263),
Offset(10.571908024680148, 50.90199743528123),
Offset(10.556100830038979, 50.91067436255417),
Offset(10.550301900803326, 50.913857526194576),
Offset(10.552191547658389, 50.91282025622321),
Offset(10.559731500666238, 50.90868140518888),
Offset(10.571123995611522, 50.9024278066003),
Offset(10.584779162835162, 50.894932175934066),
Offset(10.59928133913936, 50.886971602793345),
Offset(10.61341688827509, 50.87921227961184),
Offset(10.626051610978607, 50.872276794062344),
Offset(10.636217557081999, 50.866696475922275),
Offset(10.643035451330938, 50.862953979299974),
Offset(10.645730709997451, 50.861474490753025),
Offset(10.64574712071989, 50.861465482535664),
Offset(10.64574712071989, 50.861465482535664),
Offset(10.64574712071989, 50.861465482535664),
Offset(10.64574712071989, 50.861465482535664),
],
<Offset>[
Offset(44.63922254046772, 59.97784435071208),
Offset(44.51938558396772, 59.853568988412086),
Offset(44.39954862746772, 59.72929362611209),
Offset(44.27971167096772, 59.605018263812084),
Offset(44.15987471436772, 59.480742901412086),
Offset(44.04003775786772, 59.35646753911209),
Offset(43.92020080136772, 59.232192176812084),
Offset(43.80036384486772, 59.10791681451209),
Offset(43.68052688826772, 58.98364145221208),
Offset(43.56068993176772, 58.859366089912086),
Offset(43.440852975267724, 58.73509072751209),
Offset(43.32101601876772, 58.61081536521208),
Offset(43.20117906226772, 58.486540002912086),
Offset(43.08134210566772, 58.36226464061208),
Offset(42.96150514916772, 58.237989278312085),
Offset(42.05520843213051, 58.328931867875795),
Offset(39.36626959101571, 58.907701815198486),
Offset(36.67733074997207, 59.48647176247307),
Offset(33.98839190895726, 60.06524170979577),
Offset(31.299453067842453, 60.644011657118455),
Offset(28.610514226827643, 61.22278160434116),
Offset(25.921575385812837, 61.80155155166385),
Offset(23.945022741160813, 61.897964477626516),
Offset(22.97250713958333, 61.455950035853576),
Offset(22.812743621605573, 61.74344568343471),
Offset(22.683965242071967, 61.975183342238736),
Offset(22.58052354762983, 62.16132744639319),
Offset(22.49907888637868, 62.30788772451427),
Offset(22.435883626662548, 62.42160806784497),
Offset(22.388071767674088, 62.507645866310696),
Offset(22.353053851184047, 62.57066086939574),
Offset(22.328632378031553, 62.614607493304206),
Offset(22.312885496421565, 62.64294412474014),
Offset(22.30413963541957, 62.65868236713898),
Offset(22.300931183179518, 62.66445600098332),
Offset(22.30197669372787, 62.66257459691839),
Offset(22.306148425807166, 62.65506753378624),
Offset(22.3124517060235, 62.643724733811524),
Offset(22.32000688562418, 62.63012913151516),
Offset(22.32803070142845, 62.615690214859356),
Offset(22.33585166844596, 62.60161632611509),
Offset(22.342842252864774, 62.5890367170786),
Offset(22.348466903765352, 62.578915115642),
Offset(22.352239132600456, 62.57212696159094),
Offset(22.353730374923767, 62.56944345985745),
Offset(22.35373945470678, 62.569427120719894),
Offset(22.35373945470678, 62.569427120719894),
Offset(22.35373945470678, 62.569427120719894),
Offset(22.35373945470678, 62.569427120719894),
],
<Offset>[
Offset(50.11037695941208, 59.97784435071208),
Offset(49.99054000291208, 59.853568988412086),
Offset(49.87070304641208, 59.72929362611209),
Offset(49.75086608991208, 59.605018263812084),
Offset(49.631029133312076, 59.480742901412086),
Offset(49.51119217681208, 59.35646753911209),
Offset(49.39135522031208, 59.232192176812084),
Offset(49.27151826381208, 59.10791681451209),
Offset(49.15168130721208, 58.98364145221208),
Offset(49.03184435071208, 58.859366089912086),
Offset(48.91200739421208, 58.73509072751209),
Offset(48.79217043771208, 58.61081536521208),
Offset(48.67233348121208, 58.486540002912086),
Offset(48.55249652461208, 58.36226464061208),
Offset(48.43265956811208, 58.237989278312085),
Offset(47.886224725057794, 58.328931867875795),
Offset(46.37283467228049, 58.907701815198486),
Offset(44.859444619603074, 59.48647176247307),
Offset(43.34605456692577, 60.06524170979577),
Offset(41.83266451414846, 60.644011657118455),
Offset(40.31927446147115, 61.22278160434116),
Offset(38.80588440879385, 61.80155155166385),
Offset(37.57729680711652, 61.897964477626516),
Offset(36.80002629848357, 61.455950035853576),
Offset(36.799396511844705, 61.74344568343471),
Offset(36.79888886839874, 61.975183342238736),
Offset(36.79848110198319, 62.16132744639319),
Offset(36.798160047714276, 62.30788772451427),
Offset(36.797910932454975, 62.42160806784497),
Offset(36.7977224584507, 62.507645866310696),
Offset(36.797584418075736, 62.57066086939574),
Offset(36.797488148804206, 62.614607493304206),
Offset(36.797426074710145, 62.64294412474014),
Offset(36.79739159858898, 62.65868236713898),
Offset(36.797378950893325, 62.66445600098332),
Offset(36.79738307228839, 62.66257459691839),
Offset(36.79739951722624, 62.65506753378624),
Offset(36.797424364711524, 62.643724733811524),
Offset(36.797454147175166, 62.63012913151516),
Offset(36.797485776999366, 62.615690214859356),
Offset(36.79751660719509, 62.60161632611509),
Offset(36.79754416402861, 62.5890367170786),
Offset(36.797566336361996, 62.578915115642),
Offset(36.79758120646094, 62.57212696159094),
Offset(36.79758708492745, 62.56944345985745),
Offset(36.79758712071989, 62.569427120719894),
Offset(36.79758712071989, 62.569427120719894),
Offset(36.79758712071989, 62.569427120719894),
Offset(36.79758712071989, 62.569427120719894),
],
),
_PathCubicTo(
<Offset>[
Offset(52.571645330995544, 59.97784435071208),
Offset(52.45180837449554, 59.853568988412086),
Offset(52.33197141799555, 59.72929362611209),
Offset(52.212134461495545, 59.605018263812084),
Offset(52.09229750489554, 59.480742901412086),
Offset(51.972460548395546, 59.35646753911209),
Offset(51.852623591895544, 59.232192176812084),
Offset(51.73278663539554, 59.10791681451209),
Offset(51.612949678795545, 58.98364145221208),
Offset(51.49311272229554, 58.859366089912086),
Offset(51.37327576579555, 58.73509072751209),
Offset(51.253438809295545, 58.61081536521208),
Offset(51.13360185279554, 58.486540002912086),
Offset(51.013764896195546, 58.36226464061208),
Offset(50.893927939695544, 58.237989278312085),
Offset(50.509381535670876, 58.328931867875795),
Offset(49.524827050387316, 58.907701815198486),
Offset(48.54027256521657, 59.48647176247307),
Offset(47.55571808003301, 60.06524170979577),
Offset(46.57116359474944, 60.644011657118455),
Offset(45.586609109565885, 61.22278160434116),
Offset(44.602054624382326, 61.80155155166385),
Offset(43.70994860636296, 61.897964477626516),
Offset(43.0205115849932, 61.455950035853576),
Offset(43.091470130506, 61.74344568343471),
Offset(43.14866670842858, 61.975183342238736),
Offset(43.19461006443314, 62.16132744639319),
Offset(43.23078349611088, 62.30788772451427),
Offset(43.25885150418633, 62.42160806784497),
Offset(43.28008701536578, 62.507645866310696),
Offset(43.295640130698196, 62.57066086939574),
Offset(43.30648686364522, 62.614607493304206),
Offset(43.313480799617274, 62.64294412474014),
Offset(43.317365250734866, 62.65868236713898),
Offset(43.31879027634261, 62.66445600098332),
Offset(43.318325915587614, 62.66257459691839),
Offset(43.31647305177763, 62.65506753378624),
Offset(43.313673466479486, 62.643724733811524),
Offset(43.31031785336869, 62.63012913151516),
Offset(43.30675409672299, 62.615690214859356),
Offset(43.30328043483139, 62.60161632611509),
Offset(43.300175585182856, 62.5890367170786),
Offset(43.29767741130703, 62.578915115642),
Offset(43.296001985810214, 62.57212696159094),
Offset(43.29533965446193, 62.56944345985745),
Offset(43.295335621700225, 62.569427120719894),
Offset(43.295335621700225, 62.569427120719894),
Offset(43.295335621700225, 62.569427120719894),
Offset(43.295335621700225, 62.569427120719894),
],
<Offset>[
Offset(54.81954300555442, 59.07868993176772),
Offset(54.69970604905442, 58.95441456946772),
Offset(54.579869092554425, 58.830139207167726),
Offset(54.46003213605442, 58.70586384486772),
Offset(54.34019517945442, 58.58158848246772),
Offset(54.220358222954424, 58.457313120167726),
Offset(54.10052126645442, 58.33303775786772),
Offset(53.98068430995442, 58.208762395567724),
Offset(53.86084735335442, 58.08448703326772),
Offset(53.74101039685442, 57.96021167096772),
Offset(53.621173440354426, 57.835936308567724),
Offset(53.50133648385442, 57.71166094626772),
Offset(53.38149952735442, 57.58738558396772),
Offset(53.261662570754424, 57.46311022166772),
Offset(53.14182561425442, 57.33883485936772),
Offset(52.905133320668966, 57.37063611066451),
Offset(52.4035689294841, 57.756211019649704),
Offset(51.90200453842384, 58.14178592858207),
Offset(51.40044014733896, 58.527360837567265),
Offset(50.89887575615409, 58.91293574655245),
Offset(50.397311365069214, 59.298510655437646),
Offset(49.895746973984345, 59.68408556442283),
Offset(49.31095249426342, 59.657574510890804),
Offset(48.70173455588635, 59.18347260189332),
Offset(48.83807534383448, 59.44481548777557),
Offset(48.94797368582479, 59.65547254999196),
Offset(49.036249922511665, 59.82468358945982),
Offset(49.1057540835974, 59.957911644778676),
Offset(49.159684365136926, 60.06128713223254),
Offset(49.20048658713519, 60.13949828685408),
Offset(49.230370570581385, 60.196780972344044),
Offset(49.2512116420263, 60.236729881531545),
Offset(49.264649892971235, 60.26248880031156),
Offset(49.27211353420417, 60.276795374069565),
Offset(49.27485159926458, 60.28204379484951),
Offset(49.27395936971321, 60.28033353741787),
Offset(49.27039925006888, 60.273509373087165),
Offset(49.265020085900304, 60.2631983993235),
Offset(49.25857256175407, 60.25083955504418),
Offset(49.25172510757334, 60.237714110608444),
Offset(49.24505076277185, 60.22492048840596),
Offset(49.23908505921234, 60.21348521501477),
Offset(49.234285031362276, 60.204284350405345),
Offset(49.23106584428998, 60.198113697790454),
Offset(49.22979323114302, 60.19567430751376),
Offset(49.22978548253567, 60.19565945470678),
Offset(49.22978548253567, 60.19565945470678),
Offset(49.22978548253567, 60.19565945470678),
Offset(49.22978548253567, 60.19565945470678),
],
<Offset>[
Offset(56.549286261168945, 57.59279225720884),
Offset(56.42944930466894, 57.468516894908845),
Offset(56.30961234816895, 57.34424153260885),
Offset(56.189775391668945, 57.219966170308844),
Offset(56.06993843506894, 57.095690807908845),
Offset(55.950101478568946, 56.97141544560885),
Offset(55.830264522068944, 56.847140083308844),
Offset(55.71042756556894, 56.72286472100885),
Offset(55.590590608968945, 56.59858935870884),
Offset(55.47075365246894, 56.474313996408846),
Offset(55.35091669596895, 56.35003863400885),
Offset(55.231079739468946, 56.22576327170884),
Offset(55.111242782968944, 56.101487909408846),
Offset(54.99140582636895, 55.97721254710884),
Offset(54.871568869868945, 55.852937184808845),
Offset(54.74864939309879, 55.78700441495241),
Offset(54.6187428701751, 55.85331485483892),
Offset(54.4888363473851, 55.9196252946648),
Offset(54.358929824561415, 55.985935734551305),
Offset(54.22902330163772, 56.052246174437805),
Offset(54.09911677881403, 56.11855661422432),
Offset(53.96921025599034, 56.18486705411082),
Offset(53.620890090363176, 55.95521797286034),
Offset(53.07340023656424, 55.42808991849018),
Offset(53.26005225271882, 55.64621405687709),
Offset(53.410504273814226, 55.82203437827575),
Offset(53.53135539844182, 55.9632626809513),
Offset(53.62650734814831, 56.07445857089216),
Offset(53.700338487990095, 56.16073866631195),
Offset(53.75619717205799, 56.226015900304674),
Offset(53.79710866923288, 56.27382564410085),
Offset(53.82564032188473, 56.307168129650464),
Offset(53.84403743305162, 56.328667249267596),
Offset(53.85425523877907, 56.34060791895026),
Offset(53.85800367951774, 56.34498839885754),
Offset(53.8567822075933, 56.34356096980227),
Offset(53.85190836522106, 56.337865329915914),
Offset(53.84454423118309, 56.32925950060268),
Offset(53.83571750075847, 56.31894446083879),
Offset(53.82634326178278, 56.30798959497809),
Offset(53.81720601142316, 56.29731167730551),
Offset(53.809038898603006, 56.28776747583528),
Offset(53.80246760846087, 56.2800881749101),
Offset(53.79806050647426, 56.274937974320686),
Offset(53.79631828528544, 56.27290199044266),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
],
),
_PathCubicTo(
<Offset>[
Offset(56.549286261168945, 57.59279225720884),
Offset(56.42944930466894, 57.468516894908845),
Offset(56.30961234816895, 57.34424153260885),
Offset(56.189775391668945, 57.219966170308844),
Offset(56.06993843506894, 57.095690807908845),
Offset(55.950101478568946, 56.97141544560885),
Offset(55.830264522068944, 56.847140083308844),
Offset(55.71042756556894, 56.72286472100885),
Offset(55.590590608968945, 56.59858935870884),
Offset(55.47075365246894, 56.474313996408846),
Offset(55.35091669596895, 56.35003863400885),
Offset(55.231079739468946, 56.22576327170884),
Offset(55.111242782968944, 56.101487909408846),
Offset(54.99140582636895, 55.97721254710884),
Offset(54.871568869868945, 55.852937184808845),
Offset(54.74864939309879, 55.78700441495241),
Offset(54.6187428701751, 55.85331485483892),
Offset(54.4888363473851, 55.9196252946648),
Offset(54.358929824561415, 55.985935734551305),
Offset(54.22902330163772, 56.052246174437805),
Offset(54.09911677881403, 56.11855661422432),
Offset(53.96921025599034, 56.18486705411082),
Offset(53.620890090363176, 55.95521797286034),
Offset(53.07340023656424, 55.42808991849018),
Offset(53.26005225271882, 55.64621405687709),
Offset(53.410504273814226, 55.82203437827575),
Offset(53.53135539844182, 55.9632626809513),
Offset(53.62650734814831, 56.07445857089216),
Offset(53.700338487990095, 56.16073866631195),
Offset(53.75619717205799, 56.226015900304674),
Offset(53.79710866923288, 56.27382564410085),
Offset(53.82564032188473, 56.307168129650464),
Offset(53.84403743305162, 56.328667249267596),
Offset(53.85425523877907, 56.34060791895026),
Offset(53.85800367951774, 56.34498839885754),
Offset(53.8567822075933, 56.34356096980227),
Offset(53.85190836522106, 56.337865329915914),
Offset(53.84454423118309, 56.32925950060268),
Offset(53.83571750075847, 56.31894446083879),
Offset(53.82634326178278, 56.30798959497809),
Offset(53.81720601142316, 56.29731167730551),
Offset(53.809038898603006, 56.28776747583528),
Offset(53.80246760846087, 56.2800881749101),
Offset(53.79806050647426, 56.274937974320686),
Offset(53.79631828528544, 56.27290199044266),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
Offset(53.796307677358, 56.272889593871334),
],
<Offset>[
Offset(57.62369742449878, 56.51075365246894),
Offset(57.50386046799878, 56.386478290168945),
Offset(57.38402351149878, 56.26220292786895),
Offset(57.26418655499878, 56.137927565568944),
Offset(57.144349598398776, 56.013652203168945),
Offset(57.02451264189878, 55.88937684086895),
Offset(56.90467568539878, 55.765101478568944),
Offset(56.78483872889878, 55.64082611626895),
Offset(56.66500177229878, 55.51655075396894),
Offset(56.54516481579878, 55.392275391668946),
Offset(56.42532785929878, 55.26800002926895),
Offset(56.30549090279878, 55.14372466696894),
Offset(56.18565394629878, 55.019449304668946),
Offset(56.06581698969878, 54.89517394236894),
Offset(55.94598003319878, 54.770898580068945),
Offset(55.89372930109525, 54.63379537519879),
Offset(55.994674010747886, 54.46761572737509),
Offset(56.09561872053984, 54.3014360794851),
Offset(56.19656343029247, 54.13525643166142),
Offset(56.2975081399451, 53.969076783837714),
Offset(56.398452849697726, 53.80289713591403),
Offset(56.49939755945036, 53.63671748809034),
Offset(56.297960835674125, 53.25914221256317),
Offset(55.7888127085716, 52.69340023656424),
Offset(56.006714995568615, 52.88005225271882),
Offset(56.182356492271566, 53.03050427381422),
Offset(56.32344115337003, 53.151355398441815),
Offset(56.43452394733299, 53.246507348148306),
Offset(56.52071628832435, 53.32033848799009),
Offset(56.5859271296418, 53.376197172057985),
Offset(56.63368824673307, 53.417108669232874),
Offset(56.66699682004896, 53.445640321884724),
Offset(56.68847407317482, 53.464037433051615),
Offset(56.700402598148585, 53.474255238779065),
Offset(56.70477862272339, 53.47800367951774),
Offset(56.703352645488735, 53.476782207593295),
Offset(56.697662798567954, 53.471908365221054),
Offset(56.689065722138324, 53.46454423118309),
Offset(56.678761173675056, 53.45571750075847),
Offset(56.66781744987426, 53.446343261782786),
Offset(56.65715039258098, 53.43720601142316),
Offset(56.64761589840118, 53.429038898603004),
Offset(56.639944407998925, 53.42246760846087),
Offset(56.634799445615464, 53.418060506474255),
Offset(56.632765532511705, 53.416318285285435),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
],
<Offset>[
Offset(57.62369742449878, 56.51075365246894),
Offset(57.50386046799878, 56.386478290168945),
Offset(57.38402351149878, 56.26220292786895),
Offset(57.26418655499878, 56.137927565568944),
Offset(57.144349598398776, 56.013652203168945),
Offset(57.02451264189878, 55.88937684086895),
Offset(56.90467568539878, 55.765101478568944),
Offset(56.78483872889878, 55.64082611626895),
Offset(56.66500177229878, 55.51655075396894),
Offset(56.54516481579878, 55.392275391668946),
Offset(56.42532785929878, 55.26800002926895),
Offset(56.30549090279878, 55.14372466696894),
Offset(56.18565394629878, 55.019449304668946),
Offset(56.06581698969878, 54.89517394236894),
Offset(55.94598003319878, 54.770898580068945),
Offset(55.89372930109525, 54.63379537519879),
Offset(55.994674010747886, 54.46761572737509),
Offset(56.09561872053984, 54.3014360794851),
Offset(56.19656343029247, 54.13525643166142),
Offset(56.2975081399451, 53.969076783837714),
Offset(56.398452849697726, 53.80289713591403),
Offset(56.49939755945036, 53.63671748809034),
Offset(56.297960835674125, 53.25914221256317),
Offset(55.7888127085716, 52.69340023656424),
Offset(56.006714995568615, 52.88005225271882),
Offset(56.182356492271566, 53.03050427381422),
Offset(56.32344115337003, 53.151355398441815),
Offset(56.43452394733299, 53.246507348148306),
Offset(56.52071628832435, 53.32033848799009),
Offset(56.5859271296418, 53.376197172057985),
Offset(56.63368824673307, 53.417108669232874),
Offset(56.66699682004896, 53.445640321884724),
Offset(56.68847407317482, 53.464037433051615),
Offset(56.700402598148585, 53.474255238779065),
Offset(56.70477862272339, 53.47800367951774),
Offset(56.703352645488735, 53.476782207593295),
Offset(56.697662798567954, 53.471908365221054),
Offset(56.689065722138324, 53.46454423118309),
Offset(56.678761173675056, 53.45571750075847),
Offset(56.66781744987426, 53.446343261782786),
Offset(56.65715039258098, 53.43720601142316),
Offset(56.64761589840118, 53.429038898603004),
Offset(56.639944407998925, 53.42246760846087),
Offset(56.634799445615464, 53.418060506474255),
Offset(56.632765532511705, 53.416318285285435),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
Offset(56.63275314854878, 53.416307677358),
],
),
_PathClose(
),
_PathMoveTo(
<Offset>[
Offset(50.11037695941208, 50.11515566052058),
Offset(49.99054000291208, 49.99088029822058),
Offset(49.87070304641208, 49.866604935920584),
Offset(49.75086608991208, 49.74232957362058),
Offset(49.631029133312076, 49.61805421122058),
Offset(49.51119217681208, 49.493778848920584),
Offset(49.39135522031208, 49.36950348662058),
Offset(49.27151826381208, 49.24522812432058),
Offset(49.15168130721208, 49.12095276202058),
Offset(49.03184435071208, 48.99667739972058),
Offset(48.91200739421208, 48.87240203732058),
Offset(48.79217043771208, 48.74812667502058),
Offset(48.67233348121208, 48.62385131272058),
Offset(48.55249652461208, 48.49957595042058),
Offset(48.43265956811208, 48.37530058812058),
Offset(47.886224725057794, 48.02143406108567),
Offset(46.37283467228049, 47.32255270277091),
Offset(44.859444619603074, 46.89224005129487),
Offset(43.346054566925766, 46.73049610683733),
Offset(41.83266451414846, 46.83732086930855),
Offset(40.31927446147115, 47.21271433859731),
Offset(38.80588440879385, 47.856676514909296),
Offset(37.57729680711651, 48.70078707684129),
Offset(36.80002629848357, 49.649293275456884),
Offset(36.799396511844705, 51.39862444641657),
Offset(36.79888886839874, 52.36567493589737),
Offset(36.798481101983185, 52.561543433342045),
Offset(36.798160047714276, 52.73365619281698),
Offset(36.79791093245497, 52.88590941777883),
Offset(36.79772245845069, 53.021274808915365),
Offset(36.79758441807573, 53.14247036493895),
Offset(36.797488148804206, 53.25181934443066),
Offset(36.797426074710145, 53.351374286002354),
Offset(36.79739159858897, 53.44294120991781),
Offset(36.79737895089332, 53.52811757525859),
Offset(36.79738307228838, 53.60832148263455),
Offset(36.79739951722624, 53.68481556964314),
Offset(36.79742436471152, 53.7587295593099),
Offset(36.79745414717516, 53.83107808312845),
Offset(36.79748577699936, 53.90277982470875),
Offset(36.79751660719509, 53.97463627018816),
Offset(36.7975441640286, 54.047410713622526),
Offset(36.797566336361996, 54.121768596383546),
Offset(36.79758120646093, 54.19832738700239),
Offset(36.79758708492745, 54.27764432912612),
Offset(36.79758712071989, 54.35877252649494),
Offset(36.79758712071989, 54.439911871108684),
Offset(36.79758712071989, 54.52105121572243),
Offset(36.79758712071989, 54.522707120719886),
],
),
_PathCubicTo(
<Offset>[
Offset(50.08645981463666, 50.11515566052058),
Offset(49.96662285813666, 49.99088029822058),
Offset(49.846785901636665, 49.866604935920584),
Offset(49.72694894513666, 49.74232957362058),
Offset(49.60711198853666, 49.61805421122058),
Offset(49.48727503203666, 49.493778848920584),
Offset(49.36743807553666, 49.36950348662058),
Offset(49.24760111903666, 49.24522812432058),
Offset(49.12776416243666, 49.12095276202058),
Offset(49.00792720593666, 48.99667739972058),
Offset(48.888090249436665, 48.87240203732058),
Offset(48.76825329293666, 48.74812667502058),
Offset(48.64841633643666, 48.62385131272058),
Offset(48.528579379836664, 48.49957595042058),
Offset(48.40874242333666, 48.37530058812058),
Offset(47.748134816992604, 48.02143406108567),
Offset(45.764925398174555, 47.32255270277091),
Offset(43.63340675157196, 46.89224005129487),
Offset(41.35357887709607, 46.73049610683733),
Offset(38.92544177464233, 46.83732086930855),
Offset(36.34899544441074, 47.21271433859731),
Offset(33.624239886301304, 47.856676514909296),
Offset(31.234909988934966, 48.70078707684129),
Offset(29.494550483270046, 49.649293275456884),
Offset(28.52755585328232, 51.39862444641657),
Offset(27.99274184675299, 52.36567493589737),
Offset(27.883944986437346, 52.561543433342045),
Offset(27.788401120897287, 52.73365619281698),
Offset(27.703935759159105, 52.88590941777883),
Offset(27.628890333495022, 53.021274808915365),
Offset(27.561748066907615, 53.14247036493895),
Offset(27.50121256374279, 53.25181934443066),
Offset(27.446138611583994, 53.351374286002354),
Offset(27.39551864926441, 53.44294120991781),
Offset(27.348461571724304, 53.52811757525859),
Offset(27.30417642143305, 53.60832148263455),
Offset(27.261959043201006, 53.68481556964314),
Offset(27.22117949399494, 53.7587295593099),
Offset(27.181272090318803, 53.83107808312845),
Offset(27.141724722671132, 53.90277982470875),
Offset(27.102090684257846, 53.97463627018816),
Offset(27.061944627502523, 54.047410713622526),
Offset(27.020915803873248, 54.121768596383546),
Offset(26.978660256499385, 54.19832738700239),
Offset(26.934867640331532, 54.27764432912612),
Offset(26.890065739866895, 54.35877252649494),
Offset(26.845257628088735, 54.439911871108684),
Offset(26.80044951631057, 54.52105121572243),
Offset(26.79953506506211, 54.522707120719886),
],
<Offset>[
Offset(50.06706564959764, 50.095761495481554),
Offset(49.947228693097635, 49.97148613318156),
Offset(49.82739173659764, 49.84721077088156),
Offset(49.70755478009764, 49.722935408581556),
Offset(49.587717823497634, 49.59866004618156),
Offset(49.46788086699764, 49.47438468388156),
Offset(49.34804391049764, 49.350109321581556),
Offset(49.228206953997635, 49.22583395928156),
Offset(49.10836999739764, 49.101558596981555),
Offset(48.988533040897636, 48.97728323468156),
Offset(48.86869608439764, 48.85300787228156),
Offset(48.74885912789764, 48.728732509981555),
Offset(48.62902217139764, 48.60445714768156),
Offset(48.50918521479764, 48.48018178538155),
Offset(48.38934825829764, 48.35590642308156),
Offset(47.63616175837963, 47.909461002472696),
Offset(45.27199148434817, 46.82961878894062),
Offset(42.63925301353386, 45.89808631326019),
Offset(39.73794634587201, 45.11486357561327),
Offset(36.56807148124825, 44.47995057591447),
Offset(33.12962841986258, 43.993347314049146),
Offset(29.42261716161499, 43.65505379022298),
Offset(26.09208026716689, 43.55795735507321),
Offset(23.57078423835929, 43.725527030546125),
Offset(21.82019681522399, 44.69126540835824),
Offset(20.852132118393076, 45.225065207537455),
Offset(20.655446961021294, 45.333045407926),
Offset(20.48269090511957, 45.42794597703926),
Offset(20.329938213814554, 45.511911872434275),
Offset(20.194194600945227, 45.58657907636557),
Offset(20.07272166098446, 45.653443959015796),
Offset(19.9631788172331, 45.71378559792097),
Offset(19.86349838492615, 45.76873405934452),
Offset(19.771861154060407, 45.81928371469362),
Offset(19.686658130182877, 45.86631413371717),
Offset(19.606461097044544, 45.91060615824605),
Offset(19.529998528318746, 45.95285505476088),
Offset(19.456132860826976, 45.993682926121764),
Offset(19.38384252924341, 46.033648522053056),
Offset(19.312202675685725, 46.07325777772334),
Offset(19.240406520613522, 46.11295210654384),
Offset(19.167685822801204, 46.1531519089212),
Offset(19.093370918660103, 46.19422371115027),
Offset(19.016840503908618, 46.236507634411616),
Offset(18.93753395574349, 46.28031064453808),
Offset(18.856404467546696, 46.325111254174736),
Offset(18.775263760542785, 46.369918003542615),
Offset(18.69412305351876, 46.41472475293062),
Offset(18.692467120719886, 46.41563917637766),
],
<Offset>[
Offset(50.06706564959764, 50.07184435070614),
Offset(49.947228693097635, 49.94756898840614),
Offset(49.82739173659764, 49.82329362610614),
Offset(49.70755478009764, 49.69901826380614),
Offset(49.587717823497634, 49.57474290140614),
Offset(49.46788086699764, 49.45046753910614),
Offset(49.34804391049764, 49.32619217680614),
Offset(49.228206953997635, 49.20191681450614),
Offset(49.10836999739764, 49.07764145220614),
Offset(48.988533040897636, 48.95336608990614),
Offset(48.86869608439764, 48.82909072750614),
Offset(48.74885912789764, 48.70481536520614),
Offset(48.62902217139764, 48.58054000290614),
Offset(48.50918521479764, 48.456264640606136),
Offset(48.38934825829764, 48.33198927830614),
Offset(47.63616175837963, 47.7713707071586),
Offset(45.27199148434817, 46.22170752947073),
Offset(42.63925301353386, 44.672044351691675),
Offset(39.73794634587201, 43.12238117401274),
Offset(36.56807148124825, 41.572717996339655),
Offset(33.12962841986258, 40.02305481856121),
Offset(29.42261716161499, 38.47339164088308),
Offset(26.09208026716689, 37.21554892929752),
Offset(23.57078423835929, 36.42002629846431),
Offset(21.82019681522399, 36.41939651182523),
Offset(20.852132118393076, 36.41888886837908),
Offset(20.655446961021294, 36.41848110196339),
Offset(20.48269090511957, 36.41816004771427),
Offset(20.329938213814554, 36.41791093245497),
Offset(20.194194600945227, 36.4177224584507),
Offset(20.07272166098446, 36.41758441807573),
Offset(19.9631788172331, 36.4174881488042),
Offset(19.86349838492615, 36.417426074710136),
Offset(19.771861154060407, 36.41739159858898),
Offset(19.686658130182877, 36.417378950893315),
Offset(19.606461097044544, 36.41738307228839),
Offset(19.529998528318746, 36.41739951720605),
Offset(19.456132860826976, 36.41742436469134),
Offset(19.38384252924341, 36.417454147155),
Offset(19.312202675685725, 36.417485776979206),
Offset(19.240406520613522, 36.417516607174946),
Offset(19.167685822801204, 36.41754416400846),
Offset(19.093370918660103, 36.41756633636199),
Offset(19.016840503908618, 36.41758120646094),
Offset(18.93753395574349, 36.41758708492745),
Offset(18.856404467546696, 36.41758712071989),
Offset(18.775263760542785, 36.41758712071989),
Offset(18.69412305351876, 36.41758712071989),
Offset(18.692467120719886, 36.41758712071989),
],
),
_PathCubicTo(
<Offset>[
Offset(50.06706564959764, 50.04792720593072),
Offset(49.947228693097635, 49.92365184363072),
Offset(49.82739173659764, 49.799376481330725),
Offset(49.70755478009764, 49.675101119030714),
Offset(49.587717823497634, 49.550825756630715),
Offset(49.46788086699764, 49.42655039433072),
Offset(49.34804391049764, 49.30227503203072),
Offset(49.228206953997635, 49.177999669730724),
Offset(49.10836999739764, 49.05372430743071),
Offset(48.988533040897636, 48.929448945130716),
Offset(48.86869608439764, 48.80517358273072),
Offset(48.74885912789764, 48.68089822043072),
Offset(48.62902217139764, 48.55662285813072),
Offset(48.50918521479764, 48.43234749583071),
Offset(48.38934825829764, 48.308072133530715),
Offset(47.63616175837963, 47.63328079909341),
Offset(45.27199148434817, 45.61379825536479),
Offset(42.63925301353386, 43.44600648366056),
Offset(39.73794634587201, 41.12990548418304),
Offset(36.56807148124825, 38.66549525683352),
Offset(33.12962841986258, 36.05277580150079),
Offset(29.42261716161499, 33.29174711839053),
Offset(26.09208026716689, 30.873162111115967),
Offset(23.57078423835929, 29.11455048325078),
Offset(21.82019681522399, 28.147555853262837),
Offset(20.852132118393076, 27.612742525999973),
Offset(20.655446961021294, 27.50394701174588),
Offset(20.48269090511957, 27.408404506536804),
Offset(20.329938213814554, 27.323940514395186),
Offset(20.194194600945227, 27.2488964636637),
Offset(20.07272166098446, 27.181755574389616),
Offset(19.9631788172331, 27.121221448636877),
Offset(19.86349838492615, 27.066148872338097),
Offset(19.771861154060407, 27.015530283259928),
Offset(19.686658130182877, 26.968474575656916),
Offset(19.606461097044544, 26.924190791705026),
Offset(19.529998528318746, 26.88197477619479),
Offset(19.456132860826976, 26.841196586461674),
Offset(19.38384252924341, 26.80129053948502),
Offset(19.312202675685725, 26.761744526458134),
Offset(19.240406520613522, 26.72211184147367),
Offset(19.167685822801204, 26.68196713796564),
Offset(19.093370918660103, 26.64093966853734),
Offset(19.016840503908618, 26.598685477488807),
Offset(18.93753395574349, 26.5548942211387),
Offset(18.856404467546696, 26.5100936830677),
Offset(18.775263760542785, 26.465286933679703),
Offset(18.69412305351876, 26.42048018431182),
Offset(18.692467120719886, 26.419565760864774),
],
<Offset>[
Offset(50.08645981463666, 50.02853304089169),
Offset(49.96662285813666, 49.90425767859169),
Offset(49.846785901636665, 49.779982316291694),
Offset(49.72694894513666, 49.6557069539917),
Offset(49.60711198853666, 49.5314315915917),
Offset(49.48727503203666, 49.4071562292917),
Offset(49.36743807553666, 49.28288086699169),
Offset(49.24760111903666, 49.15860550469169),
Offset(49.12776416243666, 49.034330142391696),
Offset(49.00792720593666, 48.9100547800917),
Offset(48.888090249436665, 48.7857794176917),
Offset(48.76825329293666, 48.66150405539169),
Offset(48.64841633643666, 48.53722869309169),
Offset(48.528579379836664, 48.412953330791694),
Offset(48.40874242333666, 48.2886779684917),
Offset(47.748134816992604, 47.521307740480445),
Offset(45.76492539817846, 45.1208643415384),
Offset(43.63340675156854, 42.45185274562246),
Offset(41.35357887709607, 39.51427295295898),
Offset(38.92544177464233, 36.30812496343945),
Offset(36.34899544441074, 32.83340877695263),
Offset(33.624239886301304, 29.090124393704222),
Offset(31.234909988934966, 25.730332389347893),
Offset(29.494550483270046, 23.19078423834003),
Offset(28.52755585328232, 21.440196815204512),
Offset(27.99274184675299, 20.472132118373413),
Offset(27.883944986437346, 20.275446961001492),
Offset(27.788401120897287, 20.102690905119566),
Offset(27.703935759159105, 19.94993821381455),
Offset(27.628890333495022, 19.814194600945225),
Offset(27.56174806690762, 19.692721660984457),
Offset(27.50121256374279, 19.583178817233097),
Offset(27.446138611583994, 19.483498384926143),
Offset(27.395518649284597, 19.391861154060404),
Offset(27.348461571724304, 19.306658130182875),
Offset(27.30417642143305, 19.22646109704454),
Offset(27.261959043201006, 19.14999852829856),
Offset(27.22117949401511, 19.076132860806798),
Offset(27.1812720903188, 19.003842529223242),
Offset(27.141724722671132, 18.93220267566557),
Offset(27.102090684257846, 18.860406520593376),
Offset(27.061944627502523, 18.787685822781068),
Offset(27.020915803893374, 18.7133709186601),
Offset(26.978660256499385, 18.636840503908616),
Offset(26.934867640331532, 18.557533955743487),
Offset(26.890065739866895, 18.476404467546697),
Offset(26.84525762810885, 18.395263760542782),
Offset(26.80044951631057, 18.314123053518756),
Offset(26.79953506506211, 18.312467120719884),
],
<Offset>[
Offset(50.11037695941208, 50.02853304089169),
Offset(49.99054000291208, 49.90425767859169),
Offset(49.87070304641208, 49.779982316291694),
Offset(49.75086608991208, 49.6557069539917),
Offset(49.631029133312076, 49.5314315915917),
Offset(49.51119217681208, 49.4071562292917),
Offset(49.39135522031208, 49.28288086699169),
Offset(49.27151826381208, 49.15860550469169),
Offset(49.15168130721208, 49.034330142391696),
Offset(49.03184435071208, 48.9100547800917),
Offset(48.91200739421208, 48.7857794176917),
Offset(48.79217043771208, 48.66150405539169),
Offset(48.67233348121208, 48.53722869309169),
Offset(48.55249652461208, 48.412953330791694),
Offset(48.43265956811208, 48.2886779684917),
Offset(47.886224725057794, 47.521307740480445),
Offset(46.37283467228049, 45.1208643415384),
Offset(44.859444619603074, 42.45185274562246),
Offset(43.346054566925766, 39.51427295295898),
Offset(41.83266451414846, 36.30812496343945),
Offset(40.31927446147115, 32.83340877695263),
Offset(38.80588440879385, 29.090124393704222),
Offset(37.57729680711652, 25.730332389347893),
Offset(36.80002629848357, 23.19078423834003),
Offset(36.799396511844705, 21.440196815204512),
Offset(36.79888886839874, 20.472132118373413),
Offset(36.798481101983185, 20.275446961001492),
Offset(36.798160047714276, 20.102690905119566),
Offset(36.79791093245497, 19.94993821381455),
Offset(36.79772245845069, 19.814194600945225),
Offset(36.79758441807573, 19.692721660984457),
Offset(36.797488148804206, 19.583178817233097),
Offset(36.797426074710145, 19.483498384926143),
Offset(36.79739159858897, 19.391861154060404),
Offset(36.79737895089332, 19.306658130182875),
Offset(36.79738307228838, 19.22646109704454),
Offset(36.79739951722624, 19.14999852829856),
Offset(36.79742436471152, 19.076132860806798),
Offset(36.79745414717516, 19.003842529223242),
Offset(36.79748577699936, 18.93220267566557),
Offset(36.79751660719509, 18.860406520593376),
Offset(36.7975441640286, 18.787685822781068),
Offset(36.797566336361996, 18.7133709186601),
Offset(36.79758120646093, 18.636840503908616),
Offset(36.79758708492745, 18.557533955743487),
Offset(36.79758712071989, 18.476404467546697),
Offset(36.79758712071989, 18.395263760542782),
Offset(36.79758712071989, 18.314123053518756),
Offset(36.79758712071989, 18.312467120719884),
],
),
_PathCubicTo(
<Offset>[
Offset(50.1342941041875, 50.02853304089169),
Offset(50.014457147687494, 49.90425767859169),
Offset(49.8946201911875, 49.779982316291694),
Offset(49.7747832346875, 49.6557069539917),
Offset(49.65494627808749, 49.5314315915917),
Offset(49.5351093215875, 49.4071562292917),
Offset(49.415272365087496, 49.28288086699169),
Offset(49.295435408587494, 49.15860550469169),
Offset(49.1755984519875, 49.034330142391696),
Offset(49.055761495487495, 48.9100547800917),
Offset(48.9359245389875, 48.7857794176917),
Offset(48.8160875824875, 48.66150405539169),
Offset(48.696250625987496, 48.53722869309169),
Offset(48.5764136693875, 48.412953330791694),
Offset(48.4565767128875, 48.2886779684917),
Offset(48.02431502037189, 47.521307740480445),
Offset(46.98074593174941, 45.1208643415384),
Offset(46.08548658116816, 42.45185274562246),
Offset(45.338536968526306, 39.51427295295898),
Offset(44.73989709372328, 36.30812496343945),
Offset(44.28956695695909, 32.83340877695263),
Offset(43.9875465581158, 29.090124393704222),
Offset(43.91970523287322, 25.730332389347893),
Offset(44.10552703056539, 23.19078423834003),
Offset(45.07126540837772, 21.440196815204512),
Offset(45.60506520753746, 20.472132118373413),
Offset(45.7130454079458, 20.275446961001492),
Offset(45.80794597703926, 20.102690905119566),
Offset(45.89191187243428, 19.94993821381455),
Offset(45.96657907636557, 19.814194600945225),
Offset(46.0334439590158, 19.692721660984457),
Offset(46.09378559792097, 19.583178817233097),
Offset(46.14873405934452, 19.483498384926143),
Offset(46.19928371471381, 19.391861154060404),
Offset(46.24631413371717, 19.306658130182875),
Offset(46.29060615824605, 19.22646109704454),
Offset(46.332855054781064, 19.14999852829856),
Offset(46.37368292614194, 19.076132860806798),
Offset(46.41364852205306, 19.003842529223242),
Offset(46.453257777743495, 18.93220267566557),
Offset(46.492952106563976, 18.860406520593376),
Offset(46.533151908941335, 18.787685822781068),
Offset(46.574223711150275, 18.7133709186601),
Offset(46.61650763441162, 18.636840503908616),
Offset(46.66031064453808, 18.557533955743487),
Offset(46.70511125417474, 18.476404467546697),
Offset(46.74991800356274, 18.395263760542782),
Offset(46.79472475293062, 18.314123053518756),
Offset(46.79563917637766, 18.312467120719884),
],
<Offset>[
Offset(50.15368826922652, 50.04792720593072),
Offset(50.033851312726526, 49.92365184363072),
Offset(49.91401435622653, 49.799376481330725),
Offset(49.79417739972652, 49.675101119030714),
Offset(49.674340443126525, 49.550825756630715),
Offset(49.55450348662653, 49.42655039433072),
Offset(49.43466653012652, 49.30227503203072),
Offset(49.314829573626525, 49.177999669730724),
Offset(49.19499261702653, 49.05372430743071),
Offset(49.07515566052652, 48.929448945130716),
Offset(48.955318704026524, 48.80517358273072),
Offset(48.83548174752653, 48.68089822043072),
Offset(48.71564479102652, 48.55662285813072),
Offset(48.59580783442652, 48.43234749583071),
Offset(48.47597087792653, 48.308072133530715),
Offset(48.136288078984855, 47.63328079909341),
Offset(47.473679845580676, 45.61379825536869),
Offset(47.07964031920627, 43.44600648365714),
Offset(46.95416949975036, 41.12990548418304),
Offset(47.097267387117355, 38.66549525683352),
Offset(47.508933981507255, 36.05277580150079),
Offset(48.18916928282006, 33.29174711839053),
Offset(49.06253495466028, 30.873162111115967),
Offset(50.02929327547615, 29.114550483250788),
Offset(51.77862444643605, 28.14755585326284),
Offset(52.74567493591703, 27.612742526019634),
Offset(52.94154343336185, 27.50394701174588),
Offset(53.11365619281698, 27.408404506536808),
Offset(53.26590941777883, 27.323940514375185),
Offset(53.40127480891536, 27.24889646368377),
Offset(53.522470364938954, 27.18175557438962),
Offset(53.63181934443066, 27.121221448636877),
Offset(53.731374286002364, 27.066148872338097),
Offset(53.822941209917815, 27.015530283280118),
Offset(53.9081175752586, 26.968474575656916),
Offset(53.988321482634554, 26.924190791684836),
Offset(54.064815569663324, 26.88197477619479),
Offset(54.13872955933007, 26.841196586461674),
Offset(54.211078083148614, 26.801290539485016),
Offset(54.282779824728905, 26.761744526458138),
Offset(54.3546362702083, 26.72211184147367),
Offset(54.42741071364266, 26.681967137945506),
Offset(54.50176859638355, 26.640939668537342),
Offset(54.57832738700239, 26.598685477488804),
Offset(54.65764432912613, 26.5548942211387),
Offset(54.73877252649494, 26.510093683047582),
Offset(54.81991187110869, 26.46528693369982),
Offset(54.90105121572243, 26.42048018431182),
Offset(54.90270712071989, 26.419565760864774),
],
<Offset>[
Offset(50.15368826922652, 50.07184435070614),
Offset(50.033851312726526, 49.94756898840614),
Offset(49.91401435622653, 49.82329362610614),
Offset(49.79417739972652, 49.69901826380614),
Offset(49.674340443126525, 49.57474290140614),
Offset(49.55450348662653, 49.45046753910614),
Offset(49.43466653012652, 49.32619217680614),
Offset(49.314829573626525, 49.20191681450614),
Offset(49.19499261702653, 49.07764145220614),
Offset(49.07515566052652, 48.95336608990614),
Offset(48.955318704026524, 48.82909072750614),
Offset(48.83548174752653, 48.70481536520614),
Offset(48.71564479102652, 48.58054000290614),
Offset(48.59580783442652, 48.456264640606136),
Offset(48.47597087792653, 48.33198927830614),
Offset(48.136288078984855, 47.7713707071586),
Offset(47.473679845580676, 46.22170752947072),
Offset(47.07964031920627, 44.672044351691675),
Offset(46.95416949975036, 43.12238117401274),
Offset(47.097267387117355, 41.572717996339655),
Offset(47.508933981507255, 40.02305481856121),
Offset(48.18916928282006, 38.47339164088308),
Offset(49.06253495466028, 37.21554892929752),
Offset(50.02929327547615, 36.42002629846431),
Offset(51.77862444643605, 36.41939651182523),
Offset(52.74567493591703, 36.41888886837908),
Offset(52.94154343336185, 36.41848110196339),
Offset(53.11365619281698, 36.41816004771427),
Offset(53.26590941777883, 36.41791093245497),
Offset(53.40127480891536, 36.4177224584507),
Offset(53.522470364938954, 36.417584418075734),
Offset(53.63181934443066, 36.4174881488042),
Offset(53.731374286002364, 36.417426074710136),
Offset(53.822941209917815, 36.41739159858898),
Offset(53.9081175752586, 36.417378950893315),
Offset(53.988321482634554, 36.41738307228838),
Offset(54.064815569663324, 36.41739951720605),
Offset(54.13872955933007, 36.41742436469134),
Offset(54.211078083148614, 36.417454147155),
Offset(54.282779824728905, 36.417485776979206),
Offset(54.3546362702083, 36.417516607174946),
Offset(54.42741071364266, 36.41754416400846),
Offset(54.50176859638355, 36.41756633636199),
Offset(54.57832738700239, 36.41758120646094),
Offset(54.65764432912613, 36.41758708492745),
Offset(54.73877252649494, 36.41758712071989),
Offset(54.81991187110869, 36.41758712071989),
Offset(54.90105121572243, 36.41758712071989),
Offset(54.90270712071989, 36.41758712071989),
],
),
_PathCubicTo(
<Offset>[
Offset(50.15368826922652, 50.095761495481554),
Offset(50.033851312726526, 49.97148613318156),
Offset(49.91401435622653, 49.84721077088156),
Offset(49.79417739972652, 49.722935408581556),
Offset(49.674340443126525, 49.59866004618156),
Offset(49.55450348662653, 49.47438468388156),
Offset(49.43466653012652, 49.350109321581556),
Offset(49.314829573626525, 49.22583395928156),
Offset(49.19499261702653, 49.101558596981555),
Offset(49.07515566052652, 48.97728323468156),
Offset(48.955318704026524, 48.85300787228156),
Offset(48.83548174752653, 48.728732509981555),
Offset(48.71564479102652, 48.60445714768156),
Offset(48.59580783442652, 48.48018178538155),
Offset(48.47597087792653, 48.35590642308156),
Offset(48.136288078984855, 47.909461002472696),
Offset(47.473679845580676, 46.82961878893964),
Offset(47.07964031920627, 45.89808631325676),
Offset(46.95416949975036, 45.11486357561327),
Offset(47.097267387117355, 44.47995057591447),
Offset(47.508933981507255, 43.993347314049146),
Offset(48.18916928282006, 43.65505379020504),
Offset(49.06253495466028, 43.55795735505423),
Offset(50.02929327547615, 43.725527030546125),
Offset(51.77862444643605, 44.69126540835824),
Offset(52.74567493591703, 45.225065207517794),
Offset(52.94154343336185, 45.333045407926),
Offset(53.11365619281698, 45.42794597703926),
Offset(53.26590941777883, 45.511911872434275),
Offset(53.40127480891536, 45.58657907636557),
Offset(53.522470364938954, 45.653443959015796),
Offset(53.63181934443066, 45.71378559792097),
Offset(53.731374286002364, 45.76873405934451),
Offset(53.822941209917815, 45.819283714713805),
Offset(53.9081175752586, 45.86631413371717),
Offset(53.988321482634554, 45.91060615824605),
Offset(54.064815569663324, 45.95285505476088),
Offset(54.13872955933007, 45.993682926121764),
Offset(54.211078083148614, 46.03364852203289),
Offset(54.282779824728905, 46.07325777772334),
Offset(54.3546362702083, 46.11295210654383),
Offset(54.42741071364266, 46.1531519089212),
Offset(54.50176859638355, 46.19422371115027),
Offset(54.57832738700239, 46.236507634411616),
Offset(54.65764432912613, 46.280310644538076),
Offset(54.73877252649494, 46.32511125417474),
Offset(54.81991187110869, 46.36991800356274),
Offset(54.90105121572243, 46.41472475293062),
Offset(54.90270712071989, 46.41563917637766),
],
<Offset>[
Offset(50.1342941041875, 50.11515566052058),
Offset(50.014457147687494, 49.99088029822058),
Offset(49.8946201911875, 49.866604935920584),
Offset(49.7747832346875, 49.74232957362058),
Offset(49.65494627808749, 49.61805421122058),
Offset(49.5351093215875, 49.493778848920584),
Offset(49.415272365087496, 49.36950348662058),
Offset(49.295435408587494, 49.24522812432058),
Offset(49.1755984519875, 49.12095276202058),
Offset(49.055761495487495, 48.99667739972058),
Offset(48.9359245389875, 48.87240203732058),
Offset(48.8160875824875, 48.74812667502058),
Offset(48.696250625987496, 48.62385131272058),
Offset(48.5764136693875, 48.49957595042058),
Offset(48.4565767128875, 48.37530058812058),
Offset(48.02431502037189, 48.02143406108567),
Offset(46.980745931750384, 47.32255270277091),
Offset(46.08548658117158, 46.89224005129487),
Offset(45.3385369685263, 46.73049610683733),
Offset(44.73989709372328, 46.83732086930855),
Offset(44.28956695695909, 47.21271433859731),
Offset(43.98754655813375, 47.856676514909296),
Offset(43.919705232892206, 48.70078707684129),
Offset(44.10552703056539, 49.649293275456884),
Offset(45.07126540837772, 51.39862444641657),
Offset(45.60506520755712, 52.36567493589737),
Offset(45.7130454079458, 52.56154343334205),
Offset(45.80794597703926, 52.73365619281698),
Offset(45.89191187243428, 52.88590941777883),
Offset(45.96657907636557, 53.021274808915365),
Offset(46.0334439590158, 53.14247036493895),
Offset(46.09378559792097, 53.25181934443066),
Offset(46.14873405934452, 53.351374286002354),
Offset(46.19928371469362, 53.44294120991781),
Offset(46.24631413371717, 53.5281175752586),
Offset(46.29060615824605, 53.60832148263455),
Offset(46.332855054781064, 53.68481556964314),
Offset(46.373682926141946, 53.7587295593099),
Offset(46.413648522073224, 53.83107808312845),
Offset(46.453257777743495, 53.90277982470875),
Offset(46.49295210656398, 53.97463627018816),
Offset(46.533151908941335, 54.04741071362252),
Offset(46.574223711150275, 54.121768596383546),
Offset(46.61650763441162, 54.19832738700239),
Offset(46.660310644538086, 54.27764432912612),
Offset(46.70511125417474, 54.35877252649494),
Offset(46.74991800354262, 54.439911871108684),
Offset(46.79472475293062, 54.52105121572244),
Offset(46.79563917637766, 54.522707120719886),
],
<Offset>[
Offset(50.11037695941208, 50.11515566052058),
Offset(49.99054000291208, 49.99088029822058),
Offset(49.87070304641208, 49.866604935920584),
Offset(49.75086608991208, 49.74232957362058),
Offset(49.631029133312076, 49.61805421122058),
Offset(49.51119217681208, 49.493778848920584),
Offset(49.39135522031208, 49.36950348662058),
Offset(49.27151826381208, 49.24522812432058),
Offset(49.15168130721208, 49.12095276202058),
Offset(49.03184435071208, 48.99667739972058),
Offset(48.91200739421208, 48.87240203732058),
Offset(48.79217043771208, 48.74812667502058),
Offset(48.67233348121208, 48.62385131272058),
Offset(48.55249652461208, 48.49957595042058),
Offset(48.43265956811208, 48.37530058812058),
Offset(47.886224725057794, 48.02143406108567),
Offset(46.37283467228049, 47.32255270277091),
Offset(44.859444619603074, 46.89224005129487),
Offset(43.346054566925766, 46.73049610683733),
Offset(41.83266451414846, 46.83732086930855),
Offset(40.31927446147115, 47.21271433859731),
Offset(38.80588440879385, 47.856676514909296),
Offset(37.57729680711652, 48.70078707684129),
Offset(36.80002629848357, 49.649293275456884),
Offset(36.799396511844705, 51.39862444641657),
Offset(36.79888886839874, 52.36567493589737),
Offset(36.798481101983185, 52.56154343334205),
Offset(36.798160047714276, 52.73365619281698),
Offset(36.79791093245497, 52.88590941777883),
Offset(36.79772245845069, 53.021274808915365),
Offset(36.79758441807573, 53.14247036493895),
Offset(36.797488148804206, 53.25181934443066),
Offset(36.797426074710145, 53.351374286002354),
Offset(36.79739159858897, 53.44294120991781),
Offset(36.79737895089332, 53.5281175752586),
Offset(36.79738307228838, 53.60832148263455),
Offset(36.79739951722624, 53.68481556964314),
Offset(36.79742436471152, 53.7587295593099),
Offset(36.79745414717516, 53.83107808312845),
Offset(36.79748577699936, 53.90277982470875),
Offset(36.79751660719509, 53.97463627018816),
Offset(36.7975441640286, 54.04741071362252),
Offset(36.797566336361996, 54.121768596383546),
Offset(36.79758120646093, 54.19832738700239),
Offset(36.79758708492745, 54.27764432912612),
Offset(36.79758712071989, 54.35877252649494),
Offset(36.79758712071989, 54.439911871108684),
Offset(36.79758712071989, 54.52105121572244),
Offset(36.79758712071989, 54.522707120719886),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
],
),
_PathCubicTo(
<Offset>[
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
Offset(60.937745291919896, 54.52270224391989),
],
<Offset>[
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
],
<Offset>[
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
],
),
_PathCubicTo(
<Offset>[
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
Offset(57.739181458913336, 54.52270224391989),
],
<Offset>[
Offset(56.04837809389354, 52.900684331474906),
Offset(56.030300891829654, 52.8847340126718),
Offset(56.01222368976779, 52.86878369386669),
Offset(55.9941464877039, 52.85283337506358),
Offset(55.976069285642026, 52.83688305626048),
Offset(55.95799208358016, 52.820932737455365),
Offset(55.93991488151627, 52.80498241865226),
Offset(55.921837679454406, 52.78903209984916),
Offset(55.90376047739254, 52.77308178104404),
Offset(55.88568327532865, 52.757131462240935),
Offset(55.86760607326678, 52.741181143437835),
Offset(55.84952887120491, 52.72523082463272),
Offset(55.831451669141025, 52.70928050582962),
Offset(55.81337446707916, 52.69333018702651),
Offset(55.79529726501527, 52.6773798682214),
Offset(55.78900433275297, 52.672562732506236),
Offset(55.80942241203834, 52.69298081179161),
Offset(55.829840491323715, 52.713398891074974),
Offset(55.850258570607075, 52.73381697036035),
Offset(55.87067664989245, 52.75423504964572),
Offset(55.89109472917782, 52.77465312892909),
Offset(55.91151280846119, 52.795071208214466),
Offset(55.93193088774657, 52.81548928749984),
Offset(55.952348967031945, 52.8359073667832),
Offset(55.972767046315305, 52.856325446068574),
Offset(55.997486569314425, 52.87878031963378),
Offset(56.02633547827809, 52.9031905157102),
Offset(56.05518438724176, 52.9276007117846),
Offset(56.084033296203415, 52.952010907861016),
Offset(56.11288220516708, 52.97642110393542),
Offset(56.14173111413075, 53.00083130001184),
Offset(56.170580023094416, 53.025241496086245),
Offset(56.19942893205808, 53.04965169216266),
Offset(56.22827784102175, 53.07406188823707),
Offset(56.25712674998542, 53.098472084313485),
Offset(56.285975658949084, 53.122882280387884),
Offset(56.31482456791073, 53.1472924764643),
Offset(56.3436734768744, 53.17170267253871),
Offset(56.37252238583807, 53.196112868615124),
Offset(56.401371294801734, 53.22052306468953),
Offset(56.4302202037654, 53.24493326076595),
Offset(56.45906911272907, 53.26934345684035),
Offset(56.487918021692735, 53.29375365291677),
Offset(56.51676693065439, 53.318163848991176),
Offset(56.54561583961806, 53.342574045067586),
Offset(56.574464748581725, 53.36698424114199),
Offset(56.60331365754539, 53.39139443721841),
Offset(56.63216256650906, 53.415804633292815),
Offset(56.632751319752806, 53.41630280056001),
],
<Offset>[
Offset(56.04837809389354, 52.900684331474906),
Offset(56.030300891829654, 52.8847340126718),
Offset(56.01222368976779, 52.86878369386669),
Offset(55.9941464877039, 52.85283337506358),
Offset(55.976069285642026, 52.83688305626048),
Offset(55.95799208358016, 52.820932737455365),
Offset(55.93991488151627, 52.80498241865226),
Offset(55.921837679454406, 52.78903209984916),
Offset(55.90376047739254, 52.77308178104404),
Offset(55.88568327532865, 52.757131462240935),
Offset(55.86760607326678, 52.741181143437835),
Offset(55.84952887120491, 52.72523082463272),
Offset(55.831451669141025, 52.70928050582962),
Offset(55.81337446707916, 52.69333018702651),
Offset(55.79529726501527, 52.6773798682214),
Offset(55.78900433275297, 52.672562732506236),
Offset(55.80942241203834, 52.69298081179161),
Offset(55.829840491323715, 52.713398891074974),
Offset(55.850258570607075, 52.73381697036035),
Offset(55.87067664989245, 52.75423504964572),
Offset(55.89109472917782, 52.77465312892909),
Offset(55.91151280846119, 52.795071208214466),
Offset(55.93193088774657, 52.81548928749984),
Offset(55.952348967031945, 52.8359073667832),
Offset(55.972767046315305, 52.856325446068574),
Offset(55.997486569314425, 52.87878031963378),
Offset(56.02633547827809, 52.9031905157102),
Offset(56.05518438724176, 52.9276007117846),
Offset(56.084033296203415, 52.952010907861016),
Offset(56.11288220516708, 52.97642110393542),
Offset(56.14173111413075, 53.00083130001184),
Offset(56.170580023094416, 53.025241496086245),
Offset(56.19942893205808, 53.04965169216266),
Offset(56.22827784102175, 53.07406188823707),
Offset(56.25712674998542, 53.098472084313485),
Offset(56.285975658949084, 53.122882280387884),
Offset(56.31482456791073, 53.1472924764643),
Offset(56.3436734768744, 53.17170267253871),
Offset(56.37252238583807, 53.196112868615124),
Offset(56.401371294801734, 53.22052306468953),
Offset(56.4302202037654, 53.24493326076595),
Offset(56.45906911272907, 53.26934345684035),
Offset(56.487918021692735, 53.29375365291677),
Offset(56.51676693065439, 53.318163848991176),
Offset(56.54561583961806, 53.342574045067586),
Offset(56.574464748581725, 53.36698424114199),
Offset(56.60331365754539, 53.39139443721841),
Offset(56.63216256650906, 53.415804633292815),
Offset(56.632751319752806, 53.41630280056001),
],
),
_PathCubicTo(
<Offset>[
Offset(56.04837809389354, 52.9006440978749),
Offset(56.030300891829654, 52.8846937790718),
Offset(56.01222368976779, 52.868743460266685),
Offset(55.9941464877039, 52.85279314146358),
Offset(55.976069285642026, 52.83684282266048),
Offset(55.95799208358016, 52.82089250385536),
Offset(55.93991488151627, 52.80494218505226),
Offset(55.921837679454406, 52.788991866249155),
Offset(55.90376047739254, 52.773041547444045),
Offset(55.88568327532865, 52.75709122864094),
Offset(55.86760607326678, 52.74114090983784),
Offset(55.84952887120491, 52.72519059103272),
Offset(55.831451669141025, 52.709240272229614),
Offset(55.81337446707916, 52.693289953426515),
Offset(55.79529726501527, 52.6773396346214),
Offset(55.78900433275297, 52.67252249890623),
Offset(55.80942241203834, 52.69294057819161),
Offset(55.829840491323715, 52.71335865747497),
Offset(55.850258570607075, 52.73377673676035),
Offset(55.87067664989245, 52.754194816045725),
Offset(55.89109472917782, 52.774612895329085),
Offset(55.91151280846119, 52.79503097461446),
Offset(55.93193088774657, 52.81544905389984),
Offset(55.952348967031945, 52.8358671331832),
Offset(55.972767046315305, 52.85628521246858),
Offset(55.997486569314425, 52.87874008603378),
Offset(56.02633547827809, 52.9031502821102),
Offset(56.05518438724176, 52.9275604781846),
Offset(56.084033296203415, 52.95197067426102),
Offset(56.11288220516708, 52.976380870335426),
Offset(56.14173111413075, 53.00079106641184),
Offset(56.170580023094416, 53.02520126248624),
Offset(56.19942893205808, 53.049611458562666),
Offset(56.22827784102175, 53.074021654637065),
Offset(56.25712674998542, 53.09843185071348),
Offset(56.285975658949084, 53.12284204678789),
Offset(56.31482456791073, 53.147252242864305),
Offset(56.3436734768744, 53.17166243893871),
Offset(56.37252238583807, 53.19607263501513),
Offset(56.401371294801734, 53.22048283108953),
Offset(56.4302202037654, 53.24489302716594),
Offset(56.45906911272907, 53.26930322324035),
Offset(56.487918021692735, 53.29371341931677),
Offset(56.51676693065439, 53.31812361539117),
Offset(56.54561583961806, 53.34253381146759),
Offset(56.574464748581725, 53.366944007541996),
Offset(56.60331365754539, 53.39135420361841),
Offset(56.63216256650906, 53.41576439969282),
Offset(56.632751319752806, 53.41626256696001),
],
<Offset>[
Offset(53.21193262270276, 55.75726624798824),
Offset(53.193855420638876, 55.741315929185134),
Offset(53.17577821857701, 55.725365610380024),
Offset(53.15770101651312, 55.70941529157692),
Offset(53.139623814451255, 55.69346497277382),
Offset(53.12154661238938, 55.6775146539687),
Offset(53.1034694103255, 55.6615643351656),
Offset(53.08539220826363, 55.645614016362494),
Offset(53.06731500620175, 55.629663697557376),
Offset(53.049237804137874, 55.61371337875428),
Offset(53.031160602076, 55.59776305995117),
Offset(53.01308340001413, 55.58181274114605),
Offset(52.99500619795025, 55.56586242234295),
Offset(52.97692899588838, 55.549912103539846),
Offset(52.95885179382449, 55.533961784734736),
Offset(52.95255886156219, 55.52914464901957),
Offset(52.97297694084756, 55.54956272830495),
Offset(52.99339502013294, 55.56998080758831),
Offset(53.013813099416296, 55.590398886873686),
Offset(53.034231178701674, 55.610816966159064),
Offset(53.05464925798705, 55.63123504544242),
Offset(53.07506733727041, 55.6516531247278),
Offset(53.09548541655579, 55.67207120401317),
Offset(53.11590349584117, 55.69248928329654),
Offset(53.136321575124526, 55.712907362581916),
Offset(53.16104109812365, 55.73536223614712),
Offset(53.189890007087314, 55.759772432223535),
Offset(53.21873891605098, 55.78418262829794),
Offset(53.24758782501264, 55.80859282437436),
Offset(53.276436733976304, 55.83300302044876),
Offset(53.30528564293997, 55.857413216525174),
Offset(53.33413455190364, 55.88182341259958),
Offset(53.362983460867305, 55.906233608676),
Offset(53.39183236983097, 55.9306438047504),
Offset(53.42068127879464, 55.95505400082682),
Offset(53.449530187758306, 55.979464196901226),
Offset(53.47837909671996, 56.00387439297764),
Offset(53.50722800568363, 56.02828458905205),
Offset(53.53607691464729, 56.052694785128466),
Offset(53.56492582361096, 56.077104981202865),
Offset(53.59377473257462, 56.10151517727928),
Offset(53.6226236415383, 56.12592537335369),
Offset(53.65147255050196, 56.150335569430105),
Offset(53.68032145946361, 56.17474576550451),
Offset(53.70917036842728, 56.19915596158093),
Offset(53.73801927739095, 56.22356615765533),
Offset(53.766868186354614, 56.247976353731744),
Offset(53.79571709531828, 56.27238654980615),
Offset(53.79630584856203, 56.272884717073346),
],
<Offset>[
Offset(53.21190192690009, 55.75726624798824),
Offset(53.19382472483621, 55.741315929185134),
Offset(53.17574752277434, 55.725365610380024),
Offset(53.15767032071045, 55.70941529157692),
Offset(53.139593118648584, 55.69346497277382),
Offset(53.121515916586716, 55.6775146539687),
Offset(53.10343871452283, 55.6615643351656),
Offset(53.08536151246096, 55.645614016362494),
Offset(53.06728431039909, 55.629663697557376),
Offset(53.04920710833521, 55.61371337875428),
Offset(53.031129906273335, 55.59776305995117),
Offset(53.01305270421147, 55.58181274114605),
Offset(52.99497550214758, 55.56586242234295),
Offset(52.97689830008571, 55.549912103539846),
Offset(52.95882109802183, 55.533961784734736),
Offset(52.95252816575952, 55.52914464901957),
Offset(52.972946245044895, 55.54956272830495),
Offset(52.993364324330265, 55.56998080758831),
Offset(53.01378240361363, 55.590398886873686),
Offset(53.03420048289901, 55.610816966159064),
Offset(53.05461856218438, 55.63123504544242),
Offset(53.07503664146775, 55.6516531247278),
Offset(53.095454720753125, 55.67207120401317),
Offset(53.115872800038495, 55.69248928329654),
Offset(53.13629087932186, 55.712907362581916),
Offset(53.161010402320976, 55.73536223614712),
Offset(53.18985931128464, 55.759772432223535),
Offset(53.21870822024831, 55.78418262829794),
Offset(53.247557129209966, 55.80859282437436),
Offset(53.27640603817363, 55.83300302044876),
Offset(53.3052549471373, 55.857413216525174),
Offset(53.33410385610097, 55.88182341259958),
Offset(53.362952765064634, 55.906233608676),
Offset(53.3918016740283, 55.9306438047504),
Offset(53.42065058299197, 55.95505400082682),
Offset(53.449499491955635, 55.979464196901226),
Offset(53.47834840091729, 56.00387439297764),
Offset(53.50719730988096, 56.02828458905205),
Offset(53.536046218844625, 56.052694785128466),
Offset(53.56489512780829, 56.077104981202865),
Offset(53.59374403677196, 56.10151517727928),
Offset(53.622592945735626, 56.12592537335369),
Offset(53.65144185469929, 56.150335569430105),
Offset(53.68029076366095, 56.17474576550451),
Offset(53.709139672624616, 56.19915596158093),
Offset(53.73798858158828, 56.22356615765533),
Offset(53.76683749055195, 56.247976353731744),
Offset(53.79568639951562, 56.27238654980615),
Offset(53.796275152759364, 56.272884717073346),
],
),
_PathCubicTo(
<Offset>[
Offset(53.21190192690009, 55.75722601438824),
Offset(53.19382472483621, 55.74127569558514),
Offset(53.17574752277434, 55.72532537678002),
Offset(53.15767032071045, 55.70937505797692),
Offset(53.139593118648584, 55.693424739173814),
Offset(53.121515916586716, 55.677474420368696),
Offset(53.10343871452283, 55.6615241015656),
Offset(53.08536151246096, 55.6455737827625),
Offset(53.06728431039909, 55.62962346395738),
Offset(53.04920710833521, 55.61367314515427),
Offset(53.031129906273335, 55.59772282635117),
Offset(53.01305270421147, 55.581772507546056),
Offset(52.99497550214758, 55.565822188742956),
Offset(52.97689830008571, 55.54987186993985),
Offset(52.95882109802183, 55.53392155113473),
Offset(52.95252816575952, 55.52910441541957),
Offset(52.972946245044895, 55.549522494704945),
Offset(52.993364324330265, 55.56994057398831),
Offset(53.01378240361363, 55.59035865327368),
Offset(53.03420048289901, 55.61077673255906),
Offset(53.05461856218438, 55.63119481184242),
Offset(53.07503664146775, 55.6516128911278),
Offset(53.095454720753125, 55.672030970413175),
Offset(53.115872800038495, 55.692449049696535),
Offset(53.13629087932186, 55.71286712898191),
Offset(53.161010402320976, 55.73532200254712),
Offset(53.18985931128464, 55.75973219862353),
Offset(53.21870822024831, 55.78414239469794),
Offset(53.247557129209966, 55.808552590774354),
Offset(53.27640603817363, 55.83296278684876),
Offset(53.3052549471373, 55.85737298292518),
Offset(53.33410385610097, 55.881783178999584),
Offset(53.362952765064634, 55.906193375076),
Offset(53.3918016740283, 55.9306035711504),
Offset(53.42065058299197, 55.955013767226816),
Offset(53.449499491955635, 55.97942396330122),
Offset(53.47834840091729, 56.00383415937764),
Offset(53.50719730988096, 56.028244355452046),
Offset(53.536046218844625, 56.05265455152846),
Offset(53.56489512780829, 56.07706474760286),
Offset(53.59374403677196, 56.101474943679285),
Offset(53.622592945735626, 56.125885139753684),
Offset(53.65144185469929, 56.15029533583011),
Offset(53.68029076366095, 56.17470553190451),
Offset(53.709139672624616, 56.199115727980924),
Offset(53.73798858158828, 56.22352592405533),
Offset(53.76683749055195, 56.24793612013175),
Offset(53.79568639951562, 56.27234631620615),
Offset(53.796275152759364, 56.27284448347334),
],
<Offset>[
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856235894),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856235894),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
],
<Offset>[
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856235894),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
],
),
_PathCubicTo(
<Offset>[
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856235894),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
Offset(54.902705291919894, 57.37931485623388),
Offset(54.902705291919894, 57.379314856233876),
],
<Offset>[
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243940005),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
],
<Offset>[
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243940005),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
],
),
_PathCubicTo(
<Offset>[
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243940005),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
Offset(54.902705291919894, 60.557742243937994),
],
<Offset>[
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.53659283720165),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.5785472078895, 61.10082674001624),
Offset(57.412981276788415, 62.9439908225431),
Offset(59.24741534567123, 64.7871549050921),
Offset(61.081849414574165, 66.63031898762097),
Offset(62.91628348345698, 68.47348307014985),
Offset(64.75071755235992, 70.31664715269883),
Offset(66.58515162124274, 72.15981123522772),
Offset(68.41958569014567, 74.00297531775661),
Offset(70.2540197590285, 75.84613940028547),
Offset(72.08845382793142, 77.68930348283448),
Offset(73.03297916605787, 78.63832439892204),
Offset(73.12319212266141, 78.72896787526297),
Offset(73.21340507924484, 78.81961135158377),
Offset(73.30361803584839, 78.9102548279046),
Offset(73.39383099245194, 79.0008983042254),
Offset(73.48404394903537, 79.09154178054621),
Offset(73.5742569056389, 79.18218525686703),
Offset(73.66446986222233, 79.27282873318785),
Offset(73.75468281882588, 79.36347220952878),
Offset(73.84489577542942, 79.45411568584959),
Offset(73.93510873201285, 79.5447591621704),
Offset(74.0253216886164, 79.6354026384912),
Offset(74.11553464521995, 79.72604611481202),
Offset(74.20574760180338, 79.81668959113284),
Offset(74.29596055840692, 79.90733306745365),
Offset(74.38617351501047, 79.99797654379458),
Offset(74.4763864715939, 80.0886200201154),
Offset(74.56659942819743, 80.1792634964362),
Offset(74.65681238478088, 80.26990697275701),
Offset(74.74702534138441, 80.36055044907783),
Offset(74.83723829798797, 80.45119392539866),
Offset(74.9274512545714, 80.54183740171945),
Offset(75.01766421117493, 80.63248087806039),
Offset(75.0195052919199, 80.63433074491833),
],
<Offset>[
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.53659283720165),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.5785472078895, 61.10082674001624),
Offset(57.412981276788415, 62.9439908225431),
Offset(59.24741534567123, 64.7871549050921),
Offset(61.081849414574165, 66.63031898762097),
Offset(62.91628348345698, 68.47348307014985),
Offset(64.75071755235992, 70.31664715269883),
Offset(66.58515162124274, 72.15981123522772),
Offset(68.41958569014567, 74.00297531775661),
Offset(70.2540197590285, 75.84613940028547),
Offset(72.08845382793142, 77.68930348283448),
Offset(73.03297916605787, 78.63832439892204),
Offset(73.12319212266141, 78.72896787526297),
Offset(73.21340507924484, 78.81961135158377),
Offset(73.30361803584839, 78.9102548279046),
Offset(73.39383099245194, 79.0008983042254),
Offset(73.48404394903537, 79.09154178054621),
Offset(73.5742569056389, 79.18218525686703),
Offset(73.66446986222233, 79.27282873318785),
Offset(73.75468281882588, 79.36347220952878),
Offset(73.84489577542942, 79.45411568584959),
Offset(73.93510873201285, 79.5447591621704),
Offset(74.0253216886164, 79.6354026384912),
Offset(74.11553464521995, 79.72604611481202),
Offset(74.20574760180338, 79.81668959113284),
Offset(74.29596055840692, 79.90733306745365),
Offset(74.38617351501047, 79.99797654379458),
Offset(74.4763864715939, 80.0886200201154),
Offset(74.56659942819743, 80.1792634964362),
Offset(74.65681238478088, 80.26990697275701),
Offset(74.74702534138441, 80.36055044907783),
Offset(74.83723829798797, 80.45119392539866),
Offset(74.9274512545714, 80.54183740171945),
Offset(75.01766421117493, 80.63248087806039),
Offset(75.0195052919199, 80.63433074491833),
],
),
_PathCubicTo(
<Offset>[
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.016985758228515, 60.53659283720165),
Offset(55.016985758228515, 60.536592837199635),
Offset(55.5785472078895, 61.10082674001624),
Offset(57.412981276788415, 62.9439908225431),
Offset(59.24741534567123, 64.7871549050921),
Offset(61.081849414574165, 66.63031898762097),
Offset(62.91628348345698, 68.47348307014985),
Offset(64.75071755235992, 70.31664715269883),
Offset(66.58515162124274, 72.15981123522772),
Offset(68.41958569014567, 74.00297531775661),
Offset(70.2540197590285, 75.84613940028547),
Offset(72.08845382793142, 77.68930348283448),
Offset(73.03297916605787, 78.63832439892204),
Offset(73.12319212266141, 78.72896787526297),
Offset(73.21340507924484, 78.81961135158377),
Offset(73.30361803584839, 78.9102548279046),
Offset(73.39383099245194, 79.0008983042254),
Offset(73.48404394903537, 79.09154178054621),
Offset(73.5742569056389, 79.18218525686703),
Offset(73.66446986222233, 79.27282873318785),
Offset(73.75468281882588, 79.36347220952878),
Offset(73.84489577542942, 79.45411568584959),
Offset(73.93510873201285, 79.5447591621704),
Offset(74.0253216886164, 79.6354026384912),
Offset(74.11553464521995, 79.72604611481202),
Offset(74.20574760180338, 79.81668959113284),
Offset(74.29596055840692, 79.90733306745365),
Offset(74.38617351501047, 79.99797654379458),
Offset(74.4763864715939, 80.0886200201154),
Offset(74.56659942819743, 80.1792634964362),
Offset(74.65681238478088, 80.26990697275701),
Offset(74.74702534138441, 80.36055044907783),
Offset(74.83723829798797, 80.45119392539866),
Offset(74.9274512545714, 80.54183740171945),
Offset(75.01766421117493, 80.63248087806039),
Offset(75.0195052919199, 80.63433074491833),
],
<Offset>[
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.54176433622131),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.57337474962035, 55.105999198285396),
Offset(63.407805684985675, 56.94916641434585),
Offset(65.24223662033491, 58.79233363042842),
Offset(67.07666755572437, 60.63550084647077),
Offset(68.9110984910736, 62.47866806253323),
Offset(70.74552942644296, 64.32183527861581),
Offset(72.57996036179219, 66.16500249467828),
Offset(74.41439129716153, 68.00816971074073),
Offset(76.24882223253088, 69.85133692678309),
Offset(78.08325316790022, 71.69450414286567),
Offset(79.02777766633133, 72.64352589864858),
Offset(79.11799198534516, 72.73416801257923),
Offset(79.20820630433886, 72.82481012648977),
Offset(79.29842062333256, 72.91545224042041),
Offset(79.38863494234639, 73.00609435433094),
Offset(79.47884926134012, 73.09673646824147),
Offset(79.56906358035394, 73.18737858215201),
Offset(79.65927789932752, 73.27802069608266),
Offset(79.74949221834135, 73.36866281001332),
Offset(79.83970653735517, 73.45930492392384),
Offset(79.92992085632878, 73.5499470378545),
Offset(80.0201351753426, 73.64058915176503),
Offset(80.11034949435643, 73.73123126567556),
Offset(80.20056381333, 73.82187337960622),
Offset(80.29077813234383, 73.91251549351674),
Offset(80.38099245135766, 74.00315760744739),
Offset(80.47120677033126, 74.09379972137803),
Offset(80.56142108934507, 74.18444183528857),
Offset(80.65163540833879, 74.2750839491991),
Offset(80.74184972735262, 74.36572606310963),
Offset(80.83206404634632, 74.45636817704029),
Offset(80.92227836534002, 74.54701029095082),
Offset(81.01249268435384, 74.63765240488146),
Offset(81.01433379290023, 74.639502243938),
],
<Offset>[
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.54176433622131),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.57337474962035, 55.105999198285396),
Offset(63.407805684985675, 56.94916641434585),
Offset(65.24223662033491, 58.79233363042842),
Offset(67.07666755572437, 60.63550084647077),
Offset(68.9110984910736, 62.47866806253323),
Offset(70.74552942644296, 64.32183527861581),
Offset(72.57996036179219, 66.16500249467828),
Offset(74.41439129716153, 68.00816971074073),
Offset(76.24882223253088, 69.85133692678309),
Offset(78.08325316790022, 71.69450414286567),
Offset(79.02777766633133, 72.64352589864858),
Offset(79.11799198534516, 72.73416801257923),
Offset(79.20820630433886, 72.82481012648977),
Offset(79.29842062333256, 72.91545224042041),
Offset(79.38863494234639, 73.00609435433094),
Offset(79.47884926134012, 73.09673646824147),
Offset(79.56906358035394, 73.18737858215201),
Offset(79.65927789932752, 73.27802069608266),
Offset(79.74949221834135, 73.36866281001332),
Offset(79.83970653735517, 73.45930492392384),
Offset(79.92992085632878, 73.5499470378545),
Offset(80.0201351753426, 73.64058915176503),
Offset(80.11034949435643, 73.73123126567556),
Offset(80.20056381333, 73.82187337960622),
Offset(80.29077813234383, 73.91251549351674),
Offset(80.38099245135766, 74.00315760744739),
Offset(80.47120677033126, 74.09379972137803),
Offset(80.56142108934507, 74.18444183528857),
Offset(80.65163540833879, 74.2750839491991),
Offset(80.74184972735262, 74.36572606310963),
Offset(80.83206404634632, 74.45636817704029),
Offset(80.92227836534002, 74.54701029095082),
Offset(81.01249268435384, 74.63765240488146),
Offset(81.01433379290023, 74.639502243938),
],
),
_PathCubicTo(
<Offset>[
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.01181425920885, 54.54176433622131),
Offset(61.01181425920885, 54.5417643362193),
Offset(61.57337474962035, 55.105999198285396),
Offset(63.407805684985675, 56.94916641434585),
Offset(65.24223662033491, 58.79233363042842),
Offset(67.07666755572437, 60.63550084647077),
Offset(68.9110984910736, 62.47866806253323),
Offset(70.74552942644296, 64.32183527861581),
Offset(72.57996036179219, 66.16500249467828),
Offset(74.41439129716153, 68.00816971074073),
Offset(76.24882223253088, 69.85133692678309),
Offset(78.08325316790022, 71.69450414286567),
Offset(79.02777766633133, 72.64352589864858),
Offset(79.11799198534516, 72.73416801257923),
Offset(79.20820630433886, 72.82481012648977),
Offset(79.29842062333256, 72.91545224042041),
Offset(79.38863494234639, 73.00609435433094),
Offset(79.47884926134012, 73.09673646824147),
Offset(79.56906358035394, 73.18737858215201),
Offset(79.65927789932752, 73.27802069608266),
Offset(79.74949221834135, 73.36866281001332),
Offset(79.83970653735517, 73.45930492392384),
Offset(79.92992085632878, 73.5499470378545),
Offset(80.0201351753426, 73.64058915176503),
Offset(80.11034949435643, 73.73123126567556),
Offset(80.20056381333, 73.82187337960622),
Offset(80.29077813234383, 73.91251549351674),
Offset(80.38099245135766, 74.00315760744739),
Offset(80.47120677033126, 74.09379972137803),
Offset(80.56142108934507, 74.18444183528857),
Offset(80.65163540833879, 74.2750839491991),
Offset(80.74184972735262, 74.36572606310963),
Offset(80.83206404634632, 74.45636817704029),
Offset(80.92227836534002, 74.54701029095082),
Offset(81.01249268435384, 74.63765240488146),
Offset(81.01433379290023, 74.639502243938),
],
<Offset>[
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.522702243936166),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.937745291917885, 54.52270224394),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529189977, 54.52270224395811),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.937745291919896, 54.522702243937985),
Offset(60.937745291919896, 54.52270224395811),
Offset(60.93774529189977, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224391788),
Offset(60.93774529191989, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529191989, 54.52270224395811),
Offset(60.93774529191989, 54.522702243937985),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194001, 54.52270224391787),
Offset(60.93774529189977, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.93774529189978, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194002, 54.52270224393799),
Offset(60.93774529189978, 54.52270224393799),
Offset(60.937745291919896, 54.522702243938),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.93774529189978, 54.52270224395811),
Offset(60.93774529191989, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224391788),
Offset(60.937745291919896, 54.522702243938),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529191989, 54.52270224393799),
],
<Offset>[
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.93774529192372, 54.522702243936166),
Offset(60.93774529192372, 54.52270224393415),
Offset(60.937745291917885, 54.52270224394),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529189977, 54.52270224395811),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.937745291919896, 54.522702243937985),
Offset(60.937745291919896, 54.522702243937985),
Offset(60.93774529189977, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224391788),
Offset(60.93774529191989, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529191989, 54.52270224395811),
Offset(60.93774529191989, 54.522702243937985),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194001, 54.52270224391787),
Offset(60.93774529189977, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.93774529189978, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529194002, 54.52270224393799),
Offset(60.93774529189978, 54.52270224393799),
Offset(60.937745291919896, 54.522702243938),
Offset(60.93774529194001, 54.52270224393799),
Offset(60.93774529189978, 54.52270224395811),
Offset(60.93774529191989, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224391788),
Offset(60.937745291919896, 54.522702243938),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.937745291919896, 54.52270224393799),
Offset(60.93774529191989, 54.52270224393799),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.920754690484827),
Offset(48.0, 32.45354569710999),
Offset(48.0, 33.98010729644093),
Offset(48.0, 37.048746193280394),
Offset(48.0, 43.18831617722788),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
],
),
_PathCubicTo(
<Offset>[
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.90656),
Offset(52.445826306158004, 31.920754690484827),
Offset(52.445826306158004, 32.45354569710999),
Offset(52.445826306158004, 33.98010729644093),
Offset(52.445826306158004, 37.048746193280394),
Offset(52.445826306158004, 43.18831617722788),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
Offset(52.445826306158004, 55.9765324848),
],
<Offset>[
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.305635610355335),
Offset(56.04672, 28.319830300840163),
Offset(56.04672, 28.852621307465323),
Offset(56.04672, 30.379182906796263),
Offset(56.04672, 33.44782180363573),
Offset(56.04672, 39.587391787583215),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
Offset(56.04672, 52.37560809515534),
],
<Offset>[
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.85984),
Offset(56.04672, 23.874034690484827),
Offset(56.04672, 24.406825697109987),
Offset(56.04672, 25.933387296440927),
Offset(56.04672, 29.002026193280393),
Offset(56.04672, 35.14159617722788),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
Offset(56.04672, 47.9298124848),
],
),
_PathCubicTo(
<Offset>[
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.414013693841998),
Offset(56.04672, 19.428208384326826),
Offset(56.04672, 19.960999390951986),
Offset(56.04672, 21.487560990282926),
Offset(56.04672, 24.556199887122393),
Offset(56.04672, 30.69576987106988),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
Offset(56.04672, 43.483986178642),
],
<Offset>[
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.813119999999998),
Offset(52.445826306158004, 15.827314690484826),
Offset(52.445826306158004, 16.360105697109987),
Offset(52.445826306158004, 17.886667296440926),
Offset(52.445826306158004, 20.955306193280393),
Offset(52.445826306158004, 27.09487617722788),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
Offset(52.445826306158004, 39.8830924848),
],
<Offset>[
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.813119999999998),
Offset(48.0, 15.827314690484826),
Offset(48.0, 16.360105697109987),
Offset(48.0, 17.886667296440926),
Offset(48.0, 20.955306193280393),
Offset(48.0, 27.09487617722788),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
Offset(48.0, 39.8830924848),
],
),
_PathCubicTo(
<Offset>[
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.813119999999998),
Offset(43.554173693841996, 15.827314690484826),
Offset(43.554173693841996, 16.360105697109987),
Offset(43.554173693841996, 17.886667296440926),
Offset(43.554173693841996, 20.955306193280393),
Offset(43.554173693841996, 27.09487617722788),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
Offset(43.554173693841996, 39.8830924848),
],
<Offset>[
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.414013693841998),
Offset(39.95328, 19.428208384326826),
Offset(39.95328, 19.960999390951986),
Offset(39.95328, 21.487560990282926),
Offset(39.95328, 24.556199887122393),
Offset(39.95328, 30.69576987106988),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
Offset(39.95328, 43.483986178642),
],
<Offset>[
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.85984),
Offset(39.95328, 23.874034690484827),
Offset(39.95328, 24.406825697109987),
Offset(39.95328, 25.933387296440927),
Offset(39.95328, 29.002026193280393),
Offset(39.95328, 35.14159617722788),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
Offset(39.95328, 47.9298124848),
],
),
_PathCubicTo(
<Offset>[
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.305635610355335),
Offset(39.95328, 28.319830300840163),
Offset(39.95328, 28.852621307465323),
Offset(39.95328, 30.379182906796263),
Offset(39.95328, 33.44782180363573),
Offset(39.95328, 39.587391787583215),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
Offset(39.95328, 52.37560809515534),
],
<Offset>[
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.90656),
Offset(43.554173693841996, 31.920754690484827),
Offset(43.554173693841996, 32.45354569710999),
Offset(43.554173693841996, 33.98010729644093),
Offset(43.554173693841996, 37.048746193280394),
Offset(43.554173693841996, 43.18831617722788),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
Offset(43.554173693841996, 55.9765324848),
],
<Offset>[
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.90656),
Offset(48.0, 31.920754690484827),
Offset(48.0, 32.45354569710999),
Offset(48.0, 33.98010729644093),
Offset(48.0, 37.048746193280394),
Offset(48.0, 43.18831617722788),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
Offset(48.0, 55.9765324848),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
],
),
_PathCubicTo(
<Offset>[
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
Offset(43.554173693841996, 39.95328),
],
<Offset>[
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
Offset(39.95328, 43.554173693841996),
],
<Offset>[
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
Offset(39.95328, 48.0),
],
),
_PathCubicTo(
<Offset>[
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
Offset(39.95328, 52.445826306158004),
],
<Offset>[
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
Offset(43.554173693841996, 56.04672),
],
<Offset>[
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
Offset(48.0, 56.04672),
],
),
_PathCubicTo(
<Offset>[
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
Offset(52.445826306158004, 56.04672),
],
<Offset>[
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
Offset(56.04672, 52.445826306158004),
],
<Offset>[
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
Offset(56.04672, 48.0),
],
),
_PathCubicTo(
<Offset>[
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
Offset(56.04672, 43.554173693841996),
],
<Offset>[
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
Offset(52.445826306158004, 39.95328),
],
<Offset>[
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
Offset(48.0, 39.95328),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.07926469426482),
Offset(48.0, 63.5472012869485),
Offset(48.0, 62.02272441694872),
Offset(48.0, 58.958276167797024),
Offset(48.0, 52.827090609197995),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
],
),
_PathCubicTo(
<Offset>[
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.09344),
Offset(43.554173693841996, 64.07926469426482),
Offset(43.554173693841996, 63.5472012869485),
Offset(43.554173693841996, 62.02272441694872),
Offset(43.554173693841996, 58.958276167797024),
Offset(43.554173693841996, 52.827090609197995),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
Offset(43.554173693841996, 40.0563383664),
],
<Offset>[
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.69436438964466),
Offset(39.95328, 67.68018908390948),
Offset(39.95328, 67.14812567659317),
Offset(39.95328, 65.62364880659338),
Offset(39.95328, 62.559200557441685),
Offset(39.95328, 56.428014998842656),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
Offset(39.95328, 43.65726275604466),
],
<Offset>[
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.14016000000001),
Offset(39.95328, 72.12598469426482),
Offset(39.95328, 71.5939212869485),
Offset(39.95328, 70.06944441694873),
Offset(39.95328, 67.00499616779703),
Offset(39.95328, 60.873810609197996),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
Offset(39.95328, 48.1030583664),
],
),
_PathCubicTo(
<Offset>[
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.585986306158),
Offset(39.95328, 76.57181100042281),
Offset(39.95328, 76.0397475931065),
Offset(39.95328, 74.51527072310672),
Offset(39.95328, 71.45082247395503),
Offset(39.95328, 65.319636915356),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
Offset(39.95328, 52.548884672558),
],
<Offset>[
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.18688),
Offset(43.554173693841996, 80.17270469426481),
Offset(43.554173693841996, 79.6406412869485),
Offset(43.554173693841996, 78.11616441694872),
Offset(43.554173693841996, 75.05171616779703),
Offset(43.554173693841996, 68.920530609198),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
Offset(43.554173693841996, 56.1497783664),
],
<Offset>[
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.18688),
Offset(48.0, 80.17270469426481),
Offset(48.0, 79.6406412869485),
Offset(48.0, 78.11616441694872),
Offset(48.0, 75.05171616779703),
Offset(48.0, 68.920530609198),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
Offset(48.0, 56.1497783664),
],
),
_PathCubicTo(
<Offset>[
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.18688),
Offset(52.445826306158004, 80.17270469426481),
Offset(52.445826306158004, 79.6406412869485),
Offset(52.445826306158004, 78.11616441694872),
Offset(52.445826306158004, 75.05171616779703),
Offset(52.445826306158004, 68.920530609198),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
Offset(52.445826306158004, 56.1497783664),
],
<Offset>[
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.585986306158),
Offset(56.04672, 76.57181100042281),
Offset(56.04672, 76.0397475931065),
Offset(56.04672, 74.51527072310672),
Offset(56.04672, 71.45082247395503),
Offset(56.04672, 65.319636915356),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
Offset(56.04672, 52.548884672558),
],
<Offset>[
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.14016000000001),
Offset(56.04672, 72.12598469426482),
Offset(56.04672, 71.5939212869485),
Offset(56.04672, 70.06944441694873),
Offset(56.04672, 67.00499616779703),
Offset(56.04672, 60.873810609197996),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
Offset(56.04672, 48.1030583664),
],
),
_PathCubicTo(
<Offset>[
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.69436438964466),
Offset(56.04672, 67.68018908390948),
Offset(56.04672, 67.14812567659317),
Offset(56.04672, 65.62364880659338),
Offset(56.04672, 62.559200557441685),
Offset(56.04672, 56.428014998842656),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
Offset(56.04672, 43.65726275604466),
],
<Offset>[
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.09344),
Offset(52.445826306158004, 64.07926469426482),
Offset(52.445826306158004, 63.5472012869485),
Offset(52.445826306158004, 62.02272441694872),
Offset(52.445826306158004, 58.958276167797024),
Offset(52.445826306158004, 52.827090609197995),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
Offset(52.445826306158004, 40.0563383664),
],
<Offset>[
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.09344),
Offset(48.0, 64.07926469426482),
Offset(48.0, 63.5472012869485),
Offset(48.0, 62.02272441694872),
Offset(48.0, 58.958276167797024),
Offset(48.0, 52.827090609197995),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
Offset(48.0, 40.0563383664),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/ellipsis_search.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/ellipsis_search.g.dart",
"repo_id": "flutter",
"token_count": 154110
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'material_button.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart' show MaterialTapTargetSize;
// Examples can assume:
// late BuildContext context;
/// Used with [ButtonTheme] and [ButtonThemeData] to define a button's base
/// colors, and the defaults for the button's minimum size, internal padding,
/// and shape.
enum ButtonTextTheme {
/// Button text is black or white depending on [ThemeData.brightness].
normal,
/// Button text is [ColorScheme.secondary].
accent,
/// Button text is based on [ThemeData.primaryColor].
primary,
}
/// Used with [ButtonTheme] and [ButtonThemeData] to define how the button bar
/// should size itself with either constraints or internal padding.
enum ButtonBarLayoutBehavior {
/// Button bars will be constrained to a minimum height of 52.
///
/// This setting is require to create button bars which conform to the
/// Material Design specification.
constrained,
/// Button bars will calculate their padding from the button theme padding.
padded,
}
/// Used with [ButtonThemeData] to configure the color and geometry of buttons.
///
/// This class is planned to be deprecated in a future release.
/// Please use one or more of these buttons and associated themes instead:
///
/// * [ElevatedButton], [ElevatedButtonTheme], [ElevatedButtonThemeData],
/// * [FilledButton], [FilledButtonTheme], [FilledButtonThemeData],
/// * [OutlinedButton], [OutlinedButtonTheme], [OutlinedButtonThemeData]
/// * [TextButton], [TextButtonTheme], [TextButtonThemeData],
///
/// A button theme can be specified as part of the overall Material theme
/// using [ThemeData.buttonTheme]. The Material theme's button theme data
/// can be overridden with [ButtonTheme].
///
/// The actual appearance of buttons depends on the button theme, the
/// button's enabled state, its elevation (if any), and the overall [Theme].
///
/// See also:
///
/// * [RawMaterialButton], which can be used to configure a button that doesn't
/// depend on any inherited themes.
class ButtonTheme extends InheritedTheme {
/// Creates a button theme.
ButtonTheme({
super.key,
ButtonTextTheme textTheme = ButtonTextTheme.normal,
ButtonBarLayoutBehavior layoutBehavior = ButtonBarLayoutBehavior.padded,
double minWidth = 88.0,
double height = 36.0,
EdgeInsetsGeometry? padding,
ShapeBorder? shape,
bool alignedDropdown = false,
Color? buttonColor,
Color? disabledColor,
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
Color? splashColor,
ColorScheme? colorScheme,
MaterialTapTargetSize? materialTapTargetSize,
required super.child,
}) : assert(minWidth >= 0.0),
assert(height >= 0.0),
data = ButtonThemeData(
textTheme: textTheme,
minWidth: minWidth,
height: height,
padding: padding,
shape: shape,
alignedDropdown: alignedDropdown,
layoutBehavior: layoutBehavior,
buttonColor: buttonColor,
disabledColor: disabledColor,
focusColor: focusColor,
hoverColor: hoverColor,
highlightColor: highlightColor,
splashColor: splashColor,
colorScheme: colorScheme,
materialTapTargetSize: materialTapTargetSize,
);
/// Creates a button theme from [data].
const ButtonTheme.fromButtonThemeData({
super.key,
required this.data,
required super.child,
});
/// Specifies the color and geometry of buttons.
final ButtonThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// Typical usage is as follows:
///
/// ```dart
/// ButtonThemeData theme = ButtonTheme.of(context);
/// ```
static ButtonThemeData of(BuildContext context) {
final ButtonTheme? inheritedButtonTheme = context.dependOnInheritedWidgetOfExactType<ButtonTheme>();
ButtonThemeData? buttonTheme = inheritedButtonTheme?.data;
if (buttonTheme?.colorScheme == null) { // if buttonTheme or buttonTheme.colorScheme is null
final ThemeData theme = Theme.of(context);
buttonTheme ??= theme.buttonTheme;
if (buttonTheme.colorScheme == null) {
buttonTheme = buttonTheme.copyWith(
colorScheme: theme.buttonTheme.colorScheme ?? theme.colorScheme,
);
assert(buttonTheme.colorScheme != null);
}
}
return buttonTheme!;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ButtonTheme.fromButtonThemeData(data: data, child: child);
}
@override
bool updateShouldNotify(ButtonTheme oldWidget) => data != oldWidget.data;
}
/// Used with [ButtonTheme] to configure the color and geometry of buttons.
///
/// This class is planned to be deprecated in a future release.
/// Please use one or more of these buttons and associated themes instead:
///
/// * [TextButton], [TextButtonTheme], [TextButtonThemeData],
/// * [ElevatedButton], [ElevatedButtonTheme], [ElevatedButtonThemeData],
/// * [OutlinedButton], [OutlinedButtonTheme], [OutlinedButtonThemeData]
///
/// A button theme can be specified as part of the overall Material theme
/// using [ThemeData.buttonTheme]. The Material theme's button theme data
/// can be overridden with [ButtonTheme].
@immutable
class ButtonThemeData with Diagnosticable {
/// Create a button theme object that can be used with [ButtonTheme]
/// or [ThemeData].
///
/// The [minWidth] and [height] parameters must greater than or equal to zero.
///
/// The ButtonTheme's methods that have a [MaterialButton] parameter and
/// have a name with a `get` prefix are used to configure a
/// [RawMaterialButton].
const ButtonThemeData({
this.textTheme = ButtonTextTheme.normal,
this.minWidth = 88.0,
this.height = 36.0,
EdgeInsetsGeometry? padding,
ShapeBorder? shape,
this.layoutBehavior = ButtonBarLayoutBehavior.padded,
this.alignedDropdown = false,
Color? buttonColor,
Color? disabledColor,
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
Color? splashColor,
this.colorScheme,
MaterialTapTargetSize? materialTapTargetSize,
}) : assert(minWidth >= 0.0),
assert(height >= 0.0),
_buttonColor = buttonColor,
_disabledColor = disabledColor,
_focusColor = focusColor,
_hoverColor = hoverColor,
_highlightColor = highlightColor,
_splashColor = splashColor,
_padding = padding,
_shape = shape,
_materialTapTargetSize = materialTapTargetSize;
/// The minimum width for buttons.
///
/// The actual horizontal space allocated for a button's child is
/// at least this value less the theme's horizontal [padding].
///
/// Defaults to 88.0 logical pixels.
final double minWidth;
/// The minimum height for buttons.
///
/// Defaults to 36.0 logical pixels.
final double height;
/// Defines a button's base colors, and the defaults for the button's minimum
/// size, internal padding, and shape.
///
/// Despite the name, this property is not a [TextTheme], its value is not a
/// collection of [TextStyle]s.
final ButtonTextTheme textTheme;
/// Defines whether a [ButtonBar] should size itself with a minimum size
/// constraint or with padding.
///
/// Defaults to [ButtonBarLayoutBehavior.padded].
final ButtonBarLayoutBehavior layoutBehavior;
/// Convenience that returns [minWidth] and [height] as a
/// [BoxConstraints] object.
BoxConstraints get constraints {
return BoxConstraints(
minWidth: minWidth,
minHeight: height,
);
}
/// Padding for a button's child (typically the button's label).
///
/// Defaults to 24.0 on the left and right if [textTheme] is
/// [ButtonTextTheme.primary], 16.0 on the left and right otherwise.
///
/// See also:
///
/// * [getPadding], which is used to calculate padding for the [button]'s
/// child (typically the button's label).
EdgeInsetsGeometry get padding {
return _padding ?? switch (textTheme) {
ButtonTextTheme.normal => const EdgeInsets.symmetric(horizontal: 16.0),
ButtonTextTheme.accent => const EdgeInsets.symmetric(horizontal: 16.0),
ButtonTextTheme.primary => const EdgeInsets.symmetric(horizontal: 24.0),
};
}
final EdgeInsetsGeometry? _padding;
/// The shape of a button's material.
///
/// The button's highlight and splash are clipped to this shape. If the
/// button has an elevation, then its drop shadow is defined by this
/// shape as well.
///
/// Defaults to a rounded rectangle with circular corner radii of 4.0 if
/// [textTheme] is [ButtonTextTheme.primary], a rounded rectangle with
/// circular corner radii of 2.0 otherwise.
///
/// See also:
///
/// * [getShape], which is used to calculate the shape of the [button]'s
/// [Material].
ShapeBorder get shape {
return _shape ?? switch (textTheme) {
ButtonTextTheme.normal || ButtonTextTheme.accent =>
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2.0))),
ButtonTextTheme.primary =>
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))),
};
}
final ShapeBorder? _shape;
/// If true, then a [DropdownButton] menu's width will match the button's
/// width.
///
/// If false (the default), then the dropdown's menu will be wider than
/// its button. In either case the dropdown button will line up the leading
/// edge of the menu's value with the leading edge of the values
/// displayed by the menu items.
///
/// This property only affects [DropdownButton] and its menu.
final bool alignedDropdown;
/// The background fill color.
///
/// This property is null by default.
///
/// If the button is in the focused, hovering, or highlighted state, then the
/// [focusColor], [hoverColor], or [highlightColor] will take precedence over
/// the [buttonColor].
///
/// See also:
///
/// * [getFillColor], which is used to compute the background fill color.
final Color? _buttonColor;
/// The background fill color when disabled.
///
/// This property is null by default.
///
/// See also:
///
/// * [getDisabledFillColor], which is to compute background fill color for
/// disabled state.
final Color? _disabledColor;
/// The fill color of the button when it has the input focus.
///
/// This property is null by default.
///
/// If the button is in the hovering or highlighted state, then the [hoverColor]
/// or [highlightColor] will take precedence over the [focusColor].
///
/// See also:
///
/// * [getFocusColor], which is used to compute the fill color of the button
/// when it has input focus.
final Color? _focusColor;
/// The fill color of the button when a pointer is hovering over it.
///
/// This property is null by default.
///
/// If the button is in the highlighted state, then the [highlightColor] will
/// take precedence over the [hoverColor].
///
/// See also:
///
/// * [getHoverColor], which is used to compute the fill color of the button
/// when it has input focus.
final Color? _hoverColor;
/// The color of the overlay that appears when a button is pressed.
///
/// This property is null by default.
///
/// See also:
///
/// * [getHighlightColor], which is used to compute the color of the overlay
/// that appears when the [button] is pressed.
final Color? _highlightColor;
/// The color of the ink "splash" overlay that appears when a button is tapped.
///
/// This property is null by default.
///
/// See also:
///
/// * [getSplashColor], which is used to compute the color of the ink
/// "splash" overlay that appears when the (enabled) [button] is tapped.
final Color? _splashColor;
/// A set of thirteen colors that can be used to derive the button theme's
/// colors.
///
/// This property was added much later than the theme's set of highly specific
/// colors, like [ThemeData.highlightColor] and [ThemeData.splashColor] etc.
///
/// The colors for new button classes can be defined exclusively in terms of
/// [colorScheme]. When it's possible, the existing buttons will (continue to)
/// gradually migrate to it.
final ColorScheme? colorScheme;
// The minimum size of a button's tap target.
//
// This property is null by default.
final MaterialTapTargetSize? _materialTapTargetSize;
/// The [button]'s overall brightness.
///
/// Returns the button's [MaterialButton.colorBrightness] if it is non-null,
/// otherwise the color scheme's [ColorScheme.brightness] is returned.
Brightness getBrightness(MaterialButton button) {
return button.colorBrightness ?? colorScheme!.brightness;
}
/// Defines the [button]'s base colors, and the defaults for the button's
/// minimum size, internal padding, and shape.
///
/// Despite the name, this property is not the [TextTheme] whose
/// [TextTheme.button] is used as the button text's [TextStyle].
ButtonTextTheme getTextTheme(MaterialButton button) => button.textTheme ?? textTheme;
/// The foreground color of the [button]'s text and icon when
/// [MaterialButton.onPressed] is null (when MaterialButton.enabled is false).
///
/// Returns the button's [MaterialButton.disabledColor] if it is non-null.
/// Otherwise the color scheme's [ColorScheme.onSurface] color is returned
/// with its opacity set to 0.38.
///
/// If [MaterialButton.textColor] is a [MaterialStateProperty<Color>], it will be
/// used as the `disabledTextColor`. It will be resolved in the [MaterialState.disabled] state.
Color getDisabledTextColor(MaterialButton button) {
return button.textColor ?? button.disabledTextColor ?? colorScheme!.onSurface.withOpacity(0.38);
}
/// The [button]'s background color when [MaterialButton.onPressed] is null
/// (when [MaterialButton.enabled] is false).
///
/// Returns the button's [MaterialButton.disabledColor] if it is non-null.
///
/// Otherwise the value of the `disabledColor` constructor parameter
/// is returned, if it is non-null.
///
/// Otherwise the color scheme's [ColorScheme.onSurface] color is returned
/// with its opacity set to 0.38.
Color getDisabledFillColor(MaterialButton button) {
return button.disabledColor ?? _disabledColor ?? colorScheme!.onSurface.withOpacity(0.38);
}
/// The button's background fill color or null for buttons that don't have
/// a background color.
///
/// Returns [MaterialButton.color] if it is non-null and the button
/// is enabled.
///
/// Otherwise, returns [MaterialButton.disabledColor] if it is non-null and
/// the button is disabled.
///
/// Otherwise the fill color depends on the value of [getTextTheme].
///
/// * [ButtonTextTheme.normal] or [ButtonTextTheme.accent], the
/// color scheme's [ColorScheme.primary] color if the [button] is enabled
/// the value of [getDisabledFillColor] otherwise.
/// * [ButtonTextTheme.primary], if the [button] is enabled then the value
/// of the `buttonColor` constructor parameter if it is non-null,
/// otherwise the color scheme's ColorScheme.primary color. If the button
/// is not enabled then the colorScheme's [ColorScheme.onSurface] color
/// with opacity 0.12.
Color? getFillColor(MaterialButton button) {
final Color? fillColor = button.enabled ? button.color : button.disabledColor;
if (fillColor != null) {
return fillColor;
}
if (button.runtimeType == MaterialButton) {
return null;
}
if (button.enabled && _buttonColor != null) {
return _buttonColor;
}
switch (getTextTheme(button)) {
case ButtonTextTheme.normal:
case ButtonTextTheme.accent:
return button.enabled ? colorScheme!.primary : getDisabledFillColor(button);
case ButtonTextTheme.primary:
return button.enabled
? _buttonColor ?? colorScheme!.primary
: colorScheme!.onSurface.withOpacity(0.12);
}
}
/// The foreground color of the [button]'s text and icon.
///
/// If [button] is not [MaterialButton.enabled], the value of
/// [getDisabledTextColor] is returned. If the button is enabled and
/// [MaterialButton.textColor] is non-null, then [MaterialButton.textColor]
/// is returned.
///
/// Otherwise the text color depends on the value of [getTextTheme]
/// and [getBrightness].
///
/// * [ButtonTextTheme.normal]: [Colors.white] is used if [getBrightness]
/// resolves to [Brightness.dark]. [Colors.black87] is used if
/// [getBrightness] resolves to [Brightness.light].
/// * [ButtonTextTheme.accent]: [ColorScheme.secondary] of [colorScheme].
/// * [ButtonTextTheme.primary]: If [getFillColor] is dark then [Colors.white],
/// otherwise [Colors.black].
Color getTextColor(MaterialButton button) {
if (!button.enabled) {
return getDisabledTextColor(button);
}
if (button.textColor != null) {
return button.textColor!;
}
switch (getTextTheme(button)) {
case ButtonTextTheme.normal:
return getBrightness(button) == Brightness.dark ? Colors.white : Colors.black87;
case ButtonTextTheme.accent:
return colorScheme!.secondary;
case ButtonTextTheme.primary:
final Color? fillColor = getFillColor(button);
final bool fillIsDark = fillColor != null
? ThemeData.estimateBrightnessForColor(fillColor) == Brightness.dark
: getBrightness(button) == Brightness.dark;
return fillIsDark ? Colors.white : Colors.black;
}
}
/// The color of the ink "splash" overlay that appears when the (enabled)
/// [button] is tapped.
///
/// Returns the button's [MaterialButton.splashColor] if it is non-null.
///
/// Otherwise, returns the value of the `splashColor` constructor parameter
/// it is non-null.
///
/// Otherwise, returns the value of the `splashColor` constructor parameter
/// if it is non-null and [getTextTheme] is not [ButtonTextTheme.primary].
///
/// Otherwise, returns [getTextColor] with an opacity of 0.12.
Color getSplashColor(MaterialButton button) {
if (button.splashColor != null) {
return button.splashColor!;
}
if (_splashColor != null) {
switch (getTextTheme(button)) {
case ButtonTextTheme.normal:
case ButtonTextTheme.accent:
return _splashColor;
case ButtonTextTheme.primary:
break;
}
}
return getTextColor(button).withOpacity(0.12);
}
/// The fill color of the button when it has input focus.
///
/// Returns the button's [MaterialButton.focusColor] if it is non-null.
/// Otherwise the focus color depends on [getTextTheme]:
///
/// * [ButtonTextTheme.normal], [ButtonTextTheme.accent]: returns the
/// value of the `focusColor` constructor parameter if it is non-null,
/// otherwise the value of [getTextColor] with opacity 0.12.
/// * [ButtonTextTheme.primary], returns [Colors.transparent].
Color getFocusColor(MaterialButton button) {
return button.focusColor ?? _focusColor ?? getTextColor(button).withOpacity(0.12);
}
/// The fill color of the button when it has input focus.
///
/// Returns the button's [MaterialButton.focusColor] if it is non-null.
/// Otherwise the focus color depends on [getTextTheme]:
///
/// * [ButtonTextTheme.normal], [ButtonTextTheme.accent],
/// [ButtonTextTheme.primary]: returns the value of the `focusColor`
/// constructor parameter if it is non-null, otherwise the value of
/// [getTextColor] with opacity 0.04.
Color getHoverColor(MaterialButton button) {
return button.hoverColor ?? _hoverColor ?? getTextColor(button).withOpacity(0.04);
}
/// The color of the overlay that appears when the [button] is pressed.
///
/// Returns the button's [MaterialButton.highlightColor] if it is non-null.
/// Otherwise the highlight color depends on [getTextTheme]:
///
/// * [ButtonTextTheme.normal], [ButtonTextTheme.accent]: returns the
/// value of the `highlightColor` constructor parameter if it is non-null,
/// otherwise the value of [getTextColor] with opacity 0.16.
/// * [ButtonTextTheme.primary], returns [Colors.transparent].
Color getHighlightColor(MaterialButton button) {
if (button.highlightColor != null) {
return button.highlightColor!;
}
switch (getTextTheme(button)) {
case ButtonTextTheme.normal:
case ButtonTextTheme.accent:
return _highlightColor ?? getTextColor(button).withOpacity(0.16);
case ButtonTextTheme.primary:
return Colors.transparent;
}
}
/// The [button]'s elevation when it is enabled and has not been pressed.
///
/// Returns the button's [MaterialButton.elevation] if it is non-null,
/// otherwise it is 2.0.
double getElevation(MaterialButton button) => button.elevation ?? 2.0;
/// The [button]'s elevation when it is enabled and has focus.
///
/// Returns the button's [MaterialButton.focusElevation] if it is non-null,
/// otherwise the highlight elevation is 4.0.
double getFocusElevation(MaterialButton button) => button.focusElevation ?? 4.0;
/// The [button]'s elevation when it is enabled and has focus.
///
/// Returns the button's [MaterialButton.hoverElevation] if it is non-null,
/// otherwise the highlight elevation is 4.0.
double getHoverElevation(MaterialButton button) => button.hoverElevation ?? 4.0;
/// The [button]'s elevation when it is enabled and has been pressed.
///
/// Returns the button's [MaterialButton.highlightElevation] if it is non-null,
/// otherwise the highlight elevation is 8.0.
double getHighlightElevation(MaterialButton button) => button.highlightElevation ?? 8.0;
/// The [button]'s elevation when [MaterialButton.onPressed] is null (when
/// MaterialButton.enabled is false).
///
/// Returns the button's [MaterialButton.elevation] if it is non-null.
///
/// Otherwise the disabled elevation is 0.0.
double getDisabledElevation(MaterialButton button) => button.disabledElevation ?? 0.0;
/// Padding for the [button]'s child (typically the button's label).
///
/// Returns the button's [MaterialButton.padding] if it is non-null,
/// otherwise, returns the `padding` of the constructor parameter if it is
/// non-null.
///
/// Otherwise, returns horizontal padding of 24.0 on the left and right if
/// [getTextTheme] is [ButtonTextTheme.primary], 16.0 on the left and right
/// otherwise.
EdgeInsetsGeometry getPadding(MaterialButton button) {
return button.padding ?? _padding ?? switch (getTextTheme(button)) {
ButtonTextTheme.normal => const EdgeInsets.symmetric(horizontal: 16.0),
ButtonTextTheme.accent => const EdgeInsets.symmetric(horizontal: 16.0),
ButtonTextTheme.primary => const EdgeInsets.symmetric(horizontal: 24.0),
};
}
/// The shape of the [button]'s [Material].
///
/// Returns the button's [MaterialButton.shape] if it is non-null, otherwise
/// [shape] is returned.
ShapeBorder getShape(MaterialButton button) => button.shape ?? shape;
/// The duration of the [button]'s highlight animation.
///
/// Returns the button's [MaterialButton.animationDuration] it if is non-null,
/// otherwise 200ms.
Duration getAnimationDuration(MaterialButton button) {
return button.animationDuration ?? kThemeChangeDuration;
}
/// The [BoxConstraints] that the define the [button]'s size.
///
/// By default this method just returns [constraints]. Subclasses
/// could override this method to return a value that was,
/// for example, based on the button's type.
BoxConstraints getConstraints(MaterialButton button) => constraints;
/// The minimum size of the [button]'s tap target.
///
/// Returns the button's [MaterialButton.materialTapTargetSize] if it is non-null.
///
/// Otherwise the value of the `materialTapTargetSize` constructor
/// parameter is returned if that's non-null.
///
/// Otherwise [MaterialTapTargetSize.padded] is returned.
MaterialTapTargetSize getMaterialTapTargetSize(MaterialButton button) {
return button.materialTapTargetSize ?? _materialTapTargetSize ?? MaterialTapTargetSize.padded;
}
/// Creates a copy of this button theme data object with the matching fields
/// replaced with the non-null parameter values.
ButtonThemeData copyWith({
ButtonTextTheme? textTheme,
ButtonBarLayoutBehavior? layoutBehavior,
double? minWidth,
double? height,
EdgeInsetsGeometry? padding,
ShapeBorder? shape,
bool? alignedDropdown,
Color? buttonColor,
Color? disabledColor,
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
Color? splashColor,
ColorScheme? colorScheme,
MaterialTapTargetSize? materialTapTargetSize,
}) {
return ButtonThemeData(
textTheme: textTheme ?? this.textTheme,
layoutBehavior: layoutBehavior ?? this.layoutBehavior,
minWidth: minWidth ?? this.minWidth,
height: height ?? this.height,
padding: padding ?? this.padding,
shape: shape ?? this.shape,
alignedDropdown: alignedDropdown ?? this.alignedDropdown,
buttonColor: buttonColor ?? _buttonColor,
disabledColor: disabledColor ?? _disabledColor,
focusColor: focusColor ?? _focusColor,
hoverColor: hoverColor ?? _hoverColor,
highlightColor: highlightColor ?? _highlightColor,
splashColor: splashColor ?? _splashColor,
colorScheme: colorScheme ?? this.colorScheme,
materialTapTargetSize: materialTapTargetSize ?? _materialTapTargetSize,
);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ButtonThemeData
&& other.textTheme == textTheme
&& other.minWidth == minWidth
&& other.height == height
&& other.padding == padding
&& other.shape == shape
&& other.alignedDropdown == alignedDropdown
&& other._buttonColor == _buttonColor
&& other._disabledColor == _disabledColor
&& other._focusColor == _focusColor
&& other._hoverColor == _hoverColor
&& other._highlightColor == _highlightColor
&& other._splashColor == _splashColor
&& other.colorScheme == colorScheme
&& other._materialTapTargetSize == _materialTapTargetSize;
}
@override
int get hashCode => Object.hash(
textTheme,
minWidth,
height,
padding,
shape,
alignedDropdown,
_buttonColor,
_disabledColor,
_focusColor,
_hoverColor,
_highlightColor,
_splashColor,
colorScheme,
_materialTapTargetSize,
);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
const ButtonThemeData defaultTheme = ButtonThemeData();
properties.add(EnumProperty<ButtonTextTheme>('textTheme', textTheme, defaultValue: defaultTheme.textTheme));
properties.add(DoubleProperty('minWidth', minWidth, defaultValue: defaultTheme.minWidth));
properties.add(DoubleProperty('height', height, defaultValue: defaultTheme.height));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: defaultTheme.padding));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: defaultTheme.shape));
properties.add(FlagProperty('alignedDropdown',
value: alignedDropdown,
defaultValue: defaultTheme.alignedDropdown,
ifTrue: 'dropdown width matches button',
));
properties.add(ColorProperty('buttonColor', _buttonColor, defaultValue: null));
properties.add(ColorProperty('disabledColor', _disabledColor, defaultValue: null));
properties.add(ColorProperty('focusColor', _focusColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', _hoverColor, defaultValue: null));
properties.add(ColorProperty('highlightColor', _highlightColor, defaultValue: null));
properties.add(ColorProperty('splashColor', _splashColor, defaultValue: null));
properties.add(DiagnosticsProperty<ColorScheme>('colorScheme', colorScheme, defaultValue: defaultTheme.colorScheme));
properties.add(DiagnosticsProperty<MaterialTapTargetSize>('materialTapTargetSize', _materialTapTargetSize, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/material/button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/button_theme.dart",
"repo_id": "flutter",
"token_count": 8909
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'data_table.dart';
/// A data source for obtaining row data for [PaginatedDataTable] objects.
///
/// A data table source provides two main pieces of information:
///
/// * The number of rows in the data table ([rowCount]).
/// * The data for each row (indexed from `0` to `rowCount - 1`).
///
/// It also provides a listener API ([addListener]/[removeListener]) so that
/// consumers of the data can be notified when it changes. When the data
/// changes, call [notifyListeners] to send the notifications.
///
/// DataTableSource objects are expected to be long-lived, not recreated with
/// each build.
///
/// If a [DataTableSource] is used with a [PaginatedDataTable] that supports
/// sortable columns (see [DataColumn.onSort] and
/// [PaginatedDataTable.sortColumnIndex]), the rows reported by the data source
/// must be reported in the sorted order.
abstract class DataTableSource extends ChangeNotifier {
/// Called to obtain the data about a particular row.
///
/// Rows should be keyed so that state can be maintained when the data source
/// is sorted (e.g. in response to [DataColumn.onSort]). Keys should be
/// consistent for a given [DataRow] regardless of the sort order (i.e. the
/// key represents the data's identity, not the row position).
///
/// The [DataRow.byIndex] constructor provides a convenient way to construct
/// [DataRow] objects for this method's purposes without having to worry about
/// independently keying each row. The index passed to that constructor is the
/// index of the underlying data, which is different than the `index`
/// parameter for [getRow], which represents the _sorted_ position.
///
/// If the given index does not correspond to a row, or if no data is yet
/// available for a row, then return null. The row will be left blank and a
/// loading indicator will be displayed over the table. Once data is available
/// or once it is firmly established that the row index in question is beyond
/// the end of the table, call [notifyListeners]. (See [rowCount].)
///
/// If the underlying data changes, call [notifyListeners].
DataRow? getRow(int index);
/// Called to obtain the number of rows to tell the user are available.
///
/// If [isRowCountApproximate] is false, then this must be an accurate number,
/// and [getRow] must return a non-null value for all indices in the range 0
/// to one less than the row count.
///
/// If [isRowCountApproximate] is true, then the user will be allowed to
/// attempt to display rows up to this [rowCount], and the display will
/// indicate that the count is approximate. The row count should therefore be
/// greater than the actual number of rows if at all possible.
///
/// If the row count changes, call [notifyListeners].
int get rowCount;
/// Called to establish if [rowCount] is a precise number or might be an
/// over-estimate. If this returns true (i.e. the count is approximate), and
/// then later the exact number becomes available, then call
/// [notifyListeners].
bool get isRowCountApproximate;
/// Called to obtain the number of rows that are currently selected.
///
/// If the selected row count changes, call [notifyListeners].
///
/// Selected rows are those whose [DataRow.selected] property is set to true.
int get selectedRowCount;
}
| flutter/packages/flutter/lib/src/material/data_table_source.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/data_table_source.dart",
"repo_id": "flutter",
"token_count": 933
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'button_theme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'input_border.dart';
import 'input_decorator.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'scrollbar.dart';
import 'shadows.dart';
import 'theme.dart';
const Duration _kDropdownMenuDuration = Duration(milliseconds: 300);
const double _kMenuItemHeight = kMinInteractiveDimension;
const double _kDenseButtonHeight = 24.0;
const EdgeInsets _kMenuItemPadding = EdgeInsets.symmetric(horizontal: 16.0);
const EdgeInsetsGeometry _kAlignedButtonPadding = EdgeInsetsDirectional.only(start: 16.0, end: 4.0);
const EdgeInsets _kUnalignedButtonPadding = EdgeInsets.zero;
const EdgeInsets _kAlignedMenuMargin = EdgeInsets.zero;
const EdgeInsetsGeometry _kUnalignedMenuMargin = EdgeInsetsDirectional.only(start: 16.0, end: 24.0);
/// A builder to customize dropdown buttons.
///
/// Used by [DropdownButton.selectedItemBuilder].
typedef DropdownButtonBuilder = List<Widget> Function(BuildContext context);
class _DropdownMenuPainter extends CustomPainter {
_DropdownMenuPainter({
this.color,
this.elevation,
this.selectedIndex,
this.borderRadius,
required this.resize,
required this.getSelectedItemOffset,
}) : _painter = BoxDecoration(
// If you add an image here, you must provide a real
// configuration in the paint() function and you must provide some sort
// of onChanged callback here.
color: color,
borderRadius: borderRadius ?? const BorderRadius.all(Radius.circular(2.0)),
boxShadow: kElevationToShadow[elevation],
).createBoxPainter(),
super(repaint: resize);
final Color? color;
final int? elevation;
final int? selectedIndex;
final BorderRadius? borderRadius;
final Animation<double> resize;
final ValueGetter<double> getSelectedItemOffset;
final BoxPainter _painter;
@override
void paint(Canvas canvas, Size size) {
final double selectedItemOffset = getSelectedItemOffset();
final Tween<double> top = Tween<double>(
begin: clampDouble(selectedItemOffset, 0.0, math.max(size.height - _kMenuItemHeight, 0.0)),
end: 0.0,
);
final Tween<double> bottom = Tween<double>(
begin: clampDouble(top.begin! + _kMenuItemHeight, math.min(_kMenuItemHeight, size.height), size.height),
end: size.height,
);
final Rect rect = Rect.fromLTRB(0.0, top.evaluate(resize), size.width, bottom.evaluate(resize));
_painter.paint(canvas, rect.topLeft, ImageConfiguration(size: rect.size));
}
@override
bool shouldRepaint(_DropdownMenuPainter oldPainter) {
return oldPainter.color != color
|| oldPainter.elevation != elevation
|| oldPainter.selectedIndex != selectedIndex
|| oldPainter.borderRadius != borderRadius
|| oldPainter.resize != resize;
}
}
// The widget that is the button wrapping the menu items.
class _DropdownMenuItemButton<T> extends StatefulWidget {
const _DropdownMenuItemButton({
super.key,
this.padding,
required this.route,
required this.buttonRect,
required this.constraints,
required this.itemIndex,
required this.enableFeedback,
required this.scrollController,
});
final _DropdownRoute<T> route;
final ScrollController scrollController;
final EdgeInsets? padding;
final Rect buttonRect;
final BoxConstraints constraints;
final int itemIndex;
final bool enableFeedback;
@override
_DropdownMenuItemButtonState<T> createState() => _DropdownMenuItemButtonState<T>();
}
class _DropdownMenuItemButtonState<T> extends State<_DropdownMenuItemButton<T>> {
void _handleFocusChange(bool focused) {
final bool inTraditionalMode = switch (FocusManager.instance.highlightMode) {
FocusHighlightMode.touch => false,
FocusHighlightMode.traditional => true,
};
if (focused && inTraditionalMode) {
final _MenuLimits menuLimits = widget.route.getMenuLimits(
widget.buttonRect,
widget.constraints.maxHeight,
widget.itemIndex,
);
widget.scrollController.animateTo(
menuLimits.scrollOffset,
curve: Curves.easeInOut,
duration: const Duration(milliseconds: 100),
);
}
}
void _handleOnTap() {
final DropdownMenuItem<T> dropdownMenuItem = widget.route.items[widget.itemIndex].item!;
dropdownMenuItem.onTap?.call();
Navigator.pop(
context,
_DropdownRouteResult<T>(dropdownMenuItem.value),
);
}
static const Map<ShortcutActivator, Intent> _webShortcuts = <ShortcutActivator, Intent>{
// On the web, up/down don't change focus, *except* in a <select>
// element, which is what a dropdown emulates.
SingleActivator(LogicalKeyboardKey.arrowDown): DirectionalFocusIntent(TraversalDirection.down),
SingleActivator(LogicalKeyboardKey.arrowUp): DirectionalFocusIntent(TraversalDirection.up),
};
@override
Widget build(BuildContext context) {
final DropdownMenuItem<T> dropdownMenuItem = widget.route.items[widget.itemIndex].item!;
final CurvedAnimation opacity;
final double unit = 0.5 / (widget.route.items.length + 1.5);
if (widget.itemIndex == widget.route.selectedIndex) {
opacity = CurvedAnimation(parent: widget.route.animation!, curve: const Threshold(0.0));
} else {
final double start = clampDouble(0.5 + (widget.itemIndex + 1) * unit, 0.0, 1.0);
final double end = clampDouble(start + 1.5 * unit, 0.0, 1.0);
opacity = CurvedAnimation(parent: widget.route.animation!, curve: Interval(start, end));
}
Widget child = Container(
padding: widget.padding,
height: widget.route.itemHeight,
child: widget.route.items[widget.itemIndex],
);
// An [InkWell] is added to the item only if it is enabled
if (dropdownMenuItem.enabled) {
child = InkWell(
autofocus: widget.itemIndex == widget.route.selectedIndex,
enableFeedback: widget.enableFeedback,
onTap: _handleOnTap,
onFocusChange: _handleFocusChange,
child: child,
);
}
child = FadeTransition(opacity: opacity, child: child);
if (kIsWeb && dropdownMenuItem.enabled) {
child = Shortcuts(
shortcuts: _webShortcuts,
child: child,
);
}
return child;
}
}
class _DropdownMenu<T> extends StatefulWidget {
const _DropdownMenu({
super.key,
this.padding,
required this.route,
required this.buttonRect,
required this.constraints,
this.dropdownColor,
required this.enableFeedback,
this.borderRadius,
required this.scrollController,
});
final _DropdownRoute<T> route;
final EdgeInsets? padding;
final Rect buttonRect;
final BoxConstraints constraints;
final Color? dropdownColor;
final bool enableFeedback;
final BorderRadius? borderRadius;
final ScrollController scrollController;
@override
_DropdownMenuState<T> createState() => _DropdownMenuState<T>();
}
class _DropdownMenuState<T> extends State<_DropdownMenu<T>> {
late CurvedAnimation _fadeOpacity;
late CurvedAnimation _resize;
@override
void initState() {
super.initState();
// We need to hold these animations as state because of their curve
// direction. When the route's animation reverses, if we were to recreate
// the CurvedAnimation objects in build, we'd lose
// CurvedAnimation._curveDirection.
_fadeOpacity = CurvedAnimation(
parent: widget.route.animation!,
curve: const Interval(0.0, 0.25),
reverseCurve: const Interval(0.75, 1.0),
);
_resize = CurvedAnimation(
parent: widget.route.animation!,
curve: const Interval(0.25, 0.5),
reverseCurve: const Threshold(0.0),
);
}
@override
Widget build(BuildContext context) {
// The menu is shown in three stages (unit timing in brackets):
// [0s - 0.25s] - Fade in a rect-sized menu container with the selected item.
// [0.25s - 0.5s] - Grow the otherwise empty menu container from the center
// until it's big enough for as many items as we're going to show.
// [0.5s - 1.0s] Fade in the remaining visible items from top to bottom.
//
// When the menu is dismissed we just fade the entire thing out
// in the first 0.25s.
assert(debugCheckHasMaterialLocalizations(context));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final _DropdownRoute<T> route = widget.route;
final List<Widget> children = <Widget>[
for (int itemIndex = 0; itemIndex < route.items.length; ++itemIndex)
_DropdownMenuItemButton<T>(
route: widget.route,
padding: widget.padding,
buttonRect: widget.buttonRect,
constraints: widget.constraints,
itemIndex: itemIndex,
enableFeedback: widget.enableFeedback,
scrollController: widget.scrollController,
),
];
return FadeTransition(
opacity: _fadeOpacity,
child: CustomPaint(
painter: _DropdownMenuPainter(
color: widget.dropdownColor ?? Theme.of(context).canvasColor,
elevation: route.elevation,
selectedIndex: route.selectedIndex,
resize: _resize,
borderRadius: widget.borderRadius,
// This offset is passed as a callback, not a value, because it must
// be retrieved at paint time (after layout), not at build time.
getSelectedItemOffset: () => route.getItemOffset(route.selectedIndex),
),
child: Semantics(
scopesRoute: true,
namesRoute: true,
explicitChildNodes: true,
label: localizations.popupMenuLabel,
child: ClipRRect(
borderRadius: widget.borderRadius ?? BorderRadius.zero,
clipBehavior: widget.borderRadius != null
? Clip.antiAlias
: Clip.none,
child: Material(
type: MaterialType.transparency,
textStyle: route.style,
child: ScrollConfiguration(
// Dropdown menus should never overscroll or display an overscroll indicator.
// Scrollbars are built-in below.
// Platform must use Theme and ScrollPhysics must be Clamping.
behavior: ScrollConfiguration.of(context).copyWith(
scrollbars: false,
overscroll: false,
physics: const ClampingScrollPhysics(),
platform: Theme.of(context).platform,
),
child: PrimaryScrollController(
controller: widget.scrollController,
child: Scrollbar(
thumbVisibility: true,
child: ListView(
// Ensure this always inherits the PrimaryScrollController
primary: true,
padding: kMaterialListPadding,
shrinkWrap: true,
children: children,
),
),
),
),
),
),
),
),
);
}
}
class _DropdownMenuRouteLayout<T> extends SingleChildLayoutDelegate {
_DropdownMenuRouteLayout({
required this.buttonRect,
required this.route,
required this.textDirection,
});
final Rect buttonRect;
final _DropdownRoute<T> route;
final TextDirection? textDirection;
@override
BoxConstraints getConstraintsForChild(BoxConstraints constraints) {
// The maximum height of a simple menu should be one or more rows less than
// the view height. This ensures a tappable area outside of the simple menu
// with which to dismiss the menu.
// -- https://material.io/design/components/menus.html#usage
double maxHeight = math.max(0.0, constraints.maxHeight - 2 * _kMenuItemHeight);
if (route.menuMaxHeight != null && route.menuMaxHeight! <= maxHeight) {
maxHeight = route.menuMaxHeight!;
}
// The width of a menu should be at most the view width. This ensures that
// the menu does not extend past the left and right edges of the screen.
final double width = math.min(constraints.maxWidth, buttonRect.width);
return BoxConstraints(
minWidth: width,
maxWidth: width,
maxHeight: maxHeight,
);
}
@override
Offset getPositionForChild(Size size, Size childSize) {
final _MenuLimits menuLimits = route.getMenuLimits(buttonRect, size.height, route.selectedIndex);
assert(() {
final Rect container = Offset.zero & size;
if (container.intersect(buttonRect) == buttonRect) {
// If the button was entirely on-screen, then verify
// that the menu is also on-screen.
// If the button was a bit off-screen, then, oh well.
assert(menuLimits.top >= 0.0);
assert(menuLimits.top + menuLimits.height <= size.height);
}
return true;
}());
assert(textDirection != null);
final double left = switch (textDirection!) {
TextDirection.rtl => clampDouble(buttonRect.right, 0.0, size.width) - childSize.width,
TextDirection.ltr => clampDouble(buttonRect.left, 0.0, size.width - childSize.width),
};
return Offset(left, menuLimits.top);
}
@override
bool shouldRelayout(_DropdownMenuRouteLayout<T> oldDelegate) {
return buttonRect != oldDelegate.buttonRect || textDirection != oldDelegate.textDirection;
}
}
// We box the return value so that the return value can be null. Otherwise,
// canceling the route (which returns null) would get confused with actually
// returning a real null value.
@immutable
class _DropdownRouteResult<T> {
const _DropdownRouteResult(this.result);
final T? result;
@override
bool operator ==(Object other) {
return other is _DropdownRouteResult<T>
&& other.result == result;
}
@override
int get hashCode => result.hashCode;
}
class _MenuLimits {
const _MenuLimits(this.top, this.bottom, this.height, this.scrollOffset);
final double top;
final double bottom;
final double height;
final double scrollOffset;
}
class _DropdownRoute<T> extends PopupRoute<_DropdownRouteResult<T>> {
_DropdownRoute({
required this.items,
required this.padding,
required this.buttonRect,
required this.selectedIndex,
this.elevation = 8,
required this.capturedThemes,
required this.style,
this.barrierLabel,
this.itemHeight,
this.dropdownColor,
this.menuMaxHeight,
required this.enableFeedback,
this.borderRadius,
}) : itemHeights = List<double>.filled(items.length, itemHeight ?? kMinInteractiveDimension);
final List<_MenuItem<T>> items;
final EdgeInsetsGeometry padding;
final Rect buttonRect;
final int selectedIndex;
final int elevation;
final CapturedThemes capturedThemes;
final TextStyle style;
final double? itemHeight;
final Color? dropdownColor;
final double? menuMaxHeight;
final bool enableFeedback;
final BorderRadius? borderRadius;
final List<double> itemHeights;
@override
Duration get transitionDuration => _kDropdownMenuDuration;
@override
bool get barrierDismissible => true;
@override
Color? get barrierColor => null;
@override
final String? barrierLabel;
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
return LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return _DropdownRoutePage<T>(
route: this,
constraints: constraints,
items: items,
padding: padding,
buttonRect: buttonRect,
selectedIndex: selectedIndex,
elevation: elevation,
capturedThemes: capturedThemes,
style: style,
dropdownColor: dropdownColor,
enableFeedback: enableFeedback,
borderRadius: borderRadius,
);
},
);
}
void _dismiss() {
if (isActive) {
navigator?.removeRoute(this);
}
}
double getItemOffset(int index) {
double offset = kMaterialListPadding.top;
if (items.isNotEmpty && index > 0) {
assert(items.length == itemHeights.length);
offset += itemHeights
.sublist(0, index)
.reduce((double total, double height) => total + height);
}
return offset;
}
// Returns the vertical extent of the menu and the initial scrollOffset
// for the ListView that contains the menu items. The vertical center of the
// selected item is aligned with the button's vertical center, as far as
// that's possible given availableHeight.
_MenuLimits getMenuLimits(Rect buttonRect, double availableHeight, int index) {
double computedMaxHeight = availableHeight - 2.0 * _kMenuItemHeight;
if (menuMaxHeight != null) {
computedMaxHeight = math.min(computedMaxHeight, menuMaxHeight!);
}
final double buttonTop = buttonRect.top;
final double buttonBottom = math.min(buttonRect.bottom, availableHeight);
final double selectedItemOffset = getItemOffset(index);
// If the button is placed on the bottom or top of the screen, its top or
// bottom may be less than [_kMenuItemHeight] from the edge of the screen.
// In this case, we want to change the menu limits to align with the top
// or bottom edge of the button.
final double topLimit = math.min(_kMenuItemHeight, buttonTop);
final double bottomLimit = math.max(availableHeight - _kMenuItemHeight, buttonBottom);
double menuTop = (buttonTop - selectedItemOffset) - (itemHeights[selectedIndex] - buttonRect.height) / 2.0;
double preferredMenuHeight = kMaterialListPadding.vertical;
if (items.isNotEmpty) {
preferredMenuHeight += itemHeights.reduce((double total, double height) => total + height);
}
// If there are too many elements in the menu, we need to shrink it down
// so it is at most the computedMaxHeight.
final double menuHeight = math.min(computedMaxHeight, preferredMenuHeight);
double menuBottom = menuTop + menuHeight;
// If the computed top or bottom of the menu are outside of the range
// specified, we need to bring them into range. If the item height is larger
// than the button height and the button is at the very bottom or top of the
// screen, the menu will be aligned with the bottom or top of the button
// respectively.
if (menuTop < topLimit) {
menuTop = math.min(buttonTop, topLimit);
menuBottom = menuTop + menuHeight;
}
if (menuBottom > bottomLimit) {
menuBottom = math.max(buttonBottom, bottomLimit);
menuTop = menuBottom - menuHeight;
}
if (menuBottom - itemHeights[selectedIndex] / 2.0 < buttonBottom - buttonRect.height / 2.0) {
menuBottom = buttonBottom - buttonRect.height / 2.0 + itemHeights[selectedIndex] / 2.0;
menuTop = menuBottom - menuHeight;
}
double scrollOffset = 0;
// If all of the menu items will not fit within availableHeight then
// compute the scroll offset that will line the selected menu item up
// with the select item. This is only done when the menu is first
// shown - subsequently we leave the scroll offset where the user left
// it. This scroll offset is only accurate for fixed height menu items
// (the default).
if (preferredMenuHeight > computedMaxHeight) {
// The offset should be zero if the selected item is in view at the beginning
// of the menu. Otherwise, the scroll offset should center the item if possible.
scrollOffset = math.max(0.0, selectedItemOffset - (buttonTop - menuTop));
// If the selected item's scroll offset is greater than the maximum scroll offset,
// set it instead to the maximum allowed scroll offset.
scrollOffset = math.min(scrollOffset, preferredMenuHeight - menuHeight);
}
assert((menuBottom - menuTop - menuHeight).abs() < precisionErrorTolerance);
return _MenuLimits(menuTop, menuBottom, menuHeight, scrollOffset);
}
}
class _DropdownRoutePage<T> extends StatefulWidget {
const _DropdownRoutePage({
super.key,
required this.route,
required this.constraints,
this.items,
required this.padding,
required this.buttonRect,
required this.selectedIndex,
this.elevation = 8,
required this.capturedThemes,
this.style,
required this.dropdownColor,
required this.enableFeedback,
this.borderRadius,
});
final _DropdownRoute<T> route;
final BoxConstraints constraints;
final List<_MenuItem<T>>? items;
final EdgeInsetsGeometry padding;
final Rect buttonRect;
final int selectedIndex;
final int elevation;
final CapturedThemes capturedThemes;
final TextStyle? style;
final Color? dropdownColor;
final bool enableFeedback;
final BorderRadius? borderRadius;
@override
State<_DropdownRoutePage<T>> createState() => _DropdownRoutePageState<T>();
}
class _DropdownRoutePageState<T> extends State<_DropdownRoutePage<T>> {
late ScrollController _scrollController;
@override
void initState(){
super.initState();
// Computing the initialScrollOffset now, before the items have been laid
// out. This only works if the item heights are effectively fixed, i.e. either
// DropdownButton.itemHeight is specified or DropdownButton.itemHeight is null
// and all of the items' intrinsic heights are less than kMinInteractiveDimension.
// Otherwise the initialScrollOffset is just a rough approximation based on
// treating the items as if their heights were all equal to kMinInteractiveDimension.
final _MenuLimits menuLimits = widget.route.getMenuLimits(widget.buttonRect, widget.constraints.maxHeight, widget.selectedIndex);
_scrollController = ScrollController(initialScrollOffset: menuLimits.scrollOffset);
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
final TextDirection? textDirection = Directionality.maybeOf(context);
final Widget menu = _DropdownMenu<T>(
route: widget.route,
padding: widget.padding.resolve(textDirection),
buttonRect: widget.buttonRect,
constraints: widget.constraints,
dropdownColor: widget.dropdownColor,
enableFeedback: widget.enableFeedback,
borderRadius: widget.borderRadius,
scrollController: _scrollController,
);
return MediaQuery.removePadding(
context: context,
removeTop: true,
removeBottom: true,
removeLeft: true,
removeRight: true,
child: Builder(
builder: (BuildContext context) {
return CustomSingleChildLayout(
delegate: _DropdownMenuRouteLayout<T>(
buttonRect: widget.buttonRect,
route: widget.route,
textDirection: textDirection,
),
child: widget.capturedThemes.wrap(menu),
);
},
),
);
}
@override
void dispose() {
_scrollController.dispose();
super.dispose();
}
}
// This widget enables _DropdownRoute to look up the sizes of
// each menu item. These sizes are used to compute the offset of the selected
// item so that _DropdownRoutePage can align the vertical center of the
// selected item lines up with the vertical center of the dropdown button,
// as closely as possible.
class _MenuItem<T> extends SingleChildRenderObjectWidget {
const _MenuItem({
super.key,
required this.onLayout,
required this.item,
}) : super(child: item);
final ValueChanged<Size> onLayout;
final DropdownMenuItem<T>? item;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderMenuItem(onLayout);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderMenuItem renderObject) {
renderObject.onLayout = onLayout;
}
}
class _RenderMenuItem extends RenderProxyBox {
_RenderMenuItem(this.onLayout, [RenderBox? child]) : super(child);
ValueChanged<Size> onLayout;
@override
void performLayout() {
super.performLayout();
onLayout(size);
}
}
// The container widget for a menu item created by a [DropdownButton]. It
// provides the default configuration for [DropdownMenuItem]s, as well as a
// [DropdownButton]'s hint and disabledHint widgets.
class _DropdownMenuItemContainer extends StatelessWidget {
/// Creates an item for a dropdown menu.
///
/// The [child] argument is required.
const _DropdownMenuItemContainer({
super.key,
this.alignment = AlignmentDirectional.centerStart,
required this.child,
});
/// The widget below this widget in the tree.
///
/// Typically a [Text] widget.
final Widget child;
/// Defines how the item is positioned within the container.
///
/// Defaults to [AlignmentDirectional.centerStart].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
@override
Widget build(BuildContext context) {
return Container(
constraints: const BoxConstraints(minHeight: _kMenuItemHeight),
alignment: alignment,
child: child,
);
}
}
/// An item in a menu created by a [DropdownButton].
///
/// The type `T` is the type of the value the entry represents. All the entries
/// in a given menu must represent values with consistent types.
class DropdownMenuItem<T> extends _DropdownMenuItemContainer {
/// Creates an item for a dropdown menu.
///
/// The [child] argument is required.
const DropdownMenuItem({
super.key,
this.onTap,
this.value,
this.enabled = true,
super.alignment,
required super.child,
});
/// Called when the dropdown menu item is tapped.
final VoidCallback? onTap;
/// The value to return if the user selects this menu item.
///
/// Eventually returned in a call to [DropdownButton.onChanged].
final T? value;
/// Whether or not a user can select this menu item.
///
/// Defaults to `true`.
final bool enabled;
}
/// An inherited widget that causes any descendant [DropdownButton]
/// widgets to not include their regular underline.
///
/// This is used by [DataTable] to remove the underline from any
/// [DropdownButton] widgets placed within material data tables, as
/// required by the Material Design specification.
class DropdownButtonHideUnderline extends InheritedWidget {
/// Creates a [DropdownButtonHideUnderline]. A non-null [child] must
/// be given.
const DropdownButtonHideUnderline({
super.key,
required super.child,
});
/// Returns whether the underline of [DropdownButton] widgets should
/// be hidden.
static bool at(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<DropdownButtonHideUnderline>() != null;
}
@override
bool updateShouldNotify(DropdownButtonHideUnderline oldWidget) => false;
}
/// A Material Design button for selecting from a list of items.
///
/// A dropdown button lets the user select from a number of items. The button
/// shows the currently selected item as well as an arrow that opens a menu for
/// selecting another item.
///
/// ## Updating to [DropdownMenu]
///
/// There is a Material 3 version of this component,
/// [DropdownMenu] that is preferred for applications that are configured
/// for Material 3 (see [ThemeData.useMaterial3]).
/// The [DropdownMenu] widget's visuals
/// are a little bit different, see the Material 3 spec at
/// <https://m3.material.io/components/menus/guidelines> for
/// more details.
///
/// The [DropdownMenu] widget's API is also slightly different.
/// To update from [DropdownButton] to [DropdownMenu], you will
/// need to make the following changes:
///
/// 1. Instead of using [DropdownButton.items], which
/// takes a list of [DropdownMenuItem]s, use
/// [DropdownMenu.dropdownMenuEntries], which
/// takes a list of [DropdownMenuEntry]'s.
///
/// 2. Instead of using [DropdownButton.onChanged],
/// use [DropdownMenu.onSelected], which is also
/// a callback that is called when the user selects an entry.
///
/// 3. In [DropdownMenu] it is not required to track
/// the current selection in your app's state.
/// So, instead of tracking the current selection in
/// the [DropdownButton.value] property, you can set the
/// [DropdownMenu.initialSelection] property to the
/// item that should be selected before there is any user action.
///
/// 4. You may also need to make changes to the styling of the
/// [DropdownMenu], see the properties in the [DropdownMenu]
/// constructor for more details.
///
/// See the sample below for an example of migrating
/// from [DropdownButton] to [DropdownMenu].
///
/// ## Using [DropdownButton]
/// {@youtube 560 315 https://www.youtube.com/watch?v=ZzQ_PWrFihg}
///
/// One ancestor must be a [Material] widget and typically this is
/// provided by the app's [Scaffold].
///
/// The type `T` is the type of the [value] that each dropdown item represents.
/// All the entries in a given menu must represent values with consistent types.
/// Typically, an enum is used. Each [DropdownMenuItem] in [items] must be
/// specialized with that same type argument.
///
/// The [onChanged] callback should update a state variable that defines the
/// dropdown's value. It should also call [State.setState] to rebuild the
/// dropdown with the new value.
///
///
/// {@tool dartpad}
/// This sample shows a [DropdownButton] with a large arrow icon,
/// purple text style, and bold purple underline, whose value is one of "One",
/// "Two", "Free", or "Four".
///
/// 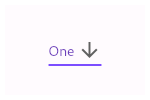
///
/// ** See code in examples/api/lib/material/dropdown/dropdown_button.0.dart **
/// {@end-tool}
///
/// If the [onChanged] callback is null or the list of [items] is null
/// then the dropdown button will be disabled, i.e. its arrow will be
/// displayed in grey and it will not respond to input. A disabled button
/// will display the [disabledHint] widget if it is non-null. However, if
/// [disabledHint] is null and [hint] is non-null, the [hint] widget will
/// instead be displayed.
///
/// {@tool dartpad}
/// This sample shows how you would rewrite the above [DropdownButton]
/// to use the [DropdownMenu].
///
/// ** See code in examples/api/lib/material/dropdown_menu/dropdown_menu.1.dart **
/// {@end-tool}
///
///
/// See also:
///
/// * [DropdownButtonFormField], which integrates with the [Form] widget.
/// * [DropdownMenuItem], the class used to represent the [items].
/// * [DropdownButtonHideUnderline], which prevents its descendant dropdown buttons
/// from displaying their underlines.
/// * [ElevatedButton], [TextButton], ordinary buttons that trigger a single action.
/// * <https://material.io/design/components/menus.html#dropdown-menu>
class DropdownButton<T> extends StatefulWidget {
/// Creates a dropdown button.
///
/// The [items] must have distinct values. If [value] isn't null then it
/// must be equal to one of the [DropdownMenuItem] values. If [items] or
/// [onChanged] is null, the button will be disabled, the down arrow
/// will be greyed out.
///
/// If [value] is null and the button is enabled, [hint] will be displayed
/// if it is non-null.
///
/// If [value] is null and the button is disabled, [disabledHint] will be displayed
/// if it is non-null. If [disabledHint] is null, then [hint] will be displayed
/// if it is non-null.
///
/// The [dropdownColor] argument specifies the background color of the
/// dropdown when it is open. If it is null, the current theme's
/// [ThemeData.canvasColor] will be used instead.
DropdownButton({
super.key,
required this.items,
this.selectedItemBuilder,
this.value,
this.hint,
this.disabledHint,
required this.onChanged,
this.onTap,
this.elevation = 8,
this.style,
this.underline,
this.icon,
this.iconDisabledColor,
this.iconEnabledColor,
this.iconSize = 24.0,
this.isDense = false,
this.isExpanded = false,
this.itemHeight = kMinInteractiveDimension,
this.focusColor,
this.focusNode,
this.autofocus = false,
this.dropdownColor,
this.menuMaxHeight,
this.enableFeedback,
this.alignment = AlignmentDirectional.centerStart,
this.borderRadius,
this.padding,
// When adding new arguments, consider adding similar arguments to
// DropdownButtonFormField.
}) : assert(items == null || items.isEmpty || value == null ||
items.where((DropdownMenuItem<T> item) {
return item.value == value;
}).length == 1,
"There should be exactly one item with [DropdownButton]'s value: "
'$value. \n'
'Either zero or 2 or more [DropdownMenuItem]s were detected '
'with the same value',
),
assert(itemHeight == null || itemHeight >= kMinInteractiveDimension),
_inputDecoration = null,
_isEmpty = false,
_isFocused = false;
DropdownButton._formField({
super.key,
required this.items,
this.selectedItemBuilder,
this.value,
this.hint,
this.disabledHint,
required this.onChanged,
this.onTap,
this.elevation = 8,
this.style,
this.underline,
this.icon,
this.iconDisabledColor,
this.iconEnabledColor,
this.iconSize = 24.0,
this.isDense = false,
this.isExpanded = false,
this.itemHeight = kMinInteractiveDimension,
this.focusColor,
this.focusNode,
this.autofocus = false,
this.dropdownColor,
this.menuMaxHeight,
this.enableFeedback,
this.alignment = AlignmentDirectional.centerStart,
this.borderRadius,
this.padding,
required InputDecoration inputDecoration,
required bool isEmpty,
required bool isFocused,
}) : assert(items == null || items.isEmpty || value == null ||
items.where((DropdownMenuItem<T> item) {
return item.value == value;
}).length == 1,
"There should be exactly one item with [DropdownButtonFormField]'s value: "
'$value. \n'
'Either zero or 2 or more [DropdownMenuItem]s were detected '
'with the same value',
),
assert(itemHeight == null || itemHeight >= kMinInteractiveDimension),
_inputDecoration = inputDecoration,
_isEmpty = isEmpty,
_isFocused = isFocused;
/// The list of items the user can select.
///
/// If the [onChanged] callback is null or the list of items is null
/// then the dropdown button will be disabled, i.e. its arrow will be
/// displayed in grey and it will not respond to input.
final List<DropdownMenuItem<T>>? items;
/// The value of the currently selected [DropdownMenuItem].
///
/// If [value] is null and the button is enabled, [hint] will be displayed
/// if it is non-null.
///
/// If [value] is null and the button is disabled, [disabledHint] will be displayed
/// if it is non-null. If [disabledHint] is null, then [hint] will be displayed
/// if it is non-null.
final T? value;
/// A placeholder widget that is displayed by the dropdown button.
///
/// If [value] is null and the dropdown is enabled ([items] and [onChanged] are non-null),
/// this widget is displayed as a placeholder for the dropdown button's value.
///
/// If [value] is null and the dropdown is disabled and [disabledHint] is null,
/// this widget is used as the placeholder.
final Widget? hint;
/// A preferred placeholder widget that is displayed when the dropdown is disabled.
///
/// If [value] is null, the dropdown is disabled ([items] or [onChanged] is null),
/// this widget is displayed as a placeholder for the dropdown button's value.
final Widget? disabledHint;
/// {@template flutter.material.dropdownButton.onChanged}
/// Called when the user selects an item.
///
/// If the [onChanged] callback is null or the list of [DropdownButton.items]
/// is null then the dropdown button will be disabled, i.e. its arrow will be
/// displayed in grey and it will not respond to input. A disabled button
/// will display the [DropdownButton.disabledHint] widget if it is non-null.
/// If [DropdownButton.disabledHint] is also null but [DropdownButton.hint] is
/// non-null, [DropdownButton.hint] will instead be displayed.
/// {@endtemplate}
final ValueChanged<T?>? onChanged;
/// Called when the dropdown button is tapped.
///
/// This is distinct from [onChanged], which is called when the user
/// selects an item from the dropdown.
///
/// The callback will not be invoked if the dropdown button is disabled.
final VoidCallback? onTap;
/// A builder to customize the dropdown buttons corresponding to the
/// [DropdownMenuItem]s in [items].
///
/// When a [DropdownMenuItem] is selected, the widget that will be displayed
/// from the list corresponds to the [DropdownMenuItem] of the same index
/// in [items].
///
/// {@tool dartpad}
/// This sample shows a `DropdownButton` with a button with [Text] that
/// corresponds to but is unique from [DropdownMenuItem].
///
/// ** See code in examples/api/lib/material/dropdown/dropdown_button.selected_item_builder.0.dart **
/// {@end-tool}
///
/// If this callback is null, the [DropdownMenuItem] from [items]
/// that matches [value] will be displayed.
final DropdownButtonBuilder? selectedItemBuilder;
/// The z-coordinate at which to place the menu when open.
///
/// The following elevations have defined shadows: 1, 2, 3, 4, 6, 8, 9, 12,
/// 16, and 24. See [kElevationToShadow].
///
/// Defaults to 8, the appropriate elevation for dropdown buttons.
final int elevation;
/// The text style to use for text in the dropdown button and the dropdown
/// menu that appears when you tap the button.
///
/// To use a separate text style for selected item when it's displayed within
/// the dropdown button, consider using [selectedItemBuilder].
///
/// {@tool dartpad}
/// This sample shows a `DropdownButton` with a dropdown button text style
/// that is different than its menu items.
///
/// ** See code in examples/api/lib/material/dropdown/dropdown_button.style.0.dart **
/// {@end-tool}
///
/// Defaults to the [TextTheme.titleMedium] value of the current
/// [ThemeData.textTheme] of the current [Theme].
final TextStyle? style;
/// The widget to use for drawing the drop-down button's underline.
///
/// Defaults to a 0.0 width bottom border with color 0xFFBDBDBD.
final Widget? underline;
/// The widget to use for the drop-down button's icon.
///
/// Defaults to an [Icon] with the [Icons.arrow_drop_down] glyph.
final Widget? icon;
/// The color of any [Icon] descendant of [icon] if this button is disabled,
/// i.e. if [onChanged] is null.
///
/// Defaults to [MaterialColor.shade400] of [Colors.grey] when the theme's
/// [ThemeData.brightness] is [Brightness.light] and to
/// [Colors.white10] when it is [Brightness.dark]
final Color? iconDisabledColor;
/// The color of any [Icon] descendant of [icon] if this button is enabled,
/// i.e. if [onChanged] is defined.
///
/// Defaults to [MaterialColor.shade700] of [Colors.grey] when the theme's
/// [ThemeData.brightness] is [Brightness.light] and to
/// [Colors.white70] when it is [Brightness.dark]
final Color? iconEnabledColor;
/// The size to use for the drop-down button's down arrow icon button.
///
/// Defaults to 24.0.
final double iconSize;
/// Reduce the button's height.
///
/// By default this button's height is the same as its menu items' heights.
/// If isDense is true, the button's height is reduced by about half. This
/// can be useful when the button is embedded in a container that adds
/// its own decorations, like [InputDecorator].
final bool isDense;
/// Set the dropdown's inner contents to horizontally fill its parent.
///
/// By default this button's inner width is the minimum size of its contents.
/// If [isExpanded] is true, the inner width is expanded to fill its
/// surrounding container.
final bool isExpanded;
/// If null, then the menu item heights will vary according to each menu item's
/// intrinsic height.
///
/// The default value is [kMinInteractiveDimension], which is also the minimum
/// height for menu items.
///
/// If this value is null and there isn't enough vertical room for the menu,
/// then the menu's initial scroll offset may not align the selected item with
/// the dropdown button. That's because, in this case, the initial scroll
/// offset is computed as if all of the menu item heights were
/// [kMinInteractiveDimension].
final double? itemHeight;
/// The color for the button's [Material] when it has the input focus.
final Color? focusColor;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// The background color of the dropdown.
///
/// If it is not provided, the theme's [ThemeData.canvasColor] will be used
/// instead.
final Color? dropdownColor;
/// Padding around the visible portion of the dropdown widget.
///
/// As the padding increases, the size of the [DropdownButton] will also
/// increase. The padding is included in the clickable area of the dropdown
/// widget, so this can make the widget easier to click.
///
/// Padding can be useful when used with a custom border. The clickable
/// area will stay flush with the border, as opposed to an external [Padding]
/// widget which will leave a non-clickable gap.
final EdgeInsetsGeometry? padding;
/// The maximum height of the menu.
///
/// The maximum height of the menu must be at least one row shorter than
/// the height of the app's view. This ensures that a tappable area
/// outside of the simple menu is present so the user can dismiss the menu.
///
/// If this property is set above the maximum allowable height threshold
/// mentioned above, then the menu defaults to being padded at the top
/// and bottom of the menu by at one menu item's height.
final double? menuMaxHeight;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// By default, platform-specific feedback is enabled.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// Defines how the hint or the selected item is positioned within the button.
///
/// Defaults to [AlignmentDirectional.centerStart].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// Defines the corner radii of the menu's rounded rectangle shape.
final BorderRadius? borderRadius;
final InputDecoration? _inputDecoration;
final bool _isEmpty;
final bool _isFocused;
@override
State<DropdownButton<T>> createState() => _DropdownButtonState<T>();
}
class _DropdownButtonState<T> extends State<DropdownButton<T>> with WidgetsBindingObserver {
int? _selectedIndex;
_DropdownRoute<T>? _dropdownRoute;
Orientation? _lastOrientation;
FocusNode? _internalNode;
FocusNode? get focusNode => widget.focusNode ?? _internalNode;
late Map<Type, Action<Intent>> _actionMap;
// Only used if needed to create _internalNode.
FocusNode _createFocusNode() {
return FocusNode(debugLabel: '${widget.runtimeType}');
}
@override
void initState() {
super.initState();
_updateSelectedIndex();
if (widget.focusNode == null) {
_internalNode ??= _createFocusNode();
}
_actionMap = <Type, Action<Intent>>{
ActivateIntent: CallbackAction<ActivateIntent>(
onInvoke: (ActivateIntent intent) => _handleTap(),
),
ButtonActivateIntent: CallbackAction<ButtonActivateIntent>(
onInvoke: (ButtonActivateIntent intent) => _handleTap(),
),
};
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
_removeDropdownRoute();
_internalNode?.dispose();
super.dispose();
}
void _removeDropdownRoute() {
_dropdownRoute?._dismiss();
_dropdownRoute = null;
_lastOrientation = null;
}
@override
void didUpdateWidget(DropdownButton<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.focusNode == null) {
_internalNode ??= _createFocusNode();
}
_updateSelectedIndex();
}
void _updateSelectedIndex() {
if (widget.items == null
|| widget.items!.isEmpty
|| (widget.value == null &&
widget.items!
.where((DropdownMenuItem<T> item) => item.enabled && item.value == widget.value)
.isEmpty)) {
_selectedIndex = null;
return;
}
assert(widget.items!.where((DropdownMenuItem<T> item) => item.value == widget.value).length == 1);
for (int itemIndex = 0; itemIndex < widget.items!.length; itemIndex++) {
if (widget.items![itemIndex].value == widget.value) {
_selectedIndex = itemIndex;
return;
}
}
}
TextStyle? get _textStyle => widget.style ?? Theme.of(context).textTheme.titleMedium;
void _handleTap() {
final TextDirection? textDirection = Directionality.maybeOf(context);
final EdgeInsetsGeometry menuMargin = ButtonTheme.of(context).alignedDropdown
? _kAlignedMenuMargin
: _kUnalignedMenuMargin;
final List<_MenuItem<T>> menuItems = <_MenuItem<T>>[
for (int index = 0; index < widget.items!.length; index += 1)
_MenuItem<T>(
item: widget.items![index],
onLayout: (Size size) {
// If [_dropdownRoute] is null and onLayout is called, this means
// that performLayout was called on a _DropdownRoute that has not
// left the widget tree but is already on its way out.
//
// Since onLayout is used primarily to collect the desired heights
// of each menu item before laying them out, not having the _DropdownRoute
// collect each item's height to lay out is fine since the route is
// already on its way out.
if (_dropdownRoute == null) {
return;
}
_dropdownRoute!.itemHeights[index] = size.height;
},
),
];
final NavigatorState navigator = Navigator.of(context);
assert(_dropdownRoute == null);
final RenderBox itemBox = context.findRenderObject()! as RenderBox;
final Rect itemRect = itemBox.localToGlobal(Offset.zero, ancestor: navigator.context.findRenderObject()) & itemBox.size;
_dropdownRoute = _DropdownRoute<T>(
items: menuItems,
buttonRect: menuMargin.resolve(textDirection).inflateRect(itemRect),
padding: _kMenuItemPadding.resolve(textDirection),
selectedIndex: _selectedIndex ?? 0,
elevation: widget.elevation,
capturedThemes: InheritedTheme.capture(from: context, to: navigator.context),
style: _textStyle!,
barrierLabel: MaterialLocalizations.of(context).modalBarrierDismissLabel,
itemHeight: widget.itemHeight,
dropdownColor: widget.dropdownColor,
menuMaxHeight: widget.menuMaxHeight,
enableFeedback: widget.enableFeedback ?? true,
borderRadius: widget.borderRadius,
);
focusNode?.requestFocus();
navigator.push(_dropdownRoute!).then<void>((_DropdownRouteResult<T>? newValue) {
_removeDropdownRoute();
if (!mounted || newValue == null) {
return;
}
widget.onChanged?.call(newValue.result);
});
widget.onTap?.call();
}
// When isDense is true, reduce the height of this button from _kMenuItemHeight to
// _kDenseButtonHeight, but don't make it smaller than the text that it contains.
// Similarly, we don't reduce the height of the button so much that its icon
// would be clipped.
double get _denseButtonHeight {
final double fontSize = _textStyle!.fontSize ?? Theme.of(context).textTheme.titleMedium!.fontSize!;
final double scaledFontSize = MediaQuery.textScalerOf(context).scale(fontSize);
return math.max(scaledFontSize, math.max(widget.iconSize, _kDenseButtonHeight));
}
Color get _iconColor {
// These colors are not defined in the Material Design spec.
final Brightness brightness = Theme.of(context).brightness;
if (_enabled) {
return widget.iconEnabledColor ?? switch (brightness) {
Brightness.light => Colors.grey.shade700,
Brightness.dark => Colors.white70,
};
} else {
return widget.iconDisabledColor ?? switch (brightness) {
Brightness.light => Colors.grey.shade400,
Brightness.dark => Colors.white10,
};
}
}
bool get _enabled => widget.items != null && widget.items!.isNotEmpty && widget.onChanged != null;
Orientation _getOrientation(BuildContext context) {
Orientation? result = MediaQuery.maybeOrientationOf(context);
if (result == null) {
// If there's no MediaQuery, then use the view aspect to determine
// orientation.
final Size size = View.of(context).physicalSize;
result = size.width > size.height ? Orientation.landscape : Orientation.portrait;
}
return result;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMaterialLocalizations(context));
final Orientation newOrientation = _getOrientation(context);
_lastOrientation ??= newOrientation;
if (newOrientation != _lastOrientation) {
_removeDropdownRoute();
_lastOrientation = newOrientation;
}
// The width of the button and the menu are defined by the widest
// item and the width of the hint.
// We should explicitly type the items list to be a list of <Widget>,
// otherwise, no explicit type adding items maybe trigger a crash/failure
// when hint and selectedItemBuilder are provided.
final List<Widget> items = widget.selectedItemBuilder == null
? (widget.items != null ? List<Widget>.of(widget.items!) : <Widget>[])
: List<Widget>.of(widget.selectedItemBuilder!(context));
int? hintIndex;
if (widget.hint != null || (!_enabled && widget.disabledHint != null)) {
final Widget displayedHint = _enabled ? widget.hint! : widget.disabledHint ?? widget.hint!;
hintIndex = items.length;
items.add(DefaultTextStyle(
style: _textStyle!.copyWith(color: Theme.of(context).hintColor),
child: IgnorePointer(
child: _DropdownMenuItemContainer(
alignment: widget.alignment,
child: displayedHint,
),
),
));
}
final EdgeInsetsGeometry padding = ButtonTheme.of(context).alignedDropdown
? _kAlignedButtonPadding
: _kUnalignedButtonPadding;
// If value is null (then _selectedIndex is null) then we
// display the hint or nothing at all.
final Widget innerItemsWidget;
if (items.isEmpty) {
innerItemsWidget = const SizedBox.shrink();
} else {
innerItemsWidget = IndexedStack(
index: _selectedIndex ?? hintIndex,
alignment: widget.alignment,
children: widget.isDense ? items : items.map((Widget item) {
return widget.itemHeight != null
? SizedBox(height: widget.itemHeight, child: item)
: Column(mainAxisSize: MainAxisSize.min, children: <Widget>[item]);
}).toList(),
);
}
const Icon defaultIcon = Icon(Icons.arrow_drop_down);
Widget result = DefaultTextStyle(
style: _enabled ? _textStyle! : _textStyle!.copyWith(color: Theme.of(context).disabledColor),
child: Container(
padding: padding.resolve(Directionality.of(context)),
height: widget.isDense ? _denseButtonHeight : null,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
if (widget.isExpanded)
Expanded(child: innerItemsWidget)
else
innerItemsWidget,
IconTheme(
data: IconThemeData(
color: _iconColor,
size: widget.iconSize,
),
child: widget.icon ?? defaultIcon,
),
],
),
),
);
if (!DropdownButtonHideUnderline.at(context)) {
final double bottom = (widget.isDense || widget.itemHeight == null) ? 0.0 : 8.0;
result = Stack(
children: <Widget>[
result,
Positioned(
left: 0.0,
right: 0.0,
bottom: bottom,
child: widget.underline ?? Container(
height: 1.0,
decoration: const BoxDecoration(
border: Border(
bottom: BorderSide(
color: Color(0xFFBDBDBD),
width: 0.0,
),
),
),
),
),
],
);
}
final MouseCursor effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor>(
MaterialStateMouseCursor.clickable,
<MaterialState>{
if (!_enabled) MaterialState.disabled,
},
);
if (widget._inputDecoration != null) {
result = InputDecorator(
decoration: widget._inputDecoration!,
isEmpty: widget._isEmpty,
isFocused: widget._isFocused,
child: result,
);
}
return Semantics(
button: true,
child: Actions(
actions: _actionMap,
child: InkWell(
mouseCursor: effectiveMouseCursor,
onTap: _enabled ? _handleTap : null,
canRequestFocus: _enabled,
borderRadius: widget.borderRadius,
focusNode: focusNode,
autofocus: widget.autofocus,
focusColor: widget.focusColor ?? Theme.of(context).focusColor,
enableFeedback: false,
child: widget.padding == null ? result : Padding(padding: widget.padding!, child: result),
),
),
);
}
}
/// A [FormField] that contains a [DropdownButton].
///
/// This is a convenience widget that wraps a [DropdownButton] widget in a
/// [FormField].
///
/// A [Form] ancestor is not required. The [Form] allows one to
/// save, reset, or validate multiple fields at once. To use without a [Form],
/// pass a [GlobalKey] to the constructor and use [GlobalKey.currentState] to
/// save or reset the form field.
///
/// The `value` parameter maps to [FormField.initialValue].
///
/// See also:
///
/// * [DropdownButton], which is the underlying text field without the [Form]
/// integration.
class DropdownButtonFormField<T> extends FormField<T> {
/// Creates a [DropdownButton] widget that is a [FormField], wrapped in an
/// [InputDecorator].
///
/// For a description of the `onSaved`, `validator`, or `autovalidateMode`
/// parameters, see [FormField]. For the rest (other than [decoration]), see
/// [DropdownButton].
DropdownButtonFormField({
super.key,
required List<DropdownMenuItem<T>>? items,
DropdownButtonBuilder? selectedItemBuilder,
T? value,
Widget? hint,
Widget? disabledHint,
required this.onChanged,
VoidCallback? onTap,
int elevation = 8,
TextStyle? style,
Widget? icon,
Color? iconDisabledColor,
Color? iconEnabledColor,
double iconSize = 24.0,
bool isDense = true,
bool isExpanded = false,
double? itemHeight,
Color? focusColor,
FocusNode? focusNode,
bool autofocus = false,
Color? dropdownColor,
InputDecoration? decoration,
super.onSaved,
super.validator,
AutovalidateMode? autovalidateMode,
double? menuMaxHeight,
bool? enableFeedback,
AlignmentGeometry alignment = AlignmentDirectional.centerStart,
BorderRadius? borderRadius,
EdgeInsetsGeometry? padding,
// When adding new arguments, consider adding similar arguments to
// DropdownButton.
}) : assert(items == null || items.isEmpty || value == null ||
items.where((DropdownMenuItem<T> item) {
return item.value == value;
}).length == 1,
"There should be exactly one item with [DropdownButton]'s value: "
'$value. \n'
'Either zero or 2 or more [DropdownMenuItem]s were detected '
'with the same value',
),
assert(itemHeight == null || itemHeight >= kMinInteractiveDimension),
decoration = decoration ?? InputDecoration(focusColor: focusColor),
super(
initialValue: value,
autovalidateMode: autovalidateMode ?? AutovalidateMode.disabled,
builder: (FormFieldState<T> field) {
final _DropdownButtonFormFieldState<T> state = field as _DropdownButtonFormFieldState<T>;
final InputDecoration decorationArg = decoration ?? InputDecoration(focusColor: focusColor);
final InputDecoration effectiveDecoration = decorationArg.applyDefaults(
Theme.of(field.context).inputDecorationTheme,
);
final bool showSelectedItem = items != null && items.where((DropdownMenuItem<T> item) => item.value == state.value).isNotEmpty;
bool isHintOrDisabledHintAvailable() {
final bool isDropdownDisabled = onChanged == null || (items == null || items.isEmpty);
if (isDropdownDisabled) {
return hint != null || disabledHint != null;
} else {
return hint != null;
}
}
final bool isEmpty = !showSelectedItem && !isHintOrDisabledHintAvailable();
final bool hasError = effectiveDecoration.errorText != null;
// An unfocusable Focus widget so that this widget can detect if its
// descendants have focus or not.
return Focus(
canRequestFocus: false,
skipTraversal: true,
child: Builder(builder: (BuildContext context) {
final bool isFocused = Focus.of(context).hasFocus;
InputBorder? resolveInputBorder() {
if (hasError) {
if (isFocused) {
return effectiveDecoration.focusedErrorBorder;
}
return effectiveDecoration.errorBorder;
}
if (isFocused) {
return effectiveDecoration.focusedBorder;
}
if (effectiveDecoration.enabled) {
return effectiveDecoration.enabledBorder;
}
return effectiveDecoration.border;
}
BorderRadius? effectiveBorderRadius() {
final InputBorder? inputBorder = resolveInputBorder();
if (inputBorder is OutlineInputBorder) {
return inputBorder.borderRadius;
}
if (inputBorder is UnderlineInputBorder) {
return inputBorder.borderRadius;
}
return null;
}
return DropdownButtonHideUnderline(
child: DropdownButton<T>._formField(
items: items,
selectedItemBuilder: selectedItemBuilder,
value: state.value,
hint: hint,
disabledHint: disabledHint,
onChanged: onChanged == null ? null : state.didChange,
onTap: onTap,
elevation: elevation,
style: style,
icon: icon,
iconDisabledColor: iconDisabledColor,
iconEnabledColor: iconEnabledColor,
iconSize: iconSize,
isDense: isDense,
isExpanded: isExpanded,
itemHeight: itemHeight,
focusColor: focusColor,
focusNode: focusNode,
autofocus: autofocus,
dropdownColor: dropdownColor,
menuMaxHeight: menuMaxHeight,
enableFeedback: enableFeedback,
alignment: alignment,
borderRadius: borderRadius ?? effectiveBorderRadius(),
inputDecoration: effectiveDecoration.copyWith(errorText: field.errorText),
isEmpty: isEmpty,
isFocused: isFocused,
padding: padding,
),
);
}),
);
},
);
/// {@macro flutter.material.dropdownButton.onChanged}
final ValueChanged<T?>? onChanged;
/// The decoration to show around the dropdown button form field.
///
/// By default, draws a horizontal line under the dropdown button field but
/// can be configured to show an icon, label, hint text, and error text.
///
/// If not specified, an [InputDecorator] with the `focusColor` set to the
/// supplied `focusColor` (if any) will be used.
final InputDecoration decoration;
@override
FormFieldState<T> createState() => _DropdownButtonFormFieldState<T>();
}
class _DropdownButtonFormFieldState<T> extends FormFieldState<T> {
DropdownButtonFormField<T> get _dropdownButtonFormField => widget as DropdownButtonFormField<T>;
@override
void didChange(T? value) {
super.didChange(value);
_dropdownButtonFormField.onChanged!(value);
}
@override
void didUpdateWidget(DropdownButtonFormField<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.initialValue != widget.initialValue) {
setValue(widget.initialValue);
}
}
@override
void reset() {
super.reset();
_dropdownButtonFormField.onChanged!(value);
}
}
| flutter/packages/flutter/lib/src/material/dropdown.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/dropdown.dart",
"repo_id": "flutter",
"token_count": 22451
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'scaffold.dart';
/// The margin that a [FloatingActionButton] should leave between it and the
/// edge of the screen.
///
/// [FloatingActionButtonLocation.endFloat] uses this to set the appropriate margin
/// between the [FloatingActionButton] and the end of the screen.
const double kFloatingActionButtonMargin = 16.0;
/// The amount of time the [FloatingActionButton] takes to transition in or out.
///
/// The [Scaffold] uses this to set the duration of [FloatingActionButton]
/// motion, entrance, and exit animations.
const Duration kFloatingActionButtonSegue = Duration(milliseconds: 200);
/// The fraction of a circle the [FloatingActionButton] should turn when it enters.
///
/// Its value corresponds to 0.125 of a full circle, equivalent to 45 degrees or pi/4 radians.
const double kFloatingActionButtonTurnInterval = 0.125;
/// If a [FloatingActionButton] is used on a [Scaffold] in certain positions,
/// it is moved [kMiniButtonOffsetAdjustment] pixels closer to the edge of the screen.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// More specifically:
/// * In the following positions, the [FloatingActionButton] is moved *horizontally*
/// closer to the edge of the screen:
/// * [FloatingActionButtonLocation.miniStartTop]
/// * [FloatingActionButtonLocation.miniStartFloat]
/// * [FloatingActionButtonLocation.miniStartDocked]
/// * [FloatingActionButtonLocation.miniEndTop]
/// * [FloatingActionButtonLocation.miniEndFloat]
/// * [FloatingActionButtonLocation.miniEndDocked]
/// * In the following positions, the [FloatingActionButton] is moved *vertically*
/// closer to the bottom of the screen:
/// * [FloatingActionButtonLocation.miniStartFloat]
/// * [FloatingActionButtonLocation.miniCenterFloat]
/// * [FloatingActionButtonLocation.miniEndFloat]
const double kMiniButtonOffsetAdjustment = 4.0;
/// An object that defines a position for the [FloatingActionButton]
/// based on the [Scaffold]'s [ScaffoldPrelayoutGeometry].
///
/// Flutter provides [FloatingActionButtonLocation]s for the common
/// [FloatingActionButton] placements in Material Design applications. These
/// locations are available as static members of this class.
///
/// ## Floating Action Button placements
///
/// The following diagrams show the available placement locations for the FloatingActionButton.
///
/// * [FloatingActionButtonLocation.centerDocked]:
///
/// 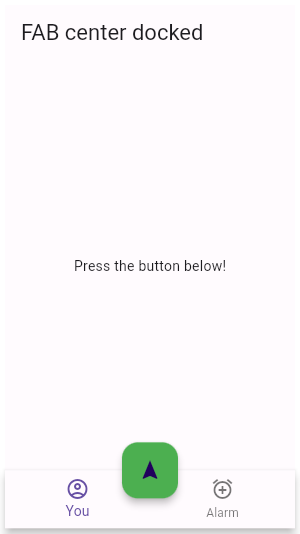
///
///
/// * [FloatingActionButtonLocation.centerFloat]:
///
/// 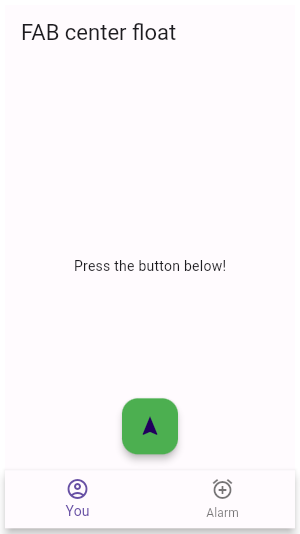
///
///
/// * [FloatingActionButtonLocation.centerTop]:
///
/// 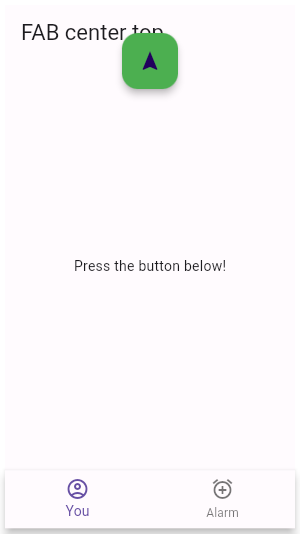
///
///
/// * [FloatingActionButtonLocation.endDocked]:
///
/// 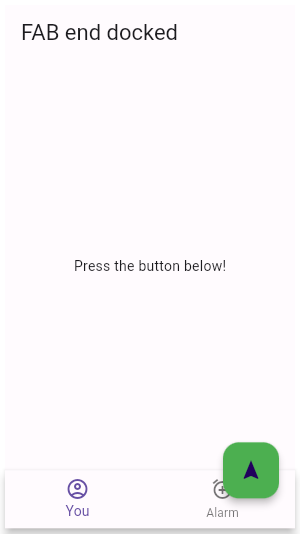
///
///
/// * [FloatingActionButtonLocation.endFloat]:
///
/// 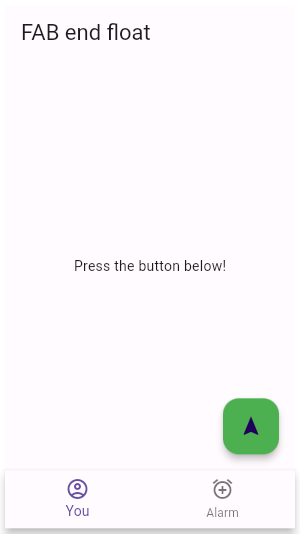
///
///
/// * [FloatingActionButtonLocation.endTop]:
///
/// 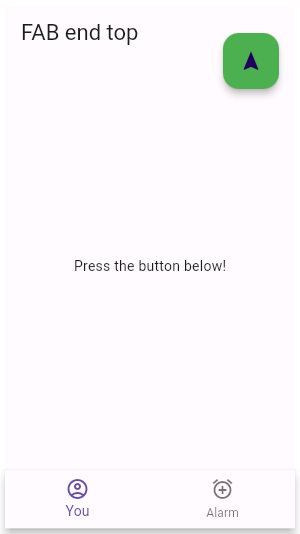
///
///
/// * [FloatingActionButtonLocation.startDocked]:
///
/// 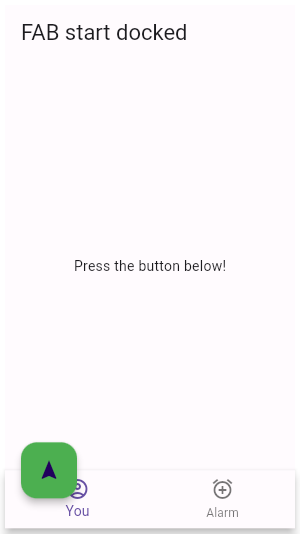
///
///
/// * [FloatingActionButtonLocation.startFloat]:
///
/// 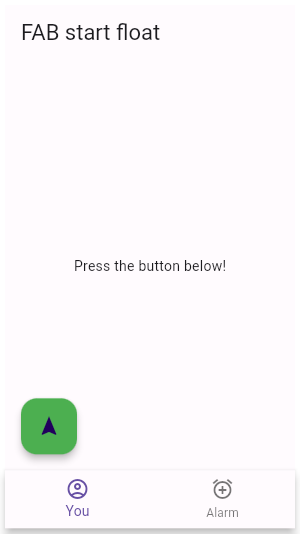
///
///
/// * [FloatingActionButtonLocation.startTop]:
///
/// 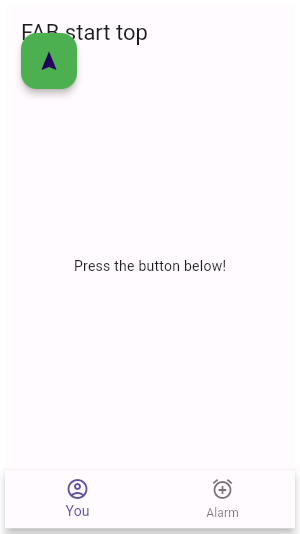
///
///
/// * [FloatingActionButtonLocation.miniCenterDocked]:
///
/// 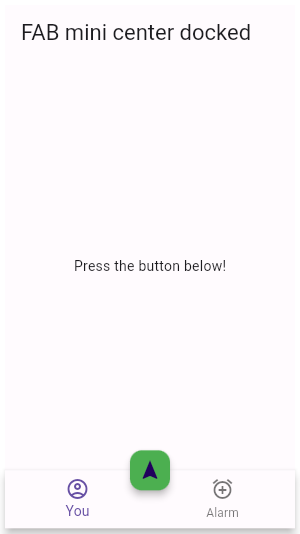
///
///
/// * [FloatingActionButtonLocation.miniCenterFloat]:
///
/// 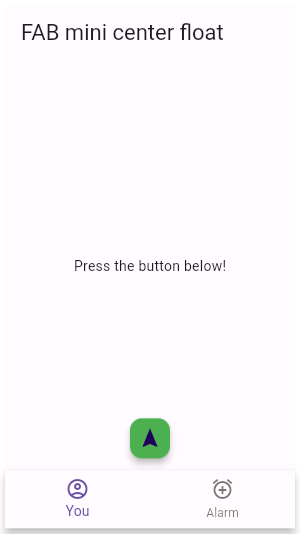
///
///
/// * [FloatingActionButtonLocation.miniCenterTop]:
///
/// 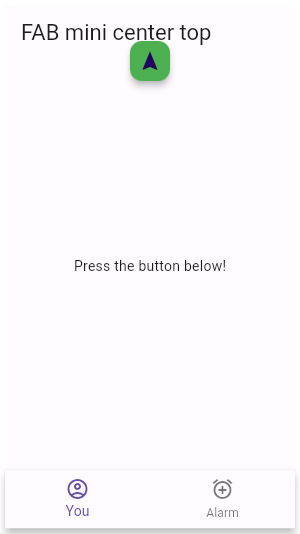
///
///
/// * [FloatingActionButtonLocation.miniEndDocked]:
///
/// 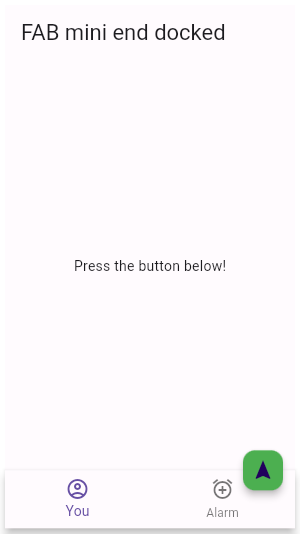
///
///
/// * [FloatingActionButtonLocation.miniEndFloat]:
///
/// 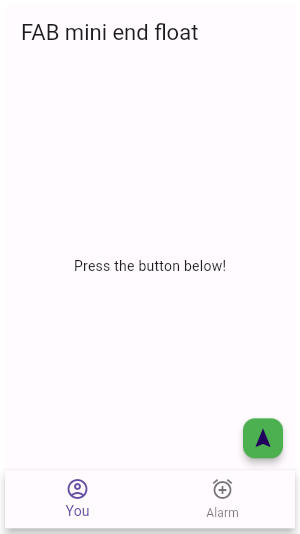
///
///
/// * [FloatingActionButtonLocation.miniEndTop]:
///
/// 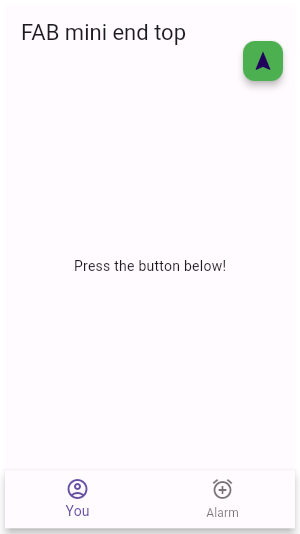
///
///
/// * [FloatingActionButtonLocation.miniStartDocked]:
///
/// 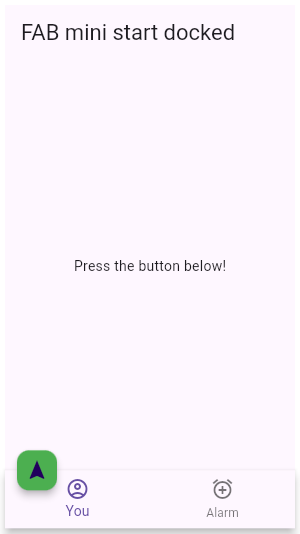
///
///
/// * [FloatingActionButtonLocation.miniStartFloat]:
///
/// 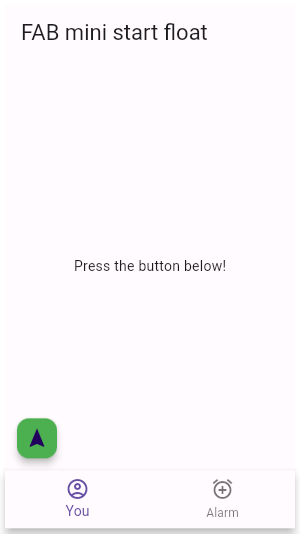
///
///
/// * [FloatingActionButtonLocation.miniStartTop]:
///
/// 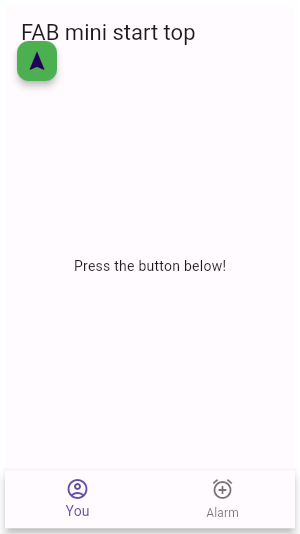
///
///
/// See also:
///
/// * [FloatingActionButton], which is a circular button typically shown in the
/// bottom right corner of the app.
/// * [FloatingActionButtonAnimator], which is used to animate the
/// [Scaffold.floatingActionButton] from one [FloatingActionButtonLocation] to
/// another.
/// * [ScaffoldPrelayoutGeometry], the geometry that
/// [FloatingActionButtonLocation]s use to position the [FloatingActionButton].
abstract class FloatingActionButtonLocation {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const FloatingActionButtonLocation();
/// Start-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartTop] and set [FloatingActionButton.mini] to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 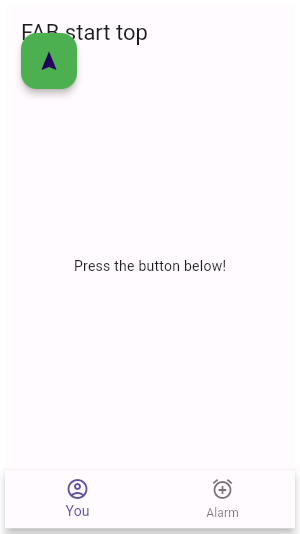
static const FloatingActionButtonLocation startTop = _StartTopFabLocation();
/// Start-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], optimized for mini floating
/// action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 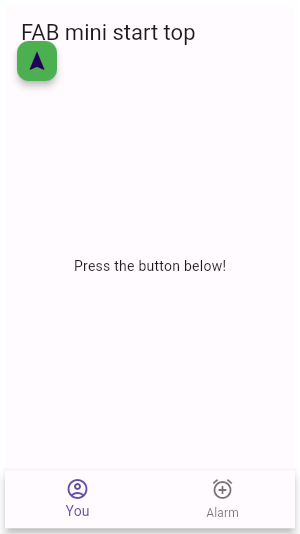
static const FloatingActionButtonLocation miniStartTop = _MiniStartTopFabLocation();
/// Centered [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 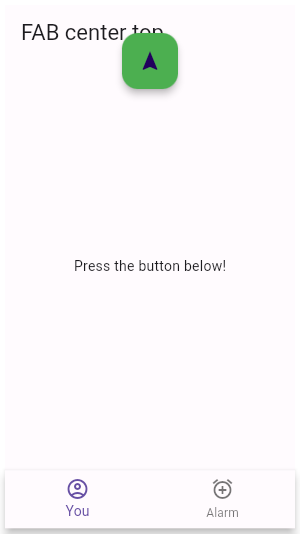
static const FloatingActionButtonLocation centerTop = _CenterTopFabLocation();
/// Centered [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], intended to be used with
/// [FloatingActionButton.mini] set to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 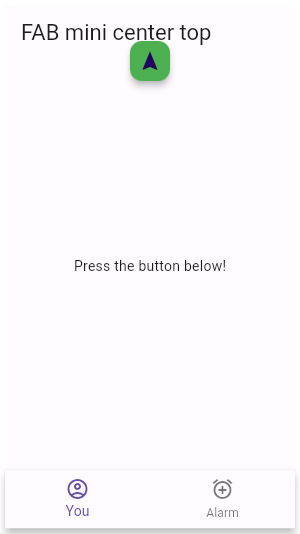
static const FloatingActionButtonLocation miniCenterTop = _MiniCenterTopFabLocation();
/// End-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndTop] and set [FloatingActionButton.mini] to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 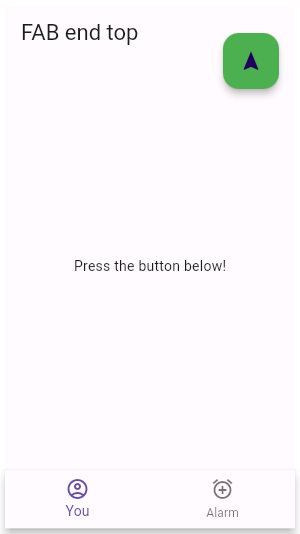
static const FloatingActionButtonLocation endTop = _EndTopFabLocation();
/// End-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], optimized for mini floating
/// action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 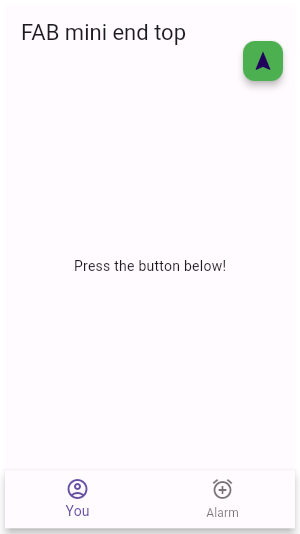
static const FloatingActionButtonLocation miniEndTop = _MiniEndTopFabLocation();
/// Start-aligned [FloatingActionButton], floating at the bottom of the screen.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartFloat] and set [FloatingActionButton.mini] to true.
///
/// 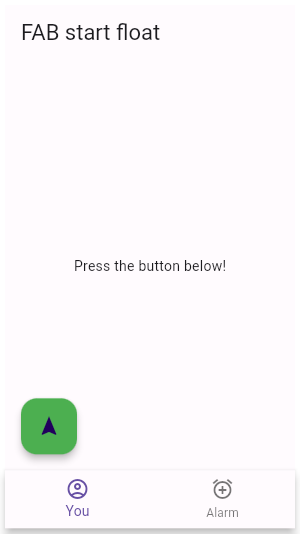
static const FloatingActionButtonLocation startFloat = _StartFloatFabLocation();
/// Start-aligned [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// Compared to [FloatingActionButtonLocation.startFloat], floating action
/// buttons using this location will move horizontally _and_ vertically
/// closer to the edges, by [kMiniButtonOffsetAdjustment] each.
///
/// 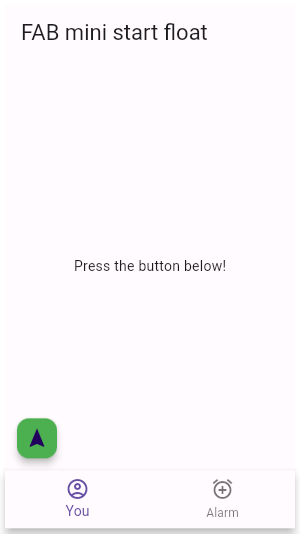
static const FloatingActionButtonLocation miniStartFloat = _MiniStartFloatFabLocation();
/// Centered [FloatingActionButton], floating at the bottom of the screen.
///
/// To position a mini floating action button, use [miniCenterFloat] and
/// set [FloatingActionButton.mini] to true.
///
/// 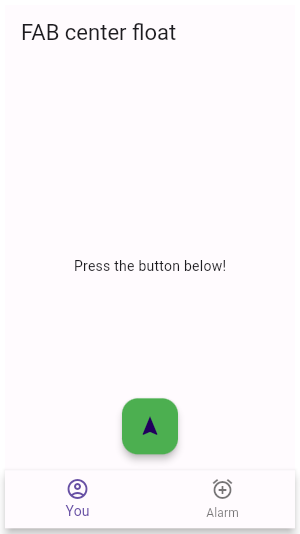
static const FloatingActionButtonLocation centerFloat = _CenterFloatFabLocation();
/// Centered [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align horizontally with
/// the locations [FloatingActionButtonLocation.miniStartFloat]
/// and [FloatingActionButtonLocation.miniEndFloat].
///
/// Compared to [FloatingActionButtonLocation.centerFloat], floating action
/// buttons using this location will move vertically down
/// by [kMiniButtonOffsetAdjustment].
///
/// 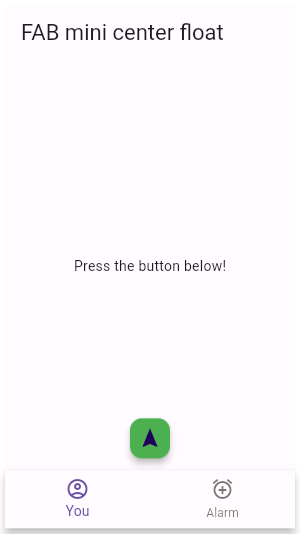
static const FloatingActionButtonLocation miniCenterFloat = _MiniCenterFloatFabLocation();
/// End-aligned [FloatingActionButton], floating at the bottom of the screen.
///
/// This is the default alignment of [FloatingActionButton]s in Material applications.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndFloat] and set [FloatingActionButton.mini] to true.
///
/// 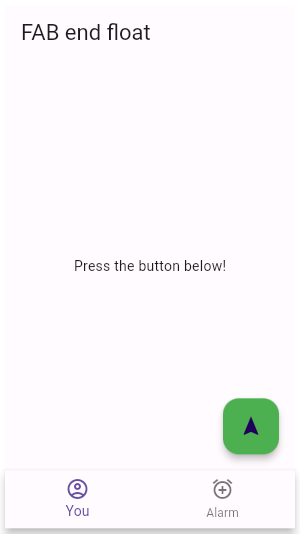
static const FloatingActionButtonLocation endFloat = _EndFloatFabLocation();
/// End-aligned [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// Compared to [FloatingActionButtonLocation.endFloat], floating action
/// buttons using this location will move horizontally _and_ vertically
/// closer to the edges, by [kMiniButtonOffsetAdjustment] each.
///
/// 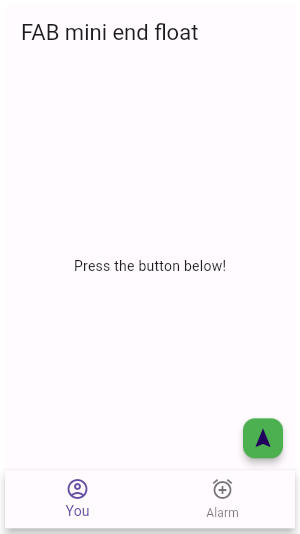
static const FloatingActionButtonLocation miniEndFloat = _MiniEndFloatFabLocation();
/// Start-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartDocked] and set [FloatingActionButton.mini] to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 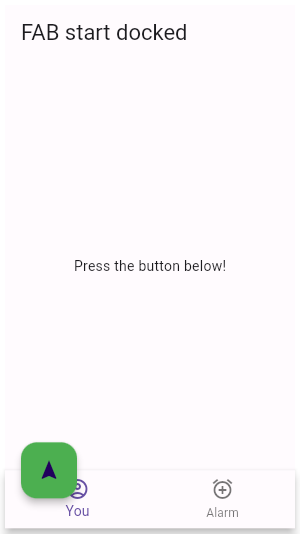
static const FloatingActionButtonLocation startDocked = _StartDockedFabLocation();
/// Start-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar,
/// optimized for mini floating action buttons.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 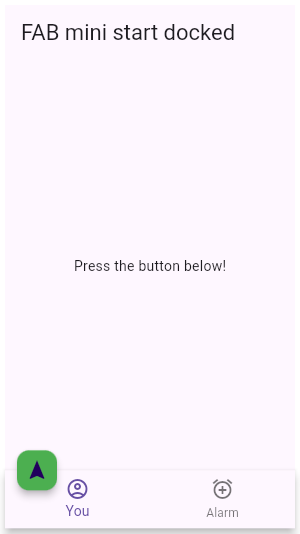
static const FloatingActionButtonLocation miniStartDocked = _MiniStartDockedFabLocation();
/// Centered [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 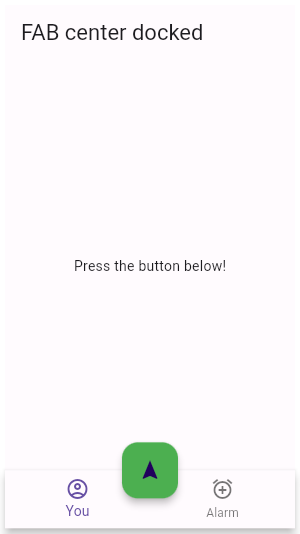
static const FloatingActionButtonLocation centerDocked = _CenterDockedFabLocation();
/// Centered [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar;
/// intended to be used with [FloatingActionButton.mini] set to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 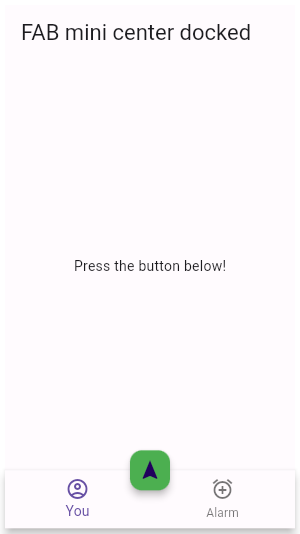
static const FloatingActionButtonLocation miniCenterDocked = _MiniCenterDockedFabLocation();
/// End-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 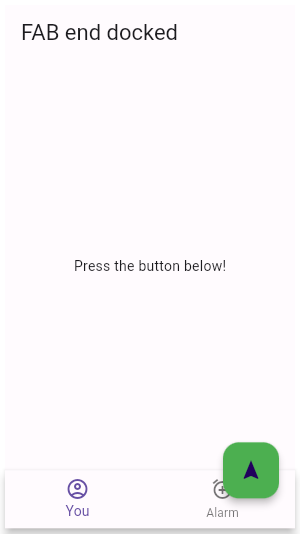
static const FloatingActionButtonLocation endDocked = _EndDockedFabLocation();
/// End-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar,
/// optimized for mini floating action buttons.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndDocked] and set [FloatingActionButton.mini] to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 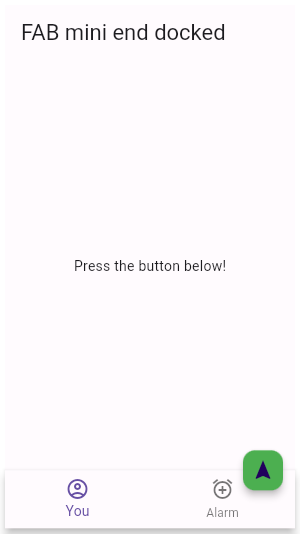
static const FloatingActionButtonLocation miniEndDocked = _MiniEndDockedFabLocation();
/// End-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the floating
/// action button lines up with the center of the bottom navigation bar.
///
/// This is unlikely to be a useful location for apps which has a [BottomNavigationBar]
/// or a non material 3 [BottomAppBar].
///
/// 
static const FloatingActionButtonLocation endContained = _EndContainedFabLocation();
/// Places the [FloatingActionButton] based on the [Scaffold]'s layout.
///
/// This uses a [ScaffoldPrelayoutGeometry], which the [Scaffold] constructs
/// during its layout phase after it has laid out every widget it can lay out
/// except the [FloatingActionButton]. The [Scaffold] uses the [Offset]
/// returned from this method to position the [FloatingActionButton] and
/// complete its layout.
Offset getOffset(ScaffoldPrelayoutGeometry scaffoldGeometry);
@override
String toString() => objectRuntimeType(this, 'FloatingActionButtonLocation');
}
/// A base class that simplifies building [FloatingActionButtonLocation]s when
/// used with mixins [FabTopOffsetY], [FabFloatOffsetY], [FabDockedOffsetY],
/// [FabStartOffsetX], [FabCenterOffsetX], [FabEndOffsetX], and [FabMiniOffsetAdjustment].
///
/// A subclass of [FloatingActionButtonLocation] which implements its [getOffset] method
/// using three other methods: [getOffsetX], [getOffsetY], and [isMini].
///
/// Different mixins on this class override different methods, so that combining
/// a set of mixins creates a floating action button location.
///
/// For example: the location [FloatingActionButtonLocation.miniEndTop]
/// is based on a class that extends [StandardFabLocation]
/// with mixins [FabMiniOffsetAdjustment], [FabEndOffsetX], and [FabTopOffsetY].
///
/// You can create your own subclass of [StandardFabLocation]
/// to implement a custom [FloatingActionButtonLocation].
///
/// {@tool dartpad}
/// This is an example of a user-defined [FloatingActionButtonLocation].
///
/// The example shows a [Scaffold] with an [AppBar], a [BottomAppBar], and a
/// [FloatingActionButton] using a custom [FloatingActionButtonLocation].
///
/// The new [FloatingActionButtonLocation] is defined
/// by extending [StandardFabLocation] with two mixins,
/// [FabEndOffsetX] and [FabFloatOffsetY], and overriding the
/// [getOffsetX] method to adjust the FAB's x-coordinate, creating a
/// [FloatingActionButtonLocation] slightly different from
/// [FloatingActionButtonLocation.endFloat].
///
/// ** See code in examples/api/lib/material/floating_action_button_location/standard_fab_location.0.dart **
/// {@end-tool}
///
abstract class StandardFabLocation extends FloatingActionButtonLocation {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const StandardFabLocation();
/// Obtains the x-offset to place the [FloatingActionButton] based on the
/// [Scaffold]'s layout.
///
/// Used by [getOffset] to compute its x-coordinate.
double getOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment);
/// Obtains the y-offset to place the [FloatingActionButton] based on the
/// [Scaffold]'s layout.
///
/// Used by [getOffset] to compute its y-coordinate.
double getOffsetY(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment);
/// A function returning whether this [StandardFabLocation] is optimized for
/// mini [FloatingActionButton]s.
bool isMini () => false;
@override
Offset getOffset(ScaffoldPrelayoutGeometry scaffoldGeometry) {
final double adjustment = isMini() ? kMiniButtonOffsetAdjustment : 0.0;
return Offset(
getOffsetX(scaffoldGeometry, adjustment),
getOffsetY(scaffoldGeometry, adjustment),
);
}
/// Calculates x-offset for left-aligned [FloatingActionButtonLocation]s.
static double _leftOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return kFloatingActionButtonMargin
+ scaffoldGeometry.minInsets.left
- adjustment;
}
/// Calculates x-offset for right-aligned [FloatingActionButtonLocation]s.
static double _rightOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return scaffoldGeometry.scaffoldSize.width
- kFloatingActionButtonMargin
- scaffoldGeometry.minInsets.right
- scaffoldGeometry.floatingActionButtonSize.width
+ adjustment;
}
}
/// Mixin for a "top" floating action button location, such as
/// [FloatingActionButtonLocation.startTop].
///
/// The `adjustment`, typically [kMiniButtonOffsetAdjustment], is ignored in the
/// Y axis of "top" positions. For "top" positions, the X offset is adjusted to
/// move closer to the edge of the screen. This is so that a minified floating
/// action button appears to align with [CircleAvatar]s in the
/// [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
mixin FabTopOffsetY on StandardFabLocation {
/// Calculates y-offset for [FloatingActionButtonLocation]s floating over
/// the transition between the [Scaffold.appBar] and the [Scaffold.body].
@override
double getOffsetY(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
if (scaffoldGeometry.contentTop > scaffoldGeometry.minViewPadding.top) {
final double fabHalfHeight = scaffoldGeometry.floatingActionButtonSize.height / 2.0;
return scaffoldGeometry.contentTop - fabHalfHeight;
}
// Otherwise, ensure we are placed within the bounds of a safe area.
return scaffoldGeometry.minViewPadding.top;
}
}
/// Mixin for a "float" floating action button location, such as [FloatingActionButtonLocation.centerFloat].
mixin FabFloatOffsetY on StandardFabLocation {
/// Calculates y-offset for [FloatingActionButtonLocation]s floating at
/// the bottom of the screen.
@override
double getOffsetY(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
final double contentBottom = scaffoldGeometry.contentBottom;
final double bottomContentHeight = scaffoldGeometry.scaffoldSize.height - contentBottom;
final double bottomSheetHeight = scaffoldGeometry.bottomSheetSize.height;
final double fabHeight = scaffoldGeometry.floatingActionButtonSize.height;
final double snackBarHeight = scaffoldGeometry.snackBarSize.height;
final double safeMargin = math.max(
kFloatingActionButtonMargin,
scaffoldGeometry.minViewPadding.bottom - bottomContentHeight + kFloatingActionButtonMargin,
);
double fabY = contentBottom - fabHeight - safeMargin;
if (snackBarHeight > 0.0) {
fabY = math.min(fabY, contentBottom - snackBarHeight - fabHeight - kFloatingActionButtonMargin);
}
if (bottomSheetHeight > 0.0) {
fabY = math.min(fabY, contentBottom - bottomSheetHeight - fabHeight / 2.0);
}
return fabY + adjustment;
}
}
/// Mixin for a "docked" floating action button location, such as [FloatingActionButtonLocation.endDocked].
mixin FabDockedOffsetY on StandardFabLocation {
/// Calculates y-offset for [FloatingActionButtonLocation]s floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
@override
double getOffsetY(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
final double contentBottom = scaffoldGeometry.contentBottom;
final double contentMargin = scaffoldGeometry.scaffoldSize.height - contentBottom;
final double bottomViewPadding = scaffoldGeometry.minViewPadding.bottom;
final double bottomSheetHeight = scaffoldGeometry.bottomSheetSize.height;
final double fabHeight = scaffoldGeometry.floatingActionButtonSize.height;
final double snackBarHeight = scaffoldGeometry.snackBarSize.height;
final double bottomMinInset = scaffoldGeometry.minInsets.bottom;
double safeMargin;
if (contentMargin > bottomMinInset + fabHeight / 2.0) {
// If contentMargin is higher than bottomMinInset enough to display the
// FAB without clipping, don't provide a margin
safeMargin = 0.0;
} else if (bottomMinInset == 0.0) {
// If bottomMinInset is zero(the software keyboard is not on the screen)
// provide bottomViewPadding as margin
safeMargin = bottomViewPadding;
} else {
// Provide a margin that would shift the FAB enough so that it stays away
// from the keyboard
safeMargin = fabHeight / 2.0 + kFloatingActionButtonMargin;
}
double fabY = contentBottom - fabHeight / 2.0 - safeMargin;
// The FAB should sit with a margin between it and the snack bar.
if (snackBarHeight > 0.0) {
fabY = math.min(fabY, contentBottom - snackBarHeight - fabHeight - kFloatingActionButtonMargin);
}
// The FAB should sit with its center in front of the top of the bottom sheet.
if (bottomSheetHeight > 0.0) {
fabY = math.min(fabY, contentBottom - bottomSheetHeight - fabHeight / 2.0);
}
final double maxFabY = scaffoldGeometry.scaffoldSize.height - fabHeight - safeMargin;
return math.min(maxFabY, fabY);
}
}
/// Mixin for a "contained" floating action button location, such as [FloatingActionButtonLocation.endContained].
mixin FabContainedOffsetY on StandardFabLocation {
/// Calculates y-offset for [FloatingActionButtonLocation]s floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the center of the bottom navigation bar.
@override
double getOffsetY(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
final double contentBottom = scaffoldGeometry.contentBottom;
final double contentMargin = scaffoldGeometry.scaffoldSize.height - contentBottom;
final double bottomViewPadding = scaffoldGeometry.minViewPadding.bottom;
final double fabHeight = scaffoldGeometry.floatingActionButtonSize.height;
double safeMargin;
if (contentMargin > bottomViewPadding + fabHeight) {
// If contentMargin is higher than bottomViewPadding enough to display the
// FAB without clipping, don't provide a margin
safeMargin = 0.0;
} else {
safeMargin = bottomViewPadding;
}
// This is to compute the distance between the content bottom to the top edge
// of the floating action button. This can be negative if content margin is
// too small.
final double contentBottomToFabTop = (contentMargin - bottomViewPadding - fabHeight) / 2.0;
final double fabY = contentBottom + contentBottomToFabTop;
final double maxFabY = scaffoldGeometry.scaffoldSize.height - fabHeight - safeMargin;
return math.min(maxFabY, fabY);
}
}
/// Mixin for a "start" floating action button location, such as [FloatingActionButtonLocation.startTop].
mixin FabStartOffsetX on StandardFabLocation {
/// Calculates x-offset for start-aligned [FloatingActionButtonLocation]s.
@override
double getOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return switch (scaffoldGeometry.textDirection) {
TextDirection.rtl => StandardFabLocation._rightOffsetX(scaffoldGeometry, adjustment),
TextDirection.ltr => StandardFabLocation._leftOffsetX(scaffoldGeometry, adjustment),
};
}
}
/// Mixin for a "center" floating action button location, such as [FloatingActionButtonLocation.centerFloat].
mixin FabCenterOffsetX on StandardFabLocation {
/// Calculates x-offset for center-aligned [FloatingActionButtonLocation]s.
@override
double getOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return (scaffoldGeometry.scaffoldSize.width - scaffoldGeometry.floatingActionButtonSize.width) / 2.0;
}
}
/// Mixin for an "end" floating action button location, such as [FloatingActionButtonLocation.endDocked].
mixin FabEndOffsetX on StandardFabLocation {
/// Calculates x-offset for end-aligned [FloatingActionButtonLocation]s.
@override
double getOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return switch (scaffoldGeometry.textDirection) {
TextDirection.rtl => StandardFabLocation._leftOffsetX(scaffoldGeometry, adjustment),
TextDirection.ltr => StandardFabLocation._rightOffsetX(scaffoldGeometry, adjustment),
};
}
}
/// Mixin for a "mini" floating action button location, such as [FloatingActionButtonLocation.miniStartTop].
mixin FabMiniOffsetAdjustment on StandardFabLocation {
@override
bool isMini () => true;
}
class _StartTopFabLocation extends StandardFabLocation
with FabStartOffsetX, FabTopOffsetY {
const _StartTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.startTop';
}
class _MiniStartTopFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabStartOffsetX, FabTopOffsetY {
const _MiniStartTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniStartTop';
}
class _CenterTopFabLocation extends StandardFabLocation
with FabCenterOffsetX, FabTopOffsetY {
const _CenterTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.centerTop';
}
class _MiniCenterTopFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabCenterOffsetX, FabTopOffsetY {
const _MiniCenterTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniCenterTop';
}
class _EndTopFabLocation extends StandardFabLocation
with FabEndOffsetX, FabTopOffsetY {
const _EndTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.endTop';
}
class _MiniEndTopFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabEndOffsetX, FabTopOffsetY {
const _MiniEndTopFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniEndTop';
}
class _StartFloatFabLocation extends StandardFabLocation
with FabStartOffsetX, FabFloatOffsetY {
const _StartFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.startFloat';
}
class _MiniStartFloatFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabStartOffsetX, FabFloatOffsetY {
const _MiniStartFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniStartFloat';
}
class _CenterFloatFabLocation extends StandardFabLocation
with FabCenterOffsetX, FabFloatOffsetY {
const _CenterFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.centerFloat';
}
class _MiniCenterFloatFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabCenterOffsetX, FabFloatOffsetY {
const _MiniCenterFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniCenterFloat';
}
class _EndFloatFabLocation extends StandardFabLocation
with FabEndOffsetX, FabFloatOffsetY {
const _EndFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.endFloat';
}
class _MiniEndFloatFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabEndOffsetX, FabFloatOffsetY {
const _MiniEndFloatFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniEndFloat';
}
class _StartDockedFabLocation extends StandardFabLocation
with FabStartOffsetX, FabDockedOffsetY {
const _StartDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.startDocked';
}
class _MiniStartDockedFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabStartOffsetX, FabDockedOffsetY {
const _MiniStartDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniStartDocked';
}
class _CenterDockedFabLocation extends StandardFabLocation
with FabCenterOffsetX, FabDockedOffsetY {
const _CenterDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.centerDocked';
}
class _MiniCenterDockedFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabCenterOffsetX, FabDockedOffsetY {
const _MiniCenterDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniCenterDocked';
}
class _EndDockedFabLocation extends StandardFabLocation
with FabEndOffsetX, FabDockedOffsetY {
const _EndDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.endDocked';
}
class _MiniEndDockedFabLocation extends StandardFabLocation
with FabMiniOffsetAdjustment, FabEndOffsetX, FabDockedOffsetY {
const _MiniEndDockedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.miniEndDocked';
}
class _EndContainedFabLocation extends StandardFabLocation
with FabEndOffsetX, FabContainedOffsetY {
const _EndContainedFabLocation();
@override
String toString() => 'FloatingActionButtonLocation.endContained';
}
/// Provider of animations to move the [FloatingActionButton] between [FloatingActionButtonLocation]s.
///
/// The [Scaffold] uses [Scaffold.floatingActionButtonAnimator] to define:
///
/// * The [Offset] of the [FloatingActionButton] between the old and new
/// [FloatingActionButtonLocation]s as part of the transition animation.
/// * An [Animation] to scale the [FloatingActionButton] during the transition.
/// * An [Animation] to rotate the [FloatingActionButton] during the transition.
/// * Where to start a new animation from if an animation is interrupted.
///
/// See also:
///
/// * [FloatingActionButton], which is a circular button typically shown in the
/// bottom right corner of the app.
/// * [FloatingActionButtonLocation], which the [Scaffold] uses to place the
/// [Scaffold.floatingActionButton] within the [Scaffold]'s layout.
abstract class FloatingActionButtonAnimator {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const FloatingActionButtonAnimator();
/// Moves the [FloatingActionButton] by scaling out and then in at a new
/// [FloatingActionButtonLocation].
///
/// This animator shrinks the [FloatingActionButton] down until it disappears, then
/// grows it back to full size at its new [FloatingActionButtonLocation].
///
/// This is the default [FloatingActionButton] motion animation.
static const FloatingActionButtonAnimator scaling = _ScalingFabMotionAnimator();
/// Gets the [FloatingActionButton]'s position relative to the origin of the
/// [Scaffold] based on [progress].
///
/// [begin] is the [Offset] provided by the previous
/// [FloatingActionButtonLocation].
///
/// [end] is the [Offset] provided by the new
/// [FloatingActionButtonLocation].
///
/// [progress] is the current progress of the transition animation.
/// When [progress] is 0.0, the returned [Offset] should be equal to [begin].
/// when [progress] is 1.0, the returned [Offset] should be equal to [end].
Offset getOffset({ required Offset begin, required Offset end, required double progress });
/// Animates the scale of the [FloatingActionButton].
///
/// The animation should both start and end with a value of 1.0.
///
/// For example, to create an animation that linearly scales out and then back in,
/// you could join animations that pass each other:
///
/// ```dart
/// @override
/// Animation<double> getScaleAnimation({required Animation<double> parent}) {
/// // The animations will cross at value 0, and the train will return to 1.0.
/// return TrainHoppingAnimation(
/// Tween<double>(begin: 1.0, end: -1.0).animate(parent),
/// Tween<double>(begin: -1.0, end: 1.0).animate(parent),
/// );
/// }
/// ```
Animation<double> getScaleAnimation({ required Animation<double> parent });
/// Animates the rotation of [Scaffold.floatingActionButton].
///
/// The animation should both start and end with a value of 0.0 or 1.0.
///
/// The animation values are a fraction of a full circle, with 0.0 and 1.0
/// corresponding to 0 and 360 degrees, while 0.5 corresponds to 180 degrees.
///
/// For example, to create a rotation animation that rotates the
/// [FloatingActionButton] through a full circle:
///
/// ```dart
/// @override
/// Animation<double> getRotationAnimation({required Animation<double> parent}) {
/// return Tween<double>(begin: 0.0, end: 1.0).animate(parent);
/// }
/// ```
Animation<double> getRotationAnimation({ required Animation<double> parent });
/// Gets the progress value to restart a motion animation from when the animation is interrupted.
///
/// [previousValue] is the value of the animation before it was interrupted.
///
/// The restart of the animation will affect all three parts of the motion animation:
/// offset animation, scale animation, and rotation animation.
///
/// An interruption triggers if the [Scaffold] is given a new [FloatingActionButtonLocation]
/// while it is still animating a transition between two previous [FloatingActionButtonLocation]s.
///
/// A sensible default is usually 0.0, which is the same as restarting
/// the animation from the beginning, regardless of the original state of the animation.
double getAnimationRestart(double previousValue) => 0.0;
@override
String toString() => objectRuntimeType(this, 'FloatingActionButtonAnimator');
}
class _ScalingFabMotionAnimator extends FloatingActionButtonAnimator {
const _ScalingFabMotionAnimator();
@override
Offset getOffset({ required Offset begin, required Offset end, required double progress }) {
if (progress < 0.5) {
return begin;
} else {
return end;
}
}
@override
Animation<double> getScaleAnimation({ required Animation<double> parent }) {
// Animate the scale down from 1 to 0 in the first half of the animation
// then from 0 back to 1 in the second half.
const Curve curve = Interval(0.5, 1.0, curve: Curves.ease);
return _AnimationSwap<double>(
ReverseAnimation(parent.drive(CurveTween(curve: curve.flipped))),
parent.drive(CurveTween(curve: curve)),
parent,
0.5,
);
}
// Because we only see the last half of the rotation tween,
// it needs to go twice as far.
static final Animatable<double> _rotationTween = Tween<double>(
begin: 1.0 - kFloatingActionButtonTurnInterval * 2.0,
end: 1.0,
);
static final Animatable<double> _thresholdCenterTween = CurveTween(curve: const Threshold(0.5));
@override
Animation<double> getRotationAnimation({ required Animation<double> parent }) {
// This rotation will turn on the way in, but not on the way out.
return _AnimationSwap<double>(
parent.drive(_rotationTween),
ReverseAnimation(parent.drive(_thresholdCenterTween)),
parent,
0.5,
);
}
// If the animation was just starting, we'll continue from where we left off.
// If the animation was finishing, we'll treat it as if we were starting at that point in reverse.
// This avoids a size jump during the animation.
@override
double getAnimationRestart(double previousValue) => math.min(1.0 - previousValue, previousValue);
}
/// An animation that swaps from one animation to the next when the [parent] passes [swapThreshold].
///
/// The [value] of this animation is the value of [first] when [parent.value] < [swapThreshold]
/// and the value of [next] otherwise.
class _AnimationSwap<T> extends CompoundAnimation<T> {
/// Creates an [_AnimationSwap].
///
/// Either argument can be an [_AnimationSwap] itself to combine multiple
/// animations.
_AnimationSwap(Animation<T> first, Animation<T> next, this.parent, this.swapThreshold) : super(first: first, next: next);
final Animation<double> parent;
final double swapThreshold;
@override
T get value => parent.value < swapThreshold ? first.value : next.value;
}
| flutter/packages/flutter/lib/src/material/floating_action_button_location.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/floating_action_button_location.dart",
"repo_id": "flutter",
"token_count": 13127
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'date.dart';
import 'date_picker_theme.dart';
import 'input_border.dart';
import 'input_decorator.dart';
import 'material_localizations.dart';
import 'text_form_field.dart';
import 'theme.dart';
/// A [TextFormField] configured to accept and validate a date entered by a user.
///
/// When the field is saved or submitted, the text will be parsed into a
/// [DateTime] according to the ambient locale's compact date format. If the
/// input text doesn't parse into a date, the [errorFormatText] message will
/// be displayed under the field.
///
/// [firstDate], [lastDate], and [selectableDayPredicate] provide constraints on
/// what days are valid. If the input date isn't in the date range or doesn't pass
/// the given predicate, then the [errorInvalidText] message will be displayed
/// under the field.
///
/// See also:
///
/// * [showDatePicker], which shows a dialog that contains a Material Design
/// date picker which includes support for text entry of dates.
/// * [MaterialLocalizations.parseCompactDate], which is used to parse the text
/// input into a [DateTime].
///
class InputDatePickerFormField extends StatefulWidget {
/// Creates a [TextFormField] configured to accept and validate a date.
///
/// If the optional [initialDate] is provided, then it will be used to populate
/// the text field. If the [fieldHintText] is provided, it will be shown.
///
/// If [initialDate] is provided, it must not be before [firstDate] or after
/// [lastDate]. If [selectableDayPredicate] is provided, it must return `true`
/// for [initialDate].
///
/// [firstDate] must be on or before [lastDate].
InputDatePickerFormField({
super.key,
DateTime? initialDate,
required DateTime firstDate,
required DateTime lastDate,
this.onDateSubmitted,
this.onDateSaved,
this.selectableDayPredicate,
this.errorFormatText,
this.errorInvalidText,
this.fieldHintText,
this.fieldLabelText,
this.keyboardType,
this.autofocus = false,
this.acceptEmptyDate = false,
this.focusNode,
}) : initialDate = initialDate != null ? DateUtils.dateOnly(initialDate) : null,
firstDate = DateUtils.dateOnly(firstDate),
lastDate = DateUtils.dateOnly(lastDate) {
assert(
!this.lastDate.isBefore(this.firstDate),
'lastDate ${this.lastDate} must be on or after firstDate ${this.firstDate}.',
);
assert(
initialDate == null || !this.initialDate!.isBefore(this.firstDate),
'initialDate ${this.initialDate} must be on or after firstDate ${this.firstDate}.',
);
assert(
initialDate == null || !this.initialDate!.isAfter(this.lastDate),
'initialDate ${this.initialDate} must be on or before lastDate ${this.lastDate}.',
);
assert(
selectableDayPredicate == null || initialDate == null || selectableDayPredicate!(this.initialDate!),
'Provided initialDate ${this.initialDate} must satisfy provided selectableDayPredicate.',
);
}
/// If provided, it will be used as the default value of the field.
final DateTime? initialDate;
/// The earliest allowable [DateTime] that the user can input.
final DateTime firstDate;
/// The latest allowable [DateTime] that the user can input.
final DateTime lastDate;
/// An optional method to call when the user indicates they are done editing
/// the text in the field. Will only be called if the input represents a valid
/// [DateTime].
final ValueChanged<DateTime>? onDateSubmitted;
/// An optional method to call with the final date when the form is
/// saved via [FormState.save]. Will only be called if the input represents
/// a valid [DateTime].
final ValueChanged<DateTime>? onDateSaved;
/// Function to provide full control over which [DateTime] can be selected.
final SelectableDayPredicate? selectableDayPredicate;
/// The error text displayed if the entered date is not in the correct format.
final String? errorFormatText;
/// The error text displayed if the date is not valid.
///
/// A date is not valid if it is earlier than [firstDate], later than
/// [lastDate], or doesn't pass the [selectableDayPredicate].
final String? errorInvalidText;
/// The hint text displayed in the [TextField].
///
/// If this is null, it will default to the date format string. For example,
/// 'mm/dd/yyyy' for en_US.
final String? fieldHintText;
/// The label text displayed in the [TextField].
///
/// If this is null, it will default to the words representing the date format
/// string. For example, 'Month, Day, Year' for en_US.
final String? fieldLabelText;
/// The keyboard type of the [TextField].
///
/// If this is null, it will default to [TextInputType.datetime]
final TextInputType? keyboardType;
/// {@macro flutter.widgets.editableText.autofocus}
final bool autofocus;
/// Determines if an empty date would show [errorFormatText] or not.
///
/// Defaults to false.
///
/// If true, [errorFormatText] is not shown when the date input field is empty.
final bool acceptEmptyDate;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
@override
State<InputDatePickerFormField> createState() => _InputDatePickerFormFieldState();
}
class _InputDatePickerFormFieldState extends State<InputDatePickerFormField> {
final TextEditingController _controller = TextEditingController();
DateTime? _selectedDate;
String? _inputText;
bool _autoSelected = false;
@override
void initState() {
super.initState();
_selectedDate = widget.initialDate;
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_updateValueForSelectedDate();
}
@override
void didUpdateWidget(InputDatePickerFormField oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.initialDate != oldWidget.initialDate) {
// Can't update the form field in the middle of a build, so do it next frame
WidgetsBinding.instance.addPostFrameCallback((Duration timeStamp) {
setState(() {
_selectedDate = widget.initialDate;
_updateValueForSelectedDate();
});
}, debugLabel: 'InputDatePickerFormField.update');
}
}
void _updateValueForSelectedDate() {
if (_selectedDate != null) {
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
_inputText = localizations.formatCompactDate(_selectedDate!);
TextEditingValue textEditingValue = TextEditingValue(text: _inputText!);
// Select the new text if we are auto focused and haven't selected the text before.
if (widget.autofocus && !_autoSelected) {
textEditingValue = textEditingValue.copyWith(selection: TextSelection(
baseOffset: 0,
extentOffset: _inputText!.length,
));
_autoSelected = true;
}
_controller.value = textEditingValue;
} else {
_inputText = '';
_controller.value = TextEditingValue(text: _inputText!);
}
}
DateTime? _parseDate(String? text) {
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
return localizations.parseCompactDate(text);
}
bool _isValidAcceptableDate(DateTime? date) {
return
date != null &&
!date.isBefore(widget.firstDate) &&
!date.isAfter(widget.lastDate) &&
(widget.selectableDayPredicate == null || widget.selectableDayPredicate!(date));
}
String? _validateDate(String? text) {
if ((text == null || text.isEmpty) && widget.acceptEmptyDate) {
return null;
}
final DateTime? date = _parseDate(text);
if (date == null) {
return widget.errorFormatText ?? MaterialLocalizations.of(context).invalidDateFormatLabel;
} else if (!_isValidAcceptableDate(date)) {
return widget.errorInvalidText ?? MaterialLocalizations.of(context).dateOutOfRangeLabel;
}
return null;
}
void _updateDate(String? text, ValueChanged<DateTime>? callback) {
final DateTime? date = _parseDate(text);
if (_isValidAcceptableDate(date)) {
_selectedDate = date;
_inputText = text;
callback?.call(_selectedDate!);
}
}
void _handleSaved(String? text) {
_updateDate(text, widget.onDateSaved);
}
void _handleSubmitted(String text) {
_updateDate(text, widget.onDateSubmitted);
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final bool useMaterial3 = theme.useMaterial3;
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final DatePickerThemeData datePickerTheme = theme.datePickerTheme;
final InputDecorationTheme inputTheme = theme.inputDecorationTheme;
final InputBorder effectiveInputBorder = datePickerTheme.inputDecorationTheme?.border
?? theme.inputDecorationTheme.border
?? (useMaterial3 ? const OutlineInputBorder() : const UnderlineInputBorder());
return Semantics(
container: true,
child: TextFormField(
decoration: InputDecoration(
hintText: widget.fieldHintText ?? localizations.dateHelpText,
labelText: widget.fieldLabelText ?? localizations.dateInputLabel,
).applyDefaults(inputTheme
.merge(datePickerTheme.inputDecorationTheme)
.copyWith(border: effectiveInputBorder),
),
validator: _validateDate,
keyboardType: widget.keyboardType ?? TextInputType.datetime,
onSaved: _handleSaved,
onFieldSubmitted: _handleSubmitted,
autofocus: widget.autofocus,
controller: _controller,
focusNode: widget.focusNode,
),
);
}
}
| flutter/packages/flutter/lib/src/material/input_date_picker_form_field.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/input_date_picker_form_field.dart",
"repo_id": "flutter",
"token_count": 3264
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/animation.dart';
// BEGIN GENERATED TOKEN PROPERTIES - Motion
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
/// The set of durations in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
abstract final class Durations {
/// The short1 duration (50ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration short1 = Duration(milliseconds: 50);
/// The short2 duration (100ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration short2 = Duration(milliseconds: 100);
/// The short3 duration (150ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration short3 = Duration(milliseconds: 150);
/// The short4 duration (200ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration short4 = Duration(milliseconds: 200);
/// The medium1 duration (250ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration medium1 = Duration(milliseconds: 250);
/// The medium2 duration (300ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration medium2 = Duration(milliseconds: 300);
/// The medium3 duration (350ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration medium3 = Duration(milliseconds: 350);
/// The medium4 duration (400ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration medium4 = Duration(milliseconds: 400);
/// The long1 duration (450ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration long1 = Duration(milliseconds: 450);
/// The long2 duration (500ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration long2 = Duration(milliseconds: 500);
/// The long3 duration (550ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration long3 = Duration(milliseconds: 550);
/// The long4 duration (600ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration long4 = Duration(milliseconds: 600);
/// The extralong1 duration (700ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration extralong1 = Duration(milliseconds: 700);
/// The extralong2 duration (800ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration extralong2 = Duration(milliseconds: 800);
/// The extralong3 duration (900ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration extralong3 = Duration(milliseconds: 900);
/// The extralong4 duration (1000ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration extralong4 = Duration(milliseconds: 1000);
}
// TODO(guidezpl): Improve with description and assets, b/289870605
/// The set of easing curves in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
/// * [Curves], for a collection of non-Material animation easing curves.
abstract final class Easing {
/// The emphasizedAccelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve emphasizedAccelerate = Cubic(0.3, 0.0, 0.8, 0.15);
/// The emphasizedDecelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve emphasizedDecelerate = Cubic(0.05, 0.7, 0.1, 1.0);
/// The linear easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve linear = Cubic(0.0, 0.0, 1.0, 1.0);
/// The standard easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve standard = Cubic(0.2, 0.0, 0.0, 1.0);
/// The standardAccelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve standardAccelerate = Cubic(0.3, 0.0, 1.0, 1.0);
/// The standardDecelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve standardDecelerate = Cubic(0.0, 0.0, 0.0, 1.0);
/// The legacyDecelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve legacyDecelerate = Cubic(0.0, 0.0, 0.2, 1.0);
/// The legacyAccelerate easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve legacyAccelerate = Cubic(0.4, 0.0, 1.0, 1.0);
/// The legacy easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve legacy = Cubic(0.4, 0.0, 0.2, 1.0);
}
// END GENERATED TOKEN PROPERTIES - Motion
| flutter/packages/flutter/lib/src/material/motion.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/motion.dart",
"repo_id": "flutter",
"token_count": 4395
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
@immutable
/// Defines the visual properties of [ProgressIndicator] widgets.
///
/// Used by [ProgressIndicatorTheme] to control the visual properties of
/// progress indicators in a widget subtree.
///
/// To obtain this configuration, use [ProgressIndicatorTheme.of] to access
/// the closest ancestor [ProgressIndicatorTheme] of the current [BuildContext].
///
/// See also:
///
/// * [ProgressIndicatorTheme], an [InheritedWidget] that propagates the
/// theme down its subtree.
/// * [ThemeData.progressIndicatorTheme], which describes the defaults for
/// any progress indicators as part of the application's [ThemeData].
class ProgressIndicatorThemeData with Diagnosticable {
/// Creates the set of properties used to configure [ProgressIndicator] widgets.
const ProgressIndicatorThemeData({
this.color,
this.linearTrackColor,
this.linearMinHeight,
this.circularTrackColor,
this.refreshBackgroundColor,
});
/// The color of the [ProgressIndicator]'s indicator.
///
/// If null, then it will use [ColorScheme.primary] of the ambient
/// [ThemeData.colorScheme].
///
/// See also:
///
/// * [ProgressIndicator.color], which specifies the indicator color for a
/// specific progress indicator.
/// * [ProgressIndicator.valueColor], which specifies the indicator color
/// a an animated color.
final Color? color;
/// {@macro flutter.material.LinearProgressIndicator.trackColor}
final Color? linearTrackColor;
/// {@macro flutter.material.LinearProgressIndicator.minHeight}
final double? linearMinHeight;
/// {@macro flutter.material.CircularProgressIndicator.trackColor}
final Color? circularTrackColor;
/// {@macro flutter.material.RefreshProgressIndicator.backgroundColor}
final Color? refreshBackgroundColor;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
ProgressIndicatorThemeData copyWith({
Color? color,
Color? linearTrackColor,
double? linearMinHeight,
Color? circularTrackColor,
Color? refreshBackgroundColor,
}) {
return ProgressIndicatorThemeData(
color: color ?? this.color,
linearTrackColor : linearTrackColor ?? this.linearTrackColor,
linearMinHeight : linearMinHeight ?? this.linearMinHeight,
circularTrackColor : circularTrackColor ?? this.circularTrackColor,
refreshBackgroundColor : refreshBackgroundColor ?? this.refreshBackgroundColor,
);
}
/// Linearly interpolate between two progress indicator themes.
///
/// If both arguments are null, then null is returned.
static ProgressIndicatorThemeData? lerp(ProgressIndicatorThemeData? a, ProgressIndicatorThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return ProgressIndicatorThemeData(
color: Color.lerp(a?.color, b?.color, t),
linearTrackColor : Color.lerp(a?.linearTrackColor, b?.linearTrackColor, t),
linearMinHeight : lerpDouble(a?.linearMinHeight, b?.linearMinHeight, t),
circularTrackColor : Color.lerp(a?.circularTrackColor, b?.circularTrackColor, t),
refreshBackgroundColor : Color.lerp(a?.refreshBackgroundColor, b?.refreshBackgroundColor, t),
);
}
@override
int get hashCode => Object.hash(
color,
linearTrackColor,
linearMinHeight,
circularTrackColor,
refreshBackgroundColor,
);
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ProgressIndicatorThemeData
&& other.color == color
&& other.linearTrackColor == linearTrackColor
&& other.linearMinHeight == linearMinHeight
&& other.circularTrackColor == circularTrackColor
&& other.refreshBackgroundColor == refreshBackgroundColor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(ColorProperty('linearTrackColor', linearTrackColor, defaultValue: null));
properties.add(DoubleProperty('linearMinHeight', linearMinHeight, defaultValue: null));
properties.add(ColorProperty('circularTrackColor', circularTrackColor, defaultValue: null));
properties.add(ColorProperty('refreshBackgroundColor', refreshBackgroundColor, defaultValue: null));
}
}
/// An inherited widget that defines the configuration for
/// [ProgressIndicator]s in this widget's subtree.
///
/// Values specified here are used for [ProgressIndicator] properties that are not
/// given an explicit non-null value.
///
/// {@tool snippet}
///
/// Here is an example of a progress indicator theme that applies a red indicator
/// color.
///
/// ```dart
/// const ProgressIndicatorTheme(
/// data: ProgressIndicatorThemeData(
/// color: Colors.red,
/// ),
/// child: LinearProgressIndicator()
/// )
/// ```
/// {@end-tool}
class ProgressIndicatorTheme extends InheritedTheme {
/// Creates a theme that controls the configurations for [ProgressIndicator]
/// widgets.
const ProgressIndicatorTheme({
super.key,
required this.data,
required super.child,
});
/// The properties for descendant [ProgressIndicator] widgets.
final ProgressIndicatorThemeData data;
/// Returns the [data] from the closest [ProgressIndicatorTheme] ancestor. If
/// there is no ancestor, it returns [ThemeData.progressIndicatorTheme].
///
/// Typical usage is as follows:
///
/// ```dart
/// ProgressIndicatorThemeData theme = ProgressIndicatorTheme.of(context);
/// ```
static ProgressIndicatorThemeData of(BuildContext context) {
final ProgressIndicatorTheme? progressIndicatorTheme = context.dependOnInheritedWidgetOfExactType<ProgressIndicatorTheme>();
return progressIndicatorTheme?.data ?? Theme.of(context).progressIndicatorTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ProgressIndicatorTheme(data: data, child: child);
}
@override
bool updateShouldNotify(ProgressIndicatorTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/progress_indicator_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/progress_indicator_theme.dart",
"repo_id": "flutter",
"token_count": 1937
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'adaptive_text_selection_toolbar.dart';
import 'desktop_text_selection.dart';
import 'feedback.dart';
import 'magnifier.dart';
import 'text_selection.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
// late FocusNode myFocusNode;
/// An eyeballed value that moves the cursor slightly left of where it is
/// rendered for text on Android so its positioning more accurately matches the
/// native iOS text cursor positioning.
///
/// This value is in device pixels, not logical pixels as is typically used
/// throughout the codebase.
const int iOSHorizontalOffset = -2;
class _TextSpanEditingController extends TextEditingController {
_TextSpanEditingController({required TextSpan textSpan}):
_textSpan = textSpan,
super(text: textSpan.toPlainText(includeSemanticsLabels: false));
final TextSpan _textSpan;
@override
TextSpan buildTextSpan({required BuildContext context, TextStyle? style, required bool withComposing}) {
// This does not care about composing.
return TextSpan(
style: style,
children: <TextSpan>[_textSpan],
);
}
@override
set text(String? newText) {
// This should never be reached.
throw UnimplementedError();
}
}
class _SelectableTextSelectionGestureDetectorBuilder extends TextSelectionGestureDetectorBuilder {
_SelectableTextSelectionGestureDetectorBuilder({
required _SelectableTextState state,
}) : _state = state,
super(delegate: state);
final _SelectableTextState _state;
/// The viewport offset pixels of any [Scrollable] containing the
/// [RenderEditable] at the last drag start.
double _dragStartScrollOffset = 0.0;
/// The viewport offset pixels of the [RenderEditable] at the last drag start.
double _dragStartViewportOffset = 0.0;
double get _scrollPosition {
final ScrollableState? scrollableState =
delegate.editableTextKey.currentContext == null
? null
: Scrollable.maybeOf(delegate.editableTextKey.currentContext!);
return scrollableState == null
? 0.0
: scrollableState.position.pixels;
}
AxisDirection? get _scrollDirection {
final ScrollableState? scrollableState =
delegate.editableTextKey.currentContext == null
? null
: Scrollable.maybeOf(delegate.editableTextKey.currentContext!);
return scrollableState?.axisDirection;
}
@override
void onForcePressStart(ForcePressDetails details) {
super.onForcePressStart(details);
if (delegate.selectionEnabled && shouldShowSelectionToolbar) {
editableText.showToolbar();
}
}
@override
void onForcePressEnd(ForcePressDetails details) {
// Not required.
}
@override
void onSingleLongTapStart(LongPressStartDetails details) {
if (!delegate.selectionEnabled) {
return;
}
renderEditable.selectWord(cause: SelectionChangedCause.longPress);
Feedback.forLongPress(_state.context);
_dragStartViewportOffset = renderEditable.offset.pixels;
_dragStartScrollOffset = _scrollPosition;
}
@override
void onSingleLongTapMoveUpdate(LongPressMoveUpdateDetails details) {
if (!delegate.selectionEnabled) {
return;
}
// Adjust the drag start offset for possible viewport offset changes.
final Offset editableOffset = renderEditable.maxLines == 1
? Offset(renderEditable.offset.pixels - _dragStartViewportOffset, 0.0)
: Offset(0.0, renderEditable.offset.pixels - _dragStartViewportOffset);
final double effectiveScrollPosition = _scrollPosition - _dragStartScrollOffset;
final bool scrollingOnVerticalAxis = _scrollDirection == AxisDirection.up || _scrollDirection == AxisDirection.down;
final Offset scrollableOffset = Offset(
!scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
);
renderEditable.selectWordsInRange(
from: details.globalPosition - details.offsetFromOrigin - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.longPress,
);
}
@override
void onSingleTapUp(TapDragUpDetails details) {
editableText.hideToolbar();
if (delegate.selectionEnabled) {
switch (Theme.of(_state.context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
renderEditable.selectWordEdge(cause: SelectionChangedCause.tap);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
}
}
_state.widget.onTap?.call();
}
}
/// A run of selectable text with a single style.
///
/// Consider using [SelectionArea] or [SelectableRegion] instead, which enable
/// selection on a widget subtree, including but not limited to [Text] widgets.
///
/// The [SelectableText] widget displays a string of text with a single style.
/// The string might break across multiple lines or might all be displayed on
/// the same line depending on the layout constraints.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ZSU3ZXOs6hc}
///
/// The [style] argument is optional. When omitted, the text will use the style
/// from the closest enclosing [DefaultTextStyle]. If the given style's
/// [TextStyle.inherit] property is true (the default), the given style will
/// be merged with the closest enclosing [DefaultTextStyle]. This merging
/// behavior is useful, for example, to make the text bold while using the
/// default font family and size.
///
/// {@macro flutter.material.textfield.wantKeepAlive}
///
/// {@tool snippet}
///
/// ```dart
/// const SelectableText(
/// 'Hello! How are you?',
/// textAlign: TextAlign.center,
/// style: TextStyle(fontWeight: FontWeight.bold),
/// )
/// ```
/// {@end-tool}
///
/// Using the [SelectableText.rich] constructor, the [SelectableText] widget can
/// display a paragraph with differently styled [TextSpan]s. The sample
/// that follows displays "Hello beautiful world" with different styles
/// for each word.
///
/// {@tool snippet}
///
/// ```dart
/// const SelectableText.rich(
/// TextSpan(
/// text: 'Hello', // default text style
/// children: <TextSpan>[
/// TextSpan(text: ' beautiful ', style: TextStyle(fontStyle: FontStyle.italic)),
/// TextSpan(text: 'world', style: TextStyle(fontWeight: FontWeight.bold)),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Interactivity
///
/// To make [SelectableText] react to touch events, use callback [onTap] to achieve
/// the desired behavior.
///
/// ## Scrolling Considerations
///
/// If this [SelectableText] is not a descendant of [Scaffold] and is being used
/// within a [Scrollable] or nested [Scrollable]s, consider placing a
/// [ScrollNotificationObserver] above the root [Scrollable] that contains this
/// [SelectableText] to ensure proper scroll coordination for [SelectableText]
/// and its components like [TextSelectionOverlay].
///
/// See also:
///
/// * [Text], which is the non selectable version of this widget.
/// * [TextField], which is the editable version of this widget.
/// * [SelectionArea], which enables the selection of multiple [Text] widgets
/// and of other widgets.
class SelectableText extends StatefulWidget {
/// Creates a selectable text widget.
///
/// If the [style] argument is null, the text will use the style from the
/// closest enclosing [DefaultTextStyle].
///
/// If the [showCursor], [autofocus], [dragStartBehavior],
/// [selectionHeightStyle], [selectionWidthStyle] and [data] arguments are
/// specified, the [maxLines] argument must be greater than zero.
const SelectableText(
String this.data, {
super.key,
this.focusNode,
this.style,
this.strutStyle,
this.textAlign,
this.textDirection,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
this.textScaleFactor,
this.textScaler,
this.showCursor = false,
this.autofocus = false,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
this.toolbarOptions,
this.minLines,
this.maxLines,
this.cursorWidth = 2.0,
this.cursorHeight,
this.cursorRadius,
this.cursorColor,
this.selectionHeightStyle = ui.BoxHeightStyle.tight,
this.selectionWidthStyle = ui.BoxWidthStyle.tight,
this.dragStartBehavior = DragStartBehavior.start,
this.enableInteractiveSelection = true,
this.selectionControls,
this.onTap,
this.scrollPhysics,
this.semanticsLabel,
this.textHeightBehavior,
this.textWidthBasis,
this.onSelectionChanged,
this.contextMenuBuilder = _defaultContextMenuBuilder,
this.magnifierConfiguration,
}) : assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
textScaler == null || textScaleFactor == null,
'textScaleFactor is deprecated and cannot be specified when textScaler is specified.',
),
textSpan = null;
/// Creates a selectable text widget with a [TextSpan].
///
/// The [TextSpan.children] attribute of the [textSpan] parameter must only
/// contain [TextSpan]s. Other types of [InlineSpan] are not allowed.
const SelectableText.rich(
TextSpan this.textSpan, {
super.key,
this.focusNode,
this.style,
this.strutStyle,
this.textAlign,
this.textDirection,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
this.textScaleFactor,
this.textScaler,
this.showCursor = false,
this.autofocus = false,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
this.toolbarOptions,
this.minLines,
this.maxLines,
this.cursorWidth = 2.0,
this.cursorHeight,
this.cursorRadius,
this.cursorColor,
this.selectionHeightStyle = ui.BoxHeightStyle.tight,
this.selectionWidthStyle = ui.BoxWidthStyle.tight,
this.dragStartBehavior = DragStartBehavior.start,
this.enableInteractiveSelection = true,
this.selectionControls,
this.onTap,
this.scrollPhysics,
this.semanticsLabel,
this.textHeightBehavior,
this.textWidthBasis,
this.onSelectionChanged,
this.contextMenuBuilder = _defaultContextMenuBuilder,
this.magnifierConfiguration,
}) : assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
textScaler == null || textScaleFactor == null,
'textScaleFactor is deprecated and cannot be specified when textScaler is specified.',
),
data = null;
/// The text to display.
///
/// This will be null if a [textSpan] is provided instead.
final String? data;
/// The text to display as a [TextSpan].
///
/// This will be null if [data] is provided instead.
final TextSpan? textSpan;
/// Defines the focus for this widget.
///
/// Text is only selectable when widget is focused.
///
/// The [focusNode] is a long-lived object that's typically managed by a
/// [StatefulWidget] parent. See [FocusNode] for more information.
///
/// To give the focus to this widget, provide a [focusNode] and then
/// use the current [FocusScope] to request the focus:
///
/// ```dart
/// FocusScope.of(context).requestFocus(myFocusNode);
/// ```
///
/// This happens automatically when the widget is tapped.
///
/// To be notified when the widget gains or loses the focus, add a listener
/// to the [focusNode]:
///
/// ```dart
/// myFocusNode.addListener(() { print(myFocusNode.hasFocus); });
/// ```
///
/// If null, this widget will create its own [FocusNode] with
/// [FocusNode.skipTraversal] parameter set to `true`, which causes the widget
/// to be skipped over during focus traversal.
final FocusNode? focusNode;
/// The style to use for the text.
///
/// If null, defaults [DefaultTextStyle] of context.
final TextStyle? style;
/// {@macro flutter.widgets.editableText.strutStyle}
final StrutStyle? strutStyle;
/// {@macro flutter.widgets.editableText.textAlign}
final TextAlign? textAlign;
/// {@macro flutter.widgets.editableText.textDirection}
final TextDirection? textDirection;
/// {@macro flutter.widgets.editableText.textScaleFactor}
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
final double? textScaleFactor;
/// {@macro flutter.painting.textPainter.textScaler}
final TextScaler? textScaler;
/// {@macro flutter.widgets.editableText.autofocus}
final bool autofocus;
/// {@macro flutter.widgets.editableText.minLines}
final int? minLines;
/// {@macro flutter.widgets.editableText.maxLines}
final int? maxLines;
/// {@macro flutter.widgets.editableText.showCursor}
final bool showCursor;
/// {@macro flutter.widgets.editableText.cursorWidth}
final double cursorWidth;
/// {@macro flutter.widgets.editableText.cursorHeight}
final double? cursorHeight;
/// {@macro flutter.widgets.editableText.cursorRadius}
final Radius? cursorRadius;
/// The color of the cursor.
///
/// The cursor indicates the current text insertion point.
///
/// If null then [DefaultSelectionStyle.cursorColor] is used. If that is also
/// null and [ThemeData.platform] is [TargetPlatform.iOS] or
/// [TargetPlatform.macOS], then [CupertinoThemeData.primaryColor] is used.
/// Otherwise [ColorScheme.primary] of [ThemeData.colorScheme] is used.
final Color? cursorColor;
/// Controls how tall the selection highlight boxes are computed to be.
///
/// See [ui.BoxHeightStyle] for details on available styles.
final ui.BoxHeightStyle selectionHeightStyle;
/// Controls how wide the selection highlight boxes are computed to be.
///
/// See [ui.BoxWidthStyle] for details on available styles.
final ui.BoxWidthStyle selectionWidthStyle;
/// {@macro flutter.widgets.editableText.enableInteractiveSelection}
final bool enableInteractiveSelection;
/// {@macro flutter.widgets.editableText.selectionControls}
final TextSelectionControls? selectionControls;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// Configuration of toolbar options.
///
/// Paste and cut will be disabled regardless.
///
/// If not set, select all and copy will be enabled by default.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
final ToolbarOptions? toolbarOptions;
/// {@macro flutter.widgets.editableText.selectionEnabled}
bool get selectionEnabled => enableInteractiveSelection;
/// Called when the user taps on this selectable text.
///
/// The selectable text builds a [GestureDetector] to handle input events like tap,
/// to trigger focus requests, to move the caret, adjust the selection, etc.
/// Handling some of those events by wrapping the selectable text with a competing
/// GestureDetector is problematic.
///
/// To unconditionally handle taps, without interfering with the selectable text's
/// internal gesture detector, provide this callback.
///
/// To be notified when the text field gains or loses the focus, provide a
/// [focusNode] and add a listener to that.
///
/// To listen to arbitrary pointer events without competing with the
/// selectable text's internal gesture detector, use a [Listener].
final GestureTapCallback? onTap;
/// {@macro flutter.widgets.editableText.scrollPhysics}
final ScrollPhysics? scrollPhysics;
/// {@macro flutter.widgets.Text.semanticsLabel}
final String? semanticsLabel;
/// {@macro dart.ui.textHeightBehavior}
final TextHeightBehavior? textHeightBehavior;
/// {@macro flutter.painting.textPainter.textWidthBasis}
final TextWidthBasis? textWidthBasis;
/// {@macro flutter.widgets.editableText.onSelectionChanged}
final SelectionChangedCallback? onSelectionChanged;
/// {@macro flutter.widgets.EditableText.contextMenuBuilder}
final EditableTextContextMenuBuilder? contextMenuBuilder;
static Widget _defaultContextMenuBuilder(BuildContext context, EditableTextState editableTextState) {
return AdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
}
/// The configuration for the magnifier used when the text is selected.
///
/// By default, builds a [CupertinoTextMagnifier] on iOS and [TextMagnifier]
/// on Android, and builds nothing on all other platforms. To suppress the
/// magnifier, consider passing [TextMagnifierConfiguration.disabled].
///
/// {@macro flutter.widgets.magnifier.intro}
final TextMagnifierConfiguration? magnifierConfiguration;
@override
State<SelectableText> createState() => _SelectableTextState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('data', data, defaultValue: null));
properties.add(DiagnosticsProperty<String>('semanticsLabel', semanticsLabel, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('style', style, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('autofocus', autofocus, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('showCursor', showCursor, defaultValue: false));
properties.add(IntProperty('minLines', minLines, defaultValue: null));
properties.add(IntProperty('maxLines', maxLines, defaultValue: null));
properties.add(EnumProperty<TextAlign>('textAlign', textAlign, defaultValue: null));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(DoubleProperty('textScaleFactor', textScaleFactor, defaultValue: null));
properties.add(DiagnosticsProperty<TextScaler>('textScaler', textScaler, defaultValue: null));
properties.add(DoubleProperty('cursorWidth', cursorWidth, defaultValue: 2.0));
properties.add(DoubleProperty('cursorHeight', cursorHeight, defaultValue: null));
properties.add(DiagnosticsProperty<Radius>('cursorRadius', cursorRadius, defaultValue: null));
properties.add(DiagnosticsProperty<Color>('cursorColor', cursorColor, defaultValue: null));
properties.add(FlagProperty('selectionEnabled', value: selectionEnabled, defaultValue: true, ifFalse: 'selection disabled'));
properties.add(DiagnosticsProperty<TextSelectionControls>('selectionControls', selectionControls, defaultValue: null));
properties.add(DiagnosticsProperty<ScrollPhysics>('scrollPhysics', scrollPhysics, defaultValue: null));
properties.add(DiagnosticsProperty<TextHeightBehavior>('textHeightBehavior', textHeightBehavior, defaultValue: null));
}
}
class _SelectableTextState extends State<SelectableText> implements TextSelectionGestureDetectorBuilderDelegate {
EditableTextState? get _editableText => editableTextKey.currentState;
late _TextSpanEditingController _controller;
FocusNode? _focusNode;
FocusNode get _effectiveFocusNode =>
widget.focusNode ?? (_focusNode ??= FocusNode(skipTraversal: true));
bool _showSelectionHandles = false;
late _SelectableTextSelectionGestureDetectorBuilder _selectionGestureDetectorBuilder;
// API for TextSelectionGestureDetectorBuilderDelegate.
@override
late bool forcePressEnabled;
@override
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
@override
bool get selectionEnabled => widget.selectionEnabled;
// End of API for TextSelectionGestureDetectorBuilderDelegate.
@override
void initState() {
super.initState();
_selectionGestureDetectorBuilder = _SelectableTextSelectionGestureDetectorBuilder(
state: this,
);
_controller = _TextSpanEditingController(
textSpan: widget.textSpan ?? TextSpan(text: widget.data),
);
_controller.addListener(_onControllerChanged);
}
@override
void didUpdateWidget(SelectableText oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.data != oldWidget.data || widget.textSpan != oldWidget.textSpan) {
_controller.removeListener(_onControllerChanged);
_controller.dispose();
_controller = _TextSpanEditingController(
textSpan: widget.textSpan ?? TextSpan(text: widget.data),
);
_controller.addListener(_onControllerChanged);
}
if (_effectiveFocusNode.hasFocus && _controller.selection.isCollapsed) {
_showSelectionHandles = false;
} else {
_showSelectionHandles = true;
}
}
@override
void dispose() {
_focusNode?.dispose();
_controller.dispose();
super.dispose();
}
void _onControllerChanged() {
final bool showSelectionHandles = !_effectiveFocusNode.hasFocus
|| !_controller.selection.isCollapsed;
if (showSelectionHandles == _showSelectionHandles) {
return;
}
setState(() {
_showSelectionHandles = showSelectionHandles;
});
}
void _handleSelectionChanged(TextSelection selection, SelectionChangedCause? cause) {
final bool willShowSelectionHandles = _shouldShowSelectionHandles(cause);
if (willShowSelectionHandles != _showSelectionHandles) {
setState(() {
_showSelectionHandles = willShowSelectionHandles;
});
}
widget.onSelectionChanged?.call(selection, cause);
switch (Theme.of(context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
if (cause == SelectionChangedCause.longPress) {
_editableText?.bringIntoView(selection.base);
}
return;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
// Do nothing.
}
}
/// Toggle the toolbar when a selection handle is tapped.
void _handleSelectionHandleTapped() {
if (_controller.selection.isCollapsed) {
_editableText!.toggleToolbar();
}
}
bool _shouldShowSelectionHandles(SelectionChangedCause? cause) {
// When the text field is activated by something that doesn't trigger the
// selection overlay, we shouldn't show the handles either.
if (!_selectionGestureDetectorBuilder.shouldShowSelectionToolbar) {
return false;
}
if (_controller.selection.isCollapsed) {
return false;
}
if (cause == SelectionChangedCause.keyboard) {
return false;
}
if (cause == SelectionChangedCause.longPress) {
return true;
}
if (_controller.text.isNotEmpty) {
return true;
}
return false;
}
@override
Widget build(BuildContext context) {
// TODO(garyq): Assert to block WidgetSpans from being used here are removed,
// but we still do not yet have nice handling of things like carets, clipboard,
// and other features. We should add proper support. Currently, caret handling
// is blocked on SkParagraph switch and https://github.com/flutter/engine/pull/27010
// should be landed in SkParagraph after the switch is complete.
assert(debugCheckHasMediaQuery(context));
assert(debugCheckHasDirectionality(context));
assert(
!(widget.style != null && !widget.style!.inherit &&
(widget.style!.fontSize == null || widget.style!.textBaseline == null)),
'inherit false style must supply fontSize and textBaseline',
);
final ThemeData theme = Theme.of(context);
final DefaultSelectionStyle selectionStyle = DefaultSelectionStyle.of(context);
final FocusNode focusNode = _effectiveFocusNode;
TextSelectionControls? textSelectionControls = widget.selectionControls;
final bool paintCursorAboveText;
final bool cursorOpacityAnimates;
Offset? cursorOffset;
final Color cursorColor;
final Color selectionColor;
Radius? cursorRadius = widget.cursorRadius;
switch (theme.platform) {
case TargetPlatform.iOS:
final CupertinoThemeData cupertinoTheme = CupertinoTheme.of(context);
forcePressEnabled = true;
textSelectionControls ??= cupertinoTextSelectionHandleControls;
paintCursorAboveText = true;
cursorOpacityAnimates = true;
cursorColor = widget.cursorColor ?? selectionStyle.cursorColor ?? cupertinoTheme.primaryColor;
selectionColor = selectionStyle.selectionColor ?? cupertinoTheme.primaryColor.withOpacity(0.40);
cursorRadius ??= const Radius.circular(2.0);
cursorOffset = Offset(iOSHorizontalOffset / MediaQuery.devicePixelRatioOf(context), 0);
case TargetPlatform.macOS:
final CupertinoThemeData cupertinoTheme = CupertinoTheme.of(context);
forcePressEnabled = false;
textSelectionControls ??= cupertinoDesktopTextSelectionHandleControls;
paintCursorAboveText = true;
cursorOpacityAnimates = true;
cursorColor = widget.cursorColor ?? selectionStyle.cursorColor ?? cupertinoTheme.primaryColor;
selectionColor = selectionStyle.selectionColor ?? cupertinoTheme.primaryColor.withOpacity(0.40);
cursorRadius ??= const Radius.circular(2.0);
cursorOffset = Offset(iOSHorizontalOffset / MediaQuery.devicePixelRatioOf(context), 0);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
forcePressEnabled = false;
textSelectionControls ??= materialTextSelectionHandleControls;
paintCursorAboveText = false;
cursorOpacityAnimates = false;
cursorColor = widget.cursorColor ?? selectionStyle.cursorColor ?? theme.colorScheme.primary;
selectionColor = selectionStyle.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
case TargetPlatform.linux:
case TargetPlatform.windows:
forcePressEnabled = false;
textSelectionControls ??= desktopTextSelectionHandleControls;
paintCursorAboveText = false;
cursorOpacityAnimates = false;
cursorColor = widget.cursorColor ?? selectionStyle.cursorColor ?? theme.colorScheme.primary;
selectionColor = selectionStyle.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
}
final DefaultTextStyle defaultTextStyle = DefaultTextStyle.of(context);
TextStyle? effectiveTextStyle = widget.style;
if (effectiveTextStyle == null || effectiveTextStyle.inherit) {
effectiveTextStyle = defaultTextStyle.style.merge(widget.style ?? _controller._textSpan.style);
}
final TextScaler? effectiveScaler = widget.textScaler ?? switch (widget.textScaleFactor) {
null => null,
final double textScaleFactor => TextScaler.linear(textScaleFactor),
};
final Widget child = RepaintBoundary(
child: EditableText(
key: editableTextKey,
style: effectiveTextStyle,
readOnly: true,
toolbarOptions: widget.toolbarOptions,
textWidthBasis: widget.textWidthBasis ?? defaultTextStyle.textWidthBasis,
textHeightBehavior: widget.textHeightBehavior ?? defaultTextStyle.textHeightBehavior,
showSelectionHandles: _showSelectionHandles,
showCursor: widget.showCursor,
controller: _controller,
focusNode: focusNode,
strutStyle: widget.strutStyle ?? const StrutStyle(),
textAlign: widget.textAlign ?? defaultTextStyle.textAlign ?? TextAlign.start,
textDirection: widget.textDirection,
textScaler: effectiveScaler,
autofocus: widget.autofocus,
forceLine: false,
minLines: widget.minLines,
maxLines: widget.maxLines ?? defaultTextStyle.maxLines,
selectionColor: selectionColor,
selectionControls: widget.selectionEnabled ? textSelectionControls : null,
onSelectionChanged: _handleSelectionChanged,
onSelectionHandleTapped: _handleSelectionHandleTapped,
rendererIgnoresPointer: true,
cursorWidth: widget.cursorWidth,
cursorHeight: widget.cursorHeight,
cursorRadius: cursorRadius,
cursorColor: cursorColor,
selectionHeightStyle: widget.selectionHeightStyle,
selectionWidthStyle: widget.selectionWidthStyle,
cursorOpacityAnimates: cursorOpacityAnimates,
cursorOffset: cursorOffset,
paintCursorAboveText: paintCursorAboveText,
backgroundCursorColor: CupertinoColors.inactiveGray,
enableInteractiveSelection: widget.enableInteractiveSelection,
magnifierConfiguration: widget.magnifierConfiguration ?? TextMagnifier.adaptiveMagnifierConfiguration,
dragStartBehavior: widget.dragStartBehavior,
scrollPhysics: widget.scrollPhysics,
autofillHints: null,
contextMenuBuilder: widget.contextMenuBuilder,
),
);
return Semantics(
label: widget.semanticsLabel,
excludeSemantics: widget.semanticsLabel != null,
onLongPress: () {
_effectiveFocusNode.requestFocus();
},
child: _selectionGestureDetectorBuilder.buildGestureDetector(
behavior: HitTestBehavior.translucent,
child: child,
),
);
}
}
| flutter/packages/flutter/lib/src/material/selectable_text.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/selectable_text.dart",
"repo_id": "flutter",
"token_count": 10089
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'colors.dart';
/// Used with [TabBar.indicator] to draw a horizontal line below the
/// selected tab.
///
/// The selected tab underline is inset from the tab's boundary by [insets].
/// The [borderSide] defines the line's color and weight.
///
/// The [TabBar.indicatorSize] property can be used to define the indicator's
/// bounds in terms of its (centered) widget with [TabBarIndicatorSize.label],
/// or the entire tab with [TabBarIndicatorSize.tab].
class UnderlineTabIndicator extends Decoration {
/// Create an underline style selected tab indicator.
const UnderlineTabIndicator({
this.borderRadius,
this.borderSide = const BorderSide(width: 2.0, color: Colors.white),
this.insets = EdgeInsets.zero,
});
/// The radius of the indicator's corners.
///
/// If this value is non-null, rounded rectangular tab indicator is
/// drawn, otherwise rectangular tab indicator is drawn.
final BorderRadius? borderRadius;
/// The color and weight of the horizontal line drawn below the selected tab.
final BorderSide borderSide;
/// Locates the selected tab's underline relative to the tab's boundary.
///
/// The [TabBar.indicatorSize] property can be used to define the tab
/// indicator's bounds in terms of its (centered) tab widget with
/// [TabBarIndicatorSize.label], or the entire tab with
/// [TabBarIndicatorSize.tab].
final EdgeInsetsGeometry insets;
@override
Decoration? lerpFrom(Decoration? a, double t) {
if (a is UnderlineTabIndicator) {
return UnderlineTabIndicator(
borderSide: BorderSide.lerp(a.borderSide, borderSide, t),
insets: EdgeInsetsGeometry.lerp(a.insets, insets, t)!,
);
}
return super.lerpFrom(a, t);
}
@override
Decoration? lerpTo(Decoration? b, double t) {
if (b is UnderlineTabIndicator) {
return UnderlineTabIndicator(
borderSide: BorderSide.lerp(borderSide, b.borderSide, t),
insets: EdgeInsetsGeometry.lerp(insets, b.insets, t)!,
);
}
return super.lerpTo(b, t);
}
@override
BoxPainter createBoxPainter([ VoidCallback? onChanged ]) {
return _UnderlinePainter(this, borderRadius, onChanged);
}
Rect _indicatorRectFor(Rect rect, TextDirection textDirection) {
final Rect indicator = insets.resolve(textDirection).deflateRect(rect);
return Rect.fromLTWH(
indicator.left,
indicator.bottom - borderSide.width,
indicator.width,
borderSide.width,
);
}
@override
Path getClipPath(Rect rect, TextDirection textDirection) {
if (borderRadius != null) {
return Path()..addRRect(
borderRadius!.toRRect(_indicatorRectFor(rect, textDirection))
);
}
return Path()..addRect(_indicatorRectFor(rect, textDirection));
}
}
class _UnderlinePainter extends BoxPainter {
_UnderlinePainter(
this.decoration,
this.borderRadius,
super.onChanged,
);
final UnderlineTabIndicator decoration;
final BorderRadius? borderRadius;
@override
void paint(Canvas canvas, Offset offset, ImageConfiguration configuration) {
assert(configuration.size != null);
final Rect rect = offset & configuration.size!;
final TextDirection textDirection = configuration.textDirection!;
final Paint paint;
if (borderRadius != null) {
paint = Paint()..color = decoration.borderSide.color;
final Rect indicator = decoration._indicatorRectFor(rect, textDirection);
final RRect rrect = RRect.fromRectAndCorners(
indicator,
topLeft: borderRadius!.topLeft,
topRight: borderRadius!.topRight,
bottomRight: borderRadius!.bottomRight,
bottomLeft: borderRadius!.bottomLeft,
);
canvas.drawRRect(rrect, paint);
} else {
paint = decoration.borderSide.toPaint()..strokeCap = StrokeCap.square;
final Rect indicator = decoration._indicatorRectFor(rect, textDirection)
.deflate(decoration.borderSide.width / 2.0);
canvas.drawLine(indicator.bottomLeft, indicator.bottomRight, paint);
}
}
}
| flutter/packages/flutter/lib/src/material/tab_indicator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/tab_indicator.dart",
"repo_id": "flutter",
"token_count": 1446
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'color_scheme.dart';
import 'constants.dart';
import 'ink_ripple.dart';
import 'material_state.dart';
import 'text_button.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'toggle_buttons_theme.dart';
// Examples can assume:
// List<bool> isSelected = <bool>[];
// void setState(dynamic arg) { }
/// A set of toggle buttons.
///
/// The list of [children] are laid out along [direction]. The state of each button
/// is controlled by [isSelected], which is a list of bools that determine
/// if a button is in an unselected or selected state. They are both
/// correlated by their index in the list. The length of [isSelected] has to
/// match the length of the [children] list.
///
/// There is a Material 3 version of this component, [SegmentedButton],
/// that's preferred for applications that are configured for Material 3
/// (see [ThemeData.useMaterial3]).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=kVEguaQWGAY}
///
/// ## Updating to [SegmentedButton]
///
/// There is a Material 3 version of this component, [SegmentedButton],
/// that's preferred for applications that are configured for Material 3
/// (see [ThemeData.useMaterial3]). The [SegmentedButton] widget's visuals
/// are a little bit different, see the Material 3 spec at
/// <https://m3.material.io/components/segmented-buttons/overview> for
/// more details. The [SegmentedButton] widget's API is also slightly different.
/// While the [ToggleButtons] widget can have list of widgets, the
/// [SegmentedButton] widget has a list of [ButtonSegment]s with
/// a type value. While the [ToggleButtons] uses a list of boolean values
/// to determine the selection state of each button, the [SegmentedButton]
/// uses a set of type values to determine the selection state of each segment.
/// The [SegmentedButton.style] is a [ButtonStyle] style field, which can be
/// used to customize the entire segmented button and the individual segments.
///
/// {@tool dartpad}
/// This sample shows how to migrate [ToggleButtons] that allows multiple
/// or no selection to [SegmentedButton] that allows multiple or no selection.
///
/// ** See code in examples/api/lib/material/toggle_buttons/toggle_buttons.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example showcase [ToggleButtons] in various configurations.
///
/// ** See code in examples/api/lib/material/toggle_buttons/toggle_buttons.0.dart **
/// {@end-tool}
///
/// ## Customizing toggle buttons
/// Each toggle's behavior can be configured by the [onPressed] callback, which
/// can update the [isSelected] list however it wants to.
///
/// {@animation 700 150 https://flutter.github.io/assets-for-api-docs/assets/material/toggle_buttons_simple.mp4}
///
/// Here is an implementation that allows for multiple buttons to be
/// simultaneously selected, while requiring none of the buttons to be
/// selected.
///
/// ```dart
/// ToggleButtons(
/// isSelected: isSelected,
/// onPressed: (int index) {
/// setState(() {
/// isSelected[index] = !isSelected[index];
/// });
/// },
/// children: const <Widget>[
/// Icon(Icons.ac_unit),
/// Icon(Icons.call),
/// Icon(Icons.cake),
/// ],
/// ),
/// ```
///
/// {@animation 700 150 https://flutter.github.io/assets-for-api-docs/assets/material/toggle_buttons_required_mutually_exclusive.mp4}
///
/// Here is an implementation that requires mutually exclusive selection while
/// requiring at least one selection. This assumes that [isSelected] was
/// properly initialized with one selection.
///
/// ```dart
/// ToggleButtons(
/// isSelected: isSelected,
/// onPressed: (int index) {
/// setState(() {
/// for (int buttonIndex = 0; buttonIndex < isSelected.length; buttonIndex++) {
/// if (buttonIndex == index) {
/// isSelected[buttonIndex] = true;
/// } else {
/// isSelected[buttonIndex] = false;
/// }
/// }
/// });
/// },
/// children: const <Widget>[
/// Icon(Icons.ac_unit),
/// Icon(Icons.call),
/// Icon(Icons.cake),
/// ],
/// ),
/// ```
///
/// {@animation 700 150 https://flutter.github.io/assets-for-api-docs/assets/material/toggle_buttons_mutually_exclusive.mp4}
///
/// Here is an implementation that requires mutually exclusive selection,
/// but allows for none of the buttons to be selected.
///
/// ```dart
/// ToggleButtons(
/// isSelected: isSelected,
/// onPressed: (int index) {
/// setState(() {
/// for (int buttonIndex = 0; buttonIndex < isSelected.length; buttonIndex++) {
/// if (buttonIndex == index) {
/// isSelected[buttonIndex] = !isSelected[buttonIndex];
/// } else {
/// isSelected[buttonIndex] = false;
/// }
/// }
/// });
/// },
/// children: const <Widget>[
/// Icon(Icons.ac_unit),
/// Icon(Icons.call),
/// Icon(Icons.cake),
/// ],
/// ),
/// ```
///
/// {@animation 700 150 https://flutter.github.io/assets-for-api-docs/assets/material/toggle_buttons_required.mp4}
///
/// Here is an implementation that allows for multiple buttons to be
/// simultaneously selected, while requiring at least one selection. This
/// assumes that [isSelected] was properly initialized with one selection.
///
/// ```dart
/// ToggleButtons(
/// isSelected: isSelected,
/// onPressed: (int index) {
/// int count = 0;
/// for (final bool value in isSelected) {
/// if (value) {
/// count += 1;
/// }
/// }
/// if (isSelected[index] && count < 2) {
/// return;
/// }
/// setState(() {
/// isSelected[index] = !isSelected[index];
/// });
/// },
/// children: const <Widget>[
/// Icon(Icons.ac_unit),
/// Icon(Icons.call),
/// Icon(Icons.cake),
/// ],
/// ),
/// ```
///
/// ## ToggleButton Borders
/// The toggle buttons, by default, have a solid, 1 logical pixel border
/// surrounding itself and separating each button. The toggle button borders'
/// color, width, and corner radii are configurable.
///
/// The [selectedBorderColor] determines the border's color when the button is
/// selected, while [disabledBorderColor] determines the border's color when
/// the button is disabled. [borderColor] is used when the button is enabled.
///
/// To remove the border, set [renderBorder] to false. Setting [borderWidth] to
/// 0.0 results in a hairline border. For more information on hairline borders,
/// see [BorderSide.width].
///
/// See also:
///
/// * <https://material.io/design/components/buttons.html#toggle-button>
class ToggleButtons extends StatelessWidget {
/// Creates a set of toggle buttons.
///
/// It displays its widgets provided in a [List] of [children] along [direction].
/// The state of each button is controlled by [isSelected], which is a list
/// of bools that determine if a button is in an active, disabled, or
/// selected state. They are both correlated by their index in the list.
/// The length of [isSelected] has to match the length of the [children]
/// list.
///
/// Both [children] and [isSelected] properties arguments are required.
///
/// The [focusNodes] argument must be null or a list of nodes. If [direction]
/// is [Axis.vertical], [verticalDirection] must not be null.
const ToggleButtons({
super.key,
required this.children,
required this.isSelected,
this.onPressed,
this.mouseCursor,
this.tapTargetSize,
this.textStyle,
this.constraints,
this.color,
this.selectedColor,
this.disabledColor,
this.fillColor,
this.focusColor,
this.highlightColor,
this.hoverColor,
this.splashColor,
this.focusNodes,
this.renderBorder = true,
this.borderColor,
this.selectedBorderColor,
this.disabledBorderColor,
this.borderRadius,
this.borderWidth,
this.direction = Axis.horizontal,
this.verticalDirection = VerticalDirection.down,
}) : assert(children.length == isSelected.length);
static const double _defaultBorderWidth = 1.0;
/// The toggle button widgets.
///
/// These are typically [Icon] or [Text] widgets. The boolean selection
/// state of each widget is defined by the corresponding [isSelected]
/// list item.
///
/// The length of children has to match the length of [isSelected]. If
/// [focusNodes] is not null, the length of children has to also match
/// the length of [focusNodes].
final List<Widget> children;
/// The corresponding selection state of each toggle button.
///
/// Each value in this list represents the selection state of the [children]
/// widget at the same index.
///
/// The length of [isSelected] has to match the length of [children].
final List<bool> isSelected;
/// The callback that is called when a button is tapped.
///
/// The index parameter of the callback is the index of the button that is
/// tapped or otherwise activated.
///
/// When the callback is null, all toggle buttons will be disabled.
final void Function(int index)? onPressed;
/// {@macro flutter.material.RawMaterialButton.mouseCursor}
///
/// If this property is null, [MaterialStateMouseCursor.clickable] will be used.
final MouseCursor? mouseCursor;
/// Configures the minimum size of the area within which the buttons may
/// be pressed.
///
/// If the [tapTargetSize] is larger than [constraints], the buttons will
/// include a transparent margin that responds to taps.
///
/// Defaults to [ThemeData.materialTapTargetSize].
final MaterialTapTargetSize? tapTargetSize;
/// The [TextStyle] to apply to any text in these toggle buttons.
///
/// [TextStyle.color] will be ignored and substituted by [color],
/// [selectedColor] or [disabledColor] depending on whether the buttons
/// are active, selected, or disabled.
final TextStyle? textStyle;
/// Defines the button's size.
///
/// Typically used to constrain the button's minimum size.
///
/// If this property is null, then
/// BoxConstraints(minWidth: 48.0, minHeight: 48.0) is be used.
final BoxConstraints? constraints;
/// The color for descendant [Text] and [Icon] widgets if the button is
/// enabled and not selected.
///
/// If [onPressed] is not null, this color will be used for values in
/// [isSelected] that are false.
///
/// If this property is null, then ToggleButtonTheme.of(context).color
/// is used. If [ToggleButtonsThemeData.color] is also null, then
/// Theme.of(context).colorScheme.onSurface is used.
final Color? color;
/// The color for descendant [Text] and [Icon] widgets if the button is
/// selected.
///
/// If [onPressed] is not null, this color will be used for values in
/// [isSelected] that are true.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).selectedColor is used. If
/// [ToggleButtonsThemeData.selectedColor] is also null, then
/// Theme.of(context).colorScheme.primary is used.
final Color? selectedColor;
/// The color for descendant [Text] and [Icon] widgets if the button is
/// disabled.
///
/// If [onPressed] is null, this color will be used.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).disabledColor is used. If
/// [ToggleButtonsThemeData.disabledColor] is also null, then
/// Theme.of(context).colorScheme.onSurface.withOpacity(0.38) is used.
final Color? disabledColor;
/// The fill color for selected toggle buttons.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).fillColor is used. If
/// [ToggleButtonsThemeData.fillColor] is also null, then
/// the fill color is null.
///
/// If fillColor is a [MaterialStateProperty<Color>], then [MaterialStateProperty.resolve]
/// is used for the following [MaterialState]s:
///
/// * [MaterialState.disabled]
/// * [MaterialState.selected]
///
final Color? fillColor;
/// The color to use for filling the button when the button has input focus.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).focusColor is used. If
/// [ToggleButtonsThemeData.focusColor] is also null, then
/// Theme.of(context).focusColor is used.
final Color? focusColor;
/// The highlight color for the button's [InkWell].
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).highlightColor is used. If
/// [ToggleButtonsThemeData.highlightColor] is also null, then
/// Theme.of(context).highlightColor is used.
final Color? highlightColor;
/// The splash color for the button's [InkWell].
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).splashColor is used. If
/// [ToggleButtonsThemeData.splashColor] is also null, then
/// Theme.of(context).splashColor is used.
final Color? splashColor;
/// The color to use for filling the button when the button has a pointer
/// hovering over it.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).hoverColor is used. If
/// [ToggleButtonsThemeData.hoverColor] is also null, then
/// Theme.of(context).hoverColor is used.
final Color? hoverColor;
/// The list of [FocusNode]s, corresponding to each toggle button.
///
/// Focus is used to determine which widget should be affected by keyboard
/// events. The focus tree keeps track of which widget is currently focused
/// on by the user.
///
/// If not null, the length of focusNodes has to match the length of
/// [children].
///
/// See [FocusNode] for more information about how focus nodes are used.
final List<FocusNode>? focusNodes;
/// Whether or not to render a border around each toggle button.
///
/// When true, a border with [borderWidth], [borderRadius] and the
/// appropriate border color will render. Otherwise, no border will be
/// rendered.
final bool renderBorder;
/// The border color to display when the toggle button is enabled and not
/// selected.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).borderColor is used. If
/// [ToggleButtonsThemeData.borderColor] is also null, then
/// Theme.of(context).colorScheme.onSurface is used.
final Color? borderColor;
/// The border color to display when the toggle button is selected.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).selectedBorderColor is used. If
/// [ToggleButtonsThemeData.selectedBorderColor] is also null, then
/// Theme.of(context).colorScheme.primary is used.
final Color? selectedBorderColor;
/// The border color to display when the toggle button is disabled.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).disabledBorderColor is used. If
/// [ToggleButtonsThemeData.disabledBorderColor] is also null, then
/// Theme.of(context).disabledBorderColor is used.
final Color? disabledBorderColor;
/// The width of the border surrounding each toggle button.
///
/// This applies to both the greater surrounding border, as well as the
/// borders rendered between toggle buttons.
///
/// To render a hairline border (one physical pixel), set borderWidth to 0.0.
/// See [BorderSide.width] for more details on hairline borders.
///
/// To omit the border entirely, set [renderBorder] to false.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).borderWidth is used. If
/// [ToggleButtonsThemeData.borderWidth] is also null, then
/// a width of 1.0 is used.
final double? borderWidth;
/// The radii of the border's corners.
///
/// If this property is null, then
/// ToggleButtonTheme.of(context).borderRadius is used. If
/// [ToggleButtonsThemeData.borderRadius] is also null, then
/// the buttons default to non-rounded borders.
final BorderRadius? borderRadius;
/// The direction along which the buttons are rendered.
///
/// Defaults to [Axis.horizontal].
final Axis direction;
/// If [direction] is [Axis.vertical], this parameter determines whether to lay out
/// the buttons starting from the first or last child from top to bottom.
final VerticalDirection verticalDirection;
// Determines if this is the first child that is being laid out
// by the render object, _not_ the order of the children in its list.
bool _isFirstButton(int index, int length, TextDirection textDirection) {
switch (direction) {
case Axis.horizontal:
return switch (textDirection) {
TextDirection.rtl => index == length - 1,
TextDirection.ltr => index == 0,
};
case Axis.vertical:
return switch (verticalDirection) {
VerticalDirection.up => index == length - 1,
VerticalDirection.down => index == 0,
};
}
}
// Determines if this is the last child that is being laid out
// by the render object, _not_ the order of the children in its list.
bool _isLastButton(int index, int length, TextDirection textDirection) {
switch (direction) {
case Axis.horizontal:
return switch (textDirection) {
TextDirection.rtl => index == 0,
TextDirection.ltr => index == length - 1,
};
case Axis.vertical:
return switch (verticalDirection) {
VerticalDirection.up => index == 0,
VerticalDirection.down => index == length - 1,
};
}
}
BorderRadius _getEdgeBorderRadius(
int index,
int length,
TextDirection textDirection,
ToggleButtonsThemeData toggleButtonsTheme,
) {
final BorderRadius resultingBorderRadius = borderRadius
?? toggleButtonsTheme.borderRadius
?? BorderRadius.zero;
if (length == 1) {
return resultingBorderRadius;
} else if (direction == Axis.horizontal) {
if (_isFirstButton(index, length, textDirection)) {
return BorderRadius.only(
topLeft: resultingBorderRadius.topLeft,
bottomLeft: resultingBorderRadius.bottomLeft,
);
} else if (_isLastButton(index, length, textDirection)) {
return BorderRadius.only(
topRight: resultingBorderRadius.topRight,
bottomRight: resultingBorderRadius.bottomRight,
);
}
} else {
if (_isFirstButton(index, length, textDirection)) {
return BorderRadius.only(
topLeft: resultingBorderRadius.topLeft,
topRight: resultingBorderRadius.topRight,
);
} else if (_isLastButton(index, length, textDirection)) {
return BorderRadius.only(
bottomLeft: resultingBorderRadius.bottomLeft,
bottomRight: resultingBorderRadius.bottomRight,
);
}
}
return BorderRadius.zero;
}
BorderRadius _getClipBorderRadius(
int index,
int length,
TextDirection textDirection,
ToggleButtonsThemeData toggleButtonsTheme,
) {
final BorderRadius resultingBorderRadius = borderRadius
?? toggleButtonsTheme.borderRadius
?? BorderRadius.zero;
final double resultingBorderWidth = borderWidth
?? toggleButtonsTheme.borderWidth
?? _defaultBorderWidth;
if (length == 1) {
return BorderRadius.only(
topLeft: resultingBorderRadius.topLeft - Radius.circular(resultingBorderWidth / 2.0),
bottomLeft: resultingBorderRadius.bottomLeft - Radius.circular(resultingBorderWidth / 2.0),
topRight: resultingBorderRadius.topRight - Radius.circular(resultingBorderWidth / 2.0),
bottomRight: resultingBorderRadius.bottomRight - Radius.circular(resultingBorderWidth / 2.0),
);
} else if (direction == Axis.horizontal) {
if (_isFirstButton(index, length, textDirection)) {
return BorderRadius.only(
topLeft: resultingBorderRadius.topLeft - Radius.circular(resultingBorderWidth / 2.0),
bottomLeft: resultingBorderRadius.bottomLeft - Radius.circular(resultingBorderWidth / 2.0),
);
} else if (_isLastButton(index, length, textDirection)) {
return BorderRadius.only(
topRight: resultingBorderRadius.topRight - Radius.circular(resultingBorderWidth / 2.0),
bottomRight: resultingBorderRadius.bottomRight - Radius.circular(resultingBorderWidth / 2.0),
);
}
} else {
if (_isFirstButton(index, length, textDirection)) {
return BorderRadius.only(
topLeft: resultingBorderRadius.topLeft - Radius.circular(resultingBorderWidth / 2.0),
topRight: resultingBorderRadius.topRight - Radius.circular(resultingBorderWidth / 2.0),
);
} else if (_isLastButton(index, length, textDirection)) {
return BorderRadius.only(
bottomLeft: resultingBorderRadius.bottomLeft - Radius.circular(resultingBorderWidth / 2.0),
bottomRight: resultingBorderRadius.bottomRight - Radius.circular(resultingBorderWidth / 2.0),
);
}
}
return BorderRadius.zero;
}
BorderSide _getLeadingBorderSide(
int index,
ThemeData theme,
ToggleButtonsThemeData toggleButtonsTheme,
) {
if (!renderBorder) {
return BorderSide.none;
}
final double resultingBorderWidth = borderWidth
?? toggleButtonsTheme.borderWidth
?? _defaultBorderWidth;
if (onPressed != null && (isSelected[index] || (index != 0 && isSelected[index - 1]))) {
return BorderSide(
color: selectedBorderColor
?? toggleButtonsTheme.selectedBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else if (onPressed != null && !isSelected[index]) {
return BorderSide(
color: borderColor
?? toggleButtonsTheme.borderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else {
return BorderSide(
color: disabledBorderColor
?? toggleButtonsTheme.disabledBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
}
}
BorderSide _getBorderSide(
int index,
ThemeData theme,
ToggleButtonsThemeData toggleButtonsTheme,
) {
if (!renderBorder) {
return BorderSide.none;
}
final double resultingBorderWidth = borderWidth
?? toggleButtonsTheme.borderWidth
?? _defaultBorderWidth;
if (onPressed != null && isSelected[index]) {
return BorderSide(
color: selectedBorderColor
?? toggleButtonsTheme.selectedBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else if (onPressed != null && !isSelected[index]) {
return BorderSide(
color: borderColor
?? toggleButtonsTheme.borderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else {
return BorderSide(
color: disabledBorderColor
?? toggleButtonsTheme.disabledBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
}
}
BorderSide _getTrailingBorderSide(
int index,
ThemeData theme,
ToggleButtonsThemeData toggleButtonsTheme,
) {
if (!renderBorder) {
return BorderSide.none;
}
if (index != children.length - 1) {
return BorderSide.none;
}
final double resultingBorderWidth = borderWidth
?? toggleButtonsTheme.borderWidth
?? _defaultBorderWidth;
if (onPressed != null && (isSelected[index])) {
return BorderSide(
color: selectedBorderColor
?? toggleButtonsTheme.selectedBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else if (onPressed != null && !isSelected[index]) {
return BorderSide(
color: borderColor
?? toggleButtonsTheme.borderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
} else {
return BorderSide(
color: disabledBorderColor
?? toggleButtonsTheme.disabledBorderColor
?? theme.colorScheme.onSurface.withOpacity(0.12),
width: resultingBorderWidth,
);
}
}
@override
Widget build(BuildContext context) {
assert(
() {
if (focusNodes != null) {
return focusNodes!.length == children.length;
}
return true;
}(),
'focusNodes.length must match children.length.\n'
'There are ${focusNodes!.length} focus nodes, while '
'there are ${children.length} children.',
);
final ThemeData theme = Theme.of(context);
final ToggleButtonsThemeData toggleButtonsTheme = ToggleButtonsTheme.of(context);
final TextDirection textDirection = Directionality.of(context);
final List<Widget> buttons = List<Widget>.generate(children.length, (int index) {
final BorderRadius edgeBorderRadius = _getEdgeBorderRadius(index, children.length, textDirection, toggleButtonsTheme);
final BorderRadius clipBorderRadius = _getClipBorderRadius(index, children.length, textDirection, toggleButtonsTheme);
final BorderSide leadingBorderSide = _getLeadingBorderSide(index, theme, toggleButtonsTheme);
final BorderSide borderSide = _getBorderSide(index, theme, toggleButtonsTheme);
final BorderSide trailingBorderSide = _getTrailingBorderSide(index, theme, toggleButtonsTheme);
final Set<MaterialState> states = <MaterialState>{
if (isSelected[index] && onPressed != null) MaterialState.selected,
if (onPressed == null) MaterialState.disabled,
};
final Color effectiveFillColor = _ResolveFillColor(fillColor
?? toggleButtonsTheme.fillColor).resolve(states)
?? _DefaultFillColor(theme.colorScheme).resolve(states);
final Color currentColor;
if (onPressed != null && isSelected[index]) {
currentColor = selectedColor
?? toggleButtonsTheme.selectedColor
?? theme.colorScheme.primary;
} else if (onPressed != null && !isSelected[index]) {
currentColor = color
?? toggleButtonsTheme.color
?? theme.colorScheme.onSurface.withOpacity(0.87);
} else {
currentColor = disabledColor
?? toggleButtonsTheme.disabledColor
?? theme.colorScheme.onSurface.withOpacity(0.38);
}
final TextStyle currentTextStyle = textStyle
?? toggleButtonsTheme.textStyle
?? theme.textTheme.bodyMedium!;
final BoxConstraints? currentConstraints = constraints
?? toggleButtonsTheme.constraints;
final Size minimumSize = currentConstraints == null
? const Size.square(kMinInteractiveDimension)
: Size(currentConstraints.minWidth, currentConstraints.minHeight);
final Size? maximumSize = currentConstraints == null
? null
: Size(currentConstraints.maxWidth, currentConstraints.maxHeight);
final Size minPaddingSize;
switch (tapTargetSize ?? theme.materialTapTargetSize) {
case MaterialTapTargetSize.padded:
if (direction == Axis.horizontal) {
minPaddingSize = const Size(
0.0,
kMinInteractiveDimension,
);
} else {
minPaddingSize = const Size(
kMinInteractiveDimension,
0.0,
);
}
assert(minPaddingSize.width >= 0.0);
assert(minPaddingSize.height >= 0.0);
case MaterialTapTargetSize.shrinkWrap:
minPaddingSize = Size.zero;
}
Widget button = _SelectToggleButton(
leadingBorderSide: leadingBorderSide,
borderSide: borderSide,
trailingBorderSide: trailingBorderSide,
borderRadius: edgeBorderRadius,
isFirstButton: index == 0,
isLastButton: index == children.length - 1,
direction: direction,
verticalDirection: verticalDirection,
child: ClipRRect(
borderRadius: clipBorderRadius,
child: TextButton(
focusNode: focusNodes != null ? focusNodes![index] : null,
style: ButtonStyle(
backgroundColor: MaterialStatePropertyAll<Color?>(effectiveFillColor),
foregroundColor: MaterialStatePropertyAll<Color?>(currentColor),
overlayColor: _ToggleButtonDefaultOverlay(
selected: onPressed != null && isSelected[index],
unselected: onPressed != null && !isSelected[index],
colorScheme: theme.colorScheme,
disabledColor: disabledColor ?? toggleButtonsTheme.disabledColor,
focusColor: focusColor ?? toggleButtonsTheme.focusColor,
highlightColor: highlightColor ?? toggleButtonsTheme.highlightColor,
hoverColor: hoverColor ?? toggleButtonsTheme.hoverColor,
splashColor: splashColor ?? toggleButtonsTheme.splashColor,
),
elevation: const MaterialStatePropertyAll<double>(0),
textStyle: MaterialStatePropertyAll<TextStyle?>(currentTextStyle.copyWith(
color: currentColor,
)),
padding: const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.zero),
minimumSize: MaterialStatePropertyAll<Size?>(minimumSize),
maximumSize: MaterialStatePropertyAll<Size?>(maximumSize),
shape: const MaterialStatePropertyAll<OutlinedBorder>(RoundedRectangleBorder()),
mouseCursor: MaterialStatePropertyAll<MouseCursor?>(mouseCursor),
visualDensity: VisualDensity.standard,
tapTargetSize: MaterialTapTargetSize.shrinkWrap,
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
splashFactory: InkRipple.splashFactory,
),
onPressed: onPressed != null
? () {onPressed!(index);}
: null,
child: children[index],
),
),
);
if (currentConstraints != null) {
button = Center(child: button);
}
return MergeSemantics(
child: Semantics(
container: true,
checked: isSelected[index],
enabled: onPressed != null,
child: _InputPadding(
minSize: minPaddingSize,
direction: direction,
child: button,
),
),
);
});
if (direction == Axis.vertical) {
return IntrinsicWidth(
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
verticalDirection: verticalDirection,
children: buttons,
),
);
}
return IntrinsicHeight(
child: Row(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: buttons,
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('disabled',
value: onPressed == null,
ifTrue: 'Buttons are disabled',
ifFalse: 'Buttons are enabled',
));
textStyle?.debugFillProperties(properties, prefix: 'textStyle.');
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(ColorProperty('selectedColor', selectedColor, defaultValue: null));
properties.add(ColorProperty('disabledColor', disabledColor, defaultValue: null));
properties.add(ColorProperty('fillColor', fillColor, defaultValue: null));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: null));
properties.add(ColorProperty('highlightColor', highlightColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: null));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: null));
properties.add(ColorProperty('borderColor', borderColor, defaultValue: null));
properties.add(ColorProperty('selectedBorderColor', selectedBorderColor, defaultValue: null));
properties.add(ColorProperty('disabledBorderColor', disabledBorderColor, defaultValue: null));
properties.add(DiagnosticsProperty<BorderRadius>('borderRadius', borderRadius, defaultValue: null));
properties.add(DoubleProperty('borderWidth', borderWidth, defaultValue: null));
properties.add(DiagnosticsProperty<Axis>('direction', direction, defaultValue: Axis.horizontal));
properties.add(DiagnosticsProperty<VerticalDirection>('verticalDirection', verticalDirection, defaultValue: VerticalDirection.down));
}
}
@immutable
class _ResolveFillColor extends MaterialStateProperty<Color?> with Diagnosticable {
_ResolveFillColor(this.primary);
final Color? primary;
@override
Color? resolve(Set<MaterialState> states) {
if (primary is MaterialStateProperty<Color>) {
return MaterialStateProperty.resolveAs<Color?>(primary, states);
}
return states.contains(MaterialState.selected) ? primary : null;
}
}
@immutable
class _DefaultFillColor extends MaterialStateProperty<Color> with Diagnosticable {
_DefaultFillColor(this.colorScheme);
final ColorScheme colorScheme;
@override
Color resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return colorScheme.primary.withOpacity(0.12);
}
return colorScheme.surface.withOpacity(0.0);
}
}
@immutable
class _ToggleButtonDefaultOverlay extends MaterialStateProperty<Color?> {
_ToggleButtonDefaultOverlay({
required this.selected,
required this.unselected,
this.colorScheme,
this.focusColor,
this.highlightColor,
this.hoverColor,
this.splashColor,
this.disabledColor,
});
final bool selected;
final bool unselected;
final ColorScheme? colorScheme;
final Color? focusColor;
final Color? highlightColor;
final Color? hoverColor;
final Color? splashColor;
final Color? disabledColor;
@override
Color? resolve(Set<MaterialState> states) {
if (selected) {
if (states.contains(MaterialState.pressed)) {
return splashColor ?? colorScheme?.primary.withOpacity(0.16);
}
if (states.contains(MaterialState.hovered)) {
return hoverColor ?? colorScheme?.primary.withOpacity(0.04);
}
if (states.contains(MaterialState.focused)) {
return focusColor ?? colorScheme?.primary.withOpacity(0.12);
}
} else if (unselected) {
if (states.contains(MaterialState.pressed)) {
return splashColor ?? highlightColor ?? colorScheme?.onSurface.withOpacity(0.16);
}
if (states.contains(MaterialState.hovered)) {
return hoverColor ?? colorScheme?.onSurface.withOpacity(0.04);
}
if (states.contains(MaterialState.focused)) {
return focusColor ?? colorScheme?.onSurface.withOpacity(0.12);
}
}
return null;
}
@override
String toString() {
return '''
{
selected:
hovered: $hoverColor, otherwise: ${colorScheme?.primary.withOpacity(0.04)},
focused: $focusColor, otherwise: ${colorScheme?.primary.withOpacity(0.12)},
pressed: $splashColor, otherwise: ${colorScheme?.primary.withOpacity(0.16)},
unselected:
hovered: $hoverColor, otherwise: ${colorScheme?.onSurface.withOpacity(0.04)},
focused: $focusColor, otherwise: ${colorScheme?.onSurface.withOpacity(0.12)},
pressed: $splashColor, otherwise: ${colorScheme?.onSurface.withOpacity(0.16)},
otherwise: null,
}
''';
}
}
class _SelectToggleButton extends SingleChildRenderObjectWidget {
const _SelectToggleButton({
required Widget super.child,
required this.leadingBorderSide,
required this.borderSide,
required this.trailingBorderSide,
required this.borderRadius,
required this.isFirstButton,
required this.isLastButton,
required this.direction,
required this.verticalDirection,
});
// The width and color of the button's leading side border.
final BorderSide leadingBorderSide;
// The width and color of the side borders.
//
// If [direction] is [Axis.horizontal], this corresponds to the width and color
// of the button's top and bottom side borders.
//
// If [direction] is [Axis.vertical], this corresponds to the width and color
// of the button's left and right side borders.
final BorderSide borderSide;
// The width and color of the button's trailing side border.
final BorderSide trailingBorderSide;
// The border radii of each corner of the button.
final BorderRadius borderRadius;
// Whether or not this toggle button is the first button in the list.
final bool isFirstButton;
// Whether or not this toggle button is the last button in the list.
final bool isLastButton;
// The direction along which the buttons are rendered.
final Axis direction;
// If [direction] is [Axis.vertical], this property defines whether or not this button in its list
// of buttons is laid out starting from top to bottom or from bottom to top.
final VerticalDirection verticalDirection;
@override
_SelectToggleButtonRenderObject createRenderObject(BuildContext context) => _SelectToggleButtonRenderObject(
leadingBorderSide,
borderSide,
trailingBorderSide,
borderRadius,
isFirstButton,
isLastButton,
direction,
verticalDirection,
Directionality.of(context),
);
@override
void updateRenderObject(BuildContext context, _SelectToggleButtonRenderObject renderObject) {
renderObject
..leadingBorderSide = leadingBorderSide
..borderSide = borderSide
..trailingBorderSide = trailingBorderSide
..borderRadius = borderRadius
..isFirstButton = isFirstButton
..isLastButton = isLastButton
..direction = direction
..verticalDirection = verticalDirection
..textDirection = Directionality.of(context);
}
}
class _SelectToggleButtonRenderObject extends RenderShiftedBox {
_SelectToggleButtonRenderObject(
this._leadingBorderSide,
this._borderSide,
this._trailingBorderSide,
this._borderRadius,
this._isFirstButton,
this._isLastButton,
this._direction,
this._verticalDirection,
this._textDirection, [
RenderBox? child,
]) : super(child);
Axis get direction => _direction;
Axis _direction;
set direction(Axis value) {
if (_direction == value) {
return;
}
_direction = value;
markNeedsLayout();
}
VerticalDirection get verticalDirection => _verticalDirection;
VerticalDirection _verticalDirection;
set verticalDirection(VerticalDirection value) {
if (_verticalDirection == value) {
return;
}
_verticalDirection = value;
markNeedsLayout();
}
// The width and color of the button's leading side border.
BorderSide get leadingBorderSide => _leadingBorderSide;
BorderSide _leadingBorderSide;
set leadingBorderSide(BorderSide value) {
if (_leadingBorderSide == value) {
return;
}
_leadingBorderSide = value;
markNeedsLayout();
}
// The width and color of the button's top and bottom side borders.
BorderSide get borderSide => _borderSide;
BorderSide _borderSide;
set borderSide(BorderSide value) {
if (_borderSide == value) {
return;
}
_borderSide = value;
markNeedsLayout();
}
// The width and color of the button's trailing side border.
BorderSide get trailingBorderSide => _trailingBorderSide;
BorderSide _trailingBorderSide;
set trailingBorderSide(BorderSide value) {
if (_trailingBorderSide == value) {
return;
}
_trailingBorderSide = value;
markNeedsLayout();
}
// The border radii of each corner of the button.
BorderRadius get borderRadius => _borderRadius;
BorderRadius _borderRadius;
set borderRadius(BorderRadius value) {
if (_borderRadius == value) {
return;
}
_borderRadius = value;
markNeedsLayout();
}
// Whether or not this toggle button is the first button in the list.
bool get isFirstButton => _isFirstButton;
bool _isFirstButton;
set isFirstButton(bool value) {
if (_isFirstButton == value) {
return;
}
_isFirstButton = value;
markNeedsLayout();
}
// Whether or not this toggle button is the last button in the list.
bool get isLastButton => _isLastButton;
bool _isLastButton;
set isLastButton(bool value) {
if (_isLastButton == value) {
return;
}
_isLastButton = value;
markNeedsLayout();
}
// The direction in which text flows for this application.
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
markNeedsLayout();
}
static double _maxHeight(RenderBox? box, double width) {
return box == null ? 0.0 : box.getMaxIntrinsicHeight(width);
}
static double _minHeight(RenderBox? box, double width) {
return box == null ? 0.0 : box.getMinIntrinsicHeight(width);
}
static double _minWidth(RenderBox? box, double height) {
return box == null ? 0.0 : box.getMinIntrinsicWidth(height);
}
static double _maxWidth(RenderBox? box, double height) {
return box == null ? 0.0 : box.getMaxIntrinsicWidth(height);
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
// The baseline of this widget is the baseline of its child
final BaselineOffset childOffset = BaselineOffset(child?.computeDistanceToActualBaseline(baseline));
return switch (direction) {
Axis.horizontal => childOffset + borderSide.width,
Axis.vertical => childOffset + leadingBorderSide.width,
}.offset;
}
@override
double computeMaxIntrinsicHeight(double width) {
return direction == Axis.horizontal
? borderSide.width * 2.0 + _maxHeight(child, width)
: leadingBorderSide.width + _maxHeight(child, width) + trailingBorderSide.width;
}
@override
double computeMinIntrinsicHeight(double width) {
return direction == Axis.horizontal
? borderSide.width * 2.0 + _minHeight(child, width)
: leadingBorderSide.width + _maxHeight(child, width) + trailingBorderSide.width;
}
@override
double computeMaxIntrinsicWidth(double height) {
return direction == Axis.horizontal
? leadingBorderSide.width + _maxWidth(child, height) + trailingBorderSide.width
: borderSide.width * 2.0 + _maxWidth(child, height);
}
@override
double computeMinIntrinsicWidth(double height) {
return direction == Axis.horizontal
? leadingBorderSide.width + _minWidth(child, height) + trailingBorderSide.width
: borderSide.width * 2.0 + _minWidth(child, height);
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.dryLayoutChild,
);
}
@override
void performLayout() {
size = _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.layoutChild,
);
if (child == null) {
return;
}
final BoxParentData childParentData = child!.parentData! as BoxParentData;
if (direction == Axis.horizontal) {
childParentData.offset = switch (textDirection) {
TextDirection.ltr => Offset(leadingBorderSide.width, borderSide.width),
TextDirection.rtl => Offset(trailingBorderSide.width, borderSide.width),
};
} else {
childParentData.offset = switch (verticalDirection) {
VerticalDirection.down => Offset(borderSide.width, leadingBorderSide.width),
VerticalDirection.up => Offset(borderSide.width, trailingBorderSide.width),
};
}
}
Size _computeSize({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
if (child == null) {
if (direction == Axis.horizontal) {
return constraints.constrain(Size(
leadingBorderSide.width + trailingBorderSide.width,
borderSide.width * 2.0,
));
} else {
return constraints.constrain(Size(
borderSide.width * 2.0,
leadingBorderSide.width + trailingBorderSide.width,
));
}
}
final double leftConstraint;
final double rightConstraint;
final double topConstraint;
final double bottomConstraint;
// It does not matter what [textDirection] or [verticalDirection] is,
// since deflating the size constraints horizontally/vertically
// and the returned size accounts for the width of both sides.
if (direction == Axis.horizontal) {
rightConstraint = trailingBorderSide.width;
leftConstraint = leadingBorderSide.width;
topConstraint = borderSide.width;
bottomConstraint = borderSide.width;
} else {
rightConstraint = borderSide.width;
leftConstraint = borderSide.width;
topConstraint = leadingBorderSide.width;
bottomConstraint = trailingBorderSide.width;
}
final BoxConstraints innerConstraints = constraints.deflate(
EdgeInsets.only(
left: leftConstraint,
top: topConstraint,
right: rightConstraint,
bottom: bottomConstraint,
),
);
final Size childSize = layoutChild(child!, innerConstraints);
return constraints.constrain(Size(
leftConstraint + childSize.width + rightConstraint,
topConstraint + childSize.height + bottomConstraint,
));
}
@override
void paint(PaintingContext context, Offset offset) {
super.paint(context, offset);
final Offset bottomRight = size.bottomRight(offset);
final Rect outer = Rect.fromLTRB(offset.dx, offset.dy, bottomRight.dx, bottomRight.dy);
final Rect center = outer.deflate(borderSide.width / 2.0);
const double sweepAngle = math.pi / 2.0;
final RRect rrect = RRect.fromRectAndCorners(
center,
topLeft: (borderRadius.topLeft.x * borderRadius.topLeft.y != 0.0) ? borderRadius.topLeft : Radius.zero,
topRight: (borderRadius.topRight.x * borderRadius.topRight.y != 0.0) ? borderRadius.topRight : Radius.zero,
bottomLeft: (borderRadius.bottomLeft.x * borderRadius.bottomLeft.y != 0.0) ? borderRadius.bottomLeft : Radius.zero,
bottomRight: (borderRadius.bottomRight.x * borderRadius.bottomRight.y != 0.0) ? borderRadius.bottomRight : Radius.zero,
).scaleRadii();
final Rect tlCorner = Rect.fromLTWH(
rrect.left,
rrect.top,
rrect.tlRadiusX * 2.0,
rrect.tlRadiusY * 2.0,
);
final Rect blCorner = Rect.fromLTWH(
rrect.left,
rrect.bottom - (rrect.blRadiusY * 2.0),
rrect.blRadiusX * 2.0,
rrect.blRadiusY * 2.0,
);
final Rect trCorner = Rect.fromLTWH(
rrect.right - (rrect.trRadiusX * 2),
rrect.top,
rrect.trRadiusX * 2,
rrect.trRadiusY * 2,
);
final Rect brCorner = Rect.fromLTWH(
rrect.right - (rrect.brRadiusX * 2),
rrect.bottom - (rrect.brRadiusY * 2),
rrect.brRadiusX * 2,
rrect.brRadiusY * 2,
);
final Paint leadingPaint = leadingBorderSide.toPaint();
// Only one button.
if (isFirstButton && isLastButton) {
final Path leadingPath = Path();
final double startX = (rrect.brRadiusX == 0.0) ? outer.right : rrect.right - rrect.brRadiusX;
leadingPath..moveTo(startX, rrect.bottom)
..lineTo(rrect.left + rrect.blRadiusX, rrect.bottom)
..addArc(blCorner, math.pi / 2.0, sweepAngle)
..lineTo(rrect.left, rrect.top + rrect.tlRadiusY)
..addArc(tlCorner, math.pi, sweepAngle)
..lineTo(rrect.right - rrect.trRadiusX, rrect.top)
..addArc(trCorner, math.pi * 3.0 / 2.0, sweepAngle)
..lineTo(rrect.right, rrect.bottom - rrect.brRadiusY)
..addArc(brCorner, 0, sweepAngle);
context.canvas.drawPath(leadingPath, leadingPaint);
return;
}
if (direction == Axis.horizontal) {
switch (textDirection) {
case TextDirection.ltr:
if (isLastButton) {
final Path leftPath = Path();
leftPath..moveTo(rrect.left, rrect.bottom + leadingBorderSide.width / 2)
..lineTo(rrect.left, rrect.top - leadingBorderSide.width / 2);
context.canvas.drawPath(leftPath, leadingPaint);
final Paint endingPaint = trailingBorderSide.toPaint();
final Path endingPath = Path();
endingPath..moveTo(rrect.left + borderSide.width / 2.0, rrect.top)
..lineTo(rrect.right - rrect.trRadiusX, rrect.top)
..addArc(trCorner, math.pi * 3.0 / 2.0, sweepAngle)
..lineTo(rrect.right, rrect.bottom - rrect.brRadiusY)
..addArc(brCorner, 0, sweepAngle)
..lineTo(rrect.left + borderSide.width / 2.0, rrect.bottom);
context.canvas.drawPath(endingPath, endingPaint);
} else if (isFirstButton) {
final Path leadingPath = Path();
leadingPath..moveTo(outer.right, rrect.bottom)
..lineTo(rrect.left + rrect.blRadiusX, rrect.bottom)
..addArc(blCorner, math.pi / 2.0, sweepAngle)
..lineTo(rrect.left, rrect.top + rrect.tlRadiusY)
..addArc(tlCorner, math.pi, sweepAngle)
..lineTo(outer.right, rrect.top);
context.canvas.drawPath(leadingPath, leadingPaint);
} else {
final Path leadingPath = Path();
leadingPath..moveTo(rrect.left, rrect.bottom + leadingBorderSide.width / 2)
..lineTo(rrect.left, rrect.top - leadingBorderSide.width / 2);
context.canvas.drawPath(leadingPath, leadingPaint);
final Paint horizontalPaint = borderSide.toPaint();
final Path horizontalPaths = Path();
horizontalPaths..moveTo(rrect.left + borderSide.width / 2.0, rrect.top)
..lineTo(outer.right - rrect.trRadiusX, rrect.top)
..moveTo(rrect.left + borderSide.width / 2.0 + rrect.tlRadiusX, rrect.bottom)
..lineTo(outer.right - rrect.trRadiusX, rrect.bottom);
context.canvas.drawPath(horizontalPaths, horizontalPaint);
}
case TextDirection.rtl:
if (isLastButton) {
final Path leadingPath = Path();
leadingPath..moveTo(rrect.right, rrect.bottom + leadingBorderSide.width / 2)
..lineTo(rrect.right, rrect.top - leadingBorderSide.width / 2);
context.canvas.drawPath(leadingPath, leadingPaint);
final Paint endingPaint = trailingBorderSide.toPaint();
final Path endingPath = Path();
endingPath..moveTo(rrect.right - borderSide.width / 2.0, rrect.top)
..lineTo(rrect.left + rrect.tlRadiusX, rrect.top)
..addArc(tlCorner, math.pi * 3.0 / 2.0, -sweepAngle)
..lineTo(rrect.left, rrect.bottom - rrect.blRadiusY)
..addArc(blCorner, math.pi, -sweepAngle)
..lineTo(rrect.right - borderSide.width / 2.0, rrect.bottom);
context.canvas.drawPath(endingPath, endingPaint);
} else if (isFirstButton) {
final Path leadingPath = Path();
leadingPath..moveTo(outer.left, rrect.bottom)
..lineTo(rrect.right - rrect.brRadiusX, rrect.bottom)
..addArc(brCorner, math.pi / 2.0, -sweepAngle)
..lineTo(rrect.right, rrect.top + rrect.trRadiusY)
..addArc(trCorner, 0, -sweepAngle)
..lineTo(outer.left, rrect.top);
context.canvas.drawPath(leadingPath, leadingPaint);
} else {
final Path leadingPath = Path();
leadingPath..moveTo(rrect.right, rrect.bottom + leadingBorderSide.width / 2)
..lineTo(rrect.right, rrect.top - leadingBorderSide.width / 2);
context.canvas.drawPath(leadingPath, leadingPaint);
final Paint horizontalPaint = borderSide.toPaint();
final Path horizontalPaths = Path();
horizontalPaths..moveTo(rrect.right - borderSide.width / 2.0, rrect.top)
..lineTo(outer.left - rrect.tlRadiusX, rrect.top)
..moveTo(rrect.right - borderSide.width / 2.0 + rrect.trRadiusX, rrect.bottom)
..lineTo(outer.left - rrect.tlRadiusX, rrect.bottom);
context.canvas.drawPath(horizontalPaths, horizontalPaint);
}
}
} else {
switch (verticalDirection) {
case VerticalDirection.down:
if (isLastButton) {
final Path topPath = Path();
topPath..moveTo(outer.left, outer.top + leadingBorderSide.width / 2)
..lineTo(outer.right, outer.top + leadingBorderSide.width / 2);
context.canvas.drawPath(topPath, leadingPaint);
final Paint endingPaint = trailingBorderSide.toPaint();
final Path endingPath = Path();
endingPath..moveTo(rrect.left, rrect.top + leadingBorderSide.width / 2.0)
..lineTo(rrect.left, rrect.bottom - rrect.blRadiusY)
..addArc(blCorner, math.pi * 3.0, -sweepAngle)
..lineTo(rrect.right - rrect.blRadiusX, rrect.bottom)
..addArc(brCorner, math.pi / 2.0, -sweepAngle)
..lineTo(rrect.right, rrect.top + leadingBorderSide.width / 2.0);
context.canvas.drawPath(endingPath, endingPaint);
} else if (isFirstButton) {
final Path leadingPath = Path();
leadingPath..moveTo(rrect.left, outer.bottom)
..lineTo(rrect.left, rrect.top + rrect.tlRadiusX)
..addArc(tlCorner, math.pi, sweepAngle)
..lineTo(rrect.right - rrect.trRadiusX, rrect.top)
..addArc(trCorner, math.pi * 3.0 / 2.0, sweepAngle)
..lineTo(rrect.right, outer.bottom);
context.canvas.drawPath(leadingPath, leadingPaint);
} else {
final Path topPath = Path();
topPath..moveTo(outer.left, outer.top + leadingBorderSide.width / 2)
..lineTo(outer.right, outer.top + leadingBorderSide.width / 2);
context.canvas.drawPath(topPath, leadingPaint);
final Paint paint = borderSide.toPaint();
final Path paths = Path(); // Left and right borders.
paths..moveTo(rrect.left, outer.top + leadingBorderSide.width)
..lineTo(rrect.left, outer.bottom)
..moveTo(rrect.right, outer.top + leadingBorderSide.width)
..lineTo(rrect.right, outer.bottom);
context.canvas.drawPath(paths, paint);
}
case VerticalDirection.up:
if (isLastButton) {
final Path bottomPath = Path();
bottomPath..moveTo(outer.left, outer.bottom - leadingBorderSide.width / 2.0)
..lineTo(outer.right, outer.bottom - leadingBorderSide.width / 2.0);
context.canvas.drawPath(bottomPath, leadingPaint);
final Paint endingPaint = trailingBorderSide.toPaint();
final Path endingPath = Path();
endingPath..moveTo(rrect.left, rrect.bottom - leadingBorderSide.width / 2.0)
..lineTo(rrect.left, rrect.top + rrect.tlRadiusY)
..addArc(tlCorner, math.pi, sweepAngle)
..lineTo(rrect.right - rrect.trRadiusX, rrect.top)
..addArc(trCorner, math.pi * 3.0 / 2.0, sweepAngle)
..lineTo(rrect.right, rrect.bottom - leadingBorderSide.width / 2.0);
context.canvas.drawPath(endingPath, endingPaint);
} else if (isFirstButton) {
final Path leadingPath = Path();
leadingPath..moveTo(rrect.left, outer.top)
..lineTo(rrect.left, rrect.bottom - rrect.blRadiusY)
..addArc(blCorner, math.pi, -sweepAngle)
..lineTo(rrect.right - rrect.brRadiusX, rrect.bottom)
..addArc(brCorner, math.pi / 2.0, -sweepAngle)
..lineTo(rrect.right, outer.top);
context.canvas.drawPath(leadingPath, leadingPaint);
} else {
final Path bottomPath = Path();
bottomPath..moveTo(outer.left, outer.bottom - leadingBorderSide.width / 2.0)
..lineTo(outer.right, outer.bottom - leadingBorderSide.width / 2.0);
context.canvas.drawPath(bottomPath, leadingPaint);
final Paint paint = borderSide.toPaint();
final Path paths = Path(); // Left and right borders.
paths..moveTo(rrect.left, outer.top)
..lineTo(rrect.left, outer.bottom - leadingBorderSide.width)
..moveTo(rrect.right, outer.top)
..lineTo(rrect.right, outer.bottom - leadingBorderSide.width);
context.canvas.drawPath(paths, paint);
}
}
}
}
}
/// A widget to pad the area around a [ToggleButtons]'s children.
///
/// This widget is based on a similar one used in [ButtonStyleButton] but it
/// only redirects taps along one axis to ensure the correct button is tapped
/// within the [ToggleButtons].
///
/// This ensures that a widget takes up at least as much space as the minSize
/// parameter to ensure adequate tap target size, while keeping the widget
/// visually smaller to the user.
class _InputPadding extends SingleChildRenderObjectWidget {
const _InputPadding({
super.child,
required this.minSize,
required this.direction,
});
final Size minSize;
final Axis direction;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderInputPadding(minSize, direction);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderInputPadding renderObject) {
renderObject.minSize = minSize;
renderObject.direction = direction;
}
}
class _RenderInputPadding extends RenderShiftedBox {
_RenderInputPadding(this._minSize, this._direction, [RenderBox? child]) : super(child);
Size get minSize => _minSize;
Size _minSize;
set minSize(Size value) {
if (_minSize == value) {
return;
}
_minSize = value;
markNeedsLayout();
}
Axis get direction => _direction;
Axis _direction;
set direction(Axis value) {
if (_direction == value) {
return;
}
_direction = value;
markNeedsLayout();
}
@override
double computeMinIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMinIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMinIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMaxIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMaxIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
Size _computeSize({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
if (child != null) {
final Size childSize = layoutChild(child!, constraints);
final double height = math.max(childSize.width, minSize.width);
final double width = math.max(childSize.height, minSize.height);
return constraints.constrain(Size(height, width));
}
return Size.zero;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.dryLayoutChild,
);
}
@override
void performLayout() {
size = _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.layoutChild,
);
if (child != null) {
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Alignment.center.alongOffset(size - child!.size as Offset);
}
}
@override
bool hitTest(BoxHitTestResult result, { required Offset position }) {
// The super.hitTest() method also checks hitTestChildren(). We don't
// want that in this case because we've padded around the children per
// tapTargetSize.
if (!size.contains(position)) {
return false;
}
// Only adjust one axis to ensure the correct button is tapped.
Offset center;
if (direction == Axis.horizontal) {
center = Offset(position.dx, child!.size.height / 2);
} else {
center = Offset(child!.size.width / 2, position.dy);
}
return result.addWithRawTransform(
transform: MatrixUtils.forceToPoint(center),
position: center,
hitTest: (BoxHitTestResult result, Offset position) {
assert(position == center);
return child!.hitTest(result, position: center);
},
);
}
}
| flutter/packages/flutter/lib/src/material/toggle_buttons.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/toggle_buttons.dart",
"repo_id": "flutter",
"token_count": 22704
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'border_radius.dart';
import 'borders.dart';
import 'edge_insets.dart';
// Examples can assume:
// late BuildContext context;
/// The shape to use when rendering a [Border] or [BoxDecoration].
///
/// Consider using [ShapeBorder] subclasses directly (with [ShapeDecoration]),
/// instead of using [BoxShape] and [Border], if the shapes will need to be
/// interpolated or animated. The [Border] class cannot interpolate between
/// different shapes.
enum BoxShape {
/// An axis-aligned, 2D rectangle. May have rounded corners (described by a
/// [BorderRadius]). The edges of the rectangle will match the edges of the box
/// into which the [Border] or [BoxDecoration] is painted.
///
/// See also:
///
/// * [RoundedRectangleBorder], the equivalent [ShapeBorder].
rectangle,
/// A circle centered in the middle of the box into which the [Border] or
/// [BoxDecoration] is painted. The diameter of the circle is the shortest
/// dimension of the box, either the width or the height, such that the circle
/// touches the edges of the box.
///
/// See also:
///
/// * [CircleBorder], the equivalent [ShapeBorder].
circle,
// Don't add more, instead create a new ShapeBorder.
}
/// Base class for box borders that can paint as rectangles, circles, or rounded
/// rectangles.
///
/// This class is extended by [Border] and [BorderDirectional] to provide
/// concrete versions of four-sided borders using different conventions for
/// specifying the sides.
///
/// The only API difference that this class introduces over [ShapeBorder] is
/// that its [paint] method takes additional arguments.
///
/// See also:
///
/// * [BorderSide], which is used to describe each side of the box.
/// * [RoundedRectangleBorder], another way of describing a box's border.
/// * [CircleBorder], another way of describing a circle border.
/// * [BoxDecoration], which uses a [BoxBorder] to describe its borders.
abstract class BoxBorder extends ShapeBorder {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const BoxBorder();
/// The top side of this border.
///
/// This getter is available on both [Border] and [BorderDirectional]. If
/// [isUniform] is true, then this is the same style as all the other sides.
BorderSide get top;
/// The bottom side of this border.
BorderSide get bottom;
/// Whether all four sides of the border are identical. Uniform borders are
/// typically more efficient to paint.
///
/// A uniform border by definition has no text direction dependency and
/// therefore could be expressed as a [Border], even if it is currently a
/// [BorderDirectional]. A uniform border can also be expressed as a
/// [RoundedRectangleBorder].
bool get isUniform;
// We override this to tighten the return value, so that callers can assume
// that we'll return a [BoxBorder].
@override
BoxBorder? add(ShapeBorder other, { bool reversed = false }) => null;
/// Linearly interpolate between two borders.
///
/// If a border is null, it is treated as having four [BorderSide.none]
/// borders.
///
/// This supports interpolating between [Border] and [BorderDirectional]
/// objects. If both objects are different types but both have sides on one or
/// both of their lateral edges (the two sides that aren't the top and bottom)
/// other than [BorderSide.none], then the sides are interpolated by reducing
/// `a`'s lateral edges to [BorderSide.none] over the first half of the
/// animation, and then bringing `b`'s lateral edges _from_ [BorderSide.none]
/// over the second half of the animation.
///
/// For a more flexible approach, consider [ShapeBorder.lerp], which would
/// instead [add] the two sets of sides and interpolate them simultaneously.
///
/// {@macro dart.ui.shadow.lerp}
static BoxBorder? lerp(BoxBorder? a, BoxBorder? b, double t) {
if (identical(a, b)) {
return a;
}
if ((a is Border?) && (b is Border?)) {
return Border.lerp(a, b, t);
}
if ((a is BorderDirectional?) && (b is BorderDirectional?)) {
return BorderDirectional.lerp(a, b, t);
}
if (b is Border && a is BorderDirectional) {
final BoxBorder c = b;
b = a;
a = c;
t = 1.0 - t;
// fall through to next case
}
if (a is Border && b is BorderDirectional) {
if (b.start == BorderSide.none && b.end == BorderSide.none) {
// The fact that b is a BorderDirectional really doesn't matter, it turns out.
return Border(
top: BorderSide.lerp(a.top, b.top, t),
right: BorderSide.lerp(a.right, BorderSide.none, t),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
left: BorderSide.lerp(a.left, BorderSide.none, t),
);
}
if (a.left == BorderSide.none && a.right == BorderSide.none) {
// The fact that a is a Border really doesn't matter, it turns out.
return BorderDirectional(
top: BorderSide.lerp(a.top, b.top, t),
start: BorderSide.lerp(BorderSide.none, b.start, t),
end: BorderSide.lerp(BorderSide.none, b.end, t),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
);
}
// Since we have to swap a visual border for a directional one,
// we speed up the horizontal sides' transitions and switch from
// one mode to the other at t=0.5.
if (t < 0.5) {
return Border(
top: BorderSide.lerp(a.top, b.top, t),
right: BorderSide.lerp(a.right, BorderSide.none, t * 2.0),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
left: BorderSide.lerp(a.left, BorderSide.none, t * 2.0),
);
}
return BorderDirectional(
top: BorderSide.lerp(a.top, b.top, t),
start: BorderSide.lerp(BorderSide.none, b.start, (t - 0.5) * 2.0),
end: BorderSide.lerp(BorderSide.none, b.end, (t - 0.5) * 2.0),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
);
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('BoxBorder.lerp can only interpolate Border and BorderDirectional classes.'),
ErrorDescription(
'BoxBorder.lerp() was called with two objects of type ${a.runtimeType} and ${b.runtimeType}:\n'
' $a\n'
' $b\n'
'However, only Border and BorderDirectional classes are supported by this method.',
),
ErrorHint('For a more general interpolation method, consider using ShapeBorder.lerp instead.'),
]);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
assert(textDirection != null, 'The textDirection argument to $runtimeType.getInnerPath must not be null.');
return Path()
..addRect(dimensions.resolve(textDirection).deflateRect(rect));
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
assert(textDirection != null, 'The textDirection argument to $runtimeType.getOuterPath must not be null.');
return Path()
..addRect(rect);
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
// For `ShapeDecoration(shape: Border.all())`, a rectangle with sharp edges
// is always painted. There is no borderRadius parameter for
// ShapeDecoration or Border, only for BoxDecoration, which doesn't call
// this method.
canvas.drawRect(rect, paint);
}
@override
bool get preferPaintInterior => true;
/// Paints the border within the given [Rect] on the given [Canvas].
///
/// This is an extension of the [ShapeBorder.paint] method. It allows
/// [BoxBorder] borders to be applied to different [BoxShape]s and with
/// different [borderRadius] parameters, without changing the [BoxBorder]
/// object itself.
///
/// The `shape` argument specifies the [BoxShape] to draw the border on.
///
/// If the `shape` is specifies a rectangular box shape
/// ([BoxShape.rectangle]), then the `borderRadius` argument describes the
/// corners of the rectangle.
///
/// The [getInnerPath] and [getOuterPath] methods do not know about the
/// `shape` and `borderRadius` arguments.
///
/// See also:
///
/// * [paintBorder], which is used if the border has non-uniform colors or styles and no borderRadius.
/// * [Border.paint], similar to this method, includes additional comments
/// and provides more details on each parameter than described here.
@override
void paint(
Canvas canvas,
Rect rect, {
TextDirection? textDirection,
BoxShape shape = BoxShape.rectangle,
BorderRadius? borderRadius,
});
static void _paintUniformBorderWithRadius(Canvas canvas, Rect rect, BorderSide side, BorderRadius borderRadius) {
assert(side.style != BorderStyle.none);
final Paint paint = Paint()
..color = side.color;
final double width = side.width;
if (width == 0.0) {
paint
..style = PaintingStyle.stroke
..strokeWidth = 0.0;
canvas.drawRRect(borderRadius.toRRect(rect), paint);
} else {
final RRect borderRect = borderRadius.toRRect(rect);
final RRect inner = borderRect.deflate(side.strokeInset);
final RRect outer = borderRect.inflate(side.strokeOutset);
canvas.drawDRRect(outer, inner, paint);
}
}
/// Paints a Border with different widths, styles and strokeAligns, on any
/// borderRadius while using a single color.
///
/// See also:
///
/// * [paintBorder], which supports multiple colors but not borderRadius.
/// * [paint], which calls this method.
static void paintNonUniformBorder(
Canvas canvas,
Rect rect, {
required BorderRadius? borderRadius,
required TextDirection? textDirection,
BoxShape shape = BoxShape.rectangle,
BorderSide top = BorderSide.none,
BorderSide right = BorderSide.none,
BorderSide bottom = BorderSide.none,
BorderSide left = BorderSide.none,
required Color color,
}) {
final RRect borderRect;
switch (shape) {
case BoxShape.rectangle:
borderRect = (borderRadius ?? BorderRadius.zero)
.resolve(textDirection)
.toRRect(rect);
case BoxShape.circle:
assert(borderRadius == null, 'A borderRadius cannot be given when shape is a BoxShape.circle.');
borderRect = RRect.fromRectAndRadius(
Rect.fromCircle(center: rect.center, radius: rect.shortestSide / 2.0),
Radius.circular(rect.width),
);
}
final Paint paint = Paint()..color = color;
final RRect inner = _deflateRRect(borderRect, EdgeInsets.fromLTRB(left.strokeInset, top.strokeInset, right.strokeInset, bottom.strokeInset));
final RRect outer = _inflateRRect(borderRect, EdgeInsets.fromLTRB(left.strokeOutset, top.strokeOutset, right.strokeOutset, bottom.strokeOutset));
canvas.drawDRRect(outer, inner, paint);
}
static RRect _inflateRRect(RRect rect, EdgeInsets insets) {
return RRect.fromLTRBAndCorners(
rect.left - insets.left,
rect.top - insets.top,
rect.right + insets.right,
rect.bottom + insets.bottom,
topLeft: (rect.tlRadius + Radius.elliptical(insets.left, insets.top)).clamp(minimum: Radius.zero),
topRight: (rect.trRadius + Radius.elliptical(insets.right, insets.top)).clamp(minimum: Radius.zero),
bottomRight: (rect.brRadius + Radius.elliptical(insets.right, insets.bottom)).clamp(minimum: Radius.zero),
bottomLeft: (rect.blRadius + Radius.elliptical(insets.left, insets.bottom)).clamp(minimum: Radius.zero),
);
}
static RRect _deflateRRect(RRect rect, EdgeInsets insets) {
return RRect.fromLTRBAndCorners(
rect.left + insets.left,
rect.top + insets.top,
rect.right - insets.right,
rect.bottom - insets.bottom,
topLeft: (rect.tlRadius - Radius.elliptical(insets.left, insets.top)).clamp(minimum: Radius.zero),
topRight: (rect.trRadius - Radius.elliptical(insets.right, insets.top)).clamp(minimum: Radius.zero),
bottomRight: (rect.brRadius - Radius.elliptical(insets.right, insets.bottom)).clamp(minimum: Radius.zero),
bottomLeft:(rect.blRadius - Radius.elliptical(insets.left, insets.bottom)).clamp(minimum: Radius.zero),
);
}
static void _paintUniformBorderWithCircle(Canvas canvas, Rect rect, BorderSide side) {
assert(side.style != BorderStyle.none);
final double radius = (rect.shortestSide + side.strokeOffset) / 2;
canvas.drawCircle(rect.center, radius, side.toPaint());
}
static void _paintUniformBorderWithRectangle(Canvas canvas, Rect rect, BorderSide side) {
assert(side.style != BorderStyle.none);
canvas.drawRect(rect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
/// A border of a box, comprised of four sides: top, right, bottom, left.
///
/// The sides are represented by [BorderSide] objects.
///
/// {@tool snippet}
///
/// All four borders the same, two-pixel wide solid white:
///
/// ```dart
/// Border.all(width: 2.0, color: const Color(0xFFFFFFFF))
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// The border for a Material Design divider:
///
/// ```dart
/// Border(bottom: BorderSide(color: Theme.of(context).dividerColor))
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// A 1990s-era "OK" button:
///
/// ```dart
/// Container(
/// decoration: const BoxDecoration(
/// border: Border(
/// top: BorderSide(color: Color(0xFFFFFFFF)),
/// left: BorderSide(color: Color(0xFFFFFFFF)),
/// right: BorderSide(),
/// bottom: BorderSide(),
/// ),
/// ),
/// child: Container(
/// padding: const EdgeInsets.symmetric(horizontal: 20.0, vertical: 2.0),
/// decoration: const BoxDecoration(
/// border: Border(
/// top: BorderSide(color: Color(0xFFDFDFDF)),
/// left: BorderSide(color: Color(0xFFDFDFDF)),
/// right: BorderSide(color: Color(0xFF7F7F7F)),
/// bottom: BorderSide(color: Color(0xFF7F7F7F)),
/// ),
/// color: Color(0xFFBFBFBF),
/// ),
/// child: const Text(
/// 'OK',
/// textAlign: TextAlign.center,
/// style: TextStyle(color: Color(0xFF000000))
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [BoxDecoration], which uses this class to describe its edge decoration.
/// * [BorderSide], which is used to describe each side of the box.
/// * [Theme], from the material layer, which can be queried to obtain appropriate colors
/// to use for borders in a [MaterialApp], as shown in the "divider" sample above.
/// * [paint], which explains the behavior of [BoxDecoration] parameters.
/// * <https://pub.dev/packages/non_uniform_border>, a package that implements
/// a Non-Uniform Border on ShapeBorder, which is used by Material Design
/// buttons and other widgets, under the "shape" field.
class Border extends BoxBorder {
/// Creates a border.
///
/// All the sides of the border default to [BorderSide.none].
const Border({
this.top = BorderSide.none,
this.right = BorderSide.none,
this.bottom = BorderSide.none,
this.left = BorderSide.none,
});
/// Creates a border whose sides are all the same.
const Border.fromBorderSide(BorderSide side)
: top = side,
right = side,
bottom = side,
left = side;
/// Creates a border with symmetrical vertical and horizontal sides.
///
/// The `vertical` argument applies to the [left] and [right] sides, and the
/// `horizontal` argument applies to the [top] and [bottom] sides.
///
/// All arguments default to [BorderSide.none].
const Border.symmetric({
BorderSide vertical = BorderSide.none,
BorderSide horizontal = BorderSide.none,
}) : left = vertical,
top = horizontal,
right = vertical,
bottom = horizontal;
/// A uniform border with all sides the same color and width.
///
/// The sides default to black solid borders, one logical pixel wide.
factory Border.all({
Color color = const Color(0xFF000000),
double width = 1.0,
BorderStyle style = BorderStyle.solid,
double strokeAlign = BorderSide.strokeAlignInside,
}) {
final BorderSide side = BorderSide(color: color, width: width, style: style, strokeAlign: strokeAlign);
return Border.fromBorderSide(side);
}
/// Creates a [Border] that represents the addition of the two given
/// [Border]s.
///
/// It is only valid to call this if [BorderSide.canMerge] returns true for
/// the pairwise combination of each side on both [Border]s.
static Border merge(Border a, Border b) {
assert(BorderSide.canMerge(a.top, b.top));
assert(BorderSide.canMerge(a.right, b.right));
assert(BorderSide.canMerge(a.bottom, b.bottom));
assert(BorderSide.canMerge(a.left, b.left));
return Border(
top: BorderSide.merge(a.top, b.top),
right: BorderSide.merge(a.right, b.right),
bottom: BorderSide.merge(a.bottom, b.bottom),
left: BorderSide.merge(a.left, b.left),
);
}
@override
final BorderSide top;
/// The right side of this border.
final BorderSide right;
@override
final BorderSide bottom;
/// The left side of this border.
final BorderSide left;
@override
EdgeInsetsGeometry get dimensions {
if (_widthIsUniform) {
return EdgeInsets.all(top.strokeInset);
}
return EdgeInsets.fromLTRB(left.strokeInset, top.strokeInset, right.strokeInset, bottom.strokeInset);
}
@override
bool get isUniform => _colorIsUniform && _widthIsUniform && _styleIsUniform && _strokeAlignIsUniform;
bool get _colorIsUniform {
final Color topColor = top.color;
return left.color == topColor && bottom.color == topColor && right.color == topColor;
}
bool get _widthIsUniform {
final double topWidth = top.width;
return left.width == topWidth && bottom.width == topWidth && right.width == topWidth;
}
bool get _styleIsUniform {
final BorderStyle topStyle = top.style;
return left.style == topStyle && bottom.style == topStyle && right.style == topStyle;
}
bool get _strokeAlignIsUniform {
final double topStrokeAlign = top.strokeAlign;
return left.strokeAlign == topStrokeAlign
&& bottom.strokeAlign == topStrokeAlign
&& right.strokeAlign == topStrokeAlign;
}
Set<Color> _distinctVisibleColors() {
final Set<Color> distinctVisibleColors = <Color>{};
if (top.style != BorderStyle.none) {
distinctVisibleColors.add(top.color);
}
if (right.style != BorderStyle.none) {
distinctVisibleColors.add(right.color);
}
if (bottom.style != BorderStyle.none) {
distinctVisibleColors.add(bottom.color);
}
if (left.style != BorderStyle.none) {
distinctVisibleColors.add(left.color);
}
return distinctVisibleColors;
}
// [BoxBorder.paintNonUniformBorder] is about 20% faster than [paintBorder],
// but [paintBorder] is able to draw hairline borders when width is zero
// and style is [BorderStyle.solid].
bool get _hasHairlineBorder =>
(top.style == BorderStyle.solid && top.width == 0.0) ||
(right.style == BorderStyle.solid && right.width == 0.0) ||
(bottom.style == BorderStyle.solid && bottom.width == 0.0) ||
(left.style == BorderStyle.solid && left.width == 0.0);
@override
Border? add(ShapeBorder other, { bool reversed = false }) {
if (other is Border &&
BorderSide.canMerge(top, other.top) &&
BorderSide.canMerge(right, other.right) &&
BorderSide.canMerge(bottom, other.bottom) &&
BorderSide.canMerge(left, other.left)) {
return Border.merge(this, other);
}
return null;
}
@override
Border scale(double t) {
return Border(
top: top.scale(t),
right: right.scale(t),
bottom: bottom.scale(t),
left: left.scale(t),
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is Border) {
return Border.lerp(a, this, t);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is Border) {
return Border.lerp(this, b, t);
}
return super.lerpTo(b, t);
}
/// Linearly interpolate between two borders.
///
/// If a border is null, it is treated as having four [BorderSide.none]
/// borders.
///
/// {@macro dart.ui.shadow.lerp}
static Border? lerp(Border? a, Border? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
return Border(
top: BorderSide.lerp(a.top, b.top, t),
right: BorderSide.lerp(a.right, b.right, t),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
left: BorderSide.lerp(a.left, b.left, t),
);
}
/// Paints the border within the given [Rect] on the given [Canvas].
///
/// Uniform borders and non-uniform borders with similar colors and styles
/// are more efficient to paint than more complex borders.
///
/// You can provide a [BoxShape] to draw the border on. If the `shape` in
/// [BoxShape.circle], there is the requirement that the border has uniform
/// color and style.
///
/// If you specify a rectangular box shape ([BoxShape.rectangle]), then you
/// may specify a [BorderRadius]. If a `borderRadius` is specified, there is
/// the requirement that the border has uniform color and style.
///
/// The [getInnerPath] and [getOuterPath] methods do not know about the
/// `shape` and `borderRadius` arguments.
///
/// The `textDirection` argument is not used by this paint method.
///
/// See also:
///
/// * [paintBorder], which is used if the border has non-uniform colors or styles and no borderRadius.
/// * <https://pub.dev/packages/non_uniform_border>, a package that implements
/// a Non-Uniform Border on ShapeBorder, which is used by Material Design
/// buttons and other widgets, under the "shape" field.
@override
void paint(
Canvas canvas,
Rect rect, {
TextDirection? textDirection,
BoxShape shape = BoxShape.rectangle,
BorderRadius? borderRadius,
}) {
if (isUniform) {
switch (top.style) {
case BorderStyle.none:
return;
case BorderStyle.solid:
switch (shape) {
case BoxShape.circle:
assert(borderRadius == null, 'A borderRadius cannot be given when shape is a BoxShape.circle.');
BoxBorder._paintUniformBorderWithCircle(canvas, rect, top);
case BoxShape.rectangle:
if (borderRadius != null && borderRadius != BorderRadius.zero) {
BoxBorder._paintUniformBorderWithRadius(canvas, rect, top, borderRadius);
return;
}
BoxBorder._paintUniformBorderWithRectangle(canvas, rect, top);
}
return;
}
}
if (_styleIsUniform && top.style == BorderStyle.none) {
return;
}
// Allow painting non-uniform borders if the visible colors are uniform.
final Set<Color> visibleColors = _distinctVisibleColors();
final bool hasHairlineBorder = _hasHairlineBorder;
// Paint a non uniform border if a single color is visible
// and (borderRadius is present) or (border is visible and width != 0.0).
if (visibleColors.length == 1 &&
!hasHairlineBorder &&
(shape == BoxShape.circle ||
(borderRadius != null && borderRadius != BorderRadius.zero))) {
BoxBorder.paintNonUniformBorder(canvas, rect,
shape: shape,
borderRadius: borderRadius,
textDirection: textDirection,
top: top.style == BorderStyle.none ? BorderSide.none : top,
right: right.style == BorderStyle.none ? BorderSide.none : right,
bottom: bottom.style == BorderStyle.none ? BorderSide.none : bottom,
left: left.style == BorderStyle.none ? BorderSide.none : left,
color: visibleColors.first);
return;
}
assert(() {
if (hasHairlineBorder) {
assert(borderRadius == null || borderRadius == BorderRadius.zero,
'A hairline border like `BorderSide(width: 0.0, style: BorderStyle.solid)` can only be drawn when BorderRadius is zero or null.');
}
if (borderRadius != null && borderRadius != BorderRadius.zero) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A borderRadius can only be given on borders with uniform colors.'),
ErrorDescription('The following is not uniform:'),
if (!_colorIsUniform) ErrorDescription('BorderSide.color'),
]);
}
return true;
}());
assert(() {
if (shape != BoxShape.rectangle) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A Border can only be drawn as a circle on borders with uniform colors.'),
ErrorDescription('The following is not uniform:'),
if (!_colorIsUniform) ErrorDescription('BorderSide.color'),
]);
}
return true;
}());
assert(() {
if (!_strokeAlignIsUniform || top.strokeAlign != BorderSide.strokeAlignInside) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A Border can only draw strokeAlign different than BorderSide.strokeAlignInside on borders with uniform colors.'),
]);
}
return true;
}());
paintBorder(canvas, rect, top: top, right: right, bottom: bottom, left: left);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is Border
&& other.top == top
&& other.right == right
&& other.bottom == bottom
&& other.left == left;
}
@override
int get hashCode => Object.hash(top, right, bottom, left);
@override
String toString() {
if (isUniform) {
return '${objectRuntimeType(this, 'Border')}.all($top)';
}
final List<String> arguments = <String>[
if (top != BorderSide.none) 'top: $top',
if (right != BorderSide.none) 'right: $right',
if (bottom != BorderSide.none) 'bottom: $bottom',
if (left != BorderSide.none) 'left: $left',
];
return '${objectRuntimeType(this, 'Border')}(${arguments.join(", ")})';
}
}
/// A border of a box, comprised of four sides, the lateral sides of which
/// flip over based on the reading direction.
///
/// The lateral sides are called [start] and [end]. When painted in
/// left-to-right environments, the [start] side will be painted on the left and
/// the [end] side on the right; in right-to-left environments, it is the
/// reverse. The other two sides are [top] and [bottom].
///
/// The sides are represented by [BorderSide] objects.
///
/// If the [start] and [end] sides are the same, then it is slightly more
/// efficient to use a [Border] object rather than a [BorderDirectional] object.
///
/// See also:
///
/// * [BoxDecoration], which uses this class to describe its edge decoration.
/// * [BorderSide], which is used to describe each side of the box.
/// * [Theme], from the material layer, which can be queried to obtain appropriate colors
/// to use for borders in a [MaterialApp], as shown in the "divider" sample above.
/// * <https://pub.dev/packages/non_uniform_border>, a package that implements
/// a Non-Uniform Border on ShapeBorder, which is used by Material Design
/// buttons and other widgets, under the "shape" field.
class BorderDirectional extends BoxBorder {
/// Creates a border.
///
/// The [start] and [end] sides represent the horizontal sides; the start side
/// is on the leading edge given the reading direction, and the end side is on
/// the trailing edge. They are resolved during [paint].
///
/// All the sides of the border default to [BorderSide.none].
const BorderDirectional({
this.top = BorderSide.none,
this.start = BorderSide.none,
this.end = BorderSide.none,
this.bottom = BorderSide.none,
});
/// Creates a [BorderDirectional] that represents the addition of the two
/// given [BorderDirectional]s.
///
/// It is only valid to call this if [BorderSide.canMerge] returns true for
/// the pairwise combination of each side on both [BorderDirectional]s.
static BorderDirectional merge(BorderDirectional a, BorderDirectional b) {
assert(BorderSide.canMerge(a.top, b.top));
assert(BorderSide.canMerge(a.start, b.start));
assert(BorderSide.canMerge(a.end, b.end));
assert(BorderSide.canMerge(a.bottom, b.bottom));
return BorderDirectional(
top: BorderSide.merge(a.top, b.top),
start: BorderSide.merge(a.start, b.start),
end: BorderSide.merge(a.end, b.end),
bottom: BorderSide.merge(a.bottom, b.bottom),
);
}
@override
final BorderSide top;
/// The start side of this border.
///
/// This is the side on the left in left-to-right text and on the right in
/// right-to-left text.
///
/// See also:
///
/// * [TextDirection], which is used to describe the reading direction.
final BorderSide start;
/// The end side of this border.
///
/// This is the side on the right in left-to-right text and on the left in
/// right-to-left text.
///
/// See also:
///
/// * [TextDirection], which is used to describe the reading direction.
final BorderSide end;
@override
final BorderSide bottom;
@override
EdgeInsetsGeometry get dimensions {
if (isUniform) {
return EdgeInsetsDirectional.all(top.strokeInset);
}
return EdgeInsetsDirectional.fromSTEB(start.strokeInset, top.strokeInset, end.strokeInset, bottom.strokeInset);
}
@override
bool get isUniform => _colorIsUniform && _widthIsUniform && _styleIsUniform && _strokeAlignIsUniform;
bool get _colorIsUniform {
final Color topColor = top.color;
return start.color == topColor && bottom.color == topColor && end.color == topColor;
}
bool get _widthIsUniform {
final double topWidth = top.width;
return start.width == topWidth && bottom.width == topWidth && end.width == topWidth;
}
bool get _styleIsUniform {
final BorderStyle topStyle = top.style;
return start.style == topStyle && bottom.style == topStyle && end.style == topStyle;
}
bool get _strokeAlignIsUniform {
final double topStrokeAlign = top.strokeAlign;
return start.strokeAlign == topStrokeAlign
&& bottom.strokeAlign == topStrokeAlign
&& end.strokeAlign == topStrokeAlign;
}
Set<Color> _distinctVisibleColors() {
final Set<Color> distinctVisibleColors = <Color>{};
if (top.style != BorderStyle.none) {
distinctVisibleColors.add(top.color);
}
if (end.style != BorderStyle.none) {
distinctVisibleColors.add(end.color);
}
if (bottom.style != BorderStyle.none) {
distinctVisibleColors.add(bottom.color);
}
if (start.style != BorderStyle.none) {
distinctVisibleColors.add(start.color);
}
return distinctVisibleColors;
}
bool get _hasHairlineBorder =>
(top.style == BorderStyle.solid && top.width == 0.0) ||
(end.style == BorderStyle.solid && end.width == 0.0) ||
(bottom.style == BorderStyle.solid && bottom.width == 0.0) ||
(start.style == BorderStyle.solid && start.width == 0.0);
@override
BoxBorder? add(ShapeBorder other, { bool reversed = false }) {
if (other is BorderDirectional) {
final BorderDirectional typedOther = other;
if (BorderSide.canMerge(top, typedOther.top) &&
BorderSide.canMerge(start, typedOther.start) &&
BorderSide.canMerge(end, typedOther.end) &&
BorderSide.canMerge(bottom, typedOther.bottom)) {
return BorderDirectional.merge(this, typedOther);
}
return null;
}
if (other is Border) {
final Border typedOther = other;
if (!BorderSide.canMerge(typedOther.top, top) ||
!BorderSide.canMerge(typedOther.bottom, bottom)) {
return null;
}
if (start != BorderSide.none ||
end != BorderSide.none) {
if (typedOther.left != BorderSide.none ||
typedOther.right != BorderSide.none) {
return null;
}
assert(typedOther.left == BorderSide.none);
assert(typedOther.right == BorderSide.none);
return BorderDirectional(
top: BorderSide.merge(typedOther.top, top),
start: start,
end: end,
bottom: BorderSide.merge(typedOther.bottom, bottom),
);
}
assert(start == BorderSide.none);
assert(end == BorderSide.none);
return Border(
top: BorderSide.merge(typedOther.top, top),
right: typedOther.right,
bottom: BorderSide.merge(typedOther.bottom, bottom),
left: typedOther.left,
);
}
return null;
}
@override
BorderDirectional scale(double t) {
return BorderDirectional(
top: top.scale(t),
start: start.scale(t),
end: end.scale(t),
bottom: bottom.scale(t),
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is BorderDirectional) {
return BorderDirectional.lerp(a, this, t);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is BorderDirectional) {
return BorderDirectional.lerp(this, b, t);
}
return super.lerpTo(b, t);
}
/// Linearly interpolate between two borders.
///
/// If a border is null, it is treated as having four [BorderSide.none]
/// borders.
///
/// {@macro dart.ui.shadow.lerp}
static BorderDirectional? lerp(BorderDirectional? a, BorderDirectional? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
return BorderDirectional(
top: BorderSide.lerp(a.top, b.top, t),
end: BorderSide.lerp(a.end, b.end, t),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
start: BorderSide.lerp(a.start, b.start, t),
);
}
/// Paints the border within the given [Rect] on the given [Canvas].
///
/// Uniform borders are more efficient to paint than more complex borders.
///
/// You can provide a [BoxShape] to draw the border on. If the `shape` in
/// [BoxShape.circle], there is the requirement that the border [isUniform].
///
/// If you specify a rectangular box shape ([BoxShape.rectangle]), then you
/// may specify a [BorderRadius]. If a `borderRadius` is specified, there is
/// the requirement that the border [isUniform].
///
/// The [getInnerPath] and [getOuterPath] methods do not know about the
/// `shape` and `borderRadius` arguments.
///
/// The `textDirection` argument is used to determine which of [start] and
/// [end] map to the left and right. For [TextDirection.ltr], the [start] is
/// the left and the [end] is the right; for [TextDirection.rtl], it is the
/// reverse.
///
/// See also:
///
/// * [paintBorder], which is used if the border has non-uniform colors or styles and no borderRadius.
@override
void paint(
Canvas canvas,
Rect rect, {
TextDirection? textDirection,
BoxShape shape = BoxShape.rectangle,
BorderRadius? borderRadius,
}) {
if (isUniform) {
switch (top.style) {
case BorderStyle.none:
return;
case BorderStyle.solid:
switch (shape) {
case BoxShape.circle:
assert(borderRadius == null, 'A borderRadius cannot be given when shape is a BoxShape.circle.');
BoxBorder._paintUniformBorderWithCircle(canvas, rect, top);
case BoxShape.rectangle:
if (borderRadius != null && borderRadius != BorderRadius.zero) {
BoxBorder._paintUniformBorderWithRadius(canvas, rect, top, borderRadius);
return;
}
BoxBorder._paintUniformBorderWithRectangle(canvas, rect, top);
}
return;
}
}
if (_styleIsUniform && top.style == BorderStyle.none) {
return;
}
assert(textDirection != null, 'Non-uniform BorderDirectional objects require a TextDirection when painting.');
final (BorderSide left, BorderSide right) = switch (textDirection!) {
TextDirection.rtl => (end, start),
TextDirection.ltr => (start, end),
};
// Allow painting non-uniform borders if the visible colors are uniform.
final Set<Color> visibleColors = _distinctVisibleColors();
final bool hasHairlineBorder = _hasHairlineBorder;
if (visibleColors.length == 1 &&
!hasHairlineBorder &&
(shape == BoxShape.circle ||
(borderRadius != null && borderRadius != BorderRadius.zero))) {
BoxBorder.paintNonUniformBorder(canvas, rect,
shape: shape,
borderRadius: borderRadius,
textDirection: textDirection,
top: top.style == BorderStyle.none ? BorderSide.none : top,
right: right.style == BorderStyle.none ? BorderSide.none : right,
bottom: bottom.style == BorderStyle.none ? BorderSide.none : bottom,
left: left.style == BorderStyle.none ? BorderSide.none : left,
color: visibleColors.first);
return;
}
if (hasHairlineBorder) {
assert(borderRadius == null || borderRadius == BorderRadius.zero, 'A side like `BorderSide(width: 0.0, style: BorderStyle.solid)` can only be drawn when BorderRadius is zero or null.');
}
assert(borderRadius == null, 'A borderRadius can only be given for borders with uniform colors.');
assert(shape == BoxShape.rectangle, 'A Border can only be drawn as a circle on borders with uniform colors.');
assert(_strokeAlignIsUniform && top.strokeAlign == BorderSide.strokeAlignInside, 'A Border can only draw strokeAlign different than strokeAlignInside on borders with uniform colors.');
paintBorder(canvas, rect, top: top, left: left, bottom: bottom, right: right);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is BorderDirectional
&& other.top == top
&& other.start == start
&& other.end == end
&& other.bottom == bottom;
}
@override
int get hashCode => Object.hash(top, start, end, bottom);
@override
String toString() {
final List<String> arguments = <String>[
if (top != BorderSide.none) 'top: $top',
if (start != BorderSide.none) 'start: $start',
if (end != BorderSide.none) 'end: $end',
if (bottom != BorderSide.none) 'bottom: $bottom',
];
return '${objectRuntimeType(this, 'BorderDirectional')}(${arguments.join(", ")})';
}
}
| flutter/packages/flutter/lib/src/painting/box_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/box_border.dart",
"repo_id": "flutter",
"token_count": 14219
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:developer';
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'image_stream.dart';
const int _kDefaultSize = 1000;
const int _kDefaultSizeBytes = 100 << 20; // 100 MiB
/// Class for caching images.
///
/// Implements a least-recently-used cache of up to 1000 images, and up to 100
/// MB. The maximum size can be adjusted using [maximumSize] and
/// [maximumSizeBytes].
///
/// The cache also holds a list of 'live' references. An image is considered
/// live if its [ImageStreamCompleter]'s listener count has never dropped to
/// zero after adding at least one listener. The cache uses
/// [ImageStreamCompleter.addOnLastListenerRemovedCallback] to determine when
/// this has happened.
///
/// The [putIfAbsent] method is the main entry-point to the cache API. It
/// returns the previously cached [ImageStreamCompleter] for the given key, if
/// available; if not, it calls the given callback to obtain it first. In either
/// case, the key is moved to the 'most recently used' position.
///
/// A caller can determine whether an image is already in the cache by using
/// [containsKey], which will return true if the image is tracked by the cache
/// in a pending or completed state. More fine grained information is available
/// by using the [statusForKey] method.
///
/// Generally this class is not used directly. The [ImageProvider] class and its
/// subclasses automatically handle the caching of images.
///
/// A shared instance of this cache is retained by [PaintingBinding] and can be
/// obtained via the [imageCache] top-level property in the [painting] library.
///
/// {@tool snippet}
///
/// This sample shows how to supply your own caching logic and replace the
/// global [imageCache] variable.
///
/// ```dart
/// /// This is the custom implementation of [ImageCache] where we can override
/// /// the logic.
/// class MyImageCache extends ImageCache {
/// @override
/// void clear() {
/// print('Clearing cache!');
/// super.clear();
/// }
/// }
///
/// class MyWidgetsBinding extends WidgetsFlutterBinding {
/// @override
/// ImageCache createImageCache() => MyImageCache();
/// }
///
/// void main() {
/// // The constructor sets global variables.
/// MyWidgetsBinding();
/// runApp(const MyApp());
/// }
///
/// class MyApp extends StatelessWidget {
/// const MyApp({super.key});
///
/// @override
/// Widget build(BuildContext context) {
/// return Container();
/// }
/// }
/// ```
/// {@end-tool}
class ImageCache {
final Map<Object, _PendingImage> _pendingImages = <Object, _PendingImage>{};
final Map<Object, _CachedImage> _cache = <Object, _CachedImage>{};
/// ImageStreamCompleters with at least one listener. These images may or may
/// not fit into the _pendingImages or _cache objects.
///
/// Unlike _cache, the [_CachedImage] for this may have a null byte size.
final Map<Object, _LiveImage> _liveImages = <Object, _LiveImage>{};
/// Maximum number of entries to store in the cache.
///
/// Once this many entries have been cached, the least-recently-used entry is
/// evicted when adding a new entry.
int get maximumSize => _maximumSize;
int _maximumSize = _kDefaultSize;
/// Changes the maximum cache size.
///
/// If the new size is smaller than the current number of elements, the
/// extraneous elements are evicted immediately. Setting this to zero and then
/// returning it to its original value will therefore immediately clear the
/// cache.
set maximumSize(int value) {
assert(value >= 0);
if (value == maximumSize) {
return;
}
TimelineTask? debugTimelineTask;
if (!kReleaseMode) {
debugTimelineTask = TimelineTask()..start(
'ImageCache.setMaximumSize',
arguments: <String, dynamic>{'value': value},
);
}
_maximumSize = value;
if (maximumSize == 0) {
clear();
} else {
_checkCacheSize(debugTimelineTask);
}
if (!kReleaseMode) {
debugTimelineTask!.finish();
}
}
/// The current number of cached entries.
int get currentSize => _cache.length;
/// Maximum size of entries to store in the cache in bytes.
///
/// Once more than this amount of bytes have been cached, the
/// least-recently-used entry is evicted until there are fewer than the
/// maximum bytes.
int get maximumSizeBytes => _maximumSizeBytes;
int _maximumSizeBytes = _kDefaultSizeBytes;
/// Changes the maximum cache bytes.
///
/// If the new size is smaller than the current size in bytes, the
/// extraneous elements are evicted immediately. Setting this to zero and then
/// returning it to its original value will therefore immediately clear the
/// cache.
set maximumSizeBytes(int value) {
assert(value >= 0);
if (value == _maximumSizeBytes) {
return;
}
TimelineTask? debugTimelineTask;
if (!kReleaseMode) {
debugTimelineTask = TimelineTask()..start(
'ImageCache.setMaximumSizeBytes',
arguments: <String, dynamic>{'value': value},
);
}
_maximumSizeBytes = value;
if (_maximumSizeBytes == 0) {
clear();
} else {
_checkCacheSize(debugTimelineTask);
}
if (!kReleaseMode) {
debugTimelineTask!.finish();
}
}
/// The current size of cached entries in bytes.
int get currentSizeBytes => _currentSizeBytes;
int _currentSizeBytes = 0;
/// Evicts all pending and keepAlive entries from the cache.
///
/// This is useful if, for instance, the root asset bundle has been updated
/// and therefore new images must be obtained.
///
/// Images which have not finished loading yet will not be removed from the
/// cache, and when they complete they will be inserted as normal.
///
/// This method does not clear live references to images, since clearing those
/// would not reduce memory pressure. Such images still have listeners in the
/// application code, and will still remain resident in memory.
///
/// To clear live references, use [clearLiveImages].
void clear() {
if (!kReleaseMode) {
Timeline.instantSync(
'ImageCache.clear',
arguments: <String, dynamic>{
'pendingImages': _pendingImages.length,
'keepAliveImages': _cache.length,
'liveImages': _liveImages.length,
'currentSizeInBytes': _currentSizeBytes,
},
);
}
for (final _CachedImage image in _cache.values) {
image.dispose();
}
_cache.clear();
for (final _PendingImage pendingImage in _pendingImages.values) {
pendingImage.removeListener();
}
_pendingImages.clear();
_currentSizeBytes = 0;
}
/// Evicts a single entry from the cache, returning true if successful.
///
/// Pending images waiting for completion are removed as well, returning true
/// if successful. When a pending image is removed the listener on it is
/// removed as well to prevent it from adding itself to the cache if it
/// eventually completes.
///
/// If this method removes a pending image, it will also remove
/// the corresponding live tracking of the image, since it is no longer clear
/// if the image will ever complete or have any listeners, and failing to
/// remove the live reference could leave the cache in a state where all
/// subsequent calls to [putIfAbsent] will return an [ImageStreamCompleter]
/// that will never complete.
///
/// If this method removes a completed image, it will _not_ remove the live
/// reference to the image, which will only be cleared when the listener
/// count on the completer drops to zero. To clear live image references,
/// whether completed or not, use [clearLiveImages].
///
/// The `key` must be equal to an object used to cache an image in
/// [ImageCache.putIfAbsent].
///
/// If the key is not immediately available, as is common, consider using
/// [ImageProvider.evict] to call this method indirectly instead.
///
/// The `includeLive` argument determines whether images that still have
/// listeners in the tree should be evicted as well. This parameter should be
/// set to true in cases where the image may be corrupted and needs to be
/// completely discarded by the cache. It should be set to false when calls
/// to evict are trying to relieve memory pressure, since an image with a
/// listener will not actually be evicted from memory, and subsequent attempts
/// to load it will end up allocating more memory for the image again.
///
/// See also:
///
/// * [ImageProvider], for providing images to the [Image] widget.
bool evict(Object key, { bool includeLive = true }) {
if (includeLive) {
// Remove from live images - the cache will not be able to mark
// it as complete, and it might be getting evicted because it
// will never complete, e.g. it was loaded in a FakeAsync zone.
// In such a case, we need to make sure subsequent calls to
// putIfAbsent don't return this image that may never complete.
final _LiveImage? image = _liveImages.remove(key);
image?.dispose();
}
final _PendingImage? pendingImage = _pendingImages.remove(key);
if (pendingImage != null) {
if (!kReleaseMode) {
Timeline.instantSync('ImageCache.evict', arguments: <String, dynamic>{
'type': 'pending',
});
}
pendingImage.removeListener();
return true;
}
final _CachedImage? image = _cache.remove(key);
if (image != null) {
if (!kReleaseMode) {
Timeline.instantSync('ImageCache.evict', arguments: <String, dynamic>{
'type': 'keepAlive',
'sizeInBytes': image.sizeBytes,
});
}
_currentSizeBytes -= image.sizeBytes!;
image.dispose();
return true;
}
if (!kReleaseMode) {
Timeline.instantSync('ImageCache.evict', arguments: <String, dynamic>{
'type': 'miss',
});
}
return false;
}
/// Updates the least recently used image cache with this image, if it is
/// less than the [maximumSizeBytes] of this cache.
///
/// Resizes the cache as appropriate to maintain the constraints of
/// [maximumSize] and [maximumSizeBytes].
void _touch(Object key, _CachedImage image, TimelineTask? timelineTask) {
if (image.sizeBytes != null && image.sizeBytes! <= maximumSizeBytes && maximumSize > 0) {
_currentSizeBytes += image.sizeBytes!;
_cache[key] = image;
_checkCacheSize(timelineTask);
} else {
image.dispose();
}
}
void _trackLiveImage(Object key, ImageStreamCompleter completer, int? sizeBytes) {
// Avoid adding unnecessary callbacks to the completer.
_liveImages.putIfAbsent(key, () {
// Even if no callers to ImageProvider.resolve have listened to the stream,
// the cache is listening to the stream and will remove itself once the
// image completes to move it from pending to keepAlive.
// Even if the cache size is 0, we still add this tracker, which will add
// a keep alive handle to the stream.
return _LiveImage(
completer,
() {
_liveImages.remove(key);
},
);
}).sizeBytes ??= sizeBytes;
}
/// Returns the previously cached [ImageStream] for the given key, if available;
/// if not, calls the given callback to obtain it first. In either case, the
/// key is moved to the 'most recently used' position.
///
/// In the event that the loader throws an exception, it will be caught only if
/// `onError` is also provided. When an exception is caught resolving an image,
/// no completers are cached and `null` is returned instead of a new
/// completer.
///
/// Images that are larger than [maximumSizeBytes] are not cached, and do not
/// cause other images in the cache to be evicted.
ImageStreamCompleter? putIfAbsent(Object key, ImageStreamCompleter Function() loader, { ImageErrorListener? onError }) {
TimelineTask? debugTimelineTask;
if (!kReleaseMode) {
debugTimelineTask = TimelineTask()..start(
'ImageCache.putIfAbsent',
arguments: <String, dynamic>{
'key': key.toString(),
},
);
}
ImageStreamCompleter? result = _pendingImages[key]?.completer;
// Nothing needs to be done because the image hasn't loaded yet.
if (result != null) {
if (!kReleaseMode) {
debugTimelineTask!.finish(arguments: <String, dynamic>{'result': 'pending'});
}
return result;
}
// Remove the provider from the list so that we can move it to the
// recently used position below.
// Don't use _touch here, which would trigger a check on cache size that is
// not needed since this is just moving an existing cache entry to the head.
final _CachedImage? image = _cache.remove(key);
if (image != null) {
if (!kReleaseMode) {
debugTimelineTask!.finish(arguments: <String, dynamic>{'result': 'keepAlive'});
}
// The image might have been keptAlive but had no listeners (so not live).
// Make sure the cache starts tracking it as live again.
_trackLiveImage(
key,
image.completer,
image.sizeBytes,
);
_cache[key] = image;
return image.completer;
}
final _LiveImage? liveImage = _liveImages[key];
if (liveImage != null) {
_touch(
key,
_CachedImage(
liveImage.completer,
sizeBytes: liveImage.sizeBytes,
),
debugTimelineTask,
);
if (!kReleaseMode) {
debugTimelineTask!.finish(arguments: <String, dynamic>{'result': 'keepAlive'});
}
return liveImage.completer;
}
try {
result = loader();
_trackLiveImage(key, result, null);
} catch (error, stackTrace) {
if (!kReleaseMode) {
debugTimelineTask!.finish(arguments: <String, dynamic>{
'result': 'error',
'error': error.toString(),
'stackTrace': stackTrace.toString(),
});
}
if (onError != null) {
onError(error, stackTrace);
return null;
} else {
rethrow;
}
}
if (!kReleaseMode) {
debugTimelineTask!.start('listener');
}
// A multi-frame provider may call the listener more than once. We need do make
// sure that some cleanup works won't run multiple times, such as finishing the
// tracing task or removing the listeners
bool listenedOnce = false;
// We shouldn't use the _pendingImages map if the cache is disabled, but we
// will have to listen to the image at least once so we don't leak it in
// the live image tracking.
final bool trackPendingImage = maximumSize > 0 && maximumSizeBytes > 0;
late _PendingImage pendingImage;
void listener(ImageInfo? info, bool syncCall) {
int? sizeBytes;
if (info != null) {
sizeBytes = info.sizeBytes;
info.dispose();
}
final _CachedImage image = _CachedImage(
result!,
sizeBytes: sizeBytes,
);
_trackLiveImage(key, result, sizeBytes);
// Only touch if the cache was enabled when resolve was initially called.
if (trackPendingImage) {
_touch(key, image, debugTimelineTask);
} else {
image.dispose();
}
_pendingImages.remove(key);
if (!listenedOnce) {
pendingImage.removeListener();
}
if (!kReleaseMode && !listenedOnce) {
debugTimelineTask!
..finish(arguments: <String, dynamic>{
'syncCall': syncCall,
'sizeInBytes': sizeBytes,
})
..finish(arguments: <String, dynamic>{
'currentSizeBytes': currentSizeBytes,
'currentSize': currentSize,
});
}
listenedOnce = true;
}
final ImageStreamListener streamListener = ImageStreamListener(listener);
pendingImage = _PendingImage(result, streamListener);
if (trackPendingImage) {
_pendingImages[key] = pendingImage;
}
// Listener is removed in [_PendingImage.removeListener].
result.addListener(streamListener);
return result;
}
/// The [ImageCacheStatus] information for the given `key`.
ImageCacheStatus statusForKey(Object key) {
return ImageCacheStatus._(
pending: _pendingImages.containsKey(key),
keepAlive: _cache.containsKey(key),
live: _liveImages.containsKey(key),
);
}
/// Returns whether this `key` has been previously added by [putIfAbsent].
bool containsKey(Object key) {
return _pendingImages[key] != null || _cache[key] != null;
}
/// The number of live images being held by the [ImageCache].
///
/// Compare with [ImageCache.currentSize] for keepAlive images.
int get liveImageCount => _liveImages.length;
/// The number of images being tracked as pending in the [ImageCache].
///
/// Compare with [ImageCache.currentSize] for keepAlive images.
int get pendingImageCount => _pendingImages.length;
/// Clears any live references to images in this cache.
///
/// An image is considered live if its [ImageStreamCompleter] has never hit
/// zero listeners after adding at least one listener. The
/// [ImageStreamCompleter.addOnLastListenerRemovedCallback] is used to
/// determine when this has happened.
///
/// This is called after a hot reload to evict any stale references to image
/// data for assets that have changed. Calling this method does not relieve
/// memory pressure, since the live image caching only tracks image instances
/// that are also being held by at least one other object.
void clearLiveImages() {
for (final _LiveImage image in _liveImages.values) {
image.dispose();
}
_liveImages.clear();
}
// Remove images from the cache until both the length and bytes are below
// maximum, or the cache is empty.
void _checkCacheSize(TimelineTask? timelineTask) {
final Map<String, dynamic> finishArgs = <String, dynamic>{};
if (!kReleaseMode) {
timelineTask!.start('checkCacheSize');
finishArgs['evictedKeys'] = <String>[];
finishArgs['currentSize'] = currentSize;
finishArgs['currentSizeBytes'] = currentSizeBytes;
}
while (_currentSizeBytes > _maximumSizeBytes || _cache.length > _maximumSize) {
final Object key = _cache.keys.first;
final _CachedImage image = _cache[key]!;
_currentSizeBytes -= image.sizeBytes!;
image.dispose();
_cache.remove(key);
if (!kReleaseMode) {
(finishArgs['evictedKeys'] as List<String>).add(key.toString());
}
}
if (!kReleaseMode) {
finishArgs['endSize'] = currentSize;
finishArgs['endSizeBytes'] = currentSizeBytes;
timelineTask!.finish(arguments: finishArgs);
}
assert(_currentSizeBytes >= 0);
assert(_cache.length <= maximumSize);
assert(_currentSizeBytes <= maximumSizeBytes);
}
}
/// Information about how the [ImageCache] is tracking an image.
///
/// A [pending] image is one that has not completed yet. It may also be tracked
/// as [live] because something is listening to it.
///
/// A [keepAlive] image is being held in the cache, which uses Least Recently
/// Used semantics to determine when to evict an image. These images are subject
/// to eviction based on [ImageCache.maximumSizeBytes] and
/// [ImageCache.maximumSize]. It may be [live], but not [pending].
///
/// A [live] image is being held until its [ImageStreamCompleter] has no more
/// listeners. It may also be [pending] or [keepAlive].
///
/// An [untracked] image is not being cached.
///
/// To obtain an [ImageCacheStatus], use [ImageCache.statusForKey] or
/// [ImageProvider.obtainCacheStatus].
@immutable
class ImageCacheStatus {
const ImageCacheStatus._({
this.pending = false,
this.keepAlive = false,
this.live = false,
}) : assert(!pending || !keepAlive);
/// An image that has been submitted to [ImageCache.putIfAbsent], but
/// not yet completed.
final bool pending;
/// An image that has been submitted to [ImageCache.putIfAbsent], has
/// completed, fits based on the sizing rules of the cache, and has not been
/// evicted.
///
/// Such images will be kept alive even if [live] is false, as long
/// as they have not been evicted from the cache based on its sizing rules.
final bool keepAlive;
/// An image that has been submitted to [ImageCache.putIfAbsent] and has at
/// least one listener on its [ImageStreamCompleter].
///
/// Such images may also be [keepAlive] if they fit in the cache based on its
/// sizing rules. They may also be [pending] if they have not yet resolved.
final bool live;
/// An image that is tracked in some way by the [ImageCache], whether
/// [pending], [keepAlive], or [live].
bool get tracked => pending || keepAlive || live;
/// An image that either has not been submitted to
/// [ImageCache.putIfAbsent] or has otherwise been evicted from the
/// [keepAlive] and [live] caches.
bool get untracked => !pending && !keepAlive && !live;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ImageCacheStatus
&& other.pending == pending
&& other.keepAlive == keepAlive
&& other.live == live;
}
@override
int get hashCode => Object.hash(pending, keepAlive, live);
@override
String toString() => '${objectRuntimeType(this, 'ImageCacheStatus')}(pending: $pending, live: $live, keepAlive: $keepAlive)';
}
/// Base class for [_CachedImage] and [_LiveImage].
///
/// Exists primarily so that a [_LiveImage] cannot be added to the
/// [ImageCache._cache].
abstract class _CachedImageBase {
_CachedImageBase(
this.completer, {
this.sizeBytes,
}) : handle = completer.keepAlive() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/painting.dart',
className: '$_CachedImageBase',
object: this,
);
}
}
final ImageStreamCompleter completer;
int? sizeBytes;
ImageStreamCompleterHandle? handle;
@mustCallSuper
void dispose() {
assert(handle != null);
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
// Give any interested parties a chance to listen to the stream before we
// potentially dispose it.
SchedulerBinding.instance.addPostFrameCallback((Duration timeStamp) {
assert(handle != null);
handle?.dispose();
handle = null;
}, debugLabel: 'CachedImage.disposeHandle');
}
}
class _CachedImage extends _CachedImageBase {
_CachedImage(super.completer, {super.sizeBytes});
}
class _LiveImage extends _CachedImageBase {
_LiveImage(ImageStreamCompleter completer, VoidCallback handleRemove, {int? sizeBytes})
: super(completer, sizeBytes: sizeBytes) {
_handleRemove = () {
handleRemove();
dispose();
};
completer.addOnLastListenerRemovedCallback(_handleRemove);
}
late VoidCallback _handleRemove;
@override
void dispose() {
completer.removeOnLastListenerRemovedCallback(_handleRemove);
super.dispose();
}
@override
String toString() => describeIdentity(this);
}
class _PendingImage {
_PendingImage(this.completer, this.listener);
final ImageStreamCompleter completer;
final ImageStreamListener listener;
void removeListener() {
completer.removeListener(listener);
}
}
| flutter/packages/flutter/lib/src/painting/image_cache.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/image_cache.dart",
"repo_id": "flutter",
"token_count": 7866
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart' show Matrix4;
import 'basic_types.dart';
import 'borders.dart';
import 'circle_border.dart';
import 'rounded_rectangle_border.dart';
import 'stadium_border.dart';
// Conversion from radians to degrees.
const double _kRadToDeg = 180 / math.pi;
// Conversion from degrees to radians.
const double _kDegToRad = math.pi / 180;
/// A border that fits a star or polygon-shaped border within the rectangle of
/// the widget it is applied to.
///
/// Typically used with a [ShapeDecoration] to draw a polygonal or star shaped
/// border.
///
/// {@tool dartpad}
/// This example serves both as a usage example, as well as an explorer for
/// determining the parameters to use with a [StarBorder]. The resulting code
/// can be copied and pasted into your app. A [Container] is just one widget
/// which takes a [ShapeBorder]. [Dialog]s, [OutlinedButton]s,
/// [ElevatedButton]s, etc. all can be shaped with a [ShapeBorder].
///
/// ** See code in examples/api/lib/painting/star_border/star_border.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [BorderSide], which is used to describe how the edge of the shape is
/// drawn.
class StarBorder extends OutlinedBorder {
/// Create a const star-shaped border with the given number [points] on the
/// star.
const StarBorder({
super.side,
this.points = 5,
double innerRadiusRatio = 0.4,
this.pointRounding = 0,
this.valleyRounding = 0,
double rotation = 0,
this.squash = 0,
}) : assert(squash >= 0),
assert(squash <= 1),
assert(pointRounding >= 0),
assert(pointRounding <= 1),
assert(valleyRounding >= 0),
assert(valleyRounding <= 1),
assert(
(valleyRounding + pointRounding) <= 1,
'The sum of valleyRounding ($valleyRounding) and '
'pointRounding ($pointRounding) must not exceed one.'),
assert(innerRadiusRatio >= 0),
assert(innerRadiusRatio <= 1),
assert(points >= 2),
_rotationRadians = rotation * _kDegToRad,
_innerRadiusRatio = innerRadiusRatio;
/// Create a const polygon border with the given number of [sides].
const StarBorder.polygon({
super.side,
double sides = 5,
this.pointRounding = 0,
double rotation = 0,
this.squash = 0,
}) : assert(squash >= 0),
assert(squash <= 1),
assert(pointRounding >= 0),
assert(pointRounding <= 1),
assert(sides >= 2),
points = sides,
valleyRounding = 0,
_rotationRadians = rotation * _kDegToRad,
_innerRadiusRatio = null;
/// The number of points in this star, or sides on a polygon.
///
/// This is a floating point number: if this is not a whole number, then an
/// additional star point or corner shorter than the others will be added to
/// finish the shape. Only whole-numbered values will yield a symmetric shape.
/// (This enables the number of points to be animated smoothly.)
///
/// For stars created with [StarBorder], this is the number of points on
/// the star. For polygons created with [StarBorder.polygon], this is the
/// number of sides on the polygon.
///
/// Must be greater than or equal to two.
final double points;
/// The ratio of the inner radius of a star with the outer radius.
///
/// When making a star using [StarBorder], this is the ratio of the inner
/// radius that to the outer radius. If it is one, then the inner radius
/// will equal the outer radius.
///
/// For polygons created with [StarBorder.polygon], getting this value will
/// return the incircle radius of the polygon (the radius of a circle
/// inscribed inside the polygon).
///
/// Defaults to 0.4 for stars, and must be between zero and one, inclusive.
double get innerRadiusRatio {
// Polygons are just a special case of a star where the inner radius is the
// incircle radius of the polygon (the radius of an inscribed circle).
return _innerRadiusRatio ?? math.cos(math.pi / points);
}
final double? _innerRadiusRatio;
/// The amount of rounding on the points of stars, or the corners of polygons.
///
/// This is a value between zero and one which describes how rounded the point
/// or corner should be. A value of zero means no rounding (sharp corners),
/// and a value of one means that the entire point or corner is a portion of a
/// circle.
///
/// Defaults to zero. The sum of [pointRounding] and [valleyRounding] must be
/// less than or equal to one.
final double pointRounding;
/// The amount of rounding of the interior corners of stars.
///
/// This is a value between zero and one which describes how rounded the inner
/// corners in a star (the "valley" between points) should be. A value of zero
/// means no rounding (sharp corners), and a value of one means that the
/// entire corner is a portion of a circle.
///
/// Defaults to zero. The sum of [pointRounding] and [valleyRounding] must be
/// less than or equal to one. For polygons created with [StarBorder.polygon],
/// this will always be zero.
final double valleyRounding;
/// The rotation in clockwise degrees around the center of the shape.
///
/// The rotation occurs before the [squash] effect is applied, so that you can
/// fine tune where the points of a star or corners of a polygon start.
///
/// Defaults to zero, meaning that the first point or corner is pointing up.
double get rotation => _rotationRadians * _kRadToDeg;
final double _rotationRadians;
/// How much of the aspect ratio of the attached widget to take on.
///
/// If [squash] is non-zero, the border will match the aspect ratio of the
/// bounding box of the widget that it is attached to, which can give a
/// squashed appearance.
///
/// The [squash] parameter lets you control how much of that aspect ratio this
/// border takes on.
///
/// A value of zero means that the border will be drawn with a square aspect
/// ratio at the size of the shortest side of the bounding rectangle, ignoring
/// the aspect ratio of the widget, and a value of one means it will be drawn
/// with the aspect ratio of the widget. The value of [squash] has no effect
/// if the widget is square to begin with.
///
/// Defaults to zero, and must be between zero and one, inclusive.
final double squash;
@override
ShapeBorder scale(double t) {
return StarBorder(
points: points,
side: side.scale(t),
rotation: rotation,
innerRadiusRatio: innerRadiusRatio,
pointRounding: pointRounding,
valleyRounding: valleyRounding,
squash: squash,
);
}
ShapeBorder? _twoPhaseLerp(
double t,
double split,
ShapeBorder? Function(double t) first,
ShapeBorder? Function(double t) second,
) {
// If the rectangle has square corners, then skip the extra lerp to round the corners.
if (t < split) {
return first(t * (1 / split));
} else {
t = (1 / (1.0 - split)) * (t - split);
return second(t);
}
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (t == 0) {
return a;
}
if (t == 1.0) {
return this;
}
if (a is StarBorder) {
return StarBorder(
side: BorderSide.lerp(a.side, side, t),
points: ui.lerpDouble(a.points, points, t)!,
rotation: ui.lerpDouble(a._rotationRadians, _rotationRadians, t)! * _kRadToDeg,
innerRadiusRatio: ui.lerpDouble(a.innerRadiusRatio, innerRadiusRatio, t)!,
pointRounding: ui.lerpDouble(a.pointRounding, pointRounding, t)!,
valleyRounding: ui.lerpDouble(a.valleyRounding, valleyRounding, t)!,
squash: ui.lerpDouble(a.squash, squash, t)!,
);
}
if (a is CircleBorder) {
if (points >= 2.5) {
final double lerpedPoints = ui.lerpDouble(points.round(), points, t)!;
return StarBorder(
side: BorderSide.lerp(a.side, side, t),
points: lerpedPoints,
squash: ui.lerpDouble(a.eccentricity, squash, t)!,
rotation: rotation,
innerRadiusRatio: ui.lerpDouble(math.cos(math.pi / lerpedPoints), innerRadiusRatio, t)!,
pointRounding: ui.lerpDouble(1.0, pointRounding, t)!,
valleyRounding: ui.lerpDouble(0.0, valleyRounding, t)!,
);
} else {
// Have a slightly different lerp for two-pointed stars, since they get
// kind of squirrelly with near-zero innerRadiusRatios.
final double lerpedPoints = ui.lerpDouble(points, 2, t)!;
return StarBorder(
side: BorderSide.lerp(a.side, side, t),
points: lerpedPoints,
squash: ui.lerpDouble(a.eccentricity, squash, t)!,
rotation: rotation,
innerRadiusRatio: ui.lerpDouble(1, innerRadiusRatio, t)!,
pointRounding: ui.lerpDouble(0.5, pointRounding, t)!,
valleyRounding: ui.lerpDouble(0.5, valleyRounding, t)!,
);
}
}
if (a is StadiumBorder) {
// Lerp from a stadium to a circle first, and from there to a star.
final BorderSide lerpedSide = BorderSide.lerp(a.side, side, t);
return _twoPhaseLerp(
t,
0.5,
(double t) => a.lerpTo(CircleBorder(side: lerpedSide), t),
(double t) => lerpFrom(CircleBorder(side: lerpedSide), t),
);
}
if (a is RoundedRectangleBorder) {
// Lerp from a rectangle to a stadium, then from a Stadium to a circle,
// then from a circle to a star.
final BorderSide lerpedSide = BorderSide.lerp(a.side, side, t);
return _twoPhaseLerp(
t,
1 / 3,
(double t) {
return StadiumBorder(side: lerpedSide).lerpFrom(a, t);
},
(double t) {
return _twoPhaseLerp(
t,
0.5,
(double t) => StadiumBorder(side: lerpedSide).lerpTo(CircleBorder(side: lerpedSide), t),
(double t) => lerpFrom(CircleBorder(side: lerpedSide), t),
);
},
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (t == 0) {
return this;
}
if (t == 1.0) {
return b;
}
if (b is StarBorder) {
return StarBorder(
side: BorderSide.lerp(side, b.side, t),
points: ui.lerpDouble(points, b.points, t)!,
rotation: ui.lerpDouble(_rotationRadians, b._rotationRadians, t)! * _kRadToDeg,
innerRadiusRatio: ui.lerpDouble(innerRadiusRatio, b.innerRadiusRatio, t)!,
pointRounding: ui.lerpDouble(pointRounding, b.pointRounding, t)!,
valleyRounding: ui.lerpDouble(valleyRounding, b.valleyRounding, t)!,
squash: ui.lerpDouble(squash, b.squash, t)!,
);
}
if (b is CircleBorder) {
// Have a slightly different lerp for two-pointed stars, since they get
// kind of squirrelly with near-zero innerRadiusRatios.
if (points >= 2.5) {
final double lerpedPoints = ui.lerpDouble(points, points.round(), t)!;
return StarBorder(
side: BorderSide.lerp(side, b.side, t),
points: lerpedPoints,
squash: ui.lerpDouble(squash, b.eccentricity, t)!,
rotation: rotation,
innerRadiusRatio: ui.lerpDouble(innerRadiusRatio, math.cos(math.pi / lerpedPoints), t)!,
pointRounding: ui.lerpDouble(pointRounding, 1.0, t)!,
valleyRounding: ui.lerpDouble(valleyRounding, 0.0, t)!,
);
} else {
final double lerpedPoints = ui.lerpDouble(points, 2, t)!;
return StarBorder(
side: BorderSide.lerp(side, b.side, t),
points: lerpedPoints,
squash: ui.lerpDouble(squash, b.eccentricity, t)!,
rotation: rotation,
innerRadiusRatio: ui.lerpDouble(innerRadiusRatio, 1, t)!,
pointRounding: ui.lerpDouble(pointRounding, 0.5, t)!,
valleyRounding: ui.lerpDouble(valleyRounding, 0.5, t)!,
);
}
}
if (b is StadiumBorder) {
// Lerp to a circle first, then to a stadium.
final BorderSide lerpedSide = BorderSide.lerp(side, b.side, t);
return _twoPhaseLerp(
t,
0.5,
(double t) => lerpTo(CircleBorder(side: lerpedSide), t),
(double t) => b.lerpFrom(CircleBorder(side: lerpedSide), t),
);
}
if (b is RoundedRectangleBorder) {
// Lerp to a circle, and then to a stadium, then to a rounded rect.
final BorderSide lerpedSide = BorderSide.lerp(side, b.side, t);
return _twoPhaseLerp(
t,
2 / 3,
(double t) {
return _twoPhaseLerp(
t,
0.5,
(double t) => lerpTo(CircleBorder(side: lerpedSide), t),
(double t) => StadiumBorder(side: lerpedSide).lerpFrom(CircleBorder(side: lerpedSide), t),
);
},
(double t) {
return StadiumBorder(side: lerpedSide).lerpTo(b, t);
},
);
}
return super.lerpTo(b, t);
}
@override
StarBorder copyWith({
BorderSide? side,
double? points,
double? innerRadiusRatio,
double? pointRounding,
double? valleyRounding,
double? rotation,
double? squash,
}) {
return StarBorder(
side: side ?? this.side,
points: points ?? this.points,
rotation: rotation ?? this.rotation,
innerRadiusRatio: innerRadiusRatio ?? this.innerRadiusRatio,
pointRounding: pointRounding ?? this.pointRounding,
valleyRounding: valleyRounding ?? this.valleyRounding,
squash: squash ?? this.squash,
);
}
@override
Path getInnerPath(Rect rect, {TextDirection? textDirection}) {
final Rect adjustedRect = rect.deflate(side.strokeInset);
return _StarGenerator(
points: points,
rotation: _rotationRadians,
innerRadiusRatio: innerRadiusRatio,
pointRounding: pointRounding,
valleyRounding: valleyRounding,
squash: squash,
).generate(adjustedRect);
}
@override
Path getOuterPath(Rect rect, {TextDirection? textDirection}) {
return _StarGenerator(
points: points,
rotation: _rotationRadians,
innerRadiusRatio: innerRadiusRatio,
pointRounding: pointRounding,
valleyRounding: valleyRounding,
squash: squash,
).generate(rect);
}
@override
void paint(Canvas canvas, Rect rect, {TextDirection? textDirection}) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final Rect adjustedRect = rect.inflate(side.strokeOffset / 2);
final Path path = _StarGenerator(
points: points,
rotation: _rotationRadians,
innerRadiusRatio: innerRadiusRatio,
pointRounding: pointRounding,
valleyRounding: valleyRounding,
squash: squash,
).generate(adjustedRect);
canvas.drawPath(path, side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is StarBorder
&& other.side == side
&& other.points == points
&& other._innerRadiusRatio == _innerRadiusRatio
&& other.pointRounding == pointRounding
&& other.valleyRounding == valleyRounding
&& other._rotationRadians == _rotationRadians
&& other.squash == squash;
}
@override
int get hashCode => side.hashCode;
@override
String toString() {
return '${objectRuntimeType(this, 'StarBorder')}($side, points: $points, innerRadiusRatio: $innerRadiusRatio)';
}
}
class _PointInfo {
_PointInfo({
required this.valley,
required this.point,
required this.valleyArc1,
required this.pointArc1,
required this.valleyArc2,
required this.pointArc2,
});
Offset valley;
Offset point;
Offset valleyArc1;
Offset pointArc1;
Offset pointArc2;
Offset valleyArc2;
}
class _StarGenerator {
const _StarGenerator({
required this.points,
required this.innerRadiusRatio,
required this.pointRounding,
required this.valleyRounding,
required this.rotation,
required this.squash,
}) : assert(points > 1),
assert(innerRadiusRatio <= 1),
assert(innerRadiusRatio >= 0),
assert(squash >= 0),
assert(squash <= 1),
assert(pointRounding >= 0),
assert(pointRounding <= 1),
assert(valleyRounding >= 0),
assert(valleyRounding <= 1),
assert(pointRounding + valleyRounding <= 1);
final double points;
final double innerRadiusRatio;
final double pointRounding;
final double valleyRounding;
final double rotation;
final double squash;
Path generate(Rect rect) {
final double radius = rect.shortestSide / 2;
final Offset center = rect.center;
// The minimum allowed inner radius ratio. Numerical instabilities occur near
// zero, so we just don't allow values in that range.
const double minInnerRadiusRatio = .002;
// Map the innerRadiusRatio so that we don't get values close to zero, since
// things get a little squirrelly there because the path thinks that the
// length of the conicTo is small enough that it can render it as a straight
// line, even though it will be scaled up later. This maps the range from
// [0, 1] to [minInnerRadiusRatio, 1].
final double mappedInnerRadiusRatio = (innerRadiusRatio * (1.0 - minInnerRadiusRatio)) + minInnerRadiusRatio;
// First, generate the "points" of the star.
final List<_PointInfo> points = <_PointInfo>[];
final double maxDiameter = 2.0 *
_generatePoints(
pointList: points,
center: center,
radius: radius,
innerRadius: radius * mappedInnerRadiusRatio,
);
// Calculate the endpoints of each of the arcs, then draw the arcs.
final Path path = Path();
_drawPoints(path, points);
Offset scale = Offset(rect.width / maxDiameter, rect.height / maxDiameter);
if (rect.shortestSide == rect.width) {
scale = Offset(scale.dx, squash * scale.dy + (1 - squash) * scale.dx);
} else {
scale = Offset(squash * scale.dx + (1 - squash) * scale.dy, scale.dy);
}
// Scale the border so that it matches the size of the widget rectangle, so
// that "rotation" of the shape doesn't affect how much of the rectangle it
// covers.
final Matrix4 squashMatrix = Matrix4.translationValues(rect.center.dx, rect.center.dy, 0);
squashMatrix.multiply(Matrix4.diagonal3Values(scale.dx, scale.dy, 1));
squashMatrix.multiply(Matrix4.rotationZ(rotation));
squashMatrix.multiply(Matrix4.translationValues(-rect.center.dx, -rect.center.dy, 0));
return path.transform(squashMatrix.storage);
}
double _generatePoints({
required List<_PointInfo> pointList,
required Offset center,
required double radius,
required double innerRadius,
}) {
final double step = math.pi / points;
// Start initial rotation one step before zero.
double angle = -math.pi / 2 - step;
Offset valley = Offset(
center.dx + math.cos(angle) * innerRadius,
center.dy + math.sin(angle) * innerRadius,
);
// In order to do overall scale properly, calculate the actual radius at the
// point, taking into account the rounding of the points and the weight of
// the corner point. This effectively is evaluating the rational quadratic
// bezier at the midpoint of the curve.
Offset getCurveMidpoint(Offset a, Offset b, Offset c, Offset a1, Offset c1) {
final double angle = _getAngle(a, b, c);
final double w = _getWeight(angle) / 2;
return (a1 / 4 + b * w + c1 / 4) / (0.5 + w);
}
double addPoint(
double pointAngle,
double pointStep,
double pointRadius,
double pointInnerRadius,
) {
pointAngle += pointStep;
final Offset point = Offset(
center.dx + math.cos(pointAngle) * pointRadius,
center.dy + math.sin(pointAngle) * pointRadius,
);
pointAngle += pointStep;
final Offset nextValley = Offset(
center.dx + math.cos(pointAngle) * pointInnerRadius,
center.dy + math.sin(pointAngle) * pointInnerRadius,
);
final Offset valleyArc1 = valley + (point - valley) * valleyRounding;
final Offset pointArc1 = point + (valley - point) * pointRounding;
final Offset pointArc2 = point + (nextValley - point) * pointRounding;
final Offset valleyArc2 = nextValley + (point - nextValley) * valleyRounding;
pointList.add(_PointInfo(
valley: valley,
point: point,
valleyArc1: valleyArc1,
pointArc1: pointArc1,
pointArc2: pointArc2,
valleyArc2: valleyArc2,
));
valley = nextValley;
return pointAngle;
}
final double remainder = points - points.truncateToDouble();
final bool hasIntegerSides = remainder < 1e-6;
final double wholeSides = points - (hasIntegerSides ? 0 : 1);
for (int i = 0; i < wholeSides; i += 1) {
angle = addPoint(angle, step, radius, innerRadius);
}
double valleyRadius = 0;
double pointRadius = 0;
final _PointInfo thisPoint = pointList[0];
final _PointInfo nextPoint = pointList[1];
final Offset pointMidpoint =
getCurveMidpoint(thisPoint.valley, thisPoint.point, nextPoint.valley, thisPoint.pointArc1, thisPoint.pointArc2);
final Offset valleyMidpoint = getCurveMidpoint(
thisPoint.point, nextPoint.valley, nextPoint.point, thisPoint.valleyArc2, nextPoint.valleyArc1);
valleyRadius = (valleyMidpoint - center).distance;
pointRadius = (pointMidpoint - center).distance;
// Add the final point to close the shape if there are fractional sides to
// account for.
if (!hasIntegerSides) {
final double effectiveInnerRadius = math.max(valleyRadius, innerRadius);
final double endingRadius = effectiveInnerRadius + remainder * (radius - effectiveInnerRadius);
addPoint(angle, step * remainder, endingRadius, innerRadius);
}
// The rounding added to the valley radius can sometimes push it outside of
// the rounding of the point, since the rounding amount can be different
// between the points and the valleys, so we have to evaluate both the
// valley and the point radii, and pick the largest. Also, since this value
// is used later to determine the scale, we need to keep it finite and
// non-zero.
return clampDouble(math.max(valleyRadius, pointRadius), double.minPositive, double.maxFinite);
}
void _drawPoints(Path path, List<_PointInfo> points) {
final Offset startingPoint = points.first.pointArc1;
path.moveTo(startingPoint.dx, startingPoint.dy);
final double pointAngle = _getAngle(points[0].valley, points[0].point, points[1].valley);
final double pointWeight = _getWeight(pointAngle);
final double valleyAngle = _getAngle(points[1].point, points[1].valley, points[0].point);
final double valleyWeight = _getWeight(valleyAngle);
for (int i = 0; i < points.length; i += 1) {
final _PointInfo point = points[i];
final _PointInfo nextPoint = points[(i + 1) % points.length];
path.lineTo(point.pointArc1.dx, point.pointArc1.dy);
if (pointAngle != 180 && pointAngle != 0) {
path.conicTo(point.point.dx, point.point.dy, point.pointArc2.dx, point.pointArc2.dy, pointWeight);
} else {
path.lineTo(point.pointArc2.dx, point.pointArc2.dy);
}
path.lineTo(point.valleyArc2.dx, point.valleyArc2.dy);
if (valleyAngle != 180 && valleyAngle != 0) {
path.conicTo(
nextPoint.valley.dx, nextPoint.valley.dy, nextPoint.valleyArc1.dx, nextPoint.valleyArc1.dy, valleyWeight);
} else {
path.lineTo(nextPoint.valleyArc1.dx, nextPoint.valleyArc1.dy);
}
}
path.close();
}
double _getWeight(double angle) {
return math.cos((angle / 2) % (math.pi / 2));
}
// Returns the included angle between points ABC in radians.
double _getAngle(Offset a, Offset b, Offset c) {
if (a == c || b == c || b == a) {
return 0;
}
final Offset u = a - b;
final Offset v = c - b;
final double dot = u.dx * v.dx + u.dy * v.dy;
final double m1 = b.dx == a.dx ? double.infinity : -u.dy / -u.dx;
final double m2 = b.dx == c.dx ? double.infinity : -v.dy / -v.dx;
double angle = math.atan2(m1 - m2, 1 + m1 * m2).abs();
if (dot < 0) {
angle += math.pi;
}
return angle;
}
}
| flutter/packages/flutter/lib/src/painting/star_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/star_border.dart",
"repo_id": "flutter",
"token_count": 9585
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'object.dart';
// For SingleChildLayoutDelegate and RenderCustomSingleChildLayoutBox, see shifted_box.dart
/// [ParentData] used by [RenderCustomMultiChildLayoutBox].
class MultiChildLayoutParentData extends ContainerBoxParentData<RenderBox> {
/// An object representing the identity of this child.
Object? id;
@override
String toString() => '${super.toString()}; id=$id';
}
/// A delegate that controls the layout of multiple children.
///
/// Used with [CustomMultiChildLayout] (in the widgets library) and
/// [RenderCustomMultiChildLayoutBox] (in the rendering library).
///
/// Delegates must be idempotent. Specifically, if two delegates are equal, then
/// they must produce the same layout. To change the layout, replace the
/// delegate with a different instance whose [shouldRelayout] returns true when
/// given the previous instance.
///
/// Override [getSize] to control the overall size of the layout. The size of
/// the layout cannot depend on layout properties of the children. This was
/// a design decision to simplify the delegate implementations: This way,
/// the delegate implementations do not have to also handle various intrinsic
/// sizing functions if the parent's size depended on the children.
/// If you want to build a custom layout where you define the size of that widget
/// based on its children, then you will have to create a custom render object.
/// See [MultiChildRenderObjectWidget] with [ContainerRenderObjectMixin] and
/// [RenderBoxContainerDefaultsMixin] to get started or [RenderStack] for an
/// example implementation.
///
/// Override [performLayout] to size and position the children. An
/// implementation of [performLayout] must call [layoutChild] exactly once for
/// each child, but it may call [layoutChild] on children in an arbitrary order.
/// Typically a delegate will use the size returned from [layoutChild] on one
/// child to determine the constraints for [performLayout] on another child or
/// to determine the offset for [positionChild] for that child or another child.
///
/// Override [shouldRelayout] to determine when the layout of the children needs
/// to be recomputed when the delegate changes.
///
/// The most efficient way to trigger a relayout is to supply a `relayout`
/// argument to the constructor of the [MultiChildLayoutDelegate]. The custom
/// layout will listen to this value and relayout whenever the Listenable
/// notifies its listeners, such as when an [Animation] ticks. This allows
/// the custom layout to avoid the build phase of the pipeline.
///
/// Each child must be wrapped in a [LayoutId] widget to assign the id that
/// identifies it to the delegate. The [LayoutId.id] needs to be unique among
/// the children that the [CustomMultiChildLayout] manages.
///
/// {@tool snippet}
///
/// Below is an example implementation of [performLayout] that causes one widget
/// (the follower) to be the same size as another (the leader):
///
/// ```dart
/// // Define your own slot numbers, depending upon the id assigned by LayoutId.
/// // Typical usage is to define an enum like the one below, and use those
/// // values as the ids.
/// enum _Slot {
/// leader,
/// follower,
/// }
///
/// class FollowTheLeader extends MultiChildLayoutDelegate {
/// @override
/// void performLayout(Size size) {
/// Size leaderSize = Size.zero;
///
/// if (hasChild(_Slot.leader)) {
/// leaderSize = layoutChild(_Slot.leader, BoxConstraints.loose(size));
/// positionChild(_Slot.leader, Offset.zero);
/// }
///
/// if (hasChild(_Slot.follower)) {
/// layoutChild(_Slot.follower, BoxConstraints.tight(leaderSize));
/// positionChild(_Slot.follower, Offset(size.width - leaderSize.width,
/// size.height - leaderSize.height));
/// }
/// }
///
/// @override
/// bool shouldRelayout(MultiChildLayoutDelegate oldDelegate) => false;
/// }
/// ```
/// {@end-tool}
///
/// The delegate gives the leader widget loose constraints, which means the
/// child determines what size to be (subject to fitting within the given size).
/// The delegate then remembers the size of that child and places it in the
/// upper left corner.
///
/// The delegate then gives the follower widget tight constraints, forcing it to
/// match the size of the leader widget. The delegate then places the follower
/// widget in the bottom right corner.
///
/// The leader and follower widget will paint in the order they appear in the
/// child list, regardless of the order in which [layoutChild] is called on
/// them.
///
/// See also:
///
/// * [CustomMultiChildLayout], the widget that uses this delegate.
/// * [RenderCustomMultiChildLayoutBox], render object that uses this
/// delegate.
abstract class MultiChildLayoutDelegate {
/// Creates a layout delegate.
///
/// The layout will update whenever [relayout] notifies its listeners.
MultiChildLayoutDelegate({ Listenable? relayout }) : _relayout = relayout;
final Listenable? _relayout;
Map<Object, RenderBox>? _idToChild;
Set<RenderBox>? _debugChildrenNeedingLayout;
/// True if a non-null LayoutChild was provided for the specified id.
///
/// Call this from the [performLayout] method to determine which children
/// are available, if the child list might vary.
///
/// This method cannot be called from [getSize] as the size is not allowed
/// to depend on the children.
bool hasChild(Object childId) => _idToChild![childId] != null;
/// Ask the child to update its layout within the limits specified by
/// the constraints parameter. The child's size is returned.
///
/// Call this from your [performLayout] function to lay out each
/// child. Every child must be laid out using this function exactly
/// once each time the [performLayout] function is called.
Size layoutChild(Object childId, BoxConstraints constraints) {
final RenderBox? child = _idToChild![childId];
assert(() {
if (child == null) {
throw FlutterError(
'The $this custom multichild layout delegate tried to lay out a non-existent child.\n'
'There is no child with the id "$childId".',
);
}
if (!_debugChildrenNeedingLayout!.remove(child)) {
throw FlutterError(
'The $this custom multichild layout delegate tried to lay out the child with id "$childId" more than once.\n'
'Each child must be laid out exactly once.',
);
}
try {
assert(constraints.debugAssertIsValid(isAppliedConstraint: true));
} on AssertionError catch (exception) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The $this custom multichild layout delegate provided invalid box constraints for the child with id "$childId".'),
DiagnosticsProperty<AssertionError>('Exception', exception, showName: false),
ErrorDescription(
'The minimum width and height must be greater than or equal to zero.\n'
'The maximum width must be greater than or equal to the minimum width.\n'
'The maximum height must be greater than or equal to the minimum height.',
),
]);
}
return true;
}());
child!.layout(constraints, parentUsesSize: true);
return child.size;
}
/// Specify the child's origin relative to this origin.
///
/// Call this from your [performLayout] function to position each
/// child. If you do not call this for a child, its position will
/// remain unchanged. Children initially have their position set to
/// (0,0), i.e. the top left of the [RenderCustomMultiChildLayoutBox].
void positionChild(Object childId, Offset offset) {
final RenderBox? child = _idToChild![childId];
assert(() {
if (child == null) {
throw FlutterError(
'The $this custom multichild layout delegate tried to position out a non-existent child:\n'
'There is no child with the id "$childId".',
);
}
return true;
}());
final MultiChildLayoutParentData childParentData = child!.parentData! as MultiChildLayoutParentData;
childParentData.offset = offset;
}
DiagnosticsNode _debugDescribeChild(RenderBox child) {
final MultiChildLayoutParentData childParentData = child.parentData! as MultiChildLayoutParentData;
return DiagnosticsProperty<RenderBox>('${childParentData.id}', child);
}
void _callPerformLayout(Size size, RenderBox? firstChild) {
// A particular layout delegate could be called reentrantly, e.g. if it used
// by both a parent and a child. So, we must restore the _idToChild map when
// we return.
final Map<Object, RenderBox>? previousIdToChild = _idToChild;
Set<RenderBox>? debugPreviousChildrenNeedingLayout;
assert(() {
debugPreviousChildrenNeedingLayout = _debugChildrenNeedingLayout;
_debugChildrenNeedingLayout = <RenderBox>{};
return true;
}());
try {
_idToChild = <Object, RenderBox>{};
RenderBox? child = firstChild;
while (child != null) {
final MultiChildLayoutParentData childParentData = child.parentData! as MultiChildLayoutParentData;
assert(() {
if (childParentData.id == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Every child of a RenderCustomMultiChildLayoutBox must have an ID in its parent data.'),
child!.describeForError('The following child has no ID'),
]);
}
return true;
}());
_idToChild![childParentData.id!] = child;
assert(() {
_debugChildrenNeedingLayout!.add(child!);
return true;
}());
child = childParentData.nextSibling;
}
performLayout(size);
assert(() {
if (_debugChildrenNeedingLayout!.isNotEmpty) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Each child must be laid out exactly once.'),
DiagnosticsBlock(
name:
'The $this custom multichild layout delegate forgot '
'to lay out the following '
'${_debugChildrenNeedingLayout!.length > 1 ? 'children' : 'child'}',
properties: _debugChildrenNeedingLayout!.map<DiagnosticsNode>(_debugDescribeChild).toList(),
),
]);
}
return true;
}());
} finally {
_idToChild = previousIdToChild;
assert(() {
_debugChildrenNeedingLayout = debugPreviousChildrenNeedingLayout;
return true;
}());
}
}
/// Override this method to return the size of this object given the
/// incoming constraints.
///
/// The size cannot reflect the sizes of the children. If this layout has a
/// fixed width or height the returned size can reflect that; the size will be
/// constrained to the given constraints.
///
/// By default, attempts to size the box to the biggest size
/// possible given the constraints.
Size getSize(BoxConstraints constraints) => constraints.biggest;
/// Override this method to lay out and position all children given this
/// widget's size.
///
/// This method must call [layoutChild] for each child. It should also specify
/// the final position of each child with [positionChild].
void performLayout(Size size);
/// Override this method to return true when the children need to be
/// laid out.
///
/// This should compare the fields of the current delegate and the given
/// `oldDelegate` and return true if the fields are such that the layout would
/// be different.
bool shouldRelayout(covariant MultiChildLayoutDelegate oldDelegate);
/// Override this method to include additional information in the
/// debugging data printed by [debugDumpRenderTree] and friends.
///
/// By default, returns the [runtimeType] of the class.
@override
String toString() => objectRuntimeType(this, 'MultiChildLayoutDelegate');
}
/// Defers the layout of multiple children to a delegate.
///
/// The delegate can determine the layout constraints for each child and can
/// decide where to position each child. The delegate can also determine the
/// size of the parent, but the size of the parent cannot depend on the sizes of
/// the children.
class RenderCustomMultiChildLayoutBox extends RenderBox
with ContainerRenderObjectMixin<RenderBox, MultiChildLayoutParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, MultiChildLayoutParentData> {
/// Creates a render object that customizes the layout of multiple children.
RenderCustomMultiChildLayoutBox({
List<RenderBox>? children,
required MultiChildLayoutDelegate delegate,
}) : _delegate = delegate {
addAll(children);
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! MultiChildLayoutParentData) {
child.parentData = MultiChildLayoutParentData();
}
}
/// The delegate that controls the layout of the children.
MultiChildLayoutDelegate get delegate => _delegate;
MultiChildLayoutDelegate _delegate;
set delegate(MultiChildLayoutDelegate newDelegate) {
if (_delegate == newDelegate) {
return;
}
final MultiChildLayoutDelegate oldDelegate = _delegate;
if (newDelegate.runtimeType != oldDelegate.runtimeType || newDelegate.shouldRelayout(oldDelegate)) {
markNeedsLayout();
}
_delegate = newDelegate;
if (attached) {
oldDelegate._relayout?.removeListener(markNeedsLayout);
newDelegate._relayout?.addListener(markNeedsLayout);
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_delegate._relayout?.addListener(markNeedsLayout);
}
@override
void detach() {
_delegate._relayout?.removeListener(markNeedsLayout);
super.detach();
}
Size _getSize(BoxConstraints constraints) {
assert(constraints.debugAssertIsValid());
return constraints.constrain(_delegate.getSize(constraints));
}
// TODO(ianh): It's a bit dubious to be using the getSize function from the delegate to
// figure out the intrinsic dimensions. We really should either not support intrinsics,
// or we should expose intrinsic delegate callbacks and throw if they're not implemented.
@override
double computeMinIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return _getSize(constraints);
}
@override
void performLayout() {
size = _getSize(constraints);
delegate._callPerformLayout(size, firstChild);
}
@override
void paint(PaintingContext context, Offset offset) {
defaultPaint(context, offset);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
return defaultHitTestChildren(result, position: position);
}
}
| flutter/packages/flutter/lib/src/rendering/custom_layout.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/custom_layout.dart",
"repo_id": "flutter",
"token_count": 4990
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:math' as math;
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle, Gradient, LineMetrics, PlaceholderAlignment, Shader, TextBox, TextHeightBehavior;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/semantics.dart';
import 'package:flutter/services.dart';
import 'box.dart';
import 'debug.dart';
import 'layer.dart';
import 'layout_helper.dart';
import 'object.dart';
import 'selection.dart';
/// The start and end positions for a word.
typedef _WordBoundaryRecord = ({TextPosition wordStart, TextPosition wordEnd});
const String _kEllipsis = '\u2026';
/// Used by the [RenderParagraph] to map its rendering children to their
/// corresponding semantics nodes.
///
/// The [RichText] uses this to tag the relation between its placeholder spans
/// and their semantics nodes.
@immutable
class PlaceholderSpanIndexSemanticsTag extends SemanticsTag {
/// Creates a semantics tag with the input `index`.
///
/// Different [PlaceholderSpanIndexSemanticsTag]s with the same `index` are
/// consider the same.
const PlaceholderSpanIndexSemanticsTag(this.index) : super('PlaceholderSpanIndexSemanticsTag($index)');
/// The index of this tag.
final int index;
@override
bool operator ==(Object other) {
return other is PlaceholderSpanIndexSemanticsTag
&& other.index == index;
}
@override
int get hashCode => Object.hash(PlaceholderSpanIndexSemanticsTag, index);
}
/// Parent data used by [RenderParagraph] and [RenderEditable] to annotate
/// inline contents (such as [WidgetSpan]s) with.
class TextParentData extends ParentData with ContainerParentDataMixin<RenderBox> {
/// The offset at which to paint the child in the parent's coordinate system.
///
/// A `null` value indicates this inline widget is not laid out. For instance,
/// when the inline widget has never been laid out, or the inline widget is
/// ellipsized away.
Offset? get offset => _offset;
Offset? _offset;
/// The [PlaceholderSpan] associated with this render child.
///
/// This field is usually set by a [ParentDataWidget], and is typically not
/// null when `performLayout` is called.
PlaceholderSpan? span;
@override
void detach() {
span = null;
_offset = null;
super.detach();
}
@override
String toString() => 'widget: $span, ${offset == null ? "not laid out" : "offset: $offset"}';
}
/// A mixin that provides useful default behaviors for text [RenderBox]es
/// ([RenderParagraph] and [RenderEditable] for example) with inline content
/// children managed by the [ContainerRenderObjectMixin] mixin.
///
/// This mixin assumes every child managed by the [ContainerRenderObjectMixin]
/// mixin corresponds to a [PlaceholderSpan], and they are organized in logical
/// order of the text (the order each [PlaceholderSpan] is encountered when the
/// user reads the text).
///
/// To use this mixin in a [RenderBox] class:
///
/// * Call [layoutInlineChildren] in the `performLayout` and `computeDryLayout`
/// implementation, and during intrinsic size calculations, to get the size
/// information of the inline widgets as a `List` of `PlaceholderDimensions`.
/// Determine the positioning of the inline widgets (which is usually done by
/// a [TextPainter] using its line break algorithm).
///
/// * Call [positionInlineChildren] with the positioning information of the
/// inline widgets.
///
/// * Implement [RenderBox.applyPaintTransform], optionally with
/// [defaultApplyPaintTransform].
///
/// * Call [paintInlineChildren] in [RenderBox.paint] to paint the inline widgets.
///
/// * Call [hitTestInlineChildren] in [RenderBox.hitTestChildren] to hit test the
/// inline widgets.
///
/// See also:
///
/// * [WidgetSpan.extractFromInlineSpan], a helper function for extracting
/// [WidgetSpan]s from an [InlineSpan] tree.
mixin RenderInlineChildrenContainerDefaults on RenderBox, ContainerRenderObjectMixin<RenderBox, TextParentData> {
@override
void setupParentData(RenderBox child) {
if (child.parentData is! TextParentData) {
child.parentData = TextParentData();
}
}
static PlaceholderDimensions _layoutChild(RenderBox child, double maxWidth, ChildLayouter layoutChild) {
final TextParentData parentData = child.parentData! as TextParentData;
final PlaceholderSpan? span = parentData.span;
assert(span != null);
return span == null
? PlaceholderDimensions.empty
: PlaceholderDimensions(
size: layoutChild(child, BoxConstraints(maxWidth: maxWidth)),
alignment: span.alignment,
baseline: span.baseline,
baselineOffset: switch (span.alignment) {
ui.PlaceholderAlignment.aboveBaseline ||
ui.PlaceholderAlignment.belowBaseline ||
ui.PlaceholderAlignment.bottom ||
ui.PlaceholderAlignment.middle ||
ui.PlaceholderAlignment.top => null,
ui.PlaceholderAlignment.baseline => child.getDistanceToBaseline(span.baseline!),
},
);
}
/// Computes the layout for every inline child using the given `layoutChild`
/// function and the `maxWidth` constraint.
///
/// Returns a list of [PlaceholderDimensions], representing the layout results
/// for each child managed by the [ContainerRenderObjectMixin] mixin.
///
/// Since this method does not impose a maximum height constraint on the
/// inline children, some children may become taller than this [RenderBox].
///
/// See also:
///
/// * [TextPainter.setPlaceholderDimensions], the method that usually takes
/// the layout results from this method as the input.
@protected
List<PlaceholderDimensions> layoutInlineChildren(double maxWidth, ChildLayouter layoutChild) {
return <PlaceholderDimensions>[
for (RenderBox? child = firstChild; child != null; child = childAfter(child))
_layoutChild(child, maxWidth, layoutChild),
];
}
/// Positions each inline child according to the coordinates provided in the
/// `boxes` list.
///
/// The `boxes` list must be in logical order, which is the order each child
/// is encountered when the user reads the text. Usually the length of the
/// list equals [childCount], but it can be less than that, when some children
/// are omitted due to ellipsing. It never exceeds [childCount].
///
/// See also:
///
/// * [TextPainter.inlinePlaceholderBoxes], the method that can be used to
/// get the input `boxes`.
@protected
void positionInlineChildren(List<ui.TextBox> boxes) {
RenderBox? child = firstChild;
for (final ui.TextBox box in boxes) {
if (child == null) {
assert(false, 'The length of boxes (${boxes.length}) should be greater than childCount ($childCount)');
return;
}
final TextParentData textParentData = child.parentData! as TextParentData;
textParentData._offset = Offset(box.left, box.top);
child = childAfter(child);
}
while (child != null) {
final TextParentData textParentData = child.parentData! as TextParentData;
textParentData._offset = null;
child = childAfter(child);
}
}
/// Applies the transform that would be applied when painting the given child
/// to the given matrix.
///
/// Render children whose [TextParentData.offset] is null zeros out the
/// `transform` to indicate they're invisible thus should not be painted.
@protected
void defaultApplyPaintTransform(RenderBox child, Matrix4 transform) {
final TextParentData childParentData = child.parentData! as TextParentData;
final Offset? offset = childParentData.offset;
if (offset == null) {
transform.setZero();
} else {
transform.translate(offset.dx, offset.dy);
}
}
/// Paints each inline child.
///
/// Render children whose [TextParentData.offset] is null will be skipped by
/// this method.
@protected
void paintInlineChildren(PaintingContext context, Offset offset) {
RenderBox? child = firstChild;
while (child != null) {
final TextParentData childParentData = child.parentData! as TextParentData;
final Offset? childOffset = childParentData.offset;
if (childOffset == null) {
return;
}
context.paintChild(child, childOffset + offset);
child = childAfter(child);
}
}
/// Performs a hit test on each inline child.
///
/// Render children whose [TextParentData.offset] is null will be skipped by
/// this method.
@protected
bool hitTestInlineChildren(BoxHitTestResult result, Offset position) {
RenderBox? child = firstChild;
while (child != null) {
final TextParentData childParentData = child.parentData! as TextParentData;
final Offset? childOffset = childParentData.offset;
if (childOffset == null) {
return false;
}
final bool isHit = result.addWithPaintOffset(
offset: childOffset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) => child!.hitTest(result, position: transformed),
);
if (isHit) {
return true;
}
child = childAfter(child);
}
return false;
}
}
/// A render object that displays a paragraph of text.
class RenderParagraph extends RenderBox with ContainerRenderObjectMixin<RenderBox, TextParentData>, RenderInlineChildrenContainerDefaults, RelayoutWhenSystemFontsChangeMixin {
/// Creates a paragraph render object.
///
/// The [maxLines] property may be null (and indeed defaults to null), but if
/// it is not null, it must be greater than zero.
RenderParagraph(InlineSpan text, {
TextAlign textAlign = TextAlign.start,
required TextDirection textDirection,
bool softWrap = true,
TextOverflow overflow = TextOverflow.clip,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double textScaleFactor = 1.0,
TextScaler textScaler = TextScaler.noScaling,
int? maxLines,
Locale? locale,
StrutStyle? strutStyle,
TextWidthBasis textWidthBasis = TextWidthBasis.parent,
ui.TextHeightBehavior? textHeightBehavior,
List<RenderBox>? children,
Color? selectionColor,
SelectionRegistrar? registrar,
}) : assert(text.debugAssertIsValid()),
assert(maxLines == null || maxLines > 0),
assert(
identical(textScaler, TextScaler.noScaling) || textScaleFactor == 1.0,
'textScaleFactor is deprecated and cannot be specified when textScaler is specified.',
),
_softWrap = softWrap,
_overflow = overflow,
_selectionColor = selectionColor,
_textPainter = TextPainter(
text: text,
textAlign: textAlign,
textDirection: textDirection,
textScaler: textScaler == TextScaler.noScaling ? TextScaler.linear(textScaleFactor) : textScaler,
maxLines: maxLines,
ellipsis: overflow == TextOverflow.ellipsis ? _kEllipsis : null,
locale: locale,
strutStyle: strutStyle,
textWidthBasis: textWidthBasis,
textHeightBehavior: textHeightBehavior,
) {
addAll(children);
this.registrar = registrar;
}
static final String _placeholderCharacter = String.fromCharCode(PlaceholderSpan.placeholderCodeUnit);
final TextPainter _textPainter;
// Currently, computing min/max intrinsic width/height will destroy state
// inside the painter. Instead of calling _layout again to get back the correct
// state, use a separate TextPainter for intrinsics calculation.
//
// TODO(abarth): Make computing the min/max intrinsic width/height a
// non-destructive operation.
TextPainter? _textIntrinsicsCache;
TextPainter get _textIntrinsics {
return (_textIntrinsicsCache ??= TextPainter())
..text = _textPainter.text
..textAlign = _textPainter.textAlign
..textDirection = _textPainter.textDirection
..textScaler = _textPainter.textScaler
..maxLines = _textPainter.maxLines
..ellipsis = _textPainter.ellipsis
..locale = _textPainter.locale
..strutStyle = _textPainter.strutStyle
..textWidthBasis = _textPainter.textWidthBasis
..textHeightBehavior = _textPainter.textHeightBehavior;
}
List<AttributedString>? _cachedAttributedLabels;
List<InlineSpanSemanticsInformation>? _cachedCombinedSemanticsInfos;
/// The text to display.
InlineSpan get text => _textPainter.text!;
set text(InlineSpan value) {
switch (_textPainter.text!.compareTo(value)) {
case RenderComparison.identical:
return;
case RenderComparison.metadata:
_textPainter.text = value;
_cachedCombinedSemanticsInfos = null;
markNeedsSemanticsUpdate();
case RenderComparison.paint:
_textPainter.text = value;
_cachedAttributedLabels = null;
_canComputeIntrinsicsCached = null;
_cachedCombinedSemanticsInfos = null;
markNeedsPaint();
markNeedsSemanticsUpdate();
case RenderComparison.layout:
_textPainter.text = value;
_overflowShader = null;
_cachedAttributedLabels = null;
_cachedCombinedSemanticsInfos = null;
_canComputeIntrinsicsCached = null;
markNeedsLayout();
_removeSelectionRegistrarSubscription();
_disposeSelectableFragments();
_updateSelectionRegistrarSubscription();
}
}
/// The ongoing selections in this paragraph.
///
/// The selection does not include selections in [PlaceholderSpan] if there
/// are any.
@visibleForTesting
List<TextSelection> get selections {
if (_lastSelectableFragments == null) {
return const <TextSelection>[];
}
final List<TextSelection> results = <TextSelection>[];
for (final _SelectableFragment fragment in _lastSelectableFragments!) {
if (fragment._textSelectionStart != null &&
fragment._textSelectionEnd != null) {
results.add(
TextSelection(
baseOffset: fragment._textSelectionStart!.offset,
extentOffset: fragment._textSelectionEnd!.offset
)
);
}
}
return results;
}
// Should be null if selection is not enabled, i.e. _registrar = null. The
// paragraph splits on [PlaceholderSpan.placeholderCodeUnit], and stores each
// fragment in this list.
List<_SelectableFragment>? _lastSelectableFragments;
/// The [SelectionRegistrar] this paragraph will be, or is, registered to.
SelectionRegistrar? get registrar => _registrar;
SelectionRegistrar? _registrar;
set registrar(SelectionRegistrar? value) {
if (value == _registrar) {
return;
}
_removeSelectionRegistrarSubscription();
_disposeSelectableFragments();
_registrar = value;
_updateSelectionRegistrarSubscription();
}
void _updateSelectionRegistrarSubscription() {
if (_registrar == null) {
return;
}
_lastSelectableFragments ??= _getSelectableFragments();
_lastSelectableFragments!.forEach(_registrar!.add);
if (_lastSelectableFragments!.isNotEmpty) {
markNeedsCompositingBitsUpdate();
}
}
void _removeSelectionRegistrarSubscription() {
if (_registrar == null || _lastSelectableFragments == null) {
return;
}
_lastSelectableFragments!.forEach(_registrar!.remove);
}
List<_SelectableFragment> _getSelectableFragments() {
final String plainText = text.toPlainText(includeSemanticsLabels: false);
final List<_SelectableFragment> result = <_SelectableFragment>[];
int start = 0;
while (start < plainText.length) {
int end = plainText.indexOf(_placeholderCharacter, start);
if (start != end) {
if (end == -1) {
end = plainText.length;
}
result.add(
_SelectableFragment(
paragraph: this,
range: TextRange(start: start, end: end),
fullText: plainText,
),
);
start = end;
}
start += 1;
}
return result;
}
void _disposeSelectableFragments() {
if (_lastSelectableFragments == null) {
return;
}
for (final _SelectableFragment fragment in _lastSelectableFragments!) {
fragment.dispose();
}
_lastSelectableFragments = null;
}
@override
bool get alwaysNeedsCompositing => _lastSelectableFragments?.isNotEmpty ?? false;
@override
void markNeedsLayout() {
_lastSelectableFragments?.forEach((_SelectableFragment element) => element.didChangeParagraphLayout());
super.markNeedsLayout();
}
@override
void dispose() {
_removeSelectionRegistrarSubscription();
_disposeSelectableFragments();
_textPainter.dispose();
_textIntrinsicsCache?.dispose();
super.dispose();
}
/// How the text should be aligned horizontally.
TextAlign get textAlign => _textPainter.textAlign;
set textAlign(TextAlign value) {
if (_textPainter.textAlign == value) {
return;
}
_textPainter.textAlign = value;
markNeedsPaint();
}
/// The directionality of the text.
///
/// This decides how the [TextAlign.start], [TextAlign.end], and
/// [TextAlign.justify] values of [textAlign] are interpreted.
///
/// This is also used to disambiguate how to render bidirectional text. For
/// example, if the [text] is an English phrase followed by a Hebrew phrase,
/// in a [TextDirection.ltr] context the English phrase will be on the left
/// and the Hebrew phrase to its right, while in a [TextDirection.rtl]
/// context, the English phrase will be on the right and the Hebrew phrase on
/// its left.
TextDirection get textDirection => _textPainter.textDirection!;
set textDirection(TextDirection value) {
if (_textPainter.textDirection == value) {
return;
}
_textPainter.textDirection = value;
markNeedsLayout();
}
/// Whether the text should break at soft line breaks.
///
/// If false, the glyphs in the text will be positioned as if there was
/// unlimited horizontal space.
///
/// If [softWrap] is false, [overflow] and [textAlign] may have unexpected
/// effects.
bool get softWrap => _softWrap;
bool _softWrap;
set softWrap(bool value) {
if (_softWrap == value) {
return;
}
_softWrap = value;
markNeedsLayout();
}
/// How visual overflow should be handled.
TextOverflow get overflow => _overflow;
TextOverflow _overflow;
set overflow(TextOverflow value) {
if (_overflow == value) {
return;
}
_overflow = value;
_textPainter.ellipsis = value == TextOverflow.ellipsis ? _kEllipsis : null;
markNeedsLayout();
}
/// Deprecated. Will be removed in a future version of Flutter. Use
/// [textScaler] instead.
///
/// The number of font pixels for each logical pixel.
///
/// For example, if the text scale factor is 1.5, text will be 50% larger than
/// the specified font size.
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double get textScaleFactor => _textPainter.textScaleFactor;
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
set textScaleFactor(double value) {
textScaler = TextScaler.linear(value);
}
/// {@macro flutter.painting.textPainter.textScaler}
TextScaler get textScaler => _textPainter.textScaler;
set textScaler(TextScaler value) {
if (_textPainter.textScaler == value) {
return;
}
_textPainter.textScaler = value;
_overflowShader = null;
markNeedsLayout();
}
/// An optional maximum number of lines for the text to span, wrapping if
/// necessary. If the text exceeds the given number of lines, it will be
/// truncated according to [overflow] and [softWrap].
int? get maxLines => _textPainter.maxLines;
/// The value may be null. If it is not null, then it must be greater than
/// zero.
set maxLines(int? value) {
assert(value == null || value > 0);
if (_textPainter.maxLines == value) {
return;
}
_textPainter.maxLines = value;
_overflowShader = null;
markNeedsLayout();
}
/// Used by this paragraph's internal [TextPainter] to select a
/// locale-specific font.
///
/// In some cases, the same Unicode character may be rendered differently
/// depending on the locale. For example, the '骨' character is rendered
/// differently in the Chinese and Japanese locales. In these cases, the
/// [locale] may be used to select a locale-specific font.
Locale? get locale => _textPainter.locale;
/// The value may be null.
set locale(Locale? value) {
if (_textPainter.locale == value) {
return;
}
_textPainter.locale = value;
_overflowShader = null;
markNeedsLayout();
}
/// {@macro flutter.painting.textPainter.strutStyle}
StrutStyle? get strutStyle => _textPainter.strutStyle;
/// The value may be null.
set strutStyle(StrutStyle? value) {
if (_textPainter.strutStyle == value) {
return;
}
_textPainter.strutStyle = value;
_overflowShader = null;
markNeedsLayout();
}
/// {@macro flutter.painting.textPainter.textWidthBasis}
TextWidthBasis get textWidthBasis => _textPainter.textWidthBasis;
set textWidthBasis(TextWidthBasis value) {
if (_textPainter.textWidthBasis == value) {
return;
}
_textPainter.textWidthBasis = value;
_overflowShader = null;
markNeedsLayout();
}
/// {@macro dart.ui.textHeightBehavior}
ui.TextHeightBehavior? get textHeightBehavior => _textPainter.textHeightBehavior;
set textHeightBehavior(ui.TextHeightBehavior? value) {
if (_textPainter.textHeightBehavior == value) {
return;
}
_textPainter.textHeightBehavior = value;
_overflowShader = null;
markNeedsLayout();
}
/// The color to use when painting the selection.
///
/// Ignored if the text is not selectable (e.g. if [registrar] is null).
Color? get selectionColor => _selectionColor;
Color? _selectionColor;
set selectionColor(Color? value) {
if (_selectionColor == value) {
return;
}
_selectionColor = value;
if (_lastSelectableFragments?.any((_SelectableFragment fragment) => fragment.value.hasSelection) ?? false) {
markNeedsPaint();
}
}
Offset _getOffsetForPosition(TextPosition position) {
return getOffsetForCaret(position, Rect.zero) + Offset(0, getFullHeightForCaret(position) ?? 0.0);
}
@override
double computeMinIntrinsicWidth(double height) {
if (!_canComputeIntrinsics()) {
return 0.0;
}
final List<PlaceholderDimensions> placeholderDimensions = layoutInlineChildren(
double.infinity,
(RenderBox child, BoxConstraints constraints) => Size(child.getMinIntrinsicWidth(double.infinity), 0.0),
);
return (_textIntrinsics..setPlaceholderDimensions(placeholderDimensions)..layout())
.minIntrinsicWidth;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (!_canComputeIntrinsics()) {
return 0.0;
}
final List<PlaceholderDimensions> placeholderDimensions = layoutInlineChildren(
double.infinity,
// Height and baseline is irrelevant as all text will be laid
// out in a single line. Therefore, using 0.0 as a dummy for the height.
(RenderBox child, BoxConstraints constraints) => Size(child.getMaxIntrinsicWidth(double.infinity), 0.0),
);
return (_textIntrinsics..setPlaceholderDimensions(placeholderDimensions)..layout())
.maxIntrinsicWidth;
}
double _computeIntrinsicHeight(double width) {
if (!_canComputeIntrinsics()) {
return 0.0;
}
return (_textIntrinsics
..setPlaceholderDimensions(layoutInlineChildren(width, ChildLayoutHelper.dryLayoutChild))
..layout(minWidth: width, maxWidth: _adjustMaxWidth(width)))
.height;
}
@override
double computeMinIntrinsicHeight(double width) {
return _computeIntrinsicHeight(width);
}
@override
double computeMaxIntrinsicHeight(double width) {
return _computeIntrinsicHeight(width);
}
@override
double computeDistanceToActualBaseline(TextBaseline baseline) {
assert(!debugNeedsLayout);
assert(constraints.debugAssertIsValid());
_layoutTextWithConstraints(constraints);
// TODO(garyq): Since our metric for ideographic baseline is currently
// inaccurate and the non-alphabetic baselines are based off of the
// alphabetic baseline, we use the alphabetic for now to produce correct
// layouts. We should eventually change this back to pass the `baseline`
// property when the ideographic baseline is properly implemented
// (https://github.com/flutter/flutter/issues/22625).
return _textPainter.computeDistanceToActualBaseline(TextBaseline.alphabetic);
}
/// Whether all inline widget children of this [RenderBox] support dry layout
/// calculation.
bool _canComputeDryLayoutForInlineWidgets() {
// Dry layout cannot be calculated without a full layout for
// alignments that require the baseline (baseline, aboveBaseline,
// belowBaseline).
return text.visitChildren((InlineSpan span) {
return (span is! PlaceholderSpan) || switch (span.alignment) {
ui.PlaceholderAlignment.baseline ||
ui.PlaceholderAlignment.aboveBaseline ||
ui.PlaceholderAlignment.belowBaseline => false,
ui.PlaceholderAlignment.top ||
ui.PlaceholderAlignment.middle ||
ui.PlaceholderAlignment.bottom => true,
};
});
}
bool? _canComputeIntrinsicsCached;
// Intrinsics cannot be calculated without a full layout for
// alignments that require the baseline (baseline, aboveBaseline,
// belowBaseline).
bool _canComputeIntrinsics() {
final bool returnValue = _canComputeIntrinsicsCached ??= _canComputeDryLayoutForInlineWidgets();
assert(
returnValue || RenderObject.debugCheckingIntrinsics,
'Intrinsics are not available for PlaceholderAlignment.baseline, '
'PlaceholderAlignment.aboveBaseline, or PlaceholderAlignment.belowBaseline.',
);
return returnValue;
}
@override
bool hitTestSelf(Offset position) => true;
@override
@protected
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final GlyphInfo? glyph = _textPainter.getClosestGlyphForOffset(position);
// The hit-test can't fall through the horizontal gaps between visually
// adjacent characters on the same line, even with a large letter-spacing or
// text justification, as graphemeClusterLayoutBounds.width is the advance
// width to the next character, so there's no gap between their
// graphemeClusterLayoutBounds rects.
final InlineSpan? spanHit = glyph != null && glyph.graphemeClusterLayoutBounds.contains(position)
? _textPainter.text!.getSpanForPosition(TextPosition(offset: glyph.graphemeClusterCodeUnitRange.start))
: null;
switch (spanHit) {
case final HitTestTarget span:
result.add(HitTestEntry(span));
return true;
case _:
return hitTestInlineChildren(result, position);
}
}
bool _needsClipping = false;
ui.Shader? _overflowShader;
/// Whether this paragraph currently has a [dart:ui.Shader] for its overflow
/// effect.
///
/// Used to test this object. Not for use in production.
@visibleForTesting
bool get debugHasOverflowShader => _overflowShader != null;
@override
void systemFontsDidChange() {
super.systemFontsDidChange();
_textPainter.markNeedsLayout();
}
// Placeholder dimensions representing the sizes of child inline widgets.
//
// These need to be cached because the text painter's placeholder dimensions
// will be overwritten during intrinsic width/height calculations and must be
// restored to the original values before final layout and painting.
List<PlaceholderDimensions>? _placeholderDimensions;
double _adjustMaxWidth(double maxWidth) {
return softWrap || overflow == TextOverflow.ellipsis ? maxWidth : double.infinity;
}
void _layoutTextWithConstraints(BoxConstraints constraints) {
_textPainter
..setPlaceholderDimensions(_placeholderDimensions)
..layout(minWidth: constraints.minWidth, maxWidth: _adjustMaxWidth(constraints.maxWidth));
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (!_canComputeIntrinsics()) {
assert(debugCannotComputeDryLayout(
reason: 'Dry layout not available for alignments that require baseline.',
));
return Size.zero;
}
final Size size = (_textIntrinsics
..setPlaceholderDimensions(layoutInlineChildren(constraints.maxWidth, ChildLayoutHelper.dryLayoutChild))
..layout(minWidth: constraints.minWidth, maxWidth: _adjustMaxWidth(constraints.maxWidth)))
.size;
return constraints.constrain(size);
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
_placeholderDimensions = layoutInlineChildren(constraints.maxWidth, ChildLayoutHelper.layoutChild);
_layoutTextWithConstraints(constraints);
positionInlineChildren(_textPainter.inlinePlaceholderBoxes!);
// We grab _textPainter.size and _textPainter.didExceedMaxLines here because
// assigning to `size` will trigger us to validate our intrinsic sizes,
// which will change _textPainter's layout because the intrinsic size
// calculations are destructive. Other _textPainter state will also be
// affected. See also RenderEditable which has a similar issue.
final Size textSize = _textPainter.size;
final bool textDidExceedMaxLines = _textPainter.didExceedMaxLines;
size = constraints.constrain(textSize);
final bool didOverflowHeight = size.height < textSize.height || textDidExceedMaxLines;
final bool didOverflowWidth = size.width < textSize.width;
// TODO(abarth): We're only measuring the sizes of the line boxes here. If
// the glyphs draw outside the line boxes, we might think that there isn't
// visual overflow when there actually is visual overflow. This can become
// a problem if we start having horizontal overflow and introduce a clip
// that affects the actual (but undetected) vertical overflow.
final bool hasVisualOverflow = didOverflowWidth || didOverflowHeight;
if (hasVisualOverflow) {
switch (_overflow) {
case TextOverflow.visible:
_needsClipping = false;
_overflowShader = null;
case TextOverflow.clip:
case TextOverflow.ellipsis:
_needsClipping = true;
_overflowShader = null;
case TextOverflow.fade:
_needsClipping = true;
final TextPainter fadeSizePainter = TextPainter(
text: TextSpan(style: _textPainter.text!.style, text: '\u2026'),
textDirection: textDirection,
textScaler: textScaler,
locale: locale,
)..layout();
if (didOverflowWidth) {
final (double fadeStart, double fadeEnd) = switch (textDirection) {
TextDirection.rtl => (fadeSizePainter.width, 0.0),
TextDirection.ltr => (size.width - fadeSizePainter.width, size.width),
};
_overflowShader = ui.Gradient.linear(
Offset(fadeStart, 0.0),
Offset(fadeEnd, 0.0),
<Color>[const Color(0xFFFFFFFF), const Color(0x00FFFFFF)],
);
} else {
final double fadeEnd = size.height;
final double fadeStart = fadeEnd - fadeSizePainter.height / 2.0;
_overflowShader = ui.Gradient.linear(
Offset(0.0, fadeStart),
Offset(0.0, fadeEnd),
<Color>[const Color(0xFFFFFFFF), const Color(0x00FFFFFF)],
);
}
fadeSizePainter.dispose();
}
} else {
_needsClipping = false;
_overflowShader = null;
}
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
defaultApplyPaintTransform(child, transform);
}
@override
void paint(PaintingContext context, Offset offset) {
// Text alignment only triggers repaint so it's possible the text layout has
// been invalidated but performLayout wasn't called at this point. Make sure
// the TextPainter has a valid layout.
_layoutTextWithConstraints(constraints);
assert(() {
if (debugRepaintTextRainbowEnabled) {
final Paint paint = Paint()
..color = debugCurrentRepaintColor.toColor();
context.canvas.drawRect(offset & size, paint);
}
return true;
}());
if (_needsClipping) {
final Rect bounds = offset & size;
if (_overflowShader != null) {
// This layer limits what the shader below blends with to be just the
// text (as opposed to the text and its background).
context.canvas.saveLayer(bounds, Paint());
} else {
context.canvas.save();
}
context.canvas.clipRect(bounds);
}
if (_lastSelectableFragments != null) {
for (final _SelectableFragment fragment in _lastSelectableFragments!) {
fragment.paint(context, offset);
}
}
_textPainter.paint(context.canvas, offset);
paintInlineChildren(context, offset);
if (_needsClipping) {
if (_overflowShader != null) {
context.canvas.translate(offset.dx, offset.dy);
final Paint paint = Paint()
..blendMode = BlendMode.modulate
..shader = _overflowShader;
context.canvas.drawRect(Offset.zero & size, paint);
}
context.canvas.restore();
}
}
/// Returns the offset at which to paint the caret.
///
/// Valid only after [layout].
Offset getOffsetForCaret(TextPosition position, Rect caretPrototype) {
assert(!debugNeedsLayout);
_layoutTextWithConstraints(constraints);
return _textPainter.getOffsetForCaret(position, caretPrototype);
}
/// {@macro flutter.painting.textPainter.getFullHeightForCaret}
///
/// Valid only after [layout].
double? getFullHeightForCaret(TextPosition position) {
assert(!debugNeedsLayout);
_layoutTextWithConstraints(constraints);
return _textPainter.getFullHeightForCaret(position, Rect.zero);
}
/// Returns a list of rects that bound the given selection.
///
/// The [boxHeightStyle] and [boxWidthStyle] arguments may be used to select
/// the shape of the [TextBox]es. These properties default to
/// [ui.BoxHeightStyle.tight] and [ui.BoxWidthStyle.tight] respectively.
///
/// A given selection might have more than one rect if the [RenderParagraph]
/// contains multiple [InlineSpan]s or bidirectional text, because logically
/// contiguous text might not be visually contiguous.
///
/// Valid only after [layout].
///
/// See also:
///
/// * [TextPainter.getBoxesForSelection], the method in TextPainter to get
/// the equivalent boxes.
List<ui.TextBox> getBoxesForSelection(
TextSelection selection, {
ui.BoxHeightStyle boxHeightStyle = ui.BoxHeightStyle.tight,
ui.BoxWidthStyle boxWidthStyle = ui.BoxWidthStyle.tight,
}) {
assert(!debugNeedsLayout);
_layoutTextWithConstraints(constraints);
return _textPainter.getBoxesForSelection(
selection,
boxHeightStyle: boxHeightStyle,
boxWidthStyle: boxWidthStyle,
);
}
/// Returns the position within the text for the given pixel offset.
///
/// Valid only after [layout].
TextPosition getPositionForOffset(Offset offset) {
assert(!debugNeedsLayout);
_layoutTextWithConstraints(constraints);
return _textPainter.getPositionForOffset(offset);
}
/// Returns the text range of the word at the given offset. Characters not
/// part of a word, such as spaces, symbols, and punctuation, have word breaks
/// on both sides. In such cases, this method will return a text range that
/// contains the given text position.
///
/// Word boundaries are defined more precisely in Unicode Standard Annex #29
/// <http://www.unicode.org/reports/tr29/#Word_Boundaries>.
///
/// Valid only after [layout].
TextRange getWordBoundary(TextPosition position) {
assert(!debugNeedsLayout);
_layoutTextWithConstraints(constraints);
return _textPainter.getWordBoundary(position);
}
TextRange _getLineAtOffset(TextPosition position) => _textPainter.getLineBoundary(position);
TextPosition _getTextPositionAbove(TextPosition position) {
// -0.5 of preferredLineHeight points to the middle of the line above.
final double preferredLineHeight = _textPainter.preferredLineHeight;
final double verticalOffset = -0.5 * preferredLineHeight;
return _getTextPositionVertical(position, verticalOffset);
}
TextPosition _getTextPositionBelow(TextPosition position) {
// 1.5 of preferredLineHeight points to the middle of the line below.
final double preferredLineHeight = _textPainter.preferredLineHeight;
final double verticalOffset = 1.5 * preferredLineHeight;
return _getTextPositionVertical(position, verticalOffset);
}
TextPosition _getTextPositionVertical(TextPosition position, double verticalOffset) {
final Offset caretOffset = _textPainter.getOffsetForCaret(position, Rect.zero);
final Offset caretOffsetTranslated = caretOffset.translate(0.0, verticalOffset);
return _textPainter.getPositionForOffset(caretOffsetTranslated);
}
/// Returns the size of the text as laid out.
///
/// This can differ from [size] if the text overflowed or if the [constraints]
/// provided by the parent [RenderObject] forced the layout to be bigger than
/// necessary for the given [text].
///
/// This returns the [TextPainter.size] of the underlying [TextPainter].
///
/// Valid only after [layout].
Size get textSize {
assert(!debugNeedsLayout);
return _textPainter.size;
}
/// Whether the text was truncated or ellipsized as laid out.
///
/// This returns the [TextPainter.didExceedMaxLines] of the underlying [TextPainter].
///
/// Valid only after [layout].
bool get didExceedMaxLines {
assert(!debugNeedsLayout);
return _textPainter.didExceedMaxLines;
}
/// Collected during [describeSemanticsConfiguration], used by
/// [assembleSemanticsNode] and [_combineSemanticsInfo].
List<InlineSpanSemanticsInformation>? _semanticsInfo;
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
_semanticsInfo = text.getSemanticsInformation();
bool needsAssembleSemanticsNode = false;
bool needsChildConfigurationsDelegate = false;
for (final InlineSpanSemanticsInformation info in _semanticsInfo!) {
if (info.recognizer != null) {
needsAssembleSemanticsNode = true;
break;
}
needsChildConfigurationsDelegate = needsChildConfigurationsDelegate || info.isPlaceholder;
}
if (needsAssembleSemanticsNode) {
config.explicitChildNodes = true;
config.isSemanticBoundary = true;
} else if (needsChildConfigurationsDelegate) {
config.childConfigurationsDelegate = _childSemanticsConfigurationsDelegate;
} else {
if (_cachedAttributedLabels == null) {
final StringBuffer buffer = StringBuffer();
int offset = 0;
final List<StringAttribute> attributes = <StringAttribute>[];
for (final InlineSpanSemanticsInformation info in _semanticsInfo!) {
final String label = info.semanticsLabel ?? info.text;
for (final StringAttribute infoAttribute in info.stringAttributes) {
final TextRange originalRange = infoAttribute.range;
attributes.add(
infoAttribute.copy(
range: TextRange(
start: offset + originalRange.start,
end: offset + originalRange.end,
),
),
);
}
buffer.write(label);
offset += label.length;
}
_cachedAttributedLabels = <AttributedString>[AttributedString(buffer.toString(), attributes: attributes)];
}
config.attributedLabel = _cachedAttributedLabels![0];
config.textDirection = textDirection;
}
}
ChildSemanticsConfigurationsResult _childSemanticsConfigurationsDelegate(List<SemanticsConfiguration> childConfigs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
int placeholderIndex = 0;
int childConfigsIndex = 0;
int attributedLabelCacheIndex = 0;
InlineSpanSemanticsInformation? seenTextInfo;
_cachedCombinedSemanticsInfos ??= combineSemanticsInfo(_semanticsInfo!);
for (final InlineSpanSemanticsInformation info in _cachedCombinedSemanticsInfos!) {
if (info.isPlaceholder) {
if (seenTextInfo != null) {
builder.markAsMergeUp(_createSemanticsConfigForTextInfo(seenTextInfo, attributedLabelCacheIndex));
attributedLabelCacheIndex += 1;
}
// Mark every childConfig belongs to this placeholder to merge up group.
while (childConfigsIndex < childConfigs.length &&
childConfigs[childConfigsIndex].tagsChildrenWith(PlaceholderSpanIndexSemanticsTag(placeholderIndex))) {
builder.markAsMergeUp(childConfigs[childConfigsIndex]);
childConfigsIndex += 1;
}
placeholderIndex += 1;
} else {
seenTextInfo = info;
}
}
// Handle plain text info at the end.
if (seenTextInfo != null) {
builder.markAsMergeUp(_createSemanticsConfigForTextInfo(seenTextInfo, attributedLabelCacheIndex));
}
return builder.build();
}
SemanticsConfiguration _createSemanticsConfigForTextInfo(InlineSpanSemanticsInformation textInfo, int cacheIndex) {
assert(!textInfo.requiresOwnNode);
final List<AttributedString> cachedStrings = _cachedAttributedLabels ??= <AttributedString>[];
assert(cacheIndex <= cachedStrings.length);
final bool hasCache = cacheIndex < cachedStrings.length;
late AttributedString attributedLabel;
if (hasCache) {
attributedLabel = cachedStrings[cacheIndex];
} else {
assert(cachedStrings.length == cacheIndex);
attributedLabel = AttributedString(
textInfo.semanticsLabel ?? textInfo.text,
attributes: textInfo.stringAttributes,
);
cachedStrings.add(attributedLabel);
}
return SemanticsConfiguration()
..textDirection = textDirection
..attributedLabel = attributedLabel;
}
// Caches [SemanticsNode]s created during [assembleSemanticsNode] so they
// can be re-used when [assembleSemanticsNode] is called again. This ensures
// stable ids for the [SemanticsNode]s of [TextSpan]s across
// [assembleSemanticsNode] invocations.
LinkedHashMap<Key, SemanticsNode>? _cachedChildNodes;
@override
void assembleSemanticsNode(SemanticsNode node, SemanticsConfiguration config, Iterable<SemanticsNode> children) {
assert(_semanticsInfo != null && _semanticsInfo!.isNotEmpty);
final List<SemanticsNode> newChildren = <SemanticsNode>[];
TextDirection currentDirection = textDirection;
Rect currentRect;
double ordinal = 0.0;
int start = 0;
int placeholderIndex = 0;
int childIndex = 0;
RenderBox? child = firstChild;
final LinkedHashMap<Key, SemanticsNode> newChildCache = LinkedHashMap<Key, SemanticsNode>();
_cachedCombinedSemanticsInfos ??= combineSemanticsInfo(_semanticsInfo!);
for (final InlineSpanSemanticsInformation info in _cachedCombinedSemanticsInfos!) {
final TextSelection selection = TextSelection(
baseOffset: start,
extentOffset: start + info.text.length,
);
start += info.text.length;
if (info.isPlaceholder) {
// A placeholder span may have 0 to multiple semantics nodes, we need
// to annotate all of the semantics nodes belong to this span.
while (children.length > childIndex &&
children.elementAt(childIndex).isTagged(PlaceholderSpanIndexSemanticsTag(placeholderIndex))) {
final SemanticsNode childNode = children.elementAt(childIndex);
final TextParentData parentData = child!.parentData! as TextParentData;
// parentData.scale may be null if the render object is truncated.
if (parentData.offset != null) {
newChildren.add(childNode);
}
childIndex += 1;
}
child = childAfter(child!);
placeholderIndex += 1;
} else {
final TextDirection initialDirection = currentDirection;
final List<ui.TextBox> rects = getBoxesForSelection(selection);
if (rects.isEmpty) {
continue;
}
Rect rect = rects.first.toRect();
currentDirection = rects.first.direction;
for (final ui.TextBox textBox in rects.skip(1)) {
rect = rect.expandToInclude(textBox.toRect());
currentDirection = textBox.direction;
}
// Any of the text boxes may have had infinite dimensions.
// We shouldn't pass infinite dimensions up to the bridges.
rect = Rect.fromLTWH(
math.max(0.0, rect.left),
math.max(0.0, rect.top),
math.min(rect.width, constraints.maxWidth),
math.min(rect.height, constraints.maxHeight),
);
// round the current rectangle to make this API testable and add some
// padding so that the accessibility rects do not overlap with the text.
currentRect = Rect.fromLTRB(
rect.left.floorToDouble() - 4.0,
rect.top.floorToDouble() - 4.0,
rect.right.ceilToDouble() + 4.0,
rect.bottom.ceilToDouble() + 4.0,
);
final SemanticsConfiguration configuration = SemanticsConfiguration()
..sortKey = OrdinalSortKey(ordinal++)
..textDirection = initialDirection
..attributedLabel = AttributedString(info.semanticsLabel ?? info.text, attributes: info.stringAttributes);
final GestureRecognizer? recognizer = info.recognizer;
if (recognizer != null) {
if (recognizer is TapGestureRecognizer) {
if (recognizer.onTap != null) {
configuration.onTap = recognizer.onTap;
configuration.isLink = true;
}
} else if (recognizer is DoubleTapGestureRecognizer) {
if (recognizer.onDoubleTap != null) {
configuration.onTap = recognizer.onDoubleTap;
configuration.isLink = true;
}
} else if (recognizer is LongPressGestureRecognizer) {
if (recognizer.onLongPress != null) {
configuration.onLongPress = recognizer.onLongPress;
}
} else {
assert(false, '${recognizer.runtimeType} is not supported.');
}
}
if (node.parentPaintClipRect != null) {
final Rect paintRect = node.parentPaintClipRect!.intersect(currentRect);
configuration.isHidden = paintRect.isEmpty && !currentRect.isEmpty;
}
final SemanticsNode newChild;
if (_cachedChildNodes?.isNotEmpty ?? false) {
newChild = _cachedChildNodes!.remove(_cachedChildNodes!.keys.first)!;
} else {
final UniqueKey key = UniqueKey();
newChild = SemanticsNode(
key: key,
showOnScreen: _createShowOnScreenFor(key),
);
}
newChild
..updateWith(config: configuration)
..rect = currentRect;
newChildCache[newChild.key!] = newChild;
newChildren.add(newChild);
}
}
// Makes sure we annotated all of the semantics children.
assert(childIndex == children.length);
assert(child == null);
_cachedChildNodes = newChildCache;
node.updateWith(config: config, childrenInInversePaintOrder: newChildren);
}
VoidCallback? _createShowOnScreenFor(Key key) {
return () {
final SemanticsNode node = _cachedChildNodes![key]!;
showOnScreen(descendant: this, rect: node.rect);
};
}
@override
void clearSemantics() {
super.clearSemantics();
_cachedChildNodes = null;
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return <DiagnosticsNode>[
text.toDiagnosticsNode(
name: 'text',
style: DiagnosticsTreeStyle.transition,
),
];
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<TextAlign>('textAlign', textAlign));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection));
properties.add(
FlagProperty(
'softWrap',
value: softWrap,
ifTrue: 'wrapping at box width',
ifFalse: 'no wrapping except at line break characters',
showName: true,
),
);
properties.add(EnumProperty<TextOverflow>('overflow', overflow));
properties.add(
DiagnosticsProperty<TextScaler>('textScaler', textScaler, defaultValue: TextScaler.noScaling),
);
properties.add(
DiagnosticsProperty<Locale>(
'locale',
locale,
defaultValue: null,
),
);
properties.add(IntProperty('maxLines', maxLines, ifNull: 'unlimited'));
}
}
/// A continuous, selectable piece of paragraph.
///
/// Since the selections in [PlaceholderSpan] are handled independently in its
/// subtree, a selection in [RenderParagraph] can't continue across a
/// [PlaceholderSpan]. The [RenderParagraph] splits itself on [PlaceholderSpan]
/// to create multiple `_SelectableFragment`s so that they can be selected
/// separately.
class _SelectableFragment with Selectable, Diagnosticable, ChangeNotifier implements TextLayoutMetrics {
_SelectableFragment({
required this.paragraph,
required this.fullText,
required this.range,
}) : assert(range.isValid && !range.isCollapsed && range.isNormalized) {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
_selectionGeometry = _getSelectionGeometry();
}
final TextRange range;
final RenderParagraph paragraph;
final String fullText;
TextPosition? _textSelectionStart;
TextPosition? _textSelectionEnd;
bool _selectableContainsOriginWord = false;
LayerLink? _startHandleLayerLink;
LayerLink? _endHandleLayerLink;
@override
SelectionGeometry get value => _selectionGeometry;
late SelectionGeometry _selectionGeometry;
void _updateSelectionGeometry() {
final SelectionGeometry newValue = _getSelectionGeometry();
if (_selectionGeometry == newValue) {
return;
}
_selectionGeometry = newValue;
notifyListeners();
}
SelectionGeometry _getSelectionGeometry() {
if (_textSelectionStart == null || _textSelectionEnd == null) {
return const SelectionGeometry(
status: SelectionStatus.none,
hasContent: true,
);
}
final int selectionStart = _textSelectionStart!.offset;
final int selectionEnd = _textSelectionEnd!.offset;
final bool isReversed = selectionStart > selectionEnd;
final Offset startOffsetInParagraphCoordinates = paragraph._getOffsetForPosition(TextPosition(offset: selectionStart));
final Offset endOffsetInParagraphCoordinates = selectionStart == selectionEnd
? startOffsetInParagraphCoordinates
: paragraph._getOffsetForPosition(TextPosition(offset: selectionEnd));
final bool flipHandles = isReversed != (TextDirection.rtl == paragraph.textDirection);
final TextSelection selection = TextSelection(
baseOffset: selectionStart,
extentOffset: selectionEnd,
);
final List<Rect> selectionRects = <Rect>[];
for (final TextBox textBox in paragraph.getBoxesForSelection(selection)) {
selectionRects.add(textBox.toRect());
}
return SelectionGeometry(
startSelectionPoint: SelectionPoint(
localPosition: startOffsetInParagraphCoordinates,
lineHeight: paragraph._textPainter.preferredLineHeight,
handleType: flipHandles ? TextSelectionHandleType.right : TextSelectionHandleType.left
),
endSelectionPoint: SelectionPoint(
localPosition: endOffsetInParagraphCoordinates,
lineHeight: paragraph._textPainter.preferredLineHeight,
handleType: flipHandles ? TextSelectionHandleType.left : TextSelectionHandleType.right,
),
selectionRects: selectionRects,
status: _textSelectionStart!.offset == _textSelectionEnd!.offset
? SelectionStatus.collapsed
: SelectionStatus.uncollapsed,
hasContent: true,
);
}
@override
SelectionResult dispatchSelectionEvent(SelectionEvent event) {
late final SelectionResult result;
final TextPosition? existingSelectionStart = _textSelectionStart;
final TextPosition? existingSelectionEnd = _textSelectionEnd;
switch (event.type) {
case SelectionEventType.startEdgeUpdate:
case SelectionEventType.endEdgeUpdate:
final SelectionEdgeUpdateEvent edgeUpdate = event as SelectionEdgeUpdateEvent;
final TextGranularity granularity = event.granularity;
switch (granularity) {
case TextGranularity.character:
result = _updateSelectionEdge(edgeUpdate.globalPosition, isEnd: edgeUpdate.type == SelectionEventType.endEdgeUpdate);
case TextGranularity.word:
result = _updateSelectionEdgeByWord(edgeUpdate.globalPosition, isEnd: edgeUpdate.type == SelectionEventType.endEdgeUpdate);
case TextGranularity.document:
case TextGranularity.line:
assert(false, 'Moving the selection edge by line or document is not supported.');
}
case SelectionEventType.clear:
result = _handleClearSelection();
case SelectionEventType.selectAll:
result = _handleSelectAll();
case SelectionEventType.selectWord:
final SelectWordSelectionEvent selectWord = event as SelectWordSelectionEvent;
result = _handleSelectWord(selectWord.globalPosition);
case SelectionEventType.granularlyExtendSelection:
final GranularlyExtendSelectionEvent granularlyExtendSelection = event as GranularlyExtendSelectionEvent;
result = _handleGranularlyExtendSelection(
granularlyExtendSelection.forward,
granularlyExtendSelection.isEnd,
granularlyExtendSelection.granularity,
);
case SelectionEventType.directionallyExtendSelection:
final DirectionallyExtendSelectionEvent directionallyExtendSelection = event as DirectionallyExtendSelectionEvent;
result = _handleDirectionallyExtendSelection(
directionallyExtendSelection.dx,
directionallyExtendSelection.isEnd,
directionallyExtendSelection.direction,
);
}
if (existingSelectionStart != _textSelectionStart ||
existingSelectionEnd != _textSelectionEnd) {
_didChangeSelection();
}
return result;
}
@override
SelectedContent? getSelectedContent() {
if (_textSelectionStart == null || _textSelectionEnd == null) {
return null;
}
final int start = math.min(_textSelectionStart!.offset, _textSelectionEnd!.offset);
final int end = math.max(_textSelectionStart!.offset, _textSelectionEnd!.offset);
return SelectedContent(
plainText: fullText.substring(start, end),
);
}
void _didChangeSelection() {
paragraph.markNeedsPaint();
_updateSelectionGeometry();
}
SelectionResult _updateSelectionEdge(Offset globalPosition, {required bool isEnd}) {
_setSelectionPosition(null, isEnd: isEnd);
final Matrix4 transform = paragraph.getTransformTo(null);
transform.invert();
final Offset localPosition = MatrixUtils.transformPoint(transform, globalPosition);
if (_rect.isEmpty) {
return SelectionUtils.getResultBasedOnRect(_rect, localPosition);
}
final Offset adjustedOffset = SelectionUtils.adjustDragOffset(
_rect,
localPosition,
direction: paragraph.textDirection,
);
final TextPosition position = _clampTextPosition(paragraph.getPositionForOffset(adjustedOffset));
_setSelectionPosition(position, isEnd: isEnd);
if (position.offset == range.end) {
return SelectionResult.next;
}
if (position.offset == range.start) {
return SelectionResult.previous;
}
// TODO(chunhtai): The geometry information should not be used to determine
// selection result. This is a workaround to RenderParagraph, where it does
// not have a way to get accurate text length if its text is truncated due to
// layout constraint.
return SelectionUtils.getResultBasedOnRect(_rect, localPosition);
}
TextPosition _closestWordBoundary(
_WordBoundaryRecord wordBoundary,
TextPosition position,
) {
final int differenceA = (position.offset - wordBoundary.wordStart.offset).abs();
final int differenceB = (position.offset - wordBoundary.wordEnd.offset).abs();
return differenceA < differenceB ? wordBoundary.wordStart : wordBoundary.wordEnd;
}
TextPosition _updateSelectionStartEdgeByWord(
_WordBoundaryRecord? wordBoundary,
TextPosition position,
TextPosition? existingSelectionStart,
TextPosition? existingSelectionEnd,
) {
TextPosition? targetPosition;
if (wordBoundary != null) {
assert(wordBoundary.wordStart.offset >= range.start && wordBoundary.wordEnd.offset <= range.end);
if (_selectableContainsOriginWord && existingSelectionStart != null && existingSelectionEnd != null) {
final bool isSamePosition = position.offset == existingSelectionEnd.offset;
final bool isSelectionInverted = existingSelectionStart.offset > existingSelectionEnd.offset;
final bool shouldSwapEdges = !isSamePosition && (isSelectionInverted != (position.offset > existingSelectionEnd.offset));
if (shouldSwapEdges) {
if (position.offset < existingSelectionEnd.offset) {
targetPosition = wordBoundary.wordStart;
} else {
targetPosition = wordBoundary.wordEnd;
}
// When the selection is inverted by the new position it is necessary to
// swap the start edge (moving edge) with the end edge (static edge) to
// maintain the origin word within the selection.
final _WordBoundaryRecord localWordBoundary = _getWordBoundaryAtPosition(existingSelectionEnd);
assert(localWordBoundary.wordStart.offset >= range.start && localWordBoundary.wordEnd.offset <= range.end);
_setSelectionPosition(existingSelectionEnd.offset == localWordBoundary.wordStart.offset ? localWordBoundary.wordEnd : localWordBoundary.wordStart, isEnd: true);
} else {
if (position.offset < existingSelectionEnd.offset) {
targetPosition = wordBoundary.wordStart;
} else if (position.offset > existingSelectionEnd.offset) {
targetPosition = wordBoundary.wordEnd;
} else {
// Keep the origin word in bounds when position is at the static edge.
targetPosition = existingSelectionStart;
}
}
} else {
if (existingSelectionEnd != null) {
// If the end edge exists and the start edge is being moved, then the
// start edge is moved to encompass the entire word at the new position.
if (position.offset < existingSelectionEnd.offset) {
targetPosition = wordBoundary.wordStart;
} else {
targetPosition = wordBoundary.wordEnd;
}
} else {
// Move the start edge to the closest word boundary.
targetPosition = _closestWordBoundary(wordBoundary, position);
}
}
} else {
// The position is not contained within the current rect. The targetPosition
// will either be at the end or beginning of the current rect. See [SelectionUtils.adjustDragOffset]
// for a more in depth explanation on this adjustment.
if (_selectableContainsOriginWord && existingSelectionStart != null && existingSelectionEnd != null) {
// When the selection is inverted by the new position it is necessary to
// swap the start edge (moving edge) with the end edge (static edge) to
// maintain the origin word within the selection.
final bool isSamePosition = position.offset == existingSelectionEnd.offset;
final bool isSelectionInverted = existingSelectionStart.offset > existingSelectionEnd.offset;
final bool shouldSwapEdges = !isSamePosition && (isSelectionInverted != (position.offset > existingSelectionEnd.offset));
if (shouldSwapEdges) {
final _WordBoundaryRecord localWordBoundary = _getWordBoundaryAtPosition(existingSelectionEnd);
assert(localWordBoundary.wordStart.offset >= range.start && localWordBoundary.wordEnd.offset <= range.end);
_setSelectionPosition(isSelectionInverted ? localWordBoundary.wordEnd : localWordBoundary.wordStart, isEnd: true);
}
}
}
return targetPosition ?? position;
}
TextPosition _updateSelectionEndEdgeByWord(
_WordBoundaryRecord? wordBoundary,
TextPosition position,
TextPosition? existingSelectionStart,
TextPosition? existingSelectionEnd,
) {
TextPosition? targetPosition;
if (wordBoundary != null) {
assert(wordBoundary.wordStart.offset >= range.start && wordBoundary.wordEnd.offset <= range.end);
if (_selectableContainsOriginWord && existingSelectionStart != null && existingSelectionEnd != null) {
final bool isSamePosition = position.offset == existingSelectionStart.offset;
final bool isSelectionInverted = existingSelectionStart.offset > existingSelectionEnd.offset;
final bool shouldSwapEdges = !isSamePosition && (isSelectionInverted != (position.offset < existingSelectionStart.offset));
if (shouldSwapEdges) {
if (position.offset < existingSelectionStart.offset) {
targetPosition = wordBoundary.wordStart;
} else {
targetPosition = wordBoundary.wordEnd;
}
// When the selection is inverted by the new position it is necessary to
// swap the end edge (moving edge) with the start edge (static edge) to
// maintain the origin word within the selection.
final _WordBoundaryRecord localWordBoundary = _getWordBoundaryAtPosition(existingSelectionStart);
assert(localWordBoundary.wordStart.offset >= range.start && localWordBoundary.wordEnd.offset <= range.end);
_setSelectionPosition(existingSelectionStart.offset == localWordBoundary.wordStart.offset ? localWordBoundary.wordEnd : localWordBoundary.wordStart, isEnd: false);
} else {
if (position.offset < existingSelectionStart.offset) {
targetPosition = wordBoundary.wordStart;
} else if (position.offset > existingSelectionStart.offset) {
targetPosition = wordBoundary.wordEnd;
} else {
// Keep the origin word in bounds when position is at the static edge.
targetPosition = existingSelectionEnd;
}
}
} else {
if (existingSelectionStart != null) {
// If the start edge exists and the end edge is being moved, then the
// end edge is moved to encompass the entire word at the new position.
if (position.offset < existingSelectionStart.offset) {
targetPosition = wordBoundary.wordStart;
} else {
targetPosition = wordBoundary.wordEnd;
}
} else {
// Move the end edge to the closest word boundary.
targetPosition = _closestWordBoundary(wordBoundary, position);
}
}
} else {
// The position is not contained within the current rect. The targetPosition
// will either be at the end or beginning of the current rect. See [SelectionUtils.adjustDragOffset]
// for a more in depth explanation on this adjustment.
if (_selectableContainsOriginWord && existingSelectionStart != null && existingSelectionEnd != null) {
// When the selection is inverted by the new position it is necessary to
// swap the end edge (moving edge) with the start edge (static edge) to
// maintain the origin word within the selection.
final bool isSamePosition = position.offset == existingSelectionStart.offset;
final bool isSelectionInverted = existingSelectionStart.offset > existingSelectionEnd.offset;
final bool shouldSwapEdges = isSelectionInverted != (position.offset < existingSelectionStart.offset) || isSamePosition;
if (shouldSwapEdges) {
final _WordBoundaryRecord localWordBoundary = _getWordBoundaryAtPosition(existingSelectionStart);
assert(localWordBoundary.wordStart.offset >= range.start && localWordBoundary.wordEnd.offset <= range.end);
_setSelectionPosition(isSelectionInverted ? localWordBoundary.wordStart : localWordBoundary.wordEnd, isEnd: false);
}
}
}
return targetPosition ?? position;
}
SelectionResult _updateSelectionEdgeByWord(Offset globalPosition, {required bool isEnd}) {
// When the start/end edges are swapped, i.e. the start is after the end, and
// the scrollable synthesizes an event for the opposite edge, this will potentially
// move the opposite edge outside of the origin word boundary and we are unable to recover.
final TextPosition? existingSelectionStart = _textSelectionStart;
final TextPosition? existingSelectionEnd = _textSelectionEnd;
_setSelectionPosition(null, isEnd: isEnd);
final Matrix4 transform = paragraph.getTransformTo(null);
transform.invert();
final Offset localPosition = MatrixUtils.transformPoint(transform, globalPosition);
if (_rect.isEmpty) {
return SelectionUtils.getResultBasedOnRect(_rect, localPosition);
}
final Offset adjustedOffset = SelectionUtils.adjustDragOffset(
_rect,
localPosition,
direction: paragraph.textDirection,
);
final TextPosition position = paragraph.getPositionForOffset(adjustedOffset);
// Check if the original local position is within the rect, if it is not then
// we do not need to look up the word boundary for that position. This is to
// maintain a selectables selection collapsed at 0 when the local position is
// not located inside its rect.
_WordBoundaryRecord? wordBoundary = _rect.contains(localPosition) ? _getWordBoundaryAtPosition(position) : null;
if (wordBoundary != null
&& (wordBoundary.wordStart.offset < range.start && wordBoundary.wordEnd.offset <= range.start
|| wordBoundary.wordStart.offset >= range.end && wordBoundary.wordEnd.offset > range.end)) {
// When the position is located at a placeholder inside of the text, then we may compute
// a word boundary that does not belong to the current selectable fragment. In this case
// we should invalidate the word boundary so that it is not taken into account when
// computing the target position.
wordBoundary = null;
}
final TextPosition targetPosition = _clampTextPosition(isEnd ? _updateSelectionEndEdgeByWord(wordBoundary, position, existingSelectionStart, existingSelectionEnd) : _updateSelectionStartEdgeByWord(wordBoundary, position, existingSelectionStart, existingSelectionEnd));
_setSelectionPosition(targetPosition, isEnd: isEnd);
if (targetPosition.offset == range.end) {
return SelectionResult.next;
}
if (targetPosition.offset == range.start) {
return SelectionResult.previous;
}
// TODO(chunhtai): The geometry information should not be used to determine
// selection result. This is a workaround to RenderParagraph, where it does
// not have a way to get accurate text length if its text is truncated due to
// layout constraint.
return SelectionUtils.getResultBasedOnRect(_rect, localPosition);
}
TextPosition _clampTextPosition(TextPosition position) {
// Affinity of range.end is upstream.
if (position.offset > range.end ||
(position.offset == range.end && position.affinity == TextAffinity.downstream)) {
return TextPosition(offset: range.end, affinity: TextAffinity.upstream);
}
if (position.offset < range.start) {
return TextPosition(offset: range.start);
}
return position;
}
void _setSelectionPosition(TextPosition? position, {required bool isEnd}) {
if (isEnd) {
_textSelectionEnd = position;
} else {
_textSelectionStart = position;
}
}
SelectionResult _handleClearSelection() {
_textSelectionStart = null;
_textSelectionEnd = null;
_selectableContainsOriginWord = false;
return SelectionResult.none;
}
SelectionResult _handleSelectAll() {
_textSelectionStart = TextPosition(offset: range.start);
_textSelectionEnd = TextPosition(offset: range.end, affinity: TextAffinity.upstream);
return SelectionResult.none;
}
SelectionResult _handleSelectWord(Offset globalPosition) {
final TextPosition position = paragraph.getPositionForOffset(paragraph.globalToLocal(globalPosition));
if (_positionIsWithinCurrentSelection(position) && _textSelectionStart != _textSelectionEnd) {
return SelectionResult.end;
}
final _WordBoundaryRecord wordBoundary = _getWordBoundaryAtPosition(position);
// This fragment may not contain the word, decide what direction the target
// fragment is located in. Because fragments are separated by placeholder
// spans, we also check if the beginning or end of the word is touching
// either edge of this fragment.
if (wordBoundary.wordStart.offset < range.start && wordBoundary.wordEnd.offset <= range.start) {
return SelectionResult.previous;
} else if (wordBoundary.wordStart.offset >= range.end && wordBoundary.wordEnd.offset > range.end) {
return SelectionResult.next;
}
// Fragments are separated by placeholder span, the word boundary shouldn't
// expand across fragments.
assert(wordBoundary.wordStart.offset >= range.start && wordBoundary.wordEnd.offset <= range.end);
_textSelectionStart = wordBoundary.wordStart;
_textSelectionEnd = wordBoundary.wordEnd;
_selectableContainsOriginWord = true;
return SelectionResult.end;
}
_WordBoundaryRecord _getWordBoundaryAtPosition(TextPosition position) {
final TextRange word = paragraph.getWordBoundary(position);
assert(word.isNormalized);
late TextPosition start;
late TextPosition end;
if (position.offset > word.end) {
start = end = TextPosition(offset: position.offset);
} else {
start = TextPosition(offset: word.start);
end = TextPosition(offset: word.end, affinity: TextAffinity.upstream);
}
return (wordStart: start, wordEnd: end);
}
SelectionResult _handleDirectionallyExtendSelection(double horizontalBaseline, bool isExtent, SelectionExtendDirection movement) {
final Matrix4 transform = paragraph.getTransformTo(null);
if (transform.invert() == 0.0) {
switch (movement) {
case SelectionExtendDirection.previousLine:
case SelectionExtendDirection.backward:
return SelectionResult.previous;
case SelectionExtendDirection.nextLine:
case SelectionExtendDirection.forward:
return SelectionResult.next;
}
}
final double baselineInParagraphCoordinates = MatrixUtils.transformPoint(transform, Offset(horizontalBaseline, 0)).dx;
assert(!baselineInParagraphCoordinates.isNaN);
final TextPosition newPosition;
final SelectionResult result;
switch (movement) {
case SelectionExtendDirection.previousLine:
case SelectionExtendDirection.nextLine:
assert(_textSelectionEnd != null && _textSelectionStart != null);
final TextPosition targetedEdge = isExtent ? _textSelectionEnd! : _textSelectionStart!;
final MapEntry<TextPosition, SelectionResult> moveResult = _handleVerticalMovement(
targetedEdge,
horizontalBaselineInParagraphCoordinates: baselineInParagraphCoordinates,
below: movement == SelectionExtendDirection.nextLine,
);
newPosition = moveResult.key;
result = moveResult.value;
case SelectionExtendDirection.forward:
case SelectionExtendDirection.backward:
_textSelectionEnd ??= movement == SelectionExtendDirection.forward
? TextPosition(offset: range.start)
: TextPosition(offset: range.end, affinity: TextAffinity.upstream);
_textSelectionStart ??= _textSelectionEnd;
final TextPosition targetedEdge = isExtent ? _textSelectionEnd! : _textSelectionStart!;
final Offset edgeOffsetInParagraphCoordinates = paragraph._getOffsetForPosition(targetedEdge);
final Offset baselineOffsetInParagraphCoordinates = Offset(
baselineInParagraphCoordinates,
// Use half of line height to point to the middle of the line.
edgeOffsetInParagraphCoordinates.dy - paragraph._textPainter.preferredLineHeight / 2,
);
newPosition = paragraph.getPositionForOffset(baselineOffsetInParagraphCoordinates);
result = SelectionResult.end;
}
if (isExtent) {
_textSelectionEnd = newPosition;
} else {
_textSelectionStart = newPosition;
}
return result;
}
SelectionResult _handleGranularlyExtendSelection(bool forward, bool isExtent, TextGranularity granularity) {
_textSelectionEnd ??= forward
? TextPosition(offset: range.start)
: TextPosition(offset: range.end, affinity: TextAffinity.upstream);
_textSelectionStart ??= _textSelectionEnd;
final TextPosition targetedEdge = isExtent ? _textSelectionEnd! : _textSelectionStart!;
if (forward && (targetedEdge.offset == range.end)) {
return SelectionResult.next;
}
if (!forward && (targetedEdge.offset == range.start)) {
return SelectionResult.previous;
}
final SelectionResult result;
final TextPosition newPosition;
switch (granularity) {
case TextGranularity.character:
final String text = range.textInside(fullText);
newPosition = _moveBeyondTextBoundaryAtDirection(targetedEdge, forward, CharacterBoundary(text));
result = SelectionResult.end;
case TextGranularity.word:
final TextBoundary textBoundary = paragraph._textPainter.wordBoundaries.moveByWordBoundary;
newPosition = _moveBeyondTextBoundaryAtDirection(targetedEdge, forward, textBoundary);
result = SelectionResult.end;
case TextGranularity.line:
newPosition = _moveToTextBoundaryAtDirection(targetedEdge, forward, LineBoundary(this));
result = SelectionResult.end;
case TextGranularity.document:
final String text = range.textInside(fullText);
newPosition = _moveBeyondTextBoundaryAtDirection(targetedEdge, forward, DocumentBoundary(text));
if (forward && newPosition.offset == range.end) {
result = SelectionResult.next;
} else if (!forward && newPosition.offset == range.start) {
result = SelectionResult.previous;
} else {
result = SelectionResult.end;
}
}
if (isExtent) {
_textSelectionEnd = newPosition;
} else {
_textSelectionStart = newPosition;
}
return result;
}
// Move **beyond** the local boundary of the given type (unless range.start or
// range.end is reached). Used for most TextGranularity types except for
// TextGranularity.line, to ensure the selection movement doesn't get stuck at
// a local fixed point.
TextPosition _moveBeyondTextBoundaryAtDirection(TextPosition end, bool forward, TextBoundary textBoundary) {
final int newOffset = forward
? textBoundary.getTrailingTextBoundaryAt(end.offset) ?? range.end
: textBoundary.getLeadingTextBoundaryAt(end.offset - 1) ?? range.start;
return TextPosition(offset: newOffset);
}
// Move **to** the local boundary of the given type. Typically used for line
// boundaries, such that performing "move to line start" more than once never
// moves the selection to the previous line.
TextPosition _moveToTextBoundaryAtDirection(TextPosition end, bool forward, TextBoundary textBoundary) {
assert(end.offset >= 0);
final int caretOffset;
switch (end.affinity) {
case TextAffinity.upstream:
if (end.offset < 1 && !forward) {
assert (end.offset == 0);
return const TextPosition(offset: 0);
}
final CharacterBoundary characterBoundary = CharacterBoundary(fullText);
caretOffset = math.max(
0,
characterBoundary.getLeadingTextBoundaryAt(range.start + end.offset) ?? range.start,
) - 1;
case TextAffinity.downstream:
caretOffset = end.offset;
}
final int offset = forward
? textBoundary.getTrailingTextBoundaryAt(caretOffset) ?? range.end
: textBoundary.getLeadingTextBoundaryAt(caretOffset) ?? range.start;
return TextPosition(offset: offset);
}
MapEntry<TextPosition, SelectionResult> _handleVerticalMovement(TextPosition position, {required double horizontalBaselineInParagraphCoordinates, required bool below}) {
final List<ui.LineMetrics> lines = paragraph._textPainter.computeLineMetrics();
final Offset offset = paragraph.getOffsetForCaret(position, Rect.zero);
int currentLine = lines.length - 1;
for (final ui.LineMetrics lineMetrics in lines) {
if (lineMetrics.baseline > offset.dy) {
currentLine = lineMetrics.lineNumber;
break;
}
}
final TextPosition newPosition;
if (below && currentLine == lines.length - 1) {
newPosition = TextPosition(offset: range.end, affinity: TextAffinity.upstream);
} else if (!below && currentLine == 0) {
newPosition = TextPosition(offset: range.start);
} else {
final int newLine = below ? currentLine + 1 : currentLine - 1;
newPosition = _clampTextPosition(
paragraph.getPositionForOffset(Offset(horizontalBaselineInParagraphCoordinates, lines[newLine].baseline))
);
}
final SelectionResult result;
if (newPosition.offset == range.start) {
result = SelectionResult.previous;
} else if (newPosition.offset == range.end) {
result = SelectionResult.next;
} else {
result = SelectionResult.end;
}
assert(result != SelectionResult.next || below);
assert(result != SelectionResult.previous || !below);
return MapEntry<TextPosition, SelectionResult>(newPosition, result);
}
/// Whether the given text position is contained in current selection
/// range.
///
/// The parameter `start` must be smaller than `end`.
bool _positionIsWithinCurrentSelection(TextPosition position) {
if (_textSelectionStart == null || _textSelectionEnd == null) {
return false;
}
// Normalize current selection.
late TextPosition currentStart;
late TextPosition currentEnd;
if (_compareTextPositions(_textSelectionStart!, _textSelectionEnd!) > 0) {
currentStart = _textSelectionStart!;
currentEnd = _textSelectionEnd!;
} else {
currentStart = _textSelectionEnd!;
currentEnd = _textSelectionStart!;
}
return _compareTextPositions(currentStart, position) >= 0 && _compareTextPositions(currentEnd, position) <= 0;
}
/// Compares two text positions.
///
/// Returns 1 if `position` < `otherPosition`, -1 if `position` > `otherPosition`,
/// or 0 if they are equal.
static int _compareTextPositions(TextPosition position, TextPosition otherPosition) {
if (position.offset < otherPosition.offset) {
return 1;
} else if (position.offset > otherPosition.offset) {
return -1;
} else if (position.affinity == otherPosition.affinity){
return 0;
} else {
return position.affinity == TextAffinity.upstream ? 1 : -1;
}
}
@override
Matrix4 getTransformTo(RenderObject? ancestor) {
return paragraph.getTransformTo(ancestor);
}
@override
void pushHandleLayers(LayerLink? startHandle, LayerLink? endHandle) {
if (!paragraph.attached) {
assert(startHandle == null && endHandle == null, 'Only clean up can be called.');
return;
}
if (_startHandleLayerLink != startHandle) {
_startHandleLayerLink = startHandle;
paragraph.markNeedsPaint();
}
if (_endHandleLayerLink != endHandle) {
_endHandleLayerLink = endHandle;
paragraph.markNeedsPaint();
}
}
List<Rect>? _cachedBoundingBoxes;
@override
List<Rect> get boundingBoxes {
if (_cachedBoundingBoxes == null) {
final List<TextBox> boxes = paragraph.getBoxesForSelection(
TextSelection(baseOffset: range.start, extentOffset: range.end),
);
if (boxes.isNotEmpty) {
_cachedBoundingBoxes = <Rect>[];
for (final TextBox textBox in boxes) {
_cachedBoundingBoxes!.add(textBox.toRect());
}
} else {
final Offset offset = paragraph._getOffsetForPosition(TextPosition(offset: range.start));
final Rect rect = Rect.fromPoints(offset, offset.translate(0, - paragraph._textPainter.preferredLineHeight));
_cachedBoundingBoxes = <Rect>[rect];
}
}
return _cachedBoundingBoxes!;
}
Rect? _cachedRect;
Rect get _rect {
if (_cachedRect == null) {
final List<TextBox> boxes = paragraph.getBoxesForSelection(
TextSelection(baseOffset: range.start, extentOffset: range.end),
);
if (boxes.isNotEmpty) {
Rect result = boxes.first.toRect();
for (int index = 1; index < boxes.length; index += 1) {
result = result.expandToInclude(boxes[index].toRect());
}
_cachedRect = result;
} else {
final Offset offset = paragraph._getOffsetForPosition(TextPosition(offset: range.start));
_cachedRect = Rect.fromPoints(offset, offset.translate(0, - paragraph._textPainter.preferredLineHeight));
}
}
return _cachedRect!;
}
void didChangeParagraphLayout() {
_cachedRect = null;
}
@override
Size get size {
return _rect.size;
}
void paint(PaintingContext context, Offset offset) {
if (_textSelectionStart == null || _textSelectionEnd == null) {
return;
}
if (paragraph.selectionColor != null) {
final TextSelection selection = TextSelection(
baseOffset: _textSelectionStart!.offset,
extentOffset: _textSelectionEnd!.offset,
);
final Paint selectionPaint = Paint()
..style = PaintingStyle.fill
..color = paragraph.selectionColor!;
for (final TextBox textBox in paragraph.getBoxesForSelection(selection)) {
context.canvas.drawRect(
textBox.toRect().shift(offset), selectionPaint);
}
}
if (_startHandleLayerLink != null && value.startSelectionPoint != null) {
context.pushLayer(
LeaderLayer(
link: _startHandleLayerLink!,
offset: offset + value.startSelectionPoint!.localPosition,
),
(PaintingContext context, Offset offset) { },
Offset.zero,
);
}
if (_endHandleLayerLink != null && value.endSelectionPoint != null) {
context.pushLayer(
LeaderLayer(
link: _endHandleLayerLink!,
offset: offset + value.endSelectionPoint!.localPosition,
),
(PaintingContext context, Offset offset) { },
Offset.zero,
);
}
}
@override
TextSelection getLineAtOffset(TextPosition position) {
final TextRange line = paragraph._getLineAtOffset(position);
final int start = line.start.clamp(range.start, range.end);
final int end = line.end.clamp(range.start, range.end);
return TextSelection(baseOffset: start, extentOffset: end);
}
@override
TextPosition getTextPositionAbove(TextPosition position) {
return _clampTextPosition(paragraph._getTextPositionAbove(position));
}
@override
TextPosition getTextPositionBelow(TextPosition position) {
return _clampTextPosition(paragraph._getTextPositionBelow(position));
}
@override
TextRange getWordBoundary(TextPosition position) => paragraph.getWordBoundary(position);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('textInsideRange', range.textInside(fullText)));
properties.add(DiagnosticsProperty<TextRange>('range', range));
properties.add(DiagnosticsProperty<String>('fullText', fullText));
}
}
| flutter/packages/flutter/lib/src/rendering/paragraph.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/paragraph.dart",
"repo_id": "flutter",
"token_count": 29736
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:vector_math/vector_math_64.dart';
import 'debug.dart';
import 'object.dart';
import 'sliver.dart';
/// Insets a [RenderSliver] by applying [resolvedPadding] on each side.
///
/// A [RenderSliverEdgeInsetsPadding] subclass wraps the [SliverGeometry.layoutExtent]
/// of its child. Any incoming [SliverConstraints.overlap] is ignored and not
/// passed on to the child.
///
/// {@template flutter.rendering.RenderSliverEdgeInsetsPadding}
/// Applying padding in the main extent of the viewport to slivers that have scroll effects is likely to have
/// undesired effects. For example, wrapping a [SliverPersistentHeader] with
/// `pinned:true` will cause only the appbar to stay pinned while the padding will scroll away.
/// {@endtemplate}
abstract class RenderSliverEdgeInsetsPadding extends RenderSliver with RenderObjectWithChildMixin<RenderSliver> {
/// The amount to pad the child in each dimension.
///
/// The offsets are specified in terms of visual edges, left, top, right, and
/// bottom. These values are not affected by the [TextDirection].
///
/// Must not be null or contain negative values when [performLayout] is called.
EdgeInsets? get resolvedPadding;
/// The padding in the scroll direction on the side nearest the 0.0 scroll direction.
///
/// Only valid after layout has started, since before layout the render object
/// doesn't know what direction it will be laid out in.
double get beforePadding {
assert(resolvedPadding != null);
return switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
AxisDirection.up => resolvedPadding!.bottom,
AxisDirection.right => resolvedPadding!.left,
AxisDirection.down => resolvedPadding!.top,
AxisDirection.left => resolvedPadding!.right,
};
}
/// The padding in the scroll direction on the side furthest from the 0.0 scroll offset.
///
/// Only valid after layout has started, since before layout the render object
/// doesn't know what direction it will be laid out in.
double get afterPadding {
assert(resolvedPadding != null);
return switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
AxisDirection.up => resolvedPadding!.top,
AxisDirection.right => resolvedPadding!.right,
AxisDirection.down => resolvedPadding!.bottom,
AxisDirection.left => resolvedPadding!.left,
};
}
/// The total padding in the [SliverConstraints.axisDirection]. (In other
/// words, for a vertical downwards-growing list, the sum of the padding on
/// the top and bottom.)
///
/// Only valid after layout has started, since before layout the render object
/// doesn't know what direction it will be laid out in.
double get mainAxisPadding {
assert(resolvedPadding != null);
return resolvedPadding!.along(constraints.axis);
}
/// The total padding in the cross-axis direction. (In other words, for a
/// vertical downwards-growing list, the sum of the padding on the left and
/// right.)
///
/// Only valid after layout has started, since before layout the render object
/// doesn't know what direction it will be laid out in.
double get crossAxisPadding {
assert(resolvedPadding != null);
return switch (constraints.axis) {
Axis.horizontal => resolvedPadding!.vertical,
Axis.vertical => resolvedPadding!.horizontal,
};
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverPhysicalParentData) {
child.parentData = SliverPhysicalParentData();
}
}
@override
void performLayout() {
final SliverConstraints constraints = this.constraints;
double paintOffset({required double from, required double to}) => calculatePaintOffset(constraints, from: from, to: to);
double cacheOffset({required double from, required double to}) => calculateCacheOffset(constraints, from: from, to: to);
assert(this.resolvedPadding != null);
final EdgeInsets resolvedPadding = this.resolvedPadding!;
final double beforePadding = this.beforePadding;
final double afterPadding = this.afterPadding;
final double mainAxisPadding = this.mainAxisPadding;
final double crossAxisPadding = this.crossAxisPadding;
if (child == null) {
final double paintExtent = paintOffset(from: 0.0, to: mainAxisPadding);
final double cacheExtent = cacheOffset(from: 0.0, to: mainAxisPadding);
geometry = SliverGeometry(
scrollExtent: mainAxisPadding,
paintExtent: math.min(paintExtent, constraints.remainingPaintExtent),
maxPaintExtent: mainAxisPadding,
cacheExtent: cacheExtent,
);
return;
}
final double beforePaddingPaintExtent = paintOffset(from: 0.0, to: beforePadding);
double overlap = constraints.overlap;
if (overlap > 0) {
overlap = math.max(0.0, constraints.overlap - beforePaddingPaintExtent);
}
child!.layout(
constraints.copyWith(
scrollOffset: math.max(0.0, constraints.scrollOffset - beforePadding),
cacheOrigin: math.min(0.0, constraints.cacheOrigin + beforePadding),
overlap: overlap,
remainingPaintExtent: constraints.remainingPaintExtent - paintOffset(from: 0.0, to: beforePadding),
remainingCacheExtent: constraints.remainingCacheExtent - cacheOffset(from: 0.0, to: beforePadding),
crossAxisExtent: math.max(0.0, constraints.crossAxisExtent - crossAxisPadding),
precedingScrollExtent: beforePadding + constraints.precedingScrollExtent,
),
parentUsesSize: true,
);
final SliverGeometry childLayoutGeometry = child!.geometry!;
if (childLayoutGeometry.scrollOffsetCorrection != null) {
geometry = SliverGeometry(
scrollOffsetCorrection: childLayoutGeometry.scrollOffsetCorrection,
);
return;
}
final double scrollExtent = childLayoutGeometry.scrollExtent;
final double beforePaddingCacheExtent = cacheOffset(from: 0.0, to: beforePadding);
final double afterPaddingCacheExtent = cacheOffset(from: beforePadding + scrollExtent, to: mainAxisPadding + scrollExtent);
final double afterPaddingPaintExtent = paintOffset(from: beforePadding + scrollExtent, to: mainAxisPadding + scrollExtent);
final double mainAxisPaddingCacheExtent = beforePaddingCacheExtent + afterPaddingCacheExtent;
final double mainAxisPaddingPaintExtent = beforePaddingPaintExtent + afterPaddingPaintExtent;
final double paintExtent = math.min(
beforePaddingPaintExtent + math.max(childLayoutGeometry.paintExtent, childLayoutGeometry.layoutExtent + afterPaddingPaintExtent),
constraints.remainingPaintExtent,
);
geometry = SliverGeometry(
paintOrigin: childLayoutGeometry.paintOrigin,
scrollExtent: mainAxisPadding + scrollExtent,
paintExtent: paintExtent,
layoutExtent: math.min(mainAxisPaddingPaintExtent + childLayoutGeometry.layoutExtent, paintExtent),
cacheExtent: math.min(mainAxisPaddingCacheExtent + childLayoutGeometry.cacheExtent, constraints.remainingCacheExtent),
maxPaintExtent: mainAxisPadding + childLayoutGeometry.maxPaintExtent,
hitTestExtent: math.max(
mainAxisPaddingPaintExtent + childLayoutGeometry.paintExtent,
beforePaddingPaintExtent + childLayoutGeometry.hitTestExtent,
),
hasVisualOverflow: childLayoutGeometry.hasVisualOverflow,
);
final double calculatedOffset = switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
AxisDirection.up => paintOffset(from: resolvedPadding.bottom + scrollExtent, to: resolvedPadding.vertical + scrollExtent),
AxisDirection.left => paintOffset(from: resolvedPadding.right + scrollExtent, to: resolvedPadding.horizontal + scrollExtent),
AxisDirection.right => paintOffset(from: 0.0, to: resolvedPadding.left),
AxisDirection.down => paintOffset(from: 0.0, to: resolvedPadding.top),
};
final SliverPhysicalParentData childParentData = child!.parentData! as SliverPhysicalParentData;
childParentData.paintOffset = switch (constraints.axis) {
Axis.horizontal => Offset(calculatedOffset, resolvedPadding.top),
Axis.vertical => Offset(resolvedPadding.left, calculatedOffset),
};
assert(beforePadding == this.beforePadding);
assert(afterPadding == this.afterPadding);
assert(mainAxisPadding == this.mainAxisPadding);
assert(crossAxisPadding == this.crossAxisPadding);
}
@override
bool hitTestChildren(SliverHitTestResult result, { required double mainAxisPosition, required double crossAxisPosition }) {
if (child != null && child!.geometry!.hitTestExtent > 0.0) {
final SliverPhysicalParentData childParentData = child!.parentData! as SliverPhysicalParentData;
result.addWithAxisOffset(
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
mainAxisOffset: childMainAxisPosition(child!),
crossAxisOffset: childCrossAxisPosition(child!),
paintOffset: childParentData.paintOffset,
hitTest: child!.hitTest,
);
}
return false;
}
@override
double childMainAxisPosition(RenderSliver child) {
assert(child == this.child);
return calculatePaintOffset(constraints, from: 0.0, to: beforePadding);
}
@override
double childCrossAxisPosition(RenderSliver child) {
assert(child == this.child);
assert(resolvedPadding != null);
return switch (constraints.axis) {
Axis.horizontal => resolvedPadding!.top,
Axis.vertical => resolvedPadding!.left,
};
}
@override
double? childScrollOffset(RenderObject child) {
assert(child.parent == this);
return beforePadding;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
assert(child == this.child);
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.applyPaintTransform(transform);
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null && child!.geometry!.visible) {
final SliverPhysicalParentData childParentData = child!.parentData! as SliverPhysicalParentData;
context.paintChild(child!, offset + childParentData.paintOffset);
}
}
@override
void debugPaint(PaintingContext context, Offset offset) {
super.debugPaint(context, offset);
assert(() {
if (debugPaintSizeEnabled) {
final Size parentSize = getAbsoluteSize();
final Rect outerRect = offset & parentSize;
Rect? innerRect;
if (child != null) {
final Size childSize = child!.getAbsoluteSize();
final SliverPhysicalParentData childParentData = child!.parentData! as SliverPhysicalParentData;
innerRect = (offset + childParentData.paintOffset) & childSize;
assert(innerRect.top >= outerRect.top);
assert(innerRect.left >= outerRect.left);
assert(innerRect.right <= outerRect.right);
assert(innerRect.bottom <= outerRect.bottom);
}
debugPaintPadding(context.canvas, outerRect, innerRect);
}
return true;
}());
}
}
/// Insets a [RenderSliver], applying padding on each side.
///
/// A [RenderSliverPadding] object wraps the [SliverGeometry.layoutExtent] of
/// its child. Any incoming [SliverConstraints.overlap] is ignored and not
/// passed on to the child.
///
/// {@macro flutter.rendering.RenderSliverEdgeInsetsPadding}
class RenderSliverPadding extends RenderSliverEdgeInsetsPadding {
/// Creates a render object that insets its child in a viewport.
///
/// The [padding] argument must have non-negative insets.
RenderSliverPadding({
required EdgeInsetsGeometry padding,
TextDirection? textDirection,
RenderSliver? child,
}) : assert(padding.isNonNegative),
_padding = padding,
_textDirection = textDirection {
this.child = child;
}
@override
EdgeInsets? get resolvedPadding => _resolvedPadding;
EdgeInsets? _resolvedPadding;
void _resolve() {
if (resolvedPadding != null) {
return;
}
_resolvedPadding = padding.resolve(textDirection);
assert(resolvedPadding!.isNonNegative);
}
void _markNeedsResolution() {
_resolvedPadding = null;
markNeedsLayout();
}
/// The amount to pad the child in each dimension.
///
/// If this is set to an [EdgeInsetsDirectional] object, then [textDirection]
/// must not be null.
EdgeInsetsGeometry get padding => _padding;
EdgeInsetsGeometry _padding;
set padding(EdgeInsetsGeometry value) {
assert(padding.isNonNegative);
if (_padding == value) {
return;
}
_padding = value;
_markNeedsResolution();
}
/// The text direction with which to resolve [padding].
///
/// This may be changed to null, but only after the [padding] has been changed
/// to a value that does not depend on the direction.
TextDirection? get textDirection => _textDirection;
TextDirection? _textDirection;
set textDirection(TextDirection? value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
_markNeedsResolution();
}
@override
void performLayout() {
_resolve();
super.performLayout();
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_padding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_padding.dart",
"repo_id": "flutter",
"token_count": 4612
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show AccessibilityFeatures, SemanticsActionEvent, SemanticsUpdateBuilder;
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'debug.dart';
export 'dart:ui' show AccessibilityFeatures, SemanticsActionEvent, SemanticsUpdateBuilder;
/// The glue between the semantics layer and the Flutter engine.
mixin SemanticsBinding on BindingBase {
@override
void initInstances() {
super.initInstances();
_instance = this;
_accessibilityFeatures = platformDispatcher.accessibilityFeatures;
platformDispatcher
..onSemanticsEnabledChanged = _handleSemanticsEnabledChanged
..onSemanticsActionEvent = _handleSemanticsActionEvent
..onAccessibilityFeaturesChanged = handleAccessibilityFeaturesChanged;
_handleSemanticsEnabledChanged();
}
/// The current [SemanticsBinding], if one has been created.
///
/// Provides access to the features exposed by this mixin. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [runApp] or [WidgetsFlutterBinding.ensureInitialized].
static SemanticsBinding get instance => BindingBase.checkInstance(_instance);
static SemanticsBinding? _instance;
/// Whether semantics information must be collected.
///
/// Returns true if either the platform has requested semantics information
/// to be generated or if [ensureSemantics] has been called otherwise.
///
/// To get notified when this value changes register a listener with
/// [addSemanticsEnabledListener].
bool get semanticsEnabled {
assert(_semanticsEnabled.value == (_outstandingHandles > 0));
return _semanticsEnabled.value;
}
late final ValueNotifier<bool> _semanticsEnabled = ValueNotifier<bool>(platformDispatcher.semanticsEnabled);
/// Adds a `listener` to be called when [semanticsEnabled] changes.
///
/// See also:
///
/// * [removeSemanticsEnabledListener] to remove the listener again.
/// * [ValueNotifier.addListener], which documents how and when listeners are
/// called.
void addSemanticsEnabledListener(VoidCallback listener) {
_semanticsEnabled.addListener(listener);
}
/// Removes a `listener` added by [addSemanticsEnabledListener].
///
/// See also:
///
/// * [ValueNotifier.removeListener], which documents how listeners are
/// removed.
void removeSemanticsEnabledListener(VoidCallback listener) {
_semanticsEnabled.removeListener(listener);
}
/// The number of clients registered to listen for semantics.
///
/// The number is increased whenever [ensureSemantics] is called and decreased
/// when [SemanticsHandle.dispose] is called.
int get debugOutstandingSemanticsHandles => _outstandingHandles;
int _outstandingHandles = 0;
/// Creates a new [SemanticsHandle] and requests the collection of semantics
/// information.
///
/// Semantics information are only collected when there are clients interested
/// in them. These clients express their interest by holding a
/// [SemanticsHandle].
///
/// Clients can close their [SemanticsHandle] by calling
/// [SemanticsHandle.dispose]. Once all outstanding [SemanticsHandle] objects
/// are closed, semantics information are no longer collected.
SemanticsHandle ensureSemantics() {
assert(_outstandingHandles >= 0);
_outstandingHandles++;
assert(_outstandingHandles > 0);
_semanticsEnabled.value = true;
return SemanticsHandle._(_didDisposeSemanticsHandle);
}
void _didDisposeSemanticsHandle() {
assert(_outstandingHandles > 0);
_outstandingHandles--;
assert(_outstandingHandles >= 0);
_semanticsEnabled.value = _outstandingHandles > 0;
}
// Handle for semantics request from the platform.
SemanticsHandle? _semanticsHandle;
void _handleSemanticsEnabledChanged() {
if (platformDispatcher.semanticsEnabled) {
_semanticsHandle ??= ensureSemantics();
} else {
_semanticsHandle?.dispose();
_semanticsHandle = null;
}
}
void _handleSemanticsActionEvent(ui.SemanticsActionEvent action) {
final Object? arguments = action.arguments;
final ui.SemanticsActionEvent decodedAction = arguments is ByteData
? action.copyWith(arguments: const StandardMessageCodec().decodeMessage(arguments))
: action;
performSemanticsAction(decodedAction);
}
/// Called whenever the platform requests an action to be performed on a
/// [SemanticsNode].
///
/// This callback is invoked when a user interacts with the app via an
/// accessibility service (e.g. TalkBack and VoiceOver) and initiates an
/// action on the focused node.
///
/// Bindings that mixin the [SemanticsBinding] must implement this method and
/// perform the given `action` on the [SemanticsNode] specified by
/// [SemanticsActionEvent.nodeId].
///
/// See [dart:ui.PlatformDispatcher.onSemanticsActionEvent].
@protected
void performSemanticsAction(ui.SemanticsActionEvent action);
/// The currently active set of [AccessibilityFeatures].
///
/// This is set when the binding is first initialized and updated whenever a
/// flag is changed.
///
/// To listen to changes to accessibility features, create a
/// [WidgetsBindingObserver] and listen to
/// [WidgetsBindingObserver.didChangeAccessibilityFeatures].
ui.AccessibilityFeatures get accessibilityFeatures => _accessibilityFeatures;
late ui.AccessibilityFeatures _accessibilityFeatures;
/// Called when the platform accessibility features change.
///
/// See [dart:ui.PlatformDispatcher.onAccessibilityFeaturesChanged].
@protected
@mustCallSuper
void handleAccessibilityFeaturesChanged() {
_accessibilityFeatures = platformDispatcher.accessibilityFeatures;
}
/// Creates an empty semantics update builder.
///
/// The caller is responsible for filling out the semantics node updates.
///
/// This method is used by the [SemanticsOwner] to create builder for all its
/// semantics updates.
ui.SemanticsUpdateBuilder createSemanticsUpdateBuilder() {
return ui.SemanticsUpdateBuilder();
}
/// The platform is requesting that animations be disabled or simplified.
///
/// This setting can be overridden for testing or debugging by setting
/// [debugSemanticsDisableAnimations].
bool get disableAnimations {
bool value = _accessibilityFeatures.disableAnimations;
assert(() {
if (debugSemanticsDisableAnimations != null) {
value = debugSemanticsDisableAnimations!;
}
return true;
}());
return value;
}
}
/// A reference to the semantics information generated by the framework.
///
/// Semantics information are only collected when there are clients interested
/// in them. These clients express their interest by holding a
/// [SemanticsHandle]. When the client no longer needs the
/// semantics information, it must call [dispose] on the [SemanticsHandle] to
/// close it. When all open [SemanticsHandle]s are disposed, the framework will
/// stop updating the semantics information.
///
/// To obtain a [SemanticsHandle], call [SemanticsBinding.ensureSemantics].
class SemanticsHandle {
SemanticsHandle._(this._onDispose) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/semantics.dart',
className: '$SemanticsHandle',
object: this,
);
}
}
final VoidCallback _onDispose;
/// Closes the semantics handle.
///
/// When all the outstanding [SemanticsHandle] objects are closed, the
/// framework will stop generating semantics information.
@mustCallSuper
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_onDispose();
}
}
| flutter/packages/flutter/lib/src/semantics/binding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/semantics/binding.dart",
"repo_id": "flutter",
"token_count": 2336
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The flavor this app was built with.
///
/// This is equivalent to the value argued to the `--flavor` option at build time.
/// This will be `null` if the `--flavor` option was not provided.
const String? appFlavor = String.fromEnvironment('FLUTTER_APP_FLAVOR') != '' ?
String.fromEnvironment('FLUTTER_APP_FLAVOR') : null;
| flutter/packages/flutter/lib/src/services/flavor.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/flavor.dart",
"repo_id": "flutter",
"token_count": 141
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'keyboard_maps.g.dart';
import 'raw_keyboard.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'keyboard_key.g.dart' show LogicalKeyboardKey, PhysicalKeyboardKey;
// Android sets the 0x80000000 bit on a character to indicate that it is a
// combining character, so we use this mask to remove that bit to make it a
// valid Unicode character again.
const int _kCombiningCharacterMask = 0x7fffffff;
/// Platform-specific key event data for Android.
///
/// This class is deprecated and will be removed. Platform specific key event
/// data will no longer be available. See [KeyEvent] for what is available.
///
/// This object contains information about key events obtained from Android's
/// `KeyEvent` interface.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyEventDataAndroid extends RawKeyEventData {
/// Creates a key event data structure specific for Android.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEventDataAndroid({
this.flags = 0,
this.codePoint = 0,
this.plainCodePoint = 0,
this.keyCode = 0,
this.scanCode = 0,
this.metaState = 0,
this.eventSource = 0,
this.vendorId = 0,
this.productId = 0,
this.deviceId = 0,
this.repeatCount = 0,
});
/// The current set of additional flags for this event.
///
/// Flags indicate things like repeat state, etc.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getFlags()>
/// for more information.
final int flags;
/// The Unicode code point represented by the key event, if any.
///
/// If there is no Unicode code point, this value is zero.
///
/// Dead keys are represented as Unicode combining characters.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getUnicodeChar()>
/// for more information.
final int codePoint;
/// The Unicode code point represented by the key event, if any, without
/// regard to any modifier keys which are currently pressed.
///
/// If there is no Unicode code point, this value is zero.
///
/// Dead keys are represented as Unicode combining characters.
///
/// This is the result of calling KeyEvent.getUnicodeChar(0) on Android.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getUnicodeChar(int)>
/// for more information.
final int plainCodePoint;
/// The hardware key code corresponding to this key event.
///
/// This is the physical key that was pressed, not the Unicode character.
/// See [codePoint] for the Unicode character.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getKeyCode()>
/// for more information.
final int keyCode;
/// The hardware scan code id corresponding to this key event.
///
/// These values are not reliable and vary from device to device, so this
/// information is mainly useful for debugging.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getScanCode()>
/// for more information.
final int scanCode;
/// The modifiers that were present when the key event occurred.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getMetaState()>
/// for the numerical values of the [metaState]. Many of these constants are
/// also replicated as static constants in this class.
///
/// See also:
///
/// * [modifiersPressed], which returns a Map of currently pressed modifiers
/// and their keyboard side.
/// * [isModifierPressed], to see if a specific modifier is pressed.
/// * [isControlPressed], to see if a CTRL key is pressed.
/// * [isShiftPressed], to see if a SHIFT key is pressed.
/// * [isAltPressed], to see if an ALT key is pressed.
/// * [isMetaPressed], to see if a META key is pressed.
final int metaState;
/// The source of the event.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent.html#getSource()>
/// for the numerical values of the `source`. Many of these constants are also
/// replicated as static constants in this class.
final int eventSource;
/// The vendor ID of the device that produced the event.
///
/// See <https://developer.android.com/reference/android/view/InputDevice.html#getVendorId()>
/// for the numerical values of the [vendorId].
final int vendorId;
/// The product ID of the device that produced the event.
///
/// See <https://developer.android.com/reference/android/view/InputDevice.html#getProductId()>
/// for the numerical values of the [productId].
final int productId;
/// The ID of the device that produced the event.
///
/// See https://developer.android.com/reference/android/view/InputDevice.html#getId()
final int deviceId;
/// The repeat count of the event.
///
/// See <https://developer.android.com/reference/android/view/KeyEvent#getRepeatCount()>
/// for more information.
final int repeatCount;
// The source code that indicates that an event came from a joystick.
// from https://developer.android.com/reference/android/view/InputDevice.html#SOURCE_JOYSTICK
static const int _sourceJoystick = 0x01000010;
// Android only reports a single code point for the key label.
@override
String get keyLabel => plainCodePoint == 0 ? '' : String.fromCharCode(plainCodePoint & _kCombiningCharacterMask);
@override
PhysicalKeyboardKey get physicalKey {
if (kAndroidToPhysicalKey.containsKey(scanCode)) {
return kAndroidToPhysicalKey[scanCode]!;
}
// Android sends DPAD_UP, etc. as the keyCode for joystick DPAD events, but
// it doesn't set the scanCode for those, so we have to detect this, and set
// our own DPAD physical keys. The logical key will still match "arrowUp",
// etc.
if (eventSource & _sourceJoystick == _sourceJoystick) {
final LogicalKeyboardKey? foundKey = kAndroidToLogicalKey[keyCode];
if (foundKey == LogicalKeyboardKey.arrowUp) {
return PhysicalKeyboardKey.arrowUp;
}
if (foundKey == LogicalKeyboardKey.arrowDown) {
return PhysicalKeyboardKey.arrowDown;
}
if (foundKey == LogicalKeyboardKey.arrowLeft) {
return PhysicalKeyboardKey.arrowLeft;
}
if (foundKey == LogicalKeyboardKey.arrowRight) {
return PhysicalKeyboardKey.arrowRight;
}
}
return PhysicalKeyboardKey(LogicalKeyboardKey.androidPlane + scanCode);
}
@override
LogicalKeyboardKey get logicalKey {
// Look to see if the keyCode is a printable number pad key, so that a
// difference between regular keys (e.g. "=") and the number pad version
// (e.g. the "=" on the number pad) can be determined.
final LogicalKeyboardKey? numPadKey = kAndroidNumPadMap[keyCode];
if (numPadKey != null) {
return numPadKey;
}
// If it has a non-control-character label, then either return the existing
// constant, or construct a new Unicode-based key from it. Don't mark it as
// autogenerated, since the label uniquely identifies an ID from the Unicode
// plane.
if (keyLabel.isNotEmpty && !LogicalKeyboardKey.isControlCharacter(keyLabel)) {
final int combinedCodePoint = plainCodePoint & _kCombiningCharacterMask;
final int keyId = LogicalKeyboardKey.unicodePlane | (combinedCodePoint & LogicalKeyboardKey.valueMask);
return LogicalKeyboardKey.findKeyByKeyId(keyId) ?? LogicalKeyboardKey(keyId);
}
// Look to see if the keyCode is one we know about and have a mapping for.
final LogicalKeyboardKey? newKey = kAndroidToLogicalKey[keyCode];
if (newKey != null) {
return newKey;
}
return LogicalKeyboardKey(keyCode | LogicalKeyboardKey.androidPlane);
}
bool _isLeftRightModifierPressed(KeyboardSide side, int anyMask, int leftMask, int rightMask) {
if (metaState & anyMask == 0) {
return false;
}
return switch (side) {
KeyboardSide.any => true,
KeyboardSide.all => (metaState & leftMask != 0) && (metaState & rightMask != 0),
KeyboardSide.left => metaState & leftMask != 0,
KeyboardSide.right => metaState & rightMask != 0,
};
}
@override
bool isModifierPressed(ModifierKey key, { KeyboardSide side = KeyboardSide.any }) {
return switch (key) {
ModifierKey.controlModifier => _isLeftRightModifierPressed(side, modifierControl, modifierLeftControl, modifierRightControl),
ModifierKey.shiftModifier => _isLeftRightModifierPressed(side, modifierShift, modifierLeftShift, modifierRightShift),
ModifierKey.altModifier => _isLeftRightModifierPressed(side, modifierAlt, modifierLeftAlt, modifierRightAlt),
ModifierKey.metaModifier => _isLeftRightModifierPressed(side, modifierMeta, modifierLeftMeta, modifierRightMeta),
ModifierKey.capsLockModifier => metaState & modifierCapsLock != 0,
ModifierKey.numLockModifier => metaState & modifierNumLock != 0,
ModifierKey.scrollLockModifier => metaState & modifierScrollLock != 0,
ModifierKey.functionModifier => metaState & modifierFunction != 0,
ModifierKey.symbolModifier => metaState & modifierSym != 0,
};
}
@override
KeyboardSide? getModifierSide(ModifierKey key) {
KeyboardSide? findSide(int anyMask, int leftMask, int rightMask) {
final int combinedMask = leftMask | rightMask;
final int combined = metaState & combinedMask;
if (combined == leftMask) {
return KeyboardSide.left;
} else if (combined == rightMask) {
return KeyboardSide.right;
} else if (combined == combinedMask) {
return KeyboardSide.all;
}
// If the platform code sets the "any" modifier, but not a specific side,
// then we return "all", assuming that there is only one of that modifier
// key on the keyboard.
if (metaState & anyMask != 0) {
return KeyboardSide.all;
}
return null;
}
switch (key) {
case ModifierKey.controlModifier:
return findSide(modifierControl, modifierLeftControl, modifierRightControl);
case ModifierKey.shiftModifier:
return findSide(modifierShift, modifierLeftShift, modifierRightShift);
case ModifierKey.altModifier:
return findSide(modifierAlt, modifierLeftAlt, modifierRightAlt);
case ModifierKey.metaModifier:
return findSide(modifierMeta, modifierLeftMeta, modifierRightMeta);
case ModifierKey.capsLockModifier:
case ModifierKey.numLockModifier:
case ModifierKey.scrollLockModifier:
case ModifierKey.functionModifier:
case ModifierKey.symbolModifier:
return KeyboardSide.all;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<int>('flags', flags));
properties.add(DiagnosticsProperty<int>('codePoint', codePoint));
properties.add(DiagnosticsProperty<int>('plainCodePoint', plainCodePoint));
properties.add(DiagnosticsProperty<int>('keyCode', keyCode));
properties.add(DiagnosticsProperty<int>('scanCode', scanCode));
properties.add(DiagnosticsProperty<int>('metaState', metaState));
}
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is RawKeyEventDataAndroid
&& other.flags == flags
&& other.codePoint == codePoint
&& other.plainCodePoint == plainCodePoint
&& other.keyCode == keyCode
&& other.scanCode == scanCode
&& other.metaState == metaState;
}
@override
int get hashCode => Object.hash(
flags,
codePoint,
plainCodePoint,
keyCode,
scanCode,
metaState,
);
// Modifier key masks.
/// No modifier keys are pressed in the [metaState] field.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierNone = 0;
/// This mask is used to check the [metaState] field to test whether one of
/// the ALT modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierAlt = 0x02;
/// This mask is used to check the [metaState] field to test whether the left
/// ALT modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftAlt = 0x10;
/// This mask is used to check the [metaState] field to test whether the right
/// ALT modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightAlt = 0x20;
/// This mask is used to check the [metaState] field to test whether one of
/// the SHIFT modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierShift = 0x01;
/// This mask is used to check the [metaState] field to test whether the left
/// SHIFT modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftShift = 0x40;
/// This mask is used to check the [metaState] field to test whether the right
/// SHIFT modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightShift = 0x80;
/// This mask is used to check the [metaState] field to test whether the SYM
/// modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierSym = 0x04;
/// This mask is used to check the [metaState] field to test whether the
/// Function modifier key (Fn) is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierFunction = 0x08;
/// This mask is used to check the [metaState] field to test whether one of
/// the CTRL modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierControl = 0x1000;
/// This mask is used to check the [metaState] field to test whether the left
/// CTRL modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftControl = 0x2000;
/// This mask is used to check the [metaState] field to test whether the right
/// CTRL modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightControl = 0x4000;
/// This mask is used to check the [metaState] field to test whether one of
/// the META modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierMeta = 0x10000;
/// This mask is used to check the [metaState] field to test whether the left
/// META modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftMeta = 0x20000;
/// This mask is used to check the [metaState] field to test whether the right
/// META modifier key is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightMeta = 0x40000;
/// This mask is used to check the [metaState] field to test whether the CAPS
/// LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierCapsLock = 0x100000;
/// This mask is used to check the [metaState] field to test whether the NUM
/// LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierNumLock = 0x200000;
/// This mask is used to check the [metaState] field to test whether the
/// SCROLL LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierScrollLock = 0x400000;
}
| flutter/packages/flutter/lib/src/services/raw_keyboard_android.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/raw_keyboard_android.dart",
"repo_id": "flutter",
"token_count": 5754
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show TextRange;
import 'package:flutter/foundation.dart';
import 'text_editing.dart';
import 'text_input.dart' show TextEditingValue;
export 'dart:ui' show TextRange;
export 'text_editing.dart' show TextSelection;
export 'text_input.dart' show TextEditingValue;
TextAffinity? _toTextAffinity(String? affinity) {
return switch (affinity) {
'TextAffinity.downstream' => TextAffinity.downstream,
'TextAffinity.upstream' => TextAffinity.upstream,
_ => null,
};
}
// Replaces a range of text in the original string with the text given in the
// replacement string.
String _replace(String originalText, String replacementText, TextRange replacementRange) {
assert(replacementRange.isValid);
return originalText.replaceRange(replacementRange.start, replacementRange.end, replacementText);
}
// Verify that the given range is within the text.
bool _debugTextRangeIsValid(TextRange range, String text) {
if (!range.isValid) {
return true;
}
return (range.start >= 0 && range.start <= text.length)
&& (range.end >= 0 && range.end <= text.length);
}
/// A structure representing a granular change that has occurred to the editing
/// state as a result of text editing.
///
/// See also:
///
/// * [TextEditingDeltaInsertion], a delta representing an insertion.
/// * [TextEditingDeltaDeletion], a delta representing a deletion.
/// * [TextEditingDeltaReplacement], a delta representing a replacement.
/// * [TextEditingDeltaNonTextUpdate], a delta representing an update to the
/// selection and/or composing region.
/// * [TextInputConfiguration], to opt-in your [DeltaTextInputClient] to receive
/// [TextEditingDelta]'s you must set [TextInputConfiguration.enableDeltaModel]
/// to true.
abstract class TextEditingDelta with Diagnosticable {
/// Creates a delta for a given change to the editing state.
const TextEditingDelta({
required this.oldText,
required this.selection,
required this.composing,
});
/// Creates an instance of this class from a JSON object by inferring the
/// type of delta based on values sent from the engine.
factory TextEditingDelta.fromJSON(Map<String, dynamic> encoded) {
// An insertion delta is one where replacement destination is collapsed.
//
// A deletion delta is one where the replacement source is empty.
//
// An insertion/deletion can still occur when the replacement destination is not
// collapsed, or the replacement source is not empty.
//
// On native platforms when composing text, the entire composing region is
// replaced on input, rather than reporting character by character
// insertion/deletion. In these cases we can detect if there was an
// insertion/deletion by checking if the text inside the original composing
// region was modified by the replacement. If the text is the same then we have
// an insertion/deletion. If the text is different then we can say we have
// a replacement.
//
// For example say we are currently composing the word: 'world'.
// Our current state is 'worl|' with the cursor at the end of 'l'. If we
// input the character 'd', the platform will tell us 'worl' was replaced
// with 'world' at range (0,4). Here we can check if the text found in the
// composing region (0,4) has been modified. We see that it hasn't because
// 'worl' == 'worl', so this means that the text in
// 'world'{replacementDestinationEnd, replacementDestinationStart + replacementSourceEnd}
// can be considered an insertion. In this case we inserted 'd'.
//
// Similarly for a deletion, say we are currently composing the word: 'worl'.
// Our current state is 'world|' with the cursor at the end of 'd'. If we
// press backspace to delete the character 'd', the platform will tell us 'world'
// was replaced with 'worl' at range (0,5). Here we can check if the text found
// in the new composing region, is the same as the replacement text. We can do this
// by using oldText{replacementDestinationStart, replacementDestinationStart + replacementSourceEnd}
// which in this case is 'worl'. We then compare 'worl' with 'worl' and
// verify that they are the same. This means that the text in
// 'world'{replacementDestinationEnd, replacementDestinationStart + replacementSourceEnd} was deleted.
// In this case the character 'd' was deleted.
//
// A replacement delta occurs when the original composing region has been
// modified.
//
// A non text update delta occurs when the selection and/or composing region
// has been changed by the platform, and there have been no changes to the
// text value.
final String oldText = encoded['oldText'] as String;
final int replacementDestinationStart = encoded['deltaStart'] as int;
final int replacementDestinationEnd = encoded['deltaEnd'] as int;
final String replacementSource = encoded['deltaText'] as String;
const int replacementSourceStart = 0;
final int replacementSourceEnd = replacementSource.length;
// This delta is explicitly a non text update.
final bool isNonTextUpdate = replacementDestinationStart == -1 && replacementDestinationStart == replacementDestinationEnd;
final TextRange newComposing = TextRange(
start: encoded['composingBase'] as int? ?? -1,
end: encoded['composingExtent'] as int? ?? -1,
);
final TextSelection newSelection = TextSelection(
baseOffset: encoded['selectionBase'] as int? ?? -1,
extentOffset: encoded['selectionExtent'] as int? ?? -1,
affinity: _toTextAffinity(encoded['selectionAffinity'] as String?) ??
TextAffinity.downstream,
isDirectional: encoded['selectionIsDirectional'] as bool? ?? false,
);
if (isNonTextUpdate) {
assert(_debugTextRangeIsValid(newSelection, oldText), 'The selection range: $newSelection is not within the bounds of text: $oldText of length: ${oldText.length}');
assert(_debugTextRangeIsValid(newComposing, oldText), 'The composing range: $newComposing is not within the bounds of text: $oldText of length: ${oldText.length}');
return TextEditingDeltaNonTextUpdate(
oldText: oldText,
selection: newSelection,
composing: newComposing,
);
}
assert(_debugTextRangeIsValid(TextRange(start: replacementDestinationStart, end: replacementDestinationEnd), oldText), 'The delta range: ${TextRange(start: replacementSourceStart, end: replacementSourceEnd)} is not within the bounds of text: $oldText of length: ${oldText.length}');
final String newText = _replace(oldText, replacementSource, TextRange(start: replacementDestinationStart, end: replacementDestinationEnd));
assert(_debugTextRangeIsValid(newSelection, newText), 'The selection range: $newSelection is not within the bounds of text: $newText of length: ${newText.length}');
assert(_debugTextRangeIsValid(newComposing, newText), 'The composing range: $newComposing is not within the bounds of text: $newText of length: ${newText.length}');
final bool isEqual = oldText == newText;
final bool isDeletionGreaterThanOne = (replacementDestinationEnd - replacementDestinationStart) - (replacementSourceEnd - replacementSourceStart) > 1;
final bool isDeletingByReplacingWithEmpty = replacementSource.isEmpty && replacementSourceStart == 0 && replacementSourceStart == replacementSourceEnd;
final bool isReplacedByShorter = isDeletionGreaterThanOne && (replacementSourceEnd - replacementSourceStart < replacementDestinationEnd - replacementDestinationStart);
final bool isReplacedByLonger = replacementSourceEnd - replacementSourceStart > replacementDestinationEnd - replacementDestinationStart;
final bool isReplacedBySame = replacementSourceEnd - replacementSourceStart == replacementDestinationEnd - replacementDestinationStart;
final bool isInsertingInsideComposingRegion = replacementDestinationStart + replacementSourceEnd > replacementDestinationEnd;
final bool isDeletingInsideComposingRegion =
!isReplacedByShorter && !isDeletingByReplacingWithEmpty && replacementDestinationStart + replacementSourceEnd < replacementDestinationEnd;
String newComposingText;
String originalComposingText;
if (isDeletingByReplacingWithEmpty || isDeletingInsideComposingRegion || isReplacedByShorter) {
newComposingText = replacementSource.substring(replacementSourceStart, replacementSourceEnd);
originalComposingText = oldText.substring(replacementDestinationStart, replacementDestinationStart + replacementSourceEnd);
} else {
newComposingText = replacementSource.substring(replacementSourceStart, replacementSourceStart + (replacementDestinationEnd - replacementDestinationStart));
originalComposingText = oldText.substring(replacementDestinationStart, replacementDestinationEnd);
}
final bool isOriginalComposingRegionTextChanged = !(originalComposingText == newComposingText);
final bool isReplaced = isOriginalComposingRegionTextChanged ||
(isReplacedByLonger || isReplacedByShorter || isReplacedBySame);
if (isEqual) {
return TextEditingDeltaNonTextUpdate(
oldText: oldText,
selection: newSelection,
composing: newComposing,
);
} else if ((isDeletingByReplacingWithEmpty || isDeletingInsideComposingRegion) &&
!isOriginalComposingRegionTextChanged) { // Deletion.
int actualStart = replacementDestinationStart;
if (!isDeletionGreaterThanOne) {
actualStart = replacementDestinationEnd - 1;
}
return TextEditingDeltaDeletion(
oldText: oldText,
deletedRange: TextRange(
start: actualStart,
end: replacementDestinationEnd,
),
selection: newSelection,
composing: newComposing,
);
} else if ((replacementDestinationStart == replacementDestinationEnd || isInsertingInsideComposingRegion) &&
!isOriginalComposingRegionTextChanged) { // Insertion.
return TextEditingDeltaInsertion(
oldText: oldText,
textInserted: replacementSource.substring(replacementDestinationEnd - replacementDestinationStart, (replacementDestinationEnd - replacementDestinationStart) + (replacementSource.length - (replacementDestinationEnd - replacementDestinationStart))),
insertionOffset: replacementDestinationEnd,
selection: newSelection,
composing: newComposing,
);
} else if (isReplaced) { // Replacement.
return TextEditingDeltaReplacement(
oldText: oldText,
replacementText: replacementSource,
replacedRange: TextRange(
start: replacementDestinationStart,
end: replacementDestinationEnd,
),
selection: newSelection,
composing: newComposing,
);
}
assert(false);
return TextEditingDeltaNonTextUpdate(
oldText: oldText,
selection: newSelection,
composing: newComposing,
);
}
/// The old text state before the delta has occurred.
final String oldText;
/// The range of text that is currently selected after the delta has been
/// applied.
final TextSelection selection;
/// The range of text that is still being composed after the delta has been
/// applied.
final TextRange composing;
/// This method will take the given [TextEditingValue] and return a new
/// [TextEditingValue] with that instance of [TextEditingDelta] applied to it.
TextEditingValue apply(TextEditingValue value);
}
/// A structure representing an insertion of a single/or contiguous sequence of
/// characters at some offset of an editing state.
@immutable
class TextEditingDeltaInsertion extends TextEditingDelta {
/// Creates an insertion delta for a given change to the editing state.
///
/// {@template flutter.services.TextEditingDelta.optIn}
/// See also:
///
/// * [TextInputConfiguration], to opt-in your [DeltaTextInputClient] to receive
/// [TextEditingDelta]'s you must set [TextInputConfiguration.enableDeltaModel]
/// to true.
/// {@endtemplate}
const TextEditingDeltaInsertion({
required super.oldText,
required this.textInserted,
required this.insertionOffset,
required super.selection,
required super.composing,
});
/// The text that is being inserted into [oldText].
final String textInserted;
/// The offset in the [oldText] where the insertion begins.
final int insertionOffset;
@override
TextEditingValue apply(TextEditingValue value) {
// To stay inline with the plain text model we should follow a last write wins
// policy and apply the delta to the oldText. This is due to the asynchronous
// nature of the connection between the framework and platform text input plugins.
String newText = oldText;
assert(_debugTextRangeIsValid(TextRange.collapsed(insertionOffset), newText), 'Applying TextEditingDeltaInsertion failed, the insertionOffset: $insertionOffset is not within the bounds of $newText of length: ${newText.length}');
newText = _replace(newText, textInserted, TextRange.collapsed(insertionOffset));
assert(_debugTextRangeIsValid(selection, newText), 'Applying TextEditingDeltaInsertion failed, the selection range: $selection is not within the bounds of $newText of length: ${newText.length}');
assert(_debugTextRangeIsValid(composing, newText), 'Applying TextEditingDeltaInsertion failed, the composing range: $composing is not within the bounds of $newText of length: ${newText.length}');
return value.copyWith(text: newText, selection: selection, composing: composing);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('oldText', oldText));
properties.add(DiagnosticsProperty<String>('textInserted', textInserted));
properties.add(DiagnosticsProperty<int>('insertionOffset', insertionOffset));
properties.add(DiagnosticsProperty<TextSelection>('selection', selection));
properties.add(DiagnosticsProperty<TextRange>('composing', composing));
}
}
/// A structure representing the deletion of a single/or contiguous sequence of
/// characters in an editing state.
@immutable
class TextEditingDeltaDeletion extends TextEditingDelta {
/// Creates a deletion delta for a given change to the editing state.
///
/// {@macro flutter.services.TextEditingDelta.optIn}
const TextEditingDeltaDeletion({
required super.oldText,
required this.deletedRange,
required super.selection,
required super.composing,
});
/// The range in [oldText] that is being deleted.
final TextRange deletedRange;
/// The text from [oldText] that is being deleted.
String get textDeleted => oldText.substring(deletedRange.start, deletedRange.end);
@override
TextEditingValue apply(TextEditingValue value) {
// To stay inline with the plain text model we should follow a last write wins
// policy and apply the delta to the oldText. This is due to the asynchronous
// nature of the connection between the framework and platform text input plugins.
String newText = oldText;
assert(_debugTextRangeIsValid(deletedRange, newText), 'Applying TextEditingDeltaDeletion failed, the deletedRange: $deletedRange is not within the bounds of $newText of length: ${newText.length}');
newText = _replace(newText, '', deletedRange);
assert(_debugTextRangeIsValid(selection, newText), 'Applying TextEditingDeltaDeletion failed, the selection range: $selection is not within the bounds of $newText of length: ${newText.length}');
assert(_debugTextRangeIsValid(composing, newText), 'Applying TextEditingDeltaDeletion failed, the composing range: $composing is not within the bounds of $newText of length: ${newText.length}');
return value.copyWith(text: newText, selection: selection, composing: composing);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('oldText', oldText));
properties.add(DiagnosticsProperty<String>('textDeleted', textDeleted));
properties.add(DiagnosticsProperty<TextRange>('deletedRange', deletedRange));
properties.add(DiagnosticsProperty<TextSelection>('selection', selection));
properties.add(DiagnosticsProperty<TextRange>('composing', composing));
}
}
/// A structure representing a replacement of a range of characters with a
/// new sequence of text.
@immutable
class TextEditingDeltaReplacement extends TextEditingDelta {
/// Creates a replacement delta for a given change to the editing state.
///
/// The range that is being replaced can either grow or shrink based on the
/// given replacement text.
///
/// A replacement can occur in cases such as auto-correct, suggestions, and
/// when a selection is replaced by a single character.
///
/// {@macro flutter.services.TextEditingDelta.optIn}
const TextEditingDeltaReplacement({
required super.oldText,
required this.replacementText,
required this.replacedRange,
required super.selection,
required super.composing,
});
/// The new text that is replacing [replacedRange] in [oldText].
final String replacementText;
/// The range in [oldText] that is being replaced.
final TextRange replacedRange;
/// The original text that is being replaced in [oldText].
String get textReplaced => oldText.substring(replacedRange.start, replacedRange.end);
@override
TextEditingValue apply(TextEditingValue value) {
// To stay inline with the plain text model we should follow a last write wins
// policy and apply the delta to the oldText. This is due to the asynchronous
// nature of the connection between the framework and platform text input plugins.
String newText = oldText;
assert(_debugTextRangeIsValid(replacedRange, newText), 'Applying TextEditingDeltaReplacement failed, the replacedRange: $replacedRange is not within the bounds of $newText of length: ${newText.length}');
newText = _replace(newText, replacementText, replacedRange);
assert(_debugTextRangeIsValid(selection, newText), 'Applying TextEditingDeltaReplacement failed, the selection range: $selection is not within the bounds of $newText of length: ${newText.length}');
assert(_debugTextRangeIsValid(composing, newText), 'Applying TextEditingDeltaReplacement failed, the composing range: $composing is not within the bounds of $newText of length: ${newText.length}');
return value.copyWith(text: newText, selection: selection, composing: composing);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('oldText', oldText));
properties.add(DiagnosticsProperty<String>('textReplaced', textReplaced));
properties.add(DiagnosticsProperty<String>('replacementText', replacementText));
properties.add(DiagnosticsProperty<TextRange>('replacedRange', replacedRange));
properties.add(DiagnosticsProperty<TextSelection>('selection', selection));
properties.add(DiagnosticsProperty<TextRange>('composing', composing));
}
}
/// A structure representing changes to the selection and/or composing regions
/// of an editing state and no changes to the text value.
@immutable
class TextEditingDeltaNonTextUpdate extends TextEditingDelta {
/// Creates a delta representing no updates to the text value of the current
/// editing state. This delta includes updates to the selection and/or composing
/// regions.
///
/// A situation where this delta would be created is when dragging the selection
/// handles. There are no changes to the text, but there are updates to the selection
/// and potentially the composing region as well.
///
/// {@macro flutter.services.TextEditingDelta.optIn}
const TextEditingDeltaNonTextUpdate({
required super.oldText,
required super.selection,
required super.composing,
});
@override
TextEditingValue apply(TextEditingValue value) {
// To stay inline with the plain text model we should follow a last write wins
// policy and apply the delta to the oldText. This is due to the asynchronous
// nature of the connection between the framework and platform text input plugins.
assert(_debugTextRangeIsValid(selection, oldText), 'Applying TextEditingDeltaNonTextUpdate failed, the selection range: $selection is not within the bounds of $oldText of length: ${oldText.length}');
assert(_debugTextRangeIsValid(composing, oldText), 'Applying TextEditingDeltaNonTextUpdate failed, the composing region: $composing is not within the bounds of $oldText of length: ${oldText.length}');
return TextEditingValue(text: oldText, selection: selection, composing: composing);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('oldText', oldText));
properties.add(DiagnosticsProperty<TextSelection>('selection', selection));
properties.add(DiagnosticsProperty<TextRange>('composing', composing));
}
}
| flutter/packages/flutter/lib/src/services/text_editing_delta.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/text_editing_delta.dart",
"repo_id": "flutter",
"token_count": 6242
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
/// Annotates a region of the layer tree with a value.
///
/// See also:
///
/// * [Layer.find], for an example of how this value is retrieved.
/// * [AnnotatedRegionLayer], the layer pushed into the layer tree.
class AnnotatedRegion<T extends Object> extends SingleChildRenderObjectWidget {
/// Creates a new annotated region to insert [value] into the layer tree.
///
/// Neither [child] nor [value] may be null.
///
/// [sized] defaults to true and controls whether the annotated region will
/// clip its child.
const AnnotatedRegion({
super.key,
required Widget super.child,
required this.value,
this.sized = true,
});
/// A value which can be retrieved using [Layer.find].
final T value;
/// If false, the layer pushed into the tree will not be provided with a size.
///
/// An [AnnotatedRegionLayer] with a size checks that the offset provided in
/// [Layer.find] is within the bounds, returning null otherwise.
///
/// See also:
///
/// * [AnnotatedRegionLayer], for a description of this behavior.
final bool sized;
@override
RenderObject createRenderObject(BuildContext context) {
return RenderAnnotatedRegion<T>(value: value, sized: sized);
}
@override
void updateRenderObject(BuildContext context, RenderAnnotatedRegion<T> renderObject) {
renderObject
..value = value
..sized = sized;
}
}
| flutter/packages/flutter/lib/src/widgets/annotated_region.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/annotated_region.dart",
"repo_id": "flutter",
"token_count": 480
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:developer' show Timeline; // to disambiguate reference in dartdocs below
import 'package:flutter/foundation.dart';
import 'basic.dart';
import 'framework.dart';
import 'localizations.dart';
import 'lookup_boundary.dart';
import 'media_query.dart';
import 'overlay.dart';
import 'table.dart';
// Examples can assume:
// late BuildContext context;
// List<Widget> children = <Widget>[];
// List<Widget> items = <Widget>[];
// Any changes to this file should be reflected in the debugAssertAllWidgetVarsUnset()
// function below.
/// Log the dirty widgets that are built each frame.
///
/// Combined with [debugPrintBuildScope] or [debugPrintBeginFrameBanner], this
/// allows you to distinguish builds triggered by the initial mounting of a
/// widget tree (e.g. in a call to [runApp]) from the regular builds triggered
/// by the pipeline.
///
/// Combined with [debugPrintScheduleBuildForStacks], this lets you watch a
/// widget's dirty/clean lifecycle.
///
/// To get similar information but showing it on the timeline available from
/// Flutter DevTools rather than getting it in the console (where it can be
/// overwhelming), consider [debugProfileBuildsEnabled].
///
/// See also:
///
/// * [WidgetsBinding.drawFrame], which pumps the build and rendering pipeline
/// to generate a frame.
bool debugPrintRebuildDirtyWidgets = false;
/// Signature for [debugOnRebuildDirtyWidget] implementations.
typedef RebuildDirtyWidgetCallback = void Function(Element e, bool builtOnce);
/// Callback invoked for every dirty widget built each frame.
///
/// This callback is only invoked in debug builds.
///
/// See also:
///
/// * [debugPrintRebuildDirtyWidgets], which does something similar but logs
/// to the console instead of invoking a callback.
/// * [debugOnProfilePaint], which does something similar for [RenderObject]
/// painting.
/// * [WidgetInspectorService], which uses the [debugOnRebuildDirtyWidget]
/// callback to generate aggregate profile statistics describing which widget
/// rebuilds occurred when the
/// `ext.flutter.inspector.trackRebuildDirtyWidgets` service extension is
/// enabled.
RebuildDirtyWidgetCallback? debugOnRebuildDirtyWidget;
/// Log all calls to [BuildOwner.buildScope].
///
/// Combined with [debugPrintScheduleBuildForStacks], this allows you to track
/// when a [State.setState] call gets serviced.
///
/// Combined with [debugPrintRebuildDirtyWidgets] or
/// [debugPrintBeginFrameBanner], this allows you to distinguish builds
/// triggered by the initial mounting of a widget tree (e.g. in a call to
/// [runApp]) from the regular builds triggered by the pipeline.
///
/// See also:
///
/// * [WidgetsBinding.drawFrame], which pumps the build and rendering pipeline
/// to generate a frame.
bool debugPrintBuildScope = false;
/// Log the call stacks that mark widgets as needing to be rebuilt.
///
/// This is called whenever [BuildOwner.scheduleBuildFor] adds an element to the
/// dirty list. Typically this is as a result of [Element.markNeedsBuild] being
/// called, which itself is usually a result of [State.setState] being called.
///
/// To see when a widget is rebuilt, see [debugPrintRebuildDirtyWidgets].
///
/// To see when the dirty list is flushed, see [debugPrintBuildScope].
///
/// To see when a frame is scheduled, see [debugPrintScheduleFrameStacks].
bool debugPrintScheduleBuildForStacks = false;
/// Log when widgets with global keys are deactivated and log when they are
/// reactivated (retaken).
///
/// This can help track down framework bugs relating to the [GlobalKey] logic.
bool debugPrintGlobalKeyedWidgetLifecycle = false;
/// Adds [Timeline] events for every Widget built.
///
/// The timing information this flag exposes is not representative of the actual
/// cost of building, because the overhead of adding timeline events is
/// significant relative to the time each object takes to build. However, it can
/// expose unexpected widget behavior in the timeline.
///
/// In debug builds, additional information is included in the trace (such as
/// the properties of widgets being built). Collecting this data is
/// expensive and further makes these traces non-representative of actual
/// performance. This data is omitted in profile builds.
///
/// For more information about performance debugging in Flutter, see
/// <https://flutter.dev/docs/perf/rendering>.
///
/// See also:
///
/// * [debugPrintRebuildDirtyWidgets], which does something similar but
/// reporting the builds to the console.
/// * [debugProfileLayoutsEnabled], which does something similar for layout,
/// and [debugPrintLayouts], its console equivalent.
/// * [debugProfilePaintsEnabled], which does something similar for painting.
/// * [debugProfileBuildsEnabledUserWidgets], which adds events for user-created
/// [Widget] build times and incurs less overhead.
/// * [debugEnhanceBuildTimelineArguments], which enhances the trace with
/// debugging information related to [Widget] builds.
bool debugProfileBuildsEnabled = false;
/// Adds [Timeline] events for every user-created [Widget] built.
///
/// A user-created [Widget] is any [Widget] that is constructed in the root
/// library. Often [Widget]s contain child [Widget]s that are constructed in
/// libraries (for example, a [TextButton] having a [RichText] child). Timeline
/// events for those children will be omitted with this flag. This works for any
/// [Widget] not just ones declared in the root library.
///
/// See also:
///
/// * [debugProfileBuildsEnabled], which functions similarly but shows events
/// for every widget and has a higher overhead cost.
/// * [debugEnhanceBuildTimelineArguments], which enhances the trace with
/// debugging information related to [Widget] builds.
bool debugProfileBuildsEnabledUserWidgets = false;
/// Adds debugging information to [Timeline] events related to [Widget] builds.
///
/// This flag will only add [Timeline] event arguments for debug builds.
/// Additional arguments will be added for the "BUILD" [Timeline] event and for
/// all [Widget] build [Timeline] events, which are the [Timeline] events that
/// are added when either of [debugProfileBuildsEnabled] and
/// [debugProfileBuildsEnabledUserWidgets] are true. The debugging information
/// that will be added in trace arguments includes stats around [Widget] dirty
/// states and [Widget] diagnostic information (i.e. [Widget] properties).
///
/// See also:
///
/// * [debugProfileBuildsEnabled], which adds [Timeline] events for every
/// [Widget] built.
/// * [debugProfileBuildsEnabledUserWidgets], which adds [Timeline] events for
/// every user-created [Widget] built.
/// * [debugEnhanceLayoutTimelineArguments], which does something similar for
/// events related to [RenderObject] layouts.
/// * [debugEnhancePaintTimelineArguments], which does something similar for
/// events related to [RenderObject] paints.
bool debugEnhanceBuildTimelineArguments = false;
/// Show banners for deprecated widgets.
bool debugHighlightDeprecatedWidgets = false;
Key? _firstNonUniqueKey(Iterable<Widget> widgets) {
final Set<Key> keySet = HashSet<Key>();
for (final Widget widget in widgets) {
if (widget.key == null) {
continue;
}
if (!keySet.add(widget.key!)) {
return widget.key;
}
}
return null;
}
/// Asserts if the given child list contains any duplicate non-null keys.
///
/// To invoke this function, use the following pattern:
///
/// ```dart
/// class MyWidget extends StatelessWidget {
/// MyWidget({ super.key, required this.children }) {
/// assert(!debugChildrenHaveDuplicateKeys(this, children));
/// }
///
/// final List<Widget> children;
///
/// // ...
/// }
/// ```
///
/// If specified, the `message` overrides the default message.
///
/// For a version of this function that can be used in contexts where
/// the list of items does not have a particular parent, see
/// [debugItemsHaveDuplicateKeys].
///
/// Does nothing if asserts are disabled. Always returns false.
bool debugChildrenHaveDuplicateKeys(Widget parent, Iterable<Widget> children, { String? message }) {
assert(() {
final Key? nonUniqueKey = _firstNonUniqueKey(children);
if (nonUniqueKey != null) {
throw FlutterError(
"${message ?? 'Duplicate keys found.\n'
'If multiple keyed widgets exist as children of another widget, they must have unique keys.'}"
'\n$parent has multiple children with key $nonUniqueKey.',
);
}
return true;
}());
return false;
}
/// Asserts if the given list of items contains any duplicate non-null keys.
///
/// To invoke this function, use the following pattern:
///
/// ```dart
/// assert(!debugItemsHaveDuplicateKeys(items));
/// ```
///
/// For a version of this function specifically intended for parents
/// checking their children lists, see [debugChildrenHaveDuplicateKeys].
///
/// Does nothing if asserts are disabled. Always returns false.
bool debugItemsHaveDuplicateKeys(Iterable<Widget> items) {
assert(() {
final Key? nonUniqueKey = _firstNonUniqueKey(items);
if (nonUniqueKey != null) {
throw FlutterError('Duplicate key found: $nonUniqueKey.');
}
return true;
}());
return false;
}
/// Asserts that the given context has a [Table] ancestor.
///
/// Used by [TableRowInkWell] to make sure that it is only used in an appropriate context.
///
/// To invoke this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasTable(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This method can be expensive (it walks the element tree).
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasTable(BuildContext context) {
assert(() {
if (context.widget is! Table && context.findAncestorWidgetOfExactType<Table>() == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Table widget found.'),
ErrorDescription('${context.widget.runtimeType} widgets require a Table widget ancestor.'),
context.describeWidget('The specific widget that could not find a Table ancestor was'),
context.describeOwnershipChain('The ownership chain for the affected widget is'),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has a [MediaQuery] ancestor.
///
/// Used by various widgets to make sure that they are only used in an
/// appropriate context.
///
/// To invoke this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasMediaQuery(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasMediaQuery(BuildContext context) {
assert(() {
if (context.widget is! MediaQuery && context.getElementForInheritedWidgetOfExactType<MediaQuery>() == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No MediaQuery widget ancestor found.'),
ErrorDescription('${context.widget.runtimeType} widgets require a MediaQuery widget ancestor.'),
context.describeWidget('The specific widget that could not find a MediaQuery ancestor was'),
context.describeOwnershipChain('The ownership chain for the affected widget is'),
ErrorHint(
'No MediaQuery ancestor could be found starting from the context '
'that was passed to MediaQuery.of(). This can happen because the '
'context used is not a descendant of a View widget, which introduces '
'a MediaQuery.'
),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has a [Directionality] ancestor.
///
/// Used by various widgets to make sure that they are only used in an
/// appropriate context.
///
/// To invoke this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasDirectionality(context));
/// ```
///
/// To improve the error messages you can add some extra color using the
/// named arguments.
///
/// * why: explain why the direction is needed, for example "to resolve
/// the 'alignment' argument". Should be an adverb phrase describing why.
/// * hint: explain why this might be happening, for example "The default
/// value of the 'alignment' argument of the $runtimeType widget is an
/// AlignmentDirectional value.". Should be a fully punctuated sentence.
/// * alternative: provide additional advice specific to the situation,
/// especially an alternative to providing a Directionality ancestor.
/// For example, "Alternatively, consider specifying the 'textDirection'
/// argument.". Should be a fully punctuated sentence.
///
/// Each one can be null, in which case it is skipped (this is the default).
/// If they are non-null, they are included in the order above, interspersed
/// with the more generic advice regarding [Directionality].
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasDirectionality(BuildContext context, { String? why, String? hint, String? alternative }) {
assert(() {
if (context.widget is! Directionality && context.getElementForInheritedWidgetOfExactType<Directionality>() == null) {
why = why == null ? '' : ' $why';
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Directionality widget found.'),
ErrorDescription('${context.widget.runtimeType} widgets require a Directionality widget ancestor$why.\n'),
if (hint != null)
ErrorHint(hint),
context.describeWidget('The specific widget that could not find a Directionality ancestor was'),
context.describeOwnershipChain('The ownership chain for the affected widget is'),
ErrorHint(
'Typically, the Directionality widget is introduced by the MaterialApp '
'or WidgetsApp widget at the top of your application widget tree. It '
'determines the ambient reading direction and is used, for example, to '
'determine how to lay out text, how to interpret "start" and "end" '
'values, and to resolve EdgeInsetsDirectional, '
'AlignmentDirectional, and other *Directional objects.',
),
if (alternative != null)
ErrorHint(alternative),
]);
}
return true;
}());
return true;
}
/// Asserts that the `built` widget is not null.
///
/// Used when the given `widget` calls a builder function to check that the
/// function returned a non-null value, as typically required.
///
/// Does nothing when asserts are disabled.
void debugWidgetBuilderValue(Widget widget, Widget? built) {
assert(() {
if (built == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A build function returned null.'),
DiagnosticsProperty<Widget>('The offending widget is', widget, style: DiagnosticsTreeStyle.errorProperty),
ErrorDescription('Build functions must never return null.'),
ErrorHint(
'To return an empty space that causes the building widget to fill available room, return "Container()". '
'To return an empty space that takes as little room as possible, return "Container(width: 0.0, height: 0.0)".',
),
]);
}
if (widget == built) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A build function returned context.widget.'),
DiagnosticsProperty<Widget>('The offending widget is', widget, style: DiagnosticsTreeStyle.errorProperty),
ErrorDescription(
'Build functions must never return their BuildContext parameter\'s widget or a child that contains "context.widget". '
'Doing so introduces a loop in the widget tree that can cause the app to crash.',
),
]);
}
return true;
}());
}
/// Asserts that the given context has a [Localizations] ancestor that contains
/// a [WidgetsLocalizations] delegate.
///
/// To call this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasWidgetsLocalizations(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasWidgetsLocalizations(BuildContext context) {
assert(() {
if (Localizations.of<WidgetsLocalizations>(context, WidgetsLocalizations) == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No WidgetsLocalizations found.'),
ErrorDescription(
'${context.widget.runtimeType} widgets require WidgetsLocalizations '
'to be provided by a Localizations widget ancestor.',
),
ErrorDescription(
'The widgets library uses Localizations to generate messages, '
'labels, and abbreviations.',
),
ErrorHint(
'To introduce a WidgetsLocalizations, either use a '
'WidgetsApp at the root of your application to include them '
'automatically, or add a Localization widget with a '
'WidgetsLocalizations delegate.',
),
...context.describeMissingAncestor(expectedAncestorType: WidgetsLocalizations),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has an [Overlay] ancestor.
///
/// To call this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasOverlay(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This method can be expensive (it walks the element tree).
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasOverlay(BuildContext context) {
assert(() {
if (LookupBoundary.findAncestorWidgetOfExactType<Overlay>(context) == null) {
final bool hiddenByBoundary = LookupBoundary.debugIsHidingAncestorWidgetOfExactType<Overlay>(context);
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Overlay widget found${hiddenByBoundary ? ' within the closest LookupBoundary' : ''}.'),
if (hiddenByBoundary)
ErrorDescription(
'There is an ancestor Overlay widget, but it is hidden by a LookupBoundary.'
),
ErrorDescription(
'${context.widget.runtimeType} widgets require an Overlay '
'widget ancestor within the closest LookupBoundary.\n'
'An overlay lets widgets float on top of other widget children.',
),
ErrorHint(
'To introduce an Overlay widget, you can either directly '
'include one, or use a widget that contains an Overlay itself, '
'such as a Navigator, WidgetApp, MaterialApp, or CupertinoApp.',
),
...context.describeMissingAncestor(expectedAncestorType: Overlay),
]);
}
return true;
}());
return true;
}
/// Returns true if none of the widget library debug variables have been changed.
///
/// This function is used by the test framework to ensure that debug variables
/// haven't been inadvertently changed.
///
/// See [the widgets library](widgets/widgets-library.html) for a complete list.
bool debugAssertAllWidgetVarsUnset(String reason) {
assert(() {
if (debugPrintRebuildDirtyWidgets ||
debugPrintBuildScope ||
debugPrintScheduleBuildForStacks ||
debugPrintGlobalKeyedWidgetLifecycle ||
debugProfileBuildsEnabled ||
debugHighlightDeprecatedWidgets ||
debugProfileBuildsEnabledUserWidgets) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
| flutter/packages/flutter/lib/src/widgets/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/debug.dart",
"repo_id": "flutter",
"token_count": 6176
} | 671 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'navigator.dart';
import 'pop_scope.dart';
import 'restoration.dart';
import 'restoration_properties.dart';
import 'routes.dart';
import 'will_pop_scope.dart';
// Duration for delay before announcement in IOS so that the announcement won't be interrupted.
const Duration _kIOSAnnouncementDelayDuration = Duration(seconds: 1);
// Examples can assume:
// late BuildContext context;
/// An optional container for grouping together multiple form field widgets
/// (e.g. [TextField] widgets).
///
/// Each individual form field should be wrapped in a [FormField] widget, with
/// the [Form] widget as a common ancestor of all of those. Call methods on
/// [FormState] to save, reset, or validate each [FormField] that is a
/// descendant of this [Form]. To obtain the [FormState], you may use [Form.of]
/// with a context whose ancestor is the [Form], or pass a [GlobalKey] to the
/// [Form] constructor and call [GlobalKey.currentState].
///
/// {@tool dartpad}
/// This example shows a [Form] with one [TextFormField] to enter an email
/// address and an [ElevatedButton] to submit the form. A [GlobalKey] is used here
/// to identify the [Form] and validate input.
///
/// 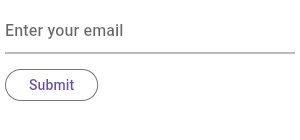
///
/// ** See code in examples/api/lib/widgets/form/form.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [GlobalKey], a key that is unique across the entire app.
/// * [FormField], a single form field widget that maintains the current state.
/// * [TextFormField], a convenience widget that wraps a [TextField] widget in a [FormField].
class Form extends StatefulWidget {
/// Creates a container for form fields.
const Form({
super.key,
required this.child,
this.canPop,
this.onPopInvoked,
@Deprecated(
'Use canPop and/or onPopInvoked instead. '
'This feature was deprecated after v3.12.0-1.0.pre.',
)
this.onWillPop,
this.onChanged,
AutovalidateMode? autovalidateMode,
}) : autovalidateMode = autovalidateMode ?? AutovalidateMode.disabled,
assert((onPopInvoked == null && canPop == null) || onWillPop == null, 'onWillPop is deprecated; use canPop and/or onPopInvoked.');
/// Returns the [FormState] of the closest [Form] widget which encloses the
/// given context, or null if none is found.
///
/// Typical usage is as follows:
///
/// ```dart
/// FormState? form = Form.maybeOf(context);
/// form?.save();
/// ```
///
/// Calling this method will create a dependency on the closest [Form] in the
/// [context], if there is one.
///
/// See also:
///
/// * [Form.of], which is similar to this method, but asserts if no [Form]
/// ancestor is found.
static FormState? maybeOf(BuildContext context) {
final _FormScope? scope = context.dependOnInheritedWidgetOfExactType<_FormScope>();
return scope?._formState;
}
/// Returns the [FormState] of the closest [Form] widget which encloses the
/// given context.
///
/// Typical usage is as follows:
///
/// ```dart
/// FormState form = Form.of(context);
/// form.save();
/// ```
///
/// If no [Form] ancestor is found, this will assert in debug mode, and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest [Form] in the
/// [context].
///
/// See also:
///
/// * [Form.maybeOf], which is similar to this method, but returns null if no
/// [Form] ancestor is found.
static FormState of(BuildContext context) {
final FormState? formState = maybeOf(context);
assert(() {
if (formState == null) {
throw FlutterError(
'Form.of() was called with a context that does not contain a Form widget.\n'
'No Form widget ancestor could be found starting from the context that '
'was passed to Form.of(). This can happen because you are using a widget '
'that looks for a Form ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return formState!;
}
/// The widget below this widget in the tree.
///
/// This is the root of the widget hierarchy that contains this form.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Enables the form to veto attempts by the user to dismiss the [ModalRoute]
/// that contains the form.
///
/// If the callback returns a Future that resolves to false, the form's route
/// will not be popped.
///
/// See also:
///
/// * [WillPopScope], another widget that provides a way to intercept the
/// back button.
@Deprecated(
'Use canPop and/or onPopInvoked instead. '
'This feature was deprecated after v3.12.0-1.0.pre.',
)
final WillPopCallback? onWillPop;
/// {@macro flutter.widgets.PopScope.canPop}
///
/// {@tool dartpad}
/// This sample demonstrates how to use this parameter to show a confirmation
/// dialog when a navigation pop would cause form data to be lost.
///
/// ** See code in examples/api/lib/widgets/form/form.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [onPopInvoked], which also comes from [PopScope] and is often used in
/// conjunction with this parameter.
/// * [PopScope.canPop], which is what [Form] delegates to internally.
final bool? canPop;
/// {@macro flutter.widgets.navigator.onPopInvoked}
///
/// {@tool dartpad}
/// This sample demonstrates how to use this parameter to show a confirmation
/// dialog when a navigation pop would cause form data to be lost.
///
/// ** See code in examples/api/lib/widgets/form/form.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [canPop], which also comes from [PopScope] and is often used in
/// conjunction with this parameter.
/// * [PopScope.onPopInvoked], which is what [Form] delegates to internally.
final PopInvokedCallback? onPopInvoked;
/// Called when one of the form fields changes.
///
/// In addition to this callback being invoked, all the form fields themselves
/// will rebuild.
final VoidCallback? onChanged;
/// Used to enable/disable form fields auto validation and update their error
/// text.
///
/// {@macro flutter.widgets.FormField.autovalidateMode}
final AutovalidateMode autovalidateMode;
@override
FormState createState() => FormState();
}
/// State associated with a [Form] widget.
///
/// A [FormState] object can be used to [save], [reset], and [validate] every
/// [FormField] that is a descendant of the associated [Form].
///
/// Typically obtained via [Form.of].
class FormState extends State<Form> {
int _generation = 0;
bool _hasInteractedByUser = false;
final Set<FormFieldState<dynamic>> _fields = <FormFieldState<dynamic>>{};
// Called when a form field has changed. This will cause all form fields
// to rebuild, useful if form fields have interdependencies.
void _fieldDidChange() {
widget.onChanged?.call();
_hasInteractedByUser = _fields
.any((FormFieldState<dynamic> field) => field._hasInteractedByUser.value);
_forceRebuild();
}
void _forceRebuild() {
setState(() {
++_generation;
});
}
void _register(FormFieldState<dynamic> field) {
_fields.add(field);
}
void _unregister(FormFieldState<dynamic> field) {
_fields.remove(field);
}
@override
Widget build(BuildContext context) {
switch (widget.autovalidateMode) {
case AutovalidateMode.always:
_validate();
case AutovalidateMode.onUserInteraction:
if (_hasInteractedByUser) {
_validate();
}
case AutovalidateMode.disabled:
break;
}
if (widget.canPop != null || widget.onPopInvoked != null) {
return PopScope(
canPop: widget.canPop ?? true,
onPopInvoked: widget.onPopInvoked,
child: _FormScope(
formState: this,
generation: _generation,
child: widget.child,
),
);
}
return WillPopScope(
onWillPop: widget.onWillPop,
child: _FormScope(
formState: this,
generation: _generation,
child: widget.child,
),
);
}
/// Saves every [FormField] that is a descendant of this [Form].
void save() {
for (final FormFieldState<dynamic> field in _fields) {
field.save();
}
}
/// Resets every [FormField] that is a descendant of this [Form] back to its
/// [FormField.initialValue].
///
/// The [Form.onChanged] callback will be called.
///
/// If the form's [Form.autovalidateMode] property is [AutovalidateMode.always],
/// the fields will all be revalidated after being reset.
void reset() {
for (final FormFieldState<dynamic> field in _fields) {
field.reset();
}
_hasInteractedByUser = false;
_fieldDidChange();
}
/// Validates every [FormField] that is a descendant of this [Form], and
/// returns true if there are no errors.
///
/// The form will rebuild to report the results.
///
/// See also:
/// * [validateGranularly], which also validates descendant [FormField]s,
/// but instead returns a [Set] of fields with errors.
bool validate() {
_hasInteractedByUser = true;
_forceRebuild();
return _validate();
}
/// Validates every [FormField] that is a descendant of this [Form], and
/// returns a [Set] of [FormFieldState] of the invalid field(s) only, if any.
///
/// This method can be useful to highlight field(s) with errors.
///
/// The form will rebuild to report the results.
///
/// See also:
/// * [validate], which also validates descendant [FormField]s,
/// and return true if there are no errors.
Set<FormFieldState<Object?>> validateGranularly() {
final Set<FormFieldState<Object?>> invalidFields = <FormFieldState<Object?>>{};
_hasInteractedByUser = true;
_forceRebuild();
_validate(invalidFields);
return invalidFields;
}
bool _validate([Set<FormFieldState<Object?>>? invalidFields]) {
bool hasError = false;
String errorMessage = '';
for (final FormFieldState<dynamic> field in _fields) {
final bool isFieldValid = field.validate();
hasError = !isFieldValid || hasError;
errorMessage += field.errorText ?? '';
if (invalidFields != null && !isFieldValid) {
invalidFields.add(field);
}
}
if (errorMessage.isNotEmpty) {
final TextDirection directionality = Directionality.of(context);
if (defaultTargetPlatform == TargetPlatform.iOS) {
unawaited(Future<void>(() async {
await Future<void>.delayed(_kIOSAnnouncementDelayDuration);
SemanticsService.announce(errorMessage, directionality, assertiveness: Assertiveness.assertive);
}));
} else {
SemanticsService.announce(errorMessage, directionality, assertiveness: Assertiveness.assertive);
}
}
return !hasError;
}
}
class _FormScope extends InheritedWidget {
const _FormScope({
required super.child,
required FormState formState,
required int generation,
}) : _formState = formState,
_generation = generation;
final FormState _formState;
/// Incremented every time a form field has changed. This lets us know when
/// to rebuild the form.
final int _generation;
/// The [Form] associated with this widget.
Form get form => _formState.widget;
@override
bool updateShouldNotify(_FormScope old) => _generation != old._generation;
}
/// Signature for validating a form field.
///
/// Returns an error string to display if the input is invalid, or null
/// otherwise.
///
/// Used by [FormField.validator].
typedef FormFieldValidator<T> = String? Function(T? value);
/// Signature for being notified when a form field changes value.
///
/// Used by [FormField.onSaved].
typedef FormFieldSetter<T> = void Function(T? newValue);
/// Signature for building the widget representing the form field.
///
/// Used by [FormField.builder].
typedef FormFieldBuilder<T> = Widget Function(FormFieldState<T> field);
/// A single form field.
///
/// This widget maintains the current state of the form field, so that updates
/// and validation errors are visually reflected in the UI.
///
/// When used inside a [Form], you can use methods on [FormState] to query or
/// manipulate the form data as a whole. For example, calling [FormState.save]
/// will invoke each [FormField]'s [onSaved] callback in turn.
///
/// Use a [GlobalKey] with [FormField] if you want to retrieve its current
/// state, for example if you want one form field to depend on another.
///
/// A [Form] ancestor is not required. The [Form] allows one to
/// save, reset, or validate multiple fields at once. To use without a [Form],
/// pass a [GlobalKey] to the constructor and use [GlobalKey.currentState] to
/// save or reset the form field.
///
/// See also:
///
/// * [Form], which is the widget that aggregates the form fields.
/// * [TextField], which is a commonly used form field for entering text.
class FormField<T> extends StatefulWidget {
/// Creates a single form field.
const FormField({
super.key,
required this.builder,
this.onSaved,
this.validator,
this.initialValue,
this.enabled = true,
AutovalidateMode? autovalidateMode,
this.restorationId,
}) : autovalidateMode = autovalidateMode ?? AutovalidateMode.disabled;
/// An optional method to call with the final value when the form is saved via
/// [FormState.save].
final FormFieldSetter<T>? onSaved;
/// An optional method that validates an input. Returns an error string to
/// display if the input is invalid, or null otherwise.
///
/// The returned value is exposed by the [FormFieldState.errorText] property.
/// The [TextFormField] uses this to override the [InputDecoration.errorText]
/// value.
///
/// Alternating between error and normal state can cause the height of the
/// [TextFormField] to change if no other subtext decoration is set on the
/// field. To create a field whose height is fixed regardless of whether or
/// not an error is displayed, either wrap the [TextFormField] in a fixed
/// height parent like [SizedBox], or set the [InputDecoration.helperText]
/// parameter to a space.
final FormFieldValidator<T>? validator;
/// Function that returns the widget representing this form field. It is
/// passed the form field state as input, containing the current value and
/// validation state of this field.
final FormFieldBuilder<T> builder;
/// An optional value to initialize the form field to, or null otherwise.
///
/// This is called `value` in the [DropdownButtonFormField] constructor to be
/// consistent with [DropdownButton].
final T? initialValue;
/// Whether the form is able to receive user input.
///
/// Defaults to true. If [autovalidateMode] is not [AutovalidateMode.disabled],
/// the field will be auto validated. Likewise, if this field is false, the widget
/// will not be validated regardless of [autovalidateMode].
final bool enabled;
/// Used to enable/disable this form field auto validation and update its
/// error text.
///
/// {@template flutter.widgets.FormField.autovalidateMode}
/// If [AutovalidateMode.onUserInteraction], this FormField will only
/// auto-validate after its content changes. If [AutovalidateMode.always], it
/// will auto-validate even without user interaction. If
/// [AutovalidateMode.disabled], auto-validation will be disabled.
///
/// Defaults to [AutovalidateMode.disabled].
/// {@endtemplate}
final AutovalidateMode autovalidateMode;
/// Restoration ID to save and restore the state of the form field.
///
/// Setting the restoration ID to a non-null value results in whether or not
/// the form field validation persists.
///
/// The state of this widget is persisted in a [RestorationBucket] claimed
/// from the surrounding [RestorationScope] using the provided restoration ID.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
final String? restorationId;
@override
FormFieldState<T> createState() => FormFieldState<T>();
}
/// The current state of a [FormField]. Passed to the [FormFieldBuilder] method
/// for use in constructing the form field's widget.
class FormFieldState<T> extends State<FormField<T>> with RestorationMixin {
late T? _value = widget.initialValue;
final RestorableStringN _errorText = RestorableStringN(null);
final RestorableBool _hasInteractedByUser = RestorableBool(false);
/// The current value of the form field.
T? get value => _value;
/// The current validation error returned by the [FormField.validator]
/// callback, or null if no errors have been triggered. This only updates when
/// [validate] is called.
String? get errorText => _errorText.value;
/// True if this field has any validation errors.
bool get hasError => _errorText.value != null;
/// Returns true if the user has modified the value of this field.
///
/// This only updates to true once [didChange] has been called and resets to
/// false when [reset] is called.
bool get hasInteractedByUser => _hasInteractedByUser.value;
/// True if the current value is valid.
///
/// This will not set [errorText] or [hasError] and it will not update
/// error display.
///
/// See also:
///
/// * [validate], which may update [errorText] and [hasError].
bool get isValid => widget.validator?.call(_value) == null;
/// Calls the [FormField]'s onSaved method with the current value.
void save() {
widget.onSaved?.call(value);
}
/// Resets the field to its initial value.
void reset() {
setState(() {
_value = widget.initialValue;
_hasInteractedByUser.value = false;
_errorText.value = null;
});
Form.maybeOf(context)?._fieldDidChange();
}
/// Calls [FormField.validator] to set the [errorText]. Returns true if there
/// were no errors.
///
/// See also:
///
/// * [isValid], which passively gets the validity without setting
/// [errorText] or [hasError].
bool validate() {
setState(() {
_validate();
});
return !hasError;
}
void _validate() {
if (widget.validator != null) {
_errorText.value = widget.validator!(_value);
} else {
_errorText.value = null;
}
}
/// Updates this field's state to the new value. Useful for responding to
/// child widget changes, e.g. [Slider]'s [Slider.onChanged] argument.
///
/// Triggers the [Form.onChanged] callback and, if [Form.autovalidateMode] is
/// [AutovalidateMode.always] or [AutovalidateMode.onUserInteraction],
/// revalidates all the fields of the form.
void didChange(T? value) {
setState(() {
_value = value;
_hasInteractedByUser.value = true;
});
Form.maybeOf(context)?._fieldDidChange();
}
/// Sets the value associated with this form field.
///
/// This method should only be called by subclasses that need to update
/// the form field value due to state changes identified during the widget
/// build phase, when calling `setState` is prohibited. In all other cases,
/// the value should be set by a call to [didChange], which ensures that
/// `setState` is called.
@protected
// ignore: use_setters_to_change_properties, (API predates enforcing the lint)
void setValue(T? value) {
_value = value;
}
@override
String? get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_errorText, 'error_text');
registerForRestoration(_hasInteractedByUser, 'has_interacted_by_user');
}
@override
void deactivate() {
Form.maybeOf(context)?._unregister(this);
super.deactivate();
}
@override
void dispose() {
_errorText.dispose();
_hasInteractedByUser.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
if (widget.enabled) {
switch (widget.autovalidateMode) {
case AutovalidateMode.always:
_validate();
case AutovalidateMode.onUserInteraction:
if (_hasInteractedByUser.value) {
_validate();
}
case AutovalidateMode.disabled:
break;
}
}
Form.maybeOf(context)?._register(this);
return widget.builder(this);
}
}
/// Used to configure the auto validation of [FormField] and [Form] widgets.
enum AutovalidateMode {
/// No auto validation will occur.
disabled,
/// Used to auto-validate [Form] and [FormField] even without user interaction.
always,
/// Used to auto-validate [Form] and [FormField] only after each user
/// interaction.
onUserInteraction,
}
| flutter/packages/flutter/lib/src/widgets/form.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/form.dart",
"repo_id": "flutter",
"token_count": 6753
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart' show clampDouble;
import 'package:flutter/gestures.dart';
import 'package:flutter/physics.dart';
import 'package:vector_math/vector_math_64.dart' show Matrix4, Quad, Vector3;
import 'basic.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'layout_builder.dart';
import 'ticker_provider.dart';
// Examples can assume:
// late BuildContext context;
// late Offset? _childWasTappedAt;
// late TransformationController _transformationController;
// Widget child = const Placeholder();
/// A signature for widget builders that take a [Quad] of the current viewport.
///
/// See also:
///
/// * [InteractiveViewer.builder], whose builder is of this type.
/// * [WidgetBuilder], which is similar, but takes no viewport.
typedef InteractiveViewerWidgetBuilder = Widget Function(BuildContext context, Quad viewport);
/// A widget that enables pan and zoom interactions with its child.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=zrn7V3bMJvg}
///
/// The user can transform the child by dragging to pan or pinching to zoom.
///
/// By default, InteractiveViewer clips its child using [Clip.hardEdge].
/// To prevent this behavior, consider setting [clipBehavior] to [Clip.none].
/// When [clipBehavior] is [Clip.none], InteractiveViewer may draw outside of
/// its original area of the screen, such as when a child is zoomed in and
/// increases in size. However, it will not receive gestures outside of its original area.
/// To prevent dead areas where InteractiveViewer does not receive gestures,
/// don't set [clipBehavior] or be sure that the InteractiveViewer widget is the
/// size of the area that should be interactive.
///
/// See also:
/// * The [Flutter Gallery's transformations demo](https://github.com/flutter/gallery/blob/main/lib/demos/reference/transformations_demo.dart),
/// which includes the use of InteractiveViewer.
/// * The [flutter-go demo](https://github.com/justinmc/flutter-go), which includes robust positioning of an InteractiveViewer child
/// that works for all screen sizes and child sizes.
/// * The [Lazy Flutter Performance Session](https://www.youtube.com/watch?v=qax_nOpgz7E), which includes the use of an InteractiveViewer to
/// performantly view subsets of a large set of widgets using the builder constructor.
///
/// {@tool dartpad}
/// This example shows a simple Container that can be panned and zoomed.
///
/// ** See code in examples/api/lib/widgets/interactive_viewer/interactive_viewer.0.dart **
/// {@end-tool}
@immutable
class InteractiveViewer extends StatefulWidget {
/// Create an InteractiveViewer.
InteractiveViewer({
super.key,
this.clipBehavior = Clip.hardEdge,
this.panAxis = PanAxis.free,
this.boundaryMargin = EdgeInsets.zero,
this.constrained = true,
// These default scale values were eyeballed as reasonable limits for common
// use cases.
this.maxScale = 2.5,
this.minScale = 0.8,
this.interactionEndFrictionCoefficient = _kDrag,
this.onInteractionEnd,
this.onInteractionStart,
this.onInteractionUpdate,
this.panEnabled = true,
this.scaleEnabled = true,
this.scaleFactor = kDefaultMouseScrollToScaleFactor,
this.transformationController,
this.alignment,
this.trackpadScrollCausesScale = false,
required Widget this.child,
}) : assert(minScale > 0),
assert(interactionEndFrictionCoefficient > 0),
assert(minScale.isFinite),
assert(maxScale > 0),
assert(!maxScale.isNaN),
assert(maxScale >= minScale),
// boundaryMargin must be either fully infinite or fully finite, but not
// a mix of both.
assert(
(boundaryMargin.horizontal.isInfinite
&& boundaryMargin.vertical.isInfinite) || (boundaryMargin.top.isFinite
&& boundaryMargin.right.isFinite && boundaryMargin.bottom.isFinite
&& boundaryMargin.left.isFinite),
),
builder = null;
/// Creates an InteractiveViewer for a child that is created on demand.
///
/// Can be used to render a child that changes in response to the current
/// transformation.
///
/// See the [builder] attribute docs for an example of using it to optimize a
/// large child.
InteractiveViewer.builder({
super.key,
this.clipBehavior = Clip.hardEdge,
this.panAxis = PanAxis.free,
this.boundaryMargin = EdgeInsets.zero,
// These default scale values were eyeballed as reasonable limits for common
// use cases.
this.maxScale = 2.5,
this.minScale = 0.8,
this.interactionEndFrictionCoefficient = _kDrag,
this.onInteractionEnd,
this.onInteractionStart,
this.onInteractionUpdate,
this.panEnabled = true,
this.scaleEnabled = true,
this.scaleFactor = 200.0,
this.transformationController,
this.alignment,
this.trackpadScrollCausesScale = false,
required InteractiveViewerWidgetBuilder this.builder,
}) : assert(minScale > 0),
assert(interactionEndFrictionCoefficient > 0),
assert(minScale.isFinite),
assert(maxScale > 0),
assert(!maxScale.isNaN),
assert(maxScale >= minScale),
// boundaryMargin must be either fully infinite or fully finite, but not
// a mix of both.
assert(
(boundaryMargin.horizontal.isInfinite && boundaryMargin.vertical.isInfinite) ||
(boundaryMargin.top.isFinite &&
boundaryMargin.right.isFinite &&
boundaryMargin.bottom.isFinite &&
boundaryMargin.left.isFinite),
),
constrained = false,
child = null;
/// The alignment of the child's origin, relative to the size of the box.
final Alignment? alignment;
/// If set to [Clip.none], the child may extend beyond the size of the InteractiveViewer,
/// but it will not receive gestures in these areas.
/// Be sure that the InteractiveViewer is the desired size when using [Clip.none].
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// When set to [PanAxis.aligned], panning is only allowed in the horizontal
/// axis or the vertical axis, diagonal panning is not allowed.
///
/// When set to [PanAxis.vertical] or [PanAxis.horizontal] panning is only
/// allowed in the specified axis. For example, if set to [PanAxis.vertical],
/// panning will only be allowed in the vertical axis. And if set to [PanAxis.horizontal],
/// panning will only be allowed in the horizontal axis.
///
/// When set to [PanAxis.free] panning is allowed in all directions.
///
/// Defaults to [PanAxis.free].
final PanAxis panAxis;
/// A margin for the visible boundaries of the child.
///
/// Any transformation that results in the viewport being able to view outside
/// of the boundaries will be stopped at the boundary. The boundaries do not
/// rotate with the rest of the scene, so they are always aligned with the
/// viewport.
///
/// To produce no boundaries at all, pass infinite [EdgeInsets], such as
/// `EdgeInsets.all(double.infinity)`.
///
/// No edge can be NaN.
///
/// Defaults to [EdgeInsets.zero], which results in boundaries that are the
/// exact same size and position as the [child].
final EdgeInsets boundaryMargin;
/// Builds the child of this widget.
///
/// Passed with the [InteractiveViewer.builder] constructor. Otherwise, the
/// [child] parameter must be passed directly, and this is null.
///
/// {@tool dartpad}
/// This example shows how to use builder to create a [Table] whose cell
/// contents are only built when they are visible. Built and remove cells are
/// logged in the console for illustration.
///
/// ** See code in examples/api/lib/widgets/interactive_viewer/interactive_viewer.builder.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListView.builder], which follows a similar pattern.
final InteractiveViewerWidgetBuilder? builder;
/// The child [Widget] that is transformed by InteractiveViewer.
///
/// If the [InteractiveViewer.builder] constructor is used, then this will be
/// null, otherwise it is required.
final Widget? child;
/// Whether the normal size constraints at this point in the widget tree are
/// applied to the child.
///
/// If set to false, then the child will be given infinite constraints. This
/// is often useful when a child should be bigger than the InteractiveViewer.
///
/// For example, for a child which is bigger than the viewport but can be
/// panned to reveal parts that were initially offscreen, [constrained] must
/// be set to false to allow it to size itself properly. If [constrained] is
/// true and the child can only size itself to the viewport, then areas
/// initially outside of the viewport will not be able to receive user
/// interaction events. If experiencing regions of the child that are not
/// receptive to user gestures, make sure [constrained] is false and the child
/// is sized properly.
///
/// Defaults to true.
///
/// {@tool dartpad}
/// This example shows how to create a pannable table. Because the table is
/// larger than the entire screen, setting [constrained] to false is necessary
/// to allow it to be drawn to its full size. The parts of the table that
/// exceed the screen size can then be panned into view.
///
/// ** See code in examples/api/lib/widgets/interactive_viewer/interactive_viewer.constrained.0.dart **
/// {@end-tool}
final bool constrained;
/// If false, the user will be prevented from panning.
///
/// Defaults to true.
///
/// See also:
///
/// * [scaleEnabled], which is similar but for scale.
final bool panEnabled;
/// If false, the user will be prevented from scaling.
///
/// Defaults to true.
///
/// See also:
///
/// * [panEnabled], which is similar but for panning.
final bool scaleEnabled;
/// {@macro flutter.gestures.scale.trackpadScrollCausesScale}
final bool trackpadScrollCausesScale;
/// Determines the amount of scale to be performed per pointer scroll.
///
/// Defaults to [kDefaultMouseScrollToScaleFactor].
///
/// Increasing this value above the default causes scaling to feel slower,
/// while decreasing it causes scaling to feel faster.
///
/// The amount of scale is calculated as the exponential function of the
/// [PointerScrollEvent.scrollDelta] to [scaleFactor] ratio. In the Flutter
/// engine, the mousewheel [PointerScrollEvent.scrollDelta] is hardcoded to 20
/// per scroll, while a trackpad scroll can be any amount.
///
/// Affects only pointer device scrolling, not pinch to zoom.
final double scaleFactor;
/// The maximum allowed scale.
///
/// The scale will be clamped between this and [minScale] inclusively.
///
/// Defaults to 2.5.
///
/// Must be greater than zero and greater than [minScale].
final double maxScale;
/// The minimum allowed scale.
///
/// The scale will be clamped between this and [maxScale] inclusively.
///
/// Scale is also affected by [boundaryMargin]. If the scale would result in
/// viewing beyond the boundary, then it will not be allowed. By default,
/// boundaryMargin is EdgeInsets.zero, so scaling below 1.0 will not be
/// allowed in most cases without first increasing the boundaryMargin.
///
/// Defaults to 0.8.
///
/// Must be a finite number greater than zero and less than [maxScale].
final double minScale;
/// Changes the deceleration behavior after a gesture.
///
/// Defaults to 0.0000135.
///
/// Must be a finite number greater than zero.
final double interactionEndFrictionCoefficient;
/// Called when the user ends a pan or scale gesture on the widget.
///
/// At the time this is called, the [TransformationController] will have
/// already been updated to reflect the change caused by the interaction,
/// though a pan may cause an inertia animation after this is called as well.
///
/// {@template flutter.widgets.InteractiveViewer.onInteractionEnd}
/// Will be called even if the interaction is disabled with [panEnabled] or
/// [scaleEnabled] for both touch gestures and mouse interactions.
///
/// A [GestureDetector] wrapping the InteractiveViewer will not respond to
/// [GestureDetector.onScaleStart], [GestureDetector.onScaleUpdate], and
/// [GestureDetector.onScaleEnd]. Use [onInteractionStart],
/// [onInteractionUpdate], and [onInteractionEnd] to respond to those
/// gestures.
/// {@endtemplate}
///
/// See also:
///
/// * [onInteractionStart], which handles the start of the same interaction.
/// * [onInteractionUpdate], which handles an update to the same interaction.
final GestureScaleEndCallback? onInteractionEnd;
/// Called when the user begins a pan or scale gesture on the widget.
///
/// At the time this is called, the [TransformationController] will not have
/// changed due to this interaction.
///
/// {@macro flutter.widgets.InteractiveViewer.onInteractionEnd}
///
/// The coordinates provided in the details' `focalPoint` and
/// `localFocalPoint` are normal Flutter event coordinates, not
/// InteractiveViewer scene coordinates. See
/// [TransformationController.toScene] for how to convert these coordinates to
/// scene coordinates relative to the child.
///
/// See also:
///
/// * [onInteractionUpdate], which handles an update to the same interaction.
/// * [onInteractionEnd], which handles the end of the same interaction.
final GestureScaleStartCallback? onInteractionStart;
/// Called when the user updates a pan or scale gesture on the widget.
///
/// At the time this is called, the [TransformationController] will have
/// already been updated to reflect the change caused by the interaction, if
/// the interaction caused the matrix to change.
///
/// {@macro flutter.widgets.InteractiveViewer.onInteractionEnd}
///
/// The coordinates provided in the details' `focalPoint` and
/// `localFocalPoint` are normal Flutter event coordinates, not
/// InteractiveViewer scene coordinates. See
/// [TransformationController.toScene] for how to convert these coordinates to
/// scene coordinates relative to the child.
///
/// See also:
///
/// * [onInteractionStart], which handles the start of the same interaction.
/// * [onInteractionEnd], which handles the end of the same interaction.
final GestureScaleUpdateCallback? onInteractionUpdate;
/// A [TransformationController] for the transformation performed on the
/// child.
///
/// Whenever the child is transformed, the [Matrix4] value is updated and all
/// listeners are notified. If the value is set, InteractiveViewer will update
/// to respect the new value.
///
/// {@tool dartpad}
/// This example shows how transformationController can be used to animate the
/// transformation back to its starting position.
///
/// ** See code in examples/api/lib/widgets/interactive_viewer/interactive_viewer.transformation_controller.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ValueNotifier], the parent class of TransformationController.
/// * [TextEditingController] for an example of another similar pattern.
final TransformationController? transformationController;
// Used as the coefficient of friction in the inertial translation animation.
// This value was eyeballed to give a feel similar to Google Photos.
static const double _kDrag = 0.0000135;
/// Returns the closest point to the given point on the given line segment.
@visibleForTesting
static Vector3 getNearestPointOnLine(Vector3 point, Vector3 l1, Vector3 l2) {
final double lengthSquared = math.pow(l2.x - l1.x, 2.0).toDouble()
+ math.pow(l2.y - l1.y, 2.0).toDouble();
// In this case, l1 == l2.
if (lengthSquared == 0) {
return l1;
}
// Calculate how far down the line segment the closest point is and return
// the point.
final Vector3 l1P = point - l1;
final Vector3 l1L2 = l2 - l1;
final double fraction = clampDouble(l1P.dot(l1L2) / lengthSquared, 0.0, 1.0);
return l1 + l1L2 * fraction;
}
/// Given a quad, return its axis aligned bounding box.
@visibleForTesting
static Quad getAxisAlignedBoundingBox(Quad quad) {
final double minX = math.min(
quad.point0.x,
math.min(
quad.point1.x,
math.min(
quad.point2.x,
quad.point3.x,
),
),
);
final double minY = math.min(
quad.point0.y,
math.min(
quad.point1.y,
math.min(
quad.point2.y,
quad.point3.y,
),
),
);
final double maxX = math.max(
quad.point0.x,
math.max(
quad.point1.x,
math.max(
quad.point2.x,
quad.point3.x,
),
),
);
final double maxY = math.max(
quad.point0.y,
math.max(
quad.point1.y,
math.max(
quad.point2.y,
quad.point3.y,
),
),
);
return Quad.points(
Vector3(minX, minY, 0),
Vector3(maxX, minY, 0),
Vector3(maxX, maxY, 0),
Vector3(minX, maxY, 0),
);
}
/// Returns true iff the point is inside the rectangle given by the Quad,
/// inclusively.
/// Algorithm from https://math.stackexchange.com/a/190373.
@visibleForTesting
static bool pointIsInside(Vector3 point, Quad quad) {
final Vector3 aM = point - quad.point0;
final Vector3 aB = quad.point1 - quad.point0;
final Vector3 aD = quad.point3 - quad.point0;
final double aMAB = aM.dot(aB);
final double aBAB = aB.dot(aB);
final double aMAD = aM.dot(aD);
final double aDAD = aD.dot(aD);
return 0 <= aMAB && aMAB <= aBAB && 0 <= aMAD && aMAD <= aDAD;
}
/// Get the point inside (inclusively) the given Quad that is nearest to the
/// given Vector3.
@visibleForTesting
static Vector3 getNearestPointInside(Vector3 point, Quad quad) {
// If the point is inside the axis aligned bounding box, then it's ok where
// it is.
if (pointIsInside(point, quad)) {
return point;
}
// Otherwise, return the nearest point on the quad.
final List<Vector3> closestPoints = <Vector3>[
InteractiveViewer.getNearestPointOnLine(point, quad.point0, quad.point1),
InteractiveViewer.getNearestPointOnLine(point, quad.point1, quad.point2),
InteractiveViewer.getNearestPointOnLine(point, quad.point2, quad.point3),
InteractiveViewer.getNearestPointOnLine(point, quad.point3, quad.point0),
];
double minDistance = double.infinity;
late Vector3 closestOverall;
for (final Vector3 closePoint in closestPoints) {
final double distance = math.sqrt(
math.pow(point.x - closePoint.x, 2) + math.pow(point.y - closePoint.y, 2),
);
if (distance < minDistance) {
minDistance = distance;
closestOverall = closePoint;
}
}
return closestOverall;
}
@override
State<InteractiveViewer> createState() => _InteractiveViewerState();
}
class _InteractiveViewerState extends State<InteractiveViewer> with TickerProviderStateMixin {
TransformationController? _transformationController;
final GlobalKey _childKey = GlobalKey();
final GlobalKey _parentKey = GlobalKey();
Animation<Offset>? _animation;
Animation<double>? _scaleAnimation;
late Offset _scaleAnimationFocalPoint;
late AnimationController _controller;
late AnimationController _scaleController;
Axis? _currentAxis; // Used with panAxis.
Offset? _referenceFocalPoint; // Point where the current gesture began.
double? _scaleStart; // Scale value at start of scaling gesture.
double? _rotationStart = 0.0; // Rotation at start of rotation gesture.
double _currentRotation = 0.0; // Rotation of _transformationController.value.
_GestureType? _gestureType;
// TODO(justinmc): Add rotateEnabled parameter to the widget and remove this
// hardcoded value when the rotation feature is implemented.
// https://github.com/flutter/flutter/issues/57698
final bool _rotateEnabled = false;
// The _boundaryRect is calculated by adding the boundaryMargin to the size of
// the child.
Rect get _boundaryRect {
assert(_childKey.currentContext != null);
assert(!widget.boundaryMargin.left.isNaN);
assert(!widget.boundaryMargin.right.isNaN);
assert(!widget.boundaryMargin.top.isNaN);
assert(!widget.boundaryMargin.bottom.isNaN);
final RenderBox childRenderBox = _childKey.currentContext!.findRenderObject()! as RenderBox;
final Size childSize = childRenderBox.size;
final Rect boundaryRect = widget.boundaryMargin.inflateRect(Offset.zero & childSize);
assert(
!boundaryRect.isEmpty,
"InteractiveViewer's child must have nonzero dimensions.",
);
// Boundaries that are partially infinite are not allowed because Matrix4's
// rotation and translation methods don't handle infinites well.
assert(
boundaryRect.isFinite ||
(boundaryRect.left.isInfinite
&& boundaryRect.top.isInfinite
&& boundaryRect.right.isInfinite
&& boundaryRect.bottom.isInfinite),
'boundaryRect must either be infinite in all directions or finite in all directions.',
);
return boundaryRect;
}
// The Rect representing the child's parent.
Rect get _viewport {
assert(_parentKey.currentContext != null);
final RenderBox parentRenderBox = _parentKey.currentContext!.findRenderObject()! as RenderBox;
return Offset.zero & parentRenderBox.size;
}
// Return a new matrix representing the given matrix after applying the given
// translation.
Matrix4 _matrixTranslate(Matrix4 matrix, Offset translation) {
if (translation == Offset.zero) {
return matrix.clone();
}
final Offset alignedTranslation;
if (_currentAxis != null) {
alignedTranslation = switch (widget.panAxis){
PanAxis.horizontal => _alignAxis(translation, Axis.horizontal),
PanAxis.vertical => _alignAxis(translation, Axis.vertical),
PanAxis.aligned => _alignAxis(translation, _currentAxis!),
PanAxis.free => translation,
};
} else {
alignedTranslation = translation;
}
final Matrix4 nextMatrix = matrix.clone()..translate(
alignedTranslation.dx,
alignedTranslation.dy,
);
// Transform the viewport to determine where its four corners will be after
// the child has been transformed.
final Quad nextViewport = _transformViewport(nextMatrix, _viewport);
// If the boundaries are infinite, then no need to check if the translation
// fits within them.
if (_boundaryRect.isInfinite) {
return nextMatrix;
}
// Expand the boundaries with rotation. This prevents the problem where a
// mismatch in orientation between the viewport and boundaries effectively
// limits translation. With this approach, all points that are visible with
// no rotation are visible after rotation.
final Quad boundariesAabbQuad = _getAxisAlignedBoundingBoxWithRotation(
_boundaryRect,
_currentRotation,
);
// If the given translation fits completely within the boundaries, allow it.
final Offset offendingDistance = _exceedsBy(boundariesAabbQuad, nextViewport);
if (offendingDistance == Offset.zero) {
return nextMatrix;
}
// Desired translation goes out of bounds, so translate to the nearest
// in-bounds point instead.
final Offset nextTotalTranslation = _getMatrixTranslation(nextMatrix);
final double currentScale = matrix.getMaxScaleOnAxis();
final Offset correctedTotalTranslation = Offset(
nextTotalTranslation.dx - offendingDistance.dx * currentScale,
nextTotalTranslation.dy - offendingDistance.dy * currentScale,
);
// TODO(justinmc): This needs some work to handle rotation properly. The
// idea is that the boundaries are axis aligned (boundariesAabbQuad), but
// calculating the translation to put the viewport inside that Quad is more
// complicated than this when rotated.
// https://github.com/flutter/flutter/issues/57698
final Matrix4 correctedMatrix = matrix.clone()..setTranslation(Vector3(
correctedTotalTranslation.dx,
correctedTotalTranslation.dy,
0.0,
));
// Double check that the corrected translation fits.
final Quad correctedViewport = _transformViewport(correctedMatrix, _viewport);
final Offset offendingCorrectedDistance = _exceedsBy(boundariesAabbQuad, correctedViewport);
if (offendingCorrectedDistance == Offset.zero) {
return correctedMatrix;
}
// If the corrected translation doesn't fit in either direction, don't allow
// any translation at all. This happens when the viewport is larger than the
// entire boundary.
if (offendingCorrectedDistance.dx != 0.0 && offendingCorrectedDistance.dy != 0.0) {
return matrix.clone();
}
// Otherwise, allow translation in only the direction that fits. This
// happens when the viewport is larger than the boundary in one direction.
final Offset unidirectionalCorrectedTotalTranslation = Offset(
offendingCorrectedDistance.dx == 0.0 ? correctedTotalTranslation.dx : 0.0,
offendingCorrectedDistance.dy == 0.0 ? correctedTotalTranslation.dy : 0.0,
);
return matrix.clone()..setTranslation(Vector3(
unidirectionalCorrectedTotalTranslation.dx,
unidirectionalCorrectedTotalTranslation.dy,
0.0,
));
}
// Return a new matrix representing the given matrix after applying the given
// scale.
Matrix4 _matrixScale(Matrix4 matrix, double scale) {
if (scale == 1.0) {
return matrix.clone();
}
assert(scale != 0.0);
// Don't allow a scale that results in an overall scale beyond min/max
// scale.
final double currentScale = _transformationController!.value.getMaxScaleOnAxis();
final double totalScale = math.max(
currentScale * scale,
// Ensure that the scale cannot make the child so big that it can't fit
// inside the boundaries (in either direction).
math.max(
_viewport.width / _boundaryRect.width,
_viewport.height / _boundaryRect.height,
),
);
final double clampedTotalScale = clampDouble(totalScale,
widget.minScale,
widget.maxScale,
);
final double clampedScale = clampedTotalScale / currentScale;
return matrix.clone()..scale(clampedScale);
}
// Return a new matrix representing the given matrix after applying the given
// rotation.
Matrix4 _matrixRotate(Matrix4 matrix, double rotation, Offset focalPoint) {
if (rotation == 0) {
return matrix.clone();
}
final Offset focalPointScene = _transformationController!.toScene(
focalPoint,
);
return matrix
.clone()
..translate(focalPointScene.dx, focalPointScene.dy)
..rotateZ(-rotation)
..translate(-focalPointScene.dx, -focalPointScene.dy);
}
// Returns true iff the given _GestureType is enabled.
bool _gestureIsSupported(_GestureType? gestureType) {
return switch (gestureType) {
_GestureType.rotate => _rotateEnabled,
_GestureType.scale => widget.scaleEnabled,
_GestureType.pan || null => widget.panEnabled,
};
}
// Decide which type of gesture this is by comparing the amount of scale
// and rotation in the gesture, if any. Scale starts at 1 and rotation
// starts at 0. Pan will have no scale and no rotation because it uses only one
// finger.
_GestureType _getGestureType(ScaleUpdateDetails details) {
final double scale = !widget.scaleEnabled ? 1.0 : details.scale;
final double rotation = !_rotateEnabled ? 0.0 : details.rotation;
if ((scale - 1).abs() > rotation.abs()) {
return _GestureType.scale;
} else if (rotation != 0.0) {
return _GestureType.rotate;
} else {
return _GestureType.pan;
}
}
// Handle the start of a gesture. All of pan, scale, and rotate are handled
// with GestureDetector's scale gesture.
void _onScaleStart(ScaleStartDetails details) {
widget.onInteractionStart?.call(details);
if (_controller.isAnimating) {
_controller.stop();
_controller.reset();
_animation?.removeListener(_onAnimate);
_animation = null;
}
if (_scaleController.isAnimating) {
_scaleController.stop();
_scaleController.reset();
_scaleAnimation?.removeListener(_onScaleAnimate);
_scaleAnimation = null;
}
_gestureType = null;
_currentAxis = null;
_scaleStart = _transformationController!.value.getMaxScaleOnAxis();
_referenceFocalPoint = _transformationController!.toScene(
details.localFocalPoint,
);
_rotationStart = _currentRotation;
}
// Handle an update to an ongoing gesture. All of pan, scale, and rotate are
// handled with GestureDetector's scale gesture.
void _onScaleUpdate(ScaleUpdateDetails details) {
final double scale = _transformationController!.value.getMaxScaleOnAxis();
_scaleAnimationFocalPoint = details.localFocalPoint;
final Offset focalPointScene = _transformationController!.toScene(
details.localFocalPoint,
);
if (_gestureType == _GestureType.pan) {
// When a gesture first starts, it sometimes has no change in scale and
// rotation despite being a two-finger gesture. Here the gesture is
// allowed to be reinterpreted as its correct type after originally
// being marked as a pan.
_gestureType = _getGestureType(details);
} else {
_gestureType ??= _getGestureType(details);
}
if (!_gestureIsSupported(_gestureType)) {
widget.onInteractionUpdate?.call(details);
return;
}
switch (_gestureType!) {
case _GestureType.scale:
assert(_scaleStart != null);
// details.scale gives us the amount to change the scale as of the
// start of this gesture, so calculate the amount to scale as of the
// previous call to _onScaleUpdate.
final double desiredScale = _scaleStart! * details.scale;
final double scaleChange = desiredScale / scale;
_transformationController!.value = _matrixScale(
_transformationController!.value,
scaleChange,
);
// While scaling, translate such that the user's two fingers stay on
// the same places in the scene. That means that the focal point of
// the scale should be on the same place in the scene before and after
// the scale.
final Offset focalPointSceneScaled = _transformationController!.toScene(
details.localFocalPoint,
);
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
focalPointSceneScaled - _referenceFocalPoint!,
);
// details.localFocalPoint should now be at the same location as the
// original _referenceFocalPoint point. If it's not, that's because
// the translate came in contact with a boundary. In that case, update
// _referenceFocalPoint so subsequent updates happen in relation to
// the new effective focal point.
final Offset focalPointSceneCheck = _transformationController!.toScene(
details.localFocalPoint,
);
if (_round(_referenceFocalPoint!) != _round(focalPointSceneCheck)) {
_referenceFocalPoint = focalPointSceneCheck;
}
case _GestureType.rotate:
if (details.rotation == 0.0) {
widget.onInteractionUpdate?.call(details);
return;
}
final double desiredRotation = _rotationStart! + details.rotation;
_transformationController!.value = _matrixRotate(
_transformationController!.value,
_currentRotation - desiredRotation,
details.localFocalPoint,
);
_currentRotation = desiredRotation;
case _GestureType.pan:
assert(_referenceFocalPoint != null);
// details may have a change in scale here when scaleEnabled is false.
// In an effort to keep the behavior similar whether or not scaleEnabled
// is true, these gestures are thrown away.
if (details.scale != 1.0) {
widget.onInteractionUpdate?.call(details);
return;
}
_currentAxis ??= _getPanAxis(_referenceFocalPoint!, focalPointScene);
// Translate so that the same point in the scene is underneath the
// focal point before and after the movement.
final Offset translationChange = focalPointScene - _referenceFocalPoint!;
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
translationChange,
);
_referenceFocalPoint = _transformationController!.toScene(
details.localFocalPoint,
);
}
widget.onInteractionUpdate?.call(details);
}
// Handle the end of a gesture of _GestureType. All of pan, scale, and rotate
// are handled with GestureDetector's scale gesture.
void _onScaleEnd(ScaleEndDetails details) {
widget.onInteractionEnd?.call(details);
_scaleStart = null;
_rotationStart = null;
_referenceFocalPoint = null;
_animation?.removeListener(_onAnimate);
_scaleAnimation?.removeListener(_onScaleAnimate);
_controller.reset();
_scaleController.reset();
if (!_gestureIsSupported(_gestureType)) {
_currentAxis = null;
return;
}
switch (_gestureType) {
case _GestureType.pan:
if (details.velocity.pixelsPerSecond.distance < kMinFlingVelocity) {
_currentAxis = null;
return;
}
final Vector3 translationVector = _transformationController!.value.getTranslation();
final Offset translation = Offset(translationVector.x, translationVector.y);
final FrictionSimulation frictionSimulationX = FrictionSimulation(
widget.interactionEndFrictionCoefficient,
translation.dx,
details.velocity.pixelsPerSecond.dx,
);
final FrictionSimulation frictionSimulationY = FrictionSimulation(
widget.interactionEndFrictionCoefficient,
translation.dy,
details.velocity.pixelsPerSecond.dy,
);
final double tFinal = _getFinalTime(
details.velocity.pixelsPerSecond.distance,
widget.interactionEndFrictionCoefficient,
);
_animation = Tween<Offset>(
begin: translation,
end: Offset(frictionSimulationX.finalX, frictionSimulationY.finalX),
).animate(CurvedAnimation(
parent: _controller,
curve: Curves.decelerate,
));
_controller.duration = Duration(milliseconds: (tFinal * 1000).round());
_animation!.addListener(_onAnimate);
_controller.forward();
case _GestureType.scale:
if (details.scaleVelocity.abs() < 0.1) {
_currentAxis = null;
return;
}
final double scale = _transformationController!.value.getMaxScaleOnAxis();
final FrictionSimulation frictionSimulation = FrictionSimulation(
widget.interactionEndFrictionCoefficient * widget.scaleFactor,
scale,
details.scaleVelocity / 10
);
final double tFinal = _getFinalTime(details.scaleVelocity.abs(), widget.interactionEndFrictionCoefficient, effectivelyMotionless: 0.1);
_scaleAnimation = Tween<double>(
begin: scale,
end: frictionSimulation.x(tFinal)
).animate(CurvedAnimation(
parent: _scaleController,
curve: Curves.decelerate
));
_scaleController.duration = Duration(milliseconds: (tFinal * 1000).round());
_scaleAnimation!.addListener(_onScaleAnimate);
_scaleController.forward();
case _GestureType.rotate || null:
break;
}
}
// Handle mousewheel and web trackpad scroll events.
void _receivedPointerSignal(PointerSignalEvent event) {
final double scaleChange;
if (event is PointerScrollEvent) {
if (event.kind == PointerDeviceKind.trackpad && !widget.trackpadScrollCausesScale) {
// Trackpad scroll, so treat it as a pan.
widget.onInteractionStart?.call(
ScaleStartDetails(
focalPoint: event.position,
localFocalPoint: event.localPosition,
),
);
final Offset localDelta = PointerEvent.transformDeltaViaPositions(
untransformedEndPosition: event.position + event.scrollDelta,
untransformedDelta: event.scrollDelta,
transform: event.transform,
);
if (!_gestureIsSupported(_GestureType.pan)) {
widget.onInteractionUpdate?.call(ScaleUpdateDetails(
focalPoint: event.position - event.scrollDelta,
localFocalPoint: event.localPosition - event.scrollDelta,
focalPointDelta: -localDelta,
));
widget.onInteractionEnd?.call(ScaleEndDetails());
return;
}
final Offset focalPointScene = _transformationController!.toScene(
event.localPosition,
);
final Offset newFocalPointScene = _transformationController!.toScene(
event.localPosition - localDelta,
);
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
newFocalPointScene - focalPointScene
);
widget.onInteractionUpdate?.call(ScaleUpdateDetails(
focalPoint: event.position - event.scrollDelta,
localFocalPoint: event.localPosition - localDelta,
focalPointDelta: -localDelta
));
widget.onInteractionEnd?.call(ScaleEndDetails());
return;
}
// Ignore left and right mouse wheel scroll.
if (event.scrollDelta.dy == 0.0) {
return;
}
scaleChange = math.exp(-event.scrollDelta.dy / widget.scaleFactor);
}
else if (event is PointerScaleEvent) {
scaleChange = event.scale;
}
else {
return;
}
widget.onInteractionStart?.call(
ScaleStartDetails(
focalPoint: event.position,
localFocalPoint: event.localPosition,
),
);
if (!_gestureIsSupported(_GestureType.scale)) {
widget.onInteractionUpdate?.call(ScaleUpdateDetails(
focalPoint: event.position,
localFocalPoint: event.localPosition,
scale: scaleChange,
));
widget.onInteractionEnd?.call(ScaleEndDetails());
return;
}
final Offset focalPointScene = _transformationController!.toScene(
event.localPosition,
);
_transformationController!.value = _matrixScale(
_transformationController!.value,
scaleChange,
);
// After scaling, translate such that the event's position is at the
// same scene point before and after the scale.
final Offset focalPointSceneScaled = _transformationController!.toScene(
event.localPosition,
);
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
focalPointSceneScaled - focalPointScene,
);
widget.onInteractionUpdate?.call(ScaleUpdateDetails(
focalPoint: event.position,
localFocalPoint: event.localPosition,
scale: scaleChange,
));
widget.onInteractionEnd?.call(ScaleEndDetails());
}
// Handle inertia drag animation.
void _onAnimate() {
if (!_controller.isAnimating) {
_currentAxis = null;
_animation?.removeListener(_onAnimate);
_animation = null;
_controller.reset();
return;
}
// Translate such that the resulting translation is _animation.value.
final Vector3 translationVector = _transformationController!.value.getTranslation();
final Offset translation = Offset(translationVector.x, translationVector.y);
final Offset translationScene = _transformationController!.toScene(
translation,
);
final Offset animationScene = _transformationController!.toScene(
_animation!.value,
);
final Offset translationChangeScene = animationScene - translationScene;
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
translationChangeScene,
);
}
// Handle inertia scale animation.
void _onScaleAnimate() {
if (!_scaleController.isAnimating) {
_currentAxis = null;
_scaleAnimation?.removeListener(_onScaleAnimate);
_scaleAnimation = null;
_scaleController.reset();
return;
}
final double desiredScale = _scaleAnimation!.value;
final double scaleChange = desiredScale / _transformationController!.value.getMaxScaleOnAxis();
final Offset referenceFocalPoint = _transformationController!.toScene(
_scaleAnimationFocalPoint,
);
_transformationController!.value = _matrixScale(
_transformationController!.value,
scaleChange,
);
// While scaling, translate such that the user's two fingers stay on
// the same places in the scene. That means that the focal point of
// the scale should be on the same place in the scene before and after
// the scale.
final Offset focalPointSceneScaled = _transformationController!.toScene(
_scaleAnimationFocalPoint,
);
_transformationController!.value = _matrixTranslate(
_transformationController!.value,
focalPointSceneScaled - referenceFocalPoint,
);
}
void _onTransformationControllerChange() {
// A change to the TransformationController's value is a change to the
// state.
setState(() {});
}
@override
void initState() {
super.initState();
_transformationController = widget.transformationController
?? TransformationController();
_transformationController!.addListener(_onTransformationControllerChange);
_controller = AnimationController(
vsync: this,
);
_scaleController = AnimationController(
vsync: this
);
}
@override
void didUpdateWidget(InteractiveViewer oldWidget) {
super.didUpdateWidget(oldWidget);
// Handle all cases of needing to dispose and initialize
// transformationControllers.
if (oldWidget.transformationController == null) {
if (widget.transformationController != null) {
_transformationController!.removeListener(_onTransformationControllerChange);
_transformationController!.dispose();
_transformationController = widget.transformationController;
_transformationController!.addListener(_onTransformationControllerChange);
}
} else {
if (widget.transformationController == null) {
_transformationController!.removeListener(_onTransformationControllerChange);
_transformationController = TransformationController();
_transformationController!.addListener(_onTransformationControllerChange);
} else if (widget.transformationController != oldWidget.transformationController) {
_transformationController!.removeListener(_onTransformationControllerChange);
_transformationController = widget.transformationController;
_transformationController!.addListener(_onTransformationControllerChange);
}
}
}
@override
void dispose() {
_controller.dispose();
_scaleController.dispose();
_transformationController!.removeListener(_onTransformationControllerChange);
if (widget.transformationController == null) {
_transformationController!.dispose();
}
super.dispose();
}
@override
Widget build(BuildContext context) {
Widget child;
if (widget.child != null) {
child = _InteractiveViewerBuilt(
childKey: _childKey,
clipBehavior: widget.clipBehavior,
constrained: widget.constrained,
matrix: _transformationController!.value,
alignment: widget.alignment,
child: widget.child!,
);
} else {
// When using InteractiveViewer.builder, then constrained is false and the
// viewport is the size of the constraints.
assert(widget.builder != null);
assert(!widget.constrained);
child = LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
final Matrix4 matrix = _transformationController!.value;
return _InteractiveViewerBuilt(
childKey: _childKey,
clipBehavior: widget.clipBehavior,
constrained: widget.constrained,
alignment: widget.alignment,
matrix: matrix,
child: widget.builder!(
context,
_transformViewport(matrix, Offset.zero & constraints.biggest),
),
);
},
);
}
return Listener(
key: _parentKey,
onPointerSignal: _receivedPointerSignal,
child: GestureDetector(
behavior: HitTestBehavior.opaque, // Necessary when panning off screen.
onScaleEnd: _onScaleEnd,
onScaleStart: _onScaleStart,
onScaleUpdate: _onScaleUpdate,
trackpadScrollCausesScale: widget.trackpadScrollCausesScale,
trackpadScrollToScaleFactor: Offset(0, -1/widget.scaleFactor),
child: child,
),
);
}
}
// This widget allows us to easily swap in and out the LayoutBuilder in
// InteractiveViewer's depending on if it's using a builder or a child.
class _InteractiveViewerBuilt extends StatelessWidget {
const _InteractiveViewerBuilt({
required this.child,
required this.childKey,
required this.clipBehavior,
required this.constrained,
required this.matrix,
required this.alignment,
});
final Widget child;
final GlobalKey childKey;
final Clip clipBehavior;
final bool constrained;
final Matrix4 matrix;
final Alignment? alignment;
@override
Widget build(BuildContext context) {
Widget child = Transform(
transform: matrix,
alignment: alignment,
child: KeyedSubtree(
key: childKey,
child: this.child,
),
);
if (!constrained) {
child = OverflowBox(
alignment: Alignment.topLeft,
minWidth: 0.0,
minHeight: 0.0,
maxWidth: double.infinity,
maxHeight: double.infinity,
child: child,
);
}
return ClipRect(
clipBehavior: clipBehavior,
child: child,
);
}
}
/// A thin wrapper on [ValueNotifier] whose value is a [Matrix4] representing a
/// transformation.
///
/// The [value] defaults to the identity matrix, which corresponds to no
/// transformation.
///
/// See also:
///
/// * [InteractiveViewer.transformationController] for detailed documentation
/// on how to use TransformationController with [InteractiveViewer].
class TransformationController extends ValueNotifier<Matrix4> {
/// Create an instance of [TransformationController].
///
/// The [value] defaults to the identity matrix, which corresponds to no
/// transformation.
TransformationController([Matrix4? value]) : super(value ?? Matrix4.identity());
/// Return the scene point at the given viewport point.
///
/// A viewport point is relative to the parent while a scene point is relative
/// to the child, regardless of transformation. Calling toScene with a
/// viewport point essentially returns the scene coordinate that lies
/// underneath the viewport point given the transform.
///
/// The viewport transforms as the inverse of the child (i.e. moving the child
/// left is equivalent to moving the viewport right).
///
/// This method is often useful when determining where an event on the parent
/// occurs on the child. This example shows how to determine where a tap on
/// the parent occurred on the child.
///
/// ```dart
/// @override
/// Widget build(BuildContext context) {
/// return GestureDetector(
/// onTapUp: (TapUpDetails details) {
/// _childWasTappedAt = _transformationController.toScene(
/// details.localPosition,
/// );
/// },
/// child: InteractiveViewer(
/// transformationController: _transformationController,
/// child: child,
/// ),
/// );
/// }
/// ```
Offset toScene(Offset viewportPoint) {
// On viewportPoint, perform the inverse transformation of the scene to get
// where the point would be in the scene before the transformation.
final Matrix4 inverseMatrix = Matrix4.inverted(value);
final Vector3 untransformed = inverseMatrix.transform3(Vector3(
viewportPoint.dx,
viewportPoint.dy,
0,
));
return Offset(untransformed.x, untransformed.y);
}
}
// A classification of relevant user gestures. Each contiguous user gesture is
// represented by exactly one _GestureType.
enum _GestureType {
pan,
scale,
rotate,
}
// Given a velocity and drag, calculate the time at which motion will come to
// a stop, within the margin of effectivelyMotionless.
double _getFinalTime(double velocity, double drag, {double effectivelyMotionless = 10}) {
return math.log(effectivelyMotionless / velocity) / math.log(drag / 100);
}
// Return the translation from the given Matrix4 as an Offset.
Offset _getMatrixTranslation(Matrix4 matrix) {
final Vector3 nextTranslation = matrix.getTranslation();
return Offset(nextTranslation.x, nextTranslation.y);
}
// Transform the four corners of the viewport by the inverse of the given
// matrix. This gives the viewport after the child has been transformed by the
// given matrix. The viewport transforms as the inverse of the child (i.e.
// moving the child left is equivalent to moving the viewport right).
Quad _transformViewport(Matrix4 matrix, Rect viewport) {
final Matrix4 inverseMatrix = matrix.clone()..invert();
return Quad.points(
inverseMatrix.transform3(Vector3(
viewport.topLeft.dx,
viewport.topLeft.dy,
0.0,
)),
inverseMatrix.transform3(Vector3(
viewport.topRight.dx,
viewport.topRight.dy,
0.0,
)),
inverseMatrix.transform3(Vector3(
viewport.bottomRight.dx,
viewport.bottomRight.dy,
0.0,
)),
inverseMatrix.transform3(Vector3(
viewport.bottomLeft.dx,
viewport.bottomLeft.dy,
0.0,
)),
);
}
// Find the axis aligned bounding box for the rect rotated about its center by
// the given amount.
Quad _getAxisAlignedBoundingBoxWithRotation(Rect rect, double rotation) {
final Matrix4 rotationMatrix = Matrix4.identity()
..translate(rect.size.width / 2, rect.size.height / 2)
..rotateZ(rotation)
..translate(-rect.size.width / 2, -rect.size.height / 2);
final Quad boundariesRotated = Quad.points(
rotationMatrix.transform3(Vector3(rect.left, rect.top, 0.0)),
rotationMatrix.transform3(Vector3(rect.right, rect.top, 0.0)),
rotationMatrix.transform3(Vector3(rect.right, rect.bottom, 0.0)),
rotationMatrix.transform3(Vector3(rect.left, rect.bottom, 0.0)),
);
return InteractiveViewer.getAxisAlignedBoundingBox(boundariesRotated);
}
// Return the amount that viewport lies outside of boundary. If the viewport
// is completely contained within the boundary (inclusively), then returns
// Offset.zero.
Offset _exceedsBy(Quad boundary, Quad viewport) {
final List<Vector3> viewportPoints = <Vector3>[
viewport.point0, viewport.point1, viewport.point2, viewport.point3,
];
Offset largestExcess = Offset.zero;
for (final Vector3 point in viewportPoints) {
final Vector3 pointInside = InteractiveViewer.getNearestPointInside(point, boundary);
final Offset excess = Offset(
pointInside.x - point.x,
pointInside.y - point.y,
);
if (excess.dx.abs() > largestExcess.dx.abs()) {
largestExcess = Offset(excess.dx, largestExcess.dy);
}
if (excess.dy.abs() > largestExcess.dy.abs()) {
largestExcess = Offset(largestExcess.dx, excess.dy);
}
}
return _round(largestExcess);
}
// Round the output values. This works around a precision problem where
// values that should have been zero were given as within 10^-10 of zero.
Offset _round(Offset offset) {
return Offset(
double.parse(offset.dx.toStringAsFixed(9)),
double.parse(offset.dy.toStringAsFixed(9)),
);
}
// Align the given offset to the given axis by allowing movement only in the
// axis direction.
Offset _alignAxis(Offset offset, Axis axis) {
return switch (axis) {
Axis.horizontal => Offset(offset.dx, 0.0),
Axis.vertical => Offset(0.0, offset.dy),
};
}
// Given two points, return the axis where the distance between the points is
// greatest. If they are equal, return null.
Axis? _getPanAxis(Offset point1, Offset point2) {
if (point1 == point2) {
return null;
}
final double x = point2.dx - point1.dx;
final double y = point2.dy - point1.dy;
return x.abs() > y.abs() ? Axis.horizontal : Axis.vertical;
}
/// This enum is used to specify the behavior of the [InteractiveViewer] when
/// the user drags the viewport.
enum PanAxis{
/// The user can only pan the viewport along the horizontal axis.
horizontal,
/// The user can only pan the viewport along the vertical axis.
vertical,
/// The user can pan the viewport along the horizontal and vertical axes
/// but not diagonally.
aligned,
/// The user can pan the viewport freely in any direction.
free,
}
| flutter/packages/flutter/lib/src/widgets/interactive_viewer.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/interactive_viewer.dart",
"repo_id": "flutter",
"token_count": 18118
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'basic.dart';
import 'framework.dart';
import 'lookup_boundary.dart';
import 'ticker_provider.dart';
const String _flutterWidgetsLibrary = 'package:flutter/widgets.dart';
// Examples can assume:
// late BuildContext context;
// * OverlayEntry Implementation
/// A place in an [Overlay] that can contain a widget.
///
/// Overlay entries are inserted into an [Overlay] using the
/// [OverlayState.insert] or [OverlayState.insertAll] functions. To find the
/// closest enclosing overlay for a given [BuildContext], use the [Overlay.of]
/// function.
///
/// An overlay entry can be in at most one overlay at a time. To remove an entry
/// from its overlay, call the [remove] function on the overlay entry.
///
/// Because an [Overlay] uses a [Stack] layout, overlay entries can use
/// [Positioned] and [AnimatedPositioned] to position themselves within the
/// overlay.
///
/// For example, [Draggable] uses an [OverlayEntry] to show the drag avatar that
/// follows the user's finger across the screen after the drag begins. Using the
/// overlay to display the drag avatar lets the avatar float over the other
/// widgets in the app. As the user's finger moves, draggable calls
/// [markNeedsBuild] on the overlay entry to cause it to rebuild. In its build,
/// the entry includes a [Positioned] with its top and left property set to
/// position the drag avatar near the user's finger. When the drag is over,
/// [Draggable] removes the entry from the overlay to remove the drag avatar
/// from view.
///
/// By default, if there is an entirely [opaque] entry over this one, then this
/// one will not be included in the widget tree (in particular, stateful widgets
/// within the overlay entry will not be instantiated). To ensure that your
/// overlay entry is still built even if it is not visible, set [maintainState]
/// to true. This is more expensive, so should be done with care. In particular,
/// if widgets in an overlay entry with [maintainState] set to true repeatedly
/// call [State.setState], the user's battery will be drained unnecessarily.
///
/// [OverlayEntry] is a [Listenable] that notifies when the widget built by
/// [builder] is mounted or unmounted, whose exact state can be queried by
/// [mounted]. After the owner of the [OverlayEntry] calls [remove] and then
/// [dispose], the widget may not be immediately removed from the widget tree.
/// As a result listeners of the [OverlayEntry] can get notified for one last
/// time after the [dispose] call, when the widget is eventually unmounted.
///
/// {@macro flutter.widgets.overlayPortalVsOverlayEntry}
///
/// See also:
///
/// * [OverlayPortal], an alternative API for inserting widgets into an
/// [Overlay] using a builder callback.
/// * [Overlay], a stack of entries that can be managed independently.
/// * [OverlayState], the current state of an Overlay.
/// * [WidgetsApp], a convenience widget that wraps a number of widgets that
/// are commonly required for an application.
/// * [MaterialApp], a convenience widget that wraps a number of widgets that
/// are commonly required for Material Design applications.
class OverlayEntry implements Listenable {
/// Creates an overlay entry.
///
/// To insert the entry into an [Overlay], first find the overlay using
/// [Overlay.of] and then call [OverlayState.insert]. To remove the entry,
/// call [remove] on the overlay entry itself.
OverlayEntry({
required this.builder,
bool opaque = false,
bool maintainState = false,
this.canSizeOverlay = false,
}) : _opaque = opaque,
_maintainState = maintainState {
if (kFlutterMemoryAllocationsEnabled) {
_maybeDispatchObjectCreation();
}
}
/// This entry will include the widget built by this builder in the overlay at
/// the entry's position.
///
/// To cause this builder to be called again, call [markNeedsBuild] on this
/// overlay entry.
final WidgetBuilder builder;
/// Whether this entry occludes the entire overlay.
///
/// If an entry claims to be opaque, then, for efficiency, the overlay will
/// skip building entries below that entry unless they have [maintainState]
/// set.
bool get opaque => _opaque;
bool _opaque;
set opaque(bool value) {
assert(!_disposedByOwner);
if (_opaque == value) {
return;
}
_opaque = value;
_overlay?._didChangeEntryOpacity();
}
/// Whether this entry must be included in the tree even if there is a fully
/// [opaque] entry above it.
///
/// By default, if there is an entirely [opaque] entry over this one, then this
/// one will not be included in the widget tree (in particular, stateful widgets
/// within the overlay entry will not be instantiated). To ensure that your
/// overlay entry is still built even if it is not visible, set [maintainState]
/// to true. This is more expensive, so should be done with care. In particular,
/// if widgets in an overlay entry with [maintainState] set to true repeatedly
/// call [State.setState], the user's battery will be drained unnecessarily.
///
/// This is used by the [Navigator] and [Route] objects to ensure that routes
/// are kept around even when in the background, so that [Future]s promised
/// from subsequent routes will be handled properly when they complete.
bool get maintainState => _maintainState;
bool _maintainState;
set maintainState(bool value) {
assert(!_disposedByOwner);
if (_maintainState == value) {
return;
}
_maintainState = value;
assert(_overlay != null);
_overlay!._didChangeEntryOpacity();
}
/// Whether the content of this [OverlayEntry] can be used to size the
/// [Overlay].
///
/// In most situations the overlay sizes itself based on its incoming
/// constraints to be as large as possible. However, if that would result in
/// an infinite size, it has to rely on one of its children to size itself. In
/// this situation, the overlay will consult the topmost non-[Positioned]
/// overlay entry that has this property set to true, lay it out with the
/// incoming [BoxConstraints] of the overlay, and force all other
/// non-[Positioned] overlay entries to have the same size. The [Positioned]
/// entries are laid out as usual based on the calculated size of the overlay.
///
/// Overlay entries that set this to true must be able to handle unconstrained
/// [BoxConstraints].
///
/// Setting this to true has no effect if the overlay entry uses a [Positioned]
/// widget to position itself in the overlay.
final bool canSizeOverlay;
/// Whether the [OverlayEntry] is currently mounted in the widget tree.
///
/// The [OverlayEntry] notifies its listeners when this value changes.
bool get mounted => _overlayEntryStateNotifier?.value != null;
/// The currently mounted `_OverlayEntryWidgetState` built using this [OverlayEntry].
ValueNotifier<_OverlayEntryWidgetState?>? _overlayEntryStateNotifier = ValueNotifier<_OverlayEntryWidgetState?>(null);
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
/// Dispatches event of object creation to [FlutterMemoryAllocations.instance].
void _maybeDispatchObjectCreation() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterWidgetsLibrary,
className: '$OverlayEntry',
object: this,
);
}
}
@override
void addListener(VoidCallback listener) {
assert(!_disposedByOwner);
_overlayEntryStateNotifier?.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
_overlayEntryStateNotifier?.removeListener(listener);
}
OverlayState? _overlay;
final GlobalKey<_OverlayEntryWidgetState> _key = GlobalKey<_OverlayEntryWidgetState>();
/// Remove this entry from the overlay.
///
/// This should only be called once.
///
/// This method removes this overlay entry from the overlay immediately. The
/// UI will be updated in the same frame if this method is called before the
/// overlay rebuild in this frame; otherwise, the UI will be updated in the
/// next frame. This means that it is safe to call during builds, but also
/// that if you do call this after the overlay rebuild, the UI will not update
/// until the next frame (i.e. many milliseconds later).
void remove() {
assert(_overlay != null);
assert(!_disposedByOwner);
final OverlayState overlay = _overlay!;
_overlay = null;
if (!overlay.mounted) {
return;
}
overlay._entries.remove(this);
if (SchedulerBinding.instance.schedulerPhase == SchedulerPhase.persistentCallbacks) {
SchedulerBinding.instance.addPostFrameCallback((Duration duration) {
overlay._markDirty();
}, debugLabel: 'OverlayEntry.markDirty');
} else {
overlay._markDirty();
}
}
/// Cause this entry to rebuild during the next pipeline flush.
///
/// You need to call this function if the output of [builder] has changed.
void markNeedsBuild() {
assert(!_disposedByOwner);
_key.currentState?._markNeedsBuild();
}
void _didUnmount() {
assert(!mounted);
if (_disposedByOwner) {
_overlayEntryStateNotifier?.dispose();
_overlayEntryStateNotifier = null;
}
}
bool _disposedByOwner = false;
/// Discards any resources used by this [OverlayEntry].
///
/// This method must be called after [remove] if the [OverlayEntry] is
/// inserted into an [Overlay].
///
/// After this is called, the object is not in a usable state and should be
/// discarded (calls to [addListener] will throw after the object is disposed).
/// However, the listeners registered may not be immediately released until
/// the widget built using this [OverlayEntry] is unmounted from the widget
/// tree.
///
/// This method should only be called by the object's owner.
void dispose() {
assert(!_disposedByOwner);
assert(_overlay == null, 'An OverlayEntry must first be removed from the Overlay before dispose is called.');
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_disposedByOwner = true;
if (!mounted) {
// If we're still mounted when disposed, then this will be disposed in
// _didUnmount, to allow notifications to occur until the entry is
// unmounted.
_overlayEntryStateNotifier?.dispose();
_overlayEntryStateNotifier = null;
}
}
@override
String toString() => '${describeIdentity(this)}(opaque: $opaque; maintainState: $maintainState)${_disposedByOwner ? "(DISPOSED)" : ""}';
}
class _OverlayEntryWidget extends StatefulWidget {
const _OverlayEntryWidget({
required Key key,
required this.entry,
required this.overlayState,
this.tickerEnabled = true,
}) : super(key: key);
final OverlayEntry entry;
final OverlayState overlayState;
final bool tickerEnabled;
@override
_OverlayEntryWidgetState createState() => _OverlayEntryWidgetState();
}
class _OverlayEntryWidgetState extends State<_OverlayEntryWidget> {
late _RenderTheater _theater;
// Manages the stack of theater children whose paint order are sorted by their
// _zOrderIndex. The children added by OverlayPortal are added to this linked
// list, and they will be shown _above_ the OverlayEntry tied to this widget.
// The children with larger zOrderIndex values (i.e. those called `show`
// recently) will be painted last.
//
// This linked list is lazily created in `_add`, and the entries are added/removed
// via `_add`/`_remove`, called by OverlayPortals lower in the tree. `_add` or
// `_remove` does not cause this widget to rebuild, the linked list will be
// read by _RenderTheater as part of its render child model. This would ideally
// be in a RenderObject but there may not be RenderObjects between
// _RenderTheater and the render subtree OverlayEntry builds.
LinkedList<_OverlayEntryLocation>? _sortedTheaterSiblings;
// Worst-case O(N), N being the number of children added to the top spot in
// the same frame. This can be a bit expensive when there's a lot of global
// key reparenting in the same frame but N is usually a small number.
void _add(_OverlayEntryLocation child) {
assert(mounted);
final LinkedList<_OverlayEntryLocation> children = _sortedTheaterSiblings ??= LinkedList<_OverlayEntryLocation>();
assert(!children.contains(child));
_OverlayEntryLocation? insertPosition = children.isEmpty ? null : children.last;
while (insertPosition != null && insertPosition._zOrderIndex > child._zOrderIndex) {
insertPosition = insertPosition.previous;
}
if (insertPosition == null) {
children.addFirst(child);
} else {
insertPosition.insertAfter(child);
}
assert(children.contains(child));
}
void _remove(_OverlayEntryLocation child) {
assert(_sortedTheaterSiblings != null);
final bool wasInCollection = _sortedTheaterSiblings?.remove(child) ?? false;
assert(wasInCollection);
}
// Returns an Iterable that traverse the children in the child model in paint
// order (from farthest to the user to the closest to the user).
//
// The iterator should be safe to use even when the child model is being
// mutated. The reason for that is it's allowed to add/remove/move deferred
// children to a _RenderTheater during performLayout, but the affected
// children don't have to be laid out in the same performLayout call.
late final Iterable<RenderBox> _paintOrderIterable = _createChildIterable(reversed: false);
// An Iterable that traverse the children in the child model in
// hit-test order (from closest to the user to the farthest to the user).
late final Iterable<RenderBox> _hitTestOrderIterable = _createChildIterable(reversed: true);
// The following uses sync* because hit-testing is lazy, and LinkedList as a
// Iterable doesn't support concurrent modification.
Iterable<RenderBox> _createChildIterable({ required bool reversed }) sync* {
final LinkedList<_OverlayEntryLocation>? children = _sortedTheaterSiblings;
if (children == null || children.isEmpty) {
return;
}
_OverlayEntryLocation? candidate = reversed ? children.last : children.first;
while (candidate != null) {
final RenderBox? renderBox = candidate._overlayChildRenderBox;
candidate = reversed ? candidate.previous : candidate.next;
if (renderBox != null) {
yield renderBox;
}
}
}
@override
void initState() {
super.initState();
widget.entry._overlayEntryStateNotifier!.value = this;
_theater = context.findAncestorRenderObjectOfType<_RenderTheater>()!;
assert(_sortedTheaterSiblings == null);
}
@override
void didUpdateWidget(_OverlayEntryWidget oldWidget) {
super.didUpdateWidget(oldWidget);
// OverlayState's build method always returns a RenderObjectWidget _Theater,
// so it's safe to assume that state equality implies render object equality.
assert(oldWidget.entry == widget.entry);
if (oldWidget.overlayState != widget.overlayState) {
final _RenderTheater newTheater = context.findAncestorRenderObjectOfType<_RenderTheater>()!;
assert(_theater != newTheater);
_theater = newTheater;
}
}
@override
void dispose() {
widget.entry._overlayEntryStateNotifier?.value = null;
widget.entry._didUnmount();
_sortedTheaterSiblings = null;
super.dispose();
}
@override
Widget build(BuildContext context) {
return TickerMode(
enabled: widget.tickerEnabled,
child: _RenderTheaterMarker(
theater: _theater,
overlayEntryWidgetState: this,
child: widget.entry.builder(context),
),
);
}
void _markNeedsBuild() {
setState(() { /* the state that changed is in the builder */ });
}
}
/// A stack of entries that can be managed independently.
///
/// Overlays let independent child widgets "float" visual elements on top of
/// other widgets by inserting them into the overlay's stack. The overlay lets
/// each of these widgets manage their participation in the overlay using
/// [OverlayEntry] objects.
///
/// Although you can create an [Overlay] directly, it's most common to use the
/// overlay created by the [Navigator] in a [WidgetsApp], [CupertinoApp] or a
/// [MaterialApp]. The navigator uses its overlay to manage the visual
/// appearance of its routes.
///
/// The [Overlay] widget uses a custom stack implementation, which is very
/// similar to the [Stack] widget. The main use case of [Overlay] is related to
/// navigation and being able to insert widgets on top of the pages in an app.
/// For layout purposes unrelated to navigation, consider using [Stack] instead.
///
/// An [Overlay] widget requires a [Directionality] widget to be in scope, so
/// that it can resolve direction-sensitive coordinates of any
/// [Positioned.directional] children.
///
/// For widgets drawn in an [OverlayEntry], do not assume that the size of the
/// [Overlay] is the size returned by [MediaQuery.sizeOf]. Nested overlays can
/// have different sizes.
///
/// {@tool dartpad}
/// This example shows how to use the [Overlay] to highlight the [NavigationBar]
/// destination.
///
/// ** See code in examples/api/lib/widgets/overlay/overlay.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [OverlayEntry], the class that is used for describing the overlay entries.
/// * [OverlayState], which is used to insert the entries into the overlay.
/// * [WidgetsApp], which inserts an [Overlay] widget indirectly via its [Navigator].
/// * [MaterialApp], which inserts an [Overlay] widget indirectly via its [Navigator].
/// * [CupertinoApp], which inserts an [Overlay] widget indirectly via its [Navigator].
/// * [Stack], which allows directly displaying a stack of widgets.
class Overlay extends StatefulWidget {
/// Creates an overlay.
///
/// The initial entries will be inserted into the overlay when its associated
/// [OverlayState] is initialized.
///
/// Rather than creating an overlay, consider using the overlay that is
/// created by the [Navigator] in a [WidgetsApp], [CupertinoApp], or a
/// [MaterialApp] for the application.
const Overlay({
super.key,
this.initialEntries = const <OverlayEntry>[],
this.clipBehavior = Clip.hardEdge,
});
/// Wrap the provided `child` in an [Overlay] to allow other visual elements
/// (packed in [OverlayEntry]s) to float on top of the child.
///
/// This is a convenience method over the regular [Overlay] constructor: It
/// creates an [Overlay] and puts the provided `child` in an [OverlayEntry]
/// at the bottom of that newly created Overlay.
static Widget wrap({
Key? key,
Clip clipBehavior = Clip.hardEdge,
required Widget child,
}) {
return _WrappingOverlay(key: key, clipBehavior: clipBehavior, child: child);
}
/// The entries to include in the overlay initially.
///
/// These entries are only used when the [OverlayState] is initialized. If you
/// are providing a new [Overlay] description for an overlay that's already in
/// the tree, then the new entries are ignored.
///
/// To add entries to an [Overlay] that is already in the tree, use
/// [Overlay.of] to obtain the [OverlayState] (or assign a [GlobalKey] to the
/// [Overlay] widget and obtain the [OverlayState] via
/// [GlobalKey.currentState]), and then use [OverlayState.insert] or
/// [OverlayState.insertAll].
///
/// To remove an entry from an [Overlay], use [OverlayEntry.remove].
final List<OverlayEntry> initialEntries;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// The [OverlayState] from the closest instance of [Overlay] that encloses
/// the given context within the closest [LookupBoundary], and, in debug mode,
/// will throw if one is not found.
///
/// In debug mode, if the `debugRequiredFor` argument is provided and an
/// overlay isn't found, then this function will throw an exception containing
/// the runtime type of the given widget in the error message. The exception
/// attempts to explain that the calling [Widget] (the one given by the
/// `debugRequiredFor` argument) needs an [Overlay] to be present to function.
/// If `debugRequiredFor` is not supplied, then the error message is more
/// generic.
///
/// Typical usage is as follows:
///
/// ```dart
/// OverlayState overlay = Overlay.of(context);
/// ```
///
/// If `rootOverlay` is set to true, the state from the furthest instance of
/// this class is given instead. Useful for installing overlay entries above
/// all subsequent instances of [Overlay].
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [Overlay.maybeOf] for a similar function that returns null if an
/// [Overlay] is not found.
static OverlayState of(
BuildContext context, {
bool rootOverlay = false,
Widget? debugRequiredFor,
}) {
final OverlayState? result = maybeOf(context, rootOverlay: rootOverlay);
assert(() {
if (result == null) {
final bool hiddenByBoundary = LookupBoundary.debugIsHidingAncestorStateOfType<OverlayState>(context);
final List<DiagnosticsNode> information = <DiagnosticsNode>[
ErrorSummary('No Overlay widget found${hiddenByBoundary ? ' within the closest LookupBoundary' : ''}.'),
if (hiddenByBoundary)
ErrorDescription(
'There is an ancestor Overlay widget, but it is hidden by a LookupBoundary.'
),
ErrorDescription('${debugRequiredFor?.runtimeType ?? 'Some'} widgets require an Overlay widget ancestor for correct operation.'),
ErrorHint('The most common way to add an Overlay to an application is to include a MaterialApp, CupertinoApp or Navigator widget in the runApp() call.'),
if (debugRequiredFor != null) DiagnosticsProperty<Widget>('The specific widget that failed to find an overlay was', debugRequiredFor, style: DiagnosticsTreeStyle.errorProperty),
if (context.widget != debugRequiredFor)
context.describeElement('The context from which that widget was searching for an overlay was'),
];
throw FlutterError.fromParts(information);
}
return true;
}());
return result!;
}
/// The [OverlayState] from the closest instance of [Overlay] that encloses
/// the given context within the closest [LookupBoundary], if any.
///
/// Typical usage is as follows:
///
/// ```dart
/// OverlayState? overlay = Overlay.maybeOf(context);
/// ```
///
/// If `rootOverlay` is set to true, the state from the furthest instance of
/// this class is given instead. Useful for installing overlay entries above
/// all subsequent instances of [Overlay].
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [Overlay.of] for a similar function that returns a non-nullable result
/// and throws if an [Overlay] is not found.
static OverlayState? maybeOf(
BuildContext context, {
bool rootOverlay = false,
}) {
return rootOverlay
? LookupBoundary.findRootAncestorStateOfType<OverlayState>(context)
: LookupBoundary.findAncestorStateOfType<OverlayState>(context);
}
@override
OverlayState createState() => OverlayState();
}
/// The current state of an [Overlay].
///
/// Used to insert [OverlayEntry]s into the overlay using the [insert] and
/// [insertAll] functions.
class OverlayState extends State<Overlay> with TickerProviderStateMixin {
final List<OverlayEntry> _entries = <OverlayEntry>[];
@override
void initState() {
super.initState();
insertAll(widget.initialEntries);
}
int _insertionIndex(OverlayEntry? below, OverlayEntry? above) {
assert(above == null || below == null);
if (below != null) {
return _entries.indexOf(below);
}
if (above != null) {
return _entries.indexOf(above) + 1;
}
return _entries.length;
}
bool _debugCanInsertEntry(OverlayEntry entry) {
final List<DiagnosticsNode> operandsInformation = <DiagnosticsNode>[
DiagnosticsProperty<OverlayEntry>('The OverlayEntry was', entry, style: DiagnosticsTreeStyle.errorProperty),
DiagnosticsProperty<OverlayState>(
'The Overlay the OverlayEntry was trying to insert to was', this, style: DiagnosticsTreeStyle.errorProperty,
),
];
if (!mounted) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Attempted to insert an OverlayEntry to an already disposed Overlay.'),
...operandsInformation,
]);
}
final OverlayState? currentOverlay = entry._overlay;
final bool alreadyContainsEntry = _entries.contains(entry);
if (alreadyContainsEntry) {
final bool inconsistentOverlayState = !identical(currentOverlay, this);
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The specified entry is already present in the target Overlay.'),
...operandsInformation,
if (inconsistentOverlayState) ErrorHint('This could be an error in the Flutter framework.')
else ErrorHint(
'Consider calling remove on the OverlayEntry before inserting it to a different Overlay, '
'or switching to the OverlayPortal API to avoid manual OverlayEntry management.'
),
if (inconsistentOverlayState) DiagnosticsProperty<OverlayState>(
"The OverlayEntry's current Overlay was", currentOverlay, style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
if (currentOverlay == null) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The specified entry is already present in a different Overlay.'),
...operandsInformation,
DiagnosticsProperty<OverlayState>("The OverlayEntry's current Overlay was", currentOverlay, style: DiagnosticsTreeStyle.errorProperty,),
ErrorHint(
'Consider calling remove on the OverlayEntry before inserting it to a different Overlay, '
'or switching to the OverlayPortal API to avoid manual OverlayEntry management.'
)
]);
}
/// Insert the given entry into the overlay.
///
/// If `below` is non-null, the entry is inserted just below `below`.
/// If `above` is non-null, the entry is inserted just above `above`.
/// Otherwise, the entry is inserted on top.
///
/// It is an error to specify both `above` and `below`.
void insert(OverlayEntry entry, { OverlayEntry? below, OverlayEntry? above }) {
assert(_debugVerifyInsertPosition(above, below));
assert(_debugCanInsertEntry(entry));
entry._overlay = this;
setState(() {
_entries.insert(_insertionIndex(below, above), entry);
});
}
/// Insert all the entries in the given iterable.
///
/// If `below` is non-null, the entries are inserted just below `below`.
/// If `above` is non-null, the entries are inserted just above `above`.
/// Otherwise, the entries are inserted on top.
///
/// It is an error to specify both `above` and `below`.
void insertAll(Iterable<OverlayEntry> entries, { OverlayEntry? below, OverlayEntry? above }) {
assert(_debugVerifyInsertPosition(above, below));
assert(entries.every(_debugCanInsertEntry));
if (entries.isEmpty) {
return;
}
for (final OverlayEntry entry in entries) {
assert(entry._overlay == null);
entry._overlay = this;
}
setState(() {
_entries.insertAll(_insertionIndex(below, above), entries);
});
}
bool _debugVerifyInsertPosition(OverlayEntry? above, OverlayEntry? below, { Iterable<OverlayEntry>? newEntries }) {
assert(
above == null || below == null,
'Only one of `above` and `below` may be specified.',
);
assert(
above == null || (above._overlay == this && _entries.contains(above) && (newEntries?.contains(above) ?? true)),
'The provided entry used for `above` must be present in the Overlay${newEntries != null ? ' and in the `newEntriesList`' : ''}.',
);
assert(
below == null || (below._overlay == this && _entries.contains(below) && (newEntries?.contains(below) ?? true)),
'The provided entry used for `below` must be present in the Overlay${newEntries != null ? ' and in the `newEntriesList`' : ''}.',
);
return true;
}
/// Remove all the entries listed in the given iterable, then reinsert them
/// into the overlay in the given order.
///
/// Entries mention in `newEntries` but absent from the overlay are inserted
/// as if with [insertAll].
///
/// Entries not mentioned in `newEntries` but present in the overlay are
/// positioned as a group in the resulting list relative to the entries that
/// were moved, as specified by one of `below` or `above`, which, if
/// specified, must be one of the entries in `newEntries`:
///
/// If `below` is non-null, the group is positioned just below `below`.
/// If `above` is non-null, the group is positioned just above `above`.
/// Otherwise, the group is left on top, with all the rearranged entries
/// below.
///
/// It is an error to specify both `above` and `below`.
void rearrange(Iterable<OverlayEntry> newEntries, { OverlayEntry? below, OverlayEntry? above }) {
final List<OverlayEntry> newEntriesList = newEntries is List<OverlayEntry> ? newEntries : newEntries.toList(growable: false);
assert(_debugVerifyInsertPosition(above, below, newEntries: newEntriesList));
assert(
newEntriesList.every((OverlayEntry entry) => entry._overlay == null || entry._overlay == this),
'One or more of the specified entries are already present in another Overlay.',
);
assert(
newEntriesList.every((OverlayEntry entry) => _entries.indexOf(entry) == _entries.lastIndexOf(entry)),
'One or more of the specified entries are specified multiple times.',
);
if (newEntriesList.isEmpty) {
return;
}
if (listEquals(_entries, newEntriesList)) {
return;
}
final LinkedHashSet<OverlayEntry> old = LinkedHashSet<OverlayEntry>.of(_entries);
for (final OverlayEntry entry in newEntriesList) {
entry._overlay ??= this;
}
setState(() {
_entries.clear();
_entries.addAll(newEntriesList);
old.removeAll(newEntriesList);
_entries.insertAll(_insertionIndex(below, above), old);
});
}
void _markDirty() {
if (mounted) {
setState(() {});
}
}
/// (DEBUG ONLY) Check whether a given entry is visible (i.e., not behind an
/// opaque entry).
///
/// This is an O(N) algorithm, and should not be necessary except for debug
/// asserts. To avoid people depending on it, this function is implemented
/// only in debug mode, and always returns false in release mode.
bool debugIsVisible(OverlayEntry entry) {
bool result = false;
assert(_entries.contains(entry));
assert(() {
for (int i = _entries.length - 1; i > 0; i -= 1) {
final OverlayEntry candidate = _entries[i];
if (candidate == entry) {
result = true;
break;
}
if (candidate.opaque) {
break;
}
}
return true;
}());
return result;
}
void _didChangeEntryOpacity() {
setState(() {
// We use the opacity of the entry in our build function, which means we
// our state has changed.
});
}
@override
Widget build(BuildContext context) {
// This list is filled backwards and then reversed below before
// it is added to the tree.
final List<_OverlayEntryWidget> children = <_OverlayEntryWidget>[];
bool onstage = true;
int onstageCount = 0;
for (final OverlayEntry entry in _entries.reversed) {
if (onstage) {
onstageCount += 1;
children.add(_OverlayEntryWidget(
key: entry._key,
overlayState: this,
entry: entry,
));
if (entry.opaque) {
onstage = false;
}
} else if (entry.maintainState) {
children.add(_OverlayEntryWidget(
key: entry._key,
overlayState: this,
entry: entry,
tickerEnabled: false,
));
}
}
return _Theater(
skipCount: children.length - onstageCount,
clipBehavior: widget.clipBehavior,
children: children.reversed.toList(growable: false),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
// TODO(jacobr): use IterableProperty instead as that would
// provide a slightly more consistent string summary of the List.
properties.add(DiagnosticsProperty<List<OverlayEntry>>('entries', _entries));
}
}
class _WrappingOverlay extends StatefulWidget {
const _WrappingOverlay({super.key, this.clipBehavior = Clip.hardEdge, required this.child});
final Clip clipBehavior;
final Widget child;
@override
State<_WrappingOverlay> createState() => _WrappingOverlayState();
}
class _WrappingOverlayState extends State<_WrappingOverlay> {
late final OverlayEntry _entry = OverlayEntry(
canSizeOverlay: true,
opaque: true,
builder: (BuildContext context) {
return widget.child;
}
);
@override
void didUpdateWidget(_WrappingOverlay oldWidget) {
super.didUpdateWidget(oldWidget);
_entry.markNeedsBuild();
}
@override
Widget build(BuildContext context) {
return Overlay(
clipBehavior: widget.clipBehavior,
initialEntries: <OverlayEntry>[_entry],
);
}
}
/// Special version of a [Stack], that doesn't layout and render the first
/// [skipCount] children.
///
/// The first [skipCount] children are considered "offstage".
class _Theater extends MultiChildRenderObjectWidget {
const _Theater({
this.skipCount = 0,
this.clipBehavior = Clip.hardEdge,
required List<_OverlayEntryWidget> super.children,
}) : assert(skipCount >= 0),
assert(children.length >= skipCount);
final int skipCount;
final Clip clipBehavior;
@override
_TheaterElement createElement() => _TheaterElement(this);
@override
_RenderTheater createRenderObject(BuildContext context) {
return _RenderTheater(
skipCount: skipCount,
textDirection: Directionality.of(context),
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, _RenderTheater renderObject) {
renderObject
..skipCount = skipCount
..textDirection = Directionality.of(context)
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('skipCount', skipCount));
}
}
class _TheaterElement extends MultiChildRenderObjectElement {
_TheaterElement(_Theater super.widget);
@override
_RenderTheater get renderObject => super.renderObject as _RenderTheater;
@override
void insertRenderObjectChild(RenderBox child, IndexedSlot<Element?> slot) {
super.insertRenderObjectChild(child, slot);
final _TheaterParentData parentData = child.parentData! as _TheaterParentData;
parentData.overlayEntry = ((widget as _Theater).children[slot.index] as _OverlayEntryWidget).entry;
assert(parentData.overlayEntry != null);
}
@override
void moveRenderObjectChild(RenderBox child, IndexedSlot<Element?> oldSlot, IndexedSlot<Element?> newSlot) {
super.moveRenderObjectChild(child, oldSlot, newSlot);
assert(() {
final _TheaterParentData parentData = child.parentData! as _TheaterParentData;
return parentData.overlayEntry == ((widget as _Theater).children[newSlot.index] as _OverlayEntryWidget).entry;
}());
}
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
final _Theater theater = widget as _Theater;
assert(children.length >= theater.skipCount);
children.skip(theater.skipCount).forEach(visitor);
}
}
// A `RenderBox` that sizes itself to its parent's size, implements the stack
// layout algorithm and renders its children in the given `theater`.
mixin _RenderTheaterMixin on RenderBox {
_RenderTheater get theater;
Iterable<RenderBox> _childrenInPaintOrder();
Iterable<RenderBox> _childrenInHitTestOrder();
@override
void setupParentData(RenderBox child) {
if (child.parentData is! StackParentData) {
child.parentData = StackParentData();
}
}
void layoutChild(RenderBox child, BoxConstraints nonPositionedChildConstraints) {
final StackParentData childParentData = child.parentData! as StackParentData;
final Alignment alignment = theater._resolvedAlignment;
if (!childParentData.isPositioned) {
child.layout(nonPositionedChildConstraints, parentUsesSize: true);
childParentData.offset = Offset.zero;
} else {
assert(child is! _RenderDeferredLayoutBox, 'all _RenderDeferredLayoutBoxes must be non-positioned children.');
RenderStack.layoutPositionedChild(child, childParentData, size, alignment);
}
assert(child.parentData == childParentData);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final Iterator<RenderBox> iterator = _childrenInHitTestOrder().iterator;
bool isHit = false;
while (!isHit && iterator.moveNext()) {
final RenderBox child = iterator.current;
final StackParentData childParentData = child.parentData! as StackParentData;
final RenderBox localChild = child;
bool childHitTest(BoxHitTestResult result, Offset position) => localChild.hitTest(result, position: position);
isHit = result.addWithPaintOffset(offset: childParentData.offset, position: position, hitTest: childHitTest);
}
return isHit;
}
@override
void paint(PaintingContext context, Offset offset) {
for (final RenderBox child in _childrenInPaintOrder()) {
final StackParentData childParentData = child.parentData! as StackParentData;
context.paintChild(child, childParentData.offset + offset);
}
}
}
class _TheaterParentData extends StackParentData {
// The OverlayEntry that directly created this child. This field is null for
// children that are created by an OverlayPortal.
OverlayEntry? overlayEntry;
/// A [OverlayPortal] makes its overlay child a render child of an ancestor
/// [Overlay]. Currently, to make sure the overlay child is painted after its
/// [OverlayPortal], and before the next [OverlayEntry] (which could be
/// something that should obstruct the overlay child, such as a [ModalRoute])
/// in the host [Overlay], the paint order of each overlay child is managed by
/// the [OverlayEntry] that hosts its [OverlayPortal].
///
/// The following methods are exposed to allow easy access to the overlay
/// children's render objects whose order is managed by [overlayEntry], in the
/// right order.
// _overlayStateMounted is set to null in _OverlayEntryWidgetState's dispose
// method. This property is only accessed during layout, paint and hit-test so
// the `value!` should be safe.
Iterator<RenderBox>? get paintOrderIterator => overlayEntry?._overlayEntryStateNotifier?.value!._paintOrderIterable.iterator;
Iterator<RenderBox>? get hitTestOrderIterator => overlayEntry?._overlayEntryStateNotifier?.value!._hitTestOrderIterable.iterator;
// A convenience method for traversing `paintOrderIterator` with a
// [RenderObjectVisitor].
void visitOverlayPortalChildrenOnOverlayEntry(RenderObjectVisitor visitor) => overlayEntry?._overlayEntryStateNotifier?.value!._paintOrderIterable.forEach(visitor);
}
class _RenderTheater extends RenderBox with ContainerRenderObjectMixin<RenderBox, StackParentData>, _RenderTheaterMixin {
_RenderTheater({
List<RenderBox>? children,
required TextDirection textDirection,
int skipCount = 0,
Clip clipBehavior = Clip.hardEdge,
}) : assert(skipCount >= 0),
_textDirection = textDirection,
_skipCount = skipCount,
_clipBehavior = clipBehavior {
addAll(children);
}
@override
_RenderTheater get theater => this;
@override
void setupParentData(RenderBox child) {
if (child.parentData is! _TheaterParentData) {
child.parentData = _TheaterParentData();
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
RenderBox? child = firstChild;
while (child != null) {
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
final Iterator<RenderBox>? iterator = childParentData.paintOrderIterator;
if (iterator != null) {
while (iterator.moveNext()) {
iterator.current.attach(owner);
}
}
child = childParentData.nextSibling;
}
}
static void _detachChild(RenderObject child) => child.detach();
@override
void detach() {
super.detach();
RenderBox? child = firstChild;
while (child != null) {
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
childParentData.visitOverlayPortalChildrenOnOverlayEntry(_detachChild);
child = childParentData.nextSibling;
}
}
@override
void redepthChildren() => visitChildren(redepthChild);
Alignment? _alignmentCache;
Alignment get _resolvedAlignment => _alignmentCache ??= AlignmentDirectional.topStart.resolve(textDirection);
void _markNeedResolution() {
_alignmentCache = null;
markNeedsLayout();
}
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
_markNeedResolution();
}
int get skipCount => _skipCount;
int _skipCount;
set skipCount(int value) {
if (_skipCount != value) {
_skipCount = value;
markNeedsLayout();
}
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
// Adding/removing deferred child does not affect the layout of other children,
// or that of the Overlay, so there's no need to invalidate the layout of the
// Overlay.
//
// When _skipMarkNeedsLayout is true, markNeedsLayout does not do anything.
bool _skipMarkNeedsLayout = false;
void _addDeferredChild(_RenderDeferredLayoutBox child) {
assert(!_skipMarkNeedsLayout);
_skipMarkNeedsLayout = true;
adoptChild(child);
// The Overlay still needs repainting when a deferred child is added. Usually
// `markNeedsLayout` implies `markNeedsPaint`, but here `markNeedsLayout` is
// skipped when the `_skipMarkNeedsLayout` flag is set.
markNeedsPaint();
_skipMarkNeedsLayout = false;
// After adding `child` to the render tree, we want to make sure it will be
// laid out in the same frame. This is done by calling markNeedsLayout on the
// layout surrogate. This ensures `child` is reachable via tree walk (see
// _RenderLayoutSurrogateProxyBox.performLayout).
child._layoutSurrogate.markNeedsLayout();
}
void _removeDeferredChild(_RenderDeferredLayoutBox child) {
assert(!_skipMarkNeedsLayout);
_skipMarkNeedsLayout = true;
dropChild(child);
// The Overlay still needs repainting when a deferred child is dropped. See
// the comment in `_addDeferredChild`.
markNeedsPaint();
_skipMarkNeedsLayout = false;
}
@override
void markNeedsLayout() {
if (!_skipMarkNeedsLayout) {
super.markNeedsLayout();
}
}
RenderBox? get _firstOnstageChild {
if (skipCount == super.childCount) {
return null;
}
RenderBox? child = super.firstChild;
for (int toSkip = skipCount; toSkip > 0; toSkip--) {
final StackParentData childParentData = child!.parentData! as StackParentData;
child = childParentData.nextSibling;
assert(child != null);
}
return child;
}
RenderBox? get _lastOnstageChild => skipCount == super.childCount ? null : lastChild;
@override
double computeMinIntrinsicWidth(double height) {
return RenderStack.getIntrinsicDimension(_firstOnstageChild, (RenderBox child) => child.getMinIntrinsicWidth(height));
}
@override
double computeMaxIntrinsicWidth(double height) {
return RenderStack.getIntrinsicDimension(_firstOnstageChild, (RenderBox child) => child.getMaxIntrinsicWidth(height));
}
@override
double computeMinIntrinsicHeight(double width) {
return RenderStack.getIntrinsicDimension(_firstOnstageChild, (RenderBox child) => child.getMinIntrinsicHeight(width));
}
@override
double computeMaxIntrinsicHeight(double width) {
return RenderStack.getIntrinsicDimension(_firstOnstageChild, (RenderBox child) => child.getMaxIntrinsicHeight(width));
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
assert(!debugNeedsLayout);
double? result;
RenderBox? child = _firstOnstageChild;
while (child != null) {
assert(!child.debugNeedsLayout);
final StackParentData childParentData = child.parentData! as StackParentData;
double? candidate = child.getDistanceToActualBaseline(baseline);
if (candidate != null) {
candidate += childParentData.offset.dy;
if (result != null) {
result = math.min(result, candidate);
} else {
result = candidate;
}
}
child = childParentData.nextSibling;
}
return result;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
if (constraints.biggest.isFinite) {
return constraints.biggest;
}
return _findSizeDeterminingChild().getDryLayout(constraints);
}
@override
// The following uses sync* because concurrent modifications should be allowed
// during layout.
Iterable<RenderBox> _childrenInPaintOrder() sync* {
RenderBox? child = _firstOnstageChild;
while (child != null) {
yield child;
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
final Iterator<RenderBox>? innerIterator = childParentData.paintOrderIterator;
if (innerIterator != null) {
while (innerIterator.moveNext()) {
yield innerIterator.current;
}
}
child = childParentData.nextSibling;
}
}
@override
// The following uses sync* because hit testing should be lazy.
Iterable<RenderBox> _childrenInHitTestOrder() sync* {
RenderBox? child = _lastOnstageChild;
int childLeft = childCount - skipCount;
while (child != null) {
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
final Iterator<RenderBox>? innerIterator = childParentData.hitTestOrderIterator;
if (innerIterator != null) {
while (innerIterator.moveNext()) {
yield innerIterator.current;
}
}
yield child;
childLeft -= 1;
child = childLeft <= 0 ? null : childParentData.previousSibling;
}
}
@override
bool get sizedByParent => false;
@override
void performLayout() {
RenderBox? sizeDeterminingChild;
if (constraints.biggest.isFinite) {
size = constraints.biggest;
} else {
sizeDeterminingChild = _findSizeDeterminingChild();
layoutChild(sizeDeterminingChild, constraints);
size = sizeDeterminingChild.size;
}
// Equivalent to BoxConstraints used by RenderStack for StackFit.expand.
final BoxConstraints nonPositionedChildConstraints = BoxConstraints.tight(size);
for (final RenderBox child in _childrenInPaintOrder()) {
if (child != sizeDeterminingChild) {
layoutChild(child, nonPositionedChildConstraints);
}
}
}
RenderBox _findSizeDeterminingChild() {
RenderBox? child = _lastOnstageChild;
while (child != null) {
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
if ((childParentData.overlayEntry?.canSizeOverlay ?? false) && !childParentData.isPositioned) {
return child;
}
child = childParentData.previousSibling;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Overlay was given infinite constraints and cannot be sized by a suitable child.'),
ErrorDescription(
'The constraints given to the overlay ($constraints) would result in an illegal '
'infinite size (${constraints.biggest}). To avoid that, the Overlay tried to size '
'itself to one of its children, but no suitable non-positioned child that belongs to an '
'OverlayEntry with canSizeOverlay set to true could be found.',
),
ErrorHint(
'Try wrapping the Overlay in a SizedBox to give it a finite size or '
'use an OverlayEntry with canSizeOverlay set to true.',
),
]);
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void paint(PaintingContext context, Offset offset) {
if (clipBehavior != Clip.none) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
super.paint,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
super.paint(context, offset);
}
}
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
@override
void visitChildren(RenderObjectVisitor visitor) {
RenderBox? child = firstChild;
while (child != null) {
visitor(child);
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
childParentData.visitOverlayPortalChildrenOnOverlayEntry(visitor);
child = childParentData.nextSibling;
}
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
RenderBox? child = _firstOnstageChild;
while (child != null) {
visitor(child);
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
child = childParentData.nextSibling;
}
}
@override
Rect? describeApproximatePaintClip(RenderObject child) {
switch (clipBehavior) {
case Clip.none:
return null;
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
return Offset.zero & size;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('skipCount', skipCount));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection));
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> offstageChildren = <DiagnosticsNode>[];
final List<DiagnosticsNode> onstageChildren = <DiagnosticsNode>[];
int count = 1;
bool onstage = false;
RenderBox? child = firstChild;
final RenderBox? firstOnstageChild = _firstOnstageChild;
while (child != null) {
final _TheaterParentData childParentData = child.parentData! as _TheaterParentData;
if (child == firstOnstageChild) {
onstage = true;
count = 1;
}
if (onstage) {
onstageChildren.add(
child.toDiagnosticsNode(
name: 'onstage $count',
),
);
} else {
offstageChildren.add(
child.toDiagnosticsNode(
name: 'offstage $count',
style: DiagnosticsTreeStyle.offstage,
),
);
}
int subcount = 1;
childParentData.visitOverlayPortalChildrenOnOverlayEntry((RenderObject renderObject) {
final RenderBox child = renderObject as RenderBox;
if (onstage) {
onstageChildren.add(
child.toDiagnosticsNode(
name: 'onstage $count - $subcount',
),
);
} else {
offstageChildren.add(
child.toDiagnosticsNode(
name: 'offstage $count - $subcount',
style: DiagnosticsTreeStyle.offstage,
),
);
}
subcount += 1;
});
child = childParentData.nextSibling;
count += 1;
}
return <DiagnosticsNode>[
...onstageChildren,
if (offstageChildren.isNotEmpty)
...offstageChildren
else
DiagnosticsNode.message(
'no offstage children',
style: DiagnosticsTreeStyle.offstage,
),
];
}
}
// * OverlayPortal Implementation
// OverlayPortal is inspired by the
// [flutter_portal](https://pub.dev/packages/flutter_portal) package.
//
// ** RenderObject hierarchy
// The widget works by inserting its overlay child's render subtree directly
// under [Overlay]'s render object (_RenderTheater).
// https://user-images.githubusercontent.com/31859944/171971838-62ed3975-4b5d-4733-a9c9-f79e263b8fcc.jpg
//
// To ensure the overlay child render subtree does not do layout twice, the
// subtree must only perform layout after both its _RenderTheater and the
// [OverlayPortal]'s render object (_RenderLayoutSurrogateProxyBox) have
// finished layout. This is handled by _RenderDeferredLayoutBox.
//
// ** Z-Index of an overlay child
// [_OverlayEntryLocation] is a (currently private) interface that allows an
// [OverlayPortal] to insert its overlay child into a specific [Overlay], as
// well as specifying the paint order between the overlay child and other
// children of the _RenderTheater.
//
// Since [OverlayPortal] is only allowed to target ancestor [Overlay]s
// (_RenderTheater must finish doing layout before _RenderDeferredLayoutBox),
// the _RenderTheater should typically be acquired using an [InheritedWidget]
// (currently, _RenderTheaterMarker) in case the [OverlayPortal] gets
// reparented.
/// A class to show, hide and bring to top an [OverlayPortal]'s overlay child
/// in the target [Overlay].
///
/// A [OverlayPortalController] can only be given to at most one [OverlayPortal]
/// at a time. When an [OverlayPortalController] is moved from one
/// [OverlayPortal] to another, its [isShowing] state does not carry over.
///
/// [OverlayPortalController.show] and [OverlayPortalController.hide] can be
/// called even before the controller is assigned to any [OverlayPortal], but
/// they typically should not be called while the widget tree is being rebuilt.
class OverlayPortalController {
/// Creates an [OverlayPortalController], optionally with a String identifier
/// `debugLabel`.
OverlayPortalController({ String? debugLabel }) : _debugLabel = debugLabel;
_OverlayPortalState? _attachTarget;
// A separate _zOrderIndex to allow `show()` or `hide()` to be called when the
// controller is not yet attached. Once this controller is attached,
// _attachTarget._zOrderIndex will be used as the source of truth, and this
// variable will be set to null.
int? _zOrderIndex;
final String? _debugLabel;
static int _wallTime = kIsWeb
? -9007199254740992 // -2^53
: -1 << 63;
// Returns a unique and monotonically increasing timestamp that represents
// now.
//
// The value this method returns increments after each call.
int _now() {
final int now = _wallTime += 1;
assert(_zOrderIndex == null || _zOrderIndex! < now);
assert(_attachTarget?._zOrderIndex == null || _attachTarget!._zOrderIndex! < now);
return now;
}
/// Show the overlay child of the [OverlayPortal] this controller is attached
/// to, at the top of the target [Overlay].
///
/// When there are more than one [OverlayPortal]s that target the same
/// [Overlay], the overlay child of the last [OverlayPortal] to have called
/// [show] appears at the top level, unobstructed.
///
/// If [isShowing] is already true, calling this method brings the overlay
/// child it controls to the top.
///
/// This method should typically not be called while the widget tree is being
/// rebuilt.
void show() {
final _OverlayPortalState? state = _attachTarget;
if (state != null) {
state.show(_now());
} else {
_zOrderIndex = _now();
}
}
/// Hide the [OverlayPortal]'s overlay child.
///
/// Once hidden, the overlay child will be removed from the widget tree the
/// next time the widget tree rebuilds, and stateful widgets in the overlay
/// child may lose states as a result.
///
/// This method should typically not be called while the widget tree is being
/// rebuilt.
void hide() {
final _OverlayPortalState? state = _attachTarget;
if (state != null) {
state.hide();
} else {
assert(_zOrderIndex != null);
_zOrderIndex = null;
}
}
/// Whether the associated [OverlayPortal] should build and show its overlay
/// child, using its `overlayChildBuilder`.
bool get isShowing {
final _OverlayPortalState? state = _attachTarget;
return state != null
? state._zOrderIndex != null
: _zOrderIndex != null;
}
/// Convenience method for toggling the current [isShowing] status.
///
/// This method should typically not be called while the widget tree is being
/// rebuilt.
void toggle() => isShowing ? hide() : show();
@override
String toString() {
final String? debugLabel = _debugLabel;
final String label = debugLabel == null ? '' : '($debugLabel)';
final String isDetached = _attachTarget != null ? '' : ' DETACHED';
return '${objectRuntimeType(this, 'OverlayPortalController')}$label$isDetached';
}
}
/// A widget that renders its overlay child on an [Overlay].
///
/// The overlay child is initially hidden until [OverlayPortalController.show]
/// is called on the associated [controller]. The [OverlayPortal] uses
/// [overlayChildBuilder] to build its overlay child and renders it on the
/// specified [Overlay] as if it was inserted using an [OverlayEntry], while it
/// can depend on the same set of [InheritedWidget]s (such as [Theme]) that this
/// widget can depend on.
///
/// This widget requires an [Overlay] ancestor in the widget tree when its
/// overlay child is showing. The overlay child is rendered by the [Overlay]
/// ancestor, not by the widget itself. This allows the overlay child to float
/// above other widgets, independent of its position in the widget tree.
///
/// When [OverlayPortalController.hide] is called, the widget built using
/// [overlayChildBuilder] will be removed from the widget tree the next time the
/// widget rebuilds. Stateful descendants in the overlay child subtree may lose
/// states as a result.
///
/// {@tool dartpad}
/// This example uses an [OverlayPortal] to build a tooltip that becomes visible
/// when the user taps on the [child] widget. There's a [DefaultTextStyle] above
/// the [OverlayPortal] controlling the [TextStyle] of both the [child] widget
/// and the widget [overlayChildBuilder] builds, which isn't otherwise doable if
/// the tooltip was added as an [OverlayEntry].
///
/// ** See code in examples/api/lib/widgets/overlay/overlay_portal.0.dart **
/// {@end-tool}
///
/// ### Paint Order
///
/// In an [Overlay], an overlay child is painted after the [OverlayEntry]
/// associated with its [OverlayPortal] (that is, the [OverlayEntry] closest to
/// the [OverlayPortal] in the widget tree, which usually represents the
/// enclosing [Route]), and before the next [OverlayEntry].
///
/// When an [OverlayEntry] has multiple associated [OverlayPortal]s, the paint
/// order between their overlay children is the order in which
/// [OverlayPortalController.show] was called. The last [OverlayPortal] to have
/// called `show` gets to paint its overlay child in the foreground.
///
/// ### Semantics
///
/// The semantics subtree generated by the overlay child is considered attached
/// to [OverlayPortal] instead of the target [Overlay]. An [OverlayPortal]'s
/// semantics subtree can be dropped from the semantics tree due to invisibility
/// while the overlay child is still visible (for example, when the
/// [OverlayPortal] is completely invisible in a [ListView] but kept alive by
/// a [KeepAlive] widget). When this happens the semantics subtree generated by
/// the overlay child is also dropped, even if the overlay child is still visible
/// on screen.
///
/// {@template flutter.widgets.overlayPortalVsOverlayEntry}
/// ### Differences between [OverlayPortal] and [OverlayEntry]
///
/// The main difference between [OverlayEntry] and [OverlayPortal] is that
/// [OverlayEntry] builds its widget subtree as a child of the target [Overlay],
/// while [OverlayPortal] uses [OverlayPortal.overlayChildBuilder] to build a
/// child widget of itself. This allows [OverlayPortal]'s overlay child to depend
/// on the same set of [InheritedWidget]s as [OverlayPortal], and it's also
/// guaranteed that the overlay child will not outlive its [OverlayPortal].
///
/// On the other hand, [OverlayPortal]'s implementation is more complex. For
/// instance, it does a bit more work than a regular widget during global key
/// reparenting. If the content to be shown on the [Overlay] doesn't benefit
/// from being a part of [OverlayPortal]'s subtree, consider using an
/// [OverlayEntry] instead.
/// {@endtemplate}
///
/// See also:
///
/// * [OverlayEntry], an alternative API for inserting widgets into an
/// [Overlay].
/// * [Positioned], which can be used to size and position the overlay child in
/// relation to the target [Overlay]'s boundaries.
/// * [CompositedTransformFollower], which can be used to position the overlay
/// child in relation to the linked [CompositedTransformTarget] widget.
class OverlayPortal extends StatefulWidget {
/// Creates an [OverlayPortal] that renders the widget [overlayChildBuilder]
/// builds on the closest [Overlay] when [OverlayPortalController.show] is
/// called.
const OverlayPortal({
super.key,
required this.controller,
required this.overlayChildBuilder,
this.child,
}) : _targetRootOverlay = false;
/// Creates an [OverlayPortal] that renders the widget [overlayChildBuilder]
/// builds on the root [Overlay] when [OverlayPortalController.show] is
/// called.
const OverlayPortal.targetsRootOverlay({
super.key,
required this.controller,
required this.overlayChildBuilder,
this.child,
}) : _targetRootOverlay = true;
/// The controller to show, hide and bring to top the overlay child.
final OverlayPortalController controller;
/// A [WidgetBuilder] used to build a widget below this widget in the tree,
/// that renders on the closest [Overlay].
///
/// The said widget will only be built and shown in the closest [Overlay] once
/// [OverlayPortalController.show] is called on the associated [controller].
/// It will be painted in front of the [OverlayEntry] closest to this widget
/// in the widget tree (which is usually the enclosing [Route]).
///
/// The built overlay child widget is inserted below this widget in the widget
/// tree, allowing it to depend on [InheritedWidget]s above it, and be
/// notified when the [InheritedWidget]s change.
///
/// Unlike [child], the built overlay child can visually extend outside the
/// bounds of this widget without being clipped, and receive hit-test events
/// outside of this widget's bounds, as long as it does not extend outside of
/// the [Overlay] on which it is rendered.
final WidgetBuilder overlayChildBuilder;
/// A widget below this widget in the tree.
final Widget? child;
final bool _targetRootOverlay;
@override
State<OverlayPortal> createState() => _OverlayPortalState();
}
class _OverlayPortalState extends State<OverlayPortal> {
int? _zOrderIndex;
// The location of the overlay child within the overlay. This object will be
// used as the slot of the overlay child widget.
//
// The developer must call `show` to reveal the overlay so we can get a unique
// timestamp of the user interaction for determining the z-index of the
// overlay child in the overlay.
//
// Avoid invalidating the cache if possible, since the framework uses `==` to
// compare slots, and _OverlayEntryLocation can't override that operator since
// it's mutable. Changing slots can be relatively slow.
bool _childModelMayHaveChanged = true;
_OverlayEntryLocation? _locationCache;
static bool _isTheSameLocation(_OverlayEntryLocation locationCache, _RenderTheaterMarker marker) {
return locationCache._childModel == marker.overlayEntryWidgetState
&& locationCache._theater == marker.theater;
}
_OverlayEntryLocation _getLocation(int zOrderIndex, bool targetRootOverlay) {
final _OverlayEntryLocation? cachedLocation = _locationCache;
late final _RenderTheaterMarker marker = _RenderTheaterMarker.of(context, targetRootOverlay: targetRootOverlay);
final bool isCacheValid = cachedLocation != null
&& (!_childModelMayHaveChanged || _isTheSameLocation(cachedLocation, marker));
_childModelMayHaveChanged = false;
if (isCacheValid) {
assert(cachedLocation._zOrderIndex == zOrderIndex);
assert(cachedLocation._debugIsLocationValid());
return cachedLocation;
}
// Otherwise invalidate the cache and create a new location.
cachedLocation?._debugMarkLocationInvalid();
final _OverlayEntryLocation newLocation = _OverlayEntryLocation(zOrderIndex, marker.overlayEntryWidgetState, marker.theater);
assert(newLocation._zOrderIndex == zOrderIndex);
return _locationCache = newLocation;
}
@override
void initState() {
super.initState();
_setupController(widget.controller);
}
void _setupController(OverlayPortalController controller) {
assert(
controller._attachTarget == null || controller._attachTarget == this,
'Failed to attach $controller to $this. It is already attached to ${controller._attachTarget}.'
);
final int? controllerZOrderIndex = controller._zOrderIndex;
final int? zOrderIndex = _zOrderIndex;
if (zOrderIndex == null || (controllerZOrderIndex != null && controllerZOrderIndex > zOrderIndex)) {
_zOrderIndex = controllerZOrderIndex;
}
controller._zOrderIndex = null;
controller._attachTarget = this;
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_childModelMayHaveChanged = true;
}
@override
void didUpdateWidget(OverlayPortal oldWidget) {
super.didUpdateWidget(oldWidget);
_childModelMayHaveChanged = _childModelMayHaveChanged || oldWidget._targetRootOverlay != widget._targetRootOverlay;
if (oldWidget.controller != widget.controller) {
oldWidget.controller._attachTarget = null;
_setupController(widget.controller);
}
}
@override
void dispose() {
assert(widget.controller._attachTarget == this);
widget.controller._attachTarget = null;
_locationCache?._debugMarkLocationInvalid();
_locationCache = null;
super.dispose();
}
void show(int zOrderIndex) {
assert(
SchedulerBinding.instance.schedulerPhase != SchedulerPhase.persistentCallbacks,
'${widget.controller.runtimeType}.show() should not be called during build.'
);
setState(() { _zOrderIndex = zOrderIndex; });
_locationCache?._debugMarkLocationInvalid();
_locationCache = null;
}
void hide() {
assert(SchedulerBinding.instance.schedulerPhase != SchedulerPhase.persistentCallbacks);
setState(() { _zOrderIndex = null; });
_locationCache?._debugMarkLocationInvalid();
_locationCache = null;
}
@override
Widget build(BuildContext context) {
final int? zOrderIndex = _zOrderIndex;
if (zOrderIndex == null) {
return _OverlayPortal(
overlayLocation: null,
overlayChild: null,
child: widget.child,
);
}
return _OverlayPortal(
overlayLocation: _getLocation(zOrderIndex, widget._targetRootOverlay),
overlayChild: _DeferredLayout(child: Builder(builder: widget.overlayChildBuilder)),
child: widget.child,
);
}
}
/// A location in an [Overlay].
///
/// An [_OverlayEntryLocation] determines the [Overlay] the associated
/// [OverlayPortal] should put its overlay child onto, as well as the overlay
/// child's paint order in relation to other contents painted on the [Overlay].
//
// An _OverlayEntryLocation is a cursor pointing to a location in a particular
// Overlay's child model, and provides methods to insert/remove/move a
// _RenderDeferredLayoutBox to/from its target _theater.
//
// The occupant (a `RenderBox`) will be painted above the associated
// [OverlayEntry], but below the [OverlayEntry] above that [OverlayEntry].
//
// Additionally, `_activate` and `_deactivate` are called when the overlay
// child's `_OverlayPortalElement` activates/deactivates (for instance, during
// global key reparenting).
// `_OverlayPortalElement` removes its overlay child's render object from the
// target `_RenderTheater` when it deactivates and puts it back on `activated`.
// These 2 methods can be used to "hide" a child in the child model without
// removing it, when the child is expensive/difficult to re-insert at the
// correct location on `activated`.
//
// ### Equality
//
// An `_OverlayEntryLocation` will be used as an Element's slot. These 3 parts
// uniquely identify a place in an overlay's child model:
// - _theater
// - _childModel (the OverlayEntry)
// - _zOrderIndex
//
// Since it can't implement operator== (it's mutable), the same `_OverlayEntryLocation`
// instance must not be used to represent more than one locations.
final class _OverlayEntryLocation extends LinkedListEntry<_OverlayEntryLocation> {
_OverlayEntryLocation(this._zOrderIndex, this._childModel, this._theater);
final int _zOrderIndex;
final _OverlayEntryWidgetState _childModel;
final _RenderTheater _theater;
_RenderDeferredLayoutBox? _overlayChildRenderBox;
void _addToChildModel(_RenderDeferredLayoutBox child) {
assert(_overlayChildRenderBox == null, 'Failed to add $child. This location ($this) is already occupied by $_overlayChildRenderBox.');
_overlayChildRenderBox = child;
_childModel._add(this);
_theater.markNeedsPaint();
_theater.markNeedsCompositingBitsUpdate();
_theater.markNeedsSemanticsUpdate();
}
void _removeFromChildModel(_RenderDeferredLayoutBox child) {
assert(child == _overlayChildRenderBox);
_overlayChildRenderBox = null;
assert(_childModel._sortedTheaterSiblings?.contains(this) ?? false);
_childModel._remove(this);
_theater.markNeedsPaint();
_theater.markNeedsCompositingBitsUpdate();
_theater.markNeedsSemanticsUpdate();
}
void _addChild(_RenderDeferredLayoutBox child) {
assert(_debugIsLocationValid());
_addToChildModel(child);
_theater._addDeferredChild(child);
assert(child.parent == _theater);
}
void _removeChild(_RenderDeferredLayoutBox child) {
// This call is allowed even when this location is disposed.
_removeFromChildModel(child);
_theater._removeDeferredChild(child);
assert(child.parent == null);
}
void _moveChild(_RenderDeferredLayoutBox child, _OverlayEntryLocation fromLocation) {
assert(fromLocation != this);
assert(_debugIsLocationValid());
final _RenderTheater fromTheater = fromLocation._theater;
final _OverlayEntryWidgetState fromModel = fromLocation._childModel;
if (fromTheater != _theater) {
fromTheater._removeDeferredChild(child);
_theater._addDeferredChild(child);
}
if (fromModel != _childModel || fromLocation._zOrderIndex != _zOrderIndex) {
fromLocation._removeFromChildModel(child);
_addToChildModel(child);
}
}
void _activate(_RenderDeferredLayoutBox child) {
// This call is allowed even when this location is invalidated.
// See _OverlayPortalElement.activate.
assert(_overlayChildRenderBox == null, '$_overlayChildRenderBox');
_theater._addDeferredChild(child);
_overlayChildRenderBox = child;
}
void _deactivate(_RenderDeferredLayoutBox child) {
// This call is allowed even when this location is invalidated.
_theater._removeDeferredChild(child);
_overlayChildRenderBox = null;
}
// Throws a StateError if this location is already invalidated and shouldn't
// be used as an OverlayPortal slot. Must be used in asserts.
//
// Generally, `assert(_debugIsLocationValid())` should be used to prevent
// invalid accesses to an invalid `_OverlayEntryLocation` object. Exceptions
// to this rule are _removeChild, _deactive, which will be called when the
// OverlayPortal is being removed from the widget tree and may use the
// location information to perform cleanup tasks.
//
// Another exception is the _activate method which is called by
// _OverlayPortalElement.activate. See the comment in _OverlayPortalElement.activate.
bool _debugIsLocationValid() {
if (_debugMarkLocationInvalidStackTrace == null) {
return true;
}
throw StateError('$this is already disposed. Stack trace: $_debugMarkLocationInvalidStackTrace');
}
// The StackTrace of the first _debugMarkLocationInvalid call. It's only for
// debugging purposes and the StackTrace will only be captured in debug builds.
//
// The effect of this method is not reversible. Once marked invalid, this
// object can't be marked as valid again.
StackTrace? _debugMarkLocationInvalidStackTrace;
@mustCallSuper
void _debugMarkLocationInvalid() {
assert(_debugIsLocationValid());
assert(() {
_debugMarkLocationInvalidStackTrace = StackTrace.current;
return true;
}());
}
@override
String toString() => '${objectRuntimeType(this, '_OverlayEntryLocation')}[${shortHash(this)}] ${_debugMarkLocationInvalidStackTrace != null ? "(INVALID)":""}';
}
class _RenderTheaterMarker extends InheritedWidget {
const _RenderTheaterMarker({
required this.theater,
required this.overlayEntryWidgetState,
required super.child,
});
final _RenderTheater theater;
final _OverlayEntryWidgetState overlayEntryWidgetState;
@override
bool updateShouldNotify(_RenderTheaterMarker oldWidget) {
return oldWidget.theater != theater
|| oldWidget.overlayEntryWidgetState != overlayEntryWidgetState;
}
static _RenderTheaterMarker of(BuildContext context, { bool targetRootOverlay = false }) {
final _RenderTheaterMarker? marker;
if (targetRootOverlay) {
final InheritedElement? ancestor = _rootRenderTheaterMarkerOf(context.getElementForInheritedWidgetOfExactType<_RenderTheaterMarker>());
assert(ancestor == null || ancestor.widget is _RenderTheaterMarker);
marker = ancestor != null ? context.dependOnInheritedElement(ancestor) as _RenderTheaterMarker? : null;
} else {
marker = context.dependOnInheritedWidgetOfExactType<_RenderTheaterMarker>();
}
if (marker != null) {
return marker;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Overlay widget found.'),
ErrorDescription(
'${context.widget.runtimeType} widgets require an Overlay widget ancestor.\n'
'An overlay lets widgets float on top of other widget children.',
),
ErrorHint(
'To introduce an Overlay widget, you can either directly '
'include one, or use a widget that contains an Overlay itself, '
'such as a Navigator, WidgetApp, MaterialApp, or CupertinoApp.',
),
...context.describeMissingAncestor(expectedAncestorType: Overlay),
]);
}
static InheritedElement? _rootRenderTheaterMarkerOf(InheritedElement? theaterMarkerElement) {
assert(theaterMarkerElement == null || theaterMarkerElement.widget is _RenderTheaterMarker);
if (theaterMarkerElement == null) {
return null;
}
InheritedElement? ancestor;
theaterMarkerElement.visitAncestorElements((Element element) {
ancestor = element.getElementForInheritedWidgetOfExactType<_RenderTheaterMarker>();
return false;
});
return ancestor == null ? theaterMarkerElement : _rootRenderTheaterMarkerOf(ancestor);
}
}
class _OverlayPortal extends RenderObjectWidget {
/// Creates a widget that renders the given [overlayChild] in the [Overlay]
/// specified by `overlayLocation`.
///
/// The `overlayLocation` parameter must not be null when [overlayChild] is not
/// null.
_OverlayPortal({
required this.overlayLocation,
required this.overlayChild,
required this.child,
}) : assert(overlayChild == null || overlayLocation != null),
assert(overlayLocation == null || overlayLocation._debugIsLocationValid());
final Widget? overlayChild;
/// A widget below this widget in the tree.
final Widget? child;
final _OverlayEntryLocation? overlayLocation;
@override
RenderObjectElement createElement() => _OverlayPortalElement(this);
@override
RenderObject createRenderObject(BuildContext context) => _RenderLayoutSurrogateProxyBox();
}
class _OverlayPortalElement extends RenderObjectElement {
_OverlayPortalElement(_OverlayPortal super.widget);
@override
_RenderLayoutSurrogateProxyBox get renderObject => super.renderObject as _RenderLayoutSurrogateProxyBox;
Element? _overlayChild;
Element? _child;
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
final _OverlayPortal widget = this.widget as _OverlayPortal;
_child = updateChild(_child, widget.child, null);
_overlayChild = updateChild(_overlayChild, widget.overlayChild, widget.overlayLocation);
}
@override
void update(_OverlayPortal newWidget) {
super.update(newWidget);
_child = updateChild(_child, newWidget.child, null);
_overlayChild = updateChild(_overlayChild, newWidget.overlayChild, newWidget.overlayLocation);
}
@override
void forgetChild(Element child) {
// The _overlayChild Element does not have a key because the _DeferredLayout
// widget does not take a Key, so only the regular _child can be taken
// during global key reparenting.
assert(child == _child);
_child = null;
super.forgetChild(child);
}
@override
void visitChildren(ElementVisitor visitor) {
final Element? child = _child;
final Element? overlayChild = _overlayChild;
if (child != null) {
visitor(child);
}
if (overlayChild != null) {
visitor(overlayChild);
}
}
@override
void activate() {
super.activate();
final Element? overlayChild = _overlayChild;
if (overlayChild != null) {
final _RenderDeferredLayoutBox? box = overlayChild.renderObject as _RenderDeferredLayoutBox?;
if (box != null) {
assert(!box.attached);
assert(renderObject._deferredLayoutChild == box);
// updateChild has not been called at this point so the RenderTheater in
// the overlay location could be detached. Adding children to a detached
// RenderObject is still allowed however this isn't the most efficient.
(overlayChild.slot! as _OverlayEntryLocation)._activate(box);
}
}
}
@override
void deactivate() {
final Element? overlayChild = _overlayChild;
// Instead of just detaching the render objects, removing them from the
// render subtree entirely. This is a workaround for the
// !renderObject.attached assert in the `super.deactivate()` method.
if (overlayChild != null) {
final _RenderDeferredLayoutBox? box = overlayChild.renderObject as _RenderDeferredLayoutBox?;
if (box != null) {
(overlayChild.slot! as _OverlayEntryLocation)._deactivate(box);
}
}
super.deactivate();
}
@override
void insertRenderObjectChild(RenderBox child, _OverlayEntryLocation? slot) {
assert(child.parent == null, "$child's parent is not null: ${child.parent}");
if (slot != null) {
renderObject._deferredLayoutChild = child as _RenderDeferredLayoutBox;
slot._addChild(child);
} else {
renderObject.child = child;
}
}
// The [_DeferredLayout] widget does not have a key so there will be no
// reparenting between _overlayChild and _child, thus the non-null-typed slots.
@override
void moveRenderObjectChild(_RenderDeferredLayoutBox child, _OverlayEntryLocation oldSlot, _OverlayEntryLocation newSlot) {
assert(newSlot._debugIsLocationValid());
newSlot._moveChild(child, oldSlot);
}
@override
void removeRenderObjectChild(RenderBox child, _OverlayEntryLocation? slot) {
if (slot == null) {
renderObject.child = null;
return;
}
assert(renderObject._deferredLayoutChild == child);
slot._removeChild(child as _RenderDeferredLayoutBox);
renderObject._deferredLayoutChild = null;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Element>('child', _child, defaultValue: null));
properties.add(DiagnosticsProperty<Element>('overlayChild', _overlayChild, defaultValue: null));
properties.add(DiagnosticsProperty<Object>('overlayLocation', _overlayChild?.slot, defaultValue: null));
}
}
class _DeferredLayout extends SingleChildRenderObjectWidget {
const _DeferredLayout({
// This widget must not be given a key: we currently do not support
// reparenting between the overlayChild and child.
required Widget child,
}) : super(child: child);
_RenderLayoutSurrogateProxyBox getLayoutParent(BuildContext context) {
return context.findAncestorRenderObjectOfType<_RenderLayoutSurrogateProxyBox>()!;
}
@override
_RenderDeferredLayoutBox createRenderObject(BuildContext context) {
final _RenderLayoutSurrogateProxyBox parent = getLayoutParent(context);
final _RenderDeferredLayoutBox renderObject = _RenderDeferredLayoutBox(parent);
parent._deferredLayoutChild = renderObject;
return renderObject;
}
@override
void updateRenderObject(BuildContext context, _RenderDeferredLayoutBox renderObject) {
assert(renderObject._layoutSurrogate == getLayoutParent(context));
assert(getLayoutParent(context)._deferredLayoutChild == renderObject);
}
}
// A `RenderProxyBox` that defers its layout until its `_layoutSurrogate` (which
// is not necessarily an ancestor of this RenderBox, but shares at least one
// `_RenderTheater` ancestor with this RenderBox) is laid out.
//
// This `RenderObject` must be a child of a `_RenderTheater`. It guarantees that:
//
// 1. It's a relayout boundary, so calling `markNeedsLayout` on it never dirties
// its `_RenderTheater`.
//
// 2. Its `layout` implementation is overridden such that `performLayout` does
// not do anything when its called from `layout`, preventing the parent
// `_RenderTheater` from laying out this subtree prematurely (but this
// `RenderObject` may still be resized). Instead, `markNeedsLayout` will be
// called from within `layout` to schedule a layout update for this relayout
// boundary when needed.
//
// 3. When invoked from `PipelineOwner.flushLayout`, or
// `_layoutSurrogate.performLayout`, this `RenderObject` behaves like an
// `Overlay` that has only one entry.
final class _RenderDeferredLayoutBox extends RenderProxyBox with _RenderTheaterMixin, LinkedListEntry<_RenderDeferredLayoutBox> {
_RenderDeferredLayoutBox(this._layoutSurrogate);
StackParentData get stackParentData => parentData! as StackParentData;
final _RenderLayoutSurrogateProxyBox _layoutSurrogate;
@override
Iterable<RenderBox> _childrenInPaintOrder() {
final RenderBox? child = this.child;
return child == null
? const Iterable<RenderBox>.empty()
: Iterable<RenderBox>.generate(1, (int i) => child);
}
@override
Iterable<RenderBox> _childrenInHitTestOrder() => _childrenInPaintOrder();
@override
_RenderTheater get theater {
final RenderObject? parent = this.parent;
return parent is _RenderTheater
? parent
: throw FlutterError('$parent of $this is not a _RenderTheater');
}
@override
void redepthChildren() {
_layoutSurrogate.redepthChild(this);
super.redepthChildren();
}
@override
bool get sizedByParent => true;
bool _needsLayout = true;
@override
void markNeedsLayout() {
_needsLayout = true;
super.markNeedsLayout();
}
@override
RenderObject? get debugLayoutParent => _layoutSurrogate;
void layoutByLayoutSurrogate() {
assert(!_theaterDoingThisLayout);
final _RenderTheater? theater = parent as _RenderTheater?;
if (theater == null || !attached) {
assert(false, '$this is not attached to parent');
return;
}
super.layout(BoxConstraints.tight(theater.constraints.biggest));
}
bool _theaterDoingThisLayout = false;
@override
void layout(Constraints constraints, { bool parentUsesSize = false }) {
assert(_needsLayout == debugNeedsLayout);
// Only _RenderTheater calls this implementation.
assert(parent != null);
final bool scheduleDeferredLayout = _needsLayout || this.constraints != constraints;
assert(!_theaterDoingThisLayout);
_theaterDoingThisLayout = true;
super.layout(constraints, parentUsesSize: parentUsesSize);
assert(_theaterDoingThisLayout);
_theaterDoingThisLayout = false;
_needsLayout = false;
assert(!debugNeedsLayout);
if (scheduleDeferredLayout) {
final _RenderTheater parent = this.parent! as _RenderTheater;
// Invoking markNeedsLayout as a layout callback allows this node to be
// merged back to the `PipelineOwner`'s dirty list in the right order, if
// it's not already dirty. Otherwise this may cause some dirty descendants
// to performLayout a second time.
parent.invokeLayoutCallback((BoxConstraints constraints) { markNeedsLayout(); });
}
}
@override
void performResize() {
size = constraints.biggest;
}
bool _debugMutationsLocked = false;
@override
void performLayout() {
assert(!_debugMutationsLocked);
if (_theaterDoingThisLayout) {
_needsLayout = false;
return;
}
assert(() {
_debugMutationsLocked = true;
return true;
}());
// This method is directly being invoked from `PipelineOwner.flushLayout`,
// or from `_layoutSurrogate`'s performLayout.
assert(parent != null);
final RenderBox? child = this.child;
if (child == null) {
_needsLayout = false;
return;
}
assert(constraints.isTight);
layoutChild(child, constraints);
assert(() {
_debugMutationsLocked = false;
return true;
}());
_needsLayout = false;
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
final BoxParentData childParentData = child.parentData! as BoxParentData;
final Offset offset = childParentData.offset;
transform.translate(offset.dx, offset.dy);
}
}
// A RenderProxyBox that makes sure its `deferredLayoutChild` has a greater
// depth than itself.
class _RenderLayoutSurrogateProxyBox extends RenderProxyBox {
_RenderDeferredLayoutBox? _deferredLayoutChild;
@override
void redepthChildren() {
super.redepthChildren();
final _RenderDeferredLayoutBox? child = _deferredLayoutChild;
// If child is not attached, this method will be invoked by child's real
// parent when it's attached.
if (child != null && child.attached) {
assert(child.attached);
redepthChild(child);
}
}
@override
void performLayout() {
super.performLayout();
// Try to layout `_deferredLayoutChild` here now that its configuration
// and constraints are up-to-date. Additionally, during the very first
// layout, this makes sure that _deferredLayoutChild is reachable via tree
// walk.
_deferredLayoutChild?.layoutByLayoutSurrogate();
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
super.visitChildrenForSemantics(visitor);
final _RenderDeferredLayoutBox? deferredChild = _deferredLayoutChild;
if (deferredChild != null) {
visitor(deferredChild);
}
}
}
| flutter/packages/flutter/lib/src/widgets/overlay.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/overlay.dart",
"repo_id": "flutter",
"token_count": 28390
} | 674 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'editable_text.dart';
import 'restoration.dart';
/// A [RestorableProperty] that makes the wrapped value accessible to the owning
/// [State] object via the [value] getter and setter.
///
/// Whenever a new [value] is set, [didUpdateValue] is called. Subclasses should
/// call [notifyListeners] from this method if the new value changes what
/// [toPrimitives] returns.
///
/// ## Using a RestorableValue
///
/// {@tool dartpad}
/// A [StatefulWidget] that has a restorable [int] property.
///
/// ** See code in examples/api/lib/widgets/restoration_properties/restorable_value.0.dart **
/// {@end-tool}
///
/// ## Creating a subclass
///
/// {@tool snippet}
/// This example shows how to create a new [RestorableValue] subclass,
/// in this case for the [Duration] class.
///
/// ```dart
/// class RestorableDuration extends RestorableValue<Duration> {
/// @override
/// Duration createDefaultValue() => Duration.zero;
///
/// @override
/// void didUpdateValue(Duration? oldValue) {
/// if (oldValue == null || oldValue.inMicroseconds != value.inMicroseconds) {
/// notifyListeners();
/// }
/// }
///
/// @override
/// Duration fromPrimitives(Object? data) {
/// if (data != null) {
/// return Duration(microseconds: data as int);
/// }
/// return Duration.zero;
/// }
///
/// @override
/// Object toPrimitives() {
/// return value.inMicroseconds;
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [RestorableProperty], which is the super class of this class.
/// * [RestorationMixin], to which a [RestorableValue] needs to be registered
/// in order to work.
/// * [RestorationManager], which provides an overview of how state restoration
/// works in Flutter.
abstract class RestorableValue<T> extends RestorableProperty<T> {
/// The current value stored in this property.
///
/// A representation of the current value is stored in the restoration data.
/// During state restoration, the property will restore the value to what it
/// was when the restoration data it is getting restored from was collected.
///
/// The [value] can only be accessed after the property has been registered
/// with a [RestorationMixin] by calling
/// [RestorationMixin.registerForRestoration].
T get value {
assert(isRegistered);
return _value as T;
}
T? _value;
set value(T newValue) {
assert(isRegistered);
if (newValue != _value) {
final T? oldValue = _value;
_value = newValue;
didUpdateValue(oldValue);
}
}
@mustCallSuper
@override
void initWithValue(T value) {
_value = value;
}
/// Called whenever a new value is assigned to [value].
///
/// The new value can be accessed via the regular [value] getter and the
/// previous value is provided as `oldValue`.
///
/// Subclasses should call [notifyListeners] from this method, if the new
/// value changes what [toPrimitives] returns.
@protected
void didUpdateValue(T? oldValue);
}
// _RestorablePrimitiveValueN and its subclasses allows for null values.
// See [_RestorablePrimitiveValue] for the non-nullable version of this class.
class _RestorablePrimitiveValueN<T extends Object?> extends RestorableValue<T> {
_RestorablePrimitiveValueN(this._defaultValue)
: assert(debugIsSerializableForRestoration(_defaultValue)),
super();
final T _defaultValue;
@override
T createDefaultValue() => _defaultValue;
@override
void didUpdateValue(T? oldValue) {
assert(debugIsSerializableForRestoration(value));
notifyListeners();
}
@override
T fromPrimitives(Object? serialized) => serialized as T;
@override
Object? toPrimitives() => value;
}
// _RestorablePrimitiveValue and its subclasses are non-nullable.
// See [_RestorablePrimitiveValueN] for the nullable version of this class.
class _RestorablePrimitiveValue<T extends Object> extends _RestorablePrimitiveValueN<T> {
_RestorablePrimitiveValue(super.defaultValue)
: assert(debugIsSerializableForRestoration(defaultValue));
@override
set value(T value) {
super.value = value;
}
@override
T fromPrimitives(Object? serialized) {
assert(serialized != null);
return super.fromPrimitives(serialized);
}
@override
Object toPrimitives() {
return super.toPrimitives()!;
}
}
/// A [RestorableProperty] that knows how to store and restore a [num].
///
/// {@template flutter.widgets.RestorableNum}
/// The current [value] of this property is stored in the restoration data.
/// During state restoration the property is restored to the value it had when
/// the restoration data it is getting restored from was collected.
///
/// If no restoration data is available, [value] is initialized to the
/// `defaultValue` given in the constructor.
/// {@endtemplate}
///
/// Instead of using the more generic [RestorableNum] directly, consider using
/// one of the more specific subclasses (e.g. [RestorableDouble] to store a
/// [double] and [RestorableInt] to store an [int]).
///
/// See also:
///
/// * [RestorableNumN] for the nullable version of this class.
class RestorableNum<T extends num> extends _RestorablePrimitiveValue<T> {
/// Creates a [RestorableNum].
///
/// {@template flutter.widgets.RestorableNum.constructor}
/// If no restoration data is available to restore the value in this property
/// from, the property will be initialized with the provided `defaultValue`.
/// {@endtemplate}
RestorableNum(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [double].
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableDoubleN] for the nullable version of this class.
class RestorableDouble extends RestorableNum<double> {
/// Creates a [RestorableDouble].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableDouble(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore an [int].
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableIntN] for the nullable version of this class.
class RestorableInt extends RestorableNum<int> {
/// Creates a [RestorableInt].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableInt(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [String].
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableStringN] for the nullable version of this class.
class RestorableString extends _RestorablePrimitiveValue<String> {
/// Creates a [RestorableString].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableString(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [bool].
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableBoolN] for the nullable version of this class.
class RestorableBool extends _RestorablePrimitiveValue<bool> {
/// Creates a [RestorableBool].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableBool(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [bool] that is
/// nullable.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableBool] for the non-nullable version of this class.
class RestorableBoolN extends _RestorablePrimitiveValueN<bool?> {
/// Creates a [RestorableBoolN].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableBoolN(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [num]
/// that is nullable.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// Instead of using the more generic [RestorableNumN] directly, consider using
/// one of the more specific subclasses (e.g. [RestorableDoubleN] to store a
/// [double] and [RestorableIntN] to store an [int]).
///
/// See also:
///
/// * [RestorableNum] for the non-nullable version of this class.
class RestorableNumN<T extends num?> extends _RestorablePrimitiveValueN<T> {
/// Creates a [RestorableNumN].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableNumN(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [double]
/// that is nullable.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableDouble] for the non-nullable version of this class.
class RestorableDoubleN extends RestorableNumN<double?> {
/// Creates a [RestorableDoubleN].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableDoubleN(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore an [int]
/// that is nullable.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableInt] for the non-nullable version of this class.
class RestorableIntN extends RestorableNumN<int?> {
/// Creates a [RestorableIntN].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableIntN(super.defaultValue);
}
/// A [RestorableProperty] that knows how to store and restore a [String]
/// that is nullable.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// See also:
///
/// * [RestorableString] for the non-nullable version of this class.
class RestorableStringN extends _RestorablePrimitiveValueN<String?> {
/// Creates a [RestorableString].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableStringN(super.defaultValue);
}
/// A [RestorableValue] that knows how to save and restore [DateTime].
///
/// {@macro flutter.widgets.RestorableNum}.
class RestorableDateTime extends RestorableValue<DateTime> {
/// Creates a [RestorableDateTime].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableDateTime(DateTime defaultValue) : _defaultValue = defaultValue;
final DateTime _defaultValue;
@override
DateTime createDefaultValue() => _defaultValue;
@override
void didUpdateValue(DateTime? oldValue) {
assert(debugIsSerializableForRestoration(value.millisecondsSinceEpoch));
notifyListeners();
}
@override
DateTime fromPrimitives(Object? data) => DateTime.fromMillisecondsSinceEpoch(data! as int);
@override
Object? toPrimitives() => value.millisecondsSinceEpoch;
}
/// A [RestorableValue] that knows how to save and restore [DateTime] that is
/// nullable.
///
/// {@macro flutter.widgets.RestorableNum}.
class RestorableDateTimeN extends RestorableValue<DateTime?> {
/// Creates a [RestorableDateTime].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableDateTimeN(DateTime? defaultValue) : _defaultValue = defaultValue;
final DateTime? _defaultValue;
@override
DateTime? createDefaultValue() => _defaultValue;
@override
void didUpdateValue(DateTime? oldValue) {
assert(debugIsSerializableForRestoration(value?.millisecondsSinceEpoch));
notifyListeners();
}
@override
DateTime? fromPrimitives(Object? data) => data != null ? DateTime.fromMillisecondsSinceEpoch(data as int) : null;
@override
Object? toPrimitives() => value?.millisecondsSinceEpoch;
}
/// A base class for creating a [RestorableProperty] that stores and restores a
/// [Listenable].
///
/// This class may be used to implement a [RestorableProperty] for a
/// [Listenable], whose information it needs to store in the restoration data
/// change whenever the [Listenable] notifies its listeners.
///
/// The [RestorationMixin] this property is registered with will call
/// [toPrimitives] whenever the wrapped [Listenable] notifies its listeners to
/// update the information that this property has stored in the restoration
/// data.
abstract class RestorableListenable<T extends Listenable> extends RestorableProperty<T> {
/// The [Listenable] stored in this property.
///
/// A representation of the current value of the [Listenable] is stored in the
/// restoration data. During state restoration, the [Listenable] returned by
/// this getter will be restored to the state it had when the restoration data
/// the property is getting restored from was collected.
///
/// The [value] can only be accessed after the property has been registered
/// with a [RestorationMixin] by calling
/// [RestorationMixin.registerForRestoration].
T get value {
assert(isRegistered);
return _value!;
}
T? _value;
@override
void initWithValue(T value) {
_value?.removeListener(notifyListeners);
_value = value;
_value!.addListener(notifyListeners);
}
@override
void dispose() {
super.dispose();
_value?.removeListener(notifyListeners);
}
}
/// A base class for creating a [RestorableProperty] that stores and restores a
/// [ChangeNotifier].
///
/// This class may be used to implement a [RestorableProperty] for a
/// [ChangeNotifier], whose information it needs to store in the restoration
/// data change whenever the [ChangeNotifier] notifies its listeners.
///
/// The [RestorationMixin] this property is registered with will call
/// [toPrimitives] whenever the wrapped [ChangeNotifier] notifies its listeners
/// to update the information that this property has stored in the restoration
/// data.
///
/// Furthermore, the property will dispose the wrapped [ChangeNotifier] when
/// either the property itself is disposed or its value is replaced with another
/// [ChangeNotifier] instance.
abstract class RestorableChangeNotifier<T extends ChangeNotifier> extends RestorableListenable<T> {
@override
void initWithValue(T value) {
_disposeOldValue();
super.initWithValue(value);
}
@override
void dispose() {
_disposeOldValue();
super.dispose();
}
void _disposeOldValue() {
if (_value != null) {
// Scheduling a microtask for dispose to give other entities a chance
// to remove their listeners first.
scheduleMicrotask(_value!.dispose);
}
}
}
/// A [RestorableProperty] that knows how to store and restore a
/// [TextEditingController].
///
/// The [TextEditingController] is accessible via the [value] getter. During
/// state restoration, the property will restore [TextEditingController.text] to
/// the value it had when the restoration data it is getting restored from was
/// collected.
class RestorableTextEditingController extends RestorableChangeNotifier<TextEditingController> {
/// Creates a [RestorableTextEditingController].
///
/// This constructor treats a null `text` argument as if it were the empty
/// string.
factory RestorableTextEditingController({String? text}) => RestorableTextEditingController.fromValue(
text == null ? TextEditingValue.empty : TextEditingValue(text: text),
);
/// Creates a [RestorableTextEditingController] from an initial
/// [TextEditingValue].
///
/// This constructor treats a null `value` argument as if it were
/// [TextEditingValue.empty].
RestorableTextEditingController.fromValue(TextEditingValue value) : _initialValue = value;
final TextEditingValue _initialValue;
@override
TextEditingController createDefaultValue() {
return TextEditingController.fromValue(_initialValue);
}
@override
TextEditingController fromPrimitives(Object? data) {
return TextEditingController(text: data! as String);
}
@override
Object toPrimitives() {
return value.text;
}
}
/// A [RestorableProperty] that knows how to store and restore a nullable [Enum]
/// type.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// The values are serialized using the name of the enum, obtained using the
/// [EnumName.name] extension accessor.
///
/// The represented value is accessible via the [value] getter. The set of
/// values in the enum are accessible via the [values] getter. Since
/// [RestorableEnumN] allows null, this set will include null.
///
/// See also:
///
/// * [RestorableEnum], a class similar to this one that knows how to store and
/// restore non-nullable [Enum] types.
class RestorableEnumN<T extends Enum> extends RestorableValue<T?> {
/// Creates a [RestorableEnumN].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableEnumN(T? defaultValue, { required Iterable<T> values })
: assert(defaultValue == null || values.contains(defaultValue),
'Default value $defaultValue not found in $T values: $values'),
_defaultValue = defaultValue,
values = values.toSet();
@override
T? createDefaultValue() => _defaultValue;
final T? _defaultValue;
@override
set value(T? newValue) {
assert(newValue == null || values.contains(newValue),
'Attempted to set an unknown enum value "$newValue" that is not null, or '
'in the valid set of enum values for the $T type: '
'${values.map<String>((T value) => value.name).toSet()}');
super.value = newValue;
}
/// The set of non-null values that this [RestorableEnumN] may represent.
///
/// This is a required field that supplies the enum values that are serialized
/// and restored.
///
/// If a value is encountered that is not null or a value in this set,
/// [fromPrimitives] will assert when restoring.
///
/// It is typically set to the `values` list of the enum type.
///
/// In addition to this set, because [RestorableEnumN] allows nullable values,
/// null is also a valid value, even though it doesn't appear in this set.
///
/// {@tool snippet} For example, to create a [RestorableEnumN] with an
/// [AxisDirection] enum value, with a default value of null, you would build
/// it like the code below:
///
/// ```dart
/// RestorableEnumN<AxisDirection> axis = RestorableEnumN<AxisDirection>(null, values: AxisDirection.values);
/// ```
/// {@end-tool}
Set<T> values;
@override
void didUpdateValue(T? oldValue) {
notifyListeners();
}
@override
T? fromPrimitives(Object? data) {
if (data == null) {
return null;
}
if (data is String) {
for (final T allowed in values) {
if (allowed.name == data) {
return allowed;
}
}
assert(false,
'Attempted to set an unknown enum value "$data" that is not null, or '
'in the valid set of enum values for the $T type: '
'${values.map<String>((T value) => value.name).toSet()}');
}
return _defaultValue;
}
@override
Object? toPrimitives() => value?.name;
}
/// A [RestorableProperty] that knows how to store and restore an [Enum]
/// type.
///
/// {@macro flutter.widgets.RestorableNum}
///
/// The values are serialized using the name of the enum, obtained using the
/// [EnumName.name] extension accessor.
///
/// The represented value is accessible via the [value] getter.
///
/// See also:
///
/// * [RestorableEnumN], a class similar to this one that knows how to store and
/// restore nullable [Enum] types.
class RestorableEnum<T extends Enum> extends RestorableValue<T> {
/// Creates a [RestorableEnum].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableEnum(T defaultValue, { required Iterable<T> values })
: assert(values.contains(defaultValue),
'Default value $defaultValue not found in $T values: $values'),
_defaultValue = defaultValue,
values = values.toSet();
@override
T createDefaultValue() => _defaultValue;
final T _defaultValue;
@override
set value(T newValue) {
assert(values.contains(newValue),
'Attempted to set an unknown enum value "$newValue" that is not in the '
'valid set of enum values for the $T type: '
'${values.map<String>((T value) => value.name).toSet()}');
super.value = newValue;
}
/// The set of values that this [RestorableEnum] may represent.
///
/// This is a required field that supplies the possible enum values that can
/// be serialized and restored.
///
/// If a value is encountered that is not in this set, [fromPrimitives] will
/// assert when restoring.
///
/// It is typically set to the `values` list of the enum type.
///
/// {@tool snippet} For example, to create a [RestorableEnum] with an
/// [AxisDirection] enum value, with a default value of [AxisDirection.up],
/// you would build it like the code below:
///
/// ```dart
/// RestorableEnum<AxisDirection> axis = RestorableEnum<AxisDirection>(AxisDirection.up, values: AxisDirection.values);
/// ```
/// {@end-tool}
Set<T> values;
@override
void didUpdateValue(T? oldValue) {
notifyListeners();
}
@override
T fromPrimitives(Object? data) {
if (data != null && data is String) {
for (final T allowed in values) {
if (allowed.name == data) {
return allowed;
}
}
assert(false,
'Attempted to restore an unknown enum value "$data" that is not in the '
'valid set of enum values for the $T type: '
'${values.map<String>((T value) => value.name).toSet()}');
}
return _defaultValue;
}
@override
Object toPrimitives() => value.name;
}
| flutter/packages/flutter/lib/src/widgets/restoration_properties.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/restoration_properties.dart",
"repo_id": "flutter",
"token_count": 6627
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/physics.dart';
/// An implementation of scroll physics that matches iOS.
///
/// See also:
///
/// * [ClampingScrollSimulation], which implements Android scroll physics.
class BouncingScrollSimulation extends Simulation {
/// Creates a simulation group for scrolling on iOS, with the given
/// parameters.
///
/// The position and velocity arguments must use the same units as will be
/// expected from the [x] and [dx] methods respectively (typically logical
/// pixels and logical pixels per second respectively).
///
/// The leading and trailing extents must use the unit of length, the same
/// unit as used for the position argument and as expected from the [x]
/// method (typically logical pixels).
///
/// The units used with the provided [SpringDescription] must similarly be
/// consistent with the other arguments. A default set of constants is used
/// for the `spring` description if it is omitted; these defaults assume
/// that the unit of length is the logical pixel.
BouncingScrollSimulation({
required double position,
required double velocity,
required this.leadingExtent,
required this.trailingExtent,
required this.spring,
double constantDeceleration = 0,
super.tolerance,
}) : assert(leadingExtent <= trailingExtent) {
if (position < leadingExtent) {
_springSimulation = _underscrollSimulation(position, velocity);
_springTime = double.negativeInfinity;
} else if (position > trailingExtent) {
_springSimulation = _overscrollSimulation(position, velocity);
_springTime = double.negativeInfinity;
} else {
// Taken from UIScrollView.decelerationRate (.normal = 0.998)
// 0.998^1000 = ~0.135
_frictionSimulation = FrictionSimulation(0.135, position, velocity, constantDeceleration: constantDeceleration);
final double finalX = _frictionSimulation.finalX;
if (velocity > 0.0 && finalX > trailingExtent) {
_springTime = _frictionSimulation.timeAtX(trailingExtent);
_springSimulation = _overscrollSimulation(
trailingExtent,
math.min(_frictionSimulation.dx(_springTime), maxSpringTransferVelocity),
);
assert(_springTime.isFinite);
} else if (velocity < 0.0 && finalX < leadingExtent) {
_springTime = _frictionSimulation.timeAtX(leadingExtent);
_springSimulation = _underscrollSimulation(
leadingExtent,
math.min(_frictionSimulation.dx(_springTime), maxSpringTransferVelocity),
);
assert(_springTime.isFinite);
} else {
_springTime = double.infinity;
}
}
}
/// The maximum velocity that can be transferred from the inertia of a ballistic
/// scroll into overscroll.
static const double maxSpringTransferVelocity = 5000.0;
/// When [x] falls below this value the simulation switches from an internal friction
/// model to a spring model which causes [x] to "spring" back to [leadingExtent].
final double leadingExtent;
/// When [x] exceeds this value the simulation switches from an internal friction
/// model to a spring model which causes [x] to "spring" back to [trailingExtent].
final double trailingExtent;
/// The spring used to return [x] to either [leadingExtent] or [trailingExtent].
final SpringDescription spring;
late FrictionSimulation _frictionSimulation;
late Simulation _springSimulation;
late double _springTime;
double _timeOffset = 0.0;
Simulation _underscrollSimulation(double x, double dx) {
return ScrollSpringSimulation(spring, x, leadingExtent, dx);
}
Simulation _overscrollSimulation(double x, double dx) {
return ScrollSpringSimulation(spring, x, trailingExtent, dx);
}
Simulation _simulation(double time) {
final Simulation simulation;
if (time > _springTime) {
_timeOffset = _springTime.isFinite ? _springTime : 0.0;
simulation = _springSimulation;
} else {
_timeOffset = 0.0;
simulation = _frictionSimulation;
}
return simulation..tolerance = tolerance;
}
@override
double x(double time) => _simulation(time).x(time - _timeOffset);
@override
double dx(double time) => _simulation(time).dx(time - _timeOffset);
@override
bool isDone(double time) => _simulation(time).isDone(time - _timeOffset);
@override
String toString() {
return '${objectRuntimeType(this, 'BouncingScrollSimulation')}(leadingExtent: $leadingExtent, trailingExtent: $trailingExtent)';
}
}
/// An implementation of scroll physics that aligns with Android.
///
/// For any value of [velocity], this travels the same total distance as the
/// Android scroll physics.
///
/// This scroll physics has been adjusted relative to Android's in order to make
/// it ballistic, meaning that the deceleration at any moment is a function only
/// of the current velocity [dx] and does not depend on how long ago the
/// simulation was started. (This is required by Flutter's scrolling protocol,
/// where [ScrollActivityDelegate.goBallistic] may restart a scroll activity
/// using only its current velocity and the scroll position's own state.)
/// Compared to this scroll physics, Android's moves faster at the very
/// beginning, then slower, and it ends at the same place but a little later.
///
/// Times are measured in seconds, and positions in logical pixels.
///
/// See also:
///
/// * [BouncingScrollSimulation], which implements iOS scroll physics.
//
// This class is based on OverScroller.java from Android:
// https://android.googlesource.com/platform/frameworks/base/+/android-13.0.0_r24/core/java/android/widget/OverScroller.java#738
// and in particular class SplineOverScroller (at the end of the file), starting
// at method "fling". (A very similar algorithm is in Scroller.java in the same
// directory, but OverScroller is what's used by RecyclerView.)
//
// In the Android implementation, times are in milliseconds, positions are in
// physical pixels, but velocity is in physical pixels per whole second.
//
// The "See..." comments below refer to SplineOverScroller methods and values.
class ClampingScrollSimulation extends Simulation {
/// Creates a scroll physics simulation that aligns with Android scrolling.
ClampingScrollSimulation({
required this.position,
required this.velocity,
this.friction = 0.015,
super.tolerance,
}) {
_duration = _flingDuration();
_distance = _flingDistance();
}
/// The position of the particle at the beginning of the simulation, in
/// logical pixels.
final double position;
/// The velocity at which the particle is traveling at the beginning of the
/// simulation, in logical pixels per second.
final double velocity;
/// The amount of friction the particle experiences as it travels.
///
/// The more friction the particle experiences, the sooner it stops and the
/// less far it travels.
///
/// The default value causes the particle to travel the same total distance
/// as in the Android scroll physics.
// See mFlingFriction.
final double friction;
/// The total time the simulation will run, in seconds.
late double _duration;
/// The total, signed, distance the simulation will travel, in logical pixels.
late double _distance;
// See DECELERATION_RATE.
static final double _kDecelerationRate = math.log(0.78) / math.log(0.9);
// See INFLEXION.
static const double _kInflexion = 0.35;
// See mPhysicalCoeff. This has a value of 0.84 times Earth gravity,
// expressed in units of logical pixels per second^2.
static const double _physicalCoeff =
9.80665 // g, in meters per second^2
* 39.37 // 1 meter / 1 inch
* 160.0 // 1 inch / 1 logical pixel
* 0.84; // "look and feel tuning"
// See getSplineFlingDuration().
double _flingDuration() {
// See getSplineDeceleration(). That function's value is
// math.log(velocity.abs() / referenceVelocity).
final double referenceVelocity = friction * _physicalCoeff / _kInflexion;
// This is the value getSplineFlingDuration() would return, but in seconds.
final double androidDuration =
math.pow(velocity.abs() / referenceVelocity,
1 / (_kDecelerationRate - 1.0)) as double;
// We finish a bit sooner than Android, in order to travel the
// same total distance.
return _kDecelerationRate * _kInflexion * androidDuration;
}
// See getSplineFlingDistance(). This returns the same value but with the
// sign of [velocity], and in logical pixels.
double _flingDistance() {
final double distance = velocity * _duration / _kDecelerationRate;
assert(() {
// This is the more complicated calculation that getSplineFlingDistance()
// actually performs, which boils down to the much simpler formula above.
final double referenceVelocity = friction * _physicalCoeff / _kInflexion;
final double logVelocity = math.log(velocity.abs() / referenceVelocity);
final double distanceAgain =
friction * _physicalCoeff
* math.exp(logVelocity * _kDecelerationRate / (_kDecelerationRate - 1.0));
return (distance.abs() - distanceAgain).abs() < tolerance.distance;
}());
return distance;
}
@override
double x(double time) {
final double t = clampDouble(time / _duration, 0.0, 1.0);
return position + _distance * (1.0 - math.pow(1.0 - t, _kDecelerationRate));
}
@override
double dx(double time) {
final double t = clampDouble(time / _duration, 0.0, 1.0);
return velocity * math.pow(1.0 - t, _kDecelerationRate - 1.0);
}
@override
bool isDone(double time) {
return time >= _duration;
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_simulation.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_simulation.dart",
"repo_id": "flutter",
"token_count": 3051
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart' show TickerProvider;
import 'framework.dart';
import 'scroll_position.dart';
import 'scrollable.dart';
/// Delegate for configuring a [SliverPersistentHeader].
abstract class SliverPersistentHeaderDelegate {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliverPersistentHeaderDelegate();
/// The widget to place inside the [SliverPersistentHeader].
///
/// The `context` is the [BuildContext] of the sliver.
///
/// The `shrinkOffset` is a distance from [maxExtent] towards [minExtent]
/// representing the current amount by which the sliver has been shrunk. When
/// the `shrinkOffset` is zero, the contents will be rendered with a dimension
/// of [maxExtent] in the main axis. When `shrinkOffset` equals the difference
/// between [maxExtent] and [minExtent] (a positive number), the contents will
/// be rendered with a dimension of [minExtent] in the main axis. The
/// `shrinkOffset` will always be a positive number in that range.
///
/// The `overlapsContent` argument is true if subsequent slivers (if any) will
/// be rendered beneath this one, and false if the sliver will not have any
/// contents below it. Typically this is used to decide whether to draw a
/// shadow to simulate the sliver being above the contents below it. Typically
/// this is true when `shrinkOffset` is at its greatest value and false
/// otherwise, but that is not guaranteed. See [NestedScrollView] for an
/// example of a case where `overlapsContent`'s value can be unrelated to
/// `shrinkOffset`.
Widget build(BuildContext context, double shrinkOffset, bool overlapsContent);
/// The smallest size to allow the header to reach, when it shrinks at the
/// start of the viewport.
///
/// This must return a value equal to or less than [maxExtent].
///
/// This value should not change over the lifetime of the delegate. It should
/// be based entirely on the constructor arguments passed to the delegate. See
/// [shouldRebuild], which must return true if a new delegate would return a
/// different value.
double get minExtent;
/// The size of the header when it is not shrinking at the top of the
/// viewport.
///
/// This must return a value equal to or greater than [minExtent].
///
/// This value should not change over the lifetime of the delegate. It should
/// be based entirely on the constructor arguments passed to the delegate. See
/// [shouldRebuild], which must return true if a new delegate would return a
/// different value.
double get maxExtent;
/// A [TickerProvider] to use when animating the header's size changes.
///
/// Must not be null if the persistent header is a floating header, and
/// [snapConfiguration] or [showOnScreenConfiguration] is not null.
TickerProvider? get vsync => null;
/// Specifies how floating headers should animate in and out of view.
///
/// If the value of this property is null, then floating headers will
/// not animate into place.
///
/// This is only used for floating headers (those with
/// [SliverPersistentHeader.floating] set to true).
///
/// Defaults to null.
FloatingHeaderSnapConfiguration? get snapConfiguration => null;
/// Specifies an [AsyncCallback] and offset for execution.
///
/// If the value of this property is null, then callback will not be
/// triggered.
///
/// This is only used for stretching headers (those with
/// [SliverAppBar.stretch] set to true).
///
/// Defaults to null.
OverScrollHeaderStretchConfiguration? get stretchConfiguration => null;
/// Specifies how floating headers and pinned headers should behave in
/// response to [RenderObject.showOnScreen] calls.
///
/// Defaults to null.
PersistentHeaderShowOnScreenConfiguration? get showOnScreenConfiguration => null;
/// Whether this delegate is meaningfully different from the old delegate.
///
/// If this returns false, then the header might not be rebuilt, even though
/// the instance of the delegate changed.
///
/// This must return true if `oldDelegate` and this object would return
/// different values for [minExtent], [maxExtent], [snapConfiguration], or
/// would return a meaningfully different widget tree from [build] for the
/// same arguments.
bool shouldRebuild(covariant SliverPersistentHeaderDelegate oldDelegate);
}
/// A sliver whose size varies when the sliver is scrolled to the edge
/// of the viewport opposite the sliver's [GrowthDirection].
///
/// In the normal case of a [CustomScrollView] with no centered sliver, this
/// sliver will vary its size when scrolled to the leading edge of the viewport.
///
/// This is the layout primitive that [SliverAppBar] uses for its
/// shrinking/growing effect.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
class SliverPersistentHeader extends StatelessWidget {
/// Creates a sliver that varies its size when it is scrolled to the start of
/// a viewport.
const SliverPersistentHeader({
super.key,
required this.delegate,
this.pinned = false,
this.floating = false,
});
/// Configuration for the sliver's layout.
///
/// The delegate provides the following information:
///
/// * The minimum and maximum dimensions of the sliver.
///
/// * The builder for generating the widgets of the sliver.
///
/// * The instructions for snapping the scroll offset, if [floating] is true.
final SliverPersistentHeaderDelegate delegate;
/// Whether to stick the header to the start of the viewport once it has
/// reached its minimum size.
///
/// If this is false, the header will continue scrolling off the screen after
/// it has shrunk to its minimum extent.
final bool pinned;
/// Whether the header should immediately grow again if the user reverses
/// scroll direction.
///
/// If this is false, the header only grows again once the user reaches the
/// part of the viewport that contains the sliver.
///
/// The [delegate]'s [SliverPersistentHeaderDelegate.snapConfiguration] is
/// ignored unless [floating] is true.
final bool floating;
@override
Widget build(BuildContext context) {
if (floating && pinned) {
return _SliverFloatingPinnedPersistentHeader(delegate: delegate);
}
if (pinned) {
return _SliverPinnedPersistentHeader(delegate: delegate);
}
if (floating) {
return _SliverFloatingPersistentHeader(delegate: delegate);
}
return _SliverScrollingPersistentHeader(delegate: delegate);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(
DiagnosticsProperty<SliverPersistentHeaderDelegate>(
'delegate',
delegate,
),
);
final List<String> flags = <String>[
if (pinned) 'pinned',
if (floating) 'floating',
];
if (flags.isEmpty) {
flags.add('normal');
}
properties.add(IterableProperty<String>('mode', flags));
}
}
class _FloatingHeader extends StatefulWidget {
const _FloatingHeader({ required this.child });
final Widget child;
@override
_FloatingHeaderState createState() => _FloatingHeaderState();
}
// A wrapper for the widget created by _SliverPersistentHeaderElement that
// starts and stops the floating app bar's snap-into-view or snap-out-of-view
// animation. It also informs the float when pointer scrolling by updating the
// last known ScrollDirection when scrolling began.
class _FloatingHeaderState extends State<_FloatingHeader> {
ScrollPosition? _position;
@override
void didChangeDependencies() {
super.didChangeDependencies();
if (_position != null) {
_position!.isScrollingNotifier.removeListener(_isScrollingListener);
}
_position = Scrollable.maybeOf(context)?.position;
if (_position != null) {
_position!.isScrollingNotifier.addListener(_isScrollingListener);
}
}
@override
void dispose() {
if (_position != null) {
_position!.isScrollingNotifier.removeListener(_isScrollingListener);
}
super.dispose();
}
RenderSliverFloatingPersistentHeader? _headerRenderer() {
return context.findAncestorRenderObjectOfType<RenderSliverFloatingPersistentHeader>();
}
void _isScrollingListener() {
assert(_position != null);
// When a scroll stops, then maybe snap the app bar into view.
// Similarly, when a scroll starts, then maybe stop the snap animation.
// Update the scrolling direction as well for pointer scrolling updates.
final RenderSliverFloatingPersistentHeader? header = _headerRenderer();
if (_position!.isScrollingNotifier.value) {
header?.updateScrollStartDirection(_position!.userScrollDirection);
// Only SliverAppBars support snapping, headers will not snap.
header?.maybeStopSnapAnimation(_position!.userScrollDirection);
} else {
// Only SliverAppBars support snapping, headers will not snap.
header?.maybeStartSnapAnimation(_position!.userScrollDirection);
}
}
@override
Widget build(BuildContext context) => widget.child;
}
class _SliverPersistentHeaderElement extends RenderObjectElement {
_SliverPersistentHeaderElement(
_SliverPersistentHeaderRenderObjectWidget super.widget, {
this.floating = false,
});
final bool floating;
@override
_RenderSliverPersistentHeaderForWidgetsMixin get renderObject => super.renderObject as _RenderSliverPersistentHeaderForWidgetsMixin;
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
renderObject._element = this;
}
@override
void unmount() {
renderObject._element = null;
super.unmount();
}
@override
void update(_SliverPersistentHeaderRenderObjectWidget newWidget) {
final _SliverPersistentHeaderRenderObjectWidget oldWidget = widget as _SliverPersistentHeaderRenderObjectWidget;
super.update(newWidget);
final SliverPersistentHeaderDelegate newDelegate = newWidget.delegate;
final SliverPersistentHeaderDelegate oldDelegate = oldWidget.delegate;
if (newDelegate != oldDelegate &&
(newDelegate.runtimeType != oldDelegate.runtimeType || newDelegate.shouldRebuild(oldDelegate))) {
renderObject.triggerRebuild();
}
}
@override
void performRebuild() {
super.performRebuild();
renderObject.triggerRebuild();
}
Element? child;
void _build(double shrinkOffset, bool overlapsContent) {
owner!.buildScope(this, () {
final _SliverPersistentHeaderRenderObjectWidget sliverPersistentHeaderRenderObjectWidget = widget as _SliverPersistentHeaderRenderObjectWidget;
child = updateChild(
child,
floating
? _FloatingHeader(child: sliverPersistentHeaderRenderObjectWidget.delegate.build(
this,
shrinkOffset,
overlapsContent
))
: sliverPersistentHeaderRenderObjectWidget.delegate.build(this, shrinkOffset, overlapsContent),
null,
);
});
}
@override
void forgetChild(Element child) {
assert(child == this.child);
this.child = null;
super.forgetChild(child);
}
@override
void insertRenderObjectChild(covariant RenderBox child, Object? slot) {
assert(renderObject.debugValidateChild(child));
renderObject.child = child;
}
@override
void moveRenderObjectChild(covariant RenderObject child, Object? oldSlot, Object? newSlot) {
assert(false);
}
@override
void removeRenderObjectChild(covariant RenderObject child, Object? slot) {
renderObject.child = null;
}
@override
void visitChildren(ElementVisitor visitor) {
if (child != null) {
visitor(child!);
}
}
}
abstract class _SliverPersistentHeaderRenderObjectWidget extends RenderObjectWidget {
const _SliverPersistentHeaderRenderObjectWidget({
required this.delegate,
this.floating = false,
});
final SliverPersistentHeaderDelegate delegate;
final bool floating;
@override
_SliverPersistentHeaderElement createElement() => _SliverPersistentHeaderElement(this, floating: floating);
@override
_RenderSliverPersistentHeaderForWidgetsMixin createRenderObject(BuildContext context);
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(
DiagnosticsProperty<SliverPersistentHeaderDelegate>(
'delegate',
delegate,
),
);
}
}
mixin _RenderSliverPersistentHeaderForWidgetsMixin on RenderSliverPersistentHeader {
_SliverPersistentHeaderElement? _element;
@override
double get minExtent => (_element!.widget as _SliverPersistentHeaderRenderObjectWidget).delegate.minExtent;
@override
double get maxExtent => (_element!.widget as _SliverPersistentHeaderRenderObjectWidget).delegate.maxExtent;
@override
void updateChild(double shrinkOffset, bool overlapsContent) {
assert(_element != null);
_element!._build(shrinkOffset, overlapsContent);
}
@protected
void triggerRebuild() {
markNeedsLayout();
}
}
class _SliverScrollingPersistentHeader extends _SliverPersistentHeaderRenderObjectWidget {
const _SliverScrollingPersistentHeader({
required super.delegate,
});
@override
_RenderSliverPersistentHeaderForWidgetsMixin createRenderObject(BuildContext context) {
return _RenderSliverScrollingPersistentHeaderForWidgets(
stretchConfiguration: delegate.stretchConfiguration,
);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderSliverScrollingPersistentHeaderForWidgets renderObject) {
renderObject.stretchConfiguration = delegate.stretchConfiguration;
}
}
class _RenderSliverScrollingPersistentHeaderForWidgets extends RenderSliverScrollingPersistentHeader
with _RenderSliverPersistentHeaderForWidgetsMixin {
_RenderSliverScrollingPersistentHeaderForWidgets({
super.stretchConfiguration,
});
}
class _SliverPinnedPersistentHeader extends _SliverPersistentHeaderRenderObjectWidget {
const _SliverPinnedPersistentHeader({
required super.delegate,
});
@override
_RenderSliverPersistentHeaderForWidgetsMixin createRenderObject(BuildContext context) {
return _RenderSliverPinnedPersistentHeaderForWidgets(
stretchConfiguration: delegate.stretchConfiguration,
showOnScreenConfiguration: delegate.showOnScreenConfiguration,
);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderSliverPinnedPersistentHeaderForWidgets renderObject) {
renderObject
..stretchConfiguration = delegate.stretchConfiguration
..showOnScreenConfiguration = delegate.showOnScreenConfiguration;
}
}
class _RenderSliverPinnedPersistentHeaderForWidgets extends RenderSliverPinnedPersistentHeader
with _RenderSliverPersistentHeaderForWidgetsMixin {
_RenderSliverPinnedPersistentHeaderForWidgets({
super.stretchConfiguration,
super.showOnScreenConfiguration,
});
}
class _SliverFloatingPersistentHeader extends _SliverPersistentHeaderRenderObjectWidget {
const _SliverFloatingPersistentHeader({
required super.delegate,
}) : super(
floating: true,
);
@override
_RenderSliverPersistentHeaderForWidgetsMixin createRenderObject(BuildContext context) {
return _RenderSliverFloatingPersistentHeaderForWidgets(
vsync: delegate.vsync,
snapConfiguration: delegate.snapConfiguration,
stretchConfiguration: delegate.stretchConfiguration,
showOnScreenConfiguration: delegate.showOnScreenConfiguration,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSliverFloatingPersistentHeaderForWidgets renderObject) {
renderObject.vsync = delegate.vsync;
renderObject.snapConfiguration = delegate.snapConfiguration;
renderObject.stretchConfiguration = delegate.stretchConfiguration;
renderObject.showOnScreenConfiguration = delegate.showOnScreenConfiguration;
}
}
class _RenderSliverFloatingPinnedPersistentHeaderForWidgets extends RenderSliverFloatingPinnedPersistentHeader
with _RenderSliverPersistentHeaderForWidgetsMixin {
_RenderSliverFloatingPinnedPersistentHeaderForWidgets({
required super.vsync,
super.snapConfiguration,
super.stretchConfiguration,
super.showOnScreenConfiguration,
});
}
class _SliverFloatingPinnedPersistentHeader extends _SliverPersistentHeaderRenderObjectWidget {
const _SliverFloatingPinnedPersistentHeader({
required super.delegate,
}) : super(
floating: true,
);
@override
_RenderSliverPersistentHeaderForWidgetsMixin createRenderObject(BuildContext context) {
return _RenderSliverFloatingPinnedPersistentHeaderForWidgets(
vsync: delegate.vsync,
snapConfiguration: delegate.snapConfiguration,
stretchConfiguration: delegate.stretchConfiguration,
showOnScreenConfiguration: delegate.showOnScreenConfiguration,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSliverFloatingPinnedPersistentHeaderForWidgets renderObject) {
renderObject.vsync = delegate.vsync;
renderObject.snapConfiguration = delegate.snapConfiguration;
renderObject.stretchConfiguration = delegate.stretchConfiguration;
renderObject.showOnScreenConfiguration = delegate.showOnScreenConfiguration;
}
}
class _RenderSliverFloatingPersistentHeaderForWidgets extends RenderSliverFloatingPersistentHeader
with _RenderSliverPersistentHeaderForWidgetsMixin {
_RenderSliverFloatingPersistentHeaderForWidgets({
required super.vsync,
super.snapConfiguration,
super.stretchConfiguration,
super.showOnScreenConfiguration,
});
}
| flutter/packages/flutter/lib/src/widgets/sliver_persistent_header.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/sliver_persistent_header.dart",
"repo_id": "flutter",
"token_count": 5334
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'framework.dart';
export 'package:flutter/scheduler.dart' show TickerProvider;
// Examples can assume:
// late BuildContext context;
/// Enables or disables tickers (and thus animation controllers) in the widget
/// subtree.
///
/// This only works if [AnimationController] objects are created using
/// widget-aware ticker providers. For example, using a
/// [TickerProviderStateMixin] or a [SingleTickerProviderStateMixin].
class TickerMode extends StatefulWidget {
/// Creates a widget that enables or disables tickers.
const TickerMode({
super.key,
required this.enabled,
required this.child,
});
/// The requested ticker mode for this subtree.
///
/// The effective ticker mode of this subtree may differ from this value
/// if there is an ancestor [TickerMode] with this field set to false.
///
/// If true and all ancestor [TickerMode]s are also enabled, then tickers in
/// this subtree will tick.
///
/// If false, then tickers in this subtree will not tick regardless of any
/// ancestor [TickerMode]s. Animations driven by such tickers are not paused,
/// they just don't call their callbacks. Time still elapses.
final bool enabled;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Whether tickers in the given subtree should be enabled or disabled.
///
/// This is used automatically by [TickerProviderStateMixin] and
/// [SingleTickerProviderStateMixin] to decide if their tickers should be
/// enabled or disabled.
///
/// In the absence of a [TickerMode] widget, this function defaults to true.
///
/// Typical usage is as follows:
///
/// ```dart
/// bool tickingEnabled = TickerMode.of(context);
/// ```
static bool of(BuildContext context) {
final _EffectiveTickerMode? widget = context.dependOnInheritedWidgetOfExactType<_EffectiveTickerMode>();
return widget?.enabled ?? true;
}
/// Obtains a [ValueListenable] from the [TickerMode] surrounding the `context`,
/// which indicates whether tickers are enabled in the given subtree.
///
/// When that [TickerMode] enabled or disabled tickers, the listenable notifies
/// its listeners.
///
/// While the [ValueListenable] is stable for the lifetime of the surrounding
/// [TickerMode], calling this method does not establish a dependency between
/// the `context` and the [TickerMode] and the widget owning the `context`
/// does not rebuild when the ticker mode changes from true to false or vice
/// versa. This is preferable when the ticker mode does not impact what is
/// currently rendered on screen, e.g. because it is only used to mute/unmute a
/// [Ticker]. Since no dependency is established, the widget owning the
/// `context` is also not informed when it is moved to a new location in the
/// tree where it may have a different [TickerMode] ancestor. When this
/// happens, the widget must manually unsubscribe from the old listenable,
/// obtain a new one from the new ancestor [TickerMode] by calling this method
/// again, and re-subscribe to it. [StatefulWidget]s can, for example, do this
/// in [State.activate], which is called after the widget has been moved to
/// a new location.
///
/// Alternatively, [of] can be used instead of this method to create a
/// dependency between the provided `context` and the ancestor [TickerMode].
/// In this case, the widget automatically rebuilds when the ticker mode
/// changes or when it is moved to a new [TickerMode] ancestor, which
/// simplifies the management cost in the widget at the expensive of some
/// potential unnecessary rebuilds.
///
/// In the absence of a [TickerMode] widget, this function returns a
/// [ValueListenable], whose [ValueListenable.value] is always true.
static ValueListenable<bool> getNotifier(BuildContext context) {
final _EffectiveTickerMode? widget = context.getInheritedWidgetOfExactType<_EffectiveTickerMode>();
return widget?.notifier ?? const _ConstantValueListenable<bool>(true);
}
@override
State<TickerMode> createState() => _TickerModeState();
}
class _TickerModeState extends State<TickerMode> {
bool _ancestorTicketMode = true;
final ValueNotifier<bool> _effectiveMode = ValueNotifier<bool>(true);
@override
void didChangeDependencies() {
super.didChangeDependencies();
_ancestorTicketMode = TickerMode.of(context);
_updateEffectiveMode();
}
@override
void didUpdateWidget(TickerMode oldWidget) {
super.didUpdateWidget(oldWidget);
_updateEffectiveMode();
}
@override
void dispose() {
_effectiveMode.dispose();
super.dispose();
}
void _updateEffectiveMode() {
_effectiveMode.value = _ancestorTicketMode && widget.enabled;
}
@override
Widget build(BuildContext context) {
return _EffectiveTickerMode(
enabled: _effectiveMode.value,
notifier: _effectiveMode,
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('requested mode', value: widget.enabled, ifTrue: 'enabled', ifFalse: 'disabled', showName: true));
}
}
class _EffectiveTickerMode extends InheritedWidget {
const _EffectiveTickerMode({
required this.enabled,
required this.notifier,
required super.child,
});
final bool enabled;
final ValueNotifier<bool> notifier;
@override
bool updateShouldNotify(_EffectiveTickerMode oldWidget) => enabled != oldWidget.enabled;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('effective mode', value: enabled, ifTrue: 'enabled', ifFalse: 'disabled', showName: true));
}
}
/// Provides a single [Ticker] that is configured to only tick while the current
/// tree is enabled, as defined by [TickerMode].
///
/// To create the [AnimationController] in a [State] that only uses a single
/// [AnimationController], mix in this class, then pass `vsync: this`
/// to the animation controller constructor.
///
/// This mixin only supports vending a single ticker. If you might have multiple
/// [AnimationController] objects over the lifetime of the [State], use a full
/// [TickerProviderStateMixin] instead.
@optionalTypeArgs
mixin SingleTickerProviderStateMixin<T extends StatefulWidget> on State<T> implements TickerProvider {
Ticker? _ticker;
@override
Ticker createTicker(TickerCallback onTick) {
assert(() {
if (_ticker == null) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType is a SingleTickerProviderStateMixin but multiple tickers were created.'),
ErrorDescription('A SingleTickerProviderStateMixin can only be used as a TickerProvider once.'),
ErrorHint(
'If a State is used for multiple AnimationController objects, or if it is passed to other '
'objects and those objects might use it more than one time in total, then instead of '
'mixing in a SingleTickerProviderStateMixin, use a regular TickerProviderStateMixin.',
),
]);
}());
_ticker = Ticker(onTick, debugLabel: kDebugMode ? 'created by ${describeIdentity(this)}' : null);
_updateTickerModeNotifier();
_updateTicker(); // Sets _ticker.mute correctly.
return _ticker!;
}
@override
void dispose() {
assert(() {
if (_ticker == null || !_ticker!.isActive) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$this was disposed with an active Ticker.'),
ErrorDescription(
'$runtimeType created a Ticker via its SingleTickerProviderStateMixin, but at the time '
'dispose() was called on the mixin, that Ticker was still active. The Ticker must '
'be disposed before calling super.dispose().',
),
ErrorHint(
'Tickers used by AnimationControllers '
'should be disposed by calling dispose() on the AnimationController itself. '
'Otherwise, the ticker will leak.',
),
_ticker!.describeForError('The offending ticker was'),
]);
}());
_tickerModeNotifier?.removeListener(_updateTicker);
_tickerModeNotifier = null;
super.dispose();
}
ValueListenable<bool>? _tickerModeNotifier;
@override
void activate() {
super.activate();
// We may have a new TickerMode ancestor.
_updateTickerModeNotifier();
_updateTicker();
}
void _updateTicker() {
if (_ticker != null) {
_ticker!.muted = !_tickerModeNotifier!.value;
}
}
void _updateTickerModeNotifier() {
final ValueListenable<bool> newNotifier = TickerMode.getNotifier(context);
if (newNotifier == _tickerModeNotifier) {
return;
}
_tickerModeNotifier?.removeListener(_updateTicker);
newNotifier.addListener(_updateTicker);
_tickerModeNotifier = newNotifier;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
String? tickerDescription;
if (_ticker != null) {
if (_ticker!.isActive && _ticker!.muted) {
tickerDescription = 'active but muted';
} else if (_ticker!.isActive) {
tickerDescription = 'active';
} else if (_ticker!.muted) {
tickerDescription = 'inactive and muted';
} else {
tickerDescription = 'inactive';
}
}
properties.add(DiagnosticsProperty<Ticker>('ticker', _ticker, description: tickerDescription, showSeparator: false, defaultValue: null));
}
}
/// Provides [Ticker] objects that are configured to only tick while the current
/// tree is enabled, as defined by [TickerMode].
///
/// To create an [AnimationController] in a class that uses this mixin, pass
/// `vsync: this` to the animation controller constructor whenever you
/// create a new animation controller.
///
/// If you only have a single [Ticker] (for example only a single
/// [AnimationController]) for the lifetime of your [State], then using a
/// [SingleTickerProviderStateMixin] is more efficient. This is the common case.
@optionalTypeArgs
mixin TickerProviderStateMixin<T extends StatefulWidget> on State<T> implements TickerProvider {
Set<Ticker>? _tickers;
@override
Ticker createTicker(TickerCallback onTick) {
if (_tickerModeNotifier == null) {
// Setup TickerMode notifier before we vend the first ticker.
_updateTickerModeNotifier();
}
assert(_tickerModeNotifier != null);
_tickers ??= <_WidgetTicker>{};
final _WidgetTicker result = _WidgetTicker(onTick, this, debugLabel: kDebugMode ? 'created by ${describeIdentity(this)}' : null)
..muted = !_tickerModeNotifier!.value;
_tickers!.add(result);
return result;
}
void _removeTicker(_WidgetTicker ticker) {
assert(_tickers != null);
assert(_tickers!.contains(ticker));
_tickers!.remove(ticker);
}
ValueListenable<bool>? _tickerModeNotifier;
@override
void activate() {
super.activate();
// We may have a new TickerMode ancestor, get its Notifier.
_updateTickerModeNotifier();
_updateTickers();
}
void _updateTickers() {
if (_tickers != null) {
final bool muted = !_tickerModeNotifier!.value;
for (final Ticker ticker in _tickers!) {
ticker.muted = muted;
}
}
}
void _updateTickerModeNotifier() {
final ValueListenable<bool> newNotifier = TickerMode.getNotifier(context);
if (newNotifier == _tickerModeNotifier) {
return;
}
_tickerModeNotifier?.removeListener(_updateTickers);
newNotifier.addListener(_updateTickers);
_tickerModeNotifier = newNotifier;
}
@override
void dispose() {
assert(() {
if (_tickers != null) {
for (final Ticker ticker in _tickers!) {
if (ticker.isActive) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$this was disposed with an active Ticker.'),
ErrorDescription(
'$runtimeType created a Ticker via its TickerProviderStateMixin, but at the time '
'dispose() was called on the mixin, that Ticker was still active. All Tickers must '
'be disposed before calling super.dispose().',
),
ErrorHint(
'Tickers used by AnimationControllers '
'should be disposed by calling dispose() on the AnimationController itself. '
'Otherwise, the ticker will leak.',
),
ticker.describeForError('The offending ticker was'),
]);
}
}
}
return true;
}());
_tickerModeNotifier?.removeListener(_updateTickers);
_tickerModeNotifier = null;
super.dispose();
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Set<Ticker>>(
'tickers',
_tickers,
description: _tickers != null ?
'tracking ${_tickers!.length} ticker${_tickers!.length == 1 ? "" : "s"}' :
null,
defaultValue: null,
));
}
}
// This class should really be called _DisposingTicker or some such, but this
// class name leaks into stack traces and error messages and that name would be
// confusing. Instead we use the less precise but more anodyne "_WidgetTicker",
// which attracts less attention.
class _WidgetTicker extends Ticker {
_WidgetTicker(super.onTick, this._creator, { super.debugLabel });
final TickerProviderStateMixin _creator;
@override
void dispose() {
_creator._removeTicker(this);
super.dispose();
}
}
class _ConstantValueListenable<T> implements ValueListenable<T> {
const _ConstantValueListenable(this.value);
@override
void addListener(VoidCallback listener) {
// Intentionally left empty: Value cannot change, so we never have to
// notify registered listeners.
}
@override
void removeListener(VoidCallback listener) {
// Intentionally left empty: Value cannot change, so we never have to
// notify registered listeners.
}
@override
final T value;
}
| flutter/packages/flutter/lib/src/widgets/ticker_provider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/ticker_provider.dart",
"repo_id": "flutter",
"token_count": 4816
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
/*
* Here lies golden tests for packages/flutter_test/lib/src/binding.dart
* because [matchesGoldenFile] does not use Skia Gold in its native package.
*/
LiveTestWidgetsFlutterBinding().framePolicy = LiveTestWidgetsFlutterBindingFramePolicy.onlyPumps;
testWidgets('Should show event indicator for pointer events', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(200, 200), allLayers: true);
addTearDown(animationSheet.dispose);
final List<Offset> taps = <Offset>[];
Widget target({bool recording = true}) => Container(
padding: const EdgeInsets.fromLTRB(20, 10, 25, 20),
child: animationSheet.record(
MaterialApp(
home: Container(
decoration: BoxDecoration(
color: const Color.fromARGB(255, 128, 128, 128),
border: Border.all(),
),
child: Center(
child: Container(
width: 40,
height: 40,
color: Colors.black,
child: GestureDetector(
onTapDown: (TapDownDetails details) {
taps.add(details.globalPosition);
},
),
),
),
),
),
recording: recording,
),
);
await tester.pumpWidget(target(recording: false));
await tester.pumpFrames(target(), const Duration(milliseconds: 50));
final TestGesture gesture1 = await tester.createGesture(pointer: 1);
await gesture1.down(tester.getCenter(find.byType(GestureDetector)) + const Offset(10, 10));
expect(taps, equals(const <Offset>[Offset(130, 120)]));
taps.clear();
await tester.pumpFrames(target(), const Duration(milliseconds: 100));
final TestGesture gesture2 = await tester.createGesture(pointer: 2);
await gesture2.down(tester.getTopLeft(find.byType(GestureDetector)) + const Offset(30, -10));
await gesture1.moveBy(const Offset(50, 50));
await tester.pumpFrames(target(), const Duration(milliseconds: 100));
await gesture1.up();
await gesture2.up();
await tester.pumpFrames(target(), const Duration(milliseconds: 50));
expect(taps, isEmpty);
await expectLater(
animationSheet.collate(6),
matchesGoldenFile('LiveBinding.press.animation.png'),
);
// Currently skipped due to daily flake: https://github.com/flutter/flutter/issues/87588
}, skip: true); // Typically skip: isBrowser https://github.com/flutter/flutter/issues/42767
testWidgets('Should show event indicator for pointer events with setSurfaceSize', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(200, 200), allLayers: true);
addTearDown(animationSheet.dispose);
final List<Offset> taps = <Offset>[];
Widget target({bool recording = true}) => Container(
padding: const EdgeInsets.fromLTRB(20, 10, 25, 20),
child: animationSheet.record(
MaterialApp(
home: Container(
decoration: BoxDecoration(
color: const Color.fromARGB(255, 128, 128, 128),
border: Border.all(),
),
child: Center(
child: Container(
width: 40,
height: 40,
color: Colors.black,
child: GestureDetector(
onTapDown: (TapDownDetails details) {
taps.add(details.globalPosition);
},
),
),
),
),
),
recording: recording,
),
);
await tester.binding.setSurfaceSize(const Size(300, 300));
await tester.pumpWidget(target(recording: false));
await tester.pumpFrames(target(), const Duration(milliseconds: 50));
final TestGesture gesture1 = await tester.createGesture(pointer: 1);
await gesture1.down(tester.getCenter(find.byType(GestureDetector)) + const Offset(10, 10));
expect(taps, equals(const <Offset>[Offset(130, 120)]));
taps.clear();
await tester.pumpFrames(target(), const Duration(milliseconds: 100));
final TestGesture gesture2 = await tester.createGesture(pointer: 2);
await gesture2.down(tester.getTopLeft(find.byType(GestureDetector)) + const Offset(30, -10));
await gesture1.moveBy(const Offset(50, 50));
await tester.pumpFrames(target(), const Duration(milliseconds: 100));
await gesture1.up();
await gesture2.up();
await tester.pumpFrames(target(), const Duration(milliseconds: 50));
expect(taps, isEmpty);
await expectLater(
animationSheet.collate(6),
matchesGoldenFile('LiveBinding.press.animation.2.png'),
);
},
skip: isBrowser, // [intended] https://github.com/flutter/flutter/issues/56001
);
}
| flutter/packages/flutter/test/animation/live_binding_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/live_binding_test.dart",
"repo_id": "flutter",
"token_count": 2139
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testWidgets('has correct backdrop filters', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbar(
anchor: Offset.zero,
children: <Widget>[
CupertinoDesktopTextSelectionToolbarButton(
child: const Text('Tap me'),
onPressed: () {},
),
],
),
),
),
);
final BackdropFilter toolbarFilter = tester.firstWidget<BackdropFilter>(
find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbar),
matching: find.byType(BackdropFilter),
),
);
expect(
toolbarFilter.filter.runtimeType,
// _ComposeImageFilter is internal so we can't test if its filters are
// for blur and saturation, but checking if it's a _ComposeImageFilter
// should be enough. Outer and inner parameters don't matter, we just need
// a new _ComposeImageFilter to get its runtimeType.
//
// As web doesn't support ImageFilter.compose, we use just blur when
// kIsWeb.
kIsWeb
? ImageFilter.blur().runtimeType
: ImageFilter.compose(
outer: ImageFilter.blur(),
inner: ImageFilter.blur(),
).runtimeType,
);
});
testWidgets('has shadow', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbar(
anchor: Offset.zero,
children: <Widget>[
CupertinoDesktopTextSelectionToolbarButton(
child: const Text('Tap me'),
onPressed: () {},
),
],
),
),
),
);
final DecoratedBox decoratedBox = tester.firstWidget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbar),
matching: find.byType(DecoratedBox),
),
);
expect(
(decoratedBox.decoration as BoxDecoration).boxShadow,
isNotNull,
);
});
testWidgets('is translucent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbar(
anchor: Offset.zero,
children: <Widget>[
CupertinoDesktopTextSelectionToolbarButton(
child: const Text('Tap me'),
onPressed: () {},
),
],
),
),
),
);
final DecoratedBox decoratedBox = tester
.widgetList<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbar),
matching: find.byType(DecoratedBox),
),
)
// The second DecoratedBox should be the one with color.
.elementAt(1);
expect(
(decoratedBox.decoration as BoxDecoration).color!.opacity,
lessThan(1.0),
);
});
testWidgets('positions itself at the anchor', (WidgetTester tester) async {
// An arbitrary point on the screen to position at.
const Offset anchor = Offset(30.0, 40.0);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbar(
anchor: anchor,
children: <Widget>[
CupertinoDesktopTextSelectionToolbarButton(
child: const Text('Tap me'),
onPressed: () {},
),
],
),
),
),
);
expect(
tester
.getTopLeft(find.byType(CupertinoDesktopTextSelectionToolbarButton)),
// Greater than due to padding internal to the toolbar.
greaterThan(anchor),
);
});
}
| flutter/packages/flutter/test/cupertino/desktop_text_selection_toolbar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/desktop_text_selection_toolbar_test.dart",
"repo_id": "flutter",
"token_count": 1911
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('!chrome')
library;
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
late FakeBuilder mockHelper;
setUp(() {
mockHelper = FakeBuilder();
});
int testListLength = 10;
SliverList buildAListOfStuff() {
return SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return SizedBox(
height: 200.0,
child: Center(child: Text(index.toString())),
);
},
childCount: testListLength,
),
);
}
void uiTestGroup() {
testWidgets("doesn't invoke anything without user interaction", (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
expect(mockHelper.invocations, isEmpty);
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
Offset.zero,
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('calls the indicator builder when starting to overscroll', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
// Drag down but not enough to trigger the refresh.
await tester.drag(find.text('0'), const Offset(0.0, 50.0), touchSlopY: 0);
await tester.pump();
// The function is referenced once while passing into CupertinoSliverRefreshControl
// and is called.
expect(mockHelper.invocations.first, matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: 50,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
));
expect(mockHelper.invocations, hasLength(1));
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
const Offset(0.0, 50.0),
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets(
"don't call the builder if overscroll doesn't move slivers like on Android",
(WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
),
);
// Drag down but not enough to trigger the refresh.
await tester.drag(find.text('0'), const Offset(0.0, 50.0));
await tester.pump();
expect(mockHelper.invocations, isEmpty);
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
Offset.zero,
);
},
variant: TargetPlatformVariant.only(TargetPlatform.android),
);
testWidgets('let the builder update as canceled drag scrolls away', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
// Drag down but not enough to trigger the refresh.
await tester.drag(find.text('0'), const Offset(0.0, 50.0), touchSlopY: 0);
await tester.pump();
await tester.pump(const Duration(milliseconds: 20));
await tester.pump(const Duration(milliseconds: 20));
await tester.pump(const Duration(seconds: 3));
expect(mockHelper.invocations, containsAllInOrder(<void>[
matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: 50,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(48.07979523362715),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(48.36801747187993),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(43.98499220391114),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(44.63031931875867),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
]));
// The builder isn't called again when the sliver completely goes away.
expect(mockHelper.invocations, hasLength(3));
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
Offset.zero,
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('drag past threshold triggers refresh task', (WidgetTester tester) async {
final List<MethodCall> platformCallLog = <MethodCall>[];
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
platformCallLog.add(methodCall);
return null;
});
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
await gesture.moveBy(const Offset(0.0, 99.0));
await tester.pump();
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
await gesture.moveBy(const Offset(0.0, -3.0));
}
else {
await gesture.moveBy(const Offset(0.0, -30.0));
}
await tester.pump();
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
await gesture.moveBy(const Offset(0.0, 90.0));
}
else {
await gesture.moveBy(const Offset(0.0, 50.0));
}
await tester.pump();
expect(mockHelper.invocations, containsAllInOrder(<void>[
matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: 99,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(96),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: moreOrLessEquals(86.78169),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(112.51104),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(105.80452021305739),
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
),
]));
// The refresh callback is triggered after the frame.
expect(mockHelper.invocations.last, const RefreshTaskInvocation());
expect(mockHelper.invocations, hasLength(4));
expect(
platformCallLog.last,
isMethodCall('HapticFeedback.vibrate', arguments: 'HapticFeedbackType.mediumImpact'),
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets(
'refreshing task keeps the sliver expanded forever until done',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0);
await tester.pump();
// Let it start snapping back.
await tester.pump(const Duration(milliseconds: 50));
expect(mockHelper.invocations, containsAllInOrder(<Matcher>[
matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150,
refreshTriggerPullDistance: 100, // Default value.
refreshIndicatorExtent: 60, // Default value.
),
equals(const RefreshTaskInvocation()),
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(124.87933920045268),
refreshTriggerPullDistance: 100, // Default value.
refreshIndicatorExtent: 60, // Default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(127.10396988577114),
refreshTriggerPullDistance: 100, // Default value.
refreshIndicatorExtent: 60, // Default value.
),
]));
// Reaches refresh state and sliver's at 60.0 in height after a while.
await tester.pump(const Duration(seconds: 1));
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.refresh,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
// Stays in that state forever until future completes.
await tester.pump(const Duration(seconds: 1000));
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
const Offset(0.0, 60.0),
);
mockHelper.refreshCompleter.complete(null);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
expect(mockHelper.invocations, hasLength(5));
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'refreshing task keeps the sliver expanded forever until completes with error',
(WidgetTester tester) async {
final FlutterError error = FlutterError('Oops');
double errorCount = 0;
final TargetPlatform? platform = debugDefaultTargetPlatformOverride; // Will not be correct within the zone.
runZonedGuarded(
() async {
mockHelper.refreshCompleter = Completer<void>.sync();
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0);
await tester.pump();
// Let it start snapping back.
await tester.pump(const Duration(milliseconds: 50));
expect(mockHelper.invocations, containsAllInOrder(<Matcher>[
matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
),
equals(const RefreshTaskInvocation()),
if (platform == TargetPlatform.macOS) matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(124.87933920045268),
refreshTriggerPullDistance: 100, // Default value.
refreshIndicatorExtent: 60, // Default value.
)
else matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: moreOrLessEquals(127.10396988577114),
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
),
]));
// Reaches refresh state and sliver's at 60.0 in height after a while.
await tester.pump(const Duration(seconds: 1));
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.refresh,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
// Stays in that state forever until future completes.
await tester.pump(const Duration(seconds: 1000));
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')),
const Offset(0.0, 60.0),
);
mockHelper.refreshCompleter.completeError(error);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
expect(mockHelper.invocations, hasLength(5));
},
(Object e, StackTrace stack) {
expect(e, error);
expect(errorCount, 0);
errorCount++;
},
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets('expanded refreshing sliver scrolls normally', (WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
// Given a box constraint of 150, the Center will occupy all that height.
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 150.0),
);
await tester.drag(find.text('0'), const Offset(0.0, -300.0), touchSlopY: 0, warnIfMissed: false); // hits the list
await tester.pump();
// Refresh indicator still being told to layout the same way.
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.refresh,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
// Now the sliver is scrolled off screen.
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(
tester.getTopLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-210.0),
);
expect(
tester.getBottomLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-150.0),
);
expect(
tester.getTopLeft(find.widgetWithText(Center, '0')).dy,
moreOrLessEquals(-150.0),
);
}
else {
expect(
tester.getTopLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-175.38461538461536),
);
expect(
tester.getBottomLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-115.38461538461536),
);
expect(
tester.getTopLeft(find.widgetWithText(Center, '0')).dy,
moreOrLessEquals(-115.38461538461536),
);
}
// Scroll the top of the refresh indicator back to overscroll, it will
// snap to the size of the refresh indicator and stay there.
await tester.drag(find.text('1'), const Offset(0.0, 200.0), warnIfMissed: false); // hits the list
await tester.pump();
await tester.pump(const Duration(seconds: 2));
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 60.0),
);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 60.0, 800.0, 260.0),
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('expanded refreshing sliver goes away when done', (WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 150.0),
);
expect(mockHelper.invocations, contains(const RefreshTaskInvocation()));
// Rebuilds the sliver with a layout extent now.
await tester.pump();
// Let it snap back to occupy the indicator's final sliver space only.
await tester.pump(const Duration(seconds: 2));
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.refresh,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 60.0),
);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 60.0, 800.0, 260.0),
);
mockHelper.refreshCompleter.complete(null);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 60,
refreshIndicatorExtent: 60, // Default value.
refreshTriggerPullDistance: 100, // Default value.
)));
await tester.pump(const Duration(seconds: 5));
expect(find.text('-1'), findsNothing);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('builder still called when sliver snapped back more than 90%', (WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 150.0),
);
expect(mockHelper.invocations, contains(const RefreshTaskInvocation()));
// Rebuilds the sliver with a layout extent now.
await tester.pump();
// Let it snap back to occupy the indicator's final sliver space only.
await tester.pump(const Duration(seconds: 2));
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.refresh,
pulledExtent: 60,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
expect(
tester.getRect(find.widgetWithText(Center, '-1')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 60.0),
);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 60.0, 800.0, 260.0),
);
mockHelper.refreshCompleter.complete(null);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 60,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
// Waiting for refresh control to reach approximately 5% of height
await tester.pump(const Duration(milliseconds: 400));
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(
tester.getRect(find.widgetWithText(Center, '0')).top,
moreOrLessEquals(3.9543032206542765, epsilon: 4e-1),
);
expect(
tester.getRect(find.widgetWithText(Center, '-1')).height,
moreOrLessEquals(3.9543032206542765, epsilon: 4e-1),
);
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.inactive,
pulledExtent: 3.9543032206542765, // ~5% of 60.0
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
}
else {
expect(
tester.getRect(find.widgetWithText(Center, '0')).top,
moreOrLessEquals(3.0, epsilon: 4e-1),
);
expect(
tester.getRect(find.widgetWithText(Center, '-1')).height,
moreOrLessEquals(3.0, epsilon: 4e-1),
);
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.inactive,
pulledExtent: 2.6980688300546443, // ~5% of 60.0
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
}
expect(find.text('-1'), findsOneWidget);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets(
'retracting sliver during done cannot be pulled to refresh again until fully retracted',
(WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), pointer: 1, touchSlopY: 0.0);
await tester.pump();
expect(mockHelper.invocations, contains(const RefreshTaskInvocation()));
mockHelper.refreshCompleter.complete(null);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 150.0, // Still overscrolled here.
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
// Let it start going away but not fully.
await tester.pump(const Duration(milliseconds: 100));
// The refresh indicator is still building.
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 90.13497854600749,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
expect(
tester.getBottomLeft(find.widgetWithText(Center, '-1')).dy,
moreOrLessEquals(90.13497854600749),
);
}
else {
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 91.31180913199277,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
expect(
tester.getBottomLeft(find.widgetWithText(Center, '-1')).dy,
moreOrLessEquals(91.311809131992776),
);
}
// Start another drag by an amount that would have been enough to
// trigger another refresh if it were in the right state.
await tester.drag(find.text('0'), const Offset(0.0, 150.0), pointer: 1, touchSlopY: 0.0, warnIfMissed: false);
await tester.pump();
// Instead, it's still in the done state because the sliver never
// fully retracted.
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 118.29756539042118,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
}
else {
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 147.3772721631821,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
}
// Now let it fully go away.
await tester.pump(const Duration(seconds: 5));
expect(find.text('-1'), findsNothing);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
// Start another drag. It's now in drag mode.
await tester.drag(find.text('0'), const Offset(0.0, 40.0), pointer: 1, touchSlopY: 0.0);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: 40,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'sliver held in overscroll when task finishes completes normally',
(WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
// Start a refresh.
await gesture.moveBy(const Offset(0.0, 150.0));
await tester.pump();
expect(mockHelper.invocations, contains(const RefreshTaskInvocation()));
// Complete the task while held down.
mockHelper.refreshCompleter.complete(null);
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 150.0, // Still overscrolled here.
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 150.0, 800.0, 350.0),
);
await gesture.up();
await tester.pump();
await tester.pump(const Duration(seconds: 5));
expect(find.text('-1'), findsNothing);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'sliver scrolled away when task completes properly removes itself',
(WidgetTester tester) async {
if (testListLength < 4) {
// This test only makes sense when the list is long enough that
// the indicator can be scrolled away while refreshing.
return;
}
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
// Start a refresh.
await tester.drag(find.text('0'), const Offset(0.0, 150.0));
await tester.pump();
expect(mockHelper.invocations, contains(const RefreshTaskInvocation()));
await tester.drag(find.text('0'), const Offset(0.0, -300.0));
await tester.pump();
// Refresh indicator still being told to layout the same way.
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 60,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
// Now the sliver is scrolled off screen.
expect(
tester.getTopLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-175.38461538461536),
);
expect(
tester.getBottomLeft(find.widgetWithText(Center, '-1', skipOffstage: false)).dy,
moreOrLessEquals(-115.38461538461536),
);
// Complete the task while scrolled away.
mockHelper.refreshCompleter.complete(null);
// The sliver is instantly gone since there is no overscroll physics
// simulation.
await tester.pump();
// The next item's position is not disturbed.
expect(
tester.getTopLeft(find.widgetWithText(Center, '0')).dy,
moreOrLessEquals(-115.38461538461536),
);
// Scrolling past the first item still results in a new overscroll.
// The layout extent is gone.
await tester.drag(find.text('1'), const Offset(0.0, 120.0));
await tester.pump();
expect(mockHelper.invocations, contains(matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: 4.615384615384642,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
)));
// Snaps away normally.
await tester.pump();
await tester.pump(const Duration(seconds: 2));
expect(find.text('-1'), findsNothing);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
"don't do anything unless it can be overscrolled at the start of the list",
(WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
buildAListOfStuff(),
CupertinoSliverRefreshControl( // it's in the middle now.
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.fling(find.byType(SizedBox).first, const Offset(0.0, 200.0), 2000.0);
await tester.fling(find.byType(SizedBox).first, const Offset(0.0, -200.0), 3000.0, warnIfMissed: false); // IgnorePointer is enabled while scroll is ballistic.
expect(mockHelper.invocations, isEmpty);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'without an onRefresh, builder is called with arm for one frame then sliver goes away',
(WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0), touchSlopY: 0.0);
await tester.pump();
expect(mockHelper.invocations.first, matchesBuilder(
refreshState: RefreshIndicatorMode.armed,
pulledExtent: 150.0,
refreshTriggerPullDistance: 100.0, // Default value.
refreshIndicatorExtent: 60.0, // Default value.
));
await tester.pump(const Duration(milliseconds: 10));
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(mockHelper.invocations.last, matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: moreOrLessEquals(148.36088180097366),
refreshTriggerPullDistance: 100.0, // Default value.
refreshIndicatorExtent: 60.0, // Default value.
));
}
else {
expect(mockHelper.invocations.last, matchesBuilder(
refreshState: RefreshIndicatorMode.done,
pulledExtent: moreOrLessEquals(148.6463892921364),
refreshTriggerPullDistance: 100.0, // Default value.
refreshIndicatorExtent: 60.0, // Default value.
));
}
await tester.pump(const Duration(seconds: 5));
expect(find.text('-1'), findsNothing);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets('Should not crash when dragged', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
physics: const BouncingScrollPhysics(),
slivers: <Widget>[
CupertinoSliverRefreshControl(
onRefresh: () async => Future<void>.delayed(const Duration(days: 2000)),
),
],
),
),
);
await tester.dragFrom(const Offset(100, 10), const Offset(0.0, 50.0), touchSlopY: 0);
await tester.pump();
await tester.dragFrom(const Offset(100, 10), const Offset(0, 500), touchSlopY: 0);
await tester.pump();
expect(tester.takeException(), isNull);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
// Test to make sure the refresh sliver's overscroll isn't eaten by the
// nav bar sliver https://github.com/flutter/flutter/issues/74516.
testWidgets(
'properly displays when the refresh sliver is behind the large title nav bar sliver',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: Text('Title'),
),
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
final double initialFirstCellY = tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy;
// Drag down but not enough to trigger the refresh.
await tester.drag(find.text('0'), const Offset(0.0, 50.0), touchSlopY: 0);
await tester.pump();
expect(mockHelper.invocations.first, matchesBuilder(
refreshState: RefreshIndicatorMode.drag,
pulledExtent: 50,
refreshTriggerPullDistance: 100, // default value.
refreshIndicatorExtent: 60, // default value.
));
expect(mockHelper.invocations, hasLength(1));
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
initialFirstCellY + 50,
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
}
void stateMachineTestGroup() {
testWidgets('starts in inactive state', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.inactive,
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('goes to drag and returns to inactive in a small drag', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 20.0));
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.drag,
);
await tester.pump(const Duration(seconds: 2));
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.inactive,
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('goes to armed the frame it passes the threshold', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
refreshTriggerPullDistance: 80.0,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
await gesture.moveBy(const Offset(0.0, 79.0));
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.drag,
);
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
await gesture.moveBy(const Offset(0.0, 20.0)); // Overscrolling, need to move more than 1px.
}
else {
await gesture.moveBy(const Offset(0.0, 3.0)); // Overscrolling, need to move more than 1px.
}
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets(
'goes to refresh the frame it crossed back the refresh threshold',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
refreshTriggerPullDistance: 90.0,
refreshIndicatorExtent: 50.0,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
await gesture.moveBy(const Offset(0.0, 90.0)); // Arm it.
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
await gesture.moveBy(const Offset(0.0, -80.0)); // Overscrolling, need to move more than -40.
await tester.pump();
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(10.0), // Below 50 now.
);
}
else {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(49.775111111111116), // Below 50 now.
);
}
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.refresh,
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'goes to done internally as soon as the task finishes',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 100.0), touchSlopY: 0.0);
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
// The sliver scroll offset correction is applied on the next frame.
await tester.pump();
await tester.pump(const Duration(seconds: 2));
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.refresh,
);
expect(
tester.getRect(find.widgetWithText(SizedBox, '0')),
const Rect.fromLTRB(0.0, 60.0, 800.0, 260.0),
);
mockHelper.refreshCompleter.complete(null);
// The task completed between frames. The internal state goes to done
// right away even though the sliver gets a new offset correction the
// next frame.
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.done,
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'goes back to inactive when retracting back past 10% of arming distance',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
await gesture.moveBy(const Offset(0.0, 150.0));
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
mockHelper.refreshCompleter.complete(null);
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.done,
);
await tester.pump();
// Now back in overscroll mode.
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
await gesture.moveBy(const Offset(0.0, -125.0));
}
else {
await gesture.moveBy(const Offset(0.0, -200.0));
}
await tester.pump();
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(25.0),
);
}
else {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(27.944444444444457),
);
}
// Need to bring it to 100 * 0.1 to reset to inactive.
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.done,
);
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
await gesture.moveBy(const Offset(0.0, -16.0));
}
else {
await gesture.moveBy(const Offset(0.0, -35.0));
}
await tester.pump();
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(9.0),
);
}
else {
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(9.313890708161875),
);
}
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.inactive,
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
'goes back to inactive if already scrolled away when task completes',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: mockHelper.builder,
onRefresh: mockHelper.refreshTask,
),
buildAListOfStuff(),
],
),
),
);
final TestGesture gesture = await tester.startGesture(Offset.zero);
await gesture.moveBy(const Offset(0.0, 150.0));
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
await tester.pump(); // Sliver scroll offset correction is applied one frame later.
await gesture.moveBy(const Offset(0.0, -300.0));
double indicatorDestinationPosition = -145.0332383665717;
if (debugDefaultTargetPlatformOverride == TargetPlatform.macOS) {
indicatorDestinationPosition = -150.0;
}
await tester.pump();
// The refresh indicator is offscreen now.
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(indicatorDestinationPosition),
);
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.refresh,
);
mockHelper.refreshCompleter.complete(null);
// The sliver layout extent is removed on next frame.
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.inactive,
);
// Nothing moved.
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(indicatorDestinationPosition),
);
await tester.pump(const Duration(seconds: 2));
// Everything stayed as is.
expect(
tester.getTopLeft(find.widgetWithText(SizedBox, '0')).dy,
moreOrLessEquals(indicatorDestinationPosition),
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets(
"don't have to build any indicators or occupy space during refresh",
(WidgetTester tester) async {
mockHelper.refreshIndicator = const Center(child: Text('-1'));
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverRefreshControl(
builder: null,
onRefresh: mockHelper.refreshTask,
refreshIndicatorExtent: 0.0,
),
buildAListOfStuff(),
],
),
),
);
await tester.drag(find.text('0'), const Offset(0.0, 150.0));
await tester.pump();
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder))),
RefreshIndicatorMode.armed,
);
await tester.pump();
await tester.pump(const Duration(seconds: 5));
// In refresh mode but has no UI.
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.refresh,
);
expect(
tester.getRect(find.widgetWithText(Center, '0')),
const Rect.fromLTRB(0.0, 0.0, 800.0, 200.0),
);
mockHelper.refreshCompleter.complete(null);
await tester.pump();
// Goes to inactive right away since the sliver is already collapsed.
expect(
CupertinoSliverRefreshControl.state(tester.element(find.byType(LayoutBuilder, skipOffstage: false))),
RefreshIndicatorMode.inactive,
);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets('buildRefreshIndicator progress', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Builder(
builder: (BuildContext context) {
return CupertinoSliverRefreshControl.buildRefreshIndicator(
context,
RefreshIndicatorMode.drag,
10, 100, 10,
);
},
),
),
);
expect(tester.widget<CupertinoActivityIndicator>(find.byType(CupertinoActivityIndicator)).progress, 10.0 / 100.0);
await tester.pumpWidget(
CupertinoApp(
home: Builder(
builder: (BuildContext context) {
return CupertinoSliverRefreshControl.buildRefreshIndicator(
context,
RefreshIndicatorMode.drag,
26, 100, 10,
);
},
),
),
);
expect(tester.widget<CupertinoActivityIndicator>(find.byType(CupertinoActivityIndicator)).progress, 26.0 / 100.0);
await tester.pumpWidget(
CupertinoApp(
home: Builder(
builder: (BuildContext context) {
return CupertinoSliverRefreshControl.buildRefreshIndicator(
context,
RefreshIndicatorMode.drag,
100, 100, 10,
);
},
),
),
);
expect(tester.widget<CupertinoActivityIndicator>(find.byType(CupertinoActivityIndicator)).progress, 100.0 / 100.0);
});
testWidgets('indicator should not become larger when overscrolled', (WidgetTester tester) async {
// test for https://github.com/flutter/flutter/issues/79841
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Builder(
builder: (BuildContext context) {
return CupertinoSliverRefreshControl.buildRefreshIndicator(
context,
RefreshIndicatorMode.done,
120, 100, 10,
);
},
),
),
);
expect(tester.widget<CupertinoActivityIndicator>(find.byType(CupertinoActivityIndicator)).radius, 14.0);
});
}
group('UI tests long list', uiTestGroup);
// Test the internal state machine directly to make sure the UI aren't just
// correct by coincidence.
group('state machine test long list', stateMachineTestGroup);
// Retest everything and make sure that it still works when the whole list
// is smaller than the viewport size.
testListLength = 2;
group('UI tests short list', uiTestGroup);
// Test the internal state machine directly to make sure the UI aren't just
// correct by coincidence.
group('state machine test short list', stateMachineTestGroup);
testWidgets(
'Does not crash when paintExtent > remainingPaintExtent',
(WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/46871.
await tester.pumpWidget(
CupertinoApp(
home: CustomScrollView(
physics: const BouncingScrollPhysics(),
slivers: <Widget>[
const CupertinoSliverRefreshControl(),
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) => const SizedBox(height: 100),
childCount: 20,
),
),
],
),
),
);
// Drag the content down far enough so that
// geometry.paintExtent > constraints.maxPaintExtent
await tester.dragFrom(const Offset(10, 10), const Offset(0, 500));
await tester.pump();
expect(tester.takeException(), isNull);
},
);
}
class FakeBuilder {
Completer<void> refreshCompleter = Completer<void>.sync();
final List<MockHelperInvocation> invocations = <MockHelperInvocation>[];
Widget refreshIndicator = Container();
Widget builder(
BuildContext context,
RefreshIndicatorMode refreshState,
double pulledExtent,
double refreshTriggerPullDistance,
double refreshIndicatorExtent,
) {
if (pulledExtent < 0.0) {
throw TestFailure('The pulledExtent should never be less than 0.0');
}
if (refreshTriggerPullDistance < 0.0) {
throw TestFailure('The refreshTriggerPullDistance should never be less than 0.0');
}
if (refreshIndicatorExtent < 0.0) {
throw TestFailure('The refreshIndicatorExtent should never be less than 0.0');
}
invocations.add(BuilderInvocation(
refreshState: refreshState,
pulledExtent: pulledExtent,
refreshTriggerPullDistance: refreshTriggerPullDistance,
refreshIndicatorExtent: refreshIndicatorExtent,
));
return refreshIndicator;
}
Future<void> refreshTask() {
invocations.add(const RefreshTaskInvocation());
return refreshCompleter.future;
}
}
abstract class MockHelperInvocation {
const MockHelperInvocation();
}
@immutable
class RefreshTaskInvocation extends MockHelperInvocation {
const RefreshTaskInvocation();
}
@immutable
class BuilderInvocation extends MockHelperInvocation {
const BuilderInvocation({
required this.refreshState,
required this.pulledExtent,
required this.refreshIndicatorExtent,
required this.refreshTriggerPullDistance,
});
final RefreshIndicatorMode refreshState;
final double pulledExtent;
final double refreshTriggerPullDistance;
final double refreshIndicatorExtent;
@override
String toString() => '{refreshState: $refreshState, pulledExtent: $pulledExtent, refreshTriggerPullDistance: $refreshTriggerPullDistance, refreshIndicatorExtent: $refreshIndicatorExtent}';
}
Matcher matchesBuilder({
required RefreshIndicatorMode refreshState,
required dynamic pulledExtent,
required dynamic refreshTriggerPullDistance,
required dynamic refreshIndicatorExtent,
}) {
return isA<BuilderInvocation>()
.having((BuilderInvocation invocation) => invocation.refreshState, 'refreshState', refreshState)
.having((BuilderInvocation invocation) => invocation.pulledExtent, 'pulledExtent', pulledExtent)
.having((BuilderInvocation invocation) => invocation.refreshTriggerPullDistance, 'refreshTriggerPullDistance', refreshTriggerPullDistance)
.having((BuilderInvocation invocation) => invocation.refreshIndicatorExtent, 'refreshIndicatorExtent', refreshIndicatorExtent);
}
| flutter/packages/flutter/test/cupertino/refresh_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/refresh_test.dart",
"repo_id": "flutter",
"token_count": 27291
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Passes textAlign to underlying CupertinoTextField', (WidgetTester tester) async {
const TextAlign alignment = TextAlign.center;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
textAlign: alignment,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.textAlign, alignment);
});
testWidgets('Passes scrollPhysics to underlying TextField', (WidgetTester tester) async {
const ScrollPhysics scrollPhysics = ScrollPhysics();
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
scrollPhysics: scrollPhysics,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.scrollPhysics, scrollPhysics);
});
testWidgets('Passes textAlignVertical to underlying CupertinoTextField', (WidgetTester tester) async {
const TextAlignVertical textAlignVertical = TextAlignVertical.bottom;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
textAlignVertical: textAlignVertical,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.textAlignVertical, textAlignVertical);
});
testWidgets('Passes textInputAction to underlying CupertinoTextField', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
textInputAction: TextInputAction.next,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.textInputAction, TextInputAction.next);
});
testWidgets('Passes onEditingComplete to underlying CupertinoTextField', (WidgetTester tester) async {
void onEditingComplete() {}
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
onEditingComplete: onEditingComplete,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.onEditingComplete, onEditingComplete);
});
testWidgets('Passes cursor attributes to underlying CupertinoTextField', (WidgetTester tester) async {
const double cursorWidth = 3.14;
const double cursorHeight = 6.28;
const Radius cursorRadius = Radius.circular(2);
const Color cursorColor = CupertinoColors.systemPurple;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
cursorWidth: cursorWidth,
cursorHeight: cursorHeight,
cursorColor: cursorColor,
),
),
),
);
final Finder textFieldFinder = find.byType(CupertinoTextField);
expect(textFieldFinder, findsOneWidget);
final CupertinoTextField textFieldWidget = tester.widget(textFieldFinder);
expect(textFieldWidget.cursorWidth, cursorWidth);
expect(textFieldWidget.cursorHeight, cursorHeight);
expect(textFieldWidget.cursorRadius, cursorRadius);
expect(textFieldWidget.cursorColor, cursorColor);
});
testWidgets('onFieldSubmit callbacks are called', (WidgetTester tester) async {
bool called = false;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
onFieldSubmitted: (String value) {
called = true;
},
),
),
),
);
await tester.showKeyboard(find.byType(CupertinoTextField));
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pump();
expect(called, true);
});
testWidgets('onChanged callbacks are called', (WidgetTester tester) async {
late String value;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
onChanged: (String v) {
value = v;
},
),
),
),
);
await tester.enterText(find.byType(CupertinoTextField), 'Soup');
await tester.pump();
expect(value, 'Soup');
});
testWidgets('autovalidateMode is passed to super', (WidgetTester tester) async {
int validateCalled = 0;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
autovalidateMode: AutovalidateMode.always,
validator: (String? value) {
validateCalled++;
return null;
},
),
),
),
);
expect(validateCalled, 1);
await tester.enterText(find.byType(CupertinoTextField), 'a');
await tester.pump();
expect(validateCalled, 2);
});
testWidgets('validate is called if widget is enabled', (WidgetTester tester) async {
int validateCalled = 0;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
enabled: true,
autovalidateMode: AutovalidateMode.always,
validator: (String? value) {
validateCalled += 1;
return null;
},
),
),
),
);
expect(validateCalled, 1);
await tester.enterText(find.byType(CupertinoTextField), 'a');
await tester.pump();
expect(validateCalled, 2);
});
testWidgets('readonly text form field will hide cursor by default', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
initialValue: 'readonly',
readOnly: true,
),
),
),
);
await tester.showKeyboard(find.byType(CupertinoTextFormFieldRow));
expect(tester.testTextInput.hasAnyClients, false);
await tester.tap(find.byType(CupertinoTextField));
await tester.pump();
expect(tester.testTextInput.hasAnyClients, false);
await tester.longPress(find.text('readonly'));
await tester.pump();
// Context menu should not have paste.
expect(find.byType(CupertinoTextSelectionToolbar), findsOneWidget);
expect(find.text('Paste'), findsNothing);
final EditableTextState editableTextState =
tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
// Make sure it does not paint caret for a period of time.
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
}, skip: isBrowser); // [intended] We do not use Flutter-rendered context menu on the Web.
testWidgets('onTap is called upon tap', (WidgetTester tester) async {
int tapCount = 0;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
onTap: () {
tapCount += 1;
},
),
),
),
);
expect(tapCount, 0);
await tester.tap(find.byType(CupertinoTextField));
// Wait a bit so they're all single taps and not double taps.
await tester.pump(const Duration(milliseconds: 300));
await tester.tap(find.byType(CupertinoTextField));
await tester.pump(const Duration(milliseconds: 300));
await tester.tap(find.byType(CupertinoTextField));
await tester.pump(const Duration(milliseconds: 300));
expect(tapCount, 3);
});
// Regression test for https://github.com/flutter/flutter/issues/54472.
testWidgets('reset resets the text fields value to the initialValue', (WidgetTester tester) async {
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
initialValue: 'initialValue',
),
),
));
await tester.enterText(find.byType(CupertinoTextFormFieldRow), 'changedValue');
final FormFieldState<String> state = tester.state<FormFieldState<String>>(find.byType(CupertinoTextFormFieldRow));
state.reset();
expect(find.text('changedValue'), findsNothing);
expect(find.text('initialValue'), findsOneWidget);
});
// Regression test for https://github.com/flutter/flutter/issues/54472.
testWidgets('didChange changes text fields value', (WidgetTester tester) async {
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
initialValue: 'initialValue',
),
),
));
expect(find.text('initialValue'), findsOneWidget);
final FormFieldState<String> state = tester
.state<FormFieldState<String>>(find.byType(CupertinoTextFormFieldRow));
state.didChange('changedValue');
expect(find.text('initialValue'), findsNothing);
expect(find.text('changedValue'), findsOneWidget);
});
testWidgets('onChanged callbacks value and FormFieldState.value are sync', (WidgetTester tester) async {
bool called = false;
late FormFieldState<String> state;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
onChanged: (String value) {
called = true;
expect(value, state.value);
},
),
),
),
);
state = tester
.state<FormFieldState<String>>(find.byType(CupertinoTextFormFieldRow));
await tester.enterText(find.byType(CupertinoTextField), 'Soup');
expect(called, true);
});
testWidgets('autofillHints is passed to super', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
autofillHints: const <String>[AutofillHints.countryName],
),
),
),
);
final CupertinoTextField widget =
tester.widget(find.byType(CupertinoTextField));
expect(widget.autofillHints, equals(const <String>[AutofillHints.countryName]));
});
testWidgets('autovalidateMode is passed to super', (WidgetTester tester) async {
int validateCalled = 0;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CupertinoTextFormFieldRow(
autovalidateMode: AutovalidateMode.onUserInteraction,
validator: (String? value) {
validateCalled++;
return null;
},
),
),
),
);
expect(validateCalled, 0);
await tester.enterText(find.byType(CupertinoTextField), 'a');
await tester.pump();
expect(validateCalled, 1);
});
testWidgets('AutovalidateMode.always mode shows error from the start', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
initialValue: 'Value',
autovalidateMode: AutovalidateMode.always,
validator: (String? value) => 'Error',
),
),
),
);
final Finder errorTextFinder = find.byType(Text);
expect(errorTextFinder, findsOneWidget);
final Text errorText = tester.widget(errorTextFinder);
expect(errorText.data, 'Error');
});
testWidgets('Shows error text upon invalid input', (WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: '');
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
controller: controller,
autovalidateMode: AutovalidateMode.onUserInteraction,
validator: (String? value) => 'Error',
),
),
),
);
expect(find.byType(Text), findsNothing);
controller.text = 'Value';
await tester.pumpAndSettle();
final Finder errorTextFinder = find.byType(Text);
expect(errorTextFinder, findsOneWidget);
final Text errorText = tester.widget(errorTextFinder);
expect(errorText.data, 'Error');
});
testWidgets('Shows prefix', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
prefix: const Text('Enter Value'),
),
),
),
);
final Finder errorTextFinder = find.byType(Text);
expect(errorTextFinder, findsOneWidget);
final Text errorText = tester.widget(errorTextFinder);
expect(errorText.data, 'Enter Value');
});
testWidgets('Passes textDirection to underlying CupertinoTextField', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
textDirection: TextDirection.ltr,
),
),
),
);
final Finder ltrTextFieldFinder = find.byType(CupertinoTextField);
expect(ltrTextFieldFinder, findsOneWidget);
final CupertinoTextField ltrTextFieldWidget = tester.widget(ltrTextFieldFinder);
expect(ltrTextFieldWidget.textDirection, TextDirection.ltr);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoTextFormFieldRow(
textDirection: TextDirection.rtl,
),
),
),
);
final Finder rtlTextFieldFinder = find.byType(CupertinoTextField);
expect(rtlTextFieldFinder, findsOneWidget);
final CupertinoTextField rtlTextFieldWidget = tester.widget(rtlTextFieldFinder);
expect(rtlTextFieldWidget.textDirection, TextDirection.rtl);
});
testWidgets(
'CupertinoTextFormFieldRow onChanged is called when the form is reset', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/123009.
final GlobalKey<FormFieldState<String>> stateKey = GlobalKey<FormFieldState<String>>();
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String value = 'initialValue';
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: Form(
key: formKey,
child: CupertinoTextFormFieldRow(
key: stateKey,
initialValue: value,
onChanged: (String newValue) {
value = newValue;
},
),
),
),
),
);
// Initial value is 'initialValue'.
expect(stateKey.currentState!.value, 'initialValue');
expect(value, 'initialValue');
// Change value to 'changedValue'.
await tester.enterText(find.byType(CupertinoTextField), 'changedValue');
expect(stateKey.currentState!.value,'changedValue');
expect(value, 'changedValue');
// Should be back to 'initialValue' when the form is reset.
formKey.currentState!.reset();
await tester.pump();
expect(stateKey.currentState!.value,'initialValue');
expect(value, 'initialValue');
});
}
| flutter/packages/flutter/test/cupertino/text_form_field_row_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/text_form_field_row_test.dart",
"repo_id": "flutter",
"token_count": 6590
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
class TestBinding extends BindingBase { }
void main() {
test('BindingBase.debugCheckZone', () async {
final BindingBase binding = TestBinding();
binding.debugCheckZone('test1');
BindingBase.debugZoneErrorsAreFatal = true;
Zone.current.fork().run(() {
try {
binding.debugCheckZone('test2');
fail('expected an exception');
} catch (error) {
expect(error, isA<FlutterError>());
expect(error.toString(),
'Zone mismatch.\n'
'The Flutter bindings were initialized in a different zone than is now being used. '
'This will likely cause confusion and bugs as any zone-specific configuration will '
'inconsistently use the configuration of the original binding initialization zone '
'or this zone based on hard-to-predict factors such as which zone was active when '
'a particular callback was set.\n'
'It is important to use the same zone when calling `ensureInitialized` on the '
'binding as when calling `test2` later.\n'
'To make this error non-fatal, set BindingBase.debugZoneErrorsAreFatal to false '
'before the bindings are initialized (i.e. as the first statement in `void main() { }`).',
);
}
});
BindingBase.debugZoneErrorsAreFatal = false;
Zone.current.fork().run(() {
bool sawError = false;
final FlutterExceptionHandler? lastHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails details) {
final Object error = details.exception;
expect(error, isA<FlutterError>());
expect(error.toString(),
'Zone mismatch.\n'
'The Flutter bindings were initialized in a different zone than is now being used. '
'This will likely cause confusion and bugs as any zone-specific configuration will '
'inconsistently use the configuration of the original binding initialization zone '
'or this zone based on hard-to-predict factors such as which zone was active when '
'a particular callback was set.\n'
'It is important to use the same zone when calling `ensureInitialized` on the '
'binding as when calling `test3` later.\n'
'To make this warning fatal, set BindingBase.debugZoneErrorsAreFatal to true '
'before the bindings are initialized (i.e. as the first statement in `void main() { }`).',
);
sawError = true;
};
binding.debugCheckZone('test3');
expect(sawError, isTrue);
FlutterError.onError = lastHandler;
});
});
}
| flutter/packages/flutter/test/foundation/binding_2_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/binding_2_test.dart",
"repo_id": "flutter",
"token_count": 1036
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('LicenseEntryWithLineBreaks - most cases', () {
// There's some trailing spaces in this string.
// To avoid IDEs stripping them, I've escaped them as \u0020.
final List<LicenseParagraph> paragraphs = const LicenseEntryWithLineBreaks(<String>[], '''
A
A
A
B
B
B
C
C
C
D
D
D
E
E
F
G
G
G
[H
H
H]
\u0020\u0020
I\u000cJ
\u000cK
K
\u000c
L
L L
L L
L L
L L
L L
M
M\u0020\u0020\u0020
M\u0020\u0020\u0020\u0020
N
O
O
P
QQQ
RR RRR RRRR RRRRR
R
S
T
U
V
W
X
\u0020\u0020\u0020\u0020\u0020\u0020
Y''').paragraphs.toList();
int index = 0;
expect(paragraphs[index].text, 'A A A');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'B B B');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'C C C');
expect(paragraphs[index].indent, 1);
index += 1;
expect(paragraphs[index].text, 'D D D');
expect(paragraphs[index].indent, LicenseParagraph.centeredIndent);
index += 1;
expect(paragraphs[index].text, 'E E');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'F');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'G G G');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, '[H H H]');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'I');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'J');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'K K');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'L L L L L L L L L L L');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'M M M ');
expect(paragraphs[index].indent, 1);
index += 1;
expect(paragraphs[index].text, 'N');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'O O');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'P');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'QQQ');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'RR RRR RRRR RRRRR R');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'S');
expect(paragraphs[index].indent, 0);
index += 1;
expect(paragraphs[index].text, 'T');
expect(paragraphs[index].indent, 1);
index += 1;
expect(paragraphs[index].text, 'U');
expect(paragraphs[index].indent, 2);
index += 1;
expect(paragraphs[index].text, 'V');
expect(paragraphs[index].indent, 3);
index += 1;
expect(paragraphs[index].text, 'W');
expect(paragraphs[index].indent, 2);
index += 1;
expect(paragraphs[index].text, 'X');
expect(paragraphs[index].indent, 2);
index += 1;
expect(paragraphs[index].text, 'Y');
expect(paragraphs[index].indent, 2);
index += 1;
expect(paragraphs, hasLength(index));
});
test('LicenseEntryWithLineBreaks - leading and trailing whitespace', () {
expect(const LicenseEntryWithLineBreaks(<String>[], ' \n\n ').paragraphs.toList(), isEmpty);
expect(const LicenseEntryWithLineBreaks(<String>[], ' \r\n\r\n ').paragraphs.toList(), isEmpty);
List<LicenseParagraph> paragraphs;
paragraphs = const LicenseEntryWithLineBreaks(<String>[], ' \nA\n ').paragraphs.toList();
expect(paragraphs[0].text, 'A');
expect(paragraphs[0].indent, 0);
expect(paragraphs, hasLength(1));
paragraphs = const LicenseEntryWithLineBreaks(<String>[], '\n\n\nA\n\n\n').paragraphs.toList();
expect(paragraphs[0].text, 'A');
expect(paragraphs[0].indent, 0);
expect(paragraphs, hasLength(1));
});
test('LicenseRegistry', () async {
expect(await LicenseRegistry.licenses.toList(), isEmpty);
LicenseRegistry.addLicense(() async* {
yield const LicenseEntryWithLineBreaks(<String>[], 'A');
yield const LicenseEntryWithLineBreaks(<String>[], 'B');
});
LicenseRegistry.addLicense(() async* {
yield const LicenseEntryWithLineBreaks(<String>[], 'C');
yield const LicenseEntryWithLineBreaks(<String>[], 'D');
});
expect(await LicenseRegistry.licenses.toList(), hasLength(4));
final List<LicenseEntry> licenses = await LicenseRegistry.licenses.toList();
expect(licenses, hasLength(4));
expect(licenses[0].paragraphs.single.text, 'A');
expect(licenses[1].paragraphs.single.text, 'B');
expect(licenses[2].paragraphs.single.text, 'C');
expect(licenses[3].paragraphs.single.text, 'D');
});
}
| flutter/packages/flutter/test/foundation/licenses_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/licenses_test.dart",
"repo_id": "flutter",
"token_count": 2112
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
import 'gesture_tester.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testGesture('Should recognize pan', (GestureTester tester) {
final PanGestureRecognizer pan = PanGestureRecognizer();
final TapGestureRecognizer tap = TapGestureRecognizer()..onTap = () {};
addTearDown(pan.dispose);
addTearDown(tap.dispose);
bool didStartPan = false;
pan.onStart = (_) {
didStartPan = true;
};
Offset? updatedScrollDelta;
pan.onUpdate = (DragUpdateDetails details) {
updatedScrollDelta = details.delta;
};
bool didEndPan = false;
pan.onEnd = (DragEndDetails details) {
didEndPan = true;
};
bool didTap = false;
tap.onTap = () {
didTap = true;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(5);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
expect(didTap, isFalse);
tester.route(down);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
expect(didTap, isFalse);
// touch should give up when it hits kTouchSlop, which was 18.0 when this test was last updated.
tester.route(pointer.move(const Offset(20.0, 20.0))); // moved 10 horizontally and 10 vertically which is 14 total
expect(didStartPan, isFalse); // 14 < 18
tester.route(pointer.move(const Offset(20.0, 30.0))); // moved 10 horizontally and 20 vertically which is 22 total
expect(didStartPan, isTrue); // 22 > 18
didStartPan = false;
expect(didEndPan, isFalse);
expect(didTap, isFalse);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(0.0, -5.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
expect(didTap, isFalse);
tester.route(pointer.up());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isTrue);
didEndPan = false;
expect(didTap, isFalse);
});
testGesture('Should report most recent point to onStart by default', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer();
final VerticalDragGestureRecognizer competingDrag = VerticalDragGestureRecognizer()
..onStart = (_) {};
addTearDown(drag.dispose);
addTearDown(competingDrag.dispose);
late Offset positionAtOnStart;
drag.onStart = (DragStartDetails details) {
positionAtOnStart = details.globalPosition;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
competingDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(30.0, 0.0)));
expect(positionAtOnStart, const Offset(30.0, 00.0));
});
testGesture('Should report most recent point to onStart with a start configuration', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer();
final VerticalDragGestureRecognizer competingDrag = VerticalDragGestureRecognizer()
..onStart = (_) {};
addTearDown(drag.dispose);
addTearDown(competingDrag.dispose);
Offset? positionAtOnStart;
drag.onStart = (DragStartDetails details) {
positionAtOnStart = details.globalPosition;
};
Offset? updateOffset;
drag.onUpdate = (DragUpdateDetails details) {
updateOffset = details.globalPosition;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
competingDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(30.0, 0.0)));
expect(positionAtOnStart, const Offset(30.0, 0.0));
expect(updateOffset, null);
});
testGesture('Should recognize drag', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
bool didStartDrag = false;
drag.onStart = (_) {
didStartDrag = true;
};
double? updatedDelta;
drag.onUpdate = (DragUpdateDetails details) {
updatedDelta = details.primaryDelta;
};
bool didEndDrag = false;
drag.onEnd = (DragEndDetails details) {
didEndDrag = true;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(down);
expect(didStartDrag, isTrue);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isTrue);
didStartDrag = false;
expect(updatedDelta, 10.0);
updatedDelta = null;
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, 0.0);
updatedDelta = null;
expect(didEndDrag, isFalse);
tester.route(pointer.up());
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isTrue);
didEndDrag = false;
});
testGesture('Should reject mouse drag when configured to ignore mouse pointers - Horizontal', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer(supportedDevices: <PointerDeviceKind>{
PointerDeviceKind.touch,
}) ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
bool didStartDrag = false;
drag.onStart = (_) {
didStartDrag = true;
};
double? updatedDelta;
drag.onUpdate = (DragUpdateDetails details) {
updatedDelta = details.primaryDelta;
};
bool didEndDrag = false;
drag.onEnd = (DragEndDetails details) {
didEndDrag = true;
};
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(down);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.up());
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
});
testGesture('Should reject mouse drag when configured to ignore mouse pointers - Vertical', (GestureTester tester) {
final VerticalDragGestureRecognizer drag = VerticalDragGestureRecognizer(supportedDevices: <PointerDeviceKind>{
PointerDeviceKind.touch,
})..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
bool didStartDrag = false;
drag.onStart = (_) {
didStartDrag = true;
};
double? updatedDelta;
drag.onUpdate = (DragUpdateDetails details) {
updatedDelta = details.primaryDelta;
};
bool didEndDrag = false;
drag.onEnd = (DragEndDetails details) {
didEndDrag = true;
};
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(down);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(25.0, 20.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.move(const Offset(25.0, 20.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(pointer.up());
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
});
testGesture('DragGestureRecognizer.onStart behavior test', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
Duration? startTimestamp;
Offset? positionAtOnStart;
drag.onStart = (DragStartDetails details) {
startTimestamp = details.sourceTimeStamp;
positionAtOnStart = details.globalPosition;
};
Duration? updatedTimestamp;
Offset? updateDelta;
drag.onUpdate = (DragUpdateDetails details) {
updatedTimestamp = details.sourceTimeStamp;
updateDelta = details.delta;
};
// No competing, dragStartBehavior == DragStartBehavior.down
final TestPointer pointer = TestPointer(5);
PointerDownEvent down = pointer.down(const Offset(10.0, 10.0), timeStamp: const Duration(milliseconds: 100));
drag.addPointer(down);
tester.closeArena(5);
expect(startTimestamp, isNull);
expect(positionAtOnStart, isNull);
expect(updatedTimestamp, isNull);
tester.route(down);
// The only horizontal drag gesture win the arena when the pointer down.
expect(startTimestamp, const Duration(milliseconds: 100));
expect(positionAtOnStart, const Offset(10.0, 10.0));
expect(updatedTimestamp, isNull);
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 200)));
expect(updatedTimestamp, const Duration(milliseconds: 200));
expect(updateDelta, const Offset(10.0, 0.0));
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 300)));
expect(updatedTimestamp, const Duration(milliseconds: 300));
expect(updateDelta, Offset.zero);
tester.route(pointer.up());
// No competing, dragStartBehavior == DragStartBehavior.start
// When there are no other gestures competing with this gesture in the arena,
// there's no difference in behavior between the two settings.
drag.dragStartBehavior = DragStartBehavior.start;
startTimestamp = null;
positionAtOnStart = null;
updatedTimestamp = null;
updateDelta = null;
down = pointer.down(const Offset(10.0, 10.0), timeStamp: const Duration(milliseconds: 400));
drag.addPointer(down);
tester.closeArena(5);
tester.route(down);
expect(startTimestamp, const Duration(milliseconds: 400));
expect(positionAtOnStart, const Offset(10.0, 10.0));
expect(updatedTimestamp, isNull);
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 500)));
expect(updatedTimestamp, const Duration(milliseconds: 500));
tester.route(pointer.up());
// With competing, dragStartBehavior == DragStartBehavior.start
startTimestamp = null;
positionAtOnStart = null;
updatedTimestamp = null;
updateDelta = null;
final VerticalDragGestureRecognizer competingDrag = VerticalDragGestureRecognizer()
..onStart = (_) {};
addTearDown(competingDrag.dispose);
down = pointer.down(const Offset(10.0, 10.0), timeStamp: const Duration(milliseconds: 600));
drag.addPointer(down);
competingDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
// The pointer down event do not trigger anything.
expect(startTimestamp, isNull);
expect(positionAtOnStart, isNull);
expect(updatedTimestamp, isNull);
tester.route(pointer.move(const Offset(30.0, 10.0), timeStamp: const Duration(milliseconds: 700)));
expect(startTimestamp, const Duration(milliseconds: 700));
// Using the position of the pointer at the time this gesture recognizer won the arena.
expect(positionAtOnStart, const Offset(30.0, 10.0));
expect(updatedTimestamp, isNull); // Do not trigger an update event.
tester.route(pointer.up());
// With competing, dragStartBehavior == DragStartBehavior.down
drag.dragStartBehavior = DragStartBehavior.down;
startTimestamp = null;
positionAtOnStart = null;
updatedTimestamp = null;
updateDelta = null;
down = pointer.down(const Offset(10.0, 10.0), timeStamp: const Duration(milliseconds: 800));
drag.addPointer(down);
competingDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
expect(startTimestamp, isNull);
expect(positionAtOnStart, isNull);
expect(updatedTimestamp, isNull);
tester.route(pointer.move(const Offset(30.0, 10.0), timeStamp: const Duration(milliseconds: 900)));
expect(startTimestamp, const Duration(milliseconds: 900));
// Using the position of the first detected down event for the pointer.
expect(positionAtOnStart, const Offset(10.0, 10.0));
expect(updatedTimestamp, const Duration(milliseconds: 900)); // Also, trigger an update event.
expect(updateDelta, const Offset(20.0, 0.0));
tester.route(pointer.up());
});
testGesture('Should report original timestamps', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
Duration? startTimestamp;
drag.onStart = (DragStartDetails details) {
startTimestamp = details.sourceTimeStamp;
};
Duration? updatedTimestamp;
drag.onUpdate = (DragUpdateDetails details) {
updatedTimestamp = details.sourceTimeStamp;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0), timeStamp: const Duration(milliseconds: 100));
drag.addPointer(down);
tester.closeArena(5);
expect(startTimestamp, isNull);
tester.route(down);
expect(startTimestamp, const Duration(milliseconds: 100));
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 200)));
expect(updatedTimestamp, const Duration(milliseconds: 200));
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 300)));
expect(updatedTimestamp, const Duration(milliseconds: 300));
});
testGesture('Should report initial down point to onStart with a down configuration', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down;
final VerticalDragGestureRecognizer competingDrag = VerticalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..onStart = (_) {};
addTearDown(drag.dispose);
addTearDown(competingDrag.dispose);
Offset? positionAtOnStart;
drag.onStart = (DragStartDetails details) {
positionAtOnStart = details.globalPosition;
};
Offset? updateOffset;
Offset? updateDelta;
drag.onUpdate = (DragUpdateDetails details) {
updateOffset = details.globalPosition;
updateDelta = details.delta;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
competingDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(30.0, 0.0)));
expect(positionAtOnStart, const Offset(10.0, 10.0));
// The drag is horizontal so we're going to ignore the vertical delta position
// when calculating the new global position.
expect(updateOffset, const Offset(30.0, 10.0));
expect(updateDelta, const Offset(20.0, 0.0));
});
testGesture('Drag with multiple pointers in down behavior - sumAllPointers', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag1 =
HorizontalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..multitouchDragStrategy = MultitouchDragStrategy.sumAllPointers;
final VerticalDragGestureRecognizer drag2 =
VerticalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..multitouchDragStrategy = MultitouchDragStrategy.sumAllPointers;
addTearDown(drag1.dispose);
addTearDown(drag2.dispose);
final List<String> log = <String>[];
drag1.onDown = (_) { log.add('drag1-down'); };
drag1.onStart = (_) { log.add('drag1-start'); };
drag1.onUpdate = (_) { log.add('drag1-update'); };
drag1.onEnd = (_) { log.add('drag1-end'); };
drag1.onCancel = () { log.add('drag1-cancel'); };
drag2.onDown = (_) { log.add('drag2-down'); };
drag2.onStart = (_) { log.add('drag2-start'); };
drag2.onUpdate = (_) { log.add('drag2-update'); };
drag2.onEnd = (_) { log.add('drag2-end'); };
drag2.onCancel = () { log.add('drag2-cancel'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(const Offset(10.0, 10.0));
drag1.addPointer(down5);
drag2.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
tester.route(pointer5.move(const Offset(100.0, 0.0)));
log.add('-b');
tester.route(pointer5.move(const Offset(50.0, 50.0)));
log.add('-c');
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(const Offset(20.0, 20.0));
drag1.addPointer(down6);
drag2.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-d');
// Check all active pointers can trigger 'drag1-update'.
tester.route(pointer5.move(const Offset(0.0, 100.0)));
log.add('-e');
tester.route(pointer5.move(const Offset(70.0, 70.0)));
log.add('-f');
tester.route(pointer6.move(const Offset(0.0, 100.0)));
log.add('-g');
tester.route(pointer6.move(const Offset(70.0, 70.0)));
log.add('-h');
tester.route(pointer5.up());
tester.route(pointer6.up());
expect(log, <String>[
'drag1-down',
'drag2-down',
'-a',
'drag2-cancel',
'drag1-start',
'drag1-update',
'-b',
'drag1-update',
'-c',
'drag2-down',
'drag2-cancel',
'-d',
'drag1-update',
'-e',
'drag1-update',
'-f',
'drag1-update',
'-g',
'drag1-update',
'-h',
'drag1-end'
]);
});
testGesture('Drag with multiple pointers in down behavior - default', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag1 =
HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
final VerticalDragGestureRecognizer drag2 =
VerticalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag1.dispose);
addTearDown(drag2.dispose);
final List<String> log = <String>[];
drag1.onDown = (_) { log.add('drag1-down'); };
drag1.onStart = (_) { log.add('drag1-start'); };
drag1.onUpdate = (_) { log.add('drag1-update'); };
drag1.onEnd = (_) { log.add('drag1-end'); };
drag1.onCancel = () { log.add('drag1-cancel'); };
drag2.onDown = (_) { log.add('drag2-down'); };
drag2.onStart = (_) { log.add('drag2-start'); };
drag2.onUpdate = (_) { log.add('drag2-update'); };
drag2.onEnd = (_) { log.add('drag2-end'); };
drag2.onCancel = () { log.add('drag2-cancel'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(const Offset(10.0, 10.0));
drag1.addPointer(down5);
drag2.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
tester.route(pointer5.move(const Offset(100.0, 0.0)));
log.add('-b');
tester.route(pointer5.move(const Offset(50.0, 50.0)));
log.add('-c');
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(const Offset(20.0, 20.0));
drag1.addPointer(down6);
drag2.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-d');
// Current active pointer is pointer6.
// Should not trigger the drag1-update.
tester.route(pointer5.move(const Offset(0.0, 100.0)));
log.add('-e');
tester.route(pointer5.move(const Offset(70.0, 70.0)));
log.add('-f');
// The active pointer can trigger the drag1-update.
tester.route(pointer6.move(const Offset(0.0, 100.0)));
log.add('-g');
tester.route(pointer6.move(const Offset(70.0, 70.0)));
log.add('-h');
// Release the active pointer.
tester.route(pointer6.up());
log.add('-i');
// Current active pointer should be pointer5.
// The active pointer can trigger the drag1-update.
tester.route(pointer5.move(const Offset(0.0, 100.0)));
log.add('-j');
tester.route(pointer5.move(const Offset(70.0, 70.0)));
log.add('-k');
tester.route(pointer5.up());
expect(log, <String>[
'drag1-down',
'drag2-down',
'-a',
'drag2-cancel',
'drag1-start',
'drag1-update',
'-b',
'drag1-update',
'-c',
'drag2-down',
'drag2-cancel',
'-d',
'-e',
'-f',
'drag1-update',
'-g',
'drag1-update',
'-h',
'-i',
'drag1-update',
'-j',
'drag1-update',
'-k',
'drag1-end'
]);
});
testGesture('Drag with multiple pointers in down behavior - latestPointer', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag1 =
HorizontalDragGestureRecognizer()
..multitouchDragStrategy = MultitouchDragStrategy.latestPointer
..dragStartBehavior = DragStartBehavior.down;
final VerticalDragGestureRecognizer drag2 =
VerticalDragGestureRecognizer()
..multitouchDragStrategy = MultitouchDragStrategy.latestPointer
..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag1.dispose);
addTearDown(drag2.dispose);
final List<String> log = <String>[];
drag1.onDown = (_) { log.add('drag1-down'); };
drag1.onStart = (_) { log.add('drag1-start'); };
drag1.onUpdate = (_) { log.add('drag1-update'); };
drag1.onEnd = (_) { log.add('drag1-end'); };
drag1.onCancel = () { log.add('drag1-cancel'); };
drag2.onDown = (_) { log.add('drag2-down'); };
drag2.onStart = (_) { log.add('drag2-start'); };
drag2.onUpdate = (_) { log.add('drag2-update'); };
drag2.onEnd = (_) { log.add('drag2-end'); };
drag2.onCancel = () { log.add('drag2-cancel'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(const Offset(10.0, 10.0));
drag1.addPointer(down5);
drag2.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
tester.route(pointer5.move(const Offset(100.0, 0.0)));
log.add('-b');
tester.route(pointer5.move(const Offset(50.0, 50.0)));
log.add('-c');
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(const Offset(20.0, 20.0));
drag1.addPointer(down6);
drag2.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-d');
// Current active pointer is pointer6.
// Should not trigger the drag1-update.
tester.route(pointer5.move(const Offset(0.0, 100.0)));
log.add('-e');
tester.route(pointer5.move(const Offset(70.0, 70.0)));
log.add('-f');
// The active pointer can trigger the drag1-update.
tester.route(pointer6.move(const Offset(0.0, 100.0)));
log.add('-g');
tester.route(pointer6.move(const Offset(70.0, 70.0)));
log.add('-h');
final TestPointer pointer7 = TestPointer(7);
final PointerDownEvent down7 = pointer7.down(const Offset(20.0, 20.0));
drag1.addPointer(down7);
drag2.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
log.add('-i');
// Current active pointer is pointer7.
// Release the active pointer.
tester.route(pointer7.up());
log.add('-j');
// Current active pointer should be pointer5 (the first accepted pointer).
// The active pointer can trigger the drag1-update.
tester.route(pointer5.move(const Offset(0.0, 100.0)));
log.add('-k');
tester.route(pointer5.move(const Offset(70.0, 70.0)));
log.add('-l');
tester.route(pointer5.up());
tester.route(pointer6.up());
expect(log, <String>[
'drag1-down',
'drag2-down',
'-a',
'drag2-cancel',
'drag1-start',
'drag1-update',
'-b',
'drag1-update',
'-c',
'drag2-down',
'drag2-cancel',
'-d',
'-e',
'-f',
'drag1-update',
'-g',
'drag1-update',
'-h',
'drag2-down',
'drag2-cancel',
'-i',
'-j',
'drag1-update',
'-k',
'drag1-update',
'-l',
'drag1-end'
]);
});
testGesture('Horizontal drag with multiple pointers - averageBoundaryPointers', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag =
HorizontalDragGestureRecognizer()
..multitouchDragStrategy = MultitouchDragStrategy.averageBoundaryPointers;
final List<String> log = <String>[];
drag.onUpdate = (DragUpdateDetails details) { log.add('drag-update (${details.delta})'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(Offset.zero);
drag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
// #5 pointer move to right 100.0, received delta should be (100.0, 0.0).
tester.route(pointer5.move(const Offset(100.0, 0.0)));
// _moveDeltaBeforeFrame = { 5: Offset(100, 0), }
// Put down the second pointer 6.
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(Offset.zero);
drag.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-b');
// #6 pointer move to right 110.0, received delta should be (10, 0.0).
tester.route(pointer6.move(const Offset(110.0, 0.0)));
// _moveDeltaBeforeFrame = { 5: Offset(100, 0), 6: Offset(110, 0),}
// Put down the second pointer 7.
final TestPointer pointer7 = TestPointer(7);
final PointerDownEvent down7 = pointer7.down(Offset.zero);
drag.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
log.add('-c');
// #7 pointer move to left 100, received delta should be (-100.0, 0.0).
tester.route(pointer7.move(const Offset(-100.0, 0.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(100, 0),
// 6: Offset(110, 0),
// 7: Offset(-100, 0),
// }
// Put down the second pointer 8.
final TestPointer pointer8= TestPointer(8);
final PointerDownEvent down8 = pointer8.down(Offset.zero);
drag.addPointer(down8);
tester.closeArena(8);
tester.route(down8);
log.add('-d');
// #8 pointer move to left 110, received delta should be (-10, 0.0).
tester.route(pointer8.move(const Offset(-110.0, 0.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(100, 0),
// 6: Offset(110, 0),
// 7: Offset(-100, 0),
// 8: Offset(-110, 0),
// }
log.add('-e');
// #5 pointer move to right 20.0, received delta should be (10.0, 0.0).
tester.route(pointer5.move(const Offset(120.0, 0.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(120, 0),
// 6: Offset(110, 0),
// 7: Offset(-100, 0),
// 8: Offset(-110, 0),
// }
log.add('-f');
// #7 pointer move to left 20, received delta should be (-10.0, 0.0).
tester.route(pointer7.move(const Offset(-120.0, 0.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(120, 0),
// 6: Offset(110, 0),
// 7: Offset(-120, 0),
// 8: Offset(-110, 0),
// }
// Trigger a new frame.
SchedulerBinding.instance.handleBeginFrame(const Duration(milliseconds: 100));
SchedulerBinding.instance.handleDrawFrame();
// _moveDeltaBeforeFrame = { }
log.add('-g');
// #6 pointer move to right 10.0, received delta should be (10, 0.0).
tester.route(pointer6.move(const Offset(120, 0.0)));
// _moveDeltaBeforeFrame = {
// 6: Offset(10, 0),
// }
log.add('-h');
// #8 pointer move to left 10, received delta should be (-10, 0.0).
tester.route(pointer8.move(const Offset(-120, 0.0)));
// _moveDeltaBeforeFrame = {
// 6: Offset(10, 0),
// 8: Offset(-10, 0),
// }
log.add('-i');
// #5 pointer move to right 10.0, received delta should be (0.0, 0.0).
tester.route(pointer5.move(const Offset(130, 0.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(10, 0),
// 6: Offset(10, 0),
// 8: Offset(-10, 0),
// }
log.add('-j');
// #7 pointer move to left 10, received delta should be (0.0, 0.0).
tester.route(pointer7.move(const Offset(-130.0, 0.0)));
tester.route(pointer5.up());
tester.route(pointer6.up());
tester.route(pointer7.up());
tester.route(pointer8.up());
// Tear down 'currentSystemFrameTimeStamp'
SchedulerBinding.instance.handleBeginFrame(Duration.zero);
SchedulerBinding.instance.handleDrawFrame();
expect(log, <String>[
'-a',
'drag-update (Offset(100.0, 0.0))',
'-b',
'drag-update (Offset(10.0, 0.0))',
'-c',
'drag-update (Offset(-100.0, 0.0))',
'-d',
'drag-update (Offset(-10.0, 0.0))',
'-e',
'drag-update (Offset(10.0, 0.0))',
'-f',
'drag-update (Offset(-10.0, 0.0))',
'-g',
'drag-update (Offset(10.0, 0.0))',
'-h',
'drag-update (Offset(-10.0, 0.0))',
'-i',
'drag-update (Offset(0.0, 0.0))',
'-j',
'drag-update (Offset(0.0, 0.0))'
]);
});
testGesture('Vertical drag with multiple pointers - averageBoundaryPointers', (GestureTester tester) {
final VerticalDragGestureRecognizer drag =
VerticalDragGestureRecognizer()
..multitouchDragStrategy = MultitouchDragStrategy.averageBoundaryPointers;
final List<String> log = <String>[];
drag.onUpdate = (DragUpdateDetails details) { log.add('drag-update (${details.delta})'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(Offset.zero);
drag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
// #5 pointer move to down 100.0, received delta should be (0.0, 100.0).
tester.route(pointer5.move(const Offset(0.0, 100.0)));
// _moveDeltaBeforeFrame = { 5: Offset(0, 100), }
// Put down the second pointer 6.
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(Offset.zero);
drag.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-b');
// #6 pointer move to down 110.0, received delta should be (0, 10.0).
tester.route(pointer6.move(const Offset(0.0, 110.0)));
// _moveDeltaBeforeFrame = { 5: Offset(0, 100), 6: Offset(0, 110),}
// Put down the second pointer 7.
final TestPointer pointer7 = TestPointer(7);
final PointerDownEvent down7 = pointer7.down(Offset.zero);
drag.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
log.add('-c');
// #7 pointer move to up 100, received delta should be (0.0, -100.0).
tester.route(pointer7.move(const Offset(0.0, -100.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(0, 100),
// 6: Offset(0, 110),
// 7: Offset(0, -100),
// }
// Put down the second pointer 8.
final TestPointer pointer8= TestPointer(8);
final PointerDownEvent down8 = pointer8.down(Offset.zero);
drag.addPointer(down8);
tester.closeArena(8);
tester.route(down8);
log.add('-d');
// #8 pointer move to up 110, received delta should be (0, -10.0).
tester.route(pointer8.move(const Offset(0.0, -110.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(0, 100),
// 6: Offset(0, 110),
// 7: Offset(0, -100),
// 8: Offset(0, -110),
// }
log.add('-e');
// #5 pointer move to down 20.0, received delta should be (0.0, 10.0).
tester.route(pointer5.move(const Offset(0.0, 120.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(0, 120),
// 6: Offset(0, 110),
// 7: Offset(0, -100),
// 8: Offset(0, -110),
// }
log.add('-f');
// #7 pointer move to up 20, received delta should be (0.0, -10.0).
tester.route(pointer7.move(const Offset(0.0, -120.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(0, 120),
// 6: Offset(0, 110),
// 7: Offset(0, -120),
// 8: Offset(0, -110),
// }
// Trigger a new frame.
SchedulerBinding.instance.handleBeginFrame(const Duration(milliseconds: 100));
SchedulerBinding.instance.handleDrawFrame();
// _moveDeltaBeforeFrame = { }
log.add('-g');
// #6 pointer move to down 10.0, received delta should be (0, 10.0).
tester.route(pointer6.move(const Offset(0, 120.0)));
// _moveDeltaBeforeFrame = {
// 6: Offset(0, 10),
// }
log.add('-h');
// #8 pointer move to up 10, received delta should be (0, -10.0).
tester.route(pointer8.move(const Offset(0, -120.0)));
// _moveDeltaBeforeFrame = {
// 6: Offset(0, 10),
// 8: Offset(0, -10),
// }
log.add('-i');
// #5 pointer move to down 10.0, received delta should be (0.0, 0.0).
tester.route(pointer5.move(const Offset(0, 130.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(0, 10),
// 6: Offset(0, 10),
// 8: Offset(0, -10),
// }
log.add('-j');
// #7 pointer move to up 10, received delta should be (0.0, 0.0).
tester.route(pointer7.move(const Offset(0.0, -130.0)));
tester.route(pointer5.up());
tester.route(pointer6.up());
tester.route(pointer7.up());
tester.route(pointer8.up());
// Tear down 'currentSystemFrameTimeStamp'
SchedulerBinding.instance.handleBeginFrame(Duration.zero);
SchedulerBinding.instance.handleDrawFrame();
expect(log, <String>[
'-a',
'drag-update (Offset(0.0, 100.0))',
'-b',
'drag-update (Offset(0.0, 10.0))',
'-c',
'drag-update (Offset(0.0, -100.0))',
'-d',
'drag-update (Offset(0.0, -10.0))',
'-e',
'drag-update (Offset(0.0, 10.0))',
'-f',
'drag-update (Offset(0.0, -10.0))',
'-g',
'drag-update (Offset(0.0, 10.0))',
'-h',
'drag-update (Offset(0.0, -10.0))',
'-i',
'drag-update (Offset(0.0, 0.0))',
'-j',
'drag-update (Offset(0.0, 0.0))'
]);
});
testGesture('Pan drag with multiple pointers - averageBoundaryPointers', (GestureTester tester) {
final PanGestureRecognizer drag =
PanGestureRecognizer()
..multitouchDragStrategy = MultitouchDragStrategy.averageBoundaryPointers;
final List<String> log = <String>[];
drag.onUpdate = (DragUpdateDetails details) { log.add('drag-update (${details.delta})'); };
final TestPointer pointer5 = TestPointer(5);
final PointerDownEvent down5 = pointer5.down(Offset.zero);
drag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
log.add('-a');
// #5 pointer move (100.0, 100.0), received delta should be (100.0, 100.0).
// offset = 100 / 1
// delta = offset - 0 (last offset)
tester.route(pointer5.move(const Offset(100.0, 100.0)));
// _moveDeltaBeforeFrame = { 5: Offset(100, 100), }
// Put down the second pointer 6.
final TestPointer pointer6 = TestPointer(6);
final PointerDownEvent down6 = pointer6.down(Offset.zero);
drag.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
log.add('-b');
// #6 pointer move (110.0, 110.0), received delta should be (5, 5).
// offset = (100 + 110) / 2
// delta = offset - 100 (last offset)
tester.route(pointer6.move(const Offset(110.0, 110.0)));
// _moveDeltaBeforeFrame = { 5: Offset(100, 100), 6: Offset(110, 110),}
// Put down the second pointer 7.
final TestPointer pointer7 = TestPointer(7);
final PointerDownEvent down7 = pointer7.down(Offset.zero);
drag.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
log.add('-c');
// #7 pointer move (-100.0, -100.0), received delta should be (-68.3, -68.3).
// offset = (100 + 110 -100) / 3
// delta = offset - 105(last offset)
tester.route(pointer7.move(const Offset(-100.0, -100.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(100, 100),
// 6: Offset(110, 110),
// 7: Offset(-100, -100),
// }
// Put down the second pointer 8.
final TestPointer pointer8= TestPointer(8);
final PointerDownEvent down8 = pointer8.down(Offset.zero);
drag.addPointer(down8);
tester.closeArena(8);
tester.route(down8);
log.add('-d');
// #8 pointer (-110.0, -110.0), received delta should be (-36.7, -36.7).
// offset = (100 + 110 -100 - 110) / 4
// delta = offset - 36.7(last offset)
tester.route(pointer8.move(const Offset(-110.0, -110.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(100, 100),
// 6: Offset(110, 110),
// 7: Offset(-100, -100),
// 8: Offset(-110, -110),
// }
log.add('-e');
// #5 pointer move (20.0, 20.0), received delta should be (5.0, 5.0).
// offset = (100 + 110 -100 - 110 + 20) / 4
// delta = offset - 0 (last offset)
tester.route(pointer5.move(const Offset(120.0, 120.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(120, 120),
// 6: Offset(110, 110),
// 7: Offset(-100, -100),
// 8: Offset(-110, -110),
// }
log.add('-f');
// #7 pointer move (-20.0, -20.0), received delta should be (-5.0, -5.0).
// offset = (120 + 110 -100 - 110 - 20) / 4
// delta = offset - 5 (last offset)
tester.route(pointer7.move(const Offset(-120.0, -120.0)));
// _moveDeltaBeforeFrame = {
// 5: Offset(120, 120),
// 6: Offset(110, 110),
// 7: Offset(-120, -120),
// 8: Offset(-110, -110),
// }
// Trigger a new frame.
SchedulerBinding.instance.handleBeginFrame(const Duration(milliseconds: 100));
SchedulerBinding.instance.handleDrawFrame();
// _moveDeltaBeforeFrame = { }
log.add('-g');
// #6 pointer move (10.0, 10.0), received delta should be (2.5, 2.5).
// offset = 10 / 4
// delta = offset - 0 (last offset)
tester.route(pointer6.move(const Offset(120, 120)));
// _moveDeltaBeforeFrame = {
// 6: Offset(10, 10),
// }
log.add('-h');
// #8 pointer move (-10.0, -10.0), received delta should be (-2.5, -2.5).
// offset = (10 - 10) / 4
// delta = offset - 2.5 (last offset)
tester.route(pointer8.move(const Offset(-120, -120)));
// _moveDeltaBeforeFrame = {
// 6: Offset(10, 10),
// 8: Offset(-10, -10),
// }
log.add('-i');
// #5 pointer move (10.0, 10.0), received delta should be (2.5, 2.5).
// offset = (10 - 10 + 10) / 4
// delta = offset - 0 (last offset)
tester.route(pointer5.move(const Offset(130, 130)));
// _moveDeltaBeforeFrame = {
// 5: Offset(10, 10),
// 6: Offset(10, 10),
// 8: Offset(-10, -10),
// }
log.add('-j');
// #7 pointer move (-10.0, -10.0), received delta should be (-2.5, -2.5).
// offset = (10 + 10 - 10 - 10) / 4
// delta = offset - 2.5 (last offset)
tester.route(pointer7.move(const Offset(-130.0, -130.0)));
tester.route(pointer5.up());
tester.route(pointer6.up());
tester.route(pointer7.up());
tester.route(pointer8.up());
// Tear down 'currentSystemFrameTimeStamp'
SchedulerBinding.instance.handleBeginFrame(Duration.zero);
SchedulerBinding.instance.handleDrawFrame();
expect(log, <String>[
'-a',
'drag-update (Offset(100.0, 100.0))',
'-b',
'drag-update (Offset(5.0, 5.0))',
'-c',
'drag-update (Offset(-68.3, -68.3))',
'-d',
'drag-update (Offset(-36.7, -36.7))',
'-e',
'drag-update (Offset(5.0, 5.0))',
'-f',
'drag-update (Offset(-5.0, -5.0))',
'-g',
'drag-update (Offset(2.5, 2.5))',
'-h',
'drag-update (Offset(-2.5, -2.5))',
'-i',
'drag-update (Offset(2.5, 2.5))',
'-j',
'drag-update (Offset(-2.5, -2.5))'
]);
});
testGesture('Clamp max velocity', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
late Velocity velocity;
double? primaryVelocity;
drag.onEnd = (DragEndDetails details) {
velocity = details.velocity;
primaryVelocity = details.primaryVelocity;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 25.0), timeStamp: const Duration(milliseconds: 10));
drag.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 10)));
tester.route(pointer.move(const Offset(30.0, 25.0), timeStamp: const Duration(milliseconds: 11)));
tester.route(pointer.move(const Offset(40.0, 25.0), timeStamp: const Duration(milliseconds: 12)));
tester.route(pointer.move(const Offset(50.0, 25.0), timeStamp: const Duration(milliseconds: 13)));
tester.route(pointer.move(const Offset(60.0, 25.0), timeStamp: const Duration(milliseconds: 14)));
tester.route(pointer.move(const Offset(70.0, 25.0), timeStamp: const Duration(milliseconds: 15)));
tester.route(pointer.move(const Offset(80.0, 25.0), timeStamp: const Duration(milliseconds: 16)));
tester.route(pointer.move(const Offset(90.0, 25.0), timeStamp: const Duration(milliseconds: 17)));
tester.route(pointer.move(const Offset(100.0, 25.0), timeStamp: const Duration(milliseconds: 18)));
tester.route(pointer.move(const Offset(110.0, 25.0), timeStamp: const Duration(milliseconds: 19)));
tester.route(pointer.move(const Offset(120.0, 25.0), timeStamp: const Duration(milliseconds: 20)));
tester.route(pointer.up(timeStamp: const Duration(milliseconds: 20)));
expect(velocity.pixelsPerSecond.dx, inInclusiveRange(0.99 * kMaxFlingVelocity, kMaxFlingVelocity));
expect(velocity.pixelsPerSecond.dy, moreOrLessEquals(0.0));
expect(primaryVelocity, velocity.pixelsPerSecond.dx);
});
/// Drag the pointer at the given velocity, and return the details
/// the recognizer passes to onEnd.
///
/// This method will mutate `recognizer.onEnd`.
DragEndDetails performDragToEnd(GestureTester tester, DragGestureRecognizer recognizer, Offset pointerVelocity) {
late DragEndDetails actual;
recognizer.onEnd = (DragEndDetails details) {
actual = details;
};
final TestPointer pointer = TestPointer();
final PointerDownEvent down = pointer.down(Offset.zero);
recognizer.addPointer(down);
tester.closeArena(pointer.pointer);
tester.route(down);
tester.route(pointer.move(pointerVelocity * 0.025, timeStamp: const Duration(milliseconds: 25)));
tester.route(pointer.move(pointerVelocity * 0.050, timeStamp: const Duration(milliseconds: 50)));
tester.route(pointer.up(timeStamp: const Duration(milliseconds: 50)));
return actual;
}
testGesture('Clamp max pan velocity in 2D, isotropically', (GestureTester tester) {
final PanGestureRecognizer recognizer = PanGestureRecognizer();
addTearDown(recognizer.dispose);
void checkDrag(Offset pointerVelocity, Offset expectedVelocity) {
final DragEndDetails actual = performDragToEnd(tester, recognizer, pointerVelocity);
expect(actual.velocity.pixelsPerSecond, offsetMoreOrLessEquals(expectedVelocity, epsilon: 0.1));
expect(actual.primaryVelocity, isNull);
}
checkDrag(const Offset( 400.0, 400.0), const Offset( 400.0, 400.0));
checkDrag(const Offset( 2000.0, -2000.0), const Offset( 2000.0, -2000.0));
checkDrag(const Offset(-8000.0, -8000.0), const Offset(-5656.9, -5656.9));
checkDrag(const Offset(-8000.0, 6000.0), const Offset(-6400.0, 4800.0));
checkDrag(const Offset(-9000.0, 0.0), const Offset(-8000.0, 0.0));
checkDrag(const Offset(-9000.0, -1000.0), const Offset(-7951.1, - 883.5));
checkDrag(const Offset(-1000.0, 9000.0), const Offset(- 883.5, 7951.1));
checkDrag(const Offset( 0.0, 9000.0), const Offset( 0.0, 8000.0));
});
testGesture('Clamp max vertical-drag velocity vertically', (GestureTester tester) {
final VerticalDragGestureRecognizer recognizer = VerticalDragGestureRecognizer();
addTearDown(recognizer.dispose);
void checkDrag(Offset pointerVelocity, double expectedVelocity) {
final DragEndDetails actual = performDragToEnd(tester, recognizer, pointerVelocity);
expect(actual.primaryVelocity, moreOrLessEquals(expectedVelocity, epsilon: 0.1));
expect(actual.velocity.pixelsPerSecond.dx, 0.0);
expect(actual.velocity.pixelsPerSecond.dy, actual.primaryVelocity);
}
checkDrag(const Offset( 500.0, 400.0), 400.0);
checkDrag(const Offset( 3000.0, -2000.0), -2000.0);
checkDrag(const Offset(-9000.0, -9000.0), -8000.0);
checkDrag(const Offset(-9000.0, 0.0), 0.0);
checkDrag(const Offset(-9000.0, 1000.0), 1000.0);
checkDrag(const Offset(-1000.0, -9000.0), -8000.0);
checkDrag(const Offset( 0.0, -9000.0), -8000.0);
});
testGesture('Clamp max horizontal-drag velocity horizontally', (GestureTester tester) {
final HorizontalDragGestureRecognizer recognizer = HorizontalDragGestureRecognizer();
addTearDown(recognizer.dispose);
void checkDrag(Offset pointerVelocity, double expectedVelocity) {
final DragEndDetails actual = performDragToEnd(tester, recognizer, pointerVelocity);
expect(actual.primaryVelocity, moreOrLessEquals(expectedVelocity, epsilon: 0.1));
expect(actual.velocity.pixelsPerSecond.dx, actual.primaryVelocity);
expect(actual.velocity.pixelsPerSecond.dy, 0.0);
}
checkDrag(const Offset( 500.0, 400.0), 500.0);
checkDrag(const Offset( 3000.0, -2000.0), 3000.0);
checkDrag(const Offset(-9000.0, -9000.0), -8000.0);
checkDrag(const Offset(-9000.0, 0.0), -8000.0);
checkDrag(const Offset(-9000.0, 1000.0), -8000.0);
checkDrag(const Offset(-1000.0, -9000.0), -1000.0);
checkDrag(const Offset( 0.0, -9000.0), 0.0);
});
testGesture('Synthesized pointer events are ignored for velocity tracking', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
late Velocity velocity;
drag.onEnd = (DragEndDetails details) {
velocity = details.velocity;
};
final TestPointer pointer = TestPointer();
final PointerDownEvent down = pointer.down(const Offset(10.0, 25.0), timeStamp: const Duration(milliseconds: 10));
drag.addPointer(down);
tester.closeArena(1);
tester.route(down);
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 20)));
tester.route(pointer.move(const Offset(30.0, 25.0), timeStamp: const Duration(milliseconds: 30)));
tester.route(pointer.move(const Offset(40.0, 25.0), timeStamp: const Duration(milliseconds: 40)));
tester.route(pointer.move(const Offset(50.0, 25.0), timeStamp: const Duration(milliseconds: 50)));
tester.route(const PointerMoveEvent(
pointer: 1,
// Simulate a small synthesized wobble which would have slowed down the
// horizontal velocity from 1 px/ms and introduced a slight vertical velocity.
position: Offset(51.0, 26.0),
timeStamp: Duration(milliseconds: 60),
synthesized: true,
));
tester.route(pointer.up(timeStamp: const Duration(milliseconds: 70)));
expect(velocity.pixelsPerSecond.dx, moreOrLessEquals(1000.0));
expect(velocity.pixelsPerSecond.dy, moreOrLessEquals(0.0));
});
/// Checks that quick flick gestures with 1 down, 2 move and 1 up pointer
/// events still have a velocity
testGesture('Quick flicks have velocity', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
late Velocity velocity;
drag.onEnd = (DragEndDetails details) {
velocity = details.velocity;
};
final TestPointer pointer = TestPointer();
final PointerDownEvent down = pointer.down(const Offset(10.0, 25.0), timeStamp: const Duration(milliseconds: 10));
drag.addPointer(down);
tester.closeArena(1);
tester.route(down);
tester.route(pointer.move(const Offset(20.0, 25.0), timeStamp: const Duration(milliseconds: 20)));
tester.route(pointer.move(const Offset(30.0, 25.0), timeStamp: const Duration(milliseconds: 30)));
tester.route(pointer.up(timeStamp: const Duration(milliseconds: 40)));
// 3 events moving by 10px every 10ms = 1000px/s.
expect(velocity.pixelsPerSecond.dx, moreOrLessEquals(1000.0));
expect(velocity.pixelsPerSecond.dy, moreOrLessEquals(0.0));
});
testGesture('Drag details', (GestureTester tester) {
expect(DragDownDetails(), hasOneLineDescription);
expect(DragStartDetails(), hasOneLineDescription);
expect(DragUpdateDetails(globalPosition: Offset.zero), hasOneLineDescription);
expect(DragEndDetails(), hasOneLineDescription);
});
testGesture('Should recognize drag', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
bool didStartDrag = false;
drag.onStart = (_) {
didStartDrag = true;
};
Offset? updateDelta;
double? updatePrimaryDelta;
drag.onUpdate = (DragUpdateDetails details) {
updateDelta = details.delta;
updatePrimaryDelta = details.primaryDelta;
};
bool didEndDrag = false;
drag.onEnd = (DragEndDetails details) {
didEndDrag = true;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updateDelta, isNull);
expect(updatePrimaryDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(down);
expect(didStartDrag, isTrue);
expect(updateDelta, isNull);
expect(updatePrimaryDelta, isNull);
expect(didEndDrag, isFalse);
didStartDrag = false;
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updateDelta, const Offset(10.0, 0.0));
expect(updatePrimaryDelta, 10.0);
expect(didEndDrag, isFalse);
updateDelta = null;
updatePrimaryDelta = null;
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updateDelta, Offset.zero);
expect(updatePrimaryDelta, 0.0);
expect(didEndDrag, isFalse);
updateDelta = null;
updatePrimaryDelta = null;
tester.route(pointer.up());
expect(didStartDrag, isFalse);
expect(updateDelta, isNull);
expect(updatePrimaryDelta, isNull);
expect(didEndDrag, isTrue);
didEndDrag = false;
});
testGesture('Should recognize drag', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer() ..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
Offset? latestGlobalPosition;
drag.onStart = (DragStartDetails details) {
latestGlobalPosition = details.globalPosition;
};
Offset? latestDelta;
drag.onUpdate = (DragUpdateDetails details) {
latestGlobalPosition = details.globalPosition;
latestDelta = details.delta;
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
tester.route(down);
expect(latestGlobalPosition, const Offset(10.0, 10.0));
expect(latestDelta, isNull);
tester.route(pointer.move(const Offset(20.0, 25.0)));
expect(latestGlobalPosition, const Offset(20.0, 25.0));
expect(latestDelta, const Offset(10.0, 0.0));
tester.route(pointer.move(const Offset(0.0, 45.0)));
expect(latestGlobalPosition, const Offset(0.0, 45.0));
expect(latestDelta, const Offset(-20.0, 0.0));
tester.route(pointer.up());
});
testGesture('Can filter drags based on device kind', (GestureTester tester) {
final HorizontalDragGestureRecognizer drag =
HorizontalDragGestureRecognizer(
supportedDevices: <PointerDeviceKind>{ PointerDeviceKind.mouse },
)
..dragStartBehavior = DragStartBehavior.down;
addTearDown(drag.dispose);
bool didStartDrag = false;
drag.onStart = (_) {
didStartDrag = true;
};
double? updatedDelta;
drag.onUpdate = (DragUpdateDetails details) {
updatedDelta = details.primaryDelta;
};
bool didEndDrag = false;
drag.onEnd = (DragEndDetails details) {
didEndDrag = true;
};
// Using a touch pointer to drag shouldn't be recognized.
final TestPointer touchPointer = TestPointer(5);
final PointerDownEvent touchDown = touchPointer.down(const Offset(10.0, 10.0));
drag.addPointer(touchDown);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(touchDown);
// Still doesn't recognize the drag because it's coming from a touch pointer.
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(touchPointer.move(const Offset(20.0, 25.0)));
// Still doesn't recognize the drag because it's coming from a touch pointer.
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(touchPointer.up());
// Still doesn't recognize the drag because it's coming from a touch pointer.
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
// Using a mouse pointer to drag should be recognized.
final TestPointer mousePointer = TestPointer(5, PointerDeviceKind.mouse);
final PointerDownEvent mouseDown = mousePointer.down(const Offset(10.0, 10.0));
drag.addPointer(mouseDown);
tester.closeArena(5);
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(mouseDown);
expect(didStartDrag, isTrue);
didStartDrag = false;
expect(updatedDelta, isNull);
expect(didEndDrag, isFalse);
tester.route(mousePointer.move(const Offset(20.0, 25.0)));
expect(didStartDrag, isFalse);
expect(updatedDelta, 10.0);
updatedDelta = null;
expect(didEndDrag, isFalse);
tester.route(mousePointer.up());
expect(didStartDrag, isFalse);
expect(updatedDelta, isNull);
expect(didEndDrag, isTrue);
didEndDrag = false;
});
group('Enforce consistent-button restriction:', () {
late PanGestureRecognizer pan;
late TapGestureRecognizer tap;
final List<String> logs = <String>[];
setUp(() {
tap = TapGestureRecognizer()
..onTap = () {}; // Need a callback to enable competition
pan = PanGestureRecognizer()
..onStart = (DragStartDetails details) {
logs.add('start');
}
..onDown = (DragDownDetails details) {
logs.add('down');
}
..onUpdate = (DragUpdateDetails details) {
logs.add('update');
}
..onCancel = () {
logs.add('cancel');
}
..onEnd = (DragEndDetails details) {
logs.add('end');
};
});
tearDown(() {
pan.dispose();
tap.dispose();
logs.clear();
});
testGesture('Button change before acceptance should lead to immediate cancel', (GestureTester tester) {
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(5);
tester.route(down);
expect(logs, <String>['down']);
// Move out of slop so make sure button changes takes priority over slops
tester.route(pointer.move(const Offset(30.0, 30.0), buttons: kSecondaryButton));
expect(logs, <String>['down', 'cancel']);
tester.route(pointer.up());
});
testGesture('Button change before acceptance should not prevent the next drag', (GestureTester tester) {
{ // First drag (which is canceled)
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(down.pointer);
tester.route(down);
tester.route(pointer.move(const Offset(10.0, 10.0), buttons: kSecondaryButton));
tester.route(pointer.up());
expect(logs, <String>['down', 'cancel']);
}
logs.clear();
final TestPointer pointer2 = TestPointer(6, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down2 = pointer2.down(const Offset(10.0, 10.0));
pan.addPointer(down2);
tap.addPointer(down2);
tester.closeArena(down2.pointer);
tester.route(down2);
expect(logs, <String>['down']);
tester.route(pointer2.move(const Offset(30.0, 30.0)));
expect(logs, <String>['down', 'start']);
tester.route(pointer2.up());
expect(logs, <String>['down', 'start', 'end']);
});
testGesture('Button change after acceptance should lead to immediate end', (GestureTester tester) {
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(down.pointer);
tester.route(down);
expect(logs, <String>['down']);
tester.route(pointer.move(const Offset(30.0, 30.0)));
expect(logs, <String>['down', 'start']);
tester.route(pointer.move(const Offset(30.0, 30.0), buttons: kSecondaryButton));
expect(logs, <String>['down', 'start', 'end']);
// Make sure no further updates are sent
tester.route(pointer.move(const Offset(50.0, 50.0)));
expect(logs, <String>['down', 'start', 'end']);
tester.route(pointer.up());
});
testGesture('Button change after acceptance should not prevent the next drag', (GestureTester tester) {
{ // First drag (which is canceled)
final TestPointer pointer = TestPointer(5, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(down.pointer);
tester.route(down);
tester.route(pointer.move(const Offset(30.0, 30.0)));
tester.route(pointer.move(const Offset(30.0, 31.0), buttons: kSecondaryButton));
tester.route(pointer.up());
expect(logs, <String>['down', 'start', 'end']);
}
logs.clear();
final TestPointer pointer2 = TestPointer(6, PointerDeviceKind.mouse, kPrimaryButton);
final PointerDownEvent down2 = pointer2.down(const Offset(10.0, 10.0));
pan.addPointer(down2);
tap.addPointer(down2);
tester.closeArena(down2.pointer);
tester.route(down2);
expect(logs, <String>['down']);
tester.route(pointer2.move(const Offset(30.0, 30.0)));
expect(logs, <String>['down', 'start']);
tester.route(pointer2.up());
expect(logs, <String>['down', 'start', 'end']);
});
});
group('Recognizers listening on different buttons do not form competition:', () {
// This test is assisted by tap recognizers. If a tap gesture has
// no competing recognizers, a pointer down event triggers its onTapDown
// immediately; if there are competitors, onTapDown is triggered after a
// timeout.
// The following tests make sure that drag recognizers do not form
// competition with a tap gesture recognizer listening on a different button.
final List<String> recognized = <String>[];
late TapGestureRecognizer tapPrimary;
late TapGestureRecognizer tapSecondary;
late PanGestureRecognizer pan;
setUp(() {
tapPrimary = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
recognized.add('tapPrimary');
};
tapSecondary = TapGestureRecognizer()
..onSecondaryTapDown = (TapDownDetails details) {
recognized.add('tapSecondary');
};
pan = PanGestureRecognizer()
..onStart = (_) {
recognized.add('drag');
};
});
tearDown(() {
recognized.clear();
tapPrimary.dispose();
tapSecondary.dispose();
pan.dispose();
});
testGesture('A primary pan recognizer does not form competition with a secondary tap recognizer', (GestureTester tester) {
final TestPointer pointer = TestPointer(
1,
PointerDeviceKind.touch,
0,
kSecondaryButton,
);
final PointerDownEvent down = pointer.down(const Offset(10, 10));
pan.addPointer(down);
tapSecondary.addPointer(down);
tester.closeArena(down.pointer);
tester.route(down);
expect(recognized, <String>['tapSecondary']);
});
testGesture('A primary pan recognizer forms competition with a primary tap recognizer', (GestureTester tester) {
final TestPointer pointer = TestPointer(
1,
PointerDeviceKind.touch,
kPrimaryButton,
);
final PointerDownEvent down = pointer.down(const Offset(10, 10));
pan.addPointer(down);
tapPrimary.addPointer(down);
tester.closeArena(down.pointer);
tester.route(down);
expect(recognized, <String>[]);
tester.route(pointer.up());
expect(recognized, <String>['tapPrimary']);
});
});
testGesture('A secondary drag should not trigger primary', (GestureTester tester) {
final List<String> recognized = <String>[];
final TapGestureRecognizer tap = TapGestureRecognizer()
..onTap = () {}; // Need a listener to enable competition.
final PanGestureRecognizer pan = PanGestureRecognizer()
..onDown = (DragDownDetails details) {
recognized.add('primaryDown');
}
..onStart = (DragStartDetails details) {
recognized.add('primaryStart');
}
..onUpdate = (DragUpdateDetails details) {
recognized.add('primaryUpdate');
}
..onEnd = (DragEndDetails details) {
recognized.add('primaryEnd');
}
..onCancel = () {
recognized.add('primaryCancel');
};
addTearDown(pan.dispose);
addTearDown(tap.dispose);
final TestPointer pointer = TestPointer(
5,
PointerDeviceKind.touch,
0,
kSecondaryButton,
);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(20.0, 30.0)));
tester.route(pointer.move(const Offset(20.0, 25.0)));
tester.route(pointer.up());
expect(recognized, <String>[]);
recognized.clear();
});
testGesture('A secondary drag should not trigger primary', (GestureTester tester) {
final List<String> recognized = <String>[];
final TapGestureRecognizer tap = TapGestureRecognizer()
..onTap = () {}; // Need a listener to enable competition.
final PanGestureRecognizer pan = PanGestureRecognizer()
..onDown = (DragDownDetails details) {
recognized.add('primaryDown');
}
..onStart = (DragStartDetails details) {
recognized.add('primaryStart');
}
..onUpdate = (DragUpdateDetails details) {
recognized.add('primaryUpdate');
}
..onEnd = (DragEndDetails details) {
recognized.add('primaryEnd');
}
..onCancel = () {
recognized.add('primaryCancel');
};
final TestPointer pointer = TestPointer(
5,
PointerDeviceKind.touch,
0,
kSecondaryButton,
);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
pan.addPointer(down);
tap.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(20.0, 30.0)));
tester.route(pointer.move(const Offset(20.0, 25.0)));
tester.route(pointer.up());
expect(recognized, <String>[]);
recognized.clear();
addTearDown(pan.dispose);
addTearDown(tap.dispose);
recognized.clear();
});
testGesture(
'On multiple pointers, DragGestureRecognizer is canceled '
'when all pointers are canceled (FIFO)',
(GestureTester tester) {
// This test simulates the following scenario:
// P1 down, P2 down, P1 up, P2 up
final List<String> logs = <String>[];
final HorizontalDragGestureRecognizer hori = HorizontalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downH'); }
..onStart = (DragStartDetails details) { logs.add('startH'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateH'); }
..onEnd = (DragEndDetails details) { logs.add('endH'); }
..onCancel = () { logs.add('cancelH'); };
// Competitor
final TapGestureRecognizer vert = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(hori.dispose);
addTearDown(vert.dispose);
final TestPointer pointer1 = TestPointer(4);
final TestPointer pointer2 = TestPointer(5);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 10.0));
hori.addPointer(down1);
vert.addPointer(down1);
tester.route(down1);
tester.closeArena(pointer1.pointer);
expect(logs, <String>['downH']);
logs.clear();
hori.addPointer(down2);
vert.addPointer(down2);
tester.route(down2);
tester.closeArena(pointer2.pointer);
expect(logs, <String>[]);
logs.clear();
tester.route(pointer1.up());
GestureBinding.instance.gestureArena.sweep(pointer1.pointer);
expect(logs, <String>['downT', 'upT']);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
expect(logs, <String>['cancelH']);
logs.clear();
},
);
testGesture(
'On multiple pointers, DragGestureRecognizer is canceled '
'when all pointers are canceled (FILO)',
(GestureTester tester) {
// This test simulates the following scenario:
// P1 down, P2 down, P1 up, P2 up
final List<String> logs = <String>[];
final HorizontalDragGestureRecognizer hori = HorizontalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downH'); }
..onStart = (DragStartDetails details) { logs.add('startH'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateH'); }
..onEnd = (DragEndDetails details) { logs.add('endH'); }
..onCancel = () { logs.add('cancelH'); };
// Competitor
final TapGestureRecognizer vert = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(hori.dispose);
addTearDown(vert.dispose);
final TestPointer pointer1 = TestPointer(4);
final TestPointer pointer2 = TestPointer(5);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 10.0));
hori.addPointer(down1);
vert.addPointer(down1);
tester.route(down1);
tester.closeArena(pointer1.pointer);
expect(logs, <String>['downH']);
logs.clear();
hori.addPointer(down2);
vert.addPointer(down2);
tester.route(down2);
tester.closeArena(pointer2.pointer);
expect(logs, <String>[]);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
// Tap is not triggered because pointer2 is not its primary pointer
expect(logs, <String>[]);
logs.clear();
tester.route(pointer1.up());
GestureBinding.instance.gestureArena.sweep(pointer1.pointer);
expect(logs, <String>['cancelH', 'downT', 'upT']);
logs.clear();
},
);
testGesture(
'On multiple pointers, DragGestureRecognizer is accepted when the '
'first pointer is accepted',
(GestureTester tester) {
// This test simulates the following scenario:
// P1 down, P2 down, P1 moves away, P2 up
final List<String> logs = <String>[];
final HorizontalDragGestureRecognizer hori = HorizontalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downH'); }
..onStart = (DragStartDetails details) { logs.add('startH'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateH'); }
..onEnd = (DragEndDetails details) { logs.add('endH'); }
..onCancel = () { logs.add('cancelH'); };
// Competitor
final TapGestureRecognizer vert = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(hori.dispose);
addTearDown(vert.dispose);
final TestPointer pointer1 = TestPointer(4);
final TestPointer pointer2 = TestPointer(5);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 10.0));
hori.addPointer(down1);
vert.addPointer(down1);
tester.route(down1);
tester.closeArena(pointer1.pointer);
expect(logs, <String>['downH']);
logs.clear();
hori.addPointer(down2);
vert.addPointer(down2);
tester.route(down2);
tester.closeArena(pointer2.pointer);
expect(logs, <String>[]);
logs.clear();
tester.route(pointer1.move(const Offset(100, 100)));
expect(logs, <String>['startH']);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
expect(logs, <String>[]);
logs.clear();
tester.route(pointer1.up());
GestureBinding.instance.gestureArena.sweep(pointer1.pointer);
expect(logs, <String>['endH']);
logs.clear();
},
);
testGesture(
'On multiple pointers, canceled pointers (due to up) do not '
'prevent later pointers getting accepted',
(GestureTester tester) {
// This test simulates the following scenario:
// P1 down, P2 down, P1 Up, P2 moves away
final List<String> logs = <String>[];
final HorizontalDragGestureRecognizer hori = HorizontalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downH'); }
..onStart = (DragStartDetails details) { logs.add('startH'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateH'); }
..onEnd = (DragEndDetails details) { logs.add('endH'); }
..onCancel = () { logs.add('cancelH'); };
// Competitor
final TapGestureRecognizer vert = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(hori.dispose);
addTearDown(vert.dispose);
final TestPointer pointer1 = TestPointer(4);
final TestPointer pointer2 = TestPointer(5);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 10.0));
hori.addPointer(down1);
vert.addPointer(down1);
tester.route(down1);
tester.closeArena(pointer1.pointer);
expect(logs, <String>['downH']);
logs.clear();
hori.addPointer(down2);
vert.addPointer(down2);
tester.route(down2);
tester.closeArena(pointer2.pointer);
expect(logs, <String>[]);
logs.clear();
tester.route(pointer1.up());
GestureBinding.instance.gestureArena.sweep(pointer1.pointer);
expect(logs, <String>['downT', 'upT']);
logs.clear();
tester.route(pointer2.move(const Offset(100, 100)));
expect(logs, <String>['startH']);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
expect(logs, <String>['endH']);
logs.clear();
},
);
testGesture(
'On multiple pointers, canceled pointers (due to buttons) do not '
'prevent later pointers getting accepted',
(GestureTester tester) {
// This test simulates the following scenario:
// P1 down, P2 down, P1 change buttons, P2 moves away
final List<String> logs = <String>[];
final HorizontalDragGestureRecognizer hori = HorizontalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downH'); }
..onStart = (DragStartDetails details) { logs.add('startH'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateH'); }
..onEnd = (DragEndDetails details) { logs.add('endH'); }
..onCancel = () { logs.add('cancelH'); };
// Competitor
final TapGestureRecognizer vert = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(hori.dispose);
addTearDown(vert.dispose);
final TestPointer pointer1 = TestPointer();
final TestPointer pointer2 = TestPointer(2);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 10.0));
hori.addPointer(down1);
vert.addPointer(down1);
tester.route(down1);
tester.closeArena(pointer1.pointer);
hori.addPointer(down2);
vert.addPointer(down2);
tester.route(down2);
tester.closeArena(pointer2.pointer);
expect(logs, <String>['downH']);
logs.clear();
// Pointer 1 changes buttons, which cancel tap, leaving drag the only
// remaining member of arena 1, therefore drag is accepted.
tester.route(pointer1.move(const Offset(9.9, 9.9), buttons: kSecondaryButton));
expect(logs, <String>['startH']);
logs.clear();
tester.route(pointer2.move(const Offset(100, 100)));
expect(logs, <String>['updateH']);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
expect(logs, <String>['endH']);
logs.clear();
},
);
testGesture(
'On multiple pointers, the last tracking pointer can be rejected by [resolvePointer] when the '
'other pointer already accepted the VerticalDragGestureRecognizer',
(GestureTester tester) {
// Regressing test for https://github.com/flutter/flutter/issues/68373
final List<String> logs = <String>[];
final VerticalDragGestureRecognizer drag = VerticalDragGestureRecognizer()
..onDown = (DragDownDetails details) { logs.add('downD'); }
..onStart = (DragStartDetails details) { logs.add('startD'); }
..onUpdate = (DragUpdateDetails details) { logs.add('updateD'); }
..onEnd = (DragEndDetails details) { logs.add('endD'); }
..onCancel = () { logs.add('cancelD'); };
// Competitor
final TapGestureRecognizer tap = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) { logs.add('downT'); }
..onTapUp = (TapUpDetails details) { logs.add('upT'); }
..onTapCancel = () {};
addTearDown(tap.dispose);
addTearDown(drag.dispose);
final TestPointer pointer1 = TestPointer();
final TestPointer pointer2 = TestPointer(2);
final TestPointer pointer3 = TestPointer(3);
final TestPointer pointer4 = TestPointer(4);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
final PointerDownEvent down2 = pointer2.down(const Offset(11.0, 11.0));
final PointerDownEvent down3 = pointer3.down(const Offset(12.0, 12.0));
final PointerDownEvent down4 = pointer4.down(const Offset(13.0, 13.0));
tap.addPointer(down1);
drag.addPointer(down1);
tester.closeArena(pointer1.pointer);
tester.route(down1);
expect(logs, <String>['downD']);
logs.clear();
tap.addPointer(down2);
drag.addPointer(down2);
tester.closeArena(pointer2.pointer);
tester.route(down2);
expect(logs, <String>[]);
tap.addPointer(down3);
drag.addPointer(down3);
tester.closeArena(pointer3.pointer);
tester.route(down3);
expect(logs, <String>[]);
drag.addPointer(down4);
tester.closeArena(pointer4.pointer);
tester.route(down4);
expect(logs, <String>['startD']);
logs.clear();
tester.route(pointer2.up());
GestureBinding.instance.gestureArena.sweep(pointer2.pointer);
expect(logs, <String>[]);
tester.route(pointer4.cancel());
expect(logs, <String>[]);
tester.route(pointer3.cancel());
expect(logs, <String>[]);
tester.route(pointer1.cancel());
expect(logs, <String>['endD']);
logs.clear();
},
);
testGesture('Does not crash when one of the 2 pointers wins by default and is then released', (GestureTester tester) {
// Regression test for https://github.com/flutter/flutter/issues/82784
bool didStartDrag = false;
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer()
..onStart = (_) { didStartDrag = true; }
..onEnd = (DragEndDetails details) {} // Crash triggers at onEnd.
..dragStartBehavior = DragStartBehavior.down;
final TapGestureRecognizer tap = TapGestureRecognizer()..onTap = () {};
final TapGestureRecognizer tap2 = TapGestureRecognizer()..onTap = () {};
// The pointer1 is caught by drag and tap.
final TestPointer pointer1 = TestPointer(5);
final PointerDownEvent down1 = pointer1.down(const Offset(10.0, 10.0));
drag.addPointer(down1);
tap.addPointer(down1);
tester.closeArena(pointer1.pointer);
tester.route(down1);
// The pointer2 is caught by drag and tap2.
final TestPointer pointer2 = TestPointer(6);
final PointerDownEvent down2 = pointer2.down(const Offset(10.0, 10.0));
drag.addPointer(down2);
tap2.addPointer(down2);
tester.closeArena(pointer2.pointer);
tester.route(down2);
// The tap is disposed, leaving drag the default winner.
tap.dispose();
// Wait for microtasks to finish, during which drag claims victory.
tester.async.flushMicrotasks();
expect(didStartDrag, true);
// The pointer1 is released, leaving pointer2 drag's only pointer.
tester.route(pointer1.up());
drag.dispose();
// Passes if no crashes here.
tap2.dispose();
});
testGesture('Should recognize pan gestures from platform', (GestureTester tester) {
final PanGestureRecognizer pan = PanGestureRecognizer();
// We need a competing gesture recognizer so that the gesture is not immediately claimed.
final PanGestureRecognizer competingPan = PanGestureRecognizer();
addTearDown(pan.dispose);
addTearDown(competingPan.dispose);
bool didStartPan = false;
pan.onStart = (_) {
didStartPan = true;
};
Offset? updatedScrollDelta;
pan.onUpdate = (DragUpdateDetails details) {
updatedScrollDelta = details.delta;
};
bool didEndPan = false;
pan.onEnd = (DragEndDetails details) {
didEndPan = true;
};
final TestPointer pointer = TestPointer(2, PointerDeviceKind.trackpad);
final PointerPanZoomStartEvent start = pointer.panZoomStart(const Offset(10.0, 10.0));
pan.addPointerPanZoom(start);
competingPan.addPointerPanZoom(start);
tester.closeArena(2);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(start);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
// Gesture will be claimed when distance reaches kPanSlop, which was 36.0 when this test was last updated.
tester.route(pointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(20.0, 20.0))); // moved 20 horizontally and 20 vertically which is 28 total
expect(didStartPan, isFalse); // 28 < 36
tester.route(pointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(30.0, 30.0))); // moved 30 horizontally and 30 vertically which is 42 total
expect(didStartPan, isTrue); // 42 > 36
didStartPan = false;
expect(didEndPan, isFalse);
tester.route(pointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(30.0, 25.0)));
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(0.0, -5.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
tester.route(pointer.panZoomEnd());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isTrue);
didEndPan = false;
});
testGesture('Pointer pan/zooms drags should allow touches to join them', (GestureTester tester) {
final PanGestureRecognizer pan = PanGestureRecognizer();
// We need a competing gesture recognizer so that the gesture is not immediately claimed.
final PanGestureRecognizer competingPan = PanGestureRecognizer();
addTearDown(pan.dispose);
addTearDown(competingPan.dispose);
bool didStartPan = false;
pan.onStart = (_) {
didStartPan = true;
};
Offset? updatedScrollDelta;
pan.onUpdate = (DragUpdateDetails details) {
updatedScrollDelta = details.delta;
};
bool didEndPan = false;
pan.onEnd = (DragEndDetails details) {
didEndPan = true;
};
final TestPointer panZoomPointer = TestPointer(2, PointerDeviceKind.trackpad);
final TestPointer touchPointer = TestPointer(3);
final PointerPanZoomStartEvent start = panZoomPointer.panZoomStart(const Offset(10.0, 10.0));
pan.addPointerPanZoom(start);
competingPan.addPointerPanZoom(start);
tester.closeArena(2);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(start);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
// Gesture will be claimed when distance reaches kPanSlop, which was 36.0 when this test was last updated.
tester.route(panZoomPointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(20.0, 20.0))); // moved 20 horizontally and 20 vertically which is 28 total
expect(didStartPan, isFalse); // 28 < 36
tester.route(panZoomPointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(30.0, 30.0))); // moved 30 horizontally and 30 vertically which is 42 total
expect(didStartPan, isTrue); // 42 > 36
didStartPan = false;
expect(didEndPan, isFalse);
tester.route(panZoomPointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(30.0, 25.0)));
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(0.0, -5.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
final PointerDownEvent touchDown = touchPointer.down(const Offset(20.0, 20.0));
pan.addPointer(touchDown);
competingPan.addPointer(touchDown);
tester.closeArena(3);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(touchDown);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(touchPointer.move(const Offset(25.0, 25.0)));
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(5.0, 5.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
tester.route(touchPointer.up());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(panZoomPointer.panZoomEnd());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isTrue);
didEndPan = false;
});
testGesture('Touch drags should allow pointer pan/zooms to join them', (GestureTester tester) {
final PanGestureRecognizer pan = PanGestureRecognizer();
// We need a competing gesture recognizer so that the gesture is not immediately claimed.
final PanGestureRecognizer competingPan = PanGestureRecognizer();
addTearDown(pan.dispose);
addTearDown(competingPan.dispose);
bool didStartPan = false;
pan.onStart = (_) {
didStartPan = true;
};
Offset? updatedScrollDelta;
pan.onUpdate = (DragUpdateDetails details) {
updatedScrollDelta = details.delta;
};
bool didEndPan = false;
pan.onEnd = (DragEndDetails details) {
didEndPan = true;
};
final TestPointer panZoomPointer = TestPointer(2, PointerDeviceKind.trackpad);
final TestPointer touchPointer = TestPointer(3);
final PointerDownEvent touchDown = touchPointer.down(const Offset(20.0, 20.0));
pan.addPointer(touchDown);
competingPan.addPointer(touchDown);
tester.closeArena(3);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(touchPointer.move(const Offset(60.0, 60.0)));
expect(didStartPan, isTrue);
didStartPan = false;
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(touchPointer.move(const Offset(70.0, 70.0)));
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(10.0, 10.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
final PointerPanZoomStartEvent start = panZoomPointer.panZoomStart(const Offset(10.0, 10.0));
pan.addPointerPanZoom(start);
competingPan.addPointerPanZoom(start);
tester.closeArena(2);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(start);
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
// Gesture will be claimed when distance reaches kPanSlop, which was 36.0 when this test was last updated.
tester.route(panZoomPointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(20.0, 20.0))); // moved 20 horizontally and 20 vertically which is 28 total
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(20.0, 20.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
tester.route(panZoomPointer.panZoomUpdate(const Offset(10.0, 10.0), pan: const Offset(30.0, 30.0))); // moved 30 horizontally and 30 vertically which is 42 total
expect(didStartPan, isFalse);
expect(updatedScrollDelta, const Offset(10.0, 10.0));
updatedScrollDelta = null;
expect(didEndPan, isFalse);
tester.route(panZoomPointer.panZoomEnd());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isFalse);
tester.route(touchPointer.up());
expect(didStartPan, isFalse);
expect(updatedScrollDelta, isNull);
expect(didEndPan, isTrue);
didEndPan = false;
});
}
| flutter/packages/flutter/test/gestures/drag_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/drag_test.dart",
"repo_id": "flutter",
"token_count": 35757
} | 685 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.