text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import '../../data/gallery_options.dart';
import '../../gallery_localizations.dart';
// BEGIN dataTableDemo
class DataTableDemo extends StatefulWidget {
const DataTableDemo({super.key});
@override
State<DataTableDemo> createState() => _DataTableDemoState();
}
class _RestorableDessertSelections extends RestorableProperty<Set<int>> {
Set<int> _dessertSelections = <int>{};
/// Returns whether or not a dessert row is selected by index.
bool isSelected(int index) => _dessertSelections.contains(index);
/// Takes a list of [_Dessert]s and saves the row indices of selected rows
/// into a [Set].
void setDessertSelections(List<_Dessert> desserts) {
final Set<int> updatedSet = <int>{};
for (int i = 0; i < desserts.length; i += 1) {
final _Dessert dessert = desserts[i];
if (dessert.selected) {
updatedSet.add(i);
}
}
_dessertSelections = updatedSet;
notifyListeners();
}
@override
Set<int> createDefaultValue() => _dessertSelections;
@override
Set<int> fromPrimitives(Object? data) {
final List<dynamic> selectedItemIndices = data! as List<dynamic>;
_dessertSelections = <int>{
...selectedItemIndices.map<int>((dynamic id) => id as int),
};
return _dessertSelections;
}
@override
void initWithValue(Set<int> value) {
_dessertSelections = value;
}
@override
Object toPrimitives() => _dessertSelections.toList();
}
class _DataTableDemoState extends State<DataTableDemo> with RestorationMixin {
final _RestorableDessertSelections _dessertSelections =
_RestorableDessertSelections();
final RestorableInt _rowIndex = RestorableInt(0);
final RestorableInt _rowsPerPage =
RestorableInt(PaginatedDataTable.defaultRowsPerPage);
final RestorableBool _sortAscending = RestorableBool(true);
final RestorableIntN _sortColumnIndex = RestorableIntN(null);
_DessertDataSource? _dessertsDataSource;
@override
String get restorationId => 'data_table_demo';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_dessertSelections, 'selected_row_indices');
registerForRestoration(_rowIndex, 'current_row_index');
registerForRestoration(_rowsPerPage, 'rows_per_page');
registerForRestoration(_sortAscending, 'sort_ascending');
registerForRestoration(_sortColumnIndex, 'sort_column_index');
_dessertsDataSource ??= _DessertDataSource(context);
switch (_sortColumnIndex.value) {
case 0:
_dessertsDataSource!._sort<String>((_Dessert d) => d.name, _sortAscending.value);
case 1:
_dessertsDataSource!
._sort<num>((_Dessert d) => d.calories, _sortAscending.value);
case 2:
_dessertsDataSource!._sort<num>((_Dessert d) => d.fat, _sortAscending.value);
case 3:
_dessertsDataSource!._sort<num>((_Dessert d) => d.carbs, _sortAscending.value);
case 4:
_dessertsDataSource!._sort<num>((_Dessert d) => d.protein, _sortAscending.value);
case 5:
_dessertsDataSource!._sort<num>((_Dessert d) => d.sodium, _sortAscending.value);
case 6:
_dessertsDataSource!._sort<num>((_Dessert d) => d.calcium, _sortAscending.value);
case 7:
_dessertsDataSource!._sort<num>((_Dessert d) => d.iron, _sortAscending.value);
}
_dessertsDataSource!.updateSelectedDesserts(_dessertSelections);
_dessertsDataSource!.addListener(_updateSelectedDessertRowListener);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_dessertsDataSource ??= _DessertDataSource(context);
_dessertsDataSource!.addListener(_updateSelectedDessertRowListener);
}
void _updateSelectedDessertRowListener() {
_dessertSelections.setDessertSelections(_dessertsDataSource!._desserts);
}
void _sort<T>(
Comparable<T> Function(_Dessert d) getField,
int columnIndex,
bool ascending,
) {
_dessertsDataSource!._sort<T>(getField, ascending);
setState(() {
_sortColumnIndex.value = columnIndex;
_sortAscending.value = ascending;
});
}
@override
void dispose() {
_rowsPerPage.dispose();
_sortColumnIndex.dispose();
_sortAscending.dispose();
_dessertsDataSource!.removeListener(_updateSelectedDessertRowListener);
_dessertsDataSource!.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: Text(localizations.demoDataTableTitle),
),
body: Scrollbar(
child: ListView(
restorationId: 'data_table_list_view',
padding: const EdgeInsets.all(16),
children: <Widget>[
PaginatedDataTable(
header: Text(localizations.dataTableHeader),
rowsPerPage: _rowsPerPage.value,
onRowsPerPageChanged: (int? value) {
setState(() {
_rowsPerPage.value = value!;
});
},
initialFirstRowIndex: _rowIndex.value,
onPageChanged: (int rowIndex) {
setState(() {
_rowIndex.value = rowIndex;
});
},
sortColumnIndex: _sortColumnIndex.value,
sortAscending: _sortAscending.value,
onSelectAll: _dessertsDataSource!._selectAll,
columns: <DataColumn>[
DataColumn(
label: Text(localizations.dataTableColumnDessert),
onSort: (int columnIndex, bool ascending) =>
_sort<String>((_Dessert d) => d.name, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnCalories),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.calories, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnFat),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.fat, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnCarbs),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.carbs, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnProtein),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.protein, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnSodium),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.sodium, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnCalcium),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.calcium, columnIndex, ascending),
),
DataColumn(
label: Text(localizations.dataTableColumnIron),
numeric: true,
onSort: (int columnIndex, bool ascending) =>
_sort<num>((_Dessert d) => d.iron, columnIndex, ascending),
),
],
source: _dessertsDataSource!,
),
],
),
),
);
}
}
class _Dessert {
_Dessert(
this.name,
this.calories,
this.fat,
this.carbs,
this.protein,
this.sodium,
this.calcium,
this.iron,
);
final String name;
final int calories;
final double fat;
final int carbs;
final double protein;
final int sodium;
final int calcium;
final int iron;
bool selected = false;
}
class _DessertDataSource extends DataTableSource {
_DessertDataSource(this.context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
_desserts = <_Dessert>[
_Dessert(
localizations.dataTableRowFrozenYogurt,
159,
6.0,
24,
4.0,
87,
14,
1,
),
_Dessert(
localizations.dataTableRowIceCreamSandwich,
237,
9.0,
37,
4.3,
129,
8,
1,
),
_Dessert(
localizations.dataTableRowEclair,
262,
16.0,
24,
6.0,
337,
6,
7,
),
_Dessert(
localizations.dataTableRowCupcake,
305,
3.7,
67,
4.3,
413,
3,
8,
),
_Dessert(
localizations.dataTableRowGingerbread,
356,
16.0,
49,
3.9,
327,
7,
16,
),
_Dessert(
localizations.dataTableRowJellyBean,
375,
0.0,
94,
0.0,
50,
0,
0,
),
_Dessert(
localizations.dataTableRowLollipop,
392,
0.2,
98,
0.0,
38,
0,
2,
),
_Dessert(
localizations.dataTableRowHoneycomb,
408,
3.2,
87,
6.5,
562,
0,
45,
),
_Dessert(
localizations.dataTableRowDonut,
452,
25.0,
51,
4.9,
326,
2,
22,
),
_Dessert(
localizations.dataTableRowApplePie,
518,
26.0,
65,
7.0,
54,
12,
6,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowFrozenYogurt,
),
168,
6.0,
26,
4.0,
87,
14,
1,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowIceCreamSandwich,
),
246,
9.0,
39,
4.3,
129,
8,
1,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowEclair,
),
271,
16.0,
26,
6.0,
337,
6,
7,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowCupcake,
),
314,
3.7,
69,
4.3,
413,
3,
8,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowGingerbread,
),
345,
16.0,
51,
3.9,
327,
7,
16,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowJellyBean,
),
364,
0.0,
96,
0.0,
50,
0,
0,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowLollipop,
),
401,
0.2,
100,
0.0,
38,
0,
2,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowHoneycomb,
),
417,
3.2,
89,
6.5,
562,
0,
45,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowDonut,
),
461,
25.0,
53,
4.9,
326,
2,
22,
),
_Dessert(
localizations.dataTableRowWithSugar(
localizations.dataTableRowApplePie,
),
527,
26.0,
67,
7.0,
54,
12,
6,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowFrozenYogurt,
),
223,
6.0,
36,
4.0,
87,
14,
1,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowIceCreamSandwich,
),
301,
9.0,
49,
4.3,
129,
8,
1,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowEclair,
),
326,
16.0,
36,
6.0,
337,
6,
7,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowCupcake,
),
369,
3.7,
79,
4.3,
413,
3,
8,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowGingerbread,
),
420,
16.0,
61,
3.9,
327,
7,
16,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowJellyBean,
),
439,
0.0,
106,
0.0,
50,
0,
0,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowLollipop,
),
456,
0.2,
110,
0.0,
38,
0,
2,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowHoneycomb,
),
472,
3.2,
99,
6.5,
562,
0,
45,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowDonut,
),
516,
25.0,
63,
4.9,
326,
2,
22,
),
_Dessert(
localizations.dataTableRowWithHoney(
localizations.dataTableRowApplePie,
),
582,
26.0,
77,
7.0,
54,
12,
6,
),
];
}
final BuildContext context;
late List<_Dessert> _desserts;
void _sort<T>(Comparable<T> Function(_Dessert d) getField, bool ascending) {
_desserts.sort((_Dessert a, _Dessert b) {
final Comparable<T> aValue = getField(a);
final Comparable<T> bValue = getField(b);
return ascending
? Comparable.compare(aValue, bValue)
: Comparable.compare(bValue, aValue);
});
notifyListeners();
}
int _selectedCount = 0;
void updateSelectedDesserts(_RestorableDessertSelections selectedRows) {
_selectedCount = 0;
for (int i = 0; i < _desserts.length; i += 1) {
final _Dessert dessert = _desserts[i];
if (selectedRows.isSelected(i)) {
dessert.selected = true;
_selectedCount += 1;
} else {
dessert.selected = false;
}
}
notifyListeners();
}
@override
DataRow? getRow(int index) {
final NumberFormat format = NumberFormat.decimalPercentPattern(
locale: GalleryOptions.of(context).locale.toString(),
decimalDigits: 0,
);
assert(index >= 0);
if (index >= _desserts.length) {
return null;
}
final _Dessert dessert = _desserts[index];
return DataRow.byIndex(
index: index,
selected: dessert.selected,
onSelectChanged: (bool? value) {
if (dessert.selected != value) {
_selectedCount += value! ? 1 : -1;
assert(_selectedCount >= 0);
dessert.selected = value;
notifyListeners();
}
},
cells: <DataCell>[
DataCell(Text(dessert.name)),
DataCell(Text('${dessert.calories}')),
DataCell(Text(dessert.fat.toStringAsFixed(1))),
DataCell(Text('${dessert.carbs}')),
DataCell(Text(dessert.protein.toStringAsFixed(1))),
DataCell(Text('${dessert.sodium}')),
DataCell(Text(format.format(dessert.calcium / 100))),
DataCell(Text(format.format(dessert.iron / 100))),
],
);
}
@override
int get rowCount => _desserts.length;
@override
bool get isRowCountApproximate => false;
@override
int get selectedRowCount => _selectedCount;
void _selectAll(bool? checked) {
for (final _Dessert dessert in _desserts) {
dessert.selected = checked ?? false;
}
_selectedCount = checked! ? _desserts.length : 0;
notifyListeners();
}
}
// END
| flutter/dev/integration_tests/new_gallery/lib/demos/material/data_table_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/demos/material/data_table_demo.dart",
"repo_id": "flutter",
"token_count": 8715
} | 550 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/scheduler.dart';
import 'animation.dart';
import 'overlay.dart';
/// [Widget] to enforce a global lock system for [FeatureDiscovery] widgets.
///
/// This widget enforces that at most one [FeatureDiscovery] widget in its
/// widget tree is shown at a time.
///
/// Users wanting to use [FeatureDiscovery] need to put this controller
/// above [FeatureDiscovery] widgets in the widget tree.
class FeatureDiscoveryController extends StatefulWidget {
const FeatureDiscoveryController(this.child, {super.key});
final Widget child;
static _FeatureDiscoveryControllerState _of(BuildContext context) {
final _FeatureDiscoveryControllerState? matchResult =
context.findAncestorStateOfType<_FeatureDiscoveryControllerState>();
if (matchResult != null) {
return matchResult;
}
throw FlutterError(
'FeatureDiscoveryController.of() called with a context that does not '
'contain a FeatureDiscoveryController.\n The context used was:\n '
'$context');
}
@override
State<FeatureDiscoveryController> createState() =>
_FeatureDiscoveryControllerState();
}
class _FeatureDiscoveryControllerState
extends State<FeatureDiscoveryController> {
bool _isLocked = false;
/// Flag to indicate whether a [FeatureDiscovery] widget descendant is
/// currently showing its overlay or not.
///
/// If true, then no other [FeatureDiscovery] widget should display its
/// overlay.
bool get isLocked => _isLocked;
/// Lock the controller.
///
/// Note we do not [setState] here because this function will be called
/// by the first [FeatureDiscovery] ready to show its overlay, and any
/// additional [FeatureDiscovery] widgets wanting to show their overlays
/// will already be scheduled to be built, so the lock change will be caught
/// in their builds.
void lock() => _isLocked = true;
/// Unlock the controller.
void unlock() => setState(() => _isLocked = false);
@override
void didChangeDependencies() {
super.didChangeDependencies();
assert(
context.findAncestorStateOfType<_FeatureDiscoveryControllerState>() ==
null,
'There should not be another ancestor of type '
'FeatureDiscoveryController in the widget tree.',
);
}
@override
Widget build(BuildContext context) => widget.child;
}
/// Widget that highlights the [child] with an overlay.
///
/// This widget loosely follows the guidelines set forth in the Material Specs:
/// https://material.io/archive/guidelines/growth-communications/feature-discovery.html.
class FeatureDiscovery extends StatefulWidget {
const FeatureDiscovery({
super.key,
required this.title,
required this.description,
required this.child,
required this.showOverlay,
this.onDismiss,
this.onTap,
this.color,
});
/// Title to be displayed in the overlay.
final String title;
/// Description to be displayed in the overlay.
final String description;
/// Icon to be promoted.
final Icon child;
/// Flag to indicate whether to show the overlay or not anchored to the
/// [child].
final bool showOverlay;
/// Callback invoked when the user dismisses an overlay.
final void Function()? onDismiss;
/// Callback invoked when the user taps on the tap target of an overlay.
final void Function()? onTap;
/// Color with which to fill the outer circle.
final Color? color;
@visibleForTesting
static const Key overlayKey = Key('overlay key');
@visibleForTesting
static const Key gestureDetectorKey = Key('gesture detector key');
@override
State<FeatureDiscovery> createState() => _FeatureDiscoveryState();
}
class _FeatureDiscoveryState extends State<FeatureDiscovery>
with TickerProviderStateMixin {
bool showOverlay = false;
FeatureDiscoveryStatus status = FeatureDiscoveryStatus.closed;
late AnimationController openController;
late AnimationController rippleController;
late AnimationController tapController;
late AnimationController dismissController;
late Animations animations;
OverlayEntry? overlay;
Widget buildOverlay(BuildContext ctx, Offset center) {
debugCheckHasMediaQuery(ctx);
debugCheckHasDirectionality(ctx);
final Size deviceSize = MediaQuery.of(ctx).size;
final Color color = widget.color ?? Theme.of(ctx).colorScheme.primary;
// Wrap in transparent [Material] to enable widgets that require one.
return Material(
key: FeatureDiscovery.overlayKey,
type: MaterialType.transparency,
child: Stack(
children: <Widget>[
MouseRegion(
cursor: SystemMouseCursors.click,
child: GestureDetector(
key: FeatureDiscovery.gestureDetectorKey,
onTap: dismiss,
child: Container(
width: double.infinity,
height: double.infinity,
color: Colors.transparent,
),
),
),
Background(
animations: animations,
status: status,
color: color,
center: center,
deviceSize: deviceSize,
textDirection: Directionality.of(ctx),
),
Content(
animations: animations,
status: status,
center: center,
deviceSize: deviceSize,
title: widget.title,
description: widget.description,
textTheme: Theme.of(ctx).textTheme,
),
Ripple(
animations: animations,
status: status,
center: center,
),
TapTarget(
animations: animations,
status: status,
center: center,
onTap: tap,
child: widget.child,
),
],
),
);
}
/// Method to handle user tap on [TapTarget].
///
/// Tapping will stop any active controller and start the [tapController].
void tap() {
openController.stop();
rippleController.stop();
dismissController.stop();
tapController.forward(from: 0.0);
}
/// Method to handle user dismissal.
///
/// Dismissal will stop any active controller and start the
/// [dismissController].
void dismiss() {
openController.stop();
rippleController.stop();
tapController.stop();
dismissController.forward(from: 0.0);
}
@override
Widget build(BuildContext context) {
return LayoutBuilder(builder: (BuildContext ctx, _) {
if (overlay != null) {
SchedulerBinding.instance.addPostFrameCallback((_) {
// [OverlayEntry] needs to be explicitly rebuilt when necessary.
overlay!.markNeedsBuild();
});
} else {
if (showOverlay && !FeatureDiscoveryController._of(ctx).isLocked) {
final OverlayEntry entry = OverlayEntry(
builder: (_) => buildOverlay(ctx, getOverlayCenter(ctx)),
);
// Lock [FeatureDiscoveryController] early in order to prevent
// another [FeatureDiscovery] widget from trying to show its
// overlay while the post frame callback and set state are not
// complete.
FeatureDiscoveryController._of(ctx).lock();
SchedulerBinding.instance.addPostFrameCallback((_) {
setState(() {
overlay = entry;
status = FeatureDiscoveryStatus.closed;
openController.forward(from: 0.0);
});
Overlay.of(context).insert(entry);
});
}
}
return widget.child;
});
}
/// Compute the center position of the overlay.
Offset getOverlayCenter(BuildContext parentCtx) {
final RenderBox box = parentCtx.findRenderObject()! as RenderBox;
final Size size = box.size;
final Offset topLeftPosition = box.localToGlobal(Offset.zero);
final Offset centerPosition = Offset(
topLeftPosition.dx + size.width / 2,
topLeftPosition.dy + size.height / 2,
);
return centerPosition;
}
static bool _featureHighlightShown = false;
@override
void initState() {
super.initState();
initAnimationControllers();
initAnimations();
showOverlay = widget.showOverlay && !_featureHighlightShown;
if (showOverlay) {
_featureHighlightShown = true;
}
}
void initAnimationControllers() {
openController = AnimationController(
duration: const Duration(milliseconds: 500),
vsync: this,
)
..addListener(() {
setState(() {});
})
..addStatusListener((AnimationStatus animationStatus) {
if (animationStatus == AnimationStatus.forward) {
setState(() => status = FeatureDiscoveryStatus.open);
} else if (animationStatus == AnimationStatus.completed) {
rippleController.forward(from: 0.0);
}
});
rippleController = AnimationController(
duration: const Duration(milliseconds: 1000),
vsync: this,
)
..addListener(() {
setState(() {});
})
..addStatusListener((AnimationStatus animationStatus) {
if (animationStatus == AnimationStatus.forward) {
setState(() => status = FeatureDiscoveryStatus.ripple);
} else if (animationStatus == AnimationStatus.completed) {
rippleController.forward(from: 0.0);
}
});
tapController = AnimationController(
duration: const Duration(milliseconds: 250),
vsync: this,
)
..addListener(() {
setState(() {});
})
..addStatusListener((AnimationStatus animationStatus) {
if (animationStatus == AnimationStatus.forward) {
setState(() => status = FeatureDiscoveryStatus.tap);
} else if (animationStatus == AnimationStatus.completed) {
widget.onTap?.call();
cleanUponOverlayClose();
}
});
dismissController = AnimationController(
duration: const Duration(milliseconds: 250),
vsync: this,
)
..addListener(() {
setState(() {});
})
..addStatusListener((AnimationStatus animationStatus) {
if (animationStatus == AnimationStatus.forward) {
setState(() => status = FeatureDiscoveryStatus.dismiss);
} else if (animationStatus == AnimationStatus.completed) {
widget.onDismiss?.call();
cleanUponOverlayClose();
}
});
}
void initAnimations() {
animations = Animations(
openController,
tapController,
rippleController,
dismissController,
);
}
/// Clean up once overlay has been dismissed or tap target has been tapped.
///
/// This is called upon [tapController] and [dismissController] end.
void cleanUponOverlayClose() {
FeatureDiscoveryController._of(context).unlock();
setState(() {
status = FeatureDiscoveryStatus.closed;
showOverlay = false;
overlay?.remove();
overlay = null;
});
}
@override
void didUpdateWidget(FeatureDiscovery oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.showOverlay != oldWidget.showOverlay) {
showOverlay = widget.showOverlay;
}
}
@override
void dispose() {
overlay?.remove();
openController.dispose();
rippleController.dispose();
tapController.dispose();
dismissController.dispose();
super.dispose();
}
}
| flutter/dev/integration_tests/new_gallery/lib/feature_discovery/feature_discovery.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/feature_discovery/feature_discovery.dart",
"repo_id": "flutter",
"token_count": 4200
} | 551 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:url_launcher/url_launcher.dart';
import '../constants.dart';
import '../data/demos.dart';
import '../data/gallery_options.dart';
import '../gallery_localizations.dart';
import '../layout/adaptive.dart';
import '../studies/crane/colors.dart';
import '../studies/crane/routes.dart' as crane_routes;
import '../studies/fortnightly/routes.dart' as fortnightly_routes;
import '../studies/rally/colors.dart';
import '../studies/rally/routes.dart' as rally_routes;
import '../studies/reply/routes.dart' as reply_routes;
import '../studies/shrine/colors.dart';
import '../studies/shrine/routes.dart' as shrine_routes;
import '../studies/starter/routes.dart' as starter_app_routes;
import 'category_list_item.dart';
import 'settings.dart';
import 'splash.dart';
const double _horizontalPadding = 32.0;
const double _horizontalDesktopPadding = 81.0;
const double _carouselHeightMin = 240.0;
const double _carouselItemDesktopMargin = 8.0;
const double _carouselItemMobileMargin = 4.0;
const double _carouselItemWidth = 296.0;
class ToggleSplashNotification extends Notification {}
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
final bool isDesktop = isDisplayDesktop(context);
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final Map<String, GalleryDemo> studyDemos = Demos.studies(localizations);
final List<Widget> carouselCards = <Widget>[
_CarouselCard(
demo: studyDemos['reply'],
asset: const AssetImage(
'assets/studies/reply_card.png',
package: 'flutter_gallery_assets',
),
assetColor: const Color(0xFF344955),
assetDark: const AssetImage(
'assets/studies/reply_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF1D2327),
textColor: Colors.white,
studyRoute: reply_routes.homeRoute,
),
_CarouselCard(
demo: studyDemos['shrine'],
asset: const AssetImage(
'assets/studies/shrine_card.png',
package: 'flutter_gallery_assets',
),
assetColor: const Color(0xFFFEDBD0),
assetDark: const AssetImage(
'assets/studies/shrine_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF543B3C),
textColor: shrineBrown900,
studyRoute: shrine_routes.loginRoute,
),
_CarouselCard(
demo: studyDemos['rally'],
textColor: RallyColors.accountColors[0],
asset: const AssetImage(
'assets/studies/rally_card.png',
package: 'flutter_gallery_assets',
),
assetColor: const Color(0xFFD1F2E6),
assetDark: const AssetImage(
'assets/studies/rally_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF253538),
studyRoute: rally_routes.loginRoute,
),
_CarouselCard(
demo: studyDemos['crane'],
asset: const AssetImage(
'assets/studies/crane_card.png',
package: 'flutter_gallery_assets',
),
assetColor: const Color(0xFFFBF6F8),
assetDark: const AssetImage(
'assets/studies/crane_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF591946),
textColor: cranePurple700,
studyRoute: crane_routes.defaultRoute,
),
_CarouselCard(
demo: studyDemos['fortnightly'],
asset: const AssetImage(
'assets/studies/fortnightly_card.png',
package: 'flutter_gallery_assets',
),
assetColor: Colors.white,
assetDark: const AssetImage(
'assets/studies/fortnightly_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF1F1F1F),
studyRoute: fortnightly_routes.defaultRoute,
),
_CarouselCard(
demo: studyDemos['starterApp'],
asset: const AssetImage(
'assets/studies/starter_card.png',
package: 'flutter_gallery_assets',
),
assetColor: const Color(0xFFFAF6FE),
assetDark: const AssetImage(
'assets/studies/starter_card_dark.png',
package: 'flutter_gallery_assets',
),
assetDarkColor: const Color(0xFF3F3D45),
textColor: Colors.black,
studyRoute: starter_app_routes.defaultRoute,
),
];
if (isDesktop) {
// Desktop layout
final List<_DesktopCategoryItem> desktopCategoryItems = <_DesktopCategoryItem>[
_DesktopCategoryItem(
category: GalleryDemoCategory.material,
asset: const AssetImage(
'assets/icons/material/material.png',
package: 'flutter_gallery_assets',
),
demos: Demos.materialDemos(localizations),
),
_DesktopCategoryItem(
category: GalleryDemoCategory.cupertino,
asset: const AssetImage(
'assets/icons/cupertino/cupertino.png',
package: 'flutter_gallery_assets',
),
demos: Demos.cupertinoDemos(localizations),
),
_DesktopCategoryItem(
category: GalleryDemoCategory.other,
asset: const AssetImage(
'assets/icons/reference/reference.png',
package: 'flutter_gallery_assets',
),
demos: Demos.otherDemos(localizations),
),
];
return Scaffold(
body: ListView(
// Makes integration tests possible.
key: const ValueKey<String>('HomeListView'),
primary: true,
padding: const EdgeInsetsDirectional.only(
top: firstHeaderDesktopTopPadding,
),
children: <Widget>[
_DesktopHomeItem(child: _GalleryHeader()),
_DesktopCarousel(
height: _carouselHeight(0.7, context),
children: carouselCards,
),
_DesktopHomeItem(child: _CategoriesHeader()),
SizedBox(
height: 585,
child: _DesktopHomeItem(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: spaceBetween(28, desktopCategoryItems),
),
),
),
const SizedBox(height: 81),
_DesktopHomeItem(
child: Row(
children: <Widget>[
MouseRegion(
cursor: SystemMouseCursors.click,
child: GestureDetector(
onTap: () async {
final Uri url = Uri.parse('https://flutter.dev');
if (await canLaunchUrl(url)) {
await launchUrl(url);
}
},
excludeFromSemantics: true,
child: FadeInImage(
image: Theme.of(context).colorScheme.brightness ==
Brightness.dark
? const AssetImage(
'assets/logo/flutter_logo.png',
package: 'flutter_gallery_assets',
)
: const AssetImage(
'assets/logo/flutter_logo_color.png',
package: 'flutter_gallery_assets',
),
placeholder: MemoryImage(kTransparentImage),
fadeInDuration: entranceAnimationDuration,
),
),
),
const Expanded(
child: Wrap(
crossAxisAlignment: WrapCrossAlignment.center,
alignment: WrapAlignment.end,
children: <Widget>[
SettingsAbout(),
SettingsFeedback(),
SettingsAttribution(),
],
),
),
],
),
),
const SizedBox(height: 109),
],
),
);
} else {
// Mobile layout
return Scaffold(
body: _AnimatedHomePage(
restorationId: 'animated_page',
isSplashPageAnimationFinished:
SplashPageAnimation.of(context)!.isFinished,
carouselCards: carouselCards,
),
);
}
}
List<Widget> spaceBetween(double paddingBetween, List<Widget> children) {
return <Widget>[
for (int index = 0; index < children.length; index++) ...<Widget>[
Flexible(
child: children[index],
),
if (index < children.length - 1) SizedBox(width: paddingBetween),
],
];
}
}
class _GalleryHeader extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Header(
color: Theme.of(context).colorScheme.primaryContainer,
text: GalleryLocalizations.of(context)!.homeHeaderGallery,
);
}
}
class _CategoriesHeader extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Header(
color: Theme.of(context).colorScheme.primary,
text: GalleryLocalizations.of(context)!.homeHeaderCategories,
);
}
}
class Header extends StatelessWidget {
const Header({super.key, required this.color, required this.text});
final Color color;
final String text;
@override
Widget build(BuildContext context) {
return Align(
alignment: AlignmentDirectional.centerStart,
child: Padding(
padding: EdgeInsets.only(
top: isDisplayDesktop(context) ? 63 : 15,
bottom: isDisplayDesktop(context) ? 21 : 11,
),
child: SelectableText(
text,
style: Theme.of(context).textTheme.headlineMedium!.apply(
color: color,
fontSizeDelta:
isDisplayDesktop(context) ? desktopDisplay1FontDelta : 0,
),
),
),
);
}
}
class _AnimatedHomePage extends StatefulWidget {
const _AnimatedHomePage({
required this.restorationId,
required this.carouselCards,
required this.isSplashPageAnimationFinished,
});
final String restorationId;
final List<Widget> carouselCards;
final bool isSplashPageAnimationFinished;
@override
_AnimatedHomePageState createState() => _AnimatedHomePageState();
}
class _AnimatedHomePageState extends State<_AnimatedHomePage>
with RestorationMixin, SingleTickerProviderStateMixin {
late AnimationController _animationController;
Timer? _launchTimer;
final RestorableBool _isMaterialListExpanded = RestorableBool(false);
final RestorableBool _isCupertinoListExpanded = RestorableBool(false);
final RestorableBool _isOtherListExpanded = RestorableBool(false);
@override
String get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_isMaterialListExpanded, 'material_list');
registerForRestoration(_isCupertinoListExpanded, 'cupertino_list');
registerForRestoration(_isOtherListExpanded, 'other_list');
}
@override
void initState() {
super.initState();
_animationController = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 800),
);
if (widget.isSplashPageAnimationFinished) {
// To avoid the animation from running when changing the window size from
// desktop to mobile, we do not animate our widget if the
// splash page animation is finished on initState.
_animationController.value = 1.0;
} else {
// Start our animation halfway through the splash page animation.
_launchTimer = Timer(
halfSplashPageAnimationDuration,
() {
_animationController.forward();
},
);
}
}
@override
void dispose() {
_animationController.dispose();
_launchTimer?.cancel();
_launchTimer = null;
_isMaterialListExpanded.dispose();
_isCupertinoListExpanded.dispose();
_isOtherListExpanded.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final bool isTestMode = GalleryOptions.of(context).isTestMode;
return Stack(
children: <Widget>[
ListView(
// Makes integration tests possible.
key: const ValueKey<String>('HomeListView'),
primary: true,
restorationId: 'home_list_view',
children: <Widget>[
const SizedBox(height: 8),
Container(
margin:
const EdgeInsets.symmetric(horizontal: _horizontalPadding),
child: _GalleryHeader(),
),
_MobileCarousel(
animationController: _animationController,
restorationId: 'home_carousel',
children: widget.carouselCards,
),
Container(
margin:
const EdgeInsets.symmetric(horizontal: _horizontalPadding),
child: _CategoriesHeader(),
),
_AnimatedCategoryItem(
startDelayFraction: 0.00,
controller: _animationController,
child: CategoryListItem(
key: const PageStorageKey<GalleryDemoCategory>(
GalleryDemoCategory.material,
),
restorationId: 'home_material_category_list',
category: GalleryDemoCategory.material,
imageString: 'assets/icons/material/material.png',
demos: Demos.materialDemos(localizations),
initiallyExpanded:
_isMaterialListExpanded.value || isTestMode,
onTap: (bool shouldOpenList) {
_isMaterialListExpanded.value = shouldOpenList;
}),
),
_AnimatedCategoryItem(
startDelayFraction: 0.05,
controller: _animationController,
child: CategoryListItem(
key: const PageStorageKey<GalleryDemoCategory>(
GalleryDemoCategory.cupertino,
),
restorationId: 'home_cupertino_category_list',
category: GalleryDemoCategory.cupertino,
imageString: 'assets/icons/cupertino/cupertino.png',
demos: Demos.cupertinoDemos(localizations),
initiallyExpanded:
_isCupertinoListExpanded.value || isTestMode,
onTap: (bool shouldOpenList) {
_isCupertinoListExpanded.value = shouldOpenList;
}),
),
_AnimatedCategoryItem(
startDelayFraction: 0.10,
controller: _animationController,
child: CategoryListItem(
key: const PageStorageKey<GalleryDemoCategory>(
GalleryDemoCategory.other,
),
restorationId: 'home_other_category_list',
category: GalleryDemoCategory.other,
imageString: 'assets/icons/reference/reference.png',
demos: Demos.otherDemos(localizations),
initiallyExpanded: _isOtherListExpanded.value || isTestMode,
onTap: (bool shouldOpenList) {
_isOtherListExpanded.value = shouldOpenList;
}),
),
],
),
Align(
alignment: Alignment.topCenter,
child: GestureDetector(
onVerticalDragEnd: (DragEndDetails details) {
if (details.velocity.pixelsPerSecond.dy > 200) {
ToggleSplashNotification().dispatch(context);
}
},
child: SafeArea(
child: Container(
height: 40,
// If we don't set the color, gestures are not detected.
color: Colors.transparent,
),
),
),
),
],
);
}
}
class _DesktopHomeItem extends StatelessWidget {
const _DesktopHomeItem({required this.child});
final Widget child;
@override
Widget build(BuildContext context) {
return Align(
child: Container(
constraints: const BoxConstraints(maxWidth: maxHomeItemWidth),
padding: const EdgeInsets.symmetric(
horizontal: _horizontalDesktopPadding,
),
child: child,
),
);
}
}
class _DesktopCategoryItem extends StatelessWidget {
const _DesktopCategoryItem({
required this.category,
required this.asset,
required this.demos,
});
final GalleryDemoCategory category;
final ImageProvider asset;
final List<GalleryDemo> demos;
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
return Material(
borderRadius: BorderRadius.circular(10),
clipBehavior: Clip.antiAlias,
color: colorScheme.surface,
child: Semantics(
container: true,
child: FocusTraversalGroup(
policy: WidgetOrderTraversalPolicy(),
child: Column(
children: <Widget>[
_DesktopCategoryHeader(
category: category,
asset: asset,
),
Divider(
height: 2,
thickness: 2,
color: colorScheme.background,
),
Flexible(
child: ListView.builder(
// Makes integration tests possible.
key: ValueKey<String>('${category.name}DemoList'),
primary: false,
itemBuilder: (BuildContext context, int index) =>
CategoryDemoItem(demo: demos[index]),
itemCount: demos.length,
),
),
],
),
),
),
);
}
}
class _DesktopCategoryHeader extends StatelessWidget {
const _DesktopCategoryHeader({
required this.category,
required this.asset,
});
final GalleryDemoCategory category;
final ImageProvider asset;
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
return Material(
// Makes integration tests possible.
key: ValueKey<String>('${category.name}CategoryHeader'),
color: colorScheme.onBackground,
child: Row(
children: <Widget>[
Padding(
padding: const EdgeInsets.all(10),
child: FadeInImage(
image: asset,
placeholder: MemoryImage(kTransparentImage),
fadeInDuration: entranceAnimationDuration,
width: 64,
height: 64,
excludeFromSemantics: true,
),
),
Flexible(
child: Padding(
padding: const EdgeInsetsDirectional.only(start: 8),
child: Semantics(
header: true,
child: SelectableText(
category.displayTitle(GalleryLocalizations.of(context)!)!,
style: Theme.of(context).textTheme.headlineSmall!.apply(
color: colorScheme.onSurface,
),
),
),
),
),
],
),
);
}
}
/// Animates the category item to stagger in. The [_AnimatedCategoryItem.startDelayFraction]
/// gives a delay in the unit of a fraction of the whole animation duration,
/// which is defined in [_AnimatedHomePageState].
class _AnimatedCategoryItem extends StatelessWidget {
_AnimatedCategoryItem({
required double startDelayFraction,
required this.controller,
required this.child,
}) : topPaddingAnimation = Tween<double>(
begin: 60.0,
end: 0.0,
).animate(
CurvedAnimation(
parent: controller,
curve: Interval(
0.000 + startDelayFraction,
0.400 + startDelayFraction,
curve: Curves.ease,
),
),
);
final Widget child;
final AnimationController controller;
final Animation<double> topPaddingAnimation;
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: controller,
builder: (BuildContext context, Widget? child) {
return Padding(
padding: EdgeInsets.only(top: topPaddingAnimation.value),
child: child,
);
},
child: child,
);
}
}
/// Animates the carousel to come in from the right.
class _AnimatedCarousel extends StatelessWidget {
_AnimatedCarousel({
required this.child,
required this.controller,
}) : startPositionAnimation = Tween<double>(
begin: 1.0,
end: 0.0,
).animate(
CurvedAnimation(
parent: controller,
curve: const Interval(
0.200,
0.800,
curve: Curves.ease,
),
),
);
final Widget child;
final AnimationController controller;
final Animation<double> startPositionAnimation;
@override
Widget build(BuildContext context) {
return LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
return Stack(
children: <Widget>[
SizedBox(height: _carouselHeight(.4, context)),
AnimatedBuilder(
animation: controller,
builder: (BuildContext context, Widget? child) {
return PositionedDirectional(
start: constraints.maxWidth * startPositionAnimation.value,
child: child!,
);
},
child: SizedBox(
height: _carouselHeight(.4, context),
width: constraints.maxWidth,
child: child,
),
),
],
);
});
}
}
/// Animates a carousel card to come in from the right.
class _AnimatedCarouselCard extends StatelessWidget {
_AnimatedCarouselCard({
required this.child,
required this.controller,
}) : startPaddingAnimation = Tween<double>(
begin: _horizontalPadding,
end: 0.0,
).animate(
CurvedAnimation(
parent: controller,
curve: const Interval(
0.900,
1.000,
curve: Curves.ease,
),
),
);
final Widget child;
final AnimationController controller;
final Animation<double> startPaddingAnimation;
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: controller,
builder: (BuildContext context, Widget? child) {
return Padding(
padding: EdgeInsetsDirectional.only(
start: startPaddingAnimation.value,
),
child: child,
);
},
child: child,
);
}
}
class _MobileCarousel extends StatefulWidget {
const _MobileCarousel({
required this.animationController,
this.restorationId,
required this.children,
});
final AnimationController animationController;
final String? restorationId;
final List<Widget> children;
@override
_MobileCarouselState createState() => _MobileCarouselState();
}
class _MobileCarouselState extends State<_MobileCarousel>
with RestorationMixin, SingleTickerProviderStateMixin {
late PageController _controller;
final RestorableInt _currentPage = RestorableInt(0);
@override
String? get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_currentPage, 'carousel_page');
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
// The viewPortFraction is calculated as the width of the device minus the
// padding.
final double width = MediaQuery.of(context).size.width;
const double padding = _carouselItemMobileMargin * 2;
_controller = PageController(
initialPage: _currentPage.value,
viewportFraction: (_carouselItemWidth + padding) / width,
);
}
@override
void dispose() {
_controller.dispose();
_currentPage.dispose();
super.dispose();
}
Widget builder(int index) {
final AnimatedBuilder carouselCard = AnimatedBuilder(
animation: _controller,
builder: (BuildContext context, Widget? child) {
double value;
if (_controller.position.haveDimensions) {
value = _controller.page! - index;
} else {
// If haveDimensions is false, use _currentPage to calculate value.
value = (_currentPage.value - index).toDouble();
}
// .3 is an approximation of the curve used in the design.
value = (1 - (value.abs() * .3)).clamp(0, 1).toDouble();
value = Curves.easeOut.transform(value);
return Transform.scale(
scale: value,
child: child,
);
},
child: widget.children[index],
);
// We only want the second card to be animated.
if (index == 1) {
return _AnimatedCarouselCard(
controller: widget.animationController,
child: carouselCard,
);
} else {
return carouselCard;
}
}
@override
Widget build(BuildContext context) {
return _AnimatedCarousel(
controller: widget.animationController,
child: PageView.builder(
// Makes integration tests possible.
key: const ValueKey<String>('studyDemoList'),
onPageChanged: (int value) {
setState(() {
_currentPage.value = value;
});
},
controller: _controller,
pageSnapping: false,
itemCount: widget.children.length,
itemBuilder: (BuildContext context, int index) => builder(index),
allowImplicitScrolling: true,
),
);
}
}
/// This creates a horizontally scrolling [ListView] of items.
///
/// This class uses a [ListView] with a custom [ScrollPhysics] to enable
/// snapping behavior. A [PageView] was considered but does not allow for
/// multiple pages visible without centering the first page.
class _DesktopCarousel extends StatefulWidget {
const _DesktopCarousel({required this.height, required this.children});
final double height;
final List<Widget> children;
@override
_DesktopCarouselState createState() => _DesktopCarouselState();
}
class _DesktopCarouselState extends State<_DesktopCarousel> {
late ScrollController _controller;
@override
void initState() {
super.initState();
_controller = ScrollController();
_controller.addListener(() {
setState(() {});
});
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
bool showPreviousButton = false;
bool showNextButton = true;
// Only check this after the _controller has been attached to the ListView.
if (_controller.hasClients) {
showPreviousButton = _controller.offset > 0;
showNextButton =
_controller.offset < _controller.position.maxScrollExtent;
}
final bool isDesktop = isDisplayDesktop(context);
return Align(
child: Container(
height: widget.height,
constraints: const BoxConstraints(maxWidth: maxHomeItemWidth),
child: Stack(
children: <Widget>[
ListView.builder(
padding: EdgeInsets.symmetric(
horizontal: isDesktop
? _horizontalDesktopPadding - _carouselItemDesktopMargin
: _horizontalPadding - _carouselItemMobileMargin,
),
scrollDirection: Axis.horizontal,
primary: false,
physics: const _SnappingScrollPhysics(),
controller: _controller,
itemExtent: _carouselItemWidth,
itemCount: widget.children.length,
itemBuilder: (BuildContext context, int index) => Padding(
padding: const EdgeInsets.symmetric(vertical: 8.0),
child: widget.children[index],
),
),
if (showPreviousButton)
_DesktopPageButton(
onTap: () {
_controller.animateTo(
_controller.offset - _carouselItemWidth,
duration: const Duration(milliseconds: 200),
curve: Curves.easeInOut,
);
},
),
if (showNextButton)
_DesktopPageButton(
isEnd: true,
onTap: () {
_controller.animateTo(
_controller.offset + _carouselItemWidth,
duration: const Duration(milliseconds: 200),
curve: Curves.easeInOut,
);
},
),
],
),
),
);
}
}
/// Scrolling physics that snaps to the new item in the [_DesktopCarousel].
class _SnappingScrollPhysics extends ScrollPhysics {
const _SnappingScrollPhysics({super.parent});
@override
_SnappingScrollPhysics applyTo(ScrollPhysics? ancestor) {
return _SnappingScrollPhysics(parent: buildParent(ancestor));
}
double _getTargetPixels(
ScrollMetrics position,
Tolerance tolerance,
double velocity,
) {
final double itemWidth = position.viewportDimension / 4;
double item = position.pixels / itemWidth;
if (velocity < -tolerance.velocity) {
item -= 0.5;
} else if (velocity > tolerance.velocity) {
item += 0.5;
}
return math.min(
item.roundToDouble() * itemWidth,
position.maxScrollExtent,
);
}
@override
Simulation? createBallisticSimulation(
ScrollMetrics position,
double velocity,
) {
if ((velocity <= 0.0 && position.pixels <= position.minScrollExtent) ||
(velocity >= 0.0 && position.pixels >= position.maxScrollExtent)) {
return super.createBallisticSimulation(position, velocity);
}
final Tolerance tolerance = toleranceFor(position);
final double target = _getTargetPixels(position, tolerance, velocity);
if (target != position.pixels) {
return ScrollSpringSimulation(
spring,
position.pixels,
target,
velocity,
tolerance: tolerance,
);
}
return null;
}
@override
bool get allowImplicitScrolling => true;
}
class _DesktopPageButton extends StatelessWidget {
const _DesktopPageButton({
this.isEnd = false,
this.onTap,
});
final bool isEnd;
final GestureTapCallback? onTap;
@override
Widget build(BuildContext context) {
const double buttonSize = 58.0;
const double padding = _horizontalDesktopPadding - buttonSize / 2;
return ExcludeSemantics(
child: Align(
alignment: isEnd
? AlignmentDirectional.centerEnd
: AlignmentDirectional.centerStart,
child: Container(
width: buttonSize,
height: buttonSize,
margin: EdgeInsetsDirectional.only(
start: isEnd ? 0 : padding,
end: isEnd ? padding : 0,
),
child: Tooltip(
message: isEnd
? MaterialLocalizations.of(context).nextPageTooltip
: MaterialLocalizations.of(context).previousPageTooltip,
child: Material(
color: Colors.black.withOpacity(0.5),
shape: const CircleBorder(),
clipBehavior: Clip.antiAlias,
child: InkWell(
onTap: onTap,
child: Icon(
isEnd ? Icons.arrow_forward_ios : Icons.arrow_back_ios,
color: Colors.white,
),
),
),
),
),
),
);
}
}
class _CarouselCard extends StatelessWidget {
const _CarouselCard({
required this.demo,
this.asset,
this.assetDark,
this.assetColor,
this.assetDarkColor,
this.textColor,
required this.studyRoute,
});
final GalleryDemo? demo;
final ImageProvider? asset;
final ImageProvider? assetDark;
final Color? assetColor;
final Color? assetDarkColor;
final Color? textColor;
final String studyRoute;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final bool isDark = Theme.of(context).colorScheme.brightness == Brightness.dark;
final ImageProvider<Object>? asset = isDark ? assetDark : this.asset;
final Color? assetColor = isDark ? assetDarkColor : this.assetColor;
final Color? textColor = isDark ? Colors.white.withOpacity(0.87) : this.textColor;
final bool isDesktop = isDisplayDesktop(context);
return Container(
padding: EdgeInsets.symmetric(
horizontal: isDesktop
? _carouselItemDesktopMargin
: _carouselItemMobileMargin),
margin: const EdgeInsets.symmetric(vertical: 16.0),
height: _carouselHeight(0.7, context),
width: _carouselItemWidth,
child: Material(
// Makes integration tests possible.
key: ValueKey<String>(demo!.describe),
color: assetColor,
elevation: 4,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
clipBehavior: Clip.antiAlias,
child: Stack(
fit: StackFit.expand,
children: <Widget>[
if (asset != null)
FadeInImage(
image: asset,
placeholder: MemoryImage(kTransparentImage),
fit: BoxFit.cover,
height: _carouselHeightMin,
fadeInDuration: entranceAnimationDuration,
),
Padding(
padding: const EdgeInsetsDirectional.fromSTEB(16, 0, 16, 16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Text(
demo!.title,
style: textTheme.bodySmall!.apply(color: textColor),
maxLines: 3,
overflow: TextOverflow.visible,
),
Text(
demo!.subtitle,
style: textTheme.labelSmall!.apply(color: textColor),
maxLines: 5,
overflow: TextOverflow.visible,
),
],
),
),
Positioned.fill(
child: Material(
color: Colors.transparent,
child: InkWell(
onTap: () {
Navigator.of(context)
.popUntil((Route<void> route) => route.settings.name == '/');
Navigator.of(context).restorablePushNamed(studyRoute);
},
),
),
),
],
),
),
);
}
}
double _carouselHeight(double scaleFactor, BuildContext context) => math.max(
_carouselHeightMin *
GalleryOptions.of(context).textScaleFactor(context) *
scaleFactor,
_carouselHeightMin);
/// Wrap the studies with this to display a back button and allow the user to
/// exit them at any time.
class StudyWrapper extends StatefulWidget {
const StudyWrapper({
super.key,
required this.study,
this.alignment = AlignmentDirectional.bottomStart,
this.hasBottomNavBar = false,
});
final Widget study;
final bool hasBottomNavBar;
final AlignmentDirectional alignment;
@override
State<StudyWrapper> createState() => _StudyWrapperState();
}
class _StudyWrapperState extends State<StudyWrapper> {
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final TextTheme textTheme = Theme.of(context).textTheme;
return ApplyTextOptions(
child: Stack(
children: <Widget>[
Semantics(
sortKey: const OrdinalSortKey(1),
child: RestorationScope(
restorationId: 'study_wrapper',
child: widget.study,
),
),
if (!isDisplayFoldable(context))
SafeArea(
child: Align(
alignment: widget.alignment,
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 16.0,
vertical: widget.hasBottomNavBar
? kBottomNavigationBarHeight + 16.0
: 16.0),
child: Semantics(
sortKey: const OrdinalSortKey(0),
label: GalleryLocalizations.of(context)!.backToGallery,
button: true,
enabled: true,
excludeSemantics: true,
child: FloatingActionButton.extended(
heroTag: _BackButtonHeroTag(),
key: const ValueKey<String>('Back'),
onPressed: () {
Navigator.of(context)
.popUntil((Route<void> route) => route.settings.name == '/');
},
icon: IconTheme(
data: IconThemeData(color: colorScheme.onPrimary),
child: const BackButtonIcon(),
),
label: Text(
MaterialLocalizations.of(context).backButtonTooltip,
style: textTheme.labelLarge!
.apply(color: colorScheme.onPrimary),
),
),
),
),
),
),
],
),
);
}
}
class _BackButtonHeroTag {}
| flutter/dev/integration_tests/new_gallery/lib/pages/home.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/pages/home.dart",
"repo_id": "flutter",
"token_count": 17866
} | 552 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../../gallery_localizations.dart';
import 'destination.dart';
List<FlyDestination> getFlyDestinations(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return <FlyDestination>[
FlyDestination(
id: 0,
destination: localizations.craneFly0,
stops: 1,
duration: const Duration(hours: 6, minutes: 15),
assetSemanticLabel: localizations.craneFly0SemanticLabel,
),
FlyDestination(
id: 1,
destination: localizations.craneFly1,
stops: 0,
duration: const Duration(hours: 13, minutes: 30),
assetSemanticLabel: localizations.craneFly1SemanticLabel,
imageAspectRatio: 400 / 410,
),
FlyDestination(
id: 2,
destination: localizations.craneFly2,
stops: 0,
duration: const Duration(hours: 5, minutes: 16),
assetSemanticLabel: localizations.craneFly2SemanticLabel,
imageAspectRatio: 400 / 394,
),
FlyDestination(
id: 3,
destination: localizations.craneFly3,
stops: 2,
duration: const Duration(hours: 19, minutes: 40),
assetSemanticLabel: localizations.craneFly3SemanticLabel,
imageAspectRatio: 400 / 377,
),
FlyDestination(
id: 4,
destination: localizations.craneFly4,
stops: 0,
duration: const Duration(hours: 8, minutes: 24),
assetSemanticLabel: localizations.craneFly4SemanticLabel,
imageAspectRatio: 400 / 308,
),
FlyDestination(
id: 5,
destination: localizations.craneFly5,
stops: 1,
duration: const Duration(hours: 14, minutes: 12),
assetSemanticLabel: localizations.craneFly5SemanticLabel,
imageAspectRatio: 400 / 418,
),
FlyDestination(
id: 6,
destination: localizations.craneFly6,
stops: 0,
duration: const Duration(hours: 5, minutes: 24),
assetSemanticLabel: localizations.craneFly6SemanticLabel,
imageAspectRatio: 400 / 345,
),
FlyDestination(
id: 7,
destination: localizations.craneFly7,
stops: 1,
duration: const Duration(hours: 5, minutes: 43),
assetSemanticLabel: localizations.craneFly7SemanticLabel,
imageAspectRatio: 400 / 408,
),
FlyDestination(
id: 8,
destination: localizations.craneFly8,
stops: 0,
duration: const Duration(hours: 8, minutes: 25),
assetSemanticLabel: localizations.craneFly8SemanticLabel,
imageAspectRatio: 400 / 399,
),
FlyDestination(
id: 9,
destination: localizations.craneFly9,
stops: 1,
duration: const Duration(hours: 15, minutes: 52),
assetSemanticLabel: localizations.craneFly9SemanticLabel,
imageAspectRatio: 400 / 379,
),
FlyDestination(
id: 10,
destination: localizations.craneFly10,
stops: 0,
duration: const Duration(hours: 5, minutes: 57),
assetSemanticLabel: localizations.craneFly10SemanticLabel,
imageAspectRatio: 400 / 307,
),
FlyDestination(
id: 11,
destination: localizations.craneFly11,
stops: 1,
duration: const Duration(hours: 13, minutes: 24),
assetSemanticLabel: localizations.craneFly11SemanticLabel,
imageAspectRatio: 400 / 369,
),
FlyDestination(
id: 12,
destination: localizations.craneFly12,
stops: 2,
duration: const Duration(hours: 10, minutes: 20),
assetSemanticLabel: localizations.craneFly12SemanticLabel,
imageAspectRatio: 400 / 394,
),
FlyDestination(
id: 13,
destination: localizations.craneFly13,
stops: 0,
duration: const Duration(hours: 7, minutes: 15),
assetSemanticLabel: localizations.craneFly13SemanticLabel,
imageAspectRatio: 400 / 433,
),
];
}
List<SleepDestination> getSleepDestinations(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return <SleepDestination>[
SleepDestination(
id: 0,
destination: localizations.craneSleep0,
total: 2241,
assetSemanticLabel: localizations.craneSleep0SemanticLabel,
imageAspectRatio: 400 / 308,
),
SleepDestination(
id: 1,
destination: localizations.craneSleep1,
total: 876,
assetSemanticLabel: localizations.craneSleep1SemanticLabel,
),
SleepDestination(
id: 2,
destination: localizations.craneSleep2,
total: 1286,
assetSemanticLabel: localizations.craneSleep2SemanticLabel,
imageAspectRatio: 400 / 377,
),
SleepDestination(
id: 3,
destination: localizations.craneSleep3,
total: 496,
assetSemanticLabel: localizations.craneSleep3SemanticLabel,
imageAspectRatio: 400 / 379,
),
SleepDestination(
id: 4,
destination: localizations.craneSleep4,
total: 390,
assetSemanticLabel: localizations.craneSleep4SemanticLabel,
imageAspectRatio: 400 / 418,
),
SleepDestination(
id: 5,
destination: localizations.craneSleep5,
total: 876,
assetSemanticLabel: localizations.craneSleep5SemanticLabel,
imageAspectRatio: 400 / 410,
),
SleepDestination(
id: 6,
destination: localizations.craneSleep6,
total: 989,
assetSemanticLabel: localizations.craneSleep6SemanticLabel,
imageAspectRatio: 400 / 394,
),
SleepDestination(
id: 7,
destination: localizations.craneSleep7,
total: 306,
assetSemanticLabel: localizations.craneSleep7SemanticLabel,
imageAspectRatio: 400 / 266,
),
SleepDestination(
id: 8,
destination: localizations.craneSleep8,
total: 385,
assetSemanticLabel: localizations.craneSleep8SemanticLabel,
imageAspectRatio: 400 / 376,
),
SleepDestination(
id: 9,
destination: localizations.craneSleep9,
total: 989,
assetSemanticLabel: localizations.craneSleep9SemanticLabel,
imageAspectRatio: 400 / 369,
),
SleepDestination(
id: 10,
destination: localizations.craneSleep10,
total: 1380,
assetSemanticLabel: localizations.craneSleep10SemanticLabel,
imageAspectRatio: 400 / 307,
),
SleepDestination(
id: 11,
destination: localizations.craneSleep11,
total: 1109,
assetSemanticLabel: localizations.craneSleep11SemanticLabel,
imageAspectRatio: 400 / 456,
),
];
}
List<EatDestination> getEatDestinations(BuildContext context) {
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return <EatDestination>[
EatDestination(
id: 0,
destination: localizations.craneEat0,
total: 354,
assetSemanticLabel: localizations.craneEat0SemanticLabel,
imageAspectRatio: 400 / 444,
),
EatDestination(
id: 1,
destination: localizations.craneEat1,
total: 623,
assetSemanticLabel: localizations.craneEat1SemanticLabel,
imageAspectRatio: 400 / 340,
),
EatDestination(
id: 2,
destination: localizations.craneEat2,
total: 124,
assetSemanticLabel: localizations.craneEat2SemanticLabel,
imageAspectRatio: 400 / 406,
),
EatDestination(
id: 3,
destination: localizations.craneEat3,
total: 495,
assetSemanticLabel: localizations.craneEat3SemanticLabel,
imageAspectRatio: 400 / 323,
),
EatDestination(
id: 4,
destination: localizations.craneEat4,
total: 683,
assetSemanticLabel: localizations.craneEat4SemanticLabel,
imageAspectRatio: 400 / 404,
),
EatDestination(
id: 5,
destination: localizations.craneEat5,
total: 786,
assetSemanticLabel: localizations.craneEat5SemanticLabel,
imageAspectRatio: 400 / 407,
),
EatDestination(
id: 6,
destination: localizations.craneEat6,
total: 323,
assetSemanticLabel: localizations.craneEat6SemanticLabel,
imageAspectRatio: 400 / 431,
),
EatDestination(
id: 7,
destination: localizations.craneEat7,
total: 285,
assetSemanticLabel: localizations.craneEat7SemanticLabel,
imageAspectRatio: 400 / 422,
),
EatDestination(
id: 8,
destination: localizations.craneEat8,
total: 323,
assetSemanticLabel: localizations.craneEat8SemanticLabel,
imageAspectRatio: 400 / 300,
),
EatDestination(
id: 9,
destination: localizations.craneEat9,
total: 1406,
assetSemanticLabel: localizations.craneEat9SemanticLabel,
imageAspectRatio: 400 / 451,
),
EatDestination(
id: 10,
destination: localizations.craneEat10,
total: 849,
assetSemanticLabel: localizations.craneEat10SemanticLabel,
imageAspectRatio: 400 / 266,
),
];
}
| flutter/dev/integration_tests/new_gallery/lib/studies/crane/model/data.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/crane/model/data.dart",
"repo_id": "flutter",
"token_count": 3698
} | 553 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import '../../data/gallery_options.dart';
/// Get the locale string for the context.
String locale(BuildContext context) =>
GalleryOptions.of(context).locale.toString();
/// Currency formatter for USD.
NumberFormat usdWithSignFormat(BuildContext context, {int decimalDigits = 2}) {
return NumberFormat.currency(
locale: locale(context),
name: r'$',
decimalDigits: decimalDigits,
);
}
/// Percent formatter with two decimal points.
NumberFormat percentFormat(BuildContext context, {int decimalDigits = 2}) {
return NumberFormat.decimalPercentPattern(
locale: locale(context),
decimalDigits: decimalDigits,
);
}
/// Date formatter with year / number month / day.
DateFormat shortDateFormat(BuildContext context) =>
DateFormat.yMd(locale(context));
/// Date formatter with year / month / day.
DateFormat longDateFormat(BuildContext context) =>
DateFormat.yMMMMd(locale(context));
/// Date formatter with abbreviated month and day.
DateFormat dateFormatAbbreviatedMonthDay(BuildContext context) =>
DateFormat.MMMd(locale(context));
/// Date formatter with year and abbreviated month.
DateFormat dateFormatMonthYear(BuildContext context) =>
DateFormat.yMMM(locale(context));
| flutter/dev/integration_tests/new_gallery/lib/studies/rally/formatters.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/rally/formatters.dart",
"repo_id": "flutter",
"token_count": 437
} | 554 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import 'model/email_model.dart';
import 'model/email_store.dart';
import 'profile_avatar.dart';
class MailViewPage extends StatelessWidget {
const MailViewPage({
super.key,
required this.id,
required this.email,
});
final int id;
final Email email;
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
bottom: false,
child: SizedBox(
height: double.infinity,
child: Material(
color: Theme.of(context).cardColor,
child: SingleChildScrollView(
padding: const EdgeInsetsDirectional.only(
top: 42,
start: 20,
end: 20,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
_MailViewHeader(email: email),
const SizedBox(height: 32),
_MailViewBody(message: email.message),
if (email.containsPictures) ...<Widget>[
const SizedBox(height: 28),
const _PictureGrid(),
],
const SizedBox(height: kToolbarHeight),
],
),
),
),
),
),
);
}
}
class _MailViewHeader extends StatelessWidget {
const _MailViewHeader({required this.email});
final Email email;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Column(
children: <Widget>[
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: SelectableText(
email.subject,
style: textTheme.headlineMedium!.copyWith(height: 1.1),
),
),
IconButton(
key: const ValueKey<String>('ReplyExit'),
icon: const Icon(Icons.keyboard_arrow_down),
onPressed: () {
Provider.of<EmailStore>(
context,
listen: false,
).selectedEmailId = -1;
Navigator.pop(context);
},
splashRadius: 20,
),
],
),
const SizedBox(height: 16),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
SelectableText('${email.sender} - ${email.time}'),
const SizedBox(height: 4),
SelectableText(
'To ${email.recipients},',
style: textTheme.bodySmall!.copyWith(
color: Theme.of(context)
.navigationRailTheme
.unselectedLabelTextStyle!
.color,
),
),
],
),
Padding(
padding: const EdgeInsetsDirectional.only(end: 4),
child: ProfileAvatar(avatar: email.avatar),
),
],
),
],
);
}
}
class _MailViewBody extends StatelessWidget {
const _MailViewBody({required this.message});
final String message;
@override
Widget build(BuildContext context) {
return SelectableText(
message,
style: Theme.of(context).textTheme.bodyMedium!.copyWith(fontSize: 16),
);
}
}
class _PictureGrid extends StatelessWidget {
const _PictureGrid();
bool _shouldShrinkImage() {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.android:
return true;
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return false;
}
}
@override
Widget build(BuildContext context) {
return GridView.builder(
shrinkWrap: true,
physics: const NeverScrollableScrollPhysics(),
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2,
crossAxisSpacing: 4,
mainAxisSpacing: 4,
),
itemCount: 4,
itemBuilder: (BuildContext context, int index) {
return Image.asset(
'reply/attachments/paris_${index + 1}.jpg',
gaplessPlayback: true,
package: 'flutter_gallery_assets',
fit: BoxFit.fill,
cacheWidth: _shouldShrinkImage() ? 500 : null,
);
},
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/reply/mail_view_page.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/reply/mail_view_page.dart",
"repo_id": "flutter",
"token_count": 2474
} | 555 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../../gallery_localizations.dart';
class Category {
const Category({
required this.name,
});
// A function taking a BuildContext as input and
// returns the internationalized name of the category.
final String Function(BuildContext) name;
}
Category categoryAll = Category(
name: (BuildContext context) => GalleryLocalizations.of(context)!.shrineCategoryNameAll,
);
Category categoryAccessories = Category(
name: (BuildContext context) =>
GalleryLocalizations.of(context)!.shrineCategoryNameAccessories,
);
Category categoryClothing = Category(
name: (BuildContext context) =>
GalleryLocalizations.of(context)!.shrineCategoryNameClothing,
);
Category categoryHome = Category(
name: (BuildContext context) => GalleryLocalizations.of(context)!.shrineCategoryNameHome,
);
List<Category> categories = <Category>[
categoryAll,
categoryAccessories,
categoryClothing,
categoryHome,
];
class Product {
const Product({
required this.category,
required this.id,
required this.isFeatured,
required this.name,
required this.price,
this.assetAspectRatio = 1,
});
final Category category;
final int id;
final bool isFeatured;
final double assetAspectRatio;
// A function taking a BuildContext as input and
// returns the internationalized name of the product.
final String Function(BuildContext) name;
final int price;
String get assetName => '$id-0.jpg';
String get assetPackage => 'shrine_images';
}
| flutter/dev/integration_tests/new_gallery/lib/studies/shrine/model/product.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/shrine/model/product.dart",
"repo_id": "flutter",
"token_count": 494
} | 556 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery_localizations.dart';
import '../../layout/adaptive.dart';
const double appBarDesktopHeight = 128.0;
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final bool isDesktop = isDisplayDesktop(context);
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
final SafeArea body = SafeArea(
child: Padding(
padding: isDesktop
? const EdgeInsets.symmetric(horizontal: 72, vertical: 48)
: const EdgeInsets.symmetric(horizontal: 16, vertical: 24),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
SelectableText(
localizations.starterAppGenericHeadline,
style: textTheme.displaySmall!.copyWith(
color: colorScheme.onSecondary,
),
),
const SizedBox(height: 10),
SelectableText(
localizations.starterAppGenericSubtitle,
style: textTheme.titleMedium,
),
const SizedBox(height: 48),
SelectableText(
localizations.starterAppGenericBody,
style: textTheme.bodyLarge,
),
],
),
),
);
if (isDesktop) {
return Row(
children: <Widget>[
const ListDrawer(),
const VerticalDivider(width: 1),
Expanded(
child: Scaffold(
appBar: const AdaptiveAppBar(
isDesktop: true,
),
body: body,
floatingActionButton: FloatingActionButton.extended(
heroTag: 'Extended Add',
onPressed: () {},
label: Text(
localizations.starterAppGenericButton,
style: TextStyle(color: colorScheme.onSecondary),
),
icon: Icon(Icons.add, color: colorScheme.onSecondary),
tooltip: localizations.starterAppTooltipAdd,
),
),
),
],
);
} else {
return Scaffold(
appBar: const AdaptiveAppBar(),
body: body,
drawer: const ListDrawer(),
floatingActionButton: FloatingActionButton(
heroTag: 'Add',
onPressed: () {},
tooltip: localizations.starterAppTooltipAdd,
child: Icon(
Icons.add,
color: Theme.of(context).colorScheme.onSecondary,
),
),
);
}
}
}
class AdaptiveAppBar extends StatelessWidget implements PreferredSizeWidget {
const AdaptiveAppBar({
super.key,
this.isDesktop = false,
});
final bool isDesktop;
@override
Size get preferredSize => isDesktop
? const Size.fromHeight(appBarDesktopHeight)
: const Size.fromHeight(kToolbarHeight);
@override
Widget build(BuildContext context) {
final ThemeData themeData = Theme.of(context);
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return AppBar(
automaticallyImplyLeading: !isDesktop,
title: isDesktop
? null
: SelectableText(localizations.starterAppGenericTitle),
bottom: isDesktop
? PreferredSize(
preferredSize: const Size.fromHeight(26),
child: Container(
alignment: AlignmentDirectional.centerStart,
margin: const EdgeInsetsDirectional.fromSTEB(72, 0, 0, 22),
child: SelectableText(
localizations.starterAppGenericTitle,
style: themeData.textTheme.titleLarge!.copyWith(
color: themeData.colorScheme.onPrimary,
),
),
),
)
: null,
actions: <Widget>[
IconButton(
icon: const Icon(Icons.share),
tooltip: localizations.starterAppTooltipShare,
onPressed: () {},
),
IconButton(
icon: const Icon(Icons.favorite),
tooltip: localizations.starterAppTooltipFavorite,
onPressed: () {},
),
IconButton(
icon: const Icon(Icons.search),
tooltip: localizations.starterAppTooltipSearch,
onPressed: () {},
),
],
);
}
}
class ListDrawer extends StatefulWidget {
const ListDrawer({super.key});
@override
State<ListDrawer> createState() => _ListDrawerState();
}
class _ListDrawerState extends State<ListDrawer> {
static const int numItems = 9;
int selectedItem = 0;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
final GalleryLocalizations localizations = GalleryLocalizations.of(context)!;
return Drawer(
child: SafeArea(
child: ListView(
children: <Widget>[
ListTile(
title: SelectableText(
localizations.starterAppTitle,
style: textTheme.titleLarge,
),
subtitle: SelectableText(
localizations.starterAppGenericSubtitle,
style: textTheme.bodyMedium,
),
),
const Divider(),
...Iterable<int>.generate(numItems).toList().map((int i) {
return ListTile(
selected: i == selectedItem,
leading: const Icon(Icons.favorite),
title: Text(
localizations.starterAppDrawerItem(i + 1),
),
onTap: () {
setState(() {
selectedItem = i;
});
},
);
}),
],
),
),
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/starter/home.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/starter/home.dart",
"repo_id": "flutter",
"token_count": 2920
} | 557 |
# platform_interaction
Integration test of platform interaction.
| flutter/dev/integration_tests/platform_interaction/README.md/0 | {
"file_path": "flutter/dev/integration_tests/platform_interaction/README.md",
"repo_id": "flutter",
"token_count": 15
} | 558 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter_driver/driver_extension.dart';
import 'src/system_navigation.dart';
import 'src/test_step.dart';
void main() {
enableFlutterDriverExtension();
runApp(const TestApp());
}
class TestApp extends StatefulWidget {
const TestApp({super.key});
@override
State<TestApp> createState() => _TestAppState();
}
class _TestAppState extends State<TestApp> {
static final List<TestStep> steps = <TestStep>[
() => systemNavigatorPop(),
];
Future<TestStepResult>? _result;
int _step = 0;
void _executeNextStep() {
setState(() {
if (_step < steps.length) {
_result = steps[_step++]();
} else {
_result = Future<TestStepResult>.value(TestStepResult.complete);
}
});
}
Widget _buildTestResultWidget(
BuildContext context,
AsyncSnapshot<TestStepResult> snapshot,
) {
return TestStepResult.fromSnapshot(snapshot).asWidget(context);
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Platform Interaction Test',
home: Scaffold(
appBar: AppBar(
title: const Text('Platform Interaction Test'),
),
body: Padding(
padding: const EdgeInsets.all(20.0),
child: FutureBuilder<TestStepResult>(
future: _result,
builder: _buildTestResultWidget,
),
),
floatingActionButton: FloatingActionButton(
key: const ValueKey<String>('step'),
onPressed: _executeNextStep,
child: const Icon(Icons.navigate_next),
),
),
);
}
}
| flutter/dev/integration_tests/platform_interaction/lib/main.dart/0 | {
"file_path": "flutter/dev/integration_tests/platform_interaction/lib/main.dart",
"repo_id": "flutter",
"token_count": 711
} | 559 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert' show utf8;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
Future<void> main() async {
const Text text = Text('Hello, world!', textDirection: TextDirection.ltr);
// These calls must not result in an error. They behave differently in
// release mode compared to debug or profile.
// The test will grep logcat for any errors emitted by Flutter.
print(text.toDiagnosticsNode());
print(text.toStringDeep());
// regression test for https://github.com/flutter/flutter/issues/49601
final List<int> computed = await compute(_utf8Encode, 'test');
print(computed);
runApp(
const Center(
child: text,
),
);
}
List<int> _utf8Encode(String data) {
return utf8.encode(data);
}
| flutter/dev/integration_tests/release_smoke_test/lib/main.dart/0 | {
"file_path": "flutter/dev/integration_tests/release_smoke_test/lib/main.dart",
"repo_id": "flutter",
"token_count": 295
} | 560 |
# Flutter UI integration tests
This project contains a collection of non-plugin-dependent UI
integration tests. The device code is in the `lib/` directory, the
driver code is in the `test_driver/` directory. They work together.
Normally they are run via the devicelab.
## keyboard\_resize
Verifies that showing and hiding the keyboard resizes the content.
## routing
Verifies that `flutter drive --route` works correctly.
| flutter/dev/integration_tests/ui/README.md/0 | {
"file_path": "flutter/dev/integration_tests/ui/README.md",
"repo_id": "flutter",
"token_count": 111
} | 561 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_driver/driver_extension.dart';
void main() {
enableFlutterDriverExtension();
runApp(const DriverTestApp());
}
class DriverTestApp extends StatefulWidget {
const DriverTestApp({super.key});
@override
State<StatefulWidget> createState() {
return DriverTestAppState();
}
}
class DriverTestAppState extends State<DriverTestApp> {
bool present = true;
Letter _selectedValue = Letter.a;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('FlutterDriver test'),
),
body: ListView(
padding: const EdgeInsets.all(5.0),
children: <Widget>[
Row(
children: <Widget>[
Expanded(
child: Text(present ? 'present' : 'absent'),
),
ElevatedButton(
child: const Text(
'toggle',
key: ValueKey<String>('togglePresent'),
),
onPressed: () {
setState(() {
present = !present;
});
},
),
],
),
Row(
children: <Widget>[
const Expanded(
child: Text('hit testability'),
),
DropdownButton<Letter>(
key: const ValueKey<String>('dropdown'),
value: _selectedValue,
onChanged: (Letter? newValue) {
setState(() {
_selectedValue = newValue!;
});
},
items: const <DropdownMenuItem<Letter>>[
DropdownMenuItem<Letter>(
value: Letter.a,
child: Text('Aaa', key: ValueKey<String>('a')),
),
DropdownMenuItem<Letter>(
value: Letter.b,
child: Text('Bbb', key: ValueKey<String>('b')),
),
DropdownMenuItem<Letter>(
value: Letter.c,
child: Text('Ccc', key: ValueKey<String>('c')),
),
],
),
],
),
const TextField(
key: ValueKey<String>('enter-text-field'),
),
],
),
),
);
}
}
enum Letter { a, b, c }
| flutter/dev/integration_tests/ui/lib/driver.dart/0 | {
"file_path": "flutter/dev/integration_tests/ui/lib/driver.dart",
"repo_id": "flutter",
"token_count": 1537
} | 562 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
// Regression test for https://github.com/flutter/flutter/issues/111285
void main() {
testWidgets('Can load asset from same package without error', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(home: Scaffold(body: Image.asset('assets/foo.png', package: 'integration_ui'))));
await tester.pumpAndSettle();
// If this asset couldn't be loaded, the exception message would be
// "asset failed to load"
expect(tester.takeException().toString(), contains('Invalid image data'));
});
}
| flutter/dev/integration_tests/ui/test/asset_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/ui/test/asset_test.dart",
"repo_id": "flutter",
"token_count": 242
} | 563 |
<!DOCTYPE HTML>
<!-- Copyright 2014 The Flutter Authors. All rights reserved.
Use of this source code is governed by a BSD-style license that can be
found in the LICENSE file. -->
<html>
<head>
<meta charset="UTF-8">
<meta content="IE=Edge" http-equiv="X-UA-Compatible">
<title>Web Test</title>
<!-- iOS meta tags & icons -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Web Test">
<!-- Favicon -->
<link rel="icon" type="image/png" href="favicon.png"/>
<link rel="manifest" href="manifest.json">
<script>
// This is to break the serviceWorker registration, and make it throw a DOMException!
// Test for issue https://github.com/flutter/flutter/issues/103972
window.navigator.serviceWorker.register = (url) => {
console.error('Failed to register/update a ServiceWorker for scope ', url,
': Storage access is restricted in this context due to user settings or private browsing mode.');
return Promise.reject(new DOMException('The operation is insecure.'));
}
</script>
<script>
// The value below is injected by flutter build, do not touch.
var serviceWorkerVersion = null;
</script>
<!-- This script adds the flutter initialization JS code -->
<script src="flutter.js" defer></script>
</head>
<body>
<script>
window.addEventListener('load', function(ev) {
// Download main.dart.js
_flutter.loader.loadEntrypoint({
serviceWorker: {
serviceWorkerVersion: serviceWorkerVersion,
}
}).then(function(engineInitializer) {
return engineInitializer.autoStart();
});
});
</script>
</body>
</html>
| flutter/dev/integration_tests/web/web/index_with_blocked_service_workers.html/0 | {
"file_path": "flutter/dev/integration_tests/web/web/index_with_blocked_service_workers.html",
"repo_id": "flutter",
"token_count": 608
} | 564 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'package:flutter_driver/flutter_driver.dart';
import 'package:integration_test/integration_test_driver_extended.dart' as test;
/// Browser screen dimensions for the FlutterDriver test.
const int _kScreenshotWidth = 1024;
const int _kScreenshotHeight = 1024;
/// Convenience wrapper around [test.integrationDriver].
///
/// Adds the capability to take test screenshots.
Future<void> runTestWithScreenshots({
int browserWidth = _kScreenshotWidth,
int browserHeight = _kScreenshotHeight,
}) async {
final WebFlutterDriver driver =
await FlutterDriver.connect() as WebFlutterDriver;
(await driver.webDriver.window).setSize(Rectangle<int>(0, 0, browserWidth, browserHeight));
test.integrationDriver(
driver: driver,
onScreenshot: (String screenshotName, List<int> screenshotBytes, [Map<String, Object?>? args]) async {
// TODO(yjbanov): implement, see https://github.com/flutter/flutter/issues/86120
return true;
},
);
}
| flutter/dev/integration_tests/web_e2e_tests/lib/screenshot_support.dart/0 | {
"file_path": "flutter/dev/integration_tests/web_e2e_tests/lib/screenshot_support.dart",
"repo_id": "flutter",
"token_count": 351
} | 565 |
# This file tracks properties of this Flutter project.
# Used by Flutter tool to assess capabilities and perform upgrades etc.
#
# This file should be version controlled.
version:
revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
channel: unknown
project_type: app
# Tracks metadata for the flutter migrate command
migration:
platforms:
- platform: root
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: android
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: ios
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: linux
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: macos
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: web
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
- platform: windows
create_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
base_revision: c865207540a1eb960aa89ac61ba89d0f0fa7bd17
# User provided section
# List of Local paths (relative to this file) that should be
# ignored by the migrate tool.
#
# Files that are not part of the templates will be ignored by default.
unmanaged_files:
- 'lib/main.dart'
- 'ios/Runner.xcodeproj/project.pbxproj'
| flutter/dev/integration_tests/windows_startup_test/.metadata/0 | {
"file_path": "flutter/dev/integration_tests/windows_startup_test/.metadata",
"repo_id": "flutter",
"token_count": 743
} | 566 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:io';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const MaterialApp(
title: 'Actions Demo',
home: FocusDemo(),
));
}
/// A class that can hold invocation information that an [UndoableAction] can
/// use to undo/redo itself.
///
/// Instances of this class are returned from [UndoableAction]s and placed on
/// the undo stack when they are invoked.
class Memento extends Object with Diagnosticable {
const Memento({
required this.name,
required this.undo,
required this.redo,
});
/// Returns true if this Memento can be used to undo.
///
/// Subclasses could override to provide their own conditions when a command is
/// undoable.
bool get canUndo => true;
/// Returns true if this Memento can be used to redo.
///
/// Subclasses could override to provide their own conditions when a command is
/// redoable.
bool get canRedo => true;
final String name;
final VoidCallback undo;
final ValueGetter<Memento> redo;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('name', name));
}
}
/// Undoable Actions
/// An [ActionDispatcher] subclass that manages the invocation of undoable
/// actions.
class UndoableActionDispatcher extends ActionDispatcher implements Listenable {
// A stack of actions that have been performed. The most recent action
// performed is at the end of the list.
final DoubleLinkedQueue<Memento> _completedActions = DoubleLinkedQueue<Memento>();
// A stack of actions that can be redone. The most recent action performed is
// at the end of the list.
final List<Memento> _undoneActions = <Memento>[];
/// The maximum number of undo levels allowed.
///
/// If this value is set to a value smaller than the number of completed
/// actions, then the stack of completed actions is truncated to only include
/// the last [maxUndoLevels] actions.
int get maxUndoLevels => 1000;
final Set<VoidCallback> _listeners = <VoidCallback>{};
@override
void addListener(VoidCallback listener) {
_listeners.add(listener);
}
@override
void removeListener(VoidCallback listener) {
_listeners.remove(listener);
}
/// Notifies listeners that the [ActionDispatcher] has changed state.
///
/// May only be called by subclasses.
@protected
void notifyListeners() {
for (final VoidCallback callback in _listeners) {
callback();
}
}
@override
Object? invokeAction(Action<Intent> action, Intent intent, [BuildContext? context]) {
final Object? result = super.invokeAction(action, intent, context);
print('Invoking ${action is UndoableAction ? 'undoable ' : ''}$intent as $action: $this ');
if (action is UndoableAction) {
_completedActions.addLast(result! as Memento);
_undoneActions.clear();
_pruneActions();
notifyListeners();
}
return result;
}
// Enforces undo level limit.
void _pruneActions() {
while (_completedActions.length > maxUndoLevels) {
_completedActions.removeFirst();
}
}
/// Returns true if there is an action on the stack that can be undone.
bool get canUndo {
if (_completedActions.isNotEmpty) {
return _completedActions.first.canUndo;
}
return false;
}
/// Returns true if an action that has been undone can be re-invoked.
bool get canRedo {
if (_undoneActions.isNotEmpty) {
return _undoneActions.first.canRedo;
}
return false;
}
/// Undoes the last action executed if possible.
///
/// Returns true if the action was successfully undone.
bool undo() {
print('Undoing. $this');
if (!canUndo) {
return false;
}
final Memento memento = _completedActions.removeLast();
memento.undo();
_undoneActions.add(memento);
notifyListeners();
return true;
}
/// Re-invokes a previously undone action, if possible.
///
/// Returns true if the action was successfully invoked.
bool redo() {
print('Redoing. $this');
if (!canRedo) {
return false;
}
final Memento memento = _undoneActions.removeLast();
final Memento replacement = memento.redo();
_completedActions.add(replacement);
_pruneActions();
notifyListeners();
return true;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('undoable items', _completedActions.length));
properties.add(IntProperty('redoable items', _undoneActions.length));
properties.add(IterableProperty<Memento>('undo stack', _completedActions));
properties.add(IterableProperty<Memento>('redo stack', _undoneActions));
}
}
class UndoIntent extends Intent {
const UndoIntent();
}
class UndoAction extends Action<UndoIntent> {
@override
bool isEnabled(UndoIntent intent) {
final BuildContext? buildContext = primaryFocus?.context ?? FocusDemo.appKey.currentContext;
if (buildContext == null) {
return false;
}
final UndoableActionDispatcher manager = Actions.of(buildContext) as UndoableActionDispatcher;
return manager.canUndo;
}
@override
void invoke(UndoIntent intent) {
final BuildContext? buildContext = primaryFocus?.context ?? FocusDemo.appKey.currentContext;
if (buildContext == null) {
return;
}
final UndoableActionDispatcher manager = Actions.of(primaryFocus?.context ?? FocusDemo.appKey.currentContext!) as UndoableActionDispatcher;
manager.undo();
}
}
class RedoIntent extends Intent {
const RedoIntent();
}
class RedoAction extends Action<RedoIntent> {
@override
bool isEnabled(RedoIntent intent) {
final BuildContext? buildContext = primaryFocus?.context ?? FocusDemo.appKey.currentContext;
if (buildContext == null) {
return false;
}
final UndoableActionDispatcher manager = Actions.of(buildContext) as UndoableActionDispatcher;
return manager.canRedo;
}
@override
RedoAction invoke(RedoIntent intent) {
final BuildContext? buildContext = primaryFocus?.context ?? FocusDemo.appKey.currentContext;
if (buildContext == null) {
return this;
}
final UndoableActionDispatcher manager = Actions.of(buildContext) as UndoableActionDispatcher;
manager.redo();
return this;
}
}
/// An action that can be undone.
abstract class UndoableAction<T extends Intent> extends Action<T> { }
class UndoableFocusActionBase<T extends Intent> extends UndoableAction<T> {
@override
@mustCallSuper
Memento invoke(T intent) {
final FocusNode? previousFocus = primaryFocus;
return Memento(name: previousFocus!.debugLabel!, undo: () {
previousFocus.requestFocus();
}, redo: () {
return invoke(intent);
});
}
}
class UndoableRequestFocusAction extends UndoableFocusActionBase<RequestFocusIntent> {
@override
Memento invoke(RequestFocusIntent intent) {
final Memento memento = super.invoke(intent);
intent.focusNode.requestFocus();
return memento;
}
}
/// Actions for manipulating focus.
class UndoableNextFocusAction extends UndoableFocusActionBase<NextFocusIntent> {
@override
Memento invoke(NextFocusIntent intent) {
final Memento memento = super.invoke(intent);
primaryFocus?.nextFocus();
return memento;
}
}
class UndoablePreviousFocusAction extends UndoableFocusActionBase<PreviousFocusIntent> {
@override
Memento invoke(PreviousFocusIntent intent) {
final Memento memento = super.invoke(intent);
primaryFocus?.previousFocus();
return memento;
}
}
class UndoableDirectionalFocusAction extends UndoableFocusActionBase<DirectionalFocusIntent> {
@override
Memento invoke(DirectionalFocusIntent intent) {
final Memento memento = super.invoke(intent);
primaryFocus?.focusInDirection(intent.direction);
return memento;
}
}
/// A button class that takes focus when clicked.
class DemoButton extends StatefulWidget {
const DemoButton({super.key, required this.name});
final String name;
@override
State<DemoButton> createState() => _DemoButtonState();
}
class _DemoButtonState extends State<DemoButton> {
late final FocusNode _focusNode = FocusNode(debugLabel: widget.name);
final GlobalKey _nameKey = GlobalKey();
void _handleOnPressed() {
print('Button ${widget.name} pressed.');
setState(() {
Actions.invoke(_nameKey.currentContext!, RequestFocusIntent(_focusNode));
});
}
@override
void dispose() {
super.dispose();
_focusNode.dispose();
}
@override
Widget build(BuildContext context) {
return TextButton(
focusNode: _focusNode,
style: ButtonStyle(
foregroundColor: const MaterialStatePropertyAll<Color>(Colors.black),
overlayColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return Colors.red;
}
if (states.contains(MaterialState.hovered)) {
return Colors.blue;
}
return Colors.transparent;
}),
),
onPressed: () => _handleOnPressed(),
child: Text(widget.name, key: _nameKey),
);
}
}
class FocusDemo extends StatefulWidget {
const FocusDemo({super.key});
static GlobalKey appKey = GlobalKey();
@override
State<FocusDemo> createState() => _FocusDemoState();
}
class _FocusDemoState extends State<FocusDemo> {
final FocusNode outlineFocus = FocusNode(debugLabel: 'Demo Focus Node');
late final UndoableActionDispatcher dispatcher = UndoableActionDispatcher();
bool canUndo = false;
bool canRedo = false;
@override
void initState() {
super.initState();
canUndo = dispatcher.canUndo;
canRedo = dispatcher.canRedo;
dispatcher.addListener(_handleUndoStateChange);
}
void _handleUndoStateChange() {
if (dispatcher.canUndo != canUndo) {
setState(() {
canUndo = dispatcher.canUndo;
});
}
if (dispatcher.canRedo != canRedo) {
setState(() {
canRedo = dispatcher.canRedo;
});
}
}
@override
void dispose() {
dispatcher.removeListener(_handleUndoStateChange);
outlineFocus.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Actions(
dispatcher: dispatcher,
actions: <Type, Action<Intent>>{
RequestFocusIntent: UndoableRequestFocusAction(),
NextFocusIntent: UndoableNextFocusAction(),
PreviousFocusIntent: UndoablePreviousFocusAction(),
DirectionalFocusIntent: UndoableDirectionalFocusAction(),
UndoIntent: UndoAction(),
RedoIntent: RedoAction(),
},
child: FocusTraversalGroup(
policy: ReadingOrderTraversalPolicy(),
child: Shortcuts(
shortcuts: <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.keyZ, meta: Platform.isMacOS, control: !Platform.isMacOS, shift: true): const RedoIntent(),
SingleActivator(LogicalKeyboardKey.keyZ, meta: Platform.isMacOS, control: !Platform.isMacOS): const UndoIntent(),
},
child: FocusScope(
key: FocusDemo.appKey,
debugLabel: 'Scope',
autofocus: true,
child: DefaultTextStyle(
style: textTheme.headlineMedium!,
child: Scaffold(
appBar: AppBar(
title: const Text('Actions Demo'),
),
body: Center(
child: Builder(builder: (BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'One'),
DemoButton(name: 'Two'),
DemoButton(name: 'Three'),
],
),
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Four'),
DemoButton(name: 'Five'),
DemoButton(name: 'Six'),
],
),
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Seven'),
DemoButton(name: 'Eight'),
DemoButton(name: 'Nine'),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(8.0),
child: ElevatedButton(
onPressed: canUndo
? () {
Actions.invoke(context, const UndoIntent());
}
: null,
child: const Text('UNDO'),
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: ElevatedButton(
onPressed: canRedo
? () {
Actions.invoke(context, const RedoIntent());
}
: null,
child: const Text('REDO'),
),
),
],
),
],
);
}),
),
),
),
),
),
),
);
}
}
| flutter/dev/manual_tests/lib/actions.dart/0 | {
"file_path": "flutter/dev/manual_tests/lib/actions.dart",
"repo_id": "flutter",
"token_count": 6185
} | 567 |
This file makes the dev/bots/analyze.dart file ignore this directory.
| flutter/dev/snippets/config/.dartignore/0 | {
"file_path": "flutter/dev/snippets/config/.dartignore",
"repo_id": "flutter",
"token_count": 19
} | 568 |
{
"version": "v0_206",
"md.comp.banner.container.color": "surfaceContainerLow",
"md.comp.banner.container.elevation": "md.sys.elevation.level1",
"md.comp.banner.container.shape": "md.sys.shape.corner.none",
"md.comp.banner.desktop.with-single-line.container.height": 52.0,
"md.comp.banner.desktop.with-three-lines.container.height": 90.0,
"md.comp.banner.desktop.with-two-lines.with-image.container.height": 72.0,
"md.comp.banner.mobile.with-single-line.container.height": 54.0,
"md.comp.banner.mobile.with-two-lines.container.height": 112.0,
"md.comp.banner.mobile.with-two-lines.with-image.container.height": 120.0,
"md.comp.banner.supporting-text.color": "onSurfaceVariant",
"md.comp.banner.supporting-text.text-style": "bodyMedium",
"md.comp.banner.with-image.image.shape": "md.sys.shape.corner.full",
"md.comp.banner.with-image.image.size": 40.0
}
| flutter/dev/tools/gen_defaults/data/banner.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/banner.json",
"repo_id": "flutter",
"token_count": 350
} | 569 |
{
"version": "v0_206",
"md.sys.color.background": "md.ref.palette.neutral6",
"md.sys.color.error": "md.ref.palette.error80",
"md.sys.color.error-container": "md.ref.palette.error30",
"md.sys.color.inverse-on-surface": "md.ref.palette.neutral20",
"md.sys.color.inverse-primary": "md.ref.palette.primary40",
"md.sys.color.inverse-surface": "md.ref.palette.neutral90",
"md.sys.color.on-background": "md.ref.palette.neutral90",
"md.sys.color.on-error": "md.ref.palette.error20",
"md.sys.color.on-error-container": "md.ref.palette.error90",
"md.sys.color.on-primary": "md.ref.palette.primary20",
"md.sys.color.on-primary-container": "md.ref.palette.primary90",
"md.sys.color.on-primary-fixed": "md.ref.palette.primary10",
"md.sys.color.on-primary-fixed-variant": "md.ref.palette.primary30",
"md.sys.color.on-secondary": "md.ref.palette.secondary20",
"md.sys.color.on-secondary-container": "md.ref.palette.secondary90",
"md.sys.color.on-secondary-fixed": "md.ref.palette.secondary10",
"md.sys.color.on-secondary-fixed-variant": "md.ref.palette.secondary30",
"md.sys.color.on-surface": "md.ref.palette.neutral90",
"md.sys.color.on-surface-variant": "md.ref.palette.neutral-variant80",
"md.sys.color.on-tertiary": "md.ref.palette.tertiary20",
"md.sys.color.on-tertiary-container": "md.ref.palette.tertiary90",
"md.sys.color.on-tertiary-fixed": "md.ref.palette.tertiary10",
"md.sys.color.on-tertiary-fixed-variant": "md.ref.palette.tertiary30",
"md.sys.color.outline": "md.ref.palette.neutral-variant60",
"md.sys.color.outline-variant": "md.ref.palette.neutral-variant30",
"md.sys.color.primary": "md.ref.palette.primary80",
"md.sys.color.primary-container": "md.ref.palette.primary30",
"md.sys.color.primary-fixed": "md.ref.palette.primary90",
"md.sys.color.primary-fixed-dim": "md.ref.palette.primary80",
"md.sys.color.scrim": "md.ref.palette.neutral0",
"md.sys.color.secondary": "md.ref.palette.secondary80",
"md.sys.color.secondary-container": "md.ref.palette.secondary30",
"md.sys.color.secondary-fixed": "md.ref.palette.secondary90",
"md.sys.color.secondary-fixed-dim": "md.ref.palette.secondary80",
"md.sys.color.shadow": "md.ref.palette.neutral0",
"md.sys.color.surface": "md.ref.palette.neutral6",
"md.sys.color.surface-bright": "md.ref.palette.neutral24",
"md.sys.color.surface-container": "md.ref.palette.neutral12",
"md.sys.color.surface-container-high": "md.ref.palette.neutral17",
"md.sys.color.surface-container-highest": "md.ref.palette.neutral22",
"md.sys.color.surface-container-low": "md.ref.palette.neutral10",
"md.sys.color.surface-container-lowest": "md.ref.palette.neutral4",
"md.sys.color.surface-dim": "md.ref.palette.neutral6",
"md.sys.color.surface-tint": "primary",
"md.sys.color.surface-variant": "md.ref.palette.neutral-variant30",
"md.sys.color.tertiary": "md.ref.palette.tertiary80",
"md.sys.color.tertiary-container": "md.ref.palette.tertiary30",
"md.sys.color.tertiary-fixed": "md.ref.palette.tertiary90",
"md.sys.color.tertiary-fixed-dim": "md.ref.palette.tertiary80"
}
| flutter/dev/tools/gen_defaults/data/color_dark.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/color_dark.json",
"repo_id": "flutter",
"token_count": 1267
} | 570 |
{
"version": "v0_206",
"md.comp.outlined-icon-button.container.height": 40.0,
"md.comp.outlined-icon-button.container.shape": "md.sys.shape.corner.full",
"md.comp.outlined-icon-button.container.width": 40.0,
"md.comp.outlined-icon-button.disabled.icon.color": "onSurface",
"md.comp.outlined-icon-button.disabled.icon.opacity": 0.38,
"md.comp.outlined-icon-button.disabled.selected.container.color": "onSurface",
"md.comp.outlined-icon-button.disabled.selected.container.opacity": 0.12,
"md.comp.outlined-icon-button.disabled.unselected.outline.color": "onSurface",
"md.comp.outlined-icon-button.disabled.unselected.outline.opacity": 0.12,
"md.comp.outlined-icon-button.focus.indicator.color": "secondary",
"md.comp.outlined-icon-button.focus.indicator.outline.offset": "md.sys.state.focus-indicator.outer-offset",
"md.comp.outlined-icon-button.focus.indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.outlined-icon-button.focus.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.outlined-icon-button.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.outlined-icon-button.icon.size": 24.0,
"md.comp.outlined-icon-button.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity",
"md.comp.outlined-icon-button.selected.container.color": "inverseSurface",
"md.comp.outlined-icon-button.selected.focus.icon.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.focus.state-layer.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.hover.icon.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.hover.state-layer.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.icon.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.pressed.icon.color": "onInverseSurface",
"md.comp.outlined-icon-button.selected.pressed.state-layer.color": "onInverseSurface",
"md.comp.outlined-icon-button.unselected.focus.icon.color": "onSurfaceVariant",
"md.comp.outlined-icon-button.unselected.focus.state-layer.color": "onSurfaceVariant",
"md.comp.outlined-icon-button.unselected.hover.icon.color": "onSurfaceVariant",
"md.comp.outlined-icon-button.unselected.hover.state-layer.color": "onSurfaceVariant",
"md.comp.outlined-icon-button.unselected.icon.color": "onSurfaceVariant",
"md.comp.outlined-icon-button.unselected.outline.color": "outline",
"md.comp.outlined-icon-button.unselected.outline.width": 1.0,
"md.comp.outlined-icon-button.unselected.pressed.icon.color": "onSurface",
"md.comp.outlined-icon-button.unselected.pressed.state-layer.color": "onSurface"
}
| flutter/dev/tools/gen_defaults/data/icon_button_outlined.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/icon_button_outlined.json",
"repo_id": "flutter",
"token_count": 988
} | 571 |
{
"version": "v0_206",
"md.sys.shape.corner.extra-large": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 28.0,
"topRight": 28.0,
"bottomLeft": 28.0,
"bottomRight": 28.0
},
"md.sys.shape.corner.extra-large.top": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 28.0,
"topRight": 28.0,
"bottomLeft": 0.0,
"bottomRight": 0.0
},
"md.sys.shape.corner.extra-small": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 4.0,
"topRight": 4.0,
"bottomLeft": 4.0,
"bottomRight": 4.0
},
"md.sys.shape.corner.extra-small.top": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 4.0,
"topRight": 4.0,
"bottomLeft": 0.0,
"bottomRight": 0.0
},
"md.sys.shape.corner.full": {
"family": "SHAPE_FAMILY_CIRCULAR"
},
"md.sys.shape.corner.large": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 16.0,
"topRight": 16.0,
"bottomLeft": 16.0,
"bottomRight": 16.0
},
"md.sys.shape.corner.large.end": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 0.0,
"topRight": 16.0,
"bottomLeft": 0.0,
"bottomRight": 16.0
},
"md.sys.shape.corner.large.start": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 16.0,
"topRight": 0.0,
"bottomLeft": 16.0,
"bottomRight": 0.0
},
"md.sys.shape.corner.large.top": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 16.0,
"topRight": 16.0,
"bottomLeft": 0.0,
"bottomRight": 0.0
},
"md.sys.shape.corner.medium": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 12.0,
"topRight": 12.0,
"bottomLeft": 12.0,
"bottomRight": 12.0
},
"md.sys.shape.corner.none": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 0.0,
"topRight": 0.0,
"bottomLeft": 0.0,
"bottomRight": 0.0
},
"md.sys.shape.corner.small": {
"family": "SHAPE_FAMILY_ROUNDED_CORNERS",
"topLeft": 8.0,
"topRight": 8.0,
"bottomLeft": 8.0,
"bottomRight": 8.0
}
}
| flutter/dev/tools/gen_defaults/data/shape.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/shape.json",
"repo_id": "flutter",
"token_count": 1038
} | 572 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class AppBarTemplate extends TokenTemplate {
const AppBarTemplate(super.blockName, super.fileName, super.tokens)
: super(
colorSchemePrefix: '_colors.',
textThemePrefix: '_textTheme.',
);
@override
String generate() => '''
class _${blockName}DefaultsM3 extends AppBarTheme {
_${blockName}DefaultsM3(this.context)
: super(
elevation: ${elevation('md.comp.top-app-bar.small.container')},
scrolledUnderElevation: ${elevation('md.comp.top-app-bar.small.on-scroll.container')},
titleSpacing: NavigationToolbar.kMiddleSpacing,
toolbarHeight: ${getToken('md.comp.top-app-bar.small.container.height')},
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
@override
Color? get backgroundColor => ${componentColor('md.comp.top-app-bar.small.container')};
@override
Color? get foregroundColor => ${color('md.comp.top-app-bar.small.headline.color')};
@override
Color? get shadowColor => ${colorOrTransparent('md.comp.top-app-bar.small.container.shadow-color')};
@override
Color? get surfaceTintColor => ${colorOrTransparent('md.comp.top-app-bar.small.container.surface-tint-layer.color')};
@override
IconThemeData? get iconTheme => IconThemeData(
color: ${componentColor('md.comp.top-app-bar.small.leading-icon')},
size: ${getToken('md.comp.top-app-bar.small.leading-icon.size')},
);
@override
IconThemeData? get actionsIconTheme => IconThemeData(
color: ${componentColor('md.comp.top-app-bar.small.trailing-icon')},
size: ${getToken('md.comp.top-app-bar.small.trailing-icon.size')},
);
@override
TextStyle? get toolbarTextStyle => _textTheme.bodyMedium;
@override
TextStyle? get titleTextStyle => ${textStyle('md.comp.top-app-bar.small.headline')};
}
// Variant configuration
class _MediumScrollUnderFlexibleConfig with _ScrollUnderFlexibleConfig {
_MediumScrollUnderFlexibleConfig(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
static const double collapsedHeight = ${getToken('md.comp.top-app-bar.small.container.height')};
static const double expandedHeight = ${getToken('md.comp.top-app-bar.medium.container.height')};
@override
TextStyle? get collapsedTextStyle =>
${textStyle('md.comp.top-app-bar.small.headline')}?.apply(color: ${color('md.comp.top-app-bar.small.headline.color')});
@override
TextStyle? get expandedTextStyle =>
${textStyle('md.comp.top-app-bar.medium.headline')}?.apply(color: ${color('md.comp.top-app-bar.medium.headline.color')});
@override
EdgeInsetsGeometry get expandedTitlePadding => const EdgeInsets.fromLTRB(16, 0, 16, 20);
}
class _LargeScrollUnderFlexibleConfig with _ScrollUnderFlexibleConfig {
_LargeScrollUnderFlexibleConfig(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
static const double collapsedHeight = ${getToken('md.comp.top-app-bar.small.container.height')};
static const double expandedHeight = ${getToken('md.comp.top-app-bar.large.container.height')};
@override
TextStyle? get collapsedTextStyle =>
${textStyle('md.comp.top-app-bar.small.headline')}?.apply(color: ${color('md.comp.top-app-bar.small.headline.color')});
@override
TextStyle? get expandedTextStyle =>
${textStyle('md.comp.top-app-bar.large.headline')}?.apply(color: ${color('md.comp.top-app-bar.large.headline.color')});
@override
EdgeInsetsGeometry get expandedTitlePadding => const EdgeInsets.fromLTRB(16, 0, 16, 28);
}
''';
}
| flutter/dev/tools/gen_defaults/lib/app_bar_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/app_bar_template.dart",
"repo_id": "flutter",
"token_count": 1371
} | 573 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class FilterChipTemplate extends TokenTemplate {
const FilterChipTemplate(super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
super.textThemePrefix = '_textTheme.'
});
static const String tokenGroup = 'md.comp.filter-chip';
static const String flatVariant = '.flat';
static const String elevatedVariant = '.elevated';
@override
String generate() => '''
class _${blockName}DefaultsM3 extends ChipThemeData {
_${blockName}DefaultsM3(
this.context,
this.isEnabled,
this.isSelected,
this._chipVariant,
) : super(
shape: ${shape("$tokenGroup.container")},
showCheckmark: true,
);
final BuildContext context;
final bool isEnabled;
final bool isSelected;
final _ChipVariant _chipVariant;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
double? get elevation => _chipVariant == _ChipVariant.flat
? ${elevation("$tokenGroup$flatVariant.container")}
: isEnabled ? ${elevation("$tokenGroup$elevatedVariant.container")} : ${elevation("$tokenGroup$elevatedVariant.disabled.container")};
@override
double? get pressElevation => ${elevation("$tokenGroup$elevatedVariant.pressed.container")};
@override
TextStyle? get labelStyle => ${textStyle("$tokenGroup.label-text")}?.copyWith(
color: isEnabled
? isSelected
? ${color("$tokenGroup.selected.label-text.color")}
: ${color("$tokenGroup.unselected.label-text.color")}
: ${color("$tokenGroup.disabled.label-text.color")},
);
@override
MaterialStateProperty<Color?>? get color =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected) && states.contains(MaterialState.disabled)) {
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.disabled.selected.container")}
: ${componentColor("$tokenGroup$elevatedVariant.disabled.container")};
}
if (states.contains(MaterialState.disabled)) {
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.disabled.unselected.container")}
: ${componentColor("$tokenGroup$elevatedVariant.disabled.container")};
}
if (states.contains(MaterialState.selected)) {
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.selected.container")}
: ${componentColor("$tokenGroup$elevatedVariant.selected.container")};
}
return _chipVariant == _ChipVariant.flat
? ${componentColor("$tokenGroup$flatVariant.container")}
: ${componentColor("$tokenGroup$elevatedVariant.unselected.container")};
});
@override
Color? get shadowColor => _chipVariant == _ChipVariant.flat
? ${colorOrTransparent("$tokenGroup$flatVariant.container.shadow-color")}
: ${colorOrTransparent("$tokenGroup$elevatedVariant.container.shadow-color")};
@override
Color? get surfaceTintColor => ${colorOrTransparent("$tokenGroup.container.surface-tint-layer.color")};
@override
Color? get checkmarkColor => isEnabled
? isSelected
? ${color("$tokenGroup.with-leading-icon.selected.leading-icon.color")}
: ${color("$tokenGroup.with-leading-icon.unselected.leading-icon.color")}
: ${color("$tokenGroup.with-leading-icon.disabled.leading-icon.color")};
@override
Color? get deleteIconColor => isEnabled
? isSelected
? ${color("$tokenGroup.with-trailing-icon.selected.trailing-icon.color")}
: ${color("$tokenGroup.with-trailing-icon.unselected.trailing-icon.color")}
: ${color("$tokenGroup.with-trailing-icon.disabled.trailing-icon.color")};
@override
BorderSide? get side => _chipVariant == _ChipVariant.flat && !isSelected
? isEnabled
? ${border('$tokenGroup$flatVariant.unselected.outline')}
: ${border('$tokenGroup$flatVariant.disabled.unselected.outline')}
: const BorderSide(color: Colors.transparent);
@override
IconThemeData? get iconTheme => IconThemeData(
color: isEnabled
? isSelected
? ${color("$tokenGroup.with-leading-icon.selected.leading-icon.color")}
: ${color("$tokenGroup.with-leading-icon.unselected.leading-icon.color")}
: ${color("$tokenGroup.with-leading-icon.disabled.leading-icon.color")},
size: ${getToken("$tokenGroup.with-icon.icon.size")},
);
@override
EdgeInsetsGeometry? get padding => const EdgeInsets.all(8.0);
/// The label padding of the chip scales with the font size specified in the
/// [labelStyle], and the system font size settings that scale font sizes
/// globally.
///
/// The chip at effective font size 14.0 starts with 8px on each side and as
/// the font size scales up to closer to 28.0, the label padding is linearly
/// interpolated from 8px to 4px. Once the label has a font size of 2 or
/// higher, label padding remains 4px.
@override
EdgeInsetsGeometry? get labelPadding {
final double fontSize = labelStyle?.fontSize ?? 14.0;
final double fontSizeRatio = MediaQuery.textScalerOf(context).scale(fontSize) / 14.0;
return EdgeInsets.lerp(
const EdgeInsets.symmetric(horizontal: 8.0),
const EdgeInsets.symmetric(horizontal: 4.0),
clampDouble(fontSizeRatio - 1.0, 0.0, 1.0),
)!;
}
}
''';
}
| flutter/dev/tools/gen_defaults/lib/filter_chip_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/filter_chip_template.dart",
"repo_id": "flutter",
"token_count": 1963
} | 574 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class SliderTemplate extends TokenTemplate {
const SliderTemplate(this.tokenGroup, super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
});
final String tokenGroup;
@override
String generate() => '''
class _${blockName}DefaultsM3 extends SliderThemeData {
_${blockName}DefaultsM3(this.context)
: super(trackHeight: ${getToken('$tokenGroup.active.track.height')});
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color? get activeTrackColor => ${componentColor('$tokenGroup.active.track')};
@override
Color? get inactiveTrackColor => ${componentColor('$tokenGroup.inactive.track')};
@override
Color? get secondaryActiveTrackColor => _colors.primary.withOpacity(0.54);
@override
Color? get disabledActiveTrackColor => ${componentColor('$tokenGroup.disabled.active.track')};
@override
Color? get disabledInactiveTrackColor => ${componentColor('$tokenGroup.disabled.inactive.track')};
@override
Color? get disabledSecondaryActiveTrackColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get activeTickMarkColor => ${componentColor('$tokenGroup.with-tick-marks.active.container')};
@override
Color? get inactiveTickMarkColor => ${componentColor('$tokenGroup.with-tick-marks.inactive.container')};
@override
Color? get disabledActiveTickMarkColor => ${componentColor('$tokenGroup.with-tick-marks.disabled.container')};
@override
Color? get disabledInactiveTickMarkColor => ${componentColor('$tokenGroup.with-tick-marks.disabled.container')};
@override
Color? get thumbColor => ${componentColor('$tokenGroup.handle')};
@override
Color? get disabledThumbColor => Color.alphaBlend(${componentColor('$tokenGroup.disabled.handle')}, _colors.surface);
@override
Color? get overlayColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.dragged)) {
return ${componentColor('$tokenGroup.pressed.state-layer')};
}
if (states.contains(MaterialState.hovered)) {
return ${componentColor('$tokenGroup.hover.state-layer')};
}
if (states.contains(MaterialState.focused)) {
return ${componentColor('$tokenGroup.focus.state-layer')};
}
return Colors.transparent;
});
@override
TextStyle? get valueIndicatorTextStyle => ${textStyle('$tokenGroup.label.label-text')}!.copyWith(
color: ${componentColor('$tokenGroup.label.label-text')},
);
@override
SliderComponentShape? get valueIndicatorShape => const DropSliderValueIndicatorShape();
}
''';
}
| flutter/dev/tools/gen_defaults/lib/slider_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/slider_template.dart",
"repo_id": "flutter",
"token_count": 874
} | 575 |
## Files
### General
| File name | Explanation |
| ---- | ---- |
| [`physical_key_data.g.json`](physical_key_data.g.json) | Contains the merged physical key data from all the other sources. This file is regenerated if "--collect" is specified for the gen_keycodes script, or used as a source otherwise. |
| [`logical_key_data.g.json`](logical_key_data.g.json) | Contains the merged logical key data from all the other sources. This file is regenerated if "--collect" is specified for the gen_keycodes script, or used as a source otherwise. |
| [`supplemental_hid_codes.inc`](supplemental_hid_codes.inc) | A supplementary HID list on top of Chromium's list of HID codes for extra physical keys. Certain entries may also overwrite Chromium's corresponding entries. |
| [`supplemental_key_data.inc`](supplemental_key_data.inc) | A supplementary key list on top of Chromium's list of keys for extra logical keys.|
| [`chromium_modifiers.json`](chromium_modifiers.json) | Maps the web's `key` for modifier keys to the names of the logical keys for these keys' left and right variations.This is used when generating logical keys to provide independent values for sided logical keys. Web uses the same `key` for modifier keys of different sides, but Flutter's logical key model treats them as different keys.|
| [`printable.json`](printable.json) | Maps Flutter key name to its printable character. This character is used as the key label.|
| [`synonyms.json`](synonyms.json) | Maps pseudo-keys that represent other keys to the sets of keys they represent. For example, this contains the "shift" key that represents either a "shiftLeft" or "shiftRight" key.|
| [`layout_goals.json`](layout_goals.json) | A list of layout goals, keys that the platform keyboard manager should find mappings for. Each key in this file is the key name of the goal, both logical and physical simultaneously, while its value represents whether the goal is mandatory. A mandatory goal must be fulfilled, and the manager will use the default value from this file if a mapping can not be found. A non-mandatory goal is suggestive, only used if the key mapping information is malformed (e.g. contains no ASCII characters.) |
### Framework
| File name | Explanation |
| ---- | ---- |
| [`keyboard_key.tmpl`](keyboard_key.tmpl) | The template for `keyboard_key.g.dart`. |
| [`keyboard_maps.tmpl`](keyboard_maps.tmpl) | The template for `keyboard_maps.g.dart`. |
### Android
| File name | Explanation |
| ---- | ---- |
| [`android_keyboard_map_java.tmpl`](android_keyboard_map_java.tmpl) | The template for `KeyboardMap.java`. |
| [`android_key_name_to_name.json`](android_key_name_to_name.json) | Maps a logical key name to the names of its corresponding keycode constants. This is used to convert logical keys.|
### iOS
| File name | Explanation |
| ---- | ---- |
| [`ios_logical_to_physical.json`](ios_logical_to_physical.json) | Maps a logical key name to the names of its corresponding physical keys. This is used to derive logical keys (from `keyCode`) that can't or shouldn't be derived from `characterIgnoringModifiers`. |
| [`ios_key_code_map_mm.tmpl`](ios_key_code_map_mm.tmpl) | The template for `KeyCodeMap.mm`.|
### Web
| File name | Explanation |
| ---- | ---- |
| [`web_key_map_dart.tmpl`](web_key_map_dart.tmpl) | The template for `key_map.dart`. |
| [`web_logical_location_mapping.json`](web_logical_location_mapping.json) | Maps a pair of the web's `key` and `location` to the name for its corresponding logical key. This is used to distinguish between logical keys with the same `key` but different `locations`. |
### Windows
| File name | Explanation |
| ---- | ---- |
| [`windows_flutter_key_map_cc.tmpl`](windows_flutter_key_map_cc.tmpl) | The template for `flutter_key_map.cc`. |
| [`windows_logical_to_window_vk.json`](windows_logical_to_window_vk.json) | Maps a logical key name to the names of its corresponding virtual keys in Win32. |
| [`windows_scancode_logical_map.json`](windows_scancode_logical_map.json) | Maps a physical key name to a logical key name. This is used to when a `keycode` maps to multiple keys (including when the `keycode` is 0), therefore can only be told apart by the scan code. |
### Linux (GTK)
| File name | Explanation |
| ---- | ---- |
| [`gtk_key_mapping_cc.tmpl`](gtk_key_mapping_cc.tmpl) | The template for `key_mapping.cc`. |
| [`gtk_lock_bit_mapping.json`](gtk_lock_bit_mapping.json) | Maps a name for GTK's modifier bit macro to Flutter's logical name (element #0) and physical name (element #1). This is used to generate checked keys that GTK should keep lock state synchronous on.|
| [`gtk_logical_name_mapping.json`](gtk_logical_name_mapping.json) | Maps a logical key name to the macro names of its corresponding `keyval`s. This is used to convert logical keys.|
| [`gtk_modifier_bit_mapping.json`](gtk_modifier_bit_mapping.json) | Maps a name for GTK's modifier bit macro to Flutter's physical name (element #0), logical name (element #1), and the logical name for the paired key (element #2). This is used to generate checked keys where GTK should keep the pressed state synchronized.|
| [`gtk_numpad_shift.json`](gtk_numpad_shift.json) | Maps the name of a `keyval` macro of a numpad key to that of the corresponding key with NumLock on. GTK uses different `keyval` for numpad keys with and without NumLock on, but Flutter's logical key model treats them as the same key.|
### Linux (GLFW)
| File name | Explanation |
| ---- | ---- |
| [`glfw_key_name_to_name.json`](glfw_key_name_to_name.json) | Maps a logical key name to the names of its GLFW macro. (Unused for now.) |
| [`glfw_keyboard_map_cc.tmpl`](glfw_keyboard_map_cc.tmpl) | The template for `keyboard_map.cc`. (Unused for now.) |
### macOS
| File name | Explanation |
| ---- | ---- |
| [`macos_key_code_map_cc.tmpl`](macos_key_code_map_cc.tmpl) | The template for `KeyCodeMap.mm`. |
| [`macos_logical_to_physical.json`](macos_logical_to_physical.json) | Maps a logical key name to the names of its corresponding physical keys. This is used to derive logical keys (from `keyCode`) that can't or shouldn't be derived from `characterIgnoringModifiers`. |
### Fuchsia
| File name | Explanation |
| ---- | ---- |
| [`fuchsia_keyboard_map_cc.tmpl`](fuchsia_keyboard_map_cc.tmpl) | The template for `keyboard_map.cc`. (Unused for now.) |
| flutter/dev/tools/gen_keycodes/data/README.md/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/README.md",
"repo_id": "flutter",
"token_count": 1973
} | 576 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
// DO NOT EDIT -- DO NOT EDIT -- DO NOT EDIT
// This file is generated by dev/tools/gen_keycodes/bin/gen_keycodes.dart and
// should not be edited directly.
//
// Edit the template dev/tools/gen_keycodes/data/keyboard_key.tmpl instead.
// See dev/tools/gen_keycodes/README.md for more information.
/// A base class for all keyboard key types.
///
/// See also:
///
/// * [PhysicalKeyboardKey], a class with static values that describe the keys
/// that are returned from [RawKeyEvent.physicalKey].
/// * [LogicalKeyboardKey], a class with static values that describe the keys
/// that are returned from [RawKeyEvent.logicalKey].
abstract class KeyboardKey with Diagnosticable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const KeyboardKey();
}
/// A class with static values that describe the keys that are returned from
/// [RawKeyEvent.logicalKey].
///
/// These represent *logical* keys, which are keys which are interpreted in the
/// context of any modifiers, modes, or keyboard layouts which may be in effect.
///
/// This is contrast to [PhysicalKeyboardKey], which represents a physical key
/// in a particular location on the keyboard, without regard for the modifier
/// state, mode, or keyboard layout.
///
/// As an example, if you wanted to implement an app where the "Q" key "quit"
/// something, you'd want to look at the logical key to detect this, since you
/// would like to have it match the key with "Q" on it, instead of always
/// looking for "the key next to the TAB key", since on a French keyboard,
/// the key next to the TAB key has an "A" on it.
///
/// Conversely, if you wanted a game where the key next to the CAPS LOCK (the
/// "A" key on a QWERTY keyboard) moved the player to the left, you'd want to
/// look at the physical key to make sure that regardless of the character the
/// key produces, you got the key that is in that location on the keyboard.
///
/// {@tool dartpad}
/// This example shows how to detect if the user has selected the logical "Q"
/// key and handle the key if they have.
///
/// ** See code in examples/api/lib/services/keyboard_key/logical_keyboard_key.0.dart **
/// {@end-tool}
/// See also:
///
/// * [RawKeyEvent], the keyboard event object received by widgets that listen
/// to keyboard events.
/// * [Focus.onKey], the handler on a widget that lets you handle key events.
/// * [RawKeyboardListener], a widget used to listen to keyboard events (but
/// not handle them).
@immutable
class LogicalKeyboardKey extends KeyboardKey {
/// Creates a new LogicalKeyboardKey object for a key ID.
const LogicalKeyboardKey(this.keyId);
/// A unique code representing this key.
///
/// This is an opaque code. It should not be unpacked to derive information
/// from it, as the representation of the code could change at any time.
final int keyId;
// Returns the bits that are not included in [valueMask], shifted to the
// right.
//
// For example, if the input is 0x12abcdabcd, then the result is 0x12.
//
// This is mostly equivalent to a right shift, resolving the problem that
// JavaScript only support 32-bit bitwise operation and needs to use division
// instead.
static int _nonValueBits(int n) {
// `n >> valueMaskWidth` is equivalent to `n / divisorForValueMask`.
const int divisorForValueMask = valueMask + 1;
const int valueMaskWidth = 32;
// Equivalent to assert(divisorForValueMask == (1 << valueMaskWidth)).
const int firstDivisorWidth = 28;
assert(divisorForValueMask ==
(1 << firstDivisorWidth) * (1 << (valueMaskWidth - firstDivisorWidth)));
// JS only supports up to 2^53 - 1, therefore non-value bits can only
// contain (maxSafeIntegerWidth - valueMaskWidth) bits.
const int maxSafeIntegerWidth = 52;
const int nonValueMask = (1 << (maxSafeIntegerWidth - valueMaskWidth)) - 1;
if (kIsWeb) {
return (n / divisorForValueMask).floor() & nonValueMask;
} else {
return (n >> valueMaskWidth) & nonValueMask;
}
}
static String? _unicodeKeyLabel(int keyId) {
if (_nonValueBits(keyId) == 0) {
return String.fromCharCode(keyId).toUpperCase();
}
return null;
}
/// A description representing the character produced by a [RawKeyEvent].
///
/// This value is useful for providing readable strings for keys or keyboard
/// shortcuts. Do not use this value to compare equality of keys; compare
/// [keyId] instead.
///
/// For printable keys, this is usually the printable character in upper case
/// ignoring modifiers or combining keys, such as 'A', '1', or '/'. This
/// might also return accented letters (such as 'Ù') for keys labeled as so,
/// but not if such character is a result from preceding combining keys ('`̀'
/// followed by key U).
///
/// For other keys, [keyLabel] looks up the full key name from a predefined
/// map, such as 'F1', 'Shift Left', or 'Media Down'. This value is an empty
/// string if there's no key label data for a key.
///
/// For the printable representation that takes into consideration the
/// modifiers and combining keys, see [RawKeyEvent.character].
///
/// {@macro flutter.services.RawKeyEventData.keyLabel}
String get keyLabel {
return _unicodeKeyLabel(keyId)
?? _keyLabels[keyId]
?? '';
}
/// The debug string to print for this keyboard key, which will be null in
/// release mode.
///
/// For printable keys, this is usually a more descriptive name related to
/// [keyLabel], such as 'Key A', 'Digit 1', 'Backslash'. This might
/// also return accented letters (such as 'Key Ù') for keys labeled as so.
///
/// For other keys, this looks up the full key name from a predefined map (the
/// same value as [keyLabel]), such as 'F1', 'Shift Left', or 'Media Down'. If
/// there's no key label data for a key, this returns a name that explains the
/// ID (such as 'Key with ID 0x00100012345').
String? get debugName {
String? result;
assert(() {
result = _keyLabels[keyId];
if (result == null) {
final String? unicodeKeyLabel = _unicodeKeyLabel(keyId);
if (unicodeKeyLabel != null) {
result = 'Key $unicodeKeyLabel';
} else {
result = 'Key with ID 0x${keyId.toRadixString(16).padLeft(11, '0')}';
}
}
return true;
}());
return result;
}
@override
int get hashCode => keyId.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is LogicalKeyboardKey
&& other.keyId == keyId;
}
/// Returns the [LogicalKeyboardKey] constant that matches the given ID, or
/// null, if not found.
static LogicalKeyboardKey? findKeyByKeyId(int keyId) => _knownLogicalKeys[keyId];
/// Returns true if the given label represents a Unicode control character.
///
/// Examples of control characters are characters like "U+000A LINE FEED (LF)"
/// or "U+001B ESCAPE (ESC)".
///
/// See <https://en.wikipedia.org/wiki/Unicode_control_characters> for more
/// information.
///
/// Used by [RawKeyEvent] subclasses to help construct IDs.
static bool isControlCharacter(String label) {
if (label.length != 1) {
return false;
}
final int codeUnit = label.codeUnitAt(0);
return (codeUnit <= 0x1f && codeUnit >= 0x00) || (codeUnit >= 0x7f && codeUnit <= 0x9f);
}
/// Returns true if the [keyId] of this object is one that is auto-generated by
/// Flutter.
///
/// Auto-generated key IDs are generated in response to platform key codes
/// which Flutter doesn't recognize, and their IDs shouldn't be used in a
/// persistent way.
///
/// Auto-generated IDs should be a rare occurrence: Flutter supports most keys.
///
/// Keys that generate Unicode characters (even if unknown to Flutter) will
/// not return true for [isAutogenerated], since they will be assigned a
/// Unicode-based code that will remain stable.
///
/// If Flutter adds support for a previously unsupported key code, the ID it
/// reports will change, but the ID will remain stable on the platform it is
/// produced on until Flutter adds support for recognizing it.
///
/// So, hypothetically, if Android added a new key code of 0xffff,
/// representing a new "do what I mean" key, then the auto-generated code
/// would be 0x1020000ffff, but once Flutter added the "doWhatIMean" key to
/// the definitions below, the new code would be 0x0020000ffff for all
/// platforms that had a "do what I mean" key from then on.
bool get isAutogenerated => (keyId & planeMask) >= startOfPlatformPlanes;
/// Returns a set of pseudo-key synonyms for the given `key`.
///
/// This allows finding the pseudo-keys that also represent a concrete `key`
/// so that a class with a key map can match pseudo-keys as well as the actual
/// generated keys.
///
/// Pseudo-keys returned in the set are typically used to represent keys which
/// appear in multiple places on the keyboard, such as the [shift], [alt],
/// [control], and [meta] keys. Pseudo-keys in the returned set won't ever be
/// generated directly, but if a more specific key event is received, then
/// this set can be used to find the more general pseudo-key. For example, if
/// this is a [shiftLeft] key, this accessor will return the set
/// `<LogicalKeyboardKey>{ shift }`.
Set<LogicalKeyboardKey> get synonyms => _synonyms[this] ?? <LogicalKeyboardKey>{};
/// Takes a set of keys, and returns the same set, but with any keys that have
/// synonyms replaced.
///
/// It is used, for example, to take sets of keys with members like
/// [controlRight] and [controlLeft] and convert that set to contain just
/// [control], so that the question "is any control key down?" can be asked.
static Set<LogicalKeyboardKey> collapseSynonyms(Set<LogicalKeyboardKey> input) {
return input.expand((LogicalKeyboardKey element) {
return _synonyms[element] ?? <LogicalKeyboardKey>{element};
}).toSet();
}
/// Returns the given set with any pseudo-keys expanded into their synonyms.
///
/// It is used, for example, to take sets of keys with members like [control]
/// and [shift] and convert that set to contain [controlLeft], [controlRight],
/// [shiftLeft], and [shiftRight].
static Set<LogicalKeyboardKey> expandSynonyms(Set<LogicalKeyboardKey> input) {
return input.expand((LogicalKeyboardKey element) {
return _reverseSynonyms[element] ?? <LogicalKeyboardKey>{element};
}).toSet();
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('keyId', '0x${keyId.toRadixString(16).padLeft(8, '0')}'));
properties.add(StringProperty('keyLabel', keyLabel));
properties.add(StringProperty('debugName', debugName, defaultValue: null));
}
@@@MASK_CONSTANTS@@@
@@@LOGICAL_KEY_DEFINITIONS@@@
/// A list of all predefined constant [LogicalKeyboardKey]s.
static Iterable<LogicalKeyboardKey> get knownLogicalKeys => _knownLogicalKeys.values;
// A list of all predefined constant LogicalKeyboardKeys so they can be
// searched.
static const Map<int, LogicalKeyboardKey> _knownLogicalKeys = <int, LogicalKeyboardKey>{
@@@LOGICAL_KEY_MAP@@@
};
// A map of keys to the pseudo-key synonym for that key.
static final Map<LogicalKeyboardKey, Set<LogicalKeyboardKey>> _synonyms = <LogicalKeyboardKey, Set<LogicalKeyboardKey>>{
@@@LOGICAL_KEY_SYNONYMS@@@ };
// A map of pseudo-key to the set of keys that are synonyms for that pseudo-key.
static final Map<LogicalKeyboardKey, Set<LogicalKeyboardKey>> _reverseSynonyms = <LogicalKeyboardKey, Set<LogicalKeyboardKey>>{
@@@LOGICAL_KEY_REVERSE_SYNONYMS@@@ };
static const Map<int, String> _keyLabels = <int, String>{
@@@LOGICAL_KEY_KEY_LABELS@@@
};
}
/// A class with static values that describe the keys that are returned from
/// [RawKeyEvent.physicalKey].
///
/// These represent *physical* keys, which are keys which represent a particular
/// key location on a QWERTY keyboard. It ignores any modifiers, modes, or
/// keyboard layouts which may be in effect. This is contrast to
/// [LogicalKeyboardKey], which represents a logical key interpreted in the
/// context of modifiers, modes, and/or keyboard layouts.
///
/// As an example, if you wanted a game where the key next to the CAPS LOCK (the
/// "A" key on a QWERTY keyboard) moved the player to the left, you'd want to
/// look at the physical key to make sure that regardless of the character the
/// key produces, you got the key that is in that location on the keyboard.
///
/// Conversely, if you wanted to implement an app where the "Q" key "quit"
/// something, you'd want to look at the logical key to detect this, since you
/// would like to have it match the key with "Q" on it, instead of always
/// looking for "the key next to the TAB key", since on a French keyboard,
/// the key next to the TAB key has an "A" on it.
///
/// {@tool dartpad}
/// This example shows how to detect if the user has selected the physical key
/// to the right of the CAPS LOCK key.
///
/// ** See code in examples/api/lib/services/keyboard_key/physical_keyboard_key.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RawKeyEvent], the keyboard event object received by widgets that listen
/// to keyboard events.
/// * [Focus.onKey], the handler on a widget that lets you handle key events.
/// * [RawKeyboardListener], a widget used to listen to keyboard events (but
/// not handle them).
@immutable
class PhysicalKeyboardKey extends KeyboardKey {
/// Creates a new PhysicalKeyboardKey object for a USB HID usage.
const PhysicalKeyboardKey(this.usbHidUsage);
/// The unique USB HID usage ID of this physical key on the keyboard.
///
/// Due to the variations in platform APIs, this may not be the actual HID
/// usage code from the hardware, but a value derived from available
/// information on the platform.
///
/// See <https://www.usb.org/sites/default/files/documents/hut1_12v2.pdf>
/// for the HID usage values and their meanings.
final int usbHidUsage;
/// The debug string to print for this keyboard key, which will be null in
/// release mode.
String? get debugName {
String? result;
assert(() {
result = _debugNames[usbHidUsage] ??
'Key with ID 0x${usbHidUsage.toRadixString(16).padLeft(8, '0')}';
return true;
}());
return result;
}
@override
int get hashCode => usbHidUsage.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is PhysicalKeyboardKey
&& other.usbHidUsage == usbHidUsage;
}
/// Finds a known [PhysicalKeyboardKey] that matches the given USB HID usage
/// code.
static PhysicalKeyboardKey? findKeyByCode(int usageCode) => _knownPhysicalKeys[usageCode];
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('usbHidUsage', '0x${usbHidUsage.toRadixString(16).padLeft(8, '0')}'));
properties.add(StringProperty('debugName', debugName, defaultValue: null));
}
// Key constants for all keyboard keys in the USB HID specification at the
// time Flutter was built.
@@@PHYSICAL_KEY_DEFINITIONS@@@
/// A list of all predefined constant [PhysicalKeyboardKey]s.
static Iterable<PhysicalKeyboardKey> get knownPhysicalKeys => _knownPhysicalKeys.values;
// A list of all the predefined constant PhysicalKeyboardKeys so that they
// can be searched.
static const Map<int, PhysicalKeyboardKey> _knownPhysicalKeys = <int, PhysicalKeyboardKey>{
@@@PHYSICAL_KEY_MAP@@@
};
static const Map<int, String> _debugNames = kReleaseMode ?
<int, String>{} :
<int, String>{
@@@PHYSICAL_KEY_DEBUG_NAMES@@@
};
}
| flutter/dev/tools/gen_keycodes/data/keyboard_key.tmpl/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/keyboard_key.tmpl",
"repo_id": "flutter",
"token_count": 5027
} | 577 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:path/path.dart' as path;
import 'base_code_gen.dart';
import 'constants.dart';
import 'logical_key_data.dart';
import 'physical_key_data.dart';
import 'utils.dart';
/// Generates the key mapping for Android, based on the information in the key
/// data structure given to it.
class AndroidCodeGenerator extends PlatformCodeGenerator {
AndroidCodeGenerator(super.keyData, super.logicalData);
/// This generates the map of Android key codes to logical keys.
String get _androidKeyCodeMap {
final StringBuffer androidKeyCodeMap = StringBuffer();
for (final LogicalKeyEntry entry in logicalData.entries) {
for (final int code in entry.androidValues) {
androidKeyCodeMap.writeln(' put(${toHex(code, digits: 10)}L, ${toHex(entry.value, digits: 10)}L); // ${entry.constantName}');
}
}
return androidKeyCodeMap.toString().trimRight();
}
/// This generates the map of Android scan codes to physical keys.
String get _androidScanCodeMap {
final StringBuffer androidScanCodeMap = StringBuffer();
for (final PhysicalKeyEntry entry in keyData.entries) {
for (final int code in entry.androidScanCodes.cast<int>()) {
androidScanCodeMap.writeln(' put(${toHex(code, digits: 10)}L, ${toHex(entry.usbHidCode, digits: 10)}L); // ${entry.constantName}');
}
}
return androidScanCodeMap.toString().trimRight();
}
String get _pressingGoals {
final OutputLines<int> lines = OutputLines<int>('Android pressing goals');
const Map<String, List<String>> goalsSource = <String, List<String>>{
'SHIFT': <String>['ShiftLeft', 'ShiftRight'],
'CTRL': <String>['ControlLeft', 'ControlRight'],
'ALT': <String>['AltLeft', 'AltRight'],
};
goalsSource.forEach((String flagName, List<String> keys) {
int? lineId;
final List<String> keysString = keys.map((String keyName) {
final PhysicalKeyEntry physicalKey = keyData.entryByName(keyName);
final LogicalKeyEntry logicalKey = logicalData.entryByName(keyName);
lineId ??= physicalKey.usbHidCode;
return ' new KeyPair(${toHex(physicalKey.usbHidCode)}L, '
'${toHex(logicalKey.value, digits: 10)}L), // ${physicalKey.name}';
}).toList();
lines.add(lineId!,
' new PressingGoal(\n'
' KeyEvent.META_${flagName}_ON,\n'
' new KeyPair[] {\n'
'${keysString.join('\n')}\n'
' }),');
});
return lines.sortedJoin().trimRight();
}
String get _togglingGoals {
final OutputLines<int> lines = OutputLines<int>('Android toggling goals');
const Map<String, String> goalsSource = <String, String>{
'CAPS_LOCK': 'CapsLock',
};
goalsSource.forEach((String flagName, String keyName) {
final PhysicalKeyEntry physicalKey = keyData.entryByName(keyName);
final LogicalKeyEntry logicalKey = logicalData.entryByName(keyName);
lines.add(physicalKey.usbHidCode,
' new TogglingGoal(KeyEvent.META_${flagName}_ON, '
'${toHex(physicalKey.usbHidCode)}L, '
'${toHex(logicalKey.value, digits: 10)}L),');
});
return lines.sortedJoin().trimRight();
}
/// This generates the mask values for the part of a key code that defines its plane.
String get _maskConstants {
final StringBuffer buffer = StringBuffer();
const List<MaskConstant> maskConstants = <MaskConstant>[
kValueMask,
kUnicodePlane,
kAndroidPlane,
];
for (final MaskConstant constant in maskConstants) {
buffer.writeln(' public static final long k${constant.upperCamelName} = ${toHex(constant.value, digits: 11)}L;');
}
return buffer.toString().trimRight();
}
@override
String get templatePath => path.join(dataRoot, 'android_keyboard_map_java.tmpl');
@override
String outputPath(String platform) => path.join(PlatformCodeGenerator.engineRoot, 'shell', 'platform',
path.join('android', 'io', 'flutter', 'embedding', 'android', 'KeyboardMap.java'));
@override
Map<String, String> mappings() {
return <String, String>{
'ANDROID_SCAN_CODE_MAP': _androidScanCodeMap,
'ANDROID_KEY_CODE_MAP': _androidKeyCodeMap,
'PRESSING_GOALS': _pressingGoals,
'TOGGLING_GOALS': _togglingGoals,
'MASK_CONSTANTS': _maskConstants,
};
}
}
| flutter/dev/tools/gen_keycodes/lib/android_code_gen.dart/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/lib/android_code_gen.dart",
"repo_id": "flutter",
"token_count": 1755
} | 578 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file/file.dart';
import 'package:file/memory.dart';
import 'package:platform/platform.dart';
import 'package:pub_semver/pub_semver.dart';
import 'package:test/test.dart';
import '../../../packages/flutter_tools/test/src/fake_process_manager.dart';
import '../create_api_docs.dart' as apidocs;
import '../dartdoc_checker.dart';
void main() {
group('FlutterInformation', () {
late FakeProcessManager fakeProcessManager;
late FakePlatform fakePlatform;
late MemoryFileSystem memoryFileSystem;
late apidocs.FlutterInformation flutterInformation;
void setUpWithEnvironment(Map<String, String> environment) {
fakePlatform = FakePlatform(environment: environment);
flutterInformation = apidocs.FlutterInformation(
filesystem: memoryFileSystem,
processManager: fakeProcessManager,
platform: fakePlatform,
);
apidocs.FlutterInformation.instance = flutterInformation;
}
setUp(() {
fakeProcessManager = FakeProcessManager.empty();
memoryFileSystem = MemoryFileSystem();
setUpWithEnvironment(<String, String>{});
});
test('getBranchName does not call git if env LUCI_BRANCH provided', () {
setUpWithEnvironment(
<String, String>{
'LUCI_BRANCH': branchName,
},
);
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['flutter', '--version', '--machine'],
stdout: testVersionInfo,
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
expect(
apidocs.FlutterInformation.instance.getBranchName(),
branchName,
);
expect(fakeProcessManager, hasNoRemainingExpectations);
});
test('getBranchName calls git if env LUCI_BRANCH not provided', () {
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['flutter', '--version', '--machine'],
stdout: testVersionInfo,
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
expect(
apidocs.FlutterInformation.instance.getBranchName(),
branchName,
);
expect(fakeProcessManager, hasNoRemainingExpectations);
});
test('getBranchName calls git if env LUCI_BRANCH is empty', () {
setUpWithEnvironment(
<String, String>{
'LUCI_BRANCH': '',
},
);
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['flutter', '--version', '--machine'],
stdout: testVersionInfo,
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
expect(
apidocs.FlutterInformation.instance.getBranchName(),
branchName,
);
expect(fakeProcessManager, hasNoRemainingExpectations);
});
test("runPubProcess doesn't use the pub binary", () {
final Platform platform = FakePlatform(
environment: <String, String>{
'FLUTTER_ROOT': '/flutter',
},
);
final ProcessManager processManager = FakeProcessManager.list(
<FakeCommand>[
const FakeCommand(
command: <String>['/flutter/bin/flutter', 'pub', '--one', '--two'],
),
],
);
apidocs.FlutterInformation.instance =
apidocs.FlutterInformation(platform: platform, processManager: processManager, filesystem: memoryFileSystem);
apidocs.runPubProcess(
arguments: <String>['--one', '--two'],
processManager: processManager,
filesystem: memoryFileSystem,
);
expect(processManager, hasNoRemainingExpectations);
});
test('calls out to flutter if FLUTTER_VERSION is not set', () async {
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['flutter', '--version', '--machine'],
stdout: testVersionInfo,
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
final Map<String, dynamic> info = flutterInformation.getFlutterInformation();
expect(fakeProcessManager, hasNoRemainingExpectations);
expect(info['frameworkVersion'], equals(Version.parse('2.5.0')));
});
test("doesn't call out to flutter if FLUTTER_VERSION is set", () async {
setUpWithEnvironment(<String, String>{
'FLUTTER_VERSION': testVersionInfo,
});
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
final Map<String, dynamic> info = flutterInformation.getFlutterInformation();
expect(fakeProcessManager, hasNoRemainingExpectations);
expect(info['frameworkVersion'], equals(Version.parse('2.5.0')));
});
test('getFlutterRoot calls out to flutter if FLUTTER_ROOT is not set', () async {
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['flutter', '--version', '--machine'],
stdout: testVersionInfo,
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
));
fakeProcessManager.addCommand(const FakeCommand(
command: <Pattern>['git', 'rev-parse', 'HEAD'],
));
final Directory root = flutterInformation.getFlutterRoot();
expect(fakeProcessManager, hasNoRemainingExpectations);
expect(root.path, equals('/home/user/flutter'));
});
test("getFlutterRoot doesn't call out to flutter if FLUTTER_ROOT is set", () async {
setUpWithEnvironment(<String, String>{'FLUTTER_ROOT': '/home/user/flutter'});
final Directory root = flutterInformation.getFlutterRoot();
expect(fakeProcessManager, hasNoRemainingExpectations);
expect(root.path, equals('/home/user/flutter'));
});
test('parses version properly', () async {
fakePlatform.environment['FLUTTER_VERSION'] = testVersionInfo;
fakeProcessManager.addCommands(<FakeCommand>[
const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
),
const FakeCommand(
command: <String>['git', 'rev-parse', 'HEAD'],
),
]);
final Map<String, dynamic> info = flutterInformation.getFlutterInformation();
expect(info['frameworkVersion'], isNotNull);
expect(info['frameworkVersion'], equals(Version.parse('2.5.0')));
expect(info['dartSdkVersion'], isNotNull);
expect(info['dartSdkVersion'], equals(Version.parse('2.14.0-360.0.dev')));
});
test('the engine realm is read from the engine.realm file', () async {
final Directory flutterHome = memoryFileSystem
.directory('/home')
.childDirectory('user')
.childDirectory('flutter')
.childDirectory('bin')
.childDirectory('internal');
flutterHome.childFile('engine.realm')
..createSync(recursive: true)
..writeAsStringSync('realm');
setUpWithEnvironment(<String, String>{'FLUTTER_ROOT': '/home/user/flutter'});
fakeProcessManager.addCommands(<FakeCommand>[
const FakeCommand(
command: <Pattern>['/home/user/flutter/bin/flutter', '--version', '--machine'],
stdout: testVersionInfo,
),
const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
),
const FakeCommand(
command: <String>['git', 'rev-parse', 'HEAD'],
),
]);
final Map<String, dynamic> info = flutterInformation.getFlutterInformation();
expect(fakeProcessManager, hasNoRemainingExpectations);
expect(info['engineRealm'], equals('realm'));
});
});
group('DartDocGenerator', () {
late apidocs.DartdocGenerator generator;
late MemoryFileSystem fs;
late FakeProcessManager processManager;
late Directory publishRoot;
setUp(() {
fs = MemoryFileSystem.test();
publishRoot = fs.directory('/path/to/publish');
processManager = FakeProcessManager.empty();
generator = apidocs.DartdocGenerator(
packageRoot: fs.directory('/path/to/package'),
publishRoot: publishRoot,
docsRoot: fs.directory('/path/to/docs'),
filesystem: fs,
processManager: processManager,
);
final Directory repoRoot = fs.directory('/flutter');
repoRoot.childDirectory('packages').createSync(recursive: true);
apidocs.FlutterInformation.instance = apidocs.FlutterInformation(
filesystem: fs,
processManager: processManager,
platform: FakePlatform(environment: <String, String>{
'FLUTTER_ROOT': repoRoot.path,
}),
);
});
test('.generateDartDoc() invokes dartdoc with the correct command line arguments', () async {
processManager.addCommands(<FakeCommand>[
const FakeCommand(command: <String>['/flutter/bin/flutter', 'pub', 'get']),
const FakeCommand(
command: <String>['/flutter/bin/flutter', '--version', '--machine'],
stdout: testVersionInfo,
),
const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
),
const FakeCommand(
command: <String>['git', 'rev-parse', 'HEAD'],
),
const FakeCommand(
command: <String>['/flutter/bin/flutter', 'pub', 'global', 'list'],
),
FakeCommand(
command: <Pattern>[
'/flutter/bin/flutter',
'pub',
'global',
'run',
'--enable-asserts',
'dartdoc',
'--output',
'/path/to/publish/flutter',
'--allow-tools',
'--json',
'--validate-links',
'--link-to-source-excludes',
'/flutter/bin/cache',
'--link-to-source-root',
'/flutter',
'--link-to-source-uri-template',
'https://github.com/flutter/flutter/blob/main/%f%#L%l%',
'--inject-html',
'--use-base-href',
'--header',
'/path/to/docs/styles.html',
'--header',
'/path/to/docs/analytics-header.html',
'--header',
'/path/to/docs/survey.html',
'--header',
'/path/to/docs/snippets.html',
'--header',
'/path/to/docs/opensearch.html',
'--footer',
'/path/to/docs/analytics-footer.html',
'--footer-text',
'/path/to/package/footer.html',
'--allow-warnings-in-packages',
// match package names
RegExp(r'^(\w+,)+(\w+)$'),
'--exclude-packages',
RegExp(r'^(\w+,)+(\w+)$'),
'--exclude',
// match dart package URIs
RegExp(r'^([\w\/:.]+,)+([\w\/:.]+)$'),
'--favicon',
'/path/to/docs/favicon.ico',
'--package-order',
'flutter,Dart,${apidocs.kPlatformIntegrationPackageName},flutter_test,flutter_driver',
'--auto-include-dependencies',
],
),
]);
// This will throw while sanity checking generated files, which is tested independently
await expectLater(
() => generator.generateDartdoc(),
throwsA(
isA<Exception>().having(
(Exception e) => e.toString(),
'message',
contains(RegExp(r'Missing .* which probably means the documentation failed to build correctly.')),
),
),
);
expect(processManager, hasNoRemainingExpectations);
});
test('sanity checks spot check generated files', () async {
processManager.addCommands(<FakeCommand>[
const FakeCommand(command: <String>['/flutter/bin/flutter', 'pub', 'get']),
const FakeCommand(
command: <String>['/flutter/bin/flutter', '--version', '--machine'],
stdout: testVersionInfo,
),
const FakeCommand(
command: <Pattern>['git', 'status', '-b', '--porcelain'],
stdout: '## $branchName',
),
const FakeCommand(
command: <String>['git', 'rev-parse', 'HEAD'],
),
const FakeCommand(
command: <String>['/flutter/bin/flutter', 'pub', 'global', 'list'],
),
FakeCommand(
command: <Pattern>[
'/flutter/bin/flutter',
'pub',
'global',
'run',
'--enable-asserts',
'dartdoc',
'--output',
'/path/to/publish/flutter',
'--allow-tools',
'--json',
'--validate-links',
'--link-to-source-excludes',
'/flutter/bin/cache',
'--link-to-source-root',
'/flutter',
'--link-to-source-uri-template',
'https://github.com/flutter/flutter/blob/main/%f%#L%l%',
'--inject-html',
'--use-base-href',
'--header',
'/path/to/docs/styles.html',
'--header',
'/path/to/docs/analytics-header.html',
'--header',
'/path/to/docs/survey.html',
'--header',
'/path/to/docs/snippets.html',
'--header',
'/path/to/docs/opensearch.html',
'--footer',
'/path/to/docs/analytics-footer.html',
'--footer-text',
'/path/to/package/footer.html',
'--allow-warnings-in-packages',
// match package names
RegExp(r'^(\w+,)+(\w+)$'),
'--exclude-packages',
RegExp(r'^(\w+,)+(\w+)$'),
'--exclude',
// match dart package URIs
RegExp(r'^([\w\/:.]+,)+([\w\/:.]+)$'),
'--favicon',
'/path/to/docs/favicon.ico',
'--package-order',
'flutter,Dart,${apidocs.kPlatformIntegrationPackageName},flutter_test,flutter_driver',
'--auto-include-dependencies',
],
onRun: (_) {
for (final File canary in generator.canaries) {
canary.createSync(recursive: true);
}
for (final String path in dartdocDirectiveCanaryFiles) {
publishRoot.childDirectory('flutter').childFile(path).createSync(recursive: true);
}
for (final String path in dartdocDirectiveCanaryLibraries) {
publishRoot.childDirectory('flutter').childDirectory(path).createSync(recursive: true);
}
publishRoot.childDirectory('flutter').childFile('index.html').createSync();
final Directory widgetsDir = publishRoot
.childDirectory('flutter')
.childDirectory('widgets')
..createSync(recursive: true);
widgetsDir.childFile('showGeneralDialog.html').writeAsStringSync('''
<pre id="longSnippet1">
<code class="language-dart">
import 'package:flutter/material.dart';
</code>
</pre>
''',
);
expect(publishRoot.childDirectory('flutter').existsSync(), isTrue);
(widgetsDir
.childDirectory('ModalRoute')
..createSync(recursive: true))
.childFile('barrierColor.html')
.writeAsStringSync('''
<pre id="sample-code">
<code class="language-dart">
class FooClass {
Color get barrierColor => FooColor();
}
</code>
</pre>
''');
const String queryParams = 'split=1&run=true&sample_id=widgets.Listener.123&channel=main';
widgetsDir.childFile('Listener-class.html').writeAsStringSync('''
<iframe class="snippet-dartpad" src="https://dartpad.dev/embed-flutter.html?$queryParams">
</iframe>
''');
}
),
]);
await generator.generateDartdoc();
});
});
}
const String branchName = 'stable';
const String testVersionInfo = '''
{
"frameworkVersion": "2.5.0",
"channel": "$branchName",
"repositoryUrl": "[email protected]:flutter/flutter.git",
"frameworkRevision": "0000000000000000000000000000000000000000",
"frameworkCommitDate": "2021-07-28 13:03:40 -0700",
"engineRevision": "0000000000000000000000000000000000000001",
"dartSdkVersion": "2.14.0 (build 2.14.0-360.0.dev)",
"flutterRoot": "/home/user/flutter"
}
''';
| flutter/dev/tools/test/create_api_docs_test.dart/0 | {
"file_path": "flutter/dev/tools/test/create_api_docs_test.dart",
"repo_id": "flutter",
"token_count": 7724
} | 579 |
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<!-- Empty SVG file -->
<svg
xmlns="http://www.w3.org/2000/svg"
id="empty_svg"
width="100px"
height="50px">
</svg>
| flutter/dev/tools/vitool/test_assets/empty_svg_2_100x50.svg/0 | {
"file_path": "flutter/dev/tools/vitool/test_assets/empty_svg_2_100x50.svg",
"repo_id": "flutter",
"token_count": 82
} | 580 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
import 'common.dart';
void main() {
initTimelineTests();
TestWidgetsFlutterBinding.ensureInitialized();
test('Image cache tracing', () async {
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter completer2 = TestImageStreamCompleter();
PaintingBinding.instance.imageCache.putIfAbsent(
'Test',
() => completer1,
);
PaintingBinding.instance.imageCache.clear();
completer2.testSetImage(ImageInfo(image: await createTestImage()));
PaintingBinding.instance.imageCache.putIfAbsent(
'Test2',
() => completer2,
);
PaintingBinding.instance.imageCache.evict('Test2');
_expectTimelineEvents(
await fetchTimelineEvents(),
<Map<String, dynamic>>[
<String, dynamic>{
'name': 'ImageCache.putIfAbsent',
'args': <String, dynamic>{
'key': 'Test',
'isolateId': isolateId,
'parentId': null,
},
},
<String, dynamic>{
'name': 'listener',
'args': <String, dynamic>{
'isolateId': isolateId,
'parentId': null,
},
},
<String, dynamic>{
'name': 'ImageCache.clear',
'args': <String, dynamic>{
'pendingImages': 1,
'keepAliveImages': 0,
'liveImages': 1,
'currentSizeInBytes': 0,
'isolateId': isolateId,
'parentId': null,
},
},
<String, dynamic>{
'name': 'ImageCache.putIfAbsent',
'args': <String, dynamic>{
'key': 'Test2',
'isolateId': isolateId,
'parentId': null,
},
},
<String, dynamic>{
'name': 'ImageCache.evict',
'args': <String, dynamic>{
'sizeInBytes': 4,
'isolateId': isolateId,
'parentId': null,
},
},
],
);
}, skip: isBrowser); // [intended] uses dart:isolate and io.
}
void _expectTimelineEvents(List<TimelineEvent> events, List<Map<String, dynamic>> expected) {
for (final TimelineEvent event in events) {
for (int index = 0; index < expected.length; index += 1) {
if (expected[index]['name'] == event.json!['name']) {
final Map<String, dynamic> expectedArgs = expected[index]['args'] as Map<String, dynamic>;
final Map<String, dynamic> args = event.json!['args'] as Map<String, dynamic>;
if (_mapsEqual(expectedArgs, args)) {
expected.removeAt(index);
}
}
}
}
if (expected.isNotEmpty) {
final String encodedEvents = jsonEncode(events);
fail('Timeline did not contain expected events: $expected\nactual: $encodedEvents');
}
}
bool _mapsEqual(Map<String, dynamic> expectedArgs, Map<String, dynamic> args) {
for (final String key in expectedArgs.keys) {
if (expectedArgs[key] != args[key]) {
return false;
}
}
return true;
}
class TestImageStreamCompleter extends ImageStreamCompleter {
void testSetImage(ImageInfo image) {
setImage(image);
}
}
| flutter/dev/tracing_tests/test/image_cache_tracing_test.dart/0 | {
"file_path": "flutter/dev/tracing_tests/test/image_cache_tracing_test.dart",
"repo_id": "flutter",
"token_count": 1476
} | 581 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
pluginManagement {
def flutterSdkPath = {
def properties = new Properties()
file("local.properties").withInputStream { properties.load(it) }
def flutterSdkPath = properties.getProperty("flutter.sdk")
assert flutterSdkPath != null, "flutter.sdk not set in local.properties"
return flutterSdkPath
}
settings.ext.flutterSdkPath = flutterSdkPath()
includeBuild("${settings.ext.flutterSdkPath}/packages/flutter_tools/gradle")
repositories {
google()
mavenCentral()
gradlePluginPortal()
}
}
buildscript {
dependencyLocking {
lockFile = file("${rootProject.projectDir}/buildscript-gradle.lockfile")
lockAllConfigurations()
}
}
plugins {
id "dev.flutter.flutter-plugin-loader" version "1.0.0"
id "com.android.application" version "7.2.0" apply false
id "org.jetbrains.kotlin.android" version "1.6.10" apply false
}
include ":app"
| flutter/examples/api/android/settings.gradle/0 | {
"file_path": "flutter/examples/api/android/settings.gradle",
"repo_id": "flutter",
"token_count": 422
} | 582 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoSliverNavigationBar].
void main() => runApp(const SliverNavBarApp());
class SliverNavBarApp extends StatelessWidget {
const SliverNavBarApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: SliverNavBarExample(),
);
}
}
class SliverNavBarExample extends StatelessWidget {
const SliverNavBarExample({super.key});
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
// A ScrollView that creates custom scroll effects using slivers.
child: CustomScrollView(
// A list of sliver widgets.
slivers: <Widget>[
const CupertinoSliverNavigationBar(
leading: Icon(CupertinoIcons.person_2),
// This title is visible in both collapsed and expanded states.
// When the "middle" parameter is omitted, the widget provided
// in the "largeTitle" parameter is used instead in the collapsed state.
largeTitle: Text('Contacts'),
trailing: Icon(CupertinoIcons.add_circled),
),
// This widget fills the remaining space in the viewport.
// Drag the scrollable area to collapse the CupertinoSliverNavigationBar.
SliverFillRemaining(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
const Text('Drag me up', textAlign: TextAlign.center),
CupertinoButton.filled(
onPressed: () {
Navigator.push(
context,
CupertinoPageRoute<Widget>(
builder: (BuildContext context) {
return const NextPage();
},
),
);
},
child: const Text('Go to Next Page'),
),
],
),
),
],
),
);
}
}
class NextPage extends StatelessWidget {
const NextPage({super.key});
@override
Widget build(BuildContext context) {
final Brightness brightness = CupertinoTheme.brightnessOf(context);
return CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
backgroundColor: CupertinoColors.systemYellow,
border: Border(
bottom: BorderSide(
color: brightness == Brightness.light ? CupertinoColors.black : CupertinoColors.white,
),
),
// The middle widget is visible in both collapsed and expanded states.
middle: const Text('Contacts Group'),
// When the "middle" parameter is implemented, the largest title is only visible
// when the CupertinoSliverNavigationBar is fully expanded.
largeTitle: const Text('Family'),
),
const SliverFillRemaining(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Text('Drag me up', textAlign: TextAlign.center),
// When the "leading" parameter is omitted on a route that has a previous page,
// the back button is automatically added to the leading position.
Text('Tap on the leading button to navigate back', textAlign: TextAlign.center),
],
),
),
],
),
);
}
}
| flutter/examples/api/lib/cupertino/nav_bar/cupertino_sliver_nav_bar.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/nav_bar/cupertino_sliver_nav_bar.0.dart",
"repo_id": "flutter",
"token_count": 1691
} | 583 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoTabController].
void main() => runApp(const TabControllerApp());
class TabControllerApp extends StatelessWidget {
const TabControllerApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: TabControllerExample(),
);
}
}
class TabControllerExample extends StatefulWidget {
const TabControllerExample({super.key});
@override
State<TabControllerExample> createState() => _TabControllerExampleState();
}
class _TabControllerExampleState extends State<TabControllerExample> {
final CupertinoTabController controller = CupertinoTabController();
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return CupertinoTabScaffold(
controller: controller,
tabBar: CupertinoTabBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(CupertinoIcons.square_grid_2x2_fill),
label: 'Browse',
),
BottomNavigationBarItem(
icon: Icon(CupertinoIcons.star_circle_fill),
label: 'Starred',
),
],
),
tabBuilder: (BuildContext context, int index) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Content of tab $index'),
const SizedBox(height: 10),
CupertinoButton(
onPressed: () => controller.index = 0,
child: const Text('Go to first tab'),
),
],
),
);
},
);
}
}
| flutter/examples/api/lib/cupertino/tab_scaffold/cupertino_tab_controller.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/tab_scaffold/cupertino_tab_controller.0.dart",
"repo_id": "flutter",
"token_count": 797
} | 584 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverAppBar].
void main() => runApp(const AppBarApp());
class AppBarApp extends StatelessWidget {
const AppBarApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: SliverAppBarExample(),
);
}
}
class SliverAppBarExample extends StatefulWidget {
const SliverAppBarExample({super.key});
@override
State<SliverAppBarExample> createState() => _SliverAppBarExampleState();
}
class _SliverAppBarExampleState extends State<SliverAppBarExample> {
bool _pinned = true;
bool _snap = false;
bool _floating = false;
// [SliverAppBar]s are typically used in [CustomScrollView.slivers], which in
// turn can be placed in a [Scaffold.body].
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: <Widget>[
SliverAppBar(
pinned: _pinned,
snap: _snap,
floating: _floating,
expandedHeight: 160.0,
flexibleSpace: const FlexibleSpaceBar(
title: Text('SliverAppBar'),
background: FlutterLogo(),
),
),
const SliverToBoxAdapter(
child: SizedBox(
height: 20,
child: Center(
child: Text('Scroll to see the SliverAppBar in effect.'),
),
),
),
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return Container(
color: index.isOdd ? Colors.white : Colors.black12,
height: 100.0,
child: Center(
child: Text('$index', textScaler: const TextScaler.linear(5)),
),
);
},
childCount: 20,
),
),
],
),
bottomNavigationBar: BottomAppBar(
child: Padding(
padding: const EdgeInsets.all(8),
child: OverflowBar(
overflowAlignment: OverflowBarAlignment.center,
children: <Widget>[
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Text('pinned'),
Switch(
onChanged: (bool val) {
setState(() {
_pinned = val;
});
},
value: _pinned,
),
],
),
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Text('snap'),
Switch(
onChanged: (bool val) {
setState(() {
_snap = val;
// Snapping only applies when the app bar is floating.
_floating = _floating || _snap;
});
},
value: _snap,
),
],
),
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Text('floating'),
Switch(
onChanged: (bool val) {
setState(() {
_floating = val;
_snap = _snap && _floating;
});
},
value: _floating,
),
],
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/app_bar/sliver_app_bar.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/app_bar/sliver_app_bar.1.dart",
"repo_id": "flutter",
"token_count": 2174
} | 585 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [showModalBottomSheet].
void main() => runApp(const BottomSheetApp());
class BottomSheetApp extends StatelessWidget {
const BottomSheetApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Bottom Sheet Sample')),
body: const BottomSheetExample(),
),
);
}
}
class BottomSheetExample extends StatelessWidget {
const BottomSheetExample({super.key});
@override
Widget build(BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('showModalBottomSheet'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
return Container(
height: 200,
color: Colors.amber,
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Text('Modal BottomSheet'),
ElevatedButton(
child: const Text('Close BottomSheet'),
onPressed: () => Navigator.pop(context),
),
],
),
),
);
},
);
},
),
);
}
}
| flutter/examples/api/lib/material/bottom_sheet/show_modal_bottom_sheet.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/bottom_sheet/show_modal_bottom_sheet.0.dart",
"repo_id": "flutter",
"token_count": 816
} | 586 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ActionChoice].
void main() => runApp(const ChipApp());
class ChipApp extends StatelessWidget {
const ChipApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(colorSchemeSeed: const Color(0xff6750a4), useMaterial3: true),
home: const ActionChoiceExample(),
);
}
}
class ActionChoiceExample extends StatefulWidget {
const ActionChoiceExample({super.key});
@override
State<ActionChoiceExample> createState() => _ActionChoiceExampleState();
}
class _ActionChoiceExampleState extends State<ActionChoiceExample> {
int? _value = 1;
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Scaffold(
appBar: AppBar(
title: const Text('ActionChoice Sample'),
),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Choose an item', style: textTheme.labelLarge),
const SizedBox(height: 10.0),
Wrap(
spacing: 5.0,
children: List<Widget>.generate(
3,
(int index) {
return ChoiceChip(
label: Text('Item $index'),
selected: _value == index,
onSelected: (bool selected) {
setState(() {
_value = selected ? index : null;
});
},
);
},
).toList(),
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/choice_chip/choice_chip.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/choice_chip/choice_chip.0.dart",
"repo_id": "flutter",
"token_count": 876
} | 587 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Dialog].
void main() => runApp(const DialogExampleApp());
class DialogExampleApp extends StatelessWidget {
const DialogExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Dialog Sample')),
body: const Center(
child: DialogExample(),
),
),
);
}
}
class DialogExample extends StatelessWidget {
const DialogExample({super.key});
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => Dialog(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('This is a typical dialog.'),
const SizedBox(height: 15),
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Close'),
),
],
),
),
),
),
child: const Text('Show Dialog'),
),
const SizedBox(height: 10),
TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => Dialog.fullscreen(
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('This is a fullscreen dialog.'),
const SizedBox(height: 15),
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Close'),
),
],
),
),
),
child: const Text('Show Fullscreen Dialog'),
),
],
);
}
}
| flutter/examples/api/lib/material/dialog/dialog.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/dialog/dialog.0.dart",
"repo_id": "flutter",
"token_count": 1326
} | 588 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ExpansionPanelList].
void main() => runApp(const ExpansionPanelListExampleApp());
class ExpansionPanelListExampleApp extends StatelessWidget {
const ExpansionPanelListExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ExpansionPanelList Sample')),
body: const ExpansionPanelListExample(),
),
);
}
}
// stores ExpansionPanel state information
class Item {
Item({
required this.expandedValue,
required this.headerValue,
this.isExpanded = false,
});
String expandedValue;
String headerValue;
bool isExpanded;
}
List<Item> generateItems(int numberOfItems) {
return List<Item>.generate(numberOfItems, (int index) {
return Item(
headerValue: 'Panel $index',
expandedValue: 'This is item number $index',
);
});
}
class ExpansionPanelListExample extends StatefulWidget {
const ExpansionPanelListExample({super.key});
@override
State<ExpansionPanelListExample> createState() => _ExpansionPanelListExampleState();
}
class _ExpansionPanelListExampleState extends State<ExpansionPanelListExample> {
final List<Item> _data = generateItems(8);
@override
Widget build(BuildContext context) {
return SingleChildScrollView(
child: Container(
child: _buildPanel(),
),
);
}
Widget _buildPanel() {
return ExpansionPanelList(
expansionCallback: (int index, bool isExpanded) {
setState(() {
_data[index].isExpanded = isExpanded;
});
},
children: _data.map<ExpansionPanel>((Item item) {
return ExpansionPanel(
headerBuilder: (BuildContext context, bool isExpanded) {
return ListTile(
title: Text(item.headerValue),
);
},
body: ListTile(
title: Text(item.expandedValue),
subtitle: const Text('To delete this panel, tap the trash can icon'),
trailing: const Icon(Icons.delete),
onTap: () {
setState(() {
_data.removeWhere((Item currentItem) => item == currentItem);
});
}),
isExpanded: item.isExpanded,
);
}).toList(),
);
}
}
| flutter/examples/api/lib/material/expansion_panel/expansion_panel_list.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/expansion_panel/expansion_panel_list.0.dart",
"repo_id": "flutter",
"token_count": 974
} | 589 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Image.frameBuilder].
void main() {
runApp(
MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Image.frameBuilder Sample')),
body: const Center(
child: ImageClipExample(
image: NetworkImage('https://flutter.github.io/assets-for-api-docs/assets/widgets/puffin.jpg'),
),
),
),
),
);
}
class ImageClipExample extends StatelessWidget {
const ImageClipExample({super.key, required this.image});
final ImageProvider image;
@override
Widget build(BuildContext context) {
return ClipRRect(
borderRadius: BorderRadius.circular(100),
child: Ink.image(
fit: BoxFit.fill,
width: 300,
height: 300,
image: image,
child: InkWell(
onTap: () {/* ... */},
child: const Align(
child: Padding(
padding: EdgeInsets.all(10.0),
child: Text(
'PUFFIN',
style: TextStyle(
fontWeight: FontWeight.w900,
color: Colors.white,
),
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/material/ink/ink.image_clip.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/ink/ink.image_clip.0.dart",
"repo_id": "flutter",
"token_count": 663
} | 590 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InputDecoration.prefixIconConstraints].
void main() => runApp(const PrefixIconConstraintsExampleApp());
class PrefixIconConstraintsExampleApp extends StatelessWidget {
const PrefixIconConstraintsExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('InputDecoration Sample')),
body: const PrefixIconConstraintsExample(),
),
);
}
}
class PrefixIconConstraintsExample extends StatelessWidget {
const PrefixIconConstraintsExample({super.key});
@override
Widget build(BuildContext context) {
return const Padding(
padding: EdgeInsets.symmetric(horizontal: 8.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextField(
decoration: InputDecoration(
hintText: 'Normal Icon Constraints',
prefixIcon: Icon(Icons.search),
),
),
SizedBox(height: 10),
TextField(
decoration: InputDecoration(
isDense: true,
hintText: 'Smaller Icon Constraints',
prefixIcon: Icon(Icons.search),
prefixIconConstraints: BoxConstraints(
minHeight: 32,
minWidth: 32,
),
),
),
],
),
);
}
}
| flutter/examples/api/lib/material/input_decorator/input_decoration.prefix_icon_constraints.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/input_decorator/input_decoration.prefix_icon_constraints.0.dart",
"repo_id": "flutter",
"token_count": 715
} | 591 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [MenuAcceleratorLabel].
void main() => runApp(const MenuAcceleratorApp());
class MyMenuBar extends StatelessWidget {
const MyMenuBar({super.key});
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Expanded(
child: MenuBar(
children: <Widget>[
SubmenuButton(
menuChildren: <Widget>[
MenuItemButton(
onPressed: () {
showAboutDialog(
context: context,
applicationName: 'MenuBar Sample',
applicationVersion: '1.0.0',
);
},
child: const MenuAcceleratorLabel('&About'),
),
MenuItemButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Saved!'),
),
);
},
child: const MenuAcceleratorLabel('&Save'),
),
MenuItemButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Quit!'),
),
);
},
child: const MenuAcceleratorLabel('&Quit'),
),
],
child: const MenuAcceleratorLabel('&File'),
),
SubmenuButton(
menuChildren: <Widget>[
MenuItemButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Magnify!'),
),
);
},
child: const MenuAcceleratorLabel('&Magnify'),
),
MenuItemButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Minify!'),
),
);
},
child: const MenuAcceleratorLabel('Mi&nify'),
),
],
child: const MenuAcceleratorLabel('&View'),
),
],
),
),
],
),
Expanded(
child: FlutterLogo(
size: MediaQuery.of(context).size.shortestSide * 0.5,
),
),
],
);
}
}
class MenuAcceleratorApp extends StatelessWidget {
const MenuAcceleratorApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Shortcuts(
shortcuts: <ShortcutActivator, Intent>{
const SingleActivator(LogicalKeyboardKey.keyT, control: true): VoidCallbackIntent(() {
debugDumpApp();
}),
},
child: const Scaffold(body: SafeArea(child: MyMenuBar())),
),
);
}
}
| flutter/examples/api/lib/material/menu_anchor/menu_accelerator_label.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/menu_anchor/menu_accelerator_label.0.dart",
"repo_id": "flutter",
"token_count": 2411
} | 592 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [PaginatedDataTable].
class MyDataSource extends DataTableSource {
@override
int get rowCount => 3;
@override
DataRow? getRow(int index) {
switch (index) {
case 0: return const DataRow(
cells: <DataCell>[
DataCell(Text('Sarah')),
DataCell(Text('19')),
DataCell(Text('Student')),
],
);
case 1: return const DataRow(
cells: <DataCell>[
DataCell(Text('Janine')),
DataCell(Text('43')),
DataCell(Text('Professor')),
],
);
case 2: return const DataRow(
cells: <DataCell>[
DataCell(Text('William')),
DataCell(Text('27')),
DataCell(Text('Associate Professor')),
],
);
default: return null;
}
}
@override
bool get isRowCountApproximate => false;
@override
int get selectedRowCount => 0;
}
final DataTableSource dataSource = MyDataSource();
void main() => runApp(const DataTableExampleApp());
class DataTableExampleApp extends StatelessWidget {
const DataTableExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: SingleChildScrollView(
padding: EdgeInsets.all(12.0),
child: DataTableExample(),
),
);
}
}
class DataTableExample extends StatelessWidget {
const DataTableExample({super.key});
@override
Widget build(BuildContext context) {
return PaginatedDataTable(
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
),
DataColumn(
label: Text('Role'),
),
],
source: dataSource,
);
}
}
| flutter/examples/api/lib/material/paginated_data_table/paginated_data_table.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/paginated_data_table/paginated_data_table.0.dart",
"repo_id": "flutter",
"token_count": 810
} | 593 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RadioListTile.toggleable].
void main() => runApp(const RadioListTileApp());
class RadioListTileApp extends StatelessWidget {
const RadioListTileApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('RadioListTile.toggleable Sample')),
body: const RadioListTileExample(),
),
);
}
}
class RadioListTileExample extends StatefulWidget {
const RadioListTileExample({super.key});
@override
State<RadioListTileExample> createState() => _RadioListTileExampleState();
}
class _RadioListTileExampleState extends State<RadioListTileExample> {
int? groupValue;
static const List<String> selections = <String>[
'Hercules Mulligan',
'Eliza Hamilton',
'Philip Schuyler',
'Maria Reynolds',
'Samuel Seabury',
];
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView.builder(
itemBuilder: (BuildContext context, int index) {
return RadioListTile<int>(
value: index,
groupValue: groupValue,
toggleable: true,
title: Text(selections[index]),
onChanged: (int? value) {
setState(() {
groupValue = value;
});
},
);
},
itemCount: selections.length,
),
);
}
}
| flutter/examples/api/lib/material/radio_list_tile/radio_list_tile.toggleable.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/radio_list_tile/radio_list_tile.toggleable.0.dart",
"repo_id": "flutter",
"token_count": 654
} | 594 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ScaffoldMessenger].
void main() => runApp(const ScaffoldMessengerExampleApp());
class ScaffoldMessengerExampleApp extends StatelessWidget {
const ScaffoldMessengerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ScaffoldMessenger Sample')),
body: const Center(
child: ScaffoldMessengerExample(),
),
),
);
}
}
class ScaffoldMessengerExample extends StatelessWidget {
const ScaffoldMessengerExample({super.key});
@override
Widget build(BuildContext context) {
return OutlinedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('A SnackBar has been shown.'),
),
);
},
child: const Text('Show SnackBar'),
);
}
}
| flutter/examples/api/lib/material/scaffold/scaffold_messenger.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/scaffold/scaffold_messenger.0.dart",
"repo_id": "flutter",
"token_count": 420
} | 595 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SegmentedButton].
void main() {
runApp(const SegmentedButtonApp());
}
class SegmentedButtonApp extends StatelessWidget {
const SegmentedButtonApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Spacer(),
Text('Single choice'),
SingleChoice(),
SizedBox(height: 20),
Text('Multiple choice'),
MultipleChoice(),
Spacer(),
],
),
),
),
);
}
}
enum Calendar { day, week, month, year }
class SingleChoice extends StatefulWidget {
const SingleChoice({super.key});
@override
State<SingleChoice> createState() => _SingleChoiceState();
}
class _SingleChoiceState extends State<SingleChoice> {
Calendar calendarView = Calendar.day;
@override
Widget build(BuildContext context) {
return SegmentedButton<Calendar>(
segments: const <ButtonSegment<Calendar>>[
ButtonSegment<Calendar>(value: Calendar.day, label: Text('Day'), icon: Icon(Icons.calendar_view_day)),
ButtonSegment<Calendar>(value: Calendar.week, label: Text('Week'), icon: Icon(Icons.calendar_view_week)),
ButtonSegment<Calendar>(value: Calendar.month, label: Text('Month'), icon: Icon(Icons.calendar_view_month)),
ButtonSegment<Calendar>(value: Calendar.year, label: Text('Year'), icon: Icon(Icons.calendar_today)),
],
selected: <Calendar>{calendarView},
onSelectionChanged: (Set<Calendar> newSelection) {
setState(() {
// By default there is only a single segment that can be
// selected at one time, so its value is always the first
// item in the selected set.
calendarView = newSelection.first;
});
},
);
}
}
enum Sizes { extraSmall, small, medium, large, extraLarge }
class MultipleChoice extends StatefulWidget {
const MultipleChoice({super.key});
@override
State<MultipleChoice> createState() => _MultipleChoiceState();
}
class _MultipleChoiceState extends State<MultipleChoice> {
Set<Sizes> selection = <Sizes>{Sizes.large, Sizes.extraLarge};
@override
Widget build(BuildContext context) {
return SegmentedButton<Sizes>(
segments: const <ButtonSegment<Sizes>>[
ButtonSegment<Sizes>(value: Sizes.extraSmall, label: Text('XS')),
ButtonSegment<Sizes>(value: Sizes.small, label: Text('S')),
ButtonSegment<Sizes>(value: Sizes.medium, label: Text('M')),
ButtonSegment<Sizes>(
value: Sizes.large,
label: Text('L'),
),
ButtonSegment<Sizes>(value: Sizes.extraLarge, label: Text('XL')),
],
selected: selection,
onSelectionChanged: (Set<Sizes> newSelection) {
setState(() {
selection = newSelection;
});
},
multiSelectionEnabled: true,
);
}
}
| flutter/examples/api/lib/material/segmented_button/segmented_button.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/segmented_button/segmented_button.0.dart",
"repo_id": "flutter",
"token_count": 1300
} | 596 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Switch].
void main() => runApp(const SwitchApp());
class SwitchApp extends StatelessWidget {
const SwitchApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('Switch Sample')),
body: const Center(
child: SwitchExample(),
),
),
);
}
}
class SwitchExample extends StatefulWidget {
const SwitchExample({super.key});
@override
State<SwitchExample> createState() => _SwitchExampleState();
}
class _SwitchExampleState extends State<SwitchExample> {
bool light = true;
@override
Widget build(BuildContext context) {
final MaterialStateProperty<Color?> trackColor = MaterialStateProperty.resolveWith<Color?>(
(Set<MaterialState> states) {
// Track color when the switch is selected.
if (states.contains(MaterialState.selected)) {
return Colors.amber;
}
// Otherwise return null to set default track color
// for remaining states such as when the switch is
// hovered, focused, or disabled.
return null;
},
);
final MaterialStateProperty<Color?> overlayColor = MaterialStateProperty.resolveWith<Color?>(
(Set<MaterialState> states) {
// Material color when switch is selected.
if (states.contains(MaterialState.selected)) {
return Colors.amber.withOpacity(0.54);
}
// Material color when switch is disabled.
if (states.contains(MaterialState.disabled)) {
return Colors.grey.shade400;
}
// Otherwise return null to set default material color
// for remaining states such as when the switch is
// hovered, or focused.
return null;
},
);
return Switch(
// This bool value toggles the switch.
value: light,
overlayColor: overlayColor,
trackColor: trackColor,
thumbColor: const MaterialStatePropertyAll<Color>(Colors.black),
onChanged: (bool value) {
// This is called when the user toggles the switch.
setState(() {
light = value;
});
},
);
}
}
| flutter/examples/api/lib/material/switch/switch.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/switch/switch.1.dart",
"repo_id": "flutter",
"token_count": 900
} | 597 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for Material Design 3 [TextField]s.
void main() {
runApp(const TextFieldExamplesApp());
}
class TextFieldExamplesApp extends StatelessWidget {
const TextFieldExamplesApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(colorSchemeSeed: const Color(0xff6750a4), useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('TextField Examples')),
body: const Column(
children: <Widget>[
Spacer(),
FilledTextFieldExample(),
OutlinedTextFieldExample(),
Spacer(),
],
),
),
);
}
}
/// An example of the filled text field type.
///
/// A filled [TextField] with default settings matching the spec:
/// https://m3.material.io/components/text-fields/specs#6d654d1d-262e-4697-858c-9a75e8e7c81d
class FilledTextFieldExample extends StatelessWidget {
const FilledTextFieldExample({super.key});
@override
Widget build(BuildContext context) {
return const TextField(
decoration: InputDecoration(
prefixIcon: Icon(Icons.search),
suffixIcon: Icon(Icons.clear),
labelText: 'Filled',
hintText: 'hint text',
helperText: 'supporting text',
filled: true,
),
);
}
}
/// An example of the outlined text field type.
///
/// A Outlined [TextField] with default settings matching the spec:
/// https://m3.material.io/components/text-fields/specs#68b00bd6-ab40-4b4f-93d9-ed1fbbc5d06e
class OutlinedTextFieldExample extends StatelessWidget {
const OutlinedTextFieldExample({super.key});
@override
Widget build(BuildContext context) {
return const TextField(
decoration: InputDecoration(
prefixIcon: Icon(Icons.search),
suffixIcon: Icon(Icons.clear),
labelText: 'Outlined',
hintText: 'hint text',
helperText: 'supporting text',
border: OutlineInputBorder(),
),
);
}
}
| flutter/examples/api/lib/material/text_field/text_field.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/text_field/text_field.2.dart",
"repo_id": "flutter",
"token_count": 845
} | 598 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// An example showing usage of [StarBorder].
import 'package:flutter/material.dart';
const int _kParameterPrecision = 2;
void main() => runApp(const StarBorderApp());
class StarBorderApp extends StatelessWidget {
const StarBorderApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('StarBorder Example'),
backgroundColor: const Color(0xff323232),
),
body: const StarBorderExample(),
),
);
}
}
class StarBorderExample extends StatefulWidget {
const StarBorderExample({super.key});
@override
State<StarBorderExample> createState() => _StarBorderExampleState();
}
class _StarBorderExampleState extends State<StarBorderExample> {
final OptionModel _model = OptionModel();
final TextEditingController _textController = TextEditingController();
@override
void initState() {
super.initState();
_model.addListener(_modelChanged);
}
@override
void dispose() {
_model.removeListener(_modelChanged);
_textController.dispose();
super.dispose();
}
void _modelChanged() {
setState(() {});
}
@override
Widget build(BuildContext context) {
return DefaultTextStyle(
style: const TextStyle(
color: Colors.black,
fontSize: 14.0,
fontFamily: 'Roboto',
fontStyle: FontStyle.normal,
),
child: ListView(
children: <Widget>[
ColoredBox(
color: Colors.grey.shade200,
child: Options(_model),
),
Padding(
padding: const EdgeInsets.all(18.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: ExampleBorder(
border: StarBorder(
side: const BorderSide(),
points: _model.points,
innerRadiusRatio: _model.innerRadiusRatio,
pointRounding: _model.pointRounding,
valleyRounding: _model.valleyRounding,
rotation: _model.rotation,
squash: _model.squash,
),
title: 'Star',
),
),
Expanded(
child: ExampleBorder(
border: StarBorder.polygon(
side: const BorderSide(),
sides: _model.points,
pointRounding: _model.pointRounding,
rotation: _model.rotation,
squash: _model.squash,
),
title: 'Polygon',
),
),
],
),
),
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: Container(
color: Colors.black12,
margin: const EdgeInsets.all(16.0),
padding: const EdgeInsets.all(16.0),
child: SelectableText(_model.starCode),
),
),
Expanded(
child: Container(
color: Colors.black12,
margin: const EdgeInsets.all(16.0),
padding: const EdgeInsets.all(16.0),
child: SelectableText(_model.polygonCode),
),
),
],
),
],
),
);
}
}
class ExampleBorder extends StatelessWidget {
const ExampleBorder({
super.key,
required this.border,
required this.title,
});
final StarBorder border;
final String title;
@override
Widget build(BuildContext context) {
return Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(20),
width: 150,
height: 100,
decoration: ShapeDecoration(
color: Colors.blue.shade100,
shape: border,
),
child: Text(title),
);
}
}
class Options extends StatefulWidget {
const Options(this.model, {super.key});
final OptionModel model;
@override
State<Options> createState() => _OptionsState();
}
class _OptionsState extends State<Options> {
@override
void initState() {
super.initState();
widget.model.addListener(_modelChanged);
}
@override
void didUpdateWidget(Options oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.model != oldWidget.model) {
oldWidget.model.removeListener(_modelChanged);
widget.model.addListener(_modelChanged);
}
}
@override
void dispose() {
super.dispose();
widget.model.removeListener(_modelChanged);
}
void _modelChanged() {
setState(() {});
}
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.fromLTRB(5.0, 0.0, 5.0, 10.0),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Row(
children: <Widget>[
Expanded(
child: ControlSlider(
label: 'Point Rounding',
value: widget.model.pointRounding,
onChanged: (double value) {
widget.model.pointRounding = value;
},
),
),
Expanded(
child: ControlSlider(
label: 'Valley Rounding',
value: widget.model.valleyRounding,
onChanged: (double value) {
widget.model.valleyRounding = value;
},
),
),
],
),
Row(
children: <Widget>[
Expanded(
child: ControlSlider(
label: 'Squash',
value: widget.model.squash,
onChanged: (double value) {
widget.model.squash = value;
},
),
),
Expanded(
child: ControlSlider(
label: 'Rotation',
value: widget.model.rotation,
max: 360,
onChanged: (double value) {
widget.model.rotation = value;
},
),
),
],
),
Row(
children: <Widget>[
Expanded(
child: Row(
children: <Widget>[
Expanded(
child: ControlSlider(
label: 'Points',
value: widget.model.points,
min: 3,
max: 20,
precision: 1,
onChanged: (double value) {
widget.model.points = value;
},
),
),
Tooltip(
message: 'Round the number of points to the nearest integer.',
child: Padding(
padding: const EdgeInsets.all(8.0),
child: OutlinedButton(
child: const Text('Nearest'),
onPressed: () {
widget.model.points = widget.model.points.roundToDouble();
},
),
),
),
],
),
),
Expanded(
child: ControlSlider(
label: 'Inner Radius',
value: widget.model.innerRadiusRatio,
onChanged: (double value) {
widget.model.innerRadiusRatio = value;
},
),
),
],
),
ElevatedButton(
onPressed: () {
widget.model.reset();
},
child: const Text('Reset'),
),
],
),
);
}
}
class OptionModel extends ChangeNotifier {
double get pointRounding => _pointRounding;
double _pointRounding = 0.0;
set pointRounding(double value) {
if (value != _pointRounding) {
_pointRounding = value;
if (_valleyRounding + _pointRounding > 1) {
_valleyRounding = 1.0 - _pointRounding;
}
notifyListeners();
}
}
double get valleyRounding => _valleyRounding;
double _valleyRounding = 0.0;
set valleyRounding(double value) {
if (value != _valleyRounding) {
_valleyRounding = value;
if (_valleyRounding + _pointRounding > 1) {
_pointRounding = 1.0 - _valleyRounding;
}
notifyListeners();
}
}
double get squash => _squash;
double _squash = 0.0;
set squash(double value) {
if (value != _squash) {
_squash = value;
notifyListeners();
}
}
double get rotation => _rotation;
double _rotation = 0.0;
set rotation(double value) {
if (value != _rotation) {
_rotation = value;
notifyListeners();
}
}
double get innerRadiusRatio => _innerRadiusRatio;
double _innerRadiusRatio = 0.4;
set innerRadiusRatio(double value) {
if (value != _innerRadiusRatio) {
_innerRadiusRatio = value.clamp(0.0001, double.infinity);
notifyListeners();
}
}
double get points => _points;
double _points = 5;
set points(double value) {
if (value != _points) {
_points = value;
notifyListeners();
}
}
String get starCode {
return 'Container(\n'
' decoration: ShapeDecoration(\n'
' shape: StarBorder(\n'
' points: ${points.toStringAsFixed(_kParameterPrecision)},\n'
' rotation: ${rotation.toStringAsFixed(_kParameterPrecision)},\n'
' innerRadiusRatio: ${innerRadiusRatio.toStringAsFixed(_kParameterPrecision)},\n'
' pointRounding: ${pointRounding.toStringAsFixed(_kParameterPrecision)},\n'
' valleyRounding: ${valleyRounding.toStringAsFixed(_kParameterPrecision)},\n'
' squash: ${squash.toStringAsFixed(_kParameterPrecision)},\n'
' ),\n'
' ),\n'
');';
}
String get polygonCode {
return 'Container(\n'
' decoration: ShapeDecoration(\n'
' shape: StarBorder.polygon(\n'
' sides: ${points.toStringAsFixed(_kParameterPrecision)},\n'
' rotation: ${rotation.toStringAsFixed(_kParameterPrecision)},\n'
' cornerRounding: ${pointRounding.toStringAsFixed(_kParameterPrecision)},\n'
' squash: ${squash.toStringAsFixed(_kParameterPrecision)},\n'
' ),\n'
' ),\n'
');';
}
void reset() {
final OptionModel defaultModel = OptionModel();
_pointRounding = defaultModel.pointRounding;
_valleyRounding = defaultModel.valleyRounding;
_rotation = defaultModel.rotation;
_squash = defaultModel.squash;
_innerRadiusRatio = defaultModel._innerRadiusRatio;
_points = defaultModel.points;
notifyListeners();
}
}
class ControlSlider extends StatelessWidget {
const ControlSlider({
super.key,
required this.label,
required this.value,
required this.onChanged,
this.min = 0.0,
this.max = 1.0,
this.precision = _kParameterPrecision,
});
final String label;
final double value;
final void Function(double value) onChanged;
final double min;
final double max;
final int precision;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(4.0),
child: Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Expanded(
flex: 2,
child: Text(
label,
textAlign: TextAlign.end,
),
),
Expanded(
flex: 5,
child: Slider(
onChanged: onChanged,
min: min,
max: max,
value: value,
),
),
Expanded(
child: Text(
value.toStringAsFixed(precision),
),
),
],
),
);
}
}
| flutter/examples/api/lib/painting/star_border/star_border.0.dart/0 | {
"file_path": "flutter/examples/api/lib/painting/star_border/star_border.0.dart",
"repo_id": "flutter",
"token_count": 6431
} | 599 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [FontFeature].
void main() => runApp(const ExampleApp());
class ExampleApp extends StatelessWidget {
const ExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: ExampleWidget(),
);
}
}
final TextStyle titleStyle = TextStyle(
fontSize: 18,
fontFeatures: const <FontFeature>[FontFeature.enable('smcp')],
color: Colors.blueGrey[600],
);
class ExampleWidget extends StatelessWidget {
const ExampleWidget({super.key});
@override
Widget build(BuildContext context) {
// The Cardo, Milonga and Raleway Dots fonts can be downloaded from Google
// Fonts (https://www.google.com/fonts).
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Spacer(flex: 5),
Text('regular numbers have their place:', style: titleStyle),
const Text('The 1972 cup final was a 1-1 draw.',
style: TextStyle(
fontFamily: 'Cardo',
fontSize: 24,
)),
const Spacer(),
Text('but old-style figures blend well with lower case:', style: titleStyle),
const Text('The 1972 cup final was a 1-1 draw.',
style: TextStyle(
fontFamily: 'Cardo', fontSize: 24, fontFeatures: <FontFeature>[FontFeature.oldstyleFigures()])),
const Spacer(),
const Divider(),
const Spacer(),
Text('fractions look better with a custom ligature:', style: titleStyle),
const Text('Add 1/2 tsp of flour and stir.',
style: TextStyle(
fontFamily: 'Milonga',
fontSize: 24,
fontFeatures: <FontFeature>[FontFeature.alternativeFractions()])),
const Spacer(),
const Divider(),
const Spacer(),
Text('multiple stylistic sets in one font:', style: titleStyle),
const Text('Raleway Dots', style: TextStyle(fontFamily: 'Raleway Dots', fontSize: 48)),
Text('Raleway Dots',
style: TextStyle(
fontFeatures: <FontFeature>[FontFeature.stylisticSet(1)],
fontFamily: 'Raleway Dots',
fontSize: 48,
)),
const Spacer(flex: 5),
],
),
),
);
}
}
| flutter/examples/api/lib/ui/text/font_feature.0.dart/0 | {
"file_path": "flutter/examples/api/lib/ui/text/font_feature.0.dart",
"repo_id": "flutter",
"token_count": 1182
} | 600 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AnimatedList].
void main() {
runApp(const AnimatedListSample());
}
class AnimatedListSample extends StatefulWidget {
const AnimatedListSample({super.key});
@override
State<AnimatedListSample> createState() => _AnimatedListSampleState();
}
class _AnimatedListSampleState extends State<AnimatedListSample> {
final GlobalKey<AnimatedListState> _listKey = GlobalKey<AnimatedListState>();
late ListModel<int> _list;
int? _selectedItem;
late int _nextItem; // The next item inserted when the user presses the '+' button.
@override
void initState() {
super.initState();
_list = ListModel<int>(
listKey: _listKey,
initialItems: <int>[0, 1, 2],
removedItemBuilder: _buildRemovedItem,
);
_nextItem = 3;
}
// Used to build list items that haven't been removed.
Widget _buildItem(BuildContext context, int index, Animation<double> animation) {
return CardItem(
animation: animation,
item: _list[index],
selected: _selectedItem == _list[index],
onTap: () {
setState(() {
_selectedItem = _selectedItem == _list[index] ? null : _list[index];
});
},
);
}
/// The builder function used to build items that have been removed.
///
/// Used to build an item after it has been removed from the list. This method
/// is needed because a removed item remains visible until its animation has
/// completed (even though it's gone as far as this ListModel is concerned).
/// The widget will be used by the [AnimatedListState.removeItem] method's
/// [AnimatedRemovedItemBuilder] parameter.
Widget _buildRemovedItem(int item, BuildContext context, Animation<double> animation) {
return CardItem(
animation: animation,
item: item,
// No gesture detector here: we don't want removed items to be interactive.
);
}
// Insert the "next item" into the list model.
void _insert() {
final int index = _selectedItem == null ? _list.length : _list.indexOf(_selectedItem!);
_list.insert(index, _nextItem++);
}
// Remove the selected item from the list model.
void _remove() {
if (_selectedItem != null) {
_list.removeAt(_list.indexOf(_selectedItem!));
setState(() {
_selectedItem = null;
});
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('AnimatedList'),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.add_circle),
onPressed: _insert,
tooltip: 'insert a new item',
),
IconButton(
icon: const Icon(Icons.remove_circle),
onPressed: _remove,
tooltip: 'remove the selected item',
),
],
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: AnimatedList(
key: _listKey,
initialItemCount: _list.length,
itemBuilder: _buildItem,
),
),
),
);
}
}
typedef RemovedItemBuilder<T> = Widget Function(T item, BuildContext context, Animation<double> animation);
/// Keeps a Dart [List] in sync with an [AnimatedList].
///
/// The [insert] and [removeAt] methods apply to both the internal list and
/// the animated list that belongs to [listKey].
///
/// This class only exposes as much of the Dart List API as is needed by the
/// sample app. More list methods are easily added, however methods that
/// mutate the list must make the same changes to the animated list in terms
/// of [AnimatedListState.insertItem] and [AnimatedList.removeItem].
class ListModel<E> {
ListModel({
required this.listKey,
required this.removedItemBuilder,
Iterable<E>? initialItems,
}) : _items = List<E>.from(initialItems ?? <E>[]);
final GlobalKey<AnimatedListState> listKey;
final RemovedItemBuilder<E> removedItemBuilder;
final List<E> _items;
AnimatedListState? get _animatedList => listKey.currentState;
void insert(int index, E item) {
_items.insert(index, item);
_animatedList!.insertItem(index);
}
E removeAt(int index) {
final E removedItem = _items.removeAt(index);
if (removedItem != null) {
_animatedList!.removeItem(
index,
(BuildContext context, Animation<double> animation) {
return removedItemBuilder(removedItem, context, animation);
},
);
}
return removedItem;
}
int get length => _items.length;
E operator [](int index) => _items[index];
int indexOf(E item) => _items.indexOf(item);
}
/// Displays its integer item as 'item N' on a Card whose color is based on
/// the item's value.
///
/// The text is displayed in bright green if [selected] is
/// true. This widget's height is based on the [animation] parameter, it
/// varies from 0 to 128 as the animation varies from 0.0 to 1.0.
class CardItem extends StatelessWidget {
const CardItem({
super.key,
this.onTap,
this.selected = false,
required this.animation,
required this.item,
}) : assert(item >= 0);
final Animation<double> animation;
final VoidCallback? onTap;
final int item;
final bool selected;
@override
Widget build(BuildContext context) {
TextStyle textStyle = Theme.of(context).textTheme.headlineMedium!;
if (selected) {
textStyle = textStyle.copyWith(color: Colors.lightGreenAccent[400]);
}
return Padding(
padding: const EdgeInsets.all(2.0),
child: SizeTransition(
sizeFactor: animation,
child: GestureDetector(
behavior: HitTestBehavior.opaque,
onTap: onTap,
child: SizedBox(
height: 80.0,
child: Card(
color: Colors.primaries[item % Colors.primaries.length],
child: Center(
child: Text('Item $item', style: textStyle),
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/animated_list/animated_list.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/animated_list/animated_list.0.dart",
"repo_id": "flutter",
"token_count": 2332
} | 601 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Offstage].
void main() => runApp(const OffstageApp());
class OffstageApp extends StatelessWidget {
const OffstageApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Offstage Sample')),
body: const Center(
child: OffstageExample(),
),
),
);
}
}
class OffstageExample extends StatefulWidget {
const OffstageExample({super.key});
@override
State<OffstageExample> createState() => _OffstageExampleState();
}
class _OffstageExampleState extends State<OffstageExample> {
final GlobalKey _key = GlobalKey();
bool _offstage = true;
Size _getFlutterLogoSize() {
final RenderBox renderLogo = _key.currentContext!.findRenderObject()! as RenderBox;
return renderLogo.size;
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Offstage(
offstage: _offstage,
child: FlutterLogo(
key: _key,
size: 150.0,
),
),
Text('Flutter logo is offstage: $_offstage'),
ElevatedButton(
child: const Text('Toggle Offstage Value'),
onPressed: () {
setState(() {
_offstage = !_offstage;
});
},
),
if (_offstage)
ElevatedButton(
child: const Text('Get Flutter Logo size'),
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text('Flutter Logo size is ${_getFlutterLogoSize()}'),
),
);
}),
],
);
}
}
| flutter/examples/api/lib/widgets/basic/offstage.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/offstage.0.dart",
"repo_id": "flutter",
"token_count": 884
} | 602 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Focus].
void main() => runApp(const FocusExampleApp());
class FocusExampleApp extends StatelessWidget {
const FocusExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: FocusExample(),
);
}
}
class FocusExample extends StatefulWidget {
const FocusExample({super.key});
@override
State<FocusExample> createState() => _FocusExampleState();
}
class _FocusExampleState extends State<FocusExample> {
int focusedChild = 0;
List<Widget> children = <Widget>[];
List<FocusNode> childFocusNodes = <FocusNode>[];
@override
void initState() {
super.initState();
// Add the first child.
_addChild();
}
@override
void dispose() {
for (final FocusNode node in childFocusNodes) {
node.dispose();
}
super.dispose();
}
void _addChild() {
// Calling requestFocus here creates a deferred request for focus, since the
// node is not yet part of the focus tree.
childFocusNodes.add(FocusNode(debugLabel: 'Child ${children.length}')..requestFocus());
children.add(Padding(
padding: const EdgeInsets.all(2.0),
child: ActionChip(
focusNode: childFocusNodes.last,
label: Text('CHILD ${children.length}'),
onPressed: () {},
),
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Wrap(
children: children,
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
focusedChild = children.length;
_addChild();
});
},
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/api/lib/widgets/focus_scope/focus.2.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/focus_scope/focus.2.dart",
"repo_id": "flutter",
"token_count": 729
} | 603 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Image.loadingBuilder].
void main() => runApp(const LoadingBuilderExampleApp());
class LoadingBuilderExampleApp extends StatelessWidget {
const LoadingBuilderExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: LoadingBuilderExample(),
);
}
}
class LoadingBuilderExample extends StatelessWidget {
const LoadingBuilderExample({super.key});
@override
Widget build(BuildContext context) {
return DecoratedBox(
decoration: BoxDecoration(
color: Colors.white,
border: Border.all(),
borderRadius: BorderRadius.circular(20),
),
child: Image.network(
'https://flutter.github.io/assets-for-api-docs/assets/widgets/falcon.jpg',
loadingBuilder: (BuildContext context, Widget child, ImageChunkEvent? loadingProgress) {
if (loadingProgress == null) {
return child;
}
return Center(
child: CircularProgressIndicator(
value: loadingProgress.expectedTotalBytes != null
? loadingProgress.cumulativeBytesLoaded / loadingProgress.expectedTotalBytes!
: null,
),
);
},
),
);
}
}
| flutter/examples/api/lib/widgets/image/image.loading_builder.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/image/image.loading_builder.0.dart",
"repo_id": "flutter",
"token_count": 555
} | 604 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() => runApp(const MagnifierExampleApp());
class MagnifierExampleApp extends StatefulWidget {
const MagnifierExampleApp({super.key});
@override
State<MagnifierExampleApp> createState() => _MagnifierExampleAppState();
}
class _MagnifierExampleAppState extends State<MagnifierExampleApp> {
Offset dragGesturePosition = Offset.zero;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Drag on the logo!'),
RepaintBoundary(
child: Stack(
children: <Widget>[
GestureDetector(
onPanUpdate: (DragUpdateDetails details) => setState(
() {
dragGesturePosition = details.localPosition;
},
),
child: const FlutterLogo(size: 200),
),
Positioned(
left: dragGesturePosition.dx,
top: dragGesturePosition.dy,
child: const RawMagnifier(
decoration: MagnifierDecoration(
shape: CircleBorder(
side: BorderSide(color: Colors.pink, width: 3),
),
),
size: Size(100, 100),
magnificationScale: 2,
),
)
],
),
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/magnifier/magnifier.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/magnifier/magnifier.0.dart",
"repo_id": "flutter",
"token_count": 1051
} | 605 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Notification].
void main() => runApp(const NotificationExampleApp());
class NotificationExampleApp extends StatelessWidget {
const NotificationExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: NotificationExample(),
);
}
}
class NotificationExample extends StatelessWidget {
const NotificationExample({super.key});
static const List<String> _tabs = <String>['Months', 'Days'];
static const List<String> _months = <String>[
'January',
'February',
'March',
];
static const List<String> _days = <String>[
'Sunday',
'Monday',
'Tuesday',
];
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: _tabs.length,
child: Scaffold(
// Listens to the scroll events and returns the current position.
body: NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification scrollNotification) {
if (scrollNotification is ScrollStartNotification) {
debugPrint('Scrolling has started');
} else if (scrollNotification is ScrollEndNotification) {
debugPrint('Scrolling has ended');
}
// Return true to cancel the notification bubbling.
return true;
},
child: NestedScrollView(
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
return <Widget>[
SliverAppBar(
title: const Text('Notification Sample'),
pinned: true,
floating: true,
bottom: TabBar(
tabs: _tabs.map((String name) => Tab(text: name)).toList(),
),
),
];
},
body: TabBarView(
children: <Widget>[
ListView.builder(
itemCount: _months.length,
itemBuilder: (BuildContext context, int index) {
return ListTile(title: Text(_months[index]));
},
),
ListView.builder(
itemCount: _days.length,
itemBuilder: (BuildContext context, int index) {
return ListTile(title: Text(_days[index]));
},
),
],
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/notification_listener/notification.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/notification_listener/notification.0.dart",
"repo_id": "flutter",
"token_count": 1214
} | 606 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ScrollNotificationObserver].
void main() => runApp(const ScrollNotificationObserverApp());
class ScrollNotificationObserverApp extends StatelessWidget {
const ScrollNotificationObserverApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
// The Scaffold widget contains a [ScrollNotificationObserver].
// This is used by [AppBar] for its scrolled under behavior.
//
// We can use [ScrollNotificationObserver.maybeOf] to get the
// state of this [ScrollNotificationObserver] from descendants
// of the Scaffold widget.
//
// If you're not using a [Scaffold] widget, you can create a [ScrollNotificationObserver]
// to notify its descendants of scroll notifications by adding it to the subtree.
home: Scaffold(
appBar: AppBar(
title: const Text('ScrollNotificationObserver Sample'),
),
body: const ScrollNotificationObserverExample(),
),
);
}
}
class ScrollNotificationObserverExample extends StatefulWidget {
const ScrollNotificationObserverExample({super.key});
@override
State<ScrollNotificationObserverExample> createState() => _ScrollNotificationObserverExampleState();
}
class _ScrollNotificationObserverExampleState extends State<ScrollNotificationObserverExample> {
ScrollNotificationObserverState? _scrollNotificationObserver;
ScrollController controller = ScrollController();
bool _scrolledDown = false;
@override
void didChangeDependencies() {
super.didChangeDependencies();
// Remove any previous listener.
_scrollNotificationObserver?.removeListener(_handleScrollNotification);
// Get the ScrollNotificationObserverState from the Scaffold widget.
_scrollNotificationObserver = ScrollNotificationObserver.maybeOf(context);
// Add a new listener.
_scrollNotificationObserver?.addListener(_handleScrollNotification);
}
@override
void dispose() {
if (_scrollNotificationObserver != null) {
_scrollNotificationObserver!.removeListener(_handleScrollNotification);
_scrollNotificationObserver = null;
}
controller.dispose();
super.dispose();
}
void _handleScrollNotification(ScrollNotification notification) {
// Check if the notification is a scroll update notification and if the
// `notification.depth` is 0. This way we only listen to the scroll
// notifications from the closest scrollable, instead of those that may be nested.
if (notification is ScrollUpdateNotification && defaultScrollNotificationPredicate(notification)) {
final ScrollMetrics metrics = notification.metrics;
// Check if the user scrolled down.
if (_scrolledDown != metrics.extentBefore > 0) {
setState(() {
_scrolledDown = metrics.extentBefore > 0;
});
}
}
}
@override
Widget build(BuildContext context) {
return Stack(
children: <Widget>[
SampleList(controller: controller),
// Show the button only if the user scrolled down.
if (_scrolledDown)
Positioned(
right: 25,
bottom: 20,
child: Center(
child: GestureDetector(
onTap: () {
// Scroll to the top when the user taps the button.
controller.animateTo(0, duration: const Duration(milliseconds: 200), curve:Curves.fastOutSlowIn);
},
child: const Card(
child: Padding(
padding: EdgeInsets.all(8.0),
child: Column(
children: <Widget>[
Icon(Icons.arrow_upward_rounded),
Text('Scroll to top')
],
),
),
),
),
),
),
],
);
}
}
class SampleList extends StatelessWidget {
const SampleList({super.key, required this.controller});
final ScrollController controller;
@override
Widget build(BuildContext context) {
return ListView.builder(
controller: controller,
itemCount: 30,
itemBuilder: (BuildContext context, int index) {
return ListTile(title: Text('Item $index'));
},
);
}
}
| flutter/examples/api/lib/widgets/scroll_notification_observer/scroll_notification_observer.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scroll_notification_observer/scroll_notification_observer.0.dart",
"repo_id": "flutter",
"token_count": 1706
} | 607 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [CharacterActivator].
void main() => runApp(const CharacterActivatorExampleApp());
class CharacterActivatorExampleApp extends StatelessWidget {
const CharacterActivatorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('CharacterActivator Sample')),
body: const Center(
child: CharacterActivatorExample(),
),
),
);
}
}
class HelpMenuIntent extends Intent {
const HelpMenuIntent();
}
class CharacterActivatorExample extends StatefulWidget {
const CharacterActivatorExample({super.key});
@override
State<CharacterActivatorExample> createState() => _CharacterActivatorExampleState();
}
class _CharacterActivatorExampleState extends State<CharacterActivatorExample> {
@override
Widget build(BuildContext context) {
return Shortcuts(
shortcuts: const <ShortcutActivator, Intent>{
CharacterActivator('?'): HelpMenuIntent(),
},
child: Actions(
actions: <Type, Action<Intent>>{
HelpMenuIntent: CallbackAction<HelpMenuIntent>(
onInvoke: (HelpMenuIntent intent) {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('Keep calm and carry on!')),
);
return null;
},
),
},
child: const Focus(
autofocus: true,
child: Column(
children: <Widget>[
Text('Press question mark for help'),
],
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/shortcuts/character_activator.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/shortcuts/character_activator.0.dart",
"repo_id": "flutter",
"token_count": 720
} | 608 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
/// Flutter code sample for [SlottedMultiChildRenderObjectWidget].
/// Slots used for the children of [Diagonal] and [RenderDiagonal].
enum DiagonalSlot {
topLeft,
bottomRight,
}
/// A widget that demonstrates the usage of
/// [SlottedMultiChildRenderObjectWidget] by providing slots for two
/// children that will be arranged diagonally.
class Diagonal extends SlottedMultiChildRenderObjectWidget<DiagonalSlot, RenderBox> {
const Diagonal({
super.key,
this.topLeft,
this.bottomRight,
this.backgroundColor,
});
final Widget? topLeft;
final Widget? bottomRight;
final Color? backgroundColor;
@override
Iterable<DiagonalSlot> get slots => DiagonalSlot.values;
@override
Widget? childForSlot(DiagonalSlot slot) {
return switch (slot) {
DiagonalSlot.topLeft => topLeft,
DiagonalSlot.bottomRight => bottomRight,
};
}
// The [createRenderObject] and [updateRenderObject] methods configure the
// [RenderObject] backing this widget with the configuration of the widget.
// They do not need to do anything with the children of the widget, though.
// The children of the widget are automatically configured on the
// [RenderObject] by [SlottedRenderObjectElement.mount] and
// [SlottedRenderObjectElement.update].
@override
SlottedContainerRenderObjectMixin<DiagonalSlot, RenderBox> createRenderObject(
BuildContext context,
) {
return RenderDiagonal(
backgroundColor: backgroundColor,
);
}
@override
void updateRenderObject(
BuildContext context,
SlottedContainerRenderObjectMixin<DiagonalSlot, RenderBox> renderObject,
) {
(renderObject as RenderDiagonal).backgroundColor = backgroundColor;
}
}
/// A render object that demonstrates the usage of
/// [SlottedContainerRenderObjectMixin] by providing slots for two children that
/// will be arranged diagonally.
class RenderDiagonal extends RenderBox
with SlottedContainerRenderObjectMixin<DiagonalSlot, RenderBox>, DebugOverflowIndicatorMixin {
RenderDiagonal({Color? backgroundColor}) : _backgroundColor = backgroundColor;
// Getters and setters to configure the [RenderObject] with the configuration
// of the [Widget]. These mostly contain boilerplate code, but depending on
// where the configuration value is used, the setter has to call
// [markNeedsLayout], [markNeedsPaint], or [markNeedsSemanticsUpdate].
Color? get backgroundColor => _backgroundColor;
Color? _backgroundColor;
set backgroundColor(Color? value) {
assert(value != null);
if (_backgroundColor == value) {
return;
}
_backgroundColor = value;
markNeedsPaint();
}
// Getters to simplify accessing the slotted children.
RenderBox? get _topLeft => childForSlot(DiagonalSlot.topLeft);
RenderBox? get _bottomRight => childForSlot(DiagonalSlot.bottomRight);
// The size this render object would have if the incoming constraints were
// unconstrained; calculated during performLayout used during paint for an
// assertion that checks for unintended overflow.
late Size _childrenSize;
// Returns children in hit test order.
@override
Iterable<RenderBox> get children {
return <RenderBox>[
if (_topLeft != null) _topLeft!,
if (_bottomRight != null) _bottomRight!,
];
}
// LAYOUT
@override
void performLayout() {
// Children are allowed to be as big as they want (= unconstrained).
const BoxConstraints childConstraints = BoxConstraints();
// Lay out the top left child and position it at offset zero.
Size topLeftSize = Size.zero;
final RenderBox? topLeft = _topLeft;
if (topLeft != null) {
topLeft.layout(childConstraints, parentUsesSize: true);
_positionChild(topLeft, Offset.zero);
topLeftSize = topLeft.size;
}
// Lay out the bottom right child and position it at the bottom right corner
// of the top left child.
Size bottomRightSize = Size.zero;
final RenderBox? bottomRight = _bottomRight;
if (bottomRight != null) {
bottomRight.layout(childConstraints, parentUsesSize: true);
_positionChild(
bottomRight,
Offset(topLeftSize.width, topLeftSize.height),
);
bottomRightSize = bottomRight.size;
}
// Calculate the overall size and constrain it to the given constraints.
// Any overflow is marked (in debug mode) during paint.
_childrenSize = Size(
topLeftSize.width + bottomRightSize.width,
topLeftSize.height + bottomRightSize.height,
);
size = constraints.constrain(_childrenSize);
}
void _positionChild(RenderBox child, Offset offset) {
(child.parentData! as BoxParentData).offset = offset;
}
// PAINT
@override
void paint(PaintingContext context, Offset offset) {
// Paint the background.
if (backgroundColor != null) {
context.canvas.drawRect(
offset & size,
Paint()..color = backgroundColor!,
);
}
void paintChild(RenderBox child, PaintingContext context, Offset offset) {
final BoxParentData childParentData = child.parentData! as BoxParentData;
context.paintChild(child, childParentData.offset + offset);
}
// Paint the children at the offset calculated during layout.
final RenderBox? topLeft = _topLeft;
if (topLeft != null) {
paintChild(topLeft, context, offset);
}
final RenderBox? bottomRight = _bottomRight;
if (bottomRight != null) {
paintChild(bottomRight, context, offset);
}
// Paint an overflow indicator in debug mode if the children want to be
// larger than the incoming constraints allow.
assert(() {
paintOverflowIndicator(
context,
offset,
Offset.zero & size,
Offset.zero & _childrenSize,
);
return true;
}());
}
// HIT TEST
@override
bool hitTestChildren(BoxHitTestResult result, {required Offset position}) {
for (final RenderBox child in children) {
final BoxParentData parentData = child.parentData! as BoxParentData;
final bool isHit = result.addWithPaintOffset(
offset: parentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - parentData.offset);
return child.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
}
return false;
}
// INTRINSICS
// Incoming height/width are ignored as children are always laid out unconstrained.
@override
double computeMinIntrinsicWidth(double height) {
final double topLeftWidth = _topLeft?.getMinIntrinsicWidth(double.infinity) ?? 0;
final double bottomRightWith = _bottomRight?.getMinIntrinsicWidth(double.infinity) ?? 0;
return topLeftWidth + bottomRightWith;
}
@override
double computeMaxIntrinsicWidth(double height) {
final double topLeftWidth = _topLeft?.getMaxIntrinsicWidth(double.infinity) ?? 0;
final double bottomRightWith = _bottomRight?.getMaxIntrinsicWidth(double.infinity) ?? 0;
return topLeftWidth + bottomRightWith;
}
@override
double computeMinIntrinsicHeight(double width) {
final double topLeftHeight = _topLeft?.getMinIntrinsicHeight(double.infinity) ?? 0;
final double bottomRightHeight = _bottomRight?.getMinIntrinsicHeight(double.infinity) ?? 0;
return topLeftHeight + bottomRightHeight;
}
@override
double computeMaxIntrinsicHeight(double width) {
final double topLeftHeight = _topLeft?.getMaxIntrinsicHeight(double.infinity) ?? 0;
final double bottomRightHeight = _bottomRight?.getMaxIntrinsicHeight(double.infinity) ?? 0;
return topLeftHeight + bottomRightHeight;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
const BoxConstraints childConstraints = BoxConstraints();
final Size topLeftSize = _topLeft?.computeDryLayout(childConstraints) ?? Size.zero;
final Size bottomRightSize = _bottomRight?.computeDryLayout(childConstraints) ?? Size.zero;
return constraints.constrain(Size(
topLeftSize.width + bottomRightSize.width,
topLeftSize.height + bottomRightSize.height,
));
}
}
class ExampleWidget extends StatelessWidget {
const ExampleWidget({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Slotted RenderObject Example')),
body: Center(
child: Diagonal(
topLeft: Container(
color: Colors.green,
height: 100,
width: 200,
child: const Center(
child: Text('topLeft'),
),
),
bottomRight: Container(
color: Colors.yellow,
height: 60,
width: 30,
child: const Center(
child: Text('bottomRight'),
),
),
backgroundColor: Colors.blue,
),
),
),
);
}
}
void main() {
runApp(const ExampleWidget());
}
| flutter/examples/api/lib/widgets/slotted_render_object_widget/slotted_multi_child_render_object_widget_mixin.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/slotted_render_object_widget/slotted_multi_child_render_object_widget_mixin.0.dart",
"repo_id": "flutter",
"token_count": 3203
} | 609 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [PositionedTransition].
void main() => runApp(const PositionedTransitionExampleApp());
class PositionedTransitionExampleApp extends StatelessWidget {
const PositionedTransitionExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: PositionedTransitionExample(),
);
}
}
class PositionedTransitionExample extends StatefulWidget {
const PositionedTransitionExample({super.key});
@override
State<PositionedTransitionExample> createState() => _PositionedTransitionExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _PositionedTransitionExampleState extends State<PositionedTransitionExample> with TickerProviderStateMixin {
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true);
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
const double smallLogo = 100;
const double bigLogo = 200;
return LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
final Size biggest = constraints.biggest;
return Stack(
children: <Widget>[
PositionedTransition(
rect: RelativeRectTween(
begin: RelativeRect.fromSize(
const Rect.fromLTWH(0, 0, smallLogo, smallLogo),
biggest,
),
end: RelativeRect.fromSize(
Rect.fromLTWH(biggest.width - bigLogo, biggest.height - bigLogo, bigLogo, bigLogo),
biggest,
),
).animate(CurvedAnimation(
parent: _controller,
curve: Curves.elasticInOut,
)),
child: const Padding(
padding: EdgeInsets.all(8),
child: FlutterLogo(),
),
),
],
);
},
);
}
}
| flutter/examples/api/lib/widgets/transitions/positioned_transition.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/positioned_transition.0.dart",
"repo_id": "flutter",
"token_count": 926
} | 610 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/route/show_cupertino_modal_popup.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tap on button displays cupertino modal dialog', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ModalPopupApp(),
);
final Finder actionOne = find.text('Action One');
expect(actionOne, findsNothing);
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(actionOne, findsOneWidget);
await tester.tap(find.text('Action One'));
await tester.pumpAndSettle();
expect(actionOne, findsNothing);
});
}
| flutter/examples/api/test/cupertino/route/show_cupertino_modal_popup.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/route/show_cupertino_modal_popup.0_test.dart",
"repo_id": "flutter",
"token_count": 303
} | 611 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
// package:flutter_goldens is not used as part of the test process for web.
Future<void> testExecutable(FutureOr<void> Function() testMain) async => testMain();
| flutter/examples/api/test/goldens_web.dart/0 | {
"file_path": "flutter/examples/api/test/goldens_web.dart",
"repo_id": "flutter",
"token_count": 97
} | 612 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/autocomplete/autocomplete.4.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('can search and find options after waiting for fake network delay and debounce delay', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'a');
await tester.pump(example.fakeAPIDuration);
// No results yet, need to also wait for the debounce duration.
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.pump(example.debounceDuration);
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsOneWidget);
expect(find.text('chameleon'), findsOneWidget);
await tester.enterText(find.byType(TextFormField), 'aa');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
});
testWidgets('debounce is reset each time a character is entered', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
await tester.enterText(find.byType(TextFormField), 'c');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'ch');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'cha');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'cham');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
// Despite the total elapsed time being greater than debounceDuration +
// fakeAPIDuration, the search has not yet completed, because the debounce
// was reset each time text input happened.
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'chame');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsOneWidget);
});
testWidgets('shows an error message for network errors', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
await tester.enterText(find.byType(TextFormField), 'chame');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsOneWidget);
InputDecorator inputDecorator = tester.widget(find.byType(InputDecorator));
expect(inputDecorator.decoration.errorText, isNull);
// Turn the network off.
await tester.tap(find.byType(Switch));
await tester.pump();
await tester.enterText(find.byType(TextFormField), 'chamel');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
inputDecorator = tester.widget(find.byType(InputDecorator));
expect(inputDecorator.decoration.errorText, isNotNull);
// Turn the network back on.
await tester.tap(find.byType(Switch));
await tester.pump();
await tester.enterText(find.byType(TextFormField), 'chamele');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsOneWidget);
inputDecorator = tester.widget(find.byType(InputDecorator));
expect(inputDecorator.decoration.errorText, isNull);
});
}
| flutter/examples/api/test/material/autocomplete/autocomplete.4_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/autocomplete/autocomplete.4_test.dart",
"repo_id": "flutter",
"token_count": 1676
} | 613 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/chip/chip_attributes.avatar_box_constraints.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('RawChip.avatarBoxConstraints updates avatar size constraints', (WidgetTester tester) async {
const double border = 1.0;
const double iconSize = 18.0;
const double padding = 8.0;
await tester.pumpWidget(
const example.AvatarBoxConstraintsApp(),
);
expect(tester.getSize(find.byType(RawChip).at(0)).width, equals(202.0));
expect(tester.getSize(find.byType(RawChip).at(0)).height, equals(58.0));
Offset chipTopLeft = tester.getTopLeft(find.byWidget(tester.widget<Material>(
find.descendant(
of: find.byType(RawChip).at(0),
matching: find.byType(Material),
),
)));
Offset avatarCenter = tester.getCenter(find.byIcon(Icons.star).at(0));
expect(chipTopLeft.dx, avatarCenter.dx - (iconSize / 2) - padding - border);
expect(tester.getSize(find.byType(RawChip).at(1)).width, equals(202.0));
expect(tester.getSize(find.byType(RawChip).at(1)).height, equals(78.0));
chipTopLeft = tester.getTopLeft(find.byWidget(tester.widget<Material>(
find.descendant(
of: find.byType(RawChip).at(1),
matching: find.byType(Material),
),
)));
avatarCenter = tester.getCenter(find.byIcon(Icons.star).at(1));
expect(chipTopLeft.dx, avatarCenter.dx - (iconSize / 2) - padding - border);
expect(tester.getSize(find.byType(RawChip).at(2)).width, equals(202.0));
expect(tester.getSize(find.byType(RawChip).at(2)).height, equals(78.0));
chipTopLeft = tester.getTopLeft(find.byWidget(tester.widget<Material>(
find.descendant(
of: find.byType(RawChip).at(2),
matching: find.byType(Material),
),
)));
avatarCenter = tester.getCenter(find.byIcon(Icons.star).at(2));
expect(chipTopLeft.dx, avatarCenter.dx - (iconSize / 2) - padding - border);
});
}
| flutter/examples/api/test/material/chip/chip_attributes.avatar_box_constraints.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/chip/chip_attributes.avatar_box_constraints.0_test.dart",
"repo_id": "flutter",
"token_count": 855
} | 614 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/expansion_panel/expansion_panel_list.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ExpansionPanel can be expanded', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ExpansionPanelListExampleApp(),
);
// Verify the first tile is collapsed.
expect(tester.widget<ExpandIcon>(find.byType(ExpandIcon).first).isExpanded, false);
// Tap to expand the first tile.
await tester.tap(find.byType(ExpandIcon).first);
await tester.pumpAndSettle();
// Verify that the first tile is expanded.
expect(tester.widget<ExpandIcon>(find.byType(ExpandIcon).first).isExpanded, true);
});
testWidgets('Tap to delete a ExpansionPanel', (WidgetTester tester) async {
const int index = 3;
await tester.pumpWidget(
const example.ExpansionPanelListExampleApp(),
);
expect(find.widgetWithText(ListTile, 'Panel $index'), findsOneWidget);
expect(tester.widget<ExpandIcon>(find.byType(ExpandIcon).at(index)).isExpanded, false);
// Tap to expand the tile at index 3.
await tester.tap(find.byType(ExpandIcon).at(index));
await tester.pumpAndSettle();
expect(tester.widget<ExpandIcon>(find.byType(ExpandIcon).at(index)).isExpanded, true);
// Tap to delete the tile at index 3.
await tester.tap(find.byIcon(Icons.delete).at(index));
await tester.pumpAndSettle();
// Verify that the tile at index 3 is deleted.
expect(find.widgetWithText(ListTile, 'Panel $index'), findsNothing);
});
testWidgets('ExpansionPanelList is scrollable', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ExpansionPanelListExampleApp(),
);
expect(find.byType(SingleChildScrollView), findsOneWidget);
// Expand all the tiles.
for (int i = 0; i < 8; i++) {
await tester.tap(find.byType(ExpandIcon).at(i));
}
await tester.pumpAndSettle();
// Check panel 3 tile position.
Offset tilePosition = tester.getBottomLeft(find.widgetWithText(ListTile, 'Panel 3'));
expect(tilePosition.dy, 656.0);
// Scroll up.
await tester.drag(find.byType(SingleChildScrollView), const Offset(0, -300));
await tester.pumpAndSettle();
// Verify panel 3 tile position is updated after scrolling.
tilePosition = tester.getBottomLeft(find.widgetWithText(ListTile, 'Panel 3'));
expect(tilePosition.dy, 376.0);
});
}
| flutter/examples/api/test/material/expansion_panel/expansion_panel_list.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/expansion_panel/expansion_panel_list.0_test.dart",
"repo_id": "flutter",
"token_count": 932
} | 615 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/input_chip/input_chip.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
final String replacementChar = String.fromCharCode(
example.ChipsInputEditingController.kObjectReplacementChar);
testWidgets('User input generates InputChips', (WidgetTester tester) async {
await tester.pumpWidget(
const example.EditableChipFieldApp(),
);
await tester.pumpAndSettle();
expect(find.byType(example.EditableChipFieldApp), findsOneWidget);
expect(find.byType(example.ChipsInput<String>), findsOneWidget);
expect(find.byType(InputChip), findsOneWidget);
example.ChipsInputState<String> state =
tester.state(find.byType(example.ChipsInput<String>));
expect(state.controller.textWithoutReplacements.isEmpty, true);
await tester.tap(find.byType(example.ChipsInput<String>));
await tester.pumpAndSettle();
expect(tester.testTextInput.isVisible, true);
// Simulating text typing on the input field.
tester.testTextInput.enterText('${replacementChar}ham');
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsOneWidget);
state = tester.state(find.byType(example.ChipsInput<String>));
await tester.pumpAndSettle();
expect(state.controller.textWithoutReplacements, 'ham');
// Add new InputChip by sending the "done" action.
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pumpAndSettle();
expect(state.controller.textWithoutReplacements.isEmpty, true);
expect(find.byType(InputChip), findsNWidgets(2));
// Simulate item deletion.
await tester.tap(find.descendant(
of: find.byType(InputChip),
matching: find.byType(InkWell).last,
));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsOneWidget);
await tester.tap(find.descendant(
of: find.byType(InputChip),
matching: find.byType(InkWell).last,
));
await tester.pumpAndSettle();
expect(find.byType(InputChip), findsNothing);
});
}
| flutter/examples/api/test/material/input_chip/input_chip.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/input_chip/input_chip.1_test.dart",
"repo_id": "flutter",
"token_count": 807
} | 616 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/material/menu_anchor/menu_anchor.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can open menu', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MenuApp(),
);
await tester.tap(find.byType(TextButton));
await tester.pump();
expect(find.text(example.MenuEntry.about.label), findsOneWidget);
expect(find.text(example.MenuEntry.showMessage.label), findsOneWidget);
expect(find.text(example.MenuEntry.hideMessage.label), findsNothing);
expect(find.text('Background Color'), findsOneWidget);
expect(find.text(example.MenuEntry.colorRed.label), findsNothing);
expect(find.text(example.MenuEntry.colorGreen.label), findsNothing);
expect(find.text(example.MenuEntry.colorBlue.label), findsNothing);
expect(find.text(example.MenuApp.kMessage), findsNothing);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pump();
expect(find.text('Background Color'), findsOneWidget);
await tester.tap(find.text('Background Color'));
await tester.pump();
expect(find.text(example.MenuEntry.colorRed.label), findsOneWidget);
expect(find.text(example.MenuEntry.colorGreen.label), findsOneWidget);
expect(find.text(example.MenuEntry.colorBlue.label), findsOneWidget);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pumpAndSettle();
expect(find.text(example.MenuApp.kMessage), findsOneWidget);
expect(find.text('Last Selected: ${example.MenuEntry.showMessage.label}'), findsOneWidget);
});
testWidgets('Shortcuts work', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MenuApp(),
);
// Open the menu so we can watch state changes resulting from the shortcuts
// firing.
await tester.tap(find.byType(TextButton));
await tester.pump();
expect(find.text(example.MenuEntry.showMessage.label), findsOneWidget);
expect(find.text(example.MenuEntry.hideMessage.label), findsNothing);
expect(find.text(example.MenuApp.kMessage), findsNothing);
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyS);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
// Need to pump twice because of the one frame delay in the notification to
// update the overlay entry.
await tester.pump();
expect(find.text(example.MenuEntry.showMessage.label), findsNothing);
expect(find.text(example.MenuEntry.hideMessage.label), findsOneWidget);
expect(find.text(example.MenuApp.kMessage), findsOneWidget);
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyS);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
await tester.pump();
expect(find.text(example.MenuEntry.showMessage.label), findsOneWidget);
expect(find.text(example.MenuEntry.hideMessage.label), findsNothing);
expect(find.text(example.MenuApp.kMessage), findsNothing);
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyR);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
expect(find.text('Last Selected: ${example.MenuEntry.colorRed.label}'), findsOneWidget);
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyG);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
expect(find.text('Last Selected: ${example.MenuEntry.colorGreen.label}'), findsOneWidget);
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyB);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
expect(find.text('Last Selected: ${example.MenuEntry.colorBlue.label}'), findsOneWidget);
});
testWidgets('MenuAnchor is wrapped in a SafeArea', (WidgetTester tester) async {
const double safeAreaPadding = 100.0;
await tester.pumpWidget(
const MediaQuery(
data: MediaQueryData(
padding: EdgeInsets.symmetric(vertical: safeAreaPadding),
),
child: example.MenuApp(),
),
);
expect(tester.getTopLeft(find.byType(MenuAnchor)), const Offset(0.0, safeAreaPadding));
});
}
| flutter/examples/api/test/material/menu_anchor/menu_anchor.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/menu_anchor/menu_anchor.0_test.dart",
"repo_id": "flutter",
"token_count": 1693
} | 617 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/reorderable_list/reorderable_list_view.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Dragged Card is elevated', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ReorderableApp(),
);
Card findCardOne() {
return tester.widget<Card>(find.ancestor(of: find.text('Card 1'), matching: find.byType(Card)));
}
// Card has default elevation when not dragged.
expect(findCardOne().elevation, null);
// Dragged card is elevated.
final TestGesture drag = await tester.startGesture(tester.getCenter(find.text('Card 1')));
await tester.pump(kLongPressTimeout + kPressTimeout);
await tester.pumpAndSettle();
expect(findCardOne().elevation, 6);
// After the drag gesture ends, the card elevation has default value.
await drag.moveTo(tester.getCenter(find.text('Card 4')));
await drag.up();
await tester.pumpAndSettle();
expect(findCardOne().elevation, null);
});
}
| flutter/examples/api/test/material/reorderable_list/reorderable_list_view.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/reorderable_list/reorderable_list_view.2_test.dart",
"repo_id": "flutter",
"token_count": 449
} | 618 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/switch/switch.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can toggle switch', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SwitchApp(),
);
final Finder switchFinder = find.byType(Switch);
Switch materialSwitch = tester.widget<Switch>(switchFinder);
expect(materialSwitch.value, true);
await tester.tap(switchFinder);
await tester.pumpAndSettle();
materialSwitch = tester.widget<Switch>(switchFinder);
expect(materialSwitch.value, false);
});
}
| flutter/examples/api/test/material/switch/switch.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/switch/switch.0_test.dart",
"repo_id": "flutter",
"token_count": 268
} | 619 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/time_picker/show_time_picker.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can open and modify time picker', (WidgetTester tester) async {
const String openPicker = 'Open time picker';
final List<String> options = <String>[
'$TimePickerEntryMode',
... TimePickerEntryMode.values.map<String>((TimePickerEntryMode value) => value.name),
'$ThemeMode',
... ThemeMode.values.map<String>((ThemeMode value) => value.name),
'$TextDirection',
... TextDirection.values.map<String>((TextDirection value) => value.name),
'$MaterialTapTargetSize',
... MaterialTapTargetSize.values.map<String>((MaterialTapTargetSize value) => value.name),
'$Orientation',
... Orientation.values.map<String>((Orientation value) => value.name),
'Time Mode',
'12-hour am/pm time',
'24-hour time',
'Material Version',
'Material 2',
'Material 3',
openPicker,
];
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.ShowTimePickerApp(),
),
),
);
for (final String option in options) {
expect(find.text(option), findsOneWidget, reason: 'Unable to find $option widget in example.');
}
// Open time picker
await tester.tap(find.text(openPicker));
await tester.pumpAndSettle();
expect(find.text('Select time'), findsOneWidget);
expect(find.text('Cancel'), findsOneWidget);
expect(find.text('OK'), findsOneWidget);
// Close time picker
await tester.tapAt(const Offset(1, 1));
await tester.pumpAndSettle();
expect(find.text('Select time'), findsNothing);
expect(find.text('Cancel'), findsNothing);
expect(find.text('OK'), findsNothing);
// Change an option.
await tester.tap(find.text('Material 2'));
await tester.pumpAndSettle();
await tester.tap(find.text(openPicker));
await tester.pumpAndSettle();
expect(find.text('SELECT TIME'), findsOneWidget);
expect(find.text('CANCEL'), findsOneWidget);
expect(find.text('OK'), findsOneWidget);
});
}
| flutter/examples/api/test/material/time_picker/show_time_picker.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/time_picker/show_time_picker.0_test.dart",
"repo_id": "flutter",
"token_count": 891
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/custom_multi_child_layout.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('has four containers', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.CustomMultiChildLayoutApp(),
),
),
);
final Finder containerFinder = find.byType(Container);
expect(containerFinder, findsNWidgets(4));
});
testWidgets('containers are the same size', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.CustomMultiChildLayoutExample(),
),
),
);
final Finder containerFinder = find.byType(Container);
const Size expectedSize = Size(100, 100);
for (int i = 0; i < 4; i += 1) {
expect(tester.getSize(containerFinder.at(i)), equals(expectedSize));
}
expect(containerFinder, findsNWidgets(4));
});
testWidgets('containers are offset', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.CustomMultiChildLayoutExample(),
),
),
);
final Finder containerFinder = find.byType(Container);
Rect previousRect = tester.getRect(containerFinder.first);
for (int i = 1; i < 4; i += 1) {
expect(
tester.getRect(containerFinder.at(i)),
equals(previousRect.shift(const Offset(100, 70))),
reason: 'Rect $i not correct size',
);
previousRect = tester.getRect(containerFinder.at(i));
}
expect(containerFinder, findsNWidgets(4));
});
}
| flutter/examples/api/test/widgets/basic/custom_multi_child_layout.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/custom_multi_child_layout.0_test.dart",
"repo_id": "flutter",
"token_count": 740
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/draggable_scrollable_sheet/draggable_scrollable_sheet.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Test DraggableScrollableSheet initial state', (WidgetTester tester) async {
await tester.pumpWidget(
const example.DraggableScrollableSheetExampleApp(),
);
final Finder sheetFinder = find.byType(DraggableScrollableSheet);
// Verify that DraggableScrollableSheet is initially present
expect(sheetFinder, findsOneWidget);
// Verify that DraggableScrollableSheet is shown initially at 50% height
final DraggableScrollableSheet draggableSheet = tester.widget(sheetFinder);
expect(draggableSheet.initialChildSize, 0.5);
});
testWidgets('Test DraggableScrollableSheet drag behavior on mobile platforms', (WidgetTester tester) async {
await tester.pumpWidget(
const example.DraggableScrollableSheetExampleApp(),
);
// Verify that ListView is visible
final Finder listViewFinder = find.byType(ListView);
expect(listViewFinder, findsOneWidget);
// Get the initial size of the ListView
final Size listViewInitialSize = tester.getSize(listViewFinder);
// Drag the sheet from anywhere inside the sheet to change the sheet position
await tester.drag(listViewFinder, const Offset(0.0, -100.0));
await tester.pump();
// Verify that the ListView is expanded
final Size listViewCurrentSize = tester.getSize(listViewFinder);
expect(listViewCurrentSize.height, greaterThan(listViewInitialSize.height));
}, variant: TargetPlatformVariant.mobile());
testWidgets('Test DraggableScrollableSheet drag behavior on desktop platforms', (WidgetTester tester) async {
await tester.pumpWidget(
const example.DraggableScrollableSheetExampleApp(),
);
// Verify that Grabber is visible
final Finder grabberFinder = find.byType(example.Grabber);
expect(grabberFinder, findsOneWidget);
// Drag the Grabber to change the sheet position
await tester.drag(grabberFinder, const Offset(0.0, -100.0));
await tester.pump();
// Verify that the DraggableScrollableSheet's initialChildSize is updated
final DraggableScrollableSheet draggableSheet = tester.widget(find.byType(DraggableScrollableSheet));
expect(draggableSheet.initialChildSize, isNot(0.5));
}, variant: TargetPlatformVariant.desktop());
}
| flutter/examples/api/test/widgets/draggable_scrollable_sheet/draggable_scrollable_sheet.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/draggable_scrollable_sheet/draggable_scrollable_sheet.0_test.dart",
"repo_id": "flutter",
"token_count": 844
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
/// Simulates a system back, like a back gesture on Android.
///
/// Sends the same platform channel message that the engine sends when it
/// receives a system back.
Future<void> simulateSystemBack() {
return TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.handlePlatformMessage(
'flutter/navigation',
const JSONMessageCodec().encodeMessage(<String, dynamic>{
'method': 'popRoute',
}),
(ByteData? _) {},
);
}
| flutter/examples/api/test/widgets/navigator_utils.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/navigator_utils.dart",
"repo_id": "flutter",
"token_count": 214
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/widgets/shortcuts/callback_shortcuts.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('CallbackShortcutsApp increments and decrements', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CallbackShortcutsApp(),
);
expect(find.text('count: 0'), findsOneWidget);
// Increment the counter.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pump();
expect(find.text('count: 1'), findsOneWidget);
// Decrement the counter.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pump();
expect(find.text('count: 0'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/shortcuts/callback_shortcuts.0.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/shortcuts/callback_shortcuts.0.dart",
"repo_id": "flutter",
"token_count": 310
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
plugins {
id("com.android.application")
id("dev.flutter.flutter-gradle-plugin")
}
android {
namespace = "io.flutter.examples.hello_world"
compileSdk = flutter.compileSdkVersion
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
defaultConfig {
applicationId = "io.flutter.examples.hello_world"
minSdk = flutter.minSdkVersion
targetSdk = flutter.targetSdkVersion
versionCode = flutter.versionCode()
versionName = flutter.versionName()
}
buildTypes {
named("release") {
// TODO: Add your own signing config for the release build.
// Signing with the debug keys for now, so `flutter run --release` works.
signingConfig = signingConfigs.getByName("debug")
}
}
}
flutter {
source = "../.."
}
| flutter/examples/hello_world/android/app/build.gradle.kts/0 | {
"file_path": "flutter/examples/hello_world/android/app/build.gradle.kts",
"repo_id": "flutter",
"token_count": 413
} | 625 |
#include "Generated.xcconfig"
| flutter/examples/layers/ios/Flutter/Release.xcconfig/0 | {
"file_path": "flutter/examples/layers/ios/Flutter/Release.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
class RenderSolidColorBox extends RenderDecoratedBox {
RenderSolidColorBox(this.backgroundColor, { this.desiredSize = Size.infinite })
: super(decoration: BoxDecoration(color: backgroundColor));
final Size desiredSize;
final Color backgroundColor;
@override
double computeMinIntrinsicWidth(double height) {
return desiredSize.width == double.infinity ? 0.0 : desiredSize.width;
}
@override
double computeMaxIntrinsicWidth(double height) {
return desiredSize.width == double.infinity ? 0.0 : desiredSize.width;
}
@override
double computeMinIntrinsicHeight(double width) {
return desiredSize.height == double.infinity ? 0.0 : desiredSize.height;
}
@override
double computeMaxIntrinsicHeight(double width) {
return desiredSize.height == double.infinity ? 0.0 : desiredSize.height;
}
@override
void performLayout() {
size = constraints.constrain(desiredSize);
}
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
if (event is PointerDownEvent) {
decoration = const BoxDecoration(color: Color(0xFFFF0000));
} else if (event is PointerUpEvent) {
decoration = BoxDecoration(color: backgroundColor);
}
}
}
| flutter/examples/layers/rendering/src/solid_color_box.dart/0 | {
"file_path": "flutter/examples/layers/rendering/src/solid_color_box.dart",
"repo_id": "flutter",
"token_count": 450
} | 627 |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="12120" systemVersion="16E195" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" colorMatched="YES" initialViewController="BYZ-38-t0r">
<device id="retina4_7" orientation="portrait">
<adaptation id="fullscreen"/>
</device>
<dependencies>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="12088"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--Flutter View Controller-->
<scene sceneID="tne-QT-ifu">
<objects>
<viewController id="BYZ-38-t0r" customClass="FlutterViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="y3c-jy-aDJ"/>
<viewControllerLayoutGuide type="bottom" id="wfy-db-euE"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="8bC-Xf-vdC">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<color key="backgroundColor" red="1" green="1" blue="1" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
</view>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="dkx-z0-nzr" sceneMemberID="firstResponder"/>
</objects>
</scene>
<!--Platform View Controller-->
<scene sceneID="qxR-Ib-h1B">
<objects>
<viewController storyboardIdentifier="PlatformView" id="l7k-TM-87m" customClass="PlatformViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="aNK-cG-KHz"/>
<viewControllerLayoutGuide type="bottom" id="KQ0-mI-uQk"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="9Pa-2h-BJY" userLabel="ParentView">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<subviews>
<stackView opaque="NO" contentMode="scaleToFill" axis="vertical" translatesAutoresizingMaskIntoConstraints="NO" id="O5w-dx-5ca">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<subviews>
<view contentMode="scaleToFill" restorationIdentifier="PVController" translatesAutoresizingMaskIntoConstraints="NO" id="PVo-OY-IKE" userLabel="Top">
<rect key="frame" x="0.0" y="0.0" width="375" height="597"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="Button tapped 0 times." textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="qaB-rs-mWp">
<rect key="frame" x="95.5" y="288" width="185" height="21.5"/>
<fontDescription key="fontDescription" type="system" pointSize="18"/>
<color key="textColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<nil key="highlightedColor"/>
</label>
<button opaque="NO" contentMode="scaleToFill" contentHorizontalAlignment="center" contentVerticalAlignment="center" lineBreakMode="middleTruncation" translatesAutoresizingMaskIntoConstraints="NO" id="K7X-Ru-0N7">
<rect key="frame" x="94" y="325.5" width="188" height="38"/>
<color key="backgroundColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<constraints>
<constraint firstAttribute="width" constant="188" id="W0o-wA-IUz"/>
<constraint firstAttribute="height" constant="38" id="tEK-W1-2uy"/>
</constraints>
<fontDescription key="fontDescription" type="system" pointSize="14"/>
<state key="normal" title="Continue in Flutter view">
<color key="titleColor" cocoaTouchSystemColor="darkTextColor"/>
</state>
<userDefinedRuntimeAttributes>
<userDefinedRuntimeAttribute type="number" keyPath="type">
<integer key="value" value="0"/>
</userDefinedRuntimeAttribute>
</userDefinedRuntimeAttributes>
<connections>
<action selector="switchToFlutterView:" destination="l7k-TM-87m" eventType="touchUpInside" id="Q9G-Ua-HnS"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstItem="qaB-rs-mWp" firstAttribute="centerX" secondItem="PVo-OY-IKE" secondAttribute="centerX" id="3XU-jp-cMf"/>
<constraint firstItem="qaB-rs-mWp" firstAttribute="centerY" secondItem="PVo-OY-IKE" secondAttribute="centerY" id="GEk-gm-nLl"/>
<constraint firstItem="K7X-Ru-0N7" firstAttribute="top" secondItem="qaB-rs-mWp" secondAttribute="bottom" constant="16" id="Gwc-TH-45z"/>
<constraint firstItem="K7X-Ru-0N7" firstAttribute="centerX" secondItem="qaB-rs-mWp" secondAttribute="centerX" id="QqH-9r-NM7"/>
</constraints>
</view>
<view contentMode="scaleToFill" restorationIdentifier="Bottom" translatesAutoresizingMaskIntoConstraints="NO" id="9iX-D6-aiy" userLabel="Bottom">
<rect key="frame" x="0.0" y="597" width="375" height="70"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="iOS" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="CTc-Jz-yjr">
<rect key="frame" x="20" y="14" width="47.5" height="36"/>
<fontDescription key="fontDescription" type="system" pointSize="30"/>
<color key="textColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<nil key="highlightedColor"/>
</label>
<button opaque="NO" contentMode="scaleToFill" contentHorizontalAlignment="center" contentVerticalAlignment="center" lineBreakMode="middleTruncation" translatesAutoresizingMaskIntoConstraints="NO" id="6yL-sX-bUL">
<rect key="frame" x="300" y="-5" width="55" height="55"/>
<color key="backgroundColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<constraints>
<constraint firstAttribute="width" constant="55" id="h4F-29-Y6n"/>
<constraint firstAttribute="height" constant="55" id="q45-t3-9DA"/>
</constraints>
<color key="tintColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<state key="normal" image="ic_add.png"/>
<userDefinedRuntimeAttributes>
<userDefinedRuntimeAttribute type="number" keyPath="type">
<integer key="value" value="2"/>
</userDefinedRuntimeAttribute>
<userDefinedRuntimeAttribute type="image" keyPath="imageNormal" value="ic_add.png"/>
<userDefinedRuntimeAttribute type="color" keyPath="rippleColor">
<color key="value" red="0.82337594749999998" green="0.83186435940000003" blue="0.83186435940000003" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
</userDefinedRuntimeAttribute>
</userDefinedRuntimeAttributes>
<connections>
<action selector="handleIncrement:" destination="l7k-TM-87m" eventType="touchUpInside" id="n9c-Ap-rPx"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstAttribute="bottom" secondItem="6yL-sX-bUL" secondAttribute="bottom" constant="20" symbolic="YES" id="RqS-NP-k0w"/>
<constraint firstAttribute="trailing" secondItem="6yL-sX-bUL" secondAttribute="trailing" constant="20" symbolic="YES" id="gvv-UZ-pAw"/>
<constraint firstAttribute="bottom" secondItem="CTc-Jz-yjr" secondAttribute="bottom" constant="20" symbolic="YES" id="p6W-U8-gLe"/>
<constraint firstAttribute="height" constant="70" id="v60-11-5Pg"/>
<constraint firstItem="CTc-Jz-yjr" firstAttribute="leading" secondItem="9iX-D6-aiy" secondAttribute="leading" constant="20" symbolic="YES" id="vmT-3b-AxF"/>
</constraints>
</view>
</subviews>
</stackView>
</subviews>
<color key="backgroundColor" red="0.66666666669999997" green="0.66666666669999997" blue="0.66666666669999997" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstItem="O5w-dx-5ca" firstAttribute="top" secondItem="9Pa-2h-BJY" secondAttribute="top" id="D5u-Ck-lxI"/>
<constraint firstItem="O5w-dx-5ca" firstAttribute="leading" secondItem="9Pa-2h-BJY" secondAttribute="leading" id="Djg-Hg-SBg"/>
<constraint firstAttribute="trailing" secondItem="O5w-dx-5ca" secondAttribute="trailing" id="RDC-2b-jUw"/>
<constraint firstAttribute="bottom" secondItem="O5w-dx-5ca" secondAttribute="bottom" id="YEU-9J-aTb"/>
</constraints>
</view>
<connections>
<outlet property="countLabel" destination="qaB-rs-mWp" id="bFY-of-WoI"/>
<outlet property="incrementButton" destination="6yL-sX-bUL" id="aQR-ap-BrT"/>
</connections>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="7qW-nP-WZA" userLabel="First Responder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="805.60000000000002" y="197.45127436281859"/>
</scene>
</scenes>
<resources>
<image name="ic_add.png" width="24" height="24"/>
</resources>
</document>
| flutter/examples/platform_view/ios/Runner/Base.lproj/Main.storyboard/0 | {
"file_path": "flutter/examples/platform_view/ios/Runner/Base.lproj/Main.storyboard",
"repo_id": "flutter",
"token_count": 7530
} | 628 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() {
runApp(
const DecoratedBox(
decoration: BoxDecoration(color: Colors.white),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
textDirection: TextDirection.ltr,
children: <Widget>[
FlutterLogo(size: 48),
Padding(
padding: EdgeInsets.all(32),
child: Text(
'This app is only meant to be run under the Flutter debugger',
textDirection: TextDirection.ltr,
textAlign: TextAlign.center,
style: TextStyle(color: Colors.black87),
),
),
],
),
),
),
);
}
| flutter/examples/splash/lib/main.dart/0 | {
"file_path": "flutter/examples/splash/lib/main.dart",
"repo_id": "flutter",
"token_count": 431
} | 629 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for the TextTheme class from the Material library. *
# For fixes to
# * AppBar: fix_app_bar.yaml
# * AppBarTheme: fix_app_bar_theme.yaml
# * ColorScheme: fix_color_scheme.yaml
# * Material (general): fix_material.yaml
# * SliverAppBar: fix_sliver_app_bar.yaml
# * ThemeData: fix_theme_data.yaml
# * WidgetState: fix_widget_state.yaml
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline1'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: display4
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline1'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline2'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: display3
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline2'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline3'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: display2
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline3'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline4'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: display1
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline4'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline5'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: headline
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline5'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'headline6'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: title
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headline6'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'subtitle1'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: subhead
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'subtitle1'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'bodyText1'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: body2
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'bodyText1'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'bodyText2'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: body1
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'bodyText2'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: "Rename to 'subtitle2'"
date: 2020-01-24
element:
uris: [ 'material.dart' ]
getter: subtitle
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'subtitle2'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: 'Rename arguments'
date: 2020-01-24
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'TextTheme'
changes:
- kind: 'renameParameter'
oldName: 'display4'
newName: 'headline1'
- kind: 'renameParameter'
oldName: 'display3'
newName: 'headline2'
- kind: 'renameParameter'
oldName: 'display2'
newName: 'headline3'
- kind: 'renameParameter'
oldName: 'display1'
newName: 'headline4'
- kind: 'renameParameter'
oldName: 'headline'
newName: 'headline5'
- kind: 'renameParameter'
oldName: 'title'
newName: 'headline6'
- kind: 'renameParameter'
oldName: 'subhead'
newName: 'subtitle1'
- kind: 'renameParameter'
oldName: 'subtitle'
newName: 'subtitle2'
- kind: 'renameParameter'
oldName: 'body2'
newName: 'bodyText1'
- kind: 'renameParameter'
oldName: 'body1'
newName: 'bodyText2'
# Changes made in https://github.com/flutter/flutter/pull/48547
- title: 'Rename arguments'
date: 2020-01-24
element:
uris: [ 'material.dart' ]
method: 'copyWith'
inClass: 'TextTheme'
changes:
- kind: 'renameParameter'
oldName: 'display4'
newName: 'headline1'
- kind: 'renameParameter'
oldName: 'display3'
newName: 'headline2'
- kind: 'renameParameter'
oldName: 'display2'
newName: 'headline3'
- kind: 'renameParameter'
oldName: 'display1'
newName: 'headline4'
- kind: 'renameParameter'
oldName: 'headline'
newName: 'headline5'
- kind: 'renameParameter'
oldName: 'title'
newName: 'headline6'
- kind: 'renameParameter'
oldName: 'subhead'
newName: 'subtitle1'
- kind: 'renameParameter'
oldName: 'subtitle'
newName: 'subtitle2'
- kind: 'renameParameter'
oldName: 'body2'
newName: 'bodyText1'
- kind: 'renameParameter'
oldName: 'body1'
newName: 'bodyText2'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'displayLarge'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline1
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'displayLarge'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'displayMedium'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline2
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'displayMedium'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'displaySmall'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline3
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'displaySmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'headlineMedium'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline4
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headlineMedium'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'headlineSmall'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline5
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'headlineSmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'titleLarge'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: headline6
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'titleLarge'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'titleMedium'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: subtitle1
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'titleMedium'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'titleSmall'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: subtitle2
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'titleSmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'bodyLarge'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: bodyText1
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'bodyLarge'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'bodyMedium'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: bodyText2
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'bodyMedium'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'bodySmall'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: caption
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'bodySmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'labelLarge'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: button
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'labelLarge'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: "Rename to 'labelSmall'"
date: 2022-08-18
element:
uris: [ 'material.dart' ]
getter: overline
inClass: 'TextTheme'
changes:
- kind: 'rename'
newName: 'labelSmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: 'Rename arguments'
date: 2022-08-18
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'TextTheme'
changes:
- kind: 'renameParameter'
oldName: 'headline1'
newName: 'displayLarge'
- kind: 'renameParameter'
oldName: 'headline2'
newName: 'displayMedium'
- kind: 'renameParameter'
oldName: 'headline3'
newName: 'displaySmall'
- kind: 'renameParameter'
oldName: 'headline4'
newName: 'headlineMedium'
- kind: 'renameParameter'
oldName: 'headline5'
newName: 'headlineSmall'
- kind: 'renameParameter'
oldName: 'headline6'
newName: 'titleLarge'
- kind: 'renameParameter'
oldName: 'subtitle1'
newName: 'titleMedium'
- kind: 'renameParameter'
oldName: 'subtitle2'
newName: 'titleSmall'
- kind: 'renameParameter'
oldName: 'bodyText1'
newName: 'bodyLarge'
- kind: 'renameParameter'
oldName: 'bodyText2'
newName: 'bodyMedium'
- kind: 'renameParameter'
oldName: 'caption'
newName: 'bodySmall'
- kind: 'renameParameter'
oldName: 'button'
newName: 'labelLarge'
- kind: 'renameParameter'
oldName: 'overline'
newName: 'labelSmall'
# Changes made in https://github.com/flutter/flutter/pull/109817
- title: 'Rename arguments'
date: 2022-08-18
element:
uris: [ 'material.dart' ]
method: 'copyWith'
inClass: 'TextTheme'
changes:
- kind: 'renameParameter'
oldName: 'headline1'
newName: 'displayLarge'
- kind: 'renameParameter'
oldName: 'headline2'
newName: 'displayMedium'
- kind: 'renameParameter'
oldName: 'headline3'
newName: 'displaySmall'
- kind: 'renameParameter'
oldName: 'headline4'
newName: 'headlineMedium'
- kind: 'renameParameter'
oldName: 'headline5'
newName: 'headlineSmall'
- kind: 'renameParameter'
oldName: 'headline6'
newName: 'titleLarge'
- kind: 'renameParameter'
oldName: 'subtitle1'
newName: 'titleMedium'
- kind: 'renameParameter'
oldName: 'subtitle2'
newName: 'titleSmall'
- kind: 'renameParameter'
oldName: 'bodyText1'
newName: 'bodyLarge'
- kind: 'renameParameter'
oldName: 'bodyText2'
newName: 'bodyMedium'
- kind: 'renameParameter'
oldName: 'caption'
newName: 'bodySmall'
- kind: 'renameParameter'
oldName: 'button'
newName: 'labelLarge'
- kind: 'renameParameter'
oldName: 'overline'
newName: 'labelSmall'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_material/fix_text_theme.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_material/fix_text_theme.yaml",
"repo_id": "flutter",
"token_count": 5780
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Core Flutter framework primitives.
///
/// The features defined in this library are the lowest-level utility
/// classes and functions used by all the other layers of the Flutter
/// framework.
library foundation;
export 'package:meta/meta.dart' show
factory,
immutable,
mustCallSuper,
nonVirtual,
optionalTypeArgs,
protected,
required,
visibleForTesting;
export 'src/foundation/annotations.dart';
export 'src/foundation/assertions.dart';
export 'src/foundation/basic_types.dart';
export 'src/foundation/binding.dart';
export 'src/foundation/bitfield.dart';
export 'src/foundation/capabilities.dart';
export 'src/foundation/change_notifier.dart';
export 'src/foundation/collections.dart';
export 'src/foundation/consolidate_response.dart';
export 'src/foundation/constants.dart';
export 'src/foundation/debug.dart';
export 'src/foundation/diagnostics.dart';
export 'src/foundation/isolates.dart';
export 'src/foundation/key.dart';
export 'src/foundation/licenses.dart';
export 'src/foundation/memory_allocations.dart';
export 'src/foundation/node.dart';
export 'src/foundation/object.dart';
export 'src/foundation/observer_list.dart';
export 'src/foundation/persistent_hash_map.dart';
export 'src/foundation/platform.dart';
export 'src/foundation/print.dart';
export 'src/foundation/serialization.dart';
export 'src/foundation/service_extensions.dart';
export 'src/foundation/stack_frame.dart';
export 'src/foundation/synchronous_future.dart';
export 'src/foundation/timeline.dart';
export 'src/foundation/unicode.dart';
| flutter/packages/flutter/lib/foundation.dart/0 | {
"file_path": "flutter/packages/flutter/lib/foundation.dart",
"repo_id": "flutter",
"token_count": 521
} | 631 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'tween.dart';
export 'tween.dart' show Animatable;
// Examples can assume:
// late AnimationController myAnimationController;
/// Enables creating an [Animation] whose value is defined by a sequence of
/// [Tween]s.
///
/// Each [TweenSequenceItem] has a weight that defines its percentage of the
/// animation's duration. Each tween defines the animation's value during the
/// interval indicated by its weight.
///
/// {@tool snippet}
/// This example defines an animation that uses an easing curve to interpolate
/// between 5.0 and 10.0 during the first 40% of the animation, remains at 10.0
/// for the next 20%, and then returns to 5.0 for the final 40%.
///
/// ```dart
/// final Animation<double> animation = TweenSequence<double>(
/// <TweenSequenceItem<double>>[
/// TweenSequenceItem<double>(
/// tween: Tween<double>(begin: 5.0, end: 10.0)
/// .chain(CurveTween(curve: Curves.ease)),
/// weight: 40.0,
/// ),
/// TweenSequenceItem<double>(
/// tween: ConstantTween<double>(10.0),
/// weight: 20.0,
/// ),
/// TweenSequenceItem<double>(
/// tween: Tween<double>(begin: 10.0, end: 5.0)
/// .chain(CurveTween(curve: Curves.ease)),
/// weight: 40.0,
/// ),
/// ],
/// ).animate(myAnimationController);
/// ```
/// {@end-tool}
class TweenSequence<T> extends Animatable<T> {
/// Construct a TweenSequence.
///
/// The [items] parameter must be a list of one or more [TweenSequenceItem]s.
///
/// There's a small cost associated with building a [TweenSequence] so it's
/// best to reuse one, rather than rebuilding it on every frame, when that's
/// possible.
TweenSequence(List<TweenSequenceItem<T>> items)
: assert(items.isNotEmpty) {
_items.addAll(items);
double totalWeight = 0.0;
for (final TweenSequenceItem<T> item in _items) {
totalWeight += item.weight;
}
assert(totalWeight > 0.0);
double start = 0.0;
for (int i = 0; i < _items.length; i += 1) {
final double end = i == _items.length - 1 ? 1.0 : start + _items[i].weight / totalWeight;
_intervals.add(_Interval(start, end));
start = end;
}
}
final List<TweenSequenceItem<T>> _items = <TweenSequenceItem<T>>[];
final List<_Interval> _intervals = <_Interval>[];
T _evaluateAt(double t, int index) {
final TweenSequenceItem<T> element = _items[index];
final double tInterval = _intervals[index].value(t);
return element.tween.transform(tInterval);
}
@override
T transform(double t) {
assert(t >= 0.0 && t <= 1.0);
if (t == 1.0) {
return _evaluateAt(t, _items.length - 1);
}
for (int index = 0; index < _items.length; index++) {
if (_intervals[index].contains(t)) {
return _evaluateAt(t, index);
}
}
// Should be unreachable.
throw StateError('TweenSequence.evaluate() could not find an interval for $t');
}
@override
String toString() => 'TweenSequence(${_items.length} items)';
}
/// Enables creating a flipped [Animation] whose value is defined by a sequence
/// of [Tween]s.
///
/// This creates a [TweenSequence] that evaluates to a result that flips the
/// tween both horizontally and vertically.
///
/// This tween sequence assumes that the evaluated result has to be a double
/// between 0.0 and 1.0.
class FlippedTweenSequence extends TweenSequence<double> {
/// Creates a flipped [TweenSequence].
///
/// The [items] parameter must be a list of one or more [TweenSequenceItem]s.
///
/// There's a small cost associated with building a `TweenSequence` so it's
/// best to reuse one, rather than rebuilding it on every frame, when that's
/// possible.
FlippedTweenSequence(super.items);
@override
double transform(double t) => 1 - super.transform(1 - t);
}
/// A simple holder for one element of a [TweenSequence].
class TweenSequenceItem<T> {
/// Construct a TweenSequenceItem.
///
/// The [weight] must be greater than 0.0.
const TweenSequenceItem({
required this.tween,
required this.weight,
}) : assert(weight > 0.0);
/// Defines the value of the [TweenSequence] for the interval within the
/// animation's duration indicated by [weight] and this item's position
/// in the list of items.
///
/// {@tool snippet}
///
/// The value of this item can be "curved" by chaining it to a [CurveTween].
/// For example to create a tween that eases from 0.0 to 10.0:
///
/// ```dart
/// Tween<double>(begin: 0.0, end: 10.0)
/// .chain(CurveTween(curve: Curves.ease))
/// ```
/// {@end-tool}
final Animatable<T> tween;
/// An arbitrary value that indicates the relative percentage of a
/// [TweenSequence] animation's duration when [tween] will be used.
///
/// The percentage for an individual item is the item's weight divided by the
/// sum of all of the items' weights.
final double weight;
}
class _Interval {
const _Interval(this.start, this.end) : assert(end > start);
final double start;
final double end;
bool contains(double t) => t >= start && t < end;
double value(double t) => (t - start) / (end - start);
@override
String toString() => '<$start, $end>';
}
| flutter/packages/flutter/lib/src/animation/tween_sequence.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/animation/tween_sequence.dart",
"repo_id": "flutter",
"token_count": 1849
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show ImageFilter;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'interface_level.dart';
import 'localizations.dart';
import 'scrollbar.dart';
import 'theme.dart';
// TODO(abarth): These constants probably belong somewhere more general.
// Used XD to flutter plugin(https://github.com/AdobeXD/xd-to-flutter-plugin/)
// to derive values of TextStyle(height and letterSpacing) from
// Adobe XD template for iOS 13, which can be found in
// Apple Design Resources(https://developer.apple.com/design/resources/).
// However the values are not exactly the same as native, so eyeballing is needed.
const TextStyle _kCupertinoDialogTitleStyle = TextStyle(
fontFamily: 'CupertinoSystemDisplay',
inherit: false,
fontSize: 17.0,
fontWeight: FontWeight.w600,
height: 1.3,
letterSpacing: -0.5,
textBaseline: TextBaseline.alphabetic,
);
const TextStyle _kCupertinoDialogContentStyle = TextStyle(
fontFamily: 'CupertinoSystemText',
inherit: false,
fontSize: 13.0,
fontWeight: FontWeight.w400,
height: 1.35,
letterSpacing: -0.2,
textBaseline: TextBaseline.alphabetic,
);
const TextStyle _kCupertinoDialogActionStyle = TextStyle(
fontFamily: 'CupertinoSystemText',
inherit: false,
fontSize: 16.8,
fontWeight: FontWeight.w400,
textBaseline: TextBaseline.alphabetic,
);
// CupertinoActionSheet-specific text styles.
const TextStyle _kActionSheetActionStyle = TextStyle(
fontFamily: 'CupertinoSystemText',
inherit: false,
fontSize: 17.0,
fontWeight: FontWeight.w400,
textBaseline: TextBaseline.alphabetic,
);
const TextStyle _kActionSheetContentStyle = TextStyle(
fontFamily: 'CupertinoSystemText',
inherit: false,
fontSize: 13.0,
fontWeight: FontWeight.w400,
color: _kActionSheetContentTextColor,
textBaseline: TextBaseline.alphabetic,
);
// Generic constants shared between Dialog and ActionSheet.
const double _kBlurAmount = 20.0;
const double _kCornerRadius = 14.0;
const double _kDividerThickness = 0.3;
// Dialog specific constants.
// iOS dialogs have a normal display width and another display width that is
// used when the device is in accessibility mode. Each of these widths are
// listed below.
const double _kCupertinoDialogWidth = 270.0;
const double _kAccessibilityCupertinoDialogWidth = 310.0;
const double _kDialogEdgePadding = 20.0;
const double _kDialogMinButtonHeight = 45.0;
const double _kDialogMinButtonFontSize = 10.0;
// ActionSheet specific constants.
const double _kActionSheetEdgeHorizontalPadding = 8.0;
const double _kActionSheetCancelButtonPadding = 8.0;
const double _kActionSheetEdgeVerticalPadding = 10.0;
const double _kActionSheetContentHorizontalPadding = 16.0;
const double _kActionSheetContentVerticalPadding = 12.0;
const double _kActionSheetButtonHeight = 56.0;
// A translucent color that is painted on top of the blurred backdrop as the
// dialog's background color
// Extracted from https://developer.apple.com/design/resources/.
const Color _kDialogColor = CupertinoDynamicColor.withBrightness(
color: Color(0xCCF2F2F2),
darkColor: Color(0xBF1E1E1E),
);
// Translucent light gray that is painted on top of the blurred backdrop as the
// background color of a pressed button.
// Eyeballed from iOS 13 beta simulator.
const Color _kPressedColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFE1E1E1),
darkColor: Color(0xFF2E2E2E),
);
const Color _kActionSheetCancelPressedColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFECECEC),
darkColor: Color(0xFF49494B),
);
// Translucent, very light gray that is painted on top of the blurred backdrop
// as the action sheet's background color.
// TODO(LongCatIsLooong): https://github.com/flutter/flutter/issues/39272. Use
// System Materials once we have them.
// Extracted from https://developer.apple.com/design/resources/.
const Color _kActionSheetBackgroundColor = CupertinoDynamicColor.withBrightness(
color: Color(0xC7F9F9F9),
darkColor: Color(0xC7252525),
);
// The gray color used for text that appears in the title area.
// Extracted from https://developer.apple.com/design/resources/.
const Color _kActionSheetContentTextColor = Color(0xFF8F8F8F);
// Translucent gray that is painted on top of the blurred backdrop in the gap
// areas between the content section and actions section, as well as between
// buttons.
// Eye-balled from iOS 13 beta simulator.
const Color _kActionSheetButtonDividerColor = _kActionSheetContentTextColor;
// The alert dialog layout policy changes depending on whether the user is using
// a "regular" font size vs a "large" font size. This is a spectrum. There are
// many "regular" font sizes and many "large" font sizes. But depending on which
// policy is currently being used, a dialog is laid out differently.
//
// Empirically, the jump from one policy to the other occurs at the following text
// scale factors:
// Largest regular scale factor: 1.3529411764705883
// Smallest large scale factor: 1.6470588235294117
//
// The following constant represents a division in text scale factor beyond which
// we want to change how the dialog is laid out.
const double _kMaxRegularTextScaleFactor = 1.4;
// Accessibility mode on iOS is determined by the text scale factor that the
// user has selected.
bool _isInAccessibilityMode(BuildContext context) {
const double defaultFontSize = 14.0;
final double? scaledFontSize = MediaQuery.maybeTextScalerOf(context)?.scale(defaultFontSize);
return scaledFontSize != null && scaledFontSize > defaultFontSize * _kMaxRegularTextScaleFactor;
}
/// An iOS-style alert dialog.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=75CsnyRXf5I}
///
/// An alert dialog informs the user about situations that require
/// acknowledgment. An alert dialog has an optional title, optional content,
/// and an optional list of actions. The title is displayed above the content
/// and the actions are displayed below the content.
///
/// This dialog styles its title and content (typically a message) to match the
/// standard iOS title and message dialog text style. These default styles can
/// be overridden by explicitly defining [TextStyle]s for [Text] widgets that
/// are part of the title or content.
///
/// To display action buttons that look like standard iOS dialog buttons,
/// provide [CupertinoDialogAction]s for the [actions] given to this dialog.
///
/// Typically passed as the child widget to [showDialog], which displays the
/// dialog.
///
/// {@tool dartpad}
/// This sample shows how to use a [CupertinoAlertDialog].
/// The [CupertinoAlertDialog] shows an alert with a set of two choices
/// when [CupertinoButton] is pressed.
///
/// ** See code in examples/api/lib/cupertino/dialog/cupertino_alert_dialog.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoPopupSurface], which is a generic iOS-style popup surface that
/// holds arbitrary content to create custom popups.
/// * [CupertinoDialogAction], which is an iOS-style dialog button.
/// * [AlertDialog], a Material Design alert dialog.
/// * <https://developer.apple.com/design/human-interface-guidelines/alerts/>
class CupertinoAlertDialog extends StatefulWidget {
/// Creates an iOS-style alert dialog.
const CupertinoAlertDialog({
super.key,
this.title,
this.content,
this.actions = const <Widget>[],
this.scrollController,
this.actionScrollController,
this.insetAnimationDuration = const Duration(milliseconds: 100),
this.insetAnimationCurve = Curves.decelerate,
});
/// The (optional) title of the dialog is displayed in a large font at the top
/// of the dialog.
///
/// Typically a [Text] widget.
final Widget? title;
/// The (optional) content of the dialog is displayed in the center of the
/// dialog in a lighter font.
///
/// Typically a [Text] widget.
final Widget? content;
/// The (optional) set of actions that are displayed at the bottom of the
/// dialog.
///
/// Typically this is a list of [CupertinoDialogAction] widgets.
final List<Widget> actions;
/// A scroll controller that can be used to control the scrolling of the
/// [content] in the dialog.
///
/// Defaults to null, and is typically not needed, since most alert messages
/// are short.
///
/// See also:
///
/// * [actionScrollController], which can be used for controlling the actions
/// section when there are many actions.
final ScrollController? scrollController;
/// A scroll controller that can be used to control the scrolling of the
/// actions in the dialog.
///
/// Defaults to null, and is typically not needed.
///
/// See also:
///
/// * [scrollController], which can be used for controlling the [content]
/// section when it is long.
final ScrollController? actionScrollController;
/// {@macro flutter.material.dialog.insetAnimationDuration}
final Duration insetAnimationDuration;
/// {@macro flutter.material.dialog.insetAnimationCurve}
final Curve insetAnimationCurve;
@override
State<CupertinoAlertDialog> createState() => _CupertinoAlertDialogState();
}
class _CupertinoAlertDialogState extends State<CupertinoAlertDialog> {
ScrollController? _backupScrollController;
ScrollController? _backupActionScrollController;
ScrollController get _effectiveScrollController =>
widget.scrollController ?? (_backupScrollController ??= ScrollController());
ScrollController get _effectiveActionScrollController =>
widget.actionScrollController ?? (_backupActionScrollController ??= ScrollController());
Widget _buildContent(BuildContext context) {
const double defaultFontSize = 14.0;
final double effectiveTextScaleFactor = MediaQuery.textScalerOf(context).scale(defaultFontSize) / defaultFontSize;
final List<Widget> children = <Widget>[
if (widget.title != null || widget.content != null)
Flexible(
flex: 3,
child: _CupertinoAlertContentSection(
title: widget.title,
message: widget.content,
scrollController: _effectiveScrollController,
titlePadding: EdgeInsets.only(
left: _kDialogEdgePadding,
right: _kDialogEdgePadding,
bottom: widget.content == null ? _kDialogEdgePadding : 1.0,
top: _kDialogEdgePadding * effectiveTextScaleFactor,
),
messagePadding: EdgeInsets.only(
left: _kDialogEdgePadding,
right: _kDialogEdgePadding,
bottom: _kDialogEdgePadding * effectiveTextScaleFactor,
top: widget.title == null ? _kDialogEdgePadding : 1.0,
),
titleTextStyle: _kCupertinoDialogTitleStyle.copyWith(
color: CupertinoDynamicColor.resolve(CupertinoColors.label, context),
),
messageTextStyle: _kCupertinoDialogContentStyle.copyWith(
color: CupertinoDynamicColor.resolve(CupertinoColors.label, context),
),
),
),
];
return ColoredBox(
color: CupertinoDynamicColor.resolve(_kDialogColor, context),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: children,
),
);
}
Widget _buildActions() {
Widget actionSection = Container(
height: 0.0,
);
if (widget.actions.isNotEmpty) {
actionSection = _CupertinoAlertActionSection(
scrollController: _effectiveActionScrollController,
children: widget.actions,
);
}
return actionSection;
}
@override
Widget build(BuildContext context) {
final CupertinoLocalizations localizations = CupertinoLocalizations.of(context);
final bool isInAccessibilityMode = _isInAccessibilityMode(context);
return CupertinoUserInterfaceLevel(
data: CupertinoUserInterfaceLevelData.elevated,
child: MediaQuery.withClampedTextScaling(
// iOS does not shrink dialog content below a 1.0 scale factor
minScaleFactor: 1.0,
child: ScrollConfiguration(
// A CupertinoScrollbar is built-in below.
behavior: ScrollConfiguration.of(context).copyWith(scrollbars: false),
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return AnimatedPadding(
padding: MediaQuery.viewInsetsOf(context) +
const EdgeInsets.symmetric(horizontal: 40.0, vertical: 24.0),
duration: widget.insetAnimationDuration,
curve: widget.insetAnimationCurve,
child: MediaQuery.removeViewInsets(
removeLeft: true,
removeTop: true,
removeRight: true,
removeBottom: true,
context: context,
child: Center(
child: Container(
margin: const EdgeInsets.symmetric(vertical: _kDialogEdgePadding),
width: isInAccessibilityMode
? _kAccessibilityCupertinoDialogWidth
: _kCupertinoDialogWidth,
child: CupertinoPopupSurface(
isSurfacePainted: false,
child: Semantics(
namesRoute: true,
scopesRoute: true,
explicitChildNodes: true,
label: localizations.alertDialogLabel,
child: _CupertinoDialogRenderWidget(
contentSection: _buildContent(context),
actionsSection: _buildActions(),
dividerColor: CupertinoColors.separator,
),
),
),
),
),
),
);
},
),
),
),
);
}
@override
void dispose() {
_backupScrollController?.dispose();
_backupActionScrollController?.dispose();
super.dispose();
}
}
/// Rounded rectangle surface that looks like an iOS popup surface, e.g., alert dialog
/// and action sheet.
///
/// A [CupertinoPopupSurface] can be configured to paint or not paint a white
/// color on top of its blurred area. Typical usage should paint white on top
/// of the blur. However, the white paint can be disabled for the purpose of
/// rendering divider gaps for a more complicated layout, e.g., [CupertinoAlertDialog].
/// Additionally, the white paint can be disabled to render a blurred rounded
/// rectangle without any color (similar to iOS's volume control popup).
///
/// See also:
///
/// * [CupertinoAlertDialog], which is a dialog with a title, content, and
/// actions.
/// * <https://developer.apple.com/design/human-interface-guidelines/alerts/>
class CupertinoPopupSurface extends StatelessWidget {
/// Creates an iOS-style rounded rectangle popup surface.
const CupertinoPopupSurface({
super.key,
this.isSurfacePainted = true,
this.child,
});
/// Whether or not to paint a translucent white on top of this surface's
/// blurred background. [isSurfacePainted] should be true for a typical popup
/// that contains content without any dividers. A popup that requires dividers
/// should set [isSurfacePainted] to false and then paint its own surface area.
///
/// Some popups, like iOS's volume control popup, choose to render a blurred
/// area without any white paint covering it. To achieve this effect,
/// [isSurfacePainted] should be set to false.
final bool isSurfacePainted;
/// The widget below this widget in the tree.
final Widget? child;
@override
Widget build(BuildContext context) {
return ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(_kCornerRadius)),
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: _kBlurAmount, sigmaY: _kBlurAmount),
child: Container(
color: isSurfacePainted ? CupertinoDynamicColor.resolve(_kDialogColor, context) : null,
child: child,
),
),
);
}
}
/// An iOS-style action sheet.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=U-ao8p4A82k}
///
/// An action sheet is a specific style of alert that presents the user
/// with a set of two or more choices related to the current context.
/// An action sheet can have a title, an additional message, and a list
/// of actions. The title is displayed above the message and the actions
/// are displayed below this content.
///
/// This action sheet styles its title and message to match standard iOS action
/// sheet title and message text style.
///
/// To display action buttons that look like standard iOS action sheet buttons,
/// provide [CupertinoActionSheetAction]s for the [actions] given to this action
/// sheet.
///
/// To include a iOS-style cancel button separate from the other buttons,
/// provide an [CupertinoActionSheetAction] for the [cancelButton] given to this
/// action sheet.
///
/// An action sheet is typically passed as the child widget to
/// [showCupertinoModalPopup], which displays the action sheet by sliding it up
/// from the bottom of the screen.
///
/// {@tool dartpad}
/// This sample shows how to use a [CupertinoActionSheet].
/// The [CupertinoActionSheet] shows a modal popup that slides in from the
/// bottom when [CupertinoButton] is pressed.
///
/// ** See code in examples/api/lib/cupertino/dialog/cupertino_action_sheet.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoActionSheetAction], which is an iOS-style action sheet button.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/views/action-sheets/>
class CupertinoActionSheet extends StatefulWidget {
/// Creates an iOS-style action sheet.
///
/// An action sheet must have a non-null value for at least one of the
/// following arguments: [actions], [title], [message], or [cancelButton].
///
/// Generally, action sheets are used to give the user a choice between
/// two or more choices for the current context.
const CupertinoActionSheet({
super.key,
this.title,
this.message,
this.actions,
this.messageScrollController,
this.actionScrollController,
this.cancelButton,
}) : assert(
actions != null || title != null || message != null || cancelButton != null,
'An action sheet must have a non-null value for at least one of the following arguments: '
'actions, title, message, or cancelButton',
);
/// An optional title of the action sheet. When the [message] is non-null,
/// the font of the [title] is bold.
///
/// Typically a [Text] widget.
final Widget? title;
/// An optional descriptive message that provides more details about the
/// reason for the alert.
///
/// Typically a [Text] widget.
final Widget? message;
/// The set of actions that are displayed for the user to select.
///
/// Typically this is a list of [CupertinoActionSheetAction] widgets.
final List<Widget>? actions;
/// A scroll controller that can be used to control the scrolling of the
/// [message] in the action sheet.
///
/// This attribute is typically not needed, as alert messages should be
/// short.
final ScrollController? messageScrollController;
/// A scroll controller that can be used to control the scrolling of the
/// [actions] in the action sheet.
///
/// This attribute is typically not needed.
final ScrollController? actionScrollController;
/// The optional cancel button that is grouped separately from the other
/// actions.
///
/// Typically this is an [CupertinoActionSheetAction] widget.
final Widget? cancelButton;
@override
State<CupertinoActionSheet> createState() => _CupertinoActionSheetState();
}
class _CupertinoActionSheetState extends State<CupertinoActionSheet> {
ScrollController? _backupMessageScrollController;
ScrollController? _backupActionScrollController;
ScrollController get _effectiveMessageScrollController =>
widget.messageScrollController ?? (_backupMessageScrollController ??= ScrollController());
ScrollController get _effectiveActionScrollController =>
widget.actionScrollController ?? (_backupActionScrollController ??= ScrollController());
@override
void dispose() {
_backupMessageScrollController?.dispose();
_backupActionScrollController?.dispose();
super.dispose();
}
Widget _buildContent(BuildContext context) {
final List<Widget> content = <Widget>[];
if (widget.title != null || widget.message != null) {
final Widget titleSection = _CupertinoAlertContentSection(
title: widget.title,
message: widget.message,
scrollController: _effectiveMessageScrollController,
titlePadding: EdgeInsets.only(
left: _kActionSheetContentHorizontalPadding,
right: _kActionSheetContentHorizontalPadding,
bottom: widget.message == null ? _kActionSheetContentVerticalPadding : 0.0,
top: _kActionSheetContentVerticalPadding,
),
messagePadding: EdgeInsets.only(
left: _kActionSheetContentHorizontalPadding,
right: _kActionSheetContentHorizontalPadding,
bottom: _kActionSheetContentVerticalPadding,
top: widget.title == null ? _kActionSheetContentVerticalPadding : 0.0,
),
titleTextStyle: widget.message == null
? _kActionSheetContentStyle
: _kActionSheetContentStyle.copyWith(fontWeight: FontWeight.w600),
messageTextStyle: widget.title == null
? _kActionSheetContentStyle.copyWith(fontWeight: FontWeight.w600)
: _kActionSheetContentStyle,
additionalPaddingBetweenTitleAndMessage: const EdgeInsets.only(top: 4.0),
);
content.add(Flexible(child: titleSection));
}
return ColoredBox(
color: CupertinoDynamicColor.resolve(_kActionSheetBackgroundColor, context),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: content,
),
);
}
Widget _buildActions() {
if (widget.actions == null || widget.actions!.isEmpty) {
return Container(
height: 0.0,
);
}
return _CupertinoAlertActionSection(
scrollController: _effectiveActionScrollController,
hasCancelButton: widget.cancelButton != null,
isActionSheet: true,
children: widget.actions!,
);
}
Widget _buildCancelButton() {
final double cancelPadding = (widget.actions != null || widget.message != null || widget.title != null)
? _kActionSheetCancelButtonPadding : 0.0;
return Padding(
padding: EdgeInsets.only(top: cancelPadding),
child: _CupertinoActionSheetCancelButton(
child: widget.cancelButton,
),
);
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
final List<Widget> children = <Widget>[
Flexible(child: ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(12.0)),
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: _kBlurAmount, sigmaY: _kBlurAmount),
child: _CupertinoDialogRenderWidget(
contentSection: Builder(builder: _buildContent),
actionsSection: _buildActions(),
dividerColor: _kActionSheetButtonDividerColor,
isActionSheet: true,
),
),
),
),
if (widget.cancelButton != null) _buildCancelButton(),
];
final Orientation orientation = MediaQuery.orientationOf(context);
final double actionSheetWidth;
if (orientation == Orientation.portrait) {
actionSheetWidth = MediaQuery.sizeOf(context).width - (_kActionSheetEdgeHorizontalPadding * 2);
} else {
actionSheetWidth = MediaQuery.sizeOf(context).height - (_kActionSheetEdgeHorizontalPadding * 2);
}
return SafeArea(
child: ScrollConfiguration(
// A CupertinoScrollbar is built-in below
behavior: ScrollConfiguration.of(context).copyWith(scrollbars: false),
child: Semantics(
namesRoute: true,
scopesRoute: true,
explicitChildNodes: true,
label: 'Alert',
child: CupertinoUserInterfaceLevel(
data: CupertinoUserInterfaceLevelData.elevated,
child: Container(
width: actionSheetWidth,
margin: const EdgeInsets.symmetric(
horizontal: _kActionSheetEdgeHorizontalPadding,
vertical: _kActionSheetEdgeVerticalPadding,
),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: children,
),
),
),
),
),
);
}
}
/// A button typically used in a [CupertinoActionSheet].
///
/// See also:
///
/// * [CupertinoActionSheet], an alert that presents the user with a set of two or
/// more choices related to the current context.
class CupertinoActionSheetAction extends StatelessWidget {
/// Creates an action for an iOS-style action sheet.
const CupertinoActionSheetAction({
super.key,
required this.onPressed,
this.isDefaultAction = false,
this.isDestructiveAction = false,
required this.child,
});
/// The callback that is called when the button is tapped.
final VoidCallback onPressed;
/// Whether this action is the default choice in the action sheet.
///
/// Default buttons have bold text.
final bool isDefaultAction;
/// Whether this action might change or delete data.
///
/// Destructive buttons have red text.
final bool isDestructiveAction;
/// The widget below this widget in the tree.
///
/// Typically a [Text] widget.
final Widget child;
@override
Widget build(BuildContext context) {
TextStyle style = _kActionSheetActionStyle.copyWith(
color: isDestructiveAction
? CupertinoDynamicColor.resolve(CupertinoColors.systemRed, context)
: CupertinoTheme.of(context).primaryColor,
);
if (isDefaultAction) {
style = style.copyWith(fontWeight: FontWeight.w600);
}
return MouseRegion(
cursor: kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
child: GestureDetector(
onTap: onPressed,
behavior: HitTestBehavior.opaque,
child: ConstrainedBox(
constraints: const BoxConstraints(
minHeight: _kActionSheetButtonHeight,
),
child: Semantics(
button: true,
child: Container(
alignment: Alignment.center,
padding: const EdgeInsets.symmetric(
vertical: 16.0,
horizontal: 10.0,
),
child: DefaultTextStyle(
style: style,
textAlign: TextAlign.center,
child: child,
),
),
),
),
),
);
}
}
class _CupertinoActionSheetCancelButton extends StatefulWidget {
const _CupertinoActionSheetCancelButton({
this.child,
});
final Widget? child;
@override
_CupertinoActionSheetCancelButtonState createState() => _CupertinoActionSheetCancelButtonState();
}
class _CupertinoActionSheetCancelButtonState extends State<_CupertinoActionSheetCancelButton> {
bool isBeingPressed = false;
void _onTapDown(TapDownDetails event) {
setState(() { isBeingPressed = true; });
}
void _onTapUp(TapUpDetails event) {
setState(() { isBeingPressed = false; });
}
void _onTapCancel() {
setState(() { isBeingPressed = false; });
}
@override
Widget build(BuildContext context) {
final Color backgroundColor = isBeingPressed
? _kActionSheetCancelPressedColor
: CupertinoColors.secondarySystemGroupedBackground;
return GestureDetector(
excludeFromSemantics: true,
onTapDown: _onTapDown,
onTapUp: _onTapUp,
onTapCancel: _onTapCancel,
child: Container(
decoration: BoxDecoration(
color: CupertinoDynamicColor.resolve(backgroundColor, context),
borderRadius: const BorderRadius.all(Radius.circular(_kCornerRadius)),
),
child: widget.child,
),
);
}
}
// iOS style layout policy widget for sizing an alert dialog's content section and
// action button section.
//
// See [_RenderCupertinoDialog] for specific layout policy details.
class _CupertinoDialogRenderWidget extends RenderObjectWidget {
const _CupertinoDialogRenderWidget({
required this.contentSection,
required this.actionsSection,
required this.dividerColor,
this.isActionSheet = false,
});
final Widget contentSection;
final Widget actionsSection;
final Color dividerColor;
final bool isActionSheet;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderCupertinoDialog(
dividerThickness: _kDividerThickness,
isInAccessibilityMode: _isInAccessibilityMode(context) && !isActionSheet,
dividerColor: CupertinoDynamicColor.resolve(dividerColor, context),
isActionSheet: isActionSheet,
);
}
@override
void updateRenderObject(BuildContext context, _RenderCupertinoDialog renderObject) {
renderObject
..isInAccessibilityMode = _isInAccessibilityMode(context) && !isActionSheet
..dividerColor = CupertinoDynamicColor.resolve(dividerColor, context)
..isActionSheet = isActionSheet;
}
@override
RenderObjectElement createElement() {
return _CupertinoDialogRenderElement(this, allowMoveRenderObjectChild: isActionSheet);
}
}
class _CupertinoDialogRenderElement extends RenderObjectElement {
_CupertinoDialogRenderElement(_CupertinoDialogRenderWidget super.widget, {this.allowMoveRenderObjectChild = false});
// Whether to allow overridden method moveRenderObjectChild call or default to super.
// CupertinoActionSheet should default to [super] but CupertinoAlertDialog not.
final bool allowMoveRenderObjectChild;
Element? _contentElement;
Element? _actionsElement;
@override
_RenderCupertinoDialog get renderObject => super.renderObject as _RenderCupertinoDialog;
@override
void visitChildren(ElementVisitor visitor) {
if (_contentElement != null) {
visitor(_contentElement!);
}
if (_actionsElement != null) {
visitor(_actionsElement!);
}
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
final _CupertinoDialogRenderWidget dialogRenderWidget = widget as _CupertinoDialogRenderWidget;
_contentElement = updateChild(_contentElement, dialogRenderWidget.contentSection, _AlertDialogSections.contentSection);
_actionsElement = updateChild(_actionsElement, dialogRenderWidget.actionsSection, _AlertDialogSections.actionsSection);
}
@override
void insertRenderObjectChild(RenderObject child, _AlertDialogSections slot) {
_placeChildInSlot(child, slot);
}
@override
void moveRenderObjectChild(RenderObject child, _AlertDialogSections oldSlot, _AlertDialogSections newSlot) {
if (!allowMoveRenderObjectChild) {
assert(false);
return;
}
_placeChildInSlot(child, newSlot);
}
@override
void update(RenderObjectWidget newWidget) {
super.update(newWidget);
final _CupertinoDialogRenderWidget dialogRenderWidget = widget as _CupertinoDialogRenderWidget;
_contentElement = updateChild(_contentElement, dialogRenderWidget.contentSection, _AlertDialogSections.contentSection);
_actionsElement = updateChild(_actionsElement, dialogRenderWidget.actionsSection, _AlertDialogSections.actionsSection);
}
@override
void forgetChild(Element child) {
assert(child == _contentElement || child == _actionsElement);
if (_contentElement == child) {
_contentElement = null;
} else {
assert(_actionsElement == child);
_actionsElement = null;
}
super.forgetChild(child);
}
@override
void removeRenderObjectChild(RenderObject child, _AlertDialogSections slot) {
assert(child == renderObject.contentSection || child == renderObject.actionsSection);
if (renderObject.contentSection == child) {
renderObject.contentSection = null;
} else {
assert(renderObject.actionsSection == child);
renderObject.actionsSection = null;
}
}
void _placeChildInSlot(RenderObject child, _AlertDialogSections slot) {
switch (slot) {
case _AlertDialogSections.contentSection:
renderObject.contentSection = child as RenderBox;
case _AlertDialogSections.actionsSection:
renderObject.actionsSection = child as RenderBox;
}
}
}
// iOS style layout policy for sizing an alert dialog's content section and action
// button section.
//
// The policy is as follows:
//
// If all content and buttons fit on screen:
// The content section and action button section are sized intrinsically and centered
// vertically on screen.
//
// If all content and buttons do not fit on screen, and iOS is NOT in accessibility mode:
// A minimum height for the action button section is calculated. The action
// button section will not be rendered shorter than this minimum. See
// [_RenderCupertinoDialogActions] for the minimum height calculation.
//
// With the minimum action button section calculated, the content section can
// take up as much space as is available, up to the point that it hits the
// minimum button height at the bottom.
//
// After the content section is laid out, the action button section is allowed
// to take up any remaining space that was not consumed by the content section.
//
// If all content and buttons do not fit on screen, and iOS IS in accessibility mode:
// The button section is given up to 50% of the available height. Then the content
// section is given whatever height remains.
class _RenderCupertinoDialog extends RenderBox {
_RenderCupertinoDialog({
RenderBox? contentSection,
RenderBox? actionsSection,
double dividerThickness = 0.0,
bool isInAccessibilityMode = false,
bool isActionSheet = false,
required Color dividerColor,
}) : _contentSection = contentSection,
_actionsSection = actionsSection,
_dividerThickness = dividerThickness,
_isInAccessibilityMode = isInAccessibilityMode,
_isActionSheet = isActionSheet,
_dividerPaint = Paint()
..color = dividerColor
..style = PaintingStyle.fill;
RenderBox? get contentSection => _contentSection;
RenderBox? _contentSection;
set contentSection(RenderBox? newContentSection) {
if (newContentSection != _contentSection) {
if (_contentSection != null) {
dropChild(_contentSection!);
}
_contentSection = newContentSection;
if (_contentSection != null) {
adoptChild(_contentSection!);
}
}
}
RenderBox? get actionsSection => _actionsSection;
RenderBox? _actionsSection;
set actionsSection(RenderBox? newActionsSection) {
if (newActionsSection != _actionsSection) {
if (null != _actionsSection) {
dropChild(_actionsSection!);
}
_actionsSection = newActionsSection;
if (null != _actionsSection) {
adoptChild(_actionsSection!);
}
}
}
bool get isInAccessibilityMode => _isInAccessibilityMode;
bool _isInAccessibilityMode;
set isInAccessibilityMode(bool newValue) {
if (newValue != _isInAccessibilityMode) {
_isInAccessibilityMode = newValue;
markNeedsLayout();
}
}
bool _isActionSheet;
bool get isActionSheet => _isActionSheet;
set isActionSheet(bool newValue) {
if (newValue != _isActionSheet) {
_isActionSheet = newValue;
markNeedsLayout();
}
}
double get _dialogWidth => isInAccessibilityMode
? _kAccessibilityCupertinoDialogWidth
: _kCupertinoDialogWidth;
final double _dividerThickness;
final Paint _dividerPaint;
Color get dividerColor => _dividerPaint.color;
set dividerColor(Color newValue) {
if (dividerColor == newValue) {
return;
}
_dividerPaint.color = newValue;
markNeedsPaint();
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
if (null != contentSection) {
contentSection!.attach(owner);
}
if (null != actionsSection) {
actionsSection!.attach(owner);
}
}
@override
void detach() {
super.detach();
if (null != contentSection) {
contentSection!.detach();
}
if (null != actionsSection) {
actionsSection!.detach();
}
}
@override
void redepthChildren() {
if (null != contentSection) {
redepthChild(contentSection!);
}
if (null != actionsSection) {
redepthChild(actionsSection!);
}
}
@override
void setupParentData(RenderBox child) {
if (!isActionSheet && child.parentData is! BoxParentData) {
child.parentData = BoxParentData();
} else if (child.parentData is! MultiChildLayoutParentData) {
child.parentData = MultiChildLayoutParentData();
}
}
@override
void visitChildren(RenderObjectVisitor visitor) {
if (contentSection != null) {
visitor(contentSection!);
}
if (actionsSection != null) {
visitor(actionsSection!);
}
}
@override
List<DiagnosticsNode> debugDescribeChildren() => <DiagnosticsNode>[
if (contentSection != null) contentSection!.toDiagnosticsNode(name: 'content'),
if (actionsSection != null) actionsSection!.toDiagnosticsNode(name: 'actions'),
];
@override
double computeMinIntrinsicWidth(double height) {
return isActionSheet ? constraints.minWidth : _dialogWidth;
}
@override
double computeMaxIntrinsicWidth(double height) {
return isActionSheet ? constraints.maxWidth : _dialogWidth;
}
@override
double computeMinIntrinsicHeight(double width) {
final double contentHeight = contentSection!.getMinIntrinsicHeight(width);
final double actionsHeight = actionsSection!.getMinIntrinsicHeight(width);
final bool hasDivider = contentHeight > 0.0 && actionsHeight > 0.0;
double height = contentHeight + (hasDivider ? _dividerThickness : 0.0) + actionsHeight;
if (isActionSheet && (actionsHeight > 0 || contentHeight > 0)) {
height -= 2 * _kActionSheetEdgeVerticalPadding;
}
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
final double contentHeight = contentSection!.getMaxIntrinsicHeight(width);
final double actionsHeight = actionsSection!.getMaxIntrinsicHeight(width);
final bool hasDivider = contentHeight > 0.0 && actionsHeight > 0.0;
double height = contentHeight + (hasDivider ? _dividerThickness : 0.0) + actionsHeight;
if (isActionSheet && (actionsHeight > 0 || contentHeight > 0)) {
height -= 2 * _kActionSheetEdgeVerticalPadding;
}
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _performLayout(
constraints: constraints,
layoutChild: ChildLayoutHelper.dryLayoutChild,
).size;
}
@override
void performLayout() {
final _AlertDialogSizes dialogSizes = _performLayout(
constraints: constraints,
layoutChild: ChildLayoutHelper.layoutChild,
);
size = dialogSizes.size;
// Set the position of the actions box to sit at the bottom of the dialog.
// The content box defaults to the top left, which is where we want it.
assert(
(!isActionSheet && actionsSection!.parentData is BoxParentData) ||
(isActionSheet && actionsSection!.parentData is MultiChildLayoutParentData),
);
if (isActionSheet) {
final MultiChildLayoutParentData actionParentData = actionsSection!.parentData! as MultiChildLayoutParentData;
actionParentData.offset = Offset(0.0, dialogSizes.contentHeight + dialogSizes.dividerThickness);
} else {
final BoxParentData actionParentData = actionsSection!.parentData! as BoxParentData;
actionParentData.offset = Offset(0.0, dialogSizes.contentHeight + dialogSizes.dividerThickness);
}
}
_AlertDialogSizes _performLayout({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
return isInAccessibilityMode
? performAccessibilityLayout(
constraints: constraints,
layoutChild: layoutChild,
) : performRegularLayout(
constraints: constraints,
layoutChild: layoutChild,
);
}
// When not in accessibility mode, an alert dialog might reduce the space
// for buttons to just over 1 button's height to make room for the content
// section.
_AlertDialogSizes performRegularLayout({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
final bool hasDivider = contentSection!.getMaxIntrinsicHeight(computeMaxIntrinsicWidth(0)) > 0.0
&& actionsSection!.getMaxIntrinsicHeight(computeMaxIntrinsicWidth(0)) > 0.0;
final double dividerThickness = hasDivider ? _dividerThickness : 0.0;
final double minActionsHeight = actionsSection!.getMinIntrinsicHeight(computeMaxIntrinsicWidth(0));
final Size contentSize = layoutChild(
contentSection!,
constraints.deflate(EdgeInsets.only(bottom: minActionsHeight + dividerThickness)),
);
final Size actionsSize = layoutChild(
actionsSection!,
constraints.deflate(EdgeInsets.only(top: contentSize.height + dividerThickness)),
);
final double dialogHeight = contentSize.height + dividerThickness + actionsSize.height;
return _AlertDialogSizes(
size: isActionSheet
? Size(constraints.maxWidth, dialogHeight)
: constraints.constrain(Size(_dialogWidth, dialogHeight)),
contentHeight: contentSize.height,
dividerThickness: dividerThickness,
);
}
// When in accessibility mode, an alert dialog will allow buttons to take
// up to 50% of the dialog height, even if the content exceeds available space.
_AlertDialogSizes performAccessibilityLayout({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
final bool hasDivider = contentSection!.getMaxIntrinsicHeight(_dialogWidth) > 0.0
&& actionsSection!.getMaxIntrinsicHeight(_dialogWidth) > 0.0;
final double dividerThickness = hasDivider ? _dividerThickness : 0.0;
final double maxContentHeight = contentSection!.getMaxIntrinsicHeight(_dialogWidth);
final double maxActionsHeight = actionsSection!.getMaxIntrinsicHeight(_dialogWidth);
final Size contentSize;
final Size actionsSize;
if (maxContentHeight + dividerThickness + maxActionsHeight > constraints.maxHeight) {
// AlertDialog: There isn't enough room for everything. Following iOS's
// accessibility dialog layout policy, first we allow the actions to take
// up to 50% of the dialog height. Second we fill the rest of the
// available space with the content section.
actionsSize = layoutChild(
actionsSection!,
constraints.deflate(EdgeInsets.only(top: constraints.maxHeight / 2.0)),
);
contentSize = layoutChild(
contentSection!,
constraints.deflate(EdgeInsets.only(bottom: actionsSize.height + dividerThickness)),
);
} else {
// Everything fits. Give content and actions all the space they want.
contentSize = layoutChild(
contentSection!,
constraints,
);
actionsSize = layoutChild(
actionsSection!,
constraints.deflate(EdgeInsets.only(top: contentSize.height)),
);
}
// Calculate overall dialog height.
final double dialogHeight = contentSize.height + dividerThickness + actionsSize.height;
return _AlertDialogSizes(
size: constraints.constrain(Size(_dialogWidth, dialogHeight)),
contentHeight: contentSize.height,
dividerThickness: dividerThickness,
);
}
@override
void paint(PaintingContext context, Offset offset) {
if (isActionSheet) {
final MultiChildLayoutParentData contentParentData = contentSection!.parentData! as MultiChildLayoutParentData;
contentSection!.paint(context, offset + contentParentData.offset);
} else {
final BoxParentData contentParentData = contentSection!.parentData! as BoxParentData;
contentSection!.paint(context, offset + contentParentData.offset);
}
final bool hasDivider = contentSection!.size.height > 0.0 && actionsSection!.size.height > 0.0;
if (hasDivider) {
_paintDividerBetweenContentAndActions(context.canvas, offset);
}
if (isActionSheet) {
final MultiChildLayoutParentData actionsParentData = actionsSection!.parentData! as MultiChildLayoutParentData;
actionsSection!.paint(context, offset + actionsParentData.offset);
} else {
final BoxParentData actionsParentData = actionsSection!.parentData! as BoxParentData;
actionsSection!.paint(context, offset + actionsParentData.offset);
}
}
void _paintDividerBetweenContentAndActions(Canvas canvas, Offset offset) {
canvas.drawRect(
Rect.fromLTWH(
offset.dx,
offset.dy + contentSection!.size.height,
size.width,
_dividerThickness,
),
_dividerPaint,
);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
if (isActionSheet) {
final MultiChildLayoutParentData contentSectionParentData = contentSection!.parentData! as MultiChildLayoutParentData;
final MultiChildLayoutParentData actionsSectionParentData = actionsSection!.parentData! as MultiChildLayoutParentData;
return result.addWithPaintOffset(
offset: contentSectionParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - contentSectionParentData.offset);
return contentSection!.hitTest(result, position: transformed);
},
) ||
result.addWithPaintOffset(
offset: actionsSectionParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - actionsSectionParentData.offset);
return actionsSection!.hitTest(result, position: transformed);
},
);
}
final BoxParentData contentSectionParentData = contentSection!.parentData! as BoxParentData;
final BoxParentData actionsSectionParentData = actionsSection!.parentData! as BoxParentData;
return result.addWithPaintOffset(
offset: contentSectionParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - contentSectionParentData.offset);
return contentSection!.hitTest(result, position: transformed);
},
) ||
result.addWithPaintOffset(
offset: actionsSectionParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - actionsSectionParentData.offset);
return actionsSection!.hitTest(result, position: transformed);
},
);
}
}
class _AlertDialogSizes {
const _AlertDialogSizes({
required this.size,
required this.contentHeight,
required this.dividerThickness,
});
final Size size;
final double contentHeight;
final double dividerThickness;
}
// Visual components of an alert dialog that need to be explicitly sized and
// laid out at runtime.
enum _AlertDialogSections {
contentSection,
actionsSection,
}
// The "content section" of a CupertinoAlertDialog.
//
// If title is missing, then only content is added. If content is
// missing, then only title is added. If both are missing, then it returns
// a SingleChildScrollView with a zero-sized Container.
class _CupertinoAlertContentSection extends StatelessWidget {
const _CupertinoAlertContentSection({
this.title,
this.message,
this.scrollController,
this.titlePadding,
this.messagePadding,
this.titleTextStyle,
this.messageTextStyle,
this.additionalPaddingBetweenTitleAndMessage,
}) : assert(title == null || titlePadding != null && titleTextStyle != null),
assert(message == null || messagePadding != null && messageTextStyle != null);
// The (optional) title of the dialog is displayed in a large font at the top
// of the dialog.
//
// Typically a Text widget.
final Widget? title;
// The (optional) message of the dialog is displayed in the center of the
// dialog in a lighter font.
//
// Typically a Text widget.
final Widget? message;
// A scroll controller that can be used to control the scrolling of the
// content in the dialog.
//
// Defaults to null, and is typically not needed, since most alert contents
// are short.
final ScrollController? scrollController;
// Paddings used around title and message.
// CupertinoAlertDialog and CupertinoActionSheet have different paddings.
final EdgeInsets? titlePadding;
final EdgeInsets? messagePadding;
// Additional padding to be inserted between title and message.
// Only used for CupertinoActionSheet.
final EdgeInsets? additionalPaddingBetweenTitleAndMessage;
// Text styles used for title and message.
// CupertinoAlertDialog and CupertinoActionSheet have different text styles.
final TextStyle? titleTextStyle;
final TextStyle? messageTextStyle;
@override
Widget build(BuildContext context) {
if (title == null && message == null) {
return SingleChildScrollView(
controller: scrollController,
child: const SizedBox.shrink(),
);
}
final List<Widget> titleContentGroup = <Widget>[
if (title != null)
Padding(
padding: titlePadding!,
child: DefaultTextStyle(
style: titleTextStyle!,
textAlign: TextAlign.center,
child: title!,
),
),
if (message != null)
Padding(
padding: messagePadding!,
child: DefaultTextStyle(
style: messageTextStyle!,
textAlign: TextAlign.center,
child: message!,
),
),
];
// Add padding between the widgets if necessary.
if (additionalPaddingBetweenTitleAndMessage != null && titleContentGroup.length > 1) {
titleContentGroup.insert(1, Padding(padding: additionalPaddingBetweenTitleAndMessage!));
}
return CupertinoScrollbar(
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: titleContentGroup,
),
),
);
}
}
// The "actions section" of a [CupertinoAlertDialog].
//
// See [_RenderCupertinoDialogActions] for details about action button sizing
// and layout.
class _CupertinoAlertActionSection extends StatelessWidget {
const _CupertinoAlertActionSection({
required this.children,
this.scrollController,
this.hasCancelButton = false,
this.isActionSheet = false,
});
final List<Widget> children;
// A scroll controller that can be used to control the scrolling of the
// actions in the dialog.
//
// Defaults to null, and is typically not needed, since most alert dialogs
// don't have many actions.
final ScrollController? scrollController;
// Used in ActionSheet to denote if ActionSheet has a separate so-called
// cancel button.
//
// Defaults to false, and is not needed in dialogs.
final bool hasCancelButton;
final bool isActionSheet;
@override
Widget build(BuildContext context) {
final List<Widget> interactiveButtons = <Widget>[];
for (int i = 0; i < children.length; i += 1) {
interactiveButtons.add(
_PressableActionButton(
child: children[i],
),
);
}
return CupertinoScrollbar(
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
child: _CupertinoDialogActionsRenderWidget(
actionButtons: interactiveButtons,
dividerThickness: _kDividerThickness,
hasCancelButton: hasCancelButton,
isActionSheet: isActionSheet,
),
),
);
}
}
// Button that updates its render state when pressed.
//
// The pressed state is forwarded to an _ActionButtonParentDataWidget. The
// corresponding _ActionButtonParentData is then interpreted and rendered
// appropriately by _RenderCupertinoDialogActions.
class _PressableActionButton extends StatefulWidget {
const _PressableActionButton({
required this.child,
});
final Widget child;
@override
_PressableActionButtonState createState() => _PressableActionButtonState();
}
class _PressableActionButtonState extends State<_PressableActionButton> {
bool _isPressed = false;
@override
Widget build(BuildContext context) {
return _ActionButtonParentDataWidget(
isPressed: _isPressed,
child: MergeSemantics(
// TODO(mattcarroll): Button press dynamics need overhaul for iOS:
// https://github.com/flutter/flutter/issues/19786
child: GestureDetector(
excludeFromSemantics: true,
behavior: HitTestBehavior.opaque,
onTapDown: (TapDownDetails details) => setState(() {
_isPressed = true;
}),
onTapUp: (TapUpDetails details) => setState(() {
_isPressed = false;
}),
// TODO(mattcarroll): Cancel is currently triggered when user moves
// past slop instead of off button: https://github.com/flutter/flutter/issues/19783
onTapCancel: () => setState(() => _isPressed = false),
child: widget.child,
),
),
);
}
}
// ParentDataWidget that updates _ActionButtonParentData for an action button.
//
// Each action button requires knowledge of whether or not it is pressed so that
// the dialog can correctly render the button. The pressed state is held within
// _ActionButtonParentData. _ActionButtonParentDataWidget is responsible for
// updating the pressed state of an _ActionButtonParentData based on the
// incoming [isPressed] property.
class _ActionButtonParentDataWidget
extends ParentDataWidget<_ActionButtonParentData> {
const _ActionButtonParentDataWidget({
required this.isPressed,
required super.child,
});
final bool isPressed;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is _ActionButtonParentData);
final _ActionButtonParentData parentData =
renderObject.parentData! as _ActionButtonParentData;
if (parentData.isPressed != isPressed) {
parentData.isPressed = isPressed;
// Force a repaint.
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsPaint();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => _CupertinoDialogActionsRenderWidget;
}
// ParentData applied to individual action buttons that report whether or not
// that button is currently pressed by the user.
class _ActionButtonParentData extends MultiChildLayoutParentData {
bool isPressed = false;
}
/// A button typically used in a [CupertinoAlertDialog].
///
/// See also:
///
/// * [CupertinoAlertDialog], a dialog that informs the user about situations
/// that require acknowledgment.
class CupertinoDialogAction extends StatelessWidget {
/// Creates an action for an iOS-style dialog.
const CupertinoDialogAction({
super.key,
this.onPressed,
this.isDefaultAction = false,
this.isDestructiveAction = false,
this.textStyle,
required this.child,
});
/// The callback that is called when the button is tapped or otherwise
/// activated.
///
/// If this is set to null, the button will be disabled.
final VoidCallback? onPressed;
/// Set to true if button is the default choice in the dialog.
///
/// Default buttons have bold text. Similar to
/// [UIAlertController.preferredAction](https://developer.apple.com/documentation/uikit/uialertcontroller/1620102-preferredaction),
/// but more than one action can have this attribute set to true in the same
/// [CupertinoAlertDialog].
///
/// This parameters defaults to false.
final bool isDefaultAction;
/// Whether this action destroys an object.
///
/// For example, an action that deletes an email is destructive.
///
/// Defaults to false.
final bool isDestructiveAction;
/// [TextStyle] to apply to any text that appears in this button.
///
/// Dialog actions have a built-in text resizing policy for long text. To
/// ensure that this resizing policy always works as expected, [textStyle]
/// must be used if a text size is desired other than that specified in
/// [_kCupertinoDialogActionStyle].
final TextStyle? textStyle;
/// The widget below this widget in the tree.
///
/// Typically a [Text] widget.
final Widget child;
/// Whether the button is enabled or disabled. Buttons are disabled by
/// default. To enable a button, set its [onPressed] property to a non-null
/// value.
bool get enabled => onPressed != null;
// Dialog action content shrinks to fit, up to a certain point, and if it still
// cannot fit at the minimum size, the text content is ellipsized.
//
// This policy only applies when the device is not in accessibility mode.
Widget _buildContentWithRegularSizingPolicy({
required BuildContext context,
required TextStyle textStyle,
required Widget content,
required double padding,
}) {
final bool isInAccessibilityMode = _isInAccessibilityMode(context);
final double dialogWidth = isInAccessibilityMode
? _kAccessibilityCupertinoDialogWidth
: _kCupertinoDialogWidth;
// The fontSizeRatio is the ratio of the current text size (including any
// iOS scale factor) vs the minimum text size that we allow in action
// buttons. This ratio information is used to automatically scale down action
// button text to fit the available space.
final double fontSizeRatio = MediaQuery.textScalerOf(context).scale(textStyle.fontSize!) / _kDialogMinButtonFontSize;
return IntrinsicHeight(
child: SizedBox(
width: double.infinity,
child: FittedBox(
fit: BoxFit.scaleDown,
child: ConstrainedBox(
constraints: BoxConstraints(
maxWidth: fontSizeRatio * (dialogWidth - (2 * padding)),
),
child: Semantics(
button: true,
onTap: onPressed,
child: DefaultTextStyle(
style: textStyle,
textAlign: TextAlign.center,
overflow: TextOverflow.ellipsis,
maxLines: 1,
child: content,
),
),
),
),
),
);
}
// Dialog action content is permitted to be as large as it wants when in
// accessibility mode. If text is used as the content, the text wraps instead
// of ellipsizing.
Widget _buildContentWithAccessibilitySizingPolicy({
required TextStyle textStyle,
required Widget content,
}) {
return DefaultTextStyle(
style: textStyle,
textAlign: TextAlign.center,
child: content,
);
}
@override
Widget build(BuildContext context) {
TextStyle style = _kCupertinoDialogActionStyle.copyWith(
color: CupertinoDynamicColor.resolve(
isDestructiveAction ? CupertinoColors.systemRed : CupertinoTheme.of(context).primaryColor,
context,
),
).merge(textStyle);
if (isDefaultAction) {
style = style.copyWith(fontWeight: FontWeight.w600);
}
if (!enabled) {
style = style.copyWith(color: style.color!.withOpacity(0.5));
}
final double fontSize = style.fontSize ?? kDefaultFontSize;
final double fontSizeToScale = fontSize == 0.0 ? kDefaultFontSize : fontSize;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(fontSizeToScale) / fontSizeToScale;
final double padding = 8.0 * effectiveTextScale;
// Apply a sizing policy to the action button's content based on whether or
// not the device is in accessibility mode.
// TODO(mattcarroll): The following logic is not entirely correct. It is also
// the case that if content text does not contain a space, it should also
// wrap instead of ellipsizing. We are consciously not implementing that
// now due to complexity.
final Widget sizedContent = _isInAccessibilityMode(context)
? _buildContentWithAccessibilitySizingPolicy(
textStyle: style,
content: child,
)
: _buildContentWithRegularSizingPolicy(
context: context,
textStyle: style,
content: child,
padding: padding,
);
return MouseRegion(
cursor: onPressed != null && kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
child: GestureDetector(
excludeFromSemantics: true,
onTap: onPressed,
behavior: HitTestBehavior.opaque,
child: ConstrainedBox(
constraints: const BoxConstraints(
minHeight: _kDialogMinButtonHeight,
),
child: Container(
alignment: Alignment.center,
padding: EdgeInsets.all(padding),
child: sizedContent,
),
),
),
);
}
}
// iOS style dialog action button layout.
//
// [_CupertinoDialogActionsRenderWidget] does not provide any scrolling
// behavior for its buttons. It only handles the sizing and layout of buttons.
// Scrolling behavior can be composed on top of this widget, if desired.
//
// See [_RenderCupertinoDialogActions] for specific layout policy details.
class _CupertinoDialogActionsRenderWidget extends MultiChildRenderObjectWidget {
const _CupertinoDialogActionsRenderWidget({
required List<Widget> actionButtons,
double dividerThickness = 0.0,
bool hasCancelButton = false,
bool isActionSheet = false,
}) : _dividerThickness = dividerThickness,
_hasCancelButton = hasCancelButton,
_isActionSheet = isActionSheet,
super(children: actionButtons);
final double _dividerThickness;
final bool _hasCancelButton;
final bool _isActionSheet;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderCupertinoDialogActions(
dialogWidth: _isActionSheet
? null
: _isInAccessibilityMode(context)
? _kAccessibilityCupertinoDialogWidth
: _kCupertinoDialogWidth,
dividerThickness: _dividerThickness,
dialogColor: CupertinoDynamicColor.resolve(_isActionSheet ? _kActionSheetBackgroundColor : _kDialogColor, context),
dialogPressedColor: CupertinoDynamicColor.resolve(_kPressedColor, context),
dividerColor: CupertinoDynamicColor.resolve(_isActionSheet ? _kActionSheetButtonDividerColor : CupertinoColors.separator, context),
hasCancelButton: _hasCancelButton,
isActionSheet: _isActionSheet,
);
}
@override
void updateRenderObject(BuildContext context, _RenderCupertinoDialogActions renderObject) {
renderObject
..dialogWidth = _isActionSheet
? null
: _isInAccessibilityMode(context)
? _kAccessibilityCupertinoDialogWidth
: _kCupertinoDialogWidth
..dividerThickness = _dividerThickness
..dialogColor = CupertinoDynamicColor.resolve(_isActionSheet ? _kActionSheetBackgroundColor : _kDialogColor, context)
..dialogPressedColor = CupertinoDynamicColor.resolve(_kPressedColor, context)
..dividerColor = CupertinoDynamicColor.resolve(_isActionSheet ? _kActionSheetButtonDividerColor : CupertinoColors.separator, context)
..hasCancelButton = _hasCancelButton
..isActionSheet = _isActionSheet;
}
}
// iOS style layout policy for sizing and positioning an alert dialog's action
// buttons.
//
// The policy is as follows:
//
// If a single action button is provided, or if 2 action buttons are provided
// that can fit side-by-side, then action buttons are sized and laid out in a
// single horizontal row. The row is exactly as wide as the dialog, and the row
// is as tall as the tallest action button. A horizontal divider is drawn above
// the button row. If 2 action buttons are provided, a vertical divider is
// drawn between them. The thickness of the divider is set by [dividerThickness].
//
// If 2 action buttons are provided but they cannot fit side-by-side, then the
// 2 buttons are stacked vertically. A horizontal divider is drawn above each
// button. The thickness of the divider is set by [dividerThickness]. The minimum
// height of this [RenderBox] in the case of 2 stacked buttons is as tall as
// the 2 buttons stacked. This is different than the 3+ button case where the
// minimum height is only 1.5 buttons tall. See the 3+ button explanation for
// more info.
//
// If 3+ action buttons are provided then they are all stacked vertically. A
// horizontal divider is drawn above each button. The thickness of the divider
// is set by [dividerThickness]. The minimum height of this [RenderBox] in the case
// of 3+ stacked buttons is as tall as the 1st button + 50% the height of the
// 2nd button. In other words, the minimum height is 1.5 buttons tall. This
// minimum height of 1.5 buttons is expected to work in tandem with a surrounding
// [ScrollView] to match the iOS dialog behavior.
//
// Each button is expected to have an _ActionButtonParentData which reports
// whether or not that button is currently pressed. If a button is pressed,
// then the dividers above and below that pressed button are not drawn - instead
// they are filled with the standard white dialog background color. The one
// exception is the very 1st divider which is always rendered. This policy comes
// from observation of native iOS dialogs.
class _RenderCupertinoDialogActions extends RenderBox
with ContainerRenderObjectMixin<RenderBox, MultiChildLayoutParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, MultiChildLayoutParentData> {
_RenderCupertinoDialogActions({
List<RenderBox>? children,
double? dialogWidth,
double dividerThickness = 0.0,
required Color dialogColor,
required Color dialogPressedColor,
required Color dividerColor,
bool hasCancelButton = false,
bool isActionSheet = false,
}) : assert(isActionSheet || dialogWidth != null),
_dialogWidth = dialogWidth,
_buttonBackgroundPaint = Paint()
..color = dialogColor
..style = PaintingStyle.fill,
_pressedButtonBackgroundPaint = Paint()
..color = dialogPressedColor
..style = PaintingStyle.fill,
_dividerPaint = Paint()
..color = dividerColor
..style = PaintingStyle.fill,
_dividerThickness = dividerThickness,
_hasCancelButton = hasCancelButton,
_isActionSheet = isActionSheet {
addAll(children);
}
double? get dialogWidth => _dialogWidth;
double? _dialogWidth;
set dialogWidth(double? newWidth) {
if (newWidth != _dialogWidth) {
_dialogWidth = newWidth;
markNeedsLayout();
}
}
// The thickness of the divider between buttons.
double get dividerThickness => _dividerThickness;
double _dividerThickness;
set dividerThickness(double newValue) {
if (newValue != _dividerThickness) {
_dividerThickness = newValue;
markNeedsLayout();
}
}
bool _hasCancelButton;
bool get hasCancelButton => _hasCancelButton;
set hasCancelButton(bool newValue) {
if (newValue == _hasCancelButton) {
return;
}
_hasCancelButton = newValue;
markNeedsLayout();
}
Color get dialogColor => _buttonBackgroundPaint.color;
final Paint _buttonBackgroundPaint;
set dialogColor(Color value) {
if (value == _buttonBackgroundPaint.color) {
return;
}
_buttonBackgroundPaint.color = value;
markNeedsPaint();
}
Color get dialogPressedColor => _pressedButtonBackgroundPaint.color;
final Paint _pressedButtonBackgroundPaint;
set dialogPressedColor(Color value) {
if (value == _pressedButtonBackgroundPaint.color) {
return;
}
_pressedButtonBackgroundPaint.color = value;
markNeedsPaint();
}
Color get dividerColor => _dividerPaint.color;
final Paint _dividerPaint;
set dividerColor(Color value) {
if (value == _dividerPaint.color) {
return;
}
_dividerPaint.color = value;
markNeedsPaint();
}
bool get isActionSheet => _isActionSheet;
bool _isActionSheet;
set isActionSheet(bool value) {
if (value == _isActionSheet) {
return;
}
_isActionSheet = value;
markNeedsPaint();
}
Iterable<RenderBox> get _pressedButtons {
final List<RenderBox> boxes = <RenderBox>[];
RenderBox? currentChild = firstChild;
while (currentChild != null) {
assert(currentChild.parentData is _ActionButtonParentData);
final _ActionButtonParentData parentData = currentChild.parentData! as _ActionButtonParentData;
if (parentData.isPressed) {
boxes.add(currentChild);
}
currentChild = childAfter(currentChild);
}
return boxes;
}
bool get _isButtonPressed {
RenderBox? currentChild = firstChild;
while (currentChild != null) {
assert(currentChild.parentData is _ActionButtonParentData);
final _ActionButtonParentData parentData = currentChild.parentData! as _ActionButtonParentData;
if (parentData.isPressed) {
return true;
}
currentChild = childAfter(currentChild);
}
return false;
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! _ActionButtonParentData) {
child.parentData = _ActionButtonParentData();
}
}
@override
double computeMinIntrinsicWidth(double height) {
return isActionSheet ? constraints.minWidth : dialogWidth!;
}
@override
double computeMaxIntrinsicWidth(double height) {
return isActionSheet ? constraints.maxWidth : dialogWidth!;
}
@override
double computeMinIntrinsicHeight(double width) {
if (childCount == 0) {
return 0.0;
} else if (isActionSheet) {
if (childCount == 1) {
return firstChild!.computeMaxIntrinsicHeight(width) + dividerThickness;
}
if (hasCancelButton && childCount < 4) {
return _computeMinIntrinsicHeightWithCancel(width);
}
return _computeMinIntrinsicHeightStacked(width);
} else if (childCount == 1) {
// If only 1 button, display the button across the entire dialog.
return _computeMinIntrinsicHeightSideBySide(width);
} else if (childCount == 2 && _isSingleButtonRow(width)) {
// The first 2 buttons fit side-by-side. Display them horizontally.
return _computeMinIntrinsicHeightSideBySide(width);
}
// 3+ buttons are always stacked. The minimum height when stacked is
// 1.5 buttons tall.
return _computeMinIntrinsicHeightStacked(width);
}
// The minimum height for more than 2-3 buttons when a cancel button is
// included is the full height of button stack.
double _computeMinIntrinsicHeightWithCancel(double width) {
assert(childCount == 2 || childCount == 3);
if (childCount == 2) {
return firstChild!.getMinIntrinsicHeight(width)
+ childAfter(firstChild!)!.getMinIntrinsicHeight(width)
+ dividerThickness;
}
return firstChild!.getMinIntrinsicHeight(width)
+ childAfter(firstChild!)!.getMinIntrinsicHeight(width)
+ childAfter(childAfter(firstChild!)!)!.getMinIntrinsicHeight(width)
+ (dividerThickness * 2);
}
// The minimum height for a single row of buttons is the larger of the buttons'
// min intrinsic heights.
double _computeMinIntrinsicHeightSideBySide(double width) {
assert(childCount >= 1 && childCount <= 2);
final double minHeight;
if (childCount == 1) {
minHeight = firstChild!.getMinIntrinsicHeight(width);
} else {
final double perButtonWidth = (width - dividerThickness) / 2.0;
minHeight = math.max(
firstChild!.getMinIntrinsicHeight(perButtonWidth),
lastChild!.getMinIntrinsicHeight(perButtonWidth),
);
}
return minHeight;
}
// Dialog: The minimum height for 2+ stacked buttons is the height of the 1st
// button + 50% the height of the 2nd button + the divider between the two.
//
// ActionSheet: The minimum height for more than 2 buttons when no cancel
// button or 4+ buttons when a cancel button is included is the height of the
// 1st button + 50% the height of the 2nd button + 2 dividers.
double _computeMinIntrinsicHeightStacked(double width) {
assert(childCount >= 2);
return firstChild!.getMinIntrinsicHeight(width)
+ dividerThickness
+ (0.5 * childAfter(firstChild!)!.getMinIntrinsicHeight(width));
}
@override
double computeMaxIntrinsicHeight(double width) {
if (childCount == 0) {
// No buttons. Zero height.
return 0.0;
} else if (isActionSheet) {
if (childCount == 1) {
return firstChild!.computeMaxIntrinsicHeight(width) + dividerThickness;
}
return _computeMaxIntrinsicHeightStacked(width);
} else if (childCount == 1) {
// One button. Our max intrinsic height is equal to the button's.
return firstChild!.getMaxIntrinsicHeight(width);
} else if (childCount == 2) {
// Two buttons...
if (_isSingleButtonRow(width)) {
// The 2 buttons fit side by side so our max intrinsic height is equal
// to the taller of the 2 buttons.
final double perButtonWidth = (width - dividerThickness) / 2.0;
return math.max(
firstChild!.getMaxIntrinsicHeight(perButtonWidth),
lastChild!.getMaxIntrinsicHeight(perButtonWidth),
);
} else {
// The 2 buttons do not fit side by side. Measure total height as a
// vertical stack.
return _computeMaxIntrinsicHeightStacked(width);
}
}
// Three+ buttons. Stack the buttons vertically with dividers and measure
// the overall height.
return _computeMaxIntrinsicHeightStacked(width);
}
// Max height of a stack of buttons is the sum of all button heights + a
// divider for each button.
double _computeMaxIntrinsicHeightStacked(double width) {
assert(childCount >= 2);
final double allDividersHeight = (childCount - 1) * dividerThickness;
double heightAccumulation = allDividersHeight;
RenderBox? button = firstChild;
while (button != null) {
heightAccumulation += button.getMaxIntrinsicHeight(width);
button = childAfter(button);
}
return heightAccumulation;
}
bool _isSingleButtonRow(double width) {
final bool isSingleButtonRow;
if (childCount == 1) {
isSingleButtonRow = true;
} else if (childCount == 2) {
// There are 2 buttons. If they can fit side-by-side then that's what
// we want to do. Otherwise, stack them vertically.
final double sideBySideWidth = firstChild!.getMaxIntrinsicWidth(double.infinity)
+ dividerThickness
+ lastChild!.getMaxIntrinsicWidth(double.infinity);
isSingleButtonRow = sideBySideWidth <= width;
} else {
isSingleButtonRow = false;
}
return isSingleButtonRow;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _performLayout(constraints: constraints, dry: true);
}
@override
void performLayout() {
size = _performLayout(constraints: constraints);
}
Size _performLayout({required BoxConstraints constraints, bool dry = false}) {
final ChildLayouter layoutChild = dry
? ChildLayoutHelper.dryLayoutChild
: ChildLayoutHelper.layoutChild;
if (!isActionSheet && _isSingleButtonRow(dialogWidth!)) {
if (childCount == 1) {
// We have 1 button. Our size is the width of the dialog and the height
// of the single button.
final Size childSize = layoutChild(
firstChild!,
constraints,
);
return constraints.constrain(
Size(dialogWidth!, childSize.height),
);
} else {
// Each button gets half the available width, minus a single divider.
final BoxConstraints perButtonConstraints = BoxConstraints(
minWidth: (constraints.minWidth - dividerThickness) / 2.0,
maxWidth: (constraints.maxWidth - dividerThickness) / 2.0,
);
// Layout the 2 buttons.
final Size firstChildSize = layoutChild(
firstChild!,
perButtonConstraints,
);
final Size lastChildSize = layoutChild(
lastChild!,
perButtonConstraints,
);
if (!dry) {
// The 2nd button needs to be offset to the right.
assert(lastChild!.parentData is MultiChildLayoutParentData);
final MultiChildLayoutParentData secondButtonParentData = lastChild!.parentData! as MultiChildLayoutParentData;
secondButtonParentData.offset = Offset(firstChildSize.width + dividerThickness, 0.0);
}
// Calculate our size based on the button sizes.
return constraints.constrain(
Size(
dialogWidth!,
math.max(
firstChildSize.height,
lastChildSize.height,
),
),
);
}
} else {
// We need to stack buttons vertically, plus dividers above each button (except the 1st).
final BoxConstraints perButtonConstraints = constraints.copyWith(
minHeight: 0.0,
maxHeight: double.infinity,
);
RenderBox? child = firstChild;
int index = 0;
double verticalOffset = 0.0;
while (child != null) {
final Size childSize = layoutChild(
child,
perButtonConstraints,
);
if (!dry) {
assert(child.parentData is MultiChildLayoutParentData);
final MultiChildLayoutParentData parentData = child.parentData! as MultiChildLayoutParentData;
parentData.offset = Offset(0.0, verticalOffset);
}
verticalOffset += childSize.height;
if (index < childCount - 1) {
// Add a gap for the next divider.
verticalOffset += dividerThickness;
}
index += 1;
child = childAfter(child);
}
// Our height is the accumulated height of all buttons and dividers.
return constraints.constrain(
Size(computeMaxIntrinsicWidth(0), verticalOffset),
);
}
}
@override
void paint(PaintingContext context, Offset offset) {
final Canvas canvas = context.canvas;
if (!isActionSheet && _isSingleButtonRow(size.width)) {
_drawButtonBackgroundsAndDividersSingleRow(canvas, offset);
} else {
_drawButtonBackgroundsAndDividersStacked(canvas, offset);
}
_drawButtons(context, offset);
}
void _drawButtonBackgroundsAndDividersSingleRow(Canvas canvas, Offset offset) {
// The vertical divider sits between the left button and right button (if
// the dialog has 2 buttons). The vertical divider is hidden if either the
// left or right button is pressed.
final Rect verticalDivider = childCount == 2 && !_isButtonPressed
? Rect.fromLTWH(
offset.dx + firstChild!.size.width,
offset.dy,
dividerThickness,
math.max(
firstChild!.size.height,
lastChild!.size.height,
),
)
: Rect.zero;
final List<Rect> pressedButtonRects = _pressedButtons.map<Rect>((RenderBox pressedButton) {
final MultiChildLayoutParentData buttonParentData = pressedButton.parentData! as MultiChildLayoutParentData;
return Rect.fromLTWH(
offset.dx + buttonParentData.offset.dx,
offset.dy + buttonParentData.offset.dy,
pressedButton.size.width,
pressedButton.size.height,
);
}).toList();
// Create the button backgrounds path and paint it.
final Path backgroundFillPath = Path()
..fillType = PathFillType.evenOdd
..addRect(Rect.fromLTWH(0.0, 0.0, size.width, size.height))
..addRect(verticalDivider);
for (int i = 0; i < pressedButtonRects.length; i += 1) {
backgroundFillPath.addRect(pressedButtonRects[i]);
}
canvas.drawPath(
backgroundFillPath,
_buttonBackgroundPaint,
);
// Create the pressed buttons background path and paint it.
final Path pressedBackgroundFillPath = Path();
for (int i = 0; i < pressedButtonRects.length; i += 1) {
pressedBackgroundFillPath.addRect(pressedButtonRects[i]);
}
canvas.drawPath(
pressedBackgroundFillPath,
_pressedButtonBackgroundPaint,
);
// Create the dividers path and paint it.
final Path dividersPath = Path()
..addRect(verticalDivider);
canvas.drawPath(
dividersPath,
_dividerPaint,
);
}
void _drawButtonBackgroundsAndDividersStacked(Canvas canvas, Offset offset) {
final Offset dividerOffset = Offset(0.0, dividerThickness);
final Path backgroundFillPath = Path()
..fillType = PathFillType.evenOdd
..addRect(Rect.fromLTWH(0.0, 0.0, size.width, size.height));
final Path pressedBackgroundFillPath = Path();
final Path dividersPath = Path();
Offset accumulatingOffset = offset;
RenderBox? child = firstChild;
RenderBox? prevChild;
while (child != null) {
assert(child.parentData is _ActionButtonParentData);
final _ActionButtonParentData currentButtonParentData = child.parentData! as _ActionButtonParentData;
final bool isButtonPressed = currentButtonParentData.isPressed;
bool isPrevButtonPressed = false;
if (prevChild != null) {
assert(prevChild.parentData is _ActionButtonParentData);
final _ActionButtonParentData previousButtonParentData = prevChild.parentData! as _ActionButtonParentData;
isPrevButtonPressed = previousButtonParentData.isPressed;
}
final bool isDividerPresent = child != firstChild;
final bool isDividerPainted = isDividerPresent && !(isButtonPressed || isPrevButtonPressed);
final Rect dividerRect = Rect.fromLTWH(
accumulatingOffset.dx,
accumulatingOffset.dy,
size.width,
dividerThickness,
);
final Rect buttonBackgroundRect = Rect.fromLTWH(
accumulatingOffset.dx,
accumulatingOffset.dy + (isDividerPresent ? dividerThickness : 0.0),
size.width,
child.size.height,
);
// If this button is pressed, then we don't want a white background to be
// painted, so we erase this button from the background path.
if (isButtonPressed) {
backgroundFillPath.addRect(buttonBackgroundRect);
pressedBackgroundFillPath.addRect(buttonBackgroundRect);
}
// If this divider is needed, then we erase the divider area from the
// background path, and on top of that we paint a translucent gray to
// darken the divider area.
if (isDividerPainted) {
backgroundFillPath.addRect(dividerRect);
dividersPath.addRect(dividerRect);
}
accumulatingOffset += (isDividerPresent ? dividerOffset : Offset.zero)
+ Offset(0.0, child.size.height);
prevChild = child;
child = childAfter(child);
}
canvas.drawPath(backgroundFillPath, _buttonBackgroundPaint);
canvas.drawPath(pressedBackgroundFillPath, _pressedButtonBackgroundPaint);
canvas.drawPath(dividersPath, _dividerPaint);
}
void _drawButtons(PaintingContext context, Offset offset) {
RenderBox? child = firstChild;
while (child != null) {
final MultiChildLayoutParentData childParentData = child.parentData! as MultiChildLayoutParentData;
context.paintChild(child, childParentData.offset + offset);
child = childAfter(child);
}
}
@override
bool hitTestChildren(BoxHitTestResult result, {required Offset position}) {
return defaultHitTestChildren(result, position: position);
}
}
| flutter/packages/flutter/lib/src/cupertino/dialog.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/dialog.dart",
"repo_id": "flutter",
"token_count": 29749
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
// All values eyeballed.
const double _kScrollbarMinLength = 36.0;
const double _kScrollbarMinOverscrollLength = 8.0;
const Duration _kScrollbarTimeToFade = Duration(milliseconds: 1200);
const Duration _kScrollbarFadeDuration = Duration(milliseconds: 250);
const Duration _kScrollbarResizeDuration = Duration(milliseconds: 100);
// Extracted from iOS 13.1 beta using Debug View Hierarchy.
const Color _kScrollbarColor = CupertinoDynamicColor.withBrightness(
color: Color(0x59000000),
darkColor: Color(0x80FFFFFF),
);
// This is the amount of space from the top of a vertical scrollbar to the
// top edge of the scrollable, measured when the vertical scrollbar overscrolls
// to the top.
// TODO(LongCatIsLooong): fix https://github.com/flutter/flutter/issues/32175
const double _kScrollbarMainAxisMargin = 3.0;
const double _kScrollbarCrossAxisMargin = 3.0;
/// An iOS style scrollbar.
///
/// To add a scrollbar to a [ScrollView], wrap the scroll view widget in
/// a [CupertinoScrollbar] widget.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=DbkIQSvwnZc}
///
/// {@macro flutter.widgets.Scrollbar}
///
/// When dragging a [CupertinoScrollbar] thumb, the thickness and radius will
/// animate from [thickness] and [radius] to [thicknessWhileDragging] and
/// [radiusWhileDragging], respectively.
///
/// {@tool dartpad}
/// This sample shows a [CupertinoScrollbar] that fades in and out of view as scrolling occurs.
/// The scrollbar will fade into view as the user scrolls, and fade out when scrolling stops.
/// The `thickness` of the scrollbar will animate from 6 pixels to the `thicknessWhileDragging` of 10
/// when it is dragged by the user. The `radius` of the scrollbar thumb corners will animate from 34
/// to the `radiusWhileDragging` of 0 when the scrollbar is being dragged by the user.
///
/// ** See code in examples/api/lib/cupertino/scrollbar/cupertino_scrollbar.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// When [thumbVisibility] is true, the scrollbar thumb will remain visible without the
/// fade animation. This requires that a [ScrollController] is provided to controller,
/// or that the [PrimaryScrollController] is available.
///
/// ** See code in examples/api/lib/cupertino/scrollbar/cupertino_scrollbar.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListView], which displays a linear, scrollable list of children.
/// * [GridView], which displays a 2 dimensional, scrollable array of children.
/// * [Scrollbar], a Material Design scrollbar.
/// * [RawScrollbar], a basic scrollbar that fades in and out, extended
/// by this class to add more animations and behaviors.
class CupertinoScrollbar extends RawScrollbar {
/// Creates an iOS style scrollbar that wraps the given [child].
///
/// The [child] should be a source of [ScrollNotification] notifications,
/// typically a [Scrollable] widget.
const CupertinoScrollbar({
super.key,
required super.child,
super.controller,
bool? thumbVisibility,
double super.thickness = defaultThickness,
this.thicknessWhileDragging = defaultThicknessWhileDragging,
Radius super.radius = defaultRadius,
this.radiusWhileDragging = defaultRadiusWhileDragging,
ScrollNotificationPredicate? notificationPredicate,
super.scrollbarOrientation,
}) : assert(thickness < double.infinity),
assert(thicknessWhileDragging < double.infinity),
super(
thumbVisibility: thumbVisibility ?? false,
fadeDuration: _kScrollbarFadeDuration,
timeToFade: _kScrollbarTimeToFade,
pressDuration: const Duration(milliseconds: 100),
notificationPredicate: notificationPredicate ?? defaultScrollNotificationPredicate,
);
/// Default value for [thickness] if it's not specified in [CupertinoScrollbar].
static const double defaultThickness = 3;
/// Default value for [thicknessWhileDragging] if it's not specified in
/// [CupertinoScrollbar].
static const double defaultThicknessWhileDragging = 8.0;
/// Default value for [radius] if it's not specified in [CupertinoScrollbar].
static const Radius defaultRadius = Radius.circular(1.5);
/// Default value for [radiusWhileDragging] if it's not specified in
/// [CupertinoScrollbar].
static const Radius defaultRadiusWhileDragging = Radius.circular(4.0);
/// The thickness of the scrollbar when it's being dragged by the user.
///
/// When the user starts dragging the scrollbar, the thickness will animate
/// from [thickness] to this value, then animate back when the user stops
/// dragging the scrollbar.
final double thicknessWhileDragging;
/// The radius of the scrollbar edges when the scrollbar is being dragged by
/// the user.
///
/// When the user starts dragging the scrollbar, the radius will animate
/// from [radius] to this value, then animate back when the user stops
/// dragging the scrollbar.
final Radius radiusWhileDragging;
@override
RawScrollbarState<CupertinoScrollbar> createState() => _CupertinoScrollbarState();
}
class _CupertinoScrollbarState extends RawScrollbarState<CupertinoScrollbar> {
late AnimationController _thicknessAnimationController;
double get _thickness {
return widget.thickness! + _thicknessAnimationController.value * (widget.thicknessWhileDragging - widget.thickness!);
}
Radius get _radius {
return Radius.lerp(widget.radius, widget.radiusWhileDragging, _thicknessAnimationController.value)!;
}
@override
void initState() {
super.initState();
_thicknessAnimationController = AnimationController(
vsync: this,
duration: _kScrollbarResizeDuration,
);
_thicknessAnimationController.addListener(() {
updateScrollbarPainter();
});
}
@override
void updateScrollbarPainter() {
scrollbarPainter
..color = CupertinoDynamicColor.resolve(_kScrollbarColor, context)
..textDirection = Directionality.of(context)
..thickness = _thickness
..mainAxisMargin = _kScrollbarMainAxisMargin
..crossAxisMargin = _kScrollbarCrossAxisMargin
..radius = _radius
..padding = MediaQuery.paddingOf(context)
..minLength = _kScrollbarMinLength
..minOverscrollLength = _kScrollbarMinOverscrollLength
..scrollbarOrientation = widget.scrollbarOrientation;
}
double _pressStartAxisPosition = 0.0;
// Long press event callbacks handle the gesture where the user long presses
// on the scrollbar thumb and then drags the scrollbar without releasing.
@override
void handleThumbPressStart(Offset localPosition) {
super.handleThumbPressStart(localPosition);
final Axis? direction = getScrollbarDirection();
if (direction == null) {
return;
}
_pressStartAxisPosition = switch (direction) {
Axis.vertical => localPosition.dy,
Axis.horizontal => localPosition.dx,
};
}
@override
void handleThumbPress() {
if (getScrollbarDirection() == null) {
return;
}
super.handleThumbPress();
_thicknessAnimationController.forward().then<void>(
(_) => HapticFeedback.mediumImpact(),
);
}
@override
void handleThumbPressEnd(Offset localPosition, Velocity velocity) {
final Axis? direction = getScrollbarDirection();
if (direction == null) {
return;
}
_thicknessAnimationController.reverse();
super.handleThumbPressEnd(localPosition, velocity);
switch (direction) {
case Axis.vertical:
if (velocity.pixelsPerSecond.dy.abs() < 10 &&
(localPosition.dy - _pressStartAxisPosition).abs() > 0) {
HapticFeedback.mediumImpact();
}
case Axis.horizontal:
if (velocity.pixelsPerSecond.dx.abs() < 10 &&
(localPosition.dx - _pressStartAxisPosition).abs() > 0) {
HapticFeedback.mediumImpact();
}
}
}
@override
void dispose() {
_thicknessAnimationController.dispose();
super.dispose();
}
}
| flutter/packages/flutter/lib/src/cupertino/scrollbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/scrollbar.dart",
"repo_id": "flutter",
"token_count": 2637
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
import 'colors.dart';
const Color _kThumbBorderColor = Color(0x0A000000);
const List<BoxShadow> _kSwitchBoxShadows = <BoxShadow> [
BoxShadow(
color: Color(0x26000000),
offset: Offset(0, 3),
blurRadius: 8.0,
),
BoxShadow(
color: Color(0x0F000000),
offset: Offset(0, 3),
blurRadius: 1.0,
),
];
const List<BoxShadow> _kSliderBoxShadows = <BoxShadow> [
BoxShadow(
color: Color(0x26000000),
offset: Offset(0, 3),
blurRadius: 8.0,
),
BoxShadow(
color: Color(0x29000000),
offset: Offset(0, 1),
blurRadius: 1.0,
),
BoxShadow(
color: Color(0x1A000000),
offset: Offset(0, 3),
blurRadius: 1.0,
),
];
/// Paints an iOS-style slider thumb or switch thumb.
///
/// Used by [CupertinoSwitch] and [CupertinoSlider].
class CupertinoThumbPainter {
/// Creates an object that paints an iOS-style slider thumb.
const CupertinoThumbPainter({
this.color = CupertinoColors.white,
this.shadows = _kSliderBoxShadows,
});
/// Creates an object that paints an iOS-style switch thumb.
const CupertinoThumbPainter.switchThumb({
Color color = CupertinoColors.white,
List<BoxShadow> shadows = _kSwitchBoxShadows,
}) : this(color: color, shadows: shadows);
/// The color of the interior of the thumb.
final Color color;
/// The list of [BoxShadow] to paint below the thumb.
final List<BoxShadow> shadows;
/// Half the default diameter of the thumb.
static const double radius = 14.0;
/// The default amount the thumb should be extended horizontally when pressed.
static const double extension = 7.0;
/// Paints the thumb onto the given canvas in the given rectangle.
///
/// Consider using [radius] and [extension] when deciding how large a
/// rectangle to use for the thumb.
void paint(Canvas canvas, Rect rect) {
final RRect rrect = RRect.fromRectAndRadius(
rect,
Radius.circular(rect.shortestSide / 2.0),
);
for (final BoxShadow shadow in shadows) {
canvas.drawRRect(rrect.shift(shadow.offset), shadow.toPaint());
}
canvas.drawRRect(
rrect.inflate(0.5),
Paint()..color = _kThumbBorderColor,
);
canvas.drawRRect(rrect, Paint()..color = color);
}
}
| flutter/packages/flutter/lib/src/cupertino/thumb_painter.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/thumb_painter.dart",
"repo_id": "flutter",
"token_count": 874
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
// COMMON SIGNATURES
/// Signature for callbacks that report that an underlying value has changed.
///
/// See also:
///
/// * [ValueSetter], for callbacks that report that a value has been set.
typedef ValueChanged<T> = void Function(T value);
/// Signature for callbacks that report that a value has been set.
///
/// This is the same signature as [ValueChanged], but is used when the
/// callback is called even if the underlying value has not changed.
/// For example, service extensions use this callback because they
/// call the callback whenever the extension is called with a
/// value, regardless of whether the given value is new or not.
///
/// See also:
///
/// * [ValueGetter], the getter equivalent of this signature.
/// * [AsyncValueSetter], an asynchronous version of this signature.
typedef ValueSetter<T> = void Function(T value);
/// Signature for callbacks that are to report a value on demand.
///
/// See also:
///
/// * [ValueSetter], the setter equivalent of this signature.
/// * [AsyncValueGetter], an asynchronous version of this signature.
typedef ValueGetter<T> = T Function();
/// Signature for callbacks that filter an iterable.
typedef IterableFilter<T> = Iterable<T> Function(Iterable<T> input);
/// Signature of callbacks that have no arguments and return no data, but that
/// return a [Future] to indicate when their work is complete.
///
/// See also:
///
/// * [VoidCallback], a synchronous version of this signature.
/// * [AsyncValueGetter], a signature for asynchronous getters.
/// * [AsyncValueSetter], a signature for asynchronous setters.
typedef AsyncCallback = Future<void> Function();
/// Signature for callbacks that report that a value has been set and return a
/// [Future] that completes when the value has been saved.
///
/// See also:
///
/// * [ValueSetter], a synchronous version of this signature.
/// * [AsyncValueGetter], the getter equivalent of this signature.
typedef AsyncValueSetter<T> = Future<void> Function(T value);
/// Signature for callbacks that are to asynchronously report a value on demand.
///
/// See also:
///
/// * [ValueGetter], a synchronous version of this signature.
/// * [AsyncValueSetter], the setter equivalent of this signature.
typedef AsyncValueGetter<T> = Future<T> Function();
// LAZY CACHING ITERATOR
/// A lazy caching version of [Iterable].
///
/// This iterable is efficient in the following ways:
///
/// * It will not walk the given iterator more than you ask for.
///
/// * If you use it twice (e.g. you check [isNotEmpty], then
/// use [single]), it will only walk the given iterator
/// once. This caching will even work efficiently if you are
/// running two side-by-side iterators on the same iterable.
///
/// * [toList] uses its EfficientLength variant to create its
/// list quickly.
///
/// It is inefficient in the following ways:
///
/// * The first iteration through has caching overhead.
///
/// * It requires more memory than a non-caching iterator.
///
/// * The [length] and [toList] properties immediately pre-cache the
/// entire list. Using these fields therefore loses the laziness of
/// the iterable. However, it still gets cached.
///
/// The caching behavior is propagated to the iterators that are
/// created by [map], [where], [expand], [take], [takeWhile], [skip],
/// and [skipWhile], and is used by the built-in methods that use an
/// iterator like [isNotEmpty] and [single].
///
/// Because a CachingIterable only walks the underlying data once, it
/// cannot be used multiple times with the underlying data changing
/// between each use. You must create a new iterable each time. This
/// also applies to any iterables derived from this one, e.g. as
/// returned by `where`.
class CachingIterable<E> extends IterableBase<E> {
/// Creates a [CachingIterable] using the given [Iterator] as the source of
/// data. The iterator must not throw exceptions.
///
/// Since the argument is an [Iterator], not an [Iterable], it is
/// guaranteed that the underlying data set will only be walked
/// once. If you have an [Iterable], you can pass its [iterator]
/// field as the argument to this constructor.
///
/// You can this with an existing `sync*` function as follows:
///
/// ```dart
/// Iterable<int> range(int start, int end) sync* {
/// for (int index = start; index <= end; index += 1) {
/// yield index;
/// }
/// }
///
/// Iterable<int> i = CachingIterable<int>(range(1, 5).iterator);
/// print(i.length); // walks the list
/// print(i.length); // efficient
/// ```
///
/// Beware that this will eagerly evaluate the `range` iterable, and because
/// of that it would be better to just implement `range` as something that
/// returns a `List` to begin with if possible.
CachingIterable(this._prefillIterator);
final Iterator<E> _prefillIterator;
final List<E> _results = <E>[];
@override
Iterator<E> get iterator {
return _LazyListIterator<E>(this);
}
@override
Iterable<T> map<T>(T Function(E e) toElement) {
return CachingIterable<T>(super.map<T>(toElement).iterator);
}
@override
Iterable<E> where(bool Function(E element) test) {
return CachingIterable<E>(super.where(test).iterator);
}
@override
Iterable<T> expand<T>(Iterable<T> Function(E element) toElements) {
return CachingIterable<T>(super.expand<T>(toElements).iterator);
}
@override
Iterable<E> take(int count) {
return CachingIterable<E>(super.take(count).iterator);
}
@override
Iterable<E> takeWhile(bool Function(E value) test) {
return CachingIterable<E>(super.takeWhile(test).iterator);
}
@override
Iterable<E> skip(int count) {
return CachingIterable<E>(super.skip(count).iterator);
}
@override
Iterable<E> skipWhile(bool Function(E value) test) {
return CachingIterable<E>(super.skipWhile(test).iterator);
}
@override
int get length {
_precacheEntireList();
return _results.length;
}
@override
List<E> toList({ bool growable = true }) {
_precacheEntireList();
return List<E>.of(_results, growable: growable);
}
void _precacheEntireList() {
while (_fillNext()) { }
}
bool _fillNext() {
if (!_prefillIterator.moveNext()) {
return false;
}
_results.add(_prefillIterator.current);
return true;
}
}
class _LazyListIterator<E> implements Iterator<E> {
_LazyListIterator(this._owner) : _index = -1;
final CachingIterable<E> _owner;
int _index;
@override
E get current {
assert(_index >= 0); // called "current" before "moveNext()"
if (_index < 0 || _index == _owner._results.length) {
throw StateError('current can not be call after moveNext has returned false');
}
return _owner._results[_index];
}
@override
bool moveNext() {
if (_index >= _owner._results.length) {
return false;
}
_index += 1;
if (_index == _owner._results.length) {
return _owner._fillNext();
}
return true;
}
}
/// A factory interface that also reports the type of the created objects.
class Factory<T> {
/// Creates a new factory.
const Factory(this.constructor);
/// Creates a new object of type T.
final ValueGetter<T> constructor;
/// The type of the objects created by this factory.
Type get type => T;
@override
String toString() {
return 'Factory(type: $type)';
}
}
/// Linearly interpolate between two `Duration`s.
Duration lerpDuration(Duration a, Duration b, double t) {
return Duration(
microseconds: (a.inMicroseconds + (b.inMicroseconds - a.inMicroseconds) * t).round(),
);
}
| flutter/packages/flutter/lib/src/foundation/basic_types.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/basic_types.dart",
"repo_id": "flutter",
"token_count": 2408
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
/// A list optimized for the observer pattern when there are small numbers of
/// observers.
///
/// Consider using an [ObserverList] instead of a [List] when the number of
/// [contains] calls dominates the number of [add] and [remove] calls.
///
/// This class will include in the [iterator] each added item in the order it
/// was added, as many times as it was added.
///
/// If there will be a large number of observers, consider using
/// [HashedObserverList] instead. It has slightly different iteration semantics,
/// but serves a similar purpose, while being more efficient for large numbers
/// of observers.
///
/// See also:
///
/// * [HashedObserverList] for a list that is optimized for larger numbers of
/// observers.
// TODO(ianh): Use DelegatingIterable, possibly moving it from the collection
// package to foundation, or to dart:collection.
class ObserverList<T> extends Iterable<T> {
final List<T> _list = <T>[];
bool _isDirty = false;
late final HashSet<T> _set = HashSet<T>();
/// Adds an item to the end of this list.
///
/// This operation has constant time complexity.
void add(T item) {
_isDirty = true;
_list.add(item);
}
/// Removes an item from the list.
///
/// This is O(N) in the number of items in the list.
///
/// Returns whether the item was present in the list.
bool remove(T item) {
_isDirty = true;
_set.clear(); // Clear the set so that we don't leak items.
return _list.remove(item);
}
/// Removes all items from the list.
void clear() {
_isDirty = false;
_list.clear();
_set.clear();
}
@override
bool contains(Object? element) {
if (_list.length < 3) {
return _list.contains(element);
}
if (_isDirty) {
_set.addAll(_list);
_isDirty = false;
}
return _set.contains(element);
}
@override
Iterator<T> get iterator => _list.iterator;
@override
bool get isEmpty => _list.isEmpty;
@override
bool get isNotEmpty => _list.isNotEmpty;
@override
List<T> toList({bool growable = true}) {
return _list.toList(growable: growable);
}
}
/// A list optimized for the observer pattern, but for larger numbers of observers.
///
/// For small numbers of observers (e.g. less than 10), use [ObserverList] instead.
///
/// The iteration semantics of the this class are slightly different from
/// [ObserverList]. This class will only return an item once in the [iterator],
/// no matter how many times it was added, although it does require that an item
/// be removed as many times as it was added for it to stop appearing in the
/// [iterator]. It will return them in the order the first instance of an item
/// was originally added.
///
/// See also:
///
/// * [ObserverList] for a list that is fast for small numbers of observers.
class HashedObserverList<T> extends Iterable<T> {
final LinkedHashMap<T, int> _map = LinkedHashMap<T, int>();
/// Adds an item to the end of this list.
///
/// This has constant time complexity.
void add(T item) {
_map[item] = (_map[item] ?? 0) + 1;
}
/// Removes an item from the list.
///
/// This operation has constant time complexity.
///
/// Returns whether the item was present in the list.
bool remove(T item) {
final int? value = _map[item];
if (value == null) {
return false;
}
if (value == 1) {
_map.remove(item);
} else {
_map[item] = value - 1;
}
return true;
}
@override
bool contains(Object? element) => _map.containsKey(element);
@override
Iterator<T> get iterator => _map.keys.iterator;
@override
bool get isEmpty => _map.isEmpty;
@override
bool get isNotEmpty => _map.isNotEmpty;
}
| flutter/packages/flutter/lib/src/foundation/observer_list.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/observer_list.dart",
"repo_id": "flutter",
"token_count": 1251
} | 637 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'velocity_tracker.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
export 'velocity_tracker.dart' show Velocity;
/// Details object for callbacks that use [GestureDragDownCallback].
///
/// See also:
///
/// * [DragGestureRecognizer.onDown], which uses [GestureDragDownCallback].
/// * [DragStartDetails], the details for [GestureDragStartCallback].
/// * [DragUpdateDetails], the details for [GestureDragUpdateCallback].
/// * [DragEndDetails], the details for [GestureDragEndCallback].
class DragDownDetails {
/// Creates details for a [GestureDragDownCallback].
DragDownDetails({
this.globalPosition = Offset.zero,
Offset? localPosition,
}) : localPosition = localPosition ?? globalPosition;
/// The global position at which the pointer contacted the screen.
///
/// Defaults to the origin if not specified in the constructor.
///
/// See also:
///
/// * [localPosition], which is the [globalPosition] transformed to the
/// coordinate space of the event receiver.
final Offset globalPosition;
/// The local position in the coordinate system of the event receiver at
/// which the pointer contacted the screen.
///
/// Defaults to [globalPosition] if not specified in the constructor.
final Offset localPosition;
@override
String toString() => '${objectRuntimeType(this, 'DragDownDetails')}($globalPosition)';
}
/// Signature for when a pointer has contacted the screen and might begin to
/// move.
///
/// The `details` object provides the position of the touch.
///
/// See [DragGestureRecognizer.onDown].
typedef GestureDragDownCallback = void Function(DragDownDetails details);
/// Details object for callbacks that use [GestureDragStartCallback].
///
/// See also:
///
/// * [DragGestureRecognizer.onStart], which uses [GestureDragStartCallback].
/// * [DragDownDetails], the details for [GestureDragDownCallback].
/// * [DragUpdateDetails], the details for [GestureDragUpdateCallback].
/// * [DragEndDetails], the details for [GestureDragEndCallback].
class DragStartDetails {
/// Creates details for a [GestureDragStartCallback].
DragStartDetails({
this.sourceTimeStamp,
this.globalPosition = Offset.zero,
Offset? localPosition,
this.kind,
}) : localPosition = localPosition ?? globalPosition;
/// Recorded timestamp of the source pointer event that triggered the drag
/// event.
///
/// Could be null if triggered from proxied events such as accessibility.
final Duration? sourceTimeStamp;
/// The global position at which the pointer contacted the screen.
///
/// Defaults to the origin if not specified in the constructor.
///
/// See also:
///
/// * [localPosition], which is the [globalPosition] transformed to the
/// coordinate space of the event receiver.
final Offset globalPosition;
/// The local position in the coordinate system of the event receiver at
/// which the pointer contacted the screen.
///
/// Defaults to [globalPosition] if not specified in the constructor.
final Offset localPosition;
/// The kind of the device that initiated the event.
final PointerDeviceKind? kind;
// TODO(ianh): Expose the current position, so that you can have a no-jump
// drag even when disambiguating (though of course it would lag the finger
// instead).
@override
String toString() => '${objectRuntimeType(this, 'DragStartDetails')}($globalPosition)';
}
/// {@template flutter.gestures.dragdetails.GestureDragStartCallback}
/// Signature for when a pointer has contacted the screen and has begun to move.
///
/// The `details` object provides the position of the touch when it first
/// touched the surface.
/// {@endtemplate}
///
/// See [DragGestureRecognizer.onStart].
typedef GestureDragStartCallback = void Function(DragStartDetails details);
/// Details object for callbacks that use [GestureDragUpdateCallback].
///
/// See also:
///
/// * [DragGestureRecognizer.onUpdate], which uses [GestureDragUpdateCallback].
/// * [DragDownDetails], the details for [GestureDragDownCallback].
/// * [DragStartDetails], the details for [GestureDragStartCallback].
/// * [DragEndDetails], the details for [GestureDragEndCallback].
class DragUpdateDetails {
/// Creates details for a [GestureDragUpdateCallback].
///
/// If [primaryDelta] is non-null, then its value must match one of the
/// coordinates of [delta] and the other coordinate must be zero.
DragUpdateDetails({
this.sourceTimeStamp,
this.delta = Offset.zero,
this.primaryDelta,
required this.globalPosition,
Offset? localPosition,
}) : assert(
primaryDelta == null
|| (primaryDelta == delta.dx && delta.dy == 0.0)
|| (primaryDelta == delta.dy && delta.dx == 0.0),
),
localPosition = localPosition ?? globalPosition;
/// Recorded timestamp of the source pointer event that triggered the drag
/// event.
///
/// Could be null if triggered from proxied events such as accessibility.
final Duration? sourceTimeStamp;
/// The amount the pointer has moved in the coordinate space of the event
/// receiver since the previous update.
///
/// If the [GestureDragUpdateCallback] is for a one-dimensional drag (e.g.,
/// a horizontal or vertical drag), then this offset contains only the delta
/// in that direction (i.e., the coordinate in the other direction is zero).
///
/// Defaults to zero if not specified in the constructor.
final Offset delta;
/// The amount the pointer has moved along the primary axis in the coordinate
/// space of the event receiver since the previous
/// update.
///
/// If the [GestureDragUpdateCallback] is for a one-dimensional drag (e.g.,
/// a horizontal or vertical drag), then this value contains the component of
/// [delta] along the primary axis (e.g., horizontal or vertical,
/// respectively). Otherwise, if the [GestureDragUpdateCallback] is for a
/// two-dimensional drag (e.g., a pan), then this value is null.
///
/// Defaults to null if not specified in the constructor.
final double? primaryDelta;
/// The pointer's global position when it triggered this update.
///
/// See also:
///
/// * [localPosition], which is the [globalPosition] transformed to the
/// coordinate space of the event receiver.
final Offset globalPosition;
/// The local position in the coordinate system of the event receiver at
/// which the pointer contacted the screen.
///
/// Defaults to [globalPosition] if not specified in the constructor.
final Offset localPosition;
@override
String toString() => '${objectRuntimeType(this, 'DragUpdateDetails')}($delta)';
}
/// {@template flutter.gestures.dragdetails.GestureDragUpdateCallback}
/// Signature for when a pointer that is in contact with the screen and moving
/// has moved again.
///
/// The `details` object provides the position of the touch and the distance it
/// has traveled since the last update.
/// {@endtemplate}
///
/// See [DragGestureRecognizer.onUpdate].
typedef GestureDragUpdateCallback = void Function(DragUpdateDetails details);
/// Details object for callbacks that use [GestureDragEndCallback].
///
/// See also:
///
/// * [DragGestureRecognizer.onEnd], which uses [GestureDragEndCallback].
/// * [DragDownDetails], the details for [GestureDragDownCallback].
/// * [DragStartDetails], the details for [GestureDragStartCallback].
/// * [DragUpdateDetails], the details for [GestureDragUpdateCallback].
class DragEndDetails {
/// Creates details for a [GestureDragEndCallback].
///
/// If [primaryVelocity] is non-null, its value must match one of the
/// coordinates of `velocity.pixelsPerSecond` and the other coordinate
/// must be zero.
DragEndDetails({
this.velocity = Velocity.zero,
this.primaryVelocity,
this.globalPosition = Offset.zero,
Offset? localPosition,
}) : assert(
primaryVelocity == null
|| (primaryVelocity == velocity.pixelsPerSecond.dx && velocity.pixelsPerSecond.dy == 0)
|| (primaryVelocity == velocity.pixelsPerSecond.dy && velocity.pixelsPerSecond.dx == 0),
),
localPosition = localPosition ?? globalPosition;
/// The velocity the pointer was moving when it stopped contacting the screen.
///
/// Defaults to zero if not specified in the constructor.
final Velocity velocity;
/// The velocity the pointer was moving along the primary axis when it stopped
/// contacting the screen, in logical pixels per second.
///
/// If the [GestureDragEndCallback] is for a one-dimensional drag (e.g., a
/// horizontal or vertical drag), then this value contains the component of
/// [velocity] along the primary axis (e.g., horizontal or vertical,
/// respectively). Otherwise, if the [GestureDragEndCallback] is for a
/// two-dimensional drag (e.g., a pan), then this value is null.
///
/// Defaults to null if not specified in the constructor.
final double? primaryVelocity;
/// The global position the pointer is located at when the drag
/// gesture has been completed.
///
/// Defaults to the origin if not specified in the constructor.
///
/// See also:
///
/// * [localPosition], which is the [globalPosition] transformed to the
/// coordinate space of the event receiver.
final Offset globalPosition;
/// The local position in the coordinate system of the event receiver when
/// the drag gesture has been completed.
///
/// Defaults to [globalPosition] if not specified in the constructor.
final Offset localPosition;
@override
String toString() => '${objectRuntimeType(this, 'DragEndDetails')}($velocity)';
}
| flutter/packages/flutter/lib/src/gestures/drag_details.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/drag_details.dart",
"repo_id": "flutter",
"token_count": 2769
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'arena.dart';
import 'constants.dart';
import 'events.dart';
import 'recognizer.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'package:vector_math/vector_math_64.dart' show Matrix4;
export 'arena.dart' show GestureDisposition;
export 'events.dart' show PointerCancelEvent, PointerDownEvent, PointerEvent, PointerUpEvent;
/// Details for [GestureTapDownCallback], such as position.
///
/// See also:
///
/// * [GestureDetector.onTapDown], which receives this information.
/// * [TapGestureRecognizer], which passes this information to one of its callbacks.
class TapDownDetails {
/// Creates details for a [GestureTapDownCallback].
TapDownDetails({
this.globalPosition = Offset.zero,
Offset? localPosition,
this.kind,
}) : localPosition = localPosition ?? globalPosition;
/// The global position at which the pointer contacted the screen.
final Offset globalPosition;
/// The kind of the device that initiated the event.
final PointerDeviceKind? kind;
/// The local position at which the pointer contacted the screen.
final Offset localPosition;
}
/// {@template flutter.gestures.tap.GestureTapDownCallback}
/// Signature for when a pointer that might cause a tap has contacted the
/// screen.
///
/// The position at which the pointer contacted the screen is available in the
/// `details`.
/// {@endtemplate}
///
/// See also:
///
/// * [GestureDetector.onTapDown], which matches this signature.
/// * [TapGestureRecognizer], which uses this signature in one of its callbacks.
typedef GestureTapDownCallback = void Function(TapDownDetails details);
/// Details for [GestureTapUpCallback], such as position.
///
/// See also:
///
/// * [GestureDetector.onTapUp], which receives this information.
/// * [TapGestureRecognizer], which passes this information to one of its callbacks.
class TapUpDetails {
/// Creates a [TapUpDetails] data object.
TapUpDetails({
required this.kind,
this.globalPosition = Offset.zero,
Offset? localPosition,
}) : localPosition = localPosition ?? globalPosition;
/// The global position at which the pointer contacted the screen.
final Offset globalPosition;
/// The local position at which the pointer contacted the screen.
final Offset localPosition;
/// The kind of the device that initiated the event.
final PointerDeviceKind kind;
}
/// {@template flutter.gestures.tap.GestureTapUpCallback}
/// Signature for when a pointer that will trigger a tap has stopped contacting
/// the screen.
///
/// The position at which the pointer stopped contacting the screen is available
/// in the `details`.
/// {@endtemplate}
///
/// See also:
///
/// * [GestureDetector.onTapUp], which matches this signature.
/// * [TapGestureRecognizer], which uses this signature in one of its callbacks.
typedef GestureTapUpCallback = void Function(TapUpDetails details);
/// Signature for when a tap has occurred.
///
/// See also:
///
/// * [GestureDetector.onTap], which matches this signature.
/// * [TapGestureRecognizer], which uses this signature in one of its callbacks.
typedef GestureTapCallback = void Function();
/// Signature for when the pointer that previously triggered a
/// [GestureTapDownCallback] will not end up causing a tap.
///
/// See also:
///
/// * [GestureDetector.onTapCancel], which matches this signature.
/// * [TapGestureRecognizer], which uses this signature in one of its callbacks.
typedef GestureTapCancelCallback = void Function();
/// A base class for gesture recognizers that recognize taps.
///
/// Gesture recognizers take part in gesture arenas to enable potential gestures
/// to be disambiguated from each other. This process is managed by a
/// [GestureArenaManager].
///
/// A tap is defined as a sequence of events that starts with a down, followed
/// by optional moves, then ends with an up. All move events must contain the
/// same `buttons` as the down event, and must not be too far from the initial
/// position. The gesture is rejected on any violation, a cancel event, or
/// if any other recognizers wins the arena. It is accepted only when it is the
/// last member of the arena.
///
/// The [BaseTapGestureRecognizer] considers all the pointers involved in the
/// pointer event sequence as contributing to one gesture. For this reason,
/// extra pointer interactions during a tap sequence are not recognized as
/// additional taps. For example, down-1, down-2, up-1, up-2 produces only one
/// tap on up-1.
///
/// The [BaseTapGestureRecognizer] can not be directly used, since it does not
/// define which buttons to accept, or what to do when a tap happens. If you
/// want to build a custom tap recognizer, extend this class by overriding
/// [isPointerAllowed] and the handler methods.
///
/// See also:
///
/// * [TapGestureRecognizer], a ready-to-use tap recognizer that recognizes
/// taps of the primary button and taps of the secondary button.
/// * [ModalBarrier], a widget that uses a custom tap recognizer that accepts
/// any buttons.
abstract class BaseTapGestureRecognizer extends PrimaryPointerGestureRecognizer {
/// Creates a tap gesture recognizer.
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
BaseTapGestureRecognizer({
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
})
: super(deadline: kPressTimeout);
bool _sentTapDown = false;
bool _wonArenaForPrimaryPointer = false;
PointerDownEvent? _down;
PointerUpEvent? _up;
/// A pointer has contacted the screen, which might be the start of a tap.
///
/// This triggers after the down event, once a short timeout ([deadline]) has
/// elapsed, or once the gesture has won the arena, whichever comes first.
///
/// The parameter `down` is the down event of the primary pointer that started
/// the tap sequence.
///
/// If this recognizer doesn't win the arena, [handleTapCancel] is called next.
/// Otherwise, [handleTapUp] is called next.
@protected
void handleTapDown({ required PointerDownEvent down });
/// A pointer has stopped contacting the screen, which is recognized as a tap.
///
/// This triggers on the up event if the recognizer wins the arena with it
/// or has previously won.
///
/// The parameter `down` is the down event of the primary pointer that started
/// the tap sequence, and `up` is the up event that ended the tap sequence.
///
/// If this recognizer doesn't win the arena, [handleTapCancel] is called
/// instead.
@protected
void handleTapUp({ required PointerDownEvent down, required PointerUpEvent up });
/// A pointer that previously triggered [handleTapDown] will not end up
/// causing a tap.
///
/// This triggers once the gesture loses the arena if [handleTapDown] has
/// been previously triggered.
///
/// The parameter `down` is the down event of the primary pointer that started
/// the tap sequence; `cancel` is the cancel event, which might be null;
/// `reason` is a short description of the cause if `cancel` is null, which
/// can be "forced" if other gestures won the arena, or "spontaneous"
/// otherwise.
///
/// If this recognizer wins the arena, [handleTapUp] is called instead.
@protected
void handleTapCancel({ required PointerDownEvent down, PointerCancelEvent? cancel, required String reason });
@override
void addAllowedPointer(PointerDownEvent event) {
if (state == GestureRecognizerState.ready) {
// If there is no result in the previous gesture arena,
// we ignore them and prepare to accept a new pointer.
if (_down != null && _up != null) {
assert(_down!.pointer == _up!.pointer);
_reset();
}
assert(_down == null && _up == null);
// `_down` must be assigned in this method instead of `handlePrimaryPointer`,
// because `acceptGesture` might be called before `handlePrimaryPointer`,
// which relies on `_down` to call `handleTapDown`.
_down = event;
}
if (_down != null) {
// This happens when this tap gesture has been rejected while the pointer
// is down (i.e. due to movement), when another allowed pointer is added,
// in which case all pointers are ignored. The `_down` being null
// means that _reset() has been called, since it is always set at the
// first allowed down event and will not be cleared except for reset(),
super.addAllowedPointer(event);
}
}
@override
@protected
void startTrackingPointer(int pointer, [Matrix4? transform]) {
// The recognizer should never track any pointers when `_down` is null,
// because calling `_checkDown` in this state will throw exception.
assert(_down != null);
super.startTrackingPointer(pointer, transform);
}
@override
void handlePrimaryPointer(PointerEvent event) {
if (event is PointerUpEvent) {
_up = event;
_checkUp();
} else if (event is PointerCancelEvent) {
resolve(GestureDisposition.rejected);
if (_sentTapDown) {
_checkCancel(event, '');
}
_reset();
} else if (event.buttons != _down!.buttons) {
resolve(GestureDisposition.rejected);
stopTrackingPointer(primaryPointer!);
}
}
@override
void resolve(GestureDisposition disposition) {
if (_wonArenaForPrimaryPointer && disposition == GestureDisposition.rejected) {
// This can happen if the gesture has been canceled. For example, when
// the pointer has exceeded the touch slop, the buttons have been changed,
// or if the recognizer is disposed.
assert(_sentTapDown);
_checkCancel(null, 'spontaneous');
_reset();
}
super.resolve(disposition);
}
@override
void didExceedDeadline() {
_checkDown();
}
@override
void acceptGesture(int pointer) {
super.acceptGesture(pointer);
if (pointer == primaryPointer) {
_checkDown();
_wonArenaForPrimaryPointer = true;
_checkUp();
}
}
@override
void rejectGesture(int pointer) {
super.rejectGesture(pointer);
if (pointer == primaryPointer) {
// Another gesture won the arena.
assert(state != GestureRecognizerState.possible);
if (_sentTapDown) {
_checkCancel(null, 'forced');
}
_reset();
}
}
void _checkDown() {
if (_sentTapDown) {
return;
}
handleTapDown(down: _down!);
_sentTapDown = true;
}
void _checkUp() {
if (!_wonArenaForPrimaryPointer || _up == null) {
return;
}
assert(_up!.pointer == _down!.pointer);
handleTapUp(down: _down!, up: _up!);
_reset();
}
void _checkCancel(PointerCancelEvent? event, String note) {
handleTapCancel(down: _down!, cancel: event, reason: note);
}
void _reset() {
_sentTapDown = false;
_wonArenaForPrimaryPointer = false;
_up = null;
_down = null;
}
@override
String get debugDescription => 'base tap';
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('wonArenaForPrimaryPointer', value: _wonArenaForPrimaryPointer, ifTrue: 'won arena'));
properties.add(DiagnosticsProperty<Offset>('finalPosition', _up?.position, defaultValue: null));
properties.add(DiagnosticsProperty<Offset>('finalLocalPosition', _up?.localPosition, defaultValue: _up?.position));
properties.add(DiagnosticsProperty<int>('button', _down?.buttons, defaultValue: null));
properties.add(FlagProperty('sentTapDown', value: _sentTapDown, ifTrue: 'sent tap down'));
}
}
/// Recognizes taps.
///
/// Gesture recognizers take part in gesture arenas to enable potential gestures
/// to be disambiguated from each other. This process is managed by a
/// [GestureArenaManager].
///
/// [TapGestureRecognizer] considers all the pointers involved in the pointer
/// event sequence as contributing to one gesture. For this reason, extra
/// pointer interactions during a tap sequence are not recognized as additional
/// taps. For example, down-1, down-2, up-1, up-2 produces only one tap on up-1.
///
/// [TapGestureRecognizer] competes on pointer events of [kPrimaryButton] only
/// when it has at least one non-null `onTap*` callback, on events of
/// [kSecondaryButton] only when it has at least one non-null `onSecondaryTap*`
/// callback, and on events of [kTertiaryButton] only when it has at least
/// one non-null `onTertiaryTap*` callback. If it has no callbacks, it is a
/// no-op.
///
/// {@template flutter.gestures.tap.TapGestureRecognizer.allowedButtonsFilter}
/// The [allowedButtonsFilter] argument only gives this recognizer the
/// ability to limit the buttons it accepts. It does not provide the
/// ability to recognize any buttons beyond the ones it already accepts:
/// kPrimaryButton, kSecondaryButton or kTertiaryButton. Therefore, a
/// combined value of `kPrimaryButton & kSecondaryButton` would be ignored,
/// but `kPrimaryButton | kSecondaryButton` would be allowed, as long as
/// only one of them is selected at a time.
/// {@endtemplate}
///
/// See also:
///
/// * [GestureDetector.onTap], which uses this recognizer.
/// * [MultiTapGestureRecognizer]
class TapGestureRecognizer extends BaseTapGestureRecognizer {
/// Creates a tap gesture recognizer.
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
TapGestureRecognizer({
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
});
/// {@template flutter.gestures.tap.TapGestureRecognizer.onTapDown}
/// A pointer has contacted the screen at a particular location with a primary
/// button, which might be the start of a tap.
/// {@endtemplate}
///
/// This triggers after the down event, once a short timeout ([deadline]) has
/// elapsed, or once the gestures has won the arena, whichever comes first.
///
/// If this recognizer doesn't win the arena, [onTapCancel] is called next.
/// Otherwise, [onTapUp] is called next.
///
/// See also:
///
/// * [kPrimaryButton], the button this callback responds to.
/// * [onSecondaryTapDown], a similar callback but for a secondary button.
/// * [onTertiaryTapDown], a similar callback but for a tertiary button.
/// * [TapDownDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onTapDown], which exposes this callback.
GestureTapDownCallback? onTapDown;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onTapUp}
/// A pointer has stopped contacting the screen at a particular location,
/// which is recognized as a tap of a primary button.
/// {@endtemplate}
///
/// This triggers on the up event, if the recognizer wins the arena with it
/// or has previously won, immediately followed by [onTap].
///
/// If this recognizer doesn't win the arena, [onTapCancel] is called instead.
///
/// See also:
///
/// * [kPrimaryButton], the button this callback responds to.
/// * [onSecondaryTapUp], a similar callback but for a secondary button.
/// * [onTertiaryTapUp], a similar callback but for a tertiary button.
/// * [TapUpDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onTapUp], which exposes this callback.
GestureTapUpCallback? onTapUp;
/// A pointer has stopped contacting the screen, which is recognized as a tap
/// of a primary button.
///
/// This triggers on the up event, if the recognizer wins the arena with it
/// or has previously won, immediately following [onTapUp].
///
/// If this recognizer doesn't win the arena, [onTapCancel] is called instead.
///
/// See also:
///
/// * [kPrimaryButton], the button this callback responds to.
/// * [onSecondaryTap], a similar callback but for a secondary button.
/// * [onTapUp], which has the same timing but with details.
/// * [GestureDetector.onTap], which exposes this callback.
GestureTapCallback? onTap;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onTapCancel}
/// A pointer that previously triggered [onTapDown] will not end up causing
/// a tap.
/// {@endtemplate}
///
/// This triggers once the gesture loses the arena if [onTapDown] has
/// previously been triggered.
///
/// If this recognizer wins the arena, [onTapUp] and [onTap] are called
/// instead.
///
/// See also:
///
/// * [kPrimaryButton], the button this callback responds to.
/// * [onSecondaryTapCancel], a similar callback but for a secondary button.
/// * [onTertiaryTapCancel], a similar callback but for a tertiary button.
/// * [GestureDetector.onTapCancel], which exposes this callback.
GestureTapCancelCallback? onTapCancel;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onSecondaryTap}
/// A pointer has stopped contacting the screen, which is recognized as a tap
/// of a secondary button.
/// {@endtemplate}
///
/// This triggers on the up event, if the recognizer wins the arena with it or
/// has previously won, immediately following [onSecondaryTapUp].
///
/// If this recognizer doesn't win the arena, [onSecondaryTapCancel] is called
/// instead.
///
/// See also:
///
/// * [kSecondaryButton], the button this callback responds to.
/// * [onSecondaryTapUp], which has the same timing but with details.
/// * [GestureDetector.onSecondaryTap], which exposes this callback.
GestureTapCallback? onSecondaryTap;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onSecondaryTapDown}
/// A pointer has contacted the screen at a particular location with a
/// secondary button, which might be the start of a secondary tap.
/// {@endtemplate}
///
/// This triggers after the down event, once a short timeout ([deadline]) has
/// elapsed, or once the gestures has won the arena, whichever comes first.
///
/// If this recognizer doesn't win the arena, [onSecondaryTapCancel] is called
/// next. Otherwise, [onSecondaryTapUp] is called next.
///
/// See also:
///
/// * [kSecondaryButton], the button this callback responds to.
/// * [onTapDown], a similar callback but for a primary button.
/// * [onTertiaryTapDown], a similar callback but for a tertiary button.
/// * [TapDownDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onSecondaryTapDown], which exposes this callback.
GestureTapDownCallback? onSecondaryTapDown;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onSecondaryTapUp}
/// A pointer has stopped contacting the screen at a particular location,
/// which is recognized as a tap of a secondary button.
/// {@endtemplate}
///
/// This triggers on the up event if the recognizer wins the arena with it
/// or has previously won.
///
/// If this recognizer doesn't win the arena, [onSecondaryTapCancel] is called
/// instead.
///
/// See also:
///
/// * [onSecondaryTap], a handler triggered right after this one that doesn't
/// pass any details about the tap.
/// * [kSecondaryButton], the button this callback responds to.
/// * [onTapUp], a similar callback but for a primary button.
/// * [onTertiaryTapUp], a similar callback but for a tertiary button.
/// * [TapUpDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onSecondaryTapUp], which exposes this callback.
GestureTapUpCallback? onSecondaryTapUp;
/// {@template flutter.gestures.tap.TapGestureRecognizer.onSecondaryTapCancel}
/// A pointer that previously triggered [onSecondaryTapDown] will not end up
/// causing a tap.
/// {@endtemplate}
///
/// This triggers once the gesture loses the arena if [onSecondaryTapDown]
/// has previously been triggered.
///
/// If this recognizer wins the arena, [onSecondaryTapUp] is called instead.
///
/// See also:
///
/// * [kSecondaryButton], the button this callback responds to.
/// * [onTapCancel], a similar callback but for a primary button.
/// * [onTertiaryTapCancel], a similar callback but for a tertiary button.
/// * [GestureDetector.onSecondaryTapCancel], which exposes this callback.
GestureTapCancelCallback? onSecondaryTapCancel;
/// A pointer has contacted the screen at a particular location with a
/// tertiary button, which might be the start of a tertiary tap.
///
/// This triggers after the down event, once a short timeout ([deadline]) has
/// elapsed, or once the gestures has won the arena, whichever comes first.
///
/// If this recognizer doesn't win the arena, [onTertiaryTapCancel] is called
/// next. Otherwise, [onTertiaryTapUp] is called next.
///
/// See also:
///
/// * [kTertiaryButton], the button this callback responds to.
/// * [onTapDown], a similar callback but for a primary button.
/// * [onSecondaryTapDown], a similar callback but for a secondary button.
/// * [TapDownDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onTertiaryTapDown], which exposes this callback.
GestureTapDownCallback? onTertiaryTapDown;
/// A pointer has stopped contacting the screen at a particular location,
/// which is recognized as a tap of a tertiary button.
///
/// This triggers on the up event if the recognizer wins the arena with it
/// or has previously won.
///
/// If this recognizer doesn't win the arena, [onTertiaryTapCancel] is called
/// instead.
///
/// See also:
///
/// * [kTertiaryButton], the button this callback responds to.
/// * [onTapUp], a similar callback but for a primary button.
/// * [onSecondaryTapUp], a similar callback but for a secondary button.
/// * [TapUpDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onTertiaryTapUp], which exposes this callback.
GestureTapUpCallback? onTertiaryTapUp;
/// A pointer that previously triggered [onTertiaryTapDown] will not end up
/// causing a tap.
///
/// This triggers once the gesture loses the arena if [onTertiaryTapDown]
/// has previously been triggered.
///
/// If this recognizer wins the arena, [onTertiaryTapUp] is called instead.
///
/// See also:
///
/// * [kSecondaryButton], the button this callback responds to.
/// * [onTapCancel], a similar callback but for a primary button.
/// * [onSecondaryTapCancel], a similar callback but for a secondary button.
/// * [GestureDetector.onTertiaryTapCancel], which exposes this callback.
GestureTapCancelCallback? onTertiaryTapCancel;
@override
bool isPointerAllowed(PointerDownEvent event) {
switch (event.buttons) {
case kPrimaryButton:
if (onTapDown == null &&
onTap == null &&
onTapUp == null &&
onTapCancel == null) {
return false;
}
case kSecondaryButton:
if (onSecondaryTap == null &&
onSecondaryTapDown == null &&
onSecondaryTapUp == null &&
onSecondaryTapCancel == null) {
return false;
}
case kTertiaryButton:
if (onTertiaryTapDown == null &&
onTertiaryTapUp == null &&
onTertiaryTapCancel == null) {
return false;
}
default:
return false;
}
return super.isPointerAllowed(event);
}
@protected
@override
void handleTapDown({required PointerDownEvent down}) {
final TapDownDetails details = TapDownDetails(
globalPosition: down.position,
localPosition: down.localPosition,
kind: getKindForPointer(down.pointer),
);
switch (down.buttons) {
case kPrimaryButton:
if (onTapDown != null) {
invokeCallback<void>('onTapDown', () => onTapDown!(details));
}
case kSecondaryButton:
if (onSecondaryTapDown != null) {
invokeCallback<void>('onSecondaryTapDown', () => onSecondaryTapDown!(details));
}
case kTertiaryButton:
if (onTertiaryTapDown != null) {
invokeCallback<void>('onTertiaryTapDown', () => onTertiaryTapDown!(details));
}
default:
}
}
@protected
@override
void handleTapUp({ required PointerDownEvent down, required PointerUpEvent up}) {
final TapUpDetails details = TapUpDetails(
kind: up.kind,
globalPosition: up.position,
localPosition: up.localPosition,
);
switch (down.buttons) {
case kPrimaryButton:
if (onTapUp != null) {
invokeCallback<void>('onTapUp', () => onTapUp!(details));
}
if (onTap != null) {
invokeCallback<void>('onTap', onTap!);
}
case kSecondaryButton:
if (onSecondaryTapUp != null) {
invokeCallback<void>('onSecondaryTapUp', () => onSecondaryTapUp!(details));
}
if (onSecondaryTap != null) {
invokeCallback<void>('onSecondaryTap', () => onSecondaryTap!());
}
case kTertiaryButton:
if (onTertiaryTapUp != null) {
invokeCallback<void>('onTertiaryTapUp', () => onTertiaryTapUp!(details));
}
default:
}
}
@protected
@override
void handleTapCancel({ required PointerDownEvent down, PointerCancelEvent? cancel, required String reason }) {
final String note = reason == '' ? reason : '$reason ';
switch (down.buttons) {
case kPrimaryButton:
if (onTapCancel != null) {
invokeCallback<void>('${note}onTapCancel', onTapCancel!);
}
case kSecondaryButton:
if (onSecondaryTapCancel != null) {
invokeCallback<void>('${note}onSecondaryTapCancel', onSecondaryTapCancel!);
}
case kTertiaryButton:
if (onTertiaryTapCancel != null) {
invokeCallback<void>('${note}onTertiaryTapCancel', onTertiaryTapCancel!);
}
default:
}
}
@override
String get debugDescription => 'tap';
}
| flutter/packages/flutter/lib/src/gestures/tap.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/tap.dart",
"repo_id": "flutter",
"token_count": 8314
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$event_add = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
0.761904761905,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(32.01015625, 23.52890625),
Offset(32.01015329719101, 23.53062658538017),
Offset(31.908309754160946, 24.86045986263573),
Offset(29.93347206506402, 28.868314694169428),
Offset(26.259363778077685, 30.74028679651635),
Offset(23.62410382175968, 30.58943972916481),
Offset(21.925014841592773, 29.92755775441251),
Offset(20.79646278990295, 29.175637008874478),
Offset(20.023435215871057, 28.454097084853878),
Offset(19.478915461565702, 27.800711501947685),
Offset(19.08488578667808, 27.224748870271775),
Offset(18.794354809526837, 26.724256833788246),
Offset(18.590826530804044, 26.293721762918267),
Offset(18.45085412812233, 25.930620946266075),
Offset(18.35542242952162, 25.63066280891873),
Offset(18.291196017351787, 25.38919913375952),
Offset(18.248665479323506, 25.201028049969374),
Offset(18.221259589085008, 25.06135592936411),
Offset(18.204520556081953, 24.965782149690927),
Offset(18.19555633054043, 24.91035753064884),
Offset(18.192643234510292, 24.891585410299637),
Offset(18.192636718750002, 24.891542968750002),
],
),
_PathCubicTo(
<Offset>[
Offset(32.01015625, 23.52890625),
Offset(32.01015329719101, 23.53062658538017),
Offset(31.908309754160946, 24.86045986263573),
Offset(29.93347206506402, 28.868314694169428),
Offset(26.259363778077685, 30.74028679651635),
Offset(23.62410382175968, 30.58943972916481),
Offset(21.925014841592773, 29.92755775441251),
Offset(20.79646278990295, 29.175637008874478),
Offset(20.023435215871057, 28.454097084853878),
Offset(19.478915461565702, 27.800711501947685),
Offset(19.08488578667808, 27.224748870271775),
Offset(18.794354809526837, 26.724256833788246),
Offset(18.590826530804044, 26.293721762918267),
Offset(18.45085412812233, 25.930620946266075),
Offset(18.35542242952162, 25.63066280891873),
Offset(18.291196017351787, 25.38919913375952),
Offset(18.248665479323506, 25.201028049969374),
Offset(18.221259589085008, 25.06135592936411),
Offset(18.204520556081953, 24.965782149690927),
Offset(18.19555633054043, 24.91035753064884),
Offset(18.192643234510292, 24.891585410299637),
Offset(18.192636718750002, 24.891542968750002),
],
<Offset>[
Offset(24.01015625, 23.52890625),
Offset(24.010258478063488, 23.528888402424656),
Offset(24.091555200930664, 23.52418084688126),
Offset(24.377115816859927, 23.6152417705347),
Offset(24.633459690010874, 23.859538100350317),
Offset(24.736069637313253, 24.1352514457875),
Offset(24.72116824601048, 24.371795772576675),
Offset(24.648015807099657, 24.549452838288758),
Offset(24.55323690381452, 24.674789163937373),
Offset(24.455385525488182, 24.759322859315567),
Offset(24.363483168967523, 24.813428460885813),
Offset(24.28176718778124, 24.845878439805734),
Offset(24.21233699329442, 24.865919213504945),
Offset(24.154550207153598, 24.87807591728),
Offset(24.107302586424357, 24.885092784958154),
Offset(24.06957428023968, 24.88885460147735),
Offset(24.040355338731057, 24.890659603706283),
Offset(24.01876825740043, 24.89137581050446),
Offset(24.004045682792036, 24.891564195943896),
Offset(23.995525876462455, 24.89156214884712),
Offset(23.9926432343554, 24.891543022538777),
Offset(23.99263671875, 24.89154296875),
],
<Offset>[
Offset(24.01015625, 23.52890625),
Offset(24.010258478063488, 23.528888402424656),
Offset(24.091555200930664, 23.52418084688126),
Offset(24.377115816859927, 23.6152417705347),
Offset(24.633459690010874, 23.859538100350317),
Offset(24.736069637313253, 24.1352514457875),
Offset(24.72116824601048, 24.371795772576675),
Offset(24.648015807099657, 24.549452838288758),
Offset(24.55323690381452, 24.674789163937373),
Offset(24.455385525488182, 24.759322859315567),
Offset(24.363483168967523, 24.813428460885813),
Offset(24.28176718778124, 24.845878439805734),
Offset(24.21233699329442, 24.865919213504945),
Offset(24.154550207153598, 24.87807591728),
Offset(24.107302586424357, 24.885092784958154),
Offset(24.06957428023968, 24.88885460147735),
Offset(24.040355338731057, 24.890659603706283),
Offset(24.01876825740043, 24.89137581050446),
Offset(24.004045682792036, 24.891564195943896),
Offset(23.995525876462455, 24.89156214884712),
Offset(23.9926432343554, 24.891543022538777),
Offset(23.99263671875, 24.89154296875),
],
),
_PathCubicTo(
<Offset>[
Offset(24.01015625, 23.52890625),
Offset(24.010258478063488, 23.528888402424656),
Offset(24.091555200930664, 23.52418084688126),
Offset(24.377115816859927, 23.6152417705347),
Offset(24.633459690010874, 23.859538100350317),
Offset(24.736069637313253, 24.1352514457875),
Offset(24.72116824601048, 24.371795772576675),
Offset(24.648015807099657, 24.549452838288758),
Offset(24.55323690381452, 24.674789163937373),
Offset(24.455385525488182, 24.759322859315567),
Offset(24.363483168967523, 24.813428460885813),
Offset(24.28176718778124, 24.845878439805734),
Offset(24.21233699329442, 24.865919213504945),
Offset(24.154550207153598, 24.87807591728),
Offset(24.107302586424357, 24.885092784958154),
Offset(24.06957428023968, 24.88885460147735),
Offset(24.040355338731057, 24.890659603706283),
Offset(24.01876825740043, 24.89137581050446),
Offset(24.004045682792036, 24.891564195943896),
Offset(23.995525876462455, 24.89156214884712),
Offset(23.9926432343554, 24.891543022538777),
Offset(23.99263671875, 24.89154296875),
],
<Offset>[
Offset(24.01015625, 31.52890625),
Offset(24.008520295107978, 31.528783221552175),
Offset(22.755276185176193, 31.340935400111544),
Offset(19.124042893225194, 29.17159801873879),
Offset(17.752710993844843, 25.48544218841713),
Offset(18.281881353935944, 23.023285630233925),
Offset(19.165406264174642, 21.57564236815897),
Offset(20.021831636513937, 20.69789982109205),
Offset(20.773928982898013, 20.14498747599391),
Offset(21.41399688285607, 19.782852795393087),
Offset(21.95216275958156, 19.534831078596365),
Offset(22.40338879379873, 19.358466061551326),
Offset(22.7845344438811, 19.244408751014564),
Offset(23.10200517816752, 19.174379838248726),
Offset(23.36173256246378, 19.133212628055418),
Offset(23.569229747957515, 19.110476338589457),
Offset(23.729986892467963, 19.098969744298735),
Offset(23.84878813854078, 19.09386714218904),
Offset(23.929827729045, 19.092039069233813),
Offset(23.976730494660732, 19.091592602925097),
Offset(23.99260084659454, 19.09154302269367),
Offset(23.99263671875, 19.09154296875),
],
<Offset>[
Offset(24.01015625, 31.52890625),
Offset(24.008520295107978, 31.528783221552175),
Offset(22.755276185176193, 31.340935400111544),
Offset(19.124042893225194, 29.17159801873879),
Offset(17.752710993844843, 25.48544218841713),
Offset(18.281881353935944, 23.023285630233925),
Offset(19.165406264174642, 21.57564236815897),
Offset(20.021831636513937, 20.69789982109205),
Offset(20.773928982898013, 20.14498747599391),
Offset(21.41399688285607, 19.782852795393087),
Offset(21.95216275958156, 19.534831078596365),
Offset(22.40338879379873, 19.358466061551326),
Offset(22.7845344438811, 19.244408751014564),
Offset(23.10200517816752, 19.174379838248726),
Offset(23.36173256246378, 19.133212628055418),
Offset(23.569229747957515, 19.110476338589457),
Offset(23.729986892467963, 19.098969744298735),
Offset(23.84878813854078, 19.09386714218904),
Offset(23.929827729045, 19.092039069233813),
Offset(23.976730494660732, 19.091592602925097),
Offset(23.99260084659454, 19.09154302269367),
Offset(23.99263671875, 19.09154296875),
],
),
_PathCubicTo(
<Offset>[
Offset(24.01015625, 31.52890625),
Offset(24.008520295107978, 31.528783221552175),
Offset(22.755276185176193, 31.340935400111544),
Offset(19.124042893225194, 29.17159801873879),
Offset(17.752710993844843, 25.48544218841713),
Offset(18.281881353935944, 23.023285630233925),
Offset(19.165406264174642, 21.57564236815897),
Offset(20.021831636513937, 20.69789982109205),
Offset(20.773928982898013, 20.14498747599391),
Offset(21.41399688285607, 19.782852795393087),
Offset(21.95216275958156, 19.534831078596365),
Offset(22.40338879379873, 19.358466061551326),
Offset(22.7845344438811, 19.244408751014564),
Offset(23.10200517816752, 19.174379838248726),
Offset(23.36173256246378, 19.133212628055418),
Offset(23.569229747957515, 19.110476338589457),
Offset(23.729986892467963, 19.098969744298735),
Offset(23.84878813854078, 19.09386714218904),
Offset(23.929827729045, 19.092039069233813),
Offset(23.976730494660732, 19.091592602925097),
Offset(23.99260084659454, 19.09154302269367),
Offset(23.99263671875, 19.09154296875),
],
<Offset>[
Offset(32.01015625, 31.52890625),
Offset(32.0084151142355, 31.530521404507688),
Offset(30.572030738406475, 32.67721441586602),
Offset(24.68039914142929, 34.424670942373524),
Offset(19.378615081911654, 32.36619088458316),
Offset(17.16991553838237, 29.477473913611234),
Offset(16.369252859756937, 27.131404349994806),
Offset(16.17027861931723, 25.32408399167777),
Offset(16.244127294954552, 23.924295396910416),
Offset(16.437526818933584, 22.824241438025204),
Offset(16.673565377292114, 21.94615148798233),
Offset(16.915976415544325, 21.236844455533838),
Offset(17.16302398139072, 20.67221130042789),
Offset(17.39830909913625, 20.226924867234807),
Offset(17.609852405561046, 19.878782652015996),
Offset(17.79085148506962, 19.61082087087162),
Offset(17.938297033060415, 19.409338190561826),
Offset(18.05127947022536, 19.26384726104869),
Offset(18.13030260233492, 19.166257022980844),
Offset(18.17676094873871, 19.11038798472682),
Offset(18.192600846749432, 19.091585410454528),
Offset(18.19263671875, 19.09154296875),
],
<Offset>[
Offset(32.01015625, 31.52890625),
Offset(32.0084151142355, 31.530521404507688),
Offset(30.572030738406475, 32.67721441586602),
Offset(24.68039914142929, 34.424670942373524),
Offset(19.378615081911654, 32.36619088458316),
Offset(17.16991553838237, 29.477473913611234),
Offset(16.369252859756937, 27.131404349994806),
Offset(16.17027861931723, 25.32408399167777),
Offset(16.244127294954552, 23.924295396910416),
Offset(16.437526818933584, 22.824241438025204),
Offset(16.673565377292114, 21.94615148798233),
Offset(16.915976415544325, 21.236844455533838),
Offset(17.16302398139072, 20.67221130042789),
Offset(17.39830909913625, 20.226924867234807),
Offset(17.609852405561046, 19.878782652015996),
Offset(17.79085148506962, 19.61082087087162),
Offset(17.938297033060415, 19.409338190561826),
Offset(18.05127947022536, 19.26384726104869),
Offset(18.13030260233492, 19.166257022980844),
Offset(18.17676094873871, 19.11038798472682),
Offset(18.192600846749432, 19.091585410454528),
Offset(18.19263671875, 19.09154296875),
],
),
_PathCubicTo(
<Offset>[
Offset(32.01015625, 31.52890625),
Offset(32.0084151142355, 31.530521404507688),
Offset(30.572030738406475, 32.67721441586602),
Offset(24.68039914142929, 34.424670942373524),
Offset(19.378615081911654, 32.36619088458316),
Offset(17.16991553838237, 29.477473913611234),
Offset(16.369252859756937, 27.131404349994806),
Offset(16.17027861931723, 25.32408399167777),
Offset(16.244127294954552, 23.924295396910416),
Offset(16.437526818933584, 22.824241438025204),
Offset(16.673565377292114, 21.94615148798233),
Offset(16.915976415544325, 21.236844455533838),
Offset(17.16302398139072, 20.67221130042789),
Offset(17.39830909913625, 20.226924867234807),
Offset(17.609852405561046, 19.878782652015996),
Offset(17.79085148506962, 19.61082087087162),
Offset(17.938297033060415, 19.409338190561826),
Offset(18.05127947022536, 19.26384726104869),
Offset(18.13030260233492, 19.166257022980844),
Offset(18.17676094873871, 19.11038798472682),
Offset(18.192600846749432, 19.091585410454528),
Offset(18.19263671875, 19.09154296875),
],
<Offset>[
Offset(32.01015625, 23.52890625),
Offset(32.01015329719101, 23.53062658538017),
Offset(31.908309754160946, 24.86045986263573),
Offset(29.93347206506402, 28.868314694169428),
Offset(26.259363778077685, 30.74028679651635),
Offset(23.62410382175968, 30.58943972916481),
Offset(21.925014841592773, 29.92755775441251),
Offset(20.79646278990295, 29.175637008874478),
Offset(20.023435215871057, 28.454097084853878),
Offset(19.478915461565702, 27.800711501947685),
Offset(19.08488578667808, 27.224748870271775),
Offset(18.794354809526837, 26.724256833788246),
Offset(18.590826530804044, 26.293721762918267),
Offset(18.45085412812233, 25.930620946266075),
Offset(18.35542242952162, 25.63066280891873),
Offset(18.291196017351787, 25.38919913375952),
Offset(18.248665479323506, 25.201028049969374),
Offset(18.221259589085008, 25.06135592936411),
Offset(18.204520556081953, 24.965782149690927),
Offset(18.19555633054043, 24.91035753064884),
Offset(18.192643234510292, 24.891585410299637),
Offset(18.192636718750002, 24.891542968750002),
],
<Offset>[
Offset(32.01015625, 23.52890625),
Offset(32.01015329719101, 23.53062658538017),
Offset(31.908309754160946, 24.86045986263573),
Offset(29.93347206506402, 28.868314694169428),
Offset(26.259363778077685, 30.74028679651635),
Offset(23.62410382175968, 30.58943972916481),
Offset(21.925014841592773, 29.92755775441251),
Offset(20.79646278990295, 29.175637008874478),
Offset(20.023435215871057, 28.454097084853878),
Offset(19.478915461565702, 27.800711501947685),
Offset(19.08488578667808, 27.224748870271775),
Offset(18.794354809526837, 26.724256833788246),
Offset(18.590826530804044, 26.293721762918267),
Offset(18.45085412812233, 25.930620946266075),
Offset(18.35542242952162, 25.63066280891873),
Offset(18.291196017351787, 25.38919913375952),
Offset(18.248665479323506, 25.201028049969374),
Offset(18.221259589085008, 25.06135592936411),
Offset(18.204520556081953, 24.965782149690927),
Offset(18.19555633054043, 24.91035753064884),
Offset(18.192643234510292, 24.891585410299637),
Offset(18.192636718750002, 24.891542968750002),
],
),
_PathClose(
),
_PathMoveTo(
<Offset>[
Offset(30.4, 6.0),
Offset(30.403826772654973, 6.001600955318109),
Offset(33.26297392821128, 7.464112893661335),
Offset(40.3252246978808, 15.636425198410986),
Offset(41.008618677528034, 25.792861690388108),
Offset(37.989766327618334, 31.726851345525642),
Offset(34.66109919246733, 34.936041005423874),
Offset(31.70815663793754, 36.68371605880273),
Offset(29.216038057712375, 37.618746191043186),
Offset(27.14455430692518, 38.09258228629963),
Offset(25.430782781823723, 38.305428372486986),
Offset(24.014543572941673, 38.36973863661014),
Offset(22.850742435528595, 38.323714848128795),
Offset(21.905079756466474, 38.2162199326415),
Offset(21.146729034109512, 38.08362328363005),
Offset(20.550519051592694, 37.94957638018356),
Offset(20.09440860715983, 37.82880891010404),
Offset(19.760567137156492, 37.73014235182733),
Offset(19.53440820396981, 37.65826086700748),
Offset(19.40409629300435, 37.614964895879226),
Offset(19.360099392114897, 37.60003390984549),
Offset(19.360000000000003, 37.6),
],
),
_PathCubicTo(
<Offset>[
Offset(30.4, 6.0),
Offset(30.403826772654973, 6.001600955318109),
Offset(33.26297392821128, 7.464112893661335),
Offset(40.3252246978808, 15.636425198410986),
Offset(41.008618677528034, 25.792861690388108),
Offset(37.989766327618334, 31.726851345525642),
Offset(34.66109919246733, 34.936041005423874),
Offset(31.70815663793754, 36.68371605880273),
Offset(29.216038057712375, 37.618746191043186),
Offset(27.14455430692518, 38.09258228629963),
Offset(25.430782781823723, 38.305428372486986),
Offset(24.014543572941673, 38.36973863661014),
Offset(22.850742435528595, 38.323714848128795),
Offset(21.905079756466474, 38.2162199326415),
Offset(21.146729034109512, 38.08362328363005),
Offset(20.550519051592694, 37.94957638018356),
Offset(20.09440860715983, 37.82880891010404),
Offset(19.760567137156492, 37.73014235182733),
Offset(19.53440820396981, 37.65826086700748),
Offset(19.40409629300435, 37.614964895879226),
Offset(19.360099392114897, 37.60003390984549),
Offset(19.360000000000003, 37.6),
],
<Offset>[
Offset(30.4, 9.2),
Offset(30.40313149947277, 9.201558882969117),
Offset(32.7284623219095, 10.590814714953448),
Offset(38.22399552842691, 17.858967697692623),
Offset(38.25631919906162, 26.44322332561483),
Offset(35.40809101426741, 31.282065019304213),
Offset(32.438794399733, 33.81757964365679),
Offset(29.857682969703255, 35.14309485192405),
Offset(27.704314889345774, 35.8068255158658),
Offset(25.92799884987233, 36.101994260730635),
Offset(24.466254618069335, 36.19398941957121),
Offset(23.263192215348667, 36.174773685308374),
Offset(22.279621415763266, 36.07511066313264),
Offset(21.484061744872044, 35.93474150102899),
Offset(20.84850102452528, 35.782871220868955),
Offset(20.35038123867983, 35.6382250750284),
Offset(19.970261228654596, 35.512132966341014),
Offset(19.692575089612635, 35.41113888450116),
Offset(19.504721022470996, 35.338450816323444),
Offset(19.39657814028366, 35.29497707751041),
Offset(19.360082437010554, 35.28003390990745),
Offset(19.36, 35.28),
],
<Offset>[
Offset(30.4, 9.2),
Offset(30.40313149947277, 9.201558882969117),
Offset(32.7284623219095, 10.590814714953448),
Offset(38.22399552842691, 17.858967697692623),
Offset(38.25631919906162, 26.44322332561483),
Offset(35.40809101426741, 31.282065019304213),
Offset(32.438794399733, 33.81757964365679),
Offset(29.857682969703255, 35.14309485192405),
Offset(27.704314889345774, 35.8068255158658),
Offset(25.92799884987233, 36.101994260730635),
Offset(24.466254618069335, 36.19398941957121),
Offset(23.263192215348667, 36.174773685308374),
Offset(22.279621415763266, 36.07511066313264),
Offset(21.484061744872044, 35.93474150102899),
Offset(20.84850102452528, 35.782871220868955),
Offset(20.35038123867983, 35.6382250750284),
Offset(19.970261228654596, 35.512132966341014),
Offset(19.692575089612635, 35.41113888450116),
Offset(19.504721022470996, 35.338450816323444),
Offset(19.39657814028366, 35.29497707751041),
Offset(19.360082437010554, 35.28003390990745),
Offset(19.36, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(30.4, 9.2),
Offset(30.40313149947277, 9.201558882969117),
Offset(32.7284623219095, 10.590814714953448),
Offset(38.22399552842691, 17.858967697692623),
Offset(38.25631919906162, 26.44322332561483),
Offset(35.40809101426741, 31.282065019304213),
Offset(32.438794399733, 33.81757964365679),
Offset(29.857682969703255, 35.14309485192405),
Offset(27.704314889345774, 35.8068255158658),
Offset(25.92799884987233, 36.101994260730635),
Offset(24.466254618069335, 36.19398941957121),
Offset(23.263192215348667, 36.174773685308374),
Offset(22.279621415763266, 36.07511066313264),
Offset(21.484061744872044, 35.93474150102899),
Offset(20.84850102452528, 35.782871220868955),
Offset(20.35038123867983, 35.6382250750284),
Offset(19.970261228654596, 35.512132966341014),
Offset(19.692575089612635, 35.41113888450116),
Offset(19.504721022470996, 35.338450816323444),
Offset(19.39657814028366, 35.29497707751041),
Offset(19.360082437010554, 35.28003390990745),
Offset(19.36, 35.28),
],
<Offset>[
Offset(17.6, 9.2),
Offset(17.60329978886874, 9.1987777902403),
Offset(20.22165503674104, 8.452768289746293),
Offset(29.333825531300356, 9.454051019877056),
Offset(35.65487265815472, 15.434025411749177),
Offset(37.18723631915313, 20.955363765900515),
Offset(36.912639846801326, 24.928360472719458),
Offset(36.02016779721799, 27.741200178986897),
Offset(34.95199759005531, 29.759932842399394),
Offset(33.8903509521483, 31.235772432519248),
Offset(32.91201042973245, 32.335876764553674),
Offset(32.04305202055572, 33.16936825493635),
Offset(31.274038155747874, 33.790626584071326),
Offset(30.609975471322077, 34.250669454651266),
Offset(30.051509275569657, 34.589959182532034),
Offset(29.595786459300463, 34.83767382337693),
Offset(29.236965003706672, 35.01554345232007),
Offset(28.96858895891731, 35.13917069432573),
Offset(28.78396122520713, 35.21970209032819),
Offset(28.6765294137589, 35.264904466627655),
Offset(28.640082436762725, 35.279966089490074),
Offset(28.64, 35.28),
],
<Offset>[
Offset(17.6, 9.2),
Offset(17.60329978886874, 9.1987777902403),
Offset(20.22165503674104, 8.452768289746293),
Offset(29.333825531300356, 9.454051019877056),
Offset(35.65487265815472, 15.434025411749177),
Offset(37.18723631915313, 20.955363765900515),
Offset(36.912639846801326, 24.928360472719458),
Offset(36.02016779721799, 27.741200178986897),
Offset(34.95199759005531, 29.759932842399394),
Offset(33.8903509521483, 31.235772432519248),
Offset(32.91201042973245, 32.335876764553674),
Offset(32.04305202055572, 33.16936825493635),
Offset(31.274038155747874, 33.790626584071326),
Offset(30.609975471322077, 34.250669454651266),
Offset(30.051509275569657, 34.589959182532034),
Offset(29.595786459300463, 34.83767382337693),
Offset(29.236965003706672, 35.01554345232007),
Offset(28.96858895891731, 35.13917069432573),
Offset(28.78396122520713, 35.21970209032819),
Offset(28.6765294137589, 35.264904466627655),
Offset(28.640082436762725, 35.279966089490074),
Offset(28.64, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(17.6, 9.2),
Offset(17.60329978886874, 9.1987777902403),
Offset(20.22165503674104, 8.452768289746293),
Offset(29.333825531300356, 9.454051019877056),
Offset(35.65487265815472, 15.434025411749177),
Offset(37.18723631915313, 20.955363765900515),
Offset(36.912639846801326, 24.928360472719458),
Offset(36.02016779721799, 27.741200178986897),
Offset(34.95199759005531, 29.759932842399394),
Offset(33.8903509521483, 31.235772432519248),
Offset(32.91201042973245, 32.335876764553674),
Offset(32.04305202055572, 33.16936825493635),
Offset(31.274038155747874, 33.790626584071326),
Offset(30.609975471322077, 34.250669454651266),
Offset(30.051509275569657, 34.589959182532034),
Offset(29.595786459300463, 34.83767382337693),
Offset(29.236965003706672, 35.01554345232007),
Offset(28.96858895891731, 35.13917069432573),
Offset(28.78396122520713, 35.21970209032819),
Offset(28.6765294137589, 35.264904466627655),
Offset(28.640082436762725, 35.279966089490074),
Offset(28.64, 35.28),
],
<Offset>[
Offset(17.6, 6.0),
Offset(17.60399506205094, 5.99881986258929),
Offset(20.75616664304283, 5.326066468454183),
Offset(31.43505470075425, 7.231508520595419),
Offset(38.40717213662114, 14.783663776522452),
Offset(39.76891163250406, 21.400150092121944),
Offset(39.13494463953566, 26.04682183448654),
Offset(37.87064146545227, 29.28182138586558),
Offset(36.463720758421914, 31.571853517576777),
Offset(35.106906409201144, 33.22636045808824),
Offset(33.876538593486835, 34.44731571746945),
Offset(32.79440337814872, 35.364333206238115),
Offset(31.845159175513203, 36.03923076906747),
Offset(31.030993482916507, 36.53214788626377),
Offset(30.349737285153886, 36.890711245293126),
Offset(29.795924272213327, 37.14902512853209),
Offset(29.36111238221191, 37.33221939608309),
Offset(29.03658100646117, 37.4581741616519),
Offset(28.81364840670594, 37.539512141012224),
Offset(28.684047566479585, 37.58489228499647),
Offset(28.64009939186707, 37.59996608942812),
Offset(28.64, 37.6),
],
<Offset>[
Offset(17.6, 6.0),
Offset(17.60399506205094, 5.99881986258929),
Offset(20.75616664304283, 5.326066468454183),
Offset(31.43505470075425, 7.231508520595419),
Offset(38.40717213662114, 14.783663776522452),
Offset(39.76891163250406, 21.400150092121944),
Offset(39.13494463953566, 26.04682183448654),
Offset(37.87064146545227, 29.28182138586558),
Offset(36.463720758421914, 31.571853517576777),
Offset(35.106906409201144, 33.22636045808824),
Offset(33.876538593486835, 34.44731571746945),
Offset(32.79440337814872, 35.364333206238115),
Offset(31.845159175513203, 36.03923076906747),
Offset(31.030993482916507, 36.53214788626377),
Offset(30.349737285153886, 36.890711245293126),
Offset(29.795924272213327, 37.14902512853209),
Offset(29.36111238221191, 37.33221939608309),
Offset(29.03658100646117, 37.4581741616519),
Offset(28.81364840670594, 37.539512141012224),
Offset(28.684047566479585, 37.58489228499647),
Offset(28.64009939186707, 37.59996608942812),
Offset(28.64, 37.6),
],
),
_PathCubicTo(
<Offset>[
Offset(17.6, 6.0),
Offset(17.60399506205094, 5.99881986258929),
Offset(20.75616664304283, 5.326066468454183),
Offset(31.43505470075425, 7.231508520595419),
Offset(38.40717213662114, 14.783663776522452),
Offset(39.76891163250406, 21.400150092121944),
Offset(39.13494463953566, 26.04682183448654),
Offset(37.87064146545227, 29.28182138586558),
Offset(36.463720758421914, 31.571853517576777),
Offset(35.106906409201144, 33.22636045808824),
Offset(33.876538593486835, 34.44731571746945),
Offset(32.79440337814872, 35.364333206238115),
Offset(31.845159175513203, 36.03923076906747),
Offset(31.030993482916507, 36.53214788626377),
Offset(30.349737285153886, 36.890711245293126),
Offset(29.795924272213327, 37.14902512853209),
Offset(29.36111238221191, 37.33221939608309),
Offset(29.03658100646117, 37.4581741616519),
Offset(28.81364840670594, 37.539512141012224),
Offset(28.684047566479585, 37.58489228499647),
Offset(28.64009939186707, 37.59996608942812),
Offset(28.64, 37.6),
],
<Offset>[
Offset(14.399999999999999, 6.0),
Offset(14.404037134399934, 5.998124589407087),
Offset(17.629464821750716, 4.791554862152392),
Offset(29.212512201472613, 5.130279351141528),
Offset(37.75681050139441, 12.03136429805604),
Offset(40.21369795872548, 18.81847477877102),
Offset(40.25340600130274, 23.824517041752205),
Offset(39.411262672330956, 27.43134771763129),
Offset(38.2756414335993, 30.060130349210176),
Offset(37.09749443477014, 32.009805001035396),
Offset(35.98797754640261, 33.48278755371506),
Offset(34.989368329450485, 34.61298184864511),
Offset(34.09376336050936, 35.468109749302144),
Offset(33.31247191452901, 36.111129874669345),
Offset(32.650489347914984, 36.5924832357089),
Offset(32.10727557736848, 36.948887315619224),
Offset(31.67778832597493, 37.20807201757785),
Offset(31.35558447378734, 37.390182114108036),
Offset(31.133458457389974, 37.50982495951341),
Offset(31.004035384848397, 37.57737413227578),
Offset(30.960099391805116, 37.59994913432378),
Offset(30.96, 37.6),
],
<Offset>[
Offset(14.399999999999999, 6.0),
Offset(14.404037134399934, 5.998124589407087),
Offset(17.629464821750716, 4.791554862152392),
Offset(29.212512201472613, 5.130279351141528),
Offset(37.75681050139441, 12.03136429805604),
Offset(40.21369795872548, 18.81847477877102),
Offset(40.25340600130274, 23.824517041752205),
Offset(39.411262672330956, 27.43134771763129),
Offset(38.2756414335993, 30.060130349210176),
Offset(37.09749443477014, 32.009805001035396),
Offset(35.98797754640261, 33.48278755371506),
Offset(34.989368329450485, 34.61298184864511),
Offset(34.09376336050936, 35.468109749302144),
Offset(33.31247191452901, 36.111129874669345),
Offset(32.650489347914984, 36.5924832357089),
Offset(32.10727557736848, 36.948887315619224),
Offset(31.67778832597493, 37.20807201757785),
Offset(31.35558447378734, 37.390182114108036),
Offset(31.133458457389974, 37.50982495951341),
Offset(31.004035384848397, 37.57737413227578),
Offset(30.960099391805116, 37.59994913432378),
Offset(30.96, 37.6),
],
),
_PathCubicTo(
<Offset>[
Offset(14.399999999999999, 6.0),
Offset(14.404037134399934, 5.998124589407087),
Offset(17.629464821750716, 4.791554862152392),
Offset(29.212512201472613, 5.130279351141528),
Offset(37.75681050139441, 12.03136429805604),
Offset(40.21369795872548, 18.81847477877102),
Offset(40.25340600130274, 23.824517041752205),
Offset(39.411262672330956, 27.43134771763129),
Offset(38.2756414335993, 30.060130349210176),
Offset(37.09749443477014, 32.009805001035396),
Offset(35.98797754640261, 33.48278755371506),
Offset(34.989368329450485, 34.61298184864511),
Offset(34.09376336050936, 35.468109749302144),
Offset(33.31247191452901, 36.111129874669345),
Offset(32.650489347914984, 36.5924832357089),
Offset(32.10727557736848, 36.948887315619224),
Offset(31.67778832597493, 37.20807201757785),
Offset(31.35558447378734, 37.390182114108036),
Offset(31.133458457389974, 37.50982495951341),
Offset(31.004035384848397, 37.57737413227578),
Offset(30.960099391805116, 37.59994913432378),
Offset(30.96, 37.6),
],
<Offset>[
Offset(14.399999999999999, 9.2),
Offset(14.40334186121773, 9.198082517058094),
Offset(17.09495321544893, 7.918256683444506),
Offset(27.11128303201872, 7.3528218504231635),
Offset(35.004511022928, 12.681725933282765),
Offset(37.63202264537456, 18.37368845254959),
Offset(38.03110120856841, 22.706055679985123),
Offset(37.56078900409667, 25.890726510752607),
Offset(36.7639182652327, 28.248209674032793),
Offset(35.88093897771729, 30.019216975466403),
Offset(35.023449382648224, 31.371348600799287),
Offset(34.238016971857476, 32.41801689734335),
Offset(33.52264234074403, 33.21950556430599),
Offset(32.891453902934586, 33.82965144305683),
Offset(32.35226133833075, 34.2917311729478),
Offset(31.90713776445562, 34.63753601046407),
Offset(31.55364094746969, 34.89139607381483),
Offset(31.287592426243478, 35.07117864678187),
Offset(31.10377127589116, 35.19001490882938),
Offset(30.996517232127708, 35.25738631390697),
Offset(30.96008243670077, 35.27994913438573),
Offset(30.96, 35.28),
],
<Offset>[
Offset(14.399999999999999, 9.2),
Offset(14.40334186121773, 9.198082517058094),
Offset(17.09495321544893, 7.918256683444506),
Offset(27.11128303201872, 7.3528218504231635),
Offset(35.004511022928, 12.681725933282765),
Offset(37.63202264537456, 18.37368845254959),
Offset(38.03110120856841, 22.706055679985123),
Offset(37.56078900409667, 25.890726510752607),
Offset(36.7639182652327, 28.248209674032793),
Offset(35.88093897771729, 30.019216975466403),
Offset(35.023449382648224, 31.371348600799287),
Offset(34.238016971857476, 32.41801689734335),
Offset(33.52264234074403, 33.21950556430599),
Offset(32.891453902934586, 33.82965144305683),
Offset(32.35226133833075, 34.2917311729478),
Offset(31.90713776445562, 34.63753601046407),
Offset(31.55364094746969, 34.89139607381483),
Offset(31.287592426243478, 35.07117864678187),
Offset(31.10377127589116, 35.19001490882938),
Offset(30.996517232127708, 35.25738631390697),
Offset(30.96008243670077, 35.27994913438573),
Offset(30.96, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(14.399999999999999, 9.2),
Offset(14.40334186121773, 9.198082517058094),
Offset(17.09495321544893, 7.918256683444506),
Offset(27.11128303201872, 7.3528218504231635),
Offset(35.004511022928, 12.681725933282765),
Offset(37.63202264537456, 18.37368845254959),
Offset(38.03110120856841, 22.706055679985123),
Offset(37.56078900409667, 25.890726510752607),
Offset(36.7639182652327, 28.248209674032793),
Offset(35.88093897771729, 30.019216975466403),
Offset(35.023449382648224, 31.371348600799287),
Offset(34.238016971857476, 32.41801689734335),
Offset(33.52264234074403, 33.21950556430599),
Offset(32.891453902934586, 33.82965144305683),
Offset(32.35226133833075, 34.2917311729478),
Offset(31.90713776445562, 34.63753601046407),
Offset(31.55364094746969, 34.89139607381483),
Offset(31.287592426243478, 35.07117864678187),
Offset(31.10377127589116, 35.19001490882938),
Offset(30.996517232127708, 35.25738631390697),
Offset(30.96008243670077, 35.27994913438573),
Offset(30.96, 35.28),
],
<Offset>[
Offset(12.799999999999999, 9.2),
Offset(12.803362897392224, 9.197734880466992),
Offset(15.531602304802872, 7.651000880293612),
Offset(26.0000117823779, 6.302207265696218),
Offset(34.67933020531464, 11.305576194049557),
Offset(37.85441580848527, 17.08285079587413),
Offset(38.590331889451946, 21.594903283617956),
Offset(38.33109960753601, 24.965489676635464),
Offset(37.669878602821385, 27.49234808984949),
Offset(36.87623299050179, 29.41093924693998),
Offset(36.07916885910612, 30.889084518922097),
Offset(35.33549944750836, 32.042341218546845),
Offset(34.646944433242105, 32.93394505442333),
Offset(34.032193118740835, 33.61914243725962),
Offset(33.502637369711294, 34.14261716815569),
Offset(33.0628134170332, 34.53746710400763),
Offset(32.7119789193512, 34.82932238456221),
Offset(32.44709415990656, 35.03718262300993),
Offset(32.263676301233176, 35.175171318079975),
Offset(32.15651114131211, 35.253627237546624),
Offset(32.12008243666979, 35.27994065683356),
Offset(32.120000000000005, 35.28),
],
<Offset>[
Offset(12.799999999999999, 9.2),
Offset(12.803362897392224, 9.197734880466992),
Offset(15.531602304802872, 7.651000880293612),
Offset(26.0000117823779, 6.302207265696218),
Offset(34.67933020531464, 11.305576194049557),
Offset(37.85441580848527, 17.08285079587413),
Offset(38.590331889451946, 21.594903283617956),
Offset(38.33109960753601, 24.965489676635464),
Offset(37.669878602821385, 27.49234808984949),
Offset(36.87623299050179, 29.41093924693998),
Offset(36.07916885910612, 30.889084518922097),
Offset(35.33549944750836, 32.042341218546845),
Offset(34.646944433242105, 32.93394505442333),
Offset(34.032193118740835, 33.61914243725962),
Offset(33.502637369711294, 34.14261716815569),
Offset(33.0628134170332, 34.53746710400763),
Offset(32.7119789193512, 34.82932238456221),
Offset(32.44709415990656, 35.03718262300993),
Offset(32.263676301233176, 35.175171318079975),
Offset(32.15651114131211, 35.253627237546624),
Offset(32.12008243666979, 35.27994065683356),
Offset(32.120000000000005, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(11.031994628903998, 9.2),
Offset(11.035380771339659, 9.197350740866831),
Offset(13.80409430047159, 7.355682320651514),
Offset(24.772053321059442, 5.141274622728069),
Offset(34.320004310241, 9.784926112551638),
Offset(38.10016100028201, 15.656470851989635),
Offset(39.20828366912931, 20.36707615556587),
Offset(39.18229541021911, 23.94309986897611),
Offset(38.670967817106856, 26.657118501948734),
Offset(37.976036215765966, 28.738790314969485),
Offset(37.24574242457374, 30.35618108951862),
Offset(36.54822126727992, 31.627218332357877),
Offset(35.88930201966152, 32.61839973239491),
Offset(35.29271378159415, 33.38652927918864),
Offset(34.773806746124365, 33.97784569229438),
Offset(34.33983889265947, 34.42689062644846),
Offset(33.99194626674555, 34.76073074956073),
Offset(33.72834746797622, 34.99961690261951),
Offset(33.545375247961886, 35.15876910047291),
Offset(33.438308304985036, 35.24947344554947),
Offset(33.40188633068016, 35.27993128910995),
Offset(33.401803894044605, 35.28),
],
<Offset>[
Offset(9.616003417967999, 10.631994628904),
Offset(9.61909704372049, 10.629018885991774),
Offset(12.181344285634644, 8.518356219904028),
Offset(22.84828784303791, 5.2060705244071706),
Offset(32.80057167595657, 8.858076892900925),
Offset(37.14168235852622, 14.315045482191389),
Offset(38.70872208508259, 18.883202806434653),
Offset(39.03593220224475, 22.434844949065443),
Offset(38.79624415881739, 25.177353691127),
Offset(38.31245942468365, 27.309683066288713),
Offset(37.74842362764679, 28.984514638765333),
Offset(37.18325875817459, 30.31250428874126),
Offset(36.6287274978085, 31.35943365120302),
Offset(36.11385686779049, 32.17927219665962),
Offset(35.65842668096787, 32.816297930813164),
Offset(35.27304416151989, 33.30400436440065),
Offset(34.96151326541533, 33.66908727918451),
Offset(34.72407380578028, 33.931780449070615),
Offset(34.55859985994813, 34.10752150024265),
Offset(34.46153218185176, 34.2079560289239),
Offset(34.42847237120061, 34.241727680595176),
Offset(34.4283975219732, 34.241803894044594),
],
<Offset>[
Offset(9.616003417967999, 12.399999999999999),
Offset(9.61871290412033, 12.397001012044338),
Offset(11.886025725992546, 10.245864224235309),
Offset(21.68735520006976, 6.434028985725625),
Offset(31.279921594458653, 9.217402787974557),
Offset(35.71530241464173, 14.069300290394656),
Offset(37.4808949570305, 18.265251026757294),
Offset(38.01354239458539, 21.58364914638235),
Offset(37.96101457091663, 24.176264476841528),
Offset(37.64031049271316, 26.209879841024538),
Offset(37.21552019824331, 27.817941073297703),
Offset(36.76813587198562, 29.0997824689697),
Offset(36.313182175780085, 30.117076064783603),
Offset(35.88124370971952, 30.918751533806304),
Offset(35.49365520510656, 31.545128554400094),
Offset(35.16246768396071, 32.02697888877437),
Offset(34.892921630413845, 32.38911993179016),
Offset(34.68650808538985, 32.65052714100095),
Offset(34.542197642341065, 32.825822553513945),
Offset(34.4573783898546, 32.92615886525098),
Offset(34.428463003477, 32.9599237865848),
Offset(34.428397521973196, 32.96),
],
),
_PathCubicTo(
<Offset>[
Offset(9.616003417967999, 12.399999999999999),
Offset(9.61871290412033, 12.397001012044338),
Offset(11.886025725992546, 10.245864224235309),
Offset(21.68735520006976, 6.434028985725625),
Offset(31.279921594458653, 9.217402787974557),
Offset(35.71530241464173, 14.069300290394656),
Offset(37.4808949570305, 18.265251026757294),
Offset(38.01354239458539, 21.58364914638235),
Offset(37.96101457091663, 24.176264476841528),
Offset(37.64031049271316, 26.209879841024538),
Offset(37.21552019824331, 27.817941073297703),
Offset(36.76813587198562, 29.0997824689697),
Offset(36.313182175780085, 30.117076064783603),
Offset(35.88124370971952, 30.918751533806304),
Offset(35.49365520510656, 31.545128554400094),
Offset(35.16246768396071, 32.02697888877437),
Offset(34.892921630413845, 32.38911993179016),
Offset(34.68650808538985, 32.65052714100095),
Offset(34.542197642341065, 32.825822553513945),
Offset(34.4573783898546, 32.92615886525098),
Offset(34.428463003477, 32.9599237865848),
Offset(34.428397521973196, 32.96),
],
<Offset>[
Offset(9.59999999999928, 34.8),
Offset(9.59784278428286, 34.79670302849285),
Offset(8.12880763309575, 32.13010384432861),
Offset(6.967635927464624, 21.981318090494952),
Offset(12.01057274235646, 13.75616979739632),
Offset(17.64579962789941, 10.942884872751186),
Offset(21.93035490934461, 10.42490759674647),
Offset(25.067931468540735, 10.790046353371297),
Offset(27.388013931066364, 11.485259520063472),
Offset(29.134377359648454, 12.26967958533492),
Offset(30.47438250198729, 13.033044731841269),
Offset(31.519653538066578, 13.731270250539573),
Offset(32.32658046011612, 14.373990554688655),
Offset(32.945527457598416, 14.946296972765026),
Offset(33.41756535579906, 15.438372656482638),
Offset(33.77306221888595, 15.846518849853458),
Offset(34.035475835072845, 16.171767455952793),
Offset(34.22216124688238, 16.41716283685671),
Offset(34.34598889992722, 16.58700373110889),
Offset(34.416353737915934, 16.68620653787546),
Offset(34.43994679577361, 16.719923702224868),
Offset(34.440000000000516, 16.72),
],
<Offset>[
Offset(9.59999999999928, 34.8),
Offset(9.59784278428286, 34.79670302849285),
Offset(8.12880763309575, 32.13010384432861),
Offset(6.967635927464624, 21.981318090494952),
Offset(12.01057274235646, 13.75616979739632),
Offset(17.64579962789941, 10.942884872751186),
Offset(21.93035490934461, 10.42490759674647),
Offset(25.067931468540735, 10.790046353371297),
Offset(27.388013931066364, 11.485259520063472),
Offset(29.134377359648454, 12.26967958533492),
Offset(30.47438250198729, 13.033044731841269),
Offset(31.519653538066578, 13.731270250539573),
Offset(32.32658046011612, 14.373990554688655),
Offset(32.945527457598416, 14.946296972765026),
Offset(33.41756535579906, 15.438372656482638),
Offset(33.77306221888595, 15.846518849853458),
Offset(34.035475835072845, 16.171767455952793),
Offset(34.22216124688238, 16.41716283685671),
Offset(34.34598889992722, 16.58700373110889),
Offset(34.416353737915934, 16.68620653787546),
Offset(34.43994679577361, 16.719923702224868),
Offset(34.440000000000516, 16.72),
],
),
_PathCubicTo(
<Offset>[
Offset(9.59999999999928, 36.568005371096),
Offset(9.5974586446827, 36.564685154545415),
Offset(7.833489073453652, 33.857611848659886),
Offset(5.8067032844964785, 23.20927655181341),
Offset(10.489922660858541, 14.115495692469953),
Offset(16.219419684014916, 10.697139680954454),
Offset(20.70252778129252, 9.806955817069111),
Offset(24.045541660881376, 9.938850550688198),
Offset(26.55278434316561, 10.484170305778001),
Offset(28.46222842767796, 11.169876360070745),
Offset(29.941479072583817, 11.866471166373643),
Offset(31.10453065187761, 12.518548430768014),
Offset(32.011035138087706, 13.131632968269235),
Offset(32.71291429952744, 13.685776309911715),
Offset(33.252793879937755, 14.16720328006957),
Offset(33.662485741326776, 14.569493374227186),
Offset(33.96688420007136, 14.891800108558444),
Offset(34.184595526491954, 15.135909528787048),
Offset(34.32958668232016, 15.305304784380185),
Offset(34.41219994591878, 15.404409374202539),
Offset(34.43993742805, 15.438119808214498),
Offset(34.440000000000516, 15.438196105955399),
],
<Offset>[
Offset(11.031994628903279, 38.0),
Offset(11.029123312699099, 37.9969720897259),
Offset(8.99348984375479, 35.49599871228041),
Offset(5.860990795973912, 25.144157116262335),
Offset(9.549309004043138, 15.638180829591539),
Offset(14.86508318012379, 11.653393915996185),
Offset(19.207540534520554, 10.300923899661631),
Offset(22.52803239611086, 10.077509007067547),
Offset(25.06545930180785, 10.349832425351934),
Offset(27.02703710229079, 10.82349808484828),
Offset(28.564988950784752, 11.353230513276403),
Offset(29.786059048943372, 11.872533770641846),
Offset(30.74921284177406, 12.380962067429417),
Offset(31.503551677244786, 12.853223394675975),
Offset(32.089754659866806, 13.271077127444466),
Offset(32.538598576444194, 13.624728880051993),
Offset(32.87461986019893, 13.910647255693526),
Offset(33.11641904008201, 14.128585696683976),
Offset(33.27819061447309, 14.280478644316606),
Offset(33.37064493049935, 14.369583080230184),
Offset(33.40173373474159, 14.399931289667558),
Offset(33.401803894045116, 14.399999999999999),
],
<Offset>[
Offset(12.79999999999928, 38.0),
Offset(12.797105438751665, 37.997356229326066),
Offset(10.720997848086075, 35.79131727192251),
Offset(7.08894925729237, 26.305089759230484),
Offset(9.90863489911677, 17.15883091108946),
Offset(14.619337988327057, 13.07977385988068),
Offset(18.589588754843195, 11.528751027713723),
Offset(21.676836593427762, 11.099898814726902),
Offset(24.064370087522377, 11.18506201325269),
Offset(25.927233877026616, 11.495647016818776),
Offset(27.398415385317126, 11.886133942679876),
Offset(28.573337229171813, 12.287656656830816),
Offset(29.50685525535464, 12.696507389457834),
Offset(30.243031014391473, 13.085836552746947),
Offset(30.818585283453736, 13.435848603305775),
Offset(31.26157310081792, 13.735305357611166),
Offset(31.594652512804586, 13.97923889069501),
Offset(31.83516573201235, 14.166151417074401),
Offset(31.99649166774438, 14.29688086192367),
Offset(32.08884776682643, 14.37373687222734),
Offset(32.119929840731224, 14.399940657391166),
Offset(32.120000000000516, 14.399999999999999),
],
),
_PathCubicTo(
<Offset>[
Offset(12.79999999999928, 38.0),
Offset(12.797105438751665, 37.997356229326066),
Offset(10.720997848086075, 35.79131727192251),
Offset(7.08894925729237, 26.305089759230484),
Offset(9.90863489911677, 17.15883091108946),
Offset(14.619337988327057, 13.07977385988068),
Offset(18.589588754843195, 11.528751027713723),
Offset(21.676836593427762, 11.099898814726902),
Offset(24.064370087522377, 11.18506201325269),
Offset(25.927233877026616, 11.495647016818776),
Offset(27.398415385317126, 11.886133942679876),
Offset(28.573337229171813, 12.287656656830816),
Offset(29.50685525535464, 12.696507389457834),
Offset(30.243031014391473, 13.085836552746947),
Offset(30.818585283453736, 13.435848603305775),
Offset(31.26157310081792, 13.735305357611166),
Offset(31.594652512804586, 13.97923889069501),
Offset(31.83516573201235, 14.166151417074401),
Offset(31.99649166774438, 14.29688086192367),
Offset(32.08884776682643, 14.37373687222734),
Offset(32.119929840731224, 14.399940657391166),
Offset(32.120000000000516, 14.399999999999999),
],
<Offset>[
Offset(35.19999999999928, 38.0),
Offset(35.19681093230872, 38.0022231416015),
Offset(32.60791059713087, 39.532898516035026),
Offset(22.646746752263834, 41.013693945407724),
Offset(14.461166345703841, 36.42492726035435),
Offset(11.505833704777048, 31.15150105333715),
Offset(10.760359222473623, 27.08488457685406),
Offset(10.892488145276982, 24.053214492366923),
Offset(11.380925361280685, 21.767124191818905),
Offset(11.99311769804367, 20.0115352161887),
Offset(12.618342714906678, 18.63783108896057),
Offset(13.208582570059477, 17.547116159981847),
Offset(13.766625960381576, 16.694354527815143),
Offset(14.272681993103916, 16.032962633907964),
Offset(14.713320844126082, 15.523444670395389),
Offset(15.082113964731814, 15.13627004800123),
Offset(15.377920906463453, 14.84827054023167),
Offset(15.602141460729166, 14.642095749881417),
Offset(15.75782131295615, 14.504691132415362),
Offset(15.84893303824477, 14.426363941272161),
Offset(15.879929841164916, 14.400059343121573),
Offset(15.88000000000052, 14.400000000000002),
],
<Offset>[
Offset(35.19999999999928, 38.0),
Offset(35.19681093230872, 38.0022231416015),
Offset(32.60791059713087, 39.532898516035026),
Offset(22.646746752263834, 41.013693945407724),
Offset(14.461166345703841, 36.42492726035435),
Offset(11.505833704777048, 31.15150105333715),
Offset(10.760359222473623, 27.08488457685406),
Offset(10.892488145276982, 24.053214492366923),
Offset(11.380925361280685, 21.767124191818905),
Offset(11.99311769804367, 20.0115352161887),
Offset(12.618342714906678, 18.63783108896057),
Offset(13.208582570059477, 17.547116159981847),
Offset(13.766625960381576, 16.694354527815143),
Offset(14.272681993103916, 16.032962633907964),
Offset(14.713320844126082, 15.523444670395389),
Offset(15.082113964731814, 15.13627004800123),
Offset(15.377920906463453, 14.84827054023167),
Offset(15.602141460729166, 14.642095749881417),
Offset(15.75782131295615, 14.504691132415362),
Offset(15.84893303824477, 14.426363941272161),
Offset(15.879929841164916, 14.400059343121573),
Offset(15.88000000000052, 14.400000000000002),
],
),
_PathCubicTo(
<Offset>[
Offset(36.96800537109528, 38.0),
Offset(36.96479305836129, 38.00260728120166),
Offset(34.33541860146215, 39.828217075677124),
Offset(23.874705213582292, 42.17462658837587),
Offset(14.820492240777476, 37.94557734185227),
Offset(11.260088512980314, 32.57788099722164),
Offset(10.142407442796266, 28.31271170490615),
Offset(10.041292342593884, 25.075604300026278),
Offset(10.379836146995213, 22.60235377971966),
Offset(10.893314472779496, 20.683684148159198),
Offset(11.45176914943905, 19.170734518364043),
Offset(11.99586075028792, 17.962239046170815),
Offset(12.524268373962157, 17.009899849843556),
Offset(13.012161330250605, 16.26557579197894),
Offset(13.442151467713014, 15.688216146256698),
Offset(13.805088489105541, 15.246846525560404),
Offset(14.097953559069104, 14.916862175233152),
Offset(14.320888152659505, 14.679661470271842),
Offset(14.476122366227445, 14.521093350022426),
Offset(14.567135874571848, 14.430517733269317),
Offset(14.598125947154546, 14.400068710845181),
Offset(14.59819610595592, 14.400000000000002),
],
<Offset>[
Offset(38.39999999999928, 36.568005371096),
Offset(38.39707999354177, 36.57094261318526),
Offset(35.97380546508267, 38.668216305375985),
Offset(25.809585778031217, 42.120339076898446),
Offset(16.34317737789906, 38.88619099866767),
Offset(12.216342748022047, 33.93221750111277),
Offset(10.636375525388786, 29.80769895167812),
Offset(10.17995079897323, 26.593113564796795),
Offset(10.245498266569147, 24.08967882107742),
Offset(10.54693619755703, 22.118875473546364),
Offset(10.938528496341814, 20.547224640163105),
Offset(11.34984609016175, 19.280710649105057),
Offset(11.773597473122338, 18.271722146157202),
Offset(12.179608415014865, 17.474938414261594),
Offset(12.546025315087908, 16.851255366327646),
Offset(12.860323994930349, 16.370733690442982),
Offset(13.116800706204186, 16.009126515105578),
Offset(13.313564320556432, 15.747837956681783),
Offset(13.451296226163867, 15.572489417869503),
Offset(13.532309580599492, 15.472072748688737),
Offset(13.559937428607608, 15.43827240415359),
Offset(13.560000000000521, 15.438196105955402),
],
<Offset>[
Offset(38.39999999999928, 34.8),
Offset(38.39746413314193, 34.802960487132694),
Offset(36.26912402472477, 36.9407083010447),
Offset(26.970518420999362, 40.89238061557998),
Offset(17.86382745939698, 38.52686510359404),
Offset(13.642722691906542, 34.1779626929095),
Offset(11.864202653440877, 30.425650731355475),
Offset(11.202340606632587, 27.444309367479892),
Offset(11.080727854469902, 25.090768035362892),
Offset(11.219085129527524, 23.218678698810542),
Offset(11.471431925745287, 21.713798205630734),
Offset(11.764968976350719, 20.493432468876616),
Offset(12.089142795150753, 19.514079732576622),
Offset(12.412221573085839, 18.735459077114903),
Offset(12.710796790949217, 18.122424742740712),
Offset(12.970900472489523, 17.647759166069257),
Offset(13.18539234120567, 17.289093862499925),
Offset(13.351130040946858, 17.029091264751443),
Offset(13.46769844377093, 16.85418836459821),
Offset(13.536463372596648, 16.753869912361658),
Offset(13.559946796331216, 16.72007629816396),
Offset(13.560000000000521, 16.720000000000002),
],
),
_PathCubicTo(
<Offset>[
Offset(38.39999999999928, 34.8),
Offset(38.39746413314193, 34.802960487132694),
Offset(36.26912402472477, 36.9407083010447),
Offset(26.970518420999362, 40.89238061557998),
Offset(17.86382745939698, 38.52686510359404),
Offset(13.642722691906542, 34.1779626929095),
Offset(11.864202653440877, 30.425650731355475),
Offset(11.202340606632587, 27.444309367479892),
Offset(11.080727854469902, 25.090768035362892),
Offset(11.219085129527524, 23.218678698810542),
Offset(11.471431925745287, 21.713798205630734),
Offset(11.764968976350719, 20.493432468876616),
Offset(12.089142795150753, 19.514079732576622),
Offset(12.412221573085839, 18.735459077114903),
Offset(12.710796790949217, 18.122424742740712),
Offset(12.970900472489523, 17.647759166069257),
Offset(13.18539234120567, 17.289093862499925),
Offset(13.351130040946858, 17.029091264751443),
Offset(13.46769844377093, 16.85418836459821),
Offset(13.536463372596648, 16.753869912361658),
Offset(13.559946796331216, 16.72007629816396),
Offset(13.560000000000521, 16.720000000000002),
],
<Offset>[
Offset(38.39999999999928, 12.399999999999999),
Offset(38.40233104541737, 12.403254993575636),
Offset(40.010705268837285, 15.05379555199991),
Offset(41.67912260717661, 25.33458312060852),
Offset(37.12992380866187, 33.974333657006966),
Offset(31.71444988536301, 37.29146697645951),
Offset(27.420336202581215, 38.25488026372505),
Offset(24.155656284272606, 38.22865781563067),
Offset(21.662790033036117, 37.77421276160458),
Offset(19.734973328897453, 37.15279487779348),
Offset(18.223129072025984, 36.49387087604118),
Offset(17.02442847950175, 35.858187127988955),
Offset(16.086989933508065, 35.25430902754969),
Offset(15.359347654246855, 34.705808098402464),
Offset(14.798392858038833, 34.227689182068374),
Offset(14.371865162879587, 33.82721830215536),
Offset(14.05442399074233, 33.50582546884106),
Offset(13.827074373753874, 33.26211553603463),
Offset(13.675508714262623, 33.09285871938644),
Offset(13.589090441641469, 32.993784640943325),
Offset(13.56006548206162, 32.96007629773027),
Offset(13.560000000000523, 32.96),
],
<Offset>[
Offset(38.39999999999928, 12.399999999999999),
Offset(38.40233104541737, 12.403254993575636),
Offset(40.010705268837285, 15.05379555199991),
Offset(41.67912260717661, 25.33458312060852),
Offset(37.12992380866187, 33.974333657006966),
Offset(31.71444988536301, 37.29146697645951),
Offset(27.420336202581215, 38.25488026372505),
Offset(24.155656284272606, 38.22865781563067),
Offset(21.662790033036117, 37.77421276160458),
Offset(19.734973328897453, 37.15279487779348),
Offset(18.223129072025984, 36.49387087604118),
Offset(17.02442847950175, 35.858187127988955),
Offset(16.086989933508065, 35.25430902754969),
Offset(15.359347654246855, 34.705808098402464),
Offset(14.798392858038833, 34.227689182068374),
Offset(14.371865162879587, 33.82721830215536),
Offset(14.05442399074233, 33.50582546884106),
Offset(13.827074373753874, 33.26211553603463),
Offset(13.675508714262623, 33.09285871938644),
Offset(13.589090441641469, 32.993784640943325),
Offset(13.56006548206162, 32.96007629773027),
Offset(13.560000000000523, 32.96),
],
),
_PathCubicTo(
<Offset>[
Offset(38.39999999999928, 10.631994628904),
Offset(38.40271518501753, 10.635272867523073),
Offset(40.30602382847938, 13.32628754766863),
Offset(42.84005525014476, 24.106624659290063),
Offset(38.65057389015979, 33.61500776193334),
Offset(33.14082982924751, 37.53721216825625),
Offset(28.648163330633302, 38.872832043402404),
Offset(25.17804609193196, 39.07985361831377),
Offset(22.498019620936873, 38.77530197589005),
Offset(20.407122260867947, 38.252598103057665),
Offset(18.756032501429452, 37.66044444150881),
Offset(17.439551365690722, 37.07090894776051),
Offset(16.402535255536478, 36.496666613969104),
Offset(15.591960812317827, 35.966328761255774),
Offset(14.96316433390014, 35.49885855848144),
Offset(14.48244164043876, 35.10424377778163),
Offset(14.123015625743813, 34.78579281623541),
Offset(13.864640094144299, 34.543368844104286),
Offset(13.691910931869685, 34.374557666115145),
Offset(13.593244233638625, 34.275581804616245),
Offset(13.560074849785229, 34.241880191740634),
Offset(13.560000000000525, 34.2418038940446),
],
<Offset>[
Offset(36.96800537109528, 9.2),
Offset(36.97105051700113, 9.202985932342585),
Offset(39.146023058178244, 11.687900684048108),
Offset(42.78576773866732, 22.171744094841138),
Offset(39.5911875469752, 32.09232262481175),
Offset(34.49516633313863, 36.58095793321451),
Offset(30.143150577405272, 38.37886396080988),
Offset(26.695555356702478, 38.941195161934424),
Offset(23.985344662294633, 38.90963985631612),
Offset(21.842313586255116, 38.59897637828013),
Offset(20.132522623228514, 38.17368509460604),
Offset(18.75802296862496, 37.71692360788668),
Offset(17.664357551850124, 37.247337514808926),
Offset(16.801323434600484, 36.79888167649151),
Offset(16.126203553971088, 36.394984711106545),
Offset(15.606328805321338, 36.04900827195683),
Offset(15.215279965616238, 35.76694566910032),
Offset(14.93281658055424, 35.55069267620736),
Offset(14.743306999716763, 35.39938380617872),
Offset(14.634799249058046, 35.3104080985886),
Offset(14.598278543093638, 35.28006871028757),
Offset(14.598196105955923, 35.28),
],
<Offset>[
Offset(35.19999999999928, 9.2),
Offset(35.20306839094856, 9.202601792742424),
Offset(37.41851505384696, 11.39258212440601),
Offset(41.557809277348866, 21.01081145187299),
Offset(39.23186165190157, 30.57167254331383),
Offset(34.74091152493536, 35.15457798933002),
Offset(30.761102357082628, 37.151036832757796),
Offset(27.54675115938558, 37.918805354275065),
Offset(24.986433876580104, 38.07441026841536),
Offset(22.94211681151929, 37.92682744630963),
Offset(21.299096188696144, 37.640781665202574),
Offset(19.970744788396516, 37.30180072169771),
Offset(18.906715138269544, 36.931792192780506),
Offset(18.061844097453793, 36.56626851842054),
Offset(17.397372930384158, 36.23021323524523),
Offset(16.88335428094761, 35.93843179439765),
Offset(16.495247313010587, 35.69835403409884),
Offset(16.214069888623904, 35.51312695581694),
Offset(16.025005946445468, 35.38298158857166),
Offset(15.916596412730968, 35.30625430659144),
Offset(15.880082437104008, 35.280059342563966),
Offset(15.880000000000523, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(35.19999999999928, 9.2),
Offset(35.20306839094856, 9.202601792742424),
Offset(37.41851505384696, 11.39258212440601),
Offset(41.557809277348866, 21.01081145187299),
Offset(39.23186165190157, 30.57167254331383),
Offset(34.74091152493536, 35.15457798933002),
Offset(30.761102357082628, 37.151036832757796),
Offset(27.54675115938558, 37.918805354275065),
Offset(24.986433876580104, 38.07441026841536),
Offset(22.94211681151929, 37.92682744630963),
Offset(21.299096188696144, 37.640781665202574),
Offset(19.970744788396516, 37.30180072169771),
Offset(18.906715138269544, 36.931792192780506),
Offset(18.061844097453793, 36.56626851842054),
Offset(17.397372930384158, 36.23021323524523),
Offset(16.88335428094761, 35.93843179439765),
Offset(16.495247313010587, 35.69835403409884),
Offset(16.214069888623904, 35.51312695581694),
Offset(16.025005946445468, 35.38298158857166),
Offset(15.916596412730968, 35.30625430659144),
Offset(15.880082437104008, 35.280059342563966),
Offset(15.880000000000523, 35.28),
],
<Offset>[
Offset(33.599999999999284, 9.2),
Offset(33.60308942712306, 9.202254156151323),
Offset(35.85516414320091, 11.125326321255116),
Offset(40.446538027708044, 19.960196867146045),
Offset(38.9066808342882, 29.195522804080625),
Offset(34.96330468804608, 33.86374033265456),
Offset(31.320333037966172, 36.03988443639063),
Offset(28.31706176282492, 36.99356852015792),
Offset(25.892394214168796, 37.31854868423206),
Offset(23.93741082430379, 37.31854971778321),
Offset(22.35481566515403, 37.15851758332538),
Offset(21.068227264047398, 36.926125042901205),
Offset(20.03101723076762, 36.64623168289785),
Offset(19.20258331326005, 36.35575951262332),
Offset(18.547748961764704, 36.08109923045312),
Offset(18.039029933525192, 35.83836288794122),
Offset(17.653585284892095, 35.63628034484623),
Offset(17.37357162228699, 35.47913093204501),
Offset(17.184910971787485, 35.36813799782225),
Offset(17.076590321915372, 35.3024952302311),
Offset(17.040082437073032, 35.280050865011795),
Offset(17.04000000000052, 35.28),
],
<Offset>[
Offset(33.599999999999284, 9.2),
Offset(33.60308942712306, 9.202254156151323),
Offset(35.85516414320091, 11.125326321255116),
Offset(40.446538027708044, 19.960196867146045),
Offset(38.9066808342882, 29.195522804080625),
Offset(34.96330468804608, 33.86374033265456),
Offset(31.320333037966172, 36.03988443639063),
Offset(28.31706176282492, 36.99356852015792),
Offset(25.892394214168796, 37.31854868423206),
Offset(23.93741082430379, 37.31854971778321),
Offset(22.35481566515403, 37.15851758332538),
Offset(21.068227264047398, 36.926125042901205),
Offset(20.03101723076762, 36.64623168289785),
Offset(19.20258331326005, 36.35575951262332),
Offset(18.547748961764704, 36.08109923045312),
Offset(18.039029933525192, 35.83836288794122),
Offset(17.653585284892095, 35.63628034484623),
Offset(17.37357162228699, 35.47913093204501),
Offset(17.184910971787485, 35.36813799782225),
Offset(17.076590321915372, 35.3024952302311),
Offset(17.040082437073032, 35.280050865011795),
Offset(17.04000000000052, 35.28),
],
),
_PathCubicTo(
<Offset>[
Offset(33.599999999999284, 9.2),
Offset(33.60308942712306, 9.202254156151323),
Offset(35.85516414320091, 11.125326321255116),
Offset(40.446538027708044, 19.960196867146045),
Offset(38.9066808342882, 29.195522804080625),
Offset(34.96330468804608, 33.86374033265456),
Offset(31.320333037966172, 36.03988443639063),
Offset(28.31706176282492, 36.99356852015792),
Offset(25.892394214168796, 37.31854868423206),
Offset(23.93741082430379, 37.31854971778321),
Offset(22.35481566515403, 37.15851758332538),
Offset(21.068227264047398, 36.926125042901205),
Offset(20.03101723076762, 36.64623168289785),
Offset(19.20258331326005, 36.35575951262332),
Offset(18.547748961764704, 36.08109923045312),
Offset(18.039029933525192, 35.83836288794122),
Offset(17.653585284892095, 35.63628034484623),
Offset(17.37357162228699, 35.47913093204501),
Offset(17.184910971787485, 35.36813799782225),
Offset(17.076590321915372, 35.3024952302311),
Offset(17.040082437073032, 35.280050865011795),
Offset(17.04000000000052, 35.28),
],
<Offset>[
Offset(33.599999999999284, 6.0),
Offset(33.60378470030526, 6.002296228500315),
Offset(36.38967574950269, 7.998624499963004),
Offset(42.54776719716194, 17.73765436786441),
Offset(41.658980312754615, 28.5451611688539),
Offset(37.54498000139701, 34.308526658875984),
Offset(33.54263783070051, 37.15834579815771),
Offset(30.167535431059207, 38.534189727036605),
Offset(27.404117382535397, 39.130469359409446),
Offset(25.153966281356634, 39.3091377433522),
Offset(23.319343828908416, 39.26995653624116),
Offset(21.819578621640403, 39.12108999420297),
Offset(20.60213825053295, 38.894835867893995),
Offset(19.62360132485448, 38.637237944235835),
Offset(18.845976971348936, 38.38185129321421),
Offset(18.239167746438056, 38.14971419309638),
Offset(17.777732663397334, 37.95295628860924),
Offset(17.441563669830845, 37.79813439937118),
Offset(17.214598153286296, 37.687948048506286),
Offset(17.08410847463606, 37.62248304859991),
Offset(17.040099392177375, 37.600050864949836),
Offset(17.040000000000525, 37.6),
],
<Offset>[
Offset(33.599999999999284, 6.0),
Offset(33.60378470030526, 6.002296228500315),
Offset(36.38967574950269, 7.998624499963004),
Offset(42.54776719716194, 17.73765436786441),
Offset(41.658980312754615, 28.5451611688539),
Offset(37.54498000139701, 34.308526658875984),
Offset(33.54263783070051, 37.15834579815771),
Offset(30.167535431059207, 38.534189727036605),
Offset(27.404117382535397, 39.130469359409446),
Offset(25.153966281356634, 39.3091377433522),
Offset(23.319343828908416, 39.26995653624116),
Offset(21.819578621640403, 39.12108999420297),
Offset(20.60213825053295, 38.894835867893995),
Offset(19.62360132485448, 38.637237944235835),
Offset(18.845976971348936, 38.38185129321421),
Offset(18.239167746438056, 38.14971419309638),
Offset(17.777732663397334, 37.95295628860924),
Offset(17.441563669830845, 37.79813439937118),
Offset(17.214598153286296, 37.687948048506286),
Offset(17.08410847463606, 37.62248304859991),
Offset(17.040099392177375, 37.600050864949836),
Offset(17.040000000000525, 37.6),
],
),
_PathCubicTo(
<Offset>[
Offset(33.599999999999284, 6.0),
Offset(33.60378470030526, 6.002296228500315),
Offset(36.38967574950269, 7.998624499963004),
Offset(42.54776719716194, 17.73765436786441),
Offset(41.658980312754615, 28.5451611688539),
Offset(37.54498000139701, 34.308526658875984),
Offset(33.54263783070051, 37.15834579815771),
Offset(30.167535431059207, 38.534189727036605),
Offset(27.404117382535397, 39.130469359409446),
Offset(25.153966281356634, 39.3091377433522),
Offset(23.319343828908416, 39.26995653624116),
Offset(21.819578621640403, 39.12108999420297),
Offset(20.60213825053295, 38.894835867893995),
Offset(19.62360132485448, 38.637237944235835),
Offset(18.845976971348936, 38.38185129321421),
Offset(18.239167746438056, 38.14971419309638),
Offset(17.777732663397334, 37.95295628860924),
Offset(17.441563669830845, 37.79813439937118),
Offset(17.214598153286296, 37.687948048506286),
Offset(17.08410847463606, 37.62248304859991),
Offset(17.040099392177375, 37.600050864949836),
Offset(17.040000000000525, 37.6),
],
<Offset>[
Offset(30.39999999999928, 6.0),
Offset(30.403826772654256, 6.001600955318109),
Offset(33.262973928210585, 7.464112893661214),
Offset(40.3252246978803, 15.636425198410514),
Offset(41.00861867752789, 25.79286169038749),
Offset(37.98976632761843, 31.726851345525063),
Offset(34.66109919246759, 34.93604100542338),
Offset(31.70815663793789, 36.68371605880232),
Offset(29.216038057712783, 37.618746191042845),
Offset(27.144554306925627, 38.092582286299354),
Offset(25.430782781824195, 38.30542837248677),
Offset(24.014543572942166, 38.369738636609966),
Offset(22.850742435529103, 38.32371484812867),
Offset(21.90507975646699, 38.2162199326414),
Offset(21.146729034110027, 38.08362328362998),
Offset(20.550519051593216, 37.949576380183515),
Offset(20.094408607160354, 37.82880891010401),
Offset(19.760567137157015, 37.73014235182732),
Offset(19.53440820397033, 37.65826086700747),
Offset(19.40409629300487, 37.61496489587922),
Offset(19.360099392115416, 37.60003390984549),
Offset(19.360000000000525, 37.6),
],
<Offset>[
Offset(30.39999999999928, 6.0),
Offset(30.403826772654256, 6.001600955318109),
Offset(33.262973928210585, 7.464112893661214),
Offset(40.3252246978803, 15.636425198410514),
Offset(41.00861867752789, 25.79286169038749),
Offset(37.98976632761843, 31.726851345525063),
Offset(34.66109919246759, 34.93604100542338),
Offset(31.70815663793789, 36.68371605880232),
Offset(29.216038057712783, 37.618746191042845),
Offset(27.144554306925627, 38.092582286299354),
Offset(25.430782781824195, 38.30542837248677),
Offset(24.014543572942166, 38.369738636609966),
Offset(22.850742435529103, 38.32371484812867),
Offset(21.90507975646699, 38.2162199326414),
Offset(21.146729034110027, 38.08362328362998),
Offset(20.550519051593216, 37.949576380183515),
Offset(20.094408607160354, 37.82880891010401),
Offset(19.760567137157015, 37.73014235182732),
Offset(19.53440820397033, 37.65826086700747),
Offset(19.40409629300487, 37.61496489587922),
Offset(19.360099392115416, 37.60003390984549),
Offset(19.360000000000525, 37.6),
],
),
_PathClose(
),
_PathMoveTo(
<Offset>[
Offset(35.2, 34.8),
Offset(35.19750620549164, 34.80226521395049),
Offset(33.142422203433355, 36.40619669474303),
Offset(24.747975921718226, 38.79115144612656),
Offset(17.213465824170402, 35.77456562512825),
Offset(14.087509018127873, 31.596287379559158),
Offset(12.982664015207707, 28.20334593862164),
Offset(12.742961813510924, 25.59383569924602),
Offset(12.89264852964688, 23.57904486699663),
Offset(13.20967315509607, 22.002123241757968),
Offset(13.58287087866059, 20.749270041876564),
Offset(13.959933927651988, 19.74208111128378),
Offset(14.3377469801464, 18.94295871281142),
Offset(14.693700004697835, 18.31444106552057),
Offset(15.011548853709796, 17.824196733156548),
Offset(15.282251777644161, 17.447621353156435),
Offset(15.50206828496817, 17.16494648399472),
Offset(15.670133508272507, 16.961099217207604),
Offset(15.78750849445444, 16.8245011830994),
Offset(15.856451190964936, 16.746351759640973),
Offset(15.879946796268738, 16.720059343059617),
Offset(15.879999999999999, 16.720000000000002),
],
),
_PathCubicTo(
<Offset>[
Offset(35.2, 34.8),
Offset(35.19750620549164, 34.80226521395049),
Offset(33.142422203433355, 36.40619669474303),
Offset(24.747975921718226, 38.79115144612656),
Offset(17.213465824170402, 35.77456562512825),
Offset(14.087509018127873, 31.596287379559158),
Offset(12.982664015207707, 28.20334593862164),
Offset(12.742961813510924, 25.59383569924602),
Offset(12.89264852964688, 23.57904486699663),
Offset(13.20967315509607, 22.002123241757968),
Offset(13.58287087866059, 20.749270041876564),
Offset(13.959933927651988, 19.74208111128378),
Offset(14.3377469801464, 18.94295871281142),
Offset(14.693700004697835, 18.31444106552057),
Offset(15.011548853709796, 17.824196733156548),
Offset(15.282251777644161, 17.447621353156435),
Offset(15.50206828496817, 17.16494648399472),
Offset(15.670133508272507, 16.961099217207604),
Offset(15.78750849445444, 16.8245011830994),
Offset(15.856451190964936, 16.746351759640973),
Offset(15.879946796268738, 16.720059343059617),
Offset(15.879999999999999, 16.720000000000002),
],
<Offset>[
Offset(12.799999999999999, 34.8),
Offset(12.797800711934586, 34.79739830167506),
Offset(11.255509454388564, 32.66461545063052),
Offset(9.190178426746762, 24.08254725994932),
Offset(12.660934377583331, 16.50846927586335),
Offset(17.201013301677882, 13.52456018610269),
Offset(20.811893547577277, 12.647212389481304),
Offset(23.527310261661704, 12.640520021606001),
Offset(25.576093255888573, 12.996982688430414),
Offset(27.143789334079017, 13.48623504238804),
Offset(28.362943549071037, 13.997572895595871),
Offset(29.324688586764324, 14.482621608132748),
Offset(30.077976275119465, 14.945111574454113),
Offset(30.664049025985392, 15.367314984359552),
Offset(31.116813293037453, 15.736600666066936),
Offset(31.46171091373027, 16.046656662766367),
Offset(31.718799891309303, 16.29591483445806),
Offset(31.903157779555688, 16.485154884400586),
Offset(32.02617884924267, 16.61669091260771),
Offset(32.0963659195466, 16.69372469059615),
Offset(32.11994679583504, 16.71994065732921),
Offset(32.12, 16.72),
],
<Offset>[
Offset(12.799999999999999, 34.8),
Offset(12.797800711934586, 34.79739830167506),
Offset(11.255509454388564, 32.66461545063052),
Offset(9.190178426746762, 24.08254725994932),
Offset(12.660934377583331, 16.50846927586335),
Offset(17.201013301677882, 13.52456018610269),
Offset(20.811893547577277, 12.647212389481304),
Offset(23.527310261661704, 12.640520021606001),
Offset(25.576093255888573, 12.996982688430414),
Offset(27.143789334079017, 13.48623504238804),
Offset(28.362943549071037, 13.997572895595871),
Offset(29.324688586764324, 14.482621608132748),
Offset(30.077976275119465, 14.945111574454113),
Offset(30.664049025985392, 15.367314984359552),
Offset(31.116813293037453, 15.736600666066936),
Offset(31.46171091373027, 16.046656662766367),
Offset(31.718799891309303, 16.29591483445806),
Offset(31.903157779555688, 16.485154884400586),
Offset(32.02617884924267, 16.61669091260771),
Offset(32.0963659195466, 16.69372469059615),
Offset(32.11994679583504, 16.71994065732921),
Offset(32.12, 16.72),
],
),
_PathCubicTo(
<Offset>[
Offset(12.799999999999999, 34.8),
Offset(12.797800711934586, 34.79739830167506),
Offset(11.255509454388564, 32.66461545063052),
Offset(9.190178426746762, 24.08254725994932),
Offset(12.660934377583331, 16.50846927586335),
Offset(17.201013301677882, 13.52456018610269),
Offset(20.811893547577277, 12.647212389481304),
Offset(23.527310261661704, 12.640520021606001),
Offset(25.576093255888573, 12.996982688430414),
Offset(27.143789334079017, 13.48623504238804),
Offset(28.362943549071037, 13.997572895595871),
Offset(29.324688586764324, 14.482621608132748),
Offset(30.077976275119465, 14.945111574454113),
Offset(30.664049025985392, 15.367314984359552),
Offset(31.116813293037453, 15.736600666066936),
Offset(31.46171091373027, 16.046656662766367),
Offset(31.718799891309303, 16.29591483445806),
Offset(31.903157779555688, 16.485154884400586),
Offset(32.02617884924267, 16.61669091260771),
Offset(32.0963659195466, 16.69372469059615),
Offset(32.11994679583504, 16.71994065732921),
Offset(32.12, 16.72),
],
<Offset>[
Offset(12.799999999999999, 17.2),
Offset(12.801624714436713, 17.19762969959451),
Offset(14.195323289048401, 15.467755433523894),
Offset(20.74693885874317, 11.858563513900311),
Offset(27.798581509148605, 12.931480282116368),
Offset(31.400227525107965, 15.970884980320555),
Offset(33.03456990761612, 18.79874987920025),
Offset(33.70491543695029, 21.113936659438757),
Offset(33.89057068190488, 22.962546401906028),
Offset(33.83484434786968, 24.434469183017498),
Offset(33.667848449720154, 25.61048713663265),
Offset(33.45712105352585, 26.55492884029244),
Offset(33.219141883828776, 27.31243459193295),
Offset(32.97964808975476, 27.915446358228348),
Offset(32.75706734575072, 28.390737011252952),
Offset(32.562468884751034, 28.759088841119738),
Offset(32.40161047308811, 29.037632525154663),
Offset(32.277114041046914, 29.239673954694513),
Offset(32.189458347486145, 29.375646191369892),
Offset(32.13771575951039, 29.453657691624603),
Offset(32.12004004890893, 29.47994065698845),
Offset(32.12, 29.479999999999997),
],
<Offset>[
Offset(12.799999999999999, 17.2),
Offset(12.801624714436713, 17.19762969959451),
Offset(14.195323289048401, 15.467755433523894),
Offset(20.74693885874317, 11.858563513900311),
Offset(27.798581509148605, 12.931480282116368),
Offset(31.400227525107965, 15.970884980320555),
Offset(33.03456990761612, 18.79874987920025),
Offset(33.70491543695029, 21.113936659438757),
Offset(33.89057068190488, 22.962546401906028),
Offset(33.83484434786968, 24.434469183017498),
Offset(33.667848449720154, 25.61048713663265),
Offset(33.45712105352585, 26.55492884029244),
Offset(33.219141883828776, 27.31243459193295),
Offset(32.97964808975476, 27.915446358228348),
Offset(32.75706734575072, 28.390737011252952),
Offset(32.562468884751034, 28.759088841119738),
Offset(32.40161047308811, 29.037632525154663),
Offset(32.277114041046914, 29.239673954694513),
Offset(32.189458347486145, 29.375646191369892),
Offset(32.13771575951039, 29.453657691624603),
Offset(32.12004004890893, 29.47994065698845),
Offset(32.12, 29.479999999999997),
],
),
_PathCubicTo(
<Offset>[
Offset(12.799999999999999, 17.2),
Offset(12.801624714436713, 17.19762969959451),
Offset(14.195323289048401, 15.467755433523894),
Offset(20.74693885874317, 11.858563513900311),
Offset(27.798581509148605, 12.931480282116368),
Offset(31.400227525107965, 15.970884980320555),
Offset(33.03456990761612, 18.79874987920025),
Offset(33.70491543695029, 21.113936659438757),
Offset(33.89057068190488, 22.962546401906028),
Offset(33.83484434786968, 24.434469183017498),
Offset(33.667848449720154, 25.61048713663265),
Offset(33.45712105352585, 26.55492884029244),
Offset(33.219141883828776, 27.31243459193295),
Offset(32.97964808975476, 27.915446358228348),
Offset(32.75706734575072, 28.390737011252952),
Offset(32.562468884751034, 28.759088841119738),
Offset(32.40161047308811, 29.037632525154663),
Offset(32.277114041046914, 29.239673954694513),
Offset(32.189458347486145, 29.375646191369892),
Offset(32.13771575951039, 29.453657691624603),
Offset(32.12004004890893, 29.47994065698845),
Offset(32.12, 29.479999999999997),
],
<Offset>[
Offset(35.2, 17.2),
Offset(35.20133020799377, 17.202496611869943),
Offset(36.08223603809319, 19.20933667763641),
Offset(36.30473635371463, 26.567167700077555),
Offset(32.35111295573567, 32.19757663138127),
Offset(28.286723241557954, 34.04261217377702),
Offset(25.205340375246543, 34.35488342834059),
Offset(22.92056698879951, 34.06725233707878),
Offset(21.20712595566319, 33.54460858047224),
Offset(19.90072816888673, 32.95035738238742),
Offset(18.887775779309706, 32.36218428291335),
Offset(18.092366394413514, 31.814388343443472),
Offset(17.478912588855714, 31.310281730290257),
Offset(17.009299068467204, 30.862572439389364),
Offset(16.651802906423065, 30.478333078342565),
Offset(16.383009748664925, 30.160053531509803),
Offset(16.184878866746974, 29.906664174691322),
Offset(16.044089769763733, 29.71561828750153),
Offset(15.950787992697913, 29.583456461861584),
Offset(15.897801030928724, 29.506284760669423),
Offset(15.880040049342629, 29.480059342718857),
Offset(15.88, 29.48),
],
<Offset>[
Offset(35.2, 17.2),
Offset(35.20133020799377, 17.202496611869943),
Offset(36.08223603809319, 19.20933667763641),
Offset(36.30473635371463, 26.567167700077555),
Offset(32.35111295573567, 32.19757663138127),
Offset(28.286723241557954, 34.04261217377702),
Offset(25.205340375246543, 34.35488342834059),
Offset(22.92056698879951, 34.06725233707878),
Offset(21.20712595566319, 33.54460858047224),
Offset(19.90072816888673, 32.95035738238742),
Offset(18.887775779309706, 32.36218428291335),
Offset(18.092366394413514, 31.814388343443472),
Offset(17.478912588855714, 31.310281730290257),
Offset(17.009299068467204, 30.862572439389364),
Offset(16.651802906423065, 30.478333078342565),
Offset(16.383009748664925, 30.160053531509803),
Offset(16.184878866746974, 29.906664174691322),
Offset(16.044089769763733, 29.71561828750153),
Offset(15.950787992697913, 29.583456461861584),
Offset(15.897801030928724, 29.506284760669423),
Offset(15.880040049342629, 29.480059342718857),
Offset(15.88, 29.48),
],
),
_PathCubicTo(
<Offset>[
Offset(35.2, 17.2),
Offset(35.20133020799377, 17.202496611869943),
Offset(36.08223603809319, 19.20933667763641),
Offset(36.30473635371463, 26.567167700077555),
Offset(32.35111295573567, 32.19757663138127),
Offset(28.286723241557954, 34.04261217377702),
Offset(25.205340375246543, 34.35488342834059),
Offset(22.92056698879951, 34.06725233707878),
Offset(21.20712595566319, 33.54460858047224),
Offset(19.90072816888673, 32.95035738238742),
Offset(18.887775779309706, 32.36218428291335),
Offset(18.092366394413514, 31.814388343443472),
Offset(17.478912588855714, 31.310281730290257),
Offset(17.009299068467204, 30.862572439389364),
Offset(16.651802906423065, 30.478333078342565),
Offset(16.383009748664925, 30.160053531509803),
Offset(16.184878866746974, 29.906664174691322),
Offset(16.044089769763733, 29.71561828750153),
Offset(15.950787992697913, 29.583456461861584),
Offset(15.897801030928724, 29.506284760669423),
Offset(15.880040049342629, 29.480059342718857),
Offset(15.88, 29.48),
],
<Offset>[
Offset(35.2, 34.8),
Offset(35.19750620549164, 34.80226521395049),
Offset(33.142422203433355, 36.40619669474303),
Offset(24.747975921718226, 38.79115144612656),
Offset(17.213465824170402, 35.77456562512825),
Offset(14.087509018127873, 31.596287379559158),
Offset(12.982664015207707, 28.20334593862164),
Offset(12.742961813510924, 25.59383569924602),
Offset(12.89264852964688, 23.57904486699663),
Offset(13.20967315509607, 22.002123241757968),
Offset(13.58287087866059, 20.749270041876564),
Offset(13.959933927651988, 19.74208111128378),
Offset(14.3377469801464, 18.94295871281142),
Offset(14.693700004697835, 18.31444106552057),
Offset(15.011548853709796, 17.824196733156548),
Offset(15.282251777644161, 17.447621353156435),
Offset(15.50206828496817, 17.16494648399472),
Offset(15.670133508272507, 16.961099217207604),
Offset(15.78750849445444, 16.8245011830994),
Offset(15.856451190964936, 16.746351759640973),
Offset(15.879946796268738, 16.720059343059617),
Offset(15.879999999999999, 16.720000000000002),
],
<Offset>[
Offset(35.2, 34.8),
Offset(35.19750620549164, 34.80226521395049),
Offset(33.142422203433355, 36.40619669474303),
Offset(24.747975921718226, 38.79115144612656),
Offset(17.213465824170402, 35.77456562512825),
Offset(14.087509018127873, 31.596287379559158),
Offset(12.982664015207707, 28.20334593862164),
Offset(12.742961813510924, 25.59383569924602),
Offset(12.89264852964688, 23.57904486699663),
Offset(13.20967315509607, 22.002123241757968),
Offset(13.58287087866059, 20.749270041876564),
Offset(13.959933927651988, 19.74208111128378),
Offset(14.3377469801464, 18.94295871281142),
Offset(14.693700004697835, 18.31444106552057),
Offset(15.011548853709796, 17.824196733156548),
Offset(15.282251777644161, 17.447621353156435),
Offset(15.50206828496817, 17.16494648399472),
Offset(15.670133508272507, 16.961099217207604),
Offset(15.78750849445444, 16.8245011830994),
Offset(15.856451190964936, 16.746351759640973),
Offset(15.879946796268738, 16.720059343059617),
Offset(15.879999999999999, 16.720000000000002),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.285714285714,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.285714285714,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 22.0),
Offset(10.000434881903109, 21.996958187115208),
Offset(10.537203766746376, 19.66951300869151),
Offset(15.2007493434278, 12.928722391574958),
Offset(22.726894381864838, 9.915284806391266),
Offset(28.34794602732309, 10.54283219457061),
Offset(32.08037023016842, 12.393638944810997),
Offset(34.49466952610868, 14.52044771427649),
Offset(36.03113891519555, 16.566851514784105),
Offset(36.98867178324178, 18.40585973475647),
Offset(37.56526083167866, 20.0020381982159),
Offset(37.893194841987366, 21.35819435185408),
Offset(38.06150889201586, 22.492031936757623),
Offset(38.13048882144999, 23.426165819354733),
Offset(38.14094176630335, 24.183755168682307),
Offset(38.12034219741291, 24.78481604721129),
Offset(38.086964642108946, 25.247969219160655),
Offset(38.052600274850896, 25.58884408148203),
Offset(38.02444615197159, 25.820689465683056),
Offset(38.00640765629513, 25.954621335624125),
Offset(38.000014616095385, 25.999897684661754),
Offset(38.0, 25.999999999999993),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.00043422098076, 22.003041907302272),
Offset(38.136820741489856, 24.387684566345136),
Offset(35.547238360668324, 32.16463632024782),
Offset(29.165901647620203, 37.16485701278654),
Offset(23.59397879929007, 38.13630598086269),
Offset(19.492625674491244, 37.4046102774286),
Offset(16.57939189493825, 36.038877661605),
Offset(14.531388217272726, 34.50454144206201),
Offset(13.097234362356085, 33.007202753941485),
Offset(12.096778906088034, 31.63631636258276),
Offset(11.402238533172989, 30.426227977976552),
Offset(10.923182521372642, 29.384871830477124),
Offset(10.595404301988683, 28.507417683425455),
Offset(10.373244457117737, 27.783058732629918),
Offset(10.224722997264447, 27.20027240995278),
Offset(10.12708256221043, 26.746299649396278),
Offset(10.064627393328172, 26.40943775873551),
Offset(10.026738643716017, 26.17898303549632),
Offset(10.006554675981915, 26.045357661563475),
Offset(10.000014616843135, 26.00010231523142),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 26.0),
Offset(37.99956511809689, 26.003041812884792),
Offset(37.46279623325363, 28.33048699130849),
Offset(32.7992506565722, 35.07127760842504),
Offset(25.273105618135162, 38.08471519360873),
Offset(19.652053972676917, 37.4571678054294),
Offset(15.919629769831586, 35.606361055189005),
Offset(13.50533047389132, 33.479552285723514),
Offset(11.968861084804454, 31.433148485215895),
Offset(11.011328216758224, 29.59414026524353),
Offset(10.43473916832134, 27.9979618017841),
Offset(10.106805158012635, 26.641805648145926),
Offset(9.938491107984142, 25.507968063242377),
Offset(9.86951117855001, 24.573834180645267),
Offset(9.859058233696649, 23.816244831317686),
Offset(9.87965780258709, 23.215183952788717),
Offset(9.913035357891056, 22.752030780839345),
Offset(9.947399725149102, 22.411155918517977),
Offset(9.97555384802841, 22.17931053431695),
Offset(9.993592343704867, 22.045378664375875),
Offset(9.999985383904612, 22.00010231533824),
Offset(10.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.999565779019244, 25.996958092697728),
Offset(9.863179258510144, 23.612315433654864),
Offset(12.452761639331678, 15.835363679752177),
Offset(18.834098352379797, 10.835142987213462),
Offset(24.406021200709937, 9.863694019137322),
Offset(28.507374325508763, 10.595389722571399),
Offset(31.42060810506175, 11.961122338395),
Offset(33.46861178272727, 13.495458557937988),
Offset(34.90276563764392, 14.992797246058515),
Offset(35.90322109391197, 16.36368363741724),
Offset(36.59776146682701, 17.573772022023455),
Offset(37.07681747862736, 18.615128169522876),
Offset(37.40459569801132, 19.492582316574545),
Offset(37.626755542882265, 20.216941267370075),
Offset(37.77527700273555, 20.799727590047226),
Offset(37.87291743778957, 21.253700350603722),
Offset(37.93537260667183, 21.590562241264497),
Offset(37.973261356283984, 21.821016964503688),
Offset(37.99344532401808, 21.954642338436525),
Offset(37.99998538315687, 21.999897684768573),
Offset(38.0, 21.999999999999993),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 10.0),
Offset(26.003041812884792, 10.000434881903109),
Offset(28.33048699130849, 10.537203766746376),
Offset(35.07127760842504, 15.2007493434278),
Offset(38.08471519360873, 22.726894381864838),
Offset(37.4571678054294, 28.34794602732309),
Offset(35.606361055189005, 32.08037023016842),
Offset(33.479552285723514, 34.49466952610868),
Offset(31.433148485215895, 36.03113891519555),
Offset(29.59414026524353, 36.98867178324178),
Offset(27.9979618017841, 37.56526083167866),
Offset(26.641805648145922, 37.893194841987366),
Offset(25.507968063242377, 38.06150889201586),
Offset(24.573834180645267, 38.13048882144999),
Offset(23.81624483131769, 38.14094176630335),
Offset(23.215183952788713, 38.12034219741291),
Offset(22.752030780839345, 38.086964642108946),
Offset(22.411155918517974, 38.0526002748509),
Offset(22.179310534316947, 38.024446151971595),
Offset(22.045378664375875, 38.00640765629513),
Offset(22.000102315338243, 38.000014616095385),
Offset(22.000000000000004, 38.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.996958092697728, 38.00043422098076),
Offset(23.612315433654864, 38.136820741489856),
Offset(15.835363679752177, 35.547238360668324),
Offset(10.835142987213462, 29.165901647620203),
Offset(9.863694019137322, 23.59397879929007),
Offset(10.595389722571403, 19.49262567449124),
Offset(11.961122338395, 16.57939189493825),
Offset(13.495458557937988, 14.531388217272726),
Offset(14.992797246058515, 13.097234362356085),
Offset(16.36368363741724, 12.096778906088034),
Offset(17.573772022023455, 11.402238533172993),
Offset(18.615128169522876, 10.923182521372642),
Offset(19.492582316574545, 10.595404301988683),
Offset(20.21694126737008, 10.373244457117734),
Offset(20.79972759004722, 10.22472299726445),
Offset(21.253700350603722, 10.12708256221043),
Offset(21.590562241264493, 10.064627393328175),
Offset(21.821016964503684, 10.02673864371602),
Offset(21.954642338436525, 10.006554675981915),
Offset(21.999897684768577, 10.000014616843131),
Offset(21.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 38.0),
Offset(21.996958187115208, 37.99956511809689),
Offset(19.66951300869151, 37.46279623325363),
Offset(12.928722391574958, 32.7992506565722),
Offset(9.915284806391266, 25.273105618135162),
Offset(10.54283219457061, 19.652053972676917),
Offset(12.393638944811, 15.919629769831582),
Offset(14.52044771427649, 13.50533047389132),
Offset(16.566851514784105, 11.968861084804454),
Offset(18.40585973475647, 11.011328216758224),
Offset(20.0020381982159, 10.43473916832134),
Offset(21.358194351854078, 10.106805158012639),
Offset(22.492031936757623, 9.938491107984142),
Offset(23.426165819354733, 9.86951117855001),
Offset(24.18375516868231, 9.859058233696645),
Offset(24.784816047211287, 9.879657802587094),
Offset(25.247969219160655, 9.913035357891056),
Offset(25.588844081482026, 9.947399725149106),
Offset(25.820689465683053, 9.975553848028413),
Offset(25.954621335624125, 9.993592343704867),
Offset(25.999897684661757, 9.999985383904608),
Offset(25.999999999999996, 9.999999999999996),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.003041907302272, 9.999565779019244),
Offset(24.387684566345136, 9.863179258510144),
Offset(32.16463632024782, 12.452761639331678),
Offset(37.16485701278654, 18.834098352379797),
Offset(38.13630598086269, 24.406021200709937),
Offset(37.4046102774286, 28.50737432550876),
Offset(36.038877661605, 31.42060810506175),
Offset(34.50454144206201, 33.46861178272727),
Offset(33.007202753941485, 34.90276563764392),
Offset(31.63631636258276, 35.90322109391197),
Offset(30.426227977976545, 36.597761466827016),
Offset(29.384871830477124, 37.07681747862736),
Offset(28.507417683425455, 37.40459569801132),
Offset(27.78305873262992, 37.62675554288226),
Offset(27.20027240995278, 37.77527700273556),
Offset(26.746299649396278, 37.87291743778957),
Offset(26.409437758735507, 37.935372606671834),
Offset(26.178983035496316, 37.973261356283984),
Offset(26.045357661563475, 37.99344532401808),
Offset(26.000102315231423, 37.99998538315686),
Offset(26.000000000000004, 38.0),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/event_add.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/event_add.g.dart",
"repo_id": "flutter",
"token_count": 98027
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'badge_theme.dart';
import 'color_scheme.dart';
import 'theme.dart';
/// A Material Design "badge".
///
/// A badge's [label] conveys a small amount of information about its
/// [child], like a count or status. If the label is null then this is
/// a "small" badge that's displayed as a [smallSize] diameter filled
/// circle. Otherwise this is a [StadiumBorder] shaped "large" badge
/// with height [largeSize].
///
/// Badges are typically used to decorate the icon within a
/// [BottomNavigationBarItem] or a [NavigationRailDestination]
/// or a button's icon, as in [TextButton.icon]. The badge's default
/// configuration is intended to work well with a default sized (24)
/// [Icon].
class Badge extends StatelessWidget {
/// Create a Badge that stacks [label] on top of [child].
///
/// If [label] is null then just a filled circle is displayed. Otherwise
/// the [label] is displayed within a [StadiumBorder] shaped area.
const Badge({
super.key,
this.backgroundColor,
this.textColor,
this.smallSize,
this.largeSize,
this.textStyle,
this.padding,
this.alignment,
this.offset,
this.label,
this.isLabelVisible = true,
this.child,
});
/// Convenience constructor for creating a badge with a numeric
/// label with 1-3 digits based on [count].
///
/// Initializes [label] with a [Text] widget that contains [count].
/// If [count] is greater than 999, then the label is '999+'.
Badge.count({
super.key,
this.backgroundColor,
this.textColor,
this.smallSize,
this.largeSize,
this.textStyle,
this.padding,
this.alignment,
this.offset,
required int count,
this.isLabelVisible = true,
this.child,
}) : label = Text(count > 999 ? '999+' : '$count');
/// The badge's fill color.
///
/// Defaults to the [BadgeTheme]'s background color, or
/// [ColorScheme.errorColor] if the theme value is null.
final Color? backgroundColor;
/// The color of the badge's [label] text.
///
/// This color overrides the color of the label's [textStyle].
///
/// Defaults to the [BadgeTheme]'s foreground color, or
/// [ColorScheme.onError] if the theme value is null.
final Color? textColor;
/// The diameter of the badge if [label] is null.
///
/// Defaults to the [BadgeTheme]'s small size, or 6 if the theme value
/// is null.
final double? smallSize;
/// The badge's height if [label] is non-null.
///
/// Defaults to the [BadgeTheme]'s large size, or 16 if the theme value
/// is null. If the default value is overridden then it may be useful to
/// also override [padding] and [alignment].
final double? largeSize;
/// The [DefaultTextStyle] for the badge's label.
///
/// The text style's color is overwritten by the [textColor].
///
/// This value is only used if [label] is non-null.
///
/// Defaults to the [BadgeTheme]'s text style, or the overall theme's
/// [TextTheme.labelSmall] if the badge theme's value is null. If
/// the default text style is overridden then it may be useful to
/// also override [largeSize], [padding], and [alignment].
final TextStyle? textStyle;
/// The padding added to the badge's label.
///
/// This value is only used if [label] is non-null.
///
/// Defaults to the [BadgeTheme]'s padding, or 4 pixels on the
/// left and right if the theme's value is null.
final EdgeInsetsGeometry? padding;
/// Combined with [offset] to determine the location of the [label]
/// relative to the [child].
///
/// The alignment positions the label in the same way a child of an
/// [Align] widget is positioned, except that, the alignment is
/// resolved as if the label was a [largeSize] square and [offset]
/// is added to the result.
///
/// This value is only used if [label] is non-null.
///
/// Defaults to the [BadgeTheme]'s alignment, or
/// [AlignmentDirectional.topEnd] if the theme's value is null.
final AlignmentGeometry? alignment;
/// Combined with [alignment] to determine the location of the [label]
/// relative to the [child].
///
/// This value is only used if [label] is non-null.
///
/// Defaults to the [BadgeTheme]'s offset, or
/// if the theme's value is null then `Offset(4, -4)` for
/// [TextDirection.ltr] or `Offset(-4, -4)` for [TextDirection.rtl].
final Offset? offset;
/// The badge's content, typically a [Text] widget that contains 1 to 4
/// characters.
///
/// If the label is null then this is a "small" badge that's
/// displayed as a [smallSize] diameter filled circle. Otherwise
/// this is a [StadiumBorder] shaped "large" badge with height [largeSize].
final Widget? label;
/// If false, the badge's [label] is not included.
///
/// This flag is true by default. It's intended to make it convenient
/// to create a badge that's only shown under certain conditions.
final bool isLabelVisible;
/// The widget that the badge is stacked on top of.
///
/// Typically this is an default sized [Icon] that's part of a
/// [BottomNavigationBarItem] or a [NavigationRailDestination].
final Widget? child;
@override
Widget build(BuildContext context) {
if (!isLabelVisible) {
return child ?? const SizedBox();
}
final BadgeThemeData badgeTheme = BadgeTheme.of(context);
final BadgeThemeData defaults = _BadgeDefaultsM3(context);
final double effectiveSmallSize = smallSize ?? badgeTheme.smallSize ?? defaults.smallSize!;
final double effectiveLargeSize = largeSize ?? badgeTheme.largeSize ?? defaults.largeSize!;
final Widget badge = DefaultTextStyle(
style: (textStyle ?? badgeTheme.textStyle ?? defaults.textStyle!).copyWith(
color: textColor ?? badgeTheme.textColor ?? defaults.textColor!,
),
child: IntrinsicWidth(
child: Container(
height: label == null ? effectiveSmallSize : effectiveLargeSize,
clipBehavior: Clip.antiAlias,
decoration: ShapeDecoration(
color: backgroundColor ?? badgeTheme.backgroundColor ?? defaults.backgroundColor!,
shape: const StadiumBorder(),
),
padding: label == null ? null : (padding ?? badgeTheme.padding ?? defaults.padding!),
alignment: label == null ? null : Alignment.center,
child: label ?? SizedBox(width: effectiveSmallSize, height: effectiveSmallSize),
),
),
);
if (child == null) {
return badge;
}
final AlignmentGeometry effectiveAlignment = alignment ?? badgeTheme.alignment ?? defaults.alignment!;
final TextDirection textDirection = Directionality.of(context);
final Offset defaultOffset = textDirection == TextDirection.ltr ? const Offset(4, -4) : const Offset(-4, -4);
final Offset effectiveOffset = offset ?? badgeTheme.offset ?? defaultOffset;
return
Stack(
clipBehavior: Clip.none,
children: <Widget>[
child!,
Positioned.fill(
child: _Badge(
alignment: effectiveAlignment,
offset: label == null ? Offset.zero : effectiveOffset,
textDirection: textDirection,
child: badge,
),
),
],
);
}
}
class _Badge extends SingleChildRenderObjectWidget {
const _Badge({
required this.alignment,
required this.offset,
required this.textDirection,
super.child, // the badge
});
final AlignmentGeometry alignment;
final Offset offset;
final TextDirection textDirection;
@override
_RenderBadge createRenderObject(BuildContext context) {
return _RenderBadge(
alignment: alignment,
offset: offset,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, _RenderBadge renderObject) {
renderObject
..alignment = alignment
..offset = offset
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DiagnosticsProperty<Offset>('offset', offset));
}
}
class _RenderBadge extends RenderAligningShiftedBox {
_RenderBadge({
super.textDirection,
super.alignment,
required Offset offset,
}) : _offset = offset;
Offset get offset => _offset;
Offset _offset;
set offset(Offset value) {
if (_offset == value) {
return;
}
_offset = value;
markNeedsLayout();
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
assert(constraints.hasBoundedWidth);
assert(constraints.hasBoundedHeight);
size = constraints.biggest;
child!.layout(const BoxConstraints(), parentUsesSize: true);
final double badgeSize = child!.size.height;
final Alignment resolvedAlignment = alignment.resolve(textDirection);
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = offset + resolvedAlignment.alongOffset(Offset(size.width - badgeSize, size.height - badgeSize));
}
}
// BEGIN GENERATED TOKEN PROPERTIES - Badge
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _BadgeDefaultsM3 extends BadgeThemeData {
_BadgeDefaultsM3(this.context) : super(
smallSize: 6.0,
largeSize: 16.0,
padding: const EdgeInsets.symmetric(horizontal: 4),
alignment: AlignmentDirectional.topEnd,
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override
Color? get backgroundColor => _colors.error;
@override
Color? get textColor => _colors.onError;
@override
TextStyle? get textStyle => Theme.of(context).textTheme.labelSmall;
}
// END GENERATED TOKEN PROPERTIES - Badge
| flutter/packages/flutter/lib/src/material/badge.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/badge.dart",
"repo_id": "flutter",
"token_count": 3380
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'date.dart';
import 'date_picker_theme.dart';
import 'debug.dart';
import 'divider.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'text_theme.dart';
import 'theme.dart';
const Duration _monthScrollDuration = Duration(milliseconds: 200);
const double _dayPickerRowHeight = 42.0;
const int _maxDayPickerRowCount = 6; // A 31 day month that starts on Saturday.
// One extra row for the day-of-week header.
const double _maxDayPickerHeight = _dayPickerRowHeight * (_maxDayPickerRowCount + 1);
const double _monthPickerHorizontalPadding = 8.0;
const int _yearPickerColumnCount = 3;
const double _yearPickerPadding = 16.0;
const double _yearPickerRowHeight = 52.0;
const double _yearPickerRowSpacing = 8.0;
const double _subHeaderHeight = 52.0;
const double _monthNavButtonsWidth = 108.0;
/// Displays a grid of days for a given month and allows the user to select a
/// date.
///
/// Days are arranged in a rectangular grid with one column for each day of the
/// week. Controls are provided to change the year and month that the grid is
/// showing.
///
/// The calendar picker widget is rarely used directly. Instead, consider using
/// [showDatePicker], which will create a dialog that uses this as well as
/// provides a text entry option.
///
/// See also:
///
/// * [showDatePicker], which creates a Dialog that contains a
/// [CalendarDatePicker] and provides an optional compact view where the
/// user can enter a date as a line of text.
/// * [showTimePicker], which shows a dialog that contains a Material Design
/// time picker.
///
class CalendarDatePicker extends StatefulWidget {
/// Creates a calendar date picker.
///
/// It will display a grid of days for the [initialDate]'s month, or, if that
/// is null, the [currentDate]'s month. The day indicated by [initialDate] will
/// be selected if it is not null.
///
/// The optional [onDisplayedMonthChanged] callback can be used to track
/// the currently displayed month.
///
/// The user interface provides a way to change the year of the month being
/// displayed. By default it will show the day grid, but this can be changed
/// to start in the year selection interface with [initialCalendarMode] set
/// to [DatePickerMode.year].
///
/// The [lastDate] must be after or equal to [firstDate].
///
/// The [initialDate], if provided, must be between [firstDate] and [lastDate]
/// or equal to one of them.
///
/// The [currentDate] represents the current day (i.e. today). This
/// date will be highlighted in the day grid. If null, the date of
/// `DateTime.now()` will be used.
///
/// If [selectableDayPredicate] and [initialDate] are both non-null,
/// [selectableDayPredicate] must return `true` for the [initialDate].
CalendarDatePicker({
super.key,
required DateTime? initialDate,
required DateTime firstDate,
required DateTime lastDate,
DateTime? currentDate,
required this.onDateChanged,
this.onDisplayedMonthChanged,
this.initialCalendarMode = DatePickerMode.day,
this.selectableDayPredicate,
}) : initialDate = initialDate == null ? null : DateUtils.dateOnly(initialDate),
firstDate = DateUtils.dateOnly(firstDate),
lastDate = DateUtils.dateOnly(lastDate),
currentDate = DateUtils.dateOnly(currentDate ?? DateTime.now()) {
assert(
!this.lastDate.isBefore(this.firstDate),
'lastDate ${this.lastDate} must be on or after firstDate ${this.firstDate}.',
);
assert(
this.initialDate == null || !this.initialDate!.isBefore(this.firstDate),
'initialDate ${this.initialDate} must be on or after firstDate ${this.firstDate}.',
);
assert(
this.initialDate == null || !this.initialDate!.isAfter(this.lastDate),
'initialDate ${this.initialDate} must be on or before lastDate ${this.lastDate}.',
);
assert(
selectableDayPredicate == null || this.initialDate == null || selectableDayPredicate!(this.initialDate!),
'Provided initialDate ${this.initialDate} must satisfy provided selectableDayPredicate.',
);
}
/// The initially selected [DateTime] that the picker should display.
///
/// Subsequently changing this has no effect. To change the selected date,
/// change the [key] to create a new instance of the [CalendarDatePicker], and
/// provide that widget the new [initialDate]. This will reset the widget's
/// interactive state.
final DateTime? initialDate;
/// The earliest allowable [DateTime] that the user can select.
final DateTime firstDate;
/// The latest allowable [DateTime] that the user can select.
final DateTime lastDate;
/// The [DateTime] representing today. It will be highlighted in the day grid.
final DateTime currentDate;
/// Called when the user selects a date in the picker.
final ValueChanged<DateTime> onDateChanged;
/// Called when the user navigates to a new month/year in the picker.
final ValueChanged<DateTime>? onDisplayedMonthChanged;
/// The initial display of the calendar picker.
///
/// Subsequently changing this has no effect. To change the calendar mode,
/// change the [key] to create a new instance of the [CalendarDatePicker], and
/// provide that widget a new [initialCalendarMode]. This will reset the
/// widget's interactive state.
final DatePickerMode initialCalendarMode;
/// Function to provide full control over which dates in the calendar can be selected.
final SelectableDayPredicate? selectableDayPredicate;
@override
State<CalendarDatePicker> createState() => _CalendarDatePickerState();
}
class _CalendarDatePickerState extends State<CalendarDatePicker> {
bool _announcedInitialDate = false;
late DatePickerMode _mode;
late DateTime _currentDisplayedMonthDate;
DateTime? _selectedDate;
final GlobalKey _monthPickerKey = GlobalKey();
final GlobalKey _yearPickerKey = GlobalKey();
late MaterialLocalizations _localizations;
late TextDirection _textDirection;
@override
void initState() {
super.initState();
_mode = widget.initialCalendarMode;
final DateTime currentDisplayedDate = widget.initialDate ?? widget.currentDate;
_currentDisplayedMonthDate = DateTime(currentDisplayedDate.year, currentDisplayedDate.month);
if (widget.initialDate != null) {
_selectedDate = widget.initialDate;
}
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMaterialLocalizations(context));
assert(debugCheckHasDirectionality(context));
_localizations = MaterialLocalizations.of(context);
_textDirection = Directionality.of(context);
if (!_announcedInitialDate && widget.initialDate != null) {
assert(_selectedDate != null);
_announcedInitialDate = true;
final bool isToday = DateUtils.isSameDay(widget.currentDate, _selectedDate);
final String semanticLabelSuffix = isToday ? ', ${_localizations.currentDateLabel}' : '';
SemanticsService.announce(
'${_localizations.formatFullDate(_selectedDate!)}$semanticLabelSuffix',
_textDirection,
);
}
}
void _vibrate() {
switch (Theme.of(context).platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
HapticFeedback.vibrate();
case TargetPlatform.iOS:
case TargetPlatform.macOS:
break;
}
}
void _handleModeChanged(DatePickerMode mode) {
_vibrate();
setState(() {
_mode = mode;
if (_selectedDate != null) {
if (_mode == DatePickerMode.day) {
SemanticsService.announce(
_localizations.formatMonthYear(_selectedDate!),
_textDirection,
);
} else {
SemanticsService.announce(
_localizations.formatYear(_selectedDate!),
_textDirection,
);
}
}
});
}
void _handleMonthChanged(DateTime date) {
setState(() {
if (_currentDisplayedMonthDate.year != date.year || _currentDisplayedMonthDate.month != date.month) {
_currentDisplayedMonthDate = DateTime(date.year, date.month);
widget.onDisplayedMonthChanged?.call(_currentDisplayedMonthDate);
}
});
}
void _handleYearChanged(DateTime value) {
_vibrate();
final int daysInMonth = DateUtils.getDaysInMonth(value.year, value.month);
final int preferredDay = math.min(_selectedDate?.day ?? 1, daysInMonth);
value = value.copyWith(day: preferredDay);
if (value.isBefore(widget.firstDate)) {
value = widget.firstDate;
} else if (value.isAfter(widget.lastDate)) {
value = widget.lastDate;
}
setState(() {
_mode = DatePickerMode.day;
_handleMonthChanged(value);
if (_isSelectable(value)) {
_selectedDate = value;
widget.onDateChanged(_selectedDate!);
}
});
}
void _handleDayChanged(DateTime value) {
_vibrate();
setState(() {
_selectedDate = value;
widget.onDateChanged(_selectedDate!);
switch (Theme.of(context).platform) {
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
final bool isToday = DateUtils.isSameDay(widget.currentDate, _selectedDate);
final String semanticLabelSuffix = isToday ? ', ${_localizations.currentDateLabel}' : '';
SemanticsService.announce(
'${_localizations.selectedDateLabel} ${_localizations.formatFullDate(_selectedDate!)}$semanticLabelSuffix',
_textDirection,
);
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
break;
}
});
}
bool _isSelectable(DateTime date) {
return widget.selectableDayPredicate == null || widget.selectableDayPredicate!.call(date);
}
Widget _buildPicker() {
switch (_mode) {
case DatePickerMode.day:
return _MonthPicker(
key: _monthPickerKey,
initialMonth: _currentDisplayedMonthDate,
currentDate: widget.currentDate,
firstDate: widget.firstDate,
lastDate: widget.lastDate,
selectedDate: _selectedDate,
onChanged: _handleDayChanged,
onDisplayedMonthChanged: _handleMonthChanged,
selectableDayPredicate: widget.selectableDayPredicate,
);
case DatePickerMode.year:
return Padding(
padding: const EdgeInsets.only(top: _subHeaderHeight),
child: YearPicker(
key: _yearPickerKey,
currentDate: widget.currentDate,
firstDate: widget.firstDate,
lastDate: widget.lastDate,
selectedDate: _currentDisplayedMonthDate,
onChanged: _handleYearChanged,
),
);
}
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMaterialLocalizations(context));
assert(debugCheckHasDirectionality(context));
return Stack(
children: <Widget>[
SizedBox(
height: _subHeaderHeight + _maxDayPickerHeight,
child: _buildPicker(),
),
// Put the mode toggle button on top so that it won't be covered up by the _MonthPicker
_DatePickerModeToggleButton(
mode: _mode,
title: _localizations.formatMonthYear(_currentDisplayedMonthDate),
onTitlePressed: () {
// Toggle the day/year mode.
_handleModeChanged(_mode == DatePickerMode.day ? DatePickerMode.year : DatePickerMode.day);
},
),
],
);
}
}
/// A button that used to toggle the [DatePickerMode] for a date picker.
///
/// This appears above the calendar grid and allows the user to toggle the
/// [DatePickerMode] to display either the calendar view or the year list.
class _DatePickerModeToggleButton extends StatefulWidget {
const _DatePickerModeToggleButton({
required this.mode,
required this.title,
required this.onTitlePressed,
});
/// The current display of the calendar picker.
final DatePickerMode mode;
/// The text that displays the current month/year being viewed.
final String title;
/// The callback when the title is pressed.
final VoidCallback onTitlePressed;
@override
_DatePickerModeToggleButtonState createState() => _DatePickerModeToggleButtonState();
}
class _DatePickerModeToggleButtonState extends State<_DatePickerModeToggleButton> with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
value: widget.mode == DatePickerMode.year ? 0.5 : 0,
upperBound: 0.5,
duration: const Duration(milliseconds: 200),
vsync: this,
);
}
@override
void didUpdateWidget(_DatePickerModeToggleButton oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.mode == widget.mode) {
return;
}
if (widget.mode == DatePickerMode.year) {
_controller.forward();
} else {
_controller.reverse();
}
}
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final TextTheme textTheme = Theme.of(context).textTheme;
final Color controlColor = colorScheme.onSurface.withOpacity(0.60);
return Container(
padding: const EdgeInsetsDirectional.only(start: 16, end: 4),
height: _subHeaderHeight,
child: Row(
children: <Widget>[
Flexible(
child: Semantics(
label: MaterialLocalizations.of(context).selectYearSemanticsLabel,
excludeSemantics: true,
button: true,
container: true,
child: SizedBox(
height: _subHeaderHeight,
child: InkWell(
onTap: widget.onTitlePressed,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 8),
child: Row(
children: <Widget>[
Flexible(
child: Text(
widget.title,
overflow: TextOverflow.ellipsis,
style: textTheme.titleSmall?.copyWith(
color: controlColor,
),
),
),
RotationTransition(
turns: _controller,
child: Icon(
Icons.arrow_drop_down,
color: controlColor,
),
),
],
),
),
),
),
),
),
if (widget.mode == DatePickerMode.day)
// Give space for the prev/next month buttons that are underneath this row
const SizedBox(width: _monthNavButtonsWidth),
],
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
class _MonthPicker extends StatefulWidget {
/// Creates a month picker.
_MonthPicker({
super.key,
required this.initialMonth,
required this.currentDate,
required this.firstDate,
required this.lastDate,
required this.selectedDate,
required this.onChanged,
required this.onDisplayedMonthChanged,
this.selectableDayPredicate,
}) : assert(!firstDate.isAfter(lastDate)),
assert(selectedDate == null || !selectedDate.isBefore(firstDate)),
assert(selectedDate == null || !selectedDate.isAfter(lastDate));
/// The initial month to display.
///
/// Subsequently changing this has no effect. To change the selected month,
/// change the [key] to create a new instance of the [_MonthPicker], and
/// provide that widget the new [initialMonth]. This will reset the widget's
/// interactive state.
final DateTime initialMonth;
/// The current date.
///
/// This date is subtly highlighted in the picker.
final DateTime currentDate;
/// The earliest date the user is permitted to pick.
///
/// This date must be on or before the [lastDate].
final DateTime firstDate;
/// The latest date the user is permitted to pick.
///
/// This date must be on or after the [firstDate].
final DateTime lastDate;
/// The currently selected date.
///
/// This date is highlighted in the picker.
final DateTime? selectedDate;
/// Called when the user picks a day.
final ValueChanged<DateTime> onChanged;
/// Called when the user navigates to a new month.
final ValueChanged<DateTime> onDisplayedMonthChanged;
/// Optional user supplied predicate function to customize selectable days.
final SelectableDayPredicate? selectableDayPredicate;
@override
_MonthPickerState createState() => _MonthPickerState();
}
class _MonthPickerState extends State<_MonthPicker> {
final GlobalKey _pageViewKey = GlobalKey();
late DateTime _currentMonth;
late PageController _pageController;
late MaterialLocalizations _localizations;
late TextDirection _textDirection;
Map<ShortcutActivator, Intent>? _shortcutMap;
Map<Type, Action<Intent>>? _actionMap;
late FocusNode _dayGridFocus;
DateTime? _focusedDay;
@override
void initState() {
super.initState();
_currentMonth = widget.initialMonth;
_pageController = PageController(initialPage: DateUtils.monthDelta(widget.firstDate, _currentMonth));
_shortcutMap = const <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.arrowLeft): DirectionalFocusIntent(TraversalDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight): DirectionalFocusIntent(TraversalDirection.right),
SingleActivator(LogicalKeyboardKey.arrowDown): DirectionalFocusIntent(TraversalDirection.down),
SingleActivator(LogicalKeyboardKey.arrowUp): DirectionalFocusIntent(TraversalDirection.up),
};
_actionMap = <Type, Action<Intent>>{
NextFocusIntent: CallbackAction<NextFocusIntent>(onInvoke: _handleGridNextFocus),
PreviousFocusIntent: CallbackAction<PreviousFocusIntent>(onInvoke: _handleGridPreviousFocus),
DirectionalFocusIntent: CallbackAction<DirectionalFocusIntent>(onInvoke: _handleDirectionFocus),
};
_dayGridFocus = FocusNode(debugLabel: 'Day Grid');
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_localizations = MaterialLocalizations.of(context);
_textDirection = Directionality.of(context);
}
@override
void dispose() {
_pageController.dispose();
_dayGridFocus.dispose();
super.dispose();
}
void _handleDateSelected(DateTime selectedDate) {
_focusedDay = selectedDate;
widget.onChanged(selectedDate);
}
void _handleMonthPageChanged(int monthPage) {
setState(() {
final DateTime monthDate = DateUtils.addMonthsToMonthDate(widget.firstDate, monthPage);
if (!DateUtils.isSameMonth(_currentMonth, monthDate)) {
_currentMonth = DateTime(monthDate.year, monthDate.month);
widget.onDisplayedMonthChanged(_currentMonth);
if (_focusedDay != null && !DateUtils.isSameMonth(_focusedDay, _currentMonth)) {
// We have navigated to a new month with the grid focused, but the
// focused day is not in this month. Choose a new one trying to keep
// the same day of the month.
_focusedDay = _focusableDayForMonth(_currentMonth, _focusedDay!.day);
}
SemanticsService.announce(
_localizations.formatMonthYear(_currentMonth),
_textDirection,
);
}
});
}
/// Returns a focusable date for the given month.
///
/// If the preferredDay is available in the month it will be returned,
/// otherwise the first selectable day in the month will be returned. If
/// no dates are selectable in the month, then it will return null.
DateTime? _focusableDayForMonth(DateTime month, int preferredDay) {
final int daysInMonth = DateUtils.getDaysInMonth(month.year, month.month);
// Can we use the preferred day in this month?
if (preferredDay <= daysInMonth) {
final DateTime newFocus = DateTime(month.year, month.month, preferredDay);
if (_isSelectable(newFocus)) {
return newFocus;
}
}
// Start at the 1st and take the first selectable date.
for (int day = 1; day <= daysInMonth; day++) {
final DateTime newFocus = DateTime(month.year, month.month, day);
if (_isSelectable(newFocus)) {
return newFocus;
}
}
return null;
}
/// Navigate to the next month.
void _handleNextMonth() {
if (!_isDisplayingLastMonth) {
_pageController.nextPage(
duration: _monthScrollDuration,
curve: Curves.ease,
);
}
}
/// Navigate to the previous month.
void _handlePreviousMonth() {
if (!_isDisplayingFirstMonth) {
_pageController.previousPage(
duration: _monthScrollDuration,
curve: Curves.ease,
);
}
}
/// Navigate to the given month.
void _showMonth(DateTime month, { bool jump = false}) {
final int monthPage = DateUtils.monthDelta(widget.firstDate, month);
if (jump) {
_pageController.jumpToPage(monthPage);
} else {
_pageController.animateToPage(
monthPage,
duration: _monthScrollDuration,
curve: Curves.ease,
);
}
}
/// True if the earliest allowable month is displayed.
bool get _isDisplayingFirstMonth {
return !_currentMonth.isAfter(
DateTime(widget.firstDate.year, widget.firstDate.month),
);
}
/// True if the latest allowable month is displayed.
bool get _isDisplayingLastMonth {
return !_currentMonth.isBefore(
DateTime(widget.lastDate.year, widget.lastDate.month),
);
}
/// Handler for when the overall day grid obtains or loses focus.
void _handleGridFocusChange(bool focused) {
setState(() {
if (focused && _focusedDay == null) {
if (DateUtils.isSameMonth(widget.selectedDate, _currentMonth)) {
_focusedDay = widget.selectedDate;
} else if (DateUtils.isSameMonth(widget.currentDate, _currentMonth)) {
_focusedDay = _focusableDayForMonth(_currentMonth, widget.currentDate.day);
} else {
_focusedDay = _focusableDayForMonth(_currentMonth, 1);
}
}
});
}
/// Move focus to the next element after the day grid.
void _handleGridNextFocus(NextFocusIntent intent) {
_dayGridFocus.requestFocus();
_dayGridFocus.nextFocus();
}
/// Move focus to the previous element before the day grid.
void _handleGridPreviousFocus(PreviousFocusIntent intent) {
_dayGridFocus.requestFocus();
_dayGridFocus.previousFocus();
}
/// Move the internal focus date in the direction of the given intent.
///
/// This will attempt to move the focused day to the next selectable day in
/// the given direction. If the new date is not in the current month, then
/// the page view will be scrolled to show the new date's month.
///
/// For horizontal directions, it will move forward or backward a day (depending
/// on the current [TextDirection]). For vertical directions it will move up and
/// down a week at a time.
void _handleDirectionFocus(DirectionalFocusIntent intent) {
assert(_focusedDay != null);
setState(() {
final DateTime? nextDate = _nextDateInDirection(_focusedDay!, intent.direction);
if (nextDate != null) {
_focusedDay = nextDate;
if (!DateUtils.isSameMonth(_focusedDay, _currentMonth)) {
_showMonth(_focusedDay!);
}
}
});
}
static const Map<TraversalDirection, int> _directionOffset = <TraversalDirection, int>{
TraversalDirection.up: -DateTime.daysPerWeek,
TraversalDirection.right: 1,
TraversalDirection.down: DateTime.daysPerWeek,
TraversalDirection.left: -1,
};
int _dayDirectionOffset(TraversalDirection traversalDirection, TextDirection textDirection) {
// Swap left and right if the text direction if RTL
if (textDirection == TextDirection.rtl) {
if (traversalDirection == TraversalDirection.left) {
traversalDirection = TraversalDirection.right;
} else if (traversalDirection == TraversalDirection.right) {
traversalDirection = TraversalDirection.left;
}
}
return _directionOffset[traversalDirection]!;
}
DateTime? _nextDateInDirection(DateTime date, TraversalDirection direction) {
final TextDirection textDirection = Directionality.of(context);
DateTime nextDate = DateUtils.addDaysToDate(date, _dayDirectionOffset(direction, textDirection));
while (!nextDate.isBefore(widget.firstDate) && !nextDate.isAfter(widget.lastDate)) {
if (_isSelectable(nextDate)) {
return nextDate;
}
nextDate = DateUtils.addDaysToDate(nextDate, _dayDirectionOffset(direction, textDirection));
}
return null;
}
bool _isSelectable(DateTime date) {
return widget.selectableDayPredicate == null || widget.selectableDayPredicate!.call(date);
}
Widget _buildItems(BuildContext context, int index) {
final DateTime month = DateUtils.addMonthsToMonthDate(widget.firstDate, index);
return _DayPicker(
key: ValueKey<DateTime>(month),
selectedDate: widget.selectedDate,
currentDate: widget.currentDate,
onChanged: _handleDateSelected,
firstDate: widget.firstDate,
lastDate: widget.lastDate,
displayedMonth: month,
selectableDayPredicate: widget.selectableDayPredicate,
);
}
@override
Widget build(BuildContext context) {
final Color controlColor = Theme.of(context).colorScheme.onSurface.withOpacity(0.60);
return Semantics(
child: Column(
children: <Widget>[
Container(
padding: const EdgeInsetsDirectional.only(start: 16, end: 4),
height: _subHeaderHeight,
child: Row(
children: <Widget>[
const Spacer(),
IconButton(
icon: const Icon(Icons.chevron_left),
color: controlColor,
tooltip: _isDisplayingFirstMonth ? null : _localizations.previousMonthTooltip,
onPressed: _isDisplayingFirstMonth ? null : _handlePreviousMonth,
),
IconButton(
icon: const Icon(Icons.chevron_right),
color: controlColor,
tooltip: _isDisplayingLastMonth ? null : _localizations.nextMonthTooltip,
onPressed: _isDisplayingLastMonth ? null : _handleNextMonth,
),
],
),
),
Expanded(
child: FocusableActionDetector(
shortcuts: _shortcutMap,
actions: _actionMap,
focusNode: _dayGridFocus,
onFocusChange: _handleGridFocusChange,
child: _FocusedDate(
date: _dayGridFocus.hasFocus ? _focusedDay : null,
child: PageView.builder(
key: _pageViewKey,
controller: _pageController,
itemBuilder: _buildItems,
itemCount: DateUtils.monthDelta(widget.firstDate, widget.lastDate) + 1,
onPageChanged: _handleMonthPageChanged,
),
),
),
),
],
),
);
}
}
/// InheritedWidget indicating what the current focused date is for its children.
///
/// This is used by the [_MonthPicker] to let its children [_DayPicker]s know
/// what the currently focused date (if any) should be.
class _FocusedDate extends InheritedWidget {
const _FocusedDate({
required super.child,
this.date,
});
final DateTime? date;
@override
bool updateShouldNotify(_FocusedDate oldWidget) {
return !DateUtils.isSameDay(date, oldWidget.date);
}
static DateTime? maybeOf(BuildContext context) {
final _FocusedDate? focusedDate = context.dependOnInheritedWidgetOfExactType<_FocusedDate>();
return focusedDate?.date;
}
}
/// Displays the days of a given month and allows choosing a day.
///
/// The days are arranged in a rectangular grid with one column for each day of
/// the week.
class _DayPicker extends StatefulWidget {
/// Creates a day picker.
_DayPicker({
super.key,
required this.currentDate,
required this.displayedMonth,
required this.firstDate,
required this.lastDate,
required this.selectedDate,
required this.onChanged,
this.selectableDayPredicate,
}) : assert(!firstDate.isAfter(lastDate)),
assert(selectedDate == null || !selectedDate.isBefore(firstDate)),
assert(selectedDate == null || !selectedDate.isAfter(lastDate));
/// The currently selected date.
///
/// This date is highlighted in the picker.
final DateTime? selectedDate;
/// The current date at the time the picker is displayed.
final DateTime currentDate;
/// Called when the user picks a day.
final ValueChanged<DateTime> onChanged;
/// The earliest date the user is permitted to pick.
///
/// This date must be on or before the [lastDate].
final DateTime firstDate;
/// The latest date the user is permitted to pick.
///
/// This date must be on or after the [firstDate].
final DateTime lastDate;
/// The month whose days are displayed by this picker.
final DateTime displayedMonth;
/// Optional user supplied predicate function to customize selectable days.
final SelectableDayPredicate? selectableDayPredicate;
@override
_DayPickerState createState() => _DayPickerState();
}
class _DayPickerState extends State<_DayPicker> {
/// List of [FocusNode]s, one for each day of the month.
late List<FocusNode> _dayFocusNodes;
@override
void initState() {
super.initState();
final int daysInMonth = DateUtils.getDaysInMonth(widget.displayedMonth.year, widget.displayedMonth.month);
_dayFocusNodes = List<FocusNode>.generate(
daysInMonth,
(int index) => FocusNode(skipTraversal: true, debugLabel: 'Day ${index + 1}'),
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
// Check to see if the focused date is in this month, if so focus it.
final DateTime? focusedDate = _FocusedDate.maybeOf(context);
if (focusedDate != null && DateUtils.isSameMonth(widget.displayedMonth, focusedDate)) {
_dayFocusNodes[focusedDate.day - 1].requestFocus();
}
}
@override
void dispose() {
for (final FocusNode node in _dayFocusNodes) {
node.dispose();
}
super.dispose();
}
/// Builds widgets showing abbreviated days of week. The first widget in the
/// returned list corresponds to the first day of week for the current locale.
///
/// Examples:
///
/// ┌ Sunday is the first day of week in the US (en_US)
/// |
/// S M T W T F S ← the returned list contains these widgets
/// _ _ _ _ _ 1 2
/// 3 4 5 6 7 8 9
///
/// ┌ But it's Monday in the UK (en_GB)
/// |
/// M T W T F S S ← the returned list contains these widgets
/// _ _ _ _ 1 2 3
/// 4 5 6 7 8 9 10
///
List<Widget> _dayHeaders(TextStyle? headerStyle, MaterialLocalizations localizations) {
final List<Widget> result = <Widget>[];
for (int i = localizations.firstDayOfWeekIndex; result.length < DateTime.daysPerWeek; i = (i + 1) % DateTime.daysPerWeek) {
final String weekday = localizations.narrowWeekdays[i];
result.add(ExcludeSemantics(
child: Center(child: Text(weekday, style: headerStyle)),
));
}
return result;
}
@override
Widget build(BuildContext context) {
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final DatePickerThemeData datePickerTheme = DatePickerTheme.of(context);
final DatePickerThemeData defaults = DatePickerTheme.defaults(context);
final TextStyle? weekdayStyle = datePickerTheme.weekdayStyle ?? defaults.weekdayStyle;
final int year = widget.displayedMonth.year;
final int month = widget.displayedMonth.month;
final int daysInMonth = DateUtils.getDaysInMonth(year, month);
final int dayOffset = DateUtils.firstDayOffset(year, month, localizations);
final List<Widget> dayItems = _dayHeaders(weekdayStyle, localizations);
// 1-based day of month, e.g. 1-31 for January, and 1-29 for February on
// a leap year.
int day = -dayOffset;
while (day < daysInMonth) {
day++;
if (day < 1) {
dayItems.add(Container());
} else {
final DateTime dayToBuild = DateTime(year, month, day);
final bool isDisabled =
dayToBuild.isAfter(widget.lastDate) ||
dayToBuild.isBefore(widget.firstDate) ||
(widget.selectableDayPredicate != null && !widget.selectableDayPredicate!(dayToBuild));
final bool isSelectedDay = DateUtils.isSameDay(widget.selectedDate, dayToBuild);
final bool isToday = DateUtils.isSameDay(widget.currentDate, dayToBuild);
dayItems.add(
_Day(
dayToBuild,
key: ValueKey<DateTime>(dayToBuild),
isDisabled: isDisabled,
isSelectedDay: isSelectedDay,
isToday: isToday,
onChanged: widget.onChanged,
focusNode: _dayFocusNodes[day - 1],
),
);
}
}
return Padding(
padding: const EdgeInsets.symmetric(
horizontal: _monthPickerHorizontalPadding,
),
child: GridView.custom(
physics: const ClampingScrollPhysics(),
gridDelegate: _dayPickerGridDelegate,
childrenDelegate: SliverChildListDelegate(
dayItems,
addRepaintBoundaries: false,
),
),
);
}
}
class _Day extends StatefulWidget {
const _Day(
this.day, {
super.key,
required this.isDisabled,
required this.isSelectedDay,
required this.isToday,
required this.onChanged,
required this.focusNode,
});
final DateTime day;
final bool isDisabled;
final bool isSelectedDay;
final bool isToday;
final ValueChanged<DateTime> onChanged;
final FocusNode? focusNode;
@override
State<_Day> createState() => _DayState();
}
class _DayState extends State<_Day> {
final MaterialStatesController _statesController = MaterialStatesController();
@override
Widget build(BuildContext context) {
final DatePickerThemeData defaults = DatePickerTheme.defaults(context);
final DatePickerThemeData datePickerTheme = DatePickerTheme.of(context);
final TextStyle? dayStyle = datePickerTheme.dayStyle ?? defaults.dayStyle;
T? effectiveValue<T>(T? Function(DatePickerThemeData? theme) getProperty) {
return getProperty(datePickerTheme) ?? getProperty(defaults);
}
T? resolve<T>(MaterialStateProperty<T>? Function(DatePickerThemeData? theme) getProperty, Set<MaterialState> states) {
return effectiveValue(
(DatePickerThemeData? theme) {
return getProperty(theme)?.resolve(states);
},
);
}
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final String semanticLabelSuffix = widget.isToday ? ', ${localizations.currentDateLabel}' : '';
final Set<MaterialState> states = <MaterialState>{
if (widget.isDisabled) MaterialState.disabled,
if (widget.isSelectedDay) MaterialState.selected,
};
_statesController.value = states;
final Color? dayForegroundColor = resolve<Color?>((DatePickerThemeData? theme) => widget.isToday ? theme?.todayForegroundColor : theme?.dayForegroundColor, states);
final Color? dayBackgroundColor = resolve<Color?>((DatePickerThemeData? theme) => widget.isToday ? theme?.todayBackgroundColor : theme?.dayBackgroundColor, states);
final MaterialStateProperty<Color?> dayOverlayColor = MaterialStateProperty.resolveWith<Color?>(
(Set<MaterialState> states) => effectiveValue((DatePickerThemeData? theme) => theme?.dayOverlayColor?.resolve(states)),
);
final OutlinedBorder dayShape = resolve<OutlinedBorder?>((DatePickerThemeData? theme) => theme?.dayShape, states)!;
final ShapeDecoration decoration = widget.isToday
? ShapeDecoration(
color: dayBackgroundColor,
shape: dayShape.copyWith(
side: (datePickerTheme.todayBorder ?? defaults.todayBorder!)
.copyWith(color: dayForegroundColor),
),
)
: ShapeDecoration(
color: dayBackgroundColor,
shape: dayShape,
);
Widget dayWidget = DecoratedBox(
decoration: decoration,
child: Center(
child: Text(localizations.formatDecimal(widget.day.day), style: dayStyle?.apply(color: dayForegroundColor)),
),
);
if (widget.isDisabled) {
dayWidget = ExcludeSemantics(
child: dayWidget,
);
} else {
dayWidget = InkResponse(
focusNode: widget.focusNode,
onTap: () => widget.onChanged(widget.day),
statesController: _statesController,
overlayColor: dayOverlayColor,
customBorder: dayShape,
containedInkWell: true,
child: Semantics(
// We want the day of month to be spoken first irrespective of the
// locale-specific preferences or TextDirection. This is because
// an accessibility user is more likely to be interested in the
// day of month before the rest of the date, as they are looking
// for the day of month. To do that we prepend day of month to the
// formatted full date.
label: '${localizations.formatDecimal(widget.day.day)}, ${localizations.formatFullDate(widget.day)}$semanticLabelSuffix',
// Set button to true to make the date selectable.
button: true,
selected: widget.isSelectedDay,
excludeSemantics: true,
child: dayWidget,
),
);
}
return dayWidget;
}
@override
void dispose() {
_statesController.dispose();
super.dispose();
}
}
class _DayPickerGridDelegate extends SliverGridDelegate {
const _DayPickerGridDelegate();
@override
SliverGridLayout getLayout(SliverConstraints constraints) {
const int columnCount = DateTime.daysPerWeek;
final double tileWidth = constraints.crossAxisExtent / columnCount;
final double tileHeight = math.min(
_dayPickerRowHeight,
constraints.viewportMainAxisExtent / (_maxDayPickerRowCount + 1),
);
return SliverGridRegularTileLayout(
childCrossAxisExtent: tileWidth,
childMainAxisExtent: tileHeight,
crossAxisCount: columnCount,
crossAxisStride: tileWidth,
mainAxisStride: tileHeight,
reverseCrossAxis: axisDirectionIsReversed(constraints.crossAxisDirection),
);
}
@override
bool shouldRelayout(_DayPickerGridDelegate oldDelegate) => false;
}
const _DayPickerGridDelegate _dayPickerGridDelegate = _DayPickerGridDelegate();
/// A scrollable grid of years to allow picking a year.
///
/// The year picker widget is rarely used directly. Instead, consider using
/// [CalendarDatePicker], or [showDatePicker] which create full date pickers.
///
/// See also:
///
/// * [CalendarDatePicker], which provides a Material Design date picker
/// interface.
///
/// * [showDatePicker], which shows a dialog containing a Material Design
/// date picker.
///
class YearPicker extends StatefulWidget {
/// Creates a year picker.
///
/// The [lastDate] must be after the [firstDate].
YearPicker({
super.key,
DateTime? currentDate,
required this.firstDate,
required this.lastDate,
@Deprecated(
'This parameter has no effect and can be removed. Previously it controlled '
'the month that was used in "onChanged" when a new year was selected, but '
'now that role is filled by "selectedDate" instead. '
'This feature was deprecated after v3.13.0-0.3.pre.'
)
DateTime? initialDate,
required this.selectedDate,
required this.onChanged,
this.dragStartBehavior = DragStartBehavior.start,
}) : assert(!firstDate.isAfter(lastDate)),
currentDate = DateUtils.dateOnly(currentDate ?? DateTime.now());
/// The current date.
///
/// This date is subtly highlighted in the picker.
final DateTime currentDate;
/// The earliest date the user is permitted to pick.
final DateTime firstDate;
/// The latest date the user is permitted to pick.
final DateTime lastDate;
/// The currently selected date.
///
/// This date is highlighted in the picker.
final DateTime? selectedDate;
/// Called when the user picks a year.
final ValueChanged<DateTime> onChanged;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
@override
State<YearPicker> createState() => _YearPickerState();
}
class _YearPickerState extends State<YearPicker> {
ScrollController? _scrollController;
final MaterialStatesController _statesController = MaterialStatesController();
// The approximate number of years necessary to fill the available space.
static const int minYears = 18;
@override
void initState() {
super.initState();
_scrollController = ScrollController(initialScrollOffset: _scrollOffsetForYear(widget.selectedDate ?? widget.firstDate));
}
@override
void dispose() {
_scrollController?.dispose();
_statesController.dispose();
super.dispose();
}
@override
void didUpdateWidget(YearPicker oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.selectedDate != oldWidget.selectedDate && widget.selectedDate != null) {
_scrollController!.jumpTo(_scrollOffsetForYear(widget.selectedDate!));
}
}
double _scrollOffsetForYear(DateTime date) {
final int initialYearIndex = date.year - widget.firstDate.year;
final int initialYearRow = initialYearIndex ~/ _yearPickerColumnCount;
// Move the offset down by 2 rows to approximately center it.
final int centeredYearRow = initialYearRow - 2;
return _itemCount < minYears ? 0 : centeredYearRow * _yearPickerRowHeight;
}
Widget _buildYearItem(BuildContext context, int index) {
final DatePickerThemeData datePickerTheme = DatePickerTheme.of(context);
final DatePickerThemeData defaults = DatePickerTheme.defaults(context);
T? effectiveValue<T>(T? Function(DatePickerThemeData? theme) getProperty) {
return getProperty(datePickerTheme) ?? getProperty(defaults);
}
T? resolve<T>(MaterialStateProperty<T>? Function(DatePickerThemeData? theme) getProperty, Set<MaterialState> states) {
return effectiveValue(
(DatePickerThemeData? theme) {
return getProperty(theme)?.resolve(states);
},
);
}
// Backfill the _YearPicker with disabled years if necessary.
final int offset = _itemCount < minYears ? (minYears - _itemCount) ~/ 2 : 0;
final int year = widget.firstDate.year + index - offset;
final bool isSelected = year == widget.selectedDate?.year;
final bool isCurrentYear = year == widget.currentDate.year;
final bool isDisabled = year < widget.firstDate.year || year > widget.lastDate.year;
const double decorationHeight = 36.0;
const double decorationWidth = 72.0;
final Set<MaterialState> states = <MaterialState>{
if (isDisabled) MaterialState.disabled,
if (isSelected) MaterialState.selected,
};
final Color? textColor = resolve<Color?>((DatePickerThemeData? theme) => isCurrentYear ? theme?.todayForegroundColor : theme?.yearForegroundColor, states);
final Color? background = resolve<Color?>((DatePickerThemeData? theme) => isCurrentYear ? theme?.todayBackgroundColor : theme?.yearBackgroundColor, states);
final MaterialStateProperty<Color?> overlayColor =
MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) =>
effectiveValue((DatePickerThemeData? theme) => theme?.yearOverlayColor?.resolve(states)),
);
BoxBorder? border;
if (isCurrentYear) {
final BorderSide? todayBorder = datePickerTheme.todayBorder ?? defaults.todayBorder;
if (todayBorder != null) {
border = Border.fromBorderSide(todayBorder.copyWith(color: textColor));
}
}
final BoxDecoration decoration = BoxDecoration(
border: border,
color: background,
borderRadius: BorderRadius.circular(decorationHeight / 2),
);
final TextStyle? itemStyle = (datePickerTheme.yearStyle ?? defaults.yearStyle)?.apply(color: textColor);
Widget yearItem = Center(
child: Container(
decoration: decoration,
height: decorationHeight,
width: decorationWidth,
child: Center(
child: Semantics(
selected: isSelected,
button: true,
child: Text(year.toString(), style: itemStyle),
),
),
),
);
if (isDisabled) {
yearItem = ExcludeSemantics(
child: yearItem,
);
} else {
DateTime date = DateTime(year, widget.selectedDate?.month ?? DateTime.january);
if (date.isBefore(DateTime(widget.firstDate.year, widget.firstDate.month))) {
// Ignore firstDate.day because we're just working in years and months here.
assert(date.year == widget.firstDate.year);
date = DateTime(year, widget.firstDate.month);
} else if (date.isAfter(widget.lastDate)) {
// No need to ignore the day here because it can only be bigger than what we care about.
assert(date.year == widget.lastDate.year);
date = DateTime(year, widget.lastDate.month);
}
_statesController.value = states;
yearItem = InkWell(
key: ValueKey<int>(year),
onTap: () => widget.onChanged(date),
statesController: _statesController,
overlayColor: overlayColor,
child: yearItem,
);
}
return yearItem;
}
int get _itemCount {
return widget.lastDate.year - widget.firstDate.year + 1;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
return Column(
children: <Widget>[
const Divider(),
Expanded(
child: GridView.builder(
controller: _scrollController,
dragStartBehavior: widget.dragStartBehavior,
gridDelegate: _yearPickerGridDelegate,
itemBuilder: _buildYearItem,
itemCount: math.max(_itemCount, minYears),
padding: const EdgeInsets.symmetric(horizontal: _yearPickerPadding),
),
),
const Divider(),
],
);
}
}
class _YearPickerGridDelegate extends SliverGridDelegate {
const _YearPickerGridDelegate();
@override
SliverGridLayout getLayout(SliverConstraints constraints) {
final double tileWidth =
(constraints.crossAxisExtent - (_yearPickerColumnCount - 1) * _yearPickerRowSpacing) / _yearPickerColumnCount;
return SliverGridRegularTileLayout(
childCrossAxisExtent: tileWidth,
childMainAxisExtent: _yearPickerRowHeight,
crossAxisCount: _yearPickerColumnCount,
crossAxisStride: tileWidth + _yearPickerRowSpacing,
mainAxisStride: _yearPickerRowHeight,
reverseCrossAxis: axisDirectionIsReversed(constraints.crossAxisDirection),
);
}
@override
bool shouldRelayout(_YearPickerGridDelegate oldDelegate) => false;
}
const _YearPickerGridDelegate _yearPickerGridDelegate = _YearPickerGridDelegate();
| flutter/packages/flutter/lib/src/material/calendar_date_picker.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/calendar_date_picker.dart",
"repo_id": "flutter",
"token_count": 17655
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'material_state.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [DataTable]
/// widgets.
///
/// Descendant widgets obtain the current [DataTableThemeData] object
/// using `DataTableTheme.of(context)`. Instances of
/// [DataTableThemeData] can be customized with
/// [DataTableThemeData.copyWith].
///
/// Typically a [DataTableThemeData] is specified as part of the
/// overall [Theme] with [ThemeData.dataTableTheme].
///
/// All [DataTableThemeData] properties are `null` by default. When
/// null, the [DataTable] will use the values from [ThemeData] if they exist,
/// otherwise it will provide its own defaults based on the overall [Theme]'s
/// textTheme and colorScheme. See the individual [DataTable] properties for
/// details.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class DataTableThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.dataTableTheme].
const DataTableThemeData({
this.decoration,
this.dataRowColor,
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? dataRowHeight,
double? dataRowMinHeight,
double? dataRowMaxHeight,
this.dataTextStyle,
this.headingRowColor,
this.headingRowHeight,
this.headingTextStyle,
this.horizontalMargin,
this.columnSpacing,
this.dividerThickness,
this.checkboxHorizontalMargin,
this.headingCellCursor,
this.dataRowCursor,
}) : assert(dataRowMinHeight == null || dataRowMaxHeight == null || dataRowMaxHeight >= dataRowMinHeight),
assert(dataRowHeight == null || (dataRowMinHeight == null && dataRowMaxHeight == null),
'dataRowHeight ($dataRowHeight) must not be set if dataRowMinHeight ($dataRowMinHeight) or dataRowMaxHeight ($dataRowMaxHeight) are set.'),
dataRowMinHeight = dataRowHeight ?? dataRowMinHeight,
dataRowMaxHeight = dataRowHeight ?? dataRowMaxHeight;
/// {@macro flutter.material.dataTable.decoration}
final Decoration? decoration;
/// {@macro flutter.material.dataTable.dataRowColor}
/// {@macro flutter.material.DataTable.dataRowColor}
final MaterialStateProperty<Color?>? dataRowColor;
/// {@macro flutter.material.dataTable.dataRowHeight}
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? get dataRowHeight => dataRowMinHeight == dataRowMaxHeight ? dataRowMinHeight : null;
/// {@macro flutter.material.dataTable.dataRowMinHeight}
final double? dataRowMinHeight;
/// {@macro flutter.material.dataTable.dataRowMaxHeight}
final double? dataRowMaxHeight;
/// {@macro flutter.material.dataTable.dataTextStyle}
final TextStyle? dataTextStyle;
/// {@macro flutter.material.dataTable.headingRowColor}
/// {@macro flutter.material.DataTable.headingRowColor}
final MaterialStateProperty<Color?>? headingRowColor;
/// {@macro flutter.material.dataTable.headingRowHeight}
final double? headingRowHeight;
/// {@macro flutter.material.dataTable.headingTextStyle}
final TextStyle? headingTextStyle;
/// {@macro flutter.material.dataTable.horizontalMargin}
final double? horizontalMargin;
/// {@macro flutter.material.dataTable.columnSpacing}
final double? columnSpacing;
/// {@macro flutter.material.dataTable.dividerThickness}
final double? dividerThickness;
/// {@macro flutter.material.dataTable.checkboxHorizontalMargin}
final double? checkboxHorizontalMargin;
/// If specified, overrides the default value of [DataColumn.mouseCursor].
final MaterialStateProperty<MouseCursor?>? headingCellCursor;
/// If specified, overrides the default value of [DataRow.mouseCursor].
final MaterialStateProperty<MouseCursor?>? dataRowCursor;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
DataTableThemeData copyWith({
Decoration? decoration,
MaterialStateProperty<Color?>? dataRowColor,
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? dataRowHeight,
double? dataRowMinHeight,
double? dataRowMaxHeight,
TextStyle? dataTextStyle,
MaterialStateProperty<Color?>? headingRowColor,
double? headingRowHeight,
TextStyle? headingTextStyle,
double? horizontalMargin,
double? columnSpacing,
double? dividerThickness,
double? checkboxHorizontalMargin,
MaterialStateProperty<MouseCursor?>? headingCellCursor,
MaterialStateProperty<MouseCursor?>? dataRowCursor,
}) {
assert(dataRowHeight == null || (dataRowMinHeight == null && dataRowMaxHeight == null),
'dataRowHeight ($dataRowHeight) must not be set if dataRowMinHeight ($dataRowMinHeight) or dataRowMaxHeight ($dataRowMaxHeight) are set.');
dataRowMinHeight = dataRowHeight ?? dataRowMinHeight;
dataRowMaxHeight = dataRowHeight ?? dataRowMaxHeight;
return DataTableThemeData(
decoration: decoration ?? this.decoration,
dataRowColor: dataRowColor ?? this.dataRowColor,
dataRowMinHeight: dataRowMinHeight ?? this.dataRowMinHeight,
dataRowMaxHeight: dataRowMaxHeight ?? this.dataRowMaxHeight,
dataTextStyle: dataTextStyle ?? this.dataTextStyle,
headingRowColor: headingRowColor ?? this.headingRowColor,
headingRowHeight: headingRowHeight ?? this.headingRowHeight,
headingTextStyle: headingTextStyle ?? this.headingTextStyle,
horizontalMargin: horizontalMargin ?? this.horizontalMargin,
columnSpacing: columnSpacing ?? this.columnSpacing,
dividerThickness: dividerThickness ?? this.dividerThickness,
checkboxHorizontalMargin: checkboxHorizontalMargin ?? this.checkboxHorizontalMargin,
headingCellCursor: headingCellCursor ?? this.headingCellCursor,
dataRowCursor: dataRowCursor ?? this.dataRowCursor,
);
}
/// Linearly interpolate between two [DataTableThemeData]s.
///
/// {@macro dart.ui.shadow.lerp}
static DataTableThemeData lerp(DataTableThemeData a, DataTableThemeData b, double t) {
if (identical(a, b)) {
return a;
}
return DataTableThemeData(
decoration: Decoration.lerp(a.decoration, b.decoration, t),
dataRowColor: MaterialStateProperty.lerp<Color?>(a.dataRowColor, b.dataRowColor, t, Color.lerp),
dataRowMinHeight: lerpDouble(a.dataRowMinHeight, b.dataRowMinHeight, t),
dataRowMaxHeight: lerpDouble(a.dataRowMaxHeight, b.dataRowMaxHeight, t),
dataTextStyle: TextStyle.lerp(a.dataTextStyle, b.dataTextStyle, t),
headingRowColor: MaterialStateProperty.lerp<Color?>(a.headingRowColor, b.headingRowColor, t, Color.lerp),
headingRowHeight: lerpDouble(a.headingRowHeight, b.headingRowHeight, t),
headingTextStyle: TextStyle.lerp(a.headingTextStyle, b.headingTextStyle, t),
horizontalMargin: lerpDouble(a.horizontalMargin, b.horizontalMargin, t),
columnSpacing: lerpDouble(a.columnSpacing, b.columnSpacing, t),
dividerThickness: lerpDouble(a.dividerThickness, b.dividerThickness, t),
checkboxHorizontalMargin: lerpDouble(a.checkboxHorizontalMargin, b.checkboxHorizontalMargin, t),
headingCellCursor: t < 0.5 ? a.headingCellCursor : b.headingCellCursor,
dataRowCursor: t < 0.5 ? a.dataRowCursor : b.dataRowCursor,
);
}
@override
int get hashCode => Object.hash(
decoration,
dataRowColor,
dataRowMinHeight,
dataRowMaxHeight,
dataTextStyle,
headingRowColor,
headingRowHeight,
headingTextStyle,
horizontalMargin,
columnSpacing,
dividerThickness,
checkboxHorizontalMargin,
headingCellCursor,
dataRowCursor,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is DataTableThemeData
&& other.decoration == decoration
&& other.dataRowColor == dataRowColor
&& other.dataRowMinHeight == dataRowMinHeight
&& other.dataRowMaxHeight == dataRowMaxHeight
&& other.dataTextStyle == dataTextStyle
&& other.headingRowColor == headingRowColor
&& other.headingRowHeight == headingRowHeight
&& other.headingTextStyle == headingTextStyle
&& other.horizontalMargin == horizontalMargin
&& other.columnSpacing == columnSpacing
&& other.dividerThickness == dividerThickness
&& other.checkboxHorizontalMargin == checkboxHorizontalMargin
&& other.headingCellCursor == headingCellCursor
&& other.dataRowCursor == dataRowCursor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Decoration>('decoration', decoration, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('dataRowColor', dataRowColor, defaultValue: null));
properties.add(DoubleProperty('dataRowMinHeight', dataRowMinHeight, defaultValue: null));
properties.add(DoubleProperty('dataRowMaxHeight', dataRowMaxHeight, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('dataTextStyle', dataTextStyle, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('headingRowColor', headingRowColor, defaultValue: null));
properties.add(DoubleProperty('headingRowHeight', headingRowHeight, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('headingTextStyle', headingTextStyle, defaultValue: null));
properties.add(DoubleProperty('horizontalMargin', horizontalMargin, defaultValue: null));
properties.add(DoubleProperty('columnSpacing', columnSpacing, defaultValue: null));
properties.add(DoubleProperty('dividerThickness', dividerThickness, defaultValue: null));
properties.add(DoubleProperty('checkboxHorizontalMargin', checkboxHorizontalMargin, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>?>('headingCellCursor', headingCellCursor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>?>('dataRowCursor', dataRowCursor, defaultValue: null));
}
}
/// Applies a data table theme to descendant [DataTable] widgets.
///
/// Descendant widgets obtain the current theme's [DataTableTheme] object using
/// [DataTableTheme.of]. When a widget uses [DataTableTheme.of], it is
/// automatically rebuilt if the theme later changes.
///
/// A data table theme can be specified as part of the overall Material
/// theme using [ThemeData.dataTableTheme].
///
/// See also:
///
/// * [DataTableThemeData], which describes the actual configuration
/// of a data table theme.
class DataTableTheme extends InheritedWidget {
/// Constructs a data table theme that configures all descendant
/// [DataTable] widgets.
const DataTableTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [DataTable] widgets.
final DataTableThemeData data;
/// Returns the configuration [data] from the closest
/// [DataTableTheme] ancestor. If there is no ancestor, it returns
/// [ThemeData.dataTableTheme]. Applications can assume that the
/// returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// DataTableThemeData theme = DataTableTheme.of(context);
/// ```
static DataTableThemeData of(BuildContext context) {
final DataTableTheme? dataTableTheme = context.dependOnInheritedWidgetOfExactType<DataTableTheme>();
return dataTableTheme?.data ?? Theme.of(context).dataTableTheme;
}
@override
bool updateShouldNotify(DataTableTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/data_table_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/data_table_theme.dart",
"repo_id": "flutter",
"token_count": 3953
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'dropdown_menu_theme.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'input_border.dart';
import 'input_decorator.dart';
import 'material_state.dart';
import 'menu_anchor.dart';
import 'menu_style.dart';
import 'text_field.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// late BuildContext context;
// late FocusNode myFocusNode;
/// A callback function that returns the index of the item that matches the
/// current contents of a text field.
///
/// If a match doesn't exist then null must be returned.
///
/// Used by [DropdownMenu.searchCallback].
typedef SearchCallback<T> = int? Function(List<DropdownMenuEntry<T>> entries, String query);
// Navigation shortcuts to move the selected menu items up or down.
final Map<ShortcutActivator, Intent> _kMenuTraversalShortcuts = <ShortcutActivator, Intent> {
LogicalKeySet(LogicalKeyboardKey.arrowUp): const _ArrowUpIntent(),
LogicalKeySet(LogicalKeyboardKey.arrowDown): const _ArrowDownIntent(),
};
const double _kMinimumWidth = 112.0;
const double _kDefaultHorizontalPadding = 12.0;
/// Defines a [DropdownMenu] menu button that represents one item view in the menu.
///
/// See also:
///
/// * [DropdownMenu]
class DropdownMenuEntry<T> {
/// Creates an entry that is used with [DropdownMenu.dropdownMenuEntries].
const DropdownMenuEntry({
required this.value,
required this.label,
this.labelWidget,
this.leadingIcon,
this.trailingIcon,
this.enabled = true,
this.style,
});
/// the value used to identify the entry.
///
/// This value must be unique across all entries in a [DropdownMenu].
final T value;
/// The label displayed in the center of the menu item.
final String label;
/// Overrides the default label widget which is `Text(label)`.
///
/// {@tool dartpad}
/// This sample shows how to override the default label [Text]
/// widget with one that forces the menu entry to appear on one line
/// by specifying [Text.maxLines] and [Text.overflow].
///
/// ** See code in examples/api/lib/material/dropdown_menu/dropdown_menu_entry_label_widget.0.dart **
/// {@end-tool}
final Widget? labelWidget;
/// An optional icon to display before the label.
final Widget? leadingIcon;
/// An optional icon to display after the label.
final Widget? trailingIcon;
/// Whether the menu item is enabled or disabled.
///
/// The default value is true. If true, the [DropdownMenuEntry.label] will be filled
/// out in the text field of the [DropdownMenu] when this entry is clicked; otherwise,
/// this entry is disabled.
final bool enabled;
/// Customizes this menu item's appearance.
///
/// Null by default.
final ButtonStyle? style;
}
/// A dropdown menu that can be opened from a [TextField]. The selected
/// menu item is displayed in that field.
///
/// This widget is used to help people make a choice from a menu and put the
/// selected item into the text input field. People can also filter the list based
/// on the text input or search one item in the menu list.
///
/// The menu is composed of a list of [DropdownMenuEntry]s. People can provide information,
/// such as: label, leading icon or trailing icon for each entry. The [TextField]
/// will be updated based on the selection from the menu entries. The text field
/// will stay empty if the selected entry is disabled.
///
/// The dropdown menu can be traversed by pressing the up or down key. During the
/// process, the corresponding item will be highlighted and the text field will be updated.
/// Disabled items will be skipped during traversal.
///
/// The menu can be scrollable if not all items in the list are displayed at once.
///
/// {@tool dartpad}
/// This sample shows how to display outlined [DropdownMenu] and filled [DropdownMenu].
///
/// ** See code in examples/api/lib/material/dropdown_menu/dropdown_menu.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [MenuAnchor], which is a widget used to mark the "anchor" for a set of submenus.
/// The [DropdownMenu] uses a [TextField] as the "anchor".
/// * [TextField], which is a text input widget that uses an [InputDecoration].
/// * [DropdownMenuEntry], which is used to build the [MenuItemButton] in the [DropdownMenu] list.
class DropdownMenu<T> extends StatefulWidget {
/// Creates a const [DropdownMenu].
///
/// The leading and trailing icons in the text field can be customized by using
/// [leadingIcon], [trailingIcon] and [selectedTrailingIcon] properties. They are
/// passed down to the [InputDecoration] properties, and will override values
/// in the [InputDecoration.prefixIcon] and [InputDecoration.suffixIcon].
///
/// Except leading and trailing icons, the text field can be configured by the
/// [InputDecorationTheme] property. The menu can be configured by the [menuStyle].
const DropdownMenu({
super.key,
this.enabled = true,
this.width,
this.menuHeight,
this.leadingIcon,
this.trailingIcon,
this.label,
this.hintText,
this.helperText,
this.errorText,
this.selectedTrailingIcon,
this.enableFilter = false,
this.enableSearch = true,
this.textStyle,
this.inputDecorationTheme,
this.menuStyle,
this.controller,
this.initialSelection,
this.onSelected,
this.focusNode,
this.requestFocusOnTap,
this.expandedInsets,
this.searchCallback,
required this.dropdownMenuEntries,
this.inputFormatters,
});
/// Determine if the [DropdownMenu] is enabled.
///
/// Defaults to true.
final bool enabled;
/// Determine the width of the [DropdownMenu].
///
/// If this is null, the width of the [DropdownMenu] will be the same as the width of the widest
/// menu item plus the width of the leading/trailing icon.
final double? width;
/// Determine the height of the menu.
///
/// If this is null, the menu will display as many items as possible on the screen.
final double? menuHeight;
/// An optional Icon at the front of the text input field.
///
/// Defaults to null. If this is not null, the menu items will have extra paddings to be aligned
/// with the text in the text field.
final Widget? leadingIcon;
/// An optional icon at the end of the text field.
///
/// Defaults to an [Icon] with [Icons.arrow_drop_down].
final Widget? trailingIcon;
/// Optional widget that describes the input field.
///
/// When the input field is empty and unfocused, the label is displayed on
/// top of the input field (i.e., at the same location on the screen where
/// text may be entered in the input field). When the input field receives
/// focus (or if the field is non-empty), the label moves above, either
/// vertically adjacent to, or to the center of the input field.
///
/// Defaults to null.
final Widget? label;
/// Text that suggests what sort of input the field accepts.
///
/// Defaults to null;
final String? hintText;
/// Text that provides context about the [DropdownMenu]'s value, such
/// as how the value will be used.
///
/// If non-null, the text is displayed below the input field, in
/// the same location as [errorText]. If a non-null [errorText] value is
/// specified then the helper text is not shown.
///
/// Defaults to null;
///
/// See also:
///
/// * [InputDecoration.helperText], which is the text that provides context about the [InputDecorator.child]'s value.
final String? helperText;
/// Text that appears below the input field and the border to show the error message.
///
/// If non-null, the border's color animates to red and the [helperText] is not shown.
///
/// Defaults to null;
///
/// See also:
///
/// * [InputDecoration.errorText], which is the text that appears below the [InputDecorator.child] and the border.
final String? errorText;
/// An optional icon at the end of the text field to indicate that the text
/// field is pressed.
///
/// Defaults to an [Icon] with [Icons.arrow_drop_up].
final Widget? selectedTrailingIcon;
/// Determine if the menu list can be filtered by the text input.
///
/// Defaults to false.
final bool enableFilter;
/// Determine if the first item that matches the text input can be highlighted.
///
/// Defaults to true as the search function could be commonly used.
final bool enableSearch;
/// The text style for the [TextField] of the [DropdownMenu];
///
/// Defaults to the overall theme's [TextTheme.bodyLarge]
/// if the dropdown menu theme's value is null.
final TextStyle? textStyle;
/// Defines the default appearance of [InputDecoration] to show around the text field.
///
/// By default, shows a outlined text field.
final InputDecorationTheme? inputDecorationTheme;
/// The [MenuStyle] that defines the visual attributes of the menu.
///
/// The default width of the menu is set to the width of the text field.
final MenuStyle? menuStyle;
/// Controls the text being edited or selected in the menu.
///
/// If null, this widget will create its own [TextEditingController].
final TextEditingController? controller;
/// The value used to for an initial selection.
///
/// Defaults to null.
final T? initialSelection;
/// The callback is called when a selection is made.
///
/// Defaults to null. If null, only the text field is updated.
final ValueChanged<T?>? onSelected;
/// Defines the keyboard focus for this widget.
///
/// The [focusNode] is a long-lived object that's typically managed by a
/// [StatefulWidget] parent. See [FocusNode] for more information.
///
/// To give the keyboard focus to this widget, provide a [focusNode] and then
/// use the current [FocusScope] to request the focus:
///
/// ```dart
/// FocusScope.of(context).requestFocus(myFocusNode);
/// ```
///
/// This happens automatically when the widget is tapped.
///
/// To be notified when the widget gains or loses the focus, add a listener
/// to the [focusNode]:
///
/// ```dart
/// myFocusNode.addListener(() { print(myFocusNode.hasFocus); });
/// ```
///
/// If null, this widget will create its own [FocusNode].
///
/// ## Keyboard
///
/// Requesting the focus will typically cause the keyboard to be shown
/// if it's not showing already.
///
/// On Android, the user can hide the keyboard - without changing the focus -
/// with the system back button. They can restore the keyboard's visibility
/// by tapping on a text field. The user might hide the keyboard and
/// switch to a physical keyboard, or they might just need to get it
/// out of the way for a moment, to expose something it's
/// obscuring. In this case requesting the focus again will not
/// cause the focus to change, and will not make the keyboard visible.
///
/// If this is non-null, the behaviour of [requestFocusOnTap] is overridden
/// by the [FocusNode.canRequestFocus] property.
final FocusNode? focusNode;
/// Determine if the dropdown button requests focus and the on-screen virtual
/// keyboard is shown in response to a touch event.
///
/// Ignored if a [focusNode] is explicitly provided (in which case,
/// [FocusNode.canRequestFocus] controls the behavior).
///
/// Defaults to null, which enables platform-specific behavior:
///
/// * On mobile platforms, acts as if set to false; tapping on the text
/// field and opening the menu will not cause a focus request and the
/// virtual keyboard will not appear.
///
/// * On desktop platforms, acts as if set to true; the dropdown takes the
/// focus when activated.
///
/// Set this to true or false explicitly to override the default behavior.
final bool? requestFocusOnTap;
/// Descriptions of the menu items in the [DropdownMenu].
///
/// This is a required parameter. It is recommended that at least one [DropdownMenuEntry]
/// is provided. If this is an empty list, the menu will be empty and only
/// contain space for padding.
final List<DropdownMenuEntry<T>> dropdownMenuEntries;
/// Defines the menu text field's width to be equal to its parent's width
/// plus the horizontal width of the specified insets.
///
/// If this property is null, the width of the text field will be determined
/// by the width of menu items or [DropdownMenu.width]. If this property is not null,
/// the text field's width will match the parent's width plus the specified insets.
/// If the value of this property is [EdgeInsets.zero], the width of the text field will be the same
/// as its parent's width.
///
/// The [expandedInsets]' top and bottom are ignored, only its left and right
/// properties are used.
///
/// Defaults to null.
final EdgeInsets? expandedInsets;
/// When [DropdownMenu.enableSearch] is true, this callback is used to compute
/// the index of the search result to be highlighted.
///
/// {@tool snippet}
///
/// In this example the `searchCallback` returns the index of the search result
/// that exactly matches the query.
///
/// ```dart
/// DropdownMenu<Text>(
/// searchCallback: (List<DropdownMenuEntry<Text>> entries, String query) {
/// if (query.isEmpty) {
/// return null;
/// }
/// final int index = entries.indexWhere((DropdownMenuEntry<Text> entry) => entry.label == query);
///
/// return index != -1 ? index : null;
/// },
/// dropdownMenuEntries: const <DropdownMenuEntry<Text>>[],
/// )
/// ```
/// {@end-tool}
///
/// Defaults to null. If this is null and [DropdownMenu.enableSearch] is true,
/// the default function will return the index of the first matching result
/// which contains the contents of the text input field.
final SearchCallback<T>? searchCallback;
/// Optional input validation and formatting overrides.
///
/// Formatters are run in the provided order when the user changes the text
/// this widget contains. When this parameter changes, the new formatters will
/// not be applied until the next time the user inserts or deletes text.
/// Formatters don't run when the text is changed
/// programmatically via [controller].
///
/// See also:
///
/// * [TextEditingController], which implements the [Listenable] interface
/// and notifies its listeners on [TextEditingValue] changes.
final List<TextInputFormatter>? inputFormatters;
@override
State<DropdownMenu<T>> createState() => _DropdownMenuState<T>();
}
class _DropdownMenuState<T> extends State<DropdownMenu<T>> {
final GlobalKey _anchorKey = GlobalKey();
final GlobalKey _leadingKey = GlobalKey();
late List<GlobalKey> buttonItemKeys;
final MenuController _controller = MenuController();
late bool _enableFilter;
late List<DropdownMenuEntry<T>> filteredEntries;
List<Widget>? _initialMenu;
int? currentHighlight;
double? leadingPadding;
bool _menuHasEnabledItem = false;
TextEditingController? _localTextEditingController;
TextEditingController get _textEditingController {
return widget.controller ?? (_localTextEditingController ??= TextEditingController());
}
@override
void initState() {
super.initState();
_enableFilter = widget.enableFilter;
filteredEntries = widget.dropdownMenuEntries;
buttonItemKeys = List<GlobalKey>.generate(filteredEntries.length, (int index) => GlobalKey());
_menuHasEnabledItem = filteredEntries.any((DropdownMenuEntry<T> entry) => entry.enabled);
final int index = filteredEntries.indexWhere((DropdownMenuEntry<T> entry) => entry.value == widget.initialSelection);
if (index != -1) {
_textEditingController.value = TextEditingValue(
text: filteredEntries[index].label,
selection: TextSelection.collapsed(offset: filteredEntries[index].label.length),
);
}
refreshLeadingPadding();
}
@override
void dispose() {
_localTextEditingController?.dispose();
_localTextEditingController = null;
super.dispose();
}
@override
void didUpdateWidget(DropdownMenu<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.controller != widget.controller) {
if (widget.controller != null) {
_localTextEditingController?.dispose();
_localTextEditingController = null;
}
}
if (oldWidget.enableSearch != widget.enableSearch) {
if (!widget.enableSearch) {
currentHighlight = null;
}
}
if (oldWidget.dropdownMenuEntries != widget.dropdownMenuEntries) {
currentHighlight = null;
filteredEntries = widget.dropdownMenuEntries;
buttonItemKeys = List<GlobalKey>.generate(filteredEntries.length, (int index) => GlobalKey());
_menuHasEnabledItem = filteredEntries.any((DropdownMenuEntry<T> entry) => entry.enabled);
}
if (oldWidget.leadingIcon != widget.leadingIcon) {
refreshLeadingPadding();
}
if (oldWidget.initialSelection != widget.initialSelection) {
final int index = filteredEntries.indexWhere((DropdownMenuEntry<T> entry) => entry.value == widget.initialSelection);
if (index != -1) {
_textEditingController.value = TextEditingValue(
text: filteredEntries[index].label,
selection: TextSelection.collapsed(offset: filteredEntries[index].label.length),
);
}
}
}
bool canRequestFocus() {
return widget.focusNode?.canRequestFocus ?? widget.requestFocusOnTap
?? switch (Theme.of(context).platform) {
TargetPlatform.iOS || TargetPlatform.android || TargetPlatform.fuchsia => false,
TargetPlatform.macOS || TargetPlatform.linux || TargetPlatform.windows => true,
};
}
void refreshLeadingPadding() {
WidgetsBinding.instance.addPostFrameCallback((_) {
if (!mounted) {
return;
}
setState(() {
leadingPadding = getWidth(_leadingKey);
});
}, debugLabel: 'DropdownMenu.refreshLeadingPadding');
}
void scrollToHighlight() {
WidgetsBinding.instance.addPostFrameCallback((_) {
final BuildContext? highlightContext = buttonItemKeys[currentHighlight!].currentContext;
if (highlightContext != null) {
Scrollable.ensureVisible(highlightContext);
}
}, debugLabel: 'DropdownMenu.scrollToHighlight');
}
double? getWidth(GlobalKey key) {
final BuildContext? context = key.currentContext;
if (context != null) {
final RenderBox box = context.findRenderObject()! as RenderBox;
return box.hasSize ? box.size.width : null;
}
return null;
}
List<DropdownMenuEntry<T>> filter(List<DropdownMenuEntry<T>> entries, TextEditingController textEditingController) {
final String filterText = textEditingController.text.toLowerCase();
return entries
.where((DropdownMenuEntry<T> entry) => entry.label.toLowerCase().contains(filterText))
.toList();
}
int? search(List<DropdownMenuEntry<T>> entries, TextEditingController textEditingController) {
final String searchText = textEditingController.value.text.toLowerCase();
if (searchText.isEmpty) {
return null;
}
final int index = entries.indexWhere((DropdownMenuEntry<T> entry) => entry.label.toLowerCase().contains(searchText));
return index != -1 ? index : null;
}
List<Widget> _buildButtons(
List<DropdownMenuEntry<T>> filteredEntries,
TextDirection textDirection,
{ int? focusedIndex, bool enableScrollToHighlight = true}
) {
final List<Widget> result = <Widget>[];
for (int i = 0; i < filteredEntries.length; i++) {
final DropdownMenuEntry<T> entry = filteredEntries[i];
// By default, when the text field has a leading icon but a menu entry doesn't
// have one, the label of the entry should have extra padding to be aligned
// with the text in the text input field. When both the text field and the
// menu entry have leading icons, the menu entry should remove the extra
// paddings so its leading icon will be aligned with the leading icon of
// the text field.
final double padding = entry.leadingIcon == null ? (leadingPadding ?? _kDefaultHorizontalPadding) : _kDefaultHorizontalPadding;
final ButtonStyle defaultStyle = switch (textDirection) {
TextDirection.rtl => MenuItemButton.styleFrom(padding: EdgeInsets.only(left: _kDefaultHorizontalPadding, right: padding)),
TextDirection.ltr => MenuItemButton.styleFrom(padding: EdgeInsets.only(left: padding, right: _kDefaultHorizontalPadding)),
};
ButtonStyle effectiveStyle = entry.style ?? defaultStyle;
final Color focusedBackgroundColor = effectiveStyle.foregroundColor?.resolve(<MaterialState>{MaterialState.focused})
?? Theme.of(context).colorScheme.onSurface;
Widget label = entry.labelWidget ?? Text(entry.label);
if (widget.width != null) {
final double horizontalPadding = padding + _kDefaultHorizontalPadding;
label = ConstrainedBox(
constraints: BoxConstraints(maxWidth: widget.width! - horizontalPadding),
child: label,
);
}
// Simulate the focused state because the text field should always be focused
// during traversal. If the menu item has a custom foreground color, the "focused"
// color will also change to foregroundColor.withOpacity(0.12).
effectiveStyle = entry.enabled && i == focusedIndex
? effectiveStyle.copyWith(
backgroundColor: MaterialStatePropertyAll<Color>(focusedBackgroundColor.withOpacity(0.12))
)
: effectiveStyle;
final Widget menuItemButton = MenuItemButton(
key: enableScrollToHighlight ? buttonItemKeys[i] : null,
style: effectiveStyle,
leadingIcon: entry.leadingIcon,
trailingIcon: entry.trailingIcon,
onPressed: entry.enabled
? () {
_textEditingController.value = TextEditingValue(
text: entry.label,
selection: TextSelection.collapsed(offset: entry.label.length),
);
currentHighlight = widget.enableSearch ? i : null;
widget.onSelected?.call(entry.value);
}
: null,
requestFocusOnHover: false,
child: label,
);
result.add(menuItemButton);
}
return result;
}
void handleUpKeyInvoke(_) {
setState(() {
if (!_menuHasEnabledItem || !_controller.isOpen) {
return;
}
_enableFilter = false;
currentHighlight ??= 0;
currentHighlight = (currentHighlight! - 1) % filteredEntries.length;
while (!filteredEntries[currentHighlight!].enabled) {
currentHighlight = (currentHighlight! - 1) % filteredEntries.length;
}
final String currentLabel = filteredEntries[currentHighlight!].label;
_textEditingController.value = TextEditingValue(
text: currentLabel,
selection: TextSelection.collapsed(offset: currentLabel.length),
);
});
}
void handleDownKeyInvoke(_) {
setState(() {
if (!_menuHasEnabledItem || !_controller.isOpen) {
return;
}
_enableFilter = false;
currentHighlight ??= -1;
currentHighlight = (currentHighlight! + 1) % filteredEntries.length;
while (!filteredEntries[currentHighlight!].enabled) {
currentHighlight = (currentHighlight! + 1) % filteredEntries.length;
}
final String currentLabel = filteredEntries[currentHighlight!].label;
_textEditingController.value = TextEditingValue(
text: currentLabel,
selection: TextSelection.collapsed(offset: currentLabel.length),
);
});
}
void handlePressed(MenuController controller) {
if (controller.isOpen) {
currentHighlight = null;
controller.close();
} else { // close to open
if (_textEditingController.text.isNotEmpty) {
_enableFilter = false;
}
controller.open();
}
setState(() {});
}
@override
Widget build(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
_initialMenu ??= _buildButtons(widget.dropdownMenuEntries, textDirection, enableScrollToHighlight: false);
final DropdownMenuThemeData theme = DropdownMenuTheme.of(context);
final DropdownMenuThemeData defaults = _DropdownMenuDefaultsM3(context);
if (_enableFilter) {
filteredEntries = filter(widget.dropdownMenuEntries, _textEditingController);
}
if (widget.enableSearch) {
if (widget.searchCallback != null) {
currentHighlight = widget.searchCallback!.call(filteredEntries, _textEditingController.text);
} else {
currentHighlight = search(filteredEntries, _textEditingController);
}
if (currentHighlight != null) {
scrollToHighlight();
}
}
final List<Widget> menu = _buildButtons(filteredEntries, textDirection, focusedIndex: currentHighlight);
final TextStyle? effectiveTextStyle = widget.textStyle ?? theme.textStyle ?? defaults.textStyle;
MenuStyle? effectiveMenuStyle = widget.menuStyle
?? theme.menuStyle
?? defaults.menuStyle!;
final double? anchorWidth = getWidth(_anchorKey);
if (widget.width != null) {
effectiveMenuStyle = effectiveMenuStyle.copyWith(minimumSize: MaterialStatePropertyAll<Size?>(Size(widget.width!, 0.0)));
} else if (anchorWidth != null){
effectiveMenuStyle = effectiveMenuStyle.copyWith(minimumSize: MaterialStatePropertyAll<Size?>(Size(anchorWidth, 0.0)));
}
if (widget.menuHeight != null) {
effectiveMenuStyle = effectiveMenuStyle.copyWith(maximumSize: MaterialStatePropertyAll<Size>(Size(double.infinity, widget.menuHeight!)));
}
final InputDecorationTheme effectiveInputDecorationTheme = widget.inputDecorationTheme
?? theme.inputDecorationTheme
?? defaults.inputDecorationTheme!;
final MouseCursor effectiveMouseCursor = canRequestFocus() ? SystemMouseCursors.text : SystemMouseCursors.click;
Widget menuAnchor = MenuAnchor(
style: effectiveMenuStyle,
controller: _controller,
menuChildren: menu,
crossAxisUnconstrained: false,
builder: (BuildContext context, MenuController controller, Widget? child) {
assert(_initialMenu != null);
final Widget trailingButton = Padding(
padding: const EdgeInsets.all(4.0),
child: IconButton(
isSelected: controller.isOpen,
icon: widget.trailingIcon ?? const Icon(Icons.arrow_drop_down),
selectedIcon: widget.selectedTrailingIcon ?? const Icon(Icons.arrow_drop_up),
onPressed: () {
handlePressed(controller);
},
),
);
final Widget leadingButton = Padding(
padding: const EdgeInsets.all(8.0),
child: widget.leadingIcon ?? const SizedBox()
);
final Widget textField = TextField(
key: _anchorKey,
mouseCursor: effectiveMouseCursor,
focusNode: widget.focusNode,
canRequestFocus: canRequestFocus(),
enableInteractiveSelection: canRequestFocus(),
textAlignVertical: TextAlignVertical.center,
style: effectiveTextStyle,
controller: _textEditingController,
onEditingComplete: () {
if (currentHighlight != null) {
final DropdownMenuEntry<T> entry = filteredEntries[currentHighlight!];
if (entry.enabled) {
_textEditingController.value = TextEditingValue(
text: entry.label,
selection: TextSelection.collapsed(offset: entry.label.length),
);
widget.onSelected?.call(entry.value);
}
} else {
widget.onSelected?.call(null);
}
if (!widget.enableSearch) {
currentHighlight = null;
}
controller.close();
},
onTap: () {
handlePressed(controller);
},
onChanged: (String text) {
controller.open();
setState(() {
filteredEntries = widget.dropdownMenuEntries;
_enableFilter = widget.enableFilter;
});
},
inputFormatters: widget.inputFormatters,
decoration: InputDecoration(
enabled: widget.enabled,
label: widget.label,
hintText: widget.hintText,
helperText: widget.helperText,
errorText: widget.errorText,
prefixIcon: widget.leadingIcon != null ? Container(
key: _leadingKey,
child: widget.leadingIcon
) : null,
suffixIcon: trailingButton,
).applyDefaults(effectiveInputDecorationTheme)
);
if (widget.expandedInsets != null) {
// If [expandedInsets] is not null, the width of the text field should depend
// on its parent width. So we don't need to use `_DropdownMenuBody` to
// calculate the children's width.
return textField;
}
return _DropdownMenuBody(
width: widget.width,
children: <Widget>[
textField,
for (final Widget item in _initialMenu!) item,
trailingButton,
leadingButton,
],
);
},
);
if (widget.expandedInsets != null) {
menuAnchor = Container(
alignment: AlignmentDirectional.topStart,
padding: widget.expandedInsets?.copyWith(top: 0.0, bottom: 0.0),
child: menuAnchor,
);
}
return Shortcuts(
shortcuts: _kMenuTraversalShortcuts,
child: Actions(
actions: <Type, Action<Intent>>{
_ArrowUpIntent: CallbackAction<_ArrowUpIntent>(
onInvoke: handleUpKeyInvoke,
),
_ArrowDownIntent: CallbackAction<_ArrowDownIntent>(
onInvoke: handleDownKeyInvoke,
),
},
child: menuAnchor,
),
);
}
}
class _ArrowUpIntent extends Intent {
const _ArrowUpIntent();
}
class _ArrowDownIntent extends Intent {
const _ArrowDownIntent();
}
class _DropdownMenuBody extends MultiChildRenderObjectWidget {
const _DropdownMenuBody({
super.children,
this.width,
});
final double? width;
@override
_RenderDropdownMenuBody createRenderObject(BuildContext context) {
return _RenderDropdownMenuBody(
width: width,
);
}
@override
void updateRenderObject(BuildContext context, _RenderDropdownMenuBody renderObject) {
renderObject.width = width;
}
}
class _DropdownMenuBodyParentData extends ContainerBoxParentData<RenderBox> { }
class _RenderDropdownMenuBody extends RenderBox
with ContainerRenderObjectMixin<RenderBox, _DropdownMenuBodyParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, _DropdownMenuBodyParentData> {
_RenderDropdownMenuBody({
double? width,
}) : _width = width;
double? get width => _width;
double? _width;
set width(double? value) {
if (_width == value) {
return;
}
_width = value;
markNeedsLayout();
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! _DropdownMenuBodyParentData) {
child.parentData = _DropdownMenuBodyParentData();
}
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
double maxWidth = 0.0;
double? maxHeight;
RenderBox? child = firstChild;
final BoxConstraints innerConstraints = BoxConstraints(
maxWidth: width ?? computeMaxIntrinsicWidth(constraints.maxWidth),
maxHeight: computeMaxIntrinsicHeight(constraints.maxHeight),
);
while (child != null) {
if (child == firstChild) {
child.layout(innerConstraints, parentUsesSize: true);
maxHeight ??= child.size.height;
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
continue;
}
child.layout(innerConstraints, parentUsesSize: true);
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
childParentData.offset = Offset.zero;
maxWidth = math.max(maxWidth, child.size.width);
maxHeight ??= child.size.height;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
assert(maxHeight != null);
maxWidth = math.max(_kMinimumWidth, maxWidth);
size = constraints.constrain(Size(width ?? maxWidth, maxHeight!));
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderBox? child = firstChild;
if (child != null) {
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
context.paintChild(child, offset + childParentData.offset);
}
}
@override
Size computeDryLayout(BoxConstraints constraints) {
final BoxConstraints constraints = this.constraints;
double maxWidth = 0.0;
double? maxHeight;
RenderBox? child = firstChild;
final BoxConstraints innerConstraints = BoxConstraints(
maxWidth: width ?? computeMaxIntrinsicWidth(constraints.maxWidth),
maxHeight: computeMaxIntrinsicHeight(constraints.maxHeight),
);
while (child != null) {
if (child == firstChild) {
final Size childSize = child.getDryLayout(innerConstraints);
maxHeight ??= childSize.height;
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
continue;
}
final Size childSize = child.getDryLayout(innerConstraints);
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
childParentData.offset = Offset.zero;
maxWidth = math.max(maxWidth, childSize.width);
maxHeight ??= childSize.height;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
assert(maxHeight != null);
maxWidth = math.max(_kMinimumWidth, maxWidth);
return constraints.constrain(Size(width ?? maxWidth, maxHeight!));
}
@override
double computeMinIntrinsicWidth(double height) {
RenderBox? child = firstChild;
double width = 0;
while (child != null) {
if (child == firstChild) {
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
child = childParentData.nextSibling;
continue;
}
final double maxIntrinsicWidth = child.getMinIntrinsicWidth(height);
if (child == lastChild) {
width += maxIntrinsicWidth;
}
if (child == childBefore(lastChild!)) {
width += maxIntrinsicWidth;
}
width = math.max(width, maxIntrinsicWidth);
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
child = childParentData.nextSibling;
}
return math.max(width, _kMinimumWidth);
}
@override
double computeMaxIntrinsicWidth(double height) {
RenderBox? child = firstChild;
double width = 0;
while (child != null) {
if (child == firstChild) {
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
child = childParentData.nextSibling;
continue;
}
final double maxIntrinsicWidth = child.getMaxIntrinsicWidth(height);
// Add the width of leading Icon.
if (child == lastChild) {
width += maxIntrinsicWidth;
}
// Add the width of trailing Icon.
if (child == childBefore(lastChild!)) {
width += maxIntrinsicWidth;
}
width = math.max(width, maxIntrinsicWidth);
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
child = childParentData.nextSibling;
}
return math.max(width, _kMinimumWidth);
}
@override
double computeMinIntrinsicHeight(double height) {
final RenderBox? child = firstChild;
double width = 0;
if (child != null) {
width = math.max(width, child.getMinIntrinsicHeight(height));
}
return width;
}
@override
double computeMaxIntrinsicHeight(double height) {
final RenderBox? child = firstChild;
double width = 0;
if (child != null) {
width = math.max(width, child.getMaxIntrinsicHeight(height));
}
return width;
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final RenderBox? child = firstChild;
if (child != null) {
final _DropdownMenuBodyParentData childParentData = child.parentData! as _DropdownMenuBodyParentData;
final bool isHit = result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
}
return false;
}
}
// Hand coded defaults. These will be updated once we have tokens/spec.
class _DropdownMenuDefaultsM3 extends DropdownMenuThemeData {
_DropdownMenuDefaultsM3(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
@override
TextStyle? get textStyle => _theme.textTheme.bodyLarge;
@override
MenuStyle get menuStyle {
return const MenuStyle(
minimumSize: MaterialStatePropertyAll<Size>(Size(_kMinimumWidth, 0.0)),
maximumSize: MaterialStatePropertyAll<Size>(Size.infinite),
visualDensity: VisualDensity.standard,
);
}
@override
InputDecorationTheme get inputDecorationTheme {
return const InputDecorationTheme(border: OutlineInputBorder());
}
}
| flutter/packages/flutter/lib/src/material/dropdown_menu.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/dropdown_menu.dart",
"repo_id": "flutter",
"token_count": 13296
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'material_state.dart';
/// Defines default property values for descendant [FloatingActionButton]
/// widgets.
///
/// Descendant widgets obtain the current [FloatingActionButtonThemeData] object
/// using `Theme.of(context).floatingActionButtonTheme`. Instances of
/// [FloatingActionButtonThemeData] can be customized with
/// [FloatingActionButtonThemeData.copyWith].
///
/// Typically a [FloatingActionButtonThemeData] is specified as part of the
/// overall [Theme] with [ThemeData.floatingActionButtonTheme].
///
/// All [FloatingActionButtonThemeData] properties are `null` by default.
/// When null, the [FloatingActionButton] will use the values from [ThemeData]
/// if they exist, otherwise it will provide its own defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class FloatingActionButtonThemeData with Diagnosticable {
/// Creates a theme that can be used for
/// [ThemeData.floatingActionButtonTheme].
const FloatingActionButtonThemeData({
this.foregroundColor,
this.backgroundColor,
this.focusColor,
this.hoverColor,
this.splashColor,
this.elevation,
this.focusElevation,
this.hoverElevation,
this.disabledElevation,
this.highlightElevation,
this.shape,
this.enableFeedback,
this.iconSize,
this.sizeConstraints,
this.smallSizeConstraints,
this.largeSizeConstraints,
this.extendedSizeConstraints,
this.extendedIconLabelSpacing,
this.extendedPadding,
this.extendedTextStyle,
this.mouseCursor,
});
/// Color to be used for the unselected, enabled [FloatingActionButton]'s
/// foreground.
final Color? foregroundColor;
/// Color to be used for the unselected, enabled [FloatingActionButton]'s
/// background.
final Color? backgroundColor;
/// The color to use for filling the button when the button has input focus.
final Color? focusColor;
/// The color to use for filling the button when the button has a pointer
/// hovering over it.
final Color? hoverColor;
/// The splash color for this [FloatingActionButton]'s [InkWell].
final Color? splashColor;
/// The z-coordinate to be used for the unselected, enabled
/// [FloatingActionButton]'s elevation foreground.
final double? elevation;
/// The z-coordinate at which to place this button relative to its parent when
/// the button has the input focus.
///
/// This controls the size of the shadow below the floating action button.
final double? focusElevation;
/// The z-coordinate at which to place this button relative to its parent when
/// the button is enabled and has a pointer hovering over it.
///
/// This controls the size of the shadow below the floating action button.
final double? hoverElevation;
/// The z-coordinate to be used for the disabled [FloatingActionButton]'s
/// elevation foreground.
final double? disabledElevation;
/// The z-coordinate to be used for the selected, enabled
/// [FloatingActionButton]'s elevation foreground.
final double? highlightElevation;
/// The shape to be used for the floating action button's [Material].
final ShapeBorder? shape;
/// If specified, defines the feedback property for [FloatingActionButton].
///
/// If [FloatingActionButton.enableFeedback] is provided, [enableFeedback] is
/// ignored.
final bool? enableFeedback;
/// Overrides the default icon size for the [FloatingActionButton];
final double? iconSize;
/// Overrides the default size constraints for the [FloatingActionButton].
final BoxConstraints? sizeConstraints;
/// Overrides the default size constraints for [FloatingActionButton.small].
final BoxConstraints? smallSizeConstraints;
/// Overrides the default size constraints for [FloatingActionButton.large].
final BoxConstraints? largeSizeConstraints;
/// Overrides the default size constraints for [FloatingActionButton.extended].
final BoxConstraints? extendedSizeConstraints;
/// The spacing between the icon and the label for an extended
/// [FloatingActionButton].
final double? extendedIconLabelSpacing;
/// The padding for an extended [FloatingActionButton]'s content.
final EdgeInsetsGeometry? extendedPadding;
/// The text style for an extended [FloatingActionButton]'s label.
final TextStyle? extendedTextStyle;
/// {@macro flutter.material.RawMaterialButton.mouseCursor}
///
/// If specified, overrides the default value of [FloatingActionButton.mouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
FloatingActionButtonThemeData copyWith({
Color? foregroundColor,
Color? backgroundColor,
Color? focusColor,
Color? hoverColor,
Color? splashColor,
double? elevation,
double? focusElevation,
double? hoverElevation,
double? disabledElevation,
double? highlightElevation,
ShapeBorder? shape,
bool? enableFeedback,
double? iconSize,
BoxConstraints? sizeConstraints,
BoxConstraints? smallSizeConstraints,
BoxConstraints? largeSizeConstraints,
BoxConstraints? extendedSizeConstraints,
double? extendedIconLabelSpacing,
EdgeInsetsGeometry? extendedPadding,
TextStyle? extendedTextStyle,
MaterialStateProperty<MouseCursor?>? mouseCursor,
}) {
return FloatingActionButtonThemeData(
foregroundColor: foregroundColor ?? this.foregroundColor,
backgroundColor: backgroundColor ?? this.backgroundColor,
focusColor: focusColor ?? this.focusColor,
hoverColor: hoverColor ?? this.hoverColor,
splashColor: splashColor ?? this.splashColor,
elevation: elevation ?? this.elevation,
focusElevation: focusElevation ?? this.focusElevation,
hoverElevation: hoverElevation ?? this.hoverElevation,
disabledElevation: disabledElevation ?? this.disabledElevation,
highlightElevation: highlightElevation ?? this.highlightElevation,
shape: shape ?? this.shape,
enableFeedback: enableFeedback ?? this.enableFeedback,
iconSize: iconSize ?? this.iconSize,
sizeConstraints: sizeConstraints ?? this.sizeConstraints,
smallSizeConstraints: smallSizeConstraints ?? this.smallSizeConstraints,
largeSizeConstraints: largeSizeConstraints ?? this.largeSizeConstraints,
extendedSizeConstraints: extendedSizeConstraints ?? this.extendedSizeConstraints,
extendedIconLabelSpacing: extendedIconLabelSpacing ?? this.extendedIconLabelSpacing,
extendedPadding: extendedPadding ?? this.extendedPadding,
extendedTextStyle: extendedTextStyle ?? this.extendedTextStyle,
mouseCursor: mouseCursor ?? this.mouseCursor,
);
}
/// Linearly interpolate between two floating action button themes.
///
/// If both arguments are null then null is returned.
///
/// {@macro dart.ui.shadow.lerp}
static FloatingActionButtonThemeData? lerp(FloatingActionButtonThemeData? a, FloatingActionButtonThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return FloatingActionButtonThemeData(
foregroundColor: Color.lerp(a?.foregroundColor, b?.foregroundColor, t),
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
focusColor: Color.lerp(a?.focusColor, b?.focusColor, t),
hoverColor: Color.lerp(a?.hoverColor, b?.hoverColor, t),
splashColor: Color.lerp(a?.splashColor, b?.splashColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
focusElevation: lerpDouble(a?.focusElevation, b?.focusElevation, t),
hoverElevation: lerpDouble(a?.hoverElevation, b?.hoverElevation, t),
disabledElevation: lerpDouble(a?.disabledElevation, b?.disabledElevation, t),
highlightElevation: lerpDouble(a?.highlightElevation, b?.highlightElevation, t),
shape: ShapeBorder.lerp(a?.shape, b?.shape, t),
enableFeedback: t < 0.5 ? a?.enableFeedback : b?.enableFeedback,
iconSize: lerpDouble(a?.iconSize, b?.iconSize, t),
sizeConstraints: BoxConstraints.lerp(a?.sizeConstraints, b?.sizeConstraints, t),
smallSizeConstraints: BoxConstraints.lerp(a?.smallSizeConstraints, b?.smallSizeConstraints, t),
largeSizeConstraints: BoxConstraints.lerp(a?.largeSizeConstraints, b?.largeSizeConstraints, t),
extendedSizeConstraints: BoxConstraints.lerp(a?.extendedSizeConstraints, b?.extendedSizeConstraints, t),
extendedIconLabelSpacing: lerpDouble(a?.extendedIconLabelSpacing, b?.extendedIconLabelSpacing, t),
extendedPadding: EdgeInsetsGeometry.lerp(a?.extendedPadding, b?.extendedPadding, t),
extendedTextStyle: TextStyle.lerp(a?.extendedTextStyle, b?.extendedTextStyle, t),
mouseCursor: t < 0.5 ? a?.mouseCursor : b?.mouseCursor,
);
}
@override
int get hashCode => Object.hash(
foregroundColor,
backgroundColor,
focusColor,
hoverColor,
splashColor,
elevation,
focusElevation,
hoverElevation,
disabledElevation,
highlightElevation,
shape,
enableFeedback,
iconSize,
sizeConstraints,
smallSizeConstraints,
largeSizeConstraints,
extendedSizeConstraints,
extendedIconLabelSpacing,
extendedPadding,
Object.hash(
extendedTextStyle,
mouseCursor,
),
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is FloatingActionButtonThemeData
&& other.foregroundColor == foregroundColor
&& other.backgroundColor == backgroundColor
&& other.focusColor == focusColor
&& other.hoverColor == hoverColor
&& other.splashColor == splashColor
&& other.elevation == elevation
&& other.focusElevation == focusElevation
&& other.hoverElevation == hoverElevation
&& other.disabledElevation == disabledElevation
&& other.highlightElevation == highlightElevation
&& other.shape == shape
&& other.enableFeedback == enableFeedback
&& other.iconSize == iconSize
&& other.sizeConstraints == sizeConstraints
&& other.smallSizeConstraints == smallSizeConstraints
&& other.largeSizeConstraints == largeSizeConstraints
&& other.extendedSizeConstraints == extendedSizeConstraints
&& other.extendedIconLabelSpacing == extendedIconLabelSpacing
&& other.extendedPadding == extendedPadding
&& other.extendedTextStyle == extendedTextStyle
&& other.mouseCursor == mouseCursor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('foregroundColor', foregroundColor, defaultValue: null));
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: null));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(DoubleProperty('focusElevation', focusElevation, defaultValue: null));
properties.add(DoubleProperty('hoverElevation', hoverElevation, defaultValue: null));
properties.add(DoubleProperty('disabledElevation', disabledElevation, defaultValue: null));
properties.add(DoubleProperty('highlightElevation', highlightElevation, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('enableFeedback', enableFeedback, defaultValue: null));
properties.add(DoubleProperty('iconSize', iconSize, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('sizeConstraints', sizeConstraints, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('smallSizeConstraints', smallSizeConstraints, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('largeSizeConstraints', largeSizeConstraints, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('extendedSizeConstraints', extendedSizeConstraints, defaultValue: null));
properties.add(DoubleProperty('extendedIconLabelSpacing', extendedIconLabelSpacing, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('extendedPadding', extendedPadding, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('extendedTextStyle', extendedTextStyle, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>>('mouseCursor', mouseCursor, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/material/floating_action_button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/floating_action_button_theme.dart",
"repo_id": "flutter",
"token_count": 4234
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'icon_button.dart';
import 'icon_button_theme.dart';
import 'input_border.dart';
import 'material.dart';
import 'material_state.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// late Widget _myIcon;
// The duration value extracted from:
// https://github.com/material-components/material-components-android/blob/master/lib/java/com/google/android/material/textfield/TextInputLayout.java
const Duration _kTransitionDuration = Duration(milliseconds: 167);
const Curve _kTransitionCurve = Curves.fastOutSlowIn;
const double _kFinalLabelScale = 0.75;
// The default duration for hint fade in/out transitions.
//
// Animating hint is not mentioned in the Material specification.
// The animation is kept for backward compatibility and a short duration
// is used to mitigate the UX impact.
const Duration _kHintFadeTransitionDuration = Duration(milliseconds: 20);
// Defines the gap in the InputDecorator's outline border where the
// floating label will appear.
class _InputBorderGap extends ChangeNotifier {
double? _start;
double? get start => _start;
set start(double? value) {
if (value != _start) {
_start = value;
notifyListeners();
}
}
double _extent = 0.0;
double get extent => _extent;
set extent(double value) {
if (value != _extent) {
_extent = value;
notifyListeners();
}
}
@override
// ignore: avoid_equals_and_hash_code_on_mutable_classes, this class is not used in collection
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is _InputBorderGap
&& other.start == start
&& other.extent == extent;
}
@override
// ignore: avoid_equals_and_hash_code_on_mutable_classes, this class is not used in collection
int get hashCode => Object.hash(start, extent);
@override
String toString() => describeIdentity(this);
}
// Used to interpolate between two InputBorders.
class _InputBorderTween extends Tween<InputBorder> {
_InputBorderTween({super.begin, super.end});
@override
InputBorder lerp(double t) => ShapeBorder.lerp(begin, end, t)! as InputBorder;
}
// Passes the _InputBorderGap parameters along to an InputBorder's paint method.
class _InputBorderPainter extends CustomPainter {
_InputBorderPainter({
required Listenable repaint,
required this.borderAnimation,
required this.border,
required this.gapAnimation,
required this.gap,
required this.textDirection,
required this.fillColor,
required this.hoverAnimation,
required this.hoverColorTween,
}) : super(repaint: repaint);
final Animation<double> borderAnimation;
final _InputBorderTween border;
final Animation<double> gapAnimation;
final _InputBorderGap gap;
final TextDirection textDirection;
final Color fillColor;
final ColorTween hoverColorTween;
final Animation<double> hoverAnimation;
Color get blendedColor => Color.alphaBlend(hoverColorTween.evaluate(hoverAnimation)!, fillColor);
@override
void paint(Canvas canvas, Size size) {
final InputBorder borderValue = border.evaluate(borderAnimation);
final Rect canvasRect = Offset.zero & size;
final Color blendedFillColor = blendedColor;
if (blendedFillColor.alpha > 0) {
canvas.drawPath(
borderValue.getOuterPath(canvasRect, textDirection: textDirection),
Paint()
..color = blendedFillColor
..style = PaintingStyle.fill,
);
}
borderValue.paint(
canvas,
canvasRect,
gapStart: gap.start,
gapExtent: gap.extent,
gapPercentage: gapAnimation.value,
textDirection: textDirection,
);
}
@override
bool shouldRepaint(_InputBorderPainter oldPainter) {
return borderAnimation != oldPainter.borderAnimation
|| hoverAnimation != oldPainter.hoverAnimation
|| gapAnimation != oldPainter.gapAnimation
|| border != oldPainter.border
|| gap != oldPainter.gap
|| textDirection != oldPainter.textDirection;
}
@override
String toString() => describeIdentity(this);
}
// An analog of AnimatedContainer, which can animate its shaped border, for
// _InputBorder. This specialized animated container is needed because the
// _InputBorderGap, which is computed at layout time, is required by the
// _InputBorder's paint method.
class _BorderContainer extends StatefulWidget {
const _BorderContainer({
required this.border,
required this.gap,
required this.gapAnimation,
required this.fillColor,
required this.hoverColor,
required this.isHovering,
});
final InputBorder border;
final _InputBorderGap gap;
final Animation<double> gapAnimation;
final Color fillColor;
final Color hoverColor;
final bool isHovering;
@override
_BorderContainerState createState() => _BorderContainerState();
}
class _BorderContainerState extends State<_BorderContainer> with TickerProviderStateMixin {
static const Duration _kHoverDuration = Duration(milliseconds: 15);
late AnimationController _controller;
late AnimationController _hoverColorController;
late CurvedAnimation _borderAnimation;
late _InputBorderTween _border;
late CurvedAnimation _hoverAnimation;
late ColorTween _hoverColorTween;
@override
void initState() {
super.initState();
_hoverColorController = AnimationController(
duration: _kHoverDuration,
value: widget.isHovering ? 1.0 : 0.0,
vsync: this,
);
_controller = AnimationController(
duration: _kTransitionDuration,
vsync: this,
);
_borderAnimation = CurvedAnimation(
parent: _controller,
curve: _kTransitionCurve,
reverseCurve: _kTransitionCurve.flipped,
);
_border = _InputBorderTween(
begin: widget.border,
end: widget.border,
);
_hoverAnimation = CurvedAnimation(
parent: _hoverColorController,
curve: Curves.linear,
);
_hoverColorTween = ColorTween(begin: Colors.transparent, end: widget.hoverColor);
}
@override
void dispose() {
_controller.dispose();
_hoverColorController.dispose();
_borderAnimation.dispose();
_hoverAnimation.dispose();
super.dispose();
}
@override
void didUpdateWidget(_BorderContainer oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.border != oldWidget.border) {
_border = _InputBorderTween(
begin: oldWidget.border,
end: widget.border,
);
_controller
..value = 0.0
..forward();
}
if (widget.hoverColor != oldWidget.hoverColor) {
_hoverColorTween = ColorTween(begin: Colors.transparent, end: widget.hoverColor);
}
if (widget.isHovering != oldWidget.isHovering) {
if (widget.isHovering) {
_hoverColorController.forward();
} else {
_hoverColorController.reverse();
}
}
}
@override
Widget build(BuildContext context) {
return CustomPaint(
foregroundPainter: _InputBorderPainter(
repaint: Listenable.merge(<Listenable>[
_borderAnimation,
widget.gap,
_hoverColorController,
]),
borderAnimation: _borderAnimation,
border: _border,
gapAnimation: widget.gapAnimation,
gap: widget.gap,
textDirection: Directionality.of(context),
fillColor: widget.fillColor,
hoverColorTween: _hoverColorTween,
hoverAnimation: _hoverAnimation,
),
);
}
}
// Used to "shake" the floating label to the left and right
// when the errorText first appears.
class _Shaker extends AnimatedWidget {
const _Shaker({
required Animation<double> animation,
this.child,
}) : super(listenable: animation);
final Widget? child;
Animation<double> get animation => listenable as Animation<double>;
double get translateX {
const double shakeDelta = 4.0;
final double t = animation.value;
if (t <= 0.25) {
return -t * shakeDelta;
} else if (t < 0.75) {
return (t - 0.5) * shakeDelta;
} else {
return (1.0 - t) * 4.0 * shakeDelta;
}
}
@override
Widget build(BuildContext context) {
return Transform(
transform: Matrix4.translationValues(translateX, 0.0, 0.0),
child: child,
);
}
}
// Display the helper and error text. When the error text appears
// it fades and the helper text fades out. The error text also
// slides upwards a little when it first appears.
class _HelperError extends StatefulWidget {
const _HelperError({
this.textAlign,
this.helper,
this.helperText,
this.helperStyle,
this.helperMaxLines,
this.error,
this.errorText,
this.errorStyle,
this.errorMaxLines,
});
final TextAlign? textAlign;
final Widget? helper;
final String? helperText;
final TextStyle? helperStyle;
final int? helperMaxLines;
final Widget? error;
final String? errorText;
final TextStyle? errorStyle;
final int? errorMaxLines;
@override
_HelperErrorState createState() => _HelperErrorState();
}
class _HelperErrorState extends State<_HelperError> with SingleTickerProviderStateMixin {
// If the height of this widget and the counter are zero ("empty") at
// layout time, no space is allocated for the subtext.
static const Widget empty = SizedBox.shrink();
late AnimationController _controller;
Widget? _helper;
Widget? _error;
bool get _hasHelper => widget.helperText != null || widget.helper != null;
bool get _hasError => widget.errorText != null || widget.error != null;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: _kTransitionDuration,
vsync: this,
);
if (_hasError) {
_error = _buildError();
_controller.value = 1.0;
} else if (_hasHelper) {
_helper = _buildHelper();
}
_controller.addListener(_handleChange);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
void _handleChange() {
setState(() {
// The _controller's value has changed.
});
}
@override
void didUpdateWidget(_HelperError old) {
super.didUpdateWidget(old);
final Widget? newError = widget.error;
final String? newErrorText = widget.errorText;
final Widget? newHelper = widget.helper;
final String? newHelperText = widget.helperText;
final Widget? oldError = old.error;
final String? oldErrorText = old.errorText;
final Widget? oldHelper = old.helper;
final String? oldHelperText = old.helperText;
final bool errorStateChanged = (newError != null) != (oldError != null);
final bool errorTextStateChanged = (newErrorText != null) != (oldErrorText != null);
final bool helperStateChanged = (newHelper != null) != (oldHelper != null);
final bool helperTextStateChanged = newErrorText == null && (newHelperText != null) != (oldHelperText != null);
if (errorStateChanged || errorTextStateChanged || helperStateChanged || helperTextStateChanged) {
if (newError != null || newErrorText != null) {
_error = _buildError();
_controller.forward();
} else if (newHelper != null || newHelperText != null) {
_helper = _buildHelper();
_controller.reverse();
} else {
_controller.reverse();
}
}
}
Widget _buildHelper() {
assert(widget.helper != null || widget.helperText != null);
return Semantics(
container: true,
child: FadeTransition(
opacity: Tween<double>(begin: 1.0, end: 0.0).animate(_controller),
child: widget.helper ?? Text(
widget.helperText!,
style: widget.helperStyle,
textAlign: widget.textAlign,
overflow: TextOverflow.ellipsis,
maxLines: widget.helperMaxLines,
),
),
);
}
Widget _buildError() {
assert(widget.error != null || widget.errorText != null);
return Semantics(
container: true,
child: FadeTransition(
opacity: _controller,
child: FractionalTranslation(
translation: Tween<Offset>(
begin: const Offset(0.0, -0.25),
end: Offset.zero,
).evaluate(_controller.view),
child: widget.error ?? Text(
widget.errorText!,
style: widget.errorStyle,
textAlign: widget.textAlign,
overflow: TextOverflow.ellipsis,
maxLines: widget.errorMaxLines,
),
),
),
);
}
@override
Widget build(BuildContext context) {
if (_controller.isDismissed) {
_error = null;
if (_hasHelper) {
return _helper = _buildHelper();
} else {
_helper = null;
return empty;
}
}
if (_controller.isCompleted) {
_helper = null;
if (_hasError) {
return _error = _buildError();
} else {
_error = null;
return empty;
}
}
if (_helper == null && _hasError) {
return _buildError();
}
if (_error == null && _hasHelper) {
return _buildHelper();
}
if (_hasError) {
return Stack(
children: <Widget>[
FadeTransition(
opacity: Tween<double>(begin: 1.0, end: 0.0).animate(_controller),
child: _helper,
),
_buildError(),
],
);
}
if (_hasHelper) {
return Stack(
children: <Widget>[
_buildHelper(),
FadeTransition(
opacity: _controller,
child: _error,
),
],
);
}
return empty;
}
}
/// Defines **how** the floating label should behave.
///
/// See also:
///
/// * [InputDecoration.floatingLabelBehavior] which defines the behavior for
/// [InputDecoration.label] or [InputDecoration.labelText].
/// * [FloatingLabelAlignment] which defines **where** the floating label
/// should displayed.
enum FloatingLabelBehavior {
/// The label will always be positioned within the content, or hidden.
never,
/// The label will float when the input is focused, or has content.
auto,
/// The label will always float above the content.
always,
}
/// Defines **where** the floating label should be displayed within an
/// [InputDecorator].
///
/// See also:
///
/// * [InputDecoration.floatingLabelAlignment] which defines the alignment for
/// [InputDecoration.label] or [InputDecoration.labelText].
/// * [FloatingLabelBehavior] which defines **how** the floating label should
/// behave.
@immutable
class FloatingLabelAlignment {
const FloatingLabelAlignment._(this._x) : assert(_x >= -1.0 && _x <= 1.0);
// -1 denotes start, 0 denotes center, and 1 denotes end.
final double _x;
/// Align the floating label on the leading edge of the [InputDecorator].
///
/// For left-to-right text ([TextDirection.ltr]), this is the left edge.
///
/// For right-to-left text ([TextDirection.rtl]), this is the right edge.
static const FloatingLabelAlignment start = FloatingLabelAlignment._(-1.0);
/// Aligns the floating label to the center of an [InputDecorator].
static const FloatingLabelAlignment center = FloatingLabelAlignment._(0.0);
@override
int get hashCode => _x.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is FloatingLabelAlignment
&& _x == other._x;
}
static String _stringify(double x) {
if (x == -1.0) {
return 'FloatingLabelAlignment.start';
}
if (x == 0.0) {
return 'FloatingLabelAlignment.center';
}
return 'FloatingLabelAlignment(x: ${x.toStringAsFixed(1)})';
}
@override
String toString() => _stringify(_x);
}
// Identifies the children of a _RenderDecorationElement.
enum _DecorationSlot {
icon,
input,
label,
hint,
prefix,
suffix,
prefixIcon,
suffixIcon,
helperError,
counter,
container,
}
// An analog of InputDecoration for the _Decorator widget.
@immutable
class _Decoration {
const _Decoration({
required this.contentPadding,
required this.isCollapsed,
required this.floatingLabelHeight,
required this.floatingLabelProgress,
required this.floatingLabelAlignment,
required this.border,
required this.borderGap,
required this.alignLabelWithHint,
required this.isDense,
required this.visualDensity,
this.icon,
this.input,
this.label,
this.hint,
this.prefix,
this.suffix,
this.prefixIcon,
this.suffixIcon,
this.helperError,
this.counter,
this.container,
});
final EdgeInsetsGeometry contentPadding;
final bool isCollapsed;
final double floatingLabelHeight;
final double floatingLabelProgress;
final FloatingLabelAlignment floatingLabelAlignment;
final InputBorder border;
final _InputBorderGap borderGap;
final bool alignLabelWithHint;
final bool? isDense;
final VisualDensity visualDensity;
final Widget? icon;
final Widget? input;
final Widget? label;
final Widget? hint;
final Widget? prefix;
final Widget? suffix;
final Widget? prefixIcon;
final Widget? suffixIcon;
final Widget? helperError;
final Widget? counter;
final Widget? container;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is _Decoration
&& other.contentPadding == contentPadding
&& other.isCollapsed == isCollapsed
&& other.floatingLabelHeight == floatingLabelHeight
&& other.floatingLabelProgress == floatingLabelProgress
&& other.floatingLabelAlignment == floatingLabelAlignment
&& other.border == border
&& other.borderGap == borderGap
&& other.alignLabelWithHint == alignLabelWithHint
&& other.isDense == isDense
&& other.visualDensity == visualDensity
&& other.icon == icon
&& other.input == input
&& other.label == label
&& other.hint == hint
&& other.prefix == prefix
&& other.suffix == suffix
&& other.prefixIcon == prefixIcon
&& other.suffixIcon == suffixIcon
&& other.helperError == helperError
&& other.counter == counter
&& other.container == container;
}
@override
int get hashCode => Object.hash(
contentPadding,
floatingLabelHeight,
floatingLabelProgress,
floatingLabelAlignment,
border,
borderGap,
alignLabelWithHint,
isDense,
visualDensity,
icon,
input,
label,
hint,
prefix,
suffix,
prefixIcon,
suffixIcon,
helperError,
counter,
container,
);
}
// A container for the layout values computed by _RenderDecoration._layout.
// These values are used by _RenderDecoration.performLayout to position
// all of the renderer children of a _RenderDecoration.
class _RenderDecorationLayout {
const _RenderDecorationLayout({
required this.boxToBaseline,
required this.inputBaseline, // for InputBorderType.underline
required this.outlineBaseline, // for InputBorderType.outline
required this.subtextBaseline,
required this.containerHeight,
required this.subtextHeight,
});
final Map<RenderBox?, double> boxToBaseline;
final double inputBaseline;
final double outlineBaseline;
final double subtextBaseline; // helper/error counter
final double containerHeight;
final double subtextHeight;
}
// The workhorse: layout and paint a _Decorator widget's _Decoration.
class _RenderDecoration extends RenderBox with SlottedContainerRenderObjectMixin<_DecorationSlot, RenderBox> {
_RenderDecoration({
required _Decoration decoration,
required TextDirection textDirection,
required TextBaseline textBaseline,
required bool isFocused,
required bool expands,
required bool material3,
TextAlignVertical? textAlignVertical,
}) : _decoration = decoration,
_textDirection = textDirection,
_textBaseline = textBaseline,
_textAlignVertical = textAlignVertical,
_isFocused = isFocused,
_expands = expands,
_material3 = material3;
static const double subtextGap = 8.0;
RenderBox? get icon => childForSlot(_DecorationSlot.icon);
RenderBox? get input => childForSlot(_DecorationSlot.input);
RenderBox? get label => childForSlot(_DecorationSlot.label);
RenderBox? get hint => childForSlot(_DecorationSlot.hint);
RenderBox? get prefix => childForSlot(_DecorationSlot.prefix);
RenderBox? get suffix => childForSlot(_DecorationSlot.suffix);
RenderBox? get prefixIcon => childForSlot(_DecorationSlot.prefixIcon);
RenderBox? get suffixIcon => childForSlot(_DecorationSlot.suffixIcon);
RenderBox? get helperError => childForSlot(_DecorationSlot.helperError);
RenderBox? get counter => childForSlot(_DecorationSlot.counter);
RenderBox? get container => childForSlot(_DecorationSlot.container);
// The returned list is ordered for hit testing.
@override
Iterable<RenderBox> get children {
return <RenderBox>[
if (icon != null)
icon!,
if (input != null)
input!,
if (prefixIcon != null)
prefixIcon!,
if (suffixIcon != null)
suffixIcon!,
if (prefix != null)
prefix!,
if (suffix != null)
suffix!,
if (label != null)
label!,
if (hint != null)
hint!,
if (helperError != null)
helperError!,
if (counter != null)
counter!,
if (container != null)
container!,
];
}
_Decoration get decoration => _decoration;
_Decoration _decoration;
set decoration(_Decoration value) {
if (_decoration == value) {
return;
}
_decoration = value;
markNeedsLayout();
}
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
markNeedsLayout();
}
TextBaseline get textBaseline => _textBaseline;
TextBaseline _textBaseline;
set textBaseline(TextBaseline value) {
if (_textBaseline == value) {
return;
}
_textBaseline = value;
markNeedsLayout();
}
TextAlignVertical get _defaultTextAlignVertical => _isOutlineAligned
? TextAlignVertical.center
: TextAlignVertical.top;
TextAlignVertical get textAlignVertical => _textAlignVertical ?? _defaultTextAlignVertical;
TextAlignVertical? _textAlignVertical;
set textAlignVertical(TextAlignVertical? value) {
if (_textAlignVertical == value) {
return;
}
// No need to relayout if the effective value is still the same.
if (textAlignVertical.y == (value?.y ?? _defaultTextAlignVertical.y)) {
_textAlignVertical = value;
return;
}
_textAlignVertical = value;
markNeedsLayout();
}
bool get isFocused => _isFocused;
bool _isFocused;
set isFocused(bool value) {
if (_isFocused == value) {
return;
}
_isFocused = value;
markNeedsSemanticsUpdate();
}
bool get expands => _expands;
bool _expands = false;
set expands(bool value) {
if (_expands == value) {
return;
}
_expands = value;
markNeedsLayout();
}
bool get material3 => _material3;
bool _material3 = false;
set material3(bool value) {
if (_material3 == value) {
return;
}
_material3 = value;
markNeedsLayout();
}
// Indicates that the decoration should be aligned to accommodate an outline
// border.
bool get _isOutlineAligned {
return !decoration.isCollapsed && decoration.border.isOutline;
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (icon != null) {
visitor(icon!);
}
if (prefix != null) {
visitor(prefix!);
}
if (prefixIcon != null) {
visitor(prefixIcon!);
}
if (label != null) {
visitor(label!);
}
if (hint != null) {
if (isFocused) {
visitor(hint!);
} else if (label == null) {
visitor(hint!);
}
}
if (input != null) {
visitor(input!);
}
if (suffixIcon != null) {
visitor(suffixIcon!);
}
if (suffix != null) {
visitor(suffix!);
}
if (container != null) {
visitor(container!);
}
if (helperError != null) {
visitor(helperError!);
}
if (counter != null) {
visitor(counter!);
}
}
@override
bool get sizedByParent => false;
static double _minWidth(RenderBox? box, double height) {
return box == null ? 0.0 : box.getMinIntrinsicWidth(height);
}
static double _maxWidth(RenderBox? box, double height) {
return box == null ? 0.0 : box.getMaxIntrinsicWidth(height);
}
static double _minHeight(RenderBox? box, double width) {
return box == null ? 0.0 : box.getMinIntrinsicHeight(width);
}
static Size _boxSize(RenderBox? box) => box == null ? Size.zero : box.size;
static BoxParentData _boxParentData(RenderBox box) => box.parentData! as BoxParentData;
EdgeInsets get contentPadding => decoration.contentPadding as EdgeInsets;
// Lay out the given box if needed, and return its baseline.
double _layoutLineBox(RenderBox? box, BoxConstraints constraints) {
if (box == null) {
return 0.0;
}
box.layout(constraints, parentUsesSize: true);
// Since internally, all layout is performed against the alphabetic baseline,
// (eg, ascents/descents are all relative to alphabetic, even if the font is
// an ideographic or hanging font), we should always obtain the reference
// baseline from the alphabetic baseline. The ideographic baseline is for
// use post-layout and is derived from the alphabetic baseline combined with
// the font metrics.
final double baseline = box.getDistanceToBaseline(TextBaseline.alphabetic)!;
assert(() {
if (baseline >= 0) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary("One of InputDecorator's children reported a negative baseline offset."),
ErrorDescription(
'${box.runtimeType}, of size ${box.size}, reported a negative '
'alphabetic baseline of $baseline.',
),
]);
}());
return baseline;
}
// Returns a value used by performLayout to position all of the renderers.
// This method applies layout to all of the renderers except the container.
// For convenience, the container is laid out in performLayout().
_RenderDecorationLayout _layout(BoxConstraints layoutConstraints) {
assert(
layoutConstraints.maxWidth < double.infinity,
'An InputDecorator, which is typically created by a TextField, cannot '
'have an unbounded width.\n'
'This happens when the parent widget does not provide a finite width '
'constraint. For example, if the InputDecorator is contained by a Row, '
'then its width must be constrained. An Expanded widget or a SizedBox '
'can be used to constrain the width of the InputDecorator or the '
'TextField that contains it.',
);
// Margin on each side of subtext (counter and helperError)
final Map<RenderBox?, double> boxToBaseline = <RenderBox?, double>{};
final BoxConstraints boxConstraints = layoutConstraints.loosen();
// Layout all the widgets used by InputDecorator
boxToBaseline[icon] = _layoutLineBox(icon, boxConstraints);
final BoxConstraints containerConstraints = boxConstraints.copyWith(
maxWidth: boxConstraints.maxWidth - _boxSize(icon).width,
);
boxToBaseline[prefixIcon] = _layoutLineBox(prefixIcon, containerConstraints);
boxToBaseline[suffixIcon] = _layoutLineBox(suffixIcon, containerConstraints);
final BoxConstraints contentConstraints = containerConstraints.copyWith(
maxWidth: math.max(0.0, containerConstraints.maxWidth - contentPadding.horizontal),
);
boxToBaseline[prefix] = _layoutLineBox(prefix, contentConstraints);
boxToBaseline[suffix] = _layoutLineBox(suffix, contentConstraints);
final double inputWidth = math.max(
0.0,
constraints.maxWidth - (
_boxSize(icon).width
+ (prefixIcon != null ? 0 : (textDirection == TextDirection.ltr ? contentPadding.left : contentPadding.right))
+ _boxSize(prefixIcon).width
+ _boxSize(prefix).width
+ _boxSize(suffix).width
+ _boxSize(suffixIcon).width
+ (suffixIcon != null ? 0 : (textDirection == TextDirection.ltr ? contentPadding.right : contentPadding.left))),
);
// Increase the available width for the label when it is scaled down.
final double invertedLabelScale = lerpDouble(1.00, 1 / _kFinalLabelScale, decoration.floatingLabelProgress)!;
double suffixIconWidth = _boxSize(suffixIcon).width;
if (decoration.border.isOutline) {
suffixIconWidth = lerpDouble(suffixIconWidth, 0.0, decoration.floatingLabelProgress)!;
}
final double labelWidth = math.max(
0.0,
constraints.maxWidth - (
_boxSize(icon).width
+ contentPadding.left
+ _boxSize(prefixIcon).width
+ suffixIconWidth
+ contentPadding.right),
);
boxToBaseline[label] = _layoutLineBox(
label,
boxConstraints.copyWith(maxWidth: labelWidth * invertedLabelScale),
);
boxToBaseline[hint] = _layoutLineBox(
hint,
boxConstraints.copyWith(minWidth: inputWidth, maxWidth: inputWidth),
);
boxToBaseline[counter] = _layoutLineBox(counter, contentConstraints);
// The helper or error text can occupy the full width less the space
// occupied by the icon and counter.
boxToBaseline[helperError] = _layoutLineBox(
helperError,
contentConstraints.copyWith(
maxWidth: math.max(0.0, contentConstraints.maxWidth - _boxSize(counter).width),
),
);
// The height of the input needs to accommodate label above and counter and
// helperError below, when they exist.
final double labelHeight = label == null
? 0
: decoration.floatingLabelHeight;
final double topHeight = decoration.border.isOutline
? math.max(labelHeight - boxToBaseline[label]!, 0)
: labelHeight;
final double counterHeight = counter == null
? 0
: boxToBaseline[counter]! + subtextGap;
final bool helperErrorExists = helperError?.size != null
&& helperError!.size.height > 0;
final double helperErrorHeight = !helperErrorExists
? 0
: helperError!.size.height + subtextGap;
final double bottomHeight = math.max(
counterHeight,
helperErrorHeight,
);
final Offset densityOffset = decoration.visualDensity.baseSizeAdjustment;
boxToBaseline[input] = _layoutLineBox(
input,
boxConstraints.deflate(EdgeInsets.only(
top: contentPadding.top + topHeight + densityOffset.dy / 2,
bottom: contentPadding.bottom + bottomHeight + densityOffset.dy / 2,
)).copyWith(
minWidth: inputWidth,
maxWidth: inputWidth,
),
);
// The field can be occupied by a hint or by the input itself
final double hintHeight = hint?.size.height ?? 0;
final double inputDirectHeight = input?.size.height ?? 0;
final double inputHeight = math.max(hintHeight, inputDirectHeight);
final double inputInternalBaseline = math.max(
boxToBaseline[input]!,
boxToBaseline[hint]!,
);
// Calculate the amount that prefix/suffix affects height above and below
// the input.
final double prefixHeight = prefix?.size.height ?? 0;
final double suffixHeight = suffix?.size.height ?? 0;
final double fixHeight = math.max(
boxToBaseline[prefix]!,
boxToBaseline[suffix]!,
);
final double fixAboveInput = math.max(0, fixHeight - inputInternalBaseline);
final double fixBelowBaseline = math.max(
prefixHeight - boxToBaseline[prefix]!,
suffixHeight - boxToBaseline[suffix]!,
);
// TODO(justinmc): fixBelowInput should have no effect when there is no
// prefix/suffix below the input.
// https://github.com/flutter/flutter/issues/66050
final double fixBelowInput = math.max(
0,
fixBelowBaseline - (inputHeight - inputInternalBaseline),
);
// Calculate the height of the input text container.
final double prefixIconHeight = prefixIcon?.size.height ?? 0;
final double suffixIconHeight = suffixIcon?.size.height ?? 0;
final double fixIconHeight = math.max(prefixIconHeight, suffixIconHeight);
final double contentHeight = math.max(
fixIconHeight,
topHeight
+ contentPadding.top
+ fixAboveInput
+ inputHeight
+ fixBelowInput
+ contentPadding.bottom
+ densityOffset.dy,
);
final double minContainerHeight = decoration.isDense! || decoration.isCollapsed || expands
? 0.0
: kMinInteractiveDimension;
final double maxContainerHeight = math.max(0.0, boxConstraints.maxHeight - bottomHeight);
final double containerHeight = expands
? maxContainerHeight
: math.min(math.max(contentHeight, minContainerHeight), maxContainerHeight);
// Ensure the text is vertically centered in cases where the content is
// shorter than kMinInteractiveDimension.
final double interactiveAdjustment = minContainerHeight > contentHeight
? (minContainerHeight - contentHeight) / 2.0
: 0.0;
// Try to consider the prefix/suffix as part of the text when aligning it.
// If the prefix/suffix overflows however, allow it to extend outside of the
// input and align the remaining part of the text and prefix/suffix.
final double overflow = math.max(0, contentHeight - maxContainerHeight);
// Map textAlignVertical from -1:1 to 0:1 so that it can be used to scale
// the baseline from its minimum to maximum values.
final double textAlignVerticalFactor = (textAlignVertical.y + 1.0) / 2.0;
// Adjust to try to fit top overflow inside the input on an inverse scale of
// textAlignVertical, so that top aligned text adjusts the most and bottom
// aligned text doesn't adjust at all.
final double baselineAdjustment = fixAboveInput - overflow * (1 - textAlignVerticalFactor);
// The baselines that will be used to draw the actual input text content.
final double topInputBaseline = contentPadding.top
+ topHeight
+ inputInternalBaseline
+ baselineAdjustment
+ interactiveAdjustment
+ densityOffset.dy / 2.0;
final double maxContentHeight = containerHeight - contentPadding.vertical - topHeight - densityOffset.dy;
final double alignableHeight = fixAboveInput + inputHeight + fixBelowInput;
final double maxVerticalOffset = maxContentHeight - alignableHeight;
final double textAlignVerticalOffset = maxVerticalOffset * textAlignVerticalFactor;
final double inputBaseline = topInputBaseline + textAlignVerticalOffset;
// The three main alignments for the baseline when an outline is present are
//
// * top (-1.0): topmost point considering padding.
// * center (0.0): the absolute center of the input ignoring padding but
// accommodating the border and floating label.
// * bottom (1.0): bottommost point considering padding.
//
// That means that if the padding is uneven, center is not the exact
// midpoint of top and bottom. To account for this, the above center and
// below center alignments are interpolated independently.
final double outlineCenterBaseline = inputInternalBaseline
+ baselineAdjustment / 2.0
+ (containerHeight - inputHeight) / 2.0;
final double outlineTopBaseline = topInputBaseline;
final double outlineBottomBaseline = topInputBaseline + maxVerticalOffset;
final double outlineBaseline = _interpolateThree(
outlineTopBaseline,
outlineCenterBaseline,
outlineBottomBaseline,
textAlignVertical,
);
// Find the positions of the text below the input when it exists.
double subtextCounterBaseline = 0;
double subtextHelperBaseline = 0;
double subtextCounterHeight = 0;
double subtextHelperHeight = 0;
if (counter != null) {
subtextCounterBaseline =
containerHeight + subtextGap + boxToBaseline[counter]!;
subtextCounterHeight = counter!.size.height + subtextGap;
}
if (helperErrorExists) {
subtextHelperBaseline =
containerHeight + subtextGap + boxToBaseline[helperError]!;
subtextHelperHeight = helperErrorHeight;
}
final double subtextBaseline = math.max(
subtextCounterBaseline,
subtextHelperBaseline,
);
final double subtextHeight = math.max(
subtextCounterHeight,
subtextHelperHeight,
);
return _RenderDecorationLayout(
boxToBaseline: boxToBaseline,
containerHeight: containerHeight,
inputBaseline: inputBaseline,
outlineBaseline: outlineBaseline,
subtextBaseline: subtextBaseline,
subtextHeight: subtextHeight,
);
}
// Interpolate between three stops using textAlignVertical. This is used to
// calculate the outline baseline, which ignores padding when the alignment is
// middle. When the alignment is less than zero, it interpolates between the
// centered text box's top and the top of the content padding. When the
// alignment is greater than zero, it interpolates between the centered box's
// top and the position that would align the bottom of the box with the bottom
// padding.
double _interpolateThree(double begin, double middle, double end, TextAlignVertical textAlignVertical) {
if (textAlignVertical.y <= 0) {
// It's possible for begin, middle, and end to not be in order because of
// excessive padding. Those cases are handled by using middle.
if (begin >= middle) {
return middle;
}
// Do a standard linear interpolation on the first half, between begin and
// middle.
final double t = textAlignVertical.y + 1;
return begin + (middle - begin) * t;
}
if (middle >= end) {
return middle;
}
// Do a standard linear interpolation on the second half, between middle and
// end.
final double t = textAlignVertical.y;
return middle + (end - middle) * t;
}
@override
double computeMinIntrinsicWidth(double height) {
return _minWidth(icon, height)
+ (prefixIcon != null ? 0.0 : (textDirection == TextDirection.ltr ? contentPadding.left : contentPadding.right))
+ _minWidth(prefixIcon, height)
+ _minWidth(prefix, height)
+ math.max(_minWidth(input, height), _minWidth(hint, height))
+ _minWidth(suffix, height)
+ _minWidth(suffixIcon, height)
+ (suffixIcon != null ? 0.0 : (textDirection == TextDirection.ltr ? contentPadding.right : contentPadding.left));
}
@override
double computeMaxIntrinsicWidth(double height) {
return _maxWidth(icon, height)
+ (prefixIcon != null ? 0.0 : (textDirection == TextDirection.ltr ? contentPadding.left : contentPadding.right))
+ _maxWidth(prefixIcon, height)
+ _maxWidth(prefix, height)
+ math.max(_maxWidth(input, height), _maxWidth(hint, height))
+ _maxWidth(suffix, height)
+ _maxWidth(suffixIcon, height)
+ (suffixIcon != null ? 0.0 : (textDirection == TextDirection.ltr ? contentPadding.right : contentPadding.left));
}
double _lineHeight(double width, List<RenderBox?> boxes) {
double height = 0.0;
for (final RenderBox? box in boxes) {
if (box == null) {
continue;
}
height = math.max(_minHeight(box, width), height);
}
return height;
// TODO(hansmuller): this should compute the overall line height for the
// boxes when they've been baseline-aligned.
// See https://github.com/flutter/flutter/issues/13715
}
@override
double computeMinIntrinsicHeight(double width) {
final double iconHeight = _minHeight(icon, width);
final double iconWidth = _minWidth(icon, iconHeight);
width = math.max(width - iconWidth, 0.0);
final double prefixIconHeight = _minHeight(prefixIcon, width);
final double prefixIconWidth = _minWidth(prefixIcon, prefixIconHeight);
final double suffixIconHeight = _minHeight(suffixIcon, width);
final double suffixIconWidth = _minWidth(suffixIcon, suffixIconHeight);
width = math.max(width - contentPadding.horizontal, 0.0);
final double counterHeight = _minHeight(counter, width);
final double counterWidth = _minWidth(counter, counterHeight);
final double helperErrorAvailableWidth = math.max(width - counterWidth, 0.0);
final double helperErrorHeight = _minHeight(helperError, helperErrorAvailableWidth);
double subtextHeight = math.max(counterHeight, helperErrorHeight);
if (subtextHeight > 0.0) {
subtextHeight += subtextGap;
}
final double prefixHeight = _minHeight(prefix, width);
final double prefixWidth = _minWidth(prefix, prefixHeight);
final double suffixHeight = _minHeight(suffix, width);
final double suffixWidth = _minWidth(suffix, suffixHeight);
final double availableInputWidth = math.max(width - prefixWidth - suffixWidth - prefixIconWidth - suffixIconWidth, 0.0);
final double inputHeight = _lineHeight(availableInputWidth, <RenderBox?>[input, hint]);
final double inputMaxHeight = <double>[inputHeight, prefixHeight, suffixHeight].reduce(math.max);
final Offset densityOffset = decoration.visualDensity.baseSizeAdjustment;
final double contentHeight = contentPadding.top
+ (label == null ? 0.0 : decoration.floatingLabelHeight)
+ inputMaxHeight
+ contentPadding.bottom
+ densityOffset.dy;
final double containerHeight = <double>[iconHeight, contentHeight, prefixIconHeight, suffixIconHeight].reduce(math.max);
final double minContainerHeight = decoration.isDense! || expands
? 0.0
: kMinInteractiveDimension;
return math.max(containerHeight, minContainerHeight) + subtextHeight;
}
@override
double computeMaxIntrinsicHeight(double width) {
return computeMinIntrinsicHeight(width);
}
@override
double computeDistanceToActualBaseline(TextBaseline baseline) {
final RenderBox? input = this.input;
return input == null
? 0.0
: _boxParentData(input).offset.dy + (input.computeDistanceToActualBaseline(baseline) ?? 0.0);
}
// Records where the label was painted.
Matrix4? _labelTransform;
@override
Size computeDryLayout(BoxConstraints constraints) {
assert(debugCannotComputeDryLayout(
reason: 'Layout requires baseline metrics, which are only available after a full layout.',
));
return Size.zero;
}
ChildSemanticsConfigurationsResult _childSemanticsConfigurationDelegate(List<SemanticsConfiguration> childConfigs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
List<SemanticsConfiguration>? prefixMergeGroup;
List<SemanticsConfiguration>? suffixMergeGroup;
for (final SemanticsConfiguration childConfig in childConfigs) {
if (childConfig.tagsChildrenWith(_InputDecoratorState._kPrefixSemanticsTag)) {
prefixMergeGroup ??= <SemanticsConfiguration>[];
prefixMergeGroup.add(childConfig);
} else if (childConfig.tagsChildrenWith(_InputDecoratorState._kSuffixSemanticsTag)) {
suffixMergeGroup ??= <SemanticsConfiguration>[];
suffixMergeGroup.add(childConfig);
} else {
builder.markAsMergeUp(childConfig);
}
}
if (prefixMergeGroup != null) {
builder.markAsSiblingMergeGroup(prefixMergeGroup);
}
if (suffixMergeGroup != null) {
builder.markAsSiblingMergeGroup(suffixMergeGroup);
}
return builder.build();
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
config.childConfigurationsDelegate = _childSemanticsConfigurationDelegate;
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
_labelTransform = null;
final _RenderDecorationLayout layout = _layout(constraints);
final double overallWidth = constraints.maxWidth;
final double overallHeight = layout.containerHeight + layout.subtextHeight;
final RenderBox? container = this.container;
if (container != null) {
final BoxConstraints containerConstraints = BoxConstraints.tightFor(
height: layout.containerHeight,
width: overallWidth - _boxSize(icon).width,
);
container.layout(containerConstraints, parentUsesSize: true);
final double x = switch (textDirection) {
TextDirection.rtl => 0.0,
TextDirection.ltr => _boxSize(icon).width,
};
_boxParentData(container).offset = Offset(x, 0.0);
}
late double height;
double centerLayout(RenderBox box, double x) {
_boxParentData(box).offset = Offset(x, (height - box.size.height) / 2.0);
return box.size.width;
}
late double baseline;
double baselineLayout(RenderBox box, double x) {
_boxParentData(box).offset = Offset(x, baseline - layout.boxToBaseline[box]!);
return box.size.width;
}
final double left = contentPadding.left;
final double right = overallWidth - contentPadding.right;
height = layout.containerHeight;
baseline = _isOutlineAligned ? layout.outlineBaseline : layout.inputBaseline;
if (icon != null) {
final double x = switch (textDirection) {
TextDirection.rtl => overallWidth - icon!.size.width,
TextDirection.ltr => 0.0,
};
centerLayout(icon!, x);
}
switch (textDirection) {
case TextDirection.rtl: {
double start = right - _boxSize(icon).width;
double end = left;
if (prefixIcon != null) {
start += contentPadding.right;
start -= centerLayout(prefixIcon!, start - prefixIcon!.size.width);
}
if (label != null) {
if (decoration.alignLabelWithHint) {
baselineLayout(label!, start - label!.size.width);
} else {
centerLayout(label!, start - label!.size.width);
}
}
if (prefix != null) {
start -= baselineLayout(prefix!, start - prefix!.size.width);
}
if (input != null) {
baselineLayout(input!, start - input!.size.width);
}
if (hint != null) {
baselineLayout(hint!, start - hint!.size.width);
}
if (suffixIcon != null) {
end -= contentPadding.left;
end += centerLayout(suffixIcon!, end);
}
if (suffix != null) {
end += baselineLayout(suffix!, end);
}
break;
}
case TextDirection.ltr: {
double start = left + _boxSize(icon).width;
double end = right;
if (prefixIcon != null) {
start -= contentPadding.left;
start += centerLayout(prefixIcon!, start);
}
if (label != null) {
if (decoration.alignLabelWithHint) {
baselineLayout(label!, start);
} else {
centerLayout(label!, start);
}
}
if (prefix != null) {
start += baselineLayout(prefix!, start);
}
if (input != null) {
baselineLayout(input!, start);
}
if (hint != null) {
baselineLayout(hint!, start);
}
if (suffixIcon != null) {
end += contentPadding.right;
end -= centerLayout(suffixIcon!, end - suffixIcon!.size.width);
}
if (suffix != null) {
end -= baselineLayout(suffix!, end - suffix!.size.width);
}
break;
}
}
if (helperError != null || counter != null) {
height = layout.subtextHeight;
baseline = layout.subtextBaseline;
switch (textDirection) {
case TextDirection.rtl:
if (helperError != null) {
baselineLayout(helperError!, right - helperError!.size.width - _boxSize(icon).width);
}
if (counter != null) {
baselineLayout(counter!, left);
}
case TextDirection.ltr:
if (helperError != null) {
baselineLayout(helperError!, left + _boxSize(icon).width);
}
if (counter != null) {
baselineLayout(counter!, right - counter!.size.width);
}
}
}
if (label != null) {
final double labelX = _boxParentData(label!).offset.dx;
// +1 shifts the range of x from (-1.0, 1.0) to (0.0, 2.0).
final double floatAlign = decoration.floatingLabelAlignment._x + 1;
final double floatWidth = _boxSize(label).width * _kFinalLabelScale;
// When floating label is centered, its x is relative to
// _BorderContainer's x and is independent of label's x.
switch (textDirection) {
case TextDirection.rtl:
double offsetToPrefixIcon = 0.0;
if (prefixIcon != null && !decoration.alignLabelWithHint) {
offsetToPrefixIcon = material3 ? _boxSize(prefixIcon).width - left : 0;
}
decoration.borderGap.start = lerpDouble(labelX + _boxSize(label).width + offsetToPrefixIcon,
_boxSize(container).width / 2.0 + floatWidth / 2.0,
floatAlign);
case TextDirection.ltr:
// The value of _InputBorderGap.start is relative to the origin of the
// _BorderContainer which is inset by the icon's width. Although, when
// floating label is centered, it's already relative to _BorderContainer.
double offsetToPrefixIcon = 0.0;
if (prefixIcon != null && !decoration.alignLabelWithHint) {
offsetToPrefixIcon = material3 ? (-_boxSize(prefixIcon).width + left) : 0;
}
decoration.borderGap.start = lerpDouble(labelX - _boxSize(icon).width + offsetToPrefixIcon,
_boxSize(container).width / 2.0 - floatWidth / 2.0,
floatAlign);
}
decoration.borderGap.extent = label!.size.width * _kFinalLabelScale;
} else {
decoration.borderGap.start = null;
decoration.borderGap.extent = 0.0;
}
size = constraints.constrain(Size(overallWidth, overallHeight));
assert(size.width == constraints.constrainWidth(overallWidth));
assert(size.height == constraints.constrainHeight(overallHeight));
}
void _paintLabel(PaintingContext context, Offset offset) {
context.paintChild(label!, offset);
}
@override
void paint(PaintingContext context, Offset offset) {
void doPaint(RenderBox? child) {
if (child != null) {
context.paintChild(child, _boxParentData(child).offset + offset);
}
}
doPaint(container);
if (label != null) {
final Offset labelOffset = _boxParentData(label!).offset;
final double labelHeight = _boxSize(label).height;
final double labelWidth = _boxSize(label).width;
// +1 shifts the range of x from (-1.0, 1.0) to (0.0, 2.0).
final double floatAlign = decoration.floatingLabelAlignment._x + 1;
final double floatWidth = labelWidth * _kFinalLabelScale;
final double borderWeight = decoration.border.borderSide.width;
final double t = decoration.floatingLabelProgress;
// The center of the outline border label ends up a little below the
// center of the top border line.
final bool isOutlineBorder = decoration.border.isOutline;
// Temporary opt-in fix for https://github.com/flutter/flutter/issues/54028
// Center the scaled label relative to the border.
final double floatingY = isOutlineBorder ? (-labelHeight * _kFinalLabelScale) / 2.0 + borderWeight / 2.0 : contentPadding.top;
final double scale = lerpDouble(1.0, _kFinalLabelScale, t)!;
final double centeredFloatX = _boxParentData(container!).offset.dx +
_boxSize(container).width / 2.0 - floatWidth / 2.0;
final double startX;
double floatStartX;
switch (textDirection) {
case TextDirection.rtl: // origin is on the right
startX = labelOffset.dx + labelWidth * (1.0 - scale);
floatStartX = startX;
if (prefixIcon != null && !decoration.alignLabelWithHint && isOutlineBorder) {
floatStartX += material3 ? _boxSize(prefixIcon).width - contentPadding.left : 0.0;
}
case TextDirection.ltr: // origin on the left
startX = labelOffset.dx;
floatStartX = startX;
if (prefixIcon != null && !decoration.alignLabelWithHint && isOutlineBorder) {
floatStartX += material3 ? -_boxSize(prefixIcon).width + contentPadding.left : 0.0;
}
}
final double floatEndX = lerpDouble(floatStartX, centeredFloatX, floatAlign)!;
final double dx = lerpDouble(startX, floatEndX, t)!;
final double dy = lerpDouble(0.0, floatingY - labelOffset.dy, t)!;
_labelTransform = Matrix4.identity()
..translate(dx, labelOffset.dy + dy)
..scale(scale);
layer = context.pushTransform(
needsCompositing,
offset,
_labelTransform!,
_paintLabel,
oldLayer: layer as TransformLayer?,
);
} else {
layer = null;
}
doPaint(icon);
doPaint(prefix);
doPaint(suffix);
doPaint(prefixIcon);
doPaint(suffixIcon);
doPaint(hint);
doPaint(input);
doPaint(helperError);
doPaint(counter);
}
@override
bool hitTestSelf(Offset position) => true;
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
for (final RenderBox child in children) {
// The label must be handled specially since we've transformed it.
final Offset offset = _boxParentData(child).offset;
final bool isHit = result.addWithPaintOffset(
offset: offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - offset);
return child.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
}
return false;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
if (child == label && _labelTransform != null) {
final Offset labelOffset = _boxParentData(label!).offset;
transform
..multiply(_labelTransform!)
..translate(-labelOffset.dx, -labelOffset.dy);
}
super.applyPaintTransform(child, transform);
}
}
class _Decorator extends SlottedMultiChildRenderObjectWidget<_DecorationSlot, RenderBox> {
const _Decorator({
required this.textAlignVertical,
required this.decoration,
required this.textDirection,
required this.textBaseline,
required this.isFocused,
required this.expands,
});
final _Decoration decoration;
final TextDirection textDirection;
final TextBaseline textBaseline;
final TextAlignVertical? textAlignVertical;
final bool isFocused;
final bool expands;
@override
Iterable<_DecorationSlot> get slots => _DecorationSlot.values;
@override
Widget? childForSlot(_DecorationSlot slot) {
return switch (slot) {
_DecorationSlot.icon => decoration.icon,
_DecorationSlot.input => decoration.input,
_DecorationSlot.label => decoration.label,
_DecorationSlot.hint => decoration.hint,
_DecorationSlot.prefix => decoration.prefix,
_DecorationSlot.suffix => decoration.suffix,
_DecorationSlot.prefixIcon => decoration.prefixIcon,
_DecorationSlot.suffixIcon => decoration.suffixIcon,
_DecorationSlot.helperError => decoration.helperError,
_DecorationSlot.counter => decoration.counter,
_DecorationSlot.container => decoration.container,
};
}
@override
_RenderDecoration createRenderObject(BuildContext context) {
return _RenderDecoration(
decoration: decoration,
textDirection: textDirection,
textBaseline: textBaseline,
textAlignVertical: textAlignVertical,
isFocused: isFocused,
expands: expands,
material3: Theme.of(context).useMaterial3,
);
}
@override
void updateRenderObject(BuildContext context, _RenderDecoration renderObject) {
renderObject
..decoration = decoration
..expands = expands
..isFocused = isFocused
..textAlignVertical = textAlignVertical
..textBaseline = textBaseline
..textDirection = textDirection;
}
}
class _AffixText extends StatelessWidget {
const _AffixText({
required this.labelIsFloating,
this.text,
this.style,
this.child,
this.semanticsSortKey,
required this.semanticsTag,
});
final bool labelIsFloating;
final String? text;
final TextStyle? style;
final Widget? child;
final SemanticsSortKey? semanticsSortKey;
final SemanticsTag semanticsTag;
@override
Widget build(BuildContext context) {
return DefaultTextStyle.merge(
style: style,
child: IgnorePointer(
ignoring: !labelIsFloating,
child: AnimatedOpacity(
duration: _kTransitionDuration,
curve: _kTransitionCurve,
opacity: labelIsFloating ? 1.0 : 0.0,
child: Semantics(
sortKey: semanticsSortKey,
tagForChildren: semanticsTag,
child: child ?? (text == null ? null : Text(text!, style: style)),
),
),
),
);
}
}
/// Defines the appearance of a Material Design text field.
///
/// [InputDecorator] displays the visual elements of a Material Design text
/// field around its input [child]. The visual elements themselves are defined
/// by an [InputDecoration] object and their layout and appearance depend
/// on the `baseStyle`, `textAlign`, `isFocused`, and `isEmpty` parameters.
///
/// [TextField] uses this widget to decorate its [EditableText] child.
///
/// [InputDecorator] can be used to create widgets that look and behave like a
/// [TextField] but support other kinds of input.
///
/// Requires one of its ancestors to be a [Material] widget. The [child] widget,
/// as well as the decorative widgets specified in [decoration], must have
/// non-negative baselines.
///
/// See also:
///
/// * [TextField], which uses an [InputDecorator] to display a border,
/// labels, and icons, around its [EditableText] child.
/// * [Decoration] and [DecoratedBox], for drawing arbitrary decorations
/// around other widgets.
class InputDecorator extends StatefulWidget {
/// Creates a widget that displays a border, labels, and icons,
/// for a [TextField].
///
/// The [isFocused], [isHovering], [expands], and [isEmpty] arguments must not
/// be null.
const InputDecorator({
super.key,
required this.decoration,
this.baseStyle,
this.textAlign,
this.textAlignVertical,
this.isFocused = false,
this.isHovering = false,
this.expands = false,
this.isEmpty = false,
this.child,
});
/// The text and styles to use when decorating the child.
///
/// Null [InputDecoration] properties are initialized with the corresponding
/// values from [ThemeData.inputDecorationTheme].
final InputDecoration decoration;
/// The style on which to base the label, hint, counter, and error styles
/// if the [decoration] does not provide explicit styles.
///
/// If null, [baseStyle] defaults to the `titleMedium` style from the
/// current [Theme], see [ThemeData.textTheme].
///
/// The [TextStyle.textBaseline] of the [baseStyle] is used to determine
/// the baseline used for text alignment.
final TextStyle? baseStyle;
/// How the text in the decoration should be aligned horizontally.
final TextAlign? textAlign;
/// {@template flutter.material.InputDecorator.textAlignVertical}
/// How the text should be aligned vertically.
///
/// Determines the alignment of the baseline within the available space of
/// the input (typically a TextField). For example, TextAlignVertical.top will
/// place the baseline such that the text, and any attached decoration like
/// prefix and suffix, is as close to the top of the input as possible without
/// overflowing. The heights of the prefix and suffix are similarly included
/// for other alignment values. If the height is greater than the height
/// available, then the prefix and suffix will be allowed to overflow first
/// before the text scrolls.
/// {@endtemplate}
final TextAlignVertical? textAlignVertical;
/// Whether the input field has focus.
///
/// Determines the position of the label text and the color and weight of the
/// border.
///
/// Defaults to false.
///
/// See also:
///
/// * [InputDecoration.hoverColor], which is also blended into the focus
/// color and fill color when the [isHovering] is true to produce the final
/// color.
final bool isFocused;
/// Whether the input field is being hovered over by a mouse pointer.
///
/// Determines the container fill color, which is a blend of
/// [InputDecoration.hoverColor] with [InputDecoration.fillColor] when
/// true, and [InputDecoration.fillColor] when not.
///
/// Defaults to false.
final bool isHovering;
/// If true, the height of the input field will be as large as possible.
///
/// If wrapped in a widget that constrains its child's height, like Expanded
/// or SizedBox, the input field will only be affected if [expands] is set to
/// true.
///
/// See [TextField.minLines] and [TextField.maxLines] for related ways to
/// affect the height of an input. When [expands] is true, both must be null
/// in order to avoid ambiguity in determining the height.
///
/// Defaults to false.
final bool expands;
/// Whether the input field is empty.
///
/// Determines the position of the label text and whether to display the hint
/// text.
///
/// Defaults to false.
final bool isEmpty;
/// The widget below this widget in the tree.
///
/// Typically an [EditableText], [DropdownButton], or [InkWell].
final Widget? child;
/// Whether the label needs to get out of the way of the input, either by
/// floating or disappearing.
///
/// Will withdraw when not empty, or when focused while enabled.
bool get _labelShouldWithdraw => !isEmpty || (isFocused && decoration.enabled);
@override
State<InputDecorator> createState() => _InputDecoratorState();
/// The RenderBox that defines this decorator's "container". That's the
/// area which is filled if [InputDecoration.filled] is true. It's the area
/// adjacent to [InputDecoration.icon] and above the widgets that contain
/// [InputDecoration.helperText], [InputDecoration.errorText], and
/// [InputDecoration.counterText].
///
/// [TextField] renders ink splashes within the container.
static RenderBox? containerOf(BuildContext context) {
final _RenderDecoration? result = context.findAncestorRenderObjectOfType<_RenderDecoration>();
return result?.container;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<InputDecoration>('decoration', decoration));
properties.add(DiagnosticsProperty<TextStyle>('baseStyle', baseStyle, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('isFocused', isFocused));
properties.add(DiagnosticsProperty<bool>('expands', expands, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('isEmpty', isEmpty));
}
}
class _InputDecoratorState extends State<InputDecorator> with TickerProviderStateMixin {
late final AnimationController _floatingLabelController;
late final CurvedAnimation _floatingLabelAnimation;
late final AnimationController _shakingLabelController;
final _InputBorderGap _borderGap = _InputBorderGap();
static const OrdinalSortKey _kPrefixSemanticsSortOrder = OrdinalSortKey(0);
static const OrdinalSortKey _kInputSemanticsSortOrder = OrdinalSortKey(1);
static const OrdinalSortKey _kSuffixSemanticsSortOrder = OrdinalSortKey(2);
static const SemanticsTag _kPrefixSemanticsTag = SemanticsTag('_InputDecoratorState.prefix');
static const SemanticsTag _kSuffixSemanticsTag = SemanticsTag('_InputDecoratorState.suffix');
@override
void initState() {
super.initState();
final bool labelIsInitiallyFloating = widget.decoration.floatingLabelBehavior == FloatingLabelBehavior.always
|| (widget.decoration.floatingLabelBehavior != FloatingLabelBehavior.never &&
widget._labelShouldWithdraw);
_floatingLabelController = AnimationController(
duration: _kTransitionDuration,
vsync: this,
value: labelIsInitiallyFloating ? 1.0 : 0.0,
);
_floatingLabelController.addListener(_handleChange);
_floatingLabelAnimation = CurvedAnimation(
parent: _floatingLabelController,
curve: _kTransitionCurve,
reverseCurve: _kTransitionCurve.flipped,
);
_shakingLabelController = AnimationController(
duration: _kTransitionDuration,
vsync: this,
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_effectiveDecoration = null;
}
@override
void dispose() {
_floatingLabelController.dispose();
_floatingLabelAnimation.dispose();
_shakingLabelController.dispose();
_borderGap.dispose();
super.dispose();
}
void _handleChange() {
setState(() {
// The _floatingLabelController's value has changed.
});
}
InputDecoration? _effectiveDecoration;
InputDecoration get decoration => _effectiveDecoration ??= widget.decoration.applyDefaults(Theme.of(context).inputDecorationTheme);
TextAlign? get textAlign => widget.textAlign;
bool get isFocused => widget.isFocused;
bool get _hasError => decoration.errorText != null || decoration.error != null;
bool get isHovering => widget.isHovering && decoration.enabled;
bool get isEmpty => widget.isEmpty;
bool get _floatingLabelEnabled {
return decoration.floatingLabelBehavior != FloatingLabelBehavior.never;
}
@override
void didUpdateWidget(InputDecorator old) {
super.didUpdateWidget(old);
if (widget.decoration != old.decoration) {
_effectiveDecoration = null;
}
final bool floatBehaviorChanged = widget.decoration.floatingLabelBehavior != old.decoration.floatingLabelBehavior;
if (widget._labelShouldWithdraw != old._labelShouldWithdraw || floatBehaviorChanged) {
if (_floatingLabelEnabled
&& (widget._labelShouldWithdraw || widget.decoration.floatingLabelBehavior == FloatingLabelBehavior.always)) {
_floatingLabelController.forward();
} else {
_floatingLabelController.reverse();
}
}
final String? errorText = decoration.errorText;
final String? oldErrorText = old.decoration.errorText;
if (_floatingLabelController.isCompleted && errorText != null && errorText != oldErrorText) {
_shakingLabelController
..value = 0.0
..forward();
}
}
Color _getDefaultM2BorderColor(ThemeData themeData) {
if (!decoration.enabled && !isFocused) {
return ((decoration.filled ?? false) && !(decoration.border?.isOutline ?? false))
? Colors.transparent
: themeData.disabledColor;
}
if (_hasError) {
return themeData.colorScheme.error;
}
if (isFocused) {
return themeData.colorScheme.primary;
}
if (decoration.filled!) {
return themeData.hintColor;
}
final Color enabledColor = themeData.colorScheme.onSurface.withOpacity(0.38);
if (isHovering) {
final Color hoverColor = decoration.hoverColor ?? themeData.inputDecorationTheme.hoverColor ?? themeData.hoverColor;
return Color.alphaBlend(hoverColor.withOpacity(0.12), enabledColor);
}
return enabledColor;
}
Color _getFillColor(ThemeData themeData, InputDecorationTheme defaults) {
if (decoration.filled != true) { // filled == null same as filled == false
return Colors.transparent;
}
if (decoration.fillColor != null) {
return MaterialStateProperty.resolveAs(decoration.fillColor!, materialState);
}
return MaterialStateProperty.resolveAs(defaults.fillColor!, materialState);
}
Color _getHoverColor(ThemeData themeData) {
if (decoration.filled == null || !decoration.filled! || isFocused || !decoration.enabled) {
return Colors.transparent;
}
return decoration.hoverColor ?? themeData.inputDecorationTheme.hoverColor ?? themeData.hoverColor;
}
Color _getIconColor(ThemeData themeData, InputDecorationTheme defaults) {
return MaterialStateProperty.resolveAs(decoration.iconColor, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.iconColor, materialState)
?? MaterialStateProperty.resolveAs(defaults.iconColor!, materialState);
}
Color _getPrefixIconColor(ThemeData themeData, InputDecorationTheme defaults) {
return MaterialStateProperty.resolveAs(decoration.prefixIconColor, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.prefixIconColor, materialState)
?? MaterialStateProperty.resolveAs(defaults.prefixIconColor!, materialState);
}
Color _getSuffixIconColor(ThemeData themeData, InputDecorationTheme defaults) {
return MaterialStateProperty.resolveAs(decoration.suffixIconColor, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.suffixIconColor, materialState)
?? MaterialStateProperty.resolveAs(defaults.suffixIconColor!, materialState);
}
// True if the label will be shown and the hint will not.
// If we're not focused, there's no value, labelText was provided, and
// floatingLabelBehavior isn't set to always, then the label appears where the
// hint would.
bool get _hasInlineLabel {
return !widget._labelShouldWithdraw
&& (decoration.labelText != null || decoration.label != null)
&& decoration.floatingLabelBehavior != FloatingLabelBehavior.always;
}
// If the label is a floating placeholder, it's always shown.
bool get _shouldShowLabel => _hasInlineLabel || _floatingLabelEnabled;
// The base style for the inline label when they're displayed "inline",
// i.e. when they appear in place of the empty text field.
TextStyle _getInlineLabelStyle(ThemeData themeData, InputDecorationTheme defaults) {
final TextStyle defaultStyle = MaterialStateProperty.resolveAs(defaults.labelStyle!, materialState);
final TextStyle? style = MaterialStateProperty.resolveAs(decoration.labelStyle, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.labelStyle, materialState);
return themeData.textTheme.titleMedium!
.merge(widget.baseStyle)
.merge(defaultStyle)
.merge(style)
.copyWith(height: 1);
}
// The base style for the inline hint when they're displayed "inline",
// i.e. when they appear in place of the empty text field.
TextStyle _getInlineHintStyle(ThemeData themeData, InputDecorationTheme defaults) {
final TextStyle defaultStyle = MaterialStateProperty.resolveAs(defaults.hintStyle!, materialState);
final TextStyle? style = MaterialStateProperty.resolveAs(decoration.hintStyle, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.hintStyle, materialState);
return themeData.textTheme.titleMedium!
.merge(widget.baseStyle)
.merge(defaultStyle)
.merge(style);
}
TextStyle _getFloatingLabelStyle(ThemeData themeData, InputDecorationTheme defaults) {
TextStyle defaultTextStyle = MaterialStateProperty.resolveAs(defaults.floatingLabelStyle!, materialState);
if (_hasError && decoration.errorStyle?.color != null) {
defaultTextStyle = defaultTextStyle.copyWith(color: decoration.errorStyle?.color);
}
defaultTextStyle = defaultTextStyle.merge(decoration.floatingLabelStyle ?? decoration.labelStyle);
final TextStyle? style = MaterialStateProperty.resolveAs(decoration.floatingLabelStyle, materialState)
?? MaterialStateProperty.resolveAs(themeData.inputDecorationTheme.floatingLabelStyle, materialState);
return themeData.textTheme.titleMedium!
.merge(widget.baseStyle)
.merge(defaultTextStyle)
.merge(style)
.copyWith(height: 1);
}
TextStyle _getHelperStyle(ThemeData themeData, InputDecorationTheme defaults) {
return MaterialStateProperty.resolveAs(defaults.helperStyle!, materialState)
.merge(MaterialStateProperty.resolveAs(decoration.helperStyle, materialState));
}
TextStyle _getErrorStyle(ThemeData themeData, InputDecorationTheme defaults) {
return MaterialStateProperty.resolveAs(defaults.errorStyle!, materialState)
.merge(decoration.errorStyle);
}
Set<MaterialState> get materialState {
return <MaterialState>{
if (!decoration.enabled) MaterialState.disabled,
if (isFocused) MaterialState.focused,
if (isHovering) MaterialState.hovered,
if (_hasError) MaterialState.error,
};
}
InputBorder _getDefaultBorder(ThemeData themeData, InputDecorationTheme defaults) {
final InputBorder border = MaterialStateProperty.resolveAs(decoration.border, materialState)
?? const UnderlineInputBorder();
if (decoration.border is MaterialStateProperty<InputBorder>) {
return border;
}
if (border.borderSide == BorderSide.none) {
return border;
}
if (themeData.useMaterial3) {
if (decoration.filled!) {
return border.copyWith(
borderSide: MaterialStateProperty.resolveAs(defaults.activeIndicatorBorder, materialState),
);
} else {
return border.copyWith(
borderSide: MaterialStateProperty.resolveAs(defaults.outlineBorder, materialState),
);
}
}
else{
return border.copyWith(
borderSide: BorderSide(
color: _getDefaultM2BorderColor(themeData),
width: (
(decoration.isCollapsed ?? themeData.inputDecorationTheme.isCollapsed)
|| decoration.border == InputBorder.none
|| !decoration.enabled)
? 0.0
: isFocused ? 2.0 : 1.0,
),
);
}
}
@override
Widget build(BuildContext context) {
final ThemeData themeData = Theme.of(context);
final InputDecorationTheme defaults =
Theme.of(context).useMaterial3 ? _InputDecoratorDefaultsM3(context) : _InputDecoratorDefaultsM2(context);
final TextStyle labelStyle = _getInlineLabelStyle(themeData, defaults);
final TextBaseline textBaseline = labelStyle.textBaseline!;
final TextStyle hintStyle = _getInlineHintStyle(themeData, defaults);
final String? hintText = decoration.hintText;
final Widget? hint = hintText == null ? null : AnimatedOpacity(
opacity: (isEmpty && !_hasInlineLabel) ? 1.0 : 0.0,
duration: decoration.hintFadeDuration ?? _kHintFadeTransitionDuration,
curve: _kTransitionCurve,
child: Text(
hintText,
style: hintStyle,
textDirection: decoration.hintTextDirection,
overflow: hintStyle.overflow ?? TextOverflow.ellipsis,
textAlign: textAlign,
maxLines: decoration.hintMaxLines,
),
);
InputBorder? border;
if (!decoration.enabled) {
border = _hasError ? decoration.errorBorder : decoration.disabledBorder;
} else if (isFocused) {
border = _hasError ? decoration.focusedErrorBorder : decoration.focusedBorder;
} else {
border = _hasError ? decoration.errorBorder : decoration.enabledBorder;
}
border ??= _getDefaultBorder(themeData, defaults);
final Widget container = _BorderContainer(
border: border,
gap: _borderGap,
gapAnimation: _floatingLabelAnimation,
fillColor: _getFillColor(themeData, defaults),
hoverColor: _getHoverColor(themeData),
isHovering: isHovering,
);
final Widget? label = decoration.labelText == null && decoration.label == null ? null : _Shaker(
animation: _shakingLabelController.view,
child: AnimatedOpacity(
duration: _kTransitionDuration,
curve: _kTransitionCurve,
opacity: _shouldShowLabel ? 1.0 : 0.0,
child: AnimatedDefaultTextStyle(
duration:_kTransitionDuration,
curve: _kTransitionCurve,
style: widget._labelShouldWithdraw
? _getFloatingLabelStyle(themeData, defaults)
: labelStyle,
child: decoration.label ?? Text(
decoration.labelText!,
overflow: TextOverflow.ellipsis,
textAlign: textAlign,
),
),
),
);
final bool hasPrefix = decoration.prefix != null || decoration.prefixText != null;
final bool hasSuffix = decoration.suffix != null || decoration.suffixText != null;
Widget? input = widget.child;
// If at least two out of the three are visible, it needs semantics sort
// order.
final bool needsSemanticsSortOrder = widget._labelShouldWithdraw && (input != null ? (hasPrefix || hasSuffix) : (hasPrefix && hasSuffix));
final Widget? prefix = hasPrefix
? _AffixText(
labelIsFloating: widget._labelShouldWithdraw,
text: decoration.prefixText,
style: MaterialStateProperty.resolveAs(decoration.prefixStyle, materialState) ?? hintStyle,
semanticsSortKey: needsSemanticsSortOrder ? _kPrefixSemanticsSortOrder : null,
semanticsTag: _kPrefixSemanticsTag,
child: decoration.prefix,
)
: null;
final Widget? suffix = hasSuffix
? _AffixText(
labelIsFloating: widget._labelShouldWithdraw,
text: decoration.suffixText,
style: MaterialStateProperty.resolveAs(decoration.suffixStyle, materialState) ?? hintStyle,
semanticsSortKey: needsSemanticsSortOrder ? _kSuffixSemanticsSortOrder : null,
semanticsTag: _kSuffixSemanticsTag,
child: decoration.suffix,
)
: null;
if (input != null && needsSemanticsSortOrder) {
input = Semantics(
sortKey: _kInputSemanticsSortOrder,
child: input,
);
}
final bool decorationIsDense = decoration.isDense ?? false;
final double iconSize = decorationIsDense ? 18.0 : 24.0;
final Widget? icon = decoration.icon == null ? null :
MouseRegion(
cursor: SystemMouseCursors.basic,
child: Padding(
padding: const EdgeInsetsDirectional.only(end: 16.0),
child: IconTheme.merge(
data: IconThemeData(
color: _getIconColor(themeData, defaults),
size: iconSize,
),
child: decoration.icon!,
),
),
);
final Widget? prefixIcon = decoration.prefixIcon == null ? null :
Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: MouseRegion(
cursor: SystemMouseCursors.basic,
child: ConstrainedBox(
constraints: decoration.prefixIconConstraints ??
themeData.visualDensity.effectiveConstraints(
const BoxConstraints(
minWidth: kMinInteractiveDimension,
minHeight: kMinInteractiveDimension,
),
),
child: IconTheme.merge(
data: IconThemeData(
color: _getPrefixIconColor(themeData, defaults),
size: iconSize,
),
child: IconButtonTheme(
data: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: _getPrefixIconColor(themeData, defaults),
iconSize: iconSize,
),
),
child: Semantics(
child: decoration.prefixIcon,
),
),
),
),
),
);
final Widget? suffixIcon = decoration.suffixIcon == null ? null :
Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: MouseRegion(
cursor: SystemMouseCursors.basic,
child: ConstrainedBox(
constraints: decoration.suffixIconConstraints ??
themeData.visualDensity.effectiveConstraints(
const BoxConstraints(
minWidth: kMinInteractiveDimension,
minHeight: kMinInteractiveDimension,
),
),
child: IconTheme.merge(
data: IconThemeData(
color: _getSuffixIconColor(themeData, defaults),
size: iconSize,
),
child: IconButtonTheme(
data: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: _getSuffixIconColor(themeData, defaults),
iconSize: iconSize,
),
),
child: Semantics(
child: decoration.suffixIcon,
),
),
),
),
),
);
final Widget helperError = _HelperError(
textAlign: textAlign,
helper: decoration.helper,
helperText: decoration.helperText,
helperStyle: _getHelperStyle(themeData, defaults),
helperMaxLines: decoration.helperMaxLines,
error: decoration.error,
errorText: decoration.errorText,
errorStyle: _getErrorStyle(themeData, defaults),
errorMaxLines: decoration.errorMaxLines,
);
Widget? counter;
if (decoration.counter != null) {
counter = decoration.counter;
} else if (decoration.counterText != null && decoration.counterText != '') {
counter = Semantics(
container: true,
liveRegion: isFocused,
child: Text(
decoration.counterText!,
style: _getHelperStyle(themeData, defaults).merge(MaterialStateProperty.resolveAs(decoration.counterStyle, materialState)),
overflow: TextOverflow.ellipsis,
semanticsLabel: decoration.semanticCounterText,
),
);
}
// The _Decoration widget and _RenderDecoration assume that contentPadding
// has been resolved to EdgeInsets.
final TextDirection textDirection = Directionality.of(context);
final EdgeInsets? decorationContentPadding = decoration.contentPadding?.resolve(textDirection);
final EdgeInsets contentPadding;
final double floatingLabelHeight;
if (decoration.isCollapsed ?? themeData.inputDecorationTheme.isCollapsed) {
floatingLabelHeight = 0.0;
contentPadding = decorationContentPadding ?? EdgeInsets.zero;
} else if (!border.isOutline) {
// 4.0: the vertical gap between the inline elements and the floating label.
floatingLabelHeight = MediaQuery.textScalerOf(context).scale(4.0 + 0.75 * labelStyle.fontSize!);
if (decoration.filled ?? false) {
contentPadding = decorationContentPadding ?? (Theme.of(context).useMaterial3
? decorationIsDense
? const EdgeInsets.fromLTRB(12.0, 4.0, 12.0, 4.0)
: const EdgeInsets.fromLTRB(12.0, 8.0, 12.0, 8.0)
: decorationIsDense
? const EdgeInsets.fromLTRB(12.0, 8.0, 12.0, 8.0)
: const EdgeInsets.fromLTRB(12.0, 12.0, 12.0, 12.0));
} else {
// No left or right padding for underline borders that aren't filled
// is a small concession to backwards compatibility. This eliminates
// the most noticeable layout change introduced by #13734.
contentPadding = decorationContentPadding ?? (Theme.of(context).useMaterial3
? decorationIsDense
? const EdgeInsets.fromLTRB(0.0, 4.0, 0.0, 4.0)
: const EdgeInsets.fromLTRB(0.0, 8.0, 0.0, 8.0)
: decorationIsDense
? const EdgeInsets.fromLTRB(0.0, 8.0, 0.0, 8.0)
: const EdgeInsets.fromLTRB(0.0, 12.0, 0.0, 12.0));
}
} else {
floatingLabelHeight = 0.0;
contentPadding = decorationContentPadding ?? (Theme.of(context).useMaterial3
? decorationIsDense
? const EdgeInsets.fromLTRB(12.0, 16.0, 12.0, 8.0)
: const EdgeInsets.fromLTRB(12.0, 20.0, 12.0, 12.0)
: decorationIsDense
? const EdgeInsets.fromLTRB(12.0, 20.0, 12.0, 12.0)
: const EdgeInsets.fromLTRB(12.0, 24.0, 12.0, 16.0));
}
final _Decorator decorator = _Decorator(
decoration: _Decoration(
contentPadding: contentPadding,
isCollapsed: decoration.isCollapsed ?? themeData.inputDecorationTheme.isCollapsed,
floatingLabelHeight: floatingLabelHeight,
floatingLabelAlignment: decoration.floatingLabelAlignment!,
floatingLabelProgress: _floatingLabelAnimation.value,
border: border,
borderGap: _borderGap,
alignLabelWithHint: decoration.alignLabelWithHint ?? false,
isDense: decoration.isDense,
visualDensity: themeData.visualDensity,
icon: icon,
input: input,
label: label,
hint: hint,
prefix: prefix,
suffix: suffix,
prefixIcon: prefixIcon,
suffixIcon: suffixIcon,
helperError: helperError,
counter: counter,
container: container
),
textDirection: textDirection,
textBaseline: textBaseline,
textAlignVertical: widget.textAlignVertical,
isFocused: isFocused,
expands: widget.expands,
);
final BoxConstraints? constraints = decoration.constraints ?? themeData.inputDecorationTheme.constraints;
if (constraints != null) {
return ConstrainedBox(
constraints: constraints,
child: decorator,
);
}
return decorator;
}
}
/// The border, labels, icons, and styles used to decorate a Material
/// Design text field.
///
/// The [TextField] and [InputDecorator] classes use [InputDecoration] objects
/// to describe their decoration. (In fact, this class is merely the
/// configuration of an [InputDecorator], which does all the heavy lifting.)
///
/// {@tool dartpad}
/// This sample shows how to style a `TextField` using an `InputDecorator`. The
/// TextField displays a "send message" icon to the left of the input area,
/// which is surrounded by a border an all sides. It displays the `hintText`
/// inside the input area to help the user understand what input is required. It
/// displays the `helperText` and `counterText` below the input area.
///
/// 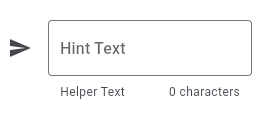
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to style a "collapsed" `TextField` using an
/// `InputDecorator`. The collapsed `TextField` surrounds the hint text and
/// input area with a border, but does not add padding around them.
///
/// 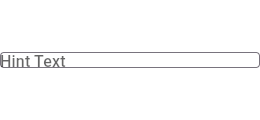
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to create a `TextField` with hint text, a red border
/// on all sides, and an error message. To display a red border and error
/// message, provide `errorText` to the [InputDecoration] constructor.
///
/// 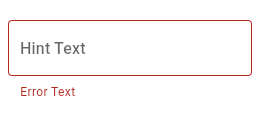
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to style a `TextField` with a round border and
/// additional text before and after the input area. It displays "Prefix" before
/// the input area, and "Suffix" after the input area.
///
/// 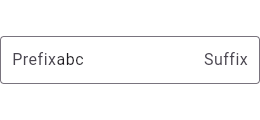
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.3.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to style a `TextField` with a prefixIcon that changes color
/// based on the `MaterialState`. The color defaults to gray, be blue while focused
/// and red if in an error state.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.material_state.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to style a `TextField` with a prefixIcon that changes color
/// based on the `MaterialState` through the use of `ThemeData`. The color defaults
/// to gray, be blue while focused and red if in an error state.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.material_state.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TextField], which is a text input widget that uses an
/// [InputDecoration].
/// * [InputDecorator], which is a widget that draws an [InputDecoration]
/// around an input child widget.
/// * [Decoration] and [DecoratedBox], for drawing borders and backgrounds
/// around a child widget.
@immutable
class InputDecoration {
/// Creates a bundle of the border, labels, icons, and styles used to
/// decorate a Material Design text field.
///
/// Unless specified by [ThemeData.inputDecorationTheme], [InputDecorator]
/// defaults [isDense] to false and [filled] to false. The default border is
/// an instance of [UnderlineInputBorder]. If [border] is [InputBorder.none]
/// then no border is drawn.
///
/// Only one of [prefix] and [prefixText] can be specified.
///
/// Similarly, only one of [suffix] and [suffixText] can be specified.
const InputDecoration({
this.icon,
this.iconColor,
this.label,
this.labelText,
this.labelStyle,
this.floatingLabelStyle,
this.helper,
this.helperText,
this.helperStyle,
this.helperMaxLines,
this.hintText,
this.hintStyle,
this.hintTextDirection,
this.hintMaxLines,
this.hintFadeDuration,
this.error,
this.errorText,
this.errorStyle,
this.errorMaxLines,
this.floatingLabelBehavior,
this.floatingLabelAlignment,
this.isCollapsed,
this.isDense,
this.contentPadding,
this.prefixIcon,
this.prefixIconConstraints,
this.prefix,
this.prefixText,
this.prefixStyle,
this.prefixIconColor,
this.suffixIcon,
this.suffix,
this.suffixText,
this.suffixStyle,
this.suffixIconColor,
this.suffixIconConstraints,
this.counter,
this.counterText,
this.counterStyle,
this.filled,
this.fillColor,
this.focusColor,
this.hoverColor,
this.errorBorder,
this.focusedBorder,
this.focusedErrorBorder,
this.disabledBorder,
this.enabledBorder,
this.border,
this.enabled = true,
this.semanticCounterText,
this.alignLabelWithHint,
this.constraints,
}) : assert(!(label != null && labelText != null), 'Declaring both label and labelText is not supported.'),
assert(!(helper != null && helperText != null), 'Declaring both helper and helperText is not supported.'),
assert(!(prefix != null && prefixText != null), 'Declaring both prefix and prefixText is not supported.'),
assert(!(suffix != null && suffixText != null), 'Declaring both suffix and suffixText is not supported.'),
assert(!(error != null && errorText != null), 'Declaring both error and errorText is not supported.');
/// Defines an [InputDecorator] that is the same size as the input field.
///
/// This type of input decoration does not include a border by default.
///
/// Sets the [isCollapsed] property to true.
const InputDecoration.collapsed({
required this.hintText,
this.floatingLabelBehavior,
this.floatingLabelAlignment,
this.hintStyle,
this.hintTextDirection,
this.filled = false,
this.fillColor,
this.focusColor,
this.hoverColor,
this.border = InputBorder.none,
this.enabled = true,
}) : icon = null,
iconColor = null,
label = null,
labelText = null,
labelStyle = null,
floatingLabelStyle = null,
helper = null,
helperText = null,
helperStyle = null,
helperMaxLines = null,
hintMaxLines = null,
hintFadeDuration = null,
error = null,
errorText = null,
errorStyle = null,
errorMaxLines = null,
isDense = false,
contentPadding = EdgeInsets.zero,
isCollapsed = true,
prefixIcon = null,
prefix = null,
prefixText = null,
prefixStyle = null,
prefixIconColor = null,
prefixIconConstraints = null,
suffix = null,
suffixIcon = null,
suffixText = null,
suffixStyle = null,
suffixIconColor = null,
suffixIconConstraints = null,
counter = null,
counterText = null,
counterStyle = null,
errorBorder = null,
focusedBorder = null,
focusedErrorBorder = null,
disabledBorder = null,
enabledBorder = null,
semanticCounterText = null,
alignLabelWithHint = false,
constraints = null;
/// An icon to show before the input field and outside of the decoration's
/// container.
///
/// The size and color of the icon is configured automatically using an
/// [IconTheme] and therefore does not need to be explicitly given in the
/// icon widget.
///
/// The trailing edge of the icon is padded by 16dps.
///
/// The decoration's container is the area which is filled if [filled] is
/// true and bordered per the [border]. It's the area adjacent to
/// [icon] and above the widgets that contain [helperText],
/// [errorText], and [counterText].
///
/// See [Icon], [ImageIcon].
final Widget? icon;
/// The color of the [icon].
///
/// If [iconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
final Color? iconColor;
/// Optional widget that describes the input field.
///
/// {@template flutter.material.inputDecoration.label}
/// When the input field is empty and unfocused, the label is displayed on
/// top of the input field (i.e., at the same location on the screen where
/// text may be entered in the input field). When the input field receives
/// focus (or if the field is non-empty), depending on [floatingLabelAlignment],
/// the label moves above, either vertically adjacent to, or to the center of
/// the input field.
/// {@endtemplate}
///
/// This can be used, for example, to add multiple [TextStyle]'s to a label that would
/// otherwise be specified using [labelText], which only takes one [TextStyle].
///
/// {@tool dartpad}
/// This example shows a `TextField` with a [Text.rich] widget as the [label].
/// The widget contains multiple [Text] widgets with different [TextStyle]'s.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.label.0.dart **
/// {@end-tool}
///
/// Only one of [label] and [labelText] can be specified.
final Widget? label;
/// Optional text that describes the input field.
///
/// {@macro flutter.material.inputDecoration.label}
///
/// If a more elaborate label is required, consider using [label] instead.
/// Only one of [label] and [labelText] can be specified.
final String? labelText;
/// {@template flutter.material.inputDecoration.labelStyle}
/// The style to use for [InputDecoration.labelText] when the label is on top
/// of the input field.
///
/// If [labelStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// When the [InputDecoration.labelText] is above (i.e., vertically adjacent to)
/// the input field, the text uses the [floatingLabelStyle] instead.
///
/// If null, defaults to a value derived from the base [TextStyle] for the
/// input field and the current [Theme].
///
/// Specifying this style will override the default behavior
/// of [InputDecoration] that changes the color of the label to the
/// [InputDecoration.errorStyle] color or [ColorScheme.error].
///
/// {@tool dartpad}
/// It's possible to override the label style for just the error state, or
/// just the default state, or both.
///
/// In this example the [labelStyle] is specified with a [MaterialStateProperty]
/// which resolves to a text style whose color depends on the decorator's
/// error state.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.label_style_error.0.dart **
/// {@end-tool}
/// {@endtemplate}
final TextStyle? labelStyle;
/// {@template flutter.material.inputDecoration.floatingLabelStyle}
/// The style to use for [InputDecoration.labelText] when the label is
/// above (i.e., vertically adjacent to) the input field.
///
/// When the [InputDecoration.labelText] is on top of the input field, the
/// text uses the [labelStyle] instead.
///
/// If [floatingLabelStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to [labelStyle].
///
/// Specifying this style will override the default behavior
/// of [InputDecoration] that changes the color of the label to the
/// [InputDecoration.errorStyle] color or [ColorScheme.error].
///
/// {@tool dartpad}
/// It's possible to override the label style for just the error state, or
/// just the default state, or both.
///
/// In this example the [floatingLabelStyle] is specified with a
/// [MaterialStateProperty] which resolves to a text style whose color depends
/// on the decorator's error state.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.floating_label_style_error.0.dart **
/// {@end-tool}
/// {@endtemplate}
final TextStyle? floatingLabelStyle;
/// Optional widget that appears below the [InputDecorator.child].
///
/// If non-null, the [helper] is displayed below the [InputDecorator.child], in
/// the same location as [error]. If a non-null [error] or [errorText] value is
/// specified then the [helper] is not shown.
///
/// {@tool dartpad}
/// This example shows a `TextField` with a [Text.rich] widget as the [helper].
/// The widget contains [Text] and [Icon] widgets with different styles.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.helper.0.dart **
/// {@end-tool}
///
/// Only one of [helper] and [helperText] can be specified.
final Widget? helper;
/// Text that provides context about the [InputDecorator.child]'s value, such
/// as how the value will be used.
///
/// If non-null, the text is displayed below the [InputDecorator.child], in
/// the same location as [errorText]. If a non-null [errorText] value is
/// specified then the helper text is not shown.
///
/// If a more elaborate helper text is required, consider using [helper] instead.
///
/// Only one of [helper] and [helperText] can be specified.
final String? helperText;
/// The style to use for the [helperText].
///
/// If [helperStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
final TextStyle? helperStyle;
/// The maximum number of lines the [helperText] can occupy.
///
/// Defaults to null, which means that the [helperText] will be limited
/// to a single line with [TextOverflow.ellipsis].
///
/// This value is passed along to the [Text.maxLines] attribute
/// of the [Text] widget used to display the helper.
///
/// See also:
///
/// * [errorMaxLines], the equivalent but for the [errorText].
final int? helperMaxLines;
/// Text that suggests what sort of input the field accepts.
///
/// Displayed on top of the [InputDecorator.child] (i.e., at the same location
/// on the screen where text may be entered in the [InputDecorator.child])
/// when the input [isEmpty] and either (a) [labelText] is null or (b) the
/// input has the focus.
final String? hintText;
/// The style to use for the [hintText].
///
/// If [hintStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// Also used for the [labelText] when the [labelText] is displayed on
/// top of the input field (i.e., at the same location on the screen where
/// text may be entered in the [InputDecorator.child]).
///
/// If null, defaults to a value derived from the base [TextStyle] for the
/// input field and the current [Theme].
final TextStyle? hintStyle;
/// The direction to use for the [hintText].
///
/// If null, defaults to a value derived from [Directionality] for the
/// input field and the current context.
final TextDirection? hintTextDirection;
/// The maximum number of lines the [hintText] can occupy.
///
/// Defaults to the value of [TextField.maxLines] attribute.
///
/// This value is passed along to the [Text.maxLines] attribute
/// of the [Text] widget used to display the hint text. [TextOverflow.ellipsis] is
/// used to handle the overflow when it is limited to single line.
final int? hintMaxLines;
/// The duration of the [hintText] fade in and fade out animations.
///
/// If null, defaults to [InputDecorationTheme.hintFadeDuration].
/// If [InputDecorationTheme.hintFadeDuration] is null defaults to 20ms.
final Duration? hintFadeDuration;
/// Optional widget that appears below the [InputDecorator.child] and the border.
///
/// If non-null, the border's color animates to red and the [helperText] is not shown.
///
/// Only one of [error] and [errorText] can be specified.
final Widget? error;
/// Text that appears below the [InputDecorator.child] and the border.
///
/// If non-null, the border's color animates to red and the [helperText] is
/// not shown.
///
/// In a [TextFormField], this is overridden by the value returned from
/// [TextFormField.validator], if that is not null.
///
/// If a more elaborate error is required, consider using [error] instead.
///
/// Only one of [error] and [errorText] can be specified.
final String? errorText;
/// {@template flutter.material.inputDecoration.errorStyle}
/// The style to use for the [InputDecoration.errorText].
///
/// If null, defaults of a value derived from the base [TextStyle] for the
/// input field and the current [Theme].
///
/// By default the color of style will be used by the label of
/// [InputDecoration] if [InputDecoration.errorText] is not null. See
/// [InputDecoration.labelStyle] or [InputDecoration.floatingLabelStyle] for
/// an example of how to replicate this behavior when specifying those
/// styles.
/// {@endtemplate}
final TextStyle? errorStyle;
/// The maximum number of lines the [errorText] can occupy.
///
/// Defaults to null, which means that the [errorText] will be limited
/// to a single line with [TextOverflow.ellipsis].
///
/// This value is passed along to the [Text.maxLines] attribute
/// of the [Text] widget used to display the error.
///
/// See also:
///
/// * [helperMaxLines], the equivalent but for the [helperText].
final int? errorMaxLines;
/// {@template flutter.material.inputDecoration.floatingLabelBehavior}
/// Defines **how** the floating label should behave.
///
/// When [FloatingLabelBehavior.auto] the label will float to the top only when
/// the field is focused or has some text content, otherwise it will appear
/// in the field in place of the content.
///
/// When [FloatingLabelBehavior.always] the label will always float at the top
/// of the field above the content.
///
/// When [FloatingLabelBehavior.never] the label will always appear in an empty
/// field in place of the content.
/// {@endtemplate}
///
/// If null, [InputDecorationTheme.floatingLabelBehavior] will be used.
///
/// See also:
///
/// * [floatingLabelAlignment] which defines **where** the floating label
/// should be displayed.
final FloatingLabelBehavior? floatingLabelBehavior;
/// {@template flutter.material.inputDecoration.floatingLabelAlignment}
/// Defines **where** the floating label should be displayed.
///
/// [FloatingLabelAlignment.start] aligns the floating label to the leftmost
/// (when [TextDirection.ltr]) or rightmost (when [TextDirection.rtl]),
/// possible position, which is vertically adjacent to the label, on top of
/// the field.
///
/// [FloatingLabelAlignment.center] aligns the floating label to the center on
/// top of the field.
/// {@endtemplate}
///
/// If null, [InputDecorationTheme.floatingLabelAlignment] will be used.
///
/// See also:
///
/// * [floatingLabelBehavior] which defines **how** the floating label should
/// behave.
final FloatingLabelAlignment? floatingLabelAlignment;
/// Whether the [InputDecorator.child] is part of a dense form (i.e., uses less vertical
/// space).
///
/// Defaults to false.
final bool? isDense;
/// The padding for the input decoration's container.
///
/// {@macro flutter.material.input_decorator.container_description}
///
/// By default the [contentPadding] reflects [isDense] and the type of the
/// [border].
///
/// If [isCollapsed] is true then [contentPadding] is [EdgeInsets.zero].
///
/// ### Material 3 default content padding
///
/// If `isOutline` property of [border] is false and if [filled] is true then
/// [contentPadding] is `EdgeInsets.fromLTRB(12, 4, 12, 4)` when [isDense]
/// is true and `EdgeInsets.fromLTRB(12, 8, 12, 8)` when [isDense] is false.
///
/// If `isOutline` property of [border] is false and if [filled] is false then
/// [contentPadding] is `EdgeInsets.fromLTRB(0, 4, 0, 4)` when [isDense] is
/// true and `EdgeInsets.fromLTRB(0, 8, 0, 8)` when [isDense] is false.
///
/// If `isOutline` property of [border] is true then [contentPadding] is
/// `EdgeInsets.fromLTRB(12, 16, 12, 8)` when [isDense] is true
/// and `EdgeInsets.fromLTRB(12, 20, 12, 12)` when [isDense] is false.
///
/// ### Material 2 default content padding
///
/// If `isOutline` property of [border] is false and if [filled] is true then
/// [contentPadding] is `EdgeInsets.fromLTRB(12, 8, 12, 8)` when [isDense]
/// is true and `EdgeInsets.fromLTRB(12, 12, 12, 12)` when [isDense] is false.
///
/// If `isOutline` property of [border] is false and if [filled] is false then
/// [contentPadding] is `EdgeInsets.fromLTRB(0, 8, 0, 8)` when [isDense] is
/// true and `EdgeInsets.fromLTRB(0, 12, 0, 12)` when [isDense] is false.
///
/// If `isOutline` property of [border] is true then [contentPadding] is
/// `EdgeInsets.fromLTRB(12, 20, 12, 12)` when [isDense] is true
/// and `EdgeInsets.fromLTRB(12, 24, 12, 16)` when [isDense] is false.
final EdgeInsetsGeometry? contentPadding;
/// Whether the decoration is the same size as the input field.
///
/// A collapsed decoration cannot have [labelText], [errorText], an [icon].
///
/// To create a collapsed input decoration, use [InputDecoration.collapsed].
final bool? isCollapsed;
/// An icon that appears before the [prefix] or [prefixText] and before
/// the editable part of the text field, within the decoration's container.
///
/// The size and color of the prefix icon is configured automatically using an
/// [IconTheme] and therefore does not need to be explicitly given in the
/// icon widget.
///
/// The prefix icon is constrained with a minimum size of 48px by 48px, but
/// can be expanded beyond that. Anything larger than 24px will require
/// additional padding to ensure it matches the Material Design spec of 12px
/// padding between the left edge of the input and leading edge of the prefix
/// icon. The following snippet shows how to pad the leading edge of the
/// prefix icon:
///
/// ```dart
/// prefixIcon: Padding(
/// padding: const EdgeInsetsDirectional.only(start: 12.0),
/// child: _myIcon, // _myIcon is a 48px-wide widget.
/// )
/// ```
///
/// {@macro flutter.material.input_decorator.container_description}
///
/// The prefix icon alignment can be changed using [Align] with a fixed `widthFactor` and
/// `heightFactor`.
///
/// {@tool dartpad}
/// This example shows how the prefix icon alignment can be changed using [Align] with
/// a fixed `widthFactor` and `heightFactor`.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.prefix_icon.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Icon] and [ImageIcon], which are typically used to show icons.
/// * [prefix] and [prefixText], which are other ways to show content
/// before the text field (but after the icon).
/// * [suffixIcon], which is the same but on the trailing edge.
/// * [Align] A widget that aligns its child within itself and optionally
/// sizes itself based on the child's size.
final Widget? prefixIcon;
/// The constraints for the prefix icon.
///
/// This can be used to modify the [BoxConstraints] surrounding [prefixIcon].
///
/// This property is particularly useful for getting the decoration's height
/// less than 48px. This can be achieved by setting [isDense] to true and
/// setting the constraints' minimum height and width to a value lower than
/// 48px.
///
/// {@tool dartpad}
/// This example shows the differences between two `TextField` widgets when
/// [prefixIconConstraints] is set to the default value and when one is not.
///
/// The [isDense] property must be set to true to be able to
/// set the constraints smaller than 48px.
///
/// If null, [BoxConstraints] with a minimum width and height of 48px is
/// used.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.prefix_icon_constraints.0.dart **
/// {@end-tool}
final BoxConstraints? prefixIconConstraints;
/// Optional widget to place on the line before the input.
///
/// This can be used, for example, to add some padding to text that would
/// otherwise be specified using [prefixText], or to add a custom widget in
/// front of the input. The widget's baseline is lined up with the input
/// baseline.
///
/// Only one of [prefix] and [prefixText] can be specified.
///
/// The [prefix] appears after the [prefixIcon], if both are specified.
///
/// See also:
///
/// * [suffix], the equivalent but on the trailing edge.
final Widget? prefix;
/// Optional text prefix to place on the line before the input.
///
/// Uses the [prefixStyle]. Uses [hintStyle] if [prefixStyle] isn't specified.
/// The prefix text is not returned as part of the user's input.
///
/// If a more elaborate prefix is required, consider using [prefix] instead.
/// Only one of [prefix] and [prefixText] can be specified.
///
/// The [prefixText] appears after the [prefixIcon], if both are specified.
///
/// See also:
///
/// * [suffixText], the equivalent but on the trailing edge.
final String? prefixText;
/// The style to use for the [prefixText].
///
/// If [prefixStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [hintStyle].
///
/// See also:
///
/// * [suffixStyle], the equivalent but on the trailing edge.
final TextStyle? prefixStyle;
/// Optional color of the prefixIcon
///
/// Defaults to [iconColor]
///
/// If [prefixIconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
final Color? prefixIconColor;
/// An icon that appears after the editable part of the text field and
/// after the [suffix] or [suffixText], within the decoration's container.
///
/// The size and color of the suffix icon is configured automatically using an
/// [IconTheme] and therefore does not need to be explicitly given in the
/// icon widget.
///
/// The suffix icon is constrained with a minimum size of 48px by 48px, but
/// can be expanded beyond that. Anything larger than 24px will require
/// additional padding to ensure it matches the Material Design spec of 12px
/// padding between the right edge of the input and trailing edge of the
/// prefix icon. The following snippet shows how to pad the trailing edge of
/// the suffix icon:
///
/// ```dart
/// suffixIcon: Padding(
/// padding: const EdgeInsetsDirectional.only(end: 12.0),
/// child: _myIcon, // myIcon is a 48px-wide widget.
/// )
/// ```
///
/// The decoration's container is the area which is filled if [filled] is
/// true and bordered per the [border]. It's the area adjacent to
/// [icon] and above the widgets that contain [helperText],
/// [errorText], and [counterText].
///
/// The suffix icon alignment can be changed using [Align] with a fixed `widthFactor` and
/// `heightFactor`.
///
/// {@tool dartpad}
/// This example shows how the suffix icon alignment can be changed using [Align] with
/// a fixed `widthFactor` and `heightFactor`.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.suffix_icon.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Icon] and [ImageIcon], which are typically used to show icons.
/// * [suffix] and [suffixText], which are other ways to show content
/// after the text field (but before the icon).
/// * [prefixIcon], which is the same but on the leading edge.
/// * [Align] A widget that aligns its child within itself and optionally
/// sizes itself based on the child's size.
final Widget? suffixIcon;
/// Optional widget to place on the line after the input.
///
/// This can be used, for example, to add some padding to the text that would
/// otherwise be specified using [suffixText], or to add a custom widget after
/// the input. The widget's baseline is lined up with the input baseline.
///
/// Only one of [suffix] and [suffixText] can be specified.
///
/// The [suffix] appears before the [suffixIcon], if both are specified.
///
/// See also:
///
/// * [prefix], the equivalent but on the leading edge.
final Widget? suffix;
/// Optional text suffix to place on the line after the input.
///
/// Uses the [suffixStyle]. Uses [hintStyle] if [suffixStyle] isn't specified.
/// The suffix text is not returned as part of the user's input.
///
/// If a more elaborate suffix is required, consider using [suffix] instead.
/// Only one of [suffix] and [suffixText] can be specified.
///
/// The [suffixText] appears before the [suffixIcon], if both are specified.
///
/// See also:
///
/// * [prefixText], the equivalent but on the leading edge.
final String? suffixText;
/// The style to use for the [suffixText].
///
/// If [suffixStyle] is a [MaterialStateTextStyle], then the effective text
/// style can depend on the [MaterialState.focused] state, i.e. if the
/// [TextField] is focused or not.
///
/// If null, defaults to the [hintStyle].
///
/// See also:
///
/// * [prefixStyle], the equivalent but on the leading edge.
final TextStyle? suffixStyle;
/// Optional color of the [suffixIcon].
///
/// Defaults to [iconColor]
///
/// If [suffixIconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
final Color? suffixIconColor;
/// The constraints for the suffix icon.
///
/// This can be used to modify the [BoxConstraints] surrounding [suffixIcon].
///
/// This property is particularly useful for getting the decoration's height
/// less than 48px. This can be achieved by setting [isDense] to true and
/// setting the constraints' minimum height and width to a value lower than
/// 48px.
///
/// If null, a [BoxConstraints] with a minimum width and height of 48px is
/// used.
///
/// {@tool dartpad}
/// This example shows the differences between two `TextField` widgets when
/// [suffixIconConstraints] is set to the default value and when one is not.
///
/// The [isDense] property must be set to true to be able to
/// set the constraints smaller than 48px.
///
/// If null, [BoxConstraints] with a minimum width and height of 48px is
/// used.
///
/// ** See code in examples/api/lib/material/input_decorator/input_decoration.suffix_icon_constraints.0.dart **
/// {@end-tool}
final BoxConstraints? suffixIconConstraints;
/// Optional text to place below the line as a character count.
///
/// Rendered using [counterStyle]. Uses [helperStyle] if [counterStyle] is
/// null.
///
/// The semantic label can be replaced by providing a [semanticCounterText].
///
/// If null or an empty string and [counter] isn't specified, then nothing
/// will appear in the counter's location.
final String? counterText;
/// Optional custom counter widget to go in the place otherwise occupied by
/// [counterText]. If this property is non null, then [counterText] is
/// ignored.
final Widget? counter;
/// The style to use for the [counterText].
///
/// If [counterStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [helperStyle].
final TextStyle? counterStyle;
/// If true the decoration's container is filled with [fillColor].
///
/// When [InputDecorator.isHovering] is true, the [hoverColor] is also blended
/// into the final fill color.
///
/// Typically this field set to true if [border] is an [UnderlineInputBorder].
///
/// {@template flutter.material.input_decorator.container_description}
/// The decoration's container is the area which is filled if [filled] is true
/// and bordered per the [border]. It's the area adjacent to [icon] and above
/// the widgets that contain [helperText], [errorText], and [counterText].
/// {@endtemplate}
///
/// This property is false by default.
final bool? filled;
/// The base fill color of the decoration's container color.
///
/// When [InputDecorator.isHovering] is true, the [hoverColor] is also blended
/// into the final fill color.
///
/// By default the [fillColor] is based on the current
/// [InputDecorationTheme.fillColor].
///
/// {@macro flutter.material.input_decorator.container_description}
final Color? fillColor;
/// The fill color of the decoration's container when it has the input focus.
///
/// By default the [focusColor] is based on the current
/// [InputDecorationTheme.focusColor].
///
/// This [focusColor] is ignored by [TextField] and [TextFormField] because
/// they don't respond to focus changes by changing their decorator's
/// container color, they respond by changing their border to the
/// [focusedBorder], which you can change the color of.
///
/// {@macro flutter.material.input_decorator.container_description}
final Color? focusColor;
/// The color of the highlight for the decoration shown if the container
/// is being hovered over by a mouse.
///
/// If [filled] is true, the [hoverColor] is blended with [fillColor] and
/// fills the decoration's container.
///
/// If [filled] is false, and [InputDecorator.isFocused] is false, the color
/// is blended over the [enabledBorder]'s color.
///
/// By default the [hoverColor] is based on the current [Theme].
///
/// {@macro flutter.material.input_decorator.container_description}
final Color? hoverColor;
/// The border to display when the [InputDecorator] does not have the focus and
/// is showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? errorBorder;
/// The border to display when the [InputDecorator] has the focus and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? focusedBorder;
/// The border to display when the [InputDecorator] has the focus and is
/// showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? focusedErrorBorder;
/// The border to display when the [InputDecorator] is disabled and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecoration.enabled], which is false if the [InputDecorator] is disabled.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? disabledBorder;
/// The border to display when the [InputDecorator] is enabled and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecoration.enabled], which is false if the [InputDecorator] is disabled.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
final InputBorder? enabledBorder;
/// The shape of the border to draw around the decoration's container.
///
/// If [border] is a [MaterialStateUnderlineInputBorder]
/// or [MaterialStateOutlineInputBorder], then the effective border can depend on
/// the [MaterialState.focused] state, i.e. if the [TextField] is focused or not.
///
/// If [border] derives from [InputBorder] the border's [InputBorder.borderSide],
/// i.e. the border's color and width, will be overridden to reflect the input
/// decorator's state. Only the border's shape is used. If custom [BorderSide]
/// values are desired for a given state, all four borders – [errorBorder],
/// [focusedBorder], [enabledBorder], [disabledBorder] – must be set.
///
/// The decoration's container is the area which is filled if [filled] is
/// true and bordered per the [border]. It's the area adjacent to
/// [InputDecoration.icon] and above the widgets that contain
/// [InputDecoration.helperText], [InputDecoration.errorText], and
/// [InputDecoration.counterText].
///
/// The border's bounds, i.e. the value of `border.getOuterPath()`, define
/// the area to be filled.
///
/// This property is only used when the appropriate one of [errorBorder],
/// [focusedBorder], [focusedErrorBorder], [disabledBorder], or [enabledBorder]
/// is not specified. This border's [InputBorder.borderSide] property is
/// configured by the InputDecorator, depending on the values of
/// [InputDecoration.errorText], [InputDecoration.enabled],
/// [InputDecorator.isFocused] and the current [Theme].
///
/// Typically one of [UnderlineInputBorder] or [OutlineInputBorder].
/// If null, InputDecorator's default is `const UnderlineInputBorder()`.
///
/// See also:
///
/// * [InputBorder.none], which doesn't draw a border.
/// * [UnderlineInputBorder], which draws a horizontal line at the
/// bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
final InputBorder? border;
/// If false [helperText],[errorText], and [counterText] are not displayed,
/// and the opacity of the remaining visual elements is reduced.
///
/// This property is true by default.
final bool enabled;
/// A semantic label for the [counterText].
///
/// Defaults to null.
///
/// If provided, this replaces the semantic label of the [counterText].
final String? semanticCounterText;
/// Typically set to true when the [InputDecorator] contains a multiline
/// [TextField] ([TextField.maxLines] is null or > 1) to override the default
/// behavior of aligning the label with the center of the [TextField].
///
/// Defaults to false.
final bool? alignLabelWithHint;
/// Defines minimum and maximum sizes for the [InputDecorator].
///
/// Typically the decorator will fill the horizontal space it is given. For
/// larger screens, it may be useful to have the maximum width clamped to
/// a given value so it doesn't fill the whole screen. This property
/// allows you to control how big the decorator will be in its available
/// space.
///
/// If null, then the ambient [ThemeData.inputDecorationTheme]'s
/// [InputDecorationTheme.constraints] will be used. If that
/// is null then the decorator will fill the available width with
/// a default height based on text size.
final BoxConstraints? constraints;
/// Creates a copy of this input decoration with the given fields replaced
/// by the new values.
InputDecoration copyWith({
Widget? icon,
Color? iconColor,
Widget? label,
String? labelText,
TextStyle? labelStyle,
TextStyle? floatingLabelStyle,
Widget? helper,
String? helperText,
TextStyle? helperStyle,
int? helperMaxLines,
String? hintText,
TextStyle? hintStyle,
TextDirection? hintTextDirection,
Duration? hintFadeDuration,
int? hintMaxLines,
Widget? error,
String? errorText,
TextStyle? errorStyle,
int? errorMaxLines,
FloatingLabelBehavior? floatingLabelBehavior,
FloatingLabelAlignment? floatingLabelAlignment,
bool? isCollapsed,
bool? isDense,
EdgeInsetsGeometry? contentPadding,
Widget? prefixIcon,
Widget? prefix,
String? prefixText,
BoxConstraints? prefixIconConstraints,
TextStyle? prefixStyle,
Color? prefixIconColor,
Widget? suffixIcon,
Widget? suffix,
String? suffixText,
TextStyle? suffixStyle,
Color? suffixIconColor,
BoxConstraints? suffixIconConstraints,
Widget? counter,
String? counterText,
TextStyle? counterStyle,
bool? filled,
Color? fillColor,
Color? focusColor,
Color? hoverColor,
InputBorder? errorBorder,
InputBorder? focusedBorder,
InputBorder? focusedErrorBorder,
InputBorder? disabledBorder,
InputBorder? enabledBorder,
InputBorder? border,
bool? enabled,
String? semanticCounterText,
bool? alignLabelWithHint,
BoxConstraints? constraints,
}) {
return InputDecoration(
icon: icon ?? this.icon,
iconColor: iconColor ?? this.iconColor,
label: label ?? this.label,
labelText: labelText ?? this.labelText,
labelStyle: labelStyle ?? this.labelStyle,
floatingLabelStyle: floatingLabelStyle ?? this.floatingLabelStyle,
helper: helper ?? this.helper,
helperText: helperText ?? this.helperText,
helperStyle: helperStyle ?? this.helperStyle,
helperMaxLines : helperMaxLines ?? this.helperMaxLines,
hintText: hintText ?? this.hintText,
hintStyle: hintStyle ?? this.hintStyle,
hintTextDirection: hintTextDirection ?? this.hintTextDirection,
hintMaxLines: hintMaxLines ?? this.hintMaxLines,
hintFadeDuration: hintFadeDuration ?? this.hintFadeDuration,
error: error ?? this.error,
errorText: errorText ?? this.errorText,
errorStyle: errorStyle ?? this.errorStyle,
errorMaxLines: errorMaxLines ?? this.errorMaxLines,
floatingLabelBehavior: floatingLabelBehavior ?? this.floatingLabelBehavior,
floatingLabelAlignment: floatingLabelAlignment ?? this.floatingLabelAlignment,
isCollapsed: isCollapsed ?? this.isCollapsed,
isDense: isDense ?? this.isDense,
contentPadding: contentPadding ?? this.contentPadding,
prefixIcon: prefixIcon ?? this.prefixIcon,
prefix: prefix ?? this.prefix,
prefixText: prefixText ?? this.prefixText,
prefixStyle: prefixStyle ?? this.prefixStyle,
prefixIconColor: prefixIconColor ?? this.prefixIconColor,
prefixIconConstraints: prefixIconConstraints ?? this.prefixIconConstraints,
suffixIcon: suffixIcon ?? this.suffixIcon,
suffix: suffix ?? this.suffix,
suffixText: suffixText ?? this.suffixText,
suffixStyle: suffixStyle ?? this.suffixStyle,
suffixIconColor: suffixIconColor ?? this.suffixIconColor,
suffixIconConstraints: suffixIconConstraints ?? this.suffixIconConstraints,
counter: counter ?? this.counter,
counterText: counterText ?? this.counterText,
counterStyle: counterStyle ?? this.counterStyle,
filled: filled ?? this.filled,
fillColor: fillColor ?? this.fillColor,
focusColor: focusColor ?? this.focusColor,
hoverColor: hoverColor ?? this.hoverColor,
errorBorder: errorBorder ?? this.errorBorder,
focusedBorder: focusedBorder ?? this.focusedBorder,
focusedErrorBorder: focusedErrorBorder ?? this.focusedErrorBorder,
disabledBorder: disabledBorder ?? this.disabledBorder,
enabledBorder: enabledBorder ?? this.enabledBorder,
border: border ?? this.border,
enabled: enabled ?? this.enabled,
semanticCounterText: semanticCounterText ?? this.semanticCounterText,
alignLabelWithHint: alignLabelWithHint ?? this.alignLabelWithHint,
constraints: constraints ?? this.constraints,
);
}
/// Used by widgets like [TextField] and [InputDecorator] to create a new
/// [InputDecoration] with default values taken from the [theme].
///
/// Only null valued properties from this [InputDecoration] are replaced
/// by the corresponding values from [theme].
InputDecoration applyDefaults(InputDecorationTheme theme) {
return copyWith(
labelStyle: labelStyle ?? theme.labelStyle,
floatingLabelStyle: floatingLabelStyle ?? theme.floatingLabelStyle,
helperStyle: helperStyle ?? theme.helperStyle,
helperMaxLines : helperMaxLines ?? theme.helperMaxLines,
hintStyle: hintStyle ?? theme.hintStyle,
hintFadeDuration: hintFadeDuration ?? theme.hintFadeDuration,
errorStyle: errorStyle ?? theme.errorStyle,
errorMaxLines: errorMaxLines ?? theme.errorMaxLines,
floatingLabelBehavior: floatingLabelBehavior ?? theme.floatingLabelBehavior,
floatingLabelAlignment: floatingLabelAlignment ?? theme.floatingLabelAlignment,
isDense: isDense ?? theme.isDense,
contentPadding: contentPadding ?? theme.contentPadding,
isCollapsed: isCollapsed ?? theme.isCollapsed,
iconColor: iconColor ?? theme.iconColor,
prefixStyle: prefixStyle ?? theme.prefixStyle,
prefixIconColor: prefixIconColor ?? theme.prefixIconColor,
suffixStyle: suffixStyle ?? theme.suffixStyle,
suffixIconColor: suffixIconColor ?? theme.suffixIconColor,
counterStyle: counterStyle ?? theme.counterStyle,
filled: filled ?? theme.filled,
fillColor: fillColor ?? theme.fillColor,
focusColor: focusColor ?? theme.focusColor,
hoverColor: hoverColor ?? theme.hoverColor,
errorBorder: errorBorder ?? theme.errorBorder,
focusedBorder: focusedBorder ?? theme.focusedBorder,
focusedErrorBorder: focusedErrorBorder ?? theme.focusedErrorBorder,
disabledBorder: disabledBorder ?? theme.disabledBorder,
enabledBorder: enabledBorder ?? theme.enabledBorder,
border: border ?? theme.border,
alignLabelWithHint: alignLabelWithHint ?? theme.alignLabelWithHint,
constraints: constraints ?? theme.constraints,
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is InputDecoration
&& other.icon == icon
&& other.iconColor == iconColor
&& other.label == label
&& other.labelText == labelText
&& other.labelStyle == labelStyle
&& other.floatingLabelStyle == floatingLabelStyle
&& other.helper == helper
&& other.helperText == helperText
&& other.helperStyle == helperStyle
&& other.helperMaxLines == helperMaxLines
&& other.hintText == hintText
&& other.hintStyle == hintStyle
&& other.hintTextDirection == hintTextDirection
&& other.hintMaxLines == hintMaxLines
&& other.hintFadeDuration == hintFadeDuration
&& other.error == error
&& other.errorText == errorText
&& other.errorStyle == errorStyle
&& other.errorMaxLines == errorMaxLines
&& other.floatingLabelBehavior == floatingLabelBehavior
&& other.floatingLabelAlignment == floatingLabelAlignment
&& other.isDense == isDense
&& other.contentPadding == contentPadding
&& other.isCollapsed == isCollapsed
&& other.prefixIcon == prefixIcon
&& other.prefixIconColor == prefixIconColor
&& other.prefix == prefix
&& other.prefixText == prefixText
&& other.prefixStyle == prefixStyle
&& other.prefixIconConstraints == prefixIconConstraints
&& other.suffixIcon == suffixIcon
&& other.suffixIconColor == suffixIconColor
&& other.suffix == suffix
&& other.suffixText == suffixText
&& other.suffixStyle == suffixStyle
&& other.suffixIconConstraints == suffixIconConstraints
&& other.counter == counter
&& other.counterText == counterText
&& other.counterStyle == counterStyle
&& other.filled == filled
&& other.fillColor == fillColor
&& other.focusColor == focusColor
&& other.hoverColor == hoverColor
&& other.errorBorder == errorBorder
&& other.focusedBorder == focusedBorder
&& other.focusedErrorBorder == focusedErrorBorder
&& other.disabledBorder == disabledBorder
&& other.enabledBorder == enabledBorder
&& other.border == border
&& other.enabled == enabled
&& other.semanticCounterText == semanticCounterText
&& other.alignLabelWithHint == alignLabelWithHint
&& other.constraints == constraints;
}
@override
int get hashCode {
final List<Object?> values = <Object?>[
icon,
iconColor,
label,
labelText,
floatingLabelStyle,
labelStyle,
helper,
helperText,
helperStyle,
helperMaxLines,
hintText,
hintStyle,
hintTextDirection,
hintMaxLines,
hintFadeDuration,
error,
errorText,
errorStyle,
errorMaxLines,
floatingLabelBehavior,
floatingLabelAlignment,
isDense,
contentPadding,
isCollapsed,
filled,
fillColor,
focusColor,
hoverColor,
prefixIcon,
prefixIconColor,
prefix,
prefixText,
prefixStyle,
prefixIconConstraints,
suffixIcon,
suffixIconColor,
suffix,
suffixText,
suffixStyle,
suffixIconConstraints,
counter,
counterText,
counterStyle,
errorBorder,
focusedBorder,
focusedErrorBorder,
disabledBorder,
enabledBorder,
border,
enabled,
semanticCounterText,
alignLabelWithHint,
constraints,
];
return Object.hashAll(values);
}
@override
String toString() {
final List<String> description = <String>[
if (icon != null) 'icon: $icon',
if (iconColor != null) 'iconColor: $iconColor',
if (label != null) 'label: $label',
if (labelText != null) 'labelText: "$labelText"',
if (floatingLabelStyle != null) 'floatingLabelStyle: "$floatingLabelStyle"',
if (helper != null) 'helper: "$helper"',
if (helperText != null) 'helperText: "$helperText"',
if (helperMaxLines != null) 'helperMaxLines: "$helperMaxLines"',
if (hintText != null) 'hintText: "$hintText"',
if (hintMaxLines != null) 'hintMaxLines: "$hintMaxLines"',
if (hintFadeDuration != null) 'hintFadeDuration: "$hintFadeDuration"',
if (error != null) 'error: "$error"',
if (errorText != null) 'errorText: "$errorText"',
if (errorStyle != null) 'errorStyle: "$errorStyle"',
if (errorMaxLines != null) 'errorMaxLines: "$errorMaxLines"',
if (floatingLabelBehavior != null) 'floatingLabelBehavior: $floatingLabelBehavior',
if (floatingLabelAlignment != null) 'floatingLabelAlignment: $floatingLabelAlignment',
if (isDense ?? false) 'isDense: $isDense',
if (contentPadding != null) 'contentPadding: $contentPadding',
if (isCollapsed ?? false) 'isCollapsed: $isCollapsed',
if (prefixIcon != null) 'prefixIcon: $prefixIcon',
if (prefixIconColor != null) 'prefixIconColor: $prefixIconColor',
if (prefix != null) 'prefix: $prefix',
if (prefixText != null) 'prefixText: $prefixText',
if (prefixStyle != null) 'prefixStyle: $prefixStyle',
if (prefixIconConstraints != null) 'prefixIconConstraints: $prefixIconConstraints',
if (suffixIcon != null) 'suffixIcon: $suffixIcon',
if (suffixIconColor != null) 'suffixIconColor: $suffixIconColor',
if (suffix != null) 'suffix: $suffix',
if (suffixText != null) 'suffixText: $suffixText',
if (suffixStyle != null) 'suffixStyle: $suffixStyle',
if (suffixIconConstraints != null) 'suffixIconConstraints: $suffixIconConstraints',
if (counter != null) 'counter: $counter',
if (counterText != null) 'counterText: $counterText',
if (counterStyle != null) 'counterStyle: $counterStyle',
if (filled ?? false) 'filled: true',
if (fillColor != null) 'fillColor: $fillColor',
if (focusColor != null) 'focusColor: $focusColor',
if (hoverColor != null) 'hoverColor: $hoverColor',
if (errorBorder != null) 'errorBorder: $errorBorder',
if (focusedBorder != null) 'focusedBorder: $focusedBorder',
if (focusedErrorBorder != null) 'focusedErrorBorder: $focusedErrorBorder',
if (disabledBorder != null) 'disabledBorder: $disabledBorder',
if (enabledBorder != null) 'enabledBorder: $enabledBorder',
if (border != null) 'border: $border',
if (!enabled) 'enabled: false',
if (semanticCounterText != null) 'semanticCounterText: $semanticCounterText',
if (alignLabelWithHint != null) 'alignLabelWithHint: $alignLabelWithHint',
if (constraints != null) 'constraints: $constraints',
];
return 'InputDecoration(${description.join(', ')})';
}
}
/// Defines the default appearance of [InputDecorator]s.
///
/// This class is used to define the value of [ThemeData.inputDecorationTheme].
/// The [InputDecorator], [TextField], and [TextFormField] widgets use
/// the current input decoration theme to initialize null [InputDecoration]
/// properties.
///
/// The [InputDecoration.applyDefaults] method is used to combine a input
/// decoration theme with an [InputDecoration] object.
@immutable
class InputDecorationTheme with Diagnosticable {
/// Creates a value for [ThemeData.inputDecorationTheme] that
/// defines default values for [InputDecorator].
const InputDecorationTheme({
this.labelStyle,
this.floatingLabelStyle,
this.helperStyle,
this.helperMaxLines,
this.hintStyle,
this.hintFadeDuration,
this.errorStyle,
this.errorMaxLines,
this.floatingLabelBehavior = FloatingLabelBehavior.auto,
this.floatingLabelAlignment = FloatingLabelAlignment.start,
this.isDense = false,
this.contentPadding,
this.isCollapsed = false,
this.iconColor,
this.prefixStyle,
this.prefixIconColor,
this.suffixStyle,
this.suffixIconColor,
this.counterStyle,
this.filled = false,
this.fillColor,
this.activeIndicatorBorder,
this.outlineBorder,
this.focusColor,
this.hoverColor,
this.errorBorder,
this.focusedBorder,
this.focusedErrorBorder,
this.disabledBorder,
this.enabledBorder,
this.border,
this.alignLabelWithHint = false,
this.constraints,
});
/// {@macro flutter.material.inputDecoration.labelStyle}
final TextStyle? labelStyle;
/// {@macro flutter.material.inputDecoration.floatingLabelStyle}
final TextStyle? floatingLabelStyle;
/// The style to use for [InputDecoration.helperText].
///
/// If [helperStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
final TextStyle? helperStyle;
/// The maximum number of lines the [InputDecoration.helperText] can occupy.
///
/// Defaults to null, which means that the [InputDecoration.helperText] will
/// be limited to a single line with [TextOverflow.ellipsis].
///
/// This value is passed along to the [Text.maxLines] attribute
/// of the [Text] widget used to display the helper.
///
/// See also:
///
/// * [errorMaxLines], the equivalent but for the [InputDecoration.errorText].
final int? helperMaxLines;
/// The style to use for the [InputDecoration.hintText].
///
/// If [hintStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// Also used for the [InputDecoration.labelText] when the
/// [InputDecoration.labelText] is displayed on top of the input field (i.e.,
/// at the same location on the screen where text may be entered in the input
/// field).
///
/// If null, defaults to a value derived from the base [TextStyle] for the
/// input field and the current [Theme].
final TextStyle? hintStyle;
/// The duration of the [InputDecoration.hintText] fade in and fade out animations.
final Duration? hintFadeDuration;
/// {@macro flutter.material.inputDecoration.errorStyle}
final TextStyle? errorStyle;
/// The maximum number of lines the [InputDecoration.errorText] can occupy.
///
/// Defaults to null, which means that the [InputDecoration.errorText] will be
/// limited to a single line with [TextOverflow.ellipsis].
///
/// This value is passed along to the [Text.maxLines] attribute
/// of the [Text] widget used to display the error.
///
/// See also:
///
/// * [helperMaxLines], the equivalent but for the [InputDecoration.helperText].
final int? errorMaxLines;
/// {@macro flutter.material.inputDecoration.floatingLabelBehavior}
///
/// Defaults to [FloatingLabelBehavior.auto].
final FloatingLabelBehavior floatingLabelBehavior;
/// {@macro flutter.material.inputDecoration.floatingLabelAlignment}
///
/// Defaults to [FloatingLabelAlignment.start].
final FloatingLabelAlignment floatingLabelAlignment;
/// Whether the input decorator's child is part of a dense form (i.e., uses
/// less vertical space).
///
/// Defaults to false.
final bool isDense;
/// The padding for the input decoration's container.
///
/// The decoration's container is the area which is filled if
/// [InputDecoration.filled] is true and bordered per the [border].
/// It's the area adjacent to [InputDecoration.icon] and above the
/// [InputDecoration.icon] and above the widgets that contain
/// [InputDecoration.helperText], [InputDecoration.errorText], and
/// [InputDecoration.counterText].
///
/// By default the [contentPadding] reflects [isDense] and the type of the
/// [border]. If [isCollapsed] is true then [contentPadding] is
/// [EdgeInsets.zero].
final EdgeInsetsGeometry? contentPadding;
/// Whether the decoration is the same size as the input field.
///
/// A collapsed decoration cannot have [InputDecoration.labelText],
/// [InputDecoration.errorText], or an [InputDecoration.icon].
final bool isCollapsed;
/// The Color to use for the [InputDecoration.icon].
///
/// If [iconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [ColorScheme.primary].
final Color? iconColor;
/// The style to use for the [InputDecoration.prefixText].
///
/// If [prefixStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [hintStyle].
final TextStyle? prefixStyle;
/// The Color to use for the [InputDecoration.prefixIcon].
///
/// If [prefixIconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [ColorScheme.primary].
final Color? prefixIconColor;
/// The style to use for the [InputDecoration.suffixText].
///
/// If [suffixStyle] is a [MaterialStateTextStyle], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [hintStyle].
final TextStyle? suffixStyle;
/// The Color to use for the [InputDecoration.suffixIcon].
///
/// If [suffixIconColor] is a [MaterialStateColor], then the effective
/// color can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [ColorScheme.primary].
final Color? suffixIconColor;
/// The style to use for the [InputDecoration.counterText].
///
/// If [counterStyle] is a [MaterialStateTextStyle], then the effective
/// text style can depend on the [MaterialState.focused] state, i.e.
/// if the [TextField] is focused or not.
///
/// If null, defaults to the [helperStyle].
final TextStyle? counterStyle;
/// If true the decoration's container is filled with [fillColor].
///
/// Typically this field set to true if [border] is an
/// [UnderlineInputBorder].
///
/// The decoration's container is the area, defined by the border's
/// [InputBorder.getOuterPath], which is filled if [filled] is
/// true and bordered per the [border].
///
/// This property is false by default.
final bool filled;
/// The color to fill the decoration's container with, if [filled] is true.
///
/// By default the fillColor is based on the current [Theme].
///
/// The decoration's container is the area, defined by the border's
/// [InputBorder.getOuterPath], which is filled if [filled] is
/// true and bordered per the [border].
final Color? fillColor;
/// The borderSide of the OutlineInputBorder with `color` and `weight`.
final BorderSide? outlineBorder;
/// The borderSide of the UnderlineInputBorder with `color` and `weight`.
final BorderSide? activeIndicatorBorder;
/// The color to blend with the decoration's [fillColor] with, if [filled] is
/// true and the container has the input focus.
///
/// By default the [focusColor] is based on the current [Theme].
///
/// The decoration's container is the area, defined by the border's
/// [InputBorder.getOuterPath], which is filled if [filled] is
/// true and bordered per the [border].
final Color? focusColor;
/// The color to blend with the decoration's [fillColor] with, if the
/// decoration is being hovered over by a mouse pointer.
///
/// By default the [hoverColor] is based on the current [Theme].
///
/// The decoration's container is the area, defined by the border's
/// [InputBorder.getOuterPath], which is filled if [filled] is
/// true and bordered per the [border].
///
/// The container will be filled when hovered over even if [filled] is false.
final Color? hoverColor;
/// The border to display when the [InputDecorator] does not have the focus and
/// is showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? errorBorder;
/// The border to display when the [InputDecorator] has the focus and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? focusedBorder;
/// The border to display when the [InputDecorator] has the focus and is
/// showing an error.
///
/// See also:
///
/// * [InputDecorator.isFocused], which is true if the [InputDecorator]'s child
/// has the focus.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? focusedErrorBorder;
/// The border to display when the [InputDecorator] is disabled and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecoration.enabled], which is false if the [InputDecorator] is disabled.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [enabledBorder], displayed when [InputDecoration.enabled] is true
/// and [InputDecoration.errorText] is null.
final InputBorder? disabledBorder;
/// The border to display when the [InputDecorator] is enabled and is not
/// showing an error.
///
/// See also:
///
/// * [InputDecoration.enabled], which is false if the [InputDecorator] is disabled.
/// * [InputDecoration.errorText], the error shown by the [InputDecorator], if non-null.
/// * [border], for a description of where the [InputDecorator] border appears.
/// * [UnderlineInputBorder], an [InputDecorator] border which draws a horizontal
/// line at the bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
/// * [InputBorder.none], which doesn't draw a border.
/// * [errorBorder], displayed when [InputDecorator.isFocused] is false
/// and [InputDecoration.errorText] is non-null.
/// * [focusedBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is null.
/// * [focusedErrorBorder], displayed when [InputDecorator.isFocused] is true
/// and [InputDecoration.errorText] is non-null.
/// * [disabledBorder], displayed when [InputDecoration.enabled] is false
/// and [InputDecoration.errorText] is null.
final InputBorder? enabledBorder;
/// The shape of the border to draw around the decoration's container.
///
/// If [border] is a [MaterialStateUnderlineInputBorder]
/// or [MaterialStateOutlineInputBorder], then the effective border can depend on
/// the [MaterialState.focused] state, i.e. if the [TextField] is focused or not.
///
/// The decoration's container is the area which is filled if [filled] is
/// true and bordered per the [border]. It's the area adjacent to
/// [InputDecoration.icon] and above the widgets that contain
/// [InputDecoration.helperText], [InputDecoration.errorText], and
/// [InputDecoration.counterText].
///
/// The border's bounds, i.e. the value of `border.getOuterPath()`, define
/// the area to be filled.
///
/// This property is only used when the appropriate one of [errorBorder],
/// [focusedBorder], [focusedErrorBorder], [disabledBorder], or [enabledBorder]
/// is not specified. This border's [InputBorder.borderSide] property is
/// configured by the InputDecorator, depending on the values of
/// [InputDecoration.errorText], [InputDecoration.enabled],
/// [InputDecorator.isFocused] and the current [Theme].
///
/// Typically one of [UnderlineInputBorder] or [OutlineInputBorder].
/// If null, InputDecorator's default is `const UnderlineInputBorder()`.
///
/// See also:
///
/// * [InputBorder.none], which doesn't draw a border.
/// * [UnderlineInputBorder], which draws a horizontal line at the
/// bottom of the input decorator's container.
/// * [OutlineInputBorder], an [InputDecorator] border which draws a
/// rounded rectangle around the input decorator's container.
final InputBorder? border;
/// Typically set to true when the [InputDecorator] contains a multiline
/// [TextField] ([TextField.maxLines] is null or > 1) to override the default
/// behavior of aligning the label with the center of the [TextField].
final bool alignLabelWithHint;
/// Defines minimum and maximum sizes for the [InputDecorator].
///
/// Typically the decorator will fill the horizontal space it is given. For
/// larger screens, it may be useful to have the maximum width clamped to
/// a given value so it doesn't fill the whole screen. This property
/// allows you to control how big the decorator will be in its available
/// space.
///
/// If null, then the decorator will fill the available width with
/// a default height based on text size.
///
/// See also:
///
/// * [InputDecoration.constraints], which can override this setting for a
/// given decorator.
final BoxConstraints? constraints;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
InputDecorationTheme copyWith({
TextStyle? labelStyle,
TextStyle? floatingLabelStyle,
TextStyle? helperStyle,
int? helperMaxLines,
TextStyle? hintStyle,
Duration? hintFadeDuration,
TextStyle? errorStyle,
int? errorMaxLines,
FloatingLabelBehavior? floatingLabelBehavior,
FloatingLabelAlignment? floatingLabelAlignment,
bool? isDense,
EdgeInsetsGeometry? contentPadding,
bool? isCollapsed,
Color? iconColor,
TextStyle? prefixStyle,
Color? prefixIconColor,
TextStyle? suffixStyle,
Color? suffixIconColor,
TextStyle? counterStyle,
bool? filled,
Color? fillColor,
BorderSide? activeIndicatorBorder,
BorderSide? outlineBorder,
Color? focusColor,
Color? hoverColor,
InputBorder? errorBorder,
InputBorder? focusedBorder,
InputBorder? focusedErrorBorder,
InputBorder? disabledBorder,
InputBorder? enabledBorder,
InputBorder? border,
bool? alignLabelWithHint,
BoxConstraints? constraints,
}) {
return InputDecorationTheme(
labelStyle: labelStyle ?? this.labelStyle,
floatingLabelStyle: floatingLabelStyle ?? this.floatingLabelStyle,
helperStyle: helperStyle ?? this.helperStyle,
helperMaxLines: helperMaxLines ?? this.helperMaxLines,
hintStyle: hintStyle ?? this.hintStyle,
hintFadeDuration: hintFadeDuration ?? this.hintFadeDuration,
errorStyle: errorStyle ?? this.errorStyle,
errorMaxLines: errorMaxLines ?? this.errorMaxLines,
floatingLabelBehavior: floatingLabelBehavior ?? this.floatingLabelBehavior,
floatingLabelAlignment: floatingLabelAlignment ?? this.floatingLabelAlignment,
isDense: isDense ?? this.isDense,
contentPadding: contentPadding ?? this.contentPadding,
iconColor: iconColor ?? this.iconColor,
isCollapsed: isCollapsed ?? this.isCollapsed,
prefixStyle: prefixStyle ?? this.prefixStyle,
prefixIconColor: prefixIconColor ?? this.prefixIconColor,
suffixStyle: suffixStyle ?? this.suffixStyle,
suffixIconColor: suffixIconColor ?? this.suffixIconColor,
counterStyle: counterStyle ?? this.counterStyle,
filled: filled ?? this.filled,
fillColor: fillColor ?? this.fillColor,
activeIndicatorBorder: activeIndicatorBorder ?? this.activeIndicatorBorder,
outlineBorder: outlineBorder ?? this.outlineBorder,
focusColor: focusColor ?? this.focusColor,
hoverColor: hoverColor ?? this.hoverColor,
errorBorder: errorBorder ?? this.errorBorder,
focusedBorder: focusedBorder ?? this.focusedBorder,
focusedErrorBorder: focusedErrorBorder ?? this.focusedErrorBorder,
disabledBorder: disabledBorder ?? this.disabledBorder,
enabledBorder: enabledBorder ?? this.enabledBorder,
border: border ?? this.border,
alignLabelWithHint: alignLabelWithHint ?? this.alignLabelWithHint,
constraints: constraints ?? this.constraints,
);
}
/// Returns a copy of this InputDecorationTheme where the non-null fields in
/// the given InputDecorationTheme override the corresponding nullable fields
/// in this InputDecorationTheme.
///
/// The non-nullable fields of InputDecorationTheme, such as [floatingLabelBehavior],
/// [isDense], [isCollapsed], [filled], and [alignLabelWithHint] cannot be overridden.
///
/// In other words, the fields of the provided [InputDecorationTheme] are used to
/// fill in the unspecified and nullable fields of this InputDecorationTheme.
InputDecorationTheme merge(InputDecorationTheme? inputDecorationTheme) {
if (inputDecorationTheme == null) {
return this;
}
return copyWith(
labelStyle: labelStyle ?? inputDecorationTheme.labelStyle,
floatingLabelStyle: floatingLabelStyle ?? inputDecorationTheme.floatingLabelStyle,
helperStyle: helperStyle ?? inputDecorationTheme.helperStyle,
helperMaxLines: helperMaxLines ?? inputDecorationTheme.helperMaxLines,
hintStyle: hintStyle ?? inputDecorationTheme.hintStyle,
hintFadeDuration: hintFadeDuration ?? inputDecorationTheme.hintFadeDuration,
errorStyle: errorStyle ?? inputDecorationTheme.errorStyle,
errorMaxLines: errorMaxLines ?? inputDecorationTheme.errorMaxLines,
contentPadding: contentPadding ?? inputDecorationTheme.contentPadding,
iconColor: iconColor ?? inputDecorationTheme.iconColor,
prefixStyle: prefixStyle ?? inputDecorationTheme.prefixStyle,
prefixIconColor: prefixIconColor ?? inputDecorationTheme.prefixIconColor,
suffixStyle: suffixStyle ?? inputDecorationTheme.suffixStyle,
suffixIconColor: suffixIconColor ?? inputDecorationTheme.suffixIconColor,
counterStyle: counterStyle ?? inputDecorationTheme.counterStyle,
fillColor: fillColor ?? inputDecorationTheme.fillColor,
activeIndicatorBorder: activeIndicatorBorder ?? inputDecorationTheme.activeIndicatorBorder,
outlineBorder: outlineBorder ?? inputDecorationTheme.outlineBorder,
focusColor: focusColor ?? inputDecorationTheme.focusColor,
hoverColor: hoverColor ?? inputDecorationTheme.hoverColor,
errorBorder: errorBorder ?? inputDecorationTheme.errorBorder,
focusedBorder: focusedBorder ?? inputDecorationTheme.focusedBorder,
focusedErrorBorder: focusedErrorBorder ?? inputDecorationTheme.focusedErrorBorder,
disabledBorder: disabledBorder ?? inputDecorationTheme.disabledBorder,
enabledBorder: enabledBorder ?? inputDecorationTheme.enabledBorder,
border: border ?? inputDecorationTheme.border,
constraints: constraints ?? inputDecorationTheme.constraints,
);
}
@override
int get hashCode => Object.hash(
labelStyle,
floatingLabelStyle,
helperStyle,
helperMaxLines,
hintStyle,
errorStyle,
errorMaxLines,
floatingLabelBehavior,
floatingLabelAlignment,
isDense,
contentPadding,
isCollapsed,
iconColor,
prefixStyle,
prefixIconColor,
suffixStyle,
suffixIconColor,
counterStyle,
filled,
Object.hash(
fillColor,
activeIndicatorBorder,
outlineBorder,
focusColor,
hoverColor,
errorBorder,
focusedBorder,
focusedErrorBorder,
disabledBorder,
enabledBorder,
border,
alignLabelWithHint,
constraints,
hintFadeDuration,
),
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is InputDecorationTheme
&& other.labelStyle == labelStyle
&& other.floatingLabelStyle == floatingLabelStyle
&& other.helperStyle == helperStyle
&& other.helperMaxLines == helperMaxLines
&& other.hintStyle == hintStyle
&& other.hintFadeDuration == hintFadeDuration
&& other.errorStyle == errorStyle
&& other.errorMaxLines == errorMaxLines
&& other.isDense == isDense
&& other.contentPadding == contentPadding
&& other.isCollapsed == isCollapsed
&& other.iconColor == iconColor
&& other.prefixStyle == prefixStyle
&& other.prefixIconColor == prefixIconColor
&& other.suffixStyle == suffixStyle
&& other.suffixIconColor == suffixIconColor
&& other.counterStyle == counterStyle
&& other.floatingLabelBehavior == floatingLabelBehavior
&& other.floatingLabelAlignment == floatingLabelAlignment
&& other.filled == filled
&& other.fillColor == fillColor
&& other.activeIndicatorBorder == activeIndicatorBorder
&& other.outlineBorder == outlineBorder
&& other.focusColor == focusColor
&& other.hoverColor == hoverColor
&& other.errorBorder == errorBorder
&& other.focusedBorder == focusedBorder
&& other.focusedErrorBorder == focusedErrorBorder
&& other.disabledBorder == disabledBorder
&& other.enabledBorder == enabledBorder
&& other.border == border
&& other.alignLabelWithHint == alignLabelWithHint
&& other.constraints == constraints
&& other.disabledBorder == disabledBorder;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
const InputDecorationTheme defaultTheme = InputDecorationTheme();
properties.add(DiagnosticsProperty<TextStyle>('labelStyle', labelStyle, defaultValue: defaultTheme.labelStyle));
properties.add(DiagnosticsProperty<TextStyle>('floatingLabelStyle', floatingLabelStyle, defaultValue: defaultTheme.floatingLabelStyle));
properties.add(DiagnosticsProperty<TextStyle>('helperStyle', helperStyle, defaultValue: defaultTheme.helperStyle));
properties.add(IntProperty('helperMaxLines', helperMaxLines, defaultValue: defaultTheme.helperMaxLines));
properties.add(DiagnosticsProperty<TextStyle>('hintStyle', hintStyle, defaultValue: defaultTheme.hintStyle));
properties.add(DiagnosticsProperty<Duration>('hintFadeDuration', hintFadeDuration, defaultValue: defaultTheme.hintFadeDuration));
properties.add(DiagnosticsProperty<TextStyle>('errorStyle', errorStyle, defaultValue: defaultTheme.errorStyle));
properties.add(IntProperty('errorMaxLines', errorMaxLines, defaultValue: defaultTheme.errorMaxLines));
properties.add(DiagnosticsProperty<FloatingLabelBehavior>('floatingLabelBehavior', floatingLabelBehavior, defaultValue: defaultTheme.floatingLabelBehavior));
properties.add(DiagnosticsProperty<FloatingLabelAlignment>('floatingLabelAlignment', floatingLabelAlignment, defaultValue: defaultTheme.floatingLabelAlignment));
properties.add(DiagnosticsProperty<bool>('isDense', isDense, defaultValue: defaultTheme.isDense));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('contentPadding', contentPadding, defaultValue: defaultTheme.contentPadding));
properties.add(DiagnosticsProperty<bool>('isCollapsed', isCollapsed, defaultValue: defaultTheme.isCollapsed));
properties.add(DiagnosticsProperty<Color>('iconColor', iconColor, defaultValue: defaultTheme.iconColor));
properties.add(DiagnosticsProperty<Color>('prefixIconColor', prefixIconColor, defaultValue: defaultTheme.prefixIconColor));
properties.add(DiagnosticsProperty<TextStyle>('prefixStyle', prefixStyle, defaultValue: defaultTheme.prefixStyle));
properties.add(DiagnosticsProperty<Color>('suffixIconColor', suffixIconColor, defaultValue: defaultTheme.suffixIconColor));
properties.add(DiagnosticsProperty<TextStyle>('suffixStyle', suffixStyle, defaultValue: defaultTheme.suffixStyle));
properties.add(DiagnosticsProperty<TextStyle>('counterStyle', counterStyle, defaultValue: defaultTheme.counterStyle));
properties.add(DiagnosticsProperty<bool>('filled', filled, defaultValue: defaultTheme.filled));
properties.add(ColorProperty('fillColor', fillColor, defaultValue: defaultTheme.fillColor));
properties.add(DiagnosticsProperty<BorderSide>('activeIndicatorBorder', activeIndicatorBorder, defaultValue: defaultTheme.activeIndicatorBorder));
properties.add(DiagnosticsProperty<BorderSide>('outlineBorder', outlineBorder, defaultValue: defaultTheme.outlineBorder));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: defaultTheme.focusColor));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: defaultTheme.hoverColor));
properties.add(DiagnosticsProperty<InputBorder>('errorBorder', errorBorder, defaultValue: defaultTheme.errorBorder));
properties.add(DiagnosticsProperty<InputBorder>('focusedBorder', focusedBorder, defaultValue: defaultTheme.focusedErrorBorder));
properties.add(DiagnosticsProperty<InputBorder>('focusedErrorBorder', focusedErrorBorder, defaultValue: defaultTheme.focusedErrorBorder));
properties.add(DiagnosticsProperty<InputBorder>('disabledBorder', disabledBorder, defaultValue: defaultTheme.disabledBorder));
properties.add(DiagnosticsProperty<InputBorder>('enabledBorder', enabledBorder, defaultValue: defaultTheme.enabledBorder));
properties.add(DiagnosticsProperty<InputBorder>('border', border, defaultValue: defaultTheme.border));
properties.add(DiagnosticsProperty<bool>('alignLabelWithHint', alignLabelWithHint, defaultValue: defaultTheme.alignLabelWithHint));
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: defaultTheme.constraints));
}
}
class _InputDecoratorDefaultsM2 extends InputDecorationTheme {
const _InputDecoratorDefaultsM2(this.context)
: super();
final BuildContext context;
@override
TextStyle? get hintStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: Theme.of(context).disabledColor);
}
return TextStyle(color: Theme.of(context).hintColor);
});
@override
TextStyle? get labelStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: Theme.of(context).disabledColor);
}
return TextStyle(color: Theme.of(context).hintColor);
});
@override
TextStyle? get floatingLabelStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: Theme.of(context).disabledColor);
}
if (states.contains(MaterialState.error)) {
return TextStyle(color: Theme.of(context).colorScheme.error);
}
if (states.contains(MaterialState.focused)) {
return TextStyle(color: Theme.of(context).colorScheme.primary);
}
return TextStyle(color: Theme.of(context).hintColor);
});
@override
TextStyle? get helperStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final ThemeData themeData= Theme.of(context);
if (states.contains(MaterialState.disabled)) {
return themeData.textTheme.bodySmall!.copyWith(color: Colors.transparent);
}
return themeData.textTheme.bodySmall!.copyWith(color: themeData.hintColor);
});
@override
TextStyle? get errorStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final ThemeData themeData= Theme.of(context);
if (states.contains(MaterialState.disabled)) {
return themeData.textTheme.bodySmall!.copyWith(color: Colors.transparent);
}
return themeData.textTheme.bodySmall!.copyWith(color: themeData.colorScheme.error);
});
@override
Color? get fillColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
return switch ((Theme.of(context).brightness, states.contains(MaterialState.disabled))) {
(Brightness.dark, true) => const Color(0x0DFFFFFF), // 5% white
(Brightness.dark, false) => const Color(0x1AFFFFFF), // 10% white
(Brightness.light, true) => const Color(0x05000000), // 2% black
(Brightness.light, false) => const Color(0x0A000000), // 4% black
};
});
@override
Color? get iconColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled) && !states.contains(MaterialState.focused)) {
return Theme.of(context).disabledColor;
}
if (states.contains(MaterialState.focused)) {
return Theme.of(context).colorScheme.primary;
}
return switch (Theme.of(context).brightness) {
Brightness.dark => Colors.white70,
Brightness.light => Colors.black45,
};
});
@override
Color? get prefixIconColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled) && !states.contains(MaterialState.focused)) {
return Theme.of(context).disabledColor;
}
if (states.contains(MaterialState.focused)) {
return Theme.of(context).colorScheme.primary;
}
return switch (Theme.of(context).brightness) {
Brightness.dark => Colors.white70,
Brightness.light => Colors.black45,
};
});
@override
Color? get suffixIconColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled) && !states.contains(MaterialState.focused)) {
return Theme.of(context).disabledColor;
}
if (states.contains(MaterialState.focused)) {
return Theme.of(context).colorScheme.primary;
}
return switch (Theme.of(context).brightness) {
Brightness.dark => Colors.white70,
Brightness.light => Colors.black45,
};
});
}
// BEGIN GENERATED TOKEN PROPERTIES - InputDecorator
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _InputDecoratorDefaultsM3 extends InputDecorationTheme {
_InputDecoratorDefaultsM3(this.context)
: super();
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
TextStyle? get hintStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: Theme.of(context).disabledColor);
}
return TextStyle(color: Theme.of(context).hintColor);
});
@override
Color? get fillColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.04);
}
return _colors.surfaceContainerHighest;
});
@override
BorderSide? get activeIndicatorBorder => MaterialStateBorderSide.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return BorderSide(color: _colors.onSurface.withOpacity(0.38));
}
if (states.contains(MaterialState.error)) {
if (states.contains(MaterialState.hovered)) {
return BorderSide(color: _colors.onErrorContainer);
}
if (states.contains(MaterialState.focused)) {
return BorderSide(color: _colors.error, width: 2.0);
}
return BorderSide(color: _colors.error);
}
if (states.contains(MaterialState.hovered)) {
return BorderSide(color: _colors.onSurface);
}
if (states.contains(MaterialState.focused)) {
return BorderSide(color: _colors.primary, width: 2.0);
}
return BorderSide(color: _colors.onSurfaceVariant);
});
@override
BorderSide? get outlineBorder => MaterialStateBorderSide.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return BorderSide(color: _colors.onSurface.withOpacity(0.12));
}
if (states.contains(MaterialState.error)) {
if (states.contains(MaterialState.hovered)) {
return BorderSide(color: _colors.onErrorContainer);
}
if (states.contains(MaterialState.focused)) {
return BorderSide(color: _colors.error, width: 2.0);
}
return BorderSide(color: _colors.error);
}
if (states.contains(MaterialState.hovered)) {
return BorderSide(color: _colors.onSurface);
}
if (states.contains(MaterialState.focused)) {
return BorderSide(color: _colors.primary, width: 2.0);
}
return BorderSide(color: _colors.outline);
});
@override
Color? get iconColor => _colors.onSurfaceVariant;
@override
Color? get prefixIconColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
return _colors.onSurfaceVariant;
});
@override
Color? get suffixIconColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.error)) {
return _colors.error;
}
return _colors.onSurfaceVariant;
});
@override
TextStyle? get labelStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final TextStyle textStyle = _textTheme.bodyLarge ?? const TextStyle();
if (states.contains(MaterialState.disabled)) {
return textStyle.copyWith(color: _colors.onSurface.withOpacity(0.38));
}
if (states.contains(MaterialState.error)) {
if (states.contains(MaterialState.hovered)) {
return textStyle.copyWith(color: _colors.onErrorContainer);
}
if (states.contains(MaterialState.focused)) {
return textStyle.copyWith(color: _colors.error);
}
return textStyle.copyWith(color: _colors.error);
}
if (states.contains(MaterialState.hovered)) {
return textStyle.copyWith(color: _colors.onSurfaceVariant);
}
if (states.contains(MaterialState.focused)) {
return textStyle.copyWith(color: _colors.primary);
}
return textStyle.copyWith(color: _colors.onSurfaceVariant);
});
@override
TextStyle? get floatingLabelStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final TextStyle textStyle = _textTheme.bodyLarge ?? const TextStyle();
if (states.contains(MaterialState.disabled)) {
return textStyle.copyWith(color: _colors.onSurface.withOpacity(0.38));
}
if (states.contains(MaterialState.error)) {
if (states.contains(MaterialState.hovered)) {
return textStyle.copyWith(color: _colors.onErrorContainer);
}
if (states.contains(MaterialState.focused)) {
return textStyle.copyWith(color: _colors.error);
}
return textStyle.copyWith(color: _colors.error);
}
if (states.contains(MaterialState.hovered)) {
return textStyle.copyWith(color: _colors.onSurfaceVariant);
}
if (states.contains(MaterialState.focused)) {
return textStyle.copyWith(color: _colors.primary);
}
return textStyle.copyWith(color: _colors.onSurfaceVariant);
});
@override
TextStyle? get helperStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final TextStyle textStyle = _textTheme.bodySmall ?? const TextStyle();
if (states.contains(MaterialState.disabled)) {
return textStyle.copyWith(color: _colors.onSurface.withOpacity(0.38));
}
return textStyle.copyWith(color: _colors.onSurfaceVariant);
});
@override
TextStyle? get errorStyle => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final TextStyle textStyle = _textTheme.bodySmall ?? const TextStyle();
return textStyle.copyWith(color: _colors.error);
});
}
// END GENERATED TOKEN PROPERTIES - InputDecorator
| flutter/packages/flutter/lib/src/material/input_decorator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/input_decorator.dart",
"repo_id": "flutter",
"token_count": 63016
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'elevation_overlay.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'navigation_bar_theme.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'tooltip.dart';
const double _kIndicatorHeight = 32;
const double _kIndicatorWidth = 64;
const double _kMaxLabelTextScaleFactor = 1.3;
// Examples can assume:
// late BuildContext context;
// late bool _isDrawerOpen;
/// Material 3 Navigation Bar component.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=DVGYddFaLv0}
///
/// Navigation bars offer a persistent and convenient way to switch between
/// primary destinations in an app.
///
/// This widget does not adjust its size with the [ThemeData.visualDensity].
///
/// The [MediaQueryData.textScaler] does not adjust the size of this widget but
/// rather the size of the [Tooltip]s displayed on long presses of the
/// destinations.
///
/// The style for the icons and text are not affected by parent
/// [DefaultTextStyle]s or [IconTheme]s but rather controlled by parameters or
/// the [NavigationBarThemeData].
///
/// This widget holds a collection of destinations (usually
/// [NavigationDestination]s).
///
/// {@tool dartpad}
/// This example shows a [NavigationBar] as it is used within a [Scaffold]
/// widget. The [NavigationBar] has three [NavigationDestination] widgets and
/// the initial [selectedIndex] is set to index 0. The [onDestinationSelected]
/// callback changes the selected item's index and displays a corresponding
/// widget in the body of the [Scaffold].
///
/// ** See code in examples/api/lib/material/navigation_bar/navigation_bar.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example showcases [NavigationBar] label behaviors. When tapping on one
/// of the label behavior options, the [labelBehavior] of the [NavigationBar]
/// will be updated.
///
/// ** See code in examples/api/lib/material/navigation_bar/navigation_bar.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows a [NavigationBar] within a main [Scaffold]
/// widget that's used to control the visibility of destination pages.
/// Each destination has its own scaffold and a nested navigator that
/// provides local navigation. The example's [NavigationBar] has four
/// [NavigationDestination] widgets with different color schemes. Its
/// [onDestinationSelected] callback changes the selected
/// destination's index and displays a corresponding page with its own
/// local navigator and scaffold - all within the body of the main
/// scaffold. The destination pages are organized in a [Stack] and
/// switching destinations fades out the current page and
/// fades in the new one. Destinations that aren't visible or animating
/// are kept [Offstage].
///
/// ** See code in examples/api/lib/material/navigation_bar/navigation_bar.2.dart **
/// {@end-tool}
/// See also:
///
/// * [NavigationDestination]
/// * [BottomNavigationBar]
/// * <https://api.flutter.dev/flutter/material/NavigationDestination-class.html>
/// * <https://m3.material.io/components/navigation-bar>
class NavigationBar extends StatelessWidget {
/// Creates a Material 3 Navigation Bar component.
///
/// The value of [destinations] must be a list of two or more
/// [NavigationDestination] values.
// TODO(goderbauer): This class cannot be const constructed, https://github.com/dart-lang/linter/issues/3366.
// ignore: prefer_const_constructors_in_immutables
NavigationBar({
super.key,
this.animationDuration,
this.selectedIndex = 0,
required this.destinations,
this.onDestinationSelected,
this.backgroundColor,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.indicatorColor,
this.indicatorShape,
this.height,
this.labelBehavior,
this.overlayColor,
}) : assert(destinations.length >= 2),
assert(0 <= selectedIndex && selectedIndex < destinations.length);
/// Determines the transition time for each destination as it goes between
/// selected and unselected.
final Duration? animationDuration;
/// Determines which one of the [destinations] is currently selected.
///
/// When this is updated, the destination (from [destinations]) at
/// [selectedIndex] goes from unselected to selected.
final int selectedIndex;
/// The list of destinations (usually [NavigationDestination]s) in this
/// [NavigationBar].
///
/// When [selectedIndex] is updated, the destination from this list at
/// [selectedIndex] will animate from 0 (unselected) to 1.0 (selected). When
/// the animation is increasing or completed, the destination is considered
/// selected, when the animation is decreasing or dismissed, the destination
/// is considered unselected.
final List<Widget> destinations;
/// Called when one of the [destinations] is selected.
///
/// This callback usually updates the int passed to [selectedIndex].
///
/// Upon updating [selectedIndex], the [NavigationBar] will be rebuilt.
final ValueChanged<int>? onDestinationSelected;
/// The color of the [NavigationBar] itself.
///
/// If null, [NavigationBarThemeData.backgroundColor] is used. If that
/// is also null, then if [ThemeData.useMaterial3] is true, the value is
/// [ColorScheme.surfaceContainer]. If that is false, the default blends [ColorScheme.surface]
/// and [ColorScheme.onSurface] using an [ElevationOverlay].
final Color? backgroundColor;
/// The elevation of the [NavigationBar] itself.
///
/// If null, [NavigationBarThemeData.elevation] is used. If that
/// is also null, then if [ThemeData.useMaterial3] is true then it will
/// be 3.0 otherwise 0.0.
final double? elevation;
/// The color used for the drop shadow to indicate elevation.
///
/// If null, [NavigationBarThemeData.shadowColor] is used. If that
/// is also null, the default value is [Colors.transparent] which
/// indicates that no drop shadow will be displayed.
///
/// See [Material.shadowColor] for more details on drop shadows.
final Color? shadowColor;
/// The color used as an overlay on [backgroundColor] to indicate elevation.
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// If null, [NavigationBarThemeData.surfaceTintColor] is used. If that
/// is also null, the default value is [Colors.transparent].
///
/// See [Material.surfaceTintColor] for more details on how this
/// overlay is applied.
final Color? surfaceTintColor;
/// The color of the [indicatorShape] when this destination is selected.
///
/// If null, [NavigationBarThemeData.indicatorColor] is used. If that
/// is also null and [ThemeData.useMaterial3] is true, [ColorScheme.secondaryContainer]
/// is used. Otherwise, [ColorScheme.secondary] with an opacity of 0.24 is used.
final Color? indicatorColor;
/// The shape of the selected indicator.
///
/// If null, [NavigationBarThemeData.indicatorShape] is used. If that
/// is also null and [ThemeData.useMaterial3] is true, [StadiumBorder] is used.
/// Otherwise, [RoundedRectangleBorder] with a circular border radius of 16 is used.
final ShapeBorder? indicatorShape;
/// The height of the [NavigationBar] itself.
///
/// If this is used in [Scaffold.bottomNavigationBar] and the scaffold is
/// full-screen, the safe area padding is also added to the height
/// automatically.
///
/// The height does not adjust with [ThemeData.visualDensity] or
/// [MediaQueryData.textScaler] as this component loses usability at
/// larger and smaller sizes due to the truncating of labels or smaller tap
/// targets.
///
/// If null, [NavigationBarThemeData.height] is used. If that
/// is also null, the default is 80.
final double? height;
/// Defines how the [destinations]' labels will be laid out and when they'll
/// be displayed.
///
/// Can be used to show all labels, show only the selected label, or hide all
/// labels.
///
/// If null, [NavigationBarThemeData.labelBehavior] is used. If that
/// is also null, the default is
/// [NavigationDestinationLabelBehavior.alwaysShow].
final NavigationDestinationLabelBehavior? labelBehavior;
/// The highlight color that's typically used to indicate that
/// the [NavigationDestination] is focused, hovered, or pressed.
final MaterialStateProperty<Color?>? overlayColor;
VoidCallback _handleTap(int index) {
return onDestinationSelected != null
? () => onDestinationSelected!(index)
: () {};
}
@override
Widget build(BuildContext context) {
final NavigationBarThemeData defaults = _defaultsFor(context);
final NavigationBarThemeData navigationBarTheme = NavigationBarTheme.of(context);
final double effectiveHeight = height ?? navigationBarTheme.height ?? defaults.height!;
final NavigationDestinationLabelBehavior effectiveLabelBehavior = labelBehavior
?? navigationBarTheme.labelBehavior
?? defaults.labelBehavior!;
return Material(
color: backgroundColor
?? navigationBarTheme.backgroundColor
?? defaults.backgroundColor!,
elevation: elevation ?? navigationBarTheme.elevation ?? defaults.elevation!,
shadowColor: shadowColor ?? navigationBarTheme.shadowColor ?? defaults.shadowColor,
surfaceTintColor: surfaceTintColor ?? navigationBarTheme.surfaceTintColor ?? defaults.surfaceTintColor,
child: SafeArea(
child: SizedBox(
height: effectiveHeight,
child: Row(
children: <Widget>[
for (int i = 0; i < destinations.length; i++)
Expanded(
child: _SelectableAnimatedBuilder(
duration: animationDuration ?? const Duration(milliseconds: 500),
isSelected: i == selectedIndex,
builder: (BuildContext context, Animation<double> animation) {
return _NavigationDestinationInfo(
index: i,
selectedIndex: selectedIndex,
totalNumberOfDestinations: destinations.length,
selectedAnimation: animation,
labelBehavior: effectiveLabelBehavior,
indicatorColor: indicatorColor,
indicatorShape: indicatorShape,
overlayColor: overlayColor,
onTap: _handleTap(i),
child: destinations[i],
);
},
),
),
],
),
),
),
);
}
}
/// Specifies when each [NavigationDestination]'s label should appear.
///
/// This is used to determine the behavior of [NavigationBar]'s destinations.
enum NavigationDestinationLabelBehavior {
/// Always shows all of the labels under each navigation bar destination,
/// selected and unselected.
alwaysShow,
/// Never shows any of the labels under the navigation bar destinations,
/// regardless of selected vs unselected.
alwaysHide,
/// Only shows the labels of the selected navigation bar destination.
///
/// When a destination is unselected, the label will be faded out, and the
/// icon will be centered.
///
/// When a destination is selected, the label will fade in and the label and
/// icon will slide up so that they are both centered.
onlyShowSelected,
}
/// A Material 3 [NavigationBar] destination.
///
/// Displays a label below an icon. Use with [NavigationBar.destinations].
///
/// See also:
///
/// * [NavigationBar], for an interactive code sample.
class NavigationDestination extends StatelessWidget {
/// Creates a navigation bar destination with an icon and a label, to be used
/// in the [NavigationBar.destinations].
const NavigationDestination({
super.key,
required this.icon,
this.selectedIcon,
required this.label,
this.tooltip,
this.enabled = true,
});
/// The [Widget] (usually an [Icon]) that's displayed for this
/// [NavigationDestination].
///
/// The icon will use [NavigationBarThemeData.iconTheme]. If this is
/// null, the default [IconThemeData] would use a size of 24.0 and
/// [ColorScheme.onSurface].
final Widget icon;
/// The optional [Widget] (usually an [Icon]) that's displayed when this
/// [NavigationDestination] is selected.
///
/// If [selectedIcon] is non-null, the destination will fade from
/// [icon] to [selectedIcon] when this destination goes from unselected to
/// selected.
///
/// The icon will use [NavigationBarThemeData.iconTheme] with
/// [MaterialState.selected]. If this is null, the default [IconThemeData]
/// would use a size of 24.0 and [ColorScheme.onSurface].
final Widget? selectedIcon;
/// The text label that appears below the icon of this
/// [NavigationDestination].
///
/// The accompanying [Text] widget will use
/// [NavigationBarThemeData.labelTextStyle]. If this are null, the default
/// text style would use [TextTheme.labelSmall] with [ColorScheme.onSurface].
final String label;
/// The text to display in the tooltip for this [NavigationDestination], when
/// the user long presses the destination.
///
/// If [tooltip] is an empty string, no tooltip will be used.
///
/// Defaults to null, in which case the [label] text will be used.
final String? tooltip;
/// Indicates that this destination is selectable.
///
/// Defaults to true.
final bool enabled;
@override
Widget build(BuildContext context) {
final _NavigationDestinationInfo info = _NavigationDestinationInfo.of(context);
const Set<MaterialState> selectedState = <MaterialState>{MaterialState.selected};
const Set<MaterialState> unselectedState = <MaterialState>{};
const Set<MaterialState> disabledState = <MaterialState>{MaterialState.disabled};
final NavigationBarThemeData navigationBarTheme = NavigationBarTheme.of(context);
final NavigationBarThemeData defaults = _defaultsFor(context);
final Animation<double> animation = info.selectedAnimation;
return _NavigationDestinationBuilder(
label: label,
tooltip: tooltip,
enabled: enabled,
buildIcon: (BuildContext context) {
final IconThemeData selectedIconTheme =
navigationBarTheme.iconTheme?.resolve(selectedState)
?? defaults.iconTheme!.resolve(selectedState)!;
final IconThemeData unselectedIconTheme =
navigationBarTheme.iconTheme?.resolve(unselectedState)
?? defaults.iconTheme!.resolve(unselectedState)!;
final IconThemeData disabledIconTheme =
navigationBarTheme.iconTheme?.resolve(disabledState)
?? defaults.iconTheme!.resolve(disabledState)!;
final Widget selectedIconWidget = IconTheme.merge(
data: enabled ? selectedIconTheme : disabledIconTheme,
child: selectedIcon ?? icon,
);
final Widget unselectedIconWidget = IconTheme.merge(
data: enabled ? unselectedIconTheme : disabledIconTheme,
child: icon,
);
return Stack(
alignment: Alignment.center,
children: <Widget>[
NavigationIndicator(
animation: animation,
color: info.indicatorColor ?? navigationBarTheme.indicatorColor ?? defaults.indicatorColor!,
shape: info.indicatorShape ?? navigationBarTheme.indicatorShape ?? defaults.indicatorShape!
),
_StatusTransitionWidgetBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return _isForwardOrCompleted(animation)
? selectedIconWidget
: unselectedIconWidget;
},
),
],
);
},
buildLabel: (BuildContext context) {
final TextStyle? effectiveSelectedLabelTextStyle = navigationBarTheme.labelTextStyle?.resolve(selectedState)
?? defaults.labelTextStyle!.resolve(selectedState);
final TextStyle? effectiveUnselectedLabelTextStyle = navigationBarTheme.labelTextStyle?.resolve(unselectedState)
?? defaults.labelTextStyle!.resolve(unselectedState);
final TextStyle? effectiveDisabledLabelTextStyle = navigationBarTheme.labelTextStyle?.resolve(disabledState)
?? defaults.labelTextStyle!.resolve(disabledState);
final TextStyle? textStyle = enabled
? _isForwardOrCompleted(animation)
? effectiveSelectedLabelTextStyle
: effectiveUnselectedLabelTextStyle
: effectiveDisabledLabelTextStyle;
return Padding(
padding: const EdgeInsets.only(top: 4),
child: MediaQuery.withClampedTextScaling(
// Set maximum text scale factor to _kMaxLabelTextScaleFactor for the
// label to keep the visual hierarchy the same even with larger font
// sizes. To opt out, wrap the [label] widget in a [MediaQuery] widget
// with a different `TextScaler`.
maxScaleFactor: _kMaxLabelTextScaleFactor,
child: Text(label, style: textStyle),
),
);
},
);
}
}
/// Widget that handles the semantics and layout of a navigation bar
/// destination.
///
/// Prefer [NavigationDestination] over this widget, as it is a simpler
/// (although less customizable) way to get navigation bar destinations.
///
/// The icon and label of this destination are built with [buildIcon] and
/// [buildLabel]. They should build the unselected and selected icon and label
/// according to [_NavigationDestinationInfo.selectedAnimation], where an
/// animation value of 0 is unselected and 1 is selected.
///
/// See [NavigationDestination] for an example.
class _NavigationDestinationBuilder extends StatefulWidget {
/// Builds a destination (icon + label) to use in a Material 3 [NavigationBar].
const _NavigationDestinationBuilder({
required this.buildIcon,
required this.buildLabel,
required this.label,
this.tooltip,
this.enabled = true,
});
/// Builds the icon for a destination in a [NavigationBar].
///
/// To animate between unselected and selected, build the icon based on
/// [_NavigationDestinationInfo.selectedAnimation]. When the animation is 0,
/// the destination is unselected, when the animation is 1, the destination is
/// selected.
///
/// The destination is considered selected as soon as the animation is
/// increasing or completed, and it is considered unselected as soon as the
/// animation is decreasing or dismissed.
final WidgetBuilder buildIcon;
/// Builds the label for a destination in a [NavigationBar].
///
/// To animate between unselected and selected, build the icon based on
/// [_NavigationDestinationInfo.selectedAnimation]. When the animation is
/// 0, the destination is unselected, when the animation is 1, the destination
/// is selected.
///
/// The destination is considered selected as soon as the animation is
/// increasing or completed, and it is considered unselected as soon as the
/// animation is decreasing or dismissed.
final WidgetBuilder buildLabel;
/// The text value of what is in the label widget, this is required for
/// semantics so that screen readers and tooltips can read the proper label.
final String label;
/// The text to display in the tooltip for this [NavigationDestination], when
/// the user long presses the destination.
///
/// If [tooltip] is an empty string, no tooltip will be used.
///
/// Defaults to null, in which case the [label] text will be used.
final String? tooltip;
/// Indicates that this destination is selectable.
///
/// Defaults to true.
final bool enabled;
@override
State<_NavigationDestinationBuilder> createState() => _NavigationDestinationBuilderState();
}
class _NavigationDestinationBuilderState extends State<_NavigationDestinationBuilder> {
final GlobalKey iconKey = GlobalKey();
@override
Widget build(BuildContext context) {
final _NavigationDestinationInfo info = _NavigationDestinationInfo.of(context);
final NavigationBarThemeData navigationBarTheme = NavigationBarTheme.of(context);
final NavigationBarThemeData defaults = _defaultsFor(context);
return _NavigationBarDestinationSemantics(
child: _NavigationBarDestinationTooltip(
message: widget.tooltip ?? widget.label,
child: _IndicatorInkWell(
iconKey: iconKey,
labelBehavior: info.labelBehavior,
customBorder: info.indicatorShape ?? navigationBarTheme.indicatorShape ?? defaults.indicatorShape,
overlayColor: info.overlayColor ?? navigationBarTheme.overlayColor,
onTap: widget.enabled ? info.onTap : null,
child: Row(
children: <Widget>[
Expanded(
child: _NavigationBarDestinationLayout(
icon: widget.buildIcon(context),
iconKey: iconKey,
label: widget.buildLabel(context),
),
),
],
),
),
),
);
}
}
class _IndicatorInkWell extends InkResponse {
const _IndicatorInkWell({
required this.iconKey,
required this.labelBehavior,
super.overlayColor,
super.customBorder,
super.onTap,
super.child,
}) : super(
containedInkWell: true,
highlightColor: Colors.transparent,
);
final GlobalKey iconKey;
final NavigationDestinationLabelBehavior labelBehavior;
@override
RectCallback? getRectCallback(RenderBox referenceBox) {
return () {
final RenderBox iconBox = iconKey.currentContext!.findRenderObject()! as RenderBox;
final Rect iconRect = iconBox.localToGlobal(Offset.zero) & iconBox.size;
return referenceBox.globalToLocal(iconRect.topLeft) & iconBox.size;
};
}
}
/// Inherited widget for passing data from the [NavigationBar] to the
/// [NavigationBar.destinations] children widgets.
///
/// Useful for building navigation destinations using:
/// `_NavigationDestinationInfo.of(context)`.
class _NavigationDestinationInfo extends InheritedWidget {
/// Adds the information needed to build a navigation destination to the
/// [child] and descendants.
const _NavigationDestinationInfo({
required this.index,
required this.selectedIndex,
required this.totalNumberOfDestinations,
required this.selectedAnimation,
required this.labelBehavior,
required this.indicatorColor,
required this.indicatorShape,
required this.overlayColor,
required this.onTap,
required super.child,
});
/// Which destination index is this in the navigation bar.
///
/// For example:
///
/// ```dart
/// NavigationBar(
/// destinations: const <Widget>[
/// NavigationDestination(
/// // This is destination index 0.
/// icon: Icon(Icons.surfing),
/// label: 'Surfing',
/// ),
/// NavigationDestination(
/// // This is destination index 1.
/// icon: Icon(Icons.support),
/// label: 'Support',
/// ),
/// NavigationDestination(
/// // This is destination index 2.
/// icon: Icon(Icons.local_hospital),
/// label: 'Hospital',
/// ),
/// ]
/// )
/// ```
///
/// This is required for semantics, so that each destination can have a label
/// "Tab 1 of 3", for example.
final int index;
/// This is the index of the currently selected destination.
///
/// This is required for `_IndicatorInkWell` to apply label padding to ripple animations
/// when label behavior is [NavigationDestinationLabelBehavior.onlyShowSelected].
final int selectedIndex;
/// How many total destinations are in this navigation bar.
///
/// This is required for semantics, so that each destination can have a label
/// "Tab 1 of 4", for example.
final int totalNumberOfDestinations;
/// Indicates whether or not this destination is selected, from 0 (unselected)
/// to 1 (selected).
final Animation<double> selectedAnimation;
/// Determines the behavior for how the labels will layout.
///
/// Can be used to show all labels (the default), show only the selected
/// label, or hide all labels.
final NavigationDestinationLabelBehavior labelBehavior;
/// The color of the selection indicator.
///
/// This is used by destinations to override the indicator color.
final Color? indicatorColor;
/// The shape of the selection indicator.
///
/// This is used by destinations to override the indicator shape.
final ShapeBorder? indicatorShape;
/// The highlight color that's typically used to indicate that
/// the [NavigationDestination] is focused, hovered, or pressed.
///
/// This is used by destinations to override the overlay color.
final MaterialStateProperty<Color?>? overlayColor;
/// The callback that should be called when this destination is tapped.
///
/// This is computed by calling [NavigationBar.onDestinationSelected]
/// with [index] passed in.
final VoidCallback onTap;
/// Returns a non null [_NavigationDestinationInfo].
///
/// This will return an error if called with no [_NavigationDestinationInfo]
/// ancestor.
///
/// Used by widgets that are implementing a navigation destination info to
/// get information like the selected animation and destination number.
static _NavigationDestinationInfo of(BuildContext context) {
final _NavigationDestinationInfo? result = context.dependOnInheritedWidgetOfExactType<_NavigationDestinationInfo>();
assert(
result != null,
'Navigation destinations need a _NavigationDestinationInfo parent, '
'which is usually provided by NavigationBar.',
);
return result!;
}
@override
bool updateShouldNotify(_NavigationDestinationInfo oldWidget) {
return index != oldWidget.index
|| totalNumberOfDestinations != oldWidget.totalNumberOfDestinations
|| selectedAnimation != oldWidget.selectedAnimation
|| labelBehavior != oldWidget.labelBehavior
|| onTap != oldWidget.onTap;
}
}
/// Selection Indicator for the Material 3 [NavigationBar] and [NavigationRail]
/// components.
///
/// When [animation] is 0, the indicator is not present. As [animation] grows
/// from 0 to 1, the indicator scales in on the x axis.
///
/// Used in a [Stack] widget behind the icons in the Material 3 Navigation Bar
/// to illuminate the selected destination.
class NavigationIndicator extends StatelessWidget {
/// Builds an indicator, usually used in a stack behind the icon of a
/// navigation bar destination.
const NavigationIndicator({
super.key,
required this.animation,
this.color,
this.width = _kIndicatorWidth,
this.height = _kIndicatorHeight,
this.borderRadius = const BorderRadius.all(Radius.circular(16)),
this.shape,
});
/// Determines the scale of the indicator.
///
/// When [animation] is 0, the indicator is not present. The indicator scales
/// in as [animation] grows from 0 to 1.
final Animation<double> animation;
/// The fill color of this indicator.
///
/// If null, defaults to [ColorScheme.secondary].
final Color? color;
/// The width of this indicator.
///
/// Defaults to `64`.
final double width;
/// The height of this indicator.
///
/// Defaults to `32`.
final double height;
/// The border radius of the shape of the indicator.
///
/// This is used to create a [RoundedRectangleBorder] shape for the indicator.
/// This is ignored if [shape] is non-null.
///
/// Defaults to `BorderRadius.circular(16)`.
final BorderRadius borderRadius;
/// The shape of the indicator.
///
/// If non-null this is used as the shape used to draw the background
/// of the indicator. If null then a [RoundedRectangleBorder] with the
/// [borderRadius] is used.
final ShapeBorder? shape;
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
// The scale should be 0 when the animation is unselected, as soon as
// the animation starts, the scale jumps to 40%, and then animates to
// 100% along a curve.
final double scale = animation.isDismissed
? 0.0
: Tween<double>(begin: .4, end: 1.0).transform(
CurveTween(curve: Curves.easeInOutCubicEmphasized).transform(animation.value));
return Transform(
alignment: Alignment.center,
// Scale in the X direction only.
transform: Matrix4.diagonal3Values(
scale,
1.0,
1.0,
),
child: child,
);
},
// Fade should be a 100ms animation whenever the parent animation changes
// direction.
child: _StatusTransitionWidgetBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return _SelectableAnimatedBuilder(
isSelected: _isForwardOrCompleted(animation),
duration: const Duration(milliseconds: 100),
alwaysDoFullAnimation: true,
builder: (BuildContext context, Animation<double> fadeAnimation) {
return FadeTransition(
opacity: fadeAnimation,
child: Container(
width: width,
height: height,
decoration: ShapeDecoration(
shape: shape ?? RoundedRectangleBorder(borderRadius: borderRadius),
color: color ?? Theme.of(context).colorScheme.secondary,
),
),
);
},
);
},
),
);
}
}
/// Widget that handles the layout of the icon + label in a navigation bar
/// destination, based on [_NavigationDestinationInfo.labelBehavior] and
/// [_NavigationDestinationInfo.selectedAnimation].
///
/// Depending on the [_NavigationDestinationInfo.labelBehavior], the labels
/// will shift and fade accordingly.
class _NavigationBarDestinationLayout extends StatelessWidget {
/// Builds a widget to layout an icon + label for a destination in a Material
/// 3 [NavigationBar].
const _NavigationBarDestinationLayout({
required this.icon,
required this.iconKey,
required this.label,
});
/// The icon widget that sits on top of the label.
///
/// See [NavigationDestination.icon].
final Widget icon;
/// The global key for the icon of this destination.
///
/// This is used to determine the position of the icon.
final GlobalKey iconKey;
/// The label widget that sits below the icon.
///
/// This widget will sometimes be faded out, depending on
/// [_NavigationDestinationInfo.selectedAnimation].
///
/// See [NavigationDestination.label].
final Widget label;
static final Key _labelKey = UniqueKey();
@override
Widget build(BuildContext context) {
return _DestinationLayoutAnimationBuilder(
builder: (BuildContext context, Animation<double> animation) {
return CustomMultiChildLayout(
delegate: _NavigationDestinationLayoutDelegate(
animation: animation,
),
children: <Widget>[
LayoutId(
id: _NavigationDestinationLayoutDelegate.iconId,
child: RepaintBoundary(
key: iconKey,
child: icon,
),
),
LayoutId(
id: _NavigationDestinationLayoutDelegate.labelId,
child: FadeTransition(
alwaysIncludeSemantics: true,
opacity: animation,
child: RepaintBoundary(
key: _labelKey,
child: label,
),
),
),
],
);
},
);
}
}
/// Determines the appropriate [Curve] and [Animation] to use for laying out the
/// [NavigationDestination], based on
/// [_NavigationDestinationInfo.labelBehavior].
///
/// The animation controlling the position and fade of the labels differs
/// from the selection animation, depending on the
/// [NavigationDestinationLabelBehavior]. This widget determines what
/// animation should be used for the position and fade of the labels.
class _DestinationLayoutAnimationBuilder extends StatelessWidget {
/// Builds a child with the appropriate animation [Curve] based on the
/// [_NavigationDestinationInfo.labelBehavior].
const _DestinationLayoutAnimationBuilder({required this.builder});
/// Builds the child of this widget.
///
/// The [Animation] will be the appropriate [Animation] to use for the layout
/// and fade of the [NavigationDestination], either a curve, always
/// showing (1), or always hiding (0).
final Widget Function(BuildContext, Animation<double>) builder;
@override
Widget build(BuildContext context) {
final _NavigationDestinationInfo info = _NavigationDestinationInfo.of(context);
switch (info.labelBehavior) {
case NavigationDestinationLabelBehavior.alwaysShow:
return builder(context, kAlwaysCompleteAnimation);
case NavigationDestinationLabelBehavior.alwaysHide:
return builder(context, kAlwaysDismissedAnimation);
case NavigationDestinationLabelBehavior.onlyShowSelected:
return _CurvedAnimationBuilder(
animation: info.selectedAnimation,
curve: Curves.easeInOutCubicEmphasized,
reverseCurve: Curves.easeInOutCubicEmphasized.flipped,
builder: (BuildContext context, Animation<double> curvedAnimation) {
return builder(context, curvedAnimation);
},
);
}
}
}
/// Semantics widget for a navigation bar destination.
///
/// Requires a [_NavigationDestinationInfo] parent (normally provided by the
/// [NavigationBar] by default).
///
/// Provides localized semantic labels to the destination, for example, it will
/// read "Home, Tab 1 of 3".
///
/// Used by [_NavigationDestinationBuilder].
class _NavigationBarDestinationSemantics extends StatelessWidget {
/// Adds the appropriate semantics for navigation bar destinations to the
/// [child].
const _NavigationBarDestinationSemantics({
required this.child,
});
/// The widget that should receive the destination semantics.
final Widget child;
@override
Widget build(BuildContext context) {
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final _NavigationDestinationInfo destinationInfo = _NavigationDestinationInfo.of(context);
// The AnimationStatusBuilder will make sure that the semantics update to
// "selected" when the animation status changes.
return _StatusTransitionWidgetBuilder(
animation: destinationInfo.selectedAnimation,
builder: (BuildContext context, Widget? child) {
return Semantics(
selected: _isForwardOrCompleted(destinationInfo.selectedAnimation),
container: true,
child: child,
);
},
child: Stack(
alignment: Alignment.center,
children: <Widget>[
child,
Semantics(
label: localizations.tabLabel(
tabIndex: destinationInfo.index + 1,
tabCount: destinationInfo.totalNumberOfDestinations,
),
),
],
),
);
}
}
/// Tooltip widget for use in a [NavigationBar].
///
/// It appears just above the navigation bar when one of the destinations is
/// long pressed.
class _NavigationBarDestinationTooltip extends StatelessWidget {
/// Adds a tooltip to the [child] widget.
const _NavigationBarDestinationTooltip({
required this.message,
required this.child,
});
/// The text that is rendered in the tooltip when it appears.
final String message;
/// The widget that, when pressed, will show a tooltip.
final Widget child;
@override
Widget build(BuildContext context) {
return Tooltip(
message: message,
// TODO(johnsonmh): Make this value configurable/themable.
verticalOffset: 42,
excludeFromSemantics: true,
preferBelow: false,
child: child,
);
}
}
/// Custom layout delegate for shifting navigation bar destinations.
///
/// This will lay out the icon + label according to the [animation].
///
/// When the [animation] is 0, the icon will be centered, and the label will be
/// positioned directly below it.
///
/// When the [animation] is 1, the label will still be positioned directly below
/// the icon, but the icon + label combination will be centered.
///
/// Used in a [CustomMultiChildLayout] widget in the
/// [_NavigationDestinationBuilder].
class _NavigationDestinationLayoutDelegate extends MultiChildLayoutDelegate {
_NavigationDestinationLayoutDelegate({required this.animation}) : super(relayout: animation);
/// The selection animation that indicates whether or not this destination is
/// selected.
///
/// See [_NavigationDestinationInfo.selectedAnimation].
final Animation<double> animation;
/// ID for the icon widget child.
///
/// This is used by the [LayoutId] when this delegate is used in a
/// [CustomMultiChildLayout].
///
/// See [_NavigationDestinationBuilder].
static const int iconId = 1;
/// ID for the label widget child.
///
/// This is used by the [LayoutId] when this delegate is used in a
/// [CustomMultiChildLayout].
///
/// See [_NavigationDestinationBuilder].
static const int labelId = 2;
@override
void performLayout(Size size) {
double halfWidth(Size size) => size.width / 2;
double halfHeight(Size size) => size.height / 2;
final Size iconSize = layoutChild(iconId, BoxConstraints.loose(size));
final Size labelSize = layoutChild(labelId, BoxConstraints.loose(size));
final double yPositionOffset = Tween<double>(
// When unselected, the icon is centered vertically.
begin: halfHeight(iconSize),
// When selected, the icon and label are centered vertically.
end: halfHeight(iconSize) + halfHeight(labelSize),
).transform(animation.value);
final double iconYPosition = halfHeight(size) - yPositionOffset;
// Position the icon.
positionChild(
iconId,
Offset(
// Center the icon horizontally.
halfWidth(size) - halfWidth(iconSize),
iconYPosition,
),
);
// Position the label.
positionChild(
labelId,
Offset(
// Center the label horizontally.
halfWidth(size) - halfWidth(labelSize),
// Label always appears directly below the icon.
iconYPosition + iconSize.height,
),
);
}
@override
bool shouldRelayout(_NavigationDestinationLayoutDelegate oldDelegate) {
return oldDelegate.animation != animation;
}
}
/// Widget that listens to an animation, and rebuilds when the animation changes
/// [AnimationStatus].
///
/// This can be more efficient than just using an [AnimatedBuilder] when you
/// only need to rebuild when the [Animation.status] changes, since
/// [AnimatedBuilder] rebuilds every time the animation ticks.
class _StatusTransitionWidgetBuilder extends StatusTransitionWidget {
/// Creates a widget that rebuilds when the given animation changes status.
const _StatusTransitionWidgetBuilder({
required super.animation,
required this.builder,
this.child,
});
/// Called every time the [animation] changes [AnimationStatus].
final TransitionBuilder builder;
/// The child widget to pass to the [builder].
///
/// If a [builder] callback's return value contains a subtree that does not
/// depend on the animation, it's more efficient to build that subtree once
/// instead of rebuilding it on every animation status change.
///
/// Using this pre-built child is entirely optional, but can improve
/// performance in some cases and is therefore a good practice.
///
/// See: [AnimatedBuilder.child]
final Widget? child;
@override
Widget build(BuildContext context) => builder(context, child);
}
// Builder widget for widgets that need to be animated from 0 (unselected) to
// 1.0 (selected).
//
// This widget creates and manages an [AnimationController] that it passes down
// to the child through the [builder] function.
//
// When [isSelected] is `true`, the animation controller will animate from
// 0 to 1 (for [duration] time).
//
// When [isSelected] is `false`, the animation controller will animate from
// 1 to 0 (for [duration] time).
//
// If [isSelected] is updated while the widget is animating, the animation will
// be reversed until it is either 0 or 1 again. If [alwaysDoFullAnimation] is
// true, the animation will reset to 0 or 1 before beginning the animation, so
// that the full animation is done.
//
// Usage:
// ```dart
// _SelectableAnimatedBuilder(
// isSelected: _isDrawerOpen,
// builder: (context, animation) {
// return AnimatedIcon(
// icon: AnimatedIcons.menu_arrow,
// progress: animation,
// semanticLabel: 'Show menu',
// );
// }
// )
// ```
class _SelectableAnimatedBuilder extends StatefulWidget {
/// Builds and maintains an [AnimationController] that will animate from 0 to
/// 1 and back depending on when [isSelected] is true.
const _SelectableAnimatedBuilder({
required this.isSelected,
this.duration = const Duration(milliseconds: 200),
this.alwaysDoFullAnimation = false,
required this.builder,
});
/// When true, the widget will animate an animation controller from 0 to 1.
///
/// The animation controller is passed to the child widget through [builder].
final bool isSelected;
/// How long the animation controller should animate for when [isSelected] is
/// updated.
///
/// If the animation is currently running and [isSelected] is updated, only
/// the [duration] left to finish the animation will be run.
final Duration duration;
/// If true, the animation will always go all the way from 0 to 1 when
/// [isSelected] is true, and from 1 to 0 when [isSelected] is false, even
/// when the status changes mid animation.
///
/// If this is false and the status changes mid animation, the animation will
/// reverse direction from it's current point.
///
/// Defaults to false.
final bool alwaysDoFullAnimation;
/// Builds the child widget based on the current animation status.
///
/// When [isSelected] is updated to true, this builder will be called and the
/// animation will animate up to 1. When [isSelected] is updated to
/// `false`, this will be called and the animation will animate down to 0.
final Widget Function(BuildContext, Animation<double>) builder;
@override
_SelectableAnimatedBuilderState createState() =>
_SelectableAnimatedBuilderState();
}
/// State that manages the [AnimationController] that is passed to
/// [_SelectableAnimatedBuilder.builder].
class _SelectableAnimatedBuilderState extends State<_SelectableAnimatedBuilder>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(vsync: this);
_controller.duration = widget.duration;
_controller.value = widget.isSelected ? 1.0 : 0.0;
}
@override
void didUpdateWidget(_SelectableAnimatedBuilder oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.duration != widget.duration) {
_controller.duration = widget.duration;
}
if (oldWidget.isSelected != widget.isSelected) {
if (widget.isSelected) {
_controller.forward(from: widget.alwaysDoFullAnimation ? 0 : null);
} else {
_controller.reverse(from: widget.alwaysDoFullAnimation ? 1 : null);
}
}
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return widget.builder(
context,
_controller,
);
}
}
/// Watches [animation] and calls [builder] with the appropriate [Curve]
/// depending on the direction of the [animation] status.
///
/// If [animation.status] is forward or complete, [curve] is used. If
/// [animation.status] is reverse or dismissed, [reverseCurve] is used.
///
/// If the [animation] changes direction while it is already running, the curve
/// used will not change, this will keep the animations smooth until it
/// completes.
///
/// This is similar to [CurvedAnimation] except the animation status listeners
/// are removed when this widget is disposed.
class _CurvedAnimationBuilder extends StatefulWidget {
const _CurvedAnimationBuilder({
required this.animation,
required this.curve,
required this.reverseCurve,
required this.builder,
});
final Animation<double> animation;
final Curve curve;
final Curve reverseCurve;
final Widget Function(BuildContext, Animation<double>) builder;
@override
_CurvedAnimationBuilderState createState() => _CurvedAnimationBuilderState();
}
class _CurvedAnimationBuilderState extends State<_CurvedAnimationBuilder> {
late AnimationStatus _animationDirection;
AnimationStatus? _preservedDirection;
@override
void initState() {
super.initState();
_animationDirection = widget.animation.status;
_updateStatus(widget.animation.status);
widget.animation.addStatusListener(_updateStatus);
}
@override
void dispose() {
widget.animation.removeStatusListener(_updateStatus);
super.dispose();
}
// Keeps track of the current animation status, as well as the "preserved
// direction" when the animation changes direction mid animation.
//
// The preserved direction is reset when the animation finishes in either
// direction.
void _updateStatus(AnimationStatus status) {
if (_animationDirection != status) {
setState(() {
_animationDirection = status;
});
}
switch (status) {
case AnimationStatus.forward || AnimationStatus.reverse when _preservedDirection != null:
break;
case AnimationStatus.forward || AnimationStatus.reverse:
setState(() { _preservedDirection = status; });
case AnimationStatus.completed || AnimationStatus.dismissed:
setState(() { _preservedDirection = null; });
}
}
@override
Widget build(BuildContext context) {
final bool shouldUseForwardCurve = (_preservedDirection ?? _animationDirection) != AnimationStatus.reverse;
final Animation<double> curvedAnimation = CurveTween(
curve: shouldUseForwardCurve ? widget.curve : widget.reverseCurve,
).animate(widget.animation);
return widget.builder(context, curvedAnimation);
}
}
/// Returns `true` if this animation is ticking forward, or has completed,
/// based on [status].
bool _isForwardOrCompleted(Animation<double> animation) {
return animation.status == AnimationStatus.forward
|| animation.status == AnimationStatus.completed;
}
NavigationBarThemeData _defaultsFor(BuildContext context) {
return Theme.of(context).useMaterial3 ? _NavigationBarDefaultsM3(context) : _NavigationBarDefaultsM2(context);
}
// Hand coded defaults based on Material Design 2.
class _NavigationBarDefaultsM2 extends NavigationBarThemeData {
_NavigationBarDefaultsM2(BuildContext context)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme,
super(
height: 80.0,
elevation: 0.0,
indicatorShape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(16))),
labelBehavior: NavigationDestinationLabelBehavior.alwaysShow,
);
final ThemeData _theme;
final ColorScheme _colors;
// With Material 2, the NavigationBar uses an overlay blend for the
// default color regardless of light/dark mode.
@override Color? get backgroundColor => ElevationOverlay.colorWithOverlay(_colors.surface, _colors.onSurface, 3.0);
@override MaterialStateProperty<IconThemeData?>? get iconTheme {
return MaterialStatePropertyAll<IconThemeData>(IconThemeData(
size: 24,
color: _colors.onSurface,
));
}
@override Color? get indicatorColor => _colors.secondary.withOpacity(0.24);
@override MaterialStateProperty<TextStyle?>? get labelTextStyle => MaterialStatePropertyAll<TextStyle?>(_theme.textTheme.labelSmall!.copyWith(color: _colors.onSurface));
}
// BEGIN GENERATED TOKEN PROPERTIES - NavigationBar
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _NavigationBarDefaultsM3 extends NavigationBarThemeData {
_NavigationBarDefaultsM3(this.context)
: super(
height: 80.0,
elevation: 3.0,
labelBehavior: NavigationDestinationLabelBehavior.alwaysShow,
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override Color? get backgroundColor => _colors.surfaceContainer;
@override Color? get shadowColor => Colors.transparent;
@override Color? get surfaceTintColor => Colors.transparent;
@override MaterialStateProperty<IconThemeData?>? get iconTheme {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
return IconThemeData(
size: 24.0,
color: states.contains(MaterialState.disabled)
? _colors.onSurfaceVariant.withOpacity(0.38)
: states.contains(MaterialState.selected)
? _colors.onSecondaryContainer
: _colors.onSurfaceVariant,
);
});
}
@override Color? get indicatorColor => _colors.secondaryContainer;
@override ShapeBorder? get indicatorShape => const StadiumBorder();
@override MaterialStateProperty<TextStyle?>? get labelTextStyle {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
final TextStyle style = _textTheme.labelMedium!;
return style.apply(
color: states.contains(MaterialState.disabled)
? _colors.onSurfaceVariant.withOpacity(0.38)
: states.contains(MaterialState.selected)
? _colors.onSurface
: _colors.onSurfaceVariant
);
});
}
}
// END GENERATED TOKEN PROPERTIES - NavigationBar
| flutter/packages/flutter/lib/src/material/navigation_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/navigation_bar.dart",
"repo_id": "flutter",
"token_count": 16427
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'material_state.dart';
import 'radio_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'toggleable.dart';
// Examples can assume:
// late BuildContext context;
// enum SingingCharacter { lafayette }
// late SingingCharacter? _character;
// late StateSetter setState;
enum _RadioType { material, adaptive }
const double _kOuterRadius = 8.0;
const double _kInnerRadius = 4.5;
/// A Material Design radio button.
///
/// Used to select between a number of mutually exclusive values. When one radio
/// button in a group is selected, the other radio buttons in the group cease to
/// be selected. The values are of type `T`, the type parameter of the [Radio]
/// class. Enums are commonly used for this purpose.
///
/// The radio button itself does not maintain any state. Instead, selecting the
/// radio invokes the [onChanged] callback, passing [value] as a parameter. If
/// [groupValue] and [value] match, this radio will be selected. Most widgets
/// will respond to [onChanged] by calling [State.setState] to update the
/// radio button's [groupValue].
///
/// {@tool dartpad}
/// Here is an example of Radio widgets wrapped in ListTiles, which is similar
/// to what you could get with the RadioListTile widget.
///
/// The currently selected character is passed into `groupValue`, which is
/// maintained by the example's `State`. In this case, the first [Radio]
/// will start off selected because `_character` is initialized to
/// `SingingCharacter.lafayette`.
///
/// If the second radio button is pressed, the example's state is updated
/// with `setState`, updating `_character` to `SingingCharacter.jefferson`.
/// This causes the buttons to rebuild with the updated `groupValue`, and
/// therefore the selection of the second button.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// ** See code in examples/api/lib/material/radio/radio.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RadioListTile], which combines this widget with a [ListTile] so that
/// you can give the radio button a label.
/// * [Slider], for selecting a value in a range.
/// * [Checkbox] and [Switch], for toggling a particular value on or off.
/// * <https://material.io/design/components/selection-controls.html#radio-buttons>
class Radio<T> extends StatefulWidget {
/// Creates a Material Design radio button.
///
/// The radio button itself does not maintain any state. Instead, when the
/// radio button is selected, the widget calls the [onChanged] callback. Most
/// widgets that use a radio button will listen for the [onChanged] callback
/// and rebuild the radio button with a new [groupValue] to update the visual
/// appearance of the radio button.
///
/// The following arguments are required:
///
/// * [value] and [groupValue] together determine whether the radio button is
/// selected.
/// * [onChanged] is called when the user selects this radio button.
const Radio({
super.key,
required this.value,
required this.groupValue,
required this.onChanged,
this.mouseCursor,
this.toggleable = false,
this.activeColor,
this.fillColor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
}) : _radioType = _RadioType.material,
useCupertinoCheckmarkStyle = false;
/// Creates an adaptive [Radio] based on whether the target platform is iOS
/// or macOS, following Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// On iOS and macOS, this constructor creates a [CupertinoRadio], which has
/// matching functionality and presentation as Material checkboxes, and are the
/// graphics expected on iOS. On other platforms, this creates a Material
/// design [Radio].
///
/// If a [CupertinoRadio] is created, the following parameters are ignored:
/// [mouseCursor], [fillColor], [hoverColor], [overlayColor], [splashRadius],
/// [materialTapTargetSize], [visualDensity].
///
/// [useCupertinoCheckmarkStyle] is used only if a [CupertinoRadio] is created.
///
/// The target platform is based on the current [Theme]: [ThemeData.platform].
const Radio.adaptive({
super.key,
required this.value,
required this.groupValue,
required this.onChanged,
this.mouseCursor,
this.toggleable = false,
this.activeColor,
this.fillColor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
this.useCupertinoCheckmarkStyle = false
}) : _radioType = _RadioType.adaptive;
/// The value represented by this radio button.
final T value;
/// The currently selected value for a group of radio buttons.
///
/// This radio button is considered selected if its [value] matches the
/// [groupValue].
final T? groupValue;
/// Called when the user selects this radio button.
///
/// The radio button passes [value] as a parameter to this callback. The radio
/// button does not actually change state until the parent widget rebuilds the
/// radio button with the new [groupValue].
///
/// If null, the radio button will be displayed as disabled.
///
/// The provided callback will not be invoked if this radio button is already
/// selected.
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// ```dart
/// Radio<SingingCharacter>(
/// value: SingingCharacter.lafayette,
/// groupValue: _character,
/// onChanged: (SingingCharacter? newValue) {
/// setState(() {
/// _character = newValue;
/// });
/// },
/// )
/// ```
final ValueChanged<T?>? onChanged;
/// {@template flutter.material.radio.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// If null, then the value of [RadioThemeData.mouseCursor] is used.
/// If that is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], a [MouseCursor] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept
/// either a [MouseCursor] or a [MaterialStateProperty<MouseCursor>].
final MouseCursor? mouseCursor;
/// Set to true if this radio button is allowed to be returned to an
/// indeterminate state by selecting it again when selected.
///
/// To indicate returning to an indeterminate state, [onChanged] will be
/// called with null.
///
/// If true, [onChanged] can be called with [value] when selected while
/// [groupValue] != [value], or with null when selected again while
/// [groupValue] == [value].
///
/// If false, [onChanged] will be called with [value] when it is selected
/// while [groupValue] != [value], and only by selecting another radio button
/// in the group (i.e. changing the value of [groupValue]) can this radio
/// button be unselected.
///
/// The default is false.
///
/// {@tool dartpad}
/// This example shows how to enable deselecting a radio button by setting the
/// [toggleable] attribute.
///
/// ** See code in examples/api/lib/material/radio/radio.toggleable.0.dart **
/// {@end-tool}
final bool toggleable;
/// The color to use when this radio button is selected.
///
/// Defaults to [ColorScheme.secondary].
///
/// If [fillColor] returns a non-null color in the [MaterialState.selected]
/// state, it will be used instead of this color.
final Color? activeColor;
/// {@template flutter.material.radio.fillColor}
/// The color that fills the radio button, in all [MaterialState]s.
///
/// Resolves in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [fillColor] based on the current [MaterialState]
/// of the [Radio], providing a different [Color] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Radio<int>(
/// value: 1,
/// groupValue: 1,
/// onChanged: (_){},
/// fillColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return Colors.orange.withOpacity(.32);
/// }
/// return Colors.orange;
/// })
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// If null, then the value of [activeColor] is used in the selected state. If
/// that is also null, then the value of [RadioThemeData.fillColor] is used.
/// If that is also null and [ThemeData.useMaterial3] is false, then
/// [ThemeData.disabledColor] is used in the disabled state, [ColorScheme.secondary]
/// is used in the selected state, and [ThemeData.unselectedWidgetColor] is used in the
/// default state; if [ThemeData.useMaterial3] is true, then [ColorScheme.onSurface]
/// is used in the disabled state, [ColorScheme.primary] is used in the
/// selected state and [ColorScheme.onSurfaceVariant] is used in the default state.
final MaterialStateProperty<Color?>? fillColor;
/// {@template flutter.material.radio.materialTapTargetSize}
/// Configures the minimum size of the tap target.
/// {@endtemplate}
///
/// If null, then the value of [RadioThemeData.materialTapTargetSize] is used.
/// If that is also null, then the value of [ThemeData.materialTapTargetSize]
/// is used.
///
/// See also:
///
/// * [MaterialTapTargetSize], for a description of how this affects tap targets.
final MaterialTapTargetSize? materialTapTargetSize;
/// {@template flutter.material.radio.visualDensity}
/// Defines how compact the radio's layout will be.
/// {@endtemplate}
///
/// {@macro flutter.material.themedata.visualDensity}
///
/// If null, then the value of [RadioThemeData.visualDensity] is used. If that
/// is also null, then the value of [ThemeData.visualDensity] is used.
///
/// See also:
///
/// * [ThemeData.visualDensity], which specifies the [visualDensity] for all
/// widgets within a [Theme].
final VisualDensity? visualDensity;
/// The color for the radio's [Material] when it has the input focus.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.focused]
/// state, it will be used instead.
///
/// If null, then the value of [RadioThemeData.overlayColor] is used in the
/// focused state. If that is also null, then the value of
/// [ThemeData.focusColor] is used.
final Color? focusColor;
/// {@template flutter.material.radio.hoverColor}
/// The color for the radio's [Material] when a pointer is hovering over it.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.hovered]
/// state, it will be used instead.
/// {@endtemplate}
///
/// If null, then the value of [RadioThemeData.overlayColor] is used in the
/// hovered state. If that is also null, then the value of
/// [ThemeData.hoverColor] is used.
final Color? hoverColor;
/// {@template flutter.material.radio.overlayColor}
/// The color for the radio's [Material].
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// {@endtemplate}
///
/// If null, then the value of [activeColor] with alpha
/// [kRadialReactionAlpha], [focusColor] and [hoverColor] is used in the
/// pressed, focused and hovered state. If that is also null,
/// the value of [RadioThemeData.overlayColor] is used. If that is also null,
/// then in Material 2, the value of [ColorScheme.secondary] with alpha
/// [kRadialReactionAlpha], [ThemeData.focusColor] and [ThemeData.hoverColor]
/// is used in the pressed, focused and hovered state. In Material3, the default
/// values are:
/// * selected
/// * pressed - Theme.colorScheme.onSurface(0.1)
/// * hovered - Theme.colorScheme.primary(0.08)
/// * focused - Theme.colorScheme.primary(0.1)
/// * pressed - Theme.colorScheme.primary(0.1)
/// * hovered - Theme.colorScheme.onSurface(0.08)
/// * focused - Theme.colorScheme.onSurface(0.1)
final MaterialStateProperty<Color?>? overlayColor;
/// {@template flutter.material.radio.splashRadius}
/// The splash radius of the circular [Material] ink response.
/// {@endtemplate}
///
/// If null, then the value of [RadioThemeData.splashRadius] is used. If that
/// is also null, then [kRadialReactionRadius] is used.
final double? splashRadius;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// Controls whether the checkmark style is used in an iOS-style radio.
///
/// Only usable under the [Radio.adaptive] constructor. If set to true, on
/// Apple platforms the radio button will appear as an iOS styled checkmark.
/// Controls the [CupertinoRadio] through [CupertinoRadio.useCheckmarkStyle].
///
/// Defaults to false.
final bool useCupertinoCheckmarkStyle;
final _RadioType _radioType;
bool get _selected => value == groupValue;
@override
State<Radio<T>> createState() => _RadioState<T>();
}
class _RadioState<T> extends State<Radio<T>> with TickerProviderStateMixin, ToggleableStateMixin {
final _RadioPainter _painter = _RadioPainter();
void _handleChanged(bool? selected) {
if (selected == null) {
widget.onChanged!(null);
return;
}
if (selected) {
widget.onChanged!(widget.value);
}
}
@override
void didUpdateWidget(Radio<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget._selected != oldWidget._selected) {
animateToValue();
}
}
@override
void dispose() {
_painter.dispose();
super.dispose();
}
@override
ValueChanged<bool?>? get onChanged => widget.onChanged != null ? _handleChanged : null;
@override
bool get tristate => widget.toggleable;
@override
bool? get value => widget._selected;
MaterialStateProperty<Color?> get _widgetFillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return null;
}
if (states.contains(MaterialState.selected)) {
return widget.activeColor;
}
return null;
});
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
switch (widget._radioType) {
case _RadioType.material:
break;
case _RadioType.adaptive:
final ThemeData theme = Theme.of(context);
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return CupertinoRadio<T>(
value: widget.value,
groupValue: widget.groupValue,
onChanged: widget.onChanged,
toggleable: widget.toggleable,
activeColor: widget.activeColor,
focusColor: widget.focusColor,
focusNode: widget.focusNode,
autofocus: widget.autofocus,
useCheckmarkStyle: widget.useCupertinoCheckmarkStyle,
);
}
}
final RadioThemeData radioTheme = RadioTheme.of(context);
final RadioThemeData defaults = Theme.of(context).useMaterial3 ? _RadioDefaultsM3(context) : _RadioDefaultsM2(context);
final MaterialTapTargetSize effectiveMaterialTapTargetSize = widget.materialTapTargetSize
?? radioTheme.materialTapTargetSize
?? defaults.materialTapTargetSize!;
final VisualDensity effectiveVisualDensity = widget.visualDensity
?? radioTheme.visualDensity
?? defaults.visualDensity!;
Size size = switch (effectiveMaterialTapTargetSize) {
MaterialTapTargetSize.padded => const Size(kMinInteractiveDimension, kMinInteractiveDimension),
MaterialTapTargetSize.shrinkWrap => const Size(kMinInteractiveDimension - 8.0, kMinInteractiveDimension - 8.0),
};
size += effectiveVisualDensity.baseSizeAdjustment;
final MaterialStateProperty<MouseCursor> effectiveMouseCursor = MaterialStateProperty.resolveWith<MouseCursor>((Set<MaterialState> states) {
return MaterialStateProperty.resolveAs<MouseCursor?>(widget.mouseCursor, states)
?? radioTheme.mouseCursor?.resolve(states)
?? MaterialStateProperty.resolveAs<MouseCursor>(MaterialStateMouseCursor.clickable, states);
});
// Colors need to be resolved in selected and non selected states separately
// so that they can be lerped between.
final Set<MaterialState> activeStates = states..add(MaterialState.selected);
final Set<MaterialState> inactiveStates = states..remove(MaterialState.selected);
final Color? activeColor = widget.fillColor?.resolve(activeStates)
?? _widgetFillColor.resolve(activeStates)
?? radioTheme.fillColor?.resolve(activeStates);
final Color effectiveActiveColor = activeColor ?? defaults.fillColor!.resolve(activeStates)!;
final Color? inactiveColor = widget.fillColor?.resolve(inactiveStates)
?? _widgetFillColor.resolve(inactiveStates)
?? radioTheme.fillColor?.resolve(inactiveStates);
final Color effectiveInactiveColor = inactiveColor ?? defaults.fillColor!.resolve(inactiveStates)!;
final Set<MaterialState> focusedStates = states..add(MaterialState.focused);
Color effectiveFocusOverlayColor = widget.overlayColor?.resolve(focusedStates)
?? widget.focusColor
?? radioTheme.overlayColor?.resolve(focusedStates)
?? defaults.overlayColor!.resolve(focusedStates)!;
final Set<MaterialState> hoveredStates = states..add(MaterialState.hovered);
Color effectiveHoverOverlayColor = widget.overlayColor?.resolve(hoveredStates)
?? widget.hoverColor
?? radioTheme.overlayColor?.resolve(hoveredStates)
?? defaults.overlayColor!.resolve(hoveredStates)!;
final Set<MaterialState> activePressedStates = activeStates..add(MaterialState.pressed);
final Color effectiveActivePressedOverlayColor = widget.overlayColor?.resolve(activePressedStates)
?? radioTheme.overlayColor?.resolve(activePressedStates)
?? activeColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(activePressedStates)!;
final Set<MaterialState> inactivePressedStates = inactiveStates..add(MaterialState.pressed);
final Color effectiveInactivePressedOverlayColor = widget.overlayColor?.resolve(inactivePressedStates)
?? radioTheme.overlayColor?.resolve(inactivePressedStates)
?? inactiveColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(inactivePressedStates)!;
if (downPosition != null) {
effectiveHoverOverlayColor = states.contains(MaterialState.selected)
? effectiveActivePressedOverlayColor
: effectiveInactivePressedOverlayColor;
effectiveFocusOverlayColor = states.contains(MaterialState.selected)
? effectiveActivePressedOverlayColor
: effectiveInactivePressedOverlayColor;
}
final bool? accessibilitySelected;
// Apple devices also use `selected` to annotate radio button's semantics
// state.
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
accessibilitySelected = null;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
accessibilitySelected = widget._selected;
}
return Semantics(
inMutuallyExclusiveGroup: true,
checked: widget._selected,
selected: accessibilitySelected,
child: buildToggleable(
focusNode: widget.focusNode,
autofocus: widget.autofocus,
mouseCursor: effectiveMouseCursor,
size: size,
painter: _painter
..position = position
..reaction = reaction
..reactionFocusFade = reactionFocusFade
..reactionHoverFade = reactionHoverFade
..inactiveReactionColor = effectiveInactivePressedOverlayColor
..reactionColor = effectiveActivePressedOverlayColor
..hoverColor = effectiveHoverOverlayColor
..focusColor = effectiveFocusOverlayColor
..splashRadius = widget.splashRadius ?? radioTheme.splashRadius ?? kRadialReactionRadius
..downPosition = downPosition
..isFocused = states.contains(MaterialState.focused)
..isHovered = states.contains(MaterialState.hovered)
..activeColor = effectiveActiveColor
..inactiveColor = effectiveInactiveColor,
),
);
}
}
class _RadioPainter extends ToggleablePainter {
@override
void paint(Canvas canvas, Size size) {
paintRadialReaction(canvas: canvas, origin: size.center(Offset.zero));
final Offset center = (Offset.zero & size).center;
// Outer circle
final Paint paint = Paint()
..color = Color.lerp(inactiveColor, activeColor, position.value)!
..style = PaintingStyle.stroke
..strokeWidth = 2.0;
canvas.drawCircle(center, _kOuterRadius, paint);
// Inner circle
if (!position.isDismissed) {
paint.style = PaintingStyle.fill;
canvas.drawCircle(center, _kInnerRadius * position.value, paint);
}
}
}
// Hand coded defaults based on Material Design 2.
class _RadioDefaultsM2 extends RadioThemeData {
_RadioDefaultsM2(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override
MaterialStateProperty<Color> get fillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _theme.disabledColor;
}
if (states.contains(MaterialState.selected)) {
return _colors.secondary;
}
return _theme.unselectedWidgetColor;
});
}
@override
MaterialStateProperty<Color> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return fillColor.resolve(states).withAlpha(kRadialReactionAlpha);
}
if (states.contains(MaterialState.hovered)) {
return _theme.hoverColor;
}
if (states.contains(MaterialState.focused)) {
return _theme.focusColor;
}
return Colors.transparent;
});
}
@override
MaterialTapTargetSize get materialTapTargetSize => _theme.materialTapTargetSize;
@override
VisualDensity get visualDensity => _theme.visualDensity;
}
// BEGIN GENERATED TOKEN PROPERTIES - Radio<T>
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _RadioDefaultsM3 extends RadioThemeData {
_RadioDefaultsM3(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override
MaterialStateProperty<Color> get fillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.pressed)) {
return _colors.primary;
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary;
}
if (states.contains(MaterialState.focused)) {
return _colors.primary;
}
return _colors.primary;
}
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface;
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface;
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface;
}
return _colors.onSurfaceVariant;
});
}
@override
MaterialStateProperty<Color> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return Colors.transparent;
}
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return Colors.transparent;
});
}
@override
MaterialTapTargetSize get materialTapTargetSize => _theme.materialTapTargetSize;
@override
VisualDensity get visualDensity => _theme.visualDensity;
}
// END GENERATED TOKEN PROPERTIES - Radio<T>
| flutter/packages/flutter/lib/src/material/radio.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/radio.dart",
"repo_id": "flutter",
"token_count": 8876
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/rendering.dart';
import 'adaptive_text_selection_toolbar.dart';
import 'debug.dart';
import 'desktop_text_selection.dart';
import 'magnifier.dart';
import 'text_selection.dart';
import 'theme.dart';
/// A widget that introduces an area for user selections with adaptive selection
/// controls.
///
/// This widget creates a [SelectableRegion] with platform-adaptive selection
/// controls.
///
/// Flutter widgets are not selectable by default. To enable selection for
/// a specific screen, consider wrapping the body of the [Route] with a
/// [SelectionArea].
///
/// The [SelectionArea] widget must have a [Localizations] ancestor that
/// contains a [MaterialLocalizations] delegate; using the [MaterialApp] widget
/// ensures that such an ancestor is present.
///
/// {@tool dartpad}
/// This example shows how to make a screen selectable.
///
/// ** See code in examples/api/lib/material/selection_area/selection_area.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SelectableRegion], which provides an overview of the selection system.
/// * [SelectableText], which enables selection on a single run of text.
class SelectionArea extends StatefulWidget {
/// Creates a [SelectionArea].
///
/// If [selectionControls] is null, a platform specific one is used.
const SelectionArea({
super.key,
this.focusNode,
this.selectionControls,
this.contextMenuBuilder = _defaultContextMenuBuilder,
this.magnifierConfiguration,
this.onSelectionChanged,
required this.child,
});
/// The configuration for the magnifier in the selection region.
///
/// By default, builds a [CupertinoTextMagnifier] on iOS and [TextMagnifier]
/// on Android, and builds nothing on all other platforms. To suppress the
/// magnifier, consider passing [TextMagnifierConfiguration.disabled].
///
/// {@macro flutter.widgets.magnifier.intro}
final TextMagnifierConfiguration? magnifierConfiguration;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// The delegate to build the selection handles and toolbar.
///
/// If it is null, the platform specific selection control is used.
final TextSelectionControls? selectionControls;
/// {@macro flutter.widgets.EditableText.contextMenuBuilder}
///
/// If not provided, will build a default menu based on the ambient
/// [ThemeData.platform].
///
/// {@tool dartpad}
/// This example shows how to build a custom context menu for any selected
/// content in a SelectionArea.
///
/// ** See code in examples/api/lib/material/context_menu/selectable_region_toolbar_builder.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar], which is built by default.
final SelectableRegionContextMenuBuilder? contextMenuBuilder;
/// Called when the selected content changes.
final ValueChanged<SelectedContent?>? onSelectionChanged;
/// The child widget this selection area applies to.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
static Widget _defaultContextMenuBuilder(BuildContext context, SelectableRegionState selectableRegionState) {
return AdaptiveTextSelectionToolbar.selectableRegion(
selectableRegionState: selectableRegionState,
);
}
@override
State<StatefulWidget> createState() => _SelectionAreaState();
}
class _SelectionAreaState extends State<SelectionArea> {
FocusNode get _effectiveFocusNode => widget.focusNode ?? (_internalNode ??= FocusNode());
FocusNode? _internalNode;
@override
void dispose() {
_internalNode?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final TextSelectionControls controls = widget.selectionControls ?? switch (Theme.of(context).platform) {
TargetPlatform.android || TargetPlatform.fuchsia => materialTextSelectionHandleControls,
TargetPlatform.linux || TargetPlatform.windows => desktopTextSelectionHandleControls,
TargetPlatform.iOS => cupertinoTextSelectionHandleControls,
TargetPlatform.macOS => cupertinoDesktopTextSelectionHandleControls,
};
return SelectableRegion(
selectionControls: controls,
focusNode: _effectiveFocusNode,
contextMenuBuilder: widget.contextMenuBuilder,
magnifierConfiguration: widget.magnifierConfiguration ?? TextMagnifier.adaptiveMagnifierConfiguration,
onSelectionChanged: widget.onSelectionChanged,
child: widget.child,
);
}
}
| flutter/packages/flutter/lib/src/material/selection_area.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/selection_area.dart",
"repo_id": "flutter",
"token_count": 1436
} | 649 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.