text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'app_bar.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'tab_bar_theme.dart';
import 'tab_controller.dart';
import 'tab_indicator.dart';
import 'text_theme.dart';
import 'theme.dart';
const double _kTabHeight = 46.0;
const double _kTextAndIconTabHeight = 72.0;
const double _kStartOffset = 52.0;
/// Defines how the bounds of the selected tab indicator are computed.
///
/// See also:
///
/// * [TabBar], which displays a row of tabs.
/// * [TabBarView], which displays a widget for the currently selected tab.
/// * [TabBar.indicator], which defines the appearance of the selected tab
/// indicator relative to the tab's bounds.
enum TabBarIndicatorSize {
/// The tab indicator's bounds are as wide as the space occupied by the tab
/// in the tab bar: from the right edge of the previous tab to the left edge
/// of the next tab.
tab,
/// The tab's bounds are only as wide as the (centered) tab widget itself.
///
/// This value is used to align the tab's label, typically a [Tab]
/// widget's text or icon, with the selected tab indicator.
label,
}
/// Defines how tabs are aligned horizontally in a [TabBar].
///
/// See also:
///
/// * [TabBar], which displays a row of tabs.
/// * [TabBarView], which displays a widget for the currently selected tab.
/// * [TabBar.tabAlignment], which defines the horizontal alignment of the
/// tabs within the [TabBar].
enum TabAlignment {
// TODO(tahatesser): Add a link to the Material Design spec for
// horizontal offset when it is available.
// It's currently sourced from androidx/compose/material3/TabRow.kt.
/// If [TabBar.isScrollable] is true, tabs are aligned to the
/// start of the [TabBar]. Otherwise throws an exception.
///
/// It is not recommended to set [TabAlignment.start] when
/// [ThemeData.useMaterial3] is false.
start,
/// If [TabBar.isScrollable] is true, tabs are aligned to the
/// start of the [TabBar] with an offset of 52.0 pixels.
/// Otherwise throws an exception.
///
/// It is not recommended to set [TabAlignment.startOffset] when
/// [ThemeData.useMaterial3] is false.
startOffset,
/// If [TabBar.isScrollable] is false, tabs are stretched to fill the
/// [TabBar]. Otherwise throws an exception.
fill,
/// Tabs are aligned to the center of the [TabBar].
center,
}
/// A Material Design [TabBar] tab.
///
/// If both [icon] and [text] are provided, the text is displayed below
/// the icon.
///
/// See also:
///
/// * [TabBar], which displays a row of tabs.
/// * [TabBarView], which displays a widget for the currently selected tab.
/// * [TabController], which coordinates tab selection between a [TabBar] and a [TabBarView].
/// * <https://material.io/design/components/tabs.html>
class Tab extends StatelessWidget implements PreferredSizeWidget {
/// Creates a Material Design [TabBar] tab.
///
/// At least one of [text], [icon], and [child] must be non-null. The [text]
/// and [child] arguments must not be used at the same time. The
/// [iconMargin] is only useful when [icon] and either one of [text] or
/// [child] is non-null.
const Tab({
super.key,
this.text,
this.icon,
this.iconMargin,
this.height,
this.child,
}) : assert(text != null || child != null || icon != null),
assert(text == null || child == null);
/// The text to display as the tab's label.
///
/// Must not be used in combination with [child].
final String? text;
/// The widget to be used as the tab's label.
///
/// Usually a [Text] widget, possibly wrapped in a [Semantics] widget.
///
/// Must not be used in combination with [text].
final Widget? child;
/// An icon to display as the tab's label.
final Widget? icon;
/// The margin added around the tab's icon.
///
/// Only useful when used in combination with [icon], and either one of
/// [text] or [child] is non-null.
///
/// Defaults to 2 pixels of bottom margin. If [ThemeData.useMaterial3] is false,
/// then defaults to 10 pixels of bottom margin.
final EdgeInsetsGeometry? iconMargin;
/// The height of the [Tab].
///
/// If null, the height will be calculated based on the content of the [Tab]. When `icon` is not
/// null along with `child` or `text`, the default height is 72.0 pixels. Without an `icon`, the
/// height is 46.0 pixels.
final double? height;
Widget _buildLabelText() {
return child ?? Text(text!, softWrap: false, overflow: TextOverflow.fade);
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
final double calculatedHeight;
final Widget label;
if (icon == null) {
calculatedHeight = _kTabHeight;
label = _buildLabelText();
} else if (text == null && child == null) {
calculatedHeight = _kTabHeight;
label = icon!;
} else {
calculatedHeight = _kTextAndIconTabHeight;
final EdgeInsetsGeometry effectiveIconMargin = iconMargin ??
(Theme.of(context).useMaterial3
? _TabsPrimaryDefaultsM3.iconMargin
: _TabsDefaultsM2.iconMargin);
label = Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Container(
margin: effectiveIconMargin,
child: icon,
),
_buildLabelText(),
],
);
}
return SizedBox(
height: height ?? calculatedHeight,
child: Center(
widthFactor: 1.0,
child: label,
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('text', text, defaultValue: null));
}
@override
Size get preferredSize {
if (height != null) {
return Size.fromHeight(height!);
} else if ((text != null || child != null) && icon != null) {
return const Size.fromHeight(_kTextAndIconTabHeight);
} else {
return const Size.fromHeight(_kTabHeight);
}
}
}
class _TabStyle extends AnimatedWidget {
const _TabStyle({
required Animation<double> animation,
required this.isSelected,
required this.isPrimary,
required this.labelColor,
required this.unselectedLabelColor,
required this.labelStyle,
required this.unselectedLabelStyle,
required this.defaults,
required this.child,
}) : super(listenable: animation);
final TextStyle? labelStyle;
final TextStyle? unselectedLabelStyle;
final bool isSelected;
final bool isPrimary;
final Color? labelColor;
final Color? unselectedLabelColor;
final TabBarTheme defaults;
final Widget child;
MaterialStateColor _resolveWithLabelColor(BuildContext context) {
final ThemeData themeData = Theme.of(context);
final TabBarTheme tabBarTheme = TabBarTheme.of(context);
final Animation<double> animation = listenable as Animation<double>;
// labelStyle.color (and tabBarTheme.labelStyle.color) is not considered
// as it'll be a breaking change without a possible migration plan. for
// details: https://github.com/flutter/flutter/pull/109541#issuecomment-1294241417
Color selectedColor = labelColor
?? tabBarTheme.labelColor
?? labelStyle?.color
?? tabBarTheme.labelStyle?.color
?? defaults.labelColor!;
final Color unselectedColor;
if (selectedColor is MaterialStateColor) {
unselectedColor = selectedColor.resolve(const <MaterialState>{});
selectedColor = selectedColor.resolve(const <MaterialState>{MaterialState.selected});
} else {
// unselectedLabelColor and tabBarTheme.unselectedLabelColor are ignored
// when labelColor is a MaterialStateColor.
unselectedColor = unselectedLabelColor
?? tabBarTheme.unselectedLabelColor
?? unselectedLabelStyle?.color
?? tabBarTheme.unselectedLabelStyle?.color
?? (themeData.useMaterial3
? defaults.unselectedLabelColor!
: selectedColor.withAlpha(0xB2)); // 70% alpha
}
return MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Color.lerp(selectedColor, unselectedColor, animation.value)!;
}
return Color.lerp(unselectedColor, selectedColor, animation.value)!;
});
}
@override
Widget build(BuildContext context) {
final TabBarTheme tabBarTheme = TabBarTheme.of(context);
final Animation<double> animation = listenable as Animation<double>;
final Set<MaterialState> states = isSelected
? const <MaterialState>{MaterialState.selected}
: const <MaterialState>{};
// To enable TextStyle.lerp(style1, style2, value), both styles must have
// the same value of inherit. Force that to be inherit=true here.
final TextStyle selectedStyle = (labelStyle
?? tabBarTheme.labelStyle
?? defaults.labelStyle!
).copyWith(inherit: true);
final TextStyle unselectedStyle = (unselectedLabelStyle
?? tabBarTheme.unselectedLabelStyle
?? labelStyle
?? defaults.unselectedLabelStyle!
).copyWith(inherit: true);
final TextStyle textStyle = isSelected
? TextStyle.lerp(selectedStyle, unselectedStyle, animation.value)!
: TextStyle.lerp(unselectedStyle, selectedStyle, animation.value)!;
final Color color = _resolveWithLabelColor(context).resolve(states);
return DefaultTextStyle(
style: textStyle.copyWith(color: color),
child: IconTheme.merge(
data: IconThemeData(
size: 24.0,
color: color,
),
child: child,
),
);
}
}
typedef _LayoutCallback = void Function(List<double> xOffsets, TextDirection textDirection, double width);
class _TabLabelBarRenderer extends RenderFlex {
_TabLabelBarRenderer({
required super.direction,
required super.mainAxisSize,
required super.mainAxisAlignment,
required super.crossAxisAlignment,
required TextDirection super.textDirection,
required super.verticalDirection,
required this.onPerformLayout,
});
_LayoutCallback onPerformLayout;
@override
void performLayout() {
super.performLayout();
// xOffsets will contain childCount+1 values, giving the offsets of the
// leading edge of the first tab as the first value, of the leading edge of
// the each subsequent tab as each subsequent value, and of the trailing
// edge of the last tab as the last value.
RenderBox? child = firstChild;
final List<double> xOffsets = <double>[];
while (child != null) {
final FlexParentData childParentData = child.parentData! as FlexParentData;
xOffsets.add(childParentData.offset.dx);
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
assert(textDirection != null);
switch (textDirection!) {
case TextDirection.rtl:
xOffsets.insert(0, size.width);
case TextDirection.ltr:
xOffsets.add(size.width);
}
onPerformLayout(xOffsets, textDirection!, size.width);
}
}
// This class and its renderer class only exist to report the widths of the tabs
// upon layout. The tab widths are only used at paint time (see _IndicatorPainter)
// or in response to input.
class _TabLabelBar extends Flex {
const _TabLabelBar({
super.children,
required this.onPerformLayout,
required super.mainAxisSize,
}) : super(
direction: Axis.horizontal,
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.center,
verticalDirection: VerticalDirection.down,
);
final _LayoutCallback onPerformLayout;
@override
RenderFlex createRenderObject(BuildContext context) {
return _TabLabelBarRenderer(
direction: direction,
mainAxisAlignment: mainAxisAlignment,
mainAxisSize: mainAxisSize,
crossAxisAlignment: crossAxisAlignment,
textDirection: getEffectiveTextDirection(context)!,
verticalDirection: verticalDirection,
onPerformLayout: onPerformLayout,
);
}
@override
void updateRenderObject(BuildContext context, _TabLabelBarRenderer renderObject) {
super.updateRenderObject(context, renderObject);
renderObject.onPerformLayout = onPerformLayout;
}
}
double _indexChangeProgress(TabController controller) {
final double controllerValue = controller.animation!.value;
final double previousIndex = controller.previousIndex.toDouble();
final double currentIndex = controller.index.toDouble();
// The controller's offset is changing because the user is dragging the
// TabBarView's PageView to the left or right.
if (!controller.indexIsChanging) {
return clampDouble((currentIndex - controllerValue).abs(), 0.0, 1.0);
}
// The TabController animation's value is changing from previousIndex to currentIndex.
return (controllerValue - currentIndex).abs() / (currentIndex - previousIndex).abs();
}
class _DividerPainter extends CustomPainter {
_DividerPainter({
required this.dividerColor,
required this.dividerHeight,
});
final Color dividerColor;
final double dividerHeight;
@override
void paint(Canvas canvas, Size size) {
if (dividerHeight <= 0.0) {
return;
}
final Paint paint = Paint()
..color = dividerColor
..strokeWidth = dividerHeight;
canvas.drawLine(
Offset(0, size.height - (paint.strokeWidth / 2)),
Offset(size.width, size.height - (paint.strokeWidth / 2)),
paint,
);
}
@override
bool shouldRepaint(_DividerPainter oldDelegate) {
return oldDelegate.dividerColor != dividerColor
|| oldDelegate.dividerHeight != dividerHeight;
}
}
class _IndicatorPainter extends CustomPainter {
_IndicatorPainter({
required this.controller,
required this.indicator,
required this.indicatorSize,
required this.tabKeys,
required _IndicatorPainter? old,
required this.indicatorPadding,
required this.labelPaddings,
this.dividerColor,
this.dividerHeight,
required this.showDivider,
}) : super(repaint: controller.animation) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/material.dart',
className: '$_IndicatorPainter',
object: this,
);
}
if (old != null) {
saveTabOffsets(old._currentTabOffsets, old._currentTextDirection);
}
}
final TabController controller;
final Decoration indicator;
final TabBarIndicatorSize indicatorSize;
final EdgeInsetsGeometry indicatorPadding;
final List<GlobalKey> tabKeys;
final List<EdgeInsetsGeometry> labelPaddings;
final Color? dividerColor;
final double? dividerHeight;
final bool showDivider;
// _currentTabOffsets and _currentTextDirection are set each time TabBar
// layout is completed. These values can be null when TabBar contains no
// tabs, since there are nothing to lay out.
List<double>? _currentTabOffsets;
TextDirection? _currentTextDirection;
Rect? _currentRect;
BoxPainter? _painter;
bool _needsPaint = false;
void markNeedsPaint() {
_needsPaint = true;
}
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_painter?.dispose();
}
void saveTabOffsets(List<double>? tabOffsets, TextDirection? textDirection) {
_currentTabOffsets = tabOffsets;
_currentTextDirection = textDirection;
}
// _currentTabOffsets[index] is the offset of the start edge of the tab at index, and
// _currentTabOffsets[_currentTabOffsets.length] is the end edge of the last tab.
int get maxTabIndex => _currentTabOffsets!.length - 2;
double centerOf(int tabIndex) {
assert(_currentTabOffsets != null);
assert(_currentTabOffsets!.isNotEmpty);
assert(tabIndex >= 0);
assert(tabIndex <= maxTabIndex);
return (_currentTabOffsets![tabIndex] + _currentTabOffsets![tabIndex + 1]) / 2.0;
}
Rect indicatorRect(Size tabBarSize, int tabIndex) {
assert(_currentTabOffsets != null);
assert(_currentTextDirection != null);
assert(_currentTabOffsets!.isNotEmpty);
assert(tabIndex >= 0);
assert(tabIndex <= maxTabIndex);
double tabLeft, tabRight;
(tabLeft, tabRight) = switch (_currentTextDirection!) {
TextDirection.rtl => (_currentTabOffsets![tabIndex + 1], _currentTabOffsets![tabIndex]),
TextDirection.ltr => (_currentTabOffsets![tabIndex], _currentTabOffsets![tabIndex + 1]),
};
if (indicatorSize == TabBarIndicatorSize.label) {
final double tabWidth = tabKeys[tabIndex].currentContext!.size!.width;
final EdgeInsetsGeometry labelPadding = labelPaddings[tabIndex];
final EdgeInsets insets = labelPadding.resolve(_currentTextDirection);
final double delta = ((tabRight - tabLeft) - (tabWidth + insets.horizontal)) / 2.0;
tabLeft += delta + insets.left;
tabRight = tabLeft + tabWidth;
}
final EdgeInsets insets = indicatorPadding.resolve(_currentTextDirection);
final Rect rect = Rect.fromLTWH(tabLeft, 0.0, tabRight - tabLeft, tabBarSize.height);
if (!(rect.size >= insets.collapsedSize)) {
throw FlutterError(
'indicatorPadding insets should be less than Tab Size\n'
'Rect Size : ${rect.size}, Insets: $insets',
);
}
return insets.deflateRect(rect);
}
@override
void paint(Canvas canvas, Size size) {
_needsPaint = false;
_painter ??= indicator.createBoxPainter(markNeedsPaint);
final double index = controller.index.toDouble();
final double value = controller.animation!.value;
final bool ltr = index > value;
final int from = (ltr ? value.floor() : value.ceil()).clamp(0, maxTabIndex);
final int to = (ltr ? from + 1 : from - 1).clamp(0, maxTabIndex);
final Rect fromRect = indicatorRect(size, from);
final Rect toRect = indicatorRect(size, to);
_currentRect = Rect.lerp(fromRect, toRect, (value - from).abs());
_currentRect = switch (indicatorSize) {
TabBarIndicatorSize.label => _applyStretchEffect(_currentRect!),
// Do nothing.
TabBarIndicatorSize.tab => _currentRect,
};
assert(_currentRect != null);
final ImageConfiguration configuration = ImageConfiguration(
size: _currentRect!.size,
textDirection: _currentTextDirection,
);
if (showDivider && dividerHeight !> 0) {
final Paint dividerPaint = Paint()..color = dividerColor!..strokeWidth = dividerHeight!;
final Offset dividerP1 = Offset(0, size.height - (dividerPaint.strokeWidth / 2));
final Offset dividerP2 = Offset(size.width, size.height - (dividerPaint.strokeWidth / 2));
canvas.drawLine(dividerP1, dividerP2, dividerPaint);
}
_painter!.paint(canvas, _currentRect!.topLeft, configuration);
}
/// Applies the stretch effect to the indicator.
Rect _applyStretchEffect(Rect rect) {
// If the tab animation is completed, there is no need to stretch the indicator
// This only works for the tab change animation via tab index, not when
// dragging a [TabBarView], but it's still ok, to avoid unnecessary calculations.
if (controller.animation!.status == AnimationStatus.completed) {
return rect;
}
final double index = controller.index.toDouble();
final double value = controller.animation!.value;
// The progress of the animation from 0 to 1.
late double tabChangeProgress;
// If we are changing tabs via index, we want to map the progress between 0 and 1.
if (controller.indexIsChanging) {
double progressLeft = (index - value).abs();
final int tabsDelta = (controller.index - controller.previousIndex).abs();
if (tabsDelta != 0) {
progressLeft /= tabsDelta;
}
tabChangeProgress = 1 - clampDouble(progressLeft, 0.0, 1.0);
} else {
// Otherwise, the progress is how close we are to the current tab.
tabChangeProgress = (index - value).abs();
}
// If the animation has finished, there is no need to apply the stretch effect.
if (tabChangeProgress == 1.0) {
return rect;
}
// The maximum amount of extra width to add to the indicator.
final double stretchSize = rect.width;
final double inflationPerSide = stretchSize * _stretchAnimation.transform(tabChangeProgress) / 2;
final Rect stretchedRect = _inflateRectHorizontally(rect, inflationPerSide);
return stretchedRect;
}
/// The animatable that stretches the indicator horizontally when changing tabs.
/// Value range is from 0 to 1, so we can multiply it by an stretch factor.
///
/// Animation starts with no stretch, then quickly goes to the max stretch amount
/// and then goes back to no stretch.
late final Animatable<double> _stretchAnimation = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.0, end: 1.0),
weight: 20,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 1.0, end: 0.0),
weight: 80,
),
],
);
/// Same as [Rect.inflate], but only inflates in the horizontal direction.
Rect _inflateRectHorizontally(Rect r, double delta) {
return Rect.fromLTRB(r.left - delta, r.top, r.right + delta, r.bottom);
}
@override
bool shouldRepaint(_IndicatorPainter old) {
return _needsPaint
|| controller != old.controller
|| indicator != old.indicator
|| tabKeys.length != old.tabKeys.length
|| (!listEquals(_currentTabOffsets, old._currentTabOffsets))
|| _currentTextDirection != old._currentTextDirection;
}
}
class _ChangeAnimation extends Animation<double> with AnimationWithParentMixin<double> {
_ChangeAnimation(this.controller);
final TabController controller;
@override
Animation<double> get parent => controller.animation!;
@override
void removeStatusListener(AnimationStatusListener listener) {
if (controller.animation != null) {
super.removeStatusListener(listener);
}
}
@override
void removeListener(VoidCallback listener) {
if (controller.animation != null) {
super.removeListener(listener);
}
}
@override
double get value => _indexChangeProgress(controller);
}
class _DragAnimation extends Animation<double> with AnimationWithParentMixin<double> {
_DragAnimation(this.controller, this.index);
final TabController controller;
final int index;
@override
Animation<double> get parent => controller.animation!;
@override
void removeStatusListener(AnimationStatusListener listener) {
if (controller.animation != null) {
super.removeStatusListener(listener);
}
}
@override
void removeListener(VoidCallback listener) {
if (controller.animation != null) {
super.removeListener(listener);
}
}
@override
double get value {
assert(!controller.indexIsChanging);
final double controllerMaxValue = (controller.length - 1).toDouble();
final double controllerValue = clampDouble(controller.animation!.value, 0.0, controllerMaxValue);
return clampDouble((controllerValue - index.toDouble()).abs(), 0.0, 1.0);
}
}
// This class, and TabBarScrollController, only exist to handle the case
// where a scrollable TabBar has a non-zero initialIndex. In that case we can
// only compute the scroll position's initial scroll offset (the "correct"
// pixels value) after the TabBar viewport width and scroll limits are known.
class _TabBarScrollPosition extends ScrollPositionWithSingleContext {
_TabBarScrollPosition({
required super.physics,
required super.context,
required super.oldPosition,
required this.tabBar,
}) : super(
initialPixels: null,
);
final _TabBarState tabBar;
bool _viewportDimensionWasNonZero = false;
// The scroll position should be adjusted at least once.
bool _needsPixelsCorrection = true;
@override
bool applyContentDimensions(double minScrollExtent, double maxScrollExtent) {
bool result = true;
if (!_viewportDimensionWasNonZero) {
_viewportDimensionWasNonZero = viewportDimension != 0.0;
}
// If the viewport never had a non-zero dimension, we just want to jump
// to the initial scroll position to avoid strange scrolling effects in
// release mode: the viewport temporarily may have a dimension of zero
// before the actual dimension is calculated. In that scenario, setting
// the actual dimension would cause a strange scroll effect without this
// guard because the super call below would start a ballistic scroll activity.
if (!_viewportDimensionWasNonZero || _needsPixelsCorrection) {
_needsPixelsCorrection = false;
correctPixels(tabBar._initialScrollOffset(viewportDimension, minScrollExtent, maxScrollExtent));
result = false;
}
return super.applyContentDimensions(minScrollExtent, maxScrollExtent) && result;
}
void markNeedsPixelsCorrection() {
_needsPixelsCorrection = true;
}
}
// This class, and TabBarScrollPosition, only exist to handle the case
// where a scrollable TabBar has a non-zero initialIndex.
class _TabBarScrollController extends ScrollController {
_TabBarScrollController(this.tabBar);
final _TabBarState tabBar;
@override
ScrollPosition createScrollPosition(ScrollPhysics physics, ScrollContext context, ScrollPosition? oldPosition) {
return _TabBarScrollPosition(
physics: physics,
context: context,
oldPosition: oldPosition,
tabBar: tabBar,
);
}
}
/// A Material Design primary tab bar.
///
/// Primary tabs are placed at the top of the content pane under a top app bar.
/// They display the main content destinations.
///
/// Typically created as the [AppBar.bottom] part of an [AppBar] and in
/// conjunction with a [TabBarView].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=POtoEH-5l40}
///
/// If a [TabController] is not provided, then a [DefaultTabController] ancestor
/// must be provided instead. The tab controller's [TabController.length] must
/// equal the length of the [tabs] list and the length of the
/// [TabBarView.children] list.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// Uses values from [TabBarTheme] if it is set in the current context.
///
/// {@tool dartpad}
/// This sample shows the implementation of [TabBar] and [TabBarView] using a [DefaultTabController].
/// Each [Tab] corresponds to a child of the [TabBarView] in the order they are written.
///
/// ** See code in examples/api/lib/material/tabs/tab_bar.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// [TabBar] can also be implemented by using a [TabController] which provides more options
/// to control the behavior of the [TabBar] and [TabBarView]. This can be used instead of
/// a [DefaultTabController], demonstrated below.
///
/// ** See code in examples/api/lib/material/tabs/tab_bar.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample showcases nested Material 3 [TabBar]s. It consists of a primary
/// [TabBar] with nested a secondary [TabBar]. The primary [TabBar] uses a
/// [DefaultTabController] while the secondary [TabBar] uses a [TabController].
///
/// ** See code in examples/api/lib/material/tabs/tab_bar.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TabBar.secondary], for a secondary tab bar.
/// * [TabBarView], which displays page views that correspond to each tab.
/// * [TabController], which coordinates tab selection between a [TabBar] and a [TabBarView].
/// * https://m3.material.io/components/tabs/overview, the Material 3
/// tab bar specification.
class TabBar extends StatefulWidget implements PreferredSizeWidget {
/// Creates a Material Design primary tab bar.
///
/// The length of the [tabs] argument must match the [controller]'s
/// [TabController.length].
///
/// If a [TabController] is not provided, then there must be a
/// [DefaultTabController] ancestor.
///
/// The [indicatorWeight] parameter defaults to 2.
///
/// The [indicatorPadding] parameter defaults to [EdgeInsets.zero].
///
/// If [indicator] is not null or provided from [TabBarTheme],
/// then [indicatorWeight] and [indicatorColor] are ignored.
const TabBar({
super.key,
required this.tabs,
this.controller,
this.isScrollable = false,
this.padding,
this.indicatorColor,
this.automaticIndicatorColorAdjustment = true,
this.indicatorWeight = 2.0,
this.indicatorPadding = EdgeInsets.zero,
this.indicator,
this.indicatorSize,
this.dividerColor,
this.dividerHeight,
this.labelColor,
this.labelStyle,
this.labelPadding,
this.unselectedLabelColor,
this.unselectedLabelStyle,
this.dragStartBehavior = DragStartBehavior.start,
this.overlayColor,
this.mouseCursor,
this.enableFeedback,
this.onTap,
this.physics,
this.splashFactory,
this.splashBorderRadius,
this.tabAlignment,
}) : _isPrimary = true,
assert(indicator != null || (indicatorWeight > 0.0));
/// Creates a Material Design secondary tab bar.
///
/// Secondary tabs are used within a content area to further separate related
/// content and establish hierarchy.
///
/// {@tool dartpad}
/// This sample showcases nested Material 3 [TabBar]s. It consists of a primary
/// [TabBar] with nested a secondary [TabBar]. The primary [TabBar] uses a
/// [DefaultTabController] while the secondary [TabBar] uses a [TabController].
///
/// ** See code in examples/api/lib/material/tabs/tab_bar.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TabBar], for a primary tab bar.
/// * [TabBarView], which displays page views that correspond to each tab.
/// * [TabController], which coordinates tab selection between a [TabBar] and a [TabBarView].
/// * https://m3.material.io/components/tabs/overview, the Material 3
/// tab bar specification.
const TabBar.secondary({
super.key,
required this.tabs,
this.controller,
this.isScrollable = false,
this.padding,
this.indicatorColor,
this.automaticIndicatorColorAdjustment = true,
this.indicatorWeight = 2.0,
this.indicatorPadding = EdgeInsets.zero,
this.indicator,
this.indicatorSize,
this.dividerColor,
this.dividerHeight,
this.labelColor,
this.labelStyle,
this.labelPadding,
this.unselectedLabelColor,
this.unselectedLabelStyle,
this.dragStartBehavior = DragStartBehavior.start,
this.overlayColor,
this.mouseCursor,
this.enableFeedback,
this.onTap,
this.physics,
this.splashFactory,
this.splashBorderRadius,
this.tabAlignment,
}) : _isPrimary = false,
assert(indicator != null || (indicatorWeight > 0.0));
/// Typically a list of two or more [Tab] widgets.
///
/// The length of this list must match the [controller]'s [TabController.length]
/// and the length of the [TabBarView.children] list.
final List<Widget> tabs;
/// This widget's selection and animation state.
///
/// If [TabController] is not provided, then the value of [DefaultTabController.of]
/// will be used.
final TabController? controller;
/// Whether this tab bar can be scrolled horizontally.
///
/// If [isScrollable] is true, then each tab is as wide as needed for its label
/// and the entire [TabBar] is scrollable. Otherwise each tab gets an equal
/// share of the available space.
final bool isScrollable;
/// The amount of space by which to inset the tab bar.
///
/// When [isScrollable] is false, this will yield the same result as if [TabBar] was wrapped
/// in a [Padding] widget. When [isScrollable] is true, the scrollable itself is inset,
/// allowing the padding to scroll with the tab bar, rather than enclosing it.
final EdgeInsetsGeometry? padding;
/// The color of the line that appears below the selected tab.
///
/// If this parameter is null, then the value of the Theme's indicatorColor
/// property is used.
///
/// If [indicator] is specified or provided from [TabBarTheme],
/// this property is ignored.
final Color? indicatorColor;
/// The thickness of the line that appears below the selected tab.
///
/// The value of this parameter must be greater than zero.
///
/// If [ThemeData.useMaterial3] is true and [TabBar] is used to create a
/// primary tab bar, the default value is 3.0. If the provided value is less
/// than 3.0, the default value is used.
///
/// If [ThemeData.useMaterial3] is true and [TabBar.secondary] is used to
/// create a secondary tab bar, the default value is 2.0.
///
/// If [ThemeData.useMaterial3] is false, the default value is 2.0.
///
/// If [indicator] is specified or provided from [TabBarTheme],
/// this property is ignored.
final double indicatorWeight;
/// The padding for the indicator.
///
/// The default value of this property is [EdgeInsets.zero].
///
/// For [isScrollable] tab bars, specifying [kTabLabelPadding] will align
/// the indicator with the tab's text for [Tab] widgets and all but the
/// shortest [Tab.text] values.
final EdgeInsetsGeometry indicatorPadding;
/// Defines the appearance of the selected tab indicator.
///
/// If [indicator] is specified or provided from [TabBarTheme],
/// the [indicatorColor] and [indicatorWeight] properties are ignored.
///
/// The default, underline-style, selected tab indicator can be defined with
/// [UnderlineTabIndicator].
///
/// The indicator's size is based on the tab's bounds. If [indicatorSize]
/// is [TabBarIndicatorSize.tab] the tab's bounds are as wide as the space
/// occupied by the tab in the tab bar. If [indicatorSize] is
/// [TabBarIndicatorSize.label], then the tab's bounds are only as wide as
/// the tab widget itself.
///
/// See also:
///
/// * [splashBorderRadius], which defines the clipping radius of the splash
/// and is generally used with [BoxDecoration.borderRadius].
final Decoration? indicator;
/// Whether this tab bar should automatically adjust the [indicatorColor].
///
/// The default value of this property is true.
///
/// If [automaticIndicatorColorAdjustment] is true,
/// then the [indicatorColor] will be automatically adjusted to [Colors.white]
/// when the [indicatorColor] is same as [Material.color] of the [Material]
/// parent widget.
final bool automaticIndicatorColorAdjustment;
/// Defines how the selected tab indicator's size is computed.
///
/// The size of the selected tab indicator is defined relative to the
/// tab's overall bounds if [indicatorSize] is [TabBarIndicatorSize.tab]
/// (the default) or relative to the bounds of the tab's widget if
/// [indicatorSize] is [TabBarIndicatorSize.label].
///
/// The selected tab's location appearance can be refined further with
/// the [indicatorColor], [indicatorWeight], [indicatorPadding], and
/// [indicator] properties.
final TabBarIndicatorSize? indicatorSize;
/// The color of the divider.
///
/// If null and [ThemeData.useMaterial3] is false, [TabBarTheme.dividerColor]
/// color is used. If that is null and [ThemeData.useMaterial3] is true,
/// [ColorScheme.outlineVariant] will be used, otherwise divider will not be drawn.
final Color? dividerColor;
/// The height of the divider.
///
/// If null and [ThemeData.useMaterial3] is true, [TabBarTheme.dividerHeight] is used.
/// If that is also null and [ThemeData.useMaterial3] is true, 1.0 will be used.
/// Otherwise divider will not be drawn.
final double? dividerHeight;
/// The color of selected tab labels.
///
/// If null, then [TabBarTheme.labelColor] is used. If that is also null and
/// [ThemeData.useMaterial3] is true, [ColorScheme.primary] will be used,
/// otherwise the color of the [ThemeData.primaryTextTheme]'s
/// [TextTheme.bodyLarge] text color is used.
///
/// If [labelColor] (or, if null, [TabBarTheme.labelColor]) is a
/// [MaterialStateColor], then the effective tab color will depend on the
/// [MaterialState.selected] state, i.e. if the [Tab] is selected or not,
/// ignoring [unselectedLabelColor] even if it's non-null.
///
/// When this color or the [TabBarTheme.labelColor] is specified, it overrides
/// the [TextStyle.color] specified for the [labelStyle] or the
/// [TabBarTheme.labelStyle].
///
/// See also:
///
/// * [unselectedLabelColor], for color of unselected tab labels.
final Color? labelColor;
/// The color of unselected tab labels.
///
/// If [labelColor] (or, if null, [TabBarTheme.labelColor]) is a
/// [MaterialStateColor], then the unselected tabs are rendered with
/// that [MaterialStateColor]'s resolved color for unselected state, even if
/// [unselectedLabelColor] is non-null.
///
/// If null, then [TabBarTheme.unselectedLabelColor] is used. If that is also
/// null and [ThemeData.useMaterial3] is true, [ColorScheme.onSurfaceVariant]
/// will be used, otherwise unselected tab labels are rendered with
/// [labelColor] at 70% opacity.
///
/// When this color or the [TabBarTheme.unselectedLabelColor] is specified, it
/// overrides the [TextStyle.color] specified for the [unselectedLabelStyle]
/// or the [TabBarTheme.unselectedLabelStyle].
///
/// See also:
///
/// * [labelColor], for color of selected tab labels.
final Color? unselectedLabelColor;
/// The text style of the selected tab labels.
///
/// The color specified in [labelStyle] and [TabBarTheme.labelStyle] is used
/// to style the label when [labelColor] or [TabBarTheme.labelColor] are not
/// specified.
///
/// If [unselectedLabelStyle] is null, then this text style will be used for
/// both selected and unselected label styles.
///
/// If this property is null, then [TabBarTheme.labelStyle] will be used.
///
/// If that is also null and [ThemeData.useMaterial3] is true, [TextTheme.titleSmall]
/// will be used, otherwise the text style of the [ThemeData.primaryTextTheme]'s
/// [TextTheme.bodyLarge] definition is used.
final TextStyle? labelStyle;
/// The text style of the unselected tab labels.
///
/// The color specified in [unselectedLabelStyle] and [TabBarTheme.unselectedLabelStyle]
/// is used to style the label when [unselectedLabelColor] or [TabBarTheme.unselectedLabelColor]
/// are not specified.
///
/// If this property is null, then [TabBarTheme.unselectedLabelStyle] will be used.
///
/// If that is also null and [ThemeData.useMaterial3] is true, [TextTheme.titleSmall]
/// will be used, otherwise then the [labelStyle] value is used. If [labelStyle] is null,
/// the text style of the [ThemeData.primaryTextTheme]'s [TextTheme.bodyLarge]
/// definition is used.
final TextStyle? unselectedLabelStyle;
/// The padding added to each of the tab labels.
///
/// If there are few tabs with both icon and text and few
/// tabs with only icon or text, this padding is vertically
/// adjusted to provide uniform padding to all tabs.
///
/// If this property is null, then [kTabLabelPadding] is used.
final EdgeInsetsGeometry? labelPadding;
/// Defines the ink response focus, hover, and splash colors.
///
/// If non-null, it is resolved against one of [MaterialState.focused],
/// [MaterialState.hovered], and [MaterialState.pressed].
///
/// [MaterialState.pressed] triggers a ripple (an ink splash), per
/// the current Material Design spec.
///
/// If the overlay color is null or resolves to null, then if [ThemeData.useMaterial3] is
/// false, the default values for [InkResponse.focusColor], [InkResponse.hoverColor], [InkResponse.splashColor],
/// and [InkResponse.highlightColor] will be used instead. If [ThemeData.useMaterial3]
/// if true, the default values are:
/// * selected:
/// * pressed - ThemeData.colorScheme.primary(0.1)
/// * hovered - ThemeData.colorScheme.primary(0.08)
/// * focused - ThemeData.colorScheme.primary(0.1)
/// * pressed - ThemeData.colorScheme.primary(0.1)
/// * hovered - ThemeData.colorScheme.onSurface(0.08)
/// * focused - ThemeData.colorScheme.onSurface(0.1)
final MaterialStateProperty<Color?>? overlayColor;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@template flutter.material.tabs.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// individual tab widgets.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// {@endtemplate}
///
/// If null, then the value of [TabBarTheme.mouseCursor] is used. If
/// that is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], which can be used to create a [MouseCursor]
/// that is also a [MaterialStateProperty<MouseCursor>].
final MouseCursor? mouseCursor;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a long-press
/// will produce a short vibration, when feedback is enabled.
///
/// Defaults to true.
final bool? enableFeedback;
/// An optional callback that's called when the [TabBar] is tapped.
///
/// The callback is applied to the index of the tab where the tap occurred.
///
/// This callback has no effect on the default handling of taps. It's for
/// applications that want to do a little extra work when a tab is tapped,
/// even if the tap doesn't change the TabController's index. TabBar [onTap]
/// callbacks should not make changes to the TabController since that would
/// interfere with the default tap handler.
final ValueChanged<int>? onTap;
/// How the [TabBar]'s scroll view should respond to user input.
///
/// For example, determines how the scroll view continues to animate after the
/// user stops dragging the scroll view.
///
/// Defaults to matching platform conventions.
final ScrollPhysics? physics;
/// Creates the tab bar's [InkWell] splash factory, which defines
/// the appearance of "ink" splashes that occur in response to taps.
///
/// Use [NoSplash.splashFactory] to defeat ink splash rendering. For example
/// to defeat both the splash and the hover/pressed overlay, but not the
/// keyboard focused overlay:
///
/// ```dart
/// TabBar(
/// splashFactory: NoSplash.splashFactory,
/// overlayColor: MaterialStateProperty.resolveWith<Color?>(
/// (Set<MaterialState> states) {
/// return states.contains(MaterialState.focused) ? null : Colors.transparent;
/// },
/// ),
/// tabs: const <Widget>[
/// // ...
/// ],
/// )
/// ```
final InteractiveInkFeatureFactory? splashFactory;
/// Defines the clipping radius of splashes that extend outside the bounds of the tab.
///
/// This can be useful to match the [BoxDecoration.borderRadius] provided as [indicator].
///
/// ```dart
/// TabBar(
/// indicator: BoxDecoration(
/// borderRadius: BorderRadius.circular(40),
/// ),
/// splashBorderRadius: BorderRadius.circular(40),
/// tabs: const <Widget>[
/// // ...
/// ],
/// )
/// ```
///
/// If this property is null, it is interpreted as [BorderRadius.zero].
final BorderRadius? splashBorderRadius;
/// Specifies the horizontal alignment of the tabs within a [TabBar].
///
/// If [TabBar.isScrollable] is false, only [TabAlignment.fill] and
/// [TabAlignment.center] are supported. Otherwise an exception is thrown.
///
/// If [TabBar.isScrollable] is true, only [TabAlignment.start], [TabAlignment.startOffset],
/// and [TabAlignment.center] are supported. Otherwise an exception is thrown.
///
/// If this is null, then the value of [TabBarTheme.tabAlignment] is used.
///
/// If [TabBarTheme.tabAlignment] is null and [ThemeData.useMaterial3] is true,
/// then [TabAlignment.startOffset] is used if [isScrollable] is true,
/// otherwise [TabAlignment.fill] is used.
///
/// If [TabBarTheme.tabAlignment] is null and [ThemeData.useMaterial3] is false,
/// then [TabAlignment.center] is used if [isScrollable] is true,
/// otherwise [TabAlignment.fill] is used.
final TabAlignment? tabAlignment;
/// A size whose height depends on if the tabs have both icons and text.
///
/// [AppBar] uses this size to compute its own preferred size.
@override
Size get preferredSize {
double maxHeight = _kTabHeight;
for (final Widget item in tabs) {
if (item is PreferredSizeWidget) {
final double itemHeight = item.preferredSize.height;
maxHeight = math.max(itemHeight, maxHeight);
}
}
return Size.fromHeight(maxHeight + indicatorWeight);
}
/// Returns whether the [TabBar] contains a tab with both text and icon.
///
/// [TabBar] uses this to give uniform padding to all tabs in cases where
/// there are some tabs with both text and icon and some which contain only
/// text or icon.
bool get tabHasTextAndIcon {
for (final Widget item in tabs) {
if (item is PreferredSizeWidget) {
if (item.preferredSize.height == _kTextAndIconTabHeight) {
return true;
}
}
}
return false;
}
/// Whether this tab bar is a primary tab bar.
///
/// Otherwise, it is a secondary tab bar.
final bool _isPrimary;
@override
State<TabBar> createState() => _TabBarState();
}
class _TabBarState extends State<TabBar> {
ScrollController? _scrollController;
TabController? _controller;
_IndicatorPainter? _indicatorPainter;
int? _currentIndex;
late double _tabStripWidth;
late List<GlobalKey> _tabKeys;
late List<EdgeInsetsGeometry> _labelPaddings;
bool _debugHasScheduledValidTabsCountCheck = false;
@override
void initState() {
super.initState();
// If indicatorSize is TabIndicatorSize.label, _tabKeys[i] is used to find
// the width of tab widget i. See _IndicatorPainter.indicatorRect().
_tabKeys = widget.tabs.map((Widget tab) => GlobalKey()).toList();
_labelPaddings = List<EdgeInsetsGeometry>.filled(widget.tabs.length, EdgeInsets.zero, growable: true);
}
TabBarTheme get _defaults {
if (Theme.of(context).useMaterial3) {
return widget._isPrimary
? _TabsPrimaryDefaultsM3(context, widget.isScrollable)
: _TabsSecondaryDefaultsM3(context, widget.isScrollable);
} else {
return _TabsDefaultsM2(context, widget.isScrollable);
}
}
Decoration _getIndicator(TabBarIndicatorSize indicatorSize) {
final ThemeData theme = Theme.of(context);
final TabBarTheme tabBarTheme = TabBarTheme.of(context);
if (widget.indicator != null) {
return widget.indicator!;
}
if (tabBarTheme.indicator != null) {
return tabBarTheme.indicator!;
}
Color color = widget.indicatorColor ?? tabBarTheme.indicatorColor ?? _defaults.indicatorColor!;
// ThemeData tries to avoid this by having indicatorColor avoid being the
// primaryColor. However, it's possible that the tab bar is on a
// Material that isn't the primaryColor. In that case, if the indicator
// color ends up matching the material's color, then this overrides it.
// When that happens, automatic transitions of the theme will likely look
// ugly as the indicator color suddenly snaps to white at one end, but it's
// not clear how to avoid that any further.
//
// The material's color might be null (if it's a transparency). In that case
// there's no good way for us to find out what the color is so we don't.
//
// TODO(xu-baolin): Remove automatic adjustment to white color indicator
// with a better long-term solution.
// https://github.com/flutter/flutter/pull/68171#pullrequestreview-517753917
if (widget.automaticIndicatorColorAdjustment &&
color.value == Material.maybeOf(context)?.color?.value) {
color = Colors.white;
}
final bool primaryWithLabelIndicator = widget._isPrimary && indicatorSize == TabBarIndicatorSize.label;
final double effectiveIndicatorWeight = theme.useMaterial3 && primaryWithLabelIndicator
? math.max(widget.indicatorWeight, _TabsPrimaryDefaultsM3.indicatorWeight)
: widget.indicatorWeight;
// Only Material 3 primary TabBar with label indicatorSize should be rounded.
final BorderRadius? effectiveBorderRadius = theme.useMaterial3 && primaryWithLabelIndicator
? BorderRadius.only(
topLeft: Radius.circular(effectiveIndicatorWeight),
topRight: Radius.circular(effectiveIndicatorWeight),
)
: null;
return UnderlineTabIndicator(
borderRadius: effectiveBorderRadius,
borderSide: BorderSide(
// TODO(tahatesser): Make sure this value matches Material 3 Tabs spec
// when `preferredSize`and `indicatorWeight` are updated to support Material 3
// https://m3.material.io/components/tabs/specs#149a189f-9039-4195-99da-15c205d20e30,
// https://github.com/flutter/flutter/issues/116136
width: effectiveIndicatorWeight,
color: color,
),
);
}
// If the TabBar is rebuilt with a new tab controller, the caller should
// dispose the old one. In that case the old controller's animation will be
// null and should not be accessed.
bool get _controllerIsValid => _controller?.animation != null;
void _updateTabController() {
final TabController? newController = widget.controller ?? DefaultTabController.maybeOf(context);
assert(() {
if (newController == null) {
throw FlutterError(
'No TabController for ${widget.runtimeType}.\n'
'When creating a ${widget.runtimeType}, you must either provide an explicit '
'TabController using the "controller" property, or you must ensure that there '
'is a DefaultTabController above the ${widget.runtimeType}.\n'
'In this case, there was neither an explicit controller nor a default controller.',
);
}
return true;
}());
if (newController == _controller) {
return;
}
if (_controllerIsValid) {
_controller!.animation!.removeListener(_handleTabControllerAnimationTick);
_controller!.removeListener(_handleTabControllerTick);
}
_controller = newController;
if (_controller != null) {
_controller!.animation!.addListener(_handleTabControllerAnimationTick);
_controller!.addListener(_handleTabControllerTick);
_currentIndex = _controller!.index;
}
}
void _initIndicatorPainter() {
final ThemeData theme = Theme.of(context);
final TabBarTheme tabBarTheme = TabBarTheme.of(context);
final TabBarIndicatorSize indicatorSize = widget.indicatorSize
?? tabBarTheme.indicatorSize
?? _defaults.indicatorSize!;
_indicatorPainter = !_controllerIsValid ? null : _IndicatorPainter(
controller: _controller!,
indicator: _getIndicator(indicatorSize),
indicatorSize: indicatorSize,
indicatorPadding: widget.indicatorPadding,
tabKeys: _tabKeys,
old: _indicatorPainter,
labelPaddings: _labelPaddings,
dividerColor: widget.dividerColor ?? tabBarTheme.dividerColor ?? _defaults.dividerColor,
dividerHeight: widget.dividerHeight ?? tabBarTheme.dividerHeight ?? _defaults.dividerHeight,
showDivider: theme.useMaterial3 && !widget.isScrollable,
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
assert(debugCheckHasMaterial(context));
_updateTabController();
_initIndicatorPainter();
}
@override
void didUpdateWidget(TabBar oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.controller != oldWidget.controller) {
_updateTabController();
_initIndicatorPainter();
// Adjust scroll position.
if (_scrollController != null && _scrollController!.hasClients) {
final ScrollPosition position = _scrollController!.position;
if (position is _TabBarScrollPosition) {
position.markNeedsPixelsCorrection();
}
}
} else if (widget.indicatorColor != oldWidget.indicatorColor ||
widget.indicatorWeight != oldWidget.indicatorWeight ||
widget.indicatorSize != oldWidget.indicatorSize ||
widget.indicatorPadding != oldWidget.indicatorPadding ||
widget.indicator != oldWidget.indicator ||
widget.dividerColor != oldWidget.dividerColor ||
widget.dividerHeight != oldWidget.dividerHeight) {
_initIndicatorPainter();
}
if (widget.tabs.length > _tabKeys.length) {
final int delta = widget.tabs.length - _tabKeys.length;
_tabKeys.addAll(List<GlobalKey>.generate(delta, (int n) => GlobalKey()));
_labelPaddings.addAll(List<EdgeInsetsGeometry>.filled(delta, EdgeInsets.zero));
} else if (widget.tabs.length < _tabKeys.length) {
_tabKeys.removeRange(widget.tabs.length, _tabKeys.length);
_labelPaddings.removeRange(widget.tabs.length, _tabKeys.length);
}
}
@override
void dispose() {
_indicatorPainter!.dispose();
if (_controllerIsValid) {
_controller!.animation!.removeListener(_handleTabControllerAnimationTick);
_controller!.removeListener(_handleTabControllerTick);
}
_controller = null;
_scrollController?.dispose();
// We don't own the _controller Animation, so it's not disposed here.
super.dispose();
}
int get maxTabIndex => _indicatorPainter!.maxTabIndex;
double _tabScrollOffset(int index, double viewportWidth, double minExtent, double maxExtent) {
if (!widget.isScrollable) {
return 0.0;
}
double tabCenter = _indicatorPainter!.centerOf(index);
double paddingStart;
switch (Directionality.of(context)) {
case TextDirection.rtl:
paddingStart = widget.padding?.resolve(TextDirection.rtl).right ?? 0;
tabCenter = _tabStripWidth - tabCenter;
case TextDirection.ltr:
paddingStart = widget.padding?.resolve(TextDirection.ltr).left ?? 0;
}
return clampDouble(tabCenter + paddingStart - viewportWidth / 2.0, minExtent, maxExtent);
}
double _tabCenteredScrollOffset(int index) {
final ScrollPosition position = _scrollController!.position;
return _tabScrollOffset(index, position.viewportDimension, position.minScrollExtent, position.maxScrollExtent);
}
double _initialScrollOffset(double viewportWidth, double minExtent, double maxExtent) {
return _tabScrollOffset(_currentIndex!, viewportWidth, minExtent, maxExtent);
}
void _scrollToCurrentIndex() {
final double offset = _tabCenteredScrollOffset(_currentIndex!);
_scrollController!.animateTo(offset, duration: kTabScrollDuration, curve: Curves.ease);
}
void _scrollToControllerValue() {
final double? leadingPosition = _currentIndex! > 0 ? _tabCenteredScrollOffset(_currentIndex! - 1) : null;
final double middlePosition = _tabCenteredScrollOffset(_currentIndex!);
final double? trailingPosition = _currentIndex! < maxTabIndex ? _tabCenteredScrollOffset(_currentIndex! + 1) : null;
final double index = _controller!.index.toDouble();
final double value = _controller!.animation!.value;
final double offset;
if (value == index - 1.0) {
offset = leadingPosition ?? middlePosition;
} else if (value == index + 1.0) {
offset = trailingPosition ?? middlePosition;
} else if (value == index) {
offset = middlePosition;
} else if (value < index) {
offset = leadingPosition == null ? middlePosition : lerpDouble(middlePosition, leadingPosition, index - value)!;
} else {
offset = trailingPosition == null ? middlePosition : lerpDouble(middlePosition, trailingPosition, value - index)!;
}
_scrollController!.jumpTo(offset);
}
void _handleTabControllerAnimationTick() {
assert(mounted);
if (!_controller!.indexIsChanging && widget.isScrollable) {
// Sync the TabBar's scroll position with the TabBarView's PageView.
_currentIndex = _controller!.index;
_scrollToControllerValue();
}
}
void _handleTabControllerTick() {
if (_controller!.index != _currentIndex) {
_currentIndex = _controller!.index;
if (widget.isScrollable) {
_scrollToCurrentIndex();
}
}
setState(() {
// Rebuild the tabs after a (potentially animated) index change
// has completed.
});
}
// Called each time layout completes.
void _saveTabOffsets(List<double> tabOffsets, TextDirection textDirection, double width) {
_tabStripWidth = width;
_indicatorPainter?.saveTabOffsets(tabOffsets, textDirection);
}
void _handleTap(int index) {
assert(index >= 0 && index < widget.tabs.length);
_controller!.animateTo(index);
widget.onTap?.call(index);
}
Widget _buildStyledTab(Widget child, bool isSelected, Animation<double> animation, TabBarTheme defaults) {
return _TabStyle(
animation: animation,
isSelected: isSelected,
isPrimary: widget._isPrimary,
labelColor: widget.labelColor,
unselectedLabelColor: widget.unselectedLabelColor,
labelStyle: widget.labelStyle,
unselectedLabelStyle: widget.unselectedLabelStyle,
defaults: defaults,
child: child,
);
}
bool _debugScheduleCheckHasValidTabsCount() {
if (_debugHasScheduledValidTabsCountCheck) {
return true;
}
WidgetsBinding.instance.addPostFrameCallback((Duration duration) {
_debugHasScheduledValidTabsCountCheck = false;
if (!mounted) {
return;
}
assert(() {
if (_controller!.length != widget.tabs.length) {
throw FlutterError(
"Controller's length property (${_controller!.length}) does not match the "
"number of tabs (${widget.tabs.length}) present in TabBar's tabs property.",
);
}
return true;
}());
}, debugLabel: 'TabBar.tabsCountCheck');
_debugHasScheduledValidTabsCountCheck = true;
return true;
}
bool _debugTabAlignmentIsValid(TabAlignment tabAlignment) {
assert(() {
if (widget.isScrollable && tabAlignment == TabAlignment.fill) {
throw FlutterError(
'$tabAlignment is only valid for non-scrollable tab bars.',
);
}
if (!widget.isScrollable
&& (tabAlignment == TabAlignment.start
|| tabAlignment == TabAlignment.startOffset)) {
throw FlutterError(
'$tabAlignment is only valid for scrollable tab bars.',
);
}
return true;
}());
return true;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
assert(_debugScheduleCheckHasValidTabsCount());
final ThemeData theme = Theme.of(context);
final TabBarTheme tabBarTheme = TabBarTheme.of(context);
final TabAlignment effectiveTabAlignment = widget.tabAlignment ?? tabBarTheme.tabAlignment ?? _defaults.tabAlignment!;
assert(_debugTabAlignmentIsValid(effectiveTabAlignment));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
if (_controller!.length == 0) {
return Container(
height: _kTabHeight + widget.indicatorWeight,
);
}
final List<Widget> wrappedTabs = List<Widget>.generate(widget.tabs.length, (int index) {
const double verticalAdjustment = (_kTextAndIconTabHeight - _kTabHeight)/2.0;
EdgeInsetsGeometry? adjustedPadding;
if (widget.tabs[index] is PreferredSizeWidget) {
final PreferredSizeWidget tab = widget.tabs[index] as PreferredSizeWidget;
if (widget.tabHasTextAndIcon && tab.preferredSize.height == _kTabHeight) {
if (widget.labelPadding != null || tabBarTheme.labelPadding != null) {
adjustedPadding = (widget.labelPadding ?? tabBarTheme.labelPadding!).add(const EdgeInsets.symmetric(vertical: verticalAdjustment));
}
else {
adjustedPadding = const EdgeInsets.symmetric(vertical: verticalAdjustment, horizontal: 16.0);
}
}
}
_labelPaddings[index] = adjustedPadding ?? widget.labelPadding ?? tabBarTheme.labelPadding ?? kTabLabelPadding;
return Center(
heightFactor: 1.0,
child: Padding(
padding: _labelPaddings[index],
child: KeyedSubtree(
key: _tabKeys[index],
child: widget.tabs[index],
),
),
);
});
// If the controller was provided by DefaultTabController and we're part
// of a Hero (typically the AppBar), then we will not be able to find the
// controller during a Hero transition. See https://github.com/flutter/flutter/issues/213.
if (_controller != null) {
final int previousIndex = _controller!.previousIndex;
if (_controller!.indexIsChanging) {
// The user tapped on a tab, the tab controller's animation is running.
assert(_currentIndex != previousIndex);
final Animation<double> animation = _ChangeAnimation(_controller!);
wrappedTabs[_currentIndex!] = _buildStyledTab(wrappedTabs[_currentIndex!], true, animation, _defaults);
wrappedTabs[previousIndex] = _buildStyledTab(wrappedTabs[previousIndex], false, animation, _defaults);
} else {
// The user is dragging the TabBarView's PageView left or right.
final int tabIndex = _currentIndex!;
final Animation<double> centerAnimation = _DragAnimation(_controller!, tabIndex);
wrappedTabs[tabIndex] = _buildStyledTab(wrappedTabs[tabIndex], true, centerAnimation, _defaults);
if (_currentIndex! > 0) {
final int tabIndex = _currentIndex! - 1;
final Animation<double> previousAnimation = ReverseAnimation(_DragAnimation(_controller!, tabIndex));
wrappedTabs[tabIndex] = _buildStyledTab(wrappedTabs[tabIndex], false, previousAnimation, _defaults);
}
if (_currentIndex! < widget.tabs.length - 1) {
final int tabIndex = _currentIndex! + 1;
final Animation<double> nextAnimation = ReverseAnimation(_DragAnimation(_controller!, tabIndex));
wrappedTabs[tabIndex] = _buildStyledTab(wrappedTabs[tabIndex], false, nextAnimation, _defaults);
}
}
}
// Add the tap handler to each tab. If the tab bar is not scrollable,
// then give all of the tabs equal flexibility so that they each occupy
// the same share of the tab bar's overall width.
final int tabCount = widget.tabs.length;
for (int index = 0; index < tabCount; index += 1) {
final Set<MaterialState> selectedState = <MaterialState>{
if (index == _currentIndex) MaterialState.selected,
};
final MouseCursor effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor?>(widget.mouseCursor, selectedState)
?? tabBarTheme.mouseCursor?.resolve(selectedState)
?? MaterialStateMouseCursor.clickable.resolve(selectedState);
final MaterialStateProperty<Color?> defaultOverlay = MaterialStateProperty.resolveWith<Color?>(
(Set<MaterialState> states) {
final Set<MaterialState> effectiveStates = selectedState..addAll(states);
return _defaults.overlayColor?.resolve(effectiveStates);
},
);
wrappedTabs[index] = InkWell(
mouseCursor: effectiveMouseCursor,
onTap: () { _handleTap(index); },
enableFeedback: widget.enableFeedback ?? true,
overlayColor: widget.overlayColor ?? tabBarTheme.overlayColor ?? defaultOverlay,
splashFactory: widget.splashFactory ?? tabBarTheme.splashFactory ?? _defaults.splashFactory,
borderRadius: widget.splashBorderRadius,
child: Padding(
padding: EdgeInsets.only(bottom: widget.indicatorWeight),
child: Stack(
children: <Widget>[
wrappedTabs[index],
Semantics(
selected: index == _currentIndex,
label: localizations.tabLabel(tabIndex: index + 1, tabCount: tabCount),
),
],
),
),
);
if (!widget.isScrollable && effectiveTabAlignment == TabAlignment.fill) {
wrappedTabs[index] = Expanded(child: wrappedTabs[index]);
}
}
Widget tabBar = CustomPaint(
painter: _indicatorPainter,
child: _TabStyle(
animation: kAlwaysDismissedAnimation,
isSelected: false,
isPrimary: widget._isPrimary,
labelColor: widget.labelColor,
unselectedLabelColor: widget.unselectedLabelColor,
labelStyle: widget.labelStyle,
unselectedLabelStyle: widget.unselectedLabelStyle,
defaults: _defaults,
child: _TabLabelBar(
onPerformLayout: _saveTabOffsets,
mainAxisSize: effectiveTabAlignment == TabAlignment.fill ? MainAxisSize.max : MainAxisSize.min,
children: wrappedTabs,
),
),
);
if (widget.isScrollable) {
final EdgeInsetsGeometry? effectivePadding = effectiveTabAlignment == TabAlignment.startOffset
? const EdgeInsetsDirectional.only(start: _kStartOffset).add(widget.padding ?? EdgeInsets.zero)
: widget.padding;
_scrollController ??= _TabBarScrollController(this);
tabBar = ScrollConfiguration(
// The scrolling tabs should not show an overscroll indicator.
behavior: ScrollConfiguration.of(context).copyWith(overscroll: false),
child: SingleChildScrollView(
dragStartBehavior: widget.dragStartBehavior,
scrollDirection: Axis.horizontal,
controller: _scrollController,
padding: effectivePadding,
physics: widget.physics,
child: tabBar,
),
);
if (theme.useMaterial3) {
final AlignmentGeometry effectiveAlignment = switch (effectiveTabAlignment) {
TabAlignment.center => Alignment.center,
TabAlignment.start || TabAlignment.startOffset || TabAlignment.fill => AlignmentDirectional.centerStart,
};
final Color dividerColor = widget.dividerColor ?? tabBarTheme.dividerColor ?? _defaults.dividerColor!;
final double dividerHeight = widget.dividerHeight ?? tabBarTheme.dividerHeight ?? _defaults.dividerHeight!;
final bool showDivider = dividerColor != Colors.transparent && dividerHeight > 0;
tabBar = Align(
heightFactor: 1.0,
widthFactor: showDivider ? null : 1.0,
alignment: effectiveAlignment,
child: tabBar,
);
if (showDivider) {
tabBar = CustomPaint(
painter: _DividerPainter(
dividerColor: widget.dividerColor ?? tabBarTheme.dividerColor ?? _defaults.dividerColor!,
dividerHeight: widget.dividerHeight ?? tabBarTheme.dividerHeight ?? _defaults.dividerHeight!,
),
child: tabBar,
);
}
}
} else if (widget.padding != null) {
tabBar = Padding(
padding: widget.padding!,
child: tabBar,
);
}
return tabBar;
}
}
/// A page view that displays the widget which corresponds to the currently
/// selected tab.
///
/// This widget is typically used in conjunction with a [TabBar].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=POtoEH-5l40}
///
/// If a [TabController] is not provided, then there must be a [DefaultTabController]
/// ancestor.
///
/// The tab controller's [TabController.length] must equal the length of the
/// [children] list and the length of the [TabBar.tabs] list.
///
/// To see a sample implementation, visit the [TabController] documentation.
class TabBarView extends StatefulWidget {
/// Creates a page view with one child per tab.
///
/// The length of [children] must be the same as the [controller]'s length.
const TabBarView({
super.key,
required this.children,
this.controller,
this.physics,
this.dragStartBehavior = DragStartBehavior.start,
this.viewportFraction = 1.0,
this.clipBehavior = Clip.hardEdge,
});
/// This widget's selection and animation state.
///
/// If [TabController] is not provided, then the value of [DefaultTabController.of]
/// will be used.
final TabController? controller;
/// One widget per tab.
///
/// Its length must match the length of the [TabBar.tabs]
/// list, as well as the [controller]'s [TabController.length].
final List<Widget> children;
/// How the page view should respond to user input.
///
/// For example, determines how the page view continues to animate after the
/// user stops dragging the page view.
///
/// The physics are modified to snap to page boundaries using
/// [PageScrollPhysics] prior to being used.
///
/// Defaults to matching platform conventions.
final ScrollPhysics? physics;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@macro flutter.widgets.pageview.viewportFraction}
final double viewportFraction;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
@override
State<TabBarView> createState() => _TabBarViewState();
}
class _TabBarViewState extends State<TabBarView> {
TabController? _controller;
PageController? _pageController;
late List<Widget> _childrenWithKey;
int? _currentIndex;
int _warpUnderwayCount = 0;
int _scrollUnderwayCount = 0;
bool _debugHasScheduledValidChildrenCountCheck = false;
// If the TabBarView is rebuilt with a new tab controller, the caller should
// dispose the old one. In that case the old controller's animation will be
// null and should not be accessed.
bool get _controllerIsValid => _controller?.animation != null;
void _updateTabController() {
final TabController? newController = widget.controller ?? DefaultTabController.maybeOf(context);
assert(() {
if (newController == null) {
throw FlutterError(
'No TabController for ${widget.runtimeType}.\n'
'When creating a ${widget.runtimeType}, you must either provide an explicit '
'TabController using the "controller" property, or you must ensure that there '
'is a DefaultTabController above the ${widget.runtimeType}.\n'
'In this case, there was neither an explicit controller nor a default controller.',
);
}
return true;
}());
if (newController == _controller) {
return;
}
if (_controllerIsValid) {
_controller!.animation!.removeListener(_handleTabControllerAnimationTick);
}
_controller = newController;
if (_controller != null) {
_controller!.animation!.addListener(_handleTabControllerAnimationTick);
}
}
void _jumpToPage(int page) {
_warpUnderwayCount += 1;
_pageController!.jumpToPage(page);
_warpUnderwayCount -= 1;
}
Future<void> _animateToPage(
int page, {
required Duration duration,
required Curve curve,
}) async {
_warpUnderwayCount += 1;
await _pageController!.animateToPage(page, duration: duration, curve: curve);
_warpUnderwayCount -= 1;
}
@override
void initState() {
super.initState();
_updateChildren();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_updateTabController();
_currentIndex = _controller!.index;
if (_pageController == null) {
_pageController = PageController(
initialPage: _currentIndex!,
viewportFraction: widget.viewportFraction,
);
} else {
_pageController!.jumpToPage(_currentIndex!);
}
}
@override
void didUpdateWidget(TabBarView oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.controller != oldWidget.controller) {
_updateTabController();
_currentIndex = _controller!.index;
_jumpToPage(_currentIndex!);
}
if (widget.viewportFraction != oldWidget.viewportFraction) {
_pageController?.dispose();
_pageController = PageController(
initialPage: _currentIndex!,
viewportFraction: widget.viewportFraction,
);
}
// While a warp is under way, we stop updating the tab page contents.
// This is tracked in https://github.com/flutter/flutter/issues/31269.
if (widget.children != oldWidget.children && _warpUnderwayCount == 0) {
_updateChildren();
}
}
@override
void dispose() {
if (_controllerIsValid) {
_controller!.animation!.removeListener(_handleTabControllerAnimationTick);
}
_controller = null;
_pageController?.dispose();
// We don't own the _controller Animation, so it's not disposed here.
super.dispose();
}
void _updateChildren() {
_childrenWithKey = KeyedSubtree.ensureUniqueKeysForList(widget.children);
}
void _handleTabControllerAnimationTick() {
if (_scrollUnderwayCount > 0 || !_controller!.indexIsChanging) {
return;
} // This widget is driving the controller's animation.
if (_controller!.index != _currentIndex) {
_currentIndex = _controller!.index;
_warpToCurrentIndex();
}
}
void _warpToCurrentIndex() {
if (!mounted || _pageController!.page == _currentIndex!.toDouble()) {
return;
}
final bool adjacentDestination = (_currentIndex! - _controller!.previousIndex).abs() == 1;
if (adjacentDestination) {
_warpToAdjacentTab(_controller!.animationDuration);
} else {
_warpToNonAdjacentTab(_controller!.animationDuration);
}
}
Future<void> _warpToAdjacentTab(Duration duration) async {
if (duration == Duration.zero) {
_jumpToPage(_currentIndex!);
} else {
await _animateToPage(_currentIndex!, duration: duration, curve: Curves.ease);
}
if (mounted) {
setState(() { _updateChildren(); });
}
return Future<void>.value();
}
Future<void> _warpToNonAdjacentTab(Duration duration) async {
final int previousIndex = _controller!.previousIndex;
assert((_currentIndex! - previousIndex).abs() > 1);
// initialPage defines which page is shown when starting the animation.
// This page is adjacent to the destination page.
final int initialPage = _currentIndex! > previousIndex
? _currentIndex! - 1
: _currentIndex! + 1;
setState(() {
// Needed for `RenderSliverMultiBoxAdaptor.move` and kept alive children.
// For motivation, see https://github.com/flutter/flutter/pull/29188 and
// https://github.com/flutter/flutter/issues/27010#issuecomment-486475152.
_childrenWithKey = List<Widget>.of(_childrenWithKey, growable: false);
final Widget temp = _childrenWithKey[initialPage];
_childrenWithKey[initialPage] = _childrenWithKey[previousIndex];
_childrenWithKey[previousIndex] = temp;
});
// Make a first jump to the adjacent page.
_jumpToPage(initialPage);
// Jump or animate to the destination page.
if (duration == Duration.zero) {
_jumpToPage(_currentIndex!);
} else {
await _animateToPage(_currentIndex!, duration: duration, curve: Curves.ease);
}
if (mounted) {
setState(() { _updateChildren(); });
}
}
void _syncControllerOffset() {
_controller!.offset = clampDouble(_pageController!.page! - _controller!.index, -1.0, 1.0);
}
// Called when the PageView scrolls
bool _handleScrollNotification(ScrollNotification notification) {
if (_warpUnderwayCount > 0 || _scrollUnderwayCount > 0) {
return false;
}
if (notification.depth != 0) {
return false;
}
if (!_controllerIsValid) {
return false;
}
_scrollUnderwayCount += 1;
final double page = _pageController!.page!;
if (notification is ScrollUpdateNotification && !_controller!.indexIsChanging) {
final bool pageChanged = (page - _controller!.index).abs() > 1.0;
if (pageChanged) {
_controller!.index = page.round();
_currentIndex =_controller!.index;
}
_syncControllerOffset();
} else if (notification is ScrollEndNotification) {
_controller!.index = page.round();
_currentIndex = _controller!.index;
if (!_controller!.indexIsChanging) {
_syncControllerOffset();
}
}
_scrollUnderwayCount -= 1;
return false;
}
bool _debugScheduleCheckHasValidChildrenCount() {
if (_debugHasScheduledValidChildrenCountCheck) {
return true;
}
WidgetsBinding.instance.addPostFrameCallback((Duration duration) {
_debugHasScheduledValidChildrenCountCheck = false;
if (!mounted) {
return;
}
assert(() {
if (_controller!.length != widget.children.length) {
throw FlutterError(
"Controller's length property (${_controller!.length}) does not match the "
"number of children (${widget.children.length}) present in TabBarView's children property.",
);
}
return true;
}());
}, debugLabel: 'TabBarView.validChildrenCountCheck');
_debugHasScheduledValidChildrenCountCheck = true;
return true;
}
@override
Widget build(BuildContext context) {
assert(_debugScheduleCheckHasValidChildrenCount());
return NotificationListener<ScrollNotification>(
onNotification: _handleScrollNotification,
child: PageView(
dragStartBehavior: widget.dragStartBehavior,
clipBehavior: widget.clipBehavior,
controller: _pageController,
physics: widget.physics == null
? const PageScrollPhysics().applyTo(const ClampingScrollPhysics())
: const PageScrollPhysics().applyTo(widget.physics),
children: _childrenWithKey,
),
);
}
}
/// Displays a single circle with the specified size, border style, border color
/// and background colors.
///
/// Used by [TabPageSelector] to indicate the selected page.
class TabPageSelectorIndicator extends StatelessWidget {
/// Creates an indicator used by [TabPageSelector].
const TabPageSelectorIndicator({
super.key,
required this.backgroundColor,
required this.borderColor,
required this.size,
this.borderStyle = BorderStyle.solid,
});
/// The indicator circle's background color.
final Color backgroundColor;
/// The indicator circle's border color.
final Color borderColor;
/// The indicator circle's diameter.
final double size;
/// The indicator circle's border style.
///
/// Defaults to [BorderStyle.solid] if value is not specified.
final BorderStyle borderStyle;
@override
Widget build(BuildContext context) {
return Container(
width: size,
height: size,
margin: const EdgeInsets.all(4.0),
decoration: BoxDecoration(
color: backgroundColor,
border: Border.all(color: borderColor, style: borderStyle),
shape: BoxShape.circle,
),
);
}
}
/// Uses [TabPageSelectorIndicator] to display a row of small circular
/// indicators, one per tab.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=Q628ue9Cq7U}
///
/// The selected tab's indicator is highlighted. Often used in conjunction with
/// a [TabBarView].
///
/// If a [TabController] is not provided, then there must be a
/// [DefaultTabController] ancestor.
class TabPageSelector extends StatelessWidget {
/// Creates a compact widget that indicates which tab has been selected.
const TabPageSelector({
super.key,
this.controller,
this.indicatorSize = 12.0,
this.color,
this.selectedColor,
this.borderStyle,
}) : assert(indicatorSize > 0.0);
/// This widget's selection and animation state.
///
/// If [TabController] is not provided, then the value of
/// [DefaultTabController.of] will be used.
final TabController? controller;
/// The indicator circle's diameter (the default value is 12.0).
final double indicatorSize;
/// The indicator circle's fill color for unselected pages.
///
/// If this parameter is null, then the indicator is filled with [Colors.transparent].
final Color? color;
/// The indicator circle's fill color for selected pages and border color
/// for all indicator circles.
///
/// If this parameter is null, then the indicator is filled with the theme's
/// [ColorScheme.secondary].
final Color? selectedColor;
/// The indicator circle's border style.
///
/// Defaults to [BorderStyle.solid] if value is not specified.
final BorderStyle? borderStyle;
Widget _buildTabIndicator(
int tabIndex,
TabController tabController,
ColorTween selectedColorTween,
ColorTween previousColorTween,
) {
final Color background;
if (tabController.indexIsChanging) {
// The selection's animation is animating from previousValue to value.
final double t = 1.0 - _indexChangeProgress(tabController);
if (tabController.index == tabIndex) {
background = selectedColorTween.lerp(t)!;
} else if (tabController.previousIndex == tabIndex) {
background = previousColorTween.lerp(t)!;
} else {
background = selectedColorTween.begin!;
}
} else {
// The selection's offset reflects how far the TabBarView has / been dragged
// to the previous page (-1.0 to 0.0) or the next page (0.0 to 1.0).
final double offset = tabController.offset;
if (tabController.index == tabIndex) {
background = selectedColorTween.lerp(1.0 - offset.abs())!;
} else if (tabController.index == tabIndex - 1 && offset > 0.0) {
background = selectedColorTween.lerp(offset)!;
} else if (tabController.index == tabIndex + 1 && offset < 0.0) {
background = selectedColorTween.lerp(-offset)!;
} else {
background = selectedColorTween.begin!;
}
}
return TabPageSelectorIndicator(
backgroundColor: background,
borderColor: selectedColorTween.end!,
size: indicatorSize,
borderStyle: borderStyle ?? BorderStyle.solid,
);
}
@override
Widget build(BuildContext context) {
final Color fixColor = color ?? Colors.transparent;
final Color fixSelectedColor = selectedColor ?? Theme.of(context).colorScheme.secondary;
final ColorTween selectedColorTween = ColorTween(begin: fixColor, end: fixSelectedColor);
final ColorTween previousColorTween = ColorTween(begin: fixSelectedColor, end: fixColor);
final TabController? tabController = controller ?? DefaultTabController.maybeOf(context);
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
assert(() {
if (tabController == null) {
throw FlutterError(
'No TabController for $runtimeType.\n'
'When creating a $runtimeType, you must either provide an explicit TabController '
'using the "controller" property, or you must ensure that there is a '
'DefaultTabController above the $runtimeType.\n'
'In this case, there was neither an explicit controller nor a default controller.',
);
}
return true;
}());
final Animation<double> animation = CurvedAnimation(
parent: tabController!.animation!,
curve: Curves.fastOutSlowIn,
);
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return Semantics(
label: localizations.tabLabel(tabIndex: tabController.index + 1, tabCount: tabController.length),
child: Row(
mainAxisSize: MainAxisSize.min,
children: List<Widget>.generate(tabController.length, (int tabIndex) {
return _buildTabIndicator(tabIndex, tabController, selectedColorTween, previousColorTween);
}).toList(),
),
);
},
);
}
}
// Hand coded defaults based on Material Design 2.
class _TabsDefaultsM2 extends TabBarTheme {
const _TabsDefaultsM2(this.context, this.isScrollable)
: super(indicatorSize: TabBarIndicatorSize.tab);
final BuildContext context;
final bool isScrollable;
@override
Color? get indicatorColor => Theme.of(context).indicatorColor;
@override
Color? get labelColor => Theme.of(context).primaryTextTheme.bodyLarge!.color!;
@override
TextStyle? get labelStyle => Theme.of(context).primaryTextTheme.bodyLarge;
@override
TextStyle? get unselectedLabelStyle => Theme.of(context).primaryTextTheme.bodyLarge;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
@override
TabAlignment? get tabAlignment => isScrollable ? TabAlignment.start : TabAlignment.fill;
static const EdgeInsetsGeometry iconMargin = EdgeInsets.only(bottom: 10);
}
// BEGIN GENERATED TOKEN PROPERTIES - Tabs
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _TabsPrimaryDefaultsM3 extends TabBarTheme {
_TabsPrimaryDefaultsM3(this.context, this.isScrollable)
: super(indicatorSize: TabBarIndicatorSize.label);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
final bool isScrollable;
// This value comes from Divider widget defaults. Token db deprecated 'primary-navigation-tab.divider.color' token.
@override
Color? get dividerColor => _colors.outlineVariant;
// This value comes from Divider widget defaults. Token db deprecated 'primary-navigation-tab.divider.height' token.
@override
double? get dividerHeight => 1.0;
@override
Color? get indicatorColor => _colors.primary;
@override
Color? get labelColor => _colors.primary;
@override
TextStyle? get labelStyle => _textTheme.titleSmall;
@override
Color? get unselectedLabelColor => _colors.onSurfaceVariant;
@override
TextStyle? get unselectedLabelStyle => _textTheme.titleSmall;
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return null;
}
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return null;
});
}
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
@override
TabAlignment? get tabAlignment => isScrollable ? TabAlignment.startOffset : TabAlignment.fill;
static double indicatorWeight = 3.0;
// TODO(davidmartos96): This value doesn't currently exist in
// https://m3.material.io/components/tabs/specs
// Update this when the token is available.
static const EdgeInsetsGeometry iconMargin = EdgeInsets.only(bottom: 2);
}
class _TabsSecondaryDefaultsM3 extends TabBarTheme {
_TabsSecondaryDefaultsM3(this.context, this.isScrollable)
: super(indicatorSize: TabBarIndicatorSize.tab);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
final bool isScrollable;
// This value comes from Divider widget defaults. Token db deprecated 'secondary-navigation-tab.divider.color' token.
@override
Color? get dividerColor => _colors.outlineVariant;
// This value comes from Divider widget defaults. Token db deprecated 'secondary-navigation-tab.divider.height' token.
@override
double? get dividerHeight => 1.0;
@override
Color? get indicatorColor => _colors.primary;
@override
Color? get labelColor => _colors.onSurface;
@override
TextStyle? get labelStyle => _textTheme.titleSmall;
@override
Color? get unselectedLabelColor => _colors.onSurfaceVariant;
@override
TextStyle? get unselectedLabelStyle => _textTheme.titleSmall;
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return null;
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return null;
});
}
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
@override
TabAlignment? get tabAlignment => isScrollable ? TabAlignment.startOffset : TabAlignment.fill;
}
// END GENERATED TOKEN PROPERTIES - Tabs
| flutter/packages/flutter/lib/src/material/tabs.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/tabs.dart",
"repo_id": "flutter",
"token_count": 30446
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the color and border properties of [ToggleButtons] widgets.
///
/// Used by [ToggleButtonsTheme] to control the color and border properties
/// of toggle buttons in a widget subtree.
///
/// To obtain the current [ToggleButtonsTheme], use [ToggleButtonsTheme.of].
///
/// Values specified here are used for [ToggleButtons] properties that are not
/// given an explicit non-null value.
///
/// See also:
///
/// * [ToggleButtonsTheme], which describes the actual configuration of a
/// toggle buttons theme.
@immutable
class ToggleButtonsThemeData with Diagnosticable {
/// Creates the set of color and border properties used to configure
/// [ToggleButtons].
const ToggleButtonsThemeData({
this.textStyle,
this.constraints,
this.color,
this.selectedColor,
this.disabledColor,
this.fillColor,
this.focusColor,
this.highlightColor,
this.hoverColor,
this.splashColor,
this.borderColor,
this.selectedBorderColor,
this.disabledBorderColor,
this.borderRadius,
this.borderWidth,
});
/// The default text style for [ToggleButtons.children].
///
/// [TextStyle.color] will be ignored and substituted by [color],
/// [selectedColor] or [disabledColor] depending on whether the buttons
/// are active, selected, or disabled.
final TextStyle? textStyle;
/// Defines the button's size.
///
/// Typically used to constrain the button's minimum size.
final BoxConstraints? constraints;
/// The color for descendant [Text] and [Icon] widgets if the toggle button
/// is enabled.
final Color? color;
/// The color for descendant [Text] and [Icon] widgets if the toggle button
/// is selected.
final Color? selectedColor;
/// The color for descendant [Text] and [Icon] widgets if the toggle button
/// is disabled.
final Color? disabledColor;
/// The fill color for selected toggle buttons.
final Color? fillColor;
/// The color to use for filling the button when the button has input focus.
final Color? focusColor;
/// The highlight color for the toggle button's [InkWell].
final Color? highlightColor;
/// The splash color for the toggle button's [InkWell].
final Color? splashColor;
/// The color to use for filling the toggle button when the button has a
/// pointer hovering over it.
final Color? hoverColor;
/// The border color to display when the toggle button is enabled.
final Color? borderColor;
/// The border color to display when the toggle button is selected.
final Color? selectedBorderColor;
/// The border color to display when the toggle button is disabled.
final Color? disabledBorderColor;
/// The width of the border surrounding each toggle button.
///
/// This applies to both the greater surrounding border, as well as the
/// borders dividing each toggle button.
///
/// To render a hairline border (one physical pixel), set borderWidth to 0.0.
/// See [BorderSide.width] for more details on hairline borders.
final double? borderWidth;
/// The radii of the border's corners.
final BorderRadius? borderRadius;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
ToggleButtonsThemeData copyWith({
TextStyle? textStyle,
BoxConstraints? constraints,
Color? color,
Color? selectedColor,
Color? disabledColor,
Color? fillColor,
Color? focusColor,
Color? highlightColor,
Color? hoverColor,
Color? splashColor,
Color? borderColor,
Color? selectedBorderColor,
Color? disabledBorderColor,
BorderRadius? borderRadius,
double? borderWidth,
}) {
return ToggleButtonsThemeData(
textStyle: textStyle ?? this.textStyle,
constraints: constraints ?? this.constraints,
color: color ?? this.color,
selectedColor: selectedColor ?? this.selectedColor,
disabledColor: disabledColor ?? this.disabledColor,
fillColor: fillColor ?? this.fillColor,
focusColor: focusColor ?? this.focusColor,
highlightColor: highlightColor ?? this.highlightColor,
hoverColor: hoverColor ?? this.hoverColor,
splashColor: splashColor ?? this.splashColor,
borderColor: borderColor ?? this.borderColor,
selectedBorderColor: selectedBorderColor ?? this.selectedBorderColor,
disabledBorderColor: disabledBorderColor ?? this.disabledBorderColor,
borderRadius: borderRadius ?? this.borderRadius,
borderWidth: borderWidth ?? this.borderWidth,
);
}
/// Linearly interpolate between two toggle buttons themes.
static ToggleButtonsThemeData? lerp(ToggleButtonsThemeData? a, ToggleButtonsThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return ToggleButtonsThemeData(
textStyle: TextStyle.lerp(a?.textStyle, b?.textStyle, t),
constraints: BoxConstraints.lerp(a?.constraints, b?.constraints, t),
color: Color.lerp(a?.color, b?.color, t),
selectedColor: Color.lerp(a?.selectedColor, b?.selectedColor, t),
disabledColor: Color.lerp(a?.disabledColor, b?.disabledColor, t),
fillColor: Color.lerp(a?.fillColor, b?.fillColor, t),
focusColor: Color.lerp(a?.focusColor, b?.focusColor, t),
highlightColor: Color.lerp(a?.highlightColor, b?.highlightColor, t),
hoverColor: Color.lerp(a?.hoverColor, b?.hoverColor, t),
splashColor: Color.lerp(a?.splashColor, b?.splashColor, t),
borderColor: Color.lerp(a?.borderColor, b?.borderColor, t),
selectedBorderColor: Color.lerp(a?.selectedBorderColor, b?.selectedBorderColor, t),
disabledBorderColor: Color.lerp(a?.disabledBorderColor, b?.disabledBorderColor, t),
borderRadius: BorderRadius.lerp(a?.borderRadius, b?.borderRadius, t),
borderWidth: lerpDouble(a?.borderWidth, b?.borderWidth, t),
);
}
@override
int get hashCode => Object.hash(
textStyle,
constraints,
color,
selectedColor,
disabledColor,
fillColor,
focusColor,
highlightColor,
hoverColor,
splashColor,
borderColor,
selectedBorderColor,
disabledBorderColor,
borderRadius,
borderWidth,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ToggleButtonsThemeData
&& other.textStyle == textStyle
&& other.constraints == constraints
&& other.color == color
&& other.selectedColor == selectedColor
&& other.disabledColor == disabledColor
&& other.fillColor == fillColor
&& other.focusColor == focusColor
&& other.highlightColor == highlightColor
&& other.hoverColor == hoverColor
&& other.splashColor == splashColor
&& other.borderColor == borderColor
&& other.selectedBorderColor == selectedBorderColor
&& other.disabledBorderColor == disabledBorderColor
&& other.borderRadius == borderRadius
&& other.borderWidth == borderWidth;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
textStyle?.debugFillProperties(properties, prefix: 'textStyle.');
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: null));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(ColorProperty('selectedColor', selectedColor, defaultValue: null));
properties.add(ColorProperty('disabledColor', disabledColor, defaultValue: null));
properties.add(ColorProperty('fillColor', fillColor, defaultValue: null));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: null));
properties.add(ColorProperty('highlightColor', highlightColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: null));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: null));
properties.add(ColorProperty('borderColor', borderColor, defaultValue: null));
properties.add(ColorProperty('selectedBorderColor', selectedBorderColor, defaultValue: null));
properties.add(ColorProperty('disabledBorderColor', disabledBorderColor, defaultValue: null));
properties.add(DiagnosticsProperty<BorderRadius>('borderRadius', borderRadius, defaultValue: null));
properties.add(DoubleProperty('borderWidth', borderWidth, defaultValue: null));
}
}
/// An inherited widget that defines color and border parameters for
/// [ToggleButtons] in this widget's subtree.
///
/// Values specified here are used for [ToggleButtons] properties that are not
/// given an explicit non-null value.
class ToggleButtonsTheme extends InheritedTheme {
/// Creates a toggle buttons theme that controls the color and border
/// parameters for [ToggleButtons].
const ToggleButtonsTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the color and border values for descendant [ToggleButtons] widgets.
final ToggleButtonsThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [ToggleButtonsTheme] widget, then
/// [ThemeData.toggleButtonsTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// ToggleButtonsThemeData theme = ToggleButtonsTheme.of(context);
/// ```
static ToggleButtonsThemeData of(BuildContext context) {
final ToggleButtonsTheme? toggleButtonsTheme = context.dependOnInheritedWidgetOfExactType<ToggleButtonsTheme>();
return toggleButtonsTheme?.data ?? Theme.of(context).toggleButtonsTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ToggleButtonsTheme(data: data, child: child);
}
@override
bool updateShouldNotify(ToggleButtonsTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/toggle_buttons_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/toggle_buttons_theme.dart",
"repo_id": "flutter",
"token_count": 3227
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'border_radius.dart';
import 'box_border.dart';
import 'box_shadow.dart';
import 'colors.dart';
import 'decoration.dart';
import 'decoration_image.dart';
import 'edge_insets.dart';
import 'gradient.dart';
import 'image_provider.dart';
/// An immutable description of how to paint a box.
///
/// The [BoxDecoration] class provides a variety of ways to draw a box.
///
/// The box has a [border], a body, and may cast a [boxShadow].
///
/// The [shape] of the box can be a circle or a rectangle. If it is a rectangle,
/// then the [borderRadius] property controls the roundness of the corners.
///
/// The body of the box is painted in layers. The bottom-most layer is the
/// [color], which fills the box. Above that is the [gradient], which also fills
/// the box. Finally there is the [image], the precise alignment of which is
/// controlled by the [DecorationImage] class.
///
/// The [border] paints over the body; the [boxShadow], naturally, paints below it.
///
/// {@tool snippet}
///
/// The following applies a [BoxDecoration] to a [Container] widget to draw an
/// [image] of an owl with a thick black [border] and rounded corners.
///
/// 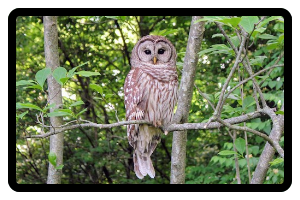
///
/// ```dart
/// Container(
/// decoration: BoxDecoration(
/// color: const Color(0xff7c94b6),
/// image: const DecorationImage(
/// image: NetworkImage('https://flutter.github.io/assets-for-api-docs/assets/widgets/owl-2.jpg'),
/// fit: BoxFit.cover,
/// ),
/// border: Border.all(
/// width: 8,
/// ),
/// borderRadius: BorderRadius.circular(12),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@template flutter.painting.BoxDecoration.clip}
/// The [shape] or the [borderRadius] won't clip the children of the
/// decorated [Container]. If the clip is required, insert a clip widget
/// (e.g., [ClipRect], [ClipRRect], [ClipPath]) as the child of the [Container].
/// Be aware that clipping may be costly in terms of performance.
/// {@endtemplate}
///
/// See also:
///
/// * [DecoratedBox] and [Container], widgets that can be configured with
/// [BoxDecoration] objects.
/// * [DecoratedSliver], a widget that can be configured with a [BoxDecoration]
/// that is converted to render with slivers.
/// * [CustomPaint], a widget that lets you draw arbitrary graphics.
/// * [Decoration], the base class which lets you define other decorations.
class BoxDecoration extends Decoration {
/// Creates a box decoration.
///
/// * If [color] is null, this decoration does not paint a background color.
/// * If [image] is null, this decoration does not paint a background image.
/// * If [border] is null, this decoration does not paint a border.
/// * If [borderRadius] is null, this decoration uses more efficient background
/// painting commands. The [borderRadius] argument must be null if [shape] is
/// [BoxShape.circle].
/// * If [boxShadow] is null, this decoration does not paint a shadow.
/// * If [gradient] is null, this decoration does not paint gradients.
/// * If [backgroundBlendMode] is null, this decoration paints with [BlendMode.srcOver]
const BoxDecoration({
this.color,
this.image,
this.border,
this.borderRadius,
this.boxShadow,
this.gradient,
this.backgroundBlendMode,
this.shape = BoxShape.rectangle,
}) : assert(
backgroundBlendMode == null || color != null || gradient != null,
"backgroundBlendMode applies to BoxDecoration's background color or "
'gradient, but no color or gradient was provided.',
);
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
BoxDecoration copyWith({
Color? color,
DecorationImage? image,
BoxBorder? border,
BorderRadiusGeometry? borderRadius,
List<BoxShadow>? boxShadow,
Gradient? gradient,
BlendMode? backgroundBlendMode,
BoxShape? shape,
}) {
return BoxDecoration(
color: color ?? this.color,
image: image ?? this.image,
border: border ?? this.border,
borderRadius: borderRadius ?? this.borderRadius,
boxShadow: boxShadow ?? this.boxShadow,
gradient: gradient ?? this.gradient,
backgroundBlendMode: backgroundBlendMode ?? this.backgroundBlendMode,
shape: shape ?? this.shape,
);
}
@override
bool debugAssertIsValid() {
assert(shape != BoxShape.circle || borderRadius == null); // Can't have a border radius if you're a circle.
return super.debugAssertIsValid();
}
/// The color to fill in the background of the box.
///
/// The color is filled into the [shape] of the box (e.g., either a rectangle,
/// potentially with a [borderRadius], or a circle).
///
/// This is ignored if [gradient] is non-null.
///
/// The [color] is drawn under the [image].
final Color? color;
/// An image to paint above the background [color] or [gradient].
///
/// If [shape] is [BoxShape.circle] then the image is clipped to the circle's
/// boundary; if [borderRadius] is non-null then the image is clipped to the
/// given radii.
final DecorationImage? image;
/// A border to draw above the background [color], [gradient], or [image].
///
/// Follows the [shape] and [borderRadius].
///
/// Use [Border] objects to describe borders that do not depend on the reading
/// direction.
///
/// Use [BoxBorder] objects to describe borders that should flip their left
/// and right edges based on whether the text is being read left-to-right or
/// right-to-left.
final BoxBorder? border;
/// If non-null, the corners of this box are rounded by this [BorderRadius].
///
/// Applies only to boxes with rectangular shapes; ignored if [shape] is not
/// [BoxShape.rectangle].
///
/// {@macro flutter.painting.BoxDecoration.clip}
final BorderRadiusGeometry? borderRadius;
/// A list of shadows cast by this box behind the box.
///
/// The shadow follows the [shape] of the box.
///
/// See also:
///
/// * [kElevationToShadow], for some predefined shadows used in Material
/// Design.
/// * [PhysicalModel], a widget for showing shadows.
final List<BoxShadow>? boxShadow;
/// A gradient to use when filling the box.
///
/// If this is specified, [color] has no effect.
///
/// The [gradient] is drawn under the [image].
final Gradient? gradient;
/// The blend mode applied to the [color] or [gradient] background of the box.
///
/// If no [backgroundBlendMode] is provided then the default painting blend
/// mode is used.
///
/// If no [color] or [gradient] is provided then the blend mode has no impact.
final BlendMode? backgroundBlendMode;
/// The shape to fill the background [color], [gradient], and [image] into and
/// to cast as the [boxShadow].
///
/// If this is [BoxShape.circle] then [borderRadius] is ignored.
///
/// The [shape] cannot be interpolated; animating between two [BoxDecoration]s
/// with different [shape]s will result in a discontinuity in the rendering.
/// To interpolate between two shapes, consider using [ShapeDecoration] and
/// different [ShapeBorder]s; in particular, [CircleBorder] instead of
/// [BoxShape.circle] and [RoundedRectangleBorder] instead of
/// [BoxShape.rectangle].
///
/// {@macro flutter.painting.BoxDecoration.clip}
final BoxShape shape;
@override
EdgeInsetsGeometry get padding => border?.dimensions ?? EdgeInsets.zero;
@override
Path getClipPath(Rect rect, TextDirection textDirection) {
switch (shape) {
case BoxShape.circle:
final Offset center = rect.center;
final double radius = rect.shortestSide / 2.0;
final Rect square = Rect.fromCircle(center: center, radius: radius);
return Path()..addOval(square);
case BoxShape.rectangle:
if (borderRadius != null) {
return Path()..addRRect(borderRadius!.resolve(textDirection).toRRect(rect));
}
return Path()..addRect(rect);
}
}
/// Returns a new box decoration that is scaled by the given factor.
BoxDecoration scale(double factor) {
return BoxDecoration(
color: Color.lerp(null, color, factor),
image: DecorationImage.lerp(null, image, factor),
border: BoxBorder.lerp(null, border, factor),
borderRadius: BorderRadiusGeometry.lerp(null, borderRadius, factor),
boxShadow: BoxShadow.lerpList(null, boxShadow, factor),
gradient: gradient?.scale(factor),
shape: shape,
);
}
@override
bool get isComplex => boxShadow != null;
@override
BoxDecoration? lerpFrom(Decoration? a, double t) {
if (a == null) {
return scale(t);
}
if (a is BoxDecoration) {
return BoxDecoration.lerp(a, this, t);
}
return super.lerpFrom(a, t) as BoxDecoration?;
}
@override
BoxDecoration? lerpTo(Decoration? b, double t) {
if (b == null) {
return scale(1.0 - t);
}
if (b is BoxDecoration) {
return BoxDecoration.lerp(this, b, t);
}
return super.lerpTo(b, t) as BoxDecoration?;
}
/// Linearly interpolate between two box decorations.
///
/// Interpolates each parameter of the box decoration separately.
///
/// The [shape] is not interpolated. To interpolate the shape, consider using
/// a [ShapeDecoration] with different border shapes.
///
/// If both values are null, this returns null. Otherwise, it returns a
/// non-null value. If one of the values is null, then the result is obtained
/// by applying [scale] to the other value. If neither value is null and `t ==
/// 0.0`, then `a` is returned unmodified; if `t == 1.0` then `b` is returned
/// unmodified. Otherwise, the values are computed by interpolating the
/// properties appropriately.
///
/// {@macro dart.ui.shadow.lerp}
///
/// See also:
///
/// * [Decoration.lerp], which can interpolate between any two types of
/// [Decoration]s, not just [BoxDecoration]s.
/// * [lerpFrom] and [lerpTo], which are used to implement [Decoration.lerp]
/// and which use [BoxDecoration.lerp] when interpolating two
/// [BoxDecoration]s or a [BoxDecoration] to or from null.
static BoxDecoration? lerp(BoxDecoration? a, BoxDecoration? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
if (t == 0.0) {
return a;
}
if (t == 1.0) {
return b;
}
return BoxDecoration(
color: Color.lerp(a.color, b.color, t),
image: DecorationImage.lerp(a.image, b.image, t),
border: BoxBorder.lerp(a.border, b.border, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, b.borderRadius, t),
boxShadow: BoxShadow.lerpList(a.boxShadow, b.boxShadow, t),
gradient: Gradient.lerp(a.gradient, b.gradient, t),
shape: t < 0.5 ? a.shape : b.shape,
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is BoxDecoration
&& other.color == color
&& other.image == image
&& other.border == border
&& other.borderRadius == borderRadius
&& listEquals<BoxShadow>(other.boxShadow, boxShadow)
&& other.gradient == gradient
&& other.backgroundBlendMode == backgroundBlendMode
&& other.shape == shape;
}
@override
int get hashCode => Object.hash(
color,
image,
border,
borderRadius,
boxShadow == null ? null : Object.hashAll(boxShadow!),
gradient,
backgroundBlendMode,
shape,
);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties
..defaultDiagnosticsTreeStyle = DiagnosticsTreeStyle.whitespace
..emptyBodyDescription = '<no decorations specified>';
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(DiagnosticsProperty<DecorationImage>('image', image, defaultValue: null));
properties.add(DiagnosticsProperty<BoxBorder>('border', border, defaultValue: null));
properties.add(DiagnosticsProperty<BorderRadiusGeometry>('borderRadius', borderRadius, defaultValue: null));
properties.add(IterableProperty<BoxShadow>('boxShadow', boxShadow, defaultValue: null, style: DiagnosticsTreeStyle.whitespace));
properties.add(DiagnosticsProperty<Gradient>('gradient', gradient, defaultValue: null));
properties.add(EnumProperty<BoxShape>('shape', shape, defaultValue: BoxShape.rectangle));
}
@override
bool hitTest(Size size, Offset position, { TextDirection? textDirection }) {
assert((Offset.zero & size).contains(position));
switch (shape) {
case BoxShape.rectangle:
if (borderRadius != null) {
final RRect bounds = borderRadius!.resolve(textDirection).toRRect(Offset.zero & size);
return bounds.contains(position);
}
return true;
case BoxShape.circle:
// Circles are inscribed into our smallest dimension.
final Offset center = size.center(Offset.zero);
final double distance = (position - center).distance;
return distance <= math.min(size.width, size.height) / 2.0;
}
}
@override
BoxPainter createBoxPainter([ VoidCallback? onChanged ]) {
assert(onChanged != null || image == null);
return _BoxDecorationPainter(this, onChanged);
}
}
/// An object that paints a [BoxDecoration] into a canvas.
class _BoxDecorationPainter extends BoxPainter {
_BoxDecorationPainter(this._decoration, super.onChanged);
final BoxDecoration _decoration;
Paint? _cachedBackgroundPaint;
Rect? _rectForCachedBackgroundPaint;
Paint _getBackgroundPaint(Rect rect, TextDirection? textDirection) {
assert(_decoration.gradient != null || _rectForCachedBackgroundPaint == null);
if (_cachedBackgroundPaint == null ||
(_decoration.gradient != null && _rectForCachedBackgroundPaint != rect)) {
final Paint paint = Paint();
if (_decoration.backgroundBlendMode != null) {
paint.blendMode = _decoration.backgroundBlendMode!;
}
if (_decoration.color != null) {
paint.color = _decoration.color!;
}
if (_decoration.gradient != null) {
paint.shader = _decoration.gradient!.createShader(rect, textDirection: textDirection);
_rectForCachedBackgroundPaint = rect;
}
_cachedBackgroundPaint = paint;
}
return _cachedBackgroundPaint!;
}
void _paintBox(Canvas canvas, Rect rect, Paint paint, TextDirection? textDirection) {
switch (_decoration.shape) {
case BoxShape.circle:
assert(_decoration.borderRadius == null);
final Offset center = rect.center;
final double radius = rect.shortestSide / 2.0;
canvas.drawCircle(center, radius, paint);
case BoxShape.rectangle:
if (_decoration.borderRadius == null || _decoration.borderRadius == BorderRadius.zero) {
canvas.drawRect(rect, paint);
} else {
canvas.drawRRect(_decoration.borderRadius!.resolve(textDirection).toRRect(rect), paint);
}
}
}
void _paintShadows(Canvas canvas, Rect rect, TextDirection? textDirection) {
if (_decoration.boxShadow == null) {
return;
}
for (final BoxShadow boxShadow in _decoration.boxShadow!) {
final Paint paint = boxShadow.toPaint();
final Rect bounds = rect.shift(boxShadow.offset).inflate(boxShadow.spreadRadius);
_paintBox(canvas, bounds, paint, textDirection);
}
}
void _paintBackgroundColor(Canvas canvas, Rect rect, TextDirection? textDirection) {
if (_decoration.color != null || _decoration.gradient != null) {
_paintBox(canvas, rect, _getBackgroundPaint(rect, textDirection), textDirection);
}
}
DecorationImagePainter? _imagePainter;
void _paintBackgroundImage(Canvas canvas, Rect rect, ImageConfiguration configuration) {
if (_decoration.image == null) {
return;
}
_imagePainter ??= _decoration.image!.createPainter(onChanged!);
Path? clipPath;
switch (_decoration.shape) {
case BoxShape.circle:
assert(_decoration.borderRadius == null);
final Offset center = rect.center;
final double radius = rect.shortestSide / 2.0;
final Rect square = Rect.fromCircle(center: center, radius: radius);
clipPath = Path()..addOval(square);
case BoxShape.rectangle:
if (_decoration.borderRadius != null) {
clipPath = Path()..addRRect(_decoration.borderRadius!.resolve(configuration.textDirection).toRRect(rect));
}
}
_imagePainter!.paint(canvas, rect, clipPath, configuration);
}
@override
void dispose() {
_imagePainter?.dispose();
super.dispose();
}
/// Paint the box decoration into the given location on the given canvas.
@override
void paint(Canvas canvas, Offset offset, ImageConfiguration configuration) {
assert(configuration.size != null);
final Rect rect = offset & configuration.size!;
final TextDirection? textDirection = configuration.textDirection;
_paintShadows(canvas, rect, textDirection);
_paintBackgroundColor(canvas, rect, textDirection);
_paintBackgroundImage(canvas, rect, configuration);
_decoration.border?.paint(
canvas,
rect,
shape: _decoration.shape,
borderRadius: _decoration.borderRadius?.resolve(textDirection),
textDirection: configuration.textDirection,
);
}
@override
String toString() {
return 'BoxPainter for $_decoration';
}
}
| flutter/packages/flutter/lib/src/painting/box_decoration.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/box_decoration.dart",
"repo_id": "flutter",
"token_count": 6182
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'dart:ui' as ui show Codec, FrameInfo, Image, ImmutableBuffer;
import 'binding.dart';
/// Creates an image from a list of bytes.
///
/// This function attempts to interpret the given bytes an image. If successful,
/// the returned [Future] resolves to the decoded image. Otherwise, the [Future]
/// resolves to null.
///
/// If the image is animated, this returns the first frame. Consider
/// [instantiateImageCodec] if support for animated images is necessary.
///
/// This function differs from [ui.decodeImageFromList] in that it defers to
/// [PaintingBinding.instantiateImageCodecWithSize], and therefore can be mocked
/// in tests.
Future<ui.Image> decodeImageFromList(Uint8List bytes) async {
final ui.ImmutableBuffer buffer = await ui.ImmutableBuffer.fromUint8List(bytes);
final ui.Codec codec = await PaintingBinding.instance.instantiateImageCodecWithSize(buffer);
final ui.FrameInfo frameInfo = await codec.getNextFrame();
return frameInfo.image;
}
| flutter/packages/flutter/lib/src/painting/image_decoder.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/image_decoder.dart",
"repo_id": "flutter",
"token_count": 330
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'text_style.dart';
/// Defines the strut, which sets the minimum height a line can be
/// relative to the baseline.
///
/// Strut applies to all lines in the paragraph. Strut is a feature that
/// allows minimum line heights to be set. The effect is as if a zero
/// width space was included at the beginning of each line in the
/// paragraph. This imaginary space is 'shaped' according the properties
/// defined in this class. Flutter's strut is based on
/// [typesetting strut](https://en.wikipedia.org/wiki/Strut_(typesetting))
/// and CSS's [line-height](https://www.w3.org/TR/CSS2/visudet.html#line-height).
///
/// No lines may be shorter than the strut. The ascent and descent of the
/// strut are calculated, and any laid out text that has a shorter ascent or
/// descent than the strut's ascent or descent will take the ascent and
/// descent of the strut. Text with ascents or descents larger than the
/// strut's ascent or descent will layout as normal and extend past the strut.
///
/// Strut is defined independently from any text content or [TextStyle]s.
///
/// The vertical components of strut are as follows:
///
/// * Half the font-defined leading
/// * `ascent * height`
/// * `descent * height`
/// * Half the font-defined leading
///
/// The sum of these four values is the total height of the line.
///
/// Ascent is the font's spacing above the baseline without leading and
/// descent is the spacing below the baseline without leading. Leading is
/// split evenly between the top and bottom. The values for `ascent` and
/// `descent` are provided by the font named by [fontFamily]. If no
/// [fontFamily] or [fontFamilyFallback] is provided, then the platform's
/// default family will be used. Many fonts will have leading values of
/// zero, so in practice, the leading component is often irrelevant.
///
/// When [height] is omitted or null, then the font defined ascent and descent
/// will be used. The font's combined ascent and descent may be taller or
/// shorter than the [fontSize]. When [height] is provided, the line's EM-square
/// ascent and descent (which sums to [fontSize]) will be scaled by [height] to
/// achieve a final line height of `fontSize * height + fontSize * leading`
/// logical pixels. The proportion of ascent:descent with [height] specified
/// is the same as the font metrics defined ascent:descent ratio.
///
/// 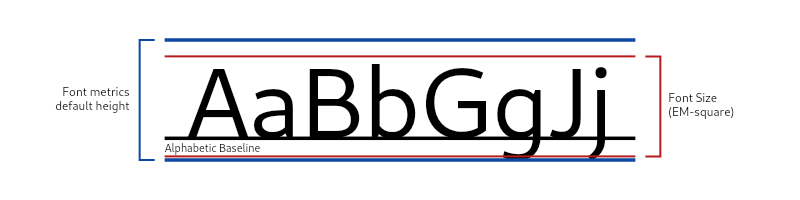
///
/// Each line's spacing above the baseline will be at least as tall as the
/// half leading plus ascent. Each line's spacing below the baseline will
/// be at least as tall as the half leading plus descent.
///
/// See also:
///
/// * [StrutStyle](dart-ui/StrutStyle-class.html), the class in the [dart:ui] library.
///
/// ### Fields and their default values.
// ///////////////////////////////////////////////////////////////////////////
// The defaults are noted here for convenience. The actual place where they //
// are defined is in the engine paragraph_style.h of LibTxt. The values here//
// should be updated should it change in the engine. The engine specifies //
// the defaults in order to reduce the amount of data we pass to native as //
// strut will usually be unspecified. //
// ///////////////////////////////////////////////////////////////////////////
///
/// Omitted or null properties will take the default values specified below:
///
/// * [fontFamily]: the name of the font to use when calculating the strut
/// (e.g., Roboto). No glyphs from the font will be drawn and the font will
/// be used purely for metrics.
///
/// * [fontFamilyFallback]: an ordered list of font family names that will
/// be searched for when the font in [fontFamily] cannot be found. When
/// all specified font families have been exhausted an no match was found,
/// the default platform font will be used.
///
/// * [fontSize]: the size of the ascent plus descent in logical pixels. This
/// is also used as the basis of the custom leading calculation. This value
/// cannot be negative.
/// Default is 14 logical pixels.
///
/// * [height]: the multiple of [fontSize] the line's height should be.
/// The line's height will take the font's ascent and descent values if
/// [height] is omitted or null. If provided, the EM-square ascent and
/// descent (which sum to [fontSize]) is scaled by [height].
/// The [height] will impact the spacing above and below the baseline
/// differently depending on the ratios between the font's ascent and
/// descent. This property is separate from the leading multiplier, which
/// is controlled through [leading].
/// Default is null.
///
/// * [leading]: the custom leading to apply to the strut as a multiple of
/// [fontSize]. Leading is additional spacing between lines. Half of the
/// leading is added to the top and the other half to the bottom of the
/// line height. This differs from [height] since the spacing is equally
/// distributed above and below the baseline.
/// Default is null, which will use the font-specified leading.
///
/// * [fontWeight]: the typeface thickness to use when calculating the strut
/// (e.g., bold).
/// Default is [FontWeight.w400].
///
/// * [fontStyle]: the typeface variant to use when calculating the strut
/// (e.g., italic).
/// Default is [FontStyle.normal].
///
/// * [forceStrutHeight]: when true, all lines will be laid out with the
/// height of the strut. All line and run-specific metrics will be
/// ignored/overridden and only strut metrics will be used instead.
/// This property guarantees uniform line spacing, however text in
/// adjacent lines may overlap. This property should be enabled with
/// caution as it bypasses a large portion of the vertical layout system.
/// The default value is false.
///
/// ### Examples
///
/// {@tool snippet}
/// In this simple case, the text will be rendered at font size 10, however,
/// the vertical height of each line will be the strut height (Roboto in
/// font size 30 * 1.5) as the text itself is shorter than the strut.
///
/// ```dart
/// const Text(
/// 'Hello, world!\nSecond line!',
/// style: TextStyle(
/// fontSize: 10,
/// fontFamily: 'Raleway',
/// ),
/// strutStyle: StrutStyle(
/// fontFamily: 'Roboto',
/// fontSize: 30,
/// height: 1.5,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// Here, strut is used to absorb the additional line height in the second line.
/// The strut [height] was defined as 1.5 (the default font size is 14), which
/// caused all lines to be laid out taller than without strut. This extra space
/// was able to accommodate the larger font size of `Second line!` without
/// causing the line height to change for the second line only. All lines in
/// this example are thus the same height (`14 * 1.5`).
///
/// ```dart
/// const Text.rich(
/// TextSpan(
/// text: 'First line!\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Roboto'
/// ),
/// children: <TextSpan>[
/// TextSpan(
/// text: 'Second line!\n',
/// style: TextStyle(
/// fontSize: 16,
/// fontFamily: 'Roboto',
/// ),
/// ),
/// TextSpan(
/// text: 'Third line!\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Roboto',
/// ),
/// ),
/// ],
/// ),
/// strutStyle: StrutStyle(
/// fontFamily: 'Roboto',
/// height: 1.5,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// Here, strut is used to enable strange and overlapping text to achieve unique
/// effects. The `M`s in lines 2 and 3 are able to extend above their lines and
/// fill empty space in lines above. The [forceStrutHeight] is enabled and functions
/// as a 'grid' for the glyphs to draw on.
///
/// 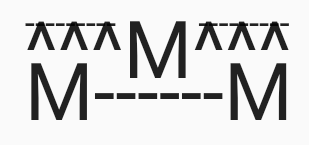
///
/// ```dart
/// const Text.rich(
/// TextSpan(
/// text: '--------- ---------\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Roboto',
/// ),
/// children: <TextSpan>[
/// TextSpan(
/// text: '^^^M^^^\n',
/// style: TextStyle(
/// fontSize: 30,
/// fontFamily: 'Roboto',
/// ),
/// ),
/// TextSpan(
/// text: 'M------M\n',
/// style: TextStyle(
/// fontSize: 30,
/// fontFamily: 'Roboto',
/// ),
/// ),
/// ],
/// ),
/// strutStyle: StrutStyle(
/// fontFamily: 'Roboto',
/// fontSize: 14,
/// height: 1,
/// forceStrutHeight: true,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example uses forceStrutHeight to create a 'drop cap' for the 'T' in 'The'.
/// By locking the line heights to the metrics of the 14pt serif font, we are able
/// to lay out a large 37pt 'T' on the second line to take up space on both the first
/// and second lines.
///
/// 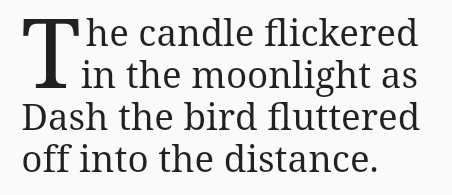
///
/// ```dart
/// const Text.rich(
/// TextSpan(
/// text: ' he candle flickered\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Serif'
/// ),
/// children: <TextSpan>[
/// TextSpan(
/// text: 'T',
/// style: TextStyle(
/// fontSize: 37,
/// fontFamily: 'Serif'
/// ),
/// ),
/// TextSpan(
/// text: 'in the moonlight as\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Serif'
/// ),
/// ),
/// TextSpan(
/// text: 'Dash the bird fluttered\n',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Serif'
/// ),
/// ),
/// TextSpan(
/// text: 'off into the distance.',
/// style: TextStyle(
/// fontSize: 14,
/// fontFamily: 'Serif'
/// ),
/// ),
/// ],
/// ),
/// strutStyle: StrutStyle(
/// fontFamily: 'Serif',
/// fontSize: 14,
/// forceStrutHeight: true,
/// ),
/// )
/// ```
/// {@end-tool}
///
@immutable
class StrutStyle with Diagnosticable {
/// Creates a strut style.
///
/// The `package` argument must be non-null if the font family is defined in a
/// package. It is combined with the `fontFamily` argument to set the
/// [fontFamily] property.
///
/// If provided, fontSize must be positive and non-zero, leading must be
/// zero or positive.
const StrutStyle({
String? fontFamily,
List<String>? fontFamilyFallback,
this.fontSize,
this.height,
this.leadingDistribution,
this.leading,
this.fontWeight,
this.fontStyle,
this.forceStrutHeight,
this.debugLabel,
String? package,
}) : fontFamily = package == null ? fontFamily : 'packages/$package/$fontFamily',
_fontFamilyFallback = fontFamilyFallback,
_package = package,
assert(fontSize == null || fontSize > 0),
assert(leading == null || leading >= 0),
assert(package == null || (fontFamily != null || fontFamilyFallback != null));
/// Builds a StrutStyle that contains values of the equivalent properties in
/// the provided [textStyle].
///
/// The named parameters override the [textStyle]'s argument's properties.
/// Since TextStyle does not contain [leading] or [forceStrutHeight], these
/// values will take on default values (null and false) unless otherwise
/// specified.
///
/// If provided, fontSize must be positive and non-zero, leading must be
/// zero or positive.
///
/// When [textStyle] has a package and a new [package] is also specified,
/// the entire font family fallback list should be redefined since the
/// [textStyle]'s package data is inherited by being prepended onto the
/// font family names. If [fontFamilyFallback] is meant to be empty, pass
/// an empty list instead of null. This prevents the previous package name
/// from being prepended twice.
StrutStyle.fromTextStyle(
TextStyle textStyle, {
String? fontFamily,
List<String>? fontFamilyFallback,
double? fontSize,
double? height,
TextLeadingDistribution? leadingDistribution,
this.leading, // TextStyle does not have an equivalent (yet).
FontWeight? fontWeight,
FontStyle? fontStyle,
this.forceStrutHeight,
String? debugLabel,
String? package,
}) : assert(fontSize == null || fontSize > 0),
assert(leading == null || leading >= 0),
assert(package == null || fontFamily != null || fontFamilyFallback != null),
fontFamily = fontFamily != null ? (package == null ? fontFamily : 'packages/$package/$fontFamily') : textStyle.fontFamily,
_fontFamilyFallback = fontFamilyFallback ?? textStyle.fontFamilyFallback,
height = height ?? textStyle.height,
leadingDistribution = leadingDistribution ?? textStyle.leadingDistribution,
fontSize = fontSize ?? textStyle.fontSize,
fontWeight = fontWeight ?? textStyle.fontWeight,
fontStyle = fontStyle ?? textStyle.fontStyle,
debugLabel = debugLabel ?? textStyle.debugLabel,
_package = package; // the textStyle._package data is embedded in the
// fontFamily names, so we no longer need it.
/// A [StrutStyle] that will have no impact on the text layout.
///
/// Equivalent to having no strut at all. All lines will be laid out according to
/// the properties defined in [TextStyle].
static const StrutStyle disabled = StrutStyle(
height: 0.0,
leading: 0.0,
);
/// The name of the font to use when calculating the strut (e.g., Roboto). If
/// the font is defined in a package, this will be prefixed with
/// 'packages/package_name/' (e.g. 'packages/cool_fonts/Roboto'). The
/// prefixing is done by the constructor when the `package` argument is
/// provided.
///
/// The value provided in [fontFamily] will act as the preferred/first font
/// family that will be searched for, followed in order by the font families
/// in [fontFamilyFallback]. If all font families are exhausted and no match
/// was found, the default platform font family will be used instead. Unlike
/// [TextStyle.fontFamilyFallback], the font does not need to contain the
/// desired glyphs to match.
final String? fontFamily;
/// The ordered list of font families to fall back on when a higher priority
/// font family cannot be found.
///
/// The value provided in [fontFamily] will act as the preferred/first font
/// family that will be searched for, followed in order by the font families
/// in [fontFamilyFallback]. If all font families are exhausted and no match
/// was found, the default platform font family will be used instead. Unlike
/// [TextStyle.fontFamilyFallback], the font does not need to contain the
/// desired glyphs to match.
///
/// When [fontFamily] is null or not provided, the first value in [fontFamilyFallback]
/// acts as the preferred/first font family. When neither is provided, then
/// the default platform font will be used. Providing and empty list or null
/// for this property is the same as omitting it.
///
/// If the font is defined in a package, each font family in the list will be
/// prefixed with 'packages/package_name/' (e.g. 'packages/cool_fonts/Roboto').
/// The package name should be provided by the `package` argument in the
/// constructor.
List<String>? get fontFamilyFallback {
if (_package != null && _fontFamilyFallback != null) {
return _fontFamilyFallback.map((String family) => 'packages/$_package/$family').toList();
}
return _fontFamilyFallback;
}
final List<String>? _fontFamilyFallback;
// This is stored in order to prefix the fontFamilies in _fontFamilyFallback
// in the [fontFamilyFallback] getter.
final String? _package;
/// The size of text (in logical pixels) to use when obtaining metrics from the font.
///
/// The [fontSize] is used to get the base set of metrics that are then used to calculated
/// the metrics of strut. The height and leading are expressed as a multiple of
/// [fontSize].
///
/// The default fontSize is 14 logical pixels.
final double? fontSize;
/// The minimum height of the strut, as a multiple of [fontSize].
///
/// When [height] is omitted or null, then the strut's height will be the sum
/// of the strut's font-defined ascent, its font-defined descent, and its
/// [leading]. The font's combined ascent and descent may be taller or shorter
/// than the [fontSize].
///
/// When [height] is provided, the line's EM-square ascent and descent (which
/// sums to [fontSize]) will be scaled by [height] to achieve a final strut
/// height of `fontSize * height + fontSize * leading` logical pixels. The
/// following diagram illustrates the differences between the font metrics
/// defined height and the EM-square height:
///
/// 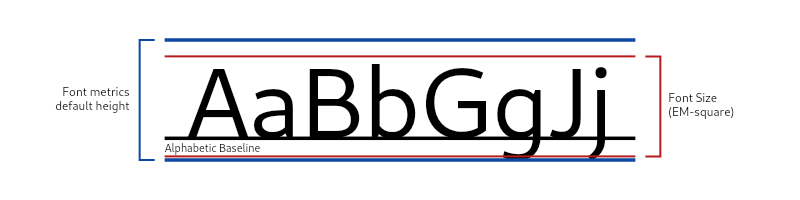
///
/// The ratio of ascent:descent with [height] specified is the same as the
/// font metrics defined ascent:descent ratio when [height] is null or omitted.
///
/// See [TextStyle.height], which works in a similar manner.
///
/// The default height is null.
final double? height;
/// How the vertical space added by the [height] multiplier should be
/// distributed over and under the strut.
///
/// When a non-null [height] is specified, after accommodating the imaginary
/// strut glyph, the remaining vertical space from the allotted
/// `fontSize * height` logical pixels will be distributed over and under the
/// strut, according to the [leadingDistribution] property.
///
/// The additional leading introduced by the [leading] property applies
/// independently of [leadingDistribution]: it will always be distributed
/// evenly over and under the strut, regardless of [leadingDistribution].
///
/// Defaults to null, which defers to the paragraph's
/// `ParagraphStyle.textHeightBehavior`'s [leadingDistribution].
final TextLeadingDistribution? leadingDistribution;
/// The typeface thickness to use when calculating the strut (e.g., bold).
///
/// The default fontWeight is [FontWeight.w400].
final FontWeight? fontWeight;
/// The typeface variant to use when calculating the strut (e.g., italics).
///
/// The default fontStyle is [FontStyle.normal].
final FontStyle? fontStyle;
/// The additional leading to apply to the strut as a multiple of [fontSize],
/// independent of [height] and [leadingDistribution].
///
/// Leading is additional spacing between lines. Half of the leading is added
/// to the top and the other half to the bottom of the line. This differs
/// from [height] since the spacing is always equally distributed above and
/// below the baseline, regardless of [leadingDistribution].
///
/// The default leading is null, which will use the font-specified leading.
final double? leading;
/// Whether the strut height should be forced.
///
/// When true, all lines will be laid out with the height of the
/// strut. All line and run-specific metrics will be ignored/overridden
/// and only strut metrics will be used instead. This property guarantees
/// uniform line spacing, however text in adjacent lines may overlap.
///
/// This property should be enabled with caution as
/// it bypasses a large portion of the vertical layout system.
///
/// This is equivalent to setting [TextStyle.height] to zero for all [TextStyle]s
/// in the paragraph. Since the height of each line is calculated as a max of the
/// metrics of each run of text, zero height [TextStyle]s cause the minimums
/// defined by strut to always manifest, resulting in all lines having the height
/// of the strut.
///
/// The default is false.
final bool? forceStrutHeight;
/// A human-readable description of this strut style.
///
/// This property is maintained only in debug builds.
///
/// This property is not considered when comparing strut styles using `==` or
/// [compareTo], and it does not affect [hashCode].
final String? debugLabel;
/// Describe the difference between this style and another, in terms of how
/// much damage it will make to the rendering.
///
/// See also:
///
/// * [TextSpan.compareTo], which does the same thing for entire [TextSpan]s.
RenderComparison compareTo(StrutStyle other) {
if (identical(this, other)) {
return RenderComparison.identical;
}
if (fontFamily != other.fontFamily ||
fontSize != other.fontSize ||
fontWeight != other.fontWeight ||
fontStyle != other.fontStyle ||
height != other.height ||
leading != other.leading ||
forceStrutHeight != other.forceStrutHeight ||
!listEquals(fontFamilyFallback, other.fontFamilyFallback)) {
return RenderComparison.layout;
}
return RenderComparison.identical;
}
/// Returns a new strut style that inherits its null values from
/// corresponding properties in the [other] [TextStyle].
///
/// The "missing" properties of the this strut style are _filled_ by
/// the properties of the provided [TextStyle]. This is possible because
/// [StrutStyle] shares many of the same basic properties as [TextStyle].
///
/// If the given text style is null, returns this strut style.
StrutStyle inheritFromTextStyle(TextStyle? other) {
if (other == null) {
return this;
}
return StrutStyle(
fontFamily: fontFamily ?? other.fontFamily,
fontFamilyFallback: fontFamilyFallback ?? other.fontFamilyFallback,
fontSize: fontSize ?? other.fontSize,
height: height ?? other.height,
leading: leading, // No equivalent property in TextStyle yet.
fontWeight: fontWeight ?? other.fontWeight,
fontStyle: fontStyle ?? other.fontStyle,
forceStrutHeight: forceStrutHeight, // StrutStyle-unique property.
debugLabel: debugLabel ?? other.debugLabel,
// Package is embedded within the getters for fontFamilyFallback.
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is StrutStyle
&& other.fontFamily == fontFamily
&& other.fontSize == fontSize
&& other.fontWeight == fontWeight
&& other.fontStyle == fontStyle
&& other.height == height
&& other.leading == leading
&& other.forceStrutHeight == forceStrutHeight;
}
@override
int get hashCode => Object.hash(
fontFamily,
fontSize,
fontWeight,
fontStyle,
height,
leading,
forceStrutHeight,
);
@override
String toStringShort() => objectRuntimeType(this, 'StrutStyle');
/// Adds all properties prefixing property names with the optional `prefix`.
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties, { String prefix = '' }) {
super.debugFillProperties(properties);
if (debugLabel != null) {
properties.add(MessageProperty('${prefix}debugLabel', debugLabel!));
}
final List<DiagnosticsNode> styles = <DiagnosticsNode>[
StringProperty('${prefix}family', fontFamily, defaultValue: null, quoted: false),
IterableProperty<String>('${prefix}familyFallback', fontFamilyFallback, defaultValue: null),
DoubleProperty('${prefix}size', fontSize, defaultValue: null),
];
String? weightDescription;
if (fontWeight != null) {
weightDescription = 'w${fontWeight!.index + 1}00';
}
// TODO(jacobr): switch this to use enumProperty which will either cause the
// weight description to change to w600 from 600 or require existing
// enumProperty to handle this special case.
styles.add(DiagnosticsProperty<FontWeight>(
'${prefix}weight',
fontWeight,
description: weightDescription,
defaultValue: null,
));
styles.add(EnumProperty<FontStyle>('${prefix}style', fontStyle, defaultValue: null));
styles.add(DoubleProperty('${prefix}height', height, unit: 'x', defaultValue: null));
styles.add(FlagProperty('${prefix}forceStrutHeight', value: forceStrutHeight, ifTrue: '$prefix<strut height forced>', ifFalse: '$prefix<strut height normal>'));
final bool styleSpecified = styles.any((DiagnosticsNode n) => !n.isFiltered(DiagnosticLevel.info));
styles.forEach(properties.add);
if (!styleSpecified) {
properties.add(FlagProperty('forceStrutHeight', value: forceStrutHeight, ifTrue: '$prefix<strut height forced>', ifFalse: '$prefix<strut height normal>'));
}
}
}
| flutter/packages/flutter/lib/src/painting/strut_style.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/strut_style.dart",
"repo_id": "flutter",
"token_count": 7902
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'package:flutter/semantics.dart';
import 'box.dart';
import 'object.dart';
import 'proxy_box.dart';
/// Signature of the function returned by [CustomPainter.semanticsBuilder].
///
/// Builds semantics information describing the picture drawn by a
/// [CustomPainter]. Each [CustomPainterSemantics] in the returned list is
/// converted into a [SemanticsNode] by copying its properties.
///
/// The returned list must not be mutated after this function completes. To
/// change the semantic information, the function must return a new list
/// instead.
typedef SemanticsBuilderCallback = List<CustomPainterSemantics> Function(Size size);
/// The interface used by [CustomPaint] (in the widgets library) and
/// [RenderCustomPaint] (in the rendering library).
///
/// To implement a custom painter, either subclass or implement this interface
/// to define your custom paint delegate. [CustomPainter] subclasses must
/// implement the [paint] and [shouldRepaint] methods, and may optionally also
/// implement the [hitTest] and [shouldRebuildSemantics] methods, and the
/// [semanticsBuilder] getter.
///
/// The [paint] method is called whenever the custom object needs to be repainted.
///
/// The [shouldRepaint] method is called when a new instance of the class
/// is provided, to check if the new instance actually represents different
/// information.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=vvI_NUXK00s}
///
/// The most efficient way to trigger a repaint is to either:
///
/// * Extend this class and supply a `repaint` argument to the constructor of
/// the [CustomPainter], where that object notifies its listeners when it is
/// time to repaint.
/// * Extend [Listenable] (e.g. via [ChangeNotifier]) and implement
/// [CustomPainter], so that the object itself provides the notifications
/// directly.
///
/// In either case, the [CustomPaint] widget or [RenderCustomPaint]
/// render object will listen to the [Listenable] and repaint whenever the
/// animation ticks, avoiding both the build and layout phases of the pipeline.
///
/// The [hitTest] method is called when the user interacts with the underlying
/// render object, to determine if the user hit the object or missed it.
///
/// The [semanticsBuilder] is called whenever the custom object needs to rebuild
/// its semantics information.
///
/// The [shouldRebuildSemantics] method is called when a new instance of the
/// class is provided, to check if the new instance contains different
/// information that affects the semantics tree.
///
/// {@tool snippet}
///
/// This sample extends the same code shown for [RadialGradient] to create a
/// custom painter that paints a sky.
///
/// ```dart
/// class Sky extends CustomPainter {
/// @override
/// void paint(Canvas canvas, Size size) {
/// final Rect rect = Offset.zero & size;
/// const RadialGradient gradient = RadialGradient(
/// center: Alignment(0.7, -0.6),
/// radius: 0.2,
/// colors: <Color>[Color(0xFFFFFF00), Color(0xFF0099FF)],
/// stops: <double>[0.4, 1.0],
/// );
/// canvas.drawRect(
/// rect,
/// Paint()..shader = gradient.createShader(rect),
/// );
/// }
///
/// @override
/// SemanticsBuilderCallback get semanticsBuilder {
/// return (Size size) {
/// // Annotate a rectangle containing the picture of the sun
/// // with the label "Sun". When text to speech feature is enabled on the
/// // device, a user will be able to locate the sun on this picture by
/// // touch.
/// Rect rect = Offset.zero & size;
/// final double width = size.shortestSide * 0.4;
/// rect = const Alignment(0.8, -0.9).inscribe(Size(width, width), rect);
/// return <CustomPainterSemantics>[
/// CustomPainterSemantics(
/// rect: rect,
/// properties: const SemanticsProperties(
/// label: 'Sun',
/// textDirection: TextDirection.ltr,
/// ),
/// ),
/// ];
/// };
/// }
///
/// // Since this Sky painter has no fields, it always paints
/// // the same thing and semantics information is the same.
/// // Therefore we return false here. If we had fields (set
/// // from the constructor) then we would return true if any
/// // of them differed from the same fields on the oldDelegate.
/// @override
/// bool shouldRepaint(Sky oldDelegate) => false;
/// @override
/// bool shouldRebuildSemantics(Sky oldDelegate) => false;
/// }
/// ```
/// {@end-tool}
///
/// ## Composition and the sharing of canvases
///
/// Widgets (or rather, render objects) are composited together using a minimum
/// number of [Canvas]es, for performance reasons. As a result, a
/// [CustomPainter]'s [Canvas] may be the same as that used by other widgets
/// (including other [CustomPaint] widgets).
///
/// This is mostly unnoticeable, except when using unusual [BlendMode]s. For
/// example, trying to use [BlendMode.dstOut] to "punch a hole" through a
/// previously-drawn image may erase more than was intended, because previous
/// widgets will have been painted onto the same canvas.
///
/// To avoid this issue, consider using [Canvas.saveLayer] and
/// [Canvas.restore] when using such blend modes. Creating new layers is
/// relatively expensive, however, and should be done sparingly to avoid
/// introducing jank.
///
/// See also:
///
/// * [Canvas], the class that a custom painter uses to paint.
/// * [CustomPaint], the widget that uses [CustomPainter], and whose sample
/// code shows how to use the above `Sky` class.
/// * [RadialGradient], whose sample code section shows a different take
/// on the sample code above.
abstract class CustomPainter extends Listenable {
/// Creates a custom painter.
///
/// The painter will repaint whenever `repaint` notifies its listeners.
const CustomPainter({ Listenable? repaint }) : _repaint = repaint;
final Listenable? _repaint;
/// Register a closure to be notified when it is time to repaint.
///
/// The [CustomPainter] implementation merely forwards to the same method on
/// the [Listenable] provided to the constructor in the `repaint` argument, if
/// it was not null.
@override
void addListener(VoidCallback listener) => _repaint?.addListener(listener);
/// Remove a previously registered closure from the list of closures that the
/// object notifies when it is time to repaint.
///
/// The [CustomPainter] implementation merely forwards to the same method on
/// the [Listenable] provided to the constructor in the `repaint` argument, if
/// it was not null.
@override
void removeListener(VoidCallback listener) => _repaint?.removeListener(listener);
/// Called whenever the object needs to paint. The given [Canvas] has its
/// coordinate space configured such that the origin is at the top left of the
/// box. The area of the box is the size of the [size] argument.
///
/// Paint operations should remain inside the given area. Graphical
/// operations outside the bounds may be silently ignored, clipped, or not
/// clipped. It may sometimes be difficult to guarantee that a certain
/// operation is inside the bounds (e.g., drawing a rectangle whose size is
/// determined by user inputs). In that case, consider calling
/// [Canvas.clipRect] at the beginning of [paint] so everything that follows
/// will be guaranteed to only draw within the clipped area.
///
/// Implementations should be wary of correctly pairing any calls to
/// [Canvas.save]/[Canvas.saveLayer] and [Canvas.restore], otherwise all
/// subsequent painting on this canvas may be affected, with potentially
/// hilarious but confusing results.
///
/// To paint text on a [Canvas], use a [TextPainter].
///
/// To paint an image on a [Canvas]:
///
/// 1. Obtain an [ImageStream], for example by calling [ImageProvider.resolve]
/// on an [AssetImage] or [NetworkImage] object.
///
/// 2. Whenever the [ImageStream]'s underlying [ImageInfo] object changes
/// (see [ImageStream.addListener]), create a new instance of your custom
/// paint delegate, giving it the new [ImageInfo] object.
///
/// 3. In your delegate's [paint] method, call the [Canvas.drawImage],
/// [Canvas.drawImageRect], or [Canvas.drawImageNine] methods to paint the
/// [ImageInfo.image] object, applying the [ImageInfo.scale] value to
/// obtain the correct rendering size.
void paint(Canvas canvas, Size size);
/// Returns a function that builds semantic information for the picture drawn
/// by this painter.
///
/// If the returned function is null, this painter will not contribute new
/// [SemanticsNode]s to the semantics tree and the [CustomPaint] corresponding
/// to this painter will not create a semantics boundary. However, if the
/// child of a [CustomPaint] is not null, the child may contribute
/// [SemanticsNode]s to the tree.
///
/// See also:
///
/// * [SemanticsConfiguration.isSemanticBoundary], which causes new
/// [SemanticsNode]s to be added to the semantics tree.
/// * [RenderCustomPaint], which uses this getter to build semantics.
SemanticsBuilderCallback? get semanticsBuilder => null;
/// Called whenever a new instance of the custom painter delegate class is
/// provided to the [RenderCustomPaint] object, or any time that a new
/// [CustomPaint] object is created with a new instance of the custom painter
/// delegate class (which amounts to the same thing, because the latter is
/// implemented in terms of the former).
///
/// If the new instance would cause [semanticsBuilder] to create different
/// semantics information, then this method should return true, otherwise it
/// should return false.
///
/// If the method returns false, then the [semanticsBuilder] call might be
/// optimized away.
///
/// It's possible that the [semanticsBuilder] will get called even if
/// [shouldRebuildSemantics] would return false. For example, it is called
/// when the [CustomPaint] is rendered for the very first time, or when the
/// box changes its size.
///
/// By default this method delegates to [shouldRepaint] under the assumption
/// that in most cases semantics change when something new is drawn.
bool shouldRebuildSemantics(covariant CustomPainter oldDelegate) => shouldRepaint(oldDelegate);
/// Called whenever a new instance of the custom painter delegate class is
/// provided to the [RenderCustomPaint] object, or any time that a new
/// [CustomPaint] object is created with a new instance of the custom painter
/// delegate class (which amounts to the same thing, because the latter is
/// implemented in terms of the former).
///
/// If the new instance represents different information than the old
/// instance, then the method should return true, otherwise it should return
/// false.
///
/// If the method returns false, then the [paint] call might be optimized
/// away.
///
/// It's possible that the [paint] method will get called even if
/// [shouldRepaint] returns false (e.g. if an ancestor or descendant needed to
/// be repainted). It's also possible that the [paint] method will get called
/// without [shouldRepaint] being called at all (e.g. if the box changes
/// size).
///
/// If a custom delegate has a particularly expensive paint function such that
/// repaints should be avoided as much as possible, a [RepaintBoundary] or
/// [RenderRepaintBoundary] (or other render object with
/// [RenderObject.isRepaintBoundary] set to true) might be helpful.
///
/// The `oldDelegate` argument will never be null.
bool shouldRepaint(covariant CustomPainter oldDelegate);
/// Called whenever a hit test is being performed on an object that is using
/// this custom paint delegate.
///
/// The given point is relative to the same coordinate space as the last
/// [paint] call.
///
/// The default behavior is to consider all points to be hits for
/// background painters, and no points to be hits for foreground painters.
///
/// Return true if the given position corresponds to a point on the drawn
/// image that should be considered a "hit", false if it corresponds to a
/// point that should be considered outside the painted image, and null to use
/// the default behavior.
bool? hitTest(Offset position) => null;
@override
String toString() => '${describeIdentity(this)}(${ _repaint?.toString() ?? "" })';
}
/// Contains properties describing information drawn in a rectangle contained by
/// the [Canvas] used by a [CustomPaint].
///
/// This information is used, for example, by assistive technologies to improve
/// the accessibility of applications.
///
/// Implement [CustomPainter.semanticsBuilder] to build the semantic
/// description of the whole picture drawn by a [CustomPaint], rather than one
/// particular rectangle.
///
/// See also:
///
/// * [SemanticsNode], which is created using the properties of this class.
/// * [CustomPainter], which creates instances of this class.
@immutable
class CustomPainterSemantics {
/// Creates semantics information describing a rectangle on a canvas.
const CustomPainterSemantics({
this.key,
required this.rect,
required this.properties,
this.transform,
this.tags,
});
/// Identifies this object in a list of siblings.
///
/// [SemanticsNode] inherits this key, so that when the list of nodes is
/// updated, its nodes are updated from [CustomPainterSemantics] with matching
/// keys.
///
/// If this is null, the update algorithm does not guarantee which
/// [SemanticsNode] will be updated using this instance.
///
/// This value is assigned to [SemanticsNode.key] during update.
final Key? key;
/// The location and size of the box on the canvas where this piece of semantic
/// information applies.
///
/// This value is assigned to [SemanticsNode.rect] during update.
final Rect rect;
/// The transform from the canvas' coordinate system to its parent's
/// coordinate system.
///
/// This value is assigned to [SemanticsNode.transform] during update.
final Matrix4? transform;
/// Contains properties that are assigned to the [SemanticsNode] created or
/// updated from this object.
///
/// See also:
///
/// * [Semantics], which is a widget that also uses [SemanticsProperties] to
/// annotate.
final SemanticsProperties properties;
/// Tags used by the parent [SemanticsNode] to determine the layout of the
/// semantics tree.
///
/// This value is assigned to [SemanticsNode.tags] during update.
final Set<SemanticsTag>? tags;
}
/// Provides a canvas on which to draw during the paint phase.
///
/// When asked to paint, [RenderCustomPaint] first asks its [painter] to paint
/// on the current canvas, then it paints its child, and then, after painting
/// its child, it asks its [foregroundPainter] to paint. The coordinate system of
/// the canvas matches the coordinate system of the [CustomPaint] object. The
/// painters are expected to paint within a rectangle starting at the origin and
/// encompassing a region of the given size. (If the painters paint outside
/// those bounds, there might be insufficient memory allocated to rasterize the
/// painting commands and the resulting behavior is undefined.)
///
/// Painters are implemented by subclassing or implementing [CustomPainter].
///
/// Because custom paint calls its painters during paint, you cannot mark the
/// tree as needing a new layout during the callback (the layout for this frame
/// has already happened).
///
/// Custom painters normally size themselves to their child. If they do not have
/// a child, they attempt to size themselves to the [preferredSize], which
/// defaults to [Size.zero].
///
/// See also:
///
/// * [CustomPainter], the class that custom painter delegates should extend.
/// * [Canvas], the API provided to custom painter delegates.
class RenderCustomPaint extends RenderProxyBox {
/// Creates a render object that delegates its painting.
RenderCustomPaint({
CustomPainter? painter,
CustomPainter? foregroundPainter,
Size preferredSize = Size.zero,
this.isComplex = false,
this.willChange = false,
RenderBox? child,
}) : _painter = painter,
_foregroundPainter = foregroundPainter,
_preferredSize = preferredSize,
super(child);
/// The background custom paint delegate.
///
/// This painter, if non-null, is called to paint behind the children.
CustomPainter? get painter => _painter;
CustomPainter? _painter;
/// Set a new background custom paint delegate.
///
/// If the new delegate is the same as the previous one, this does nothing.
///
/// If the new delegate is the same class as the previous one, then the new
/// delegate has its [CustomPainter.shouldRepaint] called; if the result is
/// true, then the delegate will be called.
///
/// If the new delegate is a different class than the previous one, then the
/// delegate will be called.
///
/// If the new value is null, then there is no background custom painter.
set painter(CustomPainter? value) {
if (_painter == value) {
return;
}
final CustomPainter? oldPainter = _painter;
_painter = value;
_didUpdatePainter(_painter, oldPainter);
}
/// The foreground custom paint delegate.
///
/// This painter, if non-null, is called to paint in front of the children.
CustomPainter? get foregroundPainter => _foregroundPainter;
CustomPainter? _foregroundPainter;
/// Set a new foreground custom paint delegate.
///
/// If the new delegate is the same as the previous one, this does nothing.
///
/// If the new delegate is the same class as the previous one, then the new
/// delegate has its [CustomPainter.shouldRepaint] called; if the result is
/// true, then the delegate will be called.
///
/// If the new delegate is a different class than the previous one, then the
/// delegate will be called.
///
/// If the new value is null, then there is no foreground custom painter.
set foregroundPainter(CustomPainter? value) {
if (_foregroundPainter == value) {
return;
}
final CustomPainter? oldPainter = _foregroundPainter;
_foregroundPainter = value;
_didUpdatePainter(_foregroundPainter, oldPainter);
}
void _didUpdatePainter(CustomPainter? newPainter, CustomPainter? oldPainter) {
// Check if we need to repaint.
if (newPainter == null) {
assert(oldPainter != null); // We should be called only for changes.
markNeedsPaint();
} else if (oldPainter == null ||
newPainter.runtimeType != oldPainter.runtimeType ||
newPainter.shouldRepaint(oldPainter)) {
markNeedsPaint();
}
if (attached) {
oldPainter?.removeListener(markNeedsPaint);
newPainter?.addListener(markNeedsPaint);
}
// Check if we need to rebuild semantics.
if (newPainter == null) {
assert(oldPainter != null); // We should be called only for changes.
if (attached) {
markNeedsSemanticsUpdate();
}
} else if (oldPainter == null ||
newPainter.runtimeType != oldPainter.runtimeType ||
newPainter.shouldRebuildSemantics(oldPainter)) {
markNeedsSemanticsUpdate();
}
}
/// The size that this [RenderCustomPaint] should aim for, given the layout
/// constraints, if there is no child.
///
/// Defaults to [Size.zero].
///
/// If there's a child, this is ignored, and the size of the child is used
/// instead.
Size get preferredSize => _preferredSize;
Size _preferredSize;
set preferredSize(Size value) {
if (preferredSize == value) {
return;
}
_preferredSize = value;
markNeedsLayout();
}
/// Whether to hint that this layer's painting should be cached.
///
/// The compositor contains a raster cache that holds bitmaps of layers in
/// order to avoid the cost of repeatedly rendering those layers on each
/// frame. If this flag is not set, then the compositor will apply its own
/// heuristics to decide whether the layer containing this render object is
/// complex enough to benefit from caching.
bool isComplex;
/// Whether the raster cache should be told that this painting is likely
/// to change in the next frame.
///
/// This hint tells the compositor not to cache the layer containing this
/// render object because the cache will not be used in the future. If this
/// hint is not set, the compositor will apply its own heuristics to decide
/// whether this layer is likely to be reused in the future.
bool willChange;
@override
double computeMinIntrinsicWidth(double height) {
if (child == null) {
return preferredSize.width.isFinite ? preferredSize.width : 0;
}
return super.computeMinIntrinsicWidth(height);
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child == null) {
return preferredSize.width.isFinite ? preferredSize.width : 0;
}
return super.computeMaxIntrinsicWidth(height);
}
@override
double computeMinIntrinsicHeight(double width) {
if (child == null) {
return preferredSize.height.isFinite ? preferredSize.height : 0;
}
return super.computeMinIntrinsicHeight(width);
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child == null) {
return preferredSize.height.isFinite ? preferredSize.height : 0;
}
return super.computeMaxIntrinsicHeight(width);
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_painter?.addListener(markNeedsPaint);
_foregroundPainter?.addListener(markNeedsPaint);
}
@override
void detach() {
_painter?.removeListener(markNeedsPaint);
_foregroundPainter?.removeListener(markNeedsPaint);
super.detach();
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
if (_foregroundPainter != null && (_foregroundPainter!.hitTest(position) ?? false)) {
return true;
}
return super.hitTestChildren(result, position: position);
}
@override
bool hitTestSelf(Offset position) {
return _painter != null && (_painter!.hitTest(position) ?? true);
}
@override
void performLayout() {
super.performLayout();
markNeedsSemanticsUpdate();
}
@override
Size computeSizeForNoChild(BoxConstraints constraints) {
return constraints.constrain(preferredSize);
}
void _paintWithPainter(Canvas canvas, Offset offset, CustomPainter painter) {
late int debugPreviousCanvasSaveCount;
canvas.save();
assert(() {
debugPreviousCanvasSaveCount = canvas.getSaveCount();
return true;
}());
if (offset != Offset.zero) {
canvas.translate(offset.dx, offset.dy);
}
painter.paint(canvas, size);
assert(() {
// This isn't perfect. For example, we can't catch the case of
// someone first restoring, then setting a transform or whatnot,
// then saving.
// If this becomes a real problem, we could add logic to the
// Canvas class to lock the canvas at a particular save count
// such that restore() fails if it would take the lock count
// below that number.
final int debugNewCanvasSaveCount = canvas.getSaveCount();
if (debugNewCanvasSaveCount > debugPreviousCanvasSaveCount) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The $painter custom painter called canvas.save() or canvas.saveLayer() at least '
'${debugNewCanvasSaveCount - debugPreviousCanvasSaveCount} more '
'time${debugNewCanvasSaveCount - debugPreviousCanvasSaveCount == 1 ? '' : 's' } '
'than it called canvas.restore().',
),
ErrorDescription('This leaves the canvas in an inconsistent state and will probably result in a broken display.'),
ErrorHint('You must pair each call to save()/saveLayer() with a later matching call to restore().'),
]);
}
if (debugNewCanvasSaveCount < debugPreviousCanvasSaveCount) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The $painter custom painter called canvas.restore() '
'${debugPreviousCanvasSaveCount - debugNewCanvasSaveCount} more '
'time${debugPreviousCanvasSaveCount - debugNewCanvasSaveCount == 1 ? '' : 's' } '
'than it called canvas.save() or canvas.saveLayer().',
),
ErrorDescription('This leaves the canvas in an inconsistent state and will result in a broken display.'),
ErrorHint('You should only call restore() if you first called save() or saveLayer().'),
]);
}
return debugNewCanvasSaveCount == debugPreviousCanvasSaveCount;
}());
canvas.restore();
}
@override
void paint(PaintingContext context, Offset offset) {
if (_painter != null) {
_paintWithPainter(context.canvas, offset, _painter!);
_setRasterCacheHints(context);
}
super.paint(context, offset);
if (_foregroundPainter != null) {
_paintWithPainter(context.canvas, offset, _foregroundPainter!);
_setRasterCacheHints(context);
}
}
void _setRasterCacheHints(PaintingContext context) {
if (isComplex) {
context.setIsComplexHint();
}
if (willChange) {
context.setWillChangeHint();
}
}
/// Builds semantics for the picture drawn by [painter].
SemanticsBuilderCallback? _backgroundSemanticsBuilder;
/// Builds semantics for the picture drawn by [foregroundPainter].
SemanticsBuilderCallback? _foregroundSemanticsBuilder;
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
_backgroundSemanticsBuilder = painter?.semanticsBuilder;
_foregroundSemanticsBuilder = foregroundPainter?.semanticsBuilder;
config.isSemanticBoundary = _backgroundSemanticsBuilder != null || _foregroundSemanticsBuilder != null;
}
/// Describe the semantics of the picture painted by the [painter].
List<SemanticsNode>? _backgroundSemanticsNodes;
/// Describe the semantics of the picture painted by the [foregroundPainter].
List<SemanticsNode>? _foregroundSemanticsNodes;
@override
void assembleSemanticsNode(
SemanticsNode node,
SemanticsConfiguration config,
Iterable<SemanticsNode> children,
) {
assert(() {
if (child == null && children.isNotEmpty) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'$runtimeType does not have a child widget but received a non-empty list of child SemanticsNode:\n'
'${children.join('\n')}',
),
]);
}
return true;
}());
final List<CustomPainterSemantics> backgroundSemantics = _backgroundSemanticsBuilder != null
? _backgroundSemanticsBuilder!(size)
: const <CustomPainterSemantics>[];
_backgroundSemanticsNodes = _updateSemanticsChildren(_backgroundSemanticsNodes, backgroundSemantics);
final List<CustomPainterSemantics> foregroundSemantics = _foregroundSemanticsBuilder != null
? _foregroundSemanticsBuilder!(size)
: const <CustomPainterSemantics>[];
_foregroundSemanticsNodes = _updateSemanticsChildren(_foregroundSemanticsNodes, foregroundSemantics);
final bool hasBackgroundSemantics = _backgroundSemanticsNodes != null && _backgroundSemanticsNodes!.isNotEmpty;
final bool hasForegroundSemantics = _foregroundSemanticsNodes != null && _foregroundSemanticsNodes!.isNotEmpty;
final List<SemanticsNode> finalChildren = <SemanticsNode>[
if (hasBackgroundSemantics) ..._backgroundSemanticsNodes!,
...children,
if (hasForegroundSemantics) ..._foregroundSemanticsNodes!,
];
super.assembleSemanticsNode(node, config, finalChildren);
}
@override
void clearSemantics() {
super.clearSemantics();
_backgroundSemanticsNodes = null;
_foregroundSemanticsNodes = null;
}
/// Updates the nodes of `oldSemantics` using data in `newChildSemantics`, and
/// returns a new list containing child nodes sorted according to the order
/// specified by `newChildSemantics`.
///
/// [SemanticsNode]s that match [CustomPainterSemantics] by [Key]s preserve
/// their [SemanticsNode.key] field. If a node with the same key appears in
/// a different position in the list, it is moved to the new position, but the
/// same object is reused.
///
/// [SemanticsNode]s whose `key` is null may be updated from
/// [CustomPainterSemantics] whose `key` is also null. However, the algorithm
/// does not guarantee it. If your semantics require that specific nodes are
/// updated from specific [CustomPainterSemantics], it is recommended to match
/// them by specifying non-null keys.
///
/// The algorithm tries to be as close to [RenderObjectElement.updateChildren]
/// as possible, deviating only where the concepts diverge between widgets and
/// semantics. For example, a [SemanticsNode] can be updated from a
/// [CustomPainterSemantics] based on `Key` alone; their types are not
/// considered because there is only one type of [SemanticsNode]. There is no
/// concept of a "forgotten" node in semantics, deactivated nodes, or global
/// keys.
static List<SemanticsNode> _updateSemanticsChildren(
List<SemanticsNode>? oldSemantics,
List<CustomPainterSemantics>? newChildSemantics,
) {
oldSemantics = oldSemantics ?? const <SemanticsNode>[];
newChildSemantics = newChildSemantics ?? const <CustomPainterSemantics>[];
assert(() {
final Map<Key, int> keys = HashMap<Key, int>();
final List<DiagnosticsNode> information = <DiagnosticsNode>[];
for (int i = 0; i < newChildSemantics!.length; i += 1) {
final CustomPainterSemantics child = newChildSemantics[i];
if (child.key != null) {
if (keys.containsKey(child.key)) {
information.add(ErrorDescription('- duplicate key ${child.key} found at position $i'));
}
keys[child.key!] = i;
}
}
if (information.isNotEmpty) {
information.insert(0, ErrorSummary('Failed to update the list of CustomPainterSemantics:'));
throw FlutterError.fromParts(information);
}
return true;
}());
int newChildrenTop = 0;
int oldChildrenTop = 0;
int newChildrenBottom = newChildSemantics.length - 1;
int oldChildrenBottom = oldSemantics.length - 1;
final List<SemanticsNode?> newChildren = List<SemanticsNode?>.filled(newChildSemantics.length, null);
// Update the top of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final SemanticsNode oldChild = oldSemantics[oldChildrenTop];
final CustomPainterSemantics newSemantics = newChildSemantics[newChildrenTop];
if (!_canUpdateSemanticsChild(oldChild, newSemantics)) {
break;
}
final SemanticsNode newChild = _updateSemanticsChild(oldChild, newSemantics);
newChildren[newChildrenTop] = newChild;
newChildrenTop += 1;
oldChildrenTop += 1;
}
// Scan the bottom of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final SemanticsNode oldChild = oldSemantics[oldChildrenBottom];
final CustomPainterSemantics newChild = newChildSemantics[newChildrenBottom];
if (!_canUpdateSemanticsChild(oldChild, newChild)) {
break;
}
oldChildrenBottom -= 1;
newChildrenBottom -= 1;
}
// Scan the old children in the middle of the list.
final bool haveOldChildren = oldChildrenTop <= oldChildrenBottom;
late final Map<Key, SemanticsNode> oldKeyedChildren;
if (haveOldChildren) {
oldKeyedChildren = <Key, SemanticsNode>{};
while (oldChildrenTop <= oldChildrenBottom) {
final SemanticsNode oldChild = oldSemantics[oldChildrenTop];
if (oldChild.key != null) {
oldKeyedChildren[oldChild.key!] = oldChild;
}
oldChildrenTop += 1;
}
}
// Update the middle of the list.
while (newChildrenTop <= newChildrenBottom) {
SemanticsNode? oldChild;
final CustomPainterSemantics newSemantics = newChildSemantics[newChildrenTop];
if (haveOldChildren) {
final Key? key = newSemantics.key;
if (key != null) {
oldChild = oldKeyedChildren[key];
if (oldChild != null) {
if (_canUpdateSemanticsChild(oldChild, newSemantics)) {
// we found a match!
// remove it from oldKeyedChildren so we don't unsync it later
oldKeyedChildren.remove(key);
} else {
// Not a match, let's pretend we didn't see it for now.
oldChild = null;
}
}
}
}
assert(oldChild == null || _canUpdateSemanticsChild(oldChild, newSemantics));
final SemanticsNode newChild = _updateSemanticsChild(oldChild, newSemantics);
assert(oldChild == newChild || oldChild == null);
newChildren[newChildrenTop] = newChild;
newChildrenTop += 1;
}
// We've scanned the whole list.
assert(oldChildrenTop == oldChildrenBottom + 1);
assert(newChildrenTop == newChildrenBottom + 1);
assert(newChildSemantics.length - newChildrenTop == oldSemantics.length - oldChildrenTop);
newChildrenBottom = newChildSemantics.length - 1;
oldChildrenBottom = oldSemantics.length - 1;
// Update the bottom of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final SemanticsNode oldChild = oldSemantics[oldChildrenTop];
final CustomPainterSemantics newSemantics = newChildSemantics[newChildrenTop];
assert(_canUpdateSemanticsChild(oldChild, newSemantics));
final SemanticsNode newChild = _updateSemanticsChild(oldChild, newSemantics);
assert(oldChild == newChild);
newChildren[newChildrenTop] = newChild;
newChildrenTop += 1;
oldChildrenTop += 1;
}
assert(() {
for (final SemanticsNode? node in newChildren) {
assert(node != null);
}
return true;
}());
return newChildren.cast<SemanticsNode>();
}
/// Whether `oldChild` can be updated with properties from `newSemantics`.
///
/// If `oldChild` can be updated, it is updated using [_updateSemanticsChild].
/// Otherwise, the node is replaced by a new instance of [SemanticsNode].
static bool _canUpdateSemanticsChild(SemanticsNode oldChild, CustomPainterSemantics newSemantics) {
return oldChild.key == newSemantics.key;
}
/// Updates `oldChild` using the properties of `newSemantics`.
///
/// This method requires that `_canUpdateSemanticsChild(oldChild, newSemantics)`
/// is true prior to calling it.
static SemanticsNode _updateSemanticsChild(SemanticsNode? oldChild, CustomPainterSemantics newSemantics) {
assert(oldChild == null || _canUpdateSemanticsChild(oldChild, newSemantics));
final SemanticsNode newChild = oldChild ?? SemanticsNode(
key: newSemantics.key,
);
final SemanticsProperties properties = newSemantics.properties;
final SemanticsConfiguration config = SemanticsConfiguration();
if (properties.sortKey != null) {
config.sortKey = properties.sortKey;
}
if (properties.checked != null) {
config.isChecked = properties.checked;
}
if (properties.mixed != null) {
config.isCheckStateMixed = properties.mixed;
}
if (properties.selected != null) {
config.isSelected = properties.selected!;
}
if (properties.button != null) {
config.isButton = properties.button!;
}
if (properties.expanded != null) {
config.isExpanded = properties.expanded;
}
if (properties.link != null) {
config.isLink = properties.link!;
}
if (properties.textField != null) {
config.isTextField = properties.textField!;
}
if (properties.slider != null) {
config.isSlider = properties.slider!;
}
if (properties.keyboardKey != null) {
config.isKeyboardKey = properties.keyboardKey!;
}
if (properties.readOnly != null) {
config.isReadOnly = properties.readOnly!;
}
if (properties.focusable != null) {
config.isFocusable = properties.focusable!;
}
if (properties.focused != null) {
config.isFocused = properties.focused!;
}
if (properties.enabled != null) {
config.isEnabled = properties.enabled;
}
if (properties.inMutuallyExclusiveGroup != null) {
config.isInMutuallyExclusiveGroup = properties.inMutuallyExclusiveGroup!;
}
if (properties.obscured != null) {
config.isObscured = properties.obscured!;
}
if (properties.multiline != null) {
config.isMultiline = properties.multiline!;
}
if (properties.hidden != null) {
config.isHidden = properties.hidden!;
}
if (properties.header != null) {
config.isHeader = properties.header!;
}
if (properties.scopesRoute != null) {
config.scopesRoute = properties.scopesRoute!;
}
if (properties.namesRoute != null) {
config.namesRoute = properties.namesRoute!;
}
if (properties.liveRegion != null) {
config.liveRegion = properties.liveRegion!;
}
if (properties.maxValueLength != null) {
config.maxValueLength = properties.maxValueLength;
}
if (properties.currentValueLength != null) {
config.currentValueLength = properties.currentValueLength;
}
if (properties.toggled != null) {
config.isToggled = properties.toggled;
}
if (properties.image != null) {
config.isImage = properties.image!;
}
if (properties.label != null) {
config.label = properties.label!;
}
if (properties.value != null) {
config.value = properties.value!;
}
if (properties.increasedValue != null) {
config.increasedValue = properties.increasedValue!;
}
if (properties.decreasedValue != null) {
config.decreasedValue = properties.decreasedValue!;
}
if (properties.hint != null) {
config.hint = properties.hint!;
}
if (properties.textDirection != null) {
config.textDirection = properties.textDirection;
}
if (properties.onTap != null) {
config.onTap = properties.onTap;
}
if (properties.onLongPress != null) {
config.onLongPress = properties.onLongPress;
}
if (properties.onScrollLeft != null) {
config.onScrollLeft = properties.onScrollLeft;
}
if (properties.onScrollRight != null) {
config.onScrollRight = properties.onScrollRight;
}
if (properties.onScrollUp != null) {
config.onScrollUp = properties.onScrollUp;
}
if (properties.onScrollDown != null) {
config.onScrollDown = properties.onScrollDown;
}
if (properties.onIncrease != null) {
config.onIncrease = properties.onIncrease;
}
if (properties.onDecrease != null) {
config.onDecrease = properties.onDecrease;
}
if (properties.onCopy != null) {
config.onCopy = properties.onCopy;
}
if (properties.onCut != null) {
config.onCut = properties.onCut;
}
if (properties.onPaste != null) {
config.onPaste = properties.onPaste;
}
if (properties.onMoveCursorForwardByCharacter != null) {
config.onMoveCursorForwardByCharacter = properties.onMoveCursorForwardByCharacter;
}
if (properties.onMoveCursorBackwardByCharacter != null) {
config.onMoveCursorBackwardByCharacter = properties.onMoveCursorBackwardByCharacter;
}
if (properties.onMoveCursorForwardByWord != null) {
config.onMoveCursorForwardByWord = properties.onMoveCursorForwardByWord;
}
if (properties.onMoveCursorBackwardByWord != null) {
config.onMoveCursorBackwardByWord = properties.onMoveCursorBackwardByWord;
}
if (properties.onSetSelection != null) {
config.onSetSelection = properties.onSetSelection;
}
if (properties.onSetText != null) {
config.onSetText = properties.onSetText;
}
if (properties.onDidGainAccessibilityFocus != null) {
config.onDidGainAccessibilityFocus = properties.onDidGainAccessibilityFocus;
}
if (properties.onDidLoseAccessibilityFocus != null) {
config.onDidLoseAccessibilityFocus = properties.onDidLoseAccessibilityFocus;
}
if (properties.onDismiss != null) {
config.onDismiss = properties.onDismiss;
}
newChild.updateWith(
config: config,
// As of now CustomPainter does not support multiple tree levels.
childrenInInversePaintOrder: const <SemanticsNode>[],
);
newChild
..rect = newSemantics.rect
..transform = newSemantics.transform
..tags = newSemantics.tags;
return newChild;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(MessageProperty('painter', '$painter'));
properties.add(MessageProperty('foregroundPainter', '$foregroundPainter', level: foregroundPainter != null ? DiagnosticLevel.info : DiagnosticLevel.fine));
properties.add(DiagnosticsProperty<Size>('preferredSize', preferredSize, defaultValue: Size.zero));
properties.add(DiagnosticsProperty<bool>('isComplex', isComplex, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('willChange', willChange, defaultValue: false));
}
}
| flutter/packages/flutter/lib/src/rendering/custom_paint.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/custom_paint.dart",
"repo_id": "flutter",
"token_count": 13320
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'layer.dart';
import 'object.dart';
/// The options that control whether the performance overlay displays certain
/// aspects of the compositor.
enum PerformanceOverlayOption {
// these must be in the order needed for their index values to match the
// constants in //engine/src/sky/compositor/performance_overlay_layer.h
/// Display the frame time and FPS of the last frame rendered. This field is
/// updated every frame.
///
/// This is the time spent by the rasterizer as it tries
/// to convert the layer tree obtained from the widgets into OpenGL commands
/// and tries to flush them onto the screen. When the total time taken by this
/// step exceeds the frame slice, a frame is lost.
displayRasterizerStatistics,
/// Display the rasterizer frame times as they change over a set period of
/// time in the form of a graph. The y axis of the graph denotes the total
/// time spent by the rasterizer as a fraction of the total frame slice. When
/// the bar turns red, a frame is lost.
visualizeRasterizerStatistics,
/// Display the frame time and FPS at which the interface can construct a
/// layer tree for the rasterizer (whose behavior is described above) to
/// consume.
///
/// This involves all layout, animations, etc. When the total time taken by
/// this step exceeds the frame slice, a frame is lost.
displayEngineStatistics,
/// Display the engine frame times as they change over a set period of time
/// in the form of a graph. The y axis of the graph denotes the total time
/// spent by the engine as a fraction of the total frame slice. When the bar
/// turns red, a frame is lost.
visualizeEngineStatistics,
}
/// Displays performance statistics.
///
/// The overlay shows two time series. The first shows how much time was
/// required on this thread to produce each frame. The second shows how much
/// time was required on the raster thread (formerly known as the GPU thread)
/// to produce each frame. Ideally, both these values would be less than
/// the total frame budget for the hardware on which the app is running.
/// For example, if the hardware has a screen that updates at 60 Hz, each
/// thread should ideally spend less than 16ms producing each frame.
/// This ideal condition is indicated by a green vertical line for each thread.
/// Otherwise, the performance overlay shows a red vertical line.
///
/// The simplest way to show the performance overlay is to set
/// [MaterialApp.showPerformanceOverlay] or [WidgetsApp.showPerformanceOverlay]
/// to true.
class RenderPerformanceOverlay extends RenderBox {
/// Creates a performance overlay render object.
RenderPerformanceOverlay({
int optionsMask = 0,
int rasterizerThreshold = 0,
bool checkerboardRasterCacheImages = false,
bool checkerboardOffscreenLayers = false,
}) : _optionsMask = optionsMask,
_rasterizerThreshold = rasterizerThreshold,
_checkerboardRasterCacheImages = checkerboardRasterCacheImages,
_checkerboardOffscreenLayers = checkerboardOffscreenLayers;
/// The mask is created by shifting 1 by the index of the specific
/// [PerformanceOverlayOption] to enable.
int get optionsMask => _optionsMask;
int _optionsMask;
set optionsMask(int value) {
if (value == _optionsMask) {
return;
}
_optionsMask = value;
markNeedsPaint();
}
/// The rasterizer threshold is an integer specifying the number of frame
/// intervals that the rasterizer must miss before it decides that the frame
/// is suitable for capturing an SkPicture trace for further analysis.
int get rasterizerThreshold => _rasterizerThreshold;
int _rasterizerThreshold;
set rasterizerThreshold(int value) {
if (value == _rasterizerThreshold) {
return;
}
_rasterizerThreshold = value;
markNeedsPaint();
}
/// Whether the raster cache should checkerboard cached entries.
bool get checkerboardRasterCacheImages => _checkerboardRasterCacheImages;
bool _checkerboardRasterCacheImages;
set checkerboardRasterCacheImages(bool value) {
if (value == _checkerboardRasterCacheImages) {
return;
}
_checkerboardRasterCacheImages = value;
markNeedsPaint();
}
/// Whether the compositor should checkerboard layers rendered to offscreen bitmaps.
bool get checkerboardOffscreenLayers => _checkerboardOffscreenLayers;
bool _checkerboardOffscreenLayers;
set checkerboardOffscreenLayers(bool value) {
if (value == _checkerboardOffscreenLayers) {
return;
}
_checkerboardOffscreenLayers = value;
markNeedsPaint();
}
@override
bool get sizedByParent => true;
@override
bool get alwaysNeedsCompositing => true;
@override
double computeMinIntrinsicWidth(double height) {
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
return 0.0;
}
double get _intrinsicHeight {
const double kDefaultGraphHeight = 80.0;
double result = 0.0;
if ((optionsMask | (1 << PerformanceOverlayOption.displayRasterizerStatistics.index) > 0) ||
(optionsMask | (1 << PerformanceOverlayOption.visualizeRasterizerStatistics.index) > 0)) {
result += kDefaultGraphHeight;
}
if ((optionsMask | (1 << PerformanceOverlayOption.displayEngineStatistics.index) > 0) ||
(optionsMask | (1 << PerformanceOverlayOption.visualizeEngineStatistics.index) > 0)) {
result += kDefaultGraphHeight;
}
return result;
}
@override
double computeMinIntrinsicHeight(double width) {
return _intrinsicHeight;
}
@override
double computeMaxIntrinsicHeight(double width) {
return _intrinsicHeight;
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return constraints.constrain(Size(double.infinity, _intrinsicHeight));
}
@override
void paint(PaintingContext context, Offset offset) {
assert(needsCompositing);
context.addLayer(PerformanceOverlayLayer(
overlayRect: Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height),
optionsMask: optionsMask,
rasterizerThreshold: rasterizerThreshold,
checkerboardRasterCacheImages: checkerboardRasterCacheImages,
checkerboardOffscreenLayers: checkerboardOffscreenLayers,
));
}
}
| flutter/packages/flutter/lib/src/rendering/performance_overlay.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/performance_overlay.dart",
"repo_id": "flutter",
"token_count": 1922
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/semantics.dart';
import 'box.dart';
import 'object.dart';
import 'sliver.dart';
import 'viewport.dart';
import 'viewport_offset.dart';
// Trims the specified edges of the given `Rect` [original], so that they do not
// exceed the given values.
Rect? _trim(
Rect? original, {
double top = -double.infinity,
double right = double.infinity,
double bottom = double.infinity,
double left = -double.infinity,
}) => original?.intersect(Rect.fromLTRB(left, top, right, bottom));
/// Specifies how a stretched header is to trigger an [AsyncCallback].
///
/// See also:
///
/// * [SliverAppBar], which creates a header that can be stretched into an
/// overscroll area and trigger a callback function.
class OverScrollHeaderStretchConfiguration {
/// Creates an object that specifies how a stretched header may activate an
/// [AsyncCallback].
OverScrollHeaderStretchConfiguration({
this.stretchTriggerOffset = 100.0,
this.onStretchTrigger,
});
/// The offset of overscroll required to trigger the [onStretchTrigger].
final double stretchTriggerOffset;
/// The callback function to be executed when a user over-scrolls to the
/// offset specified by [stretchTriggerOffset].
final AsyncCallback? onStretchTrigger;
}
/// {@template flutter.rendering.PersistentHeaderShowOnScreenConfiguration}
/// Specifies how a pinned header or a floating header should react to
/// [RenderObject.showOnScreen] calls.
/// {@endtemplate}
@immutable
class PersistentHeaderShowOnScreenConfiguration {
/// Creates an object that specifies how a pinned or floating persistent header
/// should behave in response to [RenderObject.showOnScreen] calls.
const PersistentHeaderShowOnScreenConfiguration({
this.minShowOnScreenExtent = double.negativeInfinity,
this.maxShowOnScreenExtent = double.infinity,
}) : assert(minShowOnScreenExtent <= maxShowOnScreenExtent);
/// The smallest the floating header can expand to in the main axis direction,
/// in response to a [RenderObject.showOnScreen] call, in addition to its
/// [RenderSliverPersistentHeader.minExtent].
///
/// When a floating persistent header is told to show a [Rect] on screen, it
/// may expand itself to accommodate the [Rect]. The minimum extent that is
/// allowed for such expansion is either
/// [RenderSliverPersistentHeader.minExtent] or [minShowOnScreenExtent],
/// whichever is larger. If the persistent header's current extent is already
/// larger than that maximum extent, it will remain unchanged.
///
/// This parameter can be set to the persistent header's `maxExtent` (or
/// `double.infinity`) so the persistent header will always try to expand when
/// [RenderObject.showOnScreen] is called on it.
///
/// Defaults to [double.negativeInfinity], must be less than or equal to
/// [maxShowOnScreenExtent]. Has no effect unless the persistent header is a
/// floating header.
final double minShowOnScreenExtent;
/// The biggest the floating header can expand to in the main axis direction,
/// in response to a [RenderObject.showOnScreen] call, in addition to its
/// [RenderSliverPersistentHeader.maxExtent].
///
/// When a floating persistent header is told to show a [Rect] on screen, it
/// may expand itself to accommodate the [Rect]. The maximum extent that is
/// allowed for such expansion is either
/// [RenderSliverPersistentHeader.maxExtent] or [maxShowOnScreenExtent],
/// whichever is smaller. If the persistent header's current extent is already
/// larger than that maximum extent, it will remain unchanged.
///
/// This parameter can be set to the persistent header's `minExtent` (or
/// `double.negativeInfinity`) so the persistent header will never try to
/// expand when [RenderObject.showOnScreen] is called on it.
///
/// Defaults to [double.infinity], must be greater than or equal to
/// [minShowOnScreenExtent]. Has no effect unless the persistent header is a
/// floating header.
final double maxShowOnScreenExtent;
}
/// A base class for slivers that have a [RenderBox] child which scrolls
/// normally, except that when it hits the leading edge (typically the top) of
/// the viewport, it shrinks to a minimum size ([minExtent]).
///
/// This class primarily provides helpers for managing the child, in particular:
///
/// * [layoutChild], which applies min and max extents and a scroll offset to
/// lay out the child. This is normally called from [performLayout].
///
/// * [childExtent], to convert the child's box layout dimensions to the sliver
/// geometry model.
///
/// * hit testing, painting, and other details of the sliver protocol.
///
/// Subclasses must implement [performLayout], [minExtent], and [maxExtent], and
/// typically also will implement [updateChild].
abstract class RenderSliverPersistentHeader extends RenderSliver with RenderObjectWithChildMixin<RenderBox>, RenderSliverHelpers {
/// Creates a sliver that changes its size when scrolled to the start of the
/// viewport.
///
/// This is an abstract class; this constructor only initializes the [child].
RenderSliverPersistentHeader({
RenderBox? child,
this.stretchConfiguration,
}) {
this.child = child;
}
late double _lastStretchOffset;
/// The biggest that this render object can become, in the main axis direction.
///
/// This value should not be based on the child. If it changes, call
/// [markNeedsLayout].
double get maxExtent;
/// The smallest that this render object can become, in the main axis direction.
///
/// If this is based on the intrinsic dimensions of the child, the child
/// should be measured during [updateChild] and the value cached and returned
/// here. The [updateChild] method will automatically be invoked any time the
/// child changes its intrinsic dimensions.
double get minExtent;
/// The dimension of the child in the main axis.
@protected
double get childExtent {
if (child == null) {
return 0.0;
}
assert(child!.hasSize);
return switch (constraints.axis) {
Axis.vertical => child!.size.height,
Axis.horizontal => child!.size.width,
};
}
bool _needsUpdateChild = true;
double _lastShrinkOffset = 0.0;
bool _lastOverlapsContent = false;
/// Defines the parameters used to execute an [AsyncCallback] when a
/// stretching header over-scrolls.
///
/// If [stretchConfiguration] is null then callback is not triggered.
///
/// See also:
///
/// * [SliverAppBar], which creates a header that can stretched into an
/// overscroll area and trigger a callback function.
OverScrollHeaderStretchConfiguration? stretchConfiguration;
/// Update the child render object if necessary.
///
/// Called before the first layout, any time [markNeedsLayout] is called, and
/// any time the scroll offset changes. The `shrinkOffset` is the difference
/// between the [maxExtent] and the current size. Zero means the header is
/// fully expanded, any greater number up to [maxExtent] means that the header
/// has been scrolled by that much. The `overlapsContent` argument is true if
/// the sliver's leading edge is beyond its normal place in the viewport
/// contents, and false otherwise. It may still paint beyond its normal place
/// if the [minExtent] after this call is greater than the amount of space that
/// would normally be left.
///
/// The render object will size itself to the larger of (a) the [maxExtent]
/// minus the child's intrinsic height and (b) the [maxExtent] minus the
/// shrink offset.
///
/// When this method is called by [layoutChild], the [child] can be set,
/// mutated, or replaced. (It should not be called outside [layoutChild].)
///
/// Any time this method would mutate the child, call [markNeedsLayout].
@protected
void updateChild(double shrinkOffset, bool overlapsContent) { }
@override
void markNeedsLayout() {
// This is automatically called whenever the child's intrinsic dimensions
// change, at which point we should remeasure them during the next layout.
_needsUpdateChild = true;
super.markNeedsLayout();
}
/// Lays out the [child].
///
/// This is called by [performLayout]. It applies the given `scrollOffset`
/// (which need not match the offset given by the [constraints]) and the
/// `maxExtent` (which need not match the value returned by the [maxExtent]
/// getter).
///
/// The `overlapsContent` argument is passed to [updateChild].
@protected
void layoutChild(double scrollOffset, double maxExtent, { bool overlapsContent = false }) {
final double shrinkOffset = math.min(scrollOffset, maxExtent);
if (_needsUpdateChild || _lastShrinkOffset != shrinkOffset || _lastOverlapsContent != overlapsContent) {
invokeLayoutCallback<SliverConstraints>((SliverConstraints constraints) {
assert(constraints == this.constraints);
updateChild(shrinkOffset, overlapsContent);
});
_lastShrinkOffset = shrinkOffset;
_lastOverlapsContent = overlapsContent;
_needsUpdateChild = false;
}
assert(() {
if (minExtent <= maxExtent) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The maxExtent for this $runtimeType is less than its minExtent.'),
DoubleProperty('The specified maxExtent was', maxExtent),
DoubleProperty('The specified minExtent was', minExtent),
]);
}());
double stretchOffset = 0.0;
if (stretchConfiguration != null && constraints.scrollOffset == 0.0) {
stretchOffset += constraints.overlap.abs();
}
child?.layout(
constraints.asBoxConstraints(
maxExtent: math.max(minExtent, maxExtent - shrinkOffset) + stretchOffset,
),
parentUsesSize: true,
);
if (stretchConfiguration != null &&
stretchConfiguration!.onStretchTrigger != null &&
stretchOffset >= stretchConfiguration!.stretchTriggerOffset &&
_lastStretchOffset <= stretchConfiguration!.stretchTriggerOffset) {
stretchConfiguration!.onStretchTrigger!();
}
_lastStretchOffset = stretchOffset;
}
/// Returns the distance from the leading _visible_ edge of the sliver to the
/// side of the child closest to that edge, in the scroll axis direction.
///
/// For example, if the [constraints] describe this sliver as having an axis
/// direction of [AxisDirection.down], then this is the distance from the top
/// of the visible portion of the sliver to the top of the child. If the child
/// is scrolled partially off the top of the viewport, then this will be
/// negative. On the other hand, if the [constraints] describe this sliver as
/// having an axis direction of [AxisDirection.up], then this is the distance
/// from the bottom of the visible portion of the sliver to the bottom of the
/// child. In both cases, this is the direction of increasing
/// [SliverConstraints.scrollOffset].
///
/// Calling this when the child is not visible is not valid.
///
/// The argument must be the value of the [child] property.
///
/// This must be implemented by [RenderSliverPersistentHeader] subclasses.
///
/// If there is no child, this should return 0.0.
@override
double childMainAxisPosition(covariant RenderObject child) => super.childMainAxisPosition(child);
@override
bool hitTestChildren(SliverHitTestResult result, { required double mainAxisPosition, required double crossAxisPosition }) {
assert(geometry!.hitTestExtent > 0.0);
if (child != null) {
return hitTestBoxChild(BoxHitTestResult.wrap(result), child!, mainAxisPosition: mainAxisPosition, crossAxisPosition: crossAxisPosition);
}
return false;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
assert(child == this.child);
applyPaintTransformForBoxChild(child as RenderBox, transform);
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null && geometry!.visible) {
offset += switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
AxisDirection.up => Offset(0.0, geometry!.paintExtent - childMainAxisPosition(child!) - childExtent),
AxisDirection.left => Offset(geometry!.paintExtent - childMainAxisPosition(child!) - childExtent, 0.0),
AxisDirection.right => Offset(childMainAxisPosition(child!), 0.0),
AxisDirection.down => Offset(0.0, childMainAxisPosition(child!)),
};
context.paintChild(child!, offset);
}
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.addTagForChildren(RenderViewport.excludeFromScrolling);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty.lazy('maxExtent', () => maxExtent));
properties.add(DoubleProperty.lazy('child position', () => childMainAxisPosition(child!)));
}
}
/// A sliver with a [RenderBox] child which scrolls normally, except that when
/// it hits the leading edge (typically the top) of the viewport, it shrinks to
/// a minimum size before continuing to scroll.
///
/// This sliver makes no effort to avoid overlapping other content.
abstract class RenderSliverScrollingPersistentHeader extends RenderSliverPersistentHeader {
/// Creates a sliver that shrinks when it hits the start of the viewport, then
/// scrolls off.
RenderSliverScrollingPersistentHeader({
super.child,
super.stretchConfiguration,
});
// Distance from our leading edge to the child's leading edge, in the axis
// direction. Negative if we're scrolled off the top.
double? _childPosition;
/// Updates [geometry], and returns the new value for [childMainAxisPosition].
///
/// This is used by [performLayout].
@protected
double updateGeometry() {
double stretchOffset = 0.0;
if (stretchConfiguration != null) {
stretchOffset += constraints.overlap.abs();
}
final double maxExtent = this.maxExtent;
final double paintExtent = maxExtent - constraints.scrollOffset;
final double cacheExtent = calculateCacheOffset(
constraints,
from: 0.0,
to: maxExtent,
);
geometry = SliverGeometry(
cacheExtent: cacheExtent,
scrollExtent: maxExtent,
paintOrigin: math.min(constraints.overlap, 0.0),
paintExtent: clampDouble(paintExtent, 0.0, constraints.remainingPaintExtent),
maxPaintExtent: maxExtent + stretchOffset,
hasVisualOverflow: true, // Conservatively say we do have overflow to avoid complexity.
);
return stretchOffset > 0 ? 0.0 : math.min(0.0, paintExtent - childExtent);
}
@override
void performLayout() {
final SliverConstraints constraints = this.constraints;
final double maxExtent = this.maxExtent;
layoutChild(constraints.scrollOffset, maxExtent);
final double paintExtent = maxExtent - constraints.scrollOffset;
geometry = SliverGeometry(
scrollExtent: maxExtent,
paintOrigin: math.min(constraints.overlap, 0.0),
paintExtent: clampDouble(paintExtent, 0.0, constraints.remainingPaintExtent),
maxPaintExtent: maxExtent,
hasVisualOverflow: true, // Conservatively say we do have overflow to avoid complexity.
);
_childPosition = updateGeometry();
}
@override
double childMainAxisPosition(RenderBox child) {
assert(child == this.child);
assert(_childPosition != null);
return _childPosition!;
}
}
/// A sliver with a [RenderBox] child which never scrolls off the viewport in
/// the positive scroll direction, and which first scrolls on at a full size but
/// then shrinks as the viewport continues to scroll.
///
/// This sliver avoids overlapping other earlier slivers where possible.
abstract class RenderSliverPinnedPersistentHeader extends RenderSliverPersistentHeader {
/// Creates a sliver that shrinks when it hits the start of the viewport, then
/// stays pinned there.
RenderSliverPinnedPersistentHeader({
super.child,
super.stretchConfiguration,
this.showOnScreenConfiguration = const PersistentHeaderShowOnScreenConfiguration(),
});
/// Specifies the persistent header's behavior when `showOnScreen` is called.
///
/// If set to null, the persistent header will delegate the `showOnScreen` call
/// to it's parent [RenderObject].
PersistentHeaderShowOnScreenConfiguration? showOnScreenConfiguration;
@override
void performLayout() {
final SliverConstraints constraints = this.constraints;
final double maxExtent = this.maxExtent;
final bool overlapsContent = constraints.overlap > 0.0;
layoutChild(constraints.scrollOffset, maxExtent, overlapsContent: overlapsContent);
final double effectiveRemainingPaintExtent = math.max(0, constraints.remainingPaintExtent - constraints.overlap);
final double layoutExtent = clampDouble(maxExtent - constraints.scrollOffset, 0.0, effectiveRemainingPaintExtent);
final double stretchOffset = stretchConfiguration != null ?
constraints.overlap.abs() :
0.0;
geometry = SliverGeometry(
scrollExtent: maxExtent,
paintOrigin: constraints.overlap,
paintExtent: math.min(childExtent, effectiveRemainingPaintExtent),
layoutExtent: layoutExtent,
maxPaintExtent: maxExtent + stretchOffset,
maxScrollObstructionExtent: minExtent,
cacheExtent: layoutExtent > 0.0 ? -constraints.cacheOrigin + layoutExtent : layoutExtent,
hasVisualOverflow: true, // Conservatively say we do have overflow to avoid complexity.
);
}
@override
double childMainAxisPosition(RenderBox child) => 0.0;
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
final Rect? localBounds = descendant != null
? MatrixUtils.transformRect(descendant.getTransformTo(this), rect ?? descendant.paintBounds)
: rect;
final Rect? newRect = switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
AxisDirection.up => _trim(localBounds, bottom: childExtent),
AxisDirection.left => _trim(localBounds, right: childExtent),
AxisDirection.right => _trim(localBounds, left: 0),
AxisDirection.down => _trim(localBounds, top: 0),
};
super.showOnScreen(
descendant: this,
rect: newRect,
duration: duration,
curve: curve,
);
}
}
/// Specifies how a floating header is to be "snapped" (animated) into or out
/// of view.
///
/// See also:
///
/// * [RenderSliverFloatingPersistentHeader.maybeStartSnapAnimation] and
/// [RenderSliverFloatingPersistentHeader.maybeStopSnapAnimation], which
/// start or stop the floating header's animation.
/// * [SliverAppBar], which creates a header that can be pinned, floating,
/// and snapped into view via the corresponding parameters.
class FloatingHeaderSnapConfiguration {
/// Creates an object that specifies how a floating header is to be "snapped"
/// (animated) into or out of view.
FloatingHeaderSnapConfiguration({
this.curve = Curves.ease,
this.duration = const Duration(milliseconds: 300),
});
/// The snap animation curve.
final Curve curve;
/// The snap animation's duration.
final Duration duration;
}
/// A sliver with a [RenderBox] child which shrinks and scrolls like a
/// [RenderSliverScrollingPersistentHeader], but immediately comes back when the
/// user scrolls in the reverse direction.
///
/// See also:
///
/// * [RenderSliverFloatingPinnedPersistentHeader], which is similar but sticks
/// to the start of the viewport rather than scrolling off.
abstract class RenderSliverFloatingPersistentHeader extends RenderSliverPersistentHeader {
/// Creates a sliver that shrinks when it hits the start of the viewport, then
/// scrolls off, and comes back immediately when the user reverses the scroll
/// direction.
RenderSliverFloatingPersistentHeader({
super.child,
TickerProvider? vsync,
this.snapConfiguration,
super.stretchConfiguration,
required this.showOnScreenConfiguration,
}) : _vsync = vsync;
AnimationController? _controller;
late Animation<double> _animation;
double? _lastActualScrollOffset;
double? _effectiveScrollOffset;
// Important for pointer scrolling, which does not have the same concept of
// a hold and release scroll movement, like dragging.
// This keeps track of the last ScrollDirection when scrolling started.
ScrollDirection? _lastStartedScrollDirection;
// Distance from our leading edge to the child's leading edge, in the axis
// direction. Negative if we're scrolled off the top.
double? _childPosition;
@override
void detach() {
_controller?.dispose();
_controller = null; // lazily recreated if we're reattached.
super.detach();
}
/// A [TickerProvider] to use when animating the scroll position.
TickerProvider? get vsync => _vsync;
TickerProvider? _vsync;
set vsync(TickerProvider? value) {
if (value == _vsync) {
return;
}
_vsync = value;
if (value == null) {
_controller?.dispose();
_controller = null;
} else {
_controller?.resync(value);
}
}
/// Defines the parameters used to snap (animate) the floating header in and
/// out of view.
///
/// If [snapConfiguration] is null then the floating header does not snap.
///
/// See also:
///
/// * [RenderSliverFloatingPersistentHeader.maybeStartSnapAnimation] and
/// [RenderSliverFloatingPersistentHeader.maybeStopSnapAnimation], which
/// start or stop the floating header's animation.
/// * [SliverAppBar], which creates a header that can be pinned, floating,
/// and snapped into view via the corresponding parameters.
FloatingHeaderSnapConfiguration? snapConfiguration;
/// {@macro flutter.rendering.PersistentHeaderShowOnScreenConfiguration}
///
/// If set to null, the persistent header will delegate the `showOnScreen` call
/// to it's parent [RenderObject].
PersistentHeaderShowOnScreenConfiguration? showOnScreenConfiguration;
/// Updates [geometry], and returns the new value for [childMainAxisPosition].
///
/// This is used by [performLayout].
@protected
double updateGeometry() {
double stretchOffset = 0.0;
if (stretchConfiguration != null) {
stretchOffset += constraints.overlap.abs();
}
final double maxExtent = this.maxExtent;
final double paintExtent = maxExtent - _effectiveScrollOffset!;
final double layoutExtent = maxExtent - constraints.scrollOffset;
geometry = SliverGeometry(
scrollExtent: maxExtent,
paintOrigin: math.min(constraints.overlap, 0.0),
paintExtent: clampDouble(paintExtent, 0.0, constraints.remainingPaintExtent),
layoutExtent: clampDouble(layoutExtent, 0.0, constraints.remainingPaintExtent),
maxPaintExtent: maxExtent + stretchOffset,
hasVisualOverflow: true, // Conservatively say we do have overflow to avoid complexity.
);
return stretchOffset > 0 ? 0.0 : math.min(0.0, paintExtent - childExtent);
}
void _updateAnimation(Duration duration, double endValue, Curve curve) {
assert(
vsync != null,
'vsync must not be null if the floating header changes size animatedly.',
);
final AnimationController effectiveController =
_controller ??= AnimationController(vsync: vsync!, duration: duration)
..addListener(() {
if (_effectiveScrollOffset == _animation.value) {
return;
}
_effectiveScrollOffset = _animation.value;
markNeedsLayout();
});
_animation = effectiveController.drive(
Tween<double>(
begin: _effectiveScrollOffset,
end: endValue,
).chain(CurveTween(curve: curve)),
);
}
/// Update the last known ScrollDirection when scrolling began.
// ignore: use_setters_to_change_properties, (API predates enforcing the lint)
void updateScrollStartDirection(ScrollDirection direction) {
_lastStartedScrollDirection = direction;
}
/// If the header isn't already fully exposed, then scroll it into view.
void maybeStartSnapAnimation(ScrollDirection direction) {
final FloatingHeaderSnapConfiguration? snap = snapConfiguration;
if (snap == null) {
return;
}
if (direction == ScrollDirection.forward && _effectiveScrollOffset! <= 0.0) {
return;
}
if (direction == ScrollDirection.reverse && _effectiveScrollOffset! >= maxExtent) {
return;
}
_updateAnimation(
snap.duration,
direction == ScrollDirection.forward ? 0.0 : maxExtent,
snap.curve,
);
_controller?.forward(from: 0.0);
}
/// If a header snap animation or a [showOnScreen] expand animation is underway
/// then stop it.
void maybeStopSnapAnimation(ScrollDirection direction) {
_controller?.stop();
}
@override
void performLayout() {
final SliverConstraints constraints = this.constraints;
final double maxExtent = this.maxExtent;
if (_lastActualScrollOffset != null && // We've laid out at least once to get an initial position, and either
((constraints.scrollOffset < _lastActualScrollOffset!) || // we are scrolling back, so should reveal, or
(_effectiveScrollOffset! < maxExtent))) { // some part of it is visible, so should shrink or reveal as appropriate.
double delta = _lastActualScrollOffset! - constraints.scrollOffset;
final bool allowFloatingExpansion = constraints.userScrollDirection == ScrollDirection.forward
|| (_lastStartedScrollDirection != null && _lastStartedScrollDirection == ScrollDirection.forward);
if (allowFloatingExpansion) {
if (_effectiveScrollOffset! > maxExtent) {
// We're scrolled off-screen, but should reveal, so pretend we're just at the limit.
_effectiveScrollOffset = maxExtent;
}
} else {
if (delta > 0.0) {
// Disallow the expansion. (But allow shrinking, i.e. delta < 0.0 is fine.)
delta = 0.0;
}
}
_effectiveScrollOffset = clampDouble(_effectiveScrollOffset! - delta, 0.0, constraints.scrollOffset);
} else {
_effectiveScrollOffset = constraints.scrollOffset;
}
final bool overlapsContent = _effectiveScrollOffset! < constraints.scrollOffset;
layoutChild(
_effectiveScrollOffset!,
maxExtent,
overlapsContent: overlapsContent,
);
_childPosition = updateGeometry();
_lastActualScrollOffset = constraints.scrollOffset;
}
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
final PersistentHeaderShowOnScreenConfiguration? showOnScreen = showOnScreenConfiguration;
if (showOnScreen == null) {
return super.showOnScreen(descendant: descendant, rect: rect, duration: duration, curve: curve);
}
assert(child != null || descendant == null);
// We prefer the child's coordinate space (instead of the sliver's) because
// it's easier for us to convert the target rect into target extents: when
// the sliver is sitting above the leading edge (not possible with pinned
// headers), the leading edge of the sliver and the leading edge of the child
// will not be aligned. The only exception is when child is null (and thus
// descendant == null).
final Rect? childBounds = descendant != null
? MatrixUtils.transformRect(descendant.getTransformTo(child), rect ?? descendant.paintBounds)
: rect;
double targetExtent;
Rect? targetRect;
switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
case AxisDirection.up:
targetExtent = childExtent - (childBounds?.top ?? 0);
targetRect = _trim(childBounds, bottom: childExtent);
case AxisDirection.right:
targetExtent = childBounds?.right ?? childExtent;
targetRect = _trim(childBounds, left: 0);
case AxisDirection.down:
targetExtent = childBounds?.bottom ?? childExtent;
targetRect = _trim(childBounds, top: 0);
case AxisDirection.left:
targetExtent = childExtent - (childBounds?.left ?? 0);
targetRect = _trim(childBounds, right: childExtent);
}
// A stretch header can have a bigger childExtent than maxExtent.
final double effectiveMaxExtent = math.max(childExtent, maxExtent);
targetExtent = clampDouble(
clampDouble(
targetExtent,
showOnScreen.minShowOnScreenExtent,
showOnScreen.maxShowOnScreenExtent,
),
// Clamp the value back to the valid range after applying additional
// constraints. Contracting is not allowed.
childExtent,
effectiveMaxExtent);
// Expands the header if needed, with animation.
if (targetExtent > childExtent && _controller?.status != AnimationStatus.forward) {
final double targetScrollOffset = maxExtent - targetExtent;
assert(
vsync != null,
'vsync must not be null if the floating header changes size animatedly.',
);
_updateAnimation(duration, targetScrollOffset, curve);
_controller?.forward(from: 0.0);
}
super.showOnScreen(
descendant: descendant == null ? this : child,
rect: targetRect,
duration: duration,
curve: curve,
);
}
@override
double childMainAxisPosition(RenderBox child) {
assert(child == this.child);
return _childPosition ?? 0.0;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('effective scroll offset', _effectiveScrollOffset));
}
}
/// A sliver with a [RenderBox] child which shrinks and then remains pinned to
/// the start of the viewport like a [RenderSliverPinnedPersistentHeader], but
/// immediately grows when the user scrolls in the reverse direction.
///
/// See also:
///
/// * [RenderSliverFloatingPersistentHeader], which is similar but scrolls off
/// the top rather than sticking to it.
abstract class RenderSliverFloatingPinnedPersistentHeader extends RenderSliverFloatingPersistentHeader {
/// Creates a sliver that shrinks when it hits the start of the viewport, then
/// stays pinned there, and grows immediately when the user reverses the
/// scroll direction.
RenderSliverFloatingPinnedPersistentHeader({
super.child,
super.vsync,
super.snapConfiguration,
super.stretchConfiguration,
super.showOnScreenConfiguration,
});
@override
double updateGeometry() {
final double minExtent = this.minExtent;
final double minAllowedExtent = constraints.remainingPaintExtent > minExtent ?
minExtent :
constraints.remainingPaintExtent;
final double maxExtent = this.maxExtent;
final double paintExtent = maxExtent - _effectiveScrollOffset!;
final double clampedPaintExtent = clampDouble(paintExtent,
minAllowedExtent,
constraints.remainingPaintExtent,
);
final double layoutExtent = maxExtent - constraints.scrollOffset;
final double stretchOffset = stretchConfiguration != null ?
constraints.overlap.abs() :
0.0;
geometry = SliverGeometry(
scrollExtent: maxExtent,
paintOrigin: math.min(constraints.overlap, 0.0),
paintExtent: clampedPaintExtent,
layoutExtent: clampDouble(layoutExtent, 0.0, clampedPaintExtent),
maxPaintExtent: maxExtent + stretchOffset,
maxScrollObstructionExtent: minExtent,
hasVisualOverflow: true, // Conservatively say we do have overflow to avoid complexity.
);
return 0.0;
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_persistent_header.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_persistent_header.dart",
"repo_id": "flutter",
"token_count": 10050
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Overrides the setting of [SemanticsBinding.disableAnimations] for debugging
/// and testing.
///
/// This value is ignored in non-debug builds.
bool? debugSemanticsDisableAnimations;
| flutter/packages/flutter/lib/src/semantics/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/semantics/debug.dart",
"repo_id": "flutter",
"token_count": 91
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
export 'dart:typed_data' show ByteData;
/// A class that enables the dynamic loading of fonts at runtime.
///
/// The [FontLoader] class provides a builder pattern, where the caller builds
/// up the assets that make up a font family, then calls [load] to load the
/// entire font family into a running Flutter application.
class FontLoader {
/// Creates a new [FontLoader] that will load font assets for the specified
/// [family].
///
/// The font family will not be available for use until [load] has been
/// called.
FontLoader(this.family)
: _loaded = false,
_fontFutures = <Future<Uint8List>>[];
/// The font family being loaded.
///
/// The family groups a series of related font assets, each of which defines
/// how to render a specific [FontWeight] and [FontStyle] within the family.
final String family;
/// Registers a font asset to be loaded by this font loader.
///
/// The [bytes] argument specifies the actual font asset bytes. Currently,
/// only OpenType (OTF) and TrueType (TTF) fonts are supported.
void addFont(Future<ByteData> bytes) {
if (_loaded) {
throw StateError('FontLoader is already loaded');
}
_fontFutures.add(bytes.then(
(ByteData data) => Uint8List.view(data.buffer, data.offsetInBytes, data.lengthInBytes),
));
}
/// Loads this font loader's font [family] and all of its associated assets
/// into the Flutter engine, making the font available to the current
/// application.
///
/// This method should only be called once per font loader. Attempts to
/// load fonts from the same loader more than once will cause a [StateError]
/// to be thrown.
///
/// The returned future will complete with an error if any of the font asset
/// futures yield an error.
Future<void> load() async {
if (_loaded) {
throw StateError('FontLoader is already loaded');
}
_loaded = true;
final Iterable<Future<void>> loadFutures = _fontFutures.map(
(Future<Uint8List> f) => f.then<void>(
(Uint8List list) => loadFont(list, family),
),
);
await Future.wait(loadFutures.toList());
}
/// Hook called to load a font asset into the engine.
///
/// Subclasses may override this to replace the default loading logic with
/// custom logic (for example, to mock the underlying engine API in tests).
@protected
@visibleForTesting
Future<void> loadFont(Uint8List list, String family) {
return loadFontFromList(list, fontFamily: family);
}
bool _loaded;
final List<Future<Uint8List>> _fontFutures;
}
| flutter/packages/flutter/lib/src/services/font_loader.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/font_loader.dart",
"repo_id": "flutter",
"token_count": 860
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'keyboard_maps.g.dart';
import 'raw_keyboard.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'keyboard_key.g.dart' show LogicalKeyboardKey, PhysicalKeyboardKey;
/// Platform-specific key event data for Fuchsia.
///
/// This class is deprecated and will be removed. Platform specific key event
/// data will no longer be available. See [KeyEvent] for what is available.
///
/// This object contains information about key events obtained from Fuchsia's
/// `KeyData` interface.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyEventDataFuchsia extends RawKeyEventData {
/// Creates a key event data structure specific for Fuchsia.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEventDataFuchsia({
this.hidUsage = 0,
this.codePoint = 0,
this.modifiers = 0,
});
/// The USB HID usage.
///
/// See <http://www.usb.org/developers/hidpage/Hut1_12v2.pdf> for more
/// information.
final int hidUsage;
/// The Unicode code point represented by the key event, if any.
///
/// If there is no Unicode code point, this value is zero.
///
/// Dead keys are represented as Unicode combining characters.
final int codePoint;
/// The modifiers that were present when the key event occurred.
///
/// See <https://fuchsia.googlesource.com/garnet/+/master/public/fidl/fuchsia.ui.input/input_event_constants.fidl>
/// for the numerical values of the modifiers. Many of these are also
/// replicated as static constants in this class.
///
/// See also:
///
/// * [modifiersPressed], which returns a Map of currently pressed modifiers
/// and their keyboard side.
/// * [isModifierPressed], to see if a specific modifier is pressed.
/// * [isControlPressed], to see if a CTRL key is pressed.
/// * [isShiftPressed], to see if a SHIFT key is pressed.
/// * [isAltPressed], to see if an ALT key is pressed.
/// * [isMetaPressed], to see if a META key is pressed.
final int modifiers;
// Fuchsia only reports a single code point for the key label.
@override
String get keyLabel => codePoint == 0 ? '' : String.fromCharCode(codePoint);
@override
LogicalKeyboardKey get logicalKey {
// If the key has a printable representation, then make a logical key based
// on that.
if (codePoint != 0) {
final int flutterId = LogicalKeyboardKey.unicodePlane | codePoint & LogicalKeyboardKey.valueMask;
return kFuchsiaToLogicalKey[flutterId] ?? LogicalKeyboardKey(LogicalKeyboardKey.unicodePlane | codePoint & LogicalKeyboardKey.valueMask);
}
// Look to see if the hidUsage is one we know about and have a mapping for.
final LogicalKeyboardKey? newKey = kFuchsiaToLogicalKey[hidUsage | LogicalKeyboardKey.fuchsiaPlane];
if (newKey != null) {
return newKey;
}
// This is a non-printable key that we don't know about, so we mint a new
// code.
return LogicalKeyboardKey(hidUsage | LogicalKeyboardKey.fuchsiaPlane);
}
@override
PhysicalKeyboardKey get physicalKey => kFuchsiaToPhysicalKey[hidUsage] ?? PhysicalKeyboardKey(LogicalKeyboardKey.fuchsiaPlane + hidUsage);
bool _isLeftRightModifierPressed(KeyboardSide side, int anyMask, int leftMask, int rightMask) {
if (modifiers & anyMask == 0) {
return false;
}
return switch (side) {
KeyboardSide.any => true,
KeyboardSide.all => (modifiers & leftMask != 0) && (modifiers & rightMask != 0),
KeyboardSide.left => modifiers & leftMask != 0,
KeyboardSide.right => modifiers & rightMask != 0,
};
}
@override
bool isModifierPressed(ModifierKey key, { KeyboardSide side = KeyboardSide.any }) {
switch (key) {
case ModifierKey.controlModifier:
return _isLeftRightModifierPressed(side, modifierControl, modifierLeftControl, modifierRightControl);
case ModifierKey.shiftModifier:
return _isLeftRightModifierPressed(side, modifierShift, modifierLeftShift, modifierRightShift);
case ModifierKey.altModifier:
return _isLeftRightModifierPressed(side, modifierAlt, modifierLeftAlt, modifierRightAlt);
case ModifierKey.metaModifier:
return _isLeftRightModifierPressed(side, modifierMeta, modifierLeftMeta, modifierRightMeta);
case ModifierKey.capsLockModifier:
return modifiers & modifierCapsLock != 0;
case ModifierKey.numLockModifier:
case ModifierKey.scrollLockModifier:
case ModifierKey.functionModifier:
case ModifierKey.symbolModifier:
// Fuchsia doesn't have masks for these keys (yet).
return false;
}
}
@override
KeyboardSide? getModifierSide(ModifierKey key) {
KeyboardSide? findSide(int anyMask, int leftMask, int rightMask) {
final int combined = modifiers & anyMask;
if (combined == leftMask) {
return KeyboardSide.left;
} else if (combined == rightMask) {
return KeyboardSide.right;
} else if (combined == anyMask) {
return KeyboardSide.all;
}
return null;
}
switch (key) {
case ModifierKey.controlModifier:
return findSide(modifierControl, modifierLeftControl, modifierRightControl, );
case ModifierKey.shiftModifier:
return findSide(modifierShift, modifierLeftShift, modifierRightShift);
case ModifierKey.altModifier:
return findSide(modifierAlt, modifierLeftAlt, modifierRightAlt);
case ModifierKey.metaModifier:
return findSide(modifierMeta, modifierLeftMeta, modifierRightMeta);
case ModifierKey.capsLockModifier:
return (modifiers & modifierCapsLock == 0) ? null : KeyboardSide.all;
case ModifierKey.numLockModifier:
case ModifierKey.scrollLockModifier:
case ModifierKey.functionModifier:
case ModifierKey.symbolModifier:
// Fuchsia doesn't support these modifiers, so they can't be pressed.
return null;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<int>('hidUsage', hidUsage));
properties.add(DiagnosticsProperty<int>('codePoint', codePoint));
properties.add(DiagnosticsProperty<int>('modifiers', modifiers));
}
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is RawKeyEventDataFuchsia
&& other.hidUsage == hidUsage
&& other.codePoint == codePoint
&& other.modifiers == modifiers;
}
@override
int get hashCode => Object.hash(
hidUsage,
codePoint,
modifiers,
);
// Keyboard modifier masks for Fuchsia modifiers.
/// The [modifiers] field indicates that no modifier keys are pressed if it
/// equals this value.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierNone = 0x0;
/// This mask is used to check the [modifiers] field to test whether the CAPS
/// LOCK modifier key is on.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierCapsLock = 0x1;
/// This mask is used to check the [modifiers] field to test whether the left
/// SHIFT modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftShift = 0x2;
/// This mask is used to check the [modifiers] field to test whether the right
/// SHIFT modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightShift = 0x4;
/// This mask is used to check the [modifiers] field to test whether one of
/// the SHIFT modifier keys is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierShift = modifierLeftShift | modifierRightShift;
/// This mask is used to check the [modifiers] field to test whether the left
/// CTRL modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftControl = 0x8;
/// This mask is used to check the [modifiers] field to test whether the right
/// CTRL modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightControl = 0x10;
/// This mask is used to check the [modifiers] field to test whether one of
/// the CTRL modifier keys is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierControl = modifierLeftControl | modifierRightControl;
/// This mask is used to check the [modifiers] field to test whether the left
/// ALT modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftAlt = 0x20;
/// This mask is used to check the [modifiers] field to test whether the right
/// ALT modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightAlt = 0x40;
/// This mask is used to check the [modifiers] field to test whether one of
/// the ALT modifier keys is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierAlt = modifierLeftAlt | modifierRightAlt;
/// This mask is used to check the [modifiers] field to test whether the left
/// META modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierLeftMeta = 0x80;
/// This mask is used to check the [modifiers] field to test whether the right
/// META modifier key is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierRightMeta = 0x100;
/// This mask is used to check the [modifiers] field to test whether one of
/// the META modifier keys is pressed.
///
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierMeta = modifierLeftMeta | modifierRightMeta;
}
| flutter/packages/flutter/lib/src/services/raw_keyboard_fuchsia.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/raw_keyboard_fuchsia.dart",
"repo_id": "flutter",
"token_count": 3829
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:characters/characters.dart';
import 'package:flutter/foundation.dart';
import 'text_input.dart';
export 'package:flutter/foundation.dart' show TargetPlatform;
export 'text_input.dart' show TextEditingValue;
// Examples can assume:
// late RegExp _pattern;
/// Mechanisms for enforcing maximum length limits.
///
/// This is used by [TextField] to specify how the [TextField.maxLength] should
/// be applied.
///
/// {@template flutter.services.textFormatter.maxLengthEnforcement}
/// ### [MaxLengthEnforcement.enforced] versus
/// [MaxLengthEnforcement.truncateAfterCompositionEnds]
///
/// Both [MaxLengthEnforcement.enforced] and
/// [MaxLengthEnforcement.truncateAfterCompositionEnds] make sure the final
/// length of the text does not exceed the max length specified. The difference
/// is that [MaxLengthEnforcement.enforced] truncates all text while
/// [MaxLengthEnforcement.truncateAfterCompositionEnds] allows composing text to
/// exceed the limit. Allowing this "placeholder" composing text to exceed the
/// limit may provide a better user experience on some platforms for entering
/// ideographic characters (e.g. CJK characters) via composing on phonetic
/// keyboards.
///
/// Some input methods (Gboard on Android for example) initiate text composition
/// even for Latin characters, in which case the best experience may be to
/// truncate those composing characters with [MaxLengthEnforcement.enforced].
///
/// In fields that strictly support only a small subset of characters, such as
/// verification code fields, [MaxLengthEnforcement.enforced] may provide the
/// best experience.
/// {@endtemplate}
///
/// See also:
///
/// * [TextField.maxLengthEnforcement] which is used in conjunction with
/// [TextField.maxLength] to limit the length of user input. [TextField] also
/// provides a character counter to provide visual feedback.
enum MaxLengthEnforcement {
/// No enforcement applied to the editing value. It's possible to exceed the
/// max length.
none,
/// Keep the length of the text input from exceeding the max length even when
/// the text has an unfinished composing region.
enforced,
/// Users can still input text if the current value is composing even after
/// reaching the max length limit. After composing ends, the value will be
/// truncated.
truncateAfterCompositionEnds,
}
/// A [TextInputFormatter] can be optionally injected into an [EditableText]
/// to provide as-you-type validation and formatting of the text being edited.
///
/// Text modification should only be applied when text is being committed by the
/// IME and not on text under composition (i.e., only when
/// [TextEditingValue.composing] is collapsed).
///
/// See also the [FilteringTextInputFormatter], a subclass that
/// removes characters that the user tries to enter if they do, or do
/// not, match a given pattern (as applicable).
///
/// To create custom formatters, extend the [TextInputFormatter] class and
/// implement the [formatEditUpdate] method.
///
/// ## Handling emojis and other complex characters
/// {@macro flutter.widgets.EditableText.onChanged}
///
/// See also:
///
/// * [EditableText] on which the formatting apply.
/// * [FilteringTextInputFormatter], a provided formatter for filtering
/// characters.
abstract class TextInputFormatter {
/// This constructor enables subclasses to provide const constructors so that they can be used in const expressions.
const TextInputFormatter();
/// A shorthand to creating a custom [TextInputFormatter] which formats
/// incoming text input changes with the given function.
const factory TextInputFormatter.withFunction(TextInputFormatFunction formatFunction) = _SimpleTextInputFormatter;
/// Called when text is being typed or cut/copy/pasted in the [EditableText].
///
/// You can override the resulting text based on the previous text value and
/// the incoming new text value.
///
/// When formatters are chained, `oldValue` reflects the initial value of
/// [TextEditingValue] at the beginning of the chain.
TextEditingValue formatEditUpdate(
TextEditingValue oldValue,
TextEditingValue newValue,
);
}
/// Function signature expected for creating custom [TextInputFormatter]
/// shorthands via [TextInputFormatter.withFunction].
typedef TextInputFormatFunction = TextEditingValue Function(
TextEditingValue oldValue,
TextEditingValue newValue,
);
/// Wiring for [TextInputFormatter.withFunction].
class _SimpleTextInputFormatter extends TextInputFormatter {
const _SimpleTextInputFormatter(this.formatFunction);
final TextInputFormatFunction formatFunction;
@override
TextEditingValue formatEditUpdate(
TextEditingValue oldValue,
TextEditingValue newValue,
) {
return formatFunction(oldValue, newValue);
}
}
// A mutable, half-open range [`base`, `extent`) within a string.
class _MutableTextRange {
_MutableTextRange(this.base, this.extent);
static _MutableTextRange? fromComposingRange(TextRange range) {
return range.isValid && !range.isCollapsed
? _MutableTextRange(range.start, range.end)
: null;
}
static _MutableTextRange? fromTextSelection(TextSelection selection) {
return selection.isValid
? _MutableTextRange(selection.baseOffset, selection.extentOffset)
: null;
}
/// The start index of the range, inclusive.
///
/// The value of [base] should always be greater than or equal to 0, and can
/// be larger than, smaller than, or equal to [extent].
int base;
/// The end index of the range, exclusive.
///
/// The value of [extent] should always be greater than or equal to 0, and can
/// be larger than, smaller than, or equal to [base].
int extent;
}
// The intermediate state of a [FilteringTextInputFormatter] when it's
// formatting a new user input.
class _TextEditingValueAccumulator {
_TextEditingValueAccumulator(this.inputValue)
: selection = _MutableTextRange.fromTextSelection(inputValue.selection),
composingRegion = _MutableTextRange.fromComposingRange(inputValue.composing);
// The original string that was sent to the [FilteringTextInputFormatter] as
// input.
final TextEditingValue inputValue;
/// The [StringBuffer] that contains the string which has already been
/// formatted.
///
/// In a [FilteringTextInputFormatter], typically the replacement string,
/// instead of the original string within the given range, is written to this
/// [StringBuffer].
final StringBuffer stringBuffer = StringBuffer();
/// The updated selection, as well as the original selection from the input
/// [TextEditingValue] of the [FilteringTextInputFormatter].
///
/// This parameter will be null if the input [TextEditingValue.selection] is
/// invalid.
final _MutableTextRange? selection;
/// The updated composing region, as well as the original composing region
/// from the input [TextEditingValue] of the [FilteringTextInputFormatter].
///
/// This parameter will be null if the input [TextEditingValue.composing] is
/// invalid or collapsed.
final _MutableTextRange? composingRegion;
// Whether this state object has reached its end-of-life.
bool debugFinalized = false;
TextEditingValue finalize() {
debugFinalized = true;
final _MutableTextRange? selection = this.selection;
final _MutableTextRange? composingRegion = this.composingRegion;
return TextEditingValue(
text: stringBuffer.toString(),
composing: composingRegion == null || composingRegion.base == composingRegion.extent
? TextRange.empty
: TextRange(start: composingRegion.base, end: composingRegion.extent),
selection: selection == null
? const TextSelection.collapsed(offset: -1)
: TextSelection(
baseOffset: selection.base,
extentOffset: selection.extent,
// Try to preserve the selection affinity and isDirectional. This
// may not make sense if the selection has changed.
affinity: inputValue.selection.affinity,
isDirectional: inputValue.selection.isDirectional,
),
);
}
}
/// A [TextInputFormatter] that prevents the insertion of characters matching
/// (or not matching) a particular pattern, by replacing the characters with the
/// given [replacementString].
///
/// Instances of filtered characters found in the new [TextEditingValue]s
/// will be replaced by the [replacementString] which defaults to the empty
/// string, and the current [TextEditingValue.selection] and
/// [TextEditingValue.composing] region will be adjusted to account for the
/// replacement.
///
/// This formatter is typically used to match potentially recurring [Pattern]s
/// in the new [TextEditingValue]. It never completely rejects the new
/// [TextEditingValue] and falls back to the current [TextEditingValue] when the
/// given [filterPattern] fails to match. Consider using a different
/// [TextInputFormatter] such as:
///
/// ```dart
/// // _pattern is a RegExp or other Pattern object
/// TextInputFormatter.withFunction(
/// (TextEditingValue oldValue, TextEditingValue newValue) {
/// return _pattern.hasMatch(newValue.text) ? newValue : oldValue;
/// },
/// ),
/// ```
///
/// for accepting/rejecting new input based on a predicate on the full string.
/// As an example, [FilteringTextInputFormatter] typically shouldn't be used
/// with [RegExp]s that contain positional matchers (`^` or `$`) since these
/// patterns are usually meant for matching the whole string.
///
/// ### Quote characters on iOS
///
/// When filtering single (`'`) or double (`"`) quote characters, be aware that
/// the default iOS keyboard actually inserts special directional versions of
/// these characters (`‘` and `’` for single quote, and `“` and `”` for double
/// quote). Consider including all three variants in your regular expressions to
/// support iOS.
class FilteringTextInputFormatter extends TextInputFormatter {
/// Creates a formatter that replaces banned patterns with the given
/// [replacementString].
///
/// If [allow] is true, then the filter pattern is an allow list,
/// and characters must match the pattern to be accepted. See also
/// the [FilteringTextInputFormatter.allow()] constructor.
///
/// If [allow] is false, then the filter pattern is a deny list,
/// and characters that match the pattern are rejected. See also
/// the [FilteringTextInputFormatter.deny] constructor.
FilteringTextInputFormatter(
this.filterPattern, {
required this.allow,
this.replacementString = '',
});
/// Creates a formatter that only allows characters matching a pattern.
FilteringTextInputFormatter.allow(
Pattern filterPattern, {
String replacementString = '',
}) : this(filterPattern, allow: true, replacementString: replacementString);
/// Creates a formatter that blocks characters matching a pattern.
FilteringTextInputFormatter.deny(
Pattern filterPattern, {
String replacementString = '',
}) : this(filterPattern, allow: false, replacementString: replacementString);
/// A [Pattern] to match or replace in incoming [TextEditingValue]s.
///
/// The behavior of the pattern depends on the [allow] property. If
/// it is true, then this is an allow list, specifying a pattern that
/// characters must match to be accepted. Otherwise, it is a deny list,
/// specifying a pattern that characters must not match to be accepted.
///
/// {@tool snippet}
/// Typically the pattern is a regular expression, as in:
///
/// ```dart
/// FilteringTextInputFormatter onlyDigits = FilteringTextInputFormatter.allow(RegExp(r'[0-9]'));
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// If the pattern is a single character, a pattern consisting of a
/// [String] can be used:
///
/// ```dart
/// FilteringTextInputFormatter noTabs = FilteringTextInputFormatter.deny('\t');
/// ```
/// {@end-tool}
final Pattern filterPattern;
/// Whether the pattern is an allow list or not.
///
/// When true, [filterPattern] denotes an allow list: characters
/// must match the filter to be allowed.
///
/// When false, [filterPattern] denotes a deny list: characters
/// that match the filter are disallowed.
final bool allow;
/// String used to replace banned patterns.
///
/// For deny lists ([allow] is false), each match of the
/// [filterPattern] is replaced with this string. If [filterPattern]
/// can match more than one character at a time, then this can
/// result in multiple characters being replaced by a single
/// instance of this [replacementString].
///
/// For allow lists ([allow] is true), sequences between matches of
/// [filterPattern] are replaced as one, regardless of the number of
/// characters.
///
/// For example, consider a [filterPattern] consisting of just the
/// letter "o", applied to text field whose initial value is the
/// string "Into The Woods", with the [replacementString] set to
/// `*`.
///
/// If [allow] is true, then the result will be "*o*oo*". Each
/// sequence of characters not matching the pattern is replaced by
/// its own single copy of the replacement string, regardless of how
/// many characters are in that sequence.
///
/// If [allow] is false, then the result will be "Int* the W**ds".
/// Every matching sequence is replaced, and each "o" matches the
/// pattern separately.
///
/// If the pattern was the [RegExp] `o+`, the result would be the
/// same in the case where [allow] is true, but in the case where
/// [allow] is false, the result would be "Int* the W*ds" (with the
/// two "o"s replaced by a single occurrence of the replacement
/// string) because both of the "o"s would be matched simultaneously
/// by the pattern.
///
/// The filter may adjust the selection and the composing region of the text
/// after applying the text replacement, such that they still cover the same
/// text. For instance, if the pattern was `o+` and the last character "s" was
/// selected: "Into The Wood|s|", then the result will be "Into The W*d|s|",
/// with the selection still around the same character "s" despite that it is
/// now the 12th character.
///
/// In the case where one end point of the selection (or the composing region)
/// is strictly inside the banned pattern (for example, "Into The |Wo|ods"),
/// that endpoint will be moved to the end of the replacement string (it will
/// become "Into The |W*|ds" if the pattern was `o+` and the original text and
/// selection were "Into The |Wo|ods").
final String replacementString;
@override
TextEditingValue formatEditUpdate(
TextEditingValue oldValue, // unused.
TextEditingValue newValue,
) {
final _TextEditingValueAccumulator formatState = _TextEditingValueAccumulator(newValue);
assert(!formatState.debugFinalized);
final Iterable<Match> matches = filterPattern.allMatches(newValue.text);
Match? previousMatch;
for (final Match match in matches) {
assert(match.end >= match.start);
// Compute the non-match region between this `Match` and the previous
// `Match`. Depending on the value of `allow`, either the match region or
// the non-match region is the banned pattern.
//
// The non-matching region.
_processRegion(allow, previousMatch?.end ?? 0, match.start, formatState);
assert(!formatState.debugFinalized);
// The matched region.
_processRegion(!allow, match.start, match.end, formatState);
assert(!formatState.debugFinalized);
previousMatch = match;
}
// Handle the last non-matching region between the last match region and the
// end of the text.
_processRegion(allow, previousMatch?.end ?? 0, newValue.text.length, formatState);
assert(!formatState.debugFinalized);
return formatState.finalize();
}
void _processRegion(bool isBannedRegion, int regionStart, int regionEnd, _TextEditingValueAccumulator state) {
final String replacementString = isBannedRegion
? (regionStart == regionEnd ? '' : this.replacementString)
: state.inputValue.text.substring(regionStart, regionEnd);
state.stringBuffer.write(replacementString);
if (replacementString.length == regionEnd - regionStart) {
// We don't have to adjust the indices if the replaced string and the
// replacement string have the same length.
return;
}
int adjustIndex(int originalIndex) {
// The length added by adding the replacementString.
final int replacedLength = originalIndex <= regionStart && originalIndex < regionEnd ? 0 : replacementString.length;
// The length removed by removing the replacementRange.
final int removedLength = originalIndex.clamp(regionStart, regionEnd) - regionStart;
return replacedLength - removedLength;
}
state.selection?.base += adjustIndex(state.inputValue.selection.baseOffset);
state.selection?.extent += adjustIndex(state.inputValue.selection.extentOffset);
state.composingRegion?.base += adjustIndex(state.inputValue.composing.start);
state.composingRegion?.extent += adjustIndex(state.inputValue.composing.end);
}
/// A [TextInputFormatter] that forces input to be a single line.
static final TextInputFormatter singleLineFormatter = FilteringTextInputFormatter.deny('\n');
/// A [TextInputFormatter] that takes in digits `[0-9]` only.
static final TextInputFormatter digitsOnly = FilteringTextInputFormatter.allow(RegExp(r'[0-9]'));
}
/// A [TextInputFormatter] that prevents the insertion of more characters
/// than allowed.
///
/// Since this formatter only prevents new characters from being added to the
/// text, it preserves the existing [TextEditingValue.selection].
///
/// Characters are counted as user-perceived characters using the
/// [characters](https://pub.dev/packages/characters) package, so even complex
/// characters like extended grapheme clusters and surrogate pairs are counted
/// as single characters.
///
/// See also:
/// * [maxLength], which discusses the precise meaning of "number of
/// characters".
class LengthLimitingTextInputFormatter extends TextInputFormatter {
/// Creates a formatter that prevents the insertion of more characters than a
/// limit.
///
/// The [maxLength] must be null, -1 or greater than zero. If it is null or -1
/// then no limit is enforced.
LengthLimitingTextInputFormatter(
this.maxLength, {
this.maxLengthEnforcement,
}) : assert(maxLength == null || maxLength == -1 || maxLength > 0);
/// The limit on the number of user-perceived characters that this formatter
/// will allow.
///
/// The value must be null or greater than zero. If it is null or -1, then no
/// limit is enforced.
///
/// {@template flutter.services.lengthLimitingTextInputFormatter.maxLength}
/// ## Characters
///
/// For a specific definition of what is considered a character, see the
/// [characters](https://pub.dev/packages/characters) package on Pub, which is
/// what Flutter uses to delineate characters. In general, even complex
/// characters like surrogate pairs and extended grapheme clusters are
/// correctly interpreted by Flutter as each being a single user-perceived
/// character.
///
/// For instance, the character "ö" can be represented as '\u{006F}\u{0308}',
/// which is the letter "o" followed by a composed diaeresis "¨", or it can
/// be represented as '\u{00F6}', which is the Unicode scalar value "LATIN
/// SMALL LETTER O WITH DIAERESIS". It will be counted as a single character
/// in both cases.
///
/// Similarly, some emoji are represented by multiple scalar values. The
/// Unicode "THUMBS UP SIGN + MEDIUM SKIN TONE MODIFIER", "👍🏽"is counted as
/// a single character, even though it is a combination of two Unicode scalar
/// values, '\u{1F44D}\u{1F3FD}'.
/// {@endtemplate}
///
/// ### Composing text behaviors
///
/// There is no guarantee for the final value before the composing ends.
/// So while the value is composing, the constraint of [maxLength] will be
/// temporary lifted until the composing ends.
///
/// In addition, if the current value already reached the [maxLength],
/// composing is not allowed.
final int? maxLength;
/// Determines how the [maxLength] limit should be enforced.
///
/// Defaults to [MaxLengthEnforcement.enforced].
///
/// {@macro flutter.services.textFormatter.maxLengthEnforcement}
final MaxLengthEnforcement? maxLengthEnforcement;
/// Returns a [MaxLengthEnforcement] that follows the specified [platform]'s
/// convention.
///
/// {@template flutter.services.textFormatter.effectiveMaxLengthEnforcement}
/// ### Platform specific behaviors
///
/// Different platforms follow different behaviors by default, according to
/// their native behavior.
/// * Android, Windows: [MaxLengthEnforcement.enforced]. The native behavior
/// of these platforms is enforced. The composing will be handled by the
/// IME while users are entering CJK characters.
/// * iOS: [MaxLengthEnforcement.truncateAfterCompositionEnds]. iOS has no
/// default behavior and it requires users implement the behavior
/// themselves. Allow the composition to exceed to avoid breaking CJK input.
/// * Web, macOS, linux, fuchsia:
/// [MaxLengthEnforcement.truncateAfterCompositionEnds]. These platforms
/// allow the composition to exceed by default.
/// {@endtemplate}
static MaxLengthEnforcement getDefaultMaxLengthEnforcement([
TargetPlatform? platform,
]) {
if (kIsWeb) {
return MaxLengthEnforcement.truncateAfterCompositionEnds;
} else {
switch (platform ?? defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.windows:
return MaxLengthEnforcement.enforced;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.fuchsia:
return MaxLengthEnforcement.truncateAfterCompositionEnds;
}
}
}
/// Truncate the given TextEditingValue to maxLength user-perceived
/// characters.
///
/// See also:
/// * [Dart's characters package](https://pub.dev/packages/characters).
/// * [Dart's documentation on runes and grapheme clusters](https://dart.dev/guides/language/language-tour#runes-and-grapheme-clusters).
@visibleForTesting
static TextEditingValue truncate(TextEditingValue value, int maxLength) {
final CharacterRange iterator = CharacterRange(value.text);
if (value.text.characters.length > maxLength) {
iterator.expandNext(maxLength);
}
final String truncated = iterator.current;
return TextEditingValue(
text: truncated,
selection: value.selection.copyWith(
baseOffset: math.min(value.selection.start, truncated.length),
extentOffset: math.min(value.selection.end, truncated.length),
),
composing: !value.composing.isCollapsed && truncated.length > value.composing.start
? TextRange(
start: value.composing.start,
end: math.min(value.composing.end, truncated.length),
)
: TextRange.empty,
);
}
@override
TextEditingValue formatEditUpdate(
TextEditingValue oldValue,
TextEditingValue newValue,
) {
final int? maxLength = this.maxLength;
if (maxLength == null ||
maxLength == -1 ||
newValue.text.characters.length <= maxLength) {
return newValue;
}
assert(maxLength > 0);
switch (maxLengthEnforcement ?? getDefaultMaxLengthEnforcement()) {
case MaxLengthEnforcement.none:
return newValue;
case MaxLengthEnforcement.enforced:
// If already at the maximum and tried to enter even more, and has no
// selection, keep the old value.
if (oldValue.text.characters.length == maxLength && oldValue.selection.isCollapsed) {
return oldValue;
}
// Enforced to return a truncated value.
return truncate(newValue, maxLength);
case MaxLengthEnforcement.truncateAfterCompositionEnds:
// If already at the maximum and tried to enter even more, and the old
// value is not composing, keep the old value.
if (oldValue.text.characters.length == maxLength &&
!oldValue.composing.isValid) {
return oldValue;
}
// Temporarily exempt `newValue` from the maxLength limit if it has a
// composing text going and no enforcement to the composing value, until
// the composing is finished.
if (newValue.composing.isValid) {
return newValue;
}
return truncate(newValue, maxLength);
}
}
}
| flutter/packages/flutter/lib/src/services/text_formatter.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/text_formatter.dart",
"repo_id": "flutter",
"token_count": 7364
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection' show HashMap;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'actions.dart';
import 'banner.dart';
import 'basic.dart';
import 'binding.dart';
import 'default_text_editing_shortcuts.dart';
import 'focus_scope.dart';
import 'focus_traversal.dart';
import 'framework.dart';
import 'localizations.dart';
import 'media_query.dart';
import 'navigator.dart';
import 'notification_listener.dart';
import 'pages.dart';
import 'performance_overlay.dart';
import 'restoration.dart';
import 'router.dart';
import 'scrollable_helpers.dart';
import 'semantics_debugger.dart';
import 'shared_app_data.dart';
import 'shortcuts.dart';
import 'tap_region.dart';
import 'text.dart';
import 'title.dart';
import 'value_listenable_builder.dart';
import 'widget_inspector.dart';
export 'dart:ui' show Locale;
// Examples can assume:
// late Widget myWidget;
/// The signature of [WidgetsApp.localeListResolutionCallback].
///
/// A [LocaleListResolutionCallback] is responsible for computing the locale of the app's
/// [Localizations] object when the app starts and when user changes the list of
/// locales for the device.
///
/// The [locales] list is the device's preferred locales when the app started, or the
/// device's preferred locales the user selected after the app was started. This list
/// is in order of preference. If this list is null or empty, then Flutter has not yet
/// received the locale information from the platform. The [supportedLocales] parameter
/// is just the value of [WidgetsApp.supportedLocales].
///
/// See also:
///
/// * [LocaleResolutionCallback], which takes only one default locale (instead of a list)
/// and is attempted only after this callback fails or is null. [LocaleListResolutionCallback]
/// is recommended over [LocaleResolutionCallback].
typedef LocaleListResolutionCallback = Locale? Function(List<Locale>? locales, Iterable<Locale> supportedLocales);
/// {@template flutter.widgets.LocaleResolutionCallback}
/// The signature of [WidgetsApp.localeResolutionCallback].
///
/// It is recommended to provide a [LocaleListResolutionCallback] instead of a
/// [LocaleResolutionCallback] when possible, as [LocaleResolutionCallback] only
/// receives a subset of the information provided in [LocaleListResolutionCallback].
///
/// A [LocaleResolutionCallback] is responsible for computing the locale of the app's
/// [Localizations] object when the app starts and when user changes the default
/// locale for the device after [LocaleListResolutionCallback] fails or is not provided.
///
/// This callback is also used if the app is created with a specific locale using
/// the [WidgetsApp.new] `locale` parameter.
///
/// The [locale] is either the value of [WidgetsApp.locale], or the device's default
/// locale when the app started, or the device locale the user selected after the app
/// was started. The default locale is the first locale in the list of preferred
/// locales. If [locale] is null, then Flutter has not yet received the locale
/// information from the platform. The [supportedLocales] parameter is just the value of
/// [WidgetsApp.supportedLocales].
///
/// See also:
///
/// * [LocaleListResolutionCallback], which takes a list of preferred locales (instead of one locale).
/// Resolutions by [LocaleListResolutionCallback] take precedence over [LocaleResolutionCallback].
/// {@endtemplate}
typedef LocaleResolutionCallback = Locale? Function(Locale? locale, Iterable<Locale> supportedLocales);
/// The default locale resolution algorithm.
///
/// Custom resolution algorithms can be provided through
/// [WidgetsApp.localeListResolutionCallback] or
/// [WidgetsApp.localeResolutionCallback].
///
/// When no custom locale resolution algorithms are provided or if both fail
/// to resolve, Flutter will default to calling this algorithm.
///
/// This algorithm prioritizes speed at the cost of slightly less appropriate
/// resolutions for edge cases.
///
/// This algorithm will resolve to the earliest preferred locale that
/// matches the most fields, prioritizing in the order of perfect match,
/// languageCode+countryCode, languageCode+scriptCode, languageCode-only.
///
/// In the case where a locale is matched by languageCode-only and is not the
/// default (first) locale, the next preferred locale with a
/// perfect match can supersede the languageCode-only match if it exists.
///
/// When a preferredLocale matches more than one supported locale, it will
/// resolve to the first matching locale listed in the supportedLocales.
///
/// When all preferred locales have been exhausted without a match, the first
/// countryCode only match will be returned.
///
/// When no match at all is found, the first (default) locale in
/// [supportedLocales] will be returned.
///
/// To summarize, the main matching priority is:
///
/// 1. [Locale.languageCode], [Locale.scriptCode], and [Locale.countryCode]
/// 2. [Locale.languageCode] and [Locale.scriptCode] only
/// 3. [Locale.languageCode] and [Locale.countryCode] only
/// 4. [Locale.languageCode] only (with caveats, see above)
/// 5. [Locale.countryCode] only when all [preferredLocales] fail to match
/// 6. Returns the first element of [supportedLocales] as a fallback
///
/// This algorithm does not take language distance (how similar languages are to each other)
/// into account, and will not handle edge cases such as resolving `de` to `fr` rather than `zh`
/// when `de` is not supported and `zh` is listed before `fr` (German is closer to French
/// than Chinese).
Locale basicLocaleListResolution(List<Locale>? preferredLocales, Iterable<Locale> supportedLocales) {
// preferredLocales can be null when called before the platform has had a chance to
// initialize the locales. Platforms without locale passing support will provide an empty list.
// We default to the first supported locale in these cases.
if (preferredLocales == null || preferredLocales.isEmpty) {
return supportedLocales.first;
}
// Hash the supported locales because apps can support many locales and would
// be expensive to search through them many times.
final Map<String, Locale> allSupportedLocales = HashMap<String, Locale>();
final Map<String, Locale> languageAndCountryLocales = HashMap<String, Locale>();
final Map<String, Locale> languageAndScriptLocales = HashMap<String, Locale>();
final Map<String, Locale> languageLocales = HashMap<String, Locale>();
final Map<String?, Locale> countryLocales = HashMap<String?, Locale>();
for (final Locale locale in supportedLocales) {
allSupportedLocales['${locale.languageCode}_${locale.scriptCode}_${locale.countryCode}'] ??= locale;
languageAndScriptLocales['${locale.languageCode}_${locale.scriptCode}'] ??= locale;
languageAndCountryLocales['${locale.languageCode}_${locale.countryCode}'] ??= locale;
languageLocales[locale.languageCode] ??= locale;
countryLocales[locale.countryCode] ??= locale;
}
// Since languageCode-only matches are possibly low quality, we don't return
// it instantly when we find such a match. We check to see if the next
// preferred locale in the list has a high accuracy match, and only return
// the languageCode-only match when a higher accuracy match in the next
// preferred locale cannot be found.
Locale? matchesLanguageCode;
Locale? matchesCountryCode;
// Loop over user's preferred locales
for (int localeIndex = 0; localeIndex < preferredLocales.length; localeIndex += 1) {
final Locale userLocale = preferredLocales[localeIndex];
// Look for perfect match.
if (allSupportedLocales.containsKey('${userLocale.languageCode}_${userLocale.scriptCode}_${userLocale.countryCode}')) {
return userLocale;
}
// Look for language+script match.
if (userLocale.scriptCode != null) {
final Locale? match = languageAndScriptLocales['${userLocale.languageCode}_${userLocale.scriptCode}'];
if (match != null) {
return match;
}
}
// Look for language+country match.
if (userLocale.countryCode != null) {
final Locale? match = languageAndCountryLocales['${userLocale.languageCode}_${userLocale.countryCode}'];
if (match != null) {
return match;
}
}
// If there was a languageCode-only match in the previous iteration's higher
// ranked preferred locale, we return it if the current userLocale does not
// have a better match.
if (matchesLanguageCode != null) {
return matchesLanguageCode;
}
// Look and store language-only match.
Locale? match = languageLocales[userLocale.languageCode];
if (match != null) {
matchesLanguageCode = match;
// Since first (default) locale is usually highly preferred, we will allow
// a languageCode-only match to be instantly matched. If the next preferred
// languageCode is the same, we defer hastily returning until the next iteration
// since at worst it is the same and at best an improved match.
if (localeIndex == 0 &&
!(localeIndex + 1 < preferredLocales.length && preferredLocales[localeIndex + 1].languageCode == userLocale.languageCode)) {
return matchesLanguageCode;
}
}
// countryCode-only match. When all else except default supported locale fails,
// attempt to match by country only, as a user is likely to be familiar with a
// language from their listed country.
if (matchesCountryCode == null && userLocale.countryCode != null) {
match = countryLocales[userLocale.countryCode];
if (match != null) {
matchesCountryCode = match;
}
}
}
// When there is no languageCode-only match. Fallback to matching countryCode only. Country
// fallback only applies on iOS. When there is no countryCode-only match, we return first
// supported locale.
final Locale resolvedLocale = matchesLanguageCode ?? matchesCountryCode ?? supportedLocales.first;
return resolvedLocale;
}
/// The signature of [WidgetsApp.onGenerateTitle].
///
/// Used to generate a value for the app's [Title.title], which the device uses
/// to identify the app for the user. The `context` includes the [WidgetsApp]'s
/// [Localizations] widget so that this method can be used to produce a
/// localized title.
///
/// This function must not return null.
typedef GenerateAppTitle = String Function(BuildContext context);
/// The signature of [WidgetsApp.pageRouteBuilder].
///
/// Creates a [PageRoute] using the given [RouteSettings] and [WidgetBuilder].
typedef PageRouteFactory = PageRoute<T> Function<T>(RouteSettings settings, WidgetBuilder builder);
/// The signature of [WidgetsApp.onGenerateInitialRoutes].
///
/// Creates a series of one or more initial routes.
typedef InitialRouteListFactory = List<Route<dynamic>> Function(String initialRoute);
/// A convenience widget that wraps a number of widgets that are commonly
/// required for an application.
///
/// One of the primary roles that [WidgetsApp] provides is binding the system
/// back button to popping the [Navigator] or quitting the application.
///
/// It is used by both [MaterialApp] and [CupertinoApp] to implement base
/// functionality for an app.
///
/// Find references to many of the widgets that [WidgetsApp] wraps in the "See
/// also" section.
///
/// See also:
///
/// * [CheckedModeBanner], which displays a [Banner] saying "DEBUG" when
/// running in debug mode.
/// * [DefaultTextStyle], the text style to apply to descendant [Text] widgets
/// without an explicit style.
/// * [MediaQuery], which establishes a subtree in which media queries resolve
/// to a [MediaQueryData].
/// * [Localizations], which defines the [Locale] for its `child`.
/// * [Title], a widget that describes this app in the operating system.
/// * [Navigator], a widget that manages a set of child widgets with a stack
/// discipline.
/// * [Overlay], a widget that manages a [Stack] of entries that can be managed
/// independently.
/// * [SemanticsDebugger], a widget that visualizes the semantics for the child.
class WidgetsApp extends StatefulWidget {
/// Creates a widget that wraps a number of widgets that are commonly
/// required for an application.
///
/// Most callers will want to use the [home] or [routes] parameters, or both.
/// The [home] parameter is a convenience for the following [routes] map:
///
/// ```dart
/// <String, WidgetBuilder>{ '/': (BuildContext context) => myWidget }
/// ```
///
/// It is possible to specify both [home] and [routes], but only if [routes] does
/// _not_ contain an entry for `'/'`. Conversely, if [home] is omitted, [routes]
/// _must_ contain an entry for `'/'`.
///
/// If [home] or [routes] are not null, the routing implementation needs to know how
/// appropriately build [PageRoute]s. This can be achieved by supplying the
/// [pageRouteBuilder] parameter. The [pageRouteBuilder] is used by [MaterialApp]
/// and [CupertinoApp] to create [MaterialPageRoute]s and [CupertinoPageRoute],
/// respectively.
///
/// The [builder] parameter is designed to provide the ability to wrap the visible
/// content of the app in some other widget. It is recommended that you use [home]
/// rather than [builder] if you intend to only display a single route in your app.
///
/// [WidgetsApp] is also possible to provide a custom implementation of routing via the
/// [onGenerateRoute] and [onUnknownRoute] parameters. These parameters correspond
/// to [Navigator.onGenerateRoute] and [Navigator.onUnknownRoute]. If [home], [routes],
/// and [builder] are null, or if they fail to create a requested route,
/// [onGenerateRoute] will be invoked. If that fails, [onUnknownRoute] will be invoked.
///
/// The [pageRouteBuilder] is called to create a [PageRoute] that wraps newly built routes.
/// If the [builder] is non-null and the [onGenerateRoute] argument is null, then the
/// [builder] will be provided only with the context and the child widget, whereas
/// the [pageRouteBuilder] will be provided with [RouteSettings]; in that configuration,
/// the [navigatorKey], [onUnknownRoute], [navigatorObservers], and
/// [initialRoute] properties must have their default values, as they will have no effect.
///
/// The `supportedLocales` argument must be a list of one or more elements.
/// By default supportedLocales is `[const Locale('en', 'US')]`.
///
/// {@tool dartpad}
/// This sample shows a basic Flutter application using [WidgetsApp].
///
/// ** See code in examples/api/lib/widgets/app/widgets_app.widgets_app.0.dart **
/// {@end-tool}
WidgetsApp({ // can't be const because the asserts use methods on Iterable :-(
super.key,
this.navigatorKey,
this.onGenerateRoute,
this.onGenerateInitialRoutes,
this.onUnknownRoute,
this.onNavigationNotification,
List<NavigatorObserver> this.navigatorObservers = const <NavigatorObserver>[],
this.initialRoute,
this.pageRouteBuilder,
this.home,
Map<String, WidgetBuilder> this.routes = const <String, WidgetBuilder>{},
this.builder,
this.title = '',
this.onGenerateTitle,
this.textStyle,
required this.color,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowWidgetInspector = false,
this.debugShowCheckedModeBanner = true,
this.inspectorSelectButtonBuilder,
this.shortcuts,
this.actions,
this.restorationScopeId,
@Deprecated(
'Remove this parameter as it is now ignored. '
'WidgetsApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
}) : assert(
home == null ||
onGenerateInitialRoutes == null,
'If onGenerateInitialRoutes is specified, the home argument will be '
'redundant.',
),
assert(
home == null ||
!routes.containsKey(Navigator.defaultRouteName),
'If the home property is specified, the routes table '
'cannot include an entry for "/", since it would be redundant.',
),
assert(
builder != null ||
home != null ||
routes.containsKey(Navigator.defaultRouteName) ||
onGenerateRoute != null ||
onUnknownRoute != null,
'Either the home property must be specified, '
'or the routes table must include an entry for "/", '
'or there must be on onGenerateRoute callback specified, '
'or there must be an onUnknownRoute callback specified, '
'or the builder property must be specified, '
'because otherwise there is nothing to fall back on if the '
'app is started with an intent that specifies an unknown route.',
),
assert(
(home != null ||
routes.isNotEmpty ||
onGenerateRoute != null ||
onUnknownRoute != null)
||
(builder != null &&
navigatorKey == null &&
initialRoute == null &&
navigatorObservers.isEmpty),
'If no route is provided using '
'home, routes, onGenerateRoute, or onUnknownRoute, '
'a non-null callback for the builder property must be provided, '
'and the other navigator-related properties, '
'navigatorKey, initialRoute, and navigatorObservers, '
'must have their initial values '
'(null, null, and the empty list, respectively).',
),
assert(
builder != null ||
onGenerateRoute != null ||
pageRouteBuilder != null,
'If neither builder nor onGenerateRoute are provided, the '
'pageRouteBuilder must be specified so that the default handler '
'will know what kind of PageRoute transition to build.',
),
assert(supportedLocales.isNotEmpty),
routeInformationProvider = null,
routeInformationParser = null,
routerDelegate = null,
backButtonDispatcher = null,
routerConfig = null;
/// Creates a [WidgetsApp] that uses the [Router] instead of a [Navigator].
///
/// {@template flutter.widgets.WidgetsApp.router}
/// If the [routerConfig] is provided, the other router related delegates,
/// [routeInformationParser], [routeInformationProvider], [routerDelegate],
/// and [backButtonDispatcher], must all be null.
/// {@endtemplate}
WidgetsApp.router({
super.key,
this.routeInformationProvider,
this.routeInformationParser,
this.routerDelegate,
this.routerConfig,
this.backButtonDispatcher,
this.builder,
this.title = '',
this.onGenerateTitle,
this.onNavigationNotification,
this.textStyle,
required this.color,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowWidgetInspector = false,
this.debugShowCheckedModeBanner = true,
this.inspectorSelectButtonBuilder,
this.shortcuts,
this.actions,
this.restorationScopeId,
@Deprecated(
'Remove this parameter as it is now ignored. '
'WidgetsApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
}) : assert((){
if (routerConfig != null) {
assert(
(routeInformationProvider ?? routeInformationParser ?? routerDelegate ?? backButtonDispatcher) == null,
'If the routerConfig is provided, all the other router delegates must not be provided',
);
return true;
}
assert(routerDelegate != null, 'Either one of routerDelegate or routerConfig must be provided');
assert(
routeInformationProvider == null || routeInformationParser != null,
'If routeInformationProvider is provided, routeInformationParser must also be provided',
);
return true;
}()),
assert(supportedLocales.isNotEmpty),
navigatorObservers = null,
navigatorKey = null,
onGenerateRoute = null,
pageRouteBuilder = null,
home = null,
onGenerateInitialRoutes = null,
onUnknownRoute = null,
routes = null,
initialRoute = null;
/// {@template flutter.widgets.widgetsApp.navigatorKey}
/// A key to use when building the [Navigator].
///
/// If a [navigatorKey] is specified, the [Navigator] can be directly
/// manipulated without first obtaining it from a [BuildContext] via
/// [Navigator.of]: from the [navigatorKey], use the [GlobalKey.currentState]
/// getter.
///
/// If this is changed, a new [Navigator] will be created, losing all the
/// application state in the process; in that case, the [navigatorObservers]
/// must also be changed, since the previous observers will be attached to the
/// previous navigator.
///
/// The [Navigator] is only built if [onGenerateRoute] is not null; if it is
/// null, [navigatorKey] must also be null.
/// {@endtemplate}
final GlobalKey<NavigatorState>? navigatorKey;
/// {@template flutter.widgets.widgetsApp.onGenerateRoute}
/// The route generator callback used when the app is navigated to a
/// named route.
///
/// If this returns null when building the routes to handle the specified
/// [initialRoute], then all the routes are discarded and
/// [Navigator.defaultRouteName] is used instead (`/`). See [initialRoute].
///
/// During normal app operation, the [onGenerateRoute] callback will only be
/// applied to route names pushed by the application, and so should never
/// return null.
///
/// This is used if [routes] does not contain the requested route.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [builder] must not be null.
/// {@endtemplate}
///
/// If this property is not set, either the [routes] or [home] properties must
/// be set, and the [pageRouteBuilder] must also be set so that the
/// default handler will know what routes and [PageRoute]s to build.
final RouteFactory? onGenerateRoute;
/// {@template flutter.widgets.widgetsApp.onGenerateInitialRoutes}
/// The routes generator callback used for generating initial routes if
/// [initialRoute] is provided.
///
/// If this property is not set, the underlying
/// [Navigator.onGenerateInitialRoutes] will default to
/// [Navigator.defaultGenerateInitialRoutes].
/// {@endtemplate}
final InitialRouteListFactory? onGenerateInitialRoutes;
/// The [PageRoute] generator callback used when the app is navigated to a
/// named route.
///
/// A [PageRoute] represents the page in a [Navigator], so that it can
/// correctly animate between pages, and to represent the "return value" of
/// a route (e.g. which button a user selected in a modal dialog).
///
/// This callback can be used, for example, to specify that a [MaterialPageRoute]
/// or a [CupertinoPageRoute] should be used for building page transitions.
///
/// The [PageRouteFactory] type is generic, meaning the provided function must
/// itself be generic. For example (with special emphasis on the `<T>` at the
/// start of the closure):
///
/// ```dart
/// pageRouteBuilder: <T>(RouteSettings settings, WidgetBuilder builder) => PageRouteBuilder<T>(
/// settings: settings,
/// pageBuilder: (BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) => builder(context),
/// ),
/// ```
final PageRouteFactory? pageRouteBuilder;
/// {@template flutter.widgets.widgetsApp.routeInformationParser}
/// A delegate to parse the route information from the
/// [routeInformationProvider] into a generic data type to be processed by
/// the [routerDelegate] at a later stage.
///
/// This object will be used by the underlying [Router].
///
/// The generic type `T` must match the generic type of the [routerDelegate].
///
/// See also:
///
/// * [Router.routeInformationParser], which receives this object when this
/// widget builds the [Router].
/// {@endtemplate}
final RouteInformationParser<Object>? routeInformationParser;
/// {@template flutter.widgets.widgetsApp.routerDelegate}
/// A delegate that configures a widget, typically a [Navigator], with
/// parsed result from the [routeInformationParser].
///
/// This object will be used by the underlying [Router].
///
/// The generic type `T` must match the generic type of the
/// [routeInformationParser].
///
/// See also:
///
/// * [Router.routerDelegate], which receives this object when this widget
/// builds the [Router].
/// {@endtemplate}
final RouterDelegate<Object>? routerDelegate;
/// {@template flutter.widgets.widgetsApp.backButtonDispatcher}
/// A delegate that decide whether to handle the Android back button intent.
///
/// This object will be used by the underlying [Router].
///
/// If this is not provided, the widgets app will create a
/// [RootBackButtonDispatcher] by default.
///
/// See also:
///
/// * [Router.backButtonDispatcher], which receives this object when this
/// widget builds the [Router].
/// {@endtemplate}
final BackButtonDispatcher? backButtonDispatcher;
/// {@template flutter.widgets.widgetsApp.routeInformationProvider}
/// A object that provides route information through the
/// [RouteInformationProvider.value] and notifies its listener when its value
/// changes.
///
/// This object will be used by the underlying [Router].
///
/// If this is not provided, the widgets app will create a
/// [PlatformRouteInformationProvider] with initial route name equal to the
/// [dart:ui.PlatformDispatcher.defaultRouteName] by default.
///
/// See also:
///
/// * [Router.routeInformationProvider], which receives this object when this
/// widget builds the [Router].
/// {@endtemplate}
final RouteInformationProvider? routeInformationProvider;
/// {@template flutter.widgets.widgetsApp.routerConfig}
/// An object to configure the underlying [Router].
///
/// If the [routerConfig] is provided, the other router related delegates,
/// [routeInformationParser], [routeInformationProvider], [routerDelegate],
/// and [backButtonDispatcher], must all be null.
///
/// See also:
///
/// * [Router.withConfig], which receives this object when this
/// widget builds the [Router].
/// {@endtemplate}
final RouterConfig<Object>? routerConfig;
/// {@template flutter.widgets.widgetsApp.home}
/// The widget for the default route of the app ([Navigator.defaultRouteName],
/// which is `/`).
///
/// This is the route that is displayed first when the application is started
/// normally, unless [initialRoute] is specified. It's also the route that's
/// displayed if the [initialRoute] can't be displayed.
///
/// To be able to directly call [Theme.of], [MediaQuery.of], etc, in the code
/// that sets the [home] argument in the constructor, you can use a [Builder]
/// widget to get a [BuildContext].
///
/// If [home] is specified, then [routes] must not include an entry for `/`,
/// as [home] takes its place.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [builder] must not be null.
///
/// The difference between using [home] and using [builder] is that the [home]
/// subtree is inserted into the application below a [Navigator] (and thus
/// below an [Overlay], which [Navigator] uses). With [home], therefore,
/// dialog boxes will work automatically, the [routes] table will be used, and
/// APIs such as [Navigator.push] and [Navigator.pop] will work as expected.
/// In contrast, the widget returned from [builder] is inserted _above_ the
/// app's [Navigator] (if any).
/// {@endtemplate}
///
/// If this property is set, the [pageRouteBuilder] property must also be set
/// so that the default route handler will know what kind of [PageRoute]s to
/// build.
final Widget? home;
/// The application's top-level routing table.
///
/// When a named route is pushed with [Navigator.pushNamed], the route name is
/// looked up in this map. If the name is present, the associated
/// [widgets.WidgetBuilder] is used to construct a [PageRoute] specified by
/// [pageRouteBuilder] to perform an appropriate transition, including [Hero]
/// animations, to the new route.
///
/// {@template flutter.widgets.widgetsApp.routes}
/// If the app only has one page, then you can specify it using [home] instead.
///
/// If [home] is specified, then it implies an entry in this table for the
/// [Navigator.defaultRouteName] route (`/`), and it is an error to
/// redundantly provide such a route in the [routes] table.
///
/// If a route is requested that is not specified in this table (or by
/// [home]), then the [onGenerateRoute] callback is called to build the page
/// instead.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [builder] must not be null.
/// {@endtemplate}
///
/// If the routes map is not empty, the [pageRouteBuilder] property must be set
/// so that the default route handler will know what kind of [PageRoute]s to
/// build.
final Map<String, WidgetBuilder>? routes;
/// {@template flutter.widgets.widgetsApp.onUnknownRoute}
/// Called when [onGenerateRoute] fails to generate a route, except for the
/// [initialRoute].
///
/// This callback is typically used for error handling. For example, this
/// callback might always generate a "not found" page that describes the route
/// that wasn't found.
///
/// Unknown routes can arise either from errors in the app or from external
/// requests to push routes, such as from Android intents.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [builder] must not be null.
/// {@endtemplate}
final RouteFactory? onUnknownRoute;
/// {@template flutter.widgets.widgetsApp.onNavigationNotification}
/// The callback to use when receiving a [NavigationNotification].
///
/// By default this updates the engine with the navigation status and stops
/// bubbling the notification.
///
/// See also:
///
/// * [NotificationListener.onNotification], which uses this callback.
/// {@endtemplate}
final NotificationListenerCallback<NavigationNotification>? onNavigationNotification;
/// {@template flutter.widgets.widgetsApp.initialRoute}
/// The name of the first route to show, if a [Navigator] is built.
///
/// Defaults to [dart:ui.PlatformDispatcher.defaultRouteName], which may be
/// overridden by the code that launched the application.
///
/// If the route name starts with a slash, then it is treated as a "deep link",
/// and before this route is pushed, the routes leading to this one are pushed
/// also. For example, if the route was `/a/b/c`, then the app would start
/// with the four routes `/`, `/a`, `/a/b`, and `/a/b/c` loaded, in that order.
/// Even if the route was just `/a`, the app would start with `/` and `/a`
/// loaded. You can use the [onGenerateInitialRoutes] property to override
/// this behavior.
///
/// Intermediate routes aren't required to exist. In the example above, `/a`
/// and `/a/b` could be skipped if they have no matching route. But `/a/b/c` is
/// required to have a route, else [initialRoute] is ignored and
/// [Navigator.defaultRouteName] is used instead (`/`). This can happen if the
/// app is started with an intent that specifies a non-existent route.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [initialRoute] must be null and [builder] must not be null.
///
/// Changing the [initialRoute] will have no effect, as it only controls the
/// _initial_ route. To change the route while the application is running, use
/// the [Navigator] or [Router] APIs.
///
/// See also:
///
/// * [Navigator.initialRoute], which is used to implement this property.
/// * [Navigator.push], for pushing additional routes.
/// * [Navigator.pop], for removing a route from the stack.
///
/// {@endtemplate}
final String? initialRoute;
/// {@template flutter.widgets.widgetsApp.navigatorObservers}
/// The list of observers for the [Navigator] created for this app.
///
/// This list must be replaced by a list of newly-created observers if the
/// [navigatorKey] is changed.
///
/// The [Navigator] is only built if routes are provided (either via [home],
/// [routes], [onGenerateRoute], or [onUnknownRoute]); if they are not,
/// [navigatorObservers] must be the empty list and [builder] must not be null.
/// {@endtemplate}
final List<NavigatorObserver>? navigatorObservers;
/// {@template flutter.widgets.widgetsApp.builder}
/// A builder for inserting widgets above the [Navigator] or - when the
/// [WidgetsApp.router] constructor is used - above the [Router] but below the
/// other widgets created by the [WidgetsApp] widget, or for replacing the
/// [Navigator]/[Router] entirely.
///
/// For example, from the [BuildContext] passed to this method, the
/// [Directionality], [Localizations], [DefaultTextStyle], [MediaQuery], etc,
/// are all available. They can also be overridden in a way that impacts all
/// the routes in the [Navigator] or [Router].
///
/// This is rarely useful, but can be used in applications that wish to
/// override those defaults, e.g. to force the application into right-to-left
/// mode despite being in English, or to override the [MediaQuery] metrics
/// (e.g. to leave a gap for advertisements shown by a plugin from OEM code).
///
/// For specifically overriding the [title] with a value based on the
/// [Localizations], consider [onGenerateTitle] instead.
///
/// The [builder] callback is passed two arguments, the [BuildContext] (as
/// `context`) and a [Navigator] or [Router] widget (as `child`).
///
/// If no routes are provided to the regular [WidgetsApp] constructor using
/// [home], [routes], [onGenerateRoute], or [onUnknownRoute], the `child` will
/// be null, and it is the responsibility of the [builder] to provide the
/// application's routing machinery.
///
/// If routes _are_ provided to the regular [WidgetsApp] constructor using one
/// or more of those properties or if the [WidgetsApp.router] constructor is
/// used, then `child` is not null, and the returned value should include the
/// `child` in the widget subtree; if it does not, then the application will
/// have no [Navigator] or [Router] and the routing related properties (i.e.
/// [navigatorKey], [home], [routes], [onGenerateRoute], [onUnknownRoute],
/// [initialRoute], [navigatorObservers], [routeInformationProvider],
/// [backButtonDispatcher], [routerDelegate], and [routeInformationParser])
/// are ignored.
///
/// If [builder] is null, it is as if a builder was specified that returned
/// the `child` directly. If it is null, routes must be provided using one of
/// the other properties listed above.
///
/// Unless a [Navigator] is provided, either implicitly from [builder] being
/// null, or by a [builder] including its `child` argument, or by a [builder]
/// explicitly providing a [Navigator] of its own, or by the [routerDelegate]
/// building one, widgets and APIs such as [Hero], [Navigator.push] and
/// [Navigator.pop], will not function.
/// {@endtemplate}
final TransitionBuilder? builder;
/// {@template flutter.widgets.widgetsApp.title}
/// A one-line description used by the device to identify the app for the user.
///
/// On Android the titles appear above the task manager's app snapshots which are
/// displayed when the user presses the "recent apps" button. On iOS this
/// value cannot be used. `CFBundleDisplayName` from the app's `Info.plist` is
/// referred to instead whenever present, `CFBundleName` otherwise.
/// On the web it is used as the page title, which shows up in the browser's list of open tabs.
///
/// To provide a localized title instead, use [onGenerateTitle].
/// {@endtemplate}
final String title;
/// {@template flutter.widgets.widgetsApp.onGenerateTitle}
/// If non-null this callback function is called to produce the app's
/// title string, otherwise [title] is used.
///
/// The [onGenerateTitle] `context` parameter includes the [WidgetsApp]'s
/// [Localizations] widget so that this callback can be used to produce a
/// localized title.
///
/// This callback function must not return null.
///
/// The [onGenerateTitle] callback is called each time the [WidgetsApp]
/// rebuilds.
/// {@endtemplate}
final GenerateAppTitle? onGenerateTitle;
/// The default text style for [Text] in the application.
final TextStyle? textStyle;
/// {@template flutter.widgets.widgetsApp.color}
/// The primary color to use for the application in the operating system
/// interface.
///
/// For example, on Android this is the color used for the application in the
/// application switcher.
/// {@endtemplate}
final Color color;
/// {@template flutter.widgets.widgetsApp.locale}
/// The initial locale for this app's [Localizations] widget is based
/// on this value.
///
/// If the 'locale' is null then the system's locale value is used.
///
/// The value of [Localizations.locale] will equal this locale if
/// it matches one of the [supportedLocales]. Otherwise it will be
/// the first element of [supportedLocales].
/// {@endtemplate}
///
/// See also:
///
/// * [localeResolutionCallback], which can override the default
/// [supportedLocales] matching algorithm.
/// * [localizationsDelegates], which collectively define all of the localized
/// resources used by this app.
final Locale? locale;
/// {@template flutter.widgets.widgetsApp.localizationsDelegates}
/// The delegates for this app's [Localizations] widget.
///
/// The delegates collectively define all of the localized resources
/// for this application's [Localizations] widget.
/// {@endtemplate}
final Iterable<LocalizationsDelegate<dynamic>>? localizationsDelegates;
/// {@template flutter.widgets.widgetsApp.localeListResolutionCallback}
/// This callback is responsible for choosing the app's locale
/// when the app is started, and when the user changes the
/// device's locale.
///
/// When a [localeListResolutionCallback] is provided, Flutter will first
/// attempt to resolve the locale with the provided
/// [localeListResolutionCallback]. If the callback or result is null, it will
/// fallback to trying the [localeResolutionCallback]. If both
/// [localeResolutionCallback] and [localeListResolutionCallback] are left
/// null or fail to resolve (return null), basic fallback algorithm will
/// be used.
///
/// The priority of each available fallback is:
///
/// 1. [localeListResolutionCallback] is attempted.
/// 2. [localeResolutionCallback] is attempted.
/// 3. Flutter's basic resolution algorithm, as described in
/// [supportedLocales], is attempted last.
///
/// Properly localized projects should provide a more advanced algorithm than
/// the basic method from [supportedLocales], as it does not implement a
/// complete algorithm (such as the one defined in
/// [Unicode TR35](https://unicode.org/reports/tr35/#LanguageMatching))
/// and is optimized for speed at the detriment of some uncommon edge-cases.
/// {@endtemplate}
///
/// This callback considers the entire list of preferred locales.
///
/// This algorithm should be able to handle a null or empty list of preferred locales,
/// which indicates Flutter has not yet received locale information from the platform.
///
/// See also:
///
/// * [MaterialApp.localeListResolutionCallback], which sets the callback of the
/// [WidgetsApp] it creates.
/// * [basicLocaleListResolution], the default locale resolution algorithm.
final LocaleListResolutionCallback? localeListResolutionCallback;
/// {@macro flutter.widgets.widgetsApp.localeListResolutionCallback}
///
/// This callback considers only the default locale, which is the first locale
/// in the preferred locales list. It is preferred to set [localeListResolutionCallback]
/// over [localeResolutionCallback] as it provides the full preferred locales list.
///
/// This algorithm should be able to handle a null locale, which indicates
/// Flutter has not yet received locale information from the platform.
///
/// See also:
///
/// * [MaterialApp.localeResolutionCallback], which sets the callback of the
/// [WidgetsApp] it creates.
/// * [basicLocaleListResolution], the default locale resolution algorithm.
final LocaleResolutionCallback? localeResolutionCallback;
/// {@template flutter.widgets.widgetsApp.supportedLocales}
/// The list of locales that this app has been localized for.
///
/// By default only the American English locale is supported. Apps should
/// configure this list to match the locales they support.
///
/// This list must not null. Its default value is just
/// `[const Locale('en', 'US')]`.
///
/// The order of the list matters. The default locale resolution algorithm,
/// [basicLocaleListResolution], attempts to match by the following priority:
///
/// 1. [Locale.languageCode], [Locale.scriptCode], and [Locale.countryCode]
/// 2. [Locale.languageCode] and [Locale.scriptCode] only
/// 3. [Locale.languageCode] and [Locale.countryCode] only
/// 4. [Locale.languageCode] only
/// 5. [Locale.countryCode] only when all preferred locales fail to match
/// 6. Returns the first element of [supportedLocales] as a fallback
///
/// When more than one supported locale matches one of these criteria, only
/// the first matching locale is returned.
///
/// The default locale resolution algorithm can be overridden by providing a
/// value for [localeListResolutionCallback]. The provided
/// [basicLocaleListResolution] is optimized for speed and does not implement
/// a full algorithm (such as the one defined in
/// [Unicode TR35](https://unicode.org/reports/tr35/#LanguageMatching)) that
/// takes distances between languages into account.
///
/// When supporting languages with more than one script, it is recommended
/// to specify the [Locale.scriptCode] explicitly. Locales may also be defined without
/// [Locale.countryCode] to specify a generic fallback for a particular script.
///
/// A fully supported language with multiple scripts should define a generic language-only
/// locale (e.g. 'zh'), language+script only locales (e.g. 'zh_Hans' and 'zh_Hant'),
/// and any language+script+country locales (e.g. 'zh_Hans_CN'). Fully defining all of
/// these locales as supported is not strictly required but allows for proper locale resolution in
/// the most number of cases. These locales can be specified with the [Locale.fromSubtags]
/// constructor:
///
/// ```dart
/// // Full Chinese support for CN, TW, and HK
/// supportedLocales: <Locale>[
/// const Locale.fromSubtags(languageCode: 'zh'), // generic Chinese 'zh'
/// const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hans'), // generic simplified Chinese 'zh_Hans'
/// const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hant'), // generic traditional Chinese 'zh_Hant'
/// const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hans', countryCode: 'CN'), // 'zh_Hans_CN'
/// const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hant', countryCode: 'TW'), // 'zh_Hant_TW'
/// const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hant', countryCode: 'HK'), // 'zh_Hant_HK'
/// ],
/// ```
///
/// Omitting some these fallbacks may result in improperly resolved
/// edge-cases, for example, a simplified Chinese user in Taiwan ('zh_Hans_TW')
/// may resolve to traditional Chinese if 'zh_Hans' and 'zh_Hans_CN' are
/// omitted.
/// {@endtemplate}
///
/// See also:
///
/// * [MaterialApp.supportedLocales], which sets the `supportedLocales`
/// of the [WidgetsApp] it creates.
/// * [localeResolutionCallback], an app callback that resolves the app's locale
/// when the device's locale changes.
/// * [localizationsDelegates], which collectively define all of the localized
/// resources used by this app.
/// * [basicLocaleListResolution], the default locale resolution algorithm.
final Iterable<Locale> supportedLocales;
/// Turns on a performance overlay.
///
/// See also:
///
/// * <https://flutter.dev/debugging/#performance-overlay>
final bool showPerformanceOverlay;
/// Checkerboards raster cache images.
///
/// See [PerformanceOverlay.checkerboardRasterCacheImages].
final bool checkerboardRasterCacheImages;
/// Checkerboards layers rendered to offscreen bitmaps.
///
/// See [PerformanceOverlay.checkerboardOffscreenLayers].
final bool checkerboardOffscreenLayers;
/// Turns on an overlay that shows the accessibility information
/// reported by the framework.
final bool showSemanticsDebugger;
/// Turns on an overlay that enables inspecting the widget tree.
///
/// The inspector is only available in debug mode as it depends on
/// [RenderObject.debugDescribeChildren] which should not be called outside of
/// debug mode.
final bool debugShowWidgetInspector;
/// Builds the widget the [WidgetInspector] uses to switch between view and
/// inspect modes.
///
/// This lets [MaterialApp] to use a Material Design button to toggle the
/// inspector select mode without requiring [WidgetInspector] to depend on the
/// material package.
final InspectorSelectButtonBuilder? inspectorSelectButtonBuilder;
/// {@template flutter.widgets.widgetsApp.debugShowCheckedModeBanner}
/// Turns on a little "DEBUG" banner in debug mode to indicate
/// that the app is in debug mode. This is on by default (in
/// debug mode), to turn it off, set the constructor argument to
/// false. In release mode this has no effect.
///
/// To get this banner in your application if you're not using
/// WidgetsApp, include a [CheckedModeBanner] widget in your app.
///
/// This banner is intended to deter people from complaining that your
/// app is slow when it's in debug mode. In debug mode, Flutter
/// enables a large number of expensive diagnostics to aid in
/// development, and so performance in debug mode is not
/// representative of what will happen in release mode.
/// {@endtemplate}
final bool debugShowCheckedModeBanner;
/// {@template flutter.widgets.widgetsApp.shortcuts}
/// The default map of keyboard shortcuts to intents for the application.
///
/// By default, this is set to [WidgetsApp.defaultShortcuts].
///
/// Passing this will not replace [DefaultTextEditingShortcuts]. These can be
/// overridden by using a [Shortcuts] widget lower in the widget tree.
/// {@endtemplate}
///
/// {@tool snippet}
/// This example shows how to add a single shortcut for
/// [LogicalKeyboardKey.select] to the default shortcuts without needing to
/// add your own [Shortcuts] widget.
///
/// Alternatively, you could insert a [Shortcuts] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// shortcuts: <ShortcutActivator, Intent>{
/// ... WidgetsApp.defaultShortcuts,
/// const SingleActivator(LogicalKeyboardKey.select): const ActivateIntent(),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
///
/// {@template flutter.widgets.widgetsApp.shortcuts.seeAlso}
/// See also:
///
/// * [SingleActivator], which defines shortcut key combination of a single
/// key and modifiers, such as "Delete" or "Control+C".
/// * The [Shortcuts] widget, which defines a keyboard mapping.
/// * The [Actions] widget, which defines the mapping from intent to action.
/// * The [Intent] and [Action] classes, which allow definition of new
/// actions.
/// {@endtemplate}
final Map<ShortcutActivator, Intent>? shortcuts;
/// {@template flutter.widgets.widgetsApp.actions}
/// The default map of intent keys to actions for the application.
///
/// By default, this is the output of [WidgetsApp.defaultActions], called with
/// [defaultTargetPlatform]. Specifying [actions] for an app overrides the
/// default, so if you wish to modify the default [actions], you can call
/// [WidgetsApp.defaultActions] and modify the resulting map, passing it as
/// the [actions] for this app. You may also add to the bindings, or override
/// specific bindings for a widget subtree, by adding your own [Actions]
/// widget.
/// {@endtemplate}
///
/// {@tool snippet}
/// This example shows how to add a single action handling an
/// [ActivateAction] to the default actions without needing to
/// add your own [Actions] widget.
///
/// Alternatively, you could insert a [Actions] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// actions: <Type, Action<Intent>>{
/// ... WidgetsApp.defaultActions,
/// ActivateAction: CallbackAction<Intent>(
/// onInvoke: (Intent intent) {
/// // Do something here...
/// return null;
/// },
/// ),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
///
/// {@template flutter.widgets.widgetsApp.actions.seeAlso}
/// See also:
///
/// * The [shortcuts] parameter, which defines the default set of shortcuts
/// for the application.
/// * The [Shortcuts] widget, which defines a keyboard mapping.
/// * The [Actions] widget, which defines the mapping from intent to action.
/// * The [Intent] and [Action] classes, which allow definition of new
/// actions.
/// {@endtemplate}
final Map<Type, Action<Intent>>? actions;
/// {@template flutter.widgets.widgetsApp.restorationScopeId}
/// The identifier to use for state restoration of this app.
///
/// Providing a restoration ID inserts a [RootRestorationScope] into the
/// widget hierarchy, which enables state restoration for descendant widgets.
///
/// Providing a restoration ID also enables the [Navigator] or [Router] built
/// by the [WidgetsApp] to restore its state (i.e. to restore the history
/// stack of active [Route]s). See the documentation on [Navigator] for more
/// details around state restoration of [Route]s.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
/// {@endtemplate}
final String? restorationScopeId;
/// {@template flutter.widgets.widgetsApp.useInheritedMediaQuery}
/// Deprecated. This setting is now ignored.
///
/// The widget never introduces its own [MediaQuery]; the [View] widget takes
/// care of that.
/// {@endtemplate}
@Deprecated(
'This setting is now ignored. '
'WidgetsApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
final bool useInheritedMediaQuery;
/// If true, forces the performance overlay to be visible in all instances.
///
/// Used by the `showPerformanceOverlay` VM service extension.
static bool showPerformanceOverlayOverride = false;
/// If false, prevents the debug banner from being visible.
///
/// Used by the `debugAllowBanner` VM service extension.
///
/// This is how `flutter run` turns off the banner when you take a screen shot
/// with "s".
static bool debugAllowBannerOverride = true;
static const Map<ShortcutActivator, Intent> _defaultShortcuts = <ShortcutActivator, Intent>{
// Activation
SingleActivator(LogicalKeyboardKey.enter): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.numpadEnter): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.space): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.gameButtonA): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.select): ActivateIntent(),
// Dismissal
SingleActivator(LogicalKeyboardKey.escape): DismissIntent(),
// Keyboard traversal.
SingleActivator(LogicalKeyboardKey.tab): NextFocusIntent(),
SingleActivator(LogicalKeyboardKey.tab, shift: true): PreviousFocusIntent(),
SingleActivator(LogicalKeyboardKey.arrowLeft): DirectionalFocusIntent(TraversalDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight): DirectionalFocusIntent(TraversalDirection.right),
SingleActivator(LogicalKeyboardKey.arrowDown): DirectionalFocusIntent(TraversalDirection.down),
SingleActivator(LogicalKeyboardKey.arrowUp): DirectionalFocusIntent(TraversalDirection.up),
// Scrolling
SingleActivator(LogicalKeyboardKey.arrowUp, control: true): ScrollIntent(direction: AxisDirection.up),
SingleActivator(LogicalKeyboardKey.arrowDown, control: true): ScrollIntent(direction: AxisDirection.down),
SingleActivator(LogicalKeyboardKey.arrowLeft, control: true): ScrollIntent(direction: AxisDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight, control: true): ScrollIntent(direction: AxisDirection.right),
SingleActivator(LogicalKeyboardKey.pageUp): ScrollIntent(direction: AxisDirection.up, type: ScrollIncrementType.page),
SingleActivator(LogicalKeyboardKey.pageDown): ScrollIntent(direction: AxisDirection.down, type: ScrollIncrementType.page),
};
// Default shortcuts for the web platform.
static const Map<ShortcutActivator, Intent> _defaultWebShortcuts = <ShortcutActivator, Intent>{
// Activation
SingleActivator(LogicalKeyboardKey.space): PrioritizedIntents(
orderedIntents: <Intent>[
ActivateIntent(),
ScrollIntent(direction: AxisDirection.down, type: ScrollIncrementType.page),
],
),
// On the web, enter activates buttons, but not other controls.
SingleActivator(LogicalKeyboardKey.enter): ButtonActivateIntent(),
SingleActivator(LogicalKeyboardKey.numpadEnter): ButtonActivateIntent(),
// Dismissal
SingleActivator(LogicalKeyboardKey.escape): DismissIntent(),
// Keyboard traversal.
SingleActivator(LogicalKeyboardKey.tab): NextFocusIntent(),
SingleActivator(LogicalKeyboardKey.tab, shift: true): PreviousFocusIntent(),
// Scrolling
SingleActivator(LogicalKeyboardKey.arrowUp): ScrollIntent(direction: AxisDirection.up),
SingleActivator(LogicalKeyboardKey.arrowDown): ScrollIntent(direction: AxisDirection.down),
SingleActivator(LogicalKeyboardKey.arrowLeft): ScrollIntent(direction: AxisDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight): ScrollIntent(direction: AxisDirection.right),
SingleActivator(LogicalKeyboardKey.pageUp): ScrollIntent(direction: AxisDirection.up, type: ScrollIncrementType.page),
SingleActivator(LogicalKeyboardKey.pageDown): ScrollIntent(direction: AxisDirection.down, type: ScrollIncrementType.page),
};
// Default shortcuts for the macOS platform.
static const Map<ShortcutActivator, Intent> _defaultAppleOsShortcuts = <ShortcutActivator, Intent>{
// Activation
SingleActivator(LogicalKeyboardKey.enter): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.numpadEnter): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.space): ActivateIntent(),
// Dismissal
SingleActivator(LogicalKeyboardKey.escape): DismissIntent(),
// Keyboard traversal
SingleActivator(LogicalKeyboardKey.tab): NextFocusIntent(),
SingleActivator(LogicalKeyboardKey.tab, shift: true): PreviousFocusIntent(),
SingleActivator(LogicalKeyboardKey.arrowLeft): DirectionalFocusIntent(TraversalDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight): DirectionalFocusIntent(TraversalDirection.right),
SingleActivator(LogicalKeyboardKey.arrowDown): DirectionalFocusIntent(TraversalDirection.down),
SingleActivator(LogicalKeyboardKey.arrowUp): DirectionalFocusIntent(TraversalDirection.up),
// Scrolling
SingleActivator(LogicalKeyboardKey.arrowUp, meta: true): ScrollIntent(direction: AxisDirection.up),
SingleActivator(LogicalKeyboardKey.arrowDown, meta: true): ScrollIntent(direction: AxisDirection.down),
SingleActivator(LogicalKeyboardKey.arrowLeft, meta: true): ScrollIntent(direction: AxisDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight, meta: true): ScrollIntent(direction: AxisDirection.right),
SingleActivator(LogicalKeyboardKey.pageUp): ScrollIntent(direction: AxisDirection.up, type: ScrollIncrementType.page),
SingleActivator(LogicalKeyboardKey.pageDown): ScrollIntent(direction: AxisDirection.down, type: ScrollIncrementType.page),
};
/// Generates the default shortcut key bindings based on the
/// [defaultTargetPlatform].
///
/// Used by [WidgetsApp] to assign a default value to [WidgetsApp.shortcuts].
static Map<ShortcutActivator, Intent> get defaultShortcuts {
if (kIsWeb) {
return _defaultWebShortcuts;
}
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return _defaultShortcuts;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return _defaultAppleOsShortcuts;
}
}
/// The default value of [WidgetsApp.actions].
static Map<Type, Action<Intent>> defaultActions = <Type, Action<Intent>>{
DoNothingIntent: DoNothingAction(),
DoNothingAndStopPropagationIntent: DoNothingAction(consumesKey: false),
RequestFocusIntent: RequestFocusAction(),
NextFocusIntent: NextFocusAction(),
PreviousFocusIntent: PreviousFocusAction(),
DirectionalFocusIntent: DirectionalFocusAction(),
ScrollIntent: ScrollAction(),
PrioritizedIntents: PrioritizedAction(),
VoidCallbackIntent: VoidCallbackAction(),
};
@override
State<WidgetsApp> createState() => _WidgetsAppState();
}
class _WidgetsAppState extends State<WidgetsApp> with WidgetsBindingObserver {
// STATE LIFECYCLE
// If window.defaultRouteName isn't '/', we should assume it was set
// intentionally via `setInitialRoute`, and should override whatever is in
// [widget.initialRoute].
String get _initialRouteName => WidgetsBinding.instance.platformDispatcher.defaultRouteName != Navigator.defaultRouteName
? WidgetsBinding.instance.platformDispatcher.defaultRouteName
: widget.initialRoute ?? WidgetsBinding.instance.platformDispatcher.defaultRouteName;
AppLifecycleState? _appLifecycleState;
/// The default value for [onNavigationNotification].
///
/// Does nothing and stops bubbling if the app is detached. Otherwise, updates
/// the platform with [NavigationNotification.canHandlePop] and stops
/// bubbling.
bool _defaultOnNavigationNotification(NavigationNotification notification) {
switch (_appLifecycleState) {
case null:
case AppLifecycleState.detached:
case AppLifecycleState.inactive:
// Avoid updating the engine when the app isn't ready.
return true;
case AppLifecycleState.resumed:
case AppLifecycleState.hidden:
case AppLifecycleState.paused:
SystemNavigator.setFrameworkHandlesBack(notification.canHandlePop);
return true;
}
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
_appLifecycleState = state;
super.didChangeAppLifecycleState(state);
}
@override
void initState() {
super.initState();
_updateRouting();
_locale = _resolveLocales(WidgetsBinding.instance.platformDispatcher.locales, widget.supportedLocales);
WidgetsBinding.instance.addObserver(this);
_appLifecycleState = WidgetsBinding.instance.lifecycleState;
}
@override
void didUpdateWidget(WidgetsApp oldWidget) {
super.didUpdateWidget(oldWidget);
_updateRouting(oldWidget: oldWidget);
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
_defaultRouteInformationProvider?.dispose();
super.dispose();
}
void _clearRouterResource() {
_defaultRouteInformationProvider?.dispose();
_defaultRouteInformationProvider = null;
_defaultBackButtonDispatcher = null;
}
void _clearNavigatorResource() {
_navigator = null;
}
void _updateRouting({WidgetsApp? oldWidget}) {
if (_usesRouterWithDelegates) {
assert(!_usesNavigator && !_usesRouterWithConfig);
_clearNavigatorResource();
if (widget.routeInformationProvider == null && widget.routeInformationParser != null) {
_defaultRouteInformationProvider ??= PlatformRouteInformationProvider(
initialRouteInformation: RouteInformation(
uri: Uri.parse(_initialRouteName),
),
);
} else {
_defaultRouteInformationProvider?.dispose();
_defaultRouteInformationProvider = null;
}
if (widget.backButtonDispatcher == null) {
_defaultBackButtonDispatcher ??= RootBackButtonDispatcher();
}
} else if (_usesNavigator) {
assert(!_usesRouterWithDelegates && !_usesRouterWithConfig);
_clearRouterResource();
if (_navigator == null || widget.navigatorKey != oldWidget!.navigatorKey) {
_navigator = widget.navigatorKey ?? GlobalObjectKey<NavigatorState>(this);
}
assert(_navigator != null);
} else {
assert(widget.builder != null || _usesRouterWithConfig);
assert(!_usesRouterWithDelegates && !_usesNavigator);
_clearRouterResource();
_clearNavigatorResource();
}
// If we use a navigator, we have a navigator key.
assert(_usesNavigator == (_navigator != null));
}
bool get _usesRouterWithDelegates => widget.routerDelegate != null;
bool get _usesRouterWithConfig => widget.routerConfig != null;
bool get _usesNavigator => widget.home != null
|| (widget.routes?.isNotEmpty ?? false)
|| widget.onGenerateRoute != null
|| widget.onUnknownRoute != null;
// ROUTER
RouteInformationProvider? get _effectiveRouteInformationProvider => widget.routeInformationProvider ?? _defaultRouteInformationProvider;
PlatformRouteInformationProvider? _defaultRouteInformationProvider;
BackButtonDispatcher get _effectiveBackButtonDispatcher => widget.backButtonDispatcher ?? _defaultBackButtonDispatcher!;
RootBackButtonDispatcher? _defaultBackButtonDispatcher;
// NAVIGATOR
GlobalKey<NavigatorState>? _navigator;
Route<dynamic>? _onGenerateRoute(RouteSettings settings) {
final String? name = settings.name;
final WidgetBuilder? pageContentBuilder = name == Navigator.defaultRouteName && widget.home != null
? (BuildContext context) => widget.home!
: widget.routes![name];
if (pageContentBuilder != null) {
assert(
widget.pageRouteBuilder != null,
'The default onGenerateRoute handler for WidgetsApp must have a '
'pageRouteBuilder set if the home or routes properties are set.',
);
final Route<dynamic> route = widget.pageRouteBuilder!<dynamic>(
settings,
pageContentBuilder,
);
return route;
}
if (widget.onGenerateRoute != null) {
return widget.onGenerateRoute!(settings);
}
return null;
}
Route<dynamic> _onUnknownRoute(RouteSettings settings) {
assert(() {
if (widget.onUnknownRoute == null) {
throw FlutterError(
'Could not find a generator for route $settings in the $runtimeType.\n'
'Make sure your root app widget has provided a way to generate \n'
'this route.\n'
'Generators for routes are searched for in the following order:\n'
' 1. For the "/" route, the "home" property, if non-null, is used.\n'
' 2. Otherwise, the "routes" table is used, if it has an entry for '
'the route.\n'
' 3. Otherwise, onGenerateRoute is called. It should return a '
'non-null value for any valid route not handled by "home" and "routes".\n'
' 4. Finally if all else fails onUnknownRoute is called.\n'
'Unfortunately, onUnknownRoute was not set.',
);
}
return true;
}());
final Route<dynamic>? result = widget.onUnknownRoute!(settings);
assert(() {
if (result == null) {
throw FlutterError(
'The onUnknownRoute callback returned null.\n'
'When the $runtimeType requested the route $settings from its '
'onUnknownRoute callback, the callback returned null. Such callbacks '
'must never return null.',
);
}
return true;
}());
return result!;
}
// On Android: the user has pressed the back button.
@override
Future<bool> didPopRoute() async {
assert(mounted);
// The back button dispatcher should handle the pop route if we use a
// router.
if (_usesRouterWithDelegates) {
return false;
}
final NavigatorState? navigator = _navigator?.currentState;
if (navigator == null) {
return false;
}
return navigator.maybePop();
}
@override
Future<bool> didPushRouteInformation(RouteInformation routeInformation) async {
assert(mounted);
// The route name provider should handle the push route if we uses a
// router.
if (_usesRouterWithDelegates) {
return false;
}
final NavigatorState? navigator = _navigator?.currentState;
if (navigator == null) {
return false;
}
final Uri uri = routeInformation.uri;
navigator.pushNamed(
Uri.decodeComponent(
Uri(
path: uri.path.isEmpty ? '/' : uri.path,
queryParameters: uri.queryParametersAll.isEmpty ? null : uri.queryParametersAll,
fragment: uri.fragment.isEmpty ? null : uri.fragment,
).toString(),
),
);
return true;
}
// LOCALIZATION
/// This is the resolved locale, and is one of the supportedLocales.
Locale? _locale;
Locale _resolveLocales(List<Locale>? preferredLocales, Iterable<Locale> supportedLocales) {
// Attempt to use localeListResolutionCallback.
if (widget.localeListResolutionCallback != null) {
final Locale? locale = widget.localeListResolutionCallback!(preferredLocales, widget.supportedLocales);
if (locale != null) {
return locale;
}
}
// localeListResolutionCallback failed, falling back to localeResolutionCallback.
if (widget.localeResolutionCallback != null) {
final Locale? locale = widget.localeResolutionCallback!(
preferredLocales != null && preferredLocales.isNotEmpty ? preferredLocales.first : null,
widget.supportedLocales,
);
if (locale != null) {
return locale;
}
}
// Both callbacks failed, falling back to default algorithm.
return basicLocaleListResolution(preferredLocales, supportedLocales);
}
@override
void didChangeLocales(List<Locale>? locales) {
final Locale newLocale = _resolveLocales(locales, widget.supportedLocales);
if (newLocale != _locale) {
setState(() {
_locale = newLocale;
});
}
}
// Combine the Localizations for Widgets with the ones contributed
// by the localizationsDelegates parameter, if any. Only the first delegate
// of a particular LocalizationsDelegate.type is loaded so the
// localizationsDelegate parameter can be used to override
// WidgetsLocalizations.delegate.
Iterable<LocalizationsDelegate<dynamic>> get _localizationsDelegates {
return <LocalizationsDelegate<dynamic>>[
if (widget.localizationsDelegates != null)
...widget.localizationsDelegates!,
DefaultWidgetsLocalizations.delegate,
];
}
// BUILDER
bool _debugCheckLocalizations(Locale appLocale) {
assert(() {
final Set<Type> unsupportedTypes =
_localizationsDelegates.map<Type>((LocalizationsDelegate<dynamic> delegate) => delegate.type).toSet();
for (final LocalizationsDelegate<dynamic> delegate in _localizationsDelegates) {
if (!unsupportedTypes.contains(delegate.type)) {
continue;
}
if (delegate.isSupported(appLocale)) {
unsupportedTypes.remove(delegate.type);
}
}
if (unsupportedTypes.isEmpty) {
return true;
}
FlutterError.reportError(FlutterErrorDetails(
exception: "Warning: This application's locale, $appLocale, is not supported by all of its localization delegates.",
library: 'widgets',
informationCollector: () => <DiagnosticsNode>[
for (final Type unsupportedType in unsupportedTypes)
ErrorDescription(
'• A $unsupportedType delegate that supports the $appLocale locale was not found.',
),
ErrorSpacer(),
if (unsupportedTypes.length == 1 && unsupportedTypes.single.toString() == 'CupertinoLocalizations')
// We previously explicitly avoided checking for this class so it's not uncommon for applications
// to have omitted importing the required delegate.
...<DiagnosticsNode>[
ErrorHint(
'If the application is built using GlobalMaterialLocalizations.delegate, consider using '
'GlobalMaterialLocalizations.delegates (plural) instead, as that will automatically declare '
'the appropriate Cupertino localizations.'
),
ErrorSpacer(),
],
ErrorHint(
'The declared supported locales for this app are: ${widget.supportedLocales.join(", ")}'
),
ErrorSpacer(),
ErrorDescription(
'See https://flutter.dev/tutorials/internationalization/ for more '
"information about configuring an app's locale, supportedLocales, "
'and localizationsDelegates parameters.',
),
],
));
return true;
}());
return true;
}
@override
Widget build(BuildContext context) {
Widget? routing;
if (_usesRouterWithDelegates) {
routing = Router<Object>(
restorationScopeId: 'router',
routeInformationProvider: _effectiveRouteInformationProvider,
routeInformationParser: widget.routeInformationParser,
routerDelegate: widget.routerDelegate!,
backButtonDispatcher: _effectiveBackButtonDispatcher,
);
} else if (_usesNavigator) {
assert(_navigator != null);
routing = FocusScope(
debugLabel: 'Navigator Scope',
autofocus: true,
child: Navigator(
clipBehavior: Clip.none,
restorationScopeId: 'nav',
key: _navigator,
initialRoute: _initialRouteName,
onGenerateRoute: _onGenerateRoute,
onGenerateInitialRoutes: widget.onGenerateInitialRoutes == null
? Navigator.defaultGenerateInitialRoutes
: (NavigatorState navigator, String initialRouteName) {
return widget.onGenerateInitialRoutes!(initialRouteName);
},
onUnknownRoute: _onUnknownRoute,
observers: widget.navigatorObservers!,
routeTraversalEdgeBehavior: kIsWeb ? TraversalEdgeBehavior.leaveFlutterView : TraversalEdgeBehavior.parentScope,
reportsRouteUpdateToEngine: true,
),
);
} else if (_usesRouterWithConfig) {
routing = Router<Object>.withConfig(
restorationScopeId: 'router',
config: widget.routerConfig!,
);
}
Widget result;
if (widget.builder != null) {
result = Builder(
builder: (BuildContext context) {
return widget.builder!(context, routing);
},
);
} else {
assert(routing != null);
result = routing!;
}
if (widget.textStyle != null) {
result = DefaultTextStyle(
style: widget.textStyle!,
child: result,
);
}
PerformanceOverlay? performanceOverlay;
// We need to push a performance overlay if any of the display or checkerboarding
// options are set.
if (widget.showPerformanceOverlay || WidgetsApp.showPerformanceOverlayOverride) {
performanceOverlay = PerformanceOverlay.allEnabled(
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
);
} else if (widget.checkerboardRasterCacheImages || widget.checkerboardOffscreenLayers) {
performanceOverlay = PerformanceOverlay(
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
);
}
if (performanceOverlay != null) {
result = Stack(
children: <Widget>[
result,
Positioned(top: 0.0, left: 0.0, right: 0.0, child: performanceOverlay),
],
);
}
if (widget.showSemanticsDebugger) {
result = SemanticsDebugger(
child: result,
);
}
assert(() {
result = ValueListenableBuilder<bool>(
valueListenable: WidgetsBinding.instance.debugShowWidgetInspectorOverrideNotifier,
builder: (BuildContext context, bool debugShowWidgetInspectorOverride, Widget? child) {
if (widget.debugShowWidgetInspector || debugShowWidgetInspectorOverride) {
return WidgetInspector(
selectButtonBuilder: widget.inspectorSelectButtonBuilder,
child: child!,
);
}
return child!;
},
child: result,
);
if (widget.debugShowCheckedModeBanner && WidgetsApp.debugAllowBannerOverride) {
result = CheckedModeBanner(
child: result,
);
}
return true;
}());
final Widget title;
if (widget.onGenerateTitle != null) {
title = Builder(
// This Builder exists to provide a context below the Localizations widget.
// The onGenerateTitle callback can refer to Localizations via its context
// parameter.
builder: (BuildContext context) {
final String title = widget.onGenerateTitle!(context);
return Title(
title: title,
color: widget.color.withOpacity(1.0),
child: result,
);
},
);
} else {
title = Title(
title: widget.title,
color: widget.color.withOpacity(1.0),
child: result,
);
}
final Locale appLocale = widget.locale != null
? _resolveLocales(<Locale>[widget.locale!], widget.supportedLocales)
: _locale!;
assert(_debugCheckLocalizations(appLocale));
return RootRestorationScope(
restorationId: widget.restorationScopeId,
child: SharedAppData(
child: NotificationListener<NavigationNotification>(
onNotification: widget.onNavigationNotification ?? _defaultOnNavigationNotification,
child: Shortcuts(
debugLabel: '<Default WidgetsApp Shortcuts>',
shortcuts: widget.shortcuts ?? WidgetsApp.defaultShortcuts,
// DefaultTextEditingShortcuts is nested inside Shortcuts so that it can
// fall through to the defaultShortcuts.
child: DefaultTextEditingShortcuts(
child: Actions(
actions: widget.actions ?? <Type, Action<Intent>>{
...WidgetsApp.defaultActions,
ScrollIntent: Action<ScrollIntent>.overridable(context: context, defaultAction: ScrollAction()),
},
child: FocusTraversalGroup(
policy: ReadingOrderTraversalPolicy(),
child: TapRegionSurface(
child: ShortcutRegistrar(
child: Localizations(
locale: appLocale,
delegates: _localizationsDelegates.toList(),
child: title,
),
),
),
),
),
),
),
),
),
);
}
}
| flutter/packages/flutter/lib/src/widgets/app.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/app.dart",
"repo_id": "flutter",
"token_count": 24908
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'image.dart';
/// A sliver widget that paints a [Decoration] either before or after its child
/// paints.
///
/// Unlike [DecoratedBox], this widget expects its child to be a sliver, and
/// must be placed in a widget that expects a sliver.
///
/// If the child sliver has infinite [SliverGeometry.scrollExtent], then we only
/// draw the decoration down to the bottom [SliverGeometry.cacheExtent], and
/// it is necessary to ensure that the bottom border does not creep
/// above the top of the bottom cache. This can happen if the bottom has a
/// border radius larger than the extent of the cache area.
///
/// Commonly used with [BoxDecoration].
///
/// The [child] is not clipped. To clip a child to the shape of a particular
/// [ShapeDecoration], consider using a [ClipPath] widget.
///
/// {@tool dartpad}
/// This sample shows a radial gradient that draws a moon on a night sky:
///
/// ** See code in examples/api/lib/widgets/sliver/decorated_sliver.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [DecoratedBox], the version of this class that works with RenderBox widgets.
/// * [Decoration], which you can extend to provide other effects with
/// [DecoratedSliver].
/// * [CustomPaint], another way to draw custom effects from the widget layer.
class DecoratedSliver extends SingleChildRenderObjectWidget {
/// Creates a widget that paints a [Decoration].
///
/// By default the decoration paints behind the child.
const DecoratedSliver({
super.key,
required this.decoration,
this.position = DecorationPosition.background,
Widget? sliver,
}) : super(child: sliver);
/// What decoration to paint.
///
/// Commonly a [BoxDecoration].
final Decoration decoration;
/// Whether to paint the box decoration behind or in front of the child.
final DecorationPosition position;
@override
RenderDecoratedSliver createRenderObject(BuildContext context) {
return RenderDecoratedSliver(
decoration: decoration,
position: position,
configuration: createLocalImageConfiguration(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderDecoratedSliver renderObject) {
renderObject
..decoration = decoration
..position = position
..configuration = createLocalImageConfiguration(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final String label = switch (position) {
DecorationPosition.background => 'bg',
DecorationPosition.foreground => 'fg',
};
properties.add(EnumProperty<DecorationPosition>('position', position, level: DiagnosticLevel.hidden));
properties.add(DiagnosticsProperty<Decoration>(label, decoration));
}
}
| flutter/packages/flutter/lib/src/widgets/decorated_sliver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/decorated_sliver.dart",
"repo_id": "flutter",
"token_count": 877
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'binding.dart';
import 'debug.dart';
import 'focus_manager.dart';
import 'inherited_model.dart';
import 'notification_listener.dart';
import 'widget_inspector.dart';
export 'package:flutter/foundation.dart' show
factory,
immutable,
mustCallSuper,
optionalTypeArgs,
protected,
required,
visibleForTesting;
export 'package:flutter/foundation.dart' show ErrorDescription, ErrorHint, ErrorSummary, FlutterError, debugPrint, debugPrintStack;
export 'package:flutter/foundation.dart' show ValueChanged, ValueGetter, ValueSetter, VoidCallback;
export 'package:flutter/foundation.dart' show DiagnosticLevel, DiagnosticsNode;
export 'package:flutter/foundation.dart' show Key, LocalKey, ValueKey;
export 'package:flutter/rendering.dart' show RenderBox, RenderObject, debugDumpLayerTree, debugDumpRenderTree;
// Examples can assume:
// late BuildContext context;
// void setState(VoidCallback fn) { }
// abstract class RenderFrogJar extends RenderObject { }
// abstract class FrogJar extends RenderObjectWidget { const FrogJar({super.key}); }
// abstract class FrogJarParentData extends ParentData { late Size size; }
// abstract class SomeWidget extends StatefulWidget { const SomeWidget({super.key}); }
// typedef ChildWidget = Placeholder;
// class _SomeWidgetState extends State<SomeWidget> { @override Widget build(BuildContext context) => widget; }
// abstract class RenderFoo extends RenderObject { }
// abstract class Foo extends RenderObjectWidget { const Foo({super.key}); }
// abstract class StatefulWidgetX { const StatefulWidgetX({this.key}); final Key? key; Widget build(BuildContext context, State state); }
// class SpecialWidget extends StatelessWidget { const SpecialWidget({ super.key, this.handler }); final VoidCallback? handler; @override Widget build(BuildContext context) => this; }
// late Object? _myState, newValue;
// int _counter = 0;
// Future<Directory> getApplicationDocumentsDirectory() async => Directory('');
// late AnimationController animation;
// An annotation used by test_analysis package to verify patterns are followed
// that allow for tree-shaking of both fields and their initializers. This
// annotation has no impact on code by itself, but indicates the following pattern
// should be followed for a given field:
//
// ```dart
// class Foo {
// final bar = kDebugMode ? Object() : null;
// }
// ```
class _DebugOnly {
const _DebugOnly();
}
const _DebugOnly _debugOnly = _DebugOnly();
// KEYS
/// A key that takes its identity from the object used as its value.
///
/// Used to tie the identity of a widget to the identity of an object used to
/// generate that widget.
///
/// See also:
///
/// * [Key], the base class for all keys.
/// * The discussion at [Widget.key] for more information about how widgets use
/// keys.
class ObjectKey extends LocalKey {
/// Creates a key that uses [identical] on [value] for its [operator==].
const ObjectKey(this.value);
/// The object whose identity is used by this key's [operator==].
final Object? value;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ObjectKey
&& identical(other.value, value);
}
@override
int get hashCode => Object.hash(runtimeType, identityHashCode(value));
@override
String toString() {
if (runtimeType == ObjectKey) {
return '[${describeIdentity(value)}]';
}
return '[${objectRuntimeType(this, 'ObjectKey')} ${describeIdentity(value)}]';
}
}
/// A key that is unique across the entire app.
///
/// Global keys uniquely identify elements. Global keys provide access to other
/// objects that are associated with those elements, such as [BuildContext].
/// For [StatefulWidget]s, global keys also provide access to [State].
///
/// Widgets that have global keys reparent their subtrees when they are moved
/// from one location in the tree to another location in the tree. In order to
/// reparent its subtree, a widget must arrive at its new location in the tree
/// in the same animation frame in which it was removed from its old location in
/// the tree.
///
/// Reparenting an [Element] using a global key is relatively expensive, as
/// this operation will trigger a call to [State.deactivate] on the associated
/// [State] and all of its descendants; then force all widgets that depends
/// on an [InheritedWidget] to rebuild.
///
/// If you don't need any of the features listed above, consider using a [Key],
/// [ValueKey], [ObjectKey], or [UniqueKey] instead.
///
/// You cannot simultaneously include two widgets in the tree with the same
/// global key. Attempting to do so will assert at runtime.
///
/// ## Pitfalls
///
/// GlobalKeys should not be re-created on every build. They should usually be
/// long-lived objects owned by a [State] object, for example.
///
/// Creating a new GlobalKey on every build will throw away the state of the
/// subtree associated with the old key and create a new fresh subtree for the
/// new key. Besides harming performance, this can also cause unexpected
/// behavior in widgets in the subtree. For example, a [GestureDetector] in the
/// subtree will be unable to track ongoing gestures since it will be recreated
/// on each build.
///
/// Instead, a good practice is to let a State object own the GlobalKey, and
/// instantiate it outside the build method, such as in [State.initState].
///
/// See also:
///
/// * The discussion at [Widget.key] for more information about how widgets use
/// keys.
@optionalTypeArgs
abstract class GlobalKey<T extends State<StatefulWidget>> extends Key {
/// Creates a [LabeledGlobalKey], which is a [GlobalKey] with a label used for
/// debugging.
///
/// The label is purely for debugging and not used for comparing the identity
/// of the key.
factory GlobalKey({ String? debugLabel }) => LabeledGlobalKey<T>(debugLabel);
/// Creates a global key without a label.
///
/// Used by subclasses because the factory constructor shadows the implicit
/// constructor.
const GlobalKey.constructor() : super.empty();
Element? get _currentElement => WidgetsBinding.instance.buildOwner!._globalKeyRegistry[this];
/// The build context in which the widget with this key builds.
///
/// The current context is null if there is no widget in the tree that matches
/// this global key.
BuildContext? get currentContext => _currentElement;
/// The widget in the tree that currently has this global key.
///
/// The current widget is null if there is no widget in the tree that matches
/// this global key.
Widget? get currentWidget => _currentElement?.widget;
/// The [State] for the widget in the tree that currently has this global key.
///
/// The current state is null if (1) there is no widget in the tree that
/// matches this global key, (2) that widget is not a [StatefulWidget], or the
/// associated [State] object is not a subtype of `T`.
T? get currentState {
final Element? element = _currentElement;
if (element is StatefulElement) {
final StatefulElement statefulElement = element;
final State state = statefulElement.state;
if (state is T) {
return state;
}
}
return null;
}
}
/// A global key with a debugging label.
///
/// The debug label is useful for documentation and for debugging. The label
/// does not affect the key's identity.
@optionalTypeArgs
class LabeledGlobalKey<T extends State<StatefulWidget>> extends GlobalKey<T> {
/// Creates a global key with a debugging label.
///
/// The label does not affect the key's identity.
// ignore: prefer_const_constructors_in_immutables , never use const for this class
LabeledGlobalKey(this._debugLabel) : super.constructor();
final String? _debugLabel;
@override
String toString() {
final String label = _debugLabel != null ? ' $_debugLabel' : '';
if (runtimeType == LabeledGlobalKey) {
return '[GlobalKey#${shortHash(this)}$label]';
}
return '[${describeIdentity(this)}$label]';
}
}
/// A global key that takes its identity from the object used as its value.
///
/// Used to tie the identity of a widget to the identity of an object used to
/// generate that widget.
///
/// Any [GlobalObjectKey] created for the same object will match.
///
/// If the object is not private, then it is possible that collisions will occur
/// where independent widgets will reuse the same object as their
/// [GlobalObjectKey] value in a different part of the tree, leading to a global
/// key conflict. To avoid this problem, create a private [GlobalObjectKey]
/// subclass, as in:
///
/// ```dart
/// class _MyKey extends GlobalObjectKey {
/// const _MyKey(super.value);
/// }
/// ```
///
/// Since the [runtimeType] of the key is part of its identity, this will
/// prevent clashes with other [GlobalObjectKey]s even if they have the same
/// value.
@optionalTypeArgs
class GlobalObjectKey<T extends State<StatefulWidget>> extends GlobalKey<T> {
/// Creates a global key that uses [identical] on [value] for its [operator==].
const GlobalObjectKey(this.value) : super.constructor();
/// The object whose identity is used by this key's [operator==].
final Object value;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is GlobalObjectKey<T>
&& identical(other.value, value);
}
@override
int get hashCode => identityHashCode(value);
@override
String toString() {
String selfType = objectRuntimeType(this, 'GlobalObjectKey');
// The runtimeType string of a GlobalObjectKey() returns 'GlobalObjectKey<State<StatefulWidget>>'
// because GlobalObjectKey is instantiated to its bounds. To avoid cluttering the output
// we remove the suffix.
const String suffix = '<State<StatefulWidget>>';
if (selfType.endsWith(suffix)) {
selfType = selfType.substring(0, selfType.length - suffix.length);
}
return '[$selfType ${describeIdentity(value)}]';
}
}
/// Describes the configuration for an [Element].
///
/// Widgets are the central class hierarchy in the Flutter framework. A widget
/// is an immutable description of part of a user interface. Widgets can be
/// inflated into elements, which manage the underlying render tree.
///
/// Widgets themselves have no mutable state (all their fields must be final).
/// If you wish to associate mutable state with a widget, consider using a
/// [StatefulWidget], which creates a [State] object (via
/// [StatefulWidget.createState]) whenever it is inflated into an element and
/// incorporated into the tree.
///
/// A given widget can be included in the tree zero or more times. In particular
/// a given widget can be placed in the tree multiple times. Each time a widget
/// is placed in the tree, it is inflated into an [Element], which means a
/// widget that is incorporated into the tree multiple times will be inflated
/// multiple times.
///
/// The [key] property controls how one widget replaces another widget in the
/// tree. If the [runtimeType] and [key] properties of the two widgets are
/// [operator==], respectively, then the new widget replaces the old widget by
/// updating the underlying element (i.e., by calling [Element.update] with the
/// new widget). Otherwise, the old element is removed from the tree, the new
/// widget is inflated into an element, and the new element is inserted into the
/// tree.
///
/// See also:
///
/// * [StatefulWidget] and [State], for widgets that can build differently
/// several times over their lifetime.
/// * [InheritedWidget], for widgets that introduce ambient state that can
/// be read by descendant widgets.
/// * [StatelessWidget], for widgets that always build the same way given a
/// particular configuration and ambient state.
@immutable
abstract class Widget extends DiagnosticableTree {
/// Initializes [key] for subclasses.
const Widget({ this.key });
/// Controls how one widget replaces another widget in the tree.
///
/// If the [runtimeType] and [key] properties of the two widgets are
/// [operator==], respectively, then the new widget replaces the old widget by
/// updating the underlying element (i.e., by calling [Element.update] with the
/// new widget). Otherwise, the old element is removed from the tree, the new
/// widget is inflated into an element, and the new element is inserted into the
/// tree.
///
/// In addition, using a [GlobalKey] as the widget's [key] allows the element
/// to be moved around the tree (changing parent) without losing state. When a
/// new widget is found (its key and type do not match a previous widget in
/// the same location), but there was a widget with that same global key
/// elsewhere in the tree in the previous frame, then that widget's element is
/// moved to the new location.
///
/// Generally, a widget that is the only child of another widget does not need
/// an explicit key.
///
/// See also:
///
/// * The discussions at [Key] and [GlobalKey].
final Key? key;
/// Inflates this configuration to a concrete instance.
///
/// A given widget can be included in the tree zero or more times. In particular
/// a given widget can be placed in the tree multiple times. Each time a widget
/// is placed in the tree, it is inflated into an [Element], which means a
/// widget that is incorporated into the tree multiple times will be inflated
/// multiple times.
@protected
@factory
Element createElement();
/// A short, textual description of this widget.
@override
String toStringShort() {
final String type = objectRuntimeType(this, 'Widget');
return key == null ? type : '$type-$key';
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.defaultDiagnosticsTreeStyle = DiagnosticsTreeStyle.dense;
}
@override
@nonVirtual
bool operator ==(Object other) => super == other;
@override
@nonVirtual
int get hashCode => super.hashCode;
/// Whether the `newWidget` can be used to update an [Element] that currently
/// has the `oldWidget` as its configuration.
///
/// An element that uses a given widget as its configuration can be updated to
/// use another widget as its configuration if, and only if, the two widgets
/// have [runtimeType] and [key] properties that are [operator==].
///
/// If the widgets have no key (their key is null), then they are considered a
/// match if they have the same type, even if their children are completely
/// different.
static bool canUpdate(Widget oldWidget, Widget newWidget) {
return oldWidget.runtimeType == newWidget.runtimeType
&& oldWidget.key == newWidget.key;
}
// Return a numeric encoding of the specific `Widget` concrete subtype.
// This is used in `Element.updateChild` to determine if a hot reload modified the
// superclass of a mounted element's configuration. The encoding of each `Widget`
// must match the corresponding `Element` encoding in `Element._debugConcreteSubtype`.
static int _debugConcreteSubtype(Widget widget) {
return widget is StatefulWidget ? 1 :
widget is StatelessWidget ? 2 :
0;
}
}
/// A widget that does not require mutable state.
///
/// A stateless widget is a widget that describes part of the user interface by
/// building a constellation of other widgets that describe the user interface
/// more concretely. The building process continues recursively until the
/// description of the user interface is fully concrete (e.g., consists
/// entirely of [RenderObjectWidget]s, which describe concrete [RenderObject]s).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=wE7khGHVkYY}
///
/// Stateless widget are useful when the part of the user interface you are
/// describing does not depend on anything other than the configuration
/// information in the object itself and the [BuildContext] in which the widget
/// is inflated. For compositions that can change dynamically, e.g. due to
/// having an internal clock-driven state, or depending on some system state,
/// consider using [StatefulWidget].
///
/// ## Performance considerations
///
/// The [build] method of a stateless widget is typically only called in three
/// situations: the first time the widget is inserted in the tree, when the
/// widget's parent changes its configuration (see [Element.rebuild]), and when
/// an [InheritedWidget] it depends on changes.
///
/// If a widget's parent will regularly change the widget's configuration, or if
/// it depends on inherited widgets that frequently change, then it is important
/// to optimize the performance of the [build] method to maintain a fluid
/// rendering performance.
///
/// There are several techniques one can use to minimize the impact of
/// rebuilding a stateless widget:
///
/// * Minimize the number of nodes transitively created by the build method and
/// any widgets it creates. For example, instead of an elaborate arrangement
/// of [Row]s, [Column]s, [Padding]s, and [SizedBox]es to position a single
/// child in a particularly fancy manner, consider using just an [Align] or a
/// [CustomSingleChildLayout]. Instead of an intricate layering of multiple
/// [Container]s and with [Decoration]s to draw just the right graphical
/// effect, consider a single [CustomPaint] widget.
///
/// * Use `const` widgets where possible, and provide a `const` constructor for
/// the widget so that users of the widget can also do so.
///
/// * Consider refactoring the stateless widget into a stateful widget so that
/// it can use some of the techniques described at [StatefulWidget], such as
/// caching common parts of subtrees and using [GlobalKey]s when changing the
/// tree structure.
///
/// * If the widget is likely to get rebuilt frequently due to the use of
/// [InheritedWidget]s, consider refactoring the stateless widget into
/// multiple widgets, with the parts of the tree that change being pushed to
/// the leaves. For example instead of building a tree with four widgets, the
/// inner-most widget depending on the [Theme], consider factoring out the
/// part of the build function that builds the inner-most widget into its own
/// widget, so that only the inner-most widget needs to be rebuilt when the
/// theme changes.
/// {@template flutter.flutter.widgets.framework.prefer_const_over_helper}
/// * When trying to create a reusable piece of UI, prefer using a widget
/// rather than a helper method. For example, if there was a function used to
/// build a widget, a [State.setState] call would require Flutter to entirely
/// rebuild the returned wrapping widget. If a [Widget] was used instead,
/// Flutter would be able to efficiently re-render only those parts that
/// really need to be updated. Even better, if the created widget is `const`,
/// Flutter would short-circuit most of the rebuild work.
/// {@endtemplate}
///
/// This video gives more explanations on why `const` constructors are important
/// and why a [Widget] is better than a helper method.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=IOyq-eTRhvo}
///
/// {@tool snippet}
///
/// The following is a skeleton of a stateless widget subclass called `GreenFrog`.
///
/// Normally, widgets have more constructor arguments, each of which corresponds
/// to a `final` property.
///
/// ```dart
/// class GreenFrog extends StatelessWidget {
/// const GreenFrog({ super.key });
///
/// @override
/// Widget build(BuildContext context) {
/// return Container(color: const Color(0xFF2DBD3A));
/// }
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// This next example shows the more generic widget `Frog` which can be given
/// a color and a child:
///
/// ```dart
/// class Frog extends StatelessWidget {
/// const Frog({
/// super.key,
/// this.color = const Color(0xFF2DBD3A),
/// this.child,
/// });
///
/// final Color color;
/// final Widget? child;
///
/// @override
/// Widget build(BuildContext context) {
/// return ColoredBox(color: color, child: child);
/// }
/// }
/// ```
/// {@end-tool}
///
/// By convention, widget constructors only use named arguments. Also by
/// convention, the first argument is [key], and the last argument is `child`,
/// `children`, or the equivalent.
///
/// See also:
///
/// * [StatefulWidget] and [State], for widgets that can build differently
/// several times over their lifetime.
/// * [InheritedWidget], for widgets that introduce ambient state that can
/// be read by descendant widgets.
abstract class StatelessWidget extends Widget {
/// Initializes [key] for subclasses.
const StatelessWidget({ super.key });
/// Creates a [StatelessElement] to manage this widget's location in the tree.
///
/// It is uncommon for subclasses to override this method.
@override
StatelessElement createElement() => StatelessElement(this);
/// Describes the part of the user interface represented by this widget.
///
/// The framework calls this method when this widget is inserted into the tree
/// in a given [BuildContext] and when the dependencies of this widget change
/// (e.g., an [InheritedWidget] referenced by this widget changes). This
/// method can potentially be called in every frame and should not have any side
/// effects beyond building a widget.
///
/// The framework replaces the subtree below this widget with the widget
/// returned by this method, either by updating the existing subtree or by
/// removing the subtree and inflating a new subtree, depending on whether the
/// widget returned by this method can update the root of the existing
/// subtree, as determined by calling [Widget.canUpdate].
///
/// Typically implementations return a newly created constellation of widgets
/// that are configured with information from this widget's constructor and
/// from the given [BuildContext].
///
/// The given [BuildContext] contains information about the location in the
/// tree at which this widget is being built. For example, the context
/// provides the set of inherited widgets for this location in the tree. A
/// given widget might be built with multiple different [BuildContext]
/// arguments over time if the widget is moved around the tree or if the
/// widget is inserted into the tree in multiple places at once.
///
/// The implementation of this method must only depend on:
///
/// * the fields of the widget, which themselves must not change over time,
/// and
/// * any ambient state obtained from the `context` using
/// [BuildContext.dependOnInheritedWidgetOfExactType].
///
/// If a widget's [build] method is to depend on anything else, use a
/// [StatefulWidget] instead.
///
/// See also:
///
/// * [StatelessWidget], which contains the discussion on performance considerations.
@protected
Widget build(BuildContext context);
}
/// A widget that has mutable state.
///
/// State is information that (1) can be read synchronously when the widget is
/// built and (2) might change during the lifetime of the widget. It is the
/// responsibility of the widget implementer to ensure that the [State] is
/// promptly notified when such state changes, using [State.setState].
///
/// A stateful widget is a widget that describes part of the user interface by
/// building a constellation of other widgets that describe the user interface
/// more concretely. The building process continues recursively until the
/// description of the user interface is fully concrete (e.g., consists
/// entirely of [RenderObjectWidget]s, which describe concrete [RenderObject]s).
///
/// Stateful widgets are useful when the part of the user interface you are
/// describing can change dynamically, e.g. due to having an internal
/// clock-driven state, or depending on some system state. For compositions that
/// depend only on the configuration information in the object itself and the
/// [BuildContext] in which the widget is inflated, consider using
/// [StatelessWidget].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=AqCMFXEmf3w}
///
/// [StatefulWidget] instances themselves are immutable and store their mutable
/// state either in separate [State] objects that are created by the
/// [createState] method, or in objects to which that [State] subscribes, for
/// example [Stream] or [ChangeNotifier] objects, to which references are stored
/// in final fields on the [StatefulWidget] itself.
///
/// The framework calls [createState] whenever it inflates a
/// [StatefulWidget], which means that multiple [State] objects might be
/// associated with the same [StatefulWidget] if that widget has been inserted
/// into the tree in multiple places. Similarly, if a [StatefulWidget] is
/// removed from the tree and later inserted in to the tree again, the framework
/// will call [createState] again to create a fresh [State] object, simplifying
/// the lifecycle of [State] objects.
///
/// A [StatefulWidget] keeps the same [State] object when moving from one
/// location in the tree to another if its creator used a [GlobalKey] for its
/// [key]. Because a widget with a [GlobalKey] can be used in at most one
/// location in the tree, a widget that uses a [GlobalKey] has at most one
/// associated element. The framework takes advantage of this property when
/// moving a widget with a global key from one location in the tree to another
/// by grafting the (unique) subtree associated with that widget from the old
/// location to the new location (instead of recreating the subtree at the new
/// location). The [State] objects associated with [StatefulWidget] are grafted
/// along with the rest of the subtree, which means the [State] object is reused
/// (instead of being recreated) in the new location. However, in order to be
/// eligible for grafting, the widget must be inserted into the new location in
/// the same animation frame in which it was removed from the old location.
///
/// ## Performance considerations
///
/// There are two primary categories of [StatefulWidget]s.
///
/// The first is one which allocates resources in [State.initState] and disposes
/// of them in [State.dispose], but which does not depend on [InheritedWidget]s
/// or call [State.setState]. Such widgets are commonly used at the root of an
/// application or page, and communicate with subwidgets via [ChangeNotifier]s,
/// [Stream]s, or other such objects. Stateful widgets following such a pattern
/// are relatively cheap (in terms of CPU and GPU cycles), because they are
/// built once then never update. They can, therefore, have somewhat complicated
/// and deep build methods.
///
/// The second category is widgets that use [State.setState] or depend on
/// [InheritedWidget]s. These will typically rebuild many times during the
/// application's lifetime, and it is therefore important to minimize the impact
/// of rebuilding such a widget. (They may also use [State.initState] or
/// [State.didChangeDependencies] and allocate resources, but the important part
/// is that they rebuild.)
///
/// There are several techniques one can use to minimize the impact of
/// rebuilding a stateful widget:
///
/// * Push the state to the leaves. For example, if your page has a ticking
/// clock, rather than putting the state at the top of the page and
/// rebuilding the entire page each time the clock ticks, create a dedicated
/// clock widget that only updates itself.
///
/// * Minimize the number of nodes transitively created by the build method and
/// any widgets it creates. Ideally, a stateful widget would only create a
/// single widget, and that widget would be a [RenderObjectWidget].
/// (Obviously this isn't always practical, but the closer a widget gets to
/// this ideal, the more efficient it will be.)
///
/// * If a subtree does not change, cache the widget that represents that
/// subtree and re-use it each time it can be used. To do this, assign
/// a widget to a `final` state variable and re-use it in the build method. It
/// is massively more efficient for a widget to be re-used than for a new (but
/// identically-configured) widget to be created. Another caching strategy
/// consists in extracting the mutable part of the widget into a [StatefulWidget]
/// which accepts a child parameter.
///
/// * Use `const` widgets where possible. (This is equivalent to caching a
/// widget and re-using it.)
///
/// * Avoid changing the depth of any created subtrees or changing the type of
/// any widgets in the subtree. For example, rather than returning either the
/// child or the child wrapped in an [IgnorePointer], always wrap the child
/// widget in an [IgnorePointer] and control the [IgnorePointer.ignoring]
/// property. This is because changing the depth of the subtree requires
/// rebuilding, laying out, and painting the entire subtree, whereas just
/// changing the property will require the least possible change to the
/// render tree (in the case of [IgnorePointer], for example, no layout or
/// repaint is necessary at all).
///
/// * If the depth must be changed for some reason, consider wrapping the
/// common parts of the subtrees in widgets that have a [GlobalKey] that
/// remains consistent for the life of the stateful widget. (The
/// [KeyedSubtree] widget may be useful for this purpose if no other widget
/// can conveniently be assigned the key.)
///
/// {@macro flutter.flutter.widgets.framework.prefer_const_over_helper}
///
/// This video gives more explanations on why `const` constructors are important
/// and why a [Widget] is better than a helper method.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=IOyq-eTRhvo}
///
/// For more details on the mechanics of rebuilding a widget, see
/// the discussion at [Element.rebuild].
///
/// {@tool snippet}
///
/// This is a skeleton of a stateful widget subclass called `YellowBird`.
///
/// In this example, the [State] has no actual state. State is normally
/// represented as private member fields. Also, normally widgets have more
/// constructor arguments, each of which corresponds to a `final` property.
///
/// ```dart
/// class YellowBird extends StatefulWidget {
/// const YellowBird({ super.key });
///
/// @override
/// State<YellowBird> createState() => _YellowBirdState();
/// }
///
/// class _YellowBirdState extends State<YellowBird> {
/// @override
/// Widget build(BuildContext context) {
/// return Container(color: const Color(0xFFFFE306));
/// }
/// }
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// This example shows the more generic widget `Bird` which can be given a
/// color and a child, and which has some internal state with a method that
/// can be called to mutate it:
///
/// ```dart
/// class Bird extends StatefulWidget {
/// const Bird({
/// super.key,
/// this.color = const Color(0xFFFFE306),
/// this.child,
/// });
///
/// final Color color;
/// final Widget? child;
///
/// @override
/// State<Bird> createState() => _BirdState();
/// }
///
/// class _BirdState extends State<Bird> {
/// double _size = 1.0;
///
/// void grow() {
/// setState(() { _size += 0.1; });
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Container(
/// color: widget.color,
/// transform: Matrix4.diagonal3Values(_size, _size, 1.0),
/// child: widget.child,
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// By convention, widget constructors only use named arguments. Also by
/// convention, the first argument is [key], and the last argument is `child`,
/// `children`, or the equivalent.
///
/// See also:
///
/// * [State], where the logic behind a [StatefulWidget] is hosted.
/// * [StatelessWidget], for widgets that always build the same way given a
/// particular configuration and ambient state.
/// * [InheritedWidget], for widgets that introduce ambient state that can
/// be read by descendant widgets.
abstract class StatefulWidget extends Widget {
/// Initializes [key] for subclasses.
const StatefulWidget({ super.key });
/// Creates a [StatefulElement] to manage this widget's location in the tree.
///
/// It is uncommon for subclasses to override this method.
@override
StatefulElement createElement() => StatefulElement(this);
/// Creates the mutable state for this widget at a given location in the tree.
///
/// Subclasses should override this method to return a newly created
/// instance of their associated [State] subclass:
///
/// ```dart
/// @override
/// State<SomeWidget> createState() => _SomeWidgetState();
/// ```
///
/// The framework can call this method multiple times over the lifetime of
/// a [StatefulWidget]. For example, if the widget is inserted into the tree
/// in multiple locations, the framework will create a separate [State] object
/// for each location. Similarly, if the widget is removed from the tree and
/// later inserted into the tree again, the framework will call [createState]
/// again to create a fresh [State] object, simplifying the lifecycle of
/// [State] objects.
@protected
@factory
State createState();
}
/// Tracks the lifecycle of [State] objects when asserts are enabled.
enum _StateLifecycle {
/// The [State] object has been created. [State.initState] is called at this
/// time.
created,
/// The [State.initState] method has been called but the [State] object is
/// not yet ready to build. [State.didChangeDependencies] is called at this time.
initialized,
/// The [State] object is ready to build and [State.dispose] has not yet been
/// called.
ready,
/// The [State.dispose] method has been called and the [State] object is
/// no longer able to build.
defunct,
}
/// The signature of [State.setState] functions.
typedef StateSetter = void Function(VoidCallback fn);
const String _flutterWidgetsLibrary = 'package:flutter/widgets.dart';
/// The logic and internal state for a [StatefulWidget].
///
/// State is information that (1) can be read synchronously when the widget is
/// built and (2) might change during the lifetime of the widget. It is the
/// responsibility of the widget implementer to ensure that the [State] is
/// promptly notified when such state changes, using [State.setState].
///
/// [State] objects are created by the framework by calling the
/// [StatefulWidget.createState] method when inflating a [StatefulWidget] to
/// insert it into the tree. Because a given [StatefulWidget] instance can be
/// inflated multiple times (e.g., the widget is incorporated into the tree in
/// multiple places at once), there might be more than one [State] object
/// associated with a given [StatefulWidget] instance. Similarly, if a
/// [StatefulWidget] is removed from the tree and later inserted in to the tree
/// again, the framework will call [StatefulWidget.createState] again to create
/// a fresh [State] object, simplifying the lifecycle of [State] objects.
///
/// [State] objects have the following lifecycle:
///
/// * The framework creates a [State] object by calling
/// [StatefulWidget.createState].
/// * The newly created [State] object is associated with a [BuildContext].
/// This association is permanent: the [State] object will never change its
/// [BuildContext]. However, the [BuildContext] itself can be moved around
/// the tree along with its subtree. At this point, the [State] object is
/// considered [mounted].
/// * The framework calls [initState]. Subclasses of [State] should override
/// [initState] to perform one-time initialization that depends on the
/// [BuildContext] or the widget, which are available as the [context] and
/// [widget] properties, respectively, when the [initState] method is
/// called.
/// * The framework calls [didChangeDependencies]. Subclasses of [State] should
/// override [didChangeDependencies] to perform initialization involving
/// [InheritedWidget]s. If [BuildContext.dependOnInheritedWidgetOfExactType] is
/// called, the [didChangeDependencies] method will be called again if the
/// inherited widgets subsequently change or if the widget moves in the tree.
/// * At this point, the [State] object is fully initialized and the framework
/// might call its [build] method any number of times to obtain a
/// description of the user interface for this subtree. [State] objects can
/// spontaneously request to rebuild their subtree by calling their
/// [setState] method, which indicates that some of their internal state
/// has changed in a way that might impact the user interface in this
/// subtree.
/// * During this time, a parent widget might rebuild and request that this
/// location in the tree update to display a new widget with the same
/// [runtimeType] and [Widget.key]. When this happens, the framework will
/// update the [widget] property to refer to the new widget and then call the
/// [didUpdateWidget] method with the previous widget as an argument. [State]
/// objects should override [didUpdateWidget] to respond to changes in their
/// associated widget (e.g., to start implicit animations). The framework
/// always calls [build] after calling [didUpdateWidget], which means any
/// calls to [setState] in [didUpdateWidget] are redundant. (See also the
/// discussion at [Element.rebuild].)
/// * During development, if a hot reload occurs (whether initiated from the
/// command line `flutter` tool by pressing `r`, or from an IDE), the
/// [reassemble] method is called. This provides an opportunity to
/// reinitialize any data that was prepared in the [initState] method.
/// * If the subtree containing the [State] object is removed from the tree
/// (e.g., because the parent built a widget with a different [runtimeType]
/// or [Widget.key]), the framework calls the [deactivate] method. Subclasses
/// should override this method to clean up any links between this object
/// and other elements in the tree (e.g. if you have provided an ancestor
/// with a pointer to a descendant's [RenderObject]).
/// * At this point, the framework might reinsert this subtree into another
/// part of the tree. If that happens, the framework will ensure that it
/// calls [build] to give the [State] object a chance to adapt to its new
/// location in the tree. If the framework does reinsert this subtree, it
/// will do so before the end of the animation frame in which the subtree was
/// removed from the tree. For this reason, [State] objects can defer
/// releasing most resources until the framework calls their [dispose]
/// method.
/// * If the framework does not reinsert this subtree by the end of the current
/// animation frame, the framework will call [dispose], which indicates that
/// this [State] object will never build again. Subclasses should override
/// this method to release any resources retained by this object (e.g.,
/// stop any active animations).
/// * After the framework calls [dispose], the [State] object is considered
/// unmounted and the [mounted] property is false. It is an error to call
/// [setState] at this point. This stage of the lifecycle is terminal: there
/// is no way to remount a [State] object that has been disposed.
///
/// See also:
///
/// * [StatefulWidget], where the current configuration of a [State] is hosted,
/// and whose documentation has sample code for [State].
/// * [StatelessWidget], for widgets that always build the same way given a
/// particular configuration and ambient state.
/// * [InheritedWidget], for widgets that introduce ambient state that can
/// be read by descendant widgets.
/// * [Widget], for an overview of widgets in general.
@optionalTypeArgs
abstract class State<T extends StatefulWidget> with Diagnosticable {
/// The current configuration.
///
/// A [State] object's configuration is the corresponding [StatefulWidget]
/// instance. This property is initialized by the framework before calling
/// [initState]. If the parent updates this location in the tree to a new
/// widget with the same [runtimeType] and [Widget.key] as the current
/// configuration, the framework will update this property to refer to the new
/// widget and then call [didUpdateWidget], passing the old configuration as
/// an argument.
T get widget => _widget!;
T? _widget;
/// The current stage in the lifecycle for this state object.
///
/// This field is used by the framework when asserts are enabled to verify
/// that [State] objects move through their lifecycle in an orderly fashion.
_StateLifecycle _debugLifecycleState = _StateLifecycle.created;
/// Verifies that the [State] that was created is one that expects to be
/// created for that particular [Widget].
bool _debugTypesAreRight(Widget widget) => widget is T;
/// The location in the tree where this widget builds.
///
/// The framework associates [State] objects with a [BuildContext] after
/// creating them with [StatefulWidget.createState] and before calling
/// [initState]. The association is permanent: the [State] object will never
/// change its [BuildContext]. However, the [BuildContext] itself can be moved
/// around the tree.
///
/// After calling [dispose], the framework severs the [State] object's
/// connection with the [BuildContext].
BuildContext get context {
assert(() {
if (_element == null) {
throw FlutterError(
'This widget has been unmounted, so the State no longer has a context (and should be considered defunct). \n'
'Consider canceling any active work during "dispose" or using the "mounted" getter to determine if the State is still active.',
);
}
return true;
}());
return _element!;
}
StatefulElement? _element;
/// Whether this [State] object is currently in a tree.
///
/// After creating a [State] object and before calling [initState], the
/// framework "mounts" the [State] object by associating it with a
/// [BuildContext]. The [State] object remains mounted until the framework
/// calls [dispose], after which time the framework will never ask the [State]
/// object to [build] again.
///
/// It is an error to call [setState] unless [mounted] is true.
bool get mounted => _element != null;
/// Called when this object is inserted into the tree.
///
/// The framework will call this method exactly once for each [State] object
/// it creates.
///
/// Override this method to perform initialization that depends on the
/// location at which this object was inserted into the tree (i.e., [context])
/// or on the widget used to configure this object (i.e., [widget]).
///
/// {@template flutter.widgets.State.initState}
/// If a [State]'s [build] method depends on an object that can itself
/// change state, for example a [ChangeNotifier] or [Stream], or some
/// other object to which one can subscribe to receive notifications, then
/// be sure to subscribe and unsubscribe properly in [initState],
/// [didUpdateWidget], and [dispose]:
///
/// * In [initState], subscribe to the object.
/// * In [didUpdateWidget] unsubscribe from the old object and subscribe
/// to the new one if the updated widget configuration requires
/// replacing the object.
/// * In [dispose], unsubscribe from the object.
///
/// {@endtemplate}
///
/// You should not use [BuildContext.dependOnInheritedWidgetOfExactType] from this
/// method. However, [didChangeDependencies] will be called immediately
/// following this method, and [BuildContext.dependOnInheritedWidgetOfExactType] can
/// be used there.
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.initState()`.
@protected
@mustCallSuper
void initState() {
assert(_debugLifecycleState == _StateLifecycle.created);
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterWidgetsLibrary,
className: '$State',
object: this,
);
}
}
/// Called whenever the widget configuration changes.
///
/// If the parent widget rebuilds and requests that this location in the tree
/// update to display a new widget with the same [runtimeType] and
/// [Widget.key], the framework will update the [widget] property of this
/// [State] object to refer to the new widget and then call this method
/// with the previous widget as an argument.
///
/// Override this method to respond when the [widget] changes (e.g., to start
/// implicit animations).
///
/// The framework always calls [build] after calling [didUpdateWidget], which
/// means any calls to [setState] in [didUpdateWidget] are redundant.
///
/// {@macro flutter.widgets.State.initState}
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.didUpdateWidget(oldWidget)`.
///
/// _See the discussion at [Element.rebuild] for more information on when this
/// method is called._
@mustCallSuper
@protected
void didUpdateWidget(covariant T oldWidget) { }
/// {@macro flutter.widgets.Element.reassemble}
///
/// In addition to this method being invoked, it is guaranteed that the
/// [build] method will be invoked when a reassemble is signaled. Most
/// widgets therefore do not need to do anything in the [reassemble] method.
///
/// See also:
///
/// * [Element.reassemble]
/// * [BindingBase.reassembleApplication]
/// * [Image], which uses this to reload images.
@protected
@mustCallSuper
void reassemble() { }
/// Notify the framework that the internal state of this object has changed.
///
/// Whenever you change the internal state of a [State] object, make the
/// change in a function that you pass to [setState]:
///
/// ```dart
/// setState(() { _myState = newValue; });
/// ```
///
/// The provided callback is immediately called synchronously. It must not
/// return a future (the callback cannot be `async`), since then it would be
/// unclear when the state was actually being set.
///
/// Calling [setState] notifies the framework that the internal state of this
/// object has changed in a way that might impact the user interface in this
/// subtree, which causes the framework to schedule a [build] for this [State]
/// object.
///
/// If you just change the state directly without calling [setState], the
/// framework might not schedule a [build] and the user interface for this
/// subtree might not be updated to reflect the new state.
///
/// Generally it is recommended that the [setState] method only be used to
/// wrap the actual changes to the state, not any computation that might be
/// associated with the change. For example, here a value used by the [build]
/// function is incremented, and then the change is written to disk, but only
/// the increment is wrapped in the [setState]:
///
/// ```dart
/// Future<void> _incrementCounter() async {
/// setState(() {
/// _counter++;
/// });
/// Directory directory = await getApplicationDocumentsDirectory(); // from path_provider package
/// final String dirName = directory.path;
/// await File('$dirName/counter.txt').writeAsString('$_counter');
/// }
/// ```
///
/// Sometimes, the changed state is in some other object not owned by the
/// widget [State], but the widget nonetheless needs to be updated to react to
/// the new state. This is especially common with [Listenable]s, such as
/// [AnimationController]s.
///
/// In such cases, it is good practice to leave a comment in the callback
/// passed to [setState] that explains what state changed:
///
/// ```dart
/// void _update() {
/// setState(() { /* The animation changed. */ });
/// }
/// //...
/// animation.addListener(_update);
/// ```
///
/// It is an error to call this method after the framework calls [dispose].
/// You can determine whether it is legal to call this method by checking
/// whether the [mounted] property is true. That said, it is better practice
/// to cancel whatever work might trigger the [setState] rather than merely
/// checking for [mounted] before calling [setState], as otherwise CPU cycles
/// will be wasted.
///
/// ## Design discussion
///
/// The original version of this API was a method called `markNeedsBuild`, for
/// consistency with [RenderObject.markNeedsLayout],
/// [RenderObject.markNeedsPaint], _et al_.
///
/// However, early user testing of the Flutter framework revealed that people
/// would call `markNeedsBuild()` much more often than necessary. Essentially,
/// people used it like a good luck charm, any time they weren't sure if they
/// needed to call it, they would call it, just in case.
///
/// Naturally, this led to performance issues in applications.
///
/// When the API was changed to take a callback instead, this practice was
/// greatly reduced. One hypothesis is that prompting developers to actually
/// update their state in a callback caused developers to think more carefully
/// about what exactly was being updated, and thus improved their understanding
/// of the appropriate times to call the method.
///
/// In practice, the [setState] method's implementation is trivial: it calls
/// the provided callback synchronously, then calls [Element.markNeedsBuild].
///
/// ## Performance considerations
///
/// There is minimal _direct_ overhead to calling this function, and as it is
/// expected to be called at most once per frame, the overhead is irrelevant
/// anyway. Nonetheless, it is best to avoid calling this function redundantly
/// (e.g. in a tight loop), as it does involve creating a closure and calling
/// it. The method is idempotent, there is no benefit to calling it more than
/// once per [State] per frame.
///
/// The _indirect_ cost of causing this function, however, is high: it causes
/// the widget to rebuild, possibly triggering rebuilds for the entire subtree
/// rooted at this widget, and further triggering a relayout and repaint of
/// the entire corresponding [RenderObject] subtree.
///
/// For this reason, this method should only be called when the [build] method
/// will, as a result of whatever state change was detected, change its result
/// meaningfully.
///
/// See also:
///
/// * [StatefulWidget], the API documentation for which has a section on
/// performance considerations that are relevant here.
@protected
void setState(VoidCallback fn) {
assert(() {
if (_debugLifecycleState == _StateLifecycle.defunct) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('setState() called after dispose(): $this'),
ErrorDescription(
'This error happens if you call setState() on a State object for a widget that '
'no longer appears in the widget tree (e.g., whose parent widget no longer '
'includes the widget in its build). This error can occur when code calls '
'setState() from a timer or an animation callback.',
),
ErrorHint(
'The preferred solution is '
'to cancel the timer or stop listening to the animation in the dispose() '
'callback. Another solution is to check the "mounted" property of this '
'object before calling setState() to ensure the object is still in the '
'tree.',
),
ErrorHint(
'This error might indicate a memory leak if setState() is being called '
'because another object is retaining a reference to this State object '
'after it has been removed from the tree. To avoid memory leaks, '
'consider breaking the reference to this object during dispose().',
),
]);
}
if (_debugLifecycleState == _StateLifecycle.created && !mounted) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('setState() called in constructor: $this'),
ErrorHint(
'This happens when you call setState() on a State object for a widget that '
"hasn't been inserted into the widget tree yet. It is not necessary to call "
'setState() in the constructor, since the state is already assumed to be dirty '
'when it is initially created.',
),
]);
}
return true;
}());
final Object? result = fn() as dynamic;
assert(() {
if (result is Future) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('setState() callback argument returned a Future.'),
ErrorDescription(
'The setState() method on $this was called with a closure or method that '
'returned a Future. Maybe it is marked as "async".',
),
ErrorHint(
'Instead of performing asynchronous work inside a call to setState(), first '
'execute the work (without updating the widget state), and then synchronously '
'update the state inside a call to setState().',
),
]);
}
// We ignore other types of return values so that you can do things like:
// setState(() => x = 3);
return true;
}());
_element!.markNeedsBuild();
}
/// Called when this object is removed from the tree.
///
/// The framework calls this method whenever it removes this [State] object
/// from the tree. In some cases, the framework will reinsert the [State]
/// object into another part of the tree (e.g., if the subtree containing this
/// [State] object is grafted from one location in the tree to another due to
/// the use of a [GlobalKey]). If that happens, the framework will call
/// [activate] to give the [State] object a chance to reacquire any resources
/// that it released in [deactivate]. It will then also call [build] to give
/// the [State] object a chance to adapt to its new location in the tree. If
/// the framework does reinsert this subtree, it will do so before the end of
/// the animation frame in which the subtree was removed from the tree. For
/// this reason, [State] objects can defer releasing most resources until the
/// framework calls their [dispose] method.
///
/// Subclasses should override this method to clean up any links between
/// this object and other elements in the tree (e.g. if you have provided an
/// ancestor with a pointer to a descendant's [RenderObject]).
///
/// Implementations of this method should end with a call to the inherited
/// method, as in `super.deactivate()`.
///
/// See also:
///
/// * [dispose], which is called after [deactivate] if the widget is removed
/// from the tree permanently.
@protected
@mustCallSuper
void deactivate() { }
/// Called when this object is reinserted into the tree after having been
/// removed via [deactivate].
///
/// In most cases, after a [State] object has been deactivated, it is _not_
/// reinserted into the tree, and its [dispose] method will be called to
/// signal that it is ready to be garbage collected.
///
/// In some cases, however, after a [State] object has been deactivated, the
/// framework will reinsert it into another part of the tree (e.g., if the
/// subtree containing this [State] object is grafted from one location in
/// the tree to another due to the use of a [GlobalKey]). If that happens,
/// the framework will call [activate] to give the [State] object a chance to
/// reacquire any resources that it released in [deactivate]. It will then
/// also call [build] to give the object a chance to adapt to its new
/// location in the tree. If the framework does reinsert this subtree, it
/// will do so before the end of the animation frame in which the subtree was
/// removed from the tree. For this reason, [State] objects can defer
/// releasing most resources until the framework calls their [dispose] method.
///
/// The framework does not call this method the first time a [State] object
/// is inserted into the tree. Instead, the framework calls [initState] in
/// that situation.
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.activate()`.
///
/// See also:
///
/// * [Element.activate], the corresponding method when an element
/// transitions from the "inactive" to the "active" lifecycle state.
@protected
@mustCallSuper
void activate() { }
/// Called when this object is removed from the tree permanently.
///
/// The framework calls this method when this [State] object will never
/// build again. After the framework calls [dispose], the [State] object is
/// considered unmounted and the [mounted] property is false. It is an error
/// to call [setState] at this point. This stage of the lifecycle is terminal:
/// there is no way to remount a [State] object that has been disposed.
///
/// Subclasses should override this method to release any resources retained
/// by this object (e.g., stop any active animations).
///
/// {@macro flutter.widgets.State.initState}
///
/// Implementations of this method should end with a call to the inherited
/// method, as in `super.dispose()`.
///
/// ## Caveats
///
/// This method is _not_ invoked at times where a developer might otherwise
/// expect it, such as application shutdown or dismissal via platform
/// native methods.
///
/// ### Application shutdown
///
/// There is no way to predict when application shutdown will happen. For
/// example, a user's battery could catch fire, or the user could drop the
/// device into a swimming pool, or the operating system could unilaterally
/// terminate the application process due to memory pressure.
///
/// Applications are responsible for ensuring that they are well-behaved
/// even in the face of a rapid unscheduled termination.
///
/// To artificially cause the entire widget tree to be disposed, consider
/// calling [runApp] with a widget such as [SizedBox.shrink].
///
/// To listen for platform shutdown messages (and other lifecycle changes),
/// consider the [AppLifecycleListener] API.
///
/// {@macro flutter.widgets.runApp.dismissal}
///
/// See the method used to bootstrap the app (e.g. [runApp] or [runWidget])
/// for suggestions on how to release resources more eagerly.
///
/// See also:
///
/// * [deactivate], which is called prior to [dispose].
@protected
@mustCallSuper
void dispose() {
assert(_debugLifecycleState == _StateLifecycle.ready);
assert(() {
_debugLifecycleState = _StateLifecycle.defunct;
return true;
}());
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
}
/// Describes the part of the user interface represented by this widget.
///
/// The framework calls this method in a number of different situations. For
/// example:
///
/// * After calling [initState].
/// * After calling [didUpdateWidget].
/// * After receiving a call to [setState].
/// * After a dependency of this [State] object changes (e.g., an
/// [InheritedWidget] referenced by the previous [build] changes).
/// * After calling [deactivate] and then reinserting the [State] object into
/// the tree at another location.
///
/// This method can potentially be called in every frame and should not have
/// any side effects beyond building a widget.
///
/// The framework replaces the subtree below this widget with the widget
/// returned by this method, either by updating the existing subtree or by
/// removing the subtree and inflating a new subtree, depending on whether the
/// widget returned by this method can update the root of the existing
/// subtree, as determined by calling [Widget.canUpdate].
///
/// Typically implementations return a newly created constellation of widgets
/// that are configured with information from this widget's constructor, the
/// given [BuildContext], and the internal state of this [State] object.
///
/// The given [BuildContext] contains information about the location in the
/// tree at which this widget is being built. For example, the context
/// provides the set of inherited widgets for this location in the tree. The
/// [BuildContext] argument is always the same as the [context] property of
/// this [State] object and will remain the same for the lifetime of this
/// object. The [BuildContext] argument is provided redundantly here so that
/// this method matches the signature for a [WidgetBuilder].
///
/// ## Design discussion
///
/// ### Why is the [build] method on [State], and not [StatefulWidget]?
///
/// Putting a `Widget build(BuildContext context)` method on [State] rather
/// than putting a `Widget build(BuildContext context, State state)` method
/// on [StatefulWidget] gives developers more flexibility when subclassing
/// [StatefulWidget].
///
/// For example, [AnimatedWidget] is a subclass of [StatefulWidget] that
/// introduces an abstract `Widget build(BuildContext context)` method for its
/// subclasses to implement. If [StatefulWidget] already had a [build] method
/// that took a [State] argument, [AnimatedWidget] would be forced to provide
/// its [State] object to subclasses even though its [State] object is an
/// internal implementation detail of [AnimatedWidget].
///
/// Conceptually, [StatelessWidget] could also be implemented as a subclass of
/// [StatefulWidget] in a similar manner. If the [build] method were on
/// [StatefulWidget] rather than [State], that would not be possible anymore.
///
/// Putting the [build] function on [State] rather than [StatefulWidget] also
/// helps avoid a category of bugs related to closures implicitly capturing
/// `this`. If you defined a closure in a [build] function on a
/// [StatefulWidget], that closure would implicitly capture `this`, which is
/// the current widget instance, and would have the (immutable) fields of that
/// instance in scope:
///
/// ```dart
/// // (this is not valid Flutter code)
/// class MyButton extends StatefulWidgetX {
/// MyButton({super.key, required this.color});
///
/// final Color color;
///
/// @override
/// Widget build(BuildContext context, State state) {
/// return SpecialWidget(
/// handler: () { print('color: $color'); },
/// );
/// }
/// }
/// ```
///
/// For example, suppose the parent builds `MyButton` with `color` being blue,
/// the `$color` in the print function refers to blue, as expected. Now,
/// suppose the parent rebuilds `MyButton` with green. The closure created by
/// the first build still implicitly refers to the original widget and the
/// `$color` still prints blue even through the widget has been updated to
/// green; should that closure outlive its widget, it would print outdated
/// information.
///
/// In contrast, with the [build] function on the [State] object, closures
/// created during [build] implicitly capture the [State] instance instead of
/// the widget instance:
///
/// ```dart
/// class MyButton extends StatefulWidget {
/// const MyButton({super.key, this.color = Colors.teal});
///
/// final Color color;
/// // ...
/// }
///
/// class MyButtonState extends State<MyButton> {
/// // ...
/// @override
/// Widget build(BuildContext context) {
/// return SpecialWidget(
/// handler: () { print('color: ${widget.color}'); },
/// );
/// }
/// }
/// ```
///
/// Now when the parent rebuilds `MyButton` with green, the closure created by
/// the first build still refers to [State] object, which is preserved across
/// rebuilds, but the framework has updated that [State] object's [widget]
/// property to refer to the new `MyButton` instance and `${widget.color}`
/// prints green, as expected.
///
/// See also:
///
/// * [StatefulWidget], which contains the discussion on performance considerations.
@protected
Widget build(BuildContext context);
/// Called when a dependency of this [State] object changes.
///
/// For example, if the previous call to [build] referenced an
/// [InheritedWidget] that later changed, the framework would call this
/// method to notify this object about the change.
///
/// This method is also called immediately after [initState]. It is safe to
/// call [BuildContext.dependOnInheritedWidgetOfExactType] from this method.
///
/// Subclasses rarely override this method because the framework always
/// calls [build] after a dependency changes. Some subclasses do override
/// this method because they need to do some expensive work (e.g., network
/// fetches) when their dependencies change, and that work would be too
/// expensive to do for every build.
@protected
@mustCallSuper
void didChangeDependencies() { }
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
assert(() {
properties.add(EnumProperty<_StateLifecycle>('lifecycle state', _debugLifecycleState, defaultValue: _StateLifecycle.ready));
return true;
}());
properties.add(ObjectFlagProperty<T>('_widget', _widget, ifNull: 'no widget'));
properties.add(ObjectFlagProperty<StatefulElement>('_element', _element, ifNull: 'not mounted'));
}
}
/// A widget that has a child widget provided to it, instead of building a new
/// widget.
///
/// Useful as a base class for other widgets, such as [InheritedWidget] and
/// [ParentDataWidget].
///
/// See also:
///
/// * [InheritedWidget], for widgets that introduce ambient state that can
/// be read by descendant widgets.
/// * [ParentDataWidget], for widgets that populate the
/// [RenderObject.parentData] slot of their child's [RenderObject] to
/// configure the parent widget's layout.
/// * [StatefulWidget] and [State], for widgets that can build differently
/// several times over their lifetime.
/// * [StatelessWidget], for widgets that always build the same way given a
/// particular configuration and ambient state.
/// * [Widget], for an overview of widgets in general.
abstract class ProxyWidget extends Widget {
/// Creates a widget that has exactly one child widget.
const ProxyWidget({ super.key, required this.child });
/// The widget below this widget in the tree.
///
/// {@template flutter.widgets.ProxyWidget.child}
/// This widget can only have one child. To lay out multiple children, let this
/// widget's child be a widget such as [Row], [Column], or [Stack], which have a
/// `children` property, and then provide the children to that widget.
/// {@endtemplate}
final Widget child;
}
/// Base class for widgets that hook [ParentData] information to children of
/// [RenderObjectWidget]s.
///
/// This can be used to provide per-child configuration for
/// [RenderObjectWidget]s with more than one child. For example, [Stack] uses
/// the [Positioned] parent data widget to position each child.
///
/// A [ParentDataWidget] is specific to a particular kind of [ParentData]. That
/// class is `T`, the [ParentData] type argument.
///
/// {@tool snippet}
///
/// This example shows how you would build a [ParentDataWidget] to configure a
/// `FrogJar` widget's children by specifying a [Size] for each one.
///
/// ```dart
/// class FrogSize extends ParentDataWidget<FrogJarParentData> {
/// const FrogSize({
/// super.key,
/// required this.size,
/// required super.child,
/// });
///
/// final Size size;
///
/// @override
/// void applyParentData(RenderObject renderObject) {
/// final FrogJarParentData parentData = renderObject.parentData! as FrogJarParentData;
/// if (parentData.size != size) {
/// parentData.size = size;
/// final RenderFrogJar targetParent = renderObject.parent! as RenderFrogJar;
/// targetParent.markNeedsLayout();
/// }
/// }
///
/// @override
/// Type get debugTypicalAncestorWidgetClass => FrogJar;
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [RenderObject], the superclass for layout algorithms.
/// * [RenderObject.parentData], the slot that this class configures.
/// * [ParentData], the superclass of the data that will be placed in
/// [RenderObject.parentData] slots. The `T` type parameter for
/// [ParentDataWidget] is a [ParentData].
/// * [RenderObjectWidget], the class for widgets that wrap [RenderObject]s.
/// * [StatefulWidget] and [State], for widgets that can build differently
/// several times over their lifetime.
abstract class ParentDataWidget<T extends ParentData> extends ProxyWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const ParentDataWidget({ super.key, required super.child });
@override
ParentDataElement<T> createElement() => ParentDataElement<T>(this);
/// Checks if this widget can apply its parent data to the provided
/// `renderObject`.
///
/// The [RenderObject.parentData] of the provided `renderObject` is
/// typically set up by an ancestor [RenderObjectWidget] of the type returned
/// by [debugTypicalAncestorWidgetClass].
///
/// This is called just before [applyParentData] is invoked with the same
/// [RenderObject] provided to that method.
bool debugIsValidRenderObject(RenderObject renderObject) {
assert(T != dynamic);
assert(T != ParentData);
return renderObject.parentData is T;
}
/// Describes the [RenderObjectWidget] that is typically used to set up the
/// [ParentData] that [applyParentData] will write to.
///
/// This is only used in error messages to tell users what widget typically
/// wraps this [ParentDataWidget] through
/// [debugTypicalAncestorWidgetDescription].
///
/// ## Implementations
///
/// The returned Type should describe a subclass of `RenderObjectWidget`. If
/// more than one Type is supported, use
/// [debugTypicalAncestorWidgetDescription], which typically inserts this
/// value but can be overridden to describe more than one Type.
///
/// ```dart
/// @override
/// Type get debugTypicalAncestorWidgetClass => FrogJar;
/// ```
///
/// If the "typical" parent is generic (`Foo<T>`), consider specifying either
/// a typical type argument (e.g. `Foo<int>` if `int` is typically how the
/// type is specialized), or specifying the upper bound (e.g. `Foo<Object?>`).
Type get debugTypicalAncestorWidgetClass;
/// Describes the [RenderObjectWidget] that is typically used to set up the
/// [ParentData] that [applyParentData] will write to.
///
/// This is only used in error messages to tell users what widget typically
/// wraps this [ParentDataWidget].
///
/// Returns [debugTypicalAncestorWidgetClass] by default as a String. This can
/// be overridden to describe more than one Type of valid parent.
String get debugTypicalAncestorWidgetDescription => '$debugTypicalAncestorWidgetClass';
Iterable<DiagnosticsNode> _debugDescribeIncorrectParentDataType({
required ParentData? parentData,
RenderObjectWidget? parentDataCreator,
DiagnosticsNode? ownershipChain,
}) {
assert(T != dynamic);
assert(T != ParentData);
final String description = 'The ParentDataWidget $this wants to apply ParentData of type $T to a RenderObject';
return <DiagnosticsNode>[
if (parentData == null)
ErrorDescription(
'$description, which has not been set up to receive any ParentData.',
)
else
ErrorDescription(
'$description, which has been set up to accept ParentData of incompatible type ${parentData.runtimeType}.',
),
ErrorHint(
'Usually, this means that the $runtimeType widget has the wrong ancestor RenderObjectWidget. '
'Typically, $runtimeType widgets are placed directly inside $debugTypicalAncestorWidgetDescription widgets.',
),
if (parentDataCreator != null)
ErrorHint(
'The offending $runtimeType is currently placed inside a ${parentDataCreator.runtimeType} widget.',
),
if (ownershipChain != null)
ErrorDescription(
'The ownership chain for the RenderObject that received the incompatible parent data was:\n $ownershipChain',
),
];
}
/// Write the data from this widget into the given render object's parent data.
///
/// The framework calls this function whenever it detects that the
/// [RenderObject] associated with the [child] has outdated
/// [RenderObject.parentData]. For example, if the render object was recently
/// inserted into the render tree, the render object's parent data might not
/// match the data in this widget.
///
/// Subclasses are expected to override this function to copy data from their
/// fields into the [RenderObject.parentData] field of the given render
/// object. The render object's parent is guaranteed to have been created by a
/// widget of type `T`, which usually means that this function can assume that
/// the render object's parent data object inherits from a particular class.
///
/// If this function modifies data that can change the parent's layout or
/// painting, this function is responsible for calling
/// [RenderObject.markNeedsLayout] or [RenderObject.markNeedsPaint] on the
/// parent, as appropriate.
@protected
void applyParentData(RenderObject renderObject);
/// Whether the [ParentDataElement.applyWidgetOutOfTurn] method is allowed
/// with this widget.
///
/// This should only return true if this widget represents a [ParentData]
/// configuration that will have no impact on the layout or paint phase.
///
/// See also:
///
/// * [ParentDataElement.applyWidgetOutOfTurn], which verifies this in debug
/// mode.
@protected
bool debugCanApplyOutOfTurn() => false;
}
/// Base class for widgets that efficiently propagate information down the tree.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=og-vJqLzg2c}
///
/// To obtain the nearest instance of a particular type of inherited widget from
/// a build context, use [BuildContext.dependOnInheritedWidgetOfExactType].
///
/// Inherited widgets, when referenced in this way, will cause the consumer to
/// rebuild when the inherited widget itself changes state.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=Zbm3hjPjQMk}
///
/// {@tool snippet}
///
/// The following is a skeleton of an inherited widget called `FrogColor`:
///
/// ```dart
/// class FrogColor extends InheritedWidget {
/// const FrogColor({
/// super.key,
/// required this.color,
/// required super.child,
/// });
///
/// final Color color;
///
/// static FrogColor? maybeOf(BuildContext context) {
/// return context.dependOnInheritedWidgetOfExactType<FrogColor>();
/// }
///
/// static FrogColor of(BuildContext context) {
/// final FrogColor? result = maybeOf(context);
/// assert(result != null, 'No FrogColor found in context');
/// return result!;
/// }
///
/// @override
/// bool updateShouldNotify(FrogColor oldWidget) => color != oldWidget.color;
/// }
/// ```
/// {@end-tool}
///
/// ## Implementing the `of` and `maybeOf` methods
///
/// The convention is to provide two static methods, `of` and `maybeOf`, on the
/// [InheritedWidget] which call
/// [BuildContext.dependOnInheritedWidgetOfExactType]. This allows the class to
/// define its own fallback logic in case there isn't a widget in scope.
///
/// The `of` method typically returns a non-nullable instance and asserts if the
/// [InheritedWidget] isn't found, and the `maybeOf` method returns a nullable
/// instance, and returns null if the [InheritedWidget] isn't found. The `of`
/// method is typically implemented by calling `maybeOf` internally.
///
/// Sometimes, the `of` and `maybeOf` methods return some data rather than the
/// inherited widget itself; for example, in this case it could have returned a
/// [Color] instead of the `FrogColor` widget.
///
/// Occasionally, the inherited widget is an implementation detail of another
/// class, and is therefore private. The `of` and `maybeOf` methods in that case
/// are typically implemented on the public class instead. For example, [Theme]
/// is implemented as a [StatelessWidget] that builds a private inherited
/// widget; [Theme.of] looks for that private inherited widget using
/// [BuildContext.dependOnInheritedWidgetOfExactType] and then returns the
/// [ThemeData] inside it.
///
/// ## Calling the `of` or `maybeOf` methods
///
/// When using the `of` or `maybeOf` methods, the `context` must be a descendant
/// of the [InheritedWidget], meaning it must be "below" the [InheritedWidget]
/// in the tree.
///
/// {@tool snippet}
///
/// In this example, the `context` used is the one from the [Builder], which is
/// a child of the `FrogColor` widget, so this works.
///
/// ```dart
/// // continuing from previous example...
/// class MyPage extends StatelessWidget {
/// const MyPage({super.key});
///
/// @override
/// Widget build(BuildContext context) {
/// return Scaffold(
/// body: FrogColor(
/// color: Colors.green,
/// child: Builder(
/// builder: (BuildContext innerContext) {
/// return Text(
/// 'Hello Frog',
/// style: TextStyle(color: FrogColor.of(innerContext).color),
/// );
/// },
/// ),
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// In this example, the `context` used is the one from the `MyOtherPage`
/// widget, which is a parent of the `FrogColor` widget, so this does not work,
/// and will assert when `FrogColor.of` is called.
///
/// ```dart
/// // continuing from previous example...
///
/// class MyOtherPage extends StatelessWidget {
/// const MyOtherPage({super.key});
///
/// @override
/// Widget build(BuildContext context) {
/// return Scaffold(
/// body: FrogColor(
/// color: Colors.green,
/// child: Text(
/// 'Hello Frog',
/// style: TextStyle(color: FrogColor.of(context).color),
/// ),
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool} {@youtube 560 315 https://www.youtube.com/watch?v=1t-8rBCGBYw}
///
/// See also:
///
/// * [StatefulWidget] and [State], for widgets that can build differently
/// several times over their lifetime.
/// * [StatelessWidget], for widgets that always build the same way given a
/// particular configuration and ambient state.
/// * [Widget], for an overview of widgets in general.
/// * [InheritedNotifier], an inherited widget whose value can be a
/// [Listenable], and which will notify dependents whenever the value sends
/// notifications.
/// * [InheritedModel], an inherited widget that allows clients to subscribe to
/// changes for subparts of the value.
abstract class InheritedWidget extends ProxyWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const InheritedWidget({ super.key, required super.child });
@override
InheritedElement createElement() => InheritedElement(this);
/// Whether the framework should notify widgets that inherit from this widget.
///
/// When this widget is rebuilt, sometimes we need to rebuild the widgets that
/// inherit from this widget but sometimes we do not. For example, if the data
/// held by this widget is the same as the data held by `oldWidget`, then we
/// do not need to rebuild the widgets that inherited the data held by
/// `oldWidget`.
///
/// The framework distinguishes these cases by calling this function with the
/// widget that previously occupied this location in the tree as an argument.
/// The given widget is guaranteed to have the same [runtimeType] as this
/// object.
@protected
bool updateShouldNotify(covariant InheritedWidget oldWidget);
}
/// [RenderObjectWidget]s provide the configuration for [RenderObjectElement]s,
/// which wrap [RenderObject]s, which provide the actual rendering of the
/// application.
///
/// Usually, rather than subclassing [RenderObjectWidget] directly, render
/// object widgets subclass one of:
///
/// * [LeafRenderObjectWidget], if the widget has no children.
/// * [SingleChildRenderObjectElement], if the widget has exactly one child.
/// * [MultiChildRenderObjectWidget], if the widget takes a list of children.
/// * [SlottedMultiChildRenderObjectWidget], if the widget organizes its
/// children in different named slots.
///
/// Subclasses must implement [createRenderObject] and [updateRenderObject].
abstract class RenderObjectWidget extends Widget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RenderObjectWidget({ super.key });
/// RenderObjectWidgets always inflate to a [RenderObjectElement] subclass.
@override
@factory
RenderObjectElement createElement();
/// Creates an instance of the [RenderObject] class that this
/// [RenderObjectWidget] represents, using the configuration described by this
/// [RenderObjectWidget].
///
/// This method should not do anything with the children of the render object.
/// That should instead be handled by the method that overrides
/// [RenderObjectElement.mount] in the object rendered by this object's
/// [createElement] method. See, for example,
/// [SingleChildRenderObjectElement.mount].
@protected
@factory
RenderObject createRenderObject(BuildContext context);
/// Copies the configuration described by this [RenderObjectWidget] to the
/// given [RenderObject], which will be of the same type as returned by this
/// object's [createRenderObject].
///
/// This method should not do anything to update the children of the render
/// object. That should instead be handled by the method that overrides
/// [RenderObjectElement.update] in the object rendered by this object's
/// [createElement] method. See, for example,
/// [SingleChildRenderObjectElement.update].
@protected
void updateRenderObject(BuildContext context, covariant RenderObject renderObject) { }
/// A render object previously associated with this widget has been removed
/// from the tree. The given [RenderObject] will be of the same type as
/// returned by this object's [createRenderObject].
@protected
void didUnmountRenderObject(covariant RenderObject renderObject) { }
}
/// A superclass for [RenderObjectWidget]s that configure [RenderObject] subclasses
/// that have no children.
///
/// Subclasses must implement [createRenderObject] and [updateRenderObject].
abstract class LeafRenderObjectWidget extends RenderObjectWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const LeafRenderObjectWidget({ super.key });
@override
LeafRenderObjectElement createElement() => LeafRenderObjectElement(this);
}
/// A superclass for [RenderObjectWidget]s that configure [RenderObject] subclasses
/// that have a single child slot.
///
/// The render object assigned to this widget should make use of
/// [RenderObjectWithChildMixin] to implement a single-child model. The mixin
/// exposes a [RenderObjectWithChildMixin.child] property that allows retrieving
/// the render object belonging to the [child] widget.
///
/// Subclasses must implement [createRenderObject] and [updateRenderObject].
abstract class SingleChildRenderObjectWidget extends RenderObjectWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SingleChildRenderObjectWidget({ super.key, this.child });
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
@override
SingleChildRenderObjectElement createElement() => SingleChildRenderObjectElement(this);
}
/// A superclass for [RenderObjectWidget]s that configure [RenderObject] subclasses
/// that have a single list of children. (This superclass only provides the
/// storage for that child list, it doesn't actually provide the updating
/// logic.)
///
/// Subclasses must use a [RenderObject] that mixes in
/// [ContainerRenderObjectMixin], which provides the necessary functionality to
/// visit the children of the container render object (the render object
/// belonging to the [children] widgets). Typically, subclasses will use a
/// [RenderBox] that mixes in both [ContainerRenderObjectMixin] and
/// [RenderBoxContainerDefaultsMixin].
///
/// Subclasses must implement [createRenderObject] and [updateRenderObject].
///
/// See also:
///
/// * [Stack], which uses [MultiChildRenderObjectWidget].
/// * [RenderStack], for an example implementation of the associated render
/// object.
/// * [SlottedMultiChildRenderObjectWidget], which configures a
/// [RenderObject] that instead of having a single list of children organizes
/// its children in named slots.
abstract class MultiChildRenderObjectWidget extends RenderObjectWidget {
/// Initializes fields for subclasses.
const MultiChildRenderObjectWidget({ super.key, this.children = const <Widget>[] });
/// The widgets below this widget in the tree.
///
/// If this list is going to be mutated, it is usually wise to put a [Key] on
/// each of the child widgets, so that the framework can match old
/// configurations to new configurations and maintain the underlying render
/// objects.
///
/// Also, a [Widget] in Flutter is immutable, so directly modifying the
/// [children] such as `someMultiChildRenderObjectWidget.children.add(...)` or
/// as the example code below will result in incorrect behaviors. Whenever the
/// children list is modified, a new list object should be provided.
///
/// ```dart
/// // This code is incorrect.
/// class SomeWidgetState extends State<SomeWidget> {
/// final List<Widget> _children = <Widget>[];
///
/// void someHandler() {
/// setState(() {
/// _children.add(const ChildWidget());
/// });
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// // Reusing `List<Widget> _children` here is problematic.
/// return Row(children: _children);
/// }
/// }
/// ```
///
/// The following code corrects the problem mentioned above.
///
/// ```dart
/// class SomeWidgetState extends State<SomeWidget> {
/// final List<Widget> _children = <Widget>[];
///
/// void someHandler() {
/// setState(() {
/// // The key here allows Flutter to reuse the underlying render
/// // objects even if the children list is recreated.
/// _children.add(ChildWidget(key: UniqueKey()));
/// });
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// // Always create a new list of children as a Widget is immutable.
/// return Row(children: _children.toList());
/// }
/// }
/// ```
final List<Widget> children;
@override
MultiChildRenderObjectElement createElement() => MultiChildRenderObjectElement(this);
}
// ELEMENTS
enum _ElementLifecycle {
initial,
active,
inactive,
defunct,
}
class _InactiveElements {
bool _locked = false;
final Set<Element> _elements = HashSet<Element>();
void _unmount(Element element) {
assert(element._lifecycleState == _ElementLifecycle.inactive);
assert(() {
if (debugPrintGlobalKeyedWidgetLifecycle) {
if (element.widget.key is GlobalKey) {
debugPrint('Discarding $element from inactive elements list.');
}
}
return true;
}());
element.visitChildren((Element child) {
assert(child._parent == element);
_unmount(child);
});
element.unmount();
assert(element._lifecycleState == _ElementLifecycle.defunct);
}
void _unmountAll() {
_locked = true;
final List<Element> elements = _elements.toList()..sort(Element._sort);
_elements.clear();
try {
elements.reversed.forEach(_unmount);
} finally {
assert(_elements.isEmpty);
_locked = false;
}
}
static void _deactivateRecursively(Element element) {
assert(element._lifecycleState == _ElementLifecycle.active);
element.deactivate();
assert(element._lifecycleState == _ElementLifecycle.inactive);
element.visitChildren(_deactivateRecursively);
assert(() {
element.debugDeactivated();
return true;
}());
}
void add(Element element) {
assert(!_locked);
assert(!_elements.contains(element));
assert(element._parent == null);
if (element._lifecycleState == _ElementLifecycle.active) {
_deactivateRecursively(element);
}
_elements.add(element);
}
void remove(Element element) {
assert(!_locked);
assert(_elements.contains(element));
assert(element._parent == null);
_elements.remove(element);
assert(element._lifecycleState != _ElementLifecycle.active);
}
bool debugContains(Element element) {
late bool result;
assert(() {
result = _elements.contains(element);
return true;
}());
return result;
}
}
/// Signature for the callback to [BuildContext.visitChildElements].
///
/// The argument is the child being visited.
///
/// It is safe to call `element.visitChildElements` reentrantly within
/// this callback.
typedef ElementVisitor = void Function(Element element);
/// Signature for the callback to [BuildContext.visitAncestorElements].
///
/// The argument is the ancestor being visited.
///
/// Return false to stop the walk.
typedef ConditionalElementVisitor = bool Function(Element element);
/// A handle to the location of a widget in the widget tree.
///
/// This class presents a set of methods that can be used from
/// [StatelessWidget.build] methods and from methods on [State] objects.
///
/// [BuildContext] objects are passed to [WidgetBuilder] functions (such as
/// [StatelessWidget.build]), and are available from the [State.context] member.
/// Some static functions (e.g. [showDialog], [Theme.of], and so forth) also
/// take build contexts so that they can act on behalf of the calling widget, or
/// obtain data specifically for the given context.
///
/// Each widget has its own [BuildContext], which becomes the parent of the
/// widget returned by the [StatelessWidget.build] or [State.build] function.
/// (And similarly, the parent of any children for [RenderObjectWidget]s.)
///
/// In particular, this means that within a build method, the build context of
/// the widget of the build method is not the same as the build context of the
/// widgets returned by that build method. This can lead to some tricky cases.
/// For example, [Theme.of(context)] looks for the nearest enclosing [Theme] of
/// the given build context. If a build method for a widget Q includes a [Theme]
/// within its returned widget tree, and attempts to use [Theme.of] passing its
/// own context, the build method for Q will not find that [Theme] object. It
/// will instead find whatever [Theme] was an ancestor to the widget Q. If the
/// build context for a subpart of the returned tree is needed, a [Builder]
/// widget can be used: the build context passed to the [Builder.builder]
/// callback will be that of the [Builder] itself.
///
/// For example, in the following snippet, the [ScaffoldState.showBottomSheet]
/// method is called on the [Scaffold] widget that the build method itself
/// creates. If a [Builder] had not been used, and instead the `context`
/// argument of the build method itself had been used, no [Scaffold] would have
/// been found, and the [Scaffold.of] function would have returned null.
///
/// ```dart
/// @override
/// Widget build(BuildContext context) {
/// // here, Scaffold.of(context) returns null
/// return Scaffold(
/// appBar: AppBar(title: const Text('Demo')),
/// body: Builder(
/// builder: (BuildContext context) {
/// return TextButton(
/// child: const Text('BUTTON'),
/// onPressed: () {
/// Scaffold.of(context).showBottomSheet(
/// (BuildContext context) {
/// return Container(
/// alignment: Alignment.center,
/// height: 200,
/// color: Colors.amber,
/// child: Center(
/// child: Column(
/// mainAxisSize: MainAxisSize.min,
/// children: <Widget>[
/// const Text('BottomSheet'),
/// ElevatedButton(
/// child: const Text('Close BottomSheet'),
/// onPressed: () {
/// Navigator.pop(context);
/// },
/// )
/// ],
/// ),
/// ),
/// );
/// },
/// );
/// },
/// );
/// },
/// )
/// );
/// }
/// ```
///
/// The [BuildContext] for a particular widget can change location over time as
/// the widget is moved around the tree. Because of this, values returned from
/// the methods on this class should not be cached beyond the execution of a
/// single synchronous function.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=rIaaH87z1-g}
///
/// Avoid storing instances of [BuildContext]s because they may become invalid
/// if the widget they are associated with is unmounted from the widget tree.
/// {@template flutter.widgets.BuildContext.asynchronous_gap}
/// If a [BuildContext] is used across an asynchronous gap (i.e. after performing
/// an asynchronous operation), consider checking [mounted] to determine whether
/// the context is still valid before interacting with it:
///
/// ```dart
/// @override
/// Widget build(BuildContext context) {
/// return OutlinedButton(
/// onPressed: () async {
/// await Future<void>.delayed(const Duration(seconds: 1));
/// if (context.mounted) {
/// Navigator.of(context).pop();
/// }
/// },
/// child: const Text('Delayed pop'),
/// );
/// }
/// ```
/// {@endtemplate}
///
/// [BuildContext] objects are actually [Element] objects. The [BuildContext]
/// interface is used to discourage direct manipulation of [Element] objects.
abstract class BuildContext {
/// The current configuration of the [Element] that is this [BuildContext].
Widget get widget;
/// The [BuildOwner] for this context. The [BuildOwner] is in charge of
/// managing the rendering pipeline for this context.
BuildOwner? get owner;
/// Whether the [Widget] this context is associated with is currently
/// mounted in the widget tree.
///
/// Accessing the properties of the [BuildContext] or calling any methods on
/// it is only valid while mounted is true. If mounted is false, assertions
/// will trigger.
///
/// Once unmounted, a given [BuildContext] will never become mounted again.
///
/// {@macro flutter.widgets.BuildContext.asynchronous_gap}
bool get mounted;
/// Whether the [widget] is currently updating the widget or render tree.
///
/// For [StatefulWidget]s and [StatelessWidget]s this flag is true while
/// their respective build methods are executing.
/// [RenderObjectWidget]s set this to true while creating or configuring their
/// associated [RenderObject]s.
/// Other [Widget] types may set this to true for conceptually similar phases
/// of their lifecycle.
///
/// When this is true, it is safe for [widget] to establish a dependency to an
/// [InheritedWidget] by calling [dependOnInheritedElement] or
/// [dependOnInheritedWidgetOfExactType].
///
/// Accessing this flag in release mode is not valid.
bool get debugDoingBuild;
/// The current [RenderObject] for the widget. If the widget is a
/// [RenderObjectWidget], this is the render object that the widget created
/// for itself. Otherwise, it is the render object of the first descendant
/// [RenderObjectWidget].
///
/// This method will only return a valid result after the build phase is
/// complete. It is therefore not valid to call this from a build method.
/// It should only be called from interaction event handlers (e.g.
/// gesture callbacks) or layout or paint callbacks. It is also not valid to
/// call if [State.mounted] returns false.
///
/// If the render object is a [RenderBox], which is the common case, then the
/// size of the render object can be obtained from the [size] getter. This is
/// only valid after the layout phase, and should therefore only be examined
/// from paint callbacks or interaction event handlers (e.g. gesture
/// callbacks).
///
/// For details on the different phases of a frame, see the discussion at
/// [WidgetsBinding.drawFrame].
///
/// Calling this method is theoretically relatively expensive (O(N) in the
/// depth of the tree), but in practice is usually cheap because the tree
/// usually has many render objects and therefore the distance to the nearest
/// render object is usually short.
RenderObject? findRenderObject();
/// The size of the [RenderBox] returned by [findRenderObject].
///
/// This getter will only return a valid result after the layout phase is
/// complete. It is therefore not valid to call this from a build method.
/// It should only be called from paint callbacks or interaction event
/// handlers (e.g. gesture callbacks).
///
/// For details on the different phases of a frame, see the discussion at
/// [WidgetsBinding.drawFrame].
///
/// This getter will only return a valid result if [findRenderObject] actually
/// returns a [RenderBox]. If [findRenderObject] returns a render object that
/// is not a subtype of [RenderBox] (e.g., [RenderView]), this getter will
/// throw an exception in debug mode and will return null in release mode.
///
/// Calling this getter is theoretically relatively expensive (O(N) in the
/// depth of the tree), but in practice is usually cheap because the tree
/// usually has many render objects and therefore the distance to the nearest
/// render object is usually short.
Size? get size;
/// Registers this build context with [ancestor] such that when
/// [ancestor]'s widget changes this build context is rebuilt.
///
/// Returns `ancestor.widget`.
///
/// This method is rarely called directly. Most applications should use
/// [dependOnInheritedWidgetOfExactType], which calls this method after finding
/// the appropriate [InheritedElement] ancestor.
///
/// All of the qualifications about when [dependOnInheritedWidgetOfExactType] can
/// be called apply to this method as well.
InheritedWidget dependOnInheritedElement(InheritedElement ancestor, { Object? aspect });
/// Returns the nearest widget of the given type `T` and creates a dependency
/// on it, or null if no appropriate widget is found.
///
/// The widget found will be a concrete [InheritedWidget] subclass, and
/// calling [dependOnInheritedWidgetOfExactType] registers this build context
/// with the returned widget. When that widget changes (or a new widget of
/// that type is introduced, or the widget goes away), this build context is
/// rebuilt so that it can obtain new values from that widget.
///
/// {@template flutter.widgets.BuildContext.dependOnInheritedWidgetOfExactType}
/// This is typically called implicitly from `of()` static methods, e.g.
/// [Theme.of].
///
/// This method should not be called from widget constructors or from
/// [State.initState] methods, because those methods would not get called
/// again if the inherited value were to change. To ensure that the widget
/// correctly updates itself when the inherited value changes, only call this
/// (directly or indirectly) from build methods, layout and paint callbacks,
/// or from [State.didChangeDependencies] (which is called immediately after
/// [State.initState]).
///
/// This method should not be called from [State.dispose] because the element
/// tree is no longer stable at that time. To refer to an ancestor from that
/// method, save a reference to the ancestor in [State.didChangeDependencies].
/// It is safe to use this method from [State.deactivate], which is called
/// whenever the widget is removed from the tree.
///
/// It is also possible to call this method from interaction event handlers
/// (e.g. gesture callbacks) or timers, to obtain a value once, as long as
/// that value is not cached and/or reused later.
///
/// Calling this method is O(1) with a small constant factor, but will lead to
/// the widget being rebuilt more often.
///
/// Once a widget registers a dependency on a particular type by calling this
/// method, it will be rebuilt, and [State.didChangeDependencies] will be
/// called, whenever changes occur relating to that widget until the next time
/// the widget or one of its ancestors is moved (for example, because an
/// ancestor is added or removed).
///
/// The [aspect] parameter is only used when `T` is an
/// [InheritedWidget] subclasses that supports partial updates, like
/// [InheritedModel]. It specifies what "aspect" of the inherited
/// widget this context depends on.
/// {@endtemplate}
T? dependOnInheritedWidgetOfExactType<T extends InheritedWidget>({ Object? aspect });
/// Returns the nearest widget of the given [InheritedWidget] subclass `T` or
/// null if an appropriate ancestor is not found.
///
/// This method does not introduce a dependency the way that the more typical
/// [dependOnInheritedWidgetOfExactType] does, so this context will not be
/// rebuilt if the [InheritedWidget] changes. This function is meant for those
/// uncommon use cases where a dependency is undesirable.
///
/// This method should not be called from [State.dispose] because the element
/// tree is no longer stable at that time. To refer to an ancestor from that
/// method, save a reference to the ancestor in [State.didChangeDependencies].
/// It is safe to use this method from [State.deactivate], which is called
/// whenever the widget is removed from the tree.
///
/// It is also possible to call this method from interaction event handlers
/// (e.g. gesture callbacks) or timers, to obtain a value once, as long as
/// that value is not cached and/or reused later.
///
/// Calling this method is O(1) with a small constant factor.
T? getInheritedWidgetOfExactType<T extends InheritedWidget>();
/// Obtains the element corresponding to the nearest widget of the given type `T`,
/// which must be the type of a concrete [InheritedWidget] subclass.
///
/// Returns null if no such element is found.
///
/// {@template flutter.widgets.BuildContext.getElementForInheritedWidgetOfExactType}
/// Calling this method is O(1) with a small constant factor.
///
/// This method does not establish a relationship with the target in the way
/// that [dependOnInheritedWidgetOfExactType] does.
///
/// This method should not be called from [State.dispose] because the element
/// tree is no longer stable at that time. To refer to an ancestor from that
/// method, save a reference to the ancestor by calling
/// [dependOnInheritedWidgetOfExactType] in [State.didChangeDependencies]. It is
/// safe to use this method from [State.deactivate], which is called whenever
/// the widget is removed from the tree.
/// {@endtemplate}
InheritedElement? getElementForInheritedWidgetOfExactType<T extends InheritedWidget>();
/// Returns the nearest ancestor widget of the given type `T`, which must be the
/// type of a concrete [Widget] subclass.
///
/// {@template flutter.widgets.BuildContext.findAncestorWidgetOfExactType}
/// In general, [dependOnInheritedWidgetOfExactType] is more useful, since
/// inherited widgets will trigger consumers to rebuild when they change. This
/// method is appropriate when used in interaction event handlers (e.g.
/// gesture callbacks) or for performing one-off tasks such as asserting that
/// you have or don't have a widget of a specific type as an ancestor. The
/// return value of a Widget's build method should not depend on the value
/// returned by this method, because the build context will not rebuild if the
/// return value of this method changes. This could lead to a situation where
/// data used in the build method changes, but the widget is not rebuilt.
///
/// Calling this method is relatively expensive (O(N) in the depth of the
/// tree). Only call this method if the distance from this widget to the
/// desired ancestor is known to be small and bounded.
///
/// This method should not be called from [State.deactivate] or [State.dispose]
/// because the widget tree is no longer stable at that time. To refer to
/// an ancestor from one of those methods, save a reference to the ancestor
/// by calling [findAncestorWidgetOfExactType] in [State.didChangeDependencies].
///
/// Returns null if a widget of the requested type does not appear in the
/// ancestors of this context.
/// {@endtemplate}
T? findAncestorWidgetOfExactType<T extends Widget>();
/// Returns the [State] object of the nearest ancestor [StatefulWidget] widget
/// that is an instance of the given type `T`.
///
/// {@template flutter.widgets.BuildContext.findAncestorStateOfType}
/// This should not be used from build methods, because the build context will
/// not be rebuilt if the value that would be returned by this method changes.
/// In general, [dependOnInheritedWidgetOfExactType] is more appropriate for such
/// cases. This method is useful for changing the state of an ancestor widget in
/// a one-off manner, for example, to cause an ancestor scrolling list to
/// scroll this build context's widget into view, or to move the focus in
/// response to user interaction.
///
/// In general, though, consider using a callback that triggers a stateful
/// change in the ancestor rather than using the imperative style implied by
/// this method. This will usually lead to more maintainable and reusable code
/// since it decouples widgets from each other.
///
/// Calling this method is relatively expensive (O(N) in the depth of the
/// tree). Only call this method if the distance from this widget to the
/// desired ancestor is known to be small and bounded.
///
/// This method should not be called from [State.deactivate] or [State.dispose]
/// because the widget tree is no longer stable at that time. To refer to
/// an ancestor from one of those methods, save a reference to the ancestor
/// by calling [findAncestorStateOfType] in [State.didChangeDependencies].
/// {@endtemplate}
///
/// {@tool snippet}
///
/// ```dart
/// ScrollableState? scrollable = context.findAncestorStateOfType<ScrollableState>();
/// ```
/// {@end-tool}
T? findAncestorStateOfType<T extends State>();
/// Returns the [State] object of the furthest ancestor [StatefulWidget] widget
/// that is an instance of the given type `T`.
///
/// {@template flutter.widgets.BuildContext.findRootAncestorStateOfType}
/// Functions the same way as [findAncestorStateOfType] but keeps visiting subsequent
/// ancestors until there are none of the type instance of `T` remaining.
/// Then returns the last one found.
///
/// This operation is O(N) as well though N is the entire widget tree rather than
/// a subtree.
/// {@endtemplate}
T? findRootAncestorStateOfType<T extends State>();
/// Returns the [RenderObject] object of the nearest ancestor [RenderObjectWidget] widget
/// that is an instance of the given type `T`.
///
/// {@template flutter.widgets.BuildContext.findAncestorRenderObjectOfType}
/// This should not be used from build methods, because the build context will
/// not be rebuilt if the value that would be returned by this method changes.
/// In general, [dependOnInheritedWidgetOfExactType] is more appropriate for such
/// cases. This method is useful only in esoteric cases where a widget needs
/// to cause an ancestor to change its layout or paint behavior. For example,
/// it is used by [Material] so that [InkWell] widgets can trigger the ink
/// splash on the [Material]'s actual render object.
///
/// Calling this method is relatively expensive (O(N) in the depth of the
/// tree). Only call this method if the distance from this widget to the
/// desired ancestor is known to be small and bounded.
///
/// This method should not be called from [State.deactivate] or [State.dispose]
/// because the widget tree is no longer stable at that time. To refer to
/// an ancestor from one of those methods, save a reference to the ancestor
/// by calling [findAncestorRenderObjectOfType] in [State.didChangeDependencies].
/// {@endtemplate}
T? findAncestorRenderObjectOfType<T extends RenderObject>();
/// Walks the ancestor chain, starting with the parent of this build context's
/// widget, invoking the argument for each ancestor.
///
/// {@template flutter.widgets.BuildContext.visitAncestorElements}
/// The callback is given a reference to the ancestor widget's corresponding
/// [Element] object. The walk stops when it reaches the root widget or when
/// the callback returns false. The callback must not return null.
///
/// This is useful for inspecting the widget tree.
///
/// Calling this method is relatively expensive (O(N) in the depth of the tree).
///
/// This method should not be called from [State.deactivate] or [State.dispose]
/// because the element tree is no longer stable at that time. To refer to
/// an ancestor from one of those methods, save a reference to the ancestor
/// by calling [visitAncestorElements] in [State.didChangeDependencies].
/// {@endtemplate}
void visitAncestorElements(ConditionalElementVisitor visitor);
/// Walks the children of this widget.
///
/// {@template flutter.widgets.BuildContext.visitChildElements}
/// This is useful for applying changes to children after they are built
/// without waiting for the next frame, especially if the children are known,
/// and especially if there is exactly one child (as is always the case for
/// [StatefulWidget]s or [StatelessWidget]s).
///
/// Calling this method is very cheap for build contexts that correspond to
/// [StatefulWidget]s or [StatelessWidget]s (O(1), since there's only one
/// child).
///
/// Calling this method is potentially expensive for build contexts that
/// correspond to [RenderObjectWidget]s (O(N) in the number of children).
///
/// Calling this method recursively is extremely expensive (O(N) in the number
/// of descendants), and should be avoided if possible. Generally it is
/// significantly cheaper to use an [InheritedWidget] and have the descendants
/// pull data down, than it is to use [visitChildElements] recursively to push
/// data down to them.
/// {@endtemplate}
void visitChildElements(ElementVisitor visitor);
/// Start bubbling this notification at the given build context.
///
/// The notification will be delivered to any [NotificationListener] widgets
/// with the appropriate type parameters that are ancestors of the given
/// [BuildContext].
void dispatchNotification(Notification notification);
/// Returns a description of the [Element] associated with the current build context.
///
/// The `name` is typically something like "The element being rebuilt was".
///
/// See also:
///
/// * [Element.describeElements], which can be used to describe a list of elements.
DiagnosticsNode describeElement(String name, {DiagnosticsTreeStyle style = DiagnosticsTreeStyle.errorProperty});
/// Returns a description of the [Widget] associated with the current build context.
///
/// The `name` is typically something like "The widget being rebuilt was".
DiagnosticsNode describeWidget(String name, {DiagnosticsTreeStyle style = DiagnosticsTreeStyle.errorProperty});
/// Adds a description of a specific type of widget missing from the current
/// build context's ancestry tree.
///
/// You can find an example of using this method in [debugCheckHasMaterial].
List<DiagnosticsNode> describeMissingAncestor({ required Type expectedAncestorType });
/// Adds a description of the ownership chain from a specific [Element]
/// to the error report.
///
/// The ownership chain is useful for debugging the source of an element.
DiagnosticsNode describeOwnershipChain(String name);
}
/// Manager class for the widgets framework.
///
/// This class tracks which widgets need rebuilding, and handles other tasks
/// that apply to widget trees as a whole, such as managing the inactive element
/// list for the tree and triggering the "reassemble" command when necessary
/// during hot reload when debugging.
///
/// The main build owner is typically owned by the [WidgetsBinding], and is
/// driven from the operating system along with the rest of the
/// build/layout/paint pipeline.
///
/// Additional build owners can be built to manage off-screen widget trees.
///
/// To assign a build owner to a tree, use the
/// [RootElementMixin.assignOwner] method on the root element of the
/// widget tree.
///
/// {@tool dartpad}
/// This example shows how to build an off-screen widget tree used to measure
/// the layout size of the rendered tree. For some use cases, the simpler
/// [Offstage] widget may be a better alternative to this approach.
///
/// ** See code in examples/api/lib/widgets/framework/build_owner.0.dart **
/// {@end-tool}
class BuildOwner {
/// Creates an object that manages widgets.
///
/// If the `focusManager` argument is not specified or is null, this will
/// construct a new [FocusManager] and register its global input handlers
/// via [FocusManager.registerGlobalHandlers], which will modify static
/// state. Callers wishing to avoid altering this state can explicitly pass
/// a focus manager here.
BuildOwner({ this.onBuildScheduled, FocusManager? focusManager }) :
focusManager = focusManager ?? (FocusManager()..registerGlobalHandlers());
/// Called on each build pass when the first buildable element is marked
/// dirty.
VoidCallback? onBuildScheduled;
final _InactiveElements _inactiveElements = _InactiveElements();
final List<Element> _dirtyElements = <Element>[];
bool _scheduledFlushDirtyElements = false;
/// Whether [_dirtyElements] need to be sorted again as a result of more
/// elements becoming dirty during the build.
///
/// This is necessary to preserve the sort order defined by [Element._sort].
///
/// This field is set to null when [buildScope] is not actively rebuilding
/// the widget tree.
bool? _dirtyElementsNeedsResorting;
/// Whether [buildScope] is actively rebuilding the widget tree.
///
/// [scheduleBuildFor] should only be called when this value is true.
bool get _debugIsInBuildScope => _dirtyElementsNeedsResorting != null;
/// The object in charge of the focus tree.
///
/// Rarely used directly. Instead, consider using [FocusScope.of] to obtain
/// the [FocusScopeNode] for a given [BuildContext].
///
/// See [FocusManager] for more details.
///
/// This field will default to a [FocusManager] that has registered its
/// global input handlers via [FocusManager.registerGlobalHandlers]. Callers
/// wishing to avoid registering those handlers (and modifying the associated
/// static state) can explicitly pass a focus manager to the [BuildOwner.new]
/// constructor.
FocusManager focusManager;
/// Adds an element to the dirty elements list so that it will be rebuilt
/// when [WidgetsBinding.drawFrame] calls [buildScope].
void scheduleBuildFor(Element element) {
assert(element.owner == this);
assert(() {
if (debugPrintScheduleBuildForStacks) {
debugPrintStack(label: 'scheduleBuildFor() called for $element${_dirtyElements.contains(element) ? " (ALREADY IN LIST)" : ""}');
}
if (!element.dirty) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('scheduleBuildFor() called for a widget that is not marked as dirty.'),
element.describeElement('The method was called for the following element'),
ErrorDescription(
'This element is not current marked as dirty. Make sure to set the dirty flag before '
'calling scheduleBuildFor().',
),
ErrorHint(
'If you did not attempt to call scheduleBuildFor() yourself, then this probably '
'indicates a bug in the widgets framework. Please report it:\n'
' https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]);
}
return true;
}());
if (element._inDirtyList) {
assert(() {
if (debugPrintScheduleBuildForStacks) {
debugPrintStack(label: 'BuildOwner.scheduleBuildFor() called; _dirtyElementsNeedsResorting was $_dirtyElementsNeedsResorting (now true); dirty list is: $_dirtyElements');
}
if (!_debugIsInBuildScope) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('BuildOwner.scheduleBuildFor() called inappropriately.'),
ErrorHint(
'The BuildOwner.scheduleBuildFor() method should only be called while the '
'buildScope() method is actively rebuilding the widget tree.',
),
]);
}
return true;
}());
_dirtyElementsNeedsResorting = true;
return;
}
if (!_scheduledFlushDirtyElements && onBuildScheduled != null) {
_scheduledFlushDirtyElements = true;
onBuildScheduled!();
}
_dirtyElements.add(element);
element._inDirtyList = true;
assert(() {
if (debugPrintScheduleBuildForStacks) {
debugPrint('...dirty list is now: $_dirtyElements');
}
return true;
}());
}
int _debugStateLockLevel = 0;
bool get _debugStateLocked => _debugStateLockLevel > 0;
/// Whether this widget tree is in the build phase.
///
/// Only valid when asserts are enabled.
bool get debugBuilding => _debugBuilding;
bool _debugBuilding = false;
Element? _debugCurrentBuildTarget;
/// Establishes a scope in which calls to [State.setState] are forbidden, and
/// calls the given `callback`.
///
/// This mechanism is used to ensure that, for instance, [State.dispose] does
/// not call [State.setState].
void lockState(VoidCallback callback) {
assert(_debugStateLockLevel >= 0);
assert(() {
_debugStateLockLevel += 1;
return true;
}());
try {
callback();
} finally {
assert(() {
_debugStateLockLevel -= 1;
return true;
}());
}
assert(_debugStateLockLevel >= 0);
}
/// Establishes a scope for updating the widget tree, and calls the given
/// `callback`, if any. Then, builds all the elements that were marked as
/// dirty using [scheduleBuildFor], in depth order.
///
/// This mechanism prevents build methods from transitively requiring other
/// build methods to run, potentially causing infinite loops.
///
/// The dirty list is processed after `callback` returns, building all the
/// elements that were marked as dirty using [scheduleBuildFor], in depth
/// order. If elements are marked as dirty while this method is running, they
/// must be deeper than the `context` node, and deeper than any
/// previously-built node in this pass.
///
/// To flush the current dirty list without performing any other work, this
/// function can be called with no callback. This is what the framework does
/// each frame, in [WidgetsBinding.drawFrame].
///
/// Only one [buildScope] can be active at a time.
///
/// A [buildScope] implies a [lockState] scope as well.
///
/// To print a console message every time this method is called, set
/// [debugPrintBuildScope] to true. This is useful when debugging problems
/// involving widgets not getting marked dirty, or getting marked dirty too
/// often.
@pragma('vm:notify-debugger-on-exception')
void buildScope(Element context, [ VoidCallback? callback ]) {
if (callback == null && _dirtyElements.isEmpty) {
return;
}
assert(_debugStateLockLevel >= 0);
assert(!_debugBuilding);
assert(() {
if (debugPrintBuildScope) {
debugPrint('buildScope called with context $context; dirty list is: $_dirtyElements');
}
_debugStateLockLevel += 1;
_debugBuilding = true;
return true;
}());
if (!kReleaseMode) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (debugEnhanceBuildTimelineArguments) {
debugTimelineArguments = <String, String>{
'dirty count': '${_dirtyElements.length}',
'dirty list': '$_dirtyElements',
'lock level': '$_debugStateLockLevel',
'scope context': '$context',
};
}
return true;
}());
FlutterTimeline.startSync(
'BUILD',
arguments: debugTimelineArguments
);
}
try {
_scheduledFlushDirtyElements = true;
if (callback != null) {
assert(_debugStateLocked);
Element? debugPreviousBuildTarget;
assert(() {
debugPreviousBuildTarget = _debugCurrentBuildTarget;
_debugCurrentBuildTarget = context;
return true;
}());
_dirtyElementsNeedsResorting = false;
try {
callback();
} finally {
assert(() {
assert(_debugCurrentBuildTarget == context);
_debugCurrentBuildTarget = debugPreviousBuildTarget;
_debugElementWasRebuilt(context);
return true;
}());
}
}
_dirtyElements.sort(Element._sort);
_dirtyElementsNeedsResorting = false;
int dirtyCount = _dirtyElements.length;
int index = 0;
while (index < dirtyCount) {
final Element element = _dirtyElements[index];
assert(element._inDirtyList);
assert(() {
if (element._lifecycleState == _ElementLifecycle.active && !element._debugIsInScope(context)) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Tried to build dirty widget in the wrong build scope.'),
ErrorDescription(
'A widget which was marked as dirty and is still active was scheduled to be built, '
'but the current build scope unexpectedly does not contain that widget.',
),
ErrorHint(
'Sometimes this is detected when an element is removed from the widget tree, but the '
'element somehow did not get marked as inactive. In that case, it might be caused by '
'an ancestor element failing to implement visitChildren correctly, thus preventing '
'some or all of its descendants from being correctly deactivated.',
),
DiagnosticsProperty<Element>(
'The root of the build scope was',
context,
style: DiagnosticsTreeStyle.errorProperty,
),
DiagnosticsProperty<Element>(
'The offending element (which does not appear to be a descendant of the root of the build scope) was',
element,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
return true;
}());
final bool isTimelineTracked = !kReleaseMode && _isProfileBuildsEnabledFor(element.widget);
if (isTimelineTracked) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (kDebugMode && debugEnhanceBuildTimelineArguments) {
debugTimelineArguments = element.widget.toDiagnosticsNode().toTimelineArguments();
}
return true;
}());
FlutterTimeline.startSync(
'${element.widget.runtimeType}',
arguments: debugTimelineArguments,
);
}
try {
element.rebuild();
} catch (e, stack) {
_reportException(
ErrorDescription('while rebuilding dirty elements'),
e,
stack,
informationCollector: () => <DiagnosticsNode>[
if (kDebugMode && index < _dirtyElements.length)
DiagnosticsDebugCreator(DebugCreator(element)),
if (index < _dirtyElements.length)
element.describeElement('The element being rebuilt at the time was index $index of $dirtyCount')
else
ErrorHint('The element being rebuilt at the time was index $index of $dirtyCount, but _dirtyElements only had ${_dirtyElements.length} entries. This suggests some confusion in the framework internals.'),
],
);
}
if (isTimelineTracked) {
FlutterTimeline.finishSync();
}
index += 1;
if (dirtyCount < _dirtyElements.length || _dirtyElementsNeedsResorting!) {
_dirtyElements.sort(Element._sort);
_dirtyElementsNeedsResorting = false;
dirtyCount = _dirtyElements.length;
while (index > 0 && _dirtyElements[index - 1].dirty) {
// It is possible for previously dirty but inactive widgets to move right in the list.
// We therefore have to move the index left in the list to account for this.
// We don't know how many could have moved. However, we do know that the only possible
// change to the list is that nodes that were previously to the left of the index have
// now moved to be to the right of the right-most cleaned node, and we do know that
// all the clean nodes were to the left of the index. So we move the index left
// until just after the right-most clean node.
index -= 1;
}
}
}
assert(() {
if (_dirtyElements.any((Element element) => element._lifecycleState == _ElementLifecycle.active && element.dirty)) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('buildScope missed some dirty elements.'),
ErrorHint('This probably indicates that the dirty list should have been resorted but was not.'),
Element.describeElements('The list of dirty elements at the end of the buildScope call was', _dirtyElements),
]);
}
return true;
}());
} finally {
for (final Element element in _dirtyElements) {
assert(element._inDirtyList);
element._inDirtyList = false;
}
_dirtyElements.clear();
_scheduledFlushDirtyElements = false;
_dirtyElementsNeedsResorting = null;
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
assert(_debugBuilding);
assert(() {
_debugBuilding = false;
_debugStateLockLevel -= 1;
if (debugPrintBuildScope) {
debugPrint('buildScope finished');
}
return true;
}());
}
assert(_debugStateLockLevel >= 0);
}
Map<Element, Set<GlobalKey>>? _debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans;
void _debugTrackElementThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans(Element node, GlobalKey key) {
_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans ??= HashMap<Element, Set<GlobalKey>>();
final Set<GlobalKey> keys = _debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans!
.putIfAbsent(node, () => HashSet<GlobalKey>());
keys.add(key);
}
void _debugElementWasRebuilt(Element node) {
_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans?.remove(node);
}
final Map<GlobalKey, Element> _globalKeyRegistry = <GlobalKey, Element>{};
// In Profile/Release mode this field is initialized to `null`. The Dart compiler can
// eliminate unused fields, but not their initializers.
@_debugOnly
final Set<Element>? _debugIllFatedElements = kDebugMode ? HashSet<Element>() : null;
// This map keeps track which child reserves the global key with the parent.
// Parent, child -> global key.
// This provides us a way to remove old reservation while parent rebuilds the
// child in the same slot.
//
// In Profile/Release mode this field is initialized to `null`. The Dart compiler can
// eliminate unused fields, but not their initializers.
@_debugOnly
final Map<Element, Map<Element, GlobalKey>>? _debugGlobalKeyReservations = kDebugMode ? <Element, Map<Element, GlobalKey>>{} : null;
/// The number of [GlobalKey] instances that are currently associated with
/// [Element]s that have been built by this build owner.
int get globalKeyCount => _globalKeyRegistry.length;
void _debugRemoveGlobalKeyReservationFor(Element parent, Element child) {
assert(() {
_debugGlobalKeyReservations?[parent]?.remove(child);
return true;
}());
}
void _registerGlobalKey(GlobalKey key, Element element) {
assert(() {
if (_globalKeyRegistry.containsKey(key)) {
final Element oldElement = _globalKeyRegistry[key]!;
assert(element.widget.runtimeType != oldElement.widget.runtimeType);
_debugIllFatedElements?.add(oldElement);
}
return true;
}());
_globalKeyRegistry[key] = element;
}
void _unregisterGlobalKey(GlobalKey key, Element element) {
assert(() {
if (_globalKeyRegistry.containsKey(key) && _globalKeyRegistry[key] != element) {
final Element oldElement = _globalKeyRegistry[key]!;
assert(element.widget.runtimeType != oldElement.widget.runtimeType);
}
return true;
}());
if (_globalKeyRegistry[key] == element) {
_globalKeyRegistry.remove(key);
}
}
void _debugReserveGlobalKeyFor(Element parent, Element child, GlobalKey key) {
assert(() {
_debugGlobalKeyReservations?[parent] ??= <Element, GlobalKey>{};
_debugGlobalKeyReservations?[parent]![child] = key;
return true;
}());
}
void _debugVerifyGlobalKeyReservation() {
assert(() {
final Map<GlobalKey, Element> keyToParent = <GlobalKey, Element>{};
_debugGlobalKeyReservations?.forEach((Element parent, Map<Element, GlobalKey> childToKey) {
// We ignore parent that are unmounted or detached.
if (parent._lifecycleState == _ElementLifecycle.defunct || parent.renderObject?.attached == false) {
return;
}
childToKey.forEach((Element child, GlobalKey key) {
// If parent = null, the node is deactivated by its parent and is
// not re-attached to other part of the tree. We should ignore this
// node.
if (child._parent == null) {
return;
}
// It is possible the same key registers to the same parent twice
// with different children. That is illegal, but it is not in the
// scope of this check. Such error will be detected in
// _debugVerifyIllFatedPopulation or
// _debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans.
if (keyToParent.containsKey(key) && keyToParent[key] != parent) {
// We have duplication reservations for the same global key.
final Element older = keyToParent[key]!;
final Element newer = parent;
final FlutterError error;
if (older.toString() != newer.toString()) {
error = FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Multiple widgets used the same GlobalKey.'),
ErrorDescription(
'The key $key was used by multiple widgets. The parents of those widgets were:\n'
'- $older\n'
'- $newer\n'
'A GlobalKey can only be specified on one widget at a time in the widget tree.',
),
]);
} else {
error = FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Multiple widgets used the same GlobalKey.'),
ErrorDescription(
'The key $key was used by multiple widgets. The parents of those widgets were '
'different widgets that both had the following description:\n'
' $parent\n'
'A GlobalKey can only be specified on one widget at a time in the widget tree.',
),
]);
}
// Fix the tree by removing the duplicated child from one of its
// parents to resolve the duplicated key issue. This allows us to
// tear down the tree during testing without producing additional
// misleading exceptions.
if (child._parent != older) {
older.visitChildren((Element currentChild) {
if (currentChild == child) {
older.forgetChild(child);
}
});
}
if (child._parent != newer) {
newer.visitChildren((Element currentChild) {
if (currentChild == child) {
newer.forgetChild(child);
}
});
}
throw error;
} else {
keyToParent[key] = parent;
}
});
});
_debugGlobalKeyReservations?.clear();
return true;
}());
}
void _debugVerifyIllFatedPopulation() {
assert(() {
Map<GlobalKey, Set<Element>>? duplicates;
for (final Element element in _debugIllFatedElements ?? const <Element>{}) {
if (element._lifecycleState != _ElementLifecycle.defunct) {
assert(element.widget.key != null);
final GlobalKey key = element.widget.key! as GlobalKey;
assert(_globalKeyRegistry.containsKey(key));
duplicates ??= <GlobalKey, Set<Element>>{};
// Uses ordered set to produce consistent error message.
final Set<Element> elements = duplicates.putIfAbsent(key, () => <Element>{});
elements.add(element);
elements.add(_globalKeyRegistry[key]!);
}
}
_debugIllFatedElements?.clear();
if (duplicates != null) {
final List<DiagnosticsNode> information = <DiagnosticsNode>[];
information.add(ErrorSummary('Multiple widgets used the same GlobalKey.'));
for (final GlobalKey key in duplicates.keys) {
final Set<Element> elements = duplicates[key]!;
// TODO(jacobr): this will omit the '- ' before each widget name and
// use the more standard whitespace style instead. Please let me know
// if the '- ' style is a feature we want to maintain and we can add
// another tree style that supports it. I also see '* ' in some places
// so it would be nice to unify and normalize.
information.add(Element.describeElements('The key $key was used by ${elements.length} widgets', elements));
}
information.add(ErrorDescription('A GlobalKey can only be specified on one widget at a time in the widget tree.'));
throw FlutterError.fromParts(information);
}
return true;
}());
}
/// Complete the element build pass by unmounting any elements that are no
/// longer active.
///
/// This is called by [WidgetsBinding.drawFrame].
///
/// In debug mode, this also runs some sanity checks, for example checking for
/// duplicate global keys.
///
/// After the current call stack unwinds, a microtask that notifies listeners
/// about changes to global keys will run.
@pragma('vm:notify-debugger-on-exception')
void finalizeTree() {
if (!kReleaseMode) {
FlutterTimeline.startSync('FINALIZE TREE');
}
try {
lockState(_inactiveElements._unmountAll); // this unregisters the GlobalKeys
assert(() {
try {
_debugVerifyGlobalKeyReservation();
_debugVerifyIllFatedPopulation();
if (_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans != null &&
_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans!.isNotEmpty) {
final Set<GlobalKey> keys = HashSet<GlobalKey>();
for (final Element element in _debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans!.keys) {
if (element._lifecycleState != _ElementLifecycle.defunct) {
keys.addAll(_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans![element]!);
}
}
if (keys.isNotEmpty) {
final Map<String, int> keyStringCount = HashMap<String, int>();
for (final String key in keys.map<String>((GlobalKey key) => key.toString())) {
if (keyStringCount.containsKey(key)) {
keyStringCount.update(key, (int value) => value + 1);
} else {
keyStringCount[key] = 1;
}
}
final List<String> keyLabels = <String>[];
keyStringCount.forEach((String key, int count) {
if (count == 1) {
keyLabels.add(key);
} else {
keyLabels.add('$key ($count different affected keys had this toString representation)');
}
});
final Iterable<Element> elements = _debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans!.keys;
final Map<String, int> elementStringCount = HashMap<String, int>();
for (final String element in elements.map<String>((Element element) => element.toString())) {
if (elementStringCount.containsKey(element)) {
elementStringCount.update(element, (int value) => value + 1);
} else {
elementStringCount[element] = 1;
}
}
final List<String> elementLabels = <String>[];
elementStringCount.forEach((String element, int count) {
if (count == 1) {
elementLabels.add(element);
} else {
elementLabels.add('$element ($count different affected elements had this toString representation)');
}
});
assert(keyLabels.isNotEmpty);
final String the = keys.length == 1 ? ' the' : '';
final String s = keys.length == 1 ? '' : 's';
final String were = keys.length == 1 ? 'was' : 'were';
final String their = keys.length == 1 ? 'its' : 'their';
final String respective = elementLabels.length == 1 ? '' : ' respective';
final String those = keys.length == 1 ? 'that' : 'those';
final String s2 = elementLabels.length == 1 ? '' : 's';
final String those2 = elementLabels.length == 1 ? 'that' : 'those';
final String they = elementLabels.length == 1 ? 'it' : 'they';
final String think = elementLabels.length == 1 ? 'thinks' : 'think';
final String are = elementLabels.length == 1 ? 'is' : 'are';
// TODO(jacobr): make this error more structured to better expose which widgets had problems.
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Duplicate GlobalKey$s detected in widget tree.'),
// TODO(jacobr): refactor this code so the elements are clickable
// in GUI debug tools.
ErrorDescription(
'The following GlobalKey$s $were specified multiple times in the widget tree. This will lead to '
'parts of the widget tree being truncated unexpectedly, because the second time a key is seen, '
'the previous instance is moved to the new location. The key$s $were:\n'
'- ${keyLabels.join("\n ")}\n'
'This was determined by noticing that after$the widget$s with the above global key$s $were moved '
'out of $their$respective previous parent$s2, $those2 previous parent$s2 never updated during this frame, meaning '
'that $they either did not update at all or updated before the widget$s $were moved, in either case '
'implying that $they still $think that $they should have a child with $those global key$s.\n'
'The specific parent$s2 that did not update after having one or more children forcibly removed '
'due to GlobalKey reparenting $are:\n'
'- ${elementLabels.join("\n ")}'
'\nA GlobalKey can only be specified on one widget at a time in the widget tree.',
),
]);
}
}
} finally {
_debugElementsThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans?.clear();
}
return true;
}());
} catch (e, stack) {
// Catching the exception directly to avoid activating the ErrorWidget.
// Since the tree is in a broken state, adding the ErrorWidget would
// cause more exceptions.
_reportException(ErrorSummary('while finalizing the widget tree'), e, stack);
} finally {
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
}
/// Cause the entire subtree rooted at the given [Element] to be entirely
/// rebuilt. This is used by development tools when the application code has
/// changed and is being hot-reloaded, to cause the widget tree to pick up any
/// changed implementations.
///
/// This is expensive and should not be called except during development.
void reassemble(Element root) {
if (!kReleaseMode) {
FlutterTimeline.startSync('Preparing Hot Reload (widgets)');
}
try {
assert(root._parent == null);
assert(root.owner == this);
root.reassemble();
} finally {
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
}
}
/// Mixin this class to allow receiving [Notification] objects dispatched by
/// child elements.
///
/// See also:
/// * [NotificationListener], for a widget that allows consuming notifications.
mixin NotifiableElementMixin on Element {
/// Called when a notification of the appropriate type arrives at this
/// location in the tree.
///
/// Return true to cancel the notification bubbling. Return false to
/// allow the notification to continue to be dispatched to further ancestors.
bool onNotification(Notification notification);
@override
void attachNotificationTree() {
_notificationTree = _NotificationNode(_parent?._notificationTree, this);
}
}
class _NotificationNode {
_NotificationNode(this.parent, this.current);
NotifiableElementMixin? current;
_NotificationNode? parent;
void dispatchNotification(Notification notification) {
if (current?.onNotification(notification) ?? true) {
return;
}
parent?.dispatchNotification(notification);
}
}
bool _isProfileBuildsEnabledFor(Widget widget) {
return debugProfileBuildsEnabled ||
(debugProfileBuildsEnabledUserWidgets &&
debugIsWidgetLocalCreation(widget));
}
/// An instantiation of a [Widget] at a particular location in the tree.
///
/// Widgets describe how to configure a subtree but the same widget can be used
/// to configure multiple subtrees simultaneously because widgets are immutable.
/// An [Element] represents the use of a widget to configure a specific location
/// in the tree. Over time, the widget associated with a given element can
/// change, for example, if the parent widget rebuilds and creates a new widget
/// for this location.
///
/// Elements form a tree. Most elements have a unique child, but some widgets
/// (e.g., subclasses of [RenderObjectElement]) can have multiple children.
///
/// Elements have the following lifecycle:
///
/// * The framework creates an element by calling [Widget.createElement] on the
/// widget that will be used as the element's initial configuration.
/// * The framework calls [mount] to add the newly created element to the tree
/// at a given slot in a given parent. The [mount] method is responsible for
/// inflating any child widgets and calling [attachRenderObject] as
/// necessary to attach any associated render objects to the render tree.
/// * At this point, the element is considered "active" and might appear on
/// screen.
/// * At some point, the parent might decide to change the widget used to
/// configure this element, for example because the parent rebuilt with new
/// state. When this happens, the framework will call [update] with the new
/// widget. The new widget will always have the same [runtimeType] and key as
/// old widget. If the parent wishes to change the [runtimeType] or key of
/// the widget at this location in the tree, it can do so by unmounting this
/// element and inflating the new widget at this location.
/// * At some point, an ancestor might decide to remove this element (or an
/// intermediate ancestor) from the tree, which the ancestor does by calling
/// [deactivateChild] on itself. Deactivating the intermediate ancestor will
/// remove that element's render object from the render tree and add this
/// element to the [owner]'s list of inactive elements, causing the framework
/// to call [deactivate] on this element.
/// * At this point, the element is considered "inactive" and will not appear
/// on screen. An element can remain in the inactive state only until
/// the end of the current animation frame. At the end of the animation
/// frame, any elements that are still inactive will be unmounted.
/// * If the element gets reincorporated into the tree (e.g., because it or one
/// of its ancestors has a global key that is reused), the framework will
/// remove the element from the [owner]'s list of inactive elements, call
/// [activate] on the element, and reattach the element's render object to
/// the render tree. (At this point, the element is again considered "active"
/// and might appear on screen.)
/// * If the element does not get reincorporated into the tree by the end of
/// the current animation frame, the framework will call [unmount] on the
/// element.
/// * At this point, the element is considered "defunct" and will not be
/// incorporated into the tree in the future.
abstract class Element extends DiagnosticableTree implements BuildContext {
/// Creates an element that uses the given widget as its configuration.
///
/// Typically called by an override of [Widget.createElement].
Element(Widget widget)
: _widget = widget {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterWidgetsLibrary,
className: '$Element',
object: this,
);
}
}
Element? _parent;
_NotificationNode? _notificationTree;
/// Compare two widgets for equality.
///
/// When a widget is rebuilt with another that compares equal according
/// to `operator ==`, it is assumed that the update is redundant and the
/// work to update that branch of the tree is skipped.
///
/// It is generally discouraged to override `operator ==` on any widget that
/// has children, since a correct implementation would have to defer to the
/// children's equality operator also, and that is an O(N²) operation: each
/// child would need to itself walk all its children, each step of the tree.
///
/// It is sometimes reasonable for a leaf widget (one with no children) to
/// implement this method, if rebuilding the widget is known to be much more
/// expensive than checking the widgets' parameters for equality and if the
/// widget is expected to often be rebuilt with identical parameters.
///
/// In general, however, it is more efficient to cache the widgets used
/// in a build method if it is known that they will not change.
@nonVirtual
@override
// ignore: avoid_equals_and_hash_code_on_mutable_classes, hash_and_equals
bool operator ==(Object other) => identical(this, other);
/// Information set by parent to define where this child fits in its parent's
/// child list.
///
/// A child widget's slot is determined when the parent's [updateChild] method
/// is called to inflate the child widget. See [RenderObjectElement] for more
/// details on slots.
Object? get slot => _slot;
Object? _slot;
/// An integer that is guaranteed to be greater than the parent's, if any.
/// The element at the root of the tree must have a depth greater than 0.
int get depth {
assert(() {
if (_lifecycleState == _ElementLifecycle.initial) {
throw FlutterError('Depth is only available when element has been mounted.');
}
return true;
}());
return _depth;
}
late int _depth;
/// Returns result < 0 when [a] < [b], result == 0 when [a] == [b], result > 0
/// when [a] > [b].
static int _sort(Element a, Element b) {
final int diff = a.depth - b.depth;
// If depths are not equal, return the difference.
if (diff != 0) {
return diff;
}
// If the `dirty` values are not equal, sort with non-dirty elements being
// less than dirty elements.
final bool isBDirty = b.dirty;
if (a.dirty != isBDirty) {
return isBDirty ? -1 : 1;
}
// Otherwise, `depth`s and `dirty`s are equal.
return 0;
}
// Return a numeric encoding of the specific `Element` concrete subtype.
// This is used in `Element.updateChild` to determine if a hot reload modified the
// superclass of a mounted element's configuration. The encoding of each `Element`
// must match the corresponding `Widget` encoding in `Widget._debugConcreteSubtype`.
static int _debugConcreteSubtype(Element element) {
return element is StatefulElement ? 1 :
element is StatelessElement ? 2 :
0;
}
/// The configuration for this element.
///
/// Avoid overriding this field on [Element] subtypes to provide a more
/// specific widget type (i.e. [StatelessElement] and [StatelessWidget]).
/// Instead, cast at any call sites where the more specific type is required.
/// This avoids significant cast overhead on the getter which is accessed
/// throughout the framework internals during the build phase - and for which
/// the more specific type information is not used.
@override
Widget get widget => _widget!;
Widget? _widget;
@override
bool get mounted => _widget != null;
/// Returns true if the Element is defunct.
///
/// This getter always returns false in profile and release builds.
/// See the lifecycle documentation for [Element] for additional information.
bool get debugIsDefunct {
bool isDefunct = false;
assert(() {
isDefunct = _lifecycleState == _ElementLifecycle.defunct;
return true;
}());
return isDefunct;
}
/// Returns true if the Element is active.
///
/// This getter always returns false in profile and release builds.
/// See the lifecycle documentation for [Element] for additional information.
bool get debugIsActive {
bool isActive = false;
assert(() {
isActive = _lifecycleState == _ElementLifecycle.active;
return true;
}());
return isActive;
}
/// The object that manages the lifecycle of this element.
@override
BuildOwner? get owner => _owner;
BuildOwner? _owner;
/// {@template flutter.widgets.Element.reassemble}
/// Called whenever the application is reassembled during debugging, for
/// example during hot reload.
///
/// This method should rerun any initialization logic that depends on global
/// state, for example, image loading from asset bundles (since the asset
/// bundle may have changed).
///
/// This function will only be called during development. In release builds,
/// the `ext.flutter.reassemble` hook is not available, and so this code will
/// never execute.
///
/// Implementers should not rely on any ordering for hot reload source update,
/// reassemble, and build methods after a hot reload has been initiated. It is
/// possible that a [Timer] (e.g. an [Animation]) or a debugging session
/// attached to the isolate could trigger a build with reloaded code _before_
/// reassemble is called. Code that expects preconditions to be set by
/// reassemble after a hot reload must be resilient to being called out of
/// order, e.g. by fizzling instead of throwing. That said, once reassemble is
/// called, build will be called after it at least once.
/// {@endtemplate}
///
/// See also:
///
/// * [State.reassemble]
/// * [BindingBase.reassembleApplication]
/// * [Image], which uses this to reload images.
@mustCallSuper
@protected
void reassemble() {
markNeedsBuild();
visitChildren((Element child) {
child.reassemble();
});
}
bool _debugIsInScope(Element target) {
Element? current = this;
while (current != null) {
if (target == current) {
return true;
}
current = current._parent;
}
return false;
}
/// The render object at (or below) this location in the tree.
///
/// If this object is a [RenderObjectElement], the render object is the one at
/// this location in the tree. Otherwise, this getter will walk down the tree
/// until it finds a [RenderObjectElement].
///
/// Some locations in the tree are not backed by a render object. In those
/// cases, this getter returns null. This can happen, if the element is
/// located outside of a [View] since only the element subtree rooted in a
/// view has a render tree associated with it.
RenderObject? get renderObject {
Element? current = this;
while (current != null) {
if (current._lifecycleState == _ElementLifecycle.defunct) {
break;
} else if (current is RenderObjectElement) {
return current.renderObject;
} else {
current = current.renderObjectAttachingChild;
}
}
return null;
}
/// Returns the child of this [Element] that will insert a [RenderObject] into
/// an ancestor of this Element to construct the render tree.
///
/// Returns null if this Element doesn't have any children who need to attach
/// a [RenderObject] to an ancestor of this [Element]. A [RenderObjectElement]
/// will therefore return null because its children insert their
/// [RenderObject]s into the [RenderObjectElement] itself and not into an
/// ancestor of the [RenderObjectElement].
///
/// Furthermore, this may return null for [Element]s that hoist their own
/// independent render tree and do not extend the ancestor render tree.
@protected
Element? get renderObjectAttachingChild {
Element? next;
visitChildren((Element child) {
assert(next == null); // This verifies that there's only one child.
next = child;
});
return next;
}
@override
List<DiagnosticsNode> describeMissingAncestor({ required Type expectedAncestorType }) {
final List<DiagnosticsNode> information = <DiagnosticsNode>[];
final List<Element> ancestors = <Element>[];
visitAncestorElements((Element element) {
ancestors.add(element);
return true;
});
information.add(DiagnosticsProperty<Element>(
'The specific widget that could not find a $expectedAncestorType ancestor was',
this,
style: DiagnosticsTreeStyle.errorProperty,
));
if (ancestors.isNotEmpty) {
information.add(describeElements('The ancestors of this widget were', ancestors));
} else {
information.add(ErrorDescription(
'This widget is the root of the tree, so it has no '
'ancestors, let alone a "$expectedAncestorType" ancestor.',
));
}
return information;
}
/// Returns a list of [Element]s from the current build context to the error report.
static DiagnosticsNode describeElements(String name, Iterable<Element> elements) {
return DiagnosticsBlock(
name: name,
children: elements.map<DiagnosticsNode>((Element element) => DiagnosticsProperty<Element>('', element)).toList(),
allowTruncate: true,
);
}
@override
DiagnosticsNode describeElement(String name, {DiagnosticsTreeStyle style = DiagnosticsTreeStyle.errorProperty}) {
return DiagnosticsProperty<Element>(name, this, style: style);
}
@override
DiagnosticsNode describeWidget(String name, {DiagnosticsTreeStyle style = DiagnosticsTreeStyle.errorProperty}) {
return DiagnosticsProperty<Element>(name, this, style: style);
}
@override
DiagnosticsNode describeOwnershipChain(String name) {
// TODO(jacobr): make this structured so clients can support clicks on
// individual entries. For example, is this an iterable with arrows as
// separators?
return StringProperty(name, debugGetCreatorChain(10));
}
// This is used to verify that Element objects move through life in an
// orderly fashion.
_ElementLifecycle _lifecycleState = _ElementLifecycle.initial;
/// Calls the argument for each child. Must be overridden by subclasses that
/// support having children.
///
/// There is no guaranteed order in which the children will be visited, though
/// it should be consistent over time.
///
/// Calling this during build is dangerous: the child list might still be
/// being updated at that point, so the children might not be constructed yet,
/// or might be old children that are going to be replaced. This method should
/// only be called if it is provable that the children are available.
void visitChildren(ElementVisitor visitor) { }
/// Calls the argument for each child considered onstage.
///
/// Classes like [Offstage] and [Overlay] override this method to hide their
/// children.
///
/// Being onstage affects the element's discoverability during testing when
/// you use Flutter's [Finder] objects. For example, when you instruct the
/// test framework to tap on a widget, by default the finder will look for
/// onstage elements and ignore the offstage ones.
///
/// The default implementation defers to [visitChildren] and therefore treats
/// the element as onstage.
///
/// See also:
///
/// * [Offstage] widget that hides its children.
/// * [Finder] that skips offstage widgets by default.
/// * [RenderObject.visitChildrenForSemantics], in contrast to this method,
/// designed specifically for excluding parts of the UI from the semantics
/// tree.
void debugVisitOnstageChildren(ElementVisitor visitor) => visitChildren(visitor);
/// Wrapper around [visitChildren] for [BuildContext].
@override
void visitChildElements(ElementVisitor visitor) {
assert(() {
if (owner == null || !owner!._debugStateLocked) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('visitChildElements() called during build.'),
ErrorDescription(
"The BuildContext.visitChildElements() method can't be called during "
'build because the child list is still being updated at that point, '
'so the children might not be constructed yet, or might be old children '
'that are going to be replaced.',
),
]);
}());
visitChildren(visitor);
}
/// Update the given child with the given new configuration.
///
/// This method is the core of the widgets system. It is called each time we
/// are to add, update, or remove a child based on an updated configuration.
///
/// The `newSlot` argument specifies the new value for this element's [slot].
///
/// If the `child` is null, and the `newWidget` is not null, then we have a new
/// child for which we need to create an [Element], configured with `newWidget`.
///
/// If the `newWidget` is null, and the `child` is not null, then we need to
/// remove it because it no longer has a configuration.
///
/// If neither are null, then we need to update the `child`'s configuration to
/// be the new configuration given by `newWidget`. If `newWidget` can be given
/// to the existing child (as determined by [Widget.canUpdate]), then it is so
/// given. Otherwise, the old child needs to be disposed and a new child
/// created for the new configuration.
///
/// If both are null, then we don't have a child and won't have a child, so we
/// do nothing.
///
/// The [updateChild] method returns the new child, if it had to create one,
/// or the child that was passed in, if it just had to update the child, or
/// null, if it removed the child and did not replace it.
///
/// The following table summarizes the above:
///
/// | | **newWidget == null** | **newWidget != null** |
/// | :-----------------: | :--------------------- | :---------------------- |
/// | **child == null** | Returns null. | Returns new [Element]. |
/// | **child != null** | Old child is removed, returns null. | Old child updated if possible, returns child or new [Element]. |
///
/// The `newSlot` argument is used only if `newWidget` is not null. If `child`
/// is null (or if the old child cannot be updated), then the `newSlot` is
/// given to the new [Element] that is created for the child, via
/// [inflateWidget]. If `child` is not null (and the old child _can_ be
/// updated), then the `newSlot` is given to [updateSlotForChild] to update
/// its slot, in case it has moved around since it was last built.
///
/// See the [RenderObjectElement] documentation for more information on slots.
@protected
@pragma('dart2js:tryInline')
@pragma('vm:prefer-inline')
@pragma('wasm:prefer-inline')
Element? updateChild(Element? child, Widget? newWidget, Object? newSlot) {
if (newWidget == null) {
if (child != null) {
deactivateChild(child);
}
return null;
}
final Element newChild;
if (child != null) {
bool hasSameSuperclass = true;
// When the type of a widget is changed between Stateful and Stateless via
// hot reload, the element tree will end up in a partially invalid state.
// That is, if the widget was a StatefulWidget and is now a StatelessWidget,
// then the element tree currently contains a StatefulElement that is incorrectly
// referencing a StatelessWidget (and likewise with StatelessElement).
//
// To avoid crashing due to type errors, we need to gently guide the invalid
// element out of the tree. To do so, we ensure that the `hasSameSuperclass` condition
// returns false which prevents us from trying to update the existing element
// incorrectly.
//
// For the case where the widget becomes Stateful, we also need to avoid
// accessing `StatelessElement.widget` as the cast on the getter will
// cause a type error to be thrown. Here we avoid that by short-circuiting
// the `Widget.canUpdate` check once `hasSameSuperclass` is false.
assert(() {
final int oldElementClass = Element._debugConcreteSubtype(child);
final int newWidgetClass = Widget._debugConcreteSubtype(newWidget);
hasSameSuperclass = oldElementClass == newWidgetClass;
return true;
}());
if (hasSameSuperclass && child.widget == newWidget) {
// We don't insert a timeline event here, because otherwise it's
// confusing that widgets that "don't update" (because they didn't
// change) get "charged" on the timeline.
if (child.slot != newSlot) {
updateSlotForChild(child, newSlot);
}
newChild = child;
} else if (hasSameSuperclass && Widget.canUpdate(child.widget, newWidget)) {
if (child.slot != newSlot) {
updateSlotForChild(child, newSlot);
}
final bool isTimelineTracked = !kReleaseMode && _isProfileBuildsEnabledFor(newWidget);
if (isTimelineTracked) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (kDebugMode && debugEnhanceBuildTimelineArguments) {
debugTimelineArguments = newWidget.toDiagnosticsNode().toTimelineArguments();
}
return true;
}());
FlutterTimeline.startSync(
'${newWidget.runtimeType}',
arguments: debugTimelineArguments,
);
}
child.update(newWidget);
if (isTimelineTracked) {
FlutterTimeline.finishSync();
}
assert(child.widget == newWidget);
assert(() {
child.owner!._debugElementWasRebuilt(child);
return true;
}());
newChild = child;
} else {
deactivateChild(child);
assert(child._parent == null);
// The [debugProfileBuildsEnabled] code for this branch is inside
// [inflateWidget], since some [Element]s call [inflateWidget] directly
// instead of going through [updateChild].
newChild = inflateWidget(newWidget, newSlot);
}
} else {
// The [debugProfileBuildsEnabled] code for this branch is inside
// [inflateWidget], since some [Element]s call [inflateWidget] directly
// instead of going through [updateChild].
newChild = inflateWidget(newWidget, newSlot);
}
assert(() {
if (child != null) {
_debugRemoveGlobalKeyReservation(child);
}
final Key? key = newWidget.key;
if (key is GlobalKey) {
assert(owner != null);
owner!._debugReserveGlobalKeyFor(this, newChild, key);
}
return true;
}());
return newChild;
}
/// Updates the children of this element to use new widgets.
///
/// Attempts to update the given old children list using the given new
/// widgets, removing obsolete elements and introducing new ones as necessary,
/// and then returns the new child list.
///
/// During this function the `oldChildren` list must not be modified. If the
/// caller wishes to remove elements from `oldChildren` reentrantly while
/// this function is on the stack, the caller can supply a `forgottenChildren`
/// argument, which can be modified while this function is on the stack.
/// Whenever this function reads from `oldChildren`, this function first
/// checks whether the child is in `forgottenChildren`. If it is, the function
/// acts as if the child was not in `oldChildren`.
///
/// This function is a convenience wrapper around [updateChild], which updates
/// each individual child. If `slots` is non-null, the value for the `newSlot`
/// argument of [updateChild] is retrieved from that list using the index that
/// the currently processed `child` corresponds to in the `newWidgets` list
/// (`newWidgets` and `slots` must have the same length). If `slots` is null,
/// an [IndexedSlot<Element>] is used as the value for the `newSlot` argument.
/// In that case, [IndexedSlot.index] is set to the index that the currently
/// processed `child` corresponds to in the `newWidgets` list and
/// [IndexedSlot.value] is set to the [Element] of the previous widget in that
/// list (or null if it is the first child).
///
/// When the [slot] value of an [Element] changes, its
/// associated [renderObject] needs to move to a new position in the child
/// list of its parents. If that [RenderObject] organizes its children in a
/// linked list (as is done by the [ContainerRenderObjectMixin]) this can
/// be implemented by re-inserting the child [RenderObject] into the
/// list after the [RenderObject] associated with the [Element] provided as
/// [IndexedSlot.value] in the [slot] object.
///
/// Using the previous sibling as a [slot] is not enough, though, because
/// child [RenderObject]s are only moved around when the [slot] of their
/// associated [RenderObjectElement]s is updated. When the order of child
/// [Element]s is changed, some elements in the list may move to a new index
/// but still have the same previous sibling. For example, when
/// `[e1, e2, e3, e4]` is changed to `[e1, e3, e4, e2]` the element e4
/// continues to have e3 as a previous sibling even though its index in the list
/// has changed and its [RenderObject] needs to move to come before e2's
/// [RenderObject]. In order to trigger this move, a new [slot] value needs to
/// be assigned to its [Element] whenever its index in its
/// parent's child list changes. Using an [IndexedSlot<Element>] achieves
/// exactly that and also ensures that the underlying parent [RenderObject]
/// knows where a child needs to move to in a linked list by providing its new
/// previous sibling.
@protected
List<Element> updateChildren(List<Element> oldChildren, List<Widget> newWidgets, { Set<Element>? forgottenChildren, List<Object?>? slots }) {
assert(slots == null || newWidgets.length == slots.length);
Element? replaceWithNullIfForgotten(Element child) {
return forgottenChildren != null && forgottenChildren.contains(child) ? null : child;
}
Object? slotFor(int newChildIndex, Element? previousChild) {
return slots != null
? slots[newChildIndex]
: IndexedSlot<Element?>(newChildIndex, previousChild);
}
// This attempts to diff the new child list (newWidgets) with
// the old child list (oldChildren), and produce a new list of elements to
// be the new list of child elements of this element. The called of this
// method is expected to update this render object accordingly.
// The cases it tries to optimize for are:
// - the old list is empty
// - the lists are identical
// - there is an insertion or removal of one or more widgets in
// only one place in the list
// If a widget with a key is in both lists, it will be synced.
// Widgets without keys might be synced but there is no guarantee.
// The general approach is to sync the entire new list backwards, as follows:
// 1. Walk the lists from the top, syncing nodes, until you no longer have
// matching nodes.
// 2. Walk the lists from the bottom, without syncing nodes, until you no
// longer have matching nodes. We'll sync these nodes at the end. We
// don't sync them now because we want to sync all the nodes in order
// from beginning to end.
// At this point we narrowed the old and new lists to the point
// where the nodes no longer match.
// 3. Walk the narrowed part of the old list to get the list of
// keys and sync null with non-keyed items.
// 4. Walk the narrowed part of the new list forwards:
// * Sync non-keyed items with null
// * Sync keyed items with the source if it exists, else with null.
// 5. Walk the bottom of the list again, syncing the nodes.
// 6. Sync null with any items in the list of keys that are still
// mounted.
int newChildrenTop = 0;
int oldChildrenTop = 0;
int newChildrenBottom = newWidgets.length - 1;
int oldChildrenBottom = oldChildren.length - 1;
final List<Element> newChildren = List<Element>.filled(newWidgets.length, _NullElement.instance);
Element? previousChild;
// Update the top of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final Element? oldChild = replaceWithNullIfForgotten(oldChildren[oldChildrenTop]);
final Widget newWidget = newWidgets[newChildrenTop];
assert(oldChild == null || oldChild._lifecycleState == _ElementLifecycle.active);
if (oldChild == null || !Widget.canUpdate(oldChild.widget, newWidget)) {
break;
}
final Element newChild = updateChild(oldChild, newWidget, slotFor(newChildrenTop, previousChild))!;
assert(newChild._lifecycleState == _ElementLifecycle.active);
newChildren[newChildrenTop] = newChild;
previousChild = newChild;
newChildrenTop += 1;
oldChildrenTop += 1;
}
// Scan the bottom of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final Element? oldChild = replaceWithNullIfForgotten(oldChildren[oldChildrenBottom]);
final Widget newWidget = newWidgets[newChildrenBottom];
assert(oldChild == null || oldChild._lifecycleState == _ElementLifecycle.active);
if (oldChild == null || !Widget.canUpdate(oldChild.widget, newWidget)) {
break;
}
oldChildrenBottom -= 1;
newChildrenBottom -= 1;
}
// Scan the old children in the middle of the list.
final bool haveOldChildren = oldChildrenTop <= oldChildrenBottom;
Map<Key, Element>? oldKeyedChildren;
if (haveOldChildren) {
oldKeyedChildren = <Key, Element>{};
while (oldChildrenTop <= oldChildrenBottom) {
final Element? oldChild = replaceWithNullIfForgotten(oldChildren[oldChildrenTop]);
assert(oldChild == null || oldChild._lifecycleState == _ElementLifecycle.active);
if (oldChild != null) {
if (oldChild.widget.key != null) {
oldKeyedChildren[oldChild.widget.key!] = oldChild;
} else {
deactivateChild(oldChild);
}
}
oldChildrenTop += 1;
}
}
// Update the middle of the list.
while (newChildrenTop <= newChildrenBottom) {
Element? oldChild;
final Widget newWidget = newWidgets[newChildrenTop];
if (haveOldChildren) {
final Key? key = newWidget.key;
if (key != null) {
oldChild = oldKeyedChildren![key];
if (oldChild != null) {
if (Widget.canUpdate(oldChild.widget, newWidget)) {
// we found a match!
// remove it from oldKeyedChildren so we don't unsync it later
oldKeyedChildren.remove(key);
} else {
// Not a match, let's pretend we didn't see it for now.
oldChild = null;
}
}
}
}
assert(oldChild == null || Widget.canUpdate(oldChild.widget, newWidget));
final Element newChild = updateChild(oldChild, newWidget, slotFor(newChildrenTop, previousChild))!;
assert(newChild._lifecycleState == _ElementLifecycle.active);
assert(oldChild == newChild || oldChild == null || oldChild._lifecycleState != _ElementLifecycle.active);
newChildren[newChildrenTop] = newChild;
previousChild = newChild;
newChildrenTop += 1;
}
// We've scanned the whole list.
assert(oldChildrenTop == oldChildrenBottom + 1);
assert(newChildrenTop == newChildrenBottom + 1);
assert(newWidgets.length - newChildrenTop == oldChildren.length - oldChildrenTop);
newChildrenBottom = newWidgets.length - 1;
oldChildrenBottom = oldChildren.length - 1;
// Update the bottom of the list.
while ((oldChildrenTop <= oldChildrenBottom) && (newChildrenTop <= newChildrenBottom)) {
final Element oldChild = oldChildren[oldChildrenTop];
assert(replaceWithNullIfForgotten(oldChild) != null);
assert(oldChild._lifecycleState == _ElementLifecycle.active);
final Widget newWidget = newWidgets[newChildrenTop];
assert(Widget.canUpdate(oldChild.widget, newWidget));
final Element newChild = updateChild(oldChild, newWidget, slotFor(newChildrenTop, previousChild))!;
assert(newChild._lifecycleState == _ElementLifecycle.active);
assert(oldChild == newChild || oldChild._lifecycleState != _ElementLifecycle.active);
newChildren[newChildrenTop] = newChild;
previousChild = newChild;
newChildrenTop += 1;
oldChildrenTop += 1;
}
// Clean up any of the remaining middle nodes from the old list.
if (haveOldChildren && oldKeyedChildren!.isNotEmpty) {
for (final Element oldChild in oldKeyedChildren.values) {
if (forgottenChildren == null || !forgottenChildren.contains(oldChild)) {
deactivateChild(oldChild);
}
}
}
assert(newChildren.every((Element element) => element is! _NullElement));
return newChildren;
}
/// Add this element to the tree in the given slot of the given parent.
///
/// The framework calls this function when a newly created element is added to
/// the tree for the first time. Use this method to initialize state that
/// depends on having a parent. State that is independent of the parent can
/// more easily be initialized in the constructor.
///
/// This method transitions the element from the "initial" lifecycle state to
/// the "active" lifecycle state.
///
/// Subclasses that override this method are likely to want to also override
/// [update], [visitChildren], [RenderObjectElement.insertRenderObjectChild],
/// [RenderObjectElement.moveRenderObjectChild], and
/// [RenderObjectElement.removeRenderObjectChild].
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.mount(parent, newSlot)`.
@mustCallSuper
void mount(Element? parent, Object? newSlot) {
assert(_lifecycleState == _ElementLifecycle.initial);
assert(_parent == null);
assert(parent == null || parent._lifecycleState == _ElementLifecycle.active);
assert(slot == null);
_parent = parent;
_slot = newSlot;
_lifecycleState = _ElementLifecycle.active;
_depth = _parent != null ? _parent!.depth + 1 : 1;
if (parent != null) {
// Only assign ownership if the parent is non-null. If parent is null
// (the root node), the owner should have already been assigned.
// See RootRenderObjectElement.assignOwner().
_owner = parent.owner;
}
assert(owner != null);
final Key? key = widget.key;
if (key is GlobalKey) {
owner!._registerGlobalKey(key, this);
}
_updateInheritance();
attachNotificationTree();
}
void _debugRemoveGlobalKeyReservation(Element child) {
assert(owner != null);
owner!._debugRemoveGlobalKeyReservationFor(this, child);
}
/// Change the widget used to configure this element.
///
/// The framework calls this function when the parent wishes to use a
/// different widget to configure this element. The new widget is guaranteed
/// to have the same [runtimeType] as the old widget.
///
/// This function is called only during the "active" lifecycle state.
@mustCallSuper
void update(covariant Widget newWidget) {
// This code is hot when hot reloading, so we try to
// only call _AssertionError._evaluateAssertion once.
assert(
_lifecycleState == _ElementLifecycle.active
&& newWidget != widget
&& Widget.canUpdate(widget, newWidget),
);
// This Element was told to update and we can now release all the global key
// reservations of forgotten children. We cannot do this earlier because the
// forgotten children still represent global key duplications if the element
// never updates (the forgotten children are not removed from the tree
// until the call to update happens)
assert(() {
_debugForgottenChildrenWithGlobalKey?.forEach(_debugRemoveGlobalKeyReservation);
_debugForgottenChildrenWithGlobalKey?.clear();
return true;
}());
_widget = newWidget;
}
/// Change the slot that the given child occupies in its parent.
///
/// Called by [MultiChildRenderObjectElement], and other [RenderObjectElement]
/// subclasses that have multiple children, when child moves from one position
/// to another in this element's child list.
@protected
void updateSlotForChild(Element child, Object? newSlot) {
assert(_lifecycleState == _ElementLifecycle.active);
assert(child._parent == this);
void visit(Element element) {
element.updateSlot(newSlot);
final Element? descendant = element.renderObjectAttachingChild;
if (descendant != null) {
visit(descendant);
}
}
visit(child);
}
/// Called by [updateSlotForChild] when the framework needs to change the slot
/// that this [Element] occupies in its ancestor.
@protected
@mustCallSuper
void updateSlot(Object? newSlot) {
assert(_lifecycleState == _ElementLifecycle.active);
assert(_parent != null);
assert(_parent!._lifecycleState == _ElementLifecycle.active);
_slot = newSlot;
}
void _updateDepth(int parentDepth) {
final int expectedDepth = parentDepth + 1;
if (_depth < expectedDepth) {
_depth = expectedDepth;
visitChildren((Element child) {
child._updateDepth(expectedDepth);
});
}
}
/// Remove [renderObject] from the render tree.
///
/// The default implementation of this function calls
/// [detachRenderObject] recursively on each child. The
/// [RenderObjectElement.detachRenderObject] override does the actual work of
/// removing [renderObject] from the render tree.
///
/// This is called by [deactivateChild].
void detachRenderObject() {
visitChildren((Element child) {
child.detachRenderObject();
});
_slot = null;
}
/// Add [renderObject] to the render tree at the location specified by `newSlot`.
///
/// The default implementation of this function calls
/// [attachRenderObject] recursively on each child. The
/// [RenderObjectElement.attachRenderObject] override does the actual work of
/// adding [renderObject] to the render tree.
///
/// The `newSlot` argument specifies the new value for this element's [slot].
void attachRenderObject(Object? newSlot) {
assert(slot == null);
visitChildren((Element child) {
child.attachRenderObject(newSlot);
});
_slot = newSlot;
}
Element? _retakeInactiveElement(GlobalKey key, Widget newWidget) {
// The "inactivity" of the element being retaken here may be forward-looking: if
// we are taking an element with a GlobalKey from an element that currently has
// it as a child, then we know that element will soon no longer have that
// element as a child. The only way that assumption could be false is if the
// global key is being duplicated, and we'll try to track that using the
// _debugTrackElementThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans call below.
final Element? element = key._currentElement;
if (element == null) {
return null;
}
if (!Widget.canUpdate(element.widget, newWidget)) {
return null;
}
assert(() {
if (debugPrintGlobalKeyedWidgetLifecycle) {
debugPrint('Attempting to take $element from ${element._parent ?? "inactive elements list"} to put in $this.');
}
return true;
}());
final Element? parent = element._parent;
if (parent != null) {
assert(() {
if (parent == this) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary("A GlobalKey was used multiple times inside one widget's child list."),
DiagnosticsProperty<GlobalKey>('The offending GlobalKey was', key),
parent.describeElement('The parent of the widgets with that key was'),
element.describeElement('The first child to get instantiated with that key became'),
DiagnosticsProperty<Widget>('The second child that was to be instantiated with that key was', widget, style: DiagnosticsTreeStyle.errorProperty),
ErrorDescription('A GlobalKey can only be specified on one widget at a time in the widget tree.'),
]);
}
parent.owner!._debugTrackElementThatWillNeedToBeRebuiltDueToGlobalKeyShenanigans(
parent,
key,
);
return true;
}());
parent.forgetChild(element);
parent.deactivateChild(element);
}
assert(element._parent == null);
owner!._inactiveElements.remove(element);
return element;
}
/// Create an element for the given widget and add it as a child of this
/// element in the given slot.
///
/// This method is typically called by [updateChild] but can be called
/// directly by subclasses that need finer-grained control over creating
/// elements.
///
/// If the given widget has a global key and an element already exists that
/// has a widget with that global key, this function will reuse that element
/// (potentially grafting it from another location in the tree or reactivating
/// it from the list of inactive elements) rather than creating a new element.
///
/// The `newSlot` argument specifies the new value for this element's [slot].
///
/// The element returned by this function will already have been mounted and
/// will be in the "active" lifecycle state.
@protected
@pragma('dart2js:tryInline')
@pragma('vm:prefer-inline')
@pragma('wasm:prefer-inline')
Element inflateWidget(Widget newWidget, Object? newSlot) {
final bool isTimelineTracked = !kReleaseMode && _isProfileBuildsEnabledFor(newWidget);
if (isTimelineTracked) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (kDebugMode && debugEnhanceBuildTimelineArguments) {
debugTimelineArguments = newWidget.toDiagnosticsNode().toTimelineArguments();
}
return true;
}());
FlutterTimeline.startSync(
'${newWidget.runtimeType}',
arguments: debugTimelineArguments,
);
}
try {
final Key? key = newWidget.key;
if (key is GlobalKey) {
final Element? newChild = _retakeInactiveElement(key, newWidget);
if (newChild != null) {
assert(newChild._parent == null);
assert(() {
_debugCheckForCycles(newChild);
return true;
}());
try {
newChild._activateWithParent(this, newSlot);
} catch (_) {
// Attempt to do some clean-up if activation fails to leave tree in a reasonable state.
try {
deactivateChild(newChild);
} catch (_) {
// Clean-up failed. Only surface original exception.
}
rethrow;
}
final Element? updatedChild = updateChild(newChild, newWidget, newSlot);
assert(newChild == updatedChild);
return updatedChild!;
}
}
final Element newChild = newWidget.createElement();
assert(() {
_debugCheckForCycles(newChild);
return true;
}());
newChild.mount(this, newSlot);
assert(newChild._lifecycleState == _ElementLifecycle.active);
return newChild;
} finally {
if (isTimelineTracked) {
FlutterTimeline.finishSync();
}
}
}
void _debugCheckForCycles(Element newChild) {
assert(newChild._parent == null);
assert(() {
Element node = this;
while (node._parent != null) {
node = node._parent!;
}
assert(node != newChild); // indicates we are about to create a cycle
return true;
}());
}
/// Move the given element to the list of inactive elements and detach its
/// render object from the render tree.
///
/// This method stops the given element from being a child of this element by
/// detaching its render object from the render tree and moving the element to
/// the list of inactive elements.
///
/// This method (indirectly) calls [deactivate] on the child.
///
/// The caller is responsible for removing the child from its child model.
/// Typically [deactivateChild] is called by the element itself while it is
/// updating its child model; however, during [GlobalKey] reparenting, the new
/// parent proactively calls the old parent's [deactivateChild], first using
/// [forgetChild] to cause the old parent to update its child model.
@protected
void deactivateChild(Element child) {
assert(child._parent == this);
child._parent = null;
child.detachRenderObject();
owner!._inactiveElements.add(child); // this eventually calls child.deactivate()
assert(() {
if (debugPrintGlobalKeyedWidgetLifecycle) {
if (child.widget.key is GlobalKey) {
debugPrint('Deactivated $child (keyed child of $this)');
}
}
return true;
}());
}
// The children that have been forgotten by forgetChild. This will be used in
// [update] to remove the global key reservations of forgotten children.
//
// In Profile/Release mode this field is initialized to `null`. The Dart compiler can
// eliminate unused fields, but not their initializers.
@_debugOnly
final Set<Element>? _debugForgottenChildrenWithGlobalKey = kDebugMode ? HashSet<Element>() : null;
/// Remove the given child from the element's child list, in preparation for
/// the child being reused elsewhere in the element tree.
///
/// This updates the child model such that, e.g., [visitChildren] does not
/// walk that child anymore.
///
/// The element will still have a valid parent when this is called, and the
/// child's [Element.slot] value will be valid in the context of that parent.
/// After this is called, [deactivateChild] is called to sever the link to
/// this object.
///
/// The [update] is responsible for updating or creating the new child that
/// will replace this [child].
@protected
@mustCallSuper
void forgetChild(Element child) {
// This method is called on the old parent when the given child (with a
// global key) is given a new parent. We cannot remove the global key
// reservation directly in this method because the forgotten child is not
// removed from the tree until this Element is updated in [update]. If
// [update] is never called, the forgotten child still represents a global
// key duplication that we need to catch.
assert(() {
if (child.widget.key is GlobalKey) {
_debugForgottenChildrenWithGlobalKey?.add(child);
}
return true;
}());
}
void _activateWithParent(Element parent, Object? newSlot) {
assert(_lifecycleState == _ElementLifecycle.inactive);
_parent = parent;
assert(() {
if (debugPrintGlobalKeyedWidgetLifecycle) {
debugPrint('Reactivating $this (now child of $_parent).');
}
return true;
}());
_updateDepth(_parent!.depth);
_activateRecursively(this);
attachRenderObject(newSlot);
assert(_lifecycleState == _ElementLifecycle.active);
}
static void _activateRecursively(Element element) {
assert(element._lifecycleState == _ElementLifecycle.inactive);
element.activate();
assert(element._lifecycleState == _ElementLifecycle.active);
element.visitChildren(_activateRecursively);
}
/// Transition from the "inactive" to the "active" lifecycle state.
///
/// The framework calls this method when a previously deactivated element has
/// been reincorporated into the tree. The framework does not call this method
/// the first time an element becomes active (i.e., from the "initial"
/// lifecycle state). Instead, the framework calls [mount] in that situation.
///
/// See the lifecycle documentation for [Element] for additional information.
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.activate()`.
@mustCallSuper
void activate() {
assert(_lifecycleState == _ElementLifecycle.inactive);
assert(owner != null);
final bool hadDependencies = (_dependencies != null && _dependencies!.isNotEmpty) || _hadUnsatisfiedDependencies;
_lifecycleState = _ElementLifecycle.active;
// We unregistered our dependencies in deactivate, but never cleared the list.
// Since we're going to be reused, let's clear our list now.
_dependencies?.clear();
_hadUnsatisfiedDependencies = false;
_updateInheritance();
attachNotificationTree();
if (_dirty) {
owner!.scheduleBuildFor(this);
}
if (hadDependencies) {
didChangeDependencies();
}
}
/// Transition from the "active" to the "inactive" lifecycle state.
///
/// The framework calls this method when a previously active element is moved
/// to the list of inactive elements. While in the inactive state, the element
/// will not appear on screen. The element can remain in the inactive state
/// only until the end of the current animation frame. At the end of the
/// animation frame, if the element has not be reactivated, the framework will
/// unmount the element.
///
/// This is (indirectly) called by [deactivateChild].
///
/// See the lifecycle documentation for [Element] for additional information.
///
/// Implementations of this method should end with a call to the inherited
/// method, as in `super.deactivate()`.
@mustCallSuper
void deactivate() {
assert(_lifecycleState == _ElementLifecycle.active);
assert(_widget != null); // Use the private property to avoid a CastError during hot reload.
if (_dependencies != null && _dependencies!.isNotEmpty) {
for (final InheritedElement dependency in _dependencies!) {
dependency.removeDependent(this);
}
// For expediency, we don't actually clear the list here, even though it's
// no longer representative of what we are registered with. If we never
// get re-used, it doesn't matter. If we do, then we'll clear the list in
// activate(). The benefit of this is that it allows Element's activate()
// implementation to decide whether to rebuild based on whether we had
// dependencies here.
}
_inheritedElements = null;
_lifecycleState = _ElementLifecycle.inactive;
}
/// Called, in debug mode, after children have been deactivated (see [deactivate]).
///
/// This method is not called in release builds.
@mustCallSuper
void debugDeactivated() {
assert(_lifecycleState == _ElementLifecycle.inactive);
}
/// Transition from the "inactive" to the "defunct" lifecycle state.
///
/// Called when the framework determines that an inactive element will never
/// be reactivated. At the end of each animation frame, the framework calls
/// [unmount] on any remaining inactive elements, preventing inactive elements
/// from remaining inactive for longer than a single animation frame.
///
/// After this function is called, the element will not be incorporated into
/// the tree again.
///
/// Any resources this element holds should be released at this point. For
/// example, [RenderObjectElement.unmount] calls [RenderObject.dispose] and
/// nulls out its reference to the render object.
///
/// See the lifecycle documentation for [Element] for additional information.
///
/// Implementations of this method should end with a call to the inherited
/// method, as in `super.unmount()`.
@mustCallSuper
void unmount() {
assert(_lifecycleState == _ElementLifecycle.inactive);
assert(_widget != null); // Use the private property to avoid a CastError during hot reload.
assert(owner != null);
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
// Use the private property to avoid a CastError during hot reload.
final Key? key = _widget?.key;
if (key is GlobalKey) {
owner!._unregisterGlobalKey(key, this);
}
// Release resources to reduce the severity of memory leaks caused by
// defunct, but accidentally retained Elements.
_widget = null;
_dependencies = null;
_lifecycleState = _ElementLifecycle.defunct;
}
/// Whether the child in the provided `slot` (or one of its descendants) must
/// insert a [RenderObject] into its ancestor [RenderObjectElement] by calling
/// [RenderObjectElement.insertRenderObjectChild] on it.
///
/// This method is used to define non-rendering zones in the element tree (see
/// [WidgetsBinding] for an explanation of rendering and non-rendering zones):
///
/// Most branches of the [Element] tree are expected to eventually insert a
/// [RenderObject] into their [RenderObjectElement] ancestor to construct the
/// render tree. However, there is a notable exception: an [Element] may
/// expect that the occupant of a certain child slot creates a new independent
/// render tree and therefore is not allowed to insert a render object into
/// the existing render tree. Those elements must return false from this
/// method for the slot in question to signal to the child in that slot that
/// it must not call [RenderObjectElement.insertRenderObjectChild] on its
/// ancestor.
///
/// As an example, the element backing the [ViewAnchor] returns false from
/// this method for the [ViewAnchor.view] slot to enforce that it is occupied
/// by e.g. a [View] widget, which will ultimately bootstrap a separate
/// render tree for that view. Another example is the [ViewCollection] widget,
/// which returns false for all its slots for the same reason.
///
/// Overriding this method is not common, as elements behaving in the way
/// described above are rare.
bool debugExpectsRenderObjectForSlot(Object? slot) => true;
@override
RenderObject? findRenderObject() {
assert(() {
if (_lifecycleState != _ElementLifecycle.active) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get renderObject of inactive element.'),
ErrorDescription(
'In order for an element to have a valid renderObject, it must be '
'active, which means it is part of the tree.\n'
'Instead, this element is in the $_lifecycleState state.\n'
'If you called this method from a State object, consider guarding '
'it with State.mounted.',
),
describeElement('The findRenderObject() method was called for the following element'),
]);
}
return true;
}());
return renderObject;
}
@override
Size? get size {
assert(() {
if (_lifecycleState != _ElementLifecycle.active) {
// TODO(jacobr): is this a good separation into contract and violation?
// I have added a line of white space.
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size of inactive element.'),
ErrorDescription(
'In order for an element to have a valid size, the element must be '
'active, which means it is part of the tree.\n'
'Instead, this element is in the $_lifecycleState state.',
),
describeElement('The size getter was called for the following element'),
]);
}
if (owner!._debugBuilding) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size during build.'),
ErrorDescription(
'The size of this render object has not yet been determined because '
'the framework is still in the process of building widgets, which '
'means the render tree for this frame has not yet been determined. '
'The size getter should only be called from paint callbacks or '
'interaction event handlers (e.g. gesture callbacks).',
),
ErrorSpacer(),
ErrorHint(
'If you need some sizing information during build to decide which '
'widgets to build, consider using a LayoutBuilder widget, which can '
'tell you the layout constraints at a given location in the tree. See '
'<https://api.flutter.dev/flutter/widgets/LayoutBuilder-class.html> '
'for more details.',
),
ErrorSpacer(),
describeElement('The size getter was called for the following element'),
]);
}
return true;
}());
final RenderObject? renderObject = findRenderObject();
assert(() {
if (renderObject == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size without a render object.'),
ErrorHint(
'In order for an element to have a valid size, the element must have '
'an associated render object. This element does not have an associated '
'render object, which typically means that the size getter was called '
'too early in the pipeline (e.g., during the build phase) before the '
'framework has created the render tree.',
),
describeElement('The size getter was called for the following element'),
]);
}
if (renderObject is RenderSliver) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size from a RenderSliver.'),
ErrorHint(
'The render object associated with this element is a '
'${renderObject.runtimeType}, which is a subtype of RenderSliver. '
'Slivers do not have a size per se. They have a more elaborate '
'geometry description, which can be accessed by calling '
'findRenderObject and then using the "geometry" getter on the '
'resulting object.',
),
describeElement('The size getter was called for the following element'),
renderObject.describeForError('The associated render sliver was'),
]);
}
if (renderObject is! RenderBox) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size from a render object that is not a RenderBox.'),
ErrorHint(
'Instead of being a subtype of RenderBox, the render object associated '
'with this element is a ${renderObject.runtimeType}. If this type of '
'render object does have a size, consider calling findRenderObject '
'and extracting its size manually.',
),
describeElement('The size getter was called for the following element'),
renderObject.describeForError('The associated render object was'),
]);
}
final RenderBox box = renderObject;
if (!box.hasSize) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size from a render object that has not been through layout.'),
ErrorHint(
'The size of this render object has not yet been determined because '
'this render object has not yet been through layout, which typically '
'means that the size getter was called too early in the pipeline '
'(e.g., during the build phase) before the framework has determined '
'the size and position of the render objects during layout.',
),
describeElement('The size getter was called for the following element'),
box.describeForError('The render object from which the size was to be obtained was'),
]);
}
if (box.debugNeedsLayout) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot get size from a render object that has been marked dirty for layout.'),
ErrorHint(
'The size of this render object is ambiguous because this render object has '
'been modified since it was last laid out, which typically means that the size '
'getter was called too early in the pipeline (e.g., during the build phase) '
'before the framework has determined the size and position of the render '
'objects during layout.',
),
describeElement('The size getter was called for the following element'),
box.describeForError('The render object from which the size was to be obtained was'),
ErrorHint(
'Consider using debugPrintMarkNeedsLayoutStacks to determine why the render '
'object in question is dirty, if you did not expect this.',
),
]);
}
return true;
}());
if (renderObject is RenderBox) {
return renderObject.size;
}
return null;
}
PersistentHashMap<Type, InheritedElement>? _inheritedElements;
Set<InheritedElement>? _dependencies;
bool _hadUnsatisfiedDependencies = false;
bool _debugCheckStateIsActiveForAncestorLookup() {
assert(() {
if (_lifecycleState != _ElementLifecycle.active) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary("Looking up a deactivated widget's ancestor is unsafe."),
ErrorDescription(
"At this point the state of the widget's element tree is no longer "
'stable.',
),
ErrorHint(
"To safely refer to a widget's ancestor in its dispose() method, "
'save a reference to the ancestor by calling dependOnInheritedWidgetOfExactType() '
"in the widget's didChangeDependencies() method.",
),
]);
}
return true;
}());
return true;
}
/// Returns `true` if [dependOnInheritedElement] was previously called with [ancestor].
@protected
bool doesDependOnInheritedElement(InheritedElement ancestor) =>
_dependencies != null && _dependencies!.contains(ancestor);
@override
InheritedWidget dependOnInheritedElement(InheritedElement ancestor, { Object? aspect }) {
_dependencies ??= HashSet<InheritedElement>();
_dependencies!.add(ancestor);
ancestor.updateDependencies(this, aspect);
return ancestor.widget as InheritedWidget;
}
@override
T? dependOnInheritedWidgetOfExactType<T extends InheritedWidget>({Object? aspect}) {
assert(_debugCheckStateIsActiveForAncestorLookup());
final InheritedElement? ancestor = _inheritedElements == null ? null : _inheritedElements![T];
if (ancestor != null) {
return dependOnInheritedElement(ancestor, aspect: aspect) as T;
}
_hadUnsatisfiedDependencies = true;
return null;
}
@override
T? getInheritedWidgetOfExactType<T extends InheritedWidget>() {
return getElementForInheritedWidgetOfExactType<T>()?.widget as T?;
}
@override
InheritedElement? getElementForInheritedWidgetOfExactType<T extends InheritedWidget>() {
assert(_debugCheckStateIsActiveForAncestorLookup());
final InheritedElement? ancestor = _inheritedElements == null ? null : _inheritedElements![T];
return ancestor;
}
/// Called in [Element.mount] and [Element.activate] to register this element in
/// the notification tree.
///
/// This method is only exposed so that [NotifiableElementMixin] can be implemented.
/// Subclasses of [Element] that wish to respond to notifications should mix that
/// in instead.
///
/// See also:
/// * [NotificationListener], a widget that allows listening to notifications.
@protected
void attachNotificationTree() {
_notificationTree = _parent?._notificationTree;
}
void _updateInheritance() {
assert(_lifecycleState == _ElementLifecycle.active);
_inheritedElements = _parent?._inheritedElements;
}
@override
T? findAncestorWidgetOfExactType<T extends Widget>() {
assert(_debugCheckStateIsActiveForAncestorLookup());
Element? ancestor = _parent;
while (ancestor != null && ancestor.widget.runtimeType != T) {
ancestor = ancestor._parent;
}
return ancestor?.widget as T?;
}
@override
T? findAncestorStateOfType<T extends State<StatefulWidget>>() {
assert(_debugCheckStateIsActiveForAncestorLookup());
Element? ancestor = _parent;
while (ancestor != null) {
if (ancestor is StatefulElement && ancestor.state is T) {
break;
}
ancestor = ancestor._parent;
}
final StatefulElement? statefulAncestor = ancestor as StatefulElement?;
return statefulAncestor?.state as T?;
}
@override
T? findRootAncestorStateOfType<T extends State<StatefulWidget>>() {
assert(_debugCheckStateIsActiveForAncestorLookup());
Element? ancestor = _parent;
StatefulElement? statefulAncestor;
while (ancestor != null) {
if (ancestor is StatefulElement && ancestor.state is T) {
statefulAncestor = ancestor;
}
ancestor = ancestor._parent;
}
return statefulAncestor?.state as T?;
}
@override
T? findAncestorRenderObjectOfType<T extends RenderObject>() {
assert(_debugCheckStateIsActiveForAncestorLookup());
Element? ancestor = _parent;
while (ancestor != null) {
if (ancestor is RenderObjectElement && ancestor.renderObject is T) {
return ancestor.renderObject as T;
}
ancestor = ancestor._parent;
}
return null;
}
@override
void visitAncestorElements(ConditionalElementVisitor visitor) {
assert(_debugCheckStateIsActiveForAncestorLookup());
Element? ancestor = _parent;
while (ancestor != null && visitor(ancestor)) {
ancestor = ancestor._parent;
}
}
/// Called when a dependency of this element changes.
///
/// The [dependOnInheritedWidgetOfExactType] registers this element as depending on
/// inherited information of the given type. When the information of that type
/// changes at this location in the tree (e.g., because the [InheritedElement]
/// updated to a new [InheritedWidget] and
/// [InheritedWidget.updateShouldNotify] returned true), the framework calls
/// this function to notify this element of the change.
@mustCallSuper
void didChangeDependencies() {
assert(_lifecycleState == _ElementLifecycle.active); // otherwise markNeedsBuild is a no-op
assert(_debugCheckOwnerBuildTargetExists('didChangeDependencies'));
markNeedsBuild();
}
bool _debugCheckOwnerBuildTargetExists(String methodName) {
assert(() {
if (owner!._debugCurrentBuildTarget == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'$methodName for ${widget.runtimeType} was called at an '
'inappropriate time.',
),
ErrorDescription('It may only be called while the widgets are being built.'),
ErrorHint(
'A possible cause of this error is when $methodName is called during '
'one of:\n'
' * network I/O event\n'
' * file I/O event\n'
' * timer\n'
' * microtask (caused by Future.then, async/await, scheduleMicrotask)',
),
]);
}
return true;
}());
return true;
}
/// Returns a description of what caused this element to be created.
///
/// Useful for debugging the source of an element.
String debugGetCreatorChain(int limit) {
final List<String> chain = <String>[];
Element? node = this;
while (chain.length < limit && node != null) {
chain.add(node.toStringShort());
node = node._parent;
}
if (node != null) {
chain.add('\u22EF');
}
return chain.join(' \u2190 ');
}
/// Returns the parent chain from this element back to the root of the tree.
///
/// Useful for debug display of a tree of Elements with only nodes in the path
/// from the root to this Element expanded.
List<Element> debugGetDiagnosticChain() {
final List<Element> chain = <Element>[this];
Element? node = _parent;
while (node != null) {
chain.add(node);
node = node._parent;
}
return chain;
}
@override
void dispatchNotification(Notification notification) {
_notificationTree?.dispatchNotification(notification);
}
/// A short, textual description of this element.
@override
String toStringShort() => _widget?.toStringShort() ?? '${describeIdentity(this)}(DEFUNCT)';
@override
DiagnosticsNode toDiagnosticsNode({ String? name, DiagnosticsTreeStyle? style }) {
return _ElementDiagnosticableTreeNode(
name: name,
value: this,
style: style,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.defaultDiagnosticsTreeStyle= DiagnosticsTreeStyle.dense;
if (_lifecycleState != _ElementLifecycle.initial) {
properties.add(ObjectFlagProperty<int>('depth', depth, ifNull: 'no depth'));
}
properties.add(ObjectFlagProperty<Widget>('widget', _widget, ifNull: 'no widget'));
properties.add(DiagnosticsProperty<Key>('key', _widget?.key, showName: false, defaultValue: null, level: DiagnosticLevel.hidden));
_widget?.debugFillProperties(properties);
properties.add(FlagProperty('dirty', value: dirty, ifTrue: 'dirty'));
final Set<InheritedElement>? deps = _dependencies;
if (deps != null && deps.isNotEmpty) {
final List<InheritedElement> sortedDependencies = deps.toList()
..sort((InheritedElement a, InheritedElement b) =>
a.toStringShort().compareTo(b.toStringShort()));
final List<DiagnosticsNode> diagnosticsDependencies = sortedDependencies
.map((InheritedElement element) => element.widget.toDiagnosticsNode(style: DiagnosticsTreeStyle.sparse))
.toList();
properties.add(DiagnosticsProperty<Set<InheritedElement>>('dependencies', deps, description: diagnosticsDependencies.toString()));
}
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> children = <DiagnosticsNode>[];
visitChildren((Element child) {
children.add(child.toDiagnosticsNode());
});
return children;
}
/// Returns true if the element has been marked as needing rebuilding.
///
/// The flag is true when the element is first created and after
/// [markNeedsBuild] has been called. The flag is reset to false in the
/// [performRebuild] implementation.
bool get dirty => _dirty;
bool _dirty = true;
// Whether this is in owner._dirtyElements. This is used to know whether we
// should be adding the element back into the list when it's reactivated.
bool _inDirtyList = false;
// Whether we've already built or not. Set in [rebuild].
bool _debugBuiltOnce = false;
/// Marks the element as dirty and adds it to the global list of widgets to
/// rebuild in the next frame.
///
/// Since it is inefficient to build an element twice in one frame,
/// applications and widgets should be structured so as to only mark
/// widgets dirty during event handlers before the frame begins, not during
/// the build itself.
void markNeedsBuild() {
assert(_lifecycleState != _ElementLifecycle.defunct);
if (_lifecycleState != _ElementLifecycle.active) {
return;
}
assert(owner != null);
assert(_lifecycleState == _ElementLifecycle.active);
assert(() {
if (owner!._debugBuilding) {
assert(owner!._debugCurrentBuildTarget != null);
assert(owner!._debugStateLocked);
if (_debugIsInScope(owner!._debugCurrentBuildTarget!)) {
return true;
}
final List<DiagnosticsNode> information = <DiagnosticsNode>[
ErrorSummary('setState() or markNeedsBuild() called during build.'),
ErrorDescription(
'This ${widget.runtimeType} widget cannot be marked as needing to build because the framework '
'is already in the process of building widgets. A widget can be marked as '
'needing to be built during the build phase only if one of its ancestors '
'is currently building. This exception is allowed because the framework '
'builds parent widgets before children, which means a dirty descendant '
'will always be built. Otherwise, the framework might not visit this '
'widget during this build phase.',
),
describeElement('The widget on which setState() or markNeedsBuild() was called was'),
];
if (owner!._debugCurrentBuildTarget != null) {
information.add(owner!._debugCurrentBuildTarget!.describeWidget('The widget which was currently being built when the offending call was made was'));
}
throw FlutterError.fromParts(information);
} else if (owner!._debugStateLocked) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('setState() or markNeedsBuild() called when widget tree was locked.'),
ErrorDescription(
'This ${widget.runtimeType} widget cannot be marked as needing to build '
'because the framework is locked.',
),
describeElement('The widget on which setState() or markNeedsBuild() was called was'),
]);
}
return true;
}());
if (dirty) {
return;
}
_dirty = true;
owner!.scheduleBuildFor(this);
}
/// Cause the widget to update itself. In debug builds, also verify various
/// invariants.
///
/// Called by the [BuildOwner] when [BuildOwner.scheduleBuildFor] has been
/// called to mark this element dirty, by [mount] when the element is first
/// built, and by [update] when the widget has changed.
///
/// The method will only rebuild if [dirty] is true. To rebuild regardless
/// of the [dirty] flag, set `force` to true. Forcing a rebuild is convenient
/// from [update], during which [dirty] is false.
///
/// ## When rebuilds happen
///
/// ### Terminology
///
/// [Widget]s represent the configuration of [Element]s. Each [Element] has a
/// widget, specified in [Element.widget]. The term "widget" is often used
/// when strictly speaking "element" would be more correct.
///
/// While an [Element] has a current [Widget], over time, that widget may be
/// replaced by others. For example, the element backing a [ColoredBox] may
/// first have as its widget a [ColoredBox] whose [ColoredBox.color] is blue,
/// then later be given a new [ColoredBox] whose color is green.
///
/// At any particular time, multiple [Element]s in the same tree may have the
/// same [Widget]. For example, the same [ColoredBox] with the green color may
/// be used in multiple places in the widget tree at the same time, each being
/// backed by a different [Element].
///
/// ### Marking an element dirty
///
/// An [Element] can be marked dirty between frames. This can happen for various
/// reasons, including the following:
///
/// * The [State] of a [StatefulWidget] can cause its [Element] to be marked
/// dirty by calling the [State.setState] method.
///
/// * When an [InheritedWidget] changes, descendants that have previously
/// subscribed to it will be marked dirty.
///
/// * During a hot reload, every element is marked dirty (using [Element.reassemble]).
///
/// ### Rebuilding
///
/// Dirty elements are rebuilt during the next frame. Precisely how this is
/// done depends on the kind of element. A [StatelessElement] rebuilds by
/// using its widget's [StatelessWidget.build] method. A [StatefulElement]
/// rebuilds by using its widget's state's [State.build] method. A
/// [RenderObjectElement] rebuilds by updating its [RenderObject].
///
/// In many cases, the end result of rebuilding is a single child widget
/// or (for [MultiChildRenderObjectElement]s) a list of children widgets.
///
/// These child widgets are used to update the [widget] property of the
/// element's child (or children) elements. The new [Widget] is considered to
/// correspond to an existing [Element] if it has the same [Type] and [Key].
/// (In the case of [MultiChildRenderObjectElement]s, some effort is put into
/// tracking widgets even when they change order; see
/// [RenderObjectElement.updateChildren].)
///
/// If there was no corresponding previous child, this results in a new
/// [Element] being created (using [Widget.createElement]); that element is
/// then itself built, recursively.
///
/// If there was a child previously but the build did not provide a
/// corresponding child to update it, then the old child is discarded (or, in
/// cases involving [GlobalKey] reparenting, reused elsewhere in the element
/// tree).
///
/// The most common case, however, is that there was a corresponding previous
/// child. This is handled by asking the child [Element] to update itself
/// using the new child [Widget]. In the case of [StatefulElement]s, this
/// is what triggers [State.didUpdateWidget].
///
/// ### Not rebuilding
///
/// Before an [Element] is told to update itself with a new [Widget], the old
/// and new objects are compared using `operator ==`.
///
/// In general, this is equivalent to doing a comparison using [identical] to
/// see if the two objects are in fact the exact same instance. If they are,
/// and if the element is not already marked dirty for other reasons, then the
/// element skips updating itself as it can determine with certainty that
/// there would be no value in updating itself or its descendants.
///
/// It is strongly advised to avoid overriding `operator ==` on [Widget]
/// objects. While doing so seems like it could improve performance, in
/// practice, for non-leaf widgets, it results in O(N²) behavior. This is
/// because by necessity the comparison would have to include comparing child
/// widgets, and if those child widgets also implement `operator ==`, it
/// ultimately results in a complete walk of the widget tree... which is then
/// repeated at each level of the tree. In practice, just rebuilding is
/// cheaper. (Additionally, if _any_ subclass of [Widget] used in an
/// application implements `operator ==`, then the compiler cannot inline the
/// comparison anywhere, because it has to treat the call as virtual just in
/// case the instance happens to be one that has an overridden operator.)
///
/// Instead, the best way to avoid unnecessary rebuilds is to cache the
/// widgets that are returned from [State.build], so that each frame the same
/// widgets are used until such time as they change. Several mechanisms exist
/// to encourage this: `const` widgets, for example, are a form of automatic
/// caching (if a widget is constructed using the `const` keyword, the same
/// instance is returned each time it is constructed with the same arguments).
///
/// Another example is the [AnimatedBuilder.child] property, which allows the
/// non-animating parts of a subtree to remain static even as the
/// [AnimatedBuilder.builder] callback recreates the other components.
@pragma('dart2js:tryInline')
@pragma('vm:prefer-inline')
@pragma('wasm:prefer-inline')
void rebuild({bool force = false}) {
assert(_lifecycleState != _ElementLifecycle.initial);
if (_lifecycleState != _ElementLifecycle.active || (!_dirty && !force)) {
return;
}
assert(() {
debugOnRebuildDirtyWidget?.call(this, _debugBuiltOnce);
if (debugPrintRebuildDirtyWidgets) {
if (!_debugBuiltOnce) {
debugPrint('Building $this');
_debugBuiltOnce = true;
} else {
debugPrint('Rebuilding $this');
}
}
return true;
}());
assert(_lifecycleState == _ElementLifecycle.active);
assert(owner!._debugStateLocked);
Element? debugPreviousBuildTarget;
assert(() {
debugPreviousBuildTarget = owner!._debugCurrentBuildTarget;
owner!._debugCurrentBuildTarget = this;
return true;
}());
try {
performRebuild();
} finally {
assert(() {
owner!._debugElementWasRebuilt(this);
assert(owner!._debugCurrentBuildTarget == this);
owner!._debugCurrentBuildTarget = debugPreviousBuildTarget;
return true;
}());
}
assert(!_dirty);
}
/// Cause the widget to update itself.
///
/// Called by [rebuild] after the appropriate checks have been made.
///
/// The base implementation only clears the [dirty] flag.
@protected
@mustCallSuper
void performRebuild() {
_dirty = false;
}
}
class _ElementDiagnosticableTreeNode extends DiagnosticableTreeNode {
_ElementDiagnosticableTreeNode({
super.name,
required Element super.value,
required super.style,
this.stateful = false,
});
final bool stateful;
@override
Map<String, Object?> toJsonMap(DiagnosticsSerializationDelegate delegate) {
final Map<String, Object?> json = super.toJsonMap(delegate);
final Element element = value as Element;
if (!element.debugIsDefunct) {
json['widgetRuntimeType'] = element.widget.runtimeType.toString();
}
json['stateful'] = stateful;
return json;
}
}
/// Signature for the constructor that is called when an error occurs while
/// building a widget.
///
/// The argument provides information regarding the cause of the error.
///
/// See also:
///
/// * [ErrorWidget.builder], which can be set to override the default
/// [ErrorWidget] builder.
/// * [FlutterError.reportError], which is typically called with the same
/// [FlutterErrorDetails] object immediately prior to [ErrorWidget.builder]
/// being called.
typedef ErrorWidgetBuilder = Widget Function(FlutterErrorDetails details);
/// A widget that renders an exception's message.
///
/// This widget is used when a build method fails, to help with determining
/// where the problem lies. Exceptions are also logged to the console, which you
/// can read using `flutter logs`. The console will also include additional
/// information such as the stack trace for the exception.
///
/// It is possible to override this widget.
///
/// {@tool dartpad}
/// This example shows how to override the standard error widget builder in release
/// mode, but use the standard one in debug mode.
///
/// The error occurs when you click the "Error Prone" button.
///
/// ** See code in examples/api/lib/widgets/framework/error_widget.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [FlutterError.onError], which can be set to a method that exits the
/// application if that is preferable to showing an error message.
/// * <https://flutter.dev/docs/testing/errors>, more information about error
/// handling in Flutter.
class ErrorWidget extends LeafRenderObjectWidget {
/// Creates a widget that displays the given exception.
///
/// The message will be the stringification of the given exception, unless
/// computing that value itself throws an exception, in which case it will
/// be the string "Error".
///
/// If this object is inspected from an IDE or the devtools, and the original
/// exception is a [FlutterError] object, the original exception itself will
/// be shown in the inspection output.
ErrorWidget(Object exception)
: message = _stringify(exception),
_flutterError = exception is FlutterError ? exception : null,
super(key: UniqueKey());
/// Creates a widget that displays the given error message.
///
/// An explicit [FlutterError] can be provided to be reported to inspection
/// tools. It need not match the message.
ErrorWidget.withDetails({ this.message = '', FlutterError? error })
: _flutterError = error,
super(key: UniqueKey());
/// The configurable factory for [ErrorWidget].
///
/// When an error occurs while building a widget, the broken widget is
/// replaced by the widget returned by this function. By default, an
/// [ErrorWidget] is returned.
///
/// The system is typically in an unstable state when this function is called.
/// An exception has just been thrown in the middle of build (and possibly
/// layout), so surrounding widgets and render objects may be in a rather
/// fragile state. The framework itself (especially the [BuildOwner]) may also
/// be confused, and additional exceptions are quite likely to be thrown.
///
/// Because of this, it is highly recommended that the widget returned from
/// this function perform the least amount of work possible. A
/// [LeafRenderObjectWidget] is the best choice, especially one that
/// corresponds to a [RenderBox] that can handle the most absurd of incoming
/// constraints. The default constructor maps to a [RenderErrorBox].
///
/// The default behavior is to show the exception's message in debug mode,
/// and to show nothing but a gray background in release builds.
///
/// See also:
///
/// * [FlutterError.onError], which is typically called with the same
/// [FlutterErrorDetails] object immediately prior to this callback being
/// invoked, and which can also be configured to control how errors are
/// reported.
/// * <https://flutter.dev/docs/testing/errors>, more information about error
/// handling in Flutter.
static ErrorWidgetBuilder builder = _defaultErrorWidgetBuilder;
static Widget _defaultErrorWidgetBuilder(FlutterErrorDetails details) {
String message = '';
assert(() {
message = '${_stringify(details.exception)}\nSee also: https://flutter.dev/docs/testing/errors';
return true;
}());
final Object exception = details.exception;
return ErrorWidget.withDetails(message: message, error: exception is FlutterError ? exception : null);
}
static String _stringify(Object? exception) {
try {
return exception.toString();
} catch (error) {
// If we get here, it means things have really gone off the rails, and we're better
// off just returning a simple string and letting the developer find out what the
// root cause of all their problems are by looking at the console logs.
}
return 'Error';
}
/// The message to display.
final String message;
final FlutterError? _flutterError;
@override
RenderBox createRenderObject(BuildContext context) => RenderErrorBox(message);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
if (_flutterError == null) {
properties.add(StringProperty('message', message, quoted: false));
} else {
properties.add(_flutterError.toDiagnosticsNode(style: DiagnosticsTreeStyle.whitespace));
}
}
}
/// Signature for a function that creates a widget, e.g. [StatelessWidget.build]
/// or [State.build].
///
/// Used by [Builder.builder], [OverlayEntry.builder], etc.
///
/// See also:
///
/// * [IndexedWidgetBuilder], which is similar but also takes an index.
/// * [TransitionBuilder], which is similar but also takes a child.
/// * [ValueWidgetBuilder], which is similar but takes a value and a child.
typedef WidgetBuilder = Widget Function(BuildContext context);
/// Signature for a function that creates a widget for a given index, e.g., in a
/// list.
///
/// Used by [ListView.builder] and other APIs that use lazily-generated widgets.
///
/// See also:
///
/// * [WidgetBuilder], which is similar but only takes a [BuildContext].
/// * [TransitionBuilder], which is similar but also takes a child.
/// * [NullableIndexedWidgetBuilder], which is similar but may return null.
typedef IndexedWidgetBuilder = Widget Function(BuildContext context, int index);
/// Signature for a function that creates a widget for a given index, e.g., in a
/// list, but may return null.
///
/// Used by [SliverChildBuilderDelegate.builder] and other APIs that
/// use lazily-generated widgets where the child count is not known
/// ahead of time.
///
/// Unlike most builders, this callback can return null, indicating the index
/// is out of range. Whether and when this is valid depends on the semantics
/// of the builder. For example, [SliverChildBuilderDelegate.builder] returns
/// null when the index is out of range, where the range is defined by the
/// [SliverChildBuilderDelegate.childCount]; so in that case the `index`
/// parameter's value may determine whether returning null is valid or not.
///
/// See also:
///
/// * [WidgetBuilder], which is similar but only takes a [BuildContext].
/// * [TransitionBuilder], which is similar but also takes a child.
/// * [IndexedWidgetBuilder], which is similar but not nullable.
typedef NullableIndexedWidgetBuilder = Widget? Function(BuildContext context, int index);
/// A builder that builds a widget given a child.
///
/// The child should typically be part of the returned widget tree.
///
/// Used by [AnimatedBuilder.builder], [ListenableBuilder.builder],
/// [WidgetsApp.builder], and [MaterialApp.builder].
///
/// See also:
///
/// * [WidgetBuilder], which is similar but only takes a [BuildContext].
/// * [IndexedWidgetBuilder], which is similar but also takes an index.
/// * [ValueWidgetBuilder], which is similar but takes a value and a child.
typedef TransitionBuilder = Widget Function(BuildContext context, Widget? child);
/// An [Element] that composes other [Element]s.
///
/// Rather than creating a [RenderObject] directly, a [ComponentElement] creates
/// [RenderObject]s indirectly by creating other [Element]s.
///
/// Contrast with [RenderObjectElement].
abstract class ComponentElement extends Element {
/// Creates an element that uses the given widget as its configuration.
ComponentElement(super.widget);
Element? _child;
bool _debugDoingBuild = false;
@override
bool get debugDoingBuild => _debugDoingBuild;
@override
Element? get renderObjectAttachingChild => _child;
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
assert(_child == null);
assert(_lifecycleState == _ElementLifecycle.active);
_firstBuild();
assert(_child != null);
}
void _firstBuild() {
// StatefulElement overrides this to also call state.didChangeDependencies.
rebuild(); // This eventually calls performRebuild.
}
/// Calls the [StatelessWidget.build] method of the [StatelessWidget] object
/// (for stateless widgets) or the [State.build] method of the [State] object
/// (for stateful widgets) and then updates the widget tree.
///
/// Called automatically during [mount] to generate the first build, and by
/// [rebuild] when the element needs updating.
@override
@pragma('vm:notify-debugger-on-exception')
void performRebuild() {
Widget? built;
try {
assert(() {
_debugDoingBuild = true;
return true;
}());
built = build();
assert(() {
_debugDoingBuild = false;
return true;
}());
debugWidgetBuilderValue(widget, built);
} catch (e, stack) {
_debugDoingBuild = false;
built = ErrorWidget.builder(
_reportException(
ErrorDescription('building $this'),
e,
stack,
informationCollector: () => <DiagnosticsNode>[
if (kDebugMode)
DiagnosticsDebugCreator(DebugCreator(this)),
],
),
);
} finally {
// We delay marking the element as clean until after calling build() so
// that attempts to markNeedsBuild() during build() will be ignored.
super.performRebuild(); // clears the "dirty" flag
}
try {
_child = updateChild(_child, built, slot);
assert(_child != null);
} catch (e, stack) {
built = ErrorWidget.builder(
_reportException(
ErrorDescription('building $this'),
e,
stack,
informationCollector: () => <DiagnosticsNode>[
if (kDebugMode)
DiagnosticsDebugCreator(DebugCreator(this)),
],
),
);
_child = updateChild(null, built, slot);
}
}
/// Subclasses should override this function to actually call the appropriate
/// `build` function (e.g., [StatelessWidget.build] or [State.build]) for
/// their widget.
@protected
Widget build();
@override
void visitChildren(ElementVisitor visitor) {
if (_child != null) {
visitor(_child!);
}
}
@override
void forgetChild(Element child) {
assert(child == _child);
_child = null;
super.forgetChild(child);
}
}
/// An [Element] that uses a [StatelessWidget] as its configuration.
class StatelessElement extends ComponentElement {
/// Creates an element that uses the given widget as its configuration.
StatelessElement(StatelessWidget super.widget);
@override
Widget build() => (widget as StatelessWidget).build(this);
@override
void update(StatelessWidget newWidget) {
super.update(newWidget);
assert(widget == newWidget);
rebuild(force: true);
}
}
/// An [Element] that uses a [StatefulWidget] as its configuration.
class StatefulElement extends ComponentElement {
/// Creates an element that uses the given widget as its configuration.
StatefulElement(StatefulWidget widget)
: _state = widget.createState(),
super(widget) {
assert(() {
if (!state._debugTypesAreRight(widget)) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('StatefulWidget.createState must return a subtype of State<${widget.runtimeType}>'),
ErrorDescription(
'The createState function for ${widget.runtimeType} returned a state '
'of type ${state.runtimeType}, which is not a subtype of '
'State<${widget.runtimeType}>, violating the contract for createState.',
),
]);
}
return true;
}());
assert(state._element == null);
state._element = this;
assert(
state._widget == null,
'The createState function for $widget returned an old or invalid state '
'instance: ${state._widget}, which is not null, violating the contract '
'for createState.',
);
state._widget = widget;
assert(state._debugLifecycleState == _StateLifecycle.created);
}
@override
Widget build() => state.build(this);
/// The [State] instance associated with this location in the tree.
///
/// There is a one-to-one relationship between [State] objects and the
/// [StatefulElement] objects that hold them. The [State] objects are created
/// by [StatefulElement] in [mount].
State<StatefulWidget> get state => _state!;
State<StatefulWidget>? _state;
@override
void reassemble() {
state.reassemble();
super.reassemble();
}
@override
void _firstBuild() {
assert(state._debugLifecycleState == _StateLifecycle.created);
final Object? debugCheckForReturnedFuture = state.initState() as dynamic;
assert(() {
if (debugCheckForReturnedFuture is Future) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('${state.runtimeType}.initState() returned a Future.'),
ErrorDescription('State.initState() must be a void method without an `async` keyword.'),
ErrorHint(
'Rather than awaiting on asynchronous work directly inside of initState, '
'call a separate method to do this work without awaiting it.',
),
]);
}
return true;
}());
assert(() {
state._debugLifecycleState = _StateLifecycle.initialized;
return true;
}());
state.didChangeDependencies();
assert(() {
state._debugLifecycleState = _StateLifecycle.ready;
return true;
}());
super._firstBuild();
}
@override
void performRebuild() {
if (_didChangeDependencies) {
state.didChangeDependencies();
_didChangeDependencies = false;
}
super.performRebuild();
}
@override
void update(StatefulWidget newWidget) {
super.update(newWidget);
assert(widget == newWidget);
final StatefulWidget oldWidget = state._widget!;
state._widget = widget as StatefulWidget;
final Object? debugCheckForReturnedFuture = state.didUpdateWidget(oldWidget) as dynamic;
assert(() {
if (debugCheckForReturnedFuture is Future) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('${state.runtimeType}.didUpdateWidget() returned a Future.'),
ErrorDescription( 'State.didUpdateWidget() must be a void method without an `async` keyword.'),
ErrorHint(
'Rather than awaiting on asynchronous work directly inside of didUpdateWidget, '
'call a separate method to do this work without awaiting it.',
),
]);
}
return true;
}());
rebuild(force: true);
}
@override
void activate() {
super.activate();
state.activate();
// Since the State could have observed the deactivate() and thus disposed of
// resources allocated in the build method, we have to rebuild the widget
// so that its State can reallocate its resources.
assert(_lifecycleState == _ElementLifecycle.active); // otherwise markNeedsBuild is a no-op
markNeedsBuild();
}
@override
void deactivate() {
state.deactivate();
super.deactivate();
}
@override
void unmount() {
super.unmount();
state.dispose();
assert(() {
if (state._debugLifecycleState == _StateLifecycle.defunct) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('${state.runtimeType}.dispose failed to call super.dispose.'),
ErrorDescription(
'dispose() implementations must always call their superclass dispose() method, to ensure '
'that all the resources used by the widget are fully released.',
),
]);
}());
state._element = null;
// Release resources to reduce the severity of memory leaks caused by
// defunct, but accidentally retained Elements.
_state = null;
}
@override
InheritedWidget dependOnInheritedElement(Element ancestor, { Object? aspect }) {
assert(() {
final Type targetType = ancestor.widget.runtimeType;
if (state._debugLifecycleState == _StateLifecycle.created) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('dependOnInheritedWidgetOfExactType<$targetType>() or dependOnInheritedElement() was called before ${state.runtimeType}.initState() completed.'),
ErrorDescription(
'When an inherited widget changes, for example if the value of Theme.of() changes, '
"its dependent widgets are rebuilt. If the dependent widget's reference to "
'the inherited widget is in a constructor or an initState() method, '
'then the rebuilt dependent widget will not reflect the changes in the '
'inherited widget.',
),
ErrorHint(
'Typically references to inherited widgets should occur in widget build() methods. Alternatively, '
'initialization based on inherited widgets can be placed in the didChangeDependencies method, which '
'is called after initState and whenever the dependencies change thereafter.',
),
]);
}
if (state._debugLifecycleState == _StateLifecycle.defunct) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('dependOnInheritedWidgetOfExactType<$targetType>() or dependOnInheritedElement() was called after dispose(): $this'),
ErrorDescription(
'This error happens if you call dependOnInheritedWidgetOfExactType() on the '
'BuildContext for a widget that no longer appears in the widget tree '
'(e.g., whose parent widget no longer includes the widget in its '
'build). This error can occur when code calls '
'dependOnInheritedWidgetOfExactType() from a timer or an animation callback.',
),
ErrorHint(
'The preferred solution is to cancel the timer or stop listening to the '
'animation in the dispose() callback. Another solution is to check the '
'"mounted" property of this object before calling '
'dependOnInheritedWidgetOfExactType() to ensure the object is still in the '
'tree.',
),
ErrorHint(
'This error might indicate a memory leak if '
'dependOnInheritedWidgetOfExactType() is being called because another object '
'is retaining a reference to this State object after it has been '
'removed from the tree. To avoid memory leaks, consider breaking the '
'reference to this object during dispose().',
),
]);
}
return true;
}());
return super.dependOnInheritedElement(ancestor as InheritedElement, aspect: aspect);
}
/// This controls whether we should call [State.didChangeDependencies] from
/// the start of [build], to avoid calls when the [State] will not get built.
/// This can happen when the widget has dropped out of the tree, but depends
/// on an [InheritedWidget] that is still in the tree.
///
/// It is set initially to false, since [_firstBuild] makes the initial call
/// on the [state]. When it is true, [build] will call
/// `state.didChangeDependencies` and then sets it to false. Subsequent calls
/// to [didChangeDependencies] set it to true.
bool _didChangeDependencies = false;
@override
void didChangeDependencies() {
super.didChangeDependencies();
_didChangeDependencies = true;
}
@override
DiagnosticsNode toDiagnosticsNode({ String? name, DiagnosticsTreeStyle? style }) {
return _ElementDiagnosticableTreeNode(
name: name,
value: this,
style: style,
stateful: true,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<State<StatefulWidget>>('state', _state, defaultValue: null));
}
}
/// An [Element] that uses a [ProxyWidget] as its configuration.
abstract class ProxyElement extends ComponentElement {
/// Initializes fields for subclasses.
ProxyElement(ProxyWidget super.widget);
@override
Widget build() => (widget as ProxyWidget).child;
@override
void update(ProxyWidget newWidget) {
final ProxyWidget oldWidget = widget as ProxyWidget;
assert(widget != newWidget);
super.update(newWidget);
assert(widget == newWidget);
updated(oldWidget);
rebuild(force: true);
}
/// Called during build when the [widget] has changed.
///
/// By default, calls [notifyClients]. Subclasses may override this method to
/// avoid calling [notifyClients] unnecessarily (e.g. if the old and new
/// widgets are equivalent).
@protected
void updated(covariant ProxyWidget oldWidget) {
notifyClients(oldWidget);
}
/// Notify other objects that the widget associated with this element has
/// changed.
///
/// Called during [update] (via [updated]) after changing the widget
/// associated with this element but before rebuilding this element.
@protected
void notifyClients(covariant ProxyWidget oldWidget);
}
/// An [Element] that uses a [ParentDataWidget] as its configuration.
class ParentDataElement<T extends ParentData> extends ProxyElement {
/// Creates an element that uses the given widget as its configuration.
ParentDataElement(ParentDataWidget<T> super.widget);
/// Returns the [Type] of [ParentData] that this element has been configured
/// for.
///
/// This is only available in debug mode. It will throw in profile and
/// release modes.
Type get debugParentDataType {
Type? type;
assert(() {
type = T;
return true;
}());
if (type != null) {
return type!;
}
throw UnsupportedError('debugParentDataType is only supported in debug builds');
}
void _applyParentData(ParentDataWidget<T> widget) {
void applyParentDataToChild(Element child) {
if (child is RenderObjectElement) {
child._updateParentData(widget);
} else if (child.renderObjectAttachingChild != null) {
applyParentDataToChild(child.renderObjectAttachingChild!);
}
}
if (renderObjectAttachingChild != null) {
applyParentDataToChild(renderObjectAttachingChild!);
}
}
/// Calls [ParentDataWidget.applyParentData] on the given widget, passing it
/// the [RenderObject] whose parent data this element is ultimately
/// responsible for.
///
/// This allows a render object's [RenderObject.parentData] to be modified
/// without triggering a build. This is generally ill-advised, but makes sense
/// in situations such as the following:
///
/// * Build and layout are currently under way, but the [ParentData] in question
/// does not affect layout, and the value to be applied could not be
/// determined before build and layout (e.g. it depends on the layout of a
/// descendant).
///
/// * Paint is currently under way, but the [ParentData] in question does not
/// affect layout or paint, and the value to be applied could not be
/// determined before paint (e.g. it depends on the compositing phase).
///
/// In either case, the next build is expected to cause this element to be
/// configured with the given new widget (or a widget with equivalent data).
///
/// Only [ParentDataWidget]s that return true for
/// [ParentDataWidget.debugCanApplyOutOfTurn] can be applied this way.
///
/// The new widget must have the same child as the current widget.
///
/// An example of when this is used is the [AutomaticKeepAlive] widget. If it
/// receives a notification during the build of one of its descendants saying
/// that its child must be kept alive, it will apply a [KeepAlive] widget out
/// of turn. This is safe, because by definition the child is already alive,
/// and therefore this will not change the behavior of the parent this frame.
/// It is more efficient than requesting an additional frame just for the
/// purpose of updating the [KeepAlive] widget.
void applyWidgetOutOfTurn(ParentDataWidget<T> newWidget) {
assert(newWidget.debugCanApplyOutOfTurn());
assert(newWidget.child == (widget as ParentDataWidget<T>).child);
_applyParentData(newWidget);
}
@override
void notifyClients(ParentDataWidget<T> oldWidget) {
_applyParentData(widget as ParentDataWidget<T>);
}
}
/// An [Element] that uses an [InheritedWidget] as its configuration.
class InheritedElement extends ProxyElement {
/// Creates an element that uses the given widget as its configuration.
InheritedElement(InheritedWidget super.widget);
final Map<Element, Object?> _dependents = HashMap<Element, Object?>();
@override
void _updateInheritance() {
assert(_lifecycleState == _ElementLifecycle.active);
final PersistentHashMap<Type, InheritedElement> incomingWidgets =
_parent?._inheritedElements ?? const PersistentHashMap<Type, InheritedElement>.empty();
_inheritedElements = incomingWidgets.put(widget.runtimeType, this);
}
@override
void debugDeactivated() {
assert(() {
assert(_dependents.isEmpty);
return true;
}());
super.debugDeactivated();
}
/// Returns the dependencies value recorded for [dependent]
/// with [setDependencies].
///
/// Each dependent element is mapped to a single object value
/// which represents how the element depends on this
/// [InheritedElement]. This value is null by default and by default
/// dependent elements are rebuilt unconditionally.
///
/// Subclasses can manage these values with [updateDependencies]
/// so that they can selectively rebuild dependents in
/// [notifyDependent].
///
/// This method is typically only called in overrides of [updateDependencies].
///
/// See also:
///
/// * [updateDependencies], which is called each time a dependency is
/// created with [dependOnInheritedWidgetOfExactType].
/// * [setDependencies], which sets dependencies value for a dependent
/// element.
/// * [notifyDependent], which can be overridden to use a dependent's
/// dependencies value to decide if the dependent needs to be rebuilt.
/// * [InheritedModel], which is an example of a class that uses this method
/// to manage dependency values.
@protected
Object? getDependencies(Element dependent) {
return _dependents[dependent];
}
/// Sets the value returned by [getDependencies] value for [dependent].
///
/// Each dependent element is mapped to a single object value
/// which represents how the element depends on this
/// [InheritedElement]. The [updateDependencies] method sets this value to
/// null by default so that dependent elements are rebuilt unconditionally.
///
/// Subclasses can manage these values with [updateDependencies]
/// so that they can selectively rebuild dependents in [notifyDependent].
///
/// This method is typically only called in overrides of [updateDependencies].
///
/// See also:
///
/// * [updateDependencies], which is called each time a dependency is
/// created with [dependOnInheritedWidgetOfExactType].
/// * [getDependencies], which returns the current value for a dependent
/// element.
/// * [notifyDependent], which can be overridden to use a dependent's
/// [getDependencies] value to decide if the dependent needs to be rebuilt.
/// * [InheritedModel], which is an example of a class that uses this method
/// to manage dependency values.
@protected
void setDependencies(Element dependent, Object? value) {
_dependents[dependent] = value;
}
/// Called by [dependOnInheritedWidgetOfExactType] when a new [dependent] is added.
///
/// Each dependent element can be mapped to a single object value with
/// [setDependencies]. This method can lookup the existing dependencies with
/// [getDependencies].
///
/// By default this method sets the inherited dependencies for [dependent]
/// to null. This only serves to record an unconditional dependency on
/// [dependent].
///
/// Subclasses can manage their own dependencies values so that they
/// can selectively rebuild dependents in [notifyDependent].
///
/// See also:
///
/// * [getDependencies], which returns the current value for a dependent
/// element.
/// * [setDependencies], which sets the value for a dependent element.
/// * [notifyDependent], which can be overridden to use a dependent's
/// dependencies value to decide if the dependent needs to be rebuilt.
/// * [InheritedModel], which is an example of a class that uses this method
/// to manage dependency values.
@protected
void updateDependencies(Element dependent, Object? aspect) {
setDependencies(dependent, null);
}
/// Called by [notifyClients] for each dependent.
///
/// Calls `dependent.didChangeDependencies()` by default.
///
/// Subclasses can override this method to selectively call
/// [didChangeDependencies] based on the value of [getDependencies].
///
/// See also:
///
/// * [updateDependencies], which is called each time a dependency is
/// created with [dependOnInheritedWidgetOfExactType].
/// * [getDependencies], which returns the current value for a dependent
/// element.
/// * [setDependencies], which sets the value for a dependent element.
/// * [InheritedModel], which is an example of a class that uses this method
/// to manage dependency values.
@protected
void notifyDependent(covariant InheritedWidget oldWidget, Element dependent) {
dependent.didChangeDependencies();
}
/// Called by [Element.deactivate] to remove the provided `dependent` [Element] from this [InheritedElement].
///
/// After the dependent is removed, [Element.didChangeDependencies] will no
/// longer be called on it when this [InheritedElement] notifies its dependents.
///
/// Subclasses can override this method to release any resources retained for
/// a given [dependent].
@protected
@mustCallSuper
void removeDependent(Element dependent) {
_dependents.remove(dependent);
}
/// Calls [Element.didChangeDependencies] of all dependent elements, if
/// [InheritedWidget.updateShouldNotify] returns true.
///
/// Called by [update], immediately prior to [build].
///
/// Calls [notifyClients] to actually trigger the notifications.
@override
void updated(InheritedWidget oldWidget) {
if ((widget as InheritedWidget).updateShouldNotify(oldWidget)) {
super.updated(oldWidget);
}
}
/// Notifies all dependent elements that this inherited widget has changed, by
/// calling [Element.didChangeDependencies].
///
/// This method must only be called during the build phase. Usually this
/// method is called automatically when an inherited widget is rebuilt, e.g.
/// as a result of calling [State.setState] above the inherited widget.
///
/// See also:
///
/// * [InheritedNotifier], a subclass of [InheritedWidget] that also calls
/// this method when its [Listenable] sends a notification.
@override
void notifyClients(InheritedWidget oldWidget) {
assert(_debugCheckOwnerBuildTargetExists('notifyClients'));
for (final Element dependent in _dependents.keys) {
assert(() {
// check that it really is our descendant
Element? ancestor = dependent._parent;
while (ancestor != this && ancestor != null) {
ancestor = ancestor._parent;
}
return ancestor == this;
}());
// check that it really depends on us
assert(dependent._dependencies!.contains(this));
notifyDependent(oldWidget, dependent);
}
}
}
/// An [Element] that uses a [RenderObjectWidget] as its configuration.
///
/// [RenderObjectElement] objects have an associated [RenderObject] widget in
/// the render tree, which handles concrete operations like laying out,
/// painting, and hit testing.
///
/// Contrast with [ComponentElement].
///
/// For details on the lifecycle of an element, see the discussion at [Element].
///
/// ## Writing a RenderObjectElement subclass
///
/// There are three common child models used by most [RenderObject]s:
///
/// * Leaf render objects, with no children: The [LeafRenderObjectElement] class
/// handles this case.
///
/// * A single child: The [SingleChildRenderObjectElement] class handles this
/// case.
///
/// * A linked list of children: The [MultiChildRenderObjectElement] class
/// handles this case.
///
/// Sometimes, however, a render object's child model is more complicated. Maybe
/// it has a two-dimensional array of children. Maybe it constructs children on
/// demand. Maybe it features multiple lists. In such situations, the
/// corresponding [Element] for the [Widget] that configures that [RenderObject]
/// will be a new subclass of [RenderObjectElement].
///
/// Such a subclass is responsible for managing children, specifically the
/// [Element] children of this object, and the [RenderObject] children of its
/// corresponding [RenderObject].
///
/// ### Specializing the getters
///
/// [RenderObjectElement] objects spend much of their time acting as
/// intermediaries between their [widget] and their [renderObject]. It is
/// generally recommended against specializing the [widget] getter and
/// instead casting at the various call sites to avoid adding overhead
/// outside of this particular implementation.
///
/// ```dart
/// class FooElement extends RenderObjectElement {
/// FooElement(super.widget);
///
/// // Specializing the renderObject getter is fine because
/// // it is not performance sensitive.
/// @override
/// RenderFoo get renderObject => super.renderObject as RenderFoo;
///
/// void _foo() {
/// // For the widget getter, though, we prefer to cast locally
/// // since that results in better overall performance where the
/// // casting isn't needed:
/// final Foo foo = widget as Foo;
/// // ...
/// }
///
/// // ...
/// }
/// ```
///
/// ### Slots
///
/// Each child [Element] corresponds to a [RenderObject] which should be
/// attached to this element's render object as a child.
///
/// However, the immediate children of the element may not be the ones that
/// eventually produce the actual [RenderObject] that they correspond to. For
/// example, a [StatelessElement] (the element of a [StatelessWidget])
/// corresponds to whatever [RenderObject] its child (the element returned by
/// its [StatelessWidget.build] method) corresponds to.
///
/// Each child is therefore assigned a _[slot]_ token. This is an identifier whose
/// meaning is private to this [RenderObjectElement] node. When the descendant
/// that finally produces the [RenderObject] is ready to attach it to this
/// node's render object, it passes that slot token back to this node, and that
/// allows this node to cheaply identify where to put the child render object
/// relative to the others in the parent render object.
///
/// A child's [slot] is determined when the parent calls [updateChild] to
/// inflate the child (see the next section). It can be updated by calling
/// [updateSlotForChild].
///
/// ### Updating children
///
/// Early in the lifecycle of an element, the framework calls the [mount]
/// method. This method should call [updateChild] for each child, passing in
/// the widget for that child, and the slot for that child, thus obtaining a
/// list of child [Element]s.
///
/// Subsequently, the framework will call the [update] method. In this method,
/// the [RenderObjectElement] should call [updateChild] for each child, passing
/// in the [Element] that was obtained during [mount] or the last time [update]
/// was run (whichever happened most recently), the new [Widget], and the slot.
/// This provides the object with a new list of [Element] objects.
///
/// Where possible, the [update] method should attempt to map the elements from
/// the last pass to the widgets in the new pass. For example, if one of the
/// elements from the last pass was configured with a particular [Key], and one
/// of the widgets in this new pass has that same key, they should be paired up,
/// and the old element should be updated with the widget (and the slot
/// corresponding to the new widget's new position, also). The [updateChildren]
/// method may be useful in this regard.
///
/// [updateChild] should be called for children in their logical order. The
/// order can matter; for example, if two of the children use [PageStorage]'s
/// `writeState` feature in their build method (and neither has a [Widget.key]),
/// then the state written by the first will be overwritten by the second.
///
/// #### Dynamically determining the children during the build phase
///
/// The child widgets need not necessarily come from this element's widget
/// verbatim. They could be generated dynamically from a callback, or generated
/// in other more creative ways.
///
/// #### Dynamically determining the children during layout
///
/// If the widgets are to be generated at layout time, then generating them in
/// the [mount] and [update] methods won't work: layout of this element's render
/// object hasn't started yet at that point. Instead, the [update] method can
/// mark the render object as needing layout (see
/// [RenderObject.markNeedsLayout]), and then the render object's
/// [RenderObject.performLayout] method can call back to the element to have it
/// generate the widgets and call [updateChild] accordingly.
///
/// For a render object to call an element during layout, it must use
/// [RenderObject.invokeLayoutCallback]. For an element to call [updateChild]
/// outside of its [update] method, it must use [BuildOwner.buildScope].
///
/// The framework provides many more checks in normal operation than it does
/// when doing a build during layout. For this reason, creating widgets with
/// layout-time build semantics should be done with great care.
///
/// #### Handling errors when building
///
/// If an element calls a builder function to obtain widgets for its children,
/// it may find that the build throws an exception. Such exceptions should be
/// caught and reported using [FlutterError.reportError]. If a child is needed
/// but a builder has failed in this way, an instance of [ErrorWidget] can be
/// used instead.
///
/// ### Detaching children
///
/// It is possible, when using [GlobalKey]s, for a child to be proactively
/// removed by another element before this element has been updated.
/// (Specifically, this happens when the subtree rooted at a widget with a
/// particular [GlobalKey] is being moved from this element to an element
/// processed earlier in the build phase.) When this happens, this element's
/// [forgetChild] method will be called with a reference to the affected child
/// element.
///
/// The [forgetChild] method of a [RenderObjectElement] subclass must remove the
/// child element from its child list, so that when it next [update]s its
/// children, the removed child is not considered.
///
/// For performance reasons, if there are many elements, it may be quicker to
/// track which elements were forgotten by storing them in a [Set], rather than
/// proactively mutating the local record of the child list and the identities
/// of all the slots. For example, see the implementation of
/// [MultiChildRenderObjectElement].
///
/// ### Maintaining the render object tree
///
/// Once a descendant produces a render object, it will call
/// [insertRenderObjectChild]. If the descendant's slot changes identity, it
/// will call [moveRenderObjectChild]. If a descendant goes away, it will call
/// [removeRenderObjectChild].
///
/// These three methods should update the render tree accordingly, attaching,
/// moving, and detaching the given child render object from this element's own
/// render object respectively.
///
/// ### Walking the children
///
/// If a [RenderObjectElement] object has any children [Element]s, it must
/// expose them in its implementation of the [visitChildren] method. This method
/// is used by many of the framework's internal mechanisms, and so should be
/// fast. It is also used by the test framework and [debugDumpApp].
abstract class RenderObjectElement extends Element {
/// Creates an element that uses the given widget as its configuration.
RenderObjectElement(RenderObjectWidget super.widget);
/// The underlying [RenderObject] for this element.
///
/// If this element has been [unmount]ed, this getter will throw.
@override
RenderObject get renderObject {
assert(_renderObject != null, '$runtimeType unmounted');
return _renderObject!;
}
RenderObject? _renderObject;
@override
Element? get renderObjectAttachingChild => null;
bool _debugDoingBuild = false;
@override
bool get debugDoingBuild => _debugDoingBuild;
RenderObjectElement? _ancestorRenderObjectElement;
RenderObjectElement? _findAncestorRenderObjectElement() {
Element? ancestor = _parent;
while (ancestor != null && ancestor is! RenderObjectElement) {
// In debug mode we check whether the ancestor accepts RenderObjects to
// produce a better error message in attachRenderObject. In release mode,
// we assume only correct trees are built (i.e.
// debugExpectsRenderObjectForSlot always returns true) and don't check
// explicitly.
assert(() {
if (!ancestor!.debugExpectsRenderObjectForSlot(slot)) {
ancestor = null;
}
return true;
}());
ancestor = ancestor?._parent;
}
assert(() {
if (ancestor?.debugExpectsRenderObjectForSlot(slot) == false) {
ancestor = null;
}
return true;
}());
return ancestor as RenderObjectElement?;
}
void _debugCheckCompetingAncestors(
List<ParentDataElement<ParentData>> result,
Set<Type> debugAncestorTypes,
Set<Type> debugParentDataTypes,
List<Type> debugAncestorCulprits,
) {
assert(() {
// Check that no other ParentDataWidgets of the same
// type want to provide parent data.
if (debugAncestorTypes.length != result.length || debugParentDataTypes.length != result.length) {
// This can only occur if the Sets of ancestors and parent data types was
// provided a dupe and did not add it.
assert(debugAncestorTypes.length < result.length || debugParentDataTypes.length < result.length);
try {
// We explicitly throw here (even though we immediately redirect the
// exception elsewhere) so that debuggers will notice it when they
// have "break on exception" enabled.
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Incorrect use of ParentDataWidget.'),
ErrorDescription(
'Competing ParentDataWidgets are providing parent data to the '
'same RenderObject:'
),
for (final ParentDataElement<ParentData> ancestor in result.where((ParentDataElement<ParentData> ancestor) {
return debugAncestorCulprits.contains(ancestor.runtimeType);
}))
ErrorDescription(
'- ${ancestor.widget}, which writes ParentData of type '
'${ancestor.debugParentDataType}, (typically placed directly '
'inside a '
'${(ancestor.widget as ParentDataWidget<ParentData>).debugTypicalAncestorWidgetClass} '
'widget)'
),
ErrorDescription(
'A RenderObject can receive parent data from multiple '
'ParentDataWidgets, but the Type of ParentData must be unique to '
'prevent one overwriting another.'
),
ErrorHint(
'Usually, this indicates that one or more of the offending '
"ParentDataWidgets listed above isn't placed inside a dedicated "
"compatible ancestor widget that it isn't sharing with another "
'ParentDataWidget of the same type.'
),
ErrorHint(
'Otherwise, separating aspects of ParentData to prevent '
'conflicts can be done using mixins, mixing them all in on the '
'full ParentData Object, such as KeepAlive does with '
'KeepAliveParentDataMixin.'
),
ErrorDescription(
'The ownership chain for the RenderObject that received the '
'parent data was:\n ${debugGetCreatorChain(10)}'
),
]);
} on FlutterError catch (error) {
_reportException(
ErrorSummary('while looking for parent data.'),
error,
error.stackTrace,
);
}
}
return true;
}());
}
List<ParentDataElement<ParentData>> _findAncestorParentDataElements() {
Element? ancestor = _parent;
final List<ParentDataElement<ParentData>> result = <ParentDataElement<ParentData>>[];
final Set<Type> debugAncestorTypes = <Type>{};
final Set<Type> debugParentDataTypes = <Type>{};
final List<Type> debugAncestorCulprits = <Type>[];
// More than one ParentDataWidget can contribute ParentData, but there are
// some constraints.
// 1. ParentData can only be written by unique ParentDataWidget types.
// For example, two KeepAlive ParentDataWidgets trying to write to the
// same child is not allowed.
// 2. Each contributing ParentDataWidget must contribute to a unique
// ParentData type, less ParentData be overwritten.
// For example, there cannot be two ParentDataWidgets that both write
// ParentData of type KeepAliveParentDataMixin, if the first check was
// subverted by a subclassing of the KeepAlive ParentDataWidget.
// 3. The ParentData itself must be compatible with all ParentDataWidgets
// writing to it.
// For example, TwoDimensionalViewportParentData uses the
// KeepAliveParentDataMixin, so it could be compatible with both
// KeepAlive, and another ParentDataWidget with ParentData type
// TwoDimensionalViewportParentData or a subclass thereof.
// The first and second cases are verified here. The third is verified in
// debugIsValidRenderObject.
while (ancestor != null && ancestor is! RenderObjectElement) {
if (ancestor is ParentDataElement<ParentData>) {
assert((ParentDataElement<ParentData> ancestor) {
if (!debugAncestorTypes.add(ancestor.runtimeType) || !debugParentDataTypes.add(ancestor.debugParentDataType)) {
debugAncestorCulprits.add(ancestor.runtimeType);
}
return true;
}(ancestor));
result.add(ancestor);
}
ancestor = ancestor._parent;
}
assert(() {
if (result.isEmpty || ancestor == null) {
return true;
}
// Validate points 1 and 2 from above.
_debugCheckCompetingAncestors(
result,
debugAncestorTypes,
debugParentDataTypes,
debugAncestorCulprits,
);
return true;
}());
return result;
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
assert(() {
_debugDoingBuild = true;
return true;
}());
_renderObject = (widget as RenderObjectWidget).createRenderObject(this);
assert(!_renderObject!.debugDisposed!);
assert(() {
_debugDoingBuild = false;
return true;
}());
assert(() {
_debugUpdateRenderObjectOwner();
return true;
}());
assert(slot == newSlot);
attachRenderObject(newSlot);
super.performRebuild(); // clears the "dirty" flag
}
@override
void update(covariant RenderObjectWidget newWidget) {
super.update(newWidget);
assert(widget == newWidget);
assert(() {
_debugUpdateRenderObjectOwner();
return true;
}());
_performRebuild(); // calls widget.updateRenderObject()
}
void _debugUpdateRenderObjectOwner() {
assert(() {
renderObject.debugCreator = DebugCreator(this);
return true;
}());
}
@override
void performRebuild() { // ignore: must_call_super, _performRebuild calls super.
_performRebuild(); // calls widget.updateRenderObject()
}
@pragma('dart2js:tryInline')
@pragma('vm:prefer-inline')
@pragma('wasm:prefer-inline')
void _performRebuild() {
assert(() {
_debugDoingBuild = true;
return true;
}());
(widget as RenderObjectWidget).updateRenderObject(this, renderObject);
assert(() {
_debugDoingBuild = false;
return true;
}());
super.performRebuild(); // clears the "dirty" flag
}
@override
void deactivate() {
super.deactivate();
assert(
!renderObject.attached,
'A RenderObject was still attached when attempting to deactivate its '
'RenderObjectElement: $renderObject',
);
}
@override
void unmount() {
assert(
!renderObject.debugDisposed!,
'A RenderObject was disposed prior to its owning element being unmounted: '
'$renderObject',
);
final RenderObjectWidget oldWidget = widget as RenderObjectWidget;
super.unmount();
assert(
!renderObject.attached,
'A RenderObject was still attached when attempting to unmount its '
'RenderObjectElement: $renderObject',
);
oldWidget.didUnmountRenderObject(renderObject);
_renderObject!.dispose();
_renderObject = null;
}
void _updateParentData(ParentDataWidget<ParentData> parentDataWidget) {
bool applyParentData = true;
assert(() {
try {
if (!parentDataWidget.debugIsValidRenderObject(renderObject)) {
applyParentData = false;
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Incorrect use of ParentDataWidget.'),
...parentDataWidget._debugDescribeIncorrectParentDataType(
parentData: renderObject.parentData,
parentDataCreator: _ancestorRenderObjectElement?.widget as RenderObjectWidget?,
ownershipChain: ErrorDescription(debugGetCreatorChain(10)),
),
]);
}
} on FlutterError catch (e) {
// We catch the exception directly to avoid activating the ErrorWidget,
// while still allowing debuggers to break on exception. Since the tree
// is in a broken state, adding the ErrorWidget would likely cause more
// exceptions, which is not good for the debugging experience.
_reportException(ErrorSummary('while applying parent data.'), e, e.stackTrace);
}
return true;
}());
if (applyParentData) {
parentDataWidget.applyParentData(renderObject);
}
}
@override
void updateSlot(Object? newSlot) {
final Object? oldSlot = slot;
assert(oldSlot != newSlot);
super.updateSlot(newSlot);
assert(slot == newSlot);
assert(_ancestorRenderObjectElement == _findAncestorRenderObjectElement());
_ancestorRenderObjectElement?.moveRenderObjectChild(renderObject, oldSlot, slot);
}
@override
void attachRenderObject(Object? newSlot) {
assert(_ancestorRenderObjectElement == null);
_slot = newSlot;
_ancestorRenderObjectElement = _findAncestorRenderObjectElement();
assert(() {
if (_ancestorRenderObjectElement == null) {
FlutterError.reportError(FlutterErrorDetails(exception: FlutterError.fromParts(
<DiagnosticsNode>[
ErrorSummary(
'The render object for ${toStringShort()} cannot find ancestor render object to attach to.',
),
ErrorDescription(
'The ownership chain for the RenderObject in question was:\n ${debugGetCreatorChain(10)}',
),
ErrorHint(
'Try wrapping your widget in a View widget or any other widget that is backed by '
'a $RenderTreeRootElement to serve as the root of the render tree.',
),
]
)));
}
return true;
}());
_ancestorRenderObjectElement?.insertRenderObjectChild(renderObject, newSlot);
final List<ParentDataElement<ParentData>> parentDataElements = _findAncestorParentDataElements();
for (final ParentDataElement<ParentData> parentDataElement in parentDataElements) {
_updateParentData(parentDataElement.widget as ParentDataWidget<ParentData>);
}
}
@override
void detachRenderObject() {
if (_ancestorRenderObjectElement != null) {
_ancestorRenderObjectElement!.removeRenderObjectChild(renderObject, slot);
_ancestorRenderObjectElement = null;
}
_slot = null;
}
/// Insert the given child into [renderObject] at the given slot.
///
/// {@template flutter.widgets.RenderObjectElement.insertRenderObjectChild}
/// The semantics of `slot` are determined by this element. For example, if
/// this element has a single child, the slot should always be null. If this
/// element has a list of children, the previous sibling element wrapped in an
/// [IndexedSlot] is a convenient value for the slot.
/// {@endtemplate}
@protected
void insertRenderObjectChild(covariant RenderObject child, covariant Object? slot);
/// Move the given child from the given old slot to the given new slot.
///
/// The given child is guaranteed to have [renderObject] as its parent.
///
/// {@macro flutter.widgets.RenderObjectElement.insertRenderObjectChild}
///
/// This method is only ever called if [updateChild] can end up being called
/// with an existing [Element] child and a `slot` that differs from the slot
/// that element was previously given. [MultiChildRenderObjectElement] does this,
/// for example. [SingleChildRenderObjectElement] does not (since the `slot` is
/// always null). An [Element] that has a specific set of slots with each child
/// always having the same slot (and where children in different slots are never
/// compared against each other for the purposes of updating one slot with the
/// element from another slot) would never call this.
@protected
void moveRenderObjectChild(covariant RenderObject child, covariant Object? oldSlot, covariant Object? newSlot);
/// Remove the given child from [renderObject].
///
/// The given child is guaranteed to have been inserted at the given `slot`
/// and have [renderObject] as its parent.
@protected
void removeRenderObjectChild(covariant RenderObject child, covariant Object? slot);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<RenderObject>('renderObject', _renderObject, defaultValue: null));
}
}
/// Deprecated. Unused in the framework and will be removed in a future version
/// of Flutter.
///
/// Classes that extend this class can extend [RenderObjectElement] and mixin
/// [RootElementMixin] instead.
@Deprecated(
'Use RootElementMixin instead. '
'This feature was deprecated after v3.9.0-16.0.pre.'
)
abstract class RootRenderObjectElement extends RenderObjectElement with RootElementMixin {
/// Initializes fields for subclasses.
@Deprecated(
'Use RootElementMixin instead. '
'This feature was deprecated after v3.9.0-16.0.pre.'
)
RootRenderObjectElement(super.widget);
}
/// Mixin for the element at the root of the tree.
///
/// Only root elements may have their owner set explicitly. All other
/// elements inherit their owner from their parent.
mixin RootElementMixin on Element {
/// Set the owner of the element. The owner will be propagated to all the
/// descendants of this element.
///
/// The owner manages the dirty elements list.
///
/// The [WidgetsBinding] introduces the primary owner,
/// [WidgetsBinding.buildOwner], and assigns it to the widget tree in the call
/// to [runApp]. The binding is responsible for driving the build pipeline by
/// calling the build owner's [BuildOwner.buildScope] method. See
/// [WidgetsBinding.drawFrame].
// ignore: use_setters_to_change_properties, (API predates enforcing the lint)
void assignOwner(BuildOwner owner) {
_owner = owner;
}
@override
void mount(Element? parent, Object? newSlot) {
// Root elements should never have parents.
assert(parent == null);
assert(newSlot == null);
super.mount(parent, newSlot);
}
}
/// An [Element] that uses a [LeafRenderObjectWidget] as its configuration.
class LeafRenderObjectElement extends RenderObjectElement {
/// Creates an element that uses the given widget as its configuration.
LeafRenderObjectElement(LeafRenderObjectWidget super.widget);
@override
void forgetChild(Element child) {
assert(false);
super.forgetChild(child);
}
@override
void insertRenderObjectChild(RenderObject child, Object? slot) {
assert(false);
}
@override
void moveRenderObjectChild(RenderObject child, Object? oldSlot, Object? newSlot) {
assert(false);
}
@override
void removeRenderObjectChild(RenderObject child, Object? slot) {
assert(false);
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return widget.debugDescribeChildren();
}
}
/// An [Element] that uses a [SingleChildRenderObjectWidget] as its configuration.
///
/// The child is optional.
///
/// This element subclass can be used for [RenderObjectWidget]s whose
/// [RenderObject]s use the [RenderObjectWithChildMixin] mixin. Such widgets are
/// expected to inherit from [SingleChildRenderObjectWidget].
class SingleChildRenderObjectElement extends RenderObjectElement {
/// Creates an element that uses the given widget as its configuration.
SingleChildRenderObjectElement(SingleChildRenderObjectWidget super.widget);
Element? _child;
@override
void visitChildren(ElementVisitor visitor) {
if (_child != null) {
visitor(_child!);
}
}
@override
void forgetChild(Element child) {
assert(child == _child);
_child = null;
super.forgetChild(child);
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
_child = updateChild(_child, (widget as SingleChildRenderObjectWidget).child, null);
}
@override
void update(SingleChildRenderObjectWidget newWidget) {
super.update(newWidget);
assert(widget == newWidget);
_child = updateChild(_child, (widget as SingleChildRenderObjectWidget).child, null);
}
@override
void insertRenderObjectChild(RenderObject child, Object? slot) {
final RenderObjectWithChildMixin<RenderObject> renderObject = this.renderObject as RenderObjectWithChildMixin<RenderObject>;
assert(slot == null);
assert(renderObject.debugValidateChild(child));
renderObject.child = child;
assert(renderObject == this.renderObject);
}
@override
void moveRenderObjectChild(RenderObject child, Object? oldSlot, Object? newSlot) {
assert(false);
}
@override
void removeRenderObjectChild(RenderObject child, Object? slot) {
final RenderObjectWithChildMixin<RenderObject> renderObject = this.renderObject as RenderObjectWithChildMixin<RenderObject>;
assert(slot == null);
assert(renderObject.child == child);
renderObject.child = null;
assert(renderObject == this.renderObject);
}
}
/// An [Element] that uses a [MultiChildRenderObjectWidget] as its configuration.
///
/// This element subclass can be used for [RenderObjectWidget]s whose
/// [RenderObject]s use the [ContainerRenderObjectMixin] mixin with a parent data
/// type that implements [ContainerParentDataMixin<RenderObject>]. Such widgets
/// are expected to inherit from [MultiChildRenderObjectWidget].
///
/// See also:
///
/// * [IndexedSlot], which is used as [Element.slot]s for the children of a
/// [MultiChildRenderObjectElement].
/// * [RenderObjectElement.updateChildren], which discusses why [IndexedSlot]
/// is used for the slots of the children.
class MultiChildRenderObjectElement extends RenderObjectElement {
/// Creates an element that uses the given widget as its configuration.
MultiChildRenderObjectElement(MultiChildRenderObjectWidget super.widget)
: assert(!debugChildrenHaveDuplicateKeys(widget, widget.children));
@override
ContainerRenderObjectMixin<RenderObject, ContainerParentDataMixin<RenderObject>> get renderObject {
return super.renderObject as ContainerRenderObjectMixin<RenderObject, ContainerParentDataMixin<RenderObject>>;
}
/// The current list of children of this element.
///
/// This list is filtered to hide elements that have been forgotten (using
/// [forgetChild]).
@protected
@visibleForTesting
Iterable<Element> get children => _children.where((Element child) => !_forgottenChildren.contains(child));
late List<Element> _children;
// We keep a set of forgotten children to avoid O(n^2) work walking _children
// repeatedly to remove children.
final Set<Element> _forgottenChildren = HashSet<Element>();
@override
void insertRenderObjectChild(RenderObject child, IndexedSlot<Element?> slot) {
final ContainerRenderObjectMixin<RenderObject, ContainerParentDataMixin<RenderObject>> renderObject = this.renderObject;
assert(renderObject.debugValidateChild(child));
renderObject.insert(child, after: slot.value?.renderObject);
assert(renderObject == this.renderObject);
}
@override
void moveRenderObjectChild(RenderObject child, IndexedSlot<Element?> oldSlot, IndexedSlot<Element?> newSlot) {
final ContainerRenderObjectMixin<RenderObject, ContainerParentDataMixin<RenderObject>> renderObject = this.renderObject;
assert(child.parent == renderObject);
renderObject.move(child, after: newSlot.value?.renderObject);
assert(renderObject == this.renderObject);
}
@override
void removeRenderObjectChild(RenderObject child, Object? slot) {
final ContainerRenderObjectMixin<RenderObject, ContainerParentDataMixin<RenderObject>> renderObject = this.renderObject;
assert(child.parent == renderObject);
renderObject.remove(child);
assert(renderObject == this.renderObject);
}
@override
void visitChildren(ElementVisitor visitor) {
for (final Element child in _children) {
if (!_forgottenChildren.contains(child)) {
visitor(child);
}
}
}
@override
void forgetChild(Element child) {
assert(_children.contains(child));
assert(!_forgottenChildren.contains(child));
_forgottenChildren.add(child);
super.forgetChild(child);
}
bool _debugCheckHasAssociatedRenderObject(Element newChild) {
assert(() {
if (newChild.renderObject == null) {
FlutterError.reportError(
FlutterErrorDetails(
exception: FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The children of `MultiChildRenderObjectElement` must each has an associated render object.'),
ErrorHint(
'This typically means that the `${newChild.widget}` or its children\n'
'are not a subtype of `RenderObjectWidget`.',
),
newChild.describeElement('The following element does not have an associated render object'),
DiagnosticsDebugCreator(DebugCreator(newChild)),
]),
),
);
}
return true;
}());
return true;
}
@override
Element inflateWidget(Widget newWidget, Object? newSlot) {
final Element newChild = super.inflateWidget(newWidget, newSlot);
assert(_debugCheckHasAssociatedRenderObject(newChild));
return newChild;
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
final MultiChildRenderObjectWidget multiChildRenderObjectWidget = widget as MultiChildRenderObjectWidget;
final List<Element> children = List<Element>.filled(multiChildRenderObjectWidget.children.length, _NullElement.instance);
Element? previousChild;
for (int i = 0; i < children.length; i += 1) {
final Element newChild = inflateWidget(multiChildRenderObjectWidget.children[i], IndexedSlot<Element?>(i, previousChild));
children[i] = newChild;
previousChild = newChild;
}
_children = children;
}
@override
void update(MultiChildRenderObjectWidget newWidget) {
super.update(newWidget);
final MultiChildRenderObjectWidget multiChildRenderObjectWidget = widget as MultiChildRenderObjectWidget;
assert(widget == newWidget);
assert(!debugChildrenHaveDuplicateKeys(widget, multiChildRenderObjectWidget.children));
_children = updateChildren(_children, multiChildRenderObjectWidget.children, forgottenChildren: _forgottenChildren);
_forgottenChildren.clear();
}
}
/// A [RenderObjectElement] used to manage the root of a render tree.
///
/// Unlike any other render object element this element does not attempt to
/// attach its [renderObject] to the closest ancestor [RenderObjectElement].
/// Instead, subclasses must override [attachRenderObject] and
/// [detachRenderObject] to attach/detach the [renderObject] to whatever
/// instance manages the render tree (e.g. by assigning it to
/// [PipelineOwner.rootNode]).
abstract class RenderTreeRootElement extends RenderObjectElement {
/// Creates an element that uses the given widget as its configuration.
RenderTreeRootElement(super.widget);
@override
@mustCallSuper
void attachRenderObject(Object? newSlot) {
_slot = newSlot;
assert(_debugCheckMustNotAttachRenderObjectToAncestor());
}
@override
@mustCallSuper
void detachRenderObject() {
_slot = null;
}
@override
void updateSlot(Object? newSlot) {
super.updateSlot(newSlot);
assert(_debugCheckMustNotAttachRenderObjectToAncestor());
}
bool _debugCheckMustNotAttachRenderObjectToAncestor() {
if (!kDebugMode) {
return true;
}
if (_findAncestorRenderObjectElement() != null) {
throw FlutterError.fromParts(
<DiagnosticsNode>[
ErrorSummary(
'The RenderObject for ${toStringShort()} cannot maintain an independent render tree at its current location.',
),
ErrorDescription(
'The ownership chain for the RenderObject in question was:\n ${debugGetCreatorChain(10)}',
),
ErrorDescription(
'This RenderObject is the root of an independent render tree and it cannot '
'attach itself to an ancestor in an existing tree. The ancestor RenderObject, '
'however, expects that a child will be attached.',
),
ErrorHint(
'Try moving the subtree that contains the ${toStringShort()} widget '
'to a location where it is not expected to attach its RenderObject '
'to a parent. This could mean moving the subtree into the view '
'property of a "ViewAnchor" widget or - if the subtree is the root of '
'your widget tree - passing it to "runWidget" instead of "runApp".',
),
ErrorHint(
'If you are seeing this error in a test and the subtree containing '
'the ${toStringShort()} widget is passed to "WidgetTester.pumpWidget", '
'consider setting the "wrapWithView" parameter of that method to false.'
),
],
);
}
return true;
}
}
/// A wrapper class for the [Element] that is the creator of a [RenderObject].
///
/// Setting a [DebugCreator] as [RenderObject.debugCreator] will lead to better
/// error messages.
class DebugCreator {
/// Create a [DebugCreator] instance with input [Element].
DebugCreator(this.element);
/// The creator of the [RenderObject].
final Element element;
@override
String toString() => element.debugGetCreatorChain(12);
}
FlutterErrorDetails _reportException(
DiagnosticsNode context,
Object exception,
StackTrace? stack, {
InformationCollector? informationCollector,
}) {
final FlutterErrorDetails details = FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets library',
context: context,
informationCollector: informationCollector,
);
FlutterError.reportError(details);
return details;
}
/// A value for [Element.slot] used for children of
/// [MultiChildRenderObjectElement]s.
///
/// A slot for a [MultiChildRenderObjectElement] consists of an [index]
/// identifying where the child occupying this slot is located in the
/// [MultiChildRenderObjectElement]'s child list and an arbitrary [value] that
/// can further define where the child occupying this slot fits in its
/// parent's child list.
///
/// See also:
///
/// * [RenderObjectElement.updateChildren], which discusses why this class is
/// used as slot values for the children of a [MultiChildRenderObjectElement].
@immutable
class IndexedSlot<T extends Element?> {
/// Creates an [IndexedSlot] with the provided [index] and slot [value].
const IndexedSlot(this.index, this.value);
/// Information to define where the child occupying this slot fits in its
/// parent's child list.
final T value;
/// The index of this slot in the parent's child list.
final int index;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is IndexedSlot
&& index == other.index
&& value == other.value;
}
@override
int get hashCode => Object.hash(index, value);
}
/// Used as a placeholder in [List<Element>] objects when the actual
/// elements are not yet determined.
class _NullElement extends Element {
_NullElement() : super(const _NullWidget());
static _NullElement instance = _NullElement();
@override
bool get debugDoingBuild => throw UnimplementedError();
}
class _NullWidget extends Widget {
const _NullWidget();
@override
Element createElement() => throw UnimplementedError();
}
| flutter/packages/flutter/lib/src/widgets/framework.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/framework.dart",
"repo_id": "flutter",
"token_count": 91672
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
export 'package:flutter/services.dart' show KeyEvent;
/// A widget that calls a callback whenever the user presses or releases a key
/// on a keyboard.
///
/// A [KeyboardListener] is useful for listening to key events and
/// hardware buttons that are represented as keys. Typically used by games and
/// other apps that use keyboards for purposes other than text entry.
///
/// For text entry, consider using a [EditableText], which integrates with
/// on-screen keyboards and input method editors (IMEs).
///
/// See also:
///
/// * [EditableText], which should be used instead of this widget for text
/// entry.
class KeyboardListener extends StatelessWidget {
/// Creates a widget that receives keyboard events.
///
/// For text entry, consider using a [EditableText], which integrates with
/// on-screen keyboards and input method editors (IMEs).
///
/// The `key` is an identifier for widgets, and is unrelated to keyboards.
/// See [Widget.key].
const KeyboardListener({
super.key,
required this.focusNode,
this.autofocus = false,
this.includeSemantics = true,
this.onKeyEvent,
required this.child,
});
/// Controls whether this widget has keyboard focus.
final FocusNode focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.widgets.Focus.includeSemantics}
final bool includeSemantics;
/// Called whenever this widget receives a keyboard event.
final ValueChanged<KeyEvent>? onKeyEvent;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
Widget build(BuildContext context) {
return Focus(
focusNode: focusNode,
autofocus: autofocus,
includeSemantics: includeSemantics,
onKeyEvent: (FocusNode node, KeyEvent event) {
onKeyEvent?.call(event);
return KeyEventResult.ignored;
},
child: child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode));
}
}
| flutter/packages/flutter/lib/src/widgets/keyboard_listener.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/keyboard_listener.dart",
"repo_id": "flutter",
"token_count": 754
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async' show Timer;
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/physics.dart' show Tolerance, nearEqual;
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'basic.dart';
import 'framework.dart';
import 'media_query.dart';
import 'notification_listener.dart';
import 'scroll_notification.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
/// A visual indication that a scroll view has overscrolled.
///
/// A [GlowingOverscrollIndicator] listens for [ScrollNotification]s in order
/// to control the overscroll indication. These notifications are typically
/// generated by a [ScrollView], such as a [ListView] or a [GridView].
///
/// [GlowingOverscrollIndicator] generates [OverscrollIndicatorNotification]
/// before showing an overscroll indication. To prevent the indicator from
/// showing the indication, call
/// [OverscrollIndicatorNotification.disallowIndicator] on the notification.
///
/// Created automatically by [ScrollBehavior.buildOverscrollIndicator] on platforms
/// (e.g., Android) that commonly use this type of overscroll indication.
///
/// In a [MaterialApp], the edge glow color is the overall theme's
/// [ColorScheme.secondary] color.
///
/// ## Customizing the Glow Position for Advanced Scroll Views
///
/// When building a [CustomScrollView] with a [GlowingOverscrollIndicator], the
/// indicator will apply to the entire scrollable area, regardless of what
/// slivers the CustomScrollView contains.
///
/// For example, if your CustomScrollView contains a SliverAppBar in the first
/// position, the GlowingOverscrollIndicator will overlay the SliverAppBar. To
/// manipulate the position of the GlowingOverscrollIndicator in this case,
/// you can either make use of a [NotificationListener] and provide a
/// [OverscrollIndicatorNotification.paintOffset] to the
/// notification, or use a [NestedScrollView].
///
/// {@tool dartpad}
/// This example demonstrates how to use a [NotificationListener] to manipulate
/// the placement of a [GlowingOverscrollIndicator] when building a
/// [CustomScrollView]. Drag the scrollable to see the bounds of the overscroll
/// indicator.
///
/// ** See code in examples/api/lib/widgets/overscroll_indicator/glowing_overscroll_indicator.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example demonstrates how to use a [NestedScrollView] to manipulate the
/// placement of a [GlowingOverscrollIndicator] when building a
/// [CustomScrollView]. Drag the scrollable to see the bounds of the overscroll
/// indicator.
///
/// ** See code in examples/api/lib/widgets/overscroll_indicator/glowing_overscroll_indicator.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [OverscrollIndicatorNotification], which can be used to manipulate the
/// glow position or prevent the glow from being painted at all.
/// * [NotificationListener], to listen for the
/// [OverscrollIndicatorNotification].
/// * [StretchingOverscrollIndicator], a Material Design overscroll indicator.
class GlowingOverscrollIndicator extends StatefulWidget {
/// Creates a visual indication that a scroll view has overscrolled.
///
/// In order for this widget to display an overscroll indication, the [child]
/// widget must contain a widget that generates a [ScrollNotification], such
/// as a [ListView] or a [GridView].
const GlowingOverscrollIndicator({
super.key,
this.showLeading = true,
this.showTrailing = true,
required this.axisDirection,
required this.color,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.child,
});
/// Whether to show the overscroll glow on the side with negative scroll
/// offsets.
///
/// For a vertical downwards viewport, this is the top side.
///
/// Defaults to true.
///
/// See [showTrailing] for the corresponding control on the other side of the
/// viewport.
final bool showLeading;
/// Whether to show the overscroll glow on the side with positive scroll
/// offsets.
///
/// For a vertical downwards viewport, this is the bottom side.
///
/// Defaults to true.
///
/// See [showLeading] for the corresponding control on the other side of the
/// viewport.
final bool showTrailing;
/// {@template flutter.overscroll.axisDirection}
/// The direction of positive scroll offsets in the [Scrollable] whose
/// overscrolls are to be visualized.
/// {@endtemplate}
final AxisDirection axisDirection;
/// {@template flutter.overscroll.axis}
/// The axis along which scrolling occurs in the [Scrollable] whose
/// overscrolls are to be visualized.
/// {@endtemplate}
Axis get axis => axisDirectionToAxis(axisDirection);
/// The color of the glow. The alpha channel is ignored.
final Color color;
/// {@template flutter.overscroll.notificationPredicate}
/// A check that specifies whether a [ScrollNotification] should be
/// handled by this widget.
///
/// By default, checks whether `notification.depth == 0`. Set it to something
/// else for more complicated layouts, such as nested [ScrollView]s.
/// {@endtemplate}
final ScrollNotificationPredicate notificationPredicate;
/// The widget below this widget in the tree.
///
/// The overscroll indicator will paint on top of this child. This child (and its
/// subtree) should include a source of [ScrollNotification] notifications.
///
/// Typically a [GlowingOverscrollIndicator] is created by a
/// [ScrollBehavior.buildOverscrollIndicator] method, in which case
/// the child is usually the one provided as an argument to that method.
final Widget? child;
@override
State<GlowingOverscrollIndicator> createState() => _GlowingOverscrollIndicatorState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<AxisDirection>('axisDirection', axisDirection));
final String showDescription;
if (showLeading && showTrailing) {
showDescription = 'both sides';
} else if (showLeading) {
showDescription = 'leading side only';
} else if (showTrailing) {
showDescription = 'trailing side only';
} else {
showDescription = 'neither side (!)';
}
properties.add(MessageProperty('show', showDescription));
properties.add(ColorProperty('color', color, showName: false));
}
}
class _GlowingOverscrollIndicatorState extends State<GlowingOverscrollIndicator> with TickerProviderStateMixin {
_GlowController? _leadingController;
_GlowController? _trailingController;
Listenable? _leadingAndTrailingListener;
@override
void initState() {
super.initState();
_leadingController = _GlowController(vsync: this, color: widget.color, axis: widget.axis);
_trailingController = _GlowController(vsync: this, color: widget.color, axis: widget.axis);
_leadingAndTrailingListener = Listenable.merge(<Listenable>[_leadingController!, _trailingController!]);
}
@override
void didUpdateWidget(GlowingOverscrollIndicator oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.color != widget.color || oldWidget.axis != widget.axis) {
_leadingController!.color = widget.color;
_leadingController!.axis = widget.axis;
_trailingController!.color = widget.color;
_trailingController!.axis = widget.axis;
}
}
Type? _lastNotificationType;
final Map<bool, bool> _accepted = <bool, bool>{false: true, true: true};
bool _handleScrollNotification(ScrollNotification notification) {
if (!widget.notificationPredicate(notification)) {
return false;
}
if (notification.metrics.axis != widget.axis) {
// This widget is explicitly configured to one axis. If a notification
// from a different axis bubbles up, do nothing.
return false;
}
// Update the paint offset with the current scroll position. This makes
// sure that the glow effect correctly scrolls in line with the current
// scroll, e.g. when scrolling in the opposite direction again to hide
// the glow. Otherwise, the glow would always stay in a fixed position,
// even if the top of the content already scrolled away.
// For example (CustomScrollView with sliver before center), the scroll
// extent is [-200.0, 300.0], scroll in the opposite direction with 10.0 pixels
// before glow disappears, so the current pixels is -190.0,
// in this case, we should move the glow up 10.0 pixels and should not
// overflow the scrollable widget's edge. https://github.com/flutter/flutter/issues/64149.
_leadingController!._paintOffsetScrollPixels =
-math.min(notification.metrics.pixels - notification.metrics.minScrollExtent, _leadingController!._paintOffset);
_trailingController!._paintOffsetScrollPixels =
-math.min(notification.metrics.maxScrollExtent - notification.metrics.pixels, _trailingController!._paintOffset);
if (notification is OverscrollNotification) {
_GlowController? controller;
if (notification.overscroll < 0.0) {
controller = _leadingController;
} else if (notification.overscroll > 0.0) {
controller = _trailingController;
} else {
assert(false);
}
final bool isLeading = controller == _leadingController;
if (_lastNotificationType is! OverscrollNotification) {
final OverscrollIndicatorNotification confirmationNotification = OverscrollIndicatorNotification(leading: isLeading);
confirmationNotification.dispatch(context);
_accepted[isLeading] = confirmationNotification.accepted;
if (_accepted[isLeading]!) {
controller!._paintOffset = confirmationNotification.paintOffset;
}
}
assert(controller != null);
if (_accepted[isLeading]!) {
if (notification.velocity != 0.0) {
assert(notification.dragDetails == null);
controller!.absorbImpact(notification.velocity.abs());
} else {
assert(notification.overscroll != 0.0);
if (notification.dragDetails != null) {
final RenderBox renderer = notification.context!.findRenderObject()! as RenderBox;
assert(renderer.hasSize);
final Size size = renderer.size;
final Offset position = renderer.globalToLocal(notification.dragDetails!.globalPosition);
switch (notification.metrics.axis) {
case Axis.horizontal:
controller!.pull(notification.overscroll.abs(), size.width, clampDouble(position.dy, 0.0, size.height), size.height);
case Axis.vertical:
controller!.pull(notification.overscroll.abs(), size.height, clampDouble(position.dx, 0.0, size.width), size.width);
}
}
}
}
} else if ((notification is ScrollEndNotification && notification.dragDetails != null) ||
(notification is ScrollUpdateNotification && notification.dragDetails != null)) {
_leadingController!.scrollEnd();
_trailingController!.scrollEnd();
}
_lastNotificationType = notification.runtimeType;
return false;
}
@override
void dispose() {
_leadingController!.dispose();
_trailingController!.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return NotificationListener<ScrollNotification>(
onNotification: _handleScrollNotification,
child: RepaintBoundary(
child: CustomPaint(
foregroundPainter: _GlowingOverscrollIndicatorPainter(
leadingController: widget.showLeading ? _leadingController : null,
trailingController: widget.showTrailing ? _trailingController : null,
axisDirection: widget.axisDirection,
repaint: _leadingAndTrailingListener,
),
child: RepaintBoundary(
child: widget.child,
),
),
),
);
}
}
// The Glow logic is a port of the logic in the following file:
// https://android.googlesource.com/platform/frameworks/base/+/master/core/java/android/widget/EdgeEffect.java
// as of December 2016.
enum _GlowState { idle, absorb, pull, recede }
class _GlowController extends ChangeNotifier {
_GlowController({
required TickerProvider vsync,
required Color color,
required Axis axis,
}) : _color = color,
_axis = axis {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
_glowController = AnimationController(vsync: vsync)
..addStatusListener(_changePhase);
_decelerator = CurvedAnimation(
parent: _glowController,
curve: Curves.decelerate,
)..addListener(notifyListeners);
_glowOpacity = _decelerator.drive(_glowOpacityTween);
_glowSize = _decelerator.drive(_glowSizeTween);
_displacementTicker = vsync.createTicker(_tickDisplacement);
}
// animation of the main axis direction
_GlowState _state = _GlowState.idle;
late final AnimationController _glowController;
Timer? _pullRecedeTimer;
double _paintOffset = 0.0;
double _paintOffsetScrollPixels = 0.0;
// animation values
late final CurvedAnimation _decelerator;
final Tween<double> _glowOpacityTween = Tween<double>(begin: 0.0, end: 0.0);
late final Animation<double> _glowOpacity;
final Tween<double> _glowSizeTween = Tween<double>(begin: 0.0, end: 0.0);
late final Animation<double> _glowSize;
// animation of the cross axis position
late final Ticker _displacementTicker;
Duration? _displacementTickerLastElapsed;
double _displacementTarget = 0.5;
double _displacement = 0.5;
// tracking the pull distance
double _pullDistance = 0.0;
Color get color => _color;
Color _color;
set color(Color value) {
if (color == value) {
return;
}
_color = value;
notifyListeners();
}
Axis get axis => _axis;
Axis _axis;
set axis(Axis value) {
if (axis == value) {
return;
}
_axis = value;
notifyListeners();
}
static const Duration _recedeTime = Duration(milliseconds: 600);
static const Duration _pullTime = Duration(milliseconds: 167);
static const Duration _pullHoldTime = Duration(milliseconds: 167);
static const Duration _pullDecayTime = Duration(milliseconds: 2000);
static final Duration _crossAxisHalfTime = Duration(microseconds: (Duration.microsecondsPerSecond / 60.0).round());
static const double _maxOpacity = 0.5;
static const double _pullOpacityGlowFactor = 0.8;
static const double _velocityGlowFactor = 0.00006;
static const double _sqrt3 = 1.73205080757; // const math.sqrt(3)
static const double _widthToHeightFactor = (3.0 / 4.0) * (2.0 - _sqrt3);
// absorbed velocities are clamped to the range _minVelocity.._maxVelocity
static const double _minVelocity = 100.0; // logical pixels per second
static const double _maxVelocity = 10000.0; // logical pixels per second
@override
void dispose() {
_glowController.dispose();
_decelerator.dispose();
_displacementTicker.dispose();
_pullRecedeTimer?.cancel();
super.dispose();
}
/// Handle a scroll slamming into the edge at a particular velocity.
///
/// The velocity must be positive.
void absorbImpact(double velocity) {
assert(velocity >= 0.0);
_pullRecedeTimer?.cancel();
_pullRecedeTimer = null;
velocity = clampDouble(velocity, _minVelocity, _maxVelocity);
_glowOpacityTween.begin = _state == _GlowState.idle ? 0.3 : _glowOpacity.value;
_glowOpacityTween.end = clampDouble(velocity * _velocityGlowFactor, _glowOpacityTween.begin!, _maxOpacity);
_glowSizeTween.begin = _glowSize.value;
_glowSizeTween.end = math.min(0.025 + 7.5e-7 * velocity * velocity, 1.0);
_glowController.duration = Duration(milliseconds: (0.15 + velocity * 0.02).round());
_glowController.forward(from: 0.0);
_displacement = 0.5;
_state = _GlowState.absorb;
}
/// Handle a user-driven overscroll.
///
/// The `overscroll` argument should be the scroll distance in logical pixels,
/// the `extent` argument should be the total dimension of the viewport in the
/// main axis in logical pixels, the `crossAxisOffset` argument should be the
/// distance from the leading (left or top) edge of the cross axis of the
/// viewport, and the `crossExtent` should be the size of the cross axis. For
/// example, a pull of 50 pixels up the middle of a 200 pixel high and 100
/// pixel wide vertical viewport should result in a call of `pull(50.0, 200.0,
/// 50.0, 100.0)`. The `overscroll` value should be positive regardless of the
/// direction.
void pull(double overscroll, double extent, double crossAxisOffset, double crossExtent) {
_pullRecedeTimer?.cancel();
_pullDistance += overscroll / 200.0; // This factor is magic. Not clear why we need it to match Android.
_glowOpacityTween.begin = _glowOpacity.value;
_glowOpacityTween.end = math.min(_glowOpacity.value + overscroll / extent * _pullOpacityGlowFactor, _maxOpacity);
final double height = math.min(extent, crossExtent * _widthToHeightFactor);
_glowSizeTween.begin = _glowSize.value;
_glowSizeTween.end = math.max(1.0 - 1.0 / (0.7 * math.sqrt(_pullDistance * height)), _glowSize.value);
_displacementTarget = crossAxisOffset / crossExtent;
if (_displacementTarget != _displacement) {
if (!_displacementTicker.isTicking) {
assert(_displacementTickerLastElapsed == null);
_displacementTicker.start();
}
} else {
_displacementTicker.stop();
_displacementTickerLastElapsed = null;
}
_glowController.duration = _pullTime;
if (_state != _GlowState.pull) {
_glowController.forward(from: 0.0);
_state = _GlowState.pull;
} else {
if (!_glowController.isAnimating) {
assert(_glowController.value == 1.0);
notifyListeners();
}
}
_pullRecedeTimer = Timer(_pullHoldTime, () => _recede(_pullDecayTime));
}
void scrollEnd() {
if (_state == _GlowState.pull) {
_recede(_recedeTime);
}
}
void _changePhase(AnimationStatus status) {
if (status != AnimationStatus.completed) {
return;
}
switch (_state) {
case _GlowState.absorb:
_recede(_recedeTime);
case _GlowState.recede:
_state = _GlowState.idle;
_pullDistance = 0.0;
case _GlowState.pull:
case _GlowState.idle:
break;
}
}
void _recede(Duration duration) {
if (_state == _GlowState.recede || _state == _GlowState.idle) {
return;
}
_pullRecedeTimer?.cancel();
_pullRecedeTimer = null;
_glowOpacityTween.begin = _glowOpacity.value;
_glowOpacityTween.end = 0.0;
_glowSizeTween.begin = _glowSize.value;
_glowSizeTween.end = 0.0;
_glowController.duration = duration;
_glowController.forward(from: 0.0);
_state = _GlowState.recede;
}
void _tickDisplacement(Duration elapsed) {
if (_displacementTickerLastElapsed != null) {
final double t = (elapsed.inMicroseconds - _displacementTickerLastElapsed!.inMicroseconds).toDouble();
_displacement = _displacementTarget - (_displacementTarget - _displacement) * math.pow(2.0, -t / _crossAxisHalfTime.inMicroseconds);
notifyListeners();
}
if (nearEqual(_displacementTarget, _displacement, Tolerance.defaultTolerance.distance)) {
_displacementTicker.stop();
_displacementTickerLastElapsed = null;
} else {
_displacementTickerLastElapsed = elapsed;
}
}
void paint(Canvas canvas, Size size) {
if (_glowOpacity.value == 0.0) {
return;
}
final double baseGlowScale = size.width > size.height ? size.height / size.width : 1.0;
final double radius = size.width * 3.0 / 2.0;
final double height = math.min(size.height, size.width * _widthToHeightFactor);
final double scaleY = _glowSize.value * baseGlowScale;
final Rect rect = Rect.fromLTWH(0.0, 0.0, size.width, height);
final Offset center = Offset((size.width / 2.0) * (0.5 + _displacement), height - radius);
final Paint paint = Paint()..color = color.withOpacity(_glowOpacity.value);
canvas.save();
canvas.translate(0.0, _paintOffset + _paintOffsetScrollPixels);
canvas.scale(1.0, scaleY);
canvas.clipRect(rect);
canvas.drawCircle(center, radius, paint);
canvas.restore();
}
@override
String toString() {
return '_GlowController(color: $color, axis: ${axis.name})';
}
}
class _GlowingOverscrollIndicatorPainter extends CustomPainter {
_GlowingOverscrollIndicatorPainter({
this.leadingController,
this.trailingController,
required this.axisDirection,
super.repaint,
});
/// The controller for the overscroll glow on the side with negative scroll offsets.
///
/// For a vertical downwards viewport, this is the top side.
final _GlowController? leadingController;
/// The controller for the overscroll glow on the side with positive scroll offsets.
///
/// For a vertical downwards viewport, this is the bottom side.
final _GlowController? trailingController;
/// The direction of the viewport.
final AxisDirection axisDirection;
static const double piOver2 = math.pi / 2.0;
void _paintSide(Canvas canvas, Size size, _GlowController? controller, AxisDirection axisDirection, GrowthDirection growthDirection) {
if (controller == null) {
return;
}
switch (applyGrowthDirectionToAxisDirection(axisDirection, growthDirection)) {
case AxisDirection.up:
controller.paint(canvas, size);
case AxisDirection.down:
canvas.save();
canvas.translate(0.0, size.height);
canvas.scale(1.0, -1.0);
controller.paint(canvas, size);
canvas.restore();
case AxisDirection.left:
canvas.save();
canvas.rotate(piOver2);
canvas.scale(1.0, -1.0);
controller.paint(canvas, Size(size.height, size.width));
canvas.restore();
case AxisDirection.right:
canvas.save();
canvas.translate(size.width, 0.0);
canvas.rotate(piOver2);
controller.paint(canvas, Size(size.height, size.width));
canvas.restore();
}
}
@override
void paint(Canvas canvas, Size size) {
_paintSide(canvas, size, leadingController, axisDirection, GrowthDirection.reverse);
_paintSide(canvas, size, trailingController, axisDirection, GrowthDirection.forward);
}
@override
bool shouldRepaint(_GlowingOverscrollIndicatorPainter oldDelegate) {
return oldDelegate.leadingController != leadingController
|| oldDelegate.trailingController != trailingController;
}
@override
String toString() {
return '_GlowingOverscrollIndicatorPainter($leadingController, $trailingController)';
}
}
enum _StretchDirection {
/// The [trailing] direction indicates that the content will be stretched toward
/// the trailing edge.
trailing,
/// The [leading] direction indicates that the content will be stretched toward
/// the leading edge.
leading,
}
/// A Material Design visual indication that a scroll view has overscrolled.
///
/// A [StretchingOverscrollIndicator] listens for [ScrollNotification]s in order
/// to stretch the content of the [Scrollable]. These notifications are typically
/// generated by a [ScrollView], such as a [ListView] or a [GridView].
///
/// When triggered, the [StretchingOverscrollIndicator] generates an
/// [OverscrollIndicatorNotification] before showing an overscroll indication.
/// To prevent the indicator from showing the indication, call
/// [OverscrollIndicatorNotification.disallowIndicator] on the notification.
///
/// Created by [MaterialScrollBehavior.buildOverscrollIndicator] on platforms
/// (e.g., Android) that commonly use this type of overscroll indication when
/// [ThemeData.useMaterial3] is true. Otherwise, when [ThemeData.useMaterial3]
/// is false, a [GlowingOverscrollIndicator] is used instead.=
///
/// See also:
///
/// * [OverscrollIndicatorNotification], which can be used to prevent the
/// stretch effect from being applied at all.
/// * [NotificationListener], to listen for the
/// [OverscrollIndicatorNotification].
/// * [GlowingOverscrollIndicator], the default overscroll indicator for
/// [TargetPlatform.android] and [TargetPlatform.fuchsia].
class StretchingOverscrollIndicator extends StatefulWidget {
/// Creates a visual indication that a scroll view has overscrolled by
/// applying a stretch transformation to the content.
///
/// In order for this widget to display an overscroll indication, the [child]
/// widget must contain a widget that generates a [ScrollNotification], such
/// as a [ListView] or a [GridView].
const StretchingOverscrollIndicator({
super.key,
required this.axisDirection,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.clipBehavior = Clip.hardEdge,
this.child,
});
/// {@macro flutter.overscroll.axisDirection}
final AxisDirection axisDirection;
/// {@macro flutter.overscroll.axis}
Axis get axis => axisDirectionToAxis(axisDirection);
/// {@macro flutter.overscroll.notificationPredicate}
final ScrollNotificationPredicate notificationPredicate;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// The widget below this widget in the tree.
///
/// The overscroll indicator will apply a stretch effect to this child. This
/// child (and its subtree) should include a source of [ScrollNotification]
/// notifications.
final Widget? child;
@override
State<StretchingOverscrollIndicator> createState() => _StretchingOverscrollIndicatorState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<AxisDirection>('axisDirection', axisDirection));
}
}
class _StretchingOverscrollIndicatorState extends State<StretchingOverscrollIndicator> with TickerProviderStateMixin {
late final _StretchController _stretchController = _StretchController(vsync: this);
ScrollNotification? _lastNotification;
OverscrollNotification? _lastOverscrollNotification;
double _totalOverscroll = 0.0;
bool _accepted = true;
bool _handleScrollNotification(ScrollNotification notification) {
if (!widget.notificationPredicate(notification)) {
return false;
}
if (notification.metrics.axis != widget.axis) {
// This widget is explicitly configured to one axis. If a notification
// from a different axis bubbles up, do nothing.
return false;
}
if (notification is OverscrollNotification) {
_lastOverscrollNotification = notification;
if (_lastNotification.runtimeType is! OverscrollNotification) {
final OverscrollIndicatorNotification confirmationNotification = OverscrollIndicatorNotification(leading: notification.overscroll < 0.0);
confirmationNotification.dispatch(context);
_accepted = confirmationNotification.accepted;
}
if (_accepted) {
_totalOverscroll += notification.overscroll;
if (notification.velocity != 0.0) {
assert(notification.dragDetails == null);
_stretchController.absorbImpact(notification.velocity.abs(), _totalOverscroll);
} else {
assert(notification.overscroll != 0.0);
if (notification.dragDetails != null) {
// We clamp the overscroll amount relative to the length of the viewport,
// which is the furthest distance a single pointer could pull on the
// screen. This is because more than one pointer will multiply the
// amount of overscroll - https://github.com/flutter/flutter/issues/11884
final double viewportDimension = notification.metrics.viewportDimension;
final double distanceForPull = _totalOverscroll.abs() / viewportDimension;
final double clampedOverscroll = clampDouble(distanceForPull, 0, 1.0);
_stretchController.pull(clampedOverscroll, _totalOverscroll);
}
}
}
} else if (notification is ScrollEndNotification || notification is ScrollUpdateNotification) {
// Since the overscrolling ended, we reset the total overscroll amount.
_totalOverscroll = 0;
_stretchController.scrollEnd();
}
_lastNotification = notification;
return false;
}
AlignmentGeometry _getAlignmentForAxisDirection(_StretchDirection stretchDirection) {
// Accounts for reversed scrollables by checking the AxisDirection
final AxisDirection direction = switch (stretchDirection) {
_StretchDirection.trailing => widget.axisDirection,
_StretchDirection.leading => flipAxisDirection(widget.axisDirection),
};
return switch (direction) {
AxisDirection.up => AlignmentDirectional.topCenter,
AxisDirection.down => AlignmentDirectional.bottomCenter,
AxisDirection.left => Alignment.centerLeft,
AxisDirection.right => Alignment.centerRight,
};
}
@override
void dispose() {
_stretchController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final Size size = MediaQuery.sizeOf(context);
double mainAxisSize;
return NotificationListener<ScrollNotification>(
onNotification: _handleScrollNotification,
child: AnimatedBuilder(
animation: _stretchController,
builder: (BuildContext context, Widget? child) {
final double stretch = _stretchController.value;
double x = 1.0;
double y = 1.0;
switch (widget.axis) {
case Axis.horizontal:
x += stretch;
mainAxisSize = size.width;
case Axis.vertical:
y += stretch;
mainAxisSize = size.height;
}
final AlignmentGeometry alignment = _getAlignmentForAxisDirection(
_stretchController.stretchDirection,
);
final double viewportDimension = _lastOverscrollNotification?.metrics.viewportDimension ?? mainAxisSize;
final Widget transform = Transform(
alignment: alignment,
transform: Matrix4.diagonal3Values(x, y, 1.0),
filterQuality: stretch == 0 ? null : FilterQuality.low,
child: widget.child,
);
// Only clip if the viewport dimension is smaller than that of the
// screen size in the main axis. If the viewport takes up the whole
// screen, overflow from transforming the viewport is irrelevant.
return ClipRect(
clipBehavior: stretch != 0.0 && viewportDimension != mainAxisSize
? widget.clipBehavior
: Clip.none,
child: transform,
);
},
),
);
}
}
enum _StretchState {
idle,
absorb,
pull,
recede,
}
class _StretchController extends ChangeNotifier {
_StretchController({ required TickerProvider vsync }) {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
_stretchController = AnimationController(vsync: vsync)
..addStatusListener(_changePhase);
_decelerator = CurvedAnimation(
parent: _stretchController,
curve: Curves.decelerate,
)..addListener(notifyListeners);
_stretchSize = _decelerator.drive(_stretchSizeTween);
}
late final AnimationController _stretchController;
late final Animation<double> _stretchSize;
late final CurvedAnimation _decelerator;
final Tween<double> _stretchSizeTween = Tween<double>(begin: 0.0, end: 0.0);
_StretchState _state = _StretchState.idle;
double get pullDistance => _pullDistance;
double _pullDistance = 0.0;
_StretchDirection get stretchDirection => _stretchDirection;
_StretchDirection _stretchDirection = _StretchDirection.trailing;
// Constants from Android.
static const double _exponentialScalar = math.e / 0.33;
static const double _stretchIntensity = 0.016;
static const double _flingFriction = 1.01;
static const Duration _stretchDuration = Duration(milliseconds: 400);
double get value => _stretchSize.value;
/// Handle a fling to the edge of the viewport at a particular velocity.
///
/// The velocity must be positive.
void absorbImpact(double velocity, double totalOverscroll) {
assert(velocity >= 0.0);
velocity = clampDouble(velocity, 1, 10000);
_stretchSizeTween.begin = _stretchSize.value;
_stretchSizeTween.end = math.min(_stretchIntensity + (_flingFriction / velocity), 1.0);
_stretchController.duration = Duration(milliseconds: (velocity * 0.02).round());
_stretchController.forward(from: 0.0);
_state = _StretchState.absorb;
_stretchDirection = totalOverscroll > 0 ? _StretchDirection.trailing : _StretchDirection.leading;
}
/// Handle a user-driven overscroll.
///
/// The `normalizedOverscroll` argument should be the absolute value of the
/// scroll distance in logical pixels, divided by the extent of the viewport
/// in the main axis.
void pull(double normalizedOverscroll, double totalOverscroll) {
assert(normalizedOverscroll >= 0.0);
final _StretchDirection newStretchDirection = totalOverscroll > 0 ? _StretchDirection.trailing : _StretchDirection.leading;
if (_stretchDirection != newStretchDirection && _state == _StretchState.recede) {
// When the stretch direction changes while we are in the recede state, we need to ignore the change.
// If we don't, the stretch will instantly jump to the new direction with the recede animation still playing, which causes
// a unwanted visual abnormality (https://github.com/flutter/flutter/pull/116548#issuecomment-1414872567).
// By ignoring the directional change until the recede state is finished, we can avoid this.
return;
}
_stretchDirection = newStretchDirection;
_pullDistance = normalizedOverscroll;
_stretchSizeTween.begin = _stretchSize.value;
final double linearIntensity =_stretchIntensity * _pullDistance;
final double exponentialIntensity = _stretchIntensity * (1 - math.exp(-_pullDistance * _exponentialScalar));
_stretchSizeTween.end = linearIntensity + exponentialIntensity;
_stretchController.duration = _stretchDuration;
if (_state != _StretchState.pull) {
_stretchController.forward(from: 0.0);
_state = _StretchState.pull;
} else {
if (!_stretchController.isAnimating) {
assert(_stretchController.value == 1.0);
notifyListeners();
}
}
}
void scrollEnd() {
if (_state == _StretchState.pull) {
_recede(_stretchDuration);
}
}
void _changePhase(AnimationStatus status) {
if (status != AnimationStatus.completed) {
return;
}
switch (_state) {
case _StretchState.absorb:
_recede(_stretchDuration);
case _StretchState.recede:
_state = _StretchState.idle;
_pullDistance = 0.0;
case _StretchState.pull:
case _StretchState.idle:
break;
}
}
void _recede(Duration duration) {
if (_state == _StretchState.recede || _state == _StretchState.idle) {
return;
}
_stretchSizeTween.begin = _stretchSize.value;
_stretchSizeTween.end = 0.0;
_stretchController.duration = duration;
_stretchController.forward(from: 0.0);
_state = _StretchState.recede;
}
@override
void dispose() {
_stretchController.dispose();
_decelerator.dispose();
super.dispose();
}
@override
String toString() => '_StretchController()';
}
/// A notification that either a [GlowingOverscrollIndicator] or a
/// [StretchingOverscrollIndicator] will start showing an overscroll indication.
///
/// To prevent the indicator from showing the indication, call
/// [disallowIndicator] on the notification.
///
/// See also:
///
/// * [GlowingOverscrollIndicator], which generates this type of notification
/// by painting an indicator over the child content.
/// * [StretchingOverscrollIndicator], which generates this type of
/// notification by applying a stretch transformation to the child content.
class OverscrollIndicatorNotification extends Notification with ViewportNotificationMixin {
/// Creates a notification that an [GlowingOverscrollIndicator] or a
/// [StretchingOverscrollIndicator] will start showing an overscroll indication.
OverscrollIndicatorNotification({
required this.leading,
});
/// Whether the indication will be shown on the leading edge of the scroll
/// view.
final bool leading;
/// Controls at which offset a [GlowingOverscrollIndicator] draws.
///
/// A positive offset will move the glow away from its edge,
/// i.e. for a vertical, [leading] indicator, a [paintOffset] of 100.0 will
/// draw the indicator 100.0 pixels from the top of the edge.
/// For a vertical indicator with [leading] set to `false`, a [paintOffset]
/// of 100.0 will draw the indicator 100.0 pixels from the bottom instead.
///
/// A negative [paintOffset] is generally not useful, since the glow will be
/// clipped.
///
/// This has no effect on a [StretchingOverscrollIndicator].
double paintOffset = 0.0;
@protected
@visibleForTesting
/// Whether the current overscroll event will allow for the indicator to be
/// shown.
///
/// Calling [disallowIndicator] sets this to false, preventing the over scroll
/// indicator from showing.
///
/// Defaults to true.
bool accepted = true;
/// Call this method if the overscroll indicator should be prevented.
void disallowIndicator() {
accepted = false;
}
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('side: ${leading ? "leading edge" : "trailing edge"}');
}
}
| flutter/packages/flutter/lib/src/widgets/overscroll_indicator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/overscroll_indicator.dart",
"repo_id": "flutter",
"token_count": 12897
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'package:collection/collection.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'binding.dart';
import 'framework.dart';
import 'navigator.dart';
import 'restoration.dart';
import 'restoration_properties.dart';
/// A piece of routing information.
///
/// The route information consists of a location string of the application and
/// a state object that configures the application in that location.
///
/// This information flows two ways, from the [RouteInformationProvider] to the
/// [Router] or from the [Router] to [RouteInformationProvider].
///
/// In the former case, the [RouteInformationProvider] notifies the [Router]
/// widget when a new [RouteInformation] is available. The [Router] widget takes
/// these information and navigates accordingly.
///
/// The latter case happens in web application where the [Router] reports route
/// changes back to the web engine.
///
/// The current [RouteInformation] of an application is also used for state
/// restoration purposes. Before an application is killed, the [Router] converts
/// its current configurations into a [RouteInformation] object utilizing the
/// [RouteInformationProvider]. The [RouteInformation] object is then serialized
/// out and persisted. During state restoration, the object is deserialized and
/// passed back to the [RouteInformationProvider], which turns it into a
/// configuration for the [Router] again to restore its state from.
class RouteInformation {
/// Creates a route information object.
///
/// Either `location` or `uri` must not be null.
const RouteInformation({
@Deprecated(
'Pass Uri.parse(location) to uri parameter instead. '
'This feature was deprecated after v3.8.0-3.0.pre.'
)
String? location,
Uri? uri,
this.state,
}) : _location = location,
_uri = uri,
assert((location != null) != (uri != null));
/// The location of the application.
///
/// The string is usually in the format of multiple string identifiers with
/// slashes in between. ex: `/`, `/path`, `/path/to/the/app`.
@Deprecated(
'Use uri instead. '
'This feature was deprecated after v3.8.0-3.0.pre.'
)
String get location {
return _location ?? Uri.decodeComponent(
Uri(
path: uri.path.isEmpty ? '/' : uri.path,
queryParameters: uri.queryParametersAll.isEmpty ? null : uri.queryParametersAll,
fragment: uri.fragment.isEmpty ? null : uri.fragment,
).toString(),
);
}
final String? _location;
/// The uri location of the application.
///
/// The host and scheme will not be empty if this object is created from a
/// deep link request. They represents the website that redirect the deep
/// link.
///
/// In web platform, the host and scheme are always empty.
Uri get uri {
if (_uri != null){
return _uri;
}
return Uri.parse(_location!);
}
final Uri? _uri;
/// The state of the application in the [uri].
///
/// The app can have different states even in the same location. For example,
/// the text inside a [TextField] or the scroll position in a [ScrollView].
/// These widget states can be stored in the [state].
///
/// On the web, this information is stored in the browser history when the
/// [Router] reports this route information back to the web engine
/// through the [PlatformRouteInformationProvider]. The information
/// is then passed back, along with the [uri], when the user
/// clicks the back or forward buttons.
///
/// This information is also serialized and persisted alongside the
/// [uri] for state restoration purposes. During state restoration,
/// the information is made available again to the [Router] so it can restore
/// its configuration to the previous state.
///
/// The state must be serializable.
final Object? state;
}
/// A convenient bundle to configure a [Router] widget.
///
/// To configure a [Router] widget, one needs to provide several delegates,
/// [RouteInformationProvider], [RouteInformationParser], [RouterDelegate],
/// and [BackButtonDispatcher]. This abstract class provides way to bundle these
/// delegates into a single object to configure a [Router].
///
/// The [backButtonDispatcher], [routeInformationProvider], and
/// [routeInformationProvider] are optional.
///
/// The [routeInformationProvider] and [routeInformationParser] must
/// both be provided or both not provided.
class RouterConfig<T> {
/// Creates a [RouterConfig].
///
/// The [backButtonDispatcher], [routeInformationProvider], and
/// [routeInformationParser] are optional.
///
/// The [routeInformationProvider] and [routeInformationParser] must both be
/// provided or both not provided.
const RouterConfig({
this.routeInformationProvider,
this.routeInformationParser,
required this.routerDelegate,
this.backButtonDispatcher,
}) : assert((routeInformationProvider == null) == (routeInformationParser == null));
/// The [RouteInformationProvider] that is used to configure the [Router].
final RouteInformationProvider? routeInformationProvider;
/// The [RouteInformationParser] that is used to configure the [Router].
final RouteInformationParser<T>? routeInformationParser;
/// The [RouterDelegate] that is used to configure the [Router].
final RouterDelegate<T> routerDelegate;
/// The [BackButtonDispatcher] that is used to configure the [Router].
final BackButtonDispatcher? backButtonDispatcher;
}
/// The dispatcher for opening and closing pages of an application.
///
/// This widget listens for routing information from the operating system (e.g.
/// an initial route provided on app startup, a new route obtained when an
/// intent is received, or a notification that the user hit the system back
/// button), parses route information into data of type `T`, and then converts
/// that data into [Page] objects that it passes to a [Navigator].
///
/// Each part of this process can be overridden and configured as desired.
///
/// The [routeInformationProvider] can be overridden to change how the name of
/// the route is obtained. The [RouteInformationProvider.value] is used as the
/// initial route when the [Router] is first created. Subsequent notifications
/// from the [RouteInformationProvider] to its listeners are treated as
/// notifications that the route information has changed.
///
/// The [backButtonDispatcher] can be overridden to change how back button
/// notifications are received. This must be a [BackButtonDispatcher], which is
/// an object where callbacks can be registered, and which can be chained so
/// that back button presses are delegated to subsidiary routers. The callbacks
/// are invoked to indicate that the user is trying to close the current route
/// (by pressing the system back button); the [Router] ensures that when this
/// callback is invoked, the message is passed to the [routerDelegate] and its
/// result is provided back to the [backButtonDispatcher]. Some platforms don't
/// have back buttons (e.g. iOS and desktop platforms); on those platforms this
/// notification is never sent. Typically, the [backButtonDispatcher] for the
/// root router is an instance of [RootBackButtonDispatcher], which uses a
/// [WidgetsBindingObserver] to listen to the `popRoute` notifications from
/// [SystemChannels.navigation]. Nested [Router]s typically use a
/// [ChildBackButtonDispatcher], which must be provided the
/// [BackButtonDispatcher] of its ancestor [Router] (available via [Router.of]).
///
/// The [routeInformationParser] can be overridden to change how names obtained
/// from the [routeInformationProvider] are interpreted. It must implement the
/// [RouteInformationParser] interface, specialized with the same type as the
/// [Router] itself. This type, `T`, represents the data type that the
/// [routeInformationParser] will generate.
///
/// The [routerDelegate] can be overridden to change how the output of the
/// [routeInformationParser] is interpreted. It must implement the
/// [RouterDelegate] interface, also specialized with `T`; it takes as input
/// the data (of type `T`) from the [routeInformationParser], and is responsible
/// for providing a navigating widget to insert into the widget tree. The
/// [RouterDelegate] interface is also [Listenable]; notifications are taken
/// to mean that the [Router] needs to rebuild.
///
/// ## Concerns regarding asynchrony
///
/// Some of the APIs (notably those involving [RouteInformationParser] and
/// [RouterDelegate]) are asynchronous.
///
/// When developing objects implementing these APIs, if the work can be done
/// entirely synchronously, then consider using [SynchronousFuture] for the
/// future returned from the relevant methods. This will allow the [Router] to
/// proceed in a completely synchronous way, which removes a number of
/// complications.
///
/// Using asynchronous computation is entirely reasonable, however, and the API
/// is designed to support it. For example, maybe a set of images need to be
/// loaded before a route can be shown; waiting for those images to be loaded
/// before [RouterDelegate.setNewRoutePath] returns is a reasonable approach to
/// handle this case.
///
/// If an asynchronous operation is ongoing when a new one is to be started, the
/// precise behavior will depend on the exact circumstances, as follows:
///
/// If the active operation is a [routeInformationParser] parsing a new route information:
/// that operation's result, if it ever completes, will be discarded.
///
/// If the active operation is a [routerDelegate] handling a pop request:
/// the previous pop is immediately completed with "false", claiming that the
/// previous pop was not handled (this may cause the application to close).
///
/// If the active operation is a [routerDelegate] handling an initial route
/// or a pushed route, the result depends on the new operation. If the new
/// operation is a pop request, then the original operation's result, if it ever
/// completes, will be discarded. If the new operation is a push request,
/// however, the [routeInformationParser] will be requested to start the parsing, and
/// only if that finishes before the original [routerDelegate] request
/// completes will that original request's result be discarded.
///
/// If the identity of the [Router] widget's delegates change while an
/// asynchronous operation is in progress, to keep matters simple, all active
/// asynchronous operations will have their results discarded. It is generally
/// considered unusual for these delegates to change during the lifetime of the
/// [Router].
///
/// If the [Router] itself is disposed while an asynchronous operation is in
/// progress, all active asynchronous operations will have their results
/// discarded also.
///
/// No explicit signals are provided to the [routeInformationParser] or
/// [routerDelegate] to indicate when any of the above happens, so it is
/// strongly recommended that [RouteInformationParser] and [RouterDelegate]
/// implementations not perform extensive computation.
///
/// ## Application architectural design
///
/// An application can have zero, one, or many [Router] widgets, depending on
/// its needs.
///
/// An application might have no [Router] widgets if it has only one "screen",
/// or if the facilities provided by [Navigator] are sufficient. This is common
/// for desktop applications, where subsidiary "screens" are represented using
/// different windows rather than changing the active interface.
///
/// A particularly elaborate application might have multiple [Router] widgets,
/// in a tree configuration, with the first handling the entire route parsing
/// and making the result available for routers in the subtree. The routers in
/// the subtree do not participate in route information parsing but merely take the
/// result from the first router to build their sub routes.
///
/// Most applications only need a single [Router].
///
/// ## URL updates for web applications
///
/// In the web platform, keeping the URL in the browser's location bar up to
/// date with the application state ensures that the browser constructs its
/// history entry correctly, allowing its back and forward buttons to function
/// as the user expects.
///
/// If an app state change leads to the [Router] rebuilding, the [Router] will
/// retrieve the new route information from the [routerDelegate]'s
/// [RouterDelegate.currentConfiguration] method and the
/// [routeInformationParser]'s [RouteInformationParser.restoreRouteInformation]
/// method.
///
/// If the location in the new route information is different from the
/// current location, this is considered to be a navigation event, the
/// [PlatformRouteInformationProvider.routerReportsNewRouteInformation] method
/// calls [SystemNavigator.routeInformationUpdated] with `replace = false` to
/// notify the engine, and through that the browser, to create a history entry
/// with the new url. Otherwise,
/// [PlatformRouteInformationProvider.routerReportsNewRouteInformation] calls
/// [SystemNavigator.routeInformationUpdated] with `replace = true` to update
/// the current history entry with the latest [RouteInformation].
///
/// One can force the [Router] to report new route information as navigation
/// event to the [routeInformationProvider] (and thus the browser) even if the
/// [RouteInformation.uri] has not changed by calling the [Router.navigate]
/// method with a callback that performs the state change. This causes [Router]
/// to call the [RouteInformationProvider.routerReportsNewRouteInformation] with
/// [RouteInformationReportingType.navigate], and thus causes
/// [PlatformRouteInformationProvider] to push a new history entry regardlessly.
/// This allows one to support the browser's back and forward buttons without
/// changing the URL. For example, the scroll position of a scroll view may be
/// saved in the [RouteInformation.state]. Using [Router.navigate] to update the
/// scroll position causes the browser to create a new history entry with the
/// [RouteInformation.state] that stores this new scroll position. When the user
/// clicks the back button, the app will go back to the previous scroll position
/// without changing the URL in the location bar.
///
/// One can also force the [Router] to ignore a navigation event by making
/// those changes during a callback passed to [Router.neglect]. The [Router]
/// calls the [RouteInformationProvider.routerReportsNewRouteInformation] with
/// [RouteInformationReportingType.neglect], and thus causes
/// [PlatformRouteInformationProvider] to replace the current history entry
/// regardlessly even if it detects location change.
///
/// To opt out of URL updates entirely, pass null for [routeInformationProvider]
/// and [routeInformationParser]. This is not recommended in general, but may be
/// appropriate in the following cases:
///
/// * The application does not target the web platform.
///
/// * There are multiple router widgets in the application. Only one [Router]
/// widget should update the URL (typically the top-most one created by the
/// [WidgetsApp.router], [MaterialApp.router], or [CupertinoApp.router]).
///
/// * The application does not need to implement in-app navigation using the
/// browser's back and forward buttons.
///
/// In other cases, it is strongly recommended to implement the
/// [RouterDelegate.currentConfiguration] and
/// [RouteInformationParser.restoreRouteInformation] APIs to provide an optimal
/// user experience when running on the web platform.
///
/// ## State Restoration
///
/// The [Router] will restore the current configuration of the [routerDelegate]
/// during state restoration if it is configured with a [restorationScopeId] and
/// state restoration is enabled for the subtree. For that, the value of
/// [RouterDelegate.currentConfiguration] is serialized and persisted before the
/// app is killed by the operating system. After the app is restarted, the value
/// is deserialized and passed back to the [RouterDelegate] via a call to
/// [RouterDelegate.setRestoredRoutePath] (which by default just calls
/// [RouterDelegate.setNewRoutePath]). It is the responsibility of the
/// [RouterDelegate] to use the configuration information provided to restore
/// its internal state.
///
/// To serialize [RouterDelegate.currentConfiguration] and to deserialize it
/// again, the [Router] calls [RouteInformationParser.restoreRouteInformation]
/// and [RouteInformationParser.parseRouteInformation], respectively. Therefore,
/// if a [restorationScopeId] is provided, a [routeInformationParser] must be
/// configured as well.
class Router<T> extends StatefulWidget {
/// Creates a router.
///
/// The [routeInformationProvider] and [routeInformationParser] can be null if this
/// router does not depend on route information. A common example is a sub router
/// that builds its content completely based on the app state.
///
/// The [routeInformationProvider] and [routeInformationParser] must
/// both be provided or not provided.
const Router({
super.key,
this.routeInformationProvider,
this.routeInformationParser,
required this.routerDelegate,
this.backButtonDispatcher,
this.restorationScopeId,
}) : assert(
routeInformationProvider == null || routeInformationParser != null,
'A routeInformationParser must be provided when a routeInformationProvider is specified.',
);
/// Creates a router with a [RouterConfig].
///
/// The [RouterConfig.routeInformationProvider] and
/// [RouterConfig.routeInformationParser] can be null if this router does not
/// depend on route information. A common example is a sub router that builds
/// its content completely based on the app state.
///
/// If the [RouterConfig.routeInformationProvider] is not null, then
/// [RouterConfig.routeInformationParser] must also not be
/// null.
factory Router.withConfig({
Key? key,
required RouterConfig<T> config,
String? restorationScopeId,
}) {
return Router<T>(
key: key,
routeInformationProvider: config.routeInformationProvider,
routeInformationParser: config.routeInformationParser,
routerDelegate: config.routerDelegate,
backButtonDispatcher: config.backButtonDispatcher,
restorationScopeId: restorationScopeId,
);
}
/// The route information provider for the router.
///
/// The value at the time of first build will be used as the initial route.
/// The [Router] listens to this provider and rebuilds with new names when
/// it notifies.
///
/// This can be null if this router does not rely on the route information
/// to build its content. In such case, the [routeInformationParser] must also
/// be null.
final RouteInformationProvider? routeInformationProvider;
/// The route information parser for the router.
///
/// When the [Router] gets a new route information from the [routeInformationProvider],
/// the [Router] uses this delegate to parse the route information and produce a
/// configuration. The configuration will be used by [routerDelegate] and
/// eventually rebuilds the [Router] widget.
///
/// Since this delegate is the primary consumer of the [routeInformationProvider],
/// it must not be null if [routeInformationProvider] is not null.
final RouteInformationParser<T>? routeInformationParser;
/// The router delegate for the router.
///
/// This delegate consumes the configuration from [routeInformationParser] and
/// builds a navigating widget for the [Router].
///
/// It is also the primary respondent for the [backButtonDispatcher]. The
/// [Router] relies on [RouterDelegate.popRoute] to handle the back
/// button.
///
/// If the [RouterDelegate.currentConfiguration] returns a non-null object,
/// this [Router] will opt for URL updates.
final RouterDelegate<T> routerDelegate;
/// The back button dispatcher for the router.
///
/// The two common alternatives are the [RootBackButtonDispatcher] for root
/// router, or the [ChildBackButtonDispatcher] for other routers.
final BackButtonDispatcher? backButtonDispatcher;
/// Restoration ID to save and restore the state of the [Router].
///
/// If non-null, the [Router] will persist the [RouterDelegate]'s current
/// configuration (i.e. [RouterDelegate.currentConfiguration]). During state
/// restoration, the [Router] informs the [RouterDelegate] of the previous
/// configuration by calling [RouterDelegate.setRestoredRoutePath] (which by
/// default just calls [RouterDelegate.setNewRoutePath]). It is the
/// responsibility of the [RouterDelegate] to restore its internal state based
/// on the provided configuration.
///
/// The router uses the [RouteInformationParser] to serialize and deserialize
/// [RouterDelegate.currentConfiguration]. Therefore, a
/// [routeInformationParser] must be provided when [restorationScopeId] is
/// non-null.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
final String? restorationScopeId;
/// Retrieves the immediate [Router] ancestor from the given context.
///
/// This method provides access to the delegates in the [Router]. For example,
/// this can be used to access the [backButtonDispatcher] of the parent router
/// when creating a [ChildBackButtonDispatcher] for a nested [Router].
///
/// If no [Router] ancestor exists for the given context, this will assert in
/// debug mode, and throw an exception in release mode.
///
/// See also:
///
/// * [maybeOf], which is a similar function, but it will return null instead
/// of throwing an exception if no [Router] ancestor exists.
static Router<T> of<T extends Object?>(BuildContext context) {
final _RouterScope? scope = context.dependOnInheritedWidgetOfExactType<_RouterScope>();
assert(() {
if (scope == null) {
throw FlutterError(
'Router operation requested with a context that does not include a Router.\n'
'The context used to retrieve the Router must be that of a widget that '
'is a descendant of a Router widget.',
);
}
return true;
}());
return scope!.routerState.widget as Router<T>;
}
/// Retrieves the immediate [Router] ancestor from the given context.
///
/// This method provides access to the delegates in the [Router]. For example,
/// this can be used to access the [backButtonDispatcher] of the parent router
/// when creating a [ChildBackButtonDispatcher] for a nested [Router].
///
/// If no `Router` ancestor exists for the given context, this will return
/// null.
///
/// See also:
///
/// * [of], a similar method that returns a non-nullable value, and will
/// throw if no [Router] ancestor exists.
static Router<T>? maybeOf<T extends Object?>(BuildContext context) {
final _RouterScope? scope = context.dependOnInheritedWidgetOfExactType<_RouterScope>();
return scope?.routerState.widget as Router<T>?;
}
/// Forces the [Router] to run the [callback] and create a new history
/// entry in the browser.
///
/// The web application relies on the [Router] to report new route information
/// in order to create browser history entry. The [Router] will only report
/// them if it detects the [RouteInformation.uri] changes. Use this
/// method if you want the [Router] to report the route information even if
/// the location does not change. This can be useful when you want to
/// support the browser backward and forward button without changing the URL.
///
/// For example, you can store certain state such as the scroll position into
/// the [RouteInformation.state]. If you use this method to update the
/// scroll position multiple times with the same URL, the browser will create
/// a stack of new history entries with the same URL but different
/// [RouteInformation.state]s that store the new scroll positions. If the user
/// click the backward button in the browser, the browser will restore the
/// scroll positions saved in history entries without changing the URL.
///
/// See also:
///
/// * [Router]: see the "URL updates for web applications" section for more
/// information about route information reporting.
/// * [neglect]: which forces the [Router] to not create a new history entry
/// even if location does change.
static void navigate(BuildContext context, VoidCallback callback) {
final _RouterScope scope = context
.getElementForInheritedWidgetOfExactType<_RouterScope>()!
.widget as _RouterScope;
scope.routerState._setStateWithExplicitReportStatus(RouteInformationReportingType.navigate, callback);
}
/// Forces the [Router] to run the [callback] without creating a new history
/// entry in the browser.
///
/// The web application relies on the [Router] to report new route information
/// in order to create browser history entry. The [Router] will report them
/// automatically if it detects the [RouteInformation.uri] changes.
///
/// Creating a new route history entry makes users feel they have visited a
/// new page, and the browser back button brings them back to previous history
/// entry. Use this method if you don't want the [Router] to create a new
/// route information even if it detects changes as a result of running the
/// [callback].
///
/// Using this method will still update the URL and state in current history
/// entry.
///
/// See also:
///
/// * [Router]: see the "URL updates for web applications" section for more
/// information about route information reporting.
/// * [navigate]: which forces the [Router] to create a new history entry
/// even if location does not change.
static void neglect(BuildContext context, VoidCallback callback) {
final _RouterScope scope = context
.getElementForInheritedWidgetOfExactType<_RouterScope>()!
.widget as _RouterScope;
scope.routerState._setStateWithExplicitReportStatus(RouteInformationReportingType.neglect, callback);
}
@override
State<Router<T>> createState() => _RouterState<T>();
}
typedef _AsyncPassthrough<Q> = Future<Q> Function(Q);
typedef _RouteSetter<T> = Future<void> Function(T);
/// The [Router]'s intention when it reports a new [RouteInformation] to the
/// [RouteInformationProvider].
///
/// See also:
///
/// * [RouteInformationProvider.routerReportsNewRouteInformation]: which is
/// called by the router when it has a new route information to report.
enum RouteInformationReportingType {
/// Router does not have a specific intention.
///
/// The router generates a new route information every time it detects route
/// information may have change due to a rebuild. This is the default type if
/// neither [Router.neglect] nor [Router.navigate] was used during the
/// rebuild.
none,
/// The accompanying [RouteInformation] were generated during a
/// [Router.neglect] call.
neglect,
/// The accompanying [RouteInformation] were generated during a
/// [Router.navigate] call.
navigate,
}
class _RouterState<T> extends State<Router<T>> with RestorationMixin {
Object? _currentRouterTransaction;
RouteInformationReportingType? _currentIntentionToReport;
final _RestorableRouteInformation _routeInformation = _RestorableRouteInformation();
late bool _routeParsePending;
@override
String? get restorationId => widget.restorationScopeId;
@override
void initState() {
super.initState();
widget.routeInformationProvider?.addListener(_handleRouteInformationProviderNotification);
widget.backButtonDispatcher?.addCallback(_handleBackButtonDispatcherNotification);
widget.routerDelegate.addListener(_handleRouterDelegateNotification);
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_routeInformation, 'route');
if (_routeInformation.value != null) {
assert(widget.routeInformationParser != null);
_processRouteInformation(_routeInformation.value!, () => widget.routerDelegate.setRestoredRoutePath);
} else if (widget.routeInformationProvider != null) {
_processRouteInformation(widget.routeInformationProvider!.value, () => widget.routerDelegate.setInitialRoutePath);
}
}
bool _routeInformationReportingTaskScheduled = false;
void _scheduleRouteInformationReportingTask() {
if (_routeInformationReportingTaskScheduled || widget.routeInformationProvider == null) {
return;
}
assert(_currentIntentionToReport != null);
_routeInformationReportingTaskScheduled = true;
SchedulerBinding.instance.addPostFrameCallback(
_reportRouteInformation,
debugLabel: 'Router.reportRouteInfo',
);
}
void _reportRouteInformation(Duration timestamp) {
if (!mounted) {
return;
}
assert(_routeInformationReportingTaskScheduled);
_routeInformationReportingTaskScheduled = false;
if (_routeInformation.value != null) {
final RouteInformation currentRouteInformation = _routeInformation.value!;
assert(_currentIntentionToReport != null);
widget.routeInformationProvider!.routerReportsNewRouteInformation(currentRouteInformation, type: _currentIntentionToReport!);
}
_currentIntentionToReport = RouteInformationReportingType.none;
}
RouteInformation? _retrieveNewRouteInformation() {
final T? configuration = widget.routerDelegate.currentConfiguration;
if (configuration == null) {
return null;
}
return widget.routeInformationParser?.restoreRouteInformation(configuration);
}
void _setStateWithExplicitReportStatus(
RouteInformationReportingType status,
VoidCallback fn,
) {
assert(status.index >= RouteInformationReportingType.neglect.index);
assert(() {
if (_currentIntentionToReport != null &&
_currentIntentionToReport != RouteInformationReportingType.none &&
_currentIntentionToReport != status) {
FlutterError.reportError(
const FlutterErrorDetails(
exception:
'Both Router.navigate and Router.neglect have been called in this '
'build cycle, and the Router cannot decide whether to report the '
'route information. Please make sure only one of them is called '
'within the same build cycle.',
),
);
}
return true;
}());
_currentIntentionToReport = status;
_scheduleRouteInformationReportingTask();
fn();
}
void _maybeNeedToReportRouteInformation() {
_routeInformation.value = _retrieveNewRouteInformation();
_currentIntentionToReport ??= RouteInformationReportingType.none;
_scheduleRouteInformationReportingTask();
}
@override
void didChangeDependencies() {
_routeParsePending = true;
super.didChangeDependencies();
// The super.didChangeDependencies may have parsed the route information.
// This can happen if the didChangeDependencies is triggered by state
// restoration or first build.
if (widget.routeInformationProvider != null && _routeParsePending) {
_processRouteInformation(widget.routeInformationProvider!.value, () => widget.routerDelegate.setNewRoutePath);
}
_routeParsePending = false;
_maybeNeedToReportRouteInformation();
}
@override
void didUpdateWidget(Router<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.routeInformationProvider != oldWidget.routeInformationProvider ||
widget.backButtonDispatcher != oldWidget.backButtonDispatcher ||
widget.routeInformationParser != oldWidget.routeInformationParser ||
widget.routerDelegate != oldWidget.routerDelegate) {
_currentRouterTransaction = Object();
}
if (widget.routeInformationProvider != oldWidget.routeInformationProvider) {
oldWidget.routeInformationProvider?.removeListener(_handleRouteInformationProviderNotification);
widget.routeInformationProvider?.addListener(_handleRouteInformationProviderNotification);
if (oldWidget.routeInformationProvider?.value != widget.routeInformationProvider?.value) {
_handleRouteInformationProviderNotification();
}
}
if (widget.backButtonDispatcher != oldWidget.backButtonDispatcher) {
oldWidget.backButtonDispatcher?.removeCallback(_handleBackButtonDispatcherNotification);
widget.backButtonDispatcher?.addCallback(_handleBackButtonDispatcherNotification);
}
if (widget.routerDelegate != oldWidget.routerDelegate) {
oldWidget.routerDelegate.removeListener(_handleRouterDelegateNotification);
widget.routerDelegate.addListener(_handleRouterDelegateNotification);
_maybeNeedToReportRouteInformation();
}
}
@override
void dispose() {
_routeInformation.dispose();
widget.routeInformationProvider?.removeListener(_handleRouteInformationProviderNotification);
widget.backButtonDispatcher?.removeCallback(_handleBackButtonDispatcherNotification);
widget.routerDelegate.removeListener(_handleRouterDelegateNotification);
_currentRouterTransaction = null;
super.dispose();
}
void _processRouteInformation(RouteInformation information, ValueGetter<_RouteSetter<T>> delegateRouteSetter) {
assert(_routeParsePending);
_routeParsePending = false;
_currentRouterTransaction = Object();
widget.routeInformationParser!
.parseRouteInformationWithDependencies(information, context)
.then<void>(_processParsedRouteInformation(_currentRouterTransaction, delegateRouteSetter));
}
_RouteSetter<T> _processParsedRouteInformation(Object? transaction, ValueGetter<_RouteSetter<T>> delegateRouteSetter) {
return (T data) async {
if (_currentRouterTransaction != transaction) {
return;
}
await delegateRouteSetter()(data);
if (_currentRouterTransaction == transaction) {
_rebuild();
}
};
}
void _handleRouteInformationProviderNotification() {
_routeParsePending = true;
_processRouteInformation(widget.routeInformationProvider!.value, () => widget.routerDelegate.setNewRoutePath);
}
Future<bool> _handleBackButtonDispatcherNotification() {
_currentRouterTransaction = Object();
return widget.routerDelegate
.popRoute()
.then<bool>(_handleRoutePopped(_currentRouterTransaction));
}
_AsyncPassthrough<bool> _handleRoutePopped(Object? transaction) {
return (bool data) {
if (transaction != _currentRouterTransaction) {
// A rebuilt was trigger from a different source. Returns true to
// prevent bubbling.
return SynchronousFuture<bool>(true);
}
_rebuild();
return SynchronousFuture<bool>(data);
};
}
Future<void> _rebuild([void value]) {
setState(() {/* routerDelegate is ready to rebuild */});
_maybeNeedToReportRouteInformation();
return SynchronousFuture<void>(value);
}
void _handleRouterDelegateNotification() {
setState(() {/* routerDelegate wants to rebuild */});
_maybeNeedToReportRouteInformation();
}
@override
Widget build(BuildContext context) {
return UnmanagedRestorationScope(
bucket: bucket,
child: _RouterScope(
routeInformationProvider: widget.routeInformationProvider,
backButtonDispatcher: widget.backButtonDispatcher,
routeInformationParser: widget.routeInformationParser,
routerDelegate: widget.routerDelegate,
routerState: this,
child: Builder(
// Use a Builder so that the build method below will have a
// BuildContext that contains the _RouterScope. This also prevents
// dependencies look ups in routerDelegate from rebuilding Router
// widget that may result in re-parsing the route information.
builder: widget.routerDelegate.build,
),
),
);
}
}
class _RouterScope extends InheritedWidget {
const _RouterScope({
required this.routeInformationProvider,
required this.backButtonDispatcher,
required this.routeInformationParser,
required this.routerDelegate,
required this.routerState,
required super.child,
}) : assert(routeInformationProvider == null || routeInformationParser != null);
final ValueListenable<RouteInformation?>? routeInformationProvider;
final BackButtonDispatcher? backButtonDispatcher;
final RouteInformationParser<Object?>? routeInformationParser;
final RouterDelegate<Object?> routerDelegate;
final _RouterState<Object?> routerState;
@override
bool updateShouldNotify(_RouterScope oldWidget) {
return routeInformationProvider != oldWidget.routeInformationProvider ||
backButtonDispatcher != oldWidget.backButtonDispatcher ||
routeInformationParser != oldWidget.routeInformationParser ||
routerDelegate != oldWidget.routerDelegate ||
routerState != oldWidget.routerState;
}
}
/// A class that can be extended or mixed in that invokes a single callback,
/// which then returns a value.
///
/// While multiple callbacks can be registered, when a notification is
/// dispatched there must be only a single callback. The return values of
/// multiple callbacks are not aggregated.
///
/// `T` is the return value expected from the callback.
///
/// See also:
///
/// * [Listenable] and its subclasses, which provide a similar mechanism for
/// one-way signaling.
class _CallbackHookProvider<T> {
final ObserverList<ValueGetter<T>> _callbacks = ObserverList<ValueGetter<T>>();
/// Whether a callback is currently registered.
@protected
bool get hasCallbacks => _callbacks.isNotEmpty;
/// Register the callback to be called when the object changes.
///
/// If other callbacks have already been registered, they must be removed
/// (with [removeCallback]) before the callback is next called.
void addCallback(ValueGetter<T> callback) => _callbacks.add(callback);
/// Remove a previously registered callback.
///
/// If the given callback is not registered, the call is ignored.
void removeCallback(ValueGetter<T> callback) => _callbacks.remove(callback);
/// Calls the (single) registered callback and returns its result.
///
/// If no callback is registered, or if the callback throws, returns
/// `defaultValue`.
///
/// Call this method whenever the callback is to be invoked. If there is more
/// than one callback registered, this method will throw a [StateError].
///
/// Exceptions thrown by callbacks will be caught and reported using
/// [FlutterError.reportError].
@protected
@pragma('vm:notify-debugger-on-exception')
T invokeCallback(T defaultValue) {
if (_callbacks.isEmpty) {
return defaultValue;
}
try {
return _callbacks.single();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widget library',
context: ErrorDescription('while invoking the callback for $runtimeType'),
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<_CallbackHookProvider<T>>(
'The $runtimeType that invoked the callback was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
],
));
return defaultValue;
}
}
}
/// Report to a [Router] when the user taps the back button on platforms that
/// support back buttons (such as Android).
///
/// When [Router] widgets are nested, consider using a
/// [ChildBackButtonDispatcher], passing it the parent [BackButtonDispatcher],
/// so that the back button requests get dispatched to the appropriate [Router].
/// To make this work properly, it's important that whenever a [Router] thinks
/// it should get the back button messages (e.g. after the user taps inside it),
/// it calls [takePriority] on its [BackButtonDispatcher] (or
/// [ChildBackButtonDispatcher]) instance.
///
/// The class takes a single callback, which must return a [Future<bool>]. The
/// callback's semantics match [WidgetsBindingObserver.didPopRoute]'s, namely,
/// the callback should return a future that completes to true if it can handle
/// the pop request, and a future that completes to false otherwise.
abstract class BackButtonDispatcher extends _CallbackHookProvider<Future<bool>> {
late final LinkedHashSet<ChildBackButtonDispatcher> _children =
<ChildBackButtonDispatcher>{} as LinkedHashSet<ChildBackButtonDispatcher>;
@override
bool get hasCallbacks => super.hasCallbacks || (_children.isNotEmpty);
/// Handles a pop route request.
///
/// This method prioritizes the children list in reverse order and calls
/// [ChildBackButtonDispatcher.notifiedByParent] on them. If any of them
/// handles the request (by returning a future with true), it exits this
/// method by returning this future. Otherwise, it keeps moving on to the next
/// child until a child handles the request. If none of the children handles
/// the request, this back button dispatcher will then try to handle the request
/// by itself. This back button dispatcher handles the request by notifying the
/// router which in turn calls the [RouterDelegate.popRoute] and returns its
/// result.
///
/// To decide whether this back button dispatcher will handle the pop route
/// request, you can override the [RouterDelegate.popRoute] of the router
/// delegate you pass into the router with this back button dispatcher to
/// return a future of true or false.
@override
Future<bool> invokeCallback(Future<bool> defaultValue) {
if (_children.isNotEmpty) {
final List<ChildBackButtonDispatcher> children = _children.toList();
int childIndex = children.length - 1;
Future<bool> notifyNextChild(bool result) {
// If the previous child handles the callback, we return the result.
if (result) {
return SynchronousFuture<bool>(result);
}
// If the previous child did not handle the callback, we ask the next
// child to handle the it.
if (childIndex > 0) {
childIndex -= 1;
return children[childIndex]
.notifiedByParent(defaultValue)
.then<bool>(notifyNextChild);
}
// If none of the child handles the callback, the parent will then handle it.
return super.invokeCallback(defaultValue);
}
return children[childIndex]
.notifiedByParent(defaultValue)
.then<bool>(notifyNextChild);
}
return super.invokeCallback(defaultValue);
}
/// Creates a [ChildBackButtonDispatcher] that is a direct descendant of this
/// back button dispatcher.
///
/// To participate in handling the pop route request, call the [takePriority]
/// on the [ChildBackButtonDispatcher] created from this method.
///
/// When the pop route request is handled by this back button dispatcher, it
/// propagate the request to its direct descendants that have called the
/// [takePriority] method. If there are multiple candidates, the latest one
/// that called the [takePriority] wins the right to handle the request. If
/// the latest one does not handle the request (by returning a future of
/// false in [ChildBackButtonDispatcher.notifiedByParent]), the second latest
/// one will then have the right to handle the request. This dispatcher
/// continues finding the next candidate until there are no more candidates
/// and finally handles the request itself.
ChildBackButtonDispatcher createChildBackButtonDispatcher() {
return ChildBackButtonDispatcher(this);
}
/// Make this [BackButtonDispatcher] take priority among its peers.
///
/// This has no effect when a [BackButtonDispatcher] has no parents and no
/// children. If a [BackButtonDispatcher] does have parents or children,
/// however, it causes this object to be the one to dispatch the notification
/// when the parent would normally notify its callback.
///
/// The [BackButtonDispatcher] must have a listener registered before it can
/// be told to take priority.
void takePriority() => _children.clear();
/// Mark the given child as taking priority over this object and the other
/// children.
///
/// This causes [invokeCallback] to defer to the given child instead of
/// calling this object's callback.
///
/// Children are stored in a list, so that if the current child is removed
/// using [forget], a previous child will return to take its place. When
/// [takePriority] is called, the list is cleared.
///
/// Calling this again without first calling [forget] moves the child back to
/// the head of the list.
///
/// The [BackButtonDispatcher] must have a listener registered before it can
/// be told to defer to a child.
void deferTo(ChildBackButtonDispatcher child) {
assert(hasCallbacks);
_children.remove(child); // child may or may not be in the set already
_children.add(child);
}
/// Causes the given child to be removed from the list of children to which
/// this object might defer, as if [deferTo] had never been called for that
/// child.
///
/// This should only be called once per child, even if [deferTo] was called
/// multiple times for that child.
///
/// If no children are left in the list, this object will stop deferring to
/// its children. (This is not the same as calling [takePriority], since, if
/// this object itself is a [ChildBackButtonDispatcher], [takePriority] would
/// additionally attempt to claim priority from its parent, whereas removing
/// the last child does not.)
void forget(ChildBackButtonDispatcher child) => _children.remove(child);
}
/// The default implementation of back button dispatcher for the root router.
///
/// This dispatcher listens to platform pop route notifications. When the
/// platform wants to pop the current route, this dispatcher calls the
/// [BackButtonDispatcher.invokeCallback] method to handle the request.
class RootBackButtonDispatcher extends BackButtonDispatcher with WidgetsBindingObserver {
/// Create a root back button dispatcher.
RootBackButtonDispatcher();
@override
void addCallback(ValueGetter<Future<bool>> callback) {
if (!hasCallbacks) {
WidgetsBinding.instance.addObserver(this);
}
super.addCallback(callback);
}
@override
void removeCallback(ValueGetter<Future<bool>> callback) {
super.removeCallback(callback);
if (!hasCallbacks) {
WidgetsBinding.instance.removeObserver(this);
}
}
@override
Future<bool> didPopRoute() => invokeCallback(Future<bool>.value(false));
}
/// A variant of [BackButtonDispatcher] which listens to notifications from a
/// parent back button dispatcher, and can take priority from its parent for the
/// handling of such notifications.
///
/// Useful when [Router]s are being nested within each other.
///
/// Use [Router.of] to obtain a reference to the nearest ancestor [Router], from
/// which the [Router.backButtonDispatcher] can be found, and then used as the
/// [parent] of the [ChildBackButtonDispatcher].
class ChildBackButtonDispatcher extends BackButtonDispatcher {
/// Creates a back button dispatcher that acts as the child of another.
ChildBackButtonDispatcher(this.parent);
/// The back button dispatcher that this object will attempt to take priority
/// over when [takePriority] is called.
///
/// The parent must have a listener registered before this child object can
/// have its [takePriority] or [deferTo] methods used.
final BackButtonDispatcher parent;
/// The parent of this child back button dispatcher decide to let this
/// child to handle the invoke the callback request in
/// [BackButtonDispatcher.invokeCallback].
///
/// Return a boolean future with true if this child will handle the request;
/// otherwise, return a boolean future with false.
@protected
Future<bool> notifiedByParent(Future<bool> defaultValue) {
return invokeCallback(defaultValue);
}
@override
void takePriority() {
parent.deferTo(this);
super.takePriority();
}
@override
void deferTo(ChildBackButtonDispatcher child) {
assert(hasCallbacks);
parent.deferTo(this);
super.deferTo(child);
}
@override
void removeCallback(ValueGetter<Future<bool>> callback) {
super.removeCallback(callback);
if (!hasCallbacks) {
parent.forget(this);
}
}
}
/// A convenience widget that registers a callback for when the back button is pressed.
///
/// In order to use this widget, there must be an ancestor [Router] widget in the tree
/// that has a [RootBackButtonDispatcher]. e.g. The [Router] widget created by the
/// [MaterialApp.router] has a built-in [RootBackButtonDispatcher] by default.
///
/// It only applies to platforms that accept back button clicks, such as Android.
///
/// It can be useful for scenarios, in which you create a different state in your
/// screen but don't want to use a new page for that.
class BackButtonListener extends StatefulWidget {
/// Creates a BackButtonListener widget .
const BackButtonListener({
super.key,
required this.child,
required this.onBackButtonPressed,
});
/// The widget below this widget in the tree.
final Widget child;
/// The callback function that will be called when the back button is pressed.
///
/// It must return a boolean future with true if this child will handle the request;
/// otherwise, return a boolean future with false.
final ValueGetter<Future<bool>> onBackButtonPressed;
@override
State<BackButtonListener> createState() => _BackButtonListenerState();
}
class _BackButtonListenerState extends State<BackButtonListener> {
BackButtonDispatcher? dispatcher;
@override
void didChangeDependencies() {
dispatcher?.removeCallback(widget.onBackButtonPressed);
final BackButtonDispatcher? rootBackDispatcher = Router.of(context).backButtonDispatcher;
assert(rootBackDispatcher != null, 'The parent router must have a backButtonDispatcher to use this widget');
dispatcher = rootBackDispatcher!.createChildBackButtonDispatcher()
..addCallback(widget.onBackButtonPressed)
..takePriority();
super.didChangeDependencies();
}
@override
void didUpdateWidget(covariant BackButtonListener oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.onBackButtonPressed != widget.onBackButtonPressed) {
dispatcher?.removeCallback(oldWidget.onBackButtonPressed);
dispatcher?.addCallback(widget.onBackButtonPressed);
dispatcher?.takePriority();
}
}
@override
void dispose() {
dispatcher?.removeCallback(widget.onBackButtonPressed);
super.dispose();
}
@override
Widget build(BuildContext context) => widget.child;
}
/// A delegate that is used by the [Router] widget to parse a route information
/// into a configuration of type T.
///
/// This delegate is used when the [Router] widget is first built with initial
/// route information from [Router.routeInformationProvider] and any subsequent
/// new route notifications from it. The [Router] widget calls the [parseRouteInformation]
/// with the route information from [Router.routeInformationProvider].
///
/// One of the [parseRouteInformation] or
/// [parseRouteInformationWithDependencies] must be implemented, otherwise a
/// runtime error will be thrown.
abstract class RouteInformationParser<T> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RouteInformationParser();
/// {@template flutter.widgets.RouteInformationParser.parseRouteInformation}
/// Converts the given route information into parsed data to pass to a
/// [RouterDelegate].
///
/// The method should return a future which completes when the parsing is
/// complete. The parsing may be asynchronous if, e.g., the parser needs to
/// communicate with the OEM thread to obtain additional data about the route.
///
/// Consider using a [SynchronousFuture] if the result can be computed
/// synchronously, so that the [Router] does not need to wait for the next
/// microtask to pass the data to the [RouterDelegate].
/// {@endtemplate}
///
/// One can implement [parseRouteInformationWithDependencies] instead if
/// the parsing depends on other dependencies from the [BuildContext].
Future<T> parseRouteInformation(RouteInformation routeInformation) {
throw UnimplementedError(
'One of the parseRouteInformation or '
'parseRouteInformationWithDependencies must be implemented'
);
}
/// {@macro flutter.widgets.RouteInformationParser.parseRouteInformation}
///
/// The input [BuildContext] can be used for looking up [InheritedWidget]s
/// If one uses [BuildContext.dependOnInheritedWidgetOfExactType], a
/// dependency will be created. The [Router] will re-parse the
/// [RouteInformation] from its [RouteInformationProvider] if the dependency
/// notifies its listeners.
///
/// One can also use [BuildContext.getElementForInheritedWidgetOfExactType] to
/// look up [InheritedWidget]s without creating dependencies.
Future<T> parseRouteInformationWithDependencies(RouteInformation routeInformation, BuildContext context) {
return parseRouteInformation(routeInformation);
}
/// Restore the route information from the given configuration.
///
/// This may return null, in which case the browser history will not be
/// updated and state restoration is disabled. See [Router]'s documentation
/// for details.
///
/// The [parseRouteInformation] method must produce an equivalent
/// configuration when passed this method's return value.
RouteInformation? restoreRouteInformation(T configuration) => null;
}
/// A delegate that is used by the [Router] widget to build and configure a
/// navigating widget.
///
/// This delegate is the core piece of the [Router] widget. It responds to
/// push route and pop route intents from the engine and notifies the [Router]
/// to rebuild. It also acts as a builder for the [Router] widget and builds a
/// navigating widget, typically a [Navigator], when the [Router] widget
/// builds.
///
/// When the engine pushes a new route, the route information is parsed by the
/// [RouteInformationParser] to produce a configuration of type T. The router
/// delegate receives the configuration through [setInitialRoutePath] or
/// [setNewRoutePath] to configure itself and builds the latest navigating
/// widget when asked ([build]).
///
/// When implementing subclasses, consider defining a [Listenable] app state object to be
/// used for building the navigating widget. The router delegate would update
/// the app state accordingly and notify its own listeners when the app state has
/// changed and when it receive route related engine intents (e.g.
/// [setNewRoutePath], [setInitialRoutePath], or [popRoute]).
///
/// All subclass must implement [setNewRoutePath], [popRoute], and [build].
///
/// ## State Restoration
///
/// If the [Router] owning this delegate is configured for state restoration, it
/// will persist and restore the configuration of this [RouterDelegate] using
/// the following mechanism: Before the app is killed by the operating system,
/// the value of [currentConfiguration] is serialized out and persisted. After
/// the app has restarted, the value is deserialized and passed back to the
/// [RouterDelegate] via a call to [setRestoredRoutePath] (which by default just
/// calls [setNewRoutePath]). It is the responsibility of the [RouterDelegate]
/// to use the configuration information provided to restore its internal state.
///
/// See also:
///
/// * [RouteInformationParser], which is responsible for parsing the route
/// information to a configuration before passing in to router delegate.
/// * [Router], which is the widget that wires all the delegates together to
/// provide a fully functional routing solution.
abstract class RouterDelegate<T> extends Listenable {
/// Called by the [Router] at startup with the structure that the
/// [RouteInformationParser] obtained from parsing the initial route.
///
/// This should configure the [RouterDelegate] so that when [build] is
/// invoked, it will create a widget tree that matches the initial route.
///
/// By default, this method forwards the [configuration] to [setNewRoutePath].
///
/// Consider using a [SynchronousFuture] if the result can be computed
/// synchronously, so that the [Router] does not need to wait for the next
/// microtask to schedule a build.
///
/// See also:
///
/// * [setRestoredRoutePath], which is called instead of this method during
/// state restoration.
Future<void> setInitialRoutePath(T configuration) {
return setNewRoutePath(configuration);
}
/// Called by the [Router] during state restoration.
///
/// When the [Router] is configured for state restoration, it will persist
/// the value of [currentConfiguration] during state serialization. During
/// state restoration, the [Router] calls this method (instead of
/// [setInitialRoutePath]) to pass the previous configuration back to the
/// delegate. It is the responsibility of the delegate to restore its internal
/// state based on the provided configuration.
///
/// By default, this method forwards the `configuration` to [setNewRoutePath].
Future<void> setRestoredRoutePath(T configuration) {
return setNewRoutePath(configuration);
}
/// Called by the [Router] when the [Router.routeInformationProvider] reports that a
/// new route has been pushed to the application by the operating system.
///
/// Consider using a [SynchronousFuture] if the result can be computed
/// synchronously, so that the [Router] does not need to wait for the next
/// microtask to schedule a build.
Future<void> setNewRoutePath(T configuration);
/// Called by the [Router] when the [Router.backButtonDispatcher] reports that
/// the operating system is requesting that the current route be popped.
///
/// The method should return a boolean [Future] to indicate whether this
/// delegate handles the request. Returning false will cause the entire app
/// to be popped.
///
/// Consider using a [SynchronousFuture] if the result can be computed
/// synchronously, so that the [Router] does not need to wait for the next
/// microtask to schedule a build.
Future<bool> popRoute();
/// Called by the [Router] when it detects a route information may have
/// changed as a result of rebuild.
///
/// If this getter returns non-null, the [Router] will start to report new
/// route information back to the engine. In web applications, the new
/// route information is used for populating browser history in order to
/// support the forward and the backward buttons.
///
/// When overriding this method, the configuration returned by this getter
/// must be able to construct the current app state and build the widget
/// with the same configuration in the [build] method if it is passed back
/// to the [setNewRoutePath]. Otherwise, the browser backward and forward
/// buttons will not work properly.
///
/// By default, this getter returns null, which prevents the [Router] from
/// reporting the route information. To opt in, a subclass can override this
/// getter to return the current configuration.
///
/// At most one [Router] can opt in to route information reporting. Typically,
/// only the top-most [Router] created by [WidgetsApp.router] should opt for
/// route information reporting.
///
/// ## State Restoration
///
/// This getter is also used by the [Router] to implement state restoration.
/// During state serialization, the [Router] will persist the current
/// configuration and during state restoration pass it back to the delegate
/// by calling [setRestoredRoutePath].
T? get currentConfiguration => null;
/// Called by the [Router] to obtain the widget tree that represents the
/// current state.
///
/// This is called whenever the [Future]s returned by [setInitialRoutePath],
/// [setNewRoutePath], or [setRestoredRoutePath] complete as well as when this
/// notifies its clients (see the [Listenable] interface, which this interface
/// includes). In addition, it may be called at other times. It is important,
/// therefore, that the methods above do not update the state that the [build]
/// method uses before they complete their respective futures.
///
/// Typically this method returns a suitably-configured [Navigator]. If you do
/// plan to create a navigator, consider using the
/// [PopNavigatorRouterDelegateMixin]. If state restoration is enabled for the
/// [Router] using this delegate, consider providing a non-null
/// [Navigator.restorationScopeId] to the [Navigator] returned by this method.
///
/// This method must not return null.
///
/// The `context` is the [Router]'s build context.
Widget build(BuildContext context);
}
/// A route information provider that provides route information for the
/// [Router] widget
///
/// This provider is responsible for handing the route information through [value]
/// getter and notifies listeners, typically the [Router] widget, when a new
/// route information is available.
///
/// When the router opts for route information reporting (by overriding the
/// [RouterDelegate.currentConfiguration] to return non-null), override the
/// [routerReportsNewRouteInformation] method to process the route information.
///
/// See also:
///
/// * [PlatformRouteInformationProvider], which wires up the itself with the
/// [WidgetsBindingObserver.didPushRoute] to propagate platform push route
/// intent to the [Router] widget, as well as reports new route information
/// from the [Router] back to the engine by overriding the
/// [routerReportsNewRouteInformation].
abstract class RouteInformationProvider extends ValueListenable<RouteInformation> {
/// A callback called when the [Router] widget reports new route information
///
/// The subclasses can override this method to update theirs values or trigger
/// other side effects. For example, the [PlatformRouteInformationProvider]
/// overrides this method to report the route information back to the engine.
///
/// The `routeInformation` is the new route information generated by the
/// Router rebuild, and it can be the same or different from the
/// [value].
///
/// The `type` denotes the [Router]'s intention when it reports this
/// `routeInformation`. It is useful when deciding how to update the internal
/// state of [RouteInformationProvider] subclass with the `routeInformation`.
/// For example, [PlatformRouteInformationProvider] uses this property to
/// decide whether to push or replace the browser history entry with the new
/// `routeInformation`.
///
/// For more information on how [Router] determines a navigation event, see
/// the "URL updates for web applications" section in the [Router]
/// documentation.
void routerReportsNewRouteInformation(RouteInformation routeInformation, {RouteInformationReportingType type = RouteInformationReportingType.none}) {}
}
/// The route information provider that propagates the platform route information changes.
///
/// This provider also reports the new route information from the [Router] widget
/// back to engine using message channel method, the
/// [SystemNavigator.routeInformationUpdated].
///
/// Each time [SystemNavigator.routeInformationUpdated] is called, the
/// [SystemNavigator.selectMultiEntryHistory] method is also called. This
/// overrides the initialization behavior of
/// [Navigator.reportsRouteUpdateToEngine].
class PlatformRouteInformationProvider extends RouteInformationProvider with WidgetsBindingObserver, ChangeNotifier {
/// Create a platform route information provider.
///
/// Use the [initialRouteInformation] to set the default route information for this
/// provider.
PlatformRouteInformationProvider({
required RouteInformation initialRouteInformation,
}) : _value = initialRouteInformation {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
static bool _equals(Uri a, Uri b) {
return a.path == b.path
&& a.fragment == b.fragment
&& const DeepCollectionEquality.unordered().equals(a.queryParametersAll, b.queryParametersAll);
}
@override
void routerReportsNewRouteInformation(RouteInformation routeInformation, {RouteInformationReportingType type = RouteInformationReportingType.none}) {
final bool replace =
type == RouteInformationReportingType.neglect ||
(type == RouteInformationReportingType.none &&
_equals(_valueInEngine.uri, routeInformation.uri));
SystemNavigator.selectMultiEntryHistory();
SystemNavigator.routeInformationUpdated(
uri: routeInformation.uri,
state: routeInformation.state,
replace: replace,
);
_value = routeInformation;
_valueInEngine = routeInformation;
}
@override
RouteInformation get value => _value;
RouteInformation _value;
RouteInformation _valueInEngine = RouteInformation(uri: Uri.parse(WidgetsBinding.instance.platformDispatcher.defaultRouteName));
void _platformReportsNewRouteInformation(RouteInformation routeInformation) {
if (_value == routeInformation) {
return;
}
_value = routeInformation;
_valueInEngine = routeInformation;
notifyListeners();
}
@override
void addListener(VoidCallback listener) {
if (!hasListeners) {
WidgetsBinding.instance.addObserver(this);
}
super.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
super.removeListener(listener);
if (!hasListeners) {
WidgetsBinding.instance.removeObserver(this);
}
}
@override
void dispose() {
// In practice, this will rarely be called. We assume that the listeners
// will be added and removed in a coherent fashion such that when the object
// is no longer being used, there's no listener, and so it will get garbage
// collected.
if (hasListeners) {
WidgetsBinding.instance.removeObserver(this);
}
super.dispose();
}
@override
Future<bool> didPushRouteInformation(RouteInformation routeInformation) async {
assert(hasListeners);
_platformReportsNewRouteInformation(routeInformation);
return true;
}
}
/// A mixin that wires [RouterDelegate.popRoute] to the [Navigator] it builds.
///
/// This mixin calls [Navigator.maybePop] when it receives an Android back
/// button intent through the [RouterDelegate.popRoute]. Using this mixin
/// guarantees that the back button still respects pageless routes in the
/// navigator.
///
/// Only use this mixin if you plan to build a navigator in the
/// [RouterDelegate.build].
mixin PopNavigatorRouterDelegateMixin<T> on RouterDelegate<T> {
/// The key used for retrieving the current navigator.
///
/// When using this mixin, be sure to use this key to create the navigator.
GlobalKey<NavigatorState>? get navigatorKey;
@override
Future<bool> popRoute() {
final NavigatorState? navigator = navigatorKey?.currentState;
return navigator?.maybePop() ?? SynchronousFuture<bool>(false);
}
}
class _RestorableRouteInformation extends RestorableValue<RouteInformation?> {
@override
RouteInformation? createDefaultValue() => null;
@override
void didUpdateValue(RouteInformation? oldValue) {
notifyListeners();
}
@override
RouteInformation? fromPrimitives(Object? data) {
if (data == null) {
return null;
}
assert(data is List<Object?> && data.length == 2);
final List<Object?> castedData = data as List<Object?>;
final String? uri = castedData.first as String?;
if (uri == null) {
return null;
}
return RouteInformation(uri: Uri.parse(uri), state: castedData.last);
}
@override
Object? toPrimitives() {
return value == null ? null : <Object?>[value!.uri.toString(), value!.state];
}
}
| flutter/packages/flutter/lib/src/widgets/router.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/router.dart",
"repo_id": "flutter",
"token_count": 18823
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'debug.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'media_query.dart';
import 'notification_listener.dart';
import 'primary_scroll_controller.dart';
import 'scroll_configuration.dart';
import 'scroll_controller.dart';
import 'scroll_delegate.dart';
import 'scroll_notification.dart';
import 'scroll_physics.dart';
import 'scrollable.dart';
import 'scrollable_helpers.dart';
import 'sliver.dart';
import 'sliver_prototype_extent_list.dart';
import 'sliver_varied_extent_list.dart';
import 'viewport.dart';
// Examples can assume:
// late int itemCount;
/// A representation of how a [ScrollView] should dismiss the on-screen
/// keyboard.
enum ScrollViewKeyboardDismissBehavior {
/// `manual` means there is no automatic dismissal of the on-screen keyboard.
/// It is up to the client to dismiss the keyboard.
manual,
/// `onDrag` means that the [ScrollView] will dismiss an on-screen keyboard
/// when a drag begins.
onDrag,
}
/// A widget that combines a [Scrollable] and a [Viewport] to create an
/// interactive scrolling pane of content in one dimension.
///
/// Scrollable widgets consist of three pieces:
///
/// 1. A [Scrollable] widget, which listens for various user gestures and
/// implements the interaction design for scrolling.
/// 2. A viewport widget, such as [Viewport] or [ShrinkWrappingViewport], which
/// implements the visual design for scrolling by displaying only a portion
/// of the widgets inside the scroll view.
/// 3. One or more slivers, which are widgets that can be composed to created
/// various scrolling effects, such as lists, grids, and expanding headers.
///
/// [ScrollView] helps orchestrate these pieces by creating the [Scrollable] and
/// the viewport and deferring to its subclass to create the slivers.
///
/// To learn more about slivers, see [CustomScrollView.slivers].
///
/// To control the initial scroll offset of the scroll view, provide a
/// [controller] with its [ScrollController.initialScrollOffset] property set.
///
/// {@template flutter.widgets.ScrollView.PageStorage}
/// ## Persisting the scroll position during a session
///
/// Scroll views attempt to persist their scroll position using [PageStorage].
/// This can be disabled by setting [ScrollController.keepScrollOffset] to false
/// on the [controller]. If it is enabled, using a [PageStorageKey] for the
/// [key] of this widget is recommended to help disambiguate different scroll
/// views from each other.
/// {@endtemplate}
///
/// See also:
///
/// * [ListView], which is a commonly used [ScrollView] that displays a
/// scrolling, linear list of child widgets.
/// * [PageView], which is a scrolling list of child widgets that are each the
/// size of the viewport.
/// * [GridView], which is a [ScrollView] that displays a scrolling, 2D array
/// of child widgets.
/// * [CustomScrollView], which is a [ScrollView] that creates custom scroll
/// effects using slivers.
/// * [ScrollNotification] and [NotificationListener], which can be used to watch
/// the scroll position without using a [ScrollController].
/// * [TwoDimensionalScrollView], which is a similar widget [ScrollView] that
/// scrolls in two dimensions.
abstract class ScrollView extends StatelessWidget {
/// Creates a widget that scrolls.
///
/// The [ScrollView.primary] argument defaults to true for vertical
/// scroll views if no [controller] has been provided. The [controller] argument
/// must be null if [primary] is explicitly set to true. If [primary] is true,
/// the nearest [PrimaryScrollController] surrounding the widget is attached
/// to this scroll view.
///
/// If the [shrinkWrap] argument is true, the [center] argument must be null.
///
/// The [anchor] argument must be in the range zero to one, inclusive.
const ScrollView({
super.key,
this.scrollDirection = Axis.vertical,
this.reverse = false,
this.controller,
this.primary,
ScrollPhysics? physics,
this.scrollBehavior,
this.shrinkWrap = false,
this.center,
this.anchor = 0.0,
this.cacheExtent,
this.semanticChildCount,
this.dragStartBehavior = DragStartBehavior.start,
this.keyboardDismissBehavior = ScrollViewKeyboardDismissBehavior.manual,
this.restorationId,
this.clipBehavior = Clip.hardEdge,
}) : assert(
!(controller != null && (primary ?? false)),
'Primary ScrollViews obtain their ScrollController via inheritance '
'from a PrimaryScrollController widget. You cannot both set primary to '
'true and pass an explicit controller.',
),
assert(!shrinkWrap || center == null),
assert(anchor >= 0.0 && anchor <= 1.0),
assert(semanticChildCount == null || semanticChildCount >= 0),
physics = physics ?? ((primary ?? false) || (primary == null && controller == null && identical(scrollDirection, Axis.vertical)) ? const AlwaysScrollableScrollPhysics() : null);
/// {@template flutter.widgets.scroll_view.scrollDirection}
/// The [Axis] along which the scroll view's offset increases.
///
/// For the direction in which active scrolling may be occurring, see
/// [ScrollDirection].
///
/// Defaults to [Axis.vertical].
/// {@endtemplate}
final Axis scrollDirection;
/// {@template flutter.widgets.scroll_view.reverse}
/// Whether the scroll view scrolls in the reading direction.
///
/// For example, if the reading direction is left-to-right and
/// [scrollDirection] is [Axis.horizontal], then the scroll view scrolls from
/// left to right when [reverse] is false and from right to left when
/// [reverse] is true.
///
/// Similarly, if [scrollDirection] is [Axis.vertical], then the scroll view
/// scrolls from top to bottom when [reverse] is false and from bottom to top
/// when [reverse] is true.
///
/// Defaults to false.
/// {@endtemplate}
final bool reverse;
/// {@template flutter.widgets.scroll_view.controller}
/// An object that can be used to control the position to which this scroll
/// view is scrolled.
///
/// Must be null if [primary] is true.
///
/// A [ScrollController] serves several purposes. It can be used to control
/// the initial scroll position (see [ScrollController.initialScrollOffset]).
/// It can be used to control whether the scroll view should automatically
/// save and restore its scroll position in the [PageStorage] (see
/// [ScrollController.keepScrollOffset]). It can be used to read the current
/// scroll position (see [ScrollController.offset]), or change it (see
/// [ScrollController.animateTo]).
/// {@endtemplate}
final ScrollController? controller;
/// {@template flutter.widgets.scroll_view.primary}
/// Whether this is the primary scroll view associated with the parent
/// [PrimaryScrollController].
///
/// When this is true, the scroll view is scrollable even if it does not have
/// sufficient content to actually scroll. Otherwise, by default the user can
/// only scroll the view if it has sufficient content. See [physics].
///
/// Also when true, the scroll view is used for default [ScrollAction]s. If a
/// ScrollAction is not handled by an otherwise focused part of the application,
/// the ScrollAction will be evaluated using this scroll view, for example,
/// when executing [Shortcuts] key events like page up and down.
///
/// On iOS, this also identifies the scroll view that will scroll to top in
/// response to a tap in the status bar.
///
/// Cannot be true while a [ScrollController] is provided to `controller`,
/// only one ScrollController can be associated with a ScrollView.
///
/// Setting to false will explicitly prevent inheriting any
/// [PrimaryScrollController].
///
/// Defaults to null. When null, and a controller is not provided,
/// [PrimaryScrollController.shouldInherit] is used to decide automatic
/// inheritance.
///
/// By default, the [PrimaryScrollController] that is injected by each
/// [ModalRoute] is configured to automatically be inherited on
/// [TargetPlatformVariant.mobile] for ScrollViews in the [Axis.vertical]
/// scroll direction. Adding another to your app will override the
/// PrimaryScrollController above it.
///
/// The following video contains more information about scroll controllers,
/// the PrimaryScrollController widget, and their impact on your apps:
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=33_0ABjFJUU}
///
/// {@endtemplate}
final bool? primary;
/// {@template flutter.widgets.scroll_view.physics}
/// How the scroll view should respond to user input.
///
/// For example, determines how the scroll view continues to animate after the
/// user stops dragging the scroll view.
///
/// Defaults to matching platform conventions. Furthermore, if [primary] is
/// false, then the user cannot scroll if there is insufficient content to
/// scroll, while if [primary] is true, they can always attempt to scroll.
///
/// To force the scroll view to always be scrollable even if there is
/// insufficient content, as if [primary] was true but without necessarily
/// setting it to true, provide an [AlwaysScrollableScrollPhysics] physics
/// object, as in:
///
/// ```dart
/// physics: const AlwaysScrollableScrollPhysics(),
/// ```
///
/// To force the scroll view to use the default platform conventions and not
/// be scrollable if there is insufficient content, regardless of the value of
/// [primary], provide an explicit [ScrollPhysics] object, as in:
///
/// ```dart
/// physics: const ScrollPhysics(),
/// ```
///
/// The physics can be changed dynamically (by providing a new object in a
/// subsequent build), but new physics will only take effect if the _class_ of
/// the provided object changes. Merely constructing a new instance with a
/// different configuration is insufficient to cause the physics to be
/// reapplied. (This is because the final object used is generated
/// dynamically, which can be relatively expensive, and it would be
/// inefficient to speculatively create this object each frame to see if the
/// physics should be updated.)
/// {@endtemplate}
///
/// If an explicit [ScrollBehavior] is provided to [scrollBehavior], the
/// [ScrollPhysics] provided by that behavior will take precedence after
/// [physics].
final ScrollPhysics? physics;
/// {@macro flutter.widgets.shadow.scrollBehavior}
///
/// [ScrollBehavior]s also provide [ScrollPhysics]. If an explicit
/// [ScrollPhysics] is provided in [physics], it will take precedence,
/// followed by [scrollBehavior], and then the inherited ancestor
/// [ScrollBehavior].
final ScrollBehavior? scrollBehavior;
/// {@template flutter.widgets.scroll_view.shrinkWrap}
/// Whether the extent of the scroll view in the [scrollDirection] should be
/// determined by the contents being viewed.
///
/// If the scroll view does not shrink wrap, then the scroll view will expand
/// to the maximum allowed size in the [scrollDirection]. If the scroll view
/// has unbounded constraints in the [scrollDirection], then [shrinkWrap] must
/// be true.
///
/// Shrink wrapping the content of the scroll view is significantly more
/// expensive than expanding to the maximum allowed size because the content
/// can expand and contract during scrolling, which means the size of the
/// scroll view needs to be recomputed whenever the scroll position changes.
///
/// Defaults to false.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=LUqDNnv_dh0}
/// {@endtemplate}
final bool shrinkWrap;
/// The first child in the [GrowthDirection.forward] growth direction.
///
/// Children after [center] will be placed in the [AxisDirection] determined
/// by [scrollDirection] and [reverse] relative to the [center]. Children
/// before [center] will be placed in the opposite of the axis direction
/// relative to the [center]. This makes the [center] the inflection point of
/// the growth direction.
///
/// The [center] must be the key of one of the slivers built by [buildSlivers].
///
/// Of the built-in subclasses of [ScrollView], only [CustomScrollView]
/// supports [center]; for that class, the given key must be the key of one of
/// the slivers in the [CustomScrollView.slivers] list.
///
/// Most scroll views by default are ordered [GrowthDirection.forward].
/// Changing the default values of [ScrollView.anchor],
/// [ScrollView.center], or both, can configure a scroll view for
/// [GrowthDirection.reverse].
///
/// {@tool dartpad}
/// This sample shows a [CustomScrollView], with [Radio] buttons in the
/// [AppBar.bottom] that change the [AxisDirection] to illustrate different
/// configurations. The [CustomScrollView.anchor] and [CustomScrollView.center]
/// properties are also set to have the 0 scroll offset positioned in the middle
/// of the viewport, with [GrowthDirection.forward] and [GrowthDirection.reverse]
/// illustrated on either side. The sliver that shares the
/// [CustomScrollView.center] key is positioned at the [CustomScrollView.anchor].
///
/// ** See code in examples/api/lib/rendering/growth_direction/growth_direction.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [anchor], which controls where the [center] as aligned in the viewport.
final Key? center;
/// {@template flutter.widgets.scroll_view.anchor}
/// The relative position of the zero scroll offset.
///
/// For example, if [anchor] is 0.5 and the [AxisDirection] determined by
/// [scrollDirection] and [reverse] is [AxisDirection.down] or
/// [AxisDirection.up], then the zero scroll offset is vertically centered
/// within the viewport. If the [anchor] is 1.0, and the axis direction is
/// [AxisDirection.right], then the zero scroll offset is on the left edge of
/// the viewport.
///
/// Most scroll views by default are ordered [GrowthDirection.forward].
/// Changing the default values of [ScrollView.anchor],
/// [ScrollView.center], or both, can configure a scroll view for
/// [GrowthDirection.reverse].
///
/// {@tool dartpad}
/// This sample shows a [CustomScrollView], with [Radio] buttons in the
/// [AppBar.bottom] that change the [AxisDirection] to illustrate different
/// configurations. The [CustomScrollView.anchor] and [CustomScrollView.center]
/// properties are also set to have the 0 scroll offset positioned in the middle
/// of the viewport, with [GrowthDirection.forward] and [GrowthDirection.reverse]
/// illustrated on either side. The sliver that shares the
/// [CustomScrollView.center] key is positioned at the [CustomScrollView.anchor].
///
/// ** See code in examples/api/lib/rendering/growth_direction/growth_direction.0.dart **
/// {@end-tool}
/// {@endtemplate}
final double anchor;
/// {@macro flutter.rendering.RenderViewportBase.cacheExtent}
final double? cacheExtent;
/// The number of children that will contribute semantic information.
///
/// Some subtypes of [ScrollView] can infer this value automatically. For
/// example [ListView] will use the number of widgets in the child list,
/// while the [ListView.separated] constructor will use half that amount.
///
/// For [CustomScrollView] and other types which do not receive a builder
/// or list of widgets, the child count must be explicitly provided. If the
/// number is unknown or unbounded this should be left unset or set to null.
///
/// See also:
///
/// * [SemanticsConfiguration.scrollChildCount], the corresponding semantics property.
final int? semanticChildCount;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@template flutter.widgets.scroll_view.keyboardDismissBehavior}
/// [ScrollViewKeyboardDismissBehavior] the defines how this [ScrollView] will
/// dismiss the keyboard automatically.
/// {@endtemplate}
final ScrollViewKeyboardDismissBehavior keyboardDismissBehavior;
/// {@macro flutter.widgets.scrollable.restorationId}
final String? restorationId;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// Returns the [AxisDirection] in which the scroll view scrolls.
///
/// Combines the [scrollDirection] with the [reverse] boolean to obtain the
/// concrete [AxisDirection].
///
/// If the [scrollDirection] is [Axis.horizontal], the ambient
/// [Directionality] is also considered when selecting the concrete
/// [AxisDirection]. For example, if the ambient [Directionality] is
/// [TextDirection.rtl], then the non-reversed [AxisDirection] is
/// [AxisDirection.left] and the reversed [AxisDirection] is
/// [AxisDirection.right].
@protected
AxisDirection getDirection(BuildContext context) {
return getAxisDirectionFromAxisReverseAndDirectionality(context, scrollDirection, reverse);
}
/// Build the list of widgets to place inside the viewport.
///
/// Subclasses should override this method to build the slivers for the inside
/// of the viewport.
///
/// To learn more about slivers, see [CustomScrollView.slivers].
@protected
List<Widget> buildSlivers(BuildContext context);
/// Build the viewport.
///
/// Subclasses may override this method to change how the viewport is built.
/// The default implementation uses a [ShrinkWrappingViewport] if [shrinkWrap]
/// is true, and a regular [Viewport] otherwise.
///
/// The `offset` argument is the value obtained from
/// [Scrollable.viewportBuilder].
///
/// The `axisDirection` argument is the value obtained from [getDirection],
/// which by default uses [scrollDirection] and [reverse].
///
/// The `slivers` argument is the value obtained from [buildSlivers].
@protected
Widget buildViewport(
BuildContext context,
ViewportOffset offset,
AxisDirection axisDirection,
List<Widget> slivers,
) {
assert(() {
switch (axisDirection) {
case AxisDirection.up:
case AxisDirection.down:
return debugCheckHasDirectionality(
context,
why: 'to determine the cross-axis direction of the scroll view',
hint: 'Vertical scroll views create Viewport widgets that try to determine their cross axis direction '
'from the ambient Directionality.',
);
case AxisDirection.left:
case AxisDirection.right:
return true;
}
}());
if (shrinkWrap) {
return ShrinkWrappingViewport(
axisDirection: axisDirection,
offset: offset,
slivers: slivers,
clipBehavior: clipBehavior,
);
}
return Viewport(
axisDirection: axisDirection,
offset: offset,
slivers: slivers,
cacheExtent: cacheExtent,
center: center,
anchor: anchor,
clipBehavior: clipBehavior,
);
}
@override
Widget build(BuildContext context) {
final List<Widget> slivers = buildSlivers(context);
final AxisDirection axisDirection = getDirection(context);
final bool effectivePrimary = primary
?? controller == null && PrimaryScrollController.shouldInherit(context, scrollDirection);
final ScrollController? scrollController = effectivePrimary
? PrimaryScrollController.maybeOf(context)
: controller;
final Scrollable scrollable = Scrollable(
dragStartBehavior: dragStartBehavior,
axisDirection: axisDirection,
controller: scrollController,
physics: physics,
scrollBehavior: scrollBehavior,
semanticChildCount: semanticChildCount,
restorationId: restorationId,
viewportBuilder: (BuildContext context, ViewportOffset offset) {
return buildViewport(context, offset, axisDirection, slivers);
},
clipBehavior: clipBehavior,
);
final Widget scrollableResult = effectivePrimary && scrollController != null
// Further descendant ScrollViews will not inherit the same PrimaryScrollController
? PrimaryScrollController.none(child: scrollable)
: scrollable;
if (keyboardDismissBehavior == ScrollViewKeyboardDismissBehavior.onDrag) {
return NotificationListener<ScrollUpdateNotification>(
child: scrollableResult,
onNotification: (ScrollUpdateNotification notification) {
final FocusScopeNode focusScope = FocusScope.of(context);
if (notification.dragDetails != null && focusScope.hasFocus) {
focusScope.unfocus();
}
return false;
},
);
} else {
return scrollableResult;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<Axis>('scrollDirection', scrollDirection));
properties.add(FlagProperty('reverse', value: reverse, ifTrue: 'reversed', showName: true));
properties.add(DiagnosticsProperty<ScrollController>('controller', controller, showName: false, defaultValue: null));
properties.add(FlagProperty('primary', value: primary, ifTrue: 'using primary controller', showName: true));
properties.add(DiagnosticsProperty<ScrollPhysics>('physics', physics, showName: false, defaultValue: null));
properties.add(FlagProperty('shrinkWrap', value: shrinkWrap, ifTrue: 'shrink-wrapping', showName: true));
}
}
/// A [ScrollView] that creates custom scroll effects using [slivers].
///
/// A [CustomScrollView] lets you supply [slivers] directly to create various
/// scrolling effects, such as lists, grids, and expanding headers. For example,
/// to create a scroll view that contains an expanding app bar followed by a
/// list and a grid, use a list of three slivers: [SliverAppBar], [SliverList],
/// and [SliverGrid].
///
/// [Widget]s in these [slivers] must produce [RenderSliver] objects.
///
/// To control the initial scroll offset of the scroll view, provide a
/// [controller] with its [ScrollController.initialScrollOffset] property set.
///
/// {@animation 400 376 https://flutter.github.io/assets-for-api-docs/assets/widgets/custom_scroll_view.mp4}
///
/// {@tool snippet}
///
/// This sample code shows a scroll view that contains a flexible pinned app
/// bar, a grid, and an infinite list.
///
/// ```dart
/// CustomScrollView(
/// slivers: <Widget>[
/// const SliverAppBar(
/// pinned: true,
/// expandedHeight: 250.0,
/// flexibleSpace: FlexibleSpaceBar(
/// title: Text('Demo'),
/// ),
/// ),
/// SliverGrid(
/// gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
/// maxCrossAxisExtent: 200.0,
/// mainAxisSpacing: 10.0,
/// crossAxisSpacing: 10.0,
/// childAspectRatio: 4.0,
/// ),
/// delegate: SliverChildBuilderDelegate(
/// (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.teal[100 * (index % 9)],
/// child: Text('Grid Item $index'),
/// );
/// },
/// childCount: 20,
/// ),
/// ),
/// SliverFixedExtentList(
/// itemExtent: 50.0,
/// delegate: SliverChildBuilderDelegate(
/// (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('List Item $index'),
/// );
/// },
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// By default, if items are inserted at the "top" of a scrolling container like
/// [ListView] or [CustomScrollView], the top item and all of the items below it
/// are scrolled downwards. In some applications, it's preferable to have the
/// top of the list just grow upwards, without changing the scroll position.
/// This example demonstrates how to do that with a [CustomScrollView] with
/// two [SliverList] children, and the [CustomScrollView.center] set to the key
/// of the bottom SliverList. The top one SliverList will grow upwards, and the
/// bottom SliverList will grow downwards.
///
/// ** See code in examples/api/lib/widgets/scroll_view/custom_scroll_view.1.dart **
/// {@end-tool}
///
/// ## Accessibility
///
/// A [CustomScrollView] can allow Talkback/VoiceOver to make announcements
/// to the user when the scroll state changes. For example, on Android an
/// announcement might be read as "showing items 1 to 10 of 23". To produce
/// this announcement, the scroll view needs three pieces of information:
///
/// * The first visible child index.
/// * The total number of children.
/// * The total number of visible children.
///
/// The last value can be computed exactly by the framework, however the first
/// two must be provided. Most of the higher-level scrollable widgets provide
/// this information automatically. For example, [ListView] provides each child
/// widget with a semantic index automatically and sets the semantic child
/// count to the length of the list.
///
/// To determine visible indexes, the scroll view needs a way to associate the
/// generated semantics of each scrollable item with a semantic index. This can
/// be done by wrapping the child widgets in an [IndexedSemantics].
///
/// This semantic index is not necessarily the same as the index of the widget in
/// the scrollable, because some widgets may not contribute semantic
/// information. Consider a [ListView.separated]: every other widget is a
/// divider with no semantic information. In this case, only odd numbered
/// widgets have a semantic index (equal to the index ~/ 2). Furthermore, the
/// total number of children in this example would be half the number of
/// widgets. (The [ListView.separated] constructor handles this
/// automatically; this is only used here as an example.)
///
/// The total number of visible children can be provided by the constructor
/// parameter `semanticChildCount`. This should always be the same as the
/// number of widgets wrapped in [IndexedSemantics].
///
/// {@macro flutter.widgets.ScrollView.PageStorage}
///
/// See also:
///
/// * [SliverList], which is a sliver that displays linear list of children.
/// * [SliverFixedExtentList], which is a more efficient sliver that displays
/// linear list of children that have the same extent along the scroll axis.
/// * [SliverGrid], which is a sliver that displays a 2D array of children.
/// * [SliverPadding], which is a sliver that adds blank space around another
/// sliver.
/// * [SliverAppBar], which is a sliver that displays a header that can expand
/// and float as the scroll view scrolls.
/// * [ScrollNotification] and [NotificationListener], which can be used to watch
/// the scroll position without using a [ScrollController].
/// * [IndexedSemantics], which allows annotating child lists with an index
/// for scroll announcements.
class CustomScrollView extends ScrollView {
/// Creates a [ScrollView] that creates custom scroll effects using slivers.
///
/// See the [ScrollView] constructor for more details on these arguments.
const CustomScrollView({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.scrollBehavior,
super.shrinkWrap,
super.center,
super.anchor,
super.cacheExtent,
this.slivers = const <Widget>[],
super.semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
});
/// The slivers to place inside the viewport.
///
/// ## What is a sliver?
///
/// > _**sliver** (noun): a small, thin piece of something._
///
/// A _sliver_ is a widget backed by a [RenderSliver] subclass, i.e. one that
/// implements the constraint/geometry protocol that uses [SliverConstraints]
/// and [SliverGeometry].
///
/// This is as distinct from those widgets that are backed by [RenderBox]
/// subclasses, which use [BoxConstraints] and [Size] respectively, and are
/// known as box widgets. (Widgets like [Container], [Row], and [SizedBox] are
/// box widgets.)
///
/// While boxes are much more straightforward (implementing a simple
/// two-dimensional Cartesian layout system), slivers are much more powerful,
/// and are optimized for one-axis scrolling environments.
///
/// Slivers are hosted in viewports, also known as scroll views, most notably
/// [CustomScrollView].
///
/// ## Examples of slivers
///
/// The Flutter framework has many built-in sliver widgets, and custom widgets
/// can be created in the same manner. By convention, sliver widgets always
/// start with the prefix `Sliver` and are always used in properties called
/// `sliver` or `slivers` (as opposed to `child` and `children` which are used
/// for box widgets).
///
/// Examples of widgets unique to the sliver world include:
///
/// * [SliverList], a lazily-loading list of variably-sized box widgets.
/// * [SliverFixedExtentList], a lazily-loading list of box widgets that are
/// all forced to the same height.
/// * [SliverPrototypeExtentList], a lazily-loading list of box widgets that
/// are all forced to the same height as a given prototype widget.
/// * [SliverGrid], a lazily-loading grid of box widgets.
/// * [SliverAnimatedList] and [SliverAnimatedGrid], animated variants of
/// [SliverList] and [SliverGrid].
/// * [SliverFillRemaining], a widget that fills all remaining space in a
/// scroll view, and lays a box widget out inside that space.
/// * [SliverFillViewport], a widget that lays a list of boxes out, each
/// being sized to fit the whole viewport.
/// * [SliverPersistentHeader], a sliver that implements pinned and floating
/// headers, e.g. used to implement [SliverAppBar].
/// * [SliverToBoxAdapter], a sliver that wraps a box widget.
///
/// Examples of sliver variants of common box widgets include:
///
/// * [SliverOpacity], [SliverAnimatedOpacity], and [SliverFadeTransition],
/// sliver versions of [Opacity], [AnimatedOpacity], and [FadeTransition].
/// * [SliverIgnorePointer], a sliver version of [IgnorePointer].
/// * [SliverLayoutBuilder], a sliver version of [LayoutBuilder].
/// * [SliverOffstage], a sliver version of [Offstage].
/// * [SliverPadding], a sliver version of [Padding].
/// * [SliverReorderableList], a sliver version of [ReorderableList]
/// * [SliverSafeArea], a sliver version of [SafeArea].
/// * [SliverVisibility], a sliver version of [Visibility].
///
/// ## Benefits of slivers over boxes
///
/// The sliver protocol ([SliverConstraints] and [SliverGeometry]) enables
/// _scroll effects_, such as floating app bars, widgets that expand and
/// shrink during scroll, section headers that are pinned only while the
/// section's children are visible, etc.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=Mz3kHQxBjGg}
///
/// ## Mixing slivers and boxes
///
/// In general, slivers always wrap box widgets to actually render anything
/// (for example, there is no sliver equivalent of [Text] or [Container]);
/// the sliver part of the equation is mostly about how these boxes should
/// be laid out in a viewport (i.e. when scrolling).
///
/// Typically, the simplest way to combine boxes into a sliver environment is
/// to use a [SliverList] (maybe using a [ListView, which is a convenient
/// combination of a [CustomScrollView] and a [SliverList]). In rare cases,
/// e.g. if a single [Divider] widget is needed between two [SliverGrid]s,
/// a [SliverToBoxAdapter] can be used to wrap the box widgets.
///
/// ## Performance considerations
///
/// Because the purpose of scroll views is to, well, scroll, it is common
/// for scroll views to contain more contents than are rendered on the screen
/// at any particular time.
///
/// To improve the performance of scroll views, the content can be rendered in
/// _lazy_ widgets, notably [SliverList] and [SliverGrid] (and their variants,
/// such as [SliverFixedExtentList] and [SliverAnimatedGrid]). These widgets
/// ensure that only the portion of their child lists that are actually
/// visible get built, laid out, and painted.
///
/// The [ListView] and [GridView] widgets provide a convenient way to combine
/// a [CustomScrollView] and a [SliverList] or [SliverGrid] (respectively).
final List<Widget> slivers;
@override
List<Widget> buildSlivers(BuildContext context) => slivers;
}
/// A [ScrollView] that uses a single child layout model.
///
/// {@template flutter.widgets.BoxScroll.scrollBehaviour}
/// [ScrollView]s are often decorated with [Scrollbar]s and overscroll indicators,
/// which are managed by the inherited [ScrollBehavior]. Placing a
/// [ScrollConfiguration] above a ScrollView can modify these behaviors for that
/// ScrollView, or can be managed app-wide by providing a ScrollBehavior to
/// [MaterialApp.scrollBehavior] or [CupertinoApp.scrollBehavior].
/// {@endtemplate}
///
/// See also:
///
/// * [ListView], which is a [BoxScrollView] that uses a linear layout model.
/// * [GridView], which is a [BoxScrollView] that uses a 2D layout model.
/// * [CustomScrollView], which can combine multiple child layout models into a
/// single scroll view.
abstract class BoxScrollView extends ScrollView {
/// Creates a [ScrollView] uses a single child layout model.
///
/// If the [primary] argument is true, the [controller] must be null.
const BoxScrollView({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
this.padding,
super.cacheExtent,
super.semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
});
/// The amount of space by which to inset the children.
final EdgeInsetsGeometry? padding;
@override
List<Widget> buildSlivers(BuildContext context) {
Widget sliver = buildChildLayout(context);
EdgeInsetsGeometry? effectivePadding = padding;
if (padding == null) {
final MediaQueryData? mediaQuery = MediaQuery.maybeOf(context);
if (mediaQuery != null) {
// Automatically pad sliver with padding from MediaQuery.
final EdgeInsets mediaQueryHorizontalPadding =
mediaQuery.padding.copyWith(top: 0.0, bottom: 0.0);
final EdgeInsets mediaQueryVerticalPadding =
mediaQuery.padding.copyWith(left: 0.0, right: 0.0);
// Consume the main axis padding with SliverPadding.
effectivePadding = scrollDirection == Axis.vertical
? mediaQueryVerticalPadding
: mediaQueryHorizontalPadding;
// Leave behind the cross axis padding.
sliver = MediaQuery(
data: mediaQuery.copyWith(
padding: scrollDirection == Axis.vertical
? mediaQueryHorizontalPadding
: mediaQueryVerticalPadding,
),
child: sliver,
);
}
}
if (effectivePadding != null) {
sliver = SliverPadding(padding: effectivePadding, sliver: sliver);
}
return <Widget>[ sliver ];
}
/// Subclasses should override this method to build the layout model.
@protected
Widget buildChildLayout(BuildContext context);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
}
}
/// A scrollable list of widgets arranged linearly.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=KJpkjHGiI5A}
///
/// [ListView] is the most commonly used scrolling widget. It displays its
/// children one after another in the scroll direction. In the cross axis, the
/// children are required to fill the [ListView].
///
/// If non-null, the [itemExtent] forces the children to have the given extent
/// in the scroll direction.
///
/// If non-null, the [prototypeItem] forces the children to have the same extent
/// as the given widget in the scroll direction.
///
/// Specifying an [itemExtent] or an [prototypeItem] is more efficient than
/// letting the children determine their own extent because the scrolling
/// machinery can make use of the foreknowledge of the children's extent to save
/// work, for example when the scroll position changes drastically.
///
/// You can't specify both [itemExtent] and [prototypeItem], only one or none of
/// them.
///
/// There are four options for constructing a [ListView]:
///
/// 1. The default constructor takes an explicit [List<Widget>] of children. This
/// constructor is appropriate for list views with a small number of
/// children because constructing the [List] requires doing work for every
/// child that could possibly be displayed in the list view instead of just
/// those children that are actually visible.
///
/// 2. The [ListView.builder] constructor takes an [IndexedWidgetBuilder], which
/// builds the children on demand. This constructor is appropriate for list views
/// with a large (or infinite) number of children because the builder is called
/// only for those children that are actually visible.
///
/// 3. The [ListView.separated] constructor takes two [IndexedWidgetBuilder]s:
/// `itemBuilder` builds child items on demand, and `separatorBuilder`
/// similarly builds separator children which appear in between the child items.
/// This constructor is appropriate for list views with a fixed number of children.
///
/// 4. The [ListView.custom] constructor takes a [SliverChildDelegate], which provides
/// the ability to customize additional aspects of the child model. For example,
/// a [SliverChildDelegate] can control the algorithm used to estimate the
/// size of children that are not actually visible.
///
/// To control the initial scroll offset of the scroll view, provide a
/// [controller] with its [ScrollController.initialScrollOffset] property set.
///
/// By default, [ListView] will automatically pad the list's scrollable
/// extremities to avoid partial obstructions indicated by [MediaQuery]'s
/// padding. To avoid this behavior, override with a zero [padding] property.
///
/// {@tool snippet}
/// This example uses the default constructor for [ListView] which takes an
/// explicit [List<Widget>] of children. This [ListView]'s children are made up
/// of [Container]s with [Text].
///
/// 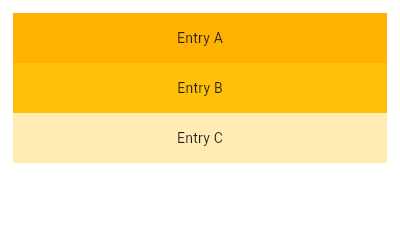
///
/// ```dart
/// ListView(
/// padding: const EdgeInsets.all(8),
/// children: <Widget>[
/// Container(
/// height: 50,
/// color: Colors.amber[600],
/// child: const Center(child: Text('Entry A')),
/// ),
/// Container(
/// height: 50,
/// color: Colors.amber[500],
/// child: const Center(child: Text('Entry B')),
/// ),
/// Container(
/// height: 50,
/// color: Colors.amber[100],
/// child: const Center(child: Text('Entry C')),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example mirrors the previous one, creating the same list using the
/// [ListView.builder] constructor. Using the [IndexedWidgetBuilder], children
/// are built lazily and can be infinite in number.
///
/// 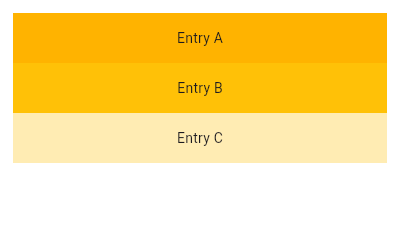
///
/// ```dart
/// final List<String> entries = <String>['A', 'B', 'C'];
/// final List<int> colorCodes = <int>[600, 500, 100];
///
/// Widget build(BuildContext context) {
/// return ListView.builder(
/// padding: const EdgeInsets.all(8),
/// itemCount: entries.length,
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// height: 50,
/// color: Colors.amber[colorCodes[index]],
/// child: Center(child: Text('Entry ${entries[index]}')),
/// );
/// }
/// );
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example continues to build from our the previous ones, creating a
/// similar list using [ListView.separated]. Here, a [Divider] is used as a
/// separator.
///
/// 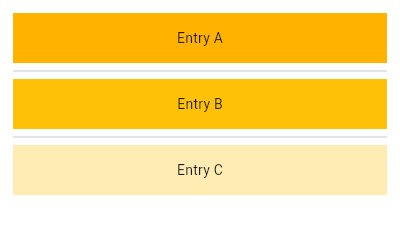
///
/// ```dart
/// final List<String> entries = <String>['A', 'B', 'C'];
/// final List<int> colorCodes = <int>[600, 500, 100];
///
/// Widget build(BuildContext context) {
/// return ListView.separated(
/// padding: const EdgeInsets.all(8),
/// itemCount: entries.length,
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// height: 50,
/// color: Colors.amber[colorCodes[index]],
/// child: Center(child: Text('Entry ${entries[index]}')),
/// );
/// },
/// separatorBuilder: (BuildContext context, int index) => const Divider(),
/// );
/// }
/// ```
/// {@end-tool}
///
/// ## Child elements' lifecycle
///
/// ### Creation
///
/// While laying out the list, visible children's elements, states and render
/// objects will be created lazily based on existing widgets (such as when using
/// the default constructor) or lazily provided ones (such as when using the
/// [ListView.builder] constructor).
///
/// ### Destruction
///
/// When a child is scrolled out of view, the associated element subtree,
/// states and render objects are destroyed. A new child at the same position
/// in the list will be lazily recreated along with new elements, states and
/// render objects when it is scrolled back.
///
/// ### Destruction mitigation
///
/// In order to preserve state as child elements are scrolled in and out of
/// view, the following options are possible:
///
/// * Moving the ownership of non-trivial UI-state-driving business logic
/// out of the list child subtree. For instance, if a list contains posts
/// with their number of upvotes coming from a cached network response, store
/// the list of posts and upvote number in a data model outside the list. Let
/// the list child UI subtree be easily recreate-able from the
/// source-of-truth model object. Use [StatefulWidget]s in the child
/// widget subtree to store instantaneous UI state only.
///
/// * Letting [KeepAlive] be the root widget of the list child widget subtree
/// that needs to be preserved. The [KeepAlive] widget marks the child
/// subtree's top render object child for keepalive. When the associated top
/// render object is scrolled out of view, the list keeps the child's render
/// object (and by extension, its associated elements and states) in a cache
/// list instead of destroying them. When scrolled back into view, the render
/// object is repainted as-is (if it wasn't marked dirty in the interim).
///
/// This only works if `addAutomaticKeepAlives` and `addRepaintBoundaries`
/// are false since those parameters cause the [ListView] to wrap each child
/// widget subtree with other widgets.
///
/// * Using [AutomaticKeepAlive] widgets (inserted by default when
/// `addAutomaticKeepAlives` is true). [AutomaticKeepAlive] allows descendant
/// widgets to control whether the subtree is actually kept alive or not.
/// This behavior is in contrast with [KeepAlive], which will unconditionally keep
/// the subtree alive.
///
/// As an example, the [EditableText] widget signals its list child element
/// subtree to stay alive while its text field has input focus. If it doesn't
/// have focus and no other descendants signaled for keepalive via a
/// [KeepAliveNotification], the list child element subtree will be destroyed
/// when scrolled away.
///
/// [AutomaticKeepAlive] descendants typically signal it to be kept alive
/// by using the [AutomaticKeepAliveClientMixin], then implementing the
/// [AutomaticKeepAliveClientMixin.wantKeepAlive] getter and calling
/// [AutomaticKeepAliveClientMixin.updateKeepAlive].
///
/// ## Transitioning to [CustomScrollView]
///
/// A [ListView] is basically a [CustomScrollView] with a single [SliverList] in
/// its [CustomScrollView.slivers] property.
///
/// If [ListView] is no longer sufficient, for example because the scroll view
/// is to have both a list and a grid, or because the list is to be combined
/// with a [SliverAppBar], etc, it is straight-forward to port code from using
/// [ListView] to using [CustomScrollView] directly.
///
/// The [key], [scrollDirection], [reverse], [controller], [primary], [physics],
/// and [shrinkWrap] properties on [ListView] map directly to the identically
/// named properties on [CustomScrollView].
///
/// The [CustomScrollView.slivers] property should be a list containing either:
/// * a [SliverList] if both [itemExtent] and [prototypeItem] were null;
/// * a [SliverFixedExtentList] if [itemExtent] was not null; or
/// * a [SliverPrototypeExtentList] if [prototypeItem] was not null.
///
/// The [childrenDelegate] property on [ListView] corresponds to the
/// [SliverList.delegate] (or [SliverFixedExtentList.delegate]) property. The
/// [ListView] constructor's `children` argument corresponds to the
/// [childrenDelegate] being a [SliverChildListDelegate] with that same
/// argument. The [ListView.builder] constructor's `itemBuilder` and
/// `itemCount` arguments correspond to the [childrenDelegate] being a
/// [SliverChildBuilderDelegate] with the equivalent arguments.
///
/// The [padding] property corresponds to having a [SliverPadding] in the
/// [CustomScrollView.slivers] property instead of the list itself, and having
/// the [SliverList] instead be a child of the [SliverPadding].
///
/// [CustomScrollView]s don't automatically avoid obstructions from [MediaQuery]
/// like [ListView]s do. To reproduce the behavior, wrap the slivers in
/// [SliverSafeArea]s.
///
/// Once code has been ported to use [CustomScrollView], other slivers, such as
/// [SliverGrid] or [SliverAppBar], can be put in the [CustomScrollView.slivers]
/// list.
///
/// {@tool snippet}
///
/// Here are two brief snippets showing a [ListView] and its equivalent using
/// [CustomScrollView]:
///
/// ```dart
/// ListView(
/// padding: const EdgeInsets.all(20.0),
/// children: const <Widget>[
/// Text("I'm dedicating every day to you"),
/// Text('Domestic life was never quite my style'),
/// Text('When you smile, you knock me out, I fall apart'),
/// Text('And I thought I was so smart'),
/// ],
/// )
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// ```dart
/// CustomScrollView(
/// slivers: <Widget>[
/// SliverPadding(
/// padding: const EdgeInsets.all(20.0),
/// sliver: SliverList(
/// delegate: SliverChildListDelegate(
/// <Widget>[
/// const Text("I'm dedicating every day to you"),
/// const Text('Domestic life was never quite my style'),
/// const Text('When you smile, you knock me out, I fall apart'),
/// const Text('And I thought I was so smart'),
/// ],
/// ),
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// ## Special handling for an empty list
///
/// A common design pattern is to have a custom UI for an empty list. The best
/// way to achieve this in Flutter is just conditionally replacing the
/// [ListView] at build time with whatever widgets you need to show for the
/// empty list state:
///
/// {@tool snippet}
///
/// Example of simple empty list interface:
///
/// ```dart
/// Widget build(BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(title: const Text('Empty List Test')),
/// body: itemCount > 0
/// ? ListView.builder(
/// itemCount: itemCount,
/// itemBuilder: (BuildContext context, int index) {
/// return ListTile(
/// title: Text('Item ${index + 1}'),
/// );
/// },
/// )
/// : const Center(child: Text('No items')),
/// );
/// }
/// ```
/// {@end-tool}
///
/// ## Selection of list items
///
/// [ListView] has no built-in notion of a selected item or items. For a small
/// example of how a caller might wire up basic item selection, see
/// [ListTile.selected].
///
/// {@tool dartpad}
/// This example shows a custom implementation of [ListTile] selection in a [ListView] or [GridView].
/// Long press any [ListTile] to enable selection mode.
///
/// ** See code in examples/api/lib/widgets/scroll_view/list_view.0.dart **
/// {@end-tool}
///
/// {@macro flutter.widgets.BoxScroll.scrollBehaviour}
///
/// {@macro flutter.widgets.ScrollView.PageStorage}
///
/// See also:
///
/// * [SingleChildScrollView], which is a scrollable widget that has a single
/// child.
/// * [PageView], which is a scrolling list of child widgets that are each the
/// size of the viewport.
/// * [GridView], which is a scrollable, 2D array of widgets.
/// * [CustomScrollView], which is a scrollable widget that creates custom
/// scroll effects using slivers.
/// * [ListBody], which arranges its children in a similar manner, but without
/// scrolling.
/// * [ScrollNotification] and [NotificationListener], which can be used to watch
/// the scroll position without using a [ScrollController].
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
/// * Cookbook: [Use lists](https://flutter.dev/docs/cookbook/lists/basic-list)
/// * Cookbook: [Work with long lists](https://flutter.dev/docs/cookbook/lists/long-lists)
/// * Cookbook: [Create a horizontal list](https://flutter.dev/docs/cookbook/lists/horizontal-list)
/// * Cookbook: [Create lists with different types of items](https://flutter.dev/docs/cookbook/lists/mixed-list)
/// * Cookbook: [Implement swipe to dismiss](https://flutter.dev/docs/cookbook/gestures/dismissible)
class ListView extends BoxScrollView {
/// Creates a scrollable, linear array of widgets from an explicit [List].
///
/// This constructor is appropriate for list views with a small number of
/// children because constructing the [List] requires doing work for every
/// child that could possibly be displayed in the list view instead of just
/// those children that are actually visible.
///
/// Like other widgets in the framework, this widget expects that
/// the [children] list will not be mutated after it has been passed in here.
/// See the documentation at [SliverChildListDelegate.children] for more details.
///
/// It is usually more efficient to create children on demand using
/// [ListView.builder] because it will create the widget children lazily as necessary.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildListDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildListDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildListDelegate.addSemanticIndexes] property. None
/// may be null.
ListView({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
this.itemExtent,
this.itemExtentBuilder,
this.prototypeItem,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
List<Widget> children = const <Widget>[],
int? semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : assert(
(itemExtent == null && prototypeItem == null) ||
(itemExtent == null && itemExtentBuilder == null) ||
(prototypeItem == null && itemExtentBuilder == null),
'You can only pass one of itemExtent, prototypeItem and itemExtentBuilder.',
),
childrenDelegate = SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? children.length,
);
/// Creates a scrollable, linear array of widgets that are created on demand.
///
/// This constructor is appropriate for list views with a large (or infinite)
/// number of children because the builder is called only for those children
/// that are actually visible.
///
/// Providing a non-null `itemCount` improves the ability of the [ListView] to
/// estimate the maximum scroll extent.
///
/// The `itemBuilder` callback will be called only with indices greater than
/// or equal to zero and less than `itemCount`.
///
/// {@template flutter.widgets.ListView.builder.itemBuilder}
/// It is legal for `itemBuilder` to return `null`. If it does, the scroll view
/// will stop calling `itemBuilder`, even if it has yet to reach `itemCount`.
/// By returning `null`, the [ScrollPosition.maxScrollExtent] will not be accurate
/// unless the user has reached the end of the [ScrollView]. This can also cause the
/// [Scrollbar] to grow as the user scrolls.
///
/// For more accurate [ScrollMetrics], consider specifying `itemCount`.
/// {@endtemplate}
///
/// The `itemBuilder` should always create the widget instances when called.
/// Avoid using a builder that returns a previously-constructed widget; if the
/// list view's children are created in advance, or all at once when the
/// [ListView] itself is created, it is more efficient to use the [ListView]
/// constructor. Even more efficient, however, is to create the instances on
/// demand using this constructor's `itemBuilder` callback.
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property. None may be
/// null.
ListView.builder({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
this.itemExtent,
this.itemExtentBuilder,
this.prototypeItem,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
int? semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : assert(itemCount == null || itemCount >= 0),
assert(semanticChildCount == null || semanticChildCount <= itemCount!),
assert(
(itemExtent == null && prototypeItem == null) ||
(itemExtent == null && itemExtentBuilder == null) ||
(prototypeItem == null && itemExtentBuilder == null),
'You can only pass one of itemExtent, prototypeItem and itemExtentBuilder.',
),
childrenDelegate = SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? itemCount,
);
/// Creates a fixed-length scrollable linear array of list "items" separated
/// by list item "separators".
///
/// This constructor is appropriate for list views with a large number of
/// item and separator children because the builders are only called for
/// the children that are actually visible.
///
/// The `itemBuilder` callback will be called with indices greater than
/// or equal to zero and less than `itemCount`.
///
/// Separators only appear between list items: separator 0 appears after item
/// 0 and the last separator appears before the last item.
///
/// The `separatorBuilder` callback will be called with indices greater than
/// or equal to zero and less than `itemCount - 1`.
///
/// The `itemBuilder` and `separatorBuilder` callbacks should always
/// actually create widget instances when called. Avoid using a builder that
/// returns a previously-constructed widget; if the list view's children are
/// created in advance, or all at once when the [ListView] itself is created,
/// it is more efficient to use the [ListView] constructor.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// {@tool snippet}
///
/// This example shows how to create [ListView] whose [ListTile] list items
/// are separated by [Divider]s.
///
/// ```dart
/// ListView.separated(
/// itemCount: 25,
/// separatorBuilder: (BuildContext context, int index) => const Divider(),
/// itemBuilder: (BuildContext context, int index) {
/// return ListTile(
/// title: Text('item $index'),
/// );
/// },
/// )
/// ```
/// {@end-tool}
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property. None may be
/// null.
ListView.separated({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
required IndexedWidgetBuilder separatorBuilder,
required int itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : assert(itemCount >= 0),
itemExtent = null,
itemExtentBuilder = null,
prototypeItem = null,
childrenDelegate = SliverChildBuilderDelegate(
(BuildContext context, int index) {
final int itemIndex = index ~/ 2;
if (index.isEven) {
return itemBuilder(context, itemIndex);
}
return separatorBuilder(context, itemIndex);
},
findChildIndexCallback: findChildIndexCallback,
childCount: _computeActualChildCount(itemCount),
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
semanticIndexCallback: (Widget widget, int index) {
return index.isEven ? index ~/ 2 : null;
},
),
super(
semanticChildCount: itemCount,
);
/// Creates a scrollable, linear array of widgets with a custom child model.
///
/// For example, a custom child model can control the algorithm used to
/// estimate the size of children that are not actually visible.
///
/// {@tool dartpad}
/// This example shows a [ListView] that uses a custom [SliverChildBuilderDelegate] to support child
/// reordering.
///
/// ** See code in examples/api/lib/widgets/scroll_view/list_view.1.dart **
/// {@end-tool}
const ListView.custom({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
this.itemExtent,
this.prototypeItem,
this.itemExtentBuilder,
required this.childrenDelegate,
super.cacheExtent,
super.semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : assert(
(itemExtent == null && prototypeItem == null) ||
(itemExtent == null && itemExtentBuilder == null) ||
(prototypeItem == null && itemExtentBuilder == null),
'You can only pass one of itemExtent, prototypeItem and itemExtentBuilder.',
);
/// {@template flutter.widgets.list_view.itemExtent}
/// If non-null, forces the children to have the given extent in the scroll
/// direction.
///
/// Specifying an [itemExtent] is more efficient than letting the children
/// determine their own extent because the scrolling machinery can make use of
/// the foreknowledge of the children's extent to save work, for example when
/// the scroll position changes drastically.
///
/// See also:
///
/// * [SliverFixedExtentList], the sliver used internally when this property
/// is provided. It constrains its box children to have a specific given
/// extent along the main axis.
/// * The [prototypeItem] property, which allows forcing the children's
/// extent to be the same as the given widget.
/// * The [itemExtentBuilder] property, which allows forcing the children's
/// extent to be the value returned by the callback.
/// {@endtemplate}
final double? itemExtent;
/// {@template flutter.widgets.list_view.itemExtentBuilder}
/// If non-null, forces the children to have the corresponding extent returned
/// by the builder.
///
/// Specifying an [itemExtentBuilder] is more efficient than letting the children
/// determine their own extent because the scrolling machinery can make use of
/// the foreknowledge of the children's extent to save work, for example when
/// the scroll position changes drastically.
///
/// This will be called multiple times during the layout phase of a frame to find
/// the items that should be loaded by the lazy loading process.
///
/// Should return null if asked to build an item extent with a greater index than
/// exists.
///
/// Unlike [itemExtent] or [prototypeItem], this allows children to have
/// different extents.
///
/// See also:
///
/// * [SliverVariedExtentList], the sliver used internally when this property
/// is provided. It constrains its box children to have a specific given
/// extent along the main axis.
/// * The [itemExtent] property, which allows forcing the children's extent
/// to a given value.
/// * The [prototypeItem] property, which allows forcing the children's
/// extent to be the same as the given widget.
/// {@endtemplate}
final ItemExtentBuilder? itemExtentBuilder;
/// {@template flutter.widgets.list_view.prototypeItem}
/// If non-null, forces the children to have the same extent as the given
/// widget in the scroll direction.
///
/// Specifying an [prototypeItem] is more efficient than letting the children
/// determine their own extent because the scrolling machinery can make use of
/// the foreknowledge of the children's extent to save work, for example when
/// the scroll position changes drastically.
///
/// See also:
///
/// * [SliverPrototypeExtentList], the sliver used internally when this
/// property is provided. It constrains its box children to have the same
/// extent as a prototype item along the main axis.
/// * The [itemExtent] property, which allows forcing the children's extent
/// to a given value.
/// * The [itemExtentBuilder] property, which allows forcing the children's
/// extent to be the value returned by the callback.
/// {@endtemplate}
final Widget? prototypeItem;
/// A delegate that provides the children for the [ListView].
///
/// The [ListView.custom] constructor lets you specify this delegate
/// explicitly. The [ListView] and [ListView.builder] constructors create a
/// [childrenDelegate] that wraps the given [List] and [IndexedWidgetBuilder],
/// respectively.
final SliverChildDelegate childrenDelegate;
@override
Widget buildChildLayout(BuildContext context) {
if (itemExtent != null) {
return SliverFixedExtentList(
delegate: childrenDelegate,
itemExtent: itemExtent!,
);
} else if (itemExtentBuilder != null) {
return SliverVariedExtentList(
delegate: childrenDelegate,
itemExtentBuilder: itemExtentBuilder!,
);
} else if (prototypeItem != null) {
return SliverPrototypeExtentList(
delegate: childrenDelegate,
prototypeItem: prototypeItem!,
);
}
return SliverList(delegate: childrenDelegate);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('itemExtent', itemExtent, defaultValue: null));
}
// Helper method to compute the actual child count for the separated constructor.
static int _computeActualChildCount(int itemCount) {
return math.max(0, itemCount * 2 - 1);
}
}
/// A scrollable, 2D array of widgets.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=bLOtZDTm4H8}
///
/// The main axis direction of a grid is the direction in which it scrolls (the
/// [scrollDirection]).
///
/// The most commonly used grid layouts are [GridView.count], which creates a
/// layout with a fixed number of tiles in the cross axis, and
/// [GridView.extent], which creates a layout with tiles that have a maximum
/// cross-axis extent. A custom [SliverGridDelegate] can produce an arbitrary 2D
/// arrangement of children, including arrangements that are unaligned or
/// overlapping.
///
/// To create a grid with a large (or infinite) number of children, use the
/// [GridView.builder] constructor with either a
/// [SliverGridDelegateWithFixedCrossAxisCount] or a
/// [SliverGridDelegateWithMaxCrossAxisExtent] for the [gridDelegate].
///
/// To use a custom [SliverChildDelegate], use [GridView.custom].
///
/// To create a linear array of children, use a [ListView].
///
/// To control the initial scroll offset of the scroll view, provide a
/// [controller] with its [ScrollController.initialScrollOffset] property set.
///
/// ## Transitioning to [CustomScrollView]
///
/// A [GridView] is basically a [CustomScrollView] with a single [SliverGrid] in
/// its [CustomScrollView.slivers] property.
///
/// If [GridView] is no longer sufficient, for example because the scroll view
/// is to have both a grid and a list, or because the grid is to be combined
/// with a [SliverAppBar], etc, it is straight-forward to port code from using
/// [GridView] to using [CustomScrollView] directly.
///
/// The [key], [scrollDirection], [reverse], [controller], [primary], [physics],
/// and [shrinkWrap] properties on [GridView] map directly to the identically
/// named properties on [CustomScrollView].
///
/// The [CustomScrollView.slivers] property should be a list containing just a
/// [SliverGrid].
///
/// The [childrenDelegate] property on [GridView] corresponds to the
/// [SliverGrid.delegate] property, and the [gridDelegate] property on the
/// [GridView] corresponds to the [SliverGrid.gridDelegate] property.
///
/// The [GridView], [GridView.count], and [GridView.extent]
/// constructors' `children` arguments correspond to the [childrenDelegate]
/// being a [SliverChildListDelegate] with that same argument. The
/// [GridView.builder] constructor's `itemBuilder` and `childCount` arguments
/// correspond to the [childrenDelegate] being a [SliverChildBuilderDelegate]
/// with the matching arguments.
///
/// The [GridView.count] and [GridView.extent] constructors create
/// custom grid delegates, and have equivalently named constructors on
/// [SliverGrid] to ease the transition: [SliverGrid.count] and
/// [SliverGrid.extent] respectively.
///
/// The [padding] property corresponds to having a [SliverPadding] in the
/// [CustomScrollView.slivers] property instead of the grid itself, and having
/// the [SliverGrid] instead be a child of the [SliverPadding].
///
/// Once code has been ported to use [CustomScrollView], other slivers, such as
/// [SliverList] or [SliverAppBar], can be put in the [CustomScrollView.slivers]
/// list.
///
/// {@macro flutter.widgets.ScrollView.PageStorage}
///
/// ## Examples
///
/// {@tool snippet}
/// This example demonstrates how to create a [GridView] with two columns. The
/// children are spaced apart using the `crossAxisSpacing` and `mainAxisSpacing`
/// properties.
///
/// 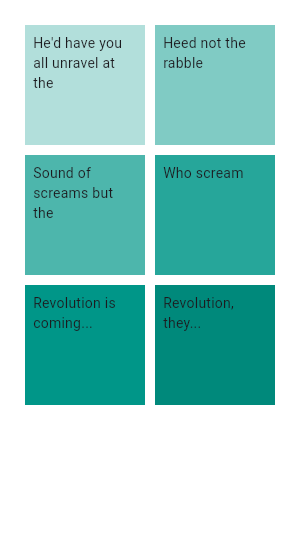
///
/// ```dart
/// GridView.count(
/// primary: false,
/// padding: const EdgeInsets.all(20),
/// crossAxisSpacing: 10,
/// mainAxisSpacing: 10,
/// crossAxisCount: 2,
/// children: <Widget>[
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[100],
/// child: const Text("He'd have you all unravel at the"),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[200],
/// child: const Text('Heed not the rabble'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[300],
/// child: const Text('Sound of screams but the'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[400],
/// child: const Text('Who scream'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[500],
/// child: const Text('Revolution is coming...'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.teal[600],
/// child: const Text('Revolution, they...'),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example shows how to create the same grid as the previous example
/// using a [CustomScrollView] and a [SliverGrid].
///
/// 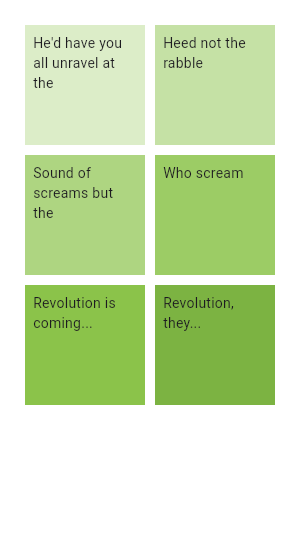
///
/// ```dart
/// CustomScrollView(
/// primary: false,
/// slivers: <Widget>[
/// SliverPadding(
/// padding: const EdgeInsets.all(20),
/// sliver: SliverGrid.count(
/// crossAxisSpacing: 10,
/// mainAxisSpacing: 10,
/// crossAxisCount: 2,
/// children: <Widget>[
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[100],
/// child: const Text("He'd have you all unravel at the"),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[200],
/// child: const Text('Heed not the rabble'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[300],
/// child: const Text('Sound of screams but the'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[400],
/// child: const Text('Who scream'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[500],
/// child: const Text('Revolution is coming...'),
/// ),
/// Container(
/// padding: const EdgeInsets.all(8),
/// color: Colors.green[600],
/// child: const Text('Revolution, they...'),
/// ),
/// ],
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows a custom implementation of selection in list and grid views.
/// Use the button in the top right (possibly hidden under the DEBUG banner) to toggle between
/// [ListView] and [GridView].
/// Long press any [ListTile] or [GridTile] to enable selection mode.
///
/// ** See code in examples/api/lib/widgets/scroll_view/list_view.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows a custom [SliverGridDelegate].
///
/// ** See code in examples/api/lib/widgets/scroll_view/grid_view.0.dart **
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Padding
///
/// By default, [GridView] will automatically pad the limits of the
/// grid's scrollable to avoid partial obstructions indicated by
/// [MediaQuery]'s padding. To avoid this behavior, override with a
/// zero [padding] property.
///
/// {@tool snippet}
/// The following example demonstrates how to override the default top padding
/// using [MediaQuery.removePadding].
///
/// ```dart
/// Widget myWidget(BuildContext context) {
/// return MediaQuery.removePadding(
/// context: context,
/// removeTop: true,
/// child: GridView.builder(
/// gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
/// crossAxisCount: 3,
/// ),
/// itemCount: 300,
/// itemBuilder: (BuildContext context, int index) {
/// return Card(
/// color: Colors.amber,
/// child: Center(child: Text('$index')),
/// );
/// }
/// ),
/// );
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [SingleChildScrollView], which is a scrollable widget that has a single
/// child.
/// * [ListView], which is scrollable, linear list of widgets.
/// * [PageView], which is a scrolling list of child widgets that are each the
/// size of the viewport.
/// * [CustomScrollView], which is a scrollable widget that creates custom
/// scroll effects using slivers.
/// * [SliverGridDelegateWithFixedCrossAxisCount], which creates a layout with
/// a fixed number of tiles in the cross axis.
/// * [SliverGridDelegateWithMaxCrossAxisExtent], which creates a layout with
/// tiles that have a maximum cross-axis extent.
/// * [ScrollNotification] and [NotificationListener], which can be used to watch
/// the scroll position without using a [ScrollController].
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class GridView extends BoxScrollView {
/// Creates a scrollable, 2D array of widgets with a custom
/// [SliverGridDelegate].
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildListDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildListDelegate.addRepaintBoundaries] property. Both must not be
/// null.
GridView({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required this.gridDelegate,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
List<Widget> children = const <Widget>[],
int? semanticChildCount,
super.dragStartBehavior,
super.clipBehavior,
super.keyboardDismissBehavior,
super.restorationId,
}) : childrenDelegate = SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? children.length,
);
/// Creates a scrollable, 2D array of widgets that are created on demand.
///
/// This constructor is appropriate for grid views with a large (or infinite)
/// number of children because the builder is called only for those children
/// that are actually visible.
///
/// Providing a non-null `itemCount` improves the ability of the [GridView] to
/// estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The [gridDelegate] argument is required.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
GridView.builder({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required this.gridDelegate,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
int? semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : childrenDelegate = SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? itemCount,
);
/// Creates a scrollable, 2D array of widgets with both a custom
/// [SliverGridDelegate] and a custom [SliverChildDelegate].
///
/// To use an [IndexedWidgetBuilder] callback to build children, either use
/// a [SliverChildBuilderDelegate] or use the [GridView.builder] constructor.
const GridView.custom({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required this.gridDelegate,
required this.childrenDelegate,
super.cacheExtent,
super.semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
});
/// Creates a scrollable, 2D array of widgets with a fixed number of tiles in
/// the cross axis.
///
/// Uses a [SliverGridDelegateWithFixedCrossAxisCount] as the [gridDelegate].
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildListDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildListDelegate.addRepaintBoundaries] property. Both must not be
/// null.
///
/// See also:
///
/// * [SliverGrid.count], the equivalent constructor for [SliverGrid].
GridView.count({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required int crossAxisCount,
double mainAxisSpacing = 0.0,
double crossAxisSpacing = 0.0,
double childAspectRatio = 1.0,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
List<Widget> children = const <Widget>[],
int? semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : gridDelegate = SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: crossAxisCount,
mainAxisSpacing: mainAxisSpacing,
crossAxisSpacing: crossAxisSpacing,
childAspectRatio: childAspectRatio,
),
childrenDelegate = SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? children.length,
);
/// Creates a scrollable, 2D array of widgets with tiles that each have a
/// maximum cross-axis extent.
///
/// Uses a [SliverGridDelegateWithMaxCrossAxisExtent] as the [gridDelegate].
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildListDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildListDelegate.addRepaintBoundaries] property. Both must not be
/// null.
///
/// See also:
///
/// * [SliverGrid.extent], the equivalent constructor for [SliverGrid].
GridView.extent({
super.key,
super.scrollDirection,
super.reverse,
super.controller,
super.primary,
super.physics,
super.shrinkWrap,
super.padding,
required double maxCrossAxisExtent,
double mainAxisSpacing = 0.0,
double crossAxisSpacing = 0.0,
double childAspectRatio = 1.0,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
super.cacheExtent,
List<Widget> children = const <Widget>[],
int? semanticChildCount,
super.dragStartBehavior,
super.keyboardDismissBehavior,
super.restorationId,
super.clipBehavior,
}) : gridDelegate = SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: maxCrossAxisExtent,
mainAxisSpacing: mainAxisSpacing,
crossAxisSpacing: crossAxisSpacing,
childAspectRatio: childAspectRatio,
),
childrenDelegate = SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
),
super(
semanticChildCount: semanticChildCount ?? children.length,
);
/// A delegate that controls the layout of the children within the [GridView].
///
/// The [GridView], [GridView.builder], and [GridView.custom] constructors let you specify this
/// delegate explicitly. The other constructors create a [gridDelegate]
/// implicitly.
final SliverGridDelegate gridDelegate;
/// A delegate that provides the children for the [GridView].
///
/// The [GridView.custom] constructor lets you specify this delegate
/// explicitly. The other constructors create a [childrenDelegate] that wraps
/// the given child list.
final SliverChildDelegate childrenDelegate;
@override
Widget buildChildLayout(BuildContext context) {
return SliverGrid(
delegate: childrenDelegate,
gridDelegate: gridDelegate,
);
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_view.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_view.dart",
"repo_id": "flutter",
"token_count": 26359
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
import 'scroll_delegate.dart';
import 'sliver.dart';
/// A sliver that places its box children in a linear array and constrains them
/// to have the same extent as a prototype item along the main axis.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
///
/// [SliverPrototypeExtentList] arranges its children in a line along
/// the main axis starting at offset zero and without gaps. Each child is
/// constrained to the same extent as the [prototypeItem] along the main axis
/// and the [SliverConstraints.crossAxisExtent] along the cross axis.
///
/// [SliverPrototypeExtentList] is more efficient than [SliverList] because
/// [SliverPrototypeExtentList] does not need to lay out its children to obtain
/// their extent along the main axis. It's a little more flexible than
/// [SliverFixedExtentList] because there's no need to determine the appropriate
/// item extent in pixels.
///
/// See also:
///
/// * [SliverFixedExtentList], whose children are forced to a given pixel
/// extent.
/// * [SliverVariedExtentList], which supports children with varying (but known
/// upfront) extents.
/// * [SliverList], which does not require its children to have the same
/// extent in the main axis.
/// * [SliverFillViewport], which sizes its children based on the
/// size of the viewport, regardless of what else is in the scroll view.
class SliverPrototypeExtentList extends SliverMultiBoxAdaptorWidget {
/// Creates a sliver that places its box children in a linear array and
/// constrains them to have the same extent as a prototype item along
/// the main axis.
const SliverPrototypeExtentList({
super.key,
required super.delegate,
required this.prototypeItem,
});
/// A sliver that places its box children in a linear array and constrains them
/// to have the same extent as a prototype item along the main axis.
///
/// This constructor is appropriate for sliver lists with a large (or
/// infinite) number of children whose extent is already determined.
///
/// Providing a non-null `itemCount` improves the ability of the [SliverGrid]
/// to estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// The `prototypeItem` argument is used to determine the extent of each item.
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverPrototypeExtentList.builder(
/// prototypeItem: Container(
/// alignment: Alignment.center,
/// child: const Text('list item prototype'),
/// ),
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('list item $index'),
/// );
/// },
/// )
/// ```
/// {@end-tool}
SliverPrototypeExtentList.builder({
super.key,
required NullableIndexedWidgetBuilder itemBuilder,
required this.prototypeItem,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// This constructor uses a list of [Widget]s to build the sliver.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverPrototypeExtentList.list(
/// prototypeItem: const Text('Hello'),
/// children: const <Widget>[
/// Text('Hello'),
/// Text('World!'),
/// ],
/// );
/// ```
/// {@end-tool}
SliverPrototypeExtentList.list({
super.key,
required List<Widget> children,
required this.prototypeItem,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// Defines the main axis extent of all of this sliver's children.
///
/// The [prototypeItem] is laid out before the rest of the sliver's children
/// and its size along the main axis fixes the size of each child. The
/// [prototypeItem] is essentially [Offstage]: it is not painted and it
/// cannot respond to input.
final Widget prototypeItem;
@override
RenderSliverMultiBoxAdaptor createRenderObject(BuildContext context) {
final _SliverPrototypeExtentListElement element = context as _SliverPrototypeExtentListElement;
return _RenderSliverPrototypeExtentList(childManager: element);
}
@override
SliverMultiBoxAdaptorElement createElement() => _SliverPrototypeExtentListElement(this);
}
class _SliverPrototypeExtentListElement extends SliverMultiBoxAdaptorElement {
_SliverPrototypeExtentListElement(SliverPrototypeExtentList super.widget);
@override
_RenderSliverPrototypeExtentList get renderObject => super.renderObject as _RenderSliverPrototypeExtentList;
Element? _prototype;
static final Object _prototypeSlot = Object();
@override
void insertRenderObjectChild(covariant RenderObject child, covariant Object slot) {
if (slot == _prototypeSlot) {
assert(child is RenderBox);
renderObject.child = child as RenderBox;
} else {
super.insertRenderObjectChild(child, slot as int);
}
}
@override
void didAdoptChild(RenderBox child) {
if (child != renderObject.child) {
super.didAdoptChild(child);
}
}
@override
void moveRenderObjectChild(RenderBox child, Object oldSlot, Object newSlot) {
if (newSlot == _prototypeSlot) {
// There's only one prototype child so it cannot be moved.
assert(false);
} else {
super.moveRenderObjectChild(child, oldSlot as int, newSlot as int);
}
}
@override
void removeRenderObjectChild(RenderBox child, Object slot) {
if (renderObject.child == child) {
renderObject.child = null;
} else {
super.removeRenderObjectChild(child, slot as int);
}
}
@override
void visitChildren(ElementVisitor visitor) {
if (_prototype != null) {
visitor(_prototype!);
}
super.visitChildren(visitor);
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
_prototype = updateChild(_prototype, (widget as SliverPrototypeExtentList).prototypeItem, _prototypeSlot);
}
@override
void update(SliverPrototypeExtentList newWidget) {
super.update(newWidget);
assert(widget == newWidget);
_prototype = updateChild(_prototype, (widget as SliverPrototypeExtentList).prototypeItem, _prototypeSlot);
}
}
class _RenderSliverPrototypeExtentList extends RenderSliverFixedExtentBoxAdaptor {
_RenderSliverPrototypeExtentList({
required _SliverPrototypeExtentListElement childManager,
}) : super(childManager: childManager);
RenderBox? _child;
RenderBox? get child => _child;
set child(RenderBox? value) {
if (_child != null) {
dropChild(_child!);
}
_child = value;
if (_child != null) {
adoptChild(_child!);
}
markNeedsLayout();
}
@override
void performLayout() {
child!.layout(constraints.asBoxConstraints(), parentUsesSize: true);
super.performLayout();
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
if (_child != null) {
_child!.attach(owner);
}
}
@override
void detach() {
super.detach();
if (_child != null) {
_child!.detach();
}
}
@override
void redepthChildren() {
if (_child != null) {
redepthChild(_child!);
}
super.redepthChildren();
}
@override
void visitChildren(RenderObjectVisitor visitor) {
if (_child != null) {
visitor(_child!);
}
super.visitChildren(visitor);
}
@override
double get itemExtent {
assert(child != null && child!.hasSize);
return constraints.axis == Axis.vertical ? child!.size.height : child!.size.width;
}
}
| flutter/packages/flutter/lib/src/widgets/sliver_prototype_extent_list.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/sliver_prototype_extent_list.dart",
"repo_id": "flutter",
"token_count": 3168
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'framework.dart';
/// A widget that describes this app in the operating system.
class Title extends StatelessWidget {
/// Creates a widget that describes this app to the Android operating system.
///
/// [title] will default to the empty string if not supplied.
/// [color] must be an opaque color (i.e. color.alpha must be 255 (0xFF)).
/// [color] and [child] are required arguments.
Title({
super.key,
this.title = '',
required this.color,
required this.child,
}) : assert(color.alpha == 0xFF);
/// A one-line description of this app for use in the window manager.
final String title;
/// A color that the window manager should use to identify this app. Must be
/// an opaque color (i.e. color.alpha must be 255 (0xFF)), and must not be
/// null.
final Color color;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
Widget build(BuildContext context) {
SystemChrome.setApplicationSwitcherDescription(
ApplicationSwitcherDescription(
label: title,
primaryColor: color.value,
),
);
return child;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('title', title, defaultValue: ''));
properties.add(ColorProperty('color', color, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/title.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/title.dart",
"repo_id": "flutter",
"token_count": 527
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The Flutter widgets framework.
///
/// To use, import `package:flutter/widgets.dart`.
///
/// See also:
///
/// * [flutter.dev/widgets](https://flutter.dev/widgets/)
/// for a catalog of commonly-used Flutter widgets.
library widgets;
export 'package:characters/characters.dart';
export 'package:vector_math/vector_math_64.dart' show Matrix4;
export 'foundation.dart' show UniqueKey;
export 'rendering.dart' show TextSelectionHandleType;
export 'src/widgets/actions.dart';
export 'src/widgets/adapter.dart';
export 'src/widgets/animated_cross_fade.dart';
export 'src/widgets/animated_scroll_view.dart';
export 'src/widgets/animated_size.dart';
export 'src/widgets/animated_switcher.dart';
export 'src/widgets/annotated_region.dart';
export 'src/widgets/app.dart';
export 'src/widgets/app_lifecycle_listener.dart';
export 'src/widgets/async.dart';
export 'src/widgets/autocomplete.dart';
export 'src/widgets/autofill.dart';
export 'src/widgets/automatic_keep_alive.dart';
export 'src/widgets/banner.dart';
export 'src/widgets/basic.dart';
export 'src/widgets/binding.dart';
export 'src/widgets/bottom_navigation_bar_item.dart';
export 'src/widgets/color_filter.dart';
export 'src/widgets/container.dart';
export 'src/widgets/context_menu_button_item.dart';
export 'src/widgets/context_menu_controller.dart';
export 'src/widgets/debug.dart';
export 'src/widgets/decorated_sliver.dart';
export 'src/widgets/default_selection_style.dart';
export 'src/widgets/default_text_editing_shortcuts.dart';
export 'src/widgets/desktop_text_selection_toolbar_layout_delegate.dart';
export 'src/widgets/dismissible.dart';
export 'src/widgets/display_feature_sub_screen.dart';
export 'src/widgets/disposable_build_context.dart';
export 'src/widgets/drag_target.dart';
export 'src/widgets/draggable_scrollable_sheet.dart';
export 'src/widgets/dual_transition_builder.dart';
export 'src/widgets/editable_text.dart';
export 'src/widgets/fade_in_image.dart';
export 'src/widgets/focus_manager.dart';
export 'src/widgets/focus_scope.dart';
export 'src/widgets/focus_traversal.dart';
export 'src/widgets/form.dart';
export 'src/widgets/framework.dart';
export 'src/widgets/gesture_detector.dart';
export 'src/widgets/grid_paper.dart';
export 'src/widgets/heroes.dart';
export 'src/widgets/icon.dart';
export 'src/widgets/icon_data.dart';
export 'src/widgets/icon_theme.dart';
export 'src/widgets/icon_theme_data.dart';
export 'src/widgets/image.dart';
export 'src/widgets/image_filter.dart';
export 'src/widgets/image_icon.dart';
export 'src/widgets/implicit_animations.dart';
export 'src/widgets/inherited_model.dart';
export 'src/widgets/inherited_notifier.dart';
export 'src/widgets/inherited_theme.dart';
export 'src/widgets/interactive_viewer.dart';
export 'src/widgets/keyboard_listener.dart';
export 'src/widgets/layout_builder.dart';
export 'src/widgets/list_wheel_scroll_view.dart';
export 'src/widgets/localizations.dart';
export 'src/widgets/lookup_boundary.dart';
export 'src/widgets/magnifier.dart';
export 'src/widgets/media_query.dart';
export 'src/widgets/modal_barrier.dart';
export 'src/widgets/navigation_toolbar.dart';
export 'src/widgets/navigator.dart';
export 'src/widgets/navigator_pop_handler.dart';
export 'src/widgets/nested_scroll_view.dart';
export 'src/widgets/notification_listener.dart';
export 'src/widgets/orientation_builder.dart';
export 'src/widgets/overflow_bar.dart';
export 'src/widgets/overlay.dart';
export 'src/widgets/overscroll_indicator.dart';
export 'src/widgets/page_storage.dart';
export 'src/widgets/page_view.dart';
export 'src/widgets/pages.dart';
export 'src/widgets/performance_overlay.dart';
export 'src/widgets/placeholder.dart';
export 'src/widgets/platform_menu_bar.dart';
export 'src/widgets/platform_selectable_region_context_menu.dart';
export 'src/widgets/platform_view.dart';
export 'src/widgets/pop_scope.dart';
export 'src/widgets/preferred_size.dart';
export 'src/widgets/primary_scroll_controller.dart';
export 'src/widgets/raw_keyboard_listener.dart';
export 'src/widgets/reorderable_list.dart';
export 'src/widgets/restoration.dart';
export 'src/widgets/restoration_properties.dart';
export 'src/widgets/router.dart';
export 'src/widgets/routes.dart';
export 'src/widgets/safe_area.dart';
export 'src/widgets/scroll_activity.dart';
export 'src/widgets/scroll_aware_image_provider.dart';
export 'src/widgets/scroll_configuration.dart';
export 'src/widgets/scroll_context.dart';
export 'src/widgets/scroll_controller.dart';
export 'src/widgets/scroll_delegate.dart';
export 'src/widgets/scroll_metrics.dart';
export 'src/widgets/scroll_notification.dart';
export 'src/widgets/scroll_notification_observer.dart';
export 'src/widgets/scroll_physics.dart';
export 'src/widgets/scroll_position.dart';
export 'src/widgets/scroll_position_with_single_context.dart';
export 'src/widgets/scroll_simulation.dart';
export 'src/widgets/scroll_view.dart';
export 'src/widgets/scrollable.dart';
export 'src/widgets/scrollable_helpers.dart';
export 'src/widgets/scrollbar.dart';
export 'src/widgets/selectable_region.dart';
export 'src/widgets/selection_container.dart';
export 'src/widgets/semantics_debugger.dart';
export 'src/widgets/service_extensions.dart';
export 'src/widgets/shared_app_data.dart';
export 'src/widgets/shortcuts.dart';
export 'src/widgets/single_child_scroll_view.dart';
export 'src/widgets/size_changed_layout_notifier.dart';
export 'src/widgets/sliver.dart';
export 'src/widgets/sliver_fill.dart';
export 'src/widgets/sliver_layout_builder.dart';
export 'src/widgets/sliver_persistent_header.dart';
export 'src/widgets/sliver_prototype_extent_list.dart';
export 'src/widgets/sliver_varied_extent_list.dart';
export 'src/widgets/slotted_render_object_widget.dart';
export 'src/widgets/snapshot_widget.dart';
export 'src/widgets/spacer.dart';
export 'src/widgets/spell_check.dart';
export 'src/widgets/status_transitions.dart';
export 'src/widgets/table.dart';
export 'src/widgets/tap_region.dart';
export 'src/widgets/text.dart';
export 'src/widgets/text_editing_intents.dart';
export 'src/widgets/text_selection.dart';
export 'src/widgets/text_selection_toolbar_anchors.dart';
export 'src/widgets/text_selection_toolbar_layout_delegate.dart';
export 'src/widgets/texture.dart';
export 'src/widgets/ticker_provider.dart';
export 'src/widgets/title.dart';
export 'src/widgets/transitions.dart';
export 'src/widgets/tween_animation_builder.dart';
export 'src/widgets/two_dimensional_scroll_view.dart';
export 'src/widgets/two_dimensional_viewport.dart';
export 'src/widgets/undo_history.dart';
export 'src/widgets/unique_widget.dart';
export 'src/widgets/value_listenable_builder.dart';
export 'src/widgets/view.dart';
export 'src/widgets/viewport.dart';
export 'src/widgets/visibility.dart';
export 'src/widgets/widget_inspector.dart';
export 'src/widgets/widget_span.dart';
export 'src/widgets/widget_state.dart';
export 'src/widgets/will_pop_scope.dart';
| flutter/packages/flutter/lib/widgets.dart/0 | {
"file_path": "flutter/packages/flutter/lib/widgets.dart",
"repo_id": "flutter",
"token_count": 2612
} | 671 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
const String kApiDocsLink = 'See "Types with special considerations" at https://api.flutter.dev/flutter/animation/Tween-class.html for more information.';
void main() {
test('throws flutter error when tweening types that do not fully satisfy tween requirements - Object', () {
final Tween<Object> objectTween = Tween<Object>(
begin: Object(),
end: Object(),
);
expect(
() => objectTween.transform(0.1),
throwsA(isA<FlutterError>().having(
(FlutterError error) => error.diagnostics.map((DiagnosticsNode node) => node.toString()),
'diagnostics',
<String>[
'Cannot lerp between "Instance of \'Object\'" and "Instance of \'Object\'".',
'The type Object might not fully implement `+`, `-`, and/or `*`. $kApiDocsLink',
'There may be a dedicated "ObjectTween" for this type, or you may need to create one.',
],
)),
);
});
test('throws flutter error when tweening types that do not fully satisfy tween requirements - Color', () {
final Tween<Color> colorTween = Tween<Color>(
begin: const Color(0xFF000000),
end: const Color(0xFFFFFFFF),
);
expect(
() => colorTween.transform(0.1),
throwsA(isA<FlutterError>().having(
(FlutterError error) => error.diagnostics.map((DiagnosticsNode node) => node.toString()),
'diagnostics',
<String>[
'Cannot lerp between "Color(0xff000000)" and "Color(0xffffffff)".',
'The type Color might not fully implement `+`, `-`, and/or `*`. $kApiDocsLink',
'To lerp colors, consider ColorTween instead.',
],
)),
);
});
test('throws flutter error when tweening types that do not fully satisfy tween requirements - Rect', () {
final Tween<Rect> rectTween = Tween<Rect>(
begin: const Rect.fromLTWH(0, 0, 10, 10),
end: const Rect.fromLTWH(2, 2, 2, 2),
);
expect(
() => rectTween.transform(0.1),
throwsA(isA<FlutterError>().having(
(FlutterError error) => error.diagnostics.map((DiagnosticsNode node) => node.toString()),
'diagnostics',
<String>[
'Cannot lerp between "Rect.fromLTRB(0.0, 0.0, 10.0, 10.0)" and "Rect.fromLTRB(2.0, 2.0, 4.0, 4.0)".',
'The type Rect might not fully implement `+`, `-`, and/or `*`. $kApiDocsLink',
'To lerp rects, consider RectTween instead.',
],
)),
);
});
test('throws flutter error when tweening types that do not fully satisfy tween requirements - int', () {
final Tween<int> colorTween = Tween<int>(
begin: 0,
end: 1,
);
expect(
() => colorTween.transform(0.1),
throwsA(isA<FlutterError>().having(
(FlutterError error) => error.diagnostics.map((DiagnosticsNode node) => node.toString()),
'diagnostics',
<String>[
'Cannot lerp between "0" and "1".',
'The type int returned a double after multiplication with a double value. $kApiDocsLink',
'To lerp int values, consider IntTween or StepTween instead.',
],
)),
);
});
test('Can chain tweens', () {
final Tween<double> tween = Tween<double>(begin: 0.30, end: 0.50);
expect(tween, hasOneLineDescription);
final Animatable<double> chain = tween.chain(Tween<double>(begin: 0.50, end: 1.0));
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
expect(chain.evaluate(controller), 0.40);
expect(chain, hasOneLineDescription);
});
test('Can animate tweens', () {
final Tween<double> tween = Tween<double>(begin: 0.30, end: 0.50);
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final Animation<double> animation = tween.animate(controller);
controller.value = 0.50;
expect(animation.value, 0.40);
expect(animation, hasOneLineDescription);
expect(animation.toStringDetails(), hasOneLineDescription);
});
test('Can drive tweens', () {
final Tween<double> tween = Tween<double>(begin: 0.30, end: 0.50);
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final Animation<double> animation = controller.drive(tween);
controller.value = 0.50;
expect(animation.value, 0.40);
expect(animation, hasOneLineDescription);
expect(animation.toStringDetails(), hasOneLineDescription);
});
test('BorderTween nullable test', () {
BorderTween tween = BorderTween();
expect(tween.lerp(0.0), null);
expect(tween.lerp(1.0), null);
tween = BorderTween(end: const Border(top: BorderSide()));
expect(tween.lerp(0.0), const Border());
expect(tween.lerp(0.5), const Border(top: BorderSide(width: 0.5)));
expect(tween.lerp(1.0), const Border(top: BorderSide()));
});
test('SizeTween', () {
final SizeTween tween = SizeTween(begin: Size.zero, end: const Size(20.0, 30.0));
expect(tween.lerp(0.5), equals(const Size(10.0, 15.0)));
expect(tween, hasOneLineDescription);
});
test('IntTween', () {
final IntTween tween = IntTween(begin: 5, end: 9);
expect(tween.lerp(0.5), 7);
expect(tween.lerp(0.7), 8);
});
test('RectTween', () {
const Rect a = Rect.fromLTWH(5.0, 3.0, 7.0, 11.0);
const Rect b = Rect.fromLTWH(8.0, 12.0, 14.0, 18.0);
final RectTween tween = RectTween(begin: a, end: b);
expect(tween.lerp(0.5), equals(Rect.lerp(a, b, 0.5)));
expect(tween, hasOneLineDescription);
});
test('Matrix4Tween', () {
final Matrix4 a = Matrix4.identity();
final Matrix4 b = a.clone()..translate(6.0, -8.0)..scale(0.5, 1.0, 5.0);
final Matrix4Tween tween = Matrix4Tween(begin: a, end: b);
expect(tween.lerp(0.0), equals(a));
expect(tween.lerp(1.0), equals(b));
expect(
tween.lerp(0.5),
equals(a.clone()..translate(3.0, -4.0)..scale(0.75, 1.0, 3.0)),
);
final Matrix4 c = a.clone()..rotateZ(1.0);
final Matrix4Tween rotationTween = Matrix4Tween(begin: a, end: c);
expect(rotationTween.lerp(0.0), equals(a));
expect(rotationTween.lerp(1.0), equals(c));
expect(
rotationTween.lerp(0.5).absoluteError(a.clone()..rotateZ(0.5)),
moreOrLessEquals(0.0),
);
});
test('ConstantTween', () {
final ConstantTween<double> tween = ConstantTween<double>(100.0);
expect(tween.begin, 100.0);
expect(tween.end, 100.0);
expect(tween.lerp(0.0), 100.0);
expect(tween.lerp(0.5), 100.0);
expect(tween.lerp(1.0), 100.0);
});
test('ReverseTween', () {
final ReverseTween<int> tween = ReverseTween<int>(IntTween(begin: 5, end: 9));
expect(tween.lerp(0.5), 7);
expect(tween.lerp(0.7), 6);
});
test('ColorTween', () {
final ColorTween tween = ColorTween(
begin: const Color(0xff000000),
end: const Color(0xffffffff),
);
expect(tween.lerp(0.0), const Color(0xff000000));
expect(tween.lerp(0.5), const Color(0xff7f7f7f));
expect(tween.lerp(0.7), const Color(0xffb2b2b2));
expect(tween.lerp(1.0), const Color(0xffffffff));
});
test('StepTween', () {
final StepTween tween = StepTween(begin: 5, end: 9);
expect(tween.lerp(0.5), 7);
expect(tween.lerp(0.7), 7);
});
test('CurveTween', () {
final CurveTween tween = CurveTween(curve: Curves.easeIn);
expect(tween.transform(0.0), 0.0);
expect(tween.transform(0.5), 0.31640625);
expect(tween.transform(1.0), 1.0);
});
test('BorderRadiusTween nullable test', () {
final BorderRadiusTween tween = BorderRadiusTween();
expect(tween.transform(0.0), null);
expect(tween.transform(1.0), null);
expect(tween.lerp(0.0), null);
});
}
| flutter/packages/flutter/test/animation/tween_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/tween_test.dart",
"repo_id": "flutter",
"token_count": 3284
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:math';
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('Alert dialog control test', (WidgetTester tester) async {
bool didDelete = false;
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The title'),
content: const Text('The content'),
actions: <Widget>[
const CupertinoDialogAction(
child: Text('Cancel'),
),
CupertinoDialogAction(
isDestructiveAction: true,
onPressed: () {
didDelete = true;
Navigator.pop(context);
},
child: const Text('Delete'),
),
],
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
expect(didDelete, isFalse);
await tester.tap(find.text('Delete'));
await tester.pump();
expect(didDelete, isTrue);
expect(find.text('Delete'), findsNothing);
});
testWidgets('Dialog not barrier dismissible by default', (WidgetTester tester) async {
await tester.pumpWidget(createAppWithCenteredButton(const Text('Go')));
final BuildContext context = tester.element(find.text('Go'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return Container(
width: 100.0,
height: 100.0,
alignment: Alignment.center,
child: const Text('Dialog'),
);
},
);
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text('Dialog'), findsOneWidget);
// Tap on the barrier, which shouldn't do anything this time.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text('Dialog'), findsOneWidget);
});
testWidgets('Dialog configurable to be barrier dismissible', (WidgetTester tester) async {
await tester.pumpWidget(createAppWithCenteredButton(const Text('Go')));
final BuildContext context = tester.element(find.text('Go'));
showCupertinoDialog<void>(
context: context,
barrierDismissible: true,
builder: (BuildContext context) {
return Container(
width: 100.0,
height: 100.0,
alignment: Alignment.center,
child: const Text('Dialog'),
);
},
);
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text('Dialog'), findsOneWidget);
// Tap off the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text('Dialog'), findsNothing);
});
testWidgets('Dialog destructive action style', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(const CupertinoDialogAction(
isDestructiveAction: true,
child: Text('Ok'),
)));
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.color!.withAlpha(255), CupertinoColors.systemRed.color);
});
testWidgets('Dialog default action style', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoTheme(
data: const CupertinoThemeData(
primaryColor: CupertinoColors.systemGreen,
),
child: boilerplate(const CupertinoDialogAction(
child: Text('Ok'),
)),
),
);
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.color!.withAlpha(255), CupertinoColors.systemGreen.color);
});
testWidgets('Dialog dark theme', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(platformBrightness: Brightness.dark),
child: CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('Content'),
actions: <Widget>[
CupertinoDialogAction(
isDefaultAction: true,
onPressed: () {},
child: const Text('Cancel'),
),
const CupertinoDialogAction(child: Text('OK')),
],
),
),
),
);
final RichText cancelText = tester.widget<RichText>(
find.descendant(of: find.text('Cancel'), matching: find.byType(RichText)),
);
expect(
cancelText.text.style!.color!.value,
0xFF0A84FF, // dark elevated color of systemBlue.
);
expect(
find.byType(CupertinoAlertDialog),
paints..rect(color: const Color(0xBF1E1E1E)),
);
});
testWidgets('Has semantic annotations', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(const MaterialApp(home: Material(
child: CupertinoAlertDialog(
title: Text('The Title'),
content: Text('Content'),
actions: <Widget>[
CupertinoDialogAction(child: Text('Cancel')),
CupertinoDialogAction(child: Text('OK')),
],
),
)));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute, SemanticsFlag.namesRoute],
label: 'Alert',
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
children: <TestSemantics>[
TestSemantics(label: 'The Title'),
TestSemantics(label: 'Content'),
],
),
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isButton],
label: 'Cancel',
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isButton],
label: 'OK',
),
],
),
],
),
],
),
],
),
],
),
],
),
ignoreId: true,
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
});
testWidgets('Dialog default action style', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(const CupertinoDialogAction(
isDefaultAction: true,
child: Text('Ok'),
)));
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.fontWeight, equals(FontWeight.w600));
});
testWidgets('Dialog default and destructive action styles', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(const CupertinoDialogAction(
isDefaultAction: true,
isDestructiveAction: true,
child: Text('Ok'),
)));
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.color!.withAlpha(255), CupertinoColors.systemRed.color);
expect(widget.style.fontWeight, equals(FontWeight.w600));
});
testWidgets('Dialog disabled action style', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(const CupertinoDialogAction(
child: Text('Ok'),
)));
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.color!.opacity, greaterThanOrEqualTo(127 / 255));
expect(widget.style.color!.opacity, lessThanOrEqualTo(128 / 255));
});
testWidgets('Dialog enabled action style', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(CupertinoDialogAction(
child: const Text('Ok'),
onPressed: () {},
)));
final DefaultTextStyle widget = tester.widget(find.byType(DefaultTextStyle));
expect(widget.style.color!.opacity, equals(1.0));
});
testWidgets('Message is scrollable, has correct padding with large text sizes', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: CupertinoAlertDialog(
title: const Text('The Title'),
content: Text('Very long content ' * 20),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('Cancel'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('OK'),
),
],
scrollController: scrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
scrollController.jumpTo(100.0);
expect(scrollController.offset, 100.0);
// Set the scroll position back to zero.
scrollController.jumpTo(0.0);
await tester.pumpAndSettle();
// Expect the modal dialog box to take all available height.
expect(
tester.getSize(find.byType(ClipRRect)),
equals(const Size(310.0, 560.0 - 24.0 * 2)),
);
// Check sizes/locations of the text. The text is large so these 2 buttons are stacked.
// Visually the "Cancel" button and "OK" button are the same height when using the
// regular font. However, when using the test font, "Cancel" becomes 2 lines which
// is why the height we're verifying for "Cancel" is larger than "OK".
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(tester.getSize(find.text('The Title')), equals(const Size(270.0, 132.0)));
}
expect(tester.getTopLeft(find.text('The Title')), equals(const Offset(265.0, 80.0 + 24.0)));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Cancel')), equals(const Size(310.0, 148.0)));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'OK')), equals(const Size(310.0, 98.0)));
});
testWidgets('Dialog respects small constraints.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return Center(
child: ConstrainedBox(
// Constrain the dialog to a tiny size and ensure it respects
// these exact constraints.
constraints: BoxConstraints.tight(const Size(200.0, 100.0)),
child: CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('Option 1'),
),
CupertinoDialogAction(
child: Text('Option 2'),
),
CupertinoDialogAction(
child: Text('Option 3'),
),
],
scrollController: scrollController,
),
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
const double topAndBottomMargin = 40.0;
const double topAndBottomPadding = 24.0 * 2;
const double leftAndRightPadding = 40.0 * 2;
final Finder modalFinder = find.byType(ClipRRect);
expect(
tester.getSize(modalFinder),
equals(const Size(200.0 - leftAndRightPadding, 100.0 - topAndBottomMargin - topAndBottomPadding)),
);
});
testWidgets('Button list is scrollable, has correct position with large text sizes.', (WidgetTester tester) async {
final ScrollController actionScrollController = ScrollController();
addTearDown(actionScrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: CupertinoAlertDialog(
title: const Text('The title'),
content: const Text('The content.'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('One'),
),
CupertinoDialogAction(
child: Text('Two'),
),
CupertinoDialogAction(
child: Text('Three'),
),
CupertinoDialogAction(
child: Text('Chocolate Brownies'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('Cancel'),
),
],
actionScrollController: actionScrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
// Check that the action buttons list is scrollable.
expect(actionScrollController.offset, 0.0);
actionScrollController.jumpTo(100.0);
expect(actionScrollController.offset, 100.0);
actionScrollController.jumpTo(0.0);
// Check that the action buttons are aligned vertically.
expect(tester.getCenter(find.widgetWithText(CupertinoDialogAction, 'One')).dx, equals(400.0));
expect(tester.getCenter(find.widgetWithText(CupertinoDialogAction, 'Two')).dx, equals(400.0));
expect(tester.getCenter(find.widgetWithText(CupertinoDialogAction, 'Three')).dx, equals(400.0));
expect(tester.getCenter(find.widgetWithText(CupertinoDialogAction, 'Chocolate Brownies')).dx, equals(400.0));
expect(tester.getCenter(find.widgetWithText(CupertinoDialogAction, 'Cancel')).dx, equals(400.0));
// Check that the action buttons are the correct heights.
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'One')).height, equals(98.0));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Two')).height, equals(98.0));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Three')).height, equals(98.0));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Chocolate Brownies')).height, equals(248.0));
expect(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Cancel')).height, equals(148.0));
});
testWidgets('Title Section is empty, Button section is not empty.', (WidgetTester tester) async {
final ScrollController actionScrollController = ScrollController();
addTearDown(actionScrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withNoTextScaling(
child: CupertinoAlertDialog(
actions: const <Widget>[
CupertinoDialogAction(
child: Text('One'),
),
CupertinoDialogAction(
child: Text('Two'),
),
],
actionScrollController: actionScrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
// Check that the dialog size is the same as the actions section size. This
// ensures that an empty content section doesn't accidentally render some
// empty space in the dialog.
final Finder contentSectionFinder = find.byElementPredicate((Element element) {
return element.widget.runtimeType.toString() == '_CupertinoAlertActionSection';
});
final Finder modalBoundaryFinder = find.byType(ClipRRect);
expect(
tester.getSize(contentSectionFinder),
tester.getSize(modalBoundaryFinder),
);
// Check that the title/message section is not displayed
expect(actionScrollController.offset, 0.0);
expect(tester.getTopLeft(find.widgetWithText(CupertinoDialogAction, 'One')).dy, equals(277.5));
// Check that the button's vertical size is the same.
expect(
tester.getSize(find.widgetWithText(CupertinoDialogAction, 'One')).height,
equals(tester.getSize(find.widgetWithText(CupertinoDialogAction, 'Two')).height),
);
});
testWidgets('Button section is empty, Title section is not empty.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withNoTextScaling(
child: CupertinoAlertDialog(
title: const Text('The title'),
content: const Text('The content.'),
scrollController: scrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
// Check that there's no button action section.
expect(scrollController.offset, 0.0);
expect(find.widgetWithText(CupertinoDialogAction, 'One'), findsNothing);
// Check that the dialog size is the same as the content section size. This
// ensures that an empty button section doesn't accidentally render some
// empty space in the dialog.
final Finder contentSectionFinder = find.byElementPredicate((Element element) {
return element.widget.runtimeType.toString() == '_CupertinoAlertContentSection';
});
final Finder modalBoundaryFinder = find.byType(ClipRRect);
expect(
tester.getSize(contentSectionFinder),
tester.getSize(modalBoundaryFinder),
);
});
testWidgets('Actions section height for 1 button is height of button.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('OK'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
final RenderBox okButtonBox = findActionButtonRenderBoxByTitle(tester, 'OK');
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
expect(okButtonBox.size.width, actionsSectionBox.size.width);
expect(okButtonBox.size.height, actionsSectionBox.size.height);
});
testWidgets('Actions section height for 2 side-by-side buttons is height of tallest button.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
late double dividerWidth; // Will be set when the dialog builder runs. Needs a BuildContext.
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
dividerWidth = 0.3;
return CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('OK'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('Cancel'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
final RenderBox okButtonBox = findActionButtonRenderBoxByTitle(tester, 'OK');
final RenderBox cancelButtonBox = findActionButtonRenderBoxByTitle(tester, 'Cancel');
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
expect(okButtonBox.size.width, cancelButtonBox.size.width);
expect(
actionsSectionBox.size.width,
okButtonBox.size.width + cancelButtonBox.size.width + dividerWidth,
);
expect(
actionsSectionBox.size.height,
max(okButtonBox.size.height, cancelButtonBox.size.height),
);
});
testWidgets('Actions section height for 2 stacked buttons with enough room is height of both buttons.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
const double dividerThickness = 0.3;
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('OK'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('This is too long to fit'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
final RenderBox okButtonBox = findActionButtonRenderBoxByTitle(tester, 'OK');
final RenderBox longButtonBox = findActionButtonRenderBoxByTitle(tester, 'This is too long to fit');
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
expect(okButtonBox.size.width, longButtonBox.size.width);
expect(okButtonBox.size.width, actionsSectionBox.size.width);
expect(
okButtonBox.size.height + dividerThickness + longButtonBox.size.height,
actionsSectionBox.size.height,
);
});
testWidgets('Actions section height for 2 stacked buttons without enough room and regular font is 1.5 buttons tall.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: Text('The message\n' * 40),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('OK'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('This is too long to fit'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
expect(
actionsSectionBox.size.height,
67.80000000000001,
);
});
testWidgets('Actions section height for 2 stacked buttons without enough room and large accessibility font is 50% of dialog height.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: CupertinoAlertDialog(
title: const Text('The Title'),
content: Text('The message\n' * 20),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('This button is multi line'),
),
CupertinoDialogAction(
isDestructiveAction: true,
child: Text('This button is multi line'),
),
],
scrollController: scrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
// The two multiline buttons with large text are taller than 50% of the
// dialog height, but with the accessibility layout policy, the 2 buttons
// should be in a scrollable area equal to half the dialog height.
expect(
actionsSectionBox.size.height,
280.0 - 24.0,
);
});
testWidgets('Actions section height for 3 buttons without enough room is 1.5 buttons tall.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: Text('The message\n' * 40),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('Option 1'),
),
CupertinoDialogAction(
child: Text('Option 2'),
),
CupertinoDialogAction(
child: Text('Option 3'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
await tester.pumpAndSettle();
final RenderBox option1ButtonBox = findActionButtonRenderBoxByTitle(tester, 'Option 1');
final RenderBox option2ButtonBox = findActionButtonRenderBoxByTitle(tester, 'Option 2');
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
expect(option1ButtonBox.size.width, option2ButtonBox.size.width);
expect(option1ButtonBox.size.width, actionsSectionBox.size.width);
// Expected Height = button 1 + divider + 1/2 button 2 = 67.80000000000001
const double expectedHeight = 67.80000000000001;
expect(
actionsSectionBox.size.height,
moreOrLessEquals(expectedHeight),
);
});
testWidgets('Actions section overscroll is painted white.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('Option 1'),
),
CupertinoDialogAction(
child: Text('Option 2'),
),
CupertinoDialogAction(
child: Text('Option 3'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
// The way that overscroll white is accomplished in a scrollable action
// section is that the custom RenderBox that lays out the buttons and draws
// the dividers also paints a white background the size of Rect.largest.
// That background ends up being clipped by the containing ScrollView.
//
// Here we test that the Rect(0.0, 0.0, renderBox.size.width, renderBox.size.height)
// is contained within the painted Path.
// We don't test for exclusion because for some reason the Path is reporting
// that even points beyond Rect.largest are within the Path. That's not an
// issue for our use-case, so we don't worry about it.
expect(actionsSectionBox, paints..path(
includes: <Offset>[
Offset.zero,
Offset(actionsSectionBox.size.width, actionsSectionBox.size.height),
],
));
});
testWidgets('Pressed button changes appearance and dividers disappear.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
const double dividerThickness = 0.3;
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The Title'),
content: const Text('The message'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('Option 1'),
),
CupertinoDialogAction(
child: Text('Option 2'),
),
CupertinoDialogAction(
child: Text('Option 3'),
),
],
scrollController: scrollController,
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
const Color normalButtonBackgroundColor = Color(0xCCF2F2F2);
const Color pressedButtonBackgroundColor = Color(0xFFE1E1E1);
final RenderBox firstButtonBox = findActionButtonRenderBoxByTitle(tester, 'Option 1');
final RenderBox secondButtonBox = findActionButtonRenderBoxByTitle(tester, 'Option 2');
final RenderBox actionsSectionBox = findScrollableActionsSectionRenderBox(tester);
final Offset pressedButtonCenter = Offset(
secondButtonBox.size.width / 2.0,
firstButtonBox.size.height + dividerThickness + (secondButtonBox.size.height / 2.0),
);
final Offset topDividerCenter = Offset(
secondButtonBox.size.width / 2.0,
firstButtonBox.size.height + (0.5 * dividerThickness),
);
final Offset bottomDividerCenter = Offset(
secondButtonBox.size.width / 2.0,
firstButtonBox.size.height +
dividerThickness +
secondButtonBox.size.height +
(0.5 * dividerThickness),
);
// Before pressing the button, verify following expectations:
// - Background includes the button that will be pressed
// - Background excludes the divider above and below the button that will be pressed
// - Pressed button background does NOT include the button that will be pressed
expect(actionsSectionBox, paints
..path(
color: normalButtonBackgroundColor,
includes: <Offset>[
pressedButtonCenter,
],
excludes: <Offset>[
topDividerCenter,
bottomDividerCenter,
],
)
..path(
color: pressedButtonBackgroundColor,
excludes: <Offset>[
pressedButtonCenter,
],
),
);
// Press down on the button.
final TestGesture gesture = await tester.press(find.widgetWithText(CupertinoDialogAction, 'Option 2'));
await tester.pump();
// While pressing the button, verify following expectations:
// - Background excludes the pressed button
// - Background includes the divider above and below the pressed button
// - Pressed button background includes the pressed
expect(actionsSectionBox, paints
..path(
color: normalButtonBackgroundColor,
// The background should contain the divider above and below the pressed
// button. While pressed, surrounding dividers disappear, which means
// they become part of the background.
includes: <Offset>[
topDividerCenter,
bottomDividerCenter,
],
// The background path should not include the tapped button background...
excludes: <Offset>[
pressedButtonCenter,
],
)
// For a pressed button, a dedicated path is painted with a pressed button
// background color...
..path(
color: pressedButtonBackgroundColor,
includes: <Offset>[
pressedButtonCenter,
],
),
);
// We must explicitly cause an "up" gesture to avoid a crash.
// TODO(mattcarroll): remove this call, https://github.com/flutter/flutter/issues/19540
await gesture.up();
});
testWidgets('ScaleTransition animation for showCupertinoDialog()', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: Builder(
builder: (BuildContext context) {
return CupertinoButton(
onPressed: () {
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The title'),
content: const Text('The content'),
actions: <Widget>[
const CupertinoDialogAction(
child: Text('Cancel'),
),
CupertinoDialogAction(
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context);
},
child: const Text('Delete'),
),
],
);
},
);
},
child: const Text('Go'),
);
},
),
),
),
);
await tester.tap(find.text('Go'));
// Enter animation.
await tester.pump();
Transform transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.3, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.145, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.044, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.013, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.003, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.000, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transform = tester.widget(find.byType(Transform));
expect(transform.transform[0], moreOrLessEquals(1.000, epsilon: 0.001));
await tester.tap(find.text('Delete'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
// No scaling on exit animation.
expect(find.byType(Transform), findsNothing);
});
testWidgets('FadeTransition animation for showCupertinoDialog()', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: Builder(
builder: (BuildContext context) {
return CupertinoButton(
onPressed: () {
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return CupertinoAlertDialog(
title: const Text('The title'),
content: const Text('The content'),
actions: <Widget>[
const CupertinoDialogAction(
child: Text('Cancel'),
),
CupertinoDialogAction(
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context);
},
child: const Text('Delete'),
),
],
);
},
);
},
child: const Text('Go'),
);
},
),
),
),
);
await tester.tap(find.text('Go'));
// Enter animation.
await tester.pump();
final Finder fadeTransitionFinder = find.ancestor(of: find.byType(CupertinoAlertDialog), matching: find.byType(FadeTransition));
FadeTransition transition = tester.firstWidget(fadeTransitionFinder);
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.081, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.332, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.667, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.918, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(1.0, epsilon: 0.001));
await tester.tap(find.text('Delete'));
// Exit animation, look at reverse FadeTransition.
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(1.0, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.918, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.667, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.332, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.081, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(fadeTransitionFinder);
expect(transition.opacity.value, moreOrLessEquals(0.0, epsilon: 0.001));
});
testWidgets('Actions are accessible by key', (WidgetTester tester) async {
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return const CupertinoAlertDialog(
title: Text('The Title'),
content: Text('The message'),
actions: <Widget>[
CupertinoDialogAction(
key: Key('option_1'),
child: Text('Option 1'),
),
CupertinoDialogAction(
key: Key('option_2'),
child: Text('Option 2'),
),
],
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
expect(find.byKey(const Key('option_1')), findsOneWidget);
expect(find.byKey(const Key('option_2')), findsOneWidget);
expect(find.byKey(const Key('option_3')), findsNothing);
});
testWidgets('Dialog widget insets by MediaQuery viewInsets', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: MediaQuery(
data: MediaQueryData(),
child: CupertinoAlertDialog(content: Placeholder(fallbackHeight: 200.0)),
),
),
);
final Rect placeholderRectWithoutInsets = tester.getRect(find.byType(Placeholder));
await tester.pumpWidget(
const MaterialApp(
home: MediaQuery(
data: MediaQueryData(viewInsets: EdgeInsets.fromLTRB(40.0, 30.0, 20.0, 10.0)),
child: CupertinoAlertDialog(content: Placeholder(fallbackHeight: 200.0)),
),
),
);
// no change yet because padding is animated
expect(tester.getRect(find.byType(Placeholder)), placeholderRectWithoutInsets);
await tester.pump(const Duration(seconds: 1));
// once animation settles the dialog is padded by the new viewInsets
expect(tester.getRect(find.byType(Placeholder)), placeholderRectWithoutInsets.translate(10, 10));
});
testWidgets('Material2 - Default cupertino dialog golden', (WidgetTester tester) async {
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
useMaterial3: false,
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: const RepaintBoundary(
child: CupertinoAlertDialog(
title: Text('Title'),
content: Text('text'),
actions: <Widget>[
CupertinoDialogAction(child: Text('No')),
CupertinoDialogAction(child: Text('OK')),
],
),
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
await expectLater(
find.byType(CupertinoAlertDialog),
matchesGoldenFile('m2_dialog_test.cupertino.default.png'),
);
});
testWidgets('Material3 - Default cupertino dialog golden', (WidgetTester tester) async {
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
useMaterial3: true,
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: const RepaintBoundary(
child: CupertinoAlertDialog(
title: Text('Title'),
content: Text('text'),
actions: <Widget>[
CupertinoDialogAction(child: Text('No')),
CupertinoDialogAction(child: Text('OK')),
],
),
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
await expectLater(
find.byType(CupertinoAlertDialog),
matchesGoldenFile('m3_dialog_test.cupertino.default.png'),
);
});
testWidgets('showCupertinoDialog - custom barrierLabel', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
CupertinoApp(
home: Builder(
builder: (BuildContext context) {
return Center(
child: CupertinoButton(
child: const Text('X'),
onPressed: () {
showCupertinoDialog<void>(
context: context,
barrierLabel: 'Custom label',
builder: (BuildContext context) {
return const CupertinoAlertDialog(
title: Text('Title'),
content: Text('Content'),
actions: <Widget>[
CupertinoDialogAction(child: Text('Yes')),
CupertinoDialogAction(child: Text('No')),
],
);
},
);
},
),
);
},
),
),
);
expect(semantics, isNot(includesNodeWith(
label: 'Custom label',
flags: <SemanticsFlag>[SemanticsFlag.namesRoute],
)));
semantics.dispose();
});
testWidgets('CupertinoDialogRoute is state restorable', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'app',
home: _RestorableDialogTestWidget(),
),
);
expect(find.byType(CupertinoAlertDialog), findsNothing);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(CupertinoAlertDialog), findsOneWidget);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
expect(find.byType(CupertinoAlertDialog), findsOneWidget);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(find.byType(CupertinoAlertDialog), findsNothing);
await tester.restoreFrom(restorationData);
expect(find.byType(CupertinoAlertDialog), findsOneWidget);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('Conflicting scrollbars are not applied by ScrollBehavior to CupertinoAlertDialog', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/83819
final ScrollController actionScrollController = ScrollController();
addTearDown(actionScrollController.dispose);
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withNoTextScaling(
child: CupertinoAlertDialog(
title: const Text('Test Title'),
content: const Text('Test Content'),
actions: const <Widget>[
CupertinoDialogAction(
child: Text('One'),
),
CupertinoDialogAction(
child: Text('Two'),
),
],
actionScrollController: actionScrollController,
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pump();
// The inherited ScrollBehavior should not apply Scrollbars since they are
// already built in to the widget.
expect(find.byType(Scrollbar), findsNothing);
expect(find.byType(RawScrollbar), findsNothing);
// Built in CupertinoScrollbars should only number 2: one for the actions,
// one for the content.
expect(find.byType(CupertinoScrollbar), findsNWidgets(2));
}, variant: TargetPlatformVariant.all());
testWidgets('CupertinoAlertDialog scrollbars controllers should be different', (WidgetTester tester) async {
// https://github.com/flutter/flutter/pull/81278
await tester.pumpWidget(
const MaterialApp(
home: MediaQuery(
data: MediaQueryData(),
child: CupertinoAlertDialog(
actions: <Widget>[
CupertinoDialogAction(child: Text('OK')),
],
content: Placeholder(fallbackHeight: 200.0),
),
),
),
);
final List<CupertinoScrollbar> scrollbars =
find.descendant(
of: find.byType(CupertinoAlertDialog),
matching: find.byType(CupertinoScrollbar),
).evaluate().map((Element e) => e.widget as CupertinoScrollbar).toList();
expect(scrollbars.length, 2);
expect(scrollbars[0].controller != scrollbars[1].controller, isTrue);
});
group('showCupertinoDialog avoids overlapping display features', () {
testWidgets('positioning using anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(Placeholder)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(800.0, 600.0));
});
testWidgets('positioning using Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(Placeholder)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(800.0, 600.0));
});
testWidgets('default positioning', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(Placeholder)), Offset.zero);
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(390.0, 600.0));
});
});
testWidgets('Hovering over Cupertino alert dialog action updates cursor to clickable on Web', (WidgetTester tester) async {
await tester.pumpWidget(
createAppWithButtonThatLaunchesDialog(
dialogBuilder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 3.0,
maxScaleFactor: 3.0,
child: RepaintBoundary(
child: CupertinoAlertDialog(
title: const Text('Title'),
content: const Text('text'),
actions: <Widget>[
CupertinoDialogAction(
onPressed: () {},
child: const Text('NO'),
),
CupertinoDialogAction(
onPressed: () {},
child: const Text('OK'),
),
],
),
),
);
},
),
);
await tester.tap(find.text('Go'));
await tester.pumpAndSettle();
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: const Offset(10, 10));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
final Offset dialogAction = tester.getCenter(find.text('OK'));
await gesture.moveTo(dialogAction);
await tester.pumpAndSettle();
expect(
RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic,
);
});
}
RenderBox findActionButtonRenderBoxByTitle(WidgetTester tester, String title) {
final RenderObject buttonBox = tester.renderObject(find.widgetWithText(CupertinoDialogAction, title));
assert(buttonBox is RenderBox);
return buttonBox as RenderBox;
}
RenderBox findScrollableActionsSectionRenderBox(WidgetTester tester) {
final RenderObject actionsSection = tester.renderObject(find.byElementPredicate((Element element) {
return element.widget.runtimeType.toString() == '_CupertinoAlertActionSection';
}));
assert(actionsSection is RenderBox);
return actionsSection as RenderBox;
}
Widget createAppWithButtonThatLaunchesDialog({
required WidgetBuilder dialogBuilder,
bool? useMaterial3,
}) {
return MaterialApp(
theme: ThemeData(useMaterial3: useMaterial3),
home: Material(
child: Center(
child: Builder(builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
showDialog<void>(
context: context,
builder: dialogBuilder,
);
},
child: const Text('Go'),
);
}),
),
),
);
}
Widget boilerplate(Widget child) {
return Directionality(
textDirection: TextDirection.ltr,
child: child,
);
}
Widget createAppWithCenteredButton(Widget child) {
return MaterialApp(
home: Material(
child: Center(
child: ElevatedButton(
onPressed: null,
child: child,
),
),
),
);
}
class _RestorableDialogTestWidget extends StatelessWidget {
const _RestorableDialogTestWidget();
@pragma('vm:entry-point')
static Route<Object?> _dialogBuilder(BuildContext context, Object? arguments) {
return CupertinoDialogRoute<void>(
context: context,
builder: (BuildContext context) {
return const CupertinoAlertDialog(
title: Text('Title'),
content: Text('Content'),
actions: <Widget>[
CupertinoDialogAction(child: Text('Yes')),
CupertinoDialogAction(child: Text('No')),
],
);
},
);
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('Home'),
),
child: Center(child: CupertinoButton(
onPressed: () {
Navigator.of(context).restorablePush(_dialogBuilder);
},
child: const Text('X'),
)),
);
}
}
| flutter/packages/flutter/test/cupertino/dialog_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/dialog_test.dart",
"repo_id": "flutter",
"token_count": 25176
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('!chrome')
library;
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
late MockNavigatorObserver navigatorObserver;
setUp(() {
navigatorObserver = MockNavigatorObserver();
});
testWidgets('Middle auto-populates with title', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// There should be a Text widget with the title in the nav bar even though
// we didn't specify anything in the nav bar constructor.
expect(find.widgetWithText(CupertinoNavigationBar, 'An iPod'), findsOneWidget);
// As a title, it should also be centered.
expect(tester.getCenter(find.text('An iPod')).dx, 400.0);
});
testWidgets('Large title auto-populates with title', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(),
],
),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// There should be 2 Text widget with the title in the nav bar. One in the
// large title position and one in the middle position (though the middle
// position Text is initially invisible while the sliver is expanded).
expect(
find.widgetWithText(CupertinoSliverNavigationBar, 'An iPod'),
findsNWidgets(2),
);
final List<Element> titles = tester.elementList(find.text('An iPod'))
.toList()
..sort((Element a, Element b) {
final RenderParagraph aParagraph = a.renderObject! as RenderParagraph;
final RenderParagraph bParagraph = b.renderObject! as RenderParagraph;
return aParagraph.text.style!.fontSize!.compareTo(
bParagraph.text.style!.fontSize!,
);
});
final Iterable<double> opacities = titles.map<double>((Element element) {
final RenderAnimatedOpacity renderOpacity =
element.findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
return renderOpacity.opacity.value;
});
expect(opacities, <double> [
0.0, // Initially the smaller font title is invisible.
1.0, // The larger font title is visible.
]);
// Check that the large font title is at the right spot.
expect(
tester.getTopLeft(find.byWidget(titles[1].widget)),
const Offset(16.0, 54.0),
);
// The smaller, initially invisible title, should still be positioned in the
// center.
expect(tester.getCenter(find.byWidget(titles[0].widget)).dx, 400.0);
});
testWidgets('Leading auto-populates with back button with previous title', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'A Phone',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.widgetWithText(CupertinoNavigationBar, 'A Phone'), findsOneWidget);
expect(tester.getCenter(find.text('A Phone')).dx, 400.0);
// Also shows the previous page's title next to the back button.
expect(find.widgetWithText(CupertinoButton, 'An iPod'), findsOneWidget);
// 3 paddings + 1 test font character at font size 34.0.
// The epsilon is needed since the text theme has a negative letter spacing thus.
expect(tester.getTopLeft(find.text('An iPod')).dx, moreOrLessEquals(8.0 + 4.0 + 34.0 + 6.0, epsilon: 0.5));
});
testWidgets('Previous title is correct on first transition frame', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'A Phone',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
// Trigger the route push
await tester.pump();
// Draw the first frame.
await tester.pump();
// Also shows the previous page's title next to the back button.
expect(find.widgetWithText(CupertinoButton, 'An iPod'), findsOneWidget);
});
testWidgets('Previous title stays up to date with changing routes', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
final CupertinoPageRoute<void> route2 = CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
);
final CupertinoPageRoute<void> route3 = CupertinoPageRoute<void>(
title: 'A Phone',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
);
tester.state<NavigatorState>(find.byType(Navigator)).push(route2);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
tester.state<NavigatorState>(find.byType(Navigator)).push(route3);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
tester.state<NavigatorState>(find.byType(Navigator)).replace(
oldRoute: route2,
newRoute: CupertinoPageRoute<void>(
title: 'An Internet communicator',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.widgetWithText(CupertinoNavigationBar, 'A Phone'), findsOneWidget);
expect(tester.getCenter(find.text('A Phone')).dx, 400.0);
// After swapping the route behind the top one, the previous label changes
// from An iPod to Back (since An Internet communicator is too long to
// fit in the back button).
expect(find.widgetWithText(CupertinoButton, 'Back'), findsOneWidget);
// The epsilon is needed since the text theme has a negative letter spacing thus.
expect(tester.getTopLeft(find.text('Back')).dx, moreOrLessEquals(8.0 + 4.0 + 34.0 + 6.0, epsilon: 0.5));
});
testWidgets('Back swipe dismiss interrupted by route push', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/28728
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: scaffoldKey,
child: Center(
child: CupertinoButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('route')),
);
},
));
},
child: const Text('push'),
),
),
),
),
);
// Check the basic iOS back-swipe dismiss transition. Dragging the pushed
// route halfway across the screen will trigger the iOS dismiss animation
await tester.tap(find.text('push'));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0));
await gesture.up();
await tester.pump();
expect( // The 'route' route has been dragged to the right, halfway across the screen
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(CupertinoPageScaffold))),
const Offset(400, 0),
);
expect( // The 'push' route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('push'), matching: find.byType(CupertinoPageScaffold))).dx,
lessThan(0),
);
await tester.pumpAndSettle();
expect(find.text('push'), findsOneWidget);
expect(
tester.getTopLeft(find.ancestor(of: find.text('push'), matching: find.byType(CupertinoPageScaffold))),
Offset.zero,
);
expect(find.text('route'), findsNothing);
// Run the dismiss animation 60%, which exposes the route "push" button,
// and then press the button.
await tester.tap(find.text('push'));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0)); // Drag halfway.
await gesture.up();
// Trigger the snapping animation.
// Since the back swipe drag was brought to >=50% of the screen, it will
// self snap to finish the pop transition as the gesture is lifted.
//
// This drag drop animation is 400ms when dropped exactly halfway
// (800 / [pixel distance remaining], see
// _CupertinoBackGestureController.dragEnd). It follows a curve that is very
// steep initially.
await tester.pump();
expect(
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(CupertinoPageScaffold))),
const Offset(400, 0),
);
// Let the dismissing snapping animation go 60%.
await tester.pump(const Duration(milliseconds: 240));
expect(
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(CupertinoPageScaffold))).dx,
moreOrLessEquals(798, epsilon: 1),
);
// Use the navigator to push a route instead of tapping the 'push' button.
// The topmost route (the one that's animating away), ignores input while
// the pop is underway because route.navigator.userGestureInProgress.
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('route')),
);
},
));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
false,
);
});
testWidgets('Back swipe less than halfway is interrupted by route pop', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/141268
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: scaffoldKey,
child: Center(
child: Column(
children: <Widget>[
const Text('Page 1'),
CupertinoButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 2')),
);
},
));
},
child: const Text('Push Page 2'),
),
],
),
),
),
),
);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
await tester.tap(find.text('Push Page 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsOneWidget);
// Start a back gesture and move it less than 50% across the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 300.0));
await gesture.moveBy(const Offset(100.0, 0.0));
await tester.pump();
expect( // The second route has been dragged to the right.
tester.getTopLeft(find.ancestor(of: find.text('Page 2'), matching: find.byType(CupertinoPageScaffold))),
const Offset(100.0, 0.0),
);
expect( // The first route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))).dx,
lessThan(0),
);
// Programmatically pop and observe that Page 2 was popped as if there were
// no back gesture.
Navigator.pop<void>(scaffoldKey.currentContext!);
await tester.pumpAndSettle();
expect(
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))),
Offset.zero,
);
expect(find.text('Page 2'), findsNothing);
});
testWidgets('Back swipe more than halfway is interrupted by route pop', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/141268
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: scaffoldKey,
child: Center(
child: Column(
children: <Widget>[
const Text('Page 1'),
CupertinoButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 2')),
);
},
));
},
child: const Text('Push Page 2'),
),
],
),
),
),
),
);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
await tester.tap(find.text('Push Page 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsOneWidget);
// Start a back gesture and move it more than 50% across the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 300.0));
await gesture.moveBy(const Offset(500.0, 0.0));
await tester.pump();
expect( // The second route has been dragged to the right.
tester.getTopLeft(find.ancestor(of: find.text('Page 2'), matching: find.byType(CupertinoPageScaffold))),
const Offset(500.0, 0.0),
);
expect( // The first route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))).dx,
lessThan(0),
);
// Programmatically pop and observe that Page 2 was popped as if there were
// no back gesture.
Navigator.pop<void>(scaffoldKey.currentContext!);
await tester.pumpAndSettle();
expect(
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))),
Offset.zero,
);
expect(find.text('Page 2'), findsNothing);
});
testWidgets('Back swipe less than halfway is interrupted by route push', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/141268
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: scaffoldKey,
child: Center(
child: Column(
children: <Widget>[
const Text('Page 1'),
CupertinoButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 2')),
);
},
));
},
child: const Text('Push Page 2'),
),
],
),
),
),
),
);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
await tester.tap(find.text('Push Page 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsOneWidget);
// Start a back gesture and move it less than 50% across the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 300.0));
await gesture.moveBy(const Offset(100.0, 0.0));
await tester.pump();
expect( // The second route has been dragged to the right.
tester.getTopLeft(find.ancestor(of: find.text('Page 2'), matching: find.byType(CupertinoPageScaffold))),
const Offset(100.0, 0.0),
);
expect( // The first route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))).dx,
lessThan(0),
);
// Programmatically push and observe that Page 3 was pushed as if there were
// no back gesture.
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 3')),
);
},
));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsNothing);
expect(
tester.getTopLeft(find.ancestor(of: find.text('Page 3'), matching: find.byType(CupertinoPageScaffold))),
Offset.zero,
);
});
testWidgets('Back swipe more than halfway is interrupted by route push', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/141268
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: scaffoldKey,
child: Center(
child: Column(
children: <Widget>[
const Text('Page 1'),
CupertinoButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 2')),
);
},
));
},
child: const Text('Push Page 2'),
),
],
),
),
),
),
);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
await tester.tap(find.text('Push Page 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsOneWidget);
// Start a back gesture and move it more than 50% across the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 300.0));
await gesture.moveBy(const Offset(500.0, 0.0));
await tester.pump();
expect( // The second route has been dragged to the right.
tester.getTopLeft(find.ancestor(of: find.text('Page 2'), matching: find.byType(CupertinoPageScaffold))),
const Offset(500.0, 0.0),
);
expect( // The first route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('Page 1'), matching: find.byType(CupertinoPageScaffold))).dx,
lessThan(0),
);
// Programmatically push and observe that Page 3 was pushed as if there were
// no back gesture.
Navigator.push<void>(scaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Center(child: Text('Page 3')),
);
},
));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsNothing);
expect(
tester.getTopLeft(find.ancestor(of: find.text('Page 3'), matching: find.byType(CupertinoPageScaffold))),
Offset.zero,
);
});
testWidgets('Fullscreen route animates correct transform values over time', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Builder(
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('Button'),
onPressed: () {
Navigator.push<void>(context, CupertinoPageRoute<void>(
fullscreenDialog: true,
builder: (BuildContext context) {
return Column(
children: <Widget>[
const Placeholder(),
CupertinoButton(
child: const Text('Close'),
onPressed: () {
Navigator.pop<void>(context);
},
),
],
);
},
));
},
);
},
),
),
);
// Enter animation.
await tester.tap(find.text('Button'));
await tester.pump();
// We use a higher number of intervals since the animation has to scale the
// entire screen.
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(475.6, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(350.0, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(237.4, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(149.2, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(89.5, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(54.4, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(33.2, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(20.4, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(12.6, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(7.4, epsilon: 0.1));
// Exit animation
await tester.tap(find.text('Close'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(411.03, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(484.35, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(530.67, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(557.61, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(573.88, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(583.86, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(590.26, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(594.58, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(597.66, epsilon: 0.1));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dy, moreOrLessEquals(600.0, epsilon: 0.1));
});
Future<void> testParallax(WidgetTester tester, {required bool fromFullscreenDialog}) async {
await tester.pumpWidget(
CupertinoApp(
onGenerateRoute: (RouteSettings settings) => CupertinoPageRoute<void>(
fullscreenDialog: fromFullscreenDialog,
settings: settings,
builder: (BuildContext context) {
return Column(
children: <Widget>[
const Placeholder(),
CupertinoButton(
child: const Text('Button'),
onPressed: () {
Navigator.push<void>(context, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('Close'),
onPressed: () {
Navigator.pop<void>(context);
},
);
},
));
},
),
],
);
},
),
),
);
// Enter animation.
await tester.tap(find.text('Button'));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(0.0, epsilon: 0.1));
await tester.pump();
// We use a higher number of intervals since the animation has to scale the
// entire screen.
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-55.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-111.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-161.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-200.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-226.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-242.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-251.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-257.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-261.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-263.0, epsilon: 1.0));
// Exit animation
await tester.tap(find.text('Close'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-83.0, epsilon: 1.0));
await tester.pump(const Duration(milliseconds: 360));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(-0.0, epsilon: 1.0));
}
testWidgets('CupertinoPageRoute has parallax when non fullscreenDialog route is pushed on top', (WidgetTester tester) async {
await testParallax(tester, fromFullscreenDialog: false);
});
testWidgets('FullscreenDialog CupertinoPageRoute has parallax when non fullscreenDialog route is pushed on top', (WidgetTester tester) async {
await testParallax(tester, fromFullscreenDialog: true);
});
Future<void> testNoParallax(WidgetTester tester, {required bool fromFullscreenDialog}) async{
await tester.pumpWidget(
CupertinoApp(
onGenerateRoute: (RouteSettings settings) => CupertinoPageRoute<void>(
fullscreenDialog: fromFullscreenDialog,
builder: (BuildContext context) {
return Column(
children: <Widget>[
const Placeholder(),
CupertinoButton(
child: const Text('Button'),
onPressed: () {
Navigator.push<void>(context, CupertinoPageRoute<void>(
fullscreenDialog: true,
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('Close'),
onPressed: () {
Navigator.pop<void>(context);
},
);
},
));
},
),
],
);
},
),
),
);
// Enter animation.
await tester.tap(find.text('Button'));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, moreOrLessEquals(0.0, epsilon: 0.1));
await tester.pump();
// We use a higher number of intervals since the animation has to scale the
// entire screen.
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
// Exit animation
await tester.tap(find.text('Close'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
await tester.pump(const Duration(milliseconds: 360));
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
}
testWidgets('CupertinoPageRoute has no parallax when fullscreenDialog route is pushed on top', (WidgetTester tester) async {
await testNoParallax(tester, fromFullscreenDialog: false);
});
testWidgets('FullscreenDialog CupertinoPageRoute has no parallax when fullscreenDialog route is pushed on top', (WidgetTester tester) async {
await testNoParallax(tester, fromFullscreenDialog: true);
});
testWidgets('Animated push/pop is not linear', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Text('1'),
),
);
final CupertinoPageRoute<void> route2 = CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Text('2'),
);
},
);
tester.state<NavigatorState>(find.byType(Navigator)).push(route2);
// The whole transition is 500ms based on CupertinoPageRoute.transitionDuration.
// Break it up into small chunks.
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-69, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(609, epsilon: 1));
await tester.pump(const Duration(milliseconds: 50));
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-136, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(362, epsilon: 1));
await tester.pump(const Duration(milliseconds: 50));
// Translation slows down as time goes on.
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-191, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(192, epsilon: 1));
// Finish the rest of the animation
await tester.pump(const Duration(milliseconds: 350));
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-197, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(190, epsilon: 1));
await tester.pump(const Duration(milliseconds: 50));
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-129, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(437, epsilon: 1));
await tester.pump(const Duration(milliseconds: 50));
// Translation slows down as time goes on.
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-74, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(607, epsilon: 1));
});
testWidgets('Dragged pop gesture is linear', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Text('1'),
),
);
final CupertinoPageRoute<void> route2 = CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Text('2'),
);
},
);
tester.state<NavigatorState>(find.byType(Navigator)).push(route2);
await tester.pumpAndSettle();
expect(find.text('1'), findsNothing);
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(0));
final TestGesture swipeGesture = await tester.startGesture(const Offset(5, 100));
await swipeGesture.moveBy(const Offset(100, 0));
await tester.pump();
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-233, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(100));
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
true,
);
await swipeGesture.moveBy(const Offset(100, 0));
await tester.pump();
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-200));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(200));
// Moving by the same distance each time produces linear movements on both
// routes.
await swipeGesture.moveBy(const Offset(100, 0));
await tester.pump();
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-166, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(300));
});
// Regression test for https://github.com/flutter/flutter/issues/137033.
testWidgets('Update pages during a drag gesture will not stuck', (WidgetTester tester) async {
await tester.pumpWidget(const _TestPageUpdate());
// Tap this button will update the pages in two seconds.
await tester.tap(find.text('Update Pages'));
await tester.pump();
// Start swiping.
final TestGesture swipeGesture = await tester.startGesture(const Offset(5, 100));
await swipeGesture.moveBy(const Offset(100, 0));
await tester.pump();
expect(
tester.stateList<NavigatorState>(find.byType(Navigator)).last.userGestureInProgress,
true,
);
// Wait for pages to update.
await tester.pump(const Duration(seconds: 3));
// Verify pages are updated.
expect(
find.text('New page'),
findsOneWidget,
);
// Verify `userGestureInProgress` is set to false.
expect(
tester.stateList<NavigatorState>(find.byType(Navigator)).last.userGestureInProgress,
false,
);
});
testWidgets('Pop gesture snapping is not linear', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Text('1'),
),
);
final CupertinoPageRoute<void> route2 = CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Text('2'),
);
},
);
tester.state<NavigatorState>(find.byType(Navigator)).push(route2);
await tester.pumpAndSettle();
final TestGesture swipeGesture = await tester.startGesture(const Offset(5, 100));
await swipeGesture.moveBy(const Offset(500, 0));
await swipeGesture.up();
await tester.pump();
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-100));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(500));
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
true,
);
await tester.pump(const Duration(milliseconds: 50));
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-19, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(744, epsilon: 1));
await tester.pump(const Duration(milliseconds: 50));
// Rate of change is slowing down.
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(-4, epsilon: 1));
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(787, epsilon: 1));
await tester.pumpAndSettle();
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
false,
);
});
testWidgets('Snapped drags forwards and backwards should signal didStart/StopUserGesture', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigatorKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
navigatorObservers: <NavigatorObserver>[navigatorObserver],
navigatorKey: navigatorKey,
home: const Text('1'),
),
);
final CupertinoPageRoute<void> route2 = CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
child: Text('2'),
);
},
);
navigatorKey.currentState!.push(route2);
await tester.pumpAndSettle();
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didPush);
await tester.dragFrom(const Offset(5, 100), const Offset(100, 0));
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStartUserGesture);
await tester.pump();
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(100));
expect(navigatorKey.currentState!.userGestureInProgress, true);
// Didn't drag far enough to snap into dismissing this route.
// Each 100px distance takes 100ms to snap back.
await tester.pump(const Duration(milliseconds: 101));
// Back to the page covering the whole screen.
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(0));
expect(navigatorKey.currentState!.userGestureInProgress, false);
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStopUserGesture);
expect(navigatorObserver.invocations.removeLast(), isNot(NavigatorInvocation.didPop));
await tester.dragFrom(const Offset(5, 100), const Offset(500, 0));
await tester.pump();
expect(tester.getTopLeft(find.text('2')).dx, moreOrLessEquals(500));
expect(navigatorKey.currentState!.userGestureInProgress, true);
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didPop);
// Did go far enough to snap out of this route.
await tester.pump(const Duration(milliseconds: 301));
// Back to the page covering the whole screen.
expect(find.text('2'), findsNothing);
// First route covers the whole screen.
expect(tester.getTopLeft(find.text('1')).dx, moreOrLessEquals(0));
expect(navigatorKey.currentState!.userGestureInProgress, false);
});
/// Regression test for https://github.com/flutter/flutter/issues/29596.
testWidgets('test edge swipe then drop back at ending point works', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
navigatorObservers: <NavigatorObserver>[navigatorObserver],
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
final TestGesture gesture = await tester.startGesture(const Offset(5, 200));
// The width of the page.
await gesture.moveBy(const Offset(800, 0));
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStartUserGesture);
await gesture.up();
await tester.pump();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStopUserGesture);
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didPop);
});
testWidgets('test edge swipe then drop back at starting point works', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
navigatorObservers: <NavigatorObserver>[navigatorObserver],
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
final TestGesture gesture = await tester.startGesture(const Offset(5, 200));
// Move right a bit
await gesture.moveBy(const Offset(300, 0));
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStartUserGesture);
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
true,
);
await tester.pump();
// Move back to where we started.
await gesture.moveBy(const Offset(-300, 0));
await gesture.up();
await tester.pump();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
expect(navigatorObserver.invocations.removeLast(), NavigatorInvocation.didStopUserGesture);
expect(navigatorObserver.invocations.removeLast(), isNot(NavigatorInvocation.didPop));
expect(
tester.state<NavigatorState>(find.byType(Navigator)).userGestureInProgress,
false,
);
});
group('Cupertino page transitions', () {
CupertinoPageRoute<void> buildRoute({required bool fullscreenDialog}) {
return CupertinoPageRoute<void>(
fullscreenDialog: fullscreenDialog,
builder: (_) => const SizedBox(),
);
}
testWidgets('when route is not fullscreenDialog, it has a barrierColor', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: SizedBox.expand(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
buildRoute(fullscreenDialog: false),
);
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, const Color(0x18000000));
});
testWidgets('when route is a fullscreenDialog, it has no barrierColor', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: SizedBox.expand(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
buildRoute(fullscreenDialog: true),
);
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, isNull);
});
testWidgets('when route is not fullscreenDialog, it has a _CupertinoEdgeShadowDecoration', (WidgetTester tester) async {
PaintPattern paintsShadowRect({required double dx, required Color color}) {
return paints..everything((Symbol methodName, List<dynamic> arguments) {
if (methodName != #drawRect) {
return true;
}
final Rect rect = arguments[0] as Rect;
final Color paintColor = (arguments[1] as Paint).color;
// _CupertinoEdgeShadowDecoration draws the shadows with a series of
// differently colored 1px-wide rects. Skip rects that aren't being
// drawn by the _CupertinoEdgeShadowDecoration.
if (rect.top != 0 || rect.width != 1.0 || rect.height != 600) {
return true;
}
// Skip calls for rects until the one with the given position offset
if ((rect.left - dx).abs() >= 1) {
return true;
}
if (paintColor.value == color.value) {
return true;
}
throw '''
For a rect with an expected left-side position: $dx (drawn at ${rect.left}):
Expected a rect with color: $color,
And drew a rect with color: $paintColor.
''';
});
}
await tester.pumpWidget(
const MaterialApp(
home: SizedBox.expand(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
buildRoute(fullscreenDialog: false),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 1));
final RenderBox box = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
// Animation starts with effectively no shadow
expect(box, paintsShadowRect(dx: 795, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 785, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 775, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 765, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 755, color: const Color(0x00000000)));
await tester.pump(const Duration(milliseconds: 100));
// Part-way through the transition, the shadow is approaching the full gradient
expect(box, paintsShadowRect(dx: 296, color: const Color(0x03000000)));
expect(box, paintsShadowRect(dx: 286, color: const Color(0x02000000)));
expect(box, paintsShadowRect(dx: 276, color: const Color(0x01000000)));
expect(box, paintsShadowRect(dx: 266, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 266, color: const Color(0x00000000)));
await tester.pumpAndSettle();
// At the end of the transition, the shadow is a gradient between
// 0x04000000 and 0x00000000 and is now offscreen
expect(box, paintsShadowRect(dx: -1, color: const Color(0x04000000)));
expect(box, paintsShadowRect(dx: -10, color: const Color(0x03000000)));
expect(box, paintsShadowRect(dx: -20, color: const Color(0x02000000)));
expect(box, paintsShadowRect(dx: -30, color: const Color(0x01000000)));
expect(box, paintsShadowRect(dx: -40, color: const Color(0x00000000)));
// Start animation in reverse
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(box, paintsShadowRect(dx: 498, color: const Color(0x04000000)));
expect(box, paintsShadowRect(dx: 488, color: const Color(0x03000000)));
expect(box, paintsShadowRect(dx: 478, color: const Color(0x02000000)));
expect(box, paintsShadowRect(dx: 468, color: const Color(0x01000000)));
expect(box, paintsShadowRect(dx: 458, color: const Color(0x00000000)));
await tester.pump(const Duration(milliseconds: 150));
// At the end of the animation, the shadow approaches full transparency
expect(box, paintsShadowRect(dx: 794, color: const Color(0x01000000)));
expect(box, paintsShadowRect(dx: 784, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 774, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 764, color: const Color(0x00000000)));
expect(box, paintsShadowRect(dx: 754, color: const Color(0x00000000)));
});
testWidgets('when route is fullscreenDialog, it has no visible _CupertinoEdgeShadowDecoration', (WidgetTester tester) async {
PaintPattern paintsNoShadows() {
return paints..everything((Symbol methodName, List<dynamic> arguments) {
if (methodName != #drawRect) {
return true;
}
final Rect rect = arguments[0] as Rect;
// _CupertinoEdgeShadowDecoration draws the shadows with a series of
// differently colored 1px rects. Skip all rects not drawn by a
// _CupertinoEdgeShadowDecoration.
if (rect.width != 1.0) {
return true;
}
throw '''
Expected: no rects with a width of 1px.
Found: $rect.
''';
});
}
await tester.pumpWidget(
const MaterialApp(
home: SizedBox.expand(),
),
);
final RenderBox box = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
tester.state<NavigatorState>(find.byType(Navigator)).push(
buildRoute(fullscreenDialog: true),
);
await tester.pumpAndSettle();
expect(box, paintsNoShadows());
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(box, paintsNoShadows());
});
});
testWidgets('ModalPopup overlay dark mode', (WidgetTester tester) async {
late StateSetter stateSetter;
Brightness brightness = Brightness.light;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
stateSetter = setter;
return CupertinoApp(
theme: CupertinoThemeData(brightness: brightness),
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
}),
),
);
},
),
);
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(
tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color!.value,
0x33000000,
);
stateSetter(() { brightness = Brightness.dark; });
await tester.pump();
// TODO(LongCatIsLooong): The background overlay SHOULD switch to dark color.
expect(
tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color!.value,
0x33000000,
);
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
}),
),
),
);
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(
tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color!.value,
0x7A000000,
);
});
testWidgets('During back swipe the route ignores input', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/39989
final GlobalKey homeScaffoldKey = GlobalKey();
final GlobalKey pageScaffoldKey = GlobalKey();
int homeTapCount = 0;
int pageTapCount = 0;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
key: homeScaffoldKey,
child: GestureDetector(
onTap: () {
homeTapCount += 1;
},
),
),
),
);
await tester.tap(find.byKey(homeScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 0);
Navigator.push<void>(homeScaffoldKey.currentContext!, CupertinoPageRoute<void>(
builder: (BuildContext context) {
return CupertinoPageScaffold(
key: pageScaffoldKey,
child: Padding(
padding: const EdgeInsets.all(16),
child: GestureDetector(
onTap: () {
pageTapCount += 1;
},
),
),
);
},
));
await tester.pumpAndSettle();
await tester.tap(find.byKey(pageScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 1);
// Start the basic iOS back-swipe dismiss transition. Drag the pushed
// "page" route halfway across the screen. The underlying "home" will
// start sliding in from the left.
final TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0));
await tester.pump();
expect(tester.getTopLeft(find.byKey(pageScaffoldKey)), const Offset(400, 0));
expect(tester.getTopLeft(find.byKey(homeScaffoldKey)).dx, lessThan(0));
// Tapping on the "page" route doesn't trigger the GestureDetector because
// it's being dragged.
await tester.tap(find.byKey(pageScaffoldKey), warnIfMissed: false);
expect(homeTapCount, 1);
expect(pageTapCount, 1);
});
testWidgets('showCupertinoModalPopup uses root navigator by default', (WidgetTester tester) async {
final PopupObserver rootObserver = PopupObserver();
final PopupObserver nestedObserver = PopupObserver();
await tester.pumpWidget(CupertinoApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.text('tap'));
expect(rootObserver.popupCount, 1);
expect(nestedObserver.popupCount, 0);
});
testWidgets('back swipe to screen edges does not dismiss the hero animation', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigator = GlobalKey<NavigatorState>();
final UniqueKey container = UniqueKey();
await tester.pumpWidget(CupertinoApp(
navigatorKey: navigator,
routes: <String, WidgetBuilder>{
'/': (BuildContext context) {
return CupertinoPageScaffold(
child: Center(
child: Hero(
tag: 'tag',
transitionOnUserGestures: true,
child: SizedBox(key: container, height: 150.0, width: 150.0),
),
),
);
},
'/page2': (BuildContext context) {
return CupertinoPageScaffold(
child: Center(
child: Padding(
padding: const EdgeInsets.fromLTRB(100.0, 0.0, 0.0, 0.0),
child: Hero(
tag: 'tag',
transitionOnUserGestures: true,
child: SizedBox(key: container, height: 150.0, width: 150.0),
),
),
),
);
},
},
));
RenderBox box = tester.renderObject(find.byKey(container)) as RenderBox;
final double initialPosition = box.localToGlobal(Offset.zero).dx;
navigator.currentState!.pushNamed('/page2');
await tester.pumpAndSettle();
box = tester.renderObject(find.byKey(container)) as RenderBox;
final double finalPosition = box.localToGlobal(Offset.zero).dx;
final TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(200, 0));
await tester.pump();
box = tester.renderObject(find.byKey(container)) as RenderBox;
final double firstPosition = box.localToGlobal(Offset.zero).dx;
// Checks the hero is in-transit.
expect(finalPosition, greaterThan(firstPosition));
expect(firstPosition, greaterThan(initialPosition));
// Goes back to final position.
await gesture.moveBy(const Offset(-200, 0));
await tester.pump();
box = tester.renderObject(find.byKey(container)) as RenderBox;
final double secondPosition = box.localToGlobal(Offset.zero).dx;
// There will be a small difference.
expect(finalPosition - secondPosition, lessThan(0.001));
await gesture.moveBy(const Offset(400, 0));
await tester.pump();
box = tester.renderObject(find.byKey(container)) as RenderBox;
final double thirdPosition = box.localToGlobal(Offset.zero).dx;
// Checks the hero is still in-transit and moves further away from the first
// position.
expect(finalPosition, greaterThan(thirdPosition));
expect(thirdPosition, greaterThan(initialPosition));
expect(firstPosition, greaterThan(thirdPosition));
});
testWidgets('showCupertinoModalPopup uses nested navigator if useRootNavigator is false', (WidgetTester tester) async {
final PopupObserver rootObserver = PopupObserver();
final PopupObserver nestedObserver = PopupObserver();
await tester.pumpWidget(CupertinoApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
useRootNavigator: false,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.text('tap'));
expect(rootObserver.popupCount, 0);
expect(nestedObserver.popupCount, 1);
});
testWidgets('showCupertinoDialog uses root navigator by default', (WidgetTester tester) async {
final DialogObserver rootObserver = DialogObserver();
final DialogObserver nestedObserver = DialogObserver();
await tester.pumpWidget(CupertinoApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.text('tap'));
expect(rootObserver.dialogCount, 1);
expect(nestedObserver.dialogCount, 0);
});
testWidgets('showCupertinoDialog uses nested navigator if useRootNavigator is false', (WidgetTester tester) async {
final DialogObserver rootObserver = DialogObserver();
final DialogObserver nestedObserver = DialogObserver();
await tester.pumpWidget(CupertinoApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoDialog<void>(
context: context,
useRootNavigator: false,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.text('tap'));
expect(rootObserver.dialogCount, 0);
expect(nestedObserver.dialogCount, 1);
});
testWidgets('showCupertinoModalPopup does not allow for semantics dismiss by default', (WidgetTester tester) async {
debugDefaultTargetPlatformOverride = TargetPlatform.iOS;
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(CupertinoApp(
home: Navigator(
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Push the route.
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(semantics, isNot(includesNodeWith(
actions: <SemanticsAction>[SemanticsAction.tap],
label: 'Dismiss',
)));
debugDefaultTargetPlatformOverride = null;
semantics.dispose();
});
testWidgets('showCupertinoModalPopup allows for semantics dismiss when set', (WidgetTester tester) async {
debugDefaultTargetPlatformOverride = TargetPlatform.iOS;
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(CupertinoApp(
home: Navigator(
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
semanticsDismissible: true,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Push the route.
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(semantics, includesNodeWith(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Dismiss',
));
debugDefaultTargetPlatformOverride = null;
semantics.dispose();
});
testWidgets('showCupertinoModalPopup passes RouteSettings to PopupRoute', (WidgetTester tester) async {
final RouteSettingsObserver routeSettingsObserver = RouteSettingsObserver();
await tester.pumpWidget(CupertinoApp(
navigatorObservers: <NavigatorObserver>[routeSettingsObserver],
home: Navigator(
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<dynamic>(
pageBuilder: (BuildContext context, Animation<double> _, Animation<double> __) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
routeSettings: const RouteSettings(name: '/modal'),
);
},
child: const Text('tap'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.text('tap'));
expect(routeSettingsObserver.routeName, '/modal');
});
testWidgets('showCupertinoModalPopup transparent barrier color is transparent', (WidgetTester tester) async {
const Color kTransparentColor = Color(0x00000000);
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
barrierColor: kTransparentColor,
);
},
child: const Text('tap'),
);
}),
),
));
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, null);
});
testWidgets('showCupertinoModalPopup null barrier color must be default gray barrier color', (WidgetTester tester) async {
// Barrier color for a Cupertino modal barrier.
// Extracted from https://developer.apple.com/design/resources/.
const Color kModalBarrierColor = CupertinoDynamicColor.withBrightness(
color: Color(0x33000000),
darkColor: Color(0x7A000000),
);
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
);
},
child: const Text('tap'),
);
}),
),
));
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, kModalBarrierColor);
});
testWidgets('showCupertinoModalPopup custom barrier color', (WidgetTester tester) async {
const Color customColor = Color(0x11223344);
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const SizedBox(),
barrierColor: customColor,
);
},
child: const Text('tap'),
);
}),
),
));
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, customColor);
});
testWidgets('showCupertinoModalPopup barrier dismissible', (WidgetTester tester) async {
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const Text('Visible'),
);
},
child: const Text('tap'),
);
}),
),
));
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
await tester.tapAt(tester.getTopLeft(find.ancestor(of: find.text('tap'), matching: find.byType(CupertinoPageScaffold))));
await tester.pumpAndSettle();
expect(find.text('Visible'), findsNothing);
});
testWidgets('showCupertinoModalPopup barrier not dismissible', (WidgetTester tester) async {
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Builder(builder: (BuildContext context) {
return GestureDetector(
onTap: () async {
await showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => const Text('Visible'),
barrierDismissible: false,
);
},
child: const Text('tap'),
);
}),
),
));
await tester.tap(find.text('tap'));
await tester.pumpAndSettle();
await tester.tapAt(tester.getTopLeft(find.ancestor(of: find.text('tap'), matching: find.byType(CupertinoPageScaffold))));
await tester.pumpAndSettle();
expect(find.text('Visible'), findsOneWidget);
});
testWidgets('CupertinoPage works', (WidgetTester tester) async {
final LocalKey pageKey = UniqueKey();
final TransitionDetector detector = TransitionDetector();
List<Page<void>> myPages = <Page<void>>[
CupertinoPage<void>(
key: pageKey,
title: 'title one',
child: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(key: UniqueKey()),
child: const Text('first'),
),
),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test shouldn't call this.
return true;
},
transitionDelegate: detector,
),
);
expect(detector.hasTransition, isFalse);
expect(find.widgetWithText(CupertinoNavigationBar, 'title one'), findsOneWidget);
expect(find.text('first'), findsOneWidget);
myPages = <Page<void>>[
CupertinoPage<void>(
key: pageKey,
title: 'title two',
child: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(key: UniqueKey()),
child: const Text('second'),
),
),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test shouldn't call this.
return true;
},
transitionDelegate: detector,
),
);
// There should be no transition because the page has the same key.
expect(detector.hasTransition, isFalse);
// The content does update.
expect(find.text('first'), findsNothing);
expect(find.widgetWithText(CupertinoNavigationBar, 'title one'), findsNothing);
expect(find.text('second'), findsOneWidget);
expect(find.widgetWithText(CupertinoNavigationBar, 'title two'), findsOneWidget);
});
testWidgets('CupertinoPage can toggle MaintainState', (WidgetTester tester) async {
final LocalKey pageKeyOne = UniqueKey();
final LocalKey pageKeyTwo = UniqueKey();
final TransitionDetector detector = TransitionDetector();
List<Page<void>> myPages = <Page<void>>[
CupertinoPage<void>(key: pageKeyOne, maintainState: false, child: const Text('first')),
CupertinoPage<void>(key: pageKeyTwo, child: const Text('second')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test shouldn't call this.
return true;
},
transitionDelegate: detector,
),
);
expect(detector.hasTransition, isFalse);
// Page one does not maintain state.
expect(find.text('first', skipOffstage: false), findsNothing);
expect(find.text('second'), findsOneWidget);
myPages = <Page<void>>[
CupertinoPage<void>(key: pageKeyOne, child: const Text('first')),
CupertinoPage<void>(key: pageKeyTwo, child: const Text('second')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test shouldn't call this.
return true;
},
transitionDelegate: detector,
),
);
// There should be no transition because the page has the same key.
expect(detector.hasTransition, isFalse);
// Page one sets the maintain state to be true, its widget tree should be
// built.
expect(find.text('first', skipOffstage: false), findsOneWidget);
expect(find.text('second'), findsOneWidget);
});
testWidgets('Popping routes should cancel down events', (WidgetTester tester) async {
await tester.pumpWidget(const _TestPostRouteCancel());
final TestGesture gesture = await tester.createGesture();
await gesture.down(tester.getCenter(find.text('PointerCancelEvents: 0')));
await gesture.up();
await tester.pumpAndSettle();
expect(find.byType(CupertinoButton), findsNothing);
expect(find.text('Hold'), findsOneWidget);
await gesture.down(tester.getCenter(find.text('Hold')));
await tester.pump(const Duration(seconds: 2));
await tester.pumpAndSettle();
expect(find.text('Hold'), findsNothing);
expect(find.byType(CupertinoButton), findsOneWidget);
expect(find.text('PointerCancelEvents: 1'), findsOneWidget);
});
testWidgets('Popping routes during back swipe should not crash', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/63984#issuecomment-675679939
final CupertinoPageRoute<void> r = CupertinoPageRoute<void>(builder: (BuildContext context) {
return const Scaffold(
body: Center(
child: Text('child'),
),
);
});
late NavigatorState navigator;
await tester.pumpWidget(CupertinoApp(
home: Center(
child: Builder(builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Home'),
onPressed: () {
navigator = Navigator.of(context);
navigator.push<void>(r);
},
);
}),
),
));
final TestGesture gesture = await tester.createGesture();
await gesture.down(tester.getCenter(find.byType(ElevatedButton)));
await gesture.up();
await tester.pumpAndSettle();
await gesture.down(const Offset(3, 300));
// Need 2 events to form a valid drag
await tester.pump(const Duration(milliseconds: 100));
await gesture.moveTo(const Offset(30, 300), timeStamp: const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 200));
await gesture.moveTo(const Offset(50, 300), timeStamp: const Duration(milliseconds: 200));
// Pause a while so that the route is popped when the drag is canceled
await tester.pump(const Duration(milliseconds: 1000));
await gesture.moveTo(const Offset(51, 300), timeStamp: const Duration(milliseconds: 1200));
// Remove the drag
navigator.removeRoute(r);
await tester.pump();
});
testWidgets('CupertinoModalPopupRoute is state restorable', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'app',
home: _RestorableModalTestWidget(),
),
);
expect(find.byType(CupertinoActionSheet), findsNothing);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(CupertinoActionSheet), findsOneWidget);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
expect(find.byType(CupertinoActionSheet), findsOneWidget);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(find.byType(CupertinoActionSheet), findsNothing);
await tester.restoreFrom(restorationData);
expect(find.byType(CupertinoActionSheet), findsOneWidget);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
group('showCupertinoDialog avoids overlapping display features', () {
testWidgets('positioning with anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(Placeholder)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(800.0, 600.0));
});
testWidgets('positioning with Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// Since this is RTL, it should place the dialog on the right screen
expect(tester.getTopLeft(find.byType(Placeholder)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(800.0, 600.0));
});
testWidgets('positioning by default', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoDialog<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(Placeholder)), Offset.zero);
expect(tester.getBottomRight(find.byType(Placeholder)), const Offset(390.0, 600.0));
});
});
group('showCupertinoModalPopup avoids overlapping display features', () {
testWidgets('positioning using anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 410);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 800);
});
testWidgets('positioning using Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// This is RTL, so it should place the dialog on the right screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 410);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 800);
});
testWidgets('default positioning', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 390.0);
});
});
}
class MockNavigatorObserver extends NavigatorObserver {
final List<NavigatorInvocation> invocations = <NavigatorInvocation>[];
@override
void didStartUserGesture(Route<dynamic> route, Route<dynamic>? previousRoute) {
invocations.add(NavigatorInvocation.didStartUserGesture);
}
@override
void didPop(Route<dynamic> route, Route<dynamic>? previousRoute) {
invocations.add(NavigatorInvocation.didPop);
}
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
invocations.add(NavigatorInvocation.didPush);
}
@override
void didStopUserGesture() {
invocations.add(NavigatorInvocation.didStopUserGesture);
}
}
enum NavigatorInvocation {
didStartUserGesture,
didPop,
didPush,
didStopUserGesture,
}
class PopupObserver extends NavigatorObserver {
int popupCount = 0;
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is CupertinoModalPopupRoute) {
popupCount++;
}
super.didPush(route, previousRoute);
}
}
class DialogObserver extends NavigatorObserver {
int dialogCount = 0;
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is CupertinoDialogRoute) {
dialogCount++;
}
super.didPush(route, previousRoute);
}
}
class RouteSettingsObserver extends NavigatorObserver {
String? routeName;
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is CupertinoModalPopupRoute) {
routeName = route.settings.name;
}
super.didPush(route, previousRoute);
}
}
class TransitionDetector extends DefaultTransitionDelegate<void> {
bool hasTransition = false;
@override
Iterable<RouteTransitionRecord> resolve({
required List<RouteTransitionRecord> newPageRouteHistory,
required Map<RouteTransitionRecord?, RouteTransitionRecord> locationToExitingPageRoute,
required Map<RouteTransitionRecord?, List<RouteTransitionRecord>> pageRouteToPagelessRoutes,
}) {
hasTransition = true;
return super.resolve(
newPageRouteHistory: newPageRouteHistory,
locationToExitingPageRoute: locationToExitingPageRoute,
pageRouteToPagelessRoutes: pageRouteToPagelessRoutes,
);
}
}
Widget buildNavigator({
required List<Page<dynamic>> pages,
required FlutterView view,
PopPageCallback? onPopPage,
GlobalKey<NavigatorState>? key,
TransitionDelegate<dynamic>? transitionDelegate,
}) {
return MediaQuery(
data: MediaQueryData.fromView(view),
child: Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultCupertinoLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: Navigator(
key: key,
pages: pages,
onPopPage: onPopPage,
transitionDelegate: transitionDelegate ?? const DefaultTransitionDelegate<dynamic>(),
),
),
),
);
}
// A test target to updating pages in navigator.
//
// It contains 3 routes:
//
// * The initial route, 'home'.
// * The 'old' route, displays a button showing 'Update pages'. Tap the button
// will update pages.
// * The 'new' route, displays the new page.
class _TestPageUpdate extends StatefulWidget {
const _TestPageUpdate();
@override
State<StatefulWidget> createState() => _TestPageUpdateState();
}
class _TestPageUpdateState extends State<_TestPageUpdate> {
bool updatePages = false;
@override
Widget build(BuildContext context) {
final GlobalKey<State<StatefulWidget>> navKey = GlobalKey();
return MaterialApp(
home: Navigator(
key: navKey,
pages: updatePages
? <Page<dynamic>>[
const CupertinoPage<dynamic>(name: '/home', child: Text('home')),
const CupertinoPage<dynamic>(name: '/home/new', child: Text('New page')),
]
: <Page<dynamic>>[
const CupertinoPage<dynamic>(name: '/home', child: Text('home')),
CupertinoPage<dynamic>(name: '/home/old', child: buildMainPage()),
],
onPopPage: (_, __) {
return false;
},
),
);
}
Widget buildMainPage() {
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Main'),
ElevatedButton(
onPressed: () {
Future<void>.delayed(const Duration(seconds: 2), () {
setState(() {
updatePages = true;
});
});
},
child: const Text('Update Pages'),
),
],
),
),
);
}
}
// A test target for post-route cancel events.
//
// It contains 2 routes:
//
// * The initial route, 'home', displays a button showing 'PointerCancelEvents: #',
// where # is the number of cancel events received. Tapping the button pushes
// route 'sub'.
// * The 'sub' route, displays a text showing 'Hold'. Holding the button (a down
// event) will pop this route after 1 second.
//
// Holding the 'Hold' button at the moment of popping will force the navigator to
// cancel the down event, increasing the Home counter by 1.
class _TestPostRouteCancel extends StatefulWidget {
const _TestPostRouteCancel();
@override
State<StatefulWidget> createState() => _TestPostRouteCancelState();
}
class _TestPostRouteCancelState extends State<_TestPostRouteCancel> {
int counter = 0;
Widget _buildHome(BuildContext context) {
return Center(
child: CupertinoButton(
child: Text('PointerCancelEvents: $counter'),
onPressed: () => Navigator.pushNamed<void>(context, 'sub'),
),
);
}
Widget _buildSub(BuildContext context) {
return Listener(
onPointerDown: (_) {
Future<void>.delayed(const Duration(seconds: 1)).then((_) {
Navigator.pop(context);
});
},
onPointerCancel: (_) {
setState(() {
counter += 1;
});
},
child: const Center(
child: Text('Hold', style: TextStyle(color: Colors.blue)),
),
);
}
@override
Widget build(BuildContext context) {
return CupertinoApp(
initialRoute: 'home',
onGenerateRoute: (RouteSettings settings) => CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) => switch (settings.name) {
'home' => _buildHome(context),
'sub' => _buildSub(context),
_ => throw UnimplementedError(),
},
),
);
}
}
class _RestorableModalTestWidget extends StatelessWidget {
const _RestorableModalTestWidget();
@pragma('vm:entry-point')
static Route<void> _modalBuilder(BuildContext context, Object? arguments) {
return CupertinoModalPopupRoute<void>(
builder: (BuildContext context) {
return CupertinoActionSheet(
title: const Text('Title'),
message: const Text('Message'),
actions: <Widget>[
CupertinoActionSheetAction(
child: const Text('Action One'),
onPressed: () {
Navigator.pop(context);
},
),
CupertinoActionSheetAction(
child: const Text('Action Two'),
onPressed: () {
Navigator.pop(context);
},
),
],
);
},
);
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('Home'),
),
child: Center(child: CupertinoButton(
onPressed: () {
Navigator.of(context).restorablePush(_modalBuilder);
},
child: const Text('X'),
)),
);
}
}
| flutter/packages/flutter/test/cupertino/route_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/route_test.dart",
"repo_id": "flutter",
"token_count": 40737
} | 674 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:ui' as ui show BoxHeightStyle;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/clipboard_utils.dart';
import '../widgets/editable_text_utils.dart' show findRenderEditable, textOffsetToPosition;
class _LongCupertinoLocalizationsDelegate extends LocalizationsDelegate<CupertinoLocalizations> {
const _LongCupertinoLocalizationsDelegate();
@override
bool isSupported(Locale locale) => locale.languageCode == 'en';
@override
Future<_LongCupertinoLocalizations> load(Locale locale) => _LongCupertinoLocalizations.load(locale);
@override
bool shouldReload(_LongCupertinoLocalizationsDelegate old) => false;
@override
String toString() => '_LongCupertinoLocalizations.delegate(en_US)';
}
class _LongCupertinoLocalizations extends DefaultCupertinoLocalizations {
const _LongCupertinoLocalizations();
@override
String get cutButtonLabel => 'Cutttttttttttttttttttttttttttttttttttttttttttt';
@override
String get copyButtonLabel => 'Copyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyyy';
@override
String get pasteButtonLabel => 'Pasteeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee';
@override
String get selectAllButtonLabel => 'Select Allllllllllllllllllllllllllllllll';
static Future<_LongCupertinoLocalizations> load(Locale locale) {
return SynchronousFuture<_LongCupertinoLocalizations>(const _LongCupertinoLocalizations());
}
static const LocalizationsDelegate<CupertinoLocalizations> delegate = _LongCupertinoLocalizationsDelegate();
}
const _LongCupertinoLocalizations _longLocalizations = _LongCupertinoLocalizations();
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final MockClipboard mockClipboard = MockClipboard();
List<TextSelectionPoint> globalize(Iterable<TextSelectionPoint> points, RenderBox box) {
return points.map<TextSelectionPoint>((TextSelectionPoint point) {
return TextSelectionPoint(
box.localToGlobal(point.point),
point.direction,
);
}).toList();
}
setUp(() async {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(
SystemChannels.platform,
mockClipboard.handleMethodCall,
);
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
});
tearDown(() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(
SystemChannels.platform,
null,
);
});
group('canSelectAll', () {
Widget createEditableText({
Key? key,
String? text,
TextSelection? selection,
}) {
final TextEditingController controller = TextEditingController(text: text)
..selection = selection ?? const TextSelection.collapsed(offset: -1);
addTearDown(controller.dispose);
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
return CupertinoApp(
home: EditableText(
key: key,
controller: controller,
focusNode: focusNode,
style: const TextStyle(),
cursorColor: const Color.fromARGB(0, 0, 0, 0),
backgroundCursorColor: const Color.fromARGB(0, 0, 0, 0),
),
);
}
testWidgets('should return false when there is no text', (WidgetTester tester) async {
final GlobalKey<EditableTextState> key = GlobalKey();
await tester.pumpWidget(createEditableText(key: key));
expect(cupertinoTextSelectionControls.canSelectAll(key.currentState!), false);
});
testWidgets('should return true when there is text and collapsed selection', (WidgetTester tester) async {
final GlobalKey<EditableTextState> key = GlobalKey();
await tester.pumpWidget(createEditableText(
key: key,
text: '123',
));
expect(cupertinoTextSelectionControls.canSelectAll(key.currentState!), true);
});
testWidgets('should return false when there is text and partial uncollapsed selection', (WidgetTester tester) async {
final GlobalKey<EditableTextState> key = GlobalKey();
await tester.pumpWidget(createEditableText(
key: key,
text: '123',
selection: const TextSelection(baseOffset: 1, extentOffset: 2),
));
expect(cupertinoTextSelectionControls.canSelectAll(key.currentState!), false);
});
testWidgets('should return false when there is text and full selection', (WidgetTester tester) async {
final GlobalKey<EditableTextState> key = GlobalKey();
await tester.pumpWidget(createEditableText(
key: key,
text: '123',
selection: const TextSelection(baseOffset: 0, extentOffset: 3),
));
expect(cupertinoTextSelectionControls.canSelectAll(key.currentState!), false);
});
});
group('cupertino handles', () {
testWidgets('draws transparent handle correctly', (WidgetTester tester) async {
await tester.pumpWidget(RepaintBoundary(
child: CupertinoTheme(
data: const CupertinoThemeData(
primaryColor: Color(0x550000AA),
),
child: Builder(
builder: (BuildContext context) {
return Container(
color: CupertinoColors.white,
height: 800,
width: 800,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 250),
child: FittedBox(
child: cupertinoTextSelectionControls.buildHandle(
context,
TextSelectionHandleType.right,
10.0,
),
),
),
);
},
),
),
));
await expectLater(
find.byType(RepaintBoundary),
matchesGoldenFile('text_selection.handle.transparent.png'),
);
});
});
group('Text selection menu overflow (iOS)', () {
Finder findOverflowNextButton() {
return find.byWidgetPredicate((Widget widget) =>
widget is CustomPaint &&
'${widget.painter?.runtimeType}' == '_RightCupertinoChevronPainter',
);
}
Finder findOverflowBackButton() => find.byWidgetPredicate((Widget widget) =>
widget is CustomPaint &&
'${widget.painter?.runtimeType}' == '_LeftCupertinoChevronPainter',
);
testWidgets('All menu items show when they fit.', (WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'abc def ghi');
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: Center(
child: CupertinoTextField(
autofocus: true,
controller: controller,
),
),
),
),
));
// This extra pump is so autofocus can propagate to renderEditable.
await tester.pump();
// Initially, the menu isn't shown at all.
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Long press on an empty space to show the selection menu.
await tester.longPressAt(textOffsetToPosition(tester, 4));
await tester.pumpAndSettle();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Double tap to select a word and show the full selection menu.
final Offset textOffset = textOffsetToPosition(tester, 1);
await tester.tapAt(textOffset);
await tester.pump(const Duration(milliseconds: 200));
await tester.tapAt(textOffset);
await tester.pumpAndSettle();
// The full menu is shown without the navigation buttons.
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets("When a menu item doesn't fit, a second page is used.", (WidgetTester tester) async {
// Set the screen size to more narrow, so that Paste can't fit.
tester.view.physicalSize = const Size(1000, 800);
addTearDown(tester.view.reset);
final TextEditingController controller = TextEditingController(text: 'abc def ghi');
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: Center(
child: CupertinoTextField(
controller: controller,
),
),
),
),
));
Future<void> tapNextButton() async {
await tester.tapAt(tester.getCenter(findOverflowNextButton()));
await tester.pumpAndSettle();
}
Future<void> tapBackButton() async {
await tester.tapAt(tester.getCenter(findOverflowBackButton()));
await tester.pumpAndSettle();
}
// Initially, the menu isn't shown at all.
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Double tap to select a word and show the selection menu.
final Offset textOffset = textOffsetToPosition(tester, 1);
await tester.tapAt(textOffset);
await tester.pump(const Duration(milliseconds: 200));
await tester.tapAt(textOffset);
await tester.pumpAndSettle();
// The last button is missing, and a next button is shown.
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button shows both the overflow, back, and next buttons.
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button shows the next, back, and Look Up button
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button shows the back and Search Web button
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsOneWidget);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the back button thrice shows the first page again with the next button.
await tapBackButton();
await tapBackButton();
await tapBackButton();
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets('A smaller menu puts each button on its own page.', (WidgetTester tester) async {
// Set the screen size to more narrow, so that two buttons can't fit on
// the same page.
tester.view.physicalSize = const Size(640, 800);
addTearDown(tester.view.reset);
final TextEditingController controller = TextEditingController(text: 'abc def ghi');
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: Center(
child: CupertinoTextField(
controller: controller,
),
),
),
),
));
Future<void> tapNextButton() async {
await tester.tapAt(tester.getCenter(findOverflowNextButton()));
await tester.pumpAndSettle();
}
Future<void> tapBackButton() async {
await tester.tapAt(tester.getCenter(findOverflowBackButton()));
await tester.pumpAndSettle();
}
// Initially, the menu isn't shown at all.
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Double tap to select a word and show the selection menu.
final Offset textOffset = textOffsetToPosition(tester, 1);
await tester.tapAt(textOffset);
await tester.pump(const Duration(milliseconds: 200));
await tester.tapAt(textOffset);
await tester.pumpAndSettle();
// Only the first button fits, and a next button is shown.
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(2));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button shows Copy.
await tapNextButton();
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(3));
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button again shows Paste
await tapNextButton();
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(3));
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button again shows the Look Up Button.
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button again shows the Search Web Button.
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tapping the next button again shows the last page and the Share button
await tapNextButton();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsOneWidget);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsNothing);
// Tapping the back button 5 times shows the first page again.
await tapBackButton();
await tapBackButton();
await tapBackButton();
await tapBackButton();
await tapBackButton();
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(2));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(find.text('Look Up'), findsNothing);
expect(find.text('Search Web'), findsNothing);
expect(find.text('Share...'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets('Handles very long locale strings', (WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'abc def ghi');
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
locale: const Locale('en', 'us'),
localizationsDelegates: const <LocalizationsDelegate<dynamic>>[
_LongCupertinoLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
home: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: Center(
child: CupertinoTextField(
autofocus: true,
controller: controller,
),
),
),
),
));
// This extra pump is so autofocus can propagate to renderEditable.
await tester.pump();
// Initially, the menu isn't shown at all.
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Long press on an empty space to show the selection menu, with only the
// paste button visible.
await tester.longPressAt(textOffsetToPosition(tester, 4));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
// Tap next to go to the second and final page.
await tester.tapAt(tester.getCenter(findOverflowNextButton()));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsOneWidget);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsNothing);
// Tap select all to show the full selection menu.
await tester.tap(find.text(_longLocalizations.selectAllButtonLabel));
await tester.pumpAndSettle();
// Only one button fits on each page.
expect(find.text(_longLocalizations.cutButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
// Tap next to go to the second page.
await tester.tapAt(tester.getCenter(findOverflowNextButton()));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tap twice to go to the third page.
await tester.tapAt(tester.getCenter(findOverflowNextButton()));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tap back to go to the second page again.
await tester.tapAt(tester.getCenter(findOverflowBackButton()));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsNothing);
expect(find.text(_longLocalizations.copyButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsOneWidget);
expect(findOverflowNextButton(), findsOneWidget);
// Tap back to go to the first page again.
await tester.tapAt(tester.getCenter(findOverflowBackButton()));
await tester.pumpAndSettle();
expect(find.text(_longLocalizations.cutButtonLabel), findsOneWidget);
expect(find.text(_longLocalizations.copyButtonLabel), findsNothing);
expect(find.text(_longLocalizations.pasteButtonLabel), findsNothing);
expect(find.text(_longLocalizations.selectAllButtonLabel), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsOneWidget);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets(
'When selecting multiple lines over max lines',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'abc\ndef\nghi\njkl\nmno\npqr');
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: Center(
child: CupertinoTextField(
autofocus: true,
padding: const EdgeInsets.all(8.0),
controller: controller,
maxLines: 2,
),
),
),
),
));
// This extra pump is so autofocus can propagate to renderEditable.
await tester.pump();
// Initially, the menu isn't shown at all.
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select All'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Long press on an space to show the selection menu.
await tester.longPressAt(textOffsetToPosition(tester, 1));
await tester.pumpAndSettle();
expect(find.text('Cut'), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// Tap to select all.
await tester.tap(find.text('Select All'));
await tester.pumpAndSettle();
// Only Cut, Copy, and Paste are shown.
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsNothing);
expect(findOverflowBackButton(), findsNothing);
expect(findOverflowNextButton(), findsNothing);
// The menu appears at the top of the visible selection.
final Offset selectionOffset = tester
.getTopLeft(find.byType(CupertinoTextSelectionToolbarButton).first);
final Offset textFieldOffset =
tester.getTopLeft(find.byType(CupertinoTextField));
// 7.0 + 44.0 + 8.0 - 8.0 = _kToolbarArrowSize + text_button_height + _kToolbarContentDistance - padding
expect(selectionOffset.dy + 7.0 + 44.0 + 8.0 - 8.0, equals(textFieldOffset.dy));
},
skip: isBrowser, // [intended] the selection menu isn't required by web
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
});
testWidgets('iOS selection handles scale with rich text (selection style 1)', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: SelectableText.rich(
TextSpan(
children: <InlineSpan>[
TextSpan(text: 'abc ', style: TextStyle(fontSize: 100.0)),
TextSpan(text: 'def ', style: TextStyle(fontSize: 50.0)),
TextSpan(text: 'hij', style: TextStyle(fontSize: 25.0)),
],
),
),
),
),
);
final EditableText editableTextWidget = tester.widget(find.byType(EditableText));
final EditableTextState editableTextState = tester.state(find.byType(EditableText));
final TextEditingController controller = editableTextWidget.controller;
// Double tap to select the second word.
const int index = 4;
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pumpAndSettle();
expect(editableTextState.selectionOverlay!.handlesAreVisible, isTrue);
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 7);
// Drag the right handle 2 letters to the right. Placing the end handle on
// the third word. We use a small offset because the endpoint is on the very
// corner of the handle.
final TextSelection selection = controller.selection;
final RenderEditable renderEditable = findRenderEditable(tester);
final List<TextSelectionPoint> endpoints = globalize(
renderEditable.getEndpointsForSelection(selection),
renderEditable,
);
expect(endpoints.length, 2);
final Offset handlePos = endpoints[1].point + const Offset(1.0, 1.0);
final Offset newHandlePos = textOffsetToPosition(tester, 11);
final TestGesture gesture = await tester.startGesture(handlePos, pointer: 7);
await tester.pump();
await gesture.moveTo(newHandlePos);
await tester.pump();
await gesture.up();
await tester.pump();
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 11);
// Find start and end handles and verify their sizes.
expect(find.byType(Overlay), findsOneWidget);
expect(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
), findsNWidgets(2));
final Iterable<RenderBox> handles = tester.renderObjectList(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
));
// The handle height is determined by the formula:
// textLineHeight + _kSelectionHandleRadius * 2 - _kSelectionHandleOverlap .
// The text line height will be the value of the fontSize.
// The constant _kSelectionHandleRadius has the value of 6.
// The constant _kSelectionHandleOverlap has the value of 1.5.
// In the case of the start handle, which is located on the word 'def',
// 50.0 + 6 * 2 - 1.5 = 60.5 .
expect(handles.first.size.height, 60.5);
expect(handles.last.size.height, 35.5);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets('iOS selection handles scale with rich text (selection style 2)', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: SelectableText.rich(
TextSpan(
children: <InlineSpan>[
TextSpan(text: 'abc ', style: TextStyle(fontSize: 100.0)),
TextSpan(text: 'def ', style: TextStyle(fontSize: 50.0)),
TextSpan(text: 'hij', style: TextStyle(fontSize: 25.0)),
],
),
selectionHeightStyle: ui.BoxHeightStyle.max,
),
),
),
);
final EditableText editableTextWidget = tester.widget(find.byType(EditableText));
final EditableTextState editableTextState = tester.state(find.byType(EditableText));
final TextEditingController controller = editableTextWidget.controller;
// Double tap to select the second word.
const int index = 4;
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pumpAndSettle();
expect(editableTextState.selectionOverlay!.handlesAreVisible, isTrue);
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 7);
// Drag the right handle 2 letters to the right. Placing the end handle on
// the third word. We use a small offset because the endpoint is on the very
// corner of the handle.
final TextSelection selection = controller.selection;
final RenderEditable renderEditable = findRenderEditable(tester);
final List<TextSelectionPoint> endpoints = globalize(
renderEditable.getEndpointsForSelection(selection),
renderEditable,
);
expect(endpoints.length, 2);
final Offset handlePos = endpoints[1].point + const Offset(1.0, 1.0);
final Offset newHandlePos = textOffsetToPosition(tester, 11);
final TestGesture gesture = await tester.startGesture(handlePos, pointer: 7);
await tester.pump();
await gesture.moveTo(newHandlePos);
await tester.pump();
await gesture.up();
await tester.pump();
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 11);
// Find start and end handles and verify their sizes.
expect(find.byType(Overlay), findsOneWidget);
expect(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
), findsNWidgets(2));
final Iterable<RenderBox> handles = tester.renderObjectList(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
));
// The handle height is determined by the formula:
// textLineHeight + _kSelectionHandleRadius * 2 - _kSelectionHandleOverlap .
// The text line height will be the value of the fontSize, of the largest word on the line.
// The constant _kSelectionHandleRadius has the value of 6.
// The constant _kSelectionHandleOverlap has the value of 1.5.
// In the case of the start handle, which is located on the word 'def',
// 100 + 6 * 2 - 1.5 = 110.5 .
// In this case both selection handles are the same size because the selection
// height style is set to BoxHeightStyle.max which means that the height of
// the selection highlight will be the height of the largest word on the line.
expect(handles.first.size.height, 110.5);
expect(handles.last.size.height, 110.5);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets('iOS selection handles scale with rich text (grapheme clusters)', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: SelectableText.rich(
TextSpan(
children: <InlineSpan>[
TextSpan(text: 'abc ', style: TextStyle(fontSize: 100.0)),
TextSpan(text: 'def ', style: TextStyle(fontSize: 50.0)),
TextSpan(text: '👨👩👦 ', style: TextStyle(fontSize: 35.0)),
TextSpan(text: 'hij', style: TextStyle(fontSize: 25.0)),
],
),
),
),
),
);
final EditableText editableTextWidget = tester.widget(find.byType(EditableText));
final EditableTextState editableTextState = tester.state(find.byType(EditableText));
final TextEditingController controller = editableTextWidget.controller;
// Double tap to select the second word.
const int index = 4;
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pumpAndSettle();
expect(editableTextState.selectionOverlay!.handlesAreVisible, isTrue);
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 7);
// Drag the right handle 2 letters to the right. Placing the end handle on
// the third word. We use a small offset because the endpoint is on the very
// corner of the handle.
final TextSelection selection = controller.selection;
final RenderEditable renderEditable = findRenderEditable(tester);
final List<TextSelectionPoint> endpoints = globalize(
renderEditable.getEndpointsForSelection(selection),
renderEditable,
);
expect(endpoints.length, 2);
final Offset handlePos = endpoints[1].point + const Offset(1.0, 1.0);
final Offset newHandlePos = textOffsetToPosition(tester, 16);
final TestGesture gesture = await tester.startGesture(handlePos, pointer: 7);
await tester.pump();
await gesture.moveTo(newHandlePos);
await tester.pump();
await gesture.up();
await tester.pump();
expect(controller.selection.baseOffset, 4);
expect(controller.selection.extentOffset, 16);
// Find start and end handles and verify their sizes.
expect(find.byType(Overlay), findsOneWidget);
expect(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
), findsNWidgets(2));
final Iterable<RenderBox> handles = tester.renderObjectList(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
));
// The handle height is determined by the formula:
// textLineHeight + _kSelectionHandleRadius * 2 - _kSelectionHandleOverlap .
// The text line height will be the value of the fontSize.
// The constant _kSelectionHandleRadius has the value of 6.
// The constant _kSelectionHandleOverlap has the value of 1.5.
// In the case of the end handle, which is located on the grapheme cluster '👨👩👦',
// 35.0 + 6 * 2 - 1.5 = 45.5 .
expect(handles.first.size.height, 60.5);
expect(handles.last.size.height, 45.5);
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
testWidgets(
'iOS selection handles scaling falls back to preferredLineHeight when the current frame does not match the previous', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: SelectableText.rich(
TextSpan(
children: <InlineSpan>[
TextSpan(text: 'abc', style: TextStyle(fontSize: 40.0)),
TextSpan(text: 'def', style: TextStyle(fontSize: 50.0)),
],
),
),
),
),
);
final EditableText editableTextWidget = tester.widget(find.byType(EditableText));
final EditableTextState editableTextState = tester.state(find.byType(EditableText));
final TextEditingController controller = editableTextWidget.controller;
// Double tap to select the second word.
const int index = 4;
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(textOffsetToPosition(tester, index));
await tester.pumpAndSettle();
expect(editableTextState.selectionOverlay!.handlesAreVisible, isTrue);
expect(controller.selection.baseOffset, 0);
expect(controller.selection.extentOffset, 6);
// Drag the right handle 2 letters to the right. Placing the end handle on
// the third word. We use a small offset because the endpoint is on the very
// corner of the handle.
final TextSelection selection = controller.selection;
final RenderEditable renderEditable = findRenderEditable(tester);
final List<TextSelectionPoint> endpoints = globalize(
renderEditable.getEndpointsForSelection(selection),
renderEditable,
);
expect(endpoints.length, 2);
final Offset handlePos = endpoints[1].point + const Offset(1.0, 1.0);
final Offset newHandlePos = textOffsetToPosition(tester, 3);
final TestGesture gesture = await tester.startGesture(handlePos, pointer: 7);
await tester.pump();
await gesture.moveTo(newHandlePos);
await tester.pump();
await gesture.up();
await tester.pump();
expect(controller.selection.baseOffset, 0);
expect(controller.selection.extentOffset, 3);
// Find start and end handles and verify their sizes.
expect(find.byType(Overlay), findsOneWidget);
expect(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
), findsNWidgets(2));
final Iterable<RenderBox> handles = tester.renderObjectList(find.descendant(
of: find.byType(Overlay),
matching: find.byType(CustomPaint),
));
// The handle height is determined by the formula:
// textLineHeight + _kSelectionHandleRadius * 2 - _kSelectionHandleOverlap .
// The text line height will be the value of the fontSize.
// The constant _kSelectionHandleRadius has the value of 6.
// The constant _kSelectionHandleOverlap has the value of 1.5.
// In the case of the start handle, which is located on the word 'abc',
// 40.0 + 6 * 2 - 1.5 = 50.5 .
//
// We are now using the current frames selection and text in order to
// calculate the start and end handle heights (we fall back to preferredLineHeight
// when the current frame differs from the previous frame), where previously
// we would be using a mix of the previous and current frame. This could
// result in the start and end handle heights being calculated inaccurately
// if one of the handles falls between two varying text styles.
expect(handles.first.size.height, 50.5);
expect(handles.last.size.height, 50.5); // This is 60.5 with the previous frame.
},
skip: isBrowser, // [intended] We do not use Flutter-rendered context menu on the Web.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }),
);
}
| flutter/packages/flutter/test/cupertino/text_selection_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/text_selection_test.dart",
"repo_id": "flutter",
"token_count": 16131
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
// based on the sample code in foundation/binding.dart
mixin FooBinding on BindingBase {
@override
void initInstances() {
super.initInstances();
_instance = this;
}
static FooBinding get instance => BindingBase.checkInstance(_instance);
static FooBinding? _instance;
}
class FooLibraryBinding extends BindingBase with FooBinding {
static FooBinding ensureInitialized() {
if (FooBinding._instance == null) {
FooLibraryBinding();
}
return FooBinding.instance;
}
}
void main() {
test('BindingBase.debugBindingType', () async {
expect(BindingBase.debugBindingType(), isNull);
FooLibraryBinding.ensureInitialized();
expect(BindingBase.debugBindingType(), FooLibraryBinding);
});
}
| flutter/packages/flutter/test/foundation/binding_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/binding_test.dart",
"repo_id": "flutter",
"token_count": 314
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('clampDouble', () {
expect(clampDouble(-1.0, 0.0, 1.0), equals(0.0));
expect(clampDouble(2.0, 0.0, 1.0), equals(1.0));
expect(clampDouble(double.infinity, 0.0, 1.0), equals(1.0));
expect(clampDouble(-double.infinity, 0.0, 1.0), equals(0.0));
expect(clampDouble(double.nan, 0.0, double.infinity), equals(double.infinity));
});
}
| flutter/packages/flutter/test/foundation/math_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/math_test.dart",
"repo_id": "flutter",
"token_count": 238
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:vector_math/vector_math_64.dart';
import 'gesture_tester.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testGesture('toString control tests', (GestureTester tester) {
expect(const PointerDownEvent(), hasOneLineDescription);
expect(const PointerDownEvent().toStringFull(), hasOneLineDescription);
});
testGesture('nthMouseButton control tests', (GestureTester tester) {
expect(nthMouseButton(2), kSecondaryMouseButton);
expect(nthStylusButton(2), kSecondaryStylusButton);
});
testGesture('smallestButton tests', (GestureTester tester) {
expect(smallestButton(0x0), equals(0x0));
expect(smallestButton(0x1), equals(0x1));
expect(smallestButton(0x200), equals(0x200));
expect(smallestButton(0x220), equals(0x20));
});
testGesture('isSingleButton tests', (GestureTester tester) {
expect(isSingleButton(0x0), isFalse);
expect(isSingleButton(0x1), isTrue);
expect(isSingleButton(0x200), isTrue);
expect(isSingleButton(0x220), isFalse);
});
test('computed hit slop values are based on pointer device kind', () {
expect(computeHitSlop(PointerDeviceKind.mouse, null), kPrecisePointerHitSlop);
expect(computeHitSlop(PointerDeviceKind.stylus, null), kTouchSlop);
expect(computeHitSlop(PointerDeviceKind.invertedStylus, null), kTouchSlop);
expect(computeHitSlop(PointerDeviceKind.touch, null), kTouchSlop);
expect(computeHitSlop(PointerDeviceKind.unknown, null), kTouchSlop);
expect(computePanSlop(PointerDeviceKind.mouse, null), kPrecisePointerPanSlop);
expect(computePanSlop(PointerDeviceKind.stylus, null), kPanSlop);
expect(computePanSlop(PointerDeviceKind.invertedStylus, null), kPanSlop);
expect(computePanSlop(PointerDeviceKind.touch, null), kPanSlop);
expect(computePanSlop(PointerDeviceKind.unknown, null), kPanSlop);
expect(computeScaleSlop(PointerDeviceKind.mouse), kPrecisePointerScaleSlop);
expect(computeScaleSlop(PointerDeviceKind.stylus), kScaleSlop);
expect(computeScaleSlop(PointerDeviceKind.invertedStylus), kScaleSlop);
expect(computeScaleSlop(PointerDeviceKind.touch), kScaleSlop);
expect(computeScaleSlop(PointerDeviceKind.unknown), kScaleSlop);
});
test('computed hit slop values defer to device value when pointer kind is touch', () {
const DeviceGestureSettings settings = DeviceGestureSettings(touchSlop: 1);
expect(computeHitSlop(PointerDeviceKind.mouse, settings), kPrecisePointerHitSlop);
expect(computeHitSlop(PointerDeviceKind.stylus, settings), 1);
expect(computeHitSlop(PointerDeviceKind.invertedStylus, settings), 1);
expect(computeHitSlop(PointerDeviceKind.touch, settings), 1);
expect(computeHitSlop(PointerDeviceKind.unknown, settings), 1);
expect(computePanSlop(PointerDeviceKind.mouse, settings), kPrecisePointerPanSlop);
// Pan slop is 2x touch slop
expect(computePanSlop(PointerDeviceKind.stylus, settings), 2);
expect(computePanSlop(PointerDeviceKind.invertedStylus, settings), 2);
expect(computePanSlop(PointerDeviceKind.touch, settings), 2);
expect(computePanSlop(PointerDeviceKind.unknown, settings), 2);
});
group('fromMouseEvent', () {
const PointerEvent hover = PointerHoverEvent(
timeStamp: Duration(days: 1),
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distance: 11,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
synthesized: true,
);
const PointerEvent move = PointerMoveEvent(
timeStamp: Duration(days: 1),
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
synthesized: true,
);
test('PointerEnterEvent.fromMouseEvent(hover)', () {
final PointerEnterEvent event = PointerEnterEvent.fromMouseEvent(hover);
const PointerEnterEvent empty = PointerEnterEvent();
expect(event.timeStamp, hover.timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, hover.kind);
expect(event.device, hover.device);
expect(event.position, hover.position);
expect(event.buttons, hover.buttons);
expect(event.down, empty.down);
expect(event.obscured, hover.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, hover.pressureMin);
expect(event.pressureMax, hover.pressureMax);
expect(event.distance, hover.distance);
expect(event.distanceMax, hover.distanceMax);
expect(event.distanceMax, hover.distanceMax);
expect(event.size, hover.size);
expect(event.radiusMajor, hover.radiusMajor);
expect(event.radiusMinor, hover.radiusMinor);
expect(event.radiusMin, hover.radiusMin);
expect(event.radiusMax, hover.radiusMax);
expect(event.orientation, hover.orientation);
expect(event.tilt, hover.tilt);
expect(event.synthesized, hover.synthesized);
});
test('PointerExitEvent.fromMouseEvent(hover)', () {
final PointerExitEvent event = PointerExitEvent.fromMouseEvent(hover);
const PointerExitEvent empty = PointerExitEvent();
expect(event.timeStamp, hover.timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, hover.kind);
expect(event.device, hover.device);
expect(event.position, hover.position);
expect(event.buttons, hover.buttons);
expect(event.down, empty.down);
expect(event.obscured, hover.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, hover.pressureMin);
expect(event.pressureMax, hover.pressureMax);
expect(event.distance, hover.distance);
expect(event.distanceMax, hover.distanceMax);
expect(event.distanceMax, hover.distanceMax);
expect(event.size, hover.size);
expect(event.radiusMajor, hover.radiusMajor);
expect(event.radiusMinor, hover.radiusMinor);
expect(event.radiusMin, hover.radiusMin);
expect(event.radiusMax, hover.radiusMax);
expect(event.orientation, hover.orientation);
expect(event.tilt, hover.tilt);
expect(event.synthesized, hover.synthesized);
});
test('PointerEnterEvent.fromMouseEvent(move)', () {
final PointerEnterEvent event = PointerEnterEvent.fromMouseEvent(move);
const PointerEnterEvent empty = PointerEnterEvent();
expect(event.timeStamp, move.timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, move.kind);
expect(event.device, move.device);
expect(event.position, move.position);
expect(event.buttons, move.buttons);
expect(event.down, move.down);
expect(event.obscured, move.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, move.pressureMin);
expect(event.pressureMax, move.pressureMax);
expect(event.distance, move.distance);
expect(event.distanceMax, move.distanceMax);
expect(event.distanceMax, move.distanceMax);
expect(event.size, move.size);
expect(event.radiusMajor, move.radiusMajor);
expect(event.radiusMinor, move.radiusMinor);
expect(event.radiusMin, move.radiusMin);
expect(event.radiusMax, move.radiusMax);
expect(event.orientation, move.orientation);
expect(event.tilt, move.tilt);
expect(event.synthesized, move.synthesized);
});
test('PointerExitEvent.fromMouseEvent(move)', () {
final PointerExitEvent event = PointerExitEvent.fromMouseEvent(move);
const PointerExitEvent empty = PointerExitEvent();
expect(event.timeStamp, move.timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, move.kind);
expect(event.device, move.device);
expect(event.position, move.position);
expect(event.buttons, move.buttons);
expect(event.down, move.down);
expect(event.obscured, move.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, move.pressureMin);
expect(event.pressureMax, move.pressureMax);
expect(event.distance, move.distance);
expect(event.distanceMax, move.distanceMax);
expect(event.distanceMax, move.distanceMax);
expect(event.size, move.size);
expect(event.radiusMajor, move.radiusMajor);
expect(event.radiusMinor, move.radiusMinor);
expect(event.radiusMin, move.radiusMin);
expect(event.radiusMax, move.radiusMax);
expect(event.orientation, move.orientation);
expect(event.tilt, move.tilt);
expect(event.synthesized, move.synthesized);
});
});
group('Default values of PointerEvents:', () {
// Some parameters are intentionally set to a non-trivial value.
test('PointerDownEvent', () {
const PointerDownEvent event = PointerDownEvent();
expect(event.buttons, kPrimaryButton);
});
test('PointerMoveEvent', () {
const PointerMoveEvent event = PointerMoveEvent();
expect(event.buttons, kPrimaryButton);
});
});
test('paintTransformToPointerEventTransform', () {
Matrix4 original = Matrix4.identity();
Matrix4 changed = PointerEvent.removePerspectiveTransform(original);
expect(changed, original);
original = Matrix4.identity()..scale(3.0);
changed = PointerEvent.removePerspectiveTransform(original);
expect(changed, isNot(original));
original
..setColumn(2, Vector4(0, 0, 1, 0))
..setRow(2, Vector4(0, 0, 1, 0));
expect(changed, original);
});
test('transformPosition', () {
const Offset position = Offset(20, 30);
expect(PointerEvent.transformPosition(null, position), position);
expect(PointerEvent.transformPosition(Matrix4.identity(), position), position);
final Matrix4 transform = Matrix4.translationValues(10, 20, 0);
expect(PointerEvent.transformPosition(transform, position), const Offset(20.0 + 10.0, 30.0 + 20.0));
});
test('transformDeltaViaPositions', () {
Offset transformedDelta = PointerEvent.transformDeltaViaPositions(
untransformedEndPosition: const Offset(20, 30),
untransformedDelta: const Offset(5, 5),
transform: Matrix4.identity()..scale(2.0, 2.0, 1.0),
);
expect(transformedDelta, const Offset(10.0, 10.0));
transformedDelta = PointerEvent.transformDeltaViaPositions(
untransformedEndPosition: const Offset(20, 30),
transformedEndPosition: const Offset(40, 60),
untransformedDelta: const Offset(5, 5),
transform: Matrix4.identity()..scale(2.0, 2.0, 1.0),
);
expect(transformedDelta, const Offset(10.0, 10.0));
transformedDelta = PointerEvent.transformDeltaViaPositions(
untransformedEndPosition: const Offset(20, 30),
transformedEndPosition: const Offset(40, 60),
untransformedDelta: const Offset(5, 5),
transform: null,
);
expect(transformedDelta, const Offset(5, 5));
});
test('transforming events', () {
final Matrix4 transform = (Matrix4.identity()..scale(2.0, 2.0, 1.0)).multiplied(Matrix4.translationValues(10.0, 20.0, 0.0));
const Offset localPosition = Offset(60, 100);
const Offset localDelta = Offset(10, 10);
const PointerAddedEvent added = PointerAddedEvent(
timeStamp: Duration(seconds: 2),
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
obscured: true,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
);
_expectTransformedEvent(
original: added,
transform: transform,
localPosition: localPosition,
);
const PointerCancelEvent cancel = PointerCancelEvent(
timeStamp: Duration(seconds: 2),
pointer: 45,
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
buttons: 4,
obscured: true,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
);
_expectTransformedEvent(
original: cancel,
transform: transform,
localPosition: localPosition,
);
const PointerDownEvent down = PointerDownEvent(
timeStamp: Duration(seconds: 2),
pointer: 45,
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
buttons: 4,
obscured: true,
pressure: 34,
pressureMin: 10,
pressureMax: 60,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
);
_expectTransformedEvent(
original: down,
transform: transform,
localPosition: localPosition,
);
const PointerEnterEvent enter = PointerEnterEvent(
timeStamp: Duration(seconds: 2),
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
delta: Offset(5, 5),
buttons: 4,
obscured: true,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
synthesized: true,
);
_expectTransformedEvent(
original: enter,
transform: transform,
localPosition: localPosition,
localDelta: localDelta,
);
const PointerExitEvent exit = PointerExitEvent(
timeStamp: Duration(seconds: 2),
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
delta: Offset(5, 5),
buttons: 4,
obscured: true,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
synthesized: true,
);
_expectTransformedEvent(
original: exit,
transform: transform,
localPosition: localPosition,
localDelta: localDelta,
);
const PointerHoverEvent hover = PointerHoverEvent(
timeStamp: Duration(seconds: 2),
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
delta: Offset(5, 5),
buttons: 4,
obscured: true,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
synthesized: true,
);
_expectTransformedEvent(
original: hover,
transform: transform,
localPosition: localPosition,
localDelta: localDelta,
);
const PointerMoveEvent move = PointerMoveEvent(
timeStamp: Duration(seconds: 2),
pointer: 45,
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
delta: Offset(5, 5),
buttons: 4,
obscured: true,
pressure: 34,
pressureMin: 10,
pressureMax: 60,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
platformData: 10,
synthesized: true,
);
_expectTransformedEvent(
original: move,
transform: transform,
localPosition: localPosition,
localDelta: localDelta,
);
const PointerRemovedEvent removed = PointerRemovedEvent(
timeStamp: Duration(seconds: 2),
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
obscured: true,
pressureMin: 10,
pressureMax: 60,
distanceMax: 24,
radiusMin: 10,
radiusMax: 50,
);
_expectTransformedEvent(
original: removed,
transform: transform,
localPosition: localPosition,
);
const PointerScrollEvent scroll = PointerScrollEvent(
timeStamp: Duration(seconds: 2),
device: 1,
position: Offset(20, 30),
);
_expectTransformedEvent(
original: scroll,
transform: transform,
localPosition: localPosition,
);
const PointerPanZoomStartEvent panZoomStart = PointerPanZoomStartEvent(
timeStamp: Duration(seconds: 2),
device: 1,
position: Offset(20, 30),
);
_expectTransformedEvent(
original: panZoomStart,
transform: transform,
localPosition: localPosition,
);
const PointerPanZoomUpdateEvent panZoomUpdate = PointerPanZoomUpdateEvent(
timeStamp: Duration(seconds: 2),
device: 1,
position: Offset(20, 30),
);
_expectTransformedEvent(
original: panZoomUpdate,
transform: transform,
localPosition: localPosition,
);
const PointerPanZoomEndEvent panZoomEnd = PointerPanZoomEndEvent(
timeStamp: Duration(seconds: 2),
device: 1,
position: Offset(20, 30),
);
_expectTransformedEvent(
original: panZoomEnd,
transform: transform,
localPosition: localPosition,
);
const PointerUpEvent up = PointerUpEvent(
timeStamp: Duration(seconds: 2),
pointer: 45,
kind: PointerDeviceKind.mouse,
device: 1,
position: Offset(20, 30),
buttons: 4,
obscured: true,
pressure: 34,
pressureMin: 10,
pressureMax: 60,
distance: 12,
distanceMax: 24,
size: 10,
radiusMajor: 33,
radiusMinor: 44,
radiusMin: 10,
radiusMax: 50,
orientation: 2,
tilt: 4,
);
_expectTransformedEvent(
original: up,
transform: transform,
localPosition: localPosition,
);
});
group('copyWith', () {
const PointerEvent added = PointerAddedEvent(
timeStamp: Duration(days: 1),
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distance: 11,
distanceMax: 110,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
);
const PointerEvent hover = PointerHoverEvent(
timeStamp: Duration(days: 1),
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distance: 11,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
synthesized: true,
);
const PointerEvent down = PointerDownEvent(
timeStamp: Duration(days: 1),
pointer: 1,
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
);
const PointerEvent move = PointerMoveEvent(
timeStamp: Duration(days: 1),
pointer: 1,
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
delta: Offset(1.0, 2.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
synthesized: true,
);
const PointerEvent up = PointerUpEvent(
timeStamp: Duration(days: 1),
pointer: 1,
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
buttons: 7,
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distanceMax: 110,
size: 11,
radiusMajor: 11,
radiusMinor: 9,
radiusMin: 1.1,
radiusMax: 22,
orientation: 1.1,
tilt: 1.1,
);
const PointerEvent removed = PointerRemovedEvent(
timeStamp: Duration(days: 1),
kind: PointerDeviceKind.unknown,
device: 10,
position: Offset(101.0, 202.0),
obscured: true,
pressureMax: 2.1,
pressureMin: 1.1,
distanceMax: 110,
radiusMin: 1.1,
radiusMax: 22,
);
const Offset position = Offset.zero;
const Duration timeStamp = Duration(days: 2);
test('PointerAddedEvent.copyWith()', () {
final PointerEvent event = added.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerAddedEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, added.kind);
expect(event.device, added.device);
expect(event.position, position);
expect(event.delta, empty.delta);
expect(event.buttons, added.buttons);
expect(event.down, empty.down);
expect(event.obscured, added.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, added.pressureMin);
expect(event.pressureMax, added.pressureMax);
expect(event.distance, added.distance);
expect(event.distanceMax, added.distanceMax);
expect(event.distanceMax, added.distanceMax);
expect(event.size, empty.size);
expect(event.radiusMajor, empty.radiusMajor);
expect(event.radiusMinor, empty.radiusMinor);
expect(event.radiusMin, added.radiusMin);
expect(event.radiusMax, added.radiusMax);
expect(event.orientation, added.orientation);
expect(event.tilt, added.tilt);
expect(event.synthesized, empty.synthesized);
});
test('PointerHoverEvent.copyWith()', () {
final PointerEvent event = hover.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerHoverEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, hover.kind);
expect(event.device, hover.device);
expect(event.position, position);
expect(event.delta, empty.delta);
expect(event.buttons, hover.buttons);
expect(event.down, empty.down);
expect(event.obscured, hover.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, hover.pressureMin);
expect(event.pressureMax, hover.pressureMax);
expect(event.distance, hover.distance);
expect(event.distanceMax, hover.distanceMax);
expect(event.distanceMax, hover.distanceMax);
expect(event.size, hover.size);
expect(event.radiusMajor, hover.radiusMajor);
expect(event.radiusMinor, hover.radiusMinor);
expect(event.radiusMin, hover.radiusMin);
expect(event.radiusMax, hover.radiusMax);
expect(event.orientation, hover.orientation);
expect(event.tilt, hover.tilt);
expect(event.synthesized, hover.synthesized);
});
test('PointerDownEvent.copyWith()', () {
final PointerEvent event = down.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerDownEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, down.pointer);
expect(event.kind, down.kind);
expect(event.device, down.device);
expect(event.position, position);
expect(event.delta, empty.delta);
expect(event.buttons, down.buttons);
expect(event.down, empty.down);
expect(event.obscured, down.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, down.pressureMin);
expect(event.pressureMax, down.pressureMax);
expect(event.distance, down.distance);
expect(event.distanceMax, down.distanceMax);
expect(event.distanceMax, down.distanceMax);
expect(event.size, down.size);
expect(event.radiusMajor, down.radiusMajor);
expect(event.radiusMinor, down.radiusMinor);
expect(event.radiusMin, down.radiusMin);
expect(event.radiusMax, down.radiusMax);
expect(event.orientation, down.orientation);
expect(event.tilt, down.tilt);
expect(event.synthesized, empty.synthesized);
});
test('PointerMoveEvent.copyWith()', () {
final PointerEvent event = move.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerMoveEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, move.pointer);
expect(event.kind, move.kind);
expect(event.device, move.device);
expect(event.position, position);
expect(event.delta, move.delta);
expect(event.buttons, move.buttons);
expect(event.down, move.down);
expect(event.obscured, move.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, move.pressureMin);
expect(event.pressureMax, move.pressureMax);
expect(event.distance, move.distance);
expect(event.distanceMax, move.distanceMax);
expect(event.distanceMax, move.distanceMax);
expect(event.size, move.size);
expect(event.radiusMajor, move.radiusMajor);
expect(event.radiusMinor, move.radiusMinor);
expect(event.radiusMin, move.radiusMin);
expect(event.radiusMax, move.radiusMax);
expect(event.orientation, move.orientation);
expect(event.tilt, move.tilt);
expect(event.synthesized, move.synthesized);
});
test('PointerUpEvent.copyWith()', () {
final PointerEvent event = up.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerUpEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, up.pointer);
expect(event.kind, up.kind);
expect(event.device, up.device);
expect(event.position, position);
expect(event.delta, up.delta);
expect(event.buttons, up.buttons);
expect(event.down, empty.down);
expect(event.obscured, up.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, up.pressureMin);
expect(event.pressureMax, up.pressureMax);
expect(event.distance, up.distance);
expect(event.distanceMax, up.distanceMax);
expect(event.distanceMax, up.distanceMax);
expect(event.size, up.size);
expect(event.radiusMajor, up.radiusMajor);
expect(event.radiusMinor, up.radiusMinor);
expect(event.radiusMin, up.radiusMin);
expect(event.radiusMax, up.radiusMax);
expect(event.orientation, up.orientation);
expect(event.tilt, up.tilt);
expect(event.synthesized, empty.synthesized);
});
test('PointerRemovedEvent.copyWith()', () {
final PointerEvent event = removed.copyWith(position: position, timeStamp: timeStamp);
const PointerEvent empty = PointerRemovedEvent();
expect(event.timeStamp, timeStamp);
expect(event.pointer, empty.pointer);
expect(event.kind, removed.kind);
expect(event.device, removed.device);
expect(event.position, position);
expect(event.delta, empty.delta);
expect(event.buttons, removed.buttons);
expect(event.down, empty.down);
expect(event.obscured, removed.obscured);
expect(event.pressure, empty.pressure);
expect(event.pressureMin, removed.pressureMin);
expect(event.pressureMax, removed.pressureMax);
expect(event.distance, empty.distance);
expect(event.distanceMax, removed.distanceMax);
expect(event.distanceMax, removed.distanceMax);
expect(event.size, empty.size);
expect(event.radiusMajor, empty.radiusMajor);
expect(event.radiusMinor, empty.radiusMinor);
expect(event.radiusMin, removed.radiusMin);
expect(event.radiusMax, removed.radiusMax);
expect(event.orientation, empty.orientation);
expect(event.tilt, empty.tilt);
expect(event.synthesized, empty.synthesized);
});
});
test('Ensure certain event types are allowed', () {
// Regression test for https://github.com/flutter/flutter/issues/107962
expect(const PointerHoverEvent(kind: PointerDeviceKind.trackpad), isNotNull);
// Regression test for https://github.com/flutter/flutter/issues/108176
expect(const PointerScrollInertiaCancelEvent(kind: PointerDeviceKind.trackpad), isNotNull);
expect(const PointerScrollEvent(kind: PointerDeviceKind.trackpad), isNotNull);
// The test passes if it compiles.
});
test('Ensure certain event types are not allowed', () {
expect(() => PointerDownEvent(kind: PointerDeviceKind.trackpad), throwsAssertionError);
expect(() => PointerMoveEvent(kind: PointerDeviceKind.trackpad), throwsAssertionError);
expect(() => PointerUpEvent(kind: PointerDeviceKind.trackpad), throwsAssertionError);
});
}
void _expectTransformedEvent({
required PointerEvent original,
required Matrix4 transform,
Offset? localDelta,
Offset? localPosition,
}) {
expect(original.position, original.localPosition);
expect(original.delta, original.localDelta);
expect(original.original, isNull);
expect(original.transform, isNull);
final PointerEvent transformed = original.transformed(transform);
expect(transformed.original, same(original));
expect(transformed.transform, transform);
expect(transformed.localDelta, localDelta ?? original.localDelta);
expect(transformed.localPosition, localPosition ?? original.localPosition);
expect(transformed.buttons, original.buttons);
expect(transformed.delta, original.delta);
expect(transformed.device, original.device);
expect(transformed.distance, original.distance);
expect(transformed.distanceMax, original.distanceMax);
expect(transformed.distanceMin, original.distanceMin);
expect(transformed.down, original.down);
expect(transformed.kind, original.kind);
expect(transformed.obscured, original.obscured);
expect(transformed.orientation, original.orientation);
expect(transformed.platformData, original.platformData);
expect(transformed.pointer, original.pointer);
expect(transformed.position, original.position);
expect(transformed.pressure, original.pressure);
expect(transformed.pressureMax, original.pressureMax);
expect(transformed.pressureMin, original.pressureMin);
expect(transformed.radiusMajor, original.radiusMajor);
expect(transformed.radiusMax, original.radiusMax);
expect(transformed.radiusMin, original.radiusMin);
expect(transformed.radiusMinor, original.radiusMinor);
expect(transformed.size, original.size);
expect(transformed.synthesized, original.synthesized);
expect(transformed.tilt, original.tilt);
expect(transformed.timeStamp, original.timeStamp);
}
| flutter/packages/flutter/test/gestures/events_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/events_test.dart",
"repo_id": "flutter",
"token_count": 12915
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show VoidCallback;
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
import 'gesture_tester.dart';
// Down/move/up pair 1: normal tap sequence
const PointerDownEvent down = PointerDownEvent(
pointer: 5,
position: Offset(10, 10),
);
const PointerMoveEvent move = PointerMoveEvent(
pointer: 5,
position: Offset(15, 15),
);
const PointerUpEvent up = PointerUpEvent(
pointer: 5,
position: Offset(15, 15),
);
// Down/move/up pair 2: tap sequence with a large move in the middle
const PointerDownEvent down2 = PointerDownEvent(
pointer: 6,
position: Offset(10, 10),
);
const PointerMoveEvent move2 = PointerMoveEvent(
pointer: 6,
position: Offset(100, 200),
);
const PointerUpEvent up2 = PointerUpEvent(
pointer: 6,
position: Offset(100, 200),
);
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
test('GestureRecognizer smoketest', () {
final TestGestureRecognizer recognizer = TestGestureRecognizer(debugOwner: 0);
expect(recognizer, hasAGoodToStringDeep);
});
test('GestureRecognizer dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => TestGestureRecognizer().dispose(),
TestGestureRecognizer
,),
areCreateAndDispose,
);
});
test('OffsetPair', () {
const OffsetPair offset1 = OffsetPair(
local: Offset(10, 20),
global: Offset(30, 40),
);
expect(offset1.local, const Offset(10, 20));
expect(offset1.global, const Offset(30, 40));
const OffsetPair offset2 = OffsetPair(
local: Offset(50, 60),
global: Offset(70, 80),
);
final OffsetPair sum = offset2 + offset1;
expect(sum.local, const Offset(60, 80));
expect(sum.global, const Offset(100, 120));
final OffsetPair difference = offset2 - offset1;
expect(difference.local, const Offset(40, 40));
expect(difference.global, const Offset(40, 40));
});
group('PrimaryPointerGestureRecognizer', () {
testGesture('cleans up state after winning arena', (GestureTester tester) {
final List<String> resolutions = <String>[];
final IndefiniteGestureRecognizer indefinite = IndefiniteGestureRecognizer();
final TestPrimaryPointerGestureRecognizer<PointerUpEvent> accepting = TestPrimaryPointerGestureRecognizer<PointerUpEvent>(
GestureDisposition.accepted,
onAcceptGesture: () => resolutions.add('accepted'),
onRejectGesture: () => resolutions.add('rejected'),
);
expect(accepting.state, GestureRecognizerState.ready);
expect(accepting.primaryPointer, isNull);
expect(accepting.initialPosition, isNull);
expect(resolutions, <String>[]);
indefinite.addPointer(down);
accepting.addPointer(down);
expect(accepting.state, GestureRecognizerState.possible);
expect(accepting.primaryPointer, 5);
expect(accepting.initialPosition!.global, down.position);
expect(accepting.initialPosition!.local, down.localPosition);
expect(resolutions, <String>[]);
tester.closeArena(5);
tester.async.flushMicrotasks();
tester.route(down);
tester.route(up);
expect(accepting.state, GestureRecognizerState.ready);
expect(accepting.primaryPointer, 5);
expect(accepting.initialPosition, isNull);
expect(resolutions, <String>['accepted']);
});
testGesture('cleans up state after losing arena', (GestureTester tester) {
final List<String> resolutions = <String>[];
final IndefiniteGestureRecognizer indefinite = IndefiniteGestureRecognizer();
final TestPrimaryPointerGestureRecognizer<PointerMoveEvent> rejecting = TestPrimaryPointerGestureRecognizer<PointerMoveEvent>(
GestureDisposition.rejected,
onAcceptGesture: () => resolutions.add('accepted'),
onRejectGesture: () => resolutions.add('rejected'),
);
expect(rejecting.state, GestureRecognizerState.ready);
expect(rejecting.primaryPointer, isNull);
expect(rejecting.initialPosition, isNull);
expect(resolutions, <String>[]);
indefinite.addPointer(down);
rejecting.addPointer(down);
expect(rejecting.state, GestureRecognizerState.possible);
expect(rejecting.primaryPointer, 5);
expect(rejecting.initialPosition!.global, down.position);
expect(rejecting.initialPosition!.local, down.localPosition);
expect(resolutions, <String>[]);
tester.closeArena(5);
tester.async.flushMicrotasks();
tester.route(down);
tester.route(move);
expect(rejecting.state, GestureRecognizerState.defunct);
expect(rejecting.primaryPointer, 5);
expect(rejecting.initialPosition!.global, down.position);
expect(rejecting.initialPosition!.local, down.localPosition);
expect(resolutions, <String>['rejected']);
tester.route(up);
expect(rejecting.state, GestureRecognizerState.ready);
expect(rejecting.primaryPointer, 5);
expect(rejecting.initialPosition, isNull);
expect(resolutions, <String>['rejected']);
});
testGesture('works properly when recycled', (GestureTester tester) {
final List<String> resolutions = <String>[];
final IndefiniteGestureRecognizer indefinite = IndefiniteGestureRecognizer();
final TestPrimaryPointerGestureRecognizer<PointerUpEvent> accepting = TestPrimaryPointerGestureRecognizer<PointerUpEvent>(
GestureDisposition.accepted,
preAcceptSlopTolerance: 15,
postAcceptSlopTolerance: 1000,
onAcceptGesture: () => resolutions.add('accepted'),
onRejectGesture: () => resolutions.add('rejected'),
);
// Send one complete pointer sequence
indefinite.addPointer(down);
accepting.addPointer(down);
tester.closeArena(5);
tester.async.flushMicrotasks();
tester.route(down);
tester.route(up);
expect(resolutions, <String>['accepted']);
resolutions.clear();
// Send a follow-on sequence that breaks preAcceptSlopTolerance
indefinite.addPointer(down2);
accepting.addPointer(down2);
tester.closeArena(6);
tester.async.flushMicrotasks();
tester.route(down2);
tester.route(move2);
expect(resolutions, <String>['rejected']);
tester.route(up2);
expect(resolutions, <String>['rejected']);
});
});
}
class TestGestureRecognizer extends GestureRecognizer {
TestGestureRecognizer({ super.debugOwner });
@override
String get debugDescription => 'debugDescription content';
@override
void addPointer(PointerDownEvent event) { }
@override
void acceptGesture(int pointer) { }
@override
void rejectGesture(int pointer) { }
}
/// Gesture recognizer that adds itself to the gesture arena but never
/// resolves itself.
class IndefiniteGestureRecognizer extends GestureRecognizer {
@override
void addAllowedPointer(PointerDownEvent event) {
GestureBinding.instance.gestureArena.add(event.pointer, this);
}
@override
void acceptGesture(int pointer) { }
@override
void rejectGesture(int pointer) { }
@override
String get debugDescription => 'Unresolving';
}
/// Gesture recognizer that resolves with [resolution] when it handles an event
/// on the primary pointer of type [T]
class TestPrimaryPointerGestureRecognizer<T extends PointerEvent> extends PrimaryPointerGestureRecognizer {
TestPrimaryPointerGestureRecognizer(
this.resolution, {
this.onAcceptGesture,
this.onRejectGesture,
super.preAcceptSlopTolerance,
super.postAcceptSlopTolerance,
});
final GestureDisposition resolution;
final VoidCallback? onAcceptGesture;
final VoidCallback? onRejectGesture;
@override
void acceptGesture(int pointer) {
super.acceptGesture(pointer);
if (onAcceptGesture != null) {
onAcceptGesture!();
}
}
@override
void rejectGesture(int pointer) {
super.rejectGesture(pointer);
if (onRejectGesture != null) {
onRejectGesture!();
}
}
@override
void handlePrimaryPointer(PointerEvent event) {
if (event is T) {
resolve(resolution);
}
}
@override
String get debugDescription => 'TestPrimaryPointer';
}
| flutter/packages/flutter/test/gestures/recognizer_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/recognizer_test.dart",
"repo_id": "flutter",
"token_count": 3127
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' as io;
import 'package:flutter/foundation.dart';
/// Whether or not Flutter CI has configured Impeller for this test run.
///
/// This is intended only to be used for a migration effort to enable Impeller
/// on Flutter CI.
///
/// See also: https://github.com/flutter/flutter/issues/143616
bool get impellerEnabled {
if (kIsWeb) {
return false;
}
return io.Platform.environment.containsKey('FLUTTER_TEST_IMPELLER');
}
| flutter/packages/flutter/test/impeller_test_helpers.dart/0 | {
"file_path": "flutter/packages/flutter/test/impeller_test_helpers.dart",
"repo_id": "flutter",
"token_count": 189
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('BadgeThemeData copyWith, ==, hashCode basics', () {
expect(const BadgeThemeData(), const BadgeThemeData().copyWith());
expect(const BadgeThemeData().hashCode, const BadgeThemeData().copyWith().hashCode);
});
test('BadgeThemeData lerp special cases', () {
expect(BadgeThemeData.lerp(null, null, 0), const BadgeThemeData());
const BadgeThemeData data = BadgeThemeData();
expect(identical(BadgeThemeData.lerp(data, data, 0.5), data), true);
});
test('BadgeThemeData defaults', () {
const BadgeThemeData themeData = BadgeThemeData();
expect(themeData.backgroundColor, null);
expect(themeData.textColor, null);
expect(themeData.smallSize, null);
expect(themeData.largeSize, null);
expect(themeData.textStyle, null);
expect(themeData.padding, null);
expect(themeData.alignment, null);
expect(themeData.offset, null);
});
testWidgets('Default BadgeThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const BadgeThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('BadgeThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const BadgeThemeData(
backgroundColor: Color(0xfffffff0),
textColor: Color(0xfffffff1),
smallSize: 1,
largeSize: 2,
textStyle: TextStyle(fontSize: 4),
padding: EdgeInsets.all(5),
alignment: AlignmentDirectional(6, 7),
offset: Offset.zero,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'backgroundColor: Color(0xfffffff0)',
'textColor: Color(0xfffffff1)',
'smallSize: 1.0',
'largeSize: 2.0',
'textStyle: TextStyle(inherit: true, size: 4.0)',
'padding: EdgeInsets.all(5.0)',
'alignment: AlignmentDirectional(6.0, 7.0)',
'offset: Offset(0.0, 0.0)'
]);
});
testWidgets('Badge uses ThemeData badge theme', (WidgetTester tester) async {
const Color green = Color(0xff00ff00);
const Color black = Color(0xff000000);
const BadgeThemeData badgeTheme = BadgeThemeData(
backgroundColor: green,
textColor: black,
smallSize: 5,
largeSize: 20,
textStyle: TextStyle(fontSize: 12),
padding: EdgeInsets.symmetric(horizontal: 5),
alignment: Alignment.topRight,
offset: Offset(24, 0),
);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.light(useMaterial3: true).copyWith(
badgeTheme: badgeTheme,
),
home: const Scaffold(
body: Badge(
label: Text('1234'),
child: Icon(Icons.add),
),
),
),
);
// text width = 48 = fontSize * 4, text height = fontSize
expect(tester.getSize(find.text('1234')), const Size(48, 12));
expect(tester.getTopLeft(find.text('1234')), const Offset(33, 4));
expect(tester.getSize(find.byType(Badge)), const Size(24, 24)); // default Icon size
expect(tester.getTopLeft(find.byType(Badge)), Offset.zero);
final TextStyle textStyle = tester.renderObject<RenderParagraph>(find.text('1234')).text.style!;
expect(textStyle.fontSize, 12);
expect(textStyle.color, black);
final RenderBox box = tester.renderObject(find.byType(Badge));
expect(box, paints..rrect(rrect: RRect.fromLTRBR(28, 0, 86, 20, const Radius.circular(10)), color: green));
});
// This test is essentially the same as 'Badge uses ThemeData badge theme'. In
// this case the theme is introduced with the BadgeTheme widget instead of
// ThemeData.badgeTheme.
testWidgets('Badge uses BadgeTheme', (WidgetTester tester) async {
const Color green = Color(0xff00ff00);
const Color black = Color(0xff000000);
const BadgeThemeData badgeTheme = BadgeThemeData(
backgroundColor: green,
textColor: black,
smallSize: 5,
largeSize: 20,
textStyle: TextStyle(fontSize: 12),
padding: EdgeInsets.symmetric(horizontal: 5),
alignment: Alignment.topRight,
offset: Offset(24, 0),
);
await tester.pumpWidget(
const MaterialApp(
home: BadgeTheme(
data: badgeTheme,
child: Scaffold(
body: Badge(
label: Text('1234'),
child: Icon(Icons.add),
),
),
),
),
);
expect(tester.getSize(find.text('1234')), const Size(48, 12));
expect(tester.getTopLeft(find.text('1234')), const Offset(33, 4));
expect(tester.getSize(find.byType(Badge)), const Size(24, 24)); // default Icon size
expect(tester.getTopLeft(find.byType(Badge)), Offset.zero);
final TextStyle textStyle = tester.renderObject<RenderParagraph>(find.text('1234')).text.style!;
expect(textStyle.fontSize, 12);
expect(textStyle.color, black);
final RenderBox box = tester.renderObject(find.byType(Badge));
expect(box, paints..rrect(rrect: RRect.fromLTRBR(28, 0, 86, 20, const Radius.circular(10)), color: green));
});
}
| flutter/packages/flutter/test/material/badge_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/badge_theme_test.dart",
"repo_id": "flutter",
"token_count": 2245
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'feedback_tester.dart';
Widget wrap({ required Widget child }) {
return MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Material(child: child),
),
);
}
void main() {
testWidgets('CheckboxListTile control test', (WidgetTester tester) async {
final List<dynamic> log = <dynamic>[];
await tester.pumpWidget(wrap(
child: CheckboxListTile(
value: true,
onChanged: (bool? value) { log.add(value); },
title: const Text('Hello'),
),
));
await tester.tap(find.text('Hello'));
log.add('-');
await tester.tap(find.byType(Checkbox));
expect(log, equals(<dynamic>[false, '-', false]));
});
testWidgets('Material2 - CheckboxListTile checkColor test', (WidgetTester tester) async {
const Color checkBoxBorderColor = Color(0xff2196f3);
Color checkBoxCheckColor = const Color(0xffFFFFFF);
Widget buildFrame(Color? color) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: CheckboxListTile(
value: true,
checkColor: color,
onChanged: (bool? value) {},
),
),
);
}
RenderBox getCheckboxListTileRenderer() {
return tester.renderObject<RenderBox>(find.byType(CheckboxListTile));
}
await tester.pumpWidget(buildFrame(null));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: checkBoxBorderColor)..path(color: checkBoxCheckColor));
checkBoxCheckColor = const Color(0xFF000000);
await tester.pumpWidget(buildFrame(checkBoxCheckColor));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: checkBoxBorderColor)..path(color: checkBoxCheckColor));
});
testWidgets('Material3 - CheckboxListTile checkColor test', (WidgetTester tester) async {
const Color checkBoxBorderColor = Color(0xff6750a4);
Color checkBoxCheckColor = const Color(0xffFFFFFF);
Widget buildFrame(Color? color) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: CheckboxListTile(
value: true,
checkColor: color,
onChanged: (bool? value) {},
),
),
);
}
RenderBox getCheckboxListTileRenderer() {
return tester.renderObject<RenderBox>(find.byType(CheckboxListTile));
}
await tester.pumpWidget(buildFrame(null));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: checkBoxBorderColor)..path(color: checkBoxCheckColor));
checkBoxCheckColor = const Color(0xFF000000);
await tester.pumpWidget(buildFrame(checkBoxCheckColor));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: checkBoxBorderColor)..path(color: checkBoxCheckColor));
});
testWidgets('CheckboxListTile activeColor test', (WidgetTester tester) async {
Widget buildFrame(Color? themeColor, Color? activeColor) {
return wrap(
child: Theme(
data: ThemeData(
checkboxTheme: CheckboxThemeData(
fillColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
return states.contains(MaterialState.selected) ? themeColor : null;
}),
),
),
child: CheckboxListTile(
value: true,
activeColor: activeColor,
onChanged: (bool? value) {},
),
),
);
}
RenderBox getCheckboxListTileRenderer() {
return tester.renderObject<RenderBox>(find.byType(CheckboxListTile));
}
await tester.pumpWidget(buildFrame(const Color(0xFF000000), null));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: const Color(0xFF000000)));
await tester.pumpWidget(buildFrame(const Color(0xFF000000), const Color(0xFFFFFFFF)));
await tester.pumpAndSettle();
expect(getCheckboxListTileRenderer(), paints..path(color: const Color(0xFFFFFFFF)));
});
testWidgets('CheckboxListTile can autofocus unless disabled.', (WidgetTester tester) async {
final GlobalKey childKey = GlobalKey();
await tester.pumpWidget(
wrap(
child: CheckboxListTile(
value: true,
onChanged: (_) {},
title: Text('Hello', key: childKey),
autofocus: true,
),
),
);
await tester.pump();
expect(Focus.maybeOf(childKey.currentContext!)!.hasPrimaryFocus, isTrue);
await tester.pumpWidget(
wrap(
child: CheckboxListTile(
value: true,
onChanged: null,
title: Text('Hello', key: childKey),
autofocus: true,
),
),
);
await tester.pump();
expect(Focus.maybeOf(childKey.currentContext!)!.hasPrimaryFocus, isFalse);
});
testWidgets('CheckboxListTile contentPadding test', (WidgetTester tester) async {
await tester.pumpWidget(
wrap(
child: const Center(
child: CheckboxListTile(
value: false,
onChanged: null,
title: Text('Title'),
contentPadding: EdgeInsets.fromLTRB(10, 18, 4, 2),
),
),
),
);
final Rect paddingRect = tester.getRect(find.byType(SafeArea));
final Rect checkboxRect = tester.getRect(find.byType(Checkbox));
final Rect titleRect = tester.getRect(find.text('Title'));
final Rect tallerWidget = checkboxRect.height > titleRect.height ? checkboxRect : titleRect;
// Check the offsets of Checkbox and title after padding is applied.
expect(paddingRect.right, checkboxRect.right + 4);
expect(paddingRect.left, titleRect.left - 10);
// Calculate the remaining height from the default ListTile height.
final double remainingHeight = 56 - tallerWidget.height;
expect(paddingRect.top, tallerWidget.top - remainingHeight / 2 - 18);
expect(paddingRect.bottom, tallerWidget.bottom + remainingHeight / 2 + 2);
});
testWidgets('CheckboxListTile tristate test', (WidgetTester tester) async {
bool? value = false;
bool tristate = false;
await tester.pumpWidget(
Material(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return wrap(
child: CheckboxListTile(
title: const Text('Title'),
tristate: tristate,
value: value,
onChanged: (bool? v) {
setState(() {
value = v;
});
},
),
);
},
),
),
);
expect(tester.widget<Checkbox>(find.byType(Checkbox)).value, false);
// Tap the checkbox when tristate is disabled.
await tester.tap(find.byType(Checkbox));
await tester.pumpAndSettle();
expect(value, true);
await tester.tap(find.byType(Checkbox));
await tester.pumpAndSettle();
expect(value, false);
// Tap the listTile when tristate is disabled.
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
expect(value, true);
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
expect(value, false);
// Enable tristate
tristate = true;
await tester.pumpAndSettle();
expect(tester.widget<Checkbox>(find.byType(Checkbox)).value, false);
// Tap the checkbox when tristate is enabled.
await tester.tap(find.byType(Checkbox));
await tester.pumpAndSettle();
expect(value, true);
await tester.tap(find.byType(Checkbox));
await tester.pumpAndSettle();
expect(value, null);
await tester.tap(find.byType(Checkbox));
await tester.pumpAndSettle();
expect(value, false);
// Tap the listTile when tristate is enabled.
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
expect(value, true);
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
expect(value, null);
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
expect(value, false);
});
testWidgets('CheckboxListTile respects shape', (WidgetTester tester) async {
const ShapeBorder shapeBorder = RoundedRectangleBorder(
borderRadius: BorderRadius.horizontal(right: Radius.circular(100)),
);
await tester.pumpWidget(wrap(
child: const CheckboxListTile(
value: false,
onChanged: null,
title: Text('Title'),
shape: shapeBorder,
),
));
expect(tester.widget<InkWell>(find.byType(InkWell)).customBorder, shapeBorder);
});
testWidgets('CheckboxListTile respects tileColor', (WidgetTester tester) async {
final Color tileColor = Colors.red.shade500;
await tester.pumpWidget(
wrap(
child: Center(
child: CheckboxListTile(
value: false,
onChanged: null,
title: const Text('Title'),
tileColor: tileColor,
),
),
),
);
expect(find.byType(Material), paints..rect(color: tileColor));
});
testWidgets('CheckboxListTile respects selectedTileColor', (WidgetTester tester) async {
final Color selectedTileColor = Colors.green.shade500;
await tester.pumpWidget(
wrap(
child: Center(
child: CheckboxListTile(
value: false,
onChanged: null,
title: const Text('Title'),
selected: true,
selectedTileColor: selectedTileColor,
),
),
),
);
expect(find.byType(Material), paints..rect(color: selectedTileColor));
});
testWidgets('CheckboxListTile selected item text Color', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/pull/76908
const Color activeColor = Color(0xff00ff00);
Widget buildFrame({ Color? activeColor, Color? fillColor }) {
return MaterialApp(
theme: ThemeData.light().copyWith(
checkboxTheme: CheckboxThemeData(
fillColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
return states.contains(MaterialState.selected) ? fillColor : null;
}),
),
),
home: Scaffold(
body: Center(
child: CheckboxListTile(
activeColor: activeColor,
selected: true,
title: const Text('title'),
value: true,
onChanged: (bool? value) { },
),
),
),
);
}
Color? textColor(String text) {
return tester.renderObject<RenderParagraph>(find.text(text)).text.style?.color;
}
await tester.pumpWidget(buildFrame(fillColor: activeColor));
expect(textColor('title'), activeColor);
await tester.pumpWidget(buildFrame(activeColor: activeColor));
expect(textColor('title'), activeColor);
});
testWidgets('CheckboxListTile respects checkbox shape and side', (WidgetTester tester) async {
Widget buildApp(BorderSide side, OutlinedBorder shape) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile(
value: false,
onChanged: (bool? newValue) {},
side: side,
checkboxShape: shape,
);
}),
),
),
);
}
const RoundedRectangleBorder border1 = RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(5)));
const BorderSide side1 = BorderSide(
color: Color(0xfff44336),
);
await tester.pumpWidget(buildApp(side1, border1));
expect(tester.widget<CheckboxListTile>(find.byType(CheckboxListTile)).side, side1);
expect(tester.widget<CheckboxListTile>(find.byType(CheckboxListTile)).checkboxShape, border1);
expect(tester.widget<Checkbox>(find.byType(Checkbox)).side, side1);
expect(tester.widget<Checkbox>(find.byType(Checkbox)).shape, border1);
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..drrect(
color: const Color(0xfff44336),
outer: RRect.fromLTRBR(11.0, 11.0, 29.0, 29.0, const Radius.circular(5)),
inner: RRect.fromLTRBR(12.0, 12.0, 28.0, 28.0, const Radius.circular(4)),
),
);
const RoundedRectangleBorder border2 = RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(5)));
const BorderSide side2 = BorderSide(
width: 4.0,
color: Color(0xff424242),
);
await tester.pumpWidget(buildApp(side2, border2));
expect(tester.widget<Checkbox>(find.byType(Checkbox)).side, side2);
expect(tester.widget<Checkbox>(find.byType(Checkbox)).shape, border2);
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..drrect(
color: const Color(0xff424242),
outer: RRect.fromLTRBR(11.0, 11.0, 29.0, 29.0, const Radius.circular(5)),
inner: RRect.fromLTRBR(15.0, 15.0, 25.0, 25.0, const Radius.circular(1)),
),
);
});
testWidgets('CheckboxListTile respects visualDensity', (WidgetTester tester) async {
const Key key = Key('test');
Future<void> buildTest(VisualDensity visualDensity) async {
return tester.pumpWidget(
wrap(
child: Center(
child: CheckboxListTile(
key: key,
value: false,
onChanged: (bool? value) {},
autofocus: true,
visualDensity: visualDensity,
),
),
),
);
}
await buildTest(VisualDensity.standard);
final RenderBox box = tester.renderObject(find.byKey(key));
await tester.pumpAndSettle();
expect(box.size, equals(const Size(800, 56)));
});
testWidgets('CheckboxListTile respects focusNode', (WidgetTester tester) async {
final GlobalKey childKey = GlobalKey();
await tester.pumpWidget(
wrap(
child: Center(
child: CheckboxListTile(
value: false,
title: Text('A', key: childKey),
onChanged: (bool? value) {},
),
),
),
);
await tester.pump();
final FocusNode tileNode = Focus.of(childKey.currentContext!);
tileNode.requestFocus();
await tester.pump(); // Let the focus take effect.
expect(Focus.of(childKey.currentContext!).hasPrimaryFocus, isTrue);
expect(tileNode.hasPrimaryFocus, isTrue);
});
testWidgets('CheckboxListTile onFocusChange callback', (WidgetTester tester) async {
final FocusNode node = FocusNode(debugLabel: 'CheckboxListTile onFocusChange');
bool gotFocus = false;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: CheckboxListTile(
value: true,
focusNode: node,
onFocusChange: (bool focused) {
gotFocus = focused;
},
onChanged: (bool? value) {},
),
),
),
);
node.requestFocus();
await tester.pump();
expect(gotFocus, isTrue);
expect(node.hasFocus, isTrue);
node.unfocus();
await tester.pump();
expect(gotFocus, isFalse);
expect(node.hasFocus, isFalse);
node.dispose();
});
testWidgets('CheckboxListTile can be disabled', (WidgetTester tester) async {
bool? value = false;
bool enabled = true;
await tester.pumpWidget(
Material(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return wrap(
child: CheckboxListTile(
title: const Text('Title'),
enabled: enabled,
value: value,
onChanged: (bool? v) {
setState(() {
value = v;
enabled = !enabled;
});
},
),
);
},
),
),
);
final Finder checkbox = find.byType(Checkbox);
// verify initial values
expect(tester.widget<Checkbox>(checkbox).value, false);
expect(enabled, true);
// Tap the checkbox to disable CheckboxListTile
await tester.tap(checkbox);
await tester.pumpAndSettle();
expect(tester.widget<Checkbox>(checkbox).value, true);
expect(enabled, false);
await tester.tap(checkbox);
await tester.pumpAndSettle();
expect(tester.widget<Checkbox>(checkbox).value, true);
});
testWidgets('CheckboxListTile respects mouseCursor when hovered', (WidgetTester tester) async {
// Test Checkbox() constructor
await tester.pumpWidget(
wrap(
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: CheckboxListTile(
mouseCursor: SystemMouseCursors.text,
value: true,
onChanged: (_) {},
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: tester.getCenter(find.byType(Checkbox)));
await tester.pump();
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Test default cursor
await tester.pumpWidget(
wrap(
child: CheckboxListTile(
value: true,
onChanged: (_) {},
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
// Test default cursor when disabled
await tester.pumpWidget(
wrap(
child: const MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: CheckboxListTile(
value: true,
onChanged: null,
),
),
),
);
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
// Test cursor when tristate
await tester.pumpWidget(
wrap(
child: const MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: CheckboxListTile(
value: null,
tristate: true,
onChanged: null,
mouseCursor: _SelectedGrabMouseCursor(),
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.grab);
await tester.pumpAndSettle();
});
testWidgets('CheckboxListTile respects fillColor in enabled/disabled states', (WidgetTester tester) async {
const Color activeEnabledFillColor = Color(0xFF000001);
const Color activeDisabledFillColor = Color(0xFF000002);
Color getFillColor(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return activeDisabledFillColor;
}
return activeEnabledFillColor;
}
final MaterialStateProperty<Color> fillColor = MaterialStateColor.resolveWith(getFillColor);
Widget buildFrame({required bool enabled}) {
return wrap(
child: CheckboxListTile(
value: true,
fillColor: fillColor,
onChanged: enabled ? (bool? value) { } : null,
)
);
}
RenderBox getCheckboxRenderer() {
return tester.renderObject<RenderBox>(find.byType(Checkbox));
}
await tester.pumpWidget(buildFrame(enabled: true));
await tester.pumpAndSettle();
expect(getCheckboxRenderer(), paints..path(color: activeEnabledFillColor));
await tester.pumpWidget(buildFrame(enabled: false));
await tester.pumpAndSettle();
expect(getCheckboxRenderer(), paints..path(color: activeDisabledFillColor));
});
testWidgets('CheckboxListTile respects fillColor in hovered state', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color hoveredFillColor = Color(0xFF000001);
Color getFillColor(Set<MaterialState> states) {
if (states.contains(MaterialState.hovered)) {
return hoveredFillColor;
}
return Colors.transparent;
}
final MaterialStateProperty<Color> fillColor =
MaterialStateColor.resolveWith(getFillColor);
Widget buildFrame() {
return wrap(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile(
value: true,
fillColor: fillColor,
onChanged: (bool? value) { },
);
},
),
);
}
RenderBox getCheckboxRenderer() {
return tester.renderObject<RenderBox>(find.byType(Checkbox));
}
await tester.pumpWidget(buildFrame());
await tester.pumpAndSettle();
// Start hovering
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(getCheckboxRenderer(), paints..path(color: hoveredFillColor));
});
testWidgets('CheckboxListTile respects hoverColor', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
bool? value = true;
Widget buildApp({bool enabled = true}) {
return wrap(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile(
value: value,
onChanged: enabled ? (bool? newValue) {
setState(() {
value = newValue;
});
} : null,
hoverColor: Colors.orange[500],
);
}),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..path(style: PaintingStyle.fill)
..path(style: PaintingStyle.stroke, strokeWidth: 2.0),
);
// Start hovering
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..circle(color: Colors.orange[500])
..path(style: PaintingStyle.fill)
..path(style: PaintingStyle.stroke, strokeWidth: 2.0),
);
// Check what happens when disabled.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..path(style: PaintingStyle.fill)
..path(style: PaintingStyle.stroke, strokeWidth: 2.0),
);
});
testWidgets('Material2 - CheckboxListTile respects overlayColor in active/pressed/hovered states', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color fillColor = Color(0xFF000000);
const Color activePressedOverlayColor = Color(0xFF000001);
const Color inactivePressedOverlayColor = Color(0xFF000002);
const Color hoverOverlayColor = Color(0xFF000003);
const Color hoverColor = Color(0xFF000005);
Color? getOverlayColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
if (states.contains(MaterialState.selected)) {
return activePressedOverlayColor;
}
return inactivePressedOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverOverlayColor;
}
return null;
}
const double splashRadius = 24.0;
Widget buildCheckbox({bool active = false, bool useOverlay = true}) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: CheckboxListTile(
value: active,
onChanged: (_) { },
fillColor: const MaterialStatePropertyAll<Color>(fillColor),
overlayColor: useOverlay ? MaterialStateProperty.resolveWith(getOverlayColor) : null,
hoverColor: hoverColor,
splashRadius: splashRadius,
),
),
);
}
await tester.pumpWidget(buildCheckbox(useOverlay: false));
final TestGesture gesture1 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle()
..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
),
reason: 'Default inactive pressed Checkbox should have overlay color from fillColor',
);
await tester.pumpWidget(buildCheckbox(active: true, useOverlay: false));
final TestGesture gesture2 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle()
..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
),
reason: 'Default active pressed Checkbox should have overlay color from fillColor',
);
await tester.pumpWidget(buildCheckbox());
final TestGesture gesture3 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle()
..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
),
reason: 'Inactive pressed Checkbox should have overlay color: $inactivePressedOverlayColor',
);
await tester.pumpWidget(buildCheckbox(active: true));
final TestGesture gesture4 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle()
..circle(
color: activePressedOverlayColor,
radius: splashRadius,
),
reason: 'Active pressed Checkbox should have overlay color: $activePressedOverlayColor',
);
// Start hovering
await tester.pumpWidget(Container());
await tester.pumpWidget(buildCheckbox());
await tester.pumpAndSettle();
final TestGesture gesture5 = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture5.addPointer();
await gesture5.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..circle(
color: hoverOverlayColor,
radius: splashRadius,
),
reason: 'Hovered Checkbox should use overlay color $hoverOverlayColor over $hoverColor',
);
// Finish gestures to release resources.
await gesture1.up();
await gesture2.up();
await gesture3.up();
await gesture4.up();
await tester.pumpAndSettle();
});
testWidgets('Material3 - CheckboxListTile respects overlayColor in active/pressed/hovered states', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color fillColor = Color(0xFF000000);
const Color activePressedOverlayColor = Color(0xFF000001);
const Color inactivePressedOverlayColor = Color(0xFF000002);
const Color hoverOverlayColor = Color(0xFF000003);
const Color hoverColor = Color(0xFF000005);
Color? getOverlayColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
if (states.contains(MaterialState.selected)) {
return activePressedOverlayColor;
}
return inactivePressedOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverOverlayColor;
}
return null;
}
const double splashRadius = 24.0;
Widget buildCheckbox({bool active = false, bool useOverlay = true}) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: CheckboxListTile(
value: active,
onChanged: (_) { },
fillColor: const MaterialStatePropertyAll<Color>(fillColor),
overlayColor: useOverlay ? MaterialStateProperty.resolveWith(getOverlayColor) : null,
hoverColor: hoverColor,
splashRadius: splashRadius,
),
),
);
}
await tester.pumpWidget(buildCheckbox(useOverlay: false));
final TestGesture gesture1 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
kIsWeb ? (paints..circle()..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
)) : (paints..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
)),
reason: 'Default inactive pressed Checkbox should have overlay color from fillColor',
);
await tester.pumpWidget(buildCheckbox(active: true, useOverlay: false));
final TestGesture gesture2 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
kIsWeb ? (paints..circle()..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
)) : (paints..circle(
color: fillColor.withAlpha(kRadialReactionAlpha),
radius: splashRadius,
)),
reason: 'Default active pressed Checkbox should have overlay color from fillColor',
);
await tester.pumpWidget(buildCheckbox());
final TestGesture gesture3 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
kIsWeb ? (paints..circle()..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
)) : (paints..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
)),
reason: 'Inactive pressed Checkbox should have overlay color: $inactivePressedOverlayColor',
);
await tester.pumpWidget(buildCheckbox(active: true));
final TestGesture gesture4 = await tester.startGesture(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
kIsWeb ? (paints..circle()..circle(
color: activePressedOverlayColor,
radius: splashRadius,
)) : (paints..circle(
color: activePressedOverlayColor,
radius: splashRadius,
)),
reason: 'Active pressed Checkbox should have overlay color: $activePressedOverlayColor',
);
// Start hovering
await tester.pumpWidget(Container());
await tester.pumpWidget(buildCheckbox());
await tester.pumpAndSettle();
final TestGesture gesture5 = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture5.addPointer();
await gesture5.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle(
color: hoverOverlayColor,
radius: splashRadius,
),
reason: 'Hovered Checkbox should use overlay color $hoverOverlayColor over $hoverColor',
);
// Finish gestures to release resources.
await gesture1.up();
await gesture2.up();
await gesture3.up();
await gesture4.up();
await tester.pumpAndSettle();
});
testWidgets('CheckboxListTile respects splashRadius', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const double splashRadius = 30;
Widget buildApp() {
return wrap(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile(
value: false,
onChanged: (bool? newValue) {},
hoverColor: Colors.orange[500],
splashRadius: splashRadius,
);
}),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..circle(color: Colors.orange[500], radius: splashRadius),
);
});
testWidgets('CheckboxListTile respects materialTapTargetSize', (WidgetTester tester) async {
await tester.pumpWidget(
wrap(
child: CheckboxListTile(
value: true,
onChanged: (bool? newValue) { },
),
),
);
// default test
expect(tester.getSize(find.byType(Checkbox)), const Size(40.0, 40.0));
await tester.pumpWidget(
wrap(
child: CheckboxListTile(
materialTapTargetSize: MaterialTapTargetSize.padded,
value: true,
onChanged: (bool? newValue) { },
),
),
);
expect(tester.getSize(find.byType(Checkbox)), const Size(48.0, 48.0));
});
testWidgets('Material3 - CheckboxListTile respects isError', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: true);
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
bool? value = true;
Widget buildApp() {
return MaterialApp(
theme: themeData,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile(
isError: true,
value: value,
onChanged: (bool? newValue) {
setState(() {
value = newValue;
});
},
);
}),
),
),
);
}
// Default color
await tester.pumpWidget(Container());
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints..path(color: themeData.colorScheme.error)..path(color: themeData.colorScheme.onError)
);
// Start hovering
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Checkbox)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Checkbox))),
paints
..circle(color: themeData.colorScheme.error.withOpacity(0.08))
..path(color: themeData.colorScheme.error)
);
});
testWidgets('CheckboxListTile.adaptive shows the correct checkbox platform widget', (WidgetTester tester) async {
Widget buildApp(TargetPlatform platform) {
return MaterialApp(
theme: ThemeData(platform: platform),
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return CheckboxListTile.adaptive(
value: false,
onChanged: (bool? newValue) {},
);
}),
),
),
);
}
for (final TargetPlatform platform in <TargetPlatform>[ TargetPlatform.iOS, TargetPlatform.macOS ]) {
await tester.pumpWidget(buildApp(platform));
await tester.pumpAndSettle();
expect(find.byType(CupertinoCheckbox), findsOneWidget);
}
for (final TargetPlatform platform in <TargetPlatform>[ TargetPlatform.android, TargetPlatform.fuchsia, TargetPlatform.linux, TargetPlatform.windows ]) {
await tester.pumpWidget(buildApp(platform));
await tester.pumpAndSettle();
expect(find.byType(CupertinoCheckbox), findsNothing);
}
});
group('feedback', () {
late FeedbackTester feedback;
setUp(() {
feedback = FeedbackTester();
});
tearDown(() {
feedback.dispose();
});
testWidgets('CheckboxListTile respects enableFeedback', (WidgetTester tester) async {
Future<void> buildTest(bool enableFeedback) async {
return tester.pumpWidget(
wrap(
child: Center(
child: CheckboxListTile(
value: false,
onChanged: (bool? value) {},
enableFeedback: enableFeedback,
),
),
),
);
}
await buildTest(false);
await tester.tap(find.byType(CheckboxListTile));
await tester.pump(const Duration(seconds: 1));
expect(feedback.clickSoundCount, 0);
expect(feedback.hapticCount, 0);
await buildTest(true);
await tester.tap(find.byType(CheckboxListTile));
await tester.pump(const Duration(seconds: 1));
expect(feedback.clickSoundCount, 1);
expect(feedback.hapticCount, 0);
});
});
testWidgets('CheckboxListTile has proper semantics', (WidgetTester tester) async {
final List<dynamic> log = <dynamic>[];
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(wrap(
child: CheckboxListTile(
value: true,
onChanged: (bool? value) { log.add(value); },
title: const Text('Hello'),
checkboxSemanticLabel: 'there',
),
));
expect(tester.getSemantics(find.byType(CheckboxListTile)), matchesSemantics(
hasCheckedState: true,
isChecked: true,
hasEnabledState: true,
isEnabled: true,
hasTapAction: true,
isFocusable: true,
label: 'Hello\nthere',
));
handle.dispose();
});
testWidgets('CheckboxListTile.control widget should not request focus on traversal', (WidgetTester tester) async {
final GlobalKey firstChildKey = GlobalKey();
final GlobalKey secondChildKey = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Column(
children: <Widget>[
CheckboxListTile(
value: true,
onChanged: (bool? value) {},
title: Text('Hey', key: firstChildKey),
),
CheckboxListTile(
value: true,
onChanged: (bool? value) {},
title: Text('There', key: secondChildKey),
),
],
),
),
),
);
await tester.pump();
Focus.of(firstChildKey.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(firstChildKey.currentContext!).hasPrimaryFocus, isTrue);
Focus.of(firstChildKey.currentContext!).nextFocus();
await tester.pump();
expect(Focus.of(firstChildKey.currentContext!).hasPrimaryFocus, isFalse);
expect(Focus.of(secondChildKey.currentContext!).hasPrimaryFocus, isTrue);
});
}
class _SelectedGrabMouseCursor extends MaterialStateMouseCursor {
const _SelectedGrabMouseCursor();
@override
MouseCursor resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return SystemMouseCursors.grab;
}
return SystemMouseCursors.basic;
}
@override
String get debugDescription => '_SelectedGrabMouseCursor()';
}
| flutter/packages/flutter/test/material/checkbox_list_tile_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/checkbox_list_tile_test.dart",
"repo_id": "flutter",
"token_count": 16835
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testWidgets('can press', (WidgetTester tester) async {
bool pressed = false;
await tester.pumpWidget(
MaterialApp(
home: Center(
child: DesktopTextSelectionToolbarButton(
onPressed: () {
pressed = true;
},
child: const Text('Tap me'),
),
),
),
);
expect(pressed, false);
await tester.tap(find.byType(DesktopTextSelectionToolbarButton));
expect(pressed, true);
});
testWidgets('passing null to onPressed disables the button', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Center(
child: DesktopTextSelectionToolbarButton(
onPressed: null,
child: Text('Cannot tap me'),
),
),
),
);
expect(find.byType(TextButton), findsOneWidget);
final TextButton button = tester.widget(find.byType(TextButton));
expect(button.enabled, isFalse);
});
}
| flutter/packages/flutter/test/material/desktop_text_selection_toolbar_button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/desktop_text_selection_toolbar_button_test.dart",
"repo_id": "flutter",
"token_count": 533
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
Widget wrap({ required Widget child, ThemeData? theme }) {
return MaterialApp(
theme: theme,
home: Center(
child: Material(child: child),
),
);
}
void main() {
testWidgets('ExpandIcon test', (WidgetTester tester) async {
bool expanded = false;
IconTheme iconTheme;
// Light mode tests
await tester.pumpWidget(wrap(
child: ExpandIcon(
onPressed: (bool isExpanded) {
expanded = !expanded;
},
),
));
await tester.pumpAndSettle();
expect(expanded, isFalse);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.black54));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
expect(expanded, isTrue);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.black54));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
expect(expanded, isFalse);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.black54));
// Dark mode tests
await tester.pumpWidget(wrap(
child: ExpandIcon(
onPressed: (bool isExpanded) {
expanded = !expanded;
},
),
theme: ThemeData(brightness: Brightness.dark),
));
await tester.pumpAndSettle();
expect(expanded, isFalse);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.white60));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
expect(expanded, isTrue);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.white60));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
expect(expanded, isFalse);
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.white60));
});
testWidgets('Material2 - ExpandIcon disabled', (WidgetTester tester) async {
IconTheme iconTheme;
// Test light mode.
await tester.pumpWidget(wrap(
theme: ThemeData(useMaterial3: false),
child: const ExpandIcon(onPressed: null),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.black38));
// Test dark mode.
await tester.pumpWidget(wrap(
child: const ExpandIcon(onPressed: null),
theme: ThemeData(useMaterial3: false, brightness: Brightness.dark),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.white38));
});
testWidgets('Material3 - ExpandIcon disabled', (WidgetTester tester) async {
ThemeData theme = ThemeData();
IconTheme iconTheme;
// Test light mode.
await tester.pumpWidget(wrap(
theme: theme,
child: const ExpandIcon(onPressed: null),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(
iconTheme.data.color,
equals(theme.colorScheme.onSurface.withOpacity(0.38)),
);
theme = ThemeData(brightness: Brightness.dark);
// Test dark mode.
await tester.pumpWidget(wrap(
theme: theme,
child: const ExpandIcon(onPressed: null),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(
iconTheme.data.color,
equals(theme.colorScheme.onSurface.withOpacity(0.38)),
);
});
testWidgets('ExpandIcon test isExpanded does not trigger callback', (WidgetTester tester) async {
bool expanded = false;
await tester.pumpWidget(wrap(
child: ExpandIcon(
onPressed: (bool isExpanded) {
expanded = !expanded;
},
),
));
await tester.pumpWidget(wrap(
child: ExpandIcon(
isExpanded: true,
onPressed: (bool isExpanded) {
expanded = !expanded;
},
),
));
expect(expanded, isFalse);
});
testWidgets('ExpandIcon is rotated initially if isExpanded is true on first build', (WidgetTester tester) async {
bool expanded = true;
await tester.pumpWidget(wrap(
child: ExpandIcon(
isExpanded: expanded,
onPressed: (bool isExpanded) {
expanded = !isExpanded;
},
),
));
final RotationTransition rotation = tester.firstWidget(find.byType(RotationTransition));
expect(rotation.turns.value, 0.5);
});
testWidgets('ExpandIcon default size is 24', (WidgetTester tester) async {
final ExpandIcon expandIcon = ExpandIcon(
onPressed: (bool isExpanded) {},
);
await tester.pumpWidget(wrap(
child: expandIcon,
));
final ExpandIcon icon = tester.firstWidget(find.byWidget(expandIcon));
expect(icon.size, 24);
});
testWidgets('ExpandIcon has the correct given size', (WidgetTester tester) async {
ExpandIcon expandIcon = ExpandIcon(
size: 36,
onPressed: (bool isExpanded) {},
);
await tester.pumpWidget(wrap(
child: expandIcon,
));
ExpandIcon icon = tester.firstWidget(find.byWidget(expandIcon));
expect(icon.size, 36);
expandIcon = ExpandIcon(
size: 48,
onPressed: (bool isExpanded) {},
);
await tester.pumpWidget(wrap(
child: expandIcon,
));
icon = tester.firstWidget(find.byWidget(expandIcon));
expect(icon.size, 48);
});
testWidgets('Material2 - ExpandIcon has correct semantic hints', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
const DefaultMaterialLocalizations localizations = DefaultMaterialLocalizations();
await tester.pumpWidget(wrap(
theme: ThemeData(useMaterial3: false),
child: ExpandIcon(
isExpanded: true,
onPressed: (bool _) { },
),
));
expect(tester.getSemantics(find.byType(ExpandIcon)), matchesSemantics(
hasTapAction: true,
hasEnabledState: true,
isEnabled: true,
isFocusable: true,
isButton: true,
onTapHint: localizations.expandedIconTapHint,
));
await tester.pumpWidget(wrap(
theme: ThemeData(useMaterial3: false),
child: ExpandIcon(
onPressed: (bool _) { },
),
));
expect(tester.getSemantics(find.byType(ExpandIcon)), matchesSemantics(
hasTapAction: true,
hasEnabledState: true,
isEnabled: true,
isFocusable: true,
isButton: true,
onTapHint: localizations.collapsedIconTapHint,
));
handle.dispose();
});
testWidgets('Material3 - ExpandIcon has correct semantic hints', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
const DefaultMaterialLocalizations localizations = DefaultMaterialLocalizations();
await tester.pumpWidget(wrap(
child: ExpandIcon(
isExpanded: true,
onPressed: (bool _) { },
),
));
expect(tester.getSemantics(find.byType(ExpandIcon)), matchesSemantics(
onTapHint: localizations.expandedIconTapHint,
children: <Matcher>[
matchesSemantics(
hasTapAction: true,
hasEnabledState: true,
isEnabled: true,
isFocusable: true,
isButton: true,
),
],
));
await tester.pumpWidget(wrap(
child: ExpandIcon(
onPressed: (bool _) { },
),
));
expect(tester.getSemantics(find.byType(ExpandIcon)), matchesSemantics(
onTapHint: localizations.collapsedIconTapHint,
children: <Matcher>[
matchesSemantics(
hasTapAction: true,
hasEnabledState: true,
isEnabled: true,
isFocusable: true,
isButton: true,
),
],
));
handle.dispose();
});
testWidgets('ExpandIcon uses custom icon color and expanded icon color', (WidgetTester tester) async {
bool expanded = false;
IconTheme iconTheme;
await tester.pumpWidget(wrap(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return ExpandIcon(
isExpanded: expanded,
onPressed: (bool isExpanded) {
setState(() {
expanded = !isExpanded;
});
},
color: Colors.indigo,
);
},
),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.indigo));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.indigo));
expanded = false;
await tester.pumpWidget(wrap(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return ExpandIcon(
isExpanded: expanded,
onPressed: (bool isExpanded) {
setState(() {
expanded = !isExpanded;
});
},
color: Colors.indigo,
expandedColor: Colors.teal,
);
},
),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.indigo));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.teal));
await tester.tap(find.byType(ExpandIcon));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.indigo));
});
testWidgets('ExpandIcon uses custom disabled icon color', (WidgetTester tester) async {
IconTheme iconTheme;
await tester.pumpWidget(wrap(
child: const ExpandIcon(
onPressed: null,
disabledColor: Colors.cyan,
),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.cyan));
await tester.pumpWidget(wrap(
child: const ExpandIcon(
onPressed: null,
color: Colors.indigo,
disabledColor: Colors.cyan,
),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.cyan));
await tester.pumpWidget(wrap(
child: const ExpandIcon(
isExpanded: true,
onPressed: null,
disabledColor: Colors.cyan,
),
));
await tester.pumpWidget(wrap(
child: const ExpandIcon(
isExpanded: true,
onPressed: null,
expandedColor: Colors.teal,
disabledColor: Colors.cyan,
),
));
await tester.pumpAndSettle();
iconTheme = tester.firstWidget(find.byType(IconTheme).last);
expect(iconTheme.data.color, equals(Colors.cyan));
});
}
| flutter/packages/flutter/test/material/expand_icon_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/expand_icon_test.dart",
"repo_id": "flutter",
"token_count": 4698
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('GridTile control test', (WidgetTester tester) async {
final Key headerKey = UniqueKey();
final Key footerKey = UniqueKey();
await tester.pumpWidget(MaterialApp(
home: GridTile(
header: GridTileBar(
key: headerKey,
leading: const Icon(Icons.thumb_up),
title: const Text('Header'),
subtitle: const Text('Subtitle'),
trailing: const Icon(Icons.thumb_up),
),
footer: GridTileBar(
key: footerKey,
title: const Text('Footer'),
backgroundColor: Colors.black38,
),
child: DecoratedBox(
decoration: BoxDecoration(
color: Colors.green[500],
),
),
),
));
expect(find.text('Header'), findsOneWidget);
expect(find.text('Footer'), findsOneWidget);
expect(
tester.getBottomLeft(find.byKey(headerKey)).dy,
lessThan(tester.getTopLeft(find.byKey(footerKey)).dy),
);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: GridTile(child: Text('Simple')),
),
);
expect(find.text('Simple'), findsOneWidget);
});
}
| flutter/packages/flutter/test/material/grid_title_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/grid_title_test.dart",
"repo_id": "flutter",
"token_count": 618
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
setUp(() {
debugResetSemanticsIdCounter();
});
testWidgets('MaterialButton defaults', (WidgetTester tester) async {
final Finder rawButtonMaterial = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(Material),
);
// Enabled MaterialButton
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: () { },
child: const Text('button'),
),
),
),
);
Material material = tester.widget<Material>(rawButtonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderOnForeground, true);
expect(material.borderRadius, null);
expect(material.clipBehavior, Clip.none);
expect(material.color, null);
expect(material.elevation, 2.0);
expect(material.shadowColor, null);
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2.0))));
expect(material.textStyle!.color, const Color(0xdd000000));
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
expect(material.type, MaterialType.transparency);
final Offset center = tester.getCenter(find.byType(MaterialButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
// Only elevation changes when enabled and pressed.
material = tester.widget<Material>(rawButtonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderOnForeground, true);
expect(material.borderRadius, null);
expect(material.clipBehavior, Clip.none);
expect(material.color, null);
expect(material.elevation, 8.0);
expect(material.shadowColor, null);
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2.0))));
expect(material.textStyle!.color, const Color(0xdd000000));
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
expect(material.type, MaterialType.transparency);
// Disabled MaterialButton
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: const Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: null,
child: Text('button'),
),
),
),
);
material = tester.widget<Material>(rawButtonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderOnForeground, true);
expect(material.borderRadius, null);
expect(material.clipBehavior, Clip.none);
expect(material.color, null);
expect(material.elevation, 0.0);
expect(material.shadowColor, null);
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2.0))));
expect(material.textStyle!.color, const Color(0x61000000));
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
expect(material.type, MaterialType.transparency);
// Finish gesture to release resources.
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Does MaterialButton work with hover', (WidgetTester tester) async {
const Color hoverColor = Color(0xff001122);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
hoverColor: hoverColor,
onPressed: () { },
child: const Text('button'),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(MaterialButton)));
await tester.pumpAndSettle();
final RenderObject inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect(color: hoverColor));
});
testWidgets('Does MaterialButton work with focus', (WidgetTester tester) async {
const Color focusColor = Color(0xff001122);
final FocusNode focusNode = FocusNode(debugLabel: 'MaterialButton Node');
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
focusColor: focusColor,
focusNode: focusNode,
onPressed: () { },
child: const Text('button'),
),
),
);
FocusManager.instance.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
focusNode.requestFocus();
await tester.pumpAndSettle();
final RenderObject inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect(color: focusColor));
focusNode.dispose();
});
testWidgets('MaterialButton elevation and colors have proper precedence', (WidgetTester tester) async {
const double elevation = 10.0;
const double focusElevation = 11.0;
const double hoverElevation = 12.0;
const double highlightElevation = 13.0;
const Color focusColor = Color(0xff001122);
const Color hoverColor = Color(0xff112233);
const Color highlightColor = Color(0xff223344);
final Finder rawButtonMaterial = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(Material),
);
final FocusNode focusNode = FocusNode(debugLabel: 'MaterialButton Node');
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
focusColor: focusColor,
hoverColor: hoverColor,
highlightColor: highlightColor,
elevation: elevation,
focusElevation: focusElevation,
hoverElevation: hoverElevation,
highlightElevation: highlightElevation,
focusNode: focusNode,
onPressed: () { },
child: const Text('button'),
),
),
);
await tester.pumpAndSettle();
FocusManager.instance.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
// Base elevation
Material material = tester.widget<Material>(rawButtonMaterial);
expect(material.elevation, equals(elevation));
// Focus elevation overrides base
focusNode.requestFocus();
await tester.pumpAndSettle();
material = tester.widget<Material>(rawButtonMaterial);
RenderObject inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect(color: focusColor));
expect(focusNode.hasPrimaryFocus, isTrue);
expect(material.elevation, equals(focusElevation));
// Hover elevation overrides focus
TestGesture? gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
addTearDown(() => gesture?.removePointer());
await gesture.moveTo(tester.getCenter(find.byType(MaterialButton)));
await tester.pumpAndSettle();
material = tester.widget<Material>(rawButtonMaterial);
inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect(color: focusColor)..rect(color: hoverColor));
expect(material.elevation, equals(hoverElevation));
await gesture.removePointer();
gesture = null;
// Highlight elevation overrides hover
final TestGesture gesture2 = await tester.startGesture(tester.getCenter(find.byType(MaterialButton)));
addTearDown(gesture2.removePointer);
await tester.pumpAndSettle();
material = tester.widget<Material>(rawButtonMaterial);
inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect(color: focusColor)..rect(color: highlightColor));
expect(material.elevation, equals(highlightElevation));
await gesture2.up();
focusNode.dispose();
});
testWidgets("MaterialButton's disabledColor takes precedence over its default disabled color.", (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/30012.
final Finder rawButtonMaterial = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(Material),
);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
disabledColor: Color(0xff00ff00),
onPressed: null,
child: Text('button'),
),
),
);
final Material material = tester.widget<Material>(rawButtonMaterial);
expect(material.color, const Color(0xff00ff00));
});
testWidgets('Default MaterialButton meets a11y contrast guidelines', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: MaterialButton(
child: const Text('MaterialButton'),
onPressed: () { },
),
),
),
),
);
// Default, not disabled.
await expectLater(tester, meetsGuideline(textContrastGuideline));
// Highlighted (pressed).
final Offset center = tester.getCenter(find.byType(MaterialButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pump(); // Start the splash and highlight animations.
await tester.pump(const Duration(milliseconds: 800)); // Wait for splash and highlight to be well under way.
await expectLater(tester, meetsGuideline(textContrastGuideline));
// Finish gesture to release resources.
await gesture.up();
await tester.pumpAndSettle();
},
skip: isBrowser, // https://github.com/flutter/flutter/issues/44115
);
testWidgets('MaterialButton gets focus when autofocus is set.', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'MaterialButton');
await tester.pumpWidget(
MaterialApp(
home: Center(
child: MaterialButton(
focusNode: focusNode,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isFalse);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: MaterialButton(
autofocus: true,
focusNode: focusNode,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isTrue);
focusNode.dispose();
});
testWidgets('MaterialButton onPressed and onLongPress callbacks are correctly called when non-null', (WidgetTester tester) async {
bool wasPressed;
Finder materialButton;
Widget buildFrame({ VoidCallback? onPressed, VoidCallback? onLongPress }) {
return Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: onPressed,
onLongPress: onLongPress,
child: const Text('button'),
),
);
}
// onPressed not null, onLongPress null.
wasPressed = false;
await tester.pumpWidget(
buildFrame(onPressed: () { wasPressed = true; }),
);
materialButton = find.byType(MaterialButton);
expect(tester.widget<MaterialButton>(materialButton).enabled, true);
await tester.tap(materialButton);
expect(wasPressed, true);
// onPressed null, onLongPress not null.
wasPressed = false;
await tester.pumpWidget(
buildFrame(onLongPress: () { wasPressed = true; }),
);
materialButton = find.byType(MaterialButton);
expect(tester.widget<MaterialButton>(materialButton).enabled, true);
await tester.longPress(materialButton);
expect(wasPressed, true);
// onPressed null, onLongPress null.
await tester.pumpWidget(
buildFrame(),
);
materialButton = find.byType(MaterialButton);
expect(tester.widget<MaterialButton>(materialButton).enabled, false);
});
testWidgets('MaterialButton onPressed and onLongPress callbacks are distinctly recognized', (WidgetTester tester) async {
bool didPressButton = false;
bool didLongPressButton = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: () {
didPressButton = true;
},
onLongPress: () {
didLongPressButton = true;
},
child: const Text('button'),
),
),
);
final Finder materialButton = find.byType(MaterialButton);
expect(tester.widget<MaterialButton>(materialButton).enabled, true);
expect(didPressButton, isFalse);
await tester.tap(materialButton);
expect(didPressButton, isTrue);
expect(didLongPressButton, isFalse);
await tester.longPress(materialButton);
expect(didLongPressButton, isTrue);
});
testWidgets('MaterialButton changes mouse cursor when hovered', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: MaterialButton(
onPressed: () {},
mouseCursor: SystemMouseCursors.text,
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: Offset.zero);
await tester.pump();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Test default cursor
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: MaterialButton(
onPressed: () {},
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
// Test default cursor when disabled
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: MaterialButton(
onPressed: null,
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
});
// This test is very similar to the '...explicit splashColor and highlightColor' test
// in icon_button_test.dart. If you change this one, you may want to also change that one.
testWidgets('MaterialButton with explicit splashColor and highlightColor', (WidgetTester tester) async {
const Color directSplashColor = Color(0xFF000011);
const Color directHighlightColor = Color(0xFF000011);
Widget buttonWidget = Center(
child: MaterialButton(
splashColor: directSplashColor,
highlightColor: directHighlightColor,
onPressed: () { /* to make sure the button is enabled */ },
clipBehavior: Clip.antiAlias,
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Theme(
data: ThemeData(
useMaterial3: false,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
),
child: buttonWidget,
),
),
);
final Offset center = tester.getCenter(find.byType(MaterialButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pump(); // start gesture
await tester.pump(const Duration(milliseconds: 200)); // wait for splash to be well under way
// Painter is translated to the center by the Center widget and not
// the Material widget.
const Rect expectedClipRect = Rect.fromLTRB(0.0, 0.0, 88.0, 36.0);
final Path expectedClipPath = Path()
..addRRect(RRect.fromRectAndRadius(
expectedClipRect,
const Radius.circular(2.0),
));
expect(
Material.of(tester.element(find.byType(InkWell))),
paints
..clipPath(pathMatcher: coversSameAreaAs(
expectedClipPath,
areaToCompare: expectedClipRect.inflate(10.0),
))
..circle(color: directSplashColor)
..rect(color: directHighlightColor),
);
const Color themeSplashColor1 = Color(0xFF001100);
const Color themeHighlightColor1 = Color(0xFF001100);
buttonWidget = Center(
child: MaterialButton(
onPressed: () { /* to make sure the button is enabled */ },
clipBehavior: Clip.antiAlias,
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Theme(
data: ThemeData(
useMaterial3: false,
highlightColor: themeHighlightColor1,
splashColor: themeSplashColor1,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
),
child: buttonWidget,
),
),
);
expect(
Material.of(tester.element(find.byType(InkWell))),
paints
..clipPath(pathMatcher: coversSameAreaAs(
expectedClipPath,
areaToCompare: expectedClipRect.inflate(10.0),
))
..circle(color: themeSplashColor1)
..rect(color: themeHighlightColor1),
);
const Color themeSplashColor2 = Color(0xFF002200);
const Color themeHighlightColor2 = Color(0xFF002200);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Theme(
data: ThemeData(
useMaterial3: false,
highlightColor: themeHighlightColor2,
splashColor: themeSplashColor2,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
),
child: buttonWidget, // same widget, so does not get updated because of us
),
),
);
expect(
Material.of(tester.element(find.byType(InkWell))),
paints
..circle(color: themeSplashColor2)
..rect(color: themeHighlightColor2),
);
await gesture.up();
});
testWidgets('MaterialButton has no clip by default', (WidgetTester tester) async {
final GlobalKey buttonKey = GlobalKey();
final Widget buttonWidget = Center(
child: MaterialButton(
key: buttonKey,
onPressed: () { /* to make sure the button is enabled */ },
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Theme(
data: ThemeData(
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
),
child: buttonWidget,
),
),
);
expect(
tester.renderObject(find.byKey(buttonKey)),
paintsExactlyCountTimes(#clipPath, 0),
);
});
testWidgets('Disabled MaterialButton has same semantic size as enabled and exposes disabled semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
const Rect expectedButtonSize = Rect.fromLTRB(0.0, 0.0, 116.0, 48.0);
// Button is in center of screen
final Matrix4 expectedButtonTransform = Matrix4.identity()
..translate(
TestSemantics.fullScreen.width / 2 - expectedButtonSize.width /2,
TestSemantics.fullScreen.height / 2 - expectedButtonSize.height /2,
);
// enabled button
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: MaterialButton(
child: const Text('Button'),
onPressed: () { /* to make sure the button is enabled */ },
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
id: 1,
rect: expectedButtonSize,
transform: expectedButtonTransform,
label: 'Button',
actions: <SemanticsAction>[
SemanticsAction.tap,
],
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isButton,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
],
),
],
),
));
// disabled button
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: MaterialButton(
onPressed: null, // button is disabled
child: Text('Button'),
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
id: 1,
rect: expectedButtonSize,
transform: expectedButtonTransform,
label: 'Button',
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isButton,
SemanticsFlag.isFocusable,
],
),
],
),
));
semantics.dispose();
});
testWidgets('MaterialButton minWidth and height parameters', (WidgetTester tester) async {
Widget buildFrame({ double? minWidth, double? height, EdgeInsets padding = EdgeInsets.zero, Widget? child }) {
return Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: MaterialButton(
padding: padding,
minWidth: minWidth,
height: height,
onPressed: null,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
child: child,
),
),
);
}
await tester.pumpWidget(buildFrame(minWidth: 8.0, height: 24.0));
expect(tester.getSize(find.byType(MaterialButton)), const Size(8.0, 24.0));
await tester.pumpWidget(buildFrame(minWidth: 8.0));
// Default minHeight constraint is 36, see RawMaterialButton.
expect(tester.getSize(find.byType(MaterialButton)), const Size(8.0, 36.0));
await tester.pumpWidget(buildFrame(height: 8.0));
// Default minWidth constraint is 88, see RawMaterialButton.
expect(tester.getSize(find.byType(MaterialButton)), const Size(88.0, 8.0));
await tester.pumpWidget(buildFrame());
expect(tester.getSize(find.byType(MaterialButton)), const Size(88.0, 36.0));
await tester.pumpWidget(buildFrame(padding: const EdgeInsets.all(4.0)));
expect(tester.getSize(find.byType(MaterialButton)), const Size(88.0, 36.0));
// Size is defined by the padding.
await tester.pumpWidget(
buildFrame(
minWidth: 0.0,
height: 0.0,
padding: const EdgeInsets.all(4.0),
),
);
expect(tester.getSize(find.byType(MaterialButton)), const Size(8.0, 8.0));
// Size is defined by the padded child.
await tester.pumpWidget(
buildFrame(
minWidth: 0.0,
height: 0.0,
padding: const EdgeInsets.all(4.0),
child: const SizedBox(width: 8.0, height: 8.0),
),
);
expect(tester.getSize(find.byType(MaterialButton)), const Size(16.0, 16.0));
// Size is defined by the minWidth, height constraints.
await tester.pumpWidget(
buildFrame(
minWidth: 18.0,
height: 18.0,
padding: const EdgeInsets.all(4.0),
child: const SizedBox(width: 8.0, height: 8.0),
),
);
expect(tester.getSize(find.byType(MaterialButton)), const Size(18.0, 18.0));
});
testWidgets('MaterialButton size is configurable by ThemeData.materialTapTargetSize', (WidgetTester tester) async {
final Key key1 = UniqueKey();
await tester.pumpWidget(
Theme(
data: ThemeData(materialTapTargetSize: MaterialTapTargetSize.padded),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: MaterialButton(
key: key1,
child: const SizedBox(width: 50.0, height: 8.0),
onPressed: () { },
),
),
),
),
);
expect(tester.getSize(find.byKey(key1)), const Size(88.0, 48.0));
final Key key2 = UniqueKey();
await tester.pumpWidget(
Theme(
data: ThemeData(materialTapTargetSize: MaterialTapTargetSize.shrinkWrap),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: MaterialButton(
key: key2,
child: const SizedBox(width: 50.0, height: 8.0),
onPressed: () { },
),
),
),
),
);
expect(tester.getSize(find.byKey(key2)), const Size(88.0, 36.0));
});
testWidgets('MaterialButton shape overrides ButtonTheme shape', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/29146
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: () { },
shape: const StadiumBorder(),
child: const Text('button'),
),
),
);
final Finder rawButtonMaterial = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(Material),
);
expect(tester.widget<Material>(rawButtonMaterial).shape, const StadiumBorder());
});
testWidgets('MaterialButton responds to density changes.', (WidgetTester tester) async {
const Key key = Key('test');
const Key childKey = Key('test child');
Future<void> buildTest(VisualDensity visualDensity, {bool useText = false}) async {
return tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: MaterialButton(
visualDensity: visualDensity,
key: key,
onPressed: () {},
child: useText ? const Text('Text', key: childKey) : Container(key: childKey, width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
),
);
}
await buildTest(VisualDensity.standard);
final RenderBox box = tester.renderObject(find.byKey(key));
Rect childRect = tester.getRect(find.byKey(childKey));
await tester.pumpAndSettle();
expect(box.size, equals(const Size(132, 100)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(const VisualDensity(horizontal: 3.0, vertical: 3.0));
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(156, 124)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(const VisualDensity(horizontal: -3.0, vertical: -3.0));
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(108, 100)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(VisualDensity.standard, useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(88, 48)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
await buildTest(const VisualDensity(horizontal: 3.0, vertical: 3.0), useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(112, 60)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
await buildTest(const VisualDensity(horizontal: -3.0, vertical: -3.0), useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(76, 36)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
});
testWidgets('disabledElevation is passed to RawMaterialButton', (WidgetTester tester) async {
const double disabledElevation = 16;
final Finder rawMaterialButtonFinder = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(RawMaterialButton),
);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
disabledElevation: disabledElevation,
onPressed: null, // disabled button
child: Text('button'),
),
),
);
final RawMaterialButton rawMaterialButton = tester.widget(rawMaterialButtonFinder);
expect(rawMaterialButton.disabledElevation, equals(disabledElevation));
});
testWidgets('MaterialButton.disabledElevation defaults to 0.0 when not provided', (WidgetTester tester) async {
final Finder rawMaterialButtonFinder = find.descendant(
of: find.byType(MaterialButton),
matching: find.byType(RawMaterialButton),
);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MaterialButton(
onPressed: null, // disabled button
child: Text('button'),
),
),
);
final RawMaterialButton rawMaterialButton = tester.widget(rawMaterialButtonFinder);
expect(rawMaterialButton.disabledElevation, equals(0.0));
});
}
| flutter/packages/flutter/test/material/material_button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/material_button_test.dart",
"repo_id": "flutter",
"token_count": 12292
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('copyWith, ==, hashCode basics', () {
expect(const NavigationRailThemeData(), const NavigationRailThemeData().copyWith());
expect(const NavigationRailThemeData().hashCode, const NavigationRailThemeData().copyWith().hashCode);
});
testWidgets('Material3 - Default values are used when no NavigationRail or NavigationRailThemeData properties are specified', (WidgetTester tester) async {
final ThemeData theme = ThemeData.light(useMaterial3: true);
// Material 3 defaults
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
body: NavigationRail(
selectedIndex: 0,
destinations: _destinations(),
),
),
),
);
expect(_railMaterial(tester).color, theme.colorScheme.surface);
expect(_railMaterial(tester).elevation, 0);
expect(_destinationSize(tester).width, 80.0);
expect(_selectedIconTheme(tester).size, 24.0);
expect(_selectedIconTheme(tester).color, theme.colorScheme.onSecondaryContainer);
expect(_selectedIconTheme(tester).opacity, null);
expect(_unselectedIconTheme(tester).size, 24.0);
expect(_unselectedIconTheme(tester).color, theme.colorScheme.onSurfaceVariant);
expect(_unselectedIconTheme(tester).opacity, null);
expect(_selectedLabelStyle(tester).fontSize, 14.0);
expect(_unselectedLabelStyle(tester).fontSize, 14.0);
expect(_destinationsAlign(tester).alignment, Alignment.topCenter);
expect(_labelType(tester), NavigationRailLabelType.none);
expect(find.byType(NavigationIndicator), findsWidgets);
expect(_indicatorDecoration(tester)?.color, theme.colorScheme.secondaryContainer);
expect(_indicatorDecoration(tester)?.shape, const StadiumBorder());
final InkResponse inkResponse = tester.allWidgets.firstWhere((Widget object) => object.runtimeType.toString() == '_IndicatorInkWell') as InkResponse;
expect(inkResponse.customBorder, const StadiumBorder());
});
testWidgets('Material2 - Default values are used when no NavigationRail or NavigationRailThemeData properties are specified', (WidgetTester tester) async {
// This test can be removed when `useMaterial3` is deprecated.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.light().copyWith(useMaterial3: false),
home: Scaffold(
body: NavigationRail(
selectedIndex: 0,
destinations: _destinations(),
),
),
),
);
expect(_railMaterial(tester).color, ThemeData().colorScheme.surface);
expect(_railMaterial(tester).elevation, 0);
expect(_destinationSize(tester).width, 72.0);
expect(_selectedIconTheme(tester).size, 24.0);
expect(_selectedIconTheme(tester).color, ThemeData().colorScheme.primary);
expect(_selectedIconTheme(tester).opacity, 1.0);
expect(_unselectedIconTheme(tester).size, 24.0);
expect(_unselectedIconTheme(tester).color, ThemeData().colorScheme.onSurface);
expect(_unselectedIconTheme(tester).opacity, 0.64);
expect(_selectedLabelStyle(tester).fontSize, 14.0);
expect(_unselectedLabelStyle(tester).fontSize, 14.0);
expect(_destinationsAlign(tester).alignment, Alignment.topCenter);
expect(_labelType(tester), NavigationRailLabelType.none);
expect(find.byType(NavigationIndicator), findsNothing);
});
testWidgets('NavigationRailThemeData values are used when no NavigationRail properties are specified', (WidgetTester tester) async {
const Color backgroundColor = Color(0x00000001);
const double elevation = 7.0;
const double selectedIconSize = 25.0;
const double unselectedIconSize = 23.0;
const Color selectedIconColor = Color(0x00000002);
const Color unselectedIconColor = Color(0x00000003);
const double selectedIconOpacity = 0.99;
const double unselectedIconOpacity = 0.98;
const double selectedLabelFontSize = 13.0;
const double unselectedLabelFontSize = 11.0;
const double groupAlignment = 0.0;
const NavigationRailLabelType labelType = NavigationRailLabelType.all;
const bool useIndicator = true;
const Color indicatorColor = Color(0x00000004);
const ShapeBorder indicatorShape = RoundedRectangleBorder();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: NavigationRailTheme(
data: const NavigationRailThemeData(
backgroundColor: backgroundColor,
elevation: elevation,
selectedIconTheme: IconThemeData(
size: selectedIconSize,
color: selectedIconColor,
opacity: selectedIconOpacity,
),
unselectedIconTheme: IconThemeData(
size: unselectedIconSize,
color: unselectedIconColor,
opacity: unselectedIconOpacity,
),
selectedLabelTextStyle: TextStyle(fontSize: selectedLabelFontSize),
unselectedLabelTextStyle: TextStyle(fontSize: unselectedLabelFontSize),
groupAlignment: groupAlignment,
labelType: labelType,
useIndicator: useIndicator,
indicatorColor: indicatorColor,
indicatorShape: indicatorShape,
),
child: NavigationRail(
selectedIndex: 0,
destinations: _destinations(),
),
),
),
),
);
expect(_railMaterial(tester).color, backgroundColor);
expect(_railMaterial(tester).elevation, elevation);
expect(_selectedIconTheme(tester).size, selectedIconSize);
expect(_selectedIconTheme(tester).color, selectedIconColor);
expect(_selectedIconTheme(tester).opacity, selectedIconOpacity);
expect(_unselectedIconTheme(tester).size, unselectedIconSize);
expect(_unselectedIconTheme(tester).color, unselectedIconColor);
expect(_unselectedIconTheme(tester).opacity, unselectedIconOpacity);
expect(_selectedLabelStyle(tester).fontSize, selectedLabelFontSize);
expect(_unselectedLabelStyle(tester).fontSize, unselectedLabelFontSize);
expect(_destinationsAlign(tester).alignment, Alignment.center);
expect(_labelType(tester), labelType);
expect(find.byType(NavigationIndicator), findsWidgets);
expect(_indicatorDecoration(tester)?.color, indicatorColor);
expect(_indicatorDecoration(tester)?.shape, indicatorShape);
});
testWidgets('NavigationRail values take priority over NavigationRailThemeData values when both properties are specified', (WidgetTester tester) async {
const Color backgroundColor = Color(0x00000001);
const double elevation = 7.0;
const double selectedIconSize = 25.0;
const double unselectedIconSize = 23.0;
const Color selectedIconColor = Color(0x00000002);
const Color unselectedIconColor = Color(0x00000003);
const double selectedIconOpacity = 0.99;
const double unselectedIconOpacity = 0.98;
const double selectedLabelFontSize = 13.0;
const double unselectedLabelFontSize = 11.0;
const double groupAlignment = 0.0;
const NavigationRailLabelType labelType = NavigationRailLabelType.all;
const bool useIndicator = true;
const Color indicatorColor = Color(0x00000004);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: NavigationRailTheme(
data: const NavigationRailThemeData(
backgroundColor: Color(0x00000099),
elevation: 5,
selectedIconTheme: IconThemeData(
size: 31.0,
color: Color(0x00000098),
opacity: 0.81,
),
unselectedIconTheme: IconThemeData(
size: 37.0,
color: Color(0x00000097),
opacity: 0.82,
),
selectedLabelTextStyle: TextStyle(fontSize: 9.0),
unselectedLabelTextStyle: TextStyle(fontSize: 7.0),
groupAlignment: 1.0,
labelType: NavigationRailLabelType.selected,
useIndicator: false,
indicatorColor: Color(0x00000096),
),
child: NavigationRail(
selectedIndex: 0,
destinations: _destinations(),
backgroundColor: backgroundColor,
elevation: elevation,
selectedIconTheme: const IconThemeData(
size: selectedIconSize,
color: selectedIconColor,
opacity: selectedIconOpacity,
),
unselectedIconTheme: const IconThemeData(
size: unselectedIconSize,
color: unselectedIconColor,
opacity: unselectedIconOpacity,
),
selectedLabelTextStyle: const TextStyle(fontSize: selectedLabelFontSize),
unselectedLabelTextStyle: const TextStyle(fontSize: unselectedLabelFontSize),
groupAlignment: groupAlignment,
labelType: labelType,
useIndicator: useIndicator,
indicatorColor: indicatorColor,
),
),
),
),
);
expect(_railMaterial(tester).color, backgroundColor);
expect(_railMaterial(tester).elevation, elevation);
expect(_selectedIconTheme(tester).size, selectedIconSize);
expect(_selectedIconTheme(tester).color, selectedIconColor);
expect(_selectedIconTheme(tester).opacity, selectedIconOpacity);
expect(_unselectedIconTheme(tester).size, unselectedIconSize);
expect(_unselectedIconTheme(tester).color, unselectedIconColor);
expect(_unselectedIconTheme(tester).opacity, unselectedIconOpacity);
expect(_selectedLabelStyle(tester).fontSize, selectedLabelFontSize);
expect(_unselectedLabelStyle(tester).fontSize, unselectedLabelFontSize);
expect(_destinationsAlign(tester).alignment, Alignment.center);
expect(_labelType(tester), labelType);
expect(find.byType(NavigationIndicator), findsWidgets);
expect(_indicatorDecoration(tester)?.color, indicatorColor);
});
// Regression test for https://github.com/flutter/flutter/issues/118618.
testWidgets('NavigationRailThemeData lerps correctly with null iconThemes', (WidgetTester tester) async {
final NavigationRailThemeData lerp = NavigationRailThemeData.lerp(const NavigationRailThemeData(), const NavigationRailThemeData(), 0.5)!;
expect(lerp.selectedIconTheme, isNull);
expect(lerp.unselectedIconTheme, isNull);
});
testWidgets('Default debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const NavigationRailThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('Custom debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const NavigationRailThemeData(
backgroundColor: Color(0x00000099),
elevation: 5,
selectedIconTheme: IconThemeData(color: Color(0x00000098)),
unselectedIconTheme: IconThemeData(color: Color(0x00000097)),
selectedLabelTextStyle: TextStyle(fontSize: 9.0),
unselectedLabelTextStyle: TextStyle(fontSize: 7.0),
groupAlignment: 1.0,
labelType: NavigationRailLabelType.selected,
useIndicator: true,
indicatorColor: Color(0x00000096),
indicatorShape: CircleBorder(),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description[0], 'backgroundColor: Color(0x00000099)');
expect(description[1], 'elevation: 5.0');
expect(description[2], 'unselectedLabelTextStyle: TextStyle(inherit: true, size: 7.0)');
expect(description[3], 'selectedLabelTextStyle: TextStyle(inherit: true, size: 9.0)');
// Ignore instance address for IconThemeData.
expect(description[4].contains('unselectedIconTheme: IconThemeData'), isTrue);
expect(description[4].contains('(color: Color(0x00000097))'), isTrue);
expect(description[5].contains('selectedIconTheme: IconThemeData'), isTrue);
expect(description[5].contains('(color: Color(0x00000098))'), isTrue);
expect(description[6], 'groupAlignment: 1.0');
expect(description[7], 'labelType: NavigationRailLabelType.selected');
expect(description[8], 'useIndicator: true');
expect(description[9], 'indicatorColor: Color(0x00000096)');
expect(description[10], 'indicatorShape: CircleBorder(BorderSide(width: 0.0, style: none))');
});
}
List<NavigationRailDestination> _destinations() {
return const <NavigationRailDestination>[
NavigationRailDestination(
icon: Icon(Icons.favorite_border),
selectedIcon: Icon(Icons.favorite),
label: Text('Abc'),
),
NavigationRailDestination(
icon: Icon(Icons.star_border),
selectedIcon: Icon(Icons.star),
label: Text('Def'),
),
];
}
Material _railMaterial(WidgetTester tester) {
// The first material is for the rail, and the rest are for the destinations.
return tester.firstWidget<Material>(
find.descendant(
of: find.byType(NavigationRail),
matching: find.byType(Material),
),
);
}
ShapeDecoration? _indicatorDecoration(WidgetTester tester) {
return tester.firstWidget<Container>(
find.descendant(
of: find.byType(NavigationIndicator),
matching: find.byType(Container),
),
).decoration as ShapeDecoration?;
}
IconThemeData _selectedIconTheme(WidgetTester tester) {
return _iconTheme(tester, Icons.favorite);
}
IconThemeData _unselectedIconTheme(WidgetTester tester) {
return _iconTheme(tester, Icons.star_border);
}
IconThemeData _iconTheme(WidgetTester tester, IconData icon) {
// The first IconTheme is the one added by the navigation rail.
return tester.firstWidget<IconTheme>(
find.ancestor(
of: find.byIcon(icon),
matching: find.byType(IconTheme),
),
).data;
}
TextStyle _selectedLabelStyle(WidgetTester tester) {
return tester.widget<RichText>(
find.descendant(
of: find.text('Abc'),
matching: find.byType(RichText),
),
).text.style!;
}
TextStyle _unselectedLabelStyle(WidgetTester tester) {
return tester.widget<RichText>(
find.descendant(
of: find.text('Def'),
matching: find.byType(RichText),
),
).text.style!;
}
Size _destinationSize(WidgetTester tester) {
return tester.getSize(
find.ancestor(
of: find.byIcon(Icons.favorite),
matching: find.byType(Material),
)
.first
);
}
Align _destinationsAlign(WidgetTester tester) {
// The first Expanded widget is the one within the main Column for the rail
// content.
return tester.firstWidget<Align>(
find.descendant(
of: find.byType(Expanded),
matching: find.byType(Align),
),
);
}
NavigationRailLabelType _labelType(WidgetTester tester) {
if (_visibilityAboveLabel('Abc').evaluate().isNotEmpty && _visibilityAboveLabel('Def').evaluate().isNotEmpty) {
return _labelVisibility(tester, 'Abc') ? NavigationRailLabelType.selected : NavigationRailLabelType.none;
} else {
return NavigationRailLabelType.all;
}
}
Finder _visibilityAboveLabel(String text) {
return find.ancestor(
of: find.text(text),
matching: find.byType(Visibility),
);
}
// Only valid when labelType != all.
bool _labelVisibility(WidgetTester tester, String text) {
final Visibility visibilityWidget = tester.widget<Visibility>(
find.ancestor(
of: find.text(text),
matching: find.byType(Visibility),
),
);
return visibilityWidget.visible;
}
| flutter/packages/flutter/test/material/navigation_rail_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/navigation_rail_theme_test.dart",
"repo_id": "flutter",
"token_count": 5997
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/src/services/keyboard_key.g.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('RawMaterialButton responds when tapped', (WidgetTester tester) async {
bool pressed = false;
const Color splashColor = Color(0xff00ff00);
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: RawMaterialButton(
splashColor: splashColor,
onPressed: () { pressed = true; },
child: const Text('BUTTON'),
),
),
),
),
);
await tester.tap(find.text('BUTTON'));
await tester.pump(const Duration(milliseconds: 10));
final RenderBox splash = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
expect(splash, paints..circle(color: splashColor));
await tester.pumpAndSettle();
expect(pressed, isTrue);
});
testWidgets('RawMaterialButton responds to shortcut when activated', (WidgetTester tester) async {
bool pressed = false;
final FocusNode focusNode = FocusNode(debugLabel: 'Test Button');
const Color splashColor = Color(0xff00ff00);
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Shortcuts(
shortcuts: const <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.enter): ActivateIntent(),
SingleActivator(LogicalKeyboardKey.space): ActivateIntent(),
},
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: RawMaterialButton(
splashColor: splashColor,
focusNode: focusNode,
onPressed: () { pressed = true; },
child: const Text('BUTTON'),
),
),
),
),
),
);
focusNode.requestFocus();
await tester.pump();
// Web doesn't react to enter, just space.
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump(const Duration(milliseconds: 10));
if (!kIsWeb) {
final RenderBox splash = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
expect(splash, paints..circle(color: splashColor));
}
await tester.pumpAndSettle();
expect(pressed, isTrue);
pressed = false;
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
expect(pressed, isTrue);
pressed = false;
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pump(const Duration(milliseconds: 10));
final RenderBox splash = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
expect(splash, paints..circle(color: splashColor));
await tester.pumpAndSettle();
expect(pressed, isTrue);
pressed = false;
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
expect(pressed, isTrue);
focusNode.dispose();
});
testWidgets('materialTapTargetSize.padded expands hit test area', (WidgetTester tester) async {
int pressed = 0;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: RawMaterialButton(
onPressed: () {
pressed++;
},
constraints: BoxConstraints.tight(const Size(10.0, 10.0)),
materialTapTargetSize: MaterialTapTargetSize.padded,
child: const Text('+'),
),
),
);
await tester.tapAt(const Offset(40.0, 400.0));
expect(pressed, 1);
});
testWidgets('materialTapTargetSize.padded expands semantics area', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: RawMaterialButton(
onPressed: () { },
constraints: BoxConstraints.tight(const Size(10.0, 10.0)),
materialTapTargetSize: MaterialTapTargetSize.padded,
child: const Text('+'),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isButton,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
label: '+',
textDirection: TextDirection.ltr,
rect: const Rect.fromLTRB(0.0, 0.0, 48.0, 48.0),
children: <TestSemantics>[],
),
],
),
ignoreTransform: true,
));
semantics.dispose();
});
testWidgets('Ink splash from center tap originates in correct location', (WidgetTester tester) async {
const Color highlightColor = Color(0xAAFF0000);
const Color splashColor = Color(0xAA0000FF);
const Color fillColor = Color(0xFFEF5350);
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: RawMaterialButton(
materialTapTargetSize: MaterialTapTargetSize.padded,
onPressed: () { },
fillColor: fillColor,
highlightColor: highlightColor,
splashColor: splashColor,
child: const SizedBox(),
),
),
),
),
);
final Offset center = tester.getCenter(find.byType(InkWell));
final TestGesture gesture = await tester.startGesture(center);
await tester.pump(); // start gesture
await tester.pump(const Duration(milliseconds: 200)); // wait for splash to be well under way
final RenderBox box = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
// centered in material button.
expect(box, paints..circle(x: 44.0, y: 18.0, color: splashColor));
await gesture.up();
});
testWidgets('Ink splash from tap above material originates in correct location', (WidgetTester tester) async {
const Color highlightColor = Color(0xAAFF0000);
const Color splashColor = Color(0xAA0000FF);
const Color fillColor = Color(0xFFEF5350);
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: false),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: RawMaterialButton(
materialTapTargetSize: MaterialTapTargetSize.padded,
onPressed: () { },
fillColor: fillColor,
highlightColor: highlightColor,
splashColor: splashColor,
child: const SizedBox(),
),
),
),
),
);
final Offset top = tester.getRect(find.byType(InkWell)).topCenter;
final TestGesture gesture = await tester.startGesture(top);
await tester.pump(); // start gesture
await tester.pump(const Duration(milliseconds: 200)); // wait for splash to be well under way
final RenderBox box = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
// paints above material
expect(box, paints..circle(x: 44.0, y: 0.0, color: splashColor));
await gesture.up();
});
testWidgets('off-center child is hit testable', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Column(
children: <Widget>[
RawMaterialButton(
materialTapTargetSize: MaterialTapTargetSize.padded,
onPressed: () { },
child: const SizedBox(
width: 400.0,
height: 400.0,
child: Column(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
SizedBox(
height: 50.0,
width: 400.0,
child: Text('Material'),
),
],
),
),
),
],
),
),
);
expect(find.text('Material').hitTestable(), findsOneWidget);
});
testWidgets('smaller child is hit testable', (WidgetTester tester) async {
const Key key = Key('test');
await tester.pumpWidget(
MaterialApp(
home: Column(
children: <Widget>[
RawMaterialButton(
materialTapTargetSize: MaterialTapTargetSize.padded,
onPressed: () { },
child: SizedBox(
key: key,
width: 8.0,
height: 8.0,
child: Container(
color: const Color(0xFFAABBCC),
),
),
),
],
),
),
);
expect(find.byKey(key).hitTestable(), findsOneWidget);
});
testWidgets('RawMaterialButton can be expanded by parent constraints', (WidgetTester tester) async {
const Key key = Key('test');
await tester.pumpWidget(
MaterialApp(
home: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
RawMaterialButton(
key: key,
onPressed: () { },
child: const SizedBox(),
),
],
),
),
);
expect(tester.getSize(find.byKey(key)), const Size(800.0, 48.0));
});
testWidgets('RawMaterialButton handles focus', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Button Focus');
const Key key = Key('test');
const Color focusColor = Color(0xff00ff00);
FocusManager.instance.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
await tester.pumpWidget(
MaterialApp(
home: Center(
child: RawMaterialButton(
key: key,
focusNode: focusNode,
focusColor: focusColor,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
final RenderBox box = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
expect(box, isNot(paints..rect(color: focusColor)));
focusNode.requestFocus();
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(box, paints..rect(color: focusColor));
focusNode.dispose();
});
testWidgets('RawMaterialButton loses focus when disabled.', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'RawMaterialButton');
await tester.pumpWidget(
MaterialApp(
home: Center(
child: RawMaterialButton(
autofocus: true,
focusNode: focusNode,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: RawMaterialButton(
focusNode: focusNode,
onPressed: null,
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isFalse);
focusNode.dispose();
});
testWidgets("Disabled RawMaterialButton can't be traversed to.", (WidgetTester tester) async {
final FocusNode focusNode1 = FocusNode(debugLabel: '$RawMaterialButton 1');
final FocusNode focusNode2 = FocusNode(debugLabel: '$RawMaterialButton 2');
await tester.pumpWidget(
MaterialApp(
home: FocusScope(
child: Center(
child: Column(
children: <Widget>[
RawMaterialButton(
autofocus: true,
focusNode: focusNode1,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
RawMaterialButton(
autofocus: true,
focusNode: focusNode2,
onPressed: null,
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
],
),
),
),
),
);
await tester.pump();
expect(focusNode1.hasPrimaryFocus, isTrue);
expect(focusNode2.hasPrimaryFocus, isFalse);
expect(focusNode1.nextFocus(), isFalse);
await tester.pump();
expect(focusNode1.hasPrimaryFocus, isTrue);
expect(focusNode2.hasPrimaryFocus, isFalse);
focusNode1.dispose();
focusNode2.dispose();
});
testWidgets('RawMaterialButton handles hover', (WidgetTester tester) async {
const Key key = Key('test');
const Color hoverColor = Color(0xff00ff00);
FocusManager.instance.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
await tester.pumpWidget(
MaterialApp(
home: Center(
child: RawMaterialButton(
key: key,
hoverColor: hoverColor,
hoverElevation: 10.5,
onPressed: () {},
child: Container(width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
);
final RenderBox box = Material.of(tester.element(find.byType(InkWell))) as RenderBox;
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
expect(box, isNot(paints..rect(color: hoverColor)));
await gesture.moveTo(tester.getCenter(find.byType(RawMaterialButton)));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(box, paints..rect(color: hoverColor));
});
testWidgets('RawMaterialButton onPressed and onLongPress callbacks are correctly called when non-null', (WidgetTester tester) async {
bool wasPressed;
Finder rawMaterialButton;
Widget buildFrame({ VoidCallback? onPressed, VoidCallback? onLongPress }) {
return Directionality(
textDirection: TextDirection.ltr,
child: RawMaterialButton(
onPressed: onPressed,
onLongPress: onLongPress,
child: const Text('button'),
),
);
}
// onPressed not null, onLongPress null.
wasPressed = false;
await tester.pumpWidget(
buildFrame(onPressed: () { wasPressed = true; }),
);
rawMaterialButton = find.byType(RawMaterialButton);
expect(tester.widget<RawMaterialButton>(rawMaterialButton).enabled, true);
await tester.tap(rawMaterialButton);
expect(wasPressed, true);
// onPressed null, onLongPress not null.
wasPressed = false;
await tester.pumpWidget(
buildFrame(onLongPress: () { wasPressed = true; }),
);
rawMaterialButton = find.byType(RawMaterialButton);
expect(tester.widget<RawMaterialButton>(rawMaterialButton).enabled, true);
await tester.longPress(rawMaterialButton);
expect(wasPressed, true);
// onPressed null, onLongPress null.
await tester.pumpWidget(
buildFrame(),
);
rawMaterialButton = find.byType(RawMaterialButton);
expect(tester.widget<RawMaterialButton>(rawMaterialButton).enabled, false);
});
testWidgets('RawMaterialButton onPressed and onLongPress callbacks are distinctly recognized', (WidgetTester tester) async {
bool didPressButton = false;
bool didLongPressButton = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: RawMaterialButton(
onPressed: () {
didPressButton = true;
},
onLongPress: () {
didLongPressButton = true;
},
child: const Text('button'),
),
),
);
final Finder rawMaterialButton = find.byType(RawMaterialButton);
expect(tester.widget<RawMaterialButton>(rawMaterialButton).enabled, true);
expect(didPressButton, isFalse);
await tester.tap(rawMaterialButton);
expect(didPressButton, isTrue);
expect(didLongPressButton, isFalse);
await tester.longPress(rawMaterialButton);
expect(didLongPressButton, isTrue);
});
testWidgets('RawMaterialButton responds to density changes.', (WidgetTester tester) async {
const Key key = Key('test');
const Key childKey = Key('test child');
Future<void> buildTest(VisualDensity visualDensity, {bool useText = false}) async {
return tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: RawMaterialButton(
visualDensity: visualDensity,
key: key,
onPressed: () {},
child: useText ? const Text('Text', key: childKey) : Container(key: childKey, width: 100, height: 100, color: const Color(0xffff0000)),
),
),
),
),
);
}
await buildTest(VisualDensity.standard);
final RenderBox box = tester.renderObject(find.byKey(key));
Rect childRect = tester.getRect(find.byKey(childKey));
await tester.pumpAndSettle();
expect(box.size, equals(const Size(100, 100)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(const VisualDensity(horizontal: 3.0, vertical: 3.0));
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(124, 124)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(const VisualDensity(horizontal: -3.0, vertical: -3.0));
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(100, 100)));
expect(childRect, equals(const Rect.fromLTRB(350, 250, 450, 350)));
await buildTest(VisualDensity.standard, useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(88, 48)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
await buildTest(const VisualDensity(horizontal: 3.0, vertical: 3.0), useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(100, 60)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
await buildTest(const VisualDensity(horizontal: -3.0, vertical: -3.0), useText: true);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byKey(childKey));
expect(box.size, equals(const Size(76, 36)));
expect(childRect, equals(const Rect.fromLTRB(372.0, 293.0, 428.0, 307.0)));
});
testWidgets('RawMaterialButton changes mouse cursor when hovered', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: RawMaterialButton(
onPressed: () {},
mouseCursor: SystemMouseCursors.text,
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: Offset.zero);
await tester.pump();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Test default cursor
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: RawMaterialButton(
onPressed: () {},
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
// Test default cursor when disabled
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: RawMaterialButton(
onPressed: null,
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
});
}
| flutter/packages/flutter/test/material/raw_material_button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/raw_material_button_test.dart",
"repo_id": "flutter",
"token_count": 9069
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:async';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('SnackBar control test', (WidgetTester tester) async {
const String helloSnackBar = 'Hello SnackBar';
const Key tapTarget = Key('tap-target');
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text(helloSnackBar),
duration: Duration(seconds: 2),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text(helloSnackBar), findsNothing);
await tester.tap(find.byKey(tapTarget));
expect(find.text(helloSnackBar), findsNothing);
await tester.pump(); // schedule animation
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; two second timer starts here
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 1.50s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 2.25s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 3.00s // timer triggers to dismiss snackbar, reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text(helloSnackBar), findsOneWidget); // frame 0 of dismiss animation
await tester.pump(const Duration(milliseconds: 750)); // 3.75s // last frame of animation, snackbar removed from build
expect(find.text(helloSnackBar), findsNothing);
});
testWidgets('SnackBar twice test', (WidgetTester tester) async {
int snackBarCount = 0;
const Key tapTarget = Key('tap-target');
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
snackBarCount += 1;
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('bar$snackBarCount'),
duration: const Duration(seconds: 2),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // queue bar1
await tester.tap(find.byKey(tapTarget)); // queue bar2
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // schedule animation for bar1
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // begin animation
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; two second timer starts here
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 1.50s
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 2.25s
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 3.00s // timer triggers to dismiss snackbar, reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 3.75s // last frame of animation, snackbar removed from build, new snack bar put in its place
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 4.50s // animation last frame; two second timer starts here
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 5.25s
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 6.00s
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 6.75s // timer triggers to dismiss snackbar, reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 7.50s // last frame of animation, snackbar removed from build, new snack bar put in its place
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
});
testWidgets('SnackBar cancel test', (WidgetTester tester) async {
int snackBarCount = 0;
const Key tapTarget = Key('tap-target');
late int time;
late ScaffoldFeatureController<SnackBar, SnackBarClosedReason> lastController;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
snackBarCount += 1;
lastController = ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('bar$snackBarCount'),
duration: Duration(seconds: time),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
time = 1000;
await tester.tap(find.byKey(tapTarget)); // queue bar1
final ScaffoldFeatureController<SnackBar, SnackBarClosedReason> firstController = lastController;
time = 2;
await tester.tap(find.byKey(tapTarget)); // queue bar2
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // schedule animation for bar1
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // begin animation
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; two second timer starts here
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 1.50s
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 2.25s
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 10000)); // 12.25s
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
firstController.close(); // snackbar is manually dismissed
await tester.pump(const Duration(milliseconds: 750)); // 13.00s // reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 13.75s // last frame of animation, snackbar removed from build, new snack bar put in its place
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 14.50s // animation last frame; two second timer starts here
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 15.25s
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 16.00s
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 16.75s // timer triggers to dismiss snackbar, reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 17.50s // last frame of animation, snackbar removed from build, new snack bar put in its place
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
});
testWidgets('SnackBar dismiss test', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
late DismissDirection dismissDirection;
late double width;
int snackBarCount = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
width = MediaQuery.sizeOf(context).width;
return GestureDetector(
key: tapTarget,
onTap: () {
snackBarCount += 1;
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text('bar$snackBarCount'),
duration: const Duration(seconds: 2),
dismissDirection: dismissDirection,
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
await _testSnackBarDismiss(
tester: tester,
tapTarget: tapTarget,
scaffoldWidth: width,
onDismissDirectionChange: (DismissDirection dir) => dismissDirection = dir,
onDragGestureChange: () => snackBarCount = 0,
);
});
testWidgets('SnackBar dismissDirection can be customised from SnackBarThemeData', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
late double width;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
snackBarTheme: const SnackBarThemeData(
dismissDirection: DismissDirection.startToEnd,
)
),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
width = MediaQuery.sizeOf(context).width;
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('swipe ltr'),
duration: Duration(seconds: 2),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('swipe ltr'), findsNothing);
await tester.tap(find.byKey(tapTarget));
expect(find.text('swipe ltr'), findsNothing);
await tester.pump(); // schedule animation for snack bar
expect(find.text('swipe ltr'), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text('swipe ltr'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750));
await tester.drag(find.text('swipe ltr'), Offset(width, 0.0));
await tester.pump(); // snack bar dismissed
expect(find.text('swipe ltr'), findsNothing);
});
testWidgets('dismissDirection from SnackBar should be preferred over SnackBarThemeData', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
late double width;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
snackBarTheme: const SnackBarThemeData(
dismissDirection: DismissDirection.startToEnd,
)
),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
width = MediaQuery.sizeOf(context).width;
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('swipe rtl'),
duration: Duration(seconds: 2),
dismissDirection: DismissDirection.endToStart,
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('swipe rtl'), findsNothing);
await tester.tap(find.byKey(tapTarget));
expect(find.text('swipe rtl'), findsNothing);
await tester.pump(); // schedule animation for snack bar
expect(find.text('swipe rtl'), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text('swipe rtl'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750));
await tester.drag(find.text('swipe rtl'), Offset(-width, 0.0));
await tester.pump(); // snack bar dismissed
expect(find.text('swipe rtl'), findsNothing);
});
testWidgets('SnackBar cannot be tapped twice', (WidgetTester tester) async {
int tapCount = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () {
++tapCount;
},
),
));
},
child: const Text('X'),
);
},
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
expect(tapCount, equals(0));
await tester.tap(find.text('ACTION'));
expect(tapCount, equals(1));
await tester.tap(find.text('ACTION'));
expect(tapCount, equals(1));
await tester.pump();
await tester.tap(find.text('ACTION'));
expect(tapCount, equals(1));
});
testWidgets('Material2 - Light theme SnackBar has dark background', (WidgetTester tester) async {
final ThemeData lightTheme = ThemeData.light(useMaterial3: false);
await tester.pumpWidget(
MaterialApp(
theme: lightTheme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final RenderPhysicalModel renderModel = tester.renderObject(
find.widgetWithText(Material, 'I am a snack bar.').first,
);
// There is a somewhat complicated background color calculation based
// off of the surface color. For the default light theme it
// should be this value.
expect(renderModel.color, equals(const Color(0xFF333333)));
});
testWidgets('Material3 - Light theme SnackBar has dark background', (WidgetTester tester) async {
final ThemeData lightTheme = ThemeData.light(useMaterial3: true);
await tester.pumpWidget(
MaterialApp(
theme: lightTheme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder material = find.widgetWithText(Material, 'I am a snack bar.').first;
final RenderPhysicalModel renderModel = tester.renderObject(material);
expect(renderModel.color, equals(lightTheme.colorScheme.inverseSurface));
});
testWidgets('Dark theme SnackBar has light background', (WidgetTester tester) async {
final ThemeData darkTheme = ThemeData.dark();
await tester.pumpWidget(
MaterialApp(
theme: darkTheme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final RenderPhysicalModel renderModel = tester.renderObject(
find.widgetWithText(Material, 'I am a snack bar.').first,
);
expect(renderModel.color, equals(darkTheme.colorScheme.onSurface));
});
testWidgets('Material2 - Dark theme SnackBar has primary text buttons', (WidgetTester tester) async {
final ThemeData darkTheme = ThemeData.dark(useMaterial3: false);
await tester.pumpWidget(
MaterialApp(
theme: darkTheme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final TextStyle buttonTextStyle = tester.widget<RichText>(
find.descendant(of: find.text('ACTION'), matching: find.byType(RichText))
).text.style!;
expect(buttonTextStyle.color, equals(darkTheme.colorScheme.primary));
});
testWidgets('Material3 - Dark theme SnackBar has primary text buttons', (WidgetTester tester) async {
final ThemeData darkTheme = ThemeData.dark(useMaterial3: true);
await tester.pumpWidget(
MaterialApp(
theme: darkTheme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final TextStyle buttonTextStyle = tester.widget<RichText>(
find.descendant(of: find.text('ACTION'), matching: find.byType(RichText))
).text.style!;
expect(buttonTextStyle.color, equals(darkTheme.colorScheme.inversePrimary));
});
testWidgets('SnackBar should inherit theme data from its ancestor.', (WidgetTester tester) async {
final SliderThemeData sliderTheme = SliderThemeData.fromPrimaryColors(
primaryColor: Colors.black,
primaryColorDark: Colors.black,
primaryColorLight: Colors.black,
valueIndicatorTextStyle: const TextStyle(color: Colors.black),
);
final ChipThemeData chipTheme = ChipThemeData.fromDefaults(
primaryColor: Colors.black,
secondaryColor: Colors.white,
labelStyle: const TextStyle(color: Colors.black),
);
const PageTransitionsTheme pageTransitionTheme = PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
TargetPlatform.iOS: CupertinoPageTransitionsBuilder(),
TargetPlatform.macOS: CupertinoPageTransitionsBuilder(),
},
);
final ThemeData theme = ThemeData.light().copyWith(
visualDensity: VisualDensity.standard,
primaryColor: Colors.black,
primaryColorLight: Colors.black,
primaryColorDark: Colors.black,
canvasColor: Colors.black,
shadowColor: Colors.black,
scaffoldBackgroundColor: Colors.black,
cardColor: Colors.black,
dividerColor: Colors.black,
focusColor: Colors.black,
hoverColor: Colors.black,
highlightColor: Colors.black,
splashColor: Colors.black,
splashFactory: InkRipple.splashFactory,
unselectedWidgetColor: Colors.black,
disabledColor: Colors.black,
buttonTheme: const ButtonThemeData(colorScheme: ColorScheme.dark()),
toggleButtonsTheme: const ToggleButtonsThemeData(textStyle: TextStyle(color: Colors.black)),
secondaryHeaderColor: Colors.black,
dialogBackgroundColor: Colors.black,
indicatorColor: Colors.black,
hintColor: Colors.black,
textTheme: ThemeData.dark().textTheme,
primaryTextTheme: ThemeData.dark().textTheme,
inputDecorationTheme: ThemeData.dark().inputDecorationTheme.copyWith(border: const OutlineInputBorder()),
iconTheme: ThemeData.dark().iconTheme,
primaryIconTheme: ThemeData.dark().iconTheme,
sliderTheme: sliderTheme,
tabBarTheme: const TabBarTheme(labelColor: Colors.black),
tooltipTheme: const TooltipThemeData(height: 100),
cardTheme: const CardTheme(color: Colors.black),
chipTheme: chipTheme,
platform: TargetPlatform.iOS,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
applyElevationOverlayColor: false,
pageTransitionsTheme: pageTransitionTheme,
appBarTheme: const AppBarTheme(backgroundColor: Colors.black),
scrollbarTheme: const ScrollbarThemeData(radius: Radius.circular(10.0)),
bottomAppBarTheme: const BottomAppBarTheme(color: Colors.black),
colorScheme: const ColorScheme.light(),
dialogTheme: const DialogTheme(backgroundColor: Colors.black),
floatingActionButtonTheme: const FloatingActionButtonThemeData(backgroundColor: Colors.black),
navigationRailTheme: const NavigationRailThemeData(backgroundColor: Colors.black),
typography: Typography.material2018(),
snackBarTheme: const SnackBarThemeData(backgroundColor: Colors.black),
bottomSheetTheme: const BottomSheetThemeData(backgroundColor: Colors.black),
popupMenuTheme: const PopupMenuThemeData(color: Colors.black),
bannerTheme: const MaterialBannerThemeData(backgroundColor: Colors.black),
dividerTheme: const DividerThemeData(color: Colors.black),
bottomNavigationBarTheme: const BottomNavigationBarThemeData(type: BottomNavigationBarType.fixed),
timePickerTheme: const TimePickerThemeData(backgroundColor: Colors.black),
textButtonTheme: TextButtonThemeData(style: TextButton.styleFrom(foregroundColor: Colors.red)),
elevatedButtonTheme: ElevatedButtonThemeData(style: ElevatedButton.styleFrom(backgroundColor: Colors.green)),
outlinedButtonTheme: OutlinedButtonThemeData(style: OutlinedButton.styleFrom(foregroundColor: Colors.blue)),
textSelectionTheme: const TextSelectionThemeData(cursorColor: Colors.black),
dataTableTheme: const DataTableThemeData(),
checkboxTheme: const CheckboxThemeData(),
radioTheme: const RadioThemeData(),
switchTheme: const SwitchThemeData(),
progressIndicatorTheme: const ProgressIndicatorThemeData(),
);
ThemeData? themeBeforeSnackBar;
ThemeData? themeAfterSnackBar;
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
themeBeforeSnackBar = Theme.of(context);
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Builder(
builder: (BuildContext context) {
themeAfterSnackBar = Theme.of(context);
return const Text('I am a snack bar.');
},
),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final ThemeData comparedTheme = themeBeforeSnackBar!.copyWith(
colorScheme: themeAfterSnackBar!.colorScheme,
); // Fields replaced by SnackBar.
expect(comparedTheme, themeAfterSnackBar);
});
testWidgets('Snackbar margin can be customized', (WidgetTester tester) async {
const double padding = 20.0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('I am a snack bar.'),
margin: EdgeInsets.all(padding),
behavior: SnackBarBehavior.floating,
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarBottomRight = tester.getBottomRight(materialFinder);
expect(snackBarBottomLeft.dx, padding);
expect(snackBarBottomLeft.dy, 600 - padding); // Device height is 600.
expect(snackBarBottomRight.dx, 800 - padding); // Device width is 800.
});
testWidgets('SnackbarBehavior.floating is positioned within safe area', (WidgetTester tester) async {
const double viewPadding = 50.0;
const double floatingSnackBarDefaultBottomMargin = 10.0;
await tester.pumpWidget(
MaterialApp(
home: MediaQuery(
data: const MediaQueryData(
// Simulate non-safe area.
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('I am a snack bar.'),
behavior: SnackBarBehavior.floating,
),
);
},
child: const Text('X'),
);
},
),
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // Start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
expect(
snackBarBottomLeft.dy,
// Device height is 600.
600 - viewPadding - floatingSnackBarDefaultBottomMargin,
);
});
testWidgets('Snackbar padding can be customized', (WidgetTester tester) async {
const double padding = 20.0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('I am a snack bar.'),
padding: EdgeInsets.all(padding),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder textFinder = find.text('I am a snack bar.');
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset textBottomLeft = tester.getBottomLeft(textFinder);
final Offset textTopRight = tester.getTopRight(textFinder);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarTopRight = tester.getTopRight(materialFinder);
expect(textBottomLeft.dx - snackBarBottomLeft.dx, padding);
expect(snackBarTopRight.dx - textTopRight.dx, padding);
expect(snackBarBottomLeft.dy - textBottomLeft.dy, padding);
expect(textTopRight.dy - snackBarTopRight.dy, padding);
});
testWidgets('Snackbar width can be customized', (WidgetTester tester) async {
const double width = 200.0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('I am a snack bar.'),
width: width,
behavior: SnackBarBehavior.floating,
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarBottomRight = tester.getBottomRight(materialFinder);
expect(snackBarBottomLeft.dx, (800 - width) / 2); // Device width is 800.
expect(snackBarBottomRight.dx, (800 + width) / 2); // Device width is 800.
});
testWidgets('Snackbar width can be customized from ThemeData',
(WidgetTester tester) async {
const double width = 200.0;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
snackBarTheme: const SnackBarThemeData(
width: width, behavior: SnackBarBehavior.floating),
),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Feeling snackish'),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarBottomRight = tester.getBottomRight(materialFinder);
expect(snackBarBottomLeft.dx, (800 - width) / 2); // Device width is 800.
expect(snackBarBottomRight.dx, (800 + width) / 2); // Device width is 800.
});
testWidgets('Snackbar width customization takes preference of widget over theme', (WidgetTester tester) async {
const double themeWidth = 200.0;
const double widgetWidth = 400.0;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
snackBarTheme: const SnackBarThemeData(
width: themeWidth, behavior: SnackBarBehavior.floating),
),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text('Feeling super snackish'),
width: widgetWidth,
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarBottomRight = tester.getBottomRight(materialFinder);
expect(snackBarBottomLeft.dx, (800 - widgetWidth) / 2); // Device width is 800.
expect(snackBarBottomRight.dx, (800 + widgetWidth) / 2); // Device width is 800.
});
testWidgets('Material2 - Snackbar labels can be colored as MaterialColor', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
textColor: Colors.lightBlue,
disabledTextColor: Colors.red,
label: 'ACTION',
onPressed: () { },
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Element actionTextBox = tester.element(find.text('ACTION'));
final Widget textWidget = actionTextBox.widget;
final DefaultTextStyle defaultTextStyle = DefaultTextStyle.of(actionTextBox);
if (textWidget is Text) {
final TextStyle effectiveStyle = defaultTextStyle.style.merge(textWidget.style);
expect(effectiveStyle.color, Colors.lightBlue);
} else {
expect(false, true);
}
});
testWidgets('Material3 - Snackbar labels can be colored as MaterialColor', (WidgetTester tester) async {
const MaterialColor usedColor = Colors.teal;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
textColor: usedColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Element actionTextButton =
tester.element(find.widgetWithText(TextButton, 'ACTION'));
final Widget textButton = actionTextButton.widget;
if (textButton is TextButton) {
final ButtonStyle buttonStyle = textButton.style!;
if (buttonStyle.foregroundColor is MaterialStateColor) {
// Same color when resolved
expect(buttonStyle.foregroundColor!.resolve(<MaterialState>{}), usedColor);
} else {
expect(false, true);
}
} else {
expect(false, true);
}
});
testWidgets('Snackbar labels can be colored as MaterialStateColor (Material 3)',
(WidgetTester tester) async {
const _TestMaterialStateColor usedColor = _TestMaterialStateColor();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
textColor: usedColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Element actionTextButton =
tester.element(find.widgetWithText(TextButton, 'ACTION'));
final Widget textButton = actionTextButton.widget;
if (textButton is TextButton) {
final ButtonStyle buttonStyle = textButton.style!;
if (buttonStyle.foregroundColor is MaterialStateColor) {
// Exactly the same object
expect(buttonStyle.foregroundColor, usedColor);
} else {
expect(false, true);
}
} else {
expect(false, true);
}
});
testWidgets('Material2 - SnackBar button text alignment', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () { }),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 24.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 17.0 + 40.0); // margin + bottom padding
expect(actionTextBottomLeft.dx - textBottomRight.dx, 24.0 + 12.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 24.0 + 12.0 + 30.0); // action (padding + margin) + right padding
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 17.0 + 40.0); // margin + bottom padding
});
testWidgets('Material3 - SnackBar button text alignment', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () { }),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 24.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 14.0 + 40.0); // margin + bottom padding
expect(actionTextBottomLeft.dx - textBottomRight.dx, 24.0 + 12.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 24.0 + 12.0 + 30.0); // action (padding + margin) + right padding
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 14.0 + 40.0); // margin + bottom padding
});
testWidgets(
'Material2 - Custom padding between SnackBar and its contents when set to SnackBarBehavior.fixed',
(WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.favorite), label: 'Animutation'),
BottomNavigationBarItem(icon: Icon(Icons.block), label: 'Zombo.com'),
],
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 24.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 17.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 24.0 + 12.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 24.0 + 12.0 + 30.0); // action (padding + margin) + right padding
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 17.0); // margin (with no bottom padding)
},
);
testWidgets(
'Material3 - Custom padding between SnackBar and its contents when set to SnackBarBehavior.fixed',
(WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.favorite), label: 'Animutation'),
BottomNavigationBarItem(icon: Icon(Icons.block), label: 'Zombo.com'),
],
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 24.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 14.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 24.0 + 12.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 24.0 + 12.0 + 30.0); // action (padding + margin) + right padding
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 14.0); // margin (with no bottom padding)
},
);
testWidgets('SnackBar should push FloatingActionButton above', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
// Get the Rect of the FAB to compare after the SnackBar appears.
final Rect originalFabRect = tester.getRect(find.byType(FloatingActionButton));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Rect fabRect = tester.getRect(find.byType(FloatingActionButton));
// FAB should shift upwards after SnackBar appears.
expect(fabRect.center.dy, lessThan(originalFabRect.center.dy));
final Offset snackBarTopRight = tester.getTopRight(find.byType(SnackBar));
// FAB's surrounding padding is set to [kFloatingActionButtonMargin] in floating_action_button_location.dart by default.
const int defaultFabPadding = 16;
// FAB should be positioned above the SnackBar by the default padding.
expect(fabRect.bottomRight.dy, snackBarTopRight.dy - defaultFabPadding);
});
testWidgets('Material2 - Floating SnackBar button text alignment', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: false,
snackBarTheme: const SnackBarThemeData(behavior: SnackBarBehavior.floating),
),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 31.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 27.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 16.0 + 8.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 31.0 + 30.0 + 8.0); // margin + right (padding + margin)
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 27.0); // margin (with no bottom padding)
});
testWidgets('Material3 - Floating SnackBar button text alignment', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
snackBarTheme: const SnackBarThemeData(behavior: SnackBarBehavior.floating),
),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 31.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 24.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 16.0 + 8.0); // action padding + margin
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 31.0 + 30.0 + 8.0); // margin + right (padding + margin)
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 24.0); // margin (with no bottom padding)
});
testWidgets(
'Material2 - Custom padding between SnackBar and its contents when set to SnackBarBehavior.floating',
(WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: false,
snackBarTheme: const SnackBarThemeData(behavior: SnackBarBehavior.floating),
),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.favorite), label: 'Animutation'),
BottomNavigationBarItem(icon: Icon(Icons.block), label: 'Zombo.com'),
],
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 31.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 27.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 16.0 + 8.0); // action (margin + padding)
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 31.0 + 30.0 + 8.0); // margin + right (padding + margin)
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 27.0); // margin (with no bottom padding)
},
);
testWidgets(
'Material3 - Custom padding between SnackBar and its contents when set to SnackBarBehavior.floating',
(WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
snackBarTheme: const SnackBarThemeData(behavior: SnackBarBehavior.floating),
),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Scaffold(
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.favorite), label: 'Animutation'),
BottomNavigationBarItem(icon: Icon(Icons.block), label: 'Zombo.com'),
],
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
));
},
child: const Text('X'),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750)); // Animation last frame.
final Offset textBottomLeft = tester.getBottomLeft(find.text('I am a snack bar.'));
final Offset textBottomRight = tester.getBottomRight(find.text('I am a snack bar.'));
final Offset actionTextBottomLeft = tester.getBottomLeft(find.text('ACTION'));
final Offset actionTextBottomRight = tester.getBottomRight(find.text('ACTION'));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(textBottomLeft.dx - snackBarBottomLeft.dx, 31.0 + 10.0); // margin + left padding
expect(snackBarBottomLeft.dy - textBottomLeft.dy, 24.0); // margin (with no bottom padding)
expect(actionTextBottomLeft.dx - textBottomRight.dx, 16.0 + 8.0); // action (margin + padding)
expect(snackBarBottomRight.dx - actionTextBottomRight.dx, 31.0 + 30.0 + 8.0); // margin + right (padding + margin)
expect(snackBarBottomRight.dy - actionTextBottomRight.dy, 24.0); // margin (with no bottom padding)
},
);
testWidgets('SnackBarClosedReason', (WidgetTester tester) async {
final GlobalKey<ScaffoldMessengerState> scaffoldMessengerKey = GlobalKey<ScaffoldMessengerState>();
bool actionPressed = false;
SnackBarClosedReason? closedReason;
await tester.pumpWidget(MaterialApp(
scaffoldMessengerKey: scaffoldMessengerKey,
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('snack'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
label: 'ACTION',
onPressed: () {
actionPressed = true;
},
),
)).closed.then<void>((SnackBarClosedReason reason) {
closedReason = reason;
});
},
child: const Text('X'),
);
},
),
),
));
// Pop up the snack bar and then press its action button.
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
expect(actionPressed, isFalse);
await tester.tap(find.text('ACTION'));
expect(actionPressed, isTrue);
// Closed reason is only set when the animation is complete.
await tester.pump(const Duration(milliseconds: 250));
expect(closedReason, isNull);
// Wait for animation to complete.
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(closedReason, equals(SnackBarClosedReason.action));
// Pop up the snack bar and then swipe downwards to dismiss it.
await tester.tap(find.text('X'));
await tester.pump(const Duration(milliseconds: 750));
await tester.pump(const Duration(milliseconds: 750));
await tester.drag(find.text('snack'), const Offset(0.0, 50.0));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(closedReason, equals(SnackBarClosedReason.swipe));
// Pop up the snack bar and then remove it.
await tester.tap(find.text('X'));
await tester.pump(const Duration(milliseconds: 750));
scaffoldMessengerKey.currentState!.removeCurrentSnackBar();
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(closedReason, equals(SnackBarClosedReason.remove));
// Pop up the snack bar and then hide it.
await tester.tap(find.text('X'));
await tester.pump(const Duration(milliseconds: 750));
scaffoldMessengerKey.currentState!.hideCurrentSnackBar();
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(closedReason, equals(SnackBarClosedReason.hide));
// Pop up the snack bar and then let it time out.
await tester.tap(find.text('X'));
await tester.pump(const Duration(milliseconds: 750));
await tester.pump(const Duration(milliseconds: 750));
await tester.pump(const Duration(milliseconds: 1500));
await tester.pump(); // begin animation
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(closedReason, equals(SnackBarClosedReason.timeout));
});
testWidgets('accessible navigation behavior with action', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(accessibleNavigation: true),
child: ScaffoldMessenger(
child: Builder(
builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
body: GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('snack'),
duration: const Duration(seconds: 1),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
));
},
child: const Text('X'),
),
);
},
),
),
),
));
await tester.tap(find.text('X'));
await tester.pump();
// Find action immediately
expect(find.text('ACTION'), findsOneWidget);
// Snackbar doesn't close
await tester.pump(const Duration(seconds: 10));
expect(find.text('ACTION'), findsOneWidget);
await tester.tap(find.text('ACTION'));
await tester.pump();
// Snackbar closes immediately
expect(find.text('ACTION'), findsNothing);
});
testWidgets('contributes dismiss semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(accessibleNavigation: true),
child: ScaffoldMessenger(
child: Builder(builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
body: GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('snack'),
duration: const Duration(seconds: 1),
action: SnackBarAction(
label: 'ACTION',
onPressed: () { },
),
));
},
child: const Text('X'),
),
);
}),
),
),
));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getSemantics(find.text('snack')), matchesSemantics(
isLiveRegion: true,
hasDismissAction: true,
hasScrollDownAction: true,
hasScrollUpAction: true,
label: 'snack',
textDirection: TextDirection.ltr,
));
handle.dispose();
});
testWidgets('SnackBar default display duration test', (WidgetTester tester) async {
const String helloSnackBar = 'Hello SnackBar';
const Key tapTarget = Key('tap-target');
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text(helloSnackBar),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text(helloSnackBar), findsNothing);
await tester.tap(find.byKey(tapTarget));
expect(find.text(helloSnackBar), findsNothing);
await tester.pump(); // schedule animation
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; four second timer starts here
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 1.50s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 2.25s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 3.00s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 3.75s
expect(find.text(helloSnackBar), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1000)); // 4.75s // timer triggers to dismiss snackbar, reverse animation is scheduled
await tester.pump(); // begin animation
expect(find.text(helloSnackBar), findsOneWidget); // frame 0 of dismiss animation
await tester.pump(const Duration(milliseconds: 750)); // 5.50s // last frame of animation, snackbar removed from build
expect(find.text(helloSnackBar), findsNothing);
});
testWidgets('SnackBar handles updates to accessibleNavigation', (WidgetTester tester) async {
Future<void> boilerplate({ required bool accessibleNavigation }) {
return tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: MediaQueryData(accessibleNavigation: accessibleNavigation),
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('test'),
action: SnackBarAction(label: 'foo', onPressed: () { }),
));
},
behavior: HitTestBehavior.opaque,
child: const Text('X'),
);
},
),
),
),
));
}
await boilerplate(accessibleNavigation: false);
expect(find.text('test'), findsNothing);
await tester.tap(find.text('X'));
await tester.pump(); // schedule animation
expect(find.text('test'), findsOneWidget);
await tester.pump(); // begin animation
await tester.pump(const Duration(milliseconds: 4750)); // 4.75s
expect(find.text('test'), findsOneWidget);
// Enabled accessible navigation
await boilerplate(accessibleNavigation: true);
await tester.pump(const Duration(milliseconds: 4000)); // 8.75s
await tester.pump();
expect(find.text('test'), findsOneWidget);
// disable accessible navigation
await boilerplate(accessibleNavigation: false);
await tester.pumpAndSettle(const Duration(milliseconds: 5750));
expect(find.text('test'), findsNothing);
});
testWidgets('Snackbar calls onVisible once', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
int called = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('hello'),
duration: const Duration(seconds: 1),
onVisible: () {
called += 1;
},
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
await tester.tap(find.byKey(tapTarget));
await tester.pump(); // start animation
await tester.pumpAndSettle();
expect(find.text('hello'), findsOneWidget);
expect(called, 1);
});
testWidgets('Snackbar does not call onVisible when it is queued', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
int called = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('hello'),
duration: const Duration(seconds: 1),
onVisible: () {
called += 1;
},
));
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('hello 2'),
duration: const Duration(seconds: 1),
onVisible: () {
called += 1;
},
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
await tester.tap(find.byKey(tapTarget));
await tester.pump(); // start animation
await tester.pumpAndSettle();
expect(find.text('hello'), findsOneWidget);
expect(called, 1);
});
group('SnackBar position', () {
for (final SnackBarBehavior behavior in SnackBarBehavior.values) {
final SnackBar snackBar = SnackBar(
content: const Text('SnackBar text'),
behavior: behavior,
);
testWidgets(
'$behavior should align SnackBar with the bottom of Scaffold '
'when Scaffold has no other elements',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(snackBar);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset scaffoldBottomRight = tester.getBottomRight(find.byType(Scaffold));
expect(snackBarBottomRight, equals(scaffoldBottomRight));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset scaffoldBottomLeft = tester.getBottomLeft(find.byType(Scaffold));
expect(snackBarBottomLeft, equals(scaffoldBottomLeft));
},
);
testWidgets(
'$behavior should align SnackBar with the top of BottomNavigationBar '
'when Scaffold has no FloatingActionButton',
(WidgetTester tester) async {
final UniqueKey boxKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
bottomNavigationBar: SizedBox(key: boxKey, width: 800, height: 60),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(snackBar);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset bottomNavigationBarTopRight = tester.getTopRight(find.byKey(boxKey));
expect(snackBarBottomRight, equals(bottomNavigationBarTopRight));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset bottomNavigationBarTopLeft = tester.getTopLeft(find.byKey(boxKey));
expect(snackBarBottomLeft, equals(bottomNavigationBarTopLeft));
},
);
}
testWidgets(
'Padding of ${SnackBarBehavior.fixed} is not consumed by viewInsets',
(WidgetTester tester) async {
final Widget child = MaterialApp(
home: Scaffold(
resizeToAvoidBottomInset: false,
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.fixed,
),
);
},
child: const Text('X'),
);
},
),
),
);
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(bottom: 20.0),
),
child: child,
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(); // Show snackbar
final Offset initialBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset initialBottomRight = tester.getBottomRight(find.byType(SnackBar));
// Consume bottom padding - as if by the keyboard opening
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(
viewPadding: EdgeInsets.all(20),
viewInsets: EdgeInsets.all(100),
),
child: child,
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset finalBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset finalBottomRight = tester.getBottomRight(find.byType(SnackBar));
expect(initialBottomLeft, finalBottomLeft);
expect(initialBottomRight, finalBottomRight);
},
);
testWidgets(
'${SnackBarBehavior.fixed} should align SnackBar with the bottom of Scaffold '
'when Scaffold has a FloatingActionButton',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('Snackbar text'),
behavior: SnackBarBehavior.fixed,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset scaffoldBottomRight = tester.getBottomRight(find.byType(Scaffold));
expect(snackBarBottomRight, equals(scaffoldBottomRight));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset scaffoldBottomLeft = tester.getBottomLeft(find.byType(Scaffold));
expect(snackBarBottomLeft, equals(scaffoldBottomLeft));
},
);
testWidgets(
'${SnackBarBehavior.floating} should align SnackBar with the top of FloatingActionButton when Scaffold has a FloatingActionButton',
(WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
},
child: const Text('X'),
);
},
),
),
));
await tester.tap(find.text('X'));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset floatingActionButtonTopLeft = tester.getTopLeft(
find.byType(FloatingActionButton),
);
// Since padding between the SnackBar and the FAB is created by the SnackBar,
// the bottom offset of the SnackBar should be equal to the top offset of the FAB
expect(snackBarBottomLeft.dy, floatingActionButtonTopLeft.dy);
},
);
testWidgets(
'${SnackBarBehavior.floating} should not align SnackBar with the top of FloatingActionButton '
'when Scaffold has a FloatingActionButton and floatingActionButtonLocation is set to a top position',
(WidgetTester tester) async {
Future<void> pumpApp({required FloatingActionButtonLocation fabLocation}) async {
return tester.pumpWidget(MaterialApp(
home: Scaffold(
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
floatingActionButtonLocation: fabLocation,
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
},
child: const Text('X'),
);
},
),
),
));
}
const List<FloatingActionButtonLocation> topLocations = <FloatingActionButtonLocation>[
FloatingActionButtonLocation.startTop,
FloatingActionButtonLocation.centerTop,
FloatingActionButtonLocation.endTop,
FloatingActionButtonLocation.miniStartTop,
FloatingActionButtonLocation.miniCenterTop,
FloatingActionButtonLocation.miniEndTop,
];
for (final FloatingActionButtonLocation location in topLocations) {
await pumpApp(fabLocation: location);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
expect(snackBarBottomLeft.dy, 600); // Device height is 600.
}
},
);
testWidgets(
'${SnackBarBehavior.floating} should align SnackBar with the top of FloatingActionButton '
'when Scaffold has a FloatingActionButton and floatingActionButtonLocation is not set to a top position',
(WidgetTester tester) async {
Future<void> pumpApp({required FloatingActionButtonLocation fabLocation}) async {
return tester.pumpWidget(MaterialApp(
home: Scaffold(
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
floatingActionButtonLocation: fabLocation,
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
},
child: const Text('X'),
);
},
),
),
));
}
const List<FloatingActionButtonLocation> nonTopLocations = <FloatingActionButtonLocation>[
FloatingActionButtonLocation.startDocked,
FloatingActionButtonLocation.startFloat,
FloatingActionButtonLocation.centerDocked,
FloatingActionButtonLocation.centerFloat,
FloatingActionButtonLocation.endContained,
FloatingActionButtonLocation.endDocked,
FloatingActionButtonLocation.endFloat,
FloatingActionButtonLocation.miniStartDocked,
FloatingActionButtonLocation.miniStartFloat,
FloatingActionButtonLocation.miniCenterDocked,
FloatingActionButtonLocation.miniCenterFloat,
FloatingActionButtonLocation.miniEndDocked,
FloatingActionButtonLocation.miniEndFloat,
// Regression test related to https://github.com/flutter/flutter/pull/131303.
_CustomFloatingActionButtonLocation(),
];
for (final FloatingActionButtonLocation location in nonTopLocations) {
await pumpApp(fabLocation: location);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset floatingActionButtonTopLeft = tester.getTopLeft(
find.byType(FloatingActionButton),
);
// Since padding between the SnackBar and the FAB is created by the SnackBar,
// the bottom offset of the SnackBar should be equal to the top offset of the FAB
expect(snackBarBottomLeft.dy, floatingActionButtonTopLeft.dy);
}
},
);
testWidgets(
'${SnackBarBehavior.fixed} should align SnackBar with the top of BottomNavigationBar '
'when Scaffold has a BottomNavigationBar and FloatingActionButton',
(WidgetTester tester) async {
final UniqueKey boxKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
bottomNavigationBar: SizedBox(key: boxKey, width: 800, height: 60),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('SnackBar text'),
behavior: SnackBarBehavior.fixed,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset bottomNavigationBarTopRight = tester.getTopRight(find.byKey(boxKey));
expect(snackBarBottomRight, equals(bottomNavigationBarTopRight));
final Offset snackBarBottomLeft = tester.getBottomLeft(find.byType(SnackBar));
final Offset bottomNavigationBarTopLeft = tester.getTopLeft(find.byKey(boxKey));
expect(snackBarBottomLeft, equals(bottomNavigationBarTopLeft));
},
);
testWidgets(
'${SnackBarBehavior.floating} should align SnackBar with the top of FloatingActionButton '
'when Scaffold has BottomNavigationBar and FloatingActionButton',
(WidgetTester tester) async {
final UniqueKey boxKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
bottomNavigationBar: SizedBox(key: boxKey, width: 800, height: 60),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('SnackBar text'),
behavior: SnackBarBehavior.floating,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset fabTopRight = tester.getTopRight(find.byType(FloatingActionButton));
expect(snackBarBottomRight.dy, equals(fabTopRight.dy));
},
);
testWidgets(
'${SnackBarBehavior.floating} should align SnackBar with the top of BottomNavigationBar '
'when Scaffold has both BottomNavigationBar and FloatingActionButton and '
'BottomNavigationBar.top is higher than FloatingActionButton.top',
(WidgetTester tester) async {
final UniqueKey boxKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
bottomNavigationBar: SizedBox(key: boxKey, width: 800, height: 200),
floatingActionButton: FloatingActionButton(onPressed: () {}),
floatingActionButtonLocation: FloatingActionButtonLocation.endContained,
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('SnackBar text'),
behavior: SnackBarBehavior.floating,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final Offset snackBarBottomRight = tester.getBottomRight(find.byType(SnackBar));
final Offset fabTopRight = tester.getTopRight(find.byType(FloatingActionButton));
final Offset navBarTopRight = tester.getTopRight(find.byKey(boxKey));
// Test the top of the navigation bar is higher than the top of the floating action button.
expect(fabTopRight.dy, greaterThan(navBarTopRight.dy));
expect(snackBarBottomRight.dy, equals(navBarTopRight.dy));
},
);
Future<void> openFloatingSnackBar(WidgetTester tester) async {
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('SnackBar text'),
behavior: SnackBarBehavior.floating,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
}
const String offScreenMessage = 'Floating SnackBar presented off screen.\n'
'A SnackBar with behavior property set to SnackBarBehavior.floating is fully '
'or partially off screen because some or all the widgets provided to '
'Scaffold.floatingActionButton, Scaffold.persistentFooterButtons and '
'Scaffold.bottomNavigationBar take up too much vertical space.\n'
'Consider constraining the size of these widgets to allow room for the SnackBar to be visible.';
testWidgets('Snackbar with SnackBarBehavior.floating will assert when offset too high by a large Scaffold.floatingActionButton', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84263
Future<void> boilerplate({required double? fabHeight}) {
return tester.pumpWidget(
MaterialApp(
home: Scaffold(
floatingActionButton: Container(height: fabHeight),
),
),
);
}
// Run once with a visible SnackBar to compute the empty space above SnackBar.
const double mediumFabHeight = 100;
await boilerplate(fabHeight: mediumFabHeight);
await openFloatingSnackBar(tester);
expect(tester.takeException(), isNull);
final double spaceAboveSnackBar = tester.getTopLeft(find.byType(SnackBar)).dy;
// Run with the Snackbar fully off screen.
await boilerplate(fabHeight: spaceAboveSnackBar + mediumFabHeight * 2);
await openFloatingSnackBar(tester);
AssertionError exception = tester.takeException() as AssertionError;
expect(exception.message, offScreenMessage);
// Run with the Snackbar partially off screen.
await boilerplate(fabHeight: spaceAboveSnackBar + mediumFabHeight + 10);
await openFloatingSnackBar(tester);
exception = tester.takeException() as AssertionError;
expect(exception.message, offScreenMessage);
// Run with the Snackbar fully visible right on the top of the screen.
await boilerplate(fabHeight: spaceAboveSnackBar + mediumFabHeight);
await openFloatingSnackBar(tester);
expect(tester.takeException(), isNull);
});
testWidgets('Material2 - Snackbar with SnackBarBehavior.floating will assert when offset too high by a large Scaffold.persistentFooterButtons', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84263
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
persistentFooterButtons: <Widget>[SizedBox(height: 1000)],
),
),
);
await openFloatingSnackBar(tester);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final AssertionError exception = tester.takeException() as AssertionError;
expect(exception.message, offScreenMessage);
});
testWidgets('Material3 - Snackbar with SnackBarBehavior.floating will assert when offset too high by a large Scaffold.persistentFooterButtons', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84263
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(
persistentFooterButtons: <Widget>[SizedBox(height: 1000)],
),
),
);
final FlutterExceptionHandler? handler = FlutterError.onError;
final List<String> errorMessages = <String>[];
FlutterError.onError = (FlutterErrorDetails details) {
errorMessages.add(details.exceptionAsString());
};
addTearDown(() => FlutterError.onError = handler);
await openFloatingSnackBar(tester);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
expect(errorMessages.contains(offScreenMessage), isTrue);
});
testWidgets('Material2 - Snackbar with SnackBarBehavior.floating will assert when offset too high by a large Scaffold.bottomNavigationBar', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84263
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
bottomNavigationBar: SizedBox(height: 1000),
),
),
);
await openFloatingSnackBar(tester);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
final AssertionError exception = tester.takeException() as AssertionError;
expect(exception.message, offScreenMessage);
});
testWidgets('Material3 - Snackbar with SnackBarBehavior.floating will assert when offset too high by a large Scaffold.bottomNavigationBar', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84263
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(
bottomNavigationBar: SizedBox(height: 1000),
),
),
);
final FlutterExceptionHandler? handler = FlutterError.onError;
final List<String> errorMessages = <String>[];
FlutterError.onError = (FlutterErrorDetails details) {
errorMessages.add(details.exceptionAsString());
};
addTearDown(() => FlutterError.onError = handler);
await openFloatingSnackBar(tester);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
expect(errorMessages.contains(offScreenMessage), isTrue);
});
testWidgets(
'SnackBar has correct end padding when it contains an action with fixed behavior',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('Some content'),
behavior: SnackBarBehavior.fixed,
action: SnackBarAction(
label: 'ACTION',
onPressed: () {},
),
));
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
final Offset snackBarTopRight = tester.getTopRight(find.byType(SnackBar));
final Offset actionTopRight = tester.getTopRight(find.byType(SnackBarAction));
expect(snackBarTopRight.dx - actionTopRight.dx, 12.0);
},
);
testWidgets(
'SnackBar has correct end padding when it contains an action with floating behavior',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('Some content'),
behavior: SnackBarBehavior.floating,
action: SnackBarAction(
label: 'ACTION',
onPressed: () {},
),
));
},
child: const Text('X'),
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
final Offset snackBarTopRight = tester.getTopRight(find.byType(SnackBar));
final Offset actionTopRight = tester.getTopRight(find.byType(SnackBarAction));
expect(snackBarTopRight.dx - actionTopRight.dx, 8.0 + 15.0); // button margin + horizontal scaffold outside margin
},
);
testWidgets('Floating snackbar with custom width is centered when text direction is rtl', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/140125.
const double customWidth = 400.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
behavior: SnackBarBehavior.floating,
width: customWidth,
content: Text('Feeling super snackish'),
),
);
},
child: const Text('X'),
);
},
),
),
),
),
);
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final Finder materialFinder = find.descendant(
of: find.byType(SnackBar),
matching: find.byType(Material),
);
final Offset snackBarBottomLeft = tester.getBottomLeft(materialFinder);
final Offset snackBarBottomRight = tester.getBottomRight(materialFinder);
expect(snackBarBottomLeft.dx, (800 - customWidth) / 2); // Device width is 800.
expect(snackBarBottomRight.dx, (800 + customWidth) / 2); // Device width is 800.
});
});
testWidgets('SnackBars hero across transitions when using ScaffoldMessenger', (WidgetTester tester) async {
const String snackBarText = 'hello snackbar';
const String firstHeader = 'home';
const String secondHeader = 'second';
const Key snackTarget = Key('snack-target');
const Key transitionTarget = Key('transition-target');
Widget buildApp() {
return MaterialApp(
routes: <String, WidgetBuilder> {
'/': (BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text(firstHeader)),
body: Center(
child: ElevatedButton(
key: transitionTarget,
child: const Text('PUSH'),
onPressed: () {
Navigator.of(context).pushNamed('/second');
},
),
),
floatingActionButton: FloatingActionButton(
key: snackTarget,
onPressed: () async {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text(snackBarText),
),
);
},
child: const Text('X'),
),
);
},
'/second': (BuildContext context) => Scaffold(appBar: AppBar(title: const Text(secondHeader))),
},
);
}
await tester.pumpWidget(buildApp());
expect(find.text(snackBarText), findsNothing);
expect(find.text(firstHeader), findsOneWidget);
expect(find.text(secondHeader), findsNothing);
// Present SnackBar
await tester.tap(find.byKey(snackTarget));
await tester.pump(); // schedule animation
expect(find.text(snackBarText), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text(snackBarText), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750));
expect(find.text(snackBarText), findsOneWidget);
// Push new route
await tester.tap(find.byKey(transitionTarget));
await tester.pump();
expect(find.text(snackBarText), findsOneWidget);
expect(find.text(firstHeader), findsOneWidget);
expect(find.text(secondHeader, skipOffstage: false), findsOneWidget);
await tester.pump();
expect(find.text(snackBarText), findsOneWidget);
expect(find.text(firstHeader), findsOneWidget);
expect(find.text(secondHeader), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750));
expect(find.text(snackBarText), findsOneWidget);
expect(find.text(firstHeader), findsNothing);
expect(find.text(secondHeader), findsOneWidget);
});
testWidgets('Should have only one SnackBar during back swipe navigation',
(WidgetTester tester) async {
const String snackBarText = 'hello snackbar';
const Key snackTarget = Key('snack-target');
const Key transitionTarget = Key('transition-target');
Widget buildApp() {
final PageTransitionsTheme pageTransitionTheme = PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
for (final TargetPlatform platform in TargetPlatform.values)
platform: const CupertinoPageTransitionsBuilder(),
},
);
return MaterialApp(
theme: ThemeData(pageTransitionsTheme: pageTransitionTheme),
initialRoute: '/',
routes: <String, WidgetBuilder> {
'/': (BuildContext context) {
return Scaffold(
body: Center(
child: ElevatedButton(
key: transitionTarget,
child: const Text('PUSH'),
onPressed: () {
Navigator.of(context).pushNamed('/second');
},
),
),
);
},
'/second': (BuildContext context) {
return Scaffold(
floatingActionButton: FloatingActionButton(
key: snackTarget,
onPressed: () async {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text(snackBarText),
),
);
},
child: const Text('X'),
),
);
},
},
);
}
await tester.pumpWidget(buildApp());
// Transition to second page.
await tester.tap(find.byKey(transitionTarget));
await tester.pumpAndSettle();
// Present SnackBar
await tester.tap(find.byKey(snackTarget));
await tester.pump(); // schedule animation
expect(find.text(snackBarText), findsOneWidget);
await tester.pump(); // begin animation
expect(find.text(snackBarText), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750));
expect(find.text(snackBarText), findsOneWidget);
// Start the gesture at the edge of the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 200.0));
// Trigger the swipe.
await gesture.moveBy(const Offset(100.0, 0.0));
// Back gestures should trigger and draw the hero transition in the very same
// frame (since the "from" route has already moved to reveal the "to" route).
await tester.pump();
// We should have only one SnackBar displayed on the screen.
expect(find.text(snackBarText), findsOneWidget);
});
testWidgets('Material2 - SnackBars should be shown above the bottomSheet', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('I love Flutter!'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.workWithBottomSheet.png'));
});
testWidgets('Material3 - SnackBars should be shown above the bottomSheet', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('I love Flutter!'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.workWithBottomSheet.png'));
});
testWidgets('ScaffoldMessenger does not duplicate a SnackBar when presenting a MaterialBanner.', (WidgetTester tester) async {
const Key materialBannerTapTarget = Key('materialbanner-tap-target');
const Key snackBarTapTarget = Key('snackbar-tap-target');
const String snackBarText = 'SnackBar';
const String materialBannerText = 'MaterialBanner';
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return Column(
children: <Widget>[
GestureDetector(
key: snackBarTapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text(snackBarText),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
),
GestureDetector(
key: materialBannerTapTarget,
onTap: () {
ScaffoldMessenger.of(context).showMaterialBanner(MaterialBanner(
content: const Text(materialBannerText),
actions: <Widget>[
TextButton(
child: const Text('DISMISS'),
onPressed: () => ScaffoldMessenger.of(context).hideCurrentMaterialBanner(),
),
],
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
),
],
);
},
),
),
));
await tester.tap(find.byKey(snackBarTapTarget));
await tester.tap(find.byKey(materialBannerTapTarget));
await tester.pumpAndSettle();
expect(find.text(snackBarText), findsOneWidget);
expect(find.text(materialBannerText), findsOneWidget);
});
testWidgets('Material2 - ScaffoldMessenger presents SnackBars to only the root Scaffold when Scaffolds are nested.', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: Scaffold(
body: const Scaffold(),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state<ScaffoldMessengerState>(
find.byType(ScaffoldMessenger),
);
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('ScaffoldMessenger'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle();
expect(find.byType(SnackBar), findsOneWidget);
// The FloatingActionButton helps us identify which Scaffold has the
// SnackBar here. Since the outer Scaffold contains a FAB, the SnackBar
// should be above it. If the inner Scaffold had the SnackBar, it would be
// overlapping the FAB.
await expectLater(
find.byType(MaterialApp),
matchesGoldenFile('m2_snack_bar.scaffold.nested.png'),
);
final Offset snackBarTopRight = tester.getTopRight(find.byType(SnackBar));
expect(snackBarTopRight.dy, 465.0);
});
testWidgets('Material3 - ScaffoldMessenger presents SnackBars to only the root Scaffold when Scaffolds are nested.', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: Scaffold(
body: const Scaffold(),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state<ScaffoldMessengerState>(
find.byType(ScaffoldMessenger),
);
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('ScaffoldMessenger'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle();
expect(find.byType(SnackBar), findsOneWidget);
// The FloatingActionButton helps us identify which Scaffold has the
// SnackBar here. Since the outer Scaffold contains a FAB, the SnackBar
// should be above it. If the inner Scaffold had the SnackBar, it would be
// overlapping the FAB.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.scaffold.nested.png'));
final Offset snackBarTopRight = tester.getTopRight(find.byType(SnackBar));
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(snackBarTopRight.dy, 465.0);
}
});
testWidgets('ScaffoldMessengerState clearSnackBars works as expected', (WidgetTester tester) async {
final List<String> snackBars = <String>['Hello Snackbar', 'Hi Snackbar', 'Bye Snackbar'];
int snackBarCounter = 0;
const Key tapTarget = Key('tap-target');
final GlobalKey<ScaffoldMessengerState> scaffoldMessengerKey = GlobalKey();
await tester.pumpWidget(MaterialApp(
home: ScaffoldMessenger(
key: scaffoldMessengerKey,
child: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text(snackBars[snackBarCounter++]),
duration: const Duration(seconds: 2),
));
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
),
));
expect(find.text(snackBars[0]), findsNothing);
expect(find.text(snackBars[1]), findsNothing);
expect(find.text(snackBars[2]), findsNothing);
await tester.tap(find.byKey(tapTarget));
await tester.tap(find.byKey(tapTarget));
await tester.tap(find.byKey(tapTarget));
expect(find.text(snackBars[0]), findsNothing);
expect(find.text(snackBars[1]), findsNothing);
expect(find.text(snackBars[2]), findsNothing);
await tester.pump(); // schedule animation
expect(find.text(snackBars[0]), findsOneWidget);
scaffoldMessengerKey.currentState!.clearSnackBars();
expect(find.text(snackBars[0]), findsOneWidget);
await tester.pump(const Duration(seconds: 2));
expect(find.text(snackBars[0]), findsNothing);
expect(find.text(snackBars[1]), findsNothing);
expect(find.text(snackBars[2]), findsNothing);
});
Widget doBuildApp({
required SnackBarBehavior? behavior,
EdgeInsetsGeometry? margin,
double? width,
double? actionOverflowThreshold,
}) {
return MaterialApp(
home: Scaffold(
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.send),
onPressed: () {},
),
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
behavior: behavior,
margin: margin,
width: width,
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
actionOverflowThreshold: actionOverflowThreshold,
));
},
child: const Text('X'),
);
},
),
),
);
}
testWidgets('Setting SnackBarBehavior.fixed will still assert for margin', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84935
await tester.pumpWidget(doBuildApp(
behavior: SnackBarBehavior.fixed,
margin: const EdgeInsets.all(8.0),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final AssertionError exception = tester.takeException() as AssertionError;
expect(
exception.message,
'Margin can only be used with floating behavior. SnackBarBehavior.fixed '
'was set in the SnackBar constructor.',
);
});
testWidgets('Default SnackBarBehavior will still assert for margin', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84935
await tester.pumpWidget(doBuildApp(
behavior: null,
margin: const EdgeInsets.all(8.0),
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final AssertionError exception = tester.takeException() as AssertionError;
expect(
exception.message,
'Margin can only be used with floating behavior. SnackBarBehavior.fixed '
'was set by default.',
);
});
testWidgets('Setting SnackBarBehavior.fixed will still assert for width', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84935
await tester.pumpWidget(doBuildApp(
behavior: SnackBarBehavior.fixed,
width: 5.0,
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final AssertionError exception = tester.takeException() as AssertionError;
expect(
exception.message,
'Width can only be used with floating behavior. SnackBarBehavior.fixed '
'was set in the SnackBar constructor.',
);
});
testWidgets('Default SnackBarBehavior will still assert for width', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/84935
await tester.pumpWidget(doBuildApp(
behavior: null,
width: 5.0,
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final AssertionError exception = tester.takeException() as AssertionError;
expect(
exception.message,
'Width can only be used with floating behavior. SnackBarBehavior.fixed '
'was set by default.',
);
});
for (final double overflowThreshold in <double>[-1.0, -.0001, 1.000001, 5]) {
testWidgets('SnackBar will assert for actionOverflowThreshold outside of 0-1 range', (WidgetTester tester) async {
await tester.pumpWidget(doBuildApp(
actionOverflowThreshold: overflowThreshold,
behavior: SnackBarBehavior.fixed,
));
await tester.tap(find.text('X'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
final AssertionError exception = tester.takeException() as AssertionError;
expect(exception.message, 'Action overflow threshold must be between 0 and 1 inclusive');
});
}
testWidgets('Material2 - Snackbar by default clips BackdropFilter', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/98205
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: Scaffold(
body: const Scaffold(),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state<ScaffoldMessengerState>(
find.byType(ScaffoldMessenger),
);
scaffoldMessengerState.showSnackBar(SnackBar(
backgroundColor: Colors.transparent,
content: BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 20.0,
sigmaY: 20.0,
),
child: const Text('I am a snack bar.'),
),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.fixed,
));
await tester.pumpAndSettle();
await tester.tap(find.text('I am a snack bar.'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.backdropFilter.png'));
});
testWidgets('Material3 - Snackbar by default clips BackdropFilter', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/98205
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: Scaffold(
body: const Scaffold(),
floatingActionButton: FloatingActionButton(onPressed: () {}),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state<ScaffoldMessengerState>(
find.byType(ScaffoldMessenger),
);
scaffoldMessengerState.showSnackBar(SnackBar(
backgroundColor: Colors.transparent,
content: BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 20.0,
sigmaY: 20.0,
),
child: const Text('I am a snack bar.'),
),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.fixed,
));
await tester.pumpAndSettle();
await tester.tap(find.text('I am a snack bar.'));
await tester.pump(); // start animation
await tester.pump(const Duration(milliseconds: 750));
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.backdropFilter.png'));
});
testWidgets('Floating snackbar can display optional icon', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
SnackBar(
content: const Text('Feeling snackish'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
showCloseIcon: true,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(
find.byType(MaterialApp),
matchesGoldenFile(
'snack_bar.goldenTest.floatingWithActionWithIcon.png'));
});
testWidgets('SnackBar has tooltip for Close Button', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/143793
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
SnackBar(
content: const Text('Snackbar with close button'),
duration: const Duration(days: 365),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
behavior: SnackBarBehavior.floating,
showCloseIcon: true,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate in.
expect(
find.byTooltip(MaterialLocalizations.of(scaffoldMessengerState.context).closeButtonLabel),
findsOneWidget
);
});
testWidgets('Material2 - Fixed width snackbar can display optional icon', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('Go get a snack'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
showCloseIcon: true,
behavior: SnackBarBehavior.fixed,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.fixedWithActionWithIcon.png'));
});
testWidgets('Material3 - Fixed width snackbar can display optional icon', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(SnackBar(
content: const Text('Go get a snack'),
duration: const Duration(seconds: 2),
action: SnackBarAction(label: 'ACTION', onPressed: () {}),
showCloseIcon: true,
behavior: SnackBarBehavior.fixed,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.fixedWithActionWithIcon.png'));
});
testWidgets('Material2 - Fixed snackbar can display optional icon without action', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('I wonder if there are snacks nearby?'),
duration: Duration(seconds: 2),
behavior: SnackBarBehavior.fixed,
showCloseIcon: true,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.fixedWithIcon.png'));
});
testWidgets('Material3 - Fixed snackbar can display optional icon without action', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('I wonder if there are snacks nearby?'),
duration: Duration(seconds: 2),
behavior: SnackBarBehavior.fixed,
showCloseIcon: true,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.fixedWithIcon.png'));
});
testWidgets('Material2 - Floating width snackbar can display optional icon without action', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text('Must go get a snack!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.floatingWithIcon.png'));
});
testWidgets('Material3 - Floating width snackbar can display optional icon without action', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text('Must go get a snack!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.floatingWithIcon.png'));
});
testWidgets('Material2 - Floating multi-line snackbar with icon is aligned correctly', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text(
'This is a really long snackbar message. So long, it spans across more than one line!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m2_snack_bar.goldenTest.multiLineWithIcon.png'));
});
testWidgets('Material3 - Floating multi-line snackbar with icon is aligned correctly', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text(
'This is a really long snackbar message. So long, it spans across more than one line!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
await expectLater(find.byType(MaterialApp), matchesGoldenFile('m3_snack_bar.goldenTest.multiLineWithIcon.png'));
});
testWidgets('Material2 - Floating multi-line snackbar with icon and actionOverflowThreshold=1 is aligned correctly', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text('This is a really long snackbar message. So long, it spans across more than one line!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
actionOverflowThreshold: 1,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate in.
await expectLater(
find.byType(MaterialApp),
matchesGoldenFile('m2_snack_bar.goldenTest.multiLineWithIconWithZeroActionOverflowThreshold.png'),
);
});
testWidgets('Material3 - Floating multi-line snackbar with icon and actionOverflowThreshold=1 is aligned correctly', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
home: const Scaffold(
bottomSheet: SizedBox(
width: 200,
height: 50,
child: ColoredBox(
color: Colors.pink,
),
),
),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text('This is a really long snackbar message. So long, it spans across more than one line!'),
duration: Duration(seconds: 2),
showCloseIcon: true,
behavior: SnackBarBehavior.floating,
actionOverflowThreshold: 1,
));
await tester.pumpAndSettle(); // Have the SnackBar fully animate in.
await expectLater(
find.byType(MaterialApp),
matchesGoldenFile('m3_snack_bar.goldenTest.multiLineWithIconWithZeroActionOverflowThreshold.png'),
);
});
testWidgets(
'ScaffoldMessenger will alert for snackbars that cannot be presented', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/103004
await tester.pumpWidget(const MaterialApp(
home: Center(),
));
final ScaffoldMessengerState scaffoldMessengerState = tester.state<ScaffoldMessengerState>(
find.byType(ScaffoldMessenger),
);
expect(
() {
scaffoldMessengerState.showSnackBar(const SnackBar(
content: Text('SnackBar'),
));
},
throwsA(
isA<AssertionError>().having(
(AssertionError error) => error.toString(),
'description',
contains(
'ScaffoldMessenger.showSnackBar was called, but there are currently '
'no descendant Scaffolds to present to.'
)
),
),
);
});
testWidgets('SnackBarAction backgroundColor works as a Color', (WidgetTester tester) async {
const Color backgroundColor = Colors.blue;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
backgroundColor: backgroundColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('Tap'),
);
},
),
),
),
);
await tester.tap(find.text('Tap'));
await tester.pumpAndSettle();
final Material materialBeforeDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialBeforeDismissed.color, backgroundColor);
await tester.tap(find.text('ACTION'));
await tester.pump();
final Material materialAfterDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialAfterDismissed.color, Colors.transparent);
});
testWidgets('SnackBarAction backgroundColor works as a MaterialStateColor', (WidgetTester tester) async {
final MaterialStateColor backgroundColor = MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return Colors.blue;
}
return Colors.purple;
});
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
backgroundColor: backgroundColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('Tap'),
);
},
),
),
),
);
await tester.tap(find.text('Tap'));
await tester.pumpAndSettle();
final Material materialBeforeDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialBeforeDismissed.color, Colors.purple);
await tester.tap(find.text('ACTION'));
await tester.pump();
final Material materialAfterDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialAfterDismissed.color, Colors.blue);
});
testWidgets('SnackBarAction disabledBackgroundColor works as expected', (WidgetTester tester) async {
const Color backgroundColor = Colors.blue;
const Color disabledBackgroundColor = Colors.red;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
backgroundColor: backgroundColor,
disabledBackgroundColor: disabledBackgroundColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('Tap'),
);
},
),
),
),
);
await tester.tap(find.text('Tap'));
await tester.pumpAndSettle();
final Material materialBeforeDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialBeforeDismissed.color, backgroundColor);
await tester.tap(find.text('ACTION'));
await tester.pump();
final Material materialAfterDismissed = tester.widget<Material>(find.descendant(
of: find.widgetWithText(TextButton, 'ACTION'),
matching: find.byType(Material),
));
expect(materialAfterDismissed.color, disabledBackgroundColor);
});
testWidgets('SnackBarAction asserts when backgroundColor is a MaterialStateColor and disabledBackgroundColor is also provided', (WidgetTester tester) async {
final Color backgroundColor = MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return Colors.blue;
}
return Colors.purple;
});
const Color disabledBackgroundColor = Colors.red;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: const Text('I am a snack bar.'),
duration: const Duration(seconds: 2),
action: SnackBarAction(
backgroundColor: backgroundColor,
disabledBackgroundColor: disabledBackgroundColor,
label: 'ACTION',
onPressed: () {},
),
),
);
},
child: const Text('Tap'),
);
},
),
),
),
);
await tester.tap(find.text('Tap'));
await tester.pumpAndSettle();
expect(tester.takeException(), isAssertionError.having(
(AssertionError e) => e.toString(),
'description',
contains('disabledBackgroundColor must not be provided when background color is a MaterialStateColor'))
);
});
testWidgets('SnackBar material applies SnackBar.clipBehavior', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Container(),
),
),
);
final ScaffoldMessengerState scaffoldMessengerState = tester.state(find.byType(ScaffoldMessenger));
scaffoldMessengerState.showSnackBar(
const SnackBar(content: Text('I am a snack bar.')),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
Material material = tester.widget<Material>(
find.descendant(of: find.byType(SnackBar),
matching: find.byType(Material))
);
expect(material.clipBehavior, Clip.hardEdge);
scaffoldMessengerState.hideCurrentSnackBar(); // Hide the SnackBar.
await tester.pumpAndSettle(); // Have the SnackBar fully animate out.
scaffoldMessengerState.showSnackBar(
const SnackBar(
content: Text('I am a snack bar.'),
clipBehavior: Clip.antiAlias,
),
);
await tester.pumpAndSettle(); // Have the SnackBar fully animate in.
material = tester.widget<Material>(
find.descendant(of: find.byType(SnackBar),
matching: find.byType(Material))
);
expect(material.clipBehavior, Clip.antiAlias);
});
testWidgets('Tap on button behind snack bar defined by width', (WidgetTester tester) async {
tester.view.physicalSize = const Size.square(200);
tester.view.devicePixelRatio = 1;
addTearDown(tester.view.resetPhysicalSize);
addTearDown(tester.view.resetDevicePixelRatio);
const String buttonText = 'Show snackbar';
const String snackbarContent = 'Snackbar';
const String buttonText2 = 'Try press me';
final Completer<void> completer = Completer<void>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
behavior: SnackBarBehavior.floating,
width: 100,
content: Text(snackbarContent),
),
);
},
child: const Text(buttonText),
),
ElevatedButton(
onPressed: () {
completer.complete();
},
child: const Text(buttonText2),
),
],
);
},
),
),
));
await tester.tap(find.text(buttonText));
await tester.pumpAndSettle();
expect(find.text(snackbarContent), findsOneWidget);
await tester.tapAt(tester.getTopLeft(find.text(buttonText2)));
expect(find.text(snackbarContent), findsOneWidget);
expect(completer.isCompleted, true);
});
testWidgets('Tap on button behind snack bar defined by margin', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/78537.
tester.view.physicalSize = const Size.square(200);
tester.view.devicePixelRatio = 1;
addTearDown(tester.view.resetPhysicalSize);
addTearDown(tester.view.resetDevicePixelRatio);
const String buttonText = 'Show snackbar';
const String snackbarContent = 'Snackbar';
const String buttonText2 = 'Try press me';
final Completer<void> completer = Completer<void>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
behavior: SnackBarBehavior.floating,
margin: EdgeInsets.only(left: 100),
content: Text(snackbarContent),
),
);
},
child: const Text(buttonText),
),
ElevatedButton(
onPressed: () {
completer.complete();
},
child: const Text(buttonText2),
),
],
);
},
),
),
));
await tester.tap(find.text(buttonText));
await tester.pumpAndSettle();
expect(find.text(snackbarContent), findsOneWidget);
await tester.tapAt(tester.getTopLeft(find.text(buttonText2)));
expect(find.text(snackbarContent), findsOneWidget);
expect(completer.isCompleted, true);
});
testWidgets("Can't tap on button behind snack bar defined by margin and HitTestBehavior.opaque", (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/78537.
tester.view.physicalSize = const Size.square(200);
tester.view.devicePixelRatio = 1;
addTearDown(tester.view.resetPhysicalSize);
addTearDown(tester.view.resetDevicePixelRatio);
const String buttonText = 'Show snackbar';
const String snackbarContent = 'Snackbar';
const String buttonText2 = 'Try press me';
final Completer<void> completer = Completer<void>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
hitTestBehavior: HitTestBehavior.opaque,
behavior: SnackBarBehavior.floating,
margin: EdgeInsets.only(left: 100),
content: Text(snackbarContent),
),
);
},
child: const Text(buttonText),
),
ElevatedButton(
onPressed: () {
completer.complete();
},
child: const Text(buttonText2),
),
],
);
},
),
),
));
await tester.tap(find.text(buttonText));
await tester.pumpAndSettle();
expect(find.text(snackbarContent), findsOneWidget);
await tester.tapAt(tester.getTopLeft(find.text(buttonText2)));
expect(find.text(snackbarContent), findsOneWidget);
expect(completer.isCompleted, false);
});
}
/// Start test for "SnackBar dismiss test".
Future<void> _testSnackBarDismiss({
required WidgetTester tester,
required Key tapTarget,
required double scaffoldWidth,
required ValueChanged<DismissDirection> onDismissDirectionChange,
required VoidCallback onDragGestureChange,
}) async {
final Map<DismissDirection, List<Offset>> dragGestures = _getDragGesturesOfDismissDirections(scaffoldWidth);
for (final DismissDirection key in dragGestures.keys) {
onDismissDirectionChange(key);
for (final Offset dragGesture in dragGestures[key]!) {
onDragGestureChange();
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // queue bar1
await tester.tap(find.byKey(tapTarget)); // queue bar2
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // schedule animation for bar1
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(); // begin animation
expect(find.text('bar1'), findsOneWidget);
expect(find.text('bar2'), findsNothing);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; two second timer starts here
await tester.drag(find.text('bar1'), dragGesture);
await tester.pump(); // bar1 dismissed, bar2 begins animating
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 750)); // 0.75s // animation last frame; two second timer starts here
await tester.drag(find.text('bar2'), dragGesture);
await tester.pump(); // bar2 dismissed
expect(find.text('bar1'), findsNothing);
expect(find.text('bar2'), findsNothing);
}
}
}
/// Create drag gestures for DismissDirections.
Map<DismissDirection, List<Offset>> _getDragGesturesOfDismissDirections(double scaffoldWidth) {
final Map<DismissDirection, List<Offset>> dragGestures = <DismissDirection, List<Offset>>{};
for (final DismissDirection val in DismissDirection.values) {
switch (val) {
case DismissDirection.down:
dragGestures[val] = <Offset>[const Offset(0.0, 50.0)]; // drag to bottom gesture
case DismissDirection.up:
dragGestures[val] = <Offset>[const Offset(0.0, -50.0)]; // drag to top gesture
case DismissDirection.vertical:
dragGestures[val] = <Offset>[
const Offset(0.0, 50.0), // drag to bottom gesture
const Offset(0.0, -50.0), // drag to top gesture
];
case DismissDirection.startToEnd:
dragGestures[val] = <Offset>[Offset(scaffoldWidth, 0.0)]; // drag to right gesture
case DismissDirection.endToStart:
dragGestures[val] = <Offset>[Offset(-scaffoldWidth, 0.0)]; // drag to left gesture
case DismissDirection.horizontal:
dragGestures[val] = <Offset>[
Offset(scaffoldWidth, 0.0), // drag to right gesture
Offset(-scaffoldWidth, 0.0), // drag to left gesture
];
case DismissDirection.none:
break;
}
}
return dragGestures;
}
class _TestMaterialStateColor extends MaterialStateColor {
const _TestMaterialStateColor() : super(_colorRed);
static const int _colorRed = 0xFFF44336;
static const int _colorBlue = 0xFF2196F3;
@override
Color resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return const Color(_colorBlue);
}
return const Color(_colorRed);
}
}
class _CustomFloatingActionButtonLocation extends StandardFabLocation
with FabEndOffsetX, FabFloatOffsetY {
const _CustomFloatingActionButtonLocation();
}
| flutter/packages/flutter/test/material/snack_bar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/snack_bar_test.dart",
"repo_id": "flutter",
"token_count": 69620
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('TextField works correctly when changing helperText', (WidgetTester tester) async {
await tester.pumpWidget(const MaterialApp(home: Material(child: TextField(decoration: InputDecoration(helperText: 'Awesome')))));
expect(find.text('Awesome'), findsNWidgets(1));
await tester.pump(const Duration(milliseconds: 100));
expect(find.text('Awesome'), findsNWidgets(1));
await tester.pumpWidget(const MaterialApp(home: Material(child: TextField(decoration: InputDecoration(errorText: 'Awesome')))));
expect(find.text('Awesome'), findsNWidgets(2));
});
}
| flutter/packages/flutter/test/material/text_field_helper_text_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/text_field_helper_text_test.dart",
"repo_id": "flutter",
"token_count": 267
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('TimeOfDay.format', () {
testWidgets('respects alwaysUse24HourFormat option', (WidgetTester tester) async {
Future<String> pumpTest(bool alwaysUse24HourFormat) async {
late String formattedValue;
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: MediaQueryData(alwaysUse24HourFormat: alwaysUse24HourFormat),
child: Builder(builder: (BuildContext context) {
formattedValue = const TimeOfDay(hour: 7, minute: 0).format(context);
return Container();
}),
),
));
return formattedValue;
}
expect(await pumpTest(false), '7:00 AM');
expect(await pumpTest(true), '07:00');
});
});
testWidgets('hourOfPeriod returns correct value', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/59158.
expect(const TimeOfDay(minute: 0, hour: 0).hourOfPeriod, 12);
expect(const TimeOfDay(minute: 0, hour: 1).hourOfPeriod, 1);
expect(const TimeOfDay(minute: 0, hour: 2).hourOfPeriod, 2);
expect(const TimeOfDay(minute: 0, hour: 3).hourOfPeriod, 3);
expect(const TimeOfDay(minute: 0, hour: 4).hourOfPeriod, 4);
expect(const TimeOfDay(minute: 0, hour: 5).hourOfPeriod, 5);
expect(const TimeOfDay(minute: 0, hour: 6).hourOfPeriod, 6);
expect(const TimeOfDay(minute: 0, hour: 7).hourOfPeriod, 7);
expect(const TimeOfDay(minute: 0, hour: 8).hourOfPeriod, 8);
expect(const TimeOfDay(minute: 0, hour: 9).hourOfPeriod, 9);
expect(const TimeOfDay(minute: 0, hour: 10).hourOfPeriod, 10);
expect(const TimeOfDay(minute: 0, hour: 11).hourOfPeriod, 11);
expect(const TimeOfDay(minute: 0, hour: 12).hourOfPeriod, 12);
expect(const TimeOfDay(minute: 0, hour: 13).hourOfPeriod, 1);
expect(const TimeOfDay(minute: 0, hour: 14).hourOfPeriod, 2);
expect(const TimeOfDay(minute: 0, hour: 15).hourOfPeriod, 3);
expect(const TimeOfDay(minute: 0, hour: 16).hourOfPeriod, 4);
expect(const TimeOfDay(minute: 0, hour: 17).hourOfPeriod, 5);
expect(const TimeOfDay(minute: 0, hour: 18).hourOfPeriod, 6);
expect(const TimeOfDay(minute: 0, hour: 19).hourOfPeriod, 7);
expect(const TimeOfDay(minute: 0, hour: 20).hourOfPeriod, 8);
expect(const TimeOfDay(minute: 0, hour: 21).hourOfPeriod, 9);
expect(const TimeOfDay(minute: 0, hour: 22).hourOfPeriod, 10);
expect(const TimeOfDay(minute: 0, hour: 23).hourOfPeriod, 11);
});
group('RestorableTimeOfDay tests', () {
testWidgets('value is not accessible when not registered', (WidgetTester tester) async {
final RestorableTimeOfDay property = RestorableTimeOfDay(const TimeOfDay(hour: 20, minute: 4));
addTearDown(property.dispose);
expect(() => property.value, throwsAssertionError);
});
testWidgets('work when not in restoration scope', (WidgetTester tester) async {
await tester.pumpWidget(const _RestorableWidget());
final _RestorableWidgetState state = tester.state(find.byType(_RestorableWidget));
// Initialized to default values.
expect(state.timeOfDay.value, const TimeOfDay(hour: 10, minute: 5));
// Modify values.
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 2, minute: 2);
});
await tester.pump();
expect(state.timeOfDay.value, const TimeOfDay(hour: 2, minute: 2));
});
testWidgets('restart and restore', (WidgetTester tester) async {
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root-child',
child: _RestorableWidget(),
));
_RestorableWidgetState state = tester.state(find.byType(_RestorableWidget));
// Initialized to default values.
expect(state.timeOfDay.value, const TimeOfDay(hour: 10, minute: 5));
// Modify values.
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 2, minute: 2);
});
await tester.pump();
expect(state.timeOfDay.value, const TimeOfDay(hour: 2, minute: 2));
// Restores to previous values.
await tester.restartAndRestore();
final _RestorableWidgetState oldState = state;
state = tester.state(find.byType(_RestorableWidget));
expect(state, isNot(same(oldState)));
expect(state.timeOfDay.value, const TimeOfDay(hour: 2, minute: 2));
});
testWidgets('restore to older state', (WidgetTester tester) async {
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root-child',
child: _RestorableWidget(),
));
final _RestorableWidgetState state = tester.state(find.byType(_RestorableWidget));
// Modify values.
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 2, minute: 2);
});
await tester.pump();
final TestRestorationData restorationData = await tester.getRestorationData();
// Modify values.
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 4, minute: 4);
});
await tester.pump();
// Restore to previous.
await tester.restoreFrom(restorationData);
expect(state.timeOfDay.value, const TimeOfDay(hour: 2, minute: 2));
// Restore to empty data will re-initialize to default values.
await tester.restoreFrom(TestRestorationData.empty);
expect(state.timeOfDay.value, const TimeOfDay(hour: 10, minute: 5));
});
testWidgets('call notifiers when value changes', (WidgetTester tester) async {
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root-child',
child: _RestorableWidget(),
));
final _RestorableWidgetState state = tester.state(find.byType(_RestorableWidget));
final List<String> notifyLog = <String>[];
state.timeOfDay.addListener(() {
notifyLog.add('hello world');
});
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 2, minute: 2);
});
expect(notifyLog.single, 'hello world');
notifyLog.clear();
await tester.pump();
// Does not notify when set to same value.
state.setProperties(() {
state.timeOfDay.value = const TimeOfDay(hour: 2, minute: 2);
});
expect(notifyLog, isEmpty);
});
});
}
class _RestorableWidget extends StatefulWidget {
const _RestorableWidget();
@override
State<_RestorableWidget> createState() => _RestorableWidgetState();
}
class _RestorableWidgetState extends State<_RestorableWidget> with RestorationMixin {
final RestorableTimeOfDay timeOfDay = RestorableTimeOfDay(const TimeOfDay(hour: 10, minute: 5));
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(timeOfDay, 'time_of_day');
}
void setProperties(VoidCallback callback) {
setState(callback);
}
@override
Widget build(BuildContext context) {
return const SizedBox();
}
@override
String get restorationId => 'widget';
@override
void dispose() {
timeOfDay.dispose();
super.dispose();
}
}
| flutter/packages/flutter/test/material/time_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/time_test.dart",
"repo_id": "flutter",
"token_count": 2827
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('BorderRadius control test', () {
const Rect rect = Rect.fromLTRB(19.0, 23.0, 29.0, 31.0);
BorderRadius borderRadius;
borderRadius = const BorderRadius.all(Radius.elliptical(5.0, 7.0));
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.topRight, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.bottomLeft, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.bottomRight, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.toRRect(rect), RRect.fromRectXY(rect, 5.0, 7.0));
borderRadius = BorderRadius.circular(3.0);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.topRight, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.bottomLeft, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.bottomRight, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.toRRect(rect), RRect.fromRectXY(rect, 3.0, 3.0));
const Radius radius1 = Radius.elliptical(89.0, 87.0);
const Radius radius2 = Radius.elliptical(103.0, 107.0);
borderRadius = const BorderRadius.vertical(top: radius1, bottom: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, radius1);
expect(borderRadius.topRight, radius1);
expect(borderRadius.bottomLeft, radius2);
expect(borderRadius.bottomRight, radius2);
expect(borderRadius.toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
topRight: radius1,
bottomLeft: radius2,
bottomRight: radius2,
));
borderRadius = const BorderRadius.horizontal(left: radius1, right: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, radius1);
expect(borderRadius.topRight, radius2);
expect(borderRadius.bottomLeft, radius1);
expect(borderRadius.bottomRight, radius2);
expect(borderRadius.toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
topRight: radius2,
bottomLeft: radius1,
bottomRight: radius2,
));
borderRadius = BorderRadius.zero;
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, Radius.zero);
expect(borderRadius.topRight, Radius.zero);
expect(borderRadius.bottomLeft, Radius.zero);
expect(borderRadius.bottomRight, Radius.zero);
expect(borderRadius.toRRect(rect), RRect.fromRectAndCorners(rect));
borderRadius = const BorderRadius.only(topRight: radius1, bottomRight: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topLeft, Radius.zero);
expect(borderRadius.topRight, radius1);
expect(borderRadius.bottomLeft, Radius.zero);
expect(borderRadius.bottomRight, radius2);
expect(borderRadius.toRRect(rect), RRect.fromRectAndCorners(
rect,
topRight: radius1,
bottomRight: radius2,
));
expect(
const BorderRadius.only(topLeft: Radius.elliptical(1.0, 2.0)).subtract(const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0))),
const BorderRadius.only(topLeft: Radius.elliptical(-2.0, -3.0)),
);
expect(
const BorderRadius.only(topRight: Radius.elliptical(1.0, 2.0)).add(const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0))),
const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0), topRight: Radius.elliptical(1.0, 2.0)),
);
expect(
const BorderRadius.only(topLeft: Radius.elliptical(1.0, 2.0)) - const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0)),
const BorderRadius.only(topLeft: Radius.elliptical(-2.0, -3.0)),
);
expect(
const BorderRadius.only(topRight: Radius.elliptical(1.0, 2.0)) + const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0)),
const BorderRadius.only(topLeft: Radius.elliptical(3.0, 5.0), topRight: Radius.elliptical(1.0, 2.0)),
);
expect(
-const BorderRadius.only(topLeft: Radius.elliptical(1.0, 2.0)),
const BorderRadius.only(topLeft: Radius.elliptical(-1.0, -2.0)),
);
expect(
const BorderRadius.only(
topLeft: radius1,
topRight: radius2,
bottomLeft: radius2,
bottomRight: radius1,
) * 0.0,
BorderRadius.zero,
);
expect(
BorderRadius.circular(15.0) / 10.0,
BorderRadius.circular(1.5),
);
expect(
BorderRadius.circular(15.0) ~/ 10.0,
BorderRadius.circular(1.0),
);
expect(
BorderRadius.circular(15.0) % 10.0,
BorderRadius.circular(5.0),
);
});
test('BorderRadius.lerp() invariants', () {
final BorderRadius a = BorderRadius.circular(10.0);
final BorderRadius b = BorderRadius.circular(20.0);
expect(BorderRadius.lerp(a, b, 0.25), equals(a * 1.25));
expect(BorderRadius.lerp(a, b, 0.25), equals(b * 0.625));
expect(BorderRadius.lerp(a, b, 0.25), equals(a + BorderRadius.circular(2.5)));
expect(BorderRadius.lerp(a, b, 0.25), equals(b - BorderRadius.circular(7.5)));
expect(BorderRadius.lerp(null, null, 0.25), isNull);
expect(BorderRadius.lerp(null, b, 0.25), equals(b * 0.25));
expect(BorderRadius.lerp(a, null, 0.25), equals(a * 0.75));
});
test('BorderRadius.lerp identical a,b', () {
expect(BorderRadius.lerp(null, null, 0), null);
const BorderRadius border = BorderRadius.zero;
expect(identical(BorderRadius.lerp(border, border, 0.5), border), true);
});
test('BorderRadius.lerp() crazy', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadius b = BorderRadius.only(
topRight: Radius.elliptical(100.0, 110.0),
bottomLeft: Radius.elliptical(120.0, 130.0),
bottomRight: Radius.elliptical(140.0, 150.0),
);
const BorderRadius c = BorderRadius.only(
topLeft: Radius.elliptical(5.0, 10.0), // 10,20 -> 0
topRight: Radius.elliptical(65.0, 75.0), // 30,40 -> 100,110
bottomLeft: Radius.elliptical(85.0, 95.0), // 50,60 -> 120,130
bottomRight: Radius.elliptical(70.0, 75.0), // 0,0 -> 140,150
);
expect(BorderRadius.lerp(a, b, 0.5), c);
});
test('BorderRadiusDirectional control test', () {
const Rect rect = Rect.fromLTRB(19.0, 23.0, 29.0, 31.0);
BorderRadiusDirectional borderRadius;
borderRadius = const BorderRadiusDirectional.all(Radius.elliptical(5.0, 7.0));
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.topEnd, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.bottomStart, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.bottomEnd, const Radius.elliptical(5.0, 7.0));
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectXY(rect, 5.0, 7.0));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectXY(rect, 5.0, 7.0));
borderRadius = BorderRadiusDirectional.circular(3.0);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.topEnd, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.bottomStart, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.bottomEnd, const Radius.elliptical(3.0, 3.0));
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectXY(rect, 3.0, 3.0));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectXY(rect, 3.0, 3.0));
const Radius radius1 = Radius.elliptical(89.0, 87.0);
const Radius radius2 = Radius.elliptical(103.0, 107.0);
borderRadius = const BorderRadiusDirectional.vertical(top: radius1, bottom: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, radius1);
expect(borderRadius.topEnd, radius1);
expect(borderRadius.bottomStart, radius2);
expect(borderRadius.bottomEnd, radius2);
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
topRight: radius1,
bottomLeft: radius2,
bottomRight: radius2,
));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
topRight: radius1,
bottomLeft: radius2,
bottomRight: radius2,
));
borderRadius = const BorderRadiusDirectional.horizontal(start: radius1, end: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, radius1);
expect(borderRadius.topEnd, radius2);
expect(borderRadius.bottomStart, radius1);
expect(borderRadius.bottomEnd, radius2);
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
topRight: radius2,
bottomLeft: radius1,
bottomRight: radius2,
));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius2,
topRight: radius1,
bottomLeft: radius2,
bottomRight: radius1,
));
borderRadius = BorderRadiusDirectional.zero;
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, Radius.zero);
expect(borderRadius.topEnd, Radius.zero);
expect(borderRadius.bottomStart, Radius.zero);
expect(borderRadius.bottomEnd, Radius.zero);
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectAndCorners(rect));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectAndCorners(rect));
borderRadius = const BorderRadiusDirectional.only(topEnd: radius1, bottomEnd: radius2);
expect(borderRadius, hasOneLineDescription);
expect(borderRadius.topStart, Radius.zero);
expect(borderRadius.topEnd, radius1);
expect(borderRadius.bottomStart, Radius.zero);
expect(borderRadius.bottomEnd, radius2);
expect(borderRadius.resolve(TextDirection.ltr).toRRect(rect), RRect.fromRectAndCorners(
rect,
topRight: radius1,
bottomRight: radius2,
));
expect(borderRadius.resolve(TextDirection.rtl).toRRect(rect), RRect.fromRectAndCorners(
rect,
topLeft: radius1,
bottomLeft: radius2,
));
expect(
const BorderRadiusDirectional.only(topStart: Radius.elliptical(1.0, 2.0)).subtract(const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0))),
const BorderRadiusDirectional.only(topStart: Radius.elliptical(-2.0, -3.0)),
);
expect(
const BorderRadiusDirectional.only(topEnd: Radius.elliptical(1.0, 2.0)).add(const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0))),
const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0), topEnd: Radius.elliptical(1.0, 2.0)),
);
expect(
const BorderRadiusDirectional.only(topStart: Radius.elliptical(1.0, 2.0)) - const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0)),
const BorderRadiusDirectional.only(topStart: Radius.elliptical(-2.0, -3.0)),
);
expect(
const BorderRadiusDirectional.only(topEnd: Radius.elliptical(1.0, 2.0)) + const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0)),
const BorderRadiusDirectional.only(topStart: Radius.elliptical(3.0, 5.0), topEnd: Radius.elliptical(1.0, 2.0)),
);
expect(
-const BorderRadiusDirectional.only(topStart: Radius.elliptical(1.0, 2.0)),
const BorderRadiusDirectional.only(topStart: Radius.elliptical(-1.0, -2.0)),
);
expect(
const BorderRadiusDirectional.only(
topStart: radius1,
topEnd: radius2,
bottomStart: radius2,
bottomEnd: radius1,
) * 0.0,
BorderRadiusDirectional.zero,
);
expect(
BorderRadiusDirectional.circular(15.0) / 10.0,
BorderRadiusDirectional.circular(1.5),
);
expect(
BorderRadiusDirectional.circular(15.0) ~/ 10.0,
BorderRadiusDirectional.circular(1.0),
);
expect(
BorderRadiusDirectional.circular(15.0) % 10.0,
BorderRadiusDirectional.circular(5.0),
);
});
test('BorderRadiusDirectional.lerp() invariants', () {
final BorderRadiusDirectional a = BorderRadiusDirectional.circular(10.0);
final BorderRadiusDirectional b = BorderRadiusDirectional.circular(20.0);
expect(BorderRadiusDirectional.lerp(a, b, 0.25), equals(a * 1.25));
expect(BorderRadiusDirectional.lerp(a, b, 0.25), equals(b * 0.625));
expect(BorderRadiusDirectional.lerp(a, b, 0.25), equals(a + BorderRadiusDirectional.circular(2.5)));
expect(BorderRadiusDirectional.lerp(a, b, 0.25), equals(b - BorderRadiusDirectional.circular(7.5)));
expect(BorderRadiusDirectional.lerp(null, null, 0.25), isNull);
expect(BorderRadiusDirectional.lerp(null, b, 0.25), equals(b * 0.25));
expect(BorderRadiusDirectional.lerp(a, null, 0.25), equals(a * 0.75));
});
test('BorderRadiusDirectional.lerp identical a,b', () {
expect(BorderRadiusDirectional.lerp(null, null, 0), null);
const BorderRadiusDirectional border = BorderRadiusDirectional.zero;
expect(identical(BorderRadiusDirectional.lerp(border, border, 0.5), border), true);
});
test('BorderRadiusDirectional.lerp() crazy', () {
const BorderRadiusDirectional a = BorderRadiusDirectional.only(
topStart: Radius.elliptical(10.0, 20.0),
topEnd: Radius.elliptical(30.0, 40.0),
bottomStart: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
const BorderRadiusDirectional c = BorderRadiusDirectional.only(
topStart: Radius.elliptical(5.0, 10.0), // 10,20 -> 0
topEnd: Radius.elliptical(65.0, 75.0), // 30,40 -> 100,110
bottomStart: Radius.elliptical(85.0, 95.0), // 50,60 -> 120,130
bottomEnd: Radius.elliptical(70.0, 75.0), // 0,0 -> 140,150
);
expect(BorderRadiusDirectional.lerp(a, b, 0.5), c);
});
test('BorderRadiusGeometry.lerp()', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
const BorderRadius ltr = BorderRadius.only(
topLeft: Radius.elliptical(5.0, 10.0), // 10,20 -> 0
topRight: Radius.elliptical(65.0, 75.0), // 30,40 -> 100,110
bottomLeft: Radius.elliptical(85.0, 95.0), // 50,60 -> 120,130
bottomRight: Radius.elliptical(70.0, 75.0), // 0,0 -> 140,150
);
const BorderRadius rtl = BorderRadius.only(
topLeft: Radius.elliptical(55.0, 65.0), // 10,20 -> 100,110
topRight: Radius.elliptical(15.0, 20.0), // 30,40 -> 0,0
bottomLeft: Radius.elliptical(95.0, 105.0), // 50,60 -> 140,150
bottomRight: Radius.elliptical(60.0, 65.0), // 0,0 -> 120,130
);
expect(BorderRadiusGeometry.lerp(a, b, 0.5)!.resolve(TextDirection.ltr), ltr);
expect(BorderRadiusGeometry.lerp(a, b, 0.5)!.resolve(TextDirection.rtl), rtl);
expect(BorderRadiusGeometry.lerp(a, b, 0.0)!.resolve(TextDirection.ltr), a);
expect(BorderRadiusGeometry.lerp(a, b, 1.0)!.resolve(TextDirection.rtl), b.resolve(TextDirection.rtl));
});
test('BorderRadiusGeometry.lerp identical a,b', () {
expect(BorderRadiusDirectional.lerp(null, null, 0), null);
const BorderRadiusGeometry border = BorderRadius.zero;
expect(identical(BorderRadiusGeometry.lerp(border, border, 0.5), border), true);
});
test('BorderRadiusGeometry subtract', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
expect(a.subtract(b).resolve(TextDirection.ltr), BorderRadius.only(
topLeft: const Radius.elliptical(10.0, 20.0) - Radius.zero,
topRight: const Radius.elliptical(30.0, 40.0) - const Radius.elliptical(100.0, 110.0),
bottomLeft: const Radius.elliptical(50.0, 60.0) - const Radius.elliptical(120.0, 130.0),
bottomRight: Radius.zero - const Radius.elliptical(140.0, 150.0),
));
expect(a.subtract(b).resolve(TextDirection.rtl), BorderRadius.only(
topLeft: const Radius.elliptical(10.0, 20.0) - const Radius.elliptical(100.0, 110.0),
topRight: const Radius.elliptical(30.0, 40.0) - Radius.zero,
bottomLeft: const Radius.elliptical(50.0, 60.0) - const Radius.elliptical(140.0, 150.0),
bottomRight: Radius.zero - const Radius.elliptical(120.0, 130.0),
));
});
test('BorderRadiusGeometry add', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
expect(a.add(b).resolve(TextDirection.ltr), BorderRadius.only(
topLeft: const Radius.elliptical(10.0, 20.0) + Radius.zero,
topRight: const Radius.elliptical(30.0, 40.0) + const Radius.elliptical(100.0, 110.0),
bottomLeft: const Radius.elliptical(50.0, 60.0) + const Radius.elliptical(120.0, 130.0),
bottomRight: Radius.zero + const Radius.elliptical(140.0, 150.0),
));
expect(a.add(b).resolve(TextDirection.rtl), BorderRadius.only(
topLeft: const Radius.elliptical(10.0, 20.0) + const Radius.elliptical(100.0, 110.0),
topRight: const Radius.elliptical(30.0, 40.0) + Radius.zero,
bottomLeft: const Radius.elliptical(50.0, 60.0) + const Radius.elliptical(140.0, 150.0),
bottomRight: Radius.zero + const Radius.elliptical(120.0, 130.0),
));
});
test('BorderRadiusGeometry add and multiply', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
expect((a.add(b) * 0.5).resolve(TextDirection.ltr), BorderRadius.only(
topLeft: (const Radius.elliptical(10.0, 20.0) + Radius.zero) / 2.0,
topRight: (const Radius.elliptical(30.0, 40.0) + const Radius.elliptical(100.0, 110.0)) / 2.0,
bottomLeft: (const Radius.elliptical(50.0, 60.0) + const Radius.elliptical(120.0, 130.0)) / 2.0,
bottomRight: (Radius.zero + const Radius.elliptical(140.0, 150.0)) / 2.0,
));
expect((a.add(b) * 0.5).resolve(TextDirection.rtl), BorderRadius.only(
topLeft: (const Radius.elliptical(10.0, 20.0) + const Radius.elliptical(100.0, 110.0)) / 2.0,
topRight: (const Radius.elliptical(30.0, 40.0) + Radius.zero) / 2.0,
bottomLeft: (const Radius.elliptical(50.0, 60.0) + const Radius.elliptical(140.0, 150.0)) / 2.0,
bottomRight: (Radius.zero + const Radius.elliptical(120.0, 130.0)) / 2.0,
));
});
test('BorderRadiusGeometry add and subtract', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(300.0, 500.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(30.0, 50.0),
);
const BorderRadius c = BorderRadius.only(
bottomLeft: Radius.elliptical(3.0, 5.0),
);
const BorderRadius ltr = BorderRadius.only(
topLeft: Radius.elliptical(300.0, 500.0), // tL + 0 - 0
topRight: Radius.elliptical(30.0, 50.0), // 0 + tE - 0
bottomLeft: Radius.elliptical(-3.0, -5.0), // 0 + 0 - 0
);
const BorderRadius rtl = BorderRadius.only(
topLeft: Radius.elliptical(330.0, 550.0), // 0 + 0 - 0
bottomLeft: Radius.elliptical(-3.0, -5.0), // 0 + 0 - 0
);
expect(a.add(b.subtract(c)).resolve(TextDirection.ltr), ltr);
expect(a.add(b.subtract(c)).resolve(TextDirection.rtl), rtl);
});
test('BorderRadiusGeometry add and subtract, more', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(300.0, 300.0),
topRight: Radius.elliptical(500.0, 500.0),
bottomLeft: Radius.elliptical(700.0, 700.0),
bottomRight: Radius.elliptical(900.0, 900.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topStart: Radius.elliptical(30.0, 30.0),
topEnd: Radius.elliptical(50.0, 50.0),
bottomStart: Radius.elliptical(70.0, 70.0),
bottomEnd: Radius.elliptical(90.0, 90.0),
);
const BorderRadius c = BorderRadius.only(
topLeft: Radius.elliptical(3.0, 3.0),
topRight: Radius.elliptical(5.0, 5.0),
bottomLeft: Radius.elliptical(7.0, 7.0),
bottomRight: Radius.elliptical(9.0, 9.0),
);
const BorderRadius ltr = BorderRadius.only(
topLeft: Radius.elliptical(327.0, 327.0), // tL + tS - tL
topRight: Radius.elliptical(545.0, 545.0), // tR + tE - tR
bottomLeft: Radius.elliptical(763.0, 763.0), // bL + bS - bL
bottomRight: Radius.elliptical(981.0, 981.0), // bR + bE - bR
);
const BorderRadius rtl = BorderRadius.only(
topLeft: Radius.elliptical(347.0, 347.0), // tL + tE - tL
topRight: Radius.elliptical(525.0, 525.0), // tR + TS - tR
bottomLeft: Radius.elliptical(783.0, 783.0), // bL + bE + bL
bottomRight: Radius.elliptical(961.0, 961.0), // bR + bS - bR
);
expect(a.add(b.subtract(c)).resolve(TextDirection.ltr), ltr);
expect(a.add(b.subtract(c)).resolve(TextDirection.rtl), rtl);
});
test('BorderRadiusGeometry operators', () {
const BorderRadius a = BorderRadius.only(
topLeft: Radius.elliptical(10.0, 20.0),
topRight: Radius.elliptical(30.0, 40.0),
bottomLeft: Radius.elliptical(50.0, 60.0),
);
const BorderRadiusDirectional b = BorderRadiusDirectional.only(
topEnd: Radius.elliptical(100.0, 110.0),
bottomStart: Radius.elliptical(120.0, 130.0),
bottomEnd: Radius.elliptical(140.0, 150.0),
);
const BorderRadius ltr = BorderRadius.only(
topLeft: Radius.elliptical(5.0, 10.0), // 10,20 -> 0
topRight: Radius.elliptical(65.0, 75.0), // 30,40 -> 100,110
bottomLeft: Radius.elliptical(85.0, 95.0), // 50,60 -> 120,130
bottomRight: Radius.elliptical(70.0, 75.0), // 0,0 -> 140,150
);
const BorderRadius rtl = BorderRadius.only(
topLeft: Radius.elliptical(55.0, 65.0), // 10,20 -> 100,110
topRight: Radius.elliptical(15.0, 20.0), // 30,40 -> 0,0
bottomLeft: Radius.elliptical(95.0, 105.0), // 50,60 -> 140,150
bottomRight: Radius.elliptical(60.0, 65.0), // 0,0 -> 120,130
);
expect(a.add(b.subtract(a) * 0.5).resolve(TextDirection.ltr), ltr);
expect(a.add(b.subtract(a) * 0.5).resolve(TextDirection.rtl), rtl);
expect(a.add(b.subtract(a) * 0.0).resolve(TextDirection.ltr), a);
expect(a.add(b.subtract(a) * 1.0).resolve(TextDirection.rtl), b.resolve(TextDirection.rtl));
});
test('BorderRadius copyWith, merge, ==, hashCode basics', () {
const BorderRadius firstRadius = BorderRadius.all(Radius.circular(5.0));
final BorderRadius secondRadius = firstRadius.copyWith();
expect(firstRadius, secondRadius);
expect(firstRadius.hashCode, secondRadius.hashCode);
});
test('BorderRadius copyWith parameters', () {
const Radius radius = Radius.circular(10);
const BorderRadius borderRadius = BorderRadius.all(radius);
expect(borderRadius.copyWith(topLeft: Radius.zero).topLeft, Radius.zero);
expect(borderRadius.copyWith(topLeft: Radius.zero).copyWith(topLeft: radius), borderRadius);
expect(borderRadius.copyWith(topRight: Radius.zero).topRight, Radius.zero);
expect(borderRadius.copyWith(topRight: Radius.zero).copyWith(topRight: radius), borderRadius);
expect(borderRadius.copyWith(bottomLeft: Radius.zero).bottomLeft, Radius.zero);
expect(borderRadius.copyWith(bottomLeft: Radius.zero).copyWith(bottomLeft: radius), borderRadius);
expect(borderRadius.copyWith(bottomRight: Radius.zero).bottomRight, Radius.zero);
expect(borderRadius.copyWith(bottomRight: Radius.zero).copyWith(bottomRight: radius), borderRadius);
});
}
| flutter/packages/flutter/test/painting/border_radius_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/border_radius_test.dart",
"repo_id": "flutter",
"token_count": 10566
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
// Here and below, see: https://github.com/dart-lang/sdk/issues/26980
const FlutterLogoDecoration start = FlutterLogoDecoration(
textColor: Color(0xFFD4F144),
style: FlutterLogoStyle.stacked,
margin: EdgeInsets.all(10.0),
);
const FlutterLogoDecoration end = FlutterLogoDecoration(
textColor: Color(0xFF81D4FA),
style: FlutterLogoStyle.stacked,
margin: EdgeInsets.all(10.0),
);
test('FlutterLogoDecoration lerp from null to null is null', () {
final FlutterLogoDecoration? logo = FlutterLogoDecoration.lerp(null, null, 0.5);
expect(logo, isNull);
});
test('FlutterLogoDecoration.lerp identical a,b', () {
expect(FlutterLogoDecoration.lerp(null, null, 0), null);
const FlutterLogoDecoration logo = FlutterLogoDecoration();
expect(identical(FlutterLogoDecoration.lerp(logo, logo, 0.5), logo), true);
});
test('FlutterLogoDecoration lerp from non-null to null lerps margin', () {
final FlutterLogoDecoration logo = FlutterLogoDecoration.lerp(start, null, 0.4)!;
expect(logo.textColor, start.textColor);
expect(logo.style, start.style);
expect(logo.margin, start.margin * 0.4);
});
test('FlutterLogoDecoration lerp from null to non-null lerps margin', () {
final FlutterLogoDecoration logo = FlutterLogoDecoration.lerp(null, end, 0.6)!;
expect(logo.textColor, end.textColor);
expect(logo.style, end.style);
expect(logo.margin, end.margin * 0.6);
});
test('FlutterLogoDecoration lerps colors and margins', () {
final FlutterLogoDecoration logo = FlutterLogoDecoration.lerp(start, end, 0.5)!;
expect(logo.textColor, Color.lerp(start.textColor, end.textColor, 0.5));
expect(logo.margin, EdgeInsets.lerp(start.margin, end.margin, 0.5));
});
test('FlutterLogoDecoration.lerpFrom and FlutterLogoDecoration.lerpTo', () {
expect(Decoration.lerp(start, const BoxDecoration(), 0.0), start);
expect(Decoration.lerp(start, const BoxDecoration(), 1.0), const BoxDecoration());
expect(Decoration.lerp(const BoxDecoration(), end, 0.0), const BoxDecoration());
expect(Decoration.lerp(const BoxDecoration(), end, 1.0), end);
});
test('FlutterLogoDecoration lerp changes styles at 0.5', () {
FlutterLogoDecoration logo = FlutterLogoDecoration.lerp(start, end, 0.4)!;
expect(logo.style, start.style);
logo = FlutterLogoDecoration.lerp(start, end, 0.5)!;
expect(logo.style, end.style);
});
test('FlutterLogoDecoration toString', () {
expect(
start.toString(),
equals(
'FlutterLogoDecoration(textColor: Color(0xffd4f144), style: stacked)',
),
);
expect(
FlutterLogoDecoration.lerp(null, end, 0.5).toString(),
equals(
'FlutterLogoDecoration(textColor: Color(0xff81d4fa), style: stacked, transition -1.0:0.5)',
),
);
});
testWidgets('Flutter Logo golden test', (WidgetTester tester) async {
final Key logo = UniqueKey();
await tester.pumpWidget(Container(
key: logo,
decoration: const FlutterLogoDecoration(),
));
await expectLater(
find.byKey(logo),
matchesGoldenFile('flutter_logo.png'),
);
});
}
| flutter/packages/flutter/test/painting/flutter_logo_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/flutter_logo_test.dart",
"repo_id": "flutter",
"token_count": 1355
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart';
class TestImageProvider extends ImageProvider<TestImageProvider> {
TestImageProvider(this.testImage);
final ui.Image testImage;
final Completer<ImageInfo> _completer = Completer<ImageInfo>.sync();
ImageConfiguration? configuration;
int loadCallCount = 0;
@override
Future<TestImageProvider> obtainKey(ImageConfiguration configuration) {
return SynchronousFuture<TestImageProvider>(this);
}
@override
void resolveStreamForKey(ImageConfiguration config, ImageStream stream, TestImageProvider key, ImageErrorListener handleError) {
configuration = config;
super.resolveStreamForKey(config, stream, key, handleError);
}
@override
ImageStreamCompleter loadBuffer(TestImageProvider key, DecoderBufferCallback decode) {
throw UnsupportedError('Use ImageProvider.loadImage instead.');
}
@override
ImageStreamCompleter loadImage(TestImageProvider key, ImageDecoderCallback decode) {
loadCallCount += 1;
return OneFrameImageStreamCompleter(_completer.future);
}
ImageInfo complete() {
final ImageInfo imageInfo = ImageInfo(image: testImage);
_completer.complete(imageInfo);
return imageInfo;
}
@override
String toString() => '${describeIdentity(this)}()';
}
| flutter/packages/flutter/test/painting/image_test_utils.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/image_test_utils.dart",
"repo_id": "flutter",
"token_count": 453
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
Future<void> verifyMarkedNeedsLayoutDuringTransientCallbacksPhase(WidgetTester tester, RenderObject renderObject) async {
assert(!renderObject.debugNeedsLayout);
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
final Completer<bool> animation = Completer<bool>();
tester.binding.scheduleFrameCallback((Duration timeStamp) {
animation.complete(renderObject.debugNeedsLayout);
});
// The fonts change does not mark the render object as needing layout
// immediately.
expect(renderObject.debugNeedsLayout, isFalse);
await tester.pump();
expect(await animation.future, isTrue);
}
void main() {
// TODO(polina-c): clean up leaks, https://github.com/flutter/flutter/issues/134787 [leaks-to-clean]
LeakTesting.settings = LeakTesting.settings.withIgnored(classes: <String>['CurvedAnimation']);
testWidgets('RenderParagraph relayout upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Text('text widget'),
),
);
final RenderObject renderObject = tester.renderObject(find.text('text widget'));
await verifyMarkedNeedsLayoutDuringTransientCallbacksPhase(tester, renderObject);
});
testWidgets(
'Safe to query a RelayoutWhenSystemFontsChangeMixin for text layout after system fonts changes',
(WidgetTester tester) async {
final _RenderCustomRelayoutWhenSystemFontsChange child = _RenderCustomRelayoutWhenSystemFontsChange();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: WidgetToRenderBoxAdapter(renderBox: child),
),
);
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
expect(child.hasValidTextLayout, isTrue);
},
);
testWidgets('RenderEditable relayout upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: SelectableText('text widget'),
),
);
final EditableTextState state = tester.state(find.byType(EditableText));
await verifyMarkedNeedsLayoutDuringTransientCallbacksPhase(tester, state.renderEditable);
});
testWidgets('Banner repaint upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
const Banner(
message: 'message',
location: BannerLocation.topStart,
textDirection: TextDirection.ltr,
layoutDirection: TextDirection.ltr,
),
);
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
final RenderObject renderObject = tester.renderObject(find.byType(Banner));
expect(renderObject.debugNeedsPaint, isTrue);
});
testWidgets('CupertinoDatePicker reset cache upon system fonts change - date time mode', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
onDateTimeChanged: (DateTime dateTime) { },
),
),
);
final dynamic state = tester.state(find.byType(CupertinoDatePicker));
// ignore: avoid_dynamic_calls
final Map<int, double> cache = state.estimatedColumnWidths as Map<int, double>;
expect(cache.isNotEmpty, isTrue);
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
// Cache should be cleaned.
expect(cache.isEmpty, isTrue);
final Element element = tester.element(find.byType(CupertinoDatePicker));
expect(element.dirty, isTrue);
}, skip: isBrowser); // TODO(yjbanov): cupertino does not work on the Web yet: https://github.com/flutter/flutter/issues/41920
testWidgets('CupertinoDatePicker reset cache upon system fonts change - date mode', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime dateTime) { },
),
),
);
final dynamic state = tester.state(find.byType(CupertinoDatePicker));
// ignore: avoid_dynamic_calls
final Map<int, double> cache = state.estimatedColumnWidths as Map<int, double>;
// Simulates font missing.
cache.clear();
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
// Cache should be replenished
expect(cache.isNotEmpty, isTrue);
final Element element = tester.element(find.byType(CupertinoDatePicker));
expect(element.dirty, isTrue);
}, skip: isBrowser); // TODO(yjbanov): cupertino does not work on the Web yet: https://github.com/flutter/flutter/issues/41920
testWidgets('CupertinoDatePicker reset cache upon system fonts change - time mode', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
onTimerDurationChanged: (Duration d) { },
),
),
);
final dynamic state = tester.state(find.byType(CupertinoTimerPicker));
// Simulates wrong metrics due to font missing.
// ignore: avoid_dynamic_calls
state.numberLabelWidth = 0.0;
// ignore: avoid_dynamic_calls
state.numberLabelHeight = 0.0;
// ignore: avoid_dynamic_calls
state.numberLabelBaseline = 0.0;
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
// Metrics should be refreshed
// ignore: avoid_dynamic_calls
expect(state.numberLabelWidth, lessThan(46.0 + precisionErrorTolerance));
// ignore: avoid_dynamic_calls
expect(state.numberLabelHeight, lessThan(23.0 + precisionErrorTolerance));
// ignore: avoid_dynamic_calls
expect(state.numberLabelBaseline, lessThan(18.400070190429688 + precisionErrorTolerance));
final Element element = tester.element(find.byType(CupertinoTimerPicker));
expect(element.dirty, isTrue);
}, skip: isBrowser); // TODO(yjbanov): cupertino does not work on the Web yet: https://github.com/flutter/flutter/issues/41920
testWidgets('RangeSlider relayout upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: RangeSlider(
values: const RangeValues(0.0, 1.0),
onChanged: (RangeValues values) { },
),
),
),
);
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
final RenderObject renderObject = tester.renderObject(find.byWidgetPredicate((Widget widget) => widget.runtimeType.toString() == '_RangeSliderRenderObjectWidget'));
await verifyMarkedNeedsLayoutDuringTransientCallbacksPhase(tester, renderObject);
});
testWidgets('Slider relayout upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Slider(
value: 0.0,
onChanged: (double value) { },
),
),
),
);
// _RenderSlider is the last render object in the tree.
final RenderObject renderObject = tester.allRenderObjects.last;
await verifyMarkedNeedsLayoutDuringTransientCallbacksPhase(tester, renderObject);
});
testWidgets('TimePicker relayout upon system fonts changes', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
builder: (BuildContext context, Widget? child) {
return Directionality(
key: const Key('parent'),
textDirection: TextDirection.ltr,
child: child!,
);
},
);
},
);
},
),
),
),
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
const Map<String, dynamic> data = <String, dynamic>{
'type': 'fontsChange',
};
await tester.binding.defaultBinaryMessenger.handlePlatformMessage(
'flutter/system',
SystemChannels.system.codec.encodeMessage(data),
(ByteData? data) { },
);
final RenderObject renderObject = tester.renderObject(
find.descendant(
of: find.byKey(const Key('parent')),
matching: find.byKey(const ValueKey<String>('time-picker-dial')),
),
);
expect(renderObject.debugNeedsPaint, isTrue);
});
}
class _RenderCustomRelayoutWhenSystemFontsChange extends RenderBox with RelayoutWhenSystemFontsChangeMixin {
bool hasValidTextLayout = false;
@override
void systemFontsDidChange() {
super.systemFontsDidChange();
hasValidTextLayout = false;
}
@override
void performLayout() {
size = constraints.biggest;
hasValidTextLayout = true;
}
}
| flutter/packages/flutter/test/painting/system_fonts_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/system_fonts_test.dart",
"repo_id": "flutter",
"token_count": 4302
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('RenderBaseline', () {
RenderBaseline parent;
RenderSizedBox child;
final RenderBox root = RenderPositionedBox(
alignment: Alignment.topLeft,
child: parent = RenderBaseline(
baseline: 0.0,
baselineType: TextBaseline.alphabetic,
child: child = RenderSizedBox(const Size(100.0, 100.0)),
),
);
final BoxParentData childParentData = child.parentData! as BoxParentData;
layout(root);
expect(childParentData.offset.dx, equals(0.0));
expect(childParentData.offset.dy, equals(-100.0));
expect(parent.size, equals(const Size(100.0, 0.0)));
parent.baseline = 25.0;
pumpFrame();
expect(childParentData.offset.dx, equals(0.0));
expect(childParentData.offset.dy, equals(-75.0));
expect(parent.size, equals(const Size(100.0, 25.0)));
parent.baseline = 90.0;
pumpFrame();
expect(childParentData.offset.dx, equals(0.0));
expect(childParentData.offset.dy, equals(-10.0));
expect(parent.size, equals(const Size(100.0, 90.0)));
parent.baseline = 100.0;
pumpFrame();
expect(childParentData.offset.dx, equals(0.0));
expect(childParentData.offset.dy, equals(0.0));
expect(parent.size, equals(const Size(100.0, 100.0)));
parent.baseline = 110.0;
pumpFrame();
expect(childParentData.offset.dx, equals(0.0));
expect(childParentData.offset.dy, equals(10.0));
expect(parent.size, equals(const Size(100.0, 110.0)));
});
test('RenderFlex and RenderIgnoreBaseline (control test -- with baseline)', () {
final RenderBox a, b;
final RenderBox root = RenderFlex(
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: TextBaseline.alphabetic,
textDirection: TextDirection.ltr,
children: <RenderBox>[
a = RenderParagraph(
const TextSpan(text: 'a', style: TextStyle(fontSize: 128.0, fontFamily: 'FlutterTest')), // places baseline at y=96
textDirection: TextDirection.ltr,
),
b = RenderParagraph(
const TextSpan(text: 'b', style: TextStyle(fontSize: 32.0, fontFamily: 'FlutterTest')), // 24 above baseline, 8 below baseline
textDirection: TextDirection.ltr,
),
],
);
layout(root);
final Offset aPos = a.localToGlobal(Offset.zero);
final Offset bPos = b.localToGlobal(Offset.zero);
expect(aPos.dy, 0.0);
expect(bPos.dy, 96.0 - 24.0);
});
test('RenderFlex and RenderIgnoreBaseline (with ignored baseline)', () {
final RenderBox a, b;
final RenderBox root = RenderFlex(
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: TextBaseline.alphabetic,
textDirection: TextDirection.ltr,
children: <RenderBox>[
RenderIgnoreBaseline(
child: a = RenderParagraph(
const TextSpan(text: 'a', style: TextStyle(fontSize: 128.0, fontFamily: 'FlutterTest')),
textDirection: TextDirection.ltr,
),
),
b = RenderParagraph(
const TextSpan(text: 'b', style: TextStyle(fontSize: 32.0, fontFamily: 'FlutterTest')),
textDirection: TextDirection.ltr,
),
],
);
layout(root);
final Offset aPos = a.localToGlobal(Offset.zero);
final Offset bPos = b.localToGlobal(Offset.zero);
expect(aPos.dy, 0.0);
expect(bPos.dy, 0.0);
});
}
| flutter/packages/flutter/test/rendering/baseline_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/baseline_test.dart",
"repo_id": "flutter",
"token_count": 1490
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Flex overflow indicator', (WidgetTester tester) async {
await tester.pumpWidget(
const Center(
child: Column(
children: <Widget>[
SizedBox(width: 200.0, height: 200.0),
],
),
),
);
expect(find.byType(Column), isNot(paints..rect()));
await tester.pumpWidget(
const Center(
child: SizedBox(
height: 100.0,
child: Column(
children: <Widget>[
SizedBox(width: 200.0, height: 200.0),
],
),
),
),
);
expect(tester.takeException(), isNotNull);
expect(find.byType(Column), paints..rect());
await tester.pumpWidget(
const Center(
child: SizedBox(
height: 0.0,
child: Column(
children: <Widget>[
SizedBox(width: 200.0, height: 200.0),
],
),
),
),
);
expect(find.byType(Column), isNot(paints..rect()));
});
}
| flutter/packages/flutter/test/rendering/flex_overflow_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/flex_overflow_test.dart",
"repo_id": "flutter",
"token_count": 605
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tracks picture layers accurately when painting is interleaved with a pushLayer', (WidgetTester tester) async {
// Creates a RenderObject that will paint into multiple picture layers.
// Asserts that both layers get a handle, and that all layers get correctly
// released.
final GlobalKey key = GlobalKey();
await tester.pumpWidget(RepaintBoundary(
child: CustomPaint(
key: key,
painter: SimplePainter(),
foregroundPainter: SimplePainter(),
child: const RepaintBoundary(child: Placeholder()),
),
));
final List<Layer> layers = tester.binding.renderView.debugLayer!.depthFirstIterateChildren();
final RenderObject renderObject = key.currentContext!.findRenderObject()!;
for (final Layer layer in layers) {
expect(layer.debugDisposed, false);
}
await tester.pumpWidget(const SizedBox());
for (final Layer layer in layers) {
expect(layer.debugDisposed, true);
}
expect(renderObject.debugDisposed, true);
});
}
class SimplePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
canvas.drawPaint(Paint());
}
@override
bool shouldRepaint(SimplePainter oldDelegate) => true;
}
| flutter/packages/flutter/test/rendering/object_paint_dispose_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/object_paint_dispose_test.dart",
"repo_id": "flutter",
"token_count": 512
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('relayout boundary change does not trigger relayout', (WidgetTester tester) async {
final RenderLayoutCount renderLayoutCount = RenderLayoutCount();
final Widget layoutCounter = Center(
key: GlobalKey(),
child: WidgetToRenderBoxAdapter(renderBox: renderLayoutCount),
);
await tester.pumpWidget(
Center(
child: SizedBox(
width: 100,
height: 100,
child: Center(
child: SizedBox(
width: 100,
height: 100,
child: Center(
child: layoutCounter,
),
),
),
),
),
);
expect(renderLayoutCount.layoutCount, 1);
await tester.pumpWidget(
Center(
child: SizedBox(
width: 100,
height: 100,
child: layoutCounter,
),
),
);
expect(renderLayoutCount.layoutCount, 1);
});
}
// This class is needed because LayoutBuilder's RenderObject does not always
// call the builder method in its PerformLayout method.
class RenderLayoutCount extends RenderBox {
int layoutCount = 0;
@override
void performLayout() {
layoutCount += 1;
size = constraints.biggest;
}
}
| flutter/packages/flutter/test/rendering/relayout_boundary_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/relayout_boundary_test.dart",
"repo_id": "flutter",
"token_count": 617
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('Stack can layout with top, right, bottom, left 0.0', () {
final RenderBox size = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox red = RenderDecoratedBox(
decoration: const BoxDecoration(
color: Color(0xFFFF0000),
),
child: size,
);
final RenderBox green = RenderDecoratedBox(
decoration: const BoxDecoration(
color: Color(0xFFFF0000),
),
);
final RenderBox stack = RenderStack(
textDirection: TextDirection.ltr,
children: <RenderBox>[red, green],
);
final StackParentData greenParentData = green.parentData! as StackParentData;
greenParentData
..top = 0.0
..right = 0.0
..bottom = 0.0
..left = 0.0;
layout(stack, constraints: const BoxConstraints());
expect(stack.size.width, equals(100.0));
expect(stack.size.height, equals(100.0));
expect(red.size.width, equals(100.0));
expect(red.size.height, equals(100.0));
expect(green.size.width, equals(100.0));
expect(green.size.height, equals(100.0));
});
test('Stack can layout with no children', () {
final RenderBox stack = RenderStack(
textDirection: TextDirection.ltr,
children: <RenderBox>[],
);
layout(stack, constraints: BoxConstraints.tight(const Size(100.0, 100.0)));
expect(stack.size.width, equals(100.0));
expect(stack.size.height, equals(100.0));
});
test('Stack has correct clipBehavior', () {
const BoxConstraints viewport = BoxConstraints(maxHeight: 100.0, maxWidth: 100.0);
for (final Clip? clip in <Clip?>[null, ...Clip.values]) {
final TestClipPaintingContext context = TestClipPaintingContext();
final RenderBox child = box200x200;
final RenderStack stack;
switch (clip){
case Clip.none:
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
stack = RenderStack(
textDirection: TextDirection.ltr,
children: <RenderBox>[child],
clipBehavior: clip!,
);
case null:
stack = RenderStack(
textDirection: TextDirection.ltr,
children: <RenderBox>[child],
);
}
{ // Make sure that the child is positioned so the stack will consider it as overflowed.
final StackParentData parentData = child.parentData! as StackParentData;
parentData.left = parentData.right = 0;
}
layout(stack, constraints: viewport, phase: EnginePhase.composite, onErrors: expectNoFlutterErrors);
context.paintChild(stack, Offset.zero);
// By default, clipBehavior should be Clip.hardEdge
expect(context.clipBehavior, equals(clip ?? Clip.hardEdge), reason: 'for $clip');
}
});
group('RenderIndexedStack', () {
test('visitChildrenForSemantics only visits displayed child', () {
final RenderBox child1 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child2 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child3 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox stack = RenderIndexedStack(
index: 1,
textDirection: TextDirection.ltr,
children: <RenderBox>[child1, child2, child3],
);
final List<RenderObject> visitedChildren = <RenderObject>[];
void visitor(RenderObject child) {
visitedChildren.add(child);
}
layout(stack);
stack.visitChildrenForSemantics(visitor);
expect(visitedChildren, hasLength(1));
expect(visitedChildren.first, child2);
});
test('debugDescribeChildren marks invisible children as offstage', () {
final RenderBox child1 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child2 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child3 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox stack = RenderIndexedStack(
index: 2,
children: <RenderBox>[child1, child2, child3],
);
final List<DiagnosticsNode> diagnosticNodes = stack.debugDescribeChildren();
expect(diagnosticNodes[0].name, 'child 1');
expect(diagnosticNodes[0].style, DiagnosticsTreeStyle.offstage);
expect(diagnosticNodes[1].name, 'child 2');
expect(diagnosticNodes[1].style, DiagnosticsTreeStyle.offstage);
expect(diagnosticNodes[2].name, 'child 3');
expect(diagnosticNodes[2].style, DiagnosticsTreeStyle.sparse);
});
test('debugDescribeChildren handles a null index', () {
final RenderBox child1 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child2 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox child3 = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
final RenderBox stack = RenderIndexedStack(
index: null,
children: <RenderBox>[child1, child2, child3],
);
final List<DiagnosticsNode> diagnosticNodes = stack.debugDescribeChildren();
expect(diagnosticNodes[0].name, 'child 1');
expect(diagnosticNodes[0].style, DiagnosticsTreeStyle.offstage);
expect(diagnosticNodes[1].name, 'child 2');
expect(diagnosticNodes[1].style, DiagnosticsTreeStyle.offstage);
expect(diagnosticNodes[2].name, 'child 3');
expect(diagnosticNodes[2].style, DiagnosticsTreeStyle.offstage);
});
});
test('Stack in Flex can layout with no children', () {
// Render an empty Stack in a Flex
final RenderFlex flex = RenderFlex(
textDirection: TextDirection.ltr,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <RenderBox>[
RenderStack(
textDirection: TextDirection.ltr,
children: <RenderBox>[],
),
]
);
bool stackFlutterErrorThrown = false;
layout(
flex,
constraints: BoxConstraints.tight(const Size(100.0, 100.0)),
onErrors: () {
stackFlutterErrorThrown = true;
}
);
expect(stackFlutterErrorThrown, false);
});
// More tests in ../widgets/stack_test.dart
}
| flutter/packages/flutter/test/rendering/stack_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/stack_test.dart",
"repo_id": "flutter",
"token_count": 2752
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'scheduler_tester.dart';
class TestSchedulerBinding extends BindingBase with SchedulerBinding, ServicesBinding {
final Map<String, List<Map<String, dynamic>>> eventsDispatched = <String, List<Map<String, dynamic>>>{};
VoidCallback? additionalHandleBeginFrame;
VoidCallback? additionalHandleDrawFrame;
@override
void handleBeginFrame(Duration? rawTimeStamp) {
additionalHandleBeginFrame?.call();
super.handleBeginFrame(rawTimeStamp);
}
@override
void handleDrawFrame() {
additionalHandleDrawFrame?.call();
super.handleDrawFrame();
}
@override
void postEvent(String eventKind, Map<String, dynamic> eventData) {
getEventsDispatched(eventKind).add(eventData);
}
List<Map<String, dynamic>> getEventsDispatched(String eventKind) {
return eventsDispatched.putIfAbsent(eventKind, () => <Map<String, dynamic>>[]);
}
}
class TestStrategy {
int allowedPriority = 10000;
bool shouldRunTaskWithPriority({ required int priority, required SchedulerBinding scheduler }) {
return priority >= allowedPriority;
}
}
void main() {
late TestSchedulerBinding scheduler;
setUpAll(() {
scheduler = TestSchedulerBinding();
});
tearDown(() {
scheduler.additionalHandleBeginFrame = null;
scheduler.additionalHandleDrawFrame = null;
});
test('Tasks are executed in the right order', () {
final TestStrategy strategy = TestStrategy();
scheduler.schedulingStrategy = strategy.shouldRunTaskWithPriority;
final List<int> input = <int>[2, 23, 23, 11, 0, 80, 3];
final List<int> executedTasks = <int>[];
void scheduleAddingTask(int x) {
scheduler.scheduleTask(() { executedTasks.add(x); }, Priority.idle + x);
}
input.forEach(scheduleAddingTask);
strategy.allowedPriority = 100;
for (int i = 0; i < 3; i += 1) {
expect(scheduler.handleEventLoopCallback(), isFalse);
}
expect(executedTasks.isEmpty, isTrue);
strategy.allowedPriority = 50;
for (int i = 0; i < 3; i += 1) {
expect(scheduler.handleEventLoopCallback(), i == 0 ? isTrue : isFalse);
}
expect(executedTasks, hasLength(1));
expect(executedTasks.single, equals(80));
executedTasks.clear();
strategy.allowedPriority = 20;
for (int i = 0; i < 3; i += 1) {
expect(scheduler.handleEventLoopCallback(), i < 2 ? isTrue : isFalse);
}
expect(executedTasks, hasLength(2));
expect(executedTasks[0], equals(23));
expect(executedTasks[1], equals(23));
executedTasks.clear();
scheduleAddingTask(99);
scheduleAddingTask(19);
scheduleAddingTask(5);
scheduleAddingTask(97);
for (int i = 0; i < 3; i += 1) {
expect(scheduler.handleEventLoopCallback(), i < 2 ? isTrue : isFalse);
}
expect(executedTasks, hasLength(2));
expect(executedTasks[0], equals(99));
expect(executedTasks[1], equals(97));
executedTasks.clear();
strategy.allowedPriority = 10;
for (int i = 0; i < 3; i += 1) {
expect(scheduler.handleEventLoopCallback(), i < 2 ? isTrue : isFalse);
}
expect(executedTasks, hasLength(2));
expect(executedTasks[0], equals(19));
expect(executedTasks[1], equals(11));
executedTasks.clear();
strategy.allowedPriority = 1;
for (int i = 0; i < 4; i += 1) {
expect(scheduler.handleEventLoopCallback(), i < 3 ? isTrue : isFalse);
}
expect(executedTasks, hasLength(3));
expect(executedTasks[0], equals(5));
expect(executedTasks[1], equals(3));
expect(executedTasks[2], equals(2));
executedTasks.clear();
strategy.allowedPriority = 0;
expect(scheduler.handleEventLoopCallback(), isFalse);
expect(executedTasks, hasLength(1));
expect(executedTasks[0], equals(0));
});
test('scheduleWarmUpFrame should flush microtasks between callbacks', () async {
addTearDown(() => scheduler.handleEventLoopCallback());
bool microtaskDone = false;
final Completer<void> drawFrameDone = Completer<void>();
scheduler.additionalHandleBeginFrame = () {
expect(microtaskDone, false);
scheduleMicrotask(() {
microtaskDone = true;
});
};
scheduler.additionalHandleDrawFrame = () {
expect(microtaskDone, true);
drawFrameDone.complete();
};
scheduler.scheduleWarmUpFrame();
await drawFrameDone.future;
});
test('2 calls to scheduleWarmUpFrame just schedules it once', () {
final List<VoidCallback> timerQueueTasks = <VoidCallback>[];
bool taskExecuted = false;
runZoned<void>(
() {
// Run it twice without processing the queued tasks.
scheduler.scheduleWarmUpFrame();
scheduler.scheduleWarmUpFrame();
scheduler.scheduleTask(() { taskExecuted = true; }, Priority.touch);
},
zoneSpecification: ZoneSpecification(
createTimer: (Zone self, ZoneDelegate parent, Zone zone, Duration duration, void Function() f) {
// Don't actually run the tasks, just record that it was scheduled.
timerQueueTasks.add(f);
return DummyTimer();
},
),
);
// scheduleWarmUpFrame scheduled 2 Timers, scheduleTask scheduled 0 because
// events are locked.
expect(timerQueueTasks.length, 2);
expect(taskExecuted, false);
// Run the timers so that the scheduler is no longer in warm-up state.
for (final VoidCallback timer in timerQueueTasks) {
timer();
}
// As events are locked, make scheduleTask execute after the test or it
// will execute during following tests and risk failure.
addTearDown(() => scheduler.handleEventLoopCallback());
});
test('Flutter.Frame event fired', () async {
SchedulerBinding.instance.platformDispatcher.onReportTimings!(<FrameTiming>[
FrameTiming(
vsyncStart: 5000,
buildStart: 10000,
buildFinish: 15000,
rasterStart: 16000,
rasterFinish: 20000,
rasterFinishWallTime: 20010,
frameNumber: 1991,
),
]);
final List<Map<String, dynamic>> events = scheduler.getEventsDispatched('Flutter.Frame');
expect(events, hasLength(1));
final Map<String, dynamic> event = events.first;
expect(event['number'], 1991);
expect(event['startTime'], 10000);
expect(event['elapsed'], 15000);
expect(event['build'], 5000);
expect(event['raster'], 4000);
expect(event['vsyncOverhead'], 5000);
});
test('TimingsCallback exceptions are caught', () {
FlutterErrorDetails? errorCaught;
FlutterError.onError = (FlutterErrorDetails details) {
errorCaught = details;
};
SchedulerBinding.instance.addTimingsCallback((List<FrameTiming> timings) {
throw Exception('Test');
});
SchedulerBinding.instance.platformDispatcher.onReportTimings!(<FrameTiming>[]);
expect(errorCaught!.exceptionAsString(), equals('Exception: Test'));
});
test('currentSystemFrameTimeStamp is the raw timestamp', () {
// Undo epoch set by previous tests.
scheduler.resetEpoch();
late Duration lastTimeStamp;
late Duration lastSystemTimeStamp;
void frameCallback(Duration timeStamp) {
expect(timeStamp, scheduler.currentFrameTimeStamp);
lastTimeStamp = scheduler.currentFrameTimeStamp;
lastSystemTimeStamp = scheduler.currentSystemFrameTimeStamp;
}
scheduler.scheduleFrameCallback(frameCallback);
tick(const Duration(seconds: 2));
expect(lastTimeStamp, Duration.zero);
expect(lastSystemTimeStamp, const Duration(seconds: 2));
scheduler.scheduleFrameCallback(frameCallback);
tick(const Duration(seconds: 4));
expect(lastTimeStamp, const Duration(seconds: 2));
expect(lastSystemTimeStamp, const Duration(seconds: 4));
timeDilation = 2;
scheduler.scheduleFrameCallback(frameCallback);
tick(const Duration(seconds: 6));
expect(lastTimeStamp, const Duration(seconds: 2)); // timeDilation calls SchedulerBinding.resetEpoch
expect(lastSystemTimeStamp, const Duration(seconds: 6));
scheduler.scheduleFrameCallback(frameCallback);
tick(const Duration(seconds: 8));
expect(lastTimeStamp, const Duration(seconds: 3)); // 2s + (8 - 6)s / 2
expect(lastSystemTimeStamp, const Duration(seconds: 8));
timeDilation = 1.0; // restore time dilation, or it will affect other tests
});
test('Animation frame scheduled in the middle of the warm-up frame', () {
expect(scheduler.schedulerPhase, SchedulerPhase.idle);
final List<VoidCallback> timers = <VoidCallback>[];
final ZoneSpecification timerInterceptor = ZoneSpecification(
createTimer: (Zone self, ZoneDelegate parent, Zone zone, Duration duration, void Function() callback) {
timers.add(callback);
return DummyTimer();
},
);
// Schedule a warm-up frame.
// Expect two timers, one for begin frame, and one for draw frame.
runZoned<void>(scheduler.scheduleWarmUpFrame, zoneSpecification: timerInterceptor);
expect(timers.length, 2);
final VoidCallback warmUpBeginFrame = timers.first;
final VoidCallback warmUpDrawFrame = timers.last;
timers.clear();
warmUpBeginFrame();
// Simulate an animation frame firing between warm-up begin frame and warm-up draw frame.
// Expect a timer that reschedules the frame.
expect(scheduler.hasScheduledFrame, isFalse);
SchedulerBinding.instance.platformDispatcher.onBeginFrame!(Duration.zero);
expect(scheduler.hasScheduledFrame, isFalse);
SchedulerBinding.instance.platformDispatcher.onDrawFrame!();
expect(scheduler.hasScheduledFrame, isFalse);
// The draw frame part of the warm-up frame will run the post-frame
// callback that reschedules the engine frame.
warmUpDrawFrame();
expect(scheduler.hasScheduledFrame, isTrue);
});
test('Can schedule futures to completion', () async {
bool isCompleted = false;
// `Future` is disallowed in this file due to the import of
// scheduler_tester.dart so annotations cannot be specified.
// ignore: always_specify_types
final result = scheduler.scheduleTask(
() async {
// Yield, so if awaiting `result` did not wait for completion of this
// task, the assertion on `isCompleted` will fail.
await null;
await null;
isCompleted = true;
return 1;
},
Priority.idle,
);
scheduler.handleEventLoopCallback();
await result;
expect(isCompleted, true);
});
}
class DummyTimer implements Timer {
@override
void cancel() {}
@override
bool get isActive => false;
@override
int get tick => 0;
}
| flutter/packages/flutter/test/scheduler/scheduler_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/scheduler/scheduler_test.dart",
"repo_id": "flutter",
"token_count": 3846
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
const String license1 = '''
L1Package1
L1Package2
L1Package3
L1Paragraph1
L1Paragraph2
L1Paragraph3''';
const String license2 = '''
L2Package1
L2Package2
L2Package3
L2Paragraph1
L2Paragraph2
L2Paragraph3''';
const String combinedLicenses = '''
$license1
--------------------------------------------------------------------------------
$license2
''';
class TestBinding extends BindingBase with SchedulerBinding, ServicesBinding {
@override
TestDefaultBinaryMessenger get defaultBinaryMessenger => super.defaultBinaryMessenger as TestDefaultBinaryMessenger;
@override
TestDefaultBinaryMessenger createBinaryMessenger() {
Future<ByteData?> keyboardHandler(ByteData? message) async {
return const StandardMethodCodec().encodeSuccessEnvelope(<int, int>{1:1});
}
return TestDefaultBinaryMessenger(
super.createBinaryMessenger(),
outboundHandlers: <String, MessageHandler>{'flutter/keyboard': keyboardHandler},
);
}
}
void main() {
final TestBinding binding = TestBinding();
test('Adds rootBundle LICENSES to LicenseRegistry', () async {
binding.defaultBinaryMessenger.setMockMessageHandler('flutter/assets', (ByteData? message) async {
if (const StringCodec().decodeMessage(message) == 'NOTICES.Z' && !kIsWeb) {
return Uint8List.fromList(gzip.encode(utf8.encode(combinedLicenses))).buffer.asByteData();
}
if (const StringCodec().decodeMessage(message) == 'NOTICES' && kIsWeb) {
return const StringCodec().encodeMessage(combinedLicenses);
}
return null;
});
final List<LicenseEntry> licenses = await LicenseRegistry.licenses.toList();
expect(licenses[0].packages, equals(<String>['L1Package1', 'L1Package2', 'L1Package3']));
expect(
licenses[0].paragraphs.map((LicenseParagraph p) => p.text),
equals(<String>['L1Paragraph1', 'L1Paragraph2', 'L1Paragraph3']),
);
expect(licenses[1].packages, equals(<String>['L2Package1', 'L2Package2', 'L2Package3']));
expect(
licenses[1].paragraphs.map((LicenseParagraph p) => p.text),
equals(<String>['L2Paragraph1', 'L2Paragraph2', 'L2Paragraph3']),
);
});
test('didHaveMemoryPressure clears asset caches', () async {
int flutterAssetsCallCount = 0;
binding.defaultBinaryMessenger.setMockMessageHandler('flutter/assets', (ByteData? message) async {
flutterAssetsCallCount += 1;
return ByteData.sublistView(utf8.encode('test_asset_data'));
});
await rootBundle.loadString('test_asset');
expect(flutterAssetsCallCount, 1);
await rootBundle.loadString('test_asset2');
expect(flutterAssetsCallCount, 2);
await rootBundle.loadString('test_asset');
expect(flutterAssetsCallCount, 2);
await rootBundle.loadString('test_asset2');
expect(flutterAssetsCallCount, 2);
final ByteData message = const JSONMessageCodec().encodeMessage(<String, dynamic>{'type': 'memoryPressure'})!;
await binding.defaultBinaryMessenger.handlePlatformMessage('flutter/system', message, (_) { });
await rootBundle.loadString('test_asset');
expect(flutterAssetsCallCount, 3);
await rootBundle.loadString('test_asset2');
expect(flutterAssetsCallCount, 4);
await rootBundle.loadString('test_asset');
expect(flutterAssetsCallCount, 4);
await rootBundle.loadString('test_asset2');
expect(flutterAssetsCallCount, 4);
});
test('initInstances sets a default method call handler for SystemChannels.textInput', () async {
final ByteData message = const JSONMessageCodec().encodeMessage(<String, dynamic>{'method': 'TextInput.requestElementsInRect', 'args': null})!;
await binding.defaultBinaryMessenger.handlePlatformMessage('flutter/textinput', message, (ByteData? data) {
expect(data, isNotNull);
});
});
test('Calling exitApplication sends a method call to the engine', () async {
bool sentMessage = false;
MethodCall? methodCall;
binding.defaultBinaryMessenger.setMockMessageHandler('flutter/platform', (ByteData? message) async {
methodCall = const JSONMethodCodec().decodeMethodCall(message);
sentMessage = true;
return const JSONMethodCodec().encodeSuccessEnvelope(<String, String>{'response': 'cancel'});
});
final AppExitResponse response = await binding.exitApplication(AppExitType.required);
expect(sentMessage, isTrue);
expect(methodCall, isNotNull);
expect((methodCall!.arguments as Map<String, dynamic>)['type'], equals('required'));
expect(response, equals(AppExitResponse.cancel));
});
test('Default handleRequestAppExit returns exit', () async {
const MethodCall incomingCall = MethodCall('System.requestAppExit', <dynamic>[<String, dynamic>{'type': 'cancelable'}]);
bool receivedReply = false;
Map<String, dynamic>? result;
await binding.defaultBinaryMessenger.handlePlatformMessage('flutter/platform', const JSONMethodCodec().encodeMethodCall(incomingCall),
(ByteData? message) async {
result = (const JSONMessageCodec().decodeMessage(message) as List<dynamic>)[0] as Map<String, dynamic>;
receivedReply = true;
},
);
expect(receivedReply, isTrue);
expect(result, isNotNull);
expect(result!['response'], equals('exit'));
});
test('initInstances synchronizes keyboard state', () async {
final Set<PhysicalKeyboardKey> physicalKeys = HardwareKeyboard.instance.physicalKeysPressed;
final Set<LogicalKeyboardKey> logicalKeys = HardwareKeyboard.instance.logicalKeysPressed;
expect(physicalKeys.length, 1);
expect(logicalKeys.length, 1);
expect(physicalKeys.first, const PhysicalKeyboardKey(1));
expect(logicalKeys.first, const LogicalKeyboardKey(1));
});
}
| flutter/packages/flutter/test/services/binding_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/binding_test.dart",
"repo_id": "flutter",
"token_count": 2075
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('!chrome')
library;
import 'dart:typed_data';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'message_codecs_testing.dart';
void main() {
group('JSON message codec', () {
const MessageCodec<dynamic> json = JSONMessageCodec();
test('should encode and decode big numbers', () {
checkEncodeDecode<dynamic>(json, 9223372036854775807);
checkEncodeDecode<dynamic>(json, -9223372036854775807);
});
test('should encode and decode list with a big number', () {
final List<dynamic> message = <dynamic>[-7000000000000000007]; // ignore: avoid_js_rounded_ints, since we check for round-tripping, the actual value doesn't matter!
checkEncodeDecode<dynamic>(json, message);
});
});
group('Standard message codec', () {
const MessageCodec<dynamic> standard = StandardMessageCodec();
test('should encode integers correctly at boundary cases', () {
checkEncoding<dynamic>(
standard,
-0x7fffffff - 1,
<int>[3, 0x00, 0x00, 0x00, 0x80],
);
checkEncoding<dynamic>(
standard,
-0x7fffffff - 2,
<int>[4, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xff, 0xff],
);
checkEncoding<dynamic>(
standard,
0x7fffffff,
<int>[3, 0xff, 0xff, 0xff, 0x7f],
);
checkEncoding<dynamic>(
standard,
0x7fffffff + 1,
<int>[4, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0x00],
);
checkEncoding<dynamic>(
standard,
-0x7fffffffffffffff - 1,
<int>[4, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x80],
);
checkEncoding<dynamic>(
standard,
-0x7fffffffffffffff - 2,
<int>[4, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x7f],
);
checkEncoding<dynamic>(
standard,
0x7fffffffffffffff,
<int>[4, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x7f],
);
checkEncoding<dynamic>(
standard,
0x7fffffffffffffff + 1,
<int>[4, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x80],
);
});
test('should encode and decode big numbers', () {
checkEncodeDecode<dynamic>(standard, 9223372036854775807);
checkEncodeDecode<dynamic>(standard, -9223372036854775807);
});
test('should encode and decode a list containing big numbers', () {
final List<dynamic> message = <dynamic>[
-7000000000000000007, // ignore: avoid_js_rounded_ints, browsers are skipped below
Int64List.fromList(<int>[-0x7fffffffffffffff - 1, 0, 0x7fffffffffffffff]),
];
checkEncodeDecode<dynamic>(standard, message);
});
}, skip: isBrowser); // [intended] Javascript can't handle the big integer literals used here.
}
| flutter/packages/flutter/test/services/message_codecs_vm_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/message_codecs_vm_test.dart",
"repo_id": "flutter",
"token_count": 1291
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
class TestTextInputFormatter extends TextInputFormatter {
const TestTextInputFormatter();
@override
void noSuchMethod(Invocation invocation) {
super.noSuchMethod(invocation);
}
}
void main() {
TextEditingValue testOldValue = TextEditingValue.empty;
TextEditingValue testNewValue = TextEditingValue.empty;
test('test const constructor', () {
const TestTextInputFormatter testValue1 = TestTextInputFormatter();
const TestTextInputFormatter testValue2 = TestTextInputFormatter();
expect(testValue1, same(testValue2));
});
test('withFunction wraps formatting function', () {
testOldValue = TextEditingValue.empty;
testNewValue = TextEditingValue.empty;
late TextEditingValue calledOldValue;
late TextEditingValue calledNewValue;
final TextInputFormatter formatterUnderTest = TextInputFormatter.withFunction(
(TextEditingValue oldValue, TextEditingValue newValue) {
calledOldValue = oldValue;
calledNewValue = newValue;
return TextEditingValue.empty;
},
);
formatterUnderTest.formatEditUpdate(testOldValue, testNewValue);
expect(calledOldValue, equals(testOldValue));
expect(calledNewValue, equals(testNewValue));
});
group('test provided formatters', () {
setUp(() {
// a1b(2c3
// d4)e5f6
// where the parentheses are the selection range.
testNewValue = const TextEditingValue(
text: 'a1b2c3\nd4e5f6',
selection: TextSelection(
baseOffset: 3,
extentOffset: 9,
),
);
});
test('test filtering formatter example', () {
const TextEditingValue intoTheWoods = TextEditingValue(text: 'Into the Woods');
expect(
FilteringTextInputFormatter('o', allow: true, replacementString: '*').formatEditUpdate(testOldValue, intoTheWoods),
const TextEditingValue(text: '*o*oo*'),
);
expect(
FilteringTextInputFormatter('o', allow: false, replacementString: '*').formatEditUpdate(testOldValue, intoTheWoods),
const TextEditingValue(text: 'Int* the W**ds'),
);
expect(
FilteringTextInputFormatter(RegExp('o+'), allow: true, replacementString: '*').formatEditUpdate(testOldValue, intoTheWoods),
const TextEditingValue(text: '*o*oo*'),
);
expect(
FilteringTextInputFormatter(RegExp('o+'), allow: false, replacementString: '*').formatEditUpdate(testOldValue, intoTheWoods),
const TextEditingValue(text: 'Int* the W*ds'),
);
// "Into the Wo|ods|"
const TextEditingValue selectedIntoTheWoods = TextEditingValue(text: 'Into the Woods', selection: TextSelection(baseOffset: 11, extentOffset: 14));
expect(
FilteringTextInputFormatter('o', allow: true, replacementString: '*').formatEditUpdate(testOldValue, selectedIntoTheWoods),
const TextEditingValue(text: '*o*oo*', selection: TextSelection(baseOffset: 4, extentOffset: 6)),
);
expect(
FilteringTextInputFormatter('o', allow: false, replacementString: '*').formatEditUpdate(testOldValue, selectedIntoTheWoods),
const TextEditingValue(text: 'Int* the W**ds', selection: TextSelection(baseOffset: 11, extentOffset: 14)),
);
expect(
FilteringTextInputFormatter(RegExp('o+'), allow: true, replacementString: '*').formatEditUpdate(testOldValue, selectedIntoTheWoods),
const TextEditingValue(text: '*o*oo*', selection: TextSelection(baseOffset: 4, extentOffset: 6)),
);
expect(
FilteringTextInputFormatter(RegExp('o+'), allow: false, replacementString: '*').formatEditUpdate(testOldValue, selectedIntoTheWoods),
const TextEditingValue(text: 'Int* the W*ds', selection: TextSelection(baseOffset: 11, extentOffset: 13)),
);
});
test('test filtering formatter, deny mode', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.deny(RegExp(r'[a-z]'))
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// 1(23
// 4)56
expect(actualValue, const TextEditingValue(
text: '123\n456',
selection: TextSelection(
baseOffset: 1,
extentOffset: 5,
),
));
});
test('test filtering formatter, deny mode (deprecated names)', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.deny(RegExp(r'[a-z]'))
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// 1(23
// 4)56
expect(actualValue, const TextEditingValue(
text: '123\n456',
selection: TextSelection(
baseOffset: 1,
extentOffset: 5,
),
));
});
test('test single line formatter', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.singleLineFormatter
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// a1b(2c3d4)e5f6
expect(actualValue, const TextEditingValue(
text: 'a1b2c3d4e5f6',
selection: TextSelection(
baseOffset: 3,
extentOffset: 8,
),
));
});
test('test single line formatter (deprecated names)', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.singleLineFormatter
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// a1b(2c3d4)e5f6
expect(actualValue, const TextEditingValue(
text: 'a1b2c3d4e5f6',
selection: TextSelection(
baseOffset: 3,
extentOffset: 8,
),
));
});
test('test filtering formatter, allow mode', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.allow(RegExp(r'[a-c]'))
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// ab(c)
expect(actualValue, const TextEditingValue(
text: 'abc',
selection: TextSelection(
baseOffset: 2,
extentOffset: 3,
),
));
});
test('test filtering formatter, allow mode (deprecated names)', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.allow(RegExp(r'[a-c]'))
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// ab(c)
expect(actualValue, const TextEditingValue(
text: 'abc',
selection: TextSelection(
baseOffset: 2,
extentOffset: 3,
),
));
});
test('test digits only formatter', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.digitsOnly
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// 1(234)56
expect(actualValue, const TextEditingValue(
text: '123456',
selection: TextSelection(
baseOffset: 1,
extentOffset: 4,
),
));
});
test('test digits only formatter (deprecated names)', () {
final TextEditingValue actualValue =
FilteringTextInputFormatter.digitsOnly
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// 1(234)56
expect(actualValue, const TextEditingValue(
text: '123456',
selection: TextSelection(
baseOffset: 1,
extentOffset: 4,
),
));
});
test('test length limiting formatter', () {
final TextEditingValue actualValue =
LengthLimitingTextInputFormatter(6)
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// a1b(2c3)
expect(actualValue, const TextEditingValue(
text: 'a1b2c3',
selection: TextSelection(
baseOffset: 3,
extentOffset: 6,
),
));
});
test('test length limiting formatter with zero-length string', () {
testNewValue = const TextEditingValue(
selection: TextSelection(
baseOffset: 0,
extentOffset: 0,
),
);
final TextEditingValue actualValue =
LengthLimitingTextInputFormatter(1)
.formatEditUpdate(testOldValue, testNewValue);
// Expecting the empty string.
expect(actualValue, const TextEditingValue(
selection: TextSelection(
baseOffset: 0,
extentOffset: 0,
),
));
});
test('test length limiting formatter with non-BMP Unicode scalar values', () {
testNewValue = const TextEditingValue(
text: '\u{1f984}\u{1f984}\u{1f984}\u{1f984}', // Unicode U+1f984 (UNICORN FACE)
selection: TextSelection(
// Caret is at the end of the string.
baseOffset: 8,
extentOffset: 8,
),
);
final TextEditingValue actualValue =
LengthLimitingTextInputFormatter(2)
.formatEditUpdate(testOldValue, testNewValue);
// Expecting two characters, with the caret moved to the new end of the
// string.
expect(actualValue, const TextEditingValue(
text: '\u{1f984}\u{1f984}',
selection: TextSelection(
baseOffset: 4,
extentOffset: 4,
),
));
});
test('test length limiting formatter with complex Unicode characters', () {
// TODO(gspencer): Test additional strings. We can do this once the
// formatter supports Unicode grapheme clusters.
//
// A formatter with max length 1 should accept:
// - The '\u{1F3F3}\u{FE0F}\u{200D}\u{1F308}' sequence (flag followed by
// a variation selector, a zero-width joiner, and a rainbow to make a rainbow
// flag).
// - The sequence '\u{0058}\u{0346}\u{0361}\u{035E}\u{032A}\u{031C}\u{0333}\u{0326}\u{031D}\u{0332}'
// (Latin X with many composed characters).
//
// A formatter should not count as a character:
// * The '\u{0000}\u{FEFF}' sequence. (NULL followed by zero-width no-break space).
//
// A formatter with max length 1 should truncate this to one character:
// * The '\u{1F3F3}\u{FE0F}\u{1F308}' sequence (flag with ignored variation
// selector followed by rainbow, should truncate to just flag).
// The U+1F984 U+0020 sequence: Unicorn face followed by a space should
// yield only the unicorn face.
testNewValue = const TextEditingValue(
text: '\u{1F984}\u{0020}',
selection: TextSelection(
baseOffset: 1,
extentOffset: 1,
),
);
TextEditingValue actualValue = LengthLimitingTextInputFormatter(1).formatEditUpdate(testOldValue, testNewValue);
expect(actualValue, const TextEditingValue(
text: '\u{1F984}',
selection: TextSelection(
baseOffset: 1,
extentOffset: 1,
),
));
// The U+0058 U+0059 sequence: Latin X followed by Latin Y, should yield
// Latin X.
testNewValue = const TextEditingValue(
text: '\u{0058}\u{0059}',
selection: TextSelection(
baseOffset: 1,
extentOffset: 1,
),
);
actualValue = LengthLimitingTextInputFormatter(1).formatEditUpdate(testOldValue, testNewValue);
expect(actualValue, const TextEditingValue(
text: '\u{0058}',
selection: TextSelection(
baseOffset: 1,
extentOffset: 1,
),
));
});
test('test length limiting formatter when selection is off the end', () {
final TextEditingValue actualValue =
LengthLimitingTextInputFormatter(2)
.formatEditUpdate(testOldValue, testNewValue);
// Expecting
// a1()
expect(actualValue, const TextEditingValue(
text: 'a1',
selection: TextSelection(
baseOffset: 2,
extentOffset: 2,
),
));
});
});
group('LengthLimitingTextInputFormatter', () {
group('truncate', () {
test('Removes characters from the end', () async {
const TextEditingValue value = TextEditingValue(
text: '01234567890',
);
final TextEditingValue truncated = LengthLimitingTextInputFormatter
.truncate(value, 10);
expect(truncated.text, '0123456789');
});
test('Counts surrogate pairs as single characters', () async {
const String stringOverflowing = '😆01234567890';
const TextEditingValue value = TextEditingValue(
text: stringOverflowing,
// Put the cursor at the end of the overflowing string to test if it
// ends up at the end of the new string after truncation.
selection: TextSelection.collapsed(offset: stringOverflowing.length),
);
final TextEditingValue truncated = LengthLimitingTextInputFormatter
.truncate(value, 10);
const String stringTruncated = '😆012345678';
expect(truncated.text, stringTruncated);
expect(truncated.selection.baseOffset, stringTruncated.length);
expect(truncated.selection.extentOffset, stringTruncated.length);
});
test('Counts grapheme clusters as single characters', () async {
const String stringOverflowing = '👨👩👦01234567890';
const TextEditingValue value = TextEditingValue(
text: stringOverflowing,
// Put the cursor at the end of the overflowing string to test if it
// ends up at the end of the new string after truncation.
selection: TextSelection.collapsed(offset: stringOverflowing.length),
);
final TextEditingValue truncated = LengthLimitingTextInputFormatter
.truncate(value, 10);
const String stringTruncated = '👨👩👦012345678';
expect(truncated.text, stringTruncated);
expect(truncated.selection.baseOffset, stringTruncated.length);
expect(truncated.selection.extentOffset, stringTruncated.length);
});
});
group('formatEditUpdate', () {
const int maxLength = 10;
test('Passes through when under limit', () async {
const TextEditingValue oldValue = TextEditingValue(
text: 'aaa',
);
const TextEditingValue newValue = TextEditingValue(
text: 'aaab',
);
final LengthLimitingTextInputFormatter formatter =
LengthLimitingTextInputFormatter(maxLength);
final TextEditingValue formatted = formatter.formatEditUpdate(
oldValue,
newValue,
);
expect(formatted.text, newValue.text);
});
test('Uses old value when at the limit', () async {
const TextEditingValue oldValue = TextEditingValue(
text: 'aaaaaaaaaa',
);
const TextEditingValue newValue = TextEditingValue(
text: 'aaaaabbbbbaaaaa',
);
final LengthLimitingTextInputFormatter formatter =
LengthLimitingTextInputFormatter(maxLength);
final TextEditingValue formatted = formatter.formatEditUpdate(
oldValue,
newValue,
);
expect(formatted.text, oldValue.text);
});
test('Truncates newValue when oldValue already over limit', () async {
const TextEditingValue oldValue = TextEditingValue(
text: 'aaaaaaaaaaaaaaaaaaaa',
);
const TextEditingValue newValue = TextEditingValue(
text: 'bbbbbbbbbbbbbbbbbbbb',
);
final LengthLimitingTextInputFormatter formatter =
LengthLimitingTextInputFormatter(maxLength);
final TextEditingValue formatted = formatter.formatEditUpdate(
oldValue,
newValue,
);
expect(formatted.text, 'bbbbbbbbbb');
});
});
group('get enforcement from target platform', () {
// The enforcement on Web will be always `MaxLengthEnforcement.truncateAfterCompositionEnds`
test('with TargetPlatform.windows', () async {
final MaxLengthEnforcement enforcement = LengthLimitingTextInputFormatter.getDefaultMaxLengthEnforcement(
TargetPlatform.windows,
);
if (kIsWeb) {
expect(enforcement, MaxLengthEnforcement.truncateAfterCompositionEnds);
} else {
expect(enforcement, MaxLengthEnforcement.enforced);
}
});
test('with TargetPlatform.macOS', () async {
final MaxLengthEnforcement enforcement = LengthLimitingTextInputFormatter.getDefaultMaxLengthEnforcement(
TargetPlatform.macOS,
);
expect(enforcement, MaxLengthEnforcement.truncateAfterCompositionEnds);
});
});
});
test('FilteringTextInputFormatter should return the old value if new value contains non-white-listed character', () {
const TextEditingValue oldValue = TextEditingValue(text: '12345');
const TextEditingValue newValue = TextEditingValue(text: '12345@');
final TextInputFormatter formatter = FilteringTextInputFormatter.digitsOnly;
final TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('12345'));
// The new value is always the oldValue plus a non-digit character (user press @)
expect(newValue.text, equals('12345@'));
// we expect that the formatted value returns the oldValue only since the newValue does not
// satisfy the formatter condition (which is, in this case, digitsOnly)
expect(formatted.text, equals('12345'));
});
test('FilteringTextInputFormatter should move the cursor to the right position', () {
TextEditingValue collapsedValue(String text, int offset) =>
TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: offset),
);
TextEditingValue oldValue = collapsedValue('123', 0);
TextEditingValue newValue = collapsedValue('123456', 6);
final TextInputFormatter formatter = FilteringTextInputFormatter.digitsOnly;
TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('123'));
// assert that we are passing digits only at the second time
expect(newValue.text, equals('123456'));
// assert that cursor is at the end of the text
expect(formatted.selection.baseOffset, equals(6));
// move cursor at the middle of the text and then add the number 9.
oldValue = newValue.copyWith(selection: const TextSelection.collapsed(offset: 4));
newValue = oldValue.copyWith(text: '1239456');
formatted = formatter.formatEditUpdate(oldValue, newValue);
// cursor must be now at fourth position (right after the number 9)
expect(formatted.selection.baseOffset, equals(4));
});
test('FilteringTextInputFormatter should remove non-allowed characters', () {
const TextEditingValue oldValue = TextEditingValue(text: '12345');
const TextEditingValue newValue = TextEditingValue(text: '12345@');
final TextInputFormatter formatter = FilteringTextInputFormatter.digitsOnly;
final TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('12345'));
// The new value is always the oldValue plus a non-digit character (user press @)
expect(newValue.text, equals('12345@'));
// we expect that the formatted value returns the oldValue only since the difference
// between the oldValue and the newValue is only material that isn't allowed
expect(formatted.text, equals('12345'));
});
test('WhitelistingTextInputFormatter should return the old value if new value contains non-allowed character', () {
const TextEditingValue oldValue = TextEditingValue(text: '12345');
const TextEditingValue newValue = TextEditingValue(text: '12345@');
final TextInputFormatter formatter = FilteringTextInputFormatter.digitsOnly;
final TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('12345'));
// The new value is always the oldValue plus a non-digit character (user press @)
expect(newValue.text, equals('12345@'));
// we expect that the formatted value returns the oldValue only since the newValue does not
// satisfy the formatter condition (which is, in this case, digitsOnly)
expect(formatted.text, equals('12345'));
});
test('FilteringTextInputFormatter should move the cursor to the right position', () {
TextEditingValue collapsedValue(String text, int offset) =>
TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: offset),
);
TextEditingValue oldValue = collapsedValue('123', 0);
TextEditingValue newValue = collapsedValue('123456', 6);
final TextInputFormatter formatter =
FilteringTextInputFormatter.digitsOnly;
TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('123'));
// assert that we are passing digits only at the second time
expect(newValue.text, equals('123456'));
// assert that cursor is at the end of the text
expect(formatted.selection.baseOffset, equals(6));
// move cursor at the middle of the text and then add the number 9.
oldValue = newValue.copyWith(selection: const TextSelection.collapsed(offset: 4));
newValue = oldValue.copyWith(text: '1239456');
formatted = formatter.formatEditUpdate(oldValue, newValue);
// cursor must be now at fourth position (right after the number 9)
expect(formatted.selection.baseOffset, equals(4));
});
test('WhitelistingTextInputFormatter should move the cursor to the right position', () {
TextEditingValue collapsedValue(String text, int offset) =>
TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: offset),
);
TextEditingValue oldValue = collapsedValue('123', 0);
TextEditingValue newValue = collapsedValue('123456', 6);
final TextInputFormatter formatter =
FilteringTextInputFormatter.digitsOnly;
TextEditingValue formatted = formatter.formatEditUpdate(oldValue, newValue);
// assert that we are passing digits only at the first time
expect(oldValue.text, equals('123'));
// assert that we are passing digits only at the second time
expect(newValue.text, equals('123456'));
// assert that cursor is at the end of the text
expect(formatted.selection.baseOffset, equals(6));
// move cursor at the middle of the text and then add the number 9.
oldValue = newValue.copyWith(selection: const TextSelection.collapsed(offset: 4));
newValue = oldValue.copyWith(text: '1239456');
formatted = formatter.formatEditUpdate(oldValue, newValue);
// cursor must be now at fourth position (right after the number 9)
expect(formatted.selection.baseOffset, equals(4));
});
test('FilteringTextInputFormatter should filter independent of selection', () {
// Regression test for https://github.com/flutter/flutter/issues/80842.
final TextInputFormatter formatter = FilteringTextInputFormatter.deny('abc', replacementString: '*');
const TextEditingValue oldValue = TextEditingValue.empty;
const TextEditingValue newValue = TextEditingValue(text: 'abcabcabc');
final String filteredText = formatter.formatEditUpdate(oldValue, newValue).text;
for (int i = 0; i < newValue.text.length; i += 1) {
final String text = formatter.formatEditUpdate(
oldValue,
newValue.copyWith(selection: TextSelection.collapsed(offset: i)),
).text;
expect(filteredText, text);
}
});
test('FilteringTextInputFormatter should filter independent of composingRegion', () {
final TextInputFormatter formatter = FilteringTextInputFormatter.deny('abc', replacementString: '*');
const TextEditingValue oldValue = TextEditingValue.empty;
const TextEditingValue newValue = TextEditingValue(text: 'abcabcabc');
final String filteredText = formatter.formatEditUpdate(oldValue, newValue).text;
for (int i = 0; i < newValue.text.length; i += 1) {
final String text = formatter.formatEditUpdate(
oldValue,
newValue.copyWith(composing: TextRange.collapsed(i)),
).text;
expect(filteredText, text);
}
});
test('FilteringTextInputFormatter basic filtering test', () {
final RegExp filter = RegExp('[A-Za-z0-9.@-]*');
final TextInputFormatter formatter = FilteringTextInputFormatter.allow(filter);
const TextEditingValue oldValue = TextEditingValue.empty;
const TextEditingValue newValue = TextEditingValue(text: 'ab&&ca@bcabc');
expect(formatter.formatEditUpdate(oldValue, newValue).text, 'abca@bcabc');
});
group('FilteringTextInputFormatter region', () {
const TextEditingValue oldValue = TextEditingValue.empty;
test('Preserves selection region', () {
const TextEditingValue newValue = TextEditingValue(text: 'AAABBBCCC');
// AAA | BBB | CCC => AAA | **** | CCC
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 6, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 7, extentOffset: 3),
);
// AAA | BBB CCC | => AAA | **** CCC |
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 9, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 10, extentOffset: 3),
);
// AAA BBB | CCC | => AAA **** | CCC |
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 9, extentOffset: 6),
),
).selection,
const TextSelection(baseOffset: 10, extentOffset: 7),
);
// AAAB | B | BCCC => AAA***|CCC
// Same length replacement, keep the selection at where it is.
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '***').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 5, extentOffset: 4),
),
).selection,
const TextSelection(baseOffset: 5, extentOffset: 4),
);
// AAA | BBB | CCC => AAA | CCC
expect(
FilteringTextInputFormatter.deny('BBB').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 6, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 3, extentOffset: 3),
);
expect(
FilteringTextInputFormatter.deny('BBB').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 6, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 3, extentOffset: 3),
);
// The unfortunate case, we don't know for sure where to put the selection
// so put it after the replacement string.
// AAAB|B|BCCC => AAA****|CCC
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 5, extentOffset: 4),
),
).selection,
const TextSelection(baseOffset: 7, extentOffset: 7),
);
});
test('Preserves selection region, allow', () {
const TextEditingValue newValue = TextEditingValue(text: 'AAABBBCCC');
// AAA | BBB | CCC => **** | BBB | ****
expect(
FilteringTextInputFormatter.allow('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 6, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 7, extentOffset: 4),
);
// | AAABBBCCC | => | ****BBB**** |
expect(
FilteringTextInputFormatter.allow('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 9, extentOffset: 0),
),
).selection,
const TextSelection(baseOffset: 11, extentOffset: 0),
);
// AAABBB | CCC | => ****BBB | **** |
expect(
FilteringTextInputFormatter.allow('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
selection: const TextSelection(baseOffset: 9, extentOffset: 6),
),
).selection,
const TextSelection(baseOffset: 11, extentOffset: 7),
);
// Overlapping matches: AAA | BBBBB | CCC => | BBB |
expect(
FilteringTextInputFormatter.allow('BBB').formatEditUpdate(
oldValue,
const TextEditingValue(
text: 'AAABBBBBCCC',
selection: TextSelection(baseOffset: 8, extentOffset: 3),
),
).selection,
const TextSelection(baseOffset: 3, extentOffset: 0),
);
});
test('Preserves composing region', () {
const TextEditingValue newValue = TextEditingValue(text: 'AAABBBCCC');
// AAA | BBB | CCC => AAA | **** | CCC
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
composing: const TextRange(start: 3, end: 6),
),
).composing,
const TextRange(start: 3, end: 7),
);
// AAA | BBB CCC | => AAA | **** CCC |
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
composing: const TextRange(start: 3, end: 9),
),
).composing,
const TextRange(start: 3, end: 10),
);
// AAA BBB | CCC | => AAA **** | CCC |
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '****').formatEditUpdate(
oldValue,
newValue.copyWith(
composing: const TextRange(start: 6, end: 9),
),
).composing,
const TextRange(start: 7, end: 10),
);
// AAAB | B | BCCC => AAA*** | CCC
// Same length replacement, don't move the composing region.
expect(
FilteringTextInputFormatter.deny('BBB', replacementString: '***').formatEditUpdate(
oldValue,
newValue.copyWith(
composing: const TextRange(start: 4, end: 5),
),
).composing,
const TextRange(start: 4, end: 5),
);
// AAA | BBB | CCC => | AAA CCC
expect(
FilteringTextInputFormatter.deny('BBB').formatEditUpdate(
oldValue,
newValue.copyWith(
composing: const TextRange(start: 3, end: 6),
),
).composing,
TextRange.empty,
);
});
});
}
| flutter/packages/flutter/test/services/text_formatter_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/text_formatter_test.dart",
"repo_id": "flutter",
"token_count": 12488
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AnimatedPositioned.fromRect control test', (WidgetTester tester) async {
final AnimatedPositioned positioned = AnimatedPositioned.fromRect(
rect: const Rect.fromLTWH(7.0, 5.0, 12.0, 16.0),
duration: const Duration(milliseconds: 200),
child: Container(),
);
expect(positioned.left, equals(7.0));
expect(positioned.top, equals(5.0));
expect(positioned.width, equals(12.0));
expect(positioned.height, equals(16.0));
expect(positioned, hasOneLineDescription);
});
testWidgets('AnimatedPositioned - basics (VISUAL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 50.0,
top: 30.0,
width: 70.0,
height: 110.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 37.0,
top: 31.0,
width: 59.0,
height: 71.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
const Offset first = Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0);
const Offset last = Offset(37.0 + 59.0 / 2.0, 31.0 + 71.0 / 2.0);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(first));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(Offset.lerp(first, last, 0.5)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(last));
expect(box, hasAGoodToStringDeep);
expect(
box.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderLimitedBox#00000\n'
' │ parentData: top=31.0; left=37.0; width=59.0; height=71.0;\n'
' │ offset=Offset(37.0, 31.0) (can use size)\n'
' │ constraints: BoxConstraints(w=59.0, h=71.0)\n'
' │ size: Size(59.0, 71.0)\n'
' │ maxWidth: 0.0\n'
' │ maxHeight: 0.0\n'
' │\n'
' └─child: RenderConstrainedBox#00000\n'
' parentData: <none> (can use size)\n'
' constraints: BoxConstraints(w=59.0, h=71.0)\n'
' size: Size(59.0, 71.0)\n'
' additionalConstraints: BoxConstraints(biggest)\n',
),
);
});
testWidgets('AnimatedPositionedDirectional - basics (LTR)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 50.0,
top: 30.0,
width: 70.0,
height: 110.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 37.0,
top: 31.0,
width: 59.0,
height: 71.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
const Offset first = Offset(50.0 + 70.0 / 2.0, 30.0 + 110.0 / 2.0);
const Offset last = Offset(37.0 + 59.0 / 2.0, 31.0 + 71.0 / 2.0);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(first));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(Offset.lerp(first, last, 0.5)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(last));
expect(box, hasAGoodToStringDeep);
expect(
box.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderLimitedBox#00000\n'
' │ parentData: top=31.0; left=37.0; width=59.0; height=71.0;\n'
' │ offset=Offset(37.0, 31.0) (can use size)\n'
' │ constraints: BoxConstraints(w=59.0, h=71.0)\n'
' │ size: Size(59.0, 71.0)\n'
' │ maxWidth: 0.0\n'
' │ maxHeight: 0.0\n'
' │\n'
' └─child: RenderConstrainedBox#00000\n'
' parentData: <none> (can use size)\n'
' constraints: BoxConstraints(w=59.0, h=71.0)\n'
' size: Size(59.0, 71.0)\n'
' additionalConstraints: BoxConstraints(biggest)\n',
),
);
});
testWidgets('AnimatedPositionedDirectional - basics (RTL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 50.0,
top: 30.0,
width: 70.0,
height: 110.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(800.0 - 50.0 - 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(800.0 - 50.0 - 70.0 / 2.0, 30.0 + 110.0 / 2.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 37.0,
top: 31.0,
width: 59.0,
height: 71.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
const Offset first = Offset(800.0 - 50.0 - 70.0 / 2.0, 30.0 + 110.0 / 2.0);
const Offset last = Offset(800.0 - 37.0 - 59.0 / 2.0, 31.0 + 71.0 / 2.0);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(first));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(Offset.lerp(first, last, 0.5)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(last));
expect(box, hasAGoodToStringDeep);
expect(
box.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderLimitedBox#00000\n'
' │ parentData: top=31.0; right=37.0; width=59.0; height=71.0;\n'
' │ offset=Offset(704.0, 31.0) (can use size)\n'
' │ constraints: BoxConstraints(w=59.0, h=71.0)\n'
' │ size: Size(59.0, 71.0)\n'
' │ maxWidth: 0.0\n'
' │ maxHeight: 0.0\n'
' │\n'
' └─child: RenderConstrainedBox#00000\n'
' parentData: <none> (can use size)\n'
' constraints: BoxConstraints(w=59.0, h=71.0)\n'
' size: Size(59.0, 71.0)\n'
' additionalConstraints: BoxConstraints(biggest)\n',
),
);
});
testWidgets('AnimatedPositioned - interrupted animation (VISUAL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 100.0,
top: 100.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(100.0, 100.0)));
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 150.0,
top: 150.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(100.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(150.0, 150.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(200.0, 200.0)));
});
testWidgets('AnimatedPositioned - switching variables (VISUAL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pumpWidget(
Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
AnimatedPositioned(
left: 0.0,
top: 100.0,
right: 100.0, // 700.0 from the left
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 150.0)));
});
testWidgets('AnimatedPositionedDirectional - interrupted animation (LTR)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 100.0,
top: 100.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(100.0, 100.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 150.0,
top: 150.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(100.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(150.0, 150.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(200.0, 200.0)));
});
testWidgets('AnimatedPositionedDirectional - switching variables (LTR)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(50.0, 50.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 100.0,
end: 100.0, // 700.0 from the start
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(350.0, 150.0)));
});
testWidgets('AnimatedPositionedDirectional - interrupted animation (RTL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(750.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(750.0, 50.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 100.0,
top: 100.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(750.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(700.0, 100.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 150.0,
top: 150.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(700.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(650.0, 150.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(600.0, 200.0)));
});
testWidgets('AnimatedPositionedDirectional - switching variables (RTL)', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
RenderBox box;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 0.0,
width: 100.0,
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(750.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(750.0, 50.0)));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Stack(
children: <Widget>[
AnimatedPositionedDirectional(
start: 0.0,
top: 100.0,
end: 100.0, // 700.0 from the start
height: 100.0,
duration: const Duration(seconds: 2),
child: Container(key: key),
),
],
),
),
);
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(450.0, 50.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(450.0, 100.0)));
await tester.pump(const Duration(seconds: 1));
box = key.currentContext!.findRenderObject()! as RenderBox;
expect(box.localToGlobal(box.size.center(Offset.zero)), equals(const Offset(450.0, 150.0)));
});
}
| flutter/packages/flutter/test/widgets/animated_positioned_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/animated_positioned_test.dart",
"repo_id": "flutter",
"token_count": 10742
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
class TestCanvas implements Canvas {
final List<Invocation> invocations = <Invocation>[];
@override
void noSuchMethod(Invocation invocation) {
invocations.add(invocation);
}
}
void main() {
// the textDirection values below are intentionally sometimes different and
// sometimes the same as the layoutDirection, to make sure that they don't
// affect the layout.
test('A Banner with a location of topStart paints in the top left (LTR)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.rtl,
location: BannerLocation.topStart,
layoutDirection: TextDirection.ltr,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], lessThan(100.0));
expect(translateCommand.positionalArguments[1], lessThan(100.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(-math.pi / 4.0));
});
test('A Banner with a location of topStart paints in the top right (RTL)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.ltr,
location: BannerLocation.topStart,
layoutDirection: TextDirection.rtl,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], greaterThan(900.0));
expect(translateCommand.positionalArguments[1], lessThan(100.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(math.pi / 4.0));
});
test('A Banner with a location of topEnd paints in the top right (LTR)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.ltr,
location: BannerLocation.topEnd,
layoutDirection: TextDirection.ltr,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], greaterThan(900.0));
expect(translateCommand.positionalArguments[1], lessThan(100.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(math.pi / 4.0));
});
test('A Banner with a location of topEnd paints in the top left (RTL)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.rtl,
location: BannerLocation.topEnd,
layoutDirection: TextDirection.rtl,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], lessThan(100.0));
expect(translateCommand.positionalArguments[1], lessThan(100.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(-math.pi / 4.0));
});
test('A Banner with a location of bottomStart paints in the bottom left (LTR)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.ltr,
location: BannerLocation.bottomStart,
layoutDirection: TextDirection.ltr,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], lessThan(100.0));
expect(translateCommand.positionalArguments[1], greaterThan(900.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(math.pi / 4.0));
});
test('A Banner with a location of bottomStart paints in the bottom right (RTL)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.rtl,
location: BannerLocation.bottomStart,
layoutDirection: TextDirection.rtl,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], greaterThan(900.0));
expect(translateCommand.positionalArguments[1], greaterThan(900.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(-math.pi / 4.0));
});
test('A Banner with a location of bottomEnd paints in the bottom right (LTR)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.rtl,
location: BannerLocation.bottomEnd,
layoutDirection: TextDirection.ltr,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], greaterThan(900.0));
expect(translateCommand.positionalArguments[1], greaterThan(900.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(-math.pi / 4.0));
});
test('A Banner with a location of bottomEnd paints in the bottom left (RTL)', () {
final BannerPainter bannerPainter = BannerPainter(
message: 'foo',
textDirection: TextDirection.ltr,
location: BannerLocation.bottomEnd,
layoutDirection: TextDirection.rtl,
);
final TestCanvas canvas = TestCanvas();
bannerPainter.paint(canvas, const Size(1000.0, 1000.0));
final Invocation translateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #translate;
});
expect(translateCommand, isNotNull);
expect(translateCommand.positionalArguments[0], lessThan(100.0));
expect(translateCommand.positionalArguments[1], greaterThan(900.0));
final Invocation rotateCommand = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #rotate;
});
expect(rotateCommand, isNotNull);
expect(rotateCommand.positionalArguments[0], equals(math.pi / 4.0));
});
testWidgets('Banner widget', (WidgetTester tester) async {
debugDisableShadows = false;
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Banner(message: 'Hello', location: BannerLocation.topEnd),
),
);
expect(find.byType(CustomPaint), paints
..save()
..translate(x: 800.0, y: 0.0)
..rotate(angle: math.pi / 4.0)
..rect(rect: const Rect.fromLTRB(-40.0, 28.0, 40.0, 40.0), color: const Color(0x7f000000), hasMaskFilter: true)
..rect(rect: const Rect.fromLTRB(-40.0, 28.0, 40.0, 40.0), color: const Color(0xa0b71c1c), hasMaskFilter: false)
..paragraph(offset: const Offset(-40.0, 29.0))
..restore(),
);
debugDisableShadows = true;
});
testWidgets('Banner widget in MaterialApp', (WidgetTester tester) async {
debugDisableShadows = false;
await tester.pumpWidget(const MaterialApp(home: Placeholder()));
expect(find.byType(CheckedModeBanner), paints
..save()
..translate(x: 800.0, y: 0.0)
..rotate(angle: math.pi / 4.0)
..rect(rect: const Rect.fromLTRB(-40.0, 28.0, 40.0, 40.0), color: const Color(0x7f000000), hasMaskFilter: true)
..rect(rect: const Rect.fromLTRB(-40.0, 28.0, 40.0, 40.0), color: const Color(0xa0b71c1c), hasMaskFilter: false)
..paragraph(offset: const Offset(-40.0, 29.0))
..restore(),
);
debugDisableShadows = true;
});
test('BannerPainter dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => BannerPainter(
message: 'foo',
textDirection: TextDirection.rtl,
location: BannerLocation.topStart,
layoutDirection: TextDirection.ltr,
).dispose(),
BannerPainter,
),
areCreateAndDispose,
);
});
}
| flutter/packages/flutter/test/widgets/banner_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/banner_test.dart",
"repo_id": "flutter",
"token_count": 3728
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can be placed in an infinite box', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(children: const <Widget>[Center()]),
),
);
});
}
| flutter/packages/flutter/test/widgets/center_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/center_test.dart",
"repo_id": "flutter",
"token_count": 183
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('debugChildrenHaveDuplicateKeys control test', () {
const Key key = Key('key');
final List<Widget> children = <Widget>[
Container(key: key),
Container(key: key),
];
final Widget widget = Flex(
direction: Axis.vertical,
children: children,
);
late FlutterError error;
try {
debugChildrenHaveDuplicateKeys(widget, children);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' Duplicate keys found.\n'
' If multiple keyed widgets exist as children of another widget,\n'
' they must have unique keys.\n'
' Flex(direction: vertical, mainAxisAlignment: start,\n'
' crossAxisAlignment: center) has multiple children with key\n'
" [<'key'>].\n",
),
);
}
});
test('debugItemsHaveDuplicateKeys control test', () {
const Key key = Key('key');
final List<Widget> items = <Widget>[
Container(key: key),
Container(key: key),
];
late FlutterError error;
try {
debugItemsHaveDuplicateKeys(items);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
" Duplicate key found: [<'key'>].\n",
),
);
}
});
testWidgets('debugCheckHasTable control test', (WidgetTester tester) async {
await tester.pumpWidget(
Builder(
builder: (BuildContext context) {
late FlutterError error;
try {
debugCheckHasTable(context);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error.diagnostics.length, 4);
expect(error.diagnostics[2], isA<DiagnosticsProperty<Element>>());
expect(
error.toStringDeep(),
startsWith(
'FlutterError\n'
' No Table widget found.\n'
' Builder widgets require a Table widget ancestor.\n'
' The specific widget that could not find a Table ancestor was:\n'
' Builder\n'
' The ownership chain for the affected widget is: "Builder ←', // End of ownership chain omitted, not relevant for test.
),
);
}
return Container();
},
),
);
});
testWidgets('debugCheckHasMediaQuery control test', (WidgetTester tester) async {
// Cannot use tester.pumpWidget here because it wraps the widget in a View,
// which introduces a MediaQuery ancestor.
await tester.pumpWidget(
wrapWithView: false,
Builder(
builder: (BuildContext context) {
late FlutterError error;
try {
debugCheckHasMediaQuery(context);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error.diagnostics.length, 5);
expect(error.diagnostics[2], isA<DiagnosticsProperty<Element>>());
expect(error.diagnostics.last.level, DiagnosticLevel.hint);
expect(
error.diagnostics.last.toStringDeep(),
equalsIgnoringHashCodes(
'No MediaQuery ancestor could be found starting from the context\n'
'that was passed to MediaQuery.of(). This can happen because the\n'
'context used is not a descendant of a View widget, which\n'
'introduces a MediaQuery.\n'
),
);
expect(
error.toStringDeep(),
startsWith(
'FlutterError\n'
' No MediaQuery widget ancestor found.\n'
' Builder widgets require a MediaQuery widget ancestor.\n'
' The specific widget that could not find a MediaQuery ancestor\n'
' was:\n'
' Builder\n'
' The ownership chain for the affected widget is: "Builder ←' // Full chain omitted, not relevant for test.
),
);
expect(
error.toStringDeep(),
endsWith(
'[root]"\n' // End of ownership chain.
' No MediaQuery ancestor could be found starting from the context\n'
' that was passed to MediaQuery.of(). This can happen because the\n'
' context used is not a descendant of a View widget, which\n'
' introduces a MediaQuery.\n'
),
);
}
return View(
view: tester.view,
child: const SizedBox(),
);
},
),
);
});
test('debugWidgetBuilderValue control test', () {
final Widget widget = Container();
FlutterError? error;
try {
debugWidgetBuilderValue(widget, null);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error!.diagnostics.length, 4);
expect(error.diagnostics[1], isA<DiagnosticsProperty<Widget>>());
expect(error.diagnostics[1].style, DiagnosticsTreeStyle.errorProperty);
expect(
error.diagnostics[1].toStringDeep(),
equalsIgnoringHashCodes(
'The offending widget is:\n'
' Container\n',
),
);
expect(error.diagnostics[2].level, DiagnosticLevel.info);
expect(error.diagnostics[3].level, DiagnosticLevel.hint);
expect(
error.diagnostics[3].toStringDeep(),
equalsIgnoringHashCodes(
'To return an empty space that causes the building widget to fill\n'
'available room, return "Container()". To return an empty space\n'
'that takes as little room as possible, return "Container(width:\n'
'0.0, height: 0.0)".\n',
),
);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' A build function returned null.\n'
' The offending widget is:\n'
' Container\n'
' Build functions must never return null.\n'
' To return an empty space that causes the building widget to fill\n'
' available room, return "Container()". To return an empty space\n'
' that takes as little room as possible, return "Container(width:\n'
' 0.0, height: 0.0)".\n',
),
);
error = null;
}
try {
debugWidgetBuilderValue(widget, widget);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error!.diagnostics.length, 3);
expect(error.diagnostics[1], isA<DiagnosticsProperty<Widget>>());
expect(error.diagnostics[1].style, DiagnosticsTreeStyle.errorProperty);
expect(
error.diagnostics[1].toStringDeep(),
equalsIgnoringHashCodes(
'The offending widget is:\n'
' Container\n',
),
);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' A build function returned context.widget.\n'
' The offending widget is:\n'
' Container\n'
" Build functions must never return their BuildContext parameter's\n"
' widget or a child that contains "context.widget". Doing so\n'
' introduces a loop in the widget tree that can cause the app to\n'
' crash.\n',
),
);
}
});
testWidgets('debugCheckHasWidgetsLocalizations throws', (WidgetTester tester) async {
final GlobalKey noLocalizationsAvailable = GlobalKey();
final GlobalKey localizationsAvailable = GlobalKey();
await tester.pumpWidget(
Container(
key: noLocalizationsAvailable,
child: WidgetsApp(
builder: (BuildContext context, Widget? child) {
return Container(
key: localizationsAvailable,
);
},
color: const Color(0xFF4CAF50),
),
),
);
expect(
() => debugCheckHasWidgetsLocalizations(noLocalizationsAvailable.currentContext!),
throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
contains('No WidgetsLocalizations found'),
)),
);
expect(debugCheckHasWidgetsLocalizations(localizationsAvailable.currentContext!), isTrue);
});
test('debugAssertAllWidgetVarsUnset', () {
debugHighlightDeprecatedWidgets = true;
late FlutterError error;
try {
debugAssertAllWidgetVarsUnset('The value of a widget debug variable was changed by the test.');
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error.diagnostics.length, 1);
expect(
error.toStringDeep(),
'FlutterError\n'
' The value of a widget debug variable was changed by the test.\n',
);
}
debugHighlightDeprecatedWidgets = false;
});
testWidgets('debugCreator of layers should not be null', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Stack(
children: <Widget>[
const ColorFiltered(
colorFilter: ColorFilter.mode(Color(0xFFFF0000), BlendMode.color),
child: Placeholder(),
),
const Opacity(
opacity: 0.9,
child: Placeholder(),
),
ImageFiltered(
imageFilter: ImageFilter.blur(sigmaX: 10.0, sigmaY: 10.0),
child: const Placeholder(),
),
BackdropFilter(
filter: ImageFilter.blur(sigmaX: 10.0, sigmaY: 10.0),
child: const Placeholder(),
),
ShaderMask(
shaderCallback: (Rect bounds) => const RadialGradient(
radius: 0.05,
colors: <Color>[Color(0xFFFF0000), Color(0xFF00FF00)],
tileMode: TileMode.mirror,
).createShader(bounds),
child: const Placeholder(),
),
CompositedTransformFollower(
link: LayerLink(),
),
],
),
),
),
),
);
RenderObject renderObject;
renderObject = tester.firstRenderObject(find.byType(Opacity));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
renderObject = tester.firstRenderObject(find.byType(ColorFiltered));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
renderObject = tester.firstRenderObject(find.byType(ImageFiltered));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
renderObject = tester.firstRenderObject(find.byType(BackdropFilter));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
renderObject = tester.firstRenderObject(find.byType(ShaderMask));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
renderObject = tester.firstRenderObject(find.byType(CompositedTransformFollower));
expect(renderObject.debugLayer?.debugCreator, isNotNull);
});
}
| flutter/packages/flutter/test/widgets/debug_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/debug_test.dart",
"repo_id": "flutter",
"token_count": 5413
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
@TestOn('!chrome')
library;
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'editable_text_utils.dart';
const TextStyle textStyle = TextStyle();
const Color cursorColor = Color.fromARGB(0xFF, 0xFF, 0x00, 0x00);
void main() {
late TextEditingController controller;
late FocusNode focusNode;
late FocusScopeNode focusScopeNode;
setUp(() async {
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
controller = TextEditingController();
focusNode = FocusNode();
focusScopeNode = FocusScopeNode();
});
tearDown(() {
controller.dispose();
focusNode.dispose();
focusScopeNode.dispose();
});
testWidgets('cursor has expected width, height, and radius', (WidgetTester tester) async {
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
cursorWidth: 10.0,
cursorHeight: 10.0,
cursorRadius: const Radius.circular(2.0),
),
),
),
);
final EditableText editableText = tester.firstWidget(find.byType(EditableText));
expect(editableText.cursorWidth, 10.0);
expect(editableText.cursorHeight, 10.0);
expect(editableText.cursorRadius!.x, 2.0);
});
testWidgets('cursor layout has correct width', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
late String changedValue;
final Widget widget = MaterialApp(
home: RepaintBoundary(
key: const ValueKey<int>(1),
child: EditableText(
backgroundCursorColor: Colors.grey,
key: editableTextKey,
controller: controller,
focusNode: focusNode,
style: Typography.material2018().black.titleMedium!,
cursorColor: Colors.blue,
selectionControls: materialTextSelectionControls,
keyboardType: TextInputType.text,
onChanged: (String value) {
changedValue = value;
},
cursorWidth: 15.0,
),
),
);
await tester.pumpWidget(widget);
// Populate a fake clipboard.
const String clipboardContent = ' ';
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'Clipboard.getData') {
return const <String, dynamic>{'text': clipboardContent};
}
if (methodCall.method == 'Clipboard.hasStrings') {
return <String, dynamic>{'value': clipboardContent.isNotEmpty};
}
return null;
});
// Long-press to bring up the text editing controls.
final Finder textFinder = find.byKey(editableTextKey);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
await tester.tap(find.text('Paste'));
await tester.pump();
expect(changedValue, clipboardContent);
await expectLater(
find.byKey(const ValueKey<int>(1)),
matchesGoldenFile('editable_text_test.0.png'),
);
EditableText.debugDeterministicCursor = false;
});
testWidgets('cursor layout has correct radius', (WidgetTester tester) async {
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
late String changedValue;
final Widget widget = MaterialApp(
home: RepaintBoundary(
key: const ValueKey<int>(1),
child: EditableText(
backgroundCursorColor: Colors.grey,
key: editableTextKey,
controller: controller,
focusNode: focusNode,
style: Typography.material2018().black.titleMedium!,
cursorColor: Colors.blue,
selectionControls: materialTextSelectionControls,
keyboardType: TextInputType.text,
onChanged: (String value) {
changedValue = value;
},
cursorWidth: 15.0,
cursorRadius: const Radius.circular(3.0),
),
),
);
await tester.pumpWidget(widget);
// Populate a fake clipboard.
const String clipboardContent = ' ';
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'Clipboard.getData') {
return const <String, dynamic>{'text': clipboardContent};
}
if (methodCall.method == 'Clipboard.hasStrings') {
return <String, dynamic>{'value': clipboardContent.isNotEmpty};
}
return null;
});
// Long-press to bring up the text editing controls.
final Finder textFinder = find.byKey(editableTextKey);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
await tester.tap(find.text('Paste'));
await tester.pump();
expect(changedValue, clipboardContent);
await expectLater(
find.byKey(const ValueKey<int>(1)),
matchesGoldenFile('editable_text_test.1.png'),
);
});
testWidgets('Cursor animates on iOS', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: TextField(),
),
),
);
final Finder textFinder = find.byType(TextField);
await tester.tap(textFinder);
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor!.opacity, 1.0);
int walltimeMicrosecond = 0;
double lastVerifiedOpacity = 1.0;
Future<void> verifyKeyFrame({ required double opacity, required int at }) async {
const int delta = 1;
assert(at - delta > walltimeMicrosecond);
await tester.pump(Duration(microseconds: at - delta - walltimeMicrosecond));
// Instead of verifying the opacity at each key frame, this function
// verifies the opacity immediately *before* each key frame to avoid
// fp precision issues.
expect(
renderEditable.cursorColor!.opacity,
closeTo(lastVerifiedOpacity, 0.01),
reason: 'opacity at ${at-delta} microseconds',
);
walltimeMicrosecond = at - delta;
lastVerifiedOpacity = opacity;
}
await verifyKeyFrame(opacity: 1.0, at: 500000);
await verifyKeyFrame(opacity: 0.75, at: 537500);
await verifyKeyFrame(opacity: 0.5, at: 575000);
await verifyKeyFrame(opacity: 0.25, at: 612500);
await verifyKeyFrame(opacity: 0.0, at: 650000);
await verifyKeyFrame(opacity: 0.0, at: 850000);
await verifyKeyFrame(opacity: 0.25, at: 887500);
await verifyKeyFrame(opacity: 0.5, at: 925000);
await verifyKeyFrame(opacity: 0.75, at: 962500);
await verifyKeyFrame(opacity: 1.0, at: 1000000);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS }));
testWidgets('Cursor does not animate on non-iOS platforms', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(child: TextField(maxLines: 3)),
),
);
await tester.tap(find.byType(TextField));
await tester.pump();
// Wait for the current animation to finish. If the cursor never stops its
// blinking animation the test will timeout.
await tester.pumpAndSettle();
for (int i = 0; i < 40; i += 1) {
await tester.pump(const Duration(milliseconds: 100));
expect(tester.hasRunningAnimations, false);
}
}, variant: TargetPlatformVariant.all(excluding: <TargetPlatform>{ TargetPlatform.iOS }));
testWidgets('Cursor does not animate on Android', (WidgetTester tester) async {
final Color defaultCursorColor = Color(ThemeData.fallback().colorScheme.primary.value);
const Widget widget = MaterialApp(
home: Material(
child: TextField(
maxLines: 3,
),
),
);
await tester.pumpWidget(widget);
await tester.tap(find.byType(TextField));
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
await tester.pump();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
// Android cursor goes from exactly on to exactly off on the 500ms dot.
await tester.pump(const Duration(milliseconds: 499));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
await tester.pump(const Duration(milliseconds: 1));
expect(renderEditable.cursorColor!.alpha, 0);
// Don't try to draw the cursor.
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
await tester.pump(const Duration(milliseconds: 500));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
await tester.pump(const Duration(milliseconds: 500));
expect(renderEditable.cursorColor!.alpha, 0);
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
});
testWidgets('Cursor does not animates when debugDeterministicCursor is set', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
final Color defaultCursorColor = Color(ThemeData.fallback().colorScheme.primary.value);
const Widget widget = MaterialApp(
home: Material(
child: TextField(
maxLines: 3,
),
),
);
await tester.pumpWidget(widget);
await tester.tap(find.byType(TextField));
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor!.alpha, 255);
await tester.pump();
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rrect(color: defaultCursorColor));
// Cursor draw never changes.
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rrect(color: defaultCursorColor));
// No more transient calls.
await tester.pumpAndSettle();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rrect(color: defaultCursorColor));
EditableText.debugDeterministicCursor = false;
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets('Cursor does not animate on Android when debugDeterministicCursor is set', (WidgetTester tester) async {
final Color defaultCursorColor = Color(ThemeData.fallback().colorScheme.primary.value);
EditableText.debugDeterministicCursor = true;
const Widget widget = MaterialApp(
home: Material(
child: TextField(
maxLines: 3,
),
),
);
await tester.pumpWidget(widget);
await tester.tap(find.byType(TextField));
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
await tester.pump();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
await tester.pump(const Duration(milliseconds: 500));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
// Cursor draw never changes.
await tester.pump(const Duration(milliseconds: 500));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
// No more transient calls.
await tester.pumpAndSettle();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: defaultCursorColor));
EditableText.debugDeterministicCursor = false;
});
testWidgets('Cursor animation restarts when it is moved using keys on desktop', (WidgetTester tester) async {
debugDefaultTargetPlatformOverride = TargetPlatform.macOS;
const String testText = 'Some text long enough to move the cursor around';
controller.text = testText;
final Widget widget = MaterialApp(
home: EditableText(
controller: controller,
focusNode: focusNode,
style: const TextStyle(fontSize: 20.0),
cursorColor: Colors.blue,
backgroundCursorColor: Colors.grey,
selectionControls: materialTextSelectionControls,
keyboardType: TextInputType.text,
textAlign: TextAlign.left,
),
);
await tester.pumpWidget(widget);
await tester.tap(find.byType(EditableText));
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
await tester.pump();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
// Android cursor goes from exactly on to exactly off on the 500ms dot.
await tester.pump(const Duration(milliseconds: 499));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.sendKeyDownEvent(LogicalKeyboardKey.arrowLeft);
await tester.pump();
await tester.sendKeyUpEvent(LogicalKeyboardKey.arrowLeft);
await tester.pump();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.pump(const Duration(milliseconds: 299));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.sendKeyDownEvent(LogicalKeyboardKey.arrowRight);
await tester.pump();
await tester.sendKeyUpEvent(LogicalKeyboardKey.arrowRight);
await tester.pump();
await tester.sendKeyDownEvent(LogicalKeyboardKey.arrowRight);
await tester.pump();
await tester.sendKeyUpEvent(LogicalKeyboardKey.arrowRight);
await tester.pump();
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.pump(const Duration(milliseconds: 299));
expect(renderEditable.cursorColor!.alpha, 255);
expect(renderEditable, paints..rect(color: const Color(0xff2196f3)));
await tester.pump(const Duration(milliseconds: 1));
expect(renderEditable.cursorColor!.alpha, 0);
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
debugDefaultTargetPlatformOverride = null;
},
variant: KeySimulatorTransitModeVariant.all(),
);
testWidgets('Cursor does not show when showCursor set to false', (WidgetTester tester) async {
const Widget widget = MaterialApp(
home: Material(
child: TextField(
showCursor: false,
maxLines: 3,
),
),
);
await tester.pumpWidget(widget);
await tester.tap(find.byType(TextField));
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
// Make sure it does not paint for a period of time.
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
await tester.pump(const Duration(milliseconds: 200));
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
});
testWidgets('Cursor does not show when not focused', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/106512 .
await tester.pumpWidget(
MaterialApp(
home: Material(
child: TextField(focusNode: focusNode, autofocus: true),
),
),
);
assert(focusNode.hasFocus);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
focusNode.unfocus();
await tester.pump();
for (int i = 0; i < 10; i += 10) {
// Make sure it does not paint for a period of time.
expect(renderEditable, paintsExactlyCountTimes(#drawRect, 0));
expect(tester.hasRunningAnimations, isFalse);
await tester.pump(const Duration(milliseconds: 29));
}
// Refocus and it should paint the caret.
focusNode.requestFocus();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(renderEditable, isNot(paintsExactlyCountTimes(#drawRect, 0)));
});
testWidgets('Cursor radius is 2.0', (WidgetTester tester) async {
const Widget widget = MaterialApp(
home: Material(
child: TextField(
maxLines: 3,
),
),
);
await tester.pumpWidget(widget);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorRadius, const Radius.circular(2.0));
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Cursor gets placed correctly after going out of bounds', (WidgetTester tester) async {
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
await tester.tap(find.byType(EditableText));
final RenderEditable renderEditable = findRenderEditable(tester);
renderEditable.selection = const TextSelection(baseOffset: 29, extentOffset: 29);
expect(controller.selection.baseOffset, 29);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
// Sets the origin.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Start, offset: const Offset(20, 20)));
expect(controller.selection.baseOffset, 29);
// Moves the cursor super far right
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(2090, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(2100, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(2090, 20),
));
// After peaking the cursor, we move in the opposite direction.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(1400, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
// The cursor has been set.
expect(controller.selection.baseOffset, 8);
// Go in the other direction.
// Sets the origin.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Start, offset: const Offset(20, 20)));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-5000, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-5010, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-5000, 20),
));
// Move back in the opposite direction only a few hundred.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-4850, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
expect(controller.selection.baseOffset, 10);
});
testWidgets('Updating the floating cursor correctly moves the cursor', (WidgetTester tester) async {
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
await tester.tap(find.byType(EditableText));
final RenderEditable renderEditable = findRenderEditable(tester);
renderEditable.selection = const TextSelection(baseOffset: 29, extentOffset: 29);
expect(controller.selection.baseOffset, 29);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
// Sets the origin.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Start,
offset: const Offset(20, 20),
));
expect(controller.selection.baseOffset, 29);
// Moves the cursor right a few characters.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-250, 20),
));
// But we have not yet set the offset because the user is not done placing the cursor.
expect(controller.selection.baseOffset, 29);
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
// The cursor has been set.
expect(controller.selection.baseOffset, 10);
});
testWidgets('Updating the floating cursor can end without update', (WidgetTester tester) async {
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
await tester.tap(find.byType(EditableText));
final RenderEditable renderEditable = findRenderEditable(tester);
renderEditable.selection = const TextSelection(baseOffset: 29, extentOffset: 29);
expect(controller.selection.baseOffset, 29);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Start));
expect(controller.selection.baseOffset, 29);
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
// The cursor did not change.
expect(controller.selection.baseOffset, 29);
expect(tester.takeException(), null);
});
testWidgets("Drag the floating cursor, it won't blink.", (WidgetTester tester) async {
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
final EditableTextState editableText = tester.state(find.byType(EditableText));
// Check that the cursor visibility toggles after each blink interval.
// Or if it's not blinking at all, it stays on.
Future<void> checkCursorBlinking({ bool isBlinking = true }) async {
bool initialShowCursor = true;
if (isBlinking) {
initialShowCursor = editableText.cursorCurrentlyVisible;
}
await tester.pump(editableText.cursorBlinkInterval);
expect(editableText.cursorCurrentlyVisible, equals(isBlinking ? !initialShowCursor : initialShowCursor));
await tester.pump(editableText.cursorBlinkInterval);
expect(editableText.cursorCurrentlyVisible, equals(initialShowCursor));
await tester.pump(editableText.cursorBlinkInterval ~/ 10);
expect(editableText.cursorCurrentlyVisible, equals(initialShowCursor));
await tester.pump(editableText.cursorBlinkInterval);
expect(editableText.cursorCurrentlyVisible, equals(isBlinking ? !initialShowCursor : initialShowCursor));
await tester.pump(editableText.cursorBlinkInterval);
expect(editableText.cursorCurrentlyVisible, equals(initialShowCursor));
}
final Offset textfieldStart = tester.getTopLeft(find.byType(EditableText));
await tester.tapAt(textfieldStart + const Offset(50.0, 9.0));
await tester.pumpAndSettle();
// Before dragging, the cursor should blink.
await checkCursorBlinking();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Start));
// When drag cursor, the cursor shouldn't blink.
await checkCursorBlinking(isBlinking: false);
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
// After dragging, the cursor should blink.
await checkCursorBlinking();
});
testWidgets('Turning showCursor off stops the cursor', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/108187.
final bool debugDeterministicCursor = EditableText.debugDeterministicCursor;
// This doesn't really matter.
EditableText.debugDeterministicCursor = false;
addTearDown(() { EditableText.debugDeterministicCursor = debugDeterministicCursor; });
const Key key = Key('EditableText');
Widget buildEditableText({ required bool showCursor }) {
return MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: EditableText(
key: key,
backgroundCursorColor: Colors.grey,
// Use animation controller to animate cursor blink for testing.
cursorOpacityAnimates: true,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
showCursor: showCursor,
),
),
);
}
late final EditableTextState editableTextState = tester.state(find.byKey(key));
await tester.pumpWidget(buildEditableText(showCursor: false));
await tester.tap(find.byKey(key));
await tester.pump();
// No cursor even when focused.
expect(editableTextState.cursorCurrentlyVisible, false);
// The EditableText still has focus, so the cursor should starts blinking.
await tester.pumpWidget(buildEditableText(showCursor: true));
expect(editableTextState.cursorCurrentlyVisible, true);
await tester.pump();
expect(editableTextState.cursorCurrentlyVisible, true);
// readOnly disables blinking cursor.
await tester.pumpWidget(buildEditableText(showCursor: false));
expect(editableTextState.cursorCurrentlyVisible, false);
await tester.pump();
expect(editableTextState.cursorCurrentlyVisible, false);
});
// Regression test for https://github.com/flutter/flutter/pull/30475.
testWidgets('Trying to select with the floating cursor does not crash', (WidgetTester tester) async {
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
await tester.tap(find.byType(EditableText));
final RenderEditable renderEditable = findRenderEditable(tester);
renderEditable.selection = const TextSelection(baseOffset: 29, extentOffset: 29);
expect(controller.selection.baseOffset, 29);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
// Sets the origin.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Start,
offset: const Offset(20, 20),
));
expect(controller.selection.baseOffset, 29);
// Moves the cursor right a few characters.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-250, 20),
));
// But we have not yet set the offset because the user is not done placing the cursor.
expect(controller.selection.baseOffset, 29);
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
// Immediately start a new floating cursor, in the same way as happens when
// the user tries to select text in trackpad mode.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Start, offset: const Offset(20, 20)));
await tester.pumpAndSettle();
// Set and move the second cursor like a selection. Previously, the second
// Update here caused a crash.
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-250, 20),
));
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
});
testWidgets('autofocus sets cursor to the end of text', (WidgetTester tester) async {
const String text = 'hello world';
controller.text = text;
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: FocusScope(
node: focusScopeNode,
autofocus: true,
child: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
autofocus: true,
style: textStyle,
cursorColor: cursorColor,
),
),
),
),
);
expect(focusNode.hasFocus, true);
expect(controller.selection.isCollapsed, true);
expect(controller.selection.baseOffset, text.length);
});
testWidgets('Floating cursor is painted', (WidgetTester tester) async {
const TextStyle textStyle = TextStyle();
const String text = 'hello world this is fun and cool and awesome!';
controller.text = text;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Padding(
padding: const EdgeInsets.only(top: 0.25),
child: Material(
child: TextField(
controller: controller,
focusNode: focusNode,
style: textStyle,
),
),
),
),
);
await tester.tap(find.byType(EditableText));
final RenderEditable editable = findRenderEditable(tester);
editable.selection = const TextSelection(baseOffset: 29, extentOffset: 29);
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
editableTextState.updateFloatingCursor(
RawFloatingCursorPoint(state: FloatingCursorDragState.Start, offset: const Offset(20, 20)),
);
await tester.pump();
expect(editable, paints
..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(463.8333435058594, -0.916666666666668, 466.8333435058594, 19.083333969116211),
const Radius.circular(1.0),
),
color: const Color(0xbf2196f3),
),
);
// Moves the cursor right a few characters.
editableTextState.updateFloatingCursor(
RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(-250, 20),
),
);
expect(find.byType(EditableText), paints
..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(193.83334350585938, -0.916666666666668, 3.0, 20.0),
const Radius.circular(1.0),
),
color: const Color(0xbf2196f3),
),
);
// Move the cursor away from characters, this will show the regular cursor.
editableTextState.updateFloatingCursor(
RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: const Offset(800, 0),
),
);
expect(find.byType(EditableText), paints
..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(719.3333333333333, -0.9166666666666679, 2.0, 18.0),
const Radius.circular(2.0),
),
color: const Color(0xff999999),
)
..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(800.5, -5.0, 803.5, 15.0),
const Radius.circular(1.0),
),
color: const Color(0xbf2196f3),
),
);
editableTextState.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
debugDefaultTargetPlatformOverride = null;
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }),
);
testWidgets('cursor layout', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
late String changedValue;
final Widget widget = MaterialApp(
home: RepaintBoundary(
key: const ValueKey<int>(1),
child: Column(
children: <Widget>[
const SizedBox(width: 10, height: 10),
EditableText(
backgroundCursorColor: Colors.grey,
key: editableTextKey,
controller: controller,
focusNode: focusNode,
style: Typography.material2018(platform: TargetPlatform.iOS).black.titleMedium!,
cursorColor: Colors.blue,
selectionControls: materialTextSelectionControls,
keyboardType: TextInputType.text,
onChanged: (String value) {
changedValue = value;
},
cursorWidth: 15.0,
),
],
),
),
);
await tester.pumpWidget(widget);
// Populate a fake clipboard.
const String clipboardContent = 'Hello world!';
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'Clipboard.getData') {
return const <String, dynamic>{'text': clipboardContent};
}
if (methodCall.method == 'Clipboard.hasStrings') {
return <String, dynamic>{'value': clipboardContent.isNotEmpty};
}
return null;
});
// Long-press to bring up the text editing controls.
final Finder textFinder = find.byKey(editableTextKey);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
await tester.tap(find.text('Paste'));
await tester.pump();
expect(changedValue, clipboardContent);
await expectLater(
find.byKey(const ValueKey<int>(1)),
matchesGoldenFile('editable_text_test.2.png'),
);
EditableText.debugDeterministicCursor = false;
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('cursor layout has correct height', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
late String changedValue;
final Widget widget = MaterialApp(
home: RepaintBoundary(
key: const ValueKey<int>(1),
child: Column(
children: <Widget>[
const SizedBox(width: 10, height: 10),
EditableText(
backgroundCursorColor: Colors.grey,
key: editableTextKey,
controller: controller,
focusNode: focusNode,
style: Typography.material2018(platform: TargetPlatform.iOS).black.titleMedium!,
cursorColor: Colors.blue,
selectionControls: materialTextSelectionControls,
keyboardType: TextInputType.text,
onChanged: (String value) {
changedValue = value;
},
cursorWidth: 15.0,
cursorHeight: 30.0,
),
],
),
),
);
await tester.pumpWidget(widget);
// Populate a fake clipboard.
const String clipboardContent = 'Hello world!';
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'Clipboard.getData') {
return const <String, dynamic>{'text': clipboardContent};
}
if (methodCall.method == 'Clipboard.hasStrings') {
return <String, dynamic>{'value': clipboardContent.isNotEmpty};
}
return null;
});
// Long-press to bring up the text editing controls.
final Finder textFinder = find.byKey(editableTextKey);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
await tester.tap(find.text('Paste'));
await tester.pump();
expect(changedValue, clipboardContent);
await expectLater(
find.byKey(const ValueKey<int>(1)),
matchesGoldenFile('editable_text_test.3.png'),
);
EditableText.debugDeterministicCursor = false;
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('password briefly does not show last character when disabled by system', (WidgetTester tester) async {
final bool debugDeterministicCursor = EditableText.debugDeterministicCursor;
EditableText.debugDeterministicCursor = false;
addTearDown(() {
EditableText.debugDeterministicCursor = debugDeterministicCursor;
});
await tester.pumpWidget(MaterialApp(
home: EditableText(
backgroundCursorColor: Colors.grey,
controller: controller,
obscureText: true,
focusNode: focusNode,
style: textStyle,
cursorColor: cursorColor,
),
));
await tester.enterText(find.byType(EditableText), 'AA');
await tester.pump();
await tester.enterText(find.byType(EditableText), 'AAA');
await tester.pump();
tester.binding.platformDispatcher.brieflyShowPasswordTestValue = false;
addTearDown(() {
tester.binding.platformDispatcher.brieflyShowPasswordTestValue = true;
});
expect((findRenderEditable(tester).text! as TextSpan).text, '••A');
await tester.pump(const Duration(milliseconds: 500));
expect((findRenderEditable(tester).text! as TextSpan).text, '•••');
await tester.pump(const Duration(milliseconds: 500));
await tester.pump(const Duration(milliseconds: 500));
await tester.pump(const Duration(milliseconds: 500));
expect((findRenderEditable(tester).text! as TextSpan).text, '•••');
});
testWidgets('getLocalRectForCaret with empty text', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
addTearDown(() { EditableText.debugDeterministicCursor = false; });
const String text = '12';
final TextEditingController controller = TextEditingController.fromValue(
const TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: text.length),
),
);
addTearDown(controller.dispose);
final Widget widget = EditableText(
autofocus: true,
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: const TextStyle(fontSize: 20),
textAlign: TextAlign.center,
keyboardType: TextInputType.text,
cursorColor: cursorColor,
maxLines: null,
);
await tester.pumpWidget(MaterialApp(home: widget));
final EditableTextState editableTextState = tester.firstState(find.byWidget(widget));
final RenderEditable renderEditable = editableTextState.renderEditable;
final Rect initialLocalCaretRect = renderEditable.getLocalRectForCaret(const TextPosition(offset: text.length));
for (int i = 0; i < 3; i++) {
Actions.invoke(primaryFocus!.context!, const DeleteCharacterIntent(forward: false));
await tester.pump();
expect(controller.text.length, math.max(0, text.length - 1 - i));
final Rect localRect = renderEditable.getLocalRectForCaret(
TextPosition(offset: controller.text.length),
);
expect(localRect.size, initialLocalCaretRect.size);
expect(localRect.top, initialLocalCaretRect.top);
expect(localRect.left, lessThan(initialLocalCaretRect.left));
}
expect(controller.text, isEmpty);
});
testWidgets('Caret center space test', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
addTearDown(() { EditableText.debugDeterministicCursor = false; });
final String text = 'test${' ' * 1000}';
final TextEditingController controller = TextEditingController.fromValue(
TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: text.length, affinity: TextAffinity.upstream),
),
);
addTearDown(controller.dispose);
final Widget widget = EditableText(
autofocus: true,
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: const TextStyle(),
textAlign: TextAlign.center,
keyboardType: TextInputType.text,
cursorColor: cursorColor,
cursorWidth: 13.0,
cursorHeight: 17.0,
maxLines: null,
);
await tester.pumpWidget(MaterialApp(home: widget));
final EditableTextState editableTextState = tester.firstState(find.byWidget(widget));
final Rect editableTextRect = tester.getRect(find.byWidget(widget));
final RenderEditable renderEditable = editableTextState.renderEditable;
// The trailing whitespaces are not line break opportunities.
expect(renderEditable.getLineAtOffset(TextPosition(offset: text.length)).start, 0);
// The caretRect shouldn't be outside of the RenderEditable.
final Rect caretRect = Rect.fromLTWH(
editableTextRect.right - 13.0 - 1.0,
editableTextRect.top,
13.0,
17.0,
);
expect(
renderEditable,
paints..rect(color: cursorColor, rect: caretRect),
);
},
skip: isBrowser && !isCanvasKit, // https://github.com/flutter/flutter/issues/56308
);
testWidgets('getLocalRectForCaret reports the real caret Rect', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
addTearDown(() { EditableText.debugDeterministicCursor = false; });
final String text = 'test${' ' * 50}\n'
'2nd line\n'
'\n';
final TextEditingController controller = TextEditingController.fromValue(TextEditingValue(
text: text,
selection: const TextSelection.collapsed(offset: 0),
));
addTearDown(controller.dispose);
final Widget widget = EditableText(
autofocus: true,
backgroundCursorColor: Colors.grey,
controller: controller,
focusNode: focusNode,
style: const TextStyle(fontSize: 20),
textAlign: TextAlign.center,
keyboardType: TextInputType.text,
cursorColor: cursorColor,
maxLines: null,
);
await tester.pumpWidget(MaterialApp(home: widget));
final EditableTextState editableTextState = tester.firstState(find.byWidget(widget));
final Rect editableTextRect = tester.getRect(find.byWidget(widget));
final RenderEditable renderEditable = editableTextState.renderEditable;
final Iterable<TextPosition> positions = List<int>
.generate(text.length + 1, (int index) => index)
.expand((int i) => <TextPosition>[TextPosition(offset: i, affinity: TextAffinity.upstream), TextPosition(offset: i)]);
for (final TextPosition position in positions) {
controller.selection = TextSelection.fromPosition(position);
await tester.pump();
final Rect localRect = renderEditable.getLocalRectForCaret(position);
expect(
renderEditable,
paints..rect(color: cursorColor, rect: localRect.shift(editableTextRect.topLeft)),
);
}
},
variant: TargetPlatformVariant.all(),
);
testWidgets('Floating cursor showing with local position', (WidgetTester tester) async {
EditableText.debugDeterministicCursor = true;
final GlobalKey key = GlobalKey();
controller.text = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ\n1234567890';
controller.selection = const TextSelection.collapsed(offset: 0);
await tester.pumpWidget(
MaterialApp(
home: EditableText(
key: key,
autofocus: true,
controller: controller,
focusNode: focusNode,
style: textStyle,
cursorColor: Colors.blue,
backgroundCursorColor: Colors.grey,
cursorOpacityAnimates: true,
maxLines: 2,
),
),
);
final EditableTextState state = tester.state(find.byType(EditableText));
state.updateFloatingCursor(
RawFloatingCursorPoint(
state: FloatingCursorDragState.Start,
offset: Offset.zero,
startLocation: (Offset.zero, TextPosition(offset: controller.selection.baseOffset, affinity: controller.selection.affinity))
)
);
await tester.pump();
expect(key.currentContext!.findRenderObject(), paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(-0.5, -3.0, 3, 12),
const Radius.circular(1)
)
));
state.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Update, offset: const Offset(51, 0)));
await tester.pump();
expect(key.currentContext!.findRenderObject(), paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(50.5, -3.0, 3, 12),
const Radius.circular(1)
)
));
state.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
state.updateFloatingCursor(
RawFloatingCursorPoint(
state: FloatingCursorDragState.Start,
offset: Offset.zero,
startLocation: (const Offset(800, 10), TextPosition(offset: controller.selection.baseOffset, affinity: controller.selection.affinity))
)
);
await tester.pump();
expect(key.currentContext!.findRenderObject(), paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(799.5, 4.0, 3, 12),
const Radius.circular(1)
)
));
state.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.Update, offset: const Offset(100, 10)));
await tester.pump();
expect(key.currentContext!.findRenderObject(), paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTWH(800.5, 14.0, 3, 12),
const Radius.circular(1)
)
));
state.updateFloatingCursor(RawFloatingCursorPoint(state: FloatingCursorDragState.End));
await tester.pumpAndSettle();
EditableText.debugDeterministicCursor = false;
});
}
| flutter/packages/flutter/test/widgets/editable_text_cursor_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/editable_text_cursor_test.dart",
"repo_id": "flutter",
"token_count": 19297
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('onSaved callback is called', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String? fieldValue;
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: TextFormField(
onSaved: (String? value) { fieldValue = value; },
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(fieldValue, isNull);
Future<void> checkText(String testValue) async {
await tester.enterText(find.byType(TextFormField), testValue);
formKey.currentState!.save();
// Pumping is unnecessary because callback happens regardless of frames.
expect(fieldValue, equals(testValue));
}
await checkText('Test');
await checkText('');
});
testWidgets('onChanged callback is called', (WidgetTester tester) async {
String? fieldValue;
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: TextField(
onChanged: (String value) { fieldValue = value; },
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(fieldValue, isNull);
Future<void> checkText(String testValue) async {
await tester.enterText(find.byType(TextField), testValue);
// Pumping is unnecessary because callback happens regardless of frames.
expect(fieldValue, equals(testValue));
}
await checkText('Test');
await checkText('');
});
testWidgets('Validator sets the error text only when validate is called', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String? errorText(String? value) => '${value ?? ''}/error';
Widget builder(AutovalidateMode autovalidateMode) {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
autovalidateMode: autovalidateMode,
child: TextFormField(
validator: errorText,
),
),
),
),
),
),
);
}
// Start off not autovalidating.
await tester.pumpWidget(builder(AutovalidateMode.disabled));
Future<void> checkErrorText(String testValue) async {
formKey.currentState!.reset();
await tester.pumpWidget(builder(AutovalidateMode.disabled));
await tester.enterText(find.byType(TextFormField), testValue);
await tester.pump();
// We have to manually validate if we're not autovalidating.
expect(find.text(errorText(testValue)!), findsNothing);
formKey.currentState!.validate();
await tester.pump();
expect(find.text(errorText(testValue)!), findsOneWidget);
// Try again with autovalidation. Should validate immediately.
formKey.currentState!.reset();
await tester.pumpWidget(builder(AutovalidateMode.always));
await tester.enterText(find.byType(TextFormField), testValue);
await tester.pump();
expect(find.text(errorText(testValue)!), findsOneWidget);
}
await checkErrorText('Test');
await checkErrorText('');
});
testWidgets('Should announce error text when validate returns error', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
await tester.pumpWidget(
MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: TextFormField(
validator: (_)=> 'error',
),
),
),
),
),
),
),
);
formKey.currentState!.reset();
await tester.enterText(find.byType(TextFormField), '');
await tester.pump();
// Manually validate.
expect(find.text('error'), findsNothing);
formKey.currentState!.validate();
await tester.pump();
expect(find.text('error'), findsOneWidget);
final CapturedAccessibilityAnnouncement announcement = tester.takeAnnouncements().single;
expect(announcement.message, 'error');
expect(announcement.textDirection, TextDirection.ltr);
expect(announcement.assertiveness, Assertiveness.assertive);
});
testWidgets('isValid returns true when a field is valid', (WidgetTester tester) async {
final GlobalKey<FormFieldState<String>> fieldKey1 = GlobalKey<FormFieldState<String>>();
final GlobalKey<FormFieldState<String>> fieldKey2 = GlobalKey<FormFieldState<String>>();
const String validString = 'Valid string';
String? validator(String? s) => s == validString ? null : 'Error text';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: ListView(
children: <Widget>[
TextFormField(
key: fieldKey1,
initialValue: validString,
validator: validator,
autovalidateMode: AutovalidateMode.always,
),
TextFormField(
key: fieldKey2,
initialValue: validString,
validator: validator,
autovalidateMode: AutovalidateMode.always,
),
],
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(fieldKey1.currentState!.isValid, isTrue);
expect(fieldKey2.currentState!.isValid, isTrue);
});
testWidgets(
'isValid returns false when the field is invalid and does not change error display',
(WidgetTester tester) async {
final GlobalKey<FormFieldState<String>> fieldKey1 = GlobalKey<FormFieldState<String>>();
final GlobalKey<FormFieldState<String>> fieldKey2 = GlobalKey<FormFieldState<String>>();
const String validString = 'Valid string';
String? validator(String? s) => s == validString ? null : 'Error text';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: ListView(
children: <Widget>[
TextFormField(
key: fieldKey1,
initialValue: validString,
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
TextFormField(
key: fieldKey2,
initialValue: '',
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
],
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(fieldKey1.currentState!.isValid, isTrue);
expect(fieldKey2.currentState!.isValid, isFalse);
expect(fieldKey2.currentState!.hasError, isFalse);
},
);
testWidgets(
'validateGranularly returns a set containing all, and only, invalid fields',
(WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
final UniqueKey validFieldsKey = UniqueKey();
final UniqueKey invalidFieldsKey = UniqueKey();
const String validString = 'Valid string';
const String invalidString = 'Invalid string';
String? validator(String? s) => s == validString ? null : 'Error text';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: ListView(
children: <Widget>[
TextFormField(
key: validFieldsKey,
initialValue: validString,
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
TextFormField(
key: invalidFieldsKey,
initialValue: invalidString,
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
TextFormField(
key: invalidFieldsKey,
initialValue: invalidString,
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
],
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
final Set<FormFieldState<dynamic>> validationResult = formKey.currentState!.validateGranularly();
expect(validationResult.length, equals(2));
expect(validationResult.where((FormFieldState<dynamic> field) => field.widget.key == invalidFieldsKey).length, equals(2));
expect(validationResult.where((FormFieldState<dynamic> field) => field.widget.key == validFieldsKey).length, equals(0));
},
);
testWidgets(
'Should announce error text when validateGranularly is called',
(WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
const String validString = 'Valid string';
String? validator(String? s) => s == validString ? null : 'error';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: ListView(
children: <Widget>[
TextFormField(
initialValue: validString,
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
TextFormField(
initialValue: '',
validator: validator,
autovalidateMode: AutovalidateMode.disabled,
),
],
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(find.text('error'), findsNothing);
formKey.currentState!.validateGranularly();
await tester.pump();
expect(find.text('error'), findsOneWidget);
final CapturedAccessibilityAnnouncement announcement = tester.takeAnnouncements().single;
expect(announcement.message, 'error');
expect(announcement.textDirection, TextDirection.ltr);
expect(announcement.assertiveness, Assertiveness.assertive);
},
);
testWidgets('Multiple TextFormFields communicate', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
final GlobalKey<FormFieldState<String>> fieldKey = GlobalKey<FormFieldState<String>>();
// Input 2's validator depends on a input 1's value.
String? errorText(String? input) => '${fieldKey.currentState!.value}/error';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
autovalidateMode: AutovalidateMode.always,
child: ListView(
children: <Widget>[
TextFormField(
key: fieldKey,
),
TextFormField(
validator: errorText,
),
],
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
Future<void> checkErrorText(String testValue) async {
await tester.enterText(find.byType(TextFormField).first, testValue);
await tester.pump();
// Check for a new Text widget with our error text.
expect(find.text('$testValue/error'), findsOneWidget);
return;
}
await checkErrorText('Test');
await checkErrorText('');
});
testWidgets('Provide initial value to input when no controller is specified', (WidgetTester tester) async {
const String initialValue = 'hello';
final GlobalKey<FormFieldState<String>> inputKey = GlobalKey<FormFieldState<String>>();
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: TextFormField(
key: inputKey,
initialValue: 'hello',
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
await tester.showKeyboard(find.byType(TextFormField));
// initial value should be loaded into keyboard editing state
expect(tester.testTextInput.editingState, isNotNull);
expect(tester.testTextInput.editingState!['text'], equals(initialValue));
// initial value should also be visible in the raw input line
final EditableTextState editableText = tester.state(find.byType(EditableText));
expect(editableText.widget.controller.text, equals(initialValue));
// sanity check, make sure we can still edit the text and everything updates
expect(inputKey.currentState!.value, equals(initialValue));
await tester.enterText(find.byType(TextFormField), 'world');
await tester.pump();
expect(inputKey.currentState!.value, equals('world'));
expect(editableText.widget.controller.text, equals('world'));
});
testWidgets('Controller defines initial value', (WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'hello');
addTearDown(controller.dispose);
const String initialValue = 'hello';
final GlobalKey<FormFieldState<String>> inputKey = GlobalKey<FormFieldState<String>>();
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: TextFormField(
key: inputKey,
controller: controller,
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
await tester.showKeyboard(find.byType(TextFormField));
// initial value should be loaded into keyboard editing state
expect(tester.testTextInput.editingState, isNotNull);
expect(tester.testTextInput.editingState!['text'], equals(initialValue));
// initial value should also be visible in the raw input line
final EditableTextState editableText = tester.state(find.byType(EditableText));
expect(editableText.widget.controller.text, equals(initialValue));
expect(controller.text, equals(initialValue));
// sanity check, make sure we can still edit the text and everything updates
expect(inputKey.currentState!.value, equals(initialValue));
await tester.enterText(find.byType(TextFormField), 'world');
await tester.pump();
expect(inputKey.currentState!.value, equals('world'));
expect(editableText.widget.controller.text, equals('world'));
expect(controller.text, equals('world'));
});
testWidgets('TextFormField resets to its initial value', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
final GlobalKey<FormFieldState<String>> inputKey = GlobalKey<FormFieldState<String>>();
final TextEditingController controller = TextEditingController(text: 'Plover');
addTearDown(controller.dispose);
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: TextFormField(
key: inputKey,
controller: controller,
// initialValue is 'Plover'
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
await tester.showKeyboard(find.byType(TextFormField));
final EditableTextState editableText = tester.state(find.byType(EditableText));
// overwrite initial value.
controller.text = 'Xyzzy';
await tester.idle();
expect(editableText.widget.controller.text, equals('Xyzzy'));
expect(inputKey.currentState!.value, equals('Xyzzy'));
expect(controller.text, equals('Xyzzy'));
// verify value resets to initialValue on reset.
formKey.currentState!.reset();
await tester.idle();
expect(inputKey.currentState!.value, equals('Plover'));
expect(editableText.widget.controller.text, equals('Plover'));
expect(controller.text, equals('Plover'));
});
testWidgets('TextEditingController updates to/from form field value', (WidgetTester tester) async {
final TextEditingController controller1 = TextEditingController(text: 'Foo');
addTearDown(controller1.dispose);
final TextEditingController controller2 = TextEditingController(text: 'Bar');
addTearDown(controller2.dispose);
final GlobalKey<FormFieldState<String>> inputKey = GlobalKey<FormFieldState<String>>();
TextEditingController? currentController;
late StateSetter setState;
Widget builder() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
child: TextFormField(
key: inputKey,
controller: currentController,
),
),
),
),
),
),
);
},
);
}
await tester.pumpWidget(builder());
await tester.showKeyboard(find.byType(TextFormField));
// verify initially empty.
expect(tester.testTextInput.editingState, isNotNull);
expect(tester.testTextInput.editingState!['text'], isEmpty);
final EditableTextState editableText = tester.state(find.byType(EditableText));
expect(editableText.widget.controller.text, isEmpty);
// verify changing the controller from null to controller1 sets the value.
setState(() {
currentController = controller1;
});
await tester.pump();
expect(editableText.widget.controller.text, equals('Foo'));
expect(inputKey.currentState!.value, equals('Foo'));
// verify changes to controller1 text are visible in text field and set in form value.
controller1.text = 'Wobble';
await tester.idle();
expect(editableText.widget.controller.text, equals('Wobble'));
expect(inputKey.currentState!.value, equals('Wobble'));
// verify changes to the field text update the form value and controller1.
await tester.enterText(find.byType(TextFormField), 'Wibble');
await tester.pump();
expect(inputKey.currentState!.value, equals('Wibble'));
expect(editableText.widget.controller.text, equals('Wibble'));
expect(controller1.text, equals('Wibble'));
// verify that switching from controller1 to controller2 is handled.
setState(() {
currentController = controller2;
});
await tester.pump();
expect(inputKey.currentState!.value, equals('Bar'));
expect(editableText.widget.controller.text, equals('Bar'));
expect(controller2.text, equals('Bar'));
expect(controller1.text, equals('Wibble'));
// verify changes to controller2 text are visible in text field and set in form value.
controller2.text = 'Xyzzy';
await tester.idle();
expect(editableText.widget.controller.text, equals('Xyzzy'));
expect(inputKey.currentState!.value, equals('Xyzzy'));
expect(controller1.text, equals('Wibble'));
// verify changes to controller1 text are not visible in text field or set in form value.
controller1.text = 'Plugh';
await tester.idle();
expect(editableText.widget.controller.text, equals('Xyzzy'));
expect(inputKey.currentState!.value, equals('Xyzzy'));
expect(controller1.text, equals('Plugh'));
// verify that switching from controller2 to null is handled.
setState(() {
currentController = null;
});
await tester.pump();
expect(inputKey.currentState!.value, equals('Xyzzy'));
expect(editableText.widget.controller.text, equals('Xyzzy'));
expect(controller2.text, equals('Xyzzy'));
expect(controller1.text, equals('Plugh'));
// verify that changes to the field text update the form value but not the previous controllers.
await tester.enterText(find.byType(TextFormField), 'Plover');
await tester.pump();
expect(inputKey.currentState!.value, equals('Plover'));
expect(editableText.widget.controller.text, equals('Plover'));
expect(controller1.text, equals('Plugh'));
expect(controller2.text, equals('Xyzzy'));
});
testWidgets('No crash when a TextFormField is removed from the tree', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String? fieldValue;
Widget builder(bool remove) {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: remove ? Container() : TextFormField(
autofocus: true,
onSaved: (String? value) { fieldValue = value; },
validator: (String? value) { return (value == null || value.isEmpty) ? null : 'yes'; },
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder(false));
expect(fieldValue, isNull);
expect(formKey.currentState!.validate(), isTrue);
await tester.enterText(find.byType(TextFormField), 'Test');
await tester.pumpWidget(builder(false));
// Form wasn't saved yet.
expect(fieldValue, null);
expect(formKey.currentState!.validate(), isFalse);
formKey.currentState!.save();
// Now fieldValue is saved.
expect(fieldValue, 'Test');
expect(formKey.currentState!.validate(), isFalse);
// Now remove the field with an error.
await tester.pumpWidget(builder(true));
// Reset the form. Should not crash.
formKey.currentState!.reset();
formKey.currentState!.save();
expect(formKey.currentState!.validate(), isTrue);
});
testWidgets('Does not auto-validate before value changes when autovalidateMode is set to onUserInteraction', (WidgetTester tester) async {
late FormFieldState<String> formFieldState;
String? errorText(String? value) => '$value/error';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: FormField<String>(
initialValue: 'foo',
autovalidateMode: AutovalidateMode.onUserInteraction,
builder: (FormFieldState<String> state) {
formFieldState = state;
return Container();
},
validator: errorText,
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
// The form field has no error.
expect(formFieldState.hasError, isFalse);
// No error widget is visible.
expect(find.text(errorText('foo')!), findsNothing);
});
testWidgets('auto-validate before value changes if autovalidateMode was set to always', (WidgetTester tester) async {
late FormFieldState<String> formFieldState;
String? errorText(String? value) => '$value/error';
Widget builder() {
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: FormField<String>(
initialValue: 'foo',
autovalidateMode: AutovalidateMode.always,
builder: (FormFieldState<String> state) {
formFieldState = state;
return Container();
},
validator: errorText,
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
expect(formFieldState.hasError, isTrue);
});
testWidgets('Form auto-validates form fields only after one of them changes if autovalidateMode is onUserInteraction', (WidgetTester tester) async {
const String initialValue = 'foo';
String? errorText(String? value) => 'error/$value';
Widget builder() {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
autovalidateMode: AutovalidateMode.onUserInteraction,
child: Column(
children: <Widget>[
TextFormField(
initialValue: initialValue,
validator: errorText,
),
TextFormField(
initialValue: initialValue,
validator: errorText,
),
TextFormField(
initialValue: initialValue,
validator: errorText,
),
],
),
),
),
),
),
);
}
// Makes sure the Form widget won't auto-validate the form fields
// after rebuilds if there is not user interaction.
await tester.pumpWidget(builder());
await tester.pumpWidget(builder());
// We expect no validation error text being shown.
expect(find.text(errorText(initialValue)!), findsNothing);
// Set a empty string into the first form field to
// trigger the fields validators.
await tester.enterText(find.byType(TextFormField).first, '');
await tester.pump();
// Now we expect the errors to be shown for the first Text Field and
// for the next two form fields that have their contents unchanged.
expect(find.text(errorText('')!), findsOneWidget);
expect(find.text(errorText(initialValue)!), findsNWidgets(2));
});
testWidgets('Form auto-validates form fields even before any have changed if autovalidateMode is set to always', (WidgetTester tester) async {
String? errorText(String? value) => 'error/$value';
Widget builder() {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
autovalidateMode: AutovalidateMode.always,
child: TextFormField(
validator: errorText,
),
),
),
),
),
);
}
// The issue only happens on the second build so we
// need to rebuild the tree twice.
await tester.pumpWidget(builder());
await tester.pumpWidget(builder());
// We expect validation error text being shown.
expect(find.text(errorText('')!), findsOneWidget);
});
testWidgets('Form.reset() resets form fields, and auto validation will only happen on the next user interaction if autovalidateMode is onUserInteraction', (WidgetTester tester) async {
final GlobalKey<FormState> formState = GlobalKey<FormState>();
String? errorText(String? value) => '$value/error';
Widget builder() {
return MaterialApp(
theme: ThemeData(),
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Form(
key: formState,
autovalidateMode: AutovalidateMode.onUserInteraction,
child: Material(
child: TextFormField(
initialValue: 'foo',
validator: errorText,
),
),
),
),
),
),
);
}
await tester.pumpWidget(builder());
// No error text is visible yet.
expect(find.text(errorText('foo')!), findsNothing);
await tester.enterText(find.byType(TextFormField), 'bar');
await tester.pumpAndSettle();
await tester.pump();
expect(find.text(errorText('bar')!), findsOneWidget);
// Resetting the form state should remove the error text.
formState.currentState!.reset();
await tester.pump();
expect(find.text(errorText('bar')!), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/63753.
testWidgets('Validate form should return correct validation if the value is composing', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String? fieldValue;
final Widget widget = MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: TextFormField(
maxLength: 5,
maxLengthEnforcement: MaxLengthEnforcement.truncateAfterCompositionEnds,
onSaved: (String? value) { fieldValue = value; },
validator: (String? value) => (value != null && value.length > 5) ? 'Exceeded' : null,
),
),
),
),
),
),
);
await tester.pumpWidget(widget);
final EditableTextState editableText = tester.state<EditableTextState>(find.byType(EditableText));
editableText.updateEditingValue(const TextEditingValue(text: '123456', composing: TextRange(start: 2, end: 5)));
expect(editableText.currentTextEditingValue.composing, const TextRange(start: 2, end: 5));
formKey.currentState!.save();
expect(fieldValue, '123456');
expect(formKey.currentState!.validate(), isFalse);
});
testWidgets('hasInteractedByUser returns false when the input has not changed', (WidgetTester tester) async {
final GlobalKey<FormFieldState<String>> fieldKey = GlobalKey<FormFieldState<String>>();
final Widget widget = MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: TextFormField(
key: fieldKey,
),
),
),
),
),
);
await tester.pumpWidget(widget);
expect(fieldKey.currentState!.hasInteractedByUser, isFalse);
});
testWidgets('hasInteractedByUser returns true after the input has changed', (WidgetTester tester) async {
final GlobalKey<FormFieldState<String>> fieldKey = GlobalKey<FormFieldState<String>>();
final Widget widget = MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: TextFormField(
key: fieldKey,
),
),
),
),
),
);
await tester.pumpWidget(widget);
// initially, the field has not been interacted with
expect(fieldKey.currentState!.hasInteractedByUser, isFalse);
// after entering text, the field has been interacted with
await tester.enterText(find.byType(TextFormField), 'foo');
expect(fieldKey.currentState!.hasInteractedByUser, isTrue);
});
testWidgets('hasInteractedByUser returns false after the field is reset', (WidgetTester tester) async {
final GlobalKey<FormFieldState<String>> fieldKey = GlobalKey<FormFieldState<String>>();
final Widget widget = MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: TextFormField(
key: fieldKey,
),
),
),
),
),
);
await tester.pumpWidget(widget);
// initially, the field has not been interacted with
expect(fieldKey.currentState!.hasInteractedByUser, isFalse);
// after entering text, the field has been interacted with
await tester.enterText(find.byType(TextFormField), 'foo');
expect(fieldKey.currentState!.hasInteractedByUser, isTrue);
// after resetting the field, it has not been interacted with again
fieldKey.currentState!.reset();
expect(fieldKey.currentState!.hasInteractedByUser, isFalse);
});
testWidgets('Validator is nullified and error text behaves accordingly',
(WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
bool useValidator = false;
late StateSetter setState;
String? validator(String? value) {
if (value == null || value.isEmpty) {
return 'test_error';
}
return null;
}
Widget builder() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return MaterialApp(
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Material(
child: Form(
key: formKey,
child: TextFormField(
validator: useValidator ? validator : null,
),
),
),
),
),
),
);
},
);
}
await tester.pumpWidget(builder());
// Start with no validator.
await tester.enterText(find.byType(TextFormField), '');
await tester.pump();
formKey.currentState!.validate();
await tester.pump();
expect(find.text('test_error'), findsNothing);
// Now use the validator.
setState(() {
useValidator = true;
});
await tester.pump();
formKey.currentState!.validate();
await tester.pump();
expect(find.text('test_error'), findsOneWidget);
// Remove the validator again and expect the error to disappear.
setState(() {
useValidator = false;
});
await tester.pump();
formKey.currentState!.validate();
await tester.pump();
expect(find.text('test_error'), findsNothing);
});
}
| flutter/packages/flutter/test/widgets/form_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/form_test.dart",
"repo_id": "flutter",
"token_count": 17225
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('IconDataDiagnosticsProperty includes valueProperties in JSON', () {
IconDataProperty property = IconDataProperty('foo', const IconData(101010));
final Map<String, Object> valueProperties = property.toJsonMap(const DiagnosticsSerializationDelegate())['valueProperties']! as Map<String, Object>;
expect(valueProperties['codePoint'], 101010);
property = IconDataProperty('foo', null);
final Map<String, Object?> json = property.toJsonMap(const DiagnosticsSerializationDelegate());
expect(json.containsKey('valueProperties'), isFalse);
});
}
| flutter/packages/flutter/test/widgets/icon_data_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/icon_data_test.dart",
"repo_id": "flutter",
"token_count": 275
} | 711 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'test_widgets.dart';
class TestInherited extends InheritedWidget {
const TestInherited({ super.key, required super.child, this.shouldNotify = true });
final bool shouldNotify;
@override
bool updateShouldNotify(InheritedWidget oldWidget) {
return shouldNotify;
}
}
class ValueInherited extends InheritedWidget {
const ValueInherited({ super.key, required super.child, required this.value });
final int value;
@override
bool updateShouldNotify(ValueInherited oldWidget) => value != oldWidget.value;
}
class ExpectFail extends StatefulWidget {
const ExpectFail(this.onError, { super.key });
final VoidCallback onError;
@override
ExpectFailState createState() => ExpectFailState();
}
class ExpectFailState extends State<ExpectFail> {
@override
void initState() {
super.initState();
try {
context.dependOnInheritedWidgetOfExactType<TestInherited>(); // should fail
} catch (e) {
widget.onError();
}
}
@override
Widget build(BuildContext context) => Container();
}
class ChangeNotifierInherited extends InheritedNotifier<ChangeNotifier> {
const ChangeNotifierInherited({ super.key, required super.child, super.notifier });
}
void main() {
testWidgets('Inherited notifies dependents', (WidgetTester tester) async {
final List<TestInherited> log = <TestInherited>[];
final Builder builder = Builder(
builder: (BuildContext context) {
log.add(context.dependOnInheritedWidgetOfExactType<TestInherited>()!);
return Container();
},
);
final TestInherited first = TestInherited(child: builder);
await tester.pumpWidget(first);
expect(log, equals(<TestInherited>[first]));
final TestInherited second = TestInherited(shouldNotify: false, child: builder);
await tester.pumpWidget(second);
expect(log, equals(<TestInherited>[first]));
final TestInherited third = TestInherited(child: builder);
await tester.pumpWidget(third);
expect(log, equals(<TestInherited>[first, third]));
});
testWidgets('Update inherited when reparenting state', (WidgetTester tester) async {
final GlobalKey globalKey = GlobalKey();
final List<TestInherited> log = <TestInherited>[];
TestInherited build() {
return TestInherited(
key: UniqueKey(),
child: Container(
key: globalKey,
child: Builder(
builder: (BuildContext context) {
log.add(context.dependOnInheritedWidgetOfExactType<TestInherited>()!);
return Container();
},
),
),
);
}
final TestInherited first = build();
await tester.pumpWidget(first);
expect(log, equals(<TestInherited>[first]));
final TestInherited second = build();
await tester.pumpWidget(second);
expect(log, equals(<TestInherited>[first, second]));
});
testWidgets('Update inherited when removing node', (WidgetTester tester) async {
final List<String> log = <String>[];
await tester.pumpWidget(
ValueInherited(
value: 1,
child: FlipWidget(
left: ValueInherited(
value: 2,
child: ValueInherited(
value: 3,
child: Builder(
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add('a: ${v.value}');
return const Text('', textDirection: TextDirection.ltr);
},
),
),
),
right: ValueInherited(
value: 2,
child: Builder(
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add('b: ${v.value}');
return const Text('', textDirection: TextDirection.ltr);
},
),
),
),
),
);
expect(log, equals(<String>['a: 3']));
log.clear();
await tester.pump();
expect(log, equals(<String>[]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<String>['b: 2']));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<String>['a: 3']));
log.clear();
});
testWidgets('Update inherited when removing node and child has global key', (WidgetTester tester) async {
final List<String> log = <String>[];
final Key key = GlobalKey();
await tester.pumpWidget(
ValueInherited(
value: 1,
child: FlipWidget(
left: ValueInherited(
value: 2,
child: ValueInherited(
value: 3,
child: Container(
key: key,
child: Builder(
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add('a: ${v.value}');
return const Text('', textDirection: TextDirection.ltr);
},
),
),
),
),
right: ValueInherited(
value: 2,
child: Container(
key: key,
child: Builder(
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add('b: ${v.value}');
return const Text('', textDirection: TextDirection.ltr);
},
),
),
),
),
),
);
expect(log, equals(<String>['a: 3']));
log.clear();
await tester.pump();
expect(log, equals(<String>[]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<String>['b: 2']));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<String>['a: 3']));
log.clear();
});
testWidgets('Update inherited when removing node and child has global key with constant child', (WidgetTester tester) async {
final List<int> log = <int>[];
final Key key = GlobalKey();
final Widget child = Builder(
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add(v.value);
return const Text('', textDirection: TextDirection.ltr);
},
);
await tester.pumpWidget(
ValueInherited(
value: 1,
child: FlipWidget(
left: ValueInherited(
value: 2,
child: ValueInherited(
value: 3,
child: Container(
key: key,
child: child,
),
),
),
right: ValueInherited(
value: 2,
child: Container(
key: key,
child: child,
),
),
),
),
);
expect(log, equals(<int>[3]));
log.clear();
await tester.pump();
expect(log, equals(<int>[]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<int>[2]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<int>[3]));
log.clear();
});
testWidgets('Update inherited when removing node and child has global key with constant child, minimised', (WidgetTester tester) async {
final List<int> log = <int>[];
final Widget child = Builder(
key: GlobalKey(),
builder: (BuildContext context) {
final ValueInherited v = context.dependOnInheritedWidgetOfExactType<ValueInherited>()!;
log.add(v.value);
return const Text('', textDirection: TextDirection.ltr);
},
);
await tester.pumpWidget(
ValueInherited(
value: 2,
child: FlipWidget(
left: ValueInherited(
value: 3,
child: child,
),
right: child,
),
),
);
expect(log, equals(<int>[3]));
log.clear();
await tester.pump();
expect(log, equals(<int>[]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<int>[2]));
log.clear();
flipStatefulWidget(tester);
await tester.pump();
expect(log, equals(<int>[3]));
log.clear();
});
testWidgets('Inherited widget notifies descendants when descendant previously failed to find a match', (WidgetTester tester) async {
int? inheritedValue = -1;
final Widget inner = Container(
key: GlobalKey(),
child: Builder(
builder: (BuildContext context) {
final ValueInherited? widget = context.dependOnInheritedWidgetOfExactType<ValueInherited>();
inheritedValue = widget?.value;
return Container();
},
),
);
await tester.pumpWidget(
inner,
);
expect(inheritedValue, isNull);
inheritedValue = -2;
await tester.pumpWidget(
ValueInherited(
value: 3,
child: inner,
),
);
expect(inheritedValue, equals(3));
});
testWidgets("Inherited widget doesn't notify descendants when descendant did not previously fail to find a match and had no dependencies", (WidgetTester tester) async {
int buildCount = 0;
final Widget inner = Container(
key: GlobalKey(),
child: Builder(
builder: (BuildContext context) {
buildCount += 1;
return Container();
},
),
);
await tester.pumpWidget(
inner,
);
expect(buildCount, equals(1));
await tester.pumpWidget(
ValueInherited(
value: 3,
child: inner,
),
);
expect(buildCount, equals(1));
});
testWidgets('Inherited widget does notify descendants when descendant did not previously fail to find a match but did have other dependencies', (WidgetTester tester) async {
int buildCount = 0;
final Widget inner = Container(
key: GlobalKey(),
child: TestInherited(
shouldNotify: false,
child: Builder(
builder: (BuildContext context) {
context.dependOnInheritedWidgetOfExactType<TestInherited>();
buildCount += 1;
return Container();
},
),
),
);
await tester.pumpWidget(
inner,
);
expect(buildCount, equals(1));
await tester.pumpWidget(
ValueInherited(
value: 3,
child: inner,
),
);
expect(buildCount, equals(2));
});
testWidgets("BuildContext.getInheritedWidgetOfExactType doesn't create a dependency", (WidgetTester tester) async {
int buildCount = 0;
final GlobalKey<void> inheritedKey = GlobalKey();
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
final Widget builder = Builder(
builder: (BuildContext context) {
expect(context.getInheritedWidgetOfExactType<ChangeNotifierInherited>(), equals(inheritedKey.currentWidget));
buildCount += 1;
return Container();
},
);
final Widget inner = ChangeNotifierInherited(
key: inheritedKey,
notifier: notifier,
child: builder,
);
await tester.pumpWidget(inner);
expect(buildCount, equals(1));
notifier.notifyListeners();
await tester.pumpWidget(inner);
expect(buildCount, equals(1));
});
testWidgets('initState() dependency on Inherited asserts', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/5491
bool exceptionCaught = false;
final TestInherited parent = TestInherited(child: ExpectFail(() {
exceptionCaught = true;
}));
await tester.pumpWidget(parent);
expect(exceptionCaught, isTrue);
});
testWidgets('InheritedNotifier', (WidgetTester tester) async {
int buildCount = 0;
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
final Widget builder = Builder(
builder: (BuildContext context) {
context.dependOnInheritedWidgetOfExactType<ChangeNotifierInherited>();
buildCount += 1;
return Container();
},
);
final Widget inner = ChangeNotifierInherited(
notifier: notifier,
child: builder,
);
await tester.pumpWidget(inner);
expect(buildCount, equals(1));
await tester.pumpWidget(inner);
expect(buildCount, equals(1));
await tester.pump();
expect(buildCount, equals(1));
notifier.notifyListeners();
await tester.pump();
expect(buildCount, equals(2));
await tester.pumpWidget(inner);
expect(buildCount, equals(2));
await tester.pumpWidget(ChangeNotifierInherited(
child: builder,
));
expect(buildCount, equals(3));
});
}
| flutter/packages/flutter/test/widgets/inherited_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/inherited_test.dart",
"repo_id": "flutter",
"token_count": 5659
} | 712 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/src/foundation/assertions.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
const List<Widget> children = <Widget>[
SizedBox(width: 200.0, height: 150.0),
SizedBox(width: 200.0, height: 150.0),
SizedBox(width: 200.0, height: 150.0),
SizedBox(width: 200.0, height: 150.0),
];
void expectRects(WidgetTester tester, List<Rect> expected) {
final Finder finder = find.byType(SizedBox);
final List<Rect> actual = <Rect>[];
finder.runCached(() {
for (int i = 0; i < expected.length; ++i) {
final Finder current = finder.at(i);
expect(current, findsOneWidget);
actual.add(tester.getRect(finder.at(i)));
}
});
expect(() => finder.at(expected.length), throwsRangeError);
expect(actual, equals(expected));
}
void main() {
testWidgets('ListBody down', (WidgetTester tester) async {
await tester.pumpWidget(const Flex(
direction: Axis.vertical,
children: <Widget>[ ListBody(children: children) ],
));
expectRects(
tester,
<Rect>[
const Rect.fromLTWH(0.0, 0.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 150.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 300.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 450.0, 800.0, 150.0),
],
);
});
testWidgets('ListBody up', (WidgetTester tester) async {
await tester.pumpWidget(const Flex(
direction: Axis.vertical,
children: <Widget>[ ListBody(reverse: true, children: children) ],
));
expectRects(
tester,
<Rect>[
const Rect.fromLTWH(0.0, 450.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 300.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 150.0, 800.0, 150.0),
const Rect.fromLTWH(0.0, 0.0, 800.0, 150.0),
],
);
});
testWidgets('ListBody right', (WidgetTester tester) async {
await tester.pumpWidget(const Flex(
textDirection: TextDirection.ltr,
direction: Axis.horizontal,
children: <Widget>[
Directionality(
textDirection: TextDirection.ltr,
child: ListBody(mainAxis: Axis.horizontal, children: children),
),
],
));
expectRects(
tester,
<Rect>[
const Rect.fromLTWH(0.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(200.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(400.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(600.0, 0.0, 200.0, 600.0),
],
);
});
testWidgets('ListBody left', (WidgetTester tester) async {
await tester.pumpWidget(const Flex(
textDirection: TextDirection.ltr,
direction: Axis.horizontal,
children: <Widget>[
Directionality(
textDirection: TextDirection.rtl,
child: ListBody(mainAxis: Axis.horizontal, children: children),
),
],
));
expectRects(
tester,
<Rect>[
const Rect.fromLTWH(600.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(400.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(200.0, 0.0, 200.0, 600.0),
const Rect.fromLTWH(0.0, 0.0, 200.0, 600.0),
],
);
});
testWidgets('Limited space along main axis error', (WidgetTester tester) async {
final FlutterExceptionHandler oldHandler = FlutterError.onError!;
final List<FlutterErrorDetails> errors = <FlutterErrorDetails>[];
FlutterError.onError = (FlutterErrorDetails error) => errors.add(error);
try {
await tester.pumpWidget(
const SizedBox(
width: 100,
height: 100,
child: Directionality(
textDirection: TextDirection.rtl,
child: ListBody(
mainAxis: Axis.horizontal,
children: children,
),
),
),
);
} finally {
FlutterError.onError = oldHandler;
}
expect(errors, isNotEmpty);
expect(errors.first.exception, isFlutterError);
expect((errors.first.exception as FlutterError).toStringDeep(), equalsIgnoringHashCodes(
'FlutterError\n'
' RenderListBody must have unlimited space along its main axis.\n'
' RenderListBody does not clip or resize its children, so it must\n'
' be placed in a parent that does not constrain the main axis.\n'
' You probably want to put the RenderListBody inside a\n'
' RenderViewport with a matching main axis.\n',
));
});
testWidgets('Nested ListBody unbounded cross axis error', (WidgetTester tester) async {
final FlutterExceptionHandler oldHandler = FlutterError.onError!;
final List<FlutterErrorDetails> errors = <FlutterErrorDetails>[];
FlutterError.onError = (FlutterErrorDetails error) => errors.add(error);
try {
await tester.pumpWidget(
const Flex(
textDirection: TextDirection.ltr,
direction: Axis.horizontal,
children: <Widget>[
Directionality(
textDirection: TextDirection.ltr,
child: ListBody(
mainAxis: Axis.horizontal,
children: <Widget>[
Flex(
textDirection: TextDirection.ltr,
direction: Axis.vertical,
children: <Widget>[
Directionality(
textDirection: TextDirection.ltr,
child: ListBody(
children: children,
),
),
],
),
],
),
),
],
),
);
} finally {
FlutterError.onError = oldHandler;
}
expect(errors, isNotEmpty);
expect(errors.first.exception, isFlutterError);
expect((errors.first.exception as FlutterError).toStringDeep(), equalsIgnoringHashCodes(
'FlutterError\n'
' RenderListBody must have a bounded constraint for its cross axis.\n'
' RenderListBody forces its children to expand to fit the\n'
" RenderListBody's container, so it must be placed in a parent that\n"
' constrains the cross axis to a finite dimension.\n'
' If you are attempting to nest a RenderListBody with one direction\n'
' inside one of another direction, you will want to wrap the inner\n'
' one inside a box that fixes the dimension in that direction, for\n'
' example, a RenderIntrinsicWidth or RenderIntrinsicHeight object.\n'
' This is relatively expensive, however.\n',
));
});
}
| flutter/packages/flutter/test/widgets/list_body_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/list_body_test.dart",
"repo_id": "flutter",
"token_count": 2987
} | 713 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final TestAutomatedTestWidgetsFlutterBinding binding = TestAutomatedTestWidgetsFlutterBinding();
testWidgets('Locale is available when Localizations widget stops deferring frames', (WidgetTester tester) async {
final FakeLocalizationsDelegate delegate = FakeLocalizationsDelegate();
await tester.pumpWidget(Localizations(
locale: const Locale('fo'),
delegates: <LocalizationsDelegate<dynamic>>[
WidgetsLocalizationsDelegate(),
delegate,
],
child: const Text('loaded'),
));
final dynamic state = tester.state(find.byType(Localizations));
// ignore: avoid_dynamic_calls
expect(state!.locale, isNull);
expect(find.text('loaded'), findsNothing);
late Locale locale;
binding.onAllowFrame = () {
// ignore: avoid_dynamic_calls
locale = state.locale as Locale;
};
delegate.completer.complete('foo');
await tester.idle();
expect(locale, const Locale('fo'));
await tester.pump();
expect(find.text('loaded'), findsOneWidget);
});
testWidgets('Localizations.localeOf throws when no localizations exist', (WidgetTester tester) async {
final GlobalKey contextKey = GlobalKey(debugLabel: 'Test Key');
await tester.pumpWidget(Container(key: contextKey));
expect(() => Localizations.localeOf(contextKey.currentContext!), throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
contains('does not include a Localizations ancestor'),
)));
});
testWidgets('Localizations.maybeLocaleOf returns null when no localizations exist', (WidgetTester tester) async {
final GlobalKey contextKey = GlobalKey(debugLabel: 'Test Key');
await tester.pumpWidget(Container(key: contextKey));
expect(Localizations.maybeLocaleOf(contextKey.currentContext!), isNull);
});
}
class FakeLocalizationsDelegate extends LocalizationsDelegate<String> {
final Completer<String> completer = Completer<String>();
@override
bool isSupported(Locale locale) => true;
@override
Future<String> load(Locale locale) => completer.future;
@override
bool shouldReload(LocalizationsDelegate<String> old) => false;
}
class TestAutomatedTestWidgetsFlutterBinding extends AutomatedTestWidgetsFlutterBinding {
VoidCallback? onAllowFrame;
@override
void allowFirstFrame() {
onAllowFrame?.call();
super.allowFirstFrame();
}
}
class WidgetsLocalizationsDelegate extends LocalizationsDelegate<WidgetsLocalizations> {
@override
bool isSupported(Locale locale) => true;
@override
Future<WidgetsLocalizations> load(Locale locale) => DefaultWidgetsLocalizations.load(locale);
@override
bool shouldReload(WidgetsLocalizationsDelegate old) => false;
}
| flutter/packages/flutter/test/widgets/localizations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/localizations_test.dart",
"repo_id": "flutter",
"token_count": 986
} | 714 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Restoration Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', count: 1), findsOneWidget);
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 2), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 3), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', count: 2), findsOneWidget);
});
testWidgets('restorablePushNamed', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo', arguments: 3);
await tester.pumpAndSettle();
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 0, arguments: 3), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2, arguments: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar', arguments: 4);
await tester.pumpAndSettle();
expect(findRoute('Bar', arguments: 4), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
});
testWidgets('restorablePushReplacementNamed', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacementNamed('Foo', arguments: 3);
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, arguments: 3), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2, arguments: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar', arguments: 4);
await tester.pumpAndSettle();
expect(findRoute('Bar', arguments: 4), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
});
testWidgets('restorablePopAndPushNamed', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePopAndPushNamed('Foo', arguments: 3);
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, arguments: 3), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2, arguments: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar', arguments: 4);
await tester.pumpAndSettle();
expect(findRoute('Bar', arguments: 4), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
});
testWidgets('restorablePushNamedAndRemoveUntil', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamedAndRemoveUntil('Foo', (Route<dynamic> _) => false, arguments: 3);
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, arguments: 3), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2, arguments: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar', arguments: 4);
await tester.pumpAndSettle();
expect(findRoute('Bar', arguments: 4), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1, arguments: 3), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
});
testWidgets('restorablePush', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeBuilder, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 0), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 1), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 1), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('restorablePush adds route on all platforms', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeBuilder, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
});
testWidgets('restorablePushReplacement', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacement(_routeBuilder, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('restorablePushReplacement adds route on all platforms', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacement(_routeBuilder, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
});
testWidgets('restorablePushAndRemoveUntil', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushAndRemoveUntil(_routeBuilder, (Route<dynamic> _) => false, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('restorablePushAndRemoveUntil adds route on all platforms', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushAndRemoveUntil(_routeBuilder, (Route<dynamic> _) => false, arguments: 'Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
});
testWidgets('restorableReplace', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
final Route<Object> oldRoute = ModalRoute.of(tester.element(find.text('Route: home')))!;
expect(oldRoute.settings.name, 'home');
tester.state<NavigatorState>(find.byType(Navigator)).restorableReplace(newRouteBuilder: _routeBuilder, arguments: 'Foo', oldRoute: oldRoute);
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0), findsOneWidget);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 1), findsOneWidget);
expect(findRoute('Bar'), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('restorableReplace adds route on all platforms', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
final Route<Object> oldRoute = ModalRoute.of(tester.element(find.text('Route: home')))!;
expect(oldRoute.settings.name, 'home');
tester.state<NavigatorState>(find.byType(Navigator)).restorableReplace(newRouteBuilder: _routeBuilder, arguments: 'Foo', oldRoute: oldRoute);
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
});
testWidgets('restorableReplaceRouteBelow', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Anchor');
await tester.pumpAndSettle();
await tapRouteCounter('Anchor', tester);
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 0, skipOffstage: false), findsOneWidget);
expect(findRoute('Anchor', count: 1), findsOneWidget);
final Route<Object> anchor = ModalRoute.of(tester.element(find.text('Route: Anchor')))!;
expect(anchor.settings.name, 'Anchor');
tester.state<NavigatorState>(find.byType(Navigator)).restorableReplaceRouteBelow(newRouteBuilder: _routeBuilder, arguments: 'Foo', anchorRoute: anchor);
await tester.pumpAndSettle();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, skipOffstage: false), findsOneWidget);
expect(findRoute('Anchor', count: 1), findsOneWidget);
await tapRouteCounter('Anchor', tester);
expect(findRoute('Anchor', count: 2), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, skipOffstage: false), findsOneWidget);
expect(findRoute('Anchor', count: 2), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Anchor', tester);
expect(findRoute('Anchor', count: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('home', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 0, skipOffstage: false), findsOneWidget);
expect(findRoute('Anchor', count: 2), findsOneWidget);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('restorableReplaceRouteBelow adds route on all platforms', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Anchor');
await tester.pumpAndSettle();
await tapRouteCounter('Anchor', tester);
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 0, skipOffstage: false), findsOneWidget);
expect(findRoute('Anchor', count: 1), findsOneWidget);
final Route<Object> anchor = ModalRoute.of(tester.element(find.text('Route: Anchor')))!;
expect(anchor.settings.name, 'Anchor');
tester.state<NavigatorState>(find.byType(Navigator)).restorableReplaceRouteBelow(newRouteBuilder: _routeBuilder, arguments: 'Foo', anchorRoute: anchor);
await tester.pumpAndSettle();
expect(findRoute('Foo', skipOffstage: false), findsOneWidget);
});
testWidgets('restoring a popped route', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
await tapRouteCounter('Foo', tester);
await tapRouteCounter('Foo', tester);
expect(findRoute('home'), findsNothing);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 2), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 3), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsNothing);
await tester.restoreFrom(data);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('Foo', count: 2), findsOneWidget);
});
testWidgets('popped routes are not restored', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('Bar'), findsNothing);
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('Bar'), findsNothing);
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
});
testWidgets('routes that are in the process of push are restored', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pump();
await tester.pump();
expect(findRoute('Foo'), findsOneWidget);
// Push is in progress.
final ModalRoute<Object> route1 = ModalRoute.of(tester.element(find.text('Route: Foo')))!;
final String route1id = route1.restorationScopeId.value!;
expect(route1id, isNotNull);
expect(route1.settings.name, 'Foo');
expect(route1.animation!.isCompleted, isFalse);
expect(route1.animation!.isDismissed, isFalse);
expect(route1.isActive, isTrue);
await tester.restartAndRestore();
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
final ModalRoute<Object> route2 = ModalRoute.of(tester.element(find.text('Route: Foo')))!;
expect(route2, isNot(same(route1)));
expect(route1.restorationScopeId.value, route1id);
expect(route2.animation!.isCompleted, isTrue);
expect(route2.isActive, isTrue);
});
testWidgets('routes that are in the process of pop are not restored', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
final ModalRoute<Object> route1 = ModalRoute.of(tester.element(find.text('Route: Foo')))!;
int notifyCount = 0;
route1.restorationScopeId.addListener(() {
notifyCount++;
});
expect(route1.isActive, isTrue);
expect(route1.restorationScopeId.value, isNotNull);
expect(route1.animation!.isCompleted, isTrue);
expect(notifyCount, 0);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
expect(notifyCount, 1);
await tester.pump();
await tester.pump();
// Pop is in progress.
expect(route1.restorationScopeId.value, isNull);
expect(route1.settings.name, 'Foo');
expect(route1.animation!.isCompleted, isFalse);
expect(route1.animation!.isDismissed, isFalse);
expect(route1.isActive, isFalse);
await tester.restartAndRestore();
expect(findRoute('Foo', skipOffstage: false), findsNothing);
expect(findRoute('home', count: 1), findsOneWidget);
expect(notifyCount, 1);
});
testWidgets('routes are restored in the right order', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route1');
await tester.pumpAndSettle();
expect(findRoute('route1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route2');
await tester.pumpAndSettle();
expect(findRoute('route2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route3');
await tester.pumpAndSettle();
expect(findRoute('route3'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route4');
await tester.pumpAndSettle();
expect(findRoute('route4'), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('route4'), findsOneWidget);
expect(findRoute('route3', skipOffstage: false), findsOneWidget);
expect(findRoute('route2', skipOffstage: false), findsOneWidget);
expect(findRoute('route1', skipOffstage: false), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('route3'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('route2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('route1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('home'), findsOneWidget);
});
testWidgets('all routes up to first unrestorable are restored', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route1');
await tester.pumpAndSettle();
expect(findRoute('route1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route2');
await tester.pumpAndSettle();
expect(findRoute('route2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('route3');
await tester.pumpAndSettle();
expect(findRoute('route3'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route4');
await tester.pumpAndSettle();
expect(findRoute('route4'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeBuilder, arguments: 'route5');
await tester.pumpAndSettle();
expect(findRoute('route5'), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('route5', skipOffstage: false), findsNothing);
expect(findRoute('route4', skipOffstage: false), findsNothing);
expect(findRoute('route3', skipOffstage: false), findsNothing);
expect(findRoute('route2'), findsOneWidget);
expect(findRoute('route1', skipOffstage: false), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
});
testWidgets('removing unrestorable routes restores all of them', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route1');
await tester.pumpAndSettle();
expect(findRoute('route1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route2');
await tester.pumpAndSettle();
expect(findRoute('route2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('route3');
await tester.pumpAndSettle();
expect(findRoute('route3'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route4');
await tester.pumpAndSettle();
expect(findRoute('route4'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('route5');
await tester.pumpAndSettle();
expect(findRoute('route5'), findsOneWidget);
final Route<Object> route = ModalRoute.of(tester.element(find.text('Route: route3', skipOffstage: false)))!;
expect(route.settings.name, 'route3');
tester.state<NavigatorState>(find.byType(Navigator)).removeRoute(route);
await tester.pumpAndSettle();
await tester.restartAndRestore();
expect(findRoute('route5'), findsOneWidget);
expect(findRoute('route4', skipOffstage: false), findsOneWidget);
expect(findRoute('route3', skipOffstage: false), findsNothing);
expect(findRoute('route2', skipOffstage: false), findsOneWidget);
expect(findRoute('route1', skipOffstage: false), findsOneWidget);
expect(findRoute('home', skipOffstage: false), findsOneWidget);
});
testWidgets('RestorableRouteFuture', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeFutureBuilder);
await tester.pumpAndSettle();
expect(find.text('Return value: null'), findsOneWidget);
final RestorableRouteFuture<int> routeFuture = tester
.state<RouteFutureWidgetState>(find.byType(RouteFutureWidget))
.routeFuture;
expect(routeFuture.route, isNull);
expect(routeFuture.isPresent, isFalse);
expect(routeFuture.enabled, isFalse);
routeFuture.present('Foo');
await tester.pumpAndSettle();
expect(find.text('Route: Foo'), findsOneWidget);
expect(routeFuture.route!.settings.name, 'Foo');
expect(routeFuture.isPresent, isTrue);
expect(routeFuture.enabled, isTrue);
await tester.restartAndRestore();
expect(find.text('Route: Foo'), findsOneWidget);
final RestorableRouteFuture<int> restoredRouteFuture = tester
.state<RouteFutureWidgetState>(find.byType(RouteFutureWidget, skipOffstage: false))
.routeFuture;
expect(restoredRouteFuture.route!.settings.name, 'Foo');
expect(restoredRouteFuture.isPresent, isTrue);
expect(restoredRouteFuture.enabled, isTrue);
tester.state<NavigatorState>(find.byType(Navigator)).pop(10);
await tester.pumpAndSettle();
expect(find.text('Return value: 10'), findsOneWidget);
expect(restoredRouteFuture.route, isNull);
expect(restoredRouteFuture.isPresent, isFalse);
expect(restoredRouteFuture.enabled, isFalse);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('RestorableRouteFuture in unrestorable context', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
expect(findRoute('home'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('unrestorable');
await tester.pumpAndSettle();
expect(findRoute('unrestorable'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeFutureBuilder);
await tester.pumpAndSettle();
expect(find.text('Return value: null'), findsOneWidget);
final RestorableRouteFuture<int> routeFuture = tester
.state<RouteFutureWidgetState>(find.byType(RouteFutureWidget))
.routeFuture;
expect(routeFuture.route, isNull);
expect(routeFuture.isPresent, isFalse);
expect(routeFuture.enabled, isFalse);
routeFuture.present('Foo');
await tester.pumpAndSettle();
expect(find.text('Route: Foo'), findsOneWidget);
expect(routeFuture.route!.settings.name, 'Foo');
expect(routeFuture.isPresent, isTrue);
expect(routeFuture.enabled, isFalse);
await tester.restartAndRestore();
expect(findRoute('home'), findsOneWidget);
});
testWidgets('Illegal arguments throw', (WidgetTester tester) async {
await tester.pumpWidget(const TestWidget());
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Bar');
await tester.pumpAndSettle();
final Route<Object> oldRoute = ModalRoute.of(tester.element(find.text('Route: Bar')))!;
expect(oldRoute.settings.name, 'Bar');
final Matcher throwsArgumentsAssertionError = throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
'The arguments object must be serializable via the StandardMessageCodec.',
));
final Matcher throwsBuilderAssertionError = throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
'The provided routeBuilder must be a static function.',
));
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo', arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacementNamed('Foo', arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePopAndPushNamed('Foo', arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamedAndRemoveUntil('Foo', (Route<Object?> _) => false, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePush(_routeBuilder, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacement(_routeBuilder, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushAndRemoveUntil(_routeBuilder, (Route<Object?> _) => false, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorableReplace(newRouteBuilder: _routeBuilder, oldRoute: oldRoute, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorableReplaceRouteBelow(newRouteBuilder: _routeBuilder, anchorRoute: oldRoute, arguments: Object()),
throwsArgumentsAssertionError,
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePush((BuildContext _, Object? __) => FakeRoute()),
throwsBuilderAssertionError,
skip: isBrowser, // https://github.com/flutter/flutter/issues/33615
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushReplacement((BuildContext _, Object? __) => FakeRoute()),
throwsBuilderAssertionError,
skip: isBrowser, // https://github.com/flutter/flutter/issues/33615
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorablePushAndRemoveUntil((BuildContext _, Object? __) => FakeRoute(), (Route<Object?> _) => false),
throwsBuilderAssertionError,
skip: isBrowser, // https://github.com/flutter/flutter/issues/33615
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorableReplace(newRouteBuilder: (BuildContext _, Object? __) => FakeRoute(), oldRoute: oldRoute),
throwsBuilderAssertionError,
skip: isBrowser, // https://github.com/flutter/flutter/issues/33615
);
expect(
() => tester.state<NavigatorState>(find.byType(Navigator)).restorableReplaceRouteBelow(newRouteBuilder: (BuildContext _, Object? __) => FakeRoute(), anchorRoute: oldRoute),
throwsBuilderAssertionError,
skip: isBrowser, // https://github.com/flutter/flutter/issues/33615
);
});
testWidgets('Moving scopes', (WidgetTester tester) async {
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root',
child: TestWidget(
restorationId: null,
),
));
await tapRouteCounter('home', tester);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
// Nothing is restored.
await tester.restartAndRestore();
expect(findRoute('Foo'), findsNothing);
expect(findRoute('home', count: 0), findsOneWidget);
await tapRouteCounter('home', tester);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
// Move navigator into restoration scope.
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root',
child: TestWidget(),
));
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
// Everything is restored.
await tester.restartAndRestore();
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
// Move navigator out of restoration scope.
await tester.pumpWidget(const RootRestorationScope(
restorationId: 'root',
child: TestWidget(
restorationId: null,
),
));
expect(findRoute('Foo'), findsOneWidget);
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
// Nothing is restored.
await tester.restartAndRestore();
expect(findRoute('Foo'), findsNothing);
expect(findRoute('home', count: 0), findsOneWidget);
});
testWidgets('Restoring pages', (WidgetTester tester) async {
await tester.pumpWidget(const PagedTestWidget());
expect(findRoute('home', count: 0), findsOneWidget);
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
await tapRouteCounter('Foo', tester);
await tapRouteCounter('Foo', tester);
expect(findRoute('Foo', count: 2), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.restartAndRestore();
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('bar');
await tester.pumpAndSettle();
await tapRouteCounter('bar', tester);
expect(findRoute('bar', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('Foo');
await tester.pumpAndSettle();
expect(findRoute('Foo', count: 0), findsOneWidget);
await tester.restoreFrom(data);
expect(findRoute('bar', skipOffstage: false), findsNothing);
expect(findRoute('Foo', count: 2), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('bar');
await tester.pumpAndSettle();
expect(findRoute('bar', count: 0), findsOneWidget);
});
testWidgets('Unrestorable pages', (WidgetTester tester) async {
await tester.pumpWidget(const PagedTestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p1');
await tester.pumpAndSettle();
await tapRouteCounter('p1', tester);
expect(findRoute('p1', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('r1');
await tester.pumpAndSettle();
await tapRouteCounter('r1', tester);
expect(findRoute('r1', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p2', restoreState: false);
await tester.pumpAndSettle();
await tapRouteCounter('p2', tester);
expect(findRoute('p2', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('r2');
await tester.pumpAndSettle();
await tapRouteCounter('r2', tester);
expect(findRoute('r2', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p3');
await tester.pumpAndSettle();
await tapRouteCounter('p3', tester);
expect(findRoute('p3', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('r3');
await tester.pumpAndSettle();
await tapRouteCounter('r3', tester);
expect(findRoute('r3', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('r2', skipOffstage: false), findsNothing);
expect(findRoute('r3', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('p3', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('p2', count: 0), findsOneWidget); // Page did not restore its state!
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('r1', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('p1', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(findRoute('home', count: 1), findsOneWidget);
});
testWidgets('removed page is not restored', (WidgetTester tester) async {
await tester.pumpWidget(const PagedTestWidget());
await tapRouteCounter('home', tester);
expect(findRoute('home', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p1');
await tester.pumpAndSettle();
await tapRouteCounter('p1', tester);
expect(findRoute('p1', count: 1), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('r1');
await tester.pumpAndSettle();
await tapRouteCounter('r1', tester);
expect(findRoute('r1', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p2');
await tester.pumpAndSettle();
await tapRouteCounter('p2', tester);
expect(findRoute('p2', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).removePage('p1');
await tester.pumpAndSettle();
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('p1', count: 1, skipOffstage: false), findsNothing);
expect(findRoute('r1', count: 1, skipOffstage: false), findsNothing);
expect(findRoute('p2', count: 1), findsOneWidget);
await tester.restartAndRestore();
expect(findRoute('home', count: 1, skipOffstage: false), findsOneWidget);
expect(findRoute('p1', count: 1, skipOffstage: false), findsNothing);
expect(findRoute('r1', count: 1, skipOffstage: false), findsNothing);
expect(findRoute('p2', count: 1), findsOneWidget);
tester.state<PagedTestNavigatorState>(find.byType(PagedTestNavigator)).addPage('p1');
await tester.pumpAndSettle();
expect(findRoute('p1', count: 0), findsOneWidget);
});
testWidgets('Helpful assert thrown all routes in onGenerateInitialRoutes are not restorable', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'material_app',
initialRoute: '/',
routes: <String, WidgetBuilder>{
'/': (BuildContext context) => Container(),
},
onGenerateInitialRoutes: (String initialRoute) {
return <MaterialPageRoute<void>>[
MaterialPageRoute<void>(
builder: (BuildContext context) => Container(),
),
];
},
),
);
await tester.restartAndRestore();
final dynamic exception = tester.takeException();
expect(exception, isAssertionError);
expect(
(exception as AssertionError).message,
contains('All routes returned by onGenerateInitialRoutes are not restorable.'),
);
// The previous assert leaves the widget tree in a broken state, so the
// following code catches any remaining exceptions from attempting to build
// new widget tree.
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
dynamic remainingException;
FlutterError.onError = (FlutterErrorDetails details) {
remainingException ??= details.exception;
};
await tester.pumpWidget(Container(key: UniqueKey()));
FlutterError.onError = oldHandler;
expect(remainingException, isAssertionError);
});
}
@pragma('vm:entry-point')
Route<void> _routeBuilder(BuildContext context, Object? arguments) {
return MaterialPageRoute<void>(
builder: (BuildContext context) {
return RouteWidget(
name: arguments! as String,
);
},
);
}
@pragma('vm:entry-point')
Route<void> _routeFutureBuilder(BuildContext context, Object? arguments) {
return MaterialPageRoute<void>(
builder: (BuildContext context) {
return const RouteFutureWidget();
},
);
}
class PagedTestWidget extends StatelessWidget {
const PagedTestWidget({super.key, this.restorationId = 'app'});
final String restorationId;
@override
Widget build(BuildContext context) {
return RootRestorationScope(
restorationId: restorationId,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData.fromView(View.of(context)),
child: const PagedTestNavigator(),
),
),
);
}
}
class PagedTestNavigator extends StatefulWidget {
const PagedTestNavigator({super.key});
@override
State<PagedTestNavigator> createState() => PagedTestNavigatorState();
}
class PagedTestNavigatorState extends State<PagedTestNavigator> with RestorationMixin {
final RestorableString _routes = RestorableString('r-home');
void addPage(String name, {bool restoreState = true, int? index}) {
assert(!name.contains(','));
assert(!name.startsWith('r-'));
final List<String> routes = _routes.value.split(',');
name = restoreState ? 'r-$name' : name;
if (index != null) {
routes.insert(index, name);
} else {
routes.add(name);
}
setState(() {
_routes.value = routes.join(',');
});
}
bool removePage(String name) {
final List<String> routes = _routes.value.split(',');
if (routes.remove(name) || routes.remove('r-$name')) {
setState(() {
_routes.value = routes.join(',');
});
return true;
}
return false;
}
@override
Widget build(BuildContext context) {
return Navigator(
restorationScopeId: 'nav',
onPopPage: (Route<dynamic> route, dynamic result) {
if (route.didPop(result)) {
removePage(route.settings.name!);
return true;
}
return false;
},
pages: _routes.value.isEmpty ? const <Page<Object?>>[] : _routes.value.split(',').map((String name) {
if (name.startsWith('r-')) {
name = name.substring(2);
return TestPage(
name: name,
restorationId: name,
key: ValueKey<String>(name),
);
}
return TestPage(
name: name,
key: ValueKey<String>(name),
);
}).toList(),
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<int>(
settings: settings,
builder: (BuildContext context) {
return RouteWidget(
name: settings.name!,
arguments: settings.arguments,
);
},
);
},
);
}
@override
String get restorationId => 'router';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_routes, 'routes');
}
@override
void dispose() {
super.dispose();
_routes.dispose();
}
}
class TestPage extends Page<void> {
const TestPage({super.key, required String super.name, super.restorationId});
@override
Route<void> createRoute(BuildContext context) {
return MaterialPageRoute<void>(
settings: this,
builder: (BuildContext context) {
return RouteWidget(
name: name!,
);
},
);
}
}
class TestWidget extends StatelessWidget {
const TestWidget({super.key, this.restorationId = 'app'});
final String? restorationId;
@override
Widget build(BuildContext context) {
return RootRestorationScope(
restorationId: restorationId,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData.fromView(View.of(context)),
child: Navigator(
initialRoute: 'home',
restorationScopeId: 'app',
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<int>(
settings: settings,
builder: (BuildContext context) {
return RouteWidget(
name: settings.name!,
arguments: settings.arguments,
);
},
);
},
),
),
),
);
}
}
class RouteWidget extends StatefulWidget {
const RouteWidget({super.key, required this.name, this.arguments});
final String name;
final Object? arguments;
@override
State<RouteWidget> createState() => RouteWidgetState();
}
class RouteWidgetState extends State<RouteWidget> with RestorationMixin {
final RestorableInt counter = RestorableInt(0);
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(counter, 'counter');
}
@override
String get restorationId => 'stateful';
@override
void dispose() {
super.dispose();
counter.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Column(
children: <Widget>[
GestureDetector(
child: Text('Route: ${widget.name}'),
onTap: () {
setState(() {
counter.value++;
});
},
),
if (widget.arguments != null)
Text('Arguments(home): ${widget.arguments}'),
Text('Counter(${widget.name}): ${counter.value}'),
],
),
);
}
}
class RouteFutureWidget extends StatefulWidget {
const RouteFutureWidget({super.key});
@override
State<RouteFutureWidget> createState() => RouteFutureWidgetState();
}
class RouteFutureWidgetState extends State<RouteFutureWidget> with RestorationMixin {
late RestorableRouteFuture<int> routeFuture;
int? value;
@override
void initState() {
super.initState();
routeFuture = RestorableRouteFuture<int>(
onPresent: (NavigatorState navigatorState, Object? arguments) {
return navigatorState.restorablePushNamed(arguments! as String);
},
onComplete: (int i) {
setState(() {
value = i;
});
},
);
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(routeFuture, 'routeFuture');
}
@override
String get restorationId => 'routefuturewidget';
@override
void dispose() {
super.dispose();
routeFuture.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Text('Return value: $value'),
);
}
}
Finder findRoute(String name, { Object? arguments, int? count, bool skipOffstage = true }) => _RouteFinder(name, arguments: arguments, count: count, skipOffstage: skipOffstage);
Future<void> tapRouteCounter(String name, WidgetTester tester) async {
await tester.tap(find.text('Route: $name'));
await tester.pump();
}
class _RouteFinder extends MatchFinder {
_RouteFinder(this.name, { this.arguments, this.count, super.skipOffstage });
final String name;
final Object? arguments;
final int? count;
@override
String get description {
String result = 'Route(name: $name';
if (arguments != null) {
result += ', arguments: $arguments';
}
if (count != null) {
result += ', count: $count';
}
return result;
}
@override
bool matches(Element candidate) {
final Widget widget = candidate.widget;
if (widget is RouteWidget) {
if (widget.name != name) {
return false;
}
if (arguments != null && widget.arguments != arguments) {
return false;
}
final RouteWidgetState state = (candidate as StatefulElement).state as RouteWidgetState;
if (count != null && state.counter.value != count) {
return false;
}
return true;
}
return false;
}
}
class FakeRoute extends Fake implements Route<void> { }
| flutter/packages/flutter/test/widgets/navigator_restoration_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/navigator_restoration_test.dart",
"repo_id": "flutter",
"token_count": 18164
} | 715 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
class TestPage extends StatelessWidget {
const TestPage({ super.key, this.useMaterial3 });
final bool? useMaterial3;
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Test',
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
theme: ThemeData(
useMaterial3: useMaterial3,
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<StatefulWidget> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
void _presentModalPage() {
Navigator.of(context).push(PageRouteBuilder<void>(
barrierColor: Colors.black54,
opaque: false,
pageBuilder: (BuildContext context, _, __) {
return const ModalPage();
},
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: const Center(
child: Text('Test Home'),
),
floatingActionButton: FloatingActionButton(
onPressed: _presentModalPage,
child: const Icon(Icons.add),
),
);
}
}
class ModalPage extends StatelessWidget {
const ModalPage({super.key});
@override
Widget build(BuildContext context) {
return Material(
type: MaterialType.transparency,
child: SafeArea(
child: Stack(
children: <Widget>[
InkWell(
highlightColor: Colors.transparent,
splashColor: Colors.transparent,
onTap: () {
Navigator.of(context).pop();
},
child: const SizedBox.expand(),
),
Align(
alignment: Alignment.bottomCenter,
child: Container(
height: 150,
color: Colors.teal,
),
),
],
),
),
);
}
}
void main() {
testWidgets('Material2 - Barriers show when using PageRouteBuilder', (WidgetTester tester) async {
await tester.pumpWidget(const TestPage(useMaterial3: false));
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
await expectLater(
find.byType(TestPage),
matchesGoldenFile('m2_page_route_builder.barrier.png'),
);
});
testWidgets('Material3 - Barriers show when using PageRouteBuilder', (WidgetTester tester) async {
await tester.pumpWidget(const TestPage(useMaterial3: true));
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
await expectLater(
find.byType(TestPage),
matchesGoldenFile('m3_page_route_builder.barrier.png'),
);
});
}
| flutter/packages/flutter/test/widgets/page_route_builder_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/page_route_builder_test.dart",
"repo_id": "flutter",
"token_count": 1275
} | 716 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('reassemble does not crash', (WidgetTester tester) async {
await tester.pumpWidget(const MaterialApp(
home: Text('Hello World'),
));
await tester.pump();
tester.binding.reassembleApplication();
await tester.pump();
});
}
| flutter/packages/flutter/test/widgets/reassemble_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/reassemble_test.dart",
"repo_id": "flutter",
"token_count": 177
} | 717 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Router state restoration without RouteInformationProvider', (WidgetTester tester) async {
final UniqueKey router = UniqueKey();
_TestRouterDelegate delegate() => tester.widget<Router<Object?>>(find.byKey(router)).routerDelegate as _TestRouterDelegate;
await tester.pumpWidget(_TestWidget(routerKey: router));
expect(find.text('Current config: null'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, isEmpty);
delegate().currentConfiguration = '/foo';
await tester.pumpAndSettle();
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, isEmpty);
await tester.restartAndRestore();
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, <String>['/foo']);
final TestRestorationData restorationData = await tester.getRestorationData();
delegate().currentConfiguration = '/bar';
await tester.pumpAndSettle();
expect(find.text('Current config: /bar'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, <String>['/foo']);
await tester.restoreFrom(restorationData);
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, <String>['/foo', '/foo']);
});
testWidgets('Router state restoration with RouteInformationProvider', (WidgetTester tester) async {
final UniqueKey router = UniqueKey();
_TestRouterDelegate delegate() => tester.widget<Router<Object?>>(find.byKey(router)).routerDelegate as _TestRouterDelegate;
_TestRouteInformationProvider provider() => tester.widget<Router<Object?>>(find.byKey(router)).routeInformationProvider! as _TestRouteInformationProvider;
await tester.pumpWidget(_TestWidget(routerKey: router, withInformationProvider: true));
expect(find.text('Current config: /home'), findsOneWidget);
expect(delegate().newRoutePaths, <String>['/home']);
expect(delegate().restoredRoutePaths, isEmpty);
provider().value = RouteInformation(uri: Uri(path: '/foo'));
await tester.pumpAndSettle();
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, <String>['/home', '/foo']);
expect(delegate().restoredRoutePaths, isEmpty);
await tester.restartAndRestore();
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, isEmpty);
expect(delegate().restoredRoutePaths, <String>['/foo']);
final TestRestorationData restorationData = await tester.getRestorationData();
provider().value = RouteInformation(uri: Uri.parse('/bar'));
await tester.pumpAndSettle();
expect(find.text('Current config: /bar'), findsOneWidget);
expect(delegate().newRoutePaths, <String>['/bar']);
expect(delegate().restoredRoutePaths, <String>['/foo']);
await tester.restoreFrom(restorationData);
expect(find.text('Current config: /foo'), findsOneWidget);
expect(delegate().newRoutePaths, <String>['/bar']);
expect(delegate().restoredRoutePaths, <String>['/foo', '/foo']);
});
}
class _TestRouteInformationParser extends RouteInformationParser<String> {
@override
Future<String> parseRouteInformation(RouteInformation routeInformation) {
return SynchronousFuture<String>(routeInformation.uri.toString());
}
@override
RouteInformation? restoreRouteInformation(String configuration) {
return RouteInformation(uri: Uri.parse(configuration));
}
}
class _TestRouterDelegate extends RouterDelegate<String> with ChangeNotifier {
_TestRouterDelegate() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
final List<String> newRoutePaths = <String>[];
final List<String> restoredRoutePaths = <String>[];
@override
String? get currentConfiguration => _currentConfiguration;
String? _currentConfiguration;
set currentConfiguration(String? value) {
if (value == _currentConfiguration) {
return;
}
_currentConfiguration = value;
notifyListeners();
}
@override
Future<void> setNewRoutePath(String configuration) {
_currentConfiguration = configuration;
newRoutePaths.add(configuration);
return SynchronousFuture<void>(null);
}
@override
Future<void> setRestoredRoutePath(String configuration) {
_currentConfiguration = configuration;
restoredRoutePaths.add(configuration);
return SynchronousFuture<void>(null);
}
@override
Widget build(BuildContext context) {
return Text('Current config: $currentConfiguration', textDirection: TextDirection.ltr);
}
@override
Future<bool> popRoute() async => throw UnimplementedError();
}
class _TestRouteInformationProvider extends RouteInformationProvider with ChangeNotifier {
_TestRouteInformationProvider() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
@override
RouteInformation get value => _value;
RouteInformation _value = RouteInformation(uri: Uri.parse('/home'));
set value(RouteInformation value) {
if (value == _value) {
return;
}
_value = value;
notifyListeners();
}
}
class _TestWidget extends StatefulWidget {
const _TestWidget({this.withInformationProvider = false, this.routerKey});
final bool withInformationProvider;
final Key? routerKey;
@override
State<_TestWidget> createState() => _TestWidgetState();
}
class _TestWidgetState extends State<_TestWidget> {
final _TestRouterDelegate _delegate = _TestRouterDelegate();
final _TestRouteInformationProvider _routeInformationProvider = _TestRouteInformationProvider();
@override
void dispose() {
_delegate.dispose();
_routeInformationProvider.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return RootRestorationScope(
restorationId: 'root',
child: Router<String>(
key: widget.routerKey,
restorationScopeId: 'router',
routerDelegate: _delegate,
routeInformationParser: _TestRouteInformationParser(),
routeInformationProvider: widget.withInformationProvider ? _routeInformationProvider : null,
),
);
}
}
| flutter/packages/flutter/test/widgets/router_restoration_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/router_restoration_test.dart",
"repo_id": "flutter",
"token_count": 2155
} | 718 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
ScrollController _controller = ScrollController(
initialScrollOffset: 110.0,
);
class ThePositiveNumbers extends StatelessWidget {
const ThePositiveNumbers({
super.key,
required this.from,
});
final int from;
@override
Widget build(BuildContext context) {
return ListView.builder(
key: const PageStorageKey<String>('ThePositiveNumbers'),
itemExtent: 100.0,
controller: _controller,
itemBuilder: (BuildContext context, int index) {
return Text('${index + from}', key: ValueKey<int>(index));
},
);
}
}
Future<void> performTest(WidgetTester tester, bool maintainState) async {
final GlobalKey<NavigatorState> navigatorKey = GlobalKey<NavigatorState>();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData.fromView(tester.view),
child: Navigator(
key: navigatorKey,
onGenerateRoute: (RouteSettings settings) {
if (settings.name == '/') {
return MaterialPageRoute<void>(
settings: settings,
builder: (_) => const ThePositiveNumbers(from: 0),
maintainState: maintainState,
);
} else if (settings.name == '/second') {
return MaterialPageRoute<void>(
settings: settings,
builder: (_) => const ThePositiveNumbers(from: 10000),
maintainState: maintainState,
);
}
return null;
},
),
),
),
);
// we're 600 pixels high, each item is 100 pixels high, scroll position is
// 110.0, so we should have 7 items, 1..7.
expect(find.text('0'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('1'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('2'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('3'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('4'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('5'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('6'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('7'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('8'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('100'), findsNothing, reason: 'with maintainState: $maintainState');
tester.state<ScrollableState>(find.byType(Scrollable)).position.jumpTo(1000.0);
await tester.pump(const Duration(seconds: 1));
// we're 600 pixels high, each item is 100 pixels high, scroll position is
// 1000, so we should have exactly 6 items, 10..15.
expect(find.text('0'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('1'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('8'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('9'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('11'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('12'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('13'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('14'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('15'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('16'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('100'), findsNothing, reason: 'with maintainState: $maintainState');
navigatorKey.currentState!.pushNamed('/second');
await tester.pump(); // navigating always takes two frames, one to start...
await tester.pump(const Duration(seconds: 1)); // ...and one to end the transition
// the second list is now visible, starting at 10001
expect(find.text('0'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('1'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('11'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10000'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10001'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10002'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10003'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10004'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10005'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10006'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10007'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('10008'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10010'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10100'), findsNothing, reason: 'with maintainState: $maintainState');
navigatorKey.currentState!.pop();
await tester.pump(); // again, navigating always takes two frames
// Ensure we don't clamp the scroll offset even during the navigation.
// https://github.com/flutter/flutter/issues/4883
final ScrollableState state = tester.state(find.byType(Scrollable).first);
expect(state.position.pixels, equals(1000.0), reason: 'with maintainState: $maintainState');
await tester.pump(const Duration(seconds: 1));
// we're 600 pixels high, each item is 100 pixels high, scroll position is
// 1000, so we should have exactly 6 items, 10..15.
expect(find.text('0'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('1'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('8'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('9'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('10'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('11'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('12'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('13'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('14'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('15'), findsOneWidget, reason: 'with maintainState: $maintainState');
expect(find.text('16'), findsNothing, reason: 'with maintainState: $maintainState');
expect(find.text('100'), findsNothing, reason: 'with maintainState: $maintainState');
}
void main() {
testWidgets("ScrollPosition jumpTo() doesn't call notifyListeners twice", (WidgetTester tester) async {
int count = 0;
await tester.pumpWidget(MaterialApp(
home: ListView.builder(
itemBuilder: (BuildContext context, int index) {
return Text('$index', textDirection: TextDirection.ltr);
},
),
));
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
position.addListener(() {
count++;
});
position.jumpTo(100);
expect(count, 1);
});
testWidgets('whether we remember our scroll position', (WidgetTester tester) async {
await performTest(tester, true);
await performTest(tester, false);
});
testWidgets('scroll alignment is honored by ensureVisible', (WidgetTester tester) async {
final List<int> items = List<int>.generate(11, (int index) => index).toList();
final List<FocusNode> nodes = List<FocusNode>.generate(11, (int index) => FocusNode(debugLabel: 'Item ${index + 1}')).toList();
addTearDown(() {
for (final FocusNode node in nodes) {
node.dispose();
}
});
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: ListView(
controller: controller,
children: items.map<Widget>((int item) {
return Focus(
key: ValueKey<int>(item),
focusNode: nodes[item],
child: Container(height: 110),
);
}).toList(),
),
),
);
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(0))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
expect(controller.position.pixels, equals(0.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(1))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
expect(controller.position.pixels, equals(0.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(1))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
);
expect(controller.position.pixels, equals(0.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(4))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
expect(controller.position.pixels, equals(0.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(5))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
expect(controller.position.pixels, equals(0.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(5))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
);
expect(controller.position.pixels, equals(60.0));
controller.position.ensureVisible(
tester.renderObject(find.byKey(const ValueKey<int>(0))),
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
expect(controller.position.pixels, equals(0.0));
});
testWidgets('jumpTo recommends deferred loading', (WidgetTester tester) async {
int loadedWithDeferral = 0;
int buildCount = 0;
const double height = 500;
await tester.pumpWidget(MaterialApp(
home: ListView.builder(
itemBuilder: (BuildContext context, int index) {
buildCount += 1;
if (Scrollable.recommendDeferredLoadingForContext(context)) {
loadedWithDeferral += 1;
}
return const SizedBox(height: height);
},
),
));
// The two visible on screen should have loaded without deferral.
expect(buildCount, 2);
expect(loadedWithDeferral, 0);
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
position.jumpTo(height * 100);
await tester.pump();
// All but the first two that were loaded normally should have gotten a
// recommendation to defer.
expect(buildCount, 102);
expect(loadedWithDeferral, 100);
position.jumpTo(height * 102);
await tester.pump();
// The smaller jump should not have recommended deferral.
expect(buildCount, 104);
expect(loadedWithDeferral, 100);
});
}
| flutter/packages/flutter/test/widgets/scroll_position_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/scroll_position_test.dart",
"repo_id": "flutter",
"token_count": 4075
} | 719 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/src/physics/utils.dart' show nearEqual;
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
const Color _kScrollbarColor = Color(0xFF123456);
const double _kThickness = 2.5;
const double _kMinThumbExtent = 18.0;
const Duration _kScrollbarFadeDuration = Duration(milliseconds: 300);
const Duration _kScrollbarTimeToFade = Duration(milliseconds: 600);
ScrollbarPainter _buildPainter({
TextDirection textDirection = TextDirection.ltr,
EdgeInsets padding = EdgeInsets.zero,
Color color = _kScrollbarColor,
double thickness = _kThickness,
double mainAxisMargin = 0.0,
double crossAxisMargin = 0.0,
Radius? radius,
Radius? trackRadius,
double minLength = _kMinThumbExtent,
double? minOverscrollLength,
ScrollbarOrientation? scrollbarOrientation,
required ScrollMetrics scrollMetrics,
}) {
return ScrollbarPainter(
color: color,
textDirection: textDirection,
thickness: thickness,
padding: padding,
mainAxisMargin: mainAxisMargin,
crossAxisMargin: crossAxisMargin,
radius: radius,
trackRadius: trackRadius,
minLength: minLength,
minOverscrollLength: minOverscrollLength ?? minLength,
fadeoutOpacityAnimation: kAlwaysCompleteAnimation,
scrollbarOrientation: scrollbarOrientation,
)..update(scrollMetrics, scrollMetrics.axisDirection);
}
class _DrawRectOnceCanvas extends Fake implements Canvas {
List<Rect> rects = <Rect>[];
List<RRect> rrects = <RRect>[];
@override
void drawRect(Rect rect, Paint paint) {
rects.add(rect);
}
@override
void drawRRect(ui.RRect rrect, ui.Paint paint) {
rrects.add(rrect);
}
@override
void drawLine(Offset p1, Offset p2, Paint paint) {}
}
void main() {
final _DrawRectOnceCanvas testCanvas = _DrawRectOnceCanvas();
ScrollbarPainter painter;
Rect captureRect() => testCanvas.rects.removeLast();
RRect captureRRect() => testCanvas.rrects.removeLast();
tearDown(() {
testCanvas.rects.clear();
testCanvas.rrects.clear();
});
final ScrollMetrics defaultMetrics = FixedScrollMetrics(
minScrollExtent: 0,
maxScrollExtent: 0,
pixels: 0,
viewportDimension: 100,
axisDirection: AxisDirection.down,
devicePixelRatio: 3.0,
);
test(
'Scrollbar is not smaller than minLength with large scroll views, '
'if minLength is small ',
() {
const double minLen = 3.5;
const Size size = Size(600, 10);
final ScrollMetrics metrics = defaultMetrics.copyWith(
maxScrollExtent: 100000,
viewportDimension: size.height,
);
// When overscroll.
painter = _buildPainter(
minLength: minLen,
minOverscrollLength: minLen,
scrollMetrics: metrics,
);
painter.paint(testCanvas, size);
final Rect rect0 = captureRect();
expect(rect0.top, 0);
expect(rect0.left, size.width - _kThickness);
expect(rect0.width, _kThickness);
expect(rect0.height >= minLen, true);
// When scroll normally.
const double newPixels = 1.0;
painter.update(metrics.copyWith(pixels: newPixels), metrics.axisDirection);
painter.paint(testCanvas, size);
final Rect rect1 = captureRect();
expect(rect1.left, size.width - _kThickness);
expect(rect1.width, _kThickness);
expect(rect1.height >= minLen, true);
},
);
test(
'When scrolling normally (no overscrolling), the size of the scrollbar stays the same, '
'and it scrolls evenly',
() {
const double viewportDimension = 23;
const double maxExtent = 100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
maxScrollExtent: maxExtent,
viewportDimension: viewportDimension,
);
const Size size = Size(600, viewportDimension);
const double minLen = 0;
painter = _buildPainter(
minLength: minLen,
minOverscrollLength: minLen,
scrollMetrics: defaultMetrics,
);
final List<ScrollMetrics> metricsList = <ScrollMetrics> [
startingMetrics.copyWith(pixels: 0.01),
...List<ScrollMetrics>.generate(
(maxExtent / viewportDimension).round(),
(int index) => startingMetrics.copyWith(pixels: (index + 1) * viewportDimension),
).where((ScrollMetrics metrics) => !metrics.outOfRange),
startingMetrics.copyWith(pixels: maxExtent - 0.01),
];
late double lastCoefficient;
for (final ScrollMetrics metrics in metricsList) {
painter.update(metrics, metrics.axisDirection);
painter.paint(testCanvas, size);
final Rect rect = captureRect();
final double newCoefficient = metrics.pixels/rect.top;
lastCoefficient = newCoefficient;
expect(rect.top >= 0, true);
expect(rect.bottom <= maxExtent, true);
expect(rect.left, size.width - _kThickness);
expect(rect.width, _kThickness);
expect(nearEqual(rect.height, viewportDimension * viewportDimension / (viewportDimension + maxExtent), 0.001), true);
expect(nearEqual(lastCoefficient, newCoefficient, 0.001), true);
}
},
);
test(
'mainAxisMargin is respected',
() {
const double viewportDimension = 23;
const double maxExtent = 100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
maxScrollExtent: maxExtent,
viewportDimension: viewportDimension,
);
const Size size = Size(600, viewportDimension);
const double minLen = 0;
const List<double> margins = <double> [-10, 1, viewportDimension/2 - 0.01];
for (final double margin in margins) {
painter = _buildPainter(
mainAxisMargin: margin,
minLength: minLen,
scrollMetrics: defaultMetrics,
);
// Overscroll to double.negativeInfinity (top).
painter.update(
startingMetrics.copyWith(pixels: double.negativeInfinity),
startingMetrics.axisDirection,
);
painter.paint(testCanvas, size);
expect(captureRect().top, margin);
// Overscroll to double.infinity (down).
painter.update(
startingMetrics.copyWith(pixels: double.infinity),
startingMetrics.axisDirection,
);
painter.paint(testCanvas, size);
expect(size.height - captureRect().bottom, margin);
}
},
);
test(
'crossAxisMargin & text direction are respected',
() {
const double viewportDimension = 23;
const double maxExtent = 100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
maxScrollExtent: maxExtent,
viewportDimension: viewportDimension,
);
const Size size = Size(600, viewportDimension);
const double margin = 4;
for (final TextDirection textDirection in TextDirection.values) {
painter = _buildPainter(
crossAxisMargin: margin,
scrollMetrics: startingMetrics,
textDirection: textDirection,
);
for (final AxisDirection direction in AxisDirection.values) {
painter.update(
startingMetrics.copyWith(axisDirection: direction),
direction,
);
painter.paint(testCanvas, size);
final Rect rect = captureRect();
switch (axisDirectionToAxis(direction)) {
case Axis.vertical:
expect(margin, switch (textDirection) {
TextDirection.ltr => size.width - rect.right,
TextDirection.rtl => rect.left,
});
case Axis.horizontal:
expect(margin, size.height - rect.bottom);
}
}
}
},
);
test('scrollbarOrientation are respected', () {
const double viewportDimension = 23;
const double maxExtent = 100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
maxScrollExtent: maxExtent,
viewportDimension: viewportDimension,
);
const Size size = Size(600, viewportDimension);
for (final ScrollbarOrientation scrollbarOrientation in ScrollbarOrientation.values) {
final AxisDirection axisDirection;
if (scrollbarOrientation == ScrollbarOrientation.left || scrollbarOrientation == ScrollbarOrientation.right) {
axisDirection = AxisDirection.down;
} else {
axisDirection = AxisDirection.right;
}
painter = _buildPainter(
scrollMetrics: startingMetrics,
scrollbarOrientation: scrollbarOrientation,
);
painter.update(
startingMetrics.copyWith(axisDirection: axisDirection),
axisDirection
);
painter.paint(testCanvas, size);
final Rect rect = captureRect();
switch (scrollbarOrientation) {
case ScrollbarOrientation.left:
expect(rect.left, 0);
expect(rect.top, 0);
expect(rect.right, _kThickness);
expect(rect.bottom, _kMinThumbExtent);
case ScrollbarOrientation.right:
expect(rect.left, 600 - _kThickness);
expect(rect.top, 0);
expect(rect.right, 600);
expect(rect.bottom, _kMinThumbExtent);
case ScrollbarOrientation.top:
expect(rect.left, 0);
expect(rect.top, 0);
expect(rect.right, _kMinThumbExtent);
expect(rect.bottom, _kThickness);
case ScrollbarOrientation.bottom:
expect(rect.left, 0);
expect(rect.top, 23 - _kThickness);
expect(rect.right, _kMinThumbExtent);
expect(rect.bottom, 23);
}
}
});
test('scrollbarOrientation default values are correct', () {
const double viewportDimension = 23;
const double maxExtent = 100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
maxScrollExtent: maxExtent,
viewportDimension: viewportDimension,
);
const Size size = Size(600, viewportDimension);
Rect rect;
// Vertical scroll with TextDirection.ltr
painter = _buildPainter(
scrollMetrics: startingMetrics,
);
painter.update(
startingMetrics.copyWith(axisDirection: AxisDirection.down),
AxisDirection.down
);
painter.paint(testCanvas, size);
rect = captureRect();
expect(rect.left, 600 - _kThickness);
expect(rect.top, 0);
expect(rect.right, 600);
expect(rect.bottom, _kMinThumbExtent);
// Vertical scroll with TextDirection.rtl
painter = _buildPainter(
scrollMetrics: startingMetrics,
textDirection: TextDirection.rtl,
);
painter.update(
startingMetrics.copyWith(axisDirection: AxisDirection.down),
AxisDirection.down
);
painter.paint(testCanvas, size);
rect = captureRect();
expect(rect.left, 0);
expect(rect.top, 0);
expect(rect.right, _kThickness);
expect(rect.bottom, _kMinThumbExtent);
// Horizontal scroll
painter = _buildPainter(
scrollMetrics: startingMetrics,
);
painter.update(
startingMetrics.copyWith(axisDirection: AxisDirection.right),
AxisDirection.right,
);
painter.paint(testCanvas, size);
rect = captureRect();
expect(rect.left, 0);
expect(rect.top, 23 - _kThickness);
expect(rect.right, _kMinThumbExtent);
expect(rect.bottom, 23);
});
group('Padding works for all scroll directions', () {
const EdgeInsets padding = EdgeInsets.fromLTRB(1, 2, 3, 4);
const Size size = Size(60, 80);
final ScrollMetrics metrics = defaultMetrics.copyWith(
minScrollExtent: -100,
maxScrollExtent: 240,
axisDirection: AxisDirection.down,
);
final ScrollbarPainter painter = _buildPainter(
padding: padding,
scrollMetrics: metrics,
);
testWidgets('down', (WidgetTester tester) async {
painter.update(
metrics.copyWith(
viewportDimension: size.height,
pixels: double.negativeInfinity,
),
AxisDirection.down,
);
// Top overscroll.
painter.paint(testCanvas, size);
final Rect rect0 = captureRect();
expect(rect0.top, padding.top);
expect(size.width - rect0.right, padding.right);
// Bottom overscroll.
painter.update(
metrics.copyWith(
viewportDimension: size.height,
pixels: double.infinity,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
final Rect rect1 = captureRect();
expect(size.height - rect1.bottom, padding.bottom);
expect(size.width - rect1.right, padding.right);
});
testWidgets('up', (WidgetTester tester) async {
painter.update(
metrics.copyWith(
viewportDimension: size.height,
pixels: double.infinity,
axisDirection: AxisDirection.up,
),
AxisDirection.up,
);
// Top overscroll.
painter.paint(testCanvas, size);
final Rect rect0 = captureRect();
expect(rect0.top, padding.top);
expect(size.width - rect0.right, padding.right);
// Bottom overscroll.
painter.update(
metrics.copyWith(
viewportDimension: size.height,
pixels: double.negativeInfinity,
axisDirection: AxisDirection.up,
),
AxisDirection.up,
);
painter.paint(testCanvas, size);
final Rect rect1 = captureRect();
expect(size.height - rect1.bottom, padding.bottom);
expect(size.width - rect1.right, padding.right);
});
testWidgets('left', (WidgetTester tester) async {
painter.update(
metrics.copyWith(
viewportDimension: size.width,
pixels: double.negativeInfinity,
axisDirection: AxisDirection.left,
),
AxisDirection.left,
);
// Right overscroll.
painter.paint(testCanvas, size);
final Rect rect0 = captureRect();
expect(size.height - rect0.bottom, padding.bottom);
expect(size.width - rect0.right, padding.right);
// Left overscroll.
painter.update(
metrics.copyWith(
viewportDimension: size.width,
pixels: double.infinity,
axisDirection: AxisDirection.left,
),
AxisDirection.left,
);
painter.paint(testCanvas, size);
final Rect rect1 = captureRect();
expect(size.height - rect1.bottom, padding.bottom);
expect(rect1.left, padding.left);
});
testWidgets('right', (WidgetTester tester) async {
painter.update(
metrics.copyWith(
viewportDimension: size.width,
pixels: double.infinity,
axisDirection: AxisDirection.right,
),
AxisDirection.right,
);
// Right overscroll.
painter.paint(testCanvas, size);
final Rect rect0 = captureRect();
expect(size.height - rect0.bottom, padding.bottom);
expect(size.width - rect0.right, padding.right);
// Left overscroll.
painter.update(
metrics.copyWith(
viewportDimension: size.width,
pixels: double.negativeInfinity,
axisDirection: AxisDirection.right,
),
AxisDirection.right,
);
painter.paint(testCanvas, size);
final Rect rect1 = captureRect();
expect(size.height - rect1.bottom, padding.bottom);
expect(rect1.left, padding.left);
});
});
testWidgets('thumb resizes gradually on overscroll', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.fromLTRB(1, 2, 3, 4);
const Size size = Size(60, 300);
final double scrollExtent = size.height * 10;
final ScrollMetrics metrics = defaultMetrics.copyWith(
minScrollExtent: 0,
maxScrollExtent: scrollExtent,
axisDirection: AxisDirection.down,
viewportDimension: size.height,
);
const double minOverscrollLength = 8.0;
final ScrollbarPainter painter = _buildPainter(
padding: padding,
scrollMetrics: metrics,
minLength: 36.0,
minOverscrollLength: 8.0,
);
// No overscroll gives a full sized thumb.
painter.update(
metrics.copyWith(
pixels: 0.0,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
final double fullThumbExtent = captureRect().height;
expect(fullThumbExtent, greaterThan(_kMinThumbExtent));
// Scrolling to the middle also gives a full sized thumb.
painter.update(
metrics.copyWith(
pixels: scrollExtent / 2,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
expect(captureRect().height, moreOrLessEquals(fullThumbExtent, epsilon: 1e-6));
// Scrolling just to the very end also gives a full sized thumb.
painter.update(
metrics.copyWith(
pixels: scrollExtent,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
expect(captureRect().height, moreOrLessEquals(fullThumbExtent, epsilon: 1e-6));
// Scrolling just past the end shrinks the thumb slightly.
painter.update(
metrics.copyWith(
pixels: scrollExtent * 1.001,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
expect(captureRect().height, moreOrLessEquals(fullThumbExtent, epsilon: 2.0));
// Scrolling way past the end shrinks the thumb to minimum.
painter.update(
metrics.copyWith(
pixels: double.infinity,
),
AxisDirection.down,
);
painter.paint(testCanvas, size);
expect(captureRect().height, minOverscrollLength);
});
test('should scroll towards the right direction',
() {
const Size size = Size(60, 80);
const double maxScrollExtent = 240;
const double minScrollExtent = -100;
final ScrollMetrics startingMetrics = defaultMetrics.copyWith(
minScrollExtent: minScrollExtent,
maxScrollExtent: maxScrollExtent,
axisDirection: AxisDirection.down,
viewportDimension: size.height,
);
for (final double minLength in <double>[_kMinThumbExtent, double.infinity]) {
// Disregard `minLength` and `minOverscrollLength` to keep
// scroll direction correct, if needed
painter = _buildPainter(
minLength: minLength,
minOverscrollLength: minLength,
scrollMetrics: startingMetrics,
);
final Iterable<ScrollMetrics> metricsList = Iterable<ScrollMetrics>.generate(
9999,
(int index) => startingMetrics.copyWith(pixels: minScrollExtent + index * size.height / 3),
)
.takeWhile((ScrollMetrics metrics) => !metrics.outOfRange);
Rect? previousRect;
for (final ScrollMetrics metrics in metricsList) {
painter.update(metrics, metrics.axisDirection);
painter.paint(testCanvas, size);
final Rect rect = captureRect();
if (previousRect != null) {
if (rect.height == size.height) {
// Size of the scrollbar is too large for the view port
expect(previousRect.top <= rect.top, true);
expect(previousRect.bottom <= rect.bottom, true);
} else {
// The scrollbar can fit in the view port.
expect(previousRect.top < rect.top, true);
expect(previousRect.bottom < rect.bottom, true);
}
}
previousRect = rect;
}
}
},
);
test('trackRadius and radius is respected', () {
const double minLen = 3.5;
const Size size = Size(600, 10);
final ScrollMetrics metrics = defaultMetrics.copyWith(
maxScrollExtent: 100000,
viewportDimension: size.height,
);
painter = _buildPainter(
trackRadius: const Radius.circular(2.0),
radius: const Radius.circular(3.0),
minLength: minLen,
minOverscrollLength: minLen,
scrollMetrics: metrics,
);
painter.paint(testCanvas, size);
final RRect thumbRRect = captureRRect(); // thumb
expect(thumbRRect.blRadius, const Radius.circular(3.0));
expect(thumbRRect.brRadius, const Radius.circular(3.0));
expect(thumbRRect.tlRadius, const Radius.circular(3.0));
expect(thumbRRect.trRadius, const Radius.circular(3.0));
final RRect trackRRect = captureRRect(); // track
expect(trackRRect.blRadius, const Radius.circular(2.0));
expect(trackRRect.brRadius, const Radius.circular(2.0));
expect(trackRRect.tlRadius, const Radius.circular(2.0));
expect(trackRRect.trRadius, const Radius.circular(2.0));
});
testWidgets('ScrollbarPainter asserts if no TextDirection has been provided', (WidgetTester tester) async {
final ScrollbarPainter painter = ScrollbarPainter(
color: _kScrollbarColor,
fadeoutOpacityAnimation: kAlwaysCompleteAnimation,
);
const Size size = Size(60, 80);
final ScrollMetrics scrollMetrics = defaultMetrics.copyWith(
maxScrollExtent: 100000,
viewportDimension: size.height,
);
painter.update(scrollMetrics, scrollMetrics.axisDirection);
// Try to paint the scrollbar
try {
painter.paint(testCanvas, size);
} on AssertionError catch (error) {
expect(error.message, 'A TextDirection must be provided before a Scrollbar can be painted.');
}
});
testWidgets('Tapping the track area pages the Scroll View', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 1000.0),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 360.0),
color: const Color(0x66BCBCBC),
),
);
// Tap on the track area below the thumb.
await tester.tapAt(const Offset(796.0, 550.0));
await tester.pumpAndSettle();
expect(scrollController.offset, 400.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 240.0, 800.0, 600.0),
color: const Color(0x66BCBCBC),
),
);
// Tap on the track area above the thumb.
await tester.tapAt(const Offset(796.0, 50.0));
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 360.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar never goes away until finger lift', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: RawScrollbar(
child: SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -20.0));
await tester.pump();
// Scrollbar fully showing
await tester.pump(const Duration(milliseconds: 500));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
await tester.pump(const Duration(seconds: 3));
await tester.pump(const Duration(seconds: 3));
// Still there.
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
await gesture.up();
await tester.pump(_kScrollbarTimeToFade);
await tester.pump(_kScrollbarFadeDuration * 0.5);
// Opacity going down now.
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x4fbcbcbc),
),
);
});
testWidgets('Scrollbar does not fade away while hovering', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: RawScrollbar(
child: SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -20.0));
await tester.pump();
// Scrollbar fully showing
await tester.pump(const Duration(milliseconds: 500));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
final TestPointer testPointer = TestPointer(1, ui.PointerDeviceKind.mouse);
// Hover over the thumb to prevent the scrollbar from fading out.
testPointer.hover(const Offset(790.0, 5.0));
await gesture.up();
await tester.pump(const Duration(seconds: 3));
// Still there.
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar will fade back in when hovering over known track area', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: RawScrollbar(
child: SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -20.0));
await tester.pump();
// Scrollbar fully showing
await tester.pump(const Duration(milliseconds: 500));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
await gesture.up();
await tester.pump(_kScrollbarTimeToFade);
await tester.pump(_kScrollbarFadeDuration * 0.5);
// Scrollbar is fading out
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x4fbcbcbc),
),
);
// Hover over scrollbar with mouse to bring opacity back up
final TestGesture mouseGesture = await tester.createGesture(kind: ui.PointerDeviceKind.mouse);
await mouseGesture.addPointer();
addTearDown(mouseGesture.removePointer);
await mouseGesture.moveTo(const Offset(794.0, 5.0));
await tester.pumpAndSettle();
// Scrollbar should be visible
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 3.0, 800.0, 93.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar will show on hover without needing to scroll first for metrics', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: RawScrollbar(
child: SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
await tester.pump();
// Hover over scrollbar with mouse. Even though we have not scrolled, the
// ScrollMetricsNotification will have informed the Scrollbar's hit testing.
final TestGesture mouseGesture = await tester.createGesture(kind: ui.PointerDeviceKind.mouse);
await mouseGesture.addPointer();
addTearDown(mouseGesture.removePointer);
await mouseGesture.moveTo(const Offset(794.0, 5.0));
await tester.pumpAndSettle();
// Scrollbar should have appeared in response to hover event.
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar thumb can be dragged', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// Drag the thumb down to scroll down.
const double scrollAmount = 10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 10.0, 800.0, 100.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar thumb cannot be dragged into overscroll if the physics do not allow', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// Try to drag the thumb into overscroll.
const double scrollAmount = -10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
// The physics should not have allowed us to enter overscroll.
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('Scrollbar thumb cannot be dragged into overscroll if the platform does not allow it', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
// Don't apply a scrollbar automatically for this test.
behavior: const ScrollBehavior().copyWith(
scrollbars: false,
physics: const AlwaysScrollableScrollPhysics(),
),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
primary: true,
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// Try to drag the thumb into overscroll.
const double scrollAmount = -10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
// The platform drag handling should not have allowed us to enter overscroll.
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
}, variant: const TargetPlatformVariant(<TargetPlatform>{
TargetPlatform.macOS,
TargetPlatform.linux,
TargetPlatform.windows,
TargetPlatform.fuchsia,
}));
testWidgets('Scrollbar thumb can be dragged into overscroll if the platform allows it', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
// Don't apply a scrollbar automatically for this test.
behavior: const ScrollBehavior().copyWith(
scrollbars: false,
physics: const AlwaysScrollableScrollPhysics(),
),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// Try to drag the thumb into overscroll.
const double scrollAmount = -10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
// The platform drag handling should have allowed us to enter overscroll.
expect(scrollController.offset, lessThan(-66.0));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
// The size of the scrollbar thumb shrinks when overscrolling
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 80.0),
color: const Color(0x66BCBCBC),
),
);
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
}, variant: const TargetPlatformVariant(<TargetPlatform>{
TargetPlatform.android,
TargetPlatform.iOS,
}));
// Regression test for https://github.com/flutter/flutter/issues/66444
testWidgets("RawScrollbar doesn't show when scroll the inner scrollable widget", (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey();
final GlobalKey key2 = GlobalKey();
final GlobalKey outerKey = GlobalKey();
final GlobalKey innerKey = GlobalKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
key: key2,
thumbColor: const Color(0x11111111),
child: SingleChildScrollView(
key: outerKey,
child: SizedBox(
height: 1000.0,
width: double.infinity,
child: Column(
children: <Widget>[
RawScrollbar(
key: key1,
thumbColor: const Color(0x22222222),
child: SizedBox(
height: 300.0,
width: double.infinity,
child: SingleChildScrollView(
key: innerKey,
child: const SizedBox(
key: Key('Inner scrollable'),
height: 1000.0,
width: double.infinity,
),
),
),
),
],
),
),
),
),
),
),
);
// Drag the inner scrollable widget.
await tester.drag(find.byKey(innerKey), const Offset(0.0, -25.0));
await tester.pump();
// Scrollbar fully showing.
await tester.pump(const Duration(milliseconds: 500));
expect(
tester.renderObject(find.byKey(key2)),
paintsExactlyCountTimes(#drawRect, 2), // Each bar will call [drawRect] twice.
);
expect(
tester.renderObject(find.byKey(key1)),
paintsExactlyCountTimes(#drawRect, 2),
);
});
testWidgets('Scrollbar hit test area adjusts for PointerDeviceKind', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// Drag the scrollbar just outside of the painted thumb with touch input.
// The hit test area is padded to meet the minimum interactive size.
const double scrollAmount = 10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(790.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
// The scrollbar moved by scrollAmount, and the scrollOffset moved forward.
expect(scrollController.offset, greaterThan(0.0));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 10.0, 800.0, 100.0),
color: const Color(0x66BCBCBC),
),
);
// Move back to reset.
await dragScrollbarGesture.moveBy(const Offset(0.0, -scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
// The same should not be possible with a mouse since it is more precise,
// the padding it not necessary.
final TestGesture gesture = await tester.createGesture(kind: ui.PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.down(const Offset(790.0, 45.0));
await tester.pump();
await gesture.moveTo(const Offset(790.0, 55.0));
await gesture.up();
await tester.pumpAndSettle();
// The scrollbar/scrollable should not have moved.
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('hit test', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/99324
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
bool onTap = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
trackVisibility: true,
thumbVisibility: true,
controller: scrollController,
child: SingleChildScrollView(
child: GestureDetector(
onTap: () => onTap = true,
child: const SizedBox(
width: 4000.0,
height: 4000.0,
child: ColoredBox(color: Color(0x00000000)),
),
),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(onTap, false);
// Tap on track area.
await tester.tapAt(const Offset(795.0, 550.0));
await tester.pumpAndSettle();
expect(onTap, false);
// Tap on thumb area.
await tester.tapAt(const Offset(795.0, 10.0));
await tester.pumpAndSettle();
expect(onTap, false);
// Tap on content area.
await tester.tapAt(const Offset(400.0, 300.0));
await tester.pumpAndSettle();
expect(onTap, true);
});
testWidgets('RawScrollbar.thumbVisibility asserts that a ScrollPosition is attached', (WidgetTester tester) async {
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
thumbVisibility: true,
controller: controller,
thumbColor: const Color(0x11111111),
child: const SingleChildScrollView(
child: SizedBox(
height: 1000.0,
width: 50.0,
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(error, isNotNull);
final AssertionError exception = error!.exception as AssertionError;
expect(
exception.message,
contains("The Scrollbar's ScrollController has no ScrollPosition attached."),
);
FlutterError.onError = handler;
});
testWidgets('RawScrollbar.thumbVisibility asserts that a ScrollPosition is attached', (WidgetTester tester) async {
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
thumbVisibility: true,
controller: controller,
thumbColor: const Color(0x11111111),
child: const SingleChildScrollView(
child: SizedBox(
height: 1000.0,
width: 50.0,
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(error, isNotNull);
final AssertionError exception = error!.exception as AssertionError;
expect(
exception.message,
contains("The Scrollbar's ScrollController has no ScrollPosition attached."),
);
FlutterError.onError = handler;
});
testWidgets('Interactive scrollbars should have a valid scroll controller', (WidgetTester tester) async {
final ScrollController primaryScrollController = ScrollController();
addTearDown(primaryScrollController.dispose);
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: primaryScrollController,
child: RawScrollbar(
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(
height: 1000.0,
width: 1000.0,
),
),
),
),
),
),
);
await tester.pumpAndSettle();
AssertionError? exception = tester.takeException() as AssertionError?;
// The scrollbar is not visible and cannot be interacted with, so no assertion.
expect(exception, isNull);
// Scroll to trigger the scrollbar to come into view.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -20.0));
exception = tester.takeException() as AssertionError;
expect(exception, isAssertionError);
expect(
exception.message,
'''
The Scrollbar's ScrollController has no ScrollPosition attached.
A Scrollbar cannot be painted without a ScrollPosition.
The Scrollbar attempted to use the PrimaryScrollController. This ScrollController should be associated with the ScrollView that the Scrollbar is being applied to.
If a ScrollController has not been provided, the PrimaryScrollController is used by default on mobile platforms for ScrollViews with an Axis.vertical scroll direction.
To use the PrimaryScrollController explicitly, set ScrollView.primary to true on the Scrollable widget.''',
);
});
testWidgets('Scrollbars assert on multiple scroll positions', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: Row(
children: <Widget>[
RawScrollbar(
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 10.0, height: 4000.0),
),
),
RawScrollbar(
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 10.0, height: 4000.0),
),
),
],
),
),
),
),
);
await tester.pumpAndSettle();
AssertionError? exception = tester.takeException() as AssertionError?;
// The scrollbar is not visible and cannot be interacted with, so no assertion.
expect(exception, isNull);
// Scroll to trigger the scrollbar to come into view.
final Finder scrollViews = find.byType(SingleChildScrollView);
final TestGesture gesture = await tester.startGesture(tester.getCenter(scrollViews.first));
await gesture.moveBy(const Offset(0.0, -20.0));
exception = tester.takeException() as AssertionError;
expect(exception, isAssertionError);
expect(
exception.message,
'''
The provided ScrollController is attached to more than one ScrollPosition.
The Scrollbar requires a single ScrollPosition in order to be painted.
When the scrollbar is interactive, the associated ScrollController must only have one ScrollPosition attached.
The provided ScrollController cannot be shared by multiple ScrollView widgets.''',
);
});
testWidgets('Simultaneous dragging and pointer scrolling does not cause a crash', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/70105
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66bcbcbc),
),
);
// Drag the thumb down to scroll down.
const double scrollAmount = 10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
// Drag color
color: const Color(0x66bcbcbc),
),
);
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
expect(scrollController.offset, greaterThan(10.0));
final double previousOffset = scrollController.offset;
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 10.0, 800.0, 100.0),
color: const Color(0x66bcbcbc),
),
);
// Execute a pointer scroll while dragging (drag gesture has not come up yet)
final TestPointer pointer = TestPointer(1, ui.PointerDeviceKind.mouse);
pointer.hover(const Offset(798.0, 15.0));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, 20.0)));
await tester.pumpAndSettle();
if (!kIsWeb) {
// Scrolling while holding the drag on the scrollbar and still hovered over
// the scrollbar should not have changed the scroll offset.
expect(pointer.location, const Offset(798.0, 15.0));
expect(scrollController.offset, previousOffset);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 10.0, 800.0, 100.0),
color: const Color(0x66bcbcbc),
),
);
} else {
expect(pointer.location, const Offset(798.0, 15.0));
expect(scrollController.offset, previousOffset + 20.0);
}
// Drag is still being held, move pointer to be hovering over another area
// of the scrollable (not over the scrollbar) and execute another pointer scroll
pointer.hover(tester.getCenter(find.byType(SingleChildScrollView)));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, -90.0)));
await tester.pumpAndSettle();
// Scrolling while holding the drag on the scrollbar changed the offset
expect(pointer.location, const Offset(400.0, 300.0));
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66bcbcbc),
),
);
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66bcbcbc),
),
);
});
testWidgets('Scrollbar thumb can be dragged in reverse', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
reverse: true,
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 510.0, 800.0, 600.0),
color: const Color(0x66BCBCBC),
),
);
// Drag the thumb up to scroll up.
const double scrollAmount = 10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 550.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, -scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 500.0, 800.0, 590.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('ScrollbarPainter asserts if scrollbarOrientation is used with wrong axisDirection', (WidgetTester tester) async {
final ScrollbarPainter painter = ScrollbarPainter(
color: _kScrollbarColor,
fadeoutOpacityAnimation: kAlwaysCompleteAnimation,
textDirection: TextDirection.ltr,
scrollbarOrientation: ScrollbarOrientation.left,
);
const Size size = Size(60, 80);
final ScrollMetrics scrollMetrics = defaultMetrics.copyWith(
maxScrollExtent: 100,
viewportDimension: size.height,
axisDirection: AxisDirection.right,
);
painter.update(scrollMetrics, scrollMetrics.axisDirection);
expect(() => painter.paint(testCanvas, size), throwsA(isA<AssertionError>()));
});
testWidgets('RawScrollbar mainAxisMargin property works properly', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
mainAxisMargin: 10,
thumbVisibility: true,
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 1000.0),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(rect: const Rect.fromLTRB(794.0, 10.0, 800.0, 358.0))
);
});
testWidgets('shape property of RawScrollbar can draw a BeveledRectangleBorder', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
shape: const BeveledRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0))
),
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(height: 1000.0),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..path(
includes: const <Offset>[
Offset(797.0, 0.0),
Offset(797.0, 18.0),
],
excludes: const <Offset>[
Offset(796.0, 0.0),
Offset(798.0, 0.0),
],
),
);
});
testWidgets('minThumbLength property of RawScrollbar is respected', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
minThumbLength: 21,
minOverscrollLength: 8,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 50000.0),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0)) // track
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 21.0))); // thumb
});
testWidgets('shape property of RawScrollbar can draw a CircleBorder', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
shape: const CircleBorder(side: BorderSide(width: 2.0)),
thickness: 36.0,
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(height: 1000.0, width: 1000),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..path(
includes: const <Offset>[
Offset(782.0, 180.0),
Offset(782.0, 180.0 - 18.0),
Offset(782.0 + 18.0, 180),
Offset(782.0, 180.0 + 18.0),
Offset(782.0 - 18.0, 180),
],
)
..circle(x: 782.0, y: 180.0, radius: 17.0, strokeWidth: 2.0)
);
});
testWidgets('crossAxisMargin property of RawScrollbar is respected', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
crossAxisMargin: 30,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 1000.0),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(734.0, 0.0, 800.0, 600.0))
..rect(rect: const Rect.fromLTRB(764.0, 0.0, 770.0, 360.0)));
});
testWidgets('shape property of RawScrollbar can draw a RoundedRectangleBorder', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
thickness: 20,
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.only(topLeft: Radius.circular(8))),
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(height: 1000.0, width: 1000.0),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(780.0, 0.0, 800.0, 600.0))
..path(
includes: const <Offset>[
Offset(800.0, 0.0),
],
excludes: const <Offset>[
Offset(780.0, 0.0),
],
),
);
});
testWidgets('minOverscrollLength property of RawScrollbar is respected', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
thumbVisibility: true,
minOverscrollLength: 8.0,
minThumbLength: 36.0,
child: SingleChildScrollView(
physics: const BouncingScrollPhysics(),
controller: scrollController,
child: const SizedBox(height: 10000),
)
),
)
)
);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(RawScrollbar)));
await gesture.moveBy(const Offset(0, 1000));
await tester.pump();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 8.0)));
});
testWidgets('not passing any shape or radius to RawScrollbar will draw the usual rectangular thumb', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(height: 1000.0),
),
),
)));
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 360.0))
);
});
testWidgets('The bar can show or hide when the viewport size change', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildFrame(double height) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: SizedBox(width: double.infinity, height: height)
),
),
),
);
}
await tester.pumpWidget(buildFrame(600.0));
await tester.pumpAndSettle();
expect(find.byType(RawScrollbar), isNot(paints..rect())); // Not shown.
await tester.pumpWidget(buildFrame(600.1));
await tester.pumpAndSettle();
expect(find.byType(RawScrollbar), paints..rect()); // Show the bar.
await tester.pumpWidget(buildFrame(600.0));
await tester.pumpAndSettle();
expect(find.byType(RawScrollbar), isNot(paints..rect())); // Hide the bar.
});
testWidgets('The bar can show or hide when the view size change', (WidgetTester tester) async {
addTearDown(tester.view.reset);
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildFrame() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(
width: double.infinity,
height: 600.0,
),
),
),
),
),
);
}
tester.view.physicalSize = const Size(800.0, 600.0);
tester.view.devicePixelRatio = 1;
await tester.pumpWidget(buildFrame());
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(find.byType(RawScrollbar), isNot(paints..rect())); // Not shown.
tester.view.physicalSize = const Size(800.0, 599.0);
await tester.pumpAndSettle();
expect(find.byType(RawScrollbar), paints..rect()..rect()); // Show the bar.
tester.view.physicalSize = const Size(800.0, 600.0);
await tester.pumpAndSettle();
expect(find.byType(RawScrollbar), isNot(paints..rect())); // Not shown.
});
testWidgets('Scrollbar will not flip axes based on notification is there is a scroll controller', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/87697
final ScrollController verticalScrollController = ScrollController();
addTearDown(verticalScrollController.dispose);
final ScrollController horizontalScrollController = ScrollController();
addTearDown(horizontalScrollController.dispose);
Widget buildFrame() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
thumbVisibility: true,
controller: verticalScrollController,
// This scrollbar will receive scroll notifications from both nested
// scroll views of opposite axes, but should stay on the vertical
// axis that its scroll controller is associated with.
notificationPredicate: (ScrollNotification notification) => notification.depth <= 1,
child: SingleChildScrollView(
controller: verticalScrollController,
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
controller: horizontalScrollController,
child: const SizedBox(
width: 1000.0,
height: 1000.0,
),
),
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pumpAndSettle();
expect(verticalScrollController.offset, 0.0);
expect(horizontalScrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 360.0),
color: const Color(0x66BCBCBC),
),
);
// Move the horizontal scroll view. The vertical scrollbar should not flip.
horizontalScrollController.jumpTo(10.0);
expect(verticalScrollController.offset, 0.0);
expect(horizontalScrollController.offset, 10.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 360.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('notificationPredicate depth test.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
final List<int> depths = <int>[];
Widget buildFrame() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
notificationPredicate: (ScrollNotification notification) {
depths.add(notification.depth);
return notification.depth == 0;
},
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SingleChildScrollView(),
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pumpAndSettle();
// `notificationPredicate` should be called twice with different `depth`
// because there are two scrollable widgets.
expect(depths.length, 2);
expect(depths[0], 1);
expect(depths[1], 0);
});
// Regression test for https://github.com/flutter/flutter/issues/92262
testWidgets('Do not crash when resize from scrollable to non-scrollable.', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildFrame(double height) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: RawScrollbar(
controller: scrollController,
interactive: true,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: Container(
width: 100.0,
height: height,
color: const Color(0xFF000000),
),
),
),
),
);
}
await tester.pumpWidget(buildFrame(700.0));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(600.0));
await tester.pumpAndSettle();
// Try to drag the thumb.
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(798.0, 5.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, 5.0));
await tester.pumpAndSettle();
});
testWidgets('Scrollbar thumb can be dragged when the scrollable widget has a negative minScrollExtent - desktop', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/95840
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
final UniqueKey uniqueKey = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(
scrollbars: false,
),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: CustomScrollView(
primary: true,
center: uniqueKey,
slivers: <Widget>[
SliverToBoxAdapter(
child: Container(
height: 600.0,
),
),
SliverToBoxAdapter(
key: uniqueKey,
child: Container(
height: 600.0,
),
),
SliverToBoxAdapter(
child: Container(
height: 600.0,
),
),
],
),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 200.0, 800.0, 400.0),
color: const Color(0x66BCBCBC),
),
);
// Drag the thumb up to scroll up.
const double scrollAmount = -10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 300.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, lessThan(scrollAmount * 2));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 190.0, 800.0, 390.0),
color: const Color(0x66BCBCBC),
),
);
}, variant: TargetPlatformVariant.desktop());
testWidgets('Scrollbar thumb can be dragged when the scrollable widget has a negative minScrollExtent - mobile', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/95840
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
final UniqueKey uniqueKey = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(
scrollbars: false,
),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: CustomScrollView(
center: uniqueKey,
slivers: <Widget>[
SliverToBoxAdapter(
child: Container(
height: 600.0,
),
),
SliverToBoxAdapter(
key: uniqueKey,
child: Container(
height: 600.0,
),
),
SliverToBoxAdapter(
child: Container(
height: 600.0,
),
),
],
),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 200.0, 800.0, 400.0),
color: const Color(0x66BCBCBC),
),
);
// Drag the thumb up to scroll up.
const double scrollAmount = -10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 300.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, lessThan(scrollAmount * 2));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 190.0, 800.0, 390.0),
color: const Color(0x66BCBCBC),
),
);
}, variant: TargetPlatformVariant.mobile());
test('ScrollbarPainter.shouldRepaint returns true when any of the properties changes', () {
ScrollbarPainter createPainter({
Color color = const Color(0xFF000000),
Animation<double> fadeoutOpacityAnimation = kAlwaysCompleteAnimation,
Color trackColor = const Color(0x00000000),
Color trackBorderColor = const Color(0x00000000),
TextDirection textDirection = TextDirection.ltr,
double thickness = _kThickness,
EdgeInsets padding = EdgeInsets.zero,
double mainAxisMargin = 0.0,
double crossAxisMargin = 0.0,
Radius? radius,
Radius? trackRadius,
OutlinedBorder? shape,
double minLength = _kMinThumbExtent,
double? minOverscrollLength,
ScrollbarOrientation scrollbarOrientation = ScrollbarOrientation.top,
}) {
return ScrollbarPainter(
color: color,
fadeoutOpacityAnimation: fadeoutOpacityAnimation,
trackColor: trackColor,
trackBorderColor: trackBorderColor,
textDirection: textDirection,
thickness: thickness,
padding: padding,
mainAxisMargin: mainAxisMargin,
crossAxisMargin: crossAxisMargin,
radius: radius,
trackRadius: trackRadius,
shape: shape,
minLength: minLength,
minOverscrollLength: minOverscrollLength,
scrollbarOrientation: scrollbarOrientation,
);
}
final ScrollbarPainter painter = createPainter();
expect(painter.shouldRepaint(createPainter()), false);
expect(painter.shouldRepaint(createPainter(color: const Color(0xFFFFFFFF))), true);
expect(painter.shouldRepaint(createPainter(fadeoutOpacityAnimation: kAlwaysDismissedAnimation)), true);
expect(painter.shouldRepaint(createPainter(trackColor: const Color(0xFFFFFFFF))), true);
expect(painter.shouldRepaint(createPainter(trackBorderColor: const Color(0xFFFFFFFF))), true);
expect(painter.shouldRepaint(createPainter(textDirection: TextDirection.rtl)), true);
expect(painter.shouldRepaint(createPainter(thickness: _kThickness + 1.0)), true);
expect(painter.shouldRepaint(createPainter(padding: const EdgeInsets.all(1.0))), true);
expect(painter.shouldRepaint(createPainter(mainAxisMargin: 1.0)), true);
expect(painter.shouldRepaint(createPainter(crossAxisMargin: 1.0)), true);
expect(painter.shouldRepaint(createPainter(radius: const Radius.circular(1.0))), true);
expect(painter.shouldRepaint(createPainter(trackRadius: const Radius.circular(1.0))), true);
expect(painter.shouldRepaint(createPainter(shape: const CircleBorder(side: BorderSide(width: 2.0)))), true);
expect(painter.shouldRepaint(createPainter(minLength: _kMinThumbExtent + 1.0)), true);
expect(painter.shouldRepaint(createPainter(minOverscrollLength: 1.0)), true);
expect(painter.shouldRepaint(createPainter(scrollbarOrientation: ScrollbarOrientation.bottom)), true);
});
testWidgets('Scrollbar track can be drawn', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
trackVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0x08000000),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x1a000000),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('RawScrollbar correctly assigns colors', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
thumbColor: const Color(0xFFF44336),
trackVisibility: true,
trackColor: const Color(0xFF2196F3),
trackBorderColor: const Color(0xFFFFEB3B),
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0),
color: const Color(0xFF2196F3),
)
..line(
p1: const Offset(794.0, 0.0),
p2: const Offset(794.0, 600.0),
strokeWidth: 1.0,
color: const Color(0xFFFFEB3B),
)
..rect(
rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 90.0),
color: const Color(0xFFF44336),
),
);
});
testWidgets('trackRadius and radius properties of RawScrollbar can draw RoundedRectangularRect', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
trackVisibility: true,
trackRadius: const Radius.circular(1.0),
radius: const Radius.circular(2.0),
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(RawScrollbar),
paints
..rrect(
rrect: RRect.fromLTRBR(794.0, 0.0, 800.0, 600.0, const Radius.circular(1.0)),
color: const Color(0x08000000),
)
..rrect(
rrect: RRect.fromLTRBR(794.0, 0.0, 800.0, 90.0, const Radius.circular(2.0)),
color: const Color(0x66bcbcbc),
)
);
});
testWidgets('Scrollbar asserts that a visible track has a visible thumb', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildApp() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: false,
trackVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
}
expect(() => tester.pumpWidget(buildApp()), throwsAssertionError);
});
testWidgets('Skip the ScrollPosition check if the bar was unmounted', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/103939
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildApp(bool buildBar) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(
invertColors: buildBar, // Trigger a post frame check before unmount.
),
child: PrimaryScrollController(
controller: scrollController,
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
Widget content = const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
);
if (buildBar) {
content = RawScrollbar(
thumbVisibility: true,
child: content,
);
}
return content;
},
),
),
),
);
}
await tester.pumpWidget(buildApp(true));
await tester.pumpWidget(buildApp(false));
// Go without throw.
});
testWidgets('Track offset respects MediaQuery padding', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/106834
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.all(50.0),
),
child: RawScrollbar(
controller: scrollController,
minThumbLength: 21,
minOverscrollLength: 8,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 50000.0),
),
),
)
)
);
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(744.0, 50.0, 750.0, 550.0)) // track
..rect(rect: const Rect.fromLTRB(744.0, 50.0, 750.0, 71.0)) // thumb
); // thumb
});
testWidgets('RawScrollbar.padding replaces MediaQueryData.padding', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.all(50.0),
),
child: RawScrollbar(
controller: scrollController,
minThumbLength: 21,
minOverscrollLength: 8,
thumbVisibility: true,
padding: const EdgeInsets.all(100),
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 50000.0),
),
),
)
)
);
await tester.pumpAndSettle();
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(694.0, 100.0, 700.0, 500.0)) // track
..rect(rect: const Rect.fromLTRB(694.0, 100.0, 700.0, 121.0)) // thumb
); // thumb
});
testWidgets('Scrollbar respect the NeverScrollableScrollPhysics physics', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
physics: NeverScrollableScrollPhysics(),
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
// Drag the thumb down to scroll down.
const double scrollAmount = 10.0;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 45.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
// Tap on the track area below the thumb.
await tester.tapAt(const Offset(797.0, 550.0));
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
});
testWidgets('The thumb should follow the pointer when the scroll metrics changed during dragging', (WidgetTester tester) async {
// Regressing test for https://github.com/flutter/flutter/issues/112072
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
final double height;
if (index < 10) {
height = 100;
} else {
height = 500;
}
return SizedBox(
height: height,
child: Text('$index'),
);
},
childCount: 100,
),
),
],
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
// Drag the thumb down to scroll down.
const double scrollAmount = 100;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 5.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan((100.0 * 10 + 500.0 * 90) / 3));
expect(
find.byType(RawScrollbar),
paints
..rect(rect: const Rect.fromLTRB(794.0, 0.0, 800.0, 600.0))
..rect(
rect: const Rect.fromLTRB(794.0, 200.0, 800.0, 218.0),
color: const Color(0x66BCBCBC),
),
);
});
testWidgets('The scrollable should not stutter when the scroll metrics shrink during dragging', (WidgetTester tester) async {
// Regressing test for https://github.com/flutter/flutter/issues/121574
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
final double height;
if (index < 10) {
height = 500;
} else {
height = 100;
}
return SizedBox(
height: height,
child: Text('$index'),
);
},
childCount: 100,
),
),
],
),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
// Drag the thumb down to scroll down.
const double scrollAmount = 100;
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(797.0, 5.0));
await tester.pumpAndSettle();
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
final double lastPosition = scrollController.offset;
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(lastPosition, greaterThan((100.0 * 10 + 500.0 * 90) / 6));
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
expect(scrollController.offset, greaterThan(lastPosition));
});
testWidgets('The bar supports mouse wheel event', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/pull/109659
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
Widget buildFrame() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: RawScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
primary: true,
child: SizedBox(
width: double.infinity,
height: 1200.0,
),
),
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
// Execute a pointer scroll hover on the scroll bar
final TestPointer pointer = TestPointer(1, ui.PointerDeviceKind.mouse);
pointer.hover(const Offset(798.0, 15.0));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, 30.0)));
await tester.pumpAndSettle();
expect(scrollController.offset, 30.0);
// Execute a pointer scroll outside the scroll bar
pointer.hover(const Offset(198.0, 15.0));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, 70.0)));
await tester.pumpAndSettle();
expect(scrollController.offset, 100.0);
}, variant: TargetPlatformVariant.all());
}
| flutter/packages/flutter/test/widgets/scrollbar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/scrollbar_test.dart",
"repo_id": "flutter",
"token_count": 45479
} | 720 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'semantics_tester.dart';
void main() {
testWidgets('Semantics can merge sibling group', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
const SemanticsTag first = SemanticsTag('1');
const SemanticsTag second = SemanticsTag('2');
const SemanticsTag third = SemanticsTag('3');
ChildSemanticsConfigurationsResult delegate(List<SemanticsConfiguration> configs) {
expect(configs.length, 3);
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
final List<SemanticsConfiguration> sibling = <SemanticsConfiguration>[];
// Merge first and third
for (final SemanticsConfiguration config in configs) {
if (config.tagsChildrenWith(first) || config.tagsChildrenWith(third)) {
sibling.add(config);
} else {
builder.markAsMergeUp(config);
}
}
builder.markAsSiblingMergeGroup(sibling);
return builder.build();
}
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Semantics(
label: 'parent',
child: TestConfigDelegate(
delegate: delegate,
child: Column(
children: <Widget>[
Semantics(
label: '1',
tagForChildren: first,
child: const SizedBox(width: 100, height: 100),
// this tests that empty nodes disappear
),
Semantics(
label: '2',
tagForChildren: second,
child: const SizedBox(width: 100, height: 100),
),
Semantics(
label: '3',
tagForChildren: third,
child: const SizedBox(width: 100, height: 100),
),
],
),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
label: 'parent\n2',
),
TestSemantics.rootChild(
label: '1\n3',
),
],
), ignoreId: true, ignoreRect: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('Semantics can drop semantics config', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
const SemanticsTag first = SemanticsTag('1');
const SemanticsTag second = SemanticsTag('2');
const SemanticsTag third = SemanticsTag('3');
ChildSemanticsConfigurationsResult delegate(List<SemanticsConfiguration> configs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
// Merge first and third
for (final SemanticsConfiguration config in configs) {
if (config.tagsChildrenWith(first) || config.tagsChildrenWith(third)) {
continue;
}
builder.markAsMergeUp(config);
}
return builder.build();
}
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Semantics(
label: 'parent',
child: TestConfigDelegate(
delegate: delegate,
child: Column(
children: <Widget>[
Semantics(
label: '1',
tagForChildren: first,
child: const SizedBox(width: 100, height: 100),
// this tests that empty nodes disappear
),
Semantics(
label: '2',
tagForChildren: second,
child: const SizedBox(width: 100, height: 100),
),
Semantics(
label: '3',
tagForChildren: third,
child: const SizedBox(width: 100, height: 100),
),
],
),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
label: 'parent\n2',
),
],
), ignoreId: true, ignoreRect: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('Semantics throws when mark the same config twice case 1', (WidgetTester tester) async {
const SemanticsTag first = SemanticsTag('1');
const SemanticsTag second = SemanticsTag('2');
const SemanticsTag third = SemanticsTag('3');
ChildSemanticsConfigurationsResult delegate(List<SemanticsConfiguration> configs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
// Marks the same one twice.
builder.markAsMergeUp(configs.first);
builder.markAsMergeUp(configs.first);
return builder.build();
}
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Semantics(
label: 'parent',
child: TestConfigDelegate(
delegate: delegate,
child: Column(
children: <Widget>[
Semantics(
label: '1',
tagForChildren: first,
child: const SizedBox(width: 100, height: 100),
// this tests that empty nodes disappear
),
Semantics(
label: '2',
tagForChildren: second,
child: const SizedBox(width: 100, height: 100),
),
Semantics(
label: '3',
tagForChildren: third,
child: const SizedBox(width: 100, height: 100),
),
],
),
),
),
),
);
expect(tester.takeException(), isAssertionError);
});
testWidgets('Semantics throws when mark the same config twice case 2', (WidgetTester tester) async {
const SemanticsTag first = SemanticsTag('1');
const SemanticsTag second = SemanticsTag('2');
const SemanticsTag third = SemanticsTag('3');
ChildSemanticsConfigurationsResult delegate(List<SemanticsConfiguration> configs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
// Marks the same one twice.
builder.markAsMergeUp(configs.first);
builder.markAsSiblingMergeGroup(<SemanticsConfiguration>[configs.first]);
return builder.build();
}
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Semantics(
label: 'parent',
child: TestConfigDelegate(
delegate: delegate,
child: Column(
children: <Widget>[
Semantics(
label: '1',
tagForChildren: first,
child: const SizedBox(width: 100, height: 100),
// this tests that empty nodes disappear
),
Semantics(
label: '2',
tagForChildren: second,
child: const SizedBox(width: 100, height: 100),
),
Semantics(
label: '3',
tagForChildren: third,
child: const SizedBox(width: 100, height: 100),
),
],
),
),
),
),
);
expect(tester.takeException(), isAssertionError);
});
testWidgets('RenderObject with semantics child delegate will mark correct boundary dirty', (WidgetTester tester) async {
final UniqueKey inner = UniqueKey();
final UniqueKey boundaryParent = UniqueKey();
final UniqueKey grandBoundaryParent = UniqueKey();
ChildSemanticsConfigurationsResult delegate(List<SemanticsConfiguration> configs) {
final ChildSemanticsConfigurationsResultBuilder builder = ChildSemanticsConfigurationsResultBuilder();
configs.forEach(builder.markAsMergeUp);
return builder.build();
}
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MarkSemanticsDirtySpy(
key: grandBoundaryParent,
child: MarkSemanticsDirtySpy(
key: boundaryParent,
child: TestConfigDelegate(
delegate: delegate,
child: Column(
children: <Widget>[
Semantics(
label: 'label',
child: MarkSemanticsDirtySpy(
key: inner,
child: const Text('inner'),
),
),
],
),
),
),
),
),
);
final RenderMarkSemanticsDirtySpy innerObject = tester.renderObject<RenderMarkSemanticsDirtySpy>(find.byKey(inner));
final RenderTestConfigDelegate objectWithDelegate = tester.renderObject<RenderTestConfigDelegate>(find.byType(TestConfigDelegate));
final RenderMarkSemanticsDirtySpy boundaryParentObject = tester.renderObject<RenderMarkSemanticsDirtySpy>(find.byKey(boundaryParent));
final RenderMarkSemanticsDirtySpy grandBoundaryParentObject = tester.renderObject<RenderMarkSemanticsDirtySpy>(find.byKey(grandBoundaryParent));
void resetBuildState() {
innerObject.hasRebuildSemantics = false;
boundaryParentObject.hasRebuildSemantics = false;
grandBoundaryParentObject.hasRebuildSemantics = false;
}
// Sanity check
expect(innerObject.hasRebuildSemantics, isTrue);
expect(boundaryParentObject.hasRebuildSemantics, isTrue);
expect(grandBoundaryParentObject.hasRebuildSemantics, isTrue);
resetBuildState();
innerObject.markNeedsSemanticsUpdate();
await tester.pump();
// Inner boundary should not trigger rebuild above it.
expect(innerObject.hasRebuildSemantics, isTrue);
expect(boundaryParentObject.hasRebuildSemantics, isFalse);
expect(grandBoundaryParentObject.hasRebuildSemantics, isFalse);
resetBuildState();
objectWithDelegate.markNeedsSemanticsUpdate();
await tester.pump();
// object with delegate rebuilds up to grand parent boundary;
expect(innerObject.hasRebuildSemantics, isTrue);
expect(boundaryParentObject.hasRebuildSemantics, isTrue);
expect(grandBoundaryParentObject.hasRebuildSemantics, isTrue);
resetBuildState();
boundaryParentObject.markNeedsSemanticsUpdate();
await tester.pump();
// Render objects in between child delegate and grand boundary parent does
// not mark the grand boundary parent dirty because it should not change the
// generated sibling nodes.
expect(innerObject.hasRebuildSemantics, isTrue);
expect(boundaryParentObject.hasRebuildSemantics, isTrue);
expect(grandBoundaryParentObject.hasRebuildSemantics, isFalse);
});
}
class MarkSemanticsDirtySpy extends SingleChildRenderObjectWidget {
const MarkSemanticsDirtySpy({super.key, super.child});
@override
RenderMarkSemanticsDirtySpy createRenderObject(BuildContext context) => RenderMarkSemanticsDirtySpy();
}
class RenderMarkSemanticsDirtySpy extends RenderProxyBox {
RenderMarkSemanticsDirtySpy();
bool hasRebuildSemantics = false;
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
config.isSemanticBoundary = true;
}
@override
void assembleSemanticsNode(
SemanticsNode node,
SemanticsConfiguration config,
Iterable<SemanticsNode> children,
) {
hasRebuildSemantics = true;
}
}
class TestConfigDelegate extends SingleChildRenderObjectWidget {
const TestConfigDelegate({super.key, required this.delegate, super.child});
final ChildSemanticsConfigurationsDelegate delegate;
@override
RenderTestConfigDelegate createRenderObject(BuildContext context) => RenderTestConfigDelegate(
delegate: delegate,
);
@override
void updateRenderObject(BuildContext context, RenderTestConfigDelegate renderObject) {
renderObject.delegate = delegate;
}
}
class RenderTestConfigDelegate extends RenderProxyBox {
RenderTestConfigDelegate({
ChildSemanticsConfigurationsDelegate? delegate,
}) : _delegate = delegate;
ChildSemanticsConfigurationsDelegate? get delegate => _delegate;
ChildSemanticsConfigurationsDelegate? _delegate;
set delegate(ChildSemanticsConfigurationsDelegate? value) {
if (value != _delegate) {
markNeedsSemanticsUpdate();
}
_delegate = value;
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
config.childConfigurationsDelegate = delegate;
}
}
| flutter/packages/flutter/test/widgets/semantics_child_configs_delegate_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/semantics_child_configs_delegate_test.dart",
"repo_id": "flutter",
"token_count": 5412
} | 721 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
class BadWidget extends StatefulWidget {
const BadWidget({ super.key });
@override
State<StatefulWidget> createState() => BadWidgetState();
}
class BadWidgetState extends State<BadWidget> {
BadWidgetState() {
setState(() {
_count = 1;
});
}
int _count = 0;
@override
Widget build(BuildContext context) {
return Text(_count.toString());
}
}
void main() {
testWidgets('setState() catches being used inside a constructor', (WidgetTester tester) async {
await tester.pumpWidget(const BadWidget());
expect(tester.takeException(), isFlutterError);
});
}
| flutter/packages/flutter/test/widgets/set_state_5_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/set_state_5_test.dart",
"repo_id": "flutter",
"token_count": 276
} | 722 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('SliverFillViewport control test', (WidgetTester tester) async {
final List<Widget> children = List<Widget>.generate(20, (int i) {
return ColoredBox(color: Colors.green, child: Text('$i', textDirection: TextDirection.ltr));
});
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverFillViewport(
delegate: SliverChildListDelegate(children, addAutomaticKeepAlives: false, addSemanticIndexes: false),
),
],
),
),
);
final RenderBox box = tester.renderObject<RenderBox>(find.byType(ColoredBox).first);
expect(box.size.height, equals(600.0));
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
expect(find.text('2'), findsNothing);
await tester.drag(find.byType(Scrollable), const Offset(0.0, -700.0));
await tester.pump();
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
expect(find.text('2'), findsOneWidget);
expect(find.text('3'), findsNothing);
expect(find.text('4'), findsNothing);
await tester.drag(find.byType(Scrollable), const Offset(0.0, 200.0));
await tester.pump();
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsOneWidget);
expect(find.text('2'), findsNothing);
expect(find.text('3'), findsNothing);
await tester.drag(find.byType(Scrollable), const Offset(0.0, 700.0));
await tester.pump();
final RenderBox box2 = tester.renderObject<RenderBox>(find.byType(ColoredBox).first);
expect(box2.size.height, equals(600.0));
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
expect(find.text('2'), findsNothing);
expect(find.text('3'), findsNothing);
final RenderObject viewport = tester.renderObject<RenderObject>(find.byType(SliverFillViewport).first);
expect(viewport, hasAGoodToStringDeep);
expect(
viewport.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'_RenderSliverFractionalPadding#00000 relayoutBoundary=up1\n'
' │ needs compositing\n'
' │ parentData: paintOffset=Offset(0.0, 0.0) (can use size)\n'
' │ constraints: SliverConstraints(AxisDirection.down,\n'
' │ GrowthDirection.forward, ScrollDirection.idle, scrollOffset:\n'
' │ 0.0, precedingScrollExtent: 0.0, remainingPaintExtent: 600.0,\n'
' │ crossAxisExtent: 800.0, crossAxisDirection:\n'
' │ AxisDirection.right, viewportMainAxisExtent: 600.0,\n'
' │ remainingCacheExtent: 850.0, cacheOrigin: 0.0)\n'
' │ geometry: SliverGeometry(scrollExtent: 12000.0, paintExtent:\n'
' │ 600.0, maxPaintExtent: 12000.0, hasVisualOverflow: true,\n'
' │ cacheExtent: 850.0)\n'
' │\n'
' └─child: RenderSliverFillViewport#00000 relayoutBoundary=up2\n'
' │ needs compositing\n'
' │ parentData: paintOffset=Offset(0.0, 0.0) (can use size)\n'
' │ constraints: SliverConstraints(AxisDirection.down,\n'
' │ GrowthDirection.forward, ScrollDirection.idle, scrollOffset:\n'
' │ 0.0, precedingScrollExtent: 0.0, remainingPaintExtent: 600.0,\n'
' │ crossAxisExtent: 800.0, crossAxisDirection:\n'
' │ AxisDirection.right, viewportMainAxisExtent: 600.0,\n'
' │ remainingCacheExtent: 850.0, cacheOrigin: 0.0)\n'
' │ geometry: SliverGeometry(scrollExtent: 12000.0, paintExtent:\n'
' │ 600.0, maxPaintExtent: 12000.0, hasVisualOverflow: true,\n'
' │ cacheExtent: 850.0)\n'
' │ currently live children: 0 to 1\n'
' │\n'
' ├─child with index 0: RenderRepaintBoundary#00000\n'
' │ │ needs compositing\n'
' │ │ parentData: index=0; layoutOffset=0.0\n'
' │ │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ │ layer: OffsetLayer#00000\n'
' │ │ size: Size(800.0, 600.0)\n'
' │ │ metrics: 66.7% useful (1 bad vs 2 good)\n'
' │ │ diagnosis: insufficient data to draw conclusion (less than five\n'
' │ │ repaints)\n'
' │ │\n'
' │ └─child: _RenderColoredBox#00000\n'
' │ │ parentData: <none> (can use size)\n'
' │ │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ │ size: Size(800.0, 600.0)\n'
' │ │ behavior: opaque\n'
' │ │\n'
' │ └─child: RenderParagraph#00000\n'
' │ │ parentData: <none> (can use size)\n'
' │ │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ │ semantics node: SemanticsNode#2\n'
' │ │ size: Size(800.0, 600.0)\n'
' │ │ textAlign: start\n'
' │ │ textDirection: ltr\n'
' │ │ softWrap: wrapping at box width\n'
' │ │ overflow: clip\n'
' │ │ maxLines: unlimited\n'
' │ ╘═╦══ text ═══\n'
' │ ║ TextSpan:\n'
' │ ║ <all styles inherited>\n'
' │ ║ "0"\n'
' │ ╚═══════════\n'
' └─child with index 1: RenderRepaintBoundary#00000\n'
' │ needs compositing\n'
' │ parentData: index=1; layoutOffset=600.0\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ layer: OffsetLayer#00000 DETACHED\n'
' │ size: Size(800.0, 600.0)\n'
' │ metrics: 50.0% useful (1 bad vs 1 good)\n'
' │ diagnosis: insufficient data to draw conclusion (less than five\n'
' │ repaints)\n'
' │\n'
' └─child: _RenderColoredBox#00000\n'
' │ parentData: <none> (can use size)\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ size: Size(800.0, 600.0)\n'
' │ behavior: opaque\n'
' │\n'
' └─child: RenderParagraph#00000\n'
' │ parentData: <none> (can use size)\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ semantics node: SemanticsNode#3\n'
' │ size: Size(800.0, 600.0)\n'
' │ textAlign: start\n'
' │ textDirection: ltr\n'
' │ softWrap: wrapping at box width\n'
' │ overflow: clip\n'
' │ maxLines: unlimited\n'
' ╘═╦══ text ═══\n'
' ║ TextSpan:\n'
' ║ <all styles inherited>\n'
' ║ "1"\n'
' ╚═══════════\n',
),
);
});
testWidgets('SliverFillViewport padding test', (WidgetTester tester) async {
final SliverChildListDelegate delegate = SliverChildListDelegate(
<Widget>[
const Text('0'),
],
addAutomaticKeepAlives: false,
addSemanticIndexes: false,
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverFillViewport(
viewportFraction: 0.5,
delegate: delegate,
),
],
),
),
);
final RenderSliver boxWithPadding = tester.renderObject<RenderSliver>(find.byType(SliverFillViewport));
expect(boxWithPadding.geometry!.paintExtent, equals(600.0));
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverFillViewport(
padEnds: false,
viewportFraction: 0.5,
delegate: delegate,
),
],
),
),
);
final RenderSliver boxWithoutPadding = tester.renderObject<RenderSliver>(find.byType(SliverFillViewport));
expect(boxWithoutPadding.geometry!.paintExtent, equals(300.0));
});
}
| flutter/packages/flutter/test/widgets/sliver_fill_viewport_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/sliver_fill_viewport_test.dart",
"repo_id": "flutter",
"token_count": 4034
} | 723 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
class _MockRenderSliver extends RenderSliver {
@override
void performLayout() {
geometry = const SliverGeometry(
paintOrigin: 10,
paintExtent: 10,
maxPaintExtent: 10,
);
}
}
Future<void> test(WidgetTester tester, double offset, EdgeInsetsGeometry padding, AxisDirection axisDirection, TextDirection textDirection) {
final ViewportOffset viewportOffset = ViewportOffset.fixed(offset);
addTearDown(viewportOffset.dispose);
return tester.pumpWidget(
Directionality(
textDirection: textDirection,
child: Viewport(
offset: viewportOffset,
axisDirection: axisDirection,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(width: 400.0, height: 400.0, child: Text('before'))),
SliverPadding(
padding: padding,
sliver: const SliverToBoxAdapter(child: SizedBox(width: 400.0, height: 400.0, child: Text('padded'))),
),
const SliverToBoxAdapter(child: SizedBox(width: 400.0, height: 400.0, child: Text('after'))),
],
),
),
);
}
void verify(WidgetTester tester, List<Rect> answerKey) {
final List<Rect> testAnswers = tester.renderObjectList<RenderBox>(find.byType(SizedBox, skipOffstage: false)).map<Rect>(
(RenderBox target) {
final Offset topLeft = target.localToGlobal(Offset.zero);
final Offset bottomRight = target.localToGlobal(target.size.bottomRight(Offset.zero));
return Rect.fromPoints(topLeft, bottomRight);
},
).toList();
expect(testAnswers, equals(answerKey));
}
void main() {
testWidgets('Viewport+SliverPadding basic test (VISUAL)', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.fromLTRB(25.0, 20.0, 15.0, 35.0);
await test(tester, 0.0, padding, AxisDirection.down, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, 0.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 420.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 855.0, 800.0, 400.0),
]);
await test(tester, 200.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -200.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 220.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 655.0, 800.0, 400.0),
]);
await test(tester, 390.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -390.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 30.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 465.0, 800.0, 400.0),
]);
await test(tester, 490.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -490.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, -70.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 365.0, 800.0, 400.0),
]);
await test(tester, 10000.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -10000.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, -9580.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, -9145.0, 800.0, 400.0),
]);
});
testWidgets('Viewport+SliverPadding basic test (LTR)', (WidgetTester tester) async {
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.fromSTEB(25.0, 20.0, 15.0, 35.0);
await test(tester, 0.0, padding, AxisDirection.down, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, 0.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 420.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 855.0, 800.0, 400.0),
]);
await test(tester, 200.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -200.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 220.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 655.0, 800.0, 400.0),
]);
await test(tester, 390.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -390.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, 30.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 465.0, 800.0, 400.0),
]);
await test(tester, 490.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -490.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, -70.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 365.0, 800.0, 400.0),
]);
await test(tester, 10000.0, padding, AxisDirection.down, TextDirection.ltr);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -10000.0, 800.0, 400.0),
const Rect.fromLTWH(25.0, -9580.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, -9145.0, 800.0, 400.0),
]);
});
testWidgets('Viewport+SliverPadding basic test (RTL)', (WidgetTester tester) async {
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.fromSTEB(25.0, 20.0, 15.0, 35.0);
await test(tester, 0.0, padding, AxisDirection.down, TextDirection.rtl);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, 0.0, 800.0, 400.0),
const Rect.fromLTWH(15.0, 420.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 855.0, 800.0, 400.0),
]);
await test(tester, 200.0, padding, AxisDirection.down, TextDirection.rtl);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -200.0, 800.0, 400.0),
const Rect.fromLTWH(15.0, 220.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 655.0, 800.0, 400.0),
]);
await test(tester, 390.0, padding, AxisDirection.down, TextDirection.rtl);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -390.0, 800.0, 400.0),
const Rect.fromLTWH(15.0, 30.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 465.0, 800.0, 400.0),
]);
await test(tester, 490.0, padding, AxisDirection.down, TextDirection.rtl);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -490.0, 800.0, 400.0),
const Rect.fromLTWH(15.0, -70.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, 365.0, 800.0, 400.0),
]);
await test(tester, 10000.0, padding, AxisDirection.down, TextDirection.rtl);
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -10000.0, 800.0, 400.0),
const Rect.fromLTWH(15.0, -9580.0, 760.0, 400.0),
const Rect.fromLTWH(0.0, -9145.0, 800.0, 400.0),
]);
});
testWidgets('Viewport+SliverPadding hit testing', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.all(30.0);
await test(tester, 350.0, padding, AxisDirection.down, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, -350.0, 800.0, 400.0),
const Rect.fromLTWH(30.0, 80.0, 740.0, 400.0),
const Rect.fromLTWH(0.0, 510.0, 800.0, 400.0),
]);
HitTestResult result;
result = tester.hitTestOnBinding(const Offset(10.0, 10.0));
hitsText(result, 'before');
result = tester.hitTestOnBinding(const Offset(10.0, 60.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(100.0, 100.0));
hitsText(result, 'padded');
result = tester.hitTestOnBinding(const Offset(100.0, 490.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(10.0, 520.0));
hitsText(result, 'after');
});
testWidgets('Viewport+SliverPadding hit testing up', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.all(30.0);
await test(tester, 350.0, padding, AxisDirection.up, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(0.0, 600.0+350.0-400.0, 800.0, 400.0),
const Rect.fromLTWH(30.0, 600.0-80.0-400.0, 740.0, 400.0),
const Rect.fromLTWH(0.0, 600.0-510.0-400.0, 800.0, 400.0),
]);
HitTestResult result;
result = tester.hitTestOnBinding(const Offset(10.0, 600.0-10.0));
hitsText(result, 'before');
result = tester.hitTestOnBinding(const Offset(10.0, 600.0-60.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(100.0, 600.0-100.0));
hitsText(result, 'padded');
result = tester.hitTestOnBinding(const Offset(100.0, 600.0-490.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(10.0, 600.0-520.0));
hitsText(result, 'after');
});
testWidgets('Viewport+SliverPadding hit testing left', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.all(30.0);
await test(tester, 350.0, padding, AxisDirection.left, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(800.0+350.0-400.0, 0.0, 400.0, 600.0),
const Rect.fromLTWH(800.0-80.0-400.0, 30.0, 400.0, 540.0),
const Rect.fromLTWH(800.0-510.0-400.0, 0.0, 400.0, 600.0),
]);
HitTestResult result;
result = tester.hitTestOnBinding(const Offset(800.0-10.0, 10.0));
hitsText(result, 'before');
result = tester.hitTestOnBinding(const Offset(800.0-60.0, 10.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(800.0-100.0, 100.0));
hitsText(result, 'padded');
result = tester.hitTestOnBinding(const Offset(800.0-490.0, 100.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(800.0-520.0, 10.0));
hitsText(result, 'after');
});
testWidgets('Viewport+SliverPadding hit testing right', (WidgetTester tester) async {
const EdgeInsets padding = EdgeInsets.all(30.0);
await test(tester, 350.0, padding, AxisDirection.right, TextDirection.ltr);
expect(tester.renderObject<RenderBox>(find.byType(Viewport)).size, equals(const Size(800.0, 600.0)));
verify(tester, <Rect>[
const Rect.fromLTWH(-350.0, 0.0, 400.0, 600.0),
const Rect.fromLTWH(80.0, 30.0, 400.0, 540.0),
const Rect.fromLTWH(510.0, 0.0, 400.0, 600.0),
]);
HitTestResult result;
result = tester.hitTestOnBinding(const Offset(10.0, 10.0));
hitsText(result, 'before');
result = tester.hitTestOnBinding(const Offset(60.0, 10.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(100.0, 100.0));
hitsText(result, 'padded');
result = tester.hitTestOnBinding(const Offset(490.0, 100.0));
expect(result.path.first.target, isA<RenderView>());
result = tester.hitTestOnBinding(const Offset(520.0, 10.0));
hitsText(result, 'after');
});
testWidgets('Viewport+SliverPadding no child', (WidgetTester tester) async {
final ViewportOffset offset = ViewportOffset.fixed(0.0);
addTearDown(offset.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
offset: offset,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.all(100.0)),
SliverToBoxAdapter(child: SizedBox(width: 400.0, height: 400.0, child: Text('x'))),
],
),
),
);
expect(tester.renderObject<RenderBox>(find.text('x')).localToGlobal(Offset.zero), const Offset(0.0, 200.0));
});
testWidgets('SliverPadding with no child reports correct geometry as scroll offset changes', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/64506
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
controller: controller,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.all(100.0)),
SliverToBoxAdapter(child: SizedBox(width: 400.0, height: 400.0, child: Text('x'))),
],
),
),
);
expect(tester.renderObject<RenderBox>(find.text('x')).localToGlobal(Offset.zero), const Offset(0.0, 200.0));
expect(
tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).geometry!.paintExtent,
200.0,
);
controller.jumpTo(50.0);
await tester.pump();
expect(
tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).geometry!.paintExtent,
150.0,
);
});
testWidgets('Viewport+SliverPadding changing padding', (WidgetTester tester) async {
final ViewportOffset offset1 = ViewportOffset.fixed(0.0);
addTearDown(offset1.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.left,
offset: offset1,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(90.0, 1.0, 110.0, 2.0)),
SliverToBoxAdapter(child: SizedBox(width: 201.0, child: Text('x'))),
],
),
),
);
expect(tester.renderObject<RenderBox>(find.text('x')).localToGlobal(Offset.zero), const Offset(399.0, 0.0));
final ViewportOffset offset2 = ViewportOffset.fixed(0.0);
addTearDown(offset2.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.left,
offset: offset2,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(110.0, 1.0, 80.0, 2.0)),
SliverToBoxAdapter(child: SizedBox(width: 201.0, child: Text('x'))),
],
),
),
);
expect(tester.renderObject<RenderBox>(find.text('x')).localToGlobal(Offset.zero), const Offset(409.0, 0.0));
});
testWidgets('Viewport+SliverPadding changing direction', (WidgetTester tester) async {
final ViewportOffset offset1 = ViewportOffset.fixed(0.0);
addTearDown(offset1.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.up,
offset: offset1,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(1.0, 2.0, 4.0, 8.0)),
],
),
),
);
expect(tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).afterPadding, 2.0);
final ViewportOffset offset2 = ViewportOffset.fixed(0.0);
addTearDown(offset2.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
offset: offset2,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(1.0, 2.0, 4.0, 8.0)),
],
),
),
);
expect(tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).afterPadding, 8.0);
final ViewportOffset offset3 = ViewportOffset.fixed(0.0);
addTearDown(offset3.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.right,
offset: offset3,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(1.0, 2.0, 4.0, 8.0)),
],
),
),
);
expect(tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).afterPadding, 4.0);
final ViewportOffset offset4 = ViewportOffset.fixed(0.0);
addTearDown(offset4.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.left,
offset: offset4,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(1.0, 2.0, 4.0, 8.0)),
],
),
),
);
expect(tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding)).afterPadding, 1.0);
final ViewportOffset offset5 = ViewportOffset.fixed(99999.9);
addTearDown(offset5.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Viewport(
axisDirection: AxisDirection.left,
offset: offset5,
slivers: const <Widget>[
SliverPadding(padding: EdgeInsets.fromLTRB(1.0, 2.0, 4.0, 8.0)),
],
),
),
);
expect(tester.renderObject<RenderSliverPadding>(find.byType(SliverPadding, skipOffstage: false)).afterPadding, 1.0);
});
testWidgets('SliverPadding propagates geometry offset corrections', (WidgetTester tester) async {
Widget listBuilder(IndexedWidgetBuilder sliverChildBuilder) {
return Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
cacheExtent: 0.0,
slivers: <Widget>[
SliverPadding(
padding: EdgeInsets.zero,
sliver: SliverList(
delegate: SliverChildBuilderDelegate(
sliverChildBuilder,
childCount: 10,
),
),
),
],
),
);
}
await tester.pumpWidget(
listBuilder(
(BuildContext context, int index) {
return SizedBox(
height: 200.0,
child: Center(
child: Text(index.toString()),
),
);
},
),
);
await tester.drag(find.text('2'), const Offset(0.0, -300.0));
await tester.pump();
expect(
tester.getRect(find.widgetWithText(SizedBox, '2')),
const Rect.fromLTRB(0.0, 100.0, 800.0, 300.0),
);
// Now item 0 is 400.0px and going back will underflow.
await tester.pumpWidget(
listBuilder(
(BuildContext context, int index) {
return SizedBox(
height: index == 0 ? 400.0 : 200.0,
child: Center(
child: Text(index.toString()),
),
);
},
),
);
await tester.drag(find.text('2'), const Offset(0.0, 300.0));
// On this one frame, the scroll correction must properly propagate.
await tester.pump();
expect(
tester.getRect(find.widgetWithText(SizedBox, '0')),
const Rect.fromLTRB(0.0, -200.0, 800.0, 200.0),
);
});
testWidgets('SliverPadding includes preceding padding in the precedingScrollExtent provided to child', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/49195
final UniqueKey key = UniqueKey();
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverPadding(
padding: const EdgeInsets.only(top: 30),
sliver: SliverFillRemaining(
hasScrollBody: false,
child: Container(
key: key,
color: Colors.red,
),
),
),
],
),
));
await tester.pump();
// The value of 570 is expected since SliverFillRemaining will fill all of
// the space available to it. In this test, the extent of the viewport is
// 600 pixels. If the SliverPadding widget provides the right constraints
// to SliverFillRemaining, with 30 pixels preceding it, it should only have
// a height of 570.
expect(
tester.renderObject<RenderBox>(find.byKey(key)).size.height,
equals(570),
);
});
testWidgets("SliverPadding consumes only its padding from the overlap of its parent's constraints", (WidgetTester tester) async {
final _MockRenderSliver mock = _MockRenderSliver();
addTearDown(mock.dispose);
final RenderSliverPadding renderObject = RenderSliverPadding(
padding: const EdgeInsets.only(top: 20),
);
addTearDown(renderObject.dispose);
renderObject.child = mock;
renderObject.layout(const SliverConstraints(
viewportMainAxisExtent: 100.0,
overlap: 100.0,
cacheOrigin: 0.0,
scrollOffset: 0.0,
axisDirection: AxisDirection.down,
growthDirection: GrowthDirection.forward,
crossAxisExtent: 100.0,
crossAxisDirection: AxisDirection.right,
userScrollDirection: ScrollDirection.idle,
remainingPaintExtent: 100.0,
remainingCacheExtent: 100.0,
precedingScrollExtent: 0.0,
),
parentUsesSize: true,
);
expect(mock.constraints.overlap, 80.0);
});
testWidgets("SliverPadding passes the overlap to the child if it's negative", (WidgetTester tester) async {
final _MockRenderSliver mock = _MockRenderSliver();
addTearDown(mock.dispose);
final RenderSliverPadding renderObject = RenderSliverPadding(
padding: const EdgeInsets.only(top: 20),
);
addTearDown(renderObject.dispose);
renderObject.child = mock;
renderObject.layout(const SliverConstraints(
viewportMainAxisExtent: 100.0,
overlap: -100.0,
cacheOrigin: 0.0,
scrollOffset: 0.0,
axisDirection: AxisDirection.down,
growthDirection: GrowthDirection.forward,
crossAxisExtent: 100.0,
crossAxisDirection: AxisDirection.right,
userScrollDirection: ScrollDirection.idle,
remainingPaintExtent: 100.0,
remainingCacheExtent: 100.0,
precedingScrollExtent: 0.0,
),
parentUsesSize: true,
);
expect(mock.constraints.overlap, -100.0);
});
testWidgets('SliverPadding passes the paintOrigin of the child on', (WidgetTester tester) async {
final _MockRenderSliver mock = _MockRenderSliver();
addTearDown(mock.dispose);
final RenderSliverPadding renderObject = RenderSliverPadding(
padding: const EdgeInsets.only(top: 20),
);
addTearDown(renderObject.dispose);
renderObject.child = mock;
renderObject.layout(const SliverConstraints(
viewportMainAxisExtent: 100.0,
overlap: 100.0,
cacheOrigin: 0.0,
scrollOffset: 0.0,
axisDirection: AxisDirection.down,
growthDirection: GrowthDirection.forward,
crossAxisExtent: 100.0,
crossAxisDirection: AxisDirection.right,
userScrollDirection: ScrollDirection.idle,
remainingPaintExtent: 100.0,
remainingCacheExtent: 100.0,
precedingScrollExtent: 0.0,
),
parentUsesSize: true,
);
expect(renderObject.geometry!.paintOrigin, 10.0);
});
}
void hitsText(HitTestResult hitTestResult, String text) {
switch (hitTestResult.path.first.target) {
case final TextSpan span:
expect(span.text, text);
case final RenderParagraph paragraph:
final InlineSpan span = paragraph.text;
expect(span, isA<TextSpan>());
expect((span as TextSpan).text, text);
case final HitTestTarget target:
fail('$target is not a TextSpan or a RenderParagraph.');
}
}
| flutter/packages/flutter/test/widgets/slivers_padding_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/slivers_padding_test.dart",
"repo_id": "flutter",
"token_count": 10396
} | 724 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
typedef Logger = void Function(String caller);
class TestBorder extends ShapeBorder {
const TestBorder(this.onLog);
final Logger onLog;
@override
EdgeInsetsGeometry get dimensions => const EdgeInsetsDirectional.only(start: 1.0);
@override
ShapeBorder scale(double t) => TestBorder(onLog);
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
onLog('getInnerPath $rect $textDirection');
return Path();
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
onLog('getOuterPath $rect $textDirection');
return Path();
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
onLog('paint $rect $textDirection');
}
}
| flutter/packages/flutter/test/widgets/test_border.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/test_border.dart",
"repo_id": "flutter",
"token_count": 305
} | 725 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('toString control test', (WidgetTester tester) async {
const Widget widget = FadeTransition(
opacity: kAlwaysCompleteAnimation,
child: Text('Ready', textDirection: TextDirection.ltr),
);
expect(widget.toString, isNot(throwsException));
});
group('DecoratedBoxTransition test', () {
final DecorationTween decorationTween = DecorationTween(
begin: BoxDecoration(
color: const Color(0xFFFFFFFF),
border: Border.all(
width: 4.0,
),
borderRadius: BorderRadius.zero,
boxShadow: const <BoxShadow>[
BoxShadow(
color: Color(0x66000000),
blurRadius: 10.0,
spreadRadius: 4.0,
),
],
),
end: BoxDecoration(
color: const Color(0xFF000000),
border: Border.all(
color: const Color(0xFF202020),
),
borderRadius: const BorderRadius.all(Radius.circular(10.0)),
// No shadow.
),
);
late AnimationController controller;
setUp(() {
controller = AnimationController(vsync: const TestVSync());
});
testWidgets('decoration test', (WidgetTester tester) async {
final DecoratedBoxTransition transitionUnderTest =
DecoratedBoxTransition(
decoration: decorationTween.animate(controller),
child: const Text(
"Doesn't matter",
textDirection: TextDirection.ltr,
),
);
await tester.pumpWidget(transitionUnderTest);
RenderDecoratedBox actualBox = tester.renderObject(find.byType(DecoratedBox));
BoxDecoration actualDecoration = actualBox.decoration as BoxDecoration;
expect(actualDecoration.color, const Color(0xFFFFFFFF));
expect(actualDecoration.boxShadow![0].blurRadius, 10.0);
expect(actualDecoration.boxShadow![0].spreadRadius, 4.0);
expect(actualDecoration.boxShadow![0].color, const Color(0x66000000));
controller.value = 0.5;
await tester.pump();
actualBox = tester.renderObject(find.byType(DecoratedBox));
actualDecoration = actualBox.decoration as BoxDecoration;
expect(actualDecoration.color, const Color(0xFF7F7F7F));
expect(actualDecoration.border, isA<Border>());
final Border border = actualDecoration.border! as Border;
expect(border.left.width, 2.5);
expect(border.left.style, BorderStyle.solid);
expect(border.left.color, const Color(0xFF101010));
expect(actualDecoration.borderRadius, const BorderRadius.all(Radius.circular(5.0)));
expect(actualDecoration.shape, BoxShape.rectangle);
expect(actualDecoration.boxShadow![0].blurRadius, 5.0);
expect(actualDecoration.boxShadow![0].spreadRadius, 2.0);
// Scaling a shadow doesn't change the color.
expect(actualDecoration.boxShadow![0].color, const Color(0x66000000));
controller.value = 1.0;
await tester.pump();
actualBox = tester.renderObject(find.byType(DecoratedBox));
actualDecoration = actualBox.decoration as BoxDecoration;
expect(actualDecoration.color, const Color(0xFF000000));
expect(actualDecoration.boxShadow, null);
});
testWidgets('animations work with curves test', (WidgetTester tester) async {
final Animation<Decoration> curvedDecorationAnimation =
decorationTween.animate(CurvedAnimation(
parent: controller,
curve: Curves.easeOut,
));
final DecoratedBoxTransition transitionUnderTest = DecoratedBoxTransition(
decoration: curvedDecorationAnimation,
position: DecorationPosition.foreground,
child: const Text(
"Doesn't matter",
textDirection: TextDirection.ltr,
),
);
await tester.pumpWidget(transitionUnderTest);
RenderDecoratedBox actualBox = tester.renderObject(find.byType(DecoratedBox));
BoxDecoration actualDecoration = actualBox.decoration as BoxDecoration;
expect(actualDecoration.color, const Color(0xFFFFFFFF));
expect(actualDecoration.boxShadow![0].blurRadius, 10.0);
expect(actualDecoration.boxShadow![0].spreadRadius, 4.0);
expect(actualDecoration.boxShadow![0].color, const Color(0x66000000));
controller.value = 0.5;
await tester.pump();
actualBox = tester.renderObject(find.byType(DecoratedBox));
actualDecoration = actualBox.decoration as BoxDecoration;
// Same as the test above but the values should be much closer to the
// tween's end values given the easeOut curve.
expect(actualDecoration.color, const Color(0xFF505050));
expect(actualDecoration.border, isA<Border>());
final Border border = actualDecoration.border! as Border;
expect(border.left.width, moreOrLessEquals(1.9, epsilon: 0.1));
expect(border.left.style, BorderStyle.solid);
expect(border.left.color, const Color(0xFF151515));
expect(actualDecoration.borderRadius!.resolve(TextDirection.ltr).topLeft.x, moreOrLessEquals(6.8, epsilon: 0.1));
expect(actualDecoration.shape, BoxShape.rectangle);
expect(actualDecoration.boxShadow![0].blurRadius, moreOrLessEquals(3.1, epsilon: 0.1));
expect(actualDecoration.boxShadow![0].spreadRadius, moreOrLessEquals(1.2, epsilon: 0.1));
// Scaling a shadow doesn't change the color.
expect(actualDecoration.boxShadow![0].color, const Color(0x66000000));
});
});
testWidgets('AlignTransition animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<Alignment> alignmentTween = AlignmentTween(
begin: Alignment.centerLeft,
end: Alignment.bottomRight,
).animate(controller);
final Widget widget = AlignTransition(
alignment: alignmentTween,
child: const Text('Ready', textDirection: TextDirection.ltr),
);
await tester.pumpWidget(widget);
final RenderPositionedBox actualPositionedBox = tester.renderObject(find.byType(Align));
Alignment actualAlignment = actualPositionedBox.alignment as Alignment;
expect(actualAlignment, Alignment.centerLeft);
controller.value = 0.5;
await tester.pump();
actualAlignment = actualPositionedBox.alignment as Alignment;
expect(actualAlignment, const Alignment(0.0, 0.5));
});
testWidgets('RelativePositionedTransition animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<Rect?> rectTween = RectTween(
begin: const Rect.fromLTWH(0, 0, 30, 40),
end: const Rect.fromLTWH(100, 200, 100, 200),
).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.rtl,
child: Stack(
alignment: Alignment.centerLeft,
children: <Widget>[
RelativePositionedTransition(
size: const Size(200, 300),
rect: rectTween,
child: const Placeholder(),
),
],
),
);
await tester.pumpWidget(widget);
final Positioned actualPositioned = tester.widget(find.byType(Positioned));
final RenderBox renderBox = tester.renderObject(find.byType(Placeholder));
Rect actualRect = Rect.fromLTRB(
actualPositioned.left!,
actualPositioned.top!,
actualPositioned.right ?? 0.0,
actualPositioned.bottom ?? 0.0,
);
expect(actualRect, equals(const Rect.fromLTRB(0, 0, 170, 260)));
expect(renderBox.size, equals(const Size(630, 340)));
controller.value = 0.5;
await tester.pump();
actualRect = Rect.fromLTRB(
actualPositioned.left!,
actualPositioned.top!,
actualPositioned.right ?? 0.0,
actualPositioned.bottom ?? 0.0,
);
expect(actualRect, equals(const Rect.fromLTWH(0, 0, 170, 260)));
expect(renderBox.size, equals(const Size(665, 420)));
});
testWidgets('AlignTransition keeps width and height factors', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<Alignment> alignmentTween = AlignmentTween(
begin: Alignment.centerLeft,
end: Alignment.bottomRight,
).animate(controller);
final Widget widget = AlignTransition(
alignment: alignmentTween,
widthFactor: 0.3,
heightFactor: 0.4,
child: const Text('Ready', textDirection: TextDirection.ltr),
);
await tester.pumpWidget(widget);
final Align actualAlign = tester.widget(find.byType(Align));
expect(actualAlign.widthFactor, 0.3);
expect(actualAlign.heightFactor, 0.4);
});
testWidgets('SizeTransition clamps negative size factors - vertical axis', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: -1.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: SizeTransition(
sizeFactor: animation,
fixedCrossAxisSizeFactor: 2.0,
child: const Text('Ready'),
),
);
await tester.pumpWidget(widget);
final RenderPositionedBox actualPositionedBox = tester.renderObject(find.byType(Align));
expect(actualPositionedBox.heightFactor, 0.0);
expect(actualPositionedBox.widthFactor, 2.0);
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.0);
expect(actualPositionedBox.widthFactor, 2.0);
controller.value = 0.75;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.5);
expect(actualPositionedBox.widthFactor, 2.0);
controller.value = 1.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 2.0);
});
testWidgets('SizeTransition clamps negative size factors - horizontal axis', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: -1.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: SizeTransition(
axis: Axis.horizontal,
sizeFactor: animation,
fixedCrossAxisSizeFactor: 1.0,
child: const Text('Ready'),
),
);
await tester.pumpWidget(widget);
final RenderPositionedBox actualPositionedBox = tester.renderObject(find.byType(Align));
expect(actualPositionedBox.widthFactor, 0.0);
expect(actualPositionedBox.heightFactor, 1.0);
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.widthFactor, 0.0);
expect(actualPositionedBox.heightFactor, 1.0);
controller.value = 0.75;
await tester.pump();
expect(actualPositionedBox.widthFactor, 0.5);
expect(actualPositionedBox.heightFactor, 1.0);
controller.value = 1.0;
await tester.pump();
expect(actualPositionedBox.widthFactor, 1.0);
expect(actualPositionedBox.heightFactor, 1.0);
});
testWidgets('SizeTransition with fixedCrossAxisSizeFactor should size its cross axis from its children - vertical axis', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0, end: 1.0).animate(controller);
const Key key = Key('key');
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
key: key,
child: SizeTransition(
sizeFactor: animation,
fixedCrossAxisSizeFactor: 1.0,
child: const SizedBox.square(dimension: 100),
),
),
),
);
await tester.pumpWidget(widget);
final RenderPositionedBox actualPositionedBox = tester.renderObject(find.byType(Align));
expect(actualPositionedBox.heightFactor, 0.0);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size(100, 0));
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.0);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size(100, 0));
controller.value = 0.5;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.5);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size(100, 50));
controller.value = 1.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size.square(100));
controller.value = 0.5;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.5);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size(100, 50));
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 0.0);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size(100, 0));
});
testWidgets('SizeTransition with fixedCrossAxisSizeFactor should size its cross axis from its children - horizontal axis', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
const Key key = Key('key');
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
key: key,
child: SizeTransition(
axis: Axis.horizontal,
sizeFactor: animation,
fixedCrossAxisSizeFactor: 1.0,
child: const SizedBox.square(dimension: 100),
),
),
),
);
await tester.pumpWidget(widget);
final RenderPositionedBox actualPositionedBox = tester.renderObject(find.byType(Align));
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 0.0);
expect(tester.getSize(find.byKey(key)), const Size(0, 100));
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 0.0);
expect(tester.getSize(find.byKey(key)), const Size(0, 100));
controller.value = 0.5;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 0.5);
expect(tester.getSize(find.byKey(key)), const Size(50, 100));
controller.value = 1.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 1.0);
expect(tester.getSize(find.byKey(key)), const Size.square(100));
controller.value = 0.5;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 0.5);
expect(tester.getSize(find.byKey(key)), const Size(50, 100));
controller.value = 0.0;
await tester.pump();
expect(actualPositionedBox.heightFactor, 1.0);
expect(actualPositionedBox.widthFactor, 0.0);
expect(tester.getSize(find.byKey(key)), const Size(0, 100));
});
testWidgets('MatrixTransition animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Widget widget = MatrixTransition(
alignment: Alignment.topRight,
onTransform: (double value) => Matrix4.translationValues(value, value, value),
animation: controller,
child: const Text(
'Matrix',
textDirection: TextDirection.ltr,
),
);
await tester.pumpWidget(widget);
Transform actualTransformedBox = tester.widget(find.byType(Transform));
Matrix4 actualTransform = actualTransformedBox.transform;
expect(actualTransform, equals(Matrix4.rotationZ(0.0)));
controller.value = 0.5;
await tester.pump();
actualTransformedBox = tester.widget(find.byType(Transform));
actualTransform = actualTransformedBox.transform;
expect(actualTransform, Matrix4.fromList(<double>[
1.0, 0.0, 0.0, 0.5,
0.0, 1.0, 0.0, 0.5,
0.0, 0.0, 1.0, 0.5,
0.0, 0.0, 0.0, 1.0,
])..transpose());
controller.value = 0.75;
await tester.pump();
actualTransformedBox = tester.widget(find.byType(Transform));
actualTransform = actualTransformedBox.transform;
expect(actualTransform, Matrix4.fromList(<double>[
1.0, 0.0, 0.0, 0.75,
0.0, 1.0, 0.0, 0.75,
0.0, 0.0, 1.0, 0.75,
0.0, 0.0, 0.0, 1.0,
])..transpose());
});
testWidgets('MatrixTransition maintains chosen alignment during animation', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Widget widget = MatrixTransition(
alignment: Alignment.topRight,
onTransform: (double value) => Matrix4.identity(),
animation: controller,
child: const Text('Matrix', textDirection: TextDirection.ltr),
);
await tester.pumpWidget(widget);
MatrixTransition actualTransformedBox = tester.widget(find.byType(MatrixTransition));
Alignment actualAlignment = actualTransformedBox.alignment;
expect(actualAlignment, Alignment.topRight);
controller.value = 0.5;
await tester.pump();
actualTransformedBox = tester.widget(find.byType(MatrixTransition));
actualAlignment = actualTransformedBox.alignment;
expect(actualAlignment, Alignment.topRight);
});
testWidgets('RotationTransition animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Widget widget = RotationTransition(
alignment: Alignment.topRight,
turns: controller,
child: const Text(
'Rotation',
textDirection: TextDirection.ltr,
),
);
await tester.pumpWidget(widget);
Transform actualRotatedBox = tester.widget(find.byType(Transform));
Matrix4 actualTurns = actualRotatedBox.transform;
expect(actualTurns, equals(Matrix4.rotationZ(0.0)));
controller.value = 0.5;
await tester.pump();
actualRotatedBox = tester.widget(find.byType(Transform));
actualTurns = actualRotatedBox.transform;
expect(actualTurns, matrixMoreOrLessEquals(Matrix4.fromList(<double>[
-1.0, 0.0, 0.0, 0.0,
0.0, -1.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
])..transpose()));
controller.value = 0.75;
await tester.pump();
actualRotatedBox = tester.widget(find.byType(Transform));
actualTurns = actualRotatedBox.transform;
expect(actualTurns, matrixMoreOrLessEquals(Matrix4.fromList(<double>[
0.0, 1.0, 0.0, 0.0,
-1.0, 0.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
])..transpose()));
});
testWidgets('RotationTransition maintains chosen alignment during animation', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Widget widget = RotationTransition(
alignment: Alignment.topRight,
turns: controller,
child: const Text('Rotation', textDirection: TextDirection.ltr),
);
await tester.pumpWidget(widget);
RotationTransition actualRotatedBox = tester.widget(find.byType(RotationTransition));
Alignment actualAlignment = actualRotatedBox.alignment;
expect(actualAlignment, Alignment.topRight);
controller.value = 0.5;
await tester.pump();
actualRotatedBox = tester.widget(find.byType(RotationTransition));
actualAlignment = actualRotatedBox.alignment;
expect(actualAlignment, Alignment.topRight);
});
group('FadeTransition', () {
double getOpacity(WidgetTester tester, String textValue) {
final FadeTransition opacityWidget = tester.widget<FadeTransition>(
find.ancestor(
of: find.text(textValue),
matching: find.byType(FadeTransition),
).first,
);
return opacityWidget.opacity.value;
}
testWidgets('animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: FadeTransition(
opacity: animation,
child: const Text('Fade In'),
),
);
await tester.pumpWidget(widget);
expect(getOpacity(tester, 'Fade In'), 0.0);
controller.value = 0.25;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.25);
controller.value = 0.5;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.5);
controller.value = 0.75;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.75);
controller.value = 1.0;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 1.0);
});
});
group('SliverFadeTransition', () {
double getOpacity(WidgetTester tester, String textValue) {
final SliverFadeTransition opacityWidget = tester.widget<SliverFadeTransition>(
find.ancestor(
of: find.text(textValue),
matching: find.byType(SliverFadeTransition),
).first,
);
return opacityWidget.opacity.value;
}
testWidgets('animates', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
final Widget widget = Localizations(
locale: const Locale('en', 'us'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
slivers: <Widget>[
SliverFadeTransition(
opacity: animation,
sliver: const SliverToBoxAdapter(
child: Text('Fade In'),
),
),
],
),
),
),
);
await tester.pumpWidget(widget);
expect(getOpacity(tester, 'Fade In'), 0.0);
controller.value = 0.25;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.25);
controller.value = 0.5;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.5);
controller.value = 0.75;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 0.75);
controller.value = 1.0;
await tester.pump();
expect(getOpacity(tester, 'Fade In'), 1.0);
});
});
group('MatrixTransition', () {
testWidgets('uses ImageFilter when provided with FilterQuality argument', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: MatrixTransition(
animation: animation,
onTransform: (double value) => Matrix4.identity(),
filterQuality: FilterQuality.none,
child: const Text('Matrix Transition'),
),
);
await tester.pumpWidget(widget);
// Validate that expensive layer is not left in tree before animation has started.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
controller.value = 0.25;
await tester.pump();
expect(
tester.layers,
contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)),
);
controller.value = 0.5;
await tester.pump();
expect(
tester.layers,
contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)),
);
controller.value = 0.75;
await tester.pump();
expect(
tester.layers,
contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)),
);
controller.value = 1;
await tester.pump();
// Validate that expensive layer is not left in tree after animation has finished.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
});
});
group('ScaleTransition', () {
testWidgets('uses ImageFilter when provided with FilterQuality argument', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: ScaleTransition(
scale: animation,
filterQuality: FilterQuality.none,
child: const Text('Scale Transition'),
),
);
await tester.pumpWidget(widget);
// Validate that expensive layer is not left in tree before animation has started.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
controller.value = 0.25;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 0.5;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 0.75;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 1;
await tester.pump();
// Validate that expensive layer is not left in tree after animation has finished.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
});
});
group('RotationTransition', () {
testWidgets('uses ImageFilter when provided with FilterQuality argument', (WidgetTester tester) async {
final AnimationController controller = AnimationController(vsync: const TestVSync());
addTearDown(controller.dispose);
final Animation<double> animation = Tween<double>(begin: 0.0, end: 1.0).animate(controller);
final Widget widget = Directionality(
textDirection: TextDirection.ltr,
child: RotationTransition(
turns: animation,
filterQuality: FilterQuality.none,
child: const Text('Scale Transition'),
),
);
await tester.pumpWidget(widget);
// Validate that expensive layer is not left in tree before animation has started.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
controller.value = 0.25;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 0.5;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 0.75;
await tester.pump();
expect(tester.layers, contains(isA<ImageFilterLayer>().having(
(ImageFilterLayer layer) => layer.imageFilter.toString(),
'image filter',
startsWith('ImageFilter.matrix('),
)));
controller.value = 1;
await tester.pump();
// Validate that expensive layer is not left in tree after animation has finished.
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
});
});
group('Builders', () {
testWidgets('AnimatedBuilder rebuilds when changed', (WidgetTester tester) async {
final GlobalKey<RedrawCounterState> redrawKey = GlobalKey<RedrawCounterState>();
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: AnimatedBuilder(
animation: notifier,
builder: (BuildContext context, Widget? child) {
return RedrawCounter(key: redrawKey, child: child);
},
),
),
);
expect(redrawKey.currentState!.redraws, equals(1));
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(1));
notifier.notifyListeners();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
// Pump a few more times to make sure that we don't rebuild unnecessarily.
await tester.pump();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
});
testWidgets("AnimatedBuilder doesn't rebuild the child", (WidgetTester tester) async {
final GlobalKey<RedrawCounterState> redrawKey = GlobalKey<RedrawCounterState>();
final GlobalKey<RedrawCounterState> redrawKeyChild = GlobalKey<RedrawCounterState>();
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: AnimatedBuilder(
animation: notifier,
builder: (BuildContext context, Widget? child) {
return RedrawCounter(key: redrawKey, child: child);
},
child: RedrawCounter(key: redrawKeyChild),
),
),
);
expect(redrawKey.currentState!.redraws, equals(1));
expect(redrawKeyChild.currentState!.redraws, equals(1));
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(1));
expect(redrawKeyChild.currentState!.redraws, equals(1));
notifier.notifyListeners();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
expect(redrawKeyChild.currentState!.redraws, equals(1));
// Pump a few more times to make sure that we don't rebuild unnecessarily.
await tester.pump();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
expect(redrawKeyChild.currentState!.redraws, equals(1));
});
testWidgets('ListenableBuilder rebuilds when changed', (WidgetTester tester) async {
final GlobalKey<RedrawCounterState> redrawKey = GlobalKey<RedrawCounterState>();
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListenableBuilder(
listenable: notifier,
builder: (BuildContext context, Widget? child) {
return RedrawCounter(key: redrawKey, child: child);
},
),
),
);
expect(redrawKey.currentState!.redraws, equals(1));
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(1));
notifier.notifyListeners();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
// Pump a few more times to make sure that we don't rebuild unnecessarily.
await tester.pump();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
});
testWidgets("ListenableBuilder doesn't rebuild the child", (WidgetTester tester) async {
final GlobalKey<RedrawCounterState> redrawKey = GlobalKey<RedrawCounterState>();
final GlobalKey<RedrawCounterState> redrawKeyChild = GlobalKey<RedrawCounterState>();
final ChangeNotifier notifier = ChangeNotifier();
addTearDown(notifier.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListenableBuilder(
listenable: notifier,
builder: (BuildContext context, Widget? child) {
return RedrawCounter(key: redrawKey, child: child);
},
child: RedrawCounter(key: redrawKeyChild),
),
),
);
expect(redrawKey.currentState!.redraws, equals(1));
expect(redrawKeyChild.currentState!.redraws, equals(1));
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(1));
expect(redrawKeyChild.currentState!.redraws, equals(1));
notifier.notifyListeners();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
expect(redrawKeyChild.currentState!.redraws, equals(1));
// Pump a few more times to make sure that we don't rebuild unnecessarily.
await tester.pump();
await tester.pump();
expect(redrawKey.currentState!.redraws, equals(2));
expect(redrawKeyChild.currentState!.redraws, equals(1));
});
});
}
class RedrawCounter extends StatefulWidget {
const RedrawCounter({ super.key, this.child });
final Widget? child;
@override
State<RedrawCounter> createState() => RedrawCounterState();
}
class RedrawCounterState extends State<RedrawCounter> {
int redraws = 0;
@override
Widget build(BuildContext context) {
redraws += 1;
return SizedBox(child: widget.child);
}
}
| flutter/packages/flutter/test/widgets/transitions_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/transitions_test.dart",
"repo_id": "flutter",
"token_count": 13857
} | 726 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
void main() {
test('WidgetStatesController constructor', () {
expect(WidgetStatesController().value, <WidgetState>{});
expect(WidgetStatesController(<WidgetState>{}).value, <WidgetState>{});
expect(WidgetStatesController(<WidgetState>{WidgetState.selected}).value, <WidgetState>{WidgetState.selected});
});
test('WidgetStatesController dispatches memory events', () async {
await expectLater(
await memoryEvents(() => WidgetStatesController().dispose(), WidgetStatesController),
areCreateAndDispose,
);
});
test('WidgetStatesController update, listener', () {
int count = 0;
void valueChanged() {
count += 1;
}
final WidgetStatesController controller = WidgetStatesController();
controller.addListener(valueChanged);
controller.update(WidgetState.selected, true);
expect(controller.value, <WidgetState>{WidgetState.selected});
expect(count, 1);
controller.update(WidgetState.selected, true);
expect(controller.value, <WidgetState>{WidgetState.selected});
expect(count, 1);
controller.update(WidgetState.hovered, false);
expect(count, 1);
expect(controller.value, <WidgetState>{WidgetState.selected});
controller.update(WidgetState.selected, false);
expect(count, 2);
expect(controller.value, <WidgetState>{});
controller.update(WidgetState.hovered, true);
expect(controller.value, <WidgetState>{WidgetState.hovered});
expect(count, 3);
controller.update(WidgetState.hovered, true);
expect(controller.value, <WidgetState>{WidgetState.hovered});
expect(count, 3);
controller.update(WidgetState.pressed, true);
expect(controller.value, <WidgetState>{WidgetState.hovered, WidgetState.pressed});
expect(count, 4);
controller.update(WidgetState.selected, true);
expect(controller.value, <WidgetState>{WidgetState.hovered, WidgetState.pressed, WidgetState.selected});
expect(count, 5);
controller.update(WidgetState.selected, false);
expect(controller.value, <WidgetState>{WidgetState.hovered, WidgetState.pressed});
expect(count, 6);
controller.update(WidgetState.selected, false);
expect(controller.value, <WidgetState>{WidgetState.hovered, WidgetState.pressed});
expect(count, 6);
controller.update(WidgetState.pressed, false);
expect(controller.value, <WidgetState>{WidgetState.hovered});
expect(count, 7);
controller.update(WidgetState.hovered, false);
expect(controller.value, <WidgetState>{});
expect(count, 8);
controller.removeListener(valueChanged);
controller.update(WidgetState.selected, true);
expect(controller.value, <WidgetState>{WidgetState.selected});
expect(count, 8);
});
test('WidgetStatesController const initial value', () {
int count = 0;
void valueChanged() {
count += 1;
}
final WidgetStatesController controller = WidgetStatesController(const <WidgetState>{WidgetState.selected});
controller.addListener(valueChanged);
controller.update(WidgetState.selected, true);
expect(controller.value, <WidgetState>{WidgetState.selected});
expect(count, 0);
controller.update(WidgetState.selected, false);
expect(controller.value, <WidgetState>{});
expect(count, 1);
});
}
| flutter/packages/flutter/test/widgets/widget_states_controller_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/widget_states_controller_test.dart",
"repo_id": "flutter",
"token_count": 1152
} | 727 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() {
// Changes made in https://github.com/flutter/flutter/pull/93427
ColorScheme colorScheme = ColorScheme();
colorScheme = ColorScheme();
colorScheme = ColorScheme.light();
colorScheme = ColorScheme.dark();
colorScheme = ColorScheme.highContrastLight();
colorScheme = ColorScheme.highContrastDark();
colorScheme = colorScheme.copyWith();
colorScheme.primaryContainer; // Removing field reference not supported.
colorScheme.secondaryContainer;
// Changes made in https://github.com/flutter/flutter/pull/138521
ColorScheme colorScheme = ColorScheme();
colorScheme = ColorScheme(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = ColorScheme(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme = ColorScheme.light(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = ColorScheme.light(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme = ColorScheme.dark(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = ColorScheme.dark(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme = ColorScheme.highContrastLight(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = ColorScheme.highContrastLight(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme = ColorScheme.highContrastDark(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = ColorScheme.highContrastDark(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme = colorScheme.copyWith(surface: Colors.black, onSurface: Colors.white, surfaceContainerHighest: Colors.red);
colorScheme = colorScheme.copyWith(surface: Colors.orange, onSurface: Colors.yellow, surfaceContainerHighest: Colors.blue);
colorScheme.surface;
colorScheme.onSurface;
colorScheme.surfaceContainerHighest;
}
| flutter/packages/flutter/test_fixes/material/color_scheme.dart.expect/0 | {
"file_path": "flutter/packages/flutter/test_fixes/material/color_scheme.dart.expect",
"repo_id": "flutter",
"token_count": 697
} | 728 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
void main() {
// Changes made in https://github.com/flutter/flutter/pull/66305
RenderStack renderStack = RenderStack();
renderStack = RenderStack(clipBehavior: Clip.none);
renderStack = RenderStack(clipBehavior: Clip.hardEdge);
renderStack = RenderStack(error: '');
renderStack.clipBehavior;
// Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
RenderListWheelViewport renderListWheelViewport = RenderListWheelViewport();
renderListWheelViewport = RenderListWheelViewport(clipBehavior: Clip.hardEdge);
renderListWheelViewport = RenderListWheelViewport(clipBehavior: Clip.none);
renderListWheelViewport = RenderListWheelViewport(error: '');
renderListWheelViewport.clipBehavior;
// Change made in https://github.com/flutter/flutter/pull/128522
RenderParagraph(textScaler: TextScaler.linear(math.min(123, 456)));
RenderParagraph();
RenderEditable(textScaler: TextScaler.linear(math.min(123, 456)));
RenderEditable();
}
| flutter/packages/flutter/test_fixes/rendering/rendering.dart.expect/0 | {
"file_path": "flutter/packages/flutter/test_fixes/rendering/rendering.dart.expect",
"repo_id": "flutter",
"token_count": 355
} | 729 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final FlutterMemoryAllocations ma = FlutterMemoryAllocations.instance;
setUp(() {
assert(!ma.hasListeners);
_checkSdkHandlersNotSet();
});
test('kFlutterMemoryAllocationsEnabled is false in release mode.', () {
expect(kFlutterMemoryAllocationsEnabled, isFalse);
});
testWidgets(
'$FlutterMemoryAllocations is noop when kFlutterMemoryAllocationsEnabled is false.',
(WidgetTester tester) async {
ObjectEvent? receivedEvent;
ObjectEvent listener(ObjectEvent event) => receivedEvent = event;
ma.addListener(listener);
_checkSdkHandlersNotSet();
expect(ma.hasListeners, isFalse);
await _activateFlutterObjects(tester);
_checkSdkHandlersNotSet();
expect(receivedEvent, isNull);
expect(ma.hasListeners, isFalse);
ma.removeListener(listener);
_checkSdkHandlersNotSet();
},
);
}
void _checkSdkHandlersNotSet() {
expect(Image.onCreate, isNull);
expect(Picture.onCreate, isNull);
expect(Image.onDispose, isNull);
expect(Picture.onDispose, isNull);
}
/// Create and dispose Flutter objects to fire memory allocation events.
Future<void> _activateFlutterObjects(WidgetTester tester) async {
final ValueNotifier<bool> valueNotifier = ValueNotifier<bool>(true);
final ChangeNotifier changeNotifier = ChangeNotifier()..addListener(() {});
final Picture picture = _createPicture();
valueNotifier.dispose();
changeNotifier.dispose();
picture.dispose();
final Image image = await _createImage();
image.dispose();
}
Future<Image> _createImage() async {
final Picture picture = _createPicture();
final Image result = await picture.toImage(10, 10);
picture.dispose();
return result;
}
Picture _createPicture() {
final PictureRecorder recorder = PictureRecorder();
final Canvas canvas = Canvas(recorder);
const Rect rect = Rect.fromLTWH(0.0, 0.0, 100.0, 100.0);
canvas.clipRect(rect);
return recorder.endRecording();
}
| flutter/packages/flutter/test_release/foundation/memory_allocations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test_release/foundation/memory_allocations_test.dart",
"repo_id": "flutter",
"token_count": 738
} | 730 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'message.dart';
/// A Flutter Driver command that enables or disables the FrameSync mechanism.
class SetFrameSync extends Command {
/// Creates a command to toggle the FrameSync mechanism.
const SetFrameSync(this.enabled, { super.timeout });
/// Deserializes this command from the value generated by [serialize].
SetFrameSync.deserialize(super.json)
: enabled = json['enabled']!.toLowerCase() == 'true',
super.deserialize();
/// Whether frameSync should be enabled or disabled.
final bool enabled;
@override
String get kind => 'set_frame_sync';
@override
Map<String, String> serialize() => super.serialize()..addAll(<String, String>{
'enabled': '$enabled',
});
}
| flutter/packages/flutter_driver/lib/src/common/frame_sync.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/common/frame_sync.dart",
"repo_id": "flutter",
"token_count": 253
} | 731 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'percentile_utils.dart';
import 'timeline.dart';
/// Event name for frame request pending timeline events.
const String kFrameRequestPendingEvent = 'Frame Request Pending';
/// Summarizes [TimelineEvents]s corresponding to [kFrameRequestPendingEvent] events.
///
/// `FrameRequestPendingLatency` is the time between `Animator::RequestFrame`
/// and `Animator::BeginFrame` for each frame built by the Flutter engine.
class FrameRequestPendingLatencySummarizer {
/// Creates a FrameRequestPendingLatencySummarizer given the timeline events.
FrameRequestPendingLatencySummarizer(this.frameRequestPendingEvents);
/// Timeline events with names in [kFrameRequestPendingTimelineEventNames].
final List<TimelineEvent> frameRequestPendingEvents;
/// Computes the average `FrameRequestPendingLatency` over the period of the timeline.
double computeAverageFrameRequestPendingLatency() {
final List<double> frameRequestPendingLatencies =
_computeFrameRequestPendingLatencies();
if (frameRequestPendingLatencies.isEmpty) {
return 0;
}
final double total = frameRequestPendingLatencies.reduce((double a, double b) => a + b);
return total / frameRequestPendingLatencies.length;
}
/// Computes the [percentile]-th percentile `FrameRequestPendingLatency` over the
/// period of the timeline.
double computePercentileFrameRequestPendingLatency(double percentile) {
final List<double> frameRequestPendingLatencies =
_computeFrameRequestPendingLatencies();
if (frameRequestPendingLatencies.isEmpty) {
return 0;
}
return findPercentile(frameRequestPendingLatencies, percentile);
}
List<double> _computeFrameRequestPendingLatencies() {
final List<double> result = <double>[];
final Map<String, int> starts = <String, int>{};
for (int i = 0; i < frameRequestPendingEvents.length; i++) {
final TimelineEvent event = frameRequestPendingEvents[i];
if (event.phase == 'b') {
final String? id = event.json['id'] as String?;
if (id != null) {
starts[id] = event.timestampMicros!;
}
} else if (event.phase == 'e') {
final int? start = starts[event.json['id']];
if (start != null) {
result.add((event.timestampMicros! - start).toDouble());
}
}
}
return result;
}
}
| flutter/packages/flutter_driver/lib/src/driver/frame_request_pending_latency_summarizer.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/driver/frame_request_pending_latency_summarizer.dart",
"repo_id": "flutter",
"token_count": 820
} | 732 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart' show RendererBinding;
import 'package:flutter/scheduler.dart';
import 'package:flutter/semantics.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import '../common/deserialization_factory.dart';
import '../common/error.dart';
import '../common/find.dart';
import '../common/handler_factory.dart';
import '../common/message.dart';
import '_extension_io.dart' if (dart.library.html) '_extension_web.dart';
const String _extensionMethodName = 'driver';
/// Signature for the handler passed to [enableFlutterDriverExtension].
///
/// Messages are described in string form and should return a [Future] which
/// eventually completes to a string response.
typedef DataHandler = Future<String> Function(String? message);
class _DriverBinding extends BindingBase with SchedulerBinding, ServicesBinding, GestureBinding, PaintingBinding, SemanticsBinding, RendererBinding, WidgetsBinding, TestDefaultBinaryMessengerBinding {
_DriverBinding(this._handler, this._silenceErrors, this._enableTextEntryEmulation, this.finders, this.commands);
final DataHandler? _handler;
final bool _silenceErrors;
final bool _enableTextEntryEmulation;
final List<FinderExtension>? finders;
final List<CommandExtension>? commands;
// Because you can't really control which zone a driver test uses,
// we override the test for zones here.
@override
bool debugCheckZone(String entryPoint) { return true; }
@override
void initServiceExtensions() {
super.initServiceExtensions();
final FlutterDriverExtension extension = FlutterDriverExtension(_handler, _silenceErrors, _enableTextEntryEmulation, finders: finders ?? const <FinderExtension>[], commands: commands ?? const <CommandExtension>[]);
registerServiceExtension(
name: _extensionMethodName,
callback: extension.call,
);
if (kIsWeb) {
registerWebServiceExtension(extension.call);
}
}
}
// Examples can assume:
// import 'package:flutter_driver/flutter_driver.dart';
// import 'package:flutter/widgets.dart';
// import 'package:flutter_driver/driver_extension.dart';
// import 'package:flutter_test/flutter_test.dart' hide find;
// import 'package:flutter_test/flutter_test.dart' as flutter_test;
// typedef MyHomeWidget = Placeholder;
// abstract class SomeWidget extends StatelessWidget { const SomeWidget({super.key, required this.title}); final String title; }
// late FlutterDriver driver;
// abstract class StubNestedCommand { int get times; SerializableFinder get finder; }
// class StubCommandResult extends Result { const StubCommandResult(this.arg); final String arg; @override Map<String, dynamic> toJson() => <String, dynamic>{}; }
// abstract class StubProberCommand { int get times; SerializableFinder get finder; }
/// Enables Flutter Driver VM service extension.
///
/// This extension is required for tests that use `package:flutter_driver` to
/// drive applications from a separate process. In order to allow the driver
/// to interact with the application, this method changes the behavior of the
/// framework in several ways - including keyboard interaction and text
/// editing. Applications intended for release should never include this
/// method.
///
/// Call this function prior to running your application, e.g. before you call
/// `runApp`.
///
/// Optionally you can pass a [DataHandler] callback. It will be called if the
/// test calls [FlutterDriver.requestData].
///
/// `silenceErrors` will prevent exceptions from being logged. This is useful
/// for tests where exceptions are expected. Defaults to false. Any errors
/// will still be returned in the `response` field of the result JSON along
/// with an `isError` boolean.
///
/// The `enableTextEntryEmulation` parameter controls whether the application interacts
/// with the system's text entry methods or a mocked out version used by Flutter Driver.
/// If it is set to false, [FlutterDriver.enterText] will fail,
/// but testing the application with real keyboard input is possible.
/// This value may be updated during a test by calling [FlutterDriver.setTextEntryEmulation].
///
/// The `finders` and `commands` parameters are optional and used to add custom
/// finders or commands, as in the following example.
///
/// ```dart
/// void main() {
/// enableFlutterDriverExtension(
/// finders: <FinderExtension>[ SomeFinderExtension() ],
/// commands: <CommandExtension>[ SomeCommandExtension() ],
/// );
///
/// runApp(const MyHomeWidget());
/// }
///
/// class SomeFinderExtension extends FinderExtension {
/// @override
/// String get finderType => 'SomeFinder';
///
/// @override
/// SerializableFinder deserialize(Map<String, String> params, DeserializeFinderFactory finderFactory) {
/// return SomeFinder(params['title']!);
/// }
///
/// @override
/// Finder createFinder(SerializableFinder finder, CreateFinderFactory finderFactory) {
/// final SomeFinder someFinder = finder as SomeFinder;
///
/// return flutter_test.find.byElementPredicate((Element element) {
/// final Widget widget = element.widget;
/// if (widget is SomeWidget) {
/// return widget.title == someFinder.title;
/// }
/// return false;
/// });
/// }
/// }
///
/// // Use this class in a test anywhere where a SerializableFinder is expected.
/// class SomeFinder extends SerializableFinder {
/// const SomeFinder(this.title);
///
/// final String title;
///
/// @override
/// String get finderType => 'SomeFinder';
///
/// @override
/// Map<String, String> serialize() => super.serialize()..addAll(<String, String>{
/// 'title': title,
/// });
/// }
///
/// class SomeCommandExtension extends CommandExtension {
/// @override
/// String get commandKind => 'SomeCommand';
///
/// @override
/// Future<Result> call(Command command, WidgetController prober, CreateFinderFactory finderFactory, CommandHandlerFactory handlerFactory) async {
/// final SomeCommand someCommand = command as SomeCommand;
///
/// // Deserialize [Finder]:
/// final Finder finder = finderFactory.createFinder(someCommand.finder);
///
/// // Wait for [Element]:
/// handlerFactory.waitForElement(finder);
///
/// // Alternatively, wait for [Element] absence:
/// handlerFactory.waitForAbsentElement(finder);
///
/// // Submit known [Command]s:
/// for (int i = 0; i < someCommand.times; i++) {
/// await handlerFactory.handleCommand(Tap(someCommand.finder), prober, finderFactory);
/// }
///
/// // Alternatively, use [WidgetController]:
/// for (int i = 0; i < someCommand.times; i++) {
/// await prober.tap(finder);
/// }
///
/// return const SomeCommandResult('foo bar');
/// }
///
/// @override
/// Command deserialize(Map<String, String> params, DeserializeFinderFactory finderFactory, DeserializeCommandFactory commandFactory) {
/// return SomeCommand.deserialize(params, finderFactory);
/// }
/// }
///
/// // Pass an instance of this class to `FlutterDriver.sendCommand` to invoke
/// // the custom command during a test.
/// class SomeCommand extends CommandWithTarget {
/// SomeCommand(super.finder, this.times, {super.timeout});
///
/// SomeCommand.deserialize(super.json, super.finderFactory)
/// : times = int.parse(json['times']!),
/// super.deserialize();
///
/// @override
/// Map<String, String> serialize() {
/// return super.serialize()..addAll(<String, String>{'times': '$times'});
/// }
///
/// @override
/// String get kind => 'SomeCommand';
///
/// final int times;
/// }
///
/// class SomeCommandResult extends Result {
/// const SomeCommandResult(this.resultParam);
///
/// final String resultParam;
///
/// @override
/// Map<String, dynamic> toJson() {
/// return <String, dynamic>{
/// 'resultParam': resultParam,
/// };
/// }
/// }
/// ```
void enableFlutterDriverExtension({ DataHandler? handler, bool silenceErrors = false, bool enableTextEntryEmulation = true, List<FinderExtension>? finders, List<CommandExtension>? commands}) {
_DriverBinding(handler, silenceErrors, enableTextEntryEmulation, finders ?? <FinderExtension>[], commands ?? <CommandExtension>[]);
assert(WidgetsBinding.instance is _DriverBinding);
}
/// Signature for functions that handle a command and return a result.
typedef CommandHandlerCallback = Future<Result?> Function(Command c);
/// Signature for functions that deserialize a JSON map to a command object.
typedef CommandDeserializerCallback = Command Function(Map<String, String> params);
/// Used to expand the new [Finder].
abstract class FinderExtension {
/// Identifies the type of finder to be used by the driver extension.
String get finderType;
/// Deserializes the finder from JSON generated by [SerializableFinder.serialize].
///
/// Use [finderFactory] to deserialize nested [Finder]s.
///
/// See also:
/// * [Ancestor], a finder that uses other [Finder]s as parameters.
SerializableFinder deserialize(Map<String, String> params, DeserializeFinderFactory finderFactory);
/// Signature for functions that run the given finder and return the [Element]
/// found, if any, or null otherwise.
///
/// Call [finderFactory] to create known, nested [Finder]s from [SerializableFinder]s.
Finder createFinder(SerializableFinder finder, CreateFinderFactory finderFactory);
}
/// Used to expand the new [Command].
///
/// See also:
/// * [CommandWithTarget], a base class for [Command]s with [Finder]s.
abstract class CommandExtension {
/// Identifies the type of command to be used by the driver extension.
String get commandKind;
/// Deserializes the command from JSON generated by [Command.serialize].
///
/// Use [finderFactory] to deserialize nested [Finder]s.
/// Usually used for [CommandWithTarget]s.
///
/// Call [commandFactory] to deserialize commands specified as parameters.
///
/// See also:
/// * [CommandWithTarget], a base class for commands with target finders.
/// * [Tap], a command that uses [Finder]s as parameter.
Command deserialize(Map<String, String> params, DeserializeFinderFactory finderFactory, DeserializeCommandFactory commandFactory);
/// Calls action for given [command].
/// Returns action [Result].
/// Invoke [prober] functions to perform widget actions.
/// Use [finderFactory] to create [Finder]s from [SerializableFinder].
/// Call [handlerFactory] to invoke other [Command]s or [CommandWithTarget]s.
///
/// The following example shows invoking nested command with [handlerFactory].
///
/// ```dart
/// @override
/// Future<Result> call(Command command, WidgetController prober, CreateFinderFactory finderFactory, CommandHandlerFactory handlerFactory) async {
/// final StubNestedCommand stubCommand = command as StubNestedCommand;
/// for (int i = 0; i < stubCommand.times; i++) {
/// await handlerFactory.handleCommand(Tap(stubCommand.finder), prober, finderFactory);
/// }
/// return const StubCommandResult('stub response');
/// }
/// ```
///
/// Check the example below for direct [WidgetController] usage with [prober]:
///
/// ```dart
/// @override
/// Future<Result> call(Command command, WidgetController prober, CreateFinderFactory finderFactory, CommandHandlerFactory handlerFactory) async {
/// final StubProberCommand stubCommand = command as StubProberCommand;
/// for (int i = 0; i < stubCommand.times; i++) {
/// await prober.tap(finderFactory.createFinder(stubCommand.finder));
/// }
/// return const StubCommandResult('stub response');
/// }
/// ```
Future<Result> call(Command command, WidgetController prober, CreateFinderFactory finderFactory, CommandHandlerFactory handlerFactory);
}
/// The class that manages communication between a Flutter Driver test and the
/// application being remote-controlled, on the application side.
///
/// This is not normally used directly. It is instantiated automatically when
/// calling [enableFlutterDriverExtension].
@visibleForTesting
class FlutterDriverExtension with DeserializeFinderFactory, CreateFinderFactory, DeserializeCommandFactory, CommandHandlerFactory {
/// Creates an object to manage a Flutter Driver connection.
FlutterDriverExtension(
this._requestDataHandler,
this._silenceErrors,
this._enableTextEntryEmulation, {
List<FinderExtension> finders = const <FinderExtension>[],
List<CommandExtension> commands = const <CommandExtension>[],
}) {
if (_enableTextEntryEmulation) {
registerTextInput();
}
for (final FinderExtension finder in finders) {
_finderExtensions[finder.finderType] = finder;
}
for (final CommandExtension command in commands) {
_commandExtensions[command.commandKind] = command;
}
}
final WidgetController _prober = LiveWidgetController(WidgetsBinding.instance);
final DataHandler? _requestDataHandler;
final bool _silenceErrors;
final bool _enableTextEntryEmulation;
void _log(String message) {
driverLog('FlutterDriverExtension', message);
}
final Map<String, FinderExtension> _finderExtensions = <String, FinderExtension>{};
final Map<String, CommandExtension> _commandExtensions = <String, CommandExtension>{};
/// Processes a driver command configured by [params] and returns a result
/// as an arbitrary JSON object.
///
/// [params] must contain key "command" whose value is a string that
/// identifies the kind of the command and its corresponding
/// [CommandDeserializerCallback]. Other keys and values are specific to the
/// concrete implementation of [Command] and [CommandDeserializerCallback].
///
/// The returned JSON is command specific. Generally the caller deserializes
/// the result into a subclass of [Result], but that's not strictly required.
@visibleForTesting
Future<Map<String, dynamic>> call(Map<String, String> params) async {
final String commandKind = params['command']!;
try {
final Command command = deserializeCommand(params, this);
assert(WidgetsBinding.instance.isRootWidgetAttached || !command.requiresRootWidgetAttached,
'No root widget is attached; have you remembered to call runApp()?');
Future<Result> responseFuture = handleCommand(command, _prober, this);
if (command.timeout != null) {
responseFuture = responseFuture.timeout(command.timeout!);
}
final Result response = await responseFuture;
return _makeResponse(response.toJson());
} on TimeoutException catch (error, stackTrace) {
final String message = 'Timeout while executing $commandKind: $error\n$stackTrace';
_log(message);
return _makeResponse(message, isError: true);
} catch (error, stackTrace) {
final String message = 'Uncaught extension error while executing $commandKind: $error\n$stackTrace';
if (!_silenceErrors) {
_log(message);
}
return _makeResponse(message, isError: true);
}
}
Map<String, dynamic> _makeResponse(dynamic response, { bool isError = false }) {
return <String, dynamic>{
'isError': isError,
'response': response,
};
}
@override
SerializableFinder deserializeFinder(Map<String, String> json) {
final String? finderType = json['finderType'];
if (_finderExtensions.containsKey(finderType)) {
return _finderExtensions[finderType]!.deserialize(json, this);
}
return super.deserializeFinder(json);
}
@override
Finder createFinder(SerializableFinder finder) {
final String finderType = finder.finderType;
if (_finderExtensions.containsKey(finderType)) {
return _finderExtensions[finderType]!.createFinder(finder, this);
}
return super.createFinder(finder);
}
@override
Command deserializeCommand(Map<String, String> params, DeserializeFinderFactory finderFactory) {
final String? kind = params['command'];
if (_commandExtensions.containsKey(kind)) {
return _commandExtensions[kind]!.deserialize(params, finderFactory, this);
}
return super.deserializeCommand(params, finderFactory);
}
@override
@protected
DataHandler? getDataHandler() {
return _requestDataHandler;
}
@override
Future<Result> handleCommand(Command command, WidgetController prober, CreateFinderFactory finderFactory) {
final String kind = command.kind;
if (_commandExtensions.containsKey(kind)) {
return _commandExtensions[kind]!.call(command, prober, finderFactory, this);
}
return super.handleCommand(command, prober, finderFactory);
}
}
| flutter/packages/flutter_driver/lib/src/extension/extension.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/extension/extension.dart",
"repo_id": "flutter",
"token_count": 5168
} | 733 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert' show json;
import 'dart:math';
import 'package:file/file.dart';
import 'package:flutter_driver/flutter_driver.dart';
import 'package:flutter_driver/src/driver/profiling_summarizer.dart';
import 'package:flutter_driver/src/driver/refresh_rate_summarizer.dart';
import 'package:flutter_driver/src/driver/scene_display_lag_summarizer.dart';
import 'package:flutter_driver/src/driver/vsync_frame_lag_summarizer.dart';
import 'package:path/path.dart' as path;
import '../../common.dart';
void main() {
group('TimelineSummary', () {
TimelineSummary summarize(List<Map<String, dynamic>> testEvents) {
return TimelineSummary.summarize(Timeline.fromJson(<String, dynamic>{
'traceEvents': testEvents,
}));
}
Map<String, dynamic> frameBegin(int timeStamp) => <String, dynamic>{
'name': 'Frame',
'ph': 'B',
'ts': timeStamp,
};
Map<String, dynamic> frameEnd(int timeStamp) => <String, dynamic>{
'name': 'Frame',
'ph': 'E',
'ts': timeStamp,
};
Map<String, dynamic> begin(int timeStamp) => <String, dynamic>{
'name': 'GPURasterizer::Draw',
'ph': 'B',
'ts': timeStamp,
};
Map<String, dynamic> end(int timeStamp) => <String, dynamic>{
'name': 'GPURasterizer::Draw',
'ph': 'E',
'ts': timeStamp,
};
Map<String, dynamic> lagBegin(int timeStamp, int vsyncsMissed) => <String, dynamic>{
'name': 'SceneDisplayLag',
'ph': 'b',
'ts': timeStamp,
'args': <String, String>{
'vsync_transitions_missed': vsyncsMissed.toString(),
},
};
Map<String, dynamic> lagEnd(int timeStamp, int vsyncsMissed) => <String, dynamic>{
'name': 'SceneDisplayLag',
'ph': 'e',
'ts': timeStamp,
'args': <String, String>{
'vsync_transitions_missed': vsyncsMissed.toString(),
},
};
Map<String, dynamic> cpuUsage(int timeStamp, double cpuUsage) => <String, dynamic>{
'cat': 'embedder',
'name': 'CpuUsage',
'ts': timeStamp,
'args': <String, String>{
'total_cpu_usage': cpuUsage.toString(),
},
};
Map<String, dynamic> memoryUsage(int timeStamp, double dirty, double shared) => <String, dynamic>{
'cat': 'embedder',
'name': 'MemoryUsage',
'ts': timeStamp,
'args': <String, String>{
'owned_shared_memory_usage': shared.toString(),
'dirty_memory_usage': dirty.toString(),
},
};
Map<String, dynamic> platformVsync(int timeStamp) => <String, dynamic>{
'name': 'VSYNC',
'ph': 'B',
'ts': timeStamp,
};
Map<String, dynamic> vsyncCallback(int timeStamp, {String phase = 'B', String startTime = '2750850055428', String endTime = '2750866722095'}) => <String, dynamic>{
'name': 'VsyncProcessCallback',
'ph': phase,
'ts': timeStamp,
'args': <String, dynamic>{
'StartTime': startTime,
'TargetTime': endTime,
},
};
List<Map<String, dynamic>> genGC(String name, int count, int startTime, int timeDiff) {
int ts = startTime;
bool begin = true;
final List<Map<String, dynamic>> ret = <Map<String, dynamic>>[];
for (int i = 0; i < count; i++) {
ret.add(<String, dynamic>{
'name': name,
'cat': 'GC',
'tid': 19695,
'pid': 19650,
'ts': ts,
'tts': ts,
'ph': begin ? 'B' : 'E',
'args': <String, dynamic>{
'isolateGroupId': 'isolateGroups/10824099774666259225',
},
});
ts = ts + timeDiff;
begin = !begin;
}
return ret;
}
List<Map<String, dynamic>> newGenGC(int count, int startTime, int timeDiff) {
return genGC('CollectNewGeneration', count, startTime, timeDiff);
}
List<Map<String, dynamic>> oldGenGC(int count, int startTime, int timeDiff) {
return genGC('CollectOldGeneration', count, startTime, timeDiff);
}
List<Map<String, dynamic>> rasterizeTimeSequenceInMillis(List<int> sequence) {
final List<Map<String, dynamic>> result = <Map<String, dynamic>>[];
int t = 0;
for (final int duration in sequence) {
result.add(begin(t));
t += duration * 1000;
result.add(end(t));
}
return result;
}
Map<String, dynamic> frameRequestPendingStart(String id, int timeStamp) => <String, dynamic>{
'name': 'Frame Request Pending',
'ph': 'b',
'id': id,
'ts': timeStamp,
};
Map<String, dynamic> frameRequestPendingEnd(String id, int timeStamp) => <String, dynamic>{
'name': 'Frame Request Pending',
'ph': 'e',
'id': id,
'ts': timeStamp,
};
group('frame_count', () {
test('counts frames', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(1000), frameEnd(2000),
frameBegin(3000), frameEnd(5000),
]).countFrames(),
2,
);
});
});
group('average_frame_build_time_millis', () {
test('throws when there is no data', () {
expect(
() => summarize(<Map<String, dynamic>>[]).computeAverageFrameBuildTimeMillis(),
throwsA(
isA<StateError>()
.having((StateError e) => e.message,
'message',
contains('The TimelineSummary had no events to summarize.'),
)),
);
});
test('computes average frame build time in milliseconds', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(1000), frameEnd(2000),
frameBegin(3000), frameEnd(5000),
]).computeAverageFrameBuildTimeMillis(),
1.5,
);
});
test('skips leading "end" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameEnd(1000),
frameBegin(2000), frameEnd(4000),
]).computeAverageFrameBuildTimeMillis(),
2.0,
);
});
test('skips trailing "begin" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(2000), frameEnd(4000),
frameBegin(5000),
]).computeAverageFrameBuildTimeMillis(),
2.0,
);
});
// see https://github.com/flutter/flutter/issues/54095.
test('ignore multiple "end" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(2000), frameEnd(4000),
frameEnd(4300), // rogue frame end.
frameBegin(5000), frameEnd(6000),
]).computeAverageFrameBuildTimeMillis(),
1.5,
);
});
test('pick latest when there are multiple "begin" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(1000), // rogue frame begin.
frameBegin(2000), frameEnd(4000),
frameEnd(4300), // rogue frame end.
frameBegin(4400), // rogue frame begin.
frameBegin(5000), frameEnd(6000),
]).computeAverageFrameBuildTimeMillis(),
1.5,
);
});
});
group('worst_frame_build_time_millis', () {
test('throws when there is no data', () {
expect(
() => summarize(<Map<String, dynamic>>[]).computeWorstFrameBuildTimeMillis(),
throwsA(
isA<StateError>()
.having((StateError e) => e.message,
'message',
contains('The TimelineSummary had no events to summarize.'),
)),
);
});
test('computes worst frame build time in milliseconds', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(1000), frameEnd(2000),
frameBegin(3000), frameEnd(5000),
]).computeWorstFrameBuildTimeMillis(),
2.0,
);
expect(
summarize(<Map<String, dynamic>>[
frameBegin(3000), frameEnd(5000),
frameBegin(1000), frameEnd(2000),
]).computeWorstFrameBuildTimeMillis(),
2.0,
);
});
test('skips leading "end" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameEnd(1000),
frameBegin(2000), frameEnd(4000),
]).computeWorstFrameBuildTimeMillis(),
2.0,
);
});
test('skips trailing "begin" events', () {
expect(
summarize(<Map<String, dynamic>>[
frameBegin(2000), frameEnd(4000),
frameBegin(5000),
]).computeWorstFrameBuildTimeMillis(),
2.0,
);
});
});
group('computeMissedFrameBuildBudgetCount', () {
test('computes the number of missed build budgets', () {
final TimelineSummary summary = summarize(<Map<String, dynamic>>[
frameBegin(1000), frameEnd(18000),
frameBegin(19000), frameEnd(28000),
frameBegin(29000), frameEnd(47000),
]);
expect(summary.countFrames(), 3);
expect(summary.computeMissedFrameBuildBudgetCount(), 2);
});
});
group('average_frame_rasterizer_time_millis', () {
test('throws when there is no data', () {
expect(
() => summarize(<Map<String, dynamic>>[]).computeAverageFrameRasterizerTimeMillis(),
throwsA(
isA<StateError>()
.having((StateError e) => e.message,
'message',
contains('The TimelineSummary had no events to summarize.'),
)),
);
});
test('computes average frame rasterizer time in milliseconds', () {
expect(
summarize(<Map<String, dynamic>>[
begin(1000), end(2000),
begin(3000), end(5000),
]).computeAverageFrameRasterizerTimeMillis(),
1.5,
);
});
test('skips leading "end" events', () {
expect(
summarize(<Map<String, dynamic>>[
end(1000),
begin(2000), end(4000),
]).computeAverageFrameRasterizerTimeMillis(),
2.0,
);
});
test('skips trailing "begin" events', () {
expect(
summarize(<Map<String, dynamic>>[
begin(2000), end(4000),
begin(5000),
]).computeAverageFrameRasterizerTimeMillis(),
2.0,
);
});
});
group('worst_frame_rasterizer_time_millis', () {
test('throws when there is no data', () {
expect(
() => summarize(<Map<String, dynamic>>[]).computeWorstFrameRasterizerTimeMillis(),
throwsA(
isA<StateError>()
.having((StateError e) => e.message,
'message',
contains('The TimelineSummary had no events to summarize.'),
)),
);
});
test('computes worst frame rasterizer time in milliseconds', () {
expect(
summarize(<Map<String, dynamic>>[
begin(1000), end(2000),
begin(3000), end(5000),
]).computeWorstFrameRasterizerTimeMillis(),
2.0,
);
expect(
summarize(<Map<String, dynamic>>[
begin(3000), end(5000),
begin(1000), end(2000),
]).computeWorstFrameRasterizerTimeMillis(),
2.0,
);
});
test('skips leading "end" events', () {
expect(
summarize(<Map<String, dynamic>>[
end(1000),
begin(2000), end(4000),
]).computeWorstFrameRasterizerTimeMillis(),
2.0,
);
});
test('skips trailing "begin" events', () {
expect(
summarize(<Map<String, dynamic>>[
begin(2000), end(4000),
begin(5000),
]).computeWorstFrameRasterizerTimeMillis(),
2.0,
);
});
});
group('percentile_frame_rasterizer_time_millis', () {
test('throws when there is no data', () {
expect(
() => summarize(<Map<String, dynamic>>[]).computePercentileFrameRasterizerTimeMillis(90.0),
throwsA(
isA<StateError>()
.having((StateError e) => e.message,
'message',
contains('The TimelineSummary had no events to summarize.'),
)),
);
});
const List<List<int>> sequences = <List<int>>[
<int>[1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
<int>[1, 2, 3, 4, 5],
<int>[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20],
];
const List<int> p90s = <int>[
9,
5,
18,
];
test('computes 90th frame rasterizer time in milliseconds', () {
for (int i = 0; i < sequences.length; ++i) {
expect(
summarize(rasterizeTimeSequenceInMillis(sequences[i])).computePercentileFrameRasterizerTimeMillis(90.0),
p90s[i],
);
}
});
test('compute 99th frame rasterizer time in milliseconds', () {
final List<int> sequence = <int>[];
for (int i = 1; i <= 100; ++i) {
sequence.add(i);
}
expect(
summarize(rasterizeTimeSequenceInMillis(sequence)).computePercentileFrameRasterizerTimeMillis(99.0),
99,
);
});
});
group('computeMissedFrameRasterizerBudgetCount', () {
test('computes the number of missed rasterizer budgets', () {
final TimelineSummary summary = summarize(<Map<String, dynamic>>[
begin(1000), end(18000),
begin(19000), end(28000),
begin(29000), end(47000),
]);
expect(summary.computeMissedFrameRasterizerBudgetCount(), 2);
});
});
group('summaryJson', () {
test('computes and returns summary as JSON', () {
expect(
summarize(<Map<String, dynamic>>[
begin(1000), end(19000),
begin(19001), end(29001),
begin(29002), end(49002),
...newGenGC(4, 10, 100),
...oldGenGC(5, 10000, 100),
frameBegin(1000), frameEnd(18000),
frameBegin(19000), frameEnd(28000),
frameBegin(29000), frameEnd(48000),
frameRequestPendingStart('1', 1000),
frameRequestPendingEnd('1', 2000),
frameRequestPendingStart('2', 3000),
frameRequestPendingEnd('2', 5000),
frameRequestPendingStart('3', 6000),
frameRequestPendingEnd('3', 9000),
]).summaryJson,
<String, dynamic>{
'average_frame_build_time_millis': 15.0,
'90th_percentile_frame_build_time_millis': 19.0,
'99th_percentile_frame_build_time_millis': 19.0,
'worst_frame_build_time_millis': 19.0,
'missed_frame_build_budget_count': 2,
'average_frame_rasterizer_time_millis': 16.0,
'stddev_frame_rasterizer_time_millis': 4.0,
'90th_percentile_frame_rasterizer_time_millis': 20.0,
'99th_percentile_frame_rasterizer_time_millis': 20.0,
'worst_frame_rasterizer_time_millis': 20.0,
'missed_frame_rasterizer_budget_count': 2,
'frame_count': 3,
'frame_rasterizer_count': 3,
'new_gen_gc_count': 4,
'old_gen_gc_count': 5,
'frame_build_times': <int>[17000, 9000, 19000],
'frame_rasterizer_times': <int>[18000, 10000, 20000],
'frame_begin_times': <int>[0, 18000, 28000],
'frame_rasterizer_begin_times': <int>[0, 18001, 28002],
'average_vsync_transitions_missed': 0.0,
'90th_percentile_vsync_transitions_missed': 0.0,
'99th_percentile_vsync_transitions_missed': 0.0,
'average_vsync_frame_lag': 0.0,
'90th_percentile_vsync_frame_lag': 0.0,
'99th_percentile_vsync_frame_lag': 0.0,
'average_layer_cache_count': 0.0,
'90th_percentile_layer_cache_count': 0.0,
'99th_percentile_layer_cache_count': 0.0,
'worst_layer_cache_count': 0.0,
'average_layer_cache_memory': 0.0,
'90th_percentile_layer_cache_memory': 0.0,
'99th_percentile_layer_cache_memory': 0.0,
'worst_layer_cache_memory': 0.0,
'average_picture_cache_count': 0.0,
'90th_percentile_picture_cache_count': 0.0,
'99th_percentile_picture_cache_count': 0.0,
'worst_picture_cache_count': 0.0,
'average_picture_cache_memory': 0.0,
'90th_percentile_picture_cache_memory': 0.0,
'99th_percentile_picture_cache_memory': 0.0,
'worst_picture_cache_memory': 0.0,
'total_ui_gc_time': 0.4,
'30hz_frame_percentage': 0,
'60hz_frame_percentage': 0,
'80hz_frame_percentage': 0,
'90hz_frame_percentage': 0,
'120hz_frame_percentage': 0,
'illegal_refresh_rate_frame_count': 0,
'average_frame_request_pending_latency': 2000.0,
'90th_percentile_frame_request_pending_latency': 3000.0,
'99th_percentile_frame_request_pending_latency': 3000.0,
'average_gpu_frame_time': 0,
'90th_percentile_gpu_frame_time': 0,
'99th_percentile_gpu_frame_time': 0,
'worst_gpu_frame_time': 0,
'average_gpu_memory_mb': 0,
'90th_percentile_gpu_memory_mb': 0,
'99th_percentile_gpu_memory_mb': 0,
'worst_gpu_memory_mb': 0,
},
);
});
});
group('writeTimelineToFile', () {
late Directory tempDir;
setUp(() {
useMemoryFileSystemForTesting();
tempDir = fs.systemTempDirectory.createTempSync('flutter_driver_test.');
});
tearDown(() {
tryToDelete(tempDir);
restoreFileSystem();
});
test('writes timeline to JSON file without summary', () async {
await summarize(<Map<String, String>>[<String, String>{'foo': 'bar'}])
.writeTimelineToFile('test', destinationDirectory: tempDir.path, includeSummary: false);
final String written =
await fs.file(path.join(tempDir.path, 'test.timeline.json')).readAsString();
expect(written, '{"traceEvents":[{"foo":"bar"}]}');
});
test('writes timeline to JSON file with summary', () async {
await summarize(<Map<String, dynamic>>[
<String, String>{'foo': 'bar'},
begin(1000), end(19000),
frameBegin(1000), frameEnd(18000),
]).writeTimelineToFile(
'test',
destinationDirectory: tempDir.path,
);
final String written =
await fs.file(path.join(tempDir.path, 'test.timeline.json')).readAsString();
expect(
written,
'{"traceEvents":[{"foo":"bar"},'
'{"name":"GPURasterizer::Draw","ph":"B","ts":1000},'
'{"name":"GPURasterizer::Draw","ph":"E","ts":19000},'
'{"name":"Frame","ph":"B","ts":1000},'
'{"name":"Frame","ph":"E","ts":18000}]}',
);
});
test('writes summary to JSON file', () async {
await summarize(<Map<String, dynamic>>[
begin(1000), end(19000),
begin(19001), end(29001),
begin(29002), end(49002),
frameBegin(1000), frameEnd(18000),
frameBegin(19000), frameEnd(28000),
frameBegin(29000), frameEnd(48000),
lagBegin(1000, 4), lagEnd(2000, 4),
lagBegin(1200, 12), lagEnd(2400, 12),
lagBegin(4200, 8), lagEnd(9400, 8),
...newGenGC(4, 10, 100),
...oldGenGC(5, 10000, 100),
cpuUsage(5000, 20), cpuUsage(5010, 60),
memoryUsage(6000, 20, 40), memoryUsage(6100, 30, 45),
platformVsync(7000), vsyncCallback(7500),
frameRequestPendingStart('1', 1000),
frameRequestPendingEnd('1', 2000),
frameRequestPendingStart('2', 3000),
frameRequestPendingEnd('2', 5000),
frameRequestPendingStart('3', 6000),
frameRequestPendingEnd('3', 9000),
]).writeTimelineToFile('test', destinationDirectory: tempDir.path);
final String written =
await fs.file(path.join(tempDir.path, 'test.timeline_summary.json')).readAsString();
expect(json.decode(written), <String, dynamic>{
'average_frame_build_time_millis': 15.0,
'worst_frame_build_time_millis': 19.0,
'90th_percentile_frame_build_time_millis': 19.0,
'99th_percentile_frame_build_time_millis': 19.0,
'missed_frame_build_budget_count': 2,
'average_frame_rasterizer_time_millis': 16.0,
'stddev_frame_rasterizer_time_millis': 4.0,
'90th_percentile_frame_rasterizer_time_millis': 20.0,
'99th_percentile_frame_rasterizer_time_millis': 20.0,
'worst_frame_rasterizer_time_millis': 20.0,
'missed_frame_rasterizer_budget_count': 2,
'frame_count': 3,
'frame_rasterizer_count': 3,
'new_gen_gc_count': 4,
'old_gen_gc_count': 5,
'frame_build_times': <int>[17000, 9000, 19000],
'frame_rasterizer_times': <int>[18000, 10000, 20000],
'frame_begin_times': <int>[0, 18000, 28000],
'frame_rasterizer_begin_times': <int>[0, 18001, 28002],
'average_vsync_transitions_missed': 8.0,
'90th_percentile_vsync_transitions_missed': 12.0,
'99th_percentile_vsync_transitions_missed': 12.0,
'average_vsync_frame_lag': 500.0,
'90th_percentile_vsync_frame_lag': 500.0,
'99th_percentile_vsync_frame_lag': 500.0,
'average_cpu_usage': 40.0,
'90th_percentile_cpu_usage': 60.0,
'99th_percentile_cpu_usage': 60.0,
'average_memory_usage': 67.5,
'90th_percentile_memory_usage': 75.0,
'99th_percentile_memory_usage': 75.0,
'average_layer_cache_count': 0.0,
'90th_percentile_layer_cache_count': 0.0,
'99th_percentile_layer_cache_count': 0.0,
'worst_layer_cache_count': 0.0,
'average_layer_cache_memory': 0.0,
'90th_percentile_layer_cache_memory': 0.0,
'99th_percentile_layer_cache_memory': 0.0,
'worst_layer_cache_memory': 0.0,
'average_picture_cache_count': 0.0,
'90th_percentile_picture_cache_count': 0.0,
'99th_percentile_picture_cache_count': 0.0,
'worst_picture_cache_count': 0.0,
'average_picture_cache_memory': 0.0,
'90th_percentile_picture_cache_memory': 0.0,
'99th_percentile_picture_cache_memory': 0.0,
'worst_picture_cache_memory': 0.0,
'total_ui_gc_time': 0.4,
'30hz_frame_percentage': 0,
'60hz_frame_percentage': 100,
'80hz_frame_percentage': 0,
'90hz_frame_percentage': 0,
'120hz_frame_percentage': 0,
'illegal_refresh_rate_frame_count': 0,
'average_frame_request_pending_latency': 2000.0,
'90th_percentile_frame_request_pending_latency': 3000.0,
'99th_percentile_frame_request_pending_latency': 3000.0,
'average_gpu_frame_time': 0,
'90th_percentile_gpu_frame_time': 0,
'99th_percentile_gpu_frame_time': 0,
'worst_gpu_frame_time': 0,
'average_gpu_memory_mb': 0,
'90th_percentile_gpu_memory_mb': 0,
'99th_percentile_gpu_memory_mb': 0,
'worst_gpu_memory_mb': 0,
});
});
});
group('SceneDisplayLagSummarizer tests', () {
SceneDisplayLagSummarizer summarize(List<Map<String, dynamic>> traceEvents) {
final Timeline timeline = Timeline.fromJson(<String, dynamic>{
'traceEvents': traceEvents,
});
return SceneDisplayLagSummarizer(timeline.events!);
}
test('average_vsyncs_missed', () async {
final SceneDisplayLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
lagBegin(1000, 4), lagEnd(2000, 4),
lagBegin(1200, 12), lagEnd(2400, 12),
lagBegin(4200, 8), lagEnd(9400, 8),
]);
expect(summarizer.computeAverageVsyncTransitionsMissed(), 8.0);
});
test('all stats are 0 for 0 missed transitions', () async {
final SceneDisplayLagSummarizer summarizer = summarize(<Map<String, dynamic>>[]);
expect(summarizer.computeAverageVsyncTransitionsMissed(), 0.0);
expect(summarizer.computePercentileVsyncTransitionsMissed(90.0), 0.0);
expect(summarizer.computePercentileVsyncTransitionsMissed(99.0), 0.0);
});
test('90th_percentile_vsyncs_missed', () async {
final SceneDisplayLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
lagBegin(1000, 4), lagEnd(2000, 4),
lagBegin(1200, 12), lagEnd(2400, 12),
lagBegin(4200, 8), lagEnd(9400, 8),
lagBegin(6100, 14), lagEnd(11000, 14),
lagBegin(7100, 16), lagEnd(11500, 16),
lagBegin(7400, 11), lagEnd(13000, 11),
lagBegin(8200, 27), lagEnd(14100, 27),
lagBegin(8700, 7), lagEnd(14300, 7),
lagBegin(24200, 4187), lagEnd(39400, 4187),
]);
expect(summarizer.computePercentileVsyncTransitionsMissed(90), 27.0);
});
test('99th_percentile_vsyncs_missed', () async {
final SceneDisplayLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
lagBegin(1000, 4), lagEnd(2000, 4),
lagBegin(1200, 12), lagEnd(2400, 12),
lagBegin(4200, 8), lagEnd(9400, 8),
lagBegin(6100, 14), lagEnd(11000, 14),
lagBegin(24200, 4187), lagEnd(39400, 4187),
]);
expect(summarizer.computePercentileVsyncTransitionsMissed(99), 4187.0);
});
});
group('ProfilingSummarizer tests', () {
ProfilingSummarizer summarize(List<Map<String, dynamic>> traceEvents) {
final Timeline timeline = Timeline.fromJson(<String, dynamic>{
'traceEvents': traceEvents,
});
return ProfilingSummarizer.fromEvents(timeline.events!);
}
test('has_both_cpu_and_memory_usage', () async {
final ProfilingSummarizer summarizer = summarize(<Map<String, dynamic>>[
cpuUsage(0, 10),
memoryUsage(0, 6, 10),
cpuUsage(0, 12),
memoryUsage(0, 8, 40),
]);
expect(summarizer.computeAverage(ProfileType.CPU), 11.0);
expect(summarizer.computeAverage(ProfileType.Memory), 32.0);
});
test('has_only_memory_usage', () async {
final ProfilingSummarizer summarizer = summarize(<Map<String, dynamic>>[
memoryUsage(0, 6, 10),
memoryUsage(0, 8, 40),
]);
expect(summarizer.computeAverage(ProfileType.Memory), 32.0);
expect(summarizer.summarize().containsKey('average_cpu_usage'), false);
});
test('90th_percentile_cpu_usage', () async {
final ProfilingSummarizer summarizer = summarize(<Map<String, dynamic>>[
cpuUsage(0, 10), cpuUsage(1, 20),
cpuUsage(2, 20), cpuUsage(3, 80),
cpuUsage(4, 70), cpuUsage(4, 72),
cpuUsage(4, 85), cpuUsage(4, 100),
]);
expect(summarizer.computePercentile(ProfileType.CPU, 90), 85.0);
});
});
group('VsyncFrameLagSummarizer tests', () {
VsyncFrameLagSummarizer summarize(List<Map<String, dynamic>> traceEvents) {
final Timeline timeline = Timeline.fromJson(<String, dynamic>{
'traceEvents': traceEvents,
});
return VsyncFrameLagSummarizer(timeline.events!);
}
test('average_vsync_frame_lag', () async {
final VsyncFrameLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
platformVsync(10),
vsyncCallback(12),
platformVsync(16),
vsyncCallback(29),
]);
expect(summarizer.computeAverageVsyncFrameLag(), 7.5);
});
test('malformed_event_ordering', () async {
final VsyncFrameLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
vsyncCallback(10),
platformVsync(10),
]);
expect(summarizer.computeAverageVsyncFrameLag(), 0);
expect(summarizer.computePercentileVsyncFrameLag(80), 0);
});
test('penalize_consecutive_vsyncs', () async {
final VsyncFrameLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
platformVsync(10),
platformVsync(12),
]);
expect(summarizer.computeAverageVsyncFrameLag(), 2);
});
test('pick_nearest_platform_vsync', () async {
final VsyncFrameLagSummarizer summarizer = summarize(<Map<String, dynamic>>[
platformVsync(10),
platformVsync(12),
vsyncCallback(18),
]);
expect(summarizer.computeAverageVsyncFrameLag(), 4);
});
test('percentile_vsync_frame_lag', () async {
final List<Map<String, dynamic>> events = <Map<String, dynamic>>[];
int ts = 100;
for (int i = 0; i < 100; i++) {
events.add(platformVsync(ts));
ts = ts + 10 * (i + 1);
events.add(vsyncCallback(ts));
}
final VsyncFrameLagSummarizer summarizer = summarize(events);
expect(summarizer.computePercentileVsyncFrameLag(90), 890);
expect(summarizer.computePercentileVsyncFrameLag(99), 990);
});
});
group('RefreshRateSummarizer tests', () {
const double kCompareDelta = 0.01;
RefreshRateSummary summarizeRefresh(List<Map<String, dynamic>> traceEvents) {
final Timeline timeline = Timeline.fromJson(<String, dynamic>{
'traceEvents': traceEvents,
});
return RefreshRateSummary(vsyncEvents: timeline.events!);
}
List<Map<String, dynamic>> populateEvents({required int numberOfEvents, required int startTime, required int interval, required int margin}) {
final List<Map<String, dynamic>> events = <Map<String, dynamic>>[];
int startTimeInNanoseconds = startTime;
for (int i = 0; i < numberOfEvents; i ++) {
final int randomMargin = margin >= 1 ? (-margin + Random().nextInt(margin*2)) : 0;
final int endTime = startTimeInNanoseconds + interval + randomMargin;
events.add(vsyncCallback(0, startTime: startTimeInNanoseconds.toString(), endTime: endTime.toString()));
startTimeInNanoseconds = endTime;
}
return events;
}
test('Recognize 30 hz frames.', () async {
const int startTimeInNanoseconds = 2750850055430;
const int intervalInNanoseconds = 33333333;
// allow some margins
const int margin = 3000000;
final List<Map<String, dynamic>> events = populateEvents(numberOfEvents: 100,
startTime: startTimeInNanoseconds,
interval: intervalInNanoseconds,
margin: margin,
);
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, closeTo(100, kCompareDelta));
expect(summary.percentageOf60HzFrames, 0);
expect(summary.percentageOf90HzFrames, 0);
expect(summary.percentageOf120HzFrames, 0);
expect(summary.framesWithIllegalRefreshRate, isEmpty);
});
test('Recognize 60 hz frames.', () async {
const int startTimeInNanoseconds = 2750850055430;
const int intervalInNanoseconds = 16666666;
// allow some margins
const int margin = 1200000;
final List<Map<String, dynamic>> events = populateEvents(numberOfEvents: 100,
startTime: startTimeInNanoseconds,
interval: intervalInNanoseconds,
margin: margin,
);
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, 0);
expect(summary.percentageOf60HzFrames, closeTo(100, kCompareDelta));
expect(summary.percentageOf90HzFrames, 0);
expect(summary.percentageOf120HzFrames, 0);
expect(summary.framesWithIllegalRefreshRate, isEmpty);
});
test('Recognize 90 hz frames.', () async {
const int startTimeInNanoseconds = 2750850055430;
const int intervalInNanoseconds = 11111111;
// allow some margins
const int margin = 500000;
final List<Map<String, dynamic>> events = populateEvents(numberOfEvents: 100,
startTime: startTimeInNanoseconds,
interval: intervalInNanoseconds,
margin: margin,
);
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, 0);
expect(summary.percentageOf60HzFrames, 0);
expect(summary.percentageOf90HzFrames, closeTo(100, kCompareDelta));
expect(summary.percentageOf120HzFrames, 0);
expect(summary.framesWithIllegalRefreshRate, isEmpty);
});
test('Recognize 120 hz frames.', () async {
const int startTimeInNanoseconds = 2750850055430;
const int intervalInNanoseconds = 8333333;
// allow some margins
const int margin = 300000;
final List<Map<String, dynamic>> events = populateEvents(numberOfEvents: 100,
startTime: startTimeInNanoseconds,
interval: intervalInNanoseconds,
margin: margin,
);
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, 0);
expect(summary.percentageOf60HzFrames, 0);
expect(summary.percentageOf90HzFrames, 0);
expect(summary.percentageOf120HzFrames, closeTo(100, kCompareDelta));
expect(summary.framesWithIllegalRefreshRate, isEmpty);
});
test('Identify illegal refresh rates.', () async {
const int startTimeInNanoseconds = 2750850055430;
const int intervalInNanoseconds = 10000000;
final List<Map<String, dynamic>> events = populateEvents(numberOfEvents: 1,
startTime: startTimeInNanoseconds,
interval: intervalInNanoseconds,
margin: 0,
);
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, 0);
expect(summary.percentageOf60HzFrames, 0);
expect(summary.percentageOf90HzFrames, 0);
expect(summary.percentageOf120HzFrames, 0);
expect(summary.framesWithIllegalRefreshRate, isNotEmpty);
expect(summary.framesWithIllegalRefreshRate.first, closeTo(100, kCompareDelta));
});
test('Mixed refresh rates.', () async {
final List<Map<String, dynamic>> events = <Map<String, dynamic>>[];
const int num30Hz = 10;
const int num60Hz = 20;
const int num80Hz = 20;
const int num90Hz = 20;
const int num120Hz = 40;
const int numIllegal = 10;
const int totalFrames = num30Hz + num60Hz + num80Hz + num90Hz + num120Hz + numIllegal;
// Add 30hz frames
events.addAll(populateEvents(numberOfEvents: num30Hz,
startTime: 0,
interval: 32000000,
margin: 0,
));
// Add 60hz frames
events.addAll(populateEvents(numberOfEvents: num60Hz,
startTime: 0,
interval: 16000000,
margin: 0,
));
// Add 80hz frames
events.addAll(populateEvents(numberOfEvents: num80Hz,
startTime: 0,
interval: 12000000,
margin: 0,
));
// Add 90hz frames
events.addAll(populateEvents(numberOfEvents: num90Hz,
startTime: 0,
interval: 11000000,
margin: 0,
));
// Add 120hz frames
events.addAll(populateEvents(numberOfEvents: num120Hz,
startTime: 0,
interval: 8000000,
margin: 0,
));
// Add illegal refresh rate frames
events.addAll(populateEvents(numberOfEvents: numIllegal,
startTime: 0,
interval: 60000,
margin: 0,
));
final RefreshRateSummary summary = summarizeRefresh(events);
expect(summary.percentageOf30HzFrames, closeTo(num30Hz/totalFrames*100, kCompareDelta));
expect(summary.percentageOf60HzFrames, closeTo(num60Hz/totalFrames*100, kCompareDelta));
expect(summary.percentageOf80HzFrames, closeTo(num80Hz/totalFrames*100, kCompareDelta));
expect(summary.percentageOf90HzFrames, closeTo(num90Hz/totalFrames*100, kCompareDelta));
expect(summary.percentageOf120HzFrames, closeTo(num120Hz/totalFrames*100, kCompareDelta));
expect(summary.framesWithIllegalRefreshRate, isNotEmpty);
expect(summary.framesWithIllegalRefreshRate.length, 10);
});
});
});
}
| flutter/packages/flutter_driver/test/src/real_tests/timeline_summary_test.dart/0 | {
"file_path": "flutter/packages/flutter_driver/test/src/real_tests/timeline_summary_test.dart",
"repo_id": "flutter",
"token_count": 19072
} | 734 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:ffi' show Abi;
import 'dart:io' as io;
import 'package:crypto/crypto.dart';
import 'package:file/file.dart';
import 'package:file/local.dart';
import 'package:path/path.dart' as path;
import 'package:platform/platform.dart';
import 'package:process/process.dart';
// If you are here trying to figure out how to use golden files in the Flutter
// repo itself, consider reading this wiki page:
// https://github.com/flutter/flutter/wiki/Writing-a-golden-file-test-for-package%3Aflutter
const String _kFlutterRootKey = 'FLUTTER_ROOT';
const String _kGoldctlKey = 'GOLDCTL';
const String _kTestBrowserKey = 'FLUTTER_TEST_BROWSER';
const String _kWebRendererKey = 'FLUTTER_WEB_RENDERER';
const String _kImpellerKey = 'FLUTTER_TEST_IMPELLER';
/// Signature of callbacks used to inject [print] replacements.
typedef LogCallback = void Function(String);
/// Exception thrown when an error is returned from the [SkiaClient].
class SkiaException implements Exception {
/// Creates a new `SkiaException` with a required error [message].
const SkiaException(this.message);
/// A message describing the error.
final String message;
/// Returns a description of the Skia exception.
///
/// The description always contains the [message].
@override
String toString() => 'SkiaException: $message';
}
/// A client for uploading image tests and making baseline requests to the
/// Flutter Gold Dashboard.
class SkiaGoldClient {
/// Creates a [SkiaGoldClient] with the given [workDirectory].
///
/// All other parameters are optional. They may be provided in tests to
/// override the defaults for [fs], [process], [platform], and [httpClient].
SkiaGoldClient(
this.workDirectory, {
this.fs = const LocalFileSystem(),
this.process = const LocalProcessManager(),
this.platform = const LocalPlatform(),
Abi? abi,
io.HttpClient? httpClient,
required this.log,
}) : httpClient = httpClient ?? io.HttpClient(),
abi = abi ?? Abi.current();
/// The file system to use for storing the local clone of the repository.
///
/// This is useful in tests, where a local file system (the default) can be
/// replaced by a memory file system.
final FileSystem fs;
/// A wrapper for the [dart:io.Platform] API.
///
/// This is useful in tests, where the system platform (the default) can be
/// replaced by a mock platform instance.
final Platform platform;
/// A controller for launching sub-processes.
///
/// This is useful in tests, where the real process manager (the default) can
/// be replaced by a mock process manager that doesn't really create
/// sub-processes.
final ProcessManager process;
/// A client for making Http requests to the Flutter Gold dashboard.
final io.HttpClient httpClient;
/// The ABI of the current host platform.
///
/// If not overridden for testing, defaults to [Abi.current];
final Abi abi;
/// The local [Directory] within the [comparisonRoot] for the current test
/// context. In this directory, the client will create image and JSON files
/// for the goldctl tool to use.
///
/// This is informed by the [FlutterGoldenFileComparator] [basedir]. It cannot
/// be null.
final Directory workDirectory;
/// The logging function to use when reporting messages to the console.
final LogCallback log;
/// The local [Directory] where the Flutter repository is hosted.
///
/// Uses the [fs] file system.
Directory get _flutterRoot => fs.directory(platform.environment[_kFlutterRootKey]);
/// The path to the local [Directory] where the goldctl tool is hosted.
///
/// Uses the [platform] environment in this implementation.
String get _goldctl => platform.environment[_kGoldctlKey]!;
/// Prepares the local work space for golden file testing and calls the
/// goldctl `auth` command.
///
/// This ensures that the goldctl tool is authorized and ready for testing.
/// Used by the [FlutterPostSubmitFileComparator] and the
/// [FlutterPreSubmitFileComparator].
Future<void> auth() async {
if (await clientIsAuthorized()) {
return;
}
final List<String> authCommand = <String>[
_goldctl,
'auth',
'--work-dir', workDirectory
.childDirectory('temp')
.path,
'--luci',
];
final io.ProcessResult result = await process.run(authCommand);
if (result.exitCode != 0) {
final StringBuffer buf = StringBuffer()
..writeln('Skia Gold authorization failed.')
..writeln('Luci environments authenticate using the file provided '
'by LUCI_CONTEXT. There may be an error with this file or Gold '
'authentication.')
..writeln('Debug information for Gold --------------------------------')
..writeln('stdout: ${result.stdout}')
..writeln('stderr: ${result.stderr}');
throw SkiaException(buf.toString());
}
}
/// Signals if this client is initialized for uploading images to the Gold
/// service.
///
/// Since Flutter framework tests are executed in parallel, and in random
/// order, this will signal is this instance of the Gold client has been
/// initialized.
bool _initialized = false;
/// Executes the `imgtest init` command in the goldctl tool.
///
/// The `imgtest` command collects and uploads test results to the Skia Gold
/// backend, the `init` argument initializes the current test. Used by the
/// [FlutterPostSubmitFileComparator].
Future<void> imgtestInit() async {
// This client has already been initialized
if (_initialized) {
return;
}
final File keys = workDirectory.childFile('keys.json');
final File failures = workDirectory.childFile('failures.json');
await keys.writeAsString(_getKeysJSON());
await failures.create();
final String commitHash = await _getCurrentCommit();
final List<String> imgtestInitCommand = <String>[
_goldctl,
'imgtest', 'init',
'--instance', 'flutter',
'--work-dir', workDirectory
.childDirectory('temp')
.path,
'--commit', commitHash,
'--keys-file', keys.path,
'--failure-file', failures.path,
'--passfail',
];
if (imgtestInitCommand.contains(null)) {
final StringBuffer buf = StringBuffer()
..writeln('A null argument was provided for Skia Gold imgtest init.')
..writeln('Please confirm the settings of your golden file test.')
..writeln('Arguments provided:');
imgtestInitCommand.forEach(buf.writeln);
throw SkiaException(buf.toString());
}
final io.ProcessResult result = await process.run(imgtestInitCommand);
if (result.exitCode != 0) {
_initialized = false;
final StringBuffer buf = StringBuffer()
..writeln('Skia Gold imgtest init failed.')
..writeln('An error occurred when initializing golden file test with ')
..writeln('goldctl.')
..writeln()
..writeln('Debug information for Gold --------------------------------')
..writeln('stdout: ${result.stdout}')
..writeln('stderr: ${result.stderr}');
throw SkiaException(buf.toString());
}
_initialized = true;
}
/// Executes the `imgtest add` command in the goldctl tool.
///
/// The `imgtest` command collects and uploads test results to the Skia Gold
/// backend, the `add` argument uploads the current image test. A response is
/// returned from the invocation of this command that indicates a pass or fail
/// result.
///
/// The [testName] and [goldenFile] parameters reference the current
/// comparison being evaluated by the [FlutterPostSubmitFileComparator].
Future<bool> imgtestAdd(String testName, File goldenFile) async {
final List<String> imgtestCommand = <String>[
_goldctl,
'imgtest', 'add',
'--work-dir', workDirectory
.childDirectory('temp')
.path,
'--test-name', cleanTestName(testName),
'--png-file', goldenFile.path,
'--passfail',
..._getPixelMatchingArguments(),
];
final io.ProcessResult result = await process.run(imgtestCommand);
if (result.exitCode != 0) {
// If an unapproved image has made it to post-submit, throw to close the
// tree.
String? resultContents;
final File resultFile = workDirectory.childFile(fs.path.join(
'result-state.json',
));
if (await resultFile.exists()) {
resultContents = await resultFile.readAsString();
}
final StringBuffer buf = StringBuffer()
..writeln('Skia Gold received an unapproved image in post-submit ')
..writeln('testing. Golden file images in flutter/flutter are triaged ')
..writeln('in pre-submit during code review for the given PR.')
..writeln()
..writeln('Visit https://flutter-gold.skia.org/ to view and approve ')
..writeln('the image(s), or revert the associated change. For more ')
..writeln('information, visit the wiki: ')
..writeln('https://github.com/flutter/flutter/wiki/Writing-a-golden-file-test-for-package:flutter')
..writeln()
..writeln('Debug information for Gold --------------------------------')
..writeln('stdout: ${result.stdout}')
..writeln('stderr: ${result.stderr}')
..writeln()
..writeln('result-state.json: ${resultContents ?? 'No result file found.'}');
throw SkiaException(buf.toString());
}
return true;
}
/// Signals if this client is initialized for uploading tryjobs to the Gold
/// service.
///
/// Since Flutter framework tests are executed in parallel, and in random
/// order, this will signal is this instance of the Gold client has been
/// initialized for tryjobs.
bool _tryjobInitialized = false;
/// Executes the `imgtest init` command in the goldctl tool for tryjobs.
///
/// The `imgtest` command collects and uploads test results to the Skia Gold
/// backend, the `init` argument initializes the current tryjob. Used by the
/// [FlutterPreSubmitFileComparator].
Future<void> tryjobInit() async {
// This client has already been initialized
if (_tryjobInitialized) {
return;
}
final File keys = workDirectory.childFile('keys.json');
final File failures = workDirectory.childFile('failures.json');
await keys.writeAsString(_getKeysJSON());
await failures.create();
final String commitHash = await _getCurrentCommit();
final List<String> imgtestInitCommand = <String>[
_goldctl,
'imgtest', 'init',
'--instance', 'flutter',
'--work-dir', workDirectory
.childDirectory('temp')
.path,
'--commit', commitHash,
'--keys-file', keys.path,
'--failure-file', failures.path,
'--passfail',
'--crs', 'github',
'--patchset_id', commitHash,
...getCIArguments(),
];
if (imgtestInitCommand.contains(null)) {
final StringBuffer buf = StringBuffer()
..writeln('A null argument was provided for Skia Gold tryjob init.')
..writeln('Please confirm the settings of your golden file test.')
..writeln('Arguments provided:');
imgtestInitCommand.forEach(buf.writeln);
throw SkiaException(buf.toString());
}
final io.ProcessResult result = await process.run(imgtestInitCommand);
if (result.exitCode != 0) {
_tryjobInitialized = false;
final StringBuffer buf = StringBuffer()
..writeln('Skia Gold tryjobInit failure.')
..writeln('An error occurred when initializing golden file tryjob with ')
..writeln('goldctl.')
..writeln()
..writeln('Debug information for Gold --------------------------------')
..writeln('stdout: ${result.stdout}')
..writeln('stderr: ${result.stderr}');
throw SkiaException(buf.toString());
}
_tryjobInitialized = true;
}
/// Executes the `imgtest add` command in the goldctl tool for tryjobs.
///
/// The `imgtest` command collects and uploads test results to the Skia Gold
/// backend, the `add` argument uploads the current image test. A response is
/// returned from the invocation of this command that indicates a pass or fail
/// result for the tryjob.
///
/// The [testName] and [goldenFile] parameters reference the current
/// comparison being evaluated by the [FlutterPreSubmitFileComparator].
Future<void> tryjobAdd(String testName, File goldenFile) async {
final List<String> imgtestCommand = <String>[
_goldctl,
'imgtest', 'add',
'--work-dir', workDirectory
.childDirectory('temp')
.path,
'--test-name', cleanTestName(testName),
'--png-file', goldenFile.path,
..._getPixelMatchingArguments(),
];
final io.ProcessResult result = await process.run(imgtestCommand);
final String/*!*/ resultStdout = result.stdout.toString();
if (result.exitCode != 0 &&
!(resultStdout.contains('Untriaged') || resultStdout.contains('negative image'))) {
String? resultContents;
final File resultFile = workDirectory.childFile(fs.path.join(
'result-state.json',
));
if (await resultFile.exists()) {
resultContents = await resultFile.readAsString();
}
final StringBuffer buf = StringBuffer()
..writeln('Unexpected Gold tryjobAdd failure.')
..writeln('Tryjob execution for golden file test $testName failed for')
..writeln('a reason unrelated to pixel comparison.')
..writeln()
..writeln('Debug information for Gold --------------------------------')
..writeln('stdout: ${result.stdout}')
..writeln('stderr: ${result.stderr}')
..writeln()
..writeln()
..writeln('result-state.json: ${resultContents ?? 'No result file found.'}');
throw SkiaException(buf.toString());
}
}
// Constructs arguments for `goldctl` for controlling how pixels are compared.
//
// For AOT and CanvasKit exact pixel matching is used. For the HTML renderer
// on the web a fuzzy matching algorithm is used that allows very small deltas
// because Chromium cannot exactly reproduce the same golden on all computers.
// It seems to depend on the hardware/OS/driver combination. However, those
// differences are very small (typically not noticeable to human eye).
List<String> _getPixelMatchingArguments() {
// Only use fuzzy pixel matching in the HTML renderer.
if (!_isBrowserTest || _isBrowserCanvasKitTest) {
return const <String>[];
}
// The algorithm to be used when matching images. The available options are:
// - "fuzzy": Allows for customizing the thresholds of pixel differences.
// - "sobel": Same as "fuzzy" but performs edge detection before performing
// a fuzzy match.
const String algorithm = 'fuzzy';
// The number of pixels in this image that are allowed to differ from the
// baseline.
//
// The chosen number - 20 - is arbitrary. Even for a small golden file, say
// 50 x 50, it would be less than 1% of the total number of pixels. This
// number should not grow too much. If it's growing, it is probably due to a
// larger issue that needs to be addressed at the infra level.
const int maxDifferentPixels = 20;
// The maximum acceptable difference per pixel.
//
// Uses the Manhattan distance using the RGBA color components as
// coordinates. The chosen number - 4 - is arbitrary. It's small enough to
// both not be noticeable and not trigger test flakes due to sub-pixel
// golden deltas. This number should not grow too much. If it's growing, it
// is probably due to a larger issue that needs to be addressed at the infra
// level.
const int pixelDeltaThreshold = 4;
return <String>[
'--add-test-optional-key', 'image_matching_algorithm:$algorithm',
'--add-test-optional-key', 'fuzzy_max_different_pixels:$maxDifferentPixels',
'--add-test-optional-key', 'fuzzy_pixel_delta_threshold:$pixelDeltaThreshold',
];
}
/// Returns the latest positive digest for the given test known to Flutter
/// Gold at head.
Future<String?> getExpectationForTest(String testName) async {
late String? expectation;
final String traceID = getTraceID(testName);
await io.HttpOverrides.runWithHttpOverrides<Future<void>>(() async {
final Uri requestForExpectations = Uri.parse(
'https://flutter-gold.skia.org/json/v2/latestpositivedigest/$traceID'
);
late String rawResponse;
try {
final io.HttpClientRequest request = await httpClient.getUrl(requestForExpectations);
final io.HttpClientResponse response = await request.close();
rawResponse = await utf8.decodeStream(response);
final dynamic jsonResponse = json.decode(rawResponse);
if (jsonResponse is! Map<String, dynamic>) {
throw const FormatException('Skia gold expectations do not match expected format.');
}
expectation = jsonResponse['digest'] as String?;
} on FormatException catch (error) {
log(
'Formatting error detected requesting expectations from Flutter Gold.\n'
'error: $error\n'
'url: $requestForExpectations\n'
'response: $rawResponse'
);
rethrow;
}
},
SkiaGoldHttpOverrides(),
);
return expectation;
}
/// Returns a list of bytes representing the golden image retrieved from the
/// Flutter Gold dashboard.
///
/// The provided image hash represents an expectation from Flutter Gold.
Future<List<int>>getImageBytes(String imageHash) async {
final List<int> imageBytes = <int>[];
await io.HttpOverrides.runWithHttpOverrides<Future<void>>(() async {
final Uri requestForImage = Uri.parse(
'https://flutter-gold.skia.org/img/images/$imageHash.png',
);
final io.HttpClientRequest request = await httpClient.getUrl(requestForImage);
final io.HttpClientResponse response = await request.close();
await response.forEach((List<int> bytes) => imageBytes.addAll(bytes));
},
SkiaGoldHttpOverrides(),
);
return imageBytes;
}
/// Returns the current commit hash of the Flutter repository.
Future<String> _getCurrentCommit() async {
if (!_flutterRoot.existsSync()) {
throw SkiaException('Flutter root could not be found: $_flutterRoot\n');
} else {
final io.ProcessResult revParse = await process.run(
<String>['git', 'rev-parse', 'HEAD'],
workingDirectory: _flutterRoot.path,
);
if (revParse.exitCode != 0) {
throw const SkiaException('Current commit of Flutter can not be found.');
}
return (revParse.stdout as String/*!*/).trim();
}
}
/// Returns a JSON String with keys value pairs used to uniquely identify the
/// configuration that generated the given golden file.
///
/// Currently, the only key value pairs being tracked is the platform the
/// image was rendered on, and for web tests, the browser the image was
/// rendered on.
String _getKeysJSON() {
final Map<String, dynamic> keys = <String, dynamic>{
'Platform' : platform.operatingSystem,
'Abi': abi.toString(),
'CI' : 'luci',
if (_isImpeller)
'impeller': 'swiftshader',
};
if (_isBrowserTest) {
keys['Browser'] = _browserKey;
keys['Platform'] = '${keys['Platform']}-browser';
if (_isBrowserCanvasKitTest) {
keys['WebRenderer'] = 'canvaskit';
}
}
return json.encode(keys);
}
/// Removes the file extension from the [fileName] to represent the test name
/// properly.
String cleanTestName(String fileName) {
return fileName.split(path.extension(fileName))[0];
}
/// Returns a boolean value to prevent the client from re-authorizing itself
/// for multiple tests.
Future<bool> clientIsAuthorized() async {
final File authFile = workDirectory.childFile(fs.path.join(
'temp',
'auth_opt.json',
))/*!*/;
if (await authFile.exists()) {
final String contents = await authFile.readAsString();
final Map<String, dynamic> decoded = json.decode(contents) as Map<String, dynamic>;
return !(decoded['GSUtil'] as bool/*!*/);
}
return false;
}
/// Returns a list of arguments for initializing a tryjob based on the testing
/// environment.
List<String> getCIArguments() {
final String jobId = platform.environment['LOGDOG_STREAM_PREFIX']!.split('/').last;
final List<String> refs = platform.environment['GOLD_TRYJOB']!.split('/');
final String pullRequest = refs[refs.length - 2];
return <String>[
'--changelist', pullRequest,
'--cis', 'buildbucket',
'--jobid', jobId,
];
}
bool get _isBrowserTest {
return platform.environment[_kTestBrowserKey] != null;
}
bool get _isBrowserCanvasKitTest {
return _isBrowserTest && platform.environment[_kWebRendererKey] == 'canvaskit';
}
bool get _isImpeller {
return (platform.environment[_kImpellerKey] != null);
}
String get _browserKey {
assert(_isBrowserTest);
return platform.environment[_kTestBrowserKey]!;
}
/// Returns a trace id based on the current testing environment to lookup
/// the latest positive digest on Flutter Gold with a hex-encoded md5 hash of
/// the image keys.
String getTraceID(String testName) {
final Map<String, Object?> keys = <String, Object?>{
if (_isBrowserTest)
'Browser' : _browserKey,
if (_isBrowserCanvasKitTest)
'WebRenderer' : 'canvaskit',
'CI' : 'luci',
'Platform' : platform.operatingSystem,
'Abi': abi.toString(),
'name' : testName,
'source_type' : 'flutter',
if (_isImpeller)
'impeller': 'swiftshader',
};
final String jsonTrace = json.encode(keys);
final String md5Sum = md5.convert(utf8.encode(jsonTrace)).toString();
return md5Sum;
}
}
/// Used to make HttpRequests during testing.
class SkiaGoldHttpOverrides extends io.HttpOverrides { }
| flutter/packages/flutter_goldens/lib/skia_client.dart/0 | {
"file_path": "flutter/packages/flutter_goldens/lib/skia_client.dart",
"repo_id": "flutter",
"token_count": 7577
} | 735 |
{
"datePickerHourSemanticsLabelOne": "$hourটা বাজে",
"datePickerHourSemanticsLabelOther": "$hourটা বাজে",
"datePickerMinuteSemanticsLabelOne": "১ মিনিট",
"datePickerMinuteSemanticsLabelOther": "$minute মিনিট",
"datePickerDateOrder": "mdy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"todayLabel": "আজ",
"alertDialogLabel": "সতর্কতা",
"timerPickerHourLabelOne": "ঘণ্টা",
"timerPickerHourLabelOther": "ঘণ্টা",
"timerPickerMinuteLabelOne": "মিনিট।",
"timerPickerMinuteLabelOther": "মিনিট।",
"timerPickerSecondLabelOne": "সেকেন্ড।",
"timerPickerSecondLabelOther": "সেকেন্ড।",
"cutButtonLabel": "কাট করুন",
"copyButtonLabel": "কপি করুন",
"pasteButtonLabel": "পেস্ট করুন",
"clearButtonLabel": "Clear",
"selectAllButtonLabel": "সব বেছে নিন",
"tabSemanticsLabel": "$tabCount-এর মধ্যে $tabIndex নম্বর ট্যাব",
"modalBarrierDismissLabel": "খারিজ করুন",
"searchTextFieldPlaceholderLabel": "সার্চ করুন",
"noSpellCheckReplacementsLabel": "কোনও বিকল্প বানান দেখানো হয়নি",
"menuDismissLabel": "বাতিল করার মেনু",
"lookUpButtonLabel": "লুক-আপ",
"searchWebButtonLabel": "ওয়েবে সার্চ করুন",
"shareButtonLabel": "শেয়ার করুন..."
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_bn.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_bn.arb",
"repo_id": "flutter",
"token_count": 829
} | 736 |
{
"datePickerHourSemanticsLabelOne": "$hour વાગ્યો છે",
"datePickerHourSemanticsLabelOther": "$hour વાગ્યા છે",
"datePickerMinuteSemanticsLabelOne": "1 મિનિટ",
"datePickerMinuteSemanticsLabelOther": "$minute મિનિટ",
"datePickerDateOrder": "dmy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"todayLabel": "આજે",
"alertDialogLabel": "અલર્ટ",
"timerPickerHourLabelOne": "કલાક",
"timerPickerHourLabelOther": "કલાક",
"timerPickerMinuteLabelOne": "મિનિટ",
"timerPickerMinuteLabelOther": "મિનિટ",
"timerPickerSecondLabelOne": "સેકન્ડ",
"timerPickerSecondLabelOther": "સેકન્ડ",
"cutButtonLabel": "કાપો",
"copyButtonLabel": "કૉપિ કરો",
"pasteButtonLabel": "પેસ્ટ કરો",
"selectAllButtonLabel": "બધા પસંદ કરો",
"tabSemanticsLabel": "$tabCountમાંથી $tabIndex ટૅબ",
"modalBarrierDismissLabel": "છોડી દો",
"searchTextFieldPlaceholderLabel": "શોધો",
"noSpellCheckReplacementsLabel": "બદલવા માટે કોઈ શબ્દ મળ્યો નથી",
"menuDismissLabel": "મેનૂ છોડી દો",
"lookUpButtonLabel": "શોધો",
"searchWebButtonLabel": "વેબ પર શોધો",
"shareButtonLabel": "શેર કરો…",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_gu.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_gu.arb",
"repo_id": "flutter",
"token_count": 872
} | 737 |
{
"datePickerHourSemanticsLabelOne": "$hour ໂມງກົງ",
"datePickerHourSemanticsLabelOther": "$hour ໂມງກົງ",
"datePickerMinuteSemanticsLabelOne": "1 ນາທີ",
"datePickerMinuteSemanticsLabelOther": "$minute ນາທີ",
"datePickerDateOrder": "mdy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "ກ່ອນທ່ຽງ",
"postMeridiemAbbreviation": "ຫຼັງທ່ຽງ",
"todayLabel": "ມື້ນີ້",
"alertDialogLabel": "ການເຕືອນ",
"timerPickerHourLabelOne": "ຊົ່ວໂມງ",
"timerPickerHourLabelOther": "ຊົ່ວໂມງ",
"timerPickerMinuteLabelOne": "ນທ.",
"timerPickerMinuteLabelOther": "ນທ.",
"timerPickerSecondLabelOne": "ວິ.",
"timerPickerSecondLabelOther": "ວິ.",
"cutButtonLabel": "ຕັດ",
"copyButtonLabel": "ສຳເນົາ",
"pasteButtonLabel": "ວາງ",
"selectAllButtonLabel": "ເລືອກທັງໝົດ",
"tabSemanticsLabel": "ແຖບທີ $tabIndex ຈາກທັງໝົດ $tabCount",
"modalBarrierDismissLabel": "ປິດໄວ້",
"searchTextFieldPlaceholderLabel": "ຊອກຫາ",
"noSpellCheckReplacementsLabel": "ບໍ່ພົບການແທນທີ່",
"menuDismissLabel": "ປິດເມນູ",
"lookUpButtonLabel": "ຊອກຫາຂໍ້ມູນ",
"searchWebButtonLabel": "ຊອກຫາຢູ່ອິນເຕີເນັດ",
"shareButtonLabel": "ແບ່ງປັນ...",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_lo.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_lo.arb",
"repo_id": "flutter",
"token_count": 896
} | 738 |
{
"datePickerHourSemanticsLabelOne": "$hour hora",
"datePickerHourSemanticsLabelOther": "$hour horas",
"datePickerMinuteSemanticsLabelOne": "1 minuto",
"datePickerMinuteSemanticsLabelOther": "$minute minutos",
"datePickerDateOrder": "dmy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"todayLabel": "Hoje",
"alertDialogLabel": "Alerta",
"timerPickerHourLabelOne": "hora",
"timerPickerHourLabelOther": "horas",
"timerPickerMinuteLabelOne": "min",
"timerPickerMinuteLabelOther": "min",
"timerPickerSecondLabelOne": "s",
"timerPickerSecondLabelOther": "s",
"cutButtonLabel": "Cortar",
"copyButtonLabel": "Copiar",
"pasteButtonLabel": "Colar",
"selectAllButtonLabel": "Selecionar tudo",
"tabSemanticsLabel": "Guia $tabIndex de $tabCount",
"modalBarrierDismissLabel": "Dispensar",
"searchTextFieldPlaceholderLabel": "Pesquisar",
"noSpellCheckReplacementsLabel": "Nenhuma alternativa encontrada",
"menuDismissLabel": "Dispensar menu",
"lookUpButtonLabel": "Pesquisar",
"searchWebButtonLabel": "Pesquisar na Web",
"shareButtonLabel": "Compartilhar…",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_pt.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_pt.arb",
"repo_id": "flutter",
"token_count": 438
} | 739 |
{
"datePickerHourSemanticsLabelOne": "Saat $hour",
"datePickerHourSemanticsLabelOther": "Saat $hour",
"datePickerMinuteSemanticsLabelOne": "1 dakika",
"datePickerMinuteSemanticsLabelOther": "$minute dakika",
"datePickerDateOrder": "dmy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "ÖÖ",
"postMeridiemAbbreviation": "ÖS",
"todayLabel": "Bugün",
"alertDialogLabel": "Uyarı",
"timerPickerHourLabelOne": "saat",
"timerPickerHourLabelOther": "saat",
"timerPickerMinuteLabelOne": "dk.",
"timerPickerMinuteLabelOther": "dk.",
"timerPickerSecondLabelOne": "sn.",
"timerPickerSecondLabelOther": "sn.",
"cutButtonLabel": "Kes",
"copyButtonLabel": "Kopyala",
"pasteButtonLabel": "Yapıştır",
"selectAllButtonLabel": "Tümünü Seç",
"tabSemanticsLabel": "Sekme $tabIndex/$tabCount",
"modalBarrierDismissLabel": "Kapat",
"searchTextFieldPlaceholderLabel": "Ara",
"noSpellCheckReplacementsLabel": "Yerine Kelime Bulunamadı",
"menuDismissLabel": "Menüyü kapat",
"lookUpButtonLabel": "Ara",
"searchWebButtonLabel": "Web'de Ara",
"shareButtonLabel": "Paylaş...",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_tr.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_tr.arb",
"repo_id": "flutter",
"token_count": 445
} | 740 |
{
"scriptCategory": "English-like",
"timeOfDayFormat": "H:mm",
"openAppDrawerTooltip": "নেভিগেশ্বন মেনু খোলক",
"backButtonTooltip": "উভতি যাওক",
"closeButtonTooltip": "বন্ধ কৰক",
"deleteButtonTooltip": "মচক",
"nextMonthTooltip": "পৰৱৰ্তী মাহ",
"previousMonthTooltip": "পূৰ্বৱৰ্তী মাহ",
"nextPageTooltip": "পৰৱৰ্তী পৃষ্ঠা",
"firstPageTooltip": "প্রথম পৃষ্ঠা",
"lastPageTooltip": "অন্তিম পৃষ্ঠা",
"previousPageTooltip": "পূৰ্বৱৰ্তী পৃষ্ঠা",
"showMenuTooltip": "মেনুখন দেখুৱাওক",
"aboutListTileTitle": "$applicationNameৰ বিষয়ে",
"licensesPageTitle": "অনুজ্ঞাপত্ৰসমূহ",
"pageRowsInfoTitle": "$rowCountৰ $firstRow–$lastRow",
"pageRowsInfoTitleApproximate": "$rowCountৰ $firstRow–$lastRow",
"rowsPerPageTitle": "প্ৰতিটো পৃষ্ঠাত থকা শাৰী:",
"tabLabel": "$tabCountৰ $tabIndexটা টেব",
"selectedRowCountTitleOne": "১টা বস্তু বাছনি কৰা হ'ল",
"selectedRowCountTitleOther": "$selectedRowCountটা বস্তু বাছনি কৰা হ’ল",
"cancelButtonLabel": "বাতিল কৰক",
"closeButtonLabel": "বন্ধ কৰক",
"continueButtonLabel": "অব্যাহত ৰাখক",
"copyButtonLabel": "প্ৰতিলিপি কৰক",
"cutButtonLabel": "কাট কৰক",
"scanTextButtonLabel": "পাঠ স্কেন কৰক",
"okButtonLabel": "ঠিক আছে",
"pasteButtonLabel": "পে'ষ্ট কৰক",
"selectAllButtonLabel": "সকলো বাছনি কৰক",
"viewLicensesButtonLabel": "অনুজ্ঞাপত্ৰসমূহ চাওক",
"anteMeridiemAbbreviation": "পূৰ্বাহ্ন",
"postMeridiemAbbreviation": "অপৰাহ্ন",
"timePickerHourModeAnnouncement": "সময় বাছনি কৰক",
"timePickerMinuteModeAnnouncement": "মিনিট বাছনি কৰক",
"modalBarrierDismissLabel": "অগ্ৰাহ্য কৰক",
"signedInLabel": "ছাইন ইন কৰা হ’ল",
"hideAccountsLabel": "একাউণ্টসমূহ লুকুৱাওক",
"showAccountsLabel": "একাউণ্টসমূহ দেখুৱাওক",
"drawerLabel": "নেভিগেশ্বন মেনু",
"popupMenuLabel": "প'পআপ মেনু",
"dialogLabel": "ডায়ল'গ",
"alertDialogLabel": "সতৰ্কবাৰ্তা",
"searchFieldLabel": "সন্ধান কৰক",
"reorderItemToStart": "আৰম্ভণিলৈ স্থানান্তৰ কৰক",
"reorderItemToEnd": "শেষলৈ স্থানান্তৰ কৰক",
"reorderItemUp": "ওপৰলৈ নিয়ক",
"reorderItemDown": "তললৈ স্থানান্তৰ কৰক",
"reorderItemLeft": "বাওঁফাললৈ স্থানান্তৰ কৰক",
"reorderItemRight": "সোঁফাললৈ স্থানান্তৰ কৰক",
"expandedIconTapHint": "সংকোচন কৰক",
"collapsedIconTapHint": "বিস্তাৰ কৰক",
"remainingTextFieldCharacterCountOne": "১টা বর্ণ বাকী আছে",
"remainingTextFieldCharacterCountOther": "$remainingCountটা বর্ণ বাকী আছে",
"refreshIndicatorSemanticLabel": "ৰিফ্ৰেশ্ব কৰক",
"moreButtonTooltip": "অধিক",
"dateSeparator": "/",
"dateHelpText": "mm/dd/yyyy",
"selectYearSemanticsLabel": "বছৰ বাছনি কৰক",
"unspecifiedDate": "তাৰিখ",
"unspecifiedDateRange": "তাৰিখৰ পৰিসৰ",
"dateInputLabel": "তাৰিখটো দিয়ক",
"dateRangeStartLabel": "আৰম্ভণিৰ তাৰিখ",
"dateRangeEndLabel": "সমাপ্তিৰ তাৰিখ",
"dateRangeStartDateSemanticLabel": "আৰম্ভণিৰ তাৰিখ $fullDate",
"dateRangeEndDateSemanticLabel": "সমাপ্তিৰ তাৰিখ $fullDate",
"invalidDateFormatLabel": "অমান্য ফৰ্মেট।",
"invalidDateRangeLabel": "অমান্য পৰিসৰ।",
"dateOutOfRangeLabel": "সীমাৰ বাহিৰত।",
"saveButtonLabel": "ছেভ কৰক",
"datePickerHelpText": "তাৰিখ বাছনি কৰক",
"dateRangePickerHelpText": "পৰিসৰ বাছনি কৰক",
"calendarModeButtonLabel": "কেলেণ্ডাৰলৈ সলনি কৰক",
"inputDateModeButtonLabel": "ইনপুটলৈ সলনি কৰক",
"timePickerDialHelpText": "সময় বাছনি কৰক",
"timePickerInputHelpText": "সময় দিয়ক",
"timePickerHourLabel": "ঘণ্টা",
"timePickerMinuteLabel": "মিনিট",
"invalidTimeLabel": "এটা মান্য সময় দিয়ক",
"dialModeButtonLabel": "ডায়েল বাছনিকৰ্তাৰ ম’ডলৈ সলনি কৰক",
"inputTimeModeButtonLabel": "পাঠ ইনপুটৰ ম’ডলৈ সলনি কৰক",
"licensesPackageDetailTextZero": "No licenses",
"licensesPackageDetailTextOne": "১ খন অনুজ্ঞাপত্ৰ",
"licensesPackageDetailTextOther": "$licenseCount খন অনুজ্ঞাপত্ৰ",
"keyboardKeyAlt": "Alt",
"keyboardKeyAltGraph": "AltGr",
"keyboardKeyBackspace": "বেকস্পেচ",
"keyboardKeyCapsLock": "কেপ্ছ লক",
"keyboardKeyChannelDown": "চেনেল ডাউন",
"keyboardKeyChannelUp": "চেনেল আপ",
"keyboardKeyControl": "Ctrl",
"keyboardKeyDelete": "Del",
"keyboardKeyEject": "ইজেক্ট",
"keyboardKeyEnd": "End",
"keyboardKeyEscape": "Esc",
"keyboardKeyFn": "Fn",
"keyboardKeyHome": "Home",
"keyboardKeyInsert": "Insert",
"keyboardKeyMeta": "মেটা",
"keyboardKeyNumLock": "Num Lock",
"keyboardKeyNumpad1": "নং ১",
"keyboardKeyNumpad2": "নং ২",
"keyboardKeyNumpad3": "নং ৩",
"keyboardKeyNumpad4": "নং ৪",
"keyboardKeyNumpad5": "নং ৫",
"keyboardKeyNumpad6": "নং ৬",
"keyboardKeyNumpad7": "নং ৭",
"keyboardKeyNumpad8": "নং ৮",
"keyboardKeyNumpad9": "নং ৯",
"keyboardKeyNumpad0": "নং ০",
"keyboardKeyNumpadAdd": "নং +",
"keyboardKeyNumpadComma": "নং ,",
"keyboardKeyNumpadDecimal": "নং .",
"keyboardKeyNumpadDivide": "নং /",
"keyboardKeyNumpadEnter": "Num Enter",
"keyboardKeyNumpadEqual": "নং =",
"keyboardKeyNumpadMultiply": "নং *",
"keyboardKeyNumpadParenLeft": "নং (",
"keyboardKeyNumpadParenRight": "নং )",
"keyboardKeyNumpadSubtract": "নং -",
"keyboardKeyPageDown": "PgDown",
"keyboardKeyPageUp": "PgUp",
"keyboardKeyPower": "পাৱাৰ",
"keyboardKeyPowerOff": "পাৱাৰ অফ",
"keyboardKeyPrintScreen": "প্ৰিণ্ট স্ক্ৰীন",
"keyboardKeyScrollLock": "স্ক্ৰ’ল লক",
"keyboardKeySelect": "ছিলেক্ট",
"keyboardKeySpace": "স্পেচ",
"keyboardKeyMetaMacOs": "Command",
"keyboardKeyMetaWindows": "Win",
"menuBarMenuLabel": "মেনু বাৰ মেনু",
"currentDateLabel": "আজি",
"scrimLabel": "স্ক্ৰিম",
"bottomSheetLabel": "তলৰ শ্বীট",
"scrimOnTapHint": "$modalRouteContentName বন্ধ কৰক",
"keyboardKeyShift": "শ্বিফ্ট",
"expansionTileExpandedHint": "সংকোচন কৰিবলৈ দুবাৰ টিপক",
"expansionTileCollapsedHint": "বিস্তাৰ কৰিবলৈ দুবাৰ টিপক",
"expansionTileExpandedTapHint": "সংকোচন কৰক",
"expansionTileCollapsedTapHint": "অধিক সবিশেষ জানিবলৈ বিস্তাৰ কৰক",
"expandedHint": "সংকোচন কৰা আছে",
"collapsedHint": "বিস্তাৰ কৰা আছে",
"menuDismissLabel": "অগ্ৰাহ্য কৰাৰ মেনু",
"lookUpButtonLabel": "ওপৰলৈ চাওক",
"searchWebButtonLabel": "ৱেবত সন্ধান কৰক",
"shareButtonLabel": "শ্বেয়াৰ কৰক…",
"clearButtonTooltip": "Clear text",
"selectedDateLabel": "Selected"
}
| flutter/packages/flutter_localizations/lib/src/l10n/material_as.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/material_as.arb",
"repo_id": "flutter",
"token_count": 4797
} | 741 |
{
"searchWebButtonLabel": "Search Web",
"shareButtonLabel": "Share...",
"scanTextButtonLabel": "Scan text",
"lookUpButtonLabel": "Look up",
"menuDismissLabel": "Dismiss menu",
"expansionTileExpandedHint": "double-tap to collapse",
"expansionTileCollapsedHint": "double-tap to expand",
"expansionTileExpandedTapHint": "Collapse",
"expansionTileCollapsedTapHint": "Expand for more details",
"expandedHint": "Collapsed",
"collapsedHint": "Expanded",
"scrimLabel": "Scrim",
"bottomSheetLabel": "Bottom sheet",
"scrimOnTapHint": "Close $modalRouteContentName",
"currentDateLabel": "Today",
"keyboardKeyShift": "Shift",
"menuBarMenuLabel": "Menu bar menu",
"keyboardKeyNumpad8": "Num 8",
"keyboardKeyCapsLock": "Caps lock",
"keyboardKeyAltGraph": "AltGr",
"keyboardKeyNumpad4": "Num 4",
"keyboardKeyNumpad5": "Num 5",
"keyboardKeyNumpad6": "Num 6",
"keyboardKeyNumpad7": "Num 7",
"keyboardKeyNumpad2": "Num 2",
"keyboardKeyNumpad0": "Num 0",
"keyboardKeyNumpadAdd": "Num +",
"keyboardKeyNumpadComma": "Num ,",
"keyboardKeyNumpadDecimal": "Num .",
"keyboardKeyNumpadDivide": "Num /",
"keyboardKeyNumpadEqual": "Num =",
"keyboardKeyNumpadMultiply": "Num *",
"keyboardKeyNumpadParenLeft": "Num (",
"keyboardKeyInsert": "Insert",
"keyboardKeyHome": "Home",
"keyboardKeyEject": "Eject",
"keyboardKeyNumpadParenRight": "Num )",
"keyboardKeyFn": "Fn",
"keyboardKeyEscape": "Esc",
"keyboardKeyEnd": "End",
"keyboardKeyDelete": "Del",
"keyboardKeyControl": "Ctrl",
"keyboardKeyChannelUp": "Channel up",
"keyboardKeyNumpadSubtract": "Num -",
"keyboardKeyChannelDown": "Channel down",
"keyboardKeyBackspace": "Backspace",
"keyboardKeySelect": "Select",
"keyboardKeyAlt": "Alt",
"keyboardKeyMeta": "Meta",
"keyboardKeyMetaMacOs": "Command",
"keyboardKeyMetaWindows": "Win",
"keyboardKeyNumLock": "Num lock",
"keyboardKeyNumpad1": "Num 1",
"keyboardKeyPageDown": "PgDown",
"keyboardKeySpace": "Space",
"keyboardKeyNumpad3": "Num 3",
"keyboardKeyScrollLock": "Scroll lock",
"keyboardKeyNumpad9": "Num 9",
"keyboardKeyPrintScreen": "Print screen",
"keyboardKeyPowerOff": "Power off",
"keyboardKeyPower": "Power",
"keyboardKeyPageUp": "PgUp",
"keyboardKeyNumpadEnter": "Num enter",
"inputTimeModeButtonLabel": "Switch to text input mode",
"timePickerDialHelpText": "Select time",
"timePickerHourLabel": "Hour",
"timePickerMinuteLabel": "Minute",
"invalidTimeLabel": "Enter a valid time",
"dialModeButtonLabel": "Switch to dial picker mode",
"timePickerInputHelpText": "Enter time",
"dateSeparator": "/",
"dateInputLabel": "Enter date",
"calendarModeButtonLabel": "Switch to calendar",
"dateRangePickerHelpText": "Select range",
"datePickerHelpText": "Select date",
"saveButtonLabel": "Save",
"dateOutOfRangeLabel": "Out of range.",
"invalidDateRangeLabel": "Invalid range.",
"invalidDateFormatLabel": "Invalid format.",
"dateRangeEndDateSemanticLabel": "End date $fullDate",
"dateRangeStartDateSemanticLabel": "Start date $fullDate",
"dateRangeEndLabel": "End date",
"dateRangeStartLabel": "Start date",
"inputDateModeButtonLabel": "Switch to input",
"unspecifiedDateRange": "Date range",
"unspecifiedDate": "Date",
"selectYearSemanticsLabel": "Select year",
"dateHelpText": "dd/mm/yyyy",
"moreButtonTooltip": "More",
"tabLabel": "Tab $tabIndex of $tabCount",
"showAccountsLabel": "Show accounts",
"hideAccountsLabel": "Hide accounts",
"signedInLabel": "Signed in",
"timePickerMinuteModeAnnouncement": "Select minutes",
"timePickerHourModeAnnouncement": "Select hours",
"scriptCategory": "English-like",
"timeOfDayFormat": "HH:mm",
"nextMonthTooltip": "Next month",
"pageRowsInfoTitleApproximate": "$firstRow–$lastRow of about $rowCount",
"copyButtonLabel": "Copy",
"closeButtonTooltip": "Close",
"deleteButtonTooltip": "Delete",
"selectAllButtonLabel": "Select all",
"viewLicensesButtonLabel": "View licences",
"rowsPerPageTitle": "Rows per page:",
"aboutListTileTitle": "About $applicationName",
"backButtonTooltip": "Back",
"licensesPageTitle": "Licences",
"licensesPackageDetailTextZero": "No licences",
"licensesPackageDetailTextOne": "1 licence",
"licensesPackageDetailTextOther": "$licenseCount licences",
"okButtonLabel": "OK",
"pasteButtonLabel": "Paste",
"previousMonthTooltip": "Previous month",
"closeButtonLabel": "Close",
"cutButtonLabel": "Cut",
"continueButtonLabel": "Continue",
"nextPageTooltip": "Next page",
"openAppDrawerTooltip": "Open navigation menu",
"previousPageTooltip": "Previous page",
"firstPageTooltip": "First page",
"lastPageTooltip": "Last page",
"cancelButtonLabel": "Cancel",
"pageRowsInfoTitle": "$firstRow–$lastRow of $rowCount",
"selectedRowCountTitleOne": "1 item selected",
"selectedRowCountTitleOther": "$selectedRowCount items selected",
"showMenuTooltip": "Show menu",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"modalBarrierDismissLabel": "Dismiss",
"drawerLabel": "Navigation menu",
"popupMenuLabel": "Pop-up menu",
"dialogLabel": "Dialogue",
"alertDialogLabel": "Alert",
"searchFieldLabel": "Search",
"reorderItemToStart": "Move to the start",
"reorderItemToEnd": "Move to the end",
"reorderItemUp": "Move up",
"reorderItemDown": "Move down",
"reorderItemLeft": "Move to the left",
"reorderItemRight": "Move to the right",
"expandedIconTapHint": "Collapse",
"collapsedIconTapHint": "Expand",
"remainingTextFieldCharacterCountZero": "No characters remaining",
"remainingTextFieldCharacterCountOne": "1 character remaining",
"remainingTextFieldCharacterCountOther": "$remainingCount characters remaining",
"refreshIndicatorSemanticLabel": "Refresh"
}
| flutter/packages/flutter_localizations/lib/src/l10n/material_en_GB.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/material_en_GB.arb",
"repo_id": "flutter",
"token_count": 1994
} | 742 |
{
"scriptCategory": "English-like",
"timeOfDayFormat": "HH:mm",
"openAppDrawerTooltip": "Ouvrir le menu de navigation",
"backButtonTooltip": "Retour",
"closeButtonTooltip": "Fermer",
"deleteButtonTooltip": "Supprimer",
"nextMonthTooltip": "Mois suivant",
"previousMonthTooltip": "Mois précédent",
"nextPageTooltip": "Page suivante",
"previousPageTooltip": "Page précédente",
"firstPageTooltip": "Première page",
"lastPageTooltip": "Dernière page",
"showMenuTooltip": "Afficher le menu",
"aboutListTileTitle": "À propos de $applicationName",
"licensesPageTitle": "Licences",
"pageRowsInfoTitle": "$firstRow – $lastRow sur $rowCount",
"pageRowsInfoTitleApproximate": "$firstRow – $lastRow sur environ $rowCount",
"rowsPerPageTitle": "Lignes par page :",
"tabLabel": "Onglet $tabIndex sur $tabCount",
"selectedRowCountTitleZero": "Aucun élément sélectionné",
"selectedRowCountTitleOne": "1 élément sélectionné",
"selectedRowCountTitleOther": "$selectedRowCount éléments sélectionnés",
"cancelButtonLabel": "Annuler",
"closeButtonLabel": "Fermer",
"continueButtonLabel": "Continuer",
"copyButtonLabel": "Copier",
"cutButtonLabel": "Couper",
"scanTextButtonLabel": "Scanner du texte",
"okButtonLabel": "OK",
"pasteButtonLabel": "Coller",
"selectAllButtonLabel": "Tout sélectionner",
"viewLicensesButtonLabel": "Afficher les licences",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"timePickerHourModeAnnouncement": "Sélectionner une heure",
"timePickerMinuteModeAnnouncement": "Sélectionner des minutes",
"signedInLabel": "Connecté",
"hideAccountsLabel": "Masquer les comptes",
"showAccountsLabel": "Afficher les comptes",
"modalBarrierDismissLabel": "Ignorer",
"drawerLabel": "Menu de navigation",
"popupMenuLabel": "Menu contextuel",
"dialogLabel": "Boîte de dialogue",
"alertDialogLabel": "Alerte",
"searchFieldLabel": "Rechercher",
"reorderItemToStart": "Déplacer vers le début",
"reorderItemToEnd": "Déplacer vers la fin",
"reorderItemUp": "Déplacer vers le haut",
"reorderItemDown": "Déplacer vers le bas",
"reorderItemLeft": "Déplacer vers la gauche",
"reorderItemRight": "Déplacer vers la droite",
"expandedIconTapHint": "Réduire",
"collapsedIconTapHint": "Développer",
"remainingTextFieldCharacterCountOne": "1 caractère restant",
"remainingTextFieldCharacterCountOther": "$remainingCount caractères restants",
"refreshIndicatorSemanticLabel": "Actualiser",
"moreButtonTooltip": "Plus",
"dateSeparator": "/",
"dateHelpText": "jj/mm/aaaa",
"selectYearSemanticsLabel": "Sélectionner une année",
"unspecifiedDate": "Date",
"unspecifiedDateRange": "Plage de dates",
"dateInputLabel": "Saisir une date",
"dateRangeStartLabel": "Date de début",
"dateRangeEndLabel": "Date de fin",
"dateRangeStartDateSemanticLabel": "Date de début : $fullDate",
"dateRangeEndDateSemanticLabel": "Date de fin : $fullDate",
"invalidDateFormatLabel": "Format non valide.",
"invalidDateRangeLabel": "Plage non valide.",
"dateOutOfRangeLabel": "Hors de portée.",
"saveButtonLabel": "Enregistrer",
"datePickerHelpText": "Sélectionner une date",
"dateRangePickerHelpText": "Sélectionner une plage",
"calendarModeButtonLabel": "Passer à l'agenda",
"inputDateModeButtonLabel": "Passer à la saisie",
"timePickerDialHelpText": "Sélectionner une heure",
"timePickerInputHelpText": "Saisir une heure",
"timePickerHourLabel": "Heure",
"timePickerMinuteLabel": "Minute",
"invalidTimeLabel": "Veuillez indiquer une heure valide",
"dialModeButtonLabel": "Passer au mode de sélection via le cadran",
"inputTimeModeButtonLabel": "Passer au mode de saisie au format texte",
"licensesPackageDetailTextZero": "No licenses",
"licensesPackageDetailTextOne": "1 licence",
"licensesPackageDetailTextOther": "$licenseCount licences",
"keyboardKeyAlt": "Alt",
"keyboardKeyAltGraph": "Alt Gr",
"keyboardKeyBackspace": "Retour arrière",
"keyboardKeyCapsLock": "Verr Maj",
"keyboardKeyChannelDown": "Chaîne suivante",
"keyboardKeyChannelUp": "Chaîne précédente",
"keyboardKeyControl": "Ctrl",
"keyboardKeyDelete": "Suppr",
"keyboardKeyEject": "Éjecter",
"keyboardKeyEnd": "Fin",
"keyboardKeyEscape": "Échap",
"keyboardKeyFn": "Fn",
"keyboardKeyHome": "Accueil",
"keyboardKeyInsert": "Insérer",
"keyboardKeyMeta": "Méta",
"keyboardKeyNumLock": "Verr Num",
"keyboardKeyNumpad1": "Num 1",
"keyboardKeyNumpad2": "Num 2",
"keyboardKeyNumpad3": "Num 3",
"keyboardKeyNumpad4": "Num 4",
"keyboardKeyNumpad5": "Num 5",
"keyboardKeyNumpad6": "Num 6",
"keyboardKeyNumpad7": "Num 7",
"keyboardKeyNumpad8": "Num 8",
"keyboardKeyNumpad9": "Num 9",
"keyboardKeyNumpad0": "Num 0",
"keyboardKeyNumpadAdd": "Num +",
"keyboardKeyNumpadComma": "Num ,",
"keyboardKeyNumpadDecimal": "Num .",
"keyboardKeyNumpadDivide": "Num /",
"keyboardKeyNumpadEnter": "Num Entrée",
"keyboardKeyNumpadEqual": "Num =",
"keyboardKeyNumpadMultiply": "Num *",
"keyboardKeyNumpadParenLeft": "Num (",
"keyboardKeyNumpadParenRight": "Num )",
"keyboardKeyNumpadSubtract": "Num -",
"keyboardKeyPageDown": "PgSuiv",
"keyboardKeyPageUp": "PgPréc",
"keyboardKeyPower": "Puissance",
"keyboardKeyPowerOff": "Éteindre",
"keyboardKeyPrintScreen": "Impr. écran",
"keyboardKeyScrollLock": "Arrêt défil",
"keyboardKeySelect": "Sélectionner",
"keyboardKeySpace": "Espace",
"keyboardKeyMetaMacOs": "Commande",
"keyboardKeyMetaWindows": "Win",
"menuBarMenuLabel": "Menu de la barre de menu",
"currentDateLabel": "Aujourd'hui",
"scrimLabel": "Fond",
"bottomSheetLabel": "Bottom sheet",
"scrimOnTapHint": "Fermer $modalRouteContentName",
"keyboardKeyShift": "Maj",
"expansionTileExpandedHint": "appuyez deux fois pour réduire",
"expansionTileCollapsedHint": "appuyez deux fois pour développer",
"expansionTileExpandedTapHint": "Réduire",
"expansionTileCollapsedTapHint": "Développer pour en savoir plus",
"expandedHint": "Réduit",
"collapsedHint": "Développé",
"menuDismissLabel": "Fermer le menu",
"lookUpButtonLabel": "Recherche visuelle",
"searchWebButtonLabel": "Rechercher sur le Web",
"shareButtonLabel": "Partager…",
"clearButtonTooltip": "Clear text",
"selectedDateLabel": "Selected"
}
| flutter/packages/flutter_localizations/lib/src/l10n/material_fr.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/material_fr.arb",
"repo_id": "flutter",
"token_count": 2347
} | 743 |
{
"scriptCategory": "dense",
"timeOfDayFormat": "h:mm a",
"openAppDrawerTooltip": "បើកម៉ឺនុយរុករក",
"backButtonTooltip": "ថយក្រោយ",
"closeButtonTooltip": "បិទ",
"deleteButtonTooltip": "លុប",
"nextMonthTooltip": "ខែក្រោយ",
"previousMonthTooltip": "ខែមុន",
"nextPageTooltip": "ទំព័របន្ទាប់",
"previousPageTooltip": "ទំព័រមុន",
"firstPageTooltip": "ទំព័រដំបូង",
"lastPageTooltip": "ទំព័រចុងក្រោយ",
"showMenuTooltip": "បង្ហាញម៉ឺនុយ",
"aboutListTileTitle": "អំពី $applicationName",
"licensesPageTitle": "អាជ្ញាបណ្ណ",
"pageRowsInfoTitle": "$firstRow–$lastRow ក្នុងចំណោម $rowCount",
"pageRowsInfoTitleApproximate": "$firstRow–$lastRow ក្នុងចំណោមប្រហែល $rowCount",
"rowsPerPageTitle": "ជួរដេកក្នុងមួយទំព័រ៖",
"tabLabel": "ផ្ទាំង $tabIndex ក្នុងចំណោម $tabCount",
"selectedRowCountTitleOne": "បានជ្រើសរើសធាតុ 1",
"selectedRowCountTitleOther": "បានជ្រើសរើសធាតុ $selectedRowCount",
"cancelButtonLabel": "បោះបង់",
"closeButtonLabel": "បិទ",
"continueButtonLabel": "បន្ត",
"copyButtonLabel": "ចម្លង",
"cutButtonLabel": "កាត់",
"scanTextButtonLabel": "ស្កេនអក្សរ",
"okButtonLabel": "យល់ព្រម",
"pasteButtonLabel": "ដាក់ចូល",
"selectAllButtonLabel": "ជ្រើសរើសទាំងអស់",
"viewLicensesButtonLabel": "មើលអាជ្ញាបណ្ណ",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"timePickerHourModeAnnouncement": "ជ្រើសរើសម៉ោង",
"timePickerMinuteModeAnnouncement": "ជ្រើសរើសនាទី",
"signedInLabel": "បានចូលគណនី",
"hideAccountsLabel": "លាក់គណនី",
"showAccountsLabel": "បង្ហាញគណនី",
"modalBarrierDismissLabel": "ច្រានចោល",
"drawerLabel": "ម៉ឺនុយរុករក",
"popupMenuLabel": "ម៉ឺនុយលោតឡើង",
"dialogLabel": "ប្រអប់",
"alertDialogLabel": "ជូនដំណឹង",
"searchFieldLabel": "ស្វែងរក",
"reorderItemToStart": "ផ្លាស់ទីទៅចំណុចចាប់ផ្ដើម",
"reorderItemToEnd": "ផ្លាស់ទីទៅចំណុចបញ្ចប់",
"reorderItemUp": "ផ្លាស់ទីឡើងលើ",
"reorderItemDown": "ផ្លាស់ទីចុះក្រោម",
"reorderItemLeft": "ផ្លាស់ទីទៅឆ្វេង",
"reorderItemRight": "ផ្លាស់ទីទៅស្តាំ",
"expandedIconTapHint": "បង្រួម",
"collapsedIconTapHint": "ពង្រីក",
"remainingTextFieldCharacterCountOne": "នៅសល់ 1 តួទៀត",
"remainingTextFieldCharacterCountOther": "នៅសល់ $remainingCount តួទៀត",
"refreshIndicatorSemanticLabel": "ផ្ទុកឡើងវិញ",
"moreButtonTooltip": "ច្រើនទៀត",
"dateSeparator": "/",
"dateHelpText": "ថ្ងៃ/ខែ/ឆ្នាំ",
"selectYearSemanticsLabel": "ជ្រើសរើសឆ្នាំ",
"unspecifiedDate": "កាលបរិច្ឆេទ",
"unspecifiedDateRange": "ចន្លោះកាលបរិច្ឆេទ",
"dateInputLabel": "បញ្ចូលកាលបរិច្ឆេទ",
"dateRangeStartLabel": "កាលបរិច្ឆេទចាប់ផ្ដើម",
"dateRangeEndLabel": "កាលបរិច្ឆេទបញ្ចប់",
"dateRangeStartDateSemanticLabel": "កាលបរិច្ឆេទចាប់ផ្ដើម $fullDate",
"dateRangeEndDateSemanticLabel": "កាលបរិច្ឆេទបញ្ចប់ $fullDate",
"invalidDateFormatLabel": "ទម្រង់មិនត្រឹមត្រូវទេ។",
"invalidDateRangeLabel": "ចន្លោះមិនត្រឹមត្រូវទេ។",
"dateOutOfRangeLabel": "ក្រៅចន្លោះ។",
"saveButtonLabel": "រក្សាទុក",
"datePickerHelpText": "ជ្រើសរើសកាលបរិច្ឆេទ",
"dateRangePickerHelpText": "ជ្រើសរើសចន្លោះ",
"calendarModeButtonLabel": "ប្ដូរទៅប្រតិទិន",
"inputDateModeButtonLabel": "ប្ដូរទៅការបញ្ចូល",
"timePickerDialHelpText": "ជ្រើសរើសម៉ោង",
"timePickerInputHelpText": "បញ្ចូលម៉ោង",
"timePickerHourLabel": "ម៉ោង",
"timePickerMinuteLabel": "នាទី",
"invalidTimeLabel": "បញ្ចូលពេលវេលាដែលត្រឹមត្រូវ",
"dialModeButtonLabel": "ប្ដូរទៅមុខងារផ្ទាំងជ្រើសរើសលេខ",
"inputTimeModeButtonLabel": "ប្ដូរទៅមុខងារបញ្ចូលអក្សរ",
"licensesPackageDetailTextZero": "No licenses",
"licensesPackageDetailTextOne": "អាជ្ញាបណ្ណ 1",
"licensesPackageDetailTextOther": "អាជ្ញាបណ្ណ $licenseCount",
"keyboardKeyAlt": "Alt",
"keyboardKeyAltGraph": "AltGr",
"keyboardKeyBackspace": "Backspace",
"keyboardKeyCapsLock": "Caps Lock",
"keyboardKeyChannelDown": "Channel Down",
"keyboardKeyChannelUp": "Channel Up",
"keyboardKeyControl": "Ctrl",
"keyboardKeyDelete": "Del",
"keyboardKeyEject": "Eject",
"keyboardKeyEnd": "End",
"keyboardKeyEscape": "Esc",
"keyboardKeyFn": "Fn",
"keyboardKeyHome": "Home",
"keyboardKeyInsert": "Insert",
"keyboardKeyMeta": "Meta",
"keyboardKeyNumLock": "Num Lock",
"keyboardKeyNumpad1": "Num 1",
"keyboardKeyNumpad2": "Num 2",
"keyboardKeyNumpad3": "Num 3",
"keyboardKeyNumpad4": "Num 4",
"keyboardKeyNumpad5": "Num 5",
"keyboardKeyNumpad6": "Num 6",
"keyboardKeyNumpad7": "Num 7",
"keyboardKeyNumpad8": "Num 8",
"keyboardKeyNumpad9": "Num 9",
"keyboardKeyNumpad0": "Num 0",
"keyboardKeyNumpadAdd": "Num +",
"keyboardKeyNumpadComma": "Num ,",
"keyboardKeyNumpadDecimal": "Num .",
"keyboardKeyNumpadDivide": "Num /",
"keyboardKeyNumpadEnter": "Num Enter",
"keyboardKeyNumpadEqual": "Num =",
"keyboardKeyNumpadMultiply": "Num *",
"keyboardKeyNumpadParenLeft": "Num (",
"keyboardKeyNumpadParenRight": "Num )",
"keyboardKeyNumpadSubtract": "Num -",
"keyboardKeyPageDown": "PgDown",
"keyboardKeyPageUp": "PgUp",
"keyboardKeyPower": "Power",
"keyboardKeyPowerOff": "Power Off",
"keyboardKeyPrintScreen": "Print Screen",
"keyboardKeyScrollLock": "Scroll Lock",
"keyboardKeySelect": "Select",
"keyboardKeySpace": "Space",
"keyboardKeyMetaMacOs": "Command",
"keyboardKeyMetaWindows": "Win",
"menuBarMenuLabel": "ម៉ឺនុយរបារម៉ឺនុយ",
"currentDateLabel": "ថ្ងៃនេះ",
"scrimLabel": "ផ្ទាំងស្រអាប់",
"bottomSheetLabel": "សន្លឹកខាងក្រោម",
"scrimOnTapHint": "បិទ $modalRouteContentName",
"keyboardKeyShift": "Shift",
"expansionTileExpandedHint": "ចុចពីរដង ដើម្បីបង្រួម",
"expansionTileCollapsedHint": "ចុចពីរដង ដើម្បីពង្រីក",
"expansionTileExpandedTapHint": "បង្រួម",
"expansionTileCollapsedTapHint": "ពង្រីកដើម្បីទទួលបានព័ត៌មានលម្អិតបន្ថែម",
"expandedHint": "បានបង្រួម",
"collapsedHint": "បានពង្រីក",
"menuDismissLabel": "ច្រានចោលម៉ឺនុយ",
"lookUpButtonLabel": "រកមើល",
"searchWebButtonLabel": "ស្វែងរកលើបណ្ដាញ",
"shareButtonLabel": "ចែករំលែក...",
"clearButtonTooltip": "Clear text",
"selectedDateLabel": "Selected"
}
| flutter/packages/flutter_localizations/lib/src/l10n/material_km.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/material_km.arb",
"repo_id": "flutter",
"token_count": 5260
} | 744 |
{
"scriptCategory": "English-like",
"timeOfDayFormat": "h:mm a",
"openAppDrawerTooltip": "Fungua menyu ya kusogeza",
"backButtonTooltip": "Rudi Nyuma",
"closeButtonTooltip": "Funga",
"deleteButtonTooltip": "Futa",
"nextMonthTooltip": "Mwezi ujao",
"previousMonthTooltip": "Mwezi uliopita",
"nextPageTooltip": "Ukurasa unaofuata",
"previousPageTooltip": "Ukurasa uliotangulia",
"firstPageTooltip": "Ukurasa wa kwanza",
"lastPageTooltip": "Ukurasa wa mwisho",
"showMenuTooltip": "Onyesha menyu",
"aboutListTileTitle": "Kuhusu $applicationName",
"licensesPageTitle": "Leseni",
"pageRowsInfoTitle": "$firstRow hadi $lastRow kati ya $rowCount",
"pageRowsInfoTitleApproximate": "$firstRow hadi $lastRow kati ya takriban $rowCount",
"rowsPerPageTitle": "Safu mlalo kwa kila ukurasa:",
"tabLabel": "Kichupo cha $tabIndex kati ya $tabCount",
"selectedRowCountTitleZero": "Hamna kilicho chaguliwa",
"selectedRowCountTitleOne": "Umechagua kipengee 1",
"selectedRowCountTitleOther": "Umechagua vipengee $selectedRowCount",
"cancelButtonLabel": "Ghairi",
"closeButtonLabel": "Funga",
"continueButtonLabel": "Endelea",
"copyButtonLabel": "Nakili",
"cutButtonLabel": "Kata",
"scanTextButtonLabel": "Changanua maandishi",
"okButtonLabel": "Sawa",
"pasteButtonLabel": "Bandika",
"selectAllButtonLabel": "Chagua vyote",
"viewLicensesButtonLabel": "Angalia leseni",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"timePickerHourModeAnnouncement": "Chagua saa",
"timePickerMinuteModeAnnouncement": "Chagua dakika",
"modalBarrierDismissLabel": "Ondoa",
"signedInLabel": "Umeingia katika akaunti",
"hideAccountsLabel": "Ficha akaunti",
"showAccountsLabel": "Onyesha akaunti",
"drawerLabel": "Menyu ya kusogeza",
"popupMenuLabel": "Menyu ibukizi",
"dialogLabel": "Kidirisha",
"alertDialogLabel": "Arifa",
"searchFieldLabel": "Tafuta",
"reorderItemToStart": "Sogeza hadi mwanzo",
"reorderItemToEnd": "Sogeza hadi mwisho",
"reorderItemUp": "Sogeza juu",
"reorderItemDown": "Sogeza chini",
"reorderItemLeft": "Sogeza kushoto",
"reorderItemRight": "Sogeza kulia",
"expandedIconTapHint": "Kunja",
"collapsedIconTapHint": "Panua",
"remainingTextFieldCharacterCountZero": "Hapana herufi zilizo baki",
"remainingTextFieldCharacterCountOne": "Imesalia herufi 1",
"remainingTextFieldCharacterCountOther": "Zimesalia herufi $remainingCount",
"refreshIndicatorSemanticLabel": "Onyesha upya",
"moreButtonTooltip": "Zaidi",
"dateSeparator": "/",
"dateHelpText": "dd/mm/yyyy",
"selectYearSemanticsLabel": "Chagua mwaka",
"unspecifiedDate": "Tarehe",
"unspecifiedDateRange": "Kipindi",
"dateInputLabel": "Weka Tarehe",
"dateRangeStartLabel": "Tarehe ya Kuanza",
"dateRangeEndLabel": "Tarehe ya Kumalizika",
"dateRangeStartDateSemanticLabel": "Tarehe ya kuanza $fullDate",
"dateRangeEndDateSemanticLabel": "Tarehe ya kumalizika $fullDate",
"invalidDateFormatLabel": "Muundo si sahihi.",
"invalidDateRangeLabel": "Kipindi si sahihi.",
"dateOutOfRangeLabel": "Umechagua tarehe iliyo nje ya kipindi.",
"saveButtonLabel": "Hifadhi",
"datePickerHelpText": "Chagua tarehe",
"dateRangePickerHelpText": "Chagua kipindi",
"calendarModeButtonLabel": "Badili utumie hali ya kalenda",
"inputDateModeButtonLabel": "Badili utumie hali ya kuweka maandishi",
"timePickerDialHelpText": "Chagua muda",
"timePickerInputHelpText": "Weka muda",
"timePickerHourLabel": "Saa",
"timePickerMinuteLabel": "Dakika",
"invalidTimeLabel": "Weka saa sahihi",
"dialModeButtonLabel": "Badilisha ili utumie hali ya kiteuzi cha kupiga simu",
"inputTimeModeButtonLabel": "Tumia programu ya kuingiza data ya maandishi",
"licensesPackageDetailTextZero": "No licenses",
"licensesPackageDetailTextOne": "Leseni moja",
"licensesPackageDetailTextOther": "Leseni $licenseCount",
"keyboardKeyAlt": "Alt",
"keyboardKeyAltGraph": "AltGr",
"keyboardKeyBackspace": "Backspace",
"keyboardKeyCapsLock": "Caps Lock",
"keyboardKeyChannelDown": "Channel Down",
"keyboardKeyChannelUp": "Channel Up",
"keyboardKeyControl": "Ctrl",
"keyboardKeyDelete": "Del",
"keyboardKeyEject": "Eject",
"keyboardKeyEnd": "End",
"keyboardKeyEscape": "Esc",
"keyboardKeyFn": "Fn",
"keyboardKeyHome": "Home",
"keyboardKeyInsert": "Insert",
"keyboardKeyMeta": "Meta",
"keyboardKeyNumLock": "Num Lock",
"keyboardKeyNumpad1": "Num 1",
"keyboardKeyNumpad2": "Num 2",
"keyboardKeyNumpad3": "Num 3",
"keyboardKeyNumpad4": "Num 4",
"keyboardKeyNumpad5": "Num 5",
"keyboardKeyNumpad6": "Num 6",
"keyboardKeyNumpad7": "Num 7",
"keyboardKeyNumpad8": "Num 8",
"keyboardKeyNumpad9": "Num 9",
"keyboardKeyNumpad0": "Num 0",
"keyboardKeyNumpadAdd": "Num +",
"keyboardKeyNumpadComma": "Num ,",
"keyboardKeyNumpadDecimal": "Num .",
"keyboardKeyNumpadDivide": "Num /",
"keyboardKeyNumpadEnter": "Num Enter",
"keyboardKeyNumpadEqual": "Num =",
"keyboardKeyNumpadMultiply": "Num *",
"keyboardKeyNumpadParenLeft": "Num (",
"keyboardKeyNumpadParenRight": "Num )",
"keyboardKeyNumpadSubtract": "Num -",
"keyboardKeyPageDown": "Pg-down",
"keyboardKeyPageUp": "PgUp",
"keyboardKeyPower": "Power",
"keyboardKeyPowerOff": "Power Off",
"keyboardKeyPrintScreen": "Print Screen",
"keyboardKeyScrollLock": "Scroll Lock",
"keyboardKeySelect": "Select",
"keyboardKeySpace": "Space",
"keyboardKeyMetaMacOs": "Command",
"keyboardKeyMetaWindows": "Win",
"menuBarMenuLabel": "Menyu ya upau wa menyu",
"currentDateLabel": "Leo",
"scrimLabel": "Scrim",
"bottomSheetLabel": "Safu ya Chini",
"scrimOnTapHint": "Funga $modalRouteContentName",
"keyboardKeyShift": "Shift",
"expansionTileExpandedHint": "gusa mara mbili ili ukunje",
"expansionTileCollapsedHint": "gusa mara mbili ili upanue",
"expansionTileExpandedTapHint": "Kunja",
"expansionTileCollapsedTapHint": "Panua ili upate maelezo zaidi",
"expandedHint": "Imekunjwa",
"collapsedHint": "Imepanuliwa",
"menuDismissLabel": "Ondoa menyu",
"lookUpButtonLabel": "Tafuta",
"searchWebButtonLabel": "Tafuta kwenye Wavuti",
"shareButtonLabel": "Shiriki...",
"clearButtonTooltip": "Clear text",
"selectedDateLabel": "Selected"
}
| flutter/packages/flutter_localizations/lib/src/l10n/material_sw.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/material_sw.arb",
"repo_id": "flutter",
"token_count": 2407
} | 745 |
{
"reorderItemToStart": "نقل إلى بداية القائمة",
"reorderItemToEnd": "نقل إلى نهاية القائمة",
"reorderItemUp": "نقل لأعلى",
"reorderItemDown": "نقل لأسفل",
"reorderItemLeft": "نقل لليمين",
"reorderItemRight": "نقل لليسار"
}
| flutter/packages/flutter_localizations/lib/src/l10n/widgets_ar.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/widgets_ar.arb",
"repo_id": "flutter",
"token_count": 156
} | 746 |
{
"reorderItemToStart": "Ilipat sa simula",
"reorderItemToEnd": "Ilipat sa dulo",
"reorderItemUp": "Ilipat pataas",
"reorderItemDown": "Ilipat pababa",
"reorderItemLeft": "Ilipat pakaliwa",
"reorderItemRight": "Ilipat pakanan"
}
| flutter/packages/flutter_localizations/lib/src/l10n/widgets_fil.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/widgets_fil.arb",
"repo_id": "flutter",
"token_count": 103
} | 747 |
{
"reorderItemToStart": "Басына өту",
"reorderItemToEnd": "Соңына өту",
"reorderItemUp": "Жоғарыға жылжыту",
"reorderItemDown": "Төменге жылжыту",
"reorderItemLeft": "Солға жылжыту",
"reorderItemRight": "Оңға жылжыту"
}
| flutter/packages/flutter_localizations/lib/src/l10n/widgets_kk.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/widgets_kk.arb",
"repo_id": "flutter",
"token_count": 162
} | 748 |
{
"reorderItemToStart": "Naar het begin verplaatsen",
"reorderItemToEnd": "Naar het einde verplaatsen",
"reorderItemUp": "Omhoog verplaatsen",
"reorderItemDown": "Omlaag verplaatsen",
"reorderItemLeft": "Naar links verplaatsen",
"reorderItemRight": "Naar rechts verplaatsen"
}
| flutter/packages/flutter_localizations/lib/src/l10n/widgets_nl.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/widgets_nl.arb",
"repo_id": "flutter",
"token_count": 124
} | 749 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.