description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
listlengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
# Do you speak retsec?
You and your friends want to play undercover agents. In order to exchange your *secret* messages, you've come up with the following system: you take the word, cut it in half, and place the first half behind the latter. If the word has an uneven number of characters, you leave the middle at its previous place:

That way, you'll be able to exchange your messages in private.
# Task
You're given a single word. Your task is to swap the halves. If the word has an uneven length, leave the character in the middle at that position and swap the chunks around it.
## Examples
```javascript
reverseByCenter("secret") == "retsec" // no center character
reverseByCenter("agent") == "nteag" // center character is "e"
```
```ruby
reverse_by_center("secret") == "retsec" # no center character
reverse_by_center("agent") == "nteag" # center character is "e"
```
```python
reverse_by_center("secret") == "retsec" # no center character
reverse_by_center("agent") == "nteag" # center character is "e"
```
```haskell
reverseByCenter "secret" `shouldBe` "retsec" -- no center character
reverseByCenter "agent" `shouldBe` "nteag" -- center character 'e'
```
## Remarks
Don't use this to actually exchange messages in private. | reference | def reverse_by_center(s): n = len(s) / / 2; return s[- n:] + s[n: - n] + s[: n]
| Do you speak retsec? | 5516ab668915478845000780 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5516ab668915478845000780 | 7 kyu |
# Task
Write a function that returns `true` if a given point `(x,y)` is inside of a unit circle (that is, a "normal" circle with a radius of one) centered at the origin `(0,0)` and returns `false` if the point is outside.
# Input
* `x`: The first coordinate of the given point.
* `y`: The second coordinate of the given point.
# Notes
* The region bounded by the circle is considered to be an `open disk`, so points on the boundary of the circle should return `false`.
* We are using the euclidean metric.
| reference | def point_in_circle(x, y):
return (x * x + y * y) < 1
| Point in a unit circle | 58da7ae9b340a2440500009c | [
"Mathematics",
"Fundamentals"
]
| https://www.codewars.com/kata/58da7ae9b340a2440500009c | 7 kyu |
In your class, you have started lessons about "arithmetic progression". Because you are also a programmer, you have decided to write a function.
This function, arithmetic_sequence_sum(a, r, n), should return the sum of the first (n) elements of a sequence in which each element is the sum of the given integer (a), and a number of occurences of the given integer (r), based on the element's position within the sequence.
For example:
arithmetic_sequence_sum(2, 3, 5) should return 40:
```
1 2 3 4 5
a + (a+r) + (a+r+r) + (a+r+r+r) + (a+r+r+r+r)
2 + (2+3) + (2+3+3) + (2+3+3+3) + (2+3+3+3+3) = 40
```
| reference | def arithmetic_sequence_sum(a, r, n):
return n * (a + a + (n - 1) * r) / 2
| Arithmetic sequence - sum of n elements | 55cb0597e12e896ab6000099 | [
"Fundamentals"
]
| https://www.codewars.com/kata/55cb0597e12e896ab6000099 | 7 kyu |
Create a rigged dice function that 22% of the time returns the number 6. The rest of the time it returns the integers 1,2,3,4,5 uniformly.
---
About the test case
There will only be one test case which calls the throw_rigged function 100k times and checks that 6 is returned in the range of 21700-22300 (inclusive) times. The test does not check that 1-5 is returned uniformly or randomly, but it does check that your function returns integers in the range 1-6 (inclusive).
The test works roughly 98% of the time, so you might want to run it twice if you are confident your solution is correct.
```if:javascript
In JS version, test for:
- return value should between 1-6;
- return value should be randomly;
- run your code 100000 times should produce 21700-22300 numbers 6
```
Good Luck!
| refactoring | import random
def throw_rigged():
return 6 if random . random() <= .22 else random . randrange(1, 6)
| Rigged Dice | 573acc8cffc3d13f61000533 | [
"Probability",
"Refactoring"
]
| https://www.codewars.com/kata/573acc8cffc3d13f61000533 | 7 kyu |
You found a magic dice which has infinitely many faces showing all the numbers 1,2,3,4,5,....
By rolling the magic dice infinitely many times, you find out that the probability to get the number n is 1/2^n.
You meet your friend who tells you that he has found another magic dice
with infinitely many faces 0,1,2,3,4,5 .. But he does not know the probabilities for finding a certain number.
Your friend wants to test his new dice and suggests to play a simple game: Each player rolls his dice three times. Each player takes
the maximal value of his three results. The player with the larger number wins.
Your friend rolls his dice three times.
You are clever and you know that you can, in principle, calculate
the probability to win the game. But how?
INPUT: an integer number n>=0
OUTPUT: two integers a and b, the numerator and denominator of the probability written as a/b (with the smallest possible b) that you will win the game with your three attempts
In Python output as tuple:
```python
return (a, b)
```
-------------------
EXAMPLE:
For n=1 the output should be 7 and 8. The probability to roll three times 1 is (1/2)^3=1/8. Any other combination wins, and the result is
a/b=1-1/8=7/8.
| games | def magicdice(n):
return ((2 * * n) * * 3 - (2 * * n - 1) * * 3, (2 * * n) * * 3)
| Magic Dice: Who wins? | 589e2af835999cbe2f000229 | [
"Puzzles"
]
| https://www.codewars.com/kata/589e2af835999cbe2f000229 | 6 kyu |
Given an integer, return a string with dash `'-'` marks before and after each odd digit, but do not begin or end the string with a dash mark.
Ex:
```javascript
274 -> '2-7-4'
6815 -> '68-1-5'
``` | reference | import re
def dashatize(num):
try:
return '' . join(['-' + i + '-' if int(i) % 2 else i for i in str(abs(num))]). replace('--', '-'). strip('-')
except:
return 'None'
| Dashatize it | 58223370aef9fc03fd000071 | [
"Strings",
"Arrays",
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/58223370aef9fc03fd000071 | 6 kyu |
Given a string, swap the case for each of the letters.
### Examples
```
"" --> ""
"CodeWars" --> "cODEwARS"
"abc" --> "ABC"
"ABC" --> "abc"
"123235" --> "123235"
``` | reference | def swap(string_):
return string_ . swapcase()
| Case Swapping | 5590961e6620c0825000008f | [
"Fundamentals"
]
| https://www.codewars.com/kata/5590961e6620c0825000008f | 7 kyu |
*Are you a file extension master? Let's find out by checking if Bill's files are images or audio files. Please use regex if available natively for your language.*
You will create 2 string methods:
- **isAudio/is_audio**, matching 1 or + uppercase/lowercase letter(s) (combination possible), with the extension .mp3, .flac, .alac, or .aac.
- **isImage/is_image**, matching 1 or + uppercase/lowercase letter(s) (combination possible), with the extension .jpg, .jpeg, .png, .bmp, or .gif.
*Note that this is not a generic image/audio files checker. It's meant to be a test for Bill's files only. Bill doesn't like punctuation. He doesn't like numbers, neither. Thus, his filenames are letter-only*
**Rules**
1. It should return true or false, simply.
2. File extensions should consist of lowercase letters and numbers only.
3. File names should consist of letters only (uppercase, lowercase, or both)
Good luck! | reference | def is_audio(filename):
name, ext = filename . split('.')
return name . isalpha() and ext in {'mp3', 'flac', 'alac', 'aac'}
def is_img(filename):
name, ext = filename . split('.')
return name . isalpha() and ext in {'jpg', 'jpeg', 'png', 'bmp', 'gif'}
| Master of Files | 574bd867d277832448000adf | [
"Strings",
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/574bd867d277832448000adf | 6 kyu |
Each floating-point number should be formatted that only the first two decimal places are returned. You don't need to check whether the input is a valid number because only valid numbers are used in the tests.
Don't round the numbers! Just cut them after two decimal places!
```
Right examples:
32.8493 is 32.84
14.3286 is 14.32
Incorrect examples (e.g. if you round the numbers):
32.8493 is 32.85
14.3286 is 14.33
``` | reference | def two_decimal_places(number):
return int(number * 100) / 100.0
| Formatting decimal places #1 | 5641c3f809bf31f008000042 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5641c3f809bf31f008000042 | 7 kyu |
## Task
Remove all exclamation marks from the end of words. Words are separated by a single space. There are no exclamation marks within a word.
### Examples
```
remove("Hi!") === "Hi"
remove("Hi!!!") === "Hi"
remove("!Hi") === "!Hi"
remove("!Hi!") === "!Hi"
remove("Hi! Hi!") === "Hi Hi"
remove("!!!Hi !!hi!!! !hi") === "!!!Hi !!hi !hi"
``` | reference | def remove(s):
return ' ' . join(w . rstrip('!') or w for w in s . split())
| Exclamation marks series #5: Remove all exclamation marks from the end of words | 57faf32df815ebd49e000117 | [
"Strings",
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/57faf32df815ebd49e000117 | 7 kyu |
Complete the method which accepts an array of integers, and returns one of the following:
* `"yes, ascending"` - if the numbers in the array are sorted in an ascending order
* `"yes, descending"` - if the numbers in the array are sorted in a descending order
* `"no"` - otherwise
You can assume the array will always be valid, and there will always be one correct answer. | reference | def is_sorted_and_how(arr):
if arr == sorted(arr):
return 'yes, ascending'
elif arr == sorted(arr)[:: - 1]:
return 'yes, descending'
else:
return 'no'
| Sorted? yes? no? how? | 580a4734d6df748060000045 | [
"Arrays",
"Sorting",
"Fundamentals"
]
| https://www.codewars.com/kata/580a4734d6df748060000045 | 7 kyu |
Your friend Rick is trying to send you a message, but he is concerned that it would get intercepted by his partner. He came up with a solution:
1) Add digits in random places within the message.
2) Split the resulting message in two. He wrote down every second character on one page, and the remaining ones on another. He then dispatched the two messages separately.
Write a function interweave(s1, s2) that reverses this operation to decode his message!
Example 1: interweave("hlo", "el") -> "hello"
Example 2: interweave("h3lo", "el4") -> "hello"
Rick's a bit peculiar about his formats. He would feel ashamed if he found out his message led to extra white spaces hanging around the edges of his message... | reference | def interweave(s1, s2):
s = [''] * (len(s1) + len(s2))
s[:: 2], s[1:: 2] = s1, s2
return '' . join(c for c in s if not c . isdigit()). strip()
| Interweaving strings and removing digits | 588a7d45019c42be61000009 | [
"Fundamentals"
]
| https://www.codewars.com/kata/588a7d45019c42be61000009 | 7 kyu |
<div style="border:1px solid; padding:1ex;">Inspired by <a href="/kata/55d1d6d5955ec6365400006d">Round to the next 5</a>.
<strong>Warning!</strong> This kata contains spoilers on the mentioned one. Solve that one first!</div>
# The Coins of Ter
Ter is a small country, located between Brelnam and the Orange juice ocean. It uses many different coins and bills for payment. However, one day, the leaders of Ter decide that there are too many small coins. Therefore, they ban the small coins. But no one knows _which_ coins they'll ban, so they ask you to provide a tool that can recalculate a price. After all, if one does not have a 1 Terrek bill and can only give a 2 Terrek bill, one needs to adjust the oddly priced items.
# Task
Write a function `adjust`, that takes a two integers: the lowest currency unit that's still allowed, and the price/debt that needs to get adjusted. All prices are going up, and debts are remitted. The lowest currency will always be positive.
In other words:`adjust` takes two integers, `b` and `n`, and returns the smallest number `k`, such that `n <= k` and `k % b == 0 `.
# Examples
```haskell
adjust 3 0 `shouldBe` 0
adjust 3 1 `shouldBe` 3
adjust 3 (-2) `shouldBe` 0
adjust 3 (-4) `shouldBe` (-3)
adjust 3 3 `shouldBe` 3
adjust 6 3 `shouldBe` 6
adjust 7 3 `shouldBe` 9
```
```javascript
adjust( 3, 0 ) === 0
adjust( 3, 1 ) === 3
adjust( 3, -2) === 0
adjust( 3, -4) === -3
adjust( 3, 3 ) === 3
adjust( 3, 6 ) === 6
adjust( 3, 7 ) === 9
```
```coffeescript
adjust( 3, 0 ) === 0
adjust( 3, 1 ) === 3
adjust( 3, -2) === 0
adjust( 3, -4) === -3
adjust( 3, 3 ) === 3
adjust( 3, 6 ) === 6
adjust( 3, 7 ) === 9
```
```python
adjust( 3, 0 ) == 0
adjust( 3, 1 ) == 3
adjust( 3, -2) == 0
adjust( 3, -4) == -3
adjust( 3, 3 ) == 3
adjust( 3, 6 ) == 6
adjust( 3, 7 ) == 9
```
```ruby
adjust( 3, 0 ) == 0
adjust( 3, 1 ) == 3
adjust( 3, -2) == 0
adjust( 3, -4) == -3
adjust( 3, 3 ) == 3
adjust( 3, 6 ) == 6
adjust( 3, 7 ) == 9
```
<div style="border:1px solid; padding:1ex;">
<strong>Translator notice:</strong> Make sure that you provide about the same random tests, so that a user can get feedback during "Run tests", and not only during "Submit".</div> | games | def adjust(coin, price):
return price + (coin - price) % coin
| The Coins of Ter | Round to the Next N | 55d38b959f9c33f3fb00007d | [
"Puzzles"
]
| https://www.codewars.com/kata/55d38b959f9c33f3fb00007d | 7 kyu |
Given an integer as input, can you round it to the next (meaning, "greater than or equal") multiple of 5?
Examples:
input: output:
0 -> 0
2 -> 5
3 -> 5
12 -> 15
21 -> 25
30 -> 30
-2 -> 0
-5 -> -5
etc.
Input may be any positive or negative integer (including 0).
You can assume that all inputs are valid integers.
| reference | def round_to_next5(n):
return n + (5 - n) % 5
| Round up to the next multiple of 5 | 55d1d6d5955ec6365400006d | [
"Fundamentals"
]
| https://www.codewars.com/kata/55d1d6d5955ec6365400006d | 7 kyu |
Given a credit card number we can determine who the issuer/vendor is with a few basic knowns.
```if:python
Complete the function `get_issuer()` that will use the values shown below to determine the card issuer for a given card number. If the number cannot be matched then the function should return the string `Unknown`.
```
```if-not:python
Complete the function `getIssuer()` that will use the values shown below to determine the card issuer for a given card number. If the number cannot be matched then the function should return the string `Unknown`.
```
```if:typescript
Where `Issuer` is defined with the following enum type.
~~~typescript
enum Issuer {
VISA = 'VISA',
AMEX = 'AMEX',
Mastercard = 'Mastercard',
Discover = 'Discover',
Unknown = 'Unknown',
}
~~~
```
```markdown
| Card Type | Begins With | Number Length |
|------------|----------------------|---------------|
| AMEX | 34 or 37 | 15 |
| Discover | 6011 | 16 |
| Mastercard | 51, 52, 53, 54 or 55 | 16 |
| VISA | 4 | 13 or 16 |
```
```if:c,cpp
**C/C++ note:** The return value in C is not freed.
```
## Examples
```if-not:python
~~~js
getIssuer(4111111111111111) == "VISA"
getIssuer(4111111111111) == "VISA"
getIssuer(4012888888881881) == "VISA"
getIssuer(378282246310005) == "AMEX"
getIssuer(6011111111111117) == "Discover"
getIssuer(5105105105105100) == "Mastercard"
getIssuer(5105105105105106) == "Mastercard"
getIssuer(9111111111111111) == "Unknown"
~~~
```
```if:python
~~~py
get_issuer(4111111111111111) == "VISA"
get_issuer(4111111111111) == "VISA"
get_issuer(4012888888881881) == "VISA"
get_issuer(378282246310005) == "AMEX"
get_issuer(6011111111111117) == "Discover"
get_issuer(5105105105105100) == "Mastercard"
get_issuer(5105105105105106) == "Mastercard"
get_issuer(9111111111111111) == "Unknown"
~~~
``` | algorithms | def get_issuer(number):
s = str(number)
return ("AMEX" if len(s) == 15 and s[: 2] in ("34", "37") else
"Discover" if len(s) == 16 and s . startswith("6011") else
"Mastercard" if len(s) == 16 and s[0] == "5" and s[1] in "12345" else
"VISA" if len(s) in [13, 16] and s[0] == '4' else
"Unknown")
| Credit card issuer checking | 5701e43f86306a615c001868 | [
"Algorithms"
]
| https://www.codewars.com/kata/5701e43f86306a615c001868 | 7 kyu |
# Valid HK Phone Number
## Overview
In Hong Kong, a valid phone number has the format ```xxxx xxxx``` where ```x``` is a decimal digit (0-9). For example:
```javascript
"1234 5678" // is valid
"2359 1478" // is valid
"85748475" // invalid, as there are no spaces separating the first 4 and last 4 digits
"3857 4756" // invalid; there should be exactly 1 whitespace separating the first 4 and last 4 digits respectively
"sklfjsdklfjsf" // clearly invalid
" 1234 5678 " // is NOT a valid phone number but CONTAINS a valid phone number
"skldfjs287389274329dklfj84239029043820942904823480924293042904820482409209438dslfdjs9345 8234sdklfjsdkfjskl28394723987jsfss2343242kldjf23423423SDLKFJSLKsdklf" // also contains a valid HK phone number (9345 8234)
```
## Task
Define two functions, ```isValidHKPhoneNumber``` and ```hasValidHKPhoneNumber```, that ```return```s whether a given string is a valid HK phone number and contains a valid HK phone number respectively (i.e. ```true/false``` values).
If in doubt please refer to the example tests. | reference | import re
HK_PHONE_NUMBER = '\d{4} \d{4}'
def is_valid_HK_phone_number(number):
return bool(re . match(HK_PHONE_NUMBER + '\Z', number))
def has_valid_HK_phone_number(number):
return bool(re . search(HK_PHONE_NUMBER, number))
| Valid HK Phone Number | 56f54d45af5b1fec4b000cce | [
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/56f54d45af5b1fec4b000cce | 7 kyu |
Write a function that takes one or more arrays and returns a new array of unique values in the order of the original provided arrays.
In other words, all values present from all arrays should be included in their original order, but with no duplicates in the final array.
The unique numbers should be sorted by their original order, but the final array should not be sorted in numerical order.
Check the assertion tests for examples.
*Courtesy of [FreeCodeCamp](https://www.freecodecamp.com/challenges/sorted-union), a great place to learn web-dev; plus, its founder Quincy Larson is pretty cool and amicable. I made the original one slightly more tricky ;)* | algorithms | def unite_unique(* arg):
res = []
for arr in arg:
for val in arr:
if not val in res:
res . append(val)
return res
| Sorted Union | 5729c30961cecadc4f001878 | [
"Arrays",
"Lists",
"Algorithms",
"Sorting"
]
| https://www.codewars.com/kata/5729c30961cecadc4f001878 | 7 kyu |
Your job is to build a function which determines whether or not there are double characters in a string (including whitespace characters). For example ```aa```, ``!!`` or ``` ```.
You want the function to return true if the string contains double characters and false if not. The test should not be case sensitive; for example both ```aa``` & ```aA``` return true.
Examples:
```python
double_check("abca")
#returns False
double_check("aabc")
#returns True
double_check("a 11 c d")
#returns True
double_check("AabBcC")
#returns True
double_check("a b c")
#returns True
double_check("a b c d e f g h i h k")
#returns False
double_check("2020")
#returns False
double_check("a!@€£#$%^&*()_-+=}]{[|\"':;?/>.<,~")
#returns False
```
```ruby
double_check("abca")
#returns false
double_check("aabc")
#returns true
double_check("a 11 c d")
#returns true
double_check("AabBcC")
#returns true
double_check("a b c")
#returns true
double_check("a b c d e f g h i h k")
#returns false
double_check("2020")
#returns false
double_check("a!@#$%^&*()_-+=}]{[|\"':;?/>.<,~")
#returns false
```
```haskell
doubleCheck "abca"
--returns False
doubleCheck "aabc"
--returns True
doubleCheck "a 11 c d"
--returns True
doubleCheck "AabBcC"
--returns True
doubleCheck "a b c"
--returns True
doubleCheck "a b c d e f g h i h k"
--returns False
doubleCheck "2020"
--returns False
doubleCheck "a!@€£#$%^&*()_-+=}]{[|\"':;?/>.<,~"
--returns False
```
```coffeescript
doubleCheck "abca"
#returns false
doubleCheck "aabc"
#returns true
doubleCheck "a 11 c d"
#returns true
doubleCheck "AabBcC"
#returns true
doubleCheck "a b c"
#returns true
doubleCheck "a b c d e f g h i h k"
#returns false
doubleCheck "2020"
#returns false
doubleCheck "a!@€£#$%^&*()_-+=}]{[|\"':;?/>.<,~"
#returns false
```
```javascript
doubleCheck("abca")
//returns false
doubleCheck("aabc")
//returns true
doubleCheck("a 11 c d")
//returns true
doubleCheck("AabBcC")
//returns true
doubleCheck("a b c")
//returns true
doubleCheck("a b c d e f g h i h k")
//returns false
doubleCheck("2020")
//returns false
doubleCheck("a!@€£#$%^&*()_-+=}]{[|\"':;?/>.<,~")
//returns false
```
| reference | import re
def double_check(str):
return bool(re . search(r"(.)\1", str . lower()))
| Are there doubles? | 56a24b4d9f3671584d000039 | [
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/56a24b4d9f3671584d000039 | 7 kyu |
No Story
No Description
Only by Thinking and Testing
Look at result of testcase, guess the code!
# #Series:<br>
<a href="http://www.codewars.com/kata/56d904db9963e9cf5000037d">01:A and B?</a><br>
<a href="http://www.codewars.com/kata/56d9292cc11bcc3629000533">02:Incomplete string</a><br>
<a href="http://www.codewars.com/kata/56d931ecc443d475d5000003">03:True or False</a><br>
<a href="http://www.codewars.com/kata/56d93f249c844788bc000002">04:Something capitalized</a><br>
<a href="http://www.codewars.com/kata/56d949281b5fdc7666000004">05:Uniq or not Uniq</a> <br>
<a href="http://www.codewars.com/kata/56d98b555492513acf00077d">06:Spatiotemporal index</a><br>
<a href="http://www.codewars.com/kata/56d9b46113f38864b8000c5a">07:Math of Primary School</a><br>
<a href="http://www.codewars.com/kata/56d9c274c550b4a5c2000d92">08:Math of Middle school</a><br>
<a href="http://www.codewars.com/kata/56d9cfd3f3928b4edd000021">09:From nothingness To nothingness</a><br>
<a href="http://www.codewars.com/kata/56dae2913cb6f5d428000f77">10:Not perfect? Throw away!</a> <br>
<a href="http://www.codewars.com/kata/56db19703cb6f5ec3e001393">11:Welcome to take the bus</a><br>
<a href="http://www.codewars.com/kata/56dc41173e5dd65179001167">12:A happy day will come</a><br>
<a href="http://www.codewars.com/kata/56dc5a773e5dd6dcf7001356">13:Sum of 15(Hetu Luosliu)</a><br>
<a href="http://www.codewars.com/kata/56dd3dd94c9055a413000b22">14:Nebula or Vortex</a><br>
<a href="http://www.codewars.com/kata/56dd927e4c9055f8470013a5">15:Sport Star</a><br>
<a href="http://www.codewars.com/kata/56de38c1c54a9248dd0006e4">16:Falsetto Rap Concert</a><br>
<a href="http://www.codewars.com/kata/56de4d58301c1156170008ff">17:Wind whispers</a><br>
<a href="http://www.codewars.com/kata/56de82fb9905a1c3e6000b52">18:Mobile phone simulator</a><br>
<a href="http://www.codewars.com/kata/56dfce76b832927775000027">19:Join but not join</a><br>
<a href="http://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7">20:I hate big and small</a><br>
<a href="http://www.codewars.com/kata/56e0e065ef93568edb000731">21:I want to become diabetic ;-)</a><br>
<a href="http://www.codewars.com/kata/56e0f1dc09eb083b07000028">22:How many blocks?</a><br>
<a href="http://www.codewars.com/kata/56e1161fef93568228000aad">23:Operator hidden in a string</a><br>
<a href="http://www.codewars.com/kata/56e127d4ef93568228000be2">24:Substring Magic</a><br>
<a href="http://www.codewars.com/kata/56eccc08b9d9274c300019b9">25:Report about something</a><br>
<a href="http://www.codewars.com/kata/56ee0448588cbb60740013b9">26:Retention and discard I</a><br>
<a href="http://www.codewars.com/kata/56eee006ff32e1b5b0000c32">27:Retention and discard II</a><br>
<a href="http://www.codewars.com/kata/56eff1e64794404a720002d2">28:How many "word"?</a><br>
<a href="http://www.codewars.com/kata/56f167455b913928a8000c49">29:Hail and Waterfall</a><br>
<a href="http://www.codewars.com/kata/56f214580cd8bc66a5001a0f">30:Love Forever</a><br>
<a href="http://www.codewars.com/kata/56f25b17e40b7014170002bd">31:Digital swimming pool</a><br>
<a href="http://www.codewars.com/kata/56f4202199b3861b880013e0">32:Archery contest</a><br>
<a href="http://www.codewars.com/kata/56f606236b88de2103000267">33:The repair of parchment</a><br>
<a href="http://www.codewars.com/kata/56f6b4369400f51c8e000d64">34:Who are you?</a><br>
<a href="http://www.codewars.com/kata/56f7eb14f749ba513b0009c3">35:Safe position</a><br>
<br>
# #Special recommendation
Another series, innovative and interesting, medium difficulty. People who like to challenge, can try these kata:
<a href="http://www.codewars.com/kata/56c85eebfd8fc02551000281">Play Tetris : Shape anastomosis</a><br>
<a href="http://www.codewars.com/kata/56cd5d09aa4ac772e3000323">Play FlappyBird : Advance Bravely</a><br>
| games | def testit(act, s):
dic = {("run", "_"): "_", ("run", "|"): "/",
("jump", "|"): "|", ("jump", "_"): "x"}
return "" . join([dic[act[i], s[i]] for i in range(len(s))])
| Thinking & Testing : Sport Star | 56dd927e4c9055f8470013a5 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56dd927e4c9055f8470013a5 | 7 kyu |
No Story
No Description
Only by Thinking and Testing
Look at result of testcase, guess the code!
## Series
01. [A and B?](http://www.codewars.com/kata/56d904db9963e9cf5000037d)
02. [Incomplete string](http://www.codewars.com/kata/56d9292cc11bcc3629000533)
03. [True or False](http://www.codewars.com/kata/56d931ecc443d475d5000003)
04. [Something capitalized](http://www.codewars.com/kata/56d93f249c844788bc000002)
05. [Uniq or not Uniq](http://www.codewars.com/kata/56d949281b5fdc7666000004)
06. [Spatiotemporal index](http://www.codewars.com/kata/56d98b555492513acf00077d)
07. [Math of Primary School](http://www.codewars.com/kata/56d9b46113f38864b8000c5a)
08. [Math of Middle school](http://www.codewars.com/kata/56d9c274c550b4a5c2000d92)
09. [From nothingness To nothingness](http://www.codewars.com/kata/56d9cfd3f3928b4edd000021)
10. [Not perfect? Throw away!](http://www.codewars.com/kata/56dae2913cb6f5d428000f77)
11. [Welcome to take the bus](http://www.codewars.com/kata/56db19703cb6f5ec3e001393)
12. [A happy day will come](http://www.codewars.com/kata/56dc41173e5dd65179001167)
13. [Sum of 15(Hetu Luosliu)](http://www.codewars.com/kata/56dc5a773e5dd6dcf7001356)
14. [Nebula or Vortex](http://www.codewars.com/kata/56dd3dd94c9055a413000b22)
15. [Sport Star](http://www.codewars.com/kata/56dd927e4c9055f8470013a5)
16. [Falsetto Rap Concert](http://www.codewars.com/kata/56de38c1c54a9248dd0006e4)
17. [Wind whispers](http://www.codewars.com/kata/56de4d58301c1156170008ff)
18. [Mobile phone simulator](http://www.codewars.com/kata/56de82fb9905a1c3e6000b52)
19. [Join but not join](http://www.codewars.com/kata/56dfce76b832927775000027)
20. [I hate big and small](http://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7)
21. [I want to become diabetic ;-)](http://www.codewars.com/kata/56e0e065ef93568edb000731)
22. [How many blocks?](http://www.codewars.com/kata/56e0f1dc09eb083b07000028)
23. [Operator hidden in a string](http://www.codewars.com/kata/56e1161fef93568228000aad)
24. [Substring Magic](http://www.codewars.com/kata/56e127d4ef93568228000be2)
25. [Report about something](http://www.codewars.com/kata/56eccc08b9d9274c300019b9)
26. [Retention and discard I](http://www.codewars.com/kata/56ee0448588cbb60740013b9)
27. [Retention and discard II](http://www.codewars.com/kata/56eee006ff32e1b5b0000c32)
28. [How many "word"?](http://www.codewars.com/kata/56eff1e64794404a720002d2)
29. [Hail and Waterfall](http://www.codewars.com/kata/56f167455b913928a8000c49)
30. [Love Forever](http://www.codewars.com/kata/56f214580cd8bc66a5001a0f)
31. [Digital swimming pool](http://www.codewars.com/kata/56f25b17e40b7014170002bd)
32. [Archery contest](http://www.codewars.com/kata/56f4202199b3861b880013e0)
33. [The repair of parchment](http://www.codewars.com/kata/56f606236b88de2103000267)
34. [Who are you?](http://www.codewars.com/kata/56f6b4369400f51c8e000d64)
35. [Safe position](http://www.codewars.com/kata/56f7eb14f749ba513b0009c3)
## Special recommendation
Another series, innovative and interesting, medium difficulty. People who like to challenge, can try these kata:
* [Play Tetris : Shape anastomosis](http://www.codewars.com/kata/56c85eebfd8fc02551000281)
* [Play FlappyBird : Advance Bravely](http://www.codewars.com/kata/56cd5d09aa4ac772e3000323)
| games | def testit(a, b):
return sorted(list(set(a)) + list(set(b)))
| Thinking & Testing : Uniq or not Uniq | 56d949281b5fdc7666000004 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56d949281b5fdc7666000004 | 7 kyu |
Implement `String#ipv4_address?`, which should return true if given object is an IPv4 address - four numbers (0-255) separated by dots.
It should only accept addresses in canonical representation, so no leading `0`s, spaces etc. | reference | from re import compile, match
REGEX = compile(r'((\d|[1-9]\d|1\d\d|2[0-4]\d|25[0-5])\.){4}$')
def ipv4_address(address):
# refactored thanks to @leonoverweel on CodeWars
return bool(match(REGEX, address + '.'))
| Regexp Basics - is it IPv4 address? | 567fe8b50c201947bc000056 | [
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/567fe8b50c201947bc000056 | 6 kyu |
For every positive integer N, there exists a unique sequence starting with 1 and ending with N and such that every number in the sequence is either the double of the preceeding number or the double plus 1.
For example, given N = 13, the sequence is [1, 3, 6, 13], because . . . :
```
3 = 2*1 +1
6 = 2*3
13 = 2*6 +1
```
Write a function that returns this sequence given a number N. Try generating the elements of the resulting list in ascending order, i.e., without resorting to a list reversal or prependig the elements to a list. | reference | # If we write the decision tree for the problem we can see
# that we can traverse upwards from any node simply by
# dividing by two and taking the floor. This would require
# a reversal of the list generated since we're building it
# backwards.
#
# If we examine the successor function (x -> {2x, 2x + 1})
# we can deduce that the binary representation of any number
# gives us the steps to follow to generate it: if the n-th (LTR)
# bit is set, we use 2x + 1 for the next element, otherwise we
# choose 2x. This allows us to build the sequence in order.
def climb(n):
res = []
cur = 1
mask = 1 << max(0, n . bit_length() - 2)
while cur <= n:
res . append(cur)
cur = 2 * cur + (1 if (n & mask) != 0 else 0)
mask >>= 1
return res
| Number climber | 559760bae64c31556c00006b | [
"Fundamentals"
]
| https://www.codewars.com/kata/559760bae64c31556c00006b | 7 kyu |
# Task
Let's consider a table consisting of `n` rows and `n` columns. The cell located at the intersection of the i-th row and the j-th column contains number i × j. The rows and columns are numbered starting from 1.
You are given a positive integer `x`. Your task is to count the number of cells in a table that contain number `x`.
# Example
For `n = 5 and x = 5`, the result should be `2`.
The table looks like:
```
1 2 3 4 (5)
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
(5) 10 15 20 25```
There are two number `5` in it.
For `n = 10 and x = 5`, the result should be 2.
For `n = 6 and x = 12`, the result should be 4.
```
1 2 3 4 5 6
2 4 6 8 10 (12)
3 6 9 (12) 15 18
4 8 (12) 16 20 24
5 10 15 20 25 30
6 (12) 18 24 30 36
```
# Input/Output
- `[input]` integer `n`
`1 ≤ n ≤ 10^5.`
- `[input]` integer `x`
`1 ≤ x ≤ 10^9.`
- `[output]` an integer
The number of times `x` occurs in the table. | algorithms | def count_number(n, x):
return len([j for j in range(1, n + 1) if x % j == 0 and x / j <= n])
| Simple Fun #172: Count Number | 58b635903e78b34958000056 | [
"Algorithms"
]
| https://www.codewars.com/kata/58b635903e78b34958000056 | 7 kyu |
Welcome.
In this kata you are required to, given a string, replace every letter with its position in the alphabet.
If anything in the text isn't a letter, ignore it and don't return it.
`"a" = 1`, `"b" = 2`, etc.
## Example <!-- unlisted languages will use the first entry. please keep python up top. -->
```python
alphabet_position("The sunset sets at twelve o' clock.")
```
```javascript
alphabetPosition("The sunset sets at twelve o' clock.")
```
```scala
alphabetPosition("The sunset sets at twelve o' clock.")
```
```haskell
alphabetPosition "The sunset sets at twelve o' clock."
```
```cobol
AlphabetPosition("The sunset sets at twelve o' clock.")
```
```groovy
Kata.alphabetPosition("The sunset sets at twelve o' clock.")
```
```crystal
alphabet_position("The sunset sets at twelve o' clock.")
```
```julia
alphabetposition("The sunset sets at twelve o' clock.")
```
```coffeescript
alphabetPosition("The sunset sets at twelve o' clock.")
```
```crystal
alphabet_position("The sunset sets at twelve o' clock.")
```
```coffeescript
alphabetPosition("The sunset sets at twelve o' clock.")
```
```julia
alphabetposition("The sunset sets at twelve o' clock.")
```
```coffeescript
alphabetPosition("The sunset sets at twelve o' clock.")
```
```csharp
Kata.AlphabetPosition("The sunset sets at twelve o' clock.")
```
```nasm
text: db "The sunset sets at twelve o' clock.",0h0
main:
mov rdi, text
call alphabet_position
```
Should return `"20 8 5 19 21 14 19 5 20 19 5 20 19 1 20 20 23 5 12 22 5 15 3 12 15 3 11"` ( as a string )
| reference | def alphabet_position(text):
return ' ' . join(str(ord(c) - 96) for c in text . lower() if c . isalpha())
| Replace With Alphabet Position | 546f922b54af40e1e90001da | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/546f922b54af40e1e90001da | 6 kyu |
Write a function that returns the number of occurrences of an element in an array.
~~~if:javascript
This function will be defined as a property of Array with the help of the method `Object.defineProperty`, which allows to define a new method **directly** on the object (more info about that you can find on [MDN](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/defineProperty)).
~~~
### Examples
```javascript
var arr = [0, 1, 2, 2, 3];
arr.numberOfOccurrences(0) === 1;
arr.numberOfOccurrences(4) === 0;
arr.numberOfOccurrences(2) === 2;
arr.numberOfOccurrences(3) === 1;
```
```haskell
let sample = [0, 1, 2, 2, 3]
numberOfOccurrences 0 sample `shouldBe` 1
numberOfOccurrences 4 sample `shouldBe` 0
numberOfOccurrences 2 sample `shouldBe` 2
```
```python
sample = [0, 1, 2, 2, 3]
number_of_occurrences(0, sample) == 1
number_of_occurrences(4, sample) == 0
number_of_occurrences(2, sample) == 2
number_of_occurrences(3, sample) == 1
```
```csharp
var sample = { 1, 0, 2, 2, 3 };
NumberOfOccurrences(0, sample) == 1;
NumberOfOccurrences(4, sample) == 0;
NumberOfOccurrences(2, sample) == 2;
NumberOfOccurrences(3, sample) == 1;
```
```cfml
var sample = [ 1, 0, 2, 2, 3 ]
numberOfOccurences(0, sample) == 1
numberOfOccurences(4, sample) == 0
numberOfOccurences(2, sample) == 2
numberOfOccurences(3, sample) == 1
```
```prolog
sample = [ 1, 0, 2, 2, 3 ].
number_of_occurrences(0, sample, 1).
number_of_occurrences(4, sample, 0).
number_of_occurrences(2, sample, 2).
number_of_occurrences(3, sample, 1).
``` | reference | def number_of_occurrences(s, xs):
return xs . count(s)
| Number Of Occurrences | 52829c5fe08baf7edc00122b | [
"Arrays",
"Fundamentals"
]
| https://www.codewars.com/kata/52829c5fe08baf7edc00122b | 7 kyu |
# Task
We define the weakness of number `n` as the number of positive integers smaller than `n` that have more divisors than `n`. It follows that the weaker the number, the greater overall weakness it has.
For the given integer `n`, you need to answer two questions:
* what is the weakness of the weakest numbers in the range `[1, n]`?
* how many numbers in the range [1, n] have this weakness?
Return the answer as a tuple ( where applicable: array ) of two elements, where the first element is the answer to the first question, and the second element is the answer to the second question.
# Example
For `n = 9`, the output should be `[2, 2]`
Here are the number of divisors and the specific weaknesses of each number in range `[1, 9]`:
```
1: d(1) = 1, weakness(1) = 0;
2: d(2) = 2, weakness(2) = 0;
3: d(3) = 2, weakness(3) = 0;
4: d(4) = 3, weakness(4) = 0;
5: d(5) = 2, weakness(5) = 1;
6: d(6) = 4, weakness(6) = 0;
7: d(7) = 2, weakness(7) = 2;
8: d(8) = 4, weakness(8) = 0;
9: d(9) = 3, weakness(9) = 2.
```
As you can see, the maximum weakness is `2`, and there are `2` numbers with that weakness.
# Input/Output
- `[input]` integer `n`
Constraints: `1 ≤ n ≤ 1000`
- `[output]` a pair of integers ( see example tests what encoding is actually required )
the weakness of the weakest number, and the number of integers in range `[1, n]` with this weakness | games | def weak_numbers(n):
d = [0] + [len([1 for i in range(1, j + 1) if j % i == 0])
for j in range(1, n + 1)]
w = [len([1 for i in range(1, j) if d[i] > d[j]]) for j in range(1, n + 1)]
m = max(w)
return [m, w . count(m)]
| Simple Fun #26: Weak Numbers | 5886dea04703f1712d000051 | [
"Puzzles"
]
| https://www.codewars.com/kata/5886dea04703f1712d000051 | 5 kyu |
No Story
No Description
Only by Thinking and Testing
Look at result of testcase, guess the code!
# #Series:<br>
<a href="http://www.codewars.com/kata/56d904db9963e9cf5000037d">01:A and B?</a><br>
<a href="http://www.codewars.com/kata/56d9292cc11bcc3629000533">02:Incomplete string</a><br>
<a href="http://www.codewars.com/kata/56d931ecc443d475d5000003">03:True or False</a><br>
<a href="http://www.codewars.com/kata/56d93f249c844788bc000002">04:Something capitalized</a><br>
<a href="http://www.codewars.com/kata/56d949281b5fdc7666000004">05:Uniq or not Uniq</a> <br>
<a href="http://www.codewars.com/kata/56d98b555492513acf00077d">06:Spatiotemporal index</a><br>
<a href="http://www.codewars.com/kata/56d9b46113f38864b8000c5a">07:Math of Primary School</a><br>
<a href="http://www.codewars.com/kata/56d9c274c550b4a5c2000d92">08:Math of Middle school</a><br>
<a href="http://www.codewars.com/kata/56d9cfd3f3928b4edd000021">09:From nothingness To nothingness</a><br>
<a href="http://www.codewars.com/kata/56dae2913cb6f5d428000f77">10:Not perfect? Throw away!</a> <br>
<a href="http://www.codewars.com/kata/56db19703cb6f5ec3e001393">11:Welcome to take the bus</a><br>
<a href="http://www.codewars.com/kata/56dc41173e5dd65179001167">12:A happy day will come</a><br>
<a href="http://www.codewars.com/kata/56dc5a773e5dd6dcf7001356">13:Sum of 15(Hetu Luosliu)</a><br>
<a href="http://www.codewars.com/kata/56dd3dd94c9055a413000b22">14:Nebula or Vortex</a><br>
<a href="http://www.codewars.com/kata/56dd927e4c9055f8470013a5">15:Sport Star</a><br>
<a href="http://www.codewars.com/kata/56de38c1c54a9248dd0006e4">16:Falsetto Rap Concert</a><br>
<a href="http://www.codewars.com/kata/56de4d58301c1156170008ff">17:Wind whispers</a><br>
<a href="http://www.codewars.com/kata/56de82fb9905a1c3e6000b52">18:Mobile phone simulator</a><br>
<a href="http://www.codewars.com/kata/56dfce76b832927775000027">19:Join but not join</a><br>
<a href="http://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7">20:I hate big and small</a><br>
<a href="http://www.codewars.com/kata/56e0e065ef93568edb000731">21:I want to become diabetic ;-)</a><br>
<a href="http://www.codewars.com/kata/56e0f1dc09eb083b07000028">22:How many blocks?</a><br>
<a href="http://www.codewars.com/kata/56e1161fef93568228000aad">23:Operator hidden in a string</a><br>
<a href="http://www.codewars.com/kata/56e127d4ef93568228000be2">24:Substring Magic</a><br>
<a href="http://www.codewars.com/kata/56eccc08b9d9274c300019b9">25:Report about something</a><br>
<a href="http://www.codewars.com/kata/56ee0448588cbb60740013b9">26:Retention and discard I</a><br>
<a href="http://www.codewars.com/kata/56eee006ff32e1b5b0000c32">27:Retention and discard II</a><br>
<a href="http://www.codewars.com/kata/56eff1e64794404a720002d2">28:How many "word"?</a><br>
<a href="http://www.codewars.com/kata/56f167455b913928a8000c49">29:Hail and Waterfall</a><br>
<a href="http://www.codewars.com/kata/56f214580cd8bc66a5001a0f">30:Love Forever</a><br>
<a href="http://www.codewars.com/kata/56f25b17e40b7014170002bd">31:Digital swimming pool</a><br>
<a href="http://www.codewars.com/kata/56f4202199b3861b880013e0">32:Archery contest</a><br>
<a href="http://www.codewars.com/kata/56f606236b88de2103000267">33:The repair of parchment</a><br>
<a href="http://www.codewars.com/kata/56f6b4369400f51c8e000d64">34:Who are you?</a><br>
<a href="http://www.codewars.com/kata/56f7eb14f749ba513b0009c3">35:Safe position</a><br>
<br>
# #Special recommendation
Another series, innovative and interesting, medium difficulty. People who like to challenge, can try these kata:
<a href="http://www.codewars.com/kata/56c85eebfd8fc02551000281">Play Tetris : Shape anastomosis</a><br>
<a href="http://www.codewars.com/kata/56cd5d09aa4ac772e3000323">Play FlappyBird : Advance Bravely</a><br>
| games | def mystery((a, b, c, d), (e, f, g, h)):
return [a * e + b * g, a * f + b * h, c * e + d * g, c * f + d * h]
| Thinking & Testing : Math of Middle school | 56d9c274c550b4a5c2000d92 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56d9c274c550b4a5c2000d92 | 7 kyu |
No Story
No Description
Only by Thinking and Testing
Look at the results of the testcases, and guess the code!
---
## Series:
01. [A and B?](http://www.codewars.com/kata/56d904db9963e9cf5000037d)
02. [Incomplete string](http://www.codewars.com/kata/56d9292cc11bcc3629000533)
03. [True or False](http://www.codewars.com/kata/56d931ecc443d475d5000003)
04. [Something capitalized](http://www.codewars.com/kata/56d93f249c844788bc000002)
05. [Uniq or not Uniq](http://www.codewars.com/kata/56d949281b5fdc7666000004)
06. [Spatiotemporal index](http://www.codewars.com/kata/56d98b555492513acf00077d)
07. [Math of Primary School](http://www.codewars.com/kata/56d9b46113f38864b8000c5a)
08. [Math of Middle school](http://www.codewars.com/kata/56d9c274c550b4a5c2000d92)
09. [From nothingness To nothingness](http://www.codewars.com/kata/56d9cfd3f3928b4edd000021)
10. [Not perfect? Throw away!](http://www.codewars.com/kata/56dae2913cb6f5d428000f77)
11. [Welcome to take the bus](http://www.codewars.com/kata/56db19703cb6f5ec3e001393)
12. [A happy day will come](http://www.codewars.com/kata/56dc41173e5dd65179001167)
13. [Sum of 15(Hetu Luosliu)](http://www.codewars.com/kata/56dc5a773e5dd6dcf7001356)
14. [Nebula or Vortex](http://www.codewars.com/kata/56dd3dd94c9055a413000b22)
15. [Sport Star](http://www.codewars.com/kata/56dd927e4c9055f8470013a5)
16. [Falsetto Rap Concert](http://www.codewars.com/kata/56de38c1c54a9248dd0006e4)
17. [Wind whispers](http://www.codewars.com/kata/56de4d58301c1156170008ff)
18. [Mobile phone simulator](http://www.codewars.com/kata/56de82fb9905a1c3e6000b52)
19. [Join but not join](http://www.codewars.com/kata/56dfce76b832927775000027)
20. [I hate big and small](http://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7)
21. [I want to become diabetic ;-)](http://www.codewars.com/kata/56e0e065ef93568edb000731)
22. [How many blocks?](http://www.codewars.com/kata/56e0f1dc09eb083b07000028)
23. [Operator hidden in a string](http://www.codewars.com/kata/56e1161fef93568228000aad)
24. [Substring Magic](http://www.codewars.com/kata/56e127d4ef93568228000be2)
25. [Report about something](http://www.codewars.com/kata/56eccc08b9d9274c300019b9)
26. [Retention and discard I](http://www.codewars.com/kata/56ee0448588cbb60740013b9)
27. [Retention and discard II](http://www.codewars.com/kata/56eee006ff32e1b5b0000c32)
28. [How many "word"?](http://www.codewars.com/kata/56eff1e64794404a720002d2)
29. [Hail and Waterfall](http://www.codewars.com/kata/56f167455b913928a8000c49)
30. [Love Forever](http://www.codewars.com/kata/56f214580cd8bc66a5001a0f)
31. [Digital swimming pool](http://www.codewars.com/kata/56f25b17e40b7014170002bd)
32. [Archery contest](http://www.codewars.com/kata/56f4202199b3861b880013e0)
33. [The repair of parchment](http://www.codewars.com/kata/56f606236b88de2103000267)
34. [Who are you?](http://www.codewars.com/kata/56f6b4369400f51c8e000d64)
35. [Safe position](http://www.codewars.com/kata/56f7eb14f749ba513b0009c3)
---
## Special recommendation
Another series, innovative and interesting, medium difficulty. People who like challenges, can try these kata:
* [Play Tetris : Shape anastomosis](http://www.codewars.com/kata/56c85eebfd8fc02551000281)
* [Play FlappyBird : Advance Bravely](http://www.codewars.com/kata/56cd5d09aa4ac772e3000323) | games | def mystery(n):
return [i for i in range(1, n + 1, 2) if n % i == 0]
| Thinking & Testing : Retention and discard | 56ee0448588cbb60740013b9 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56ee0448588cbb60740013b9 | 7 kyu |
No Story
No Description
Only by Thinking and Testing
Look at result of testcase, guess the code!
# #Series:<br>
<a href="http://www.codewars.com/kata/56d904db9963e9cf5000037d">01:A and B?</a><br>
<a href="http://www.codewars.com/kata/56d9292cc11bcc3629000533">02:Incomplete string</a><br>
<a href="http://www.codewars.com/kata/56d931ecc443d475d5000003">03:True or False</a><br>
<a href="http://www.codewars.com/kata/56d93f249c844788bc000002">04:Something capitalized</a><br>
<a href="http://www.codewars.com/kata/56d949281b5fdc7666000004">05:Uniq or not Uniq</a> <br>
<a href="http://www.codewars.com/kata/56d98b555492513acf00077d">06:Spatiotemporal index</a><br>
<a href="http://www.codewars.com/kata/56d9b46113f38864b8000c5a">07:Math of Primary School</a><br>
<a href="http://www.codewars.com/kata/56d9c274c550b4a5c2000d92">08:Math of Middle school</a><br>
<a href="http://www.codewars.com/kata/56d9cfd3f3928b4edd000021">09:From nothingness To nothingness</a><br>
<a href="http://www.codewars.com/kata/56dae2913cb6f5d428000f77">10:Not perfect? Throw away!</a> <br>
<a href="http://www.codewars.com/kata/56db19703cb6f5ec3e001393">11:Welcome to take the bus</a><br>
<a href="http://www.codewars.com/kata/56dc41173e5dd65179001167">12:A happy day will come</a><br>
<a href="http://www.codewars.com/kata/56dc5a773e5dd6dcf7001356">13:Sum of 15(Hetu Luosliu)</a><br>
<a href="http://www.codewars.com/kata/56dd3dd94c9055a413000b22">14:Nebula or Vortex</a><br>
<a href="http://www.codewars.com/kata/56dd927e4c9055f8470013a5">15:Sport Star</a><br>
<a href="http://www.codewars.com/kata/56de38c1c54a9248dd0006e4">16:Falsetto Rap Concert</a><br>
<a href="http://www.codewars.com/kata/56de4d58301c1156170008ff">17:Wind whispers</a><br>
<a href="http://www.codewars.com/kata/56de82fb9905a1c3e6000b52">18:Mobile phone simulator</a><br>
<a href="http://www.codewars.com/kata/56dfce76b832927775000027">19:Join but not join</a><br>
<a href="http://www.codewars.com/kata/56dfd5dfd28ffd52c6000bb7">20:I hate big and small</a><br>
<a href="http://www.codewars.com/kata/56e0e065ef93568edb000731">21:I want to become diabetic ;-)</a><br>
<a href="http://www.codewars.com/kata/56e0f1dc09eb083b07000028">22:How many blocks?</a><br>
<a href="http://www.codewars.com/kata/56e1161fef93568228000aad">23:Operator hidden in a string</a><br>
<a href="http://www.codewars.com/kata/56e127d4ef93568228000be2">24:Substring Magic</a><br>
<a href="http://www.codewars.com/kata/56eccc08b9d9274c300019b9">25:Report about something</a><br>
<a href="http://www.codewars.com/kata/56ee0448588cbb60740013b9">26:Retention and discard I</a><br>
<a href="http://www.codewars.com/kata/56eee006ff32e1b5b0000c32">27:Retention and discard II</a><br>
<a href="http://www.codewars.com/kata/56eff1e64794404a720002d2">28:How many "word"?</a><br>
<a href="http://www.codewars.com/kata/56f167455b913928a8000c49">29:Hail and Waterfall</a><br>
<a href="http://www.codewars.com/kata/56f214580cd8bc66a5001a0f">30:Love Forever</a><br>
<a href="http://www.codewars.com/kata/56f25b17e40b7014170002bd">31:Digital swimming pool</a><br>
<a href="http://www.codewars.com/kata/56f4202199b3861b880013e0">32:Archery contest</a><br>
<a href="http://www.codewars.com/kata/56f606236b88de2103000267">33:The repair of parchment</a><br>
<a href="http://www.codewars.com/kata/56f6b4369400f51c8e000d64">34:Who are you?</a><br>
<a href="http://www.codewars.com/kata/56f7eb14f749ba513b0009c3">35:Safe position</a><br>
<br>
# #Special recommendation
Another series, innovative and interesting, medium difficulty. People who like to challenge, can try these kata:
<a href="http://www.codewars.com/kata/56c85eebfd8fc02551000281">Play Tetris : Shape anastomosis</a><br>
<a href="http://www.codewars.com/kata/56cd5d09aa4ac772e3000323">Play FlappyBird : Advance Bravely</a><br>
| games | from itertools import compress
def mystery(s, n):
return '' . join(compress(s, map(int, format(n, 'b'))))
| Thinking & Testing : Retention and discard II | 56eee006ff32e1b5b0000c32 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56eee006ff32e1b5b0000c32 | 7 kyu |
# Task
Initially a number `1` is written on a board. It is possible to do the following operations with it:
```
multiply the number by 3;
increase the number by 5.```
Your task is to determine that using this two operations step by step, is it possible to obtain number `n`?
# Example
For `n = 1`, the result should be `true`.
`1 = 1`
For `n = 2`, the result should be `false`.
For `n = 3`, the result should be `true`.
`1 x 3 = 3`
For `n = 4`, the result should be `false`.
For `n = 5`, the result should be `false`.
For `n = 6`, the result should be `true`.
`1 + 5 = 6`
For `n = 18`, the result should be `true`.
`1 + 5 = 6 --> 6 x 3 = 18`
For `n = 32`, the result should be `true`.
`1 x 3 x 3 x 3 = 27 --> 27 + 5 = 32`
For `n = 100`, the result should be `false`.
For `n = 101`, the result should be `true`.
`1 + 5 + 5 + 5 ... +5 = 101`
# Input / Output
- `[input]` integer n
positive integer, n ≤ 100000
- `[output]` a boolean value
`true` if N can be obtained using given operations, `false` otherwise. | games | def number_increasing(n):
return n not in {2, 4, 7, 12, 17, 22} and n % 5 != 0
| Simple Fun #113: Number Increasing | 589d1e88e8afb7a85e00004e | [
"Puzzles"
]
| https://www.codewars.com/kata/589d1e88e8afb7a85e00004e | 7 kyu |
# Task
Call two arms equally strong if the heaviest weights they each are able to lift are equal.
Call two people equally strong if their strongest arms are equally strong (the strongest arm can be both the right and the left), and so are their weakest arms.
Given your and your friend's arms' lifting capabilities find out if you two are equally strong.
# Example
For `yourLeft = 10, yourRight = 15, friendsLeft = 15 and friendsRight = 10`, the output should be `true`;
For `yourLeft = 15, yourRight = 10, friendsLeft = 15 and friendsRight = 10`, the output should be `true`;
For `yourLeft = 15, yourRight = 10, friendsLeft = 15 and friendsRight = 9,` the output should be `false`.
# Input/Output
- `[input]` integer `yourLeft`
A non-negative integer representing the heaviest weight you can lift with your left arm.
- `[input]` integer `yourRight`
A non-negative integer representing the heaviest weight you can lift with your right arm.
- `[input]` integer `friendsLeft`
A non-negative integer representing the heaviest weight your friend can lift with his or her left arm.
- `[input]` integer `friendsRight`
A non-negative integer representing the heaviest weight your friend can lift with his or her right arm.
- `[output]` a boolean value | games | def are_equally_strong(your_left, your_right, friends_left, friends_right):
return sorted([your_left, your_right]) == sorted([friends_left, friends_right])
| Simple Fun #69: Are Equally Strong? | 5894017082b9fb62c50000df | [
"Puzzles"
]
| https://www.codewars.com/kata/5894017082b9fb62c50000df | 7 kyu |
# Task
Let's call two integers A and B friends if each integer from the array divisors is either a divisor of both A and B or neither A nor B. If two integers are friends, they are said to be in the same clan. How many clans are the integers from 1 to k, inclusive, broken into?
# Example
For `divisors = [2, 3] and k = 6`, the output should be `4`
The numbers 1 and 5 are friends and form a clan, 2 and 4 are friends and form a clan, and 3 and 6 do not have friends and each is a clan by itself. So the numbers 1 through 6 are broken into 4 clans.
# Input/Output
- `[input]` integer array `divisors`
A non-empty array of positive integers.
Constraints: `2 ≤ divisors.length < 10, 1 ≤ divisors[i] ≤ 10`.
- `[input]` integer `k`
A positive integer.
Constraints: `5 ≤ k ≤ 50`.
- `[output]` an integer | games | def number_of_clans(divisors, k):
return len({tuple(d for d in divisors if not n % d) for n in range(1, k + 1)})
| Simple Fun #21: Number Of Clans | 5886cab0a95e17e61600009d | [
"Puzzles"
]
| https://www.codewars.com/kata/5886cab0a95e17e61600009d | 7 kyu |
We are still with squared integers.
Given 4 integers `a, b, c, d` we form the sum of the squares of `a` and `b`
and then the sum of the squares of `c` and `d`. We multiply the two sums hence a number `n` and we try to
decompose `n` in a sum of two squares `e` and `f` (e and f integers >= 0) so that `n = e² + f²`.
More: `e` and `f` must result only from sums (or differences) of products between on the one hand `(a, b)` and on the other `(c, d)` each of `a, b, c, d` taken only once.
For example,
prod2sum(1, 2, 1, 3) should return [[1, 7], [5, 5]])
because
```
1==1*3-1*2
7==2*3+1*1
5==1*2+1*3
```
Suppose we have `a = 1, b = 2, c = 1, d = 3`. First we calculate the sums
`1² + 2² = 5 and 1² + 3² = 10` hence `n = 50`.
`50 = 1² + 7² or 50 = 7² + 1²` (we'll consider that these two solutions are the same)
or `50 = 5² + 5²`.
The return of our function will be an array of subarrays (in C an array of Pairs) sorted on the first elements of the subarrays. In each subarray the lower element should be the first.
`prod2sum(1, 2, 1, 3) should return [[1, 7], [5, 5]]`
`prod2sum(2, 3, 4, 5) should return [[2, 23], [7, 22]]`
because `(2² + 3²) * (4² + 5²) = 533 = (7² + 22²) = (23² + 2²)`
`prod2sum(1, 2, 2, 3) should return [[1, 8], [4, 7]]`
`prod2sum(1, 1, 3, 5) should return [[2, 8]]` (there are not always 2 solutions).
##Hint
Take a sheet of paper and with a bit of algebra try to write the product of squared numbers in another way. | reference | def prod2sum(a, b, c, d):
e = sorted([abs(a * d - b * c), abs(a * c + b * d)])
f = sorted([abs(a * c - b * d), abs(a * d + b * c)])
if e == f:
return [e]
else:
return sorted([e, f])
| Integers: Recreation Two | 55e86e212fce2aae75000060 | [
"Fundamentals",
"Mathematics",
"Puzzles"
]
| https://www.codewars.com/kata/55e86e212fce2aae75000060 | 6 kyu |
# Task
You are given a sequence of positive ints where every element appears `three times`, except one that appears only `once` (let's call it `x`) and one that appears only `twice` (let's call it `y`).
Your task is to find `x * x * y`.
# Example
For `arr=[1, 1, 1, 2, 2, 3]`, the result should be `18`
`3 x 3 x 2 = 18`
For `arr=[6, 5, 4, 100, 6, 5, 4, 100, 6, 5, 4, 200]`, the result should be `4000000`
`200 x 200 x 100 = 4000000`
# Input/Output
- `[input]` integer array `arr`
an array contains positive integers.
- `[output]` an integer
The value of `x * x * y` | games | def missing_values(seq):
a, b = sorted(seq, key=seq . count)[: 2]
return a * a * b
| Simple Fun #136: Missing Values | 58a66c208b88b2de660000c3 | [
"Puzzles"
]
| https://www.codewars.com/kata/58a66c208b88b2de660000c3 | 7 kyu |
In this kata we have to create a function that will give us some specific information of a data base. We have a sequence of postive integers that is registered by OEIS with the code [001055](https://oeis.org/A001055). This sequence give us the amount of ways that a number may be expressed as a product of its factors (including the number itself multiplied by one.)
The first terms of this sequence are shown below:
```python
n-th term a(n)
1 1
2 1
3 1
4 2
5 1
6 2
7 1
8 3
9 2
10 2
11 1
12 4
13 1
14 2
15 2
16 5
```
In the preloaded section you are given a hash table named `A001055` (Python and JavaScript) or `$a001055` (Ruby), where keys are the numbers from `1` to `1006`, and the values are the respective terms of the aforementioned sequence.
You have to create the function that receives three arguments:
1. An array with 2 elements that represent the numbers in range `[a, b]` to be considered.
2. A string with five possible valid values: `"equals to"`, `"higher than"`, `"lower than"`, `"higher and equals to"`, and `"lower and equals to"` (any other value is considered invalid).
3. The element of the `A001055` sequence.
The function should return the amount of numbers that fulfill our conditions and a sorted list of these numbers.
## Examples
1) We want to know about the numbers between `10` and `21` (included) that have more than `1` multiplicative partition:
```
range = [10, 21]
str = "higher than"
val = 1
result = (8, [10, 12, 14, 15, 16, 18, 20, 21])
```
2) We want to know the numbers between `25` and `75` that have more than or equals to `5` multiplicative partitions:
```
range = [25, 75]
str = "higher and equals to"
val = 5
result = (13, [30, 32, 36, 40, 42, 48, 54, 56, 60, 64, 66, 70, 72])
```
3) If the string command is wrong, the function will return `"wrong constraint"`:
```
range = [25, 75]
str = "qwerty"
val = 5
result = "wrong constraint"
```
Enjoy it!! | reference | import operator
def inf_database(range_, str_, val):
ops = {"higher than": operator . gt, "lower than": operator . lt, "equals to": operator . eq,
"higher and equals to": operator . ge, "lower and equals to": operator . le}
if str_ not in ops:
return "wrong constraint"
result = [i for i in range(range_[0], range_[- 1] + 1)
if ops[str_](A001055[i], val)]
return (len(result), result)
| Working with Dictionaries | 5639302a802494696d000077 | [
"Fundamentals",
"Algorithms",
"Data Structures"
]
| https://www.codewars.com/kata/5639302a802494696d000077 | 7 kyu |
# Task
Reversing an array can be a tough task, especially for a novice programmer. Mary just started coding, so she would like to start with something basic at first. Instead of reversing the array entirely, she wants to swap just its first and last elements.
Given an array `arr`, swap its `first` and `last` elements and return the resulting array.
# Example
For arr = [1, 2, 3, 4, 5], the output should be `[5, 2, 3, 4, 1]`
# Input/Output
- `[input]` integer array `arr`
Constraints: `0 ≤ arr.length ≤ 50, -1000 ≤ arr[i] ≤ 1000`
- `[output]` an integer array
Array arr with its first and its last elements swapped. | games | def first_reverse_try(arr):
if arr:
arr[0], arr[- 1] = arr[- 1], arr[0]
return arr
| Simple Fun #20: First Reverse Try | 5886c6b2f3b6ae33dd0000be | [
"Puzzles"
]
| https://www.codewars.com/kata/5886c6b2f3b6ae33dd0000be | 7 kyu |
# Task
We define the middle of the array `arr` as follows:
if `arr` contains an odd number of elements, its middle is the element whose index number is the same when counting from the beginning of the array and from its end;
if `arr` contains an even number of elements, its middle is the sum of the two elements whose index numbers when counting from the beginning and from the end of the array differ by one.
An array is called smooth if its first and its last elements are equal to one another and to the middle. Given an array arr, determine if it is smooth or not.
# Example
For arr = [7, 2, 2, 5, 10, 7], the output should be `true`
The first and the last elements of arr are equal to 7, and its middle also equals 2 + 5 = 7. Thus, the array is smooth and the output is true.
For arr = [-5, -5, 10], the output should be `false`
The first and middle elements are equal to -5, but the last element equals 10. Thus, arr is not smooth and the output is false.
# Input/Output
- `[input]` integer array `arr`
The given array.
Constraints: 2 ≤ arr.length ≤ 10<sup>3</sup>, -10<sup>3</sup> ≤ arr[i] ≤ 10<sup>3</sup>.
- `[output]` a boolean value
`true` if arr is smooth, `false` otherwise. | games | def is_smooth(arr):
d, m = divmod(len(arr), 2)
return arr[0] == arr[- 1] == arr[d] + arr[d - 1] * (m == 0)
| Simple Fun #22: Is Smooth? | 5886d35d4703f125a6000008 | [
"Puzzles"
]
| https://www.codewars.com/kata/5886d35d4703f125a6000008 | 7 kyu |
# Task
You are implementing your own HTML editor. To make it more comfortable for developers you would like to add an auto-completion feature to it.
Given the starting HTML tag, find the appropriate end tag which your editor should propose.
# Example
For startTag = "<button type='button' disabled>", the output should be "</button>";
For startTag = "<i>", the output should be "</i>".
# Input/Output
- `[input]` string `startTag`/`start_tag`
- `[output]` a string | games | def html_end_tag_by_start_tag(start_tag):
return "</" + start_tag[1: - 1]. split(" ")[0] + ">"
| Simple Fun #28: Html End Tag By Start Tag | 5886f3713a111b620f0000dc | [
"Puzzles"
]
| https://www.codewars.com/kata/5886f3713a111b620f0000dc | 7 kyu |
# Task
How many strings equal to A can be constructed using letters from the string B? Each letter can be used only once and in one string only.
# Example
For `A = "abc" and B = "abccba"`, the output should be `2`.
We can construct 2 strings `A` with letters from `B`.
# Input/Output
- `[input]` string `A`
String to construct, A contains only lowercase English letters.
Constraints: `3 ≤ A.length ≤ 9`.
- `[input]` string `B`
String containing needed letters, `B` contains only lowercase English letters.
Constraints: `3 ≤ B.length ≤ 50`.
- `[output]` an integer | games | def strings_construction(A, B):
return min(B . count(i) / / A . count(i) for i in set(A))
| Simple Fun #30: Strings Construction | 58870402c81516bbdb000088 | [
"Puzzles"
]
| https://www.codewars.com/kata/58870402c81516bbdb000088 | 7 kyu |
# Task
Elections are in progress!
Given an array of numbers representing votes given to each of the candidates, and an integer which is equal to the number of voters who haven't cast their vote yet, find the number of candidates who still have a chance to win the election.
The winner of the election must secure strictly more votes than any other candidate. If two or more candidates receive the same (maximum) number of votes, assume there is no winner at all.
**Note**: big arrays will be tested.
# Examples
```
votes = [2, 3, 5, 2]
voters = 3
result = 2
```
* Candidate `#3` may win, because he's already leading.
* Candidate `#2` may win, because with 3 additional votes he may become the new leader.
* Candidates `#1` and `#4` have no chance, because in the best case scenario each of them can only tie with the candidate `#3`.
___
```
votes = [3, 1, 1, 3, 1]
voters = 2
result = 2
```
* Candidate `#1` and `#4` can become leaders if any of them gets the votes.
* If any other candidate gets the votes, they will get tied with candidates `#1` and `#4`.
___
```
votes = [1, 3, 3, 1, 1]
voters = 0
result = 0
```
* There're no additional votes to cast, and there's already a tie. | games | def elections_winners(votes, k):
m = max(votes)
return sum(x + k > m for x in votes) or votes . count(m) == 1
| Simple Fun #41: Elections Winners | 58881b859ab1e053240000cc | [
"Puzzles"
]
| https://www.codewars.com/kata/58881b859ab1e053240000cc | 7 kyu |
## Your story
You've always loved both <a href="https://en.wikipedia.org/wiki/Fizz_buzz">Fizz Buzz</a> katas and <a href="https://en.wikipedia.org/wiki/Cuckoo_clock">cuckoo clocks</a>, and when you walked by a garage sale and saw an ornate cuckoo clock with a missing pendulum, and a "Beyond-Ultimate <a href="https://en.wikipedia.org/wiki/Raspberry_Pi">Raspberry Pi</a> Starter Kit" filled with all sorts of sensors and motors and other components, it's like you were suddenly hit by a beam of light and knew that it was your mission to combine the two to create a computerized Fizz Buzz cuckoo clock!
You took them home and set up shop on the kitchen table, getting more and more excited as you got everything working together just perfectly. Soon the only task remaining was to write a function to select from the sounds you had recorded depending on what time it was:
## Your plan
* When a minute is evenly divisible by three, the clock will say the word "Fizz".
* When a minute is evenly divisible by five, the clock will say the word "Buzz".
* When a minute is evenly divisible by both, the clock will say "Fizz Buzz", with two exceptions:
1. On the hour, instead of "Fizz Buzz", the clock door will open, and the cuckoo bird will come out and "Cuckoo" between one and twelve times depending on the hour.
2. On the half hour, instead of "Fizz Buzz", the clock door will open, and the cuckoo will come out and "Cuckoo" just once.
* With minutes that are not evenly divisible by either three or five, at first you had intended to have the clock just say the numbers ala Fizz Buzz, but then you decided at least for version 1.0 to just have the clock make a quiet, subtle "tick" sound for a little more clock nature and a little less noise.
Your input will be a string containing hour and minute values in 24-hour time, separated by a colon, and with leading zeros. For example, 1:34 pm would be `"13:34"`.
Your return value will be a string containing the combination of Fizz, Buzz, Cuckoo, and/or tick sounds that the clock needs to make at that time, separated by spaces. Note that although the input is in 24-hour time, cuckoo clocks' cuckoos are in 12-hour time.
## Some examples
```
"13:34" "tick"
"21:00" "Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo"
"11:15" "Fizz Buzz"
"03:03" "Fizz"
"14:30" "Cuckoo"
"08:55" "Buzz"
"00:00" "Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo"
"12:00" "Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo Cuckoo"
```
Have fun!
| reference | def fizz_buzz_cuckoo_clock(time):
hh, mm = map(int, time . split(":"))
if mm == 0:
return " " . join(["Cuckoo"] * (hh % 12 or 12))
elif mm == 30:
return "Cuckoo"
elif mm % 15 == 0:
return "Fizz Buzz"
elif mm % 3 == 0:
return "Fizz"
elif mm % 5 == 0:
return "Buzz"
else:
return "tick"
| Fizz Buzz Cuckoo Clock | 58485a43d750d23bad0000e6 | [
"Fundamentals"
]
| https://www.codewars.com/kata/58485a43d750d23bad0000e6 | 7 kyu |
You're familiar with [list slicing](https://docs.python.org/3/library/functions.html#slice) in Python and know, for example, that:
```python
>>> ages = [12, 14, 63, 72, 55, 24]
>>> ages[2:4]
[63, 72]
>>> ages[2:]
[63, 72, 55, 24]
>>> ages[:3]
[12, 14, 63]
```
Write a function `inverse_slice` that takes three arguments: a list `items`, an integer `a` and an integer `b`. The function should return a new list with the slice specified by `items[a:b]` __*excluded*__.
For example:
```python
>>>inverse_slice([12, 14, 63, 72, 55, 24], 2, 4)
[12, 14, 55, 24]
```
```rust
inverse_slice(&[12, 14, 63, 72, 55, 24], 2, 4) == [12, 14, 55, 24]
```
Input domain:
- `items` will always be a valid sequence.
- `b` will always be greater than `a`.
- `a` will always be greater than or equal to zero.
- `a` will always be less than the length of `items`.
- `b` may be greater than the length of `items`.
```if:cobol
#### Note for COBOL:
Remember COBOL's tables use 1-based indexing, so `a` and `b` will always be superior or equal to 1, and indexes need to be shifted by 1 in comparison with other languages:
InverseSlice [12, 14, 63, 72, 55, 24], 3, 5 => [12, 14, 55, 24]
``` | reference | def inverse_slice(items, a, b):
return items[: a] + items[b:]
| Thinkful - List and Loop Drills: Inverse Slicer | 586ec0b8d098206cce001141 | [
"Fundamentals",
"Lists"
]
| https://www.codewars.com/kata/586ec0b8d098206cce001141 | 7 kyu |
# Task
You are given a digital `number` written down on a sheet of paper.
Your task is to figure out if you rotate the given sheet of paper by 180 degrees would the number still look exactly the same.
Note: You can assume that the digital number is written like the following image:
<svg xmlns="http://www.w3.org/2000/svg" width="453.333" height="320" version="1.0" viewBox="0 0 340 240"><g fill="red"><path d="M85.2 26.6c-2.9 2-2.8 3.8.3 5.8 1.9 1.3 5.3 1.6 16.8 1.6 13.7 0 14.4-.1 16.5-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-16.8-2.2-11.5 0-14.9.3-16.7 1.6zM149 27c-2.6 2.6-2.5 3.5.2 5.4 1.8 1.3 5.2 1.6 16.8 1.6 14 0 14.7-.1 16.8-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-17-2.2-13.4 0-14.9.2-16.7 2zM278.2 26.6c-2.7 1.8-2.8 3.6-.4 5.7 1.6 1.4 4.3 1.7 16.9 1.7 14.3 0 15-.1 17.1-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-16.8-2.2-11.5 0-14.9.3-16.7 1.6zM119.7 32.8c-1.4 1.6-1.7 4.3-1.7 17 0 14.1.2 15.4 2.1 17.3l2.1 2.1 2.4-2.3c2.3-2.2 2.4-2.7 2.4-16.9 0-14-.1-14.7-2.3-16.8-2.8-2.7-3-2.7-5-.4zM207.2 33.2c-2.1 2.3-2.2 3.3-2.2 17.5 0 13.3.2 15.2 1.8 16.6 2.4 2.2 2.7 2.1 5.2-.5 1.8-2 2-3.5 2-16.2 0-13.1-.2-14.3-2.3-16.9l-2.2-2.8-2.3 2.3zM271.2 33.3c-2.1 2.2-2.2 3.3-2.2 17.4 0 13.3.2 15.2 1.8 16.6 2.4 2.2 3.5 2.1 5.5-.1 1.4-1.6 1.7-4.3 1.7-16.9 0-14.3-.1-15-2.3-17.1l-2.3-2.2-2.2 2.3zM41.2 34.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 11.5.3 14.9 1.6 16.7 1.8 2.7 3.6 2.8 5.7.4 1.4-1.6 1.7-4.3 1.7-16.9 0-14.3-.1-15-2.3-17.1L43.4 32l-2.2 2.3zM183.7 33.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 13.3.2 15.2 1.8 16.6 2.5 2.3 3.8 2.1 5.6-.5 2.3-3.3 2.3-28.8 0-32.3-1.9-3-3.5-3.1-5.7-.7zM247.7 33.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 13.3.2 15.2 1.8 16.6 2.4 2.2 3.4 2.1 5.5-.3 2.4-2.8 2.5-29.4.1-32.8-1.8-2.7-3.6-2.8-5.7-.4zM84.9 68.6l-2.4 2.6 2.8 2.4c2.7 2.3 3.5 2.4 16.6 2.4h13.7l2.8-2.9 2.7-2.8-2.7-2.2c-2.4-1.9-3.9-2.1-16.9-2.1-13.9 0-14.2 0-16.6 2.6zM213 68.5l-2.4 2.6 2.9 2.4c2.8 2.3 3.7 2.4 16.7 2.5 13.1 0 13.8-.1 16.5-2.4l2.8-2.4-2.4-2.6C244.7 66 244.5 66 230 66c-14.4 0-14.7 0-17 2.5zM277.2 68.3l-2.2 2.3 2.8 2.7c2.8 2.7 3 2.7 16.8 2.7 13.7 0 14.1-.1 16.4-2.5l2.3-2.5-2.3-2.5c-2.3-2.4-2.7-2.5-17-2.5-14 0-14.7.1-16.8 2.3zM148.5 69c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 16.5 2.5c13 0 14.5-.2 16.9-2.1l2.7-2.1-2.2-2.4c-2.2-2.3-2.7-2.4-17.4-2.4-13.9 0-15.3.2-17 2zM184.2 74.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM248.2 74.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM41.2 75.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7L43.4 73l-2.2 2.3zM77.7 74.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 14.3.1 15 2.3 17.1l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7-2.8-2.7-3-2.7-5-.4zM312.7 74.8c-1.4 1.6-1.7 4.3-1.7 17.4 0 14.2.2 15.6 2 17.3 2 1.8 2.1 1.8 4.5-.5s2.5-2.7 2.5-16.5c0-12.9-.2-14.5-2.1-16.9-2.4-3.1-3.1-3.2-5.2-.8zM84.5 110c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 17 2.5c14 0 14.7-.1 16.8-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-17.4-2.2-13.7 0-15.1.2-16.8 2zM148.8 110.1c-2.3 2.4-2.2 3.2 0 5.2 1.6 1.4 4.3 1.7 16.9 1.7 14.3 0 15-.1 17.1-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.2-2.2-17.3-2.1-13.3.1-15.1.3-16.6 2zM277.2 110.3l-2.2 2.3 2.3 2.2c2.2 2.1 3.3 2.2 17.4 2.2 13.7 0 15.1-.2 16.8-2 1.8-2 1.8-2.1-.5-4.5s-2.7-2.5-17-2.5c-14 0-14.7.1-16.8 2.3zM20.2 135.3l-2.2 2.3 2.3 2.2c2.2 2.1 3.3 2.2 16.8 2.2 13.9 0 14.6-.1 16.7-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-16.8-2.2-13.9 0-14.6.1-16.7 2.3zM84.2 135.3l-2.2 2.3 2.3 2.2c2.2 2.1 3.3 2.2 16.8 2.2 13.9 0 14.6-.1 16.7-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-16.8-2.2-13.9 0-14.6.1-16.7 2.3zM148.5 135c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 17 2.5c14 0 14.7-.1 16.8-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-17.4-2.2-13.7 0-15.1.2-16.8 2zM213.2 135.3l-2.2 2.3 2.3 2.2c2.2 2.1 3.3 2.2 16.8 2.2 13.9 0 14.6-.1 16.7-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-16.8-2.2-13.9 0-14.6.1-16.7 2.3zM277.5 135c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 17 2.5c14 0 14.7-.1 16.8-2.3l2.2-2.3-2.3-2.2c-2.2-2.1-3.3-2.2-17.4-2.2-13.7 0-15.1.2-16.8 2zM14.2 141.3c-2.1 2.2-2.2 3.3-2.2 17.4 0 13.7.2 15.1 2 16.8 2 1.8 2.1 1.8 4.5-.5s2.5-2.7 2.5-16.4c-.1-13.1-.2-14.3-2.3-16.9l-2.3-2.7-2.2 2.3zM120.2 141.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM142.2 141.3c-2 2.2-2.2 3.3-2.2 16.5 0 11.5.3 14.8 1.6 16.7 1.9 3 3.5 3.1 5.7.7 1.4-1.6 1.7-4.3 1.7-16.9 0-14.3-.1-15-2.3-17.1l-2.3-2.2-2.2 2.3zM248.2 141.7c-2 2.5-2.2 3.8-2.2 16.8 0 13.8.1 14.2 2.5 16.5l2.5 2.3 2.5-2.3c2.4-2.3 2.5-2.7 2.5-16.5v-14.1l-2.8-2.7-2.8-2.7-2.2 2.7zM313.2 141.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM183.7 141.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 13.7.2 15.1 2 16.8 2 1.8 2.1 1.8 4.5-.5s2.5-2.7 2.5-16.5c0-13.5-.1-14.3-2.3-16.3-2.8-2.7-3-2.7-5-.4zM206.7 141.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 13.7.2 15.1 2 16.8 2 1.8 2.1 1.8 4.5-.5s2.5-2.7 2.5-16.5c0-13.5-.1-14.3-2.3-16.3-2.8-2.7-3-2.7-5-.4zM271.2 142.3c-2 2.2-2.2 3.3-2.2 16.3 0 13.7.1 14.1 2.5 16.4l2.5 2.3 2.5-2.3c2.4-2.3 2.5-2.7 2.5-16.5 0-13.5-.1-14.3-2.3-16.3-2.9-2.7-2.9-2.7-5.5.1zM20 176.5l-2.4 2.6 2.9 2.4c2.8 2.3 3.7 2.4 16.5 2.5 13.1 0 13.6-.1 16.5-2.6l3-2.6-2.8-2.4c-2.7-2.3-3.4-2.4-17.1-2.4-14 0-14.3 0-16.6 2.5zM148.9 176.6l-2.4 2.6 2.8 2.4c2.7 2.3 3.5 2.4 16.6 2.4h13.7l2.8-2.9 2.7-2.8-2.7-2.2c-2.4-1.9-3.9-2.1-16.9-2.1-13.9 0-14.2 0-16.6 2.6zM213 176.5l-2.3 2.5 2.9 2.5c2.8 2.4 3.4 2.5 16.6 2.5 13 0 13.8-.1 16.5-2.4l2.8-2.4-2.4-2.6c-2.4-2.6-2.6-2.6-17.1-2.6-14.3 0-14.7.1-17 2.5zM14.2 182.3c-2.1 2.2-2.2 3.3-2.2 17.4 0 14.8 0 15.1 2.4 16.6 2.2 1.5 2.5 1.4 4.5-.7 1.9-2.1 2.1-3.3 2.1-16.7 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM55.7 181.8c-1.3 1.4-1.6 4.6-1.8 17-.2 14.5-.1 15.5 1.9 17.5l2.2 2.1 2.5-2.4c2.4-2.3 2.5-2.6 2.5-16.4-.1-13.5-.2-14.2-2.5-16.9-2.8-3.2-2.8-3.2-4.8-.9zM119.7 181.8c-1.4 1.6-1.7 4.3-1.7 16.9 0 14.3.1 15 2.3 17.1l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7-2.8-2.7-3-2.7-5-.4zM142.2 182.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 13.9.1 14.6 2.3 16.7l2.3 2.2 2.2-2.3c2.1-2.2 2.2-3.3 2.2-16.8 0-13.9-.1-14.6-2.3-16.7l-2.3-2.2-2.2 2.3zM183.7 181.8c-1.4 1.6-1.7 4.3-1.7 17.4 0 14.2.2 15.6 2 17.3 2 1.8 2.1 1.8 4.5-.5s2.5-2.7 2.5-17c0-14-.1-14.7-2.3-16.8-2.8-2.7-3-2.7-5-.4zM248.2 182.3c-2.1 2.2-2.2 3.3-2.2 16.8 0 14.1.1 14.6 2.4 16.8l2.5 2.3 2.5-2.5c2.5-2.6 2.6-2.9 2.6-16.5v-13.8l-2.8-2.7-2.8-2.7-2.2 2.3zM313.2 182.2c-2.1 2.3-2.2 3.3-2.2 17.5 0 13.3.2 15.2 1.8 16.6 2.4 2.2 3.5 2.1 5.5-.1 1.4-1.6 1.7-4.3 1.7-16.5 0-13.7-.1-14.7-2.3-17.2l-2.2-2.6-2.3 2.3zM271.1 183.6c-1.9 2.4-2.1 4-2.1 16.4 0 13.3.1 13.7 2.5 16l2.5 2.3 2.5-2.3c2.4-2.3 2.5-2.7 2.5-16.5 0-13.5-.1-14.3-2.3-16.3-2.9-2.8-3.1-2.8-5.6.4zM164.2 215.8c-11.7.2-13.6.5-15.5 2.3l-2.1 2 2.8 2.4c2.8 2.4 3.5 2.5 17.1 2.5 13.8 0 14.2-.1 16.5-2.5l2.4-2.5-2.2-2.2c-2.4-2.5-1.5-2.4-19-2zM19.5 218c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 16.6 2.5c13.6 0 14.4-.1 16.8-2.3l2.6-2.4-2.3-2.1c-2.2-2.1-3.3-2.2-17.4-2.2-13.7 0-15.1.2-16.8 2zM213.5 218c-1.8 2-1.8 2.1.5 4.5s2.7 2.5 16.5 2.5 14.2-.1 16.5-2.5c2.3-2.4 2.3-2.5.5-4.5-1.7-1.8-3.1-2-17-2s-15.3.2-17 2zM278.3 217.9l-2.2 1.8 2.8 2.7c2.7 2.6 2.9 2.6 16.7 2.6 13.7 0 14.1-.1 16.4-2.5 2.3-2.4 2.3-2.5.5-4.5-1.7-1.8-3-2-16.9-2-13.1.1-15.4.3-17.3 1.9z"/></g></svg>
# Example
For `number = "1"`, the result should be `false`
For `number = "29562"`, the result should be `true`.
For `number = "77"`, the result should be `false`.
# Input/Output
- `[input]` string `number`
sequence of digital digits given as a string.
- `[output]` a boolean value
`true` if you rotate the given sheet of paper by 180 degrees then the number still look exactly the same. `false` otherwise. | games | def rotate_paper(number):
return number == number . translate(str . maketrans('69', '96', '1347'))[:: - 1]
| Simple Fun #156: Rotate Paper By 180 Degrees | 58ad230ce0201e17080001c5 | [
"Puzzles"
]
| https://www.codewars.com/kata/58ad230ce0201e17080001c5 | 6 kyu |
# Task
John loves encryption. He can encrypt any string by the following algorithm:
```
take the first and the last letters of the word;
replace the letters between them with their number;
replace this number with the sum of it digits
until a single digit is obtained.```
Given two strings(`s1` and `s2`), return `true` if their encryption is the same, or `false` otherwise.
# Example
For `s1 = "EbnhGfjklmjhgz" and s2 = "Eabcz"`, the result should be `true`.
```
"EbnhGfjklmjhgz" --> "E12z" --> "E3z"
"Eabcz" --> "E3z"
Their encryption is the same.```
# Input/Output
- `[input]` string `s1`
The first string to be encrypted.
`s1.length >= 3`
- `[input]` string `s2`
The second string to be encrypted.
`s2.length >= 3`
- `[output]` a boolean value
`true` if encryption is the same, `false` otherwise. | algorithms | def same_encryption(s1, s2):
return (s1[0], s1[- 1], len(s1) % 9) == (s2[0], s2[- 1], len(s2) % 9)
| Simple Fun #175: Same Encryption | 58b6c403a38abaaf6c00006b | [
"Puzzles",
"Algorithms"
]
| https://www.codewars.com/kata/58b6c403a38abaaf6c00006b | 7 kyu |
# Task
Given some sticks by an array `V` of positive integers, where V[i] represents the length of the sticks, find the number of ways we can choose three of them to form a triangle.
# Example
For `V = [2, 3, 7, 4]`, the result should be `1`.
There is only `(2, 3, 4)` can form a triangle.
For `V = [5, 6, 7, 8]`, the result should be `4`.
`(5, 6, 7), (5, 6, 8), (5, 7, 8), (6, 7, 8)`
# Input/Output
- `[input]` integer array `V`
stick lengths
`3 <= V.length <= 100`
`0 < V[i] <=100`
- `[output]` an integer
number of ways we can choose 3 sticks to form a triangle. | algorithms | from itertools import combinations
def counting_triangles(v):
v . sort()
return sum(a + b > c for a, b, c in combinations(v, 3))
| Simple Fun #157: Counting Triangles | 58ad29bc4b852b14a4000050 | [
"Algorithms"
]
| https://www.codewars.com/kata/58ad29bc4b852b14a4000050 | 7 kyu |
# Task
When a candle finishes burning it leaves a leftover. makeNew leftovers can be combined to make a new candle, which, when burning down, will in turn leave another leftover.
You have candlesNumber candles in your possession. What's the total number of candles you can burn, assuming that you create new candles as soon as you have enough leftovers?
# Example
For candlesNumber = 5 and makeNew = 2, the output should be `9`.
Here is what you can do to burn 9 candles:
```
burn 5 candles, obtain 5 leftovers;
create 2 more candles, using 4 leftovers (1 leftover remains);
burn 2 candles, end up with 3 leftovers;
create another candle using 2 leftovers (1 leftover remains);
burn the created candle, which gives another leftover (2 leftovers in total);
create a candle from the remaining leftovers;
burn the last candle.
Thus, you can burn 5 + 2 + 1 + 1 = 9 candles, which is the answer.
```
# Input/Output
- `[input]` integer `candlesNumber`
The number of candles you have in your possession.
Constraints: 1 ≤ candlesNumber ≤ 50.
- `[input]` integer `makeNew`
The number of leftovers that you can use up to create a new candle.
Constraints: 2 ≤ makeNew ≤ 5.
- `[output]` an integer | games | def candles(candles, make_new):
return candles + (candles - 1) / / (make_new - 1)
| Simple Fun #18: Candles | 5884731139a9b4b7a8000002 | [
"Puzzles"
]
| https://www.codewars.com/kata/5884731139a9b4b7a8000002 | 7 kyu |
# Task
We want to turn the given integer into a number that has only one non-zero digit using a tail rounding approach. This means that at each step we take the last non 0 digit of the number and round it to 0 or to 10. If it's less than 5 we round it to 0 if it's larger than or equal to 5 we round it to 10 (rounding to 10 means increasing the next significant digit by 1). The process stops immediately once there is only one non-zero digit left.
# Example
For value = 15, the output should be `20`
For value = 1234, the output should be `1000`.
1234 -> 1230 -> 1200 -> 1000.
For value = 1445, the output should be `2000`.
1445 -> 1450 -> 1500 -> 2000.
# Input/Output
- `[input]` integer `value`
A positive integer.
Constraints: 1 ≤ value ≤ 10<sup>8</sup>
- `[output]` an integer
The rounded number. | reference | def rounders(value):
mag = 1
while value > 10:
value, r = divmod(value, 10)
value += r > 4
mag *= 10
return value * mag
| Simple Fun #17: Rounders | 58846d50f54f021d90000012 | [
"Puzzles",
"Fundamentals"
]
| https://www.codewars.com/kata/58846d50f54f021d90000012 | 7 kyu |
# Task
There're `k` square apple boxes full of apples. If a box is of size `m`, then it contains m × m apples. You noticed two interesting properties about the boxes:
```
The smallest box is of size 1,
the next one is of size 2,...,
all the way up to size k.
Boxes that have an odd size contain only yellow apples.
Boxes that have an even size contain only red apples.
```
Your task is to calculate the difference between the number of red apples and the number of yellow apples.
# Example
For k = 5, the output should be `-15`
There are 1 + 3 × 3 + 5 × 5 = 35 yellow apples and 2 × 2 + 4 × 4 = 20 red apples, thus the answer is 20 - 35 = -15.
# Input/Output
- `[input]` integer `k`
A positive integer.
Constraints: 1 ≤ k ≤ 40
- `[output]` an integer
The difference between the two types of apples. | games | def apple_boxes(k):
return sum(- (i * i) if i & 1 else i * i for i in range(1, k + 1))
| Simple Fun #16: Apple Boxes | 58846b46f4456a8919000025 | [
"Puzzles"
]
| https://www.codewars.com/kata/58846b46f4456a8919000025 | 7 kyu |
# Task
You are standing at a magical well. It has two positive integers written on it: `a` and `b`. Each time you cast a magic marble into the well, it gives you `a * b` dollars and then both `a` and `b` increase by 1. You have `n` magic marbles. How much money will you make?
# Example
For a = 1, b = 2 and n = 2, the output should be `8`
You will cast your first marble and get $2, after which the numbers will become 2 and 3. When you cast your second marble, the well will give you $6. Overall, you'll make $8. So, the output is 8.
# Input/Output
- `[input]` integer `a`
Constraints: `1 ≤ a ≤ 2000`
- `[input]` integer `b`
Constraints: `1 ≤ b ≤ 2000`
- `[input]` integer `n`
The number of magic marbles in your possession, a non-negative integer.
Constraints: `0 ≤ n ≤ 5`
- `[output]` an integer | games | def magical_well(a, b, n):
return sum((a + i) * (b + i) for i in range(n))
| Simple Fun #13: Magical Well | 588463cae61257e44600006d | [
"Puzzles"
]
| https://www.codewars.com/kata/588463cae61257e44600006d | 7 kyu |
# Task
Given integers `n`, `l` and `r`, find the number of ways to represent `n` as a sum of two integers A and B such that `l ≤ A ≤ B ≤ r`.
# Example
For n = 6, l = 2 and r = 4, the output should be `2`
There are just two ways to write 6 as A + B, where 2 ≤ A ≤ B ≤ 4:
`6 = 2 + 4` and `6 = 3 + 3`
# Input/Output
- `[input]` integer `n`
A positive integer.
Constraints: 5 ≤ n ≤ 10<sup>6</sup>.
- `[input]` integer `l`
A positive integer.
Constraints: 1 ≤ l ≤ r.
- `[input]` integer `r`
A positive integer.
Constraints: l ≤ r ≤ 10<sup>6</sup>
- `[output]` an integer | games | def count_sum_of_two_representations(n, l, r):
return max((min(r, n - l) - max(l, n - r)) / / 2 + 1, 0)
| Simple Fun #12: Count Sum of Two Representions | 5884615cbd573356ab000050 | [
"Puzzles"
]
| https://www.codewars.com/kata/5884615cbd573356ab000050 | 7 kyu |
# Task
You are given a 32-bit integer `n`. Swap each pair of adjacent bits in its binary representation and return the result as a decimal number.
The potential leading zeroes of the binary representations have to be taken into account, e.g. `0b100` as a 32-bit integer is `0b00000000000000000000000000000100` and is reversed to `0b1000`.
# Examples
For n = `13`, the output should be `14`:
13<sub>10</sub> = 1101<sub>2</sub> --> 1110<sub>2</sub> = 14<sub>10</sub>
For n = `74`, the output should be `133`:
74<sub>10</sub> = 01001010<sub>2</sub> --> 10000101<sub>2</sub> = 133<sub>10</sub>
# Input/Output
- `[input]` integer `n`
```if-not:c
` 0 ≤ n < 2^30.`
```
- `[output]` an integer
~~~if:lambdacalc
# Encodings
purity: `LetRec`<br>
numEncoding: [`BinaryScott`](https://github.com/codewars/lambda-calculus/wiki/encodings-guide#scott-binary-encoded-numerals) (leading zeroes are permitted)
~~~ | games | def swap_adjacent_bits(n):
return (n & 0x55555555) << 1 | (n & 0xaaaaaaaa) >> 1
| Simple Fun #11: Swap Adjacent Bits | 58845a92bd573378f4000035 | [
"Puzzles"
]
| https://www.codewars.com/kata/58845a92bd573378f4000035 | 7 kyu |
# Task
Let's say that number a feels comfortable with number b if a ≠ b and b lies in the segment` [a - s(a), a + s(a)]`, where `s(x)` is the sum of x's digits.
How many pairs (a, b) are there, such that a < b, both a and b lie on the segment `[L, R]`, and each number feels comfortable with the other?
# Example
For `L = 10 and R = 12`, the output should be `2`
Here are all values of s(x) to consider:
```
s(10) = 1, so 10 is comfortable with 9 and 11;
s(11) = 2, so 11 is comfortable with 9, 10, 12 and 13;
s(12) = 3, so 12 is comfortable with 9, 10, 11, 13, 14 and 15.
Thus, there are 2 pairs of numbers comfortable
with each other within the segment [10; 12]:
(10, 11) and (11, 12).
```
# Input/Output
- `[input]` integer `L`
Constraints: `1 ≤ L ≤ R ≤ 1000`
- `[input]` integer `R`
Constraints: `1 ≤ L ≤ R ≤ 1000`
- `[output]` an integer
The number of pairs satisfying all the above conditions. | games | def comfortable_numbers(l, r):
s = [sum(map(int, str(n))) for n in range(l, r + 1)]
return sum(s[i] >= i - j <= s[j] for i in range(1, len(s)) for j in range(i))
| Simple Fun #25: Comfortable Numbers | 5886dbc685d5788715000071 | [
"Puzzles"
]
| https://www.codewars.com/kata/5886dbc685d5788715000071 | 6 kyu |
We want to generate all the numbers of three digits where:
- the sum of their digits is equal to `10`
- their digits are in increasing order (the numbers may have two or more equal contiguous digits)
The numbers that fulfill these constraints are: `[118, 127, 136, 145, 226, 235, 244, 334]`. There're `8` numbers in total with `118` being the lowest and `334` being the greatest.
___
## Task
Implement a function which receives two arguments:
1. the sum of digits (`sum`)
2. the number of digits (`count`)
This function should return three values:
1. the total number of values which have `count` digits that add up to `sum` and are in increasing order
2. the lowest such value
3. the greatest such value
**Note**: if there're no values which satisfy these constaints, you should return an empty value (refer to the examples to see what exactly is expected).
## Examples
```python
find_all(10, 3) => [8, 118, 334]
find_all(27, 3) => [1, 999, 999]
find_all(84, 4) => []
```
```ruby
find_all(10, 3) => [8, 118, 334]
find_all(27, 3) => [1, 999, 999]
find_all(84, 4) => []
```
```crystal
find_all(10, 3) => [8, 118, 334]
find_all(27, 3) => [1, 999, 999]
find_all(84, 4) => []
```
```javascript
findAll(10, 3) => [8, "118", "334"]
findAll(27, 3) => [1, "999", "999"]
findAll(84, 4) => []
```
```haskell
findAll 10 3 => ( 8, Just 118, Just 334 )
findAll 27 3 => ( 1, Just 999, Just 999 )
findAll 84 4 => ( 0, Nothing, Nothing )
```
```java
// The output type is List<Long>
HowManyNumbers.findAll(10, 3) => [8, 118, 334]
HowManyNumbers.findAll(27, 3) => [1, 999, 999]
HowManyNumbers.findAll(84, 4) => []
```
```csharp
// The output type is List<long>
HowManyNumbers.FindAll(10, 3) => [8, 118, 334]
HowManyNumbers.FindAll(27, 3) => [1, 999, 999]
HowManyNumbers.FindAll(84, 4) => []
```
```cpp
// The output type is optional<tuple<uint32_t, uint64_t, uint64_t>>
find_all(10, 3) => (8, 118, 334)
find_all(27, 3) => (1, 999, 999)
find_all(84, 4) => nullopt
```
___
Features of the random tests:
* Number of tests: `112`
* Sum of digits value between `20` and `65`
* Amount of digits between `2` and `17` | reference | from itertools import combinations_with_replacement
def find_all(sum_dig, digs):
combs = combinations_with_replacement(list(range(1, 10)), digs)
target = ['' . join(str(x) for x in list(comb))
for comb in combs if sum(comb) == sum_dig]
if not target:
return []
return [len(target), int(target[0]), int(target[- 1])]
| How many numbers III? | 5877e7d568909e5ff90017e6 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic"
]
| https://www.codewars.com/kata/5877e7d568909e5ff90017e6 | 4 kyu |
# Task
A cake is sliced with `n` straight lines. Your task is to calculate the maximum number of pieces the cake can have.
# Example
For `n = 0`, the output should be `1`.
For `n = 1`, the output should be `2`.
For `n = 2`, the output should be `4`.
For `n = 3`, the output should be `7`.
See the following image to understand it:

# Input/Output
- `[input]` integer `n`
`0 ≤ n ≤ 10000`
- `[output]` an integer
The maximum number of pieces the sliced cake can have. | games | def cake_slice(n):
return (n * * 2 + n + 2) / / 2
| Simple Fun #198: Cake Slice | 58c8a41bedb423240a000007 | [
"Puzzles"
]
| https://www.codewars.com/kata/58c8a41bedb423240a000007 | 7 kyu |
Today was a sad day. Having bought a new beard trimmer, I set it to the max setting and shaved away at my joyous beard. Stupidly, I hadnt checked just how long the max setting was, and now I look like Ive just started growing it!
Your task, given a beard represented as an arrayof arrays, is to trim the beard as follows:
['|', 'J', '|', '|'],<br>
['|', '|', '|', 'J'],<br>
['...', '...', '...', '...'];<br>
To trim the beard use the following rules:<br>
trim any curled hair --> replace 'J' with '|'
trim any hair from the chin (last array) --> replace '|' or 'J' with '...'
All sub arrays will be same length. Return the corrected array of arrays | reference | def trim(beard):
return [[h . replace("J", "|") for h in b] for b in beard[: - 1]] + [["..."] * len(beard[0])]
| Shaving the Beard | 57efa1a2108d3f73f60000e9 | [
"Fundamentals",
"Arrays"
]
| https://www.codewars.com/kata/57efa1a2108d3f73f60000e9 | 7 kyu |
You would like to get the 'weight' of a name by getting the sum of the ascii values. However you believe that capital letters should be worth more than mere lowercase letters. Spaces, numbers, or any other character are worth 0.
Normally in ascii
a has a value of 97
A has a value of 65
' ' has a value of 32
0 has a value of 48
To find who has the 'weightier' name you will switch all the values so:
A will be 97
a will be 65
' ' will be 0
0 will be 0
etc...
For example Joe will have a weight of 254, instead of 286 using normal ascii values. | reference | def get_weight(name):
return sum(ord(a) for a in name . swapcase() if a . isalpha())
| Disagreeable ascii | 582cb3a637c5583f2200005d | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/582cb3a637c5583f2200005d | 7 kyu |
Finding your seat on a plane is never fun, particularly for a long haul flight... You arrive, realise again just how little leg room you get, and sort of climb into the seat covered in a pile of your own stuff.
To help confuse matters (although they claim in an effort to do the opposite) many airlines omit the letters 'I' and 'J' from their seat naming system.
the naming system consists of a number (in this case between 1-60) that denotes the section of the plane where the seat is (1-20 = front, 21-40 = middle, 40+ = back). This number is followed by a letter, A-K with the exclusions mentioned above.
Letters A-C denote seats on the left cluster, D-F the middle and G-K the right.
Given a seat number, your task is to return the seat location in the following format:
'2B' would return 'Front-Left'.
If the number is over 60, or the letter is not valid, return 'No Seat!!'. | reference | def plane_seat(a):
front, middle, back = (range(1, 21), range(21, 41), range(41, 61))
left, center, right = ('ABC', 'DEF', "GHK")
x, y = ('', '')
if int(a[: - 1]) in front:
x = 'Front-'
if int(a[: - 1]) in middle:
x = 'Middle-'
if int(a[: - 1]) in back:
x = 'Back-'
if a[- 1] in left:
y = 'Left'
if a[- 1] in center:
y = 'Middle'
if a[- 1] in right:
y = 'Right'
return x + y if all((x, y)) else 'No Seat!!'
| Holiday II - Plane Seating | 57e8f757085f7c7d6300009a | [
"Fundamentals",
"Strings",
"Arrays"
]
| https://www.codewars.com/kata/57e8f757085f7c7d6300009a | 7 kyu |
Your dad doesn't really *get* punctuation, and he's always putting extra commas in when he posts. You can tolerate the run-on sentences, as he does actually talk like that, but those extra commas have to go!
Write a function called ```dadFilter``` that takes a string as argument and returns a string with the extraneous commas removed. The returned string should not end with a comma or any trailing whitespace. | reference | from re import compile, sub
REGEX = compile(r',+')
def dad_filter(strng):
return sub(REGEX, ',', strng). rstrip(' ,')
| Dad is Commatose | 56a24b309f3671608b00003a | [
"Fundamentals",
"Strings",
"Regular Expressions"
]
| https://www.codewars.com/kata/56a24b309f3671608b00003a | 7 kyu |
**Task:** Find the number couple with the greatest difference from a list of number-couples.
**Input:** A list of number-couples, where each couple is represented as a string containing two positive integers separated by a hyphen ("-").
**Output:** The number couple with the greatest difference, or `False` if there is no difference among any of the couples or in case of an empty list.
**Additional Information:**
- All number couples will be given as strings.
- All numbers in the couples are positive integers.
- If multiple couples have the same greatest difference, return the first one encountered in the input list.
- If there is no difference (both numbers in a couple are equal), return `False`. | reference | def diff(arr):
r = arr and max(arr, key=lambda x: abs(eval(x)))
return bool(arr and eval(r)) and r
| Greatest Difference | 56b12e3ad2387de332000041 | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/56b12e3ad2387de332000041 | 7 kyu |
Implement function `createTemplate` which takes string with tags wrapped in `{{brackets}}` as input and returns closure, which can fill string with data (flat object, where keys are tag names).
```javascript
let personTmpl = createTemplate("{{name}} likes {{animalType}}");
personTmpl({ name: "John", animalType: "dogs" }); // John likes dogs
```
```clojure
((template "{{name}} likes {{animalType}}") {:name "John" :animalType "dogs"}) ;; John likes dogs
```
```python
template = create_template("{{name}} likes {{animalType}}")
template(name="John", animalType="dogs") # John likes dogs
```
```ruby
template = Template.new("{{name}} likes {{animal_type}}")
template.render(name: "John", animal_type: "dogs")
```
When key doesn't exist in the map, put there empty string. | reference | from jinja2 import Template
def create_template(template):
return lambda * * vals: Template(template). render(vals)
| Simple template | 56ae72854d005c7447000023 | [
"Strings",
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/56ae72854d005c7447000023 | 7 kyu |
Just as the title suggestes :D .
For example ->
```
largest(1); //Should return 7
largest(2); //Should return 97
....
```
Do not mind the pattern as it is just an incident ! And make sure to return false if the input is not an integer :D
This might seem simple at first, it is, but keep an eye on the performance.
Go for it ! | games | def largest(n):
return [7, 97, 997, 9973, 99991, 999983][n - 1] if type(n) == int and n > 0 else False
| Largest prime number containing n digit | 5676f07029da352ba2000065 | [
"Algorithms",
"Puzzles",
"Fundamentals",
"Performance"
]
| https://www.codewars.com/kata/5676f07029da352ba2000065 | 7 kyu |
A *perfect number* is a number in which the sum of its divisors (excluding itself) are equal to itself.
Write a function that can verify if the given integer `n` is a perfect number, and return `True` if it is, or return `False` if not.
## Examples
`n = 28` has the following divisors: `1, 2, 4, 7, 14, 28`
`1 + 2 + 4 + 7 + 14 = 28` therefore `28` is a perfect number, so you should return `True`
Another example:
`n = 25` has the following divisors: `1, 5, 25`
`1 + 5 = 6` therefore `25` is **not** a perfect number, so you should return `False`
| algorithms | def isPerfect(n):
return n in [6, 28, 496, 8128, 33550336, 8589869056, 137438691328]
| Perfect Number Verifier | 56a28c30d7eb6acef700004d | [
"Algorithms"
]
| https://www.codewars.com/kata/56a28c30d7eb6acef700004d | 7 kyu |
~~~if-not:prolog
## Write a generic function chainer
Write a generic function chainer that takes a starting value, and an array of functions to execute on it (array of symbols for Ruby).
The input for each function is the output of the previous function (except the first function, which takes the starting value as its input). Return the final value after execution is complete.
~~~
~~~if:prolog
## Write a generic predicate chainer
Write a generic predicate chainer that takes a starting value, and an array of atoms to execute on it.
The first input for each predicate is the output of the previous predicate (except the first predicate, which takes the starting value as its input). Return the final value after execution is complete.
~~~
```javascript
function add(num) {
return num + 1;
}
function mult(num) {
return num * 30;
}
chain(2, [add, mult]);
// returns 90;
```
```haskell
add = (+ 1)
mul = (* 30)
chain 2 [add, mult] -> 90
```
```ruby
def add num
num + 1
end
def mult num
num * 30
end
chain(2, [:add, :mult])
#=> returns 90
```
```csharp
double input = 2;
public static double add(double x) {
return x + 1;
}
public static double mul(double x) {
return x * 30;
}
Kata.Chain( input , new[] { (Func<double, double>)add, mul });
//=> returns 90
```
```c
int add10 (int x) { return x + 10; }
int mul30 (int x) { return x * 30; }
typedef int (*funcptr) (int);
chain(50, 2, (funcptr[2]) {add10, mul30});
// returns 1800
```
```python
def add10(x): return x + 10
def mul30(x): return x * 30
chain(50, [add10, mul30])
# returns 1800
```
```factor
: add10 ( x -- r ) 10 + ;
: mul30 ( x -- r ) 30 * ;
50 { [ add10 ] [ mul30 ] } chain
! returns 1800
```
```ocaml
let add n = n + 1 in
let mult n = n * 30 in
chain 2 [add; mult] (* -> 90 *)
```
```prolog
add(X,R):-R is X+1.
mul(X,R):-R is X*30.
?- chain(2,[add,mul],Result). % Result = 90.
```
```rust
fn add10(x) { x + 10 }
fn mul30(x) { x + 30 }
chain(50, &[&add10, &mul30]) //=> returns 1800
``` | reference | def chain(value, functions):
for f in functions:
value = f(value)
return value
| Chain me | 54fb853b2c8785dd5e000957 | [
"Fundamentals"
]
| https://www.codewars.com/kata/54fb853b2c8785dd5e000957 | 7 kyu |
Given a sequence of numbers, find the largest pair sum in the sequence.
For example
```
[10, 14, 2, 23, 19] --> 42 (= 23 + 19)
[99, 2, 2, 23, 19] --> 122 (= 99 + 23)
```
Input sequence contains minimum two elements and every element is an integer. | reference | def largest_pair_sum(numbers):
return sum(sorted(numbers)[- 2:])
| Largest pair sum in array | 556196a6091a7e7f58000018 | [
"Fundamentals"
]
| https://www.codewars.com/kata/556196a6091a7e7f58000018 | 7 kyu |
It is 2050 and romance has long gone, relationships exist solely for practicality.
MatchMyHusband is a website that matches busy working women with perfect house husbands. You have been employed by MatchMyHusband to write a function that determines who matches!!
The rules are... a match occurs providing the husband's "usefulness" rating is greater than or equal to the woman's "needs".
The husband's "usefulness" is the <strong>SUM</strong> of his cooking, cleaning and childcare abilities and takes the form of an<strong> array </strong>.
usefulness example --> [15, 26, 19] (15 + 26 + 19) = 60
Every woman that signs up, begins with a "needs" rating of <strong>100</strong>. However, it's realised that the longer women wait for their husbands, the more dissatisfied they become with our service. They also become less picky, therefore their needs are subject to exponential decay of 15% per month. https://en.wikipedia.org/wiki/Exponential_decay
Given the number of months since sign up, write a function that returns "Match!" if the husband is useful enough, or "No match!" if he's not.
| reference | def match(usefulness, months):
return "Match!" if sum(usefulness) >= 0.85 * * months * 100 else "No match!"
| Match My Husband | 5750699bcac40b3ed80001ca | [
"Fundamentals",
"Mathematics",
"Algorithms"
]
| https://www.codewars.com/kata/5750699bcac40b3ed80001ca | 7 kyu |
Apparently "Put A Pillow On Your Fridge Day is celebrated on the 29th of May each year, in Europe and the U.S. The day is all about prosperity, good fortune, and having bit of fun along the way."
All seems very weird to me.
Nevertheless, you will be given an array of two strings (s). First find out if the first string contains a fridge... (i've deemed this as being 'n', as it looks like it could hold something).
Then check that the second string has a pillow - deemed 'B' (struggled to get the obvious pillow-esque character).
If the pillow is on top of the fridge - it must be May 29th! Or a weird house... Return true; For clarity, on top means right on top, ie in the same index position.
If the pillow is anywhere else in the 'house', return false;
There may be multiple fridges, and multiple pillows. But you need at least 1 pillow ON TOP of a fridge to return true. Multiple pillows on fridges should return true also.
100 random tests | reference | def pillow(s): return ('n', 'B') in zip(* s)
| Pillow on the Fridge | 57d147bcc98a521016000320 | [
"Fundamentals",
"Strings",
"Arrays"
]
| https://www.codewars.com/kata/57d147bcc98a521016000320 | 7 kyu |
# Story
Once Mary heard a famous song, and a line from it stuck in her head. That line was "Will you still love me when I'm no longer young and beautiful?". Mary believes that a person is loved if and only if he/she is both young and beautiful, but this is quite a depressing thought, so she wants to put her belief to the test.
# Task
Knowing whether a person is young, beautiful and loved, find out if they contradict Mary's belief.
A person contradicts Mary's belief if one of the following statements is true:
```
they are young and beautiful but not loved;
they are loved but not young or not beautiful.
```
# Example
For young = true, beautiful = true and loved = true, the output should be `false`.
Young and beautiful people are loved according to Mary's belief.
For young = true, beautiful = false and loved = true, the output should be `true`.
Mary doesn't believe that not beautiful people can be loved.
# Input/Output
- `[input]` boolean `young`
- `[input]` boolean `beautiful`
- `[input]` boolean `loved`
- `[output]` a boolean value
true if the person contradicts Mary's belief, false otherwise. | games | def will_you(young, beautiful, loved):
return (young and beautiful) != loved
| Simple Fun #7: Will You? | 58844a13aa037ff143000072 | [
"Puzzles"
]
| https://www.codewars.com/kata/58844a13aa037ff143000072 | 7 kyu |
Fast & Furious Driving School's (F&F) charges for lessons are as below:
<p><table>
<tr>
<th>Time</th>
<th>Cost</th>
</tr>
<tr>
<td>Up to 1st hour</td>
<td>$30</td>
</tr>
<tr>
<td>Every subsequent half hour**</td>
<td>$10</td>
</tr>
<tr>
<td><h6>** Subsequent charges are calculated by rounding up to nearest half hour.</h6></td>
</tr>
</table>
<p>For example, if student X has a lesson for 1hr 20 minutes, he will be charged $40 (30+10) for 1 hr 30 mins and if he has a lesson for 5 minutes, he will be charged $30 for the full hour.
Out of the kindness of its heart, F&F also provides a 5 minutes grace period. So, if student X were to have a lesson for 65 minutes or 1 hr 35 mins, he will only have to pay for an hour or 1hr 30 minutes respectively.
For a given lesson time in minutes (min) , write a function <i>cost</i> to calculate how much the lesson costs. Input is always > 0.
| reference | import math
def cost(mins):
return 30 + 10 * math . ceil(max(0, mins - 60 - 5) / 30)
| Driving School Series #2 | 589b1c15081bcbfe6700017a | [
"Mathematics",
"Fundamentals"
]
| https://www.codewars.com/kata/589b1c15081bcbfe6700017a | 7 kyu |
A Narcissistic Number is a number of length `l` in which the sum of its digits to the power of `l` is equal to the original number. If this seems confusing, refer to the example below.
Ex: `153`, where `l = 3` ( the number of digits in `153` )
1<sup>3</sup> + 5<sup>3</sup> + 3<sup>3</sup> = 153
Write a function that, given `n`, returns whether or not `n` is a Narcissistic Number. | reference | def is_narcissistic(n):
num = str(n)
length = len(num)
return sum(int(a) * * length for a in num) == n
| Narcissistic Numbers | 56b22765e1007b79f2000079 | [
"Fundamentals"
]
| https://www.codewars.com/kata/56b22765e1007b79f2000079 | 7 kyu |
On the Forex Market the currency symbols for exchange between two currencies are put together in regards to their strength and weakness. The order of the currency strength is as follows:
"EUR", "GBP", "AUD", "NZD", "USD", "CAD", "CHF", "JPY"
So for AUD the currency matrix would be as follows
EURAUD, GBPAUD, AUDNZD, AUDUSD, AUDCAD, AUDCHF, AUDJPY
Your goal is to generate this currency matrix for a given currency. You can assume that the passed in currency is a valid one. | reference | def generate_currency_matrix(c):
t = ("EUR", "GBP", "AUD", "NZD", "USD", "CAD", "CHF", "JPY")
return ["%s%s" % tuple(sorted((c, o), key=t . index)) for o in t if o != c]
| Currency Matrix Generator | 57bec3bc5640aa5f3f00003e | [
"Fundamentals"
]
| https://www.codewars.com/kata/57bec3bc5640aa5f3f00003e | 7 kyu |
Note : Issues Fixed with python 2.7.6 , Use any one you like :D , ( Thanks to
Time , time , time . Your task is to write a function that will return the degrees on a analog clock from a digital time that is passed in as parameter . The digital time is type string and will be in the format 00:00 . You also need to return the degrees on the analog clock in type string and format 360:360 . Remember to round of the degrees . Remeber the basic time rules and format like 24:00 = 00:00 and 12:60 = 13:00 . Create your own validation that should return "Check your time !" in any case the time is incorrect or the format is wrong , remember this includes passing in negatives times like "-01:-10".
```
A few examples :
clock_degree("00:00") will return : "360:360"
clock_degree("01:01") will return : "30:6"
clock_degree("00:01") will return : "360:6"
clock_degree("01:00") will return : "30:360"
clock_degree("01:30") will return : "30:180"
clock_degree("24:00") will return : "Check your time !"
clock_degree("13:60") will return : "Check your time !"
clock_degree("20:34") will return : "240:204"
```
Remember that discrete hour hand movement is required - snapping to each hour position and also coterminal angles are not allowed. Goodluck and Enjoy ! | reference | def clock_degree(clock_time):
hour, minutes = (int(a) for a in clock_time . split(':'))
if not (24 > hour >= 0 and 60 > minutes >= 0):
return 'Check your time !'
return '{}:{}' . format((hour % 12) * 30 or 360, minutes * 6 or 360)
| Time Degrees | 5782a87d9fb2a5e623000158 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5782a87d9fb2a5e623000158 | 7 kyu |
Complete the function that returns a christmas tree of the given height. The height is passed through to the function and the function should return a list containing each line of the tree.
```
XMasTree(5) should return : ['____#____', '___###___', '__#####__', '_#######_', '#########', '____#____', '____#____']
XMasTree(3) should return : ['__#__', '_###_', '#####', '__#__', '__#__']
```
Pad with underscores (`_`) so each line is the same length. Also remember the trunk/stem of the tree.
## Examples
The final idea is for the tree to look like this if you decide to print each element of the list:
`n = 5` will result in:
```
____#____ 1
___###___ 2
__#####__ 3
_#######_ 4
######### -----> 5 - Height of Tree
____#____ 1
____#____ 2 - Trunk/Stem of Tree
```
`n = 3` will result in:
```
__#__ 1
_###_ 2
##### -----> 3 - Height of Tree
__#__ 1
__#__ 2 - Trunk/Stem of Tree
```
| algorithms | def xMasTree(n):
return [("#" * (x * 2 + 1)). center(n * 2 - 1, "_") for x in list(range(n)) + [0] * 2]
| Xmas Tree | 577c349edf78c178a1000108 | [
"Algorithms"
]
| https://www.codewars.com/kata/577c349edf78c178a1000108 | 7 kyu |
###BACKGROUND:
Jacob recently decided to get healthy and lose some weight. He did a lot of reading and research and after focusing on steady exercise and a healthy diet for several months, was able to shed over 50 pounds! Now he wants to share his success, and has decided to tell his friends and family how much weight they could expect to lose if they used the same plan he followed.
Lots of people are really excited about Jacob's program and they want to know how much weight they would lose if they followed his plan. Unfortunately, he's really bad at math, so he's turned to you to help write a program that will calculate the expected weight loss for a particular person, given their weight and how long they think they want to continue the plan.
###TECHNICAL DETAILS:
Jacob's weight loss protocol, if followed closely, yields loss according to a simple formulae, depending on gender. Men can expect to lose 1.5% of their current body weight each week they stay on plan. Women can expect to lose 1.2%. (Children are advised to eat whatever they want, and make sure to play outside as much as they can!)
###TASK:
Write a function that takes as input:
```
- The person's gender ('M' or 'F');
- Their current weight (in pounds);
- How long they want to stay true to the protocol (in weeks);
```
and then returns the expected weight at the end of the program.
###NOTES:
Weights (both input and output) should be decimals, rounded to the nearest tenth.
Duration (input) should be a whole number (integer). If it is not, the function should round to the nearest whole number.
When doing input parameter validity checks, evaluate them in order or your code will not pass final tests. | reference | def lose_weight(gender, weight, duration):
if not gender in ['M', 'F']:
return 'Invalid gender'
if weight <= 0:
return 'Invalid weight'
if duration <= 0:
return 'Invalid duration'
nl = 0.985 if gender == 'M' else 0.988
for i in range(duration):
weight *= nl
return round(weight, 1)
| Jacob's Weight Loss Program | 573b216f5328ffcd710013b3 | [
"Fundamentals"
]
| https://www.codewars.com/kata/573b216f5328ffcd710013b3 | 7 kyu |
Build a function that will take the length of each side of a triangle and return if it's either an Equilateral, an Isosceles, a Scalene or an invalid triangle.
It has to return a string with the type of triangle.
For example:
```javascript
typeOfTriangle(2,2,1) --> "Isosceles"
```
```coffeescript
typeOfTriangle(2,2,1) --> "Isosceles"
```
```ruby
type_of_triangle(2, 2, 1) --> "Isosceles"
```
```python
type_of_triangle(2, 2, 1) --> "Isosceles"
```
| algorithms | def type_of_triangle(a, b, c):
if any(not isinstance(x, int) for x in (a, b, c)):
return "Not a valid triangle"
a, b, c = sorted((a, b, c))
if a + b <= c:
return "Not a valid triangle"
if a == b and b == c:
return "Equilateral"
if a == b or a == c or b == c:
return "Isosceles"
return "Scalene"
| Which triangle is that? | 564d398e2ecf66cec00000a9 | [
"Geometry",
"Algorithms"
]
| https://www.codewars.com/kata/564d398e2ecf66cec00000a9 | 7 kyu |
**This Kata is intended as a small challenge for my students**
All Star Code Challenge #31
Walter has a doctor's appointment and has to leave Jesse in charge of preparing their next "cook". He left Jesse an array of Instruction objects, which contain solutions, amounts, and the appropriate scientific instrument to use for each step in the "cook". The order of the instructions must be carried out in the EXACT order they're given. However, Jesse doesn't understand JavaScript and just needs things simplified!
Create a function called helpJesse() that accepts an array of Instruction objects as an argument. The function should convert each object into a string of the following format:
"Pour {amount} mL of {solution} into a {instrument}"
It should return an array of each object-to-string conversion, in the same order given.
Walter may leave an optional "note" in the Instruction object, which should be included at the end of the string in parentheses, like so:
"Pour {amount} mL of {solution} into a {instrument} ({note})"
```javascript
function Instruction(amount, solution, instrument, note){
this.amount=amount;
this.solution=solution;
this.instrument=instrument;
if (note){
this.note = note;
}
}
var recipe = [
new Instruction(5,"Sodium Chloride","Graduated Cylinder"),
new Instruction(250,"Hydrochloric Acid","Boiling Flask"),
new Instruction(100,"Water","Erlenmeyer Flask", "Do NOT mess this step up, Jesse!")];
helpJesse(recipe);
// ["Pour 5 mL of Sodium Chloride into a Graduated Cylinder",
// "Pour 250 mL of Hydrochloric Acid into a Boiling Flask",
// "Pour 100 mL of Water into a Erlenmeyer Flask (Do NOT mess this step up, Jesse!)"]
```
```python
class Instruction:
__init__(self, amount, solution, instrument, note):
self.amount=amount
self.solution=solution
self.instrument=instrument
self.note = note
recipe = [
Instruction(5,"Sodium Chloride","Graduated Cylinder"),
Instruction(250,"Hydrochloric Acid","Boiling Flask"),
Instruction(100,"Water","Erlenmeyer Flask", "Do NOT mess this step up, Jesse!")
]
helpJesse(recipe);
# ["Pour 5 mL of Sodium Chloride into a Graduated Cylinder",
# "Pour 250 mL of Hydrochloric Acid into a Boiling Flask",
# "Pour 100 mL of Water into a Erlenmeyer Flask (Do NOT mess this step up, Jesse!)"]
```
```ruby
class Instruction
def initialize(amount, solution, instrument, note)
@amount=amount
@solution=solution
@instrument=instrument
@note = note
end
end
recipe = [
Instruction.new(5,"Sodium Chloride","Graduated Cylinder"),
Instruction.new(250,"Hydrochloric Acid","Boiling Flask"),
Instruction.new(100,"Water","Erlenmeyer Flask", "Do NOT mess this step up, Jesse!")
]
helpJesse(recipe);
# ["Pour 5 mL of Sodium Chloride into a Graduated Cylinder",
# "Pour 250 mL of Hydrochloric Acid into a Boiling Flask",
# "Pour 100 mL of Water into a Erlenmeyer Flask (Do NOT mess this step up, Jesse!)"]
```
Note:
Assume all keys in the Instruction objects are properly filled and do not need to be checked for format or value type. | reference | def help_jesse(arr):
fmt = 'Pour {a.amount} mL of {a.solution} into a {a.instrument}' . format
note = ' ({a.note})' . format
return [fmt(a=a) + (note(a=a) if hasattr(a, 'note') else '') for a in arr]
| All Star Code Challenge #31 | 5866f10311ceec6ac10001e8 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5866f10311ceec6ac10001e8 | 7 kyu |
## Task
You need to create a function, `helloWorld`, that will return the String `Hello, World!` without actually using raw strings. This includes quotes, double quotes and template strings. You can, however, use the `String` constructor and any related functions.
You cannot use the following:
```
"Hello, World!"
'Hello, World!'
`Hello, World!`
```
Good luck and try to be as creative as possible!
| games | def hello_world(): return bytes(
[72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33]). decode()
| Hello World - Without Strings | 584c7b1e2cb5e1a727000047 | [
"Strings",
"Restricted",
"Puzzles"
]
| https://www.codewars.com/kata/584c7b1e2cb5e1a727000047 | 7 kyu |
If you did Geometric Mean I ( http://www.codewars.com/kata/geometric-mean-i), you may continue with this second part.
We have seen the formula:
<a href="http://imgur.com/tD9bZui"><img src="http://i.imgur.com/tD9bZui.png?1" title="source: imgur.com" /></a>
This expression is not convenient to apply to statistical researches, specially when we have high values of the variable ```x``` and/or many entries of it, because the value of the product will be huge and an error message will appear in our codes for the calculation of the geometric mean.
In real life, most of the researches will not have so many values of a variable but each one repeated with a certain specific frequency.
This would be our situation using the above formula:
<a href="http://imgur.com/UXxrzTi"><img src="http://i.imgur.com/UXxrzTi.png?1" title="source: imgur.com" /></a>
Implementing logarithms we have:
<a href="https://imgur.com/onWZA4H"><img src="https://i.imgur.com/onWZA4H.jpg?1" title="source: imgur.com" /></a>
The logarithms more suitable for this formula are the ones with `base 10`.
Our task is to implement the function ```geometric_meanII()```(```geometricMeanII```, Javascript), that should be able to make the calculations of the geometric mean from pretty much longer arrays than the ones used for Goemetric Mean I.
The function will output the value of the geometric mean without roundings.
We will use the same criteria for invalid entries than the previous kata.
(strings and negative values of x)
```ruby
amount of entries maximum invalid entries
2 - 10 1
From 11 and above <10 % of total of entries
```
```python
amount of entries maximum invalid entries
2 - 10 1
From 11 and above 10 % of total of entries
```
```javascript
amount of entries maximum invalid entries
2 - 10 1
From 11 and above 10 % of total of entries
```
If the limit of bad entries is exceeded, the function should output 0
(When you finish this kata, Geometric Mean III will be waiting you) | reference | import math
def geometric_meanII(arr):
gm = 0
c = 0
f = 0
l = len(arr)
for a in arr:
if type(a) == int and a >= 0:
gm += math . log(a)
c += 1
else:
f += 1
if l <= 10:
if c < l - 1:
return 0
else:
if f * 100 / l > 10:
return 0
return math . exp(gm / c)
| Geometric Mean II | 56ec6016a9dfe3346e001242 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic",
"Strings"
]
| https://www.codewars.com/kata/56ec6016a9dfe3346e001242 | 6 kyu |
For a variable, ```x```, that may have different values, ```the geometric mean``` is defined as:
```math
G = \sqrt[\large{n}]{x_1 \cdot x_2 \cdot \ldots \cdot x_n}
```
Suposse that you have to calculate **the geometric mean** for a research where the amount of values of x is rather small.
Implement the function ```geometric_meanI()```, (```geometricMeanI javascript```)that receives an array with the different values of the variable and outputs the geometric mean value.
The negative values and strings will be discarded for the calculations.
Nevertheless if the amount of total invalid values is too high, the function will return ```0``` (Nothing in Haskell). The tolerance for invalid values of the variable will be as follows:
```ruby
amount of entries maximum invalid entries
2 - 10 1
From 11 and above < 10 % of total of entries
```
```javascript
amount of entries maximum invalid entries
2 - 10 1
From 11 and above 10 % of total of entries
```
```haskell
amount of entries maximum invalid entries
2 - 10 1
From 11 and above 10 % of total of entries
```
```python
amount of entries maximum invalid entries
2 - 10 1
From 11 and above 10 % of total of entries
```
You do not have to round the results.
(When you finish this kata, Geometric Mean II will be waiting you. http://www.codewars.com/kata/56ec6016a9dfe3346e001242)
| reference | # No need to reinvent the wheel
from scipy . stats . mstats import gmean
def geometric_meanI(arr):
if 0 in arr:
return 0
L = list(filter(lambda x: isinstance(x, int) and x > 0, arr))
if 9 * len(arr) > 9 * len(L) + (len(L) if len(arr) > 10 else 9):
return 0
return gmean(L)
| Geometric Mean I | 56ebcea1b9d927f9bf000544 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic"
]
| https://www.codewars.com/kata/56ebcea1b9d927f9bf000544 | 7 kyu |
Find the volume of a cone whose radius and height are provided as parameters to the function `volume`. Use the value of PI provided by your language (for example: `Math.PI` in JS, `math.pi` in Python or `Math::PI` in Ruby) and round down the volume to an Interger.
If you complete this kata and there are no issues, please remember to give it a ready vote and a difficulty rating. :) | reference | from math import pi
def volume(r, h):
return pi * r * * 2 * h / / 3
| Find the volume of a Cone. | 57b2e428d24156b312000114 | [
"Geometry",
"Fundamentals"
]
| https://www.codewars.com/kata/57b2e428d24156b312000114 | 7 kyu |
# Task
Given a rectangular matrix containing only digits, calculate the number of different `2 × 2` squares in it.
# Example
For
```
matrix = [[1, 2, 1],
[2, 2, 2],
[2, 2, 2],
[1, 2, 3],
[2, 2, 1]]
```
the output should be `6`.
Here are all 6 different 2 × 2 squares:
```
1 2
2 2
2 1
2 2
2 2
2 2
2 2
1 2
2 2
2 3
2 3
2 1
```
# Input/Output
- `[input]` 2D integer array `matrix`
Constraints:
`1 ≤ matrix.length ≤ 100,`
`1 ≤ matrix[i].length ≤ 100,`
`0 ≤ matrix[i][j] ≤ 9.`
- `[output]` an integer
The number of different `2 × 2` squares in matrix. | games | def different_squares(matrix):
s = set()
rows, cols = len(matrix), len(matrix[0])
for row in range(rows - 1):
for col in range(cols - 1):
s . add((matrix[row][col], matrix[row][col + 1],
matrix[row + 1][col], matrix[row + 1][col + 1]))
return len(s)
| Simple Fun #35: Different Squares | 588805ca44c7e8c3a100013c | [
"Puzzles"
]
| https://www.codewars.com/kata/588805ca44c7e8c3a100013c | 7 kyu |
# Task
You work in a company that prints and publishes books. You are responsible for designing the page numbering mechanism in the printer. You know how many digits a printer can print with the leftover ink.
Now you want to write a function to determine what the last page of the book is that you can number given the `current` page and `numberOfDigits`/`number_of_digits` left.
A page is considered numbered if it has the full number printed on it (e.g. if we are working with page 102 but have ink only for two digits then this page will not be considered numbered).
It's guaranteed that you can number the current page, and that you can't number the last one in the book.
# Example
For `current = 1 and numberOfDigits = 5`, the output should be `5`
The following numbers will be printed: `1, 2, 3, 4, 5`.
For `current = 21 and numberOfDigits = 5`, the output should be `22`
The following numbers will be printed: `21, 22`.
For `current = 8 and numberOfDigits = 4`, the output should be `10`
The following numbers will be printed: `8, 9, 10`.
# Input/Output
- `[input]` integer `current`
A positive integer, the number on the current page which is not yet printed.
Constraints: `1 ≤ current ≤ 1000`
- `[input]` integer `numberOfDigits`/`number_of_digits`
A positive integer, the number of digits which your printer can print.
Constraints: `1 ≤ numberOfDigits ≤ 1000` (or equivalent in PHP)
- `[output]` an integer
The last printed page number. | games | def pages_numbering_with_ink(current, digits):
while digits >= len(str(current)):
digits = digits - len(str(current))
current = current + 1
return current - 1
| Simple Fun #24: Pages Numbering with Ink | 5886da134703f125a6000033 | [
"Puzzles"
]
| https://www.codewars.com/kata/5886da134703f125a6000033 | 7 kyu |
# Task
A boy is walking a long way from school to his home. To make the walk more fun he decides to add up all the numbers of the houses that he passes by during his walk. Unfortunately, not all of the houses have numbers written on them, and on top of that the boy is regularly taking turns to change streets, so the numbers don't appear to him in any particular order.
At some point during the walk the boy encounters a house with number `0` written on it, which surprises him so much that he stops adding numbers to his total right after seeing that house.
For the given sequence of houses determine the sum that the boy will get. It is guaranteed that there will always be at least one 0 house on the path.
# Example
For `inputArray = [5, 1, 2, 3, 0, 1, 5, 0, 2]`, the output should be `11`.
The answer was obtained as `5 + 1 + 2 + 3 = 11`.
# Input/Output
- `[input]` integer array `inputArray`
Constraints: `5 ≤ inputArray.length ≤ 50, 0 ≤ inputArray[i] ≤ 10.`
- `[output]` an integer | games | def house_numbers_sum(inp):
return sum(inp[: inp . index(0)])
| Simple Fun #37: House Numbers Sum | 58880c6e79a0a3e459000004 | [
"Puzzles"
]
| https://www.codewars.com/kata/58880c6e79a0a3e459000004 | 7 kyu |
# Task
There are some people and cats in a house. You are given the number of legs they have all together. Your task is to return an array containing every possible number of people that could be in the house sorted in ascending order. It's guaranteed that each person has 2 legs and each cat has 4 legs.
# Example
For `legs = 6`, the output should be `[1, 3]`.
There could be either 1 cat and `1 person` (4 + 2 = 6) or `3 people` (2 * 3 = 6).
For `legs = 2`, the output should be `[1]`.
There can be only `1 person`.
# Input/Output
- `[input]` integer `legs`
The total number of legs in the house.
Constraints: `2 ≤ legs ≤ 100.`
- `[output]` an integer array
Every possible number of people that can be in the house. | games | def house_of_cats(legs):
return list(range(legs % 4 / / 2, legs / / 2 + 1, 2))
| Simple Fun #38: House Of Cats | 588810c99fb63e49e1000606 | [
"Puzzles"
]
| https://www.codewars.com/kata/588810c99fb63e49e1000606 | 7 kyu |
# Task
Two arrays are called similar if one can be obtained from another by swapping at most one pair of elements.
Given two arrays, check whether they are similar.
# Example
For `A = [1, 2, 3]` and `B = [1, 2, 3]`, the output should be `true;`
For `A = [1, 2, 3]` and `B = [2, 1, 3]`, the output should be `true;`
For `A = [1, 2, 2]` and `B = [2, 1, 1]`, the output should be `false.`
# Input/Output
- `[input]` integer array `A`
Array of integers.
Constraints: `3 ≤ A.length ≤ 10000, 1 ≤ A[i] ≤ 1000.`
- `[input]` integer array `B`
Array of integers of the same length as `A`.
Constraints: `B.length = A.length, 1 ≤ B[i] ≤ 1000.`
- `[output]` a boolean value
`true` if `A` and `B` are similar, `false` otherwise. | games | def are_similar(a, b):
return sorted(a) == sorted(b) and sum(i != j for i, j in zip(a, b)) in [0, 2]
| Simple Fun #42: Are Similar? | 588820169ab1e053240000e0 | [
"Puzzles"
]
| https://www.codewars.com/kata/588820169ab1e053240000e0 | 7 kyu |
# Story&Task
There are three parties in parliament. The "Conservative Party", the "Reformist Party", and a group of independants.
You are a member of the “Conservative Party” and you party is trying to pass a bill. The “Reformist Party” is trying to block it.
In order for a bill to pass, it must have a majority vote, meaning that more than half of all members must approve of a bill before it is passed . The "Conservatives" and "Reformists" always vote the same as other members of thier parties, meaning that all the members of each party will all vote yes, or all vote no .
However, independants vote individually, and the independant vote is often the determining factor as to whether a bill gets passed or not.
Your task is to find the minimum number of independents that have to vote for your party's (the Conservative Party's) bill so that it is passed .
In each test case the makeup of the Parliament will be different . In some cases your party may make up the majority of parliament, and in others it may make up the minority. If your party is the majority, you may find that you do not neeed any independants to vote in favor of your bill in order for it to pass . If your party is the minority, it may be possible that there are not enough independants for your bill to be passed . If it is impossible for your bill to pass, return `-1`.
# Input/Output
- `[input]` integer `totalMembers`
The total number of members.
- `[input]` integer `conservativePartyMembers`
The number of members in the Conservative Party.
- `[input]` integer `reformistPartyMembers`
The number of members in the Reformist Party.
- `[output]` an integer
The minimum number of independent members that have to vote as you wish so that the bill is passed, or `-1` if you can't pass it anyway.
# Example
For `n = 8, m = 3 and k = 3`, the output should be `2`.
It means:
```
Conservative Party member --> 3
Reformist Party member --> 3
the independent members --> 8 - 3 - 3 = 2
If 2 independent members change their minds
3 + 2 > 3
the bill will be passed.
If 1 independent members change their minds
perhaps the bill will be failed
(If the other independent members is against the bill).
3 + 1 <= 3 + 1
```
For `n = 13, m = 4 and k = 7`, the output should be `-1`.
```
Even if all 2 independent members support the bill
there are still not enough votes to pass the bill
4 + 2 < 7
So the output is -1
```
| games | def pass_the_bill(t, c, r):
return - 1 if t < 2 * r + 1 else max(0, t / / 2 + 1 - c)
| Simple Fun #199: Pass The Bill | 58c8a6daa7f31a623200016a | [
"Puzzles"
]
| https://www.codewars.com/kata/58c8a6daa7f31a623200016a | 7 kyu |
# Task
Given an array of 2<sup>k</sup> integers (for some integer `k`), perform the following operations until the array contains only one element:
```
On the 1st, 3rd, 5th, etc.
iterations (1-based) replace each pair of consecutive elements with their sum;
On the 2nd, 4th, 6th, etc.
iterations replace each pair of consecutive elements with their product.
```
After the algorithm has finished, there will be a single element left in the array. Return that element.
# Example
For inputArray = [1, 2, 3, 4, 5, 6, 7, 8], the output should be 186.
We have `[1, 2, 3, 4, 5, 6, 7, 8] -> [3, 7, 11, 15] -> [21, 165] -> [186]`, so the answer is 186.
# Input/Output
- `[input]` integer array `arr`
Constraints: 2<sup>1</sup> ≤ arr.length ≤ 2<sup>5</sup>, -9 ≤ arr[i] ≤ 99.
- `[output]` an integer | games | def array_conversion(arr):
sign = 0
while len(arr) > 1:
sign = 1 ^ sign
arr = list(map(lambda x, y: x + y, arr[0:: 2], arr[1:: 2])
if sign else map(lambda x, y: x * y, arr[0:: 2], arr[1:: 2]))
return arr[0]
| Simple Fun #50: Array Conversion | 588854201361435f5e0000bd | [
"Puzzles"
]
| https://www.codewars.com/kata/588854201361435f5e0000bd | 7 kyu |
# Task
Given array of integers, for each position i, search among the previous positions for the last (from the left) position that contains a smaller value. Store this value at position i in the answer. If no such value can be found, store `-1` instead.
# Example
For `items = [3, 5, 2, 4, 5]`, the output should be `[-1, 3, -1, 2, 4]`.
# Input/Output
- `[input]` integer array `arr`
Non-empty array of positive integers.
Constraints: `3 ≤ arr.length ≤ 1000, 1 ≤ arr[i] ≤ 1000.`
- `[output]` an integer array
Array containing answer values computed as described above. | games | def array_previous_less(arr):
return [next((y for y in arr[: i][:: - 1] if y < x), - 1) for i, x in enumerate(arr)]
| Simple Fun #51: Array Previous Less | 588856a82ffea640c80000cc | [
"Puzzles"
]
| https://www.codewars.com/kata/588856a82ffea640c80000cc | 7 kyu |
# Task
Your Informatics teacher at school likes coming up with new ways to help you understand the material. When you started studying numeral systems, he introduced his own numeral system, which he's convinced will help clarify things. His numeral system has base 26, and its digits are represented by English capital letters - `A for 0, B for 1, and so on`.
The teacher assigned you the following numeral system exercise: given a one-digit `number`, you should find all unordered pairs of one-digit numbers whose values add up to the `number`.
# Example
For `number = 'G'`, the output should be `["A + G", "B + F", "C + E", "D + D"]`
Translating this into the decimal numeral system we get: number = 6, so it is `["0 + 6", "1 + 5", "2 + 4", "3 + 3"]`.
# Input/Output
- `[input]` string(char in C#) `number`
A character representing a correct one-digit number in the new numeral system.
Constraints: `'A' ≤ number ≤ 'Z'.`
- `[output]` a string array
An array of strings in the format "letter1 + letter2", where "letter1" and "letter2" are correct one-digit numbers in the new numeral system. The strings should be sorted by "letter1".
Note that "letter1 + letter2" and "letter2 + letter1" are equal pairs and we don't consider them to be different. | games | def new_numeral_system(number):
outputs = {
'A': ['A + A'],
'B': ['A + B'],
'C': ['A + C', 'B + B'],
'D': ['A + D', 'B + C'],
'E': ['A + E', 'B + D', 'C + C'],
'F': ['A + F', 'B + E', 'C + D'],
'G': ['A + G', 'B + F', 'C + E', 'D + D'],
'H': ['A + H', 'B + G', 'C + F', 'D + E'],
'I': ['A + I', 'B + H', 'C + G', 'D + F', 'E + E'],
'J': ['A + J', 'B + I', 'C + H', 'D + G', 'E + F'],
'K': ['A + K', 'B + J', 'C + I', 'D + H', 'E + G', 'F + F'],
'L': ['A + L', 'B + K', 'C + J', 'D + I', 'E + H', 'F + G'],
'M': ['A + M', 'B + L', 'C + K', 'D + J', 'E + I', 'F + H', 'G + G'],
'N': ['A + N', 'B + M', 'C + L', 'D + K', 'E + J', 'F + I', 'G + H'],
'O': ['A + O', 'B + N', 'C + M', 'D + L', 'E + K', 'F + J', 'G + I', 'H + H'],
'P': ['A + P', 'B + O', 'C + N', 'D + M', 'E + L', 'F + K', 'G + J', 'H + I'],
'Q': ['A + Q', 'B + P', 'C + O', 'D + N', 'E + M', 'F + L', 'G + K', 'H + J', 'I + I'],
'R': ['A + R', 'B + Q', 'C + P', 'D + O', 'E + N', 'F + M', 'G + L', 'H + K', 'I + J'],
'S': ['A + S', 'B + R', 'C + Q', 'D + P', 'E + O', 'F + N', 'G + M', 'H + L', 'I + K', 'J + J'],
'T': ['A + T', 'B + S', 'C + R', 'D + Q', 'E + P', 'F + O', 'G + N', 'H + M', 'I + L', 'J + K'],
'U': ['A + U', 'B + T', 'C + S', 'D + R', 'E + Q', 'F + P', 'G + O', 'H + N', 'I + M', 'J + L', 'K + K'],
'V': ['A + V', 'B + U', 'C + T', 'D + S', 'E + R', 'F + Q', 'G + P', 'H + O', 'I + N', 'J + M', 'K + L'],
'W': ['A + W', 'B + V', 'C + U', 'D + T', 'E + S', 'F + R', 'G + Q', 'H + P', 'I + O', 'J + N', 'K + M', 'L + L'],
'X': ['A + X', 'B + W', 'C + V', 'D + U', 'E + T', 'F + S', 'G + R', 'H + Q', 'I + P', 'J + O', 'K + N', 'L + M'],
'Y': ['A + Y', 'B + X', 'C + W', 'D + V', 'E + U', 'F + T', 'G + S', 'H + R', 'I + Q', 'J + P', 'K + O', 'L + N', 'M + M'],
'Z': ['A + Z', 'B + Y', 'C + X', 'D + W', 'E + V', 'F + U', 'G + T', 'H + S', 'I + R', 'J + Q', 'K + P', 'L + O', 'M + N']
}
return outputs[number] # у меня нервы не в порядке
| Simple Fun #45: New Numeral System | 5888445107a0d57711000032 | [
"Puzzles"
]
| https://www.codewars.com/kata/5888445107a0d57711000032 | 7 kyu |
# Task
Below we will define what and n-interesting polygon is and your task is to find its area for a given n.
A 1-interesting polygon is just a square with a side of length 1. An n-interesting polygon is obtained by taking the n - 1-interesting polygon and appending 1-interesting polygons to its rim side by side. You can see the 1-, 2- and 3-interesting polygons in the picture below.
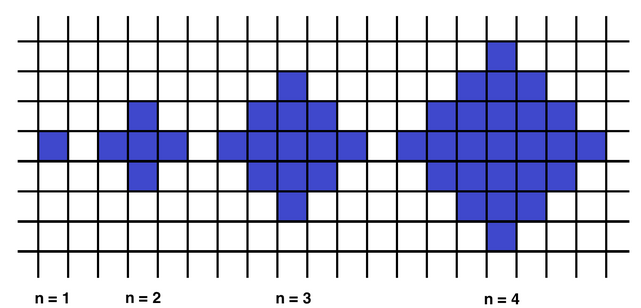
# Example
For `n = 1`, the output should be `1`;
For `n = 2`, the output should be `5`;
For `n = 3`, the output should be `13`.
# Input/Output
- `[input]` integer `n`
Constraints: `1 ≤ n < 10000.`
- `[output]` an integer
The area of the n-interesting polygon. | games | def shape_area(n):
return n * * 2 + (n - 1) * * 2
| Simple Fun #63: Shape Area | 5893e0c41a88085c330000a0 | [
"Puzzles"
]
| https://www.codewars.com/kata/5893e0c41a88085c330000a0 | 7 kyu |
# Task
Your task is to find the sum for the range `0 ... m` for all powers from `0 ... n.
# Example
For `m = 2, n = 3`, the result should be `20`
`0^0+1^0+2^0 + 0^1+1^1+2^1 + 0^2+1^2+2^2 + 0^3+1^3+2^3 = 20`
Note, that no output ever exceeds 2e9.
# Input/Output
- `[input]` integer m
`0 <= m <= 50000`
- `[input]` integer `n`
`0 <= n <= 9`
- `[output]` an integer(double in C#)
The sum value. | games | def S2N(m, n):
return sum(i * * j for i in range(m + 1) for j in range(n + 1))
| Simple Fun #137: S2N | 58a6742c14b042a042000038 | [
"Puzzles"
]
| https://www.codewars.com/kata/58a6742c14b042a042000038 | 7 kyu |
# Task
Given two integers `a` and `b`, return the sum of the numerator and the denominator of the reduced fraction `a/b`.
# Example
For `a = 2, b = 4`, the result should be `3`
Since `2/4 = 1/2 => 1 + 2 = 3`.
For `a = 10, b = 100`, the result should be `11`
Since `10/100 = 1/10 => 1 + 10 = 11`.
For `a = 5, b = 5`, the result should be `2`
Since `5/5 = 1/1 => 1 + 1 = 2`.
# Input/Output
- `[input]` integer `a`
The numerator, `1 ≤ a ≤ 2000`.
- `[input]` integer `b`
The denominator, `1 ≤ b ≤ 2000`.
- `[output]` an integer
The sum of the numerator and the denominator of the reduces fraction. | reference | from fractions import gcd
def fraction(a, b):
return (a + b) / / gcd(a, b)
| Simple Fun #179: Fraction | 58b8db810f40ea7788000126 | [
"Fundamentals"
]
| https://www.codewars.com/kata/58b8db810f40ea7788000126 | 7 kyu |
# Task
To prepare his students for an upcoming game, the sports coach decides to try some new training drills. To begin with, he lines them up and starts with the following warm-up exercise:
```
when the coach says 'L', he instructs the students to turn to the left.
Alternatively, when he says 'R', they should turn to the right.
Finally, when the coach says 'A', the students should turn around.
```
Unfortunately some students (not all of them, but at least one) can't tell left from right, meaning they always turn right when they hear 'L' and left when they hear 'R'. The coach wants to know how many times the students end up facing the same direction.
Given the list of commands the coach has given, count the number of such commands after which the students will be facing the same direction.
# Example
For commands = "LLARL", the output should be `3`.
Let's say that there are 4 students, and the second one can't tell left from right. In this case, only after the second, third and fifth commands will the students face the same direction.
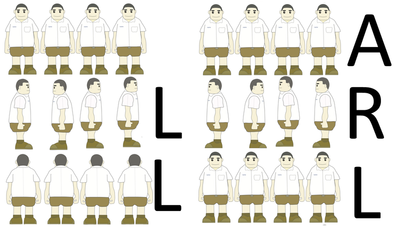
# Input/Output
- `[input]` string `commands`
String consisting of characters 'L', 'R' and 'A' only.
Constraints: 0 ≤ commands.length ≤ 35
- `[output]` an integer
The number of commands after which students face the same direction.
| games | def line_up(commands):
same = True
count = 0
for c in commands:
if c in "LR":
same = not same
if same:
count += 1
return count
| Simple Fun #14: Line Up | 5884658d02accbd0a7000039 | [
"Puzzles"
]
| https://www.codewars.com/kata/5884658d02accbd0a7000039 | 6 kyu |
# Task
You found two items in a treasure chest! The first item weighs `weight1` and is worth `value1`, and the second item weighs `weight2` and is worth `value2`. What is the total maximum value of the items you can take with you, assuming that your max weight capacity is `maxW`/`max_w` and you can't come back for the items later?
# Example
For value1 = 10, weight1 = 5, value2 = 6, weight2 = 4 and maxW = 8, the output should be
`knapsackLight(value1, weight1, value2, weight2, maxW) === 10` (or equivalent for PHP)
You can only carry the first item.
For value1 = 10, weight1 = 5, value2 = 6, weight2 = 4 and maxW = 9, the output should be
`knapsackLight(value1, weight1, value2, weight2, maxW) === 16` (or equivalent for PHP)
You're strong enough to take both of the items with you.
For value1 = 10, weight1 = 10, value2 = 6, weight2 = 10 and maxW = 9, the output should be
`knapsackLight(value1, weight1, value2, weight2, maxW) === 0` (or equivalent for PHP)
Unfortunately, you're not strong enough to take any one :(
# Input/Output
- `[input]` integer `value1`
Constraints: 2 ≤ value1 ≤ 50.
- `[input]` integer `weight1`
Constraints: 2 ≤ weight1 ≤ 30.
- `[input]` integer `value2`
Constraints: 2 ≤ value2 ≤ 50.
- `[input]` integer `weight2`
Constraints: 2 ≤ weight2 ≤ 30.
- `[input]` integer `maxW`/`max_w`
Constraints: 1 ≤ maxW ≤ 50.
- `[output]` an integer | games | def knapsack_light(v1, w1, v2, w2, W):
return max(v for v, w in [(0, 0), (v1, w1), (v2, w2), (v1 + v2, w1 + w2)] if w <= W)
| Simple Fun #5: Knapsack Light | 58842a2b4e8efb92b7000080 | [
"Puzzles"
]
| https://www.codewars.com/kata/58842a2b4e8efb92b7000080 | 7 kyu |
## Task
In order to stop the Mad Coder evil genius you need to decipher the encrypted message he sent to his minions. The message contains several numbers that, when typed into a supercomputer, will launch a missile into the sky blocking out the sun, and making all the people on Earth grumpy and sad.
You figured out that some numbers have a modified single digit in their binary representation. More specifically, in the given number `n` the `k`<sup>th</sup> bit from the right was initially set to 0, but its current value might be different. It's now up to you to write a function that will change the `k`<sup>th</sup> bit of `n` back to 0.
## Example
For n = 37 and k = 3, the output should be `33`.
37<sub>10</sub> = 100<font color='red'>1</font>01<sub>2</sub> ~> 100<font color='red'>0</font>01<sub>2</sub> = 33<sub>10</sub>
For n = 37 and k = 4, the output should be 37.
The 4<sup>th</sup> bit is 0 already (looks like the Mad Coder forgot to encrypt this number), so the answer is still 37.
## Input/Output
- `[input]` integer `n`
Constraints: 0 ≤ n ≤ 2<sup>31</sup> - 1.
- `[input]` integer `k`
The `1-based` index of the changed bit (`counting from the right`).
Constraints: 1 ≤ k ≤ 31.
- `[output]` an integer
## More Challenge
- Are you a One-Liner? Please try to complete the kata in one line(no test for it) ;-) | games | def kill_kth_bit(n, k):
return n & ~ (1 << k - 1)
| Simple Fun #8: Kill K-th Bit | 58844f1a76933b1cd0000023 | [
"Puzzles",
"Bits",
"Binary"
]
| https://www.codewars.com/kata/58844f1a76933b1cd0000023 | 7 kyu |
# Task
Given integers `a` and `b`, determine whether the following pseudocode results in an infinite loop
```javascript
while (a !== b){
a++
b--
}
```
Assume that the program is executed on a virtual machine which can store arbitrary long numbers and execute forever.
# Example
For a = 2 and b = 6, the output should be
`isInfiniteProcess(a, b) = false` (or equivalent in PHP)
For a = 2 and b = 3, the output should be
`isInfiniteProcess(a, b) = true` (or equivalent in PHP)
# Input/Output
- `[input]` integer `a`
Constraints: 0 ≤ a ≤ 100.
- `[input]` integer `b`
Constraints: 0 ≤ b ≤ 100.
- `[output]` a boolean value
true if the pseudocode will never stop, false otherwise. | games | def is_infinite_process(a, b):
return b < a or (b - a) % 2
| Simple Fun #6: Is Infinite Process? | 588431bb76933b84520000d3 | [
"Puzzles"
]
| https://www.codewars.com/kata/588431bb76933b84520000d3 | 7 kyu |
# Task
Some phone usage rate may be described as follows:
first minute of a call costs `min1` cents,
each minute from the 2nd up to 10th (inclusive) costs `min2_10` cents
each minute after 10th costs `min11` cents.
You have `s` cents on your account before the call. What is the duration of the longest call (in minutes rounded down to the nearest integer) you can have?
# Example
For min1 = 3, min2_10 = 1, min11 = 2 and s = 20, the output should be
`phoneCall(min1, min2_10, min11, s) === 14`
Here's why:
the first minute costs 3 cents, which leaves you with 20 - 3 = 17 cents;
the total cost of minutes 2 through 10 is 1 * 9 = 9, so you can talk 9 more minutes and still have 17 - 9 = 8 cents;
each next minute costs 2 cents, which means that you can talk 8 / 2 = 4 more minutes.
Thus, the longest call you can make is 1 + 9 + 4 = 14 minutes long.
# Input/Output
- `[input]` integer `min1`
Constraints: 1 ≤ min1 ≤ 10
- `[input]` integer `min2_10`
Constraints: 1 ≤ min2_10 ≤ 10
- `[input]` integer `min11`
Constraints: 1 ≤ min11 ≤ 10
- `[input]` integer `s`
Constraints: 2 ≤ s ≤ 100
- [output] an integer | games | def phone_call(min1, min2_10, min11, s):
minutes, cost = 0, min1
while s >= cost:
minutes += 1
s -= cost
if minutes == 1:
cost = min2_10
if minutes == 10:
cost = min11
return minutes
| Simple Fun #4: Phone Call | 588425ee4e8efb583d000088 | [
"Puzzles"
]
| https://www.codewars.com/kata/588425ee4e8efb583d000088 | 7 kyu |
Truncate the given string (first argument) if it is longer than the given maximum length (second argument). Return the truncated string with a `"..."` ending.
Note that inserting the three dots to the end will add to the string length.
However, if the given maximum string length num is less than or equal to 3, then the addition of the three dots does not add to the string length in determining the truncated string.
## Examples
```
('codewars', 9) ==> 'codewars'
('codewars', 7) ==> 'code...'
('codewars', 2) ==> 'co...'
```
[Taken from FCC](https://www.freecodecamp.com/challenges/truncate-a-string) | reference | def truncate_string(s, n):
return s if len(s) <= n else s[: n if n <= 3 else n - 3] + '...'
| Truncate a string! | 57af26297f75b57d1f000225 | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/57af26297f75b57d1f000225 | 7 kyu |
There are 8 balls numbered from 0 to 7.
Seven of them have the same weight. One is heavier. Your task is to find its number.
Your function ```findBall``` will receive single argument - ```scales``` object. The ```scales``` object contains an internally stored array of 8 elements (indexes 0-7), each having the same value except one, which is greater. It also has a public method named ```getWeight(left, right)``` which takes two arrays of indexes and returns -1, 0, or 1 based on the accumulation of the values found at the indexes passed are heavier, equal, or lighter.
```getWeight``` returns:
```-1``` if **left** pan is heavier
```1``` if **right** pan is heavier
```0``` if both pans weight the same
Examples of ```scales.getWeight()``` usage:
```scales.getWeight([3], [7])``` returns ```-1``` if ball 3 is heavier than ball 7, ```1``` if ball 7 is heavier, or ```0``` i these balls have the same weight.
```scales.getWeight([3, 4], [5, 2])``` returns ```-1``` if weight of balls 3 and 4 is heavier than weight of balls 5 and 2 etc.
So where's the catch, you may ask. Well - the scales is very old. You can use it only **4 TIMES** before the scale breaks.
``` if:python,ruby,crystal,c
**Note** - Use `scales.get_weight()` in the Python, Crystal, Ruby And C versions.
```
``` if:ocaml
**Note** - Use `scales#get_weight` in the OCaml version.
```
```if:c
**Note** - in C you should also pass the length of the arrays to `scales.get_weight()`.
```
Too easy ? Try higher levels:
* [conqueror](http://www.codewars.com/kata/54404a06cf36258b08000364)
* [master](http://www.codewars.com/kata/544034f426bc6adda200000e) | games | def find_ball(scales):
for i in range(0, 8, 2):
result = scales . get_weight([i], [i + 1])
if result:
return i + (result > 0)
| Find heavy ball - level: novice | 544047f0cf362503e000036e | [
"Puzzles",
"Logic",
"Riddles"
]
| https://www.codewars.com/kata/544047f0cf362503e000036e | 7 kyu |
#### Background:
<p align="center"> <img src="http://upload.wikimedia.org/wikipedia/commons/thumb/3/3a/Linear_regression.svg/438px-Linear_regression.svg.png" alt="sample linear regression, curtesy of wikipedia"></p>
A linear regression line has an equation in the form `$Y = a + bX$`, where `$X$` is the explanatory variable and `$Y$` is the dependent variable. The parameter `$b$` represents the *slope* of the line, while `$a$` is called the *intercept* (the value of `$y$` when `$x = 0$`).
For more details visit the related [wikipedia page](http://en.wikipedia.org/wiki/Simple_linear_regression).
---
---
#### Task:
The function that you have to write accepts two list/array, `$x$` and `$y$`, representing the coordinates of the points to regress (so that, for example, the first point has coordinates (`x[0], y[0]`)).
Your function should return a tuple (in Python) or an array (any other language) of two elements: `a` (intercept) and `b` (slope) in this order.
You must round your result to the first 4 decimal digits
#### Formula:
`$x_i$` and `$y_i$` is `$x$` and `$y$` co-ordinate of `$i$`-th point;
`$n$` is length of input.
`$a = \dfrac{\sum x_i^2\cdot \sum y_i - \sum x_i \cdot\sum x_iy_i}{n\sum x_i^2 - (\sum x_i)^2}$`
`$b = \dfrac{n\sum x_i y_i - \sum x_i \cdot \sum y_i}{n\sum x^2_i - (\sum x_i)^2}$`
#### Examples:
```python
regressionLine([25,30,35,40,45,50], [78,70,65,58,48,42]) == (114.381, -1.4457)
regressionLine([56,42,72,36,63,47,55,49,38,42,68,60], [147,125,160,118,149,128,150,145,115,140,152,155]) == (80.7777, 1.138)
```
```javascript
regression_line([25,30,35,40,45,50], [78,70,65,58,48,42]) === [114.381, -1.4457]
regressionLine([56,42,72,36,63,47,55,49,38,42,68,60], [147,125,160,118,149,128,150,145,115,140,152,155]) === [80.7777, 1.138]
```
```ruby
regressionLine([25,30,35,40,45,50], [78,70,65,58,48,42]) == [114.381, -1.4457]
regressionLine([56,42,72,36,63,47,55,49,38,42,68,60], [147,125,160,118,149,128,150,145,115,140,152,155]) == [80.7777, 1.138]
```
----
| reference | import numpy as np
def regressionLine(x, y):
""" Return the a (intercept)
and b (slope) of Regression Line
(Y on X).
"""
a, b = np . polyfit(x, y, 1)
return round(b, 4), round(a, 4)
| Linear Regression of Y on X | 5515395b9cd40b2c3e00116c | [
"Statistics",
"Geometry",
"Fundamentals",
"Data Science"
]
| https://www.codewars.com/kata/5515395b9cd40b2c3e00116c | 6 kyu |
This is a follow up from my kata <a href="http://www.codewars.com/kata/insert-dashes/">Insert Dashes</a>. <br/>
Write a function ```insertDashII(num)``` that will insert dashes ('-') between each two odd numbers and asterisk ('\*') between each even numbers in ```num``` <br/>
For example: <br/>
```insertDashII(454793)``` --> 4547-9-3 <br/>
```insertDashII(1012356895)``` --> 10123-56*89-5 <br/>
Zero shouldn't be considered even or odd.
| reference | import re
def insert_dash2(num):
s = str(num)
s = re . sub('(?<=[13579])(?=[13579])', '-', s)
s = re . sub('(?<=[2468])(?=[2468])', '*', s)
return s
| Insert Dashes 2 | 55c3026406402936bc000026 | [
"Fundamentals"
]
| https://www.codewars.com/kata/55c3026406402936bc000026 | 7 kyu |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.