description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
listlengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
In the previous <a title="Kata" href="https://www.codewars.com/kata/575eac1f484486d4600000b2">Kata</a> we discussed the OR case.
We will now discuss the AND case, where rather than calculating the probablility for either of two (or more) possible results, we will calculate the probability of receiving <i>all</i> of the viewed outcomes.
For example, if we want to know the probability of receiving head OR tails in two tosses of a coin, as in the last Kata we add the two probabilities together. However if we want to know the probability of receiving head AND tails, in that order, we have to work differently.
The probability of an AND event is calculated by the following rule:
`P(A ∩ B) = P(A | B) * P(B)`
or
`P(B ∩ A) = P(B | A) * P(A)`
That is, the probability of A and B both occuring is equal to the probability of A given B occuring times the probability of B occuring or vice versa.
If the events are independent, like in the case of tossing a coin, the probability of A occuring if B has occured is equal to the probability of A occuring by itself. In this case, the probability can be written as the below:
`P(A ∩ B) = P(A) * P(B)`
or
`P(B ∩ A) = P(B) * P(A)`
Applying to the heads and tails case:
`P(H ∩ T) = 0.5 * 0.5`
or
`P(H ∩ T) = 0.5 * 0.5`
<u>The task:</u>
You are given a random bag of 10 balls containing 4 colours. `Red`, `Green`, `Yellow` and `Blue`. You will also be given a sequence of 2 balls of any colour e.g. `Green` and `Red` or `Yellow` and `Yellow`.
You have to return the probability of pulling a ball at random out of the bag and it matching the first colour and then pulling a ball at random out of the bag and it matching the second colour.
You will be given a boolean value of `true` or `false` which indicates whether the balls that is taken out in the first draw is replaced in to the bag for the second draw. Hint: this will determine whether the events are independent or not.
You will receive two arrays and a boolean value. The first array contains the colours of the balls in the bag and the second contains the colour of the two balls you have to receive. As per above the final boolean value will indicate whether the balls are being replaced `true` or not `false`.
Do not mutate your inputs and do not round results.
e.g. `[["y","r","g","b","b","y","r","g","r","r"],["r","b"],false]`
<u>Other Kata in this series:</u>
<li><a title="Statistics in Kata 1: OR case - Unfair dice" href="https://www.codewars.com/kata/575eac1f484486d4600000b2">Statistics in Kata 1: OR case - Unfair dice</a></li>
| reference | def ball_probability(balls):
colors, (first, second), replaced = balls
first_choice = colors . count(first) / len(colors)
second_choice = colors . count(second) / len(colors) if replaced else (
colors . count(second) - 1 * (first == second)) / (len(colors) - 1)
return round(first_choice * second_choice, 3)
| Statistics in Kata 2: AND case - Ball bags | 576a527ea25aae70b7000c77 | [
"Statistics",
"Probability",
"Arrays",
"Fundamentals"
]
| https://www.codewars.com/kata/576a527ea25aae70b7000c77 | 6 kyu |
The year is 2070 and intelligent connected machines have replaced humans in most things. There are still a few critical jobs for mankind including machine software developer, for writing and maintaining the AI software, and machine forward deployed engineer for controlling the intelligent machines in the field.
Lorimar is a forward deployed machine engineer and at any given time he controls thousands of BOT robots through real time interfaces and intelligent automation software. These interfaces are directly connected to his own brain so at all times his mind and the robots are one. They are pre-trained to think on their own but on occasion the human will direct the robot to perform a task.
There is an issue with the ultrasonic sensor data feed from BOT785 currently connected to Lorimar through a real time interface. The data feed from sensor five should be a series of floats representing the relative distance of objects within BOT785's path. The sensor error log has been saved for triage and Lorimar needs to search through the data to determine the mean and standard deviation of the distance variables.
You will be given a list of tuples extracted from the sensor logs:
```python
sensor_data = [('Distance:', 116.28, 'Meters', 'Sensor 5 malfunction =>lorimar'), ('Distance:', 117.1, 'Meters', 'Sensor 5 malfunction =>lorimar'), ('Distance:', 123.96, 'Meters', 'Sensor 5 malfunction =>lorimar'), ('Distance:', 117.17, 'Meters', 'Sensor 5 malfunction =>lorimar')]
```
Return a tuple with the mean and standard deviation of the distance variables rounded to four decimal places. The variance should be computed using the formula for samples (dividing by N-1).
|Mean| |Standard Deviation|
```python
(118.6275, 3.5779)
```
Hint (http://math.stackexchange.com/questions/15098/sample-standard-deviation-vs-population-standard-deviation)
Please also try the other Kata in this series..
* [AD2070:Help Lorimar troubleshoot his robots-Search and Disable](https://www.codewars.com/kata/57dc0ffed8f92982af0000f6)
| algorithms | from numpy import mean, std
def sensor_analysis(sensor_data):
data = [data[1] for data in sensor_data]
return (round(mean(data), 4), round(std(data, ddof=1), 4))
| AD2070: Help Lorimar troubleshoot his robots- ultrasonic distance analysis | 57102bbfd860a3369300089c | [
"Mathematics",
"Statistics",
"Algorithms",
"Data Science"
]
| https://www.codewars.com/kata/57102bbfd860a3369300089c | 7 kyu |
The purpose of this series is developing understanding of stastical problems in AS and A level maths. Let's get started with a simple concept in statistics: Mutually exclusive events.
The probability of an OR event is calculated by the following rule:
`P(A || B) = P(A) + P(B) - P(A && B)`
The probability of event A or event B happening is equal to the probability of event A plus the probability of event B minus the probability of event A and event B happening simultaneously.
Mutually exclusive events are events that cannot happen at the same time. For example, the head and tail results of a toin coss are mutually exclusive because they can't both happen at once. Thus, the above example for a coin toss would look like this:
`P(H || T) = P(H) + P(T) - P(H && T)`
Note that the probaility of tossing a coin and the result being both head and tails is 0.
`P(H || T) = (0.5) + (0.5) - (0)`
`P(H || T) = 1`
Thus the probability of a coin toss result being a heads or a tails is 1, in other words: certain.
Your task:
You are going to have to work out the probability of one roll of a die returning two given outcomes, or rolls. Given that dice rolls are mutually exclusive, you will have to implement the above forumala. To make this interesting (this is a coding challenge after all), these dice are not fair and thus the probabilites of receiving each roll is different.
You will be given a two-dimensional array containing the number each of the results (1-6) of the die and the probability of that roll for example `[1 , 0.23]` as well as the two rolls for example `1` and `5`.
Given the two roll probabilities to calculate, return the probability of a single roll of the die returning <i>either</i>. If the total probability of the six rolls doesn't add up to one, there is a problem with the die; in this case, return null.
<i>Return your result as a string to two decimal places.</i>
Example below:
`1 : 1/6`
`2 : 1/6`
`3 : 1/6`
`4 : 1/6`
`5 : 1/6`
`6 : 1/6`
If asked for the rolls `1` and `2` then you would need to sum the probabilities, both `1/6` therefore `2/6` and return this. As above, you will need to return it as a decimal and not a fraction.
| games | def mutually_exclusive(dice, call1, call2):
dice = dict(dice)
if abs(sum(dice . values()) - 1) < 1e-12:
return '%0.2f' % (dice[call1] + dice[call2])
| Statistics in Kata 1: OR case - Unfair dice | 575eac1f484486d4600000b2 | [
"Probability",
"Statistics"
]
| https://www.codewars.com/kata/575eac1f484486d4600000b2 | 6 kyu |
We have a distribution of probability of a discrete variable (it may have only integer values)
```
x P(x)
0 0.125
1 0.375
2 0.375
3 0.125
Total = 1.000 # The sum of the probabilities for all the possible values should be one (=1)
```
The mean, ```μ```, of the values of x is:
<a href="http://imgur.com/EbDq0XT"><img src="http://i.imgur.com/EbDq0XT.png?1" title="source: imgur.com" /></a>
For our example
```
μ = 0*0.125 + 1*0.375 + 2*0.375 + 3*0.125 = 1.5
```
The variance, ```σ²``` is:
<a href="http://imgur.com/vMyeQEn"><img
src="http://i.imgur.com/vMyeQEn.png?1" title="source: imgur.com" /></a>
For our example :
```
σ² = 0.75
```
The standard deviation, ```σ``` is:
<a href="http://imgur.com/mg801Vq"><img src="http://i.imgur.com/mg801Vq.png?1" title="source: imgur.com" /></a>
Finally, for our example:
```
σ = 0.8660254037844386
```
Make the function ```stats_disc_distr()``` that receives a 2D array. Each internal array will have a pair of values: the first one, the value of the variable ```x``` and the second one its correspondent probability, ```P(x)```.
For the example given above:
```python
stats_disc_distr([[0, 0.125], [1, 0.375], [2, 0.375], [3, 0.125]]) == [1.5, 0.75, 0.8660254037844386]
```
The function should check also if it is a valid distribution.
If the sum of the probabilities is different than ```1```, the function should output an alert.
```python
stats_disc_distr([[0, 0.425], [1, 0.375], [2, 0.375], [3, 0.125]]) == "It's not a valid distribution"
```
If one of the values of ```x``` is not an integer, the function will give a specific alert:
```python
stats_disc_distr([[0.1, 0.425], [1.1, 0.375], [2, 0.375], [3, 0.125]]) == "All the variable values should be integers"
```
If the distribution has both problems will output another specific alert:
```python
stats_disc_distr([[0.1, 0.425], [1.1, 0.375], [2, 0.375], [3, 0.125]]) == "It's not a valid distribution and furthermore, one or more variable value are not integers"
```
But if a value is a float with its decimal part equals to 0 will proceed without inconveniences, (if the sum of probabilities is ```1```:
```python
stats_disc_distr([[0.0, 0.125], [1.0, 0.375], [2.0, 0.375], [3, 0.125]]) == [1.5, 0.75, 0.8660254037844386]
```
The 2Darray will not have any strings.
Enjoy it!! | reference | def stats_disc_distr(distrib):
err = check_errors(distrib)
if not err:
mean = sum(x[0] * x[1] for x in distrib)
var = sum((x[0] - mean) * * 2 * x[1] for x in distrib)
std_dev = var * * 0.5
return [mean, var, std_dev] if not err else err
def check_errors(distrib):
errors = 0
if not isclose(sum(x[1] for x in distrib), 1):
errors += 1
if not all(isinstance(x[0], int) for x in distrib):
errors += 2
if errors > 0:
return {1: "It's not a valid distribution", 2: "All the variable values should be integers",
3: "It's not a valid distribution and furthermore, one or more variable value are not integers"}[errors]
def isclose(a, b, rel_tol=1e-09, abs_tol=0.0):
return abs(a - b) <= max(rel_tol * max(abs(a), abs(b)), abs_tol)
| Mean, Variance and Standard Deviation of a Probability Distribution for Discrete Variables. | 5708ccb0fe2d01677c000565 | [
"Algorithms",
"Mathematics",
"Statistics",
"Probability"
]
| https://www.codewars.com/kata/5708ccb0fe2d01677c000565 | 6 kyu |
# Convert Improper Fraction to Mixed Number
You will need to convert an [improper fraction](https://www.mathplacementreview.com/arithmetic/fractions.php#improper-fractions) to a [mixed number](https://www.mathplacementreview.com/arithmetic/fractions.php#mixed-number). For example:
```javascript
getMixedNum('18/11'); // Should return '1 7/11'
getMixedNum('13/5'); // Should return '2 3/5'
getMixedNum('75/10'); // Should return '7 5/10'
```
```python
get_mixed_num('18/11') # Should return '1 7/11'
get_mixed_num('13/5') # Should return '2 3/5'
get_mixed_num('75/10') # Should return '7 5/10'
```
NOTE: All fractions will be greater than 0. | reference | def get_mixed_num(fraction):
n, d = [int(i) for i in fraction . split('/')]
return '{} {}/{}' . format(n / / d, n % d, d)
| Convert Improper Fraction to Mixed Number | 584acbee7d22f84dc80000e2 | [
"Fundamentals",
"Strings",
"Mathematics",
"Algorithms"
]
| https://www.codewars.com/kata/584acbee7d22f84dc80000e2 | 7 kyu |
Having two standards for a keypad layout is inconvenient!
Computer keypad's layout:
<img src="http://upload.wikimedia.org/wikipedia/commons/9/99/Numpad.svg" style="width:250px;height:250px"
alt="7 8 9 \n
4 5 6 \n
1 2 3 \n
0 \n"
/>
Cell phone keypad's layout:
<img src="http://upload.wikimedia.org/wikipedia/commons/d/dd/Mobile_phone_keyboard.svg" style="width:100px;height:70px"
alt="1 2 3\n
4 5 6\n
7 8 9\n
0\n"
/>
Solve the horror of unstandardized keypads by providing a function that converts computer input to a number as if it was typed on a phone.
Example:
"789" -> "123"
Notes:
You get a string with numbers only | games | def computer_to_phone(numbers):
return numbers . translate(str . maketrans('123789', '789123'))
| Keypad horror | 5572392fee5b0180480001ae | [
"Strings"
]
| https://www.codewars.com/kata/5572392fee5b0180480001ae | 7 kyu |
Your colleagues have been good enough(?) to buy you a birthday gift. Even though it is your birthday and not theirs, they have decided to play pass the parcel with it so that everyone has an even chance of winning. There are multiple presents, and you will receive one, but not all are nice... One even explodes and covers you in soil... strange office. To make up for this one present is a dog! Happy days! (do not buy dogs as presents, and if you do, never wrap them).
Depending on the number of passes in the game (y), and the present you unwrap (x), return as follows:
x == goodpresent --> return x with num of passes added to each charCode (turn to charCode, add y to each, turn back)<br><br>
x == crap || x == empty --> return string sorted alphabetically<br><br>
x == bang --> return string turned to char codes, each code reduced by number of passes and summed to a single figure<br><br>
x == badpresent --> return 'Take this back!'<br><br>
x == dog, return 'pass out from excitement y times' (where y is the value given for y). | reference | _RESULTS = {
'goodpresent': lambda y: '' . join(chr(ord(c) + y) for c in 'goodpresent'),
'crap': lambda y: 'acpr',
'empty': lambda y: 'empty',
'bang': lambda y: str(sum(ord(c) - y for c in 'bang')),
'badpresent': lambda y: 'Take this back!',
'dog': lambda y: 'pass out from excitement {} times' . format(y)
}
def present(x, y): return _RESULTS[x](y)
| Birthday II - Presents | 5805f0663f1f9c49be00011f | [
"Fundamentals",
"Arrays"
]
| https://www.codewars.com/kata/5805f0663f1f9c49be00011f | 7 kyu |
Mars rover is on an important mission to take pictures of Mars.
In order to take pictures of all directions it needs an algorithm to help it turn to face the correct position.
Mars rover can face 4 different directions, that would be `N`, `S`, `E`, `W`. Every time it needs to turn it will call a command `turn` passing the current position it is facing and the position it wants to face.
For example:
- if it asks `turn N E` the expected result would be `right`
- if it asks `turn N W` the expected result would be `left`
Mars rover can only make one move at a time and it will only request positions that require a single move.
Can you write an algorithm that tells if it should move `left` or `right`?
 | algorithms | def turn(frm, to):
return "right" if f" { frm }{ to } " in "NESWN" else "left"
| Turn the Mars rover to take pictures | 588e68aed4cff457d300002e | [
"Algorithms"
]
| https://www.codewars.com/kata/588e68aed4cff457d300002e | 7 kyu |
Once upon a time, more precisely upon BASIC time, there were 2 functions named LEFT$ and RIGHT$ (I wrote them uppercase because it was the custom in those early years).
These functions let you read left(/right)-most characters of a string.
* use: `LEFT$ (str, i)` -> returns `i` left-most characters of `str`.
```
- eg:
A$="ABCDEFG":PRINT LEFT$(A$,3) - prints ABC
```
and `RIGHT$ (str, i )`, of course, returns `i` right-most characters of `str`.
### So, your mission is...
...to write 2 functions ( `left$(str, i)` & `right$(str, i)` ) (`left` & `right` in **Ruby** or **Python**) that will work as the BASIC ones did,... except :
* ` i ` may be a negative integer. In this case the function returns the **whole** string **but** `i` right(/left)-most chars (respectively in left$(/right$) function).
* if ` i ` === 0 : returns an empty string;
* if **no** ` i ` is provided consider it should be `1` ( not zero ).
* if ` i ` is greater than `str` length : returns the whole str.
**and**
* if ` i ` is a `string` ( yes it can ) : returns part of `str` at left(/right) of ` i `.
+ returns `left` of **first** occurence of `i`
+ returns `right` of **last** occurence of `i`
### Examples:
```javascript
var str = 'Hello (not so) cruel World!'
left$(str,5) // -> 'Hello'
left$(str,-22) // -> 'Hello'
left$(str,1) // -> 'H'
left$(str) // -> 'H' too
left$(str,0) // -> ''
left$(str,99) // -> 'Hello (not so) cruel World!'
right$(str,6) // -> 'World!'
right$(str) // -> '!'
//== with string as 2d argument ==
left$(str,'o') // -> 'Hell'
right$(str,'o')// -> 'rld!'
left$(str,' ') // -> 'Hello' // -- string may be a space
```
```ruby
var str = 'Hello (not so) cruel World!'
left(str,5) // -> 'Hello'
left(str,-22) // -> 'Hello'
left(str,1) // -> 'H'
left(str) // -> 'H' too
left(str,0) // -> ''
left(str,99) // -> 'Hello (not so) cruel World!'
right(str,6) // -> 'World!'
right(str) // -> '!'
#== with string as 2nd argument ==
left(str,'o') // -> 'Hell'
right(str,'o')// -> 'rld!'
left(str,' ') // -> 'Hello' # -- string may be a space
```
```python
text = 'Hello (not so) cruel World!'
left(text,5) # -> 'Hello'
left(text,-22) # -> 'Hello'
left(text,1) # -> 'H'
left(text) # -> 'H' too
left(text,0) # -> ''
left(text,99) # -> 'Hello (not so) cruel World!'
right(text,6) # -> 'World!'
right(text) # -> '!'
#== with string as 2nd argument ==
left(text,'o') # -> 'Hell'
right(text,'o')# -> 'rld!'
left(text,' ') # -> 'Hello' // -- string may be a space
```
| reference | def left(string, i=1):
i = string . index(i) if type(i) == str else i
return string[: i]
def right(string, i=1):
i = string[:: - 1]. index(i[:: - 1]) if type(i) == str else i
return string[- i:] if i != 0 else ""
| Left$ and Right$ | 53f211b159c3fcec3d000efa | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/53f211b159c3fcec3d000efa | 7 kyu |
You've been collecting change all day, and it's starting to pile up in your pocket, but you're too lazy to see how much you've found.
Good thing you can code!
Create ```change_count()``` to return a dollar amount of how much change you have!
Valid types of change include:
```
penny: 0.01
nickel: 0.05
dime: 0.10
quarter: 0.25
dollar: 1.00
```
```if:python
These amounts are already preloaded as floats into the `CHANGE` dictionary for you to use!
```
```if:ruby
These amounts are already preloaded as floats into the `CHANGE` hash for you to use!
```
```if:javascript
These amounts are already preloaded as floats into the `CHANGE` object for you to use!
```
```if:php
These amounts are already preloaded as floats into the `CHANGE` (a constant) associative array for you to use!
```
You should return the total in the format ```$x.xx```.
Examples:
```python
change_count('nickel penny dime dollar') == '$1.16'
change_count('dollar dollar quarter dime dime') == '$2.45'
change_count('penny') == '$0.01'
change_count('dime') == '$0.10'
```
```javascript
changeCount('nickel penny dime dollar') == '$1.16'
changeCount('dollar dollar quarter dime dime') == '$2.45'
changeCount('penny') == '$0.01'
changeCount('dime') == '$0.10'
```
```ruby
changeCount('nickel penny dime dollar') == '$1.16'
changeCount('dollar dollar quarter dime dime') == '$2.45'
changeCount('penny') == '$0.01'
changeCount('dime') == '$0.10'
```
```php
changeCount('nickel penny dime dollar') == '$1.16'
changeCount('dollar dollar quarter dime dime') == '$2.45'
changeCount('penny') == '$0.01'
changeCount('dime') == '$0.10'
```
Warning, some change may amount to over ```$10.00```! | reference | def change_count(change):
return "$%.2f" % sum(CHANGE[coin] for coin in change . split())
| Loose Change! | 57e1857d333d8e0f76002169 | [
"Fundamentals"
]
| https://www.codewars.com/kata/57e1857d333d8e0f76002169 | 7 kyu |
2520 is the smallest number that can be divided by each of the numbers from 1 to 10 without any remainder.<br/>
<span style='font-size:20px'><b>Task:</b></span><br/>
Write
```
smallest(n)
```
that will find the smallest positive number that is evenly divisible by all of the numbers from 1 to n (n <= 40). <br/>
E.g<br/>
```javascript
smallest(5) == 60 // 1 to 5 can all divide evenly into 60
smallest(10) == 2520
```
```ruby
smallest(5) == 60 # 1 to 5 can all divide evenly into 60
smallest(10) == 2520
```
```python
smallest(5) == 60 # 1 to 5 can all divide evenly into 60
smallest(10) == 2520
``` | algorithms | from functools import reduce
from math import gcd
def smallest(n):
return reduce(lambda a, b: a * b / / gcd(a, b), range(1, n + 1))
| Satisfying numbers | 55e7d9d63bdc3caa2500007d | [
"Algorithms"
]
| https://www.codewars.com/kata/55e7d9d63bdc3caa2500007d | 7 kyu |
Create a function that takes index [0, 8] and a character. It returns a string with columns. Put character in column marked with index.
Ex.:
index = 2,
character = 'B'
```
| | |B| | | | | | |
0 1 2 3 4 5 6 7 8
```
Assume that argument index is integer [0, 8]. Assume that argument character is string with one character. | reference | def build_row_text(index, character):
a = list('|||||||||')
a[index] = "|" + character + "|"
return " " . join(a)
| Put a Letter in a Column | 563d54a7329a7af8f4000059 | [
"Fundamentals",
"Strings"
]
| https://www.codewars.com/kata/563d54a7329a7af8f4000059 | 7 kyu |
To celebrate the start of the Rio Olympics (and the return of 'the Last Leg' on C4 tonight) this is an Olympic inspired kata.
Given a string of random letters, you need to examine each. Some letters naturally have 'rings' in them. 'O' is an obvious example, but 'b', 'p', 'e', 'A', etc are all just as applicable. 'B' even has two!! Please note for this kata you can count lower case 'g' as only one ring.
Your job is to count the 'rings' in each letter and divide the total number by 2. Round the answer down. Once you have your final score:
if score is 1 or less, return 'Not even a medal!';
if score is 2, return 'Bronze!';
if score is 3, return 'Silver!';
if score is more than 3, return 'Gold!';
Dots over i's and any other letters don't count as rings.
| reference | def olympic_ring(string):
rings = string . translate(str . maketrans(
'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz',
'1201000000000011110000000011011010000000111000000000'))
score = sum(map(int, rings)) / / 2
return ['Not even a medal!', 'Bronze!', 'Silver!', 'Gold!'][(score > 1) + (score > 2) + (score > 3)]
| Olympic Rings | 57d06663eca260fe630001cc | [
"Fundamentals",
"Arrays",
"Strings"
]
| https://www.codewars.com/kata/57d06663eca260fe630001cc | 7 kyu |
>When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
John learns to play poker with his uncle. His uncle told him: Poker to be in accordance with the order of "2 3 4 5 6 7 8 9 10 J Q K A". The same suit should be put together. But his uncle did not tell him the order of the four suits.
Give you John's cards and Uncle's cards(two string `john` and `uncle`). Please reference to the order of Uncle's cards, sorting John's cards.
# Examples
```
For Python:
Suits are defined as S, D, H, C.
sort_poker("D6H2S3D5SJCQSKC7D2C5H5H10SA","S2S3S5HJHQHKC8C9C10D4D5D6D7")
should return "S3SJSKSAH2H5H10C5C7CQD2D5D6"
sort_poke("D6H2S3D5SJCQSKC7D2C5H5H10SA","C8C9C10D4D5D6D7S2S3S5HJHQHK")
should return "C5C7CQD2D5D6S3SJSKSAH2H5H10"
```
| games | def order(arr): return {card[0]: i for i, card in enumerate(arr)}
ranks = order("2 3 4 5 6 7 8 9 10 J Q K A" . split())
suit_table = {ord(suit): ' ' + suit for suit in 'SDHC'}
def split_hand(hand): return hand . translate(suit_table). split()
def sort_poker(john, uncle):
suits = order(split_hand(uncle))
return '' . join(sorted(split_hand(john),
key=lambda card: (suits[card[0]], ranks[card[1]])))
| Sorting Poker | 580ed88494291dd28c000019 | [
"Puzzles",
"Algorithms",
"Sorting"
]
| https://www.codewars.com/kata/580ed88494291dd28c000019 | 5 kyu |
You've just discovered a square (NxN) field and you notice a warning sign. The sign states that there's a single bomb in the 2D grid-like field in front of you.
Write a function `mineLocation`/`MineLocation` that accepts a 2D array, and returns the location of the mine. The mine is represented as the integer `1` in the 2D array. Areas in the 2D array that are not the mine will be represented as `0`s.
The location returned should be an array (`Tuple<int, int>` in C#, `RAX:RDX` in NASM) where the first element is the row index, and the second element is the column index of the bomb location (both should be 0 based). All 2D arrays passed into your function will be square (NxN), and there will only be one mine in the array.
### Examples:
`mineLocation( [ [1, 0, 0], [0, 0, 0], [0, 0, 0] ] )` => returns `[0, 0]` <br/>
`mineLocation( [ [0, 0, 0], [0, 1, 0], [0, 0, 0] ] )` => returns `[1, 1]` <br/>
`mineLocation( [ [0, 0, 0], [0, 0, 0], [0, 1, 0] ] )` => returns `[2, 1]` | algorithms | def mineLocation(field):
for subfield in field:
if 1 in subfield:
return [field . index(subfield), subfield . index(1)]
| Find the Mine! | 528d9adf0e03778b9e00067e | [
"Arrays",
"Algorithms"
]
| https://www.codewars.com/kata/528d9adf0e03778b9e00067e | 6 kyu |
# Task
Given an array `arr`, find the rank of the element at the ith position.
The `rank` of the arr[i] is a value equal to the number of elements `less than or equal to` arr[i] standing before arr[i], plus the number of elements `less than` arr[i] standing after arr[i].
# Example
For `arr = [2,1,2,1,2], i = 2`, the result should be `3`.
There are 2 elements `less than or equal to` arr[2] standing before arr[2]:
`arr[0] <= arr[2]`
`arr[1] <= arr[2]`
There is only 1 element `less than` arr[2] standing after arr[2]:
`arr[3] < arr[2]`
So the result is `2 + 1 = 3`.
# Input/Output
- `[input]` integer array `arr`
An array of integers.
`3 <= arr.length <= 50.`
- `[input]` integer `i`
Index of the element whose rank is to be found.
- `[output]` an integer
Rank of the element at the ith position. | games | def rank_of_element(arr, i):
return sum(x <= arr[i] if n < i else x < arr[i] for n, x in enumerate(arr))
| Simple Fun #177: Rank Of Element | 58b8cc7e8e7121740700002d | [
"Puzzles"
]
| https://www.codewars.com/kata/58b8cc7e8e7121740700002d | 7 kyu |
# Task
Imagine `n` horizontal lines and `m` vertical lines.
Some of these lines intersect, creating rectangles.
How many rectangles are there?
# Examples
For `n=2, m=2,` the result should be `1`.
there is only one 1x1 rectangle.
For `n=2, m=3`, the result should be `3`.
there are two 1x1 rectangles and one 1x2 rectangle. So `2 + 1 = 3`.
For n=3, m=3, the result should be `9`.
there are four 1x1 rectangles, two 1x2 rectangles, two 2x1 rectangles and one 2x2 rectangle. So `4 + 2 + 2 + 1 = 9`.
# Input & Output
- `[input]` integer `n`
Number of horizontal lines.
Constraints: `0 <= n <= 100`
- `[input]` integer `m`
Number of vertical lines.
Constraints: `0 <= m <= 100`
- `[output]` an integer
Number of rectangles. | games | def rectangles(n, m):
return m * n * (m - 1) * (n - 1) / 4
| Simple Fun #105: Rectangles | 589a8d9b729e7abd9a0000ed | [
"Puzzles"
]
| https://www.codewars.com/kata/589a8d9b729e7abd9a0000ed | 7 kyu |
A Cartesian coordinate system is a coordinate system that specifies each point uniquely in a plane by a pair of numerical coordinates, which are the signed distances to the point from two fixed perpendicular directed lines, measured in the same unit of length.
The сoordinates of a point in the grid are written as (x,y). Each point in a coordinate system has eight neighboring points. Provided that the grid step = 1.
It is necessary to write a function that takes a coordinate on the x-axis and y-axis and returns a list of all the neighboring points. Points inside your returned list need not be sorted (any order is valid).
For Example:
```
* Given x = 2 and y = 2, the function should return [(1,1),(1,2),(1,3),(2,1),(2,3),(3,1),(3,2),(3,3)];
* Given x = 5 and y = 7, the function should return [(6,7),(6,6),(6,8),(4,7),(4,6),(4,8),(5,6),(5,8)].
```
Note that the required data structure to contain the coordinates might not be the same between translations, so check the sample test cases provided.
| reference | from itertools import product
def cartesian_neighbor(x, y):
return [z for z in product(range(x - 1, x + 2), range(y - 1, y + 2)) if z != (x, y)]
| Cartesian neighbors | 58989a079c70093f3e00008d | [
"Fundamentals",
"Mathematics"
]
| https://www.codewars.com/kata/58989a079c70093f3e00008d | 7 kyu |
# Task
One night you go for a ride on your motorcycle. At 00:00 you start your engine, and the built-in timer automatically begins counting the length of your ride, in minutes. Off you go to explore the neighborhood.
When you finally decide to head back, you realize there's a chance the bridges on your route home are up, leaving you stranded! Unfortunately, you don't have your watch on you and don't know what time it is. All you know thanks to the bike's timer is that n minutes have passed since 00:00.
Using the bike's timer, calculate the current time. Return an answer as the sum of digits that the digital timer in the format hh:mm would show.
# Example
For n = 240, the output should be
`lateRide(n) === 4`
Since 240 minutes have passed, the current time is 04:00. The digits sum up to 0 + 4 + 0 + 0 = 4, which is the answer.
For n = 808, the output should be
`lateRide(n) === 14`
808 minutes mean that it's 13:28 now, so the answer should be 1 + 3 + 2 + 8 = 14.
# Input/Output
- `[input]` integer `n`
The duration of your ride, in minutes. It is guaranteed that you've been riding for less than a day (24 hours).
Constraints: 0 ≤ n < 60 * 24.
- `[output]` an integer
The sum of the digits the digital timer would show. | games | def late_ride(n):
return sum([int(x) for x in (str(n % 60) + str(int(n / 60)))])
| Simple Fun #3: Late Ride | 588422ba4e8efb583d00007d | [
"Puzzles"
]
| https://www.codewars.com/kata/588422ba4e8efb583d00007d | 7 kyu |
# Background
While mpg (miles per gallon) is a common unit of measurement for fuel economy in North America, for Europe the standard unit of measurement is generally liter per 100 km. Conversion between these units is somewhat hard to calculate in your head, so your task here is to implement a simple convertor in both directions.
# Functions
* `mpg2lp100km()` converts from miles per gallon to liter per 100 km.
* `lp100km2mpg()` converts in the opposite direction.
# Output
The output of both functions should be a number rounded to 2 decimal places.
# Examples
* `mpg2lp100km(42)` returns 5.6
* `lp100km2mpg( 9)` returns 26.13
# Reference
For this kata, use U.S. gallon, not imperial gallon.
* 1 gallon = 3.785411784 liters
* 1 mile = 1.609344 kilometers | algorithms | mpg2lp100km = lp100km2mpg = lambda x: round(235.214583 / x, 2)
| Fuel economy converter (mpg <-> L/100 km) | 558aa460dcfb4a94c40001d7 | [
"Algorithms"
]
| https://www.codewars.com/kata/558aa460dcfb4a94c40001d7 | 7 kyu |
Given a time in AM/PM format as a string, convert it to 24-hour [military time](https://en.wikipedia.org/wiki/24-hour_clock#Military_time) time as a string.
Midnight is `12:00:00AM` on a 12-hour clock, and `00:00:00` on a 24-hour clock. Noon is `12:00:00PM` on a 12-hour clock, and `12:00:00` on a 24-hour clock
Try not to use built-in Date/Time libraries.
## Examples
```
"07:05:45PM" --> "19:05:45"
"12:00:01AM" --> "00:00:01"
"11:46:47PM" --> "23:46:47"
``` | reference | def get_military_time(time):
if time[- 2:] == 'AM':
hour = '00' if time[0: 2] == '12' else time[0: 2]
else:
hour = '12' if time[0: 2] == '12' else str(int(time[0: 2]) + 12)
return hour + time[2: - 2]
| What time is it? | 57729a09914da60e17000329 | [
"Date Time",
"Fundamentals"
]
| https://www.codewars.com/kata/57729a09914da60e17000329 | 7 kyu |
Brief
=====
Sometimes we need information about the list/arrays we're dealing with. You'll have to write such a function in this kata. Your function must provide the following informations:
* Length of the array
* Number of integer items in the array
* Number of float items in the array
* Number of string character items in the array
* Number of whitespace items in the array
The informations will be supplied in arrays that are items of another array. Like below:
`Output array = [[array length],[no of integer items],[no of float items],[no of string chars items],[no of whitespace items]]`
Added Difficulty
----------------
If any item count in the array is zero, you'll have to replace it with a **None/nil/null** value (according to the language). And of course, if the array is empty then return **'Nothing in the array!**. For the sake of simplicity, let's just suppose that there are no nested structures.
Output
======
If you're head is spinning (just kidding!) then these examples will help you out-
```
array_info([1,2,3.33,4,5.01,'bass','kick',' '])--------->[[8],[3],[2],[2],[1]]
array_info([0.001,2,' '])------------------------------>[[3],[1],[1],[None],[1]]
array_info([])----------------------------------------->'Nothing in the array!'
array_info([' '])-------------------------------------->[[1],[None],[None],[None],[1]]
```
Remarks
-------
The input will always be arrays/lists. So no need to check the inputs.
Hint
====
See the tags!!!
Now let's get going !
| reference | def array_info(x):
if not x:
return 'Nothing in the array!'
return [
[len(x)],
[sum(isinstance(i, int) for i in x) or None],
[sum(isinstance(i, float) for i in x) or None],
[sum(isinstance(i, str) and not i . isspace() for i in x) or None],
[sum(isinstance(i, str) and i . isspace() for i in x) or None],
]
| Array Info | 57f12b4d5f2f22651c00256d | [
"Arrays",
"Fundamentals"
]
| https://www.codewars.com/kata/57f12b4d5f2f22651c00256d | 7 kyu |
# Task
You're given a substring s of some cyclic string. What's the length of the smallest possible string that can be concatenated to itself many times to obtain this cyclic string?
# Example
For` s = "cabca"`, the output should be `3`
`"cabca"` is a substring of a cycle string "ab<b><font color='red'>cabca</font></b>bcabc..." that can be obtained by concatenating `"abc"` to itself. Thus, the answer is 3.
# Input/Output
- `[input]` string `s`
Constraints: `3 ≤ s.length ≤ 15.`
- `[output]` an integer | games | def cyclic_string(s):
return next((i for i, _ in enumerate(s[1:], 1) if s . startswith(s[i:])), len(s))
| Simple Fun #55: Cyclic String | 58899594b832f80348000122 | [
"Puzzles"
]
| https://www.codewars.com/kata/58899594b832f80348000122 | 7 kyu |
#### Input
- a string `strng` of n positive numbers (n = 0 or n >= 2)
Let us call weight of a number the sum of its digits.
For example `99` will have "weight" `18`, `100` will have "weight" `1`.
Two numbers are "close" if the difference of their weights is small.
#### Task:
For each number in `strng` calculate its "weight" and then find *two* numbers
of `strng` that have:
- the smallest difference of weights ie that are the closest
- with the smallest weights
- and with the smallest indices (or ranks, numbered from 0) in `strng`
#### Output:
- an array of two arrays, each subarray in the following format:
`[number-weight, index in strng of the corresponding number, original corresponding number in `strng`]`
or a pair of two subarrays (Haskell, Clojure, FSharp) or an array of tuples (Elixir, C++)
or a (char*) in C or a string in some other languages mimicking an array of two subarrays or a string
or a matrix in R (2 rows, 3 columns, no columns names)
The two subarrays are sorted in ascending order by their number weights if these weights are different,
by their indexes in the string if they have the same weights.
#### Examples:
Let us call that function `closest`
```
strng = "103 123 4444 99 2000"
the weights are 4, 6, 16, 18, 2 (ie 2, 4, 6, 16, 18)
closest should return [[2, 4, 2000], [4, 0, 103]] (or ([2, 4, 2000], [4, 0, 103])
or [{2, 4, 2000}, {4, 0, 103}] or ... depending on the language)
because 2000 and 103 have for weight 2 and 4, their indexes in strng are 4 and 0.
The smallest difference is 2.
4 (for 103) and 6 (for 123) have a difference of 2 too but they are not
the smallest ones with a difference of 2 between their weights.
....................
strng = "80 71 62 53"
All the weights are 8.
closest should return [[8, 0, 80], [8, 1, 71]]
71 and 62 have also:
- the smallest weights (which is 8 for all)
- the smallest difference of weights (which is 0 for all pairs)
- but not the smallest indices in strng.
....................
strng = "444 2000 445 544"
the weights are 12, 2, 13, 13 (ie 2, 12, 13, 13)
closest should return [[13, 2, 445], [13, 3, 544]] or ([13, 2, 445], [13, 3, 544])
or [{13, 2, 445}, {13, 3, 544}] or ...
444 and 2000 have the smallest weights (12 and 2) but not the smallest difference of weights;
they are not the closest.
Here the smallest difference is 0 and in the result the indexes are in ascending order.
...................
closest("444 2000 445 644 2001 1002") --> [[3, 4, 2001], [3, 5, 1002]] or ([3, 4, 2001],
[3, 5, 1002]]) or [{3, 4, 2001}, {3, 5, 1002}] or ...
Here the smallest difference is 0 and in the result the indexes are in ascending order.
...................
closest("239382 162 254765 182 485944 468751 49780 108 54")
The weights are: 27, 9, 29, 11, 34, 31, 28, 9, 9.
closest should return [[9, 1, 162], [9, 7, 108]] or ([9, 1, 162], [9, 7, 108])
or [{9, 1, 162}, {9, 7, 108}] or ...
108 and 54 have the smallest difference of weights too, they also have
the smallest weights but they don't have the smallest ranks in the original string.
..................
closest("54 239382 162 254765 182 485944 468751 49780 108")
closest should return [[9, 0, 54], [9, 2, 162]] or ([9, 0, 54], [9, 2, 162])
or [{9, 0, 54}, {9, 2, 162}] or ...
```
#### Notes :
If `n == 0` `closest("")` should return []
- or ([], []) in Haskell, Clojure, FSharp
- or [{}, {}] in Elixir or '(() ()) in Racket
- or {{0,0,0}, {0,0,0}} in C++
- or "[(), ()]" in Go, Nim,
- or "{{0,0,0}, {0,0,0}}" in C, NULL in R
- or "" in Perl.
See Example tests for the format of the results in your language.
| reference | def closest(s):
wght = sorted([[sum(int(c) for c in n), i, int(n)]
for i, n in enumerate(s . split())], key=lambda k: (k[0], k[1]))
diff = [abs(a[0] - b[0]) for a, b in zip(wght, wght[1:])]
return [wght[diff . index(min(diff))], wght[diff . index(min(diff)) + 1]] if wght else []
| Closest and Smallest | 5868b2de442e3fb2bb000119 | [
"Fundamentals",
"Sorting"
]
| https://www.codewars.com/kata/5868b2de442e3fb2bb000119 | 5 kyu |
## Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Drink driving is an issue all around the world! On average 3,000 people are killed or seriously injured each year in drink drive collisions, nearly one in six of all deaths on the road involve drivers who are over the legal alcohol limit.
If you've been out drinking you may still be affected by alcohol the next day. You may feel OK, but you may still be unfit to drive or over the legal alcohol limit. It's impossible to get rid of alcohol any faster. A shower, a cup of coffee or other ways of 'sobering up' will not help. It just takes time.
</pre>
<center><img src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/drunk.png"></center>
## Description
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Your task is to write a kata that will work out if you are safe to drive! You will be given some alcoholic drinks, a time when you stopped drinking and the time you would like to drive.
You will need to return the total units (to 2 decimal places) of the alcoholic drinks and a boolean value <span style="color:#A1A85E">true</span> if you are able to drive or <span style="color:#A1A85E">false</span> if you are still unable to drive.
</pre>
## Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Create a method called <span style="color:#A1A85E">drive</span> that takes an array of drinks in the format <span style="color:#A1A85E">[[strength, volume]]</span> a time when you finished drinking and a time when you would like to drive.<br>
eg. <span style="color:#A1A85E">drive([[10.0,100]], "20:00", "21:00")</span> and it must return <span style="color:#A1A85E">[1.0, false]</span>
</pre>
## Rules
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
1. On average it takes a person <span style="color:#A1A85E">1 hour</span> for their body to remove <span style="color:#A1A85E">1 unit</span> of alcohol.
2. Units of alcohol are calculated by <span style="color:#A1A85E">strength (ABV) x volume (ml) / 1000 = units</span>. More information can be found <a href="http://www.nhs.uk/Livewell/alcohol/Pages/alcohol-units.aspx">here</a>
3. Data passed will be in the format <span style="color:#A1A85E">([[strength,volume]], finished, drive_time)</span>. Where <span style="color:#A1A85E">finished</span> is the time you stopped drinking and <span style="color:#A1A85E">drive_time</span> is the time you would like to drive.
4. If the <span style="color:#A1A85E">finished >= drive_time</span> then you can assume that it is the next day.
5. Times are passed as strings and are in 24 hour format.
6. Return total units to 2 decimal places rounded. For example <span style="color:#A1A85E">1.10 => 1.1</span> and <span style="color:#A1A85E">1.236 => 1.24</span>
7. Return <span style="color:#A1A85E">true</span> if you are able to drive and <span style="color:#A1A85E">false</span> if you are not.
</pre>
## Example
```ruby
alcohol = [[5.2,568],[5.2,568],[5.2,568],[12.0,175],[12.0,175],[12.0,175]]
drive(alcohol, "23:00", "08:15")
```
```python
alcohol = [[5.2,568],[5.2,568],[5.2,568],[12.0,175],[12.0,175],[12.0,175]]
drive(alcohol, "23:00", "08:15")
```
```javascript
alcohol = [[5.2,568],[5.2,568],[5.2,568],[12.0,175],[12.0,175],[12.0,175]];
drive(alcohol, "23:00", "08:15");
```
```csharp
double[,] alcohol = new double[,] { { 5.2, 568.0 }, { 5.2, 568.0 }, { 5.2, 568.0 }, { 12.0, 175.0 }, { 12.0, 175.0 }, { 12.0, 175.0 } };
drive(alcohol, "23:00", "08:15");
```
```swift
alcohol = [[5.2,568.0],[5.2,568.0],[5.2,568.0],[12.0,175.0],[12.0,175.0],[12.0,175.0]];
drive(alcohol, "23:00", "08:15");
```
should return
```ruby
[15.16, false]
```
```python
[15.16, False]
```
```javascript
[15.16, false]
```
```csharp
{ 15.16, false }
```
```swift
[15.16, false]
```
Good luck and enjoy!
## Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | reference | def drive(drinks, finished, drive_time):
f, d = (list(map(int, x . split(':'))) for x in [finished, drive_time])
delta = d[0] - f[0] + (d[1] - f[1]) / 60 + (24 * (f >= d))
units = sum(strength * volume for strength, volume in drinks) / 1000
return [round(units, 2), delta > units]
| Am I safe to drive? | 58ce88427e6c3f41c2000087 | [
"Fundamentals"
]
| https://www.codewars.com/kata/58ce88427e6c3f41c2000087 | 6 kyu |
This should be fairly simple. It is more of a puzzle than a programming problem.
There will be a string input in this format:
```'a+b'```
2 lower case letters (a-z) seperated by a '+'
Return the sum of the two variables.
There is one correct answer for a pair of variables.
I know the answers, it is your task to find out.
Once you crack the code for one or two of the pairs, you will have the answer for the rest.
It is like when you were in school doing math and you saw ```"11 = c+h"``` and you needed to find out what c and h were.
However you don't have an 11. You have an unknown there as well. Example:
X = a+b.
You don't know what X is, and you don't know what b is or a, but it is a puzzle and you will find out.
As part of this puzzle, there is three hints or clues on solving this. I won't tell you what the other two are, but one of them is:
```Don't overthink it. It is a simple solution ```
Given the input as a string - Return the sum of the two variables as int.
| games | def the_var(vars):
return sum(ord(c) - 96 for c in vars . split("+"))
| The unknown but known variables: Addition | 5716955a794d3013d00013f9 | [
"Puzzles"
]
| https://www.codewars.com/kata/5716955a794d3013d00013f9 | 7 kyu |
# Task
Four men, `a`, `b`, `c` and `d` are standing in a line, one behind another.
There's a wall between the first three people (`a`, `b` and `c`) and the last one (`d`).
The men `a`, `b` and `c` are lined up in order of height, so that:
* person `a` can see the backs of `b` and `c`
* person `b` can see the back of `c`
* person `c` can see just the wall
There are 4 hats, 2 black and 2 white. Each person is given a hat. None of them can see their own hat, but person `a` can see the hats of `b` and `c`, while person `b` can see the hat of person `c`. Neither `c` nor `d` can see any hats.
Once a person figures out the color of their hat, they shout it.

Your task is to return the person who will guess their hat first. You can assume that they will speak only when they reach a correct conclusion.
# Input/Output
- `[input]` string `a`
a's hat color ("white" or "black").
- `[input]` string `b`
b's hat color ("white" or "black").
- `[input]` string `c`
c's hat color ("white" or "black").
- `[input]` string `d`
d's hat color ("white" or "black").
- `[output]` an integer
The person to guess his hat's color first, `1` for `a`, `2` for `b`, `3` for `c` and `4` for `d`. | games | def guess_hat_color(a, b, c, d):
return 1 if b == c else 2
| Simple Fun #195: Guess Hat Color | 58c21c4ff130b7cab400009e | [
"Puzzles"
]
| https://www.codewars.com/kata/58c21c4ff130b7cab400009e | 7 kyu |
## Euler's Method
We want to calculate the shape of an unknown curve which starts at a given point with a given slope. This curve satisfies an ordinary differential equation (ODE):
```math
\frac{dy}{dx} = f(x, y);\\
y(x_0) = y_0
```
The starting point `$ A_0 (x_0, y_0) $` is known as well as the slope to the curve
at `$ A_0 $` and then the tangent line at `$ A_0 $` .
Take a small step along that tangent line up to a point `$ A_1 $`. Along this small step,
the slope does not change too much, so `$ A_1 $` will be close to the curve.
If we suppose that `$ A_1 $` is close enough to the curve, the same reasoning
as for the point `$ A_1 $` above can be used for other points.
After several steps, a polygonal curve `$ A_0, A_1, ..., A_n $` is computed.
The error between the two curves will be small if the step is small.
We define points `$ A_0, A_1, A_2, ..., A_n $`
whose x-coordinates are `$ x_0, x_1, ..., x_n $`
and y-coordinates are such that `$ y_{k+1} = y_k + f(x_k, y_k) \times h $`
where `$ h $` is the common step. If `$ T $` is the length `$ x_n - x_0 $` we have `$ h = T/n $`.
#### Task
For this kata we will focus on the following differential equation:
```math
\frac{dy}{dx} = 2 - e^{-4x} - 2y; \\
A_0 = (0,1)
```
with `$ x ∈ [0, 1] $`. We will then take a uniform partition of the region of `$ x $` between `$ 0 $` and `$ 1 $` and split it into `$ n $` sections (hence `$ n + 1 $` points). `$ n $` will be the input to the function `ex_euler(n)` and since `$ T $` is always 1, `$ h = 1/n $`.
We know that an exact solution is
```math
z = 1 + 0.5e^{-4x} - 0.5e^{-2x}.
```
For each `$ x_k $` we are able to calculate the `$ y_k $` as well as the values `$ z_k $` of the exact solution.
Our task is, for a given number `$ n $` of steps, to return the mean (*truncated* to 6 decimal places)
of the relative errors between the `$ y_k $` (our aproximation) and the `$ z_k $` (the exact solution).
For that we can use:
error in `$ A_k = abs(y_k - z_k) / z_k $` and then the mean is sum(errors in `$ A_k $`)/ (`$ n $` + 1)
#### Examples
```python
ex_euler(10) should return: 0.026314 (truncated from 0.026314433214799246)
with
Y = [1.0,0.9..., 0.85..., 0.83..., 0.83..., 0.85..., 0.86..., 0.88..., 0.90..., 0.91..., 0.93...]
Z = [1.0, 0.9..., 0.88..., 0.87..., 0.87..., 0.88..., 0.89..., 0.90..., 0.91..., 0.93..., 0.94...]
Relative errors = [0.0, 0.02..., 0.04..., 0.04..., 0.04..., 0.03..., 0.03..., 0.02..., 0.01..., 0.01..., 0.01...]
```
`ex_euler(17)` should return: `0.015193 (truncated from 0.015193336263370796)`.
As expected, as `$ n $` increases, our error reduces.
#### Links and graphs
<a href="https://en.wikipedia.org/wiki/Euler_method">Wiki article</a>

Below comparison between approximation (red curve) and exact solution(blue curve) for n=100:

#### Thanks to @rge123 for a better description
| algorithms | from math import floor, exp
def ex_euler(n):
# fct to study
def F(t, y): return 2 - exp(- 4 * t) - 2 * y
# initial conditions
t0 = 0
y0 = 1 # pt A0
T = 1
# variables
h = T / float(n)
X = [t0]
Y = [y0]
Z = []
R = []
# points A1 ... An
for k in range(0, n):
X . append((k + 1) * h)
Y . append(Y[k] + h * F(X[k], Y[k]))
# pts on the exact curve
for k in range(0, n + 1):
# exact solution
Z . append(1 + 0.5 * exp(- 4 * X[k]) - 0.5 * exp(- 2 * X[k]))
# relative error
R . append(abs(Y[k] - Z[k]) / float(Z[k]))
return floor((sum(R) / float(n + 1)) * 1e6) / 1e6
| Euler's method for a first-order ODE | 56347fcfd086de8f11000014 | [
"Algorithms"
]
| https://www.codewars.com/kata/56347fcfd086de8f11000014 | 5 kyu |
Given a list of unique words. Find all pairs of distinct indices (i, j) in the given list so that the concatenation of the two words, i.e. words[i] + words[j] is a palindrome.
Examples:
```ruby
["bat", "tab", "cat"] # [[0, 1], [1, 0]]
["dog", "cow", "tap", "god", "pat"] # [[0, 3], [2, 4], [3, 0], [4, 2]]
["abcd", "dcba", "lls", "s", "sssll"] # [[0, 1], [1, 0], [2, 4], [3, 2]]
```
Non-string inputs should be converted to strings.
Return an array of arrays containing pairs of distinct indices that form palindromes. Pairs should be returned in the order they appear in the original list. | reference | def palindrome_pairs(words):
indices = []
for i in range(len(words)):
for j in range(len(words)):
if i != j:
concatenation = str(words[i]) + str(words[j])
if concatenation == concatenation[:: - 1]:
indices . append([i, j])
return indices
| Palindrome Pairs | 5772ded6914da62b4b0000f8 | [
"Lists",
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/5772ded6914da62b4b0000f8 | 7 kyu |
Complete the code which should return `true` if the given object is a single ASCII letter (lower or upper case), `false` otherwise. | reference | def is_letter(s):
return len(s) == 1 and s . isalpha()
| Regexp Basics - is it a letter? | 567de72e8b3621b3c300000b | [
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/567de72e8b3621b3c300000b | 7 kyu |
A [happy number](https://en.wikipedia.org/wiki/Happy_number) is one where if you repeatedly square its digits and add them together, you eventually end up at 1.
For example:
```7 -> 49 -> 97 -> 130 -> 10 -> 1``` so ```7``` is a happy number.
```42 -> 20 -> 4 -> 16 -> 37 -> 58 -> 89 -> 145 -> 42``` so ```42``` is **not** a happy number.
This can also be done with powers other than 2.
Complete the function that receives 2 arguments: the starting number and the exponent. It should return an array of numbers containing whatever loop it encounters, or ```[1]``` if it doesn't encounter any. This array should only include the numbers in the loop, not any that lead into the loop, and should repeat the first number as the last number e.g.:
```
[42, 20, 4, 16, 37, 58, 89, 145, 42]
```
The first number in the array should be where the loop is first encountered.
All function inputs will be positive integers, with the exponent being between 2 and 4. | algorithms | def isHappy(n, pow):
nums = []
while True:
nums . append(n)
n = sum([d * * pow for d in map(int, str(n))])
if n == 1:
return [1]
if n in nums:
return nums[nums . index(n):] + [n]
| Happy numbers to the n power | 578597a122542a7d24000018 | [
"Algorithms"
]
| https://www.codewars.com/kata/578597a122542a7d24000018 | 6 kyu |
Write a function that checks if all the letters in the second string are present in the first one at least once, regardless of how many times they appear:
```
["ab", "aaa"] => true
["trances", "nectar"] => true
["compadres", "DRAPES"] => true
["parses", "parsecs"] => false
```
Function should not be case sensitive, as indicated in example #2. Note: both strings are presented as a **single argument** in the form of an array. | algorithms | def letter_check(arr):
return set(arr[1]. lower()) <= set(arr[0]. lower())
| noobCode 03: CHECK THESE LETTERS... see if letters in "String 2" are present in "String 1" | 57470efebf81fea166001627 | [
"Strings",
"Algorithms"
]
| https://www.codewars.com/kata/57470efebf81fea166001627 | 7 kyu |
Extend the Math object (JS) or module (Ruby and Python) to convert degrees to radians and viceversa. The result should be rounded to two decimal points.
Note that all methods of Math object are static.
Example:
```javascript
Math.degrees(Math.PI) //180deg
Math.radians(180) //3.14rad
```
```python
math.degrees(math.pi) //180deg
math.radians(180) //3.14rad
```
```ruby
Math.degrees(Math::PI) //180deg
Math.radians(180) //3.14rad
``` | reference | def degrees(rad):
return '%gdeg' % round(180 * rad / math . pi, 2)
def radians(deg):
return '%grad' % round(math . pi * deg / 180, 2)
math . degrees = degrees
math . radians = radians
| Convert between radians and degrees | 544e2c60908f2da03600022a | [
"Mathematics",
"Fundamentals"
]
| https://www.codewars.com/kata/544e2c60908f2da03600022a | 7 kyu |
Egg Talk.
Insert an "egg" after each consonant. If there are no consonants, there will be no eggs. Argument will consist of a string with only alphabetic characters and possibly some spaces.
eg:
hello => heggeleggleggo
eggs => egegggeggsegg
FUN KATA => FeggUNegg KeggATeggA
////
This Kata is designed for beginners to practice the basics of regular expressions. With this in mind a little bit of commenting in your solution could be very useful.
Check Eloquent Javascript p176
https://regex101.com/#javascript
| reference | import re
def heggeleggleggo(word):
return re . sub(r'(?i)([^aeiou\W])', r'\1egg', word)
| heggeleggleggo | 55ea5304685da2fb40000018 | [
"Regular Expressions",
"Fundamentals"
]
| https://www.codewars.com/kata/55ea5304685da2fb40000018 | 7 kyu |
This is the simple version of Shortest Code series. If you need some challenges, please try the [challenge version](http://www.codewars.com/kata/56f928b19982cc7a14000c9d)
## Task:
Every uppercase letter is Father, The corresponding lowercase letters is the Son.
Give you a string ```s```, If the father and son both exist, keep them. If it is a separate existence, delete them. Return the result.
For example:
```sc("Aab")``` should return ```"Aa"```
```sc("AabBc")``` should return ```"AabB"```
```sc("AaaaAaab")``` should return ```"AaaaAaa"```(father can have a lot of son)
```sc("aAAAaAAb")``` should return ```"aAAAaAA"```(son also can have a lot of father ;-)
### Series:
- [Bug in Apple](http://www.codewars.com/kata/56fe97b3cc08ca00e4000dc9)
- [Father and Son](http://www.codewars.com/kata/56fe9a0c11086cd842000008)
- [Jumping Dutch act](http://www.codewars.com/kata/570bcd9715944a2c8e000009)
- [Planting Trees](http://www.codewars.com/kata/5710443187a36a9cee0005a1)
- [Give me the equation](http://www.codewars.com/kata/56fe9b65cc08cafbc5000de3)
- [Find the murderer](http://www.codewars.com/kata/570f3fc5b29c702c5500043e)
- [Reading a Book](http://www.codewars.com/kata/570ca6a520c69f39dd0016d4)
- [Eat watermelon](http://www.codewars.com/kata/570df12ce6e9282a7d000947)
- [Special factor](http://www.codewars.com/kata/570e5d0b93214b1a950015b1)
- [Guess the Hat](http://www.codewars.com/kata/570ef7a834e61306da00035b)
- [Symmetric Sort](http://www.codewars.com/kata/5705aeb041e5befba20010ba)
- [Are they symmetrical?](http://www.codewars.com/kata/5705cc3161944b10fd0004ba)
- [Max Value](http://www.codewars.com/kata/570771871df89cf59b000742)
- [Trypophobia](http://www.codewars.com/kata/56fe9ffbc25bf33fff000f7c)
- [Virus in Apple](http://www.codewars.com/kata/5700af83d1acef83fd000048)
- [Balance Attraction](http://www.codewars.com/kata/57033601e55d30d3e0000633)
- [Remove screws I](http://www.codewars.com/kata/5710a50d336aed828100055a)
- [Remove screws II](http://www.codewars.com/kata/5710a8fd336aed00d9000594)
- [Regular expression compression](http://www.codewars.com/kata/570bae4b0237999e940016e9)
- [Collatz Array(Split or merge)](http://www.codewars.com/kata/56fe9d579b7bb6b027000001)
- [Tidy up the room](http://www.codewars.com/kata/5703ace6e55d30d3e0001029)
- [Waiting for a Bus](http://www.codewars.com/kata/57070eff924f343280000015) | games | def sc(strng):
seen = set(strng)
return '' . join(a for a in strng if a . swapcase() in seen)
| Coding 3min: Father and Son | 56fe9a0c11086cd842000008 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/56fe9a0c11086cd842000008 | 7 kyu |
Make me slow! Calling makeMeSlow() should take at least 7 seconds. | reference | def make_me_slow():
import time
time . sleep(7)
| Make Me Slow | 57f59da8d3978bb31f000152 | [
"Fundamentals"
]
| https://www.codewars.com/kata/57f59da8d3978bb31f000152 | 7 kyu |
Given
- a semi-inclusive interval `I = [l, u)` (l is in interval I but u is not)
`l` and `u` being floating numbers `(0 <= l < u)`,
- an integer `n (n > 0)`
- a function `f: x (float number) -> f(x) (float number)`
we want to return as a list the `n` values:
`f(l), f(l + 1 * d), ..., f(u -d)` where `d = (u - l) / n`
or as a string (Bash, Nim):
`"f(l), f(l + 1 * d), ..., f(u -d)"` where `d = (u - l) / n`
Call this function `interp`:
`interp(f, l, u, n) -> [f(l), f(l + 1 * d), ..., f(u - d)]`
The `n` resulting values `f(l), f(l + 1 * d), ..., f(u - d)` will be **floored** to two decimals (except Shell and Nim: see below).
For that you can use: `floor(y * 100.0) / 100.0`.
```
Examples:
interp(x -> x, 0.0, 0.9, 3) -> [0.0; 0.3; 0.6]
interp(x -> x, 0.0, 0.9, 4) -> [0.0; 0.22; 0.45; 0.67]
interp(x -> x, 0.0, 1.0, 4) -> [0.0; 0.25; 0.5; 0.75]
interp(x -> sin x, 0.0, 0.9, 3) -> [0.0; 0.29; 0.56]
```
#### Note for Shell (bash)
To avoid discussions, please do the calculations with `bc (scale = 16)` and round the result with `printf "%.2f"`.
#### Note for Nim
To avoid discussions, please round the result with something analogous to `printf "%.2f"`. | reference | from math import floor
def interp(f, l, u, n):
d = (u - l) / n
return [floor(f(l + d * i) * 100.) / 100. for i in range(n)]
| Floating-point Approximation (II) | 581ee0db1bbdd04e010002fd | [
"Fundamentals"
]
| https://www.codewars.com/kata/581ee0db1bbdd04e010002fd | 6 kyu |
Removed due to copyright infringement.
<!---
Vasya is a lazy student. He has certain amount of clean bowls and plates (lets call it `b` and `p`).
Vasya has made an eating plan for the next several days.
-----
### Vasya's eating Plan
As Vasya is lazy, he will eat exactly *1* dish per day. In order to eat a dish, he needs exactly *1 clean plate OR bowl*. We know that Vasya can cook only *2* types of dishes. He can eat:
1. Dishes of the first type from bowls
2. Dishes of the second type from either bowls or plates
When Vasya finishes eating, he leaves a dirty plate/bowl behind. His life philosophy doesn't let him eat from dirty kitchenware. So sometimes he needs to wash his plate/bowl `before eating`. Since he is lasy, so he only wash one plate or bowl each time.
-----
### Input
The input contains two integers and integer array: *b, p, dishes* :
1. `b`- the number of clean bowls `(1 ≤ b ≤ 1000)`
2. `p` - the number of clean plates `(1 ≤ p ≤ 1000)`
3. `dishes` - If `dishes[i] == 1` , then on that day, Vasya will eat the first type of dish. If `dishes[i] == 2`, then on that day Vasya will eat the second type of dish. `(1 ≤ dishes[i] ≤ 2)`
-----
### Your task
Find the minimum number of times Vasya will need to wash a plate/bowl, if he acts optimally.
### Examples:
```csharp
EatingPlan.CountClean(1,1, new int[] {1,2,1})
// Vasya will wash a bowl only on the third day, so the answer is 1
EatingPlan.CountClean(3,1,new int[] {1,1,1,1})
/* Vasya will have the first type of the dish during all four days,
and since there are only three bowls, he will wash a bowl exactly once.*/
EatingPlan.CountClean(1,2,new int[] {2, 2, 2})
/* Vasya will have the second type of dish for all three
days,and as they can be eaten from either a plate or a bowl, he will never
need to wash a plate/bowl.*/
```
```javascript
eatingPlan(1,1,[1,2,1])
//Vasya will wash a bowl only on the third day, so the answer is `1`
eatingPlan(3,1,[1, 1, 1, 1])
/* Vasya will have the first type of the dish during all four days,
and since there are only three bowls, he will wash a bowl exactly once.*/
eatingPlan(1,2,[2, 2, 2])
/* Vasya will have the second type of dish for all three
days,and as they can be eaten from either a plate or a bowl, he will never
need to wash a plate/bowl.*/
```
```ruby
eatingPlan(1,1,[1,2,1])
//Vasya will wash a bowl only on the third day, so the answer is `1`
eatingPlan(3,1,[1, 1, 1, 1])
/* Vasya will have the first type of the dish during all four days,
and since there are only three bowls, he will wash a bowl exactly once.*/
eatingPlan(1,2,[2, 2, 2])
/* Vasya will have the second type of dish for all three
days,and as they can be eaten from either a plate or a bowl, he will never
need to wash a plate/bowl.*/
```
```python
eatingPlan(1,1,[1,2,1])
//Vasya will wash a bowl only on the third day, so the answer is `1`
eatingPlan(3,1,[1, 1, 1, 1])
/* Vasya will have the first type of the dish during all four days,
and since there are only three bowls, he will wash a bowl exactly once.*/
eatingPlan(1,2,[2, 2, 2])
/* Vasya will have the second type of dish for all three
days,and as they can be eaten from either a plate or a bowl, he will never
need to wash a plate/bowl.*/
```
```coffeescript
eatingPlan(1,1,[1,2,1])
//Vasya will wash a bowl only on the third day, so the answer is `1`
eatingPlan(3,1,[1, 1, 1, 1])
/* Vasya will have the first type of the dish during all four days,
and since there are only three bowls, he will wash a bowl exactly once.*/
eatingPlan(1,2,[2, 2, 2])
/* Vasya will have the second type of dish for all three
days,and as they can be eaten from either a plate or a bowl, he will never
need to wash a plate/bowl.*/
```
---> | games | def count_clean(b, p, dishes):
dirty = max(len(dishes) - b - p, dishes . count(1) - b)
return 0 if dirty < 0 else dirty
| Vasya and Plates | 55807d36933247feff00002f | [
"Puzzles",
"Algorithms",
"Logic",
"Mathematics",
"Numbers",
"Fundamentals"
]
| https://www.codewars.com/kata/55807d36933247feff00002f | 7 kyu |
``isMatching`` checks if a string can be created by combining and rearranging the letters of two other strings (**not case sensitive**).
!**Spaces** will be ignored but **special characters** and **numbers** won't match the string and return false.
For example:
``isMatching("email box", "b aIl", "Mo x e") return true``
but
``isMatching("bouh", "0b", "uh") return false``
You need to be able to use _all_ the caracters from the two strings so:
``isMatching("kata", "kt", "aaa") return false`` | algorithms | def clean(s): return sorted(s . replace(' ', ''). lower())
def is_matching(st, st1, st2):
return clean(st) == clean(st1 + st2)
| String Matcher | 565ce4ab24ef4aee6a000074 | [
"Strings",
"Algorithms"
]
| https://www.codewars.com/kata/565ce4ab24ef4aee6a000074 | 7 kyu |
You're looking through different hex codes, and having trouble telling the difference between <a href='http://www.colorhexa.com/000001'> #000001 </a> and <a href='http://www.colorhexa.com/100000'> #100000 </a>
We need a way to tell which is red, and which is blue!
That's where you create __hex color__ !!!
It should read an RGB input, and return whichever value (red, blue, or green) is of greatest concentration!
But, if multiple colors are of equal concentration, you should return their mix!
```
red + blue = magenta
green + red = yellow
blue + green = cyan
red + blue + green = white
```
One last thing, if the string given is empty, or has all 0's, return black!
Examples:
```
codes = "087 255 054" -> "green"
codes = "181 181 170" -> "yellow"
codes = "000 000 000" -> "black"
codes = "001 001 001" -> "white"
```
| reference | colors = {
(1, 0, 0): 'red',
(0, 1, 0): 'green',
(0, 0, 1): 'blue',
(1, 0, 1): 'magenta',
(1, 1, 0): 'yellow',
(0, 1, 1): 'cyan',
(1, 1, 1): 'white',
}
def hex_color(codes):
codes = codes or '0 0 0'
items = [int(c) for c in codes . split()]
m = max(items)
return colors[tuple(i == m for i in items)] if m else 'black'
| Colored Hexes! | 57e17750621bca9e6f00006f | [
"Fundamentals"
]
| https://www.codewars.com/kata/57e17750621bca9e6f00006f | 7 kyu |
Multiplication can be thought of as repeated addition. Exponentiation can be thought of as repeated multiplication. [Tetration](https://en.wikipedia.org/wiki/Tetration) is repeated exponentiation. Just as the 4th power of 3 is `3*3*3*3`, the 4th tetration of 3 is `3^3^3^3`. By convention, we insert parentheses from right to left, so the 4th tetration of 3 is `3^(3^(3^3))) = 3^(3^27) = 3^7625597484987 = a really big number`
## Your Task
Complete the function that returns the number that is the `y`th tetration of `x`, where `x` is a positive integer, and `y` is a non-negative integer. The function should return a number (not a string that picks out the number).
**Note:** the convention is that, as with exponentiation, the 0th tetration of any number is always `1`
## Examples (inputs -> output)
```
* 4, 0 --> 1 (due to the convention mentioned above)
* 7, 1 --> 7 (7^1)
* 5, 2 --> 3125 (5^5)
* 2, 3 --> 16 (2^(2^2))
``` | reference | from functools import reduce
from itertools import repeat
def tetration(x, y):
return reduce(int . __rpow__, repeat(x, y), 1)
| Tetration | 5797bbb34be9127074000132 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5797bbb34be9127074000132 | 7 kyu |
## Description
John's each hand has five fingers(If you are surprised, please tell me how many fingers you have ;-) Even more amazing is that when he was a child, he already had 5 fingers(one hand). At that time, he often to count the number by using his fingers.
He is counting this way:
```
a - Thumb
b - Index finger
c - Middle finger
d - Ring finger
e - Little finger
a b c d e
--------------
1 2 3 4 5
9 8 7 6
10 11 12 13
17 16 15 14
18 19 20 21
.. .. .. ..
.. .. .. ..
```
So the question is: when John counting to number `n`, which is the corresponding finger?
## Task
Complete the function which accepts an integer, and returns the name of the finger on which the counting will end: `"Thumb"`, `"Index finger"`, `"Middle finger"`, `"Ring finger"`, or `"Little finger"`.
## Examples
```
10 => "Index finger"
20 => "Ring finger"
30 => "Ring finger"
50 => "Index finger"
100 => "Ring finger"
1000 => "Index finger"
10000 => "Index finger"
``` | games | a = ("Index finger", "Thumb", "Index finger", "Middle finger",
"Ring finger", "Little finger", "Ring finger", "Middle finger")
def which_finger(n):
return a[n % 8]
| T.T.T.32: Count with your fingers | 57add740e298a7a6d500000d | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/57add740e298a7a6d500000d | 7 kyu |
You have an amount of money `a0 > 0` and you deposit it with an interest rate of `p percent divided by 360` *per day* on the `1st of January 2016`. You want to have an amount `a >= a0`.
#### Task:
The function `date_nb_days` (or dateNbDays...) with parameters `a0, a, p` will return, as a string, the date on which you have just reached `a`.
#### Example:
`date_nb_days(100, 101, 0.98) --> "2017-01-01" (366 days)`
`date_nb_days(100, 150, 2.00) --> "2035-12-26" (7299 days)`
#### Notes:
- The return format of the date is `"YYYY-MM-DD"`
- The deposit is always on the `"2016-01-01"`
- Don't forget to take the rate for a day to be `p divided by 36000` since banks consider that there are `360` days in a year.
- You have: `a0 > 0, p% > 0, a >= a0` | reference | from math import log, ceil
from datetime import date, timedelta as td
def date_nb_days(a0, a, p):
n = log(a, 1 + p / 36000.0) - log(a0, 1 + p / 36000.0)
return str(date(2016, 1, 1) + td(ceil(n)))
| Target Date | 569218bc919ccba77000000b | [
"Fundamentals",
"Mathematics"
]
| https://www.codewars.com/kata/569218bc919ccba77000000b | 7 kyu |
## Task
Given a square `matrix`, your task is to reverse the order of elements on both of its longest diagonals.
The longest diagonals of a square matrix are defined as follows:
* the first longest diagonal goes from the top left corner to the bottom right one;
* the second longest diagonal goes from the top right corner to the bottom left one.
## Example
For the matrix
```
1, 2, 3
4, 5, 6
7, 8, 9
```
the output should be:
```
9, 2, 7
4, 5, 6
3, 8, 1
```
## Input/Output
- `[input]` 2D integer array `matrix`
Constraints: `1 ≤ matrix.length ≤ 10, matrix.length = matrix[i].length, 1 ≤ matrix[i][j] ≤ 1000`
- `[output]` 2D integer array
Matrix with the order of elements on its longest diagonals reversed. | games | def reverse_on_diagonals(matrix):
copy = [line[:] for line in matrix]
for i in range(len(matrix)):
copy[i][i] = matrix[- 1 - i][- 1 - i]
copy[i][- 1 - i] = matrix[- 1 - i][i]
return copy
| Simple Fun #59: Reverse On Diagonals | 5889a6b653ad4a22710000d0 | [
"Puzzles"
]
| https://www.codewars.com/kata/5889a6b653ad4a22710000d0 | 7 kyu |
# Task
You are implementing a command-line version of the Paint app. Since the command line doesn't support colors, you are using different characters to represent pixels. Your current goal is to support rectangle x1 y1 x2 y2 operation, which draws a rectangle that has an upper left corner at (x1, y1) and a lower right corner at (x2, y2). Here the x-axis points from left to right, and the y-axis points from top to bottom.
Given the initial `canvas` state and the array that represents the coordinates of the two corners, return the canvas state after the operation is applied. For the details about how rectangles are painted, see the example.
# Example
For
```
canvas = [['a', 'a', 'a', 'a', 'a', 'a', 'a', 'a'],
['a', 'a', 'a', 'a', 'a', 'a', 'a', 'a'],
['a', 'a', 'a', 'a', 'a', 'a', 'a', 'a'],
['b', 'b', 'b', 'b', 'b', 'b', 'b', 'b'],
['b', 'b', 'b', 'b', 'b', 'b', 'b', 'b']]
and rectangle = [1, 1, 4, 3]```
the output should be
```
[['a', 'a', 'a', 'a', 'a', 'a', 'a', 'a'],
['a', '*', '-', '-', '*', 'a', 'a', 'a'],
['a', '|', 'a', 'a', '|', 'a', 'a', 'a'],
['b', '*', '-', '-', '*', 'b', 'b', 'b'],
['b', 'b', 'b', 'b', 'b', 'b', 'b', 'b']]```
Note that rectangle sides are depicted as -s and |s, asterisks (*) stand for its corners and all of the other "pixels" remain the same. Color in the example is used only for illustration.
# Input/Output
- `[input]` 2D string array `canvas`
A non-empty rectangular matrix of characters.
Constraints: `2 ≤ canvas.length ≤ 10, 2 ≤ canvas[0].length ≤ 10.`
- `[input]` integer array `rectangle`
Array of four integers - [x1, y1, x2, y2].
Constraints: `0 ≤ x1 < x2 < canvas[i].length, 0 ≤ y1 < y2 < canvas.length.`
- `[output]` 2D string array | games | def draw_rectangle(canvas, rectangle):
x0, y0, x1, y1 = rectangle
for x in range(x0, x1):
canvas[y0][x] = canvas[y1][x] = '-'
for y in range(y0, y1):
canvas[y][x0] = canvas[y][x1] = '|'
canvas[y0][x0] = canvas[y0][x1] = \
canvas[y1][x0] = canvas[y1][x1] = '*'
return canvas
| Simple Fun #62: Draw Rectangle | 5889ae4f7af7f99a9a000019 | [
"Puzzles"
]
| https://www.codewars.com/kata/5889ae4f7af7f99a9a000019 | 7 kyu |
<img src=https://cdn.meme.am/instances/21245401.jpg>
Chuck Norris is the world's toughest man - he once kicked a horse in the chin. Its descendants today are known as giraffes.
Like his punches, Chuck NEVER needs more than one line of code.
Your task, to please Chuck, is to create a function that chains 4 methods on a SINGLE LINE! You can pass with multiple lines, but CHuck will pity you. Go big or go home. ONE LINE!!
Chuck expects his list of favourite items to be split, sorted, joined AND have any occurrences of the letters 'e' and 'a' removed - why, you ask? Well Nunchuks hasn't got the letters 'a' or 'e' in it has it?? Chuck says shut your mouth... and don't forget the capitals.
If anyone dares to provide Chuck with an empty string, an integer or an array, just return a description of their face once Chuck finds out: 'Broken!'
Go, go go!!! | algorithms | import re
def one_punch(s): return re . sub("[eaEA]", "", " " . join(
sorted(s . split()))) if isinstance(s, str) and s else "Broken!"
| Chuck Norris II - One Punch | 57057a035eef1f7e790009ef | [
"Fundamentals",
"Regular Expressions",
"Algorithms"
]
| https://www.codewars.com/kata/57057a035eef1f7e790009ef | 7 kyu |
I'm creating a scoreboard on my game website, but I want the score to be displayed as tally marks instead of Roman numerals. Write a function that translates the numeric score into tally marks.
My tally mark font uses the letters a, b, c, d, e to represent tally marks for 1, 2, 3, 4, 5, respectively. I want a space and line break (
) after each completed tally (5).
Assume that the score you receive will be an integer. This function should return an HTML string that I can insert into my website that represents the correct score. | algorithms | def score_to_tally(s):
return 'e <br>' * (s / / 5) + 'abcd' [s % 5 - 1: s % 5]
| Tally it up | 5630d1747935943168000013 | [
"Algorithms"
]
| https://www.codewars.com/kata/5630d1747935943168000013 | 7 kyu |
This is the simple version of Shortest Code series. If you need some challenges, please try the [challenge version](http://www.codewars.com/kata/570dff30e6e9284ba3000a8f)
## Task:
Give you a positive integer ```n```, find out the special factor.
What is the special factor? An example: if n has a factor x, we can convert them into binary string, if binary string of n contains binary string of x, we can say, x is a special factor of n.
Return an array, include all of the special factor(Ascending order)
### Example:
```
Example1:
n = 6 factor of n: 1,2,3,6
convert them to binary string:
n ==> "110"
factors: 1==>"1" 2==>"10" 3==>"11" 6==>"110"
Hmmm... It seems "110" contains all of them.
So, sc(6) should return [1,2,3,6]
Example2:
n = 15 factor of n: 1,3,5,15
convert them to binary string:
n ==> "1111"
factors: 1==>"1" 3==>"11" 5==>"101" 15==>"1111"
Hmmm... "1","11","1111" are special factors, but "101" is not.
So, sc(15) should return [1,3,15]
Example3:
n = 100 factor of n: 1,2,4,5,10,20,25,50,100
convert them to binary string:
n ==> "1100100"
factors: 1==>"1" 2==>"10" 4==>"100" 5==>"101" 10==>"1010"
20==>"10100" 25==>"11001" 50==>"110010" 100==>"1100100"
Hmmm... "1","10","100","11001","110010","1100100" are special factors,
"101","1010","10100" are not.
So, sc(100) should return [1,2,4,25,50,100]
```
### Series:
- [Bug in Apple](http://www.codewars.com/kata/56fe97b3cc08ca00e4000dc9)
- [Father and Son](http://www.codewars.com/kata/56fe9a0c11086cd842000008)
- [Jumping Dutch act](http://www.codewars.com/kata/570bcd9715944a2c8e000009)
- [Planting Trees](http://www.codewars.com/kata/5710443187a36a9cee0005a1)
- [Give me the equation](http://www.codewars.com/kata/56fe9b65cc08cafbc5000de3)
- [Find the murderer](http://www.codewars.com/kata/570f3fc5b29c702c5500043e)
- [Reading a Book](http://www.codewars.com/kata/570ca6a520c69f39dd0016d4)
- [Eat watermelon](http://www.codewars.com/kata/570df12ce6e9282a7d000947)
- [Special factor](http://www.codewars.com/kata/570e5d0b93214b1a950015b1)
- [Guess the Hat](http://www.codewars.com/kata/570ef7a834e61306da00035b)
- [Symmetric Sort](http://www.codewars.com/kata/5705aeb041e5befba20010ba)
- [Are they symmetrical?](http://www.codewars.com/kata/5705cc3161944b10fd0004ba)
- [Max Value](http://www.codewars.com/kata/570771871df89cf59b000742)
- [Trypophobia](http://www.codewars.com/kata/56fe9ffbc25bf33fff000f7c)
- [Virus in Apple](http://www.codewars.com/kata/5700af83d1acef83fd000048)
- [Balance Attraction](http://www.codewars.com/kata/57033601e55d30d3e0000633)
- [Remove screws I](http://www.codewars.com/kata/5710a50d336aed828100055a)
- [Remove screws II](http://www.codewars.com/kata/5710a8fd336aed00d9000594)
- [Regular expression compression](http://www.codewars.com/kata/570bae4b0237999e940016e9)
- [Collatz Array(Split or merge)](http://www.codewars.com/kata/56fe9d579b7bb6b027000001)
- [Tidy up the room](http://www.codewars.com/kata/5703ace6e55d30d3e0001029)
- [Waiting for a Bus](http://www.codewars.com/kata/57070eff924f343280000015) | games | def sc(n):
s = f" { n : b } "
return sorted(y for x in range(1, int(n * * .5) + 1) for y in (x, n / / x) if n % x == 0 and f" { y : b } " in s)
| Coding 3min : Special factor | 570e5d0b93214b1a950015b1 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/570e5d0b93214b1a950015b1 | 7 kyu |
The hamming distance of two equal-length strings is the number of positions, in which the two string differ. In other words, the number of character substitutions required to transform one string into the other.
For this first Kata, you will write a function ```hamming_distance(a, b)``` with two equal-length strings containing only 0s and 1s as parameters. There is no need to test the parameters for validity (but you can, if you want).The function's output should be the hamming distance of the two strings as an integer.
Example:
```python
hamming_distance('100101', '101001') == 2
hamming_distance('1010', '0101') == 4
```
```ruby
hamming_distance('100101', '101001') == 2
hamming_distance('1010', '0101') == 4
```
```javascript
hammingDistance('100101', '101001') == 2
hammingDistance('1010', '0101') == 4
```
```csharp
Kata.HammingDistance("100101', "101001") == 2
Kata.HammingDistance("1010', "0101") == 4
```
```php
hamming_distance('100101', '101001'); // => 2
hamming_distance('1010', '0101'); // => 4
```
```haskell
hammingDistance "100101" "101001" == 2
hammingDistance "1010" "0101" == 4
```
```purescript
hammingDistance "100101" "101001" == 2
hammingDistance "1010" "0101" == 4
```
```c
hamming_distance('100101', '101001'); // => 2
hamming_distance('1010', '0101'); // => 4
```
| reference | def hamming_distance(a, b):
return sum(c != d for c, d in zip(a, b))
| Hamming Distance - Part 1: Binary codes | 5624e574ec6034c3a20000e6 | [
"Fundamentals",
"Algorithms"
]
| https://www.codewars.com/kata/5624e574ec6034c3a20000e6 | 7 kyu |
The [Hamming Distance](http://en.wikipedia.org/wiki/Hamming_distance) is a measure of similarity between two strings of equal length. Complete the function so that it returns the number of positions where the input strings do not match.
### Examples:
```
a = "I like turtles"
b = "I like turkeys"
Result: 3
a = "Hello World"
b = "Hello World"
Result: 0
a = "espresso"
b = "Expresso"
Result: 2
```
You can assume that the two inputs are ASCII strings of equal length.
| algorithms | def hamming(a, b):
return sum(ch1 != ch2 for ch1, ch2 in zip(a, b))
| Hamming Distance | 5410c0e6a0e736cf5b000e69 | [
"Algorithms"
]
| https://www.codewars.com/kata/5410c0e6a0e736cf5b000e69 | 6 kyu |
# Task
The `hamming distance` between a pair of numbers is the number of binary bits that differ in their binary notation.
# Example
For `a = 25, b = 87`, the result should be `4`
```
25: 00011001
87: 01010111
```
The `hamming distance` between these two would be `4` ( the `2nd, 5th, 6th, 7th` bit ).
# Input/Output
- `[input]` integer `a`
First Number. `1 <= a <= 2^20`
- `[input]` integer `b`
Second Number. `1 <= b <= 2^20`
- `[output]` an integer
Hamming Distance
~~~if:lambdacalc
# Encoding
purity: `LetRec`
numEncoding: `BinaryScott`
~~~ | games | def hamming_distance(a, b):
return bin(a ^ b). count('1')
| Simple Fun #141: Hamming Distance | 58a6af7e8c08b1e9c40001c1 | [
"Puzzles"
]
| https://www.codewars.com/kata/58a6af7e8c08b1e9c40001c1 | 6 kyu |
>When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
Give you an array `arr` that contains some numbers(arr.length>=2), and give you a positive integer `k`. Find such two numbers m,n: m and n at least a difference of `k`, and their index is the most distant.
Returns their distance of index. If no such number found, return `-1`.
# Some Examples
```javascript
furthestDistance([8,7,1,9],5) === 2
The difference of 8 and 1, 1 and 9 is more than 5;
The index distance of (8,1) is 2;
The index distance of (1,9) is 1;
So 2 is the furthest distance of index.
furthestDistance([8,7,1,9],100) === -1
furthestDistance([1,2,3,4],1) === 3 (1 and 4)
furthestDistance([3,4,1,2],2) === 2 (3 and 1 or 4 and 2)
```
```ruby
furthest_distance([8,7,1,9],5) === 2
The difference of 8 and 1, 1 and 9 is more than 5;
The index distance of (8,1) is 2;
The index distance of (1,9) is 1;
So 2 is the furthest distance of index.
furthest_distance([8,7,1,9],100) === -1
furthest_distance([1,2,3,4],1) === 3 (1 and 4)
furthest_distance([3,4,1,2],2) === 2 (3 and 1 or 4 and 2)
```
| reference | def furthest_distance(arr, k):
# Sort the array and keep track of original indexes
srt, idx = zip(* sorted((n, i) for i, n in enumerate(arr)))
# For each index l, find the minimum index r such as arr[r] - arr[l] >= k
# Extrema are computed on the run
# As the data are sorted, r does at most 2 passes
max_dist, length, lmin, lmax = - 1, len(idx), idx[0], idx[0]
r, r_min, r_max = length - 1, [idx[- 1]] * length, [idx[- 1]] * length
for l, n in enumerate(srt):
lmin, lmax = min(lmin, idx[l]), max(lmax, idx[l])
while l < r and srt[r - 1] >= n + k:
r -= 1
r_min[r], r_max[r] = min(r_min[r + 1], idx[r]), max(r_max[r + 1], idx[r])
while r < length and srt[r] < n + k:
r += 1
if r == length or l >= r:
break
max_dist = max(max_dist, lmax - r_min[r], r_max[r] - lmin)
return max_dist
| The furthest distance of index | 581963edc929ba19e7000148 | [
"Puzzles",
"Fundamentals"
]
| https://www.codewars.com/kata/581963edc929ba19e7000148 | 6 kyu |
Given a starting list/array of data, it could make some statistical sense to know how much each value differs from the average.
If for example during a week of work you have collected 55,95,62,36,48 contacts for your business, it might be interesting to know the total (296), the average (59.2), but also how much you moved away from the average each single day.
For example on the first day you did something less than the said average (55, meaning -4.2 compared to the average), much more in the second day (95, 35.8 more than the average and so on).
The resulting list/array of differences starting from `[55, 95, 62, 36, 48]` is thus `[4.2, -35.8, -2.8, 23.2, 11.2]`.
Assuming you will only get valid inputs (ie: only arrays/lists with numbers), create a function to do that, rounding each difference to the second decimal digit (this is not needed in Haskell); extra points if you do so in some smart, clever or concise way :)
```clojure
With Clojure to round use:
(defn roundTo2 [n] (/ (Math/round (* n 100.0)) 100.0))
``` | reference | from numpy import mean
def distances_from_average(test_list):
avg = mean(test_list)
return [round(avg - x, 2) for x in test_list]
| Distance from the average | 568ff914fc7a40a18500005c | [
"Arrays",
"Lists",
"Statistics",
"Fundamentals",
"Data Science"
]
| https://www.codewars.com/kata/568ff914fc7a40a18500005c | 7 kyu |
## Task
You are given an array of integers. On each move you are allowed to increase exactly one of its element by one. Find the minimal number of moves required to obtain a strictly increasing sequence from the input.
## Example
For `arr = [1, 1, 1]`, the output should be `3`.
## Input/Output
- `[input]` integer array `arr`
Constraints:
`3 ≤ inputArray.length ≤ 100,`
`-10000 ≤ inputArray[i] ≤ 10000.`
- `[output]` an integer
The minimal number of moves needed to obtain a strictly increasing sequence from inputArray.
It's guaranteed that for the given test cases the answer always fits signed 32-bit integer type. | games | from functools import reduce
def array_change(arr): return reduce(lambda a, u: (
max(a[0] + 1, u), a[1] + max(0, a[0] - u + 1)), arr, (- 10001, 0))[1]
| Simple Fun #67: Array Change | 5893f43b779ce54da4000124 | [
"Puzzles"
]
| https://www.codewars.com/kata/5893f43b779ce54da4000124 | 7 kyu |
# Task
Given a string `s`, find out if its characters can be rearranged to form a palindrome.
# Example
For `s = "aabb"`, the output should be `true`.
We can rearrange `"aabb"` to make `"abba"`, which is a palindrome.
# Input/Output
- `[input]` string `s`
A string consisting of lowercase English letters.
Constraints:
`4 ≤ inputString.length ≤ 50.`
- `[output]` a boolean value
`true` if the characters of the inputString can be rearranged to form a palindrome, `false` otherwise.
| games | def palindrome_rearranging(s):
return sum(s . count(c) % 2 for c in set(s)) < 2
| Simple Fun #68: Palindrome Rearranging | 5893fa478a8a23ef82000031 | [
"Puzzles"
]
| https://www.codewars.com/kata/5893fa478a8a23ef82000031 | 7 kyu |
Removed due to copyright infringement.
<!---
# Task
Given a position of a knight on the standard chessboard, find the number of different moves the knight can perform.
The knight can move to a square that is two squares horizontally and one square vertically, or two squares vertically and one square horizontally away from it. The complete move therefore looks like the letter L. Check out the image below to see all valid moves for a knight piece that is placed on one of the central squares.
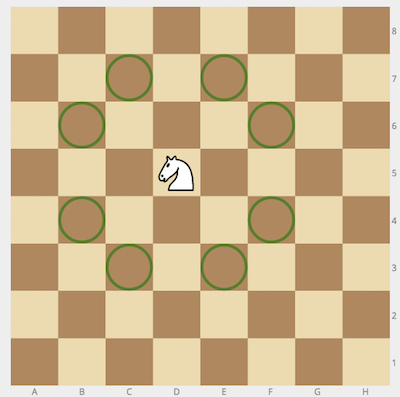
# Example
For `cell = "a1"`, the output should be `2`.
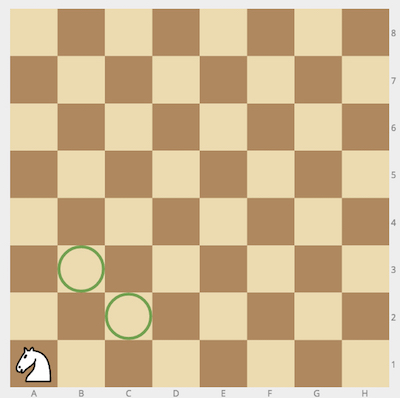
For `cell = "c2"`, the output should be `6`.
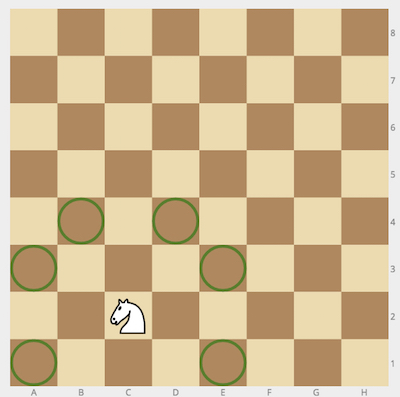
# Input/Output
- `[input]` string `cell`
String consisting of letter+number - coordinates of the knight on an 8 × 8 chessboard in chess notation.
- `[output]` an integer
---> | games | def chess_knight(cell):
x, y = (ord(c) - ord(origin) for c, origin in zip(cell, 'a1'))
return sum(0 <= x + dx < 8 and 0 <= y + dy < 8 for dx, dy in (
(- 2, - 1), (- 2, 1), (- 1, - 2), (- 1, 2), (1, - 2), (1, 2), (2, - 1), (2, 1)))
| Chess Fun #3: Chess Knight | 589433358420bf25950000b6 | [
"Puzzles",
"Games"
]
| https://www.codewars.com/kata/589433358420bf25950000b6 | 7 kyu |
## Task
Court is in session. We got a group of witnesses who have all taken an oath to tell the truth. The prosecutor is pointing at the defendants one by one and asking each witnesses a simple question - "guilty or not?". The witnesses are allowed to respond in one of the following three ways:
```
I am sure he/she is guilty.
I am sure he/she is innocent.
I have no idea.
```
The prosecutor has a hunch that one of the witnesses might not be telling the truth so she decides to cross-check all of their testimonies and see if the information gathered is consistent, i.e. there are no two witnesses A and B and a defendant C such that A says C is guilty while B says C is innocent.
## Example
For
```
evidences = [[ 0, 1, 0, 1],
[-1, 1, 0, 0],
[-1, 0, 0, 1]]
```
the output should be `true`;
For
```
evidences = [[ 1, 0],
[-1, 0],
[ 1, 1],
[ 1, 1]]
```
the output should be `false`.
# Input/Output
- `[input]` 2D integer array `evidences`
2-dimensional array of integers representing the set of testimonials. evidences[i][j] is the testimonial of the ith witness on the jth defendant. -1 means `"innocent"`, 0 means `"no idea"`, and 1 means `"guilty"`.
Constraints:
`2 ≤ evidences.length ≤ 5,`
`2 ≤ evidences[0].length ≤ 10.`
- `[output]` a boolean value
`true` if the evidence is consistent, `false` otherwise. | games | def is_information_consistent(evidences):
return all(max(l) - min(l) <= 1 for l in zip(* evidences))
| Simple Fun #86: is Information Consistent? | 58956f5ff780edf4a70000a2 | [
"Puzzles"
]
| https://www.codewars.com/kata/58956f5ff780edf4a70000a2 | 7 kyu |
# Task
Given a sequence of 0s and 1s, determine if it is a prefix of Thue-Morse sequence.
The infinite Thue-Morse sequence is obtained by first taking a sequence containing a single 0 and then repeatedly concatenating the current sequence with its binary complement.
A binary complement of a sequence X is a sequence Y of the same length such that the sum of elements X_i and Y_i on the same positions is equal to 1 for each i.
Thus the first few iterations to obtain Thue-Morse sequence are:
```
0
0 1
0 1 1 0
0 1 1 0 1 0 0 1
...
```
# Examples
For `seq=[0, 1, 1, 0, 1]`, the result should be `true`.
For `seq=[0, 1, 0, 0]`, the result should be `false`.
# Inputs & Output
- `[input]` integer array `seq`
A non-empty array.
Constraints:
`1 <= seq.length <= 1000`
`seq[i] ∈ [0,1]`
- `[output]` a boolean value
`true` if it is a prefix of Thue-Morse sequence. `false` otherwise. | games | def is_thue_morse(seq):
init_seq = [0]
while len(init_seq) < len(seq):
init_seq += [1 if n == 0 else 0 for n in init_seq]
return init_seq[: len(seq)] == seq
| Simple Fun #106: Is Thue Morse? | 589a9792ea93aae1bf00001c | [
"Puzzles"
]
| https://www.codewars.com/kata/589a9792ea93aae1bf00001c | 7 kyu |
# Task
You have set an alarm for some of the week days.
Days of the week are encoded in binary representation like this:
```
0000001 - Sunday
0000010 - Monday
0000100 - Tuesday
0001000 - Wednesday
0010000 - Thursday
0100000 - Friday
1000000 - Saturday```
For example, if your alarm is set only for Sunday and Friday, the representation will be `0100001`.
Given the current day of the week, your task is to find the day of the week when alarm will ring next time.
# Example
For `currentDay = 4, availableWeekDays = 42`, the result should be `6`.
```
currentDay = 4 means the current Day is Wednesday
availableWeekDays = 42 convert to binary is "0101010"
So the next day is 6 (Friday)
```
# Input/Output
- `[input]` integer `currentDay`
The weekdays range from 1 to 7, 1 is Sunday and 7 is Saturday
- `[input]` integer `availableWeekDays`
An integer. Days of the week are encoded in its binary representation.
- `[output]` an integer
The next day available. | games | def next_day_of_week(current_day, available_week_days):
x = 2 * * current_day
while not x & available_week_days:
x = max(1, (x * 2) % 2 * * 7)
return x . bit_length()
| Simple Fun #149: Next Day Of Week | 58aa9662c55ffbdceb000101 | [
"Puzzles"
]
| https://www.codewars.com/kata/58aa9662c55ffbdceb000101 | 7 kyu |
## Task
Consider the following algorithm for constructing 26 strings S(1) .. S(26):
```
S(1) = "a";
For i in [2, 3, ..., 26]:
S(i) = S(i - 1) + character(i) + S(i - 1).
```
For example:
```
S(1) = "a"
S(2) = S(1) + "b" + S(1) = "a" + "b" + "a" = "aba"
S(3) = S(2) + "c" + S(2) = "aba" + "c" +"aba" = "abacaba"
...
S(26) = S(25) + "z" + S(25)
```
Finally, we got a long string S(26). Your task is to find the `k`th symbol (indexing from 1) in the string S(26). All strings consist of lowercase letters only.
## Input / Output
- `[input]` integer `k`
1 ≤ k < 2<sup>26</sup>
- `[output]` a string(char in C#)
the `k`th symbol of S(26) | games | def abacaba(k):
n = 26
if k % 2:
return 'a'
while k % pow(2, n) != 0:
n -= 1
return chr(97 + n)
| Simple Fun #114: "abacaba" | 589d237fdfdef0239a00002e | [
"Puzzles"
]
| https://www.codewars.com/kata/589d237fdfdef0239a00002e | 7 kyu |
# Task
N lamps are placed in a line, some are switched on and some are off. What is the smallest number of lamps that need to be switched so that on and off lamps will alternate with each other?
You are given an array `a` of zeros and ones - `1` mean switched-on lamp and `0` means switched-off.
Your task is to find the smallest number of lamps that need to be switched.
# Example
For `a = [1, 0, 0, 1, 1, 1, 0]`, the result should be `3`.
```
a --> 1 0 0 1 1 1 0
swith --> 0 1 0
became--> 0 1 0 1 0 1 0 ```
# Input/Output
- `[input]` integer array `a`
array of zeros and ones - initial lamp setup, 1 mean switched-on lamp and 0 means switched-off.
`2 < a.length <= 1000`
- `[output]` an integer
minimum number of switches. | games | def lamps(a):
n = sum(1 for i, x in enumerate(a) if x != i % 2)
return min(n, len(a) - n)
| Simple Fun #124: Lamps | 58a3c1f12f949e21b300005c | [
"Puzzles"
]
| https://www.codewars.com/kata/58a3c1f12f949e21b300005c | 7 kyu |
# Task
After a long night (work, play, study) you find yourself sleeping on a bench in a park. As you wake up and try to figure out what happened you start counting trees.
You notice there are different tree sizes but there's always one size which is unbalanced. For example there are 2 size 2, 2 size 1 and 1 size 3. (then the size 3 is unbalanced)
Given an array representing different tree sizes. Which one is the unbalanced size.
# Notes
```
There can be any number of sizes but one is always unbalanced
The unbalanced size is always one less than the other sizes
The array is not ordered (nor the trees)
```
# Examples
For `trees = [1,1,2,2,3]`, the result should be `3`.
For `trees = [2,2,2,56,56,56,8,8]`, the result should be `8`.
For `trees = [34,76,12,99,64,99,76,12,34]`, the result should be `64`.
# Input/Output
- `[input]` integer array `trees`
Array representing different tree sizes
- `[output]` an integer
The size of the missing tree. | algorithms | def find_the_missing_tree(trees):
return sorted(trees, key=trees . count)[0]
| Simple Fun #147: Find The Missing Tree | 58aa8698ae929e1c830001c7 | [
"Algorithms"
]
| https://www.codewars.com/kata/58aa8698ae929e1c830001c7 | 7 kyu |
# Task
Round the given number `n` to the nearest multiple of `m`.
If `n` is exactly in the middle of 2 multiples of m, return `n` instead.
# Example
For `n = 20, m = 3`, the output should be `21`.
For `n = 19, m = 3`, the output should be `18`.
For `n = 50, m = 100`, the output should be `50`.
# Input/Output
- `[input]` integer `n`
`1 ≤ n < 10^9.`
- `[input]` integer `m`
`3 ≤ m < 109`.
- `[output]` an integer | reference | def rounding(n, m):
return n if n % m == m / 2 else m * round(n / m)
| Simple Fun #181: Rounding | 58bccdf56f25ff6b6d00002f | [
"Fundamentals"
]
| https://www.codewars.com/kata/58bccdf56f25ff6b6d00002f | 7 kyu |
# Task
Your task is to find the smallest number which is evenly divided by all numbers between `m` and `n` (both inclusive).
# Example
For `m = 1, n = 2`, the output should be `2`.
For `m = 2, n = 3`, the output should be `6`.
For `m = 3, n = 2`, the output should be `6` too.
For `m = 1, n = 10`, the output should be `2520`.
# Input/Output
- `[input]` integer `m`
`1 ≤ m ≤ 25`
- `[input]` integer `n`
`1 ≤ n ≤ 25`
- `[output]` an integer | reference | from numpy import lcm
from functools import reduce
def mn_lcm(* args):
a, b = sorted(args)
return reduce(lcm, range(a, b + 1))
| Simple Fun #184: LCM from m to n | 58bcdc65f6d3b11fce000045 | [
"Fundamentals"
]
| https://www.codewars.com/kata/58bcdc65f6d3b11fce000045 | 7 kyu |
# Task
Lonerz got some crazy growing plants and he wants to grow them nice and well.
Initially, the garden is completely barren.
Each morning, Lonerz can put any number of plants into the garden to grow.
And at night, each plant mutates into two plants.
Lonerz really hopes to see `n` plants in his garden.
Your task is to find the minimum number of plants Lonerz has to plant to get exactly `n` plants one day.
# Example
For `n = 5`, the output should be `2`.
Lonerz hopes to see `5` plants. He adds `1` plant on the first morning and on the third morning there would be `4` plants in the garden. He then adds `1` more and sees `5` plants.
So, Lonerz only needs to add 2 plants to his garden.
For `n = 8,` the output should be `1`.
Lonerz hopes to see `8` plants. Thus, he just needs to add `1` plant in the beginning and wait for it to double till 8.
# Input/Output
The number of plant lonerz hopes to see in his garden.
- `[input]` integer `n`
`1 <= n <= 10^7`
- `[output]` an integer
The number of plants Lonerz needs to plant. | games | def plant_doubling(n):
return bin(n). count("1")
| Simple Fun #189: Plant Doubling | 58bf97cde4a5edfd4f00008d | [
"Puzzles"
]
| https://www.codewars.com/kata/58bf97cde4a5edfd4f00008d | 7 kyu |
# Task
Consider integer numbers from 0 to n - 1 written down along the circle in such a way that the distance between any two neighbouring numbers is equal (note that 0 and n - 1 are neighbouring, too).
Given `n` and `firstNumber`/`first_number`/`first-number`, find the number which is written in the radially opposite position to firstNumber.
# Example
For n = 10 and firstNumber = 2, the output should be 7
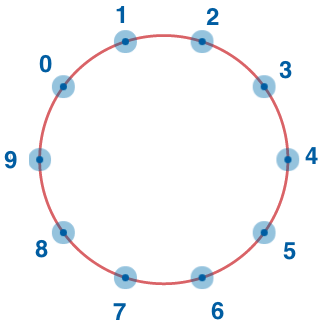
# Input/Output
- `[input]` integer `n`
A positive even integer.
Constraints: 4 ≤ n ≤ 1000.
- `[input]` integer `firstNumber`/`first_number`/`first-number`
Constraints: 0 ≤ firstNumber ≤ n - 1
- `[output]` an integer
| games | def circle_of_numbers(n, fst):
return (fst + (n / 2)) % n
| Simple Fun #2: Circle of Numbers | 58841cb52a077503c4000015 | [
"Puzzles"
]
| https://www.codewars.com/kata/58841cb52a077503c4000015 | 7 kyu |
Create a function that returns the average of an array of numbers ("scores"), rounded to the nearest whole number. You are not allowed to use any loops (including for, for/in, while, and do/while loops).
The array will never be empty. | reference | def average(array):
return round(sum(array) / len(array))
| Average Scores | 57b68bc7b69bfc8209000307 | [
"Mathematics",
"Fundamentals"
]
| https://www.codewars.com/kata/57b68bc7b69bfc8209000307 | 7 kyu |
In telecomunications we use information coding to detect and prevent errors while sending data.
A parity bit is a bit added to a string of binary code that indicates whether the number of 1-bits in the string is even or odd. Parity bits are used as the simplest form of error detecting code, and can detect a 1 bit error.
In this case we are using even parity: the parity bit is set to `0` if the number of `1`-bits is even, and is set to `1` if odd.
We are using them for the transfer of ASCII characters in binary (7-bit strings): the parity is added to the end of the 7-bit string, forming the 8th bit.
In this Kata you are to test for 1-bit errors and return a new string consisting of all of the correct ASCII caracters **in 7 bit format** (removing the parity bit), or `"error"` in place of ASCII characters in which errors were detected.
For more information on parity bits: https://en.wikipedia.org/wiki/Parity_bit
## Examples
Correct 7 bit string with an even parity bit as the 8th bit:
```
"01011001" <-- The "1" on the right is the parity bit.
```
In this example, there are three 1-bits. Three is an odd number, and the parity bit is set to `1`. No errors are detected, so return `"0101100"` (7 bits).
Example of a string of ASCII characters:
```
"01011001 01101110 01100000 01010110 10001111 01100011"
```
This should return:
```
"0101100 error 0110000 0101011 error 0110001"
``` | reference | def parity_bit(binary):
return ' ' . join(byte[: - 1] if byte . count('1') % 2 == 0 else 'error' for byte in binary . split())
| Parity bit - Error detecting code | 58409435258e102ae900030f | [
"Algorithms",
"Binary",
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/58409435258e102ae900030f | 6 kyu |
It's time to create an autocomplete function! Yay!
The autocomplete function will take in an input string and a dictionary array and return the values from the dictionary that start with the input string. If there are more than 5 matches, restrict your output to the first 5 results. If there are no matches, return an empty array.
Example:
```javascript
autocomplete('ai', ['airplane','airport','apple','ball']) = ['airplane','airport']
```
```python
autocomplete('ai', ['airplane','airport','apple','ball']) = ['airplane','airport']
```
For this kata, the dictionary will always be a valid array of strings.
Please return all results in the order given in the dictionary, even if they're not always alphabetical.
The search should NOT be case sensitive, but the case of the word should be preserved when it's returned.
For example, "Apple" and "airport" would both return for an input of 'a'. However, they should return as "Apple" and "airport" in their original cases.
<div style="background:#ffa; color:#000; padding:10px;">
<p>Important note:
<p>Any input that is NOT a letter should be treated as if it is not there. For example, an input of "$%^" should be treated as "" and an input of "ab*&1cd" should be treated as "abcd".
<p>(Thanks to wthit56 for the suggestion!)
</div>
| reference | def autocomplete(input_, dictionary):
input_ = '' . join([c for c in list(input_) if c . isalpha()])
return [x for x in dictionary if x . lower(). startswith(input_ . lower())][: 5]
| Autocomplete! Yay! | 5389864ec72ce03383000484 | [
"Strings",
"Regular Expressions",
"Arrays",
"Fundamentals"
]
| https://www.codewars.com/kata/5389864ec72ce03383000484 | 6 kyu |
Your task is to write a higher order function for chaining together
a list of unary functions. In other words, it should return a function
that does a left fold on the given functions.
```python
chained([a,b,c,d])(input)
```
Should yield the same result as
```python
d(c(b(a(input))))
``` | reference | def chained(functions):
def f(x):
for function in functions:
x = function(x)
return x
return f
| Unary function chainer | 54ca3e777120b56cb6000710 | [
"Functional Programming",
"Fundamentals"
]
| https://www.codewars.com/kata/54ca3e777120b56cb6000710 | 6 kyu |
The idea for this kata came from 9gag today:
[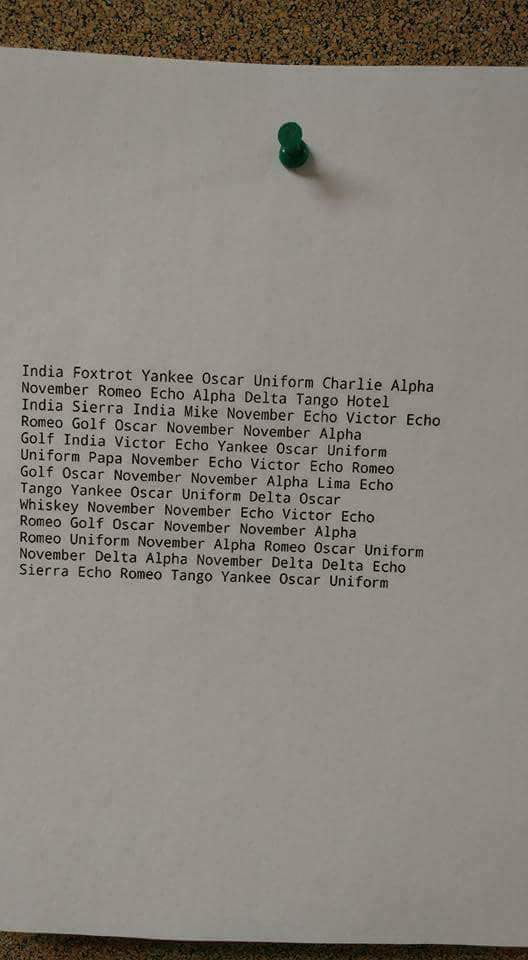](http://9gag.com/gag/amrb4r9)
## Task
You'll have to translate a string to Pilot's alphabet ([NATO phonetic alphabet](https://en.wikipedia.org/wiki/NATO_phonetic_alphabet)).
**Input:**
`If, you can read?`
**Output:**
`India Foxtrot , Yankee Oscar Uniform Charlie Alfa November Romeo Echo Alfa Delta ?`
**Note:**
* There is a preloaded dictionary that you can use, named `NATO`. It uses uppercase keys, e.g. `NATO['A']` is `"Alfa"`. See comments in the initial code to see how to access it in your language.
* The set of used punctuation is `,.!?`.
* Punctuation should be kept in your return string, but spaces should not.
* __Xray__ should not have a dash within.
* Every word and punctuation mark should be seperated by a space ' '.
* There should be no trailing whitespace
~~~if:rust
The NATO phonetic alphabet (A–Z) is preloaded:
```rust
use preloaded::NATO;
NATO[&'R']; // Romeo
NATO[&'U']; // Uniform
NATO[&'S']; // Sierra
NATO[&'T']; // Tango
```
<details>
<summary>Click to see the preloaded code</summary>
```rust
use std::collections::HashMap;
use once_cell::sync::Lazy;
#[rustfmt::skip]
pub static NATO: Lazy<HashMap<char, &'static str>> = Lazy::new(|| {
[
('A', "Alfa"), ('B', "Bravo"), ('C', "Charlie"), ('D', "Delta"),
('E', "Echo"), ('F', "Foxtrot"), ('G', "Golf"), ('H', "Hotel"),
('I', "India"), ('J', "Juliett"), ('K', "Kilo"), ('L', "Lima"),
('M', "Mike"), ('N', "November"), ('O', "Oscar"), ('P', "Papa"),
('Q', "Quebec"), ('R', "Romeo"), ('S', "Sierra"), ('T', "Tango"),
('U', "Uniform"), ('V', "Victor"), ('W', "Whiskey"), ('X', "Xray"),
('Y', "Yankee"), ('Z', "Zulu"),
]
.iter()
.copied()
.collect()
});
```
</details>
~~~ | reference | def to_nato(words):
return ' ' . join(NATO . get(char, char) for char in words . upper() if char != ' ')
| If you can read this... | 586538146b56991861000293 | [
"Fundamentals"
]
| https://www.codewars.com/kata/586538146b56991861000293 | 6 kyu |
**Kata in this series**
* [Histogram - H1](https://www.codewars.com/kata/histogram-h1/)
* [Histogram - H2](https://www.codewars.com/kata/histogram-h2/)
* [Histogram - V1](https://www.codewars.com/kata/histogram-v1/)
* [Histogram - V2](https://www.codewars.com/kata/histogram-v2/)
<h2>Background</h2>
A 6-sided die is rolled a number of times and the results are plotted as a character-based histogram.
Example:
```
10
#
#
7 #
# #
# # 5
# # #
# 3 # #
# # # #
# # # 1 #
# # # # #
-----------
1 2 3 4 5 6
```
<h2>Task</h2>
You will be passed all the dice roll results, and your task is to write the code to return a string representing a histogram, so that when it is printed it has the same format as the example.
<h2>Notes</h2>
* There are no trailing spaces on the lines
* All lines (including the last) end with a newline ```\n```
* A count is displayed above each bar (unless the count is 0)
* The number of rolls may vary but is always less than 100
| algorithms | def histogram(rolls):
hist = "-----------\n1 2 3 4 5 6\n"
for i in range(max(rolls) + 1):
hist = '' . join(['# ' if i < v else '{:<2d}' . format(
i) if i == v and v != 0 else ' ' for v in rolls]). rstrip() + '\n' + hist
return hist
| Histogram - V1 | 57c6c2e1f8392d982a0000f2 | [
"Strings",
"Algorithms"
]
| https://www.codewars.com/kata/57c6c2e1f8392d982a0000f2 | 5 kyu |
## MTV Cribs is back!

_If you haven't solved it already I recommend trying [this kata](https://www.codewars.com/kata/5834a44e44ff289b5a000075) first._
## Task
Given `n` representing the number of floors build a penthouse like this:
```
___
/___\
/_____\
| _ | 1 floor
|_|_|_|
_____
/_____\
/_______\
/_________\
/___________\
| |
| ___ | 2 floors
| | | |
|___|___|___|
_______
/_______\
/_________\
/___________\
/_____________\
/_______________\
/_________________\
| | 3 floors
| |
| _____ |
| | | |
| | | |
|_____|_____|_____|
```
**Note:** whitespace should be preserved on both sides of the roof. No invalid input tests.
Good luck! | reference | def my_crib(n):
wide = 4 + 3 + 6 * (n - 1)
door = 2 + n - 1
roof = 3 + 2 * (n - 1)
r = '{0}{1}{0}\n' . format(' ' * (wide / / 2 - n), '_' * (3 + 2 * (n - 1)))
for i in range(1, roof):
r += '{0}/{1}\\{0}\n' . format(' ' * (wide / / 2 - n - i), '_' * (3 + 2 * (n - 1) + 2 * (i - 1)))
for i in range(roof - 1 - door):
r += '|{}|\n' . format(' ' * (wide - 2))
r += '|{0}{1}{0}|\n' . format(' ' * ((wide - 1) / / 3), '_' * (1 + 2 * (n - 1)))
for i in range(1, door - 1):
r += '|{0}|{0}|{0}|\n' . format(' ' * ((wide - 2) / / 3))
return r + '|{0}|{0}|{0}|' . format('_' * ((wide - 2) / / 3))
| Now that's a crib! | 58360d112fb0ba255300008b | [
"Fundamentals",
"ASCII Art"
]
| https://www.codewars.com/kata/58360d112fb0ba255300008b | 5 kyu |
## Task
Given `n` representing the number of floors build a beautiful multi-million dollar mansions like the ones in the example below:
```
/\
/ \
/ \
/______\ number of floors 3
| |
| |
|______|
/\
/ \
/____\
| | 2 floors
|____|
/\
/__\ 1 floor
|__|
```
**Note:** whitespace should be preserved on both sides of the roof. Number of floors will go up to 30. There will be no tests with invalid input.
If you manage to complete it, you can try a harder version [here](https://www.codewars.com/kata/58360d112fb0ba255300008b).
Good luck! | reference | def my_crib(n):
roof = "\n" . join("%s/%s\\%s" % (" " * (n - i), " " *
i * 2, " " * (n - i)) for i in range(n))
ceiling = "\n/%s\\\n" % ("_" * (n * 2))
walls = ("|%s|\n" % (" " * (n * 2))) * (n - 1)
floor = "|%s|" % ("_" * (n * 2))
return roof + ceiling + walls + floor
| MTV Cribs | 5834a44e44ff289b5a000075 | [
"Fundamentals",
"ASCII Art"
]
| https://www.codewars.com/kata/5834a44e44ff289b5a000075 | 6 kyu |
Your aged grandfather is tragically optimistic about Team GB's chances in the upcoming World Cup, and has enlisted you to help him make [Union Jack](https://en.wikipedia.org/wiki/Union_Jack) flags to decorate his house with.
## Instructions
* Write a function which takes as a parameter a number which represents the dimensions of the flag. The flags will always be square, so the number 9 means you should make a flag measuring 9x9.
* It should return a *string* of the flag, with _'X' for the red/white lines and '-' for the blue background_. It should include newline characters so that it's not all on one line.
* For the sake of symmetry, treat odd and even numbers differently: odd number flags should have a central cross that is *only one 'X' thick*. Even number flags should have a central cross that is *two 'X's thick* (see examples below).
## Other points
* The smallest flag you can make without it looking silly is 7x7. If you get a number smaller than 7, simply _make the flag 7x7_.
* If you get a decimal, round it _UP to the next whole number_, e.g. 12.45 would mean making a flag that is 13x13.
* If you get something that's not a number at all, *return false*.
Translations and comments (and upvotes) welcome!

## Examples:
```javascript
unionJack(9); // (9 is odd, so the central cross is 1'X' thick)
"X---X---X
-X--X--X-
--X-X-X--
---XXX---
XXXXXXXXX
---XXX---
--X-X-X--
-X--X--X-
X---X---X"
unionJack(10); // (10 is even, so the central cross is 2 'X's thick)
'X---XX---X
-X--XX--X-
--X-XX-X--
---XXXX---
XXXXXXXXXX
XXXXXXXXXX
---XXXX---
--X-XX-X--
-X--XX--X-
X---XX---X
unionJack(1); // (the smallest possible flag is 7x7)
"X--X--X
-X-X-X-
--XXX--
XXXXXXX
--XXX--
-X-X-X-
X--X--X"
unionJack('string'); //return false.
```
```ruby
union_jack(9) # (9 is odd, so the central cross is 1'X' thick)
"X---X---X
-X--X--X-
--X-X-X--
---XXX---
XXXXXXXXX
---XXX---
--X-X-X--
-X--X--X-
X---X---X"
union_jack(10) # (10 is even, so the central cross is 2 'X's thick)
'X---XX---X
-X--XX--X-
--X-XX-X--
---XXXX---
XXXXXXXXXX
XXXXXXXXXX
---XXXX---
--X-XX-X--
-X--XX--X-
X---XX---X
union_jack(1) # (the smallest possible flag is 7x7)
"X--X--X
-X-X-X-
--XXX--
XXXXXXX
--XXX--
-X-X-X-
X--X--X"
union_jack('string') #return false.
```
```python
union_jack(9) # (9 is odd, so the central cross is 1'X' thick)
"X---X---X
-X--X--X-
--X-X-X--
---XXX---
XXXXXXXXX
---XXX---
--X-X-X--
-X--X--X-
X---X---X"
union_jack(10) # (10 is even, so the central cross is 2 'X's thick)
'X---XX---X
-X--XX--X-
--X-XX-X--
---XXXX---
XXXXXXXXXX
XXXXXXXXXX
---XXXX---
--X-XX-X--
-X--XX--X-
X---XX---X
union_jack(1) # (the smallest possible flag is 7x7)
"X--X--X
-X-X-X-
--XXX--
XXXXXXX
--XXX--
-X-X-X-
X--X--X"
union_jack('string') #return false.
``` | reference | from math import ceil
X = 'X' # X' for the red/white lines
B = '-' # '-' for the blue background
def union_jack(n):
try:
n = max(7, ceil(n))
except TypeError:
return False
half, odd = divmod(n - 1, 2)
result = []
for i in range(half):
half_row = B * i + X + B * (half - i - 1)
result . append((X * (odd + 1)). join([half_row, half_row[:: - 1]]))
return '\n' . join(result + [X * n] * (odd + 1) + result[:: - 1])
| The Union Jack | 5620281f0eeee479cd000020 | [
"Strings",
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5620281f0eeee479cd000020 | 6 kyu |
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto desired number of rows.
* Note:```Returning``` the pattern is not the same as ```Printing``` the pattern.
### Parameters:
pattern( n , x , y );
^ ^ ^
| | |
Term upto which Number of times Number of times
Basic Pattern Basic Pattern Basic Pattern
should be should be should be
created repeated repeated
horizontally vertically
* Note: `Basic Pattern` means what we created in <A HREF="http://www.codewars.com/kata/558ac25e552b51dbc60000c3">Complete The Pattern #12</A>
## Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* If `x <= 1` then the basic pattern should not be repeated horizontally.
* If `y <= 1` then the basic pattern should not be repeated vertically.
* `The length of each line is same`, and is equal to the length of longest line in the pattern.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,25]`
+ `x ∈ (-∞,10]`
+ `y ∈ (-∞,10]`
* If only two arguments are passed then the function `pattern` should run as if `y <= 1`.
* If only one argument is passed then the function `pattern` should run as if `x <= 1` & `y <= 1`.
* The function `pattern` should work when extra arguments are passed, by ignoring the extra arguments.
## Examples:
* Having Three Arguments-
+ pattern(4,3,2):
1 1 1 1
2 2 2 2 2 2
3 3 3 3 3 3
4 4 4
3 3 3 3 3 3
2 2 2 2 2 2
1 1 1 1
2 2 2 2 2 2
3 3 3 3 3 3
4 4 4
3 3 3 3 3 3
2 2 2 2 2 2
1 1 1 1
* Having Two Arguments-
+ pattern(10,2):
1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
5 5 5 5
6 6 6 6
7 7 7 7
8 8 8 8
9 9 9 9
0 0
9 9 9 9
8 8 8 8
7 7 7 7
6 6 6 6
5 5 5 5
4 4 4 4
3 3 3 3
2 2 2 2
1 1 1
* Having Only One Argument-
+ pattern(25):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
<P ALIGN="center"><FONT FACE="Impact" SIZE="5"><A HREF="http://www.codewars.com/users/curious_db97/authored">>>>LIST OF ALL MY KATAS<<<</A></FONT></P> | reference | def pattern(n: int, x: int = 1, y: int = 1, * _) - > str:
lines = []
for i in range(1, n + 1):
line = ' ' * (i - 1) + str(i % 10) + ' ' * (n - i)
line += line[- 2:: - 1]
lines . append(line + line[1:] * (x - 1))
cross = lines + lines[- 2:: - 1]
return '\n' . join(cross + (cross[1:] * (y - 1)))
| Complete The Pattern #15 | 558db3ca718883bd17000031 | [
"Arrays",
"Strings",
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/558db3ca718883bd17000031 | 6 kyu |
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto desired number of rows.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
### Parameters:
pattern( n , x );
^ ^
| |
Term upto which Number of times
Basic Pattern Basic Pattern
should be should be
created repeated
horizontally
* Note: `Basic Pattern` means what we created in [Complete the pattern #12](https://www.codewars.com/kata/558ac25e552b51dbc60000c3)
### Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* If `x <= 1` then the basic pattern should not be repeated horizontally.
* `The length of each line is same`, and is equal to the length of longest line in the pattern.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
+ `x ∈ (-∞,25]`
* If only one argument is passed then the function `pattern` should run as if `x <= 1`.
* The function `pattern` should work when extra arguments are passed, by ignoring the extra arguments.
### Examples:
* Having Two Arguments-
+ pattern(4,3):
1 1 1 1
2 2 2 2 2 2
3 3 3 3 3 3
4 4 4
3 3 3 3 3 3
2 2 2 2 2 2
1 1 1 1
+ pattern(10,2):
1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
5 5 5 5
6 6 6 6
7 7 7 7
8 8 8 8
9 9 9 9
0 0
9 9 9 9
8 8 8 8
7 7 7 7
6 6 6 6
5 5 5 5
4 4 4 4
3 3 3 3
2 2 2 2
1 1 1
* Having Only One Argument-
+ pattern(25):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
[List of all my katas]("http://www.codewars.com/users/curious_db97/authored") | reference | def pattern(n: int, x: int = 1, * _) - > str:
lines = []
for i in range(1, n + 1):
line = ' ' * (i - 1) + str(i % 10) + ' ' * (n - i)
line += line[- 2:: - 1]
lines . append(line + line[1:] * (x - 1))
return '\n' . join(lines + lines[- 2:: - 1])
| Complete The Pattern #13 | 5592e5d3ede9542ff0000057 | [
"Strings",
"Arrays",
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5592e5d3ede9542ff0000057 | 6 kyu |
<p style="text-align:left;"><font face="Impact" size="5"><A HREF="http://www.codewars.com/kata/5592e5d3ede9542ff0000057">< PREVIOUS KATA</A></font>
<span style="float:right;"><font face="Impact" size="5" ><A HREF="http://www.codewars.com/kata/558db3ca718883bd17000031">NEXT KATA ></A></font></span></p>
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto desired number of rows.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
## Parameters:
pattern( n , y );
^ ^
| |
Term upto which Number of times
Basic Pattern Basic Pattern
should be should be
created repeated
vertically
* Note: `Basic Pattern` means what we created in <A HREF="http://www.codewars.com/kata/558ac25e552b51dbc60000c3">Complete The Pattern #12</A> i.e. a `simple X`.
## Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* If `y <= 1` then the basic pattern should not be repeated vertically.
* `The length of each line is same`, and is equal to the length of longest line in the pattern.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
+ `y ∈ (-∞,25]`
* If only one argument is passed then the function `pattern` should run as if `y <= 1`.
* The function `pattern` should work when extra arguments are passed, by ignoring the extra arguments.
## Examples:
#### Having Two Arguments:
##### pattern(4,3):
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
##### pattern(10,2):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
#### Having Only One Argument:
##### pattern(25):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
<P ALIGN="center"><FONT FACE="Impact" SIZE="5"><A HREF="http://www.codewars.com/users/curious_db97/authored">>>>LIST OF ALL MY KATAS<<<</A></FONT></P> | reference | def pattern(n: int, x: int = 1, * _) - > str:
lines = []
for i in range(1, n + 1):
line = ' ' * (i - 1) + str(i % 10) + ' ' * (n - i)
lines . append(line + line[- 2:: - 1])
cross = lines + lines[- 2:: - 1]
return '\n' . join(cross + (cross[1:] * (x - 1)))
| Complete The Pattern #14 | 559379505c859be5a9000034 | [
"Strings",
"Arrays",
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/559379505c859be5a9000034 | 6 kyu |
### Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto (2n-1) rows, where n is parameter.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
#### Parameters:
pattern( n );
^
|
Term upto which
Basic Pattern(this)
should be
created
#### Rules/Note:
* If `n < 1` then it should return "" i.e. empty string.
* `The length of each line is same`, and is equal to the length of longest line in the pattern i.e (2n-1).
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,100]`
### Examples:
* pattern(5):
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
* pattern(10):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
* pattern(15):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
[List of all my katas]("http://www.codewars.com/users/curious_db97/authored") | reference | def pattern(n):
res = []
for i in range(1, n + 1):
line = ' ' * (i - 1) + str(i % 10) + ' ' * (n - i)
res . append(line + line[:: - 1][1:])
return '\n' . join(res + res[:: - 1][1:])
| Complete The Pattern #12 | 558ac25e552b51dbc60000c3 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/558ac25e552b51dbc60000c3 | 6 kyu |
Given an array `arr` of strings, complete the function by calculating the total perimeter of all the islands. Each piece of land will be marked with `'X'` while the water fields are represented as `'O'`. Consider each tile being a perfect `1 x 1` piece of land. Some examples for better visualization:
```text
['XOOXO',
'XOOXO',
'OOOXO',
'XXOXO',
'OXOOO']
```
which represents:

should return: `"Total land perimeter: 24"`.
Following input:
```text
['XOOO',
'XOXO',
'XOXO',
'OOXX',
'OOOO']
```
which represents:

should return: `"Total land perimeter: 18"` | reference | def land_perimeter(arr):
I, J = len(arr), len(arr[0])
P = 0
for i in range(I):
for j in range(J):
if arr[i][j] == 'X':
if i == 0 or arr[i - 1][j] == 'O':
P += 1
if i == I - 1 or arr[i + 1][j] == 'O':
P += 1
if j == 0 or arr[i][j - 1] == 'O':
P += 1
if j == J - 1 or arr[i][j + 1] == 'O':
P += 1
return 'Total land perimeter: ' + str(P)
| Land perimeter | 5839c48f0cf94640a20001d3 | [
"Fundamentals"
]
| https://www.codewars.com/kata/5839c48f0cf94640a20001d3 | 5 kyu |
### Task:
You have to write a function **pattern** which creates the following Pattern(See Examples) upto n(parameter) number of rows.
#### Rules/Note:
* If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.
* All the lines in the pattern have same length i.e equal to the number of characters in the last line.
* Range of n is (-∞,100]
### Examples:
pattern(5):
1
121
12321
1234321
123454321
pattern(10):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
pattern(15):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
pattern(20):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
1234567890123456543210987654321
123456789012345676543210987654321
12345678901234567876543210987654321
1234567890123456789876543210987654321
123456789012345678909876543210987654321
###Amazing Fact:
<img src="http://i1.wp.com/mathandmultimedia.com/wp-content/uploads/2010/11/amazing1.png?resize=600%2C290">
```Hint: Use \n in string to jump to next line```
| reference | def pattern(n):
output = []
for i in range(1, n + 1):
wing = ' ' * (n - i) + '' . join(str(d % 10) for d in range(1, i))
output . append(wing + str(i % 10) + wing[:: - 1])
return '\n' . join(output)
| Complete The Pattern #8 - Number Pyramid | 5575ff8c4d9c98bc96000042 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5575ff8c4d9c98bc96000042 | 6 kyu |
Write a function which takes one parameter representing the dimensions of a checkered board. The board will always be square, so 5 means you will need a 5x5 board.
The dark squares will be represented by a unicode white square, while the light squares will be represented by a unicode black square (the opposite colours ensure the board doesn't look reversed on code wars' dark background). It should return a string of the board with a space in between each square and taking into account new lines.
An even number should return a board that begins with a dark square. An odd number should return a board that begins with a light square.
### Examples
```text
Input: 5
Output:
■ □ ■ □ ■
□ ■ □ ■ □
■ □ ■ □ ■
□ ■ □ ■ □
■ □ ■ □ ■
```
**There should be no trailing white space at the end of each line, or new line characters at the end of the string.**
### Note
The squares are characters `■` and `□` with codes `\u25A0` and `\u25A1`.
Do not use HTML entities for the squares (e.g. `□` for white square) as the code doesn't consider it a valid square. A good way to check is if your solution prints a correct checker board on your local terminal.
| reference | def checkered_board(n):
return isinstance(n, int) and n > 1 and \
'\n' . join(' ' . join("■" if (x + y) % 2 ^ n %
2 else "□" for y in range(n)) for x in range(n))
| Checkered Board | 5650f1a6075b3284120000c0 | [
"Strings",
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5650f1a6075b3284120000c0 | 6 kyu |
# How much is the fish! (- Scooter )
The ocean is full of colorful fishes. We as programmers want to know the hexadecimal value of these fishes.
## Task
Take all hexadecimal valid characters (a,b,c,d,e,f) of the given name and XOR them. Return the result as an integer.
## Input
The input is always a string, which can contain spaces, upper and lower case letters but no digits.
## Example
`fisHex("redlionfish") -> e,d,f -> XOR -> 12` | reference | from functools import reduce
from operator import xor
def fisHex(name):
return reduce(xor, (int(x, 16) for x in name . lower() if 'a' <= x <= 'f'), 0)
| How much hex is the fish | 5714eb80e1bf814e53000c06 | [
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/5714eb80e1bf814e53000c06 | 6 kyu |
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto n number of rows.
* Note:```Returning``` the pattern is not the same as ```Printing``` the pattern.
### Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* `The length of each line is same`, and is equal to the number of characters in a line i.e `n`.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-50,150]`
### Examples:
+ pattern(8):
88888888
87777777
87666666
87655555
87654444
87654333
87654322
87654321
+ pattern(17):
77777777777777777
76666666666666666
76555555555555555
76544444444444444
76543333333333333
76543222222222222
76543211111111111
76543210000000000
76543210999999999
76543210988888888
76543210987777777
76543210987666666
76543210987655555
76543210987654444
76543210987654333
76543210987654322
76543210987654321
[List of all my katas]("http://www.codewars.com/users/curious_db97/authored") | reference | def pattern(n):
return "\n" . join(
"" . join(
str((n - min(j, i)) % 10) for j in range(n)
)
for i in range(max(n, 0))
)
| Complete The Pattern #16 | 55ae997d1c40a199e6000018 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/55ae997d1c40a199e6000018 | 6 kyu |
### Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto (3n-2) rows, where n is parameter.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
#### Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* `The length of each line is same`, and is equal to the length of longest line in the pattern i.e. `length = (3n-2)`.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
### Examples:
+ pattern(5) :
11111
22222
33333
44444
1234555554321
1234555554321
1234555554321
1234555554321
1234555554321
44444
33333
22222
11111
+ pattern(11):
11111111111
22222222222
33333333333
44444444444
55555555555
66666666666
77777777777
88888888888
99999999999
00000000000
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
00000000000
99999999999
88888888888
77777777777
66666666666
55555555555
44444444444
33333333333
22222222222
11111111111
<P ALIGN="center"><FONT FACE="Impact" SIZE="5"><A HREF="http://www.codewars.com/users/curious_db97/authored">>>>LIST OF ALL MY KATAS<<<</A></FONT></P>
| reference | def pattern(n):
h = '' . join(str(i % 10) for i in range(1, n))
h = h + str(n % 10) * n + h[:: - 1]
v = [(str(i % 10) * n). center(len(h)) for i in range(1, n)]
return '\n' . join(v + [h] * n + v[:: - 1])
| Complete The Pattern #11 - Plus | 5589ad588ee1db3f5e00005a | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5589ad588ee1db3f5e00005a | 6 kyu |
## Task:
You have to write a function **pattern** which returns the following Pattern(See Examples) upto (2n-1) rows, where n is parameter.
### Rules/Note:
* If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.
* All the lines in the pattern have same length i.e equal to the number of characters in the longest line.
* Range of n is (-∞,100]
## Examples:
pattern(5):
1
121
12321
1234321
123454321
1234321
12321
121
1
pattern(10):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
12345678987654321
123456787654321
1234567654321
12345654321
123454321
1234321
12321
121
1
pattern(15):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
123456789012343210987654321
1234567890123210987654321
12345678901210987654321
123456789010987654321
1234567890987654321
12345678987654321
123456787654321
1234567654321
12345654321
123454321
1234321
12321
121
1
pattern(20):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
1234567890123456543210987654321
123456789012345676543210987654321
12345678901234567876543210987654321
1234567890123456789876543210987654321
123456789012345678909876543210987654321
1234567890123456789876543210987654321
12345678901234567876543210987654321
123456789012345676543210987654321
1234567890123456543210987654321
12345678901234543210987654321
123456789012343210987654321
1234567890123210987654321
12345678901210987654321
123456789010987654321
1234567890987654321
12345678987654321
123456787654321
1234567654321
12345654321
123454321
1234321
12321
121
1
| reference | def pattern(n):
lines = []
for c in range(1, n + 1):
s = (' ' * (n - c)) + '' . join([str(s)[- 1] for s in range(1, c + 1)])
lines += [s + s[:: - 1][1:]]
lines += lines[:: - 1][1:]
return '\n' . join(str(x) for x in lines)
| Complete The Pattern #9 - Diamond | 5579e6a5256bac65e4000060 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5579e6a5256bac65e4000060 | 6 kyu |
The objective is to return all pairs of integers from a given array of integers that have a difference of 2.
The result array should be sorted in ascending order of values.
Assume there are no duplicate integers in the array. The order of the integers in the input array should not matter.
## Examples
~~~if-not:python,julia
```
[1, 2, 3, 4] should return [[1, 3], [2, 4]]
[4, 1, 2, 3] should also return [[1, 3], [2, 4]]
[1, 23, 3, 4, 7] should return [[1, 3]]
[4, 3, 1, 5, 6] should return [[1, 3], [3, 5], [4, 6]]
```
~~~
~~~if:python,julia
```
[1, 2, 3, 4] should return [(1, 3), (2, 4)]
[4, 1, 2, 3] should also return [(1, 3), (2, 4)]
[1, 23, 3, 4, 7] should return [(1, 3)]
[4, 3, 1, 5, 6] should return [(1, 3), (3, 5), (4, 6)]
```
~~~
| algorithms | def twos_difference(a):
s = set(a)
return sorted((x, x + 2) for x in a if x + 2 in s)
| Difference of 2 | 5340298112fa30e786000688 | [
"Arrays",
"Sorting",
"Algorithms"
]
| https://www.codewars.com/kata/5340298112fa30e786000688 | 6 kyu |
Three candidates take part in a TV show.
In order to decide who will take part in the final game and probably become rich, they have to roll the Wheel of Fortune!
The Wheel of Fortune is divided into 20 sections, each with a number from 5 to 100 (only mulitples of 5).
Each candidate can roll the wheel once or twice and sum up the score of each roll.
The winner one that is closest to 100 (while still being lower or equal to 100).
In case of a tie, the candidate that rolled the wheel first wins.
You receive the information about each candidate as an array of objects: each object should have a `name` and a `scores` array with the candidate roll values.
Your solution should return the name of the winner or `false` if there is no winner (all scored more than 100).
__Example:__
```javascript
var c1 = { name: "Bob", scores: [10, 65] };
var c2 = { name: "Bill", scores: [90, 5] };
var c3 = { name: "Charlie", scores: [40, 55] };
winner([c1, c2, c3]); // Returns "Bill"
```
```python
c1 = {"name": "Bob", "scores": [10, 65]}
c2 = {"name": "Bill", "scores": [90, 5]}
c3 = {"name": "Charlie", "scores": [40, 55]}
winner([c1, c2, c3]) #Returns "Bill"
```
```ruby
c1 = {"name"=>"Bob", "scores"=>[10, 65]}
c2 = {"name"=>"Bill", "scores"=>[90, 5]}
c3 = {"name"=>"Charlie", "scores"=>[40, 55]}
winner([c1, c2, c3]) #Returns "Bill"
```
Please note that inputs may be invalid: in this case, the function should return false.
Potential errors derived from the specifications are:
- More or less than three candidates take part in the game.
- A candidate did not roll the wheel or rolled it more than twice.
- Scores are not valid.
- Invalid user entry (no name or no score).
| algorithms | def winner(candidates):
try:
assert len(candidates) == 3
max_total = 0
for c in candidates:
name, scores = c['name'], c['scores']
assert 1 <= len(scores) <= 2
assert all(not s % 5 and 0 < s <= 100 for s in scores)
total = sum(scores)
if max_total < total <= 100:
selected, max_total = name, total
return selected
except:
return False
| Wheel of Fortune | 55191f78cd82ff246f000784 | [
"Games",
"Arrays",
"Algorithms"
]
| https://www.codewars.com/kata/55191f78cd82ff246f000784 | 6 kyu |
#Task#
Raj once wanted to code a program to make a plus sign using the numbers but he didn't have any idea to do so. Using `n` as a parameter in the function `pattern` complete the code and solve the pattern so that Raj fells happy.
###Rules/Note:###
* You are assured that n>1
* There are white spaces on top and bottom left corner (take help of test cases)
#Examples#
* pattern(2)
1
121
1
* pattern(5)
1
2
3
4
123454321
4
3
2
1
* pattern(12)
1
2
3
4
5
6
7
8
9
0
1
12345678901210987654321
1
0
9
8
7
6
5
4
3
2
1
| reference | def pattern(n):
s1 = '\n' . join(' ' * (n - 1) + str(i % 10) for i in range(1, n))
s2 = '' . join(str(i % 10) for i in range(1, n))
s2 = s2 + str(n % 10) + s2[:: - 1]
s3 = '\n' . join(' ' * (n - 1) + str(i % 10) for i in range(n - 1, 0, - 1))
return s1 + '\n' + s2 + '\n' + s3 + '\n'
| Numbers' Plus Pattern | 563cb92e0996a4ac0b000042 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/563cb92e0996a4ac0b000042 | 6 kyu |
Complete the function to find the count of the most frequent item of an array. You can assume that input is an array of integers. For an empty array return `0`
## Example
```python
input array: [3, -1, -1, -1, 2, 3, -1, 3, -1, 2, 4, 9, 3]
ouptut: 5
```
The most frequent number in the array is `-1` and it occurs `5` times.
| reference | def most_frequent_item_count(collection):
if collection:
return max([collection . count(item) for item in collection])
return 0
| Find Count of Most Frequent Item in an Array | 56582133c932d8239900002e | [
"Data Structures",
"Fundamentals"
]
| https://www.codewars.com/kata/56582133c932d8239900002e | 7 kyu |
## Task:
You have to write a function **pattern** which returns the following Pattern(See Examples) upto n rows, where n is parameter.
### Rules/Note:
* If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.
* The length of each line = (2n-1).
* Range of n is (-∞,100]
## Examples:
pattern(5):
12345
12345
12345
12345
12345
pattern(10):
1234567890
1234567890
1234567890
1234567890
1234567890
1234567890
1234567890
1234567890
1234567890
1234567890
pattern(15):
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
123456789012345
pattern(20):
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
12345678901234567890
| reference | def pattern(n):
nums = '1234567890'
str_nums = nums * (n / / 10) + nums[: n % 10]
return '\n' . join(' ' * (n - i - 1) + str_nums + ' ' * i for i in range(n))
| Complete The Pattern #10 - Parallelogram | 5581a7651185fe13190000ee | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5581a7651185fe13190000ee | 7 kyu |
###Task:
You have to write a function `pattern` which creates the following pattern (see examples) up to the desired number of rows.
* If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.
* If any even number is passed as argument then the pattern should last upto the largest odd number which is smaller than the passed even number.
###Examples:
pattern(9):
1
333
55555
7777777
999999999
pattern(6):
1
333
55555
```Note: There are no spaces in the pattern```
```Hint: Use \n in string to jump to next line```
| reference | def pattern(n):
return '\n' . join(str(i) * i for i in range(1, n + 1, 2))
| Complete The Pattern #6 - Odd Ladder | 5574940eae1cf7d520000076 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/5574940eae1cf7d520000076 | 7 kyu |
Create a function which checks a number for three different properties.
- is the number prime?
- is the number even?
- is the number a multiple of 10?
Each should return either true or false, which should be given as an array. Remark: The Haskell variant uses `data Property`.
### Examples
```javascript
numberProperty(7) // ==> [true, false, false]
numberProperty(10) // ==> [false, true, true]
```
```ruby
number_property(7) # ==> [true, false, false]
number_property(10) # ==> [false, true, true]
```
```python
number_property(7) # ==> [true, false, false]
number_property(10) # ==> [false, true, true]
```
```haskell
numberProperty 7 `shouldBe` Property True False False
numberProperty 10 `shouldBe` Property False True True
```
```csharp
Kata.NumberProperty(7) => new bool[] {true, false, false}
Kata.NumberProperty(10) => new bool[] {false, true, true}
```
```java
SimpleMath.numberProperty(7) => new boolean[] {true, false, false}
SimpleMath.numberProperty(10) => new boolean[] {false, true, true}
```
The number will always be an integer, either positive or negative. Note that negative numbers cannot be primes, but they can be multiples of 10:
```javascript
numberProperty(-7) // ==> [false, false, false]
numberProperty(-10) // ==> [false, true, true]
```
```ruby
number_property(-7) # ==> [false, false, false]
number_property(-10) # ==> [false, true, true]
```
```python
number_property(-7) # ==> [false, false, false]
number_property(-10) # ==> [false, true, true]
```
```haskell
numberProperty (-7) `shouldBe` Property False False False
numberProperty (-10) `shouldBe` Property False True True
```
```csharp
Kata.NumberProperty(-7) => new bool[] {false, false, false}
Kata.NumberProperty(-10) => new bool[] {false, true, true}
```
```java
SimpleMath.numberProperty(-7) => new boolean[] {false, false, false}
SimpleMath.numberProperty(-10) => new boolean[] {false, true, true}
``` | algorithms | import math
def number_property(n):
return [isPrime(n), isEven(n), isMultipleOf10(n)]
# Return isPrime? isEven? isMultipleOf10?
# your code here
def isPrime(n):
if n <= 3:
return n >= 2
if n % 2 == 0 or n % 3 == 0:
return False
for i in range(5, int(n * * 0.5) + 1, 6):
if n % i == 0 or n % (i + 2) == 0:
return False
return True
def isEven(n):
return n % 2 == 0
def isMultipleOf10(n):
return n % 10 == 0
| Simple Maths Test | 5507309481b8bd3b7e001638 | [
"Algorithms",
"Mathematics"
]
| https://www.codewars.com/kata/5507309481b8bd3b7e001638 | 7 kyu |
### Task:
You have to write a function `pattern` which creates the following pattern (See Examples) upto desired number of rows.
If the Argument is `0` or a Negative Integer then it should return `""` i.e. empty string.
### Examples:
`pattern(9)`:
123456789
234567891
345678912
456789123
567891234
678912345
789123456
891234567
912345678
`pattern(5)`:
12345
23451
34512
45123
51234
Note: There are no spaces in the pattern
Hint: Use `\n` in string to jump to next line | reference | def pattern(n):
return '\n' . join('' . join(str((x + y) % n + 1) for y in range(n)) for x in range(n))
| Complete The Pattern #7 - Cyclical Permutation | 557592fcdfc2220bed000042 | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/557592fcdfc2220bed000042 | 7 kyu |
### Task:
You have to write a function **pattern** which creates the following pattern up to `n/2` number of lines.
* If `n <= 1` then it should return `""` (i.e. empty string).
* If any odd number is passed as argument then the pattern should last up to the largest even number which is smaller than the passed odd number.
### Examples:
pattern(8):
22
4444
666666
88888888
pattern(5):
22
4444
```Note: There are no spaces in the pattern```
```Hint: Use \n in string to jump to next line```
| reference | def pattern(n):
return "\n" . join(str(i) * i for i in range(2, n + 1, 2))
| Complete The Pattern #5 - Even Ladder | 55749101ae1cf7673800003e | [
"ASCII Art",
"Fundamentals"
]
| https://www.codewars.com/kata/55749101ae1cf7673800003e | 7 kyu |
Johnny is a boy who likes to open and close lockers. He loves it so much that one day, when school was out, he snuck in just to play with the lockers.
Each locker can either be open or closed. If a locker is closed when Johnny gets to it, he opens it, and vice versa.
The lockers are numbered sequentially, starting at 1.
Starting at the first locker, Johnny runs down the row, opening each locker.
Then he runs all the way back to the beginning and runs down the row again, this time skipping to every other locker. (2,4,6, etc)
Then he runs all the way back and runs through again, this time skipping two lockers for every locker he opens or closes. (3,6,9, etc)
He continues this until he has finished running past the last locker (i.e. when the number of lockers he skips is greater than the number of lockers he has).
------
The equation could be stated as follows:
> Johnny runs down the row of lockers `n` times, starting at the first locker each run and skipping `i` lockers as he runs, where `n` is the number of lockers there are in total and `i` is the current run.
The goal of this kata is to determine which lockers are open at the end of Johnny's running.
The program accepts an integer giving the total number of lockers, and should output an array filled with the locker numbers of those which are open at the end of his run.
| games | from math import floor
# Pretty sure this is the fastest implementation; only one square root, and sqrt(n) multiplications.
# Plus, no booleans, because they're super slow.
def locker_run(l): return [i * i for i in range(1, int(floor(l * * .5)) + 1)]
| Slamming Lockers | 55a5085c1a3d379fbb000062 | [
"Algorithms",
"Fundamentals",
"Puzzles"
]
| https://www.codewars.com/kata/55a5085c1a3d379fbb000062 | 7 kyu |
### Instructions
Complete the function that returns the lyrics for the song [99 Bottles of Beer](http://en.wikipedia.org/wiki/99_Bottles_of_Beer) as **an array of strings**: each line should be a separate element - see the example at the bottom.
***Note:*** *in order to avoid hardcoded solutions, the size of your code is limited to 1000 characters*
### Lyrics
>99 bottles of beer on the wall, 99 bottles of beer.<br>
>Take one down and pass it around, 98 bottles of beer on the wall.
>
>98 bottles of beer on the wall, 98 bottles of beer.<br>
>Take one down and pass it around, 97 bottles of beer on the wall.
>
> ...and so on...
>
>3 bottles of beer on the wall, 3 bottles of beer.<br>
>Take one down and pass it around, 2 bottles of beer on the wall.
>
>2 bottles of beer on the wall, 2 bottles of beer.<br>
>Take one down and pass it around, 1 bottle of beer on the wall.
>
>1 bottle of beer on the wall, 1 bottle of beer.<br>
>Take one down and pass it around, no more bottles of beer on the wall.
>
>No more bottles of beer on the wall, no more bottles of beer.<br>
>Go to the store and buy some more, 99 bottles of beer on the wall.
### Example
```
[ "99 bottles of beer on the wall, 99 bottles of beer.",
"Take one down and pass it around, 98 bottles of beer on the wall.",
"98 bottles of beer on the wall, 98 bottles of beer.",
...and so on...
"3 bottles of beer on the wall, 3 bottles of beer.",
"Take one down and pass it around, 2 bottles of beer on the wall.",
"2 bottles of beer on the wall, 2 bottles of beer.",
"Take one down and pass it around, 1 bottle of beer on the wall.",
"1 bottle of beer on the wall, 1 bottle of beer.",
"Take one down and pass it around, no more bottles of beer on the wall.",
"No more bottles of beer on the wall, no more bottles of beer.",
"Go to the store and buy some more, 99 bottles of beer on the wall." ]
``` | algorithms | def sing():
lst = []
for i in range(97):
lst . append(
f' { 99 - i } bottles of beer on the wall, { 99 - i } bottles of beer.')
lst . append(
f'Take one down and pass it around, { 98 - i } bottles of beer on the wall.')
return lst + ['2 bottles of beer on the wall, 2 bottles of beer.', 'Take one down and pass it around, 1 bottle of beer on the wall.', '1 bottle of beer on the wall, 1 bottle of beer.', 'Take one down and pass it around, no more bottles of beer on the wall.', 'No more bottles of beer on the wall, no more bottles of beer.', 'Go to the store and buy some more, 99 bottles of beer on the wall.']
| 99 Bottles of Beer | 52a723508a4d96c6c90005ba | [
"Strings",
"Algorithms"
]
| https://www.codewars.com/kata/52a723508a4d96c6c90005ba | 7 kyu |
## Oh no!
Some really funny web dev gave you a _sequence of numbers_ from his API response as an _sequence of strings_!
You need to cast the whole array to the correct type.
Create the function that takes as a parameter a sequence of numbers represented as strings and outputs a sequence of numbers.
ie:``` ["1", "2", "3"] ``` to ``` [1, 2, 3] ```
Note that you can receive floats as well. | reference | def to_float_array(arr):
return list(map(float, arr))
| Convert an array of strings to array of numbers | 5783d8f3202c0e486c001d23 | [
"Arrays",
"Parsing",
"Strings",
"Fundamentals"
]
| https://www.codewars.com/kata/5783d8f3202c0e486c001d23 | 7 kyu |
# Esolang Interpreters #4 - Boolfuck Interpreter
## About this Kata Series
"Esolang Interpreters" is a Kata Series that originally began as three separate, independent esolang interpreter Kata authored by [@donaldsebleung](http://codewars.com/users/donaldsebleung) which all shared a similar format and were all somewhat inter-related. Under the influence of [a fellow Codewarrior](https://www.codewars.com/users/nickkwest), these three high-level inter-related Kata gradually evolved into what is known today as the "Esolang Interpreters" series.
This series is a high-level Kata Series designed to challenge the minds of bright and daring programmers by implementing interpreters for various [esoteric programming languages/Esolangs](http://esolangs.org), mainly [Brainfuck](http://esolangs.org/wiki/Brainfuck) derivatives but not limited to them, given a certain specification for a certain Esolang. Perhaps the only exception to this rule is the very first Kata in this Series which is intended as an introduction/taster to the world of esoteric programming languages and writing interpreters for them.
## The Language
Boolfuck is an [esoteric programming language (Esolang)](http://esolangs.org/wiki/Esoteric_programming_language) based on the famous [Brainfuck](http://esolangs.org/wiki/Brainfuck) (also an Esolang) which was invented in 2004 or 2005 according to [the official website](http://samuelhughes.com/boof/index.html). It is very similar to Brainfuck except for a few key differences:
- Boolfuck works with bits as opposed to bytes
- The tape for Brainfuck contains exactly 30,000 cells with the pointer starting from the very left; Boolfuck contains an infinitely long tape with the pointer starting at the "middle" (since the tape can be extended indefinitely either direction)
- Each cell in Boolfuck can only contain the values `0` or `1` (i.e. bits not bytes) as opposed to Brainfuck which has cells containing values ranging from `0` to `255` inclusive.
- The output command in Boolfuck is `;` NOT `.`
- The `-` command does **not** exist in Boolfuck since either `+` or `-` would flip a bit anyway
Anyway, here is a list of commands and their descriptions:
- `+` - Flips the value of the bit under the pointer
- `,` - Reads a bit from the input stream, storing it under the pointer. The end-user types information using characters, though. Bytes are read in little-endian order—the first bit read from the character `a`, for instance, is 1, followed by 0, 0, 0, 0, 1, 1, and finally 0. If the end-of-file has been reached, outputs a zero to the bit under the pointer.
- `;` - Outputs the bit under the pointer to the output stream. The bits get output in little-endian order, the same order in which they would be input. If the total number of bits output is not a multiple of eight at the end of the program, the last character of output gets padded with zeros on the more significant end.
- `<` - Moves the pointer left by 1 bit
- `>` - Moves the pointer right by 1 bit
- `[` - If the value under the pointer is `0` then skip to the corresponding `]`
- `]` - Jumps back to the matching `[` character, if the value under the pointer is `1`
If you haven't written an interpreter for Brainfuck yet I recommend you to complete [this Kata](https://www.codewars.com/kata/my-smallest-code-interpreter-aka-brainf-star-star-k) first.
## The Task
Write a Boolfuck interpreter which accepts up to two arguments. The first (required) argument is the Boolfuck code in the form of a string. The second (optional) argument is the input passed in by the **end-user** (i.e. as actual characters not bits) which should default to `""` if not provided. Your interpreter should return the output as actual characters (not bits!) as a string.
```javascript
function boolfuck (code, input = "")
```
```c
char* boolfuck (char *code, char *in)
```
```cpp
char* boolfuck (const char *code, const char *in)
```
```java
public static String interpret (String code, String input)
```
```fsharp
let interpret (code: string) (input: string) : string
```
```python
def boolfuck(code, input)
```
```go
func Boolfuck(code, input string) string
```
```rust
fn boolfuck(code: &str, input: Vec<u8>) -> Vec<u8>
```
```haskell
boolfuck :: String -> String -> String
```
Preloaded for you is a function `brainfuckToBoolfuck()`/`brainfuck_to_boolfuck()`/`BrainfuckToBoolfuck()` which accepts 1 required argument (the Brainfuck code) and returns its Boolfuck equivalent should you find it useful.
Please note that your interpreter should simply ignore any non-command characters. **This will be tested in the test cases.**
If in doubt, feel free to refer to the official website (link at top).
Good luck :D
## Kata in this Series
1. [Esolang Interpreters #1 - Introduction to Esolangs and My First Interpreter (MiniStringFuck)](https://www.codewars.com/kata/esolang-interpreters-number-1-introduction-to-esolangs-and-my-first-interpreter-ministringfuck)
2. [Esolang Interpreters #2 - Custom Smallfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-2-custom-smallfuck-interpreter)
3. [Esolang Interpreters #3 - Custom Paintfuck Interpreter](http://codewars.com/kata/esolang-interpreters-number-3-custom-paintf-star-star-k-interpreter)
4. **Esolang Interpreters #4 - Boolfuck Interpreter**
| algorithms | from collections import defaultdict
def boolfuck(code, input=""):
input = [int(c) for c in '' . join(
[bin(ord(c))[2:]. rjust(8, '0') for c in input][:: - 1])]
cp, p, out, bits, starts, brackets = 0, 0, [], defaultdict(int), [], {}
for i, c in enumerate(code):
if c == '[':
starts . append(i)
elif c is ']':
brackets[i] = starts . pop()
brackets[brackets[i]] = i
while cp < len(code):
if code[cp] == '[' and not bits[p]:
cp = brackets[cp]
elif code[cp] == ']':
cp = brackets[cp] - 1
elif code[cp] == '>':
p += 1
elif code[cp] == '<':
p -= 1
elif code[cp] == '+':
bits[p] = (0 if bits[p] else 1)
elif code[cp] == ',':
bits[p] = (input . pop() if input else 0)
elif code[cp] == ';':
out . append(bits[p])
cp += 1
s = ''
while out:
out, s = out[8:], s + chr(int('' . join(str(c)
for c in out[: 8][:: - 1]). rjust(8, '0'), 2))
return s
| Esolang Interpreters #4 - Boolfuck Interpreter | 5861487fdb20cff3ab000030 | [
"Esoteric Languages",
"Interpreters",
"Algorithms",
"Tutorials"
]
| https://www.codewars.com/kata/5861487fdb20cff3ab000030 | 3 kyu |
A number m of the form 10x + y is divisible by 7 if and only if x − 2y is divisible by 7. In other words, subtract twice the last digit
from the number formed by the remaining digits. Continue to do this until a number known to be divisible by 7 is obtained;
you can stop when this number has *at most* 2 digits because you are supposed to know if a number of at most 2 digits is divisible by 7 or not.
The original number is divisible by 7 if and only if the last number obtained using this procedure is divisible by 7.
#### Examples:
1 - `m = 371 -> 37 − (2×1) -> 37 − 2 = 35` ; thus, since 35 is divisible by 7, 371 is divisible by 7.
The number of steps to get the result is `1`.
2 - `m = 1603 -> 160 - (2 x 3) -> 154 -> 15 - 8 = 7` and 7 is divisible by 7.
3 - `m = 372 -> 37 − (2×2) -> 37 − 4 = 33` ; thus, since 33 is not divisible by 7, 372 is not divisible by 7.
4 - `m = 477557101->47755708->4775554->477547->47740->4774->469->28` and 28 is divisible by 7, so is 477557101.
The number of steps is 7.
#### Task:
Your task is to return to the function ```seven(m)``` (m integer >= 0) an array (or a pair, depending on the language) of numbers,
the first being the *last* number `m` with at most 2 digits obtained by your function (this last `m` will be divisible or not by 7), the second one being the number of steps to get the result.
#### Forth Note:
Return on the stack `number-of-steps, last-number-m-with-at-most-2-digits `
#### Examples:
```
seven(371) should return [35, 1]
seven(1603) should return [7, 2]
seven(477557101) should return [28, 7]
```
| reference | def seven(m, step=0):
if m < 100:
return (m, step)
x, y, step = m / / 10, m % 10, step + 1
res = x - 2 * y
return seven(res, step)
| A Rule of Divisibility by 7 | 55e6f5e58f7817808e00002e | [
"Fundamentals"
]
| https://www.codewars.com/kata/55e6f5e58f7817808e00002e | 7 kyu |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.